code
stringlengths 2.5k
150k
| kind
stringclasses 1
value |
---|---|
enzyme Using enzyme with Mocha Using enzyme with Mocha
=======================
enzyme was originally designed to work with Mocha, so getting it up and running with Mocha should be no problem at all. Simply install it and start using it:
```
npm i --save-dev enzyme
```
```
import React from 'react';
import { expect } from 'chai';
import { mount } from 'enzyme';
import { spy } from 'sinon';
import Foo from './src/Foo';
spy(Foo.prototype, 'componentDidMount');
describe('<Foo />', () => {
it('calls componentDidMount', () => {
const wrapper = mount(<Foo />);
expect(Foo.prototype.componentDidMount).to.have.property('callCount', 1);
});
});
```
enzyme Using enzyme with Tape and AVA Using enzyme with Tape and AVA
==============================
enzyme works well with [Tape](https://github.com/substack/tape) and [AVA](https://github.com/avajs/ava). Simply install it and start using it:
```
npm i --save-dev enzyme enzyme-adapter-react-16
```
Tape
-----
```
import test from 'tape';
import React from 'react';
import { shallow, mount, configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
import Foo from '../path/to/foo';
configure({ adapter: new Adapter() });
test('shallow', (t) => {
const wrapper = shallow(<Foo />);
t.equal(wrapper.contains(<span>Foo</span>), true);
});
test('mount', (t) => {
const wrapper = mount(<Foo />);
const fooInner = wrapper.find('.foo-inner');
t.equal(fooInner.is('.foo-inner'), true);
});
```
AVA
----
```
import test from 'ava';
import React from 'react';
import { shallow, mount, configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
import Foo from '../path/to/foo';
configure({ adapter: new Adapter() });
test('shallow', (t) => {
const wrapper = shallow(<Foo />);
t.is(wrapper.contains(<span>Foo</span>), true);
});
test('mount', (t) => {
const wrapper = mount(<Foo />);
const fooInner = wrapper.find('.foo-inner');
t.is(fooInner.is('.foo-inner'), true);
});
```
Example Projects
-----------------
* [enzyme-example-tape](https://github.com/TaeKimJR/enzyme-example-tape)
* [enzyme-example-ava](https://github.com/mikenikles/enzyme-example-ava)
enzyme Using enzyme with Karma Using enzyme with Karma
=======================
Karma is a popular test runner that can run tests in multiple browser environments. Depending on your Karma setup, you may have a number of options for configuring Enzyme.
Basic Enzyme setup with Karma
------------------------------
###
Configure Enzyme
Create an Enzyme setup file. This file will configure Enzyme with the appropriate React adapter. It can also be used to initialize any that you'd like available for all tests. To avoid having to import this file and Enzyme, you can re-export all Enzyme exports from this file and just import it.
```
/* test/enzyme.js */
import Enzyme from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
import jasmineEnzyme from 'jasmine-enzyme';
// Configure Enzyme for the appropriate React adapter
Enzyme.configure({ adapter: new Adapter() });
// Initialize global helpers
beforeEach(() => {
jasmineEnzyme();
});
// Re-export all enzyme exports
export * from 'enzyme';
```
###
Import Enzyme from the Enzyme setup file
Anywhere you want to use Enzyme, import the Enzyme setup file just as you would Enzyme itself.
```
/* some_test.js */
// Import anything you would normally import `from 'enzyme'` from the Enzyme setup file
import { shallow } from './test/enzyme';
// ...
```
Alternative karma-webpack setup
--------------------------------
If you're using Karma and Webpack using [karma-webpack's alternative setup](https://github.com/webpack-contrib/karma-webpack#alternative-usage), you can configure enzyme in your test entry file and import Enzyme directly in individual tests.
```
/* test/index_test.js */
import './enzyme';
const testsContext = require.context('.', true, /_test$/);
testsContext.keys().forEach(testsContext);
```
```
/* some_test.js */
// If Enzyme is configured in the test entry file, Enzyme can be imported directly
import { shallow } from 'enzyme';
// ...
```
enzyme Using enzyme with Webpack Using enzyme with Webpack
=========================
If you are using a test runner that runs code in a browser-based environment, you may be using [webpack](https://webpack.js.org/) in order to bundle your React code.
Prior to enzyme 3.0 there were some issues with conditional requires that were used to maintain backwards compatibility with React versions. With enzyme 3.0+, this should no longer be an issue. If it is, please file a GitHub issue or make a PR to this documentation with instructions on how to set it up.
enzyme Using enzyme with Jest Using enzyme with Jest
======================
Configure with Jest
--------------------
To run the setup file to configure Enzyme and the Adapter (as shown in the [Installation docs](https://airbnb.io/enzyme/docs/installation/)) with Jest, set `setupFilesAfterEnv` (previously `setupTestFrameworkScriptFile`) in your config file (check [Jest's documentation](http://jestjs.io/docs/en/configuration) for the possible locations of that config file) to literally the string `<rootDir>` and the path to your setup file.
```
{
"jest": {
"setupFilesAfterEnv": ["<rootDir>src/setupTests.js"]
}
}
```
Jest version 15 and up
-----------------------
Starting with version 15, Jest [no longer mocks modules by default](https://facebook.github.io/jest/blog/2016/09/01/jest-15.html). Because of this, you no longer have to add *any* special configuration for Jest to use it with enzyme.
Install Jest, and its Babel integrations, as recommended in the [Jest docs](https://facebook.github.io/jest/docs/en/getting-started.html). Install enzyme. Then, simply require/import React, enzyme functions, and your module at the top of a test file.
```
import React from 'react';
import { shallow, mount, render } from 'enzyme';
import Foo from '../Foo';
```
You do **not** need to include Jest's own renderer, unless you want to use it *only* for Jest snapshot testing.
Example Project for Jest version 15+
-------------------------------------
* [Example test for Jest 15+](https://github.com/vjwilson/enzyme-example-jest)
Jest prior to version 15
-------------------------
If you are using Jest 0.9 – 14.0 with enzyme and using Jest's automocking feature, you will need to mark react and enzyme to be unmocked in your `package.json`:
`package.json`:
```
{
"jest": {
"unmockedModulePathPatterns": [
"node_modules/react/",
"node_modules/enzyme/"
]
}
}
```
If you are using a previous version of Jest together with npm3, you may need to unmock [more modules](https://github.com/enzymejs/enzyme/blob/78febd90fe2fb184771b8b0356b0fcffbdad386e/docs/guides/jest.md).
enzyme Migration Guide for enzyme v2.x to v3.x Migration Guide for enzyme v2.x to v3.x
=======================================
The change from enzyme v2.x to v3.x is a more significant change than in previous major releases, due to the fact that the internal implementation of enzyme has been almost completely rewritten.
The goal of this rewrite was to address a lot of the major issues that have plagued enzyme since its initial release. It was also to simultaneously remove a lot of the dependencies that enzyme has on React internals, and to make enzyme more "pluggable", paving the way for enzyme to be used with "React-like" libraries such as Preact and Inferno.
We have done our best to make enzyme v3 as API compatible with v2.x as possible, however there are a handful of breaking changes that we decided we needed to make, intentionally, in order to support this new architecture and also improve the usability of the library long-term.
Airbnb has one of the largest enzyme test suites, coming in at around 30,000 enzyme unit tests. After upgrading enzyme to v3.x in Airbnb's code base, 99.6% of these tests succeeded with no modifications at all. Most of the tests that broke we found to be easy to fix, and some we found to actually depend on what could arguably be considered a bug in v2.x, and the breakage was actually desired.
In this guide, we will go over a couple of the most common breakages that we ran into, and how to fix them. Hopefully this will make your upgrade path that much easier. If during your upgrade you find a breakage that doesn't seem to make sense to you, feel free to file an issue.
Configuring your Adapter
-------------------------
enzyme now has an "Adapter" system. This means that you now need to install enzyme along with another module that provides the Adapter that tells enzyme how to work with your version of React (or whatever other React-like library you are using).
At the time of writing this, enzyme publishes "officially supported" adapters for React 0.13.x, 0.14.x, 15.x, and 16.x. These adapters are npm packages of the form `enzyme-adapter-react-{{version}}`.
You will want to configure enzyme with the adapter you'd like to use before using enzyme in your tests. The way to do this is with `enzyme.configure(...)`. For example, if your project depends on React 16, you would want to configure enzyme this way:
```
import Enzyme from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
Enzyme.configure({ adapter: new Adapter() });
```
The list of adapter npm packages for React semver ranges are as follows:
| enzyme Adapter Package | React semver compatibility |
| --- | --- |
| `enzyme-adapter-react-16` | `^16.4.0-0` |
| `enzyme-adapter-react-16.3` | `~16.3.0-0` |
| `enzyme-adapter-react-16.2` | `~16.2` |
| `enzyme-adapter-react-16.1` | `~16.0.0-0 \ | \ | ~16.1` |
| `enzyme-adapter-react-15` | `^15.5.0` |
| `enzyme-adapter-react-15.4` | `15.0.0-0 - 15.4.x` |
| `enzyme-adapter-react-14` | `^0.14.0` |
| `enzyme-adapter-react-13` | `^0.13.0` |
Element referential identity is no longer preserved
----------------------------------------------------
enzyme's new architecture means that the react "render tree" is transformed into an intermediate representation that is common across all react versions so that enzyme can properly traverse it independent of React's internal representations. A side effect of this is that enzyme no longer has access to the actual object references that were returned from `render` in your React components. This normally isn't much of a problem, but can manifest as a test failure in some cases.
For example, consider the following example:
```
import React from 'react';
import Icon from './path/to/Icon';
const ICONS = {
success: <Icon name="check-mark" />,
failure: <Icon name="exclamation-mark" />,
};
const StatusLabel = ({ id, label }) => <div>{ICONS[id]}{label}{ICONS[id]}</div>;
```
```
import { shallow } from 'enzyme';
import StatusLabel from './path/to/StatusLabel';
import Icon from './path/to/Icon';
const wrapper = shallow(<StatusLabel id="success" label="Success" />);
const iconCount = wrapper.find(Icon).length;
```
In v2.x, `iconCount` would be 1. In v3.x, it will be 2. This is because in v2.x it would find all of the elements matching the selector, and then remove any duplicates. Since `ICONS.success` is included twice in the render tree, but it's a constant that's reused, it would show up as a duplicate in the eyes of enzyme v2.x. In enzyme v3, the elements that are traversed are transformations of the underlying react elements, and are thus different references, resulting in two elements being found.
Although this is a breaking change, I believe the new behavior is closer to what people would actually expect and want. Having enzyme wrappers be immutable results in more deterministic tests that are less prone to flakiness from external factors.
###
Calling `props()` after a state change
In `enzyme` v2, executing an event that would change a component state (and in turn update props) would return those updated props via the `.props` method.
Now, in `enzyme` v3, you are required to re-find the component; for example:
```
class Toggler extends React.Component {
constructor(...args) {
super(...args);
this.state = { on: false };
}
toggle() {
this.setState(({ on }) => ({ on: !on }));
}
render() {
const { on } = this.state;
return (<div id="root">{on ? 'on' : 'off'}</div>);
}
}
it('passes in enzyme v2, fails in v3', () => {
const wrapper = mount(<Toggler />);
const root = wrapper.find('#root');
expect(root.text()).to.equal('off');
wrapper.instance().toggle();
expect(root.text()).to.equal('on');
});
it('passes in v2 and v3', () => {
const wrapper = mount(<Toggler />);
expect(wrapper.find('#root').text()).to.equal('off');
wrapper.instance().toggle();
expect(wrapper.find('#root').text()).to.equal('on');
});
```
`children()` now has slightly different meaning
------------------------------------------------
enzyme has a `.children()` method which is intended to return the rendered children of a wrapper.
When using `mount(...)`, it can sometimes be unclear exactly what this would mean. Consider for example the following react components:
```
class Box extends React.Component {
render() {
const { children } = this.props;
return <div className="box">{children}</div>;
}
}
class Foo extends React.Component {
render() {
return (
<Box bam>
<div className="div" />
</Box>
);
}
}
```
Now lets say we have a test which does something like:
```
const wrapper = mount(<Foo />);
```
At this point, there is an ambiguity about what `wrapper.find(Box).children()` should return. Although the `<Box ... />` element has a `children` prop of `<div className="div" />`, the actual rendered children of the element that the box component renders is a `<div className="box">...</div>` element.
Prior enzyme v3, we would observe the following behavior:
```
wrapper.find(Box).children().debug();
// => <div className="div" />
```
In enzyme v3, we now have `.children()` return the *rendered* children. In other words, it returns the element that is returned from that component's `render` function.
```
wrapper.find(Box).children().debug();
// =>
// <div className="box">
// <div className="div" />
// </div>
```
This may seem like a subtle difference, but making this change will be important for future APIs we would like to introduce.
`find()` now returns host nodes and DOM nodes
----------------------------------------------
In some cases find will return a host node and DOM node. Take the following for example:
```
const Foo = () => <div/>;
const wrapper = mount(
<div>
<Foo className="bar" />
<div className="bar"/>
</div>
);
console.log(wrapper.find('.bar').length); // 2
```
Since `<Foo/>` has the className `bar` it is returned as the *hostNode*. As expected the `<div>` with the className `bar` is also returned
To avoid this you can explicity query for the DOM node: `wrapper.find('div.bar')`. Alternatively if you would like to only find host nodes use [hostNodes()](https://airbnb.io/enzyme/docs/api/ShallowWrapper/hostNodes.html)
For `mount`, updates are sometimes required when they weren't before
---------------------------------------------------------------------
React applications are dynamic. When testing your react components, you often want to test them before *and after* certain state changes take place. When using `mount`, any react component instance in the entire render tree could register code to initiate a state change at any time.
For instance, consider the following contrived example:
```
import React from 'react';
class CurrentTime extends React.Component {
constructor(props) {
super(props);
this.state = {
now: Date.now(),
};
}
componentDidMount() {
this.tick();
}
componentWillUnmount() {
clearTimeout(this.timer);
}
tick() {
this.setState({ now: Date.now() });
this.timer = setTimeout(tick, 0);
}
render() {
const { now } = this.state;
return <span>{now}</span>;
}
}
```
In this code, there is a timer that continuously changes the rendered output of this component. This might be a reasonable thing to do in your application. The thing is, enzyme has no way of knowing that these changes are taking place, and no way to automatically update the render tree. In enzyme v2, enzyme operated *directly* on the in-memory representation of the render tree that React itself had. This means that even though enzyme couldn't know when the render tree was updated, updates would be reflected anyway, since React *does* know.
enzyme v3 architecturally created a layer where React would create an intermediate representation of the render tree at an instance in time and pass that to enzyme to traverse and inspect. This has many advantages, but one of the side effects is that now the intermediate representation does not receive automatic updates.
enzyme does attempt to automatically "update" the root wrapper in most common scenarios, but these are only the state changes that it knows about. For all other state changes, you may need to call `wrapper.update()` yourself.
The most common manifestation of this problem can be shown with the following example:
```
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
this.increment = this.increment.bind(this);
this.decrement = this.decrement.bind(this);
}
increment() {
this.setState(({ count }) => ({ count: count + 1 }));
}
decrement() {
this.setState(({ count }) => ({ count: count - 1 }));
}
render() {
const { count } = this.state;
return (
<div>
<div className="count">Count: {count}</div>
<button type="button" className="inc" onClick={this.increment}>Increment</button>
<button type="button" className="dec" onClick={this.decrement}>Decrement</button>
</div>
);
}
}
```
This is a basic "counter" component in React. Here our resulting markup is a function of `this.state.count`, which can get updated by the `increment` and `decrement` functions. Let's take a look at what some enzyme tests with this component might look like, and when we do or don't have to call `update()`.
```
const wrapper = shallow(<Counter />);
wrapper.find('.count').text(); // => "Count: 0"
```
As we can see, we can easily assert on the text and the count of this component. But we haven't caused any state changes yet. Let's see what it looks like when we simulate a `click` event on the increment and decrement buttons:
```
const wrapper = shallow(<Counter />);
wrapper.find('.count').text(); // => "Count: 0"
wrapper.find('.inc').simulate('click');
wrapper.find('.count').text(); // => "Count: 1"
wrapper.find('.inc').simulate('click');
wrapper.find('.count').text(); // => "Count: 2"
wrapper.find('.dec').simulate('click');
wrapper.find('.count').text(); // => "Count: 1"
```
In this case enzyme will automatically check for updates after an event simulation takes place, as it knows that this is a very common place for state changes to occur. In this case there is no difference between v2 and v3.
Let's consider a different way this test could have been written.
```
const wrapper = shallow(<Counter />);
wrapper.find('.count').text(); // => "Count: 0"
wrapper.instance().increment();
wrapper.find('.count').text(); // => "Count: 0" (would have been "Count: 1" in v2)
wrapper.instance().increment();
wrapper.find('.count').text(); // => "Count: 0" (would have been "Count: 2" in v2)
wrapper.instance().decrement();
wrapper.find('.count').text(); // => "Count: 0" (would have been "Count: 1" in v2)
```
The problem here is that once we grab the instance using `wrapper.instance()`, enzyme has no way of knowing if you are going to execute something that will cause a state transition, and thus does not know when to ask for an updated render tree from React. As a result, `.text()` never changes value.
The fix here is to use enzyme's `wrapper.update()` method after a state change has occurred:
```
const wrapper = shallow(<Counter />);
wrapper.find('.count').text(); // => "Count: 0"
wrapper.instance().increment();
wrapper.update();
wrapper.find('.count').text(); // => "Count: 1"
wrapper.instance().increment();
wrapper.update();
wrapper.find('.count').text(); // => "Count: 2"
wrapper.instance().decrement();
wrapper.update();
wrapper.find('.count').text(); // => "Count: 1"
```
In practice we have found that this isn't actually needed that often, and when it is it is not difficult to add. Additionally, having the enzyme wrapper automatically update alongside the real render tree can result in flaky tests when writing asynchronous tests. This breaking change was worth the architectural benefits of the new adapter system in v3, and we believe is a better choice for an assertion library to take.
`ref(refName)` now returns the actual ref instead of a wrapper
---------------------------------------------------------------
In enzyme v2, the wrapper returned from `mount(...)` had a prototype method on it `ref(refName)` that returned a wrapper around the actual element of that ref. This has now been changed to return the actual ref, which we believe is a more intuitive API.
Consider the following simple react component:
```
class Box extends React.Component {
render() {
return <div ref="abc" className="box">Hello</div>;
}
}
```
In this case we can call `.ref('abc')` on a wrapper of `Box`. In this case it will return a wrapper around the rendered div. To demonstrate, we can see that both `wrapper` and the result of `ref(...)` share the same constructor:
```
const wrapper = mount(<Box />);
// this is what would happen with enzyme v2
expect(wrapper.ref('abc')).toBeInstanceOf(wrapper.constructor);
```
In v3, the contract is slightly changed. The ref is exactly what React would assign as the ref. In this case, it would be a DOM Element:
```
const wrapper = mount(<Box />);
// this is what happens with enzyme v3
expect(wrapper.ref('abc')).toBeInstanceOf(Element);
```
Similarly, if you have a ref on a composite component, the `ref(...)` method will return an instance of that element:
```
class Bar extends React.Component {
render() {
return <Box ref="abc" />;
}
}
```
```
const wrapper = mount(<Bar />);
expect(wrapper.ref('abc')).toBeInstanceOf(Box);
```
In our experience, this is most often what people would actually want and expect out of the `.ref(...)` method.
To get the wrapper that was returned by enzyme 2:
```
const wrapper = mount(<Bar />);
const refWrapper = wrapper.findWhere((n) => n.instance() === wrapper.ref('abc'));
```
With `mount`, `.instance()` can be called at any level of the tree
-------------------------------------------------------------------
enzyme now allows for you to grab the `instance()` of a wrapper at any level of the render tree, not just at the root. This means that you can `.find(...)` a specific component, then grab its instance and call `.setState(...)` or any other methods on the instance that you'd like.
With `mount`, `.getNode()` should not be used. `.instance()` does what it used to.
-----------------------------------------------------------------------------------
For `mount` wrappers, the `.getNode()` method used to return the actual component instance. This method no longer exists, but `.instance()` is functionally equivalent to what `.getNode()` used to be.
With `shallow`, `.getNode()` should be replaced with `getElement()`
--------------------------------------------------------------------
For shallow wrappers, if you were previously using `.getNode()`, you will want to replace those calls with `.getElement()`, which is now functionally equivalent to what `.getNode()` used to do. One caveat is that previously `.getNode()` would return the actual element instance that was created in the `render` function of the component you were testing, but now it will be a structurally equal react element, but not referentially equal. Your tests will need to be updated to account for this.
Private properties and methods have been removed
-------------------------------------------------
There are several properties that are on an enzyme "wrapper" that were considered to be private and were undocumented as a result. Despite being undocumented, people may have been relying on them. In an effort to make making changes less likely to be accidentally breaking in the future, we have decided to make these properties properly "private". The following properties will no longer be accessible on enzyme `shallow` or `mount` instances:
* `.node`
* `.nodes`
* `.renderer`
* `.unrendered`
* `.root`
* `.options`
Cheerio has been updated, thus `render(...)` has been updated as well
----------------------------------------------------------------------
enzyme's top level `render` API returns a [Cheerio](https://github.com/cheeriojs/cheerio) object. The version of Cheerio that we use has been upgraded to 1.0.0. For debugging issues across enzyme v2.x and v3.x with the `render` API, we recommend checking out [Cheerio's Changelog](https://github.com/cheeriojs/cheerio/blob/48eae25c93702a29b8cd0d09c4a2dce2f912d1f4/History.md) and posting an issue on that repo instead of enzyme's unless you believe it is a bug in enzyme's use of the library.
CSS Selector
-------------
enzyme v3 now uses a real CSS selector parser rather than its own incomplete parser implementation. This is done with [rst-selector-parser](https://github.com/aweary/rst-selector-parser) a fork of [scalpel](https://github.com/gajus/scalpel/) which is a CSS parser implemented with [nearley](https://nearley.js.org/). We don't think this should cause any breakages across enzyme v2.x to v3.x, but if you believe you have found something that did indeed break, please file an issue with us. Thank you to [Brandon Dail](https://github.com/aweary) for making this happen!
CSS Selector results and `hostNodes()`
---------------------------------------
enzyme v3 now returns **all** nodes in the result set and not just html nodes. Consider this example:
```
const HelpLink = ({ text, ...rest }) => <a {...rest}>{text}</a>;
const HelpLinkContainer = ({ text, ...rest }) => (
<HelpLink text={text} {...rest} />
);
const wrapper = mount(<HelpLinkContainer aria-expanded="true" text="foo" />);
```
In enzyme v3, the expression `wrapper.find("[aria-expanded=true]").length)` will return 3 and not 1 as in previous versions. A closer look using [`debug`](../api/reactwrapper/debug) reveals:
```
// console.log(wrapper.find('[aria-expanded="true"]').debug());
<HelpLinkContainer aria-expanded={true} text="foo">
<HelpLink text="foo" aria-expanded="true">
<a aria-expanded="true">
foo
</a>
</HelpLink>
</HelpLinkContainer>
<HelpLink text="foo" aria-expanded="true">
<a aria-expanded="true">
foo
</a>
</HelpLink>
<a aria-expanded="true">
foo
</a>
```
To return only the html nodes use the [`hostNodes()`](../api/reactwrapper/hostnodes) function.
`wrapper.find("[aria-expanded=true]").hostNodes().debug()` will now return:
```
<a aria-expanded="true">foo</a>;
```
Node Equality now ignores `undefined` values
---------------------------------------------
We have updated enzyme to consider node "equality" in a semantically identical way to how react treats nodes. More specifically, we've updated enzyme's algorithms to treat `undefined` props as equivalent to the absence of a prop. Consider the following example:
```
class Foo extends React.Component {
render() {
const { foo, bar } = this.props;
return <div className={foo} id={bar} />;
}
}
```
With this component, the behavior in enzyme v2.x the behavior would have been like:
```
const wrapper = shallow(<Foo />);
wrapper.equals(<div />); // => false
wrapper.equals(<div className={undefined} id={undefined} />); // => true
```
With enzyme v3, the behavior is now as follows:
```
const wrapper = shallow(<Foo />);
wrapper.equals(<div />); // => true
wrapper.equals(<div className={undefined} id={undefined} />); // => true
```
Lifecycle methods
------------------
enzyme v2.x had an optional flag that could be passed in to all `shallow` calls which would make it so that more of the component's lifecycle methods were called (such as `componentDidMount` and `componentDidUpdate`).
With enzyme v3, we have now turned on this mode by default, instead of making it opt-in. It is now possible to *opt-out* instead. Additionally, you can now opt-out at a global level.
If you'd like to opt out globally, you can run the following:
```
import Enzyme from 'enzyme';
Enzyme.configure({ disableLifecycleMethods: true });
```
This will default enzyme back to the previous behavior globally. If instead you'd only like to opt enzyme to the previous behavior for a specific test, you can do the following:
```
import { shallow } from 'enzyme';
// ...
const wrapper = shallow(<Component />, { disableLifecycleMethods: true });
```
| programming_docs |
enzyme Using enzyme with Lab and Code Using enzyme with Lab and Code
==============================
[Lab](https://github.com/hapijs/lab) is a simple test utility for node & part of the [Hapi.js](https://github.com/hapijs/hapi) framework universe. Lab's initial code borrowed heavily from [Mocha](https://github.com/mochajs/mocha). [Code](https://github.com/hapijs/code) is Lab's standard assertion library and was created as a direct rewrite of [Chai](https://github.com/chaijs).
Example Test: enzyme + Lab + Code
=================================
```
import { shallow, mount, render } from 'enzyme';
import React from 'react';
const Code = require('code');
const Lab = require('lab');
const lab = Lab.script();
exports.lab = lab;
lab.suite('A suite', () => {
lab.test('calls componentDidMount', (done) => {
const wrapper = mount(<Foo />);
Code.expect(Foo.prototype.componentDidMount.callCount).to.equal(1);
done();
});
});
```
Example Projects
-----------------
* [enzyme-example-lab](https://github.com/gattermeier/enzyme-example-lab)
enzyme Shallow Rendering API Shallow Rendering API
=====================
Shallow rendering is useful to constrain yourself to testing a component as a unit, and to ensure that your tests aren't indirectly asserting on behavior of child components.
As of Enzyme v3, the `shallow` API does call React lifecycle methods such as `componentDidMount` and `componentDidUpdate`. You can read more about this in the [version 3 migration guide](../guides/migration-from-2-to-3#lifecycle-methods).
```
import { shallow } from 'enzyme';
import sinon from 'sinon';
import Foo from './Foo';
describe('<MyComponent />', () => {
it('renders three <Foo /> components', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo)).to.have.lengthOf(3);
});
it('renders an `.icon-star`', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.icon-star')).to.have.lengthOf(1);
});
it('renders children when passed in', () => {
const wrapper = shallow((
<MyComponent>
<div className="unique" />
</MyComponent>
));
expect(wrapper.contains(<div className="unique" />)).to.equal(true);
});
it('simulates click events', () => {
const onButtonClick = sinon.spy();
const wrapper = shallow(<Foo onButtonClick={onButtonClick} />);
wrapper.find('button').simulate('click');
expect(onButtonClick).to.have.property('callCount', 1);
});
});
```
`shallow(node[, options]) => ShallowWrapper`
---------------------------------------------
Arguments
----------
1. `node` (`ReactElement`): The node to render
2. `options` (`Object` [optional]):
* `options.context`: (`Object` [optional]): Context to be passed into the component
* `options.disableLifecycleMethods`: (`Boolean` [optional]): If set to true, `componentDidMount` is not called on the component, and `componentDidUpdate` is not called after [`setProps`](shallowwrapper/setprops) and [`setContext`](shallowwrapper/setcontext). Default to `false`.
* `options.wrappingComponent`: (`ComponentType` [optional]): A component that will render as a parent of the `node`. It can be used to provide context to the `node`, among other things. See the [`getWrappingComponent()` docs](shallowwrapper/getwrappingcomponent) for an example. **Note**: `wrappingComponent` *must* render its children.
* `options.wrappingComponentProps`: (`Object` [optional]): Initial props to pass to the `wrappingComponent` if it is specified.
* `options.suspenseFallback`: (`Boolean` [optional]): If set to true, when rendering `Suspense` enzyme will replace all the lazy components in children with `fallback` element prop. Otherwise it won't handle fallback of lazy component. Default to `true`. Note: not supported in React < 16.6.
Returns
--------
`ShallowWrapper`: The wrapper instance around the rendered output.
ShallowWrapper API
-------------------
[`.find(selector) => ShallowWrapper`](shallowwrapper/find)
-----------------------------------------------------------
Find every node in the render tree that matches the provided selector.
[`.findWhere(predicate) => ShallowWrapper`](shallowwrapper/findwhere)
----------------------------------------------------------------------
Find every node in the render tree that returns true for the provided predicate function.
[`.filter(selector) => ShallowWrapper`](shallowwrapper/filter)
---------------------------------------------------------------
Remove nodes in the current wrapper that do not match the provided selector.
[`.filterWhere(predicate) => ShallowWrapper`](shallowwrapper/filterwhere)
--------------------------------------------------------------------------
Remove nodes in the current wrapper that do not return true for the provided predicate function.
[`.hostNodes() => ShallowWrapper`](shallowwrapper/hostnodes)
-------------------------------------------------------------
Removes nodes that are not host nodes; e.g., this will only return HTML nodes.
[`.contains(nodeOrNodes) => Boolean`](shallowwrapper/contains)
---------------------------------------------------------------
Returns whether or not a given node or array of nodes is somewhere in the render tree.
[`.containsMatchingElement(node) => Boolean`](shallowwrapper/containsmatchingelement)
--------------------------------------------------------------------------------------
Returns whether or not a given react element exists in the shallow render tree.
[`.containsAllMatchingElements(nodes) => Boolean`](shallowwrapper/containsallmatchingelements)
-----------------------------------------------------------------------------------------------
Returns whether or not all the given react elements exist in the shallow render tree.
[`.containsAnyMatchingElements(nodes) => Boolean`](shallowwrapper/containsanymatchingelements)
-----------------------------------------------------------------------------------------------
Returns whether or not one of the given react elements exists in the shallow render tree.
[`.equals(node) => Boolean`](shallowwrapper/equals)
----------------------------------------------------
Returns whether or not the current render tree is equal to the given node, based on the expected value.
[`.matchesElement(node) => Boolean`](shallowwrapper/matcheselement)
--------------------------------------------------------------------
Returns whether or not a given react element matches the shallow render tree.
[`.hasClass(className) => Boolean`](shallowwrapper/hasclass)
-------------------------------------------------------------
Returns whether or not the current node has the given class name or not.
[`.is(selector) => Boolean`](shallowwrapper/is)
------------------------------------------------
Returns whether or not the current node matches a provided selector.
[`.exists([selector]) => Boolean`](shallowwrapper/exists)
----------------------------------------------------------
Returns whether or not the current node exists, or, if given a selector, whether that selector has any matching results.
[`.isEmpty() => Boolean`](shallowwrapper/isempty)
--------------------------------------------------
*Deprecated*: Use [`.exists()`](shallowwrapper/exists) instead.
[`.isEmptyRender() => Boolean`](shallowwrapper/isemptyrender)
--------------------------------------------------------------
Returns whether or not the current component returns a falsy value.
[`.not(selector) => ShallowWrapper`](shallowwrapper/not)
---------------------------------------------------------
Remove nodes in the current wrapper that match the provided selector. (inverse of `.filter()`)
[`.children([selector]) => ShallowWrapper`](shallowwrapper/children)
---------------------------------------------------------------------
Get a wrapper with all of the children nodes of the current wrapper.
[`.childAt(index) => ShallowWrapper`](shallowwrapper/childat)
--------------------------------------------------------------
Returns a new wrapper with child at the specified index.
[`.parents([selector]) => ShallowWrapper`](shallowwrapper/parents)
-------------------------------------------------------------------
Get a wrapper with all of the parents (ancestors) of the current node.
[`.parent() => ShallowWrapper`](shallowwrapper/parent)
-------------------------------------------------------
Get a wrapper with the direct parent of the current node.
[`.closest(selector) => ShallowWrapper`](shallowwrapper/closest)
-----------------------------------------------------------------
Get a wrapper with the first ancestor of the current node to match the provided selector.
[`.shallow([options]) => ShallowWrapper`](shallowwrapper/shallow)
------------------------------------------------------------------
Shallow renders the current node and returns a shallow wrapper around it.
[`.render() => CheerioWrapper`](shallowwrapper/render)
-------------------------------------------------------
Returns a CheerioWrapper of the current node's subtree.
[`.renderProp(key)() => ShallowWrapper`](shallowwrapper/renderprop)
--------------------------------------------------------------------
Returns a wrapper of the node rendered by the provided render prop.
[`.unmount() => ShallowWrapper`](shallowwrapper/unmount)
---------------------------------------------------------
A method that un-mounts the component.
[`.text() => String`](shallowwrapper/text)
-------------------------------------------
Returns a string representation of the text nodes in the current render tree.
[`.html() => String`](shallowwrapper/html)
-------------------------------------------
Returns a static HTML rendering of the current node.
[`.get(index) => ReactElement`](shallowwrapper/get)
----------------------------------------------------
Returns the node at the provided index of the current wrapper.
[`.getElement() => ReactElement`](shallowwrapper/getelement)
-------------------------------------------------------------
Returns the wrapped ReactElement.
[`.getElements() => Array<ReactElement>`](shallowwrapper/getelements)
----------------------------------------------------------------------
Returns the wrapped ReactElements.
[`.at(index) => ShallowWrapper`](shallowwrapper/at)
----------------------------------------------------
Returns a wrapper of the node at the provided index of the current wrapper.
[`.first() => ShallowWrapper`](shallowwrapper/first)
-----------------------------------------------------
Returns a wrapper of the first node of the current wrapper.
[`.last() => ShallowWrapper`](shallowwrapper/last)
---------------------------------------------------
Returns a wrapper of the last node of the current wrapper.
[`.state([key]) => Any`](shallowwrapper/state)
-----------------------------------------------
Returns the state of the root component.
[`.context([key]) => Any`](shallowwrapper/context)
---------------------------------------------------
Returns the context of the root component.
[`.props() => Object`](shallowwrapper/props)
---------------------------------------------
Returns the props of the current node.
[`.prop(key) => Any`](shallowwrapper/prop)
-------------------------------------------
Returns the named prop of the current node.
[`.key() => String`](shallowwrapper/key)
-----------------------------------------
Returns the key of the current node.
[`.invoke(propName)(...args) => Any`](shallowwrapper/invoke)
-------------------------------------------------------------
Invokes a prop function on the current node and returns the function's return value.
[`.simulate(event[, data]) => ShallowWrapper`](shallowwrapper/simulate)
------------------------------------------------------------------------
Simulates an event on the current node.
[`.setState(nextState) => ShallowWrapper`](shallowwrapper/setstate)
--------------------------------------------------------------------
Manually sets state of the root component.
[`.setProps(nextProps[, callback]) => ShallowWrapper`](shallowwrapper/setprops)
--------------------------------------------------------------------------------
Manually sets props of the root component.
[`.setContext(context) => ShallowWrapper`](shallowwrapper/setcontext)
----------------------------------------------------------------------
Manually sets context of the root component.
[`.getWrappingComponent() => ShallowWrapper`](shallowwrapper/getwrappingcomponent)
-----------------------------------------------------------------------------------
Returns a wrapper representing the `wrappingComponent`, if one was passed.
[`.instance() => ReactComponent`](shallowwrapper/instance)
-----------------------------------------------------------
Returns the instance of the root component.
[`.update() => ShallowWrapper`](shallowwrapper/update)
-------------------------------------------------------
Syncs the enzyme component tree snapshot with the react component tree.
[`.debug() => String`](shallowwrapper/debug)
---------------------------------------------
Returns a string representation of the current shallow render tree for debugging purposes.
[`.type() => String|Function|null`](shallowwrapper/type)
---------------------------------------------------------
Returns the type of the current node of the wrapper.
[`.name() => String`](shallowwrapper/name)
-------------------------------------------
Returns the name of the current node of the wrapper.
[`.forEach(fn) => ShallowWrapper`](shallowwrapper/foreach)
-----------------------------------------------------------
Iterates through each node of the current wrapper and executes the provided function
[`.map(fn) => Array`](shallowwrapper/map)
------------------------------------------
Maps the current array of nodes to another array.
[`.reduce(fn[, initialValue]) => Any`](shallowwrapper/reduce)
--------------------------------------------------------------
Reduces the current array of nodes to a value
[`.reduceRight(fn[, initialValue]) => Any`](shallowwrapper/reduceright)
------------------------------------------------------------------------
Reduces the current array of nodes to a value, from right to left.
[`.slice([begin[, end]]) => ShallowWrapper`](shallowwrapper/slice)
-------------------------------------------------------------------
Returns a new wrapper with a subset of the nodes of the original wrapper, according to the rules of `Array#slice`.
[`.tap(intercepter) => Self`](shallowwrapper/tap)
--------------------------------------------------
Taps into the wrapper method chain. Helpful for debugging.
[`.some(selector) => Boolean`](shallowwrapper/some)
----------------------------------------------------
Returns whether or not any of the nodes in the wrapper match the provided selector.
[`.someWhere(predicate) => Boolean`](shallowwrapper/somewhere)
---------------------------------------------------------------
Returns whether or not any of the nodes in the wrapper pass the provided predicate function.
[`.every(selector) => Boolean`](shallowwrapper/every)
------------------------------------------------------
Returns whether or not all of the nodes in the wrapper match the provided selector.
[`.everyWhere(predicate) => Boolean`](shallowwrapper/everywhere)
-----------------------------------------------------------------
Returns whether or not all of the nodes in the wrapper pass the provided predicate function.
[`.dive([options]) => ShallowWrapper`](shallowwrapper/dive)
------------------------------------------------------------
Shallow render the one non-DOM child of the current wrapper, and return a wrapper around the result.
enzyme Full Rendering API (mount(...)) Full Rendering API (mount(...))
===============================
Full DOM rendering is ideal for use cases where you have components that may interact with DOM APIs or need to test components that are wrapped in higher order components.
Full DOM rendering requires that a full DOM API be available at the global scope. This means that it must be run in an environment that at least “looks like” a browser environment. If you do not want to run your tests inside of a browser, the recommended approach to using `mount` is to depend on a library called [jsdom](https://github.com/tmpvar/jsdom) which is essentially a headless browser implemented completely in JS.
**Note**: unlike shallow or static rendering, full rendering actually mounts the component in the DOM, which means that tests can affect each other if they are all using the same DOM. Keep that in mind while writing your tests and, if necessary, use [`.unmount()`](reactwrapper/unmount) or something similar as cleanup.
```
import { mount } from 'enzyme';
import sinon from 'sinon';
import Foo from './Foo';
describe('<Foo />', () => {
it('calls componentDidMount', () => {
sinon.spy(Foo.prototype, 'componentDidMount');
const wrapper = mount(<Foo />);
expect(Foo.prototype.componentDidMount).to.have.property('callCount', 1);
});
it('allows us to set props', () => {
const wrapper = mount(<Foo bar="baz" />);
expect(wrapper.props().bar).to.equal('baz');
wrapper.setProps({ bar: 'foo' });
expect(wrapper.props().bar).to.equal('foo');
});
it('simulates click events', () => {
const onButtonClick = sinon.spy();
const wrapper = mount((
<Foo onButtonClick={onButtonClick} />
));
wrapper.find('button').simulate('click');
expect(onButtonClick).to.have.property('callCount', 1);
});
});
```
`mount(node[, options]) => ReactWrapper`
-----------------------------------------
Arguments
----------
1. `node` (`ReactElement`): The node to render
2. `options` (`Object` [optional]):
3. `options.context`: (`Object` [optional]): Context to be passed into the component
4. `options.attachTo`: (`DOMElement` [optional]): DOM Element to attach the component to.
5. `options.childContextTypes`: (`Object` [optional]): Merged contextTypes for all children of the wrapper.
6. `options.wrappingComponent`: (`ComponentType` [optional]): A component that will render as a parent of the `node`. It can be used to provide context to the `node`, among other things. See the [`getWrappingComponent()` docs](reactwrapper/getwrappingcomponent) for an example. **Note**: `wrappingComponent` *must* render its children.
7. `options.wrappingComponentProps`: (`Object` [optional]): Initial props to pass to the `wrappingComponent` if it is specified.
Returns
--------
`ReactWrapper`: The wrapper instance around the rendered output.
ReactWrapper API
-----------------
[`.find(selector) => ReactWrapper`](reactwrapper/find)
-------------------------------------------------------
Find every node in the render tree that matches the provided selector.
[`.findWhere(predicate) => ReactWrapper`](reactwrapper/findwhere)
------------------------------------------------------------------
Find every node in the render tree that returns true for the provided predicate function.
[`.filter(selector) => ReactWrapper`](reactwrapper/filter)
-----------------------------------------------------------
Remove nodes in the current wrapper that do not match the provided selector.
[`.filterWhere(predicate) => ReactWrapper`](reactwrapper/filterwhere)
----------------------------------------------------------------------
Remove nodes in the current wrapper that do not return true for the provided predicate function.
[`.hostNodes() => ReactWrapper`](reactwrapper/hostnodes)
---------------------------------------------------------
Removes nodes that are not host nodes; e.g., this will only return HTML nodes.
[`.contains(nodeOrNodes) => Boolean`](reactwrapper/contains)
-------------------------------------------------------------
Returns whether or not a given node or array of nodes exists in the render tree.
[`.containsMatchingElement(node) => Boolean`](reactwrapper/containsmatchingelement)
------------------------------------------------------------------------------------
Returns whether or not a given react element exists in the render tree.
[`.containsAllMatchingElements(nodes) => Boolean`](reactwrapper/containsallmatchingelements)
---------------------------------------------------------------------------------------------
Returns whether or not all the given react elements exist in the render tree.
[`.containsAnyMatchingElements(nodes) => Boolean`](reactwrapper/containsanymatchingelements)
---------------------------------------------------------------------------------------------
Returns whether or not one of the given react elements exist in the render tree.
[`.equals(node) => Boolean`](reactwrapper/equals)
--------------------------------------------------
Returns whether or not the current wrapper root node render tree looks like the one passed in.
[`.hasClass(className) => Boolean`](reactwrapper/hasclass)
-----------------------------------------------------------
Returns whether or not the current root node has the given class name or not.
[`.is(selector) => Boolean`](reactwrapper/is)
----------------------------------------------
Returns whether or not the current node matches a provided selector.
[`.exists([selector]) => Boolean`](reactwrapper/exists)
--------------------------------------------------------
Returns whether or not the current node exists, or, if given a selector, whether that selector has any matching results.
[`.isEmpty() => Boolean`](reactwrapper/isempty)
------------------------------------------------
*Deprecated*: Use [`.exists()`](reactwrapper/exists) instead.
[`.isEmptyRender() => Boolean`](reactwrapper/isemptyrender)
------------------------------------------------------------
Returns whether or not the current component returns a falsy value.
[`.not(selector) => ReactWrapper`](reactwrapper/not)
-----------------------------------------------------
Remove nodes in the current wrapper that match the provided selector. (inverse of `.filter()`)
[`.children([selector]) => ReactWrapper`](reactwrapper/children)
-----------------------------------------------------------------
Get a wrapper with all of the children nodes of the current wrapper.
[`.childAt(index) => ReactWrapper`](reactwrapper/childat)
----------------------------------------------------------
Returns a new wrapper with child at the specified index.
[`.parents([selector]) => ReactWrapper`](reactwrapper/parents)
---------------------------------------------------------------
Get a wrapper with all of the parents (ancestors) of the current node.
[`.parent() => ReactWrapper`](reactwrapper/parent)
---------------------------------------------------
Get a wrapper with the direct parent of the current node.
[`.closest(selector) => ReactWrapper`](reactwrapper/closest)
-------------------------------------------------------------
Get a wrapper with the first ancestor of the current node to match the provided selector.
[`.render() => CheerioWrapper`](reactwrapper/render)
-----------------------------------------------------
Returns a CheerioWrapper of the current node's subtree.
[`.renderProp(key)() => ReactWrapper`](reactwrapper/renderprop)
----------------------------------------------------------------
Returns a wrapper of the node rendered by the provided render prop.
[`.text() => String`](reactwrapper/text)
-----------------------------------------
Returns a string representation of the text nodes in the current render tree.
[`.html() => String`](reactwrapper/html)
-----------------------------------------
Returns a static HTML rendering of the current node.
[`.get(index) => ReactElement`](reactwrapper/get)
--------------------------------------------------
Returns the node at the provided index of the current wrapper.
[`.getDOMNode() => DOMComponent`](reactwrapper/getdomnode)
-----------------------------------------------------------
Returns the outer most DOMComponent of the current wrapper.
[`.at(index) => ReactWrapper`](reactwrapper/at)
------------------------------------------------
Returns a wrapper of the node at the provided index of the current wrapper.
[`.first() => ReactWrapper`](reactwrapper/first)
-------------------------------------------------
Returns a wrapper of the first node of the current wrapper.
[`.last() => ReactWrapper`](reactwrapper/last)
-----------------------------------------------
Returns a wrapper of the last node of the current wrapper.
[`.state([key]) => Any`](reactwrapper/state)
---------------------------------------------
Returns the state of the root component.
[`.context([key]) => Any`](reactwrapper/context)
-------------------------------------------------
Returns the context of the root component.
[`.props() => Object`](reactwrapper/props)
-------------------------------------------
Returns the props of the root component.
[`.prop(key) => Any`](reactwrapper/prop)
-----------------------------------------
Returns the named prop of the root component.
[`.invoke(propName)(...args) => Any`](reactwrapper/invoke)
-----------------------------------------------------------
Invokes a prop function on the current node and returns the function's return value.
[`.key() => String`](reactwrapper/key)
---------------------------------------
Returns the key of the root component.
[`.simulate(event[, mock]) => ReactWrapper`](reactwrapper/simulate)
--------------------------------------------------------------------
Simulates an event on the current node.
[`.setState(nextState) => ReactWrapper`](reactwrapper/setstate)
----------------------------------------------------------------
Manually sets state of the root component.
[`.setProps(nextProps[, callback]) => ReactWrapper`](reactwrapper/setprops)
----------------------------------------------------------------------------
Manually sets props of the root component.
[`.setContext(context) => ReactWrapper`](reactwrapper/setcontext)
------------------------------------------------------------------
Manually sets context of the root component.
[`.instance() => ReactComponent|DOMComponent`](reactwrapper/instance)
----------------------------------------------------------------------
Returns the wrapper's underlying instance.
[`.getWrappingComponent() => ReactWrapper`](reactwrapper/getwrappingcomponent)
-------------------------------------------------------------------------------
Returns a wrapper representing the `wrappingComponent`, if one was passed.
[`.unmount() => ReactWrapper`](reactwrapper/unmount)
-----------------------------------------------------
A method that un-mounts the component.
[`.mount() => ReactWrapper`](reactwrapper/mount)
-------------------------------------------------
A method that re-mounts the component.
[`.update() => ReactWrapper`](reactwrapper/update)
---------------------------------------------------
Syncs the enzyme component tree snapshot with the react component tree.
[`.debug() => String`](reactwrapper/debug)
-------------------------------------------
Returns a string representation of the current render tree for debugging purposes.
[`.type() => String|Function`](reactwrapper/type)
--------------------------------------------------
Returns the type of the current node of the wrapper.
[`.name() => String`](reactwrapper/name)
-----------------------------------------
Returns the name of the current node of the wrapper.
[`.forEach(fn) => ReactWrapper`](reactwrapper/foreach)
-------------------------------------------------------
Iterates through each node of the current wrapper and executes the provided function
[`.map(fn) => Array`](reactwrapper/map)
----------------------------------------
Maps the current array of nodes to another array.
[`.matchesElement(node) => Boolean`](reactwrapper/matcheselement)
------------------------------------------------------------------
Returns whether or not a given react element matches the current render tree.
[`.reduce(fn[, initialValue]) => Any`](reactwrapper/reduce)
------------------------------------------------------------
Reduces the current array of nodes to a value
[`.reduceRight(fn[, initialValue]) => Any`](reactwrapper/reduceright)
----------------------------------------------------------------------
Reduces the current array of nodes to a value, from right to left.
[`.slice([begin[, end]]) => ReactWrapper`](reactwrapper/slice)
---------------------------------------------------------------
Returns a new wrapper with a subset of the nodes of the original wrapper, according to the rules of `Array#slice`.
[`.tap(intercepter) => Self`](reactwrapper/tap)
------------------------------------------------
Taps into the wrapper method chain. Helpful for debugging.
[`.some(selector) => Boolean`](reactwrapper/some)
--------------------------------------------------
Returns whether or not any of the nodes in the wrapper match the provided selector.
[`.someWhere(predicate) => Boolean`](reactwrapper/somewhere)
-------------------------------------------------------------
Returns whether or not any of the nodes in the wrapper pass the provided predicate function.
[`.every(selector) => Boolean`](reactwrapper/every)
----------------------------------------------------
Returns whether or not all of the nodes in the wrapper match the provided selector.
[`.everyWhere(predicate) => Boolean`](reactwrapper/everywhere)
---------------------------------------------------------------
Returns whether or not all of the nodes in the wrapper pass the provided predicate function.
[`.ref(refName) => ReactComponent | HTMLElement`](reactwrapper/ref)
--------------------------------------------------------------------
Returns the node that matches the provided reference name.
[`.detach() => void`](reactwrapper/detach)
-------------------------------------------
Unmount the component from the DOM node it's attached to.
| programming_docs |
enzyme enzyme Selectors enzyme Selectors
================
Many methods in enzyme’s API accept a *selector* as an argument. You can select several different ways:
1. A Valid CSS Selector
------------------------
enzyme supports a subset of valid CSS selectors to find nodes inside a render tree. Support is as follows:
* class syntax (`.foo`, `.foo-bar`, etc.)
* element tag name syntax (`input`, `div`, `span`, etc.)
* id syntax (`#foo`, `#foo-bar`, etc.)
* attribute syntax (`[href="foo"]`, `[type="text"]`, and the other attribute selectors listed [here](https://developer.mozilla.org/en-US/docs/Learn/CSS/Introduction_to_CSS/Attribute_selectors).)
* universal syntax (`*`)
* React component name and props (`Button`, `Button[type="submit"]`, etc) - however, please note that it is strongly encouraged to find by component constructor/function and not by display name.
The attribute syntax also works by value, rather than by string. Strings, numbers, and boolean property values are supported. Example:
```
const wrapper = mount((
<div>
<span anum={3} abool={false} />
<span anum="3" abool="false" />
</div>
));
```
The selector `[anum=3]` will select the first but not the second, because there's no quotes surrounding the 3. The selector `[anum="3"]` will select the second, because it's explicitly looking for a string because of the quotes surrounding 3. The same goes for the boolean; [abool=false] will select the first but not the second, etc.
Further, enzyme supports combining any of those supported syntaxes together, as with CSS:
```
div.foo.bar
input#input-name
a[href="foo"]
.foo .bar
.foo > .bar
.foo + .bar
.foo ~ .bar
.foo input
```
**React Key and Ref Props**
While in most cases, any React prop can be used, there are exceptions. The `key` and `ref` props will never work; React uses these props internally.
**Want more CSS support?**
PRs implementing more support for CSS selectors will be accepted and is an area of development for enzyme that will likely be focused on in the future.
2. A React Component Constructor
---------------------------------
enzyme allows you to find React components based on their constructor. You can pass in the reference to the component’s constructor. Of course, this kind of selector only checks the component type; it ignores props and children.
```
function MyComponent() {
return <div />;
}
// find instances of MyComponent
const myComponents = wrapper.find(MyComponent);
```
3. A React Component’s displayName
-----------------------------------
enzyme allows you to find components based on a component’s `displayName`. If a component exists in a render tree where its `displayName` is set and has its first character as a capital letter, you can use a string to find it:
```
function MyComponent() {
return <div />;
}
MyComponent.displayName = 'My Component';
// find instances of MyComponent
const myComponents = wrapper.find('My Component');
```
NOTE: This will *only* work if the selector (and thus the component’s `displayName`) is a string starting with a capital letter. Strings starting with lower case letters will be assumed to be a CSS selector (therefore a tag name).
Selecting a HOC-wrapped component, or a component with a custom `displayName`, even with lowercase letters (for example, `withHOC(MyComponent)`) will work as well.
4. Object Property Selector
----------------------------
enzyme allows you to find components and nodes based on a subset of their properties:
```
const wrapper = mount((
<div>
<span foo={3} bar={false} title="baz" />
</div>
));
wrapper.find({ foo: 3 });
wrapper.find({ bar: false });
wrapper.find({ title: 'baz' });
```
**Undefined Properties**
Undefined properties are not allowed in the object property selector and will cause an error:
```
wrapper.find({ foo: 3, bar: undefined });
// => TypeError: Enzyme::Props can't have 'undefined' values. Try using 'findWhere()' instead.
```
If you have to search by `undefined` property value, use [`.findWhere()`](shallowwrapper/findwhere).
enzyme Static Rendering API Static Rendering API
====================
Use enzyme's `render` function to generate HTML from your React tree, and analyze the resulting HTML structure.
`render` returns a wrapper very similar to the other renderers in enzyme, [`mount`](mount) and [`shallow`](shallow); however, `render` uses a third party HTML parsing and traversal library [Cheerio](http://cheeriojs.github.io/cheerio/). We believe that Cheerio handles parsing and traversing HTML extremely well, and duplicating this functionality ourselves would be a disservice.
For the purposes of this documentation, we will refer to Cheerio's constructor as `CheerioWrapper`, which is to say that it is analogous to our `ReactWrapper` and `ShallowWrapper` constructors. You can reference the [Cheerio API docs](https://github.com/cheeriojs/cheerio#api) for methods available on a `CheerioWrapper` instance.
Example Usage
--------------
```
import React from 'react';
import { render } from 'enzyme';
import PropTypes from 'prop-types';
describe('<Foo />', () => {
it('renders three `.foo-bar`s', () => {
const wrapper = render(<Foo />);
expect(wrapper.find('.foo-bar')).to.have.lengthOf(3);
});
it('rendered the title', () => {
const wrapper = render(<Foo title="unique" />);
expect(wrapper.text()).to.contain('unique');
});
it('renders a div', () => {
const wrapper = render(<div className="myClass" />);
expect(wrapper.html()).to.contain('div');
});
it('can pass in context', () => {
function SimpleComponent(props, context) {
const { name } = context;
return <div>{name}</div>;
}
SimpleComponent.contextTypes = {
name: PropTypes.string,
};
const context = { name: 'foo' };
const wrapper = render(<SimpleComponent />, { context });
expect(wrapper.text()).to.equal('foo');
});
});
```
enzyme .setContext(context) => Self .setContext(context) => Self
============================
A method that sets the context of the root component, and re-renders. Useful for when you are wanting to test how the component behaves over time with changing contexts.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `context` (`Object`): An object containing new props to merge in with the current state
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
import React from 'react';
import PropTypes from 'prop-types';
function SimpleComponent(props, context) {
const { name } = context;
return <div>{name}</div>;
}
SimpleComponent.contextTypes = {
name: PropTypes.string,
};
```
```
const context = { name: 'foo' };
const wrapper = shallow(<SimpleComponent />, { context });
expect(wrapper.text()).to.equal('foo');
wrapper.setContext({ name: 'bar' });
expect(wrapper.text()).to.equal('bar');
wrapper.setContext({ name: 'baz' });
expect(wrapper.text()).to.equal('baz');
```
Common Gotchas
---------------
* `.setContext()` can only be used on a wrapper that was initially created with a call to `shallow()` that includes a `context` specified in the options argument.
* The root component you are rendering must have a `contextTypes` static property.
Related Methods
----------------
* [`.setState(state[, callback]) => Self`](setstate)
* [`.setProps(props[, callback]) => Self`](setprops)
enzyme .childAt(index) => ShallowWrapper .childAt(index) => ShallowWrapper
=================================
Returns a new wrapper with child at the specified index.
Arguments
----------
1. `index` (`number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the resulting node.
Examples
---------
```
const wrapper = shallow(<ToDoList items={items} />);
expect(wrapper.find('ul').childAt(0).type()).to.equal('li');
```
Related Methods
----------------
* [`.parents([selector]) => ShallowWrapper`](parents)
* [`.parent() => ShallowWrapper`](parent)
* [`.closest(selector) => ShallowWrapper`](closest)
* [`.children([selector]) => ShallowWrapper`](children)
enzyme .setState(nextState[, callback]) => Self .setState(nextState[, callback]) => Self
========================================
A method to invoke `setState()` on the root component instance, similar to how you might in the methods of the component, and re-renders. This method is useful for testing your component in hard-to-achieve states, however should be used sparingly. If possible, you should utilize your component's external API (which is often accessible via [`.instance()`](instance)) in order to get it into whatever state you want to test, in order to be as accurate of a test as possible. This is not always practical, however.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `nextState` (`Object`): An object containing new state to merge in with the current state
2. `callback` (`Function` [optional]): If provided, the callback function will be executed once setState has completed
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
class Foo extends React.Component {
constructor(props) {
super(props);
this.state = { name: 'foo' };
}
render() {
const { name } = this.state;
return (
<div className={name} />
);
}
}
```
```
const wrapper = shallow(<Foo />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(0);
wrapper.setState({ name: 'bar' });
expect(wrapper.find('.foo')).to.have.lengthOf(0);
expect(wrapper.find('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.setProps(props[, callback]) => Self`](setprops)
* [`.setContext(context) => Self`](setcontext)
enzyme .contains(nodeOrNodes) => Boolean .contains(nodeOrNodes) => Boolean
=================================
Returns whether or not all given react elements match elements in the render tree. It will determine if an element in the wrapper matches the expected element by checking if the expected element has the same props as the wrapper's element and share the same values.
Arguments
----------
1. `nodeOrNodes` (`ReactElement|Array<ReactElement>`): The node or array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has nodes anywhere in its render tree that match the ones passed in.
Example
--------
```
const wrapper = shallow((
<div>
<div data-foo="foo" data-bar="bar">Hello</div>
</div>
));
expect(wrapper.contains(<div data-foo="foo" data-bar="bar">Hello</div>)).to.equal(true);
expect(wrapper.contains(<div data-foo="foo">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="bar" data-baz="baz">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="Hello">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="bar" />)).to.equal(false);
```
```
const wrapper = shallow((
<div>
<span>Hello</span>
<div>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.contains([
<span>Hello</span>,
<div>Goodbye</div>,
])).to.equal(true);
expect(wrapper.contains([
<span>Hello</span>,
<div>World</div>,
])).to.equal(false);
```
```
const calculatedValue = 2 + 2;
const wrapper = shallow((
<div>
<div data-foo="foo" data-bar="bar">{calculatedValue}</div>
</div>
));
expect(wrapper.contains(<div data-foo="foo" data-bar="bar">{4}</div>)).to.equal(true);
```
Common Gotchas
---------------
* `.contains()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
enzyme .map(fn) => Array<Any> .map(fn) => Array<Any>
======================
Maps the current array of nodes to another array. Each node is passed in as a `ShallowWrapper` to the map function.
Arguments
----------
1. `fn` (`Function ( ShallowWrapper node, Number index ) => Any`): A mapping function to be run for every node in the collection, the results of which will be mapped to the returned array. Should expect a ShallowWrapper as the first argument, and will be run with a context of the original instance.
Returns
--------
`Array<Any>`: Returns an array of the returned values from the mapping function..
Example
--------
```
const wrapper = shallow((
<div>
<div className="foo">bax</div>
<div className="foo">bar</div>
<div className="foo">baz</div>
</div>
));
const texts = wrapper.find('.foo').map((node) => node.text());
expect(texts).to.eql(['bax', 'bar', 'baz']);
```
Related Methods
----------------
* [`.forEach(fn) => ShallowWrapper`](foreach)
* [`.reduce(fn[, initialValue]) => Any`](reduce)
* [`.reduceRight(fn[, initialValue]) => Any`](reduceright)
enzyme .children([selector]) => ShallowWrapper .children([selector]) => ShallowWrapper
=======================================
Returns a new wrapper with all of the children of the node(s) in the current wrapper. Optionally, a selector can be provided and it will filter the children by this selector
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): A selector to filter the children by.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = shallow(<ToDoList items={items} />);
expect(wrapper.find('ul').children()).to.have.lengthOf(items.length);
```
Related Methods
----------------
* [`.parents([selector]) => ShallowWrapper`](parents)
* [`.parent() => ShallowWrapper`](parent)
* [`.closest(selector) => ShallowWrapper`](closest)
enzyme .prop(key) => Any .prop(key) => Any
=================
Returns the prop value for the root node of the wrapper with the provided key. It must be a single-node wrapper.
NOTE: When called on a shallow wrapper, `.prop(key)` will return values for props on the root node that the component *renders*, not the component itself. To return the props for the entire React component, use `wrapper.instance().props`. See [`.instance() => ReactComponent`](instance)
Arguments
----------
1. `key` (`String`): The prop name, that is, `this.props[key]` or `props[key]` for the root node of the wrapper.
Example
--------
```
import PropTypes from 'prop-types';
import ValidateNumberInputComponent from './ValidateNumberInputComponent';
class MyComponent extends React.Component {
constructor(...args) {
super(...args);
this.state = {
number: 0,
};
this.onValidNumberInput = this.onValidNumberInput.bind(this);
}
onValidNumberInput(e) {
const number = e.target.value;
if (!number || typeof number === 'number') {
this.setState({ number });
}
}
render() {
const { includedProp } = this.props;
const { number } = this.state;
return (
<div className="foo bar" includedProp={includedProp}>
<ValidateNumberInputComponent onChangeHandler={onValidNumberInput} number={number} />
</div>
);
}
}
MyComponent.propTypes = {
includedProp: PropTypes.string.isRequired,
};
const wrapper = shallow(<MyComponent includedProp="Success!" excludedProp="I'm not included" />);
expect(wrapper.prop('includedProp')).to.equal('Success!');
const validInput = 1;
wrapper.find('ValidateNumberInputComponent').prop('onChangeHandler')(validInput);
expect(wrapper.state('number')).to.equal(number);
const invalidInput = 'invalid input';
wrapper.find('ValidateNumberInputComponent').prop('onChangeHandler')(invalidInput);
expect(wrapper.state('number')).to.equal(0);
// Warning: .prop(key) only returns values for props that exist in the root node.
// See the note above about wrapper.instance().props to return all props in the React component.
console.log(wrapper.prop('includedProp'));
// "Success!"
console.log(wrapper.prop('excludedProp'));
// undefined
console.log(wrapper.instance().props.excludedProp);
// "I'm not included"
```
Related Methods
----------------
* [`.props() => Object`](props)
* [`.state([key]) => Any`](state)
* [`.context([key]) => Any`](context)
enzyme .everyWhere(fn) => Boolean .everyWhere(fn) => Boolean
==========================
Returns whether or not all of the nodes in the wrapper pass the provided predicate function.
Arguments
----------
1. `predicate` (`ShallowWrapper => Boolean`): A predicate function to match the nodes.
Returns
--------
`Boolean`: True if every node in the current wrapper passed the predicate function.
Example
--------
```
const wrapper = shallow((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('foo'))).to.equal(true);
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('qoo'))).to.equal(false);
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('bar'))).to.equal(false);
```
Related Methods
----------------
* [`.some(selector) => Boolean`](some)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .text() => String .text() => String
=================
Returns a string of the rendered text of the current render tree. This function should be looked at with skepticism if being used to test what the actual HTML output of the component will be. If that is what you would like to test, use enzyme's `render` function instead.
Note: can only be called on a wrapper of a single node.
Returns
--------
`String`: The resulting string
Examples
---------
```
const wrapper = shallow(<div><b>important</b></div>);
expect(wrapper.text()).to.equal('important');
```
```
const wrapper = shallow(<div><Foo /><b>important</b></div>);
expect(wrapper.text()).to.equal('<Foo />important');
```
Related Methods
----------------
[`.html() => String`](html)
enzyme .exists([selector]) => Boolean .exists([selector]) => Boolean
==============================
Returns whether or not any nodes exist in the wrapper. Or, if a selector is passed in, whether that selector has any matches in the wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): The selector to check existence for.
Returns
--------
`Boolean`: whether or not any nodes are on the list, or the selector had any matches.
Example
--------
```
const wrapper = mount(<div className="some-class" />);
expect(wrapper.exists('.some-class')).to.equal(true);
expect(wrapper.find('.other-class').exists()).to.equal(false);
```
enzyme .last() => ShallowWrapper .last() => ShallowWrapper
=========================
Reduce the set of matched nodes to the last in the set, just like `.at(length - 1)`.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the last node in the set.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo).last().props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ShallowWrapper`](at) - retrieve a wrapper node by index
* [`.first() => ShallowWrapper`](first)
enzyme .update() => Self .update() => Self
=================
Syncs the enzyme component tree snapshot with the react component tree. Useful to run before checking the render output if something external may be updating the state of the component somewhere.
NOTE: can only be called on a wrapper instance that is also the root instance.
NOTE: this does not force a re-render. Use `wrapper.setProps({})` to force a re-render.
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
class ImpureRender extends React.Component {
constructor(props) {
super(props);
this.count = 0;
}
render() {
this.count += 1;
return <div>{this.count}</div>;
}
}
```
```
const wrapper = shallow(<ImpureRender />);
expect(wrapper.text()).to.equal('0');
wrapper.update();
expect(wrapper.text()).to.equal('1');
```
| programming_docs |
enzyme .getElements() => Array<ReactElement> .getElements() => Array<ReactElement>
=====================================
Returns the wrapped ReactElements
If the current wrapper is wrapping the root component, returns the root component's latest render output wrapped in an array.
Returns
--------
`Array<ReactElement>`: The retrieved ReactElements.
Examples
---------
```
const one = <span />;
const two = <span />;
function Test() {
return (
<div>
{one}
{two}
</div>
);
}
const wrapper = shallow(<Test />);
expect(wrapper.find('span').getElements()).to.deep.equal([one, two]);
```
Related Methods
----------------
* [`.getElement() => ReactElement`](getelement)
enzyme .shallow([options]) => ShallowWrapper .shallow([options]) => ShallowWrapper
=====================================
Shallow renders the root node and returns a shallow wrapper around it. It must be a single-node wrapper.
Arguments
----------
1. `options` (`Object` [optional]):
* `options.context`: (`Object` [optional]): Context to be passed into the component
* `options.disableLifecycleMethods`: (`Boolean` [optional]): If set to true, `componentDidMount` is not called on the component, and `componentDidUpdate` is not called after [`setProps`](shallowwrapper/setprops.md) and [`setContext`](shallowwrapper/setcontext.md). Default to `false`.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the node after it's been shallow rendered.
Examples
---------
```
function Bar() {
return (
<div>
<div className="in-bar" />
</div>
);
}
```
```
function Foo() {
return (
<div>
<Bar />
</div>
);
}
```
```
const wrapper = shallow(<Foo />);
expect(wrapper.find('.in-bar')).to.have.lengthOf(0);
expect(wrapper.find(Bar)).to.have.lengthOf(1);
expect(wrapper.find(Bar).shallow().find('.in-bar')).to.have.lengthOf(1);
```
enzyme .type() => String | Function | null .type() => String | Function | null
===================================
Returns the type of the only node of this wrapper. If it's a React component, this will be the component constructor. If it's a native DOM node, it will be a string with the tag name. If it's `null`, it will be `null`. It must be a single-node wrapper.
Returns
--------
`String | Function | null`: The type of the node
Examples
---------
```
function Foo() {
return <div />;
}
const wrapper = shallow(<Foo />);
expect(wrapper.type()).to.equal('div');
```
```
function Foo() {
return (
<div>
<button type="button" className="btn">Button</button>
</div>
);
}
const wrapper = shallow(<Foo />);
expect(wrapper.find('.btn').type()).to.equal('button');
```
```
function Foo() {
return <Bar />;
}
const wrapper = shallow(<Foo />);
expect(wrapper.type()).to.equal(Bar);
```
```
function Null() {
return null;
}
const wrapper = shallow(<Null />);
expect(wrapper.type()).to.equal(null);
```
enzyme .html() => String .html() => String
=================
Returns a string of the rendered HTML markup of the entire current render tree (not just the shallow-rendered part). It uses [static rendering](../render) internally. To see only the shallow-rendered part use [`.debug()`](debug).
Note: can only be called on a wrapper of a single node.
Returns
--------
`String`: The resulting HTML string
Examples
---------
```
function Foo() {
return (<div className="in-foo" />);
}
```
```
function Bar() {
return (
<div className="in-bar">
<Foo />
</div>
);
}
```
```
const wrapper = shallow(<Bar />);
expect(wrapper.html()).to.equal('<div class="in-bar"><div class="in-foo"></div></div>');
expect(wrapper.find(Foo).html()).to.equal('<div class="in-foo"></div>');
```
```
const wrapper = shallow(<div><b>important</b></div>);
expect(wrapper.html()).to.equal('<div><b>important</b></div>');
```
Related Methods
----------------
* [`.text() => String`](text)
* [`.debug() => String`](debug)
enzyme .invoke(invokePropName)(...args) => Any .invoke(invokePropName)(...args) => Any
=======================================
Invokes a function prop.
Arguments
----------
1. `propName` (`String`): The function prop that is invoked
2. `...args` (`Any` [optional]): Arguments that is passed to the prop function
This essentially calls wrapper.prop(propName)(...args).
Returns
--------
`Any`: Returns the value from the prop function
Example
--------
```
class Foo extends React.Component {
loadData() {
return fetch();
}
render() {
return (
<div>
<button
type="button"
onClick={() => this.loadData()}
>
Load more
</button>
</div>
);
}
}
const wrapper = shallow(<Foo />);
wrapper.find('button').invoke('onClick')().then(() => {
// expect()
});
```
enzyme .props() => Object .props() => Object
==================
Returns the props object for the root node of the wrapper. It must be a single-node wrapper.
NOTE: When called on a shallow wrapper, `.props()` will return values for props on the root node that the component *renders*, not the component itself.
This method is a reliable way of accessing the props of a node; `wrapper.instance().props` will work as well, but in React 16+, stateless functional components do not have an instance. See [`.instance() => ReactComponent`](instance)
Example
--------
```
import PropTypes from 'prop-types';
function MyComponent(props) {
const { includedProp } = props;
return (
<div className="foo bar" includedProp={includedProp}>Hello</div>
);
}
MyComponent.propTypes = {
includedProp: PropTypes.string.isRequired,
};
const wrapper = shallow(<MyComponent includedProp="Success!" excludedProp="I'm not included" />);
expect(wrapper.props().includedProp).to.equal('Success!');
// Warning: .props() only returns props that are passed to the root node,
// which does not include excludedProp in this example.
// See the note above about wrapper.instance().props.
console.log(wrapper.props());
// {children: "Hello", className: "foo bar", includedProp="Success!"}
console.log(wrapper.instance().props); // React 15.x - working as expected
// {children: "Hello", className: "foo bar", includedProp:"Success!", excludedProp: "I'm not included"}
console.log(wrapper.instance().props);
// React 16.* - Uncaught TypeError: Cannot read property 'props' of null
```
Related Methods
----------------
* [`.prop(key) => Any`](prop)
* [`.state([key]) => Any`](state)
* [`.context([key]) => Any`](context)
enzyme .isEmpty() => Boolean .isEmpty() => Boolean
=====================
**Deprecated**: Use [`.exists()`](exists) instead.
Returns whether or not the wrapper is empty.
Returns
--------
`Boolean`: whether or not the wrapper is empty.
Example
--------
```
const wrapper = shallow(<div className="some-class" />);
expect(wrapper.find('.other-class').isEmpty()).to.equal(true);
```
enzyme .at(index) => ShallowWrapper .at(index) => ShallowWrapper
============================
Returns a wrapper around the node at a given index of the current wrapper.
Arguments
----------
1. `index` (`Number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the retrieved node.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo).at(0).props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.get(index) => ReactElement`](get) - same, but returns the React node itself, with no wrapper.
* [`.first() => ShallowWrapper`](first) - same as at(0)
* [`.last() => ShallowWrapper`](last)
enzyme .simulate(event[, ...args]) => Self .simulate(event[, ...args]) => Self
===================================
Simulate events on the root node in the wrapper. It must be a single-node wrapper.
Arguments
----------
1. `event` (`String`): The event name to be simulated
2. `...args` (`Any` [optional]): A mock event object that will get passed through to the event handlers.
Returns
--------
`ShallowWrapper`: Returns itself.
Example `class component`
--------------------------
```
class Foo extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
const { count } = this.state;
return (
<div>
<div className={`clicks-${count}`}>
{count} clicks
</div>
<a href="url" onClick={() => { this.setState({ count: count + 1 }); }}>
Increment
</a>
</div>
);
}
}
const wrapper = shallow(<Foo />);
expect(wrapper.find('.clicks-0').length).to.equal(1);
wrapper.find('a').simulate('click');
expect(wrapper.find('.clicks-1').length).to.equal(1);
```
Example `functional component`
-------------------------------
```
const Foo = ({ width, height, onChange }) => (
<div>
<input name="width" value={width} onChange={onChange} />
<input name="height" value={height} onChange={onChange} />
</div>
);
Foo.propTypes = {
width: PropTypes.number.isRequired,
height: PropTypes.number.isRequired,
onChange: PropTypes.func.isRequired,
};
const testState = { width: 10, height: 20 };
const wrapper = shallow((
<Foo
width={testState.width}
height={testState.height}
onChange={(e) => {
testState[e.target.name] = e.target.value;
}}
/>
));
expect(wrapper.find('input').at(0).prop('value')).toEqual(10);
expect(wrapper.find('input').at(1).prop('value')).toEqual(20);
wrapper.find('input').at(0).simulate('change', { target: { name: 'width', value: 50 } });
wrapper.find('input').at(1).simulate('change', { target: { name: 'height', value: 70 } });
expect(testState.width).toEqual(50);
expect(testState.height).toEqual(70);
```
Common Gotchas
---------------
* Currently, event simulation for the shallow renderer does not propagate as one would normally expect in a real environment. As a result, one must call `.simulate()` on the actual node that has the event handler set.
* Even though the name would imply this simulates an actual event, `.simulate()` will in fact target the component's prop based on the event you give it. For example, `.simulate('click')` will actually get the `onClick` prop and call it.
* As noted in the function signature above passing a mock event is optional. Keep in mind that if the code you are testing uses the event for something like, calling `event.preventDefault()` or accessing any of its properties you must provide a mock event object with the properties your code requires.
enzyme .reduceRight(fn[, initialValue]) => Any .reduceRight(fn[, initialValue]) => Any
=======================================
Applies the provided reducing function to every node in the wrapper to reduce to a single value. Each node is passed in as a `ShallowWrapper`, and is processed from right to left.
Arguments
----------
1. `fn` (`Function`): A reducing function to be run for every node in the collection, with the following arguments:
* `value` (`T`): The value returned by the previous invocation of this function
* `node` (`ShallowWrapper`): A single-node wrapper around the node being processed
* `index` (`Number`): The index of the node being processed
2. `initialValue` (`T` [optional]): If provided, this will be passed in as the first argument to the first invocation of the reducing function. If omitted, the first `node` will be provided and the iteration will begin on the second node in the collection.
Returns
--------
`T`: Returns an array of the returned values from the mapping function...
Example
--------
```
function Foo() {
return (
<div>
<Bar amount={2} />
<Bar amount={4} />
<Bar amount={8} />
</div>
);
}
```
```
const wrapper = shallow(<Foo />);
const total = wrapper.find(Bar).reduceRight((amount, n) => amount + n.prop('amount'), 0);
expect(total).to.equal(14);
```
Related Methods
----------------
* [`.reduce(fn[, initialValue]) => Any`](reduce)
* [`.forEach(fn) => ShallowWrapper`](foreach)
* [`.map(fn) => Array<Any>`](map)
enzyme .renderProp(propName)(...args) => ShallowWrapper .renderProp(propName)(...args) => ShallowWrapper
================================================
Returns a function that, when called with arguments `args`, will return a new wrapper based on the render prop in the original wrapper's prop `propName`.
NOTE: can only be called on wrapper of a single non-DOM component element node.
Arguments
----------
1. `propName` (`String`):
2. `...args` (`Array<Any>`):
This essentially calls `wrapper.prop(propName)(...args)`.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the node returned from the render prop.
Examples
---------
#####
Test Setup
```
class Mouse extends React.Component {
constructor() {
super();
this.state = { x: 0, y: 0 };
}
render() {
const { render } = this.props;
return (
<div
style={{ height: '100%' }}
onMouseMove={(event) => {
this.setState({
x: event.clientX,
y: event.clientY,
});
}}
>
{render(this.state)}
</div>
);
}
}
Mouse.propTypes = {
render: PropTypes.func.isRequired,
};
```
```
const App = () => (
<div style={{ height: '100%' }}>
<Mouse
render={(x = 0, y = 0) => (
<h1>
The mouse position is ({x}, {y})
</h1>
)}
/>
</div>
);
```
#####
Testing with no arguments
```
const wrapper = shallow(<App />)
.find(Mouse)
.renderProp('render')();
expect(wrapper.equals(<h1>The mouse position is 0, 0</h1>)).to.equal(true);
```
#####
Testing with multiple arguments
```
const wrapper = shallow(<App />)
.find(Mouse)
.renderProp('render')(10, 20);
expect(wrapper.equals(<h1>The mouse position is 10, 20</h1>)).to.equal(true);
```
enzyme .context([key]) => Any .context([key]) => Any
======================
Returns the context hash for the root node of the wrapper. Optionally pass in a prop name and it will return just that value.
Arguments
----------
1. `key` (`String` [optional]): If provided, the return value will be the `this.context[key]` of the root component instance.
Example
--------
```
const wrapper = shallow(
<MyComponent />,
{ context: { foo: 10 } },
);
expect(wrapper.context().foo).to.equal(10);
expect(wrapper.context('foo')).to.equal(10);
```
Related Methods
----------------
* [`.props() => Object`](props)
* [`.prop(key) => Any`](prop)
* [`.state([key]) => Any`](state)
enzyme .simulateError(error) => Self .simulateError(error) => Self
=============================
Simulate a component throwing an error as part of its rendering lifecycle.
This is particularly useful in combination with React 16 error boundaries (ie, the `componentDidCatch` and `static getDerivedStateFromError` lifecycle methods).
Arguments
----------
1. `error` (`Any`): The error to throw.
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
function Something() {
// this is just a placeholder
return null;
}
class ErrorBoundary extends React.Component {
static getDerivedStateFromError(error) {
return {
hasError: true,
};
}
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, info) {
const { spy } = this.props;
spy(error, info);
}
render() {
const { children } = this.props;
const { hasError } = this.state;
return (
<React.Fragment>
{hasError ? 'Error' : children}
</React.Fragment>
);
}
}
ErrorBoundary.propTypes = {
children: PropTypes.node.isRequired,
spy: PropTypes.func.isRequired,
};
const spy = sinon.spy();
const wrapper = shallow(<ErrorBoundary spy={spy}><Something /></ErrorBoundary>);
const error = new Error('hi!');
wrapper.find(Something).simulateError(error);
expect(wrapper.state()).to.have.property('hasError', true);
expect(spy).to.have.property('callCount', 1);
expect(spy.args).to.deep.equal([
error,
{
componentStack: `
in Something (created by ErrorBoundary)
in ErrorBoundary (created by WrapperComponent)
in WrapperComponent`,
},
]);
```
enzyme .filterWhere(fn) => ShallowWrapper .filterWhere(fn) => ShallowWrapper
==================================
Returns a new wrapper with only the nodes of the current wrapper that, when passed into the provided predicate function, return true.
Arguments
----------
1. `predicate` (`ShallowWrapper => Boolean`): A predicate function that is passed a wrapped node.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the filtered nodes.
Example
--------
```
const wrapper = shallow(<MyComponent />);
const complexFoo = wrapper.find('.foo').filterWhere((n) => typeof n.type() !== 'string');
expect(complexFoo).to.have.lengthOf(4);
```
Related Methods
----------------
* [`.filter(selector) => ShallowWrapper`](filter)
enzyme .findWhere(fn) => ShallowWrapper .findWhere(fn) => ShallowWrapper
================================
Finds every node in the render tree that returns true for the provided predicate function.
Arguments
----------
1. `predicate` (`ShallowWrapper => Boolean`): A predicate function called with the passed in wrapped nodes.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the found nodes.
Example
--------
```
const wrapper = shallow(<MyComponent />);
const complexComponents = wrapper.findWhere((n) => n.type() !== 'string');
expect(complexComponents).to.have.lengthOf(8);
```
Related Methods
----------------
* [`.find(selector) => ShallowWrapper`](find)
enzyme .matchesElement(patternNode) => Boolean .matchesElement(patternNode) => Boolean
=======================================
Returns whether or not a given react element `patternNode` matches the wrapper's render tree. It must be a single-node wrapper, and only the root node is checked.
The `patternNode` acts like a wildcard. For it to match a node in the wrapper:
* tag names must match
* contents must match: In text nodes, leading and trailing spaces are ignored, but not space in the middle. Child elements must match according to these rules, recursively.
* `patternNode` props (attributes) must appear in the wrapper's nodes, but not the other way around. Their values must match if they do appear.
* `patternNode` style CSS properties must appear in the wrapper's node's style, but not the other way around. Their values must match if they do appear.
Arguments
----------
1. `patternNode` (`ReactElement`): The node whose presence you are detecting in the wrapper's single node.
Returns
--------
`Boolean`: whether or not the current wrapper match the one passed in.
Example
--------
```
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
// ...
}
render() {
return (
<button type="button" onClick={this.handleClick} className="foo bar">Hello</button>
);
}
}
const wrapper = shallow(<MyComponent />);
expect(wrapper.matchesElement(<button>Hello</button>)).to.equal(true);
expect(wrapper.matchesElement(<button className="foo bar">Hello</button>)).to.equal(true);
```
Common Gotchas
---------------
* `.matchesElement()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines matching based on the matching of the node's children as well.
Related Methods
----------------
* [`.containsMatchingElement() => ShallowWrapper`](containsmatchingelement) - searches all nodes in the wrapper, and searches their entire depth
enzyme .parents([selector]) => ShallowWrapper .parents([selector]) => ShallowWrapper
======================================
Returns a wrapper around all of the parents/ancestors of the single node in the wrapper. Does not include the node itself. Optionally, a selector can be provided and it will filter the parents by this selector. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): The selector to filter the parents by.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = shallow(<ToDoList />);
expect(wrapper.find('ul').parents()).to.have.lengthOf(2);
```
Related Methods
----------------
* [`.children([selector]) => ShallowWrapper`](children)
* [`.parent() => ShallowWrapper`](parent)
* [`.closest(selector) => ShallowWrapper`](closest)
| programming_docs |
enzyme .find(selector) => ShallowWrapper .find(selector) => ShallowWrapper
=================================
Finds every node in the render tree of the current wrapper that matches the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the found nodes.
Examples
---------
CSS Selectors:
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(3);
// compound selector
expect(wrapper.find('div.some-class')).to.have.lengthOf(3);
// CSS id selector
expect(wrapper.find('#foo')).to.have.lengthOf(1);
```
Component Constructors:
```
import Foo from '../components/Foo';
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo)).to.have.lengthOf(1);
```
Component Display Name:
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('Foo')).to.have.lengthOf(1);
```
Object Property Selector:
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find({ prop: 'value' })).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.findWhere(predicate) => ShallowWrapper`](findwhere)
enzyme .tap(intercepter) => Self .tap(intercepter) => Self
=========================
Invokes intercepter and returns itself. intercepter is called with itself. This is helpful when debugging nodes in method chains.
Arguments
----------
1. `intercepter` (`Self`): the current ShallowWrapper instance.
Returns
--------
`Self`: the current ShallowWrapper instance.
Example
--------
```
const result = shallow((
<ul>
<li>xxx</li>
<li>yyy</li>
<li>zzz</li>
</ul>
)).find('li')
.tap((n) => console.log(n.debug()))
.map((n) => n.text());
```
enzyme .closest(selector) => ShallowWrapper .closest(selector) => ShallowWrapper
====================================
Returns a wrapper of the first element that matches the selector by traversing up through the wrapped node's ancestors in the tree, starting with itself. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the resulting node.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo).closest('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.children([selector]) => ShallowWrapper`](children)
* [`.parent() => ShallowWrapper`](parent)
* [`.parents([selector]) => ShallowWrapper`](parents)
enzyme .dive([options]) => ShallowWrapper .dive([options]) => ShallowWrapper
==================================
Shallow render the one non-DOM child of the current wrapper, and return a wrapper around the result. It must be a single-node wrapper, and the node must be a React component.
There is no corresponding `dive` method for ReactWrappers.
NOTE: can only be called on a wrapper of a single non-DOM component element node, otherwise it will throw an error. If you have to shallow-wrap a wrapper with multiple child nodes, use [`.shallow()`](shallow).
Arguments
----------
1. `options` (`Object` [optional]):
2. `options.context`: (`Object` [optional]): Context to be passed into the component
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the current node after it's been shallow rendered.
Examples
---------
```
function Bar() {
return (
<div>
<div className="in-bar" />
</div>
);
}
```
```
function Foo() {
return (
<div>
<Bar />
</div>
);
}
```
```
const wrapper = shallow(<Foo />);
expect(wrapper.find('.in-bar')).to.have.lengthOf(0);
expect(wrapper.find(Bar)).to.have.lengthOf(1);
expect(wrapper.find(Bar).dive().find('.in-bar')).to.have.lengthOf(1);
```
enzyme .reduce(fn[, initialValue]) => Any .reduce(fn[, initialValue]) => Any
==================================
Applies the provided reducing function to every node in the wrapper to reduce to a single value. Each node is passed in as a `ShallowWrapper`, and is processed from left to right.
Arguments
----------
1. `fn` (`Function`): A reducing function to be run for every node in the collection, with the following arguments:
* `value` (`T`): The value returned by the previous invocation of this function
* `node` (`ShallowWrapper`): A wrapper around the node being processed
* `index` (`Number`): The index of the node being processed
2. `initialValue` (`T` [optional]): If provided, this will be passed in as the first argument to the first invocation of the reducing function. If omitted, the first `node` will be provided and the iteration will begin on the second node in the collection.
Returns
--------
`T`: Returns an array of the returned values from the mapping function...
Example
--------
```
function Foo() {
return (
<div>
<Bar amount={2} />
<Bar amount={4} />
<Bar amount={8} />
</div>
);
}
```
```
const wrapper = shallow(<Foo />);
const total = wrapper.find(Bar).reduce((amount, n) => amount + n.prop('amount'), 0);
expect(total).to.equal(14);
```
Related Methods
----------------
* [`.reduceRight(fn[, initialValue]) => Any`](reduceright)
* [`.forEach(fn) => ShallowWrapper`](foreach)
* [`.map(fn) => Array<Any>`](map)
enzyme .slice([begin[, end]]) => ShallowWrapper .slice([begin[, end]]) => ShallowWrapper
========================================
Returns a new wrapper with a subset of the nodes of the original wrapper, according to the rules of `Array#slice`.
Arguments
----------
1. `begin` (`Number` [optional]): Index from which to slice (defaults to `0`). If negative, this is treated as `length+begin`.
2. `end` (`Number` [optional]): Index at which to end slicing (defaults to `length`). If negative, this is treated as `length+end`.
Returns
--------
`ShallowWrapper`: A new wrapper with the subset of nodes specified.
Examples
---------
```
const wrapper = shallow((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
expect(wrapper.find('.foo').slice(1)).to.have.lengthOf(2);
expect(wrapper.find('.foo').slice(1).at(0).hasClass('bar')).to.equal(true);
expect(wrapper.find('.foo').slice(1).at(1).hasClass('baz')).to.equal(true);
```
```
const wrapper = shallow((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
expect(wrapper.find('.foo').slice(1, 2)).to.have.lengthOf(1);
expect(wrapper.find('.foo').slice(1, 2).at(0).hasClass('bar')).to.equal(true);
```
enzyme .containsMatchingElement(patternNode) => Boolean .containsMatchingElement(patternNode) => Boolean
================================================
Returns whether or not a `patternNode` react element matches any element in the render tree.
* the matches can happen anywhere in the wrapper's contents
* the wrapper can contain more than one node; all are searched
Otherwise, the match follows the same rules as `matchesElement`.
Arguments
----------
1. `patternNode` (`ReactElement`): The node whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in its render tree that matches the one passed in.
Example
--------
```
const wrapper = shallow((
<div>
<div data-foo="foo" data-bar="bar">Hello</div>
</div>
));
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar">Hello</div>)).to.equal(true);
expect(wrapper.containsMatchingElement(<div data-foo="foo">Hello</div>)).to.equal(true);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar" data-baz="baz">Hello</div>)).to.equal(false);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="Hello">Hello</div>)).to.equal(false);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar" />)).to.equal(false);
```
Common Gotchas
---------------
* `.containsMatchingElement()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
Related Methods
----------------
* [`.containsAllMatchingElements() => ShallowWrapper`](containsallmatchingelements) - must match all nodes in patternNodes
* [`.containsAnyMatchingElements() => ShallowWrapper`](containsanymatchingelements) - must match at least one in patternNodes
enzyme .some(selector) => Boolean .some(selector) => Boolean
==========================
Returns whether or not any of the nodes in the wrapper match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: True if at least one of the nodes in the current wrapper matched the provided selector.
Examples
---------
```
const wrapper = shallow((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').some('.qoo')).to.equal(true);
expect(wrapper.find('.foo').some('.foo')).to.equal(true);
expect(wrapper.find('.foo').some('.bar')).to.equal(false);
```
Related Methods
----------------
* [`.someWhere(predicate) => Boolean`](somewhere)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .instance() => ReactComponent .instance() => ReactComponent
=============================
Returns the single-node wrapper's node's underlying class instance; `this` in its methods.
NOTE: can only be called on a wrapper instance that is also the root instance. With React `16` and above, `instance()` returns `null` for stateless functional components.
Returns
--------
`ReactComponent|DOMComponent`: The retrieved instance.
Example
--------
```
function Stateless() {
return <div>Stateless</div>;
}
class Stateful extends React.Component {
render() {
return <div>Stateful</div>;
}
}
```
React 16.x
-----------
```
test('shallow wrapper instance should be null', () => {
const wrapper = shallow(<Stateless />);
const instance = wrapper.instance();
expect(instance).to.equal(null);
});
test('shallow wrapper instance should not be null', () => {
const wrapper = shallow(<Stateful />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateful);
});
```
React 15.x
-----------
```
test('shallow wrapper instance should not be null', () => {
const wrapper = shallow(<Stateless />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateless);
});
test('shallow wrapper instance should not be null', () => {
const wrapper = shallow(<Stateful />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateful);
});
```
enzyme .isEmptyRender() => Boolean .isEmptyRender() => Boolean
===========================
Returns whether or not the wrapper would ultimately render only the allowed falsy values: `false` or `null`.
Returns
--------
`Boolean`: whether the return is falsy
Example
--------
```
function Foo() {
return null;
}
const wrapper = shallow(<Foo />);
expect(wrapper.isEmptyRender()).to.equal(true);
```
enzyme .getElement() => ReactElement .getElement() => ReactElement
=============================
Returns the wrapped ReactElement.
If the current wrapper is wrapping the root component, returns the root component's latest render output.
Returns
--------
`ReactElement`: The retrieved ReactElement.
Examples
---------
```
const element = (
<div>
<span />
<span />
</div>
);
function MyComponent() {
return element;
}
const wrapper = shallow(<MyComponent />);
expect(wrapper.getElement()).to.equal(element);
```
Related Methods
----------------
* [`.getElements() => Array<ReactElement>`](getelements)
enzyme .containsAllMatchingElements(patternNodes) => Boolean .containsAllMatchingElements(patternNodes) => Boolean
=====================================================
Returns whether or not all of the given react elements in `patternNodes` match an element in the wrapper's render tree. Every single element of `patternNodes` must be matched one or more times. Matching follows the rules for `containsMatchingElement`.
Arguments
----------
1. `patternNodes` (`Array<ReactElement>`): The array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has nodes anywhere in its render tree that looks like the nodes passed in.
Example
--------
```
const style = { fontSize: 13 };
const wrapper = shallow((
<div>
<span className="foo">Hello</span>
<div style={style}>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.containsAllMatchingElements([
<span>Hello</span>,
<div>Goodbye</div>,
])).to.equal(true);
```
Common Gotchas
---------------
* `.containsAllMatchingElements()` expects an array of ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with an array of ReactElement or a JSX expression.
* Keep in mind that this method determines matching based on the matching of the node's children as well.
Related Methods
----------------
* [`.matchesElement() => ShallowWrapper`](matcheselement) - rules for matching each node
* [`.containsMatchingElement() => ShallowWrapper`](containsmatchingelement) - rules for matching whole wrapper
* [`.containsAnyMatchingElements() => ShallowWrapper`](containsanymatchingelements) - must match at least one in patternNodes
enzyme .equals(node) => Boolean .equals(node) => Boolean
========================
Returns whether or not the current wrapper root node render tree looks like the one passed in.
Arguments
----------
1. `node` (`ReactElement`): The node whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in it's render tree that looks like the one passed in.
Example
--------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.equals(<div className="foo bar" />)).to.equal(true);
```
Common Gotchas
---------------
* `.equals()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
* Following React's behavior, `.equals()` ignores properties whose values are `undefined`.
enzyme .debug([options]) => String .debug([options]) => String
===========================
Returns an HTML-like string of the wrapper for debugging purposes. Useful to print out to the console when tests are not passing when you expect them to.
Arguments
----------
`options` (`Object` [optional]):
* `options.ignoreProps`: (`Boolean` [optional]): Whether props should be omitted in the resulting string. Props are included by default.
* `options.verbose`: (`Boolean` [optional]): Whether arrays and objects passed as props should be verbosely printed.
Returns
--------
`String`: The resulting string.
Examples
---------
```
function Book({ title, pages }) {
return (
<div>
<h1 className="title">{title}</h1>
{pages && (
<NumberOfPages
pages={pages}
object={{ a: 1, b: 2 }}
/>
)}
</div>
);
}
Book.propTypes = {
title: PropTypes.string.isRequired,
pages: PropTypes.number,
};
Book.defaultProps = {
pages: null,
};
```
```
const wrapper = shallow(<Book title="Huckleberry Finn" />);
console.log(wrapper.debug());
```
Outputs to console:
```
<div>
<h1 className="title">Huckleberry Finn</h1>
</div>
```
```
const wrapper = shallow((
<Book
title="Huckleberry Finn"
pages="633 pages"
/>
));
console.log(wrapper.debug());
```
Outputs to console:
```
<div>
<h1 className="title">Huckleberry Finn</h1>
<NumberOfPages pages="633 pages" object={{...}}/>
</div>
```
```
const wrapper = shallow((
<Book
title="Huckleberry Finn"
pages="633 pages"
/>
));
console.log(wrapper.debug({ ignoreProps: true }));
```
Outputs to console:
```
<div>
<h1>Huckleberry Finn</h1>
<NumberOfPages />
</div>
```
```
const wrapper = shallow((
<Book
title="Huckleberry Finn"
pages="633 pages"
/>
));
console.log(wrapper.debug({ verbose: true }));
```
Outputs to console:
```
<div>
<h1 className="title">Huckleberry Finn</h1>
<NumberOfPages pages="633 pages" object={{ a: 1, b: 2 }}/>
</div>
```
enzyme .name() => String|null .name() => String|null
======================
Returns the name of the current node of this wrapper. If it's a composite component, this will be the name of the top-most rendered component. If it's a native DOM node, it will be a string of the tag name. If it's `null`, it will be `null`.
The order of precedence on returning the name is: `type.displayName` -> `type.name` -> `type`.
Note: can only be called on a wrapper of a single node.
Returns
--------
`String|null`: The name of the current node
Examples
---------
```
const wrapper = shallow(<div />);
expect(wrapper.name()).to.equal('div');
```
```
function SomeWrappingComponent() {
return <Foo />;
}
const wrapper = shallow(<SomeWrappingComponent />);
expect(wrapper.name()).to.equal('Foo');
```
```
Foo.displayName = 'A cool custom name';
function SomeWrappingComponent() {
return <Foo />;
}
const wrapper = shallow(<SomeWrappingComponent />);
expect(wrapper.name()).to.equal('A cool custom name');
```
enzyme .hostNodes() => ShallowWrapper .hostNodes() => ShallowWrapper
==============================
Returns a new wrapper with only host nodes. When using `react-dom`, host nodes are HTML elements rather than custom React components, e.g. `<div>` versus `<MyComponent>`.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
The following code takes a wrapper with two nodes, one a `<MyComponent>` React component, and the other a `<span>`, and filters out the React component.
```
const wrapper = shallow((
<div>
<MyComponent className="foo" />
<span className="foo" />
</div>
));
const twoNodes = wrapper.find('.foo');
expect(twoNodes.hostNodes()).to.have.lengthOf(1);
```
enzyme .not(selector) => ShallowWrapper .not(selector) => ShallowWrapper
================================
Returns a new wrapper with only the nodes of the current wrapper that don't match the provided selector.
This method is effectively the negation or inverse of [`filter`](filter).
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.foo').not('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.filterWhere(predicate) => ShallowWrapper`](filterwhere)
* [`.filter(selector) => ShallowWrapper`](filter)
enzyme .key() => String .key() => String
================
Returns the key value for the node of the current wrapper. It must be a single-node wrapper.
Example
--------
```
const wrapper = shallow((
<ul>
{['foo', 'bar'].map((s) => <li key={s}>{s}</li>)}
</ul>
)).find('li');
expect(wrapper.at(0).key()).to.equal('foo');
expect(wrapper.at(1).key()).to.equal('bar');
```
enzyme .getWrappingComponent() => ShallowWrapper .getWrappingComponent() => ShallowWrapper
=========================================
If a `wrappingComponent` was passed in `options`, this methods returns a `ShallowWrapper` around the rendered `wrappingComponent`. This `ShallowWrapper` can be used to update the `wrappingComponent`'s props, state, etc.
Returns
--------
`ShallowWrapper`: A `ShallowWrapper` around the rendered `wrappingComponent`
Examples
---------
```
import { Provider } from 'react-redux';
import { Router } from 'react-router';
import store from './my/app/store';
import mockStore from './my/app/mockStore';
function MyProvider(props) {
const { children, customStore } = props;
return (
<Provider store={customStore || store}>
<Router>
{children}
</Router>
</Provider>
);
}
MyProvider.propTypes = {
children: PropTypes.node,
customStore: PropTypes.shape({}),
};
MyProvider.defaultProps = {
children: null,
customStore: null,
};
const wrapper = shallow(<MyComponent />, {
wrappingComponent: MyProvider,
});
const provider = wrapper.getWrappingComponent();
provider.setProps({ customStore: mockStore });
```
| programming_docs |
enzyme .parent() => ShallowWrapper .parent() => ShallowWrapper
===========================
Returns a wrapper with the direct parent of the node in the current wrapper.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = shallow(<ToDoList />);
expect(wrapper.find('ul').parent().is('div')).to.equal(true);
```
Related Methods
----------------
* [`.parents([selector]) => ShallowWrapper`](parents)
* [`.children([selector]) => ShallowWrapper`](children)
* [`.closest(selector) => ShallowWrapper`](closest)
enzyme .render() => CheerioWrapper .render() => CheerioWrapper
===========================
Returns a CheerioWrapper around the rendered HTML of the single node's subtree. It must be a single-node wrapper.
Returns
--------
`CheerioWrapper`: The resulting Cheerio object
Examples
---------
```
function Foo() {
return (<div className="in-foo" />);
}
```
```
function Bar() {
return (
<div className="in-bar">
<Foo />
</div>
);
}
```
```
const wrapper = shallow(<Bar />);
expect(wrapper.find('.in-foo')).to.have.lengthOf(0);
expect(wrapper.find(Foo).render().find('.in-foo')).to.have.lengthOf(1);
```
enzyme .unmount() => Self .unmount() => Self
==================
A method that unmounts the component. This can be used to simulate a component going through an unmount/mount lifecycle.
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
import PropTypes from 'prop-types';
import sinon from 'sinon';
const spy = sinon.spy();
class Foo extends React.Component {
constructor(props) {
super(props);
this.componentWillUnmount = spy;
}
render() {
const { id } = this.props;
return (
<div className={id}>
{id}
</div>
);
}
}
Foo.propTypes = {
id: PropTypes.string.isRequired,
};
const wrapper = shallow(<Foo id="foo" />);
expect(spy).to.have.property('callCount', 0);
wrapper.unmount();
expect(spy).to.have.property('callCount', 1);
```
enzyme .every(selector) => Boolean .every(selector) => Boolean
===========================
Returns whether or not all of the nodes in the wrapper match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: True if every node in the current wrapper matched the provided selector.
Examples
---------
```
const wrapper = shallow((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').every('.foo')).to.equal(true);
expect(wrapper.find('.foo').every('.qoo')).to.equal(false);
expect(wrapper.find('.foo').every('.bar')).to.equal(false);
```
Related Methods
----------------
* [`.someWhere(predicate) => Boolean`](somewhere)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .hasClass(className) => Boolean .hasClass(className) => Boolean
===============================
Returns whether or not the wrapped node has a `className` prop including the passed in class name. It must be a single-node wrapper.
Arguments
----------
1. `className` (`String` | `RegExp`): A single class name or a regex expression.
Returns
--------
`Boolean`: whether or not the wrapped node has the class.
Example
--------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.my-button').hasClass('disabled')).to.equal(true);
```
```
// Searching using RegExp works fine when classes were injected by a jss decorator
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.my-button').hasClass(/(ComponentName)-(other)-(\d+)/)).to.equal(true);
```
Common Gotchas
---------------
* `.hasClass()` expects a class name, NOT a CSS selector. `.hasClass('.foo')` should be `.hasClass('foo')`
enzyme .state([key]) => Any .state([key]) => Any
====================
Returns the state hash for the root node of the wrapper. Optionally pass in a prop name and it will return just that value.
Arguments
----------
1. `key` (`String` [optional]): If provided, the return value will be the `this.state[key]` of the root component instance.
Example
--------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.state().foo).to.equal(10);
expect(wrapper.state('foo')).to.equal(10);
```
Related Methods
----------------
* [`.props() => Object`](props)
* [`.prop(key) => Any`](prop)
* [`.context([key]) => Any`](context)
enzyme .someWhere(fn) => Boolean .someWhere(fn) => Boolean
=========================
Returns whether or not any of the nodes in the wrapper pass the provided predicate function.
Arguments
----------
1. `predicate` (`ShallowWrapper => Boolean`): A predicate function to match the nodes.
Returns
--------
`Boolean`: True if at least one of the nodes in the current wrapper passed the predicate function.
Example
--------
```
const wrapper = shallow((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('qoo'))).to.equal(true);
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('foo'))).to.equal(true);
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('bar'))).to.equal(false);
```
Related Methods
----------------
* [`.some(selector) => Boolean`](some)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .containsAnyMatchingElements(patternNodes) => Boolean .containsAnyMatchingElements(patternNodes) => Boolean
=====================================================
Returns whether or not at least one of the given react elements in `patternNodes` matches an element in the wrapper's render tree. One or more elements of `patternNodes` must be matched one or more times. Matching follows the rules for `containsMatchingElement`.
Arguments
----------
1. `patternNodes` (`Array<ReactElement>`): The array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in its render tree that looks like one of the array passed in.
Example
--------
```
const style = { fontSize: 13 };
const wrapper = shallow((
<div>
<span className="foo">Hello</span>
<div style={style}>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.containsAnyMatchingElements([
<span>Bonjour</span>,
<div>Goodbye</div>,
])).to.equal(true);
```
Common Gotchas
---------------
* `.containsAnyMatchingElements()` expects an array of ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with an array ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
Related Methods
----------------
* [`.matchesElement() => ShallowWrapper`](matcheselement) - rules for matching each node
* [`.containsMatchingElement() => ShallowWrapper`](containsmatchingelement) - rules for matching whole wrapper
* [`.containsAllMatchingElements() => ShallowWrapper`](containsallmatchingelements) - must match all nodes in patternNodes
enzyme .get(index) => ReactElement .get(index) => ReactElement
===========================
Returns the node at a given index of the current wrapper.
Arguments
----------
1. `index` (`Number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ReactElement`: The retrieved node.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo).get(0).props.foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ShallowWrapper`](at) - same, but returns the React node in a single-node wrapper.
enzyme .is(selector) => Boolean .is(selector) => Boolean
========================
Returns whether or not the single wrapped node matches the provided selector. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: whether or not the wrapped node matches the provided selector.
Example
--------
```
const wrapper = shallow(<div className="some-class other-class" />);
expect(wrapper.is('.some-class')).to.equal(true);
```
enzyme .setProps(nextProps[, callback]) => Self .setProps(nextProps[, callback]) => Self
========================================
A method that sets the props of the root component, and re-renders. Useful for when you are wanting to test how the component behaves over time with changing props. Calling this, for instance, will call the `componentWillReceiveProps` lifecycle method.
Similar to `setState`, this method accepts a props object and will merge it in with the already existing props.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `nextProps` (`Object`): An object containing new props to merge in with the current props
2. `callback` (`Function` [optional]): If provided, the callback function will be executed once setProps has completed
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
import React from 'react';
import PropTypes from 'prop-types';
function Foo({ name }) {
return (
<div className={name} />
);
}
Foo.propTypes = {
name: PropTypes.string.isRequired,
};
```
```
const wrapper = shallow(<Foo name="foo" />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(0);
wrapper.setProps({ name: 'bar' });
expect(wrapper.find('.foo')).to.have.lengthOf(0);
expect(wrapper.find('.bar')).to.have.lengthOf(1);
```
```
import sinon from 'sinon';
const spy = sinon.spy(MyComponent.prototype, 'componentWillReceiveProps');
const wrapper = shallow(<MyComponent foo="bar" />);
expect(spy).to.have.property('callCount', 0);
wrapper.setProps({ foo: 'foo' });
expect(spy).to.have.property('callCount', 1);
```
Related Methods
----------------
* [`.setState(state) => Self`](setstate)
* [`.setContext(context) => Self`](setcontext)
enzyme .filter(selector) => ShallowWrapper .filter(selector) => ShallowWrapper
===================================
Returns a new wrapper with only the nodes of the current wrapper that match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.foo').filter('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.filterWhere(predicate) => ShallowWrapper`](filterwhere)
enzyme .forEach(fn) => Self .forEach(fn) => Self
====================
Iterates through each node of the current wrapper and executes the provided function with a wrapper around the corresponding node passed in as the first argument.
Arguments
----------
1. `fn` (`Function ( ShallowWrapper node, Number index )`): A callback to be run for every node in the collection. Should expect a ShallowWrapper as the first argument, and will be run with a context of the original instance.
Returns
--------
`ShallowWrapper`: Returns itself.
Example
--------
```
const wrapper = shallow((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
wrapper.find('.foo').forEach((node) => {
expect(node.hasClass('foo')).to.equal(true);
});
```
Related Methods
----------------
* [`.map(fn) => ShallowWrapper`](map)
enzyme .first() => ShallowWrapper .first() => ShallowWrapper
==========================
Reduce the set of matched nodes to the first in the set, just like `.at(0)`.
Returns
--------
`ShallowWrapper`: A new wrapper that wraps the first node in the set.
Examples
---------
```
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo).first().props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ShallowWrapper`](at) - retrieve any wrapper node
* [`.last() => ShallowWrapper`](last)
enzyme .setContext(context) => Self .setContext(context) => Self
============================
A method that sets the context of the root component, and re-renders. Useful for when you are wanting to test how the component behaves over time with changing contexts.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `context` (`Object`): An object containing new props to merge in with the current state
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
import React from 'react';
import PropTypes from 'prop-types';
function SimpleComponent(props, context) {
const { name } = context;
return <div>{name}</div>;
}
SimpleComponent.contextTypes = {
name: PropTypes.string,
};
```
```
const context = { name: 'foo' };
const wrapper = mount(<SimpleComponent />, { context });
expect(wrapper.text()).to.equal('foo');
wrapper.setContext({ name: 'bar' });
expect(wrapper.text()).to.equal('bar');
wrapper.setContext({ name: 'baz' });
expect(wrapper.text()).to.equal('baz');
```
Common Gotchas
---------------
* `.setContext()` can only be used on a wrapper that was initially created with a call to `mount()` that includes a `context` specified in the options argument.
* The root component you are rendering must have a `contextTypes` static property.
Related Methods
----------------
* [`.setState(state[, callback]) => Self`](setstate)
* [`.setProps(props[, callback]) => Self`](setprops)
enzyme .childAt(index) => ReactWrapper .childAt(index) => ReactWrapper
===============================
Returns a new wrapper with child at the specified index.
Arguments
----------
1. `index` (`number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the resulting node.
Examples
---------
```
const wrapper = mount(<ToDoList items={items} />);
expect(wrapper.find('ul').childAt(0).type()).to.equal('li');
```
Related Methods
----------------
* [`.parents([selector]) => ReactWrapper`](parents)
* [`.parent() => ReactWrapper`](parent)
* [`.closest(selector) => ReactWrapper`](closest)
* [`.children([selector]) => ReactWrapper`](children)
enzyme .setState(nextState[, callback]) => Self .setState(nextState[, callback]) => Self
========================================
A method to invoke `setState()` on the root component instance, similar to how you might in the methods of the component, and re-renders. This method is useful for testing your component in hard-to-achieve states, however should be used sparingly. If possible, you should utilize your component's external API (which is often accessible via [`.instance()`](instance)) in order to get it into whatever state you want to test, in order to be as accurate of a test as possible. This is not always practical, however.
NOTE: Prior to v3.8.0 of enzyme, can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `nextState` (`Object`): An object containing new state to merge in with the current state
2. `callback` (`Function` [optional]): If provided, the callback function will be executed once setState has completed
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
class Foo extends React.Component {
constructor(props) {
super(props);
this.state = { name: 'foo' };
}
render() {
const { name } = this.state;
return (
<div className={name} />
);
}
}
```
```
const wrapper = mount(<Foo />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(0);
wrapper.setState({ name: 'bar' });
expect(wrapper.find('.foo')).to.have.lengthOf(0);
expect(wrapper.find('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.setProps(props[, callback]) => Self`](setprops)
* [`.setContext(context) => Self`](setcontext)
enzyme .contains(nodeOrNodes) => Boolean .contains(nodeOrNodes) => Boolean
=================================
Returns whether or not all given react elements match elements in the render tree. It will determine if an element in the wrapper matches the expected element by checking if the expected element has the same props as the wrapper's element and share the same values.
Arguments
----------
1. `nodeOrNodes` (`ReactElement|Array<ReactElement>`): The node or array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has nodes anywhere in its render tree that match the ones passed in.
Example
--------
```
const wrapper = mount((
<div>
<div data-foo="foo" data-bar="bar">Hello</div>
</div>
));
expect(wrapper.contains(<div data-foo="foo" data-bar="bar">Hello</div>)).to.equal(true);
expect(wrapper.contains(<div data-foo="foo">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="bar" data-baz="baz">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="Hello">Hello</div>)).to.equal(false);
expect(wrapper.contains(<div data-foo="foo" data-bar="bar" />)).to.equal(false);
```
```
const wrapper = mount((
<div>
<span>Hello</span>
<div>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.contains([
<span>Hello</span>,
<div>Goodbye</div>,
])).to.equal(true);
expect(wrapper.contains([
<span>Hello</span>,
<div>World</div>,
])).to.equal(false);
```
Common Gotchas
---------------
* `.contains()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
* Every attribute of the wrapped element must be matched by the element you're checking. To permit (and ignore) additional attributes on the wrapped element, use containsMatchingElement() instead.
enzyme .map(fn) => Array<Any> .map(fn) => Array<Any>
======================
Maps the current array of nodes to another array. Each node is passed in as a `ReactWrapper` to the map function.
Arguments
----------
1. `fn` (`Function ( ReactWrapper node, Number index ) => Any`): A mapping function to be run for every node in the collection, the results of which will be mapped to the returned array. Should expect a ReactWrapper as the first argument, and will be run with a context of the original instance.
Returns
--------
`Array<Any>`: Returns an array of the returned values from the mapping function..
Example
--------
```
const wrapper = mount((
<div>
<div className="foo">bax</div>
<div className="foo">bar</div>
<div className="foo">baz</div>
</div>
));
const texts = wrapper.find('.foo').map((node) => node.text());
expect(texts).to.eql(['bax', 'bar', 'baz']);
```
Related Methods
----------------
* [`.forEach(fn) => ReactWrapper`](foreach)
* [`.reduce(fn[, initialValue]) => Any`](reduce)
* [`.reduceRight(fn[, initialValue]) => Any`](reduceright)
enzyme .children([selector]) => ReactWrapper .children([selector]) => ReactWrapper
=====================================
Returns a new wrapper with all of the children of the node(s) in the current wrapper. Optionally, a selector can be provided and it will filter the children by this selector
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): A selector to filter the children by.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = mount(<ToDoList items={items} />);
expect(wrapper.find('ul').children()).to.have.lengthOf(items.length);
```
Related Methods
----------------
* [`.parents([selector]) => ReactWrapper`](parents)
* [`.parent() => ReactWrapper`](parent)
* [`.closest(selector) => ReactWrapper`](closest)
| programming_docs |
enzyme .prop(key) => Any .prop(key) => Any
=================
Returns the prop value for the root node of the wrapper with the provided key. It must be a single-node wrapper.
Arguments
----------
1. `key` (`String`): The prop name, that is, `this.props[key]` or `props[key]` for the root node of the wrapper.
Example
--------
```
const wrapper = mount(<MyComponent foo={10} />);
expect(wrapper.prop('foo')).to.equal(10);
```
Related Methods
----------------
* [`.props() => Object`](props)
* [`.state([key]) => Any`](state)
* [`.context([key]) => Any`](context)
enzyme .everyWhere(fn) => Boolean .everyWhere(fn) => Boolean
==========================
Returns whether or not all of the nodes in the wrapper pass the provided predicate function.
Arguments
----------
1. `predicate` (`ReactWrapper => Boolean`): A predicate function to match the nodes.
Returns
--------
`Boolean`: True if every node in the current wrapper passed the predicate function.
Example
--------
```
const wrapper = mount((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('foo'))).to.equal(true);
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('qoo'))).to.equal(false);
expect(wrapper.find('.foo').everyWhere((n) => n.hasClass('bar'))).to.equal(false);
```
Related Methods
----------------
* [`.some(selector) => Boolean`](some)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .text() => String .text() => String
=================
Returns a string of the rendered text of the current render tree. This function should be looked at with skepticism if being used to test what the actual HTML output of the component will be. If that is what you would like to test, use enzyme's `render` function instead.
Note: can only be called on a wrapper of a single node.
Returns
--------
`String`: The resulting string
Examples
---------
```
const wrapper = mount(<div><b>important</b></div>);
expect(wrapper.text()).to.equal('important');
```
```
function Foo() {
return <div>This is</div>;
}
const wrapper = mount(<div><Foo /> <b>really</b> important</div>);
expect(wrapper.text()).to.equal('This is really important');
```
Related Methods
----------------
[`.html() => String`](html)
enzyme .ref(refName) => ReactComponent | HTMLElement .ref(refName) => ReactComponent | HTMLElement
=============================================
Returns the node that matches the provided reference name.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `refName` (`String`): The ref attribute of the node
Returns
--------
`ReactComponent | HTMLElement`: The node that matches the provided reference name. This can be a react component instance, or an HTML element instance.
Examples
---------
```
class Foo extends React.Component {
render() {
return (
<div>
<span ref="firstRef" amount={2}>First</span>
<span ref="secondRef" amount={4}>Second</span>
<span ref="thirdRef" amount={8}>Third</span>
</div>
);
}
}
```
```
const wrapper = mount(<Foo />);
expect(wrapper.ref('secondRef').innerText).to.equal('Second');
```
Related Methods
----------------
* [`.find(selector) => ReactWrapper`](find)
* [`.findWhere(predicate) => ReactWrapper`](findwhere)
enzyme .exists([selector]) => Boolean .exists([selector]) => Boolean
==============================
Returns whether or not any nodes exist in the wrapper. Or, if a selector is passed in, whether that selector has any matches in the wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): The selector to check existence for.
Returns
--------
`Boolean`: whether or not any nodes are on the list, or the selector had any matches.
Example
--------
```
const wrapper = mount(<div className="some-class" />);
expect(wrapper.exists('.some-class')).to.equal(true);
expect(wrapper.find('.other-class').exists()).to.equal(false);
```
enzyme .last() => ReactWrapper .last() => ReactWrapper
=======================
Reduce the set of matched nodes to the last in the set, just like `.at(length - 1)`.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the last node in the set.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo).last().props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ReactWrapper`](at) - retrieve a wrapper node by index
* [`.first() => ReactWrapper`](first)
enzyme .update() => Self .update() => Self
=================
Syncs the enzyme component tree snapshot with the react component tree. Useful to run before checking the render output if something external may be updating the state of the component somewhere.
NOTE: can only be called on a wrapper instance that is also the root instance.
NOTE: this does not force a re-render. Use `wrapper.setProps({})` to force a re-render.
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
class ImpureRender extends React.Component {
constructor(props) {
super(props);
this.count = 0;
}
render() {
this.count += 1;
return <div>{this.count}</div>;
}
}
```
```
const wrapper = mount(<ImpureRender />);
expect(wrapper.text()).to.equal('0');
wrapper.update();
expect(wrapper.text()).to.equal('1');
```
enzyme .type() => String | Function | null .type() => String | Function | null
===================================
Returns the type of the only node of this wrapper. If it's a React component, this will be the component constructor. If it's a native DOM node, it will be a string with the tag name. If it's `null`, it will be `null`. It must be a single-node wrapper.
Returns
--------
`String | Function | null`: The type of the node
Examples
---------
```
function Foo() {
return <div />;
}
const wrapper = mount(<Foo />);
expect(wrapper.type()).to.equal('div');
```
```
function Foo() {
return (
<div>
<button type="button" className="btn">Button</button>
</div>
);
}
const wrapper = mount(<Foo />);
expect(wrapper.find('.btn').type()).to.equal('button');
```
```
function Foo() {
return <Bar />;
}
const wrapper = mount(<Foo />);
expect(wrapper.type()).to.equal(Bar);
```
```
function Null() {
return null;
}
const wrapper = mount(<Null />);
expect(wrapper.type()).to.equal(null);
```
enzyme .html() => String .html() => String
=================
Returns a string of the rendered HTML markup of the current render tree. See also [`.debug()`](debug)
Note: can only be called on a wrapper of a single node.
Returns
--------
`String`: The resulting HTML string
Examples
---------
```
function Foo() {
return (<div className="in-foo" />);
}
```
```
function Bar() {
return (
<div className="in-bar">
<Foo />
</div>
);
}
```
```
const wrapper = mount(<Bar />);
expect(wrapper.html()).to.equal('<div class="in-bar"><div class="in-foo"></div></div>');
expect(wrapper.find(Foo).html()).to.equal('<div class="in-foo"></div>');
```
```
const wrapper = mount(<div><b>important</b></div>);
expect(wrapper.html()).to.equal('<div><b>important</b></div>');
```
Related Methods
----------------
[`.text() => String`](text)
enzyme .invoke(propName)(...args) => Any .invoke(propName)(...args) => Any
=================================
Invokes a function prop. Note that in React 16.8+, `.invoke` will wrap your handler with [`ReactTestUtils.act`](https://reactjs.org/docs/test-utils.html#act) and call `.update()` automatically.
Arguments
----------
1. `propName` (`String`): The function prop that is invoked
2. `...args` (`Any` [optional]): Arguments that is passed to the prop function
Returns
--------
`Any`: Returns the value from the prop function
Example
--------
```
class Foo extends React.Component {
loadData() {
return fetch();
}
render() {
return (
<div>
<button
type="button"
onClick={() => this.loadData()}
>
Load more
</button>
</div>
);
}
}
const wrapper = mount(<Foo />);
wrapper.find('button').invoke('onClick')().then(() => {
// expect()
});
```
enzyme .props() => Object .props() => Object
==================
Returns the props object for the root node of the wrapper. It must be a single-node wrapper.
This method is a reliable way of accessing the props of a node; `wrapper.instance().props` will work as well, but in React 16+, stateless functional components do not have an instance. See [`.instance() => ReactComponent`](instance)
Example
--------
```
import PropTypes from 'prop-types';
function MyComponent(props) {
const { includedProp } = props;
return (
<div className="foo bar" includedProp={includedProp}>Hello</div>
);
}
MyComponent.propTypes = {
includedProp: PropTypes.string.isRequired,
};
const wrapper = mount(<MyComponent includedProp="Success!" excludedProp="I'm not included" />);
expect(wrapper.props().includedProp).to.equal('Success!');
// Warning: .props() only returns props that are passed to the root node,
// which does not include excludedProp in this example.
// See the note above about wrapper.instance().props.
console.log(wrapper.props());
// {children: "Hello", className: "foo bar", includedProp="Success!"}
console.log(wrapper.instance().props); // React 15.x - working as expected
// {children: "Hello", className: "foo bar", includedProp:"Success!", excludedProp: "I'm not included"}
console.log(wrapper.instance().props);
// React 16.* - Uncaught TypeError: Cannot read property 'props' of null
```
Related Methods
----------------
* [`.prop(key) => Any`](prop)
* [`.state([key]) => Any`](state)
* [`.context([key]) => Any`](context)
enzyme .isEmpty() => Boolean .isEmpty() => Boolean
=====================
**Deprecated**: Use [`.exists()`](exists) instead.
Returns whether or not the wrapper is empty.
Returns
--------
`Boolean`: whether or not the wrapper is empty.
Example
--------
```
const wrapper = mount(<div className="some-class" />);
expect(wrapper.find('.other-class').isEmpty()).to.equal(true);
```
enzyme .getDOMNode() => DOMComponent .getDOMNode() => DOMComponent
=============================
Returns the outer most DOMComponent of the current wrapper.
Notes:
* can only be called on a wrapper of a single node.
* will raise if called on a wrapper of a stateless functional component.
Returns
--------
`DOMComponent`: The retrieved DOM component.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.getDOMNode()).to.have.property('className');
```
enzyme .at(index) => ReactWrapper .at(index) => ReactWrapper
==========================
Returns a wrapper around the node at a given index of the current wrapper.
Arguments
----------
1. `index` (`Number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the retrieved node.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo).at(0).props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.get(index) => ReactElement`](get) - same, but returns the React node itself, with no wrapper.
* [`.first() => ReactWrapper`](first) - same as at(0)
* [`.last() => ReactWrapper`](last)
enzyme .simulate(event[, mock]) => Self .simulate(event[, mock]) => Self
================================
Simulate events on the root node in the wrapper. It must be a single-node wrapper.
Arguments
----------
1. `event` (`String`): The event name to be simulated
2. `mock` (`Object` [optional]): A mock event object that will be merged with the event object passed to the handlers.
Returns
--------
`ReactWrapper`: Returns itself.
Example `class component`
--------------------------
```
class Foo extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
const { count } = this.state;
return (
<div>
<div className={`clicks-${count}`}>
{count} clicks
</div>
<a href="url" onClick={() => { this.setState({ count: count + 1 }); }}>
Increment
</a>
</div>
);
}
}
const wrapper = mount(<Foo />);
expect(wrapper.find('.clicks-0').length).to.equal(1);
wrapper.find('a').simulate('click');
expect(wrapper.find('.clicks-1').length).to.equal(1);
```
Example `functional component`
-------------------------------
```
const Foo = ({ width, height, onChange }) => (
<div>
<input name="width" value={width} onChange={onChange} />
<input name="height" value={height} onChange={onChange} />
</div>
);
Foo.propTypes = {
width: PropTypes.number.isRequired,
height: PropTypes.number.isRequired,
onChange: PropTypes.func.isRequired,
};
const testState = { width: 10, height: 20 };
const wrapper = mount((
<Foo
width={testState.width}
height={testState.height}
onChange={(e) => {
testState[e.target.name] = e.target.value;
}}
/>
));
expect(wrapper.find('input').at(0).prop('value')).toEqual(10);
expect(wrapper.find('input').at(1).prop('value')).toEqual(20);
wrapper.find('input').at(0).simulate('change', { target: { name: 'width', value: 50 } });
wrapper.find('input').at(1).simulate('change', { target: { name: 'height', value: 70 } });
expect(testState.width).toEqual(50);
expect(testState.height).toEqual(70);
```
Common Gotchas
---------------
* As noted in the function signature above passing a mock event is optional. It is worth noting that `ReactWrapper` will pass a `SyntheticEvent` object to the event handler in your code. Keep in mind that if the code you are testing uses properties that are not included in the `SyntheticEvent`, for instance `event.target.value`, you will need to provide a mock event like so `.simulate("change", { target: { value: "foo" }})` for it to work.
enzyme .reduceRight(fn[, initialValue]) => Any .reduceRight(fn[, initialValue]) => Any
=======================================
Applies the provided reducing function to every node in the wrapper to reduce to a single value. Each node is passed in as a `ReactWrapper`, and is processed from right to left.
Arguments
----------
1. `fn` (`Function`): A reducing function to be run for every node in the collection, with the following arguments:
* `value` (`T`): The value returned by the previous invocation of this function
* `node` (`ReactWrapper`): A single-node wrapper around the node being processed
* `index` (`Number`): The index of the node being processed
2. `initialValue` (`T` [optional]): If provided, this will be passed in as the first argument to the first invocation of the reducing function. If omitted, the first `node` will be provided and the iteration will begin on the second node in the collection.
Returns
--------
`T`: Returns an array of the returned values from the mapping function...
Example
--------
```
function Foo() {
return (
<div>
<Bar amount={2} />
<Bar amount={4} />
<Bar amount={8} />
</div>
);
}
```
```
const wrapper = mount(<Foo />);
const total = wrapper.find(Bar).reduceRight((amount, n) => amount + n.prop('amount'), 0);
expect(total).to.equal(14);
```
Related Methods
----------------
* [`.reduce(fn[, initialValue]) => Any`](reduce)
* [`.forEach(fn) => ReactWrapper`](foreach)
* [`.map(fn) => Array<Any>`](map)
enzyme .renderProp(propName)(...args) => ReactWrapper .renderProp(propName)(...args) => ReactWrapper
==============================================
Returns a function that, when called with arguments `args`, will return a new wrapper based on the render prop in the original wrapper's prop `propName`.
NOTE: can only be called on wrapper of a single non-DOM component element node.
Arguments
----------
1. `propName` (`String`):
2. `...args` (`Array<Any>`):
This essentially calls `wrapper.prop(propName)(...args)`.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the node returned from the render prop.
Examples
---------
#####
Test Setup
```
class Mouse extends React.Component {
constructor() {
super();
this.state = { x: 0, y: 0 };
}
render() {
const { render } = this.props;
return (
<div
style={{ height: '100%' }}
onMouseMove={(event) => {
this.setState({
x: event.clientX,
y: event.clientY,
});
}}
>
{render(this.state)}
</div>
);
}
}
Mouse.propTypes = {
render: PropTypes.func.isRequired,
};
```
```
const App = () => (
<div style={{ height: '100%' }}>
<Mouse
render={(x = 0, y = 0) => (
<h1>
The mouse position is ({x}, {y})
</h1>
)}
/>
</div>
);
```
#####
Testing with no arguments
```
const wrapper = mount(<App />)
.find(Mouse)
.renderProp('render')();
expect(wrapper.equals(<h1>The mouse position is 0, 0</h1>)).to.equal(true);
```
#####
Testing with multiple arguments
```
const wrapper = mount(<App />)
.find(Mouse)
.renderProp('render')(10, 20);
expect(wrapper.equals(<h1>The mouse position is 10, 20</h1>)).to.equal(true);
```
enzyme .context([key]) => Any .context([key]) => Any
======================
Returns the context hash for the root node of the wrapper. Optionally pass in a prop name and it will return just that value.
Arguments
----------
1. `key` (`String` [optional]): If provided, the return value will be the `this.context[key]` of the root component instance.
Example
--------
```
const wrapper = mount(
<MyComponent />,
{ context: { foo: 10 } },
);
expect(wrapper.context().foo).to.equal(10);
expect(wrapper.context('foo')).to.equal(10);
```
Related Methods
----------------
* [`.state([key]) => Any`](state)
* [`.props() => Object`](props)
* [`.prop(key) => Any`](prop)
enzyme .simulateError(error) => Self .simulateError(error) => Self
=============================
Simulate a component throwing an error as part of its rendering lifecycle.
This is particularly useful in combination with React 16 error boundaries (ie, the `componentDidCatch` and `static getDerivedStateFromError` lifecycle methods).
Arguments
----------
1. `error` (`Any`): The error to throw.
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
function Something() {
// this is just a placeholder
return null;
}
class ErrorBoundary extends React.Component {
static getDerivedStateFromError(error) {
return {
hasError: true,
};
}
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, info) {
const { spy } = this.props;
spy(error, info);
}
render() {
const { children } = this.props;
const { hasError } = this.state;
return (
<React.Fragment>
{hasError ? 'Error' : children}
</React.Fragment>
);
}
}
ErrorBoundary.propTypes = {
children: PropTypes.node.isRequired,
spy: PropTypes.func.isRequired,
};
const spy = sinon.spy();
const wrapper = mount(<ErrorBoundary spy={spy}><Something /></ErrorBoundary>);
const error = new Error('hi!');
wrapper.find(Something).simulateError(error);
expect(wrapper.state()).to.have.property('hasError', true);
expect(spy).to.have.property('callCount', 1);
expect(spy.args).to.deep.equal([
error,
{
componentStack: `
in Something (created by ErrorBoundary)
in ErrorBoundary (created by WrapperComponent)
in WrapperComponent`,
},
]);
```
enzyme .filterWhere(fn) => ReactWrapper .filterWhere(fn) => ReactWrapper
================================
Returns a new wrapper with only the nodes of the current wrapper that, when passed into the provided predicate function, return true.
Arguments
----------
1. `predicate` (`ReactWrapper => Boolean`): A predicate function that is passed a wrapped node.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the filtered nodes.
Example
--------
```
const wrapper = mount(<MyComponent />);
const complexComponents = wrapper.find('.foo').filterWhere((n) => typeof n.type() !== 'string');
expect(complexComponents).to.have.lengthOf(4);
```
Related Methods
----------------
* [`.filter(selector) => ReactWrapper`](filter)
| programming_docs |
enzyme .findWhere(fn) => ReactWrapper .findWhere(fn) => ReactWrapper
==============================
Finds every node in the render tree that returns true for the provided predicate function.
Arguments
----------
1. `predicate` (`ReactWrapper => Boolean`): A predicate function called with the passed in wrapped nodes.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the found nodes.
Example
--------
```
const wrapper = mount(<MyComponent />);
const complexComponents = wrapper.findWhere((n) => typeof n.type() !== 'string');
expect(complexComponents).to.have.lengthOf(8);
```
Related Methods
----------------
* [`.find(selector) => ReactWrapper`](find)
enzyme .matchesElement(patternNode) => Boolean .matchesElement(patternNode) => Boolean
=======================================
Returns whether or not a given react element `patternNode` matches the wrapper's render tree. It must be a single-node wrapper, and only the root node is checked.
The `patternNode` acts like a wildcard. For it to match a node in the wrapper:
* tag names must match
* contents must match: In text nodes, leading and trailing spaces are ignored, but not space in the middle. Child elements must match according to these rules, recursively.
* `patternNode` props (attributes) must appear in the wrapper's nodes, but not the other way around. Their values must match if they do appear.
* `patternNode` style CSS properties must appear in the wrapper's node's style, but not the other way around. Their values must match if they do appear.
Arguments
----------
1. `patternNode` (`ReactElement`): The node whose presence you are detecting in the wrapper's single node.
Returns
--------
`Boolean`: whether or not the current wrapper match the one passed in.
Example
--------
```
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
// ...
}
render() {
return (
<button type="button" onClick={this.handleClick} className="foo bar">Hello</button>
);
}
}
const wrapper = mount(<MyComponent />);
expect(wrapper.matchesElement(<button>Hello</button>)).to.equal(true);
expect(wrapper.matchesElement(<button className="foo bar">Hello</button>)).to.equal(true);
```
Common Gotchas
---------------
* `.matchesElement()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines matching based on the matching of the node's children as well.
Related Methods
----------------
* [`.containsMatchingElement() => ReactWrapper`](containsmatchingelement) - searches all nodes in the wrapper, and searches their entire depth
enzyme .parents([selector]) => ReactWrapper .parents([selector]) => ReactWrapper
====================================
Returns a wrapper around all of the parents/ancestors of the single node in the wrapper. Does not include the node itself. Optionally, a selector can be provided and it will filter the parents by this selector. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector) [optional]): The selector to filter the parents by.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = mount(<ToDoList />);
expect(wrapper.find('ul').parents()).to.have.lengthOf(2);
```
Related Methods
----------------
* [`.children([selector]) => ReactWrapper`](children)
* [`.parent() => ReactWrapper`](parent)
* [`.closest(selector) => ReactWrapper`](closest)
enzyme .find(selector) => ReactWrapper .find(selector) => ReactWrapper
===============================
Finds every node in the render tree of the current wrapper that matches the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the found nodes.
Examples
---------
CSS Selectors:
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(3);
// compound selector
expect(wrapper.find('div.some-class')).to.have.lengthOf(3);
// CSS id selector
expect(wrapper.find('#foo')).to.have.lengthOf(1);
// property selector
expect(wrapper.find('[htmlFor="checkbox"]')).to.have.lengthOf(1);
```
Component Constructors:
```
import Foo from '../components/Foo';
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo)).to.have.lengthOf(1);
```
Component Display Name:
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find('Foo')).to.have.lengthOf(1);
```
Object Property Selector:
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find({ prop: 'value' })).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.findWhere(predicate) => ReactWrapper`](findwhere)
enzyme .tap(intercepter) => Self .tap(intercepter) => Self
=========================
Invokes intercepter and returns itself. intercepter is called with itself. This is helpful when debugging nodes in method chains.
Arguments
----------
1. `intercepter` (`Self`): the current ReactWrapper instance.
Returns
--------
`Self`: the current ReactWrapper instance.
Example
--------
```
const result = mount((
<ul>
<li>xxx</li>
<li>yyy</li>
<li>zzz</li>
</ul>
)).find('li')
.tap((n) => console.log(n.debug()))
.map((n) => n.text());
```
enzyme .closest(selector) => ReactWrapper .closest(selector) => ReactWrapper
==================================
Returns a wrapper of the first element that matches the selector by traversing up through the wrapped node's ancestors in the tree, starting with itself. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the resulting node.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo).closest('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.children([selector]) => ReactWrapper`](children)
* [`.parent() => ReactWrapper`](parent)
* [`.parents([selector]) => ReactWrapper`](parents)
enzyme .detach() => void .detach() => void
=================
Detaches the react tree from the DOM. Runs `ReactDOM.unmountComponentAtNode()` under the hood.
This method will most commonly be used as a "cleanup" method if you decide to use the `attachTo` or `hydrateIn` option in `mount(node, options)`.
The method is intentionally not "fluent" (in that it doesn't return `this`) because you should not be doing anything with this wrapper after this method is called.
Using `attachTo`/`hydrateIn` is not generally recommended unless it is absolutely necessary to test something. It is your responsibility to clean up after yourself at the end of the test if you do decide to use it, though.
Examples
---------
With the `attachTo` option, you can mount components to attached DOM elements:
```
// render a component directly into document.body
const wrapper = mount(<Bar />, { attachTo: document.body });
// Or, with the `hydrateIn` option, you can mount components on top of existing DOM elements:
// hydrate a component directly onto document.body
const hydratedWrapper = mount(<Bar />, { hydrateIn: document.body });
// we can see that the component is rendered into the document
expect(wrapper.find('.in-bar')).to.have.lengthOf(1);
expect(document.body.childNodes).to.have.lengthOf(1);
// detach it to clean up after yourself
wrapper.detach();
// now we can see that
expect(document.body.childNodes).to.have.lengthOf(0);
```
Similarly, if you want to create some one-off elements for your test to mount into:
```
// create a div in the document to mount into
const div = global.document.createElement('div');
global.document.body.appendChild(div);
// div is empty. body has the div attached.
expect(document.body.childNodes).to.have.lengthOf(1);
expect(div.childNodes).to.have.lengthOf(0);
// mount a component passing div into the `attachTo` option
const wrapper = mount(<Foo />, { attachTo: div });
// or, mount a component passing div into the `hydrateIn` option
const hydratedWrapper = mount(<Foo />, { hydrateIn: div });
// we can see now the component is rendered into the document
expect(wrapper.find('.in-foo')).to.have.lengthOf(1);
expect(document.body.childNodes).to.have.lengthOf(1);
expect(div.childNodes).to.have.lengthOf(1);
// call detach to clean up
wrapper.detach();
// div is now empty, but still attached to the document
expect(document.body.childNodes).to.have.lengthOf(1);
expect(div.childNodes).to.have.lengthOf(0);
// remove div if you want
global.document.body.removeChild(div);
expect(document.body.childNodes).to.have.lengthOf(0);
expect(div.childNodes).to.have.lengthOf(0);
```
enzyme .reduce(fn[, initialValue]) => Any .reduce(fn[, initialValue]) => Any
==================================
Applies the provided reducing function to every node in the wrapper to reduce to a single value. Each node is passed in as a `ReactWrapper`, and is processed from left to right.
Arguments
----------
1. `fn` (`Function`): A reducing function to be run for every node in the collection, with the following arguments:
* `value` (`T`): The value returned by the previous invocation of this function
* `node` (`ReactWrapper`): A wrapper around the node being processed
* `index` (`Number`): The index of the node being processed
2. `initialValue` (`T` [optional]): If provided, this will be passed in as the first argument to the first invocation of the reducing function. If omitted, the first `node` will be provided and the iteration will begin on the second node in the collection.
Returns
--------
`T`: Returns an array of the returned values from the mapping function...
Example
--------
```
function Foo() {
return (
<div>
<Bar amount={2} />
<Bar amount={4} />
<Bar amount={8} />
</div>
);
}
```
```
const wrapper = mount(<Foo />);
const total = wrapper.find(Bar).reduce((amount, n) => amount + n.prop('amount'), 0);
expect(total).to.equal(14);
```
Related Methods
----------------
* [`.reduceRight(fn[, initialValue]) => Any`](reduceright)
* [`.forEach(fn) => ReactWrapper`](foreach)
* [`.map(fn) => Array<Any>`](map)
enzyme .slice([begin[, end]]) => ReactWrapper .slice([begin[, end]]) => ReactWrapper
======================================
Returns a new wrapper with a subset of the nodes of the original wrapper, according to the rules of `Array#slice`.
Arguments
----------
1. `begin` (`Number` [optional]): Index from which to slice (defaults to `0`). If negative, this is treated as `length+begin`.
2. `end` (`Number` [optional]): Index at which to end slicing (defaults to `length`). If negative, this is treated as `length+end`.
Returns
--------
`ReactWrapper`: A new wrapper with the subset of nodes specified.
Examples
---------
```
const wrapper = mount((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
expect(wrapper.find('.foo').slice(1)).to.have.lengthOf(2);
expect(wrapper.find('.foo').slice(1).at(0).hasClass('bar')).to.equal(true);
expect(wrapper.find('.foo').slice(1).at(1).hasClass('baz')).to.equal(true);
```
```
const wrapper = mount((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
expect(wrapper.find('.foo').slice(1, 2)).to.have.lengthOf(1);
expect(wrapper.find('.foo').slice(1, 2).at(0).hasClass('bar')).to.equal(true);
```
enzyme .containsMatchingElement(patternNode) => Boolean .containsMatchingElement(patternNode) => Boolean
================================================
Returns whether or not a `patternNode` react element matches any element in the render tree.
* the matches can happen anywhere in the wrapper's contents
* the wrapper can contain more than one node; all are searched
Otherwise, the match follows the same rules as `matchesElement`.
Arguments
----------
1. `patternNode` (`ReactElement`): The node whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in its render tree that matches the one passed in.
Example
--------
```
const wrapper = mount((
<div>
<div data-foo="foo" data-bar="bar">Hello</div>
</div>
));
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar">Hello</div>)).to.equal(true);
expect(wrapper.containsMatchingElement(<div data-foo="foo">Hello</div>)).to.equal(true);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar" data-baz="baz">Hello</div>)).to.equal(false);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="Hello">Hello</div>)).to.equal(false);
expect(wrapper.containsMatchingElement(<div data-foo="foo" data-bar="bar" />)).to.equal(false);
```
Common Gotchas
---------------
* `.containsMatchingElement()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
Related Methods
----------------
* [`.containsAllMatchingElements() => ReactWrapper`](containsallmatchingelements) - must match all nodes in patternNodes
* [`.containsAnyMatchingElements() => ReactWrapper`](containsanymatchingelements) - must match at least one in patternNodes
enzyme .mount() => Self .mount() => Self
================
A method that re-mounts the component, if it is not currently mounted. This can be used to simulate a component going through an unmount/mount lifecycle.
No equivalent for ShallowWrappers.
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
import PropTypes from 'prop-types';
import sinon from 'sinon';
const willMount = sinon.spy();
const didMount = sinon.spy();
const willUnmount = sinon.spy();
class Foo extends React.Component {
constructor(props) {
super(props);
this.componentWillUnmount = willUnmount;
this.componentWillMount = willMount;
this.componentDidMount = didMount;
}
render() {
const { id } = this.props;
return (
<div className={id}>
{id}
</div>
);
}
}
Foo.propTypes = {
id: PropTypes.string.isRequired,
};
const wrapper = mount(<Foo id="foo" />);
expect(willMount).to.have.property('callCount', 1);
expect(didMount).to.have.property('callCount', 1);
expect(willUnmount).to.have.property('callCount', 0);
wrapper.unmount();
expect(willMount).to.have.property('callCount', 1);
expect(didMount).to.have.property('callCount', 1);
expect(willUnmount).to.have.property('callCount', 1);
wrapper.mount();
expect(willMount).to.have.property('callCount', 2);
expect(didMount).to.have.property('callCount', 2);
expect(willUnmount).to.have.property('callCount', 1);
```
Related Methods
----------------
* [`.unmount() => Self`](unmount)
enzyme .some(selector) => Boolean .some(selector) => Boolean
==========================
Returns whether or not any of the nodes in the wrapper match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: True if at least one of the nodes in the current wrapper matched the provided selector.
Examples
---------
```
const wrapper = mount((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').some('.qoo')).to.equal(true);
expect(wrapper.find('.foo').some('.foo')).to.equal(true);
expect(wrapper.find('.foo').some('.bar')).to.equal(false);
```
Related Methods
----------------
* [`.someWhere(predicate) => Boolean`](somewhere)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .instance() => ReactComponent .instance() => ReactComponent
=============================
Returns the single-node wrapper's node's underlying class instance; `this` in its methods. It must be a single-node wrapper.
NOTE: With React `16` and above, `instance()` returns `null` for stateless functional components.
Returns
--------
`ReactComponent|DOMComponent`: The retrieved instance.
Example
--------
```
function Stateless() {
return <div>Stateless</div>;
}
class Stateful extends React.Component {
render() {
return <div>Stateful</div>;
}
}
```
React 16.x
-----------
```
test('shallow wrapper instance should be null', () => {
const wrapper = mount(<Stateless />);
const instance = wrapper.instance();
expect(instance).to.equal(null);
});
test('shallow wrapper instance should not be null', () => {
const wrapper = mount(<Stateful />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateful);
});
```
React 15.x
-----------
```
test('shallow wrapper instance should not be null', () => {
const wrapper = mount(<Stateless />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateless);
});
test('shallow wrapper instance should not be null', () => {
const wrapper = mount(<Stateful />);
const instance = wrapper.instance();
expect(instance).to.be.instanceOf(Stateful);
});
```
enzyme .isEmptyRender() => Boolean .isEmptyRender() => Boolean
===========================
Returns whether or not the wrapper would ultimately render only the allowed falsy values: `false` or `null`.
Returns
--------
`Boolean`: whether the return is falsy
Example
--------
```
function Foo() {
return null;
}
const wrapper = mount(<Foo />);
expect(wrapper.isEmptyRender()).to.equal(true);
```
enzyme .containsAllMatchingElements(patternNodes) => Boolean .containsAllMatchingElements(patternNodes) => Boolean
=====================================================
Returns whether or not all of the given react elements in `patternNodes` match an element in the wrapper's render tree. Every single element of `patternNodes` must be matched one or more times. Matching follows the rules for `containsMatchingElement`.
Arguments
----------
1. `patternNodes` (`Array<ReactElement>`): The array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has nodes anywhere in its render tree that looks like the nodes passed in.
Example
--------
```
const style = { fontSize: 13 };
const wrapper = mount((
<div>
<span className="foo">Hello</span>
<div style={style}>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.containsAllMatchingElements([
<span>Hello</span>,
<div>Goodbye</div>,
])).to.equal(true);
```
Common Gotchas
---------------
* `.containsAllMatchingElements()` expects an array of ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with an array of ReactElement or a JSX expression.
* Keep in mind that this method determines matching based on the matching of the node's children as well.
Related Methods
----------------
* [`.matchesElement() => ReactWrapper`](matcheselement) - rules for matching each node
* [`.containsMatchingElement() => ReactWrapper`](containsmatchingelement) - rules for matching whole wrapper
* [`.containsAnyMatchingElements() => ReactWrapper`](containsanymatchingelements) - must match at least one in patternNodes
enzyme .equals(node) => Boolean .equals(node) => Boolean
========================
Returns whether or not the current wrapper root node render tree looks like the one passed in.
Arguments
----------
1. `node` (`ReactElement`): The node whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in it's render tree that looks like the one passed in.
Example
--------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.equals(<div className="foo bar" />)).to.equal(true);
```
Common Gotchas
---------------
* `.equals()` expects a ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with a ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
* Following React's behavior, `.equals()` ignores properties whose values are `undefined`.
| programming_docs |
enzyme .debug([options]) => String .debug([options]) => String
===========================
Returns an HTML-like string of the wrapper for debugging purposes. Useful to print out to the console when tests are not passing when you expect them to.
Arguments
----------
`options` (`Object` [optional]):
* `options.ignoreProps`: (`Boolean` [optional]): Whether props should be omitted in the resulting string. Props are included by default.
* `options.verbose`: (`Boolean` [optional]): Whether arrays and objects passed as props should be verbosely printed.
Returns
--------
`String`: The resulting string.
Examples
---------
Say we have the following components:
```
function Foo() {
return (
<div className="foo">
<span>Foo</span>
</div>
);
}
function Bar() {
return (
<div className="bar">
<span>Non-Foo</span>
<Foo baz="bax" object={{ a: 1, b: 2 }} />
</div>
);
}
```
In this case, running:
```
console.log(mount(<Bar id="2" />).debug());
```
Would output the following to the console:
```
<Bar id="2">
<div className="bar">
<span>
Non-Foo
</span>
<Foo baz="bax" object={{...}}>
<div className="foo">
<span>
Foo
</span>
</div>
</Foo>
</div>
</Bar>
```
Likewise, running:
```
console.log(mount(<Bar id="2" />).find(Foo).debug());
```
Would output the following to the console:
```
<Foo baz="bax">
<div className="foo">
<span>
Foo
</span>
</div>
</Foo>
```
and:
```
console.log(mount(<Bar id="2" />).find(Foo).debug({ ignoreProps: true }));
```
Would output the following to the console:
```
<Foo>
<div>
<span>
Foo
</span>
</div>
</Foo>
```
and:
```
console.log(mount(<Bar id="2" />).find(Foo).debug({ verbose: true }));
```
Would output the following to the console:
```
<Foo baz="bax" object={{ a: 1, b: 2 }}>
<div className="foo">
<span>
Foo
</span>
</div>
</Foo>
```
enzyme .name() => String|null .name() => String|null
======================
Returns the name of the current node of this wrapper. If it's a composite component, this will be the name of the component. If it's a native DOM node, it will be a string of the tag name. If it's `null`, it will be `null`.
The order of precedence on returning the name is: `type.displayName` -> `type.name` -> `type`.
Note: can only be called on a wrapper of a single node.
Returns
--------
`String|null`: The name of the current node
Examples
---------
```
const wrapper = mount(<div />);
expect(wrapper.name()).to.equal('div');
```
```
const wrapper = mount(<Foo />);
expect(wrapper.name()).to.equal('Foo');
```
```
Foo.displayName = 'A cool custom name';
const wrapper = mount(<Foo />);
expect(wrapper.name()).to.equal('A cool custom name');
```
enzyme .hostNodes() => ReactWrapper .hostNodes() => ReactWrapper
============================
Returns a new wrapper with only host nodes. When using `react-dom`, host nodes are HTML elements rather than custom React components, e.g. `<div>` versus `<MyComponent>`.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
The following code takes a wrapper with two nodes, one a `<MyComponent>` React component, and the other a `<span>`, and filters out the React component.
```
const wrapper = mount((
<div>
<MyComponent className="foo" />
<span className="foo" />
</div>
));
const twoNodes = wrapper.find('.foo');
expect(twoNodes.hostNodes()).to.have.lengthOf(1);
```
enzyme .not(selector) => ReactWrapper .not(selector) => ReactWrapper
==============================
Returns a new wrapper with only the nodes of the current wrapper that don't match the provided selector.
This method is effectively the negation or inverse of [`filter`](filter).
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.foo').not('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.filterWhere(predicate) => ReactWrapper`](filterwhere)
* [`.filter(selector) => ReactWrapper`](filter)
enzyme .key() => String .key() => String
================
Returns the key value for the node of the current wrapper. It must be a single-node wrapper.
Example
--------
```
const wrapper = mount((
<ul>
{['foo', 'bar'].map((s) => <li key={s}>{s}</li>)}
</ul>
)).find('li');
expect(wrapper.at(0).key()).to.equal('foo');
expect(wrapper.at(1).key()).to.equal('bar');
```
enzyme .getWrappingComponent() => ReactWrapper .getWrappingComponent() => ReactWrapper
=======================================
If a `wrappingComponent` was passed in `options`, this methods returns a `ReactWrapper` around the rendered `wrappingComponent`. This `ReactWrapper` can be used to update the `wrappingComponent`'s props, state, etc.
Returns
--------
`ReactWrapper`: A `ReactWrapper` around the rendered `wrappingComponent`
Examples
---------
```
import { Provider } from 'react-redux';
import { Router } from 'react-router';
import store from './my/app/store';
import mockStore from './my/app/mockStore';
function MyProvider(props) {
const { children, customStore } = props;
return (
<Provider store={customStore || store}>
<Router>
{children}
</Router>
</Provider>
);
}
MyProvider.propTypes = {
children: PropTypes.node,
customStore: PropTypes.shape({}),
};
MyProvider.defaultProps = {
children: null,
customStore: null,
};
const wrapper = mount(<MyComponent />, {
wrappingComponent: MyProvider,
});
const provider = wrapper.getWrappingComponent();
provider.setProps({ customStore: mockStore });
```
enzyme .parent() => ReactWrapper .parent() => ReactWrapper
=========================
Returns a wrapper with the direct parent of the node in the current wrapper.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the resulting nodes.
Examples
---------
```
const wrapper = mount(<ToDoList />);
expect(wrapper.find('ul').parent().is('div')).to.equal(true);
```
Related Methods
----------------
* [`.parents([selector]) => ReactWrapper`](parents)
* [`.children([selector]) => ReactWrapper`](children)
* [`.closest(selector) => ReactWrapper`](closest)
enzyme .render() => CheerioWrapper .render() => CheerioWrapper
===========================
Returns a CheerioWrapper around the rendered HTML of the single node's subtree. It must be a single-node wrapper.
Returns
--------
`CheerioWrapper`: The resulting Cheerio object
Examples
---------
```
function Foo() {
return (<div className="in-foo" />);
}
```
```
function Bar() {
return (
<div className="in-bar">
<Foo />
</div>
);
}
```
```
const wrapper = mount(<Bar />);
expect(wrapper.find(Foo).render().find('.in-foo')).to.have.lengthOf(1);
```
enzyme .unmount() => Self .unmount() => Self
==================
A method that unmounts the component. This can be used to simulate a component going through an unmount/mount lifecycle.
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
import PropTypes from 'prop-types';
import sinon from 'sinon';
const willMount = sinon.spy();
const didMount = sinon.spy();
const willUnmount = sinon.spy();
class Foo extends React.Component {
constructor(props) {
super(props);
this.componentWillUnmount = willUnmount;
this.componentWillMount = willMount;
this.componentDidMount = didMount;
}
render() {
const { id } = this.props;
return (
<div className={id}>
{id}
</div>
);
}
}
Foo.propTypes = {
id: PropTypes.string.isRequired,
};
const wrapper = mount(<Foo id="foo" />);
expect(willMount).to.have.property('callCount', 1);
expect(didMount).to.have.property('callCount', 1);
expect(willUnmount).to.have.property('callCount', 0);
wrapper.unmount();
expect(willMount).to.have.property('callCount', 1);
expect(didMount).to.have.property('callCount', 1);
expect(willUnmount).to.have.property('callCount', 1);
```
Related Methods
----------------
* [`.mount() => Self`](mount)
enzyme .every(selector) => Boolean .every(selector) => Boolean
===========================
Returns whether or not all of the nodes in the wrapper match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: True if every node in the current wrapper matched the provided selector.
Examples
---------
```
const wrapper = mount((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').every('.foo')).to.equal(true);
expect(wrapper.find('.foo').every('.qoo')).to.equal(false);
expect(wrapper.find('.foo').every('.bar')).to.equal(false);
```
Related Methods
----------------
* [`.someWhere(predicate) => Boolean`](somewhere)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .hasClass(className) => Boolean .hasClass(className) => Boolean
===============================
Returns whether or not the wrapped node has a `className` prop including the passed in class name. It must be a single-node wrapper.
Arguments
----------
1. `className` (`String` | `RegExp`): A single class name or a regex expression.
Returns
--------
`Boolean`: whether or not the wrapped node has found the class name.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.my-button').hasClass('disabled')).to.equal(true);
```
```
// Searching using RegExp works fine when classes were injected by a jss decorator
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.my-button').hasClass(/(ComponentName)-(other)-(\d+)/)).to.equal(true);
```
Common Gotchas
---------------
* `.hasClass()` expects a class name, NOT a CSS selector. `.hasClass('.foo')` should be `.hasClass('foo')`
enzyme .state([key]) => Any .state([key]) => Any
====================
Returns the state hash for the root node of the wrapper. Optionally pass in a prop name and it will return just that value.
Arguments
----------
1. `key` (`String` [optional]): If provided, the return value will be the `this.state[key]` of the root component instance.
Example
--------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.state().foo).to.equal(10);
expect(wrapper.state('foo')).to.equal(10);
```
Related Methods
----------------
* [`.props() => Object`](props)
* [`.prop(key) => Any`](prop)
* [`.context([key]) => Any`](context)
enzyme .someWhere(fn) => Boolean .someWhere(fn) => Boolean
=========================
Returns whether or not any of the nodes in the wrapper pass the provided predicate function.
Arguments
----------
1. `predicate` (`ReactWrapper => Boolean`): A predicate function to match the nodes.
Returns
--------
`Boolean`: True if at least one of the nodes in the current wrapper passed the predicate function.
Example
--------
```
const wrapper = mount((
<div>
<div className="foo qoo" />
<div className="foo boo" />
<div className="foo hoo" />
</div>
));
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('qoo'))).to.equal(true);
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('foo'))).to.equal(true);
expect(wrapper.find('.foo').someWhere((n) => n.hasClass('bar'))).to.equal(false);
```
Related Methods
----------------
* [`.some(selector) => Boolean`](some)
* [`.every(selector) => Boolean`](every)
* [`.everyWhere(predicate) => Boolean`](everywhere)
enzyme .containsAnyMatchingElements(patternNodes) => Boolean .containsAnyMatchingElements(patternNodes) => Boolean
=====================================================
Returns whether or not at least one of the given react elements in `patternNodes` matches an element in the wrapper's render tree. One or more elements of `patternNodes` must be matched one or more times. Matching follows the rules for `containsMatchingElement`.
Arguments
----------
1. `patternNodes` (`Array<ReactElement>`): The array of nodes whose presence you are detecting in the current instance's render tree.
Returns
--------
`Boolean`: whether or not the current wrapper has a node anywhere in its render tree that looks like one of the array passed in.
Example
--------
```
const style = { fontSize: 13 };
const wrapper = mount((
<div>
<span className="foo">Hello</span>
<div style={style}>Goodbye</div>
<span>Again</span>
</div>
));
expect(wrapper.containsAnyMatchingElements([
<span>Bonjour</span>,
<div>Goodbye</div>,
])).to.equal(true);
```
Common Gotchas
---------------
* `.containsAnyMatchingElements()` expects an array of ReactElement, not a selector (like many other methods). Make sure that when you are calling it you are calling it with an array ReactElement or a JSX expression.
* Keep in mind that this method determines equality based on the equality of the node's children as well.
Related Methods
----------------
* [`.matchesElement() => ReactWrapper`](matcheselement) - rules for matching each node
* [`.containsMatchingElement() => ReactWrapper`](containsmatchingelement) - rules for matching whole wrapper
* [`.containsAllMatchingElements() => ReactWrapper`](containsallmatchingelements) - must match all nodes in patternNodes
enzyme .get(index) => ReactElement .get(index) => ReactElement
===========================
Returns the node at a given index of the current wrapper.
Arguments
----------
1. `index` (`Number`): A zero-based integer indicating which node to retrieve.
Returns
--------
`ReactElement`: The retrieved node.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo).get(0).props.foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ReactWrapper`](at) - same, but returns the React node in a single-node wrapper.
enzyme .is(selector) => Boolean .is(selector) => Boolean
========================
Returns whether or not the single wrapped node matches the provided selector. It must be a single-node wrapper.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`Boolean`: whether or not the wrapped node matches the provided selector.
Example
--------
```
const wrapper = mount(<div className="some-class other-class" />);
expect(wrapper.is('.some-class')).to.equal(true);
```
enzyme .setProps(nextProps[, callback]) => Self .setProps(nextProps[, callback]) => Self
========================================
A method that sets the props of the root component, and re-renders. Useful for when you are wanting to test how the component behaves over time with changing props. Calling this, for instance, will call the `componentWillReceiveProps` lifecycle method.
Similar to `setState`, this method accepts a props object and will merge it in with the already existing props.
NOTE: can only be called on a wrapper instance that is also the root instance.
Arguments
----------
1. `nextProps` (`Object`): An object containing new props to merge in with the current props
2. `callback` (`Function` [optional]): If provided, the callback function will be executed once setProps has completed
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
import React from 'react';
import PropTypes from 'prop-types';
function Foo({ name }) {
return (
<div className={name} />
);
}
Foo.propTypes = {
name: PropTypes.string.isRequired,
};
```
```
const wrapper = mount(<Foo name="foo" />);
expect(wrapper.find('.foo')).to.have.lengthOf(1);
expect(wrapper.find('.bar')).to.have.lengthOf(0);
wrapper.setProps({ name: 'bar' });
expect(wrapper.find('.foo')).to.have.lengthOf(0);
expect(wrapper.find('.bar')).to.have.lengthOf(1);
```
```
import sinon from 'sinon';
const spy = sinon.spy(MyComponent.prototype, 'componentWillReceiveProps');
const wrapper = mount(<MyComponent foo="bar" />);
expect(spy).to.have.property('callCount', 0);
wrapper.setProps({ foo: 'foo' });
expect(spy).to.have.property('callCount', 1);
```
Related Methods
----------------
* [`.setState(state) => Self`](setstate)
* [`.setContext(context) => Self`](setcontext)
enzyme .filter(selector) => ReactWrapper .filter(selector) => ReactWrapper
=================================
Returns a new wrapper with only the nodes of the current wrapper that match the provided selector.
Arguments
----------
1. `selector` ([`EnzymeSelector`](../selector)): The selector to match.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the filtered nodes.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.foo').filter('.bar')).to.have.lengthOf(1);
```
Related Methods
----------------
* [`.filterWhere(predicate) => ReactWrapper`](filterwhere)
enzyme .forEach(fn) => Self .forEach(fn) => Self
====================
Iterates through each node of the current wrapper and executes the provided function with a wrapper around the corresponding node passed in as the first argument.
Arguments
----------
1. `fn` (`Function ( ReactWrapper node, Number index )`): A callback to be run for every node in the collection. Should expect a ReactWrapper as the first argument, and will be run with a context of the original instance.
Returns
--------
`ReactWrapper`: Returns itself.
Example
--------
```
const wrapper = mount((
<div>
<div className="foo bax" />
<div className="foo bar" />
<div className="foo baz" />
</div>
));
wrapper.find('.foo').forEach((node) => {
expect(node.hasClass('foo')).to.equal(true);
});
```
Related Methods
----------------
* [`.map(fn) => ReactWrapper`](map)
enzyme .first() => ReactWrapper .first() => ReactWrapper
========================
Reduce the set of matched nodes to the first in the set, just like `.at(0)`.
Returns
--------
`ReactWrapper`: A new wrapper that wraps the first node in the set.
Examples
---------
```
const wrapper = mount(<MyComponent />);
expect(wrapper.find(Foo).first().props().foo).to.equal('bar');
```
Related Methods
----------------
* [`.at(index) => ReactWrapper`](at) - retrieve any wrapper node
* [`.last() => ReactWrapper`](last)
codeceptjs Getting Started Getting Started
================
CodeceptJS is a modern end to end testing framework with a special BDD-style syntax. The tests are written as a linear scenario of the user's action on a site.
```
Feature('CodeceptJS demo');
Scenario('check Welcome page on site', ({ I }) => {
I.amOnPage('/');
I.see('Welcome');
});
```
Tests are expected to be written in **ECMAScript 7**.
Each test is described inside a `Scenario` function with the `I` object passed into it. The `I` object is an **actor**, an abstraction for a testing user. The `I` is a proxy object for currently enabled **Helpers**.
Architecture
-------------
CodeceptJS bypasses execution commands to helpers. Depending on the helper enabled, your tests will be executed differently. If you need cross-browser support you should choose Selenium-based WebDriver or TestCafé. If you are interested in speed - you should use Chrome-based Puppeteer.
The following is a diagram of the CodeceptJS architecture:
All helpers share the same API, so it's easy to migrate tests from one backend to another. However, because of the difference in backends and their limitations, they are not guaranteed to be compatible with each other. For instance, you can't set request headers in WebDriver or Protractor, but you can do so in Puppteer or Nightmare.
**Pick one helper, as it defines how tests are executed.** If requirements change it's easy to migrate to another.
Refer to following guides to more information on:
* [▶ Playwright](https://codecept.io/playwright)
* [▶ WebDriver](https://codecept.io/webdriver)
* [▶ Puppeteer](https://codecept.io/puppeteer)
* [▶ Protractor](https://codecept.io/angular)
* [▶ Nightmare](https://codecept.io/nightmare)
* [▶ TestCafe](https://codecept.io/testcafe)
> ℹ Depending on a helper selected a list of available actions may change.
>
>
To list all available commands for the current configuration run `codeceptjs list` or enable [auto-completion by generating TypeScript definitions](#intellisense).
> 🤔 It is possible to access API of a backend you use inside a test or a [custom helper](https://codecept.io/helpers/). For instance, to use Puppeteer API inside a test use [`I.usePuppeteerTo`](https://codecept.io/helpers/Puppeteer/#usepuppeteerto) inside a test. Similar methods exist for each helper.
>
>
Writing Tests
--------------
Tests are written from a user's perspective. There is an actor (represented as `I`) which contains actions taken from helpers. A test is written as a sequence of actions performed by an actor:
```
I.amOnPage('/');
I.click('Login');
I.see('Please Login', 'h1');
// ...
```
### Opening a Page
A test should usually start by navigating the browser to a website.
Start a test by opening a page. Use the `I.amOnPage()` command for this:
```
// When "http://site.com" is url in config
I.amOnPage('/'); // -> opens http://site.com/
I.amOnPage('/about'); // -> opens http://site.com/about
I.amOnPage('https://google.com'); // -> https://google.com
```
When an URL doesn't start with a protocol (http:// or https://) it is considered to be a relative URL and will be appended to the URL which was initially set-up in the config.
> It is recommended to use a relative URL and keep the base URL in the config file, so you can easily switch between development, stage, and production environments.
>
>
### Locating Element
Element can be found by CSS or XPath locators.
```
I.seeElement('.user'); // element with CSS class user
I.seeElement('//button[contains(., "press me")]'); // button
```
By default CodeceptJS tries to guess the locator type. In order to specify the exact locator type you can pass an object called **strict locator**.
```
I.seeElement({css: 'div.user'});
I.seeElement({xpath: '//div[@class=user]'});
```
Strict locators allow to specify additional locator types:
```
// locate form element by name
I.seeElement({name: 'password'});
// locate element by React component and props
I.seeElement({react: 'user-profile', props: {name: 'davert'}});
```
In [mobile testing (opens new window)](https://codecept.io/mobile/#locating-elements) you can use `~` to specify the accessibility id to locate an element. In web application you can locate elements by their `aria-label` value.
```
// locate element by [aria-label] attribute in web
// or by accessibility id in mobile
I.seeElement('~username');
```
> [▶ Learn more about using locators in CodeceptJS](https://codecept.io/locators).
>
>
### Clicking
CodeceptJS provides a flexible syntax to specify an element to click.
By default CodeceptJS tries to find the button or link with the exact text on it
```
// search for link or button
I.click('Login');
```
If none was found, CodeceptJS tries to find a link or button containing that text. In case an image is clickable its `alt` attribute will be checked for text inclusion. Form buttons will also be searched by name.
To narrow down the results you can specify a context in the second parameter.
```
I.click('Login', '.nav'); // search only in .nav
I.click('Login', {css: 'footer'}); // search only in footer
```
> To skip guessing the locator type, pass in a strict locator - A locator starting with '#' or '.' is considered to be CSS. Locators starting with '//' or './/' are considered to be XPath.
>
>
You are not limited to buttons and links. Any element can be found by passing in valid CSS or XPath:
```
// click element by CSS
I.click('#signup');
// click element located by special test-id attribute
I.click('//dev[@test-id="myid"]');
```
> ℹ If click doesn't work in a test but works for user, it is possible that frontend application is not designed for automated testing. To overcome limitation of standard click in this edgecase use `forceClick` method. It will emulate click instead of sending native event. This command will click an element no matter if this element is visible or animating. It will send JavaScript "click" event to it.
>
>
### Filling Fields
Clicking the links is not what takes the most time during testing a web site. If your site consists only of links you can skip test automation. The most waste of time goes into the testing of forms. CodeceptJS provides several ways of doing that.
Let's submit this sample form for a test:
```
<form method="post" action="/update" id="update_form">
<label for="user_name">Name</label>
<input type="text" name="user[name]" id="user_name" /><br>
<label for="user_email">Email</label>
<input type="text" name="user[email]" id="user_email" /><br>
<label for="user_role">Role</label>
<select id="user_role" name="user[role]">
<option value="0">Admin</option>
<option value="1">User</option>
</select><br>
<input type="checkbox" id="accept" /> <label for="accept">Accept changes</label>
<div>
<input type="submit" name="submitButton" class="btn btn-primary" value="Save" />
</div>
</form>
```
We need to fill in all those fields and click the "Update" button. CodeceptJS matches form elements by their label, name, or by CSS or XPath locators.
```
// we are using label to match user_name field
I.fillField('Name', 'Miles');
// we can use input name
I.fillField('user[email]','[email protected]');
// select element by label, choose option by text
I.selectOption('Role','Admin');
// click 'Save' button, found by text
I.checkOption('Accept');
I.click('Save');
```
> ℹ `selectOption` works only with standard `<select>` HTML elements. If your selectbox is created by React, Vue, or as a component of any other framework, this method potentially won't work with it. Use `click` to manipulate it.
>
>
> ℹ `checkOption` also works only with standard `<input type="checkbox">` HTML elements. If your checkbox is created by React, Vue, or as a component of any other framework, this method potentially won't work with it. Use `click` to manipulate it.
>
>
Alternative scenario:
```
// we are using CSS
I.fillField('#user_name', 'Miles');
I.fillField('#user_email','[email protected]');
// select element by label, option by value
I.selectOption('#user_role','1');
// click 'Update' button, found by name
I.click('submitButton', '#update_form');
```
To fill in sensitive data use the `secret` function, it won't expose actual value in logs.
```
I.fillField('password', secret('123456'));
```
### Assertions
In order to verify the expected behavior of a web application, its content should be checked. CodeceptJS provides built-in assertions for that. They start with a `see` (or `dontSee`) prefix.
The most general and common assertion is `see`, which checks visilibility of a text on a page:
```
// Just a visible text on a page
I.see('Hello');
// text inside .msg element
I.see('Hello', '.msg');
// opposite
I.dontSee('Bye');
```
You should provide a text as first argument and, optionally, a locator to search for a text in a context.
You can check that specific element exists (or not) on a page, as it was described in [Locating Element](#locating-element) section.
```
I.seeElement('.notice');
I.dontSeeElement('.error');
```
Additional assertions:
```
I.seeInCurrentUrl('/user/miles');
I.seeInField('user[name]', 'Miles');
I.seeInTitle('My Website');
```
To see all possible assertions, check the helper's reference.
> ℹ If you need custom assertions, you can install an assertion libarary like `chai`, use grabbers to obtain information from a browser and perform assertions. However, it is recommended to put custom assertions into a helper for further reuse.
>
>
### Grabbing
Sometimes you need to retrieve data from a page to use it in the following steps of a scenario. Imagine the application generates a password, and you want to ensure that user can login using this password.
```
Scenario('login with generated password', async ({ I }) => {
I.fillField('email', '[email protected]');
I.click('Generate Password');
const password = await I.grabTextFrom('#password');
I.click('Login');
I.fillField('email', '[email protected]');
I.fillField('password', password);
I.click('Log in!');
I.see('Hello, Miles');
});
```
The `grabTextFrom` action is used to retrieve the text from an element. All actions starting with the `grab` prefix are expected to return data. In order to synchronize this step with a scenario you should pause the test execution with the `await` keyword of ES6. To make it work, your test should be written inside a async function (notice `async` in its definition).
```
Scenario('use page title', async ({ I }) => {
// ...
const password = await I.grabTextFrom('#password');
I.fillField('password', password);
});
```
### Waiting
In modern web applications, rendering is done on the client-side. Sometimes that may cause delays. A test may fail while trying to click an element which has not appeared on a page yet. To handle these cases, the `wait*` methods has been introduced.
```
I.waitForElement('#agree_button', 30); // secs
// clicks a button only when it is visible
I.click('#agree_button');
```
> ℹ See [helpers reference](https://codecept.io/reference) for a complete list of all available commands for the helper you use.
>
>
How It Works
-------------
Tests are written in a synchronous way. This improves the readability and maintainability of tests. While writing tests you should not think about promises, and instead should focus on the test scenario.
However, behind the scenes **all actions are wrapped in promises**, inside of the `I` object. [Global promise (opens new window)](https://github.com/codeceptjs/CodeceptJS/blob/master/lib/recorder.js) chain is initialized before each test and all `I.*` calls will be appended to it, as well as setup and teardown.
> 📺 [Learn how CodeceptJS (opens new window)](https://www.youtube.com/watch?v=MDLLpHAwy_s) works with promises by watching video on YouTube
>
>
If you want to get information from a running test you can use `await` inside the **async function**, and utilize special methods of helpers started with the `grab` prefix.
```
Scenario('try grabbers', async ({ I }) => {
let title = await I.grabTitle();
});
```
then you can use those variables in assertions:
```
var title = await I.grabTitle();
var assert = require('assert');
assert.equal(title, 'CodeceptJS');
```
Running Tests
--------------
To launch tests use the `run` command, and to execute tests in [multiple browsers](https://codecept.io/advanced/#multiple-browsers-execution) or [multiple threads](https://codecept.io/advanced/#parallel-execution) use the `run-multiple` command.
### Level of Detail
To see the step-by-step output of running tests, add the `--steps` flag:
```
npx codeceptjs run --steps
```
To see a more detailed output add the `--debug` flag:
```
npx codeceptjs run --debug
```
To see very detailed output informations use the `--verbose` flag:
```
npx codeceptjs run --verbose
```
### Filter
A single test file can be executed if you provide a relative path to such a file:
```
npx codeceptjs run github_test.js
# or
npx codeceptjs run admin/login_test.js
```
To filter a test by name use the `--grep` parameter, which will execute all tests with names matching the regex pattern.
To run all tests with the `slow` word in it:
```
npx codeceptjs run --grep "slow"
```
It is recommended to [filter tests by tags](https://codecept.io/advanced/#tags).
> For more options see [full reference of `run` command](https://codecept.io/commands/#run).
>
>
### Parallel Run
Since CodeceptJS 2.3, you can run tests in parallel by using NodeJS workers. This feature requires NodeJS >= 11.6. Use `run-workers` command with the number of workers (threads) to split tests.
```
npx codeceptjs run-workers 3
```
Tests are split by scenarios, not by files. Results are aggregated and shown up in the main process.
Configuration
--------------
Configuration is set in the `codecept.conf.js` file which was created during the `init` process. Inside the config file you can enable and configure helpers and plugins, and set bootstrap and teardown scripts.
```
exports.config = {
helpers: {
// enabled helpers with their configs
},
plugins: {
// list of used plugins
},
include: {
// current actor and page objects
}
}
```
> ▶ See complete [configuration reference](https://codecept.io/configuration).
>
>
You can have multiple configuration files for a the same project, in this case you can specify a config file to be used with `-c` when running.
```
npx codeceptjs run -c codecept.ci.conf.js
```
Tuning configuration for helpers like WebDriver, Puppeteer can be hard, as it requires good understanding of how these technologies work. Use the [`@codeceptjs/configure` (opens new window)](https://github.com/codeceptjs/configure) package with common configuration recipes.
For instance, you can set the window size or toggle headless mode, no matter of which helpers are actually used.
```
const { setHeadlessWhen, setWindowSize } = require('@codeceptjs/configure');
// run headless when CI environment variable set
setHeadlessWhen(process.env.CI);
// set window size for any helper: Puppeteer, WebDriver, TestCafe
setWindowSize(1600, 1200);
exports.config = {
// ...
}
```
> ▶ See more [configuration recipes (opens new window)](https://github.com/codeceptjs/configure)
>
>
Debug
------
CodeceptJS allows to write and debug tests on the fly while keeping your browser opened. By using the interactive shell you can stop execution at any point and type in any CodeceptJS commands.
This is especially useful while writing a new scratch. After opening a page call `pause()` to start interacting with a page:
```
I.amOnPage('/');
pause();
```
Try to perform your scenario step by step. Then copy succesful commands and insert them into a test.
### Pause
Test execution can be paused in any place of a test with `pause()` call. Variables can also be passed to `pause({data: 'hi', func: () => console.log('hello')})` which can be accessed in Interactive shell.
This launches the interactive console where you can call any action from the `I` object.
```
Interactive shell started
Press ENTER to resume test
Use JavaScript syntax to try steps in action
- Press ENTER to run the next step
- Press TAB twice to see all available commands
- Type exit + Enter to exit the interactive shell
- Prefix => to run js commands
I.
```
Type in different actions to try them, copy and paste successful ones into the test file.
Press `ENTER` to resume test execution.
To **debug test step-by-step** press Enter, the next step will be executed and interactive shell will be shown again.
To see all available commands, press TAB two times to see list of all actions included in the `I` object.
> The interactive shell can be started outside of test context by running `npx codeceptjs shell`
>
>
PageObjects and other variables can also be passed to as object:
```
pause({ loginPage, data: 'hi', func: () => console.log('hello') });
```
Inside a pause mode you can use `loginPage`, `data`, `func` variables. Arbitrary JavaScript code can be executed when used `=>` prefix:
```
I.=> loginPage.open()
I.=> func()
I.=> 2 + 5
```
### Pause on Fail
To start interactive pause automatically for a failing test you can run tests with [pauseOnFail Plugin](https://codecept.io/plugins/#pauseonfail). When a test fails, the pause mode will be activated, so you can inspect current browser session before it is closed.
> **[pauseOnFail plugin](https://codecept.io/plugins/#pauseOnFail) can be used** for new setups
>
>
To run tests with pause on fail enabled use `-p pauseOnFail` option
```
npx codeceptjs run -p pauseOnFail
```
> To enable pause after a test without a plugin you can use `After(pause)` inside a test file.
>
>
### Screenshot on Failure
By default CodeceptJS saves a screenshot of a failed test. This can be configured in [screenshotOnFail Plugin](https://codecept.io/plugins/#screenshotonfail)
> **[screenshotOnFail plugin](https://codecept.io/plugins/#screenshotonfail) is enabled by default** for new setups
>
>
### Step By Step Report
To see how the test was executed, use [stepByStepReport Plugin](https://codecept.io/plugins/#stepbystepreport). It saves a screenshot of each passed step and shows them in a nice slideshow.
Retries
--------
### Auto Retry
You can auto-retry a failed step by enabling [retryFailedStep Plugin](https://codecept.io/plugins/#retryfailedstep).
> **[retryFailedStep plugin](https://codecept.io/plugins/#retryfailedstep) is enabled by default** for new setups
>
>
### Retry Step
Unless you use retryFailedStep plugin you can manually control retries in your project.
If you have a step which often fails, you can retry execution for this single step. Use the `retry()` function before an action to ask CodeceptJS to retry it on failure:
```
I.retry().see('Welcome');
```
If you'd like to retry a step more than once, pass the amount as a parameter:
```
I.retry(3).see('Welcome');
```
Additional options can be provided to `retry`, so you can set the additional options (defined in [promise-retry (opens new window)](https://www.npmjs.com/package/promise-retry) library).
```
// retry action 3 times waiting for 0.1 second before next try
I.retry({ retries: 3, minTimeout: 100 }).see('Hello');
// retry action 3 times waiting no more than 3 seconds for last retry
I.retry({ retries: 3, maxTimeout: 3000 }).see('Hello');
// retry 2 times if error with message 'Node not visible' happens
I.retry({
retries: 2,
when: err => err.message === 'Node not visible'
}).seeElement('#user');
```
Pass a function to the `when` option to retry only when an error matches the expected one.
### Retry Scenario
When you need to rerun scenarios a few times, add the `retries` option to the `Scenario` declaration.
CodeceptJS implements retries the same way [Mocha does (opens new window)](https://mochajs.org#retry-tests); You can set the number of a retries for a feature:
```
Scenario('Really complex', ({ I }) => {
// test goes here
}).retry(2);
// alternative
Scenario('Really complex', { retries: 2 },({ I }) => {});
```
This scenario will be restarted two times on a failure. Unlike retry step, there is no `when` condition supported for retries on a scenario level.
### Retry Feature
To set this option for all scenarios in a file, add `retry` to a feature:
```
Feature('Complex JS Stuff').retry(3);
```
Every Scenario inside this feature will be rerun 3 times. You can make an exception for a specific scenario by passing the `retries` option to a Scenario.
Before
-------
Common preparation steps like opening a web page or logging in a user, can be placed in the `Before` or `Background` hooks:
```
Feature('CodeceptJS Demonstration');
Before(({ I }) => { // or Background
I.amOnPage('/documentation');
});
Scenario('test some forms', ({ I }) => {
I.click('Create User');
I.see('User is valid');
I.dontSeeInCurrentUrl('/documentation');
});
Scenario('test title', ({ I }) => {
I.seeInTitle('Example application');
});
```
Same as `Before` you can use `After` to run teardown for each scenario.
BeforeSuite
------------
If you need to run complex a setup before all tests and have to teardown this afterwards, you can use the `BeforeSuite` and `AfterSuite` functions. `BeforeSuite` and `AfterSuite` have access to the `I` object, but `BeforeSuite/AfterSuite` don't have any access to the browser, because it's not running at this moment. You can use them to execute handlers that will setup your environment. `BeforeSuite/AfterSuite` will work only for the file it was declared in (so you can declare different setups for files)
```
BeforeSuite(({ I }) => {
I.syncDown('testfolder');
});
AfterSuite(({ I }) => {
I.syncUp('testfolder');
I.clearDir('testfolder');
});
```
[Here are some ideas (opens new window)](https://github.com/codeceptjs/CodeceptJS/pull/231#issuecomment-249554933) on where to use BeforeSuite hooks.
Within
-------
To specify the exact area on a page where actions can be performed you can use the `within` function. Everything executed in its context will be narrowed to context specified by locator:
Usage: `within('section', ()=>{})`
```
I.amOnPage('https://github.com');
within('.js-signup-form', () => {
I.fillField('user[login]', 'User');
I.fillField('user[email]', '[email protected]');
I.fillField('user[password]', '[email protected]');
I.click('button');
});
I.see('There were problems creating your account.');
```
> ⚠ `within` can cause problems when used incorrectly. If you see a weird behavior of a test try to refactor it to not use `within`. It is recommended to keep within for simplest cases when possible. Since `within` returns a Promise, it may be necessary to `await` the result even when you're not intending to use the return value.
>
>
`within` can also work with IFrames. A special `frame` locator is required to locate the iframe and get into its context.
See example:
```
within({frame: "#editor"}, () => {
I.see('Page');
});
```
> ℹ IFrames can also be accessed via `I.switchTo` command of a corresponding helper.
>
>
Nested IFrames can be set by passing an array *(WebDriver, Nightmare & Puppeteer only)*:
```
within({frame: [".content", "#editor"]}, () => {
I.see('Page');
});
```
When running steps inside, a within block will be shown with a shift:
Within can return a value, which can be used in a scenario:
```
// inside async function
const val = await within('#sidebar', () => {
return I.grabTextFrom({ css: 'h1' });
});
I.fillField('Description', val);
```
Conditional Actions
--------------------
There is a way to execute unsuccessful actions to without failing a test. This might be useful when you might need to click "Accept cookie" button but probably cookies were already accepted. To handle these cases `tryTo` function was introduced:
```
tryTo(() => I.click('Accept', '.cookies'));
```
You may also use `tryTo` for cases when you deal with uncertainty on page:
* A/B testing
* soft assertions
* cookies & gdpr
`tryTo` function is enabled by default via [tryTo plugin](https://codecept.io/plugins#tryTo)
Comments
---------
There is a simple way to add additional comments to your test scenario: Use the `say` command to print information to screen:
```
I.say('I am going to publish post');
I.say('I enter title and body');
I.say('I expect post is visible on site');
```
Use the second parameter to pass in a color value (ASCII).
```
I.say('This is red', 'red'); //red is used
I.say('This is blue', 'blue'); //blue is used
I.say('This is by default'); //cyan is used
```
IntelliSense
-------------
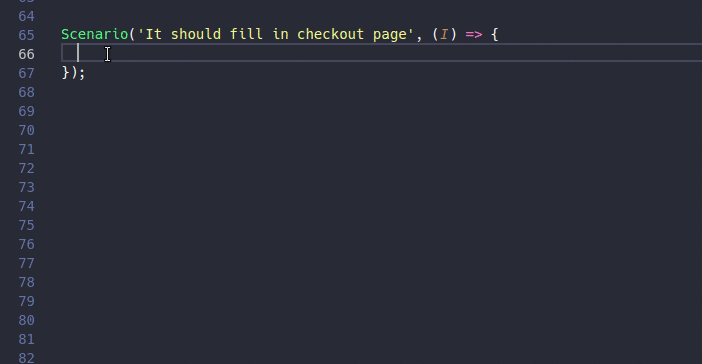
To get autocompletion when working with CodeceptJS, use Visual Studio Code or another IDE that supports TypeScript Definitions.
Generate step definitions with:
```
npx codeceptjs def
```
Create a file called `jsconfig.json` in your project root directory, unless you already have one.
```
{
"compilerOptions": {
"allowJs": true,
}
}
```
Alternatively, you can include `/// <reference path="./steps.d.ts" />` into your test files to get method autocompletion while writing tests.
Multiple Sessions
------------------
CodeceptJS allows to run several browser sessions inside a test. This can be useful for testing communication between users inside a chat or other systems. To open another browser use the `session()` function as shown in the example:
```
Scenario('test app', ({ I }) => {
I.amOnPage('/chat');
I.fillField('name', 'davert');
I.click('Sign In');
I.see('Hello, davert');
session('john', () => {
// another session started
I.amOnPage('/chat');
I.fillField('name', 'john');
I.click('Sign In');
I.see('Hello, john');
});
// switching back to default session
I.fillField('message', 'Hi, john');
// there is a message from current user
I.see('me: Hi, john', '.messages');
session('john', () => {
// let's check if john received it
I.see('davert: Hi, john', '.messages');
});
});
```
The `session` function expects the first parameter to be the name of the session. You can switch back to this session by using the same name.
You can override the configuration for the session by passing a second parameter:
```
session('john', { browser: 'firefox' } , () => {
// run this steps in firefox
I.amOnPage('/');
});
```
or just start the session without switching to it. Call `session` passing only its name:
```
Scenario('test', ({ I }) => {
// opens 3 additional browsers
session('john');
session('mary');
session('jane');
I.amOnPage('/');
// switch to session by its name
session('mary', () => {
I.amOnPage('/login');
});
}
```
`session` can return a value which can be used in a scenario:
```
// inside async function
const val = await session('john', () => {
I.amOnPage('/info');
return I.grabTextFrom({ css: 'h1' });
});
I.fillField('Description', val);
```
Functions passed into a session can use the `I` object, page objects, and any other objects declared for the scenario. This function can also be declared as async (but doesn't work as generator).
Also, you can use `within` inside a session, but you can't call session from inside `within`.
Skipping
---------
Like in Mocha you can use `x` and `only` to skip tests or to run a single test.
* `xScenario` - skips current test
* `Scenario.skip` - skips current test
* `Scenario.only` - executes only the current test
* `xFeature` - skips current suite Since 2.6.6
* `Feature.skip` - skips the current suite Since 2.6.6
Todo Test
----------
You can use `Scenario.todo` when you are planning on writing tests.
This test will be skipped like with regular `Scenario.skip` but with additional message "Test not implemented!":
Use it with a test body as a test plan:
```
Scenario.todo('Test', I => {
/**
* 1. Click to field
* 2. Fill field
*
* Result:
* 3. Field contains text
*/
});
```
Or even without a test body:
```
Scenario.todo('Test');
```
| programming_docs |
codeceptjs CodeceptUI CodeceptUI
===========
CodeceptJS has an interactive, graphical test runner. We call it CodeceptUI. It works in your browser and helps you to manage your tests.
CodeceptUI can be used for
* running tests by groups or single
* get test reports
* review tests
* edit tests and page objects
* write new tests
* reuse one browser session accross multiple test runs
* easily switch to headless/headful mode
Installation
-------------
CodeceptUI is already installed with `create-codeceptjs` command but you can install it manually via:
```
npm i @codeceptjs/ui --save
```
Usage
------
To start using CodeceptUI you need to have CodeceptJS project with a few tests written. If CodeceptUI was installed by `create-codecept` command it can be started with:
```
npm run codeceptjs:ui
```
CodeceptUI can be started in two modes:
* **Application** mode - starts Electron application in a window. Designed for desktop systems.
* **Server** mode - starts a webserver. Deigned for CI systems.
To start CodeceptUI in application mode:
```
npx codecept-ui --app
```
To start CodeceptUI in server mode:
```
npx codecept-ui
```
codeceptjs Advanced Usage Advanced Usage
===============
Data Driven Tests
------------------
Execute the same scenario on a different data set.
Let's say you want to test login for different user accounts. In this case, you need to create a datatable and fill it in with credentials. Then use `Data().Scenario` to include this data and generate multiple scenarios:
```
// Define data table inside a test or load from another module
let accounts = new DataTable(['login', 'password']); //
accounts.add(['davert', '123456']); // adding records to a table
accounts.add(['admin', '123456']);
// You can skip some data. But add them to report as skipped (just like with usual scenarios):
accounts.xadd(['admin', '23456'])
// Pass dataTable to Data()
// Use special param `current` to get current data set
Data(accounts).Scenario('Test Login', ({ I, current }) => {
I.fillField('Username', current.login); // current is reserved!
I.fillField('Password', current.password);
I.click('Sign In');
I.see('Welcome '+ current.login);
});
// Also you can set only for Data tests. It will launch executes only the current test but with all data options
Data(accounts).only.Scenario('Test Login', ({ I, current }) => {
I.fillField('Username', current.login); // current is reserved!
I.fillField('Password', current.password);
I.click('Sign In');
I.see('Welcome '+ current.login);
});
```
*Important: you can't use name `current` for pageObjects or helpers in data scenarios*
This will produce 2 tests with different data sets. Current data set is appended to a test name in output:
```
✓ Test Login | {"login":"davert","password":"123456"}
✓ Test Login | {"login":"admin","password":"123456"}
S Test Login | {"login":"admin","password":"23456"}
```
```
// You can filter your data table
Data(accounts.filter(account => account.login == 'admin')
.Scenario('Test Login', ({ I, current }) => {
I.fillField('Username', current.login);
I.fillField('Password', current.password);
I.click('Sign In');
I.see('Welcome '+ current.login);
});
```
This will limit data sets accoring passed function:
```
✓ Test Login | {"login":"admin","password":"123456"}
S Test Login | {"login":"admin","password":"23456"}
```
Data sets can also be defined with array, generator, or a function.
```
Data(function*() {
yield { user: 'davert'};
yield { user: 'andrey'};
}).Scenario() // ...
```
*HINT: If you don't use DataTable. add `toString()` method to each object added to data set, so the data could be pretty printed in a test name*
Tags
-----
Append `@tag` to your test name, so
```
Scenario('update user profile @slow')
```
Alternativly, use `tag` method of Scenario to set additional tags:
```
Scenario('update user profile', ({ }) => {
// test goes here
}).tag('@slow').tag('important');
```
All tests with `@tag` could be executed with `--grep @tag` option.
```
codeceptjs run --grep @slow
```
Use regex for more flexible filtering:
* `--grep '(?=.*@smoke2)(?=.*@smoke3)'` - run tests with @smoke2 and @smoke3 in name
* `--grep "\@smoke2|\@smoke3"` - run tests with @smoke2 or @smoke3 in name
* `--grep '((?=.*@smoke2)(?=.*@smoke3))|@smoke4'` - run tests with (@smoke2 and @smoke3) or @smoke4 in name
* `--grep '(?=.*@smoke2)^(?!.*@smoke3)'` - run tests with @smoke2 but without @smoke3 in name
* `--grep '(?=.*)^(?!.*@smoke4)'` - run all tests except @smoke4
Debug
------
CodeceptJS provides a debug mode in which additional information is printed. It can be turned on with `--debug` flag.
```
codeceptjs run --debug
```
to receive even more information turn on `--verbose` flag:
```
codeceptjs run --verbose
```
And don't forget that you can pause execution and enter **interactive console** mode by calling `pause()` inside your test.
For advanced debugging use NodeJS debugger. In WebStorm IDE:
```
node $NODE_DEBUG_OPTION ./node_modules/.bin/codeceptjs run
```
For Visual Studio Code, add the following configuration in launch.json:
```
{
"type": "node",
"request": "launch",
"name": "codeceptjs",
"args": ["run", "--grep", "@your_test_tag"],
"program": "${workspaceFolder}/node_modules/.bin/codeceptjs"
}
```
Test Options
-------------
Features and Scenarios have their options that can be set by passing a hash after their names:
```
Feature('My feature', {key: val});
Scenario('My scenario', {key: val},({ I }) => {});
```
You can use this options for build your own [plugins (opens new window)](https://codecept.io/hooks/#plugins) with [event listners (opens new window)](https://codecept.io/hooks/#api). Example:
```
// for test
event.dispatcher.on(event.test.before, (test) => {
...
if (test.opts.key) {
...
}
...
});
// or for suite
event.dispatcher.on(event.suite.before, (suite) => {
...
if (suite.opts.key) {
...
}
...
});
```
### Timeout
By default there is no timeout for tests, however you can change this value for a specific suite:
```
Feature('Stop me').timeout(5000); // set timeout to 5s
```
or for the test:
```
// set timeout to 1s
Scenario("Stop me faster",({ I }) => {
// test goes here
}).timeout(1000);
// alternative
Scenario("Stop me faster", {timeout: 1000},({ I }) => {});
// disable timeout for this scenario
Scenario("Don't stop me", {timeout: 0},({ I }) => {});
```
Dynamic Configuration
----------------------
Helpers can be reconfigured per scenario or per feature. This might be useful when some tests should be executed with different settings than others. In order to reconfigure tests use `.config()` method of `Scenario` or `Feature`.
```
Scenario('should be executed in firefox', ({ I }) => {
// I.amOnPage(..)
}).config({ browser: 'firefox' })
```
In this case `config` overrides current config of the first helper. To change config of specific helper pass two arguments: helper name and config values:
```
Scenario('should create data via v2 version of API', ({ I }) => {
// I.amOnPage(..)
}).config('REST', { endpoint: 'https://api.mysite.com/v2' })
```
Config can also be set by a function, in this case you can get a test object and specify config values based on it. This is very useful when running tests against cloud providers, like BrowserStack. This function can also be asynchronous.
```
Scenario('should report to BrowserStack', ({ I }) => {
// I.amOnPage(..)
}).config((test) => {
return { desiredCapabilities: {
project: test.suite.title,
name: test.title,
}}
});
```
Config changes can be applied to all tests in suite:
```
Feature('Admin Panel').config({ url: 'https://mysite.com/admin' });
```
Please note that some config changes can't be applied on the fly. For instance, if you set `restart: false` in your config and then changing value `browser` won't take an effect as browser is already started and won't be closed untill all tests finish.
Configuration changes will be reverted after a test or a suite.
### Rerunning Flaky Tests Multiple Times Since 2.4
End to end tests can be flaky for various reasons. Even when we can't do anything to solve this problem it we can do next two things:
* Detect flaky tests in our suite
* Fix flaky tests by rerunning them.
Both tasks can be achieved with [`run-rerun` command](https://codecept.io/commands/#run-rerun) which runs tests multiple times until all tests are passed.
You should set min and max runs boundaries so when few tests fail in a row you can rerun them until they are succeeded.
```
// inside to codecept.conf.js
exports.config = { // ...
rerun: {
// run 4 times until 1st success
minSuccess: 1,
maxReruns: 4,
}
}
```
If you want to check all your tests for stability you can set high boundaries for minimal success:
```
// inside to codecept.conf.js
exports.config = { // ...
rerun: {
// run all tests must pass exactly 5 times
minSuccess: 5,
maxReruns: 5,
}
}
```
Now execute tests with `run-rerun` command:
```
npx codeceptjs run-rerun
```
codeceptjs Configuration Configuration
==============
CodeceptJS configuration is set in `codecept.conf.js` file.
After running `codeceptjs init` it should be saved in test root.
Here is an overview of available options with their defaults:
* **tests**: `"./*_test.js"` - pattern to locate tests. Allows to enter [glob pattern (opens new window)](https://github.com/isaacs/node-glob).
* **grep**: - pattern to filter tests by name
* **include**: `{}` - actors and page objects to be registered in DI container and included in tests. Accepts objects and module `require` paths
* **timeout**: `10000` - default tests timeout
* **output**: `"./output"` - where to store failure screenshots, etc
* **helpers**: `{}` - list of enabled helpers
* **mocha**: `{}` - mocha options, [reporters (opens new window)](https://codecept.io/reports/) can be configured here
* **multiple**: `{}` - multiple options, see [Multiple Execution (opens new window)](https://codecept.io/parallel#multiple-browsers-execution)
* **bootstrap**: `"./bootstrap.js"` - an option to run code *before* tests are run. See [Hooks (opens new window)](https://codecept.io/hooks/#bootstrap-teardown)).
* **bootstrapAll**: `"./bootstrap.js"` - an option to run code *before* all test suites are run when using the run-multiple mode. See [Hooks (opens new window)](https://codecept.io/hooks/#bootstrap-teardown)).
* **teardown**: - an option to run code *after* all test suites are run when using the run-multiple mode. See [Hooks (opens new window)](https://codecept.io/hooks/#bootstrap-teardown).
* **teardownAll**: - an option to run code *after* tests are run. See [Hooks (opens new window)](https://codecept.io/hooks/#bootstrap-teardown).
* **noGlobals**: `false` - disable registering global variables like `Actor`, `Helper`, `pause`, `within`, `DataTable`
* **hooks**: - include custom listeners to plug into execution workflow. See [Custom Hooks (opens new window)](https://codecept.io/hooks/#custom-hooks)
* **translation**: - [locale (opens new window)](https://codecept.io/translation/) to be used to print s teps output, as well as used in source code.
* **require**: `[]` - array of module names to be required before codecept starts. See [Require](#require)
Require
--------
Requires described module before run. This option is useful for assertion libraries, so you may `--require should` instead of manually invoking `require('should')` within each test file. It can be used with relative paths, e.g. `"require": ["/lib/somemodule"]`, and installed packages.
You can register ts-node, so you can use Typescript in tests with ts-node package
```
exports.config = {
tests: './*_test.js',
timeout: 10000,
output: '',
helpers: {},
include: {},
bootstrap: false,
mocha: {},
// require modules
require: ["ts-node/register", "should"]
}
```
Dynamic Configuration
----------------------
By default `codecept.json` is used for configuration. You can override its values in runtime by using `--override` or `-o` option in command line, passing valid JSON as a value:
```
codeceptjs run -o '{ "helpers": {"WebDriver": {"browser": "firefox"}}}'
```
You can also switch to JS configuration format for more dynamic options. Create `codecept.conf.js` file and make it export `config` property.
See the config example:
```
exports.config = {
helpers: {
WebDriver: {
// load variables from the environment and provide defaults
url: process.env.CODECEPT_URL || 'http://localhost:3000',
user: process.env.CLOUDSERVICE_USER,
key: process.env.CLOUDSERVICE_KEY,
coloredLogs: true,
waitForTimeout: 10000
}
},
// don't build monolithic configs
mocha: require('./mocha.conf.js') || {},
include: {
I: './src/steps_file.js',
loginPage: './src/pages/login_page',
dashboardPage: new DashboardPage()
}
// here goes config as it was in codecept.json
// ....
};
```
(Don't copy-paste this config, it's just demo)
If you prefer to store your configuration files in a different location, or with a different name, you can do that with `--config` or `-c:
```
codeceptjs run --config=./path/to/my/config.js
```
Common Configuration Patterns
------------------------------
> 📺 [Watch this material (opens new window)](https://www.youtube.com/watch?v=onBnfo_rJa4&t=4s) on YouTube
>
>
[`@codeceptjs/configure` package (opens new window)](https://github.com/codeceptjs/configure) contains shared recipes for common configuration patterns. This allows to set meta-configuration, independent from a current helper enabled.
Install it and enable to easily switch to headless/window mode, change window size, etc.
```
const { setHeadlessWhen, setWindowSize } = require('@codeceptjs/configure');
setHeadlessWhen(process.env.CI);
setWindowSize(1600, 1200);
exports.config = {
// ...
}
```
Profile
--------
Using `process.env.profile` you can change the config dynamically. It provides value of `--profile` option passed to runner. Use its value to change config value on the fly.
For instance, with the config above we can change browser value using `profile` option
```
codeceptjs run --profile firefox
```
```
exports.config = {
helpers: {
WebDriver: {
url: 'http://localhost:3000',
// load value from `profile`
browser: process.env.profile || 'firefox'
}
}
};
```
codeceptjs Parallel Execution Parallel Execution
===================
CodeceptJS has two engines for running tests in parallel:
* `run-workers` - which spawns [NodeJS Worker (opens new window)](https://nodejs.org/api/worker_threads) in a thread. Tests are split by scenarios, scenarios are mixed between groups, each worker runs tests from its own group.
* `run-multiple` - which spawns a subprocess with CodeceptJS. Tests are split by files and configured in `codecept.conf.js`.
Workers are faster and simpler to start, while `run-multiple` requires additional configuration and can be used to run tests in different browsers at once.
Parallel Execution by Workers
------------------------------
It is easy to run tests in parallel if you have a lots of tests and free CPU cores. Just execute your tests using `run-workers` command specifying the number of workers to spawn:
```
npx codeceptjs run-workers 2
```
> ℹ Workers require NodeJS >= 11.7
>
>
This command is similar to `run`, however, steps output can't be shown in workers mode, as it is impossible to synchronize steps output from different processes.
Each worker spins an instance of CodeceptJS, executes a group of tests, and sends back report to the main process.
By default the tests are assigned one by one to the avaible workers this may lead to multiple execution of `BeforeSuite()`. Use the option `--suites` to assigne the suites one by one to the workers.
```
npx codeceptjs run-workers --suites 2
```
Custom Parallel Execution
--------------------------
To get a full control of parallelization create a custom execution script to match your needs. This way you can configure which tests are matched, how the groups are formed, and with which configuration each worker is executed.
Start with creating file `bin/parallel.js`.
On MacOS/Linux run following commands:
```
mkdir bin
touch bin/parallel.js
chmod +x bin/parallel.js
```
> Filename or directory can be customized. You are creating your own custom runner so take this paragraph as an example.
>
>
Create a placeholder in file:
```
#!/usr/bin/env node
const { Workers, event } = require('codeceptjs');
// here will go magic
```
Now let's see how to update this file for different parallelization modes:
### Example: Running tests in 2 browsers in 4 threads
```
const workerConfig = {
testConfig: './test/data/sandbox/codecept.customworker.js',
};
// don't initialize workers in constructor
const workers = new Workers(null, workerConfig);
// split tests by suites in 2 groups
const testGroups = workers.createGroupsOfSuites(2);
const browsers = ['firefox', 'chrome'];
const configs = browsers.map(browser => {
return helpers: {
WebDriver: { browser }
}
});
for (const config of configs) {
for (group of testGroups) {
const worker = workers.spawn();
worker.addTests(group);
worker.addConfig(config);
}
}
// Listen events for failed test
workers.on(event.test.failed, (failedTest) => {
console.log('Failed : ', failedTest.title);
});
// Listen events for passed test
workers.on(event.test.passed, (successTest) => {
console.log('Passed : ', successTest.title);
});
// test run status will also be available in event
workers.on(event.all.result, () => {
// Use printResults() to display result with standard style
workers.printResults();
});
// run workers as async function
runWorkers();
async function runWorkers() {
try {
// run bootstrapAll
await workers.bootstrapAll();
// run tests
await workers.run();
} finally {
// run teardown All
await workers.teardownAll();
}
}
```
Inside `event.all.result` you can obtain test results from all workers, so you can customize the report:
```
workers.on(event.all.result, (status, completedTests, workerStats) => {
// print output
console.log('Test status : ', status ? 'Passes' : 'Failed ');
// print stats
console.log(`Total tests : ${workerStats.tests}`);
console.log(`Passed tests : ${workerStats.passes}`);
console.log(`Failed test tests : ${workerStats.failures}`);
// If you don't want to listen for failed and passed test separately, use completedTests object
for (const test of Object.values(completedTests)) {
console.log(`Test status: ${test.err===null}, `, `Test : ${test.title}`);
}
}
```
### Example: Running Tests Split By A Custom Function
If you want your tests to split according to your need this method is suited for you. For example: If you have 4 long running test files and 4 normal test files there chance all 4 tests end up in same worker thread. For these cases custom function will be helpful.
```
/*
Define a function to split your tests.
function should return an array with this format [[file1, file2], [file3], ...]
where file1 and file2 will run in a worker thread and file3 will run in a worker thread
*/
const splitTests = () => {
const files = [
['./test/data/sandbox/guthub_test.js', './test/data/sandbox/devto_test.js'],
['./test/data/sandbox/longrunnig_test.js']
];
return files;
}
const workerConfig = {
testConfig: './test/data/sandbox/codecept.customworker.js',
by: splitTests
};
// don't initialize workers in constructor
const customWorkers = new Workers(null, workerConfig);
customWorkers.run();
// You can use event listeners similar to above example.
customWorkers.on(event.all.result, () => {
workers.printResults();
});
```
Sharing Data Between Workers
-----------------------------
NodeJS Workers can communicate between each other via messaging system. It may happen that you want to pass some data from one of workers to other. For instance, you may want to share user credentials accross all tests. Data will be appended to a container.
However, you can't access uninitialized data from a container, so to start, you need to initialized data first. Inside `bootstrap` function of the config we execute the `share` function with `local: true` to initialize value locally:
```
// inside codecept.conf.js
exports.config = {
bootstrap() {
// append empty userData to container for current worker
share({ userData: false }, { local: true });
}
}
```
Now each worker has `userData` inside a container. However, it is empty. When you obtain real data in one of tests you can this data accross tests. Use `inject` function to access data inside a container:
```
// get current value of userData
let { userData } = inject();
// if userData is still empty - update it
if (!userData) {
userData = { name: 'user', password: '123456' };
// now new userData will be shared accross all workers
share(userData);
}
```
| programming_docs |
codeceptjs Testing with Puppeteer Testing with Puppeteer
=======================
Among all Selenium alternatives the most interesting emerging ones are tools developed around Google Chrome [DevTools Protocol (opens new window)](https://chromedevtools.github.io/devtools-protocol/). And the most prominent one is [Puppeteer (opens new window)](https://github.com/GoogleChrome/puppeteer). It operates over Google Chrome directly without requiring additional tools like ChromeDriver. So tests setup with Puppeteer can be started with npm install only. If you want get faster and simpler to setup tests, Puppeteer would be your choice.
CodeceptJS uses Puppeteer to improve end to end testing experience. No need to learn the syntax of a new tool, all drivers in CodeceptJS share the same API.
Take a look at a sample test:
```
I.amOnPage('https://github.com');
I.click('Sign in', '//html/body/div[1]/header');
I.see('Sign in to GitHub', 'h1');
I.fillField('Username or email address', '[email protected]');
I.fillField('Password', '123456');
I.click('Sign in');
I.see('Incorrect username or password.', '.flash-error');
```
It's readable and simple and works using Puppeteer API!
Setup
------
To start you need CodeceptJS with Puppeteer packages installed
```
npm install codeceptjs puppeteer --save
```
Or see [alternative installation options (opens new window)](https://codecept.io/installation/)
> If you already have CodeceptJS project, just install `puppeteer` package and enable a helper it in config.
>
>
And a basic project initialized
```
npx codeceptjs init
```
You will be asked for a Helper to use, you should select Puppeteer and provide url of a website you are testing.
> Puppeteer can also work with Firefox. [Learn how to set it up](https://codecept.io/helpers/Puppeteer-firefox)
>
>
Configuring
------------
Make sure `Puppeteer` helper is enabled in `codecept.conf.js` config:
```
{ // ..
helpers: {
Puppeteer: {
url: "http://localhost",
show: true
}
}
// ..
}
```
> Turn off the `show` option if you want to run test in headless mode.
>
>
Puppeteer uses different strategies to detect if a page is loaded. In configuration use `waitForNavigation` option for that:
By default it is set to `domcontentloaded` which waits for `DOMContentLoaded` event being fired. However, for Single Page Applications it's more useful to set this value to `networkidle0` which waits for all network connections to be finished.
```
helpers: {
Puppeteer: {
url: "http://localhost",
show: true,
waitForNavigation: "networkidle0"
}
}
```
When a test runs faster than application it is recommended to increase `waitForAction` config value. It will wait for a small amount of time (100ms) by default after each user action is taken.
> ▶ More options are listed in [helper reference (opens new window)](https://codecept.io/helpers/Puppeteer/).
>
>
Writing Tests
--------------
CodeceptJS test should be created with `gt` command:
```
npx codeceptjs gt
```
As an example we will use `ToDoMvc` app for testing.
### Actions
Tests consist with a scenario of user's action taken on a page. The most widely used ones are:
* `amOnPage` - to open a webpage (accepts relative or absolute url)
* `click` - to locate a button or link and click on it
* `fillField` - to enter a text inside a field
* `selectOption`, `checkOption` - to interact with a form
* `wait*` to wait for some parts of page to be fully rendered (important for testing SPA)
* `grab*` to get values from page sources
* `see`, `dontSee` - to check for a text on a page
* `seeElement`, `dontSeeElement` - to check for elements on a page
> ℹ All actions are listed in [Puppeteer helper reference (opens new window)](https://codecept.io/helpers/Puppeteer/).\*
>
>
All actions which interact with elements **support CSS and XPath locators**. Actions like `click` or `fillField` by locate elements by their name or value on a page:
```
// search for link or button
I.click('Login');
// locate field by its label
I.fillField('Name', 'Miles');
// we can use input name
I.fillField('user[email]','[email protected]');
```
You can also specify the exact locator type with strict locators:
```
I.click({css: 'button.red'});
I.fillField({name: 'user[email]'},'[email protected]');
I.seeElement({xpath: '//body/header'});
```
### Interactive Pause
It's easy to start writing a test if you use [interactive pause](https://codecept.io/basics#debug). Just open a web page and pause execution.
```
Feature('Sample Test');
Scenario('open my website', ({ I }) => {
I.amOnPage('http://todomvc.com/examples/react/');
pause();
});
```
This is just enough to run a test, open a browser, and think what to do next to write a test case.
When you execute such test with `codeceptjs run` command you may see the browser is started
```
npx codeceptjs run --steps
```
After a page is opened a full control of a browser is given to a terminal. Type in different commands such as `click`, `see`, `fillField` to write the test. A successful commands will be saved to `./output/cli-history` file and can be copied into a test.
A complete ToDo-MVC test may look like:
```
Feature('ToDo');
Scenario('create todo item', ({ I }) => {
I.amOnPage('http://todomvc.com/examples/react/');
I.dontSeeElement('.todo-count');
I.fillField('What needs to be done?', 'Write a guide');
I.pressKey('Enter');
I.see('Write a guide', '.todo-list');
I.see('1 item left', '.todo-count');
});
```
### Grabbers
If you need to get element's value inside a test you can use `grab*` methods. They should be used with `await` operator inside `async` function:
```
const assert = require('assert');
Scenario('get value of current tasks', async ({ I }) => {
I.createTodo('do 1');
I.createTodo('do 2');
let numTodos = await I.grabTextFrom('.todo-count strong');
assert.equal(2, numTodos);
});
```
### Within
In case some actions should be taken inside one element (a container or modal window or iframe) you can use `within` block to narrow the scope. Please take a note that you can't use within inside another within in Puppeteer helper:
```
within('.todoapp', () => {
I.createTodo('my new item');
I.see('1 item left', '.todo-count');
I.click('.todo-list input.toggle');
});
I.see('0 items left', '.todo-count');
```
> [▶ Learn more about basic commands](https://codecept.io/basics#writing-tests)
>
>
CodeceptJS allows you to implement custom actions like `I.createTodo` or use **PageObjects**. Learn how to improve your tests in [PageObjects (opens new window)](https://codecept.io/pageobjects/) guide.
> [▶ Demo project is available on GitHub (opens new window)](https://github.com/DavertMik/codeceptjs-todomvc-puppeteer)
>
>
Mocking Requests
-----------------
Web application sends various requests to local services (Rest API, GraphQL) or to 3rd party services (CDNS, Google Analytics, etc). When you run tests with Puppeteer you can control those requests by mocking them. For instance, you can speed up your tests by blocking trackers, Google Analytics, and other services you don't control.
Also you can replace real request with a one explicitly defined. This is useful when you want to isolate application testing from a backend. For instance, if you don't want to save data to database, and you know the request which performs save, you can mock the request, so application will treat this as valid response, but no data will be actually saved.
To mock requests enable additional helper [MockRequest](https://codecept.io/helpers/MockRequest) (which is based on Polly.js).
```
helpers: {
Puppeteer: {
// regular Puppeteer config here
},
MockRequest: {}
}
```
And install additional packages:
```
npm i @pollyjs/core @pollyjs/adapter-puppeteer --save-dev
```
After an installation function `mockRequest` will be added to `I` object. You can use it to explicitly define which requests to block and which response they should return instead:
```
// block all Google Analytics calls
I.mockRequest('/google-analytics/*path', 200);
// return an empty successful response
I.mockRequest('GET', '/api/users', 200);
// block post requests to /api/users and return predefined object
I.mockRequest('POST', '/api/users', { user: 'davert' });
// return error request with body
I.mockRequest('GET', '/api/users/1', 404, { error: 'User not found' });
```
> See [`mockRequest` API](https://codecept.io/helpers/MockRequest#mockrequest)
>
>
To see `mockRequest` method in intellisense auto completion don't forget to run `codeceptjs def` command:
```
npx codeceptjs def
```
Mocking rules will be kept while a test is running. To stop mocking use `I.stopMocking()` command
Accessing Puppeteer API
------------------------
To get Puppeteer API inside a test use [`I.usePupepteerTo`](https://codecept.io/helpers/Puppeteer/#usepuppeteerto) method with a callback. To keep test readable provide a description of a callback inside the first parameter.
```
I.usePuppeteerTo('emulate offline mode', async ({ page, browser }) => {
await page.setOfflineMode(true);
});
```
> Puppeteer commands are asynchronous so a callback function must be async.
>
>
A Puppeteer helper is passed as argument for callback, so you can combine Puppeteer API with CodeceptJS API:
```
I.usePuppeteerTo('emulate offline mode', async (Puppeteer) => {
// access internal objects browser, page, context of helper
await Puppeteer.page.setOfflineMode(true);
// call a method of helper, await is required here
await Puppeteer.click('Reload');
});
```
Extending Helper
-----------------
To create custom `I.*` commands using Puppeteer API you need to create a custom helper.
Start with creating an `MyPuppeteer` helper using `generate:helper` or `gh` command:
```
npx codeceptjs gh
```
Then inside a Helper you can access `Puppeteer` helper of CodeceptJS. Let's say you want to create `I.renderPageToPdf` action. In this case you need to call `pdf` method of `page` object
```
// inside a MyPuppeteer helper
async renderPageToPdf() {
const page = this.helpers['Puppeteer'].page;
await page.emulateMedia('screen');
return page.pdf({path: 'page.pdf'});
}
```
The same way you can also access `browser` object to implement more actions or handle events.
> [▶ Learn more about Helpers (opens new window)](https://codecept.io/helpers/)
>
>
codeceptjs Translation
> 🌍 Since CodeceptJS 2.4.2 you can use translation for `Scenario`, `Before`, and other keywords within your tests. Please help us to update language definition files to include the best translation for your language!
>
>
Translation
============
Test output and the way tests are written can be localized. This way scenarios can be written in almost native language using UTF support of JavaScript. If you have non-English team and you work on non-English project consider enabling translation by setting translation to [one of available languages (opens new window)](https://github.com/codeceptjs/CodeceptJS/blob/master/translations).
Please refer to translated steps inside translation files and send Pull Requests to add missing.
To get autocompletion for localized method names generate definitions by running
```
npx codeceptjs def
```
Russian
--------
Add to config:
```
"translation": "ru-RU"
```
when running with `--steps` option steps output will be translated:
This also enables localized method names for actor object.
This way tests can be written in native language while it is still JavaScript:
```
Сценарий('пробую написать реферат', (Я) => {
Я.на_странице('http://yandex.ru/referats');
Я.вижу("Написать реферат по");
Я.выбираю_опцию('Психологии');
Я.кликаю("Написать реферат");
Я.вижу("Реферат по психологии");
});
```
Portuguese
-----------
To write your tests in portuguese you can enable the portuguese translation in config file like:
```
"translation": "pt-BR"
```
Now you can write test like this:
```
Scenario('Efetuar login', ({ Eu }) => {
Eu.estouNaPagina('http://minhaAplicacao.com.br');
Eu.preenchoOCampo("login", "[email protected]");
Eu.preenchoOCampo("senha", "123456");
Eu.clico("Entrar");
Eu.vejo("Seja bem vindo usuário!");
});
```
French
-------
To write your tests in French you can enable the French translation by adding to config:
```
"translation": "fr-FR"
```
Now you can write tests like this:
```
Scenario('Se connecter sur GitHub', (Je) => {
Je.suisSurLaPage('https://github.com/login');
Je.remplisLeChamp("Username or email address", "jean-dupond");
Je.remplisLeChamp("Password", "*********");
Je.cliqueSur("Sign in");
Je.vois("Learn Git and GitHub without any code!");
});
```
Italian
--------
Add to config
```
"translation": "it-IT"
```
Now you can write test like this:
```
Caratteristica('Effettuare il Login su GitHub', (io) => {
io.sono_sulla_pagina('https://github.com/login');
io.compilo_il_campo("Username or email address", "giuseppe-santoro");
io.compilo_il_campo("Password", "*********");
io.faccio_click_su("Sign in");
io.vedo("Learn Git and GitHub without any code!");
});
```
Polish
-------
Add to config
```
"translation": "pl-PL"
```
Now you can write test like this:
```
Scenario('Zakładanie konta free trial na stronie głównej GetResponse', ({ Ja }) => {
Ja.jestem_na_stronie('https://getresponse.com');
Ja.wypełniam_pole("Email address", "[email protected]");
Ja.wypełniam_pole("Password", "digital-marketing-systems");
Ja.klikam('Sign up');
Ja.czekam(1);
Ja.widzę_w_adresie_url('/account_free_created.html');
});
```
Chinese
--------
Add to config:
```
"translation": "zh-CN"
```
or
```
"translation": "zh-TW"
```
This way tests can be written in Chinese language while it is still JavaScript:
```
Feature('CodeceptJS 演示');
Scenario('成功提交表单', ({ 我 }) => {
我.在页面('/documentation')
我.填写字段('电邮', '[email protected]')
我.填写字段('密码', '123456')
我.勾选选项('激活')
我.勾选选项('男');
我.单击('创建用户')
我.看到('用户名可用')
我.在当前网址中看不到('/documentation')
});
```
or
```
Feature('CodeceptJS 演示');
Scenario('成功提交表單', ({ 我 }) => {
我.在頁面('/documentation')
我.填寫欄位('電郵', '[email protected]')
我.填寫欄位('密碼', '123456')
我.勾選選項('活化')
我.勾選選項('男');
我.單擊('建立用戶')
我.看到('用戶名可用')
我.在當前網址中看不到('/documentation')
});
```
Japanese
---------
Add to config
```
"translation": "ja-JP"
```
Now you can write test like this:
```
Scenario('ログインできる', ({ 私は }) => {
私は.ページを移動する('/login');
私は.フィールドに入力する("Eメール", "[email protected]");
私は.フィールドに入力する("パスワード", "p@ssword");
私は.クリックする('ログイン');
私は.待つ(1);
私は.URLに含まれるか確認する('/home');
});
```
Using your own translation file
--------------------------------
Create translation file like this:
```
module.exports = {
I: '',
contexts: {
Feature: 'Feature',
Scenario: 'Szenario',
Before: 'Vor',
After: 'Nach',
BeforeSuite: 'vor_der_suite',
AfterSuite: 'nach_der_suite',
},
actions: {
click: 'Klicken',
wait: 'Wartenn',
}
```
And add the file path to your config
```
"translation": "./path/to/your/translation.js"
```
codeceptjs allure allure
=======
Allure reporter
Enables Allure reporter.
#### Usage
To start please install `allure-commandline` package (which requires Java 8)
```
npm install -g allure-commandline --save-dev
```
Add this plugin to config file:
```
"plugins": {
"allure": {}
}
```
Run tests with allure plugin enabled:
```
npx codeceptjs run --plugins allure
```
By default, allure reports are saved to `output` directory. Launch Allure server and see the report like on a screenshot above:
```
allure serve output
```
#### Configuration
* `outputDir` - a directory where allure reports should be stored. Standard output directory is set by default.
* `enableScreenshotDiffPlugin` - a boolean flag for add screenshot diff to report. To attach, tou need to attach three files to the report - "diff.png", "actual.png", "expected.png". See [Allure Screenshot Plugin (opens new window)](https://github.com/allure-framework/allure2/blob/master/plugins/screen-diff-plugin/README.md)
#### Public API
There are few public API methods which can be accessed from other plugins.
```
const allure = codeceptjs.container.plugins('allure');
```
`allure` object has following methods:
* `addAttachment(name, buffer, type)` - add an attachment to current test / suite
* `addLabel(name, value)` - adds a label to current test
* `severity(value)` - adds severity label
* `epic(value)` - adds epic label
* `feature(value)` - adds feature label
* `story(value)` - adds story label
* `issue(value)` - adds issue label
* `setDescription(description, type)` - sets a description
### Parameters
* `config`
autoDelay
----------
Sometimes it takes some time for a page to respond to user's actions. Depending on app's performance this can be either slow or fast.
For instance, if you click a button and nothing happens - probably JS event is not attached to this button yet Also, if you fill field and input validation doesn't accept your input - maybe because you typed value too fast.
This plugin allows to slow down tests execution when a test running too fast. It puts a tiny delay for before and after action commands.
Commands affected (by default):
* `click`
* `fillField`
* `checkOption`
* `pressKey`
* `doubleClick`
* `rightClick`
#### Configuration
```
plugins: {
autoDelay: {
enabled: true
}
}
```
Possible config options:
* `methods`: list of affected commands. Can be overridden
* `delayBefore`: put a delay before a command. 100ms by default
* `delayAfter`: put a delay after a command. 200ms by default
### Parameters
* `config`
autoLogin
----------
Logs user in for the first test and reuses session for next tests. Works by saving cookies into memory or file. If a session expires automatically logs in again.
> For better development experience cookies can be saved into file, so a session can be reused while writing tests.
>
>
#### Usage
1. Enable this plugin and configure as described below
2. Define user session names (example: `user`, `editor`, `admin`, etc).
3. Define how users are logged in and how to check that user is logged in
4. Use `login` object inside your tests to log in:
```
// inside a test file
// use login to inject auto-login function
Before(login => {
login('user'); // login using user session
});
// Alternatively log in for one scenario
Scenario('log me in', ( {I, login} ) => {
login('admin');
I.see('I am logged in');
});
```
#### Configuration
* `saveToFile` (default: false) - save cookies to file. Allows to reuse session between execution.
* `inject` (default: `login`) - name of the login function to use
* `users` - an array containing different session names and functions to:
+ `login` - sign in into the system
+ `check` - check that user is logged in
+ `fetch` - to get current cookies (by default `I.grabCookie()`)
+ `restore` - to set cookies (by default `I.amOnPage('/'); I.setCookie(cookie)`)
#### How It Works
1. `restore` method is executed. It should open a page and set credentials.
2. `check` method is executed. It should reload a page (so cookies are applied) and check that this page belongs to logged in user.
3. If `restore` and `check` were not successful, `login` is executed
4. `login` should fill in login form
5. After successful login, `fetch` is executed to save cookies into memory or file.
#### Example: Simple login
```
autoLogin: {
enabled: true,
saveToFile: true,
inject: 'login',
users: {
admin: {
// loginAdmin function is defined in `steps_file.js`
login: (I) => I.loginAdmin(),
// if we see `Admin` on page, we assume we are logged in
check: (I) => {
I.amOnPage('/');
I.see('Admin');
}
}
}
}
```
#### Example: Multiple users
```
autoLogin: {
enabled: true,
saveToFile: true,
inject: 'loginAs', // use `loginAs` instead of login
users: {
user: {
login: (I) => {
I.amOnPage('/login');
I.fillField('email', '[email protected]');
I.fillField('password', '123456');
I.click('Login');
},
check: (I) => {
I.amOnPage('/');
I.see('User', '.navbar');
},
},
admin: {
login: (I) => {
I.amOnPage('/login');
I.fillField('email', '[email protected]');
I.fillField('password', '123456');
I.click('Login');
},
check: (I) => {
I.amOnPage('/');
I.see('Admin', '.navbar');
},
},
}
}
```
#### Example: Keep cookies between tests
If you decide to keep cookies between tests you don't need to save/retrieve cookies between tests. But you need to login once work until session expires. For this case, disable `fetch` and `restore` methods.
```
helpers: {
WebDriver: {
// config goes here
keepCookies: true; // keep cookies for all tests
}
},
plugins: {
autoLogin: {
users: {
admin: {
login: (I) => {
I.amOnPage('/login');
I.fillField('email', '[email protected]');
I.fillField('password', '123456');
I.click('Login');
},
check: (I) => {
I.amOnPage('/dashboard');
I.see('Admin', '.navbar');
},
fetch: () => {}, // empty function
restore: () => {}, // empty funciton
}
}
}
}
```
#### Example: Getting sessions from local storage
If your session is stored in local storage instead of cookies you still can obtain sessions.
```
plugins: {
autoLogin: {
admin: {
login: (I) => I.loginAsAdmin(),
check: (I) => I.see('Admin', '.navbar'),
fetch: (I) => {
return I.executeScript(() => localStorage.getItem('session_id'));
},
restore: (I, session) => {
I.amOnPage('/');
I.executeScript((session) => localStorage.setItem('session_id', session), session);
},
}
}
}
```
#### Tips: Using async function in the autoLogin
If you use async functions in the autoLogin plugin, login function should be used with `await` keyword.
```
autoLogin: {
enabled: true,
saveToFile: true,
inject: 'login',
users: {
admin: {
login: async (I) => { // If you use async function in the autoLogin plugin
const phrase = await I.grabTextFrom('#phrase')
I.fillField('username', 'admin'),
I.fillField('password', 'password')
I.fillField('phrase', phrase)
},
check: (I) => {
I.amOnPage('/');
I.see('Admin');
},
}
}
}
```
```
Scenario('login', async ( {I, login} ) => {
await login('admin') // you should use `await`
})
```
### Parameters
* `config`
commentStep
------------
Add descriptive nested steps for your tests:
```
Scenario('project update test', async (I) => {
__`Given`;
const projectId = await I.have('project');
__`When`;
projectPage.update(projectId, { title: 'new title' });
__`Then`;
projectPage.open(projectId);
I.see('new title', 'h1');
})
```
Steps prefixed with `__` will be printed as nested steps in `--steps` output:
```
Given
I have "project"
When
projectPage update
Then
projectPage open
I see "new title", "h1"
```
Also those steps will be exported to allure reports.
This plugin can be used
### Config
* `enabled` - (default: false) enable a plugin
* `regusterGlobal` - (default: false) register `__` template literal function globally. You can override function global name by providing a name as a value.
### Examples
Registering `__` globally:
```
plugins: {
commentStep: {
enabled: true,
registerGlobal: true
}
}
```
Registering `Step` globally:
```
plugins: {
commentStep: {
enabled: true,
registerGlobal: 'Step'
}
}
```
Using only local function names:
```
plugins: {
commentStep: {
enabled: true
}
}
```
Then inside a test import a comment function from a plugin. For instance, you can prepare Given/When/Then functions to use them inside tests:
```
// inside a test
const step = codeceptjs.container.plugins('commentStep');
const Given = () => step`Given`;
const When = () => step`When`;
const Then = () => step`Then`;
```
Scenario('project update test', async (I) => { Given(); const projectId = await I.have('project');
When(); projectPage.update(projectId, { title: 'new title' });
Then(); projectPage.open(projectId); I.see('new title', 'h1'); });
### Parameters
* `config`
customLocator
--------------
Creates a [custom locator (opens new window)](https://codecept.io/locators#custom-locators) by using special attributes in HTML.
If you have a convention to use `data-test-id` or `data-qa` attributes to mark active elements for e2e tests, you can enable this plugin to simplify matching elements with these attributes:
```
// replace this:
I.click({ css: '[data-test-id=register_button]');
// with this:
I.click('$register_button');
```
This plugin will create a valid XPath locator for you.
#### Configuration
* `enabled` (default: `false`) should a locator be enabled
* `prefix` (default: `$`) sets a prefix for a custom locator.
* `attribute` (default: `data-test-id`) to set an attribute to be matched.
* `strategy` (default: `xpath`) actual locator strategy to use in query (`css` or `xpath`).
* `showActual` (default: false) show in the output actually produced XPath or CSS locator. By default shows custom locator value.
#### Examples:
Using `data-test` attribute with `$` prefix:
```
// in codecept.conf.js
plugins: {
customLocator: {
enabled: true
attribute: 'data-test'
}
}
```
In a test:
```
I.seeElement('$user'); // matches => [data-test=user]
I.click('$sign-up'); // matches => [data-test=sign-up]
```
Using `data-qa` attribute with `=` prefix:
```
// in codecept.conf.js
plugins: {
customLocator: {
enabled: true
prefix: '=',
attribute: 'data-qa'
}
}
```
In a test:
```
I.seeElement('=user'); // matches => [data-qa=user]
I.click('=sign-up'); // matches => [data-qa=sign-up]
```
### Parameters
* `config`
pauseOnFail
------------
Automatically launches [interactive pause](https://codecept.io/basics/#pause) when a test fails.
Useful for debugging flaky tests on local environment. Add this plugin to config file:
```
plugins: {
pauseOnFail: {},
}
```
Unlike other plugins, `pauseOnFail` is not recommended to be enabled by default. Enable it manually on each run via `-p` option:
```
npx codeceptjs run -p pauseOnFail
```
puppeteerCoverage
------------------
Dumps puppeteers code coverage after every test.
#### Configuration
Configuration can either be taken from a corresponding helper (deprecated) or a from plugin config (recommended).
```
plugins: {
puppeteerCoverage: {
enabled: true
}
}
```
Possible config options:
* `coverageDir`: directory to dump coverage files
* `uniqueFileName`: generate a unique filename by adding uuid
First of all, your mileage may vary!
To work, you need the client javascript code to be NOT uglified. They need to be built in "development" mode. And the end of your tests, you'll get a directory full of coverage per test run. Now what? You'll need to convert the coverage code to something istanbul can read. Good news is someone wrote the code for you (see puppeteer-to-istanbul link below). Then using istanbul you need to combine the converted coverage and create a report. Good luck!
Links:
* [https://github.com/GoogleChrome/puppeteer/blob/v1.12.2/docs/api.md#class-coverage (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/v1.12.2/docs/api.md#class-coverage)
* [https://github.com/istanbuljs/puppeteer-to-istanbul (opens new window)](https://github.com/istanbuljs/puppeteer-to-istanbul)
* [https://github.com/gotwarlost/istanbul (opens new window)](https://github.com/gotwarlost/istanbul)
### Parameters
* `config`
retryFailedStep
----------------
Retries each failed step in a test.
Add this plugin to config file:
```
plugins: {
retryFailedStep: {
enabled: true
}
}
```
Run tests with plugin enabled:
```
npx codeceptjs run --plugins retryFailedStep
```
#### Configuration:
* `retries` - number of retries (by default 5),
* `when` - function, when to perform a retry (accepts error as parameter)
* `factor` - The exponential factor to use. Default is 2.
* `minTimeout` - The number of milliseconds before starting the first retry. Default is 1000.
* `maxTimeout` - The maximum number of milliseconds between two retries. Default is Infinity.
* `randomize` - Randomizes the timeouts by multiplying with a factor between 1 to 2. Default is false.
* `defaultIgnoredSteps` - an array of steps to be ignored for retry. Includes:
+ `amOnPage`
+ `wait*`
+ `send*`
+ `execute*`
+ `run*`
+ `have*`
* `ignoredSteps` - an array for custom steps to ignore on retry. Use it to append custom steps to ignored list. You can use step names or step prefixes ending with `*`. As such, `wait*` will match all steps starting with `wait`. To append your own steps to ignore list - copy and paste a default steps list. Regexp values are accepted as well.
#### Example
```
plugins: {
retryFailedStep: {
enabled: true,
ignoreSteps: [
'scroll*', // ignore all scroll steps
/Cookie/, // ignore all steps with a Cookie in it (by regexp)
]
}
}
```
#### Disable Per Test
This plugin can be disabled per test. In this case you will need to stet `I.retry()` to all flaky steps:
Use scenario configuration to disable plugin for a test
```
Scenario('scenario tite', () => {
// test goes here
}).config(test => test.disableRetryFailedStep = true)
```
### Parameters
* `config`
screenshotOnFail
-----------------
Creates screenshot on failure. Screenshot is saved into `output` directory.
Initially this functionality was part of corresponding helper but has been moved into plugin since 1.4
This plugin is **enabled by default**.
#### Configuration
Configuration can either be taken from a corresponding helper (deprecated) or a from plugin config (recommended).
```
plugins: {
screenshotOnFail: {
enabled: true
}
}
```
Possible config options:
* `uniqueScreenshotNames`: use unique names for screenshot. Default: false.
* `fullPageScreenshots`: make full page screenshots. Default: false.
### Parameters
* `config`
selenoid
---------
[Selenoid (opens new window)](https://aerokube.com/selenoid/) plugin automatically starts browsers and video recording. Works with WebDriver helper.
### Prerequisite
This plugin **requires Docker** to be installed.
> If you have issues starting Selenoid with this plugin consider using the official [Configuration Manager (opens new window)](https://aerokube.com/cm/latest/) tool from Selenoid
>
>
### Usage
Selenoid plugin can be started in two ways:
1. **Automatic** - this plugin will create and manage selenoid container for you.
2. **Manual** - you create the conatainer and configure it with a plugin (recommended).
#### Automatic
If you are new to Selenoid and you want plug and play setup use automatic mode.
Add plugin configuration in `codecept.conf.js`:
```
plugins: {
selenoid: {
enabled: true,
deletePassed: true,
autoCreate: true,
autoStart: true,
sessionTimeout: '30m',
enableVideo: true,
enableLog: true,
},
}
```
When `autoCreate` is enabled it will pull the [latest Selenoid from DockerHub (opens new window)](https://hub.docker.com/u/selenoid) and start Selenoid automatically. It will also create `browsers.json` file required by Selenoid.
In automatic mode the latest version of browser will be used for tests. It is recommended to specify exact version of each browser inside `browsers.json` file.
> **If you are using Windows machine or if `autoCreate` does not work properly, create container manually**
>
>
#### Manual
While this plugin can create containers for you for better control it is recommended to create and launch containers manually. This is especially useful for Continous Integration server as you can configure scaling for Selenoid containers.
> Use [Selenoid Configuration Manager (opens new window)](https://aerokube.com/cm/latest/) to create and start containers semi-automatically.
>
>
1. Create `browsers.json` file in the same directory `codecept.conf.js` is located [Refer to Selenoid documentation (opens new window)](https://aerokube.com/selenoid/latest/#_prepare_configuration) to know more about browsers.json.
*Sample browsers.json*
```
{
"chrome": {
"default": "latest",
"versions": {
"latest": {
"image": "selenoid/chrome:latest",
"port": "4444",
"path": "/"
}
}
}
}
```
> It is recommended to use specific versions of browsers in `browsers.json` instead of latest. This will prevent tests fail when browsers will be updated.
>
>
**⚠ At first launch selenoid plugin takes extra time to download all Docker images before tests starts**.
2. Create Selenoid container
Run the following command to create a container. To know more [refer here (opens new window)](https://aerokube.com/selenoid/latest/#_option_2_start_selenoid_container)
```
docker create \
--name selenoid \
-p 4444:4444 \
-v /var/run/docker.sock:/var/run/docker.sock \
-v `pwd`/:/etc/selenoid/:ro \
-v `pwd`/output/video/:/opt/selenoid/video/ \
-e OVERRIDE_VIDEO_OUTPUT_DIR=`pwd`/output/video/ \
aerokube/selenoid:latest-release
```
### Video Recording
This plugin allows to record and save video per each executed tests.
When `enableVideo` is `true` this plugin saves video in `output/videos` directory with each test by name To save space videos for all succesful tests are deleted. This can be changed by `deletePassed` option.
When `allure` plugin is enabled a video is attached to report automatically.
### Options:
| Param | Description |
| --- | --- |
| name | Name of the container (default : selenoid) |
| port | Port of selenium server (default : 4444) |
| autoCreate | Will automatically create container (Linux only) (default : true) |
| autoStart | If disabled start the container manually before running tests (default : true) |
| enableVideo | Enable video recording and use `video` folder of output (default: false) |
| enableLog | Enable log recording and use `logs` folder of output (default: false) |
| deletePassed | Delete video and logs of passed tests (default : true) |
| additionalParams | example: `additionalParams: '--env TEST=test'` [Refer here (opens new window)](https://docs.docker.com/engine/reference/commandline/create/) to know more |
### Parameters
* `config`
stepByStepReport
-----------------
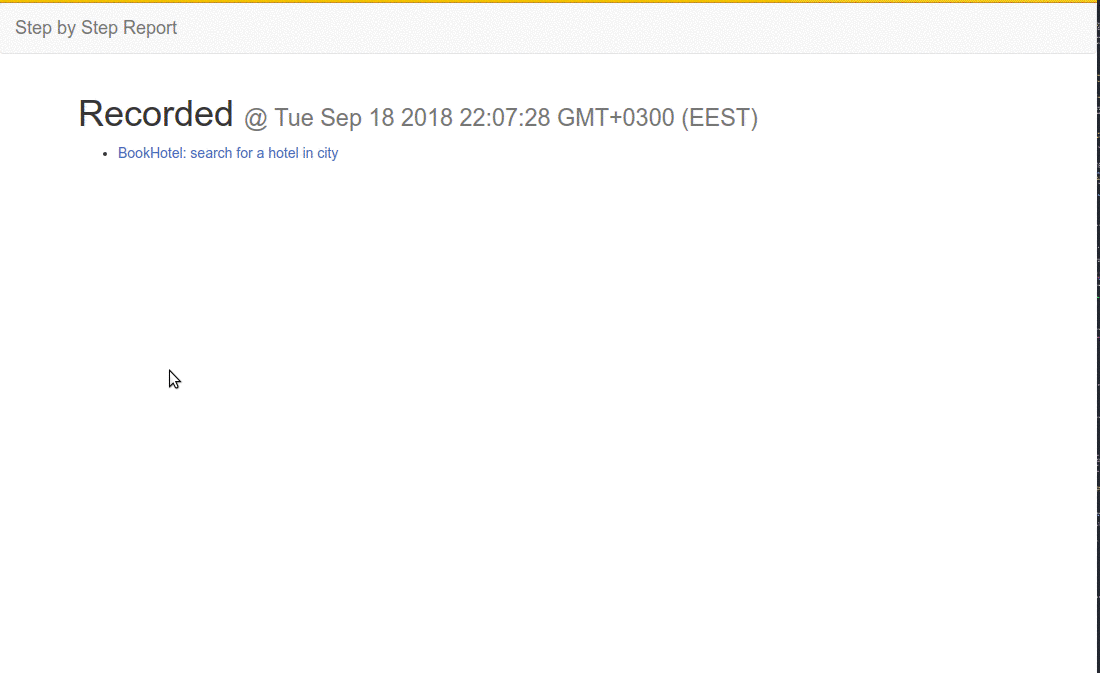
Generates step by step report for a test. After each step in a test a screenshot is created. After test executed screenshots are combined into slideshow. By default, reports are generated only for failed tests.
Run tests with plugin enabled:
```
npx codeceptjs run --plugins stepByStepReport
```
#### Configuration
```
"plugins": {
"stepByStepReport": {
"enabled": true
}
}
```
Possible config options:
* `deleteSuccessful`: do not save screenshots for successfully executed tests. Default: true.
* `animateSlides`: should animation for slides to be used. Default: true.
* `ignoreSteps`: steps to ignore in report. Array of RegExps is expected. Recommended to skip `grab*` and `wait*` steps.
* `fullPageScreenshots`: should full page screenshots be used. Default: false.
* `output`: a directory where reports should be stored. Default: `output`.
* `screenshotsForAllureReport`: If Allure plugin is enabled this plugin attaches each saved screenshot to allure report. Default: false.
### Parameters
* `config` **any**
tryTo
------
Adds global `tryTo` function inside of which all failed steps won't fail a test but will return true/false.
Enable this plugin in `codecept.conf.js` (enabled by default for new setups):
```
plugins: {
tryTo: {
enabled: true
}
}
```js
const result = await tryTo(() => I.see('Welcome'));
// if text "Welcome" is on page, result => true
// if text "Welcome" is not on page, result => false
```
Disables retryFailedStep plugin for steps inside a block;
Use this plugin if:
* you need to perform multiple assertions inside a test
* there is A/B testing on a website you test
* there is "Accept Cookie" banner which may surprisingly appear on a page.
#### Usage
#### Multiple Conditional Assertions
```
const result1 = await tryTo(() => I.see('Hello, user'));
const result2 = await tryTo(() => I.seeElement('.welcome'));
assert.ok(result1 && result2, 'Assertions were not succesful');
```
##### Optional click
```
I.amOnPage('/');
tryTo(() => I.click('Agree', '.cookies'));
```
#### Configuration
* `registerGlobal` - to register `tryTo` function globally, true by default
If `registerGlobal` is false you can use tryTo from the plugin:
```
const tryTo = codeceptjs.container.plugins('tryTo');
```
### Parameters
* `config`
wdio
-----
Webdriverio services runner.
This plugin allows to run webdriverio services like:
* selenium-standalone
* sauce
* testingbot
* browserstack
* appium
A complete list of all available services can be found on [webdriverio website (opens new window)](https://webdriver.io).
#### Setup
1. Install a webdriverio service
2. Enable `wdio` plugin in config
3. Add service name to `services` array inside wdio plugin config.
See examples below:
#### Selenium Standalone Service
Install `@wdio/selenium-standalone-service` package, as [described here (opens new window)](https://webdriver.io/docs/selenium-standalone-service). It is important to make sure it is compatible with current webdriverio version.
Enable `wdio` plugin in plugins list and add `selenium-standalone` service:
```
plugins: {
wdio: {
enabled: true,
services: ['selenium-standalone']
// additional config for service can be passed here
}
}
```
Please note, this service can be used with Protractor helper as well!
#### Sauce Service
Install `@wdio/sauce-service` package, as [described here (opens new window)](https://webdriver.io/docs/sauce-service). It is important to make sure it is compatible with current webdriverio version.
Enable `wdio` plugin in plugins list and add `sauce` service:
```
plugins: {
wdio: {
enabled: true,
services: ['sauce'],
user: ... ,// saucelabs username
key: ... // saucelabs api key
// additional config, from sauce service
}
}
```
In the same manner additional services from webdriverio can be installed, enabled, and configured.
#### Configuration
* `services` - list of enabled services
* ... - additional configuration passed into services.
### Parameters
* `config`
| programming_docs |
codeceptjs Testing with TestCafe Testing with TestCafe
======================
[TestCafe (opens new window)](https://devexpress.github.io/testcafe/) is another alternative engine for driving browsers. It is driven by unique technology which provides fast and simple cross browser testing for desktop and mobile browsers. Unlike WebDriver or Puppeteer, TestCafe doesn't control a browser at all. It is not a browser itself, like [Nightmare](https://codecept.io/nightmare) or Cypress. **TestCafe core is a proxy server** that runs behind the scene, and transforms all HTML and JS to include code that is needed for test automation.
This is very smart idea. But to use TestCafe on daily basis you need to clearly understand its benefits and limitations:
### Pros
* **Fast**. Browser is controlled from inside a web page. This makes test run inside a browser as fast as your browser can render page with no extra network requests.
* **Simple Cross-Browser Support.** Because TestCafe only launches browsers, it can **automate browser** on desktop or mobile. Unlike WebDriver, you don't need special version of browser and driver to prepare to run tests. Setup simplified. All you need is just a browser installed, and you are ready to go.
* **Stable to Execution.** Because a test is executed inside a browser, the network latency effects are reduced. Unlike WebDriver you won't hit stale element exceptions, or element not interactable exceptions, as from within a web browser all DOM elements are accessible.
Cons
-----
* **Magic.** Browsers executed in TestCafe are not aware that they run in test mode. So at some edges automation control can be broken. It's also quite hard to debug possible issues, as you don't know how actually a web page is parsed to inject automation scripts.
* **No Browser Control.** Because TestCafe do not control browser, you can't actually automate all users actions. For instance, TestCafe can't open new tabs or open a new browser window in incognito mode. There can be also some issues running tests on 3rd party servers or inside iframes.
* **Simulated Events.** Events like `click` or `doubleClick` are simulated by JavaScript internally. Inside WebDriver or Puppeteer, where those events are dispatched by a browser, called native events. Native events are closer to real user experience. So in some cases simulated events wouldn't represent actual user experience, which can lead to false positive results. For instance, a button which can't be physically clicked by a user, would be clickable inside TestCafe.
Anyway, TestCafe is a good option to start if you need cross browser testing. And here is the **reason to use TestCafe with CodeceptJS: if you hit an edge case or issue, you can easily switch your tests to WebDriver**. As all helpers in CodeceptJS share the same syntax.
CodeceptJS is a rich testing frameworks which also provides features missing in original TestCafe:
* [Cucumber integration](https://codecept.io/bdd)
* [Real Page Objects](https://codecept.io/pageobjects)
* [Data Management via API](https://codecept.io/data)
* and others
Writing Tests
--------------
To start using TestCafe with CodeceptJS install both via NPM
> If you don't have `package.json` in your project, create it with `npm init -y`.
>
>
```
npm i codeceptjs testcafe --save-dev
```
Then you need to initialize a project, selecting TestCafe when asked:
```
npx codeceptjs init
```
A first test should be created with `codeceptjs gt` command
```
npx codeceptjs gt
```
In the next example we will [TodoMVC application (opens new window)](http://todomvc.com/examples/angularjs/#/). So let's create a test which will fill in todo list:
```
Feature('TodoMVC');
Scenario('create todo item', ({ I }) => {
I.amOnPage('http://todomvc.com/examples/angularjs/#/');
I.fillField('.new-todo', todo)
I.pressKey('Enter');
I.seeNumberOfVisibleElements('.todo-list li', 1);
I.see('1 item left', '.todo-count');
});
```
Same syntax is the same for all helpers in CodeceptJS so to learn more about available commands learn [CodeceptJS Basics](https://codecept.io/basics).
> [▶ Complete list of TestCafe actions](https://codecept.io/helpers/TestCafe)
>
>
Page Objects
-------------
Multiple tests can be refactored to share some logic and locators. It is recommended to use PageObjects for this. For instance, in example above, we could create special actions for creating todos and checking them. If we move such methods in a corresponding object a test would look even clearer:
```
Scenario('Create a new todo item', async ({ I, TodosPage }) => {
I.say('Given I have an empty todo list')
I.say('When I create a todo "foo"')
TodosPage.enterTodo('foo')
I.say('Then I see the new todo on my list')
TodosPage.seeNumberOfTodos(1)
I.saveScreenshot('create-todo-item.png')
})
Scenario('Create multiple todo items', async ({ I, TodosPage }) => {
I.say('Given I have an empty todo list')
I.say('When I create todos "foo", "bar" and "baz"')
TodosPage.enterTodo('foo')
TodosPage.enterTodo('bar')
TodosPage.enterTodo('baz')
I.say('Then I have these 3 todos on my list')
TodosPage.seeNumberOfTodos(3)
I.saveScreenshot('create-multiple-todo-items.png')
})
```
> ℹ [Source code of this example (opens new window)](https://github.com/hubidu/codeceptjs-testcafe-todomvc) is available on GitHub.
>
>
A PageObject can be injected into a test by its name. Here is how TodosPage looks like:
```
// inside todos_page.js
const { I } = inject();
module.exports = {
goto() {
I.amOnPage('http://todomvc.com/examples/angularjs/#/')
},
enterTodo(todo) {
I.fillField('.new-todo', todo)
I.pressKey('Enter')
},
seeNumberOfTodos(numberOfTodos) {
I.seeNumberOfVisibleElements('.todo-list li', numberOfTodos)
},
}
```
> [▶ Read more about PageObjects in CodeceptJS](https://codecept.io/pageobjects)
>
>
Accessing TestCafe API
-----------------------
To get [testController (opens new window)](https://devexpress.github.io/testcafe/documentation/test-api/test-code-structure.html#test-controller))) inside a test use [`I.useTestCafeTo`](https://codecept.io/helpers/TestCafe/#usetestcafeto) method with a callback. To keep test readable provide a description of a callback inside the first parameter.
```
I.useTestCafeTo('do some things using native webdriverio api', async ({ t }) => {
await t.click() // use testcafe api here
});
```
Because all TestCafe commands are asynchronous a callback function must be async.
Extending
----------
If you want to use TestCafe API inside your tests you can put them into actions of `I` object. To do so you can generate a new helper, access TestCafe helper, and get the test controller.
Create a helper using `codecepjs gh` command.
```
npx codeceptjs gh
```
All methods of newly created class will be added to `I` object.
```
const Helper = codeceptjs.helper;
class MyTestCafe extends Helper {
slowlyFillField(field, text) {
// import test controller from TestCafe helper
const { t } = this.helpers.TestCafe;
// use TestCafe API here
return t.setTestSpeed(0.1)
.typeText(field, text);
}
}
```
codeceptjs TypeScript TypeScript
===========
CodeceptJS supports [type declaration (opens new window)](https://github.com/codecept-js/CodeceptJS/tree/master/typings) for [TypeScript (opens new window)](https://www.typescriptlang.org/). It means that you can write your tests in TS. Also, all of your custom steps can be written in TS
Why TypeScript?
================
With the TypeScript writing CodeceptJS tests becomes much easier. If you configure TS properly in your project as well as your IDE, you will get the following features:
* [Autocomplete (with InteliSence) (opens new window)](https://code.visualstudio.com/docs/editor/intellisense) - a tool that streamlines your work by suggesting when you typing what function or property which exists in a class, what arguments can be passed to that method, what it returns, etc. Example:
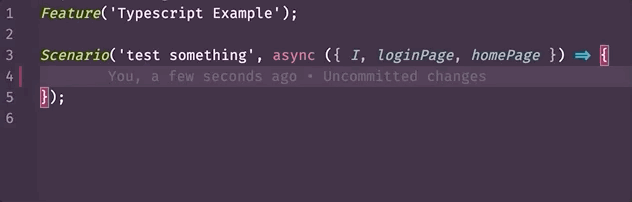
* To show additional information for a step in a test. Example:
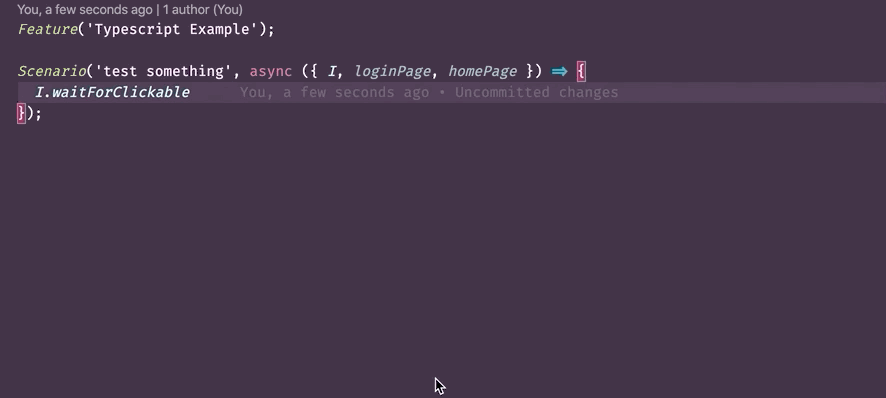
* Checks types - thanks to TypeScript support in CodeceptJS now allow to tests your tests. TypeScript can prevent some errors:
+ invalid type of variables passed to function;
+ calls no-exist method from PageObject or `I` object;
+ incorrectly used CodeceptJS features;
Getting Started
----------------
### TypeScript Boilerplate
To get started faster we prepared [typescript boilerplate project (opens new window)](https://github.com/codeceptjs/typescript-boilerplate) which can be used instead of configuring TypeScript on your own. Clone this repository into an empty folder and you are done.
Otherwise, follow next steps to introduce TypeScript into the project.
### Install TypeScipt
For writing tests in TypeScript you'll need to install `typescript` and `ts-node` into your project.
```
npm install typescript ts-node
```
### Configure codecept.conf.js
To configure TypeScript in your project, you need to add [`ts-node/register` (opens new window)](https://github.com/TypeStrong/ts-node) on first line in your config. Like in the following config file:
```
require('ts-node/register')
exports.config = {
tests: './*_test.ts',
output: './output',
helpers: {
Puppeteer: {
url: 'http://example.com',
},
},
name: 'project name',
}
```
### Configure tsconfig.json
We recommended the following configuration in a [tsconfig.json (opens new window)](https://www.typescriptlang.org/docs/handbook/tsconfig-json):
```
{
"ts-node": {
"files": true
},
"compilerOptions": {
"target": "es2018",
"lib": ["es2018", "DOM"],
"esModuleInterop": true,
"module": "commonjs",
"strictNullChecks": true,
"types": ["codeceptjs"],
},
}
```
> You can find an example project with TypeScript and CodeceptJS on our project [typescript-boilerplate (opens new window)](https://github.com/codecept-js/typescript-boilerplate).
>
>
### Set Up steps.d.ts
Configuring the `tsconfig.json` and `codecept.conf.js` is not enough, you will need to configure the `steps.d.ts` file for custom steps. Just simply do this by running this command::
`npx codeceptjs def`
As a result, a file will be created on your root folder with following content:
```
/// <reference types='codeceptjs' />
declare namespace CodeceptJS {
interface SupportObject { I: I }
interface Methods extends Puppeteer {}
interface I extends WithTranslation<Methods> {}
namespace Translation {
interface Actions {}
}
}
```
Types for custom helper or page object
---------------------------------------
If you want to get types for your [custom helper (opens new window)](https://codecept.io/helpers/#configuration), you can add their automatically with CodeceptJS command `npx codeceptjs def`.
For example, if you add the new step `printMessage` for your custom helper like this:
```
// customHelper.ts
class CustomHelper extends Helper {
printMessage(msg: string) {
console.log(msg)
}
}
export = CustomHelper
```
Then you need to add this helper to your `codecept.conf.js` like in this [docs (opens new window)](https://codecept.io/helpers/#configuration). And then run the command `npx codeceptjs def`.
As result our `steps.d.ts` file will be updated like this:
```
/// <reference types='codeceptjs' />
type CustomHelper = import('./CustomHelper');
declare namespace CodeceptJS {
interface SupportObject { I: I }
interface Methods extends Puppeteer, CustomHelper {}
interface I extends WithTranslation<Methods> {}
namespace Translation {
interface Actions {}
}
}
```
And now you can use autocomplete on your test.
Generation types for PageObject looks like for a custom helper, but `steps.d.ts` will look like:
```
/// <reference types='codeceptjs' />
type loginPage = typeof import('./loginPage');
type homePage = typeof import('./homePage');
type CustomHelper = import('./CustomHelper');
declare namespace CodeceptJS {
interface SupportObject { I: I, loginPage: loginPage, homePage: homePage }
interface Methods extends Puppeteer, CustomHelper {}
interface I extends WithTranslation<Methods> {}
namespace Translation {
interface Actions {}
}
}
```
codeceptjs vue-cli-plugin-e2e-codeceptjs vue-cli-plugin-e2e-codeceptjs
==============================
*Hey, how about some end 2 end testing for your Vue apps?* 🤔
*Let's do it together! Vue, me, [CodeceptJS (opens new window)](https://codecept.io) & [Puppeteer (opens new window)](https://pptr.dev).* 🤗
*Browser testing was never that simple. Just see it!* 😍
```
I.amOnPage('/');
I.click('My Component Button');
I.see('My Component');
I.say('I am happy!');
// that's right, this is a valid test!
```
How to try it?
---------------
**Requirements:**
* NodeJS >= 8.9
* NPM / Yarn
* Vue CLI installed globally
```
npm i vue-cli-plugin-codeceptjs-puppeteer --save-dev
```
This will install CodeceptJS, CodeceptUI & Puppeteer with Chrome browser.
To add CodeceptJS to your project invoke installer:
```
vue invoke vue-cli-plugin-codeceptjs-puppeteer
```
> You will be asked about installing a demo component. If you start a fresh project **it is recommended to agree and install a demo component**, so you could see tests passing.
>
>
Running Tests
--------------
We added npm scripts:
* `test:e2e` - will execute tests with browser opened. If you installed test component, and started a test server, running this command will show you a brower window passed test.
+ Use `--headless` option to run browser headlessly
+ Use `--serve` option to start a dev server before tests
Examples:
```
npm run test:e2e
npm run test:e2e -- --headless
npm run test:e2e -- --serve
```
> This command is a wrapper for `codecept run --steps`. You can use the [Run arguments and options](https://codecept.io/commands#run) here.
>
>
* `test:e2e:parallel` - will execute tests headlessly in parallel processes (workers). By default runs tests in 2 workers.
+ Use an argument to set number of workers
+ Use `--serve` option to start dev server before running
Examples:
```
npm run test:e2e:parallel
npm run test:e2e:parallel -- 3
npm run test:e2e:parallel -- 3 --serve
```
> This command is a wrapper for `codecept run-workers 2`. You can use the [Run arguments and options](https://codecept.io/commands#run-workers) here.
>
>
* `test:e2e:open` - this opens interactive web test runner. So you could see, review & run your tests from a browser.
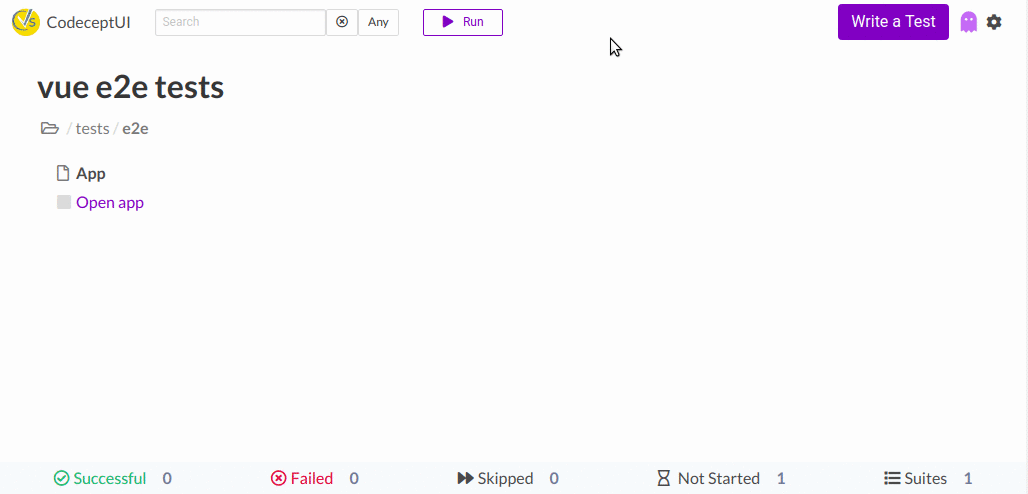
```
npm run test:e2e:open
```
Directory Structure
--------------------
Generator has created these files:
```
codecept.conf.js 👈 codeceptjs config
jsconfig.json 👈 enabling type definitons
tests
├── e2e
│ ├── app_test.js 👈 demo test, edit it!
│ ├── output 👈 temp directory for screenshots, reports, etc
│ └── support
│ └── steps_file.js 👈 common steps
└── steps.d.ts 👈 type definitions
```
If you agreed to create a demo component, you will also see `TestMe` component in `src/components` folder.
How to write tests?
--------------------
* Open `tests/e2e/app_js` and see the demo test
* Execute a test & use interactive pause to see how CodeceptJS works
* [Learn CodeceptJS basics](https://codecept.io/basics)
* [Learn how to write CodeceptJS tests with Puppeteer](https://codecept.io/puppeteer)
* [See full reference for CodeceptJS Puppeteer Helper](https://codecept.io/helpers/Puppeteer)
* Ask your questions in [Slack (opens new window)](https://bit.ly/chat-codeceptjs) & [Forum (opens new window)](https://codecept.discourse.group/)
Enjoy testing!
---------------
Testing is simple & fun, enjoy it!
With ❤ [CodeceptJS Team (opens new window)](https://codecept.io)
codeceptjs Page Objects Page Objects
=============
The UI of your web application has interaction areas which can be shared across different tests. To avoid code duplication you can put common locators and methods in one place.
Dependency Injection
---------------------
All objects described here are injected via Dependency Injection, in a similar way AngularJS does. If you want an object to be injected in a scenario by its name, you can add it to the configuration:
```
include: {
I: "./custom_steps.js",
Smth: "./pages/Smth.js",
loginPage: "./pages/Login.js",
signinFragment: "./fragments/Signin.js"
}
```
These objects can now be retrieved by the name specified in the configuration.
Required objects can be obtained via parameters in tests or via a global `inject()` call.
```
// globally inject objects by name
const { I, myPage, mySteps } = inject();
// inject objects for a test by name
Scenario('sample test', ({ I, myPage, mySteps }) => {
// ...
}
## Actor
During initialization you were asked to create a custom steps file. If you accepted this option, you are now able to use the `custom_steps.js` file to extend `I`. See how the `login` method can be added to `I`:
```js
module.exports = function() {
return actor({
login: function(email, password) {
this.fillField('Email', email);
this.fillField('Password', password);
this.click('Submit');
}
});
}
```
> ℹ Instead of `I` you should use `this` in the current context.
>
>
PageObject
-----------
If an application has different pages (login, admin, etc) you should use a page object. CodeceptJS can generate a template for it with the following command:
```
npx codeceptjs gpo
```
This will create a sample template for a page object and include it in the `codecept.json` config file.
```
const { I, otherPage } = inject();
module.exports = {
// insert your locators and methods here
}
```
As you see, the `I` object is available in scope, so you can use it just like you would do in tests. A general page object for a login page could look like this:
```
// enable I and another page object
const { I, registerPage } = inject();
module.exports = {
// setting locators
fields: {
email: '#user_basic_email',
password: '#user_basic_password'
},
submitButton: {css: '#new_user_basic input[type=submit]'},
// introducing methods
sendForm(email, password) {
I.fillField(this.fields.email, email);
I.fillField(this.fields.password, password);
I.click(this.submitButton);
},
register(email, password) {
// use another page object inside current one
registerPage.registerUser({ email, password });
}
}
```
You can include this pageobject in a test by its name (defined in `codecept.json`). If you created a `loginPage` object, it should be added to the list of arguments to be included in the test:
```
Scenario('login', ({ I, loginPage }) => {
loginPage.sendForm('[email protected]','123456');
I.see('Hello, John');
});
```
Also, you can use `async/await` inside a Page Object:
```
const { I } = inject();
module.exports = {
// setting locators
container: "//div[@class = 'numbers']",
mainItem: {
number: ".//div[contains(@class, 'numbers__main-number')]",
title: ".//div[contains(@class, 'numbers__main-title-block')]"
},
// introducing methods
async openMainArticle() {
I.waitForVisible(this.container)
let _this = this
let title;
await within(this.container, async () => {
title = await I.grabTextFrom(_this.mainItem.number);
let subtitle = await I.grabTextFrom(_this.mainItem.title);
title = title + " " + subtitle.charAt(0).toLowerCase() + subtitle.slice(1);
await I.click(_this.mainItem.title)
})
return title;
}
}
```
and use them in your tests:
```
Scenario('login2', async ({ I, loginPage, basePage }) => {
let title = await mainPage.openMainArticle()
basePage.pageShouldBeOpened(title)
});
```
Page Objects can be be functions, arrays or classes. When declared as classes you can easily extend them in other page objects.
Here is an example of declaring page object as a class:
```
const { expect } = require('chai');
const { I } = inject();
class AttachFile {
constructor() {
this.inputFileField = 'input[name=fileUpload]';
this.fileSize = '.file-size';
this.fileName = '.file-name'
}
async attachFileFrom(path) {
await I.waitForVisible(this.inputFileField)
await I.attachFile(this.inputFileField, path)
}
async hasFileSize(fileSizeText) {
await I.waitForElement(this.fileSize)
const size = await I.grabTextFrom(this.fileSize)
expect(size).toEqual(fileSizeText)
}
async hasFileSizeInPosition(fileNameText, position) {
await I.waitNumberOfVisibleElements(this.fileName, position)
const text = await I.grabTextFrom(this.fileName)
expect(text[position - 1]).toEqual(fileNameText)
}
}
// For inheritance
module.exports = new AttachFile();
module.exports.AttachFile = AttachFile;
```
Page Fragments
---------------
Similarly, CodeceptJS allows you to generate **PageFragments** and any other abstractions by running the `go` command with `--type` (or `-t`) option:
```
npx codeceptjs go --type fragment
```
Page Fragments represent autonomous parts of a page, like modal boxes, components, widgets. Technically, they are the same as PageObject but conceptually they are a bit different. For instance, it is recommended that Page Fragment includes a root locator of a component. Methods of page fragments can use `within` block to narrow scope to a root locator:
```
const { I } = inject();
// fragments/modal.js
module.exports = {
root: '#modal',
// we are clicking "Accept: inside a popup window
accept() {
within(this.root, function() {
I.click('Accept');
});
}
}
```
To use a Page Fragment within a Test Scenario, just inject it into your Scenario:
```
Scenario('failed_login', async ({ I, loginPage, modal }) => {
loginPage.sendForm('[email protected]','wrong password');
I.waitForVisible(modal.root);
within(modal.root, function () {
I.see('Login failed');
})
});
```
To use a Page Fragment within a Page Object, you can use `inject` method to get it by its name.
```
const { I, modal } = inject();
module.exports = {
doStuff() {
...
modal.accept();
...
}
}
```
> PageObject and PageFragment names are declared inside `include` section of `codecept.conf.js`. See [Dependency Injection](#dependency-injection)
>
>
StepObjects
------------
StepObjects represent complex actions which involve the usage of multiple web pages. For instance, creating users in the backend, changing permissions, etc. StepObject can be created similarly to PageObjects or PageFragments:
```
npx codeceptjs go --type step
```
Technically, they are the same as PageObjects. StepObjects can inject PageObjects and use multiple POs to make a complex scenarios:
```
const { I, userPage, permissionPage } = inject();
module.exports = {
createUser(name) {
// action composed from actions of page objects
userPage.open();
userPage.create(name);
permissionPage.activate(name);
}
};
```
Dynamic Injection
------------------
You can inject objects per test by calling `injectDependencies` function in a Scenario:
```
Scenario('search @grop', ({ I, Data }) => {
I.fillField('Username', Data.username);
I.pressKey('Enter');
}).injectDependencies({ Data: require('./data.js') });
```
This requires the `./data.js` module and assigns it to a `Data` argument in a test.
| programming_docs |
codeceptjs Protractor Testing with CodeceptJS Protractor Testing with CodeceptJS
===================================
Introduction
-------------
CodeceptJS is an acceptance testing framework. In the diversified world of JavaScript testing libraries, it aims to create a unified high-level API for end-to-end testing, powered by a variety of backends. CodeceptJS allows you to write a test and switch the execution driver via config: whether it's *wedriverio*, *puppeteer*, or *protractor* depends on you. This way you aren't bound to a specific implementation, and your acceptance tests will work no matter what framework is running them.
[Protractor (opens new window)](http://www.protractortest.org/#/) is an official tool for testing AngularJS applications. CodeceptJS should not be considered as alternative to Protractor, but rather a testing framework that leverages this powerful library.
There is no magic in testing of AngularJS application in CodeceptJS. You just execute regular Protractor commands, packaged into a simple, high-level API.
As an example, we will use the popular [TodoMVC application (opens new window)](http://todomvc.com/examples/angularjs/#/). How would we test creating a new todo item using CodeceptJS?
```
Scenario('create todo item', ({ I }) => {
I.amOnPage('/');
I.dontSeeElement('#todo-count');
I.fillField({model: 'newTodo'}, 'Write a guide');
I.pressKey('Enter');
I.see('Write a guide', {repeater: "todo in todos"});
I.see('1 item left', '#todo-count');
});
```
A similar test written using Protractor's native syntax (inherited from selenium-webdriver) would look like this:
```
it('should create todo item', (I) => {
browser.get("http://todomvc.com/examples/angularjs/#/");
expect(element(by.css("#todo-count")).isPresent()).toBeFalsy();
var inputField = element(by.model("newTodo"));
inputField.sendKeys("Write a guide");
inputField.sendKeys(protractor.Key.ENTER);
var todos = element.all(by.repeater("todo in todos"));
expect(todos.last().getText()).toEqual("Write a guide"));
element(by.css("#todo-count")).getText()).toContain('1 items left');
});
```
Compared to the API proposed by CodeceptJS, the Protractor code looks more complicated. Even more important, it's harder to read and follow the logic of the Protractor test. Readability is a crucial part of acceptance testing. Tests should be easy to modify when there are changes in the specification or design. If the test is written in Protractor, it would likely require someone familiar with Protractor to make the change, whereas CodeceptJS allows anyone to understand and modify the test. CodeceptJS provides scenario-driven approach, so a test is just a step-by-step representation of real user actions. This means you can easily read and understand the steps in a test scenario, and edit the steps when the test needs to be changed.
In this way, CodeceptJS is similar to Cucumber. If you run a test with `--steps` option you will see this output:
```
TodoMvc --
create todo item
• I am on page "/"
• I dont see element "#todo-count"
• I fill field {"model":"newTodo"}, "Write a guide"
• I press key "Enter"
• I see "Write a guide", {"repeater":"todo in todos"}
• I see "1 item left", "#todo-count"
✓ OK in 968ms
```
Unlike Cucumber, CodeceptJS is not about writing test scenarios to satisfy business rules or requirements. Instead, its **goal is to provide standard action steps you can use for testing applications**. Although it can't cover 100% of use cases, CodeceptJS aims for 90%. For the remainder, you can write your own steps inside a [custom Helper (opens new window)](https://codecept.io/helpers/) using Protractor's API.
### Setting up CodeceptJS with Protractor
To start using CodeceptJS you will need to install it via NPM and initialize it in a directory with tests.
```
npm install codeceptjs --save
npx codeceptjs init
```
You will be asked questions about the initial configuration, make sure you select the Protractor helper. If your project didn't already have the Protractor library, it **will be installed** as part of this process. Please agree to extend steps, and use `http://todomvc.com/examples/angularjs/` as the url for Protractor helper.
For TodoMVC application, you will have following config created in the `codecept.conf.js` file:
```
exports.config = { tests: './*_test.js',
timeout: 10000,
output: './output',
helpers:
{ Protractor:
{ url: 'http://todomvc.com/examples/angularjs/',
driver: 'hosted',
browser: 'chrome',
rootElement: 'body' } },
include: { I: './steps_file.js' },
bootstrap: false,
mocha: {},
name: 'todoangular'
}
```
Your first test can be generated with the `gt` command:
```
npx codeceptjs gt
```
After that, you can start writing your first CodeceptJS/Angular tests. Please refer to the [Protractor helper (opens new window)](https://codecept.io/helpers/Protractor/) documentation for a list of all available actions. You can also run the `list` command to see methods of I:
```
npx codeceptjs list
```
Starting Selenium Server
-------------------------
Protractor requires Selenium Server to be started and running. To start and stop Selenium automatically install `@wdio/selenium-standalone-service`.
```
npm i @wdio/selenium-standalone-service --save
```
Enable it in the `codecept.conf.js` file, inside the plugins section:
```
exports.config = {
// ...
// inside codecept.conf.js
plugins: {
wdio: {
enabled: true,
services: ['selenium-standalone']
}
}
}
```
Testing non-Angular Applications
---------------------------------
Protractor can also be used to test applications built without AngularJS. In this case, you need to disable the angular synchronization feature inside the config:
```
helpers: {
Protractor: {
url: "http://todomvc.com/examples/angularjs/",
driver: "hosted",
browser: "firefox",
angular: false
}
}
```
Writing Your First Test
------------------------
Your test scenario should always use the `I` object to execute commands. This is important, as all methods of `I` are running in the global promise chain. This way, CodeceptJS makes sure everything is executed in right order. To start with opening a webpage, use the `amOnPage` command for. Since we already specified the full URL to the TodoMVC app, we can pass the relative path for our url, instead of the absolute url:
```
Feature('Todo MVC');
Scenario('create todo item', ({ I }) => {
I.amOnPage('/');
});
```
All scenarios should describe actions on the site, with assertions at the end. In CodeceptJS, assertion commands have the `see` or `dontSee` prefix:
```
Feature('Todo MVC');
Scenario('create todo item', ({ I }) => {
I.amOnPage('/');
I.dontSeeElement('#todo-count');
});
```
A test can be executed with the `run` command, we recommend using the `--steps` option to print out the step-by-step execution:
```
npx codeceptjs run --steps
```
```
Test root is assumed to be /home/davert/demos/todoangular
Using the selenium server at http://localhost:4444/wd/hub
TodoMvc --
create todo item
• I am on page "/"
• I dont see element "#todo-count"
```
Running Several Scenarios
--------------------------
By now, you should have a test similar to the one shown in the beginning of this guide. We probably want to have multiple tests though, like testing the editing of todo items, checking todo items, etc.
Let's prepare our test to contain multiple scenarios. All of our test scenarios will need to to start with with the main page of application open, so `amOnPage` can be moved into the `Before` hook: Our scenarios will also probably deal with created todo items, so we can move the logic of creating a new todo into a function.
```
Feature('TodoMvc');
Before(({ I }) => {
I.amOnPage('/');
});
const createTodo = function (I, name) {
I.fillField({model: 'newTodo'}, name);
I.pressKey('Enter');
}
Scenario('create todo item', ({ I }) => {
I.dontSeeElement('#todo-count');
createTodo(I, 'Write a guide');
I.see('Write a guide', {repeater: "todo in todos"});
I.see('1 item left', '#todo-count');
});
```
and now we can add even more tests!
```
Scenario('edit todo', ({ I }) => {
createTodo(I, 'write a review');
I.see('write a review', {repeater: "todo in todos"});
I.doubleClick('write a review');
I.pressKey(['Control', 'a']);
I.pressKey('write old review');
I.pressKey('Enter');
I.see('write old review', {repeater: "todo in todos"});
});
Scenario('check todo item', ({ I }) => {
createTodo(I, 'my new item');
I.see('1 item left', '#todo-count');
I.checkOption({model: 'todo.completed'});
I.see('0 items left', '#todo-count');
});
```
> This example is [available on GitHub (opens new window)](https://github.com/DavertMik/codeceptjs-angular-todomvc).
>
>
Locators
---------
You may have noticed that CodeceptJS doesn't use `by.*` locators which are common in Protractor or Selenium Webdriver. Instead, most methods expect you to pass valid CSS selectors or XPath. If you don't want CodeceptJS to guess the locator type, then you can specify the type using *strict locators*. This is the CodeceptJS version of `by`, so you can also reuse your angular specific locators (like models, repeaters, bindings, etc):
```
{css: 'button'}
{repeater: "todo in todos"}
{binding: 'latest'}
```
When dealing with clicks, we can specify a text value. CodeceptJS will use that value to search the web page for a valid clickable element containing our specified text. This enables us to search for links and buttons by their text.
The same is true for form fields: they can be searched by field name, label, and so on.
Using smart locators makes tests easier to write, however searching an element by text is slower than searching via CSS|XPath, and is much slower than using strict locators.
Refactoring
------------
In the previous examples, we moved actions into the `createTodo` function. Is there a more elegant way of refactoring? Can we instead write a function like `I.createTodo()` which we can reuse? In fact, we can do so by editing the `steps_file.js` file created by the init command.
```
// in this file you can append custom step methods to 'I' object
module.exports = function() {
return actor({
createTodo: function(title) {
this.fillField({model: 'newTodo'}, title);
this.pressKey('Enter');
}
});
}
```
That's it, our method is now available to use as `I.createTodo(title)`:
```
Scenario('create todo item', ({ I }) => {
I.dontSeeElement('#todo-count');
I.createTodo('Write a guide');
I.see('Write a guide', {repeater: "todo in todos"});
I.see('1 item left', '#todo-count');
});
```
To learn more about refactoring options in CodeceptJS read [PageObjects guide (opens new window)](https://codecept.io/pageobjects/).
Extending
----------
What if CodeceptJS doesn't provide some specific Protractor functionality you need? If you don't know how to do something with CodeceptJS, you can simply revert back to using Protractor syntax!
Create a custom helper, define methods for it, and use it inside the I object. Your Helper can access `browser` from Protractor by accessing the Protractor helper:
```
let browser = this.helpers['Protractor'].browser;
```
or use global `element` and `by` variables to locate elements:
```
element.all(by.repeater('result in memory'));
```
This is the recommended way to implement all custom logic using low-level Protractor syntax in order to reuse it inside of test scenarios. For more information, see an [example of such a helper (opens new window)](https://codecept.io/helpers/#protractor-example).
codeceptjs Best Practices Best Practices
===============
Focus on Readability
---------------------
In CodeceptJS we encourage users to follow semantic elements on page while writing tests. Instead of CSS/XPath locators try to stick to visible keywords on page.
Take a look into the next example:
```
// it's fine but...
I.click({css: 'nav.user .user-login'});
// can be better
I.click('Login', 'nav.user');
```
If we replace raw CSS selector with a button title we can improve readability of such test. Even if the text on the button changes, it's much easier to update it.
> If your code goes beyond using `I` object or page objects, you are probably doing something wrong.
>
>
When it's hard to match text to element we recommend using [locator builder](https://codecept.io/locators#locator-builder). It allows to build complex locators via fluent API. So if you want to click an element which is not a button or a link and use its text you can use `locate()` to build a readable locator:
```
// clicks element <span class="button">Click me</span>
I.click(locate('.button').withText('Click me'));
```
Short Cuts
-----------
To write simpler and effective tests we encourage to use short cuts. Make test be focused on one feature and try to simplify everything that is not related directly to test.
* If data is required for a test, try to create that data via API. See how to do it in [Data Management](https://codecept.io/data) chapter.
* If user login is required, use [autoLogin plugin](https://codecept.io/plugins#autoLogin) instead of putting login steps inside a test.
* Break a long test into few. Long test can be fragile and complicated to follow and update.
* Use [custom steps and page objects](https://codecept.io/pageobjects) to hide steps which are not relevant to current test.
Make test as simple as:
```
Scenario('editing a metric', async ({ I, loginAs, metricPage }) => {
// login via autoLogin
loginAs('admin');
// create data with ApiDataFactory
const metric = await I.have('metric', { type: 'memory', duration: 'day' })
// use page object to open a page
metricPage.open(metric.id);
I.click('Edit');
I.see('Editing Metric');
// using a custom step
I.selectFromDropdown('duration', 'week');
I.click('Save');
I.see('Duration: Week', '.summary');
});
```
Locators
---------
* If you don't use multi-lingual website or you don't update texts often it is OK to click on links by their texts or match fields by their placeholders.
* If you don't want to rely on guessing locators, specify them manually with `{ css: 'button' }` or `{ xpath: '//button' }`. We call them strict locators. Those locators will be faster but less readable.
* Even better if you have a convention on active elements with special attributes like `data-test` or `data-qa`. Use `customLocator` plugin to easily add them to tests.
* Keep tests readable which will make them maintainable.
Page Objects
-------------
When a project is growing and more and more tests are required, it's time to think about reusing test code across the tests. Some common actions should be moved from tests to other files so to be accessible from different tests.
Here is a recommended strategy what to store where:
* Move site-wide actions into an **Actor** file (`custom_steps.js` file). Such actions like `login`, using site-wide common controls, like drop-downs, rich text editors, calendars.
* Move page-based actions and selectors into **Page Object**. All acitivities made on that page can go into methods of page object. If you test Single Page Application a PageObject should represent a screen of your application.
* When site-wide widgets are used, interactions with them should be placed in **Page Fragments**. This should be applied to global navigation, modals, widgets.
* A custom action that requires some low-level driver access, should be placed into a **Helper**. For instance, database connections, complex mouse actions, email testing, filesystem, services access.
> [Learn more](https://codecept.io/pageobjects) about different refactoring options
>
>
However, it's recommended to not overengineer and keep tests simple. If a test code doesn't require reusage at this point it should not be transformed to use page objects.
* use page objects to store common actions
* don't make page objects for every page! Only for pages shared across different tests and suites.
* use classes for page objects, this allows inheritace. Export instance of that classes.
* if a page object is focused around a form with multiple fields in it, use a flexible set of arguments in it:
```
class CheckoutForm {
fillBillingInformation(data = {}) {
// take data in a flexible format
// iterate over fields to fill them all
for (let key of Object.keys(data)) {
I.fillField(key, data[key]); // like this one
}
}
}
module.exports = new CheckoutForm();
module.exports.CheckoutForm = CheckoutForm; // for inheritance
```
* for components that are repeated accross a website (widgets) but don't belong to any page, use component objects. They are the same as page objects but focused only aroung one element:
```
class DropDownComponent {
selectFirstItem(locator) {
I.click(locator);
I.click('#dropdown-items li');
}
selectItemByName(locator, name) {
I.click(locator);
I.click(locate('li').withText(name), '#dropdown-items');
}
}
```
* another good example is datepicker component:
```
const { I } = inject();
/**
* Calendar works
*/
class DatePicker {
selectToday(locator) {
I.click(locator);
I.click('.currentDate', '.date-picker');
}
selectInNextMonth(locator, date = '15') {
I.click(locator);
I.click('show next month', '.date-picker')
I.click(date, '.date-picker')
}
}
module.exports = new DatePicker;
module.exports.DatePicker = DatePicker; // for inheritance
```
Configuration
--------------
* create multiple config files for different setups/enrionments:
+ `codecept.conf.js` - default one
+ `codecept.ci.conf.js` - for CI
+ `codecept.windows.conf.js` - for Windows, etc
* use `.env` files and dotenv package to load sensitive data
```
require('dotenv').config({ path: '.env' });
```
* move similar parts in those configs by moving them to modules and putting them to `config` dir
* when you need to load lots of page objects/components, you can get components/pageobjects file declaring them:
```
// inside config/components.js
module.exports = {
DatePicker: "./components/datePicker",
Dropdown: "./components/dropdown",
}
```
include them like this:
```
include: {
I: './steps_file',
...require('./config/pages'), // require POs and DOs for module
...require('./config/components'), // require all components
},
```
* move long helpers configuration into `config/plugins.js` and export them
* move long configuration into `config/plugins.js` and export them
* inside config files import the exact helpers or plugins needed for this setup & environment
* to pass in data from config to a test use a container:
```
// inside codecept conf file
bootstrap: () => {
codeceptjs.container.append({
testUser: {
email: '[email protected]',
password: '123456'
}
});
}
// now `testUser` can be injected into a test
```
* (alternatively) if you have more test data to pass into tests, create a separate file for them and import them similarly to page object:
```
include: {
// ...
testData: './config/testData'
}
```
* .env / different configs / different test data allows you to get configs for multiple environments
Data Access Objects
--------------------
* Concept is similar to page objects but Data access objects can act like factories or data providers for tests
* Data Objects require REST or GraphQL helpers to be enabled for data interaction
* When you need to customize access to API and go beyond what ApiDataFactory provides, implement DAO:
```
const faker = require('faker');
const { I } = inject();
const { output } = require('codeceptjs');
class InterfaceData {
async getLanguages() {
const { data } = await I.sendGetRequest('/api/languages');
const { records } = data;
output.debug(`Languages ${records.map(r => r.language)}`);
return records;
}
async getUsername() {
return faker.user.name();
}
}
module.exports = new InterfaceData;
```
| programming_docs |
codeceptjs Locators Locators
=========
CodeceptJS provides flexible strategies for locating elements:
* [CSS and XPath locators](#css-and-xpath)
* [Semantic locators](#semantic-locators): by link text, by button text, by field names, etc.
* [Locator Builder](#locator-builder)
* [ID locators](#id-locators): by CSS id or by accessibility id
* [Custom Locator Strategies](#custom-locators): by data attributes or whatever you prefer.
* [Shadow DOM](https://codecept.io/shadow): to access shadow dom elements
* [React](https://codecept.io/react): to access React elements by component names and props
Most methods in CodeceptJS use locators which can be either a string or an object.
If the locator is an object, it should have a single element, with the key signifying the locator type (`id`, `name`, `css`, `xpath`, `link`, `react`, or `class`) and the value being the locator itself. This is called a "strict" locator.
Examples:
* {permalink: /'foo'} matches `<div id="foo">`
* {name: 'foo'} matches `<div name="foo">`
* {css: 'input[type=input][value=foo]'} matches `<input type="input" value="foo">`
* {xpath: "//input[@type='submit'][contains(@value, 'foo')]"} matches `<input type="submit" value="foobar">`
* {class: 'foo'} matches `<div class="foo">`
Writing good locators can be tricky. The Mozilla team has written an excellent guide titled [Writing reliable locators for Selenium and WebDriver tests (opens new window)](https://blog.mozilla.org/webqa/2013/09/26/writing-reliable-locators-for-selenium-and-webdriver-tests/).
If you prefer, you may also pass a string for the locator. This is called a "fuzzy" locator. In this case, CodeceptJS uses a variety of heuristics (depending on the exact method called) to determine what element you're referring to. If you are locating a clickable element or an input element, CodeceptJS will use [semantic locators](#semantic-locators).
For example, here's the heuristic used for the `fillField` method:
1. Does the locator look like an ID selector (e.g. "#foo")? If so, try to find an input element matching that ID.
2. If nothing found, check if locator looks like a CSS selector. If so, run it.
3. If nothing found, check if locator looks like an XPath expression. If so, run it.
4. If nothing found, check if there is an input element with a corresponding name.
5. If nothing found, check if there is a label with specified text for input element.
6. If nothing found, throw an `ElementNotFound` exception.
> ⚠ Be warned that fuzzy locators can be significantly slower than strict locators. If speed is a concern, it's recommended you stick with explicitly specifying the locator type via object syntax.
>
>
It is recommended to avoid using implicit CSS locators in methods like `fillField` or `click`, where semantic locators are allowed. Use locator type to speed up search by various locator strategies.
```
// will search for "input[type=password]" text before trying to search by CSS
I.fillField('input[type=password]', '123456');
// replace with strict locator
I.fillField({ css: 'input[type=password]' }, '123456');
```
CSS and XPath
--------------
Both CSS and XPath is supported. Usually CodeceptJS can guess locator's type:
```
// select by CSS
I.seeElement('.user .profile');
I.seeElement('#user-name');
// select by XPath
I.seeElement('//table/tr/td[position()=3]');
```
To specify exact locator type use **strict locators**:
```
// it's not clear that 'button' is actual CSS locator
I.seeElement({ css: 'button' });
// it's not clear that 'descendant::table/tr' is actual XPath locator
I.seeElement({ xpath: 'descendant::table/tr' });
```
> ℹ Use [Locator Advicer (opens new window)](https://davertmik.github.io/locator/) to check quality of your locators.
>
>
Semantic Locators
------------------
CodeceptJS can guess an element's locator from context. For example, when clicking CodeceptJS will try to find a link or button by their text When typing into a field this field can be located by its name, placeholder.
```
I.click('Sign In');
I.fillField('Username', 'davert');
```
Various strategies are used to locate semantic elements. However, they may run slower than specifying locator by XPath or CSS.
Locator Builder
----------------
CodeceptJS provides a fluent builder to compose custom locators in JavaScript. Use `locate` function to start.
To locate `a` element inside `label` with text: 'Hello' use:
```
locate('a')
.withAttr({ href: '#' })
.inside(locate('label').withText('Hello'));
```
which will produce following XPath:
```
.//a[@href = '#'][ancestor::label[contains(., 'Hello')]]
```
Locator builder accepts both XPath and CSS as parameters but converts them to XPath as more feature-rich format. Sometimes provided locators can get very long so it's recommended to simplify the output by providing a brief description for generated XPath:
```
locate('//table')
.find('a')
.withText('Edit')
.as('edit button')
// will be printed as 'edit button'
```
`locate` has following methods:
#### find
Finds an element inside a located.
```
// find td inside a table
locate('table').find('td');
```
Switches current element to found one. Can accept another `locate` call or strict locator.
#### withAttr
Find an element with provided attributes
```
// find input with placeholder 'Type in name'
locate('input').withAttr({ placeholder: 'Type in name' });
```
#### withChild
Finds an element which contains a child element provided:
```
// finds form with <select> inside it
locate('form').withChild('select');
```
#### withDescendant
Finds an element which contains a descendant element provided:
```
// finds form with <select> which is the descendant it
locate('form').withDescendant('select');
```
#### withText
Finds element containing a text
```
locate('span').withText('Warning');
```
#### first
Get first element:
```
locate('#table td').first();
```
#### last
Get last element:
```
locate('#table td').last();
```
#### at
Get element at position:
```
// first element
locate('#table td').at(1);
// second element
locate('#table td').at(2);
// second element from end
locate('#table td').at(-2);
```
#### inside
Finds an element which contains an provided ancestor:
```
// finds `select` element inside #user_profile
locate('select').inside('form#user_profile');
```
#### before
Finds element located before the provided one
```
// finds `button` before .btn-cancel
locate('button').before('.btn-cancel');
```
#### after
Finds element located after the provided one
```
// finds `button` after .btn-cancel
locate('button').after('.btn-cancel');
```
ID Locators
------------
ID locators are best to select the exact semantic element in web and mobile testing:
* `#user` or `{ permalink: /'user' }` finds element with id="user"
* `~user` finds element with accessibility id "user" (in Mobile testing) or with `aria-label=user`.
Custom Locators
----------------
CodeceptJS allows to create custom locator strategies and use them in tests. This way you can define your own handling of elements using specially prepared attributes of elements.
What if you use special test attributes for locators such as `data-qa`, `data-test`, `test-id`, etc. We created [customLocator plugin](https://codecept.io/plugins#customlocator) to declare rules for locating element.
Instead of writing a full CSS locator like `[data-qa-id=user_name]` simplify it to `$user_name`.
```
// replace this:
I.click({ css: '[data-test-id=register_button]');
// with this:
I.click('$register_button');
```
This plugin requires two options: locator prefix and actual attribute to match.
> ℹ See [customLocator Plugin](https://codecept.io/plugins#customlocator) reference to learn how to set it up.
>
>
If you need more control over custom locators see how declare them manually without using a customLocator plugin.
#### Custom Strict Locators
If use locators of `data-element` attribute you can implement a strategy, which will allow you to use `{ data: 'my-element' }` as a valid locator.
Custom locators should be implemented in a plugin or a bootstrap script using internal CodeceptJS API:
```
// inside a plugin or a bootstrap script:
codeceptjs.locator.addFilter((providedLocator, locatorObj) => {
// providedLocator - a locator in a format it was provided
// locatorObj - a standrard locator object.
if (providedLocator.data) {
locatorObj.type = 'css';
locatorObj.value = `[data-element=${providedLocator.data}]`
}
});
```
That's all. New locator type is ready to use:
```
I.click({ data: 'user-login' });
```
#### Custom String Locators
What if we want to locators prefixed with `=` to match elements with exact text value. We can do that too:
```
// inside a plugin or a bootstrap script:
codeceptjs.locator.addFilter((providedLocator, locatorObj) => {
if (typeof providedLocator === 'string') {
// this is a string
if (providedLocator[0] === '=') {
locatorObj.value = `.//*[text()="${providedLocator.substring(1)}"]`;
locatorObj.type = 'xpath';
}
}
});
```
New locator strategy is ready to use:
```
I.click('=Login');
```
> For more details on locator object see [Locator (opens new window)](https://github.com/codeceptjs/CodeceptJS/blob/master/lib/locator.js) class implementation.
>
>
codeceptjs Installation Installation
=============
Via Installer
--------------
Creating a new project via [`create-codeceptjs` installer (opens new window)](https://github.com/codeceptjs/create-codeceptjs) is the simplest way to start
Install CodeceptJS + Playwright into current directory
```
npx create-codeceptjs .
```
Install CodeceptJS + Puppeteer into current directory
```
npx create-codeceptjs . --puppeteer
```
Install CodeceptJS + webdriverio into current directory
```
npx create-codeceptjs . --webdriverio
```
Install CodeceptJS + webdriverio into `e2e-tests` directory:
```
npx create-codeceptjs e2e-tests --webdriverio
```
Local
------
Use NPM install CodeceptJS:
```
npm install --save-dev codeceptjs
```
and started as
```
./node_modules/.bin/codeceptjs
```
To use it with WebDriver install webdriverio package:
```
npm install webdriverio --save-dev
```
To use it with Protractor install protractor package:
```
npm install protractor --save-dev
```
To use it with Nightmare install nightmare and nightmare-upload packages:
```
npm install nightmare --save-dev
```
To use it with Puppeteer install puppeteer package:
```
npm install puppeteer --save-dev
```
WebDriver
----------
WebDriver based helpers like WebDriver, Protractor, Selenium WebDriver will require [Selenium Server (opens new window)](https://codecept.io/helpers/WebDriver/#selenium-installation) or [PhantomJS (opens new window)](https://codecept.io/helpers/WebDriver/#phantomjs-installation) installed. They will also require ChromeDriver or GeckoDriver to run corresponding browsers.
We recommend to install them manually or use NPM packages:
* [Selenium Standalone (opens new window)](https://www.npmjs.com/package/selenium-standalone) to install and run Selenium, ChromeDriver, Firefox Driver with one package.
* [Phantomjs (opens new window)](https://www.npmjs.com/package/phantomjs-prebuilt): to install and execute Phantomjs
or use [Docker (opens new window)](https://github.com/SeleniumHQ/docker-selenium) for headless browser testing.
Launch Selenium with Chrome browser inside a Docker container:
```
docker run --net=host selenium/standalone-chrome
```
codeceptjs Testing with Playwright Since 2.5
Testing with Playwright Since 2.5
==================================
Playwright is a Node library to automate the [Chromium (opens new window)](https://www.chromium.org/Home), [WebKit (opens new window)](https://webkit.org/) and [Firefox (opens new window)](https://www.mozilla.org/en-US/firefox/new/) browsers with a single API. It enables **cross-browser** web automation that is **ever-green**, **capable**, **reliable** and **fast**.
Playwright was built similarly to [Puppeteer (opens new window)](https://github.com/puppeteer/puppeteer), using its API and so is very different in usage. However, Playwright has cross browser support with better design for test automaiton.
Take a look at a sample test:
```
I.amOnPage('https://github.com');
I.click('Sign in', '//html/body/div[1]/header');
I.see('Sign in to GitHub', 'h1');
I.fillField('Username or email address', '[email protected]');
I.fillField('Password', '123456');
I.click('Sign in');
I.see('Incorrect username or password.', '.flash-error');
```
It's readable and simple and working using Playwright API!
Setup
------
To start you need CodeceptJS with Playwright packages installed
```
npm install codeceptjs playwright --save
```
Or see [alternative installation options (opens new window)](https://codecept.io/installation/)
> If you already have CodeceptJS project, just install `playwright` package and enable a helper it in config.
>
>
And a basic project initialized
```
npx codeceptjs init
```
You will be asked for a Helper to use, you should select Playwright and provide url of a website you are testing.
Configuring
------------
Make sure `Playwright` helper is enabled in `codecept.conf.js` config:
```
{ // ..
helpers: {
Playwright: {
url: "http://localhost",
show: true,
browser: 'chromium'
}
}
// ..
}
```
> Turn off the `show` option if you want to run test in headless mode. If you don't specify the browser here, `chromium` will be used. Possible browsers are: `chromium`, `firefox` and `webkit`
>
>
Playwright uses different strategies to detect if a page is loaded. In configuration use `waitForNavigation` option for that:
When to consider navigation succeeded, defaults to `load`. Given an array of event strings, navigation is considered to be successful after all events have been fired. Events can be either:
* `load` - consider navigation to be finished when the load event is fired.
* `domcontentloaded` - consider navigation to be finished when the DOMContentLoaded event is fired.
* `networkidle` - consider navigation to be finished when there are no network connections for at least 500 ms.
```
helpers: {
Playwright: {
url: "http://localhost",
show: true,
browser: 'chromium',
waitForNavigation: "networkidle0"
}
}
```
When a test runs faster than application it is recommended to increase `waitForAction` config value. It will wait for a small amount of time (100ms) by default after each user action is taken.
> ▶ More options are listed in [helper reference (opens new window)](https://codecept.io/helpers/Playwright/).
>
>
Writing Tests
--------------
Additional CodeceptJS tests should be created with `gt` command:
```
npx codeceptjs gt
```
As an example we will use `ToDoMvc` app for testing.
### Actions
Tests consist with a scenario of user's action taken on a page. The most widely used ones are:
* `amOnPage` - to open a webpage (accepts relative or absolute url)
* `click` - to locate a button or link and click on it
* `fillField` - to enter a text inside a field
* `selectOption`, `checkOption` - to interact with a form
* `wait*` to wait for some parts of page to be fully rendered (important for testing SPA)
* `grab*` to get values from page sources
* `see`, `dontSee` - to check for a text on a page
* `seeElement`, `dontSeeElement` - to check for elements on a page
> ℹ All actions are listed in [Playwright helper reference (opens new window)](https://codecept.io/helpers/Playwright/).\*
>
>
All actions which interact with elements can use **[CSS or XPath locators (opens new window)](https://codecept.io/locators/#css-and-xpath)**. Actions like `click` or `fillField` can locate elements by their name or value on a page:
```
// search for link or button
I.click('Login');
// locate field by its label
I.fillField('Name', 'Miles');
// we can use input name
I.fillField('user[email]','[email protected]');
```
You can also specify the exact locator type with strict locators:
```
I.click({css: 'button.red'});
I.fillField({name: 'user[email]'},'[email protected]');
I.seeElement({xpath: '//body/header'});
```
### Interactive Pause
It's easy to start writing a test if you use [interactive pause](https://codecept.io/basics#debug). Just open a web page and pause execution.
```
Feature('Sample Test');
Scenario('open my website', ({ I }) => {
I.amOnPage('http://todomvc.com/examples/react/');
pause();
});
```
This is just enough to run a test, open a browser, and think what to do next to write a test case.
When you execute such test with `codeceptjs run` command you may see the browser is started
```
npx codeceptjs run --steps
```
After a page is opened a full control of a browser is given to a terminal. Type in different commands such as `click`, `see`, `fillField` to write the test. A successful commands will be saved to `./output/cli-history` file and can be copied into a test.
A complete ToDo-MVC test may look like:
```
Feature('ToDo');
Scenario('create todo item', ({ I }) => {
I.amOnPage('http://todomvc.com/examples/react/');
I.dontSeeElement('.todo-count');
I.fillField('What needs to be done?', 'Write a guide');
I.pressKey('Enter');
I.see('Write a guide', '.todo-list');
I.see('1 item left', '.todo-count');
});
```
### Grabbers
If you need to get element's value inside a test you can use `grab*` methods. They should be used with `await` operator inside `async` function:
```
const assert = require('assert');
Scenario('get value of current tasks', async ({ I }) => {
I.createTodo('do 1');
I.createTodo('do 2');
let numTodos = await I.grabTextFrom('.todo-count strong');
assert.equal(2, numTodos);
});
```
### Within
In case some actions should be taken inside one element (a container or modal window or iframe) you can use `within` block to narrow the scope. Please take a note that you can't use within inside another within in Playwright helper:
```
within('.todoapp', () => {
I.createTodo('my new item');
I.see('1 item left', '.todo-count');
I.click('.todo-list input.toggle');
});
I.see('0 items left', '.todo-count');
```
> [▶ Learn more about basic commands](https://codecept.io/basics#writing-tests)
>
>
CodeceptJS allows you to implement custom actions like `I.createTodo` or use **PageObjects**. Learn how to improve your tests in [PageObjects (opens new window)](https://codecept.io/pageobjects/) guide.
Multi Session Testing
----------------------
TO launch additional browser context (or incognito window) use `session` command.
```
Scenario('I try to open this site as anonymous user', () => {
I.amOnPage('/');
I.dontSee('Agree to cookies');
session('anonymous user', () => {
I.amOnPage('/');
I.see('Agree to cookies');
});
})
```
> ℹ Learn more about [multi-session testing](https://codecept.io/basics/#multiple-sessions)
>
>
Device Emulation
-----------------
Playwright can emulate browsers of mobile devices. Instead of paying for expensive devices for mobile tests you can adjust Playwright settings so it could emulate mobile browsers on iPhone, Samsung Galaxy, etc.
Device emulation can be enabled in CodeceptJS globally in a config or per session.
Playwright contains a [list of predefined devices (opens new window)](https://github.com/Microsoft/playwright/blob/master/src/deviceDescriptors.ts) to emulate, for instance this is how you can enable iPhone 6 emulation for all tests:
```
const { devices } = require('playwright');
helpers: {
Playwright: {
// regular config goes here
emulate: devices['iPhone 6'],
}
}
```
To adjust browser settings you can pass [custom options (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#browsernewcontextoptions)
```
helpers: {
Playwright: {
// regular config goes here
// put on mobile device
emulate: { isMobile: true, deviceScaleFactor: 2 }
}
}
```
To enable device emulation for a specific test, create an additional browser session and pass in config as a second parameter:
```
const { devices } = require('playwright');
Scenario('website looks nice on iPhone', () => {
session('mobile user', devices['iPhone 6'], () => {
I.amOnPage('/');
I.see('Hello, iPhone user!')
})
});
```
Configuring CI
---------------
### GitHub Actions
Playwright can be added to GitHub Actions using [official action (opens new window)](https://github.com/microsoft/playwright-github-action). Use it before starting CodeceptJS tests to install all dependencies. It is important to run tests in headless mode ([otherwise you will need to enable xvfb to emulate desktop (opens new window)](https://github.com/microsoft/playwright-github-action#run-in-headful-mode)).
```
# from workflows/tests.yml
- uses: microsoft/playwright-github-action@v1
- name: run CodeceptJS tests
run: npx codeceptjs run
```
Accessing Playwright API
-------------------------
To get [Playwright API (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md) inside a test use `I.usePlaywrightTo` method with a callback. To keep test readable provide a description of a callback inside the first parameter.
```
I.usePlaywrightTo('emulate offline mode', async ({ browser, context, page }) => {
// use browser, page, context objects inside this function
await context.setOffline(true);
});
```
Playwright commands are asynchronous so a callback function must be async.
A Playwright helper is passed as argument for callback, so you can combine Playwrigth API with CodeceptJS API:
```
I.usePlaywrightTo('emulate offline mode', async (Playwright) => {
// access internal objects browser, page, context of helper
await Playwright.context.setOffline(true);
// call a method of helper, await is required here
await Playwright.click('Reload');
});
```
Extending Helper
-----------------
To create custom `I.*` commands using Playwright API you need to create a custom helper.
Start with creating an `MyPlaywright` helper using `generate:helper` or `gh` command:
```
npx codeceptjs gh
```
Then inside a Helper you can access `Playwright` helper of CodeceptJS. Let's say you want to create `I.grabDimensionsOfCurrentPage` action. In this case you need to call `evaluate` method of `page` object
```
// inside a MyPlaywright helper
async grabDimensionsOfCurrentPage() {
const { page } = this.helpers.Playwright;
await page.goto('https://www.example.com/');
return page.evaluate(() => {
return {
width: document.documentElement.clientWidth,
height: document.documentElement.clientHeight,
deviceScaleFactor: window.devicePixelRatio
}
})
}
```
The same way you can also access `browser` object to implement more actions or handle events. For instance, you want to set the permissions, you can approach it with:
```
// inside a MyPlaywright helper
async setPermissions() {
const { browser } = this.helpers.Playwright;
const context = browser.defaultContext()
return context.setPermissions('https://html5demos.com', ['geolocation']);
}
```
> [▶ Learn more about BrowserContext (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#class-browsercontext)
>
>
> [▶ Learn more about Helpers (opens new window)](https://codecept.io/helpers/)
>
>
| programming_docs |
codeceptjs Quickstart Quickstart
===========
> CodeceptJS supports various engines for running browser tests. By default we recommend using **Playwright** which is cross-browser and performant solution.
>
>
Use [CodeceptJS all-in-one installer (opens new window)](https://github.com/codeceptjs/create-codeceptjs) to get CodeceptJS, a demo project, and Playwright.
```
npx create-codeceptjs .
```
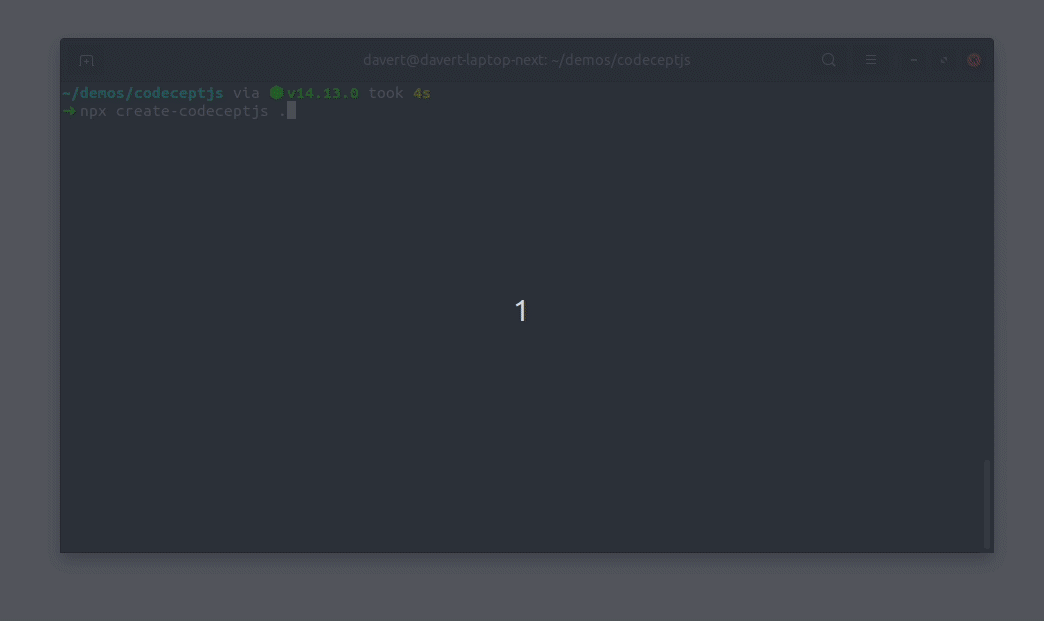
> To install codeceptjs into a different folder, like `tests` use `npx create-codeceptjs tests`
>
>
After CodeceptJS is installed, try running **demo tests** using this commands:
* `npm run codeceptjs:demo` - executes demo tests in window mode
* `npm run codeceptjs:demo:headless` - executes demo tests in headless mode
* `npm run codeceptjs:demo:ui` - open CodeceptJS UI to list and run demo tests.
[CodeceptJS UI](https://codecept.io/ui) application:
To start a new project initialize CodeceptJS to create main config file: `codecept.conf.js`.
```
npx codeceptjs init
```
Answer questions, agree on defaults, when asked to select helpers choose **Playwright**.
```
? What helpers do you want to use?
❯◉ Playwright
◯ WebDriver
◯ Protractor
◯ Puppeteer
◯ Appium
◯ Nightmare
◯ FileSystem
```
Create first feature and test when asked. Open a newly created file in your favorite JavaScript editor. The file should look like this:
```
Feature('My First Test');
Scenario('test something', ({ I }) => {
});
```
Write a simple test scenario:
```
Feature('My First Test');
Scenario('test something', ({ I }) => {
I.amOnPage('https://github.com');
I.see('GitHub');
});
```
Run a test:
```
npm run codeceptjs
```
The output should be similar to this:
```
My First Test --
test something
I am on page "https://github.com"
I see "GitHub"
✓ OK
```
To quickly execute tests use following npm scripts:
After CodeceptJS is installed, try running **demo tests** using this commands:
* `npm run codeceptjs` - executes tests in window mode
* `npm run codeceptjs:headless` - executes tests in headless mode
* `npm run codeceptjs:ui` - open CodeceptJS UI to list and run tests.
More commands available in [CodeceptJS CLI runner (opens new window)](https://codecept.io/commands/).
> [▶ Next: CodeceptJS Basics](https://codecept.io/basics/)
>
>
> [▶ Next: CodeceptJS with Playwright](https://codecept.io/playwright/)
>
>
codeceptjs Mobile Testing with Appium Mobile Testing with Appium
===========================
CodeceptJS allows to test mobile and hybrid apps in a similar manner web applications are tested. Such tests are executed using [Appium (opens new window)](http://appium.io) on emulated or physical devices. Also, Appium allows to test web application on mobile devices.
What makes CodeceptJS better for mobile testing? Take a look. Here is the sample test for a native mobile application written in CodeceptJS:
```
I.seeAppIsInstalled("io.super.app");
I.click('~startUserRegistrationCD');
I.fillField('~email of the customer', 'Nothing special'));
I.see('[email protected]', '~email of the customer'));
I.clearField('~email of the customer'));
I.dontSee('Nothing special', '~email of the customer'));
I.seeElement({
android: /'android.widget.Button',
ios: '//UIAApplication[1]/UIAWindow[1]/UIAButton[1]'
});
```
This test is easy to read and write. Also it will work both on iOS and Android devices. Doesn't it sound cool?
Setting Up
-----------
Ensure that you have [CodeceptJS installed (opens new window)](https://codecept.io/installation/). You will also need to install [Appium (opens new window)](http://appium.io/). We suggest to use [appium-doctor (opens new window)](https://www.npmjs.com/package/appium-doctor) to check if your system is ready for mobile testing.
```
npm i -g appium-doctor
```
If everything is OK, continue with installing Appium. If not, consider using cloud based alternatives like [SauceLabs (opens new window)](https://saucelabs.com) or [BrowserStack (opens new window)](http://browserstack.com). Cloud services provide hosted appium with real and emulated mobile devices.
To install Appium use npm:
```
npm i -g appium
```
Then you need to prepare application for execution. It should be packed into apk (for Android) or .ipa (for iOS) or zip.
Next, is to launch the emulator or connect physical device. Once they are prepared, launch Appium:
```
appium
```
To run mobile test you need either an device emulator (available with Android SDK or iOS), real device connected for mobile testing. Alternatively, you may execute Appium with device emulator inside Docker container.
CodeceptJS should be installed with webdriverio support:
```
npm install codeceptjs webdriverio --save
```
Configuring
------------
Initialize CodeceptJS with `init` command:
```
npx codeceptjs init
```
Select [Appium helper (opens new window)](https://codecept.io/helpers/Appium/) when asked.
```
? What helpers do you want to use?
◯ WebDriver
◯ Protractor
◯ Puppeteer
◯ Nightmare
❯◉ Appium
◯ REST
```
You will also be asked for the platform and the application package.
```
? [Appium] Application package. Path to file or url
```
Check the newly created `codecept.conf.js` configuration file. You may want to set some additional Appium settings via [desiredCapabilities (opens new window)](https://appium.io/docs/en/writing-running-appium/caps/)
```
helpers: {
Appium: {
app: "my_app.apk",
platform: "Android",
desiredCapabilities: {}
}
}
```
Once you configured Appium, create the first test by running
```
npx codeceptjs gt
```
BrowserStack Configuration
---------------------------
If you wish to use BrowserStack's [Automated Mobile App Testing (opens new window)](https://www.browserstack.com/app-automate) platform. Configure the Appium helper like this:
```
helpers: {
Appium:
app: "bs://<hashed app-id>",
host: "hub-cloud.browserstack.com",
port: 4444,
user: "BROWSERSTACK_USER",
key: "BROWSERSTACK_KEY",
device: "iPhone 7"
}
```
Here is the full list of [capabilities (opens new window)](https://www.browserstack.com/app-automate/capabilities).
You need to upload your Android app (.apk) or iOS app (.ipa) to the BrowserStack servers using the REST API before running your tests. The App URL (`bs://hashed appid`) is returned in the response of this call.
```
curl -u "USERNAME:ACCESS_KEY" \
-X POST "https://api-cloud.browserstack.com/app-automate/upload" \
-F "file=@/path/to/app/file/Application-debug.apk"
```
Writing a Test
---------------
A test is written in a scenario-driven manner, listing an actions taken by a user. This is the sample test for a native mobile application:
```
Scenario('test registration', ({ I }) => {
I.click('~startUserRegistrationCD');
I.fillField('~inputUsername', 'davert');
I.fillField('~inputEmail', '[email protected]');
I.fillField('~inputPassword', '123456');
I.hideDeviceKeyboard();
I.click('~input_preferredProgrammingLanguage');
I.click('Javascript');
I.checkOption('#io.demo.testapp:id/input_adds');
I.click('Register User (verify)');
I.swipeUp("#io.selendroid.testapp:id/LinearLayout1");
I.see('Javascript'); // see on the screen
I.see('davert', '~label_username_data'); // see in element
});
```
Mobile test is pretty similar to a web test. And it is much the same, if you test hybrid app with a web view context inside. However, mobile apps do not have URLs, Cookies, they have other features which may vary on a running platform.
There are mobile-only methods like:
* `swipeUp`, `swipeLeft`, ...
* `hideDeviceKeyboard`,
* `seeAppIsInstalled`, `installApp`, `removeApp`, `seeAppIsNotInstalled` - Android only
and [others (opens new window)](https://codecept.io/helpers/Appium/).
Locating Elements
------------------
To start writing a test it is important to understand how to locate elements for native mobile applications. In both Android and iPhone elements are defined in XML format and can be searched by XPath locators.
```
I.seeElement('//android.widget.ScrollView/android.widget.LinearLayout')'
```
> Despite showing XPath in this guide we \*\*do not recommend using XPath for testing iOS native apps. XPath runs very slow on iOS. Consider using ID or Accessibility ID locators instead.
>
>
CSS locators are not supported in native mobile apps, you need to switch to web context to use them.
Elements can also be located by their accessability id, available both at Android and iOS. Accessibility id is recommended to use for locating element, as it rarely changed.
* iOS uses [UIAccessibilityIdentification (opens new window)](https://developer.apple.com/documentation/uikit/uiaccessibilityidentification)
* Android `accessibility id` matches the content-description
* Web view uses `[aria-label]` attribute as accessibility id
* For [React Native for Android see our special guide](https://codecept.io/mobile-react-native-locators).
> If you test React Native application, consider using [Detox helper](https://codecept.io/detox) for faster tests.
>
>
Add `~` prefix to search for element by its accessibility id:
```
I.seeElement('~startUserRegistrationButton');
```
Elements can also have ids, which can be located with `#` prefix. On Android it it is important to keep full package name in id locator:
```
I.seeElement('#io.selendroid.testapp:id/inputUsername');
```
Buttons can be matched by their visible text:
```
I.tap('Click me!');
I.click('Click me!');
```
Native iOS/Android locators can be used with `android=` and `ios=` prefixes. [Learn more (opens new window)](http://webdriver.io/guide/usage/selectors.html#Mobile-Selectors).
But how to get all those locators? We recommend to use [Appium Inspector (opens new window)](https://github.com/appium/appium-desktop).
For Android you can use **UI Automator Viewer** bundled with Android SDK:
Hybrid Apps and Contexts
-------------------------
Mobile applications may have different contexts. For instance, there can be native view and web view with a browser instance in it.
To execute commands in context of a webview use `within('webview')` function:
```
I.click('~startWebView');
within('webview', () => {
I.see('Preferred car');
I.click('Send me your name!');
});
```
It will locate first available webview, switch to it, and switch back to native application after. Inside WebView all browser features are enabled: CSS locators, JavaScript, etc.
To set a specific context use `{ web: 'webview.context' }` instead:
```
within({webview: 'MyWEBVIEW_com.my.app'}, () => );
```
Alternatively use `switchToWeb` or `switchToNative` methods to switch between contexts.
```
I.click('~startWebView');
I.switchToWeb();
I.see('Preferred car');
I.click('Send me your name!');
I.switchToNative();
```
To get a list of all contexts use `grabAllContexts` method:
```
let contexts = await I.grabAllContexts();
```
Cross-Platform Testing
-----------------------
It is often happen that mobile applications behave similarly on different platforms. Can we build one test for them? Yes! CodeceptJS provides a way to specify different locators for Android and iOS platforms:
```
I.click({android: /'//android.widget.Button', ios: '//UIAApplication[1]/UIAWindow[1]/UIAButton[1]'});
```
In case some code should be executed on one platform and ignored on others use `runOnAndroid` and `runOnIOS` methods:
```
I.runOnAndroid(() => {
I.click('Hello Android');
});
I.runOnIOS(() => {
I.click('Hello iOS');
});
```
The same code can be shared for web applications as well. To execute some code in web browser only, use `I.runInWeb`:
```
I.runInWeb(() => {
I.amOnPage('/login'); // not available for mobile
I.fillField('name', 'jon');
I.fillField('password', '123456');
I.click('Login');
I.waitForElement('#success'); // no available for mobile
});
```
Just as you can specify android, and ios-specific locators, you can do so for web:
```
I.click({web: '#login', ios: '//UIAApplication[1]/UIAWindow[1]/UIAButton[1]'});
```
codeceptjs Testing with WebDriver Testing with WebDriver
=======================
How does your client, manager, or tester, or any other non-technical person, know your web application is working? By opening the browser, accessing a site, clicking on links, filling in the forms, and actually seeing the content on a web page.
End to End tests can cover standard but complex scenarios from a user's perspective. With e2e tests you can be confident that users, following all defined scenarios, won't get errors. We check **functionality of application and a user interface** (UI) as well.
What is Selenium WebDriver
---------------------------
The standard and proved way to run browser test automation over years is Selenium WebDriver. Over years this technology was standartized and works over all popular browsers and operating systems. There are cloud services like SauceLabs or BrowserStack which allow executing such browsers in the cloud. The superset of WebDriver protocol is also used to test [native and hybrid mobile applications](https://codecept.io/mobile).
Let's clarify the terms:
* Selenium - is a toolset for browser test automation
* WebDriver - a standard protocol for communicating between test framework and browsers
* JSON Wire - an older version of such protocol
We use [webdriverio (opens new window)](https://webdriver.io) library to run tests over WebDriver.
> Popular tool [Protractor](https://codecept.io/angular) also uses WebDriver for running end 2 end tests.
>
>
To proceed you need to have [CodeceptJS installed](https://codecept.io/quickstart#using-selenium-webdriver) and `WebDriver` helper selected.
Selenium WebDriver may be complicated from start, as it requires following tools to be installed and started.
1. Selenium Server - to execute and send commands to browser
2. ChromeDriver or GeckoDriver - to allow browsers to run in automated mode.
> Those tools can be easily installed via NPM. Use [selenium-standalone (opens new window)](https://www.npmjs.com/package/selenium-standalone) to automatically install them.
>
>
You can also use `@wdio/selenium-standalone-service` package, to install and start Selenium Server for your tests automatically.
```
npm i @wdio/selenium-standalone-service --save-dev
```
Enable it in config inside plugins section:
```
exports.config = {
// ...
// inside condecept.conf.js
plugins: {
wdio: {
enabled: true,
services: ['selenium-standalone']
}
}
}
```
> ⚠ It is not recommended to use wdio plugin & selenium-standalone when running tests in parallel. Consider **switching to Selenoid** if you need parallel run or using cloud services.
>
>
Configuring WebDriver
----------------------
WebDriver can be configured to run browser tests in window, headlessly, on a remote server or in a cloud.
> By default CodeceptJS is already configured to run WebDriver tests locally with Chrome or Firefox. If you just need to start running tests - proceed to the next chapter.
>
>
Configuration for WebDriver should be provided inside `codecept.conf.js` file under `helpers: WebDriver` section:
```
helpers: {
WebDriver: {
url: 'https://myapp.com',
browser: 'chrome',
host: '127.0.0.1',
port: 4444,
restart: false,
windowSize: '1920x1680',
desiredCapabilities: {
chromeOptions: {
args: [ /*"--headless",*/ "--disable-gpu", "--no-sandbox" ]
}
}
},
}
```
By default CodeceptJS runs tests in the same browser window but clears cookies and local storage after each test. This behavior can be changed with these options:
```
// change to true to restart browser between tests
restart: false,
// don't change browser state and not clear cookies between tests
keepBrowserState: true,
keepCookies: true,
```
> ▶ More config options available on [WebDriver helper reference](https://codecept.io/helpers/WebDriver#configuration)
>
>
### ChromeDriver without Selenium
If you want to run tests using raw ChromeDriver (which also supports WebDriver protocol) avoiding Selenium Server, you should provide following configuration:
```
port: 9515,
browser: 'chrome',
path: '/',
```
> If you face issues connecting to WebDriver, please check that corresponding server is running on a specified port. If host is other than `localhost` or port is other than `4444`, update the configuration.
>
>
### Selenium in Docker (Selenoid)
Browsers can be executed in Docker containers. This is useful when testing on Continous Integration server. We recommend using [Selenoid (opens new window)](https://aerokube.com/selenoid/) to run browsers in container.
CodeceptJS has [Selenoid plugin](https://codecept.io/plugins#selenoid) which can automagically load browser container setup.
### Headless Mode
It is recommended to use `@codeceptjs/configure` package to easily toggle headless mode for WebDriver:
```
// inside codecept.conf.js
const { setHeadlessWhen, setWindowSize } = require('@codeceptjs/configure');
setHeadlessWhen(process.env.HEADLESS); // enables headless mode when HEADLESS environment variable exists
```
This requires `@codeceptjs/configure` package to be installed.
Alternatively, you can enable headless mode manually via desired capabilities.
### Desired Capabilities
Additional configuration can be passed via `desiredCapabilities` option. For instance, this is how we can set to **run headless Chrome**:
```
desiredCapabilities: {
chromeOptions: {
args: [ "--headless", "--disable-gpu", "--window-size=1200,1000", "--no-sandbox" ]
}
}
```
Next popular use case for capabilities is configuring what to do with unhandled alert popups.
```
desiredCapabilities: {
// close all unexpected popups
unexpectedAlertBehaviour: 'dismiss',
}
```
### Cloud Providers
WebDriver protocol works over HTTP, so you need to have a Selenium Server to be running or any other service that will launch a browser for you. That's why you may need to specify `host`, `port`, `protocol`, and `path` parameters.
By default, those parameters are set to connect to local Selenium Server but they should be changed if you want to run tests via [Cloud Providers](https://codecept.io/helpers/WebDriver#cloud-providers). You may also need `user` and `key` parameters to authenticate on cloud service.
There are also [browser and platform specific capabilities (opens new window)](https://github.com/SeleniumHQ/selenium/wiki/DesiredCapabilities). Services like SauceLabs, BrowserStack or browser vendors can provide their own specific capabilities for more tuning.
Here is a sample BrowserStack config for running tests on iOS mobile browser:
```
helpers: {
WebDriver: {
host: 'hub.browserstack.com',
path: '/wd/hub',
url: 'http://WEBSITE:8080/renderer',
user: 'xx', // credentials
key: 'xx', // credentials
browser: 'iphone',
desiredCapabilities: {
'os_version' : '11',
'device' : 'iPhone 8', // you can select device
'real_mobile' : 'true', // real or emulated
'browserstack.local' : 'true',
'browserstack.debug' : 'true',
'browserstack.networkLogs' : 'true',
'browserstack.appium_version' : '1.9.1',
'browserstack.user' : 'xx', // credentials
'browserstack.key' : 'xx' // credentials
}
}
```
Writing Tests
--------------
CodeceptJS provides high-level API on top of WebDriver protocol. While most standard implementations focus on dealing with WebElements on page, CodeceptJS is about user scenarios and interactions. That's why you don't have a direct access to web elements inside a test, but it is proved that in majority of cases you don't need it. Tests written from user's perspective are simpler to write, understand, log and debug.
> If you come from Java, Python or Ruby don't be afraid of a new syntax. It is more flexible than you think!
>
>
A typical test case may look like this:
```
Feature('login');
Scenario('login test', ({ I }) => {
I.amOnPage('/login');
I.fillField('Username', 'john');
I.fillField('Password', '123456');
I.click('Login');
I.see('Welcome, John');
});
```
> ▶ Actions like `amOnPage`, `click`, `fillField` are not limited to WebDriver only. They work similarly for all available helpers. [Go to Basics guide to learn them](https://codecept.io/basics#writing-tests).
>
>
An empty test case can be created with `npx codeceptjs gt` command.
```
npx codeceptjs gt
```
It's easy to start writing a test if you use [interactive pause](https://codecept.io/basics#debug). Just open a web page and pause execution.
```
Feature('Sample Test');
Scenario('open my website', ({ I }) => {
I.amOnPage('/');
pause();
});
```
This is just enough to run a test, open a browser, and think what to do next to write a test case.
When you execute such test with `npx codeceptjs run` command you may see the browser is started
```
npx codeceptjs run --steps
```
After a page is opened a full control of a browser is given to a terminal. Type in different commands such as `click`, `see`, `fillField` to write the test. A successful commands will be saved to `./output/cli-history` file and can be copied into a test.
> ℹ All actions are listed in [WebDriver helper reference](https://codecept.io/helpers/WebDriver).
>
>
An interactive shell output may look like this:
```
Interactive shell started
Use JavaScript syntax to try steps in action
- Press ENTER to run the next step
- Press TAB twice to see all available commands
- Type exit + Enter to exit the interactive shell
I.fillField('.new-todo', 'Write a test')
I.pressKey('Enter')
I.
Commands have been saved to /home/davert/demos/codeceptjs/output/cli-history
```
After typing in successful commands you can copy them into a test.
Here is a test checking basic [todo application (opens new window)](http://todomvc.com/).
```
Feature('TodoMVC');
Scenario('create todo item', ({ I }) => {
I.amOnPage('/examples/vue/');
I.waitForElement('.new-todo');
I.fillField('.new-todo', 'Write a test')
I.pressKey('Enter');
I.see('1 item left', '.todo-count');
});
```
> [▶ Working example of CodeceptJS WebDriver tests (opens new window)](https://github.com/DavertMik/codeceptjs-webdriver-example) for TodoMVC application.
>
>
WebDriver helper supports standard [CSS/XPath and text locators](https://codecept.io/locators) as well as non-trivial [React locators](https://codecept.io/react) and [Shadow DOM](https://codecept.io/shadow).
Waiting
--------
Web applications do not always respond instantly. That's why WebDriver protocol has methods to wait for changes on a page. CodeceptJS provides such commands prefixed with `wait*` so you could explicitly define what effects we wait for.
Most popular "waiters" are:
* `waitForText` - wait for text to appear on a page
* `waitForElement` - wait for element to appear on a page
* `waitForInvisible` - wait element to become invisible.
By default, they will wait for 1 second. This number can be changed in WebDriver configuration:
```
// inside codecept.conf.js
exports.config = {
helpers: {
WebDriver: {
// WebDriver config goes here
// wait for 5 seconds
waitForTimeout: 5000
}
}
}
```
SmartWait
----------
It is possible to wait for elements pragmatically. If a test uses element which is not on a page yet, CodeceptJS will wait for few extra seconds before failing. This feature is based on [Implicit Wait (opens new window)](http://www.seleniumhq.org/docs/04_webdriver_advanced.jsp#implicit-waits) of Selenium. CodeceptJS enables implicit wait only when searching for a specific element and disables in all other cases. Thus, the performance of a test is not affected.
SmartWait can be enabled by setting wait option in WebDriver config. Add `smartWait: 5000` to wait for additional 5s.
```
// inside codecept.conf.js
exports.config = {
helpers: {
WebDriver: {
// WebDriver config goes here
// smart wait for 5 seconds
smartWait: 5000
}
}
}
```
SmartWait works with a CSS/XPath locators in `click`, `seeElement` and other methods. See where it is enabled and where is not:
```
I.click('Login'); // DISABLED, not a locator
I.fillField('user', 'davert'); // DISABLED, not a specific locator
I.fillField({name: 'password'}, '123456'); // ENABLED, strict locator
I.click('#login'); // ENABLED, locator is CSS ID
I.see('Hello, Davert'); // DISABLED, Not a locator
I.seeElement('#userbar'); // ENABLED
I.dontSeeElement('#login'); // DISABLED, can't wait for element to hide
I.seeNumberOfElements('button.link', 5); // DISABLED, can wait only for one element
```
SmartWait doesn't check element for visibility, so tests may fail even element is on a page.
Usage example:
```
// we use smartWait: 5000 instead of
// I.waitForElement('#click-me', 5);
// to wait for element on page
I.click('#click-me');
```
If it's hard to define what to wait, it is recommended to use [retries](https://codecept.io/basics/#retries) to rerun flaky steps.
Configuring CI
---------------
To develop tests it's fine to use local Selenium Server and window mode. Setting up WebDriver on remote CI (Continous Integration) server is different. If there is no desktop and no window mode on CI.
There are following options available:
* Use headless Chrome or Firefox.
* Use [Selenoid](https://codecept.io/plugins/selenoid) to run browsers inside Docker containers.
* Use paid [cloud services (SauceLabs, BrowserStack, TestingBot)](https://codecept.io/helpers/WebDriver#cloud-providers).
Video Recording
----------------
When [Selenoid Plugin](https://codecept.io/plugins#selenoid) is enabled video can be automatically recorded for each test.
Auto Login
-----------
To share the same user session across different tests CodeceptJS provides [autoLogin plugin](https://codecept.io/plugins#autologin). It simplifies login management and reduces time consuming login operations. Instead of filling in login form before each test it saves the cookies of a valid user session and reuses it for next tests. If a session expires or doesn't exist, logs in a user again.
This plugin requires some configuration but is very simple in use:
```
Scenario('do something with logged in user', ({ I, login) }) => {
login('user');
I.see('Dashboard','h1');
});
```
With `autoLogin` plugin you can save cookies into a file and reuse same session on different runs.
> [▶ How to set up autoLogin plugin](https://codecept.io/plugins#autologin)
>
>
Multiple Windows
-----------------
CodeceptJS allows to use several browser windows inside a test. Sometimes we are testing the functionality of websites that we cannot control, such as a closed-source managed package, and there are popups that either remain open for configuring data on the screen, or close as a result of clicking a window. We can use these functions in order to gain more control over which page is being tested with Codecept at any given time. For example:
```
const assert = require('assert');
Scenario('should open main page of configured site, open a popup, switch to main page, then switch to popup, close popup, and go back to main page', async ({ I }) => {
I.amOnPage('/');
const handleBeforePopup = await I.grabCurrentWindowHandle();
const urlBeforePopup = await I.grabCurrentUrl();
const allHandlesBeforePopup = await I.grabAllWindowHandles();
assert.equal(allHandlesBeforePopup.length, 1, 'Single Window');
await I.executeScript(() => {
window.open('https://www.w3schools.com/', 'new window', 'toolbar=yes,scrollbars=yes,resizable=yes,width=400,height=400');
});
const allHandlesAfterPopup = await I.grabAllWindowHandles();
assert.equal(allHandlesAfterPopup.length, 2, 'Two Windows');
await I.switchToWindow(allHandlesAfterPopup[1]);
const urlAfterPopup = await I.grabCurrentUrl();
assert.equal(urlAfterPopup, 'https://www.w3schools.com/', 'Expected URL: Popup');
assert.equal(handleBeforePopup, allHandlesAfterPopup[0], 'Expected Window: Main Window');
await I.switchToWindow(handleBeforePopup);
const currentURL = await I.grabCurrentUrl();
assert.equal(currentURL, urlBeforePopup, 'Expected URL: Main URL');
await I.switchToWindow(allHandlesAfterPopup[1]);
const urlAfterSwitchBack = await I.grabCurrentUrl();
assert.equal(urlAfterSwitchBack, 'https://www.w3schools.com/', 'Expected URL: Popup');
await I.closeCurrentTab();
const allHandlesAfterPopupClosed = await I.grabAllWindowHandles();
assert.equal(allHandlesAfterPopupClosed.length, 1, 'Single Window');
const currentWindowHandle = await I.grabCurrentWindowHandle();
assert.equal(currentWindowHandle, allHandlesAfterPopup[0], 'Expected Window: Main Window');
});
```
Mocking Requests
-----------------
When testing web application you can disable some of external requests calls by enabling HTTP mocking. This is useful when you want to isolate application testing from a backend. For instance, if you don't want to save data to database, and you know the request which performs save, you can mock the request, so application will treat this as valid response, but no data will be actually saved.
> **WebDriver has limited ability to mock requests**, so you can only mock only requests performed after page is loaded. This means that you can't block Google Analytics, or CDN calls, but you can mock API requests performed on user action.
>
>
To mock requests enable additional helper [MockRequest](https://codecept.io/helpers/MockRequest) (which is based on Polly.js).
```
helpers: {
WebDriver: {
// regular WebDriver config here
},
MockRequest: {}
}
```
The function `mockRequest` will be added to `I` object. You can use it to explicitly define which requests to block and which response they should return instead:
```
// block all Google Analytics calls
I.mockRequest('/google-analytics/*path', 200);
// return an empty successful response
I.mockRequest('GET', '/api/users', 200);
// block post requests to /api/users and return predefined object
I.mockRequest('POST', '/api/users', { user: 'davert' });
// return error request with body
I.mockRequest('GET', '/api/users/1', 404, { error: 'User not found' });
```
> In WebDriver mocking is disabled every time a new page is loaded. Hence, `startMocking` method should be called and the mocks should be updated, after navigating to a new page. This is a limitation of WebDriver. Consider using Puppeteer with MockRequest instead.
>
>
```
I.amOnPage('/xyz');
I.mockRequest({ ... })
I.click('Go to Next Page');
// new page is loaded, mocking is disabled now. We need to set it up again
// in WebDriver as we can't detect that the page was reloaded, so no mocking :(
```
> See [`mockRequest` API](https://codecept.io/helpers/MockRequest#mockrequest)
>
>
To see `mockRequest` method in intellisense auto completion don't forget to run `codeceptjs def` command:
```
npx codeceptjs def
```
Mocking rules will be kept while a test is running. To stop mocking use `I.stopMocking()` command
Accessing webdriverio API
--------------------------
To get [webdriverio browser API (opens new window)](https://webdriver.io/docs/api) inside a test use [`I.useWebDriverTo`](https://codecept.io/helpers/WebDriver/#usewebdriverto) method with a callback. To keep test readable provide a description of a callback inside the first parameter.
```
I.useWebDriverTo('do something with native webdriverio api', async ({ browser }) => {
// use browser object here
});
```
> webdriverio commands are asynchronous so a callback function must be async.
>
>
WebDriver helper can be obtained in this function as well. Use this to get full access to webdriverio elements inside the test.
```
I.useWebDriverTo('click all Save buttons', async (WebDriver) => {
const els = await WebDriver._locateClickable('Save');
for (let el of els) {
await el.click();
}
});
```
Extending WebDriver
--------------------
CodeceptJS doesn't aim to embrace all possible functionality of WebDriver. At some points you may find that some actions do not exist, however it is easy to add one. You will need to use WebDriver API from [webdriver.io (opens new window)](https://webdriver.io) library.
To create new actions which will be added into `I.` object you need to create a new helper. This can be done with `codeceptjs gh` command.
```
npx codeceptjs gh
```
Name a new helper "Web". Now each method of a created class can be added to I object. Be sure to enable this helper in config:
```
exports.config = {
helpers: {
WebDriver: { /* WebDriver config goes here */ },
WebHelper: {
// load custom helper
require: './web_helper.js'
}
}
}
```
> ℹ See [Custom Helper](https://codecept.io/helpers) guide to see more examples.
>
>
While implementing custom actions using WebDriver API please note that, there is two versions of protocol: WebDriver and JSON Wire. Depending on a browser version one of those protocols can be used. We can't know for sure which protocol is going to used, so we will need to implement an action using both APIs.
```
const Helper = codeceptjs.helper;
class Web extends Helper {
// method to drag an item to coordinates
async dragToPoint(el, x, y) {
// access browser object from WebDriver
const browser = this.helpers.WebDriver.browser;
await this.helpers.WebDriver.moveCursorTo(el);
if (browser.isW3C) {
// we use WebDriver protocol
return browser.performActions([
{"type": "pointerDown", "button": 0},
{"type": "pointerMove", "origin": "pointer", "duration": 1000, x, y },
{"type": "pointerUp", "button": 0}
]);
}
// we use JSON Wire protocol
await browser.buttonDown(0);
await browser.moveToElement(null, x, y);
await browser.buttonUp(0);
}
// method which restarts browser
async restartBrowser() {
const browser = this.helpers.WebDriver.browser;
await browser.reloadSession();
await browser.maximizeWindow();
}
// method which goes to previous page
async backToPreviousPage() {
const browser = this.helpers.WebDriver.browser;
await browser.back();
}
}
```
When a helper is created, regenerate your step definitions, so you could see those actions when using [intellisense](https://codecept.io/basics#intellisense):
```
npx codeceptjs def
```
| programming_docs |
codeceptjs Community Helpers Community Helpers
==================
> Share your helpers at our [Wiki Page (opens new window)](https://github.com/codeceptjs/CodeceptJS/wiki/Community-Helpers)
>
>
Here is the list of helpers created by our community. Please **add your own** by editing this page.
Email Checking
---------------
* [MailCatcher (opens new window)](https://gist.github.com/schmkr/026732dfa1627b927ff3a08dc31ee884) - to check emails via Mailcatcher locally.
* [codeceptjs-mailhog-helper (opens new window)](https://github.com/tsuemura/codeceptjs-mailhog-helper) - to check emails via Mailhog locally.
Data Sources
-------------
* [codeceptjs-httpmock (opens new window)](https://github.com/testphony/codeceptjs-httpMock) - a helper which wraps mockttp library to manage http mock in tests.
* [codeceptjs-http (opens new window)](https://github.com/testphony/codeceptjs-http) - a helper which wraps then-request library to process HTTP requests. It's alternative helper that provides more flexible request management.
* [codeceptjs-dbhelper (opens new window)](https://github.com/thiagodp/codeceptjs-dbhelper) - allows you to execute queries or commands to databases using database-js.
Cloud Providers
----------------
* [codeceptjs-saucehelper (opens new window)](https://github.com/puneet0191/codeceptjs-saucehelper/) - a helper which updates `Test Names` & `Test Results` on Saucelabs
* [codeceptjs-bshelper (opens new window)](https://github.com/PeterNgTr/codeceptjs-bshelper) - a helper which updates `Test Names` & `Test Results` on Browserstack
* [codeceptjs-tbhelper (opens new window)](https://github.com/testingbot/codeceptjs-tbhelper) - a helper which updates `Test Names` & `Test Results` on TestingBot
Integrations
-------------
* [codeceptjs-testrail (opens new window)](https://github.com/PeterNgTr/codeceptjs-testrail) - a plugin to integrate with [Testrail (opens new window)](https://www.gurock.com/testrail)
Visual-Testing
---------------
* [codeceptjs-resemblehelper (opens new window)](https://github.com/puneet0191/codeceptjs-resemblehelper) - a helper which helps with visual testing using resemble.js.
* [codeceptjs-applitoolshelper (opens new window)](https://www.npmjs.com/package/codeceptjs-applitoolshelper) - a helper which helps interaction with [Applitools (opens new window)](https://applitools.com)
Reporters
----------
* [codeceptjs-rphelper (opens new window)](https://github.com/reportportal/agent-js-codecept) is a CodeceptJS helper which can publish tests results on ReportPortal after execution.
* [codeceptjs-xray-helper (opens new window)](https://www.npmjs.com/package/codeceptjs-xray-helper) is a CodeceptJS helper which can publish tests results on [XRAY (opens new window)](https://confluence.xpand-it.com/display/XRAYCLOUD/Import+Execution+Results+-+REST).
* [codeceptjs-slack-reporter (opens new window)](https://www.npmjs.com/package/codeceptjs-slack-reporter) Get a Slack notification when one or more scenarios fail.
Page Object Code Generator
---------------------------
* [codeceptjs-CodeGenerator (opens new window)](https://github.com/senthillkumar/CodeCeptJS-PageObject) is a CodeceptJS custom wrapper which can create page class with action methods from the page object file(JSON) and project setup(Folder Structure).
Browser request control
------------------------
* [codeceptjs-resources-check (opens new window)](https://github.com/luarmr/codeceptjs-resources-check) Load a URL with Puppeteer and listen to the requests while the page is loading. Enabling count the number or check the sizes of the requests.
Assertion & Validations
------------------------
* [codeceptjs-chai (opens new window)](https://www.npmjs.com/package/codeceptjs-chai) is a CodeceptJS helper which wraps [chai (opens new window)](https://www.chaijs.com/) library to complete chai assertion steps with CodeceptJS logging.
Other
------
* [codeceptjs-cmdhelper (opens new window)](https://github.com/thiagodp/codeceptjs-cmdhelper) allows you to run commands in the terminal/console
* [eslint-plugin-codeceptjs (opens new window)](https://www.npmjs.com/package/eslint-plugin-codeceptjs) Eslint rules for CodeceptJS.
codeceptjs Commands Commands
=========
Run
----
Executes tests. Requires `codecept.conf.js` config to be present in provided path.
Run all tests from current dir
```
npx codeceptjs run
```
Load config and run tests from `test` dir
```
npx codeceptjs run -c test
```
Run only tests with "signin" word in name
```
npx codeceptjs run --grep "signin"
```
Run all tests without "@IEOnly" word in name
```
npx codeceptjs run --grep "@IEOnly" --invert
```
Run single test [path to codecept.js] [test filename]
```
npx codeceptjs run github_test.js
```
Run single test with steps printed
```
npx codeceptjs run github_test.js --steps
```
Run single test in debug mode
```
npx codeceptjs run github_test.js --debug
```
Run test with internal logs printed (global promises, and events).
```
npx codeceptjs run github_test.js --verbose
```
Select config file manually (`-c` or `--config` option)
```
npx codeceptjs run -c my.codecept.conf.js
npx codeceptjs run --config path/to/codecept.json
```
Override config on the fly. Provide valid JSON which will be merged into current config:
```
npx codeceptjs run --override '{ "helpers": {"WebDriver": {"browser": "chrome"}}}'
```
Run tests and produce xunit report:
```
npx codeceptjs run --reporter xunit
```
Use any of [Mocha reporters (opens new window)](https://github.com/mochajs/mocha/tree/master/lib/reporters) used.
Run Workers
------------
Run tests in parallel threads.
```
npx codeceptjs run-workers 3
```
Run Rerun Since 2.4
--------------------
Run tests multiple times to detect and fix flaky tests.
```
npx codeceptjs run-rerun
```
For this command configuration is required:
```
{
// inside codecept.conf.js
rerun: {
// how many times all tests should pass
minSuccess: 2,
// how many times to try to rerun all tests
maxReruns: 4,
}
}
```
Use Cases:
* `minSuccess: 1, maxReruns: 5` - run all tests no more than 5 times, until first successful run.
* `minSuccess: 3, maxReruns: 5` - run all tests no more than 5 times, until reaching 3 successfull runs.
* `minSuccess: 10, maxReruns: 10` - run all tests exactly 10 times, to check their stability.
Dry Run
--------
Prints test scenarios without executing them
```
npx codeceptjs dry-run
```
When passed `--steps` or `--debug` option runs tests, disabling all plugins and helpers, so you can get step-by-step report with no tests actually executed.
```
npx codeceptjs dry-run --steps
```
If a plugin needs to be enabled in `dry-run` mode, pass its name in `-p` option:
```
npx codeceptjs dry-run --steps -p allure
```
To enable bootstrap script in dry-run mode, pass in `--bootstrap` option when running with `--steps` or `--debug`
```
npx codeceptjs dry-run --steps --bootstrap
```
Run Multiple
-------------
Run multiple suites. Unlike `run-workers` spawns processes to execute tests. [Requires additional configuration](https://codecept.io/advanced#multiple-browsers-execution) and can be used to execute tests in multiple browsers.
```
npx codeceptjs run-multiple smoke:chrome regression:firefox
```
Init
-----
Creates `codecept.conf.js` file in current directory:
```
npx codeceptjs init
```
Or in provided path
```
npx codecept init test
```
Migrate
--------
Migrate your current `codecept.json` to `codecept.conf.js`
```
npx codeceptjs migrate
```
Shell
------
Interactive shell. Allows to try `I.` commands in runtime
```
npx codeceptjs shell
```
Generators
-----------
Create new test
```
npx codeceptjs generate:test
```
Create new pageobject
```
npx codeceptjs generate:pageobject
```
Create new helper
```
npx codeceptjs generate:helper
```
TypeScript Definitions
-----------------------
TypeScript Definitions allows IDEs to provide autocompletion when writing tests.
```
npx codeceptjs def
npx codeceptjs def --config path/to/codecept.json
```
After doing that IDE should provide autocompletion for `I` object inside `Scenario` and `within` blocks.
Add optional parameter `output` (or shortcut `-o`), if you want to place your definition file in specific folder:
```
npx codeceptjs def --output ./tests/typings
npx codeceptjs def -o ./tests/typings
```
List Commands
--------------
Prints all available methods of `I` to console
```
npx codeceptjs list
```
Local Environment Information
------------------------------
Prints debugging information concerning the local environment
```
npx codeceptjs info
```
codeceptjs Behavior Driven Development Behavior Driven Development
============================
Behavior Driven Development (BDD) is a popular software development methodology. BDD is considered an extension of TDD, and is greatly inspired by [Agile (opens new window)](http://agilemanifesto.org/) practices. The primary reason to choose BDD as your development process is to break down communication barriers between business and technical teams. BDD encourages the use of automated testing to verify all documented features of a project from the very beginning. This is why it is common to talk about BDD in the context of test frameworks (like CodeceptJS). The BDD approach, however, is about much more than testing - it is a common language for all team members to use during the development process.
What is Behavior Driven Development
------------------------------------
BDD was introduced by [Dan North (opens new window)](https://dannorth.net/introducing-bdd/). He described it as:
> outside-in, pull-based, multiple-stakeholder, multiple-scale, high-automation, agile methodology. It describes a cycle of interactions with well-defined outputs, resulting in the delivery of working, tested software that matters.
>
>
BDD has its own evolution from the days it was born, started by replacing "test" to "should" in unit tests, and moving towards powerful tools like Cucumber and Behat, which made user stories (human readable text) to be executed as an acceptance test.
The idea of story BDD can be narrowed to:
* describe features in a scenario with a formal text
* use examples to make abstract things concrete
* implement each step of a scenario for testing
* write actual code implementing the feature
By writing every feature in User Story format that is automatically executable as a test we ensure that: business, developers, QAs and managers are in the same boat.
BDD encourages exploration and debate in order to formalize the requirements and the features that needs to be implemented by requesting to write the User Stories in a way that everyone can understand.
By making tests to be a part of User Story, BDD allows non-technical personnel to write (or edit) Acceptance tests.
With this procedure we also ensure that everyone in a team knows what has been developed, what has not, what has been tested and what has not.
### Ubiquitous Language
The ubiquitous language is always referred as *common* language. That is it's main benefit. It is not a couple of our business specification's words, and not a couple of developer's technical terms. It is a common words and terms that can be understood by people for whom we are building the software and should be understood by developers. Establishing correct communication between this two groups people is vital for building successful project that will fit the domain and fulfill all business needs.
Each feature of a product should be born from a talk between
* business (analysts, product owner)
* developers
* QAs
which are known in BDD as "three amigos".
Such talks should produce written stories. There should be an actor that doing some things, the feature that should be fulfilled within the story and the result achieved.
We can try to write such simple story:
```
As a customer I want to buy several products
I put first product with $600 price to my cart
And then another one with $1000 price
When I go to checkout process
I should see that total number of products I want to buy is 2
And my order amount is $1600
```
As we can see this simple story highlights core concepts that are called *contracts*. We should fulfill those contracts to model software correctly. But how we can verify that those contracts are being satisfied? [Cucumber (opens new window)](https://cucumber.io) introduced a special language for such stories called **Gherkin**. Same story transformed to Gherkin will look like this:
```
Feature: checkout process
In order to buy products
As a customer
I want to be able to buy several products
Scenario:
Given I have product with $600 price in my cart
And I have product with $1000 price
When I go to checkout process
Then I should see that total number of products is 2
And my order amount is $1600
```
**CodeceptJS can execute this scenario step by step as an automated test**. Every step in this scenario requires a code which defines it.
Gherkin
--------
Let's learn some more about Gherkin format and then we will see how to execute it with CodeceptJS. We can enable Gherkin for current project by running `gherkin:init` command on **already initialized project**:
```
npx codeceptjs gherkin:init
```
It will add `gherkin` section to the current config. It will also prepare directories for features and step definition. And it will create the first feature file for you.
### Features
Whenever you start writing a story you are describing a specific feature of an application, with a set of scenarios and examples describing this feature. Let's open a feature file created by `gherkin:init` command, which is `feature/basic.feature`.
```
Feature: Business rules
In order to achieve my goals
As a persona
I want to be able to interact with a system
Scenario: do something
Given I have a defined step
```
This text should be rewritten to follow your buisness rules. Don't think about a web interface for a while. Think about how user interacts with your system and what goals they want to achieve. Then write interaction scenarios.
#### Scenarios
Scenarios are live examples of feature usage. Inside a feature file it should be written inside a *Feature* block. Each scenario should contain its title:
```
Feature: checkout
In order to buy product
As a customer
I need to be able to checkout the selected products
Scenario: order several products
```
Scenarios are written in step-by-step manner using Given-When-Then approach. At start, scenario should describe its context with **Given** keyword:
```
Given I have product with $600 price in my cart
And I have product with $1000 price in my cart
```
Here we also use word **And** to extend the Given and not to repeat it in each line.
This is how we described the initial conditions. Next, we perform some action. We use **When** keyword for it:
```
When I go to checkout process
```
And in the end we are verifying our expectation using **Then** keyword. The action changed the initial given state, and produced some results. Let's check that those results are what we actually expect.
```
Then I should see that total number of products is 2
And my order amount is $1600
```
This scenarios are nice as live documentation but they do not test anything yet. What we need next is to define how to run those steps. Steps can be defined by executing `gherkin:snippets` command:
```
npx codeceptjs gherkin:snippets [--path=PATH] [--feature=PATH]
```
This will produce code templates for all undefined steps in the .feature files. By default, it will scan all of the .feature files specified in the gherkin.features section of the config and produce code templates for all undefined steps. If the `--feature` option is specified, it will scan the specified .feature file(s). The stub definitions by default will be placed into the first file specified in the gherkin.steps section of the config. However, you may also use `--path` to specify a specific file in which to place all undefined steps. This file must exist and be in the gherkin.steps array of the config. Our next step will be to define those steps and transforming feature-file into a valid test.
### Step Definitions
Step definitions are placed in JavaScript file with Given/When/Then functions that map strings from feature file to functions:
```
// use I and productPage via inject() function
const { I, productPage } = inject();
// you can provide RegEx to match corresponding steps
Given(/I have product with \$(\d+) price/, (price) => {
I.amOnPage('/products');
productPage.create({ price });
I.click('Add to cart');
});
// or a simple string
When('I go to checkout process', () => {
I.click('Checkout');
});
// parameters are passed in via Cucumber expressions
Then('I should see that total number of products is {int}', (num) => {
I.see(num, '.cart');
});
Then('my order amount is ${int}', (sum) => { // eslint-disable-line
I.see('Total: ' + sum);
});
```
Steps can be either strings or regular expressions. Parameters from string are passed as function arguments. To define parameters in a string we use [Cucumber expressions (opens new window)](https://docs.cucumber.io/cucumber/cucumber-expressions/)
To list all defined steps run `gherkin:steps` command:
```
npx codeceptjs gherkin:steps
```
Use `grep` to find steps in a list (grep works on Linux & MacOS):
```
npx codeceptjs gherkin:steps | grep user
```
To run tests and see step-by step output use `--steps` optoin:
```
npx codeceptjs run --steps
```
To see not only business steps but an actual performed steps use `--debug` flag:
```
npx codeceptjs run --debug
```
Advanced Gherkin
-----------------
Let's improve our BDD suite by using the advanced features of Gherkin language.
### Background
If a group of scenarios have the same initial steps, let's that for dashboard we need always need to be logged in as administrator. We can use *Background* section to do the required preparations and not to repeat same steps across scenarios.
```
Feature: Dashboard
In order to view current state of business
As an owner
I need to be able to see reports on dashboard
Background:
Given I am logged in as administrator
And I open dashboard page
```
Steps in background are defined the same way as in scenarios.
### Tables
Scenarios can become more descriptive when you represent repeating data as tables. Instead of writing several steps "I have product with :num1 $ price in my cart" we can have one step with multiple values in it.
```
Given I have products in my cart
| name | category | price |
| Harry Potter | Books | 5 |
| iPhone 5 | Smartphones | 1200 |
| Nuclear Bomb | Weapons | 100000 |
```
Tables are the recommended way to pass arrays into test scenarios. Inside a step definition data is stored in argument passed as `DataTable` JavaScript object. You can iterate on it like this:
```
Given('I have products in my cart', (table) => { // eslint-disable-line
for (const id in table.rows) {
if (id < 1) {
continue; // skip a header of a table
}
// go by row cells
const cells = table.rows[id].cells;
// take values
const name = cells[0].value;
const category = cells[1].value;
const price = cells[2].value;
// ...
}
});
```
You can also use the `parse()` method to obtain an object that allow you to get a simple version of the table parsed by column or row, with header (or not):
* `raw()` - returns the table as a 2-D array
* `rows()` - returns the table as a 2-D array, without the first row
* `hashes()` - returns an array of objects where each row is converted to an object (column header is the key)
If we use hashes() with the previous exemple :
```
Given('I have products in my cart', (table) => { // eslint-disable-line
//parse the table by header
const tableByHeader = table.parse().hashes();
for (const row of tableByHeader) {
// take values
const name = row.name;
const category = row.category;
const price = row.price;
// ...
}
});
```
### Examples
In case scenarios represent the same logic but differ on data, we can use *Scenario Outline* to provide different examples for the same behavior. Scenario outline is just like a basic scenario with some values replaced with placeholders, which are filled from a table. Each set of values is executed as a different test.
```
Scenario Outline: order discount
Given I have product with price <price>$ in my cart
And discount for orders greater than $20 is 10 %
When I go to checkout
Then I should see overall price is "<total>" $
Examples:
| price | total |
| 10 | 10 |
| 20 | 20 |
| 21 | 18.9 |
| 30 | 27 |
| 50 | 45 |
```
### Long Strings
Text values inside a scenarios can be set inside a `"""` block:
```
Then i see in file "codecept.json"
"""
{
"output": "./output",
"helpers": {
"Puppeteer": {
"url": "http://localhost",
"restart": true,
"windowSize": "1600x1200"
}
"""
```
This string can be accessed inside a `content` property of a last argument:
```
Then('Then i see in file {string}', (file, text) => {
// file is a value of {string} from a title
const fileContent = fs.readFileSync(file).toString();
fileContent.should.include(text.content); // text.content is a value
});
```
### Tags
Gherkin scenarios and features can contain tags marked with `@`. Tags are appended to feature titles so you can easily filter by them when running tests:
```
npx codeceptjs run --grep "@important"
```
Tag should be placed before *Scenario:* or before *Feature:* keyword. In the last case all scenarios of that feature will be added to corresponding group.
Configuration
--------------
* `gherkin`
+ `features` - path to feature files
+ `steps` - array of files with step definitions
```
"gherkin": {
"features": "./features/*.feature",
"steps": [
"./step_definitions/steps.js"
]
}
```
Before
-------
You can set up some before hooks inside step definition files. Use `Before` function to do that. This function receives current test as a parameter, so you can apply additional configuration to it.
```
// inside step_definitions
Before((test) => {
// perform your code
test.retries(3); // retry test 3 times
});
```
This can be used to keep state between steps:
```
let state = {};
// inside step_definitions
Before(() => {
state = {};
});
Given('have a user', async () => {
state.user = await I.have('user');
});
When('I open account page', () => {
I.amOnPage(`/user/${state.user.slug}`);
})
```
After
------
Similarly to `Before` you can use `After` and `Fail` inside a scenario. `Fail` hook is activated on failure and receive two parameters: `test` and current `error`.
```
After(async () => {
await someService.cleanup();
});
Fail((test, err) => {
// test didn't
console.log('Failed with', err);
pause();
});
```
Tests vs Features
------------------
It is common to think that BDD scenario is equal to test. But it's actually not. Not every test should be described as a feature. Not every test is written to test real business value. For instance, regression tests or negative scenario tests are not bringing any value to business. Business analysts don't care about scenario reproducing bug #13, or what error message is displayed when user tries to enter wrong password on login screen. Writing all the tests inside a feature files creates informational overflow.
In CodeceptJS you can combine tests written in Gherkin format with classical acceptance tests. This way you can keep your feature files compact with minimal set of scenarios, and write regular tests to cover all cases. Please note, feature files will be executed before tests.
To run only features use `--features` option:
```
npx codeceptjs run --features
```
You can run a specific feature file by its filename or by grepping by name or tag.
To run only tests without features use `--tests` option:
```
npx codeceptjs run --tests
```
| programming_docs |
codeceptjs Data Management Data Management
================
> This chapter describes data management for external sources. If you are looking for using Data Sets in tests, see [Data Driven Tests (opens new window)](https://codecept.io/advanced/#data-drivern-tests) section\*
>
>
Managing data for tests is always a tricky issue. How isolate data between tests, how to prepare data for different tests, etc. There are different approaches to solve it:
1. reset database completely between tests
2. create unique non-intersecting data sets per each test
3. create and delete data for a test
The most efficient way would be to allow test to control its data, i.e. the 3rd option. However, accessing database directly is not a good idea as database vendor, schema and data are used by application internally and are out of scope of acceptance test.
Today all modern web applications have REST or GraphQL API . So it is a good idea to use it to create data for a test and delete it after. API is supposed to be a stable interface and it can be used by acceptance tests. CodeceptJS provides 4 helpers for Data Management via REST and GraphQL API.
REST
-----
[REST helper (opens new window)](https://codecept.io/helpers/REST/) allows sending raw HTTP requests to application. This is a tool to make shortcuts and create your data pragmatically via API. However, it doesn't provide tools for testing APIs, so it should be paired with WebDriver, Nightmare or Protractor helpers for browser testing.
Enable REST helper in the config. It is recommended to set `endpoint`, a base URL for all API requests. If you need some authorization you can optionally set default headers too.
See the sample config:
```
helpers: {
REST: {
endpoint: "http://localhost/api/v1/",
defaultHeaders: {
'Auth': '11111',
'Content-Type': 'application/json',
'Accept': 'application/json',
},
},
WebDriver : {
url: 'http://localhost',
browser: 'chrome'
}
}
```
REST helper provides basic methods to send requests to application:
```
I.sendGetRequest()
I.sendPostRequest()
I.sendPutRequest()
I.sendPatchRequest()
I.sendDeleteRequest()
```
As well as a method for setting headers: `haveRequestHeaders`.
Here is a usage example:
```
let postId = null;
Scenario('check post page', async ({ I }) => {
// valid access token
I.haveRequestHeaders({auth: '1111111'});
// get the first user
let user = await I.sendGetRequest('/api/users/1');
// create a post and save its Id
postId = await I.sendPostRequest('/api/posts', { author: user.id, body: 'some text' });
// open browser page of new post
I.amOnPage('/posts/2.html');
I.see('some text', 'p.body');
});
// cleanup created data
After(({ I }) => {
I.sendDeleteRequest('/api/posts/'+postId);
});
```
This can also be used to emulate Ajax requests:
```
I.sendPostRequest('/update-status', {}, { http_x_requested_with: 'xmlhttprequest' });
```
> See complete reference on [REST (opens new window)](https://codecept.io/helpers/REST) helper
>
>
GraphQL
--------
[GraphQL helper (opens new window)](https://codecept.io/helpers/GraphQL/) allows sending GraphQL queries and mutations to application, over Http. This is a tool to make shortcuts and create your data pragmatically via GraphQL endpoint. However, it doesn't provide tools for testing the endpoint, so it should be paired with WebDriver, Nightmare or Protractor helpers for browser testing.
Enable GraphQL helper in the config. It is recommended to set `endpoint`, the URL to which the requests go to. If you need some authorization you can optionally set default headers too.
See the sample config:
```
helpers: {
GraphQL: {
endpoint: "http://localhost/graphql/",
defaultHeaders: {
'Auth': '11111',
'Content-Type': 'application/json',
'Accept': 'application/json',
},
},
WebDriver : {
url: 'http://localhost',
browser: 'chrome'
}
}
```
GraphQL helper provides two basic methods to queries and mutations to application:
```
I.sendQuery()
I.sendMutation()
```
As well as a method for setting headers: `haveRequestHeaders`.
Here is a usage example:
```
let postData = null;
Scenario('check post page', async ({ I }) => {
// valid access token
I.haveRequestHeaders({auth: '1111111'});
// get the first user
let response = await I.sendQuery('{ user(id:1) { id }}');
let user = response.data;
// create a post and save its Id
response = await I.sendMutation(
'mutation createPost($input: PostInput!) { createPost(input: $input) { id }}',
{
input : {
author: user.data.id,
body: 'some text',
}
},
);
postData = response.data.data['createPost'];
// open browser page of new post
I.amOnPage(`/posts/${postData.slug}.html`);
I.see(postData.body, 'p.body');
});
// cleanup created data
After(({ I }) => {
I.sendMutation(
'mutation deletePost($permalink: /ID!) { deletePost(permalink: /$id) }',
{ permalink: /postData.id},
);
});
```
> See complete reference on [GraphQL (opens new window)](https://codecept.io/helpers/GraphQL) helper
>
>
Data Generation with Factories
-------------------------------
This concept is extended by:
* [ApiDataFactory (opens new window)](https://codecept.io/helpers/ApiDataFactory/) helper, and,
* [GraphQLDataFactory (opens new window)](https://codecept.io/helpers/GraphQLDataFactory/) helper.
These helpers build data according to defined rules and use REST API or GraphQL mutations to store them and automatically clean them up after a test.
Just define how many items of any kind you need and the data factory helper will create them for you.
To make this work some preparations are required.
At first, you need data generation libraries which are [Rosie (opens new window)](https://github.com/rosiejs/rosie) and [Faker (opens new window)](https://www.npmjs.com/package/faker). Faker can generate random names, emails, texts, and Rosie uses them to generate objects using factories.
Install rosie and faker to create a first factory:
```
npm i rosie faker --save-dev
```
Then create a module which will export a factory for an entity. And add that module as a part of the configuration for the helper.
Please look at the respective Factory sections for examples for factory modules and configuration.
### API Data Factory
This helper uses REST API to store the built data and automatically clean them up after a test, The way for setting data for a test is as simple as writing:
```
// inside async function
let post = await I.have('post');
I.haveMultiple('comment', 5, { postpermalink: /post.id});
```
After completing the preparations under 'Data Generation with Factories', create a factory module which will export a factory.
See the example providing a factory for User generation:
```
// factories/post.js
var Factory = require('rosie').Factory;
var faker = require('faker');
module.exports = new Factory()
.attr('name', () => faker.name.findName())
.attr('email', () => faker.internet.email());
```
Next is to configure helper to match factories with API:
```
ApiDataFactory: {
endpoint: "http://user.com/api",
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
},
factories: {
user: {
uri: "/users",
factory: "./factories/user"
}
}
}
```
Then, calling `I.have('user')` inside a test will create a new user for you. This is done by sending POST request to `/api/users` URL. Response is returned and can be used in tests.
At the end of a test ApiDataFactory will clean up created record for you. This is done by collecting ids from crated records and running `DELETE /api/users/{id}` requests at the end of a test. This rules can be customized in helper configuration.
> See complete reference on [ApiDataFactory (opens new window)](https://codecept.io/helpers/ApiDataFactory) helper
>
>
### GraphQL Data Factory
The helper uses GraphQL mutations to store the built data and automatically clean them up after a test. This way for setting data for a test is as simple as writing:
```
// inside async function
let post = await I.mutateData('createPost');
I.mutateMultiple('createComment', 5, { postpermalink: /post.id});
```
After completing the preparations under 'Data Generation with Factories', create a factory module which will export a factory.
The object built by the factory is sent as the variables object along with the mutation. So make sure it matches the argument type as detailed in the GraphQL schema. You may want to pass a constructor to the factory to achieve that.
See the example providing a factory for User generation:
```
// factories/post.js
var Factory = require('rosie').Factory;
var faker = require('faker');
module.exports = new Factory((buildObj) => {
return {
input: { ...buildObj },
}
})
.attr('name', () => faker.name.findName())
.attr('email', () => faker.internet.email());
```
Next is to configure helper to match factories with API:
```
GraphQLDataFactory: {
endpoint: "http://user.com/graphql",
cleanup: true,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
},
factories: {
createUser: {
query: 'mutation createUser($input: UserInput!) { createUser(input: $input) { id name }}',
factory: './factories/users',
revert: (data) => ({
query: 'mutation deleteUser($permalink: /ID!) { deleteUser(permalink: /$id) }',
variables: { id : data.id},
}),
},
}
```
Then, calling `I.mutateData('createUser')` inside a test will create a new user for you. This is done by sending a GraphQL mutation request over Http to `/graphql` endpoint. Response is returned and can be used in tests.
At the end of a test GraphQLDataFactory will clean up created record for you. This is done by collecting data from crated records, creating deletion mutation objects by passing the data to the `revert` function provided, and sending deletion mutation objects as requests at the end of a test. This behavior is according the `revert` function be customized in helper configuration. The revert function returns an object, that contains the query for deletion, and the variables object to go along with it.
> See complete reference on [GraphQLDataFactory (opens new window)](https://codecept.io/helpers/GraphQLDataFactory) helper
>
>
Requests Using Browser Session
-------------------------------
All the REST, GraphQL, GraphQLDataFactory, and ApiDataFactory helpers allow override requests before sending. This feature can be used to fetch current browser cookies and set them to REST API or GraphQL client. By doing this we can make requests within the current browser session without a need of additional authentication.
> Sharing browser session with ApiDataFactory or GraphQLDataFactory can be especially useful when you test Single Page Applications
>
>
Since CodeceptJS 2.3.3 there is a simple way to enable shared session for browser and data helpers. Install [`@codeceptjs/configure` (opens new window)](https://github.com/codeceptjs/configure) package:
```
npm i @codeceptjs/configure --save
```
Import `setSharedCookies` function and call it inside a config:
```
// in codecept.conf.js
const { setSharedCookies } = require('@codeceptjs/configure');
// share cookies between browser helpers and REST/GraphQL
setSharedCookies();
exports.config = {}
```
Without `setSharedCookies` you will need to update the config manually, so a data helper could receive cookies from a browser to make a request. If you would like to configure this process manually, here is an example of doing so:
```
let cookies; // share cookies
exports.config = {
helpers: {
ApiDataFactory: {
endpoint: 'http://local.app/api',
cleanup: true,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
},
factories: {
user: {
uri: "/users",
factory: "./factories/user",
}
},
onRequest: async (request) => {
// get a cookie if it's not obtained yet
if (cookies) cookies = await codeceptjs.container.helpers('WebDriver').grabCookie();
// add cookies to request for a current request
request.headers = { Cookie: cookies.map(c => `${c.name}=${c.value}`).join('; ') };
},
}
WebDriver: {
url: 'https://local.app/',
browser: 'chrome',
}
}
```
In this case we are accessing WebDriver helper. However, you can replace WebDriver with any helper you use.
The same can be done with GraphQLDataFactory.
The order of helpers is important! ApiDataFactory will clean up created users after a test, so it needs browser to be still opened to obtain its cookies.
codeceptjs Testing React Applications Testing React Applications
===========================
React applications require some additional love for end to end testing. At first, it is very hard to test an application which was never designed to be tested! This happens to many React application. While building components developers often forget to keep the element's semantic.
Generated HTML code may often look like this:
```
<div class="jss607 jss869 jss618 jss871 jss874 jss876" tabindex="0" role="tab" aria-selected="true" style="pointer-events: auto;">
<span class="jss877">
<span class="jss878">
<span class="jss879">Click Me!</span>
</span>
</span>
<span class="jss610"></span></div>
```
It's quite common that clickable elements are not actual `a` or `button` elements. This way `I.click('Click Me!');` won't work, as well as `fillField('name', 'value)`. Finding a correct locator for such cases turns to be almost impossible.
In this case test engineers have two options:
1. Update JSX files to change output HTML and rebuild the application
2. Test the application how it is.
We recommend for long-running projects to go with the first option. The better you write your initial HTML the cleaner and less fragile will be your tests. Replace divs with correct HTML elements, add `data-` attributes, add labels, and names to input fields to make all CodeceptJS magic like clicking link by a text to work.
However, if you can't update the code you can go to the second option. In this case, you should bind your locators to visible text on page and available semantic attribues. For instance, instead of using generated locator as this one:
```
//*[@id="document"]/div[2]/div/div[2]/div
```
use [Locator Builder](https://codecept.io/locators#locator-builder) to make clean semantic locator:
```
locate('[role=tab]').withText('Click Me!');
```
This way you can build very flexible and stable locators even on application never designed for testing.
Locators
---------
For React apps a special `react` locator is available. It allows to select an element by its component name, props and state.
```
{ react: 'MyComponent' }
{ react: 'Button', props: { title: 'Click Me' }}
{ react: 'Button', state: { some: 'state' }}
{ react: 'Input', state: 'valid'}
```
In WebDriver and Puppeteer you can use React locators in any method where locator is required:
```
I.click({ react: 'Tab', props: { title: 'Click Me!' }});
I.seeElement({ react: 't', props: { title: 'Clicked' }});
```
To find React element names and props in a tree use [React DevTools (opens new window)](https://chrome.google.com/webstore/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi) extension.
> Turn off minification for application builds otherwise component names will be uglified as well
>
>
React locators work via [resq (opens new window)](https://github.com/baruchvlz/resq) library, which handles React 16 and above.
codeceptjs Extending CodeceptJS With Custom Helpers Extending CodeceptJS With Custom Helpers
=========================================
Helper is the core concept of CodeceptJS. Helper is a wrapper on top of various libraries providing unified interface around them. When `I` object is used in tests it delegates execution of its functions to currently enabled helper classes.
Use Helpers to introduce low-level API to your tests without polluting test scenarios. Helpers can also be used to share functionality accross different project and installed as npm packages.
Development
------------
Helpers can be created by running a generator command:
```
npx codeceptjs gh
```
> or `npx codeceptjs generate:helper`
>
>
This command generates a basic helper, append it to `helpers` section of config file:
```
helpers: {
WebDriver: { },
MyHelper: {
require: './path/to/module'
}
}
```
Helpers are classes inherited from [corresponding abstract class (opens new window)](https://github.com/codeceptjs/helper). Created helper file should look like this:
```
const Helper = require('@codeceptjs/helper');
class MyHelper extends Helper {
// before/after hooks
_before() {
// remove if not used
}
_after() {
// remove if not used
}
// add custom methods here
// If you need to access other helpers
// use: this.helpers['helperName']
}
module.exports = MyHelper;
```
When the helper is enabled in config all methods of a helper class are available in `I` object. For instance, if we add a new method to helper class:
```
const Helper = require('@codeceptjs/helper');
class MyHelper extends Helper {
doAwesomeThings() {
console.log('Hello from MyHelpr');
}
}
```
We can call a new method from within `I`:
```
I.doAwesomeThings();
```
> Methods starting with `_` are considered special and won't available in `I` object.
>
>
Please note, `I` object can't be used helper class. As `I` object delegates its calls to helper classes, you can't make a circular dependency on it. Instead of calling `I` inside a helper, you can get access to other helpers by using `helpers` property of a helper. This allows you to access any other enabled helper by its name.
For instance, to perform a click with Playwright helper, do it like this:
```
doAwesomeThingsWithPlaywright() {
const { Playwright } = this.helpers;
Playwright.click('Awesome');
}
```
After a custom helper is finished you can update CodeceptJS Type Definitions by running:
```
npx codeceptjs def .
```
This way, if your tests are written with TypeScript, your IDE will be able to leverage features like autocomplete and so on.
Accessing Elements
-------------------
WebDriver, Puppeteer, Playwright, and Protractor drivers provide API for web elements. However, CodeceptJS do not expose them to tests by design, keeping test to be action focused. If you need to get access to web elements, it is recommended to implement operations for web elements in a custom helper.
To get access for elements, connect to a corresponding helper and use `_locate` function to match web elements by CSS or XPath, like you usually do:
### Acessing Elements in WebDriver
```
// inside a custom helper
async clickOnEveryElement(locator) {
const { WebDriver } = this.helpers;
const els = await WebDriver._locate(locator);
for (let el of els) {
await el.click();
}
}
```
In this case an an instance of webdriverio element is used. To get a [complete API of an element (opens new window)](https://webdriver.io/docs/api/) refer to webdriverio docs.
### Accessing Elements in Playwright & Puppeteer
Similar method can be implemented for Playwright & Puppeteer:
```
// inside a custom helper
async clickOnEveryElement(locator) {
const { Playwright } = this.helpers;
const els = await Playwright._locate(locator);
for (let el of els) {
await el.click();
}
}
```
In this case `el` will be an instance of [ElementHandle (opens new window)](https://playwright.dev/#version=master&path=docs%2Fapi.md&q=class-elementhandle) which is similar for Playwright & [Puppeteer (opens new window)](https://pptr.dev/#?product=Puppeteer&version=master&show=api-class-elementhandle).
> ℹ There are more `_locate*` methods in each helper. Take a look on documentation of a helper you use to see which exact method it exposes.
>
>
Configuration
--------------
Helpers should be enabled inside `codecept.json` or `codecept.conf.js` files. Command `generate helper` does that for you, however you can enable them manually by placing helper to `helpers` section inside config file. You can also pass additional config options to your helper from a config - **(please note, this example contains comments, while JSON format doesn't support them)**:
```
helpers: {
// here goes standard helpers:
// WebDriver, Protractor, Nightmare, etc...
// and their configuration
MyHelper: {
require: "./my_helper.js", // path to module
defaultHost: "http://mysite.com" // custom config param
}
}
```
Config values will be stored inside helper in `this.config`. To get `defaultHost` value you can use
```
this.config.defaultHost
```
in any place of your helper. You can also redefine config options inside a constructor:
```
constructor(config) {
config.defaultHost += '/api';
console.log(config.defaultHost); // http://mysite.com/api
super(config);
}
```
Hooks
------
Helpers may contain several hooks you can use to handle events of a test. Implement corresponding methods to them.
* `_init` - before all tests
* `_finishTest` - after all tests
* `_before` - before a test
* `_after` - after a test
* `_beforeStep` - before each step
* `_afterStep` - after each step
* `_beforeSuite` - before each suite
* `_afterSuite` - after each suite
* `_passed` - after a test passed
* `_failed` - after a test failed
Each implemented method should return a value as they will be added to global promise chain as well.
Conditional Retries
--------------------
It is possible to execute global conditional retries to handle unforseen errors. Lost connections and network issues are good candidates to be retried whenever they appear.
This can be done inside a helper using the global [promise recorder](https://codecept.io/hooks/#api):
Example: Retrying rendering errors in Puppeteer.
```
_before() {
const recorder = require('codeceptjs').recorder;
recorder.retry({
retries: 2,
when: err => err.message.indexOf('Cannot find context with specified id') > -1,
});
}
```
`recorder.retry` acts similarly to `I.retry()` and accepts the same parameters. It expects the `when` parameter to be set so it would handle only specific errors and not to retry for every failed step.
Retry rules are available in array `recorder.retries`. The last retry rule can be disabled by running `recorder.retries.pop()`;
Using Typescript
-----------------
With Typescript, just simply replacing `module.exports` with `export` for autocompletion.
Helper Examples
----------------
### Playwright Example
In this example we take the power of Playwright to change geolocation in our tests:
```
const Helper = require('@codeceptjs/helper');
class MyHelper extends Helper {
async setGeoLocation(longitude, latitude) {
const { browserContext } = this.helpers.Playwright;
await browserContext.setGeolocation({ longitude, latitude });
await Playwright.refreshPage();
}
}
```
### WebDriver Example
Next example demonstrates how to use WebDriver library to create your own test action. Method `seeAuthentication` will use `browser` instance of WebDriver to get access to cookies. Standard NodeJS assertion library will be used (you can use any).
```
const Helper = require('@codeceptjs/helper');
// use any assertion library you like
const assert = require('assert');
class MyHelper extends Helper {
/**
* checks that authentication cookie is set
*/
async seeAuthentication() {
// access current browser of WebDriver helper
const { WebDriver } = this.helpers
const { browser } = WebDriver;
// get all cookies according to http://webdriver.io/api/protocol/cookie.html
// any helper method should return a value in order to be added to promise chain
const res = await browser.cookie();
// get values
let cookies = res.value;
for (let k in cookies) {
// check for a cookie
if (cookies[k].name != 'logged_in') continue;
assert.equal(cookies[k].value, 'yes');
return;
}
assert.fail(cookies, 'logged_in', "Auth cookie not set");
}
}
module.exports = MyHelper;
```
### Puppeteer Example
Puppeteer has [nice and elegant API (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md) which you can use inside helpers. Accessing `page` instance via `this.helpers.Puppeteer.page` from inside a helper.
Let's see how we can use [emulate (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md#pageemulateoptions) function to emulate iPhone browser in a test.
```
const Helper = require('@codeceptjs/helper');
const puppeteer = require('puppeteer');
const iPhone = puppeteer.devices['iPhone 6'];
class MyHelper extends Helper {
async emulateIPhone() {
const { page } = this.helpers.Puppeteer;
await page.emulate(iPhone);
}
}
module.exports = MyHelper;
```
### Protractor Example
Protractor example demonstrates usage of global `element` and `by` objects. However `browser` should be accessed from a helper instance via `this.helpers['Protractor']`; We also use `chai-as-promised` library to have nice assertions with promises.
```
const Helper = require('@codeceptjs/helper');
// use any assertion library you like
const chai = require('chai');
const chaiAsPromised = require('chai-as-promised');
chai.use(chaiAsPromised);
const expect = chai.expect;
class MyHelper extends Helper {
/**
* checks that authentication cookie is set
*/
seeInHistory(historyPosition, value) {
// access browser instance from Protractor helper
this.helpers['Protractor'].browser.refresh();
// you can use `element` as well as in protractor
const history = element.all(by.repeater('result in memory'));
// use chai as promised for better assertions
// end your method with `return` to handle promises
return expect(history.get(historyPosition).getText()).to.eventually.equal(value);
}
}
module.exports = MyHelper;
```
| programming_docs |
codeceptjs GraphQLDataFactory GraphQLDataFactory
===================
**Extends Helper**
Helper for managing remote data using GraphQL queries. Uses data generators like [rosie (opens new window)](https://github.com/rosiejs/rosie) or factory girl to create new record.
By defining a factory you set the rules of how data is generated. This data will be saved on server via GraphQL queries and deleted in the end of a test.
Use Case
---------
Acceptance tests interact with a websites using UI and real browser. There is no way to create data for a specific test other than from user interface. That makes tests slow and fragile. Instead of testing a single feature you need to follow all creation/removal process.
This helper solves this problem. If a web application has GraphQL support, it can be used to create and delete test records. By combining GraphQL with Factories you can easily create records for tests:
```
I.mutateData('createUser', { name: 'davert', email: '[email protected]' });
let user = await I.mutateData('createUser', { name: 'davert'});
I.mutateMultiple('createPost', 3, {post_id: user.id});
```
To make this work you need
1. GraphQL endpoint which allows to perform create / delete requests and
2. define data generation rules
### Setup
Install [Rosie (opens new window)](https://github.com/rosiejs/rosie) and [Faker (opens new window)](https://www.npmjs.com/package/faker) libraries.
```
npm i rosie faker --save-dev
```
Create a factory file for a resource.
See the example for Users factories:
```
// tests/factories/users.js
var Factory = require('rosie').Factory;
var faker = require('faker');
// Used with a constructor function passed to Factory, so that the final build
// object matches the necessary pattern to be sent as the variables object.
module.exports = new Factory((buildObj) => ({
input: { ...buildObj },
}))
// 'attr'-id can be left out depending on the GraphQl resolvers
.attr('name', () => faker.name.findName())
.attr('email', () => faker.interact.email())
```
For more options see [rosie documentation (opens new window)](https://github.com/rosiejs/rosie).
Then configure GraphQLDataHelper to match factories and GraphQL schema:
### Configuration
GraphQLDataFactory has following config options:
* `endpoint`: URL for the GraphQL server.
* `cleanup` (default: true): should inserted records be deleted up after tests
* `factories`: list of defined factories
* `headers`: list of headers
* `GraphQL`: configuration for GraphQL requests.
See the example:
```
GraphQLDataFactory: {
endpoint: "http://user.com/graphql",
cleanup: true,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
},
factories: {
createUser: {
query: 'mutation createUser($input: UserInput!) { createUser(input: $input) { id name }}',
factory: './factories/users',
revert: (data) => ({
query: 'mutation deleteUser($id: ID!) { deleteUser(id: $id) }',
variables: { id : data.id},
}),
},
}
}
```
It is required to set GraphQL `endpoint` which is the URL to which all the queries go to. Factory file is expected to be passed via `factory` option.
This Helper uses [GraphQL (opens new window)](https://codecept.io/helpers/GraphQL/) helper and accepts its configuration in "GraphQL" section. For instance, to set timeout you should add:
```
"GraphQLDataFactory": {
"GraphQL": {
"timeout": "100000",
}
}
```
### Factory
Factory contains operations -
* `operation`: The operation/mutation that needs to be performed for creating a record in the backend.
Each operation must have the following:
* `query`: The mutation(query) string. It is expected to use variables to send data with the query.
* `factory`: The path to factory file. The object built by the factory in this file will be passed as the 'variables' object to go along with the mutation.
* `revert`: A function called with the data returned when an item is created. The object returned by this function is will be used to later delete the items created. So, make sure RELEVANT DATA IS RETURNED when a record is created by a mutation.
### Requests
Requests can be updated on the fly by using `onRequest` function. For instance, you can pass in current session from a cookie.
```
onRequest: async (request) => {
// using global codeceptjs instance
let cookie = await codeceptjs.container.helpers('WebDriver').grabCookie('session');
request.headers = { Cookie: `session=${cookie.value}` };
}
```
### Responses
By default `I.mutateData()` returns a promise with created data as specified in operation query string:
```
let client = await I.mutateData('createClient');
```
Data of created records are collected and used in the end of a test for the cleanup.
Methods
--------
### Parameters
* `config`
### \_requestCreate
Executes request to create a record to the GraphQL endpoint. Can be replaced from a custom helper.
#### Parameters
* `operation` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `variables` **any** to be sent along with the query
### \_requestDelete
Executes request to delete a record to the GraphQL endpoint. Can be replaced from a custom helper.
#### Parameters
* `operation` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `data` **any** of the record to be deleted.
### mutateData
Generates a new record using factory, sends a GraphQL mutation to store it.
```
// create a user
I.mutateData('createUser');
// create user with defined email
// and receive it when inside async function
const user = await I.mutateData('createUser', { email: '[email protected]'});
```
#### Parameters
* `operation` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to be performed
* `params` **any** predefined parameters
### mutateMultiple
Generates bunch of records and sends multiple GraphQL mutation requests to store them.
```
// create 3 users
I.mutateMultiple('createUser', 3);
// create 3 users of same age
I.mutateMultiple('createUser', 3, { age: 25 });
```
#### Parameters
* `operation` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `times` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
* `params` **any**
codeceptjs Puppeteer Puppeteer
==========
**Extends Helper**
Uses [Google Chrome's Puppeteer (opens new window)](https://github.com/GoogleChrome/puppeteer) library to run tests inside headless Chrome. Browser control is executed via DevTools Protocol (instead of Selenium). This helper works with a browser out of the box with no additional tools required to install.
Requires `puppeteer` package to be installed.
> Experimental Firefox support [can be activated (opens new window)](https://codecept.io/helpers/Puppeteer-firefox).
>
>
Configuration
--------------
This helper should be configured in codecept.json or codecept.conf.js
* `url`: base url of website to be tested
* `basicAuth`: (optional) the basic authentication to pass to base url. Example: {username: 'username', password: 'password'}
* `show`: - show Google Chrome window for debug.
* `restart`: - restart browser between tests.
* `disableScreenshots`: - don't save screenshot on failure.
* `fullPageScreenshots` - make full page screenshots on failure.
* `uniqueScreenshotNames`: - option to prevent screenshot override if you have scenarios with the same name in different suites.
* `keepBrowserState`: - keep browser state between tests when `restart` is set to false.
* `keepCookies`: - keep cookies between tests when `restart` is set to false.
* `waitForAction`: (optional) how long to wait after click, doubleClick or PressKey actions in ms. Default: 100.
* `waitForNavigation`: . When to consider navigation succeeded. Possible options: `load`, `domcontentloaded`, `networkidle0`, `networkidle2`. See [Puppeteer API (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md#pagewaitfornavigationoptions). Array values are accepted as well.
* `pressKeyDelay`: . Delay between key presses in ms. Used when calling Puppeteers page.type(...) in fillField/appendField
* `getPageTimeout` config option to set maximum navigation time in milliseconds. If the timeout is set to 0, then timeout will be disabled.
* `waitForTimeout`: (optional) default wait\* timeout in ms. Default: 1000.
* `windowSize`: (optional) default window size. Set a dimension like `640x480`.
* `userAgent`: (optional) user-agent string.
* `manualStart`: - do not start browser before a test, start it manually inside a helper with `this.helpers["Puppeteer"]._startBrowser()`.
* `browser`: - can be changed to `firefox` when using [puppeteer-firefox (opens new window)](https://codecept.io/helpers/Puppeteer-firefox).
* `chrome`: (optional) pass additional [Puppeteer run options (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md#puppeteerlaunchoptions).
#### Example #1: Wait for 0 network connections.
```
{
helpers: {
Puppeteer : {
url: "http://localhost",
restart: false,
waitForNavigation: "networkidle0",
waitForAction: 500
}
}
}
```
#### Example #2: Wait for DOMContentLoaded event and 0 network connections
```
{
helpers: {
Puppeteer : {
url: "http://localhost",
restart: false,
waitForNavigation: [ "domcontentloaded", "networkidle0" ],
waitForAction: 500
}
}
}
```
#### Example #3: Debug in window mode
```
{
helpers: {
Puppeteer : {
url: "http://localhost",
show: true
}
}
}
```
#### Example #4: Connect to remote browser by specifying [websocket endpoint (opens new window)](https://chromedevtools.github.io/devtools-protocol/#how-do-i-access-the-browser-target)
```
{
helpers: {
Puppeteer: {
url: "http://localhost",
chrome: {
browserWSEndpoint: "ws://localhost:9222/devtools/browser/c5aa6160-b5bc-4d53-bb49-6ecb36cd2e0a"
}
}
}
}
```
#### Example #5: Target URL with provided basic authentication
```
{
helpers: {
Puppeteer : {
url: 'http://localhost',
basicAuth: {username: 'username', password: 'password'},
show: true
}
}
}
```
Note: When connecting to remote browser `show` and specific `chrome` options (e.g. `headless` or `devtools`) are ignored.
Access From Helpers
--------------------
Receive Puppeteer client from a custom helper by accessing `browser` for the Browser object or `page` for the current Page object:
```
const { browser } = this.helpers.Puppeteer;
await browser.pages(); // List of pages in the browser
const { page } = this.helpers.Puppeteer;
await page.url(); // Get the url of the current page
```
Methods
--------
### Parameters
* `config`
### \_addPopupListener
Add the 'dialog' event listener to a page
#### Parameters
* `page`
### \_getPageUrl
Gets page URL including hash.
### \_locate
Get elements by different locator types, including strict locator Should be used in custom helpers:
```
const elements = await this.helpers['Puppeteer']._locate({name: 'password'});
```
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
#### Parameters
* `locator`
### \_locateCheckable
Find a checkbox by providing human readable text: NOTE: Assumes the checkable element exists
```
this.helpers['Puppeteer']._locateCheckable('I agree with terms and conditions').then // ...
```
#### Parameters
* `locator`
* `providedContext`
### \_locateClickable
Find a clickable element by providing human readable text:
```
this.helpers['Puppeteer']._locateClickable('Next page').then // ...
```
#### Parameters
* `locator`
### \_locateFields
Find field elements by providing human readable text:
```
this.helpers['Puppeteer']._locateFields('Your email').then // ...
```
#### Parameters
* `locator`
### \_setPage
Set current page
#### Parameters
* `page` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** page to set
### acceptPopup
Accepts the active JavaScript native popup window, as created by window.alert|window.confirm|window.prompt. Don't confuse popups with modal windows, as created by [various libraries (opens new window)](http://jster.net/category/windows-modals-popups).
### amAcceptingPopups
Set the automatic popup response to Accept. This must be set before a popup is triggered.
```
I.amAcceptingPopups();
I.click('#triggerPopup');
I.acceptPopup();
```
### amCancellingPopups
Set the automatic popup response to Cancel/Dismiss. This must be set before a popup is triggered.
```
I.amCancellingPopups();
I.click('#triggerPopup');
I.cancelPopup();
```
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file.> ⚠ There is an [issue with file upload in Puppeteer 2.1.0 & 2.1.1 (opens new window)](https://github.com/puppeteer/puppeteer/issues/5420), downgrade to 2.0.0 if you face it.
### cancelPopup
Dismisses the active JavaScript popup, as created by window.alert|window.confirm|window.prompt.
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `name`
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### clickLink
Performs a click on a link and waits for navigation before moving on.
```
I.clickLink('Logout', '#nav');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### closeCurrentTab
Close current tab and switches to previous.
```
I.closeCurrentTab();
```
### closeOtherTabs
Close all tabs except for the current one.
```
I.closeOtherTabs();
```
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### dontSeeInTitle
Checks that title does not contain text.
```
I.dontSeeInTitle('Error');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### downloadFile
This method is **deprecated**.
Please use `handleDownloads()` instead.
#### Parameters
* `locator`
* `customName`
### dragAndDrop
Drag an item to a destination element.
```
I.dragAndDrop('#dragHandle', '#container');
```
#### Parameters
* `srcElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `destElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### dragSlider
Drag the scrubber of a slider to a given position For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.dragSlider('#slider', 30);
I.dragSlider('#slider', -70);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** position to drag.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### executeAsyncScript
Executes async script on page. Provided function should execute a passed callback (as first argument) to signal it is finished.
Example: In Vue.js to make components completely rendered we are waiting for [nextTick (opens new window)](https://vuejs.org/v2/api/#Vue-nextTick).
```
I.executeAsyncScript(function(done) {
Vue.nextTick(done); // waiting for next tick
});
```
By passing value to `done()` function you can return values. Additional arguments can be passed as well, while `done` function is always last parameter in arguments list.
```
let val = await I.executeAsyncScript(function(url, done) {
// in browser context
$.ajax(url, { success: (data) => done(data); }
}, 'http://ajax.callback.url/');
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** Asynchronous scripts can also be executed with `executeScript` if a function returns a Promise.
### executeScript
Executes sync script on a page. Pass arguments to function as additional parameters. Will return execution result to a test. In this case you should use async function and await to receive results.
Example with jQuery DatePicker:
```
// change date of jQuery DatePicker
I.executeScript(function() {
// now we are inside browser context
$('date').datetimepicker('setDate', new Date());
});
```
Can return values. Don't forget to use `await` to get them.
```
let date = await I.executeScript(function(el) {
// only basic types can be returned
return $(el).datetimepicker('getDate').toString();
}, '#date'); // passing jquery selector
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** If a function returns a Promise It will wait for it resolution.
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### forceClick
Perform an emulated click on a link or a button, given by a locator. Unlike normal click instead of sending native event, emulates a click with JavaScript. This works on hidden, animated or inactive elements as well.
If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.forceClick('Logout');
// button of form
I.forceClick('Submit');
// CSS button
I.forceClick('#form input[type=submit]');
// XPath
I.forceClick('//form/*[@type=submit]');
// link in context
I.forceClick('Logout', '#nav');
// using strict locator
I.forceClick({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabAttributeFromAll
Retrieves an array of attributes from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let hints = await I.grabAttributeFromAll('.tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabBrowserLogs
Get JS log from browser.
```
let logs = await I.grabBrowserLogs();
console.log(JSON.stringify(logs))
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>>**
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute valueReturns cookie in JSON format. If name not passed returns all cookies for this domain.
### grabCssPropertyFrom
Grab CSS property for given locator Resumes test execution, so **should be used inside an async function with `await`** operator. If more than one element is found - value of first element is returned.
```
const value = await I.grabCssPropertyFrom('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** CSS value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabCssPropertyFromAll
Grab array of CSS properties for given locator Resumes test execution, so **should be used inside an async function with `await`** operator.
```
const values = await I.grabCssPropertyFromAll('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** CSS value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabDataFromPerformanceTiming
Grab the data from performance timing using Navigation Timing API. The returned data will contain following things in ms:
* responseEnd,
* domInteractive,
* domContentLoadedEventEnd,
* loadEventEnd Resumes test execution, so **should be used inside an async function with `await`** operator.
```
await I.amOnPage('https://example.com');
let data = await I.grabDataFromPerformanceTiming();
//Returned data
{ // all results are in [ms]
responseEnd: 23,
domInteractive: 44,
domContentLoadedEventEnd: 196,
loadEventEnd: 241
}
```
### grabElementBoundingRect
Grab the width, height, location of given locator. Provide `width` or `height`as second param to get your desired prop. Resumes test execution, so **should be used inside an async function with `await`** operator.
Returns an object with `x`, `y`, `width`, `height` keys.
```
const value = await I.grabElementBoundingRect('h3');
// value is like { x: 226.5, y: 89, width: 527, height: 220 }
```
To get only one metric use second parameter:
```
const width = await I.grabElementBoundingRect('h3', 'width');
// width == 527
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `prop`
* `elementSize` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** x, y, width or height of the given element.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<DOMRect> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>)** Element bounding rectangle
### grabHTMLFrom
Retrieves the innerHTML from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - HTML of first element is returned.
```
let postHTML = await I.grabHTMLFrom('#post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** HTML code for an element
### grabHTMLFromAll
Retrieves all the innerHTML from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let postHTMLs = await I.grabHTMLFromAll('.post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** HTML code for an element
### grabNumberOfOpenTabs
Grab number of open tabs. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let tabs = await I.grabNumberOfOpenTabs();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of open tabs
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabPopupText
Grab the text within the popup. If no popup is visible then it will return null
```
await I.grabPopupText();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | null)>**
### grabSource
Retrieves page source and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let pageSource = await I.grabSource();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** source code
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabTitle
Retrieves a page title and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let title = await I.grabTitle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** title
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves an array of value from a form located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let inputs = await I.grabValueFromAll('//form/input');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### handleDownloads
Sets a directory to where save files. Allows to test file downloads. Should be used with [FileSystem helper (opens new window)](https://codecept.io/helpers/FileSystem) to check that file were downloaded correctly.
By default files are saved to `output/downloads`. This directory is cleaned on every `handleDownloads` call, to ensure no old files are kept.
```
I.handleDownloads();
I.click('Download Avatar');
I.amInPath('output/downloads');
I.seeFile('avatar.jpg');
```
#### Parameters
* `downloadPath` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** change this parameter to set another directory for saving
### haveRequestHeaders
Set headers for all next requests
```
I.haveRequestHeaders({
'X-Sent-By': 'CodeceptJS',
});
```
#### Parameters
* `customHeaders` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** headers to set
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### openNewTab
Open new tab and switch to it
```
I.openNewTab();
```
### pressKey
Presses a key in the browser (on a focused element).
*Hint:* For populating text field or textarea, it is recommended to use [`fillField`](#fillfield).
```
I.pressKey('Backspace');
```
To press a key in combination with modifier keys, pass the sequence as an array. All modifier keys (`'Alt'`, `'Control'`, `'Meta'`, `'Shift'`) will be released afterwards.
```
I.pressKey(['Control', 'Z']);
```
For specifying operation modifier key based on operating system it is suggested to use `'CommandOrControl'`. This will press `'Command'` (also known as `'Meta'`) on macOS machines and `'Control'` on non-macOS machines.
```
I.pressKey(['CommandOrControl', 'Z']);
```
Some of the supported key names are:
* `'AltLeft'` or `'Alt'`
* `'AltRight'`
* `'ArrowDown'`
* `'ArrowLeft'`
* `'ArrowRight'`
* `'ArrowUp'`
* `'Backspace'`
* `'Clear'`
* `'ControlLeft'` or `'Control'`
* `'ControlRight'`
* `'Command'`
* `'CommandOrControl'`
* `'Delete'`
* `'End'`
* `'Enter'`
* `'Escape'`
* `'F1'` to `'F12'`
* `'Home'`
* `'Insert'`
* `'MetaLeft'` or `'Meta'`
* `'MetaRight'`
* `'Numpad0'` to `'Numpad9'`
* `'NumpadAdd'`
* `'NumpadDecimal'`
* `'NumpadDivide'`
* `'NumpadMultiply'`
* `'NumpadSubtract'`
* `'PageDown'`
* `'PageUp'`
* `'Pause'`
* `'Return'`
* `'ShiftLeft'` or `'Shift'`
* `'ShiftRight'`
* `'Space'`
* `'Tab'`
#### Parameters
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** key or array of keys to press.*Note:* Shortcuts like `'Meta'` + `'A'` do not work on macOS ([GoogleChrome/puppeteer#1313 (opens new window)](https://github.com/GoogleChrome/puppeteer/issues/1313)).
### pressKeyDown
Presses a key in the browser and leaves it in a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to press down.
### pressKeyUp
Releases a key in the browser which was previously set to a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to release.
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels.Unlike other drivers Puppeteer changes the size of a viewport, not the window! Puppeteer does not control the window of a browser so it can't adjust its real size. It also can't maximize a window.
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeAttributesOnElements
Checks that all elements with given locator have given attributes.
```
I.seeAttributesOnElements('//form', { method: "post"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `attributes` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** attributes and their values to check.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCssPropertiesOnElements
Checks that all elements with given locator have given CSS properties.
```
I.seeCssPropertiesOnElements('h3', { 'font-weight': "bold"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `cssProperties` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** object with CSS properties and their values to check.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInPopup
Checks that the active JavaScript popup, as created by `window.alert|window.confirm|window.prompt`, contains the given string.
```
I.seeInPopup('Popup text');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInTitle
Checks that title contains text.
```
I.seeInTitle('Home Page');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to check.
### seeNumberOfElements
Asserts that an element appears a given number of times in the DOM. Element is located by label or name or CSS or XPath.
```
I.seeNumberOfElements('#submitBtn', 1);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeTextEquals
Checks that text is equal to provided one.
```
I.seeTextEquals('text', 'h1');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** element value to check.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)?)** element located by CSS|XPath|strict locator.
### seeTitleEquals
Checks that title is equal to provided one.
```
I.seeTitleEquals('Test title.');
```
#### Parameters
* `text`
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.
### switchTo
Switches frame or in case of null locator reverts to parent.
```
I.switchTo('iframe'); // switch to first iframe
I.switchTo(); // switch back to main page
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### switchToNextTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToNextTab();
I.switchToNextTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
### switchToPreviousTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToPreviousTab();
I.switchToPreviousTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
### type
Types out the given text into an active field. To slow down typing use a second parameter, to set interval between key presses. *Note:* Should be used when [`fillField`](#fillfield) is not an option.
```
// passing in a string
I.type('Type this out.');
// typing values with a 100ms interval
I.type('4141555311111111', 100);
// passing in an array
I.type(['T', 'E', 'X', 'T']);
```
#### Parameters
* `keys`
* `delay` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional) delay in ms between key presses
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** or array of keys to type.
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### usePuppeteerTo
Use Puppeteer API inside a test.
First argument is a description of an action. Second argument is async function that gets this helper as parameter.
{ [`page` (opens new window)](https://github.com/puppeteer/puppeteer/blob/master/docs/api.md#class-page), [`browser` (opens new window)](https://github.com/puppeteer/puppeteer/blob/master/docs/api.md#class-browser) } from Puppeteer API are available.
```
I.usePuppeteerTo('emulate offline mode', async ({ page }) {
await page.setOfflineMode(true);
});
```
#### Parameters
* `description` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** used to show in logs.
* `fn` **[function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** async function that is executed with Puppeteer as argument
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForClickable
Waits for element to be clickable (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForClickable('.btn.continue');
I.waitForClickable('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `waitTimeout`
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForDetached
Waits for an element to become not attached to the DOM on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForDetached('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### waitForEnabled
Waits for element to become enabled (by default waits for 1sec). Element can be located by CSS or XPath.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional) time in seconds to wait, 1 by default.
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForNavigation
Waits for navigation to finish. By default takes configured `waitForNavigation` option.
See [Pupeteer's reference (opens new window)](https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md#pagewaitfornavigationoptions)
#### Parameters
* `opts` **any**
### waitForRequest
Waits for a network request.
```
I.waitForRequest('http://example.com/resource');
I.waitForRequest(request => request.url() === 'http://example.com' && request.method() === 'GET');
```
#### Parameters
* `urlOrPredicate` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))**
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** seconds to wait
### waitForResponse
Waits for a network response.
```
I.waitForResponse('http://example.com/resource');
I.waitForResponse(response => response.url() === 'http://example.com' && response.request().method() === 'GET');
```
#### Parameters
* `urlOrPredicate` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))**
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** number of seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForValue
Waits for the specified value to be in value attribute.
```
I.waitForValue('//input', "GoodValue");
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** input field.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected value.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to waitThis method accepts [React selectors (opens new window)](https://codecept.io/react).
### waitInUrl
Waiting for the part of the URL to match the expected. Useful for SPA to understand that page was changed.
```
I.waitInUrl('/info', 2);
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitNumberOfVisibleElements
Waits for a specified number of elements on the page.
```
I.waitNumberOfVisibleElements('a', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitUntil
Waits for a function to return true (waits for 1sec by default).
```
I.waitUntil(() => window.requests == 0);
I.waitUntil(() => window.requests == 0, 5);
```
#### Parameters
* `fn` **([function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function) | [string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String))** function which is executed in browser context.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `timeoutMsg` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** message to show in case of timeout fail.
* `interval` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?**
### waitUrlEquals
Waits for the entire URL to match the expected
```
I.waitUrlEquals('/info', 2);
I.waitUrlEquals('http://127.0.0.1:8000/info');
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
| programming_docs |
codeceptjs MockRequest MockRequest
============
MockRequest
------------
This helper allows to **mock requests while running tests in Puppeteer or WebDriver**. For instance, you can block calls to 3rd-party services like Google Analytics, CDNs. Another way of using is to emulate requests from server by passing prepared data.
MockRequest helper works in these [modes (opens new window)](https://netflix.github.io/pollyjs/#/configuration?id=mode):
* passthrough (default) - mock prefefined HTTP requests
* record - record all requests into a file
* replay - replay all recorded requests from a file
Combining record/replay modes allows testing websites with large datasets.
To use in passthrough mode set rules to mock requests and they will be automatically intercepted and replaced:
```
// default mode
I.mockRequest('GET', '/api/users', '[]');
```
In record-replay mode start mocking to make HTTP requests recorded/replayed, and stop when you don't need to block requests anymore:
```
// record or replay all XHR for /users page
I.startMocking();
I.amOnPage('/users');
I.stopMocking();
```
### Installations
```
npm i @codeceptjs/mock-request --save-dev
```
Requires Puppeteer helper or WebDriver helper enabled
### Configuration
#### With Puppeteer
Enable helper in config file:
```
helpers: {
Puppeteer: {
// regular Puppeteer config here
},
MockRequestHelper: {
require: '@codeceptjs/mock-request',
}
}
```
[Polly config options (opens new window)](https://netflix.github.io/pollyjs/#/configuration?id=configuration) can be passed as well:
```
// sample options
helpers: {
MockRequestHelper: {
require: '@codeceptjs/mock-request',
mode: record,
recordIfMissing: true,
recordFailedRequests: false,
expiresIn: null,
persisterOptions: {
keepUnusedRequests: false
fs: {
recordingsDir: './data/requests',
},
},
}
}
```
**TROUBLESHOOTING**: Puppeteer does not mock requests in headless mode:
Problem: request mocking does not work and in debug mode you see this in output:
```
Access to fetch at {url} from origin {url} has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
```
Solution: update Puppeteer config to include `--disable-web-security` arguments:
```
Puppeteer: {
show: false,
chrome: {
args: [
'--disable-web-security',
],
},
},
```
#### With WebDriver
This helper partially works with WebDriver. It can intercept and mock requests **only on already loaded page**.
```
helpers: {
WebDriver: {
// regular WebDriver config here
},
MockRequestHelper: {
require: '@codeceptjs/mock-request',
}
}
```
> Record/Replay mode is not tested in WebDriver but technically can work with [REST Persister (opens new window)](https://netflix.github.io/pollyjs/#/examples?id=rest-persister)
>
>
Usage
------
### 👻 Mock Requests
To intercept API requests and mock them use following API
* [startMocking()](#startMocking) - to enable request interception
* [mockRequest()](#mockRequest) - to define mock in a simple way
* [mockServer()](#mockServer) - to use PollyJS server API to define complex mocks
* [stopMocking()](#stopMocking) - to stop intercepting requests and disable mocks.
Calling `mockRequest` or `mockServer` will start mocking, if it was not enabled yet.
```
I.startMocking(); // optionally
I.mockRequest('/google-analytics/*path', 200);
// return an empty successful response
I.mockRequest('GET', '/api/users', 200);
// mock users api
I.mockServer(server => {
server.get('https://server.com/api/users*').
intercept((req, res) => { res.status(200).json(users);
});
});
I.click('Get users);
I.stopMocking();
```
### 📼 Record & Replay
> At this moment works only with Puppeteer
>
>
Record & Replay mode allows you to record all xhr & fetch requests and save them to file. On next runs those requests can be replayed. By default, it stores all passed requests, but this behavior can be customized with `I.mockServer`
Set mode via enironment variable, `replay` mode by default:
```
// enable replay mode
helpers: {
Puppeteer: {
// regular Puppeteer config here
},
MockRequest: {
require: '@codeceptjs/mock-request',
mode: process.env.MOCK_MODE || 'replay',
},
}
```
Interactions between `I.startMocking()` and `I.stopMocking()` will be recorded and saved to `data/requests` directory.
```
I.startMocking() // record requests under 'Test' name
I.startMocking('users') // record requests under 'users' name
```
Use `I.mockServer()` to customize which requests should be recorded and under which name:
```
I.startMocking();
I.mockServer((server) => {
// mock request only from ap1.com and api2.com and
// store recording into two different files
server.any('https://api1.com/*').passthrough(false).recordingName('api1');
server.any('https://api2.com/*').passthrough(false).recordingName('api2');
});
```
To stop request recording/replaying use `I.stopMocking()`.
🎥 To record HTTP interactions execute tests with MOCK\_MODE environment variable set as "record":
```
MOCK_MODE=record npx codeceptjs run --debug
```
📼 To replay them launch tests without environment variable:
```
npx codeceptjs run --debug
```
### Parameters
* `config`
### flushMocking
Waits for all requests handled by MockRequests to be resolved:
```
I.flushMocking();
```
### mockRequest
Mock response status
```
I.mockRequest('GET', '/api/users', 200);
I.mockRequest('ANY', '/secretsRoutes/*', 403);
I.mockRequest('POST', '/secrets', { secrets: 'fakeSecrets' });
I.mockRequest('GET', '/api/users/1', 404, 'User not found');
```
Multiple requests
```
I.mockRequest('GET', ['/secrets', '/v2/secrets'], 403);
```
#### Parameters
* `method` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** request method. Can be `GET`, `POST`, `PUT`, etc or `ANY`.
* `oneOrMoreUrls` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** url(s) to mock. Can be exact URL, a pattern, or an array of URLs.
* `dataOrStatusCode` **([number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number) | [string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** status code when number provided. A response body otherwise
* `additionalData` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** response body when a status code is set by previous parameter. (optional, default `null`)
### mockServer
Use PollyJS [Server Routes API (opens new window)](https://netflix.github.io/pollyjs/#/server/overview) to declare mocks via callback function:
```
// basic usage
server.get('/api/v2/users').intercept((req, res) => {
res.sendStatus(200).json({ users });
});
// passthrough requests to "/api/v2"
server.get('/api/v1').passthrough();
```
In record replay mode you can define which routes should be recorded and where to store them:
```
I.startMocking('mock');
I.mockServer((server) => {
// record requests from cdn1.com and save them to data/recording/xml
server.any('https://cdn1.com/*').passthrough(false).recordingName('xml');
// record requests from cdn2.com and save them to data/recording/svg
server.any('https://cdn2.com/*').passthrough(false).recordingName('svg');
// record requests from /api and save them to data/recording/mock (default)
server.any('/api/*').passthrough(false);
});
```
#### Parameters
* `configFn`
### passthroughMocking
Forces passthrough mode for mocking. Requires mocking to be started.
```
I.passthroughMocking();
```
### recordMocking
Forces record mode for mocking. Requires mocking to be started.
```
I.recordMocking();
```
### replayMocking
Forces replay mode for mocking. Requires mocking to be started.
```
I.replayMocking();
```
### startMocking
Starts mocking of http requests. In record mode starts recording of all requests. In replay mode blocks all requests and replaces them with saved.
If inside one test you plan to record/replay requests in several places, provide [recording name (opens new window)](https://netflix.github.io/pollyjs/#/api?id=recordingname) as the parameter.
```
// start mocking requests for a test
I.startMocking();
// start mocking requests for main page
I.startMocking('main-page');
// do actions
I.stopMocking();
I.startMocking('login-page');
```
To update [PollyJS configuration (opens new window)](https://netflix.github.io/pollyjs/#/configuration) use secon argument:
```
// change mode
I.startMocking('comments', { mode: 'replay' });
// override config
I.startMocking('users-loaded', {
recordFailedRequests: true
})
```
#### Parameters
* `title` **any** (optional, default `'Test'`)
* `config` (optional, default `{}`)
### stopMocking
Stops mocking requests. Must be called to save recorded requests into faile.
```
I.stopMocking();
```
codeceptjs TestCafe TestCafe
=========
**Extends Helper**
Uses [TestCafe (opens new window)](https://github.com/DevExpress/testcafe) library to run cross-browser tests. The browser version you want to use in tests must be installed on your system.
Requires `testcafe` package to be installed.
```
npm i testcafe --save-dev
```
Configuration
--------------
This helper should be configured in codecept.json or codecept.conf.js
* `url`: base url of website to be tested
* `show`: - show browser window.
* `windowSize`: (optional) - set browser window width and height
* `getPageTimeout` config option to set maximum navigation time in milliseconds.
* `waitForTimeout`: (optional) default wait\* timeout in ms. Default: 5000.
* `browser`: - See [https://devexpress.github.io/testcafe/documentation/using-testcafe/common-concepts/browsers/browser-support.html (opens new window)](https://devexpress.github.io/testcafe/documentation/using-testcafe/common-concepts/browsers/browser-support)
#### Example #1: Show chrome browser window
```
{
helpers: {
TestCafe : {
url: "http://localhost",
waitForTimeout: 15000,
show: true,
browser: "chrome"
}
}
}
```
To use remote device you can provide 'remote' as browser parameter this will display a link with QR Code See [https://devexpress.github.io/testcafe/documentation/recipes/test-on-remote-computers-and-mobile-devices.html (opens new window)](https://devexpress.github.io/testcafe/documentation/recipes/test-on-remote-computers-and-mobile-devices)
#### Example #2: Remote browser connection
```
{
helpers: {
TestCafe : {
url: "http://localhost",
waitForTimeout: 15000,
browser: "remote"
}
}
}
```
Access From Helpers
--------------------
Call Testcafe methods directly using the testcafe controller.
```
const testcafeTestController = this.helpers['TestCafe'].t;
const comboBox = Selector('.combo-box');
await testcafeTestController
.hover(comboBox) // hover over combo box
.click('#i-prefer-both') // click some other element
```
Methods
--------
### Parameters
* `config`
### \_locate
Get elements by different locator types, including strict locator Should be used in custom helpers:
```
const elements = await this.helpers['TestCafe']._locate('.item');
```
#### Parameters
* `locator`
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `field`
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file.
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `cookieName`
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### executeScript
Executes sync script on a page. Pass arguments to function as additional parameters. Will return execution result to a test. In this case you should use async function and await to receive results.
Example with jQuery DatePicker:
```
// change date of jQuery DatePicker
I.executeScript(function() {
// now we are inside browser context
$('date').datetimepicker('setDate', new Date());
});
```
Can return values. Don't forget to use `await` to get them.
```
let date = await I.executeScript(function(el) {
// only basic types can be returned
return $(el).datetimepicker('getDate').toString();
}, '#date'); // passing jquery selector
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
* `args` **...any** to be passed to function.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** If a function returns a Promise It will wait for it resolution.
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabAttributeFromAll
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabBrowserLogs
Get JS log from browser.
```
let logs = await I.grabBrowserLogs();
console.log(JSON.stringify(logs))
```
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute valueReturns cookie in JSON format. If name not passed returns all cookies for this domain.
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabSource
Retrieves page source and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let pageSource = await I.grabSource();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** source code
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves an array of value from a form located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let inputs = await I.grabValueFromAll('//form/input');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### pressKey
Presses a key on a focused element. Special keys like 'Enter', 'Control', [etc (opens new window)](https://code.google.com/p/selenium/wiki/JsonWireProtocol#/session/:sessionId/element/:id/value) will be replaced with corresponding unicode. If modifier key is used (Control, Command, Alt, Shift) in array, it will be released afterwards.
```
I.pressKey('Enter');
I.pressKey(['Control','a']);
```
#### Parameters
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** key or array of keys to press.
[Valid key names (opens new window)](https://w3c.github.io/webdriver/#keyboard-actions) are:
* `'Add'`,
* `'Alt'`,
* `'ArrowDown'` or `'Down arrow'`,
* `'ArrowLeft'` or `'Left arrow'`,
* `'ArrowRight'` or `'Right arrow'`,
* `'ArrowUp'` or `'Up arrow'`,
* `'Backspace'`,
* `'Command'`,
* `'Control'`,
* `'Del'`,
* `'Divide'`,
* `'End'`,
* `'Enter'`,
* `'Equals'`,
* `'Escape'`,
* `'F1 to F12'`,
* `'Home'`,
* `'Insert'`,
* `'Meta'`,
* `'Multiply'`,
* `'Numpad 0'` to `'Numpad 9'`,
* `'Pagedown'` or `'PageDown'`,
* `'Pageup'` or `'PageUp'`,
* `'Pause'`,
* `'Semicolon'`,
* `'Shift'`,
* `'Space'`,
* `'Subtract'`,
* `'Tab'`.
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels.
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeTextEquals
Checks that text is equal to provided one.
```
I.seeTextEquals('text', 'h1');
```
#### Parameters
* `text`
* `context`
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.
### switchTo
Switches frame or in case of null locator reverts to parent.
```
I.switchTo('iframe'); // switch to first iframe
I.switchTo(); // switch back to main page
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### useTestCafeTo
Use [TestCafe (opens new window)](https://devexpress.github.io/testcafe/documentation/test-api/) API inside a test.
First argument is a description of an action. Second argument is async function that gets this helper as parameter.
{ [`t` (opens new window)](https://devexpress.github.io/testcafe/documentation/test-api/test-code-structure.html#test-controller)) } object from TestCafe API is available.
```
I.useTestCafeTo('handle browser dialog', async ({ t }) {
await t.setNativeDialogHandler(() => true);
});
```
#### Parameters
* `description` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** used to show in logs.
* `fn` **[function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** async functuion that executed with TestCafe helper as argument
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitInUrl
Waiting for the part of the URL to match the expected. Useful for SPA to understand that page was changed.
```
I.waitInUrl('/info', 2);
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitNumberOfVisibleElements
Waits for a specified number of elements on the page.
```
I.waitNumberOfVisibleElements('a', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitUrlEquals
Waits for the entire URL to match the expected
```
I.waitUrlEquals('/info', 2);
I.waitUrlEquals('http://127.0.0.1:8000/info');
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
getPageUrl
-----------
Client Functions
### Parameters
* `t`
| programming_docs |
codeceptjs ApiDataFactory ApiDataFactory
===============
**Extends Helper**
Helper for managing remote data using REST API. Uses data generators like [rosie (opens new window)](https://github.com/rosiejs/rosie) or factory girl to create new record.
By defining a factory you set the rules of how data is generated. This data will be saved on server via REST API and deleted in the end of a test.
Use Case
---------
Acceptance tests interact with a websites using UI and real browser. There is no way to create data for a specific test other than from user interface. That makes tests slow and fragile. Instead of testing a single feature you need to follow all creation/removal process.
This helper solves this problem. Most of web application have API, and it can be used to create and delete test records. By combining REST API with Factories you can easily create records for tests:
```
I.have('user', { login: 'davert', email: '[email protected]' });
let id = await I.have('post', { title: 'My first post'});
I.haveMultiple('comment', 3, {post_id: id});
```
To make this work you need
1. REST API endpoint which allows to perform create / delete requests and
2. define data generation rules
### Setup
Install [Rosie (opens new window)](https://github.com/rosiejs/rosie) and [Faker (opens new window)](https://www.npmjs.com/package/faker) libraries.
```
npm i rosie faker --save-dev
```
Create a factory file for a resource.
See the example for Posts factories:
```
// tests/factories/posts.js
var Factory = require('rosie').Factory;
var faker = require('faker');
module.exports = new Factory()
// no need to set id, it will be set by REST API
.attr('author', () => faker.name.findName())
.attr('title', () => faker.lorem.sentence())
.attr('body', () => faker.lorem.paragraph());
```
For more options see [rosie documentation (opens new window)](https://github.com/rosiejs/rosie).
Then configure ApiDataHelper to match factories and REST API:
### Configuration
ApiDataFactory has following config options:
* `endpoint`: base URL for the API to send requests to.
* `cleanup` (default: true): should inserted records be deleted up after tests
* `factories`: list of defined factories
* `returnId` (default: false): return id instead of a complete response when creating items.
* `headers`: list of headers
* `REST`: configuration for REST requests
See the example:
```
ApiDataFactory: {
endpoint: "http://user.com/api",
cleanup: true,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
},
factories: {
post: {
uri: "/posts",
factory: "./factories/post",
},
comment: {
factory: "./factories/comment",
create: { post: "/comments/create" },
delete: { post: "/comments/delete/{id}" },
fetchId: (data) => data.result.id
}
}
}
```
It is required to set REST API `endpoint` which is the baseURL for all API requests. Factory file is expected to be passed via `factory` option.
This Helper uses [REST (opens new window)](https://codecept.io/helpers/REST/) helper and accepts its configuration in "REST" section. For instance, to set timeout you should add:
```
"ApiDataFactory": {
"REST": {
"timeout": "100000",
}
}
```
### Requests
By default to create a record ApiDataFactory will use endpoint and plural factory name:
* create: `POST {endpoint}/{resource} data`
* delete: `DELETE {endpoint}/{resource}/id`
Example (`endpoint`: `http://app.com/api`):
* create: POST request to `http://app.com/api/users`
* delete: DELETE request to `http://app.com/api/users/1`
This behavior can be configured with following options:
* `uri`: set different resource uri. Example: `uri: account` => `http://app.com/api/account`.
* `create`: override create options. Expected format: `{ method: uri }`. Example: `{ "post": "/users/create" }`
* `delete`: override delete options. Expected format: `{ method: uri }`. Example: `{ "post": "/users/delete/{id}" }`
Requests can also be overridden with a function which returns [axois request config (opens new window)](https://github.com/axios/axios#request-config).
```
create: (data) => ({ method: 'post', url: '/posts', data }),
delete: (id) => ({ method: 'delete', url: '/posts', data: { id } })
```
Requests can be updated on the fly by using `onRequest` function. For instance, you can pass in current session from a cookie.
```
onRequest: async (request) => {
// using global codeceptjs instance
let cookie = await codeceptjs.container.helpers('WebDriver').grabCookie('session');
request.headers = { Cookie: `session=${cookie.value}` };
}
```
### Responses
By default `I.have()` returns a promise with a created data:
```
let client = await I.have('client');
```
Ids of created records are collected and used in the end of a test for the cleanup. If you need to receive `id` instead of full response enable `returnId` in a helper config:
```
// returnId: false
let clientId = await I.have('client');
// clientId == 1
// returnId: true
let clientId = await I.have('client');
// client == { name: 'John', email: '[email protected]' }
```
By default `id` property of response is taken. This behavior can be changed by setting `fetchId` function in a factory config.
```
factories: {
post: {
uri: "/posts",
factory: "./factories/post",
fetchId: (data) => data.result.posts[0].id
}
}
```
Methods
--------
### Parameters
* `config`
### \_requestCreate
Executes request to create a record in API. Can be replaced from a in custom helper.
#### Parameters
* `factory` **any**
* `data` **any**
### \_requestDelete
Executes request to delete a record in API Can be replaced from a custom helper.
#### Parameters
* `factory` **any**
* `id` **any**
### have
Generates a new record using factory and saves API request to store it.
```
// create a user
I.have('user');
// create user with defined email
// and receive it when inside async function
const user = await I.have('user', { email: '[email protected]'});
```
#### Parameters
* `factory` **any** factory to use
* `params` **any** predefined parameters
### haveMultiple
Generates bunch of records and saves multiple API requests to store them.
```
// create 3 posts
I.haveMultiple('post', 3);
// create 3 posts by one author
I.haveMultiple('post', 3, { author: 'davert' });
```
#### Parameters
* `factory` **any**
* `times` **any**
* `params` **any**
codeceptjs FileSystem FileSystem
===========
**Extends Helper**
Helper for testing filesystem. Can be easily used to check file structures:
```
I.amInPath('test');
I.seeFile('codecept.json');
I.seeInThisFile('FileSystem');
I.dontSeeInThisFile("WebDriverIO");
```
Methods
--------
### amInPath
Enters a directory In local filesystem. Starts from a current directory
#### Parameters
* `openPath` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### dontSeeFileContentsEqual
Checks that contents of file found by `seeFile` doesn't equal to text.
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### dontSeeInThisFile
Checks that file found by `seeFile` doesn't include text.
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### grabFileNames
Returns file names in current directory.
```
I.handleDownloads();
I.click('Download Files');
I.amInPath('output/downloads');
const downloadedFileNames = I.grabFileNames();
```
### seeFile
Checks that file exists
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### seeFileContentsEqual
Checks that contents of file found by `seeFile` equal to text.
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### seeFileContentsEqualReferenceFile
Checks that contents of the file found by `seeFile` equal to contents of the file at `pathToReferenceFile`.
#### Parameters
* `pathToReferenceFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encodingReference` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### seeFileNameMatching
Checks that file with a name including given text exists in the current directory.
```
I.handleDownloads();
I.click('Download as PDF');
I.amInPath('output/downloads');
I.seeFileNameMatching('.pdf');
```
#### Parameters
* `text`
### seeInThisFile
Checks that file found by `seeFile` includes a text.
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### waitForFile
Waits for file to be present in current directory.
```
I.handleDownloads();
I.click('Download large File');
I.amInPath('output/downloads');
I.waitForFile('largeFilesName.txt', 10); // wait 10 seconds for file
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** seconds to wait
### writeToFile
Writes test to file
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
getFileContents
----------------
### Parameters
* `file` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `encoding` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
Returns **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
isFileExists
-------------
### Parameters
* `file` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `timeout` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>**
codeceptjs Playwright Playwright
===========
**Extends Helper**
Uses [Playwright (opens new window)](https://github.com/microsoft/playwright) library to run tests inside:
* Chromium
* Firefox
* Webkit (Safari)
This helper works with a browser out of the box with no additional tools required to install.
Requires `playwright` package version ^1 to be installed:
```
npm i playwright@^1 --save
```
Configuration
--------------
This helper should be configured in codecept.json or codecept.conf.js
* `url`: base url of website to be tested
* `browser`: a browser to test on, either: `chromium`, `firefox`, `webkit`. Default: chromium.
* `show`: - show browser window.
* `restart`: - restart browser between tests.
* `disableScreenshots`: - don't save screenshot on failure.
* `emulate`: launch browser in device emulation mode.
* `fullPageScreenshots` - make full page screenshots on failure.
* `uniqueScreenshotNames`: - option to prevent screenshot override if you have scenarios with the same name in different suites.
* `keepBrowserState`: - keep browser state between tests when `restart` is set to false.
* `keepCookies`: - keep cookies between tests when `restart` is set to false.
* `waitForAction`: (optional) how long to wait after click, doubleClick or PressKey actions in ms. Default: 100.
* `waitForNavigation`: . When to consider navigation succeeded. Possible options: `load`, `domcontentloaded`, `networkidle`. Choose one of those options is possible. See [Playwright API (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#pagewaitfornavigationoptions).
* `pressKeyDelay`: . Delay between key presses in ms. Used when calling Playwrights page.type(...) in fillField/appendField
* `getPageTimeout` config option to set maximum navigation time in milliseconds.
* `waitForTimeout`: (optional) default wait\* timeout in ms. Default: 1000.
* `basicAuth`: (optional) the basic authentication to pass to base url. Example: {username: 'username', password: 'password'}
* `windowSize`: (optional) default window size. Set a dimension like `640x480`.
* `userAgent`: (optional) user-agent string.
* `manualStart`: - do not start browser before a test, start it manually inside a helper with `this.helpers["Playwright"]._startBrowser()`.
* `chromium`: (optional) pass additional chromium options
#### Example #1: Wait for 0 network connections.
```
{
helpers: {
Playwright : {
url: "http://localhost",
restart: false,
waitForNavigation: "networkidle0",
waitForAction: 500
}
}
}
```
#### Example #2: Wait for DOMContentLoaded event
```
{
helpers: {
Playwright : {
url: "http://localhost",
restart: false,
waitForNavigation: "domcontentloaded",
waitForAction: 500
}
}
}
```
#### Example #3: Debug in window mode
```
{
helpers: {
Playwright : {
url: "http://localhost",
show: true
}
}
}
```
#### Example #4: Connect to remote browser by specifying [websocket endpoint (opens new window)](https://chromedevtools.github.io/devtools-protocol/#how-do-i-access-the-browser-target)
```
{
helpers: {
Playwright: {
url: "http://localhost",
chromium: {
browserWSEndpoint: "ws://localhost:9222/devtools/browser/c5aa6160-b5bc-4d53-bb49-6ecb36cd2e0a"
}
}
}
}
```
#### Example #5: Testing with Chromium extensions
[official docs (opens new window)](https://github.com/microsoft/playwright/blob/v0.11.0/docs/api.md#working-with-chrome-extensions)
```
{
helpers: {
Playwright: {
url: "http://localhost",
show: true // headless mode not supported for extensions
chromium: {
args: [
`--disable-extensions-except=${pathToExtension}`,
`--load-extension=${pathToExtension}`
]
}
}
}
}
```
#### Example #6: Launch tests emulating iPhone 6
```
const { devices } = require('playwright');
{
helpers: {
Playwright: {
url: "http://localhost",
emulate: devices['iPhone 6'],
}
}
}
```
Note: When connecting to remote browser `show` and specific `chrome` options (e.g. `headless` or `devtools`) are ignored.
Access From Helpers
--------------------
Receive Playwright client from a custom helper by accessing `browser` for the Browser object or `page` for the current Page object:
```
const { browser } = this.helpers.Playwright;
await browser.pages(); // List of pages in the browser
// get current page
const { page } = this.helpers.Playwright;
await page.url(); // Get the url of the current page
const { browserContext } = this.helpers.Playwright;
await browserContext.cookies(); // get current browser context
```
Methods
--------
### Parameters
* `config`
### \_addPopupListener
Add the 'dialog' event listener to a page
#### Parameters
* `page`
### \_getPageUrl
Gets page URL including hash.
### \_locate
Get elements by different locator types, including strict locator Should be used in custom helpers:
```
const elements = await this.helpers['Playwright']._locate({name: 'password'});
```
#### Parameters
* `locator`
### \_locateCheckable
Find a checkbox by providing human readable text: NOTE: Assumes the checkable element exists
```
this.helpers['Playwright']._locateCheckable('I agree with terms and conditions').then // ...
```
#### Parameters
* `locator`
* `providedContext`
### \_locateClickable
Find a clickable element by providing human readable text:
```
this.helpers['Playwright']._locateClickable('Next page').then // ...
```
#### Parameters
* `locator`
### \_locateFields
Find field elements by providing human readable text:
```
this.helpers['Playwright']._locateFields('Your email').then // ...
```
#### Parameters
* `locator`
### \_setPage
Set current page
#### Parameters
* `page` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** page to set
### acceptPopup
Accepts the active JavaScript native popup window, as created by window.alert|window.confirm|window.prompt. Don't confuse popups with modal windows, as created by [various libraries (opens new window)](http://jster.net/category/windows-modals-popups).
### amAcceptingPopups
Set the automatic popup response to Accept. This must be set before a popup is triggered.
```
I.amAcceptingPopups();
I.click('#triggerPopup');
I.acceptPopup();
```
### amCancellingPopups
Set the automatic popup response to Cancel/Dismiss. This must be set before a popup is triggered.
```
I.amCancellingPopups();
I.click('#triggerPopup');
I.cancelPopup();
```
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file.
### cancelPopup
Dismisses the active JavaScript popup, as created by window.alert|window.confirm|window.prompt.
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### clickLink
Clicks link and waits for navigation (deprecated)
#### Parameters
* `locator`
* `context`
### closeCurrentTab
Close current tab and switches to previous.
```
I.closeCurrentTab();
```
### closeOtherTabs
Close all tabs except for the current one.
```
I.closeOtherTabs();
```
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### dontSeeInTitle
Checks that title does not contain text.
```
I.dontSeeInTitle('Error');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### dragAndDrop
Drag an item to a destination element.
```
I.dragAndDrop('#dragHandle', '#container');
```
#### Parameters
* `srcElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `destElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### dragSlider
Drag the scrubber of a slider to a given position For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.dragSlider('#slider', 30);
I.dragSlider('#slider', -70);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** position to drag.
### executeScript
Executes a script on the page:
```
I.executeScript(() => window.alert('Hello world'));
```
Additional parameters of the function can be passed as an object argument:
```
I.executeScript(({x, y}) => x + y, {x, y});
```
You can pass only one parameter into a function but you can pass in array or object.
```
I.executeScript(([x, y]) => x + y, [x, y]);
```
If a function returns a Promise it will wait for its resolution.
#### Parameters
* `fn`
* `arg`
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
### forceClick
Force clicks an element without waiting for it to become visible and not animating.
```
I.forceClick('#hiddenButton');
I.forceClick('Click me', '#hidden');
```
#### Parameters
* `locator`
* `context`
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabAttributeFromAll
Retrieves an array of attributes from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let hints = await I.grabAttributeFromAll('.tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabBrowserLogs
Get JS log from browser.
```
let logs = await I.grabBrowserLogs();
console.log(JSON.stringify(logs))
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>>**
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute valueReturns cookie in JSON format. If name not passed returns all cookies for this domain.
### grabCssPropertyFrom
Grab CSS property for given locator Resumes test execution, so **should be used inside an async function with `await`** operator. If more than one element is found - value of first element is returned.
```
const value = await I.grabCssPropertyFrom('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** CSS value
### grabCssPropertyFromAll
Grab array of CSS properties for given locator Resumes test execution, so **should be used inside an async function with `await`** operator.
```
const values = await I.grabCssPropertyFromAll('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** CSS value
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabDataFromPerformanceTiming
Grab the data from performance timing using Navigation Timing API. The returned data will contain following things in ms:
* responseEnd,
* domInteractive,
* domContentLoadedEventEnd,
* loadEventEnd Resumes test execution, so **should be used inside an async function with `await`** operator.
```
await I.amOnPage('https://example.com');
let data = await I.grabDataFromPerformanceTiming();
//Returned data
{ // all results are in [ms]
responseEnd: 23,
domInteractive: 44,
domContentLoadedEventEnd: 196,
loadEventEnd: 241
}
```
### grabElementBoundingRect
Grab the width, height, location of given locator. Provide `width` or `height`as second param to get your desired prop. Resumes test execution, so **should be used inside an async function with `await`** operator.
Returns an object with `x`, `y`, `width`, `height` keys.
```
const value = await I.grabElementBoundingRect('h3');
// value is like { x: 226.5, y: 89, width: 527, height: 220 }
```
To get only one metric use second parameter:
```
const width = await I.grabElementBoundingRect('h3', 'width');
// width == 527
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `prop`
* `elementSize` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** x, y, width or height of the given element.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<DOMRect> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>)** Element bounding rectangle
### grabHTMLFrom
Retrieves the innerHTML from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - HTML of first element is returned.
```
let postHTML = await I.grabHTMLFrom('#post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** HTML code for an element
### grabHTMLFromAll
Retrieves all the innerHTML from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let postHTMLs = await I.grabHTMLFromAll('.post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** HTML code for an element
### grabNumberOfOpenTabs
Grab number of open tabs. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let tabs = await I.grabNumberOfOpenTabs();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of open tabs
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabPopupText
Grab the text within the popup. If no popup is visible then it will return null
```
await I.grabPopupText();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | null)>**
### grabSource
Retrieves page source and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let pageSource = await I.grabSource();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** source code
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabTitle
Retrieves a page title and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let title = await I.grabTitle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** title
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves an array of value from a form located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let inputs = await I.grabValueFromAll('//form/input');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### handleDownloads
Handles a file download.Aa file name is required to save the file on disk. Files are saved to "output" directory.
Should be used with [FileSystem helper (opens new window)](https://codecept.io/helpers/FileSystem) to check that file were downloaded correctly.
```
I.handleDownloads('downloads/avatar.jpg');
I.click('Download Avatar');
I.amInPath('output/downloads');
I.waitForFile('downloads/avatar.jpg', 5);
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** set filename for downloaded file
### haveRequestHeaders
Set headers for all next requests
```
I.haveRequestHeaders({
'X-Sent-By': 'CodeceptJS',
});
```
#### Parameters
* `customHeaders` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** headers to set
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### openNewTab
Open new tab and automatically switched to new tab
```
I.openNewTab();
```
You can pass in [page options (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#browsernewpageoptions) to emulate device on this page
```
// enable mobile
I.openNewTab({ isMobile: true });
```
#### Parameters
* `options`
### pressKey
Presses a key in the browser (on a focused element).
*Hint:* For populating text field or textarea, it is recommended to use [`fillField`](#fillfield).
```
I.pressKey('Backspace');
```
To press a key in combination with modifier keys, pass the sequence as an array. All modifier keys (`'Alt'`, `'Control'`, `'Meta'`, `'Shift'`) will be released afterwards.
```
I.pressKey(['Control', 'Z']);
```
For specifying operation modifier key based on operating system it is suggested to use `'CommandOrControl'`. This will press `'Command'` (also known as `'Meta'`) on macOS machines and `'Control'` on non-macOS machines.
```
I.pressKey(['CommandOrControl', 'Z']);
```
Some of the supported key names are:
* `'AltLeft'` or `'Alt'`
* `'AltRight'`
* `'ArrowDown'`
* `'ArrowLeft'`
* `'ArrowRight'`
* `'ArrowUp'`
* `'Backspace'`
* `'Clear'`
* `'ControlLeft'` or `'Control'`
* `'ControlRight'`
* `'Command'`
* `'CommandOrControl'`
* `'Delete'`
* `'End'`
* `'Enter'`
* `'Escape'`
* `'F1'` to `'F12'`
* `'Home'`
* `'Insert'`
* `'MetaLeft'` or `'Meta'`
* `'MetaRight'`
* `'Numpad0'` to `'Numpad9'`
* `'NumpadAdd'`
* `'NumpadDecimal'`
* `'NumpadDivide'`
* `'NumpadMultiply'`
* `'NumpadSubtract'`
* `'PageDown'`
* `'PageUp'`
* `'Pause'`
* `'Return'`
* `'ShiftLeft'` or `'Shift'`
* `'ShiftRight'`
* `'Space'`
* `'Tab'`
#### Parameters
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** key or array of keys to press.*Note:* Shortcuts like `'Meta'` + `'A'` do not work on macOS ([GoogleChrome/Puppeteer#1313 (opens new window)](https://github.com/GoogleChrome/puppeteer/issues/1313)).
### pressKeyDown
Presses a key in the browser and leaves it in a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to press down.
### pressKeyUp
Releases a key in the browser which was previously set to a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to release.
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels.Unlike other drivers Playwright changes the size of a viewport, not the window! Playwright does not control the window of a browser so it can't adjust its real size. It also can't maximize a window.Update configuration to change real window size on start:```js // inside codecept.conf.js // @codeceptjs/configure package must be installed { setWindowSize } = require('@codeceptjs/configure');
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
### seeAttributesOnElements
Checks that all elements with given locator have given attributes.
```
I.seeAttributesOnElements('//form', { method: "post"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `attributes` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** attributes and their values to check.
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCssPropertiesOnElements
Checks that all elements with given locator have given CSS properties.
```
I.seeCssPropertiesOnElements('h3', { 'font-weight': "bold"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `cssProperties` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** object with CSS properties and their values to check.
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInPopup
Checks that the active JavaScript popup, as created by `window.alert|window.confirm|window.prompt`, contains the given string.
```
I.seeInPopup('Popup text');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInTitle
Checks that title contains text.
```
I.seeInTitle('Home Page');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to check.
### seeNumberOfElements
Asserts that an element appears a given number of times in the DOM. Element is located by label or name or CSS or XPath.
```
I.seeNumberOfElements('#submitBtn', 1);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeTextEquals
Checks that text is equal to provided one.
```
I.seeTextEquals('text', 'h1');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** element value to check.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)?)** element located by CSS|XPath|strict locator.
### seeTitleEquals
Checks that title is equal to provided one.
```
I.seeTitleEquals('Test title.');
```
#### Parameters
* `text`
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.
### switchTo
Switches frame or in case of null locator reverts to parent.
```
I.switchTo('iframe'); // switch to first iframe
I.switchTo(); // switch back to main page
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### switchToNextTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToNextTab();
I.switchToNextTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
### switchToPreviousTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToPreviousTab();
I.switchToPreviousTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)**
### type
Types out the given text into an active field. To slow down typing use a second parameter, to set interval between key presses. *Note:* Should be used when [`fillField`](#fillfield) is not an option.
```
// passing in a string
I.type('Type this out.');
// typing values with a 100ms interval
I.type('4141555311111111', 100);
// passing in an array
I.type(['T', 'E', 'X', 'T']);
```
#### Parameters
* `keys`
* `delay` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional) delay in ms between key presses
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** or array of keys to type.
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### usePlaywrightTo
Use Playwright API inside a test.
First argument is a description of an action. Second argument is async function that gets this helper as parameter.
{ [`page` (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#class-page), [`context` (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#class-context) [`browser` (opens new window)](https://github.com/microsoft/playwright/blob/master/docs/api.md#class-browser) } objects from Playwright API are available.
```
I.usePlaywrightTo('emulate offline mode', async ({ context }) {
await context.setOffline(true);
});
```
#### Parameters
* `description` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** used to show in logs.
* `fn` **[function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** async functuion that executed with Playwright helper as argument
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForClickable
Waits for element to be clickable (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForClickable('.btn.continue');
I.waitForClickable('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `waitTimeout`
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForDetached
Waits for an element to become not attached to the DOM on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForDetached('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForEnabled
Waits for element to become enabled (by default waits for 1sec). Element can be located by CSS or XPath.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional) time in seconds to wait, 1 by default.
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForNavigation
Waits for navigation to finish. By default takes configured `waitForNavigation` option.
See [Pupeteer's reference (opens new window)](https://github.com/microsoft/Playwright/blob/master/docs/api.md#pagewaitfornavigationoptions)
#### Parameters
* `opts` **any**
### waitForRequest
Waits for a network request.
```
I.waitForRequest('http://example.com/resource');
I.waitForRequest(request => request.url() === 'http://example.com' && request.method() === 'GET');
```
#### Parameters
* `urlOrPredicate` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))**
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** seconds to wait
### waitForResponse
Waits for a network request.
```
I.waitForResponse('http://example.com/resource');
I.waitForResponse(request => request.url() === 'http://example.com' && request.method() === 'GET');
```
#### Parameters
* `urlOrPredicate` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))**
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** number of seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForValue
Waits for the specified value to be in value attribute.
```
I.waitForValue('//input', "GoodValue");
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** input field.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected value.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to waitThis method accepts [React selectors (opens new window)](https://codecept.io/react).
### waitInUrl
Waiting for the part of the URL to match the expected. Useful for SPA to understand that page was changed.
```
I.waitInUrl('/info', 2);
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitNumberOfVisibleElements
Waits for a specified number of elements on the page.
```
I.waitNumberOfVisibleElements('a', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitUntil
Waits for a function to return true (waits for 1sec by default).
```
I.waitUntil(() => window.requests == 0);
I.waitUntil(() => window.requests == 0, 5);
```
#### Parameters
* `fn` **([function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function) | [string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String))** function which is executed in browser context.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `timeoutMsg` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** message to show in case of timeout fail.
* `interval` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?**
### waitUrlEquals
Waits for the entire URL to match the expected
```
I.waitUrlEquals('/info', 2);
I.waitUrlEquals('http://127.0.0.1:8000/info');
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
| programming_docs |
codeceptjs Detox Detox
======
Detox
------
**Extends Helper**
This is a wrapper on top of [Detox (opens new window)](https://github.com/wix/Detox) library, aimied to unify testing experience for CodeceptJS framework. Detox provides a grey box testing for mobile applications, playing especially good for React Native apps.
Detox plays quite differently from Appium. To establish detox testing you need to build a mobile application in a special way to inject Detox code. This why **Detox is grey box testing** solution, so you need an access to application source code, and a way to build and execute it on emulator.
Comparing to Appium, Detox runs faster and more stable but requires an additional setup for build.
### Setup
1. [Install and configure Detox for iOS (opens new window)](https://github.com/wix/Detox/blob/master/docs/Introduction.GettingStarted.md) and [Android (opens new window)](https://github.com/wix/Detox/blob/master/docs/Introduction.Android.md)
2. [Build an application (opens new window)](https://github.com/wix/Detox/blob/master/docs/Introduction.GettingStarted.md#step-4-build-your-app-and-run-detox-tests) using `detox build` command.
3. Install [CodeceptJS (opens new window)](https://codecept.io) and detox-helper:
npm i @codeceptjs/detox-helper --save
Detox configuration is required in `package.json` under `detox` section.
If you completed step 1 and step 2 you should have a configuration similar this:
```
"detox": {
"configurations": {
"ios.sim.debug": {
"binaryPath": "ios/build/Build/Products/Debug-iphonesimulator/example.app",
"build": "xcodebuild -project ios/example.xcodeproj -scheme example -configuration Debug -sdk iphonesimulator -derivedDataPath ios/build",
"type": "ios.simulator",
"name": "iPhone 7"
}
}
}
```
### Configuration
Besides Detox configuration, CodeceptJS should also be configured to use Detox.
In `codecept.conf.js` enable Detox helper:
```
helpers: {
Detox: {
require: '@codeceptjs/detox-helper',
configuration: '<detox-configuration-name>',
}
}
```
It's important to specify a package name under `require` section and current detox configuration taken from `package.json`.
Options:
* `configuration` - a detox configuration name. Required.
* `reloadReactNative` - should be enabled for React Native applications.
* `reuse` - reuse application for tests. By default, Detox reinstalls and relaunches app.
* `registerGlobals` - (default: true) Register Detox helper functions `by`, `element`, `expect`, `waitFor` globally.
### Parameters
* `config`
### appendField
Appends text into the field. A field can be located by text, accessibility id, id.
```
I.appendField('name', 'davert');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### clearField
Clears a text field. A field can be located by text, accessibility id, id.
```
I.clearField('~name');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an input element to clear
### click
Clicks on an element. Element can be located by its text or id or accessibility id
The second parameter is a context (id | type | accessibility id) to narrow the search.
Same as [tap](#tap)
```
I.click('Login'); // locate by text
I.click('~nav-1'); // locate by accessibility label
I.click('#user'); // locate by id
I.click('Login', '#nav'); // locate by text inside #nav
I.click({ ios: 'Save', android: 'SAVE' }, '#main'); // different texts on iOS and Android
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** (optional, default `null`)
### clickAtPoint
Performs click on element with horizontal and vertical offset. An element is located by text, id, accessibility id.
```
I.clickAtPoint('Save', 10, 10);
I.clickAtPoint('~save', 10, 10); // locate by accessibility id
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
* `x` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** horizontal offset (optional, default `0`)
* `y` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** vertical offset (optional, default `0`)
### dontSee
Checks text not to be visible. Use second parameter to narrow down the search.
```
I.dontSee('Record created');
I.dontSee('Record updated', '#message');
I.dontSee('Record deleted', '~message');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check invisibility
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** element in which to search for text (optional, default `null`)
### dontSeeElement
Checks that element is not visible. Use second parameter to narrow down the search.
```
I.dontSeeElement('~edit'); // located by accessibility id
I.dontSeeElement('~edit', '#menu'); // element inside #menu
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** context element (optional, default `null`)
### dontSeeElementExists
Checks that element not exists. Use second parameter to narrow down the search.
```
I.dontSeeElementExist('~edit'); // located by accessibility id
I.dontSeeElementExist('~edit', '#menu'); // element inside #menu
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** context element (optional, default `null`)
### fillField
Fills in text field in an app. A field can be located by text, accessibility id, id.
```
I.fillField('Username', 'davert');
I.fillField('~name', 'davert');
I.fillField({ android: 'NAME', ios: 'name' }, 'davert');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an input element to fill in
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to fill
### goBack
Goes back on Android
```
I.goBack(); // on Android only
```
### installApp
Installs a configured application. Application is installed by default.
```
I.installApp();
```
### launchApp
Launches an application. If application instance already exists, use [relaunchApp](#relaunchApp).
```
I.launchApp();
```
### longPress
Taps an element and holds for a requested time.
```
I.longPress('Login', 2); // locate by text, hold for 2 seconds
I.longPress('~nav', 1); // locate by accessibility label, hold for second
I.longPress('Update', 2, '#menu'); // locate by text inside #menu, hold for 2 seconds
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of seconds to hold tap
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** context element (optional, default `null`)
### multiTap
Multi taps on an element. Element can be located by its text or id or accessibility id.
Set the number of taps in second argument. Optionally define the context element by third argument.
```
I.multiTap('Login', 2); // locate by text
I.multiTap('~nav', 2); // locate by accessibility label
I.multiTap('#user', 2); // locate by id
I.multiTap('Update', 2, '#menu'); // locate by id
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of taps
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** context element (optional, default `null`)
### relaunchApp
Relaunches an application.
```
I.relaunchApp();
```
### runOnAndroid
Execute code only on Android
```
I.runOnAndroid(() => {
I.click('Button');
I.see('Hi, Android');
});
```
#### Parameters
* `fn` **[Function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** a function which will be executed on android
### runOnIOS
Execute code only on iOS
```
I.runOnIOS(() => {
I.click('Button');
I.see('Hi, IOS');
});
```
#### Parameters
* `fn` **[Function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** a function which will be executed on iOS
### saveScreenshot
Saves a screenshot to the output dir
```
I.saveScreenshot('main-window.png');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
### scrollDown
Scrolls to the bottom of an element.
```
I.scrollDown('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
### scrollLeft
Scrolls to the left of an element.
```
I.scrollLeft('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
### scrollRight
Scrolls to the right of an element.
```
I.scrollRight('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
### scrollUp
Scrolls to the top of an element.
```
I.scrollUp('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
### see
Checks text to be visible. Use second parameter to narrow down the search.
```
I.see('Record created');
I.see('Record updated', '#message');
I.see('Record deleted', '~message');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check visibility
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** element inside which to search for text (optional, default `null`)
### seeElement
Checks for visibility of an element. Use second parameter to narrow down the search.
```
I.seeElement('~edit'); // located by accessibility id
I.seeElement('~edit', '#menu'); // element inside #menu
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** context element (optional, default `null`)
### seeElementExists
Checks for existence of an element. An element can be visible or not. Use second parameter to narrow down the search.
```
I.seeElementExists('~edit'); // located by accessibility id
I.seeElementExists('~edit', '#menu'); // element inside #menu
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element to locate
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** context element (optional, default `null`)
### setLandscapeOrientation
Switches device to landscape orientation
```
I.setLandscapeOrientation();
```
### setPortraitOrientation
Switches device to portrait orientation
```
I.setPortraitOrientation();
```
### shakeDevice
Shakes the device.
```
I.shakeDevice();
```
### swipeDown
Performs a swipe up inside an element. Can be `slow` or `fast` swipe.
```
I.swipeUp('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element on which to perform swipe
* `speed` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a speed to perform: `slow` or `fast`. (optional, default `'slow'`)
### swipeLeft
Performs a swipe up inside an element. Can be `slow` or `fast` swipe.
```
I.swipeUp('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element on which to perform swipe
* `speed` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a speed to perform: `slow` or `fast`. (optional, default `'slow'`)
### swipeRight
Performs a swipe up inside an element. Can be `slow` or `fast` swipe.
```
I.swipeUp('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element on which to perform swipe
* `speed` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a speed to perform: `slow` or `fast`. (optional, default `'slow'`)
### swipeUp
Performs a swipe up inside an element. Can be `slow` or `fast` swipe.
```
I.swipeUp('#container');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element on which to perform swipe
* `speed` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a speed to perform: `slow` or `fast`. (optional, default `'slow'`)
### tap
Taps on an element. Element can be located by its text or id or accessibility id.
The second parameter is a context element to narrow the search.
Same as [click](#click)
```
I.tap('Login'); // locate by text
I.tap('~nav-1'); // locate by accessibility label
I.tap('#user'); // locate by id
I.tap('Login', '#nav'); // locate by text inside #nav
I.tap({ ios: 'Save', android: 'SAVE' }, '#main'); // different texts on iOS and Android
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))**
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object) | null)** (optional, default `null`)
### wait
Waits for number of seconds
```
I.wait(2); // waits for 2 seconds
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of seconds to wait
### waitForElement
Waits for an element to exist on page.
```
I.waitForElement('#message', 1); // wait for 1 second
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element to wait for
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of seconds to wait, 5 by default (optional, default `5`)
### waitForElementVisible
Waits for an element to be visible on page.
```
I.waitForElementVisible('#message', 1); // wait for 1 second
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element to wait for
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of seconds to wait (optional, default `5`)
### waitToHide
Waits an elment to become not visible.
```
I.waitToHide('#message', 2); // wait for 2 seconds
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** an element to wait for
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of seconds to wait (optional, default `5`)
| programming_docs |
codeceptjs GraphQL GraphQL
========
**Extends Helper**
GraphQL helper allows to send additional requests to a GraphQl endpoint during acceptance tests. [Axios (opens new window)](https://github.com/axios/axios) library is used to perform requests.
Configuration
--------------
* endpoint: GraphQL base URL
* timeout: timeout for requests in milliseconds. 10000ms by default
* defaultHeaders: a list of default headers
* onRequest: a async function which can update request object.
Example
--------
```
GraphQL: {
endpoint: 'http://site.com/graphql/',
onRequest: (request) => {
request.headers.auth = '123';
}
}
```
Access From Helpers
--------------------
Send GraphQL requests by accessing `_executeQuery` method:
```
this.helpers['GraphQL']._executeQuery({
url,
data,
});
```
Methods
--------
### Parameters
* `config`
### \_executeQuery
Executes query via axios call
#### Parameters
* `request` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### \_prepareGraphQLRequest
Prepares request for axios call
#### Parameters
* `operation` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### sendMutation
Send query to GraphQL endpoint over http
```
I.sendMutation(`
mutation createUser($user: UserInput!) {
createUser(user: $user) {
id
name
email
}
}
`,
{ user: {
name: 'John Doe',
email: '[email protected]'
}
},
});
```
#### Parameters
* `mutation` **[String (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `variables` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** that may go along with the mutation
* `options` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** are additional query options
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### sendQuery
Send query to GraphQL endpoint over http. Returns a response as a promise.
```
const response = await I.sendQuery('{ users { name email }}');
// with variables
const response = await I.sendQuery(
'query getUser($id: ID) { user(id: $id) { name email }}',
{ id: 1 },
)
const user = response.data.data;
```
#### Parameters
* `query` **[String (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `variables` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** that may go along with the query
* `options` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** are additional query options
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
codeceptjs WebDriver WebDriver
==========
**Extends Helper**
WebDriver helper which wraps [webdriverio (opens new window)](http://webdriver.io/) library to manipulate browser using Selenium WebDriver or PhantomJS.
WebDriver requires Selenium Server and ChromeDriver/GeckoDriver to be installed. Those tools can be easily installed via NPM. Please check [Testing with WebDriver (opens new window)](https://codecept.io/webdriver/#testing-with-webdriver) for more details.
### Configuration
This helper should be configured in codecept.json or codecept.conf.js
* `url`: base url of website to be tested.
* `basicAuth`: (optional) the basic authentication to pass to base url. Example: {username: 'username', password: 'password'}
* `browser`: browser in which to perform testing.
* `host`: - WebDriver host to connect.
* `port`: - WebDriver port to connect.
* `protocol`: - protocol for WebDriver server.
* `path`: - path to WebDriver server,
* `restart`: - restart browser between tests.
* `smartWait`: (optional) **enables [SmartWait (opens new window)](https://codecept.io/acceptance/#smartwait)**; wait for additional milliseconds for element to appear. Enable for 5 secs: "smartWait": 5000.
* `disableScreenshots`: - don't save screenshots on failure.
* `fullPageScreenshots` - make full page screenshots on failure.
* `uniqueScreenshotNames`: - option to prevent screenshot override if you have scenarios with the same name in different suites.
* `keepBrowserState`: - keep browser state between tests when `restart` is set to false.
* `keepCookies`: - keep cookies between tests when `restart` set to false.
* `windowSize`: (optional) default window size. Set to `maximize` or a dimension in the format `640x480`.
* `waitForTimeout`: sets default wait time in *ms* for all `wait*` functions.
* `desiredCapabilities`: Selenium's [desired capabilities (opens new window)](https://github.com/SeleniumHQ/selenium/wiki/DesiredCapabilities).
* `manualStart`: - do not start browser before a test, start it manually inside a helper with `this.helpers["WebDriver"]._startBrowser()`.
* `timeouts`: [WebDriver timeouts (opens new window)](http://webdriver.io/docs/timeouts) defined as hash.
Example:
```
{
helpers: {
WebDriver : {
smartWait: 5000,
browser: "chrome",
restart: false,
windowSize: "maximize",
timeouts: {
"script": 60000,
"page load": 10000
}
}
}
}
```
Example with basic authentication
```
{
helpers: {
WebDriver : {
smartWait: 5000,
browser: "chrome",
basicAuth: {username: 'username', password: 'password'},
restart: false,
windowSize: "maximize",
timeouts: {
"script": 60000,
"page load": 10000
}
}
}
}
```
Additional configuration params can be used from [webdriverio website (opens new window)](http://webdriver.io/guide/getstarted/configuration).
### Headless Chrome
```
{
helpers: {
WebDriver : {
url: "http://localhost",
browser: "chrome",
desiredCapabilities: {
chromeOptions: {
args: [ "--headless", "--disable-gpu", "--no-sandbox" ]
}
}
}
}
}
```
### Internet Explorer
Additional configuration params can be used from [IE options (opens new window)](https://seleniumhq.github.io/selenium/docs/api/rb/Selenium/WebDriver/IE/Options)
```
{
helpers: {
WebDriver : {
url: "http://localhost",
browser: "internet explorer",
desiredCapabilities: {
ieOptions: {
"ie.browserCommandLineSwitches": "-private",
"ie.usePerProcessProxy": true,
"ie.ensureCleanSession": true,
}
}
}
}
}
```
### Selenoid Options
[Selenoid (opens new window)](https://aerokube.com/selenoid/latest/) is a modern way to run Selenium inside Docker containers. Selenoid is easy to set up and provides more features than original Selenium Server. Use `selenoidOptions` to set Selenoid capabilities
```
{
helpers: {
WebDriver : {
url: "http://localhost",
browser: "chrome",
desiredCapabilities: {
selenoidOptions: {
enableVNC: true,
}
}
}
}
}
```
### Connect Through proxy
CodeceptJS also provides flexible options when you want to execute tests to Selenium servers through proxy. You will need to update the `helpers.WebDriver.capabilities.proxy` key.
```
{
helpers: {
WebDriver: {
capabilities: {
proxy: {
"proxyType": "manual|pac",
"proxyAutoconfigUrl": "URL TO PAC FILE",
"httpProxy": "PROXY SERVER",
"sslProxy": "PROXY SERVER",
"ftpProxy": "PROXY SERVER",
"socksProxy": "PROXY SERVER",
"socksUsername": "USERNAME",
"socksPassword": "PASSWORD",
"noProxy": "BYPASS ADDRESSES"
}
}
}
}
}
```
For example,
```
{
helpers: {
WebDriver: {
capabilities: {
proxy: {
"proxyType": "manual",
"httpProxy": "http://corporate.proxy:8080",
"socksUsername": "codeceptjs",
"socksPassword": "secret",
"noProxy": "127.0.0.1,localhost"
}
}
}
}
}
```
Please refer to [Selenium - Proxy Object (opens new window)](https://github.com/SeleniumHQ/selenium/wiki/DesiredCapabilities) for more information.
### Cloud Providers
WebDriver makes it possible to execute tests against services like `Sauce Labs` `BrowserStack` `TestingBot` Check out their documentation on [available parameters (opens new window)](http://webdriver.io/guide/usage/cloudservices)
Connecting to `BrowserStack` and `Sauce Labs` is simple. All you need to do is set the `user` and `key` parameters. WebDriver automatically know which service provider to connect to.
```
{
helpers:{
WebDriver: {
url: "YOUR_DESIRED_HOST",
user: "YOUR_BROWSERSTACK_USER",
key: "YOUR_BROWSERSTACK_KEY",
capabilities: {
"browserName": "chrome",
// only set this if you're using BrowserStackLocal to test a local domain
// "browserstack.local": true,
// set this option to tell browserstack to provide addition debugging info
// "browserstack.debug": true,
}
}
}
}
```
#### SauceLabs
SauceLabs can be configured via wdio service, which should be installed additionally:
```
npm i @wdio/sauce-service --save
```
It is important to make sure it is compatible with current webdriverio version.
Enable `wdio` plugin in plugins list and add `sauce` service:
```
plugins: {
wdio: {
enabled: true,
services: ['sauce'],
user: ... ,// saucelabs username
key: ... // saucelabs api key
// additional config, from sauce service
}
}
```
See [complete reference on webdriver.io (opens new window)](https://webdriver.io/docs/sauce-service).
> Alternatively, use [codeceptjs-saucehelper (opens new window)](https://github.com/puneet0191/codeceptjs-saucehelper/) for better reporting.
>
>
#### BrowserStack
BrowserStack can be configured via wdio service, which should be installed additionally:
```
npm i @wdio/browserstack-service --save
```
It is important to make sure it is compatible with current webdriverio version.
Enable `wdio` plugin in plugins list and add `browserstack` service:
```
plugins: {
wdio: {
enabled: true,
services: ['browserstack'],
user: ... ,// browserstack username
key: ... // browserstack api key
// additional config, from browserstack service
}
}
```
See [complete reference on webdriver.io (opens new window)](https://webdriver.io/docs/browserstack-service).
> Alternatively, use [codeceptjs-bshelper (opens new window)](https://github.com/PeterNgTr/codeceptjs-bshelper) for better reporting.
>
>
#### TestingBot
> **Recommended**: use official [TestingBot Helper (opens new window)](https://github.com/testingbot/codeceptjs-tbhelper).
>
>
Alternatively, TestingBot can be configured via wdio service, which should be installed additionally:
```
npm i @wdio/testingbot-service --save
```
It is important to make sure it is compatible with current webdriverio version.
Enable `wdio` plugin in plugins list and add `testingbot` service:
```
plugins: {
wdio: {
enabled: true,
services: ['testingbot'],
user: ... ,// testingbot key
key: ... // testingbot secret
// additional config, from testingbot service
}
}
```
See [complete reference on webdriver.io (opens new window)](https://webdriver.io/docs/testingbot-service).
#### Applitools
Visual testing via Applitools service
> Use [CodeceptJS Applitools Helper (opens new window)](https://github.com/PeterNgTr/codeceptjs-applitoolshelper) with Applitools wdio service.
>
>
### Multiremote Capabilities
This is a work in progress but you can control two browsers at a time right out of the box. Individual control is something that is planned for a later version.
Here is the [webdriverio docs (opens new window)](http://webdriver.io/guide/usage/multiremote) on the subject
```
{
helpers: {
WebDriver: {
"multiremote": {
"MyChrome": {
"desiredCapabilities": {
"browserName": "chrome"
}
},
"MyFirefox": {
"desiredCapabilities": {
"browserName": "firefox"
}
}
}
}
}
}
```
Access From Helpers
--------------------
Receive a WebDriver client from a custom helper by accessing `browser` property:
```
const { WebDriver } = this.helpers;
const browser = WebDriver.browser
```
Methods
--------
### Parameters
* `config`
### \_isShadowLocator
Check if locator is type of "Shadow"
#### Parameters
* `locator` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### \_locate
Get elements by different locator types, including strict locator. Should be used in custom helpers:
```
this.helpers['WebDriver']._locate({name: 'password'}).then //...
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `smartWait`
### \_locateCheckable
Find a checkbox by providing human readable text:
```
this.helpers['WebDriver']._locateCheckable('I agree with terms and conditions').then // ...
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### \_locateClickable
Find a clickable element by providing human readable text:
```
const els = await this.helpers.WebDriver._locateClickable('Next page');
const els = await this.helpers.WebDriver._locateClickable('Next page', '.pages');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `context`
### \_locateFields
Find field elements by providing human readable text:
```
this.helpers['WebDriver']._locateFields('Your email').then // ...
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### \_locateShadow
Locate Element within the Shadow Dom
#### Parameters
* `locator` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### \_smartWait
Smart Wait to locate an element
#### Parameters
* `locator` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
### acceptPopup
Accepts the active JavaScript native popup window, as created by window.alert|window.confirm|window.prompt. Don't confuse popups with modal windows, as created by [various libraries (opens new window)](http://jster.net/category/windows-modals-popups).
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file. Appium: not tested
### cancelPopup
Dismisses the active JavaScript popup, as created by window.alert|window.confirm|window.prompt.
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator. Appium: not tested
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### closeCurrentTab
Close current tab.
```
I.closeCurrentTab();
```
### closeOtherTabs
Close all tabs except for the current one.
```
I.closeOtherTabs();
```
### defineTimeout
Set [WebDriver timeouts (opens new window)](https://webdriver.io/docs/timeouts) in realtime.
Timeouts are expected to be passed as object:
```
I.defineTimeout({ script: 5000 });
I.defineTimeout({ implicit: 10000, pageLoad: 10000, script: 5000 });
```
#### Parameters
* `timeouts` **WebdriverIO.Timeouts** WebDriver timeouts object.
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.Appium: not tested
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### dontSeeInTitle
Checks that title does not contain text.
```
I.dontSeeInTitle('Error');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### dragAndDrop
Drag an item to a destination element.
```
I.dragAndDrop('#dragHandle', '#container');
```
#### Parameters
* `srcElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `destElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.Appium: not tested
### dragSlider
Drag the scrubber of a slider to a given position For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.dragSlider('#slider', 30);
I.dragSlider('#slider', -70);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** position to drag.
### executeAsyncScript
Executes async script on page. Provided function should execute a passed callback (as first argument) to signal it is finished.
Example: In Vue.js to make components completely rendered we are waiting for [nextTick (opens new window)](https://vuejs.org/v2/api/#Vue-nextTick).
```
I.executeAsyncScript(function(done) {
Vue.nextTick(done); // waiting for next tick
});
```
By passing value to `done()` function you can return values. Additional arguments can be passed as well, while `done` function is always last parameter in arguments list.
```
let val = await I.executeAsyncScript(function(url, done) {
// in browser context
$.ajax(url, { success: (data) => done(data); }
}, 'http://ajax.callback.url/');
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>**
### executeScript
Executes sync script on a page. Pass arguments to function as additional parameters. Will return execution result to a test. In this case you should use async function and await to receive results.
Example with jQuery DatePicker:
```
// change date of jQuery DatePicker
I.executeScript(function() {
// now we are inside browser context
$('date').datetimepicker('setDate', new Date());
});
```
Can return values. Don't forget to use `await` to get them.
```
let date = await I.executeScript(function(el) {
// only basic types can be returned
return $(el).datetimepicker('getDate').toString();
}, '#date'); // passing jquery selector
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** Wraps [execute (opens new window)](http://webdriver.io/api/protocol/execute) command.
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### forceClick
Perform an emulated click on a link or a button, given by a locator. Unlike normal click instead of sending native event, emulates a click with JavaScript. This works on hidden, animated or inactive elements as well.
If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.forceClick('Logout');
// button of form
I.forceClick('Submit');
// CSS button
I.forceClick('#form input[type=submit]');
// XPath
I.forceClick('//form/*[@type=submit]');
// link in context
I.forceClick('Logout', '#nav');
// using strict locator
I.forceClick({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### forceRightClick
Emulates right click on an element. Unlike normal click instead of sending native event, emulates a click with JavaScript. This works on hidden, animated or inactive elements as well.
If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.forceRightClick('Menu');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### grabAllWindowHandles
Get all Window Handles. Useful for referencing a specific handle when calling `I.switchToWindow(handle)`
```
const windows = await I.grabAllWindowHandles();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>**
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute valueAppium: can be used for apps only with several values ("contentDescription", "text", "className", "resourceId")
### grabAttributeFromAll
Retrieves an array of attributes from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let hints = await I.grabAttributeFromAll('.tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute valueAppium: can be used for apps only with several values ("contentDescription", "text", "className", "resourceId")
### grabBrowserLogs
Get JS log from browser. Log buffer is reset after each request. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let logs = await I.grabBrowserLogs();
console.log(JSON.stringify(logs))
```
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)>> | [undefined (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/undefined))** all browser logs
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute value
### grabCssPropertyFrom
Grab CSS property for given locator Resumes test execution, so **should be used inside an async function with `await`** operator. If more than one element is found - value of first element is returned.
```
const value = await I.grabCssPropertyFrom('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** CSS value
### grabCssPropertyFromAll
Grab array of CSS properties for given locator Resumes test execution, so **should be used inside an async function with `await`** operator.
```
const values = await I.grabCssPropertyFromAll('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** CSS value
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabCurrentWindowHandle
Get the current Window Handle. Useful for referencing it when calling `I.switchToWindow(handle)`
```
const window = await I.grabCurrentWindowHandle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>**
### grabElementBoundingRect
Grab the width, height, location of given locator. Provide `width` or `height`as second param to get your desired prop. Resumes test execution, so **should be used inside an async function with `await`** operator.
Returns an object with `x`, `y`, `width`, `height` keys.
```
const value = await I.grabElementBoundingRect('h3');
// value is like { x: 226.5, y: 89, width: 527, height: 220 }
```
To get only one metric use second parameter:
```
const width = await I.grabElementBoundingRect('h3', 'width');
// width == 527
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `prop`
* `elementSize` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** x, y, width or height of the given element.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<DOMRect> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>)** Element bounding rectangle
### grabGeoLocation
Return the current geo location Resumes test execution, so **should be used inside async function with `await`** operator.
```
let geoLocation = await I.grabGeoLocation();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<{latitude: [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number), longitude: [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number), altitude: [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)}>**
### grabHTMLFrom
Retrieves the innerHTML from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - HTML of first element is returned.
```
let postHTML = await I.grabHTMLFrom('#post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** HTML code for an element
### grabHTMLFromAll
Retrieves all the innerHTML from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let postHTMLs = await I.grabHTMLFromAll('.post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** HTML code for an element
### grabNumberOfOpenTabs
Grab number of open tabs. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let tabs = await I.grabNumberOfOpenTabs();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of open tabs
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabPopupText
Grab the text within the popup. If no popup is visible then it will return null.
```
await I.grabPopupText();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>**
### grabSource
Retrieves page source and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let pageSource = await I.grabSource();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** source code
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabTitle
Retrieves a page title and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let title = await I.grabTitle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** title
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves an array of value from a form located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let inputs = await I.grabValueFromAll('//form/input');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `xOffset`
* `yOffset`
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### openNewTab
Open new tab and switch to it.
```
I.openNewTab();
```
#### Parameters
* `url`
* `windowName`
### pressKey
Presses a key in the browser (on a focused element).
*Hint:* For populating text field or textarea, it is recommended to use [`fillField`](#fillfield).
```
I.pressKey('Backspace');
```
To press a key in combination with modifier keys, pass the sequence as an array. All modifier keys (`'Alt'`, `'Control'`, `'Meta'`, `'Shift'`) will be released afterwards.
```
I.pressKey(['Control', 'Z']);
```
For specifying operation modifier key based on operating system it is suggested to use `'CommandOrControl'`. This will press `'Command'` (also known as `'Meta'`) on macOS machines and `'Control'` on non-macOS machines.
```
I.pressKey(['CommandOrControl', 'Z']);
```
Some of the supported key names are:
* `'AltLeft'` or `'Alt'`
* `'AltRight'`
* `'ArrowDown'`
* `'ArrowLeft'`
* `'ArrowRight'`
* `'ArrowUp'`
* `'Backspace'`
* `'Clear'`
* `'ControlLeft'` or `'Control'`
* `'ControlRight'`
* `'Command'`
* `'CommandOrControl'`
* `'Delete'`
* `'End'`
* `'Enter'`
* `'Escape'`
* `'F1'` to `'F12'`
* `'Home'`
* `'Insert'`
* `'MetaLeft'` or `'Meta'`
* `'MetaRight'`
* `'Numpad0'` to `'Numpad9'`
* `'NumpadAdd'`
* `'NumpadDecimal'`
* `'NumpadDivide'`
* `'NumpadMultiply'`
* `'NumpadSubtract'`
* `'PageDown'`
* `'PageUp'`
* `'Pause'`
* `'Return'`
* `'ShiftLeft'` or `'Shift'`
* `'ShiftRight'`
* `'Space'`
* `'Tab'`
#### Parameters
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** key or array of keys to press.*Note:* In case a text field or textarea is focused be aware that some browsers do not respect active modifier when combining modifier keys with other keys.
### pressKeyDown
Presses a key in the browser and leaves it in a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to press down.
### pressKeyUp
Releases a key in the browser which was previously set to a down state.
To make combinations with modifier key and user operation (e.g. `'Control'` + [`click`](#click)).
```
I.pressKeyDown('Control');
I.click('#element');
I.pressKeyUp('Control');
```
#### Parameters
* `key` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of key to release.
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels. Appium: not tested in web, in apps doesn't work
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### runInWeb
Placeholder for ~ locator only test case write once run on both Appium and WebDriver.
#### Parameters
* `fn`
### runOnAndroid
Placeholder for ~ locator only test case write once run on both Appium and WebDriver.
#### Parameters
* `caps`
* `fn`
### runOnIOS
Placeholder for ~ locator only test case write once run on both Appium and WebDriver.
#### Parameters
* `caps`
* `fn`
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollIntoView
Scroll element into viewport.
```
I.scrollIntoView('#submit');
I.scrollIntoView('#submit', true);
I.scrollIntoView('#submit', { behavior: "smooth", block: "center", inline: "center" });
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `scrollIntoViewOptions` **ScrollIntoViewOptions** see [https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollIntoView (opens new window)](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollIntoView).
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeAttributesOnElements
Checks that all elements with given locator have given attributes.
```
I.seeAttributesOnElements('//form', { method: "post"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `attributes` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** attributes and their values to check.
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.Appium: not tested
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCssPropertiesOnElements
Checks that all elements with given locator have given CSS properties.
```
I.seeCssPropertiesOnElements('h3', { 'font-weight': "bold"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `cssProperties` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** object with CSS properties and their values to check.
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInPopup
Checks that the active JavaScript popup, as created by `window.alert|window.confirm|window.prompt`, contains the given string.
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInTitle
Checks that title contains text.
```
I.seeInTitle('Home Page');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to check.
### seeNumberOfElements
Asserts that an element appears a given number of times in the DOM. Element is located by label or name or CSS or XPath.
```
I.seeNumberOfElements('#submitBtn', 1);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
This action supports [React locators (opens new window)](https://codecept.io/react#locators)
### seeTextEquals
Checks that text is equal to provided one.
```
I.seeTextEquals('text', 'h1');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** element value to check.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)?)** element located by CSS|XPath|strict locator.
### seeTitleEquals
Checks that title is equal to provided one.
```
I.seeTitleEquals('Test title.');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.Uses Selenium's JSON [cookie format (opens new window)](https://code.google.com/p/selenium/wiki/JsonWireProtocol#Cookie_JSON_Object).
### setGeoLocation
Set the current geo location
```
I.setGeoLocation(121.21, 11.56);
I.setGeoLocation(121.21, 11.56, 10);
```
#### Parameters
* `latitude` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** to set.
* `longitude` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** to set
* `altitude` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, null by default) to set
### switchTo
Switches frame or in case of null locator reverts to parent.
```
I.switchTo('iframe'); // switch to first iframe
I.switchTo(); // switch back to main page
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### switchToNextTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab.
```
I.switchToNextTab();
I.switchToNextTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional) number of tabs to switch forward, default: 1.
* `sec` **([number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number) | null)?** (optional) time in seconds to wait.
### switchToPreviousTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab.
```
I.switchToPreviousTab();
I.switchToPreviousTab(2);
```
#### Parameters
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional) number of tabs to switch backward, default: 1.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)??** (optional) time in seconds to wait.
### switchToWindow
Switch to the window with a specified handle.
```
const windows = await I.grabAllWindowHandles();
// ... do something
await I.switchToWindow( windows[0] );
const window = await I.grabCurrentWindowHandle();
// ... do something
await I.switchToWindow( window );
```
#### Parameters
* `window` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** name of window handle.
### type
Types out the given text into an active field. To slow down typing use a second parameter, to set interval between key presses. *Note:* Should be used when [`fillField`](#fillfield) is not an option.
```
// passing in a string
I.type('Type this out.');
// typing values with a 100ms interval
I.type('4141555311111111', 100);
// passing in an array
I.type(['T', 'E', 'X', 'T']);
```
#### Parameters
* `keys`
* `delay` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional) delay in ms between key presses
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** or array of keys to type.
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator. Appium: not tested
### useWebDriverTo
Use [webdriverio (opens new window)](https://webdriver.io/docs/api) API inside a test.
First argument is a description of an action. Second argument is async function that gets this helper as parameter.
{ [`browser` (opens new window)](https://webdriver.io/docs/api)) } object from WebDriver API is available.
```
I.useWebDriverTo('open multiple windows', async ({ browser }) {
// create new window
await browser.newWindow('https://webdriver.io');
});
```
#### Parameters
* `description` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** used to show in logs.
* `fn` **[function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** async functuion that executed with WebDriver helper as argument
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForClickable
Waits for element to be clickable (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForClickable('.btn.continue');
I.waitForClickable('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `waitTimeout`
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForDetached
Waits for an element to become not attached to the DOM on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForDetached('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForEnabled
Waits for element to become enabled (by default waits for 1sec). Element can be located by CSS or XPath.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional) time in seconds to wait, 1 by default.
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForValue
Waits for the specified value to be in value attribute.
```
I.waitForValue('//input', "GoodValue");
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** input field.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected value.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitInUrl
Waiting for the part of the URL to match the expected. Useful for SPA to understand that page was changed.
```
I.waitInUrl('/info', 2);
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitNumberOfVisibleElements
Waits for a specified number of elements on the page.
```
I.waitNumberOfVisibleElements('a', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitUntil
Waits for a function to return true (waits for 1sec by default).
```
I.waitUntil(() => window.requests == 0);
I.waitUntil(() => window.requests == 0, 5);
```
#### Parameters
* `fn` **([function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function) | [string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String))** function which is executed in browser context.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `timeoutMsg` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** message to show in case of timeout fail.
* `interval` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?**
### waitUrlEquals
Waits for the entire URL to match the expected
```
I.waitUrlEquals('/info', 2);
I.waitUrlEquals('http://127.0.0.1:8000/info');
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
| programming_docs |
codeceptjs Nightmare Nightmare
==========
**Extends Helper**
Nightmare helper wraps [Nightmare (opens new window)](https://github.com/segmentio/nightmare) library to provide fastest headless testing using Electron engine. Unlike Selenium-based drivers this uses Chromium-based browser with Electron with lots of client side scripts, thus should be less stable and less trusted.
Requires `nightmare` package to be installed.
Configuration
--------------
This helper should be configured in codecept.json or codecept.conf.js
* `url` - base url of website to be tested
* `restart` - restart browser between tests.
* `disableScreenshots` - don't save screenshot on failure.
* `uniqueScreenshotNames` - option to prevent screenshot override if you have scenarios with the same name in different suites.
* `fullPageScreenshots` - make full page screenshots on failure.
* `keepBrowserState` - keep browser state between tests when `restart` set to false.
* `keepCookies` - keep cookies between tests when `restart` set to false.
* `waitForAction`: (optional) how long to wait after click, doubleClick or PressKey actions in ms. Default: 500.
* `waitForTimeout`: (optional) default wait\* timeout in ms. Default: 1000.
* `windowSize`: (optional) default window size. Set a dimension like `640x480`.
* options from [Nightmare configuration (opens new window)](https://github.com/segmentio/nightmare#api)
Methods
--------
### Parameters
* `config`
### \_locate
Locate elements by different locator types, including strict locator. Should be used in custom helpers.
This method return promise with array of IDs of found elements. Actual elements can be accessed inside `evaluate` by using `codeceptjs.fetchElement()` client-side function:
```
// get an inner text of an element
let browser = this.helpers['Nightmare'].browser;
let value = this.helpers['Nightmare']._locate({name: 'password'}).then(function(els) {
return browser.evaluate(function(el) {
return codeceptjs.fetchElement(el).value;
}, els[0]);
});
```
#### Parameters
* `locator`
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)?** list of request headers can be passed
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file.Doesn't work if the Chromium DevTools panel is open (as Chromium allows only one attachment to the debugger at a time. [See more (opens new window)](https://github.com/rosshinkley/nightmare-upload#important-note-about-setting-file-upload-inputs))
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### dontSeeInTitle
Checks that title does not contain text.
```
I.dontSeeInTitle('Error');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### executeAsyncScript
Executes async script on page. Provided function should execute a passed callback (as first argument) to signal it is finished.
Example: In Vue.js to make components completely rendered we are waiting for [nextTick (opens new window)](https://vuejs.org/v2/api/#Vue-nextTick).
```
I.executeAsyncScript(function(done) {
Vue.nextTick(done); // waiting for next tick
});
```
By passing value to `done()` function you can return values. Additional arguments can be passed as well, while `done` function is always last parameter in arguments list.
```
let val = await I.executeAsyncScript(function(url, done) {
// in browser context
$.ajax(url, { success: (data) => done(data); }
}, 'http://ajax.callback.url/');
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** Wrapper for asynchronous [evaluate (opens new window)](https://github.com/segmentio/nightmare#evaluatefn-arg1-arg2). Unlike NightmareJS implementation calling `done` will return its first argument.
### executeScript
Executes sync script on a page. Pass arguments to function as additional parameters. Will return execution result to a test. In this case you should use async function and await to receive results.
Example with jQuery DatePicker:
```
// change date of jQuery DatePicker
I.executeScript(function() {
// now we are inside browser context
$('date').datetimepicker('setDate', new Date());
});
```
Can return values. Don't forget to use `await` to get them.
```
let date = await I.executeScript(function(el) {
// only basic types can be returned
return $(el).datetimepicker('getDate').toString();
}, '#date'); // passing jquery selector
```
#### Parameters
* `args` **...any** to be passed to function.
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>** Wrapper for synchronous [evaluate (opens new window)](https://github.com/segmentio/nightmare#evaluatefn-arg1-arg2)
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabAttributeFromAll
Retrieves an array of attributes from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let hints = await I.grabAttributeFromAll('.tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute valueCookie in JSON format. If name not passed returns all cookies for this domain.Multiple cookies can be received by passing query object `I.grabCookie({ secure: true});`. If you'd like get all cookies for all urls, use: `.grabCookie({ url: null }).`
### grabCssPropertyFrom
Grab CSS property for given locator Resumes test execution, so **should be used inside an async function with `await`** operator. If more than one element is found - value of first element is returned.
```
const value = await I.grabCssPropertyFrom('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** CSS value
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabElementBoundingRect
Grab the width, height, location of given locator. Provide `width` or `height`as second param to get your desired prop. Resumes test execution, so **should be used inside an async function with `await`** operator.
Returns an object with `x`, `y`, `width`, `height` keys.
```
const value = await I.grabElementBoundingRect('h3');
// value is like { x: 226.5, y: 89, width: 527, height: 220 }
```
To get only one metric use second parameter:
```
const width = await I.grabElementBoundingRect('h3', 'width');
// width == 527
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `prop`
* `elementSize` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** x, y, width or height of the given element.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<DOMRect> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>)** Element bounding rectangle
### grabHAR
Get HAR
```
let har = await I.grabHAR();
fs.writeFileSync('sample.har', JSON.stringify({log: har}));
```
### grabHTMLFrom
Retrieves the innerHTML from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - HTML of first element is returned.
```
let postHTML = await I.grabHTMLFrom('#post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** HTML code for an element
### grabHTMLFromAll
Retrieves all the innerHTML from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let postHTMLs = await I.grabHTMLFromAll('.post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** HTML code for an element
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabTitle
Retrieves a page title and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let title = await I.grabTitle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** title
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### haveHeader
Add a header override for all HTTP requests. If header is undefined, the header overrides will be reset.
```
I.haveHeader('x-my-custom-header', 'some value');
I.haveHeader(); // clear headers
```
#### Parameters
* `header`
* `value`
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### pressKey
Sends [input event (opens new window)](https://electron.atom.io/docs/api/web-contents/#webcontentssendinputeventevent) on a page. Can submit special keys like 'Enter', 'Backspace', etc
#### Parameters
* `key`
### refresh
Reload the page
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels.
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInTitle
Checks that title contains text.
```
I.seeInTitle('Home Page');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to check.
### seeNumberOfElements
Asserts that an element appears a given number of times in the DOM. Element is located by label or name or CSS or XPath.
```
I.seeNumberOfElements('#submitBtn', 1);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.Wrapper for `.cookies.set(cookie)`. [See more (opens new window)](https://github.com/segmentio/nightmare/blob/master/Readme.md#cookiessetcookie)
### triggerMouseEvent
Sends [input event (opens new window)](https://electron.atom.io/docs/api/web-contents/#contentssendinputeventevent) on a page. Should be a mouse event like: { type: 'mouseDown', x: args.x, y: args.y, button: "left" }
#### Parameters
* `event`
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForDetached
Waits for an element to become not attached to the DOM on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForDetached('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
| programming_docs |
codeceptjs Protractor Protractor
===========
**Extends Helper**
Protractor helper is based on [Protractor library (opens new window)](http://www.protractortest.org) and used for testing web applications.
Protractor requires [Selenium Server and ChromeDriver/GeckoDriver to be installed (opens new window)](https://codecept.io/quickstart/#prepare-selenium-server). To test non-Angular applications please make sure you have `angular: false` in configuration file.
### Configuration
This helper should be configured in codecept.json or codecept.conf.js
* `url` - base url of website to be tested
* `browser` - browser in which perform testing
* `angular` : disable this option to run tests for non-Angular applications.
* `driver` - which protractor driver to use (local, direct, session, hosted, sauce, browserstack). By default set to 'hosted' which requires selenium server to be started.
* `restart` - restart browser between tests.
* `smartWait`: (optional) **enables [SmartWait (opens new window)](https://codecept.io/acceptance/#smartwait)**; wait for additional milliseconds for element to appear. Enable for 5 secs: "smartWait": 5000
* `disableScreenshots` - don't save screenshot on failure
* `fullPageScreenshots` - make full page screenshots on failure.
* `uniqueScreenshotNames` - option to prevent screenshot override if you have scenarios with the same name in different suites
* `keepBrowserState` - keep browser state between tests when `restart` set to false.
* `seleniumAddress` - Selenium address to connect (default: [http://localhost:4444/wd/hub (opens new window)](http://localhost:4444/wd/hub))
* `rootElement` - Root element of AngularJS application (default: body)
* `getPageTimeout` (optional) sets default timeout for a page to be loaded. 10000 by default.
* `waitForTimeout`: (optional) sets default wait time in *ms* for all `wait*` functions. 1000 by default.
* `scriptsTimeout`: (optional) timeout in milliseconds for each script run on the browser, 10000 by default.
* `windowSize`: (optional) default window size. Set to `maximize` or a dimension in the format `640x480`.
* `manualStart` - do not start browser before a test, start it manually inside a helper with `this.helpers.WebDriver._startBrowser()`
* `capabilities`: {} - list of [Desired Capabilities (opens new window)](https://github.com/SeleniumHQ/selenium/wiki/DesiredCapabilities)
* `proxy`: set proxy settings
other options are the same as in [Protractor config (opens new window)](https://github.com/angular/protractor/blob/master/docs/referenceConf.js).
#### Sample Config
```
{
"helpers": {
"Protractor" : {
"url": "http://localhost",
"browser": "chrome",
"smartWait": 5000,
"restart": false
}
}
}
```
#### Config for Non-Angular application:
```
{
"helpers": {
"Protractor" : {
"url": "http://localhost",
"browser": "chrome",
"angular": false
}
}
}
```
#### Config for Headless Chrome
```
{
"helpers": {
"Protractor" : {
"url": "http://localhost",
"browser": "chrome",
"capabilities": {
"chromeOptions": {
"args": [ "--headless", "--disable-gpu", "--no-sandbox" ]
}
}
}
}
}
```
Access From Helpers
--------------------
Receive a WebDriverIO client from a custom helper by accessing `browser` property:
```
this.helpers['Protractor'].browser
```
Methods
--------
### Parameters
* `config`
### \_getWindowHandle
Get the window handle relative to the current handle. i.e. the next handle or the previous.
#### Parameters
* `offset` **[Number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** Offset from current handle index. i.e. offset < 0 will go to the previous handle and positive number will go to the next window handle in sequence.
### \_locate
Get elements by different locator types, including strict locator Should be used in custom helpers:
```
this.helpers['Protractor']._locate({name: 'password'}).then //...
```
To use SmartWait and wait for element to appear on a page, add `true` as second arg:
```
this.helpers['Protractor']._locate({name: 'password'}, true).then //...
```
#### Parameters
* `locator`
* `smartWait`
### \_locateCheckable
Find a checkbox by providing human readable text:
```
this.helpers['Protractor']._locateCheckable('I agree with terms and conditions').then // ...
```
#### Parameters
* `locator`
### \_locateClickable
Find a clickable element by providing human readable text:
```
this.helpers['Protractor']._locateClickable('Next page').then // ...
```
#### Parameters
* `locator`
### \_locateFields
Find field elements by providing human readable text:
```
this.helpers['Protractor']._locateFields('Your email').then // ...
```
#### Parameters
* `locator`
### acceptPopup
Accepts the active JavaScript native popup window, as created by window.alert|window.confirm|window.prompt. Don't confuse popups with modal windows, as created by [various libraries (opens new window)](http://jster.net/category/windows-modals-popups). Appium: support only web testing
### amInsideAngularApp
Enters Angular mode (switched on by default) Should be used after "amOutsideAngularApp"
### amOnPage
Opens a web page in a browser. Requires relative or absolute url. If url starts with `/`, opens a web page of a site defined in `url` config parameter.
```
I.amOnPage('/'); // opens main page of website
I.amOnPage('https://github.com'); // opens github
I.amOnPage('/login'); // opens a login page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** url path or global url.
### amOutsideAngularApp
Switch to non-Angular mode, start using WebDriver instead of Protractor in this session
### appendField
Appends text to a input field or textarea. Field is located by name, label, CSS or XPath
```
I.appendField('#myTextField', 'appended');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to append.
### attachFile
Attaches a file to element located by label, name, CSS or XPath Path to file is relative current codecept directory (where codecept.json or codecept.conf.js is located). File will be uploaded to remote system (if tests are running remotely).
```
I.attachFile('Avatar', 'data/avatar.jpg');
I.attachFile('form input[name=avatar]', 'data/avatar.jpg');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `pathToFile` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** local file path relative to codecept.json config file.
### cancelPopup
Dismisses the active JavaScript popup, as created by window.alert|window.confirm|window.prompt.
### checkOption
Selects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.checkOption('#agree');
I.checkOption('I Agree to Terms and Conditions');
I.checkOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### clearCookie
Clears a cookie by name, if none provided clears all cookies.
```
I.clearCookie();
I.clearCookie('test');
```
#### Parameters
* `cookie` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** (optional, `null` by default) cookie name
### clearField
Clears a `<textarea>` or text `<input>` element's value.
```
I.clearField('Email');
I.clearField('user[email]');
I.clearField('#email');
```
#### Parameters
* `field`
* `editable` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
### click
Perform a click on a link or a button, given by a locator. If a fuzzy locator is given, the page will be searched for a button, link, or image matching the locator string. For buttons, the "value" attribute, "name" attribute, and inner text are searched. For links, the link text is searched. For images, the "alt" attribute and inner text of any parent links are searched.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
// simple link
I.click('Logout');
// button of form
I.click('Submit');
// CSS button
I.click('#form input[type=submit]');
// XPath
I.click('//form/*[@type=submit]');
// link in context
I.click('Logout', '#nav');
// using strict locator
I.click({css: 'nav a.login'});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### closeCurrentTab
Close current tab
```
I.closeCurrentTab();
```
### closeOtherTabs
Close all tabs except for the current one.
```
I.closeOtherTabs();
```
### dontSee
Opposite to `see`. Checks that a text is not present on a page. Use context parameter to narrow down the search.
```
I.dontSee('Login'); // assume we are already logged in.
I.dontSee('Login', '.nav'); // no login inside .nav element
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** which is not present.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator in which to perfrom search.
### dontSeeCheckboxIsChecked
Verifies that the specified checkbox is not checked.
```
I.dontSeeCheckboxIsChecked('#agree'); // located by ID
I.dontSeeCheckboxIsChecked('I agree to terms'); // located by label
I.dontSeeCheckboxIsChecked('agree'); // located by name
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### dontSeeCookie
Checks that cookie with given name does not exist.
```
I.dontSeeCookie('auth'); // no auth cookie
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### dontSeeCurrentUrlEquals
Checks that current url is not equal to provided one. If a relative url provided, a configured url will be prepended to it.
```
I.dontSeeCurrentUrlEquals('/login'); // relative url are ok
I.dontSeeCurrentUrlEquals('http://mysite.com/login'); // absolute urls are also ok
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeElement
Opposite to `seeElement`. Checks that element is not visible (or in DOM)
```
I.dontSeeElement('.modal'); // modal is not shown
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeElementInDOM
Opposite to `seeElementInDOM`. Checks that element is not on page.
```
I.dontSeeElementInDOM('.nav'); // checks that element is not on page visible or not
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|Strict locator.
### dontSeeInCurrentUrl
Checks that current url does not contain a provided fragment.
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInField
Checks that value of input field or textarea doesn't equal to given value Opposite to `seeInField`.
```
I.dontSeeInField('email', '[email protected]'); // field by name
I.dontSeeInField({ css: 'form input.email' }, '[email protected]'); // field by CSS
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### dontSeeInSource
Checks that the current page does not contains the given string in its raw source code.
```
I.dontSeeInSource('<!--'); // no comments in source
```
#### Parameters
* `text`
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to check.
### dontSeeInTitle
Checks that title does not contain text.
```
I.dontSeeInTitle('Error');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### doubleClick
Performs a double-click on an element matched by link|button|label|CSS or XPath. Context can be specified as second parameter to narrow search.
```
I.doubleClick('Edit');
I.doubleClick('Edit', '.actions');
I.doubleClick({css: 'button.accept'});
I.doubleClick('.btn.edit');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable link or button located by text, or any element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element to search in CSS|XPath|Strict locator.
### dragAndDrop
Drag an item to a destination element.
```
I.dragAndDrop('#dragHandle', '#container');
```
#### Parameters
* `srcElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `destElement` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### executeAsyncScript
Executes async script on page. Provided function should execute a passed callback (as first argument) to signal it is finished.
Example: In Vue.js to make components completely rendered we are waiting for [nextTick (opens new window)](https://vuejs.org/v2/api/#Vue-nextTick).
```
I.executeAsyncScript(function(done) {
Vue.nextTick(done); // waiting for next tick
});
```
By passing value to `done()` function you can return values. Additional arguments can be passed as well, while `done` function is always last parameter in arguments list.
```
let val = await I.executeAsyncScript(function(url, done) {
// in browser context
$.ajax(url, { success: (data) => done(data); }
}, 'http://ajax.callback.url/');
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
* `args` **...any** to be passed to function.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>**
### executeScript
Executes sync script on a page. Pass arguments to function as additional parameters. Will return execution result to a test. In this case you should use async function and await to receive results.
Example with jQuery DatePicker:
```
// change date of jQuery DatePicker
I.executeScript(function() {
// now we are inside browser context
$('date').datetimepicker('setDate', new Date());
});
```
Can return values. Don't forget to use `await` to get them.
```
let date = await I.executeScript(function(el) {
// only basic types can be returned
return $(el).datetimepicker('getDate').toString();
}, '#date'); // passing jquery selector
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** function to be executed in browser context.
* `args` **...any** to be passed to function.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<any>**
### fillField
Fills a text field or textarea, after clearing its value, with the given string. Field is located by name, label, CSS, or XPath.
```
// by label
I.fillField('Email', '[email protected]');
// by name
I.fillField('password', secret('123456'));
// by CSS
I.fillField('form#login input[name=username]', 'John');
// or by strict locator
I.fillField({css: 'form#login input[name=username]'}, 'John');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to fill.
### grabAttributeFrom
Retrieves an attribute from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator. If more than one element is found - attribute of first element is returned.
```
let hint = await I.grabAttributeFrom('#tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabAttributeFromAll
Retrieves an array of attributes from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let hints = await I.grabAttributeFromAll('.tooltip', 'title');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `attr` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** attribute name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabBrowserLogs
Get JS log from browser. Log buffer is reset after each request. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let logs = await I.grabBrowserLogs();
console.log(JSON.stringify(logs))
```
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)>> | [undefined (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/undefined))** all browser logs
### grabCookie
Gets a cookie object by name. If none provided gets all cookies. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let cookie = await I.grabCookie('auth');
assert(cookie.value, '123456');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)?** cookie name.
Returns **([Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)> | [Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>)** attribute valueReturns cookie in JSON [format (opens new window)](https://code.google.com/p/selenium/wiki/JsonWireProtocol#Cookie_JSON_Object).
### grabCssPropertyFrom
Grab CSS property for given locator Resumes test execution, so **should be used inside an async function with `await`** operator. If more than one element is found - value of first element is returned.
```
const value = await I.grabCssPropertyFrom('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** CSS value
### grabCssPropertyFromAll
Grab array of CSS properties for given locator Resumes test execution, so **should be used inside an async function with `await`** operator.
```
const values = await I.grabCssPropertyFromAll('h3', 'font-weight');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `cssProperty` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** CSS property name.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** CSS value
### grabCurrentUrl
Get current URL from browser. Resumes test execution, so should be used inside an async function.
```
let url = await I.grabCurrentUrl();
console.log(`Current URL is [${url}]`);
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** current URL
### grabHTMLFrom
Retrieves the innerHTML from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - HTML of first element is returned.
```
let postHTML = await I.grabHTMLFrom('#post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** HTML code for an element
### grabHTMLFromAll
Retrieves all the innerHTML from elements located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let postHTMLs = await I.grabHTMLFromAll('.post');
```
#### Parameters
* `locator`
* `element` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** HTML code for an element
### grabNumberOfOpenTabs
Grab number of open tabs. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let tabs = await I.grabNumberOfOpenTabs();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of open tabs
### grabNumberOfVisibleElements
Grab number of visible elements by locator. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let numOfElements = await I.grabNumberOfVisibleElements('p');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)>** number of visible elements
### grabPageScrollPosition
Retrieves a page scroll position and returns it to test. Resumes test execution, so **should be used inside an async function with `await`** operator.
```
let { x, y } = await I.grabPageScrollPosition();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<PageScrollPosition>** scroll position
### grabPopupText
Grab the text within the popup. If no popup is visible then it will return null
```
await I.grabPopupText();
```
### grabSource
Retrieves page source and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let pageSource = await I.grabSource();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** source code
### grabTextFrom
Retrieves a text from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pin = await I.grabTextFrom('#pin');
```
If multiple elements found returns first element.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabTextFromAll
Retrieves all texts from an element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let pins = await I.grabTextFromAll('#pin li');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### grabTitle
Retrieves a page title and returns it to test. Resumes test execution, so **should be used inside async with `await`** operator.
```
let title = await I.grabTitle();
```
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** title
### grabValueFrom
Retrieves a value from a form element located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator. If more than one element is found - value of first element is returned.
```
let email = await I.grabValueFrom('input[name=email]');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>** attribute value
### grabValueFromAll
Retrieves an array of value from a form located by CSS or XPath and returns it to test. Resumes test execution, so **should be used inside async function with `await`** operator.
```
let inputs = await I.grabValueFromAll('//form/input');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
Returns **[Promise (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)<[Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>>** attribute value
### haveModule
Injects Angular module.
```
I.haveModule('modName', function() {
angular.module('modName', []).value('foo', 'bar');
});
```
#### Parameters
* `modName`
* `fn`
### moveCursorTo
Moves cursor to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.moveCursorTo('.tooltip');
I.moveCursorTo('#submit', 5,5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### moveTo
Moves to url
#### Parameters
* `path`
### openNewTab
Open new tab and switch to it
```
I.openNewTab();
```
### pressKey
Presses a key on a focused element. Special keys like 'Enter', 'Control', [etc (opens new window)](https://code.google.com/p/selenium/wiki/JsonWireProtocol#/session/:sessionId/element/:id/value) will be replaced with corresponding unicode. If modifier key is used (Control, Command, Alt, Shift) in array, it will be released afterwards.
```
I.pressKey('Enter');
I.pressKey(['Control','a']);
```
#### Parameters
* `key` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)>)** key or array of keys to press.
[Valid key names (opens new window)](https://w3c.github.io/webdriver/#keyboard-actions) are:
* `'Add'`,
* `'Alt'`,
* `'ArrowDown'` or `'Down arrow'`,
* `'ArrowLeft'` or `'Left arrow'`,
* `'ArrowRight'` or `'Right arrow'`,
* `'ArrowUp'` or `'Up arrow'`,
* `'Backspace'`,
* `'Command'`,
* `'Control'`,
* `'Del'`,
* `'Divide'`,
* `'End'`,
* `'Enter'`,
* `'Equals'`,
* `'Escape'`,
* `'F1 to F12'`,
* `'Home'`,
* `'Insert'`,
* `'Meta'`,
* `'Multiply'`,
* `'Numpad 0'` to `'Numpad 9'`,
* `'Pagedown'` or `'PageDown'`,
* `'Pageup'` or `'PageUp'`,
* `'Pause'`,
* `'Semicolon'`,
* `'Shift'`,
* `'Space'`,
* `'Subtract'`,
* `'Tab'`.
### refresh
Reloads page
### refreshPage
Reload the current page.
```
I.refreshPage();
```
### resetModule
Removes mocked Angular module. If modName not specified - clears all mock modules.
```
I.resetModule(); // clears all
I.resetModule('modName');
```
#### Parameters
* `modName`
### resizeWindow
Resize the current window to provided width and height. First parameter can be set to `maximize`.
#### Parameters
* `width` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** width in pixels or `maximize`.
* `height` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** height in pixels.
### rightClick
Performs right click on a clickable element matched by semantic locator, CSS or XPath.
```
// right click element with id el
I.rightClick('#el');
// right click link or button with text "Click me"
I.rightClick('Click me');
// right click button with text "Click me" inside .context
I.rightClick('Click me', '.context');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** clickable element located by CSS|XPath|strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### saveElementScreenshot
Saves screenshot of the specified locator to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder.
```
I.saveElementScreenshot(`#submit`,'debug.png');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
### saveScreenshot
Saves a screenshot to ouput folder (set in codecept.json or codecept.conf.js). Filename is relative to output folder. Optionally resize the window to the full available page `scrollHeight` and `scrollWidth` to capture the entire page by passing `true` in as the second argument.
```
I.saveScreenshot('debug.png');
I.saveScreenshot('debug.png', true) //resizes to available scrollHeight and scrollWidth before taking screenshot
```
#### Parameters
* `fileName` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** file name to save.
* `fullPage` **[boolean (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** (optional, `false` by default) flag to enable fullscreen screenshot mode.
### scrollPageToBottom
Scroll page to the bottom.
```
I.scrollPageToBottom();
```
### scrollPageToTop
Scroll page to the top.
```
I.scrollPageToTop();
```
### scrollTo
Scrolls to element matched by locator. Extra shift can be set with offsetX and offsetY options.
```
I.scrollTo('footer');
I.scrollTo('#submit', 5, 5);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `offsetX` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) X-axis offset.
* `offsetY` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `0` by default) Y-axis offset.
### see
Checks that a page contains a visible text. Use context parameter to narrow down the search.
```
I.see('Welcome'); // text welcome on a page
I.see('Welcome', '.content'); // text inside .content div
I.see('Register', {css: 'form.register'}); // use strict locator
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected on page.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|Xpath|strict locator in which to search for text.
### seeAttributesOnElements
Checks that all elements with given locator have given attributes.
```
I.seeAttributesOnElements('//form', { method: "post"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `attributes` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** attributes and their values to check.
### seeCheckboxIsChecked
Verifies that the specified checkbox is checked.
```
I.seeCheckboxIsChecked('Agree');
I.seeCheckboxIsChecked('#agree'); // I suppose user agreed to terms
I.seeCheckboxIsChecked({css: '#signup_form input[type=checkbox]'});
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
### seeCookie
Checks that cookie with given name exists.
```
I.seeCookie('Auth');
```
#### Parameters
* `name` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** cookie name.
### seeCssPropertiesOnElements
Checks that all elements with given locator have given CSS properties.
```
I.seeCssPropertiesOnElements('h3', { 'font-weight': "bold"});
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
* `cssProperties` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** object with CSS properties and their values to check.
### seeCurrentUrlEquals
Checks that current url is equal to provided one. If a relative url provided, a configured url will be prepended to it. So both examples will work:
```
I.seeCurrentUrlEquals('/register');
I.seeCurrentUrlEquals('http://my.site.com/register');
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeElement
Checks that a given Element is visible Element is located by CSS or XPath.
```
I.seeElement('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by CSS|XPath|strict locator.
### seeElementInDOM
Checks that a given Element is present in the DOM Element is located by CSS or XPath.
```
I.seeElementInDOM('#modal');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
### seeInCurrentUrl
Checks that current url contains a provided fragment.
```
I.seeInCurrentUrl('/register'); // we are on registration page
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** a fragment to check
### seeInField
Checks that the given input field or textarea equals to given value. For fuzzy locators, fields are matched by label text, the "name" attribute, CSS, and XPath.
```
I.seeInField('Username', 'davert');
I.seeInField({css: 'form textarea'},'Type your comment here');
I.seeInField('form input[type=hidden]','hidden_value');
I.seeInField('#searchform input','Search');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** located by label|name|CSS|XPath|strict locator.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInPopup
Checks that the active JavaScript popup, as created by `window.alert|window.confirm|window.prompt`, contains the given string.
```
I.seeInPopup('Popup text');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInSource
Checks that the current page contains the given string in its raw source code.
```
I.seeInSource('<h1>Green eggs & ham</h1>');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
### seeInTitle
Checks that title contains text.
```
I.seeInTitle('Home Page');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** text value to check.
### seeNumberOfElements
Asserts that an element appears a given number of times in the DOM. Element is located by label or name or CSS or XPath.
```
I.seeNumberOfElements('#submitBtn', 1);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeNumberOfVisibleElements
Asserts that an element is visible a given number of times. Element is located by CSS or XPath.
```
I.seeNumberOfVisibleElements('.buttons', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
### seeTextEquals
Checks that text is equal to provided one.
```
I.seeTextEquals('text', 'h1');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** element value to check.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)?)** element located by CSS|XPath|strict locator.
### seeTitleEquals
Checks that title is equal to provided one.
```
I.seeTitleEquals('Test title.');
```
#### Parameters
* `text`
### selectOption
Selects an option in a drop-down select. Field is searched by label | name | CSS | XPath. Option is selected by visible text or by value.
```
I.selectOption('Choose Plan', 'Monthly'); // select by label
I.selectOption('subscription', 'Monthly'); // match option by text
I.selectOption('subscription', '0'); // or by value
I.selectOption('//form/select[@name=account]','Premium');
I.selectOption('form select[name=account]', 'Premium');
I.selectOption({css: 'form select[name=account]'}, 'Premium');
```
Provide an array for the second argument to select multiple options.
```
I.selectOption('Which OS do you use?', ['Android', 'iOS']);
```
#### Parameters
* `select` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** field located by label|name|CSS|XPath|strict locator.
* `option` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any>)** visible text or value of option.
### setCookie
Sets cookie(s).
Can be a single cookie object or an array of cookies:
```
I.setCookie({name: 'auth', value: true});
// as array
I.setCookie([
{name: 'auth', value: true},
{name: 'agree', value: true}
]);
```
#### Parameters
* `cookie` **(Cookie | [Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<Cookie>)** a cookie object or array of cookie objects.
### switchTo
Switches frame or in case of null locator reverts to parent.
```
I.switchTo('iframe'); // switch to first iframe
I.switchTo(); // switch back to main page
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS|XPath|strict locator.
### switchToNextTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToNextTab();
I.switchToNextTab(2);
```
#### Parameters
* `num`
### switchToPreviousTab
Switch focus to a particular tab by its number. It waits tabs loading and then switch tab
```
I.switchToPreviousTab();
I.switchToPreviousTab(2);
```
#### Parameters
* `num`
### uncheckOption
Unselects a checkbox or radio button. Element is located by label or name or CSS or XPath.
The second parameter is a context (CSS or XPath locator) to narrow the search.
```
I.uncheckOption('#agree');
I.uncheckOption('I Agree to Terms and Conditions');
I.uncheckOption('agree', '//form');
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** checkbox located by label | name | CSS | XPath | strict locator.
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)? | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** (optional, `null` by default) element located by CSS | XPath | strict locator.
### useProtractorTo
Use [Protractor (opens new window)](https://www.protractortest.org/#/api) API inside a test.
First argument is a description of an action. Second argument is async function that gets this helper as parameter.
{ [`browser` (opens new window)](https://www.protractortest.org/#/api?view=ProtractorBrowser)) } object from Protractor API is available.
```
I.useProtractorTo('change url via in-page navigation', async ({ browser }) {
await browser.setLocation('api');
});
```
#### Parameters
* `description` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** used to show in logs.
* `fn` **[function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** async functuion that executed with Protractor helper as argument
### wait
Pauses execution for a number of seconds.
```
I.wait(2); // wait 2 secs
```
#### Parameters
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of second to wait.
### waitForClickable
Waits for element to become clickable for number of seconds.
```
I.waitForClickable('#link');
```
#### Parameters
* `locator`
* `sec`
### waitForDetached
Waits for an element to become not attached to the DOM on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForDetached('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForElement
Waits for element to be present on page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForElement('.btn.continue');
I.waitForElement('.btn.continue', 5); // wait for 5 secs
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForEnabled
Waits for element to become enabled (by default waits for 1sec). Element can be located by CSS or XPath.
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional) time in seconds to wait, 1 by default.
### waitForFunction
Waits for a function to return true (waits for 1 sec by default). Running in browser context.
```
I.waitForFunction(fn[, [args[, timeout]])
```
```
I.waitForFunction(() => window.requests == 0);
I.waitForFunction(() => window.requests == 0, 5); // waits for 5 sec
I.waitForFunction((count) => window.requests == count, [3], 5) // pass args and wait for 5 sec
```
#### Parameters
* `fn` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function))** to be executed in browser context.
* `argsOrSec` **([Array (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)<any> | [number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number))?** (optional, `1` by default) arguments for function or seconds.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?** (optional, `1` by default) time in seconds to wait
### waitForInvisible
Waits for an element to be removed or become invisible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForInvisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForText
Waits for a text to appear (by default waits for 1sec). Element can be located by CSS or XPath. Narrow down search results by providing context.
```
I.waitForText('Thank you, form has been submitted');
I.waitForText('Thank you, form has been submitted', 5, '#modal');
```
#### Parameters
* `text` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** to wait for.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `context` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))?** (optional) element located by CSS|XPath|strict locator.
### waitForValue
Waits for the specified value to be in value attribute.
```
I.waitForValue('//input', "GoodValue");
```
#### Parameters
* `field` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** input field.
* `value` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** expected value.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitForVisible
Waits for an element to become visible on a page (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitForVisible('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitInUrl
Waiting for the part of the URL to match the expected. Useful for SPA to understand that page was changed.
```
I.waitInUrl('/info', 2);
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitNumberOfVisibleElements
Waits for a specified number of elements on the page.
```
I.waitNumberOfVisibleElements('a', 3);
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `num` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** number of elements.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitToHide
Waits for an element to hide (by default waits for 1sec). Element can be located by CSS or XPath.
```
I.waitToHide('#popup');
```
#### Parameters
* `locator` **([string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) | [object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object))** element located by CSS|XPath|strict locator.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
### waitUntil
Waits for a function to return true (waits for 1sec by default).
```
I.waitUntil(() => window.requests == 0);
I.waitUntil(() => window.requests == 0, 5);
```
#### Parameters
* `fn` **([function (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function) | [string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String))** function which is executed in browser context.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
* `timeoutMsg` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** message to show in case of timeout fail.
* `interval` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)?**
### waitUrlEquals
Waits for the entire URL to match the expected
```
I.waitUrlEquals('/info', 2);
I.waitUrlEquals('http://127.0.0.1:8000/info');
```
#### Parameters
* `urlPart` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** value to check.
* `sec` **[number (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number)** (optional, `1` by default) time in seconds to wait
perform
--------
just press button if no selector is given
| programming_docs |
codeceptjs REST REST
=====
**Extends Helper**
REST helper allows to send additional requests to the REST API during acceptance tests. [Axios (opens new window)](https://github.com/axios/axios) library is used to perform requests.
Configuration
--------------
* endpoint: API base URL
* timeout: timeout for requests in milliseconds. 10000ms by default
* defaultHeaders: a list of default headers
* onRequest: a async function which can update request object.
* maxUploadFileSize: set the max content file size in MB when performing api calls.
Example
--------
```
{
helpers: {
REST: {
endpoint: 'http://site.com/api',
onRequest: (request) => {
request.headers.auth = '123';
}
}
}
```
Access From Helpers
--------------------
Send REST requests by accessing `_executeRequest` method:
```
this.helpers['REST']._executeRequest({
url,
data,
});
```
Methods
--------
### Parameters
* `config`
### \_executeRequest
Executes axios request
#### Parameters
* `request` **any**
### \_url
Generates url based on format sent (takes endpoint + url if latter lacks 'http')
#### Parameters
* `url` **any**
### sendDeleteRequest
Sends DELETE request to API.
```
I.sendDeleteRequest('/api/users/1');
```
#### Parameters
* `url` **any**
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** the headers object to be sent. By default it is sent as an empty object
### sendGetRequest
Send GET request to REST API
```
I.sendGetRequest('/api/users.json');
```
#### Parameters
* `url` **any**
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** the headers object to be sent. By default it is sent as an empty object
### sendPatchRequest
Sends PATCH request to API.
```
I.sendPatchRequest('/api/users.json', { "email": "[email protected]" });
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `payload` **any** the payload to be sent. By default it is sent as an empty object
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** the headers object to be sent. By default it is sent as an empty object
### sendPostRequest
Sends POST request to API.
```
I.sendPostRequest('/api/users.json', { "email": "[email protected]" });
```
#### Parameters
* `url` **any**
* `payload` **any** the payload to be sent. By default it is sent as an empty object
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** the headers object to be sent. By default it is sent as an empty object
### sendPutRequest
Sends PUT request to API.
```
I.sendPutRequest('/api/users.json', { "email": "[email protected]" });
```
#### Parameters
* `url` **[string (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)**
* `payload` **any** the payload to be sent. By default it is sent as an empty object
* `headers` **[object (opens new window)](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** the headers object to be sent. By default it is sent as an empty object
### setRequestTimeout
Set timeout for the request
```
I.setRequestTimeout(10000); // In milliseconds
```
#### Parameters
* `newTimeout`
codeceptjs Reporters Reporters
==========
Cli
----
By default CodeceptJS provides cli reporter with console output. Test names and failures will be printed to screen.
```
GitHub --
✓ search in 2577ms
✓ signin in 2170ms
✖ register in 1306ms
-- FAILURES:
1) GitHub: register:
Field q not found by name|text|CSS|XPath
Scenario Steps:
- I.fillField("q", "aaa") at examples/github_test.js:29:7
- I.fillField("user[password]", "[email protected]") at examples/github_test.js:28:7
- I.fillField("user[email]", "[email protected]") at examples/github_test.js:27:7
- I.fillField("user[login]", "User") at examples/github_test.js:26:7
Run with --verbose flag to see NodeJS stacktrace
```
npx codeceptjs run --stepsutput add `--steps` option to `run` command:
npx codeceptjs run --steps
```
Output:
```sh
GitHub --
search
• I am on page "https://github.com"
• I am on page "https://github.com/search"
• I fill field "Search GitHub", "CodeceptJS"
• I press key "Enter"
• I see "Codeception/CodeceptJS", "a"
✓ OK in 2681ms
signin
• I am on page "https://github.com"
• I click "Sign in"
• I see "Sign in to GitHub"
• I fill field "Username or email address", "[email protected]"
• I fill field "Password", "123456"
• I click "Sign in"
• I see "Incorrect username or password.", ".flash-error"
✓ OK in 2252ms
register
• I am on page "https://github.com"
Within .js-signup-form:
• I fill field "user[login]", "User"
• I fill field "user[email]", "[email protected]"
• I fill field "user[password]", "[email protected]"
• I fill field "q", "aaa"
✖ FAILED in 1260ms
```
To get additional information about test execution use `--debug` option.
```
npx codeceptjs run --debug
```
This will show execution steps as well as notices from test runner. To get even more information with more technical details like error stack traces, and global promises, or events use `--verbose` mode.
```
npx codeceptjs run --verbose
```
```
GitHub --
register
[1] Starting recording promises
Emitted | test.before
> WebDriver._before
[1] Queued | hook WebDriver._before()
[1] Queued | amOnPage: https://github.com
Emitted | step.before (I am on page "https://github.com")
• I am on page "https://github.com"
Emitted | step.after (I am on page "https://github.com")
Emitted | test.start ([object Object])
...
```
Please use verbose output when reporting issues to GitHub.
### Dry Run
There is a way to list all tests and their steps without actually executing them. Execute tests in `dry-run` mode to see all available tests:
```
npx codeceptjs dry-run
```
Output:
```
Tests from /home/davert/projects/codeceptjs/examples:
Business rules --
☐ do something
Google --
☐ test @123
GitHub -- /home/davert/projects/codeceptjs/examples/github_test.js
☐ Visit Home Page @retry
☐ search @grop
☐ signin @normal @important @slow
☐ signin2
☐ register
Total: 3 suites | 7 tests
--- DRY MODE: No tests were executed ---
```
Pass `--steps` or `--debug` option as in `run` command to also get steps and substeps to be printed. In this mode **tests will be executed** but all helpers and plugins disabled, so no real actions will be performed.
```
npx codecepjs dry-run --debug
```
> ℹ If you use custom JavaScript code inside tests, or rely on values from `grab*` commands, dry-run may produce error output.
>
>
Allure
-------
> ℹ We recommend using Allure reports on CI. Allure is one of the best open-source reporters designed to collect and show test reports in nicest way.
>
>
[Allure reporter (opens new window)](http://allure.qatools.ru/#) is a tool to store and display test reports. It provides nice web UI which contains all important information on test execution. CodeceptJS has built-in support for Allure reports. Inside reports you will have all steps, substeps and screenshots.
> ▶ Allure is a standalone tool. Please refer to [Allure documentation (opens new window)](https://docs.qameta.io/allure/) to learn more about using Allure reports.
>
>
Allure requires **Java 8** to work. Then Allure can be installed via NPM:
```
npm install -g allure-commandline --save-dev
```
Add [Allure plugin](https://codecept.io/plugins/#allure) in config under `plugins` section.
```
plugins: {
allure: {
}
}
```
Run tests with allure plugin enabled:
```
npx codeceptjs run --plugins allure
```
(optionally) To enable allure plugin permanently include `"enabled": true` into plugin config:
```
"plugins": {
"allure": {
"enabled": true
}
}
```
Launch Allure server and see the report like on a screenshot above:
```
allure serve output
```
Allure reporter aggregates data from other plugins like [*stepByStepReport*](https://codecept.io/plugins/#stepByStepReport) and [*screenshotOnFail*](https://codecept.io/plugins/#screenshotOnFail)
Allure reports can also be generated for `dry-run` command. So you can get the first report with no tests actually being executed. Enable allure plugin in dry-run options, and pass `--debug` option to print all tests on screen.
```
npx codeceptjs dry-run --debug -p allure
```
ReportPortal
-------------
Allure is a great reportin tool, however, if you are running tests on different machines it is hard to merge its XML result files to build a proper report. So, for enterprise grade reporting we recommend using [ReportPortal (opens new window)](https://reportportal.io).
[ReportPortal (opens new window)](https://reportportal.io) is open-source self-hosted service for aggregating test execution reports. Think of it as Kibana but for test reports.
Use official [CodeceptJS Agent for ReportPortal (opens new window)](https://github.com/reportportal/agent-js-codecept/) to start publishing your test results.
XML
----
Use default xunit reporter of Mocha to print xml reports. Provide `--reporter xunit` to get the report to screen. It is recommended to use more powerful [`mocha-junit-reporter` (opens new window)](https://www.npmjs.com/package/mocha-junit-reporter) package to get better support for Jenkins CI.
Install it via NPM (locally or globally, depending on CodeceptJS installation type):
```
npm i mocha-junit-reporter
```
Additional configuration should be added to `codecept.conf.js` to print xml report to `output` directory:
```
"mocha": {
"reporterOptions": {
"mochaFile": "output/result.xml"
}
},
```
Execute CodeceptJS with JUnit reporter:
```
codeceptjs run --reporter mocha-junit-reporter
```
Result will be located at `output/result.xml` file.
Html
-----
Best HTML reports could be produced with [mochawesome (opens new window)](https://www.npmjs.com/package/mochawesome) reporter.
Install it via NPM:
```
npm i mochawesome
```
If you get an error like this
```
"mochawesome" reporter not found
invalid reporter "mochawesome"
```
Make sure to have mocha installed or install it:
```
npm i mocha -D
```
Configure it to use `output` directory to print HTML reports:
```
"mocha": {
"reporterOptions": {
"reportDir": "output"
}
},
```
Execute CodeceptJS with HTML reporter:
```
codeceptjs run --reporter mochawesome
```
Result will be located at `output/index.html` file.
### Advanced usage
Want to have screenshots for failed tests? Then add Mochawesome helper to your config:
```
"helpers": {
"Mochawesome": {
"uniqueScreenshotNames": "true"
}
},
```
Then tests with failure will have screenshots.
### Configuration
This helper should be configured in codecept.json
* `uniqueScreenshotNames` (optional, default: false) - option to prevent screenshot override if you have scenarios with the same name in different suites. This option should be the same as in common helper.
* `disableScreenshots` (optional, default: false) - don't save screenshot on failure. This option should be the same as in common helper.
Also if you will add Mochawesome helper, then you will able to add custom context in report:
#### addMochawesomeContext
Adds context to executed test in HTML report:
```
I.addMochawesomeContext('simple string');
I.addMochawesomeContext('http://www.url.com/pathname');
I.addMochawesomeContext('http://www.url.com/screenshot-maybe.jpg');
I.addMochawesomeContext({title: 'expected output',
value: {
a: 1,
b: '2',
c: 'd'
}
});
```
##### Parameters
* `context` string, url, path to screenshot, object. See [this (opens new window)](https://www.npmjs.com/package/mochawesome#adding-test-context)
Multi Reports
--------------
Want to use several reporters in the same time? Try to use [mocha-multi (opens new window)](https://www.npmjs.com/package/mocha-multi) reporter
Install it via NPM:
```
npm i mocha-multi
```
Configure mocha-multi with reports that you want:
```
"mocha": {
"reporterOptions": {
"codeceptjs-cli-reporter": {
"stdout": "-",
"options": {
"verbose": true,
"steps": true,
}
},
"mochawesome": {
"stdout": "./output/console.log",
"options": {
"reportDir": "./output",
"reportFilename": "report"
},
"mocha-junit-reporter": {
"stdout": "./output/console.log",
"options": {
"mochaFile": "./output/result.xml"
},
"attachments": true //add screenshot for a failed test
}
}
}
```
Execute CodeceptJS with mocha-multi reporter:
```
npx codeceptjs run --reporter mocha-multi
```
This will give you cli with steps in console and HTML report in `output` directory.
rxjs RxJS Documentation RxJS Documentation
==================
RxJS is a library for composing asynchronous and event-based programs by using observable sequences. It provides one core type, the [Observable](guide/observable), satellite types (Observer, Schedulers, Subjects) and operators inspired by `Array` methods (`[map](api/index/function/map)`, `[filter](api/index/function/filter)`, `[reduce](api/index/function/reduce)`, `[every](api/index/function/every)`, etc) to allow handling asynchronous events as collections.
Think of RxJS as Lodash for events.
ReactiveX combines the [Observer pattern](https://en.wikipedia.org/wiki/Observer_pattern) with the [Iterator pattern](https://en.wikipedia.org/wiki/Iterator_pattern) and [functional programming with collections](http://martinfowler.com/articles/collection-pipeline/#NestedOperatorExpressions) to fill the need for an ideal way of managing sequences of events.
The essential concepts in RxJS which solve async event management are:
* **Observable:** represents the idea of an invokable collection of future values or events.
* **Observer:** is a collection of callbacks that knows how to listen to values delivered by the Observable.
* **Subscription:** represents the execution of an Observable, is primarily useful for cancelling the execution.
* **Operators:** are pure functions that enable a functional programming style of dealing with collections with operations like `[map](api/index/function/map)`, `[filter](api/index/function/filter)`, `[concat](api/index/function/concat)`, `[reduce](api/index/function/reduce)`, etc.
* **Subject:** is equivalent to an EventEmitter, and the only way of multicasting a value or event to multiple Observers.
* **Schedulers:** are centralized dispatchers to control concurrency, allowing us to coordinate when computation happens on e.g. `setTimeout` or `requestAnimationFrame` or others.
First examples
--------------
Normally you register event listeners.
```
document.addEventListener('click', () => console.log('Clicked!'));
```
Using RxJS you create an observable instead.
```
import { fromEvent } from 'rxjs';
fromEvent(document, 'click').subscribe(() => console.log('Clicked!'));
```
### Purity
What makes RxJS powerful is its ability to produce values using pure functions. That means your code is less prone to errors.
Normally you would create an impure function, where other pieces of your code can mess up your state.
```
let count = 0;
document.addEventListener('click', () => console.log(`Clicked ${++count} times`));
```
Using RxJS you isolate the state.
```
import { fromEvent, scan } from 'rxjs';
fromEvent(document, 'click')
.pipe(scan((count) => count + 1, 0))
.subscribe((count) => console.log(`Clicked ${count} times`));
```
The **scan** operator works just like **reduce** for arrays. It takes a value which is exposed to a callback. The returned value of the callback will then become the next value exposed the next time the callback runs.
### Flow
RxJS has a whole range of operators that helps you control how the events flow through your observables.
This is how you would allow at most one click per second, with plain JavaScript:
```
let count = 0;
let rate = 1000;
let lastClick = Date.now() - rate;
document.addEventListener('click', () => {
if (Date.now() - lastClick >= rate) {
console.log(`Clicked ${++count} times`);
lastClick = Date.now();
}
});
```
With RxJS:
```
import { fromEvent, throttleTime, scan } from 'rxjs';
fromEvent(document, 'click')
.pipe(
throttleTime(1000),
scan((count) => count + 1, 0)
)
.subscribe((count) => console.log(`Clicked ${count} times`));
```
Other flow control operators are [**filter**](api/operators/filter), [**delay**](api/operators/delay), [**debounceTime**](api/operators/debouncetime), [**take**](api/operators/take), [**takeUntil**](api/operators/takeuntil), [**distinct**](api/operators/distinct), [**distinctUntilChanged**](api/operators/distinctuntilchanged) etc.
### Values
You can transform the values passed through your observables.
Here's how you can add the current mouse x position for every click, in plain JavaScript:
```
let count = 0;
const rate = 1000;
let lastClick = Date.now() - rate;
document.addEventListener('click', (event) => {
if (Date.now() - lastClick >= rate) {
count += event.clientX;
console.log(count);
lastClick = Date.now();
}
});
```
With RxJS:
```
import { fromEvent, throttleTime, map, scan } from 'rxjs';
fromEvent(document, 'click')
.pipe(
throttleTime(1000),
map((event) => event.clientX),
scan((count, clientX) => count + clientX, 0)
)
.subscribe((count) => console.log(count));
```
Other value producing operators are [**pluck**](api/operators/pluck), [**pairwise**](api/operators/pairwise), [**sample**](api/operators/sample) etc.
rxjs Importing instructions Importing instructions
======================
There are different ways you can [install](installation) RxJS. Using/importing RxJS depends on the used RxJS version, but also depends on the used installation method.
[Pipeable operators](https://v6.rxjs.dev/guide/v6/pipeable-operators) were introduced in RxJS version 5.5. This enabled all operators to be exported from a single place. This new export site was introduced with RxJS version 6 where all pipeable operators could have been imported from `'rxjs/operators'`. For example, `import { [map](../api/index/function/map) } from 'rxjs/operators'`.
New in RxJS v7.2.0
------------------
**With RxJS v7.2.0, most operators have been moved to `['rxjs'](api#index)` export site. This means that the preferred way to import operators is from `'rxjs'`, while `'rxjs/operators'` export site has been deprecated.**
For example, instead of using:
```
import { map } from 'rxjs/operators';
```
**the preferred way** is to use:
```
import { map } from 'rxjs';
```
Although the old way of importing operators is still active, it will be removed in one of the next major versions.
Click [here to see](importing#how-to-migrate) how to migrate.
Export sites
------------
RxJS v7 exports 6 different locations out of which you can import what you need. Those are:
* `['rxjs'](api#index)` - for example: `import { [of](../api/index/function/of) } from 'rxjs';`
* `['rxjs/operators'](api#operators)` - for example: `import { [map](../api/index/function/map) } from 'rxjs/operators';`
* `['rxjs/ajax'](api#ajax)` - for example: `import { ajax } from 'rxjs/ajax';`
* `['rxjs/fetch'](api#fetch)` - for example: `import { [fromFetch](../api/fetch/fromfetch) } from 'rxjs/fetch';`
* `['rxjs/webSocket'](api#webSocket)` - for example: `import { webSocket } from 'rxjs/webSocket';`
* `['rxjs/testing'](api#testing)` - for example: `import { [TestScheduler](../api/testing/testscheduler) } from 'rxjs/testing';`
### How to migrate?
While nothing has been removed from `'rxjs/operators'`, it is strongly recommended doing the operator imports from `'rxjs'`. Almost all operator function exports have been moved to `'rxjs'`, but only a couple of old and deprecated operators have stayed in the `'rxjs/operators'`. Those operator functions are now mostly deprecated and most of them have their either static operator substitution or are kept as operators, but have a new name so that they are different to their static creation counter-part (usually ending with `With`). Those are:
| `'rxjs/operators'` Operator | Replace With Static Creation Operator | Replace With New Operator Name |
| --- | --- | --- |
| [`combineLatest`](../api/operators/combinelatest) | [`combineLatest`](../api/index/function/combinelatest) | [`combineLatestWith`](../api/index/function/combinelatestwith) |
| [`concat`](../api/operators/concat) | [`concat`](../api/index/function/concat) | [`concatWith`](../api/index/function/concatwith) |
| [`merge`](../api/operators/merge) | [`merge`](../api/index/function/merge) | [`mergeWith`](../api/index/function/mergewith) |
| [`onErrorResumeNext`](../api/operators/onerrorresumenext) | [`onErrorResumeNext`](../api/index/function/onerrorresumenext) | - |
| [`partition`](../api/operators/partition) | [`partition`](../api/index/function/partition) | - |
| [`race`](../api/operators/race) | [`race`](../api/index/function/race) | [`raceWith`](../api/index/function/racewith) |
| [`zip`](../api/operators/zip) | [`zip`](../api/index/function/zip) | [`zipWith`](../api/index/function/zipwith) |
For example, the old and deprecated way of using [`merge`](../api/operators/merge) from `'rxjs/operators'` is:
```
import { merge } from 'rxjs/operators';
a$.pipe(merge(b$)).subscribe();
```
But this should be avoided and replaced with one of the next two examples.
For example, this could be replaced by using a static creation [`merge`](../api/index/function/merge) function:
```
import { merge } from 'rxjs';
merge(a$, b$).subscribe();
```
Or it could be written using a pipeable [`mergeWith`](../api/index/function/mergewith) operator:
```
import { mergeWith } from 'rxjs';
a$.pipe(mergeWith(b$)).subscribe();
```
Depending on the preferred style, you can choose which one to follow, they are completely equal.
Since a new way of importing operators is introduced with RxJS v7.2.0, instructions will be split to prior and after this version.
### ES6 via npm
If you've installed RxJS using [ES6 via npm](installation#es6-via-npm) and installed version is:
#### v7.2.0 or later
Import only what you need:
```
import { of, map } from 'rxjs';
of(1, 2, 3).pipe(map((x) => x + '!!!')); // etc
```
To import the entire set of functionality:
```
import * as rxjs from 'rxjs';
rxjs.of(1, 2, 3).pipe(rxjs.map((x) => x + '!!!')); // etc;
```
To use with a globally imported bundle:
```
const { of, map } = rxjs;
of(1, 2, 3).pipe(map((x) => x + '!!!')); // etc
```
If you installed RxJS version:
#### v7.1.0 or older
Import only what you need:
```
import { of } from 'rxjs';
import { map } from 'rxjs/operators';
of(1, 2, 3).pipe(map((x) => x + '!!!')); // etc
```
To import the entire set of functionality:
```
import * as rxjs from 'rxjs';
import * as operators from 'rxjs';
rxjs.of(1, 2, 3).pipe(operators.map((x) => x + '!!!')); // etc;
```
To use with a globally imported bundle:
```
const { of } = rxjs;
const { map } = rxjs.operators;
of(1, 2, 3).pipe(map((x) => x + '!!!')); // etc
```
### CDN
If you installed a library [using CDN](installation#cdn), the global namespace for rxjs is `rxjs`.
#### v7.2.0 or later
```
const { range, filter, map } = rxjs;
range(1, 200)
.pipe(
filter((x) => x % 2 === 1),
map((x) => x + x)
)
.subscribe((x) => console.log(x));
```
#### v7.1.0 or older
```
const { range } = rxjs;
const { filter, map } = rxjs.operators;
range(1, 200)
.pipe(
filter((x) => x % 2 === 1),
map((x) => x + x)
)
.subscribe((x) => console.log(x));
```
| programming_docs |
rxjs Subject Subject
=======
**What is a Subject?** An RxJS Subject is a special type of Observable that allows values to be multicasted to many Observers. While plain Observables are unicast (each subscribed Observer owns an independent execution of the Observable), Subjects are multicast.
A Subject is like an Observable, but can multicast to many Observers. Subjects are like EventEmitters: they maintain a registry of many listeners.
**Every Subject is an Observable.** Given a Subject, you can `subscribe` to it, providing an Observer, which will start receiving values normally. From the perspective of the Observer, it cannot tell whether the Observable execution is coming from a plain unicast Observable or a Subject.
Internally to the Subject, `subscribe` does not invoke a new execution that delivers values. It simply registers the given Observer in a list of Observers, similarly to how `addListener` usually works in other libraries and languages.
**Every Subject is an Observer.** It is an object with the methods `next(v)`, `error(e)`, and `complete()`. To feed a new value to the Subject, just call `next(theValue)`, and it will be multicasted to the Observers registered to listen to the Subject.
In the example below, we have two Observers attached to a Subject, and we feed some values to the Subject:
```
import { Subject } from 'rxjs';
const subject = new Subject<number>();
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
subject.next(1);
subject.next(2);
// Logs:
// observerA: 1
// observerB: 1
// observerA: 2
// observerB: 2
```
Since a Subject is an Observer, this also means you may provide a Subject as the argument to the `subscribe` of any Observable, like the example below shows:
```
import { Subject, from } from 'rxjs';
const subject = new Subject<number>();
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
const observable = from([1, 2, 3]);
observable.subscribe(subject); // You can subscribe providing a Subject
// Logs:
// observerA: 1
// observerB: 1
// observerA: 2
// observerB: 2
// observerA: 3
// observerB: 3
```
With the approach above, we essentially just converted a unicast Observable execution to multicast, through the Subject. This demonstrates how Subjects are the only way of making any Observable execution be shared to multiple Observers.
There are also a few specializations of the `[Subject](../api/index/class/subject)` type: `[BehaviorSubject](../api/index/class/behaviorsubject)`, `[ReplaySubject](../api/index/class/replaysubject)`, and `[AsyncSubject](../api/index/class/asyncsubject)`.
Multicasted Observables
-----------------------
A "multicasted Observable" passes notifications through a Subject which may have many subscribers, whereas a plain "unicast Observable" only sends notifications to a single Observer.
A multicasted Observable uses a Subject under the hood to make multiple Observers see the same Observable execution.
Under the hood, this is how the `[multicast](../api/index/function/multicast)` operator works: Observers subscribe to an underlying Subject, and the Subject subscribes to the source Observable. The following example is similar to the previous example which used `observable.subscribe(subject)`:
```
import { from, Subject, multicast } from 'rxjs';
const source = from([1, 2, 3]);
const subject = new Subject();
const multicasted = source.pipe(multicast(subject));
// These are, under the hood, `subject.subscribe({...})`:
multicasted.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
multicasted.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
// This is, under the hood, `source.subscribe(subject)`:
multicasted.connect();
```
`[multicast](../api/index/function/multicast)` returns an Observable that looks like a normal Observable, but works like a Subject when it comes to subscribing. `[multicast](../api/index/function/multicast)` returns a `[ConnectableObservable](../api/index/class/connectableobservable)`, which is simply an Observable with the `[connect](../api/index/function/connect)()` method.
The `[connect](../api/index/function/connect)()` method is important to determine exactly when the shared Observable execution will start. Because `[connect](../api/index/function/connect)()` does `source.subscribe(subject)` under the hood, `[connect](../api/index/function/connect)()` returns a Subscription, which you can unsubscribe from in order to cancel the shared Observable execution.
### Reference counting
Calling `[connect](../api/index/function/connect)()` manually and handling the Subscription is often cumbersome. Usually, we want to *automatically* connect when the first Observer arrives, and automatically cancel the shared execution when the last Observer unsubscribes.
Consider the following example where subscriptions occur as outlined by this list:
1. First Observer subscribes to the multicasted Observable
2. **The multicasted Observable is connected**
3. The `next` value `0` is delivered to the first Observer
4. Second Observer subscribes to the multicasted Observable
5. The `next` value `1` is delivered to the first Observer
6. The `next` value `1` is delivered to the second Observer
7. First Observer unsubscribes from the multicasted Observable
8. The `next` value `2` is delivered to the second Observer
9. Second Observer unsubscribes from the multicasted Observable
10. **The connection to the multicasted Observable is unsubscribed**
To achieve that with explicit calls to `[connect](../api/index/function/connect)()`, we write the following code:
```
import { interval, Subject, multicast } from 'rxjs';
const source = interval(500);
const subject = new Subject();
const multicasted = source.pipe(multicast(subject));
let subscription1, subscription2, subscriptionConnect;
subscription1 = multicasted.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
// We should call `connect()` here, because the first
// subscriber to `multicasted` is interested in consuming values
subscriptionConnect = multicasted.connect();
setTimeout(() => {
subscription2 = multicasted.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
}, 600);
setTimeout(() => {
subscription1.unsubscribe();
}, 1200);
// We should unsubscribe the shared Observable execution here,
// because `multicasted` would have no more subscribers after this
setTimeout(() => {
subscription2.unsubscribe();
subscriptionConnect.unsubscribe(); // for the shared Observable execution
}, 2000);
```
If we wish to avoid explicit calls to `[connect](../api/index/function/connect)()`, we can use ConnectableObservable's `[refCount](../api/index/function/refcount)()` method (reference counting), which returns an Observable that keeps track of how many subscribers it has. When the number of subscribers increases from `0` to `1`, it will call `[connect](../api/index/function/connect)()` for us, which starts the shared execution. Only when the number of subscribers decreases from `1` to `0` will it be fully unsubscribed, stopping further execution.
`[refCount](../api/index/function/refcount)` makes the multicasted Observable automatically start executing when the first subscriber arrives, and stop executing when the last subscriber leaves.
Below is an example:
```
import { interval, Subject, multicast, refCount } from 'rxjs';
const source = interval(500);
const subject = new Subject();
const refCounted = source.pipe(multicast(subject), refCount());
let subscription1, subscription2;
// This calls `connect()`, because
// it is the first subscriber to `refCounted`
console.log('observerA subscribed');
subscription1 = refCounted.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
setTimeout(() => {
console.log('observerB subscribed');
subscription2 = refCounted.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
}, 600);
setTimeout(() => {
console.log('observerA unsubscribed');
subscription1.unsubscribe();
}, 1200);
// This is when the shared Observable execution will stop, because
// `refCounted` would have no more subscribers after this
setTimeout(() => {
console.log('observerB unsubscribed');
subscription2.unsubscribe();
}, 2000);
// Logs
// observerA subscribed
// observerA: 0
// observerB subscribed
// observerA: 1
// observerB: 1
// observerA unsubscribed
// observerB: 2
// observerB unsubscribed
```
The `[refCount](../api/index/function/refcount)()` method only exists on ConnectableObservable, and it returns an `[Observable](../api/index/class/observable)`, not another ConnectableObservable.
BehaviorSubject
---------------
One of the variants of Subjects is the `[BehaviorSubject](../api/index/class/behaviorsubject)`, which has a notion of "the current value". It stores the latest value emitted to its consumers, and whenever a new Observer subscribes, it will immediately receive the "current value" from the `[BehaviorSubject](../api/index/class/behaviorsubject)`.
BehaviorSubjects are useful for representing "values over time". For instance, an event stream of birthdays is a Subject, but the stream of a person's age would be a BehaviorSubject.
In the following example, the BehaviorSubject is initialized with the value `0` which the first Observer receives when it subscribes. The second Observer receives the value `2` even though it subscribed after the value `2` was sent.
```
import { BehaviorSubject } from 'rxjs';
const subject = new BehaviorSubject(0); // 0 is the initial value
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
subject.next(1);
subject.next(2);
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
subject.next(3);
// Logs
// observerA: 0
// observerA: 1
// observerA: 2
// observerB: 2
// observerA: 3
// observerB: 3
```
ReplaySubject
-------------
A `[ReplaySubject](../api/index/class/replaysubject)` is similar to a `[BehaviorSubject](../api/index/class/behaviorsubject)` in that it can send old values to new subscribers, but it can also *record* a part of the Observable execution.
A `[ReplaySubject](../api/index/class/replaysubject)` records multiple values from the Observable execution and replays them to new subscribers.
When creating a `[ReplaySubject](../api/index/class/replaysubject)`, you can specify how many values to replay:
```
import { ReplaySubject } from 'rxjs';
const subject = new ReplaySubject(3); // buffer 3 values for new subscribers
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
subject.next(1);
subject.next(2);
subject.next(3);
subject.next(4);
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
subject.next(5);
// Logs:
// observerA: 1
// observerA: 2
// observerA: 3
// observerA: 4
// observerB: 2
// observerB: 3
// observerB: 4
// observerA: 5
// observerB: 5
```
You can also specify a *window time* in milliseconds, besides of the buffer size, to determine how old the recorded values can be. In the following example we use a large buffer size of `100`, but a window time parameter of just `500` milliseconds.
```
import { ReplaySubject } from 'rxjs';
const subject = new ReplaySubject(100, 500 /* windowTime */);
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
let i = 1;
setInterval(() => subject.next(i++), 200);
setTimeout(() => {
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
}, 1000);
// Logs
// observerA: 1
// observerA: 2
// observerA: 3
// observerA: 4
// observerA: 5
// observerB: 3
// observerB: 4
// observerB: 5
// observerA: 6
// observerB: 6
// ...
```
AsyncSubject
------------
The AsyncSubject is a variant where only the last value of the Observable execution is sent to its observers, and only when the execution completes.
```
import { AsyncSubject } from 'rxjs';
const subject = new AsyncSubject();
subject.subscribe({
next: (v) => console.log(`observerA: ${v}`),
});
subject.next(1);
subject.next(2);
subject.next(3);
subject.next(4);
subject.subscribe({
next: (v) => console.log(`observerB: ${v}`),
});
subject.next(5);
subject.complete();
// Logs:
// observerA: 5
// observerB: 5
```
The AsyncSubject is similar to the [`last()`](../api/operators/last) operator, in that it waits for the `complete` notification in order to deliver a single value.
Void subject
------------
Sometimes the emitted value doesn't matter as much as the fact that a value was emitted.
For instance, the code below signals that one second has passed.
```
const subject = new Subject<string>();
setTimeout(() => subject.next('dummy'), 1000);
```
Passing a dummy value this way is clumsy and can confuse users.
By declaring a *void subject*, you signal that the value is irrelevant. Only the event itself matters.
```
const subject = new Subject<void>();
setTimeout(() => subject.next(), 1000);
```
A complete example with context is shown below:
```
import { Subject } from 'rxjs';
const subject = new Subject(); // Shorthand for Subject<void>
subject.subscribe({
next: () => console.log('One second has passed'),
});
setTimeout(() => subject.next(), 1000);
```
Before version 7, the default type of Subject values was `any`. `[Subject](../api/index/class/subject)<any>` disables type checking of the emitted values, whereas `[Subject](../api/index/class/subject)<void>` prevents accidental access to the emitted value. If you want the old behavior, then replace `[Subject](../api/index/class/subject)` with `[Subject](../api/index/class/subject)<any>`.
rxjs Observable Observable
==========
Observables are lazy Push collections of multiple values. They fill the missing spot in the following table:
| | Single | Multiple |
| --- | --- | --- |
| **Pull** | [`Function`](https://developer.mozilla.org/en-US/docs/Glossary/Function) | [`Iterator`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Iteration_protocols) |
| **Push** | [`Promise`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) | [`Observable`](../api/index/class/observable) |
**Example.** The following is an Observable that pushes the values `1`, `2`, `3` immediately (synchronously) when subscribed, and the value `4` after one second has passed since the subscribe call, then completes:
```
import { Observable } from 'rxjs';
const observable = new Observable(subscriber => {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
setTimeout(() => {
subscriber.next(4);
subscriber.complete();
}, 1000);
});
```
To invoke the Observable and see these values, we need to *subscribe* to it:
```
import { Observable } from 'rxjs';
const observable = new Observable(subscriber => {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
setTimeout(() => {
subscriber.next(4);
subscriber.complete();
}, 1000);
});
console.log('just before subscribe');
observable.subscribe({
next(x) { console.log('got value ' + x); },
error(err) { console.error('something wrong occurred: ' + err); },
complete() { console.log('done'); }
});
console.log('just after subscribe');
```
Which executes as such on the console:
```
just before subscribe
got value 1
got value 2
got value 3
just after subscribe
got value 4
done
```
Pull versus Push
----------------
*Pull* and *Push* are two different protocols that describe how a data *Producer* can communicate with a data *Consumer*.
**What is Pull?** In Pull systems, the Consumer determines when it receives data from the data Producer. The Producer itself is unaware of when the data will be delivered to the Consumer.
Every JavaScript Function is a Pull system. The function is a Producer of data, and the code that calls the function is consuming it by "pulling" out a *single* return value from its call.
ES2015 introduced [generator functions and iterators](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/function*) (`function*`), another type of Pull system. Code that calls `iterator.next()` is the Consumer, "pulling" out *multiple* values from the iterator (the Producer).
| | Producer | Consumer |
| --- | --- | --- |
| **Pull** | **Passive:** produces data when requested. | **Active:** decides when data is requested. |
| **Push** | **Active:** produces data at its own pace. | **Passive:** reacts to received data. |
**What is Push?** In Push systems, the Producer determines when to send data to the Consumer. The Consumer is unaware of when it will receive that data.
Promises are the most common type of Push system in JavaScript today. A Promise (the Producer) delivers a resolved value to registered callbacks (the Consumers), but unlike functions, it is the Promise which is in charge of determining precisely when that value is "pushed" to the callbacks.
RxJS introduces Observables, a new Push system for JavaScript. An Observable is a Producer of multiple values, "pushing" them to Observers (Consumers).
* A **Function** is a lazily evaluated computation that synchronously returns a single value on invocation.
* A **generator** is a lazily evaluated computation that synchronously returns zero to (potentially) infinite values on iteration.
* A **Promise** is a computation that may (or may not) eventually return a single value.
* An **Observable** is a lazily evaluated computation that can synchronously or asynchronously return zero to (potentially) infinite values from the time it's invoked onwards.
For more info about what to use when converting Observables to Promises, please refer to [this guide](https://rxjs.dev/deprecations/to-promise).
Observables as generalizations of functions
-------------------------------------------
Contrary to popular claims, Observables are not like EventEmitters nor are they like Promises for multiple values. Observables *may act* like EventEmitters in some cases, namely when they are multicasted using RxJS Subjects, but usually they don't act like EventEmitters.
Observables are like functions with zero arguments, but generalize those to allow multiple values.
Consider the following:
```
function foo() {
console.log('Hello');
return 42;
}
const x = foo.call(); // same as foo()
console.log(x);
const y = foo.call(); // same as foo()
console.log(y);
```
We expect to see as output:
```
"Hello"
42
"Hello"
42
```
You can write the same behavior above, but with Observables:
```
import { Observable } from 'rxjs';
const foo = new Observable(subscriber => {
console.log('Hello');
subscriber.next(42);
});
foo.subscribe(x => {
console.log(x);
});
foo.subscribe(y => {
console.log(y);
});
```
And the output is the same:
```
"Hello"
42
"Hello"
42
```
This happens because both functions and Observables are lazy computations. If you don't call the function, the `console.log('Hello')` won't happen. Also with Observables, if you don't "call" it (with `subscribe`), the `console.log('Hello')` won't happen. Plus, "calling" or "subscribing" is an isolated operation: two function calls trigger two separate side effects, and two Observable subscribes trigger two separate side effects. As opposed to EventEmitters which share the side effects and have eager execution regardless of the existence of subscribers, Observables have no shared execution and are lazy.
Subscribing to an Observable is analogous to calling a Function.
Some people claim that Observables are asynchronous. That is not true. If you surround a function call with logs, like this:
```
console.log('before');
console.log(foo.call());
console.log('after');
```
You will see the output:
```
"before"
"Hello"
42
"after"
```
And this is the same behavior with Observables:
```
console.log('before');
foo.subscribe(x => {
console.log(x);
});
console.log('after');
```
And the output is:
```
"before"
"Hello"
42
"after"
```
Which proves the subscription of `foo` was entirely synchronous, just like a function.
Observables are able to deliver values either synchronously or asynchronously.
What is the difference between an Observable and a function? **Observables can "return" multiple values over time**, something which functions cannot. You can't do this:
```
function foo() {
console.log('Hello');
return 42;
return 100; // dead code. will never happen
}
```
Functions can only return one value. Observables, however, can do this:
```
import { Observable } from 'rxjs';
const foo = new Observable(subscriber => {
console.log('Hello');
subscriber.next(42);
subscriber.next(100); // "return" another value
subscriber.next(200); // "return" yet another
});
console.log('before');
foo.subscribe(x => {
console.log(x);
});
console.log('after');
```
With synchronous output:
```
"before"
"Hello"
42
100
200
"after"
```
But you can also "return" values asynchronously:
```
import { Observable } from 'rxjs';
const foo = new Observable(subscriber => {
console.log('Hello');
subscriber.next(42);
subscriber.next(100);
subscriber.next(200);
setTimeout(() => {
subscriber.next(300); // happens asynchronously
}, 1000);
});
console.log('before');
foo.subscribe(x => {
console.log(x);
});
console.log('after');
```
With output:
```
"before"
"Hello"
42
100
200
"after"
300
```
Conclusion:
* `func.call()` means "*give me one value synchronously*"
* `observable.subscribe()` means "*give me any amount of values, either synchronously or asynchronously*"
Anatomy of an Observable
------------------------
Observables are **created** using `new [Observable](../api/index/class/observable)` or a creation operator, are **subscribed** to with an Observer, **execute** to deliver `next` / `error` / `complete` notifications to the Observer, and their execution may be **disposed**. These four aspects are all encoded in an Observable instance, but some of these aspects are related to other types, like Observer and Subscription.
Core Observable concerns:
* **Creating** Observables
* **Subscribing** to Observables
* **Executing** the Observable
* **Disposing** Observables
### Creating Observables
The `[Observable](../api/index/class/observable)` constructor takes one argument: the `subscribe` function.
The following example creates an Observable to emit the string `'hi'` every second to a subscriber.
```
import { Observable } from 'rxjs';
const observable = new Observable(function subscribe(subscriber) {
const id = setInterval(() => {
subscriber.next('hi')
}, 1000);
});
```
Observables can be created with `new [Observable](../api/index/class/observable)`. Most commonly, observables are created using creation functions, like `[of](../api/index/function/of)`, `[from](../api/index/function/from)`, `[interval](../api/index/function/interval)`, etc.
In the example above, the `subscribe` function is the most important piece to describe the Observable. Let's look at what subscribing means.
### Subscribing to Observables
The Observable `[observable](../api/index/const/observable)` in the example can be *subscribed* to, like this:
```
observable.subscribe(x => console.log(x));
```
It is not a coincidence that `observable.subscribe` and `subscribe` in `new [Observable](../api/index/class/observable)(function subscribe(subscriber) {...})` have the same name. In the library, they are different, but for practical purposes you can consider them conceptually equal.
This shows how `subscribe` calls are not shared among multiple Observers of the same Observable. When calling `observable.subscribe` with an Observer, the function `subscribe` in `new [Observable](../api/index/class/observable)(function subscribe(subscriber) {...})` is run for that given subscriber. Each call to `observable.subscribe` triggers its own independent setup for that given subscriber.
Subscribing to an Observable is like calling a function, providing callbacks where the data will be delivered to.
This is drastically different to event handler APIs like `addEventListener` / `removeEventListener`. With `observable.subscribe`, the given Observer is not registered as a listener in the Observable. The Observable does not even maintain a list of attached Observers.
A `subscribe` call is simply a way to start an "Observable execution" and deliver values or events to an Observer of that execution.
### Executing Observables
The code inside `new [Observable](../api/index/class/observable)(function subscribe(subscriber) {...})` represents an "Observable execution", a lazy computation that only happens for each Observer that subscribes. The execution produces multiple values over time, either synchronously or asynchronously.
There are three types of values an Observable Execution can deliver:
* "Next" notification: sends a value such as a Number, a String, an Object, etc.
* "Error" notification: sends a JavaScript Error or exception.
* "Complete" notification: does not send a value.
"Next" notifications are the most important and most common type: they represent actual data being delivered to a subscriber. "Error" and "Complete" notifications may happen only once during the Observable Execution, and there can only be either one of them.
These constraints are expressed best in the so-called *Observable Grammar* or *Contract*, written as a regular expression:
```
next*(error|complete)?
```
In an Observable Execution, zero to infinite Next notifications may be delivered. If either an Error or Complete notification is delivered, then nothing else can be delivered afterwards.
The following is an example of an Observable execution that delivers three Next notifications, then completes:
```
import { Observable } from 'rxjs';
const observable = new Observable(function subscribe(subscriber) {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
subscriber.complete();
});
```
Observables strictly adhere to the Observable Contract, so the following code would not deliver the Next notification `4`:
```
import { Observable } from 'rxjs';
const observable = new Observable(function subscribe(subscriber) {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
subscriber.complete();
subscriber.next(4); // Is not delivered because it would violate the contract
});
```
It is a good idea to wrap any code in `subscribe` with `try`/`catch` block that will deliver an Error notification if it catches an exception:
```
import { Observable } from 'rxjs';
const observable = new Observable(function subscribe(subscriber) {
try {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
subscriber.complete();
} catch (err) {
subscriber.error(err); // delivers an error if it caught one
}
});
```
### Disposing Observable Executions
Because Observable Executions may be infinite, and it's common for an Observer to want to abort execution in finite time, we need an API for canceling an execution. Since each execution is exclusive to one Observer only, once the Observer is done receiving values, it has to have a way to stop the execution, in order to avoid wasting computation power or memory resources.
When `observable.subscribe` is called, the Observer gets attached to the newly created Observable execution. This call also returns an object, the `[Subscription](../api/index/class/subscription)`:
```
const subscription = observable.subscribe(x => console.log(x));
```
The Subscription represents the ongoing execution, and has a minimal API which allows you to cancel that execution. Read more about the [`Subscription` type here](subscription). With `subscription.unsubscribe()` you can cancel the ongoing execution:
```
import { from } from 'rxjs';
const observable = from([10, 20, 30]);
const subscription = observable.subscribe(x => console.log(x));
// Later:
subscription.unsubscribe();
```
When you subscribe, you get back a Subscription, which represents the ongoing execution. Just call `unsubscribe()` to cancel the execution.
Each Observable must define how to dispose resources of that execution when we create the Observable using `create()`. You can do that by returning a custom `unsubscribe` function from within `function subscribe()`.
For instance, this is how we clear an interval execution set with `setInterval`:
```
const observable = new Observable(function subscribe(subscriber) {
// Keep track of the interval resource
const intervalId = setInterval(() => {
subscriber.next('hi');
}, 1000);
// Provide a way of canceling and disposing the interval resource
return function unsubscribe() {
clearInterval(intervalId);
};
});
```
Just like `observable.subscribe` resembles `new [Observable](../api/index/class/observable)(function subscribe() {...})`, the `unsubscribe` we return from `subscribe` is conceptually equal to `subscription.unsubscribe`. In fact, if we remove the ReactiveX types surrounding these concepts, we're left with rather straightforward JavaScript.
```
function subscribe(subscriber) {
const intervalId = setInterval(() => {
subscriber.next('hi');
}, 1000);
return function unsubscribe() {
clearInterval(intervalId);
};
}
const unsubscribe = subscribe({next: (x) => console.log(x)});
// Later:
unsubscribe(); // dispose the resources
```
The reason why we use Rx types like Observable, Observer, and Subscription is to get safety (such as the Observable Contract) and composability with Operators.
| programming_docs |
rxjs Scheduler Scheduler
=========
**What is a Scheduler?** A scheduler controls when a subscription starts and when notifications are delivered. It consists of three components.
* **A Scheduler is a data structure.** It knows how to store and queue tasks based on priority or other criteria.
* **A Scheduler is an execution context.** It denotes where and when the task is executed (e.g. immediately, or in another callback mechanism such as setTimeout or process.nextTick, or the animation frame).
* **A Scheduler has a (virtual) clock.** It provides a notion of "time" by a getter method `now()` on the scheduler. Tasks being scheduled on a particular scheduler will adhere only to the time denoted by that clock.
A Scheduler lets you define in what execution context will an Observable deliver notifications to its Observer.
In the example below, we take the usual simple Observable that emits values `1`, `2`, `3` synchronously, and use the operator `[observeOn](../api/index/function/observeon)` to specify the `[async](../api/index/const/async)` scheduler to use for delivering those values.
```
import { Observable, observeOn, asyncScheduler } from 'rxjs';
const observable = new Observable((observer) => {
observer.next(1);
observer.next(2);
observer.next(3);
observer.complete();
}).pipe(
observeOn(asyncScheduler)
);
console.log('just before subscribe');
observable.subscribe({
next(x) {
console.log('got value ' + x);
},
error(err) {
console.error('something wrong occurred: ' + err);
},
complete() {
console.log('done');
},
});
console.log('just after subscribe');
```
Which executes with the output:
```
just before subscribe
just after subscribe
got value 1
got value 2
got value 3
done
```
Notice how the notifications `got value...` were delivered after `just after subscribe`, which is different to the default behavior we have seen so far. This is because `[observeOn](../api/index/function/observeon)([asyncScheduler](../api/index/const/asyncscheduler))` introduces a proxy Observer between `new [Observable](../api/index/class/observable)` and the final Observer. Let's rename some identifiers to make that distinction obvious in the example code:
```
import { Observable, observeOn, asyncScheduler } from 'rxjs';
const observable = new Observable((proxyObserver) => {
proxyObserver.next(1);
proxyObserver.next(2);
proxyObserver.next(3);
proxyObserver.complete();
}).pipe(
observeOn(asyncScheduler)
);
const finalObserver = {
next(x) {
console.log('got value ' + x);
},
error(err) {
console.error('something wrong occurred: ' + err);
},
complete() {
console.log('done');
},
};
console.log('just before subscribe');
observable.subscribe(finalObserver);
console.log('just after subscribe');
```
The `proxyObserver` is created in `[observeOn](../api/index/function/observeon)([asyncScheduler](../api/index/const/asyncscheduler))`, and its `next(val)` function is approximately the following:
```
const proxyObserver = {
next(val) {
asyncScheduler.schedule(
(x) => finalObserver.next(x),
0 /* delay */,
val /* will be the x for the function above */
);
},
// ...
};
```
The `[async](../api/index/const/async)` Scheduler operates with a `setTimeout` or `setInterval`, even if the given `[delay](../api/index/function/delay)` was zero. As usual, in JavaScript, `setTimeout(fn, 0)` is known to run the function `fn` earliest on the next event loop iteration. This explains why `got value 1` is delivered to the `finalObserver` after `just after subscribe` happened.
The `schedule()` method of a Scheduler takes a `[delay](../api/index/function/delay)` argument, which refers to a quantity of time relative to the Scheduler's own internal clock. A Scheduler's clock need not have any relation to the actual wall-clock time. This is how temporal operators like `[delay](../api/index/function/delay)` operate not on actual time, but on time dictated by the Scheduler's clock. This is specially useful in testing, where a *virtual time Scheduler* may be used to fake wall-clock time while in reality executing scheduled tasks synchronously.
Scheduler Types
---------------
The `[async](../api/index/const/async)` Scheduler is one of the built-in schedulers provided by RxJS. Each of these can be created and returned by using static properties of the `[Scheduler](../api/index/class/scheduler)` object.
| Scheduler | Purpose |
| --- | --- |
| `null` | By not passing any scheduler, notifications are delivered synchronously and recursively. Use this for constant-time operations or tail recursive operations. |
| `[queueScheduler](../api/index/const/queuescheduler)` | Schedules on a queue in the current event frame (trampoline scheduler). Use this for iteration operations. |
| `[asapScheduler](../api/index/const/asapscheduler)` | Schedules on the micro task queue, which is the same queue used for promises. Basically after the current job, but before the next job. Use this for asynchronous conversions. |
| `[asyncScheduler](../api/index/const/asyncscheduler)` | Schedules work with `setInterval`. Use this for time-based operations. |
| `[animationFrameScheduler](../api/index/const/animationframescheduler)` | Schedules task that will happen just before next browser content repaint. Can be used to create smooth browser animations. |
Using Schedulers
----------------
You may have already used schedulers in your RxJS code without explicitly stating the type of schedulers to be used. This is because all Observable operators that deal with concurrency have optional schedulers. If you do not provide the scheduler, RxJS will pick a default scheduler by using the principle of least concurrency. This means that the scheduler which introduces the least amount of concurrency that satisfies the needs of the operator is chosen. For example, for operators returning an observable with a finite and small number of messages, RxJS uses no Scheduler, i.e. `null` or `undefined`. For operators returning a potentially large or infinite number of messages, `[queue](../api/index/const/queue)` Scheduler is used. For operators which use timers, `[async](../api/index/const/async)` is used.
Because RxJS uses the least concurrency scheduler, you can pick a different scheduler if you want to introduce concurrency for performance purpose. To specify a particular scheduler, you can use those operator methods that take a scheduler, e.g., `[from](../api/index/function/from)([10, 20, 30], [asyncScheduler](../api/index/const/asyncscheduler))`.
**Static creation operators usually take a Scheduler as argument.** For instance, `[from](../api/index/function/from)(array, scheduler)` lets you specify the Scheduler to use when delivering each notification converted from the `array`. It is usually the last argument to the operator. The following static creation operators take a Scheduler argument:
* `[bindCallback](../api/index/function/bindcallback)`
* `[bindNodeCallback](../api/index/function/bindnodecallback)`
* `[combineLatest](../api/index/function/combinelatest)`
* `[concat](../api/index/function/concat)`
* `[empty](../api/index/function/empty)`
* `[from](../api/index/function/from)`
* `fromPromise`
* `[interval](../api/index/function/interval)`
* `[merge](../api/index/function/merge)`
* `[of](../api/index/function/of)`
* `[range](../api/index/function/range)`
* `throw`
* `[timer](../api/index/function/timer)`
**Use `[subscribeOn](../api/index/function/subscribeon)` to schedule in what context will the `subscribe()` call happen.** By default, a `subscribe()` call on an Observable will happen synchronously and immediately. However, you may delay or schedule the actual subscription to happen on a given Scheduler, using the instance operator `[subscribeOn](../api/index/function/subscribeon)(scheduler)`, where `scheduler` is an argument you provide.
**Use `[observeOn](../api/index/function/observeon)` to schedule in what context will notifications be delivered.** As we saw in the examples above, instance operator `[observeOn](../api/index/function/observeon)(scheduler)` introduces a mediator Observer between the source Observable and the destination Observer, where the mediator schedules calls to the destination Observer using your given `scheduler`.
**Instance operators may take a Scheduler as argument.**
Time-related operators like `[bufferTime](../api/index/function/buffertime)`, `[debounceTime](../api/index/function/debouncetime)`, `[delay](../api/index/function/delay)`, `[auditTime](../api/index/function/audittime)`, `[sampleTime](../api/index/function/sampletime)`, `[throttleTime](../api/index/function/throttletime)`, `[timeInterval](../api/index/function/timeinterval)`, `[timeout](../api/index/function/timeout)`, `[timeoutWith](../api/index/function/timeoutwith)`, `[windowTime](../api/index/function/windowtime)` all take a Scheduler as the last argument, and otherwise operate by default on the `[asyncScheduler](../api/index/const/asyncscheduler)`.
Other instance operators that take a Scheduler as argument: `cache`, `[combineLatest](../api/index/function/combinelatest)`, `[concat](../api/index/function/concat)`, `[expand](../api/index/function/expand)`, `[merge](../api/index/function/merge)`, `[publishReplay](../api/index/function/publishreplay)`, `[startWith](../api/index/function/startwith)`.
Notice that both `cache` and `[publishReplay](../api/index/function/publishreplay)` accept a Scheduler because they utilize a ReplaySubject. The constructor of a ReplaySubjects takes an optional Scheduler as the last argument because ReplaySubject may deal with time, which only makes sense in the context of a Scheduler. By default, a ReplaySubject uses the `[queue](../api/index/const/queue)` Scheduler to provide a clock.
rxjs RxJS Operators RxJS Operators
==============
RxJS is mostly useful for its *operators*, even though the Observable is the foundation. Operators are the essential pieces that allow complex asynchronous code to be easily composed in a declarative manner.
What are operators?
-------------------
Operators are **functions**. There are two kinds of operators:
**Pipeable Operators** are the kind that can be piped to Observables using the syntax `observableInstance.pipe(operator())`. These include, [`filter(...)`](../api/operators/filter), and [`mergeMap(...)`](../api/operators/mergemap). When called, they do not *change* the existing Observable instance. Instead, they return a *new* Observable, whose subscription logic is based on the first Observable.
A Pipeable Operator is a function that takes an Observable as its input and returns another Observable. It is a pure operation: the previous Observable stays unmodified.
A Pipeable Operator is essentially a pure function which takes one Observable as input and generates another Observable as output. Subscribing to the output Observable will also subscribe to the input Observable.
**Creation Operators** are the other kind of operator, which can be called as standalone functions to create a new Observable. For example: `[of](../api/index/function/of)(1, 2, 3)` creates an observable that will emit 1, 2, and 3, one right after another. Creation operators will be discussed in more detail in a later section.
For example, the operator called [`map`](../api/operators/map) is analogous to the Array method of the same name. Just as `[1, 2, 3].map(x => x * x)` will yield `[1, 4, 9]`, the Observable created like this:
```
import { of, map } from 'rxjs';
of(1, 2, 3)
.pipe(map((x) => x * x))
.subscribe((v) => console.log(`value: ${v}`));
// Logs:
// value: 1
// value: 4
// value: 9
```
will emit `1`, `4`, `9`. Another useful operator is [`first`](../api/operators/first):
```
import { of, first } from 'rxjs';
of(1, 2, 3)
.pipe(first())
.subscribe((v) => console.log(`value: ${v}`));
// Logs:
// value: 1
```
Note that `[map](../api/index/function/map)` logically must be constructed on the fly, since it must be given the mapping function to. By contrast, `[first](../api/index/function/first)` could be a constant, but is nonetheless constructed on the fly. As a general practice, all operators are constructed, whether they need arguments or not.
Piping
------
Pipeable operators are functions, so they *could* be used like ordinary functions: `op()(obs)` — but in practice, there tend to be many of them convolved together, and quickly become unreadable: `op4()(op3()(op2()(op1()(obs))))`. For that reason, Observables have a method called `.pipe()` that accomplishes the same thing while being much easier to read:
```
obs.pipe(op1(), op2(), op3(), op4());
```
As a stylistic matter, `op()(obs)` is never used, even if there is only one operator; `obs.pipe(op())` is universally preferred.
Creation Operators
------------------
**What are creation operators?** Distinct from pipeable operators, creation operators are functions that can be used to create an Observable with some common predefined behavior or by joining other Observables.
A typical example of a creation operator would be the `[interval](../api/index/function/interval)` function. It takes a number (not an Observable) as input argument, and produces an Observable as output:
```
import { interval } from 'rxjs';
const observable = interval(1000 /* number of milliseconds */);
```
See the list of all static creation operators [here](operators#creation-operators-list).
Higher-order Observables
------------------------
Observables most commonly emit ordinary values like strings and numbers, but surprisingly often, it is necessary to handle Observables *of* Observables, so-called higher-order Observables. For example, imagine you had an Observable emitting strings that were the URLs of files you wanted to see. The code might look like this:
```
const fileObservable = urlObservable.pipe(map((url) => http.get(url)));
```
`http.get()` returns an Observable (of string or string arrays probably) for each individual URL. Now you have an Observable *of* Observables, a higher-order Observable.
But how do you work with a higher-order Observable? Typically, by *flattening*: by (somehow) converting a higher-order Observable into an ordinary Observable. For example:
```
const fileObservable = urlObservable.pipe(
map((url) => http.get(url)),
concatAll()
);
```
The [`concatAll()`](../api/operators/concatall) operator subscribes to each "inner" Observable that comes out of the "outer" Observable, and copies all the emitted values until that Observable completes, and goes on to the next one. All of the values are in that way concatenated. Other useful flattening operators (called [*join operators*](operators#join-operators)) are
* [`mergeAll()`](../api/operators/mergeall) — subscribes to each inner Observable as it arrives, then emits each value as it arrives
* [`switchAll()`](../api/operators/switchall) — subscribes to the first inner Observable when it arrives, and emits each value as it arrives, but when the next inner Observable arrives, unsubscribes to the previous one, and subscribes to the new one.
* [`exhaustAll()`](../api/operators/exhaustall) — subscribes to the first inner Observable when it arrives, and emits each value as it arrives, discarding all newly arriving inner Observables until that first one completes, then waits for the next inner Observable.
Just as many array libraries combine [`map()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) and [`flat()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/flat) (or `flatten()`) into a single [`flatMap()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/flatMap), there are mapping equivalents of all the RxJS flattening operators [`concatMap()`](../api/operators/concatmap), [`mergeMap()`](../api/operators/mergemap), [`switchMap()`](../api/operators/switchmap), and [`exhaustMap()`](../api/operators/exhaustmap).
Marble diagrams
---------------
To explain how operators work, textual descriptions are often not enough. Many operators are related to time, they may for instance delay, sample, throttle, or debounce value emissions in different ways. Diagrams are often a better tool for that. *Marble Diagrams* are visual representations of how operators work, and include the input Observable(s), the operator and its parameters, and the output Observable.
In a marble diagram, time flows to the right, and the diagram describes how values ("marbles") are emitted on the Observable execution.
Below you can see the anatomy of a marble diagram.
 Throughout this documentation site, we extensively use marble diagrams to explain how operators work. They may be really useful in other contexts too, like on a whiteboard or even in our unit tests (as ASCII diagrams).
Categories of operators
-----------------------
There are operators for different purposes, and they may be categorized as: creation, transformation, filtering, joining, multicasting, error handling, utility, etc. In the following list you will find all the operators organized in categories.
For a complete overview, see the [references page](https://rxjs.dev/api).
###
Creation Operators
* [`ajax`](../api/ajax/ajax)
* [`bindCallback`](../api/index/function/bindcallback)
* [`bindNodeCallback`](../api/index/function/bindnodecallback)
* [`defer`](../api/index/function/defer)
* [`empty`](../api/index/function/empty)
* [`from`](../api/index/function/from)
* [`fromEvent`](../api/index/function/fromevent)
* [`fromEventPattern`](../api/index/function/fromeventpattern)
* [`generate`](../api/index/function/generate)
* [`interval`](../api/index/function/interval)
* [`of`](../api/index/function/of)
* [`range`](../api/index/function/range)
* [`throwError`](../api/index/function/throwerror)
* [`timer`](../api/index/function/timer)
* [`iif`](../api/index/function/iif)
###
Join Creation Operators
These are Observable creation operators that also have join functionality -- emitting values of multiple source Observables.
* [`combineLatest`](../api/index/function/combinelatest)
* [`concat`](../api/index/function/concat)
* [`forkJoin`](../api/index/function/forkjoin)
* [`merge`](../api/index/function/merge)
* [`partition`](../api/index/function/partition)
* [`race`](../api/index/function/race)
* [`zip`](../api/index/function/zip)
### Transformation Operators
* [`buffer`](../api/operators/buffer)
* [`bufferCount`](../api/operators/buffercount)
* [`bufferTime`](../api/operators/buffertime)
* [`bufferToggle`](../api/operators/buffertoggle)
* [`bufferWhen`](../api/operators/bufferwhen)
* [`concatMap`](../api/operators/concatmap)
* [`concatMapTo`](../api/operators/concatmapto)
* [`exhaust`](../api/operators/exhaust)
* [`exhaustMap`](../api/operators/exhaustmap)
* [`expand`](../api/operators/expand)
* [`groupBy`](../api/operators/groupby)
* [`map`](../api/operators/map)
* [`mapTo`](../api/operators/mapto)
* [`mergeMap`](../api/operators/mergemap)
* [`mergeMapTo`](../api/operators/mergemapto)
* [`mergeScan`](../api/operators/mergescan)
* [`pairwise`](../api/operators/pairwise)
* [`partition`](../api/operators/partition)
* [`pluck`](../api/operators/pluck)
* [`scan`](../api/operators/scan)
* [`switchScan`](../api/operators/switchscan)
* [`switchMap`](../api/operators/switchmap)
* [`switchMapTo`](../api/operators/switchmapto)
* [`window`](../api/operators/window)
* [`windowCount`](../api/operators/windowcount)
* [`windowTime`](../api/operators/windowtime)
* [`windowToggle`](../api/operators/windowtoggle)
* [`windowWhen`](../api/operators/windowwhen)
### Filtering Operators
* [`audit`](../api/operators/audit)
* [`auditTime`](../api/operators/audittime)
* [`debounce`](../api/operators/debounce)
* [`debounceTime`](../api/operators/debouncetime)
* [`distinct`](../api/operators/distinct)
* [`distinctUntilChanged`](../api/operators/distinctuntilchanged)
* [`distinctUntilKeyChanged`](../api/operators/distinctuntilkeychanged)
* [`elementAt`](../api/operators/elementat)
* [`filter`](../api/operators/filter)
* [`first`](../api/operators/first)
* [`ignoreElements`](../api/operators/ignoreelements)
* [`last`](../api/operators/last)
* [`sample`](../api/operators/sample)
* [`sampleTime`](../api/operators/sampletime)
* [`single`](../api/operators/single)
* [`skip`](../api/operators/skip)
* [`skipLast`](../api/operators/skiplast)
* [`skipUntil`](../api/operators/skipuntil)
* [`skipWhile`](../api/operators/skipwhile)
* [`take`](../api/operators/take)
* [`takeLast`](../api/operators/takelast)
* [`takeUntil`](../api/operators/takeuntil)
* [`takeWhile`](../api/operators/takewhile)
* [`throttle`](../api/operators/throttle)
* [`throttleTime`](../api/operators/throttletime)
###
Join Operators
Also see the [Join Creation Operators](operators#join-creation-operators) section above.
* [`combineLatestAll`](../api/operators/combinelatestall)
* [`concatAll`](../api/operators/concatall)
* [`exhaustAll`](../api/operators/exhaustall)
* [`mergeAll`](../api/operators/mergeall)
* [`switchAll`](../api/operators/switchall)
* [`startWith`](../api/operators/startwith)
* [`withLatestFrom`](../api/operators/withlatestfrom)
### Multicasting Operators
* [`multicast`](../api/operators/multicast)
* [`publish`](../api/operators/publish)
* [`publishBehavior`](../api/operators/publishbehavior)
* [`publishLast`](../api/operators/publishlast)
* [`publishReplay`](../api/operators/publishreplay)
* [`share`](../api/operators/share)
### Error Handling Operators
* [`catchError`](../api/operators/catcherror)
* [`retry`](../api/operators/retry)
* [`retryWhen`](../api/operators/retrywhen)
### Utility Operators
* [`tap`](../api/operators/tap)
* [`delay`](../api/operators/delay)
* [`delayWhen`](../api/operators/delaywhen)
* [`dematerialize`](../api/operators/dematerialize)
* [`materialize`](../api/operators/materialize)
* [`observeOn`](../api/operators/observeon)
* [`subscribeOn`](../api/operators/subscribeon)
* [`timeInterval`](../api/operators/timeinterval)
* [`timestamp`](../api/operators/timestamp)
* [`timeout`](../api/operators/timeout)
* [`timeoutWith`](../api/operators/timeoutwith)
* [`toArray`](../api/operators/toarray)
### Conditional and Boolean Operators
* [`defaultIfEmpty`](../api/operators/defaultifempty)
* [`every`](../api/operators/every)
* [`find`](../api/operators/find)
* [`findIndex`](../api/operators/findindex)
* [`isEmpty`](../api/operators/isempty)
### Mathematical and Aggregate Operators
* [`count`](../api/operators/count)
* [`max`](../api/operators/max)
* [`min`](../api/operators/min)
* [`reduce`](../api/operators/reduce)
Creating custom operators
-------------------------
### Use the `[pipe](../api/index/function/pipe)()` function to make new operators
If there is a commonly used sequence of operators in your code, use the `[pipe](../api/index/function/pipe)()` function to extract the sequence into a new operator. Even if a sequence is not that common, breaking it out into a single operator can improve readability.
For example, you could make a function that discarded odd values and doubled even values like this:
```
import { pipe, filter, map } from 'rxjs';
function discardOddDoubleEven() {
return pipe(
filter((v) => !(v % 2)),
map((v) => v + v)
);
}
```
(The `[pipe](../api/index/function/pipe)()` function is analogous to, but not the same thing as, the `.pipe()` method on an Observable.)
### Creating new operators from scratch
It is more complicated, but if you have to write an operator that cannot be made from a combination of existing operators (a rare occurrance), you can write an operator from scratch using the Observable constructor, like this:
```
import { Observable, of } from 'rxjs';
function delay<T>(delayInMillis: number) {
return (observable: Observable<T>) =>
new Observable<T>((subscriber) => {
// this function will be called each time this
// Observable is subscribed to.
const allTimerIDs = new Set();
let hasCompleted = false;
const subscription = observable.subscribe({
next(value) {
// Start a timer to delay the next value
// from being pushed.
const timerID = setTimeout(() => {
subscriber.next(value);
// after we push the value, we need to clean up the timer timerID
allTimerIDs.delete(timerID);
// If the source has completed, and there are no more timers running,
// we can complete the resulting observable.
if (hasCompleted && allTimerIDs.size === 0) {
subscriber.complete();
}
}, delayInMillis);
allTimerIDs.add(timerID);
},
error(err) {
// We need to make sure we're propagating our errors through.
subscriber.error(err);
},
complete() {
hasCompleted = true;
// If we still have timers running, we don't want to complete yet.
if (allTimerIDs.size === 0) {
subscriber.complete();
}
},
});
// Return the finalization logic. This will be invoked when
// the result errors, completes, or is unsubscribed.
return () => {
subscription.unsubscribe();
// Clean up our timers.
for (const timerID of allTimerIDs) {
clearTimeout(timerID);
}
};
});
}
// Try it out!
of(1, 2, 3).pipe(delay(1000)).subscribe(console.log);
```
Note that you must
1. implement all three Observer functions, `next()`, `error()`, and `complete()` when subscribing to the input Observable.
2. implement a "finalization" function that cleans up when the Observable completes (in this case by unsubscribing and clearing any pending timeouts).
3. return that finalization function from the function passed to the Observable constructor.
Of course, this is only an example; the [`delay()`](../api/operators/delay) operator already exists.
| programming_docs |
rxjs Installation Instructions Installation Instructions
=========================
Here are different ways you can install RxJS:
ES2015 via npm
--------------
```
npm install rxjs
```
By default, RxJS 7.x will provide different variants of the code based on the consumer:
* When RxJS 7.x is used on Node.js regardless of whether it is consumed via `require` or `import`, CommonJS code targetting ES5 will be provided for execution.
* When RxJS 7.4+ is used via a bundler targeting a browser (or other non-Node.js platform) ES module code targetting ES5 will be provided by default with the option to use ES2015 code. 7.x versions prior to 7.4.0 will only provide ES5 code.
If the target browsers for a project support ES2015+ or the bundle process supports down-leveling to ES5 then the bundler can optionally be configured to allow the ES2015 RxJS code to be used instead. You can enable support for using the ES2015 RxJS code by configuring a bundler to use the `es2015` custom export condition during module resolution. Configuring a bundler to use the `es2015` custom export condition is specific to each bundler. If you are interested in using this option, please consult the documentation of your bundler for additional information. However, some general information can be found here: <https://webpack.js.org/guides/package-exports/#conditions-custom>
To import only what you need, please [check out this](importing#es6-via-npm) guide.
CommonJS via npm
----------------
If you receive an error like error TS2304: Cannot find name 'Promise' or error TS2304: Cannot find name 'Iterable' when using RxJS you may need to install a supplemental set of typings.
1. For typings users:
```
typings install es6-shim --ambient
```
2. If you're not using typings the interfaces can be copied from /es6-shim/es6-shim.d.ts.
3. Add type definition file included in tsconfig.json or CLI argument.
All Module Types (CJS/ES6/AMD/TypeScript) via npm
-------------------------------------------------
To install this library via npm version 3, use the following command:
```
npm install @reactivex/rxjs
```
If you are using npm version 2, you need to specify the library version explicitly:
```
npm install @reactivex/[email protected]
```
CDN
---
For CDN, you can use [unpkg](https://unpkg.com/):
[https://unpkg.com/rxjs@^7/dist/bundles/rxjs.umd.min.js](https://unpkg.com/rxjs@%5E7/dist/bundles/rxjs.umd.min.js)
To import what you need, please [check out this](importing#cdn) guide.
rxjs RxJS: Glossary And Semantics RxJS: Glossary And Semantics
============================
When discussing and documenting observables, it's important to have a common language and a known set of rules around what is going on. This document is an attempt to standardize these things so we can try to control the language in our docs, and hopefully other publications about RxJS, so we can discuss reactive programming with RxJS on consistent terms.
While not all of the documentation for RxJS reflects this terminology, it is a goal of the team to ensure it does, and to ensure the language and names around the library use this document as a source of truth and unified language.
Major Entities
--------------
There are high level entities that are frequently discussed. It's important to define them separately from other lower-level concepts, because they relate to the nature of observable.
### Consumer
The code that is subscribing to the observable. This is whoever is being *notified* of [nexted](glossary-and-semantics#next) values, and [errors](glossary-and-semantics#error) or [completions](glossary-and-semantics#complete).
### Producer
Any system or thing that is the source of values that are being pushed out of the observable subscription to the consumer. This can be a wide variety of things, from a `WebSocket` to a simple iteration over an `Array`. The producer is most often created during the [subscribe](glossary-and-semantics#subscribe) action, and therefor "owned" by a [subscription](glossary-and-semantics#subscription) in a 1:1 way, but that is not always the case. A producer may be shared between many subscriptions, if it is created outside of the [subscribe](glossary-and-semantics#subscribe) action, in which case it is one-to-many, resulting in a [multicast](glossary-and-semantics#multicast).
### Subscription
A contract where a [consumer](glossary-and-semantics#consumer) is [observing](glossary-and-semantics#observation) values pushed by a [producer](glossary-and-semantics#producer). The subscription (not to be confused with the `[Subscription](../api/index/class/subscription)` class or type), is an ongoing process that amounts to the function of the observable from the Consumer's perspective. Subscription starts the moment a [subscribe](glossary-and-semantics#subscribe) action is initiated, even before the [subscribe](glossary-and-semantics#subscribe) action is finished.
### Observable
The primary type in RxJS. At its highest level, an observable represents a template for connecting an [Observer](glossary-and-semantics#observer), as a [consumer](glossary-and-semantics#consumer), to a [producer](glossary-and-semantics#producer), via a [subscribe](glossary-and-semantics#subscribe) action, resulting in a [subscription](glossary-and-semantics#subscription).
### Observer
The manifestation of a [consumer](glossary-and-semantics#consumer). A type that may have some (or all) handlers for each type of [notification](glossary-and-semantics#notification): [next](glossary-and-semantics#next), [error](glossary-and-semantics#error), and [complete](glossary-and-semantics#complete). Having all three types of handlers generally gets this to be called an "observer", where if it is missing any of the notification handlers, it may be called a ["partial observer"](glossary-and-semantics#partial-observer).
Major Actions
-------------
There are specific actions and events that occur between major entities in RxJS that need to be defined. These major actions are the highest level events that occur within various parts in RxJS.
### Subscribe
The act of a [consumer](glossary-and-semantics#consumer) requesting an Observable set up a [subscription](glossary-and-semantics#subscription) so that it may [observe](glossary-and-semantics#observation) a [producer](glossary-and-semantics#producer). A subscribe action can occur with an observable via many different mechanisms. The primary mechanism is the [`subscribe` method](../api/index/class/observable#subscribe) on the [Observable class](../api/index/class/observable). Other mechanisms include the [`forEach` method](../api/index/class/observable#forEach), functions like [`lastValueFrom`](../api/index/function/lastvaluefrom), and [`firstValueFrom`](../api/index/function/firstvaluefrom), and the deprecated [`toPromise` method](../api/index/class/observable#forEach).
### Finalization
The act of cleaning up resources used by a producer. This is guaranteed to happen on `error`, `complete`, or if unsubscription occurs. This is not to be confused with [unsubscription](glossary-and-semantics#unsubscription), but it does always happen during unsubscription.
### Unsubscription
The act of a [consumer](glossary-and-semantics#consumer) telling a [producer](glossary-and-semantics#producer) is is no longer interested in receiving values. Causes [Finalization](glossary-and-semantics#finalization)
### Observation
A [consumer](glossary-and-semantics#consumer) reacting to [next](glossary-and-semantics#next), [error](glossary-and-semantics#error), or [complete](glossary-and-semantics#complete) [notifications](glossary-and-semantics#notification). This can only happen *during* [subscription](glossary-and-semantics#subscription).
### Observation Chain
When an [observable](glossary-and-semantics#observable) uses another [observable](glossary-and-semantics#observable) as a [producer](glossary-and-semantics#producer), an "observation chain" is set up. That is a chain of [observation](glossary-and-semantics#observation) such that multiple [observers](glossary-and-semantics#observer) are [notifying](glossary-and-semantics#notification) each other in a unidirectional way toward the final [consumer](glossary-and-semantics#consumer).
### Next
A value has been pushed to the [consumer](glossary-and-semantics#consumer) to be [observed](glossary-and-semantics#observation). Will only happen during [subscription](glossary-and-semantics#subscription), and cannot happen after [error](glossary-and-semantics#error), [complete](glossary-and-semantics#error), or [unsubscription](glossary-and-semantics#unsubscription). Logically, this also means it cannot happen after [finalization](glossary-and-semantics#finalization).
### Error
The [producer](glossary-and-semantics#producer) has encountered a problem and is notifying the [consumer](glossary-and-semantics#consumer). This is a notification that the [producer](glossary-and-semantics#producer) will no longer send values and will [finalize](glossary-and-semantics#finalization). This cannot occur after [complete](glossary-and-semantics#complete), any other [error](glossary-and-semantics#error), or [unsubscription](glossary-and-semantics#unsubscription). Logically, this also means it cannot happen after [finalization](glossary-and-semantics#finalization).
### Complete
The [producer](glossary-and-semantics#producer) is notifying the [consumer](glossary-and-semantics#consumer) that it is done [nexting](glossary-and-semantics#Next) values, without error, will send no more values, and it will [finalize](glossary-and-semantics#finalization). [Completion](glossary-and-semantics#complete) cannot occur after an [error](glossary-and-semantics#error), or [unsubscribe](glossary-and-semantics#unsubscription). [Complete](glossary-and-semantics#complete) cannot be called twice. [Complete](glossary-and-semantics#complete), if it occurs, will always happen before [finalization](glossary-and-semantics#finalization).
### Notification
The act of a [producer](glossary-and-semantics#producer) pushing [nexted](glossary-and-semantics#next) values, [errors](glossary-and-semantics#error) or [completions](glossary-and-semantics#complete) to a [consumer](glossary-and-semantics#consumer) to be [observed](glossary-and-semantics#observation). Not to be confused with the [`Notification` type](../api/index/class/notification), which is notification manifested as a JavaScript object.
Major Concepts
--------------
Some of what we discuss is conceptual. These are mostly common traits of behaviors that can manifest in observables or in push-based reactive systems.
### Multicast
The act of one [producer](glossary-and-semantics#producer) being [observed](glossary-and-semantics#observation) by **many** [consumers](glossary-and-semantics#consumer).
### Unicast
The act of one [producer](glossary-and-semantics#producer) being [observed](glossary-and-semantics#observation) **only one** [consumer](glossary-and-semantics#consumer). An observable is "unicast" when it only connects one [producer](glossary-and-semantics#producer) to one [consumer](glossary-and-semantics#consumer). Unicast doesn't necessarily mean ["cold"](glossary-and-semantics#cold).
### Cold
An observable is "cold" when it creates a new [producer](glossary-and-semantics#producer) during [subscribe](glossary-and-semantics#subscribe) for every new [subscription](glossary-and-semantics#subscription). As a result, a "cold" observables are *always* [unicast](glossary-and-semantics#unicast), being one [producer](glossary-and-semantics#producer) [observed](glossary-and-semantics#observation) by one [consumer](glossary-and-semantics#consumer). Cold observables can be made [hot](glossary-and-semantics#hot) but not the other way around.
### Hot
An observable is "hot", when its [producer](glossary-and-semantics#producer) was created outside of the context of the [subscribe](glossary-and-semantics#subscribe) action. This means that the "hot" observable is almost always [multicast](glossary-and-semantics#multicast). It is possible that a "hot" observable is still *technically* unicast, if it is engineered to only allow one [subscription](glossary-and-semantics#subscription) at a time, however, there is no straightforward mechanism for this in RxJS, and the scenario is a unlikely. For the purposes of discussion, all "hot" observables can be assumed to be [multicast](glossary-and-semantics#multicast). Hot observables cannot be made [cold](glossary-and-semantics#cold).
### Push
[Observables](glossary-and-semantics#observable) are a push-based type. That means rather than having the [consumer](glossary-and-semantics#consumer) call a function or perform some other action to get a value, the [consumer](glossary-and-semantics#consumer) receives values as soon as the [producer](glossary-and-semantics#producer) has produced them, via a registered [next](glossary-and-semantics#next) handler.
### Pull
Pull-based systems are the opposite of [push](glossary-and-semantics#push)-based. In a pull-based type or system, the [consumer](glossary-and-semantics#consumer) must request each value the [producer](glossary-and-semantics#producer) has produced manually, perhaps long after the [producer](glossary-and-semantics#producer) has actually done so. Examples of such systems are [Functions](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function) and [Iterators](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Iteration_protocols)
Minor Entities
--------------
### Operator
A factory function that creates an [operator function](glossary-and-semantics#operator-function). Examples of this in rxjs are functions like [`map`](../api/operators/map) and [`mergeMap`](../api/operators/mergemap), which are generally passed to [`pipe`](../api/index/class/observable#pipe). The result of calling many operators, and passing their resulting [operator functions](glossary-and-semantics#operator-function) into pipe on an observable [source](glossary-and-semantics#source) will be another [observable](glossary-and-semantics#observable), and will generally not result in [subscription](glossary-and-semantics#subscription).
### Operator Function
A function that takes an [observable](glossary-and-semantics#observable), and maps it to a new [observable](glossary-and-semantics#observable). Nothing more, nothing less. Operator functions are created by [operators](glossary-and-semantics#operator). If you were to call an rxjs operator like [map](../api/operators/map) and put the return value in a variable, the returned value would be an operator function.
### Operation
An action taken while handling a [notification](glossary-and-semantics#notification), as set up by an [operator](glossary-and-semantics#operator) and/or [operator function](glossary-and-semantics#operator-function). In RxJS, a developer can chain several [operator functions](glossary-and-semantics#operator-function) together by calling [operators](glossary-and-semantics#operator) and passing the created [operator functions](glossary-and-semantics#operator-function) to the [`pipe`](../api/index/class/observable#pipe) method of [`Observable`](../api/index/class/observable), which results in a new [observable](glossary-and-semantics#observable). During [subscription](glossary-and-semantics#subscription) to that observable, operations are performed in an order dictated by the [observation chain](glossary-and-semantics#observation-chain).
### Stream
A "stream" or "streaming" in the case of observables, refers to the collection of [operations](glossary-and-semantics#operation), as they are processed during a [subscription](glossary-and-semantics#subscription). This is not to be confused with node [Streams](https://nodejs.org/api/stream.html), and the word "stream", on its own, should be used *sparingly* in documentation and articles. Instead, prefer [observation chain](glossary-and-semantics#observation-chain), [operations](glossary-and-semantics#operation), or [subscription](glossary-and-semantics#subscription). "Streaming" is less ambiguous, and is fine to use given this defined meaning.
### Source
A [observable](glossary-and-semantics#observable) or [valid observable input](glossary-and-semantics#observable-inputs) having been converted to an observable, that will supply values to another [observable](glossary-and-semantics#observable), either as the result of an [operator](glossary-and-semantics#operator) or other function that creates one observable as another. This [source](glossary-and-semantics#source), will be the [producer](glossary-and-semantics#producer) for the resulting [observable](glossary-and-semantics#observable) and all of its [subscriptions](glossary-and-semantics#subscriptions). Sources may generally be any type of observable.
### Observable Inputs
A "observable input" ([defined as a type here](../api/index/type-alias/observableinput)), is any type that can easily converted to an [Observable](glossary-and-semantics#observable). Observable Inputs may sometimes be referred to as "valid observable sources".
### Notifier
An [observable](glossary-and-semantics#observable) that is being used to notify another [observable](glossary-and-semantics#observable) that it needs to perform some action. The action should only occur on a [next notification](glossary-and-semantics#next), and never on [error](glossary-and-semantics#error) or [complete](glossary-and-semantics#complete). Generally, notifiers are used with specific operators, such as [`takeUntil`](../api/operators/takeuntil), [`buffer`](../api/operators/buffer), or [`delayWhen`](../api/operators/delaywhen). A notifier may be passed directly, or it may be returned by a callback.
### Inner Source
One, of possibly many [sources](glossary-and-semantics#source), which are [subscribed](glossary-and-semantics#subscribe) to automatically within a single [subscription](glossary-and-semantics#subscription) to another observable. Examples of an "inner source" include the [observable inputs](glossary-and-semantics#observable-inputs) returned by the mapping function in a [mergeMap](../api/operators/mergemap) [operator](glossary-and-semantics#operator). (e.g. `source.pipe([mergeMap](../api/index/function/mergemap)(value => createInnerSource(value))))`, were `createInnerSource` returns any valid [observable input](glossary-and-semantics#observable-inputs)).
### Partial Observer
An [observer](glossary-and-semantics#observer) that lacks all necessary [notification](glossary-and-semantics#notification) handlers. Generally these are supplied by user-land [consumer](glossary-and-semantics#consumer) code. A "full observer" or "observer" would simply be an observer than had all [notification](glossary-and-semantics#notification) handlers.
Other Concepts
--------------
### Unhandled Errors
An "unhandled error" is any [error](glossary-and-semantics#error) that is not handled by a [consumer](glossary-and-semantics#consumer)-provided function, which is generally provided during the [subscribe](glossary-and-semantics#subscribe) action. If no error handler was provided, RxJS will assume the error is "unhandled" and rethrow the error on a new callstack or prevent ["producer interference"](glossary-and-semantics#producer-interface)
### Producer Interference
[Producer](glossary-and-semantics#producer) interference happens when an error is allowed to unwind the callstack the RxJS callstack during [notification](glossary-and-semantics#notification). When this happens, the error could break things like for-loops in [upstream](glossary-and-semantics#upstream-and-downstream) [sources](glossary-and-semantics#source) that are [notifying](glossary-and-semantics#notification) [consumers](glossary-and-semantics#consumer) during a [multicast](glossary-and-semantics#multicast). That would cause the other [consumers](glossary-and-semantics#consumer) in that [multicast](glossary-and-semantics#multicast) to suddenly stop receiving values without logical explanation. As of version 6, RxJS goes out of its way to prevent producer interference by ensuring that all unhandled errors are thrown on a separate callstack.
### Upstream And Downstream
The order in which [notifications](glossary-and-semantics#notification) are processed by [operations](glossary-and-semantics#operation) in a [stream](glossary-and-semantics#stream) have a directionality to them. "Upstream" refers to an [operation](glossary-and-semantics#operation) that was already processed before the current [operation](glossary-and-semantics#operation), and "downstream" refers to an [operation](glossary-and-semantics#operation) that *will* be processed *after* the current [operation](glossary-and-semantics#operation). See also: [Streaming](glossary-and-semantics#stream).
| programming_docs |
rxjs Subscription Subscription
============
**What is a Subscription?** A Subscription is an object that represents a disposable resource, usually the execution of an Observable. A Subscription has one important method, `unsubscribe`, that takes no argument and just disposes the resource held by the subscription. In previous versions of RxJS, Subscription was called "Disposable".
```
import { interval } from 'rxjs';
const observable = interval(1000);
const subscription = observable.subscribe(x => console.log(x));
// Later:
// This cancels the ongoing Observable execution which
// was started by calling subscribe with an Observer.
subscription.unsubscribe();
```
A Subscription essentially just has an `unsubscribe()` function to release resources or cancel Observable executions.
Subscriptions can also be put together, so that a call to an `unsubscribe()` of one Subscription may unsubscribe multiple Subscriptions. You can do this by "adding" one subscription into another:
```
import { interval } from 'rxjs';
const observable1 = interval(400);
const observable2 = interval(300);
const subscription = observable1.subscribe(x => console.log('first: ' + x));
const childSubscription = observable2.subscribe(x => console.log('second: ' + x));
subscription.add(childSubscription);
setTimeout(() => {
// Unsubscribes BOTH subscription and childSubscription
subscription.unsubscribe();
}, 1000);
```
When executed, we see in the console:
```
second: 0
first: 0
second: 1
first: 1
second: 2
```
Subscriptions also have a `remove(otherSubscription)` method, in order to undo the addition of a child Subscription.
rxjs Observer Observer
========
**What is an Observer?** An Observer is a consumer of values delivered by an Observable. Observers are simply a set of callbacks, one for each type of notification delivered by the Observable: `next`, `error`, and `complete`. The following is an example of a typical Observer object:
```
const observer = {
next: x => console.log('Observer got a next value: ' + x),
error: err => console.error('Observer got an error: ' + err),
complete: () => console.log('Observer got a complete notification'),
};
```
To use the Observer, provide it to the `subscribe` of an Observable:
```
observable.subscribe(observer);
```
Observers are just objects with three callbacks, one for each type of notification that an Observable may deliver.
Observers in RxJS may also be *partial*. If you don't provide one of the callbacks, the execution of the Observable will still happen normally, except some types of notifications will be ignored, because they don't have a corresponding callback in the Observer.
The example below is an `[Observer](../api/index/interface/observer)` without the `complete` callback:
```
const observer = {
next: x => console.log('Observer got a next value: ' + x),
error: err => console.error('Observer got an error: ' + err),
};
```
When subscribing to an `[Observable](../api/index/class/observable)`, you may also just provide the next callback as an argument, without being attached to an `[Observer](../api/index/interface/observer)` object, for instance like this:
```
observable.subscribe(x => console.log('Observer got a next value: ' + x));
```
Internally in `observable.subscribe`, it will create an `[Observer](../api/index/interface/observer)` object using the callback argument as the `next` handler.
rxjs Testing RxJS Code with Marble Diagrams Testing RxJS Code with Marble Diagrams
======================================
> This guide refers to usage of marble diagrams when using the new `testScheduler.run(callback)`. Some details here do not apply to using the TestScheduler manually, without using the `run()` helper.
We can test our *asynchronous* RxJS code *synchronously* and deterministically by virtualizing time using the TestScheduler. **Marble diagrams** provide a visual way for us to represent the behavior of an Observable. We can use them to assert that a particular Observable behaves as expected, as well as to create [hot and cold Observables](https://medium.com/@benlesh/hot-vs-cold-observables-f8094ed53339) we can use as mocks.
> At this time, the TestScheduler can only be used to test code that uses RxJS schedulers - `AsyncScheduler`, etc. If the code consumes a Promise, for example, it cannot be reliably tested with `[TestScheduler](../../api/testing/testscheduler)`, but instead should be tested more traditionally. See the [Known Issues](marble-testing#known-issues) section for more details.
>
>
```
import { TestScheduler } from 'rxjs/testing';
import { throttleTime } from 'rxjs';
const testScheduler = new TestScheduler((actual, expected) => {
// asserting the two objects are equal - required
// for TestScheduler assertions to work via your test framework
// e.g. using chai.
expect(actual).deep.equal(expected);
});
// This test runs synchronously.
it('generates the stream correctly', () => {
testScheduler.run((helpers) => {
const { cold, time, expectObservable, expectSubscriptions } = helpers;
const e1 = cold(' -a--b--c---|');
const e1subs = ' ^----------!';
const t = time(' ---| '); // t = 3
const expected = '-a-----c---|';
expectObservable(e1.pipe(throttleTime(t))).toBe(expected);
expectSubscriptions(e1.subscriptions).toBe(e1subs);
});
});
```
API
---
The callback function you provide to `testScheduler.run(callback)` is called with `helpers` object that contains functions you'll use to write your tests.
> When the code inside this callback is being executed, any operator that uses timers/AsyncScheduler (like delay, debounceTime, etc.,) will automatically use the TestScheduler instead, so that we have "virtual time". You do not need to pass the TestScheduler to them, like in the past.
```
testScheduler.run((helpers) => {
const { cold, hot, expectObservable, expectSubscriptions, flush, time, animate } = helpers;
// use them
});
```
Although `run()` executes entirely synchronously, the helper functions inside your callback function do not! These functions **schedule assertions** that will execute either when your callback completes or when you explicitly call `flush()`. Be wary of calling synchronous assertions, for example `expect`, from your testing library of choice, from within the callback. See [Synchronous Assertion](marble-testing#synchronous-assertion) for more information on how to do this.
* `cold(marbleDiagram: string, values?: object, error?: any)` - creates a "cold" observable whose subscription starts when the test begins.
* `hot(marbleDiagram: string, values?: object, error?: any)` - creates a "hot" observable (like a subject) that will behave as though it's already "running" when the test begins. An interesting difference is that `hot` marbles allow a `^` character to signal where the "zero frame" is. That is the point at which the subscription to observables being tested begins.
* `expectObservable(actual: [Observable](../../api/index/class/observable)<T>, subscriptionMarbles?: string).toBe(marbleDiagram: string, values?: object, error?: any)` - schedules an assertion for when the TestScheduler flushes. Give `subscriptionMarbles` as parameter to change the schedule of subscription and unsubscription. If you don't provide the `subscriptionMarbles` parameter it will subscribe at the beginning and never unsubscribe. Read below about subscription marble diagram.
* `expectSubscriptions(actualSubscriptionLogs: SubscriptionLog[]).toBe(subscriptionMarbles: string)` - like `expectObservable` schedules an assertion for when the testScheduler flushes. Both `cold()` and `hot()` return an observable with a property `subscriptions` of type `SubscriptionLog[]`. Give `subscriptions` as parameter to `expectSubscriptions` to assert whether it matches the `subscriptionsMarbles` marble diagram given in `toBe()`. Subscription marble diagrams are slightly different than Observable marble diagrams. Read more below.
* `flush()` - immediately starts virtual time. Not often used since `run()` will automatically flush for you when your callback returns, but in some cases you may wish to flush more than once or otherwise have more control.
* `time()` - converts marbles into a number indicating number of frames. It can be used by operators expecting a specific timeout. It measures time based on the position of the complete (`|`) signal:
```
testScheduler.run((helpers) => {
const { time, cold } = helpers;
const source = cold('---a--b--|');
const t = time(' --| ');
// --|
const expected = ' -----a--b|';
const result = source.pipe(delay(t));
expectObservable(result).toBe(expected);
});
```
* `animate()` - specifies when requested animation frames will be 'painted'. `animate` accepts a marble diagram and each value emission in the diagram indicates when a 'paint' occurs - at which time, any queued `requestAnimationFrame` callbacks will be executed. Call `animate` at the beginning of your test and align the marble diagrams so that it's clear when the callbacks will be executed:
```
testScheduler.run((helpers) => {
const { animate, cold } = helpers;
animate(' ---x---x---x---x');
const requests = cold('-r-------r------');
/* ... */
const expected = ' ---a-------b----';
});
```
Marble syntax
-------------
In the context of TestScheduler, a marble diagram is a string containing special syntax representing events happening over virtual time. Time progresses by *frames*. The first character of any marble string always represents the *zero frame*, or the start of time. Inside of `testScheduler.run(callback)` the frameTimeFactor is set to 1, which means one frame is equal to one virtual millisecond.
How many virtual milliseconds one frame represents depends on the value of `[TestScheduler.frameTimeFactor](../../api/testing/testscheduler#frameTimeFactor)`. For legacy reasons the value of `frameTimeFactor` is 1 *only* when your code inside the `testScheduler.run(callback)` callback is running. Outside of it, it's set to 10. This will likely change in a future version of RxJS so that it is always 1.
> IMPORTANT: This syntax guide refers to usage of marble diagrams when using the new `testScheduler.run(callback)`. The semantics of marble diagrams when using the TestScheduler manually are different, and some features like the new time progression syntax are not supported.
>
>
* `' '` whitespace: horizontal whitespace is ignored, and can be used to help vertically align multiple marble diagrams.
* `'-'` frame: 1 "frame" of virtual time passing (see above description of frames).
* `[0-9]+[ms|s|m]` time progression: the time progression syntax lets you progress virtual time by a specific amount. It's a number, followed by a time unit of `ms` (milliseconds), `s` (seconds), or `m` (minutes) without any space between them, e.g. `a 10ms b`. See [Time progression syntax](marble-testing#time-progression-syntax) for more details.
* `'|'` complete: The successful completion of an observable. This is the observable producer signaling `complete()`.
* `'#'` error: An error terminating the observable. This is the observable producer signaling `error()`.
* `[a-z0-9]` e.g. `'a'` any alphanumeric character: Represents a value being emitted by the producer signaling `next()`. Also consider that you could map this into an object or an array like this:
```
const expected = '400ms (a-b|)';
const values = {
a: 'value emitted',
b: 'another value emitted',
};
expectObservable(someStreamForTesting).toBe(expected, values);
// This would work also
const expected = '400ms (0-1|)';
const values = [
'value emitted',
'another value emitted'
];
expectObservable(someStreamForTesting).toBe(expected, values);
```
* `'()'` sync groupings: When multiple events need to be in the same frame synchronously, parentheses are used to group those events. You can group next'd values, a completion, or an error in this manner. The position of the initial `(` determines the time at which its values are emitted. While it can be counter-intuitive at first, after all the values have synchronously emitted time will progress a number of frames equal to the number of ASCII characters in the group, including the parentheses. e.g. `'(abc)'` will emit the values of a, b, and c synchronously in the same frame and then advance virtual time by 5 frames, `'(abc)'.length === 5`. This is done because it often helps you vertically align your marble diagrams, but it's a known pain point in real-world testing. [Learn more about known issues](marble-testing#known-issues).
* `'^'` subscription point: (hot observables only) shows the point at which the tested observables will be subscribed to the hot observable. This is the "zero frame" for that observable, every frame before the `^` will be negative. Negative time might seem pointless, but there are in fact advanced cases where this is necessary, usually involving ReplaySubjects.
### Time progression syntax
The new time progression syntax takes inspiration from the CSS duration syntax. It's a number (integer or floating point) immediately followed by a unit; ms (milliseconds), s (seconds), m (minutes). e.g. `100ms`, `1.4s`, `5.25m`.
When it's not the first character of the diagram it must be padded a space before/after to disambiguate it from a series of marbles. e.g. `a 1ms b` needs the spaces because `a1msb` will be interpreted as `['a', '1', 'm', 's', 'b']` where each of these characters is a value that will be next()'d as-is.
**NOTE**: You may have to subtract 1 millisecond from the time you want to progress because the alphanumeric marbles (representing an actual emitted value) *advance time 1 virtual frame* themselves already, after they emit. This can be counter-intuitive and frustrating, but for now it is indeed correct.
```
const input = ' -a-b-c|';
const expected = '-- 9ms a 9ms b 9ms (c|)';
// Depending on your personal preferences you could also
// use frame dashes to keep vertical alignment with the input.
// const input = ' -a-b-c|';
// const expected = '------- 4ms a 9ms b 9ms (c|)';
// or
// const expected = '-----------a 9ms b 9ms (c|)';
const result = cold(input).pipe(
concatMap((d) => of(d).pipe(
delay(10)
))
);
expectObservable(result).toBe(expected);
```
### Examples
`'-'` or `'------'`: Equivalent to [`NEVER`](../../api/index/const/never), or an observable that never emits or errors or completes.
`|`: Equivalent to [`EMPTY`](../../api/index/const/empty), or an observable that never emits and completes immediately.
`#`: Equivalent to [`throwError`](../../api/index/function/throwerror), or an observable that never emits and errors immediately.
`'--a--'`: An observable that waits 2 "frames", emits value `a` on frame 2 and then never completes.
`'--a--b--|'`: On frame 2 emit `a`, on frame 5 emit `b`, and on frame 8, `complete`.
`'--a--b--#'`: On frame 2 emit `a`, on frame 5 emit `b`, and on frame 8, `error`.
`'-a-^-b--|'`: In a hot observable, on frame -2 emit `a`, then on frame 2 emit `b`, and on frame 5, `complete`.
`'--(abc)-|'`: on frame 2 emit `a`, `b`, and `c`, then on frame 8, `complete`.
`'-----(a|)'`: on frame 5 emit `a` and `complete`.
`'a 9ms b 9s c|'`: on frame 0 emit `a`, on frame 10 emit `b`, on frame 9,011 emit `c`, then on frame 9,012 `complete`.
`'--a 2.5m b'`: on frame 2 emit `a`, on frame 150,003 emit `b` and never complete.
Subscription marbles
--------------------
The `expectSubscriptions` helper allows you to assert that a `cold()` or `hot()` Observable you created was subscribed/unsubscribed to at the correct point in time. The `subscriptionMarbles` parameter to `expectObservable` allows your test to defer subscription to a later virtual time, and/or unsubscribe even if the observable being tested has not yet completed.
The subscription marble syntax is slightly different to conventional marble syntax.
* `'-'` time: 1 frame time passing.
* `[0-9]+[ms|s|m]` time progression: the time progression syntax lets you progress virtual time by a specific amount. It's a number, followed by a time unit of `ms` (milliseconds), `s` (seconds), or `m` (minutes) without any space between them, e.g. `a 10ms b`. See [Time progression syntax](marble-testing#time-progression-syntax) for more details.
* `'^'` subscription point: shows the point in time at which a subscription happens.
* `'!'` unsubscription point: shows the point in time at which a subscription is unsubscribed.
There should be **at most one** `^` point in a subscription marble diagram, and **at most one** `!` point. Other than that, the `-` character is the only one allowed in a subscription marble diagram.
### Examples
`'-'` or `'------'`: no subscription ever happened.
`'--^--'`: a subscription happened after 2 "frames" of time passed, and the subscription was not unsubscribed.
`'--^--!-'`: on frame 2 a subscription happened, and on frame 5 was unsubscribed.
`'500ms ^ 1s !'`: on frame 500 a subscription happened, and on frame 1,501 was unsubscribed.
Given a hot source, test multiple subscribers that subscribe at different times:
```
testScheduler.run(({ hot, expectObservable }) => {
const source = hot('--a--a--a--a--a--a--a--');
const sub1 = ' --^-----------!';
const sub2 = ' ---------^--------!';
const expect1 = ' --a--a--a--a--';
const expect2 = ' -----------a--a--a-';
expectObservable(source, sub1).toBe(expect1);
expectObservable(source, sub2).toBe(expect2);
});
```
Manually unsubscribe from a source that will never complete:
```
it('should repeat forever', () => {
const testScheduler = createScheduler();
testScheduler.run(({ expectObservable }) => {
const foreverStream$ = interval(1).pipe(mapTo('a'));
// Omitting this arg may crash the test suite.
const unsub = '------!';
expectObservable(foreverStream$, unsub).toBe('-aaaaa');
});
});
```
Synchronous Assertion
---------------------
Sometimes, we need to assert changes in state *after* an observable stream has completed - such as when a side effect like `[tap](../../api/index/function/tap)` updates a variable. Outside of Marbles testing with TestScheduler, we might think of this as creating a delay or waiting before making our assertion.
For example:
```
let eventCount = 0;
const s1 = cold('--a--b|', { a: 'x', b: 'y' });
// side effect using 'tap' updates a variable
const result = s1.pipe(tap(() => eventCount++));
expectObservable(result).toBe('--a--b|', { a: 'x', b: 'y' });
// flush - run 'virtual time' to complete all outstanding hot or cold observables
flush();
expect(eventCount).toBe(2);
```
In the above situation we need the observable stream to complete so that we can test the variable was set to the correct value. The TestScheduler runs in 'virtual time' (synchronously), but doesn't normally run (and complete) until the testScheduler callback returns. The flush() method manually triggers the virtual time so that we can test the local variable after the observable completes.
Known issues
------------
### RxJS code that consumes Promises cannot be directly tested
If you have RxJS code that uses asynchronous scheduling - e.g. Promises, etc. - you can't reliably use marble diagrams *for that particular code*. This is because those other scheduling methods won't be virtualized or known to TestScheduler.
The solution is to test that code in isolation, with the traditional asynchronous testing methods of your testing framework. The specifics depend on your testing framework of choice, but here's a pseudo-code example:
```
// Some RxJS code that also consumes a Promise, so TestScheduler won't be able
// to correctly virtualize and the test will always be really asynchronous.
const myAsyncCode = () => from(Promise.resolve('something'));
it('has async code', (done) => {
myAsyncCode().subscribe((d) => {
assertEqual(d, 'something');
done();
});
});
```
On a related note, you also can't currently assert delays of zero, even with `AsyncScheduler`, e.g. `[delay](../../api/index/function/delay)(0)` is like saying `setTimeout(work, 0)`. This schedules a new ["task" aka "macrotask"](https://jakearchibald.com/2015/tasks-microtasks-queues-and-schedules/), so it's asynchronous, but without an explicit passage of time.
### Behavior is different outside of `testScheduler.run(callback)`
The `[TestScheduler](../../api/testing/testscheduler)` has been around since v5, but was actually intended for testing RxJS itself by the maintainers, rather than for use in regular user apps. Because of this, some of the default behaviors and features of the TestScheduler did not work well (or at all) for users. In v6 we introduced the `testScheduler.run(callback)` method which allowed us to provide new defaults and features in a non-breaking way, but it's still possible to [use the TestScheduler outside](https://github.com/ReactiveX/rxjs/blob/7113ae4b451dd8463fae71b68edab96079d089df/docs_app/content/guide/testing/internal-marble-tests.md) of `testScheduler.run(callback)`. It's important to note that if you do so, there are some major differences in how it will behave.
* `[TestScheduler](../../api/testing/testscheduler)` helper methods have more verbose names, like `testScheduler.createColdObservable()` instead of `cold()`.
* The testScheduler instance is *not* automatically used by operators that use `AsyncScheduler`, e.g. `[delay](../../api/index/function/delay)`, `[debounceTime](../../api/index/function/debouncetime)`, etc., so you have to explicitly pass it to them.
* There is NO support for time progression syntax e.g. `-a 100ms b-|`.
* 1 frame is 10 virtual milliseconds by default. i.e. `[TestScheduler.frameTimeFactor](../../api/testing/testscheduler#frameTimeFactor) = 10`.
* Each whitespace `' '` equals 1 frame, same as a hyphen `'-'`.
* There is a hard maximum number of frames set at 750 i.e. `maxFrames = 750`. After 750 they are silently ignored.
* You must explicitly flush the scheduler.
While at this time usage of the TestScheduler outside of `testScheduler.run(callback)` has not been officially deprecated, it is discouraged because it is likely to cause confusion.
| programming_docs |
rxjs webSocket webSocket
=========
`function` `stable`
Wrapper around the w3c-compatible WebSocket object provided by the browser.
### `webSocket<T>(urlConfigOrSource: string | WebSocketSubjectConfig<T>): WebSocketSubject<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `urlConfigOrSource` | `string | WebSocketSubjectConfig<T>` | The WebSocket endpoint as an url or an object with configuration and additional Observers. |
#### Returns
`[WebSocketSubject<T>](websocketsubject)`: Subject which allows to both send and receive messages via WebSocket connection.
Description
-----------
[`Subject`](../index/class/subject) that communicates with a server via WebSocket
`webSocket` is a factory function that produces a `[WebSocketSubject](websocketsubject)`, which can be used to make WebSocket connection with an arbitrary endpoint. `webSocket` accepts as an argument either a string with url of WebSocket endpoint, or an [WebSocketSubjectConfig](websocketsubjectconfig) object for providing additional configuration, as well as Observers for tracking lifecycle of WebSocket connection.
When `[WebSocketSubject](websocketsubject)` is subscribed, it attempts to make a socket connection, unless there is one made already. This means that many subscribers will always listen on the same socket, thus saving resources. If however, two instances are made of `[WebSocketSubject](websocketsubject)`, even if these two were provided with the same url, they will attempt to make separate connections. When consumer of a `[WebSocketSubject](websocketsubject)` unsubscribes, socket connection is closed, only if there are no more subscribers still listening. If after some time a consumer starts subscribing again, connection is reestablished.
Once connection is made, whenever a new message comes from the server, `[WebSocketSubject](websocketsubject)` will emit that message as a value in the stream. By default, a message from the socket is parsed via `JSON.parse`. If you want to customize how deserialization is handled (if at all), you can provide custom `resultSelector` function in [`WebSocketSubject`](websocketsubject). When connection closes, stream will complete, provided it happened without any errors. If at any point (starting, maintaining or closing a connection) there is an error, stream will also error with whatever WebSocket API has thrown.
By virtue of being a [`Subject`](../index/class/subject), `[WebSocketSubject](websocketsubject)` allows for receiving and sending messages from the server. In order to communicate with a connected endpoint, use `next`, `error` and `complete` methods. `next` sends a value to the server, so bear in mind that this value will not be serialized beforehand. Because of This, `JSON.stringify` will have to be called on a value by hand, before calling `next` with a result. Note also that if at the moment of nexting value there is no socket connection (for example no one is subscribing), those values will be buffered, and sent when connection is finally established. `complete` method closes socket connection. `error` does the same, as well as notifying the server that something went wrong via status code and string with details of what happened. Since status code is required in WebSocket API, `[WebSocketSubject](websocketsubject)` does not allow, like regular `[Subject](../index/class/subject)`, arbitrary values being passed to the `error` method. It needs to be called with an object that has `code` property with status code number and optional `reason` property with string describing details of an error.
Calling `next` does not affect subscribers of `[WebSocketSubject](websocketsubject)` - they have no information that something was sent to the server (unless of course the server responds somehow to a message). On the other hand, since calling `complete` triggers an attempt to close socket connection. If that connection is closed without any errors, stream will complete, thus notifying all subscribers. And since calling `error` closes socket connection as well, just with a different status code for the server, if closing itself proceeds without errors, subscribed Observable will not error, as one might expect, but complete as usual. In both cases (calling `complete` or `error`), if process of closing socket connection results in some errors, *then* stream will error.
**Multiplexing**
`[WebSocketSubject](websocketsubject)` has an additional operator, not found in other Subjects. It is called `multiplex` and it is used to simulate opening several socket connections, while in reality maintaining only one. For example, an application has both chat panel and real-time notifications about sport news. Since these are two distinct functions, it would make sense to have two separate connections for each. Perhaps there could even be two separate services with WebSocket endpoints, running on separate machines with only GUI combining them together. Having a socket connection for each functionality could become too resource expensive. It is a common pattern to have single WebSocket endpoint that acts as a gateway for the other services (in this case chat and sport news services). Even though there is a single connection in a client app, having the ability to manipulate streams as if it were two separate sockets is desirable. This eliminates manually registering and unregistering in a gateway for given service and filter out messages of interest. This is exactly what `multiplex` method is for.
Method accepts three parameters. First two are functions returning subscription and unsubscription messages respectively. These are messages that will be sent to the server, whenever consumer of resulting Observable subscribes and unsubscribes. Server can use them to verify that some kind of messages should start or stop being forwarded to the client. In case of the above example application, after getting subscription message with proper identifier, gateway server can decide that it should connect to real sport news service and start forwarding messages from it. Note that both messages will be sent as returned by the functions, they are by default serialized using JSON.stringify, just as messages pushed via `next`. Also bear in mind that these messages will be sent on *every* subscription and unsubscription. This is potentially dangerous, because one consumer of an Observable may unsubscribe and the server might stop sending messages, since it got unsubscription message. This needs to be handled on the server or using [`publish`](../index/function/publish) on a Observable returned from 'multiplex'.
Last argument to `multiplex` is a `messageFilter` function which should return a boolean. It is used to filter out messages sent by the server to only those that belong to simulated WebSocket stream. For example, server might mark these messages with some kind of string identifier on a message object and `messageFilter` would return `true` if there is such identifier on an object emitted by the socket. Messages which returns `false` in `messageFilter` are simply skipped, and are not passed down the stream.
Return value of `multiplex` is an Observable with messages incoming from emulated socket connection. Note that this is not a `[WebSocketSubject](websocketsubject)`, so calling `next` or `multiplex` again will fail. For pushing values to the server, use root `[WebSocketSubject](websocketsubject)`.
Examples
--------
Listening for messages from the server
```
import { webSocket } from 'rxjs/webSocket';
const subject = webSocket('ws://localhost:8081');
subject.subscribe({
next: msg => console.log('message received: ' + msg), // Called whenever there is a message from the server.
error: err => console.log(err), // Called if at any point WebSocket API signals some kind of error.
complete: () => console.log('complete') // Called when connection is closed (for whatever reason).
});
```
Pushing messages to the server
```
import { webSocket } from 'rxjs/webSocket';
const subject = webSocket('ws://localhost:8081');
subject.subscribe();
// Note that at least one consumer has to subscribe to the created subject - otherwise "nexted" values will be just buffered and not sent,
// since no connection was established!
subject.next({ message: 'some message' });
// This will send a message to the server once a connection is made. Remember value is serialized with JSON.stringify by default!
subject.complete(); // Closes the connection.
subject.error({ code: 4000, reason: 'I think our app just broke!' });
// Also closes the connection, but let's the server know that this closing is caused by some error.
```
Multiplexing WebSocket
```
import { webSocket } from 'rxjs/webSocket';
const subject = webSocket('ws://localhost:8081');
const observableA = subject.multiplex(
() => ({ subscribe: 'A' }), // When server gets this message, it will start sending messages for 'A'...
() => ({ unsubscribe: 'A' }), // ...and when gets this one, it will stop.
message => message.type === 'A' // If the function returns `true` message is passed down the stream. Skipped if the function returns false.
);
const observableB = subject.multiplex( // And the same goes for 'B'.
() => ({ subscribe: 'B' }),
() => ({ unsubscribe: 'B' }),
message => message.type === 'B'
);
const subA = observableA.subscribe(messageForA => console.log(messageForA));
// At this moment WebSocket connection is established. Server gets '{"subscribe": "A"}' message and starts sending messages for 'A',
// which we log here.
const subB = observableB.subscribe(messageForB => console.log(messageForB));
// Since we already have a connection, we just send '{"subscribe": "B"}' message to the server. It starts sending messages for 'B',
// which we log here.
subB.unsubscribe();
// Message '{"unsubscribe": "B"}' is sent to the server, which stops sending 'B' messages.
subA.unsubscribe();
// Message '{"unsubscribe": "A"}' makes the server stop sending messages for 'A'. Since there is no more subscribers to root Subject,
// socket connection closes.
```
rxjs WebSocketSubject WebSocketSubject
================
`class` `stable`
```
class WebSocketSubject<T> extends AnonymousSubject<T> {
constructor(urlConfigOrSource: string | WebSocketSubjectConfig<T> | Observable<T>, destination?: Observer<T>)
lift<R>(operator: Operator<T, R>): WebSocketSubject<R>
multiplex(subMsg: () => any, unsubMsg: () => any, messageFilter: (value: T) => boolean)
unsubscribe()
}
```
Constructor
-----------
| `constructor(urlConfigOrSource: string | WebSocketSubjectConfig<T> | Observable<T>, destination?: Observer<T>)` Parameters
| | | |
| --- | --- | --- |
| `urlConfigOrSource` | `string | WebSocketSubjectConfig<T> | [Observable](../index/class/observable)<T>` | |
| `destination` | `[Observer<T>](../index/interface/observer)` | Optional. Default is `undefined`. |
|
Methods
-------
| `lift<R>(operator: Operator<T, R>): WebSocketSubject<R>` Parameters
| | | |
| --- | --- | --- |
| `operator` | `Operator<T, R>` | |
Returns `[WebSocketSubject](websocketsubject)<R>` |
| |
| `multiplex(subMsg: () => any, unsubMsg: () => any, messageFilter: (value: T) => boolean)`
Creates an [`Observable`](../index/class/observable), that when subscribed to, sends a message, defined by the `subMsg` function, to the server over the socket to begin a subscription to data over that socket. Once data arrives, the `messageFilter` argument will be used to select the appropriate data for the resulting Observable. When finalization occurs, either due to unsubscription, completion, or error, a message defined by the `unsubMsg` argument will be sent to the server over the WebSocketSubject. Parameters
| | | |
| --- | --- | --- |
| `subMsg` | `() => any` | A function to generate the subscription message to be sent to the server. This will still be processed by the serializer in the WebSocketSubject's config. (Which defaults to JSON serialization) |
| `unsubMsg` | `() => any` | A function to generate the unsubscription message to be sent to the server at finalization. This will still be processed by the serializer in the WebSocketSubject's config. |
| `messageFilter` | `(value: T) => boolean` | A predicate for selecting the appropriate messages from the server for the output stream. |
|
| `unsubscribe()` Parameters There are no parameters. |
rxjs exhaustAll exhaustAll
==========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable by dropping inner Observables while the previous inner Observable has not yet completed.
### `exhaustAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<O, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that takes a source of Observables and propagates the first Observable exclusively until it completes before subscribing to the next.
Description
-----------
Flattens an Observable-of-Observables by dropping the next inner Observables while the current inner is still executing.
`[exhaustAll](../index/function/exhaustall)` subscribes to an Observable that emits Observables, also known as a higher-order Observable. Each time it observes one of these emitted inner Observables, the output Observable begins emitting the items emitted by that inner Observable. So far, it behaves like [`mergeAll`](mergeall). However, `[exhaustAll](../index/function/exhaustall)` ignores every new inner Observable if the previous Observable has not yet completed. Once that one completes, it will accept and flatten the next inner Observable and repeat this process.
Example
-------
Run a finite timer for each click, only if there is no currently active timer
```
import { fromEvent, map, interval, take, exhaustAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(5)))
);
const result = higherOrder.pipe(exhaustAll());
result.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`mergeAll`](mergeall)
* [`exhaustMap`](exhaustmap)
* [`zipAll`](zipall)
rxjs single single
======
`function` `stable` `operator`
Returns an observable that asserts that only one value is emitted from the observable that matches the predicate. If no predicate is provided, then it will assert that the observable only emits one value.
### `single<T>(predicate?: (value: T, index: number, source: Observable<T>) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | Optional. Default is `undefined`. A predicate function to evaluate items emitted by the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the single item emitted by the source Observable that matches the predicate.
#### Throws
`[NotFoundError](../index/interface/notfounderror)` Delivers an NotFoundError to the Observer's `error` callback if the Observable completes before any `next` notification was sent.
`[SequenceError](../index/interface/sequenceerror)` Delivers a SequenceError if more than one value is emitted that matches the provided predicate. If no predicate is provided, will deliver a SequenceError if more than one value comes from the source
Description
-----------
In the event that the observable is empty, it will throw an [`EmptyError`](../index/interface/emptyerror).
In the event that two values are found that match the predicate, or when there are two values emitted and no predicate, it will throw a [`SequenceError`](../index/interface/sequenceerror)
In the event that no values match the predicate, if one is provided, it will throw a [`NotFoundError`](../index/interface/notfounderror)
Example
-------
Expect only `name` beginning with `'B'`
```
import { of, single } from 'rxjs';
const source1 = of(
{ name: 'Ben' },
{ name: 'Tracy' },
{ name: 'Laney' },
{ name: 'Lily' }
);
source1
.pipe(single(x => x.name.startsWith('B')))
.subscribe(x => console.log(x));
// Emits 'Ben'
const source2 = of(
{ name: 'Ben' },
{ name: 'Tracy' },
{ name: 'Bradley' },
{ name: 'Lincoln' }
);
source2
.pipe(single(x => x.name.startsWith('B')))
.subscribe({ error: err => console.error(err) });
// Error emitted: SequenceError('Too many values match')
const source3 = of(
{ name: 'Laney' },
{ name: 'Tracy' },
{ name: 'Lily' },
{ name: 'Lincoln' }
);
source3
.pipe(single(x => x.name.startsWith('B')))
.subscribe({ error: err => console.error(err) });
// Error emitted: NotFoundError('No values match')
```
See Also
--------
* [`first`](first)
* [`find`](find)
* [`findIndex`](findindex)
* [`elementAt`](elementat)
rxjs multicast multicast
=========
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](../index/function/connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `multicast<T, R>(subjectOrSubjectFactory: Subject<T> | (() => Subject<T>), selector?: (source: Observable<T>) => Observable<R>): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `subjectOrSubjectFactory` | `[Subject](../index/class/subject)<T> | (() => [Subject](../index/class/subject)<T>)` | |
| `selector` | `(source: [Observable](../index/class/observable)<T>) => [Observable](../index/class/observable)<R>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
Overloads
---------
### `multicast(subject: Subject<T>): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
An operator that creates a [`ConnectableObservable`](../index/class/connectableobservable), that when connected, with the `[connect](../index/function/connect)` method, will use the provided subject to multicast the values from the source to all consumers.
#### Parameters
| | | |
| --- | --- | --- |
| `subject` | `[Subject<T>](../index/class/subject)` | The subject to multicast through. |
#### Returns
`[UnaryFunction](../index/interface/unaryfunction)<[Observable](../index/class/observable)<T>, [ConnectableObservable](../index/class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../index/class/connectableobservable)
### `multicast(subject: Subject<T>, selector: (shared: Observable<T>) => O): OperatorFunction<T, ObservedValueOf<O>>`
Because this is deprecated in favor of the [`connect`](connect) operator, and was otherwise poorly documented, rather than duplicate the effort of documenting the same behavior, please see documentation for the [`connect`](connect) operator.
#### Parameters
| | | |
| --- | --- | --- |
| `subject` | `[Subject<T>](../index/class/subject)` | The subject used to multicast. |
| `selector` | `(shared: [Observable](../index/class/observable)<T>) => O` | A setup function to setup the multicast |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an observable that mirrors the observable returned by the selector.
### `multicast(subjectFactory: () => Subject<T>): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
An operator that creates a [`ConnectableObservable`](../index/class/connectableobservable), that when connected, with the `[connect](../index/function/connect)` method, will use the provided subject to multicast the values from the source to all consumers.
#### Parameters
| | | |
| --- | --- | --- |
| `subjectFactory` | `() => [Subject](../index/class/subject)<T>` | A factory that will be called to create the subject. Passing a function here will cause the underlying subject to be "reset" on error, completion, or refCounted unsubscription of the source. |
#### Returns
`[UnaryFunction](../index/interface/unaryfunction)<[Observable](../index/class/observable)<T>, [ConnectableObservable](../index/class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../index/class/connectableobservable)
### `multicast(subjectFactory: () => Subject<T>, selector: (shared: Observable<T>) => O): OperatorFunction<T, ObservedValueOf<O>>`
Because this is deprecated in favor of the [`connect`](connect) operator, and was otherwise poorly documented, rather than duplicate the effort of documenting the same behavior, please see documentation for the [`connect`](connect) operator.
#### Parameters
| | | |
| --- | --- | --- |
| `subjectFactory` | `() => [Subject](../index/class/subject)<T>` | A factory that creates the subject used to multicast. |
| `selector` | `(shared: [Observable](../index/class/observable)<T>) => O` | A function to setup the multicast and select the output. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an observable that mirrors the observable returned by the selector.
| programming_docs |
rxjs map map
===
`function` `stable` `operator`
Applies a given `project` function to each value emitted by the source Observable, and emits the resulting values as an Observable.
### `map<T, R>(project: (value: T, index: number) => R, thisArg?: any): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => R` | The function to apply to each `value` emitted by the source Observable. The `index` parameter is the number `i` for the i-th emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to define what `this` is in the `project` function. |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`: A function that returns an Observable that emits the values from the source Observable transformed by the given `project` function.
Description
-----------
Like [Array.prototype.map()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map), it passes each source value through a transformation function to get corresponding output values.
Similar to the well known `Array.prototype.map` function, this operator applies a projection to each value and emits that projection in the output Observable.
Example
-------
Map every click to the `clientX` position of that click
```
import { fromEvent, map } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const positions = clicks.pipe(map(ev => ev.clientX));
positions.subscribe(x => console.log(x));
```
See Also
--------
* [`mapTo`](mapto)
* [`pluck`](pluck)
rxjs exhaustMap exhaustMap
==========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable only if the previous projected Observable has completed.
### `exhaustMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable containing projected Observables of each item of the source, ignoring projected Observables that start before their preceding Observable has completed.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`exhaust`](exhaust).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. When it projects a source value to an Observable, the output Observable begins emitting the items emitted by that projected Observable. However, `[exhaustMap](../index/function/exhaustmap)` ignores every new projected Observable if the previous projected Observable has not yet completed. Once that one completes, it will accept and flatten the next projected Observable and repeat this process.
Example
-------
Run a finite timer for each click, only if there is no currently active timer
```
import { fromEvent, exhaustMap, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
exhaustMap(() => interval(1000).pipe(take(5)))
);
result.subscribe(x => console.log(x));
```
Overloads
---------
### `exhaustMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `exhaustMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `exhaustMap(project: (value: T, index: number) => ObservableInput<I>, resultSelector: (outerValue: T, innerValue: I, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => [ObservableInput](../index/type-alias/observableinput)<I>` | |
| `resultSelector` | `(outerValue: T, innerValue: I, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaust`](exhaust)
* [`mergeMap`](mergemap)
* [`switchMap`](switchmap)
rxjs retry retry
=====
`function` `stable` `operator`
Returns an Observable that mirrors the source Observable with the exception of an `error`.
### `retry<T>(configOrCount: number | RetryConfig = Infinity): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `configOrCount` | `number | RetryConfig` | Optional. Default is `Infinity`. Either number of retry attempts before failing or a [RetryConfig](retryconfig) object. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that will resubscribe to the source stream when the source stream errors, at most `[count](../index/function/count)` times.
Description
-----------
If the source Observable calls `error`, this method will resubscribe to the source Observable for a maximum of `[count](../index/function/count)` resubscriptions rather than propagating the `error` call.
The number of retries is determined by the `[count](../index/function/count)` parameter. It can be set either by passing a number to `[retry](../index/function/retry)` function or by setting `[count](../index/function/count)` property when `[retry](../index/function/retry)` is configured using [RetryConfig](retryconfig). If `[count](../index/function/count)` is omitted, `[retry](../index/function/retry)` will try to resubscribe on errors infinite number of times.
Any and all items emitted by the source Observable will be emitted by the resulting Observable, even those emitted during failed subscriptions. For example, if an Observable fails at first but emits `[1, 2]` then succeeds the second time and emits: `[1, 2, 3, 4, 5, complete]` then the complete stream of emissions and notifications would be: `[1, 2, 1, 2, 3, 4, 5, complete]`.
Example
-------
```
import { interval, mergeMap, throwError, of, retry } from 'rxjs';
const source = interval(1000);
const result = source.pipe(
mergeMap(val => val > 5 ? throwError(() => 'Error!') : of(val)),
retry(2) // retry 2 times on error
);
result.subscribe({
next: value => console.log(value),
error: err => console.log(`${ err }: Retried 2 times then quit!`)
});
// Output:
// 0..1..2..3..4..5..
// 0..1..2..3..4..5..
// 0..1..2..3..4..5..
// 'Error!: Retried 2 times then quit!'
```
See Also
--------
* [`retryWhen`](retrywhen)
rxjs distinctUntilChanged distinctUntilChanged
====================
`function` `stable` `operator`
Returns a result [`Observable`](../index/class/observable) that emits all values pushed by the source observable if they are distinct in comparison to the last value the result observable emitted.
### `distinctUntilChanged<T, K>(comparator?: (previous: K, current: K) => boolean, keySelector: (value: T) => K = identity as (value: T) => K): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparator` | `(previous: K, current: K) => boolean` | Optional. Default is `undefined`. A function used to compare the previous and current keys for equality. Defaults to a `===` check. |
| `keySelector` | `(value: T) => K` | Optional. Default is `[identity](../index/function/identity) as (value: T) => K`. Used to select a key value to be passed to the `comparator`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values.
Description
-----------
When provided without parameters or with the first parameter (`[comparator](distinctuntilchanged#comparator)`), it behaves like this:
1. It will always emit the first value from the source.
2. For all subsequent values pushed by the source, they will be compared to the previously emitted values using the provided `comparator` or an `===` equality check.
3. If the value pushed by the source is determined to be unequal by this check, that value is emitted and becomes the new "previously emitted value" internally.
When the second parameter (`[keySelector](distinctuntilchanged#keySelector)`) is provided, the behavior changes:
1. It will always emit the first value from the source.
2. The `keySelector` will be run against all values, including the first value.
3. For all values after the first, the selected key will be compared against the key selected from the previously emitted value using the `comparator`.
4. If the keys are determined to be unequal by this check, the value (not the key), is emitted and the selected key from that value is saved for future comparisons against other keys.
Examples
--------
A very basic example with no `[comparator](distinctuntilchanged#comparator)`. Note that `1` is emitted more than once, because it's distinct in comparison to the *previously emitted* value, not in comparison to *all other emitted values*.
```
import { of, distinctUntilChanged } from 'rxjs';
of(1, 1, 1, 2, 2, 2, 1, 1, 3, 3)
.pipe(distinctUntilChanged())
.subscribe(console.log);
// Logs: 1, 2, 1, 3
```
With a `[comparator](distinctuntilchanged#comparator)`, you can do custom comparisons. Let's say you only want to emit a value when all of its components have changed:
```
import { of, distinctUntilChanged } from 'rxjs';
const totallyDifferentBuilds$ = of(
{ engineVersion: '1.1.0', transmissionVersion: '1.2.0' },
{ engineVersion: '1.1.0', transmissionVersion: '1.4.0' },
{ engineVersion: '1.3.0', transmissionVersion: '1.4.0' },
{ engineVersion: '1.3.0', transmissionVersion: '1.5.0' },
{ engineVersion: '2.0.0', transmissionVersion: '1.5.0' }
).pipe(
distinctUntilChanged((prev, curr) => {
return (
prev.engineVersion === curr.engineVersion ||
prev.transmissionVersion === curr.transmissionVersion
);
})
);
totallyDifferentBuilds$.subscribe(console.log);
// Logs:
// { engineVersion: '1.1.0', transmissionVersion: '1.2.0' }
// { engineVersion: '1.3.0', transmissionVersion: '1.4.0' }
// { engineVersion: '2.0.0', transmissionVersion: '1.5.0' }
```
You can also provide a custom `[comparator](distinctuntilchanged#comparator)` to check that emitted changes are only in one direction. Let's say you only want to get the next record temperature:
```
import { of, distinctUntilChanged } from 'rxjs';
const temps$ = of(30, 31, 20, 34, 33, 29, 35, 20);
const recordHighs$ = temps$.pipe(
distinctUntilChanged((prevHigh, temp) => {
// If the current temp is less than
// or the same as the previous record,
// the record hasn't changed.
return temp <= prevHigh;
})
);
recordHighs$.subscribe(console.log);
// Logs: 30, 31, 34, 35
```
Selecting update events only when the `updatedBy` field shows the account changed hands.
```
import { of, distinctUntilChanged } from 'rxjs';
// A stream of updates to a given account
const accountUpdates$ = of(
{ updatedBy: 'blesh', data: [] },
{ updatedBy: 'blesh', data: [] },
{ updatedBy: 'ncjamieson', data: [] },
{ updatedBy: 'ncjamieson', data: [] },
{ updatedBy: 'blesh', data: [] }
);
// We only want the events where it changed hands
const changedHands$ = accountUpdates$.pipe(
distinctUntilChanged(undefined, update => update.updatedBy)
);
changedHands$.subscribe(console.log);
// Logs:
// { updatedBy: 'blesh', data: Array[0] }
// { updatedBy: 'ncjamieson', data: Array[0] }
// { updatedBy: 'blesh', data: Array[0] }
```
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
rxjs findIndex findIndex
=========
`function` `stable` `operator`
Emits only the index of the first value emitted by the source Observable that meets some condition.
### `findIndex<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | A function called with each item to test for condition matching. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, number>`: A function that returns an Observable that emits the index of the first item that matches the condition.
Description
-----------
It's like [`find`](find), but emits the index of the found value, not the value itself.
`[findIndex](../index/function/findindex)` searches for the first item in the source Observable that matches the specified condition embodied by the `predicate`, and returns the (zero-based) index of the first occurrence in the source. Unlike [`first`](first), the `predicate` is required in `[findIndex](../index/function/findindex)`, and does not emit an error if a valid value is not found.
Example
-------
Emit the index of first click that happens on a DIV element
```
import { fromEvent, findIndex } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(findIndex(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `findIndex(predicate: BooleanConstructor): OperatorFunction<T, T extends Falsy ? -1 : number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T extends [Falsy](../index/type-alias/falsy) ? -1 : number>`
### `findIndex(predicate: BooleanConstructor, thisArg: any): OperatorFunction<T, T extends Falsy ? -1 : number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `thisArg` | `any` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T extends [Falsy](../index/type-alias/falsy) ? -1 : number>`
### `findIndex(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, number>`
### `findIndex(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, number>`
See Also
--------
* [`filter`](filter)
* [`find`](find)
* [`first`](first)
* [`take`](take)
rxjs take take
====
`function` `stable` `operator`
Emits only the first `[count](../index/function/count)` values emitted by the source Observable.
### `take<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `[count](../index/function/count)` | `number` | The maximum number of `next` values to emit. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits only the first `[count](../index/function/count)` values emitted by the source Observable, or all of the values from the source if the source emits fewer than `[count](../index/function/count)` values.
Description
-----------
Takes the first `[count](../index/function/count)` values from the source, then completes.
`[take](../index/function/take)` returns an Observable that emits only the first `[count](../index/function/count)` values emitted by the source Observable. If the source emits fewer than `[count](../index/function/count)` values then all of its values are emitted. After that, it completes, regardless if the source completes.
Example
-------
Take the first 5 seconds of an infinite 1-second interval Observable
```
import { interval, take } from 'rxjs';
const intervalCount = interval(1000);
const takeFive = intervalCount.pipe(take(5));
takeFive.subscribe(x => console.log(x));
// Logs:
// 0
// 1
// 2
// 3
// 4
```
See Also
--------
* [`takeLast`](takelast)
* [`takeUntil`](takeuntil)
* [`takeWhile`](takewhile)
* [`skip`](skip)
rxjs toArray toArray
=======
`function` `stable` `operator`
Collects all source emissions and emits them as an array when the source completes.
### `toArray<T>(): OperatorFunction<T, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable that emits an array of items emitted by the source Observable when source completes.
Description
-----------
Get all values inside an array when the source completes
`[toArray](../index/function/toarray)` will wait until the source Observable completes before emitting the array containing all emissions. When the source Observable errors no array will be emitted.
Example
-------
```
import { interval, take, toArray } from 'rxjs';
const source = interval(1000);
const example = source.pipe(
take(10),
toArray()
);
example.subscribe(value => console.log(value));
// output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
```
rxjs window window
======
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable whenever `windowBoundaries` emits.
### `window<T>(windowBoundaries: Observable<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowBoundaries` | `[Observable](../index/class/observable)<any>` | An Observable that completes the previous window and starts a new window. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`: A function that returns an Observable of windows, which are Observables emitting values of the source Observable.
Description
-----------
It's like [`buffer`](buffer), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits connected, non-overlapping windows. It emits the current window and opens a new one whenever the Observable `windowBoundaries` emits an item. Because each window is an Observable, the output is a higher-order Observable.
Example
-------
In every window of 1 second each, emit at most 2 click events
```
import { fromEvent, interval, window, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const sec = interval(1000);
const result = clicks.pipe(
window(sec),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`buffer`](buffer)
| programming_docs |
rxjs raceWith raceWith
========
`function` `stable` `operator`
Creates an Observable that mirrors the first source Observable to emit a next, error or complete notification from the combination of the Observable to which the operator is applied and supplied Observables.
### `raceWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Example
-------
```
import { interval, map, raceWith } from 'rxjs';
const obs1 = interval(7000).pipe(map(() => 'slow one'));
const obs2 = interval(3000).pipe(map(() => 'fast one'));
const obs3 = interval(5000).pipe(map(() => 'medium one'));
obs1
.pipe(raceWith(obs2, obs3))
.subscribe(winner => console.log(winner));
// Outputs
// a series of 'fast one'
```
rxjs last last
====
`function` `stable` `operator`
Returns an Observable that emits only the last item emitted by the source Observable. It optionally takes a predicate function as a parameter, in which case, rather than emitting the last item from the source Observable, the resulting Observable will emit the last item from the source Observable that satisfies the predicate.
### `last<T, D>(predicate?: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | Optional. Default is `undefined`. The condition any source emitted item has to satisfy. |
| `defaultValue` | `D` | Optional. Default is `undefined`. An optional default value to provide if last predicate isn't met or no values were emitted. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits only the last item satisfying the given condition from the source, or a NoSuchElementException if no such items are emitted.
#### Throws
`[EmptyError](../index/interface/emptyerror)` Delivers an EmptyError to the Observer's `error` callback if the Observable completes before any `next` notification was sent.
`Error` - Throws if no items that match the predicate are emitted by the source Observable.
Description
-----------
It will throw an error if the source completes without notification or one that matches the predicate. It returns the last value or if a predicate is provided last value that matches the predicate. It returns the given default value if no notification is emitted or matches the predicate.
Examples
--------
Last alphabet from the sequence
```
import { from, last } from 'rxjs';
const source = from(['x', 'y', 'z']);
const result = source.pipe(last());
result.subscribe(value => console.log(`Last alphabet: ${ value }`));
// Outputs
// Last alphabet: z
```
Default value when the value in the predicate is not matched
```
import { from, last } from 'rxjs';
const source = from(['x', 'y', 'z']);
const result = source.pipe(last(char => char === 'a', 'not found'));
result.subscribe(value => console.log(`'a' is ${ value }.`));
// Outputs
// 'a' is not found.
```
Overloads
---------
### `last(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `last(predicate: BooleanConstructor, defaultValue: D): OperatorFunction<T, TruthyTypesOf<T> | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T> | D>`
### `last(predicate?: null, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `null` | Optional. Default is `undefined`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`
### `last(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue?: S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => value is S` | |
| `defaultValue` | `S` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `last(predicate: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`
See Also
--------
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
* [`skipWhile`](skipwhile)
rxjs concatMapTo concatMapTo
===========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is merged multiple times in a serialized fashion on the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`concatMap`](concatmap) instead: `[concatMap](../index/function/concatmap)(() => result)`
### `concatMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable of values merged together by joining the passed Observable with itself, one after the other, for each value emitted from the source.
Description
-----------
It's like [`concatMap`](concatmap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then flattens those resulting Observables into one single Observable, which is the output Observable. Each new `innerObservable` instance emitted on the output Observable is concatenated with the previous `innerObservable` instance.
**Warning:** if source values arrive endlessly and faster than their corresponding inner Observables can complete, it will result in memory issues as inner Observables amass in an unbounded buffer waiting for their turn to be subscribed to.
Note: `[concatMapTo](../index/function/concatmapto)` is equivalent to `[mergeMapTo](../index/function/mergemapto)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, concatMapTo, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
concatMapTo(interval(1000).pipe(take(4)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
Overloads
---------
### `concatMapTo(observable: O): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `concatMapTo(observable: O, resultSelector: undefined): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `concatMapTo(observable: O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concat`](../index/function/concat)
* [`concatAll`](concatall)
* [`concatMap`](concatmap)
* [`mergeMapTo`](mergemapto)
* [`switchMapTo`](switchmapto)
rxjs merge merge
=====
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Replaced with [`mergeWith`](mergewith). Will be removed in v8.
### `merge<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `merge()`
#### Parameters
There are no parameters.
### `merge()`
#### Parameters
There are no parameters.
### `merge()`
#### Parameters
There are no parameters.
### `merge(...args: unknown[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `unknown[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, unknown>`
rxjs mergeMapTo mergeMapTo
==========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is merged multiple times in the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`mergeMap`](mergemap) instead: `[mergeMap](../index/function/mergemap)(() => result)`
### `mergeMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: number | ((outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R), concurrent: number = Infinity): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `number | ((outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R)` | Optional. Default is `undefined`. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits items from the given `innerObservable`.
Description
-----------
It's like [`mergeMap`](mergemap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then merges those resulting Observables into one single Observable, which is the output Observable.
Example
-------
For each click event, start an interval Observable ticking every 1 second
```
import { fromEvent, mergeMapTo, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(mergeMapTo(interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`concatMapTo`](concatmapto)
* [`merge`](../index/function/merge)
* [`mergeAll`](mergeall)
* [`mergeMap`](mergemap)
* [`mergeScan`](mergescan)
* [`switchMapTo`](switchmapto)
rxjs zipWith zipWith
=======
`function` `stable` `operator`
Subscribes to the source, and the observable inputs provided as arguments, and combines their values, by index, into arrays.
### `zipWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
What is meant by "combine by index": The first value from each will be made into a single array, then emitted, then the second value from each will be combined into a single array and emitted, then the third value from each will be combined into a single array and emitted, and so on.
This will continue until it is no longer able to combine values of the same index into an array.
After the last value from any one completed source is emitted in an array, the resulting observable will complete, as there is no way to continue "zipping" values together by index.
Use-cases for this operator are limited. There are memory concerns if one of the streams is emitting values at a much faster rate than the others. Usage should likely be limited to streams that emit at a similar pace, or finite streams of known length.
In many cases, authors want `[combineLatestWith](../index/function/combinelatestwith)` and not `[zipWith](../index/function/zipwith)`.
rxjs distinctUntilKeyChanged distinctUntilKeyChanged
=======================
`function` `stable` `operator`
Returns an Observable that emits all items emitted by the source Observable that are distinct by comparison from the previous item, using a property accessed by using the key provided to check if the two items are distinct.
### `distinctUntilKeyChanged<T, K extends keyof T>(key: K, compare?: (x: T[K], y: T[K]) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `K` | String key for object property lookup on each item. |
| `compare` | `(x: T[K], y: T[K]) => boolean` | Optional. Default is `undefined`. Optional comparison function called to test if an item is distinct from the previous item in the source. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values based on the key specified.
Description
-----------
If a comparator function is provided, then it will be called for each item to test for whether or not that value should be emitted.
If a comparator function is not provided, an equality check is used by default.
Examples
--------
An example comparing the name of persons
```
import { of, distinctUntilKeyChanged } from 'rxjs';
of(
{ age: 4, name: 'Foo' },
{ age: 7, name: 'Bar' },
{ age: 5, name: 'Foo' },
{ age: 6, name: 'Foo' }
).pipe(
distinctUntilKeyChanged('name')
)
.subscribe(x => console.log(x));
// displays:
// { age: 4, name: 'Foo' }
// { age: 7, name: 'Bar' }
// { age: 5, name: 'Foo' }
```
An example comparing the first letters of the name
```
import { of, distinctUntilKeyChanged } from 'rxjs';
of(
{ age: 4, name: 'Foo1' },
{ age: 7, name: 'Bar' },
{ age: 5, name: 'Foo2' },
{ age: 6, name: 'Foo3' }
).pipe(
distinctUntilKeyChanged('name', (x, y) => x.substring(0, 3) === y.substring(0, 3))
)
.subscribe(x => console.log(x));
// displays:
// { age: 4, name: 'Foo1' }
// { age: 7, name: 'Bar' }
// { age: 5, name: 'Foo2' }
```
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilChanged`](distinctuntilchanged)
rxjs zipAll zipAll
======
`function` `stable` `operator`
### `zipAll<T, R>(project?: (...values: T[]) => R)`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | Optional. Default is `undefined`. |
Overloads
---------
### `zipAll(): OperatorFunction<ObservableInput<T>, T[]>`
Collects all observable inner sources from the source, once the source completes, it will subscribe to all inner sources, combining their values by index and emitting them.
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<[ObservableInput](../index/type-alias/observableinput)<T>, T[]>`
### `zipAll(): OperatorFunction<any, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<any, T[]>`
### `zipAll(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<[ObservableInput](../index/type-alias/observableinput)<T>, R>`
### `zipAll(project: (...values: any[]) => R): OperatorFunction<any, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<any, R>`
rxjs switchScan switchScan
==========
`function` `stable` `operator`
Applies an accumulator function over the source Observable where the accumulator function itself returns an Observable, emitting values only from the most recently returned Observable.
### `switchScan<T, R, O extends ObservableInput<any>>(accumulator: (acc: R, value: T, index: number) => O, seed: R): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: R, value: T, index: number) => O` | The accumulator function called on each source value. |
| `seed` | `R` | The initial accumulation value. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an observable of the accumulated values.
Description
-----------
It's like [`mergeScan`](mergescan), but only the most recent Observable returned by the accumulator is merged into the outer Observable.
See Also
--------
* [`scan`](scan)
* [`mergeScan`](mergescan)
* [`switchMap`](switchmap)
rxjs scan scan
====
`function` `stable` `operator`
Useful for encapsulating and managing state. Applies an accumulator (or "reducer function") to each value from the source after an initial state is established -- either via a `seed` value (second argument), or from the first value from the source.
### `scan<V, A, S>(accumulator: (acc: V | A | S, value: V, index: number) => A, seed?: S): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A | S, value: V, index: number) => A` | A "reducer function". This will be called for each value after an initial state is acquired. |
| `seed` | `S` | Optional. Default is `undefined`. The initial state. If this is not provided, the first value from the source will be used as the initial state, and emitted without going through the accumulator. All subsequent values will be processed by the accumulator function. If this is provided, all values will go through the accumulator function. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, V | A>`: A function that returns an Observable of the accumulated values.
Description
-----------
It's like [`reduce`](reduce), but emits the current accumulation state after each update
This operator maintains an internal state and emits it after processing each value as follows:
1. First value arrives
* If a `seed` value was supplied (as the second argument to `[scan](../index/function/scan)`), let `state = seed` and `value = firstValue`.
* If NO `seed` value was supplied (no second argument), let `state = firstValue` and go to 3.
2. Let `state = accumulator(state, value)`.
* If an error is thrown by `accumulator`, notify the consumer of an error. The process ends.
3. Emit `state`.
4. Next value arrives, let `value = nextValue`, go to 2.
Examples
--------
An average of previous numbers. This example shows how not providing a `seed` can prime the stream with the first value from the source.
```
import { of, scan, map } from 'rxjs';
const numbers$ = of(1, 2, 3);
numbers$
.pipe(
// Get the sum of the numbers coming in.
scan((total, n) => total + n),
// Get the average by dividing the sum by the total number
// received so var (which is 1 more than the zero-based index).
map((sum, index) => sum / (index + 1))
)
.subscribe(console.log);
```
The Fibonacci sequence. This example shows how you can use a seed to prime accumulation process. Also... you know... Fibonacci. So important to like, computers and stuff that its whiteboarded in job interviews. Now you can show them the Rx version! (Please don't, haha)
```
import { interval, scan, map, startWith } from 'rxjs';
const firstTwoFibs = [0, 1];
// An endless stream of Fibonacci numbers.
const fibonacci$ = interval(1000).pipe(
// Scan to get the fibonacci numbers (after 0, 1)
scan(([a, b]) => [b, a + b], firstTwoFibs),
// Get the second number in the tuple, it's the one you calculated
map(([, n]) => n),
// Start with our first two digits :)
startWith(...firstTwoFibs)
);
fibonacci$.subscribe(console.log);
```
Overloads
---------
### `scan(accumulator: (acc: V | A, value: V, index: number) => A): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, V | A>`
### `scan(accumulator: (acc: A, value: V, index: number) => A, seed: A): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A, value: V, index: number) => A` | |
| `seed` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, A>`
### `scan(accumulator: (acc: A | S, value: V, index: number) => A, seed: S): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A | S, value: V, index: number) => A` | |
| `seed` | `S` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, A>`
See Also
--------
* [`expand`](expand)
* [`mergeScan`](mergescan)
* [`reduce`](reduce)
* [`switchScan`](switchscan)
| programming_docs |
rxjs timestamp timestamp
=========
`function` `stable` `operator`
Attaches a timestamp to each item emitted by an observable indicating when it was emitted
### `timestamp<T>(timestampProvider: TimestampProvider = dateTimestampProvider): OperatorFunction<T, Timestamp<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `timestampProvider` | `[TimestampProvider](../index/interface/timestampprovider)` | Optional. Default is `dateTimestampProvider`. An object with a `now()` method used to get the current timestamp. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Timestamp](../index/interface/timestamp)<T>>`: A function that returns an Observable that attaches a timestamp to each item emitted by the source Observable indicating when it was emitted.
Description
-----------
The `[timestamp](../index/function/timestamp)` operator maps the *source* observable stream to an object of type `{value: T, [timestamp](../index/function/timestamp): R}`. The properties are generically typed. The `value` property contains the value and type of the *source* observable. The `[timestamp](../index/function/timestamp)` is generated by the schedulers `now` function. By default, it uses the `[asyncScheduler](../index/const/asyncscheduler)` which simply returns `Date.now()` (milliseconds since 1970/01/01 00:00:00:000) and therefore is of type `number`.
Example
-------
In this example there is a timestamp attached to the document's click events
```
import { fromEvent, timestamp } from 'rxjs';
const clickWithTimestamp = fromEvent(document, 'click').pipe(
timestamp()
);
// Emits data of type { value: PointerEvent, timestamp: number }
clickWithTimestamp.subscribe(data => {
console.log(data);
});
```
rxjs windowTime windowTime
==========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable periodically in time.
### `windowTime<T>(windowTimeSpan: number, ...otherArgs: any[]): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | The amount of time, in milliseconds, to fill each window. |
| `otherArgs` | `any[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferTime`](buffertime), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable starts a new window periodically, as determined by the `windowCreationInterval` argument. It emits each window after a fixed timespan, specified by the `windowTimeSpan` argument. When the source Observable completes or encounters an error, the output Observable emits the current window and propagates the notification from the source Observable. If `windowCreationInterval` is not provided, the output Observable starts a new window when the previous window of duration `windowTimeSpan` completes. If `maxWindowCount` is provided, each window will emit at most fixed number of values. Window will complete immediately after emitting last value and next one still will open as specified by `windowTimeSpan` and `windowCreationInterval` arguments.
Examples
--------
In every window of 1 second each, emit at most 2 click events
```
import { fromEvent, windowTime, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Every 5 seconds start a window 1 second long, and emit at most 2 click events per window
```
import { fromEvent, windowTime, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000, 5000),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Same as example above but with `maxWindowCount` instead of `[take](../index/function/take)`
```
import { fromEvent, windowTime, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000, 5000, 2), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Overloads
---------
### `windowTime(windowTimeSpan: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`
### `windowTime(windowTimeSpan: number, windowCreationInterval: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `windowCreationInterval` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`
### `windowTime(windowTimeSpan: number, windowCreationInterval: number | void, maxWindowSize: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `windowCreationInterval` | `number | void` | |
| `maxWindowSize` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`bufferTime`](buffertime)
rxjs expand expand
======
`function` `stable` `operator`
Recursively projects each source value to an Observable which is merged in the output Observable.
### `expand<T, O extends ObservableInput<unknown>>(project: (value: T, index: number) => O, concurrent: number = Infinity, scheduler?: SchedulerLike): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source or the output Observable, returns an Observable. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for subscribing to each projected inner Observable. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that emits the source values and also result of applying the projection function to each value emitted on the output Observable and merging the results of the Observables obtained from this transformation.
Description
-----------
It's similar to [`mergeMap`](mergemap), but applies the projection function to every source value as well as every output value. It's recursive.
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an Observable, and then merging those resulting Observables and emitting the results of this merger. *Expand* will re-emit on the output Observable every source value. Then, each output value is given to the `project` function which returns an inner Observable to be merged on the output Observable. Those output values resulting from the projection are also given to the `project` function to produce new output values. This is how *expand* behaves recursively.
Example
-------
Start emitting the powers of two on every click, at most 10 of them
```
import { fromEvent, map, expand, of, delay, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const powersOfTwo = clicks.pipe(
map(() => 1),
expand(x => of(2 * x).pipe(delay(1000))),
take(10)
);
powersOfTwo.subscribe(x => console.log(x));
```
See Also
--------
* [`mergeMap`](mergemap)
* [`mergeScan`](mergescan)
rxjs bufferToggle bufferToggle
============
`function` `stable` `operator`
Buffers the source Observable values starting from an emission from `openings` and ending when the output of `closingSelector` emits.
### `bufferToggle<T, O>(openings: ObservableInput<O>, closingSelector: (value: O) => ObservableInput<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `openings` | `[ObservableInput](../index/type-alias/observableinput)<O>` | A Subscribable or Promise of notifications to start new buffers. |
| `closingSelector` | `(value: O) => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that takes the value emitted by the `openings` observable and returns a Subscribable or Promise, which, when it emits, signals that the associated buffer should be emitted and cleared. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array. Starts collecting only when `opening` emits, and calls the `closingSelector` function to get an Observable that tells when to close the buffer.
Buffers values from the source by opening the buffer via signals from an Observable provided to `openings`, and closing and sending the buffers when a Subscribable or Promise returned by the `closingSelector` function emits.
Example
-------
Every other second, emit the click events from the next 500ms
```
import { fromEvent, interval, bufferToggle, EMPTY } from 'rxjs';
const clicks = fromEvent(document, 'click');
const openings = interval(1000);
const buffered = clicks.pipe(bufferToggle(openings, i =>
i % 2 ? interval(500) : EMPTY
));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferWhen`](bufferwhen)
* [`windowToggle`](windowtoggle)
rxjs defaultIfEmpty defaultIfEmpty
==============
`function` `stable` `operator`
Emits a given value if the source Observable completes without emitting any `next` value, otherwise mirrors the source Observable.
### `defaultIfEmpty<T, R>(defaultValue: R): OperatorFunction<T, T | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `defaultValue` | `R` | The default value used if the source Observable is empty. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | R>`: A function that returns an Observable that emits either the specified `defaultValue` if the source Observable emits no items, or the values emitted by the source Observable.
Description
-----------
If the source Observable turns out to be empty, then this operator will emit a default value.
`[defaultIfEmpty](../index/function/defaultifempty)` emits the values emitted by the source Observable or a specified default value if the source Observable is empty (completes without having emitted any `next` value).
Example
-------
If no clicks happen in 5 seconds, then emit 'no clicks'
```
import { fromEvent, takeUntil, interval, defaultIfEmpty } from 'rxjs';
const clicks = fromEvent(document, 'click');
const clicksBeforeFive = clicks.pipe(takeUntil(interval(5000)));
const result = clicksBeforeFive.pipe(defaultIfEmpty('no clicks'));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`empty`](../index/function/empty)
* [`last`](last)
rxjs isEmpty isEmpty
=======
`function` `stable` `operator`
Emits `false` if the input Observable emits any values, or emits `true` if the input Observable completes without emitting any values.
### `isEmpty<T>(): OperatorFunction<T, boolean>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, boolean>`: A function that returns an Observable that emits boolean value indicating whether the source Observable was empty or not.
Description
-----------
Tells whether any values are emitted by an Observable.
`[isEmpty](../index/function/isempty)` transforms an Observable that emits values into an Observable that emits a single boolean value representing whether or not any values were emitted by the source Observable. As soon as the source Observable emits a value, `[isEmpty](../index/function/isempty)` will emit a `false` and complete. If the source Observable completes having not emitted anything, `[isEmpty](../index/function/isempty)` will emit a `true` and complete.
A similar effect could be achieved with [`count`](count), but `[isEmpty](../index/function/isempty)` can emit a `false` value sooner.
Examples
--------
Emit `false` for a non-empty Observable
```
import { Subject, isEmpty } from 'rxjs';
const source = new Subject<string>();
const result = source.pipe(isEmpty());
source.subscribe(x => console.log(x));
result.subscribe(x => console.log(x));
source.next('a');
source.next('b');
source.next('c');
source.complete();
// Outputs
// 'a'
// false
// 'b'
// 'c'
```
Emit `true` for an empty Observable
```
import { EMPTY, isEmpty } from 'rxjs';
const result = EMPTY.pipe(isEmpty());
result.subscribe(x => console.log(x));
// Outputs
// true
```
See Also
--------
* [`count`](count)
* [`EMPTY`](../index/const/empty)
rxjs debounceTime debounceTime
============
`function` `stable` `operator`
Emits a notification from the source Observable only after a particular time span has passed without another source emission.
### `debounceTime<T>(dueTime: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `dueTime` | `number` | The timeout duration in milliseconds (or the time unit determined internally by the optional `scheduler`) for the window of time required to wait for emission silence before emitting the most recent source value. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for managing the timers that handle the timeout for each value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified `dueTime`, and may drop some values if they occur too frequently.
Description
-----------
It's like [`delay`](delay), but passes only the most recent notification from each burst of emissions.
`[debounceTime](../index/function/debouncetime)` delays notifications emitted by the source Observable, but drops previous pending delayed emissions if a new notification arrives on the source Observable. This operator keeps track of the most recent notification from the source Observable, and emits that only when `dueTime` has passed without any other notification appearing on the source Observable. If a new value appears before `dueTime` silence occurs, the previous notification will be dropped and will not be emitted and a new `dueTime` is scheduled. If the completing event happens during `dueTime` the last cached notification is emitted before the completion event is forwarded to the output observable. If the error event happens during `dueTime` or after it only the error event is forwarded to the output observable. The cache notification is not emitted in this case.
This is a rate-limiting operator, because it is impossible for more than one notification to be emitted in any time window of duration `dueTime`, but it is also a delay-like operator since output emissions do not occur at the same time as they did on the source Observable. Optionally takes a [`SchedulerLike`](../index/interface/schedulerlike) for managing timers.
Example
-------
Emit the most recent click after a burst of clicks
```
import { fromEvent, debounceTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(debounceTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`auditTime`](audittime)
* [`debounce`](debounce)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
rxjs sampleTime sampleTime
==========
`function` `stable` `operator`
Emits the most recently emitted value from the source Observable within periodic time intervals.
### `sampleTime<T>(period: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `period` | `number` | The sampling period expressed in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for managing the timers that handle the sampling. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the results of sampling the values emitted by the source Observable at the specified time interval.
Description
-----------
Samples the source Observable at periodic time intervals, emitting what it samples.
`[sampleTime](../index/function/sampletime)` periodically looks at the source Observable and emits whichever value it has most recently emitted since the previous sampling, unless the source has not emitted anything since the previous sampling. The sampling happens periodically in time every `period` milliseconds (or the time unit defined by the optional `scheduler` argument). The sampling starts as soon as the output Observable is subscribed.
Example
-------
Every second, emit the most recent click at most once
```
import { fromEvent, sampleTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(sampleTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sample`](sample)
* [`throttleTime`](throttletime)
rxjs publish publish
=======
`function` `deprecated` `operator`
Returns a ConnectableObservable, which is a variety of Observable that waits until its connect method is called before it begins emitting items to those Observers that have subscribed to it.
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](../index/function/connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `publish<T, R>(selector?: OperatorFunction<T, R>): MonoTypeOperatorFunction<T> | OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `[OperatorFunction<T, R>](../index/interface/operatorfunction)` | Optional. Default is `undefined`. Optional selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will receive all notifications of the source from the time of the subscription on. |
#### Returns
`[MonoTypeOperatorFunction](../index/interface/monotypeoperatorfunction)<T> | [OperatorFunction](../index/interface/operatorfunction)<T, R>`: A function that returns a ConnectableObservable that upon connection causes the source Observable to emit items to its Observers.
Description
-----------
Makes a cold Observable hot
Examples
--------
Make `source$` hot by applying `[publish](../index/function/publish)` operator, then merge each inner observable into a single one and subscribe
```
import { zip, interval, of, map, publish, merge, tap } from 'rxjs';
const source$ = zip(interval(2000), of(1, 2, 3, 4, 5, 6, 7, 8, 9))
.pipe(map(([, number]) => number));
source$
.pipe(
publish(multicasted$ =>
merge(
multicasted$.pipe(tap(x => console.log('Stream 1:', x))),
multicasted$.pipe(tap(x => console.log('Stream 2:', x))),
multicasted$.pipe(tap(x => console.log('Stream 3:', x)))
)
)
)
.subscribe();
// Results every two seconds
// Stream 1: 1
// Stream 2: 1
// Stream 3: 1
// ...
// Stream 1: 9
// Stream 2: 9
// Stream 3: 9
```
See Also
--------
* [`publishLast`](publishlast)
* [`publishReplay`](publishreplay)
* [`publishBehavior`](publishbehavior)
| programming_docs |
rxjs subscribeOn subscribeOn
===========
`function` `stable` `operator`
Asynchronously subscribes Observers to this Observable on the specified [`SchedulerLike`](../index/interface/schedulerlike).
### `subscribeOn<T>(scheduler: SchedulerLike, delay: number = 0): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | The [`SchedulerLike`](../index/interface/schedulerlike) to perform subscription actions on. |
| `[delay](../index/function/delay)` | `number` | Optional. Default is `0`. A delay to pass to the scheduler to delay subscriptions |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable modified so that its subscriptions happen on the specified [`SchedulerLike`](../index/interface/schedulerlike).
Description
-----------
With `[subscribeOn](../index/function/subscribeon)` you can decide what type of scheduler a specific Observable will be using when it is subscribed to.
Schedulers control the speed and order of emissions to observers from an Observable stream.
Example
-------
Given the following code:
```
import { of, merge } from 'rxjs';
const a = of(1, 2, 3);
const b = of(4, 5, 6);
merge(a, b).subscribe(console.log);
// Outputs
// 1
// 2
// 3
// 4
// 5
// 6
```
Both Observable `a` and `b` will emit their values directly and synchronously once they are subscribed to.
If we instead use the `[subscribeOn](../index/function/subscribeon)` operator declaring that we want to use the [`asyncScheduler`](../index/const/asyncscheduler) for values emitted by Observable `a`:
```
import { of, subscribeOn, asyncScheduler, merge } from 'rxjs';
const a = of(1, 2, 3).pipe(subscribeOn(asyncScheduler));
const b = of(4, 5, 6);
merge(a, b).subscribe(console.log);
// Outputs
// 4
// 5
// 6
// 1
// 2
// 3
```
The reason for this is that Observable `b` emits its values directly and synchronously like before but the emissions from `a` are scheduled on the event loop because we are now using the [`asyncScheduler`](../index/const/asyncscheduler) for that specific Observable.
rxjs windowWhen windowWhen
==========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable using a factory function of closing Observables to determine when to start a new window.
### `windowWhen<T>(closingSelector: () => ObservableInput<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingSelector` | `() => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that takes no arguments and returns an Observable that signals (on either `next` or `complete`) when to close the previous window and start a new one. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferWhen`](bufferwhen), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits connected, non-overlapping windows. It emits the current window and opens a new one whenever the Observable produced by the specified `closingSelector` function emits an item. The first window is opened immediately when subscribing to the output Observable.
Example
-------
Emit only the first two clicks events in every window of [1-5] random seconds
```
import { fromEvent, windowWhen, interval, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowWhen(() => interval(1000 + Math.random() * 4000)),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`bufferWhen`](bufferwhen)
rxjs race race
====
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Replaced with [`raceWith`](racewith). Will be removed in v8.
### `race<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs delay delay
=====
`function` `stable` `operator`
Delays the emission of items from the source Observable by a given timeout or until a given Date.
### `delay<T>(due: number | Date, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `due` | `number | Date` | The delay duration in milliseconds (a `number`) or a `Date` until which the emission of the source items is delayed. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for managing the timers that handle the time-shift for each item. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified timeout or Date.
Description
-----------
Time shifts each item by some specified amount of milliseconds.
If the delay argument is a Number, this operator time shifts the source Observable by that amount of time expressed in milliseconds. The relative time intervals between the values are preserved.
If the delay argument is a Date, this operator time shifts the start of the Observable execution until the given date occurs.
Examples
--------
Delay each click by one second
```
import { fromEvent, delay } from 'rxjs';
const clicks = fromEvent(document, 'click');
const delayedClicks = clicks.pipe(delay(1000)); // each click emitted after 1 second
delayedClicks.subscribe(x => console.log(x));
```
Delay all clicks until a future date happens
```
import { fromEvent, delay } from 'rxjs';
const clicks = fromEvent(document, 'click');
const date = new Date('March 15, 2050 12:00:00'); // in the future
const delayedClicks = clicks.pipe(delay(date)); // click emitted only after that date
delayedClicks.subscribe(x => console.log(x));
```
See Also
--------
* [`delayWhen`](delaywhen)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
* [`debounce`](debounce)
* [`debounceTime`](debouncetime)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`audit`](audit)
* [`auditTime`](audittime)
rxjs repeat repeat
======
`function` `stable` `operator`
Returns an Observable that will resubscribe to the source stream when the source stream completes.
### `repeat<T>(countOrConfig?: number | RepeatConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `countOrConfig` | `number | RepeatConfig` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
Description
-----------
Repeats all values emitted on the source. It's like [`retry`](retry), but for non error cases.
Repeat will output values from a source until the source completes, then it will resubscribe to the source a specified number of times, with a specified delay. Repeat can be particularly useful in combination with closing operators like [`take`](take), [`takeUntil`](takeuntil), [`first`](first), or [`takeWhile`](takewhile), as it can be used to restart a source again from scratch.
Repeat is very similar to [`retry`](retry), where [`retry`](retry) will resubscribe to the source in the error case, but `[repeat](../index/function/repeat)` will resubscribe if the source completes.
Note that `[repeat](../index/function/repeat)` will *not* catch errors. Use [`retry`](retry) for that.
* `[repeat](../index/function/repeat)(0)` returns an empty observable
* `[repeat](../index/function/repeat)()` will repeat forever
* `[repeat](../index/function/repeat)({ [delay](../index/function/delay): 200 })` will repeat forever, with a delay of 200ms between repetitions.
* `[repeat](../index/function/repeat)({ [count](../index/function/count): 2, [delay](../index/function/delay): 400 })` will repeat twice, with a delay of 400ms between repetitions.
* `[repeat](../index/function/repeat)({ [delay](../index/function/delay): ([count](../index/function/count)) => [timer](../index/function/timer)([count](../index/function/count) * 1000) })` will repeat forever, but will have a delay that grows by one second for each repetition.
Example
-------
Repeat a message stream
```
import { of, repeat } from 'rxjs';
const source = of('Repeat message');
const result = source.pipe(repeat(3));
result.subscribe(x => console.log(x));
// Results
// 'Repeat message'
// 'Repeat message'
// 'Repeat message'
```
Repeat 3 values, 2 times
```
import { interval, take, repeat } from 'rxjs';
const source = interval(1000);
const result = source.pipe(take(3), repeat(2));
result.subscribe(x => console.log(x));
// Results every second
// 0
// 1
// 2
// 0
// 1
// 2
```
Defining two complex repeats with delays on the same source. Note that the second repeat cannot be called until the first repeat as exhausted it's count.
```
import { defer, of, repeat } from 'rxjs';
const source = defer(() => {
return of(`Hello, it is ${new Date()}`)
});
source.pipe(
// Repeat 3 times with a delay of 1 second between repetitions
repeat({
count: 3,
delay: 1000,
}),
// *Then* repeat forever, but with an exponential step-back
// maxing out at 1 minute.
repeat({
delay: (count) => timer(Math.min(60000, 2 ^ count * 1000))
})
)
```
See Also
--------
* [`repeatWhen`](repeatwhen)
* [`retry`](retry)
rxjs sample sample
======
`function` `stable` `operator`
Emits the most recently emitted value from the source Observable whenever another Observable, the `notifier`, emits.
### `sample<T>(notifier: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[Observable](../index/class/observable)<any>` | The Observable to use for sampling the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the results of sampling the values emitted by the source Observable whenever the notifier Observable emits value or completes.
Description
-----------
It's like [`sampleTime`](sampletime), but samples whenever the `notifier` Observable emits something.
Whenever the `notifier` Observable emits a value, `[sample](../index/function/sample)` looks at the source Observable and emits whichever value it has most recently emitted since the previous sampling, unless the source has not emitted anything since the previous sampling. The `notifier` is subscribed to as soon as the output Observable is subscribed.
Example
-------
On every click, sample the most recent `seconds` timer
```
import { fromEvent, interval, sample } from 'rxjs';
const seconds = interval(1000);
const clicks = fromEvent(document, 'click');
const result = seconds.pipe(sample(clicks));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounce`](debounce)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
rxjs combineLatest combineLatest
=============
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Replaced with [`combineLatestWith`](combinelatestwith). Will be removed in v8.
### `combineLatest<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest(...args: (Observable<any> | InteropObservable<any> | AsyncIterable<any> | PromiseLike<any> | ArrayLike<any> | Iterable<any> | ReadableStreamLike<any> | ((...values: any[]) => R))[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `([Observable](../index/class/observable)<any> | [InteropObservable](../index/interface/interopobservable)<any> | AsyncIterable<any> | PromiseLike<any> | ArrayLike<any> | Iterable<any> | [ReadableStreamLike](../index/interface/readablestreamlike)<any> | ((...values: any[]) => R))[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, unknown>`
rxjs publishLast publishLast
===========
`function` `deprecated` `operator`
Returns a connectable observable sequence that shares a single subscription to the underlying sequence containing only the last notification.
Deprecation Notes
-----------------
Will be removed in v8. To create a connectable observable with an [`AsyncSubject`](../index/class/asyncsubject) under the hood, use [`connectable`](../index/function/connectable). `source.pipe([publishLast](../index/function/publishlast)())` is equivalent to `[connectable](../index/function/connectable)(source, { connector: () => new [AsyncSubject](../index/class/asyncsubject)(), resetOnDisconnect: false })`. If you're using [`refCount`](refcount) after `[publishLast](../index/function/publishlast)`, use the [`share`](share) operator instead. `source.pipe([publishLast](../index/function/publishlast)(), [refCount](../index/function/refcount)())` is equivalent to `source.pipe([share](../index/function/share)({ connector: () => new [AsyncSubject](../index/class/asyncsubject)(), resetOnError: false, resetOnComplete: false, resetOnRefCountZero: false }))`. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishLast<T>(): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
#### Parameters
There are no parameters.
#### Returns
`[UnaryFunction](../index/interface/unaryfunction)<[Observable](../index/class/observable)<T>, [ConnectableObservable](../index/class/connectableobservable)<T>>`: A function that returns an Observable that emits elements of a sequence produced by multicasting the source sequence.
Description
-----------
Similar to [`publish`](publish), but it waits until the source observable completes and stores the last emitted value. Similarly to [`publishReplay`](publishreplay) and [`publishBehavior`](publishbehavior), this keeps storing the last value even if it has no more subscribers. If subsequent subscriptions happen, they will immediately get that last stored value and complete.
Example
-------
```
import { ConnectableObservable, interval, publishLast, tap, take } from 'rxjs';
const connectable = <ConnectableObservable<number>>interval(1000)
.pipe(
tap(x => console.log('side effect', x)),
take(3),
publishLast()
);
connectable.subscribe({
next: x => console.log('Sub. A', x),
error: err => console.log('Sub. A Error', err),
complete: () => console.log('Sub. A Complete')
});
connectable.subscribe({
next: x => console.log('Sub. B', x),
error: err => console.log('Sub. B Error', err),
complete: () => console.log('Sub. B Complete')
});
connectable.connect();
// Results:
// 'side effect 0' - after one second
// 'side effect 1' - after two seconds
// 'side effect 2' - after three seconds
// 'Sub. A 2' - immediately after 'side effect 2'
// 'Sub. B 2'
// 'Sub. A Complete'
// 'Sub. B Complete'
```
See Also
--------
* [`ConnectableObservable`](../index/class/connectableobservable)
* [`publish`](publish)
* [`publishReplay`](publishreplay)
* [`publishBehavior`](publishbehavior)
rxjs observeOn observeOn
=========
`function` `stable` `operator`
Re-emits all notifications from source Observable with specified scheduler.
### `observeOn<T>(scheduler: SchedulerLike, delay: number = 0): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Scheduler that will be used to reschedule notifications from source Observable. |
| `[delay](../index/function/delay)` | `number` | Optional. Default is `0`. Number of milliseconds that states with what delay every notification should be rescheduled. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the same notifications as the source Observable, but with provided scheduler.
Description
-----------
Ensure a specific scheduler is used, from outside of an Observable.
`[observeOn](../index/function/observeon)` is an operator that accepts a scheduler as a first parameter, which will be used to reschedule notifications emitted by the source Observable. It might be useful, if you do not have control over internal scheduler of a given Observable, but want to control when its values are emitted nevertheless.
Returned Observable emits the same notifications (nexted values, complete and error events) as the source Observable, but rescheduled with provided scheduler. Note that this doesn't mean that source Observables internal scheduler will be replaced in any way. Original scheduler still will be used, but when the source Observable emits notification, it will be immediately scheduled again - this time with scheduler passed to `[observeOn](../index/function/observeon)`. An anti-pattern would be calling `[observeOn](../index/function/observeon)` on Observable that emits lots of values synchronously, to split that emissions into asynchronous chunks. For this to happen, scheduler would have to be passed into the source Observable directly (usually into the operator that creates it). `[observeOn](../index/function/observeon)` simply delays notifications a little bit more, to ensure that they are emitted at expected moments.
As a matter of fact, `[observeOn](../index/function/observeon)` accepts second parameter, which specifies in milliseconds with what delay notifications will be emitted. The main difference between [`delay`](delay) operator and `[observeOn](../index/function/observeon)` is that `[observeOn](../index/function/observeon)` will delay all notifications - including error notifications - while `[delay](../index/function/delay)` will pass through error from source Observable immediately when it is emitted. In general it is highly recommended to use `[delay](../index/function/delay)` operator for any kind of delaying of values in the stream, while using `[observeOn](../index/function/observeon)` to specify which scheduler should be used for notification emissions in general.
Example
-------
Ensure values in subscribe are called just before browser repaint
```
import { interval, observeOn, animationFrameScheduler } from 'rxjs';
const someDiv = document.createElement('div');
someDiv.style.cssText = 'width: 200px;background: #09c';
document.body.appendChild(someDiv);
const intervals = interval(10); // Intervals are scheduled
// with async scheduler by default...
intervals.pipe(
observeOn(animationFrameScheduler) // ...but we will observe on animationFrame
) // scheduler to ensure smooth animation.
.subscribe(val => {
someDiv.style.height = val + 'px';
});
```
See Also
--------
* [`delay`](delay)
rxjs pairwise pairwise
========
`function` `stable` `operator`
Groups pairs of consecutive emissions together and emits them as an array of two values.
### `pairwise<T>(): OperatorFunction<T, [T, T]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [T, T]>`: A function that returns an Observable of pairs (as arrays) of consecutive values from the source Observable.
Description
-----------
Puts the current value and previous value together as an array, and emits that.
The Nth emission from the source Observable will cause the output Observable to emit an array [(N-1)th, Nth] of the previous and the current value, as a pair. For this reason, `[pairwise](../index/function/pairwise)` emits on the second and subsequent emissions from the source Observable, but not on the first emission, because there is no previous value in that case.
Example
-------
On every click (starting from the second), emit the relative distance to the previous click
```
import { fromEvent, pairwise, map } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const pairs = clicks.pipe(pairwise());
const distance = pairs.pipe(
map(([first, second]) => {
const x0 = first.clientX;
const y0 = first.clientY;
const x1 = second.clientX;
const y1 = second.clientY;
return Math.sqrt(Math.pow(x0 - x1, 2) + Math.pow(y0 - y1, 2));
})
);
distance.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
| programming_docs |
rxjs finalize finalize
========
`function` `stable` `operator`
Returns an Observable that mirrors the source Observable, but will call a specified function when the source terminates on complete or error. The specified function will also be called when the subscriber explicitly unsubscribes.
### `finalize<T>(callback: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `callback` | `() => void` | Function to be called when source terminates. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source, but will call the specified function on termination.
Description
-----------
Examples
--------
Execute callback function when the observable completes
```
import { interval, take, finalize } from 'rxjs';
// emit value in sequence every 1 second
const source = interval(1000);
const example = source.pipe(
take(5), //take only the first 5 values
finalize(() => console.log('Sequence complete')) // Execute when the observable completes
);
const subscribe = example.subscribe(val => console.log(val));
// results:
// 0
// 1
// 2
// 3
// 4
// 'Sequence complete'
```
Execute callback function when the subscriber explicitly unsubscribes
```
import { interval, finalize, tap, noop, timer } from 'rxjs';
const source = interval(100).pipe(
finalize(() => console.log('[finalize] Called')),
tap({
next: () => console.log('[next] Called'),
error: () => console.log('[error] Not called'),
complete: () => console.log('[tap complete] Not called')
})
);
const sub = source.subscribe({
next: x => console.log(x),
error: noop,
complete: () => console.log('[complete] Not called')
});
timer(150).subscribe(() => sub.unsubscribe());
// results:
// '[next] Called'
// 0
// '[finalize] Called'
```
rxjs mapTo mapTo
=====
`function` `deprecated` `operator`
Emits the given constant value on the output Observable every time the source Observable emits a value.
Deprecation Notes
-----------------
To be removed in v9. Use [`map`](map) instead: `[map](../index/function/map)(() => value)`.
### `mapTo<R>(value: R): OperatorFunction<unknown, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `R` | The value to map each source value to. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, R>`: A function that returns an Observable that emits the given `value` every time the source Observable emits.
Description
-----------
Like [`map`](map), but it maps every source value to the same output value every time.
Takes a constant `value` as argument, and emits that whenever the source Observable emits a value. In other words, ignores the actual source value, and simply uses the emission moment to know when to emit the given `value`.
Example
-------
Map every click to the string `'Hi'`
```
import { fromEvent, mapTo } from 'rxjs';
const clicks = fromEvent(document, 'click');
const greetings = clicks.pipe(mapTo('Hi'));
greetings.subscribe(x => console.log(x));
```
See Also
--------
* [`map`](map)
rxjs count count
=====
`function` `stable` `operator`
Counts the number of emissions on the source and emits that number when the source completes.
### `count<T>(predicate?: (value: T, index: number) => boolean): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | Optional. Default is `undefined`. A function that is used to analyze the value and the index and determine whether or not to increment the count. Return `true` to increment the count, and return `false` to keep the count the same. If the predicate is not provided, every value will be counted. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, number>`: A function that returns an Observable that emits one number that represents the count of emissions.
Description
-----------
Tells how many values were emitted, when the source completes.
`[count](../index/function/count)` transforms an Observable that emits values into an Observable that emits a single value that represents the number of values emitted by the source Observable. If the source Observable terminates with an error, `[count](../index/function/count)` will pass this error notification along without emitting a value first. If the source Observable does not terminate at all, `[count](../index/function/count)` will neither emit a value nor terminate. This operator takes an optional `predicate` function as argument, in which case the output emission will represent the number of source values that matched `true` with the `predicate`.
Examples
--------
Counts how many seconds have passed before the first click happened
```
import { interval, fromEvent, takeUntil, count } from 'rxjs';
const seconds = interval(1000);
const clicks = fromEvent(document, 'click');
const secondsBeforeClick = seconds.pipe(takeUntil(clicks));
const result = secondsBeforeClick.pipe(count());
result.subscribe(x => console.log(x));
```
Counts how many odd numbers are there between 1 and 7
```
import { range, count } from 'rxjs';
const numbers = range(1, 7);
const result = numbers.pipe(count(i => i % 2 === 1));
result.subscribe(x => console.log(x));
// Results in:
// 4
```
See Also
--------
* [`max`](max)
* [`min`](min)
* [`reduce`](reduce)
rxjs refCount refCount
========
`function` `deprecated` `operator`
Make a [`ConnectableObservable`](../index/class/connectableobservable) behave like a ordinary observable and automates the way you can connect to it.
Deprecation Notes
-----------------
Replaced with the [`share`](share) operator. How `[share](../index/function/share)` is used will depend on the connectable observable you created just prior to the `[refCount](../index/function/refcount)` operator. Details: <https://rxjs.dev/deprecations/multicasting>
### `refCount<T>(): MonoTypeOperatorFunction<T>`
#### Parameters
There are no parameters.
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that automates the connection to ConnectableObservable.
Description
-----------
Internally it counts the subscriptions to the observable and subscribes (only once) to the source if the number of subscriptions is larger than 0. If the number of subscriptions is smaller than 1, it unsubscribes from the source. This way you can make sure that everything before the *published* refCount has only a single subscription independently of the number of subscribers to the target observable.
Note that using the [`share`](share) operator is exactly the same as using the `[multicast](../index/function/multicast)(() => new [Subject](../index/class/subject)())` operator (making the observable hot) and the *refCount* operator in a sequence.
Example
-------
In the following example there are two intervals turned into connectable observables by using the *publish* operator. The first one uses the *refCount* operator, the second one does not use it. You will notice that a connectable observable does nothing until you call its connect function.
```
import { interval, tap, publish, refCount } from 'rxjs';
// Turn the interval observable into a ConnectableObservable (hot)
const refCountInterval = interval(400).pipe(
tap(num => console.log(`refCount ${ num }`)),
publish(),
refCount()
);
const publishedInterval = interval(400).pipe(
tap(num => console.log(`publish ${ num }`)),
publish()
);
refCountInterval.subscribe();
refCountInterval.subscribe();
// 'refCount 0' -----> 'refCount 1' -----> etc
// All subscriptions will receive the same value and the tap (and
// every other operator) before the `publish` operator will be executed
// only once per event independently of the number of subscriptions.
publishedInterval.subscribe();
// Nothing happens until you call .connect() on the observable.
```
See Also
--------
* [`ConnectableObservable`](../index/class/connectableobservable)
* [`share`](share)
* [`publish`](publish)
rxjs ignoreElements ignoreElements
==============
`function` `stable` `operator`
Ignores all items emitted by the source Observable and only passes calls of `complete` or `error`.
### `ignoreElements(): OperatorFunction<unknown, never>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, [never](../index/function/never)>`: A function that returns an empty Observable that only calls `complete` or `error`, based on which one is called by the source Observable.
Description
-----------
The `[ignoreElements](../index/function/ignoreelements)` operator suppresses all items emitted by the source Observable, but allows its termination notification (either `error` or `complete`) to pass through unchanged.
If you do not care about the items being emitted by an Observable, but you do want to be notified when it completes or when it terminates with an error, you can apply the `[ignoreElements](../index/function/ignoreelements)` operator to the Observable, which will ensure that it will never call its observers’ `next` handlers.
Example
-------
Ignore all `next` emissions from the source
```
import { of, ignoreElements } from 'rxjs';
of('you', 'talking', 'to', 'me')
.pipe(ignoreElements())
.subscribe({
next: word => console.log(word),
error: err => console.log('error:', err),
complete: () => console.log('the end'),
});
// result:
// 'the end'
```
rxjs shareReplay shareReplay
===========
`function` `stable` `operator`
Share source and replay specified number of emissions on subscription.
### `shareReplay<T>(configOrBufferSize?: number | ShareReplayConfig, windowTime?: number, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `configOrBufferSize` | `number | ShareReplayConfig` | Optional. Default is `undefined`. |
| `[windowTime](../index/function/windowtime)` | `number` | Optional. Default is `undefined`. Maximum time length of the replay buffer in milliseconds. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. Scheduler where connected observers within the selector function will be invoked on. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
Description
-----------
This operator is a specialization of `replay` that connects to a source observable and multicasts through a `[ReplaySubject](../index/class/replaysubject)` constructed with the specified arguments. A successfully completed source will stay cached in the `shareReplayed [observable](../index/const/observable)` forever, but an errored source can be retried.
Why use shareReplay?
--------------------
You generally want to use `[shareReplay](../index/function/sharereplay)` when you have side-effects or taxing computations that you do not wish to be executed amongst multiple subscribers. It may also be valuable in situations where you know you will have late subscribers to a stream that need access to previously emitted values. This ability to replay values on subscription is what differentiates [`share`](share) and `[shareReplay](../index/function/sharereplay)`.

Reference counting
------------------
By default `[shareReplay](../index/function/sharereplay)` will use `[refCount](../index/function/refcount)` of false, meaning that it will *not* unsubscribe the source when the reference counter drops to zero, i.e. the inner `[ReplaySubject](../index/class/replaysubject)` will *not* be unsubscribed (and potentially run for ever). This is the default as it is expected that `[shareReplay](../index/function/sharereplay)` is often used to keep around expensive to setup observables which we want to keep running instead of having to do the expensive setup again.
As of RXJS version 6.4.0 a new overload signature was added to allow for manual control over what happens when the operators internal reference counter drops to zero. If `[refCount](../index/function/refcount)` is true, the source will be unsubscribed from once the reference count drops to zero, i.e. the inner `[ReplaySubject](../index/class/replaysubject)` will be unsubscribed. All new subscribers will receive value emissions from a new `[ReplaySubject](../index/class/replaysubject)` which in turn will cause a new subscription to the source observable.
Examples
--------
Example with a third subscriber coming late to the party
```
import { interval, take, shareReplay } from 'rxjs';
const shared$ = interval(2000).pipe(
take(6),
shareReplay(3)
);
shared$.subscribe(x => console.log('sub A: ', x));
shared$.subscribe(y => console.log('sub B: ', y));
setTimeout(() => {
shared$.subscribe(y => console.log('sub C: ', y));
}, 11000);
// Logs:
// (after ~2000 ms)
// sub A: 0
// sub B: 0
// (after ~4000 ms)
// sub A: 1
// sub B: 1
// (after ~6000 ms)
// sub A: 2
// sub B: 2
// (after ~8000 ms)
// sub A: 3
// sub B: 3
// (after ~10000 ms)
// sub A: 4
// sub B: 4
// (after ~11000 ms, sub C gets the last 3 values)
// sub C: 2
// sub C: 3
// sub C: 4
// (after ~12000 ms)
// sub A: 5
// sub B: 5
// sub C: 5
```
Example for `[refCount](../index/function/refcount)` usage
```
import { Observable, tap, interval, shareReplay, take } from 'rxjs';
const log = <T>(name: string, source: Observable<T>) => source.pipe(
tap({
subscribe: () => console.log(`${ name }: subscribed`),
next: value => console.log(`${ name }: ${ value }`),
complete: () => console.log(`${ name }: completed`),
finalize: () => console.log(`${ name }: unsubscribed`)
})
);
const obs$ = log('source', interval(1000));
const shared$ = log('shared', obs$.pipe(
shareReplay({ bufferSize: 1, refCount: true }),
take(2)
));
shared$.subscribe(x => console.log('sub A: ', x));
shared$.subscribe(y => console.log('sub B: ', y));
// PRINTS:
// shared: subscribed <-- reference count = 1
// source: subscribed
// shared: subscribed <-- reference count = 2
// source: 0
// shared: 0
// sub A: 0
// shared: 0
// sub B: 0
// source: 1
// shared: 1
// sub A: 1
// shared: completed <-- take(2) completes the subscription for sub A
// shared: unsubscribed <-- reference count = 1
// shared: 1
// sub B: 1
// shared: completed <-- take(2) completes the subscription for sub B
// shared: unsubscribed <-- reference count = 0
// source: unsubscribed <-- replaySubject unsubscribes from source observable because the reference count dropped to 0 and refCount is true
// In case of refCount being false, the unsubscribe is never called on the source and the source would keep on emitting, even if no subscribers
// are listening.
// source: 2
// source: 3
// source: 4
// ...
```
See Also
--------
* [`publish`](publish)
* [`share`](share)
* [`publishReplay`](publishreplay)
rxjs combineLatestWith combineLatestWith
=================
`function` `stable` `operator`
Create an observable that combines the latest values from all passed observables and the source into arrays and emits them.
### `combineLatestWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Returns an observable, that when subscribed to, will subscribe to the source observable and all sources provided as arguments. Once all sources emit at least one value, all of the latest values will be emitted as an array. After that, every time any source emits a value, all of the latest values will be emitted as an array.
This is a useful operator for eagerly calculating values based off of changed inputs.
Example
-------
Simple concatenation of values from two inputs
```
import { fromEvent, combineLatestWith, map } from 'rxjs';
// Setup: Add two inputs to the page
const input1 = document.createElement('input');
document.body.appendChild(input1);
const input2 = document.createElement('input');
document.body.appendChild(input2);
// Get streams of changes
const input1Changes$ = fromEvent(input1, 'change');
const input2Changes$ = fromEvent(input2, 'change');
// Combine the changes by adding them together
input1Changes$.pipe(
combineLatestWith(input2Changes$),
map(([e1, e2]) => (<HTMLInputElement>e1.target).value + ' - ' + (<HTMLInputElement>e2.target).value)
)
.subscribe(x => console.log(x));
```
rxjs zip zip
===
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Replaced with [`zipWith`](zipwith). Will be removed in v8.
### `zip<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `zip()`
#### Parameters
There are no parameters.
### `zip()`
#### Parameters
There are no parameters.
### `zip()`
#### Parameters
There are no parameters.
### `zip(...sources: (Observable<any> | InteropObservable<any> | AsyncIterable<any> | PromiseLike<any> | ArrayLike<any> | Iterable<any> | ReadableStreamLike<any> | ((...values: any[]) => R))[]): OperatorFunction<T, any>`
#### Parameters
| | | |
| --- | --- | --- |
| `sources` | `([Observable](../index/class/observable)<any> | [InteropObservable](../index/interface/interopobservable)<any> | AsyncIterable<any> | PromiseLike<any> | ArrayLike<any> | Iterable<any> | [ReadableStreamLike](../index/interface/readablestreamlike)<any> | ((...values: any[]) => R))[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, any>`
rxjs publishReplay publishReplay
=============
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](../index/function/connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishReplay<T, R>(bufferSize?: number, windowTime?: number, selectorOrScheduler?: TimestampProvider | OperatorFunction<T, R>, timestampProvider?: TimestampProvider)`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | Optional. Default is `undefined`. |
| `[windowTime](../index/function/windowtime)` | `number` | Optional. Default is `undefined`. |
| `selectorOrScheduler` | `[TimestampProvider](../index/interface/timestampprovider) | [OperatorFunction](../index/interface/operatorfunction)<T, R>` | Optional. Default is `undefined`. |
| `timestampProvider` | `[TimestampProvider](../index/interface/timestampprovider)` | Optional. Default is `undefined`. |
Overloads
---------
### `publishReplay(bufferSize?: number, windowTime?: number, timestampProvider?: TimestampProvider): MonoTypeOperatorFunction<T>`
Creates a [`ConnectableObservable`](../index/class/connectableobservable) that uses a [`ReplaySubject`](../index/class/replaysubject) internally.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | Optional. Default is `undefined`. The buffer size for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `[windowTime](../index/function/windowtime)` | `number` | Optional. Default is `undefined`. The window time for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `timestampProvider` | `[TimestampProvider](../index/interface/timestampprovider)` | Optional. Default is `undefined`. The timestamp provider for the underlying [`ReplaySubject`](../index/class/replaysubject). |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `publishReplay(bufferSize: number, windowTime: number, selector: (shared: Observable<T>) => O, timestampProvider?: TimestampProvider): OperatorFunction<T, ObservedValueOf<O>>`
Creates an observable, that when subscribed to, will create a [`ReplaySubject`](../index/class/replaysubject), and pass an observable from it (using [asObservable](../index/class/subject#asObservable)) to the `selector` function, which then returns an observable that is subscribed to before "connecting" the source to the internal `[ReplaySubject](../index/class/replaysubject)`.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The buffer size for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `[windowTime](../index/function/windowtime)` | `number` | The window time for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `selector` | `(shared: [Observable](../index/class/observable)<T>) => O` | A function used to setup the multicast. |
| `timestampProvider` | `[TimestampProvider](../index/interface/timestampprovider)` | Optional. Default is `undefined`. The timestamp provider for the underlying [`ReplaySubject`](../index/class/replaysubject). |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
Since this is deprecated, for additional details see the documentation for [`connect`](connect).
### `publishReplay(bufferSize: number, windowTime: number, selector: undefined, timestampProvider: TimestampProvider): OperatorFunction<T, ObservedValueOf<O>>`
Creates a [`ConnectableObservable`](../index/class/connectableobservable) that uses a [`ReplaySubject`](../index/class/replaysubject) internally.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The buffer size for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `[windowTime](../index/function/windowtime)` | `number` | The window time for the underlying [`ReplaySubject`](../index/class/replaysubject). |
| `selector` | `undefined` | Passing `undefined` here determines that this operator will return a [`ConnectableObservable`](../index/class/connectableobservable). |
| `timestampProvider` | `[TimestampProvider](../index/interface/timestampprovider)` | The timestamp provider for the underlying [`ReplaySubject`](../index/class/replaysubject). |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
| programming_docs |
rxjs combineAll combineAll
==========
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`combineLatestAll`](combinelatestall). Will be removed in v8.
### `const combineAll: { <T>(): OperatorFunction<ObservableInput<T>, T[]>; <T>(): OperatorFunction<any, T[]>; <T, R>(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>; <R>(project: (...values: any[]) => R): OperatorFunction<...>; };`
rxjs timeout timeout
=======
`function` `stable` `operator`
Errors if Observable does not emit a value in given time span.
### `timeout<T, O extends ObservableInput<any>, M>(config: number | Date | TimeoutConfig<T, O, M>, schedulerArg?: SchedulerLike): OperatorFunction<T, T | ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../index/const/config)` | `number | Date | TimeoutConfig<T, O, M>` | |
| `schedulerArg` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that mirrors behaviour of the source Observable, unless timeout happens when it throws an error.
Description
-----------
Timeouts on Observable that doesn't emit values fast enough.
Overloads
---------
### `timeout(config: TimeoutConfig<T, O, M> & { with: (info: TimeoutInfo<T, M>) => O; }): OperatorFunction<T, T | ObservedValueOf<O>>`
If `with` is provided, this will return an observable that will switch to a different observable if the source does not push values within the specified time parameters.
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../index/const/config)` | `TimeoutConfig<T, O, M> & { with: (info: TimeoutInfo<T, M>) => O; }` | The configuration for the timeout. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
The most flexible option for creating a timeout behavior.
The first thing to know about the configuration is if you do not provide a `with` property to the configuration, when timeout conditions are met, this operator will emit a [`TimeoutError`](../index/interface/timeouterror). Otherwise, it will use the factory function provided by `with`, and switch your subscription to the result of that. Timeout conditions are provided by the settings in `[first](../index/function/first)` and `each`.
The `[first](../index/function/first)` property can be either a `Date` for a specific time, a `number` for a time period relative to the point of subscription, or it can be skipped. This property is to check timeout conditions for the arrival of the first value from the source *only*. The timings of all subsequent values from the source will be checked against the time period provided by `each`, if it was provided.
The `each` property can be either a `number` or skipped. If a value for `each` is provided, it represents the amount of time the resulting observable will wait between the arrival of values from the source before timing out. Note that if `[first](../index/function/first)` is *not* provided, the value from `each` will be used to check timeout conditions for the arrival of the first value and all subsequent values. If `[first](../index/function/first)` *is* provided, `each` will only be use to check all values after the first.
Examples
--------
Emit a custom error if there is too much time between values
```
import { interval, timeout, throwError } from 'rxjs';
class CustomTimeoutError extends Error {
constructor() {
super('It was too slow');
this.name = 'CustomTimeoutError';
}
}
const slow$ = interval(900);
slow$.pipe(
timeout({
each: 1000,
with: () => throwError(() => new CustomTimeoutError())
})
)
.subscribe({
error: console.error
});
```
Switch to a faster observable if your source is slow.
```
import { interval, timeout } from 'rxjs';
const slow$ = interval(900);
const fast$ = interval(500);
slow$.pipe(
timeout({
each: 1000,
with: () => fast$,
})
)
.subscribe(console.log);
```
### `timeout(config: any): OperatorFunction<T, T>`
Returns an observable that will error or switch to a different observable if the source does not push values within the specified time parameters.
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../index/const/config)` | `any` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T>`
The most flexible option for creating a timeout behavior.
The first thing to know about the configuration is if you do not provide a `with` property to the configuration, when timeout conditions are met, this operator will emit a [`TimeoutError`](../index/interface/timeouterror). Otherwise, it will use the factory function provided by `with`, and switch your subscription to the result of that. Timeout conditions are provided by the settings in `[first](../index/function/first)` and `each`.
The `[first](../index/function/first)` property can be either a `Date` for a specific time, a `number` for a time period relative to the point of subscription, or it can be skipped. This property is to check timeout conditions for the arrival of the first value from the source *only*. The timings of all subsequent values from the source will be checked against the time period provided by `each`, if it was provided.
The `each` property can be either a `number` or skipped. If a value for `each` is provided, it represents the amount of time the resulting observable will wait between the arrival of values from the source before timing out. Note that if `[first](../index/function/first)` is *not* provided, the value from `each` will be used to check timeout conditions for the arrival of the first value and all subsequent values. If `[first](../index/function/first)` *is* provided, `each` will only be use to check all values after the first.
#### Handling TimeoutErrors
If no `with` property was provided, subscriptions to the resulting observable may emit an error of [`TimeoutError`](../index/interface/timeouterror). The timeout error provides useful information you can examine when you're handling the error. The most common way to handle the error would be with [`catchError`](catcherror), although you could use [`tap`](tap) or just the error handler in your `subscribe` call directly, if your error handling is only a side effect (such as notifying the user, or logging).
In this case, you would check the error for `instanceof [TimeoutError](../index/interface/timeouterror)` to validate that the error was indeed from `[timeout](../index/function/timeout)`, and not from some other source. If it's not from `[timeout](../index/function/timeout)`, you should probably rethrow it if you're in a `[catchError](../index/function/catcherror)`.
Examples
--------
Emit a [`TimeoutError`](../index/interface/timeouterror) if the first value, and *only* the first value, does not arrive within 5 seconds
```
import { interval, timeout } from 'rxjs';
// A random interval that lasts between 0 and 10 seconds per tick
const source$ = interval(Math.round(Math.random() * 10_000));
source$.pipe(
timeout({ first: 5_000 })
)
.subscribe({
next: console.log,
error: console.error
});
```
Emit a [`TimeoutError`](../index/interface/timeouterror) if the source waits longer than 5 seconds between any two values or the first value and subscription.
```
import { timer, timeout, expand } from 'rxjs';
const getRandomTime = () => Math.round(Math.random() * 10_000);
// An observable that waits a random amount of time between each delivered value
const source$ = timer(getRandomTime())
.pipe(expand(() => timer(getRandomTime())));
source$
.pipe(timeout({ each: 5_000 }))
.subscribe({
next: console.log,
error: console.error
});
```
Emit a [`TimeoutError`](../index/interface/timeouterror) if the source does not emit before 7 seconds, *or* if the source waits longer than 5 seconds between any two values after the first.
```
import { timer, timeout, expand } from 'rxjs';
const getRandomTime = () => Math.round(Math.random() * 10_000);
// An observable that waits a random amount of time between each delivered value
const source$ = timer(getRandomTime())
.pipe(expand(() => timer(getRandomTime())));
source$
.pipe(timeout({ first: 7_000, each: 5_000 }))
.subscribe({
next: console.log,
error: console.error
});
```
### `timeout(first: Date, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
Returns an observable that will error if the source does not push its first value before the specified time passed as a `Date`. This is functionally the same as `[timeout](../index/function/timeout)({ [first](../index/function/first): someDate })`.
#### Parameters
| | | |
| --- | --- | --- |
| `[first](../index/function/first)` | `Date` | The date to at which the resulting observable will timeout if the source observable does not emit at least one value. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use. Defaults to [`asyncScheduler`](../index/const/asyncscheduler). |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
Errors if the first value doesn't show up before the given date and time
### `timeout(each: number, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
Returns an observable that will error if the source does not push a value within the specified time in milliseconds. This is functionally the same as `[timeout](../index/function/timeout)({ each: milliseconds })`.
#### Parameters
| | | |
| --- | --- | --- |
| `each` | `number` | The time allowed between each pushed value from the source before the resulting observable will timeout. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use. Defaults to [`asyncScheduler`](../index/const/asyncscheduler). |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
Errors if it waits too long between any value
See Also
--------
* [`timeoutWith`](timeoutwith)
rxjs sequenceEqual sequenceEqual
=============
`function` `stable` `operator`
Compares all values of two observables in sequence using an optional comparator function and returns an observable of a single boolean value representing whether or not the two sequences are equal.
### `sequenceEqual<T>(compareTo: Observable<T>, comparator: (a: T, b: T) => boolean = (a, b) => a === b): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `compareTo` | `[Observable<T>](../index/class/observable)` | The observable sequence to compare the source sequence to. |
| `comparator` | `(a: T, b: T) => boolean` | Optional. Default is `(a, b) => a === b`. An optional function to compare each value pair |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, boolean>`: A function that returns an Observable that emits a single boolean value representing whether or not the values emitted by the source Observable and provided Observable were equal in sequence.
Description
-----------
Checks to see of all values emitted by both observables are equal, in order.
`[sequenceEqual](../index/function/sequenceequal)` subscribes to two observables and buffers incoming values from each observable. Whenever either observable emits a value, the value is buffered and the buffers are shifted and compared from the bottom up; If any value pair doesn't match, the returned observable will emit `false` and complete. If one of the observables completes, the operator will wait for the other observable to complete; If the other observable emits before completing, the returned observable will emit `false` and complete. If one observable never completes or emits after the other completes, the returned observable will never complete.
Example
-------
Figure out if the Konami code matches
```
import { from, fromEvent, map, bufferCount, mergeMap, sequenceEqual } from 'rxjs';
const codes = from([
'ArrowUp',
'ArrowUp',
'ArrowDown',
'ArrowDown',
'ArrowLeft',
'ArrowRight',
'ArrowLeft',
'ArrowRight',
'KeyB',
'KeyA',
'Enter', // no start key, clearly.
]);
const keys = fromEvent<KeyboardEvent>(document, 'keyup').pipe(map(e => e.code));
const matches = keys.pipe(
bufferCount(11, 1),
mergeMap(last11 => from(last11).pipe(sequenceEqual(codes)))
);
matches.subscribe(matched => console.log('Successful cheat at Contra? ', matched));
```
See Also
--------
* [`combineLatest`](../index/function/combinelatest)
* [`zip`](../index/function/zip)
* [`withLatestFrom`](withlatestfrom)
rxjs publishBehavior publishBehavior
===============
`function` `deprecated` `operator`
Creates a [`ConnectableObservable`](../index/class/connectableobservable) that utilizes a [`BehaviorSubject`](../index/class/behaviorsubject).
Deprecation Notes
-----------------
Will be removed in v8. To create a connectable observable that uses a [`BehaviorSubject`](../index/class/behaviorsubject) under the hood, use [`connectable`](../index/function/connectable). `source.pipe([publishBehavior](../index/function/publishbehavior)(initValue))` is equivalent to `[connectable](../index/function/connectable)(source, { connector: () => new [BehaviorSubject](../index/class/behaviorsubject)(initValue), resetOnDisconnect: false })`. If you're using [`refCount`](refcount) after `[publishBehavior](../index/function/publishbehavior)`, use the [`share`](share) operator instead. `source.pipe([publishBehavior](../index/function/publishbehavior)(initValue), [refCount](../index/function/refcount)())` is equivalent to `source.pipe([share](../index/function/share)({ connector: () => new [BehaviorSubject](../index/class/behaviorsubject)(initValue), resetOnError: false, resetOnComplete: false, resetOnRefCountZero: false }))`. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishBehavior<T>(initialValue: T): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `initialValue` | `T` | The initial value passed to the [`BehaviorSubject`](../index/class/behaviorsubject). |
#### Returns
`[UnaryFunction](../index/interface/unaryfunction)<[Observable](../index/class/observable)<T>, [ConnectableObservable](../index/class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../index/class/connectableobservable)
rxjs concatMap concatMap
=========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable, in a serialized fashion waiting for each one to complete before merging the next.
### `concatMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and taking values from each projected inner Observable sequentially.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`concatAll`](concatall).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. Each new inner Observable is concatenated with the previous inner Observable.
**Warning:** if source values arrive endlessly and faster than their corresponding inner Observables can complete, it will result in memory issues as inner Observables amass in an unbounded buffer waiting for their turn to be subscribed to.
Note: `[concatMap](../index/function/concatmap)` is equivalent to `[mergeMap](../index/function/mergemap)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, concatMap, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
concatMap(ev => interval(1000).pipe(take(4)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
Overloads
---------
### `concatMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `concatMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `concatMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concat`](../index/function/concat)
* [`concatAll`](concatall)
* [`concatMapTo`](concatmapto)
* [`exhaustMap`](exhaustmap)
* [`mergeMap`](mergemap)
* [`switchMap`](switchmap)
rxjs find find
====
`function` `stable` `operator`
Emits only the first value emitted by the source Observable that meets some condition.
### `find<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | A function called with each item to test for condition matching. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | undefined>`: A function that returns an Observable that emits the first item that matches the condition.
Description
-----------
Finds the first value that passes some test and emits that.
`[find](../index/function/find)` searches for the first item in the source Observable that matches the specified condition embodied by the `predicate`, and returns the first occurrence in the source. Unlike [`first`](first), the `predicate` is required in `[find](../index/function/find)`, and does not emit an error if a valid value is not found (emits `undefined` instead).
Example
-------
Find and emit the first click that happens on a DIV element
```
import { fromEvent, find } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(find(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `find(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `find(predicate: (this: A, value: T, index: number, source: Observable<T>) => value is S, thisArg: A): OperatorFunction<T, S | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../index/class/observable)<T>) => value is S` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S | undefined>`
### `find(predicate: (value: T, index: number, source: Observable<T>) => value is S): OperatorFunction<T, S | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => value is S` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S | undefined>`
### `find(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | undefined>`
### `find(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | undefined>`
See Also
--------
* [`filter`](filter)
* [`first`](first)
* [`findIndex`](findindex)
* [`take`](take)
| programming_docs |
rxjs exhaust exhaust
=======
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`exhaustAll`](exhaustall). Will be removed in v8.
### `const exhaust: <O extends ObservableInput<any>>() => OperatorFunction<O, ObservedValueOf<O>>;`
rxjs flatMap flatMap
=======
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`mergeMap`](mergemap). Will be removed in v8.
### `const flatMap: { <T, O extends ObservableInput<any>>(project: (value: T, index: number) => O, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>; <T, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector: undefined, concurrent?: number): OperatorFunction<...>; <T, R, O extends Observabl...;`
rxjs switchMapTo switchMapTo
===========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is flattened multiple times with [`switchMap`](switchmap) in the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`switchMap`](switchmap) instead: `[switchMap](../index/function/switchmap)(() => result)`
### `switchMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits items from the given `innerObservable` (and optionally transformed through the deprecated `resultSelector`) every time a value is emitted on the source Observable, and taking only the values from the most recently projected inner Observable.
Description
-----------
It's like [`switchMap`](switchmap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then flattens those resulting Observables into one single Observable, which is the output Observable. The output Observables emits values only from the most recently emitted instance of `innerObservable`.
Example
-------
Restart an interval Observable on every click event
```
import { fromEvent, switchMapTo, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(switchMapTo(interval(1000)));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `switchMapTo(observable: O): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `switchMapTo(observable: O, resultSelector: undefined): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<unknown, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `switchMapTo(observable: O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concatMapTo`](concatmapto)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`mergeMapTo`](mergemapto)
rxjs tap tap
===
`function` `stable` `operator`
Used to perform side-effects for notifications from the source observable
### `tap<T>(observerOrNext?: Partial<TapObserver<T>> | ((value: T) => void), error?: (e: any) => void, complete?: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `observerOrNext` | `Partial<TapObserver<T>> | ((value: T) => void)` | Optional. Default is `undefined`. A next handler or partial observer |
| `error` | `(e: any) => void` | Optional. Default is `undefined`. An error handler |
| `complete` | `() => void` | Optional. Default is `undefined`. A completion handler |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable identical to the source, but runs the specified Observer or callback(s) for each item.
Description
-----------
Used when you want to affect outside state with a notification without altering the notification
Tap is designed to allow the developer a designated place to perform side effects. While you *could* perform side-effects inside of a `[map](../index/function/map)` or a `[mergeMap](../index/function/mergemap)`, that would make their mapping functions impure, which isn't always a big deal, but will make it so you can't do things like memoize those functions. The `[tap](../index/function/tap)` operator is designed solely for such side-effects to help you remove side-effects from other operations.
For any notification, next, error, or complete, `[tap](../index/function/tap)` will call the appropriate callback you have provided to it, via a function reference, or a partial observer, then pass that notification down the stream.
The observable returned by `[tap](../index/function/tap)` is an exact mirror of the source, with one exception: Any error that occurs -- synchronously -- in a handler provided to `[tap](../index/function/tap)` will be emitted as an error from the returned observable.
> Be careful! You can mutate objects as they pass through the `[tap](../index/function/tap)` operator's handlers.
>
>
The most common use of `[tap](../index/function/tap)` is actually for debugging. You can place a `[tap](../index/function/tap)(console.log)` anywhere in your observable `[pipe](../index/function/pipe)`, log out the notifications as they are emitted by the source returned by the previous operation.
Examples
--------
Check a random number before it is handled. Below is an observable that will use a random number between 0 and 1, and emit `'big'` or `'small'` depending on the size of that number. But we wanted to log what the original number was, so we have added a `[tap](../index/function/tap)(console.log)`.
```
import { of, tap, map } from 'rxjs';
of(Math.random()).pipe(
tap(console.log),
map(n => n > 0.5 ? 'big' : 'small')
).subscribe(console.log);
```
Using `[tap](../index/function/tap)` to analyze a value and force an error. Below is an observable where in our system we only want to emit numbers 3 or less we get from another source. We can force our observable to error using `[tap](../index/function/tap)`.
```
import { of, tap } from 'rxjs';
const source = of(1, 2, 3, 4, 5);
source.pipe(
tap(n => {
if (n > 3) {
throw new TypeError(`Value ${ n } is greater than 3`);
}
})
)
.subscribe({ next: console.log, error: err => console.log(err.message) });
```
We want to know when an observable completes before moving on to the next observable. The system below will emit a random series of `'X'` characters from 3 different observables in sequence. The only way we know when one observable completes and moves to the next one, in this case, is because we have added a `[tap](../index/function/tap)` with the side effect of logging to console.
```
import { of, concatMap, interval, take, map, tap } from 'rxjs';
of(1, 2, 3).pipe(
concatMap(n => interval(1000).pipe(
take(Math.round(Math.random() * 10)),
map(() => 'X'),
tap({ complete: () => console.log(`Done with ${ n }`) })
))
)
.subscribe(console.log);
```
Overloads
---------
### `tap(observer?: Partial<TapObserver<T>>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `observer` | `Partial<TapObserver<T>>` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `tap(next: (value: T) => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `tap(next?: (value: T) => void, error?: (error: any) => void, complete?: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | Optional. Default is `undefined`. |
| `error` | `(error: any) => void` | Optional. Default is `undefined`. |
| `complete` | `() => void` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
See Also
--------
* [`finalize`](finalize)
* [`Observable`](../index/class/observable#subscribe)
rxjs mergeAll mergeAll
========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable which concurrently delivers all values that are emitted on the inner Observables.
### `mergeAll<O extends ObservableInput<any>>(concurrent: number = Infinity): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of inner Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<O, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that emits values coming from all the inner Observables emitted by the source Observable.
Description
-----------
Flattens an Observable-of-Observables.
`[mergeAll](../index/function/mergeall)` subscribes to an Observable that emits Observables, also known as a higher-order Observable. Each time it observes one of these emitted inner Observables, it subscribes to that and delivers all the values from the inner Observable on the output Observable. The output Observable only completes once all inner Observables have completed. Any error delivered by a inner Observable will be immediately emitted on the output Observable.
Examples
--------
Spawn a new interval Observable for each click event, and blend their outputs as one Observable
```
import { fromEvent, map, interval, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(map(() => interval(1000)));
const firstOrder = higherOrder.pipe(mergeAll());
firstOrder.subscribe(x => console.log(x));
```
Count from 0 to 9 every second for each click, but only allow 2 concurrent timers
```
import { fromEvent, map, interval, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(10)))
);
const firstOrder = higherOrder.pipe(mergeAll(2));
firstOrder.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`exhaustAll`](exhaustall)
* [`merge`](../index/function/merge)
* [`mergeMap`](mergemap)
* [`mergeMapTo`](mergemapto)
* [`mergeScan`](mergescan)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`zipAll`](zipall)
rxjs timeoutWith timeoutWith
===========
`function` `deprecated` `operator`
When the passed timespan elapses before the source emits any given value, it will unsubscribe from the source, and switch the subscription to another observable.
Deprecation Notes
-----------------
Replaced with [`timeout`](timeout). Instead of `[timeoutWith](../index/function/timeoutwith)(100, a$, scheduler)`, use [`timeout`](timeout) with the configuration object: `[timeout](../index/function/timeout)({ each: 100, with: () => a$, scheduler })`. Instead of `[timeoutWith](../index/function/timeoutwith)(someDate, a$, scheduler)`, use [`timeout`](timeout) with the configuration object: `[timeout](../index/function/timeout)({ [first](../index/function/first): someDate, with: () => a$, scheduler })`. Will be removed in v8.
### `timeoutWith<T, R>(due: number | Date, withObservable: ObservableInput<R>, scheduler?: SchedulerLike): OperatorFunction<T, T | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `due` | `number | Date` | When passed a number, used as the time (in milliseconds) allowed between each value from the source before timeout is triggered. When passed a Date, used as the exact time at which the timeout will be triggered if the first value does not arrive. |
| `withObservable` | `[ObservableInput](../index/type-alias/observableinput)<R>` | The observable to switch to when timeout occurs. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use with time-related operations within this operator. Defaults to [`asyncScheduler`](../index/const/asyncscheduler) |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | R>`: A function that returns an Observable that mirrors behaviour of the source Observable, unless timeout happens when it starts emitting values from the `[ObservableInput](../index/type-alias/observableinput)` passed as a second parameter.
Description
-----------
Used to switch to a different observable if your source is being slow.
Useful in cases where:
* You want to switch to a different source that may be faster.
* You want to notify a user that the data stream is slow.
* You want to emit a custom error rather than the [`TimeoutError`](../index/interface/timeouterror) emitted by the default usage of [`timeout`](timeout).
If the first parameter is passed as Date and the time of the Date arrives before the first value arrives from the source, it will unsubscribe from the source and switch the subscription to another observable.
Use Date object to switch to a different observable if the first value doesn't arrive by a specific time.
Can be used to set a timeout only for the first value, however it's recommended to use the [`timeout`](timeout) operator with the `[first](../index/function/first)` configuration to get the same effect.
Examples
--------
Fallback to a faster observable
```
import { interval, timeoutWith } from 'rxjs';
const slow$ = interval(1000);
const faster$ = interval(500);
slow$
.pipe(timeoutWith(900, faster$))
.subscribe(console.log);
```
Emit your own custom timeout error
```
import { interval, timeoutWith, throwError } from 'rxjs';
class CustomTimeoutError extends Error {
constructor() {
super('It was too slow');
this.name = 'CustomTimeoutError';
}
}
const slow$ = interval(1000);
slow$
.pipe(timeoutWith(900, throwError(() => new CustomTimeoutError())))
.subscribe({
error: err => console.error(err.message)
});
```
See Also
--------
* [`timeout`](timeout)
rxjs skipLast skipLast
========
`function` `stable` `operator`
Skip a specified number of values before the completion of an observable.
### `skipLast<T>(skipCount: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `skipCount` | `number` | Number of elements to skip from the end of the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that skips the last `[count](../index/function/count)` values emitted by the source Observable.
Description
-----------
Returns an observable that will emit values as soon as it can, given a number of skipped values. For example, if you `[skipLast](../index/function/skiplast)(3)` on a source, when the source emits its fourth value, the first value the source emitted will finally be emitted from the returned observable, as it is no longer part of what needs to be skipped.
All values emitted by the result of `[skipLast](../index/function/skiplast)(N)` will be delayed by `N` emissions, as each value is held in a buffer until enough values have been emitted that that the buffered value may finally be sent to the consumer.
After subscribing, unsubscribing will not result in the emission of the buffered skipped values.
Example
-------
Skip the last 2 values of an observable with many values
```
import { of, skipLast } from 'rxjs';
const numbers = of(1, 2, 3, 4, 5);
const skipLastTwo = numbers.pipe(skipLast(2));
skipLastTwo.subscribe(x => console.log(x));
// Results in:
// 1 2 3
// (4 and 5 are skipped)
```
See Also
--------
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipWhile`](skipwhile)
* [`take`](take)
rxjs takeUntil takeUntil
=========
`function` `stable` `operator`
Emits the values emitted by the source Observable until a `notifier` Observable emits a value.
### `takeUntil<T>(notifier: ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[ObservableInput](../index/type-alias/observableinput)<any>` | The Observable whose first emitted value will cause the output Observable of `[takeUntil](../index/function/takeuntil)` to stop emitting values from the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the values from the source Observable until `notifier` emits its first value.
Description
-----------
Lets values pass until a second Observable, `notifier`, emits a value. Then, it completes.
`[takeUntil](../index/function/takeuntil)` subscribes and begins mirroring the source Observable. It also monitors a second Observable, `notifier` that you provide. If the `notifier` emits a value, the output Observable stops mirroring the source Observable and completes. If the `notifier` doesn't emit any value and completes then `[takeUntil](../index/function/takeuntil)` will pass all values.
Example
-------
Tick every second until the first click happens
```
import { interval, fromEvent, takeUntil } from 'rxjs';
const source = interval(1000);
const clicks = fromEvent(document, 'click');
const result = source.pipe(takeUntil(clicks));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`take`](take)
* [`takeLast`](takelast)
* [`takeWhile`](takewhile)
* [`skip`](skip)
rxjs repeatWhen repeatWhen
==========
`function` `deprecated` `operator`
Returns an Observable that mirrors the source Observable with the exception of a `complete`. If the source Observable calls `complete`, this method will emit to the Observable returned from `notifier`. If that Observable calls `complete` or `error`, then this method will call `complete` or `error` on the child subscription. Otherwise this method will resubscribe to the source Observable.
Deprecation Notes
-----------------
Will be removed in v9 or v10. Use [`repeat`](repeat)'s `[delay](../index/function/delay)` option instead.
### `repeatWhen<T>(notifier: (notifications: Observable<void>) => Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `(notifications: [Observable](../index/class/observable)<void>) => [Observable](../index/class/observable)<any>` | Receives an Observable of notifications with which a user can `complete` or `error`, aborting the repetition. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that that mirrors the source Observable with the exception of a `complete`.
Description
-----------
Example
-------
Repeat a message stream on click
```
import { of, fromEvent, repeatWhen } from 'rxjs';
const source = of('Repeat message');
const documentClick$ = fromEvent(document, 'click');
const result = source.pipe(repeatWhen(() => documentClick$));
result.subscribe(data => console.log(data))
```
See Also
--------
* [`repeat`](repeat)
* [`retry`](retry)
* [`retryWhen`](retrywhen)
| programming_docs |
rxjs debounce debounce
========
`function` `stable` `operator`
Emits a notification from the source Observable only after a particular time span determined by another Observable has passed without another source emission.
### `debounce<T>(durationSelector: (value: T) => ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the timeout duration for each source value, returned as an Observable or a Promise. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified duration Observable returned by `durationSelector`, and may drop some values if they occur too frequently.
Description
-----------
It's like [`debounceTime`](debouncetime), but the time span of emission silence is determined by a second Observable.
`[debounce](../index/function/debounce)` delays notifications emitted by the source Observable, but drops previous pending delayed emissions if a new notification arrives on the source Observable. This operator keeps track of the most recent notification from the source Observable, and spawns a duration Observable by calling the `durationSelector` function. The notification is emitted only when the duration Observable emits a next notification, and if no other notification was emitted on the source Observable since the duration Observable was spawned. If a new notification appears before the duration Observable emits, the previous notification will not be emitted and a new duration is scheduled from `durationSelector` is scheduled. If the completing event happens during the scheduled duration the last cached notification is emitted before the completion event is forwarded to the output observable. If the error event happens during the scheduled duration or after it only the error event is forwarded to the output observable. The cache notification is not emitted in this case.
Like [`debounceTime`](debouncetime), this is a rate-limiting operator, and also a delay-like operator since output emissions do not necessarily occur at the same time as they did on the source Observable.
Example
-------
Emit the most recent click after a burst of clicks
```
import { fromEvent, scan, debounce, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
scan(i => ++i, 1),
debounce(i => interval(200 * i))
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
rxjs reduce reduce
======
`function` `stable` `operator`
Applies an accumulator function over the source Observable, and returns the accumulated result when the source completes, given an optional seed value.
### `reduce<V, A>(accumulator: (acc: V | A, value: V, index: number) => A, seed?: any): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | The accumulator function called on each source value. |
| `seed` | `any` | Optional. Default is `undefined`. The initial accumulation value. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, V | A>`: A function that returns an Observable that emits a single value that is the result of accumulating the values emitted by the source Observable.
Description
-----------
Combines together all values emitted on the source, using an accumulator function that knows how to join a new source value into the accumulation from the past.
Like [Array.prototype.reduce()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce), `[reduce](../index/function/reduce)` applies an `accumulator` function against an accumulation and each value of the source Observable (from the past) to reduce it to a single value, emitted on the output Observable. Note that `[reduce](../index/function/reduce)` will only emit one value, only when the source Observable completes. It is equivalent to applying operator [`scan`](scan) followed by operator [`last`](last).
Returns an Observable that applies a specified `accumulator` function to each item emitted by the source Observable. If a `seed` value is specified, then that value will be used as the initial value for the accumulator. If no seed value is specified, the first item of the source is used as the seed.
Example
-------
Count the number of click events that happened in 5 seconds
```
import { fromEvent, takeUntil, interval, map, reduce } from 'rxjs';
const clicksInFiveSeconds = fromEvent(document, 'click')
.pipe(takeUntil(interval(5000)));
const ones = clicksInFiveSeconds.pipe(map(() => 1));
const seed = 0;
const count = ones.pipe(reduce((acc, one) => acc + one, seed));
count.subscribe(x => console.log(x));
```
Overloads
---------
### `reduce(accumulator: (acc: V | A, value: V, index: number) => A): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, V | A>`
### `reduce(accumulator: (acc: A, value: V, index: number) => A, seed: A): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A, value: V, index: number) => A` | |
| `seed` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, A>`
### `reduce(accumulator: (acc: A | S, value: V, index: number) => A, seed: S): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A | S, value: V, index: number) => A` | |
| `seed` | `S` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<V, A>`
See Also
--------
* [`count`](count)
* [`expand`](expand)
* [`mergeScan`](mergescan)
* [`scan`](scan)
rxjs concatAll concatAll
=========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable by concatenating the inner Observables in order.
### `concatAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<O, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable emitting values from all the inner Observables concatenated.
Description
-----------
Flattens an Observable-of-Observables by putting one inner Observable after the other.
Joins every Observable emitted by the source (a higher-order Observable), in a serial fashion. It subscribes to each inner Observable only after the previous inner Observable has completed, and merges all of their values into the returned observable.
**Warning:** If the source Observable emits Observables quickly and endlessly, and the inner Observables it emits generally complete slower than the source emits, you can run into memory issues as the incoming Observables collect in an unbounded buffer.
Note: `[concatAll](../index/function/concatall)` is equivalent to `[mergeAll](../index/function/mergeall)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, map, interval, take, concatAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(4)))
);
const firstOrder = higherOrder.pipe(concatAll());
firstOrder.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concat`](../index/function/concat)
* [`concatMap`](concatmap)
* [`concatMapTo`](concatmapto)
* [`exhaustAll`](exhaustall)
* [`mergeAll`](mergeall)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`zipAll`](zipall)
rxjs mergeWith mergeWith
=========
`function` `stable` `operator`
Merge the values from all observables to a single observable result.
### `mergeWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Creates an observable, that when subscribed to, subscribes to the source observable, and all other sources provided as arguments. All values from every source are emitted from the resulting subscription.
When all sources complete, the resulting observable will complete.
When any source errors, the resulting observable will error.
Example
-------
Joining all outputs from multiple user input event streams
```
import { fromEvent, map, mergeWith } from 'rxjs';
const clicks$ = fromEvent(document, 'click').pipe(map(() => 'click'));
const mousemoves$ = fromEvent(document, 'mousemove').pipe(map(() => 'mousemove'));
const dblclicks$ = fromEvent(document, 'dblclick').pipe(map(() => 'dblclick'));
mousemoves$
.pipe(mergeWith(clicks$, dblclicks$))
.subscribe(x => console.log(x));
// result (assuming user interactions)
// 'mousemove'
// 'mousemove'
// 'mousemove'
// 'click'
// 'click'
// 'dblclick'
```
See Also
--------
* [`merge`](../index/function/merge)
rxjs mergeMap mergeMap
========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable.
### `mergeMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: number | ((outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R), concurrent: number = Infinity): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `number | ((outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R)` | Optional. Default is `undefined`. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and merging the results of the Observables obtained from this transformation.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`mergeAll`](mergeall).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an Observable, and then merging those resulting Observables and emitting the results of this merger.
Example
-------
Map and flatten each letter to an Observable ticking every 1 second
```
import { of, mergeMap, interval, map } from 'rxjs';
const letters = of('a', 'b', 'c');
const result = letters.pipe(
mergeMap(x => interval(1000).pipe(map(i => x + i)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// a0
// b0
// c0
// a1
// b1
// c1
// continues to list a, b, c every second with respective ascending integers
```
Overloads
---------
### `mergeMap(project: (value: T, index: number) => O, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `mergeMap(project: (value: T, index: number) => O, resultSelector: undefined, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `mergeMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R, concurrent?: number): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaustMap`](exhaustmap)
* [`merge`](../index/function/merge)
* [`mergeAll`](mergeall)
* [`mergeMapTo`](mergemapto)
* [`mergeScan`](mergescan)
* [`switchMap`](switchmap)
rxjs throttle throttle
========
`function` `stable` `operator`
Emits a value from the source Observable, then ignores subsequent source values for a duration determined by another Observable, then repeats this process.
### `throttle<T>(durationSelector: (value: T) => ObservableInput<any>, config: ThrottleConfig = defaultThrottleConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the silencing duration for each source value, returned as an Observable or a Promise. |
| `[config](../index/const/config)` | `ThrottleConfig` | Optional. Default is `defaultThrottleConfig`. a configuration object to define `leading` and `trailing` behavior. Defaults to `{ leading: true, trailing: false }`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that performs the throttle operation to limit the rate of emissions from the source.
Description
-----------
It's like [`throttleTime`](throttletime), but the silencing duration is determined by a second Observable.
`[throttle](../index/function/throttle)` emits the source Observable values on the output Observable when its internal timer is disabled, and ignores source values when the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, it is forwarded to the output Observable, and then the timer is enabled by calling the `durationSelector` function with the source value, which returns the "duration" Observable. When the duration Observable emits a value, the timer is disabled, and this process repeats for the next source value.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, throttle, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(throttle(() => interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounce`](debounce)
* [`delayWhen`](delaywhen)
* [`sample`](sample)
* [`throttleTime`](throttletime)
rxjs concatWith concatWith
==========
`function` `stable` `operator`
Emits all of the values from the source observable, then, once it completes, subscribes to each observable source provided, one at a time, emitting all of their values, and not subscribing to the next one until it completes.
### `concatWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
`[concat](../index/function/concat)(a$, b$, c$)` is the same as `a$.pipe([concatWith](../index/function/concatwith)(b$, c$))`.
Example
-------
Listen for one mouse click, then listen for all mouse moves.
```
import { fromEvent, map, take, concatWith } from 'rxjs';
const clicks$ = fromEvent(document, 'click');
const moves$ = fromEvent(document, 'mousemove');
clicks$.pipe(
map(() => 'click'),
take(1),
concatWith(
moves$.pipe(
map(() => 'move')
)
)
)
.subscribe(x => console.log(x));
// 'click'
// 'move'
// 'move'
// 'move'
// ...
```
rxjs max max
===
`function` `stable` `operator`
The Max operator operates on an Observable that emits numbers (or items that can be compared with a provided function), and when source Observable completes it emits a single item: the item with the largest value.
### `max<T>(comparer?: (x: T, y: T) => number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparer` | `(x: T, y: T) => number` | Optional. Default is `undefined`. Optional comparer function that it will use instead of its default to compare the value of two items. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits item with the largest value.
Description
-----------
Examples
--------
Get the maximal value of a series of numbers
```
import { of, max } from 'rxjs';
of(5, 4, 7, 2, 8)
.pipe(max())
.subscribe(x => console.log(x));
// Outputs
// 8
```
Use a comparer function to get the maximal item
```
import { of, max } from 'rxjs';
of(
{ age: 7, name: 'Foo' },
{ age: 5, name: 'Bar' },
{ age: 9, name: 'Beer' }
).pipe(
max((a, b) => a.age < b.age ? -1 : 1)
)
.subscribe(x => console.log(x.name));
// Outputs
// 'Beer'
```
See Also
--------
* [`min`](min)
rxjs pluck pluck
=====
`function` `deprecated` `operator`
Maps each source value to its specified nested property.
Deprecation Notes
-----------------
Use [`map`](map) and optional chaining: `[pluck](../index/function/pluck)('foo', 'bar')` is `[map](../index/function/map)(x => x?.foo?.bar)`. Will be removed in v8.
### `pluck<T, R>(...properties: (string | number | symbol)[]): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `properties` | `(string | number | symbol)[]` | The nested properties to pluck from each source value. |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`: A function that returns an Observable of property values from the source values.
Description
-----------
Like [`map`](map), but meant only for picking one of the nested properties of every emitted value.
Given a list of strings or numbers describing a path to a property, retrieves the value of a specified nested property from all values in the source Observable. If a property can't be resolved, it will return `undefined` for that value.
Example
-------
Map every click to the tagName of the clicked target element
```
import { fromEvent, pluck } from 'rxjs';
const clicks = fromEvent(document, 'click');
const tagNames = clicks.pipe(pluck('target', 'tagName'));
tagNames.subscribe(x => console.log(x));
```
Overloads
---------
### `pluck(k1: K1): OperatorFunction<T, T[K1]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1]>`
### `pluck(k1: K1, k2: K2): OperatorFunction<T, T[K1][K2]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1][K2]>`
### `pluck(k1: K1, k2: K2, k3: K3): OperatorFunction<T, T[K1][K2][K3]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1][K2][K3]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4): OperatorFunction<T, T[K1][K2][K3][K4]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1][K2][K3][K4]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5): OperatorFunction<T, T[K1][K2][K3][K4][K5]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1][K2][K3][K4][K5]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5, k6: K6): OperatorFunction<T, T[K1][K2][K3][K4][K5][K6]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
| `k6` | `K6` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[K1][K2][K3][K4][K5][K6]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5, k6: K6, ...rest: string[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
| `k6` | `K6` | |
| `rest` | `string[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, unknown>`
### `pluck(...properties: string[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `properties` | `string[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, unknown>`
See Also
--------
* [`map`](map)
| programming_docs |
rxjs skipWhile skipWhile
=========
`function` `stable` `operator`
Returns an Observable that skips all items emitted by the source Observable as long as a specified condition holds true, but emits all further source items as soon as the condition becomes false.
### `skipWhile<T>(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function to test each item emitted from the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that begins emitting items emitted by the source Observable when the specified predicate becomes false.
Description
-----------
Skips all the notifications with a truthy predicate. It will not skip the notifications when the predicate is falsy. It can also be skipped using index. Once the predicate is true, it will not be called again.
Example
-------
Skip some super heroes
```
import { from, skipWhile } from 'rxjs';
const source = from(['Green Arrow', 'SuperMan', 'Flash', 'SuperGirl', 'Black Canary'])
// Skip the heroes until SuperGirl
const example = source.pipe(skipWhile(hero => hero !== 'SuperGirl'));
// output: SuperGirl, Black Canary
example.subscribe(femaleHero => console.log(femaleHero));
```
Skip values from the array until index 5
```
import { from, skipWhile } from 'rxjs';
const source = from([1, 2, 3, 4, 5, 6, 7, 9, 10]);
const example = source.pipe(skipWhile((_, i) => i !== 5));
// output: 6, 7, 9, 10
example.subscribe(value => console.log(value));
```
Overloads
---------
### `skipWhile(predicate: BooleanConstructor): OperatorFunction<T, Extract<T, Falsy> extends never ? never : T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, Extract<T, [Falsy](../index/type-alias/falsy)> extends [never](../index/function/never) ? [never](../index/function/never) : T>`
### `skipWhile(predicate: (value: T, index: number) => true): OperatorFunction<T, never>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => true` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [never](../index/function/never)>`
### `skipWhile(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
See Also
--------
* [`last`](last)
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
rxjs delayWhen delayWhen
=========
`function` `stable` `operator`
Delays the emission of items from the source Observable by a given time span determined by the emissions of another Observable.
### `delayWhen<T>(delayDurationSelector: (value: T, index: number) => Observable<any>, subscriptionDelay?: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `delayDurationSelector` | `(value: T, index: number) => [Observable](../index/class/observable)<any>` | A function that returns an Observable for each value emitted by the source Observable, which is then used to delay the emission of that item on the output Observable until the Observable returned from this function emits a value. |
| `subscriptionDelay` | `[Observable](../index/class/observable)<any>` | Optional. Default is `undefined`. An Observable that triggers the subscription to the source Observable once it emits any value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by an amount of time specified by the Observable returned by `delayDurationSelector`.
Description
-----------
It's like [`delay`](delay), but the time span of the delay duration is determined by a second Observable.
`[delayWhen](../index/function/delaywhen)` time shifts each emitted value from the source Observable by a time span determined by another Observable. When the source emits a value, the `delayDurationSelector` function is called with the source value as argument, and should return an Observable, called the "duration" Observable. The source value is emitted on the output Observable only when the duration Observable emits a value or completes. The completion of the notifier triggering the emission of the source value is deprecated behavior and will be removed in future versions.
Optionally, `[delayWhen](../index/function/delaywhen)` takes a second argument, `subscriptionDelay`, which is an Observable. When `subscriptionDelay` emits its first value or completes, the source Observable is subscribed to and starts behaving like described in the previous paragraph. If `subscriptionDelay` is not provided, `[delayWhen](../index/function/delaywhen)` will subscribe to the source Observable as soon as the output Observable is subscribed.
Example
-------
Delay each click by a random amount of time, between 0 and 5 seconds
```
import { fromEvent, delayWhen, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const delayedClicks = clicks.pipe(
delayWhen(() => interval(Math.random() * 5000))
);
delayedClicks.subscribe(x => console.log(x));
```
See Also
--------
* [`delay`](delay)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
* [`debounce`](debounce)
* [`debounceTime`](debouncetime)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`audit`](audit)
* [`auditTime`](audittime)
rxjs elementAt elementAt
=========
`function` `stable` `operator`
Emits the single value at the specified `index` in a sequence of emissions from the source Observable.
### `elementAt<T, D = T>(index: number, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `index` | `number` | Is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. The default value returned for missing indices. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits a single item, if it is found. Otherwise, it will emit the default value if given. If not, it emits an error.
#### Throws
`[ArgumentOutOfRangeError](../index/interface/argumentoutofrangeerror)` When using `[elementAt](../index/function/elementat)(i)`, it delivers an ArgumentOutOfRangeError to the Observer's `error` callback if `i < 0` or the Observable has completed before emitting the i-th `next` notification.
Description
-----------
Emits only the i-th value, then completes.
`[elementAt](../index/function/elementat)` returns an Observable that emits the item at the specified `index` in the source Observable, or a default value if that `index` is out of range and the `default` argument is provided. If the `default` argument is not given and the `index` is out of range, the output Observable will emit an `[ArgumentOutOfRangeError](../index/interface/argumentoutofrangeerror)` error.
Example
-------
Emit only the third click event
```
import { fromEvent, elementAt } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(elementAt(2));
result.subscribe(x => console.log(x));
// Results in:
// click 1 = nothing
// click 2 = nothing
// click 3 = MouseEvent object logged to console
```
See Also
--------
* [`first`](first)
* [`last`](last)
* [`skip`](skip)
* [`single`](single)
* [`take`](take)
rxjs skipUntil skipUntil
=========
`function` `stable` `operator`
Returns an Observable that skips items emitted by the source Observable until a second Observable emits an item.
### `skipUntil<T>(notifier: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[Observable](../index/class/observable)<any>` | The second Observable that has to emit an item before the source Observable's elements begin to be mirrored by the resulting Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that skips items from the source Observable until the second Observable emits an item, then emits the remaining items.
Description
-----------
The `[skipUntil](../index/function/skipuntil)` operator causes the observable stream to skip the emission of values until the passed in observable emits the first value. This can be particularly useful in combination with user interactions, responses of http requests or waiting for specific times to pass by.
Internally the `[skipUntil](../index/function/skipuntil)` operator subscribes to the passed in observable (in the following called *notifier*) in order to recognize the emission of its first value. When this happens, the operator unsubscribes from the *notifier* and starts emitting the values of the *source* observable. It will never let the *source* observable emit any values if the *notifier* completes or throws an error without emitting a value before.
Example
-------
In the following example, all emitted values of the interval observable are skipped until the user clicks anywhere within the page
```
import { interval, fromEvent, skipUntil } from 'rxjs';
const intervalObservable = interval(1000);
const click = fromEvent(document, 'click');
const emitAfterClick = intervalObservable.pipe(
skipUntil(click)
);
// clicked at 4.6s. output: 5...6...7...8........ or
// clicked at 7.3s. output: 8...9...10..11.......
emitAfterClick.subscribe(value => console.log(value));
```
See Also
--------
* [`last`](last)
* [`skip`](skip)
* [`skipWhile`](skipwhile)
* [`skipLast`](skiplast)
rxjs min min
===
`function` `stable` `operator`
The Min operator operates on an Observable that emits numbers (or items that can be compared with a provided function), and when source Observable completes it emits a single item: the item with the smallest value.
### `min<T>(comparer?: (x: T, y: T) => number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparer` | `(x: T, y: T) => number` | Optional. Default is `undefined`. Optional comparer function that it will use instead of its default to compare the value of two items. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits item with the smallest value.
Description
-----------
Examples
--------
Get the minimal value of a series of numbers
```
import { of, min } from 'rxjs';
of(5, 4, 7, 2, 8)
.pipe(min())
.subscribe(x => console.log(x));
// Outputs
// 2
```
Use a comparer function to get the minimal item
```
import { of, min } from 'rxjs';
of(
{ age: 7, name: 'Foo' },
{ age: 5, name: 'Bar' },
{ age: 9, name: 'Beer' }
).pipe(
min((a, b) => a.age < b.age ? -1 : 1)
)
.subscribe(x => console.log(x.name));
// Outputs
// 'Bar'
```
See Also
--------
* [`max`](max)
rxjs timeInterval timeInterval
============
`function` `stable` `operator`
Emits an object containing the current value, and the time that has passed between emitting the current value and the previous value, which is calculated by using the provided `scheduler`'s `now()` method to retrieve the current time at each emission, then calculating the difference. The `scheduler` defaults to [`asyncScheduler`](../index/const/asyncscheduler), so by default, the `[interval](../index/function/interval)` will be in milliseconds.
### `timeInterval<T>(scheduler: SchedulerLike = asyncScheduler): OperatorFunction<T, TimeInterval<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. Scheduler used to get the current time. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TimeInterval](../index/interface/timeinterval)<T>>`: A function that returns an Observable that emits information about value and interval.
Description
-----------
Convert an Observable that emits items into one that emits indications of the amount of time elapsed between those emissions.
Example
-------
Emit interval between current value with the last value
```
import { interval, timeInterval } from 'rxjs';
const seconds = interval(1000);
seconds
.pipe(timeInterval())
.subscribe(value => console.log(value));
// NOTE: The values will never be this precise,
// intervals created with `interval` or `setInterval`
// are non-deterministic.
// { value: 0, interval: 1000 }
// { value: 1, interval: 1000 }
// { value: 2, interval: 1000 }
```
rxjs onErrorResumeNext onErrorResumeNext
=================
`function` `stable` `operator`
### `onErrorResumeNext<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs switchMap switchMap
=========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable, emitting values only from the most recently projected Observable.
### `switchMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and taking only the values from the most recently projected inner Observable.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables.
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. Each time it observes one of these inner Observables, the output Observable begins emitting the items emitted by that inner Observable. When a new inner Observable is emitted, `[switchMap](../index/function/switchmap)` stops emitting items from the earlier-emitted inner Observable and begins emitting items from the new one. It continues to behave like this for subsequent inner Observables.
Example
-------
Generate new Observable according to source Observable values
```
import { of, switchMap } from 'rxjs';
const switched = of(1, 2, 3).pipe(switchMap(x => of(x, x ** 2, x ** 3)));
switched.subscribe(x => console.log(x));
// outputs
// 1
// 1
// 1
// 2
// 4
// 8
// 3
// 9
// 27
```
Restart an interval Observable on every click event
```
import { fromEvent, switchMap, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(switchMap(() => interval(1000)));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `switchMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `switchMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
### `switchMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../index/type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaustMap`](exhaustmap)
* [`mergeMap`](mergemap)
* [`switchAll`](switchall)
* [`switchMapTo`](switchmapto)
rxjs startWith startWith
=========
`function` `deprecated` `operator`
Deprecation Notes
-----------------
The `scheduler` parameter will be removed in v8. Use `[scheduled](../index/function/scheduled)` and `[concatAll](../index/function/concatall)`. Details: <https://rxjs.dev/deprecations/scheduler-argument>
### `startWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `startWith(value: null): OperatorFunction<T, T | null>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `null` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | null>`
### `startWith(value: undefined): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `undefined` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | undefined>`
### `startWith()`
#### Parameters
There are no parameters.
### `startWith(...values: D[]): OperatorFunction<T, T | D>`
Returns an observable that, at the moment of subscription, will synchronously emit all values provided to this operator, then subscribe to the source and mirror all of its emissions to subscribers.
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `D[]` | Items you want the modified Observable to emit first. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`: A function that returns an Observable that synchronously emits provided values before subscribing to the source Observable.
This is a useful way to know when subscription has occurred on an existing observable.
First emits its arguments in order, and then any emissions from the source.
Examples
--------
Emit a value when a timer starts.
```
import { timer, map, startWith } from 'rxjs';
timer(1000)
.pipe(
map(() => 'timer emit'),
startWith('timer start')
)
.subscribe(x => console.log(x));
// results:
// 'timer start'
// 'timer emit'
```
rxjs materialize materialize
===========
`function` `stable` `operator`
Represents all of the notifications from the source Observable as `next` emissions marked with their original types within [`Notification`](../index/class/notification) objects.
### `materialize<T>(): OperatorFunction<T, Notification<T> & ObservableNotification<T>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Notification](../index/class/notification)<T> & [ObservableNotification](../index/type-alias/observablenotification)<T>>`: A function that returns an Observable that emits [`Notification`](../index/class/notification) objects that wrap the original emissions from the source Observable with metadata.
Description
-----------
Wraps `next`, `error` and `complete` emissions in [`Notification`](../index/class/notification) objects, emitted as `next` on the output Observable.
`[materialize](../index/function/materialize)` returns an Observable that emits a `next` notification for each `next`, `error`, or `complete` emission of the source Observable. When the source Observable emits `complete`, the output Observable will emit `next` as a Notification of type "complete", and then it will emit `complete` as well. When the source Observable emits `error`, the output will emit `next` as a Notification of type "error", and then `complete`.
This operator is useful for producing metadata of the source Observable, to be consumed as `next` emissions. Use it in conjunction with [`dematerialize`](dematerialize).
Example
-------
Convert a faulty Observable to an Observable of Notifications
```
import { of, materialize, map } from 'rxjs';
const letters = of('a', 'b', 13, 'd');
const upperCase = letters.pipe(map((x: any) => x.toUpperCase()));
const materialized = upperCase.pipe(materialize());
materialized.subscribe(x => console.log(x));
// Results in the following:
// - Notification { kind: 'N', value: 'A', error: undefined, hasValue: true }
// - Notification { kind: 'N', value: 'B', error: undefined, hasValue: true }
// - Notification { kind: 'E', value: undefined, error: TypeError { message: x.toUpperCase is not a function }, hasValue: false }
```
See Also
--------
* [`Notification`](../index/class/notification)
* [`dematerialize`](dematerialize)
| programming_docs |
rxjs mergeScan mergeScan
=========
`function` `stable` `operator`
Applies an accumulator function over the source Observable where the accumulator function itself returns an Observable, then each intermediate Observable returned is merged into the output Observable.
### `mergeScan<T, R>(accumulator: (acc: R, value: T, index: number) => ObservableInput<R>, seed: R, concurrent: number = Infinity): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: R, value: T, index: number) => [ObservableInput](../index/type-alias/observableinput)<R>` | The accumulator function called on each source value. |
| `seed` | `R` | The initial accumulation value. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction<T, R>](../index/interface/operatorfunction)`: A function that returns an Observable of the accumulated values.
Description
-----------
It's like [`scan`](scan), but the Observables returned by the accumulator are merged into the outer Observable.
The first parameter of the `[mergeScan](../index/function/mergescan)` is an `accumulator` function which is being called every time the source Observable emits a value. `[mergeScan](../index/function/mergescan)` will subscribe to the value returned by the `accumulator` function and will emit values to the subscriber emitted by inner Observable.
The `accumulator` function is being called with three parameters passed to it: `acc`, `value` and `index`. The `acc` parameter is used as the state parameter whose value is initially set to the `seed` parameter (the second parameter passed to the `[mergeScan](../index/function/mergescan)` operator).
`[mergeScan](../index/function/mergescan)` internally keeps the value of the `acc` parameter: as long as the source Observable emits without inner Observable emitting, the `acc` will be set to `seed`. The next time the inner Observable emits a value, `[mergeScan](../index/function/mergescan)` will internally remember it and it will be passed to the `accumulator` function as `acc` parameter the next time source emits.
The `value` parameter of the `accumulator` function is the value emitted by the source Observable, while the `index` is a number which represent the order of the current emission by the source Observable. It starts with 0.
The last parameter to the `[mergeScan](../index/function/mergescan)` is the `concurrent` value which defaults to Infinity. It represents the maximum number of inner Observable subscriptions at a time.
Example
-------
Count the number of click events
```
import { fromEvent, map, mergeScan, of } from 'rxjs';
const click$ = fromEvent(document, 'click');
const one$ = click$.pipe(map(() => 1));
const seed = 0;
const count$ = one$.pipe(
mergeScan((acc, one) => of(acc + one), seed)
);
count$.subscribe(x => console.log(x));
// Results:
// 1
// 2
// 3
// 4
// ...and so on for each click
```
See Also
--------
* [`scan`](scan)
* [`switchScan`](switchscan)
rxjs takeWhile takeWhile
=========
`function` `stable` `operator`
Emits values emitted by the source Observable so long as each value satisfies the given `predicate`, and then completes as soon as this `predicate` is not satisfied.
### `takeWhile<T>(predicate: (value: T, index: number) => boolean, inclusive: boolean = false): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function that evaluates a value emitted by the source Observable and returns a boolean. Also takes the (zero-based) index as the second argument. |
| `inclusive` | `boolean` | Optional. Default is `false`. When set to `true` the value that caused `predicate` to return `false` will also be emitted. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits values from the source Observable so long as each value satisfies the condition defined by the `predicate`, then completes.
Description
-----------
Takes values from the source only while they pass the condition given. When the first value does not satisfy, it completes.
`[takeWhile](../index/function/takewhile)` subscribes and begins mirroring the source Observable. Each value emitted on the source is given to the `predicate` function which returns a boolean, representing a condition to be satisfied by the source values. The output Observable emits the source values until such time as the `predicate` returns false, at which point `[takeWhile](../index/function/takewhile)` stops mirroring the source Observable and completes the output Observable.
Example
-------
Emit click events only while the clientX property is greater than 200
```
import { fromEvent, takeWhile } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const result = clicks.pipe(takeWhile(ev => ev.clientX > 200));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `takeWhile(predicate: BooleanConstructor, inclusive: true): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `inclusive` | `true` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `takeWhile(predicate: BooleanConstructor, inclusive: false): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `inclusive` | `false` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `takeWhile(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `takeWhile(predicate: (value: T, index: number) => value is S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `takeWhile(predicate: (value: T, index: number) => value is S, inclusive: false): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
| `inclusive` | `false` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `takeWhile(predicate: (value: T, index: number) => boolean, inclusive?: boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
| `inclusive` | `boolean` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
See Also
--------
* [`take`](take)
* [`takeLast`](takelast)
* [`takeUntil`](takeuntil)
* [`skip`](skip)
rxjs takeLast takeLast
========
`function` `stable` `operator`
Waits for the source to complete, then emits the last N values from the source, as specified by the `[count](../index/function/count)` argument.
### `takeLast<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `[count](../index/function/count)` | `number` | The maximum number of values to emit from the end of the sequence of values emitted by the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits at most the last `[count](../index/function/count)` values emitted by the source Observable.
Description
-----------
`[takeLast](../index/function/takelast)` results in an observable that will hold values up to `[count](../index/function/count)` values in memory, until the source completes. It then pushes all values in memory to the consumer, in the order they were received from the source, then notifies the consumer that it is complete.
If for some reason the source completes before the `[count](../index/function/count)` supplied to `[takeLast](../index/function/takelast)` is reached, all values received until that point are emitted, and then completion is notified.
**Warning**: Using `[takeLast](../index/function/takelast)` with an observable that never completes will result in an observable that never emits a value.
Example
-------
Take the last 3 values of an Observable with many values
```
import { range, takeLast } from 'rxjs';
const many = range(1, 100);
const lastThree = many.pipe(takeLast(3));
lastThree.subscribe(x => console.log(x));
```
See Also
--------
* [`take`](take)
* [`takeUntil`](takeuntil)
* [`takeWhile`](takewhile)
* [`skip`](skip)
rxjs bufferCount bufferCount
===========
`function` `stable` `operator`
Buffers the source Observable values until the size hits the maximum `bufferSize` given.
### `bufferCount<T>(bufferSize: number, startBufferEvery: number = null): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The maximum size of the buffer emitted. |
| `startBufferEvery` | `number` | Optional. Default is `null`. Interval at which to start a new buffer. For example if `startBufferEvery` is `2`, then a new buffer will be started on every other value from the source. A new buffer is started at the beginning of the source by default. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array, and emits that array only when its size reaches `bufferSize`.
Buffers a number of values from the source Observable by `bufferSize` then emits the buffer and clears it, and starts a new buffer each `startBufferEvery` values. If `startBufferEvery` is not provided or is `null`, then new buffers are started immediately at the start of the source and when each buffer closes and is emitted.
Examples
--------
Emit the last two click events as an array
```
import { fromEvent, bufferCount } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferCount(2));
buffered.subscribe(x => console.log(x));
```
On every click, emit the last two click events as an array
```
import { fromEvent, bufferCount } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferCount(2, 1));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`pairwise`](pairwise)
* [`windowCount`](windowcount)
rxjs partition partition
=========
`function` `deprecated` `operator`
Splits the source Observable into two, one with values that satisfy a predicate, and another with values that don't satisfy the predicate.
Deprecation Notes
-----------------
Replaced with the `[partition](../index/function/partition)` static creation function. Will be removed in v8.
### `partition<T>(predicate: (value: T, index: number) => boolean, thisArg?: any): UnaryFunction<Observable<T>, [Observable<T>, Observable<T>]>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function that evaluates each value emitted by the source Observable. If it returns `true`, the value is emitted on the first Observable in the returned array, if `false` the value is emitted on the second Observable in the array. The `index` parameter is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[UnaryFunction](../index/interface/unaryfunction)<[Observable](../index/class/observable)<T>, [[Observable](../index/class/observable)<T>, [Observable](../index/class/observable)<T>]>`: A function that returns an array with two Observables: one with values that passed the predicate, and another with values that did not pass the predicate.
Description
-----------
It's like [`filter`](filter), but returns two Observables: one like the output of [`filter`](filter), and the other with values that did not pass the condition.
`[partition](../index/function/partition)` outputs an array with two Observables that partition the values from the source Observable through the given `predicate` function. The first Observable in that array emits source values for which the predicate argument returns true. The second Observable emits source values for which the predicate returns false. The first behaves like [`filter`](filter) and the second behaves like [`filter`](filter) with the predicate negated.
Example
-------
Partition click events into those on DIV elements and those elsewhere
```
import { fromEvent } from 'rxjs';
import { partition } from 'rxjs/operators';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const [clicksOnDivs, clicksElsewhere] = clicks.pipe(partition(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
clicksOnDivs.subscribe(x => console.log('DIV clicked: ', x));
clicksElsewhere.subscribe(x => console.log('Other clicked: ', x));
```
See Also
--------
* [`filter`](filter)
rxjs bufferTime bufferTime
==========
`function` `stable` `operator`
Buffers the source Observable values for a specific time period.
### `bufferTime<T>(bufferTimeSpan: number, ...otherArgs: any[]): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | The amount of time to fill each buffer array. |
| `otherArgs` | `any[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array, and emits those arrays periodically in time.
Buffers values from the source for a specific time duration `bufferTimeSpan`. Unless the optional argument `bufferCreationInterval` is given, it emits and resets the buffer every `bufferTimeSpan` milliseconds. If `bufferCreationInterval` is given, this operator opens the buffer every `bufferCreationInterval` milliseconds and closes (emits and resets) the buffer every `bufferTimeSpan` milliseconds. When the optional argument `maxBufferSize` is specified, the buffer will be closed either after `bufferTimeSpan` milliseconds or when it contains `maxBufferSize` elements.
Examples
--------
Every second, emit an array of the recent click events
```
import { fromEvent, bufferTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferTime(1000));
buffered.subscribe(x => console.log(x));
```
Every 5 seconds, emit the click events from the next 2 seconds
```
import { fromEvent, bufferTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferTime(2000, 5000));
buffered.subscribe(x => console.log(x));
```
Overloads
---------
### `bufferTime(bufferTimeSpan: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`
### `bufferTime(bufferTimeSpan: number, bufferCreationInterval: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `bufferCreationInterval` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`
### `bufferTime(bufferTimeSpan: number, bufferCreationInterval: number, maxBufferSize: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `bufferCreationInterval` | `number` | |
| `maxBufferSize` | `number` | |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`windowTime`](windowtime)
rxjs switchAll switchAll
=========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable producing values only from the most recent observable sequence
### `switchAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<O, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that converts a higher-order Observable into a first-order Observable producing values only from the most recent Observable sequence.
Description
-----------
Flattens an Observable-of-Observables.
`[switchAll](../index/function/switchall)` subscribes to a source that is an observable of observables, also known as a "higher-order observable" (or `[Observable](../index/class/observable)<[Observable](../index/class/observable)<T>>`). It subscribes to the most recently provided "inner observable" emitted by the source, unsubscribing from any previously subscribed to inner observable, such that only the most recent inner observable may be subscribed to at any point in time. The resulting observable returned by `[switchAll](../index/function/switchall)` will only complete if the source observable completes, *and* any currently subscribed to inner observable also has completed, if there are any.
Examples
--------
Spawn a new interval observable for each click event, but for every new click, cancel the previous interval and subscribe to the new one
```
import { fromEvent, tap, map, interval, switchAll } from 'rxjs';
const clicks = fromEvent(document, 'click').pipe(tap(() => console.log('click')));
const source = clicks.pipe(map(() => interval(1000)));
source
.pipe(switchAll())
.subscribe(x => console.log(x));
// Output
// click
// 0
// 1
// 2
// 3
// ...
// click
// 0
// 1
// 2
// ...
// click
// ...
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`exhaustAll`](exhaustall)
* [`switchMap`](switchmap)
* [`switchMapTo`](switchmapto)
* [`mergeAll`](mergeall)
rxjs bufferWhen bufferWhen
==========
`function` `stable` `operator`
Buffers the source Observable values, using a factory function of closing Observables to determine when to close, emit, and reset the buffer.
### `bufferWhen<T>(closingSelector: () => ObservableInput<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingSelector` | `() => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that takes no arguments and returns an Observable that signals buffer closure. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array. When it starts collecting values, it calls a function that returns an Observable that tells when to close the buffer and restart collecting.
Opens a buffer immediately, then closes the buffer when the observable returned by calling `closingSelector` function emits a value. When it closes the buffer, it immediately opens a new buffer and repeats the process.
Example
-------
Emit an array of the last clicks every [1-5] random seconds
```
import { fromEvent, bufferWhen, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(
bufferWhen(() => interval(1000 + Math.random() * 4000))
);
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`windowWhen`](windowwhen)
| programming_docs |
rxjs retryWhen retryWhen
=========
`function` `deprecated` `operator`
Returns an Observable that mirrors the source Observable with the exception of an `error`. If the source Observable calls `error`, this method will emit the Throwable that caused the error to the Observable returned from `notifier`. If that Observable calls `complete` or `error` then this method will call `complete` or `error` on the child subscription. Otherwise this method will resubscribe to the source Observable.
Deprecation Notes
-----------------
Will be removed in v9 or v10, use [`retry`](retry)'s `[delay](../index/function/delay)` option instead.
### `retryWhen<T>(notifier: (errors: Observable<any>) => Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `(errors: [Observable](../index/class/observable)<any>) => [Observable](../index/class/observable)<any>` | Receives an Observable of notifications with which a user can `complete` or `error`, aborting the retry. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source Observable with the exception of an `error`.
Description
-----------
Retry an observable sequence on error based on custom criteria.
Example
-------
```
import { interval, map, retryWhen, tap, delayWhen, timer } from 'rxjs';
const source = interval(1000);
const result = source.pipe(
map(value => {
if (value > 5) {
// error will be picked up by retryWhen
throw value;
}
return value;
}),
retryWhen(errors =>
errors.pipe(
// log error message
tap(value => console.log(`Value ${ value } was too high!`)),
// restart in 5 seconds
delayWhen(value => timer(value * 1000))
)
)
);
result.subscribe(value => console.log(value));
// results:
// 0
// 1
// 2
// 3
// 4
// 5
// 'Value 6 was too high!'
// - Wait 5 seconds then repeat
```
See Also
--------
* [`retry`](retry)
rxjs buffer buffer
======
`function` `stable` `operator`
Buffers the source Observable values until `closingNotifier` emits.
### `buffer<T>(closingNotifier: Observable<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingNotifier` | `[Observable](../index/class/observable)<any>` | An Observable that signals the buffer to be emitted on the output Observable. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T[]>`: A function that returns an Observable of buffers, which are arrays of values.
Description
-----------
Collects values from the past as an array, and emits that array only when another Observable emits.
Buffers the incoming Observable values until the given `closingNotifier` Observable emits a value, at which point it emits the buffer on the output Observable and starts a new buffer internally, awaiting the next time `closingNotifier` emits.
Example
-------
On every click, emit array of most recent interval events
```
import { fromEvent, interval, buffer } from 'rxjs';
const clicks = fromEvent(document, 'click');
const intervalEvents = interval(1000);
const buffered = intervalEvents.pipe(buffer(clicks));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`window`](window)
rxjs endWith endWith
=======
`function` `stable` `operator`
Returns an observable that will emit all values from the source, then synchronously emit the provided value(s) immediately after the source completes.
### `endWith<T>(...values: (SchedulerLike | T)[]): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `([SchedulerLike](../index/interface/schedulerlike) | T)[]` | Items you want the modified Observable to emit last. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits all values from the source, then synchronously emits the provided value(s) immediately after the source completes.
Description
-----------
NOTE: Passing a last argument of a Scheduler is *deprecated*, and may result in incorrect types in TypeScript.
This is useful for knowing when an observable ends. Particularly when paired with an operator like [`takeUntil`](takeuntil)
Example
-------
Emit values to know when an interval starts and stops. The interval will stop when a user clicks anywhere on the document.
```
import { interval, map, fromEvent, startWith, takeUntil, endWith } from 'rxjs';
const ticker$ = interval(5000).pipe(
map(() => 'tick')
);
const documentClicks$ = fromEvent(document, 'click');
ticker$.pipe(
startWith('interval started'),
takeUntil(documentClicks$),
endWith('interval ended by click')
)
.subscribe(x => console.log(x));
// Result (assuming a user clicks after 15 seconds)
// 'interval started'
// 'tick'
// 'tick'
// 'tick'
// 'interval ended by click'
```
Overloads
---------
### `endWith(scheduler: SchedulerLike): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `endWith(...valuesAndScheduler: [A, SchedulerLike]): OperatorFunction<T, T | ValueFromArray<A>>`
#### Parameters
| | | |
| --- | --- | --- |
| `valuesAndScheduler` | `[A, [SchedulerLike](../index/interface/schedulerlike)]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | [ValueFromArray](../index/type-alias/valuefromarray)<A>>`
### `endWith(...values: A): OperatorFunction<T, T | ValueFromArray<A>>`
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | [ValueFromArray](../index/type-alias/valuefromarray)<A>>`
See Also
--------
* [`startWith`](startwith)
* [`concat`](../index/function/concat)
* [`takeUntil`](takeuntil)
rxjs every every
=====
`function` `stable` `operator`
Returns an Observable that emits whether or not every item of the source satisfies the condition specified.
### `every<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | A function for determining if an item meets a specified condition. |
| `thisArg` | `any` | Optional. Default is `undefined`. Optional object to use for `this` in the callback. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, boolean>`: A function that returns an Observable of booleans that determines if all items of the source Observable meet the condition specified.
Description
-----------
If all values pass predicate before the source completes, emits true before completion, otherwise emit false, then complete.
Example
-------
A simple example emitting true if all elements are less than 5, false otherwise
```
import { of, every } from 'rxjs';
of(1, 2, 3, 4, 5, 6)
.pipe(every(x => x < 5))
.subscribe(x => console.log(x)); // -> false
```
Overloads
---------
### `every(predicate: BooleanConstructor): OperatorFunction<T, Exclude<T, Falsy> extends never ? false : boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, Exclude<T, [Falsy](../index/type-alias/falsy)> extends [never](../index/function/never) ? false : boolean>`
### `every(predicate: BooleanConstructor, thisArg: any): OperatorFunction<T, Exclude<T, Falsy> extends never ? false : boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `thisArg` | `any` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, Exclude<T, [Falsy](../index/type-alias/falsy)> extends [never](../index/function/never) ? false : boolean>`
### `every(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, boolean>`
### `every(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, boolean>`
rxjs windowToggle windowToggle
============
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable starting from an emission from `openings` and ending when the output of `closingSelector` emits.
### `windowToggle<T, O>(openings: ObservableInput<O>, closingSelector: (openValue: O) => ObservableInput<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `openings` | `[ObservableInput](../index/type-alias/observableinput)<O>` | An observable of notifications to start new windows. |
| `closingSelector` | `(openValue: O) => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that takes the value emitted by the `openings` observable and returns an Observable, which, when it emits a next notification, signals that the associated window should complete. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferToggle`](buffertoggle), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits windows that contain those items emitted by the source Observable between the time when the `openings` Observable emits an item and when the Observable returned by `closingSelector` emits an item.
Example
-------
Every other second, emit the click events from the next 500ms
```
import { fromEvent, interval, windowToggle, EMPTY, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const openings = interval(1000);
const result = clicks.pipe(
windowToggle(openings, i => i % 2 ? interval(500) : EMPTY),
mergeAll()
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowWhen`](windowwhen)
* [`bufferToggle`](buffertoggle)
rxjs throwIfEmpty throwIfEmpty
============
`function` `stable` `operator`
If the source observable completes without emitting a value, it will emit an error. The error will be created at that time by the optional `errorFactory` argument, otherwise, the error will be [`EmptyError`](../index/interface/emptyerror).
### `throwIfEmpty<T>(errorFactory: () => any = defaultErrorFactory): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `errorFactory` | `() => any` | Optional. Default is `defaultErrorFactory`. A factory function called to produce the error to be thrown when the source observable completes without emitting a value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that throws an error if the source Observable completed without emitting.
Description
-----------
Example
-------
Throw an error if the document wasn't clicked within 1 second
```
import { fromEvent, takeUntil, timer, throwIfEmpty } from 'rxjs';
const click$ = fromEvent(document, 'click');
click$.pipe(
takeUntil(timer(1000)),
throwIfEmpty(() => new Error('The document was not clicked within 1 second'))
)
.subscribe({
next() {
console.log('The document was clicked');
},
error(err) {
console.error(err.message);
}
});
```
rxjs concat concat
======
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Replaced with [`concatWith`](concatwith). Will be removed in v8.
### `concat<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs groupBy groupBy
=======
`function` `stable` `operator`
### `groupBy<T, K, R>(keySelector: (value: T) => K, elementOrOptions?: void | ((value: any) => any) | BasicGroupByOptions<K, T> | GroupByOptionsWithElement<K, R, T>, duration?: (grouped: GroupedObservable<any, any>) => ObservableInput<any>, connector?: () => SubjectLike<any>): OperatorFunction<T, GroupedObservable<K, R>>`
#### Parameters
| | | |
| --- | --- | --- |
| `keySelector` | `(value: T) => K` | |
| `elementOrOptions` | `void | ((value: any) => any) | BasicGroupByOptions<K, T> | GroupByOptionsWithElement<K, R, T>` | Optional. Default is `undefined`. |
| `duration` | `(grouped: GroupedObservable<any, any>) => [ObservableInput](../index/type-alias/observableinput)<any>` | Optional. Default is `undefined`. |
| `connector` | `() => [SubjectLike](../index/interface/subjectlike)<any>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, R>>`
Overloads
---------
### `groupBy(key: (value: T) => K, options: BasicGroupByOptions<K, T>): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `options` | `BasicGroupByOptions<K, T>` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, options: GroupByOptionsWithElement<K, E, T>): OperatorFunction<T, GroupedObservable<K, E>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `options` | `GroupByOptionsWithElement<K, E, T>` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, E>>`
### `groupBy(key: (value: T) => value is K): OperatorFunction<T, GroupedObservable<true, K> | GroupedObservable<false, Exclude<T, K>>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => value is K` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<true, K> | GroupedObservable<false, Exclude<T, K>>>`
### `groupBy(key: (value: T) => K): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, element: void, duration: (grouped: GroupedObservable<K, T>) => Observable<any>): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `element` | `void` | |
| `duration` | `(grouped: GroupedObservable<K, T>) => [Observable](../index/class/observable)<any>` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, element?: (value: T) => R, duration?: (grouped: GroupedObservable<K, R>) => Observable<any>): OperatorFunction<T, GroupedObservable<K, R>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `element` | `(value: T) => R` | Optional. Default is `undefined`. |
| `duration` | `(grouped: GroupedObservable<K, R>) => [Observable](../index/class/observable)<any>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, R>>`
### `groupBy(key: (value: T) => K, element?: (value: T) => R, duration?: (grouped: GroupedObservable<K, R>) => Observable<any>, connector?: () => Subject<R>): OperatorFunction<T, GroupedObservable<K, R>>`
Groups the items emitted by an Observable according to a specified criterion, and emits these grouped items as `GroupedObservables`, one [GroupedObservable](groupedobservable) per group.
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | A function that extracts the key for each item. |
| `element` | `(value: T) => R` | Optional. Default is `undefined`. A function that extracts the return element for each item. |
| `duration` | `(grouped: GroupedObservable<K, R>) => [Observable](../index/class/observable)<any>` | Optional. Default is `undefined`. A function that returns an Observable to determine how long each group should exist. |
| `connector` | `() => [Subject](../index/class/subject)<R>` | Optional. Default is `undefined`. Factory function to create an intermediate Subject through which grouped elements are emitted. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, GroupedObservable<K, R>>`: A function that returns an Observable that emits GroupedObservables, each of which corresponds to a unique key value and each of which emits those items from the source Observable that share that key value.
When the Observable emits an item, a key is computed for this item with the key function.
If a [GroupedObservable](groupedobservable) for this key exists, this [GroupedObservable](groupedobservable) emits. Otherwise, a new [GroupedObservable](groupedobservable) for this key is created and emits.
A [GroupedObservable](groupedobservable) represents values belonging to the same group represented by a common key. The common key is available as the `key` field of a [GroupedObservable](groupedobservable) instance.
The elements emitted by [GroupedObservable](groupedobservable)s are by default the items emitted by the Observable, or elements returned by the element function.
Examples
--------
Group objects by `id` and return as array
```
import { of, groupBy, mergeMap, reduce } from 'rxjs';
of(
{ id: 1, name: 'JavaScript' },
{ id: 2, name: 'Parcel' },
{ id: 2, name: 'webpack' },
{ id: 1, name: 'TypeScript' },
{ id: 3, name: 'TSLint' }
).pipe(
groupBy(p => p.id),
mergeMap(group$ => group$.pipe(reduce((acc, cur) => [...acc, cur], [])))
)
.subscribe(p => console.log(p));
// displays:
// [{ id: 1, name: 'JavaScript' }, { id: 1, name: 'TypeScript'}]
// [{ id: 2, name: 'Parcel' }, { id: 2, name: 'webpack'}]
// [{ id: 3, name: 'TSLint' }]
```
Pivot data on the `id` field
```
import { of, groupBy, mergeMap, reduce, map } from 'rxjs';
of(
{ id: 1, name: 'JavaScript' },
{ id: 2, name: 'Parcel' },
{ id: 2, name: 'webpack' },
{ id: 1, name: 'TypeScript' },
{ id: 3, name: 'TSLint' }
).pipe(
groupBy(p => p.id, { element: p => p.name }),
mergeMap(group$ => group$.pipe(reduce((acc, cur) => [...acc, cur], [`${ group$.key }`]))),
map(arr => ({ id: parseInt(arr[0], 10), values: arr.slice(1) }))
)
.subscribe(p => console.log(p));
// displays:
// { id: 1, values: [ 'JavaScript', 'TypeScript' ] }
// { id: 2, values: [ 'Parcel', 'webpack' ] }
// { id: 3, values: [ 'TSLint' ] }
```
rxjs audit audit
=====
`function` `stable` `operator`
Ignores source values for a duration determined by another Observable, then emits the most recent value from the source Observable, then repeats this process.
### `audit<T>(durationSelector: (value: T) => ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../index/type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the silencing duration, returned as an Observable or a Promise. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that performs rate-limiting of emissions from the source Observable.
Description
-----------
It's like [`auditTime`](audittime), but the silencing duration is determined by a second Observable.
`[audit](../index/function/audit)` is similar to `[throttle](../index/function/throttle)`, but emits the last value from the silenced time window, instead of the first value. `[audit](../index/function/audit)` emits the most recent value from the source Observable on the output Observable as soon as its internal timer becomes disabled, and ignores source values while the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, the timer is enabled by calling the `durationSelector` function with the source value, which returns the "duration" Observable. When the duration Observable emits a value, the timer is disabled, then the most recent source value is emitted on the output Observable, and this process repeats for the next source value.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, audit, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(audit(ev => interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounce`](debounce)
* [`delayWhen`](delaywhen)
* [`sample`](sample)
* [`throttle`](throttle)
| programming_docs |
rxjs catchError catchError
==========
`function` `stable` `operator`
Catches errors on the observable to be handled by returning a new observable or throwing an error.
### `catchError<T, O extends ObservableInput<any>>(selector: (err: any, caught: Observable<T>) => O): OperatorFunction<T, T | ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `(err: any, caught: [Observable](../index/class/observable)<T>) => O` | a function that takes as arguments `err`, which is the error, and `caught`, which is the source observable, in case you'd like to "retry" that observable by returning it again. Whatever observable is returned by the `selector` will be used to continue the observable chain. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`: A function that returns an Observable that originates from either the source or the Observable returned by the `selector` function.
Description
-----------
It only listens to the error channel and ignores notifications. Handles errors from the source observable, and maps them to a new observable. The error may also be rethrown, or a new error can be thrown to emit an error from the result. This operator handles errors, but forwards along all other events to the resulting observable. If the source observable terminates with an error, it will map that error to a new observable, subscribe to it, and forward all of its events to the resulting observable.
Examples
--------
Continue with a different Observable when there's an error
```
import { of, map, catchError } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError(err => of('I', 'II', 'III', 'IV', 'V'))
)
.subscribe(x => console.log(x));
// 1, 2, 3, I, II, III, IV, V
```
Retry the caught source Observable again in case of error, similar to `[retry](../index/function/retry)()` operator
```
import { of, map, catchError, take } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError((err, caught) => caught),
take(30)
)
.subscribe(x => console.log(x));
// 1, 2, 3, 1, 2, 3, ...
```
Throw a new error when the source Observable throws an error
```
import { of, map, catchError } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError(err => {
throw 'error in source. Details: ' + err;
})
)
.subscribe({
next: x => console.log(x),
error: err => console.log(err)
});
// 1, 2, 3, error in source. Details: four!
```
See Also
--------
* [`onErrorResumeNext`](onerrorresumenext)
* [`repeat`](repeat)
* [`repeatWhen`](repeatwhen)
* [`retry`](retry)
* [`retryWhen`](retrywhen)
rxjs connect connect
=======
`function` `stable` `operator`
Creates an observable by multicasting the source within a function that allows the developer to define the usage of the multicast prior to connection.
### `connect<T, O extends ObservableInput<unknown>>(selector: (shared: Observable<T>) => O, config: ConnectConfig<T> = DEFAULT_CONFIG): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `(shared: [Observable](../index/class/observable)<T>) => O` | A function used to set up the multicast. Gives you a multicast observable that is not yet connected. With that, you're expected to create and return and Observable, that when subscribed to, will utilize the multicast observable. After this function is executed -- and its return value subscribed to -- the operator will subscribe to the source, and the connection will be made. |
| `[config](../index/const/config)` | `ConnectConfig<T>` | Optional. Default is `DEFAULT_CONFIG`. The configuration object for `[connect](../index/function/connect)`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [ObservedValueOf](../index/type-alias/observedvalueof)<O>>`
Description
-----------
This is particularly useful if the observable source you wish to multicast could be synchronous or asynchronous. This sets it apart from [`share`](share), which, in the case of totally synchronous sources will fail to share a single subscription with multiple consumers, as by the time the subscription to the result of [`share`](share) has returned, if the source is synchronous its internal reference count will jump from 0 to 1 back to 0 and reset.
To use `[connect](../index/function/connect)`, you provide a `selector` function that will give you a multicast observable that is not yet connected. You then use that multicast observable to create a resulting observable that, when subscribed, will set up your multicast. This is generally, but not always, accomplished with [`merge`](../index/function/merge).
Note that using a [`takeUntil`](takeuntil) inside of `[connect](../index/function/connect)`'s `selector` *might* mean you were looking to use the [`takeWhile`](takewhile) operator instead.
When you subscribe to the result of `[connect](../index/function/connect)`, the `selector` function will be called. After the `selector` function returns, the observable it returns will be subscribed to, *then* the multicast will be connected to the source.
Example
-------
Sharing a totally synchronous observable
```
import { of, tap, connect, merge, map, filter } from 'rxjs';
const source$ = of(1, 2, 3, 4, 5).pipe(
tap({
subscribe: () => console.log('subscription started'),
next: n => console.log(`source emitted ${ n }`)
})
);
source$.pipe(
// Notice in here we're merging 3 subscriptions to `shared$`.
connect(shared$ => merge(
shared$.pipe(map(n => `all ${ n }`)),
shared$.pipe(filter(n => n % 2 === 0), map(n => `even ${ n }`)),
shared$.pipe(filter(n => n % 2 === 1), map(n => `odd ${ n }`))
))
)
.subscribe(console.log);
// Expected output: (notice only one subscription)
'subscription started'
'source emitted 1'
'all 1'
'odd 1'
'source emitted 2'
'all 2'
'even 2'
'source emitted 3'
'all 3'
'odd 3'
'source emitted 4'
'all 4'
'even 4'
'source emitted 5'
'all 5'
'odd 5'
```
rxjs share share
=====
`function` `stable` `operator`
Returns a new Observable that multicasts (shares) the original Observable. As long as there is at least one Subscriber this Observable will be subscribed and emitting data. When all subscribers have unsubscribed it will unsubscribe from the source Observable. Because the Observable is multicasting it makes the stream `hot`. This is an alias for `[multicast](../index/function/multicast)(() => new [Subject](../index/class/subject)()), [refCount](../index/function/refcount)()`.
### `share<T>(options: ShareConfig<T> = {}): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `options` | `ShareConfig<T>` | Optional. Default is `{}`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source.
Description
-----------
The subscription to the underlying source Observable can be reset (unsubscribe and resubscribe for new subscribers), if the subscriber count to the shared observable drops to 0, or if the source Observable errors or completes. It is possible to use notifier factories for the resets to allow for behaviors like conditional or delayed resets. Please note that resetting on error or complete of the source Observable does not behave like a transparent retry or restart of the source because the error or complete will be forwarded to all subscribers and their subscription will be closed. Only new subscribers after a reset on error or complete happened will cause a fresh subscription to the source. To achieve transparent retries or restarts pipe the source through appropriate operators before sharing.
Example
-------
Generate new multicast Observable from the `source` Observable value
```
import { interval, tap, map, take, share } from 'rxjs';
const source = interval(1000).pipe(
tap(x => console.log('Processing: ', x)),
map(x => x * x),
take(6),
share()
);
source.subscribe(x => console.log('subscription 1: ', x));
source.subscribe(x => console.log('subscription 2: ', x));
// Logs:
// Processing: 0
// subscription 1: 0
// subscription 2: 0
// Processing: 1
// subscription 1: 1
// subscription 2: 1
// Processing: 2
// subscription 1: 4
// subscription 2: 4
// Processing: 3
// subscription 1: 9
// subscription 2: 9
// Processing: 4
// subscription 1: 16
// subscription 2: 16
// Processing: 5
// subscription 1: 25
// subscription 2: 25
```
Example with notifier factory: Delayed reset
--------------------------------------------
```
import { interval, take, share, timer } from 'rxjs';
const source = interval(1000).pipe(
take(3),
share({
resetOnRefCountZero: () => timer(1000)
})
);
const subscriptionOne = source.subscribe(x => console.log('subscription 1: ', x));
setTimeout(() => subscriptionOne.unsubscribe(), 1300);
setTimeout(() => source.subscribe(x => console.log('subscription 2: ', x)), 1700);
setTimeout(() => source.subscribe(x => console.log('subscription 3: ', x)), 5000);
// Logs:
// subscription 1: 0
// (subscription 1 unsubscribes here)
// (subscription 2 subscribes here ~400ms later, source was not reset)
// subscription 2: 1
// subscription 2: 2
// (subscription 2 unsubscribes here)
// (subscription 3 subscribes here ~2000ms later, source did reset before)
// subscription 3: 0
// subscription 3: 1
// subscription 3: 2
```
See Also
--------
* [`shareReplay`](sharereplay)
rxjs combineLatestAll combineLatestAll
================
`function` `stable` `operator`
Flattens an Observable-of-Observables by applying [`combineLatest`](../index/function/combinelatest) when the Observable-of-Observables completes.
### `combineLatestAll<R>(project?: (...values: any[]) => R)`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | Optional. Default is `undefined`. optional function to map the most recent values from each inner Observable into a new result. Takes each of the most recent values from each collected inner Observable as arguments, in order. |
Description
-----------
`[combineLatestAll](../index/function/combinelatestall)` takes an Observable of Observables, and collects all Observables from it. Once the outer Observable completes, it subscribes to all collected Observables and combines their values using the [`combineLatest`](../index/function/combinelatest) strategy, such that:
* Every time an inner Observable emits, the output Observable emits
* When the returned observable emits, it emits all of the latest values by:
+ If a `project` function is provided, it is called with each recent value from each inner Observable in whatever order they arrived, and the result of the `project` function is what is emitted by the output Observable.
+ If there is no `project` function, an array of all the most recent values is emitted by the output Observable.
Example
-------
Map two click events to a finite interval Observable, then apply `[combineLatestAll](../index/function/combinelatestall)`
```
import { fromEvent, map, interval, take, combineLatestAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(Math.random() * 2000).pipe(take(3))),
take(2)
);
const result = higherOrder.pipe(combineLatestAll());
result.subscribe(x => console.log(x));
```
Overloads
---------
### `combineLatestAll(): OperatorFunction<ObservableInput<T>, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<[ObservableInput](../index/type-alias/observableinput)<T>, T[]>`
### `combineLatestAll(): OperatorFunction<any, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<any, T[]>`
### `combineLatestAll(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<[ObservableInput](../index/type-alias/observableinput)<T>, R>`
### `combineLatestAll(project: (...values: any[]) => R): OperatorFunction<any, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<any, R>`
See Also
--------
* [`combineLatest`](../index/function/combinelatest)
* [`combineLatestWith`](combinelatestwith)
* [`mergeAll`](mergeall)
rxjs throttleTime throttleTime
============
`function` `stable` `operator`
Emits a value from the source Observable, then ignores subsequent source values for `duration` milliseconds, then repeats this process.
### `throttleTime<T>(duration: number, scheduler: SchedulerLike = asyncScheduler, config: ThrottleConfig = defaultThrottleConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `duration` | `number` | Time to wait before emitting another value after emitting the last value, measured in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for managing the timers that handle the throttling. Defaults to [`asyncScheduler`](../index/const/asyncscheduler). |
| `[config](../index/const/config)` | `ThrottleConfig` | Optional. Default is `defaultThrottleConfig`. a configuration object to define `leading` and `trailing` behavior. Defaults to `{ leading: true, trailing: false }`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that performs the throttle operation to limit the rate of emissions from the source.
Description
-----------
Lets a value pass, then ignores source values for the next `duration` milliseconds.
`[throttleTime](../index/function/throttletime)` emits the source Observable values on the output Observable when its internal timer is disabled, and ignores source values when the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, it is forwarded to the output Observable, and then the timer is enabled. After `duration` milliseconds (or the time unit determined internally by the optional `scheduler`) has passed, the timer is disabled, and this process repeats for the next source value. Optionally takes a [`SchedulerLike`](../index/interface/schedulerlike) for managing timers.
Examples
--------
#### Limit click rate
Emit clicks at a rate of at most one click per second
```
import { fromEvent, throttleTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(throttleTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
rxjs skip skip
====
`function` `stable` `operator`
Returns an Observable that skips the first `[count](../index/function/count)` items emitted by the source Observable.
### `skip<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `[count](../index/function/count)` | `number` | The number of times, items emitted by source Observable should be skipped. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that skips the first `[count](../index/function/count)` values emitted by the source Observable.
Description
-----------
Skips the values until the sent notifications are equal or less than provided skip count. It raises an error if skip count is equal or more than the actual number of emits and source raises an error.
Example
-------
Skip the values before the emission
```
import { interval, skip } from 'rxjs';
// emit every half second
const source = interval(500);
// skip the first 10 emitted values
const result = source.pipe(skip(10));
result.subscribe(value => console.log(value));
// output: 10...11...12...13...
```
See Also
--------
* [`last`](last)
* [`skipWhile`](skipwhile)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
rxjs dematerialize dematerialize
=============
`function` `stable` `operator`
Converts an Observable of [`ObservableNotification`](../index/type-alias/observablenotification) objects into the emissions that they represent.
### `dematerialize<N extends ObservableNotification<any>>(): OperatorFunction<N, ValueFromNotification<N>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<N, [ValueFromNotification](../index/type-alias/valuefromnotification)<N>>`: A function that returns an Observable that emits items and notifications embedded in Notification objects emitted by the source Observable.
Description
-----------
Unwraps [`ObservableNotification`](../index/type-alias/observablenotification) objects as actual `next`, `error` and `complete` emissions. The opposite of [`materialize`](materialize).
`[dematerialize](../index/function/dematerialize)` is assumed to operate an Observable that only emits [`ObservableNotification`](../index/type-alias/observablenotification) objects as `next` emissions, and does not emit any `error`. Such Observable is the output of a `[materialize](../index/function/materialize)` operation. Those notifications are then unwrapped using the metadata they contain, and emitted as `next`, `error`, and `complete` on the output Observable.
Use this operator in conjunction with [`materialize`](materialize).
Example
-------
Convert an Observable of Notifications to an actual Observable
```
import { NextNotification, ErrorNotification, of, dematerialize } from 'rxjs';
const notifA: NextNotification<string> = { kind: 'N', value: 'A' };
const notifB: NextNotification<string> = { kind: 'N', value: 'B' };
const notifE: ErrorNotification = { kind: 'E', error: new TypeError('x.toUpperCase is not a function') };
const materialized = of(notifA, notifB, notifE);
const upperCase = materialized.pipe(dematerialize());
upperCase.subscribe({
next: x => console.log(x),
error: e => console.error(e)
});
// Results in:
// A
// B
// TypeError: x.toUpperCase is not a function
```
See Also
--------
* [`materialize`](materialize)
rxjs filter filter
======
`function` `stable` `operator`
Filter items emitted by the source Observable by only emitting those that satisfy a specified predicate.
### `filter<T>(predicate: (value: T, index: number) => boolean, thisArg?: any): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function that evaluates each value emitted by the source Observable. If it returns `true`, the value is emitted, if `false` the value is not passed to the output Observable. The `index` parameter is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable that satisfy the specified `predicate`.
Description
-----------
Like [Array.prototype.filter()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter), it only emits a value from the source if it passes a criterion function.
Similar to the well-known `Array.prototype.filter` method, this operator takes values from the source Observable, passes them through a `predicate` function and only emits those values that yielded `true`.
Example
-------
Emit only click events whose target was a DIV element
```
import { fromEvent, filter } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const clicksOnDivs = clicks.pipe(filter(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
clicksOnDivs.subscribe(x => console.log(x));
```
Overloads
---------
### `filter(predicate: (this: A, value: T, index: number) => value is S, thisArg: A): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number) => value is S` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `filter(predicate: (value: T, index: number) => value is S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `filter(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `filter(predicate: (this: A, value: T, index: number) => boolean, thisArg: A): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
### `filter(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilChanged`](distinctuntilchanged)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
* [`ignoreElements`](ignoreelements)
* [`partition`](../index/function/partition)
* [`skip`](skip)
| programming_docs |
rxjs withLatestFrom withLatestFrom
==============
`function` `stable` `operator`
Combines the source Observable with other Observables to create an Observable whose values are calculated from the latest values of each, only when the source emits.
### `withLatestFrom<T, R>(...inputs: any[]): OperatorFunction<T, R | any[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `inputs` | `any[]` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, R | any[]>`: A function that returns an Observable of projected values from the most recent values from each input Observable, or an array of the most recent values from each input Observable.
Description
-----------
Whenever the source Observable emits a value, it computes a formula using that value plus the latest values from other input Observables, then emits the output of that formula.
`[withLatestFrom](../index/function/withlatestfrom)` combines each value from the source Observable (the instance) with the latest values from the other input Observables only when the source emits a value, optionally using a `project` function to determine the value to be emitted on the output Observable. All input Observables must emit at least one value before the output Observable will emit a value.
Example
-------
On every click event, emit an array with the latest timer event plus the click event
```
import { fromEvent, interval, withLatestFrom } from 'rxjs';
const clicks = fromEvent(document, 'click');
const timer = interval(1000);
const result = clicks.pipe(withLatestFrom(timer));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatest`](../index/function/combinelatest)
rxjs first first
=====
`function` `stable` `operator`
Emits only the first value (or the first value that meets some condition) emitted by the source Observable.
### `first<T, D>(predicate?: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | Optional. Default is `undefined`. An optional function called with each item to test for condition matching. |
| `defaultValue` | `D` | Optional. Default is `undefined`. The default value emitted in case no valid value was found on the source. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits the first item that matches the condition.
#### Throws
`[EmptyError](../index/interface/emptyerror)` Delivers an EmptyError to the Observer's `error` callback if the Observable completes before any `next` notification was sent. This is how `[first](../index/function/first)()` is different from [`take`](take)(1) which completes instead.
Description
-----------
Emits only the first value. Or emits only the first value that passes some test.
If called with no arguments, `[first](../index/function/first)` emits the first value of the source Observable, then completes. If called with a `predicate` function, `[first](../index/function/first)` emits the first value of the source that matches the specified condition. Throws an error if `defaultValue` was not provided and a matching element is not found.
Examples
--------
Emit only the first click that happens on the DOM
```
import { fromEvent, first } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(first());
result.subscribe(x => console.log(x));
```
Emits the first click that happens on a DIV
```
import { fromEvent, first } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(first(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `first(predicate?: null, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `null` | Optional. Default is `undefined`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`
### `first(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T>>`
### `first(predicate: BooleanConstructor, defaultValue: D): OperatorFunction<T, TruthyTypesOf<T> | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [TruthyTypesOf](../index/type-alias/truthytypesof)<T> | D>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue?: S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => value is S` | |
| `defaultValue` | `S` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue: D): OperatorFunction<T, S | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => value is S` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, S | D>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../index/class/observable)<T>) => boolean` | |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, T | D>`
See Also
--------
* [`filter`](filter)
* [`find`](find)
* [`take`](take)
rxjs windowCount windowCount
===========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable with each nested Observable emitting at most `windowSize` values.
### `windowCount<T>(windowSize: number, startWindowEvery: number = 0): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowSize` | `number` | The maximum number of values emitted by each window. |
| `startWindowEvery` | `number` | Optional. Default is `0`. Interval at which to start a new window. For example if `startWindowEvery` is `2`, then a new window will be started on every other value from the source. A new window is started at the beginning of the source by default. |
#### Returns
`[OperatorFunction](../index/interface/operatorfunction)<T, [Observable](../index/class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observable of values.
Description
-----------
It's like [`bufferCount`](buffercount), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits windows every `startWindowEvery` items, each containing no more than `windowSize` items. When the source Observable completes or encounters an error, the output Observable emits the current window and propagates the notification from the source Observable. If `startWindowEvery` is not provided, then new windows are started immediately at the start of the source and when each window completes with size `windowSize`.
Examples
--------
Ignore every 3rd click event, starting from the first one
```
import { fromEvent, windowCount, map, skip, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowCount(3),
map(win => win.pipe(skip(1))), // skip first of every 3 clicks
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Ignore every 3rd click event, starting from the third one
```
import { fromEvent, windowCount, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowCount(2, 3),
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`bufferCount`](buffercount)
rxjs distinct distinct
========
`function` `stable` `operator`
Returns an Observable that emits all items emitted by the source Observable that are distinct by comparison from previous items.
### `distinct<T, K>(keySelector?: (value: T) => K, flushes?: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `keySelector` | `(value: T) => K` | Optional. Default is `undefined`. Optional function to select which value you want to check as distinct. |
| `flushes` | `[Observable](../index/class/observable)<any>` | Optional. Default is `undefined`. Optional Observable for flushing the internal HashSet of the operator. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values.
Description
-----------
If a `keySelector` function is provided, then it will project each value from the source observable into a new value that it will check for equality with previously projected values. If the `keySelector` function is not provided, it will use each value from the source observable directly with an equality check against previous values.
In JavaScript runtimes that support `Set`, this operator will use a `Set` to improve performance of the distinct value checking.
In other runtimes, this operator will use a minimal implementation of `Set` that relies on an `Array` and `indexOf` under the hood, so performance will degrade as more values are checked for distinction. Even in newer browsers, a long-running `[distinct](../index/function/distinct)` use might result in memory leaks. To help alleviate this in some scenarios, an optional `flushes` parameter is also provided so that the internal `Set` can be "flushed", basically clearing it of values.
Examples
--------
A simple example with numbers
```
import { of, distinct } from 'rxjs';
of(1, 1, 2, 2, 2, 1, 2, 3, 4, 3, 2, 1)
.pipe(distinct())
.subscribe(x => console.log(x));
// Outputs
// 1
// 2
// 3
// 4
```
An example using the `keySelector` function
```
import { of, distinct } from 'rxjs';
of(
{ age: 4, name: 'Foo'},
{ age: 7, name: 'Bar'},
{ age: 5, name: 'Foo'}
)
.pipe(distinct(({ name }) => name))
.subscribe(x => console.log(x));
// Outputs
// { age: 4, name: 'Foo' }
// { age: 7, name: 'Bar' }
```
See Also
--------
* [`distinctUntilChanged`](distinctuntilchanged)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
rxjs auditTime auditTime
=========
`function` `stable` `operator`
Ignores source values for `duration` milliseconds, then emits the most recent value from the source Observable, then repeats this process.
### `auditTime<T>(duration: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `duration` | `number` | Time to wait before emitting the most recent source value, measured in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../index/interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../index/const/asyncscheduler)`. The [`SchedulerLike`](../index/interface/schedulerlike) to use for managing the timers that handle the rate-limiting behavior. |
#### Returns
`[MonoTypeOperatorFunction<T>](../index/interface/monotypeoperatorfunction)`: A function that returns an Observable that performs rate-limiting of emissions from the source Observable.
Description
-----------
When it sees a source value, it ignores that plus the next ones for `duration` milliseconds, and then it emits the most recent value from the source.
`[auditTime](../index/function/audittime)` is similar to `[throttleTime](../index/function/throttletime)`, but emits the last value from the silenced time window, instead of the first value. `[auditTime](../index/function/audittime)` emits the most recent value from the source Observable on the output Observable as soon as its internal timer becomes disabled, and ignores source values while the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, the timer is enabled. After `duration` milliseconds (or the time unit determined internally by the optional `scheduler`) has passed, the timer is disabled, then the most recent source value is emitted on the output Observable, and this process repeats for the next source value. Optionally takes a [`SchedulerLike`](../index/interface/schedulerlike) for managing timers.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, auditTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(auditTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sampleTime`](sampletime)
* [`throttleTime`](throttletime)
rxjs TestScheduler TestScheduler
=============
`class` `stable`
```
class TestScheduler extends VirtualTimeScheduler {
static frameTimeFactor: 10
static parseMarblesAsSubscriptions(marbles: string, runMode: boolean = false): SubscriptionLog
static parseMarbles(marbles: string, values?: any, errorValue?: any, materializeInnerObservables: boolean = false, runMode: boolean = false): TestMessage[]
constructor(assertDeepEqual: (actual: any, expected: any) => boolean | void)
get hotObservables: HotObservable<any>[]
get coldObservables: ColdObservable<any>[]
assertDeepEqual: (actual: any, expected: any) => boolean | void
createTime(marbles: string): number
createColdObservable<T = string>(marbles: string, values?: { [marble: string]: T; }, error?: any): ColdObservable<T>
createHotObservable<T = string>(marbles: string, values?: { [marble: string]: T; }, error?: any): HotObservable<T>
expectObservable<T>(observable: Observable<T>, subscriptionMarbles: string = null)
expectSubscriptions(actualSubscriptionLogs: SubscriptionLog[]): {...}
flush()
run<T>(callback: (helpers: RunHelpers) => T): T
// inherited from index/VirtualTimeScheduler
static frameTimeFactor: 10
constructor(schedulerActionCtor: typeof AsyncAction = VirtualAction as any, maxFrames: number = Infinity)
frame: number
index: number
maxFrames: number
flush(): void
}
```
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `frameTimeFactor` | `10` | The number of virtual time units each character in a marble diagram represents. If the test scheduler is being used in "run mode", via the `run` method, this is temporarly set to `1` for the duration of the `run` block, then set back to whatever value it was. |
Static Methods
--------------
| `static parseMarblesAsSubscriptions(marbles: string, runMode: boolean = false): SubscriptionLog` Parameters
| | | |
| --- | --- | --- |
| `marbles` | `string` | |
| `runMode` | `boolean` | Optional. Default is `false`. |
Returns `SubscriptionLog` |
| `static parseMarbles(marbles: string, values?: any, errorValue?: any, materializeInnerObservables: boolean = false, runMode: boolean = false): TestMessage[]` Parameters
| | | |
| --- | --- | --- |
| `marbles` | `string` | |
| `values` | `any` | Optional. Default is `undefined`. |
| `errorValue` | `any` | Optional. Default is `undefined`. |
| `materializeInnerObservables` | `boolean` | Optional. Default is `false`. |
| `runMode` | `boolean` | Optional. Default is `false`. |
Returns `TestMessage[]` |
Constructor
-----------
| `constructor(assertDeepEqual: (actual: any, expected: any) => boolean | void)` Parameters
| | | |
| --- | --- | --- |
| `assertDeepEqual` | `(actual: any, expected: any) => boolean | void` | A function to set up your assertion for your test harness |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `hotObservables` | `HotObservable<any>[]` | Read-only. |
| `coldObservables` | `ColdObservable<any>[]` | Read-only. |
| `assertDeepEqual` | `(actual: any, expected: any) => boolean | void` | A function to set up your assertion for your test harness Declared in constructor. |
Methods
-------
| `createTime(marbles: string): number` Parameters
| | | |
| --- | --- | --- |
| `marbles` | `string` | |
Returns `number` |
| `createColdObservable<T = string>(marbles: string, values?: { [marble: string]: T; }, error?: any): ColdObservable<T>` Parameters
| | | |
| --- | --- | --- |
| `marbles` | `string` | A diagram in the marble DSL. Letters map to keys in `values` if provided. |
| `values` | `{ [marble: string]: T; }` | Optional. Default is `undefined`. Values to use for the letters in `marbles`. If ommitted, the letters themselves are used. |
| `error` | `any` | Optional. Default is `undefined`. The error to use for the `#` marble (if present). |
Returns `ColdObservable<T>` |
| `createHotObservable<T = string>(marbles: string, values?: { [marble: string]: T; }, error?: any): HotObservable<T>` Parameters
| | | |
| --- | --- | --- |
| `marbles` | `string` | A diagram in the marble DSL. Letters map to keys in `values` if provided. |
| `values` | `{ [marble: string]: T; }` | Optional. Default is `undefined`. Values to use for the letters in `marbles`. If ommitted, the letters themselves are used. |
| `error` | `any` | Optional. Default is `undefined`. The error to use for the `#` marble (if present). |
Returns `HotObservable<T>` |
| `expectObservable<T>(observable: Observable<T>, subscriptionMarbles: string = null)` Parameters
| | | |
| --- | --- | --- |
| `[observable](../index/const/observable)` | `[Observable<T>](../index/class/observable)` | |
| `subscriptionMarbles` | `string` | Optional. Default is `null`. |
|
| `expectSubscriptions(actualSubscriptionLogs: SubscriptionLog[]): {
toBe: subscriptionLogsToBeFn;
}` Parameters
| | | |
| --- | --- | --- |
| `actualSubscriptionLogs` | `SubscriptionLog[]` | |
Returns `{ toBe: subscriptionLogsToBeFn; }` |
| `flush()` Parameters There are no parameters. |
| |
| `run<T>(callback: (helpers: RunHelpers) => T): T`
The `run` method performs the test in 'run mode' - in which schedulers used within the test automatically delegate to the `[TestScheduler](testscheduler)`. That is, in 'run mode' there is no need to explicitly pass a `[TestScheduler](testscheduler)` instance to observable creators or operators. Parameters
| | | |
| --- | --- | --- |
| `callback` | `(helpers: RunHelpers) => T` | |
Returns `T` |
rxjs fromFetch fromFetch
=========
`function` `stable`
Uses [the Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) to make an HTTP request.
### `fromFetch<T>(input: string | Request, initWithSelector: RequestInit & { selector?: (response: Response) => ObservableInput<T>; } = {}): Observable<Response | T>`
#### Parameters
| | | |
| --- | --- | --- |
| `input` | `string | Request` | The resource you would like to fetch. Can be a url or a request object. |
| `initWithSelector` | `RequestInit & { selector?: (response: Response) => [ObservableInput](../index/type-alias/observableinput)<T>; }` | Optional. Default is `{}`. A configuration object for the fetch. [See MDN for more details](https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/fetch#Parameters) |
#### Returns
`[Observable](../index/class/observable)<Response | T>`: An Observable, that when subscribed to, performs an HTTP request using the native `fetch` function. The [`Subscription`](../index/class/subscription) is tied to an `AbortController` for the fetch.
Description
-----------
**WARNING** Parts of the fetch API are still experimental. `AbortController` is required for this implementation to work and use cancellation appropriately.
Will automatically set up an internal [AbortController](https://developer.mozilla.org/en-US/docs/Web/API/AbortController) in order to finalize the internal `fetch` when the subscription tears down.
If a `signal` is provided via the `init` argument, it will behave like it usually does with `fetch`. If the provided `signal` aborts, the error that `fetch` normally rejects with in that scenario will be emitted as an error from the observable.
Examples
--------
Basic use
```
import { fromFetch } from 'rxjs/fetch';
import { switchMap, of, catchError } from 'rxjs';
const data$ = fromFetch('https://api.github.com/users?per_page=5').pipe(
switchMap(response => {
if (response.ok) {
// OK return data
return response.json();
} else {
// Server is returning a status requiring the client to try something else.
return of({ error: true, message: `Error ${ response.status }` });
}
}),
catchError(err => {
// Network or other error, handle appropriately
console.error(err);
return of({ error: true, message: err.message })
})
);
data$.subscribe({
next: result => console.log(result),
complete: () => console.log('done')
});
```
#### Use with Chunked Transfer Encoding
With HTTP responses that use [chunked transfer encoding](https://tools.ietf.org/html/rfc7230#section-3.3.1), the promise returned by `fetch` will resolve as soon as the response's headers are received.
That means the `[fromFetch](fromfetch)` observable will emit a `Response` - and will then complete - before the body is received. When one of the methods on the `Response` - like `text()` or `json()` - is called, the returned promise will not resolve until the entire body has been received. Unsubscribing from any observable that uses the promise as an observable input will not abort the request.
To facilitate aborting the retrieval of responses that use chunked transfer encoding, a `selector` can be specified via the `init` parameter:
```
import { of } from 'rxjs';
import { fromFetch } from 'rxjs/fetch';
const data$ = fromFetch('https://api.github.com/users?per_page=5', {
selector: response => response.json()
});
data$.subscribe({
next: result => console.log(result),
complete: () => console.log('done')
});
```
| programming_docs |
rxjs NotificationKind NotificationKind
================
`enum` `deprecated`
Deprecation Notes
-----------------
Use a string literal instead. `[NotificationKind](notificationkind)` will be replaced with a type alias in v8. It will not be replaced with a const enum as those are not compatible with isolated modules.
```
enum NotificationKind {
NEXT: 'N'
ERROR: 'E'
COMPLETE: 'C'
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `NEXT` | `'N'` | |
| `ERROR` | `'E'` | |
| `COMPLETE` | `'C'` | |
rxjs ObservedValueTupleFromArray ObservedValueTupleFromArray
===========================
`type-alias` `stable`
Extracts a tuple of element types from an `[ObservableInput](observableinput)<any>[]`. If you have `O extends [ObservableInput](observableinput)<any>[]` and you pass in `[[Observable](../class/observable)<string>, [Observable](../class/observable)<number>]` you would get back a type of `[string, number]`.
```
type ObservedValueTupleFromArray<X> = {
[K in keyof X]: ObservedValueOf<X[K]>;
};
```
rxjs ObservableLike ObservableLike
==============
`type-alias` `deprecated`
Deprecation Notes
-----------------
Renamed to [`InteropObservable`](../interface/interopobservable). Will be removed in v8.
```
type ObservableLike<T> = InteropObservable<T>;
```
rxjs ObservableInputTuple ObservableInputTuple
====================
`type-alias` `stable`
Used to infer types from arguments to functions like [`forkJoin`](../function/forkjoin). So that you can have `[forkJoin](../function/forkjoin)([[Observable](../class/observable)<A>, PromiseLike<B>]): [Observable](../class/observable)<[A, B]>` et al.
```
type ObservableInputTuple<T> = {
[K in keyof T]: ObservableInput<T[K]>;
};
```
rxjs ObservedValuesFromArray ObservedValuesFromArray
=======================
`type-alias` `deprecated`
Deprecation Notes
-----------------
Renamed to [`ObservedValueUnionFromArray`](observedvalueunionfromarray). Will be removed in v8.
```
type ObservedValuesFromArray<X> = ObservedValueUnionFromArray<X>;
```
rxjs ObservableInput ObservableInput
===============
`type-alias` `stable`
Valid types that can be converted to observables.
```
type ObservableInput<T> = Observable<T> | InteropObservable<T> | AsyncIterable<T> | PromiseLike<T> | ArrayLike<T> | Iterable<T> | ReadableStreamLike<T>;
```
rxjs ObservableNotification ObservableNotification
======================
`type-alias` `stable`
Valid observable notification types.
```
type ObservableNotification<T> = NextNotification<T> | ErrorNotification | CompleteNotification;
```
rxjs TeardownLogic TeardownLogic
=============
`type-alias` `stable`
```
type TeardownLogic = Subscription | Unsubscribable | (() => void) | void;
```
rxjs ObservedValueUnionFromArray ObservedValueUnionFromArray
===========================
`type-alias` `stable`
Extracts a union of element types from an `[ObservableInput](observableinput)<any>[]`. If you have `O extends [ObservableInput](observableinput)<any>[]` and you pass in `[Observable](../class/observable)<string>[]` or `Promise<string>[]` you would get back a type of `string`. If you pass in `[[Observable](../class/observable)<string>, [Observable](../class/observable)<number>]` you would get back a type of `string | number`.
```
type ObservedValueUnionFromArray<X> = X extends Array<ObservableInput<infer T>> ? T : never;
```
rxjs ObservedValueOf ObservedValueOf
===============
`type-alias` `stable`
Extracts the type from an `[ObservableInput](observableinput)<any>`. If you have `O extends [ObservableInput](observableinput)<any>` and you pass in `[Observable](../class/observable)<number>`, or `Promise<number>`, etc, it will type as `number`.
```
type ObservedValueOf<O> = O extends ObservableInput<infer T> ? T : never;
```
rxjs Tail Tail
====
`type-alias` `stable`
Extracts the tail of a tuple. If you declare `[Tail](tail)<[A, B, C]>` you will get back `[B, C]`.
```
type Tail<X extends readonly, any> = [];
```
rxjs ValueFromArray ValueFromArray
==============
`type-alias` `stable`
Extracts the generic value from an Array type. If you have `T extends Array<any>`, and pass a `string[]` to it, `[ValueFromArray](valuefromarray)<T>` will return the actual type of `string`.
```
type ValueFromArray<A extends readonly, unknown> = [];
```
rxjs Falsy Falsy
=====
`type-alias` `stable`
A simple type to represent a gamut of "falsy" values... with a notable exception: `NaN` is "falsy" however, it is not and cannot be typed via TypeScript. See comments here: <https://github.com/microsoft/TypeScript/issues/28682#issuecomment-707142417>
```
type Falsy = null | undefined | false | 0 | -0 | 0n | '';
```
rxjs ValueFromNotification ValueFromNotification
=====================
`type-alias` `stable`
Gets the value type from an [`ObservableNotification`](observablenotification), if possible.
```
type ValueFromNotification<T> = T extends {
kind: 'N' | 'E' | 'C';
} ? T extends NextNotification<any> ? T extends {
value: infer V;
} ? V : undefined : never : never;
```
rxjs Head Head
====
`type-alias` `stable`
Extracts the head of a tuple. If you declare `[Head](head)<[A, B, C]>` you will get back `A`.
```
type Head<X extends readonly, any> = [];
```
rxjs PartialObserver PartialObserver
===============
`type-alias` `stable`
```
type PartialObserver<T> = NextObserver<T> | ErrorObserver<T> | CompletionObserver<T>;
```
rxjs Cons Cons
====
`type-alias` `stable`
Constructs a new tuple with the specified type at the head. If you declare `[Cons](cons)<A, [B, C]>` you will get back `[A, B, C]`.
```
type Cons<X, Y extends readonly, any> = [];
```
rxjs FactoryOrValue FactoryOrValue
==============
`type-alias` `stable`
```
type FactoryOrValue<T> = T | (() => T);
```
rxjs TruthyTypesOf TruthyTypesOf
=============
`type-alias` `stable`
```
type TruthyTypesOf<T> = T extends Falsy ? never : T;
```
rxjs SubscribableOrPromise SubscribableOrPromise
=====================
`type-alias` `deprecated`
Deprecation Notes
-----------------
Do not use. Most likely you want to use `[ObservableInput](observableinput)`. Will be removed in v8.
```
type SubscribableOrPromise<T> = Subscribable<T> | Subscribable<never> | PromiseLike<T> | InteropObservable<T>;
```
rxjs MonoTypeOperatorFunction MonoTypeOperatorFunction
========================
`interface` `stable`
```
interface MonoTypeOperatorFunction<T> extends OperatorFunction<T, T> {
// inherited from index/OperatorFunction
// inherited from index/UnaryFunction
(source: T): R
}
```
rxjs ErrorNotification ErrorNotification
=================
`interface` `stable`
A notification representing an "error" from an observable. Can be used with [`dematerialize`](../function/dematerialize).
```
interface ErrorNotification {
kind: 'E'
error: any
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `kind` | `'E'` | The kind of notification. Always "E" |
| `error` | `any` | |
rxjs SchedulerLike SchedulerLike
=============
`interface` `stable`
SCHEDULER INTERFACES
```
interface SchedulerLike extends TimestampProvider {
schedule<T>(work: (this: SchedulerAction<T>, state: T) => void, delay: number, state: T): Subscription
// inherited from index/TimestampProvider
now(): number
}
```
Class Implementations
---------------------
* `[Scheduler](../class/scheduler)`
Methods
-------
| `schedule<T>(work: (this: SchedulerAction<T>, state?: T) => void, delay: number, state?: T): Subscription` Parameters
| | | |
| --- | --- | --- |
| `work` | `(this: [SchedulerAction](scheduleraction)<T>, state?: T) => void` | |
| `[delay](../function/delay)` | `number` | |
| `state` | `T` | Optional. Default is `undefined`. |
Returns `[Subscription](../class/subscription)` |
| `schedule<T>(work: (this: SchedulerAction<T>, state?: T) => void, delay?: number, state?: T): Subscription` Parameters
| | | |
| --- | --- | --- |
| `work` | `(this: [SchedulerAction](scheduleraction)<T>, state?: T) => void` | |
| `[delay](../function/delay)` | `number` | Optional. Default is `undefined`. |
| `state` | `T` | Optional. Default is `undefined`. |
Returns `[Subscription](../class/subscription)` |
rxjs ErrorObserver ErrorObserver
=============
`interface` `stable`
```
interface ErrorObserver<T> {
closed?: boolean
next?: (value: T) => void
error: (err: any) => void
complete?: () => void
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `boolean` | |
| `next` | `(value: T) => void` | |
| `error` | `(err: any) => void` | |
| `complete` | `() => void` | |
rxjs UnsubscriptionError UnsubscriptionError
===================
`interface` `stable`
An error thrown when one or more errors have occurred during the `unsubscribe` of a [`Subscription`](../class/subscription).
```
interface UnsubscriptionError extends Error {
get errors: any[]
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `errors` | `any[]` | Read-only. |
rxjs NotFoundError NotFoundError
=============
`interface` `stable`
An error thrown when a value or values are missing from an observable sequence.
```
interface NotFoundError extends Error {
}
```
See Also
--------
* [`single`](../../operators/single)
rxjs TimeoutError TimeoutError
============
`interface` `stable` `operator`
An error thrown by the [`timeout`](../function/timeout) operator.
```
interface TimeoutError<T = unknown, M = unknown> extends Error {
info: TimeoutInfo<T, M> | null
}
```
Description
-----------
Provided so users can use as a type and do quality comparisons. We recommend you do not subclass this or create instances of this class directly. If you have need of a error representing a timeout, you should create your own error class and use that.
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `info` | `TimeoutInfo<T, M> | null` | The information provided to the error by the timeout operation that created the error. Will be `null` if used directly in non-RxJS code with an empty constructor. (Note that using this constructor directly is not recommended, you should create your own errors) |
See Also
--------
* [`timeout`](../function/timeout)
rxjs Timestamp Timestamp
=========
`interface` `stable`
A value and the time at which it was emitted.
```
interface Timestamp<T> {
value: T
timestamp: number
}
```
Description
-----------
Emitted by the `[timestamp](../function/timestamp)` operator
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `value` | `T` | |
| `[timestamp](../function/timestamp)` | `number` | The timestamp. By default, this is in epoch milliseconds. Could vary based on the timestamp provider passed to the operator. |
See Also
--------
* [`timestamp`](../function/timestamp)
rxjs SubjectLike SubjectLike
===========
`interface` `stable`
```
interface SubjectLike<T> extends Observer<T>, Subscribable<T> {
// inherited from index/Observer
next: (value: T) => void
error: (err: any) => void
complete: () => void
// inherited from index/Subscribable
subscribe(observer: Partial<Observer<T>>): Unsubscribable
}
```
rxjs CompleteNotification CompleteNotification
====================
`interface` `stable`
A notification representing a "completion" from an observable. Can be used with [`dematerialize`](../function/dematerialize).
```
interface CompleteNotification {
kind: 'C'
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `kind` | `'C'` | |
rxjs NextNotification NextNotification
================
`interface` `stable`
NOTIFICATIONS A notification representing a "next" from an observable. Can be used with [`dematerialize`](../function/dematerialize).
```
interface NextNotification<T> {
kind: 'N'
value: T
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `kind` | `'N'` | The kind of notification. Always "N" |
| `value` | `T` | The value of the notification. |
rxjs CompletionObserver CompletionObserver
==================
`interface` `stable`
```
interface CompletionObserver<T> {
closed?: boolean
next?: (value: T) => void
error?: (err: any) => void
complete: () => void
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `boolean` | |
| `next` | `(value: T) => void` | |
| `error` | `(err: any) => void` | |
| `complete` | `() => void` | |
rxjs SchedulerAction SchedulerAction
===============
`interface` `stable`
```
interface SchedulerAction<T> extends Subscription {
schedule(state?: T, delay?: number): Subscription
// inherited from index/Subscription
static EMPTY: (() => {...})
constructor(initialTeardown?: () => void)
closed: false
unsubscribe(): void
add(teardown: TeardownLogic): void
remove(teardown: Subscription | Unsubscribable | (() => void)): void
}
```
Methods
-------
| `schedule(state?: T, delay?: number): Subscription` Parameters
| | | |
| --- | --- | --- |
| `state` | `T` | Optional. Default is `undefined`. |
| `[delay](../function/delay)` | `number` | Optional. Default is `undefined`. |
Returns `[Subscription](../class/subscription)` |
rxjs Subscribable Subscribable
============
`interface` `stable`
OBSERVABLE INTERFACES
```
interface Subscribable<T> {
subscribe(observer: Partial<Observer<T>>): Unsubscribable
}
```
Child Interfaces
----------------
* `[SubjectLike](subjectlike)`
Class Implementations
---------------------
* `[Observable](../class/observable)`
+ `[ConnectableObservable](../class/connectableobservable)`
+ `[Subject](../class/subject)`
- `[BehaviorSubject](../class/behaviorsubject)`
- `[ReplaySubject](../class/replaysubject)`
- `[AsyncSubject](../class/asyncsubject)`
Methods
-------
| `subscribe(observer: Partial<Observer<T>>): Unsubscribable` Parameters
| | | |
| --- | --- | --- |
| `observer` | `Partial<[Observer](observer)<T>>` | |
Returns `[Unsubscribable](unsubscribable)` |
rxjs UnaryFunction UnaryFunction
=============
`interface` `stable`
OPERATOR INTERFACES
```
interface UnaryFunction<T, R> {
(source: T): R
}
```
Child Interfaces
----------------
* `[OperatorFunction](operatorfunction)`
+ `[MonoTypeOperatorFunction](monotypeoperatorfunction)`
Methods
-------
| `(source: T): R` Parameters
| | | |
| --- | --- | --- |
| `source` | `T` | |
Returns `R` |
rxjs SequenceError SequenceError
=============
`interface` `stable`
An error thrown when something is wrong with the sequence of values arriving on the observable.
```
interface SequenceError extends Error {
}
```
See Also
--------
* [`single`](../../operators/single)
rxjs SubscriptionLike SubscriptionLike
================
`interface` `stable`
```
interface SubscriptionLike extends Unsubscribable {
get closed: boolean
unsubscribe(): void
// inherited from index/Unsubscribable
unsubscribe(): void
}
```
Class Implementations
---------------------
* `[Subject](../class/subject)`
+ `[BehaviorSubject](../class/behaviorsubject)`
+ `[ReplaySubject](../class/replaysubject)`
+ `[AsyncSubject](../class/asyncsubject)`
* `[Subscription](../class/subscription)`
+ `[Subscriber](../class/subscriber)`
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `boolean` | Read-only. |
Methods
-------
| `unsubscribe(): void` Parameters There are no parameters. Returns `void` |
rxjs ArgumentOutOfRangeError ArgumentOutOfRangeError
=======================
`interface` `stable`
An error thrown when an element was queried at a certain index of an Observable, but no such index or position exists in that sequence.
```
interface ArgumentOutOfRangeError extends Error {
}
```
See Also
--------
* [`elementAt`](../function/elementat)
* [`take`](../function/take)
* [`takeLast`](../function/takelast)
rxjs TimestampProvider TimestampProvider
=================
`interface` `stable`
This is a type that provides a method to allow RxJS to create a numeric timestamp
```
interface TimestampProvider {
now(): number
}
```
Child Interfaces
----------------
* `[SchedulerLike](schedulerlike)`
Methods
-------
| |
| `now(): number`
Returns a timestamp as a number. Parameters There are no parameters. Returns `number` |
| This is used by types like `[ReplaySubject](../class/replaysubject)` or operators like `[timestamp](../function/timestamp)` to calculate the amount of time passed between events. |
rxjs NextObserver NextObserver
============
`interface` `stable`
OBSERVER INTERFACES
```
interface NextObserver<T> {
closed?: boolean
next: (value: T) => void
error?: (err: any) => void
complete?: () => void
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `boolean` | |
| `next` | `(value: T) => void` | |
| `error` | `(err: any) => void` | |
| `complete` | `() => void` | |
rxjs InteropObservable InteropObservable
=================
`interface` `stable`
An object that implements the `Symbol.observable` interface.
```
interface InteropObservable<T> {
__@observable: () => Subscribable<T>
}
```
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `__@[observable](../const/observable)` | `() => [Subscribable](subscribable)<T>` | |
rxjs Unsubscribable Unsubscribable
==============
`interface` `stable`
SUBSCRIPTION INTERFACES
```
interface Unsubscribable {
unsubscribe(): void
}
```
Child Interfaces
----------------
* `[SubscriptionLike](subscriptionlike)`
Methods
-------
| `unsubscribe(): void` Parameters There are no parameters. Returns `void` |
rxjs TimeInterval TimeInterval
============
`interface` `stable`
A value emitted and the amount of time since the last value was emitted.
```
interface TimeInterval<T> {
value: T
interval: number
}
```
Description
-----------
Emitted by the `[timeInterval](../function/timeinterval)` operator.
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `value` | `T` | |
| `[interval](../function/interval)` | `number` | The amount of time between this value's emission and the previous value's emission. If this is the first emitted value, then it will be the amount of time since subscription started. |
See Also
--------
* [`timeInterval`](../function/timeinterval)
rxjs Connectable Connectable
===========
`interface` `stable`
An observable with a `[connect](../function/connect)` method that is used to create a subscription to an underlying source, connecting it with all consumers via a multicast.
```
interface Connectable<T> extends Observable<T> {
connect(): Subscription
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Methods
-------
| |
| `connect(): Subscription`
(Idempotent) Calling this method will connect the underlying source observable to all subscribed consumers through an underlying [`Subject`](../class/subject). Parameters There are no parameters. Returns `[Subscription](../class/subscription)`: A subscription, that when unsubscribed, will "disconnect" the source from the connector subject, severing notifications to all consumers. |
| programming_docs |
rxjs EmptyError EmptyError
==========
`interface` `stable`
An error thrown when an Observable or a sequence was queried but has no elements.
```
interface EmptyError extends Error {
}
```
See Also
--------
* [`first`](../function/first)
* [`last`](../function/last)
* [`single`](../function/single)
* [`firstValueFrom`](../function/firstvaluefrom)
* [`lastValueFrom`](../function/lastvaluefrom)
rxjs Observer Observer
========
`interface` `stable`
```
interface Observer<T> {
next: (value: T) => void
error: (err: any) => void
complete: () => void
}
```
Child Interfaces
----------------
* `[SubjectLike](subjectlike)`
Class Implementations
---------------------
* `[Subscriber](../class/subscriber)`
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `next` | `(value: T) => void` | |
| `error` | `(err: any) => void` | |
| `complete` | `() => void` | |
rxjs ObjectUnsubscribedError ObjectUnsubscribedError
=======================
`interface` `stable`
An error thrown when an action is invalid because the object has been unsubscribed.
```
interface ObjectUnsubscribedError extends Error {
}
```
See Also
--------
* [`Subject`](../class/subject)
* [`BehaviorSubject`](../class/behaviorsubject)
rxjs OperatorFunction OperatorFunction
================
`interface` `stable`
```
interface OperatorFunction<T, R> extends UnaryFunction<Observable<T>, Observable<R>> {
// inherited from index/UnaryFunction
(source: T): R
}
```
Child Interfaces
----------------
* `[MonoTypeOperatorFunction](monotypeoperatorfunction)`
rxjs ReadableStreamLike ReadableStreamLike
==================
`interface` `stable`
The base signature RxJS will look for to identify and use a [ReadableStream](https://streams.spec.whatwg.org/#rs-class) as an [`ObservableInput`](../type-alias/observableinput) source.
```
interface ReadableStreamLike<T> {
getReader(): ReadableStreamDefaultReaderLike<T>
}
```
Methods
-------
| `getReader(): ReadableStreamDefaultReaderLike<T>` Parameters There are no parameters. Returns `ReadableStreamDefaultReaderLike<T>` |
rxjs exhaustAll exhaustAll
==========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable by dropping inner Observables while the previous inner Observable has not yet completed.
### `exhaustAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<O, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that takes a source of Observables and propagates the first Observable exclusively until it completes before subscribing to the next.
Description
-----------
Flattens an Observable-of-Observables by dropping the next inner Observables while the current inner is still executing.
`[exhaustAll](exhaustall)` subscribes to an Observable that emits Observables, also known as a higher-order Observable. Each time it observes one of these emitted inner Observables, the output Observable begins emitting the items emitted by that inner Observable. So far, it behaves like [`mergeAll`](mergeall). However, `[exhaustAll](exhaustall)` ignores every new inner Observable if the previous Observable has not yet completed. Once that one completes, it will accept and flatten the next inner Observable and repeat this process.
Example
-------
Run a finite timer for each click, only if there is no currently active timer
```
import { fromEvent, map, interval, take, exhaustAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(5)))
);
const result = higherOrder.pipe(exhaustAll());
result.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`mergeAll`](mergeall)
* [`exhaustMap`](exhaustmap)
* [`zipAll`](zipall)
rxjs single single
======
`function` `stable` `operator`
Returns an observable that asserts that only one value is emitted from the observable that matches the predicate. If no predicate is provided, then it will assert that the observable only emits one value.
### `single<T>(predicate?: (value: T, index: number, source: Observable<T>) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | Optional. Default is `undefined`. A predicate function to evaluate items emitted by the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the single item emitted by the source Observable that matches the predicate.
#### Throws
`[NotFoundError](../interface/notfounderror)` Delivers an NotFoundError to the Observer's `error` callback if the Observable completes before any `next` notification was sent.
`[SequenceError](../interface/sequenceerror)` Delivers a SequenceError if more than one value is emitted that matches the provided predicate. If no predicate is provided, will deliver a SequenceError if more than one value comes from the source
Description
-----------
In the event that the observable is empty, it will throw an [`EmptyError`](../interface/emptyerror).
In the event that two values are found that match the predicate, or when there are two values emitted and no predicate, it will throw a [`SequenceError`](../interface/sequenceerror)
In the event that no values match the predicate, if one is provided, it will throw a [`NotFoundError`](../interface/notfounderror)
Example
-------
Expect only `name` beginning with `'B'`
```
import { of, single } from 'rxjs';
const source1 = of(
{ name: 'Ben' },
{ name: 'Tracy' },
{ name: 'Laney' },
{ name: 'Lily' }
);
source1
.pipe(single(x => x.name.startsWith('B')))
.subscribe(x => console.log(x));
// Emits 'Ben'
const source2 = of(
{ name: 'Ben' },
{ name: 'Tracy' },
{ name: 'Bradley' },
{ name: 'Lincoln' }
);
source2
.pipe(single(x => x.name.startsWith('B')))
.subscribe({ error: err => console.error(err) });
// Error emitted: SequenceError('Too many values match')
const source3 = of(
{ name: 'Laney' },
{ name: 'Tracy' },
{ name: 'Lily' },
{ name: 'Lincoln' }
);
source3
.pipe(single(x => x.name.startsWith('B')))
.subscribe({ error: err => console.error(err) });
// Error emitted: NotFoundError('No values match')
```
See Also
--------
* [`first`](first)
* [`find`](find)
* [`findIndex`](findindex)
* [`elementAt`](elementat)
rxjs bindNodeCallback bindNodeCallback
================
`function` `stable`
### `bindNodeCallback<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs multicast multicast
=========
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `multicast<T, R>(subjectOrSubjectFactory: Subject<T> | (() => Subject<T>), selector?: (source: Observable<T>) => Observable<R>): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `subjectOrSubjectFactory` | `[Subject](../class/subject)<T> | (() => [Subject](../class/subject)<T>)` | |
| `selector` | `(source: [Observable](../class/observable)<T>) => [Observable](../class/observable)<R>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
Overloads
---------
### `multicast(subject: Subject<T>): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
An operator that creates a [`ConnectableObservable`](../class/connectableobservable), that when connected, with the `<connect>` method, will use the provided subject to multicast the values from the source to all consumers.
#### Parameters
| | | |
| --- | --- | --- |
| `subject` | `[Subject<T>](../class/subject)` | The subject to multicast through. |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<[Observable](../class/observable)<T>, [ConnectableObservable](../class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../class/connectableobservable)
### `multicast(subject: Subject<T>, selector: (shared: Observable<T>) => O): OperatorFunction<T, ObservedValueOf<O>>`
Because this is deprecated in favor of the [`connect`](connect) operator, and was otherwise poorly documented, rather than duplicate the effort of documenting the same behavior, please see documentation for the [`connect`](connect) operator.
#### Parameters
| | | |
| --- | --- | --- |
| `subject` | `[Subject<T>](../class/subject)` | The subject used to multicast. |
| `selector` | `(shared: [Observable](../class/observable)<T>) => O` | A setup function to setup the multicast |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an observable that mirrors the observable returned by the selector.
### `multicast(subjectFactory: () => Subject<T>): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
An operator that creates a [`ConnectableObservable`](../class/connectableobservable), that when connected, with the `<connect>` method, will use the provided subject to multicast the values from the source to all consumers.
#### Parameters
| | | |
| --- | --- | --- |
| `subjectFactory` | `() => [Subject](../class/subject)<T>` | A factory that will be called to create the subject. Passing a function here will cause the underlying subject to be "reset" on error, completion, or refCounted unsubscription of the source. |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<[Observable](../class/observable)<T>, [ConnectableObservable](../class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../class/connectableobservable)
### `multicast(subjectFactory: () => Subject<T>, selector: (shared: Observable<T>) => O): OperatorFunction<T, ObservedValueOf<O>>`
Because this is deprecated in favor of the [`connect`](connect) operator, and was otherwise poorly documented, rather than duplicate the effort of documenting the same behavior, please see documentation for the [`connect`](connect) operator.
#### Parameters
| | | |
| --- | --- | --- |
| `subjectFactory` | `() => [Subject](../class/subject)<T>` | A factory that creates the subject used to multicast. |
| `selector` | `(shared: [Observable](../class/observable)<T>) => O` | A function to setup the multicast and select the output. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an observable that mirrors the observable returned by the selector.
rxjs map map
===
`function` `stable` `operator`
Applies a given `project` function to each value emitted by the source Observable, and emits the resulting values as an Observable.
### `map<T, R>(project: (value: T, index: number) => R, thisArg?: any): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => R` | The function to apply to each `value` emitted by the source Observable. The `index` parameter is the number `i` for the i-th emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to define what `this` is in the `project` function. |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`: A function that returns an Observable that emits the values from the source Observable transformed by the given `project` function.
Description
-----------
Like [Array.prototype.map()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map), it passes each source value through a transformation function to get corresponding output values.
Similar to the well known `Array.prototype.map` function, this operator applies a projection to each value and emits that projection in the output Observable.
Example
-------
Map every click to the `clientX` position of that click
```
import { fromEvent, map } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const positions = clicks.pipe(map(ev => ev.clientX));
positions.subscribe(x => console.log(x));
```
See Also
--------
* [`mapTo`](mapto)
* [`pluck`](pluck)
rxjs exhaustMap exhaustMap
==========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable only if the previous projected Observable has completed.
### `exhaustMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable containing projected Observables of each item of the source, ignoring projected Observables that start before their preceding Observable has completed.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`exhaust`](../const/exhaust).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. When it projects a source value to an Observable, the output Observable begins emitting the items emitted by that projected Observable. However, `[exhaustMap](exhaustmap)` ignores every new projected Observable if the previous projected Observable has not yet completed. Once that one completes, it will accept and flatten the next projected Observable and repeat this process.
Example
-------
Run a finite timer for each click, only if there is no currently active timer
```
import { fromEvent, exhaustMap, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
exhaustMap(() => interval(1000).pipe(take(5)))
);
result.subscribe(x => console.log(x));
```
Overloads
---------
### `exhaustMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `exhaustMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `exhaustMap(project: (value: T, index: number) => ObservableInput<I>, resultSelector: (outerValue: T, innerValue: I, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => [ObservableInput](../type-alias/observableinput)<I>` | |
| `resultSelector` | `(outerValue: T, innerValue: I, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaust`](../const/exhaust)
* [`mergeMap`](mergemap)
* [`switchMap`](switchmap)
rxjs throwError throwError
==========
`function` `stable`
### `throwError(errorOrErrorFactory: any, scheduler?: SchedulerLike): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `errorOrErrorFactory` | `any` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<never>`
Overloads
---------
### `throwError(errorFactory: () => any): Observable<never>`
Creates an observable that will create an error instance and push it to the consumer as an error immediately upon subscription.
#### Parameters
| | | |
| --- | --- | --- |
| `errorFactory` | `() => any` | A factory function that will create the error instance that is pushed. |
#### Returns
`[Observable](../class/observable)<never>`
Just errors and does nothing else
This creation function is useful for creating an observable that will create an error and error every time it is subscribed to. Generally, inside of most operators when you might want to return an errored observable, this is unnecessary. In most cases, such as in the inner return of [`concatMap`](concatmap), [`mergeMap`](mergemap), [`defer`](defer), and many others, you can simply throw the error, and RxJS will pick that up and notify the consumer of the error.
Example
-------
Create a simple observable that will create a new error with a timestamp and log it and the message every time you subscribe to it
```
import { throwError } from 'rxjs';
let errorCount = 0;
const errorWithTimestamp$ = throwError(() => {
const error: any = new Error(`This is error number ${ ++errorCount }`);
error.timestamp = Date.now();
return error;
});
errorWithTimestamp$.subscribe({
error: err => console.log(err.timestamp, err.message)
});
errorWithTimestamp$.subscribe({
error: err => console.log(err.timestamp, err.message)
});
// Logs the timestamp and a new error message for each subscription
```
#### Unnecessary usage
Using `[throwError](throwerror)` inside of an operator or creation function with a callback, is usually not necessary
```
import { of, concatMap, timer, throwError } from 'rxjs';
const delays$ = of(1000, 2000, Infinity, 3000);
delays$.pipe(
concatMap(ms => {
if (ms < 10000) {
return timer(ms);
} else {
// This is probably overkill.
return throwError(() => new Error(`Invalid time ${ ms }`));
}
})
)
.subscribe({
next: console.log,
error: console.error
});
```
You can just throw the error instead
```
import { of, concatMap, timer } from 'rxjs';
const delays$ = of(1000, 2000, Infinity, 3000);
delays$.pipe(
concatMap(ms => {
if (ms < 10000) {
return timer(ms);
} else {
// Cleaner and easier to read for most folks.
throw new Error(`Invalid time ${ ms }`);
}
})
)
.subscribe({
next: console.log,
error: console.error
});
```
### `throwError(error: any): Observable<never>`
Returns an observable that will error with the specified error immediately upon subscription.
#### Parameters
| | | |
| --- | --- | --- |
| `error` | `any` | The error instance to emit |
#### Returns
`[Observable](../class/observable)<never>`
### `throwError(errorOrErrorFactory: any, scheduler: SchedulerLike): Observable<never>`
Notifies the consumer of an error using a given scheduler by scheduling it at delay `0` upon subscription.
#### Parameters
| | | |
| --- | --- | --- |
| `errorOrErrorFactory` | `any` | An error instance or error factory |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | A scheduler to use to schedule the error notification |
#### Returns
`[Observable](../class/observable)<never>`
| programming_docs |
rxjs retry retry
=====
`function` `stable` `operator`
Returns an Observable that mirrors the source Observable with the exception of an `error`.
### `retry<T>(configOrCount: number | RetryConfig = Infinity): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `configOrCount` | `number | RetryConfig` | Optional. Default is `Infinity`. Either number of retry attempts before failing or a [RetryConfig](retryconfig) object. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that will resubscribe to the source stream when the source stream errors, at most `<count>` times.
Description
-----------
If the source Observable calls `error`, this method will resubscribe to the source Observable for a maximum of `<count>` resubscriptions rather than propagating the `error` call.
The number of retries is determined by the `<count>` parameter. It can be set either by passing a number to `<retry>` function or by setting `<count>` property when `<retry>` is configured using [RetryConfig](retryconfig). If `<count>` is omitted, `<retry>` will try to resubscribe on errors infinite number of times.
Any and all items emitted by the source Observable will be emitted by the resulting Observable, even those emitted during failed subscriptions. For example, if an Observable fails at first but emits `[1, 2]` then succeeds the second time and emits: `[1, 2, 3, 4, 5, complete]` then the complete stream of emissions and notifications would be: `[1, 2, 1, 2, 3, 4, 5, complete]`.
Example
-------
```
import { interval, mergeMap, throwError, of, retry } from 'rxjs';
const source = interval(1000);
const result = source.pipe(
mergeMap(val => val > 5 ? throwError(() => 'Error!') : of(val)),
retry(2) // retry 2 times on error
);
result.subscribe({
next: value => console.log(value),
error: err => console.log(`${ err }: Retried 2 times then quit!`)
});
// Output:
// 0..1..2..3..4..5..
// 0..1..2..3..4..5..
// 0..1..2..3..4..5..
// 'Error!: Retried 2 times then quit!'
```
See Also
--------
* [`retryWhen`](retrywhen)
rxjs distinctUntilChanged distinctUntilChanged
====================
`function` `stable` `operator`
Returns a result [`Observable`](../class/observable) that emits all values pushed by the source observable if they are distinct in comparison to the last value the result observable emitted.
### `distinctUntilChanged<T, K>(comparator?: (previous: K, current: K) => boolean, keySelector: (value: T) => K = identity as (value: T) => K): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparator` | `(previous: K, current: K) => boolean` | Optional. Default is `undefined`. A function used to compare the previous and current keys for equality. Defaults to a `===` check. |
| `keySelector` | `(value: T) => K` | Optional. Default is `<identity> as (value: T) => K`. Used to select a key value to be passed to the `comparator`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values.
Description
-----------
When provided without parameters or with the first parameter (`[comparator](distinctuntilchanged#comparator)`), it behaves like this:
1. It will always emit the first value from the source.
2. For all subsequent values pushed by the source, they will be compared to the previously emitted values using the provided `comparator` or an `===` equality check.
3. If the value pushed by the source is determined to be unequal by this check, that value is emitted and becomes the new "previously emitted value" internally.
When the second parameter (`[keySelector](distinctuntilchanged#keySelector)`) is provided, the behavior changes:
1. It will always emit the first value from the source.
2. The `keySelector` will be run against all values, including the first value.
3. For all values after the first, the selected key will be compared against the key selected from the previously emitted value using the `comparator`.
4. If the keys are determined to be unequal by this check, the value (not the key), is emitted and the selected key from that value is saved for future comparisons against other keys.
Examples
--------
A very basic example with no `[comparator](distinctuntilchanged#comparator)`. Note that `1` is emitted more than once, because it's distinct in comparison to the *previously emitted* value, not in comparison to *all other emitted values*.
```
import { of, distinctUntilChanged } from 'rxjs';
of(1, 1, 1, 2, 2, 2, 1, 1, 3, 3)
.pipe(distinctUntilChanged())
.subscribe(console.log);
// Logs: 1, 2, 1, 3
```
With a `[comparator](distinctuntilchanged#comparator)`, you can do custom comparisons. Let's say you only want to emit a value when all of its components have changed:
```
import { of, distinctUntilChanged } from 'rxjs';
const totallyDifferentBuilds$ = of(
{ engineVersion: '1.1.0', transmissionVersion: '1.2.0' },
{ engineVersion: '1.1.0', transmissionVersion: '1.4.0' },
{ engineVersion: '1.3.0', transmissionVersion: '1.4.0' },
{ engineVersion: '1.3.0', transmissionVersion: '1.5.0' },
{ engineVersion: '2.0.0', transmissionVersion: '1.5.0' }
).pipe(
distinctUntilChanged((prev, curr) => {
return (
prev.engineVersion === curr.engineVersion ||
prev.transmissionVersion === curr.transmissionVersion
);
})
);
totallyDifferentBuilds$.subscribe(console.log);
// Logs:
// { engineVersion: '1.1.0', transmissionVersion: '1.2.0' }
// { engineVersion: '1.3.0', transmissionVersion: '1.4.0' }
// { engineVersion: '2.0.0', transmissionVersion: '1.5.0' }
```
You can also provide a custom `[comparator](distinctuntilchanged#comparator)` to check that emitted changes are only in one direction. Let's say you only want to get the next record temperature:
```
import { of, distinctUntilChanged } from 'rxjs';
const temps$ = of(30, 31, 20, 34, 33, 29, 35, 20);
const recordHighs$ = temps$.pipe(
distinctUntilChanged((prevHigh, temp) => {
// If the current temp is less than
// or the same as the previous record,
// the record hasn't changed.
return temp <= prevHigh;
})
);
recordHighs$.subscribe(console.log);
// Logs: 30, 31, 34, 35
```
Selecting update events only when the `updatedBy` field shows the account changed hands.
```
import { of, distinctUntilChanged } from 'rxjs';
// A stream of updates to a given account
const accountUpdates$ = of(
{ updatedBy: 'blesh', data: [] },
{ updatedBy: 'blesh', data: [] },
{ updatedBy: 'ncjamieson', data: [] },
{ updatedBy: 'ncjamieson', data: [] },
{ updatedBy: 'blesh', data: [] }
);
// We only want the events where it changed hands
const changedHands$ = accountUpdates$.pipe(
distinctUntilChanged(undefined, update => update.updatedBy)
);
changedHands$.subscribe(console.log);
// Logs:
// { updatedBy: 'blesh', data: Array[0] }
// { updatedBy: 'ncjamieson', data: Array[0] }
// { updatedBy: 'blesh', data: Array[0] }
```
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
rxjs findIndex findIndex
=========
`function` `stable` `operator`
Emits only the index of the first value emitted by the source Observable that meets some condition.
### `findIndex<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | A function called with each item to test for condition matching. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, number>`: A function that returns an Observable that emits the index of the first item that matches the condition.
Description
-----------
It's like [`find`](find), but emits the index of the found value, not the value itself.
`[findIndex](findindex)` searches for the first item in the source Observable that matches the specified condition embodied by the `predicate`, and returns the (zero-based) index of the first occurrence in the source. Unlike [`first`](first), the `predicate` is required in `[findIndex](findindex)`, and does not emit an error if a valid value is not found.
Example
-------
Emit the index of first click that happens on a DIV element
```
import { fromEvent, findIndex } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(findIndex(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `findIndex(predicate: BooleanConstructor): OperatorFunction<T, T extends Falsy ? -1 : number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T extends [Falsy](../type-alias/falsy) ? -1 : number>`
### `findIndex(predicate: BooleanConstructor, thisArg: any): OperatorFunction<T, T extends Falsy ? -1 : number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `thisArg` | `any` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T extends [Falsy](../type-alias/falsy) ? -1 : number>`
### `findIndex(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, number>`
### `findIndex(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, number>`
See Also
--------
* [`filter`](filter)
* [`find`](find)
* [`first`](first)
* [`take`](take)
rxjs take take
====
`function` `stable` `operator`
Emits only the first `<count>` values emitted by the source Observable.
### `take<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `<count>` | `number` | The maximum number of `next` values to emit. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits only the first `<count>` values emitted by the source Observable, or all of the values from the source if the source emits fewer than `<count>` values.
Description
-----------
Takes the first `<count>` values from the source, then completes.
`<take>` returns an Observable that emits only the first `<count>` values emitted by the source Observable. If the source emits fewer than `<count>` values then all of its values are emitted. After that, it completes, regardless if the source completes.
Example
-------
Take the first 5 seconds of an infinite 1-second interval Observable
```
import { interval, take } from 'rxjs';
const intervalCount = interval(1000);
const takeFive = intervalCount.pipe(take(5));
takeFive.subscribe(x => console.log(x));
// Logs:
// 0
// 1
// 2
// 3
// 4
```
See Also
--------
* [`takeLast`](takelast)
* [`takeUntil`](takeuntil)
* [`takeWhile`](takewhile)
* [`skip`](skip)
rxjs toArray toArray
=======
`function` `stable` `operator`
Collects all source emissions and emits them as an array when the source completes.
### `toArray<T>(): OperatorFunction<T, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable that emits an array of items emitted by the source Observable when source completes.
Description
-----------
Get all values inside an array when the source completes
`[toArray](toarray)` will wait until the source Observable completes before emitting the array containing all emissions. When the source Observable errors no array will be emitted.
Example
-------
```
import { interval, take, toArray } from 'rxjs';
const source = interval(1000);
const example = source.pipe(
take(10),
toArray()
);
example.subscribe(value => console.log(value));
// output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
```
rxjs window window
======
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable whenever `windowBoundaries` emits.
### `window<T>(windowBoundaries: Observable<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowBoundaries` | `[Observable](../class/observable)<any>` | An Observable that completes the previous window and starts a new window. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`: A function that returns an Observable of windows, which are Observables emitting values of the source Observable.
Description
-----------
It's like [`buffer`](buffer), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits connected, non-overlapping windows. It emits the current window and opens a new one whenever the Observable `windowBoundaries` emits an item. Because each window is an Observable, the output is a higher-order Observable.
Example
-------
In every window of 1 second each, emit at most 2 click events
```
import { fromEvent, interval, window, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const sec = interval(1000);
const result = clicks.pipe(
window(sec),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`buffer`](buffer)
rxjs raceWith raceWith
========
`function` `stable` `operator`
Creates an Observable that mirrors the first source Observable to emit a next, error or complete notification from the combination of the Observable to which the operator is applied and supplied Observables.
### `raceWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Example
-------
```
import { interval, map, raceWith } from 'rxjs';
const obs1 = interval(7000).pipe(map(() => 'slow one'));
const obs2 = interval(3000).pipe(map(() => 'fast one'));
const obs3 = interval(5000).pipe(map(() => 'medium one'));
obs1
.pipe(raceWith(obs2, obs3))
.subscribe(winner => console.log(winner));
// Outputs
// a series of 'fast one'
```
rxjs last last
====
`function` `stable` `operator`
Returns an Observable that emits only the last item emitted by the source Observable. It optionally takes a predicate function as a parameter, in which case, rather than emitting the last item from the source Observable, the resulting Observable will emit the last item from the source Observable that satisfies the predicate.
### `last<T, D>(predicate?: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | Optional. Default is `undefined`. The condition any source emitted item has to satisfy. |
| `defaultValue` | `D` | Optional. Default is `undefined`. An optional default value to provide if last predicate isn't met or no values were emitted. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits only the last item satisfying the given condition from the source, or a NoSuchElementException if no such items are emitted.
#### Throws
`[EmptyError](../interface/emptyerror)` Delivers an EmptyError to the Observer's `error` callback if the Observable completes before any `next` notification was sent.
`Error` - Throws if no items that match the predicate are emitted by the source Observable.
Description
-----------
It will throw an error if the source completes without notification or one that matches the predicate. It returns the last value or if a predicate is provided last value that matches the predicate. It returns the given default value if no notification is emitted or matches the predicate.
Examples
--------
Last alphabet from the sequence
```
import { from, last } from 'rxjs';
const source = from(['x', 'y', 'z']);
const result = source.pipe(last());
result.subscribe(value => console.log(`Last alphabet: ${ value }`));
// Outputs
// Last alphabet: z
```
Default value when the value in the predicate is not matched
```
import { from, last } from 'rxjs';
const source = from(['x', 'y', 'z']);
const result = source.pipe(last(char => char === 'a', 'not found'));
result.subscribe(value => console.log(`'a' is ${ value }.`));
// Outputs
// 'a' is not found.
```
Overloads
---------
### `last(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `last(predicate: BooleanConstructor, defaultValue: D): OperatorFunction<T, TruthyTypesOf<T> | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T> | D>`
### `last(predicate?: null, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `null` | Optional. Default is `undefined`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`
### `last(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue?: S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => value is S` | |
| `defaultValue` | `S` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `last(predicate: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`
See Also
--------
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
* [`skipWhile`](skipwhile)
rxjs concatMapTo concatMapTo
===========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is merged multiple times in a serialized fashion on the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`concatMap`](concatmap) instead: `[concatMap](concatmap)(() => result)`
### `concatMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable of values merged together by joining the passed Observable with itself, one after the other, for each value emitted from the source.
Description
-----------
It's like [`concatMap`](concatmap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then flattens those resulting Observables into one single Observable, which is the output Observable. Each new `innerObservable` instance emitted on the output Observable is concatenated with the previous `innerObservable` instance.
**Warning:** if source values arrive endlessly and faster than their corresponding inner Observables can complete, it will result in memory issues as inner Observables amass in an unbounded buffer waiting for their turn to be subscribed to.
Note: `[concatMapTo](concatmapto)` is equivalent to `[mergeMapTo](mergemapto)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, concatMapTo, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
concatMapTo(interval(1000).pipe(take(4)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
Overloads
---------
### `concatMapTo(observable: O): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `concatMapTo(observable: O, resultSelector: undefined): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `concatMapTo(observable: O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concat`](concat)
* [`concatAll`](concatall)
* [`concatMap`](concatmap)
* [`mergeMapTo`](mergemapto)
* [`switchMapTo`](switchmapto)
| programming_docs |
rxjs merge merge
=====
`function` `stable`
### `merge<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `merge()`
#### Parameters
There are no parameters.
### `merge()`
#### Parameters
There are no parameters.
### `merge()`
#### Parameters
There are no parameters.
### `merge(...args: (number | SchedulerLike | Observable<unknown> | InteropObservable<unknown> | AsyncIterable<unknown> | PromiseLike<unknown> | ArrayLike<unknown> | Iterable<unknown> | ReadableStreamLike<unknown>)[]): Observable<unknown>`
Creates an output Observable which concurrently emits all values from every given input Observable.
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `(number | [SchedulerLike](../interface/schedulerlike) | [Observable](../class/observable)<unknown> | [InteropObservable](../interface/interopobservable)<unknown> | AsyncIterable<unknown> | PromiseLike<unknown> | ArrayLike<unknown> | Iterable<unknown> | [ReadableStreamLike](../interface/readablestreamlike)<unknown>)[]` | |
#### Returns
`[Observable](../class/observable)<unknown>`: an Observable that emits items that are the result of every input Observable.
Flattens multiple Observables together by blending their values into one Observable.
`<merge>` subscribes to each given input Observable (as arguments), and simply forwards (without doing any transformation) all the values from all the input Observables to the output Observable. The output Observable only completes once all input Observables have completed. Any error delivered by an input Observable will be immediately emitted on the output Observable.
Examples
--------
Merge together two Observables: 1s interval and clicks
```
import { merge, fromEvent, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const timer = interval(1000);
const clicksOrTimer = merge(clicks, timer);
clicksOrTimer.subscribe(x => console.log(x));
// Results in the following:
// timer will emit ascending values, one every second(1000ms) to console
// clicks logs MouseEvents to console everytime the "document" is clicked
// Since the two streams are merged you see these happening
// as they occur.
```
Merge together 3 Observables, but run only 2 concurrently
```
import { interval, take, merge } from 'rxjs';
const timer1 = interval(1000).pipe(take(10));
const timer2 = interval(2000).pipe(take(6));
const timer3 = interval(500).pipe(take(10));
const concurrent = 2; // the argument
const merged = merge(timer1, timer2, timer3, concurrent);
merged.subscribe(x => console.log(x));
// Results in the following:
// - First timer1 and timer2 will run concurrently
// - timer1 will emit a value every 1000ms for 10 iterations
// - timer2 will emit a value every 2000ms for 6 iterations
// - after timer1 hits its max iteration, timer2 will
// continue, and timer3 will start to run concurrently with timer2
// - when timer2 hits its max iteration it terminates, and
// timer3 will continue to emit a value every 500ms until it is complete
```
rxjs mergeMapTo mergeMapTo
==========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is merged multiple times in the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`mergeMap`](mergemap) instead: `[mergeMap](mergemap)(() => result)`
### `mergeMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: number | ((outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R), concurrent: number = Infinity): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `number | ((outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R)` | Optional. Default is `undefined`. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits items from the given `innerObservable`.
Description
-----------
It's like [`mergeMap`](mergemap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then merges those resulting Observables into one single Observable, which is the output Observable.
Example
-------
For each click event, start an interval Observable ticking every 1 second
```
import { fromEvent, mergeMapTo, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(mergeMapTo(interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`concatMapTo`](concatmapto)
* [`merge`](merge)
* [`mergeAll`](mergeall)
* [`mergeMap`](mergemap)
* [`mergeScan`](mergescan)
* [`switchMapTo`](switchmapto)
rxjs fromEvent fromEvent
=========
`function` `stable`
Creates an Observable that emits events of a specific type coming from the given event target.
### `fromEvent<T>(target: any, eventName: string, options?: EventListenerOptions | ((...args: any[]) => T), resultSelector?: (...args: any[]) => T): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `any` | The DOM EventTarget, Node.js EventEmitter, JQuery-like event target, NodeList or HTMLCollection to attach the event handler to. |
| `eventName` | `string` | The event name of interest, being emitted by the `target`. |
| `options` | `EventListenerOptions | ((...args: any[]) => T)` | Optional. Default is `undefined`. Options to pass through to addEventListener |
| `resultSelector` | `(...args: any[]) => T` | Optional. Default is `undefined`. |
#### Returns
`[Observable<T>](../class/observable)`:
Description
-----------
Creates an Observable from DOM events, or Node.js EventEmitter events or others.
`[fromEvent](fromevent)` accepts as a first argument event target, which is an object with methods for registering event handler functions. As a second argument it takes string that indicates type of event we want to listen for. `[fromEvent](fromevent)` supports selected types of event targets, which are described in detail below. If your event target does not match any of the ones listed, you should use [`fromEventPattern`](fromeventpattern), which can be used on arbitrary APIs. When it comes to APIs supported by `[fromEvent](fromevent)`, their methods for adding and removing event handler functions have different names, but they all accept a string describing event type and function itself, which will be called whenever said event happens.
Every time resulting Observable is subscribed, event handler function will be registered to event target on given event type. When that event fires, value passed as a first argument to registered function will be emitted by output Observable. When Observable is unsubscribed, function will be unregistered from event target.
Note that if event target calls registered function with more than one argument, second and following arguments will not appear in resulting stream. In order to get access to them, you can pass to `[fromEvent](fromevent)` optional project function, which will be called with all arguments passed to event handler. Output Observable will then emit value returned by project function, instead of the usual value.
Remember that event targets listed below are checked via duck typing. It means that no matter what kind of object you have and no matter what environment you work in, you can safely use `[fromEvent](fromevent)` on that object if it exposes described methods (provided of course they behave as was described above). So for example if Node.js library exposes event target which has the same method names as DOM EventTarget, `[fromEvent](fromevent)` is still a good choice.
If the API you use is more callback then event handler oriented (subscribed callback function fires only once and thus there is no need to manually unregister it), you should use [`bindCallback`](bindcallback) or [`bindNodeCallback`](bindnodecallback) instead.
`[fromEvent](fromevent)` supports following types of event targets:
**DOM EventTarget**
This is an object with `addEventListener` and `removeEventListener` methods.
In the browser, `addEventListener` accepts - apart from event type string and event handler function arguments - optional third parameter, which is either an object or boolean, both used for additional configuration how and when passed function will be called. When `[fromEvent](fromevent)` is used with event target of that type, you can provide this values as third parameter as well.
**Node.js EventEmitter**
An object with `addListener` and `removeListener` methods.
**JQuery-style event target**
An object with `on` and `off` methods
**DOM NodeList**
List of DOM Nodes, returned for example by `document.querySelectorAll` or `Node.childNodes`.
Although this collection is not event target in itself, `[fromEvent](fromevent)` will iterate over all Nodes it contains and install event handler function in every of them. When returned Observable is unsubscribed, function will be removed from all Nodes.
**DOM HtmlCollection**
Just as in case of NodeList it is a collection of DOM nodes. Here as well event handler function is installed and removed in each of elements.
Examples
--------
Emit clicks happening on the DOM document
```
import { fromEvent } from 'rxjs';
const clicks = fromEvent(document, 'click');
clicks.subscribe(x => console.log(x));
// Results in:
// MouseEvent object logged to console every time a click
// occurs on the document.
```
Use `addEventListener` with capture option
```
import { fromEvent } from 'rxjs';
const clicksInDocument = fromEvent(document, 'click', true); // note optional configuration parameter
// which will be passed to addEventListener
const clicksInDiv = fromEvent(someDivInDocument, 'click');
clicksInDocument.subscribe(() => console.log('document'));
clicksInDiv.subscribe(() => console.log('div'));
// By default events bubble UP in DOM tree, so normally
// when we would click on div in document
// "div" would be logged first and then "document".
// Since we specified optional `capture` option, document
// will catch event when it goes DOWN DOM tree, so console
// will log "document" and then "div".
```
Overloads
---------
### `fromEvent(target: HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>, eventName: string): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>` | |
| `eventName` | `string` | |
#### Returns
`[Observable<T>](../class/observable)`
### `fromEvent(target: HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>, eventName: string, resultSelector: (event: T) => R): Observable<R>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>` | |
| `eventName` | `string` | |
| `resultSelector` | `(event: T) => R` | |
#### Returns
`[Observable](../class/observable)<R>`
### `fromEvent(target: HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>, eventName: string, options: EventListenerOptions): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>` | |
| `eventName` | `string` | |
| `options` | `EventListenerOptions` | |
#### Returns
`[Observable<T>](../class/observable)`
### `fromEvent(target: HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>, eventName: string, options: EventListenerOptions, resultSelector: (event: T) => R): Observable<R>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `HasEventTargetAddRemove<T> | ArrayLike<HasEventTargetAddRemove<T>>` | |
| `eventName` | `string` | |
| `options` | `EventListenerOptions` | |
| `resultSelector` | `(event: T) => R` | |
#### Returns
`[Observable](../class/observable)<R>`
### `fromEvent(target: NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>, eventName: string): Observable<unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>` | |
| `eventName` | `string` | |
#### Returns
`[Observable](../class/observable)<unknown>`
### `fromEvent(target: NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>, eventName: string): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>` | |
| `eventName` | `string` | |
#### Returns
`[Observable<T>](../class/observable)`
### `fromEvent(target: NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>, eventName: string, resultSelector: (...args: any[]) => R): Observable<R>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeStyleEventEmitter | ArrayLike<NodeStyleEventEmitter>` | |
| `eventName` | `string` | |
| `resultSelector` | `(...args: any[]) => R` | |
#### Returns
`[Observable](../class/observable)<R>`
### `fromEvent(target: NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>, eventName: string): Observable<unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>` | |
| `eventName` | `string` | |
#### Returns
`[Observable](../class/observable)<unknown>`
### `fromEvent(target: NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>, eventName: string): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>` | |
| `eventName` | `string` | |
#### Returns
`[Observable<T>](../class/observable)`
### `fromEvent(target: NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>, eventName: string, resultSelector: (...args: any[]) => R): Observable<R>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `NodeCompatibleEventEmitter | ArrayLike<NodeCompatibleEventEmitter>` | |
| `eventName` | `string` | |
| `resultSelector` | `(...args: any[]) => R` | |
#### Returns
`[Observable](../class/observable)<R>`
### `fromEvent(target: JQueryStyleEventEmitter<any, T> | ArrayLike<JQueryStyleEventEmitter<any, T>>, eventName: string): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `JQueryStyleEventEmitter<any, T> | ArrayLike<JQueryStyleEventEmitter<any, T>>` | |
| `eventName` | `string` | |
#### Returns
`[Observable<T>](../class/observable)`
### `fromEvent(target: JQueryStyleEventEmitter<any, T> | ArrayLike<JQueryStyleEventEmitter<any, T>>, eventName: string, resultSelector: (value: T, ...args: any[]) => R): Observable<R>`
#### Parameters
| | | |
| --- | --- | --- |
| `target` | `JQueryStyleEventEmitter<any, T> | ArrayLike<JQueryStyleEventEmitter<any, T>>` | |
| `eventName` | `string` | |
| `resultSelector` | `(value: T, ...args: any[]) => R` | |
#### Returns
`[Observable](../class/observable)<R>`
See Also
--------
* [`bindCallback`](bindcallback)
* [`bindNodeCallback`](bindnodecallback)
* [`fromEventPattern`](fromeventpattern)
rxjs zipWith zipWith
=======
`function` `stable` `operator`
Subscribes to the source, and the observable inputs provided as arguments, and combines their values, by index, into arrays.
### `zipWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
What is meant by "combine by index": The first value from each will be made into a single array, then emitted, then the second value from each will be combined into a single array and emitted, then the third value from each will be combined into a single array and emitted, and so on.
This will continue until it is no longer able to combine values of the same index into an array.
After the last value from any one completed source is emitted in an array, the resulting observable will complete, as there is no way to continue "zipping" values together by index.
Use-cases for this operator are limited. There are memory concerns if one of the streams is emitting values at a much faster rate than the others. Usage should likely be limited to streams that emit at a similar pace, or finite streams of known length.
In many cases, authors want `[combineLatestWith](combinelatestwith)` and not `[zipWith](zipwith)`.
rxjs distinctUntilKeyChanged distinctUntilKeyChanged
=======================
`function` `stable` `operator`
Returns an Observable that emits all items emitted by the source Observable that are distinct by comparison from the previous item, using a property accessed by using the key provided to check if the two items are distinct.
### `distinctUntilKeyChanged<T, K extends keyof T>(key: K, compare?: (x: T[K], y: T[K]) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `K` | String key for object property lookup on each item. |
| `compare` | `(x: T[K], y: T[K]) => boolean` | Optional. Default is `undefined`. Optional comparison function called to test if an item is distinct from the previous item in the source. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values based on the key specified.
Description
-----------
If a comparator function is provided, then it will be called for each item to test for whether or not that value should be emitted.
If a comparator function is not provided, an equality check is used by default.
Examples
--------
An example comparing the name of persons
```
import { of, distinctUntilKeyChanged } from 'rxjs';
of(
{ age: 4, name: 'Foo' },
{ age: 7, name: 'Bar' },
{ age: 5, name: 'Foo' },
{ age: 6, name: 'Foo' }
).pipe(
distinctUntilKeyChanged('name')
)
.subscribe(x => console.log(x));
// displays:
// { age: 4, name: 'Foo' }
// { age: 7, name: 'Bar' }
// { age: 5, name: 'Foo' }
```
An example comparing the first letters of the name
```
import { of, distinctUntilKeyChanged } from 'rxjs';
of(
{ age: 4, name: 'Foo1' },
{ age: 7, name: 'Bar' },
{ age: 5, name: 'Foo2' },
{ age: 6, name: 'Foo3' }
).pipe(
distinctUntilKeyChanged('name', (x, y) => x.substring(0, 3) === y.substring(0, 3))
)
.subscribe(x => console.log(x));
// displays:
// { age: 4, name: 'Foo1' }
// { age: 7, name: 'Bar' }
// { age: 5, name: 'Foo2' }
```
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilChanged`](distinctuntilchanged)
rxjs never never
=====
`function` `deprecated`
Deprecation Notes
-----------------
Replaced with the [`NEVER`](../const/never) constant. Will be removed in v8.
### `never()`
#### Parameters
There are no parameters.
rxjs zipAll zipAll
======
`function` `stable` `operator`
### `zipAll<T, R>(project?: (...values: T[]) => R)`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | Optional. Default is `undefined`. |
Overloads
---------
### `zipAll(): OperatorFunction<ObservableInput<T>, T[]>`
Collects all observable inner sources from the source, once the source completes, it will subscribe to all inner sources, combining their values by index and emitting them.
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<[ObservableInput](../type-alias/observableinput)<T>, T[]>`
### `zipAll(): OperatorFunction<any, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<any, T[]>`
### `zipAll(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<[ObservableInput](../type-alias/observableinput)<T>, R>`
### `zipAll(project: (...values: any[]) => R): OperatorFunction<any, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<any, R>`
| programming_docs |
rxjs firstValueFrom firstValueFrom
==============
`function` `stable`
Converts an observable to a promise by subscribing to the observable, and returning a promise that will resolve as soon as the first value arrives from the observable. The subscription will then be closed.
### `firstValueFrom<T, D>(source: Observable<T>, config?: FirstValueFromConfig<D>): Promise<T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[Observable<T>](../class/observable)` | the observable to convert to a promise |
| `[config](../const/config)` | `FirstValueFromConfig<D>` | Optional. Default is `undefined`. a configuration object to define the `defaultValue` to use if the source completes without emitting a value |
#### Returns
`Promise<T | D>`
Description
-----------
If the observable stream completes before any values were emitted, the returned promise will reject with [`EmptyError`](../interface/emptyerror) or will resolve with the default value if a default was specified.
If the observable stream emits an error, the returned promise will reject with that error.
**WARNING**: Only use this with observables you *know* will emit at least one value, *OR* complete. If the source observable does not emit one value or complete, you will end up with a promise that is hung up, and potentially all of the state of an async function hanging out in memory. To avoid this situation, look into adding something like [`timeout`](timeout), [`take`](take), [`takeWhile`](takewhile), or [`takeUntil`](takeuntil) amongst others.
Example
-------
Wait for the first value from a stream and emit it from a promise in an async function
```
import { interval, firstValueFrom } from 'rxjs';
async function execute() {
const source$ = interval(2000);
const firstNumber = await firstValueFrom(source$);
console.log(`The first number is ${ firstNumber }`);
}
execute();
// Expected output:
// 'The first number is 0'
```
See Also
--------
* [`lastValueFrom`](lastvaluefrom)
rxjs switchScan switchScan
==========
`function` `stable` `operator`
Applies an accumulator function over the source Observable where the accumulator function itself returns an Observable, emitting values only from the most recently returned Observable.
### `switchScan<T, R, O extends ObservableInput<any>>(accumulator: (acc: R, value: T, index: number) => O, seed: R): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: R, value: T, index: number) => O` | The accumulator function called on each source value. |
| `seed` | `R` | The initial accumulation value. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an observable of the accumulated values.
Description
-----------
It's like [`mergeScan`](mergescan), but only the most recent Observable returned by the accumulator is merged into the outer Observable.
See Also
--------
* [`scan`](scan)
* [`mergeScan`](mergescan)
* [`switchMap`](switchmap)
rxjs scan scan
====
`function` `stable` `operator`
Useful for encapsulating and managing state. Applies an accumulator (or "reducer function") to each value from the source after an initial state is established -- either via a `seed` value (second argument), or from the first value from the source.
### `scan<V, A, S>(accumulator: (acc: V | A | S, value: V, index: number) => A, seed?: S): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A | S, value: V, index: number) => A` | A "reducer function". This will be called for each value after an initial state is acquired. |
| `seed` | `S` | Optional. Default is `undefined`. The initial state. If this is not provided, the first value from the source will be used as the initial state, and emitted without going through the accumulator. All subsequent values will be processed by the accumulator function. If this is provided, all values will go through the accumulator function. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, V | A>`: A function that returns an Observable of the accumulated values.
Description
-----------
It's like [`reduce`](reduce), but emits the current accumulation state after each update
This operator maintains an internal state and emits it after processing each value as follows:
1. First value arrives
* If a `seed` value was supplied (as the second argument to `<scan>`), let `state = seed` and `value = firstValue`.
* If NO `seed` value was supplied (no second argument), let `state = firstValue` and go to 3.
2. Let `state = accumulator(state, value)`.
* If an error is thrown by `accumulator`, notify the consumer of an error. The process ends.
3. Emit `state`.
4. Next value arrives, let `value = nextValue`, go to 2.
Examples
--------
An average of previous numbers. This example shows how not providing a `seed` can prime the stream with the first value from the source.
```
import { of, scan, map } from 'rxjs';
const numbers$ = of(1, 2, 3);
numbers$
.pipe(
// Get the sum of the numbers coming in.
scan((total, n) => total + n),
// Get the average by dividing the sum by the total number
// received so var (which is 1 more than the zero-based index).
map((sum, index) => sum / (index + 1))
)
.subscribe(console.log);
```
The Fibonacci sequence. This example shows how you can use a seed to prime accumulation process. Also... you know... Fibonacci. So important to like, computers and stuff that its whiteboarded in job interviews. Now you can show them the Rx version! (Please don't, haha)
```
import { interval, scan, map, startWith } from 'rxjs';
const firstTwoFibs = [0, 1];
// An endless stream of Fibonacci numbers.
const fibonacci$ = interval(1000).pipe(
// Scan to get the fibonacci numbers (after 0, 1)
scan(([a, b]) => [b, a + b], firstTwoFibs),
// Get the second number in the tuple, it's the one you calculated
map(([, n]) => n),
// Start with our first two digits :)
startWith(...firstTwoFibs)
);
fibonacci$.subscribe(console.log);
```
Overloads
---------
### `scan(accumulator: (acc: V | A, value: V, index: number) => A): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, V | A>`
### `scan(accumulator: (acc: A, value: V, index: number) => A, seed: A): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A, value: V, index: number) => A` | |
| `seed` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, A>`
### `scan(accumulator: (acc: A | S, value: V, index: number) => A, seed: S): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A | S, value: V, index: number) => A` | |
| `seed` | `S` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, A>`
See Also
--------
* [`expand`](expand)
* [`mergeScan`](mergescan)
* [`reduce`](reduce)
* [`switchScan`](switchscan)
rxjs timestamp timestamp
=========
`function` `stable` `operator`
Attaches a timestamp to each item emitted by an observable indicating when it was emitted
### `timestamp<T>(timestampProvider: TimestampProvider = dateTimestampProvider): OperatorFunction<T, Timestamp<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `dateTimestampProvider`. An object with a `now()` method used to get the current timestamp. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Timestamp](../interface/timestamp)<T>>`: A function that returns an Observable that attaches a timestamp to each item emitted by the source Observable indicating when it was emitted.
Description
-----------
The `<timestamp>` operator maps the *source* observable stream to an object of type `{value: T, <timestamp>: R}`. The properties are generically typed. The `value` property contains the value and type of the *source* observable. The `<timestamp>` is generated by the schedulers `now` function. By default, it uses the `[asyncScheduler](../const/asyncscheduler)` which simply returns `Date.now()` (milliseconds since 1970/01/01 00:00:00:000) and therefore is of type `number`.
Example
-------
In this example there is a timestamp attached to the document's click events
```
import { fromEvent, timestamp } from 'rxjs';
const clickWithTimestamp = fromEvent(document, 'click').pipe(
timestamp()
);
// Emits data of type { value: PointerEvent, timestamp: number }
clickWithTimestamp.subscribe(data => {
console.log(data);
});
```
rxjs fromEventPattern fromEventPattern
================
`function` `stable`
Creates an Observable from an arbitrary API for registering event handlers.
### `fromEventPattern<T>(addHandler: (handler: NodeEventHandler) => any, removeHandler?: (handler: NodeEventHandler, signal?: any) => void, resultSelector?: (...args: any[]) => T): Observable<T | T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `addHandler` | `(handler: NodeEventHandler) => any` | A function that takes a `handler` function as argument and attaches it somehow to the actual source of events. |
| `removeHandler` | `(handler: NodeEventHandler, signal?: any) => void` | Optional. Default is `undefined`. A function that takes a `handler` function as an argument and removes it from the event source. If `addHandler` returns some kind of token, `removeHandler` function will have it as a second parameter. |
| `resultSelector` | `(...args: any[]) => T` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<T | T[]>`: Observable which, when an event happens, emits first parameter passed to registered event handler. Alternatively it emits whatever project function returns at that moment.
Description
-----------
When that method for adding event handler was something [`fromEvent`](fromevent) was not prepared for.
`[fromEventPattern](fromeventpattern)` allows you to convert into an Observable any API that supports registering handler functions for events. It is similar to [`fromEvent`](fromevent), but far more flexible. In fact, all use cases of [`fromEvent`](fromevent) could be easily handled by `[fromEventPattern](fromeventpattern)` (although in slightly more verbose way).
This operator accepts as a first argument an `addHandler` function, which will be injected with handler parameter. That handler is actually an event handler function that you now can pass to API expecting it. `addHandler` will be called whenever Observable returned by the operator is subscribed, so registering handler in API will not necessarily happen when `[fromEventPattern](fromeventpattern)` is called.
After registration, every time an event that we listen to happens, Observable returned by `[fromEventPattern](fromeventpattern)` will emit value that event handler function was called with. Note that if event handler was called with more than one argument, second and following arguments will not appear in the Observable.
If API you are using allows to unregister event handlers as well, you can pass to `[fromEventPattern](fromeventpattern)` another function - `removeHandler` - as a second parameter. It will be injected with the same handler function as before, which now you can use to unregister it from the API. `removeHandler` will be called when consumer of resulting Observable unsubscribes from it.
In some APIs unregistering is actually handled differently. Method registering an event handler returns some kind of token, which is later used to identify which function should be unregistered or it itself has method that unregisters event handler. If that is the case with your API, make sure token returned by registering method is returned by `addHandler`. Then it will be passed as a second argument to `removeHandler`, where you will be able to use it.
If you need access to all event handler parameters (not only the first one), or you need to transform them in any way, you can call `[fromEventPattern](fromeventpattern)` with optional third parameter - project function which will accept all arguments passed to event handler when it is called. Whatever is returned from project function will appear on resulting stream instead of usual event handlers first argument. This means that default project can be thought of as function that takes its first parameter and ignores the rest.
Examples
--------
Emits clicks happening on the DOM document
```
import { fromEventPattern } from 'rxjs';
function addClickHandler(handler) {
document.addEventListener('click', handler);
}
function removeClickHandler(handler) {
document.removeEventListener('click', handler);
}
const clicks = fromEventPattern(
addClickHandler,
removeClickHandler
);
clicks.subscribe(x => console.log(x));
// Whenever you click anywhere in the browser, DOM MouseEvent
// object will be logged.
```
Use with API that returns cancellation token
```
import { fromEventPattern } from 'rxjs';
const token = someAPI.registerEventHandler(function() {});
someAPI.unregisterEventHandler(token); // this APIs cancellation method accepts
// not handler itself, but special token.
const someAPIObservable = fromEventPattern(
function(handler) { return someAPI.registerEventHandler(handler); }, // Note that we return the token here...
function(handler, token) { someAPI.unregisterEventHandler(token); } // ...to then use it here.
);
```
Use with project function
```
import { fromEventPattern } from 'rxjs';
someAPI.registerEventHandler((eventType, eventMessage) => {
console.log(eventType, eventMessage); // Logs 'EVENT_TYPE' 'EVENT_MESSAGE' to console.
});
const someAPIObservable = fromEventPattern(
handler => someAPI.registerEventHandler(handler),
handler => someAPI.unregisterEventHandler(handler)
(eventType, eventMessage) => eventType + ' --- ' + eventMessage // without that function only 'EVENT_TYPE'
); // would be emitted by the Observable
someAPIObservable.subscribe(value => console.log(value));
// Logs:
// 'EVENT_TYPE --- EVENT_MESSAGE'
```
See Also
--------
* [`fromEvent`](fromevent)
* [`bindCallback`](bindcallback)
* [`bindNodeCallback`](bindnodecallback)
rxjs interval interval
========
`function` `stable`
Creates an Observable that emits sequential numbers every specified interval of time, on a specified [`SchedulerLike`](../interface/schedulerlike).
### `interval(period: number = 0, scheduler: SchedulerLike = asyncScheduler): Observable<number>`
#### Parameters
| | | |
| --- | --- | --- |
| `period` | `number` | Optional. Default is `0`. The interval size in milliseconds (by default) or the time unit determined by the scheduler's clock. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for scheduling the emission of values, and providing a notion of "time". |
#### Returns
`[Observable](../class/observable)<number>`: An Observable that emits a sequential number each time interval.
Description
-----------
Emits incremental numbers periodically in time.
`<interval>` returns an Observable that emits an infinite sequence of ascending integers, with a constant interval of time of your choosing between those emissions. The first emission is not sent immediately, but only after the first period has passed. By default, this operator uses the `[async](../const/async)` [`SchedulerLike`](../interface/schedulerlike) to provide a notion of time, but you may pass any [`SchedulerLike`](../interface/schedulerlike) to it.
Example
-------
Emits ascending numbers, one every second (1000ms) up to the number 3
```
import { interval, take } from 'rxjs';
const numbers = interval(1000);
const takeFourNumbers = numbers.pipe(take(4));
takeFourNumbers.subscribe(x => console.log('Next: ', x));
// Logs:
// Next: 0
// Next: 1
// Next: 2
// Next: 3
```
See Also
--------
* [`timer`](timer)
* [`delay`](delay)
rxjs windowTime windowTime
==========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable periodically in time.
### `windowTime<T>(windowTimeSpan: number, ...otherArgs: any[]): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | The amount of time, in milliseconds, to fill each window. |
| `otherArgs` | `any[]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferTime`](buffertime), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable starts a new window periodically, as determined by the `windowCreationInterval` argument. It emits each window after a fixed timespan, specified by the `windowTimeSpan` argument. When the source Observable completes or encounters an error, the output Observable emits the current window and propagates the notification from the source Observable. If `windowCreationInterval` is not provided, the output Observable starts a new window when the previous window of duration `windowTimeSpan` completes. If `maxWindowCount` is provided, each window will emit at most fixed number of values. Window will complete immediately after emitting last value and next one still will open as specified by `windowTimeSpan` and `windowCreationInterval` arguments.
Examples
--------
In every window of 1 second each, emit at most 2 click events
```
import { fromEvent, windowTime, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Every 5 seconds start a window 1 second long, and emit at most 2 click events per window
```
import { fromEvent, windowTime, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000, 5000),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Same as example above but with `maxWindowCount` instead of `<take>`
```
import { fromEvent, windowTime, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowTime(1000, 5000, 2), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Overloads
---------
### `windowTime(windowTimeSpan: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`
### `windowTime(windowTimeSpan: number, windowCreationInterval: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `windowCreationInterval` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`
### `windowTime(windowTimeSpan: number, windowCreationInterval: number | void, maxWindowSize: number, scheduler?: SchedulerLike): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowTimeSpan` | `number` | |
| `windowCreationInterval` | `number | void` | |
| `maxWindowSize` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`bufferTime`](buffertime)
| programming_docs |
rxjs expand expand
======
`function` `stable` `operator`
Recursively projects each source value to an Observable which is merged in the output Observable.
### `expand<T, O extends ObservableInput<unknown>>(project: (value: T, index: number) => O, concurrent: number = Infinity, scheduler?: SchedulerLike): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source or the output Observable, returns an Observable. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The [`SchedulerLike`](../interface/schedulerlike) to use for subscribing to each projected inner Observable. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that emits the source values and also result of applying the projection function to each value emitted on the output Observable and merging the results of the Observables obtained from this transformation.
Description
-----------
It's similar to [`mergeMap`](mergemap), but applies the projection function to every source value as well as every output value. It's recursive.
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an Observable, and then merging those resulting Observables and emitting the results of this merger. *Expand* will re-emit on the output Observable every source value. Then, each output value is given to the `project` function which returns an inner Observable to be merged on the output Observable. Those output values resulting from the projection are also given to the `project` function to produce new output values. This is how *expand* behaves recursively.
Example
-------
Start emitting the powers of two on every click, at most 10 of them
```
import { fromEvent, map, expand, of, delay, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const powersOfTwo = clicks.pipe(
map(() => 1),
expand(x => of(2 * x).pipe(delay(1000))),
take(10)
);
powersOfTwo.subscribe(x => console.log(x));
```
See Also
--------
* [`mergeMap`](mergemap)
* [`mergeScan`](mergescan)
rxjs bufferToggle bufferToggle
============
`function` `stable` `operator`
Buffers the source Observable values starting from an emission from `openings` and ending when the output of `closingSelector` emits.
### `bufferToggle<T, O>(openings: ObservableInput<O>, closingSelector: (value: O) => ObservableInput<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `openings` | `[ObservableInput](../type-alias/observableinput)<O>` | A Subscribable or Promise of notifications to start new buffers. |
| `closingSelector` | `(value: O) => [ObservableInput](../type-alias/observableinput)<any>` | A function that takes the value emitted by the `openings` observable and returns a Subscribable or Promise, which, when it emits, signals that the associated buffer should be emitted and cleared. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array. Starts collecting only when `opening` emits, and calls the `closingSelector` function to get an Observable that tells when to close the buffer.
Buffers values from the source by opening the buffer via signals from an Observable provided to `openings`, and closing and sending the buffers when a Subscribable or Promise returned by the `closingSelector` function emits.
Example
-------
Every other second, emit the click events from the next 500ms
```
import { fromEvent, interval, bufferToggle, EMPTY } from 'rxjs';
const clicks = fromEvent(document, 'click');
const openings = interval(1000);
const buffered = clicks.pipe(bufferToggle(openings, i =>
i % 2 ? interval(500) : EMPTY
));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferWhen`](bufferwhen)
* [`windowToggle`](windowtoggle)
rxjs defaultIfEmpty defaultIfEmpty
==============
`function` `stable` `operator`
Emits a given value if the source Observable completes without emitting any `next` value, otherwise mirrors the source Observable.
### `defaultIfEmpty<T, R>(defaultValue: R): OperatorFunction<T, T | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `defaultValue` | `R` | The default value used if the source Observable is empty. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | R>`: A function that returns an Observable that emits either the specified `defaultValue` if the source Observable emits no items, or the values emitted by the source Observable.
Description
-----------
If the source Observable turns out to be empty, then this operator will emit a default value.
`[defaultIfEmpty](defaultifempty)` emits the values emitted by the source Observable or a specified default value if the source Observable is empty (completes without having emitted any `next` value).
Example
-------
If no clicks happen in 5 seconds, then emit 'no clicks'
```
import { fromEvent, takeUntil, interval, defaultIfEmpty } from 'rxjs';
const clicks = fromEvent(document, 'click');
const clicksBeforeFive = clicks.pipe(takeUntil(interval(5000)));
const result = clicksBeforeFive.pipe(defaultIfEmpty('no clicks'));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`empty`](empty)
* [`last`](last)
rxjs isEmpty isEmpty
=======
`function` `stable` `operator`
Emits `false` if the input Observable emits any values, or emits `true` if the input Observable completes without emitting any values.
### `isEmpty<T>(): OperatorFunction<T, boolean>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, boolean>`: A function that returns an Observable that emits boolean value indicating whether the source Observable was empty or not.
Description
-----------
Tells whether any values are emitted by an Observable.
`[isEmpty](isempty)` transforms an Observable that emits values into an Observable that emits a single boolean value representing whether or not any values were emitted by the source Observable. As soon as the source Observable emits a value, `[isEmpty](isempty)` will emit a `false` and complete. If the source Observable completes having not emitted anything, `[isEmpty](isempty)` will emit a `true` and complete.
A similar effect could be achieved with [`count`](count), but `[isEmpty](isempty)` can emit a `false` value sooner.
Examples
--------
Emit `false` for a non-empty Observable
```
import { Subject, isEmpty } from 'rxjs';
const source = new Subject<string>();
const result = source.pipe(isEmpty());
source.subscribe(x => console.log(x));
result.subscribe(x => console.log(x));
source.next('a');
source.next('b');
source.next('c');
source.complete();
// Outputs
// 'a'
// false
// 'b'
// 'c'
```
Emit `true` for an empty Observable
```
import { EMPTY, isEmpty } from 'rxjs';
const result = EMPTY.pipe(isEmpty());
result.subscribe(x => console.log(x));
// Outputs
// true
```
See Also
--------
* [`count`](count)
* [`EMPTY`](../const/empty)
rxjs debounceTime debounceTime
============
`function` `stable` `operator`
Emits a notification from the source Observable only after a particular time span has passed without another source emission.
### `debounceTime<T>(dueTime: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `dueTime` | `number` | The timeout duration in milliseconds (or the time unit determined internally by the optional `scheduler`) for the window of time required to wait for emission silence before emitting the most recent source value. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for managing the timers that handle the timeout for each value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified `dueTime`, and may drop some values if they occur too frequently.
Description
-----------
It's like [`delay`](delay), but passes only the most recent notification from each burst of emissions.
`[debounceTime](debouncetime)` delays notifications emitted by the source Observable, but drops previous pending delayed emissions if a new notification arrives on the source Observable. This operator keeps track of the most recent notification from the source Observable, and emits that only when `dueTime` has passed without any other notification appearing on the source Observable. If a new value appears before `dueTime` silence occurs, the previous notification will be dropped and will not be emitted and a new `dueTime` is scheduled. If the completing event happens during `dueTime` the last cached notification is emitted before the completion event is forwarded to the output observable. If the error event happens during `dueTime` or after it only the error event is forwarded to the output observable. The cache notification is not emitted in this case.
This is a rate-limiting operator, because it is impossible for more than one notification to be emitted in any time window of duration `dueTime`, but it is also a delay-like operator since output emissions do not occur at the same time as they did on the source Observable. Optionally takes a [`SchedulerLike`](../interface/schedulerlike) for managing timers.
Example
-------
Emit the most recent click after a burst of clicks
```
import { fromEvent, debounceTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(debounceTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`auditTime`](audittime)
* [`debounce`](debounce)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
rxjs sampleTime sampleTime
==========
`function` `stable` `operator`
Emits the most recently emitted value from the source Observable within periodic time intervals.
### `sampleTime<T>(period: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `period` | `number` | The sampling period expressed in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for managing the timers that handle the sampling. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the results of sampling the values emitted by the source Observable at the specified time interval.
Description
-----------
Samples the source Observable at periodic time intervals, emitting what it samples.
`[sampleTime](sampletime)` periodically looks at the source Observable and emits whichever value it has most recently emitted since the previous sampling, unless the source has not emitted anything since the previous sampling. The sampling happens periodically in time every `period` milliseconds (or the time unit defined by the optional `scheduler` argument). The sampling starts as soon as the output Observable is subscribed.
Example
-------
Every second, emit the most recent click at most once
```
import { fromEvent, sampleTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(sampleTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sample`](sample)
* [`throttleTime`](throttletime)
rxjs iif iif
===
`function` `stable`
Checks a boolean at subscription time, and chooses between one of two observable sources
### `iif<T, F>(condition: () => boolean, trueResult: ObservableInput<T>, falseResult: ObservableInput<F>): Observable<T | F>`
#### Parameters
| | | |
| --- | --- | --- |
| `condition` | `() => boolean` | Condition which Observable should be chosen. |
| `trueResult` | `[ObservableInput](../type-alias/observableinput)<T>` | An Observable that will be subscribed if condition is true. |
| `falseResult` | `[ObservableInput](../type-alias/observableinput)<F>` | An Observable that will be subscribed if condition is false. |
#### Returns
`[Observable](../class/observable)<T | F>`: An observable that proxies to `trueResult` or `falseResult`, depending on the result of the `condition` function.
Description
-----------
`<iif>` expects a function that returns a boolean (the `condition` function), and two sources, the `trueResult` and the `falseResult`, and returns an Observable.
At the moment of subscription, the `condition` function is called. If the result is `true`, the subscription will be to the source passed as the `trueResult`, otherwise, the subscription will be to the source passed as the `falseResult`.
If you need to check more than two options to choose between more than one observable, have a look at the [`defer`](defer) creation method.
Examples
--------
Change at runtime which Observable will be subscribed
```
import { iif, of } from 'rxjs';
let subscribeToFirst;
const firstOrSecond = iif(
() => subscribeToFirst,
of('first'),
of('second')
);
subscribeToFirst = true;
firstOrSecond.subscribe(value => console.log(value));
// Logs:
// 'first'
subscribeToFirst = false;
firstOrSecond.subscribe(value => console.log(value));
// Logs:
// 'second'
```
Control access to an Observable
```
import { iif, of, EMPTY } from 'rxjs';
let accessGranted;
const observableIfYouHaveAccess = iif(
() => accessGranted,
of('It seems you have an access...'),
EMPTY
);
accessGranted = true;
observableIfYouHaveAccess.subscribe({
next: value => console.log(value),
complete: () => console.log('The end')
});
// Logs:
// 'It seems you have an access...'
// 'The end'
accessGranted = false;
observableIfYouHaveAccess.subscribe({
next: value => console.log(value),
complete: () => console.log('The end')
});
// Logs:
// 'The end'
```
See Also
--------
* [`defer`](defer)
rxjs publish publish
=======
`function` `deprecated` `operator`
Returns a ConnectableObservable, which is a variety of Observable that waits until its connect method is called before it begins emitting items to those Observers that have subscribed to it.
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `publish<T, R>(selector?: OperatorFunction<T, R>): MonoTypeOperatorFunction<T> | OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `[OperatorFunction<T, R>](../interface/operatorfunction)` | Optional. Default is `undefined`. Optional selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will receive all notifications of the source from the time of the subscription on. |
#### Returns
`[MonoTypeOperatorFunction](../interface/monotypeoperatorfunction)<T> | [OperatorFunction](../interface/operatorfunction)<T, R>`: A function that returns a ConnectableObservable that upon connection causes the source Observable to emit items to its Observers.
Description
-----------
Makes a cold Observable hot
Examples
--------
Make `source$` hot by applying `<publish>` operator, then merge each inner observable into a single one and subscribe
```
import { zip, interval, of, map, publish, merge, tap } from 'rxjs';
const source$ = zip(interval(2000), of(1, 2, 3, 4, 5, 6, 7, 8, 9))
.pipe(map(([, number]) => number));
source$
.pipe(
publish(multicasted$ =>
merge(
multicasted$.pipe(tap(x => console.log('Stream 1:', x))),
multicasted$.pipe(tap(x => console.log('Stream 2:', x))),
multicasted$.pipe(tap(x => console.log('Stream 3:', x)))
)
)
)
.subscribe();
// Results every two seconds
// Stream 1: 1
// Stream 2: 1
// Stream 3: 1
// ...
// Stream 1: 9
// Stream 2: 9
// Stream 3: 9
```
See Also
--------
* [`publishLast`](publishlast)
* [`publishReplay`](publishreplay)
* [`publishBehavior`](publishbehavior)
rxjs subscribeOn subscribeOn
===========
`function` `stable` `operator`
Asynchronously subscribes Observers to this Observable on the specified [`SchedulerLike`](../interface/schedulerlike).
### `subscribeOn<T>(scheduler: SchedulerLike, delay: number = 0): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | The [`SchedulerLike`](../interface/schedulerlike) to perform subscription actions on. |
| `<delay>` | `number` | Optional. Default is `0`. A delay to pass to the scheduler to delay subscriptions |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable modified so that its subscriptions happen on the specified [`SchedulerLike`](../interface/schedulerlike).
Description
-----------
With `[subscribeOn](subscribeon)` you can decide what type of scheduler a specific Observable will be using when it is subscribed to.
Schedulers control the speed and order of emissions to observers from an Observable stream.
Example
-------
Given the following code:
```
import { of, merge } from 'rxjs';
const a = of(1, 2, 3);
const b = of(4, 5, 6);
merge(a, b).subscribe(console.log);
// Outputs
// 1
// 2
// 3
// 4
// 5
// 6
```
Both Observable `a` and `b` will emit their values directly and synchronously once they are subscribed to.
If we instead use the `[subscribeOn](subscribeon)` operator declaring that we want to use the [`asyncScheduler`](../const/asyncscheduler) for values emitted by Observable `a`:
```
import { of, subscribeOn, asyncScheduler, merge } from 'rxjs';
const a = of(1, 2, 3).pipe(subscribeOn(asyncScheduler));
const b = of(4, 5, 6);
merge(a, b).subscribe(console.log);
// Outputs
// 4
// 5
// 6
// 1
// 2
// 3
```
The reason for this is that Observable `b` emits its values directly and synchronously like before but the emissions from `a` are scheduled on the event loop because we are now using the [`asyncScheduler`](../const/asyncscheduler) for that specific Observable.
rxjs windowWhen windowWhen
==========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable using a factory function of closing Observables to determine when to start a new window.
### `windowWhen<T>(closingSelector: () => ObservableInput<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingSelector` | `() => [ObservableInput](../type-alias/observableinput)<any>` | A function that takes no arguments and returns an Observable that signals (on either `next` or `complete`) when to close the previous window and start a new one. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferWhen`](bufferwhen), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits connected, non-overlapping windows. It emits the current window and opens a new one whenever the Observable produced by the specified `closingSelector` function emits an item. The first window is opened immediately when subscribing to the output Observable.
Example
-------
Emit only the first two clicks events in every window of [1-5] random seconds
```
import { fromEvent, windowWhen, interval, map, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowWhen(() => interval(1000 + Math.random() * 4000)),
map(win => win.pipe(take(2))), // take at most 2 emissions from each window
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`bufferWhen`](bufferwhen)
| programming_docs |
rxjs race race
====
`function` `stable`
### `race<T extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs delay delay
=====
`function` `stable` `operator`
Delays the emission of items from the source Observable by a given timeout or until a given Date.
### `delay<T>(due: number | Date, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `due` | `number | Date` | The delay duration in milliseconds (a `number`) or a `Date` until which the emission of the source items is delayed. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for managing the timers that handle the time-shift for each item. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified timeout or Date.
Description
-----------
Time shifts each item by some specified amount of milliseconds.
If the delay argument is a Number, this operator time shifts the source Observable by that amount of time expressed in milliseconds. The relative time intervals between the values are preserved.
If the delay argument is a Date, this operator time shifts the start of the Observable execution until the given date occurs.
Examples
--------
Delay each click by one second
```
import { fromEvent, delay } from 'rxjs';
const clicks = fromEvent(document, 'click');
const delayedClicks = clicks.pipe(delay(1000)); // each click emitted after 1 second
delayedClicks.subscribe(x => console.log(x));
```
Delay all clicks until a future date happens
```
import { fromEvent, delay } from 'rxjs';
const clicks = fromEvent(document, 'click');
const date = new Date('March 15, 2050 12:00:00'); // in the future
const delayedClicks = clicks.pipe(delay(date)); // click emitted only after that date
delayedClicks.subscribe(x => console.log(x));
```
See Also
--------
* [`delayWhen`](delaywhen)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
* [`debounce`](debounce)
* [`debounceTime`](debouncetime)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`audit`](audit)
* [`auditTime`](audittime)
rxjs repeat repeat
======
`function` `stable` `operator`
Returns an Observable that will resubscribe to the source stream when the source stream completes.
### `repeat<T>(countOrConfig?: number | RepeatConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `countOrConfig` | `number | RepeatConfig` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
Description
-----------
Repeats all values emitted on the source. It's like [`retry`](retry), but for non error cases.
Repeat will output values from a source until the source completes, then it will resubscribe to the source a specified number of times, with a specified delay. Repeat can be particularly useful in combination with closing operators like [`take`](take), [`takeUntil`](takeuntil), [`first`](first), or [`takeWhile`](takewhile), as it can be used to restart a source again from scratch.
Repeat is very similar to [`retry`](retry), where [`retry`](retry) will resubscribe to the source in the error case, but `<repeat>` will resubscribe if the source completes.
Note that `<repeat>` will *not* catch errors. Use [`retry`](retry) for that.
* `<repeat>(0)` returns an empty observable
* `<repeat>()` will repeat forever
* `<repeat>({ <delay>: 200 })` will repeat forever, with a delay of 200ms between repetitions.
* `<repeat>({ <count>: 2, <delay>: 400 })` will repeat twice, with a delay of 400ms between repetitions.
* `<repeat>({ <delay>: (<count>) => <timer>(<count> * 1000) })` will repeat forever, but will have a delay that grows by one second for each repetition.
Example
-------
Repeat a message stream
```
import { of, repeat } from 'rxjs';
const source = of('Repeat message');
const result = source.pipe(repeat(3));
result.subscribe(x => console.log(x));
// Results
// 'Repeat message'
// 'Repeat message'
// 'Repeat message'
```
Repeat 3 values, 2 times
```
import { interval, take, repeat } from 'rxjs';
const source = interval(1000);
const result = source.pipe(take(3), repeat(2));
result.subscribe(x => console.log(x));
// Results every second
// 0
// 1
// 2
// 0
// 1
// 2
```
Defining two complex repeats with delays on the same source. Note that the second repeat cannot be called until the first repeat as exhausted it's count.
```
import { defer, of, repeat } from 'rxjs';
const source = defer(() => {
return of(`Hello, it is ${new Date()}`)
});
source.pipe(
// Repeat 3 times with a delay of 1 second between repetitions
repeat({
count: 3,
delay: 1000,
}),
// *Then* repeat forever, but with an exponential step-back
// maxing out at 1 minute.
repeat({
delay: (count) => timer(Math.min(60000, 2 ^ count * 1000))
})
)
```
See Also
--------
* [`repeatWhen`](repeatwhen)
* [`retry`](retry)
rxjs sample sample
======
`function` `stable` `operator`
Emits the most recently emitted value from the source Observable whenever another Observable, the `notifier`, emits.
### `sample<T>(notifier: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[Observable](../class/observable)<any>` | The Observable to use for sampling the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the results of sampling the values emitted by the source Observable whenever the notifier Observable emits value or completes.
Description
-----------
It's like [`sampleTime`](sampletime), but samples whenever the `notifier` Observable emits something.
Whenever the `notifier` Observable emits a value, `<sample>` looks at the source Observable and emits whichever value it has most recently emitted since the previous sampling, unless the source has not emitted anything since the previous sampling. The `notifier` is subscribed to as soon as the output Observable is subscribed.
Example
-------
On every click, sample the most recent `seconds` timer
```
import { fromEvent, interval, sample } from 'rxjs';
const seconds = interval(1000);
const clicks = fromEvent(document, 'click');
const result = seconds.pipe(sample(clicks));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounce`](debounce)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
rxjs combineLatest combineLatest
=============
`function` `stable`
### `combineLatest<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `combineLatest(arg: T): Observable<unknown>`
You have passed `any` here, we can't figure out if it is an array or an object, so you're getting `unknown`. Use better types.
#### Parameters
| | | |
| --- | --- | --- |
| `arg` | `T` | Something typed as `any` |
#### Returns
`[Observable](../class/observable)<unknown>`
### `combineLatest(sources: []): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `sources` | `[]` | |
#### Returns
`[Observable](../class/observable)<never>`
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest()`
#### Parameters
There are no parameters.
### `combineLatest(sourcesObject: { [x: string]: never; }): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `sourcesObject` | `{ [x: string]: <never>; }` | |
#### Returns
`[Observable](../class/observable)<never>`
### `combineLatest(sourcesObject: T): Observable<{
[K in keyof T]: ObservedValueOf<T[K]>;
}>`
#### Parameters
| | | |
| --- | --- | --- |
| `sourcesObject` | `T` | |
#### Returns
`[Observable](../class/observable)<{ [K in keyof T]: [ObservedValueOf](../type-alias/observedvalueof)<T[K]>; }>`
### `combineLatest(...args: any[]): Observable<R> | Observable<ObservedValueOf<O>[]>`
Combines multiple Observables to create an Observable whose values are calculated from the latest values of each of its input Observables.
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `any[]` | |
#### Returns
`[Observable](../class/observable)<R> | [Observable](../class/observable)<[ObservedValueOf](../type-alias/observedvalueof)<O>[]>`: An Observable of projected values from the most recent values from each input Observable, or an array of the most recent values from each input Observable.
Whenever any input Observable emits a value, it computes a formula using the latest values from all the inputs, then emits the output of that formula.
`[combineLatest](combinelatest)` combines the values from all the Observables passed in the observables array. This is done by subscribing to each Observable in order and, whenever any Observable emits, collecting an array of the most recent values from each Observable. So if you pass `n` Observables to this operator, the returned Observable will always emit an array of `n` values, in an order corresponding to the order of the passed Observables (the value from the first Observable will be at index 0 of the array and so on).
Static version of `[combineLatest](combinelatest)` accepts an array of Observables. Note that an array of Observables is a good choice, if you don't know beforehand how many Observables you will combine. Passing an empty array will result in an Observable that completes immediately.
To ensure the output array always has the same length, `[combineLatest](combinelatest)` will actually wait for all input Observables to emit at least once, before it starts emitting results. This means if some Observable emits values before other Observables started emitting, all these values but the last will be lost. On the other hand, if some Observable does not emit a value but completes, resulting Observable will complete at the same moment without emitting anything, since it will now be impossible to include a value from the completed Observable in the resulting array. Also, if some input Observable does not emit any value and never completes, `[combineLatest](combinelatest)` will also never emit and never complete, since, again, it will wait for all streams to emit some value.
If at least one Observable was passed to `[combineLatest](combinelatest)` and all passed Observables emitted something, the resulting Observable will complete when all combined streams complete. So even if some Observable completes, the result of `[combineLatest](combinelatest)` will still emit values when other Observables do. In case of a completed Observable, its value from now on will always be the last emitted value. On the other hand, if any Observable errors, `[combineLatest](combinelatest)` will error immediately as well, and all other Observables will be unsubscribed.
Examples
--------
Combine two timer Observables
```
import { timer, combineLatest } from 'rxjs';
const firstTimer = timer(0, 1000); // emit 0, 1, 2... after every second, starting from now
const secondTimer = timer(500, 1000); // emit 0, 1, 2... after every second, starting 0,5s from now
const combinedTimers = combineLatest([firstTimer, secondTimer]);
combinedTimers.subscribe(value => console.log(value));
// Logs
// [0, 0] after 0.5s
// [1, 0] after 1s
// [1, 1] after 1.5s
// [2, 1] after 2s
```
Combine a dictionary of Observables
```
import { of, delay, startWith, combineLatest } from 'rxjs';
const observables = {
a: of(1).pipe(delay(1000), startWith(0)),
b: of(5).pipe(delay(5000), startWith(0)),
c: of(10).pipe(delay(10000), startWith(0))
};
const combined = combineLatest(observables);
combined.subscribe(value => console.log(value));
// Logs
// { a: 0, b: 0, c: 0 } immediately
// { a: 1, b: 0, c: 0 } after 1s
// { a: 1, b: 5, c: 0 } after 5s
// { a: 1, b: 5, c: 10 } after 10s
```
Combine an array of Observables
```
import { of, delay, startWith, combineLatest } from 'rxjs';
const observables = [1, 5, 10].map(
n => of(n).pipe(
delay(n * 1000), // emit 0 and then emit n after n seconds
startWith(0)
)
);
const combined = combineLatest(observables);
combined.subscribe(value => console.log(value));
// Logs
// [0, 0, 0] immediately
// [1, 0, 0] after 1s
// [1, 5, 0] after 5s
// [1, 5, 10] after 10s
```
Use map operator to dynamically calculate the Body-Mass Index
```
import { of, combineLatest, map } from 'rxjs';
const weight = of(70, 72, 76, 79, 75);
const height = of(1.76, 1.77, 1.78);
const bmi = combineLatest([weight, height]).pipe(
map(([w, h]) => w / (h * h)),
);
bmi.subscribe(x => console.log('BMI is ' + x));
// With output to console:
// BMI is 24.212293388429753
// BMI is 23.93948099205209
// BMI is 23.671253629592222
```
rxjs publishLast publishLast
===========
`function` `deprecated` `operator`
Returns a connectable observable sequence that shares a single subscription to the underlying sequence containing only the last notification.
Deprecation Notes
-----------------
Will be removed in v8. To create a connectable observable with an [`AsyncSubject`](../class/asyncsubject) under the hood, use [`connectable`](connectable). `source.pipe([publishLast](publishlast)())` is equivalent to `<connectable>(source, { connector: () => new [AsyncSubject](../class/asyncsubject)(), resetOnDisconnect: false })`. If you're using [`refCount`](refcount) after `[publishLast](publishlast)`, use the [`share`](share) operator instead. `source.pipe([publishLast](publishlast)(), [refCount](refcount)())` is equivalent to `source.pipe(<share>({ connector: () => new [AsyncSubject](../class/asyncsubject)(), resetOnError: false, resetOnComplete: false, resetOnRefCountZero: false }))`. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishLast<T>(): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
#### Parameters
There are no parameters.
#### Returns
`[UnaryFunction](../interface/unaryfunction)<[Observable](../class/observable)<T>, [ConnectableObservable](../class/connectableobservable)<T>>`: A function that returns an Observable that emits elements of a sequence produced by multicasting the source sequence.
Description
-----------
Similar to [`publish`](publish), but it waits until the source observable completes and stores the last emitted value. Similarly to [`publishReplay`](publishreplay) and [`publishBehavior`](publishbehavior), this keeps storing the last value even if it has no more subscribers. If subsequent subscriptions happen, they will immediately get that last stored value and complete.
Example
-------
```
import { ConnectableObservable, interval, publishLast, tap, take } from 'rxjs';
const connectable = <ConnectableObservable<number>>interval(1000)
.pipe(
tap(x => console.log('side effect', x)),
take(3),
publishLast()
);
connectable.subscribe({
next: x => console.log('Sub. A', x),
error: err => console.log('Sub. A Error', err),
complete: () => console.log('Sub. A Complete')
});
connectable.subscribe({
next: x => console.log('Sub. B', x),
error: err => console.log('Sub. B Error', err),
complete: () => console.log('Sub. B Complete')
});
connectable.connect();
// Results:
// 'side effect 0' - after one second
// 'side effect 1' - after two seconds
// 'side effect 2' - after three seconds
// 'Sub. A 2' - immediately after 'side effect 2'
// 'Sub. B 2'
// 'Sub. A Complete'
// 'Sub. B Complete'
```
See Also
--------
* [`ConnectableObservable`](../class/connectableobservable)
* [`publish`](publish)
* [`publishReplay`](publishreplay)
* [`publishBehavior`](publishbehavior)
rxjs defer defer
=====
`function` `stable`
Creates an Observable that, on subscribe, calls an Observable factory to make an Observable for each new Observer.
### `defer<R extends ObservableInput<any>>(observableFactory: () => R): Observable<ObservedValueOf<R>>`
#### Parameters
| | | |
| --- | --- | --- |
| `observableFactory` | `() => R` | The Observable factory function to invoke for each Observer that subscribes to the output Observable. May also return a Promise, which will be converted on the fly to an Observable. |
#### Returns
`[Observable](../class/observable)<[ObservedValueOf](../type-alias/observedvalueof)<R>>`: An Observable whose Observers' subscriptions trigger an invocation of the given Observable factory function.
Description
-----------
Creates the Observable lazily, that is, only when it is subscribed.
`<defer>` allows you to create an Observable only when the Observer subscribes. It waits until an Observer subscribes to it, calls the given factory function to get an Observable -- where a factory function typically generates a new Observable -- and subscribes the Observer to this Observable. In case the factory function returns a falsy value, then EMPTY is used as Observable instead. Last but not least, an exception during the factory function call is transferred to the Observer by calling `error`.
Example
-------
Subscribe to either an Observable of clicks or an Observable of interval, at random
```
import { defer, fromEvent, interval } from 'rxjs';
const clicksOrInterval = defer(() => {
return Math.random() > 0.5
? fromEvent(document, 'click')
: interval(1000);
});
clicksOrInterval.subscribe(x => console.log(x));
// Results in the following behavior:
// If the result of Math.random() is greater than 0.5 it will listen
// for clicks anywhere on the "document"; when document is clicked it
// will log a MouseEvent object to the console. If the result is less
// than 0.5 it will emit ascending numbers, one every second(1000ms).
```
See Also
--------
* [`Observable`](../class/observable)
rxjs animationFrames animationFrames
===============
`function` `stable`
An observable of animation frames
### `animationFrames(timestampProvider?: TimestampProvider)`
#### Parameters
| | | |
| --- | --- | --- |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `undefined`. An object with a `now` method that provides a numeric timestamp |
Description
-----------
Emits the amount of time elapsed since subscription and the timestamp on each animation frame. Defaults to milliseconds provided to the requestAnimationFrame's callback. Does not end on its own.
Every subscription will start a separate animation loop. Since animation frames are always scheduled by the browser to occur directly before a repaint, scheduling more than one animation frame synchronously should not be much different or have more overhead than looping over an array of events during a single animation frame. However, if for some reason the developer would like to ensure the execution of animation-related handlers are all executed during the same task by the engine, the `<share>` operator can be used.
This is useful for setting up animations with RxJS.
Examples
--------
Tweening a div to move it on the screen
```
import { animationFrames, map, takeWhile, endWith } from 'rxjs';
function tween(start: number, end: number, duration: number) {
const diff = end - start;
return animationFrames().pipe(
// Figure out what percentage of time has passed
map(({ elapsed }) => elapsed / duration),
// Take the vector while less than 100%
takeWhile(v => v < 1),
// Finish with 100%
endWith(1),
// Calculate the distance traveled between start and end
map(v => v * diff + start)
);
}
// Setup a div for us to move around
const div = document.createElement('div');
document.body.appendChild(div);
div.style.position = 'absolute';
div.style.width = '40px';
div.style.height = '40px';
div.style.backgroundColor = 'lime';
div.style.transform = 'translate3d(10px, 0, 0)';
tween(10, 200, 4000).subscribe(x => {
div.style.transform = `translate3d(${ x }px, 0, 0)`;
});
```
Providing a custom timestamp provider
```
import { animationFrames, TimestampProvider } from 'rxjs';
// A custom timestamp provider
let now = 0;
const customTSProvider: TimestampProvider = {
now() { return now++; }
};
const source$ = animationFrames(customTSProvider);
// Log increasing numbers 0...1...2... on every animation frame.
source$.subscribe(({ elapsed }) => console.log(elapsed));
```
| programming_docs |
rxjs observeOn observeOn
=========
`function` `stable` `operator`
Re-emits all notifications from source Observable with specified scheduler.
### `observeOn<T>(scheduler: SchedulerLike, delay: number = 0): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Scheduler that will be used to reschedule notifications from source Observable. |
| `<delay>` | `number` | Optional. Default is `0`. Number of milliseconds that states with what delay every notification should be rescheduled. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the same notifications as the source Observable, but with provided scheduler.
Description
-----------
Ensure a specific scheduler is used, from outside of an Observable.
`[observeOn](observeon)` is an operator that accepts a scheduler as a first parameter, which will be used to reschedule notifications emitted by the source Observable. It might be useful, if you do not have control over internal scheduler of a given Observable, but want to control when its values are emitted nevertheless.
Returned Observable emits the same notifications (nexted values, complete and error events) as the source Observable, but rescheduled with provided scheduler. Note that this doesn't mean that source Observables internal scheduler will be replaced in any way. Original scheduler still will be used, but when the source Observable emits notification, it will be immediately scheduled again - this time with scheduler passed to `[observeOn](observeon)`. An anti-pattern would be calling `[observeOn](observeon)` on Observable that emits lots of values synchronously, to split that emissions into asynchronous chunks. For this to happen, scheduler would have to be passed into the source Observable directly (usually into the operator that creates it). `[observeOn](observeon)` simply delays notifications a little bit more, to ensure that they are emitted at expected moments.
As a matter of fact, `[observeOn](observeon)` accepts second parameter, which specifies in milliseconds with what delay notifications will be emitted. The main difference between [`delay`](delay) operator and `[observeOn](observeon)` is that `[observeOn](observeon)` will delay all notifications - including error notifications - while `<delay>` will pass through error from source Observable immediately when it is emitted. In general it is highly recommended to use `<delay>` operator for any kind of delaying of values in the stream, while using `[observeOn](observeon)` to specify which scheduler should be used for notification emissions in general.
Example
-------
Ensure values in subscribe are called just before browser repaint
```
import { interval, observeOn, animationFrameScheduler } from 'rxjs';
const someDiv = document.createElement('div');
someDiv.style.cssText = 'width: 200px;background: #09c';
document.body.appendChild(someDiv);
const intervals = interval(10); // Intervals are scheduled
// with async scheduler by default...
intervals.pipe(
observeOn(animationFrameScheduler) // ...but we will observe on animationFrame
) // scheduler to ensure smooth animation.
.subscribe(val => {
someDiv.style.height = val + 'px';
});
```
See Also
--------
* [`delay`](delay)
rxjs pairwise pairwise
========
`function` `stable` `operator`
Groups pairs of consecutive emissions together and emits them as an array of two values.
### `pairwise<T>(): OperatorFunction<T, [T, T]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [T, T]>`: A function that returns an Observable of pairs (as arrays) of consecutive values from the source Observable.
Description
-----------
Puts the current value and previous value together as an array, and emits that.
The Nth emission from the source Observable will cause the output Observable to emit an array [(N-1)th, Nth] of the previous and the current value, as a pair. For this reason, `<pairwise>` emits on the second and subsequent emissions from the source Observable, but not on the first emission, because there is no previous value in that case.
Example
-------
On every click (starting from the second), emit the relative distance to the previous click
```
import { fromEvent, pairwise, map } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const pairs = clicks.pipe(pairwise());
const distance = pairs.pipe(
map(([first, second]) => {
const x0 = first.clientX;
const y0 = first.clientY;
const x1 = second.clientX;
const y1 = second.clientY;
return Math.sqrt(Math.pow(x0 - x1, 2) + Math.pow(y0 - y1, 2));
})
);
distance.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
rxjs finalize finalize
========
`function` `stable` `operator`
Returns an Observable that mirrors the source Observable, but will call a specified function when the source terminates on complete or error. The specified function will also be called when the subscriber explicitly unsubscribes.
### `finalize<T>(callback: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `callback` | `() => void` | Function to be called when source terminates. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source, but will call the specified function on termination.
Description
-----------
Examples
--------
Execute callback function when the observable completes
```
import { interval, take, finalize } from 'rxjs';
// emit value in sequence every 1 second
const source = interval(1000);
const example = source.pipe(
take(5), //take only the first 5 values
finalize(() => console.log('Sequence complete')) // Execute when the observable completes
);
const subscribe = example.subscribe(val => console.log(val));
// results:
// 0
// 1
// 2
// 3
// 4
// 'Sequence complete'
```
Execute callback function when the subscriber explicitly unsubscribes
```
import { interval, finalize, tap, noop, timer } from 'rxjs';
const source = interval(100).pipe(
finalize(() => console.log('[finalize] Called')),
tap({
next: () => console.log('[next] Called'),
error: () => console.log('[error] Not called'),
complete: () => console.log('[tap complete] Not called')
})
);
const sub = source.subscribe({
next: x => console.log(x),
error: noop,
complete: () => console.log('[complete] Not called')
});
timer(150).subscribe(() => sub.unsubscribe());
// results:
// '[next] Called'
// 0
// '[finalize] Called'
```
rxjs mapTo mapTo
=====
`function` `deprecated` `operator`
Emits the given constant value on the output Observable every time the source Observable emits a value.
Deprecation Notes
-----------------
To be removed in v9. Use [`map`](map) instead: `<map>(() => value)`.
### `mapTo<R>(value: R): OperatorFunction<unknown, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `R` | The value to map each source value to. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, R>`: A function that returns an Observable that emits the given `value` every time the source Observable emits.
Description
-----------
Like [`map`](map), but it maps every source value to the same output value every time.
Takes a constant `value` as argument, and emits that whenever the source Observable emits a value. In other words, ignores the actual source value, and simply uses the emission moment to know when to emit the given `value`.
Example
-------
Map every click to the string `'Hi'`
```
import { fromEvent, mapTo } from 'rxjs';
const clicks = fromEvent(document, 'click');
const greetings = clicks.pipe(mapTo('Hi'));
greetings.subscribe(x => console.log(x));
```
See Also
--------
* [`map`](map)
rxjs count count
=====
`function` `stable` `operator`
Counts the number of emissions on the source and emits that number when the source completes.
### `count<T>(predicate?: (value: T, index: number) => boolean): OperatorFunction<T, number>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | Optional. Default is `undefined`. A function that is used to analyze the value and the index and determine whether or not to increment the count. Return `true` to increment the count, and return `false` to keep the count the same. If the predicate is not provided, every value will be counted. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, number>`: A function that returns an Observable that emits one number that represents the count of emissions.
Description
-----------
Tells how many values were emitted, when the source completes.
`<count>` transforms an Observable that emits values into an Observable that emits a single value that represents the number of values emitted by the source Observable. If the source Observable terminates with an error, `<count>` will pass this error notification along without emitting a value first. If the source Observable does not terminate at all, `<count>` will neither emit a value nor terminate. This operator takes an optional `predicate` function as argument, in which case the output emission will represent the number of source values that matched `true` with the `predicate`.
Examples
--------
Counts how many seconds have passed before the first click happened
```
import { interval, fromEvent, takeUntil, count } from 'rxjs';
const seconds = interval(1000);
const clicks = fromEvent(document, 'click');
const secondsBeforeClick = seconds.pipe(takeUntil(clicks));
const result = secondsBeforeClick.pipe(count());
result.subscribe(x => console.log(x));
```
Counts how many odd numbers are there between 1 and 7
```
import { range, count } from 'rxjs';
const numbers = range(1, 7);
const result = numbers.pipe(count(i => i % 2 === 1));
result.subscribe(x => console.log(x));
// Results in:
// 4
```
See Also
--------
* [`max`](max)
* [`min`](min)
* [`reduce`](reduce)
rxjs refCount refCount
========
`function` `deprecated` `operator`
Make a [`ConnectableObservable`](../class/connectableobservable) behave like a ordinary observable and automates the way you can connect to it.
Deprecation Notes
-----------------
Replaced with the [`share`](share) operator. How `<share>` is used will depend on the connectable observable you created just prior to the `[refCount](refcount)` operator. Details: <https://rxjs.dev/deprecations/multicasting>
### `refCount<T>(): MonoTypeOperatorFunction<T>`
#### Parameters
There are no parameters.
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that automates the connection to ConnectableObservable.
Description
-----------
Internally it counts the subscriptions to the observable and subscribes (only once) to the source if the number of subscriptions is larger than 0. If the number of subscriptions is smaller than 1, it unsubscribes from the source. This way you can make sure that everything before the *published* refCount has only a single subscription independently of the number of subscribers to the target observable.
Note that using the [`share`](share) operator is exactly the same as using the `<multicast>(() => new [Subject](../class/subject)())` operator (making the observable hot) and the *refCount* operator in a sequence.
Example
-------
In the following example there are two intervals turned into connectable observables by using the *publish* operator. The first one uses the *refCount* operator, the second one does not use it. You will notice that a connectable observable does nothing until you call its connect function.
```
import { interval, tap, publish, refCount } from 'rxjs';
// Turn the interval observable into a ConnectableObservable (hot)
const refCountInterval = interval(400).pipe(
tap(num => console.log(`refCount ${ num }`)),
publish(),
refCount()
);
const publishedInterval = interval(400).pipe(
tap(num => console.log(`publish ${ num }`)),
publish()
);
refCountInterval.subscribe();
refCountInterval.subscribe();
// 'refCount 0' -----> 'refCount 1' -----> etc
// All subscriptions will receive the same value and the tap (and
// every other operator) before the `publish` operator will be executed
// only once per event independently of the number of subscriptions.
publishedInterval.subscribe();
// Nothing happens until you call .connect() on the observable.
```
See Also
--------
* [`ConnectableObservable`](../class/connectableobservable)
* [`share`](share)
* [`publish`](publish)
rxjs forkJoin forkJoin
========
`function` `stable`
### `forkJoin<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `forkJoin(arg: T): Observable<unknown>`
You have passed `any` here, we can't figure out if it is an array or an object, so you're getting `unknown`. Use better types.
#### Parameters
| | | |
| --- | --- | --- |
| `arg` | `T` | Something typed as `any` |
#### Returns
`[Observable](../class/observable)<unknown>`
### `forkJoin(scheduler: null): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `null` | |
#### Returns
`[Observable](../class/observable)<never>`
### `forkJoin(sources: any[]): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `sources` | `any[]` | |
#### Returns
`[Observable](../class/observable)<never>`
### `forkJoin()`
#### Parameters
There are no parameters.
### `forkJoin()`
#### Parameters
There are no parameters.
### `forkJoin()`
#### Parameters
There are no parameters.
### `forkJoin(sourcesObject: { [x: string]: never; }): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `sourcesObject` | `{ [x: string]: <never>; }` | |
#### Returns
`[Observable](../class/observable)<never>`
### `forkJoin(sourcesObject: T): Observable<{
[K in keyof T]: ObservedValueOf<T[K]>;
}>`
#### Parameters
| | | |
| --- | --- | --- |
| `sourcesObject` | `T` | |
#### Returns
`[Observable](../class/observable)<{ [K in keyof T]: [ObservedValueOf](../type-alias/observedvalueof)<T[K]>; }>`
### `forkJoin(...args: any[]): Observable<any>`
Accepts an `Array` of [`ObservableInput`](../type-alias/observableinput) or a dictionary `Object` of [`ObservableInput`](../type-alias/observableinput) and returns an [`Observable`](../class/observable) that emits either an array of values in the exact same order as the passed array, or a dictionary of values in the same shape as the passed dictionary.
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `any[]` | Any number of Observables provided either as an array or as an arguments passed directly to the operator. |
#### Returns
`[Observable](../class/observable)<any>`: Observable emitting either an array of last values emitted by passed Observables or value from project function.
Wait for Observables to complete and then combine last values they emitted; complete immediately if an empty array is passed.
`[forkJoin](forkjoin)` is an operator that takes any number of input observables which can be passed either as an array or a dictionary of input observables. If no input observables are provided (e.g. an empty array is passed), then the resulting stream will complete immediately.
`[forkJoin](forkjoin)` will wait for all passed observables to emit and complete and then it will emit an array or an object with last values from corresponding observables.
If you pass an array of `n` observables to the operator, then the resulting array will have `n` values, where the first value is the last one emitted by the first observable, second value is the last one emitted by the second observable and so on.
If you pass a dictionary of observables to the operator, then the resulting objects will have the same keys as the dictionary passed, with their last values they have emitted located at the corresponding key.
That means `[forkJoin](forkjoin)` will not emit more than once and it will complete after that. If you need to emit combined values not only at the end of the lifecycle of passed observables, but also throughout it, try out [`combineLatest`](combinelatest) or [`zip`](zip) instead.
In order for the resulting array to have the same length as the number of input observables, whenever any of the given observables completes without emitting any value, `[forkJoin](forkjoin)` will complete at that moment as well and it will not emit anything either, even if it already has some last values from other observables. Conversely, if there is an observable that never completes, `[forkJoin](forkjoin)` will never complete either, unless at any point some other observable completes without emitting a value, which brings us back to the previous case. Overall, in order for `[forkJoin](forkjoin)` to emit a value, all given observables have to emit something at least once and complete.
If any given observable errors at some point, `[forkJoin](forkjoin)` will error as well and immediately unsubscribe from the other observables.
Optionally `[forkJoin](forkjoin)` accepts a `resultSelector` function, that will be called with values which normally would land in the emitted array. Whatever is returned by the `resultSelector`, will appear in the output observable instead. This means that the default `resultSelector` can be thought of as a function that takes all its arguments and puts them into an array. Note that the `resultSelector` will be called only when `[forkJoin](forkjoin)` is supposed to emit a result.
Examples
--------
Use `[forkJoin](forkjoin)` with a dictionary of observable inputs
```
import { forkJoin, of, timer } from 'rxjs';
const observable = forkJoin({
foo: of(1, 2, 3, 4),
bar: Promise.resolve(8),
baz: timer(4000)
});
observable.subscribe({
next: value => console.log(value),
complete: () => console.log('This is how it ends!'),
});
// Logs:
// { foo: 4, bar: 8, baz: 0 } after 4 seconds
// 'This is how it ends!' immediately after
```
Use `[forkJoin](forkjoin)` with an array of observable inputs
```
import { forkJoin, of, timer } from 'rxjs';
const observable = forkJoin([
of(1, 2, 3, 4),
Promise.resolve(8),
timer(4000)
]);
observable.subscribe({
next: value => console.log(value),
complete: () => console.log('This is how it ends!'),
});
// Logs:
// [4, 8, 0] after 4 seconds
// 'This is how it ends!' immediately after
```
rxjs ignoreElements ignoreElements
==============
`function` `stable` `operator`
Ignores all items emitted by the source Observable and only passes calls of `complete` or `error`.
### `ignoreElements(): OperatorFunction<unknown, never>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, <never>>`: A function that returns an empty Observable that only calls `complete` or `error`, based on which one is called by the source Observable.
Description
-----------
The `[ignoreElements](ignoreelements)` operator suppresses all items emitted by the source Observable, but allows its termination notification (either `error` or `complete`) to pass through unchanged.
If you do not care about the items being emitted by an Observable, but you do want to be notified when it completes or when it terminates with an error, you can apply the `[ignoreElements](ignoreelements)` operator to the Observable, which will ensure that it will never call its observers’ `next` handlers.
Example
-------
Ignore all `next` emissions from the source
```
import { of, ignoreElements } from 'rxjs';
of('you', 'talking', 'to', 'me')
.pipe(ignoreElements())
.subscribe({
next: word => console.log(word),
error: err => console.log('error:', err),
complete: () => console.log('the end'),
});
// result:
// 'the end'
```
| programming_docs |
rxjs shareReplay shareReplay
===========
`function` `stable` `operator`
Share source and replay specified number of emissions on subscription.
### `shareReplay<T>(configOrBufferSize?: number | ShareReplayConfig, windowTime?: number, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `configOrBufferSize` | `number | ShareReplayConfig` | Optional. Default is `undefined`. |
| `[windowTime](windowtime)` | `number` | Optional. Default is `undefined`. Maximum time length of the replay buffer in milliseconds. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. Scheduler where connected observers within the selector function will be invoked on. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
Description
-----------
This operator is a specialization of `replay` that connects to a source observable and multicasts through a `[ReplaySubject](../class/replaysubject)` constructed with the specified arguments. A successfully completed source will stay cached in the `shareReplayed [observable](../const/observable)` forever, but an errored source can be retried.
Why use shareReplay?
--------------------
You generally want to use `[shareReplay](sharereplay)` when you have side-effects or taxing computations that you do not wish to be executed amongst multiple subscribers. It may also be valuable in situations where you know you will have late subscribers to a stream that need access to previously emitted values. This ability to replay values on subscription is what differentiates [`share`](share) and `[shareReplay](sharereplay)`.

Reference counting
------------------
By default `[shareReplay](sharereplay)` will use `[refCount](refcount)` of false, meaning that it will *not* unsubscribe the source when the reference counter drops to zero, i.e. the inner `[ReplaySubject](../class/replaysubject)` will *not* be unsubscribed (and potentially run for ever). This is the default as it is expected that `[shareReplay](sharereplay)` is often used to keep around expensive to setup observables which we want to keep running instead of having to do the expensive setup again.
As of RXJS version 6.4.0 a new overload signature was added to allow for manual control over what happens when the operators internal reference counter drops to zero. If `[refCount](refcount)` is true, the source will be unsubscribed from once the reference count drops to zero, i.e. the inner `[ReplaySubject](../class/replaysubject)` will be unsubscribed. All new subscribers will receive value emissions from a new `[ReplaySubject](../class/replaysubject)` which in turn will cause a new subscription to the source observable.
Examples
--------
Example with a third subscriber coming late to the party
```
import { interval, take, shareReplay } from 'rxjs';
const shared$ = interval(2000).pipe(
take(6),
shareReplay(3)
);
shared$.subscribe(x => console.log('sub A: ', x));
shared$.subscribe(y => console.log('sub B: ', y));
setTimeout(() => {
shared$.subscribe(y => console.log('sub C: ', y));
}, 11000);
// Logs:
// (after ~2000 ms)
// sub A: 0
// sub B: 0
// (after ~4000 ms)
// sub A: 1
// sub B: 1
// (after ~6000 ms)
// sub A: 2
// sub B: 2
// (after ~8000 ms)
// sub A: 3
// sub B: 3
// (after ~10000 ms)
// sub A: 4
// sub B: 4
// (after ~11000 ms, sub C gets the last 3 values)
// sub C: 2
// sub C: 3
// sub C: 4
// (after ~12000 ms)
// sub A: 5
// sub B: 5
// sub C: 5
```
Example for `[refCount](refcount)` usage
```
import { Observable, tap, interval, shareReplay, take } from 'rxjs';
const log = <T>(name: string, source: Observable<T>) => source.pipe(
tap({
subscribe: () => console.log(`${ name }: subscribed`),
next: value => console.log(`${ name }: ${ value }`),
complete: () => console.log(`${ name }: completed`),
finalize: () => console.log(`${ name }: unsubscribed`)
})
);
const obs$ = log('source', interval(1000));
const shared$ = log('shared', obs$.pipe(
shareReplay({ bufferSize: 1, refCount: true }),
take(2)
));
shared$.subscribe(x => console.log('sub A: ', x));
shared$.subscribe(y => console.log('sub B: ', y));
// PRINTS:
// shared: subscribed <-- reference count = 1
// source: subscribed
// shared: subscribed <-- reference count = 2
// source: 0
// shared: 0
// sub A: 0
// shared: 0
// sub B: 0
// source: 1
// shared: 1
// sub A: 1
// shared: completed <-- take(2) completes the subscription for sub A
// shared: unsubscribed <-- reference count = 1
// shared: 1
// sub B: 1
// shared: completed <-- take(2) completes the subscription for sub B
// shared: unsubscribed <-- reference count = 0
// source: unsubscribed <-- replaySubject unsubscribes from source observable because the reference count dropped to 0 and refCount is true
// In case of refCount being false, the unsubscribe is never called on the source and the source would keep on emitting, even if no subscribers
// are listening.
// source: 2
// source: 3
// source: 4
// ...
```
See Also
--------
* [`publish`](publish)
* [`share`](share)
* [`publishReplay`](publishreplay)
rxjs identity identity
========
`function` `stable`
This function takes one parameter and just returns it. Simply put, this is like `<T>(x: T): T => x`.
### `identity<T>(x: T): T`
#### Parameters
| | | |
| --- | --- | --- |
| `x` | `T` | Any value that is returned by this function |
#### Returns
`T`: The value passed as the first parameter to this function
Description
-----------
Examples
--------
This is useful in some cases when using things like `[mergeMap](mergemap)`
```
import { interval, take, map, range, mergeMap, identity } from 'rxjs';
const source$ = interval(1000).pipe(take(5));
const result$ = source$.pipe(
map(i => range(i)),
mergeMap(identity) // same as mergeMap(x => x)
);
result$.subscribe({
next: console.log
});
```
Or when you want to selectively apply an operator
```
import { interval, take, identity } from 'rxjs';
const shouldLimit = () => Math.random() < 0.5;
const source$ = interval(1000);
const result$ = source$.pipe(shouldLimit() ? take(5) : identity);
result$.subscribe({
next: console.log
});
```
rxjs combineLatestWith combineLatestWith
=================
`function` `stable` `operator`
Create an observable that combines the latest values from all passed observables and the source into arrays and emits them.
### `combineLatestWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Returns an observable, that when subscribed to, will subscribe to the source observable and all sources provided as arguments. Once all sources emit at least one value, all of the latest values will be emitted as an array. After that, every time any source emits a value, all of the latest values will be emitted as an array.
This is a useful operator for eagerly calculating values based off of changed inputs.
Example
-------
Simple concatenation of values from two inputs
```
import { fromEvent, combineLatestWith, map } from 'rxjs';
// Setup: Add two inputs to the page
const input1 = document.createElement('input');
document.body.appendChild(input1);
const input2 = document.createElement('input');
document.body.appendChild(input2);
// Get streams of changes
const input1Changes$ = fromEvent(input1, 'change');
const input2Changes$ = fromEvent(input2, 'change');
// Combine the changes by adding them together
input1Changes$.pipe(
combineLatestWith(input2Changes$),
map(([e1, e2]) => (<HTMLInputElement>e1.target).value + ' - ' + (<HTMLInputElement>e2.target).value)
)
.subscribe(x => console.log(x));
```
rxjs zip zip
===
`function` `stable`
### `zip<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `zip()`
#### Parameters
There are no parameters.
### `zip()`
#### Parameters
There are no parameters.
### `zip()`
#### Parameters
There are no parameters.
### `zip(...args: unknown[]): Observable<unknown>`
Combines multiple Observables to create an Observable whose values are calculated from the values, in order, of each of its input Observables.
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `unknown[]` | |
#### Returns
`[Observable](../class/observable)<unknown>`:
If the last parameter is a function, this function is used to compute the created value from the input values. Otherwise, an array of the input values is returned.
Example
-------
Combine age and name from different sources
```
import { of, zip, map } from 'rxjs';
const age$ = of(27, 25, 29);
const name$ = of('Foo', 'Bar', 'Beer');
const isDev$ = of(true, true, false);
zip(age$, name$, isDev$).pipe(
map(([age, name, isDev]) => ({ age, name, isDev }))
)
.subscribe(x => console.log(x));
// Outputs
// { age: 27, name: 'Foo', isDev: true }
// { age: 25, name: 'Bar', isDev: true }
// { age: 29, name: 'Beer', isDev: false }
```
rxjs generate generate
========
`function` `stable`
### `generate<T, S>(initialStateOrOptions: S | GenerateOptions<T, S>, condition?: ConditionFunc<S>, iterate?: IterateFunc<S>, resultSelectorOrScheduler?: SchedulerLike | ResultFunc<S, T>, scheduler?: SchedulerLike): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `initialStateOrOptions` | `S | GenerateOptions<T, S>` | |
| `condition` | `ConditionFunc<S>` | Optional. Default is `undefined`. |
| `iterate` | `IterateFunc<S>` | Optional. Default is `undefined`. |
| `resultSelectorOrScheduler` | `[SchedulerLike](../interface/schedulerlike) | ResultFunc<S, T>` | Optional. Default is `undefined`. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable<T>](../class/observable)`
Overloads
---------
### `generate(initialState: S, condition: ConditionFunc<S>, iterate: IterateFunc<S>, resultSelector: ResultFunc<S, T>, scheduler?: SchedulerLike): Observable<T>`
Generates an observable sequence by running a state-driven loop producing the sequence's elements, using the specified scheduler to send out observer messages.
#### Parameters
| | | |
| --- | --- | --- |
| `initialState` | `S` | Initial state. |
| `condition` | `ConditionFunc<S>` | Condition to terminate generation (upon returning false). |
| `iterate` | `IterateFunc<S>` | Iteration step function. |
| `resultSelector` | `ResultFunc<S, T>` | Selector function for results produced in the sequence. (deprecated) |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. A [`SchedulerLike`](../interface/schedulerlike) on which to run the generator loop. If not provided, defaults to emit immediately. |
#### Returns
`[Observable<T>](../class/observable)`: The generated sequence.
Examples
--------
Produces sequence of numbers
```
import { generate } from 'rxjs';
const result = generate(0, x => x < 3, x => x + 1, x => x);
result.subscribe(x => console.log(x));
// Logs:
// 0
// 1
// 2
```
Use `[asapScheduler](../const/asapscheduler)`
```
import { generate, asapScheduler } from 'rxjs';
const result = generate(1, x => x < 5, x => x * 2, x => x + 1, asapScheduler);
result.subscribe(x => console.log(x));
// Logs:
// 2
// 3
// 5
```
### `generate(initialState: S, condition: ConditionFunc<S>, iterate: IterateFunc<S>, scheduler?: SchedulerLike): Observable<S>`
Generates an Observable by running a state-driven loop that emits an element on each iteration.
#### Parameters
| | | |
| --- | --- | --- |
| `initialState` | `S` | Initial state. |
| `condition` | `ConditionFunc<S>` | Condition to terminate generation (upon returning false). |
| `iterate` | `IterateFunc<S>` | Iteration step function. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. A [`Scheduler`](../class/scheduler) on which to run the generator loop. If not provided, defaults to emitting immediately. |
#### Returns
`[Observable](../class/observable)<S>`: The generated sequence.
Use it instead of nexting values in a for loop.
`<generate>` allows you to create a stream of values generated with a loop very similar to a traditional for loop. The first argument of `<generate>` is a beginning value. The second argument is a function that accepts this value and tests if some condition still holds. If it does, then the loop continues, if not, it stops. The third value is a function which takes the previously defined value and modifies it in some way on each iteration. Note how these three parameters are direct equivalents of three expressions in a traditional for loop: the first expression initializes some state (for example, a numeric index), the second tests if the loop can perform the next iteration (for example, if the index is lower than 10) and the third states how the defined value will be modified on every step (for example, the index will be incremented by one).
Return value of a `<generate>` operator is an Observable that on each loop iteration emits a value. First of all, the condition function is ran. If it returns true, then the Observable emits the currently stored value (initial value at the first iteration) and finally updates that value with iterate function. If at some point the condition returns false, then the Observable completes at that moment.
Optionally you can pass a fourth parameter to `<generate>` - a result selector function which allows you to immediately map the value that would normally be emitted by an Observable.
If you find three anonymous functions in `<generate>` call hard to read, you can provide a single object to the operator instead where the object has the properties: `initialState`, `condition`, `iterate` and `resultSelector`, which should have respective values that you would normally pass to `<generate>`. `resultSelector` is still optional, but that form of calling `<generate>` allows you to omit `condition` as well. If you omit it, that means condition always holds, or in other words the resulting Observable will never complete.
Both forms of `<generate>` can optionally accept a scheduler. In case of a multi-parameter call, scheduler simply comes as a last argument (no matter if there is a `resultSelector` function or not). In case of a single-parameter call, you can provide it as a `scheduler` property on the object passed to the operator. In both cases, a scheduler decides when the next iteration of the loop will happen and therefore when the next value will be emitted by the Observable. For example, to ensure that each value is pushed to the Observer on a separate task in the event loop, you could use the `[async](../const/async)` scheduler. Note that by default (when no scheduler is passed) values are simply emitted synchronously.
Examples
--------
Use with condition and iterate functions
```
import { generate } from 'rxjs';
const result = generate(0, x => x < 3, x => x + 1);
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 0
// 1
// 2
// 'Complete!'
```
Use with condition, iterate and resultSelector functions
```
import { generate } from 'rxjs';
const result = generate(0, x => x < 3, x => x + 1, x => x * 1000);
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 0
// 1000
// 2000
// 'Complete!'
```
Use with options object
```
import { generate } from 'rxjs';
const result = generate({
initialState: 0,
condition(value) { return value < 3; },
iterate(value) { return value + 1; },
resultSelector(value) { return value * 1000; }
});
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 0
// 1000
// 2000
// 'Complete!'
```
Use options object without condition function
```
import { generate } from 'rxjs';
const result = generate({
initialState: 0,
iterate(value) { return value + 1; },
resultSelector(value) { return value * 1000; }
});
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!') // This will never run
});
// Logs:
// 0
// 1000
// 2000
// 3000
// ...and never stops.
```
### `generate(options: GenerateBaseOptions<S>): Observable<S>`
Generates an observable sequence by running a state-driven loop producing the sequence's elements, using the specified scheduler to send out observer messages. The overload accepts options object that might contain initial state, iterate, condition and scheduler.
#### Parameters
| | | |
| --- | --- | --- |
| `options` | `GenerateBaseOptions<S>` | Object that must contain initialState, iterate and might contain condition and scheduler. |
#### Returns
`[Observable](../class/observable)<S>`: The generated sequence.
Examples
--------
Use options object with condition function
```
import { generate } from 'rxjs';
const result = generate({
initialState: 0,
condition: x => x < 3,
iterate: x => x + 1
});
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 0
// 1
// 2
// 'Complete!'
```
### `generate(options: GenerateOptions<T, S>): Observable<T>`
Generates an observable sequence by running a state-driven loop producing the sequence's elements, using the specified scheduler to send out observer messages. The overload accepts options object that might contain initial state, iterate, condition, result selector and scheduler.
#### Parameters
| | | |
| --- | --- | --- |
| `options` | `GenerateOptions<T, S>` | Object that must contain initialState, iterate, resultSelector and might contain condition and scheduler. |
#### Returns
`[Observable<T>](../class/observable)`: The generated sequence.
Examples
--------
Use options object with condition and iterate function
```
import { generate } from 'rxjs';
const result = generate({
initialState: 0,
condition: x => x < 3,
iterate: x => x + 1,
resultSelector: x => x
});
result.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 0
// 1
// 2
// 'Complete!'
```
rxjs publishReplay publishReplay
=============
`function` `deprecated` `operator`
Deprecation Notes
-----------------
Will be removed in v8. Use the [`connectable`](connectable) observable, the [`connect`](connect) operator or the [`share`](share) operator instead. See the overloads below for equivalent replacement examples of this operator's behaviors. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishReplay<T, R>(bufferSize?: number, windowTime?: number, selectorOrScheduler?: TimestampProvider | OperatorFunction<T, R>, timestampProvider?: TimestampProvider)`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | Optional. Default is `undefined`. |
| `[windowTime](windowtime)` | `number` | Optional. Default is `undefined`. |
| `selectorOrScheduler` | `[TimestampProvider](../interface/timestampprovider) | [OperatorFunction](../interface/operatorfunction)<T, R>` | Optional. Default is `undefined`. |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `undefined`. |
Overloads
---------
### `publishReplay(bufferSize?: number, windowTime?: number, timestampProvider?: TimestampProvider): MonoTypeOperatorFunction<T>`
Creates a [`ConnectableObservable`](../class/connectableobservable) that uses a [`ReplaySubject`](../class/replaysubject) internally.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | Optional. Default is `undefined`. The buffer size for the underlying [`ReplaySubject`](../class/replaysubject). |
| `[windowTime](windowtime)` | `number` | Optional. Default is `undefined`. The window time for the underlying [`ReplaySubject`](../class/replaysubject). |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `undefined`. The timestamp provider for the underlying [`ReplaySubject`](../class/replaysubject). |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `publishReplay(bufferSize: number, windowTime: number, selector: (shared: Observable<T>) => O, timestampProvider?: TimestampProvider): OperatorFunction<T, ObservedValueOf<O>>`
Creates an observable, that when subscribed to, will create a [`ReplaySubject`](../class/replaysubject), and pass an observable from it (using [asObservable](../class/subject#asObservable)) to the `selector` function, which then returns an observable that is subscribed to before "connecting" the source to the internal `[ReplaySubject](../class/replaysubject)`.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The buffer size for the underlying [`ReplaySubject`](../class/replaysubject). |
| `[windowTime](windowtime)` | `number` | The window time for the underlying [`ReplaySubject`](../class/replaysubject). |
| `selector` | `(shared: [Observable](../class/observable)<T>) => O` | A function used to setup the multicast. |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `undefined`. The timestamp provider for the underlying [`ReplaySubject`](../class/replaysubject). |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
Since this is deprecated, for additional details see the documentation for [`connect`](connect).
### `publishReplay(bufferSize: number, windowTime: number, selector: undefined, timestampProvider: TimestampProvider): OperatorFunction<T, ObservedValueOf<O>>`
Creates a [`ConnectableObservable`](../class/connectableobservable) that uses a [`ReplaySubject`](../class/replaysubject) internally.
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The buffer size for the underlying [`ReplaySubject`](../class/replaysubject). |
| `[windowTime](windowtime)` | `number` | The window time for the underlying [`ReplaySubject`](../class/replaysubject). |
| `selector` | `undefined` | Passing `undefined` here determines that this operator will return a [`ConnectableObservable`](../class/connectableobservable). |
| `timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | The timestamp provider for the underlying [`ReplaySubject`](../class/replaysubject). |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
| programming_docs |
rxjs timeout timeout
=======
`function` `stable` `operator`
Errors if Observable does not emit a value in given time span.
### `timeout<T, O extends ObservableInput<any>, M>(config: number | Date | TimeoutConfig<T, O, M>, schedulerArg?: SchedulerLike): OperatorFunction<T, T | ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../const/config)` | `number | Date | TimeoutConfig<T, O, M>` | |
| `schedulerArg` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that mirrors behaviour of the source Observable, unless timeout happens when it throws an error.
Description
-----------
Timeouts on Observable that doesn't emit values fast enough.
Overloads
---------
### `timeout(config: TimeoutConfig<T, O, M> & { with: (info: TimeoutInfo<T, M>) => O; }): OperatorFunction<T, T | ObservedValueOf<O>>`
If `with` is provided, this will return an observable that will switch to a different observable if the source does not push values within the specified time parameters.
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../const/config)` | `TimeoutConfig<T, O, M> & { with: (info: TimeoutInfo<T, M>) => O; }` | The configuration for the timeout. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | [ObservedValueOf](../type-alias/observedvalueof)<O>>`
The most flexible option for creating a timeout behavior.
The first thing to know about the configuration is if you do not provide a `with` property to the configuration, when timeout conditions are met, this operator will emit a [`TimeoutError`](../interface/timeouterror). Otherwise, it will use the factory function provided by `with`, and switch your subscription to the result of that. Timeout conditions are provided by the settings in `<first>` and `each`.
The `<first>` property can be either a `Date` for a specific time, a `number` for a time period relative to the point of subscription, or it can be skipped. This property is to check timeout conditions for the arrival of the first value from the source *only*. The timings of all subsequent values from the source will be checked against the time period provided by `each`, if it was provided.
The `each` property can be either a `number` or skipped. If a value for `each` is provided, it represents the amount of time the resulting observable will wait between the arrival of values from the source before timing out. Note that if `<first>` is *not* provided, the value from `each` will be used to check timeout conditions for the arrival of the first value and all subsequent values. If `<first>` *is* provided, `each` will only be use to check all values after the first.
Examples
--------
Emit a custom error if there is too much time between values
```
import { interval, timeout, throwError } from 'rxjs';
class CustomTimeoutError extends Error {
constructor() {
super('It was too slow');
this.name = 'CustomTimeoutError';
}
}
const slow$ = interval(900);
slow$.pipe(
timeout({
each: 1000,
with: () => throwError(() => new CustomTimeoutError())
})
)
.subscribe({
error: console.error
});
```
Switch to a faster observable if your source is slow.
```
import { interval, timeout } from 'rxjs';
const slow$ = interval(900);
const fast$ = interval(500);
slow$.pipe(
timeout({
each: 1000,
with: () => fast$,
})
)
.subscribe(console.log);
```
### `timeout(config: any): OperatorFunction<T, T>`
Returns an observable that will error or switch to a different observable if the source does not push values within the specified time parameters.
#### Parameters
| | | |
| --- | --- | --- |
| `[config](../const/config)` | `any` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T>`
The most flexible option for creating a timeout behavior.
The first thing to know about the configuration is if you do not provide a `with` property to the configuration, when timeout conditions are met, this operator will emit a [`TimeoutError`](../interface/timeouterror). Otherwise, it will use the factory function provided by `with`, and switch your subscription to the result of that. Timeout conditions are provided by the settings in `<first>` and `each`.
The `<first>` property can be either a `Date` for a specific time, a `number` for a time period relative to the point of subscription, or it can be skipped. This property is to check timeout conditions for the arrival of the first value from the source *only*. The timings of all subsequent values from the source will be checked against the time period provided by `each`, if it was provided.
The `each` property can be either a `number` or skipped. If a value for `each` is provided, it represents the amount of time the resulting observable will wait between the arrival of values from the source before timing out. Note that if `<first>` is *not* provided, the value from `each` will be used to check timeout conditions for the arrival of the first value and all subsequent values. If `<first>` *is* provided, `each` will only be use to check all values after the first.
#### Handling TimeoutErrors
If no `with` property was provided, subscriptions to the resulting observable may emit an error of [`TimeoutError`](../interface/timeouterror). The timeout error provides useful information you can examine when you're handling the error. The most common way to handle the error would be with [`catchError`](catcherror), although you could use [`tap`](tap) or just the error handler in your `subscribe` call directly, if your error handling is only a side effect (such as notifying the user, or logging).
In this case, you would check the error for `instanceof [TimeoutError](../interface/timeouterror)` to validate that the error was indeed from `<timeout>`, and not from some other source. If it's not from `<timeout>`, you should probably rethrow it if you're in a `[catchError](catcherror)`.
Examples
--------
Emit a [`TimeoutError`](../interface/timeouterror) if the first value, and *only* the first value, does not arrive within 5 seconds
```
import { interval, timeout } from 'rxjs';
// A random interval that lasts between 0 and 10 seconds per tick
const source$ = interval(Math.round(Math.random() * 10_000));
source$.pipe(
timeout({ first: 5_000 })
)
.subscribe({
next: console.log,
error: console.error
});
```
Emit a [`TimeoutError`](../interface/timeouterror) if the source waits longer than 5 seconds between any two values or the first value and subscription.
```
import { timer, timeout, expand } from 'rxjs';
const getRandomTime = () => Math.round(Math.random() * 10_000);
// An observable that waits a random amount of time between each delivered value
const source$ = timer(getRandomTime())
.pipe(expand(() => timer(getRandomTime())));
source$
.pipe(timeout({ each: 5_000 }))
.subscribe({
next: console.log,
error: console.error
});
```
Emit a [`TimeoutError`](../interface/timeouterror) if the source does not emit before 7 seconds, *or* if the source waits longer than 5 seconds between any two values after the first.
```
import { timer, timeout, expand } from 'rxjs';
const getRandomTime = () => Math.round(Math.random() * 10_000);
// An observable that waits a random amount of time between each delivered value
const source$ = timer(getRandomTime())
.pipe(expand(() => timer(getRandomTime())));
source$
.pipe(timeout({ first: 7_000, each: 5_000 }))
.subscribe({
next: console.log,
error: console.error
});
```
### `timeout(first: Date, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
Returns an observable that will error if the source does not push its first value before the specified time passed as a `Date`. This is functionally the same as `<timeout>({ <first>: someDate })`.
#### Parameters
| | | |
| --- | --- | --- |
| `<first>` | `Date` | The date to at which the resulting observable will timeout if the source observable does not emit at least one value. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use. Defaults to [`asyncScheduler`](../const/asyncscheduler). |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
Errors if the first value doesn't show up before the given date and time
### `timeout(each: number, scheduler?: SchedulerLike): MonoTypeOperatorFunction<T>`
Returns an observable that will error if the source does not push a value within the specified time in milliseconds. This is functionally the same as `<timeout>({ each: milliseconds })`.
#### Parameters
| | | |
| --- | --- | --- |
| `each` | `number` | The time allowed between each pushed value from the source before the resulting observable will timeout. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use. Defaults to [`asyncScheduler`](../const/asyncscheduler). |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
Errors if it waits too long between any value
See Also
--------
* [`timeoutWith`](timeoutwith)
rxjs sequenceEqual sequenceEqual
=============
`function` `stable` `operator`
Compares all values of two observables in sequence using an optional comparator function and returns an observable of a single boolean value representing whether or not the two sequences are equal.
### `sequenceEqual<T>(compareTo: Observable<T>, comparator: (a: T, b: T) => boolean = (a, b) => a === b): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `compareTo` | `[Observable<T>](../class/observable)` | The observable sequence to compare the source sequence to. |
| `comparator` | `(a: T, b: T) => boolean` | Optional. Default is `(a, b) => a === b`. An optional function to compare each value pair |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, boolean>`: A function that returns an Observable that emits a single boolean value representing whether or not the values emitted by the source Observable and provided Observable were equal in sequence.
Description
-----------
Checks to see of all values emitted by both observables are equal, in order.
`[sequenceEqual](sequenceequal)` subscribes to two observables and buffers incoming values from each observable. Whenever either observable emits a value, the value is buffered and the buffers are shifted and compared from the bottom up; If any value pair doesn't match, the returned observable will emit `false` and complete. If one of the observables completes, the operator will wait for the other observable to complete; If the other observable emits before completing, the returned observable will emit `false` and complete. If one observable never completes or emits after the other completes, the returned observable will never complete.
Example
-------
Figure out if the Konami code matches
```
import { from, fromEvent, map, bufferCount, mergeMap, sequenceEqual } from 'rxjs';
const codes = from([
'ArrowUp',
'ArrowUp',
'ArrowDown',
'ArrowDown',
'ArrowLeft',
'ArrowRight',
'ArrowLeft',
'ArrowRight',
'KeyB',
'KeyA',
'Enter', // no start key, clearly.
]);
const keys = fromEvent<KeyboardEvent>(document, 'keyup').pipe(map(e => e.code));
const matches = keys.pipe(
bufferCount(11, 1),
mergeMap(last11 => from(last11).pipe(sequenceEqual(codes)))
);
matches.subscribe(matched => console.log('Successful cheat at Contra? ', matched));
```
See Also
--------
* [`combineLatest`](combinelatest)
* [`zip`](zip)
* [`withLatestFrom`](withlatestfrom)
rxjs publishBehavior publishBehavior
===============
`function` `deprecated` `operator`
Creates a [`ConnectableObservable`](../class/connectableobservable) that utilizes a [`BehaviorSubject`](../class/behaviorsubject).
Deprecation Notes
-----------------
Will be removed in v8. To create a connectable observable that uses a [`BehaviorSubject`](../class/behaviorsubject) under the hood, use [`connectable`](connectable). `source.pipe([publishBehavior](publishbehavior)(initValue))` is equivalent to `<connectable>(source, { connector: () => new [BehaviorSubject](../class/behaviorsubject)(initValue), resetOnDisconnect: false })`. If you're using [`refCount`](refcount) after `[publishBehavior](publishbehavior)`, use the [`share`](share) operator instead. `source.pipe([publishBehavior](publishbehavior)(initValue), [refCount](refcount)())` is equivalent to `source.pipe(<share>({ connector: () => new [BehaviorSubject](../class/behaviorsubject)(initValue), resetOnError: false, resetOnComplete: false, resetOnRefCountZero: false }))`. Details: <https://rxjs.dev/deprecations/multicasting>
### `publishBehavior<T>(initialValue: T): UnaryFunction<Observable<T>, ConnectableObservable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `initialValue` | `T` | The initial value passed to the [`BehaviorSubject`](../class/behaviorsubject). |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<[Observable](../class/observable)<T>, [ConnectableObservable](../class/connectableobservable)<T>>`: A function that returns a [`ConnectableObservable`](../class/connectableobservable)
rxjs timer timer
=====
`function` `stable`
### `timer(dueTime: number | Date = 0, intervalOrScheduler?: number | SchedulerLike, scheduler: SchedulerLike = asyncScheduler): Observable<number>`
#### Parameters
| | | |
| --- | --- | --- |
| `dueTime` | `number | Date` | Optional. Default is `0`. |
| `intervalOrScheduler` | `number | [SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. |
#### Returns
`[Observable](../class/observable)<number>`
Overloads
---------
### `timer(due: number | Date, scheduler?: SchedulerLike): Observable<0>`
Creates an observable that will wait for a specified time period, or exact date, before emitting the number 0.
#### Parameters
| | | |
| --- | --- | --- |
| `due` | `number | Date` | If a `number`, the amount of time in milliseconds to wait before emitting. If a `Date`, the exact time at which to emit. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use to schedule the delay. Defaults to [`asyncScheduler`](../const/asyncscheduler). |
#### Returns
`[Observable](../class/observable)<0>`
Used to emit a notification after a delay.
This observable is useful for creating delays in code, or racing against other values for ad-hoc timeouts.
The `<delay>` is specified by default in milliseconds, however providing a custom scheduler could create a different behavior.
Examples
--------
Wait 3 seconds and start another observable
You might want to use `<timer>` to delay subscription to an observable by a set amount of time. Here we use a timer with [`concatMapTo`](concatmapto) or [`concatMap`](concatmap) in order to wait a few seconds and start a subscription to a source.
```
import { of, timer, concatMap } from 'rxjs';
// This could be any observable
const source = of(1, 2, 3);
timer(3000)
.pipe(concatMap(() => source))
.subscribe(console.log);
```
Take all values until the start of the next minute
Using a `Date` as the trigger for the first emission, you can do things like wait until midnight to fire an event, or in this case, wait until a new minute starts (chosen so the example wouldn't take too long to run) in order to stop watching a stream. Leveraging [`takeUntil`](takeuntil).
```
import { interval, takeUntil, timer } from 'rxjs';
// Build a Date object that marks the
// next minute.
const currentDate = new Date();
const startOfNextMinute = new Date(
currentDate.getFullYear(),
currentDate.getMonth(),
currentDate.getDate(),
currentDate.getHours(),
currentDate.getMinutes() + 1
);
// This could be any observable stream
const source = interval(1000);
const result = source.pipe(
takeUntil(timer(startOfNextMinute))
);
result.subscribe(console.log);
```
#### Known Limitations
* The [`asyncScheduler`](../const/asyncscheduler) uses `setTimeout` which has limitations for how far in the future it can be scheduled.
* If a `scheduler` is provided that returns a timestamp other than an epoch from `now()`, and a `Date` object is passed to the `dueTime` argument, the calculation for when the first emission should occur will be incorrect. In this case, it would be best to do your own calculations ahead of time, and pass a `number` in as the `dueTime`.
### `timer(startDue: number | Date, intervalDuration: number, scheduler?: SchedulerLike): Observable<number>`
Creates an observable that starts an interval after a specified delay, emitting incrementing numbers -- starting at `0` -- on each interval after words.
#### Parameters
| | | |
| --- | --- | --- |
| `startDue` | `number | Date` | If a `number`, is the time to wait before starting the interval. If a `Date`, is the exact time at which to start the interval. |
| `intervalDuration` | `number` | The delay between each value emitted in the interval. Passing a negative number here will result in immediate completion after the first value is emitted, as though no `intervalDuration` was passed at all. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use to schedule the delay. Defaults to [`asyncScheduler`](../const/asyncscheduler). |
#### Returns
`[Observable](../class/observable)<number>`
The `<delay>` and `intervalDuration` are specified by default in milliseconds, however providing a custom scheduler could create a different behavior.
Example
-------
#### Start an interval that starts right away
Since [`interval`](interval) waits for the passed delay before starting, sometimes that's not ideal. You may want to start an interval immediately. `<timer>` works well for this. Here we have both side-by-side so you can see them in comparison.
Note that this observable will never complete.
```
import { timer, interval } from 'rxjs';
timer(0, 1000).subscribe(n => console.log('timer', n));
interval(1000).subscribe(n => console.log('interval', n));
```
#### Known Limitations
* The [`asyncScheduler`](../const/asyncscheduler) uses `setTimeout` which has limitations for how far in the future it can be scheduled.
* If a `scheduler` is provided that returns a timestamp other than an epoch from `now()`, and a `Date` object is passed to the `dueTime` argument, the calculation for when the first emission should occur will be incorrect. In this case, it would be best to do your own calculations ahead of time, and pass a `number` in as the `startDue`.
### `timer(dueTime: number | Date, unused: undefined, scheduler?: SchedulerLike): Observable<0>`
#### Parameters
| | | |
| --- | --- | --- |
| `dueTime` | `number | Date` | |
| `unused` | `undefined` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<0>`
rxjs concatMap concatMap
=========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable, in a serialized fashion waiting for each one to complete before merging the next.
### `concatMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and taking values from each projected inner Observable sequentially.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`concatAll`](concatall).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. Each new inner Observable is concatenated with the previous inner Observable.
**Warning:** if source values arrive endlessly and faster than their corresponding inner Observables can complete, it will result in memory issues as inner Observables amass in an unbounded buffer waiting for their turn to be subscribed to.
Note: `[concatMap](concatmap)` is equivalent to `[mergeMap](mergemap)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, concatMap, interval, take } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
concatMap(ev => interval(1000).pipe(take(4)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
Overloads
---------
### `concatMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `concatMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `concatMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concat`](concat)
* [`concatAll`](concatall)
* [`concatMapTo`](concatmapto)
* [`exhaustMap`](exhaustmap)
* [`mergeMap`](mergemap)
* [`switchMap`](switchmap)
| programming_docs |
rxjs pipe pipe
====
`function` `stable`
pipe() can be called on one or more functions, each of which can take one argument ("UnaryFunction") and uses it to return a value. It returns a function that takes one argument, passes it to the first UnaryFunction, and then passes the result to the next one, passes that result to the next one, and so on.
### `pipe(...fns: UnaryFunction<any, any>[]): UnaryFunction<any, any>`
#### Parameters
| | | |
| --- | --- | --- |
| `fns` | `[UnaryFunction](../interface/unaryfunction)<any, any>[]` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<any, any>`
Overloads
---------
### `pipe(): typeof identity`
#### Parameters
There are no parameters.
#### Returns
`typeof <identity>`
### `pipe(fn1: UnaryFunction<T, A>): UnaryFunction<T, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, A>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>): UnaryFunction<T, B>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, B>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>): UnaryFunction<T, C>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, C>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>): UnaryFunction<T, D>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, D>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>): UnaryFunction<T, E>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, E>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>, fn6: UnaryFunction<E, F>): UnaryFunction<T, F>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
| `fn6` | `[UnaryFunction](../interface/unaryfunction)<E, F>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, F>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>, fn6: UnaryFunction<E, F>, fn7: UnaryFunction<F, G>): UnaryFunction<T, G>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
| `fn6` | `[UnaryFunction](../interface/unaryfunction)<E, F>` | |
| `fn7` | `[UnaryFunction](../interface/unaryfunction)<F, G>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, G>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>, fn6: UnaryFunction<E, F>, fn7: UnaryFunction<F, G>, fn8: UnaryFunction<G, H>): UnaryFunction<T, H>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
| `fn6` | `[UnaryFunction](../interface/unaryfunction)<E, F>` | |
| `fn7` | `[UnaryFunction](../interface/unaryfunction)<F, G>` | |
| `fn8` | `[UnaryFunction](../interface/unaryfunction)<G, H>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, H>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>, fn6: UnaryFunction<E, F>, fn7: UnaryFunction<F, G>, fn8: UnaryFunction<G, H>, fn9: UnaryFunction<H, I>): UnaryFunction<T, I>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
| `fn6` | `[UnaryFunction](../interface/unaryfunction)<E, F>` | |
| `fn7` | `[UnaryFunction](../interface/unaryfunction)<F, G>` | |
| `fn8` | `[UnaryFunction](../interface/unaryfunction)<G, H>` | |
| `fn9` | `[UnaryFunction](../interface/unaryfunction)<H, I>` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, I>`
### `pipe(fn1: UnaryFunction<T, A>, fn2: UnaryFunction<A, B>, fn3: UnaryFunction<B, C>, fn4: UnaryFunction<C, D>, fn5: UnaryFunction<D, E>, fn6: UnaryFunction<E, F>, fn7: UnaryFunction<F, G>, fn8: UnaryFunction<G, H>, fn9: UnaryFunction<H, I>, ...fns: UnaryFunction<any, any>[]): UnaryFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `fn1` | `[UnaryFunction](../interface/unaryfunction)<T, A>` | |
| `fn2` | `[UnaryFunction](../interface/unaryfunction)<A, B>` | |
| `fn3` | `[UnaryFunction](../interface/unaryfunction)<B, C>` | |
| `fn4` | `[UnaryFunction](../interface/unaryfunction)<C, D>` | |
| `fn5` | `[UnaryFunction](../interface/unaryfunction)<D, E>` | |
| `fn6` | `[UnaryFunction](../interface/unaryfunction)<E, F>` | |
| `fn7` | `[UnaryFunction](../interface/unaryfunction)<F, G>` | |
| `fn8` | `[UnaryFunction](../interface/unaryfunction)<G, H>` | |
| `fn9` | `[UnaryFunction](../interface/unaryfunction)<H, I>` | |
| `fns` | `[UnaryFunction](../interface/unaryfunction)<any, any>[]` | |
#### Returns
`[UnaryFunction](../interface/unaryfunction)<T, unknown>`
rxjs find find
====
`function` `stable` `operator`
Emits only the first value emitted by the source Observable that meets some condition.
### `find<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | A function called with each item to test for condition matching. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | undefined>`: A function that returns an Observable that emits the first item that matches the condition.
Description
-----------
Finds the first value that passes some test and emits that.
`<find>` searches for the first item in the source Observable that matches the specified condition embodied by the `predicate`, and returns the first occurrence in the source. Unlike [`first`](first), the `predicate` is required in `<find>`, and does not emit an error if a valid value is not found (emits `undefined` instead).
Example
-------
Find and emit the first click that happens on a DIV element
```
import { fromEvent, find } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(find(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `find(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `find(predicate: (this: A, value: T, index: number, source: Observable<T>) => value is S, thisArg: A): OperatorFunction<T, S | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../class/observable)<T>) => value is S` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S | undefined>`
### `find(predicate: (value: T, index: number, source: Observable<T>) => value is S): OperatorFunction<T, S | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => value is S` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S | undefined>`
### `find(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | undefined>`
### `find(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | undefined>`
See Also
--------
* [`filter`](filter)
* [`first`](first)
* [`findIndex`](findindex)
* [`take`](take)
rxjs switchMapTo switchMapTo
===========
`function` `deprecated` `operator`
Projects each source value to the same Observable which is flattened multiple times with [`switchMap`](switchmap) in the output Observable.
Deprecation Notes
-----------------
Will be removed in v9. Use [`switchMap`](switchmap) instead: `[switchMap](switchmap)(() => result)`
### `switchMapTo<T, R, O extends ObservableInput<unknown>>(innerObservable: O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `innerObservable` | `O` | An Observable to replace each value from the source Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits items from the given `innerObservable` (and optionally transformed through the deprecated `resultSelector`) every time a value is emitted on the source Observable, and taking only the values from the most recently projected inner Observable.
Description
-----------
It's like [`switchMap`](switchmap), but maps each value always to the same inner Observable.
Maps each source value to the given Observable `innerObservable` regardless of the source value, and then flattens those resulting Observables into one single Observable, which is the output Observable. The output Observables emits values only from the most recently emitted instance of `innerObservable`.
Example
-------
Restart an interval Observable on every click event
```
import { fromEvent, switchMapTo, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(switchMapTo(interval(1000)));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `switchMapTo(observable: O): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `switchMapTo(observable: O, resultSelector: undefined): OperatorFunction<unknown, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<unknown, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `switchMapTo(observable: O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `[observable](../const/observable)` | `O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concatMapTo`](concatmapto)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`mergeMapTo`](mergemapto)
rxjs tap tap
===
`function` `stable` `operator`
Used to perform side-effects for notifications from the source observable
### `tap<T>(observerOrNext?: Partial<TapObserver<T>> | ((value: T) => void), error?: (e: any) => void, complete?: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `observerOrNext` | `Partial<TapObserver<T>> | ((value: T) => void)` | Optional. Default is `undefined`. A next handler or partial observer |
| `error` | `(e: any) => void` | Optional. Default is `undefined`. An error handler |
| `complete` | `() => void` | Optional. Default is `undefined`. A completion handler |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable identical to the source, but runs the specified Observer or callback(s) for each item.
Description
-----------
Used when you want to affect outside state with a notification without altering the notification
Tap is designed to allow the developer a designated place to perform side effects. While you *could* perform side-effects inside of a `<map>` or a `[mergeMap](mergemap)`, that would make their mapping functions impure, which isn't always a big deal, but will make it so you can't do things like memoize those functions. The `<tap>` operator is designed solely for such side-effects to help you remove side-effects from other operations.
For any notification, next, error, or complete, `<tap>` will call the appropriate callback you have provided to it, via a function reference, or a partial observer, then pass that notification down the stream.
The observable returned by `<tap>` is an exact mirror of the source, with one exception: Any error that occurs -- synchronously -- in a handler provided to `<tap>` will be emitted as an error from the returned observable.
> Be careful! You can mutate objects as they pass through the `<tap>` operator's handlers.
>
>
The most common use of `<tap>` is actually for debugging. You can place a `<tap>(console.log)` anywhere in your observable `<pipe>`, log out the notifications as they are emitted by the source returned by the previous operation.
Examples
--------
Check a random number before it is handled. Below is an observable that will use a random number between 0 and 1, and emit `'big'` or `'small'` depending on the size of that number. But we wanted to log what the original number was, so we have added a `<tap>(console.log)`.
```
import { of, tap, map } from 'rxjs';
of(Math.random()).pipe(
tap(console.log),
map(n => n > 0.5 ? 'big' : 'small')
).subscribe(console.log);
```
Using `<tap>` to analyze a value and force an error. Below is an observable where in our system we only want to emit numbers 3 or less we get from another source. We can force our observable to error using `<tap>`.
```
import { of, tap } from 'rxjs';
const source = of(1, 2, 3, 4, 5);
source.pipe(
tap(n => {
if (n > 3) {
throw new TypeError(`Value ${ n } is greater than 3`);
}
})
)
.subscribe({ next: console.log, error: err => console.log(err.message) });
```
We want to know when an observable completes before moving on to the next observable. The system below will emit a random series of `'X'` characters from 3 different observables in sequence. The only way we know when one observable completes and moves to the next one, in this case, is because we have added a `<tap>` with the side effect of logging to console.
```
import { of, concatMap, interval, take, map, tap } from 'rxjs';
of(1, 2, 3).pipe(
concatMap(n => interval(1000).pipe(
take(Math.round(Math.random() * 10)),
map(() => 'X'),
tap({ complete: () => console.log(`Done with ${ n }`) })
))
)
.subscribe(console.log);
```
Overloads
---------
### `tap(observer?: Partial<TapObserver<T>>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `observer` | `Partial<TapObserver<T>>` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `tap(next: (value: T) => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `tap(next?: (value: T) => void, error?: (error: any) => void, complete?: () => void): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | Optional. Default is `undefined`. |
| `error` | `(error: any) => void` | Optional. Default is `undefined`. |
| `complete` | `() => void` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
See Also
--------
* [`finalize`](finalize)
* [`Observable`](../class/observable#subscribe)
rxjs mergeAll mergeAll
========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable which concurrently delivers all values that are emitted on the inner Observables.
### `mergeAll<O extends ObservableInput<any>>(concurrent: number = Infinity): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of inner Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<O, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that emits values coming from all the inner Observables emitted by the source Observable.
Description
-----------
Flattens an Observable-of-Observables.
`[mergeAll](mergeall)` subscribes to an Observable that emits Observables, also known as a higher-order Observable. Each time it observes one of these emitted inner Observables, it subscribes to that and delivers all the values from the inner Observable on the output Observable. The output Observable only completes once all inner Observables have completed. Any error delivered by a inner Observable will be immediately emitted on the output Observable.
Examples
--------
Spawn a new interval Observable for each click event, and blend their outputs as one Observable
```
import { fromEvent, map, interval, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(map(() => interval(1000)));
const firstOrder = higherOrder.pipe(mergeAll());
firstOrder.subscribe(x => console.log(x));
```
Count from 0 to 9 every second for each click, but only allow 2 concurrent timers
```
import { fromEvent, map, interval, take, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(10)))
);
const firstOrder = higherOrder.pipe(mergeAll(2));
firstOrder.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`exhaustAll`](exhaustall)
* [`merge`](merge)
* [`mergeMap`](mergemap)
* [`mergeMapTo`](mergemapto)
* [`mergeScan`](mergescan)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`zipAll`](zipall)
| programming_docs |
rxjs timeoutWith timeoutWith
===========
`function` `deprecated` `operator`
When the passed timespan elapses before the source emits any given value, it will unsubscribe from the source, and switch the subscription to another observable.
Deprecation Notes
-----------------
Replaced with [`timeout`](timeout). Instead of `[timeoutWith](timeoutwith)(100, a$, scheduler)`, use [`timeout`](timeout) with the configuration object: `<timeout>({ each: 100, with: () => a$, scheduler })`. Instead of `[timeoutWith](timeoutwith)(someDate, a$, scheduler)`, use [`timeout`](timeout) with the configuration object: `<timeout>({ <first>: someDate, with: () => a$, scheduler })`. Will be removed in v8.
### `timeoutWith<T, R>(due: number | Date, withObservable: ObservableInput<R>, scheduler?: SchedulerLike): OperatorFunction<T, T | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `due` | `number | Date` | When passed a number, used as the time (in milliseconds) allowed between each value from the source before timeout is triggered. When passed a Date, used as the exact time at which the timeout will be triggered if the first value does not arrive. |
| `withObservable` | `[ObservableInput](../type-alias/observableinput)<R>` | The observable to switch to when timeout occurs. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. The scheduler to use with time-related operations within this operator. Defaults to [`asyncScheduler`](../const/asyncscheduler) |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | R>`: A function that returns an Observable that mirrors behaviour of the source Observable, unless timeout happens when it starts emitting values from the `[ObservableInput](../type-alias/observableinput)` passed as a second parameter.
Description
-----------
Used to switch to a different observable if your source is being slow.
Useful in cases where:
* You want to switch to a different source that may be faster.
* You want to notify a user that the data stream is slow.
* You want to emit a custom error rather than the [`TimeoutError`](../interface/timeouterror) emitted by the default usage of [`timeout`](timeout).
If the first parameter is passed as Date and the time of the Date arrives before the first value arrives from the source, it will unsubscribe from the source and switch the subscription to another observable.
Use Date object to switch to a different observable if the first value doesn't arrive by a specific time.
Can be used to set a timeout only for the first value, however it's recommended to use the [`timeout`](timeout) operator with the `<first>` configuration to get the same effect.
Examples
--------
Fallback to a faster observable
```
import { interval, timeoutWith } from 'rxjs';
const slow$ = interval(1000);
const faster$ = interval(500);
slow$
.pipe(timeoutWith(900, faster$))
.subscribe(console.log);
```
Emit your own custom timeout error
```
import { interval, timeoutWith, throwError } from 'rxjs';
class CustomTimeoutError extends Error {
constructor() {
super('It was too slow');
this.name = 'CustomTimeoutError';
}
}
const slow$ = interval(1000);
slow$
.pipe(timeoutWith(900, throwError(() => new CustomTimeoutError())))
.subscribe({
error: err => console.error(err.message)
});
```
See Also
--------
* [`timeout`](timeout)
rxjs skipLast skipLast
========
`function` `stable` `operator`
Skip a specified number of values before the completion of an observable.
### `skipLast<T>(skipCount: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `skipCount` | `number` | Number of elements to skip from the end of the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that skips the last `<count>` values emitted by the source Observable.
Description
-----------
Returns an observable that will emit values as soon as it can, given a number of skipped values. For example, if you `[skipLast](skiplast)(3)` on a source, when the source emits its fourth value, the first value the source emitted will finally be emitted from the returned observable, as it is no longer part of what needs to be skipped.
All values emitted by the result of `[skipLast](skiplast)(N)` will be delayed by `N` emissions, as each value is held in a buffer until enough values have been emitted that that the buffered value may finally be sent to the consumer.
After subscribing, unsubscribing will not result in the emission of the buffered skipped values.
Example
-------
Skip the last 2 values of an observable with many values
```
import { of, skipLast } from 'rxjs';
const numbers = of(1, 2, 3, 4, 5);
const skipLastTwo = numbers.pipe(skipLast(2));
skipLastTwo.subscribe(x => console.log(x));
// Results in:
// 1 2 3
// (4 and 5 are skipped)
```
See Also
--------
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipWhile`](skipwhile)
* [`take`](take)
rxjs takeUntil takeUntil
=========
`function` `stable` `operator`
Emits the values emitted by the source Observable until a `notifier` Observable emits a value.
### `takeUntil<T>(notifier: ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[ObservableInput](../type-alias/observableinput)<any>` | The Observable whose first emitted value will cause the output Observable of `[takeUntil](takeuntil)` to stop emitting values from the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits the values from the source Observable until `notifier` emits its first value.
Description
-----------
Lets values pass until a second Observable, `notifier`, emits a value. Then, it completes.
`[takeUntil](takeuntil)` subscribes and begins mirroring the source Observable. It also monitors a second Observable, `notifier` that you provide. If the `notifier` emits a value, the output Observable stops mirroring the source Observable and completes. If the `notifier` doesn't emit any value and completes then `[takeUntil](takeuntil)` will pass all values.
Example
-------
Tick every second until the first click happens
```
import { interval, fromEvent, takeUntil } from 'rxjs';
const source = interval(1000);
const clicks = fromEvent(document, 'click');
const result = source.pipe(takeUntil(clicks));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`take`](take)
* [`takeLast`](takelast)
* [`takeWhile`](takewhile)
* [`skip`](skip)
rxjs repeatWhen repeatWhen
==========
`function` `deprecated` `operator`
Returns an Observable that mirrors the source Observable with the exception of a `complete`. If the source Observable calls `complete`, this method will emit to the Observable returned from `notifier`. If that Observable calls `complete` or `error`, then this method will call `complete` or `error` on the child subscription. Otherwise this method will resubscribe to the source Observable.
Deprecation Notes
-----------------
Will be removed in v9 or v10. Use [`repeat`](repeat)'s `<delay>` option instead.
### `repeatWhen<T>(notifier: (notifications: Observable<void>) => Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `(notifications: [Observable](../class/observable)<void>) => [Observable](../class/observable)<any>` | Receives an Observable of notifications with which a user can `complete` or `error`, aborting the repetition. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that that mirrors the source Observable with the exception of a `complete`.
Description
-----------
Example
-------
Repeat a message stream on click
```
import { of, fromEvent, repeatWhen } from 'rxjs';
const source = of('Repeat message');
const documentClick$ = fromEvent(document, 'click');
const result = source.pipe(repeatWhen(() => documentClick$));
result.subscribe(data => console.log(data))
```
See Also
--------
* [`repeat`](repeat)
* [`retry`](retry)
* [`retryWhen`](retrywhen)
rxjs debounce debounce
========
`function` `stable` `operator`
Emits a notification from the source Observable only after a particular time span determined by another Observable has passed without another source emission.
### `debounce<T>(durationSelector: (value: T) => ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the timeout duration for each source value, returned as an Observable or a Promise. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by the specified duration Observable returned by `durationSelector`, and may drop some values if they occur too frequently.
Description
-----------
It's like [`debounceTime`](debouncetime), but the time span of emission silence is determined by a second Observable.
`<debounce>` delays notifications emitted by the source Observable, but drops previous pending delayed emissions if a new notification arrives on the source Observable. This operator keeps track of the most recent notification from the source Observable, and spawns a duration Observable by calling the `durationSelector` function. The notification is emitted only when the duration Observable emits a next notification, and if no other notification was emitted on the source Observable since the duration Observable was spawned. If a new notification appears before the duration Observable emits, the previous notification will not be emitted and a new duration is scheduled from `durationSelector` is scheduled. If the completing event happens during the scheduled duration the last cached notification is emitted before the completion event is forwarded to the output observable. If the error event happens during the scheduled duration or after it only the error event is forwarded to the output observable. The cache notification is not emitted in this case.
Like [`debounceTime`](debouncetime), this is a rate-limiting operator, and also a delay-like operator since output emissions do not necessarily occur at the same time as they did on the source Observable.
Example
-------
Emit the most recent click after a burst of clicks
```
import { fromEvent, scan, debounce, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
scan(i => ++i, 1),
debounce(i => interval(200 * i))
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
rxjs reduce reduce
======
`function` `stable` `operator`
Applies an accumulator function over the source Observable, and returns the accumulated result when the source completes, given an optional seed value.
### `reduce<V, A>(accumulator: (acc: V | A, value: V, index: number) => A, seed?: any): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | The accumulator function called on each source value. |
| `seed` | `any` | Optional. Default is `undefined`. The initial accumulation value. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, V | A>`: A function that returns an Observable that emits a single value that is the result of accumulating the values emitted by the source Observable.
Description
-----------
Combines together all values emitted on the source, using an accumulator function that knows how to join a new source value into the accumulation from the past.
Like [Array.prototype.reduce()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce), `<reduce>` applies an `accumulator` function against an accumulation and each value of the source Observable (from the past) to reduce it to a single value, emitted on the output Observable. Note that `<reduce>` will only emit one value, only when the source Observable completes. It is equivalent to applying operator [`scan`](scan) followed by operator [`last`](last).
Returns an Observable that applies a specified `accumulator` function to each item emitted by the source Observable. If a `seed` value is specified, then that value will be used as the initial value for the accumulator. If no seed value is specified, the first item of the source is used as the seed.
Example
-------
Count the number of click events that happened in 5 seconds
```
import { fromEvent, takeUntil, interval, map, reduce } from 'rxjs';
const clicksInFiveSeconds = fromEvent(document, 'click')
.pipe(takeUntil(interval(5000)));
const ones = clicksInFiveSeconds.pipe(map(() => 1));
const seed = 0;
const count = ones.pipe(reduce((acc, one) => acc + one, seed));
count.subscribe(x => console.log(x));
```
Overloads
---------
### `reduce(accumulator: (acc: V | A, value: V, index: number) => A): OperatorFunction<V, V | A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: V | A, value: V, index: number) => A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, V | A>`
### `reduce(accumulator: (acc: A, value: V, index: number) => A, seed: A): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A, value: V, index: number) => A` | |
| `seed` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, A>`
### `reduce(accumulator: (acc: A | S, value: V, index: number) => A, seed: S): OperatorFunction<V, A>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: A | S, value: V, index: number) => A` | |
| `seed` | `S` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<V, A>`
See Also
--------
* [`count`](count)
* [`expand`](expand)
* [`mergeScan`](mergescan)
* [`scan`](scan)
rxjs concatAll concatAll
=========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable by concatenating the inner Observables in order.
### `concatAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<O, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable emitting values from all the inner Observables concatenated.
Description
-----------
Flattens an Observable-of-Observables by putting one inner Observable after the other.
Joins every Observable emitted by the source (a higher-order Observable), in a serial fashion. It subscribes to each inner Observable only after the previous inner Observable has completed, and merges all of their values into the returned observable.
**Warning:** If the source Observable emits Observables quickly and endlessly, and the inner Observables it emits generally complete slower than the source emits, you can run into memory issues as the incoming Observables collect in an unbounded buffer.
Note: `[concatAll](concatall)` is equivalent to `[mergeAll](mergeall)` with concurrency parameter set to `1`.
Example
-------
For each click event, tick every second from 0 to 3, with no concurrency
```
import { fromEvent, map, interval, take, concatAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(1000).pipe(take(4)))
);
const firstOrder = higherOrder.pipe(concatAll());
firstOrder.subscribe(x => console.log(x));
// Results in the following:
// (results are not concurrent)
// For every click on the "document" it will emit values 0 to 3 spaced
// on a 1000ms interval
// one click = 1000ms-> 0 -1000ms-> 1 -1000ms-> 2 -1000ms-> 3
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concat`](concat)
* [`concatMap`](concatmap)
* [`concatMapTo`](concatmapto)
* [`exhaustAll`](exhaustall)
* [`mergeAll`](mergeall)
* [`switchAll`](switchall)
* [`switchMap`](switchmap)
* [`zipAll`](zipall)
rxjs mergeWith mergeWith
=========
`function` `stable` `operator`
Merge the values from all observables to a single observable result.
### `mergeWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
Creates an observable, that when subscribed to, subscribes to the source observable, and all other sources provided as arguments. All values from every source are emitted from the resulting subscription.
When all sources complete, the resulting observable will complete.
When any source errors, the resulting observable will error.
Example
-------
Joining all outputs from multiple user input event streams
```
import { fromEvent, map, mergeWith } from 'rxjs';
const clicks$ = fromEvent(document, 'click').pipe(map(() => 'click'));
const mousemoves$ = fromEvent(document, 'mousemove').pipe(map(() => 'mousemove'));
const dblclicks$ = fromEvent(document, 'dblclick').pipe(map(() => 'dblclick'));
mousemoves$
.pipe(mergeWith(clicks$, dblclicks$))
.subscribe(x => console.log(x));
// result (assuming user interactions)
// 'mousemove'
// 'mousemove'
// 'mousemove'
// 'click'
// 'click'
// 'dblclick'
```
See Also
--------
* [`merge`](merge)
rxjs mergeMap mergeMap
========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable.
### `mergeMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: number | ((outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R), concurrent: number = Infinity): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `number | ((outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R)` | Optional. Default is `undefined`. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and merging the results of the Observables obtained from this transformation.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables using [`mergeAll`](mergeall).
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an Observable, and then merging those resulting Observables and emitting the results of this merger.
Example
-------
Map and flatten each letter to an Observable ticking every 1 second
```
import { of, mergeMap, interval, map } from 'rxjs';
const letters = of('a', 'b', 'c');
const result = letters.pipe(
mergeMap(x => interval(1000).pipe(map(i => x + i)))
);
result.subscribe(x => console.log(x));
// Results in the following:
// a0
// b0
// c0
// a1
// b1
// c1
// continues to list a, b, c every second with respective ascending integers
```
Overloads
---------
### `mergeMap(project: (value: T, index: number) => O, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `mergeMap(project: (value: T, index: number) => O, resultSelector: undefined, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `mergeMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R, concurrent?: number): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
| `concurrent` | `number` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaustMap`](exhaustmap)
* [`merge`](merge)
* [`mergeAll`](mergeall)
* [`mergeMapTo`](mergemapto)
* [`mergeScan`](mergescan)
* [`switchMap`](switchmap)
| programming_docs |
rxjs throttle throttle
========
`function` `stable` `operator`
Emits a value from the source Observable, then ignores subsequent source values for a duration determined by another Observable, then repeats this process.
### `throttle<T>(durationSelector: (value: T) => ObservableInput<any>, config: ThrottleConfig = defaultThrottleConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the silencing duration for each source value, returned as an Observable or a Promise. |
| `[config](../const/config)` | `ThrottleConfig` | Optional. Default is `defaultThrottleConfig`. a configuration object to define `leading` and `trailing` behavior. Defaults to `{ leading: true, trailing: false }`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that performs the throttle operation to limit the rate of emissions from the source.
Description
-----------
It's like [`throttleTime`](throttletime), but the silencing duration is determined by a second Observable.
`<throttle>` emits the source Observable values on the output Observable when its internal timer is disabled, and ignores source values when the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, it is forwarded to the output Observable, and then the timer is enabled by calling the `durationSelector` function with the source value, which returns the "duration" Observable. When the duration Observable emits a value, the timer is disabled, and this process repeats for the next source value.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, throttle, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(throttle(() => interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounce`](debounce)
* [`delayWhen`](delaywhen)
* [`sample`](sample)
* [`throttleTime`](throttletime)
rxjs concatWith concatWith
==========
`function` `stable` `operator`
Emits all of the values from the source observable, then, once it completes, subscribes to each observable source provided, one at a time, emitting all of their values, and not subscribing to the next one until it completes.
### `concatWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Description
-----------
`<concat>(a$, b$, c$)` is the same as `a$.pipe([concatWith](concatwith)(b$, c$))`.
Example
-------
Listen for one mouse click, then listen for all mouse moves.
```
import { fromEvent, map, take, concatWith } from 'rxjs';
const clicks$ = fromEvent(document, 'click');
const moves$ = fromEvent(document, 'mousemove');
clicks$.pipe(
map(() => 'click'),
take(1),
concatWith(
moves$.pipe(
map(() => 'move')
)
)
)
.subscribe(x => console.log(x));
// 'click'
// 'move'
// 'move'
// 'move'
// ...
```
rxjs max max
===
`function` `stable` `operator`
The Max operator operates on an Observable that emits numbers (or items that can be compared with a provided function), and when source Observable completes it emits a single item: the item with the largest value.
### `max<T>(comparer?: (x: T, y: T) => number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparer` | `(x: T, y: T) => number` | Optional. Default is `undefined`. Optional comparer function that it will use instead of its default to compare the value of two items. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits item with the largest value.
Description
-----------
Examples
--------
Get the maximal value of a series of numbers
```
import { of, max } from 'rxjs';
of(5, 4, 7, 2, 8)
.pipe(max())
.subscribe(x => console.log(x));
// Outputs
// 8
```
Use a comparer function to get the maximal item
```
import { of, max } from 'rxjs';
of(
{ age: 7, name: 'Foo' },
{ age: 5, name: 'Bar' },
{ age: 9, name: 'Beer' }
).pipe(
max((a, b) => a.age < b.age ? -1 : 1)
)
.subscribe(x => console.log(x.name));
// Outputs
// 'Beer'
```
See Also
--------
* [`min`](min)
rxjs pluck pluck
=====
`function` `deprecated` `operator`
Maps each source value to its specified nested property.
Deprecation Notes
-----------------
Use [`map`](map) and optional chaining: `<pluck>('foo', 'bar')` is `<map>(x => x?.foo?.bar)`. Will be removed in v8.
### `pluck<T, R>(...properties: (string | number | symbol)[]): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `properties` | `(string | number | symbol)[]` | The nested properties to pluck from each source value. |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`: A function that returns an Observable of property values from the source values.
Description
-----------
Like [`map`](map), but meant only for picking one of the nested properties of every emitted value.
Given a list of strings or numbers describing a path to a property, retrieves the value of a specified nested property from all values in the source Observable. If a property can't be resolved, it will return `undefined` for that value.
Example
-------
Map every click to the tagName of the clicked target element
```
import { fromEvent, pluck } from 'rxjs';
const clicks = fromEvent(document, 'click');
const tagNames = clicks.pipe(pluck('target', 'tagName'));
tagNames.subscribe(x => console.log(x));
```
Overloads
---------
### `pluck(k1: K1): OperatorFunction<T, T[K1]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1]>`
### `pluck(k1: K1, k2: K2): OperatorFunction<T, T[K1][K2]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1][K2]>`
### `pluck(k1: K1, k2: K2, k3: K3): OperatorFunction<T, T[K1][K2][K3]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1][K2][K3]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4): OperatorFunction<T, T[K1][K2][K3][K4]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1][K2][K3][K4]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5): OperatorFunction<T, T[K1][K2][K3][K4][K5]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1][K2][K3][K4][K5]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5, k6: K6): OperatorFunction<T, T[K1][K2][K3][K4][K5][K6]>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
| `k6` | `K6` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[K1][K2][K3][K4][K5][K6]>`
### `pluck(k1: K1, k2: K2, k3: K3, k4: K4, k5: K5, k6: K6, ...rest: string[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `k1` | `K1` | |
| `k2` | `K2` | |
| `k3` | `K3` | |
| `k4` | `K4` | |
| `k5` | `K5` | |
| `k6` | `K6` | |
| `rest` | `string[]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, unknown>`
### `pluck(...properties: string[]): OperatorFunction<T, unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `properties` | `string[]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, unknown>`
See Also
--------
* [`map`](map)
rxjs skipWhile skipWhile
=========
`function` `stable` `operator`
Returns an Observable that skips all items emitted by the source Observable as long as a specified condition holds true, but emits all further source items as soon as the condition becomes false.
### `skipWhile<T>(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function to test each item emitted from the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that begins emitting items emitted by the source Observable when the specified predicate becomes false.
Description
-----------
Skips all the notifications with a truthy predicate. It will not skip the notifications when the predicate is falsy. It can also be skipped using index. Once the predicate is true, it will not be called again.
Example
-------
Skip some super heroes
```
import { from, skipWhile } from 'rxjs';
const source = from(['Green Arrow', 'SuperMan', 'Flash', 'SuperGirl', 'Black Canary'])
// Skip the heroes until SuperGirl
const example = source.pipe(skipWhile(hero => hero !== 'SuperGirl'));
// output: SuperGirl, Black Canary
example.subscribe(femaleHero => console.log(femaleHero));
```
Skip values from the array until index 5
```
import { from, skipWhile } from 'rxjs';
const source = from([1, 2, 3, 4, 5, 6, 7, 9, 10]);
const example = source.pipe(skipWhile((_, i) => i !== 5));
// output: 6, 7, 9, 10
example.subscribe(value => console.log(value));
```
Overloads
---------
### `skipWhile(predicate: BooleanConstructor): OperatorFunction<T, Extract<T, Falsy> extends never ? never : T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, Extract<T, [Falsy](../type-alias/falsy)> extends <never> ? <never> : T>`
### `skipWhile(predicate: (value: T, index: number) => true): OperatorFunction<T, never>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => true` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, <never>>`
### `skipWhile(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
See Also
--------
* [`last`](last)
* [`skip`](skip)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
rxjs delayWhen delayWhen
=========
`function` `stable` `operator`
Delays the emission of items from the source Observable by a given time span determined by the emissions of another Observable.
### `delayWhen<T>(delayDurationSelector: (value: T, index: number) => Observable<any>, subscriptionDelay?: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `delayDurationSelector` | `(value: T, index: number) => [Observable](../class/observable)<any>` | A function that returns an Observable for each value emitted by the source Observable, which is then used to delay the emission of that item on the output Observable until the Observable returned from this function emits a value. |
| `subscriptionDelay` | `[Observable](../class/observable)<any>` | Optional. Default is `undefined`. An Observable that triggers the subscription to the source Observable once it emits any value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that delays the emissions of the source Observable by an amount of time specified by the Observable returned by `delayDurationSelector`.
Description
-----------
It's like [`delay`](delay), but the time span of the delay duration is determined by a second Observable.
`[delayWhen](delaywhen)` time shifts each emitted value from the source Observable by a time span determined by another Observable. When the source emits a value, the `delayDurationSelector` function is called with the source value as argument, and should return an Observable, called the "duration" Observable. The source value is emitted on the output Observable only when the duration Observable emits a value or completes. The completion of the notifier triggering the emission of the source value is deprecated behavior and will be removed in future versions.
Optionally, `[delayWhen](delaywhen)` takes a second argument, `subscriptionDelay`, which is an Observable. When `subscriptionDelay` emits its first value or completes, the source Observable is subscribed to and starts behaving like described in the previous paragraph. If `subscriptionDelay` is not provided, `[delayWhen](delaywhen)` will subscribe to the source Observable as soon as the output Observable is subscribed.
Example
-------
Delay each click by a random amount of time, between 0 and 5 seconds
```
import { fromEvent, delayWhen, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const delayedClicks = clicks.pipe(
delayWhen(() => interval(Math.random() * 5000))
);
delayedClicks.subscribe(x => console.log(x));
```
See Also
--------
* [`delay`](delay)
* [`throttle`](throttle)
* [`throttleTime`](throttletime)
* [`debounce`](debounce)
* [`debounceTime`](debouncetime)
* [`sample`](sample)
* [`sampleTime`](sampletime)
* [`audit`](audit)
* [`auditTime`](audittime)
rxjs elementAt elementAt
=========
`function` `stable` `operator`
Emits the single value at the specified `index` in a sequence of emissions from the source Observable.
### `elementAt<T, D = T>(index: number, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `index` | `number` | Is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. The default value returned for missing indices. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits a single item, if it is found. Otherwise, it will emit the default value if given. If not, it emits an error.
#### Throws
`[ArgumentOutOfRangeError](../interface/argumentoutofrangeerror)` When using `[elementAt](elementat)(i)`, it delivers an ArgumentOutOfRangeError to the Observer's `error` callback if `i < 0` or the Observable has completed before emitting the i-th `next` notification.
Description
-----------
Emits only the i-th value, then completes.
`[elementAt](elementat)` returns an Observable that emits the item at the specified `index` in the source Observable, or a default value if that `index` is out of range and the `default` argument is provided. If the `default` argument is not given and the `index` is out of range, the output Observable will emit an `[ArgumentOutOfRangeError](../interface/argumentoutofrangeerror)` error.
Example
-------
Emit only the third click event
```
import { fromEvent, elementAt } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(elementAt(2));
result.subscribe(x => console.log(x));
// Results in:
// click 1 = nothing
// click 2 = nothing
// click 3 = MouseEvent object logged to console
```
See Also
--------
* [`first`](first)
* [`last`](last)
* [`skip`](skip)
* [`single`](single)
* [`take`](take)
rxjs skipUntil skipUntil
=========
`function` `stable` `operator`
Returns an Observable that skips items emitted by the source Observable until a second Observable emits an item.
### `skipUntil<T>(notifier: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `[Observable](../class/observable)<any>` | The second Observable that has to emit an item before the source Observable's elements begin to be mirrored by the resulting Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that skips items from the source Observable until the second Observable emits an item, then emits the remaining items.
Description
-----------
The `[skipUntil](skipuntil)` operator causes the observable stream to skip the emission of values until the passed in observable emits the first value. This can be particularly useful in combination with user interactions, responses of http requests or waiting for specific times to pass by.
Internally the `[skipUntil](skipuntil)` operator subscribes to the passed in observable (in the following called *notifier*) in order to recognize the emission of its first value. When this happens, the operator unsubscribes from the *notifier* and starts emitting the values of the *source* observable. It will never let the *source* observable emit any values if the *notifier* completes or throws an error without emitting a value before.
Example
-------
In the following example, all emitted values of the interval observable are skipped until the user clicks anywhere within the page
```
import { interval, fromEvent, skipUntil } from 'rxjs';
const intervalObservable = interval(1000);
const click = fromEvent(document, 'click');
const emitAfterClick = intervalObservable.pipe(
skipUntil(click)
);
// clicked at 4.6s. output: 5...6...7...8........ or
// clicked at 7.3s. output: 8...9...10..11.......
emitAfterClick.subscribe(value => console.log(value));
```
See Also
--------
* [`last`](last)
* [`skip`](skip)
* [`skipWhile`](skipwhile)
* [`skipLast`](skiplast)
rxjs min min
===
`function` `stable` `operator`
The Min operator operates on an Observable that emits numbers (or items that can be compared with a provided function), and when source Observable completes it emits a single item: the item with the smallest value.
### `min<T>(comparer?: (x: T, y: T) => number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `comparer` | `(x: T, y: T) => number` | Optional. Default is `undefined`. Optional comparer function that it will use instead of its default to compare the value of two items. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits item with the smallest value.
Description
-----------
Examples
--------
Get the minimal value of a series of numbers
```
import { of, min } from 'rxjs';
of(5, 4, 7, 2, 8)
.pipe(min())
.subscribe(x => console.log(x));
// Outputs
// 2
```
Use a comparer function to get the minimal item
```
import { of, min } from 'rxjs';
of(
{ age: 7, name: 'Foo' },
{ age: 5, name: 'Bar' },
{ age: 9, name: 'Beer' }
).pipe(
min((a, b) => a.age < b.age ? -1 : 1)
)
.subscribe(x => console.log(x.name));
// Outputs
// 'Bar'
```
See Also
--------
* [`max`](max)
rxjs isObservable isObservable
============
`function` `stable`
Tests to see if the object is an RxJS [`Observable`](../class/observable)
### `isObservable(obj: any): obj is Observable<unknown>`
#### Parameters
| | | |
| --- | --- | --- |
| `obj` | `any` | the object to test |
#### Returns
`obj is [Observable](../class/observable)<unknown>`
rxjs timeInterval timeInterval
============
`function` `stable` `operator`
Emits an object containing the current value, and the time that has passed between emitting the current value and the previous value, which is calculated by using the provided `scheduler`'s `now()` method to retrieve the current time at each emission, then calculating the difference. The `scheduler` defaults to [`asyncScheduler`](../const/asyncscheduler), so by default, the `<interval>` will be in milliseconds.
### `timeInterval<T>(scheduler: SchedulerLike = asyncScheduler): OperatorFunction<T, TimeInterval<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. Scheduler used to get the current time. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TimeInterval](../interface/timeinterval)<T>>`: A function that returns an Observable that emits information about value and interval.
Description
-----------
Convert an Observable that emits items into one that emits indications of the amount of time elapsed between those emissions.
Example
-------
Emit interval between current value with the last value
```
import { interval, timeInterval } from 'rxjs';
const seconds = interval(1000);
seconds
.pipe(timeInterval())
.subscribe(value => console.log(value));
// NOTE: The values will never be this precise,
// intervals created with `interval` or `setInterval`
// are non-deterministic.
// { value: 0, interval: 1000 }
// { value: 1, interval: 1000 }
// { value: 2, interval: 1000 }
```
| programming_docs |
rxjs connectable connectable
===========
`function` `stable`
Creates an observable that multicasts once `<connect>()` is called on it.
### `connectable<T>(source: ObservableInput<T>, config: ConnectableConfig<T> = DEFAULT_CONFIG): Connectable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | The observable source to make connectable. |
| `[config](../const/config)` | `ConnectableConfig<T>` | Optional. Default is `DEFAULT_CONFIG`. The configuration object for `<connectable>`. |
#### Returns
`[Connectable<T>](../interface/connectable)`: A "connectable" observable, that has a `<connect>()` method, that you must call to connect the source to all consumers through the subject provided as the connector.
rxjs onErrorResumeNext onErrorResumeNext
=================
`function` `stable`
### `onErrorResumeNext<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs scheduled scheduled
=========
`function` `stable`
Converts from a common [`ObservableInput`](../type-alias/observableinput) type to an observable where subscription and emissions are scheduled on the provided scheduler.
### `scheduled<T>(input: ObservableInput<T>, scheduler: SchedulerLike): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `input` | `[ObservableInput](../type-alias/observableinput)<T>` | The observable, array, promise, iterable, etc you would like to schedule |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | The scheduler to use to schedule the subscription and emissions from the returned observable. |
#### Returns
`[Observable<T>](../class/observable)`
See Also
--------
* [`from`](from)
* [`of`](of)
rxjs switchMap switchMap
=========
`function` `stable` `operator`
Projects each source value to an Observable which is merged in the output Observable, emitting values only from the most recently projected Observable.
### `switchMap<T, R, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector?: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, ObservedValueOf<O> | R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | A function that, when applied to an item emitted by the source Observable, returns an Observable. |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O> | R>`: A function that returns an Observable that emits the result of applying the projection function (and the optional deprecated `resultSelector`) to each item emitted by the source Observable and taking only the values from the most recently projected inner Observable.
Description
-----------
Maps each value to an Observable, then flattens all of these inner Observables.
Returns an Observable that emits items based on applying a function that you supply to each item emitted by the source Observable, where that function returns an (so-called "inner") Observable. Each time it observes one of these inner Observables, the output Observable begins emitting the items emitted by that inner Observable. When a new inner Observable is emitted, `[switchMap](switchmap)` stops emitting items from the earlier-emitted inner Observable and begins emitting items from the new one. It continues to behave like this for subsequent inner Observables.
Example
-------
Generate new Observable according to source Observable values
```
import { of, switchMap } from 'rxjs';
const switched = of(1, 2, 3).pipe(switchMap(x => of(x, x ** 2, x ** 3)));
switched.subscribe(x => console.log(x));
// outputs
// 1
// 1
// 1
// 2
// 4
// 8
// 3
// 9
// 27
```
Restart an interval Observable on every click event
```
import { fromEvent, switchMap, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(switchMap(() => interval(1000)));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `switchMap(project: (value: T, index: number) => O): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `switchMap(project: (value: T, index: number) => O, resultSelector: undefined): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
### `switchMap(project: (value: T, index: number) => O, resultSelector: (outerValue: T, innerValue: ObservedValueOf<O>, outerIndex: number, innerIndex: number) => R): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(value: T, index: number) => O` | |
| `resultSelector` | `(outerValue: T, innerValue: [ObservedValueOf](../type-alias/observedvalueof)<O>, outerIndex: number, innerIndex: number) => R` | |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`
See Also
--------
* [`concatMap`](concatmap)
* [`exhaustMap`](exhaustmap)
* [`mergeMap`](mergemap)
* [`switchAll`](switchall)
* [`switchMapTo`](switchmapto)
rxjs startWith startWith
=========
`function` `deprecated` `operator`
Deprecation Notes
-----------------
The `scheduler` parameter will be removed in v8. Use `<scheduled>` and `[concatAll](concatall)`. Details: <https://rxjs.dev/deprecations/scheduler-argument>
### `startWith<T, A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `startWith(value: null): OperatorFunction<T, T | null>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `null` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | null>`
### `startWith(value: undefined): OperatorFunction<T, T | undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `undefined` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | undefined>`
### `startWith()`
#### Parameters
There are no parameters.
### `startWith(...values: D[]): OperatorFunction<T, T | D>`
Returns an observable that, at the moment of subscription, will synchronously emit all values provided to this operator, then subscribe to the source and mirror all of its emissions to subscribers.
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `D[]` | Items you want the modified Observable to emit first. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`: A function that returns an Observable that synchronously emits provided values before subscribing to the source Observable.
This is a useful way to know when subscription has occurred on an existing observable.
First emits its arguments in order, and then any emissions from the source.
Examples
--------
Emit a value when a timer starts.
```
import { timer, map, startWith } from 'rxjs';
timer(1000)
.pipe(
map(() => 'timer emit'),
startWith('timer start')
)
.subscribe(x => console.log(x));
// results:
// 'timer start'
// 'timer emit'
```
rxjs materialize materialize
===========
`function` `stable` `operator`
Represents all of the notifications from the source Observable as `next` emissions marked with their original types within [`Notification`](../class/notification) objects.
### `materialize<T>(): OperatorFunction<T, Notification<T> & ObservableNotification<T>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Notification](../class/notification)<T> & [ObservableNotification](../type-alias/observablenotification)<T>>`: A function that returns an Observable that emits [`Notification`](../class/notification) objects that wrap the original emissions from the source Observable with metadata.
Description
-----------
Wraps `next`, `error` and `complete` emissions in [`Notification`](../class/notification) objects, emitted as `next` on the output Observable.
`<materialize>` returns an Observable that emits a `next` notification for each `next`, `error`, or `complete` emission of the source Observable. When the source Observable emits `complete`, the output Observable will emit `next` as a Notification of type "complete", and then it will emit `complete` as well. When the source Observable emits `error`, the output will emit `next` as a Notification of type "error", and then `complete`.
This operator is useful for producing metadata of the source Observable, to be consumed as `next` emissions. Use it in conjunction with [`dematerialize`](dematerialize).
Example
-------
Convert a faulty Observable to an Observable of Notifications
```
import { of, materialize, map } from 'rxjs';
const letters = of('a', 'b', 13, 'd');
const upperCase = letters.pipe(map((x: any) => x.toUpperCase()));
const materialized = upperCase.pipe(materialize());
materialized.subscribe(x => console.log(x));
// Results in the following:
// - Notification { kind: 'N', value: 'A', error: undefined, hasValue: true }
// - Notification { kind: 'N', value: 'B', error: undefined, hasValue: true }
// - Notification { kind: 'E', value: undefined, error: TypeError { message: x.toUpperCase is not a function }, hasValue: false }
```
See Also
--------
* [`Notification`](../class/notification)
* [`dematerialize`](dematerialize)
rxjs mergeScan mergeScan
=========
`function` `stable` `operator`
Applies an accumulator function over the source Observable where the accumulator function itself returns an Observable, then each intermediate Observable returned is merged into the output Observable.
### `mergeScan<T, R>(accumulator: (acc: R, value: T, index: number) => ObservableInput<R>, seed: R, concurrent: number = Infinity): OperatorFunction<T, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `accumulator` | `(acc: R, value: T, index: number) => [ObservableInput](../type-alias/observableinput)<R>` | The accumulator function called on each source value. |
| `seed` | `R` | The initial accumulation value. |
| `concurrent` | `number` | Optional. Default is `Infinity`. Maximum number of input Observables being subscribed to concurrently. |
#### Returns
`[OperatorFunction<T, R>](../interface/operatorfunction)`: A function that returns an Observable of the accumulated values.
Description
-----------
It's like [`scan`](scan), but the Observables returned by the accumulator are merged into the outer Observable.
The first parameter of the `[mergeScan](mergescan)` is an `accumulator` function which is being called every time the source Observable emits a value. `[mergeScan](mergescan)` will subscribe to the value returned by the `accumulator` function and will emit values to the subscriber emitted by inner Observable.
The `accumulator` function is being called with three parameters passed to it: `acc`, `value` and `index`. The `acc` parameter is used as the state parameter whose value is initially set to the `seed` parameter (the second parameter passed to the `[mergeScan](mergescan)` operator).
`[mergeScan](mergescan)` internally keeps the value of the `acc` parameter: as long as the source Observable emits without inner Observable emitting, the `acc` will be set to `seed`. The next time the inner Observable emits a value, `[mergeScan](mergescan)` will internally remember it and it will be passed to the `accumulator` function as `acc` parameter the next time source emits.
The `value` parameter of the `accumulator` function is the value emitted by the source Observable, while the `index` is a number which represent the order of the current emission by the source Observable. It starts with 0.
The last parameter to the `[mergeScan](mergescan)` is the `concurrent` value which defaults to Infinity. It represents the maximum number of inner Observable subscriptions at a time.
Example
-------
Count the number of click events
```
import { fromEvent, map, mergeScan, of } from 'rxjs';
const click$ = fromEvent(document, 'click');
const one$ = click$.pipe(map(() => 1));
const seed = 0;
const count$ = one$.pipe(
mergeScan((acc, one) => of(acc + one), seed)
);
count$.subscribe(x => console.log(x));
// Results:
// 1
// 2
// 3
// 4
// ...and so on for each click
```
See Also
--------
* [`scan`](scan)
* [`switchScan`](switchscan)
rxjs takeWhile takeWhile
=========
`function` `stable` `operator`
Emits values emitted by the source Observable so long as each value satisfies the given `predicate`, and then completes as soon as this `predicate` is not satisfied.
### `takeWhile<T>(predicate: (value: T, index: number) => boolean, inclusive: boolean = false): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function that evaluates a value emitted by the source Observable and returns a boolean. Also takes the (zero-based) index as the second argument. |
| `inclusive` | `boolean` | Optional. Default is `false`. When set to `true` the value that caused `predicate` to return `false` will also be emitted. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits values from the source Observable so long as each value satisfies the condition defined by the `predicate`, then completes.
Description
-----------
Takes values from the source only while they pass the condition given. When the first value does not satisfy, it completes.
`[takeWhile](takewhile)` subscribes and begins mirroring the source Observable. Each value emitted on the source is given to the `predicate` function which returns a boolean, representing a condition to be satisfied by the source values. The output Observable emits the source values until such time as the `predicate` returns false, at which point `[takeWhile](takewhile)` stops mirroring the source Observable and completes the output Observable.
Example
-------
Emit click events only while the clientX property is greater than 200
```
import { fromEvent, takeWhile } from 'rxjs';
const clicks = fromEvent<PointerEvent>(document, 'click');
const result = clicks.pipe(takeWhile(ev => ev.clientX > 200));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `takeWhile(predicate: BooleanConstructor, inclusive: true): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `inclusive` | `true` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `takeWhile(predicate: BooleanConstructor, inclusive: false): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `inclusive` | `false` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `takeWhile(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `takeWhile(predicate: (value: T, index: number) => value is S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `takeWhile(predicate: (value: T, index: number) => value is S, inclusive: false): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
| `inclusive` | `false` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `takeWhile(predicate: (value: T, index: number) => boolean, inclusive?: boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
| `inclusive` | `boolean` | Optional. Default is `undefined`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
See Also
--------
* [`take`](take)
* [`takeLast`](takelast)
* [`takeUntil`](takeuntil)
* [`skip`](skip)
rxjs lastValueFrom lastValueFrom
=============
`function` `stable`
Converts an observable to a promise by subscribing to the observable, waiting for it to complete, and resolving the returned promise with the last value from the observed stream.
### `lastValueFrom<T, D>(source: Observable<T>, config?: LastValueFromConfig<D>): Promise<T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[Observable<T>](../class/observable)` | the observable to convert to a promise |
| `[config](../const/config)` | `LastValueFromConfig<D>` | Optional. Default is `undefined`. a configuration object to define the `defaultValue` to use if the source completes without emitting a value |
#### Returns
`Promise<T | D>`
Description
-----------
If the observable stream completes before any values were emitted, the returned promise will reject with [`EmptyError`](../interface/emptyerror) or will resolve with the default value if a default was specified.
If the observable stream emits an error, the returned promise will reject with that error.
**WARNING**: Only use this with observables you *know* will complete. If the source observable does not complete, you will end up with a promise that is hung up, and potentially all of the state of an async function hanging out in memory. To avoid this situation, look into adding something like [`timeout`](timeout), [`take`](take), [`takeWhile`](takewhile), or [`takeUntil`](takeuntil) amongst others.
Example
-------
Wait for the last value from a stream and emit it from a promise in an async function
```
import { interval, take, lastValueFrom } from 'rxjs';
async function execute() {
const source$ = interval(2000).pipe(take(10));
const finalNumber = await lastValueFrom(source$);
console.log(`The final number is ${ finalNumber }`);
}
execute();
// Expected output:
// 'The final number is 9'
```
See Also
--------
* [`firstValueFrom`](firstvaluefrom)
rxjs takeLast takeLast
========
`function` `stable` `operator`
Waits for the source to complete, then emits the last N values from the source, as specified by the `<count>` argument.
### `takeLast<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `<count>` | `number` | The maximum number of values to emit from the end of the sequence of values emitted by the source Observable. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits at most the last `<count>` values emitted by the source Observable.
Description
-----------
`[takeLast](takelast)` results in an observable that will hold values up to `<count>` values in memory, until the source completes. It then pushes all values in memory to the consumer, in the order they were received from the source, then notifies the consumer that it is complete.
If for some reason the source completes before the `<count>` supplied to `[takeLast](takelast)` is reached, all values received until that point are emitted, and then completion is notified.
**Warning**: Using `[takeLast](takelast)` with an observable that never completes will result in an observable that never emits a value.
Example
-------
Take the last 3 values of an Observable with many values
```
import { range, takeLast } from 'rxjs';
const many = range(1, 100);
const lastThree = many.pipe(takeLast(3));
lastThree.subscribe(x => console.log(x));
```
See Also
--------
* [`take`](take)
* [`takeUntil`](takeuntil)
* [`takeWhile`](takewhile)
* [`skip`](skip)
| programming_docs |
rxjs bufferCount bufferCount
===========
`function` `stable` `operator`
Buffers the source Observable values until the size hits the maximum `bufferSize` given.
### `bufferCount<T>(bufferSize: number, startBufferEvery: number = null): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferSize` | `number` | The maximum size of the buffer emitted. |
| `startBufferEvery` | `number` | Optional. Default is `null`. Interval at which to start a new buffer. For example if `startBufferEvery` is `2`, then a new buffer will be started on every other value from the source. A new buffer is started at the beginning of the source by default. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array, and emits that array only when its size reaches `bufferSize`.
Buffers a number of values from the source Observable by `bufferSize` then emits the buffer and clears it, and starts a new buffer each `startBufferEvery` values. If `startBufferEvery` is not provided or is `null`, then new buffers are started immediately at the start of the source and when each buffer closes and is emitted.
Examples
--------
Emit the last two click events as an array
```
import { fromEvent, bufferCount } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferCount(2));
buffered.subscribe(x => console.log(x));
```
On every click, emit the last two click events as an array
```
import { fromEvent, bufferCount } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferCount(2, 1));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`pairwise`](pairwise)
* [`windowCount`](windowcount)
rxjs bindCallback bindCallback
============
`function` `stable`
### `bindCallback<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs range range
=====
`function` `stable`
Creates an Observable that emits a sequence of numbers within a specified range.
### `range(start: number, count?: number, scheduler?: SchedulerLike): Observable<number>`
#### Parameters
| | | |
| --- | --- | --- |
| `start` | `number` | The value of the first integer in the sequence. |
| `<count>` | `number` | Optional. Default is `undefined`. The number of sequential integers to generate. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. A [`SchedulerLike`](../interface/schedulerlike) to use for scheduling the emissions of the notifications. |
#### Returns
`[Observable](../class/observable)<number>`: An Observable of numbers that emits a finite range of sequential integers.
Description
-----------
Emits a sequence of numbers in a range.
`<range>` operator emits a range of sequential integers, in order, where you select the `start` of the range and its `length`. By default, uses no [`SchedulerLike`](../interface/schedulerlike) and just delivers the notifications synchronously, but may use an optional [`SchedulerLike`](../interface/schedulerlike) to regulate those deliveries.
Example
-------
Produce a range of numbers
```
import { range } from 'rxjs';
const numbers = range(1, 3);
numbers.subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// 1
// 2
// 3
// 'Complete!'
```
See Also
--------
* [`timer`](timer)
* [`interval`](interval)
rxjs partition partition
=========
`function` `stable`
Splits the source Observable into two, one with values that satisfy a predicate, and another with values that don't satisfy the predicate.
### `partition<T>(source: ObservableInput<T>, predicate: (this: any, value: T, index: number) => boolean, thisArg?: any): [Observable<T>, Observable<T>]`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `predicate` | `(this: any, value: T, index: number) => boolean` | A function that evaluates each value emitted by the source Observable. If it returns `true`, the value is emitted on the first Observable in the returned array, if `false` the value is emitted on the second Observable in the array. The `index` parameter is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[[Observable](../class/observable)<T>, [Observable](../class/observable)<T>]`: An array with two Observables: one with values that passed the predicate, and another with values that did not pass the predicate.
Description
-----------
It's like [`filter`](filter), but returns two Observables: one like the output of [`filter`](filter), and the other with values that did not pass the condition.
`<partition>` outputs an array with two Observables that partition the values from the source Observable through the given `predicate` function. The first Observable in that array emits source values for which the predicate argument returns true. The second Observable emits source values for which the predicate returns false. The first behaves like [`filter`](filter) and the second behaves like [`filter`](filter) with the predicate negated.
Example
-------
Partition a set of numbers into odds and evens observables
```
import { of, partition } from 'rxjs';
const observableValues = of(1, 2, 3, 4, 5, 6);
const [evens$, odds$] = partition(observableValues, value => value % 2 === 0);
odds$.subscribe(x => console.log('odds', x));
evens$.subscribe(x => console.log('evens', x));
// Logs:
// odds 1
// odds 3
// odds 5
// evens 2
// evens 4
// evens 6
```
Overloads
---------
### `partition(source: ObservableInput<T>, predicate: (this: A, value: T, index: number) => value is U, thisArg: A): [Observable<U>, Observable<Exclude<T, U>>]`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `predicate` | `(this: A, value: T, index: number) => value is U` | |
| `thisArg` | `A` | |
#### Returns
`[[Observable](../class/observable)<U>, [Observable](../class/observable)<Exclude<T, U>>]`
### `partition(source: ObservableInput<T>, predicate: (value: T, index: number) => value is U): [Observable<U>, Observable<Exclude<T, U>>]`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `predicate` | `(value: T, index: number) => value is U` | |
#### Returns
`[[Observable](../class/observable)<U>, [Observable](../class/observable)<Exclude<T, U>>]`
### `partition(source: ObservableInput<T>, predicate: (this: A, value: T, index: number) => boolean, thisArg: A): [Observable<T>, Observable<T>]`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `predicate` | `(this: A, value: T, index: number) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[[Observable](../class/observable)<T>, [Observable](../class/observable)<T>]`
### `partition(source: ObservableInput<T>, predicate: (value: T, index: number) => boolean): [Observable<T>, Observable<T>]`
#### Parameters
| | | |
| --- | --- | --- |
| `source` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `predicate` | `(value: T, index: number) => boolean` | |
#### Returns
`[[Observable](../class/observable)<T>, [Observable](../class/observable)<T>]`
See Also
--------
* [`filter`](filter)
rxjs bufferTime bufferTime
==========
`function` `stable` `operator`
Buffers the source Observable values for a specific time period.
### `bufferTime<T>(bufferTimeSpan: number, ...otherArgs: any[]): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | The amount of time to fill each buffer array. |
| `otherArgs` | `any[]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array, and emits those arrays periodically in time.
Buffers values from the source for a specific time duration `bufferTimeSpan`. Unless the optional argument `bufferCreationInterval` is given, it emits and resets the buffer every `bufferTimeSpan` milliseconds. If `bufferCreationInterval` is given, this operator opens the buffer every `bufferCreationInterval` milliseconds and closes (emits and resets) the buffer every `bufferTimeSpan` milliseconds. When the optional argument `maxBufferSize` is specified, the buffer will be closed either after `bufferTimeSpan` milliseconds or when it contains `maxBufferSize` elements.
Examples
--------
Every second, emit an array of the recent click events
```
import { fromEvent, bufferTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferTime(1000));
buffered.subscribe(x => console.log(x));
```
Every 5 seconds, emit the click events from the next 2 seconds
```
import { fromEvent, bufferTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(bufferTime(2000, 5000));
buffered.subscribe(x => console.log(x));
```
Overloads
---------
### `bufferTime(bufferTimeSpan: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`
### `bufferTime(bufferTimeSpan: number, bufferCreationInterval: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `bufferCreationInterval` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`
### `bufferTime(bufferTimeSpan: number, bufferCreationInterval: number, maxBufferSize: number, scheduler?: SchedulerLike): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `bufferTimeSpan` | `number` | |
| `bufferCreationInterval` | `number` | |
| `maxBufferSize` | `number` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`windowTime`](windowtime)
rxjs switchAll switchAll
=========
`function` `stable` `operator`
Converts a higher-order Observable into a first-order Observable producing values only from the most recent observable sequence
### `switchAll<O extends ObservableInput<any>>(): OperatorFunction<O, ObservedValueOf<O>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<O, [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that converts a higher-order Observable into a first-order Observable producing values only from the most recent Observable sequence.
Description
-----------
Flattens an Observable-of-Observables.
`[switchAll](switchall)` subscribes to a source that is an observable of observables, also known as a "higher-order observable" (or `[Observable](../class/observable)<[Observable](../class/observable)<T>>`). It subscribes to the most recently provided "inner observable" emitted by the source, unsubscribing from any previously subscribed to inner observable, such that only the most recent inner observable may be subscribed to at any point in time. The resulting observable returned by `[switchAll](switchall)` will only complete if the source observable completes, *and* any currently subscribed to inner observable also has completed, if there are any.
Examples
--------
Spawn a new interval observable for each click event, but for every new click, cancel the previous interval and subscribe to the new one
```
import { fromEvent, tap, map, interval, switchAll } from 'rxjs';
const clicks = fromEvent(document, 'click').pipe(tap(() => console.log('click')));
const source = clicks.pipe(map(() => interval(1000)));
source
.pipe(switchAll())
.subscribe(x => console.log(x));
// Output
// click
// 0
// 1
// 2
// 3
// ...
// click
// 0
// 1
// 2
// ...
// click
// ...
```
See Also
--------
* [`combineLatestAll`](combinelatestall)
* [`concatAll`](concatall)
* [`exhaustAll`](exhaustall)
* [`switchMap`](switchmap)
* [`switchMapTo`](switchmapto)
* [`mergeAll`](mergeall)
rxjs from from
====
`function` `stable`
Creates an Observable from an Array, an array-like object, a Promise, an iterable object, or an Observable-like object.
### `from<T>(input: ObservableInput<T>, scheduler?: SchedulerLike): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `input` | `[ObservableInput](../type-alias/observableinput)<T>` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable<T>](../class/observable)`:
Description
-----------
Converts almost anything to an Observable.
`<from>` converts various other objects and data types into Observables. It also converts a Promise, an array-like, or an [iterable](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Iteration_protocols#iterable) object into an Observable that emits the items in that promise, array, or iterable. A String, in this context, is treated as an array of characters. Observable-like objects (contains a function named with the ES2015 Symbol for Observable) can also be converted through this operator.
Examples
--------
Converts an array to an Observable
```
import { from } from 'rxjs';
const array = [10, 20, 30];
const result = from(array);
result.subscribe(x => console.log(x));
// Logs:
// 10
// 20
// 30
```
Convert an infinite iterable (from a generator) to an Observable
```
import { from, take } from 'rxjs';
function* generateDoubles(seed) {
let i = seed;
while (true) {
yield i;
i = 2 * i; // double it
}
}
const iterator = generateDoubles(3);
const result = from(iterator).pipe(take(10));
result.subscribe(x => console.log(x));
// Logs:
// 3
// 6
// 12
// 24
// 48
// 96
// 192
// 384
// 768
// 1536
```
With `[asyncScheduler](../const/asyncscheduler)`
```
import { from, asyncScheduler } from 'rxjs';
console.log('start');
const array = [10, 20, 30];
const result = from(array, asyncScheduler);
result.subscribe(x => console.log(x));
console.log('end');
// Logs:
// 'start'
// 'end'
// 10
// 20
// 30
```
See Also
--------
* [`fromEvent`](fromevent)
* [`fromEventPattern`](fromeventpattern)
rxjs of of
==
`function` `deprecated`
Deprecation Notes
-----------------
The `scheduler` parameter will be removed in v8. Use `<scheduled>`. Details: <https://rxjs.dev/deprecations/scheduler-argument>
### `of<A extends readonly, unknown>()`
#### Parameters
There are no parameters.
Overloads
---------
### `of(value: null): Observable<null>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `null` | |
#### Returns
`[Observable](../class/observable)<null>`
### `of(value: undefined): Observable<undefined>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `undefined` | |
#### Returns
`[Observable](../class/observable)<undefined>`
### `of(scheduler: SchedulerLike): Observable<never>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | |
#### Returns
`[Observable](../class/observable)<never>`
### `of(): Observable<never>`
#### Parameters
There are no parameters.
#### Returns
`[Observable](../class/observable)<never>`
### `of(): Observable<T>`
#### Parameters
There are no parameters.
#### Returns
`[Observable<T>](../class/observable)`
### `of(value: T): Observable<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
#### Returns
`[Observable<T>](../class/observable)`
### `of()`
#### Parameters
There are no parameters.
### `of(...args: (SchedulerLike | T)[]): Observable<T>`
Converts the arguments to an observable sequence.
#### Parameters
| | | |
| --- | --- | --- |
| `args` | `([SchedulerLike](../interface/schedulerlike) | T)[]` | |
#### Returns
`[Observable<T>](../class/observable)`: An Observable that emits the arguments described above and then completes.
Each argument becomes a `next` notification.
Unlike [`from`](from), it does not do any flattening and emits each argument in whole as a separate `next` notification.
Examples
--------
Emit the values `10, 20, 30`
```
import { of } from 'rxjs';
of(10, 20, 30)
.subscribe({
next: value => console.log('next:', value),
error: err => console.log('error:', err),
complete: () => console.log('the end'),
});
// Outputs
// next: 10
// next: 20
// next: 30
// the end
```
Emit the array `[1, 2, 3]`
```
import { of } from 'rxjs';
of([1, 2, 3])
.subscribe({
next: value => console.log('next:', value),
error: err => console.log('error:', err),
complete: () => console.log('the end'),
});
// Outputs
// next: [1, 2, 3]
// the end
```
rxjs bufferWhen bufferWhen
==========
`function` `stable` `operator`
Buffers the source Observable values, using a factory function of closing Observables to determine when to close, emit, and reset the buffer.
### `bufferWhen<T>(closingSelector: () => ObservableInput<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingSelector` | `() => [ObservableInput](../type-alias/observableinput)<any>` | A function that takes no arguments and returns an Observable that signals buffer closure. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable of arrays of buffered values.
Description
-----------
Collects values from the past as an array. When it starts collecting values, it calls a function that returns an Observable that tells when to close the buffer and restart collecting.
Opens a buffer immediately, then closes the buffer when the observable returned by calling `closingSelector` function emits a value. When it closes the buffer, it immediately opens a new buffer and repeats the process.
Example
-------
Emit an array of the last clicks every [1-5] random seconds
```
import { fromEvent, bufferWhen, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const buffered = clicks.pipe(
bufferWhen(() => interval(1000 + Math.random() * 4000))
);
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`buffer`](buffer)
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`windowWhen`](windowwhen)
rxjs retryWhen retryWhen
=========
`function` `deprecated` `operator`
Returns an Observable that mirrors the source Observable with the exception of an `error`. If the source Observable calls `error`, this method will emit the Throwable that caused the error to the Observable returned from `notifier`. If that Observable calls `complete` or `error` then this method will call `complete` or `error` on the child subscription. Otherwise this method will resubscribe to the source Observable.
Deprecation Notes
-----------------
Will be removed in v9 or v10, use [`retry`](retry)'s `<delay>` option instead.
### `retryWhen<T>(notifier: (errors: Observable<any>) => Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `notifier` | `(errors: [Observable](../class/observable)<any>) => [Observable](../class/observable)<any>` | Receives an Observable of notifications with which a user can `complete` or `error`, aborting the retry. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source Observable with the exception of an `error`.
Description
-----------
Retry an observable sequence on error based on custom criteria.
Example
-------
```
import { interval, map, retryWhen, tap, delayWhen, timer } from 'rxjs';
const source = interval(1000);
const result = source.pipe(
map(value => {
if (value > 5) {
// error will be picked up by retryWhen
throw value;
}
return value;
}),
retryWhen(errors =>
errors.pipe(
// log error message
tap(value => console.log(`Value ${ value } was too high!`)),
// restart in 5 seconds
delayWhen(value => timer(value * 1000))
)
)
);
result.subscribe(value => console.log(value));
// results:
// 0
// 1
// 2
// 3
// 4
// 5
// 'Value 6 was too high!'
// - Wait 5 seconds then repeat
```
See Also
--------
* [`retry`](retry)
| programming_docs |
rxjs buffer buffer
======
`function` `stable` `operator`
Buffers the source Observable values until `closingNotifier` emits.
### `buffer<T>(closingNotifier: Observable<any>): OperatorFunction<T, T[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `closingNotifier` | `[Observable](../class/observable)<any>` | An Observable that signals the buffer to be emitted on the output Observable. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T[]>`: A function that returns an Observable of buffers, which are arrays of values.
Description
-----------
Collects values from the past as an array, and emits that array only when another Observable emits.
Buffers the incoming Observable values until the given `closingNotifier` Observable emits a value, at which point it emits the buffer on the output Observable and starts a new buffer internally, awaiting the next time `closingNotifier` emits.
Example
-------
On every click, emit array of most recent interval events
```
import { fromEvent, interval, buffer } from 'rxjs';
const clicks = fromEvent(document, 'click');
const intervalEvents = interval(1000);
const buffered = intervalEvents.pipe(buffer(clicks));
buffered.subscribe(x => console.log(x));
```
See Also
--------
* [`bufferCount`](buffercount)
* [`bufferTime`](buffertime)
* [`bufferToggle`](buffertoggle)
* [`bufferWhen`](bufferwhen)
* [`window`](window)
rxjs endWith endWith
=======
`function` `stable` `operator`
Returns an observable that will emit all values from the source, then synchronously emit the provided value(s) immediately after the source completes.
### `endWith<T>(...values: (SchedulerLike | T)[]): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `([SchedulerLike](../interface/schedulerlike) | T)[]` | Items you want the modified Observable to emit last. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits all values from the source, then synchronously emits the provided value(s) immediately after the source completes.
Description
-----------
NOTE: Passing a last argument of a Scheduler is *deprecated*, and may result in incorrect types in TypeScript.
This is useful for knowing when an observable ends. Particularly when paired with an operator like [`takeUntil`](takeuntil)
Example
-------
Emit values to know when an interval starts and stops. The interval will stop when a user clicks anywhere on the document.
```
import { interval, map, fromEvent, startWith, takeUntil, endWith } from 'rxjs';
const ticker$ = interval(5000).pipe(
map(() => 'tick')
);
const documentClicks$ = fromEvent(document, 'click');
ticker$.pipe(
startWith('interval started'),
takeUntil(documentClicks$),
endWith('interval ended by click')
)
.subscribe(x => console.log(x));
// Result (assuming a user clicks after 15 seconds)
// 'interval started'
// 'tick'
// 'tick'
// 'tick'
// 'interval ended by click'
```
Overloads
---------
### `endWith(scheduler: SchedulerLike): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `endWith(...valuesAndScheduler: [A, SchedulerLike]): OperatorFunction<T, T | ValueFromArray<A>>`
#### Parameters
| | | |
| --- | --- | --- |
| `valuesAndScheduler` | `[A, [SchedulerLike](../interface/schedulerlike)]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | [ValueFromArray](../type-alias/valuefromarray)<A>>`
### `endWith(...values: A): OperatorFunction<T, T | ValueFromArray<A>>`
#### Parameters
| | | |
| --- | --- | --- |
| `values` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | [ValueFromArray](../type-alias/valuefromarray)<A>>`
See Also
--------
* [`startWith`](startwith)
* [`concat`](concat)
* [`takeUntil`](takeuntil)
rxjs every every
=====
`function` `stable` `operator`
Returns an Observable that emits whether or not every item of the source satisfies the condition specified.
### `every<T>(predicate: (value: T, index: number, source: Observable<T>) => boolean, thisArg?: any): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | A function for determining if an item meets a specified condition. |
| `thisArg` | `any` | Optional. Default is `undefined`. Optional object to use for `this` in the callback. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, boolean>`: A function that returns an Observable of booleans that determines if all items of the source Observable meet the condition specified.
Description
-----------
If all values pass predicate before the source completes, emits true before completion, otherwise emit false, then complete.
Example
-------
A simple example emitting true if all elements are less than 5, false otherwise
```
import { of, every } from 'rxjs';
of(1, 2, 3, 4, 5, 6)
.pipe(every(x => x < 5))
.subscribe(x => console.log(x)); // -> false
```
Overloads
---------
### `every(predicate: BooleanConstructor): OperatorFunction<T, Exclude<T, Falsy> extends never ? false : boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, Exclude<T, [Falsy](../type-alias/falsy)> extends <never> ? false : boolean>`
### `every(predicate: BooleanConstructor, thisArg: any): OperatorFunction<T, Exclude<T, Falsy> extends never ? false : boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `thisArg` | `any` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, Exclude<T, [Falsy](../type-alias/falsy)> extends <never> ? false : boolean>`
### `every(predicate: (this: A, value: T, index: number, source: Observable<T>) => boolean, thisArg: A): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, boolean>`
### `every(predicate: (value: T, index: number, source: Observable<T>) => boolean): OperatorFunction<T, boolean>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, boolean>`
rxjs windowToggle windowToggle
============
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable starting from an emission from `openings` and ending when the output of `closingSelector` emits.
### `windowToggle<T, O>(openings: ObservableInput<O>, closingSelector: (openValue: O) => ObservableInput<any>): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `openings` | `[ObservableInput](../type-alias/observableinput)<O>` | An observable of notifications to start new windows. |
| `closingSelector` | `(openValue: O) => [ObservableInput](../type-alias/observableinput)<any>` | A function that takes the value emitted by the `openings` observable and returns an Observable, which, when it emits a next notification, signals that the associated window should complete. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observables.
Description
-----------
It's like [`bufferToggle`](buffertoggle), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits windows that contain those items emitted by the source Observable between the time when the `openings` Observable emits an item and when the Observable returned by `closingSelector` emits an item.
Example
-------
Every other second, emit the click events from the next 500ms
```
import { fromEvent, interval, windowToggle, EMPTY, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const openings = interval(1000);
const result = clicks.pipe(
windowToggle(openings, i => i % 2 ? interval(500) : EMPTY),
mergeAll()
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowCount`](windowcount)
* [`windowTime`](windowtime)
* [`windowWhen`](windowwhen)
* [`bufferToggle`](buffertoggle)
rxjs using using
=====
`function` `stable`
Creates an Observable that uses a resource which will be disposed at the same time as the Observable.
### `using<T extends ObservableInput<any>>(resourceFactory: () => void | Unsubscribable, observableFactory: (resource: void | Unsubscribable) => void | T): Observable<ObservedValueOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `resourceFactory` | `() => void | [Unsubscribable](../interface/unsubscribable)` | A function which creates any resource object that implements `unsubscribe` method. |
| `observableFactory` | `(resource: void | [Unsubscribable](../interface/unsubscribable)) => void | T` | A function which creates an Observable, that can use injected resource object. |
#### Returns
`[Observable](../class/observable)<[ObservedValueOf](../type-alias/observedvalueof)<T>>`: An Observable that behaves the same as Observable returned by `observableFactory`, but which - when completed, errored or unsubscribed - will also call `unsubscribe` on created resource object.
Description
-----------
Use it when you catch yourself cleaning up after an Observable.
`<using>` is a factory operator, which accepts two functions. First function returns a disposable resource. It can be an arbitrary object that implements `unsubscribe` method. Second function will be injected with that object and should return an Observable. That Observable can use resource object during its execution. Both functions passed to `<using>` will be called every time someone subscribes - neither an Observable nor resource object will be shared in any way between subscriptions.
When Observable returned by `<using>` is subscribed, Observable returned from the second function will be subscribed as well. All its notifications (nexted values, completion and error events) will be emitted unchanged by the output Observable. If however someone unsubscribes from the Observable or source Observable completes or errors by itself, the `unsubscribe` method on resource object will be called. This can be used to do any necessary clean up, which otherwise would have to be handled by hand. Note that complete or error notifications are not emitted when someone cancels subscription to an Observable via `unsubscribe`, so `<using>` can be used as a hook, allowing you to make sure that all resources which need to exist during an Observable execution will be disposed at appropriate time.
See Also
--------
* [`defer`](defer)
rxjs throwIfEmpty throwIfEmpty
============
`function` `stable` `operator`
If the source observable completes without emitting a value, it will emit an error. The error will be created at that time by the optional `errorFactory` argument, otherwise, the error will be [`EmptyError`](../interface/emptyerror).
### `throwIfEmpty<T>(errorFactory: () => any = defaultErrorFactory): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `errorFactory` | `() => any` | Optional. Default is `defaultErrorFactory`. A factory function called to produce the error to be thrown when the source observable completes without emitting a value. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that throws an error if the source Observable completed without emitting.
Description
-----------
Example
-------
Throw an error if the document wasn't clicked within 1 second
```
import { fromEvent, takeUntil, timer, throwIfEmpty } from 'rxjs';
const click$ = fromEvent(document, 'click');
click$.pipe(
takeUntil(timer(1000)),
throwIfEmpty(() => new Error('The document was not clicked within 1 second'))
)
.subscribe({
next() {
console.log('The document was clicked');
},
error(err) {
console.error(err.message);
}
});
```
rxjs pairs pairs
=====
`function` `deprecated`
Convert an object into an Observable of `[key, value]` pairs.
Deprecation Notes
-----------------
Use `<from>(Object.entries(obj))` instead. Will be removed in v8.
### `pairs(obj: any, scheduler?: SchedulerLike)`
#### Parameters
| | | |
| --- | --- | --- |
| `obj` | `any` | The object to inspect and turn into an Observable sequence. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. An optional IScheduler to schedule when resulting Observable will emit values. |
Description
-----------
Turn entries of an object into a stream.
`<pairs>` takes an arbitrary object and returns an Observable that emits arrays. Each emitted array has exactly two elements - the first is a key from the object and the second is a value corresponding to that key. Keys are extracted from an object via `Object.keys` function, which means that they will be only enumerable keys that are present on an object directly - not ones inherited via prototype chain.
By default, these arrays are emitted synchronously. To change that you can pass a [`SchedulerLike`](../interface/schedulerlike) as a second argument to `<pairs>`.
Example
-------
Converts an object to an Observable
```
import { pairs } from 'rxjs';
const obj = {
foo: 42,
bar: 56,
baz: 78
};
pairs(obj).subscribe({
next: value => console.log(value),
complete: () => console.log('Complete!')
});
// Logs:
// ['foo', 42]
// ['bar', 56]
// ['baz', 78]
// 'Complete!'
```
#### Object.entries required
In IE, you will need to polyfill `Object.entries` in order to use this. [MDN has a polyfill here](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/entries)
Overloads
---------
### `pairs(arr: any, T, __2, scheduler?: SchedulerLike): Observable<[string, T]>`
#### Parameters
| | | |
| --- | --- | --- |
| `arr` | `any` | |
| `T` | | |
| `__2` | | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<[string, T]>`
### `pairs(obj: O, scheduler?: SchedulerLike): Observable<[keyof O, O[keyof O]]>`
#### Parameters
| | | |
| --- | --- | --- |
| `obj` | `O` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<[keyof O, O[keyof O]]>`
### `pairs(iterable: Iterable<T>, scheduler?: SchedulerLike): Observable<[string, T]>`
#### Parameters
| | | |
| --- | --- | --- |
| `iterable` | `Iterable<T>` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<[string, T]>`
### `pairs(n: number | bigint | boolean | symbol | ((...args: any[]) => any), scheduler?: SchedulerLike): Observable<[never, never]>`
#### Parameters
| | | |
| --- | --- | --- |
| `n` | `number | bigint | boolean | symbol | ((...args: any[]) => any)` | |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. |
#### Returns
`[Observable](../class/observable)<[<never>, <never>]>`
rxjs concat concat
======
`function` `stable`
### `concat<T extends readonly, unknown>()`
#### Parameters
There are no parameters.
rxjs groupBy groupBy
=======
`function` `stable` `operator`
### `groupBy<T, K, R>(keySelector: (value: T) => K, elementOrOptions?: void | ((value: any) => any) | BasicGroupByOptions<K, T> | GroupByOptionsWithElement<K, R, T>, duration?: (grouped: GroupedObservable<any, any>) => ObservableInput<any>, connector?: () => SubjectLike<any>): OperatorFunction<T, GroupedObservable<K, R>>`
#### Parameters
| | | |
| --- | --- | --- |
| `keySelector` | `(value: T) => K` | |
| `elementOrOptions` | `void | ((value: any) => any) | BasicGroupByOptions<K, T> | GroupByOptionsWithElement<K, R, T>` | Optional. Default is `undefined`. |
| `duration` | `(grouped: GroupedObservable<any, any>) => [ObservableInput](../type-alias/observableinput)<any>` | Optional. Default is `undefined`. |
| `connector` | `() => [SubjectLike](../interface/subjectlike)<any>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, R>>`
Overloads
---------
### `groupBy(key: (value: T) => K, options: BasicGroupByOptions<K, T>): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `options` | `BasicGroupByOptions<K, T>` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, options: GroupByOptionsWithElement<K, E, T>): OperatorFunction<T, GroupedObservable<K, E>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `options` | `GroupByOptionsWithElement<K, E, T>` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, E>>`
### `groupBy(key: (value: T) => value is K): OperatorFunction<T, GroupedObservable<true, K> | GroupedObservable<false, Exclude<T, K>>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => value is K` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<true, K> | GroupedObservable<false, Exclude<T, K>>>`
### `groupBy(key: (value: T) => K): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, element: void, duration: (grouped: GroupedObservable<K, T>) => Observable<any>): OperatorFunction<T, GroupedObservable<K, T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `element` | `void` | |
| `duration` | `(grouped: GroupedObservable<K, T>) => [Observable](../class/observable)<any>` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, T>>`
### `groupBy(key: (value: T) => K, element?: (value: T) => R, duration?: (grouped: GroupedObservable<K, R>) => Observable<any>): OperatorFunction<T, GroupedObservable<K, R>>`
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | |
| `element` | `(value: T) => R` | Optional. Default is `undefined`. |
| `duration` | `(grouped: GroupedObservable<K, R>) => [Observable](../class/observable)<any>` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, R>>`
### `groupBy(key: (value: T) => K, element?: (value: T) => R, duration?: (grouped: GroupedObservable<K, R>) => Observable<any>, connector?: () => Subject<R>): OperatorFunction<T, GroupedObservable<K, R>>`
Groups the items emitted by an Observable according to a specified criterion, and emits these grouped items as `GroupedObservables`, one [GroupedObservable](groupedobservable) per group.
#### Parameters
| | | |
| --- | --- | --- |
| `key` | `(value: T) => K` | A function that extracts the key for each item. |
| `element` | `(value: T) => R` | Optional. Default is `undefined`. A function that extracts the return element for each item. |
| `duration` | `(grouped: GroupedObservable<K, R>) => [Observable](../class/observable)<any>` | Optional. Default is `undefined`. A function that returns an Observable to determine how long each group should exist. |
| `connector` | `() => [Subject](../class/subject)<R>` | Optional. Default is `undefined`. Factory function to create an intermediate Subject through which grouped elements are emitted. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, GroupedObservable<K, R>>`: A function that returns an Observable that emits GroupedObservables, each of which corresponds to a unique key value and each of which emits those items from the source Observable that share that key value.
When the Observable emits an item, a key is computed for this item with the key function.
If a [GroupedObservable](groupedobservable) for this key exists, this [GroupedObservable](groupedobservable) emits. Otherwise, a new [GroupedObservable](groupedobservable) for this key is created and emits.
A [GroupedObservable](groupedobservable) represents values belonging to the same group represented by a common key. The common key is available as the `key` field of a [GroupedObservable](groupedobservable) instance.
The elements emitted by [GroupedObservable](groupedobservable)s are by default the items emitted by the Observable, or elements returned by the element function.
Examples
--------
Group objects by `id` and return as array
```
import { of, groupBy, mergeMap, reduce } from 'rxjs';
of(
{ id: 1, name: 'JavaScript' },
{ id: 2, name: 'Parcel' },
{ id: 2, name: 'webpack' },
{ id: 1, name: 'TypeScript' },
{ id: 3, name: 'TSLint' }
).pipe(
groupBy(p => p.id),
mergeMap(group$ => group$.pipe(reduce((acc, cur) => [...acc, cur], [])))
)
.subscribe(p => console.log(p));
// displays:
// [{ id: 1, name: 'JavaScript' }, { id: 1, name: 'TypeScript'}]
// [{ id: 2, name: 'Parcel' }, { id: 2, name: 'webpack'}]
// [{ id: 3, name: 'TSLint' }]
```
Pivot data on the `id` field
```
import { of, groupBy, mergeMap, reduce, map } from 'rxjs';
of(
{ id: 1, name: 'JavaScript' },
{ id: 2, name: 'Parcel' },
{ id: 2, name: 'webpack' },
{ id: 1, name: 'TypeScript' },
{ id: 3, name: 'TSLint' }
).pipe(
groupBy(p => p.id, { element: p => p.name }),
mergeMap(group$ => group$.pipe(reduce((acc, cur) => [...acc, cur], [`${ group$.key }`]))),
map(arr => ({ id: parseInt(arr[0], 10), values: arr.slice(1) }))
)
.subscribe(p => console.log(p));
// displays:
// { id: 1, values: [ 'JavaScript', 'TypeScript' ] }
// { id: 2, values: [ 'Parcel', 'webpack' ] }
// { id: 3, values: [ 'TSLint' ] }
```
| programming_docs |
rxjs audit audit
=====
`function` `stable` `operator`
Ignores source values for a duration determined by another Observable, then emits the most recent value from the source Observable, then repeats this process.
### `audit<T>(durationSelector: (value: T) => ObservableInput<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `durationSelector` | `(value: T) => [ObservableInput](../type-alias/observableinput)<any>` | A function that receives a value from the source Observable, for computing the silencing duration, returned as an Observable or a Promise. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that performs rate-limiting of emissions from the source Observable.
Description
-----------
It's like [`auditTime`](audittime), but the silencing duration is determined by a second Observable.
`<audit>` is similar to `<throttle>`, but emits the last value from the silenced time window, instead of the first value. `<audit>` emits the most recent value from the source Observable on the output Observable as soon as its internal timer becomes disabled, and ignores source values while the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, the timer is enabled by calling the `durationSelector` function with the source value, which returns the "duration" Observable. When the duration Observable emits a value, the timer is disabled, then the most recent source value is emitted on the output Observable, and this process repeats for the next source value.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, audit, interval } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(audit(ev => interval(1000)));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounce`](debounce)
* [`delayWhen`](delaywhen)
* [`sample`](sample)
* [`throttle`](throttle)
rxjs catchError catchError
==========
`function` `stable` `operator`
Catches errors on the observable to be handled by returning a new observable or throwing an error.
### `catchError<T, O extends ObservableInput<any>>(selector: (err: any, caught: Observable<T>) => O): OperatorFunction<T, T | ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `(err: any, caught: [Observable](../class/observable)<T>) => O` | a function that takes as arguments `err`, which is the error, and `caught`, which is the source observable, in case you'd like to "retry" that observable by returning it again. Whatever observable is returned by the `selector` will be used to continue the observable chain. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | [ObservedValueOf](../type-alias/observedvalueof)<O>>`: A function that returns an Observable that originates from either the source or the Observable returned by the `selector` function.
Description
-----------
It only listens to the error channel and ignores notifications. Handles errors from the source observable, and maps them to a new observable. The error may also be rethrown, or a new error can be thrown to emit an error from the result. This operator handles errors, but forwards along all other events to the resulting observable. If the source observable terminates with an error, it will map that error to a new observable, subscribe to it, and forward all of its events to the resulting observable.
Examples
--------
Continue with a different Observable when there's an error
```
import { of, map, catchError } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError(err => of('I', 'II', 'III', 'IV', 'V'))
)
.subscribe(x => console.log(x));
// 1, 2, 3, I, II, III, IV, V
```
Retry the caught source Observable again in case of error, similar to `<retry>()` operator
```
import { of, map, catchError, take } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError((err, caught) => caught),
take(30)
)
.subscribe(x => console.log(x));
// 1, 2, 3, 1, 2, 3, ...
```
Throw a new error when the source Observable throws an error
```
import { of, map, catchError } from 'rxjs';
of(1, 2, 3, 4, 5)
.pipe(
map(n => {
if (n === 4) {
throw 'four!';
}
return n;
}),
catchError(err => {
throw 'error in source. Details: ' + err;
})
)
.subscribe({
next: x => console.log(x),
error: err => console.log(err)
});
// 1, 2, 3, error in source. Details: four!
```
See Also
--------
* [`onErrorResumeNext`](onerrorresumenext)
* [`repeat`](repeat)
* [`repeatWhen`](repeatwhen)
* [`retry`](retry)
* [`retryWhen`](retrywhen)
rxjs connect connect
=======
`function` `stable` `operator`
Creates an observable by multicasting the source within a function that allows the developer to define the usage of the multicast prior to connection.
### `connect<T, O extends ObservableInput<unknown>>(selector: (shared: Observable<T>) => O, config: ConnectConfig<T> = DEFAULT_CONFIG): OperatorFunction<T, ObservedValueOf<O>>`
#### Parameters
| | | |
| --- | --- | --- |
| `selector` | `(shared: [Observable](../class/observable)<T>) => O` | A function used to set up the multicast. Gives you a multicast observable that is not yet connected. With that, you're expected to create and return and Observable, that when subscribed to, will utilize the multicast observable. After this function is executed -- and its return value subscribed to -- the operator will subscribe to the source, and the connection will be made. |
| `[config](../const/config)` | `ConnectConfig<T>` | Optional. Default is `DEFAULT_CONFIG`. The configuration object for `<connect>`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [ObservedValueOf](../type-alias/observedvalueof)<O>>`
Description
-----------
This is particularly useful if the observable source you wish to multicast could be synchronous or asynchronous. This sets it apart from [`share`](share), which, in the case of totally synchronous sources will fail to share a single subscription with multiple consumers, as by the time the subscription to the result of [`share`](share) has returned, if the source is synchronous its internal reference count will jump from 0 to 1 back to 0 and reset.
To use `<connect>`, you provide a `selector` function that will give you a multicast observable that is not yet connected. You then use that multicast observable to create a resulting observable that, when subscribed, will set up your multicast. This is generally, but not always, accomplished with [`merge`](merge).
Note that using a [`takeUntil`](takeuntil) inside of `<connect>`'s `selector` *might* mean you were looking to use the [`takeWhile`](takewhile) operator instead.
When you subscribe to the result of `<connect>`, the `selector` function will be called. After the `selector` function returns, the observable it returns will be subscribed to, *then* the multicast will be connected to the source.
Example
-------
Sharing a totally synchronous observable
```
import { of, tap, connect, merge, map, filter } from 'rxjs';
const source$ = of(1, 2, 3, 4, 5).pipe(
tap({
subscribe: () => console.log('subscription started'),
next: n => console.log(`source emitted ${ n }`)
})
);
source$.pipe(
// Notice in here we're merging 3 subscriptions to `shared$`.
connect(shared$ => merge(
shared$.pipe(map(n => `all ${ n }`)),
shared$.pipe(filter(n => n % 2 === 0), map(n => `even ${ n }`)),
shared$.pipe(filter(n => n % 2 === 1), map(n => `odd ${ n }`))
))
)
.subscribe(console.log);
// Expected output: (notice only one subscription)
'subscription started'
'source emitted 1'
'all 1'
'odd 1'
'source emitted 2'
'all 2'
'even 2'
'source emitted 3'
'all 3'
'odd 3'
'source emitted 4'
'all 4'
'even 4'
'source emitted 5'
'all 5'
'odd 5'
```
rxjs share share
=====
`function` `stable` `operator`
Returns a new Observable that multicasts (shares) the original Observable. As long as there is at least one Subscriber this Observable will be subscribed and emitting data. When all subscribers have unsubscribed it will unsubscribe from the source Observable. Because the Observable is multicasting it makes the stream `hot`. This is an alias for `<multicast>(() => new [Subject](../class/subject)()), [refCount](refcount)()`.
### `share<T>(options: ShareConfig<T> = {}): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `options` | `ShareConfig<T>` | Optional. Default is `{}`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that mirrors the source.
Description
-----------
The subscription to the underlying source Observable can be reset (unsubscribe and resubscribe for new subscribers), if the subscriber count to the shared observable drops to 0, or if the source Observable errors or completes. It is possible to use notifier factories for the resets to allow for behaviors like conditional or delayed resets. Please note that resetting on error or complete of the source Observable does not behave like a transparent retry or restart of the source because the error or complete will be forwarded to all subscribers and their subscription will be closed. Only new subscribers after a reset on error or complete happened will cause a fresh subscription to the source. To achieve transparent retries or restarts pipe the source through appropriate operators before sharing.
Example
-------
Generate new multicast Observable from the `source` Observable value
```
import { interval, tap, map, take, share } from 'rxjs';
const source = interval(1000).pipe(
tap(x => console.log('Processing: ', x)),
map(x => x * x),
take(6),
share()
);
source.subscribe(x => console.log('subscription 1: ', x));
source.subscribe(x => console.log('subscription 2: ', x));
// Logs:
// Processing: 0
// subscription 1: 0
// subscription 2: 0
// Processing: 1
// subscription 1: 1
// subscription 2: 1
// Processing: 2
// subscription 1: 4
// subscription 2: 4
// Processing: 3
// subscription 1: 9
// subscription 2: 9
// Processing: 4
// subscription 1: 16
// subscription 2: 16
// Processing: 5
// subscription 1: 25
// subscription 2: 25
```
Example with notifier factory: Delayed reset
--------------------------------------------
```
import { interval, take, share, timer } from 'rxjs';
const source = interval(1000).pipe(
take(3),
share({
resetOnRefCountZero: () => timer(1000)
})
);
const subscriptionOne = source.subscribe(x => console.log('subscription 1: ', x));
setTimeout(() => subscriptionOne.unsubscribe(), 1300);
setTimeout(() => source.subscribe(x => console.log('subscription 2: ', x)), 1700);
setTimeout(() => source.subscribe(x => console.log('subscription 3: ', x)), 5000);
// Logs:
// subscription 1: 0
// (subscription 1 unsubscribes here)
// (subscription 2 subscribes here ~400ms later, source was not reset)
// subscription 2: 1
// subscription 2: 2
// (subscription 2 unsubscribes here)
// (subscription 3 subscribes here ~2000ms later, source did reset before)
// subscription 3: 0
// subscription 3: 1
// subscription 3: 2
```
See Also
--------
* [`shareReplay`](sharereplay)
rxjs empty empty
=====
`function` `deprecated`
Deprecation Notes
-----------------
Replaced with the [`EMPTY`](../const/empty) constant or [`scheduled`](scheduled) (e.g. `<scheduled>([], scheduler)`). Will be removed in v8.
### `empty(scheduler?: SchedulerLike)`
#### Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `undefined`. A [`SchedulerLike`](../interface/schedulerlike) to use for scheduling the emission of the complete notification. |
rxjs combineLatestAll combineLatestAll
================
`function` `stable` `operator`
Flattens an Observable-of-Observables by applying [`combineLatest`](combinelatest) when the Observable-of-Observables completes.
### `combineLatestAll<R>(project?: (...values: any[]) => R)`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | Optional. Default is `undefined`. optional function to map the most recent values from each inner Observable into a new result. Takes each of the most recent values from each collected inner Observable as arguments, in order. |
Description
-----------
`[combineLatestAll](combinelatestall)` takes an Observable of Observables, and collects all Observables from it. Once the outer Observable completes, it subscribes to all collected Observables and combines their values using the [`combineLatest`](combinelatest) strategy, such that:
* Every time an inner Observable emits, the output Observable emits
* When the returned observable emits, it emits all of the latest values by:
+ If a `project` function is provided, it is called with each recent value from each inner Observable in whatever order they arrived, and the result of the `project` function is what is emitted by the output Observable.
+ If there is no `project` function, an array of all the most recent values is emitted by the output Observable.
Example
-------
Map two click events to a finite interval Observable, then apply `[combineLatestAll](combinelatestall)`
```
import { fromEvent, map, interval, take, combineLatestAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const higherOrder = clicks.pipe(
map(() => interval(Math.random() * 2000).pipe(take(3))),
take(2)
);
const result = higherOrder.pipe(combineLatestAll());
result.subscribe(x => console.log(x));
```
Overloads
---------
### `combineLatestAll(): OperatorFunction<ObservableInput<T>, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<[ObservableInput](../type-alias/observableinput)<T>, T[]>`
### `combineLatestAll(): OperatorFunction<any, T[]>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<any, T[]>`
### `combineLatestAll(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: T[]) => R` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<[ObservableInput](../type-alias/observableinput)<T>, R>`
### `combineLatestAll(project: (...values: any[]) => R): OperatorFunction<any, R>`
#### Parameters
| | | |
| --- | --- | --- |
| `project` | `(...values: any[]) => R` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<any, R>`
See Also
--------
* [`combineLatest`](combinelatest)
* [`combineLatestWith`](combinelatestwith)
* [`mergeAll`](mergeall)
rxjs throttleTime throttleTime
============
`function` `stable` `operator`
Emits a value from the source Observable, then ignores subsequent source values for `duration` milliseconds, then repeats this process.
### `throttleTime<T>(duration: number, scheduler: SchedulerLike = asyncScheduler, config: ThrottleConfig = defaultThrottleConfig): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `duration` | `number` | Time to wait before emitting another value after emitting the last value, measured in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for managing the timers that handle the throttling. Defaults to [`asyncScheduler`](../const/asyncscheduler). |
| `[config](../const/config)` | `ThrottleConfig` | Optional. Default is `defaultThrottleConfig`. a configuration object to define `leading` and `trailing` behavior. Defaults to `{ leading: true, trailing: false }`. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that performs the throttle operation to limit the rate of emissions from the source.
Description
-----------
Lets a value pass, then ignores source values for the next `duration` milliseconds.
`[throttleTime](throttletime)` emits the source Observable values on the output Observable when its internal timer is disabled, and ignores source values when the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, it is forwarded to the output Observable, and then the timer is enabled. After `duration` milliseconds (or the time unit determined internally by the optional `scheduler`) has passed, the timer is disabled, and this process repeats for the next source value. Optionally takes a [`SchedulerLike`](../interface/schedulerlike) for managing timers.
Examples
--------
#### Limit click rate
Emit clicks at a rate of at most one click per second
```
import { fromEvent, throttleTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(throttleTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`auditTime`](audittime)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sampleTime`](sampletime)
* [`throttle`](throttle)
rxjs skip skip
====
`function` `stable` `operator`
Returns an Observable that skips the first `<count>` items emitted by the source Observable.
### `skip<T>(count: number): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `<count>` | `number` | The number of times, items emitted by source Observable should be skipped. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that skips the first `<count>` values emitted by the source Observable.
Description
-----------
Skips the values until the sent notifications are equal or less than provided skip count. It raises an error if skip count is equal or more than the actual number of emits and source raises an error.
Example
-------
Skip the values before the emission
```
import { interval, skip } from 'rxjs';
// emit every half second
const source = interval(500);
// skip the first 10 emitted values
const result = source.pipe(skip(10));
result.subscribe(value => console.log(value));
// output: 10...11...12...13...
```
See Also
--------
* [`last`](last)
* [`skipWhile`](skipwhile)
* [`skipUntil`](skipuntil)
* [`skipLast`](skiplast)
rxjs dematerialize dematerialize
=============
`function` `stable` `operator`
Converts an Observable of [`ObservableNotification`](../type-alias/observablenotification) objects into the emissions that they represent.
### `dematerialize<N extends ObservableNotification<any>>(): OperatorFunction<N, ValueFromNotification<N>>`
#### Parameters
There are no parameters.
#### Returns
`[OperatorFunction](../interface/operatorfunction)<N, [ValueFromNotification](../type-alias/valuefromnotification)<N>>`: A function that returns an Observable that emits items and notifications embedded in Notification objects emitted by the source Observable.
Description
-----------
Unwraps [`ObservableNotification`](../type-alias/observablenotification) objects as actual `next`, `error` and `complete` emissions. The opposite of [`materialize`](materialize).
`<dematerialize>` is assumed to operate an Observable that only emits [`ObservableNotification`](../type-alias/observablenotification) objects as `next` emissions, and does not emit any `error`. Such Observable is the output of a `<materialize>` operation. Those notifications are then unwrapped using the metadata they contain, and emitted as `next`, `error`, and `complete` on the output Observable.
Use this operator in conjunction with [`materialize`](materialize).
Example
-------
Convert an Observable of Notifications to an actual Observable
```
import { NextNotification, ErrorNotification, of, dematerialize } from 'rxjs';
const notifA: NextNotification<string> = { kind: 'N', value: 'A' };
const notifB: NextNotification<string> = { kind: 'N', value: 'B' };
const notifE: ErrorNotification = { kind: 'E', error: new TypeError('x.toUpperCase is not a function') };
const materialized = of(notifA, notifB, notifE);
const upperCase = materialized.pipe(dematerialize());
upperCase.subscribe({
next: x => console.log(x),
error: e => console.error(e)
});
// Results in:
// A
// B
// TypeError: x.toUpperCase is not a function
```
See Also
--------
* [`materialize`](materialize)
| programming_docs |
rxjs filter filter
======
`function` `stable` `operator`
Filter items emitted by the source Observable by only emitting those that satisfy a specified predicate.
### `filter<T>(predicate: (value: T, index: number) => boolean, thisArg?: any): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | A function that evaluates each value emitted by the source Observable. If it returns `true`, the value is emitted, if `false` the value is not passed to the output Observable. The `index` parameter is the number `i` for the i-th source emission that has happened since the subscription, starting from the number `0`. |
| `thisArg` | `any` | Optional. Default is `undefined`. An optional argument to determine the value of `this` in the `predicate` function. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable that satisfy the specified `predicate`.
Description
-----------
Like [Array.prototype.filter()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter), it only emits a value from the source if it passes a criterion function.
Similar to the well-known `Array.prototype.filter` method, this operator takes values from the source Observable, passes them through a `predicate` function and only emits those values that yielded `true`.
Example
-------
Emit only click events whose target was a DIV element
```
import { fromEvent, filter } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const clicksOnDivs = clicks.pipe(filter(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
clicksOnDivs.subscribe(x => console.log(x));
```
Overloads
---------
### `filter(predicate: (this: A, value: T, index: number) => value is S, thisArg: A): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number) => value is S` | |
| `thisArg` | `A` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `filter(predicate: (value: T, index: number) => value is S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => value is S` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `filter(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `filter(predicate: (this: A, value: T, index: number) => boolean, thisArg: A): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(this: A, value: T, index: number) => boolean` | |
| `thisArg` | `A` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
### `filter(predicate: (value: T, index: number) => boolean): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number) => boolean` | |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`
See Also
--------
* [`distinct`](distinct)
* [`distinctUntilChanged`](distinctuntilchanged)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
* [`ignoreElements`](ignoreelements)
* [`partition`](partition)
* [`skip`](skip)
rxjs withLatestFrom withLatestFrom
==============
`function` `stable` `operator`
Combines the source Observable with other Observables to create an Observable whose values are calculated from the latest values of each, only when the source emits.
### `withLatestFrom<T, R>(...inputs: any[]): OperatorFunction<T, R | any[]>`
#### Parameters
| | | |
| --- | --- | --- |
| `inputs` | `any[]` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, R | any[]>`: A function that returns an Observable of projected values from the most recent values from each input Observable, or an array of the most recent values from each input Observable.
Description
-----------
Whenever the source Observable emits a value, it computes a formula using that value plus the latest values from other input Observables, then emits the output of that formula.
`[withLatestFrom](withlatestfrom)` combines each value from the source Observable (the instance) with the latest values from the other input Observables only when the source emits a value, optionally using a `project` function to determine the value to be emitted on the output Observable. All input Observables must emit at least one value before the output Observable will emit a value.
Example
-------
On every click event, emit an array with the latest timer event plus the click event
```
import { fromEvent, interval, withLatestFrom } from 'rxjs';
const clicks = fromEvent(document, 'click');
const timer = interval(1000);
const result = clicks.pipe(withLatestFrom(timer));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`combineLatest`](combinelatest)
rxjs first first
=====
`function` `stable` `operator`
Emits only the first value (or the first value that meets some condition) emitted by the source Observable.
### `first<T, D>(predicate?: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | Optional. Default is `undefined`. An optional function called with each item to test for condition matching. |
| `defaultValue` | `D` | Optional. Default is `undefined`. The default value emitted in case no valid value was found on the source. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`: A function that returns an Observable that emits the first item that matches the condition.
#### Throws
`[EmptyError](../interface/emptyerror)` Delivers an EmptyError to the Observer's `error` callback if the Observable completes before any `next` notification was sent. This is how `<first>()` is different from [`take`](take)(1) which completes instead.
Description
-----------
Emits only the first value. Or emits only the first value that passes some test.
If called with no arguments, `<first>` emits the first value of the source Observable, then completes. If called with a `predicate` function, `<first>` emits the first value of the source that matches the specified condition. Throws an error if `defaultValue` was not provided and a matching element is not found.
Examples
--------
Emit only the first click that happens on the DOM
```
import { fromEvent, first } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(first());
result.subscribe(x => console.log(x));
```
Emits the first click that happens on a DIV
```
import { fromEvent, first } from 'rxjs';
const div = document.createElement('div');
div.style.cssText = 'width: 200px; height: 200px; background: #09c;';
document.body.appendChild(div);
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(first(ev => (<HTMLElement>ev.target).tagName === 'DIV'));
result.subscribe(x => console.log(x));
```
Overloads
---------
### `first(predicate?: null, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `null` | Optional. Default is `undefined`. |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`
### `first(predicate: BooleanConstructor): OperatorFunction<T, TruthyTypesOf<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T>>`
### `first(predicate: BooleanConstructor, defaultValue: D): OperatorFunction<T, TruthyTypesOf<T> | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `BooleanConstructor` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [TruthyTypesOf](../type-alias/truthytypesof)<T> | D>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue?: S): OperatorFunction<T, S>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => value is S` | |
| `defaultValue` | `S` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => value is S, defaultValue: D): OperatorFunction<T, S | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => value is S` | |
| `defaultValue` | `D` | |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, S | D>`
### `first(predicate: (value: T, index: number, source: Observable<T>) => boolean, defaultValue?: D): OperatorFunction<T, T | D>`
#### Parameters
| | | |
| --- | --- | --- |
| `predicate` | `(value: T, index: number, source: [Observable](../class/observable)<T>) => boolean` | |
| `defaultValue` | `D` | Optional. Default is `undefined`. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, T | D>`
See Also
--------
* [`filter`](filter)
* [`find`](find)
* [`take`](take)
rxjs windowCount windowCount
===========
`function` `stable` `operator`
Branch out the source Observable values as a nested Observable with each nested Observable emitting at most `windowSize` values.
### `windowCount<T>(windowSize: number, startWindowEvery: number = 0): OperatorFunction<T, Observable<T>>`
#### Parameters
| | | |
| --- | --- | --- |
| `windowSize` | `number` | The maximum number of values emitted by each window. |
| `startWindowEvery` | `number` | Optional. Default is `0`. Interval at which to start a new window. For example if `startWindowEvery` is `2`, then a new window will be started on every other value from the source. A new window is started at the beginning of the source by default. |
#### Returns
`[OperatorFunction](../interface/operatorfunction)<T, [Observable](../class/observable)<T>>`: A function that returns an Observable of windows, which in turn are Observable of values.
Description
-----------
It's like [`bufferCount`](buffercount), but emits a nested Observable instead of an array.
Returns an Observable that emits windows of items it collects from the source Observable. The output Observable emits windows every `startWindowEvery` items, each containing no more than `windowSize` items. When the source Observable completes or encounters an error, the output Observable emits the current window and propagates the notification from the source Observable. If `startWindowEvery` is not provided, then new windows are started immediately at the start of the source and when each window completes with size `windowSize`.
Examples
--------
Ignore every 3rd click event, starting from the first one
```
import { fromEvent, windowCount, map, skip, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowCount(3),
map(win => win.pipe(skip(1))), // skip first of every 3 clicks
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
Ignore every 3rd click event, starting from the third one
```
import { fromEvent, windowCount, mergeAll } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(
windowCount(2, 3),
mergeAll() // flatten the Observable-of-Observables
);
result.subscribe(x => console.log(x));
```
See Also
--------
* [`window`](window)
* [`windowTime`](windowtime)
* [`windowToggle`](windowtoggle)
* [`windowWhen`](windowwhen)
* [`bufferCount`](buffercount)
rxjs distinct distinct
========
`function` `stable` `operator`
Returns an Observable that emits all items emitted by the source Observable that are distinct by comparison from previous items.
### `distinct<T, K>(keySelector?: (value: T) => K, flushes?: Observable<any>): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `keySelector` | `(value: T) => K` | Optional. Default is `undefined`. Optional function to select which value you want to check as distinct. |
| `flushes` | `[Observable](../class/observable)<any>` | Optional. Default is `undefined`. Optional Observable for flushing the internal HashSet of the operator. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that emits items from the source Observable with distinct values.
Description
-----------
If a `keySelector` function is provided, then it will project each value from the source observable into a new value that it will check for equality with previously projected values. If the `keySelector` function is not provided, it will use each value from the source observable directly with an equality check against previous values.
In JavaScript runtimes that support `Set`, this operator will use a `Set` to improve performance of the distinct value checking.
In other runtimes, this operator will use a minimal implementation of `Set` that relies on an `Array` and `indexOf` under the hood, so performance will degrade as more values are checked for distinction. Even in newer browsers, a long-running `<distinct>` use might result in memory leaks. To help alleviate this in some scenarios, an optional `flushes` parameter is also provided so that the internal `Set` can be "flushed", basically clearing it of values.
Examples
--------
A simple example with numbers
```
import { of, distinct } from 'rxjs';
of(1, 1, 2, 2, 2, 1, 2, 3, 4, 3, 2, 1)
.pipe(distinct())
.subscribe(x => console.log(x));
// Outputs
// 1
// 2
// 3
// 4
```
An example using the `keySelector` function
```
import { of, distinct } from 'rxjs';
of(
{ age: 4, name: 'Foo'},
{ age: 7, name: 'Bar'},
{ age: 5, name: 'Foo'}
)
.pipe(distinct(({ name }) => name))
.subscribe(x => console.log(x));
// Outputs
// { age: 4, name: 'Foo' }
// { age: 7, name: 'Bar' }
```
See Also
--------
* [`distinctUntilChanged`](distinctuntilchanged)
* [`distinctUntilKeyChanged`](distinctuntilkeychanged)
rxjs auditTime auditTime
=========
`function` `stable` `operator`
Ignores source values for `duration` milliseconds, then emits the most recent value from the source Observable, then repeats this process.
### `auditTime<T>(duration: number, scheduler: SchedulerLike = asyncScheduler): MonoTypeOperatorFunction<T>`
#### Parameters
| | | |
| --- | --- | --- |
| `duration` | `number` | Time to wait before emitting the most recent source value, measured in milliseconds or the time unit determined internally by the optional `scheduler`. |
| `scheduler` | `[SchedulerLike](../interface/schedulerlike)` | Optional. Default is `[asyncScheduler](../const/asyncscheduler)`. The [`SchedulerLike`](../interface/schedulerlike) to use for managing the timers that handle the rate-limiting behavior. |
#### Returns
`[MonoTypeOperatorFunction<T>](../interface/monotypeoperatorfunction)`: A function that returns an Observable that performs rate-limiting of emissions from the source Observable.
Description
-----------
When it sees a source value, it ignores that plus the next ones for `duration` milliseconds, and then it emits the most recent value from the source.
`[auditTime](audittime)` is similar to `[throttleTime](throttletime)`, but emits the last value from the silenced time window, instead of the first value. `[auditTime](audittime)` emits the most recent value from the source Observable on the output Observable as soon as its internal timer becomes disabled, and ignores source values while the timer is enabled. Initially, the timer is disabled. As soon as the first source value arrives, the timer is enabled. After `duration` milliseconds (or the time unit determined internally by the optional `scheduler`) has passed, the timer is disabled, then the most recent source value is emitted on the output Observable, and this process repeats for the next source value. Optionally takes a [`SchedulerLike`](../interface/schedulerlike) for managing timers.
Example
-------
Emit clicks at a rate of at most one click per second
```
import { fromEvent, auditTime } from 'rxjs';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(auditTime(1000));
result.subscribe(x => console.log(x));
```
See Also
--------
* [`audit`](audit)
* [`debounceTime`](debouncetime)
* [`delay`](delay)
* [`sampleTime`](sampletime)
* [`throttleTime`](throttletime)
rxjs async async
=====
`const` `deprecated`
Deprecation Notes
-----------------
Renamed to [`asyncScheduler`](asyncscheduler). Will be removed in v8.
### `const async: AsyncScheduler;`
rxjs NEVER NEVER
=====
`const` `stable`
An Observable that emits no items to the Observer and never completes.
### `const NEVER: Observable<never>;`
#### Description
A simple Observable that emits neither values nor errors nor the completion notification. It can be used for testing purposes or for composing with other Observables. Please note that by never emitting a complete notification, this Observable keeps the subscription from being disposed automatically. Subscriptions need to be manually disposed.
Example
-------
Emit the number 7, then never emit anything else (not even complete)
```
import { NEVER, startWith } from 'rxjs';
const info = () => console.log('Will not be called');
const result = NEVER.pipe(startWith(7));
result.subscribe({
next: x => console.log(x),
error: info,
complete: info
});
```
See Also
--------
* [`Observable`](../class/observable)
* [`EMPTY`](empty)
* [`of`](../function/of)
* [`throwError`](../function/throwerror)
rxjs animationFrameScheduler animationFrameScheduler
=======================
`const` `stable`
Animation Frame Scheduler
### `const animationFrameScheduler: AnimationFrameScheduler;`
#### Description
Perform task when `window.requestAnimationFrame` would fire
When `[animationFrame](animationframe)` scheduler is used with delay, it will fall back to [`asyncScheduler`](asyncscheduler) scheduler behaviour.
Without delay, `[animationFrame](animationframe)` scheduler can be used to create smooth browser animations. It makes sure scheduled task will happen just before next browser content repaint, thus performing animations as efficiently as possible.
Example
-------
Schedule div height animation
```
// html: <div style="background: #0ff;"></div>
import { animationFrameScheduler } from 'rxjs';
const div = document.querySelector('div');
animationFrameScheduler.schedule(function(height) {
div.style.height = height + "px";
this.schedule(height + 1); // `this` references currently executing Action,
// which we reschedule with new state
}, 0, 0);
// You will see a div element growing in height
```
rxjs observable observable
==========
`const` `stable`
Symbol.observable or a string "@@observable". Used for interop
### `const observable: string | symbol;`
rxjs queue queue
=====
`const` `deprecated`
Deprecation Notes
-----------------
Renamed to [`queueScheduler`](queuescheduler). Will be removed in v8.
### `const queue: QueueScheduler;`
| programming_docs |
rxjs combineAll combineAll
==========
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`combineLatestAll`](../function/combinelatestall). Will be removed in v8.
### `const combineAll: { <T>(): OperatorFunction<ObservableInput<T>, T[]>; <T>(): OperatorFunction<any, T[]>; <T, R>(project: (...values: T[]) => R): OperatorFunction<ObservableInput<T>, R>; <R>(project: (...values: any[]) => R): OperatorFunction<...>; };`
rxjs exhaust exhaust
=======
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`exhaustAll`](../function/exhaustall). Will be removed in v8.
### `const exhaust: <O extends ObservableInput<any>>() => OperatorFunction<O, ObservedValueOf<O>>;`
rxjs flatMap flatMap
=======
`const` `deprecated` `operator`
Deprecation Notes
-----------------
Renamed to [`mergeMap`](../function/mergemap). Will be removed in v8.
### `const flatMap: { <T, O extends ObservableInput<any>>(project: (value: T, index: number) => O, concurrent?: number): OperatorFunction<T, ObservedValueOf<O>>; <T, O extends ObservableInput<any>>(project: (value: T, index: number) => O, resultSelector: undefined, concurrent?: number): OperatorFunction<...>; <T, R, O extends Observabl...;`
rxjs asap asap
====
`const` `deprecated`
Deprecation Notes
-----------------
Renamed to [`asapScheduler`](asapscheduler). Will be removed in v8.
### `const asap: AsapScheduler;`
rxjs asyncScheduler asyncScheduler
==============
`const` `stable`
Async Scheduler
### `const asyncScheduler: AsyncScheduler;`
#### Description
Schedule task as if you used setTimeout(task, duration)
`<async>` scheduler schedules tasks asynchronously, by putting them on the JavaScript event loop queue. It is best used to delay tasks in time or to schedule tasks repeating in intervals.
If you just want to "defer" task, that is to perform it right after currently executing synchronous code ends (commonly achieved by `setTimeout(deferredTask, 0)`), better choice will be the [`asapScheduler`](asapscheduler) scheduler.
Examples
--------
Use async scheduler to delay task
```
import { asyncScheduler } from 'rxjs';
const task = () => console.log('it works!');
asyncScheduler.schedule(task, 2000);
// After 2 seconds logs:
// "it works!"
```
Use async scheduler to repeat task in intervals
```
import { asyncScheduler } from 'rxjs';
function task(state) {
console.log(state);
this.schedule(state + 1, 1000); // `this` references currently executing Action,
// which we reschedule with new state and delay
}
asyncScheduler.schedule(task, 3000, 0);
// Logs:
// 0 after 3s
// 1 after 4s
// 2 after 5s
// 3 after 6s
```
rxjs config config
======
`const` `stable`
The [GlobalConfig](globalconfig) object for RxJS. It is used to configure things like how to react on unhandled errors.
### `const config: GlobalConfig;`
rxjs asapScheduler asapScheduler
=============
`const` `stable`
Asap Scheduler
### `const asapScheduler: AsapScheduler;`
#### Description
Perform task as fast as it can be performed asynchronously
`<asap>` scheduler behaves the same as [`asyncScheduler`](asyncscheduler) scheduler when you use it to delay task in time. If however you set delay to `0`, `<asap>` will wait for current synchronously executing code to end and then it will try to execute given task as fast as possible.
`<asap>` scheduler will do its best to minimize time between end of currently executing code and start of scheduled task. This makes it best candidate for performing so called "deferring". Traditionally this was achieved by calling `setTimeout(deferredTask, 0)`, but that technique involves some (although minimal) unwanted delay.
Note that using `<asap>` scheduler does not necessarily mean that your task will be first to process after currently executing code. In particular, if some task was also scheduled with `<asap>` before, that task will execute first. That being said, if you need to schedule task asynchronously, but as soon as possible, `<asap>` scheduler is your best bet.
Example
-------
Compare async and asap scheduler<
```
import { asapScheduler, asyncScheduler } from 'rxjs';
asyncScheduler.schedule(() => console.log('async')); // scheduling 'async' first...
asapScheduler.schedule(() => console.log('asap'));
// Logs:
// "asap"
// "async"
// ... but 'asap' goes first!
```
rxjs animationFrame animationFrame
==============
`const` `deprecated`
Deprecation Notes
-----------------
Renamed to [`animationFrameScheduler`](animationframescheduler). Will be removed in v8.
### `const animationFrame: AnimationFrameScheduler;`
rxjs queueScheduler queueScheduler
==============
`const` `stable`
Queue Scheduler
### `const queueScheduler: QueueScheduler;`
#### Description
Put every next task on a queue, instead of executing it immediately
`<queue>` scheduler, when used with delay, behaves the same as [`asyncScheduler`](asyncscheduler) scheduler.
When used without delay, it schedules given task synchronously - executes it right when it is scheduled. However when called recursively, that is when inside the scheduled task, another task is scheduled with queue scheduler, instead of executing immediately as well, that task will be put on a queue and wait for current one to finish.
This means that when you execute task with `<queue>` scheduler, you are sure it will end before any other task scheduled with that scheduler will start.
Examples
--------
Schedule recursively first, then do something
```
import { queueScheduler } from 'rxjs';
queueScheduler.schedule(() => {
queueScheduler.schedule(() => console.log('second')); // will not happen now, but will be put on a queue
console.log('first');
});
// Logs:
// "first"
// "second"
```
Reschedule itself recursively
```
import { queueScheduler } from 'rxjs';
queueScheduler.schedule(function(state) {
if (state !== 0) {
console.log('before', state);
this.schedule(state - 1); // `this` references currently executing Action,
// which we reschedule with new state
console.log('after', state);
}
}, 0, 3);
// In scheduler that runs recursively, you would expect:
// "before", 3
// "before", 2
// "before", 1
// "after", 1
// "after", 2
// "after", 3
// But with queue it logs:
// "before", 3
// "after", 3
// "before", 2
// "after", 2
// "before", 1
// "after", 1
```
rxjs EMPTY EMPTY
=====
`const` `stable`
A simple Observable that emits no items to the Observer and immediately emits a complete notification.
### `const EMPTY: Observable<never>;`
#### Description
Just emits 'complete', and nothing else.
A simple Observable that only emits the complete notification. It can be used for composing with other Observables, such as in a [`mergeMap`](../function/mergemap).
Examples
--------
Log complete notification
```
import { EMPTY } from 'rxjs';
EMPTY.subscribe({
next: () => console.log('Next'),
complete: () => console.log('Complete!')
});
// Outputs
// Complete!
```
Emit the number 7, then complete
```
import { EMPTY, startWith } from 'rxjs';
const result = EMPTY.pipe(startWith(7));
result.subscribe(x => console.log(x));
// Outputs
// 7
```
Map and flatten only odd numbers to the sequence `'a'`, `'b'`, `'c'`
```
import { interval, mergeMap, of, EMPTY } from 'rxjs';
const interval$ = interval(1000);
const result = interval$.pipe(
mergeMap(x => x % 2 === 1 ? of('a', 'b', 'c') : EMPTY),
);
result.subscribe(x => console.log(x));
// Results in the following to the console:
// x is equal to the count on the interval, e.g. (0, 1, 2, 3, ...)
// x will occur every 1000ms
// if x % 2 is equal to 1, print a, b, c (each on its own)
// if x % 2 is not equal to 1, nothing will be output
```
See Also
--------
* [`Observable`](../class/observable)
* [`NEVER`](never)
* [`of`](../function/of)
* [`throwError`](../function/throwerror)
rxjs Subscriber Subscriber
==========
`class` `stable`
Implements the [`Observer`](../interface/observer) interface and extends the [`Subscription`](subscription) class. While the [`Observer`](../interface/observer) is the public API for consuming the values of an [`Observable`](observable), all Observers get converted to a Subscriber, in order to provide Subscription-like capabilities such as `unsubscribe`. Subscriber is a common type in RxJS, and crucial for implementing operators, but it is rarely used as a public API.
```
class Subscriber<T> extends Subscription implements Observer<T> {
static create<T>(next?: (x?: T) => void, error?: (e?: any) => void, complete?: () => void): Subscriber<T>
constructor(destination?: Subscriber<any> | Observer<any>)
protected isStopped: boolean
protected destination: Subscriber<any> | Observer<any>
next(value?: T): void
error(err?: any): void
complete(): void
unsubscribe(): void
protected _next(value: T): void
protected _error(err: any): void
protected _complete(): void
// inherited from index/Subscription
static EMPTY: (() => {...})
constructor(initialTeardown?: () => void)
closed: false
unsubscribe(): void
add(teardown: TeardownLogic): void
remove(teardown: Subscription | Unsubscribable | (() => void)): void
}
```
Static Methods
--------------
| A static factory for a Subscriber, given a (potentially partial) definition of an Observer. |
| `static create<T>(next?: (x?: T) => void, error?: (e?: any) => void, complete?: () => void): Subscriber<T>` Parameters
| | | |
| --- | --- | --- |
| `next` | `(x?: T) => void` | Optional. Default is `undefined`. The `next` callback of an Observer. |
| `error` | `(e?: any) => void` | Optional. Default is `undefined`. The `error` callback of an Observer. |
| `complete` | `() => void` | Optional. Default is `undefined`. The `complete` callback of an Observer. |
Returns `[Subscriber<T>](subscriber)`: A Subscriber wrapping the (partially defined) Observer represented by the given arguments. |
Constructor
-----------
| `constructor(destination?: Subscriber<any> | Observer<any>)` Parameters
| | | |
| --- | --- | --- |
| `destination` | `[Subscriber](subscriber)<any> | [Observer](../interface/observer)<any>` | Optional. Default is `undefined`. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `isStopped` | `boolean` | |
| `destination` | `[Subscriber](subscriber)<any> | [Observer](../interface/observer)<any>` | |
Methods
-------
| |
| `next(value?: T): void`
The [`Observer`](../interface/observer) callback to receive notifications of type `next` from the Observable, with a value. The Observable may call this method 0 or more times. Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | Optional. Default is `undefined`. The `next` value. |
Returns `void`: |
| |
| `error(err?: any): void`
The [`Observer`](../interface/observer) callback to receive notifications of type `error` from the Observable, with an attached `Error`. Notifies the Observer that the Observable has experienced an error condition. Parameters
| | | |
| --- | --- | --- |
| `err` | `any` | Optional. Default is `undefined`. The `error` exception. |
Returns `void`: |
| |
| `complete(): void`
The [`Observer`](../interface/observer) callback to receive a valueless notification of type `complete` from the Observable. Notifies the Observer that the Observable has finished sending push-based notifications. Parameters There are no parameters. Returns `void`: |
| `unsubscribe(): void` Parameters There are no parameters. Returns `void` |
| `protected _next(value: T): void` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
Returns `void` |
| `protected _error(err: any): void` Parameters
| | | |
| --- | --- | --- |
| `err` | `any` | |
Returns `void` |
| `protected _complete(): void` Parameters There are no parameters. Returns `void` |
rxjs Subject Subject
=======
`class` `stable`
A Subject is a special type of Observable that allows values to be multicasted to many Observers. Subjects are like EventEmitters.
```
class Subject<T> extends Observable<T> implements SubscriptionLike {
static create: (...args: any[]) => any
constructor()
closed: false
observers: Observer<T>[]
isStopped: false
hasError: false
thrownError: any
get observed
lift<R>(operator: Operator<T, R>): Observable<R>
next(value: T)
error(err: any)
complete()
unsubscribe()
asObservable(): Observable<T>
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Subclasses
----------
* `[BehaviorSubject](behaviorsubject)`
* `[ReplaySubject](replaysubject)`
* `[AsyncSubject](asyncsubject)`
Description
-----------
Every Subject is an Observable and an Observer. You can subscribe to a Subject, and you can call next to feed values as well as error and complete.
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `create` | `(...args: any[]) => any` | Creates a "subject" by basically gluing an observer to an observable. |
Constructor
-----------
| `constructor()` Parameters There are no parameters. |
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `false` | |
| `observers` | `[Observer](../interface/observer)<T>[]` | |
| `isStopped` | `false` | |
| `hasError` | `false` | |
| `thrownError` | `any` | |
| `observed` | | Read-only. |
Methods
-------
| `lift<R>(operator: Operator<T, R>): Observable<R>` Parameters
| | | |
| --- | --- | --- |
| `operator` | `Operator<T, R>` | |
Returns `[Observable](observable)<R>` |
| `next(value: T)` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
|
| `error(err: any)` Parameters
| | | |
| --- | --- | --- |
| `err` | `any` | |
|
| `complete()` Parameters There are no parameters. |
| `unsubscribe()` Parameters There are no parameters. |
| |
| `asObservable(): Observable<T>`
Creates a new Observable with this Subject as the source. You can do this to create customize Observer-side logic of the Subject and conceal it from code that uses the Observable. Parameters There are no parameters. Returns `[Observable<T>](observable)`: Observable that the Subject casts to |
rxjs Observable Observable
==========
`class` `stable`
A representation of any set of values over any amount of time. This is the most basic building block of RxJS.
```
class Observable<T> implements Subscribable<T> {
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Subclasses
----------
* `[ConnectableObservable](connectableobservable)`
* `[Subject](subject)`
+ `[BehaviorSubject](behaviorsubject)`
+ `[ReplaySubject](replaysubject)`
+ `[AsyncSubject](asyncsubject)`
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `create` | `(...args: any[]) => any` | Creates a new Observable by calling the Observable constructor |
Constructor
-----------
| `constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)` Parameters
| | | |
| --- | --- | --- |
| `subscribe` | `(this: [Observable](observable)<T>, subscriber: [Subscriber](subscriber)<T>) => [TeardownLogic](../type-alias/teardownlogic)` | Optional. Default is `undefined`. the function that is called when the Observable is initially subscribed to. This function is given a Subscriber, to which new values can be `next`ed, or an `error` method can be called to raise an error, or `complete` can be called to notify of a successful completion. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `source` | `[Observable](observable)<any> | undefined` | |
| `operator` | `Operator<any, T> | undefined` | |
Methods
-------
| |
| `lift<R>(operator?: Operator<T, R>): Observable<R>`
Creates a new Observable, with this Observable instance as the source, and the passed operator defined as the new observable's operator. Parameters
| | | |
| --- | --- | --- |
| `operator` | `Operator<T, R>` | Optional. Default is `undefined`. the operator defining the operation to take on the observable |
Returns `[Observable](observable)<R>`: a new observable with the Operator applied |
| |
| `subscribe(observer?: Partial<Observer<T>>): Subscription`
Invokes an execution of an Observable and registers Observer handlers for notifications it will emit. Parameters
| | | |
| --- | --- | --- |
| `observer` | `Partial<[Observer](../interface/observer)<T>>` | Optional. Default is `undefined`. |
Returns `[Subscription](subscription)` `subscribe(next: (value: T) => void): Subscription` Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | |
Returns `[Subscription](subscription)` `subscribe(next?: (value: T) => void, error?: (error: any) => void, complete?: () => void): Subscription` Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | Optional. Default is `undefined`. |
| `error` | `(error: any) => void` | Optional. Default is `undefined`. |
| `complete` | `() => void` | Optional. Default is `undefined`. |
Returns `[Subscription](subscription)` |
| Use it when you have all these Observables, but still nothing is happening. `subscribe` is not a regular operator, but a method that calls Observable's internal `subscribe` function. It might be for example a function that you passed to Observable's constructor, but most of the time it is a library implementation, which defines what will be emitted by an Observable, and when it be will emitted. This means that calling `subscribe` is actually the moment when Observable starts its work, not when it is created, as it is often the thought. Apart from starting the execution of an Observable, this method allows you to listen for values that an Observable emits, as well as for when it completes or errors. You can achieve this in two of the following ways. The first way is creating an object that implements [`Observer`](../interface/observer) interface. It should have methods defined by that interface, but note that it should be just a regular JavaScript object, which you can create yourself in any way you want (ES6 class, classic function constructor, object literal etc.). In particular, do not attempt to use any RxJS implementation details to create Observers - you don't need them. Remember also that your object does not have to implement all methods. If you find yourself creating a method that doesn't do anything, you can simply omit it. Note however, if the `error` method is not provided and an error happens, it will be thrown asynchronously. Errors thrown asynchronously cannot be caught using `try`/`catch`. Instead, use the [onUnhandledError](onunhandlederror) configuration option or use a runtime handler (like `window.onerror` or `process.on('error)`) to be notified of unhandled errors. Because of this, it's recommended that you provide an `error` method to avoid missing thrown errors. The second way is to give up on Observer object altogether and simply provide callback functions in place of its methods. This means you can provide three functions as arguments to `subscribe`, where the first function is equivalent of a `next` method, the second of an `error` method and the third of a `complete` method. Just as in case of an Observer, if you do not need to listen for something, you can omit a function by passing `undefined` or `null`, since `subscribe` recognizes these functions by where they were placed in function call. When it comes to the `error` function, as with an Observer, if not provided, errors emitted by an Observable will be thrown asynchronously. You can, however, subscribe with no parameters at all. This may be the case where you're not interested in terminal events and you also handled emissions internally by using operators (e.g. using `[tap](../function/tap)`). Whichever style of calling `subscribe` you use, in both cases it returns a Subscription object. This object allows you to call `unsubscribe` on it, which in turn will stop the work that an Observable does and will clean up all resources that an Observable used. Note that cancelling a subscription will not call `complete` callback provided to `subscribe` function, which is reserved for a regular completion signal that comes from an Observable. Remember that callbacks provided to `subscribe` are not guaranteed to be called asynchronously. It is an Observable itself that decides when these functions will be called. For example [`of`](../function/of) by default emits all its values synchronously. Always check documentation for how given Observable will behave when subscribed and if its default behavior can be modified with a `scheduler`. Examples Subscribe with an [Observer](../../../guide/observer)
```
import { of } from 'rxjs';
const sumObserver = {
sum: 0,
next(value) {
console.log('Adding: ' + value);
this.sum = this.sum + value;
},
error() {
// We actually could just remove this method,
// since we do not really care about errors right now.
},
complete() {
console.log('Sum equals: ' + this.sum);
}
};
of(1, 2, 3) // Synchronously emits 1, 2, 3 and then completes.
.subscribe(sumObserver);
// Logs:
// 'Adding: 1'
// 'Adding: 2'
// 'Adding: 3'
// 'Sum equals: 6'
```
Subscribe with functions ([deprecated](deprecations/subscribe-arguments))
```
import { of } from 'rxjs'
let sum = 0;
of(1, 2, 3).subscribe(
value => {
console.log('Adding: ' + value);
sum = sum + value;
},
undefined,
() => console.log('Sum equals: ' + sum)
);
// Logs:
// 'Adding: 1'
// 'Adding: 2'
// 'Adding: 3'
// 'Sum equals: 6'
```
Cancel a subscription
```
import { interval } from 'rxjs';
const subscription = interval(1000).subscribe({
next(num) {
console.log(num)
},
complete() {
// Will not be called, even when cancelling subscription.
console.log('completed!');
}
});
setTimeout(() => {
subscription.unsubscribe();
console.log('unsubscribed!');
}, 2500);
// Logs:
// 0 after 1s
// 1 after 2s
// 'unsubscribed!' after 2.5s
```
|
| `forEach(next: (value: T) => void): Promise<void>` Used as a NON-CANCELLABLE means of subscribing to an observable, for use with APIs that expect promises, like `[async](../const/async)/await`. You cannot unsubscribe from this. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | a handler for each value emitted by the observable |
Returns `Promise<void>`: a promise that either resolves on observable completion or rejects with the handled error |
| `forEach(next: (value: T) => void, promiseCtor: PromiseConstructorLike): Promise<void>` Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | a handler for each value emitted by the observable |
| `promiseCtor` | `PromiseConstructorLike` | a constructor function used to instantiate the Promise |
Returns `Promise<void>`: a promise that either resolves on observable completion or rejects with the handled error |
| **WARNING**: Only use this with observables you *know* will complete. If the source observable does not complete, you will end up with a promise that is hung up, and potentially all of the state of an async function hanging out in memory. To avoid this situation, look into adding something like [`timeout`](../function/timeout), [`take`](../function/take), [`takeWhile`](../function/takewhile), or [`takeUntil`](../function/takeuntil) amongst others. Example
```
import { interval, take } from 'rxjs';
const source$ = interval(1000).pipe(take(4));
async function getTotal() {
let total = 0;
await source$.forEach(value => {
total += value;
console.log('observable -> ' + value);
});
return total;
}
getTotal().then(
total => console.log('Total: ' + total)
);
// Expected:
// 'observable -> 0'
// 'observable -> 1'
// 'observable -> 2'
// 'observable -> 3'
// 'Total: 6'
```
|
| |
| `pipe(): Observable<T>`
Used to stitch together functional operators into a chain. Parameters There are no parameters. Returns `[Observable<T>](observable)` `pipe<A>(op1: OperatorFunction<T, A>): Observable<A>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
Returns `[Observable](observable)<A>` `pipe<A, B>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>): Observable<B>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
Returns `[Observable](observable)<B>` `pipe<A, B, C>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>): Observable<C>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
Returns `[Observable](observable)<C>` `pipe<A, B, C, D>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>): Observable<D>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
Returns `[Observable](observable)<D>` `pipe<A, B, C, D, E>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>): Observable<E>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
Returns `[Observable](observable)<E>` `pipe<A, B, C, D, E, F>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>, op6: OperatorFunction<E, F>): Observable<F>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
| `op6` | `[OperatorFunction](../interface/operatorfunction)<E, F>` | |
Returns `[Observable](observable)<F>` `pipe<A, B, C, D, E, F, G>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>, op6: OperatorFunction<E, F>, op7: OperatorFunction<F, G>): Observable<G>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
| `op6` | `[OperatorFunction](../interface/operatorfunction)<E, F>` | |
| `op7` | `[OperatorFunction](../interface/operatorfunction)<F, G>` | |
Returns `[Observable](observable)<G>` `pipe<A, B, C, D, E, F, G, H>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>, op6: OperatorFunction<E, F>, op7: OperatorFunction<F, G>, op8: OperatorFunction<G, H>): Observable<H>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
| `op6` | `[OperatorFunction](../interface/operatorfunction)<E, F>` | |
| `op7` | `[OperatorFunction](../interface/operatorfunction)<F, G>` | |
| `op8` | `[OperatorFunction](../interface/operatorfunction)<G, H>` | |
Returns `[Observable](observable)<H>` `pipe<A, B, C, D, E, F, G, H, I>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>, op6: OperatorFunction<E, F>, op7: OperatorFunction<F, G>, op8: OperatorFunction<G, H>, op9: OperatorFunction<H, I>): Observable<I>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
| `op6` | `[OperatorFunction](../interface/operatorfunction)<E, F>` | |
| `op7` | `[OperatorFunction](../interface/operatorfunction)<F, G>` | |
| `op8` | `[OperatorFunction](../interface/operatorfunction)<G, H>` | |
| `op9` | `[OperatorFunction](../interface/operatorfunction)<H, I>` | |
Returns `[Observable](observable)<I>` `pipe<A, B, C, D, E, F, G, H, I>(op1: OperatorFunction<T, A>, op2: OperatorFunction<A, B>, op3: OperatorFunction<B, C>, op4: OperatorFunction<C, D>, op5: OperatorFunction<D, E>, op6: OperatorFunction<E, F>, op7: OperatorFunction<F, G>, op8: OperatorFunction<G, H>, op9: OperatorFunction<H, I>, ...operations: OperatorFunction<any, any>[]): Observable<unknown>` Parameters
| | | |
| --- | --- | --- |
| `op1` | `[OperatorFunction](../interface/operatorfunction)<T, A>` | |
| `op2` | `[OperatorFunction](../interface/operatorfunction)<A, B>` | |
| `op3` | `[OperatorFunction](../interface/operatorfunction)<B, C>` | |
| `op4` | `[OperatorFunction](../interface/operatorfunction)<C, D>` | |
| `op5` | `[OperatorFunction](../interface/operatorfunction)<D, E>` | |
| `op6` | `[OperatorFunction](../interface/operatorfunction)<E, F>` | |
| `op7` | `[OperatorFunction](../interface/operatorfunction)<F, G>` | |
| `op8` | `[OperatorFunction](../interface/operatorfunction)<G, H>` | |
| `op9` | `[OperatorFunction](../interface/operatorfunction)<H, I>` | |
| `operations` | `[OperatorFunction](../interface/operatorfunction)<any, any>[]` | |
Returns `[Observable](observable)<unknown>` |
| |
| `toPromise(): Promise<T | undefined>`
Subscribe to this Observable and get a Promise resolving on `complete` with the last emission (if any). Parameters There are no parameters. Returns `Promise<T | undefined>` `toPromise(PromiseCtor: any): Promise<T | undefined>` Parameters
| | | |
| --- | --- | --- |
| `PromiseCtor` | `any` | |
Returns `Promise<T | undefined>` `toPromise(PromiseCtor: PromiseConstructorLike): Promise<T | undefined>` Parameters
| | | |
| --- | --- | --- |
| `PromiseCtor` | `PromiseConstructorLike` | |
Returns `Promise<T | undefined>` |
| **WARNING**: Only use this with observables you *know* will complete. If the source observable does not complete, you will end up with a promise that is hung up, and potentially all of the state of an async function hanging out in memory. To avoid this situation, look into adding something like [`timeout`](../function/timeout), [`take`](../function/take), [`takeWhile`](../function/takewhile), or [`takeUntil`](../function/takeuntil) amongst others. |
| programming_docs |
rxjs Notification Notification
============
`class` `deprecated`
Represents a push-based event or value that an [`Observable`](observable) can emit. This class is particularly useful for operators that manage notifications, like [`materialize`](../function/materialize), [`dematerialize`](../function/dematerialize), [`observeOn`](../function/observeon), and others. Besides wrapping the actual delivered value, it also annotates it with metadata of, for instance, what type of push message it is (`next`, `error`, or `complete`).
Deprecation Notes
-----------------
It is NOT recommended to create instances of `[Notification](notification)` directly. Rather, try to create POJOs matching the signature outlined in [`ObservableNotification`](../type-alias/observablenotification). For example: `{ kind: 'N', value: 1 }`, `{ kind: 'E', error: new Error('bad') }`, or `{ kind: 'C' }`. Will be removed in v8.
```
class Notification<T> {
static createNext<T>(value: T)
static createError(err?: any)
static createComplete(): Notification<never> & CompleteNotification
constructor(kind: "N" | "E" | "C", value?: T, error?: any)
get hasValue: boolean
get kind: 'N' | 'E' | 'C'
get value?: T
get error?: any
observe(observer: PartialObserver<T>): void
do(nextHandler: (value: T) => void, errorHandler?: (err: any) => void, completeHandler?: () => void): void
accept(nextOrObserver: NextObserver<T> | ErrorObserver<T> | CompletionObserver<T> | ((value: T) => void), error?: (err: any) => void, complete?: () => void)
toObservable(): Observable<T>
}
```
Static Methods
--------------
| A shortcut to create a Notification instance of the type `next` from a given value. |
| `static createNext<T>(value: T)` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | The `next` value. |
|
| A shortcut to create a Notification instance of the type `error` from a given error. |
| `static createError(err?: any)` Parameters
| | | |
| --- | --- | --- |
| `err` | `any` | Optional. Default is `undefined`. The `error` error. |
|
| A shortcut to create a Notification instance of the type `complete`. |
| `static createComplete(): Notification<never> & CompleteNotification` Parameters There are no parameters. Returns `[Notification](notification)<never> & [CompleteNotification](../interface/completenotification)`: The valueless "complete" Notification. |
Constructor
-----------
| `constructor(kind: "N", value?: T)` Creates a "Next" notification object. Parameters
| | | |
| --- | --- | --- |
| `kind` | `"N"` | Always `'N'` |
| `value` | `T` | Optional. Default is `undefined`. The value to notify with if observed. |
`constructor(kind: "E", value: undefined, error: any)` Creates an "Error" notification object. Parameters
| | | |
| --- | --- | --- |
| `kind` | `"E"` | Always `'E'` |
| `value` | `undefined` | Always `undefined` |
| `error` | `any` | The error to notify with if observed. |
`constructor(kind: "C")` Creates a "completion" notification object. Parameters
| | | |
| --- | --- | --- |
| `kind` | `"C"` | Always `'C'` |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `hasValue` | `boolean` | Read-only. A value signifying that the notification will "next" if observed. In truth, This is really synonymous with just checking `kind === "N"`. |
| `kind` | `'N' | 'E' | 'C'` | Read-only. Declared in constructor. |
| `value` | `T` | Read-only. Declared in constructor. |
| `error` | `any` | Read-only. Declared in constructor. |
Methods
-------
| |
| `observe(observer: PartialObserver<T>): void`
Executes the appropriate handler on a passed `observer` given the `kind` of notification. If the handler is missing it will do nothing. Even if the notification is an error, if there is no error handler on the observer, an error will not be thrown, it will noop. Parameters
| | | |
| --- | --- | --- |
| `observer` | `[PartialObserver](../type-alias/partialobserver)<T>` | The observer to notify. |
Returns `void` |
| `do(next: (value: T) => void, error: (err: any) => void, complete: () => void): void` Executes a notification on the appropriate handler from a list provided. If a handler is missing for the kind of notification, nothing is called and no error is thrown, it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | A next handler |
| `error` | `(err: any) => void` | An error handler |
| `complete` | `() => void` | A complete handler |
Returns `void` `do(next: (value: T) => void, error: (err: any) => void): void` Executes a notification on the appropriate handler from a list provided. If a handler is missing for the kind of notification, nothing is called and no error is thrown, it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | A next handler |
| `error` | `(err: any) => void` | An error handler |
Returns `void` `do(next: (value: T) => void): void` Executes the next handler if the Notification is of `kind` `"N"`. Otherwise this will not error, and it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | The next handler |
Returns `void` |
| `accept(next: (value: T) => void, error: (err: any) => void, complete: () => void): void` Executes a notification on the appropriate handler from a list provided. If a handler is missing for the kind of notification, nothing is called and no error is thrown, it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | A next handler |
| `error` | `(err: any) => void` | An error handler |
| `complete` | `() => void` | A complete handler |
Returns `void` `accept(next: (value: T) => void, error: (err: any) => void): void` Executes a notification on the appropriate handler from a list provided. If a handler is missing for the kind of notification, nothing is called and no error is thrown, it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | A next handler |
| `error` | `(err: any) => void` | An error handler |
Returns `void` `accept(next: (value: T) => void): void` Executes the next handler if the Notification is of `kind` `"N"`. Otherwise this will not error, and it will be a noop. Parameters
| | | |
| --- | --- | --- |
| `next` | `(value: T) => void` | The next handler |
Returns `void` `accept(observer: PartialObserver<T>): void` Executes the appropriate handler on a passed `observer` given the `kind` of notification. If the handler is missing it will do nothing. Even if the notification is an error, if there is no error handler on the observer, an error will not be thrown, it will noop. Parameters
| | | |
| --- | --- | --- |
| `observer` | `[PartialObserver](../type-alias/partialobserver)<T>` | The observer to notify. |
Returns `void` |
| |
| `toObservable(): Observable<T>`
Returns a simple Observable that just delivers the notification represented by this Notification instance. Parameters There are no parameters. Returns `[Observable<T>](observable)` |
See Also
--------
* [`materialize`](../function/materialize)
* [`dematerialize`](../function/dematerialize)
* [`observeOn`](../function/observeon)
rxjs AsyncSubject AsyncSubject
============
`class` `stable`
A variant of Subject that only emits a value when it completes. It will emit its latest value to all its observers on completion.
```
class AsyncSubject<T> extends Subject<T> {
next(value: T): void
complete(): void
// inherited from index/Subject
static create: (...args: any[]) => any
constructor()
closed: false
observers: Observer<T>[]
isStopped: false
hasError: false
thrownError: any
get observed
lift<R>(operator: Operator<T, R>): Observable<R>
next(value: T)
error(err: any)
complete()
unsubscribe()
asObservable(): Observable<T>
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Methods
-------
| `next(value: T): void` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
Returns `void` |
| `complete(): void` Parameters There are no parameters. Returns `void` |
rxjs Scheduler Scheduler
=========
`class` `deprecated`
An execution context and a data structure to order tasks and schedule their execution. Provides a notion of (potentially virtual) time, through the `now()` getter method.
Deprecation Notes
-----------------
Scheduler is an internal implementation detail of RxJS, and should not be used directly. Rather, create your own class and implement [`SchedulerLike`](../interface/schedulerlike). Will be made internal in v8.
```
class Scheduler implements SchedulerLike {
static now: () => number
constructor(schedulerActionCtor: typeof Action, now: () => number = Scheduler.now)
now: () => number
schedule<T>(work: (this: SchedulerAction<T>, state?: T) => void, delay: number = 0, state?: T): Subscription
}
```
Description
-----------
Each unit of work in a Scheduler is called an `Action`.
```
class Scheduler {
now(): number;
schedule(work, delay?, state?): Subscription;
}
```
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `now` | `() => number` | |
Constructor
-----------
| `constructor(schedulerActionCtor: typeof Action, now: () => number = Scheduler.now)` Parameters
| | | |
| --- | --- | --- |
| `schedulerActionCtor` | `typeof Action` | |
| `now` | `() => number` | Optional. Default is `Scheduler.now`. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `now` | `() => number` | A getter method that returns a number representing the current time (at the time this function was called) according to the scheduler's own internal clock. |
Methods
-------
| |
| `schedule<T>(work: (this: SchedulerAction<T>, state?: T) => void, delay: number = 0, state?: T): Subscription`
Schedules a function, `work`, for execution. May happen at some point in the future, according to the `[delay](../function/delay)` parameter, if specified. May be passed some context object, `state`, which will be passed to the `work` function. Parameters
| | | |
| --- | --- | --- |
| `work` | `(this: [SchedulerAction](../interface/scheduleraction)<T>, state?: T) => void` | A function representing a task, or some unit of work to be executed by the Scheduler. |
| `[delay](../function/delay)` | `number` | Optional. Default is `0`. Time to wait before executing the work, where the time unit is implicit and defined by the Scheduler itself. |
| `state` | `T` | Optional. Default is `undefined`. Some contextual data that the `work` function uses when called by the Scheduler. |
Returns `[Subscription](subscription)`: A subscription in order to be able to unsubscribe the scheduled work. |
| The given arguments will be processed an stored as an Action object in a queue of actions. |
rxjs ConnectableObservable ConnectableObservable
=====================
`class` `deprecated`
Deprecation Notes
-----------------
Will be removed in v8. Use [`connectable`](../function/connectable) to create a connectable observable. If you are using the `[refCount](../function/refcount)` method of `[ConnectableObservable](connectableobservable)`, use the [`share`](../function/share) operator instead. Details: <https://rxjs.dev/deprecations/multicasting>
```
class ConnectableObservable<T> extends Observable<T> {
constructor(source: Observable<T>, subjectFactory: () => Subject<T>)
protected _subject: Subject<T> | null
protected _refCount: number
protected _connection: Subscription | null
source: Observable<T>
protected subjectFactory: () => Subject<T>
protected getSubject(): Subject<T>
protected _teardown()
connect(): Subscription
refCount(): Observable<T>
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Constructor
-----------
| `constructor(source: Observable<T>, subjectFactory: () => Subject<T>)` Parameters
| | | |
| --- | --- | --- |
| `source` | `[Observable<T>](observable)` | The source observable |
| `subjectFactory` | `() => [Subject](subject)<T>` | The factory that creates the subject used internally. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `_subject` | `[Subject](subject)<T> | null` | |
| `_refCount` | `number` | |
| `_connection` | `[Subscription](subscription) | null` | |
| `source` | `[Observable<T>](observable)` | The source observable Declared in constructor. |
| `subjectFactory` | `() => [Subject](subject)<T>` | The factory that creates the subject used internally. Declared in constructor. |
Methods
-------
| `protected getSubject(): Subject<T>` Parameters There are no parameters. Returns `[Subject<T>](subject)` |
| `protected _teardown()` Parameters There are no parameters. |
| `connect(): Subscription` Parameters There are no parameters. Returns `[Subscription](subscription)` |
| `refCount(): Observable<T>` Parameters There are no parameters. Returns `[Observable<T>](observable)` |
rxjs ReplaySubject ReplaySubject
=============
`class` `stable`
A variant of [`Subject`](subject) that "replays" old values to new subscribers by emitting them when they first subscribe.
```
class ReplaySubject<T> extends Subject<T> {
constructor(_bufferSize: number = Infinity, _windowTime: number = Infinity, _timestampProvider: TimestampProvider = dateTimestampProvider)
next(value: T): void
// inherited from index/Subject
static create: (...args: any[]) => any
constructor()
closed: false
observers: Observer<T>[]
isStopped: false
hasError: false
thrownError: any
get observed
lift<R>(operator: Operator<T, R>): Observable<R>
next(value: T)
error(err: any)
complete()
unsubscribe()
asObservable(): Observable<T>
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Description
-----------
`[ReplaySubject](replaysubject)` has an internal buffer that will store a specified number of values that it has observed. Like `[Subject](subject)`, `[ReplaySubject](replaysubject)` "observes" values by having them passed to its `next` method. When it observes a value, it will store that value for a time determined by the configuration of the `[ReplaySubject](replaysubject)`, as passed to its constructor.
When a new subscriber subscribes to the `[ReplaySubject](replaysubject)` instance, it will synchronously emit all values in its buffer in a First-In-First-Out (FIFO) manner. The `[ReplaySubject](replaysubject)` will also complete, if it has observed completion; and it will error if it has observed an error.
There are two main configuration items to be concerned with:
1. `bufferSize` - This will determine how many items are stored in the buffer, defaults to infinite.
2. `[windowTime](../function/windowtime)` - The amount of time to hold a value in the buffer before removing it from the buffer.
Both configurations may exist simultaneously. So if you would like to buffer a maximum of 3 values, as long as the values are less than 2 seconds old, you could do so with a `new [ReplaySubject](replaysubject)(3, 2000)`.
### Differences with BehaviorSubject
`[BehaviorSubject](behaviorsubject)` is similar to `new [ReplaySubject](replaysubject)(1)`, with a couple fo exceptions:
1. `[BehaviorSubject](behaviorsubject)` comes "primed" with a single value upon construction.
2. `[ReplaySubject](replaysubject)` will replay values, even after observing an error, where `[BehaviorSubject](behaviorsubject)` will not.
Constructor
-----------
| `constructor(_bufferSize: number = Infinity, _windowTime: number = Infinity, _timestampProvider: TimestampProvider = dateTimestampProvider)` Parameters
| | | |
| --- | --- | --- |
| `_bufferSize` | `number` | Optional. Default is `Infinity`. |
| `_windowTime` | `number` | Optional. Default is `Infinity`. |
| `_timestampProvider` | `[TimestampProvider](../interface/timestampprovider)` | Optional. Default is `dateTimestampProvider`. |
|
Methods
-------
| `next(value: T): void` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
Returns `void` |
See Also
--------
* [`Subject`](subject)
* [`BehaviorSubject`](behaviorsubject)
* [`shareReplay`](../function/sharereplay)
rxjs BehaviorSubject BehaviorSubject
===============
`class` `stable`
A variant of Subject that requires an initial value and emits its current value whenever it is subscribed to.
```
class BehaviorSubject<T> extends Subject<T> {
constructor(_value: T)
get value: T
getValue(): T
next(value: T): void
// inherited from index/Subject
static create: (...args: any[]) => any
constructor()
closed: false
observers: Observer<T>[]
isStopped: false
hasError: false
thrownError: any
get observed
lift<R>(operator: Operator<T, R>): Observable<R>
next(value: T)
error(err: any)
complete()
unsubscribe()
asObservable(): Observable<T>
// inherited from index/Observable
static create: (...args: any[]) => any
constructor(subscribe?: (this: Observable<T>, subscriber: Subscriber<T>) => TeardownLogic)
source: Observable<any> | undefined
operator: Operator<any, T> | undefined
lift<R>(operator?: Operator<T, R>): Observable<R>
subscribe(observerOrNext?: Partial<Observer<T>> | ((value: T) => void), error?: (error: any) => void, complete?: () => void): Subscription
forEach(next: (value: T) => void, promiseCtor?: PromiseConstructorLike): Promise<void>
pipe(...operations: OperatorFunction<any, any>[]): Observable<any>
toPromise(promiseCtor?: PromiseConstructorLike): Promise<T | undefined>
}
```
Constructor
-----------
| `constructor(_value: T)` Parameters
| | | |
| --- | --- | --- |
| `_value` | `T` | |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `value` | `T` | Read-only. |
Methods
-------
| `getValue(): T` Parameters There are no parameters. Returns `T` |
| `next(value: T): void` Parameters
| | | |
| --- | --- | --- |
| `value` | `T` | |
Returns `void` |
rxjs VirtualTimeScheduler VirtualTimeScheduler
====================
`class` `stable`
```
class VirtualTimeScheduler extends AsyncScheduler {
static frameTimeFactor: 10
constructor(schedulerActionCtor: typeof AsyncAction = VirtualAction as any, maxFrames: number = Infinity)
frame: number
index: number
maxFrames: number
flush(): void
}
```
Subclasses
----------
* `[TestScheduler](../../testing/testscheduler)`
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `frameTimeFactor` | `10` | |
Constructor
-----------
| This creates an instance of a `[VirtualTimeScheduler](virtualtimescheduler)`. Experts only. The signature of this constructor is likely to change in the long run. |
| `constructor(schedulerActionCtor: typeof AsyncAction = VirtualAction as any, maxFrames: number = Infinity)` Parameters
| | | |
| --- | --- | --- |
| `schedulerActionCtor` | `typeof AsyncAction` | Optional. Default is `[VirtualAction](virtualaction) as any`. The type of Action to initialize when initializing actions during scheduling. |
| `maxFrames` | `number` | Optional. Default is `Infinity`. The maximum number of frames to process before stopping. Used to prevent endless flush cycles. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `frame` | `number` | The current frame for the state of the virtual scheduler instance. The the difference between two "frames" is synonymous with the passage of "virtual time units". So if you record `scheduler.frame` to be `1`, then later, observe `scheduler.frame` to be at `11`, that means `10` virtual time units have passed. |
| `index` | `number` | Used internally to examine the current virtual action index being processed. |
| `maxFrames` | `number` | The maximum number of frames to process before stopping. Used to prevent endless flush cycles. Declared in constructor. |
Methods
-------
| |
| `flush(): void`
Prompt the Scheduler to execute all of its queued actions, therefore clearing its queue. Parameters There are no parameters. Returns `void`: |
| programming_docs |
rxjs Subscription Subscription
============
`class` `stable`
Represents a disposable resource, such as the execution of an Observable. A Subscription has one important method, `unsubscribe`, that takes no argument and just disposes the resource held by the subscription.
```
class Subscription implements SubscriptionLike {
static EMPTY: (() => {...})
constructor(initialTeardown?: () => void)
closed: false
unsubscribe(): void
add(teardown: TeardownLogic): void
remove(teardown: Subscription | Unsubscribable | (() => void)): void
}
```
Subclasses
----------
* `[Subscriber](subscriber)`
Description
-----------
Additionally, subscriptions may be grouped together through the `add()` method, which will attach a child Subscription to the current Subscription. When a Subscription is unsubscribed, all its children (and its grandchildren) will be unsubscribed as well.
Static Properties
-----------------
| Property | Type | Description |
| --- | --- | --- |
| `[EMPTY](../const/empty)` | `(() => {
const [empty](../function/empty) = new [Subscription](subscription)();
empty.closed = true;
return [empty](../function/empty);
})()` | |
Constructor
-----------
| `constructor(initialTeardown?: () => void)` Parameters
| | | |
| --- | --- | --- |
| `initialTeardown` | `() => void` | Optional. Default is `undefined`. A function executed first as part of the finalization process that is kicked off when [unsubscribe](subscription#unsubscribe) is called. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `closed` | `false` | A flag to indicate whether this Subscription has already been unsubscribed. |
Methods
-------
| |
| `unsubscribe(): void`
Disposes the resources held by the subscription. May, for instance, cancel an ongoing Observable execution or cancel any other type of work that started when the Subscription was created. Parameters There are no parameters. Returns `void`: |
| |
| `add(teardown: TeardownLogic): void`
Adds a finalizer to this subscription, so that finalization will be unsubscribed/called when this subscription is unsubscribed. If this subscription is already [closed](subscription#closed), because it has already been unsubscribed, then whatever finalizer is passed to it will automatically be executed (unless the finalizer itself is also a closed subscription). Parameters
| | | |
| --- | --- | --- |
| `teardown` | `[TeardownLogic](../type-alias/teardownlogic)` | The finalization logic to add to this subscription. |
Returns `void` |
| Closed Subscriptions cannot be added as finalizers to any subscription. Adding a closed subscription to a any subscription will result in no operation. (A noop). Adding a subscription to itself, or adding `null` or `undefined` will not perform any operation at all. (A noop). `[Subscription](subscription)` instances that are added to this instance will automatically remove themselves if they are unsubscribed. Functions and [`Unsubscribable`](../interface/unsubscribable) objects that you wish to remove will need to be removed manually with [remove](subscription#remove) |
| |
| `remove(teardown: Subscription | Unsubscribable | (() => void)): void`
Removes a finalizer from this subscription that was previously added with the [add](subscription#add) method. Parameters
| | | |
| --- | --- | --- |
| `teardown` | `[Subscription](subscription) | [Unsubscribable](../interface/unsubscribable) | (() => void)` | The finalizer to remove from this subscription |
Returns `void` |
| Note that `[Subscription](subscription)` instances, when unsubscribed, will automatically remove themselves from every other `[Subscription](subscription)` they have been added to. This means that using the `remove` method is not a common thing and should be used thoughtfully. If you add the same finalizer instance of a function or an unsubscribable object to a `Subcription` instance more than once, you will need to call `remove` the same number of times to remove all instances. All finalizer instances are removed to free up memory upon unsubscription. |
rxjs VirtualAction VirtualAction
=============
`class` `stable`
```
class VirtualAction<T> extends AsyncAction<T> {
constructor(scheduler: VirtualTimeScheduler, work: (this: SchedulerAction<T>, state?: T) => void, index: number = (scheduler.index += 1))
protected active: boolean
protected scheduler: VirtualTimeScheduler
protected work: (this: SchedulerAction<T>, state?: T) => void
protected index: number
schedule(state?: T, delay: number = 0): Subscription
protected requestAsyncId(scheduler: VirtualTimeScheduler, id?: any, delay: number = 0): any
protected recycleAsyncId(scheduler: VirtualTimeScheduler, id?: any, delay: number = 0): any
protected _execute(state: T, delay: number): any
}
```
Constructor
-----------
| `constructor(scheduler: VirtualTimeScheduler, work: (this: SchedulerAction<T>, state?: T) => void, index: number = (scheduler.index += 1))` Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[VirtualTimeScheduler](virtualtimescheduler)` | |
| `work` | `(this: [SchedulerAction](../interface/scheduleraction)<T>, state?: T) => void` | |
| `index` | `number` | Optional. Default is `(scheduler.index += 1)`. |
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `active` | `boolean` | |
| `scheduler` | `[VirtualTimeScheduler](virtualtimescheduler)` | Declared in constructor. |
| `work` | `(this: [SchedulerAction](../interface/scheduleraction)<T>, state?: T) => void` | Declared in constructor. |
| `index` | `number` | Declared in constructor. |
Methods
-------
| `schedule(state?: T, delay: number = 0): Subscription` Parameters
| | | |
| --- | --- | --- |
| `state` | `T` | Optional. Default is `undefined`. |
| `[delay](../function/delay)` | `number` | Optional. Default is `0`. |
Returns `[Subscription](subscription)` |
| `protected requestAsyncId(scheduler: VirtualTimeScheduler, id?: any, delay: number = 0): any` Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[VirtualTimeScheduler](virtualtimescheduler)` | |
| `id` | `any` | Optional. Default is `undefined`. |
| `[delay](../function/delay)` | `number` | Optional. Default is `0`. |
Returns `any` |
| `protected recycleAsyncId(scheduler: VirtualTimeScheduler, id?: any, delay: number = 0): any` Parameters
| | | |
| --- | --- | --- |
| `scheduler` | `[VirtualTimeScheduler](virtualtimescheduler)` | |
| `id` | `any` | Optional. Default is `undefined`. |
| `[delay](../function/delay)` | `number` | Optional. Default is `0`. |
Returns `any` |
| `protected _execute(state: T, delay: number): any` Parameters
| | | |
| --- | --- | --- |
| `state` | `T` | |
| `[delay](../function/delay)` | `number` | |
Returns `any` |
rxjs AjaxTimeoutError AjaxTimeoutError
================
`interface` `stable`
Thrown when an AJAX request times out. Not to be confused with [`TimeoutError`](../index/interface/timeouterror).
```
interface AjaxTimeoutError extends AjaxError {
// inherited from ajax/AjaxError
xhr: XMLHttpRequest
request: AjaxRequest
status: number
responseType: XMLHttpRequestResponseType
response: any
}
```
Description
-----------
This is exported only because it is useful for checking to see if errors are an `instanceof [AjaxTimeoutError](ajaxtimeouterror)`. DO NOT use the constructor to create an instance of this type.
See Also
--------
* [`ajax`](ajax)
rxjs AjaxError AjaxError
=========
`interface` `stable`
Thrown when an error occurs during an AJAX request. This is only exported because it is useful for checking to see if an error is an `instanceof [AjaxError](ajaxerror)`. DO NOT create new instances of `[AjaxError](ajaxerror)` with the constructor.
```
interface AjaxError extends Error {
xhr: XMLHttpRequest
request: AjaxRequest
status: number
responseType: XMLHttpRequestResponseType
response: any
}
```
Child Interfaces
----------------
* `[AjaxTimeoutError](ajaxtimeouterror)`
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `xhr` | `XMLHttpRequest` | The XHR instance associated with the error. |
| `request` | `AjaxRequest` | The AjaxRequest associated with the error. |
| `status` | `number` | The HTTP status code, if the request has completed. If not, it is set to `0`. |
| `responseType` | `XMLHttpRequestResponseType` | The responseType (e.g. 'json', 'arraybuffer', or 'xml'). |
| `response` | `any` | The response data. |
See Also
--------
* [`ajax`](ajax)
rxjs AjaxResponse AjaxResponse
============
`class` `stable`
A normalized response from an AJAX request. To get the data from the response, you will want to read the `response` property.
```
class AjaxResponse<T> {
constructor(originalEvent: ProgressEvent, xhr: XMLHttpRequest, request: AjaxRequest, type: any = 'download_load')
get status: number
get response: T
get responseType: XMLHttpRequestResponseType
get loaded: number
get total: number
get responseHeaders: Record<string, string>
get originalEvent: ProgressEvent
get xhr: XMLHttpRequest
get request: AjaxRequest
get type: AjaxResponseType
}
```
Description
-----------
* DO NOT create instances of this class directly.
* DO NOT subclass this class.
It is advised not to hold this object in memory, as it has a reference to the original XHR used to make the request, as well as properties containing request and response data.
Constructor
-----------
| A normalized response from an AJAX request. To get the data from the response, you will want to read the `response` property. |
| `constructor(originalEvent: ProgressEvent, xhr: XMLHttpRequest, request: AjaxRequest, type: any = 'download_load')` Parameters
| | | |
| --- | --- | --- |
| `originalEvent` | `ProgressEvent` | The original event object from the XHR `onload` event. |
| `xhr` | `XMLHttpRequest` | The `XMLHttpRequest` object used to make the request. This is useful for examining status code, etc. |
| `request` | `AjaxRequest` | The request settings used to make the HTTP request. |
| `type` | `any` | Optional. Default is `'download_load'`. The type of the event emitted by the [`ajax`](ajax) Observable |
|
| * DO NOT create instances of this class directly.
* DO NOT subclass this class.
|
Properties
----------
| Property | Type | Description |
| --- | --- | --- |
| `status` | `number` | Read-only. The HTTP status code |
| `response` | `T` | Read-only. The response data, if any. Note that this will automatically be converted to the proper type |
| `responseType` | `XMLHttpRequestResponseType` | Read-only. The responseType set on the request. (For example: `""`, `"arraybuffer"`, `"blob"`, `"document"`, `"json"`, or `"text"`) |
| `loaded` | `number` | Read-only. The total number of bytes loaded so far. To be used with [`total`](ajaxresponse#total) while calculating progress. (You will want to set [includeDownloadProgress](includedownloadprogress) or [includeDownloadProgress](includedownloadprogress)) |
| `total` | `number` | Read-only. The total number of bytes to be loaded. To be used with [`loaded`](ajaxresponse#loaded) while calculating progress. (You will want to set [includeDownloadProgress](includedownloadprogress) or [includeDownloadProgress](includedownloadprogress)) |
| `responseHeaders` | `Record<string, string>` | Read-only. A dictionary of the response headers. |
| `originalEvent` | `ProgressEvent` | Read-only. The original event object from the raw XHR event. The original event object from the XHR `onload` event. Declared in constructor. |
| `xhr` | `XMLHttpRequest` | Read-only. The XMLHttpRequest object used to make the request. NOTE: It is advised not to hold this in memory, as it will retain references to all of it's event handlers and many other things related to the request. The `XMLHttpRequest` object used to make the request. This is useful for examining status code, etc. Declared in constructor. |
| `request` | `AjaxRequest` | Read-only. The request parameters used to make the HTTP request. The request settings used to make the HTTP request. Declared in constructor. |
| `type` | `AjaxResponseType` | Read-only. The event type. This can be used to discern between different events if you're using progress events with [includeDownloadProgress](includedownloadprogress) or [includeUploadProgress](includeuploadprogress) settings in [AjaxConfig](ajaxconfig). The event type consists of two parts: the [AjaxDirection](ajaxdirection) and the the event type. Merged with `_`, they form the `type` string. The direction can be an `upload` or a `download` direction, while an event can be `loadstart`, `progress` or `load`. `download_load` is the type of event when download has finished and the response is available. |
See Also
--------
* [`ajax`](ajax)
* [AjaxConfig](ajaxconfig)
rxjs ajax ajax
====
`const` `stable`
There is an ajax operator on the Rx object.
### `const ajax: AjaxCreationMethod;`
#### Description
It creates an observable for an Ajax request with either a request object with url, headers, etc or a string for a URL.
Examples
--------
Using `ajax()` to fetch the response object that is being returned from API
```
import { ajax } from 'rxjs/ajax';
import { map, catchError, of } from 'rxjs';
const obs$ = ajax('https://api.github.com/users?per_page=5').pipe(
map(userResponse => console.log('users: ', userResponse)),
catchError(error => {
console.log('error: ', error);
return of(error);
})
);
obs$.subscribe({
next: value => console.log(value),
error: err => console.log(err)
});
```
Using `ajax.getJSON()` to fetch data from API
```
import { ajax } from 'rxjs/ajax';
import { map, catchError, of } from 'rxjs';
const obs$ = ajax.getJSON('https://api.github.com/users?per_page=5').pipe(
map(userResponse => console.log('users: ', userResponse)),
catchError(error => {
console.log('error: ', error);
return of(error);
})
);
obs$.subscribe({
next: value => console.log(value),
error: err => console.log(err)
});
```
Using `ajax()` with object as argument and method POST with a two seconds delay
```
import { ajax } from 'rxjs/ajax';
import { map, catchError, of } from 'rxjs';
const users = ajax({
url: 'https://httpbin.org/delay/2',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'rxjs-custom-header': 'Rxjs'
},
body: {
rxjs: 'Hello World!'
}
}).pipe(
map(response => console.log('response: ', response)),
catchError(error => {
console.log('error: ', error);
return of(error);
})
);
users.subscribe({
next: value => console.log(value),
error: err => console.log(err)
});
```
Using `ajax()` to fetch. An error object that is being returned from the request
```
import { ajax } from 'rxjs/ajax';
import { map, catchError, of } from 'rxjs';
const obs$ = ajax('https://api.github.com/404').pipe(
map(userResponse => console.log('users: ', userResponse)),
catchError(error => {
console.log('error: ', error);
return of(error);
})
);
obs$.subscribe({
next: value => console.log(value),
error: err => console.log(err)
});
```
go Go Programming Language Go Programming Language
=======================
* [Sub-repositories](#subrepo)
* [Community](#community)
Standard library
----------------
| Name |
| --- |
| [tar](archive/tar/index) |
| [zip](archive/zip/index) |
| [arena](arena/index) |
| [bufio](bufio/index) |
| [builtin](builtin/index) |
| [bytes](bytes/index) |
| [compress](compress/index) |
| [bzip2](compress/bzip2/index) |
| [flate](compress/flate/index) |
| [gzip](compress/gzip/index) |
| [lzw](compress/lzw/index) |
| [zlib](compress/zlib/index) |
| [container](container/index) |
| [heap](container/heap/index) |
| [list](container/list/index) |
| [ring](container/ring/index) |
| [context](context/index) |
| [crypto](crypto/index) |
| [aes](crypto/aes/index) |
| [boring](crypto/boring/index) |
| [cipher](crypto/cipher/index) |
| [des](crypto/des/index) |
| [dsa](crypto/dsa/index) |
| [ecdh](crypto/ecdh/index) |
| [ecdsa](crypto/ecdsa/index) |
| [ed25519](crypto/ed25519/index) |
| [elliptic](crypto/elliptic/index) |
| [hmac](crypto/hmac/index) |
| [md5](crypto/md5/index) |
| [rand](crypto/rand/index) |
| [rc4](crypto/rc4/index) |
| [rsa](crypto/rsa/index) |
| [sha1](crypto/sha1/index) |
| [sha256](crypto/sha256/index) |
| [sha512](crypto/sha512/index) |
| [subtle](crypto/subtle/index) |
| [tls](crypto/tls/index) |
| [fipsonly](crypto/tls/fipsonly/index) |
| [x509](crypto/x509/index) |
| [pkix](crypto/x509/pkix/index) |
| [database](database/index) |
| [sql](database/sql/index) |
| [driver](database/sql/driver/index) |
| [debug](debug/index) |
| [buildinfo](debug/buildinfo/index) |
| [dwarf](debug/dwarf/index) |
| [elf](debug/elf/index) |
| [gosym](debug/gosym/index) |
| [macho](debug/macho/index) |
| [pe](debug/pe/index) |
| [plan9obj](debug/plan9obj/index) |
| [embed](embed/index) |
| [encoding](encoding/index) |
| [ascii85](encoding/ascii85/index) |
| [asn1](encoding/asn1/index) |
| [base32](encoding/base32/index) |
| [base64](encoding/base64/index) |
| [binary](encoding/binary/index) |
| [csv](encoding/csv/index) |
| [gob](encoding/gob/index) |
| [hex](encoding/hex/index) |
| [json](encoding/json/index) |
| [pem](encoding/pem/index) |
| [xml](encoding/xml/index) |
| [errors](errors/index) |
| [expvar](expvar/index) |
| [flag](flag/index) |
| [fmt](fmt/index) |
| [go](go/index) |
| [ast](go/ast/index) |
| [build](go/build/index) |
| [constraint](go/build/constraint/index) |
| [constant](go/constant/index) |
| [doc](go/doc/index) |
| [comment](go/doc/comment/index) |
| [format](go/format/index) |
| [importer](go/importer/index) |
| [parser](go/parser/index) |
| [printer](go/printer/index) |
| [scanner](go/scanner/index) |
| [token](go/token/index) |
| [types](go/types/index) |
| [hash](hash/index) |
| [adler32](hash/adler32/index) |
| [crc32](hash/crc32/index) |
| [crc64](hash/crc64/index) |
| [fnv](hash/fnv/index) |
| [maphash](hash/maphash/index) |
| [html](html/index) |
| [template](html/template/index) |
| [image](image/index) |
| [color](image/color/index) |
| [palette](image/color/palette/index) |
| [draw](image/draw/index) |
| [gif](image/gif/index) |
| [jpeg](image/jpeg/index) |
| [png](image/png/index) |
| [index](index/index) |
| [suffixarray](index/suffixarray/index) |
| [io](io/index) |
| [fs](io/fs/index) |
| [ioutil](io/ioutil/index) |
| [log](log/index) |
| [syslog](log/syslog/index) |
| [math](math/index) |
| [big](math/big/index) |
| [bits](math/bits/index) |
| [cmplx](math/cmplx/index) |
| [rand](math/rand/index) |
| [mime](mime/index) |
| [multipart](mime/multipart/index) |
| [quotedprintable](mime/quotedprintable/index) |
| [net](net/index) |
| [http](net/http/index) |
| [cgi](net/http/cgi/index) |
| [cookiejar](net/http/cookiejar/index) |
| [fcgi](net/http/fcgi/index) |
| [httptest](net/http/httptest/index) |
| [httptrace](net/http/httptrace/index) |
| [httputil](net/http/httputil/index) |
| [pprof](net/http/pprof/index) |
| [mail](net/mail/index) |
| [netip](net/netip/index) |
| [rpc](net/rpc/index) |
| [jsonrpc](net/rpc/jsonrpc/index) |
| [smtp](net/smtp/index) |
| [textproto](net/textproto/index) |
| [url](net/url/index) |
| [os](os/index) |
| [exec](os/exec/index) |
| [signal](os/signal/index) |
| [user](os/user/index) |
| [path](path/index) |
| [filepath](path/filepath/index) |
| [plugin](plugin/index) |
| [reflect](reflect/index) |
| [regexp](regexp/index) |
| [syntax](regexp/syntax/index) |
| [runtime](runtime/index) |
| [asan](runtime/asan/index) |
| [cgo](runtime/cgo/index) |
| [coverage](runtime/coverage/index) |
| [debug](runtime/debug/index) |
| [metrics](runtime/metrics/index) |
| msan |
| [pprof](runtime/pprof/index) |
| [race](runtime/race/index) |
| [trace](runtime/trace/index) |
| [sort](sort/index) |
| [strconv](strconv/index) |
| [strings](strings/index) |
| [sync](sync/index) |
| [atomic](sync/atomic/index) |
| [syscall](syscall/index) |
| [js](syscall/js/index) |
| [testing](testing/index) |
| [fstest](testing/fstest/index) |
| [iotest](testing/iotest/index) |
| [quick](testing/quick/index) |
| [text](text/index) |
| [scanner](text/scanner/index) |
| [tabwriter](text/tabwriter/index) |
| [template](text/template/index) |
| [parse](text/template/parse/index) |
| [time](time/index) |
| [tzdata](time/tzdata/index) |
| [unicode](unicode/index) |
| [utf16](unicode/utf16/index) |
| [utf8](unicode/utf8/index) |
| [unsafe](unsafe/index) |
Other packages
--------------
### Sub-repositories
These packages are part of the Go Project but outside the main Go tree. They are developed under looser [compatibility requirements](https://golang.org/doc/go1compat) than the Go core. Install them with "go get".
* [benchmarks](http://pkg.go.dev:6060/golang.org/x/benchmarks) — benchmarks to measure Go as it is developed.
* [blog](http://pkg.go.dev:6060/golang.org/x/blog) — [blog.golang.org](http://blog.golang.org:6060)'s implementation.
* [build](http://pkg.go.dev:6060/golang.org/x/build) — [build.golang.org](http://build.golang.org:6060)'s implementation.
* [crypto](http://pkg.go.dev:6060/golang.org/x/crypto) — additional cryptography packages.
* [debug](http://pkg.go.dev:6060/golang.org/x/debug) — an experimental debugger for Go.
* [image](http://pkg.go.dev:6060/golang.org/x/image) — additional imaging packages.
* [mobile](http://pkg.go.dev:6060/golang.org/x/mobile) — experimental support for Go on mobile platforms.
* [net](http://pkg.go.dev:6060/golang.org/x/net) — additional networking packages.
* [perf](http://pkg.go.dev:6060/golang.org/x/perf) — packages and tools for performance measurement, storage, and analysis.
* [pkgsite](http://pkg.go.dev:6060/golang.org/x/pkgsite) — home of the pkg.go.dev website.
* [review](http://pkg.go.dev:6060/golang.org/x/review) — a tool for working with Gerrit code reviews.
* [sync](http://pkg.go.dev:6060/golang.org/x/sync) — additional concurrency primitives.
* [sys](http://pkg.go.dev:6060/golang.org/x/sys) — packages for making system calls.
* [text](http://pkg.go.dev:6060/golang.org/x/text) — packages for working with text.
* [time](http://pkg.go.dev:6060/golang.org/x/time) — additional time packages.
* [tools](http://pkg.go.dev:6060/golang.org/x/tools) — godoc, goimports, gorename, and other tools.
* [tour](http://pkg.go.dev:6060/golang.org/x/tour) — [tour.golang.org](http://tour.golang.org:6060)'s implementation.
* [exp](http://pkg.go.dev:6060/golang.org/x/exp) — experimental and deprecated packages (handle with care; may change without warning).
### Community
These services can help you find Open Source packages provided by the community.
* [Pkg.go.dev](http://pkg.go.dev:6060) - the Go package discovery site.
* Projects at the Go Wiki - a curated list of Go projects.
| programming_docs |
go Package sql Package sql
============
* `import "database/sql"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package sql provides a generic interface around SQL (or SQL-like) databases.
The sql package must be used in conjunction with a database driver. See <https://golang.org/s/sqldrivers> for a list of drivers.
Drivers that do not support context cancellation will not return until after the query is completed.
For usage examples, see the wiki page at <https://golang.org/s/sqlwiki>.
#### Example (OpenDBCLI)
Code:
```
package sql_test
import (
"context"
"database/sql"
"flag"
"log"
"os"
"os/signal"
"time"
)
var pool *sql.DB // Database connection pool.
func Example_openDBCLI() {
id := flag.Int64("id", 0, "person ID to find")
dsn := flag.String("dsn", os.Getenv("DSN"), "connection data source name")
flag.Parse()
if len(*dsn) == 0 {
log.Fatal("missing dsn flag")
}
if *id == 0 {
log.Fatal("missing person ID")
}
var err error
// Opening a driver typically will not attempt to connect to the database.
pool, err = sql.Open("driver-name", *dsn)
if err != nil {
// This will not be a connection error, but a DSN parse error or
// another initialization error.
log.Fatal("unable to use data source name", err)
}
defer pool.Close()
pool.SetConnMaxLifetime(0)
pool.SetMaxIdleConns(3)
pool.SetMaxOpenConns(3)
ctx, stop := context.WithCancel(context.Background())
defer stop()
appSignal := make(chan os.Signal, 3)
signal.Notify(appSignal, os.Interrupt)
go func() {
<-appSignal
stop()
}()
Ping(ctx)
Query(ctx, *id)
}
// Ping the database to verify DSN provided by the user is valid and the
// server accessible. If the ping fails exit the program with an error.
func Ping(ctx context.Context) {
ctx, cancel := context.WithTimeout(ctx, 1*time.Second)
defer cancel()
if err := pool.PingContext(ctx); err != nil {
log.Fatalf("unable to connect to database: %v", err)
}
}
// Query the database for the information requested and prints the results.
// If the query fails exit the program with an error.
func Query(ctx context.Context, id int64) {
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
var name string
err := pool.QueryRowContext(ctx, "select p.name from people as p where p.id = :id;", sql.Named("id", id)).Scan(&name)
if err != nil {
log.Fatal("unable to execute search query", err)
}
log.Println("name=", name)
}
```
#### Example (OpenDBService)
Code:
```
package sql_test
import (
"context"
"database/sql"
"encoding/json"
"fmt"
"io"
"log"
"net/http"
"time"
)
func Example_openDBService() {
// Opening a driver typically will not attempt to connect to the database.
db, err := sql.Open("driver-name", "database=test1")
if err != nil {
// This will not be a connection error, but a DSN parse error or
// another initialization error.
log.Fatal(err)
}
db.SetConnMaxLifetime(0)
db.SetMaxIdleConns(50)
db.SetMaxOpenConns(50)
s := &Service{db: db}
http.ListenAndServe(":8080", s)
}
type Service struct {
db *sql.DB
}
func (s *Service) ServeHTTP(w http.ResponseWriter, r *http.Request) {
db := s.db
switch r.URL.Path {
default:
http.Error(w, "not found", http.StatusNotFound)
return
case "/healthz":
ctx, cancel := context.WithTimeout(r.Context(), 1*time.Second)
defer cancel()
err := s.db.PingContext(ctx)
if err != nil {
http.Error(w, fmt.Sprintf("db down: %v", err), http.StatusFailedDependency)
return
}
w.WriteHeader(http.StatusOK)
return
case "/quick-action":
// This is a short SELECT. Use the request context as the base of
// the context timeout.
ctx, cancel := context.WithTimeout(r.Context(), 3*time.Second)
defer cancel()
id := 5
org := 10
var name string
err := db.QueryRowContext(ctx, `
select
p.name
from
people as p
join organization as o on p.organization = o.id
where
p.id = :id
and o.id = :org
;`,
sql.Named("id", id),
sql.Named("org", org),
).Scan(&name)
if err != nil {
if err == sql.ErrNoRows {
http.Error(w, "not found", http.StatusNotFound)
return
}
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
io.WriteString(w, name)
return
case "/long-action":
// This is a long SELECT. Use the request context as the base of
// the context timeout, but give it some time to finish. If
// the client cancels before the query is done the query will also
// be canceled.
ctx, cancel := context.WithTimeout(r.Context(), 60*time.Second)
defer cancel()
var names []string
rows, err := db.QueryContext(ctx, "select p.name from people as p where p.active = true;")
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
for rows.Next() {
var name string
err = rows.Scan(&name)
if err != nil {
break
}
names = append(names, name)
}
// Check for errors during rows "Close".
// This may be more important if multiple statements are executed
// in a single batch and rows were written as well as read.
if closeErr := rows.Close(); closeErr != nil {
http.Error(w, closeErr.Error(), http.StatusInternalServerError)
return
}
// Check for row scan error.
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Check for errors during row iteration.
if err = rows.Err(); err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
json.NewEncoder(w).Encode(names)
return
case "/async-action":
// This action has side effects that we want to preserve
// even if the client cancels the HTTP request part way through.
// For this we do not use the http request context as a base for
// the timeout.
ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second)
defer cancel()
var orderRef = "ABC123"
tx, err := db.BeginTx(ctx, &sql.TxOptions{Isolation: sql.LevelSerializable})
_, err = tx.ExecContext(ctx, "stored_proc_name", orderRef)
if err != nil {
tx.Rollback()
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
err = tx.Commit()
if err != nil {
http.Error(w, "action in unknown state, check state before attempting again", http.StatusInternalServerError)
return
}
w.WriteHeader(http.StatusOK)
return
}
}
```
Index
-----
* [Variables](#pkg-variables)
* [func Drivers() []string](#Drivers)
* [func Register(name string, driver driver.Driver)](#Register)
* [type ColumnType](#ColumnType)
* [func (ci \*ColumnType) DatabaseTypeName() string](#ColumnType.DatabaseTypeName)
* [func (ci \*ColumnType) DecimalSize() (precision, scale int64, ok bool)](#ColumnType.DecimalSize)
* [func (ci \*ColumnType) Length() (length int64, ok bool)](#ColumnType.Length)
* [func (ci \*ColumnType) Name() string](#ColumnType.Name)
* [func (ci \*ColumnType) Nullable() (nullable, ok bool)](#ColumnType.Nullable)
* [func (ci \*ColumnType) ScanType() reflect.Type](#ColumnType.ScanType)
* [type Conn](#Conn)
* [func (c \*Conn) BeginTx(ctx context.Context, opts \*TxOptions) (\*Tx, error)](#Conn.BeginTx)
* [func (c \*Conn) Close() error](#Conn.Close)
* [func (c \*Conn) ExecContext(ctx context.Context, query string, args ...any) (Result, error)](#Conn.ExecContext)
* [func (c \*Conn) PingContext(ctx context.Context) error](#Conn.PingContext)
* [func (c \*Conn) PrepareContext(ctx context.Context, query string) (\*Stmt, error)](#Conn.PrepareContext)
* [func (c \*Conn) QueryContext(ctx context.Context, query string, args ...any) (\*Rows, error)](#Conn.QueryContext)
* [func (c \*Conn) QueryRowContext(ctx context.Context, query string, args ...any) \*Row](#Conn.QueryRowContext)
* [func (c \*Conn) Raw(f func(driverConn any) error) (err error)](#Conn.Raw)
* [type DB](#DB)
* [func Open(driverName, dataSourceName string) (\*DB, error)](#Open)
* [func OpenDB(c driver.Connector) \*DB](#OpenDB)
* [func (db \*DB) Begin() (\*Tx, error)](#DB.Begin)
* [func (db \*DB) BeginTx(ctx context.Context, opts \*TxOptions) (\*Tx, error)](#DB.BeginTx)
* [func (db \*DB) Close() error](#DB.Close)
* [func (db \*DB) Conn(ctx context.Context) (\*Conn, error)](#DB.Conn)
* [func (db \*DB) Driver() driver.Driver](#DB.Driver)
* [func (db \*DB) Exec(query string, args ...any) (Result, error)](#DB.Exec)
* [func (db \*DB) ExecContext(ctx context.Context, query string, args ...any) (Result, error)](#DB.ExecContext)
* [func (db \*DB) Ping() error](#DB.Ping)
* [func (db \*DB) PingContext(ctx context.Context) error](#DB.PingContext)
* [func (db \*DB) Prepare(query string) (\*Stmt, error)](#DB.Prepare)
* [func (db \*DB) PrepareContext(ctx context.Context, query string) (\*Stmt, error)](#DB.PrepareContext)
* [func (db \*DB) Query(query string, args ...any) (\*Rows, error)](#DB.Query)
* [func (db \*DB) QueryContext(ctx context.Context, query string, args ...any) (\*Rows, error)](#DB.QueryContext)
* [func (db \*DB) QueryRow(query string, args ...any) \*Row](#DB.QueryRow)
* [func (db \*DB) QueryRowContext(ctx context.Context, query string, args ...any) \*Row](#DB.QueryRowContext)
* [func (db \*DB) SetConnMaxIdleTime(d time.Duration)](#DB.SetConnMaxIdleTime)
* [func (db \*DB) SetConnMaxLifetime(d time.Duration)](#DB.SetConnMaxLifetime)
* [func (db \*DB) SetMaxIdleConns(n int)](#DB.SetMaxIdleConns)
* [func (db \*DB) SetMaxOpenConns(n int)](#DB.SetMaxOpenConns)
* [func (db \*DB) Stats() DBStats](#DB.Stats)
* [type DBStats](#DBStats)
* [type IsolationLevel](#IsolationLevel)
* [func (i IsolationLevel) String() string](#IsolationLevel.String)
* [type NamedArg](#NamedArg)
* [func Named(name string, value any) NamedArg](#Named)
* [type NullBool](#NullBool)
* [func (n \*NullBool) Scan(value any) error](#NullBool.Scan)
* [func (n NullBool) Value() (driver.Value, error)](#NullBool.Value)
* [type NullByte](#NullByte)
* [func (n \*NullByte) Scan(value any) error](#NullByte.Scan)
* [func (n NullByte) Value() (driver.Value, error)](#NullByte.Value)
* [type NullFloat64](#NullFloat64)
* [func (n \*NullFloat64) Scan(value any) error](#NullFloat64.Scan)
* [func (n NullFloat64) Value() (driver.Value, error)](#NullFloat64.Value)
* [type NullInt16](#NullInt16)
* [func (n \*NullInt16) Scan(value any) error](#NullInt16.Scan)
* [func (n NullInt16) Value() (driver.Value, error)](#NullInt16.Value)
* [type NullInt32](#NullInt32)
* [func (n \*NullInt32) Scan(value any) error](#NullInt32.Scan)
* [func (n NullInt32) Value() (driver.Value, error)](#NullInt32.Value)
* [type NullInt64](#NullInt64)
* [func (n \*NullInt64) Scan(value any) error](#NullInt64.Scan)
* [func (n NullInt64) Value() (driver.Value, error)](#NullInt64.Value)
* [type NullString](#NullString)
* [func (ns \*NullString) Scan(value any) error](#NullString.Scan)
* [func (ns NullString) Value() (driver.Value, error)](#NullString.Value)
* [type NullTime](#NullTime)
* [func (n \*NullTime) Scan(value any) error](#NullTime.Scan)
* [func (n NullTime) Value() (driver.Value, error)](#NullTime.Value)
* [type Out](#Out)
* [type RawBytes](#RawBytes)
* [type Result](#Result)
* [type Row](#Row)
* [func (r \*Row) Err() error](#Row.Err)
* [func (r \*Row) Scan(dest ...any) error](#Row.Scan)
* [type Rows](#Rows)
* [func (rs \*Rows) Close() error](#Rows.Close)
* [func (rs \*Rows) ColumnTypes() ([]\*ColumnType, error)](#Rows.ColumnTypes)
* [func (rs \*Rows) Columns() ([]string, error)](#Rows.Columns)
* [func (rs \*Rows) Err() error](#Rows.Err)
* [func (rs \*Rows) Next() bool](#Rows.Next)
* [func (rs \*Rows) NextResultSet() bool](#Rows.NextResultSet)
* [func (rs \*Rows) Scan(dest ...any) error](#Rows.Scan)
* [type Scanner](#Scanner)
* [type Stmt](#Stmt)
* [func (s \*Stmt) Close() error](#Stmt.Close)
* [func (s \*Stmt) Exec(args ...any) (Result, error)](#Stmt.Exec)
* [func (s \*Stmt) ExecContext(ctx context.Context, args ...any) (Result, error)](#Stmt.ExecContext)
* [func (s \*Stmt) Query(args ...any) (\*Rows, error)](#Stmt.Query)
* [func (s \*Stmt) QueryContext(ctx context.Context, args ...any) (\*Rows, error)](#Stmt.QueryContext)
* [func (s \*Stmt) QueryRow(args ...any) \*Row](#Stmt.QueryRow)
* [func (s \*Stmt) QueryRowContext(ctx context.Context, args ...any) \*Row](#Stmt.QueryRowContext)
* [type Tx](#Tx)
* [func (tx \*Tx) Commit() error](#Tx.Commit)
* [func (tx \*Tx) Exec(query string, args ...any) (Result, error)](#Tx.Exec)
* [func (tx \*Tx) ExecContext(ctx context.Context, query string, args ...any) (Result, error)](#Tx.ExecContext)
* [func (tx \*Tx) Prepare(query string) (\*Stmt, error)](#Tx.Prepare)
* [func (tx \*Tx) PrepareContext(ctx context.Context, query string) (\*Stmt, error)](#Tx.PrepareContext)
* [func (tx \*Tx) Query(query string, args ...any) (\*Rows, error)](#Tx.Query)
* [func (tx \*Tx) QueryContext(ctx context.Context, query string, args ...any) (\*Rows, error)](#Tx.QueryContext)
* [func (tx \*Tx) QueryRow(query string, args ...any) \*Row](#Tx.QueryRow)
* [func (tx \*Tx) QueryRowContext(ctx context.Context, query string, args ...any) \*Row](#Tx.QueryRowContext)
* [func (tx \*Tx) Rollback() error](#Tx.Rollback)
* [func (tx \*Tx) Stmt(stmt \*Stmt) \*Stmt](#Tx.Stmt)
* [func (tx \*Tx) StmtContext(ctx context.Context, stmt \*Stmt) \*Stmt](#Tx.StmtContext)
* [type TxOptions](#TxOptions)
### Examples
[Conn.ExecContext](#example_Conn_ExecContext) [DB.BeginTx](#example_DB_BeginTx) [DB.ExecContext](#example_DB_ExecContext) [DB.PingContext](#example_DB_PingContext) [DB.Prepare](#example_DB_Prepare) [DB.QueryContext](#example_DB_QueryContext) [DB.QueryRowContext](#example_DB_QueryRowContext) [DB.Query (MultipleResultSets)](#example_DB_Query_multipleResultSets) [Rows](#example_Rows) [Stmt](#example_Stmt) [Stmt.QueryRowContext](#example_Stmt_QueryRowContext) [Tx.ExecContext](#example_Tx_ExecContext) [Tx.Prepare](#example_Tx_Prepare) [Tx.Rollback](#example_Tx_Rollback) [Package (OpenDBCLI)](#example__openDBCLI) [Package (OpenDBService)](#example__openDBService) ### Package files
convert.go ctxutil.go sql.go
Variables
---------
ErrConnDone is returned by any operation that is performed on a connection that has already been returned to the connection pool.
```
var ErrConnDone = errors.New("sql: connection is already closed")
```
ErrNoRows is returned by Scan when QueryRow doesn't return a row. In such a case, QueryRow returns a placeholder \*Row value that defers this error until a Scan.
```
var ErrNoRows = errors.New("sql: no rows in result set")
```
ErrTxDone is returned by any operation that is performed on a transaction that has already been committed or rolled back.
```
var ErrTxDone = errors.New("sql: transaction has already been committed or rolled back")
```
func Drivers 1.4
----------------
```
func Drivers() []string
```
Drivers returns a sorted list of the names of the registered drivers.
func Register
-------------
```
func Register(name string, driver driver.Driver)
```
Register makes a database driver available by the provided name. If Register is called twice with the same name or if driver is nil, it panics.
type ColumnType 1.8
-------------------
ColumnType contains the name and type of a column.
```
type ColumnType struct {
// contains filtered or unexported fields
}
```
### func (\*ColumnType) DatabaseTypeName 1.8
```
func (ci *ColumnType) DatabaseTypeName() string
```
DatabaseTypeName returns the database system name of the column type. If an empty string is returned, then the driver type name is not supported. Consult your driver documentation for a list of driver data types. Length specifiers are not included. Common type names include "VARCHAR", "TEXT", "NVARCHAR", "DECIMAL", "BOOL", "INT", and "BIGINT".
### func (\*ColumnType) DecimalSize 1.8
```
func (ci *ColumnType) DecimalSize() (precision, scale int64, ok bool)
```
DecimalSize returns the scale and precision of a decimal type. If not applicable or if not supported ok is false.
### func (\*ColumnType) Length 1.8
```
func (ci *ColumnType) Length() (length int64, ok bool)
```
Length returns the column type length for variable length column types such as text and binary field types. If the type length is unbounded the value will be math.MaxInt64 (any database limits will still apply). If the column type is not variable length, such as an int, or if not supported by the driver ok is false.
### func (\*ColumnType) Name 1.8
```
func (ci *ColumnType) Name() string
```
Name returns the name or alias of the column.
### func (\*ColumnType) Nullable 1.8
```
func (ci *ColumnType) Nullable() (nullable, ok bool)
```
Nullable reports whether the column may be null. If a driver does not support this property ok will be false.
### func (\*ColumnType) ScanType 1.8
```
func (ci *ColumnType) ScanType() reflect.Type
```
ScanType returns a Go type suitable for scanning into using Rows.Scan. If a driver does not support this property ScanType will return the type of an empty interface.
type Conn 1.9
-------------
Conn represents a single database connection rather than a pool of database connections. Prefer running queries from DB unless there is a specific need for a continuous single database connection.
A Conn must call Close to return the connection to the database pool and may do so concurrently with a running query.
After a call to Close, all operations on the connection fail with ErrConnDone.
```
type Conn struct {
// contains filtered or unexported fields
}
```
### func (\*Conn) BeginTx 1.9
```
func (c *Conn) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
```
BeginTx starts a transaction.
The provided context is used until the transaction is committed or rolled back. If the context is canceled, the sql package will roll back the transaction. Tx.Commit will return an error if the context provided to BeginTx is canceled.
The provided TxOptions is optional and may be nil if defaults should be used. If a non-default isolation level is used that the driver doesn't support, an error will be returned.
### func (\*Conn) Close 1.9
```
func (c *Conn) Close() error
```
Close returns the connection to the connection pool. All operations after a Close will return with ErrConnDone. Close is safe to call concurrently with other operations and will block until all other operations finish. It may be useful to first cancel any used context and then call close directly after.
### func (\*Conn) ExecContext 1.9
```
func (c *Conn) ExecContext(ctx context.Context, query string, args ...any) (Result, error)
```
ExecContext executes a query without returning any rows. The args are for any placeholder parameters in the query.
#### Example
Code:
```
// A *DB is a pool of connections. Call Conn to reserve a connection for
// exclusive use.
conn, err := db.Conn(ctx)
if err != nil {
log.Fatal(err)
}
defer conn.Close() // Return the connection to the pool.
id := 41
result, err := conn.ExecContext(ctx, `UPDATE balances SET balance = balance + 10 WHERE user_id = ?;`, id)
if err != nil {
log.Fatal(err)
}
rows, err := result.RowsAffected()
if err != nil {
log.Fatal(err)
}
if rows != 1 {
log.Fatalf("expected single row affected, got %d rows affected", rows)
}
```
### func (\*Conn) PingContext 1.9
```
func (c *Conn) PingContext(ctx context.Context) error
```
PingContext verifies the connection to the database is still alive.
### func (\*Conn) PrepareContext 1.9
```
func (c *Conn) PrepareContext(ctx context.Context, query string) (*Stmt, error)
```
PrepareContext creates a prepared statement for later queries or executions. Multiple queries or executions may be run concurrently from the returned statement. The caller must call the statement's Close method when the statement is no longer needed.
The provided context is used for the preparation of the statement, not for the execution of the statement.
### func (\*Conn) QueryContext 1.9
```
func (c *Conn) QueryContext(ctx context.Context, query string, args ...any) (*Rows, error)
```
QueryContext executes a query that returns rows, typically a SELECT. The args are for any placeholder parameters in the query.
### func (\*Conn) QueryRowContext 1.9
```
func (c *Conn) QueryRowContext(ctx context.Context, query string, args ...any) *Row
```
QueryRowContext executes a query that is expected to return at most one row. QueryRowContext always returns a non-nil value. Errors are deferred until Row's Scan method is called. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
### func (\*Conn) Raw 1.13
```
func (c *Conn) Raw(f func(driverConn any) error) (err error)
```
Raw executes f exposing the underlying driver connection for the duration of f. The driverConn must not be used outside of f.
Once f returns and err is not driver.ErrBadConn, the Conn will continue to be usable until Conn.Close is called.
type DB
-------
DB is a database handle representing a pool of zero or more underlying connections. It's safe for concurrent use by multiple goroutines.
The sql package creates and frees connections automatically; it also maintains a free pool of idle connections. If the database has a concept of per-connection state, such state can be reliably observed within a transaction (Tx) or connection (Conn). Once DB.Begin is called, the returned Tx is bound to a single connection. Once Commit or Rollback is called on the transaction, that transaction's connection is returned to DB's idle connection pool. The pool size can be controlled with SetMaxIdleConns.
```
type DB struct {
// contains filtered or unexported fields
}
```
### func Open
```
func Open(driverName, dataSourceName string) (*DB, error)
```
Open opens a database specified by its database driver name and a driver-specific data source name, usually consisting of at least a database name and connection information.
Most users will open a database via a driver-specific connection helper function that returns a \*DB. No database drivers are included in the Go standard library. See <https://golang.org/s/sqldrivers> for a list of third-party drivers.
Open may just validate its arguments without creating a connection to the database. To verify that the data source name is valid, call Ping.
The returned DB is safe for concurrent use by multiple goroutines and maintains its own pool of idle connections. Thus, the Open function should be called just once. It is rarely necessary to close a DB.
### func OpenDB 1.10
```
func OpenDB(c driver.Connector) *DB
```
OpenDB opens a database using a Connector, allowing drivers to bypass a string based data source name.
Most users will open a database via a driver-specific connection helper function that returns a \*DB. No database drivers are included in the Go standard library. See <https://golang.org/s/sqldrivers> for a list of third-party drivers.
OpenDB may just validate its arguments without creating a connection to the database. To verify that the data source name is valid, call Ping.
The returned DB is safe for concurrent use by multiple goroutines and maintains its own pool of idle connections. Thus, the OpenDB function should be called just once. It is rarely necessary to close a DB.
### func (\*DB) Begin
```
func (db *DB) Begin() (*Tx, error)
```
Begin starts a transaction. The default isolation level is dependent on the driver.
Begin uses context.Background internally; to specify the context, use BeginTx.
### func (\*DB) BeginTx 1.8
```
func (db *DB) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
```
BeginTx starts a transaction.
The provided context is used until the transaction is committed or rolled back. If the context is canceled, the sql package will roll back the transaction. Tx.Commit will return an error if the context provided to BeginTx is canceled.
The provided TxOptions is optional and may be nil if defaults should be used. If a non-default isolation level is used that the driver doesn't support, an error will be returned.
#### Example
Code:
```
tx, err := db.BeginTx(ctx, &sql.TxOptions{Isolation: sql.LevelSerializable})
if err != nil {
log.Fatal(err)
}
id := 37
_, execErr := tx.Exec(`UPDATE users SET status = ? WHERE id = ?`, "paid", id)
if execErr != nil {
_ = tx.Rollback()
log.Fatal(execErr)
}
if err := tx.Commit(); err != nil {
log.Fatal(err)
}
```
### func (\*DB) Close
```
func (db *DB) Close() error
```
Close closes the database and prevents new queries from starting. Close then waits for all queries that have started processing on the server to finish.
It is rare to Close a DB, as the DB handle is meant to be long-lived and shared between many goroutines.
### func (\*DB) Conn 1.9
```
func (db *DB) Conn(ctx context.Context) (*Conn, error)
```
Conn returns a single connection by either opening a new connection or returning an existing connection from the connection pool. Conn will block until either a connection is returned or ctx is canceled. Queries run on the same Conn will be run in the same database session.
Every Conn must be returned to the database pool after use by calling Conn.Close.
### func (\*DB) Driver
```
func (db *DB) Driver() driver.Driver
```
Driver returns the database's underlying driver.
### func (\*DB) Exec
```
func (db *DB) Exec(query string, args ...any) (Result, error)
```
Exec executes a query without returning any rows. The args are for any placeholder parameters in the query.
Exec uses context.Background internally; to specify the context, use ExecContext.
### func (\*DB) ExecContext 1.8
```
func (db *DB) ExecContext(ctx context.Context, query string, args ...any) (Result, error)
```
ExecContext executes a query without returning any rows. The args are for any placeholder parameters in the query.
#### Example
Code:
```
id := 47
result, err := db.ExecContext(ctx, "UPDATE balances SET balance = balance + 10 WHERE user_id = ?", id)
if err != nil {
log.Fatal(err)
}
rows, err := result.RowsAffected()
if err != nil {
log.Fatal(err)
}
if rows != 1 {
log.Fatalf("expected to affect 1 row, affected %d", rows)
}
```
### func (\*DB) Ping 1.1
```
func (db *DB) Ping() error
```
Ping verifies a connection to the database is still alive, establishing a connection if necessary.
Ping uses context.Background internally; to specify the context, use PingContext.
### func (\*DB) PingContext 1.8
```
func (db *DB) PingContext(ctx context.Context) error
```
PingContext verifies a connection to the database is still alive, establishing a connection if necessary.
#### Example
Code:
```
// Ping and PingContext may be used to determine if communication with
// the database server is still possible.
//
// When used in a command line application Ping may be used to establish
// that further queries are possible; that the provided DSN is valid.
//
// When used in long running service Ping may be part of the health
// checking system.
ctx, cancel := context.WithTimeout(ctx, 1*time.Second)
defer cancel()
status := "up"
if err := db.PingContext(ctx); err != nil {
status = "down"
}
log.Println(status)
```
### func (\*DB) Prepare
```
func (db *DB) Prepare(query string) (*Stmt, error)
```
Prepare creates a prepared statement for later queries or executions. Multiple queries or executions may be run concurrently from the returned statement. The caller must call the statement's Close method when the statement is no longer needed.
Prepare uses context.Background internally; to specify the context, use PrepareContext.
#### Example
Code:
```
projects := []struct {
mascot string
release int
}{
{"tux", 1991},
{"duke", 1996},
{"gopher", 2009},
{"moby dock", 2013},
}
stmt, err := db.Prepare("INSERT INTO projects(id, mascot, release, category) VALUES( ?, ?, ?, ? )")
if err != nil {
log.Fatal(err)
}
defer stmt.Close() // Prepared statements take up server resources and should be closed after use.
for id, project := range projects {
if _, err := stmt.Exec(id+1, project.mascot, project.release, "open source"); err != nil {
log.Fatal(err)
}
}
```
### func (\*DB) PrepareContext 1.8
```
func (db *DB) PrepareContext(ctx context.Context, query string) (*Stmt, error)
```
PrepareContext creates a prepared statement for later queries or executions. Multiple queries or executions may be run concurrently from the returned statement. The caller must call the statement's Close method when the statement is no longer needed.
The provided context is used for the preparation of the statement, not for the execution of the statement.
### func (\*DB) Query
```
func (db *DB) Query(query string, args ...any) (*Rows, error)
```
Query executes a query that returns rows, typically a SELECT. The args are for any placeholder parameters in the query.
Query uses context.Background internally; to specify the context, use QueryContext.
#### Example (MultipleResultSets)
Code:
```
age := 27
q := `
create temp table uid (id bigint); -- Create temp table for queries.
insert into uid
select id from users where age < ?; -- Populate temp table.
-- First result set.
select
users.id, name
from
users
join uid on users.id = uid.id
;
-- Second result set.
select
ur.user, ur.role
from
user_roles as ur
join uid on uid.id = ur.user
;
`
rows, err := db.Query(q, age)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
for rows.Next() {
var (
id int64
name string
)
if err := rows.Scan(&id, &name); err != nil {
log.Fatal(err)
}
log.Printf("id %d name is %s\n", id, name)
}
if !rows.NextResultSet() {
log.Fatalf("expected more result sets: %v", rows.Err())
}
var roleMap = map[int64]string{
1: "user",
2: "admin",
3: "gopher",
}
for rows.Next() {
var (
id int64
role int64
)
if err := rows.Scan(&id, &role); err != nil {
log.Fatal(err)
}
log.Printf("id %d has role %s\n", id, roleMap[role])
}
if err := rows.Err(); err != nil {
log.Fatal(err)
}
```
### func (\*DB) QueryContext 1.8
```
func (db *DB) QueryContext(ctx context.Context, query string, args ...any) (*Rows, error)
```
QueryContext executes a query that returns rows, typically a SELECT. The args are for any placeholder parameters in the query.
#### Example
Code:
```
age := 27
rows, err := db.QueryContext(ctx, "SELECT name FROM users WHERE age=?", age)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
names := make([]string, 0)
for rows.Next() {
var name string
if err := rows.Scan(&name); err != nil {
// Check for a scan error.
// Query rows will be closed with defer.
log.Fatal(err)
}
names = append(names, name)
}
// If the database is being written to ensure to check for Close
// errors that may be returned from the driver. The query may
// encounter an auto-commit error and be forced to rollback changes.
rerr := rows.Close()
if rerr != nil {
log.Fatal(rerr)
}
// Rows.Err will report the last error encountered by Rows.Scan.
if err := rows.Err(); err != nil {
log.Fatal(err)
}
fmt.Printf("%s are %d years old", strings.Join(names, ", "), age)
```
### func (\*DB) QueryRow
```
func (db *DB) QueryRow(query string, args ...any) *Row
```
QueryRow executes a query that is expected to return at most one row. QueryRow always returns a non-nil value. Errors are deferred until Row's Scan method is called. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
QueryRow uses context.Background internally; to specify the context, use QueryRowContext.
### func (\*DB) QueryRowContext 1.8
```
func (db *DB) QueryRowContext(ctx context.Context, query string, args ...any) *Row
```
QueryRowContext executes a query that is expected to return at most one row. QueryRowContext always returns a non-nil value. Errors are deferred until Row's Scan method is called. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
#### Example
Code:
```
id := 123
var username string
var created time.Time
err := db.QueryRowContext(ctx, "SELECT username, created_at FROM users WHERE id=?", id).Scan(&username, &created)
switch {
case err == sql.ErrNoRows:
log.Printf("no user with id %d\n", id)
case err != nil:
log.Fatalf("query error: %v\n", err)
default:
log.Printf("username is %q, account created on %s\n", username, created)
}
```
### func (\*DB) SetConnMaxIdleTime 1.15
```
func (db *DB) SetConnMaxIdleTime(d time.Duration)
```
SetConnMaxIdleTime sets the maximum amount of time a connection may be idle.
Expired connections may be closed lazily before reuse.
If d <= 0, connections are not closed due to a connection's idle time.
### func (\*DB) SetConnMaxLifetime 1.6
```
func (db *DB) SetConnMaxLifetime(d time.Duration)
```
SetConnMaxLifetime sets the maximum amount of time a connection may be reused.
Expired connections may be closed lazily before reuse.
If d <= 0, connections are not closed due to a connection's age.
### func (\*DB) SetMaxIdleConns 1.1
```
func (db *DB) SetMaxIdleConns(n int)
```
SetMaxIdleConns sets the maximum number of connections in the idle connection pool.
If MaxOpenConns is greater than 0 but less than the new MaxIdleConns, then the new MaxIdleConns will be reduced to match the MaxOpenConns limit.
If n <= 0, no idle connections are retained.
The default max idle connections is currently 2. This may change in a future release.
### func (\*DB) SetMaxOpenConns 1.2
```
func (db *DB) SetMaxOpenConns(n int)
```
SetMaxOpenConns sets the maximum number of open connections to the database.
If MaxIdleConns is greater than 0 and the new MaxOpenConns is less than MaxIdleConns, then MaxIdleConns will be reduced to match the new MaxOpenConns limit.
If n <= 0, then there is no limit on the number of open connections. The default is 0 (unlimited).
### func (\*DB) Stats 1.5
```
func (db *DB) Stats() DBStats
```
Stats returns database statistics.
type DBStats 1.5
----------------
DBStats contains database statistics.
```
type DBStats struct {
MaxOpenConnections int // Maximum number of open connections to the database; added in Go 1.11
// Pool Status
OpenConnections int // The number of established connections both in use and idle.
InUse int // The number of connections currently in use; added in Go 1.11
Idle int // The number of idle connections; added in Go 1.11
// Counters
WaitCount int64 // The total number of connections waited for; added in Go 1.11
WaitDuration time.Duration // The total time blocked waiting for a new connection; added in Go 1.11
MaxIdleClosed int64 // The total number of connections closed due to SetMaxIdleConns; added in Go 1.11
MaxIdleTimeClosed int64 // The total number of connections closed due to SetConnMaxIdleTime; added in Go 1.15
MaxLifetimeClosed int64 // The total number of connections closed due to SetConnMaxLifetime; added in Go 1.11
}
```
type IsolationLevel 1.8
-----------------------
IsolationLevel is the transaction isolation level used in TxOptions.
```
type IsolationLevel int
```
Various isolation levels that drivers may support in BeginTx. If a driver does not support a given isolation level an error may be returned.
See <https://en.wikipedia.org/wiki/Isolation_(database_systems)#Isolation_levels>.
```
const (
LevelDefault IsolationLevel = iota
LevelReadUncommitted
LevelReadCommitted
LevelWriteCommitted
LevelRepeatableRead
LevelSnapshot
LevelSerializable
LevelLinearizable
)
```
### func (IsolationLevel) String 1.11
```
func (i IsolationLevel) String() string
```
String returns the name of the transaction isolation level.
type NamedArg 1.8
-----------------
A NamedArg is a named argument. NamedArg values may be used as arguments to Query or Exec and bind to the corresponding named parameter in the SQL statement.
For a more concise way to create NamedArg values, see the Named function.
```
type NamedArg struct {
// Name is the name of the parameter placeholder.
//
// If empty, the ordinal position in the argument list will be
// used.
//
// Name must omit any symbol prefix.
Name string
// Value is the value of the parameter.
// It may be assigned the same value types as the query
// arguments.
Value any
// contains filtered or unexported fields
}
```
### func Named 1.8
```
func Named(name string, value any) NamedArg
```
Named provides a more concise way to create NamedArg values.
Example usage:
```
db.ExecContext(ctx, `
delete from Invoice
where
TimeCreated < @end
and TimeCreated >= @start;`,
sql.Named("start", startTime),
sql.Named("end", endTime),
)
```
type NullBool
-------------
NullBool represents a bool that may be null. NullBool implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullBool struct {
Bool bool
Valid bool // Valid is true if Bool is not NULL
}
```
### func (\*NullBool) Scan
```
func (n *NullBool) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullBool) Value
```
func (n NullBool) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullByte 1.17
------------------
NullByte represents a byte that may be null. NullByte implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullByte struct {
Byte byte
Valid bool // Valid is true if Byte is not NULL
}
```
### func (\*NullByte) Scan 1.17
```
func (n *NullByte) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullByte) Value 1.17
```
func (n NullByte) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullFloat64
----------------
NullFloat64 represents a float64 that may be null. NullFloat64 implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullFloat64 struct {
Float64 float64
Valid bool // Valid is true if Float64 is not NULL
}
```
### func (\*NullFloat64) Scan
```
func (n *NullFloat64) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullFloat64) Value
```
func (n NullFloat64) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullInt16 1.17
-------------------
NullInt16 represents an int16 that may be null. NullInt16 implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullInt16 struct {
Int16 int16
Valid bool // Valid is true if Int16 is not NULL
}
```
### func (\*NullInt16) Scan 1.17
```
func (n *NullInt16) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullInt16) Value 1.17
```
func (n NullInt16) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullInt32 1.13
-------------------
NullInt32 represents an int32 that may be null. NullInt32 implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullInt32 struct {
Int32 int32
Valid bool // Valid is true if Int32 is not NULL
}
```
### func (\*NullInt32) Scan 1.13
```
func (n *NullInt32) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullInt32) Value 1.13
```
func (n NullInt32) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullInt64
--------------
NullInt64 represents an int64 that may be null. NullInt64 implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullInt64 struct {
Int64 int64
Valid bool // Valid is true if Int64 is not NULL
}
```
### func (\*NullInt64) Scan
```
func (n *NullInt64) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullInt64) Value
```
func (n NullInt64) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullString
---------------
NullString represents a string that may be null. NullString implements the Scanner interface so it can be used as a scan destination:
```
var s NullString
err := db.QueryRow("SELECT name FROM foo WHERE id=?", id).Scan(&s)
...
if s.Valid {
// use s.String
} else {
// NULL value
}
```
```
type NullString struct {
String string
Valid bool // Valid is true if String is not NULL
}
```
### func (\*NullString) Scan
```
func (ns *NullString) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullString) Value
```
func (ns NullString) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type NullTime 1.13
------------------
NullTime represents a time.Time that may be null. NullTime implements the Scanner interface so it can be used as a scan destination, similar to NullString.
```
type NullTime struct {
Time time.Time
Valid bool // Valid is true if Time is not NULL
}
```
### func (\*NullTime) Scan 1.13
```
func (n *NullTime) Scan(value any) error
```
Scan implements the Scanner interface.
### func (NullTime) Value 1.13
```
func (n NullTime) Value() (driver.Value, error)
```
Value implements the driver Valuer interface.
type Out 1.9
------------
Out may be used to retrieve OUTPUT value parameters from stored procedures.
Not all drivers and databases support OUTPUT value parameters.
Example usage:
```
var outArg string
_, err := db.ExecContext(ctx, "ProcName", sql.Named("Arg1", sql.Out{Dest: &outArg}))
```
```
type Out struct {
// Dest is a pointer to the value that will be set to the result of the
// stored procedure's OUTPUT parameter.
Dest any
// In is whether the parameter is an INOUT parameter. If so, the input value to the stored
// procedure is the dereferenced value of Dest's pointer, which is then replaced with
// the output value.
In bool
// contains filtered or unexported fields
}
```
type RawBytes
-------------
RawBytes is a byte slice that holds a reference to memory owned by the database itself. After a Scan into a RawBytes, the slice is only valid until the next call to Next, Scan, or Close.
```
type RawBytes []byte
```
type Result
-----------
A Result summarizes an executed SQL command.
```
type Result interface {
// LastInsertId returns the integer generated by the database
// in response to a command. Typically this will be from an
// "auto increment" column when inserting a new row. Not all
// databases support this feature, and the syntax of such
// statements varies.
LastInsertId() (int64, error)
// RowsAffected returns the number of rows affected by an
// update, insert, or delete. Not every database or database
// driver may support this.
RowsAffected() (int64, error)
}
```
type Row
--------
Row is the result of calling QueryRow to select a single row.
```
type Row struct {
// contains filtered or unexported fields
}
```
### func (\*Row) Err 1.15
```
func (r *Row) Err() error
```
Err provides a way for wrapping packages to check for query errors without calling Scan. Err returns the error, if any, that was encountered while running the query. If this error is not nil, this error will also be returned from Scan.
### func (\*Row) Scan
```
func (r *Row) Scan(dest ...any) error
```
Scan copies the columns from the matched row into the values pointed at by dest. See the documentation on Rows.Scan for details. If more than one row matches the query, Scan uses the first row and discards the rest. If no row matches the query, Scan returns ErrNoRows.
type Rows
---------
Rows is the result of a query. Its cursor starts before the first row of the result set. Use Next to advance from row to row.
```
type Rows struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
age := 27
rows, err := db.QueryContext(ctx, "SELECT name FROM users WHERE age=?", age)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
names := make([]string, 0)
for rows.Next() {
var name string
if err := rows.Scan(&name); err != nil {
log.Fatal(err)
}
names = append(names, name)
}
// Check for errors from iterating over rows.
if err := rows.Err(); err != nil {
log.Fatal(err)
}
log.Printf("%s are %d years old", strings.Join(names, ", "), age)
```
### func (\*Rows) Close
```
func (rs *Rows) Close() error
```
Close closes the Rows, preventing further enumeration. If Next is called and returns false and there are no further result sets, the Rows are closed automatically and it will suffice to check the result of Err. Close is idempotent and does not affect the result of Err.
### func (\*Rows) ColumnTypes 1.8
```
func (rs *Rows) ColumnTypes() ([]*ColumnType, error)
```
ColumnTypes returns column information such as column type, length, and nullable. Some information may not be available from some drivers.
### func (\*Rows) Columns
```
func (rs *Rows) Columns() ([]string, error)
```
Columns returns the column names. Columns returns an error if the rows are closed.
### func (\*Rows) Err
```
func (rs *Rows) Err() error
```
Err returns the error, if any, that was encountered during iteration. Err may be called after an explicit or implicit Close.
### func (\*Rows) Next
```
func (rs *Rows) Next() bool
```
Next prepares the next result row for reading with the Scan method. It returns true on success, or false if there is no next result row or an error happened while preparing it. Err should be consulted to distinguish between the two cases.
Every call to Scan, even the first one, must be preceded by a call to Next.
### func (\*Rows) NextResultSet 1.8
```
func (rs *Rows) NextResultSet() bool
```
NextResultSet prepares the next result set for reading. It reports whether there is further result sets, or false if there is no further result set or if there is an error advancing to it. The Err method should be consulted to distinguish between the two cases.
After calling NextResultSet, the Next method should always be called before scanning. If there are further result sets they may not have rows in the result set.
### func (\*Rows) Scan
```
func (rs *Rows) Scan(dest ...any) error
```
Scan copies the columns in the current row into the values pointed at by dest. The number of values in dest must be the same as the number of columns in Rows.
Scan converts columns read from the database into the following common Go types and special types provided by the sql package:
```
*string
*[]byte
*int, *int8, *int16, *int32, *int64
*uint, *uint8, *uint16, *uint32, *uint64
*bool
*float32, *float64
*interface{}
*RawBytes
*Rows (cursor value)
any type implementing Scanner (see Scanner docs)
```
In the most simple case, if the type of the value from the source column is an integer, bool or string type T and dest is of type \*T, Scan simply assigns the value through the pointer.
Scan also converts between string and numeric types, as long as no information would be lost. While Scan stringifies all numbers scanned from numeric database columns into \*string, scans into numeric types are checked for overflow. For example, a float64 with value 300 or a string with value "300" can scan into a uint16, but not into a uint8, though float64(255) or "255" can scan into a uint8. One exception is that scans of some float64 numbers to strings may lose information when stringifying. In general, scan floating point columns into \*float64.
If a dest argument has type \*[]byte, Scan saves in that argument a copy of the corresponding data. The copy is owned by the caller and can be modified and held indefinitely. The copy can be avoided by using an argument of type \*RawBytes instead; see the documentation for RawBytes for restrictions on its use.
If an argument has type \*interface{}, Scan copies the value provided by the underlying driver without conversion. When scanning from a source value of type []byte to \*interface{}, a copy of the slice is made and the caller owns the result.
Source values of type time.Time may be scanned into values of type \*time.Time, \*interface{}, \*string, or \*[]byte. When converting to the latter two, time.RFC3339Nano is used.
Source values of type bool may be scanned into types \*bool, \*interface{}, \*string, \*[]byte, or \*RawBytes.
For scanning into \*bool, the source may be true, false, 1, 0, or string inputs parseable by strconv.ParseBool.
Scan can also convert a cursor returned from a query, such as "select cursor(select \* from my\_table) from dual", into a \*Rows value that can itself be scanned from. The parent select query will close any cursor \*Rows if the parent \*Rows is closed.
If any of the first arguments implementing Scanner returns an error, that error will be wrapped in the returned error.
type Scanner
------------
Scanner is an interface used by Scan.
```
type Scanner interface {
// Scan assigns a value from a database driver.
//
// The src value will be of one of the following types:
//
// int64
// float64
// bool
// []byte
// string
// time.Time
// nil - for NULL values
//
// An error should be returned if the value cannot be stored
// without loss of information.
//
// Reference types such as []byte are only valid until the next call to Scan
// and should not be retained. Their underlying memory is owned by the driver.
// If retention is necessary, copy their values before the next call to Scan.
Scan(src any) error
}
```
type Stmt
---------
Stmt is a prepared statement. A Stmt is safe for concurrent use by multiple goroutines.
If a Stmt is prepared on a Tx or Conn, it will be bound to a single underlying connection forever. If the Tx or Conn closes, the Stmt will become unusable and all operations will return an error. If a Stmt is prepared on a DB, it will remain usable for the lifetime of the DB. When the Stmt needs to execute on a new underlying connection, it will prepare itself on the new connection automatically.
```
type Stmt struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
// In normal use, create one Stmt when your process starts.
stmt, err := db.PrepareContext(ctx, "SELECT username FROM users WHERE id = ?")
if err != nil {
log.Fatal(err)
}
defer stmt.Close()
// Then reuse it each time you need to issue the query.
id := 43
var username string
err = stmt.QueryRowContext(ctx, id).Scan(&username)
switch {
case err == sql.ErrNoRows:
log.Fatalf("no user with id %d", id)
case err != nil:
log.Fatal(err)
default:
log.Printf("username is %s\n", username)
}
```
### func (\*Stmt) Close
```
func (s *Stmt) Close() error
```
Close closes the statement.
### func (\*Stmt) Exec
```
func (s *Stmt) Exec(args ...any) (Result, error)
```
Exec executes a prepared statement with the given arguments and returns a Result summarizing the effect of the statement.
Exec uses context.Background internally; to specify the context, use ExecContext.
### func (\*Stmt) ExecContext 1.8
```
func (s *Stmt) ExecContext(ctx context.Context, args ...any) (Result, error)
```
ExecContext executes a prepared statement with the given arguments and returns a Result summarizing the effect of the statement.
### func (\*Stmt) Query
```
func (s *Stmt) Query(args ...any) (*Rows, error)
```
Query executes a prepared query statement with the given arguments and returns the query results as a \*Rows.
Query uses context.Background internally; to specify the context, use QueryContext.
### func (\*Stmt) QueryContext 1.8
```
func (s *Stmt) QueryContext(ctx context.Context, args ...any) (*Rows, error)
```
QueryContext executes a prepared query statement with the given arguments and returns the query results as a \*Rows.
### func (\*Stmt) QueryRow
```
func (s *Stmt) QueryRow(args ...any) *Row
```
QueryRow executes a prepared query statement with the given arguments. If an error occurs during the execution of the statement, that error will be returned by a call to Scan on the returned \*Row, which is always non-nil. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
Example usage:
```
var name string
err := nameByUseridStmt.QueryRow(id).Scan(&name)
```
QueryRow uses context.Background internally; to specify the context, use QueryRowContext.
### func (\*Stmt) QueryRowContext 1.8
```
func (s *Stmt) QueryRowContext(ctx context.Context, args ...any) *Row
```
QueryRowContext executes a prepared query statement with the given arguments. If an error occurs during the execution of the statement, that error will be returned by a call to Scan on the returned \*Row, which is always non-nil. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
#### Example
Code:
```
// In normal use, create one Stmt when your process starts.
stmt, err := db.PrepareContext(ctx, "SELECT username FROM users WHERE id = ?")
if err != nil {
log.Fatal(err)
}
defer stmt.Close()
// Then reuse it each time you need to issue the query.
id := 43
var username string
err = stmt.QueryRowContext(ctx, id).Scan(&username)
switch {
case err == sql.ErrNoRows:
log.Fatalf("no user with id %d", id)
case err != nil:
log.Fatal(err)
default:
log.Printf("username is %s\n", username)
}
```
type Tx
-------
Tx is an in-progress database transaction.
A transaction must end with a call to Commit or Rollback.
After a call to Commit or Rollback, all operations on the transaction fail with ErrTxDone.
The statements prepared for a transaction by calling the transaction's Prepare or Stmt methods are closed by the call to Commit or Rollback.
```
type Tx struct {
// contains filtered or unexported fields
}
```
### func (\*Tx) Commit
```
func (tx *Tx) Commit() error
```
Commit commits the transaction.
### func (\*Tx) Exec
```
func (tx *Tx) Exec(query string, args ...any) (Result, error)
```
Exec executes a query that doesn't return rows. For example: an INSERT and UPDATE.
Exec uses context.Background internally; to specify the context, use ExecContext.
### func (\*Tx) ExecContext 1.8
```
func (tx *Tx) ExecContext(ctx context.Context, query string, args ...any) (Result, error)
```
ExecContext executes a query that doesn't return rows. For example: an INSERT and UPDATE.
#### Example
Code:
```
tx, err := db.BeginTx(ctx, &sql.TxOptions{Isolation: sql.LevelSerializable})
if err != nil {
log.Fatal(err)
}
id := 37
_, execErr := tx.ExecContext(ctx, "UPDATE users SET status = ? WHERE id = ?", "paid", id)
if execErr != nil {
if rollbackErr := tx.Rollback(); rollbackErr != nil {
log.Fatalf("update failed: %v, unable to rollback: %v\n", execErr, rollbackErr)
}
log.Fatalf("update failed: %v", execErr)
}
if err := tx.Commit(); err != nil {
log.Fatal(err)
}
```
### func (\*Tx) Prepare
```
func (tx *Tx) Prepare(query string) (*Stmt, error)
```
Prepare creates a prepared statement for use within a transaction.
The returned statement operates within the transaction and will be closed when the transaction has been committed or rolled back.
To use an existing prepared statement on this transaction, see Tx.Stmt.
Prepare uses context.Background internally; to specify the context, use PrepareContext.
#### Example
Code:
```
projects := []struct {
mascot string
release int
}{
{"tux", 1991},
{"duke", 1996},
{"gopher", 2009},
{"moby dock", 2013},
}
tx, err := db.Begin()
if err != nil {
log.Fatal(err)
}
defer tx.Rollback() // The rollback will be ignored if the tx has been committed later in the function.
stmt, err := tx.Prepare("INSERT INTO projects(id, mascot, release, category) VALUES( ?, ?, ?, ? )")
if err != nil {
log.Fatal(err)
}
defer stmt.Close() // Prepared statements take up server resources and should be closed after use.
for id, project := range projects {
if _, err := stmt.Exec(id+1, project.mascot, project.release, "open source"); err != nil {
log.Fatal(err)
}
}
if err := tx.Commit(); err != nil {
log.Fatal(err)
}
```
### func (\*Tx) PrepareContext 1.8
```
func (tx *Tx) PrepareContext(ctx context.Context, query string) (*Stmt, error)
```
PrepareContext creates a prepared statement for use within a transaction.
The returned statement operates within the transaction and will be closed when the transaction has been committed or rolled back.
To use an existing prepared statement on this transaction, see Tx.Stmt.
The provided context will be used for the preparation of the context, not for the execution of the returned statement. The returned statement will run in the transaction context.
### func (\*Tx) Query
```
func (tx *Tx) Query(query string, args ...any) (*Rows, error)
```
Query executes a query that returns rows, typically a SELECT.
Query uses context.Background internally; to specify the context, use QueryContext.
### func (\*Tx) QueryContext 1.8
```
func (tx *Tx) QueryContext(ctx context.Context, query string, args ...any) (*Rows, error)
```
QueryContext executes a query that returns rows, typically a SELECT.
### func (\*Tx) QueryRow
```
func (tx *Tx) QueryRow(query string, args ...any) *Row
```
QueryRow executes a query that is expected to return at most one row. QueryRow always returns a non-nil value. Errors are deferred until Row's Scan method is called. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
QueryRow uses context.Background internally; to specify the context, use QueryRowContext.
### func (\*Tx) QueryRowContext 1.8
```
func (tx *Tx) QueryRowContext(ctx context.Context, query string, args ...any) *Row
```
QueryRowContext executes a query that is expected to return at most one row. QueryRowContext always returns a non-nil value. Errors are deferred until Row's Scan method is called. If the query selects no rows, the \*Row's Scan will return ErrNoRows. Otherwise, the \*Row's Scan scans the first selected row and discards the rest.
### func (\*Tx) Rollback
```
func (tx *Tx) Rollback() error
```
Rollback aborts the transaction.
#### Example
Code:
```
tx, err := db.BeginTx(ctx, &sql.TxOptions{Isolation: sql.LevelSerializable})
if err != nil {
log.Fatal(err)
}
id := 53
_, err = tx.ExecContext(ctx, "UPDATE drivers SET status = ? WHERE id = ?;", "assigned", id)
if err != nil {
if rollbackErr := tx.Rollback(); rollbackErr != nil {
log.Fatalf("update drivers: unable to rollback: %v", rollbackErr)
}
log.Fatal(err)
}
_, err = tx.ExecContext(ctx, "UPDATE pickups SET driver_id = $1;", id)
if err != nil {
if rollbackErr := tx.Rollback(); rollbackErr != nil {
log.Fatalf("update failed: %v, unable to back: %v", err, rollbackErr)
}
log.Fatal(err)
}
if err := tx.Commit(); err != nil {
log.Fatal(err)
}
```
### func (\*Tx) Stmt
```
func (tx *Tx) Stmt(stmt *Stmt) *Stmt
```
Stmt returns a transaction-specific prepared statement from an existing statement.
Example:
```
updateMoney, err := db.Prepare("UPDATE balance SET money=money+? WHERE id=?")
...
tx, err := db.Begin()
...
res, err := tx.Stmt(updateMoney).Exec(123.45, 98293203)
```
The returned statement operates within the transaction and will be closed when the transaction has been committed or rolled back.
Stmt uses context.Background internally; to specify the context, use StmtContext.
### func (\*Tx) StmtContext 1.8
```
func (tx *Tx) StmtContext(ctx context.Context, stmt *Stmt) *Stmt
```
StmtContext returns a transaction-specific prepared statement from an existing statement.
Example:
```
updateMoney, err := db.Prepare("UPDATE balance SET money=money+? WHERE id=?")
...
tx, err := db.Begin()
...
res, err := tx.StmtContext(ctx, updateMoney).Exec(123.45, 98293203)
```
The provided context is used for the preparation of the statement, not for the execution of the statement.
The returned statement operates within the transaction and will be closed when the transaction has been committed or rolled back.
type TxOptions 1.8
------------------
TxOptions holds the transaction options to be used in DB.BeginTx.
```
type TxOptions struct {
// Isolation is the transaction isolation level.
// If zero, the driver or database's default level is used.
Isolation IsolationLevel
ReadOnly bool
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [driver](driver/index) | Package driver defines interfaces to be implemented by database drivers as used by package sql. |
| programming_docs |
go Package driver Package driver
===============
* `import "database/sql/driver"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package driver defines interfaces to be implemented by database drivers as used by package sql.
Most code should use package sql.
The driver interface has evolved over time. Drivers should implement Connector and DriverContext interfaces. The Connector.Connect and Driver.Open methods should never return ErrBadConn. ErrBadConn should only be returned from Validator, SessionResetter, or a query method if the connection is already in an invalid (e.g. closed) state.
All Conn implementations should implement the following interfaces: Pinger, SessionResetter, and Validator.
If named parameters or context are supported, the driver's Conn should implement: ExecerContext, QueryerContext, ConnPrepareContext, and ConnBeginTx.
To support custom data types, implement NamedValueChecker. NamedValueChecker also allows queries to accept per-query options as a parameter by returning ErrRemoveArgument from CheckNamedValue.
If multiple result sets are supported, Rows should implement RowsNextResultSet. If the driver knows how to describe the types present in the returned result it should implement the following interfaces: RowsColumnTypeScanType, RowsColumnTypeDatabaseTypeName, RowsColumnTypeLength, RowsColumnTypeNullable, and RowsColumnTypePrecisionScale. A given row value may also return a Rows type, which may represent a database cursor value.
Before a connection is returned to the connection pool after use, IsValid is called if implemented. Before a connection is reused for another query, ResetSession is called if implemented. If a connection is never returned to the connection pool but immediately reused, then ResetSession is called prior to reuse but IsValid is not called.
Index
-----
* [Variables](#pkg-variables)
* [func IsScanValue(v any) bool](#IsScanValue)
* [func IsValue(v any) bool](#IsValue)
* [type ColumnConverter](#ColumnConverter)
* [type Conn](#Conn)
* [type ConnBeginTx](#ConnBeginTx)
* [type ConnPrepareContext](#ConnPrepareContext)
* [type Connector](#Connector)
* [type Driver](#Driver)
* [type DriverContext](#DriverContext)
* [type Execer](#Execer)
* [type ExecerContext](#ExecerContext)
* [type IsolationLevel](#IsolationLevel)
* [type NamedValue](#NamedValue)
* [type NamedValueChecker](#NamedValueChecker)
* [type NotNull](#NotNull)
* [func (n NotNull) ConvertValue(v any) (Value, error)](#NotNull.ConvertValue)
* [type Null](#Null)
* [func (n Null) ConvertValue(v any) (Value, error)](#Null.ConvertValue)
* [type Pinger](#Pinger)
* [type Queryer](#Queryer)
* [type QueryerContext](#QueryerContext)
* [type Result](#Result)
* [type Rows](#Rows)
* [type RowsAffected](#RowsAffected)
* [func (RowsAffected) LastInsertId() (int64, error)](#RowsAffected.LastInsertId)
* [func (v RowsAffected) RowsAffected() (int64, error)](#RowsAffected.RowsAffected)
* [type RowsColumnTypeDatabaseTypeName](#RowsColumnTypeDatabaseTypeName)
* [type RowsColumnTypeLength](#RowsColumnTypeLength)
* [type RowsColumnTypeNullable](#RowsColumnTypeNullable)
* [type RowsColumnTypePrecisionScale](#RowsColumnTypePrecisionScale)
* [type RowsColumnTypeScanType](#RowsColumnTypeScanType)
* [type RowsNextResultSet](#RowsNextResultSet)
* [type SessionResetter](#SessionResetter)
* [type Stmt](#Stmt)
* [type StmtExecContext](#StmtExecContext)
* [type StmtQueryContext](#StmtQueryContext)
* [type Tx](#Tx)
* [type TxOptions](#TxOptions)
* [type Validator](#Validator)
* [type Value](#Value)
* [type ValueConverter](#ValueConverter)
* [type Valuer](#Valuer)
### Package files
driver.go types.go
Variables
---------
Bool is a ValueConverter that converts input values to bools.
The conversion rules are:
* booleans are returned unchanged
* for integer types, 1 is true 0 is false, other integers are an error
* for strings and []byte, same rules as strconv.ParseBool
* all other types are an error
```
var Bool boolType
```
DefaultParameterConverter is the default implementation of ValueConverter that's used when a Stmt doesn't implement ColumnConverter.
DefaultParameterConverter returns its argument directly if IsValue(arg). Otherwise, if the argument implements Valuer, its Value method is used to return a Value. As a fallback, the provided argument's underlying type is used to convert it to a Value: underlying integer types are converted to int64, floats to float64, bool, string, and []byte to themselves. If the argument is a nil pointer, ConvertValue returns a nil Value. If the argument is a non-nil pointer, it is dereferenced and ConvertValue is called recursively. Other types are an error.
```
var DefaultParameterConverter defaultConverter
```
ErrBadConn should be returned by a driver to signal to the sql package that a driver.Conn is in a bad state (such as the server having earlier closed the connection) and the sql package should retry on a new connection.
To prevent duplicate operations, ErrBadConn should NOT be returned if there's a possibility that the database server might have performed the operation. Even if the server sends back an error, you shouldn't return ErrBadConn.
Errors will be checked using errors.Is. An error may wrap ErrBadConn or implement the Is(error) bool method.
```
var ErrBadConn = errors.New("driver: bad connection")
```
ErrRemoveArgument may be returned from NamedValueChecker to instruct the sql package to not pass the argument to the driver query interface. Return when accepting query specific options or structures that aren't SQL query arguments.
```
var ErrRemoveArgument = errors.New("driver: remove argument from query")
```
ErrSkip may be returned by some optional interfaces' methods to indicate at runtime that the fast path is unavailable and the sql package should continue as if the optional interface was not implemented. ErrSkip is only supported where explicitly documented.
```
var ErrSkip = errors.New("driver: skip fast-path; continue as if unimplemented")
```
Int32 is a ValueConverter that converts input values to int64, respecting the limits of an int32 value.
```
var Int32 int32Type
```
ResultNoRows is a pre-defined Result for drivers to return when a DDL command (such as a CREATE TABLE) succeeds. It returns an error for both LastInsertId and RowsAffected.
```
var ResultNoRows noRows
```
String is a ValueConverter that converts its input to a string. If the value is already a string or []byte, it's unchanged. If the value is of another type, conversion to string is done with fmt.Sprintf("%v", v).
```
var String stringType
```
func IsScanValue
----------------
```
func IsScanValue(v any) bool
```
IsScanValue is equivalent to IsValue. It exists for compatibility.
func IsValue
------------
```
func IsValue(v any) bool
```
IsValue reports whether v is a valid Value parameter type.
type ColumnConverter
--------------------
ColumnConverter may be optionally implemented by Stmt if the statement is aware of its own columns' types and can convert from any type to a driver Value.
Deprecated: Drivers should implement NamedValueChecker.
```
type ColumnConverter interface {
// ColumnConverter returns a ValueConverter for the provided
// column index. If the type of a specific column isn't known
// or shouldn't be handled specially, DefaultValueConverter
// can be returned.
ColumnConverter(idx int) ValueConverter
}
```
type Conn
---------
Conn is a connection to a database. It is not used concurrently by multiple goroutines.
Conn is assumed to be stateful.
```
type Conn interface {
// Prepare returns a prepared statement, bound to this connection.
Prepare(query string) (Stmt, error)
// Close invalidates and potentially stops any current
// prepared statements and transactions, marking this
// connection as no longer in use.
//
// Because the sql package maintains a free pool of
// connections and only calls Close when there's a surplus of
// idle connections, it shouldn't be necessary for drivers to
// do their own connection caching.
//
// Drivers must ensure all network calls made by Close
// do not block indefinitely (e.g. apply a timeout).
Close() error
// Begin starts and returns a new transaction.
//
// Deprecated: Drivers should implement ConnBeginTx instead (or additionally).
Begin() (Tx, error)
}
```
type ConnBeginTx 1.8
--------------------
ConnBeginTx enhances the Conn interface with context and TxOptions.
```
type ConnBeginTx interface {
// BeginTx starts and returns a new transaction.
// If the context is canceled by the user the sql package will
// call Tx.Rollback before discarding and closing the connection.
//
// This must check opts.Isolation to determine if there is a set
// isolation level. If the driver does not support a non-default
// level and one is set or if there is a non-default isolation level
// that is not supported, an error must be returned.
//
// This must also check opts.ReadOnly to determine if the read-only
// value is true to either set the read-only transaction property if supported
// or return an error if it is not supported.
BeginTx(ctx context.Context, opts TxOptions) (Tx, error)
}
```
type ConnPrepareContext 1.8
---------------------------
ConnPrepareContext enhances the Conn interface with context.
```
type ConnPrepareContext interface {
// PrepareContext returns a prepared statement, bound to this connection.
// context is for the preparation of the statement,
// it must not store the context within the statement itself.
PrepareContext(ctx context.Context, query string) (Stmt, error)
}
```
type Connector 1.10
-------------------
A Connector represents a driver in a fixed configuration and can create any number of equivalent Conns for use by multiple goroutines.
A Connector can be passed to sql.OpenDB, to allow drivers to implement their own sql.DB constructors, or returned by DriverContext's OpenConnector method, to allow drivers access to context and to avoid repeated parsing of driver configuration.
If a Connector implements io.Closer, the sql package's DB.Close method will call Close and return error (if any).
```
type Connector interface {
// Connect returns a connection to the database.
// Connect may return a cached connection (one previously
// closed), but doing so is unnecessary; the sql package
// maintains a pool of idle connections for efficient re-use.
//
// The provided context.Context is for dialing purposes only
// (see net.DialContext) and should not be stored or used for
// other purposes. A default timeout should still be used
// when dialing as a connection pool may call Connect
// asynchronously to any query.
//
// The returned connection is only used by one goroutine at a
// time.
Connect(context.Context) (Conn, error)
// Driver returns the underlying Driver of the Connector,
// mainly to maintain compatibility with the Driver method
// on sql.DB.
Driver() Driver
}
```
type Driver
-----------
Driver is the interface that must be implemented by a database driver.
Database drivers may implement DriverContext for access to contexts and to parse the name only once for a pool of connections, instead of once per connection.
```
type Driver interface {
// Open returns a new connection to the database.
// The name is a string in a driver-specific format.
//
// Open may return a cached connection (one previously
// closed), but doing so is unnecessary; the sql package
// maintains a pool of idle connections for efficient re-use.
//
// The returned connection is only used by one goroutine at a
// time.
Open(name string) (Conn, error)
}
```
type DriverContext 1.10
-----------------------
If a Driver implements DriverContext, then sql.DB will call OpenConnector to obtain a Connector and then invoke that Connector's Connect method to obtain each needed connection, instead of invoking the Driver's Open method for each connection. The two-step sequence allows drivers to parse the name just once and also provides access to per-Conn contexts.
```
type DriverContext interface {
// OpenConnector must parse the name in the same format that Driver.Open
// parses the name parameter.
OpenConnector(name string) (Connector, error)
}
```
type Execer
-----------
Execer is an optional interface that may be implemented by a Conn.
If a Conn implements neither ExecerContext nor Execer, the sql package's DB.Exec will first prepare a query, execute the statement, and then close the statement.
Exec may return ErrSkip.
Deprecated: Drivers should implement ExecerContext instead.
```
type Execer interface {
Exec(query string, args []Value) (Result, error)
}
```
type ExecerContext 1.8
----------------------
ExecerContext is an optional interface that may be implemented by a Conn.
If a Conn does not implement ExecerContext, the sql package's DB.Exec will fall back to Execer; if the Conn does not implement Execer either, DB.Exec will first prepare a query, execute the statement, and then close the statement.
ExecContext may return ErrSkip.
ExecContext must honor the context timeout and return when the context is canceled.
```
type ExecerContext interface {
ExecContext(ctx context.Context, query string, args []NamedValue) (Result, error)
}
```
type IsolationLevel 1.8
-----------------------
IsolationLevel is the transaction isolation level stored in TxOptions.
This type should be considered identical to sql.IsolationLevel along with any values defined on it.
```
type IsolationLevel int
```
type NamedValue 1.8
-------------------
NamedValue holds both the value name and value.
```
type NamedValue struct {
// If the Name is not empty it should be used for the parameter identifier and
// not the ordinal position.
//
// Name will not have a symbol prefix.
Name string
// Ordinal position of the parameter starting from one and is always set.
Ordinal int
// Value is the parameter value.
Value Value
}
```
type NamedValueChecker 1.9
--------------------------
NamedValueChecker may be optionally implemented by Conn or Stmt. It provides the driver more control to handle Go and database types beyond the default Values types allowed.
The sql package checks for value checkers in the following order, stopping at the first found match: Stmt.NamedValueChecker, Conn.NamedValueChecker, Stmt.ColumnConverter, DefaultParameterConverter.
If CheckNamedValue returns ErrRemoveArgument, the NamedValue will not be included in the final query arguments. This may be used to pass special options to the query itself.
If ErrSkip is returned the column converter error checking path is used for the argument. Drivers may wish to return ErrSkip after they have exhausted their own special cases.
```
type NamedValueChecker interface {
// CheckNamedValue is called before passing arguments to the driver
// and is called in place of any ColumnConverter. CheckNamedValue must do type
// validation and conversion as appropriate for the driver.
CheckNamedValue(*NamedValue) error
}
```
type NotNull
------------
NotNull is a type that implements ValueConverter by disallowing nil values but otherwise delegating to another ValueConverter.
```
type NotNull struct {
Converter ValueConverter
}
```
### func (NotNull) ConvertValue
```
func (n NotNull) ConvertValue(v any) (Value, error)
```
type Null
---------
Null is a type that implements ValueConverter by allowing nil values but otherwise delegating to another ValueConverter.
```
type Null struct {
Converter ValueConverter
}
```
### func (Null) ConvertValue
```
func (n Null) ConvertValue(v any) (Value, error)
```
type Pinger 1.8
---------------
Pinger is an optional interface that may be implemented by a Conn.
If a Conn does not implement Pinger, the sql package's DB.Ping and DB.PingContext will check if there is at least one Conn available.
If Conn.Ping returns ErrBadConn, DB.Ping and DB.PingContext will remove the Conn from pool.
```
type Pinger interface {
Ping(ctx context.Context) error
}
```
type Queryer 1.1
----------------
Queryer is an optional interface that may be implemented by a Conn.
If a Conn implements neither QueryerContext nor Queryer, the sql package's DB.Query will first prepare a query, execute the statement, and then close the statement.
Query may return ErrSkip.
Deprecated: Drivers should implement QueryerContext instead.
```
type Queryer interface {
Query(query string, args []Value) (Rows, error)
}
```
type QueryerContext 1.8
-----------------------
QueryerContext is an optional interface that may be implemented by a Conn.
If a Conn does not implement QueryerContext, the sql package's DB.Query will fall back to Queryer; if the Conn does not implement Queryer either, DB.Query will first prepare a query, execute the statement, and then close the statement.
QueryContext may return ErrSkip.
QueryContext must honor the context timeout and return when the context is canceled.
```
type QueryerContext interface {
QueryContext(ctx context.Context, query string, args []NamedValue) (Rows, error)
}
```
type Result
-----------
Result is the result of a query execution.
```
type Result interface {
// LastInsertId returns the database's auto-generated ID
// after, for example, an INSERT into a table with primary
// key.
LastInsertId() (int64, error)
// RowsAffected returns the number of rows affected by the
// query.
RowsAffected() (int64, error)
}
```
type Rows
---------
Rows is an iterator over an executed query's results.
```
type Rows interface {
// Columns returns the names of the columns. The number of
// columns of the result is inferred from the length of the
// slice. If a particular column name isn't known, an empty
// string should be returned for that entry.
Columns() []string
// Close closes the rows iterator.
Close() error
// Next is called to populate the next row of data into
// the provided slice. The provided slice will be the same
// size as the Columns() are wide.
//
// Next should return io.EOF when there are no more rows.
//
// The dest should not be written to outside of Next. Care
// should be taken when closing Rows not to modify
// a buffer held in dest.
Next(dest []Value) error
}
```
type RowsAffected
-----------------
RowsAffected implements Result for an INSERT or UPDATE operation which mutates a number of rows.
```
type RowsAffected int64
```
### func (RowsAffected) LastInsertId
```
func (RowsAffected) LastInsertId() (int64, error)
```
### func (RowsAffected) RowsAffected
```
func (v RowsAffected) RowsAffected() (int64, error)
```
type RowsColumnTypeDatabaseTypeName 1.8
---------------------------------------
RowsColumnTypeDatabaseTypeName may be implemented by Rows. It should return the database system type name without the length. Type names should be uppercase. Examples of returned types: "VARCHAR", "NVARCHAR", "VARCHAR2", "CHAR", "TEXT", "DECIMAL", "SMALLINT", "INT", "BIGINT", "BOOL", "[]BIGINT", "JSONB", "XML", "TIMESTAMP".
```
type RowsColumnTypeDatabaseTypeName interface {
Rows
ColumnTypeDatabaseTypeName(index int) string
}
```
type RowsColumnTypeLength 1.8
-----------------------------
RowsColumnTypeLength may be implemented by Rows. It should return the length of the column type if the column is a variable length type. If the column is not a variable length type ok should return false. If length is not limited other than system limits, it should return math.MaxInt64. The following are examples of returned values for various types:
```
TEXT (math.MaxInt64, true)
varchar(10) (10, true)
nvarchar(10) (10, true)
decimal (0, false)
int (0, false)
bytea(30) (30, true)
```
```
type RowsColumnTypeLength interface {
Rows
ColumnTypeLength(index int) (length int64, ok bool)
}
```
type RowsColumnTypeNullable 1.8
-------------------------------
RowsColumnTypeNullable may be implemented by Rows. The nullable value should be true if it is known the column may be null, or false if the column is known to be not nullable. If the column nullability is unknown, ok should be false.
```
type RowsColumnTypeNullable interface {
Rows
ColumnTypeNullable(index int) (nullable, ok bool)
}
```
type RowsColumnTypePrecisionScale 1.8
-------------------------------------
RowsColumnTypePrecisionScale may be implemented by Rows. It should return the precision and scale for decimal types. If not applicable, ok should be false. The following are examples of returned values for various types:
```
decimal(38, 4) (38, 4, true)
int (0, 0, false)
decimal (math.MaxInt64, math.MaxInt64, true)
```
```
type RowsColumnTypePrecisionScale interface {
Rows
ColumnTypePrecisionScale(index int) (precision, scale int64, ok bool)
}
```
type RowsColumnTypeScanType 1.8
-------------------------------
RowsColumnTypeScanType may be implemented by Rows. It should return the value type that can be used to scan types into. For example, the database column type "bigint" this should return "reflect.TypeOf(int64(0))".
```
type RowsColumnTypeScanType interface {
Rows
ColumnTypeScanType(index int) reflect.Type
}
```
type RowsNextResultSet 1.8
--------------------------
RowsNextResultSet extends the Rows interface by providing a way to signal the driver to advance to the next result set.
```
type RowsNextResultSet interface {
Rows
// HasNextResultSet is called at the end of the current result set and
// reports whether there is another result set after the current one.
HasNextResultSet() bool
// NextResultSet advances the driver to the next result set even
// if there are remaining rows in the current result set.
//
// NextResultSet should return io.EOF when there are no more result sets.
NextResultSet() error
}
```
type SessionResetter 1.10
-------------------------
SessionResetter may be implemented by Conn to allow drivers to reset the session state associated with the connection and to signal a bad connection.
```
type SessionResetter interface {
// ResetSession is called prior to executing a query on the connection
// if the connection has been used before. If the driver returns ErrBadConn
// the connection is discarded.
ResetSession(ctx context.Context) error
}
```
type Stmt
---------
Stmt is a prepared statement. It is bound to a Conn and not used by multiple goroutines concurrently.
```
type Stmt interface {
// Close closes the statement.
//
// As of Go 1.1, a Stmt will not be closed if it's in use
// by any queries.
//
// Drivers must ensure all network calls made by Close
// do not block indefinitely (e.g. apply a timeout).
Close() error
// NumInput returns the number of placeholder parameters.
//
// If NumInput returns >= 0, the sql package will sanity check
// argument counts from callers and return errors to the caller
// before the statement's Exec or Query methods are called.
//
// NumInput may also return -1, if the driver doesn't know
// its number of placeholders. In that case, the sql package
// will not sanity check Exec or Query argument counts.
NumInput() int
// Exec executes a query that doesn't return rows, such
// as an INSERT or UPDATE.
//
// Deprecated: Drivers should implement StmtExecContext instead (or additionally).
Exec(args []Value) (Result, error)
// Query executes a query that may return rows, such as a
// SELECT.
//
// Deprecated: Drivers should implement StmtQueryContext instead (or additionally).
Query(args []Value) (Rows, error)
}
```
type StmtExecContext 1.8
------------------------
StmtExecContext enhances the Stmt interface by providing Exec with context.
```
type StmtExecContext interface {
// ExecContext executes a query that doesn't return rows, such
// as an INSERT or UPDATE.
//
// ExecContext must honor the context timeout and return when it is canceled.
ExecContext(ctx context.Context, args []NamedValue) (Result, error)
}
```
type StmtQueryContext 1.8
-------------------------
StmtQueryContext enhances the Stmt interface by providing Query with context.
```
type StmtQueryContext interface {
// QueryContext executes a query that may return rows, such as a
// SELECT.
//
// QueryContext must honor the context timeout and return when it is canceled.
QueryContext(ctx context.Context, args []NamedValue) (Rows, error)
}
```
type Tx
-------
Tx is a transaction.
```
type Tx interface {
Commit() error
Rollback() error
}
```
type TxOptions 1.8
------------------
TxOptions holds the transaction options.
This type should be considered identical to sql.TxOptions.
```
type TxOptions struct {
Isolation IsolationLevel
ReadOnly bool
}
```
type Validator 1.15
-------------------
Validator may be implemented by Conn to allow drivers to signal if a connection is valid or if it should be discarded.
If implemented, drivers may return the underlying error from queries, even if the connection should be discarded by the connection pool.
```
type Validator interface {
// IsValid is called prior to placing the connection into the
// connection pool. The connection will be discarded if false is returned.
IsValid() bool
}
```
type Value
----------
Value is a value that drivers must be able to handle. It is either nil, a type handled by a database driver's NamedValueChecker interface, or an instance of one of these types:
```
int64
float64
bool
[]byte
string
time.Time
```
If the driver supports cursors, a returned Value may also implement the Rows interface in this package. This is used, for example, when a user selects a cursor such as "select cursor(select \* from my\_table) from dual". If the Rows from the select is closed, the cursor Rows will also be closed.
```
type Value any
```
type ValueConverter
-------------------
ValueConverter is the interface providing the ConvertValue method.
Various implementations of ValueConverter are provided by the driver package to provide consistent implementations of conversions between drivers. The ValueConverters have several uses:
* converting from the Value types as provided by the sql package into a database table's specific column type and making sure it fits, such as making sure a particular int64 fits in a table's uint16 column.
* converting a value as given from the database into one of the driver Value types.
* by the sql package, for converting from a driver's Value type to a user's type in a scan.
```
type ValueConverter interface {
// ConvertValue converts a value to a driver Value.
ConvertValue(v any) (Value, error)
}
```
type Valuer
-----------
Valuer is the interface providing the Value method.
Types implementing Valuer interface are able to convert themselves to a driver Value.
```
type Valuer interface {
// Value returns a driver Value.
// Value must not panic.
Value() (Value, error)
}
```
| programming_docs |
go Package bufio Package bufio
==============
* `import "bufio"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package bufio implements buffered I/O. It wraps an io.Reader or io.Writer object, creating another object (Reader or Writer) that also implements the interface but provides buffering and some help for textual I/O.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func ScanBytes(data []byte, atEOF bool) (advance int, token []byte, err error)](#ScanBytes)
* [func ScanLines(data []byte, atEOF bool) (advance int, token []byte, err error)](#ScanLines)
* [func ScanRunes(data []byte, atEOF bool) (advance int, token []byte, err error)](#ScanRunes)
* [func ScanWords(data []byte, atEOF bool) (advance int, token []byte, err error)](#ScanWords)
* [type ReadWriter](#ReadWriter)
* [func NewReadWriter(r \*Reader, w \*Writer) \*ReadWriter](#NewReadWriter)
* [type Reader](#Reader)
* [func NewReader(rd io.Reader) \*Reader](#NewReader)
* [func NewReaderSize(rd io.Reader, size int) \*Reader](#NewReaderSize)
* [func (b \*Reader) Buffered() int](#Reader.Buffered)
* [func (b \*Reader) Discard(n int) (discarded int, err error)](#Reader.Discard)
* [func (b \*Reader) Peek(n int) ([]byte, error)](#Reader.Peek)
* [func (b \*Reader) Read(p []byte) (n int, err error)](#Reader.Read)
* [func (b \*Reader) ReadByte() (byte, error)](#Reader.ReadByte)
* [func (b \*Reader) ReadBytes(delim byte) ([]byte, error)](#Reader.ReadBytes)
* [func (b \*Reader) ReadLine() (line []byte, isPrefix bool, err error)](#Reader.ReadLine)
* [func (b \*Reader) ReadRune() (r rune, size int, err error)](#Reader.ReadRune)
* [func (b \*Reader) ReadSlice(delim byte) (line []byte, err error)](#Reader.ReadSlice)
* [func (b \*Reader) ReadString(delim byte) (string, error)](#Reader.ReadString)
* [func (b \*Reader) Reset(r io.Reader)](#Reader.Reset)
* [func (b \*Reader) Size() int](#Reader.Size)
* [func (b \*Reader) UnreadByte() error](#Reader.UnreadByte)
* [func (b \*Reader) UnreadRune() error](#Reader.UnreadRune)
* [func (b \*Reader) WriteTo(w io.Writer) (n int64, err error)](#Reader.WriteTo)
* [type Scanner](#Scanner)
* [func NewScanner(r io.Reader) \*Scanner](#NewScanner)
* [func (s \*Scanner) Buffer(buf []byte, max int)](#Scanner.Buffer)
* [func (s \*Scanner) Bytes() []byte](#Scanner.Bytes)
* [func (s \*Scanner) Err() error](#Scanner.Err)
* [func (s \*Scanner) Scan() bool](#Scanner.Scan)
* [func (s \*Scanner) Split(split SplitFunc)](#Scanner.Split)
* [func (s \*Scanner) Text() string](#Scanner.Text)
* [type SplitFunc](#SplitFunc)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer) \*Writer](#NewWriter)
* [func NewWriterSize(w io.Writer, size int) \*Writer](#NewWriterSize)
* [func (b \*Writer) Available() int](#Writer.Available)
* [func (b \*Writer) AvailableBuffer() []byte](#Writer.AvailableBuffer)
* [func (b \*Writer) Buffered() int](#Writer.Buffered)
* [func (b \*Writer) Flush() error](#Writer.Flush)
* [func (b \*Writer) ReadFrom(r io.Reader) (n int64, err error)](#Writer.ReadFrom)
* [func (b \*Writer) Reset(w io.Writer)](#Writer.Reset)
* [func (b \*Writer) Size() int](#Writer.Size)
* [func (b \*Writer) Write(p []byte) (nn int, err error)](#Writer.Write)
* [func (b \*Writer) WriteByte(c byte) error](#Writer.WriteByte)
* [func (b \*Writer) WriteRune(r rune) (size int, err error)](#Writer.WriteRune)
* [func (b \*Writer) WriteString(s string) (int, error)](#Writer.WriteString)
### Examples
[Scanner.Bytes](#example_Scanner_Bytes) [Scanner (Custom)](#example_Scanner_custom) [Scanner (EmptyFinalToken)](#example_Scanner_emptyFinalToken) [Scanner (Lines)](#example_Scanner_lines) [Scanner (Words)](#example_Scanner_words) [Writer](#example_Writer) [Writer.AvailableBuffer](#example_Writer_AvailableBuffer) ### Package files
bufio.go scan.go
Constants
---------
```
const (
// MaxScanTokenSize is the maximum size used to buffer a token
// unless the user provides an explicit buffer with Scanner.Buffer.
// The actual maximum token size may be smaller as the buffer
// may need to include, for instance, a newline.
MaxScanTokenSize = 64 * 1024
)
```
Variables
---------
```
var (
ErrInvalidUnreadByte = errors.New("bufio: invalid use of UnreadByte")
ErrInvalidUnreadRune = errors.New("bufio: invalid use of UnreadRune")
ErrBufferFull = errors.New("bufio: buffer full")
ErrNegativeCount = errors.New("bufio: negative count")
)
```
Errors returned by Scanner.
```
var (
ErrTooLong = errors.New("bufio.Scanner: token too long")
ErrNegativeAdvance = errors.New("bufio.Scanner: SplitFunc returns negative advance count")
ErrAdvanceTooFar = errors.New("bufio.Scanner: SplitFunc returns advance count beyond input")
ErrBadReadCount = errors.New("bufio.Scanner: Read returned impossible count")
)
```
ErrFinalToken is a special sentinel error value. It is intended to be returned by a Split function to indicate that the token being delivered with the error is the last token and scanning should stop after this one. After ErrFinalToken is received by Scan, scanning stops with no error. The value is useful to stop processing early or when it is necessary to deliver a final empty token. One could achieve the same behavior with a custom error value but providing one here is tidier. See the emptyFinalToken example for a use of this value.
```
var ErrFinalToken = errors.New("final token")
```
func ScanBytes 1.1
------------------
```
func ScanBytes(data []byte, atEOF bool) (advance int, token []byte, err error)
```
ScanBytes is a split function for a Scanner that returns each byte as a token.
func ScanLines 1.1
------------------
```
func ScanLines(data []byte, atEOF bool) (advance int, token []byte, err error)
```
ScanLines is a split function for a Scanner that returns each line of text, stripped of any trailing end-of-line marker. The returned line may be empty. The end-of-line marker is one optional carriage return followed by one mandatory newline. In regular expression notation, it is `\r?\n`. The last non-empty line of input will be returned even if it has no newline.
func ScanRunes 1.1
------------------
```
func ScanRunes(data []byte, atEOF bool) (advance int, token []byte, err error)
```
ScanRunes is a split function for a Scanner that returns each UTF-8-encoded rune as a token. The sequence of runes returned is equivalent to that from a range loop over the input as a string, which means that erroneous UTF-8 encodings translate to U+FFFD = "\xef\xbf\xbd". Because of the Scan interface, this makes it impossible for the client to distinguish correctly encoded replacement runes from encoding errors.
func ScanWords 1.1
------------------
```
func ScanWords(data []byte, atEOF bool) (advance int, token []byte, err error)
```
ScanWords is a split function for a Scanner that returns each space-separated word of text, with surrounding spaces deleted. It will never return an empty string. The definition of space is set by unicode.IsSpace.
type ReadWriter
---------------
ReadWriter stores pointers to a Reader and a Writer. It implements io.ReadWriter.
```
type ReadWriter struct {
*Reader
*Writer
}
```
### func NewReadWriter
```
func NewReadWriter(r *Reader, w *Writer) *ReadWriter
```
NewReadWriter allocates a new ReadWriter that dispatches to r and w.
type Reader
-----------
Reader implements buffering for an io.Reader object.
```
type Reader struct {
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(rd io.Reader) *Reader
```
NewReader returns a new Reader whose buffer has the default size.
### func NewReaderSize
```
func NewReaderSize(rd io.Reader, size int) *Reader
```
NewReaderSize returns a new Reader whose buffer has at least the specified size. If the argument io.Reader is already a Reader with large enough size, it returns the underlying Reader.
### func (\*Reader) Buffered
```
func (b *Reader) Buffered() int
```
Buffered returns the number of bytes that can be read from the current buffer.
### func (\*Reader) Discard 1.5
```
func (b *Reader) Discard(n int) (discarded int, err error)
```
Discard skips the next n bytes, returning the number of bytes discarded.
If Discard skips fewer than n bytes, it also returns an error. If 0 <= n <= b.Buffered(), Discard is guaranteed to succeed without reading from the underlying io.Reader.
### func (\*Reader) Peek
```
func (b *Reader) Peek(n int) ([]byte, error)
```
Peek returns the next n bytes without advancing the reader. The bytes stop being valid at the next read call. If Peek returns fewer than n bytes, it also returns an error explaining why the read is short. The error is ErrBufferFull if n is larger than b's buffer size.
Calling Peek prevents a UnreadByte or UnreadRune call from succeeding until the next read operation.
### func (\*Reader) Read
```
func (b *Reader) Read(p []byte) (n int, err error)
```
Read reads data into p. It returns the number of bytes read into p. The bytes are taken from at most one Read on the underlying Reader, hence n may be less than len(p). To read exactly len(p) bytes, use io.ReadFull(b, p). If the underlying Reader can return a non-zero count with io.EOF, then this Read method can do so as well; see the io.Reader docs.
### func (\*Reader) ReadByte
```
func (b *Reader) ReadByte() (byte, error)
```
ReadByte reads and returns a single byte. If no byte is available, returns an error.
### func (\*Reader) ReadBytes
```
func (b *Reader) ReadBytes(delim byte) ([]byte, error)
```
ReadBytes reads until the first occurrence of delim in the input, returning a slice containing the data up to and including the delimiter. If ReadBytes encounters an error before finding a delimiter, it returns the data read before the error and the error itself (often io.EOF). ReadBytes returns err != nil if and only if the returned data does not end in delim. For simple uses, a Scanner may be more convenient.
### func (\*Reader) ReadLine
```
func (b *Reader) ReadLine() (line []byte, isPrefix bool, err error)
```
ReadLine is a low-level line-reading primitive. Most callers should use ReadBytes('\n') or ReadString('\n') instead or use a Scanner.
ReadLine tries to return a single line, not including the end-of-line bytes. If the line was too long for the buffer then isPrefix is set and the beginning of the line is returned. The rest of the line will be returned from future calls. isPrefix will be false when returning the last fragment of the line. The returned buffer is only valid until the next call to ReadLine. ReadLine either returns a non-nil line or it returns an error, never both.
The text returned from ReadLine does not include the line end ("\r\n" or "\n"). No indication or error is given if the input ends without a final line end. Calling UnreadByte after ReadLine will always unread the last byte read (possibly a character belonging to the line end) even if that byte is not part of the line returned by ReadLine.
### func (\*Reader) ReadRune
```
func (b *Reader) ReadRune() (r rune, size int, err error)
```
ReadRune reads a single UTF-8 encoded Unicode character and returns the rune and its size in bytes. If the encoded rune is invalid, it consumes one byte and returns unicode.ReplacementChar (U+FFFD) with a size of 1.
### func (\*Reader) ReadSlice
```
func (b *Reader) ReadSlice(delim byte) (line []byte, err error)
```
ReadSlice reads until the first occurrence of delim in the input, returning a slice pointing at the bytes in the buffer. The bytes stop being valid at the next read. If ReadSlice encounters an error before finding a delimiter, it returns all the data in the buffer and the error itself (often io.EOF). ReadSlice fails with error ErrBufferFull if the buffer fills without a delim. Because the data returned from ReadSlice will be overwritten by the next I/O operation, most clients should use ReadBytes or ReadString instead. ReadSlice returns err != nil if and only if line does not end in delim.
### func (\*Reader) ReadString
```
func (b *Reader) ReadString(delim byte) (string, error)
```
ReadString reads until the first occurrence of delim in the input, returning a string containing the data up to and including the delimiter. If ReadString encounters an error before finding a delimiter, it returns the data read before the error and the error itself (often io.EOF). ReadString returns err != nil if and only if the returned data does not end in delim. For simple uses, a Scanner may be more convenient.
### func (\*Reader) Reset 1.2
```
func (b *Reader) Reset(r io.Reader)
```
Reset discards any buffered data, resets all state, and switches the buffered reader to read from r. Calling Reset on the zero value of Reader initializes the internal buffer to the default size.
### func (\*Reader) Size 1.10
```
func (b *Reader) Size() int
```
Size returns the size of the underlying buffer in bytes.
### func (\*Reader) UnreadByte
```
func (b *Reader) UnreadByte() error
```
UnreadByte unreads the last byte. Only the most recently read byte can be unread.
UnreadByte returns an error if the most recent method called on the Reader was not a read operation. Notably, Peek, Discard, and WriteTo are not considered read operations.
### func (\*Reader) UnreadRune
```
func (b *Reader) UnreadRune() error
```
UnreadRune unreads the last rune. If the most recent method called on the Reader was not a ReadRune, UnreadRune returns an error. (In this regard it is stricter than UnreadByte, which will unread the last byte from any read operation.)
### func (\*Reader) WriteTo 1.1
```
func (b *Reader) WriteTo(w io.Writer) (n int64, err error)
```
WriteTo implements io.WriterTo. This may make multiple calls to the Read method of the underlying Reader. If the underlying reader supports the WriteTo method, this calls the underlying WriteTo without buffering.
type Scanner 1.1
----------------
Scanner provides a convenient interface for reading data such as a file of newline-delimited lines of text. Successive calls to the Scan method will step through the 'tokens' of a file, skipping the bytes between the tokens. The specification of a token is defined by a split function of type SplitFunc; the default split function breaks the input into lines with line termination stripped. Split functions are defined in this package for scanning a file into lines, bytes, UTF-8-encoded runes, and space-delimited words. The client may instead provide a custom split function.
Scanning stops unrecoverably at EOF, the first I/O error, or a token too large to fit in the buffer. When a scan stops, the reader may have advanced arbitrarily far past the last token. Programs that need more control over error handling or large tokens, or must run sequential scans on a reader, should use bufio.Reader instead.
```
type Scanner struct {
// contains filtered or unexported fields
}
```
#### Example (Custom)
Use a Scanner with a custom split function (built by wrapping ScanWords) to validate 32-bit decimal input.
Code:
```
// An artificial input source.
const input = "1234 5678 1234567901234567890"
scanner := bufio.NewScanner(strings.NewReader(input))
// Create a custom split function by wrapping the existing ScanWords function.
split := func(data []byte, atEOF bool) (advance int, token []byte, err error) {
advance, token, err = bufio.ScanWords(data, atEOF)
if err == nil && token != nil {
_, err = strconv.ParseInt(string(token), 10, 32)
}
return
}
// Set the split function for the scanning operation.
scanner.Split(split)
// Validate the input
for scanner.Scan() {
fmt.Printf("%s\n", scanner.Text())
}
if err := scanner.Err(); err != nil {
fmt.Printf("Invalid input: %s", err)
}
```
Output:
```
1234
5678
Invalid input: strconv.ParseInt: parsing "1234567901234567890": value out of range
```
#### Example (EmptyFinalToken)
Use a Scanner with a custom split function to parse a comma-separated list with an empty final value.
Code:
```
// Comma-separated list; last entry is empty.
const input = "1,2,3,4,"
scanner := bufio.NewScanner(strings.NewReader(input))
// Define a split function that separates on commas.
onComma := func(data []byte, atEOF bool) (advance int, token []byte, err error) {
for i := 0; i < len(data); i++ {
if data[i] == ',' {
return i + 1, data[:i], nil
}
}
if !atEOF {
return 0, nil, nil
}
// There is one final token to be delivered, which may be the empty string.
// Returning bufio.ErrFinalToken here tells Scan there are no more tokens after this
// but does not trigger an error to be returned from Scan itself.
return 0, data, bufio.ErrFinalToken
}
scanner.Split(onComma)
// Scan.
for scanner.Scan() {
fmt.Printf("%q ", scanner.Text())
}
if err := scanner.Err(); err != nil {
fmt.Fprintln(os.Stderr, "reading input:", err)
}
```
Output:
```
"1" "2" "3" "4" ""
```
#### Example (Lines)
The simplest use of a Scanner, to read standard input as a set of lines.
Code:
```
scanner := bufio.NewScanner(os.Stdin)
for scanner.Scan() {
fmt.Println(scanner.Text()) // Println will add back the final '\n'
}
if err := scanner.Err(); err != nil {
fmt.Fprintln(os.Stderr, "reading standard input:", err)
}
```
#### Example (Words)
Use a Scanner to implement a simple word-count utility by scanning the input as a sequence of space-delimited tokens.
Code:
```
// An artificial input source.
const input = "Now is the winter of our discontent,\nMade glorious summer by this sun of York.\n"
scanner := bufio.NewScanner(strings.NewReader(input))
// Set the split function for the scanning operation.
scanner.Split(bufio.ScanWords)
// Count the words.
count := 0
for scanner.Scan() {
count++
}
if err := scanner.Err(); err != nil {
fmt.Fprintln(os.Stderr, "reading input:", err)
}
fmt.Printf("%d\n", count)
```
Output:
```
15
```
### func NewScanner 1.1
```
func NewScanner(r io.Reader) *Scanner
```
NewScanner returns a new Scanner to read from r. The split function defaults to ScanLines.
### func (\*Scanner) Buffer 1.6
```
func (s *Scanner) Buffer(buf []byte, max int)
```
Buffer sets the initial buffer to use when scanning and the maximum size of buffer that may be allocated during scanning. The maximum token size is the larger of max and cap(buf). If max <= cap(buf), Scan will use this buffer only and do no allocation.
By default, Scan uses an internal buffer and sets the maximum token size to MaxScanTokenSize.
Buffer panics if it is called after scanning has started.
### func (\*Scanner) Bytes 1.1
```
func (s *Scanner) Bytes() []byte
```
Bytes returns the most recent token generated by a call to Scan. The underlying array may point to data that will be overwritten by a subsequent call to Scan. It does no allocation.
#### Example
Return the most recent call to Scan as a []byte.
Code:
```
scanner := bufio.NewScanner(strings.NewReader("gopher"))
for scanner.Scan() {
fmt.Println(len(scanner.Bytes()) == 6)
}
if err := scanner.Err(); err != nil {
fmt.Fprintln(os.Stderr, "shouldn't see an error scanning a string")
}
```
Output:
```
true
```
### func (\*Scanner) Err 1.1
```
func (s *Scanner) Err() error
```
Err returns the first non-EOF error that was encountered by the Scanner.
### func (\*Scanner) Scan 1.1
```
func (s *Scanner) Scan() bool
```
Scan advances the Scanner to the next token, which will then be available through the Bytes or Text method. It returns false when the scan stops, either by reaching the end of the input or an error. After Scan returns false, the Err method will return any error that occurred during scanning, except that if it was io.EOF, Err will return nil. Scan panics if the split function returns too many empty tokens without advancing the input. This is a common error mode for scanners.
### func (\*Scanner) Split 1.1
```
func (s *Scanner) Split(split SplitFunc)
```
Split sets the split function for the Scanner. The default split function is ScanLines.
Split panics if it is called after scanning has started.
### func (\*Scanner) Text 1.1
```
func (s *Scanner) Text() string
```
Text returns the most recent token generated by a call to Scan as a newly allocated string holding its bytes.
type SplitFunc 1.1
------------------
SplitFunc is the signature of the split function used to tokenize the input. The arguments are an initial substring of the remaining unprocessed data and a flag, atEOF, that reports whether the Reader has no more data to give. The return values are the number of bytes to advance the input and the next token to return to the user, if any, plus an error, if any.
Scanning stops if the function returns an error, in which case some of the input may be discarded. If that error is ErrFinalToken, scanning stops with no error.
Otherwise, the Scanner advances the input. If the token is not nil, the Scanner returns it to the user. If the token is nil, the Scanner reads more data and continues scanning; if there is no more data--if atEOF was true--the Scanner returns. If the data does not yet hold a complete token, for instance if it has no newline while scanning lines, a SplitFunc can return (0, nil, nil) to signal the Scanner to read more data into the slice and try again with a longer slice starting at the same point in the input.
The function is never called with an empty data slice unless atEOF is true. If atEOF is true, however, data may be non-empty and, as always, holds unprocessed text.
```
type SplitFunc func(data []byte, atEOF bool) (advance int, token []byte, err error)
```
type Writer
-----------
Writer implements buffering for an io.Writer object. If an error occurs writing to a Writer, no more data will be accepted and all subsequent writes, and Flush, will return the error. After all data has been written, the client should call the Flush method to guarantee all data has been forwarded to the underlying io.Writer.
```
type Writer struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
w := bufio.NewWriter(os.Stdout)
fmt.Fprint(w, "Hello, ")
fmt.Fprint(w, "world!")
w.Flush() // Don't forget to flush!
```
Output:
```
Hello, world!
```
### func NewWriter
```
func NewWriter(w io.Writer) *Writer
```
NewWriter returns a new Writer whose buffer has the default size. If the argument io.Writer is already a Writer with large enough buffer size, it returns the underlying Writer.
### func NewWriterSize
```
func NewWriterSize(w io.Writer, size int) *Writer
```
NewWriterSize returns a new Writer whose buffer has at least the specified size. If the argument io.Writer is already a Writer with large enough size, it returns the underlying Writer.
### func (\*Writer) Available
```
func (b *Writer) Available() int
```
Available returns how many bytes are unused in the buffer.
### func (\*Writer) AvailableBuffer 1.18
```
func (b *Writer) AvailableBuffer() []byte
```
AvailableBuffer returns an empty buffer with b.Available() capacity. This buffer is intended to be appended to and passed to an immediately succeeding Write call. The buffer is only valid until the next write operation on b.
#### Example
Code:
```
w := bufio.NewWriter(os.Stdout)
for _, i := range []int64{1, 2, 3, 4} {
b := w.AvailableBuffer()
b = strconv.AppendInt(b, i, 10)
b = append(b, ' ')
w.Write(b)
}
w.Flush()
```
Output:
```
1 2 3 4
```
### func (\*Writer) Buffered
```
func (b *Writer) Buffered() int
```
Buffered returns the number of bytes that have been written into the current buffer.
### func (\*Writer) Flush
```
func (b *Writer) Flush() error
```
Flush writes any buffered data to the underlying io.Writer.
### func (\*Writer) ReadFrom 1.1
```
func (b *Writer) ReadFrom(r io.Reader) (n int64, err error)
```
ReadFrom implements io.ReaderFrom. If the underlying writer supports the ReadFrom method, this calls the underlying ReadFrom. If there is buffered data and an underlying ReadFrom, this fills the buffer and writes it before calling ReadFrom.
### func (\*Writer) Reset 1.2
```
func (b *Writer) Reset(w io.Writer)
```
Reset discards any unflushed buffered data, clears any error, and resets b to write its output to w. Calling Reset on the zero value of Writer initializes the internal buffer to the default size.
### func (\*Writer) Size 1.10
```
func (b *Writer) Size() int
```
Size returns the size of the underlying buffer in bytes.
### func (\*Writer) Write
```
func (b *Writer) Write(p []byte) (nn int, err error)
```
Write writes the contents of p into the buffer. It returns the number of bytes written. If nn < len(p), it also returns an error explaining why the write is short.
### func (\*Writer) WriteByte
```
func (b *Writer) WriteByte(c byte) error
```
WriteByte writes a single byte.
### func (\*Writer) WriteRune
```
func (b *Writer) WriteRune(r rune) (size int, err error)
```
WriteRune writes a single Unicode code point, returning the number of bytes written and any error.
### func (\*Writer) WriteString
```
func (b *Writer) WriteString(s string) (int, error)
```
WriteString writes a string. It returns the number of bytes written. If the count is less than len(s), it also returns an error explaining why the write is short.
| programming_docs |
go Package crypto Package crypto
===============
* `import "crypto"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package crypto collects common cryptographic constants.
Index
-----
* [func RegisterHash(h Hash, f func() hash.Hash)](#RegisterHash)
* [type Decrypter](#Decrypter)
* [type DecrypterOpts](#DecrypterOpts)
* [type Hash](#Hash)
* [func (h Hash) Available() bool](#Hash.Available)
* [func (h Hash) HashFunc() Hash](#Hash.HashFunc)
* [func (h Hash) New() hash.Hash](#Hash.New)
* [func (h Hash) Size() int](#Hash.Size)
* [func (h Hash) String() string](#Hash.String)
* [type PrivateKey](#PrivateKey)
* [type PublicKey](#PublicKey)
* [type Signer](#Signer)
* [type SignerOpts](#SignerOpts)
### Package files
crypto.go
func RegisterHash
-----------------
```
func RegisterHash(h Hash, f func() hash.Hash)
```
RegisterHash registers a function that returns a new instance of the given hash function. This is intended to be called from the init function in packages that implement hash functions.
type Decrypter 1.5
------------------
Decrypter is an interface for an opaque private key that can be used for asymmetric decryption operations. An example would be an RSA key kept in a hardware module.
```
type Decrypter interface {
// Public returns the public key corresponding to the opaque,
// private key.
Public() PublicKey
// Decrypt decrypts msg. The opts argument should be appropriate for
// the primitive used. See the documentation in each implementation for
// details.
Decrypt(rand io.Reader, msg []byte, opts DecrypterOpts) (plaintext []byte, err error)
}
```
type DecrypterOpts 1.5
----------------------
```
type DecrypterOpts any
```
type Hash
---------
Hash identifies a cryptographic hash function that is implemented in another package.
```
type Hash uint
```
```
const (
MD4 Hash = 1 + iota // import golang.org/x/crypto/md4
MD5 // import crypto/md5
SHA1 // import crypto/sha1
SHA224 // import crypto/sha256
SHA256 // import crypto/sha256
SHA384 // import crypto/sha512
SHA512 // import crypto/sha512
MD5SHA1 // no implementation; MD5+SHA1 used for TLS RSA
RIPEMD160 // import golang.org/x/crypto/ripemd160
SHA3_224 // import golang.org/x/crypto/sha3
SHA3_256 // import golang.org/x/crypto/sha3
SHA3_384 // import golang.org/x/crypto/sha3
SHA3_512 // import golang.org/x/crypto/sha3
SHA512_224 // import crypto/sha512
SHA512_256 // import crypto/sha512
BLAKE2s_256 // import golang.org/x/crypto/blake2s
BLAKE2b_256 // import golang.org/x/crypto/blake2b
BLAKE2b_384 // import golang.org/x/crypto/blake2b
BLAKE2b_512 // import golang.org/x/crypto/blake2b
)
```
### func (Hash) Available
```
func (h Hash) Available() bool
```
Available reports whether the given hash function is linked into the binary.
### func (Hash) HashFunc 1.4
```
func (h Hash) HashFunc() Hash
```
HashFunc simply returns the value of h so that Hash implements SignerOpts.
### func (Hash) New
```
func (h Hash) New() hash.Hash
```
New returns a new hash.Hash calculating the given hash function. New panics if the hash function is not linked into the binary.
### func (Hash) Size
```
func (h Hash) Size() int
```
Size returns the length, in bytes, of a digest resulting from the given hash function. It doesn't require that the hash function in question be linked into the program.
### func (Hash) String 1.15
```
func (h Hash) String() string
```
type PrivateKey
---------------
PrivateKey represents a private key using an unspecified algorithm.
Although this type is an empty interface for backwards compatibility reasons, all private key types in the standard library implement the following interface
```
interface{
Public() crypto.PublicKey
Equal(x crypto.PrivateKey) bool
}
```
as well as purpose-specific interfaces such as Signer and Decrypter, which can be used for increased type safety within applications.
```
type PrivateKey any
```
type PublicKey 1.2
------------------
PublicKey represents a public key using an unspecified algorithm.
Although this type is an empty interface for backwards compatibility reasons, all public key types in the standard library implement the following interface
```
interface{
Equal(x crypto.PublicKey) bool
}
```
which can be used for increased type safety within applications.
```
type PublicKey any
```
type Signer 1.4
---------------
Signer is an interface for an opaque private key that can be used for signing operations. For example, an RSA key kept in a hardware module.
```
type Signer interface {
// Public returns the public key corresponding to the opaque,
// private key.
Public() PublicKey
// Sign signs digest with the private key, possibly using entropy from
// rand. For an RSA key, the resulting signature should be either a
// PKCS #1 v1.5 or PSS signature (as indicated by opts). For an (EC)DSA
// key, it should be a DER-serialised, ASN.1 signature structure.
//
// Hash implements the SignerOpts interface and, in most cases, one can
// simply pass in the hash function used as opts. Sign may also attempt
// to type assert opts to other types in order to obtain algorithm
// specific values. See the documentation in each package for details.
//
// Note that when a signature of a hash of a larger message is needed,
// the caller is responsible for hashing the larger message and passing
// the hash (as digest) and the hash function (as opts) to Sign.
Sign(rand io.Reader, digest []byte, opts SignerOpts) (signature []byte, err error)
}
```
type SignerOpts 1.4
-------------------
SignerOpts contains options for signing with a Signer.
```
type SignerOpts interface {
// HashFunc returns an identifier for the hash function used to produce
// the message passed to Signer.Sign, or else zero to indicate that no
// hashing was done.
HashFunc() Hash
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [aes](aes/index) | Package aes implements AES encryption (formerly Rijndael), as defined in U.S. Federal Information Processing Standards Publication 197. |
| [boring](boring/index) | Package boring exposes functions that are only available when building with Go+BoringCrypto. |
| [cipher](cipher/index) | Package cipher implements standard block cipher modes that can be wrapped around low-level block cipher implementations. |
| [des](des/index) | Package des implements the Data Encryption Standard (DES) and the Triple Data Encryption Algorithm (TDEA) as defined in U.S. Federal Information Processing Standards Publication 46-3. |
| [dsa](dsa/index) | Package dsa implements the Digital Signature Algorithm, as defined in FIPS 186-3. |
| [ecdh](ecdh/index) | Package ecdh implements Elliptic Curve Diffie-Hellman over NIST curves and Curve25519. |
| [ecdsa](ecdsa/index) | Package ecdsa implements the Elliptic Curve Digital Signature Algorithm, as defined in FIPS 186-4 and SEC 1, Version 2.0. |
| [ed25519](ed25519/index) | Package ed25519 implements the Ed25519 signature algorithm. |
| [elliptic](elliptic/index) | Package elliptic implements the standard NIST P-224, P-256, P-384, and P-521 elliptic curves over prime fields. |
| [hmac](hmac/index) | Package hmac implements the Keyed-Hash Message Authentication Code (HMAC) as defined in U.S. Federal Information Processing Standards Publication 198. |
| [md5](md5/index) | Package md5 implements the MD5 hash algorithm as defined in RFC 1321. |
| [rand](rand/index) | Package rand implements a cryptographically secure random number generator. |
| [rc4](rc4/index) | Package rc4 implements RC4 encryption, as defined in Bruce Schneier's Applied Cryptography. |
| [rsa](rsa/index) | Package rsa implements RSA encryption as specified in PKCS #1 and RFC 8017. |
| [sha1](sha1/index) | Package sha1 implements the SHA-1 hash algorithm as defined in RFC 3174. |
| [sha256](sha256/index) | Package sha256 implements the SHA224 and SHA256 hash algorithms as defined in FIPS 180-4. |
| [sha512](sha512/index) | Package sha512 implements the SHA-384, SHA-512, SHA-512/224, and SHA-512/256 hash algorithms as defined in FIPS 180-4. |
| [subtle](subtle/index) | Package subtle implements functions that are often useful in cryptographic code but require careful thought to use correctly. |
| [tls](tls/index) | Package tls partially implements TLS 1.2, as specified in RFC 5246, and TLS 1.3, as specified in RFC 8446. |
| [fipsonly](tls/fipsonly/index) | Package fipsonly restricts all TLS configuration to FIPS-approved settings. |
| [x509](x509/index) | Package x509 implements a subset of the X.509 standard. |
| [pkix](x509/pkix/index) | Package pkix contains shared, low level structures used for ASN.1 parsing and serialization of X.509 certificates, CRL and OCSP. |
go Package sha512 Package sha512
===============
* `import "crypto/sha512"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package sha512 implements the SHA-384, SHA-512, SHA-512/224, and SHA-512/256 hash algorithms as defined in FIPS 180-4.
All the hash.Hash implementations returned by this package also implement encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
Index
-----
* [Constants](#pkg-constants)
* [func New() hash.Hash](#New)
* [func New384() hash.Hash](#New384)
* [func New512\_224() hash.Hash](#New512_224)
* [func New512\_256() hash.Hash](#New512_256)
* [func Sum384(data []byte) [Size384]byte](#Sum384)
* [func Sum512(data []byte) [Size]byte](#Sum512)
* [func Sum512\_224(data []byte) [Size224]byte](#Sum512_224)
* [func Sum512\_256(data []byte) [Size256]byte](#Sum512_256)
### Package files
sha512.go sha512block.go sha512block\_amd64.go
Constants
---------
```
const (
// Size is the size, in bytes, of a SHA-512 checksum.
Size = 64
// Size224 is the size, in bytes, of a SHA-512/224 checksum.
Size224 = 28
// Size256 is the size, in bytes, of a SHA-512/256 checksum.
Size256 = 32
// Size384 is the size, in bytes, of a SHA-384 checksum.
Size384 = 48
// BlockSize is the block size, in bytes, of the SHA-512/224,
// SHA-512/256, SHA-384 and SHA-512 hash functions.
BlockSize = 128
)
```
func New
--------
```
func New() hash.Hash
```
New returns a new hash.Hash computing the SHA-512 checksum.
func New384
-----------
```
func New384() hash.Hash
```
New384 returns a new hash.Hash computing the SHA-384 checksum.
func New512\_224 1.5
--------------------
```
func New512_224() hash.Hash
```
New512\_224 returns a new hash.Hash computing the SHA-512/224 checksum.
func New512\_256 1.5
--------------------
```
func New512_256() hash.Hash
```
New512\_256 returns a new hash.Hash computing the SHA-512/256 checksum.
func Sum384 1.2
---------------
```
func Sum384(data []byte) [Size384]byte
```
Sum384 returns the SHA384 checksum of the data.
func Sum512 1.2
---------------
```
func Sum512(data []byte) [Size]byte
```
Sum512 returns the SHA512 checksum of the data.
func Sum512\_224 1.5
--------------------
```
func Sum512_224(data []byte) [Size224]byte
```
Sum512\_224 returns the Sum512/224 checksum of the data.
func Sum512\_256 1.5
--------------------
```
func Sum512_256(data []byte) [Size256]byte
```
Sum512\_256 returns the Sum512/256 checksum of the data.
go Package hmac Package hmac
=============
* `import "crypto/hmac"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package hmac implements the Keyed-Hash Message Authentication Code (HMAC) as defined in U.S. Federal Information Processing Standards Publication 198. An HMAC is a cryptographic hash that uses a key to sign a message. The receiver verifies the hash by recomputing it using the same key.
Receivers should be careful to use Equal to compare MACs in order to avoid timing side-channels:
```
// ValidMAC reports whether messageMAC is a valid HMAC tag for message.
func ValidMAC(message, messageMAC, key []byte) bool {
mac := hmac.New(sha256.New, key)
mac.Write(message)
expectedMAC := mac.Sum(nil)
return hmac.Equal(messageMAC, expectedMAC)
}
```
Index
-----
* [func Equal(mac1, mac2 []byte) bool](#Equal)
* [func New(h func() hash.Hash, key []byte) hash.Hash](#New)
### Package files
hmac.go
func Equal 1.1
--------------
```
func Equal(mac1, mac2 []byte) bool
```
Equal compares two MACs for equality without leaking timing information.
func New
--------
```
func New(h func() hash.Hash, key []byte) hash.Hash
```
New returns a new HMAC hash using the given hash.Hash type and key. New functions like sha256.New from crypto/sha256 can be used as h. h must return a new Hash every time it is called. Note that unlike other hash implementations in the standard library, the returned Hash does not implement encoding.BinaryMarshaler or encoding.BinaryUnmarshaler.
go Package md5 Package md5
============
* `import "crypto/md5"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package md5 implements the MD5 hash algorithm as defined in RFC 1321.
MD5 is cryptographically broken and should not be used for secure applications.
Index
-----
* [Constants](#pkg-constants)
* [func New() hash.Hash](#New)
* [func Sum(data []byte) [Size]byte](#Sum)
### Examples
[New](#example_New) [New (File)](#example_New_file) [Sum](#example_Sum) ### Package files
md5.go md5block.go md5block\_decl.go
Constants
---------
The blocksize of MD5 in bytes.
```
const BlockSize = 64
```
The size of an MD5 checksum in bytes.
```
const Size = 16
```
func New
--------
```
func New() hash.Hash
```
New returns a new hash.Hash computing the MD5 checksum. The Hash also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
#### Example
Code:
```
h := md5.New()
io.WriteString(h, "The fog is getting thicker!")
io.WriteString(h, "And Leon's getting laaarger!")
fmt.Printf("%x", h.Sum(nil))
```
Output:
```
e2c569be17396eca2a2e3c11578123ed
```
#### Example (File)
Code:
```
f, err := os.Open("file.txt")
if err != nil {
log.Fatal(err)
}
defer f.Close()
h := md5.New()
if _, err := io.Copy(h, f); err != nil {
log.Fatal(err)
}
fmt.Printf("%x", h.Sum(nil))
```
func Sum 1.2
------------
```
func Sum(data []byte) [Size]byte
```
Sum returns the MD5 checksum of the data.
#### Example
Code:
```
data := []byte("These pretzels are making me thirsty.")
fmt.Printf("%x", md5.Sum(data))
```
Output:
```
b0804ec967f48520697662a204f5fe72
```
go Package rc4 Package rc4
============
* `import "crypto/rc4"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package rc4 implements RC4 encryption, as defined in Bruce Schneier's Applied Cryptography.
RC4 is cryptographically broken and should not be used for secure applications.
Index
-----
* [type Cipher](#Cipher)
* [func NewCipher(key []byte) (\*Cipher, error)](#NewCipher)
* [func (c \*Cipher) Reset()](#Cipher.Reset)
* [func (c \*Cipher) XORKeyStream(dst, src []byte)](#Cipher.XORKeyStream)
* [type KeySizeError](#KeySizeError)
* [func (k KeySizeError) Error() string](#KeySizeError.Error)
### Package files
rc4.go
type Cipher
-----------
A Cipher is an instance of RC4 using a particular key.
```
type Cipher struct {
// contains filtered or unexported fields
}
```
### func NewCipher
```
func NewCipher(key []byte) (*Cipher, error)
```
NewCipher creates and returns a new Cipher. The key argument should be the RC4 key, at least 1 byte and at most 256 bytes.
### func (\*Cipher) Reset
```
func (c *Cipher) Reset()
```
Reset zeros the key data and makes the Cipher unusable.
Deprecated: Reset can't guarantee that the key will be entirely removed from the process's memory.
### func (\*Cipher) XORKeyStream
```
func (c *Cipher) XORKeyStream(dst, src []byte)
```
XORKeyStream sets dst to the result of XORing src with the key stream. Dst and src must overlap entirely or not at all.
type KeySizeError
-----------------
```
type KeySizeError int
```
### func (KeySizeError) Error
```
func (k KeySizeError) Error() string
```
go Package des Package des
============
* `import "crypto/des"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package des implements the Data Encryption Standard (DES) and the Triple Data Encryption Algorithm (TDEA) as defined in U.S. Federal Information Processing Standards Publication 46-3.
DES is cryptographically broken and should not be used for secure applications.
Index
-----
* [Constants](#pkg-constants)
* [func NewCipher(key []byte) (cipher.Block, error)](#NewCipher)
* [func NewTripleDESCipher(key []byte) (cipher.Block, error)](#NewTripleDESCipher)
* [type KeySizeError](#KeySizeError)
* [func (k KeySizeError) Error() string](#KeySizeError.Error)
### Examples
[NewTripleDESCipher](#example_NewTripleDESCipher) ### Package files
block.go cipher.go const.go
Constants
---------
The DES block size in bytes.
```
const BlockSize = 8
```
func NewCipher
--------------
```
func NewCipher(key []byte) (cipher.Block, error)
```
NewCipher creates and returns a new cipher.Block.
func NewTripleDESCipher
-----------------------
```
func NewTripleDESCipher(key []byte) (cipher.Block, error)
```
NewTripleDESCipher creates and returns a new cipher.Block.
#### Example
Code:
```
// NewTripleDESCipher can also be used when EDE2 is required by
// duplicating the first 8 bytes of the 16-byte key.
ede2Key := []byte("example key 1234")
var tripleDESKey []byte
tripleDESKey = append(tripleDESKey, ede2Key[:16]...)
tripleDESKey = append(tripleDESKey, ede2Key[:8]...)
_, err := des.NewTripleDESCipher(tripleDESKey)
if err != nil {
panic(err)
}
// See crypto/cipher for how to use a cipher.Block for encryption and
// decryption.
```
type KeySizeError
-----------------
```
type KeySizeError int
```
### func (KeySizeError) Error
```
func (k KeySizeError) Error() string
```
go Package ecdsa Package ecdsa
==============
* `import "crypto/ecdsa"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package ecdsa implements the Elliptic Curve Digital Signature Algorithm, as defined in FIPS 186-4 and SEC 1, Version 2.0.
Signatures generated by this package are not deterministic, but entropy is mixed with the private key and the message, achieving the same level of security in case of randomness source failure.
#### Example
Code:
```
privateKey, err := ecdsa.GenerateKey(elliptic.P256(), rand.Reader)
if err != nil {
panic(err)
}
msg := "hello, world"
hash := sha256.Sum256([]byte(msg))
sig, err := ecdsa.SignASN1(rand.Reader, privateKey, hash[:])
if err != nil {
panic(err)
}
fmt.Printf("signature: %x\n", sig)
valid := ecdsa.VerifyASN1(&privateKey.PublicKey, hash[:], sig)
fmt.Println("signature verified:", valid)
```
Index
-----
* [func Sign(rand io.Reader, priv \*PrivateKey, hash []byte) (r, s \*big.Int, err error)](#Sign)
* [func SignASN1(rand io.Reader, priv \*PrivateKey, hash []byte) ([]byte, error)](#SignASN1)
* [func Verify(pub \*PublicKey, hash []byte, r, s \*big.Int) bool](#Verify)
* [func VerifyASN1(pub \*PublicKey, hash, sig []byte) bool](#VerifyASN1)
* [type PrivateKey](#PrivateKey)
* [func GenerateKey(c elliptic.Curve, rand io.Reader) (\*PrivateKey, error)](#GenerateKey)
* [func (k \*PrivateKey) ECDH() (\*ecdh.PrivateKey, error)](#PrivateKey.ECDH)
* [func (priv \*PrivateKey) Equal(x crypto.PrivateKey) bool](#PrivateKey.Equal)
* [func (priv \*PrivateKey) Public() crypto.PublicKey](#PrivateKey.Public)
* [func (priv \*PrivateKey) Sign(rand io.Reader, digest []byte, opts crypto.SignerOpts) ([]byte, error)](#PrivateKey.Sign)
* [type PublicKey](#PublicKey)
* [func (k \*PublicKey) ECDH() (\*ecdh.PublicKey, error)](#PublicKey.ECDH)
* [func (pub \*PublicKey) Equal(x crypto.PublicKey) bool](#PublicKey.Equal)
### Examples
[Package](#example_) ### Package files
ecdsa.go ecdsa\_legacy.go ecdsa\_noasm.go notboring.go
func Sign
---------
```
func Sign(rand io.Reader, priv *PrivateKey, hash []byte) (r, s *big.Int, err error)
```
Sign signs a hash (which should be the result of hashing a larger message) using the private key, priv. If the hash is longer than the bit-length of the private key's curve order, the hash will be truncated to that length. It returns the signature as a pair of integers. Most applications should use SignASN1 instead of dealing directly with r, s.
func SignASN1 1.15
------------------
```
func SignASN1(rand io.Reader, priv *PrivateKey, hash []byte) ([]byte, error)
```
SignASN1 signs a hash (which should be the result of hashing a larger message) using the private key, priv. If the hash is longer than the bit-length of the private key's curve order, the hash will be truncated to that length. It returns the ASN.1 encoded signature.
func Verify
-----------
```
func Verify(pub *PublicKey, hash []byte, r, s *big.Int) bool
```
Verify verifies the signature in r, s of hash using the public key, pub. Its return value records whether the signature is valid. Most applications should use VerifyASN1 instead of dealing directly with r, s.
func VerifyASN1 1.15
--------------------
```
func VerifyASN1(pub *PublicKey, hash, sig []byte) bool
```
VerifyASN1 verifies the ASN.1 encoded signature, sig, of hash using the public key, pub. Its return value records whether the signature is valid.
type PrivateKey
---------------
PrivateKey represents an ECDSA private key.
```
type PrivateKey struct {
PublicKey
D *big.Int
}
```
### func GenerateKey
```
func GenerateKey(c elliptic.Curve, rand io.Reader) (*PrivateKey, error)
```
GenerateKey generates a public and private key pair.
### func (\*PrivateKey) ECDH 1.20
```
func (k *PrivateKey) ECDH() (*ecdh.PrivateKey, error)
```
ECDH returns k as a ecdh.PrivateKey. It returns an error if the key is invalid according to the definition of ecdh.Curve.NewPrivateKey, or if the Curve is not supported by crypto/ecdh.
### func (\*PrivateKey) Equal 1.15
```
func (priv *PrivateKey) Equal(x crypto.PrivateKey) bool
```
Equal reports whether priv and x have the same value.
See PublicKey.Equal for details on how Curve is compared.
### func (\*PrivateKey) Public 1.4
```
func (priv *PrivateKey) Public() crypto.PublicKey
```
Public returns the public key corresponding to priv.
### func (\*PrivateKey) Sign 1.4
```
func (priv *PrivateKey) Sign(rand io.Reader, digest []byte, opts crypto.SignerOpts) ([]byte, error)
```
Sign signs digest with priv, reading randomness from rand. The opts argument is not currently used but, in keeping with the crypto.Signer interface, should be the hash function used to digest the message.
This method implements crypto.Signer, which is an interface to support keys where the private part is kept in, for example, a hardware module. Common uses can use the SignASN1 function in this package directly.
type PublicKey
--------------
PublicKey represents an ECDSA public key.
```
type PublicKey struct {
elliptic.Curve
X, Y *big.Int
}
```
### func (\*PublicKey) ECDH 1.20
```
func (k *PublicKey) ECDH() (*ecdh.PublicKey, error)
```
ECDH returns k as a ecdh.PublicKey. It returns an error if the key is invalid according to the definition of ecdh.Curve.NewPublicKey, or if the Curve is not supported by crypto/ecdh.
### func (\*PublicKey) Equal 1.15
```
func (pub *PublicKey) Equal(x crypto.PublicKey) bool
```
Equal reports whether pub and x have the same value.
Two keys are only considered to have the same value if they have the same Curve value. Note that for example elliptic.P256() and elliptic.P256().Params() are different values, as the latter is a generic not constant time implementation.
| programming_docs |
go Package elliptic Package elliptic
=================
* `import "crypto/elliptic"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package elliptic implements the standard NIST P-224, P-256, P-384, and P-521 elliptic curves over prime fields.
The P224(), P256(), P384() and P521() values are necessary to use the crypto/ecdsa package. Most other uses should migrate to the more efficient and safer crypto/ecdh package.
Index
-----
* [func GenerateKey(curve Curve, rand io.Reader) (priv []byte, x, y \*big.Int, err error)](#GenerateKey)
* [func Marshal(curve Curve, x, y \*big.Int) []byte](#Marshal)
* [func MarshalCompressed(curve Curve, x, y \*big.Int) []byte](#MarshalCompressed)
* [func Unmarshal(curve Curve, data []byte) (x, y \*big.Int)](#Unmarshal)
* [func UnmarshalCompressed(curve Curve, data []byte) (x, y \*big.Int)](#UnmarshalCompressed)
* [type Curve](#Curve)
* [func P224() Curve](#P224)
* [func P256() Curve](#P256)
* [func P384() Curve](#P384)
* [func P521() Curve](#P521)
* [type CurveParams](#CurveParams)
* [func (curve \*CurveParams) Add(x1, y1, x2, y2 \*big.Int) (\*big.Int, \*big.Int)](#CurveParams.Add)
* [func (curve \*CurveParams) Double(x1, y1 \*big.Int) (\*big.Int, \*big.Int)](#CurveParams.Double)
* [func (curve \*CurveParams) IsOnCurve(x, y \*big.Int) bool](#CurveParams.IsOnCurve)
* [func (curve \*CurveParams) Params() \*CurveParams](#CurveParams.Params)
* [func (curve \*CurveParams) ScalarBaseMult(k []byte) (\*big.Int, \*big.Int)](#CurveParams.ScalarBaseMult)
* [func (curve \*CurveParams) ScalarMult(Bx, By \*big.Int, k []byte) (\*big.Int, \*big.Int)](#CurveParams.ScalarMult)
### Package files
elliptic.go nistec.go nistec\_p256.go params.go
func GenerateKey
----------------
```
func GenerateKey(curve Curve, rand io.Reader) (priv []byte, x, y *big.Int, err error)
```
GenerateKey returns a public/private key pair. The private key is generated using the given reader, which must return random data.
Note: for ECDH, use the GenerateKey methods of the crypto/ecdh package; for ECDSA, use the GenerateKey function of the crypto/ecdsa package.
func Marshal
------------
```
func Marshal(curve Curve, x, y *big.Int) []byte
```
Marshal converts a point on the curve into the uncompressed form specified in SEC 1, Version 2.0, Section 2.3.3. If the point is not on the curve (or is the conventional point at infinity), the behavior is undefined.
Note: for ECDH, use the crypto/ecdh package. This function returns an encoding equivalent to that of PublicKey.Bytes in crypto/ecdh.
func MarshalCompressed 1.15
---------------------------
```
func MarshalCompressed(curve Curve, x, y *big.Int) []byte
```
MarshalCompressed converts a point on the curve into the compressed form specified in SEC 1, Version 2.0, Section 2.3.3. If the point is not on the curve (or is the conventional point at infinity), the behavior is undefined.
func Unmarshal
--------------
```
func Unmarshal(curve Curve, data []byte) (x, y *big.Int)
```
Unmarshal converts a point, serialized by Marshal, into an x, y pair. It is an error if the point is not in uncompressed form, is not on the curve, or is the point at infinity. On error, x = nil.
Note: for ECDH, use the crypto/ecdh package. This function accepts an encoding equivalent to that of the NewPublicKey methods in crypto/ecdh.
func UnmarshalCompressed 1.15
-----------------------------
```
func UnmarshalCompressed(curve Curve, data []byte) (x, y *big.Int)
```
UnmarshalCompressed converts a point, serialized by MarshalCompressed, into an x, y pair. It is an error if the point is not in compressed form, is not on the curve, or is the point at infinity. On error, x = nil.
type Curve
----------
A Curve represents a short-form Weierstrass curve with a=-3.
The behavior of Add, Double, and ScalarMult when the input is not a point on the curve is undefined.
Note that the conventional point at infinity (0, 0) is not considered on the curve, although it can be returned by Add, Double, ScalarMult, or ScalarBaseMult (but not the Unmarshal or UnmarshalCompressed functions).
```
type Curve interface {
// Params returns the parameters for the curve.
Params() *CurveParams
// IsOnCurve reports whether the given (x,y) lies on the curve.
//
// Note: this is a low-level unsafe API. For ECDH, use the crypto/ecdh
// package. The NewPublicKey methods of NIST curves in crypto/ecdh accept
// the same encoding as the Unmarshal function, and perform on-curve checks.
IsOnCurve(x, y *big.Int) bool
// Add returns the sum of (x1,y1) and (x2,y2).
//
// Note: this is a low-level unsafe API.
Add(x1, y1, x2, y2 *big.Int) (x, y *big.Int)
// Double returns 2*(x,y).
//
// Note: this is a low-level unsafe API.
Double(x1, y1 *big.Int) (x, y *big.Int)
// ScalarMult returns k*(x,y) where k is an integer in big-endian form.
//
// Note: this is a low-level unsafe API. For ECDH, use the crypto/ecdh
// package. Most uses of ScalarMult can be replaced by a call to the ECDH
// methods of NIST curves in crypto/ecdh.
ScalarMult(x1, y1 *big.Int, k []byte) (x, y *big.Int)
// ScalarBaseMult returns k*G, where G is the base point of the group
// and k is an integer in big-endian form.
//
// Note: this is a low-level unsafe API. For ECDH, use the crypto/ecdh
// package. Most uses of ScalarBaseMult can be replaced by a call to the
// PrivateKey.PublicKey method in crypto/ecdh.
ScalarBaseMult(k []byte) (x, y *big.Int)
}
```
### func P224
```
func P224() Curve
```
P224 returns a Curve which implements NIST P-224 (FIPS 186-3, section D.2.2), also known as secp224r1. The CurveParams.Name of this Curve is "P-224".
Multiple invocations of this function will return the same value, so it can be used for equality checks and switch statements.
The cryptographic operations are implemented using constant-time algorithms.
### func P256
```
func P256() Curve
```
P256 returns a Curve which implements NIST P-256 (FIPS 186-3, section D.2.3), also known as secp256r1 or prime256v1. The CurveParams.Name of this Curve is "P-256".
Multiple invocations of this function will return the same value, so it can be used for equality checks and switch statements.
The cryptographic operations are implemented using constant-time algorithms.
### func P384
```
func P384() Curve
```
P384 returns a Curve which implements NIST P-384 (FIPS 186-3, section D.2.4), also known as secp384r1. The CurveParams.Name of this Curve is "P-384".
Multiple invocations of this function will return the same value, so it can be used for equality checks and switch statements.
The cryptographic operations are implemented using constant-time algorithms.
### func P521
```
func P521() Curve
```
P521 returns a Curve which implements NIST P-521 (FIPS 186-3, section D.2.5), also known as secp521r1. The CurveParams.Name of this Curve is "P-521".
Multiple invocations of this function will return the same value, so it can be used for equality checks and switch statements.
The cryptographic operations are implemented using constant-time algorithms.
type CurveParams
----------------
CurveParams contains the parameters of an elliptic curve and also provides a generic, non-constant time implementation of Curve.
Note: Custom curves (those not returned by P224(), P256(), P384(), and P521()) are not guaranteed to provide any security property.
```
type CurveParams struct {
P *big.Int // the order of the underlying field
N *big.Int // the order of the base point
B *big.Int // the constant of the curve equation
Gx, Gy *big.Int // (x,y) of the base point
BitSize int // the size of the underlying field
Name string // the canonical name of the curve; added in Go 1.5
}
```
### func (\*CurveParams) Add
```
func (curve *CurveParams) Add(x1, y1, x2, y2 *big.Int) (*big.Int, *big.Int)
```
Add implements Curve.Add.
Note: the CurveParams methods are not guaranteed to provide any security property. For ECDH, use the crypto/ecdh package. For ECDSA, use the crypto/ecdsa package with a Curve value returned directly from P224(), P256(), P384(), or P521().
### func (\*CurveParams) Double
```
func (curve *CurveParams) Double(x1, y1 *big.Int) (*big.Int, *big.Int)
```
Double implements Curve.Double.
Note: the CurveParams methods are not guaranteed to provide any security property. For ECDH, use the crypto/ecdh package. For ECDSA, use the crypto/ecdsa package with a Curve value returned directly from P224(), P256(), P384(), or P521().
### func (\*CurveParams) IsOnCurve
```
func (curve *CurveParams) IsOnCurve(x, y *big.Int) bool
```
IsOnCurve implements Curve.IsOnCurve.
Note: the CurveParams methods are not guaranteed to provide any security property. For ECDH, use the crypto/ecdh package. For ECDSA, use the crypto/ecdsa package with a Curve value returned directly from P224(), P256(), P384(), or P521().
### func (\*CurveParams) Params
```
func (curve *CurveParams) Params() *CurveParams
```
### func (\*CurveParams) ScalarBaseMult
```
func (curve *CurveParams) ScalarBaseMult(k []byte) (*big.Int, *big.Int)
```
ScalarBaseMult implements Curve.ScalarBaseMult.
Note: the CurveParams methods are not guaranteed to provide any security property. For ECDH, use the crypto/ecdh package. For ECDSA, use the crypto/ecdsa package with a Curve value returned directly from P224(), P256(), P384(), or P521().
### func (\*CurveParams) ScalarMult
```
func (curve *CurveParams) ScalarMult(Bx, By *big.Int, k []byte) (*big.Int, *big.Int)
```
ScalarMult implements Curve.ScalarMult.
Note: the CurveParams methods are not guaranteed to provide any security property. For ECDH, use the crypto/ecdh package. For ECDSA, use the crypto/ecdsa package with a Curve value returned directly from P224(), P256(), P384(), or P521().
go Package rsa Package rsa
============
* `import "crypto/rsa"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package rsa implements RSA encryption as specified in PKCS #1 and RFC 8017.
RSA is a single, fundamental operation that is used in this package to implement either public-key encryption or public-key signatures.
The original specification for encryption and signatures with RSA is PKCS #1 and the terms "RSA encryption" and "RSA signatures" by default refer to PKCS #1 version 1.5. However, that specification has flaws and new designs should use version 2, usually called by just OAEP and PSS, where possible.
Two sets of interfaces are included in this package. When a more abstract interface isn't necessary, there are functions for encrypting/decrypting with v1.5/OAEP and signing/verifying with v1.5/PSS. If one needs to abstract over the public key primitive, the PrivateKey type implements the Decrypter and Signer interfaces from the crypto package.
Operations in this package are implemented using constant-time algorithms, except for [GenerateKey](#GenerateKey), [PrivateKey.Precompute](#PrivateKey.Precompute), and [PrivateKey.Validate](#PrivateKey.Validate). Every other operation only leaks the bit size of the involved values, which all depend on the selected key size.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func DecryptOAEP(hash hash.Hash, random io.Reader, priv \*PrivateKey, ciphertext []byte, label []byte) ([]byte, error)](#DecryptOAEP)
* [func DecryptPKCS1v15(random io.Reader, priv \*PrivateKey, ciphertext []byte) ([]byte, error)](#DecryptPKCS1v15)
* [func DecryptPKCS1v15SessionKey(random io.Reader, priv \*PrivateKey, ciphertext []byte, key []byte) error](#DecryptPKCS1v15SessionKey)
* [func EncryptOAEP(hash hash.Hash, random io.Reader, pub \*PublicKey, msg []byte, label []byte) ([]byte, error)](#EncryptOAEP)
* [func EncryptPKCS1v15(random io.Reader, pub \*PublicKey, msg []byte) ([]byte, error)](#EncryptPKCS1v15)
* [func SignPKCS1v15(random io.Reader, priv \*PrivateKey, hash crypto.Hash, hashed []byte) ([]byte, error)](#SignPKCS1v15)
* [func SignPSS(rand io.Reader, priv \*PrivateKey, hash crypto.Hash, digest []byte, opts \*PSSOptions) ([]byte, error)](#SignPSS)
* [func VerifyPKCS1v15(pub \*PublicKey, hash crypto.Hash, hashed []byte, sig []byte) error](#VerifyPKCS1v15)
* [func VerifyPSS(pub \*PublicKey, hash crypto.Hash, digest []byte, sig []byte, opts \*PSSOptions) error](#VerifyPSS)
* [type CRTValue](#CRTValue)
* [type OAEPOptions](#OAEPOptions)
* [type PKCS1v15DecryptOptions](#PKCS1v15DecryptOptions)
* [type PSSOptions](#PSSOptions)
* [func (opts \*PSSOptions) HashFunc() crypto.Hash](#PSSOptions.HashFunc)
* [type PrecomputedValues](#PrecomputedValues)
* [type PrivateKey](#PrivateKey)
* [func GenerateKey(random io.Reader, bits int) (\*PrivateKey, error)](#GenerateKey)
* [func GenerateMultiPrimeKey(random io.Reader, nprimes int, bits int) (\*PrivateKey, error)](#GenerateMultiPrimeKey)
* [func (priv \*PrivateKey) Decrypt(rand io.Reader, ciphertext []byte, opts crypto.DecrypterOpts) (plaintext []byte, err error)](#PrivateKey.Decrypt)
* [func (priv \*PrivateKey) Equal(x crypto.PrivateKey) bool](#PrivateKey.Equal)
* [func (priv \*PrivateKey) Precompute()](#PrivateKey.Precompute)
* [func (priv \*PrivateKey) Public() crypto.PublicKey](#PrivateKey.Public)
* [func (priv \*PrivateKey) Sign(rand io.Reader, digest []byte, opts crypto.SignerOpts) ([]byte, error)](#PrivateKey.Sign)
* [func (priv \*PrivateKey) Validate() error](#PrivateKey.Validate)
* [type PublicKey](#PublicKey)
* [func (pub \*PublicKey) Equal(x crypto.PublicKey) bool](#PublicKey.Equal)
* [func (pub \*PublicKey) Size() int](#PublicKey.Size)
### Examples
[DecryptOAEP](#example_DecryptOAEP) [DecryptPKCS1v15SessionKey](#example_DecryptPKCS1v15SessionKey) [EncryptOAEP](#example_EncryptOAEP) [SignPKCS1v15](#example_SignPKCS1v15) [VerifyPKCS1v15](#example_VerifyPKCS1v15) ### Package files
notboring.go pkcs1v15.go pss.go rsa.go
Constants
---------
```
const (
// PSSSaltLengthAuto causes the salt in a PSS signature to be as large
// as possible when signing, and to be auto-detected when verifying.
PSSSaltLengthAuto = 0
// PSSSaltLengthEqualsHash causes the salt length to equal the length
// of the hash used in the signature.
PSSSaltLengthEqualsHash = -1
)
```
Variables
---------
ErrDecryption represents a failure to decrypt a message. It is deliberately vague to avoid adaptive attacks.
```
var ErrDecryption = errors.New("crypto/rsa: decryption error")
```
ErrMessageTooLong is returned when attempting to encrypt or sign a message which is too large for the size of the key. When using SignPSS, this can also be returned if the size of the salt is too large.
```
var ErrMessageTooLong = errors.New("crypto/rsa: message too long for RSA key size")
```
ErrVerification represents a failure to verify a signature. It is deliberately vague to avoid adaptive attacks.
```
var ErrVerification = errors.New("crypto/rsa: verification error")
```
func DecryptOAEP
----------------
```
func DecryptOAEP(hash hash.Hash, random io.Reader, priv *PrivateKey, ciphertext []byte, label []byte) ([]byte, error)
```
DecryptOAEP decrypts ciphertext using RSA-OAEP.
OAEP is parameterised by a hash function that is used as a random oracle. Encryption and decryption of a given message must use the same hash function and sha256.New() is a reasonable choice.
The random parameter is legacy and ignored, and it can be as nil.
The label parameter must match the value given when encrypting. See EncryptOAEP for details.
#### Example
Code:
```
ciphertext, _ := hex.DecodeString("4d1ee10e8f286390258c51a5e80802844c3e6358ad6690b7285218a7c7ed7fc3a4c7b950fbd04d4b0239cc060dcc7065ca6f84c1756deb71ca5685cadbb82be025e16449b905c568a19c088a1abfad54bf7ecc67a7df39943ec511091a34c0f2348d04e058fcff4d55644de3cd1d580791d4524b92f3e91695582e6e340a1c50b6c6d78e80b4e42c5b4d45e479b492de42bbd39cc642ebb80226bb5200020d501b24a37bcc2ec7f34e596b4fd6b063de4858dbf5a4e3dd18e262eda0ec2d19dbd8e890d672b63d368768360b20c0b6b8592a438fa275e5fa7f60bef0dd39673fd3989cc54d2cb80c08fcd19dacbc265ee1c6014616b0e04ea0328c2a04e73460")
label := []byte("orders")
plaintext, err := rsa.DecryptOAEP(sha256.New(), nil, test2048Key, ciphertext, label)
if err != nil {
fmt.Fprintf(os.Stderr, "Error from decryption: %s\n", err)
return
}
fmt.Printf("Plaintext: %s\n", string(plaintext))
// Remember that encryption only provides confidentiality. The
// ciphertext should be signed before authenticity is assumed and, even
// then, consider that messages might be reordered.
```
func DecryptPKCS1v15
--------------------
```
func DecryptPKCS1v15(random io.Reader, priv *PrivateKey, ciphertext []byte) ([]byte, error)
```
DecryptPKCS1v15 decrypts a plaintext using RSA and the padding scheme from PKCS #1 v1.5. The random parameter is legacy and ignored, and it can be as nil.
Note that whether this function returns an error or not discloses secret information. If an attacker can cause this function to run repeatedly and learn whether each instance returned an error then they can decrypt and forge signatures as if they had the private key. See DecryptPKCS1v15SessionKey for a way of solving this problem.
func DecryptPKCS1v15SessionKey
------------------------------
```
func DecryptPKCS1v15SessionKey(random io.Reader, priv *PrivateKey, ciphertext []byte, key []byte) error
```
DecryptPKCS1v15SessionKey decrypts a session key using RSA and the padding scheme from PKCS #1 v1.5. The random parameter is legacy and ignored, and it can be as nil. It returns an error if the ciphertext is the wrong length or if the ciphertext is greater than the public modulus. Otherwise, no error is returned. If the padding is valid, the resulting plaintext message is copied into key. Otherwise, key is unchanged. These alternatives occur in constant time. It is intended that the user of this function generate a random session key beforehand and continue the protocol with the resulting value. This will remove any possibility that an attacker can learn any information about the plaintext. See “Chosen Ciphertext Attacks Against Protocols Based on the RSA Encryption Standard PKCS #1”, Daniel Bleichenbacher, Advances in Cryptology (Crypto '98).
Note that if the session key is too small then it may be possible for an attacker to brute-force it. If they can do that then they can learn whether a random value was used (because it'll be different for the same ciphertext) and thus whether the padding was correct. This defeats the point of this function. Using at least a 16-byte key will protect against this attack.
#### Example
RSA is able to encrypt only a very limited amount of data. In order to encrypt reasonable amounts of data a hybrid scheme is commonly used: RSA is used to encrypt a key for a symmetric primitive like AES-GCM. Before encrypting, data is “padded” by embedding it in a known structure. This is done for a number of reasons, but the most obvious is to ensure that the value is large enough that the exponentiation is larger than the modulus. (Otherwise it could be decrypted with a square-root.) In these designs, when using PKCS #1 v1.5, it's vitally important to avoid disclosing whether the received RSA message was well-formed (that is, whether the result of decrypting is a correctly padded message) because this leaks secret information. DecryptPKCS1v15SessionKey is designed for this situation and copies the decrypted, symmetric key (if well-formed) in constant-time over a buffer that contains a random key. Thus, if the RSA result isn't well-formed, the implementation uses a random key in constant time.
Code:
```
// The hybrid scheme should use at least a 16-byte symmetric key. Here
// we read the random key that will be used if the RSA decryption isn't
// well-formed.
key := make([]byte, 32)
if _, err := rand.Read(key); err != nil {
panic("RNG failure")
}
rsaCiphertext, _ := hex.DecodeString("aabbccddeeff")
if err := rsa.DecryptPKCS1v15SessionKey(nil, rsaPrivateKey, rsaCiphertext, key); err != nil {
// Any errors that result will be “public” – meaning that they
// can be determined without any secret information. (For
// instance, if the length of key is impossible given the RSA
// public key.)
fmt.Fprintf(os.Stderr, "Error from RSA decryption: %s\n", err)
return
}
// Given the resulting key, a symmetric scheme can be used to decrypt a
// larger ciphertext.
block, err := aes.NewCipher(key)
if err != nil {
panic("aes.NewCipher failed: " + err.Error())
}
// Since the key is random, using a fixed nonce is acceptable as the
// (key, nonce) pair will still be unique, as required.
var zeroNonce [12]byte
aead, err := cipher.NewGCM(block)
if err != nil {
panic("cipher.NewGCM failed: " + err.Error())
}
ciphertext, _ := hex.DecodeString("00112233445566")
plaintext, err := aead.Open(nil, zeroNonce[:], ciphertext, nil)
if err != nil {
// The RSA ciphertext was badly formed; the decryption will
// fail here because the AES-GCM key will be incorrect.
fmt.Fprintf(os.Stderr, "Error decrypting: %s\n", err)
return
}
fmt.Printf("Plaintext: %s\n", string(plaintext))
```
func EncryptOAEP
----------------
```
func EncryptOAEP(hash hash.Hash, random io.Reader, pub *PublicKey, msg []byte, label []byte) ([]byte, error)
```
EncryptOAEP encrypts the given message with RSA-OAEP.
OAEP is parameterised by a hash function that is used as a random oracle. Encryption and decryption of a given message must use the same hash function and sha256.New() is a reasonable choice.
The random parameter is used as a source of entropy to ensure that encrypting the same message twice doesn't result in the same ciphertext.
The label parameter may contain arbitrary data that will not be encrypted, but which gives important context to the message. For example, if a given public key is used to encrypt two types of messages then distinct label values could be used to ensure that a ciphertext for one purpose cannot be used for another by an attacker. If not required it can be empty.
The message must be no longer than the length of the public modulus minus twice the hash length, minus a further 2.
#### Example
Code:
```
secretMessage := []byte("send reinforcements, we're going to advance")
label := []byte("orders")
// crypto/rand.Reader is a good source of entropy for randomizing the
// encryption function.
rng := rand.Reader
ciphertext, err := rsa.EncryptOAEP(sha256.New(), rng, &test2048Key.PublicKey, secretMessage, label)
if err != nil {
fmt.Fprintf(os.Stderr, "Error from encryption: %s\n", err)
return
}
// Since encryption is a randomized function, ciphertext will be
// different each time.
fmt.Printf("Ciphertext: %x\n", ciphertext)
```
func EncryptPKCS1v15
--------------------
```
func EncryptPKCS1v15(random io.Reader, pub *PublicKey, msg []byte) ([]byte, error)
```
EncryptPKCS1v15 encrypts the given message with RSA and the padding scheme from PKCS #1 v1.5. The message must be no longer than the length of the public modulus minus 11 bytes.
The random parameter is used as a source of entropy to ensure that encrypting the same message twice doesn't result in the same ciphertext.
WARNING: use of this function to encrypt plaintexts other than session keys is dangerous. Use RSA OAEP in new protocols.
func SignPKCS1v15
-----------------
```
func SignPKCS1v15(random io.Reader, priv *PrivateKey, hash crypto.Hash, hashed []byte) ([]byte, error)
```
SignPKCS1v15 calculates the signature of hashed using RSASSA-PKCS1-V1\_5-SIGN from RSA PKCS #1 v1.5. Note that hashed must be the result of hashing the input message using the given hash function. If hash is zero, hashed is signed directly. This isn't advisable except for interoperability.
The random parameter is legacy and ignored, and it can be as nil.
This function is deterministic. Thus, if the set of possible messages is small, an attacker may be able to build a map from messages to signatures and identify the signed messages. As ever, signatures provide authenticity, not confidentiality.
#### Example
Code:
```
message := []byte("message to be signed")
// Only small messages can be signed directly; thus the hash of a
// message, rather than the message itself, is signed. This requires
// that the hash function be collision resistant. SHA-256 is the
// least-strong hash function that should be used for this at the time
// of writing (2016).
hashed := sha256.Sum256(message)
signature, err := rsa.SignPKCS1v15(nil, rsaPrivateKey, crypto.SHA256, hashed[:])
if err != nil {
fmt.Fprintf(os.Stderr, "Error from signing: %s\n", err)
return
}
fmt.Printf("Signature: %x\n", signature)
```
func SignPSS 1.2
----------------
```
func SignPSS(rand io.Reader, priv *PrivateKey, hash crypto.Hash, digest []byte, opts *PSSOptions) ([]byte, error)
```
SignPSS calculates the signature of digest using PSS.
digest must be the result of hashing the input message using the given hash function. The opts argument may be nil, in which case sensible defaults are used. If opts.Hash is set, it overrides hash.
func VerifyPKCS1v15
-------------------
```
func VerifyPKCS1v15(pub *PublicKey, hash crypto.Hash, hashed []byte, sig []byte) error
```
VerifyPKCS1v15 verifies an RSA PKCS #1 v1.5 signature. hashed is the result of hashing the input message using the given hash function and sig is the signature. A valid signature is indicated by returning a nil error. If hash is zero then hashed is used directly. This isn't advisable except for interoperability.
#### Example
Code:
```
message := []byte("message to be signed")
signature, _ := hex.DecodeString("ad2766728615cc7a746cc553916380ca7bfa4f8983b990913bc69eb0556539a350ff0f8fe65ddfd3ebe91fe1c299c2fac135bc8c61e26be44ee259f2f80c1530")
// Only small messages can be signed directly; thus the hash of a
// message, rather than the message itself, is signed. This requires
// that the hash function be collision resistant. SHA-256 is the
// least-strong hash function that should be used for this at the time
// of writing (2016).
hashed := sha256.Sum256(message)
err := rsa.VerifyPKCS1v15(&rsaPrivateKey.PublicKey, crypto.SHA256, hashed[:], signature)
if err != nil {
fmt.Fprintf(os.Stderr, "Error from verification: %s\n", err)
return
}
// signature is a valid signature of message from the public key.
```
func VerifyPSS 1.2
------------------
```
func VerifyPSS(pub *PublicKey, hash crypto.Hash, digest []byte, sig []byte, opts *PSSOptions) error
```
VerifyPSS verifies a PSS signature.
A valid signature is indicated by returning a nil error. digest must be the result of hashing the input message using the given hash function. The opts argument may be nil, in which case sensible defaults are used. opts.Hash is ignored.
type CRTValue
-------------
CRTValue contains the precomputed Chinese remainder theorem values.
```
type CRTValue struct {
Exp *big.Int // D mod (prime-1).
Coeff *big.Int // R·Coeff ≡ 1 mod Prime.
R *big.Int // product of primes prior to this (inc p and q).
}
```
type OAEPOptions 1.5
--------------------
OAEPOptions is an interface for passing options to OAEP decryption using the crypto.Decrypter interface.
```
type OAEPOptions struct {
// Hash is the hash function that will be used when generating the mask.
Hash crypto.Hash
// MGFHash is the hash function used for MGF1.
// If zero, Hash is used instead.
MGFHash crypto.Hash // Go 1.20
// Label is an arbitrary byte string that must be equal to the value
// used when encrypting.
Label []byte
}
```
type PKCS1v15DecryptOptions 1.5
-------------------------------
PKCS1v15DecryptOptions is for passing options to PKCS #1 v1.5 decryption using the crypto.Decrypter interface.
```
type PKCS1v15DecryptOptions struct {
// SessionKeyLen is the length of the session key that is being
// decrypted. If not zero, then a padding error during decryption will
// cause a random plaintext of this length to be returned rather than
// an error. These alternatives happen in constant time.
SessionKeyLen int
}
```
type PSSOptions 1.2
-------------------
PSSOptions contains options for creating and verifying PSS signatures.
```
type PSSOptions struct {
// SaltLength controls the length of the salt used in the PSS signature. It
// can either be a positive number of bytes, or one of the special
// PSSSaltLength constants.
SaltLength int
// Hash is the hash function used to generate the message digest. If not
// zero, it overrides the hash function passed to SignPSS. It's required
// when using PrivateKey.Sign.
Hash crypto.Hash // Go 1.4
}
```
### func (\*PSSOptions) HashFunc 1.4
```
func (opts *PSSOptions) HashFunc() crypto.Hash
```
HashFunc returns opts.Hash so that PSSOptions implements crypto.SignerOpts.
type PrecomputedValues
----------------------
```
type PrecomputedValues struct {
Dp, Dq *big.Int // D mod (P-1) (or mod Q-1)
Qinv *big.Int // Q^-1 mod P
// CRTValues is used for the 3rd and subsequent primes. Due to a
// historical accident, the CRT for the first two primes is handled
// differently in PKCS #1 and interoperability is sufficiently
// important that we mirror this.
//
// Note: these values are still filled in by Precompute for
// backwards compatibility but are not used. Multi-prime RSA is very rare,
// and is implemented by this package without CRT optimizations to limit
// complexity.
CRTValues []CRTValue
// contains filtered or unexported fields
}
```
type PrivateKey
---------------
A PrivateKey represents an RSA key
```
type PrivateKey struct {
PublicKey // public part.
D *big.Int // private exponent
Primes []*big.Int // prime factors of N, has >= 2 elements.
// Precomputed contains precomputed values that speed up RSA operations,
// if available. It must be generated by calling PrivateKey.Precompute and
// must not be modified.
Precomputed PrecomputedValues
}
```
### func GenerateKey
```
func GenerateKey(random io.Reader, bits int) (*PrivateKey, error)
```
GenerateKey generates an RSA keypair of the given bit size using the random source random (for example, crypto/rand.Reader).
### func GenerateMultiPrimeKey
```
func GenerateMultiPrimeKey(random io.Reader, nprimes int, bits int) (*PrivateKey, error)
```
GenerateMultiPrimeKey generates a multi-prime RSA keypair of the given bit size and the given random source.
Table 1 in "[On the Security of Multi-prime RSA](http://www.cacr.math.uwaterloo.ca/techreports/2006/cacr2006-16.pdf)" suggests maximum numbers of primes for a given bit size.
Although the public keys are compatible (actually, indistinguishable) from the 2-prime case, the private keys are not. Thus it may not be possible to export multi-prime private keys in certain formats or to subsequently import them into other code.
This package does not implement CRT optimizations for multi-prime RSA, so the keys with more than two primes will have worse performance.
Note: The use of this function with a number of primes different from two is not recommended for the above security, compatibility, and performance reasons. Use GenerateKey instead.
### func (\*PrivateKey) Decrypt 1.5
```
func (priv *PrivateKey) Decrypt(rand io.Reader, ciphertext []byte, opts crypto.DecrypterOpts) (plaintext []byte, err error)
```
Decrypt decrypts ciphertext with priv. If opts is nil or of type \*PKCS1v15DecryptOptions then PKCS #1 v1.5 decryption is performed. Otherwise opts must have type \*OAEPOptions and OAEP decryption is done.
### func (\*PrivateKey) Equal 1.15
```
func (priv *PrivateKey) Equal(x crypto.PrivateKey) bool
```
Equal reports whether priv and x have equivalent values. It ignores Precomputed values.
### func (\*PrivateKey) Precompute
```
func (priv *PrivateKey) Precompute()
```
Precompute performs some calculations that speed up private key operations in the future.
### func (\*PrivateKey) Public 1.4
```
func (priv *PrivateKey) Public() crypto.PublicKey
```
Public returns the public key corresponding to priv.
### func (\*PrivateKey) Sign 1.4
```
func (priv *PrivateKey) Sign(rand io.Reader, digest []byte, opts crypto.SignerOpts) ([]byte, error)
```
Sign signs digest with priv, reading randomness from rand. If opts is a \*PSSOptions then the PSS algorithm will be used, otherwise PKCS #1 v1.5 will be used. digest must be the result of hashing the input message using opts.HashFunc().
This method implements crypto.Signer, which is an interface to support keys where the private part is kept in, for example, a hardware module. Common uses should use the Sign\* functions in this package directly.
### func (\*PrivateKey) Validate
```
func (priv *PrivateKey) Validate() error
```
Validate performs basic sanity checks on the key. It returns nil if the key is valid, or else an error describing a problem.
type PublicKey
--------------
A PublicKey represents the public part of an RSA key.
```
type PublicKey struct {
N *big.Int // modulus
E int // public exponent
}
```
### func (\*PublicKey) Equal 1.15
```
func (pub *PublicKey) Equal(x crypto.PublicKey) bool
```
Equal reports whether pub and x have the same value.
### func (\*PublicKey) Size 1.11
```
func (pub *PublicKey) Size() int
```
Size returns the modulus size in bytes. Raw signatures and ciphertexts for or by this public key will have the same size.
| programming_docs |
go Package cipher Package cipher
===============
* `import "crypto/cipher"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package cipher implements standard block cipher modes that can be wrapped around low-level block cipher implementations. See <https://csrc.nist.gov/groups/ST/toolkit/BCM/current_modes.html> and NIST Special Publication 800-38A.
Index
-----
* [type AEAD](#AEAD)
* [func NewGCM(cipher Block) (AEAD, error)](#NewGCM)
* [func NewGCMWithNonceSize(cipher Block, size int) (AEAD, error)](#NewGCMWithNonceSize)
* [func NewGCMWithTagSize(cipher Block, tagSize int) (AEAD, error)](#NewGCMWithTagSize)
* [type Block](#Block)
* [type BlockMode](#BlockMode)
* [func NewCBCDecrypter(b Block, iv []byte) BlockMode](#NewCBCDecrypter)
* [func NewCBCEncrypter(b Block, iv []byte) BlockMode](#NewCBCEncrypter)
* [type Stream](#Stream)
* [func NewCFBDecrypter(block Block, iv []byte) Stream](#NewCFBDecrypter)
* [func NewCFBEncrypter(block Block, iv []byte) Stream](#NewCFBEncrypter)
* [func NewCTR(block Block, iv []byte) Stream](#NewCTR)
* [func NewOFB(b Block, iv []byte) Stream](#NewOFB)
* [type StreamReader](#StreamReader)
* [func (r StreamReader) Read(dst []byte) (n int, err error)](#StreamReader.Read)
* [type StreamWriter](#StreamWriter)
* [func (w StreamWriter) Close() error](#StreamWriter.Close)
* [func (w StreamWriter) Write(src []byte) (n int, err error)](#StreamWriter.Write)
### Examples
[NewCBCDecrypter](#example_NewCBCDecrypter) [NewCBCEncrypter](#example_NewCBCEncrypter) [NewCFBDecrypter](#example_NewCFBDecrypter) [NewCFBEncrypter](#example_NewCFBEncrypter) [NewCTR](#example_NewCTR) [NewGCM (Decrypt)](#example_NewGCM_decrypt) [NewGCM (Encrypt)](#example_NewGCM_encrypt) [NewOFB](#example_NewOFB) [StreamReader](#example_StreamReader) [StreamWriter](#example_StreamWriter) ### Package files
cbc.go cfb.go cipher.go ctr.go gcm.go io.go ofb.go
type AEAD 1.2
-------------
AEAD is a cipher mode providing authenticated encryption with associated data. For a description of the methodology, see <https://en.wikipedia.org/wiki/Authenticated_encryption>.
```
type AEAD interface {
// NonceSize returns the size of the nonce that must be passed to Seal
// and Open.
NonceSize() int
// Overhead returns the maximum difference between the lengths of a
// plaintext and its ciphertext.
Overhead() int
// Seal encrypts and authenticates plaintext, authenticates the
// additional data and appends the result to dst, returning the updated
// slice. The nonce must be NonceSize() bytes long and unique for all
// time, for a given key.
//
// To reuse plaintext's storage for the encrypted output, use plaintext[:0]
// as dst. Otherwise, the remaining capacity of dst must not overlap plaintext.
Seal(dst, nonce, plaintext, additionalData []byte) []byte
// Open decrypts and authenticates ciphertext, authenticates the
// additional data and, if successful, appends the resulting plaintext
// to dst, returning the updated slice. The nonce must be NonceSize()
// bytes long and both it and the additional data must match the
// value passed to Seal.
//
// To reuse ciphertext's storage for the decrypted output, use ciphertext[:0]
// as dst. Otherwise, the remaining capacity of dst must not overlap plaintext.
//
// Even if the function fails, the contents of dst, up to its capacity,
// may be overwritten.
Open(dst, nonce, ciphertext, additionalData []byte) ([]byte, error)
}
```
### func NewGCM 1.2
```
func NewGCM(cipher Block) (AEAD, error)
```
NewGCM returns the given 128-bit, block cipher wrapped in Galois Counter Mode with the standard nonce length.
In general, the GHASH operation performed by this implementation of GCM is not constant-time. An exception is when the underlying Block was created by aes.NewCipher on systems with hardware support for AES. See the crypto/aes package documentation for details.
#### Example (Decrypt)
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// Seal/Open calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
// When decoded the key should be 16 bytes (AES-128) or 32 (AES-256).
key, _ := hex.DecodeString("6368616e676520746869732070617373776f726420746f206120736563726574")
ciphertext, _ := hex.DecodeString("c3aaa29f002ca75870806e44086700f62ce4d43e902b3888e23ceff797a7a471")
nonce, _ := hex.DecodeString("64a9433eae7ccceee2fc0eda")
block, err := aes.NewCipher(key)
if err != nil {
panic(err.Error())
}
aesgcm, err := cipher.NewGCM(block)
if err != nil {
panic(err.Error())
}
plaintext, err := aesgcm.Open(nil, nonce, ciphertext, nil)
if err != nil {
panic(err.Error())
}
fmt.Printf("%s\n", plaintext)
```
Output:
```
exampleplaintext
```
#### Example (Encrypt)
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// Seal/Open calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
// When decoded the key should be 16 bytes (AES-128) or 32 (AES-256).
key, _ := hex.DecodeString("6368616e676520746869732070617373776f726420746f206120736563726574")
plaintext := []byte("exampleplaintext")
block, err := aes.NewCipher(key)
if err != nil {
panic(err.Error())
}
// Never use more than 2^32 random nonces with a given key because of the risk of a repeat.
nonce := make([]byte, 12)
if _, err := io.ReadFull(rand.Reader, nonce); err != nil {
panic(err.Error())
}
aesgcm, err := cipher.NewGCM(block)
if err != nil {
panic(err.Error())
}
ciphertext := aesgcm.Seal(nil, nonce, plaintext, nil)
fmt.Printf("%x\n", ciphertext)
```
### func NewGCMWithNonceSize 1.5
```
func NewGCMWithNonceSize(cipher Block, size int) (AEAD, error)
```
NewGCMWithNonceSize returns the given 128-bit, block cipher wrapped in Galois Counter Mode, which accepts nonces of the given length. The length must not be zero.
Only use this function if you require compatibility with an existing cryptosystem that uses non-standard nonce lengths. All other users should use NewGCM, which is faster and more resistant to misuse.
### func NewGCMWithTagSize 1.11
```
func NewGCMWithTagSize(cipher Block, tagSize int) (AEAD, error)
```
NewGCMWithTagSize returns the given 128-bit, block cipher wrapped in Galois Counter Mode, which generates tags with the given length.
Tag sizes between 12 and 16 bytes are allowed.
Only use this function if you require compatibility with an existing cryptosystem that uses non-standard tag lengths. All other users should use NewGCM, which is more resistant to misuse.
type Block
----------
A Block represents an implementation of block cipher using a given key. It provides the capability to encrypt or decrypt individual blocks. The mode implementations extend that capability to streams of blocks.
```
type Block interface {
// BlockSize returns the cipher's block size.
BlockSize() int
// Encrypt encrypts the first block in src into dst.
// Dst and src must overlap entirely or not at all.
Encrypt(dst, src []byte)
// Decrypt decrypts the first block in src into dst.
// Dst and src must overlap entirely or not at all.
Decrypt(dst, src []byte)
}
```
type BlockMode
--------------
A BlockMode represents a block cipher running in a block-based mode (CBC, ECB etc).
```
type BlockMode interface {
// BlockSize returns the mode's block size.
BlockSize() int
// CryptBlocks encrypts or decrypts a number of blocks. The length of
// src must be a multiple of the block size. Dst and src must overlap
// entirely or not at all.
//
// If len(dst) < len(src), CryptBlocks should panic. It is acceptable
// to pass a dst bigger than src, and in that case, CryptBlocks will
// only update dst[:len(src)] and will not touch the rest of dst.
//
// Multiple calls to CryptBlocks behave as if the concatenation of
// the src buffers was passed in a single run. That is, BlockMode
// maintains state and does not reset at each CryptBlocks call.
CryptBlocks(dst, src []byte)
}
```
### func NewCBCDecrypter
```
func NewCBCDecrypter(b Block, iv []byte) BlockMode
```
NewCBCDecrypter returns a BlockMode which decrypts in cipher block chaining mode, using the given Block. The length of iv must be the same as the Block's block size and must match the iv used to encrypt the data.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
ciphertext, _ := hex.DecodeString("73c86d43a9d700a253a96c85b0f6b03ac9792e0e757f869cca306bd3cba1c62b")
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
if len(ciphertext) < aes.BlockSize {
panic("ciphertext too short")
}
iv := ciphertext[:aes.BlockSize]
ciphertext = ciphertext[aes.BlockSize:]
// CBC mode always works in whole blocks.
if len(ciphertext)%aes.BlockSize != 0 {
panic("ciphertext is not a multiple of the block size")
}
mode := cipher.NewCBCDecrypter(block, iv)
// CryptBlocks can work in-place if the two arguments are the same.
mode.CryptBlocks(ciphertext, ciphertext)
// If the original plaintext lengths are not a multiple of the block
// size, padding would have to be added when encrypting, which would be
// removed at this point. For an example, see
// https://tools.ietf.org/html/rfc5246#section-6.2.3.2. However, it's
// critical to note that ciphertexts must be authenticated (i.e. by
// using crypto/hmac) before being decrypted in order to avoid creating
// a padding oracle.
fmt.Printf("%s\n", ciphertext)
```
Output:
```
exampleplaintext
```
### func NewCBCEncrypter
```
func NewCBCEncrypter(b Block, iv []byte) BlockMode
```
NewCBCEncrypter returns a BlockMode which encrypts in cipher block chaining mode, using the given Block. The length of iv must be the same as the Block's block size.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
plaintext := []byte("exampleplaintext")
// CBC mode works on blocks so plaintexts may need to be padded to the
// next whole block. For an example of such padding, see
// https://tools.ietf.org/html/rfc5246#section-6.2.3.2. Here we'll
// assume that the plaintext is already of the correct length.
if len(plaintext)%aes.BlockSize != 0 {
panic("plaintext is not a multiple of the block size")
}
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
panic(err)
}
mode := cipher.NewCBCEncrypter(block, iv)
mode.CryptBlocks(ciphertext[aes.BlockSize:], plaintext)
// It's important to remember that ciphertexts must be authenticated
// (i.e. by using crypto/hmac) as well as being encrypted in order to
// be secure.
fmt.Printf("%x\n", ciphertext)
```
type Stream
-----------
A Stream represents a stream cipher.
```
type Stream interface {
// XORKeyStream XORs each byte in the given slice with a byte from the
// cipher's key stream. Dst and src must overlap entirely or not at all.
//
// If len(dst) < len(src), XORKeyStream should panic. It is acceptable
// to pass a dst bigger than src, and in that case, XORKeyStream will
// only update dst[:len(src)] and will not touch the rest of dst.
//
// Multiple calls to XORKeyStream behave as if the concatenation of
// the src buffers was passed in a single run. That is, Stream
// maintains state and does not reset at each XORKeyStream call.
XORKeyStream(dst, src []byte)
}
```
### func NewCFBDecrypter
```
func NewCFBDecrypter(block Block, iv []byte) Stream
```
NewCFBDecrypter returns a Stream which decrypts with cipher feedback mode, using the given Block. The iv must be the same length as the Block's block size.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
ciphertext, _ := hex.DecodeString("7dd015f06bec7f1b8f6559dad89f4131da62261786845100056b353194ad")
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
if len(ciphertext) < aes.BlockSize {
panic("ciphertext too short")
}
iv := ciphertext[:aes.BlockSize]
ciphertext = ciphertext[aes.BlockSize:]
stream := cipher.NewCFBDecrypter(block, iv)
// XORKeyStream can work in-place if the two arguments are the same.
stream.XORKeyStream(ciphertext, ciphertext)
fmt.Printf("%s", ciphertext)
```
Output:
```
some plaintext
```
### func NewCFBEncrypter
```
func NewCFBEncrypter(block Block, iv []byte) Stream
```
NewCFBEncrypter returns a Stream which encrypts with cipher feedback mode, using the given Block. The iv must be the same length as the Block's block size.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
plaintext := []byte("some plaintext")
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
panic(err)
}
stream := cipher.NewCFBEncrypter(block, iv)
stream.XORKeyStream(ciphertext[aes.BlockSize:], plaintext)
// It's important to remember that ciphertexts must be authenticated
// (i.e. by using crypto/hmac) as well as being encrypted in order to
// be secure.
fmt.Printf("%x\n", ciphertext)
```
### func NewCTR
```
func NewCTR(block Block, iv []byte) Stream
```
NewCTR returns a Stream which encrypts/decrypts using the given Block in counter mode. The length of iv must be the same as the Block's block size.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
plaintext := []byte("some plaintext")
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
panic(err)
}
stream := cipher.NewCTR(block, iv)
stream.XORKeyStream(ciphertext[aes.BlockSize:], plaintext)
// It's important to remember that ciphertexts must be authenticated
// (i.e. by using crypto/hmac) as well as being encrypted in order to
// be secure.
// CTR mode is the same for both encryption and decryption, so we can
// also decrypt that ciphertext with NewCTR.
plaintext2 := make([]byte, len(plaintext))
stream = cipher.NewCTR(block, iv)
stream.XORKeyStream(plaintext2, ciphertext[aes.BlockSize:])
fmt.Printf("%s\n", plaintext2)
```
Output:
```
some plaintext
```
### func NewOFB
```
func NewOFB(b Block, iv []byte) Stream
```
NewOFB returns a Stream that encrypts or decrypts using the block cipher b in output feedback mode. The initialization vector iv's length must be equal to b's block size.
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
plaintext := []byte("some plaintext")
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
panic(err)
}
stream := cipher.NewOFB(block, iv)
stream.XORKeyStream(ciphertext[aes.BlockSize:], plaintext)
// It's important to remember that ciphertexts must be authenticated
// (i.e. by using crypto/hmac) as well as being encrypted in order to
// be secure.
// OFB mode is the same for both encryption and decryption, so we can
// also decrypt that ciphertext with NewOFB.
plaintext2 := make([]byte, len(plaintext))
stream = cipher.NewOFB(block, iv)
stream.XORKeyStream(plaintext2, ciphertext[aes.BlockSize:])
fmt.Printf("%s\n", plaintext2)
```
Output:
```
some plaintext
```
type StreamReader
-----------------
StreamReader wraps a Stream into an io.Reader. It calls XORKeyStream to process each slice of data which passes through.
```
type StreamReader struct {
S Stream
R io.Reader
}
```
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
encrypted, _ := hex.DecodeString("cf0495cc6f75dafc23948538e79904a9")
bReader := bytes.NewReader(encrypted)
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// If the key is unique for each ciphertext, then it's ok to use a zero
// IV.
var iv [aes.BlockSize]byte
stream := cipher.NewOFB(block, iv[:])
reader := &cipher.StreamReader{S: stream, R: bReader}
// Copy the input to the output stream, decrypting as we go.
if _, err := io.Copy(os.Stdout, reader); err != nil {
panic(err)
}
// Note that this example is simplistic in that it omits any
// authentication of the encrypted data. If you were actually to use
// StreamReader in this manner, an attacker could flip arbitrary bits in
// the output.
```
Output:
```
some secret text
```
### func (StreamReader) Read
```
func (r StreamReader) Read(dst []byte) (n int, err error)
```
type StreamWriter
-----------------
StreamWriter wraps a Stream into an io.Writer. It calls XORKeyStream to process each slice of data which passes through. If any Write call returns short then the StreamWriter is out of sync and must be discarded. A StreamWriter has no internal buffering; Close does not need to be called to flush write data.
```
type StreamWriter struct {
S Stream
W io.Writer
Err error // unused
}
```
#### Example
Code:
```
// Load your secret key from a safe place and reuse it across multiple
// NewCipher calls. (Obviously don't use this example key for anything
// real.) If you want to convert a passphrase to a key, use a suitable
// package like bcrypt or scrypt.
key, _ := hex.DecodeString("6368616e676520746869732070617373")
bReader := bytes.NewReader([]byte("some secret text"))
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// If the key is unique for each ciphertext, then it's ok to use a zero
// IV.
var iv [aes.BlockSize]byte
stream := cipher.NewOFB(block, iv[:])
var out bytes.Buffer
writer := &cipher.StreamWriter{S: stream, W: &out}
// Copy the input to the output buffer, encrypting as we go.
if _, err := io.Copy(writer, bReader); err != nil {
panic(err)
}
// Note that this example is simplistic in that it omits any
// authentication of the encrypted data. If you were actually to use
// StreamReader in this manner, an attacker could flip arbitrary bits in
// the decrypted result.
fmt.Printf("%x\n", out.Bytes())
```
Output:
```
cf0495cc6f75dafc23948538e79904a9
```
### func (StreamWriter) Close
```
func (w StreamWriter) Close() error
```
Close closes the underlying Writer and returns its Close return value, if the Writer is also an io.Closer. Otherwise it returns nil.
### func (StreamWriter) Write
```
func (w StreamWriter) Write(src []byte) (n int, err error)
```
| programming_docs |
go Package dsa Package dsa
============
* `import "crypto/dsa"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package dsa implements the Digital Signature Algorithm, as defined in FIPS 186-3.
The DSA operations in this package are not implemented using constant-time algorithms.
Deprecated: DSA is a legacy algorithm, and modern alternatives such as Ed25519 (implemented by package crypto/ed25519) should be used instead. Keys with 1024-bit moduli (L1024N160 parameters) are cryptographically weak, while bigger keys are not widely supported. Note that FIPS 186-5 no longer approves DSA for signature generation.
Index
-----
* [Variables](#pkg-variables)
* [func GenerateKey(priv \*PrivateKey, rand io.Reader) error](#GenerateKey)
* [func GenerateParameters(params \*Parameters, rand io.Reader, sizes ParameterSizes) error](#GenerateParameters)
* [func Sign(rand io.Reader, priv \*PrivateKey, hash []byte) (r, s \*big.Int, err error)](#Sign)
* [func Verify(pub \*PublicKey, hash []byte, r, s \*big.Int) bool](#Verify)
* [type ParameterSizes](#ParameterSizes)
* [type Parameters](#Parameters)
* [type PrivateKey](#PrivateKey)
* [type PublicKey](#PublicKey)
### Package files
dsa.go
Variables
---------
ErrInvalidPublicKey results when a public key is not usable by this code. FIPS is quite strict about the format of DSA keys, but other code may be less so. Thus, when using keys which may have been generated by other code, this error must be handled.
```
var ErrInvalidPublicKey = errors.New("crypto/dsa: invalid public key")
```
func GenerateKey
----------------
```
func GenerateKey(priv *PrivateKey, rand io.Reader) error
```
GenerateKey generates a public&private key pair. The Parameters of the PrivateKey must already be valid (see GenerateParameters).
func GenerateParameters
-----------------------
```
func GenerateParameters(params *Parameters, rand io.Reader, sizes ParameterSizes) error
```
GenerateParameters puts a random, valid set of DSA parameters into params. This function can take many seconds, even on fast machines.
func Sign
---------
```
func Sign(rand io.Reader, priv *PrivateKey, hash []byte) (r, s *big.Int, err error)
```
Sign signs an arbitrary length hash (which should be the result of hashing a larger message) using the private key, priv. It returns the signature as a pair of integers. The security of the private key depends on the entropy of rand.
Note that FIPS 186-3 section 4.6 specifies that the hash should be truncated to the byte-length of the subgroup. This function does not perform that truncation itself.
Be aware that calling Sign with an attacker-controlled PrivateKey may require an arbitrary amount of CPU.
func Verify
-----------
```
func Verify(pub *PublicKey, hash []byte, r, s *big.Int) bool
```
Verify verifies the signature in r, s of hash using the public key, pub. It reports whether the signature is valid.
Note that FIPS 186-3 section 4.6 specifies that the hash should be truncated to the byte-length of the subgroup. This function does not perform that truncation itself.
type ParameterSizes
-------------------
ParameterSizes is an enumeration of the acceptable bit lengths of the primes in a set of DSA parameters. See FIPS 186-3, section 4.2.
```
type ParameterSizes int
```
```
const (
L1024N160 ParameterSizes = iota
L2048N224
L2048N256
L3072N256
)
```
type Parameters
---------------
Parameters represents the domain parameters for a key. These parameters can be shared across many keys. The bit length of Q must be a multiple of 8.
```
type Parameters struct {
P, Q, G *big.Int
}
```
type PrivateKey
---------------
PrivateKey represents a DSA private key.
```
type PrivateKey struct {
PublicKey
X *big.Int
}
```
type PublicKey
--------------
PublicKey represents a DSA public key.
```
type PublicKey struct {
Parameters
Y *big.Int
}
```
go Package x509 Package x509
=============
* `import "crypto/x509"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package x509 implements a subset of the X.509 standard.
It allows parsing and generating certificates, certificate signing requests, certificate revocation lists, and encoded public and private keys. It provides a certificate verifier, complete with a chain builder.
The package targets the X.509 technical profile defined by the IETF (RFC 2459/3280/5280), and as further restricted by the CA/Browser Forum Baseline Requirements. There is minimal support for features outside of these profiles, as the primary goal of the package is to provide compatibility with the publicly trusted TLS certificate ecosystem and its policies and constraints.
On macOS and Windows, certificate verification is handled by system APIs, but the package aims to apply consistent validation rules across operating systems.
Index
-----
* [Variables](#pkg-variables)
* [func CreateCertificate(rand io.Reader, template, parent \*Certificate, pub, priv any) ([]byte, error)](#CreateCertificate)
* [func CreateCertificateRequest(rand io.Reader, template \*CertificateRequest, priv any) (csr []byte, err error)](#CreateCertificateRequest)
* [func CreateRevocationList(rand io.Reader, template \*RevocationList, issuer \*Certificate, priv crypto.Signer) ([]byte, error)](#CreateRevocationList)
* [func DecryptPEMBlock(b \*pem.Block, password []byte) ([]byte, error)](#DecryptPEMBlock)
* [func EncryptPEMBlock(rand io.Reader, blockType string, data, password []byte, alg PEMCipher) (\*pem.Block, error)](#EncryptPEMBlock)
* [func IsEncryptedPEMBlock(b \*pem.Block) bool](#IsEncryptedPEMBlock)
* [func MarshalECPrivateKey(key \*ecdsa.PrivateKey) ([]byte, error)](#MarshalECPrivateKey)
* [func MarshalPKCS1PrivateKey(key \*rsa.PrivateKey) []byte](#MarshalPKCS1PrivateKey)
* [func MarshalPKCS1PublicKey(key \*rsa.PublicKey) []byte](#MarshalPKCS1PublicKey)
* [func MarshalPKCS8PrivateKey(key any) ([]byte, error)](#MarshalPKCS8PrivateKey)
* [func MarshalPKIXPublicKey(pub any) ([]byte, error)](#MarshalPKIXPublicKey)
* [func ParseCRL(crlBytes []byte) (\*pkix.CertificateList, error)](#ParseCRL)
* [func ParseDERCRL(derBytes []byte) (\*pkix.CertificateList, error)](#ParseDERCRL)
* [func ParseECPrivateKey(der []byte) (\*ecdsa.PrivateKey, error)](#ParseECPrivateKey)
* [func ParsePKCS1PrivateKey(der []byte) (\*rsa.PrivateKey, error)](#ParsePKCS1PrivateKey)
* [func ParsePKCS1PublicKey(der []byte) (\*rsa.PublicKey, error)](#ParsePKCS1PublicKey)
* [func ParsePKCS8PrivateKey(der []byte) (key any, err error)](#ParsePKCS8PrivateKey)
* [func ParsePKIXPublicKey(derBytes []byte) (pub any, err error)](#ParsePKIXPublicKey)
* [func SetFallbackRoots(roots \*CertPool)](#SetFallbackRoots)
* [type CertPool](#CertPool)
* [func NewCertPool() \*CertPool](#NewCertPool)
* [func SystemCertPool() (\*CertPool, error)](#SystemCertPool)
* [func (s \*CertPool) AddCert(cert \*Certificate)](#CertPool.AddCert)
* [func (s \*CertPool) AppendCertsFromPEM(pemCerts []byte) (ok bool)](#CertPool.AppendCertsFromPEM)
* [func (s \*CertPool) Clone() \*CertPool](#CertPool.Clone)
* [func (s \*CertPool) Equal(other \*CertPool) bool](#CertPool.Equal)
* [func (s \*CertPool) Subjects() [][]byte](#CertPool.Subjects)
* [type Certificate](#Certificate)
* [func ParseCertificate(der []byte) (\*Certificate, error)](#ParseCertificate)
* [func ParseCertificates(der []byte) ([]\*Certificate, error)](#ParseCertificates)
* [func (c \*Certificate) CheckCRLSignature(crl \*pkix.CertificateList) error](#Certificate.CheckCRLSignature)
* [func (c \*Certificate) CheckSignature(algo SignatureAlgorithm, signed, signature []byte) error](#Certificate.CheckSignature)
* [func (c \*Certificate) CheckSignatureFrom(parent \*Certificate) error](#Certificate.CheckSignatureFrom)
* [func (c \*Certificate) CreateCRL(rand io.Reader, priv any, revokedCerts []pkix.RevokedCertificate, now, expiry time.Time) (crlBytes []byte, err error)](#Certificate.CreateCRL)
* [func (c \*Certificate) Equal(other \*Certificate) bool](#Certificate.Equal)
* [func (c \*Certificate) Verify(opts VerifyOptions) (chains [][]\*Certificate, err error)](#Certificate.Verify)
* [func (c \*Certificate) VerifyHostname(h string) error](#Certificate.VerifyHostname)
* [type CertificateInvalidError](#CertificateInvalidError)
* [func (e CertificateInvalidError) Error() string](#CertificateInvalidError.Error)
* [type CertificateRequest](#CertificateRequest)
* [func ParseCertificateRequest(asn1Data []byte) (\*CertificateRequest, error)](#ParseCertificateRequest)
* [func (c \*CertificateRequest) CheckSignature() error](#CertificateRequest.CheckSignature)
* [type ConstraintViolationError](#ConstraintViolationError)
* [func (ConstraintViolationError) Error() string](#ConstraintViolationError.Error)
* [type ExtKeyUsage](#ExtKeyUsage)
* [type HostnameError](#HostnameError)
* [func (h HostnameError) Error() string](#HostnameError.Error)
* [type InsecureAlgorithmError](#InsecureAlgorithmError)
* [func (e InsecureAlgorithmError) Error() string](#InsecureAlgorithmError.Error)
* [type InvalidReason](#InvalidReason)
* [type KeyUsage](#KeyUsage)
* [type PEMCipher](#PEMCipher)
* [type PublicKeyAlgorithm](#PublicKeyAlgorithm)
* [func (algo PublicKeyAlgorithm) String() string](#PublicKeyAlgorithm.String)
* [type RevocationList](#RevocationList)
* [func ParseRevocationList(der []byte) (\*RevocationList, error)](#ParseRevocationList)
* [func (rl \*RevocationList) CheckSignatureFrom(parent \*Certificate) error](#RevocationList.CheckSignatureFrom)
* [type SignatureAlgorithm](#SignatureAlgorithm)
* [func (algo SignatureAlgorithm) String() string](#SignatureAlgorithm.String)
* [type SystemRootsError](#SystemRootsError)
* [func (se SystemRootsError) Error() string](#SystemRootsError.Error)
* [func (se SystemRootsError) Unwrap() error](#SystemRootsError.Unwrap)
* [type UnhandledCriticalExtension](#UnhandledCriticalExtension)
* [func (h UnhandledCriticalExtension) Error() string](#UnhandledCriticalExtension.Error)
* [type UnknownAuthorityError](#UnknownAuthorityError)
* [func (e UnknownAuthorityError) Error() string](#UnknownAuthorityError.Error)
* [type VerifyOptions](#VerifyOptions)
### Examples
[Certificate.Verify](#example_Certificate_Verify) [ParsePKIXPublicKey](#example_ParsePKIXPublicKey) ### Package files
cert\_pool.go notboring.go parser.go pem\_decrypt.go pkcs1.go pkcs8.go root.go root\_linux.go root\_unix.go sec1.go verify.go x509.go
Variables
---------
ErrUnsupportedAlgorithm results from attempting to perform an operation that involves algorithms that are not currently implemented.
```
var ErrUnsupportedAlgorithm = errors.New("x509: cannot verify signature: algorithm unimplemented")
```
IncorrectPasswordError is returned when an incorrect password is detected.
```
var IncorrectPasswordError = errors.New("x509: decryption password incorrect")
```
func CreateCertificate
----------------------
```
func CreateCertificate(rand io.Reader, template, parent *Certificate, pub, priv any) ([]byte, error)
```
CreateCertificate creates a new X.509 v3 certificate based on a template. The following members of template are currently used:
* AuthorityKeyId
* BasicConstraintsValid
* CRLDistributionPoints
* DNSNames
* EmailAddresses
* ExcludedDNSDomains
* ExcludedEmailAddresses
* ExcludedIPRanges
* ExcludedURIDomains
* ExtKeyUsage
* ExtraExtensions
* IPAddresses
* IsCA
* IssuingCertificateURL
* KeyUsage
* MaxPathLen
* MaxPathLenZero
* NotAfter
* NotBefore
* OCSPServer
* PermittedDNSDomains
* PermittedDNSDomainsCritical
* PermittedEmailAddresses
* PermittedIPRanges
* PermittedURIDomains
* PolicyIdentifiers
* SerialNumber
* SignatureAlgorithm
* Subject
* SubjectKeyId
* URIs
* UnknownExtKeyUsage
The certificate is signed by parent. If parent is equal to template then the certificate is self-signed. The parameter pub is the public key of the certificate to be generated and priv is the private key of the signer.
The returned slice is the certificate in DER encoding.
The currently supported key types are \*rsa.PublicKey, \*ecdsa.PublicKey and ed25519.PublicKey. pub must be a supported key type, and priv must be a crypto.Signer with a supported public key.
The AuthorityKeyId will be taken from the SubjectKeyId of parent, if any, unless the resulting certificate is self-signed. Otherwise the value from template will be used.
If SubjectKeyId from template is empty and the template is a CA, SubjectKeyId will be generated from the hash of the public key.
func CreateCertificateRequest 1.3
---------------------------------
```
func CreateCertificateRequest(rand io.Reader, template *CertificateRequest, priv any) (csr []byte, err error)
```
CreateCertificateRequest creates a new certificate request based on a template. The following members of template are used:
* SignatureAlgorithm
* Subject
* DNSNames
* EmailAddresses
* IPAddresses
* URIs
* ExtraExtensions
* Attributes (deprecated)
priv is the private key to sign the CSR with, and the corresponding public key will be included in the CSR. It must implement crypto.Signer and its Public() method must return a \*rsa.PublicKey or a \*ecdsa.PublicKey or a ed25519.PublicKey. (A \*rsa.PrivateKey, \*ecdsa.PrivateKey or ed25519.PrivateKey satisfies this.)
The returned slice is the certificate request in DER encoding.
func CreateRevocationList 1.15
------------------------------
```
func CreateRevocationList(rand io.Reader, template *RevocationList, issuer *Certificate, priv crypto.Signer) ([]byte, error)
```
CreateRevocationList creates a new X.509 v2 Certificate Revocation List, according to RFC 5280, based on template.
The CRL is signed by priv which should be the private key associated with the public key in the issuer certificate.
The issuer may not be nil, and the crlSign bit must be set in KeyUsage in order to use it as a CRL issuer.
The issuer distinguished name CRL field and authority key identifier extension are populated using the issuer certificate. issuer must have SubjectKeyId set.
func DecryptPEMBlock 1.1
------------------------
```
func DecryptPEMBlock(b *pem.Block, password []byte) ([]byte, error)
```
DecryptPEMBlock takes a PEM block encrypted according to RFC 1423 and the password used to encrypt it and returns a slice of decrypted DER encoded bytes. It inspects the DEK-Info header to determine the algorithm used for decryption. If no DEK-Info header is present, an error is returned. If an incorrect password is detected an IncorrectPasswordError is returned. Because of deficiencies in the format, it's not always possible to detect an incorrect password. In these cases no error will be returned but the decrypted DER bytes will be random noise.
Deprecated: Legacy PEM encryption as specified in RFC 1423 is insecure by design. Since it does not authenticate the ciphertext, it is vulnerable to padding oracle attacks that can let an attacker recover the plaintext.
func EncryptPEMBlock 1.1
------------------------
```
func EncryptPEMBlock(rand io.Reader, blockType string, data, password []byte, alg PEMCipher) (*pem.Block, error)
```
EncryptPEMBlock returns a PEM block of the specified type holding the given DER encoded data encrypted with the specified algorithm and password according to RFC 1423.
Deprecated: Legacy PEM encryption as specified in RFC 1423 is insecure by design. Since it does not authenticate the ciphertext, it is vulnerable to padding oracle attacks that can let an attacker recover the plaintext.
func IsEncryptedPEMBlock 1.1
----------------------------
```
func IsEncryptedPEMBlock(b *pem.Block) bool
```
IsEncryptedPEMBlock returns whether the PEM block is password encrypted according to RFC 1423.
Deprecated: Legacy PEM encryption as specified in RFC 1423 is insecure by design. Since it does not authenticate the ciphertext, it is vulnerable to padding oracle attacks that can let an attacker recover the plaintext.
func MarshalECPrivateKey 1.2
----------------------------
```
func MarshalECPrivateKey(key *ecdsa.PrivateKey) ([]byte, error)
```
MarshalECPrivateKey converts an EC private key to SEC 1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "EC PRIVATE KEY". For a more flexible key format which is not EC specific, use MarshalPKCS8PrivateKey.
func MarshalPKCS1PrivateKey
---------------------------
```
func MarshalPKCS1PrivateKey(key *rsa.PrivateKey) []byte
```
MarshalPKCS1PrivateKey converts an RSA private key to PKCS #1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "RSA PRIVATE KEY". For a more flexible key format which is not RSA specific, use MarshalPKCS8PrivateKey.
func MarshalPKCS1PublicKey 1.10
-------------------------------
```
func MarshalPKCS1PublicKey(key *rsa.PublicKey) []byte
```
MarshalPKCS1PublicKey converts an RSA public key to PKCS #1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "RSA PUBLIC KEY".
func MarshalPKCS8PrivateKey 1.10
--------------------------------
```
func MarshalPKCS8PrivateKey(key any) ([]byte, error)
```
MarshalPKCS8PrivateKey converts a private key to PKCS #8, ASN.1 DER form.
The following key types are currently supported: \*rsa.PrivateKey, \*ecdsa.PrivateKey, ed25519.PrivateKey (not a pointer), and \*ecdh.PrivateKey. Unsupported key types result in an error.
This kind of key is commonly encoded in PEM blocks of type "PRIVATE KEY".
func MarshalPKIXPublicKey
-------------------------
```
func MarshalPKIXPublicKey(pub any) ([]byte, error)
```
MarshalPKIXPublicKey converts a public key to PKIX, ASN.1 DER form. The encoded public key is a SubjectPublicKeyInfo structure (see RFC 5280, Section 4.1).
The following key types are currently supported: \*rsa.PublicKey, \*ecdsa.PublicKey, ed25519.PublicKey (not a pointer), and \*ecdh.PublicKey. Unsupported key types result in an error.
This kind of key is commonly encoded in PEM blocks of type "PUBLIC KEY".
func ParseCRL
-------------
```
func ParseCRL(crlBytes []byte) (*pkix.CertificateList, error)
```
ParseCRL parses a CRL from the given bytes. It's often the case that PEM encoded CRLs will appear where they should be DER encoded, so this function will transparently handle PEM encoding as long as there isn't any leading garbage.
Deprecated: Use ParseRevocationList instead.
func ParseDERCRL
----------------
```
func ParseDERCRL(derBytes []byte) (*pkix.CertificateList, error)
```
ParseDERCRL parses a DER encoded CRL from the given bytes.
Deprecated: Use ParseRevocationList instead.
func ParseECPrivateKey 1.1
--------------------------
```
func ParseECPrivateKey(der []byte) (*ecdsa.PrivateKey, error)
```
ParseECPrivateKey parses an EC private key in SEC 1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "EC PRIVATE KEY".
func ParsePKCS1PrivateKey
-------------------------
```
func ParsePKCS1PrivateKey(der []byte) (*rsa.PrivateKey, error)
```
ParsePKCS1PrivateKey parses an RSA private key in PKCS #1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "RSA PRIVATE KEY".
func ParsePKCS1PublicKey 1.10
-----------------------------
```
func ParsePKCS1PublicKey(der []byte) (*rsa.PublicKey, error)
```
ParsePKCS1PublicKey parses an RSA public key in PKCS #1, ASN.1 DER form.
This kind of key is commonly encoded in PEM blocks of type "RSA PUBLIC KEY".
func ParsePKCS8PrivateKey
-------------------------
```
func ParsePKCS8PrivateKey(der []byte) (key any, err error)
```
ParsePKCS8PrivateKey parses an unencrypted private key in PKCS #8, ASN.1 DER form.
It returns a \*rsa.PrivateKey, a \*ecdsa.PrivateKey, a ed25519.PrivateKey (not a pointer), or a \*ecdh.PublicKey (for X25519). More types might be supported in the future.
This kind of key is commonly encoded in PEM blocks of type "PRIVATE KEY".
func ParsePKIXPublicKey
-----------------------
```
func ParsePKIXPublicKey(derBytes []byte) (pub any, err error)
```
ParsePKIXPublicKey parses a public key in PKIX, ASN.1 DER form. The encoded public key is a SubjectPublicKeyInfo structure (see RFC 5280, Section 4.1).
It returns a \*rsa.PublicKey, \*dsa.PublicKey, \*ecdsa.PublicKey, ed25519.PublicKey (not a pointer), or \*ecdh.PublicKey (for X25519). More types might be supported in the future.
This kind of key is commonly encoded in PEM blocks of type "PUBLIC KEY".
#### Example
Code:
```
const pubPEM = `
-----BEGIN PUBLIC KEY-----
MIICIjANBgkqhkiG9w0BAQEFAAOCAg8AMIICCgKCAgEAlRuRnThUjU8/prwYxbty
WPT9pURI3lbsKMiB6Fn/VHOKE13p4D8xgOCADpdRagdT6n4etr9atzDKUSvpMtR3
CP5noNc97WiNCggBjVWhs7szEe8ugyqF23XwpHQ6uV1LKH50m92MbOWfCtjU9p/x
qhNpQQ1AZhqNy5Gevap5k8XzRmjSldNAFZMY7Yv3Gi+nyCwGwpVtBUwhuLzgNFK/
yDtw2WcWmUU7NuC8Q6MWvPebxVtCfVp/iQU6q60yyt6aGOBkhAX0LpKAEhKidixY
nP9PNVBvxgu3XZ4P36gZV6+ummKdBVnc3NqwBLu5+CcdRdusmHPHd5pHf4/38Z3/
6qU2a/fPvWzceVTEgZ47QjFMTCTmCwNt29cvi7zZeQzjtwQgn4ipN9NibRH/Ax/q
TbIzHfrJ1xa2RteWSdFjwtxi9C20HUkjXSeI4YlzQMH0fPX6KCE7aVePTOnB69I/
a9/q96DiXZajwlpq3wFctrs1oXqBp5DVrCIj8hU2wNgB7LtQ1mCtsYz//heai0K9
PhE4X6hiE0YmeAZjR0uHl8M/5aW9xCoJ72+12kKpWAa0SFRWLy6FejNYCYpkupVJ
yecLk/4L1W0l6jQQZnWErXZYe0PNFcmwGXy1Rep83kfBRNKRy5tvocalLlwXLdUk
AIU+2GKjyT3iMuzZxxFxPFMCAwEAAQ==
-----END PUBLIC KEY-----`
block, _ := pem.Decode([]byte(pubPEM))
if block == nil {
panic("failed to parse PEM block containing the public key")
}
pub, err := x509.ParsePKIXPublicKey(block.Bytes)
if err != nil {
panic("failed to parse DER encoded public key: " + err.Error())
}
switch pub := pub.(type) {
case *rsa.PublicKey:
fmt.Println("pub is of type RSA:", pub)
case *dsa.PublicKey:
fmt.Println("pub is of type DSA:", pub)
case *ecdsa.PublicKey:
fmt.Println("pub is of type ECDSA:", pub)
case ed25519.PublicKey:
fmt.Println("pub is of type Ed25519:", pub)
default:
panic("unknown type of public key")
}
```
func SetFallbackRoots 1.20
--------------------------
```
func SetFallbackRoots(roots *CertPool)
```
SetFallbackRoots sets the roots to use during certificate verification, if no custom roots are specified and a platform verifier or a system certificate pool is not available (for instance in a container which does not have a root certificate bundle). SetFallbackRoots will panic if roots is nil.
SetFallbackRoots may only be called once, if called multiple times it will panic.
The fallback behavior can be forced on all platforms, even when there is a system certificate pool, by setting GODEBUG=x509usefallbackroots=1 (note that on Windows and macOS this will disable usage of the platform verification APIs and cause the pure Go verifier to be used). Setting x509usefallbackroots=1 without calling SetFallbackRoots has no effect.
type CertPool
-------------
CertPool is a set of certificates.
```
type CertPool struct {
// contains filtered or unexported fields
}
```
### func NewCertPool
```
func NewCertPool() *CertPool
```
NewCertPool returns a new, empty CertPool.
### func SystemCertPool 1.7
```
func SystemCertPool() (*CertPool, error)
```
SystemCertPool returns a copy of the system cert pool.
On Unix systems other than macOS the environment variables SSL\_CERT\_FILE and SSL\_CERT\_DIR can be used to override the system default locations for the SSL certificate file and SSL certificate files directory, respectively. The latter can be a colon-separated list.
Any mutations to the returned pool are not written to disk and do not affect any other pool returned by SystemCertPool.
New changes in the system cert pool might not be reflected in subsequent calls.
### func (\*CertPool) AddCert
```
func (s *CertPool) AddCert(cert *Certificate)
```
AddCert adds a certificate to a pool.
### func (\*CertPool) AppendCertsFromPEM
```
func (s *CertPool) AppendCertsFromPEM(pemCerts []byte) (ok bool)
```
AppendCertsFromPEM attempts to parse a series of PEM encoded certificates. It appends any certificates found to s and reports whether any certificates were successfully parsed.
On many Linux systems, /etc/ssl/cert.pem will contain the system wide set of root CAs in a format suitable for this function.
### func (\*CertPool) Clone 1.19
```
func (s *CertPool) Clone() *CertPool
```
Clone returns a copy of s.
### func (\*CertPool) Equal 1.19
```
func (s *CertPool) Equal(other *CertPool) bool
```
Equal reports whether s and other are equal.
### func (\*CertPool) Subjects
```
func (s *CertPool) Subjects() [][]byte
```
Subjects returns a list of the DER-encoded subjects of all of the certificates in the pool.
Deprecated: if s was returned by SystemCertPool, Subjects will not include the system roots.
type Certificate
----------------
A Certificate represents an X.509 certificate.
```
type Certificate struct {
Raw []byte // Complete ASN.1 DER content (certificate, signature algorithm and signature).
RawTBSCertificate []byte // Certificate part of raw ASN.1 DER content.
RawSubjectPublicKeyInfo []byte // DER encoded SubjectPublicKeyInfo.
RawSubject []byte // DER encoded Subject
RawIssuer []byte // DER encoded Issuer
Signature []byte
SignatureAlgorithm SignatureAlgorithm
PublicKeyAlgorithm PublicKeyAlgorithm
PublicKey any
Version int
SerialNumber *big.Int
Issuer pkix.Name
Subject pkix.Name
NotBefore, NotAfter time.Time // Validity bounds.
KeyUsage KeyUsage
// Extensions contains raw X.509 extensions. When parsing certificates,
// this can be used to extract non-critical extensions that are not
// parsed by this package. When marshaling certificates, the Extensions
// field is ignored, see ExtraExtensions.
Extensions []pkix.Extension // Go 1.2
// ExtraExtensions contains extensions to be copied, raw, into any
// marshaled certificates. Values override any extensions that would
// otherwise be produced based on the other fields. The ExtraExtensions
// field is not populated when parsing certificates, see Extensions.
ExtraExtensions []pkix.Extension // Go 1.2
// UnhandledCriticalExtensions contains a list of extension IDs that
// were not (fully) processed when parsing. Verify will fail if this
// slice is non-empty, unless verification is delegated to an OS
// library which understands all the critical extensions.
//
// Users can access these extensions using Extensions and can remove
// elements from this slice if they believe that they have been
// handled.
UnhandledCriticalExtensions []asn1.ObjectIdentifier // Go 1.5
ExtKeyUsage []ExtKeyUsage // Sequence of extended key usages.
UnknownExtKeyUsage []asn1.ObjectIdentifier // Encountered extended key usages unknown to this package.
// BasicConstraintsValid indicates whether IsCA, MaxPathLen,
// and MaxPathLenZero are valid.
BasicConstraintsValid bool
IsCA bool
// MaxPathLen and MaxPathLenZero indicate the presence and
// value of the BasicConstraints' "pathLenConstraint".
//
// When parsing a certificate, a positive non-zero MaxPathLen
// means that the field was specified, -1 means it was unset,
// and MaxPathLenZero being true mean that the field was
// explicitly set to zero. The case of MaxPathLen==0 with MaxPathLenZero==false
// should be treated equivalent to -1 (unset).
//
// When generating a certificate, an unset pathLenConstraint
// can be requested with either MaxPathLen == -1 or using the
// zero value for both MaxPathLen and MaxPathLenZero.
MaxPathLen int
// MaxPathLenZero indicates that BasicConstraintsValid==true
// and MaxPathLen==0 should be interpreted as an actual
// maximum path length of zero. Otherwise, that combination is
// interpreted as MaxPathLen not being set.
MaxPathLenZero bool // Go 1.4
SubjectKeyId []byte
AuthorityKeyId []byte
// RFC 5280, 4.2.2.1 (Authority Information Access)
OCSPServer []string // Go 1.2
IssuingCertificateURL []string // Go 1.2
// Subject Alternate Name values. (Note that these values may not be valid
// if invalid values were contained within a parsed certificate. For
// example, an element of DNSNames may not be a valid DNS domain name.)
DNSNames []string
EmailAddresses []string
IPAddresses []net.IP // Go 1.1
URIs []*url.URL // Go 1.10
// Name constraints
PermittedDNSDomainsCritical bool // if true then the name constraints are marked critical.
PermittedDNSDomains []string
ExcludedDNSDomains []string // Go 1.9
PermittedIPRanges []*net.IPNet // Go 1.10
ExcludedIPRanges []*net.IPNet // Go 1.10
PermittedEmailAddresses []string // Go 1.10
ExcludedEmailAddresses []string // Go 1.10
PermittedURIDomains []string // Go 1.10
ExcludedURIDomains []string // Go 1.10
// CRL Distribution Points
CRLDistributionPoints []string // Go 1.2
PolicyIdentifiers []asn1.ObjectIdentifier
}
```
### func ParseCertificate
```
func ParseCertificate(der []byte) (*Certificate, error)
```
ParseCertificate parses a single certificate from the given ASN.1 DER data.
### func ParseCertificates
```
func ParseCertificates(der []byte) ([]*Certificate, error)
```
ParseCertificates parses one or more certificates from the given ASN.1 DER data. The certificates must be concatenated with no intermediate padding.
### func (\*Certificate) CheckCRLSignature
```
func (c *Certificate) CheckCRLSignature(crl *pkix.CertificateList) error
```
CheckCRLSignature checks that the signature in crl is from c.
Deprecated: Use RevocationList.CheckSignatureFrom instead.
### func (\*Certificate) CheckSignature
```
func (c *Certificate) CheckSignature(algo SignatureAlgorithm, signed, signature []byte) error
```
CheckSignature verifies that signature is a valid signature over signed from c's public key.
This is a low-level API that performs no validity checks on the certificate.
[MD5WithRSA](#MD5WithRSA) signatures are rejected, while [SHA1WithRSA](#SHA1WithRSA) and [ECDSAWithSHA1](#ECDSAWithSHA1) signatures are currently accepted.
### func (\*Certificate) CheckSignatureFrom
```
func (c *Certificate) CheckSignatureFrom(parent *Certificate) error
```
CheckSignatureFrom verifies that the signature on c is a valid signature from parent.
This is a low-level API that performs very limited checks, and not a full path verifier. Most users should use [Certificate.Verify](#Certificate.Verify) instead.
### func (\*Certificate) CreateCRL
```
func (c *Certificate) CreateCRL(rand io.Reader, priv any, revokedCerts []pkix.RevokedCertificate, now, expiry time.Time) (crlBytes []byte, err error)
```
CreateCRL returns a DER encoded CRL, signed by this Certificate, that contains the given list of revoked certificates.
Deprecated: this method does not generate an RFC 5280 conformant X.509 v2 CRL. To generate a standards compliant CRL, use CreateRevocationList instead.
### func (\*Certificate) Equal
```
func (c *Certificate) Equal(other *Certificate) bool
```
### func (\*Certificate) Verify
```
func (c *Certificate) Verify(opts VerifyOptions) (chains [][]*Certificate, err error)
```
Verify attempts to verify c by building one or more chains from c to a certificate in opts.Roots, using certificates in opts.Intermediates if needed. If successful, it returns one or more chains where the first element of the chain is c and the last element is from opts.Roots.
If opts.Roots is nil, the platform verifier might be used, and verification details might differ from what is described below. If system roots are unavailable the returned error will be of type SystemRootsError.
Name constraints in the intermediates will be applied to all names claimed in the chain, not just opts.DNSName. Thus it is invalid for a leaf to claim example.com if an intermediate doesn't permit it, even if example.com is not the name being validated. Note that DirectoryName constraints are not supported.
Name constraint validation follows the rules from RFC 5280, with the addition that DNS name constraints may use the leading period format defined for emails and URIs. When a constraint has a leading period it indicates that at least one additional label must be prepended to the constrained name to be considered valid.
Extended Key Usage values are enforced nested down a chain, so an intermediate or root that enumerates EKUs prevents a leaf from asserting an EKU not in that list. (While this is not specified, it is common practice in order to limit the types of certificates a CA can issue.)
Certificates that use SHA1WithRSA and ECDSAWithSHA1 signatures are not supported, and will not be used to build chains.
Certificates other than c in the returned chains should not be modified.
WARNING: this function doesn't do any revocation checking.
#### Example
Code:
```
// Verifying with a custom list of root certificates.
const rootPEM = `
-----BEGIN CERTIFICATE-----
MIIEBDCCAuygAwIBAgIDAjppMA0GCSqGSIb3DQEBBQUAMEIxCzAJBgNVBAYTAlVT
MRYwFAYDVQQKEw1HZW9UcnVzdCBJbmMuMRswGQYDVQQDExJHZW9UcnVzdCBHbG9i
YWwgQ0EwHhcNMTMwNDA1MTUxNTU1WhcNMTUwNDA0MTUxNTU1WjBJMQswCQYDVQQG
EwJVUzETMBEGA1UEChMKR29vZ2xlIEluYzElMCMGA1UEAxMcR29vZ2xlIEludGVy
bmV0IEF1dGhvcml0eSBHMjCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEB
AJwqBHdc2FCROgajguDYUEi8iT/xGXAaiEZ+4I/F8YnOIe5a/mENtzJEiaB0C1NP
VaTOgmKV7utZX8bhBYASxF6UP7xbSDj0U/ck5vuR6RXEz/RTDfRK/J9U3n2+oGtv
h8DQUB8oMANA2ghzUWx//zo8pzcGjr1LEQTrfSTe5vn8MXH7lNVg8y5Kr0LSy+rE
ahqyzFPdFUuLH8gZYR/Nnag+YyuENWllhMgZxUYi+FOVvuOAShDGKuy6lyARxzmZ
EASg8GF6lSWMTlJ14rbtCMoU/M4iarNOz0YDl5cDfsCx3nuvRTPPuj5xt970JSXC
DTWJnZ37DhF5iR43xa+OcmkCAwEAAaOB+zCB+DAfBgNVHSMEGDAWgBTAephojYn7
qwVkDBF9qn1luMrMTjAdBgNVHQ4EFgQUSt0GFhu89mi1dvWBtrtiGrpagS8wEgYD
VR0TAQH/BAgwBgEB/wIBADAOBgNVHQ8BAf8EBAMCAQYwOgYDVR0fBDMwMTAvoC2g
K4YpaHR0cDovL2NybC5nZW90cnVzdC5jb20vY3Jscy9ndGdsb2JhbC5jcmwwPQYI
KwYBBQUHAQEEMTAvMC0GCCsGAQUFBzABhiFodHRwOi8vZ3RnbG9iYWwtb2NzcC5n
ZW90cnVzdC5jb20wFwYDVR0gBBAwDjAMBgorBgEEAdZ5AgUBMA0GCSqGSIb3DQEB
BQUAA4IBAQA21waAESetKhSbOHezI6B1WLuxfoNCunLaHtiONgaX4PCVOzf9G0JY
/iLIa704XtE7JW4S615ndkZAkNoUyHgN7ZVm2o6Gb4ChulYylYbc3GrKBIxbf/a/
zG+FA1jDaFETzf3I93k9mTXwVqO94FntT0QJo544evZG0R0SnU++0ED8Vf4GXjza
HFa9llF7b1cq26KqltyMdMKVvvBulRP/F/A8rLIQjcxz++iPAsbw+zOzlTvjwsto
WHPbqCRiOwY1nQ2pM714A5AuTHhdUDqB1O6gyHA43LL5Z/qHQF1hwFGPa4NrzQU6
yuGnBXj8ytqU0CwIPX4WecigUCAkVDNx
-----END CERTIFICATE-----`
const certPEM = `
-----BEGIN CERTIFICATE-----
MIIDujCCAqKgAwIBAgIIE31FZVaPXTUwDQYJKoZIhvcNAQEFBQAwSTELMAkGA1UE
BhMCVVMxEzARBgNVBAoTCkdvb2dsZSBJbmMxJTAjBgNVBAMTHEdvb2dsZSBJbnRl
cm5ldCBBdXRob3JpdHkgRzIwHhcNMTQwMTI5MTMyNzQzWhcNMTQwNTI5MDAwMDAw
WjBpMQswCQYDVQQGEwJVUzETMBEGA1UECAwKQ2FsaWZvcm5pYTEWMBQGA1UEBwwN
TW91bnRhaW4gVmlldzETMBEGA1UECgwKR29vZ2xlIEluYzEYMBYGA1UEAwwPbWFp
bC5nb29nbGUuY29tMFkwEwYHKoZIzj0CAQYIKoZIzj0DAQcDQgAEfRrObuSW5T7q
5CnSEqefEmtH4CCv6+5EckuriNr1CjfVvqzwfAhopXkLrq45EQm8vkmf7W96XJhC
7ZM0dYi1/qOCAU8wggFLMB0GA1UdJQQWMBQGCCsGAQUFBwMBBggrBgEFBQcDAjAa
BgNVHREEEzARgg9tYWlsLmdvb2dsZS5jb20wCwYDVR0PBAQDAgeAMGgGCCsGAQUF
BwEBBFwwWjArBggrBgEFBQcwAoYfaHR0cDovL3BraS5nb29nbGUuY29tL0dJQUcy
LmNydDArBggrBgEFBQcwAYYfaHR0cDovL2NsaWVudHMxLmdvb2dsZS5jb20vb2Nz
cDAdBgNVHQ4EFgQUiJxtimAuTfwb+aUtBn5UYKreKvMwDAYDVR0TAQH/BAIwADAf
BgNVHSMEGDAWgBRK3QYWG7z2aLV29YG2u2IaulqBLzAXBgNVHSAEEDAOMAwGCisG
AQQB1nkCBQEwMAYDVR0fBCkwJzAloCOgIYYfaHR0cDovL3BraS5nb29nbGUuY29t
L0dJQUcyLmNybDANBgkqhkiG9w0BAQUFAAOCAQEAH6RYHxHdcGpMpFE3oxDoFnP+
gtuBCHan2yE2GRbJ2Cw8Lw0MmuKqHlf9RSeYfd3BXeKkj1qO6TVKwCh+0HdZk283
TZZyzmEOyclm3UGFYe82P/iDFt+CeQ3NpmBg+GoaVCuWAARJN/KfglbLyyYygcQq
0SgeDh8dRKUiaW3HQSoYvTvdTuqzwK4CXsr3b5/dAOY8uMuG/IAR3FgwTbZ1dtoW
RvOTa8hYiU6A475WuZKyEHcwnGYe57u2I2KbMgcKjPniocj4QzgYsVAVKW3IwaOh
yE+vPxsiUkvQHdO2fojCkY8jg70jxM+gu59tPDNbw3Uh/2Ij310FgTHsnGQMyA==
-----END CERTIFICATE-----`
// First, create the set of root certificates. For this example we only
// have one. It's also possible to omit this in order to use the
// default root set of the current operating system.
roots := x509.NewCertPool()
ok := roots.AppendCertsFromPEM([]byte(rootPEM))
if !ok {
panic("failed to parse root certificate")
}
block, _ := pem.Decode([]byte(certPEM))
if block == nil {
panic("failed to parse certificate PEM")
}
cert, err := x509.ParseCertificate(block.Bytes)
if err != nil {
panic("failed to parse certificate: " + err.Error())
}
opts := x509.VerifyOptions{
DNSName: "mail.google.com",
Roots: roots,
}
if _, err := cert.Verify(opts); err != nil {
panic("failed to verify certificate: " + err.Error())
}
```
### func (\*Certificate) VerifyHostname
```
func (c *Certificate) VerifyHostname(h string) error
```
VerifyHostname returns nil if c is a valid certificate for the named host. Otherwise it returns an error describing the mismatch.
IP addresses can be optionally enclosed in square brackets and are checked against the IPAddresses field. Other names are checked case insensitively against the DNSNames field. If the names are valid hostnames, the certificate fields can have a wildcard as the left-most label.
Note that the legacy Common Name field is ignored.
type CertificateInvalidError
----------------------------
CertificateInvalidError results when an odd error occurs. Users of this library probably want to handle all these errors uniformly.
```
type CertificateInvalidError struct {
Cert *Certificate
Reason InvalidReason
Detail string // Go 1.10
}
```
### func (CertificateInvalidError) Error
```
func (e CertificateInvalidError) Error() string
```
type CertificateRequest 1.3
---------------------------
CertificateRequest represents a PKCS #10, certificate signature request.
```
type CertificateRequest struct {
Raw []byte // Complete ASN.1 DER content (CSR, signature algorithm and signature).
RawTBSCertificateRequest []byte // Certificate request info part of raw ASN.1 DER content.
RawSubjectPublicKeyInfo []byte // DER encoded SubjectPublicKeyInfo.
RawSubject []byte // DER encoded Subject.
Version int
Signature []byte
SignatureAlgorithm SignatureAlgorithm
PublicKeyAlgorithm PublicKeyAlgorithm
PublicKey any
Subject pkix.Name
// Attributes contains the CSR attributes that can parse as
// pkix.AttributeTypeAndValueSET.
//
// Deprecated: Use Extensions and ExtraExtensions instead for parsing and
// generating the requestedExtensions attribute.
Attributes []pkix.AttributeTypeAndValueSET
// Extensions contains all requested extensions, in raw form. When parsing
// CSRs, this can be used to extract extensions that are not parsed by this
// package.
Extensions []pkix.Extension
// ExtraExtensions contains extensions to be copied, raw, into any CSR
// marshaled by CreateCertificateRequest. Values override any extensions
// that would otherwise be produced based on the other fields but are
// overridden by any extensions specified in Attributes.
//
// The ExtraExtensions field is not populated by ParseCertificateRequest,
// see Extensions instead.
ExtraExtensions []pkix.Extension
// Subject Alternate Name values.
DNSNames []string
EmailAddresses []string
IPAddresses []net.IP
URIs []*url.URL // Go 1.10
}
```
### func ParseCertificateRequest 1.3
```
func ParseCertificateRequest(asn1Data []byte) (*CertificateRequest, error)
```
ParseCertificateRequest parses a single certificate request from the given ASN.1 DER data.
### func (\*CertificateRequest) CheckSignature 1.5
```
func (c *CertificateRequest) CheckSignature() error
```
CheckSignature reports whether the signature on c is valid.
type ConstraintViolationError
-----------------------------
ConstraintViolationError results when a requested usage is not permitted by a certificate. For example: checking a signature when the public key isn't a certificate signing key.
```
type ConstraintViolationError struct{}
```
### func (ConstraintViolationError) Error
```
func (ConstraintViolationError) Error() string
```
type ExtKeyUsage
----------------
ExtKeyUsage represents an extended set of actions that are valid for a given key. Each of the ExtKeyUsage\* constants define a unique action.
```
type ExtKeyUsage int
```
```
const (
ExtKeyUsageAny ExtKeyUsage = iota
ExtKeyUsageServerAuth
ExtKeyUsageClientAuth
ExtKeyUsageCodeSigning
ExtKeyUsageEmailProtection
ExtKeyUsageIPSECEndSystem
ExtKeyUsageIPSECTunnel
ExtKeyUsageIPSECUser
ExtKeyUsageTimeStamping
ExtKeyUsageOCSPSigning
ExtKeyUsageMicrosoftServerGatedCrypto
ExtKeyUsageNetscapeServerGatedCrypto
ExtKeyUsageMicrosoftCommercialCodeSigning
ExtKeyUsageMicrosoftKernelCodeSigning
)
```
type HostnameError
------------------
HostnameError results when the set of authorized names doesn't match the requested name.
```
type HostnameError struct {
Certificate *Certificate
Host string
}
```
### func (HostnameError) Error
```
func (h HostnameError) Error() string
```
type InsecureAlgorithmError 1.6
-------------------------------
An InsecureAlgorithmError indicates that the SignatureAlgorithm used to generate the signature is not secure, and the signature has been rejected.
To temporarily restore support for SHA-1 signatures, include the value "x509sha1=1" in the GODEBUG environment variable. Note that this option will be removed in a future release.
```
type InsecureAlgorithmError SignatureAlgorithm
```
### func (InsecureAlgorithmError) Error 1.6
```
func (e InsecureAlgorithmError) Error() string
```
type InvalidReason
------------------
```
type InvalidReason int
```
```
const (
// NotAuthorizedToSign results when a certificate is signed by another
// which isn't marked as a CA certificate.
NotAuthorizedToSign InvalidReason = iota
// Expired results when a certificate has expired, based on the time
// given in the VerifyOptions.
Expired
// CANotAuthorizedForThisName results when an intermediate or root
// certificate has a name constraint which doesn't permit a DNS or
// other name (including IP address) in the leaf certificate.
CANotAuthorizedForThisName
// TooManyIntermediates results when a path length constraint is
// violated.
TooManyIntermediates
// IncompatibleUsage results when the certificate's key usage indicates
// that it may only be used for a different purpose.
IncompatibleUsage
// NameMismatch results when the subject name of a parent certificate
// does not match the issuer name in the child.
NameMismatch
// NameConstraintsWithoutSANs is a legacy error and is no longer returned.
NameConstraintsWithoutSANs
// UnconstrainedName results when a CA certificate contains permitted
// name constraints, but leaf certificate contains a name of an
// unsupported or unconstrained type.
UnconstrainedName
// TooManyConstraints results when the number of comparison operations
// needed to check a certificate exceeds the limit set by
// VerifyOptions.MaxConstraintComparisions. This limit exists to
// prevent pathological certificates can consuming excessive amounts of
// CPU time to verify.
TooManyConstraints
// CANotAuthorizedForExtKeyUsage results when an intermediate or root
// certificate does not permit a requested extended key usage.
CANotAuthorizedForExtKeyUsage
)
```
type KeyUsage
-------------
KeyUsage represents the set of actions that are valid for a given key. It's a bitmap of the KeyUsage\* constants.
```
type KeyUsage int
```
```
const (
KeyUsageDigitalSignature KeyUsage = 1 << iota
KeyUsageContentCommitment
KeyUsageKeyEncipherment
KeyUsageDataEncipherment
KeyUsageKeyAgreement
KeyUsageCertSign
KeyUsageCRLSign
KeyUsageEncipherOnly
KeyUsageDecipherOnly
)
```
type PEMCipher 1.1
------------------
```
type PEMCipher int
```
Possible values for the EncryptPEMBlock encryption algorithm.
```
const (
PEMCipherDES PEMCipher
PEMCipher3DES
PEMCipherAES128
PEMCipherAES192
PEMCipherAES256
)
```
type PublicKeyAlgorithm
-----------------------
```
type PublicKeyAlgorithm int
```
```
const (
UnknownPublicKeyAlgorithm PublicKeyAlgorithm = iota
RSA
DSA // Only supported for parsing.
ECDSA
Ed25519
)
```
### func (PublicKeyAlgorithm) String 1.10
```
func (algo PublicKeyAlgorithm) String() string
```
type RevocationList 1.15
------------------------
RevocationList contains the fields used to create an X.509 v2 Certificate Revocation list with CreateRevocationList.
```
type RevocationList struct {
// Raw contains the complete ASN.1 DER content of the CRL (tbsCertList,
// signatureAlgorithm, and signatureValue.)
Raw []byte // Go 1.19
// RawTBSRevocationList contains just the tbsCertList portion of the ASN.1
// DER.
RawTBSRevocationList []byte // Go 1.19
// RawIssuer contains the DER encoded Issuer.
RawIssuer []byte // Go 1.19
// Issuer contains the DN of the issuing certificate.
Issuer pkix.Name // Go 1.19
// AuthorityKeyId is used to identify the public key associated with the
// issuing certificate. It is populated from the authorityKeyIdentifier
// extension when parsing a CRL. It is ignored when creating a CRL; the
// extension is populated from the issuing certificate itself.
AuthorityKeyId []byte // Go 1.19
Signature []byte // Go 1.19
// SignatureAlgorithm is used to determine the signature algorithm to be
// used when signing the CRL. If 0 the default algorithm for the signing
// key will be used.
SignatureAlgorithm SignatureAlgorithm
// RevokedCertificates is used to populate the revokedCertificates
// sequence in the CRL, it may be empty. RevokedCertificates may be nil,
// in which case an empty CRL will be created.
RevokedCertificates []pkix.RevokedCertificate
// Number is used to populate the X.509 v2 cRLNumber extension in the CRL,
// which should be a monotonically increasing sequence number for a given
// CRL scope and CRL issuer. It is also populated from the cRLNumber
// extension when parsing a CRL.
Number *big.Int
// ThisUpdate is used to populate the thisUpdate field in the CRL, which
// indicates the issuance date of the CRL.
ThisUpdate time.Time
// NextUpdate is used to populate the nextUpdate field in the CRL, which
// indicates the date by which the next CRL will be issued. NextUpdate
// must be greater than ThisUpdate.
NextUpdate time.Time
// Extensions contains raw X.509 extensions. When creating a CRL,
// the Extensions field is ignored, see ExtraExtensions.
Extensions []pkix.Extension // Go 1.19
// ExtraExtensions contains any additional extensions to add directly to
// the CRL.
ExtraExtensions []pkix.Extension
}
```
### func ParseRevocationList 1.19
```
func ParseRevocationList(der []byte) (*RevocationList, error)
```
ParseRevocationList parses a X509 v2 Certificate Revocation List from the given ASN.1 DER data.
### func (\*RevocationList) CheckSignatureFrom 1.19
```
func (rl *RevocationList) CheckSignatureFrom(parent *Certificate) error
```
CheckSignatureFrom verifies that the signature on rl is a valid signature from issuer.
type SignatureAlgorithm
-----------------------
```
type SignatureAlgorithm int
```
```
const (
UnknownSignatureAlgorithm SignatureAlgorithm = iota
MD2WithRSA // Unsupported.
MD5WithRSA // Only supported for signing, not verification.
SHA1WithRSA // Only supported for signing, and verification of CRLs, CSRs, and OCSP responses.
SHA256WithRSA
SHA384WithRSA
SHA512WithRSA
DSAWithSHA1 // Unsupported.
DSAWithSHA256 // Unsupported.
ECDSAWithSHA1 // Only supported for signing, and verification of CRLs, CSRs, and OCSP responses.
ECDSAWithSHA256
ECDSAWithSHA384
ECDSAWithSHA512
SHA256WithRSAPSS
SHA384WithRSAPSS
SHA512WithRSAPSS
PureEd25519
)
```
### func (SignatureAlgorithm) String 1.6
```
func (algo SignatureAlgorithm) String() string
```
type SystemRootsError 1.1
-------------------------
SystemRootsError results when we fail to load the system root certificates.
```
type SystemRootsError struct {
Err error // Go 1.7
}
```
### func (SystemRootsError) Error 1.1
```
func (se SystemRootsError) Error() string
```
### func (SystemRootsError) Unwrap 1.16
```
func (se SystemRootsError) Unwrap() error
```
type UnhandledCriticalExtension
-------------------------------
```
type UnhandledCriticalExtension struct{}
```
### func (UnhandledCriticalExtension) Error
```
func (h UnhandledCriticalExtension) Error() string
```
type UnknownAuthorityError
--------------------------
UnknownAuthorityError results when the certificate issuer is unknown
```
type UnknownAuthorityError struct {
Cert *Certificate // Go 1.8
// contains filtered or unexported fields
}
```
### func (UnknownAuthorityError) Error
```
func (e UnknownAuthorityError) Error() string
```
type VerifyOptions
------------------
VerifyOptions contains parameters for Certificate.Verify.
```
type VerifyOptions struct {
// DNSName, if set, is checked against the leaf certificate with
// Certificate.VerifyHostname or the platform verifier.
DNSName string
// Intermediates is an optional pool of certificates that are not trust
// anchors, but can be used to form a chain from the leaf certificate to a
// root certificate.
Intermediates *CertPool
// Roots is the set of trusted root certificates the leaf certificate needs
// to chain up to. If nil, the system roots or the platform verifier are used.
Roots *CertPool
// CurrentTime is used to check the validity of all certificates in the
// chain. If zero, the current time is used.
CurrentTime time.Time
// KeyUsages specifies which Extended Key Usage values are acceptable. A
// chain is accepted if it allows any of the listed values. An empty list
// means ExtKeyUsageServerAuth. To accept any key usage, include ExtKeyUsageAny.
KeyUsages []ExtKeyUsage // Go 1.1
// MaxConstraintComparisions is the maximum number of comparisons to
// perform when checking a given certificate's name constraints. If
// zero, a sensible default is used. This limit prevents pathological
// certificates from consuming excessive amounts of CPU time when
// validating. It does not apply to the platform verifier.
MaxConstraintComparisions int // Go 1.10
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [pkix](pkix/index) | Package pkix contains shared, low level structures used for ASN.1 parsing and serialization of X.509 certificates, CRL and OCSP. |
| programming_docs |
go Package pkix Package pkix
=============
* `import "crypto/x509/pkix"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package pkix contains shared, low level structures used for ASN.1 parsing and serialization of X.509 certificates, CRL and OCSP.
Index
-----
* [type AlgorithmIdentifier](#AlgorithmIdentifier)
* [type AttributeTypeAndValue](#AttributeTypeAndValue)
* [type AttributeTypeAndValueSET](#AttributeTypeAndValueSET)
* [type CertificateList](#CertificateList)
* [func (certList \*CertificateList) HasExpired(now time.Time) bool](#CertificateList.HasExpired)
* [type Extension](#Extension)
* [type Name](#Name)
* [func (n \*Name) FillFromRDNSequence(rdns \*RDNSequence)](#Name.FillFromRDNSequence)
* [func (n Name) String() string](#Name.String)
* [func (n Name) ToRDNSequence() (ret RDNSequence)](#Name.ToRDNSequence)
* [type RDNSequence](#RDNSequence)
* [func (r RDNSequence) String() string](#RDNSequence.String)
* [type RelativeDistinguishedNameSET](#RelativeDistinguishedNameSET)
* [type RevokedCertificate](#RevokedCertificate)
* [type TBSCertificateList](#TBSCertificateList)
### Package files
pkix.go
type AlgorithmIdentifier
------------------------
AlgorithmIdentifier represents the ASN.1 structure of the same name. See RFC 5280, section 4.1.1.2.
```
type AlgorithmIdentifier struct {
Algorithm asn1.ObjectIdentifier
Parameters asn1.RawValue `asn1:"optional"`
}
```
type AttributeTypeAndValue
--------------------------
AttributeTypeAndValue mirrors the ASN.1 structure of the same name in RFC 5280, Section 4.1.2.4.
```
type AttributeTypeAndValue struct {
Type asn1.ObjectIdentifier
Value any
}
```
type AttributeTypeAndValueSET 1.3
---------------------------------
AttributeTypeAndValueSET represents a set of ASN.1 sequences of AttributeTypeAndValue sequences from RFC 2986 (PKCS #10).
```
type AttributeTypeAndValueSET struct {
Type asn1.ObjectIdentifier
Value [][]AttributeTypeAndValue `asn1:"set"`
}
```
type CertificateList
--------------------
CertificateList represents the ASN.1 structure of the same name. See RFC 5280, section 5.1. Use Certificate.CheckCRLSignature to verify the signature.
Deprecated: x509.RevocationList should be used instead.
```
type CertificateList struct {
TBSCertList TBSCertificateList
SignatureAlgorithm AlgorithmIdentifier
SignatureValue asn1.BitString
}
```
### func (\*CertificateList) HasExpired
```
func (certList *CertificateList) HasExpired(now time.Time) bool
```
HasExpired reports whether certList should have been updated by now.
type Extension
--------------
Extension represents the ASN.1 structure of the same name. See RFC 5280, section 4.2.
```
type Extension struct {
Id asn1.ObjectIdentifier
Critical bool `asn1:"optional"`
Value []byte
}
```
type Name
---------
Name represents an X.509 distinguished name. This only includes the common elements of a DN. Note that Name is only an approximation of the X.509 structure. If an accurate representation is needed, asn1.Unmarshal the raw subject or issuer as an RDNSequence.
```
type Name struct {
Country, Organization, OrganizationalUnit []string
Locality, Province []string
StreetAddress, PostalCode []string
SerialNumber, CommonName string
// Names contains all parsed attributes. When parsing distinguished names,
// this can be used to extract non-standard attributes that are not parsed
// by this package. When marshaling to RDNSequences, the Names field is
// ignored, see ExtraNames.
Names []AttributeTypeAndValue
// ExtraNames contains attributes to be copied, raw, into any marshaled
// distinguished names. Values override any attributes with the same OID.
// The ExtraNames field is not populated when parsing, see Names.
ExtraNames []AttributeTypeAndValue // Go 1.5
}
```
### func (\*Name) FillFromRDNSequence
```
func (n *Name) FillFromRDNSequence(rdns *RDNSequence)
```
FillFromRDNSequence populates n from the provided RDNSequence. Multi-entry RDNs are flattened, all entries are added to the relevant n fields, and the grouping is not preserved.
### func (Name) String 1.10
```
func (n Name) String() string
```
String returns the string form of n, roughly following the RFC 2253 Distinguished Names syntax.
### func (Name) ToRDNSequence
```
func (n Name) ToRDNSequence() (ret RDNSequence)
```
ToRDNSequence converts n into a single RDNSequence. The following attributes are encoded as multi-value RDNs:
* Country
* Organization
* OrganizationalUnit
* Locality
* Province
* StreetAddress
* PostalCode
Each ExtraNames entry is encoded as an individual RDN.
type RDNSequence
----------------
```
type RDNSequence []RelativeDistinguishedNameSET
```
### func (RDNSequence) String 1.10
```
func (r RDNSequence) String() string
```
String returns a string representation of the sequence r, roughly following the RFC 2253 Distinguished Names syntax.
type RelativeDistinguishedNameSET
---------------------------------
```
type RelativeDistinguishedNameSET []AttributeTypeAndValue
```
type RevokedCertificate
-----------------------
RevokedCertificate represents the ASN.1 structure of the same name. See RFC 5280, section 5.1.
```
type RevokedCertificate struct {
SerialNumber *big.Int
RevocationTime time.Time
Extensions []Extension `asn1:"optional"`
}
```
type TBSCertificateList
-----------------------
TBSCertificateList represents the ASN.1 structure of the same name. See RFC 5280, section 5.1.
Deprecated: x509.RevocationList should be used instead.
```
type TBSCertificateList struct {
Raw asn1.RawContent
Version int `asn1:"optional,default:0"`
Signature AlgorithmIdentifier
Issuer RDNSequence
ThisUpdate time.Time
NextUpdate time.Time `asn1:"optional"`
RevokedCertificates []RevokedCertificate `asn1:"optional"`
Extensions []Extension `asn1:"tag:0,optional,explicit"`
}
```
go Package tls Package tls
============
* `import "crypto/tls"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package tls partially implements TLS 1.2, as specified in RFC 5246, and TLS 1.3, as specified in RFC 8446.
Index
-----
* [Constants](#pkg-constants)
* [func CipherSuiteName(id uint16) string](#CipherSuiteName)
* [func Listen(network, laddr string, config \*Config) (net.Listener, error)](#Listen)
* [func NewListener(inner net.Listener, config \*Config) net.Listener](#NewListener)
* [type Certificate](#Certificate)
* [func LoadX509KeyPair(certFile, keyFile string) (Certificate, error)](#LoadX509KeyPair)
* [func X509KeyPair(certPEMBlock, keyPEMBlock []byte) (Certificate, error)](#X509KeyPair)
* [type CertificateRequestInfo](#CertificateRequestInfo)
* [func (c \*CertificateRequestInfo) Context() context.Context](#CertificateRequestInfo.Context)
* [func (cri \*CertificateRequestInfo) SupportsCertificate(c \*Certificate) error](#CertificateRequestInfo.SupportsCertificate)
* [type CertificateVerificationError](#CertificateVerificationError)
* [func (e \*CertificateVerificationError) Error() string](#CertificateVerificationError.Error)
* [func (e \*CertificateVerificationError) Unwrap() error](#CertificateVerificationError.Unwrap)
* [type CipherSuite](#CipherSuite)
* [func CipherSuites() []\*CipherSuite](#CipherSuites)
* [func InsecureCipherSuites() []\*CipherSuite](#InsecureCipherSuites)
* [type ClientAuthType](#ClientAuthType)
* [func (i ClientAuthType) String() string](#ClientAuthType.String)
* [type ClientHelloInfo](#ClientHelloInfo)
* [func (c \*ClientHelloInfo) Context() context.Context](#ClientHelloInfo.Context)
* [func (chi \*ClientHelloInfo) SupportsCertificate(c \*Certificate) error](#ClientHelloInfo.SupportsCertificate)
* [type ClientSessionCache](#ClientSessionCache)
* [func NewLRUClientSessionCache(capacity int) ClientSessionCache](#NewLRUClientSessionCache)
* [type ClientSessionState](#ClientSessionState)
* [type Config](#Config)
* [func (c \*Config) BuildNameToCertificate()](#Config.BuildNameToCertificate)
* [func (c \*Config) Clone() \*Config](#Config.Clone)
* [func (c \*Config) SetSessionTicketKeys(keys [][32]byte)](#Config.SetSessionTicketKeys)
* [type Conn](#Conn)
* [func Client(conn net.Conn, config \*Config) \*Conn](#Client)
* [func Dial(network, addr string, config \*Config) (\*Conn, error)](#Dial)
* [func DialWithDialer(dialer \*net.Dialer, network, addr string, config \*Config) (\*Conn, error)](#DialWithDialer)
* [func Server(conn net.Conn, config \*Config) \*Conn](#Server)
* [func (c \*Conn) Close() error](#Conn.Close)
* [func (c \*Conn) CloseWrite() error](#Conn.CloseWrite)
* [func (c \*Conn) ConnectionState() ConnectionState](#Conn.ConnectionState)
* [func (c \*Conn) Handshake() error](#Conn.Handshake)
* [func (c \*Conn) HandshakeContext(ctx context.Context) error](#Conn.HandshakeContext)
* [func (c \*Conn) LocalAddr() net.Addr](#Conn.LocalAddr)
* [func (c \*Conn) NetConn() net.Conn](#Conn.NetConn)
* [func (c \*Conn) OCSPResponse() []byte](#Conn.OCSPResponse)
* [func (c \*Conn) Read(b []byte) (int, error)](#Conn.Read)
* [func (c \*Conn) RemoteAddr() net.Addr](#Conn.RemoteAddr)
* [func (c \*Conn) SetDeadline(t time.Time) error](#Conn.SetDeadline)
* [func (c \*Conn) SetReadDeadline(t time.Time) error](#Conn.SetReadDeadline)
* [func (c \*Conn) SetWriteDeadline(t time.Time) error](#Conn.SetWriteDeadline)
* [func (c \*Conn) VerifyHostname(host string) error](#Conn.VerifyHostname)
* [func (c \*Conn) Write(b []byte) (int, error)](#Conn.Write)
* [type ConnectionState](#ConnectionState)
* [func (cs \*ConnectionState) ExportKeyingMaterial(label string, context []byte, length int) ([]byte, error)](#ConnectionState.ExportKeyingMaterial)
* [type CurveID](#CurveID)
* [func (i CurveID) String() string](#CurveID.String)
* [type Dialer](#Dialer)
* [func (d \*Dialer) Dial(network, addr string) (net.Conn, error)](#Dialer.Dial)
* [func (d \*Dialer) DialContext(ctx context.Context, network, addr string) (net.Conn, error)](#Dialer.DialContext)
* [type RecordHeaderError](#RecordHeaderError)
* [func (e RecordHeaderError) Error() string](#RecordHeaderError.Error)
* [type RenegotiationSupport](#RenegotiationSupport)
* [type SignatureScheme](#SignatureScheme)
* [func (i SignatureScheme) String() string](#SignatureScheme.String)
* [Bugs](#pkg-note-BUG)
### Examples
[Config (KeyLogWriter)](#example_Config_keyLogWriter) [Config (VerifyConnection)](#example_Config_verifyConnection) [Dial](#example_Dial) [LoadX509KeyPair](#example_LoadX509KeyPair) [X509KeyPair](#example_X509KeyPair) [X509KeyPair (HttpServer)](#example_X509KeyPair_httpServer) ### Package files
alert.go auth.go cache.go cipher\_suites.go common.go common\_string.go conn.go handshake\_client.go handshake\_client\_tls13.go handshake\_messages.go handshake\_server.go handshake\_server\_tls13.go key\_agreement.go key\_schedule.go notboring.go prf.go ticket.go tls.go
Constants
---------
A list of cipher suite IDs that are, or have been, implemented by this package.
See <https://www.iana.org/assignments/tls-parameters/tls-parameters.xml>
```
const (
// TLS 1.0 - 1.2 cipher suites.
TLS_RSA_WITH_RC4_128_SHA uint16 = 0x0005
TLS_RSA_WITH_3DES_EDE_CBC_SHA uint16 = 0x000a
TLS_RSA_WITH_AES_128_CBC_SHA uint16 = 0x002f
TLS_RSA_WITH_AES_256_CBC_SHA uint16 = 0x0035
TLS_RSA_WITH_AES_128_CBC_SHA256 uint16 = 0x003c
TLS_RSA_WITH_AES_128_GCM_SHA256 uint16 = 0x009c
TLS_RSA_WITH_AES_256_GCM_SHA384 uint16 = 0x009d
TLS_ECDHE_ECDSA_WITH_RC4_128_SHA uint16 = 0xc007
TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA uint16 = 0xc009
TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA uint16 = 0xc00a
TLS_ECDHE_RSA_WITH_RC4_128_SHA uint16 = 0xc011
TLS_ECDHE_RSA_WITH_3DES_EDE_CBC_SHA uint16 = 0xc012
TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA uint16 = 0xc013
TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA uint16 = 0xc014
TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256 uint16 = 0xc023
TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256 uint16 = 0xc027
TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256 uint16 = 0xc02f
TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256 uint16 = 0xc02b
TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384 uint16 = 0xc030
TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384 uint16 = 0xc02c
TLS_ECDHE_RSA_WITH_CHACHA20_POLY1305_SHA256 uint16 = 0xcca8
TLS_ECDHE_ECDSA_WITH_CHACHA20_POLY1305_SHA256 uint16 = 0xcca9
// TLS 1.3 cipher suites.
TLS_AES_128_GCM_SHA256 uint16 = 0x1301
TLS_AES_256_GCM_SHA384 uint16 = 0x1302
TLS_CHACHA20_POLY1305_SHA256 uint16 = 0x1303
// TLS_FALLBACK_SCSV isn't a standard cipher suite but an indicator
// that the client is doing version fallback. See RFC 7507.
TLS_FALLBACK_SCSV uint16 = 0x5600
// Legacy names for the corresponding cipher suites with the correct _SHA256
// suffix, retained for backward compatibility.
TLS_ECDHE_RSA_WITH_CHACHA20_POLY1305 = TLS_ECDHE_RSA_WITH_CHACHA20_POLY1305_SHA256
TLS_ECDHE_ECDSA_WITH_CHACHA20_POLY1305 = TLS_ECDHE_ECDSA_WITH_CHACHA20_POLY1305_SHA256
)
```
```
const (
VersionTLS10 = 0x0301
VersionTLS11 = 0x0302
VersionTLS12 = 0x0303
VersionTLS13 = 0x0304
// Deprecated: SSLv3 is cryptographically broken, and is no longer
// supported by this package. See golang.org/issue/32716.
VersionSSL30 = 0x0300
)
```
func CipherSuiteName 1.14
-------------------------
```
func CipherSuiteName(id uint16) string
```
CipherSuiteName returns the standard name for the passed cipher suite ID (e.g. "TLS\_ECDHE\_ECDSA\_WITH\_AES\_128\_GCM\_SHA256"), or a fallback representation of the ID value if the cipher suite is not implemented by this package.
func Listen
-----------
```
func Listen(network, laddr string, config *Config) (net.Listener, error)
```
Listen creates a TLS listener accepting connections on the given network address using net.Listen. The configuration config must be non-nil and must include at least one certificate or else set GetCertificate.
func NewListener
----------------
```
func NewListener(inner net.Listener, config *Config) net.Listener
```
NewListener creates a Listener which accepts connections from an inner Listener and wraps each connection with Server. The configuration config must be non-nil and must include at least one certificate or else set GetCertificate.
type Certificate
----------------
A Certificate is a chain of one or more certificates, leaf first.
```
type Certificate struct {
Certificate [][]byte
// PrivateKey contains the private key corresponding to the public key in
// Leaf. This must implement crypto.Signer with an RSA, ECDSA or Ed25519 PublicKey.
// For a server up to TLS 1.2, it can also implement crypto.Decrypter with
// an RSA PublicKey.
PrivateKey crypto.PrivateKey
// SupportedSignatureAlgorithms is an optional list restricting what
// signature algorithms the PrivateKey can be used for.
SupportedSignatureAlgorithms []SignatureScheme // Go 1.14
// OCSPStaple contains an optional OCSP response which will be served
// to clients that request it.
OCSPStaple []byte
// SignedCertificateTimestamps contains an optional list of Signed
// Certificate Timestamps which will be served to clients that request it.
SignedCertificateTimestamps [][]byte // Go 1.5
// Leaf is the parsed form of the leaf certificate, which may be initialized
// using x509.ParseCertificate to reduce per-handshake processing. If nil,
// the leaf certificate will be parsed as needed.
Leaf *x509.Certificate
}
```
### func LoadX509KeyPair
```
func LoadX509KeyPair(certFile, keyFile string) (Certificate, error)
```
LoadX509KeyPair reads and parses a public/private key pair from a pair of files. The files must contain PEM encoded data. The certificate file may contain intermediate certificates following the leaf certificate to form a certificate chain. On successful return, Certificate.Leaf will be nil because the parsed form of the certificate is not retained.
#### Example
Code:
```
cert, err := tls.LoadX509KeyPair("testdata/example-cert.pem", "testdata/example-key.pem")
if err != nil {
log.Fatal(err)
}
cfg := &tls.Config{Certificates: []tls.Certificate{cert}}
listener, err := tls.Listen("tcp", ":2000", cfg)
if err != nil {
log.Fatal(err)
}
_ = listener
```
### func X509KeyPair
```
func X509KeyPair(certPEMBlock, keyPEMBlock []byte) (Certificate, error)
```
X509KeyPair parses a public/private key pair from a pair of PEM encoded data. On successful return, Certificate.Leaf will be nil because the parsed form of the certificate is not retained.
#### Example
Code:
```
certPem := []byte(`-----BEGIN CERTIFICATE-----
MIIBhTCCASugAwIBAgIQIRi6zePL6mKjOipn+dNuaTAKBggqhkjOPQQDAjASMRAw
DgYDVQQKEwdBY21lIENvMB4XDTE3MTAyMDE5NDMwNloXDTE4MTAyMDE5NDMwNlow
EjEQMA4GA1UEChMHQWNtZSBDbzBZMBMGByqGSM49AgEGCCqGSM49AwEHA0IABD0d
7VNhbWvZLWPuj/RtHFjvtJBEwOkhbN/BnnE8rnZR8+sbwnc/KhCk3FhnpHZnQz7B
5aETbbIgmuvewdjvSBSjYzBhMA4GA1UdDwEB/wQEAwICpDATBgNVHSUEDDAKBggr
BgEFBQcDATAPBgNVHRMBAf8EBTADAQH/MCkGA1UdEQQiMCCCDmxvY2FsaG9zdDo1
NDUzgg4xMjcuMC4wLjE6NTQ1MzAKBggqhkjOPQQDAgNIADBFAiEA2zpJEPQyz6/l
Wf86aX6PepsntZv2GYlA5UpabfT2EZICICpJ5h/iI+i341gBmLiAFQOyTDT+/wQc
6MF9+Yw1Yy0t
-----END CERTIFICATE-----`)
keyPem := []byte(`-----BEGIN EC PRIVATE KEY-----
MHcCAQEEIIrYSSNQFaA2Hwf1duRSxKtLYX5CB04fSeQ6tF1aY/PuoAoGCCqGSM49
AwEHoUQDQgAEPR3tU2Fta9ktY+6P9G0cWO+0kETA6SFs38GecTyudlHz6xvCdz8q
EKTcWGekdmdDPsHloRNtsiCa697B2O9IFA==
-----END EC PRIVATE KEY-----`)
cert, err := tls.X509KeyPair(certPem, keyPem)
if err != nil {
log.Fatal(err)
}
cfg := &tls.Config{Certificates: []tls.Certificate{cert}}
listener, err := tls.Listen("tcp", ":2000", cfg)
if err != nil {
log.Fatal(err)
}
_ = listener
```
#### Example (HttpServer)
Code:
```
certPem := []byte(`-----BEGIN CERTIFICATE-----
MIIBhTCCASugAwIBAgIQIRi6zePL6mKjOipn+dNuaTAKBggqhkjOPQQDAjASMRAw
DgYDVQQKEwdBY21lIENvMB4XDTE3MTAyMDE5NDMwNloXDTE4MTAyMDE5NDMwNlow
EjEQMA4GA1UEChMHQWNtZSBDbzBZMBMGByqGSM49AgEGCCqGSM49AwEHA0IABD0d
7VNhbWvZLWPuj/RtHFjvtJBEwOkhbN/BnnE8rnZR8+sbwnc/KhCk3FhnpHZnQz7B
5aETbbIgmuvewdjvSBSjYzBhMA4GA1UdDwEB/wQEAwICpDATBgNVHSUEDDAKBggr
BgEFBQcDATAPBgNVHRMBAf8EBTADAQH/MCkGA1UdEQQiMCCCDmxvY2FsaG9zdDo1
NDUzgg4xMjcuMC4wLjE6NTQ1MzAKBggqhkjOPQQDAgNIADBFAiEA2zpJEPQyz6/l
Wf86aX6PepsntZv2GYlA5UpabfT2EZICICpJ5h/iI+i341gBmLiAFQOyTDT+/wQc
6MF9+Yw1Yy0t
-----END CERTIFICATE-----`)
keyPem := []byte(`-----BEGIN EC PRIVATE KEY-----
MHcCAQEEIIrYSSNQFaA2Hwf1duRSxKtLYX5CB04fSeQ6tF1aY/PuoAoGCCqGSM49
AwEHoUQDQgAEPR3tU2Fta9ktY+6P9G0cWO+0kETA6SFs38GecTyudlHz6xvCdz8q
EKTcWGekdmdDPsHloRNtsiCa697B2O9IFA==
-----END EC PRIVATE KEY-----`)
cert, err := tls.X509KeyPair(certPem, keyPem)
if err != nil {
log.Fatal(err)
}
cfg := &tls.Config{Certificates: []tls.Certificate{cert}}
srv := &http.Server{
TLSConfig: cfg,
ReadTimeout: time.Minute,
WriteTimeout: time.Minute,
}
log.Fatal(srv.ListenAndServeTLS("", ""))
```
type CertificateRequestInfo 1.8
-------------------------------
CertificateRequestInfo contains information from a server's CertificateRequest message, which is used to demand a certificate and proof of control from a client.
```
type CertificateRequestInfo struct {
// AcceptableCAs contains zero or more, DER-encoded, X.501
// Distinguished Names. These are the names of root or intermediate CAs
// that the server wishes the returned certificate to be signed by. An
// empty slice indicates that the server has no preference.
AcceptableCAs [][]byte
// SignatureSchemes lists the signature schemes that the server is
// willing to verify.
SignatureSchemes []SignatureScheme
// Version is the TLS version that was negotiated for this connection.
Version uint16 // Go 1.14
// contains filtered or unexported fields
}
```
### func (\*CertificateRequestInfo) Context 1.17
```
func (c *CertificateRequestInfo) Context() context.Context
```
Context returns the context of the handshake that is in progress. This context is a child of the context passed to HandshakeContext, if any, and is canceled when the handshake concludes.
### func (\*CertificateRequestInfo) SupportsCertificate 1.14
```
func (cri *CertificateRequestInfo) SupportsCertificate(c *Certificate) error
```
SupportsCertificate returns nil if the provided certificate is supported by the server that sent the CertificateRequest. Otherwise, it returns an error describing the reason for the incompatibility.
type CertificateVerificationError 1.20
--------------------------------------
CertificateVerificationError is returned when certificate verification fails during the handshake.
```
type CertificateVerificationError struct {
// UnverifiedCertificates and its contents should not be modified.
UnverifiedCertificates []*x509.Certificate
Err error
}
```
### func (\*CertificateVerificationError) Error 1.20
```
func (e *CertificateVerificationError) Error() string
```
### func (\*CertificateVerificationError) Unwrap 1.20
```
func (e *CertificateVerificationError) Unwrap() error
```
type CipherSuite 1.14
---------------------
CipherSuite is a TLS cipher suite. Note that most functions in this package accept and expose cipher suite IDs instead of this type.
```
type CipherSuite struct {
ID uint16
Name string
// Supported versions is the list of TLS protocol versions that can
// negotiate this cipher suite.
SupportedVersions []uint16
// Insecure is true if the cipher suite has known security issues
// due to its primitives, design, or implementation.
Insecure bool
}
```
### func CipherSuites 1.14
```
func CipherSuites() []*CipherSuite
```
CipherSuites returns a list of cipher suites currently implemented by this package, excluding those with security issues, which are returned by InsecureCipherSuites.
The list is sorted by ID. Note that the default cipher suites selected by this package might depend on logic that can't be captured by a static list, and might not match those returned by this function.
### func InsecureCipherSuites 1.14
```
func InsecureCipherSuites() []*CipherSuite
```
InsecureCipherSuites returns a list of cipher suites currently implemented by this package and which have security issues.
Most applications should not use the cipher suites in this list, and should only use those returned by CipherSuites.
type ClientAuthType
-------------------
ClientAuthType declares the policy the server will follow for TLS Client Authentication.
```
type ClientAuthType int
```
```
const (
// NoClientCert indicates that no client certificate should be requested
// during the handshake, and if any certificates are sent they will not
// be verified.
NoClientCert ClientAuthType = iota
// RequestClientCert indicates that a client certificate should be requested
// during the handshake, but does not require that the client send any
// certificates.
RequestClientCert
// RequireAnyClientCert indicates that a client certificate should be requested
// during the handshake, and that at least one certificate is required to be
// sent by the client, but that certificate is not required to be valid.
RequireAnyClientCert
// VerifyClientCertIfGiven indicates that a client certificate should be requested
// during the handshake, but does not require that the client sends a
// certificate. If the client does send a certificate it is required to be
// valid.
VerifyClientCertIfGiven
// RequireAndVerifyClientCert indicates that a client certificate should be requested
// during the handshake, and that at least one valid certificate is required
// to be sent by the client.
RequireAndVerifyClientCert
)
```
### func (ClientAuthType) String 1.15
```
func (i ClientAuthType) String() string
```
type ClientHelloInfo 1.4
------------------------
ClientHelloInfo contains information from a ClientHello message in order to guide application logic in the GetCertificate and GetConfigForClient callbacks.
```
type ClientHelloInfo struct {
// CipherSuites lists the CipherSuites supported by the client (e.g.
// TLS_AES_128_GCM_SHA256, TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256).
CipherSuites []uint16
// ServerName indicates the name of the server requested by the client
// in order to support virtual hosting. ServerName is only set if the
// client is using SNI (see RFC 4366, Section 3.1).
ServerName string
// SupportedCurves lists the elliptic curves supported by the client.
// SupportedCurves is set only if the Supported Elliptic Curves
// Extension is being used (see RFC 4492, Section 5.1.1).
SupportedCurves []CurveID
// SupportedPoints lists the point formats supported by the client.
// SupportedPoints is set only if the Supported Point Formats Extension
// is being used (see RFC 4492, Section 5.1.2).
SupportedPoints []uint8
// SignatureSchemes lists the signature and hash schemes that the client
// is willing to verify. SignatureSchemes is set only if the Signature
// Algorithms Extension is being used (see RFC 5246, Section 7.4.1.4.1).
SignatureSchemes []SignatureScheme // Go 1.8
// SupportedProtos lists the application protocols supported by the client.
// SupportedProtos is set only if the Application-Layer Protocol
// Negotiation Extension is being used (see RFC 7301, Section 3.1).
//
// Servers can select a protocol by setting Config.NextProtos in a
// GetConfigForClient return value.
SupportedProtos []string // Go 1.8
// SupportedVersions lists the TLS versions supported by the client.
// For TLS versions less than 1.3, this is extrapolated from the max
// version advertised by the client, so values other than the greatest
// might be rejected if used.
SupportedVersions []uint16 // Go 1.8
// Conn is the underlying net.Conn for the connection. Do not read
// from, or write to, this connection; that will cause the TLS
// connection to fail.
Conn net.Conn // Go 1.8
// contains filtered or unexported fields
}
```
### func (\*ClientHelloInfo) Context 1.17
```
func (c *ClientHelloInfo) Context() context.Context
```
Context returns the context of the handshake that is in progress. This context is a child of the context passed to HandshakeContext, if any, and is canceled when the handshake concludes.
### func (\*ClientHelloInfo) SupportsCertificate 1.14
```
func (chi *ClientHelloInfo) SupportsCertificate(c *Certificate) error
```
SupportsCertificate returns nil if the provided certificate is supported by the client that sent the ClientHello. Otherwise, it returns an error describing the reason for the incompatibility.
If this ClientHelloInfo was passed to a GetConfigForClient or GetCertificate callback, this method will take into account the associated Config. Note that if GetConfigForClient returns a different Config, the change can't be accounted for by this method.
This function will call x509.ParseCertificate unless c.Leaf is set, which can incur a significant performance cost.
type ClientSessionCache 1.3
---------------------------
ClientSessionCache is a cache of ClientSessionState objects that can be used by a client to resume a TLS session with a given server. ClientSessionCache implementations should expect to be called concurrently from different goroutines. Up to TLS 1.2, only ticket-based resumption is supported, not SessionID-based resumption. In TLS 1.3 they were merged into PSK modes, which are supported via this interface.
```
type ClientSessionCache interface {
// Get searches for a ClientSessionState associated with the given key.
// On return, ok is true if one was found.
Get(sessionKey string) (session *ClientSessionState, ok bool)
// Put adds the ClientSessionState to the cache with the given key. It might
// get called multiple times in a connection if a TLS 1.3 server provides
// more than one session ticket. If called with a nil *ClientSessionState,
// it should remove the cache entry.
Put(sessionKey string, cs *ClientSessionState)
}
```
### func NewLRUClientSessionCache 1.3
```
func NewLRUClientSessionCache(capacity int) ClientSessionCache
```
NewLRUClientSessionCache returns a ClientSessionCache with the given capacity that uses an LRU strategy. If capacity is < 1, a default capacity is used instead.
type ClientSessionState 1.3
---------------------------
ClientSessionState contains the state needed by clients to resume TLS sessions.
```
type ClientSessionState struct {
// contains filtered or unexported fields
}
```
type Config
-----------
A Config structure is used to configure a TLS client or server. After one has been passed to a TLS function it must not be modified. A Config may be reused; the tls package will also not modify it.
```
type Config struct {
// Rand provides the source of entropy for nonces and RSA blinding.
// If Rand is nil, TLS uses the cryptographic random reader in package
// crypto/rand.
// The Reader must be safe for use by multiple goroutines.
Rand io.Reader
// Time returns the current time as the number of seconds since the epoch.
// If Time is nil, TLS uses time.Now.
Time func() time.Time
// Certificates contains one or more certificate chains to present to the
// other side of the connection. The first certificate compatible with the
// peer's requirements is selected automatically.
//
// Server configurations must set one of Certificates, GetCertificate or
// GetConfigForClient. Clients doing client-authentication may set either
// Certificates or GetClientCertificate.
//
// Note: if there are multiple Certificates, and they don't have the
// optional field Leaf set, certificate selection will incur a significant
// per-handshake performance cost.
Certificates []Certificate
// NameToCertificate maps from a certificate name to an element of
// Certificates. Note that a certificate name can be of the form
// '*.example.com' and so doesn't have to be a domain name as such.
//
// Deprecated: NameToCertificate only allows associating a single
// certificate with a given name. Leave this field nil to let the library
// select the first compatible chain from Certificates.
NameToCertificate map[string]*Certificate
// GetCertificate returns a Certificate based on the given
// ClientHelloInfo. It will only be called if the client supplies SNI
// information or if Certificates is empty.
//
// If GetCertificate is nil or returns nil, then the certificate is
// retrieved from NameToCertificate. If NameToCertificate is nil, the
// best element of Certificates will be used.
//
// Once a Certificate is returned it should not be modified.
GetCertificate func(*ClientHelloInfo) (*Certificate, error) // Go 1.4
// GetClientCertificate, if not nil, is called when a server requests a
// certificate from a client. If set, the contents of Certificates will
// be ignored.
//
// If GetClientCertificate returns an error, the handshake will be
// aborted and that error will be returned. Otherwise
// GetClientCertificate must return a non-nil Certificate. If
// Certificate.Certificate is empty then no certificate will be sent to
// the server. If this is unacceptable to the server then it may abort
// the handshake.
//
// GetClientCertificate may be called multiple times for the same
// connection if renegotiation occurs or if TLS 1.3 is in use.
//
// Once a Certificate is returned it should not be modified.
GetClientCertificate func(*CertificateRequestInfo) (*Certificate, error) // Go 1.8
// GetConfigForClient, if not nil, is called after a ClientHello is
// received from a client. It may return a non-nil Config in order to
// change the Config that will be used to handle this connection. If
// the returned Config is nil, the original Config will be used. The
// Config returned by this callback may not be subsequently modified.
//
// If GetConfigForClient is nil, the Config passed to Server() will be
// used for all connections.
//
// If SessionTicketKey was explicitly set on the returned Config, or if
// SetSessionTicketKeys was called on the returned Config, those keys will
// be used. Otherwise, the original Config keys will be used (and possibly
// rotated if they are automatically managed).
GetConfigForClient func(*ClientHelloInfo) (*Config, error) // Go 1.8
// VerifyPeerCertificate, if not nil, is called after normal
// certificate verification by either a TLS client or server. It
// receives the raw ASN.1 certificates provided by the peer and also
// any verified chains that normal processing found. If it returns a
// non-nil error, the handshake is aborted and that error results.
//
// If normal verification fails then the handshake will abort before
// considering this callback. If normal verification is disabled by
// setting InsecureSkipVerify, or (for a server) when ClientAuth is
// RequestClientCert or RequireAnyClientCert, then this callback will
// be considered but the verifiedChains argument will always be nil.
//
// verifiedChains and its contents should not be modified.
VerifyPeerCertificate func(rawCerts [][]byte, verifiedChains [][]*x509.Certificate) error // Go 1.8
// VerifyConnection, if not nil, is called after normal certificate
// verification and after VerifyPeerCertificate by either a TLS client
// or server. If it returns a non-nil error, the handshake is aborted
// and that error results.
//
// If normal verification fails then the handshake will abort before
// considering this callback. This callback will run for all connections
// regardless of InsecureSkipVerify or ClientAuth settings.
VerifyConnection func(ConnectionState) error // Go 1.15
// RootCAs defines the set of root certificate authorities
// that clients use when verifying server certificates.
// If RootCAs is nil, TLS uses the host's root CA set.
RootCAs *x509.CertPool
// NextProtos is a list of supported application level protocols, in
// order of preference. If both peers support ALPN, the selected
// protocol will be one from this list, and the connection will fail
// if there is no mutually supported protocol. If NextProtos is empty
// or the peer doesn't support ALPN, the connection will succeed and
// ConnectionState.NegotiatedProtocol will be empty.
NextProtos []string
// ServerName is used to verify the hostname on the returned
// certificates unless InsecureSkipVerify is given. It is also included
// in the client's handshake to support virtual hosting unless it is
// an IP address.
ServerName string
// ClientAuth determines the server's policy for
// TLS Client Authentication. The default is NoClientCert.
ClientAuth ClientAuthType
// ClientCAs defines the set of root certificate authorities
// that servers use if required to verify a client certificate
// by the policy in ClientAuth.
ClientCAs *x509.CertPool
// InsecureSkipVerify controls whether a client verifies the server's
// certificate chain and host name. If InsecureSkipVerify is true, crypto/tls
// accepts any certificate presented by the server and any host name in that
// certificate. In this mode, TLS is susceptible to machine-in-the-middle
// attacks unless custom verification is used. This should be used only for
// testing or in combination with VerifyConnection or VerifyPeerCertificate.
InsecureSkipVerify bool
// CipherSuites is a list of enabled TLS 1.0–1.2 cipher suites. The order of
// the list is ignored. Note that TLS 1.3 ciphersuites are not configurable.
//
// If CipherSuites is nil, a safe default list is used. The default cipher
// suites might change over time.
CipherSuites []uint16
// PreferServerCipherSuites is a legacy field and has no effect.
//
// It used to control whether the server would follow the client's or the
// server's preference. Servers now select the best mutually supported
// cipher suite based on logic that takes into account inferred client
// hardware, server hardware, and security.
//
// Deprecated: PreferServerCipherSuites is ignored.
PreferServerCipherSuites bool // Go 1.1
// SessionTicketsDisabled may be set to true to disable session ticket and
// PSK (resumption) support. Note that on clients, session ticket support is
// also disabled if ClientSessionCache is nil.
SessionTicketsDisabled bool // Go 1.1
// SessionTicketKey is used by TLS servers to provide session resumption.
// See RFC 5077 and the PSK mode of RFC 8446. If zero, it will be filled
// with random data before the first server handshake.
//
// Deprecated: if this field is left at zero, session ticket keys will be
// automatically rotated every day and dropped after seven days. For
// customizing the rotation schedule or synchronizing servers that are
// terminating connections for the same host, use SetSessionTicketKeys.
SessionTicketKey [32]byte // Go 1.1
// ClientSessionCache is a cache of ClientSessionState entries for TLS
// session resumption. It is only used by clients.
ClientSessionCache ClientSessionCache // Go 1.3
// MinVersion contains the minimum TLS version that is acceptable.
//
// By default, TLS 1.2 is currently used as the minimum when acting as a
// client, and TLS 1.0 when acting as a server. TLS 1.0 is the minimum
// supported by this package, both as a client and as a server.
//
// The client-side default can temporarily be reverted to TLS 1.0 by
// including the value "x509sha1=1" in the GODEBUG environment variable.
// Note that this option will be removed in Go 1.19 (but it will still be
// possible to set this field to VersionTLS10 explicitly).
MinVersion uint16 // Go 1.2
// MaxVersion contains the maximum TLS version that is acceptable.
//
// By default, the maximum version supported by this package is used,
// which is currently TLS 1.3.
MaxVersion uint16 // Go 1.2
// CurvePreferences contains the elliptic curves that will be used in
// an ECDHE handshake, in preference order. If empty, the default will
// be used. The client will use the first preference as the type for
// its key share in TLS 1.3. This may change in the future.
CurvePreferences []CurveID // Go 1.3
// DynamicRecordSizingDisabled disables adaptive sizing of TLS records.
// When true, the largest possible TLS record size is always used. When
// false, the size of TLS records may be adjusted in an attempt to
// improve latency.
DynamicRecordSizingDisabled bool // Go 1.7
// Renegotiation controls what types of renegotiation are supported.
// The default, none, is correct for the vast majority of applications.
Renegotiation RenegotiationSupport // Go 1.7
// KeyLogWriter optionally specifies a destination for TLS master secrets
// in NSS key log format that can be used to allow external programs
// such as Wireshark to decrypt TLS connections.
// See https://developer.mozilla.org/en-US/docs/Mozilla/Projects/NSS/Key_Log_Format.
// Use of KeyLogWriter compromises security and should only be
// used for debugging.
KeyLogWriter io.Writer // Go 1.8
// contains filtered or unexported fields
}
```
#### Example (KeyLogWriter)
Code:
```
// Debugging TLS applications by decrypting a network traffic capture.
// WARNING: Use of KeyLogWriter compromises security and should only be
// used for debugging.
// Dummy test HTTP server for the example with insecure random so output is
// reproducible.
server := httptest.NewUnstartedServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {}))
server.TLS = &tls.Config{
Rand: zeroSource{}, // for example only; don't do this.
}
server.StartTLS()
defer server.Close()
// Typically the log would go to an open file:
// w, err := os.OpenFile("tls-secrets.txt", os.O_WRONLY|os.O_CREATE|os.O_TRUNC, 0600)
w := os.Stdout
client := &http.Client{
Transport: &http.Transport{
TLSClientConfig: &tls.Config{
KeyLogWriter: w,
Rand: zeroSource{}, // for reproducible output; don't do this.
InsecureSkipVerify: true, // test server certificate is not trusted.
},
},
}
resp, err := client.Get(server.URL)
if err != nil {
log.Fatalf("Failed to get URL: %v", err)
}
resp.Body.Close()
// The resulting file can be used with Wireshark to decrypt the TLS
// connection by setting (Pre)-Master-Secret log filename in SSL Protocol
// preferences.
```
#### Example (VerifyConnection)
Code:
```
// VerifyConnection can be used to replace and customize connection
// verification. This example shows a VerifyConnection implementation that
// will be approximately equivalent to what crypto/tls does normally to
// verify the peer's certificate.
// Client side configuration.
_ = &tls.Config{
// Set InsecureSkipVerify to skip the default validation we are
// replacing. This will not disable VerifyConnection.
InsecureSkipVerify: true,
VerifyConnection: func(cs tls.ConnectionState) error {
opts := x509.VerifyOptions{
DNSName: cs.ServerName,
Intermediates: x509.NewCertPool(),
}
for _, cert := range cs.PeerCertificates[1:] {
opts.Intermediates.AddCert(cert)
}
_, err := cs.PeerCertificates[0].Verify(opts)
return err
},
}
// Server side configuration.
_ = &tls.Config{
// Require client certificates (or VerifyConnection will run anyway and
// panic accessing cs.PeerCertificates[0]) but don't verify them with the
// default verifier. This will not disable VerifyConnection.
ClientAuth: tls.RequireAnyClientCert,
VerifyConnection: func(cs tls.ConnectionState) error {
opts := x509.VerifyOptions{
DNSName: cs.ServerName,
Intermediates: x509.NewCertPool(),
KeyUsages: []x509.ExtKeyUsage{x509.ExtKeyUsageClientAuth},
}
for _, cert := range cs.PeerCertificates[1:] {
opts.Intermediates.AddCert(cert)
}
_, err := cs.PeerCertificates[0].Verify(opts)
return err
},
}
// Note that when certificates are not handled by the default verifier
// ConnectionState.VerifiedChains will be nil.
```
### func (\*Config) BuildNameToCertificate
```
func (c *Config) BuildNameToCertificate()
```
BuildNameToCertificate parses c.Certificates and builds c.NameToCertificate from the CommonName and SubjectAlternateName fields of each of the leaf certificates.
Deprecated: NameToCertificate only allows associating a single certificate with a given name. Leave that field nil to let the library select the first compatible chain from Certificates.
### func (\*Config) Clone 1.8
```
func (c *Config) Clone() *Config
```
Clone returns a shallow clone of c or nil if c is nil. It is safe to clone a Config that is being used concurrently by a TLS client or server.
### func (\*Config) SetSessionTicketKeys 1.5
```
func (c *Config) SetSessionTicketKeys(keys [][32]byte)
```
SetSessionTicketKeys updates the session ticket keys for a server.
The first key will be used when creating new tickets, while all keys can be used for decrypting tickets. It is safe to call this function while the server is running in order to rotate the session ticket keys. The function will panic if keys is empty.
Calling this function will turn off automatic session ticket key rotation.
If multiple servers are terminating connections for the same host they should all have the same session ticket keys. If the session ticket keys leaks, previously recorded and future TLS connections using those keys might be compromised.
type Conn
---------
A Conn represents a secured connection. It implements the net.Conn interface.
```
type Conn struct {
// contains filtered or unexported fields
}
```
### func Client
```
func Client(conn net.Conn, config *Config) *Conn
```
Client returns a new TLS client side connection using conn as the underlying transport. The config cannot be nil: users must set either ServerName or InsecureSkipVerify in the config.
### func Dial
```
func Dial(network, addr string, config *Config) (*Conn, error)
```
Dial connects to the given network address using net.Dial and then initiates a TLS handshake, returning the resulting TLS connection. Dial interprets a nil configuration as equivalent to the zero configuration; see the documentation of Config for the defaults.
#### Example
Code:
```
// Connecting with a custom root-certificate set.
const rootPEM = `
-- GlobalSign Root R2, valid until Dec 15, 2021
-----BEGIN CERTIFICATE-----
MIIDujCCAqKgAwIBAgILBAAAAAABD4Ym5g0wDQYJKoZIhvcNAQEFBQAwTDEgMB4G
A1UECxMXR2xvYmFsU2lnbiBSb290IENBIC0gUjIxEzARBgNVBAoTCkdsb2JhbFNp
Z24xEzARBgNVBAMTCkdsb2JhbFNpZ24wHhcNMDYxMjE1MDgwMDAwWhcNMjExMjE1
MDgwMDAwWjBMMSAwHgYDVQQLExdHbG9iYWxTaWduIFJvb3QgQ0EgLSBSMjETMBEG
A1UEChMKR2xvYmFsU2lnbjETMBEGA1UEAxMKR2xvYmFsU2lnbjCCASIwDQYJKoZI
hvcNAQEBBQADggEPADCCAQoCggEBAKbPJA6+Lm8omUVCxKs+IVSbC9N/hHD6ErPL
v4dfxn+G07IwXNb9rfF73OX4YJYJkhD10FPe+3t+c4isUoh7SqbKSaZeqKeMWhG8
eoLrvozps6yWJQeXSpkqBy+0Hne/ig+1AnwblrjFuTosvNYSuetZfeLQBoZfXklq
tTleiDTsvHgMCJiEbKjNS7SgfQx5TfC4LcshytVsW33hoCmEofnTlEnLJGKRILzd
C9XZzPnqJworc5HGnRusyMvo4KD0L5CLTfuwNhv2GXqF4G3yYROIXJ/gkwpRl4pa
zq+r1feqCapgvdzZX99yqWATXgAByUr6P6TqBwMhAo6CygPCm48CAwEAAaOBnDCB
mTAOBgNVHQ8BAf8EBAMCAQYwDwYDVR0TAQH/BAUwAwEB/zAdBgNVHQ4EFgQUm+IH
V2ccHsBqBt5ZtJot39wZhi4wNgYDVR0fBC8wLTAroCmgJ4YlaHR0cDovL2NybC5n
bG9iYWxzaWduLm5ldC9yb290LXIyLmNybDAfBgNVHSMEGDAWgBSb4gdXZxwewGoG
3lm0mi3f3BmGLjANBgkqhkiG9w0BAQUFAAOCAQEAmYFThxxol4aR7OBKuEQLq4Gs
J0/WwbgcQ3izDJr86iw8bmEbTUsp9Z8FHSbBuOmDAGJFtqkIk7mpM0sYmsL4h4hO
291xNBrBVNpGP+DTKqttVCL1OmLNIG+6KYnX3ZHu01yiPqFbQfXf5WRDLenVOavS
ot+3i9DAgBkcRcAtjOj4LaR0VknFBbVPFd5uRHg5h6h+u/N5GJG79G+dwfCMNYxd
AfvDbbnvRG15RjF+Cv6pgsH/76tuIMRQyV+dTZsXjAzlAcmgQWpzU/qlULRuJQ/7
TBj0/VLZjmmx6BEP3ojY+x1J96relc8geMJgEtslQIxq/H5COEBkEveegeGTLg==
-----END CERTIFICATE-----`
// First, create the set of root certificates. For this example we only
// have one. It's also possible to omit this in order to use the
// default root set of the current operating system.
roots := x509.NewCertPool()
ok := roots.AppendCertsFromPEM([]byte(rootPEM))
if !ok {
panic("failed to parse root certificate")
}
conn, err := tls.Dial("tcp", "mail.google.com:443", &tls.Config{
RootCAs: roots,
})
if err != nil {
panic("failed to connect: " + err.Error())
}
conn.Close()
```
### func DialWithDialer 1.3
```
func DialWithDialer(dialer *net.Dialer, network, addr string, config *Config) (*Conn, error)
```
DialWithDialer connects to the given network address using dialer.Dial and then initiates a TLS handshake, returning the resulting TLS connection. Any timeout or deadline given in the dialer apply to connection and TLS handshake as a whole.
DialWithDialer interprets a nil configuration as equivalent to the zero configuration; see the documentation of Config for the defaults.
DialWithDialer uses context.Background internally; to specify the context, use Dialer.DialContext with NetDialer set to the desired dialer.
### func Server
```
func Server(conn net.Conn, config *Config) *Conn
```
Server returns a new TLS server side connection using conn as the underlying transport. The configuration config must be non-nil and must include at least one certificate or else set GetCertificate.
### func (\*Conn) Close
```
func (c *Conn) Close() error
```
Close closes the connection.
### func (\*Conn) CloseWrite 1.8
```
func (c *Conn) CloseWrite() error
```
CloseWrite shuts down the writing side of the connection. It should only be called once the handshake has completed and does not call CloseWrite on the underlying connection. Most callers should just use Close.
### func (\*Conn) ConnectionState
```
func (c *Conn) ConnectionState() ConnectionState
```
ConnectionState returns basic TLS details about the connection.
### func (\*Conn) Handshake
```
func (c *Conn) Handshake() error
```
Handshake runs the client or server handshake protocol if it has not yet been run.
Most uses of this package need not call Handshake explicitly: the first Read or Write will call it automatically.
For control over canceling or setting a timeout on a handshake, use HandshakeContext or the Dialer's DialContext method instead.
### func (\*Conn) HandshakeContext 1.17
```
func (c *Conn) HandshakeContext(ctx context.Context) error
```
HandshakeContext runs the client or server handshake protocol if it has not yet been run.
The provided Context must be non-nil. If the context is canceled before the handshake is complete, the handshake is interrupted and an error is returned. Once the handshake has completed, cancellation of the context will not affect the connection.
Most uses of this package need not call HandshakeContext explicitly: the first Read or Write will call it automatically.
### func (\*Conn) LocalAddr
```
func (c *Conn) LocalAddr() net.Addr
```
LocalAddr returns the local network address.
### func (\*Conn) NetConn 1.18
```
func (c *Conn) NetConn() net.Conn
```
NetConn returns the underlying connection that is wrapped by c. Note that writing to or reading from this connection directly will corrupt the TLS session.
### func (\*Conn) OCSPResponse
```
func (c *Conn) OCSPResponse() []byte
```
OCSPResponse returns the stapled OCSP response from the TLS server, if any. (Only valid for client connections.)
### func (\*Conn) Read
```
func (c *Conn) Read(b []byte) (int, error)
```
Read reads data from the connection.
As Read calls Handshake, in order to prevent indefinite blocking a deadline must be set for both Read and Write before Read is called when the handshake has not yet completed. See SetDeadline, SetReadDeadline, and SetWriteDeadline.
### func (\*Conn) RemoteAddr
```
func (c *Conn) RemoteAddr() net.Addr
```
RemoteAddr returns the remote network address.
### func (\*Conn) SetDeadline
```
func (c *Conn) SetDeadline(t time.Time) error
```
SetDeadline sets the read and write deadlines associated with the connection. A zero value for t means Read and Write will not time out. After a Write has timed out, the TLS state is corrupt and all future writes will return the same error.
### func (\*Conn) SetReadDeadline
```
func (c *Conn) SetReadDeadline(t time.Time) error
```
SetReadDeadline sets the read deadline on the underlying connection. A zero value for t means Read will not time out.
### func (\*Conn) SetWriteDeadline
```
func (c *Conn) SetWriteDeadline(t time.Time) error
```
SetWriteDeadline sets the write deadline on the underlying connection. A zero value for t means Write will not time out. After a Write has timed out, the TLS state is corrupt and all future writes will return the same error.
### func (\*Conn) VerifyHostname
```
func (c *Conn) VerifyHostname(host string) error
```
VerifyHostname checks that the peer certificate chain is valid for connecting to host. If so, it returns nil; if not, it returns an error describing the problem.
### func (\*Conn) Write
```
func (c *Conn) Write(b []byte) (int, error)
```
Write writes data to the connection.
As Write calls Handshake, in order to prevent indefinite blocking a deadline must be set for both Read and Write before Write is called when the handshake has not yet completed. See SetDeadline, SetReadDeadline, and SetWriteDeadline.
type ConnectionState
--------------------
ConnectionState records basic TLS details about the connection.
```
type ConnectionState struct {
// Version is the TLS version used by the connection (e.g. VersionTLS12).
Version uint16 // Go 1.3
// HandshakeComplete is true if the handshake has concluded.
HandshakeComplete bool
// DidResume is true if this connection was successfully resumed from a
// previous session with a session ticket or similar mechanism.
DidResume bool // Go 1.1
// CipherSuite is the cipher suite negotiated for the connection (e.g.
// TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256, TLS_AES_128_GCM_SHA256).
CipherSuite uint16
// NegotiatedProtocol is the application protocol negotiated with ALPN.
NegotiatedProtocol string
// NegotiatedProtocolIsMutual used to indicate a mutual NPN negotiation.
//
// Deprecated: this value is always true.
NegotiatedProtocolIsMutual bool
// ServerName is the value of the Server Name Indication extension sent by
// the client. It's available both on the server and on the client side.
ServerName string
// PeerCertificates are the parsed certificates sent by the peer, in the
// order in which they were sent. The first element is the leaf certificate
// that the connection is verified against.
//
// On the client side, it can't be empty. On the server side, it can be
// empty if Config.ClientAuth is not RequireAnyClientCert or
// RequireAndVerifyClientCert.
//
// PeerCertificates and its contents should not be modified.
PeerCertificates []*x509.Certificate
// VerifiedChains is a list of one or more chains where the first element is
// PeerCertificates[0] and the last element is from Config.RootCAs (on the
// client side) or Config.ClientCAs (on the server side).
//
// On the client side, it's set if Config.InsecureSkipVerify is false. On
// the server side, it's set if Config.ClientAuth is VerifyClientCertIfGiven
// (and the peer provided a certificate) or RequireAndVerifyClientCert.
//
// VerifiedChains and its contents should not be modified.
VerifiedChains [][]*x509.Certificate
// SignedCertificateTimestamps is a list of SCTs provided by the peer
// through the TLS handshake for the leaf certificate, if any.
SignedCertificateTimestamps [][]byte // Go 1.5
// OCSPResponse is a stapled Online Certificate Status Protocol (OCSP)
// response provided by the peer for the leaf certificate, if any.
OCSPResponse []byte // Go 1.5
// TLSUnique contains the "tls-unique" channel binding value (see RFC 5929,
// Section 3). This value will be nil for TLS 1.3 connections and for all
// resumed connections.
//
// Deprecated: there are conditions in which this value might not be unique
// to a connection. See the Security Considerations sections of RFC 5705 and
// RFC 7627, and https://mitls.org/pages/attacks/3SHAKE#channelbindings.
TLSUnique []byte // Go 1.4
// contains filtered or unexported fields
}
```
### func (\*ConnectionState) ExportKeyingMaterial 1.11
```
func (cs *ConnectionState) ExportKeyingMaterial(label string, context []byte, length int) ([]byte, error)
```
ExportKeyingMaterial returns length bytes of exported key material in a new slice as defined in RFC 5705. If context is nil, it is not used as part of the seed. If the connection was set to allow renegotiation via Config.Renegotiation, this function will return an error.
type CurveID 1.3
----------------
CurveID is the type of a TLS identifier for an elliptic curve. See <https://www.iana.org/assignments/tls-parameters/tls-parameters.xml#tls-parameters-8>.
In TLS 1.3, this type is called NamedGroup, but at this time this library only supports Elliptic Curve based groups. See RFC 8446, Section 4.2.7.
```
type CurveID uint16
```
```
const (
CurveP256 CurveID = 23
CurveP384 CurveID = 24
CurveP521 CurveID = 25
X25519 CurveID = 29
)
```
### func (CurveID) String 1.15
```
func (i CurveID) String() string
```
type Dialer 1.15
----------------
Dialer dials TLS connections given a configuration and a Dialer for the underlying connection.
```
type Dialer struct {
// NetDialer is the optional dialer to use for the TLS connections'
// underlying TCP connections.
// A nil NetDialer is equivalent to the net.Dialer zero value.
NetDialer *net.Dialer
// Config is the TLS configuration to use for new connections.
// A nil configuration is equivalent to the zero
// configuration; see the documentation of Config for the
// defaults.
Config *Config
}
```
### func (\*Dialer) Dial 1.15
```
func (d *Dialer) Dial(network, addr string) (net.Conn, error)
```
Dial connects to the given network address and initiates a TLS handshake, returning the resulting TLS connection.
The returned Conn, if any, will always be of type \*Conn.
Dial uses context.Background internally; to specify the context, use DialContext.
### func (\*Dialer) DialContext 1.15
```
func (d *Dialer) DialContext(ctx context.Context, network, addr string) (net.Conn, error)
```
DialContext connects to the given network address and initiates a TLS handshake, returning the resulting TLS connection.
The provided Context must be non-nil. If the context expires before the connection is complete, an error is returned. Once successfully connected, any expiration of the context will not affect the connection.
The returned Conn, if any, will always be of type \*Conn.
type RecordHeaderError 1.6
--------------------------
RecordHeaderError is returned when a TLS record header is invalid.
```
type RecordHeaderError struct {
// Msg contains a human readable string that describes the error.
Msg string
// RecordHeader contains the five bytes of TLS record header that
// triggered the error.
RecordHeader [5]byte
// Conn provides the underlying net.Conn in the case that a client
// sent an initial handshake that didn't look like TLS.
// It is nil if there's already been a handshake or a TLS alert has
// been written to the connection.
Conn net.Conn // Go 1.12
}
```
### func (RecordHeaderError) Error 1.6
```
func (e RecordHeaderError) Error() string
```
type RenegotiationSupport 1.7
-----------------------------
RenegotiationSupport enumerates the different levels of support for TLS renegotiation. TLS renegotiation is the act of performing subsequent handshakes on a connection after the first. This significantly complicates the state machine and has been the source of numerous, subtle security issues. Initiating a renegotiation is not supported, but support for accepting renegotiation requests may be enabled.
Even when enabled, the server may not change its identity between handshakes (i.e. the leaf certificate must be the same). Additionally, concurrent handshake and application data flow is not permitted so renegotiation can only be used with protocols that synchronise with the renegotiation, such as HTTPS.
Renegotiation is not defined in TLS 1.3.
```
type RenegotiationSupport int
```
```
const (
// RenegotiateNever disables renegotiation.
RenegotiateNever RenegotiationSupport = iota
// RenegotiateOnceAsClient allows a remote server to request
// renegotiation once per connection.
RenegotiateOnceAsClient
// RenegotiateFreelyAsClient allows a remote server to repeatedly
// request renegotiation.
RenegotiateFreelyAsClient
)
```
type SignatureScheme 1.8
------------------------
SignatureScheme identifies a signature algorithm supported by TLS. See RFC 8446, Section 4.2.3.
```
type SignatureScheme uint16
```
```
const (
// RSASSA-PKCS1-v1_5 algorithms.
PKCS1WithSHA256 SignatureScheme = 0x0401
PKCS1WithSHA384 SignatureScheme = 0x0501
PKCS1WithSHA512 SignatureScheme = 0x0601
// RSASSA-PSS algorithms with public key OID rsaEncryption.
PSSWithSHA256 SignatureScheme = 0x0804
PSSWithSHA384 SignatureScheme = 0x0805
PSSWithSHA512 SignatureScheme = 0x0806
// ECDSA algorithms. Only constrained to a specific curve in TLS 1.3.
ECDSAWithP256AndSHA256 SignatureScheme = 0x0403
ECDSAWithP384AndSHA384 SignatureScheme = 0x0503
ECDSAWithP521AndSHA512 SignatureScheme = 0x0603
// EdDSA algorithms.
Ed25519 SignatureScheme = 0x0807
// Legacy signature and hash algorithms for TLS 1.2.
PKCS1WithSHA1 SignatureScheme = 0x0201
ECDSAWithSHA1 SignatureScheme = 0x0203
)
```
### func (SignatureScheme) String 1.15
```
func (i SignatureScheme) String() string
```
Bugs
----
* ☞ The crypto/tls package only implements some countermeasures against Lucky13 attacks on CBC-mode encryption, and only on SHA1 variants. See <http://www.isg.rhul.ac.uk/tls/TLStiming.pdf> and <https://www.imperialviolet.org/2013/02/04/luckythirteen.html>.
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [fipsonly](fipsonly/index) | Package fipsonly restricts all TLS configuration to FIPS-approved settings. |
| programming_docs |
go Command fipsonly Command fipsonly
=================
Package fipsonly restricts all TLS configuration to FIPS-approved settings.
The effect is triggered by importing the package anywhere in a program, as in:
```
import _ "crypto/tls/fipsonly"
```
This package only exists when using Go compiled with GOEXPERIMENT=boringcrypto.
go Package sha256 Package sha256
===============
* `import "crypto/sha256"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package sha256 implements the SHA224 and SHA256 hash algorithms as defined in FIPS 180-4.
Index
-----
* [Constants](#pkg-constants)
* [func New() hash.Hash](#New)
* [func New224() hash.Hash](#New224)
* [func Sum224(data []byte) [Size224]byte](#Sum224)
* [func Sum256(data []byte) [Size]byte](#Sum256)
### Examples
[New](#example_New) [New (File)](#example_New_file) [Sum256](#example_Sum256) ### Package files
sha256.go sha256block.go sha256block\_amd64.go sha256block\_decl.go
Constants
---------
The blocksize of SHA256 and SHA224 in bytes.
```
const BlockSize = 64
```
The size of a SHA256 checksum in bytes.
```
const Size = 32
```
The size of a SHA224 checksum in bytes.
```
const Size224 = 28
```
func New
--------
```
func New() hash.Hash
```
New returns a new hash.Hash computing the SHA256 checksum. The Hash also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
#### Example
Code:
```
h := sha256.New()
h.Write([]byte("hello world\n"))
fmt.Printf("%x", h.Sum(nil))
```
Output:
```
a948904f2f0f479b8f8197694b30184b0d2ed1c1cd2a1ec0fb85d299a192a447
```
#### Example (File)
Code:
```
f, err := os.Open("file.txt")
if err != nil {
log.Fatal(err)
}
defer f.Close()
h := sha256.New()
if _, err := io.Copy(h, f); err != nil {
log.Fatal(err)
}
fmt.Printf("%x", h.Sum(nil))
```
func New224
-----------
```
func New224() hash.Hash
```
New224 returns a new hash.Hash computing the SHA224 checksum.
func Sum224 1.2
---------------
```
func Sum224(data []byte) [Size224]byte
```
Sum224 returns the SHA224 checksum of the data.
func Sum256 1.2
---------------
```
func Sum256(data []byte) [Size]byte
```
Sum256 returns the SHA256 checksum of the data.
#### Example
Code:
```
sum := sha256.Sum256([]byte("hello world\n"))
fmt.Printf("%x", sum)
```
Output:
```
a948904f2f0f479b8f8197694b30184b0d2ed1c1cd2a1ec0fb85d299a192a447
```
go Command boring Command boring
===============
Package boring exposes functions that are only available when building with Go+BoringCrypto. This package is available on all targets as long as the Go+BoringCrypto toolchain is used. Use the Enabled function to determine whether the BoringCrypto core is actually in use.
Any time the Go+BoringCrypto toolchain is used, the "boringcrypto" build tag is satisfied, so that applications can tag files that use this package.
go Package sha1 Package sha1
=============
* `import "crypto/sha1"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package sha1 implements the SHA-1 hash algorithm as defined in RFC 3174.
SHA-1 is cryptographically broken and should not be used for secure applications.
Index
-----
* [Constants](#pkg-constants)
* [func New() hash.Hash](#New)
* [func Sum(data []byte) [Size]byte](#Sum)
### Examples
[New](#example_New) [New (File)](#example_New_file) [Sum](#example_Sum) ### Package files
boring.go sha1.go sha1block.go sha1block\_amd64.go
Constants
---------
The blocksize of SHA-1 in bytes.
```
const BlockSize = 64
```
The size of a SHA-1 checksum in bytes.
```
const Size = 20
```
func New
--------
```
func New() hash.Hash
```
New returns a new hash.Hash computing the SHA1 checksum. The Hash also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
#### Example
Code:
```
h := sha1.New()
io.WriteString(h, "His money is twice tainted:")
io.WriteString(h, " 'taint yours and 'taint mine.")
fmt.Printf("% x", h.Sum(nil))
```
Output:
```
59 7f 6a 54 00 10 f9 4c 15 d7 18 06 a9 9a 2c 87 10 e7 47 bd
```
#### Example (File)
Code:
```
f, err := os.Open("file.txt")
if err != nil {
log.Fatal(err)
}
defer f.Close()
h := sha1.New()
if _, err := io.Copy(h, f); err != nil {
log.Fatal(err)
}
fmt.Printf("% x", h.Sum(nil))
```
func Sum 1.2
------------
```
func Sum(data []byte) [Size]byte
```
Sum returns the SHA-1 checksum of the data.
#### Example
Code:
```
data := []byte("This page intentionally left blank.")
fmt.Printf("% x", sha1.Sum(data))
```
Output:
```
af 06 49 23 bb f2 30 15 96 aa c4 c2 73 ba 32 17 8e bc 4a 96
```
go Package ecdh Package ecdh
=============
* `import "crypto/ecdh"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package ecdh implements Elliptic Curve Diffie-Hellman over NIST curves and Curve25519.
Index
-----
* [type Curve](#Curve)
* [func P256() Curve](#P256)
* [func P384() Curve](#P384)
* [func P521() Curve](#P521)
* [func X25519() Curve](#X25519)
* [type PrivateKey](#PrivateKey)
* [func (k \*PrivateKey) Bytes() []byte](#PrivateKey.Bytes)
* [func (k \*PrivateKey) Curve() Curve](#PrivateKey.Curve)
* [func (k \*PrivateKey) ECDH(remote \*PublicKey) ([]byte, error)](#PrivateKey.ECDH)
* [func (k \*PrivateKey) Equal(x crypto.PrivateKey) bool](#PrivateKey.Equal)
* [func (k \*PrivateKey) Public() crypto.PublicKey](#PrivateKey.Public)
* [func (k \*PrivateKey) PublicKey() \*PublicKey](#PrivateKey.PublicKey)
* [type PublicKey](#PublicKey)
* [func (k \*PublicKey) Bytes() []byte](#PublicKey.Bytes)
* [func (k \*PublicKey) Curve() Curve](#PublicKey.Curve)
* [func (k \*PublicKey) Equal(x crypto.PublicKey) bool](#PublicKey.Equal)
### Package files
ecdh.go nist.go x25519.go
type Curve 1.20
---------------
```
type Curve interface {
// GenerateKey generates a new PrivateKey from rand.
GenerateKey(rand io.Reader) (*PrivateKey, error)
// NewPrivateKey checks that key is valid and returns a PrivateKey.
//
// For NIST curves, this follows SEC 1, Version 2.0, Section 2.3.6, which
// amounts to decoding the bytes as a fixed length big endian integer and
// checking that the result is lower than the order of the curve. The zero
// private key is also rejected, as the encoding of the corresponding public
// key would be irregular.
//
// For X25519, this only checks the scalar length.
NewPrivateKey(key []byte) (*PrivateKey, error)
// NewPublicKey checks that key is valid and returns a PublicKey.
//
// For NIST curves, this decodes an uncompressed point according to SEC 1,
// Version 2.0, Section 2.3.4. Compressed encodings and the point at
// infinity are rejected.
//
// For X25519, this only checks the u-coordinate length. Adversarially
// selected public keys can cause ECDH to return an error.
NewPublicKey(key []byte) (*PublicKey, error)
// contains filtered or unexported methods
}
```
### func P256 1.20
```
func P256() Curve
```
P256 returns a Curve which implements NIST P-256 (FIPS 186-3, section D.2.3), also known as secp256r1 or prime256v1.
Multiple invocations of this function will return the same value, which can be used for equality checks and switch statements.
### func P384 1.20
```
func P384() Curve
```
P384 returns a Curve which implements NIST P-384 (FIPS 186-3, section D.2.4), also known as secp384r1.
Multiple invocations of this function will return the same value, which can be used for equality checks and switch statements.
### func P521 1.20
```
func P521() Curve
```
P521 returns a Curve which implements NIST P-521 (FIPS 186-3, section D.2.5), also known as secp521r1.
Multiple invocations of this function will return the same value, which can be used for equality checks and switch statements.
### func X25519 1.20
```
func X25519() Curve
```
X25519 returns a Curve which implements the X25519 function over Curve25519 (RFC 7748, Section 5).
Multiple invocations of this function will return the same value, so it can be used for equality checks and switch statements.
type PrivateKey 1.20
--------------------
PrivateKey is an ECDH private key, usually kept secret.
These keys can be parsed with crypto/x509.ParsePKCS8PrivateKey and encoded with crypto/x509.MarshalPKCS8PrivateKey. For NIST curves, they then need to be converted with crypto/ecdsa.PrivateKey.ECDH after parsing.
```
type PrivateKey struct {
// contains filtered or unexported fields
}
```
### func (\*PrivateKey) Bytes 1.20
```
func (k *PrivateKey) Bytes() []byte
```
Bytes returns a copy of the encoding of the private key.
### func (\*PrivateKey) Curve 1.20
```
func (k *PrivateKey) Curve() Curve
```
### func (\*PrivateKey) ECDH 1.20
```
func (k *PrivateKey) ECDH(remote *PublicKey) ([]byte, error)
```
ECDH performs a ECDH exchange and returns the shared secret.
For NIST curves, this performs ECDH as specified in SEC 1, Version 2.0, Section 3.3.1, and returns the x-coordinate encoded according to SEC 1, Version 2.0, Section 2.3.5. The result is never the point at infinity.
For X25519, this performs ECDH as specified in RFC 7748, Section 6.1. If the result is the all-zero value, ECDH returns an error.
### func (\*PrivateKey) Equal 1.20
```
func (k *PrivateKey) Equal(x crypto.PrivateKey) bool
```
Equal returns whether x represents the same private key as k.
Note that there can be equivalent private keys with different encodings which would return false from this check but behave the same way as inputs to ECDH.
This check is performed in constant time as long as the key types and their curve match.
### func (\*PrivateKey) Public 1.20
```
func (k *PrivateKey) Public() crypto.PublicKey
```
Public implements the implicit interface of all standard library private keys. See the docs of crypto.PrivateKey.
### func (\*PrivateKey) PublicKey 1.20
```
func (k *PrivateKey) PublicKey() *PublicKey
```
type PublicKey 1.20
-------------------
PublicKey is an ECDH public key, usually a peer's ECDH share sent over the wire.
These keys can be parsed with crypto/x509.ParsePKIXPublicKey and encoded with crypto/x509.MarshalPKIXPublicKey. For NIST curves, they then need to be converted with crypto/ecdsa.PublicKey.ECDH after parsing.
```
type PublicKey struct {
// contains filtered or unexported fields
}
```
### func (\*PublicKey) Bytes 1.20
```
func (k *PublicKey) Bytes() []byte
```
Bytes returns a copy of the encoding of the public key.
### func (\*PublicKey) Curve 1.20
```
func (k *PublicKey) Curve() Curve
```
### func (\*PublicKey) Equal 1.20
```
func (k *PublicKey) Equal(x crypto.PublicKey) bool
```
Equal returns whether x represents the same public key as k.
Note that there can be equivalent public keys with different encodings which would return false from this check but behave the same way as inputs to ECDH.
This check is performed in constant time as long as the key types and their curve match.
go Package rand Package rand
=============
* `import "crypto/rand"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package rand implements a cryptographically secure random number generator.
Index
-----
* [Variables](#pkg-variables)
* [func Int(rand io.Reader, max \*big.Int) (n \*big.Int, err error)](#Int)
* [func Prime(rand io.Reader, bits int) (\*big.Int, error)](#Prime)
* [func Read(b []byte) (n int, err error)](#Read)
### Examples
[Read](#example_Read) ### Package files
rand.go rand\_getrandom.go rand\_unix.go util.go
Variables
---------
Reader is a global, shared instance of a cryptographically secure random number generator.
On Linux, FreeBSD, Dragonfly and Solaris, Reader uses getrandom(2) if available, /dev/urandom otherwise. On OpenBSD and macOS, Reader uses getentropy(2). On other Unix-like systems, Reader reads from /dev/urandom. On Windows systems, Reader uses the RtlGenRandom API. On Wasm, Reader uses the Web Crypto API.
```
var Reader io.Reader
```
func Int
--------
```
func Int(rand io.Reader, max *big.Int) (n *big.Int, err error)
```
Int returns a uniform random value in [0, max). It panics if max <= 0.
func Prime
----------
```
func Prime(rand io.Reader, bits int) (*big.Int, error)
```
Prime returns a number of the given bit length that is prime with high probability. Prime will return error for any error returned by rand.Read or if bits < 2.
func Read
---------
```
func Read(b []byte) (n int, err error)
```
Read is a helper function that calls Reader.Read using io.ReadFull. On return, n == len(b) if and only if err == nil.
#### Example
This example reads 10 cryptographically secure pseudorandom numbers from rand.Reader and writes them to a byte slice.
Code:
```
c := 10
b := make([]byte, c)
_, err := rand.Read(b)
if err != nil {
fmt.Println("error:", err)
return
}
// The slice should now contain random bytes instead of only zeroes.
fmt.Println(bytes.Equal(b, make([]byte, c)))
```
Output:
```
false
```
go Package ed25519 Package ed25519
================
* `import "crypto/ed25519"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package ed25519 implements the Ed25519 signature algorithm. See <https://ed25519.cr.yp.to/>.
These functions are also compatible with the “Ed25519” function defined in RFC 8032. However, unlike RFC 8032's formulation, this package's private key representation includes a public key suffix to make multiple signing operations with the same key more efficient. This package refers to the RFC 8032 private key as the “seed”.
#### Example (Ed25519ctx)
Code:
```
pub, priv, err := GenerateKey(nil)
if err != nil {
log.Fatal(err)
}
msg := []byte("The quick brown fox jumps over the lazy dog")
sig, err := priv.Sign(nil, msg, &Options{
Context: "Example_ed25519ctx",
})
if err != nil {
log.Fatal(err)
}
if err := VerifyWithOptions(pub, msg, sig, &Options{
Context: "Example_ed25519ctx",
}); err != nil {
log.Fatal("invalid signature")
}
```
Index
-----
* [Constants](#pkg-constants)
* [func GenerateKey(rand io.Reader) (PublicKey, PrivateKey, error)](#GenerateKey)
* [func Sign(privateKey PrivateKey, message []byte) []byte](#Sign)
* [func Verify(publicKey PublicKey, message, sig []byte) bool](#Verify)
* [func VerifyWithOptions(publicKey PublicKey, message, sig []byte, opts \*Options) error](#VerifyWithOptions)
* [type Options](#Options)
* [func (o \*Options) HashFunc() crypto.Hash](#Options.HashFunc)
* [type PrivateKey](#PrivateKey)
* [func NewKeyFromSeed(seed []byte) PrivateKey](#NewKeyFromSeed)
* [func (priv PrivateKey) Equal(x crypto.PrivateKey) bool](#PrivateKey.Equal)
* [func (priv PrivateKey) Public() crypto.PublicKey](#PrivateKey.Public)
* [func (priv PrivateKey) Seed() []byte](#PrivateKey.Seed)
* [func (priv PrivateKey) Sign(rand io.Reader, message []byte, opts crypto.SignerOpts) (signature []byte, err error)](#PrivateKey.Sign)
* [type PublicKey](#PublicKey)
* [func (pub PublicKey) Equal(x crypto.PublicKey) bool](#PublicKey.Equal)
### Examples
[Package (Ed25519ctx)](#example__ed25519ctx) ### Package files
ed25519.go
Constants
---------
```
const (
// PublicKeySize is the size, in bytes, of public keys as used in this package.
PublicKeySize = 32
// PrivateKeySize is the size, in bytes, of private keys as used in this package.
PrivateKeySize = 64
// SignatureSize is the size, in bytes, of signatures generated and verified by this package.
SignatureSize = 64
// SeedSize is the size, in bytes, of private key seeds. These are the private key representations used by RFC 8032.
SeedSize = 32
)
```
func GenerateKey 1.13
---------------------
```
func GenerateKey(rand io.Reader) (PublicKey, PrivateKey, error)
```
GenerateKey generates a public/private key pair using entropy from rand. If rand is nil, crypto/rand.Reader will be used.
func Sign 1.13
--------------
```
func Sign(privateKey PrivateKey, message []byte) []byte
```
Sign signs the message with privateKey and returns a signature. It will panic if len(privateKey) is not [PrivateKeySize](#PrivateKeySize).
func Verify 1.13
----------------
```
func Verify(publicKey PublicKey, message, sig []byte) bool
```
Verify reports whether sig is a valid signature of message by publicKey. It will panic if len(publicKey) is not [PublicKeySize](#PublicKeySize).
func VerifyWithOptions 1.20
---------------------------
```
func VerifyWithOptions(publicKey PublicKey, message, sig []byte, opts *Options) error
```
VerifyWithOptions reports whether sig is a valid signature of message by publicKey. A valid signature is indicated by returning a nil error. It will panic if len(publicKey) is not [PublicKeySize](#PublicKeySize).
If opts.Hash is crypto.SHA512, the pre-hashed variant Ed25519ph is used and message is expected to be a SHA-512 hash, otherwise opts.Hash must be crypto.Hash(0) and the message must not be hashed, as Ed25519 performs two passes over messages to be signed.
type Options 1.20
-----------------
Options can be used with [PrivateKey.Sign](#PrivateKey.Sign) or [VerifyWithOptions](#VerifyWithOptions) to select Ed25519 variants.
```
type Options struct {
// Hash can be zero for regular Ed25519, or crypto.SHA512 for Ed25519ph.
Hash crypto.Hash
// Context, if not empty, selects Ed25519ctx or provides the context string
// for Ed25519ph. It can be at most 255 bytes in length.
Context string
}
```
### func (\*Options) HashFunc 1.20
```
func (o *Options) HashFunc() crypto.Hash
```
HashFunc returns o.Hash.
type PrivateKey 1.13
--------------------
PrivateKey is the type of Ed25519 private keys. It implements crypto.Signer.
```
type PrivateKey []byte
```
### func NewKeyFromSeed 1.13
```
func NewKeyFromSeed(seed []byte) PrivateKey
```
NewKeyFromSeed calculates a private key from a seed. It will panic if len(seed) is not [SeedSize](#SeedSize). This function is provided for interoperability with RFC 8032. RFC 8032's private keys correspond to seeds in this package.
### func (PrivateKey) Equal 1.15
```
func (priv PrivateKey) Equal(x crypto.PrivateKey) bool
```
Equal reports whether priv and x have the same value.
### func (PrivateKey) Public 1.13
```
func (priv PrivateKey) Public() crypto.PublicKey
```
Public returns the [PublicKey](#PublicKey) corresponding to priv.
### func (PrivateKey) Seed 1.13
```
func (priv PrivateKey) Seed() []byte
```
Seed returns the private key seed corresponding to priv. It is provided for interoperability with RFC 8032. RFC 8032's private keys correspond to seeds in this package.
### func (PrivateKey) Sign 1.13
```
func (priv PrivateKey) Sign(rand io.Reader, message []byte, opts crypto.SignerOpts) (signature []byte, err error)
```
Sign signs the given message with priv. rand is ignored.
If opts.HashFunc() is crypto.SHA512, the pre-hashed variant Ed25519ph is used and message is expected to be a SHA-512 hash, otherwise opts.HashFunc() must be crypto.Hash(0) and the message must not be hashed, as Ed25519 performs two passes over messages to be signed.
A value of type [Options](#Options) can be used as opts, or crypto.Hash(0) or crypto.SHA512 directly to select plain Ed25519 or Ed25519ph, respectively.
type PublicKey 1.13
-------------------
PublicKey is the type of Ed25519 public keys.
```
type PublicKey []byte
```
### func (PublicKey) Equal 1.15
```
func (pub PublicKey) Equal(x crypto.PublicKey) bool
```
Equal reports whether pub and x have the same value.
go Package subtle Package subtle
===============
* `import "crypto/subtle"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package subtle implements functions that are often useful in cryptographic code but require careful thought to use correctly.
Index
-----
* [func ConstantTimeByteEq(x, y uint8) int](#ConstantTimeByteEq)
* [func ConstantTimeCompare(x, y []byte) int](#ConstantTimeCompare)
* [func ConstantTimeCopy(v int, x, y []byte)](#ConstantTimeCopy)
* [func ConstantTimeEq(x, y int32) int](#ConstantTimeEq)
* [func ConstantTimeLessOrEq(x, y int) int](#ConstantTimeLessOrEq)
* [func ConstantTimeSelect(v, x, y int) int](#ConstantTimeSelect)
* [func XORBytes(dst, x, y []byte) int](#XORBytes)
### Package files
constant\_time.go xor.go xor\_amd64.go
func ConstantTimeByteEq
-----------------------
```
func ConstantTimeByteEq(x, y uint8) int
```
ConstantTimeByteEq returns 1 if x == y and 0 otherwise.
func ConstantTimeCompare
------------------------
```
func ConstantTimeCompare(x, y []byte) int
```
ConstantTimeCompare returns 1 if the two slices, x and y, have equal contents and 0 otherwise. The time taken is a function of the length of the slices and is independent of the contents. If the lengths of x and y do not match it returns 0 immediately.
func ConstantTimeCopy
---------------------
```
func ConstantTimeCopy(v int, x, y []byte)
```
ConstantTimeCopy copies the contents of y into x (a slice of equal length) if v == 1. If v == 0, x is left unchanged. Its behavior is undefined if v takes any other value.
func ConstantTimeEq
-------------------
```
func ConstantTimeEq(x, y int32) int
```
ConstantTimeEq returns 1 if x == y and 0 otherwise.
func ConstantTimeLessOrEq 1.2
-----------------------------
```
func ConstantTimeLessOrEq(x, y int) int
```
ConstantTimeLessOrEq returns 1 if x <= y and 0 otherwise. Its behavior is undefined if x or y are negative or > 2\*\*31 - 1.
func ConstantTimeSelect
-----------------------
```
func ConstantTimeSelect(v, x, y int) int
```
ConstantTimeSelect returns x if v == 1 and y if v == 0. Its behavior is undefined if v takes any other value.
func XORBytes 1.20
------------------
```
func XORBytes(dst, x, y []byte) int
```
XORBytes sets dst[i] = x[i] ^ y[i] for all i < n = min(len(x), len(y)), returning n, the number of bytes written to dst. If dst does not have length at least n, XORBytes panics without writing anything to dst.
| programming_docs |
go Package aes Package aes
============
* `import "crypto/aes"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package aes implements AES encryption (formerly Rijndael), as defined in U.S. Federal Information Processing Standards Publication 197.
The AES operations in this package are not implemented using constant-time algorithms. An exception is when running on systems with enabled hardware support for AES that makes these operations constant-time. Examples include amd64 systems using AES-NI extensions and s390x systems using Message-Security-Assist extensions. On such systems, when the result of NewCipher is passed to cipher.NewGCM, the GHASH operation used by GCM is also constant-time.
Index
-----
* [Constants](#pkg-constants)
* [func NewCipher(key []byte) (cipher.Block, error)](#NewCipher)
* [type KeySizeError](#KeySizeError)
* [func (k KeySizeError) Error() string](#KeySizeError.Error)
### Package files
aes\_gcm.go block.go cipher.go cipher\_asm.go const.go modes.go
Constants
---------
The AES block size in bytes.
```
const BlockSize = 16
```
func NewCipher
--------------
```
func NewCipher(key []byte) (cipher.Block, error)
```
NewCipher creates and returns a new cipher.Block. The key argument should be the AES key, either 16, 24, or 32 bytes to select AES-128, AES-192, or AES-256.
type KeySizeError
-----------------
```
type KeySizeError int
```
### func (KeySizeError) Error
```
func (k KeySizeError) Error() string
```
go Package flate Package flate
==============
* `import "compress/flate"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package flate implements the DEFLATE compressed data format, described in RFC 1951. The gzip and zlib packages implement access to DEFLATE-based file formats.
#### Example (Dictionary)
A preset dictionary can be used to improve the compression ratio. The downside to using a dictionary is that the compressor and decompressor must agree in advance what dictionary to use.
Code:
```
// The dictionary is a string of bytes. When compressing some input data,
// the compressor will attempt to substitute substrings with matches found
// in the dictionary. As such, the dictionary should only contain substrings
// that are expected to be found in the actual data stream.
const dict = `<?xml version="1.0"?>` + `<book>` + `<data>` + `<meta name="` + `" content="`
// The data to compress should (but is not required to) contain frequent
// substrings that match those in the dictionary.
const data = `<?xml version="1.0"?>
<book>
<meta name="title" content="The Go Programming Language"/>
<meta name="authors" content="Alan Donovan and Brian Kernighan"/>
<meta name="published" content="2015-10-26"/>
<meta name="isbn" content="978-0134190440"/>
<data>...</data>
</book>
`
var b bytes.Buffer
// Compress the data using the specially crafted dictionary.
zw, err := flate.NewWriterDict(&b, flate.DefaultCompression, []byte(dict))
if err != nil {
log.Fatal(err)
}
if _, err := io.Copy(zw, strings.NewReader(data)); err != nil {
log.Fatal(err)
}
if err := zw.Close(); err != nil {
log.Fatal(err)
}
// The decompressor must use the same dictionary as the compressor.
// Otherwise, the input may appear as corrupted.
fmt.Println("Decompressed output using the dictionary:")
zr := flate.NewReaderDict(bytes.NewReader(b.Bytes()), []byte(dict))
if _, err := io.Copy(os.Stdout, zr); err != nil {
log.Fatal(err)
}
if err := zr.Close(); err != nil {
log.Fatal(err)
}
fmt.Println()
// Substitute all of the bytes in the dictionary with a '#' to visually
// demonstrate the approximate effectiveness of using a preset dictionary.
fmt.Println("Substrings matched by the dictionary are marked with #:")
hashDict := []byte(dict)
for i := range hashDict {
hashDict[i] = '#'
}
zr = flate.NewReaderDict(&b, hashDict)
if _, err := io.Copy(os.Stdout, zr); err != nil {
log.Fatal(err)
}
if err := zr.Close(); err != nil {
log.Fatal(err)
}
```
Output:
```
Decompressed output using the dictionary:
<?xml version="1.0"?>
<book>
<meta name="title" content="The Go Programming Language"/>
<meta name="authors" content="Alan Donovan and Brian Kernighan"/>
<meta name="published" content="2015-10-26"/>
<meta name="isbn" content="978-0134190440"/>
<data>...</data>
</book>
Substrings matched by the dictionary are marked with #:
#####################
######
############title###########The Go Programming Language"/#
############authors###########Alan Donovan and Brian Kernighan"/#
############published###########2015-10-26"/#
############isbn###########978-0134190440"/#
######...</#####
</#####
```
#### Example (Reset)
In performance critical applications, Reset can be used to discard the current compressor or decompressor state and reinitialize them quickly by taking advantage of previously allocated memory.
Code:
```
proverbs := []string{
"Don't communicate by sharing memory, share memory by communicating.\n",
"Concurrency is not parallelism.\n",
"The bigger the interface, the weaker the abstraction.\n",
"Documentation is for users.\n",
}
var r strings.Reader
var b bytes.Buffer
buf := make([]byte, 32<<10)
zw, err := flate.NewWriter(nil, flate.DefaultCompression)
if err != nil {
log.Fatal(err)
}
zr := flate.NewReader(nil)
for _, s := range proverbs {
r.Reset(s)
b.Reset()
// Reset the compressor and encode from some input stream.
zw.Reset(&b)
if _, err := io.CopyBuffer(zw, &r, buf); err != nil {
log.Fatal(err)
}
if err := zw.Close(); err != nil {
log.Fatal(err)
}
// Reset the decompressor and decode to some output stream.
if err := zr.(flate.Resetter).Reset(&b, nil); err != nil {
log.Fatal(err)
}
if _, err := io.CopyBuffer(os.Stdout, zr, buf); err != nil {
log.Fatal(err)
}
if err := zr.Close(); err != nil {
log.Fatal(err)
}
}
```
Output:
```
Don't communicate by sharing memory, share memory by communicating.
Concurrency is not parallelism.
The bigger the interface, the weaker the abstraction.
Documentation is for users.
```
#### Example (Synchronization)
DEFLATE is suitable for transmitting compressed data across the network.
Code:
```
var wg sync.WaitGroup
defer wg.Wait()
// Use io.Pipe to simulate a network connection.
// A real network application should take care to properly close the
// underlying connection.
rp, wp := io.Pipe()
// Start a goroutine to act as the transmitter.
wg.Add(1)
go func() {
defer wg.Done()
zw, err := flate.NewWriter(wp, flate.BestSpeed)
if err != nil {
log.Fatal(err)
}
b := make([]byte, 256)
for _, m := range strings.Fields("A long time ago in a galaxy far, far away...") {
// We use a simple framing format where the first byte is the
// message length, followed the message itself.
b[0] = uint8(copy(b[1:], m))
if _, err := zw.Write(b[:1+len(m)]); err != nil {
log.Fatal(err)
}
// Flush ensures that the receiver can read all data sent so far.
if err := zw.Flush(); err != nil {
log.Fatal(err)
}
}
if err := zw.Close(); err != nil {
log.Fatal(err)
}
}()
// Start a goroutine to act as the receiver.
wg.Add(1)
go func() {
defer wg.Done()
zr := flate.NewReader(rp)
b := make([]byte, 256)
for {
// Read the message length.
// This is guaranteed to return for every corresponding
// Flush and Close on the transmitter side.
if _, err := io.ReadFull(zr, b[:1]); err != nil {
if err == io.EOF {
break // The transmitter closed the stream
}
log.Fatal(err)
}
// Read the message content.
n := int(b[0])
if _, err := io.ReadFull(zr, b[:n]); err != nil {
log.Fatal(err)
}
fmt.Printf("Received %d bytes: %s\n", n, b[:n])
}
fmt.Println()
if err := zr.Close(); err != nil {
log.Fatal(err)
}
}()
```
Output:
```
Received 1 bytes: A
Received 4 bytes: long
Received 4 bytes: time
Received 3 bytes: ago
Received 2 bytes: in
Received 1 bytes: a
Received 6 bytes: galaxy
Received 4 bytes: far,
Received 3 bytes: far
Received 7 bytes: away...
```
Index
-----
* [Constants](#pkg-constants)
* [func NewReader(r io.Reader) io.ReadCloser](#NewReader)
* [func NewReaderDict(r io.Reader, dict []byte) io.ReadCloser](#NewReaderDict)
* [type CorruptInputError](#CorruptInputError)
* [func (e CorruptInputError) Error() string](#CorruptInputError.Error)
* [type InternalError](#InternalError)
* [func (e InternalError) Error() string](#InternalError.Error)
* [type ReadError](#ReadError)
* [func (e \*ReadError) Error() string](#ReadError.Error)
* [type Reader](#Reader)
* [type Resetter](#Resetter)
* [type WriteError](#WriteError)
* [func (e \*WriteError) Error() string](#WriteError.Error)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer, level int) (\*Writer, error)](#NewWriter)
* [func NewWriterDict(w io.Writer, level int, dict []byte) (\*Writer, error)](#NewWriterDict)
* [func (w \*Writer) Close() error](#Writer.Close)
* [func (w \*Writer) Flush() error](#Writer.Flush)
* [func (w \*Writer) Reset(dst io.Writer)](#Writer.Reset)
* [func (w \*Writer) Write(data []byte) (n int, err error)](#Writer.Write)
### Examples
[Package (Dictionary)](#example__dictionary) [Package (Reset)](#example__reset) [Package (Synchronization)](#example__synchronization) ### Package files
deflate.go deflatefast.go dict\_decoder.go huffman\_bit\_writer.go huffman\_code.go inflate.go token.go
Constants
---------
```
const (
NoCompression = 0
BestSpeed = 1
BestCompression = 9
DefaultCompression = -1
// HuffmanOnly disables Lempel-Ziv match searching and only performs Huffman
// entropy encoding. This mode is useful in compressing data that has
// already been compressed with an LZ style algorithm (e.g. Snappy or LZ4)
// that lacks an entropy encoder. Compression gains are achieved when
// certain bytes in the input stream occur more frequently than others.
//
// Note that HuffmanOnly produces a compressed output that is
// RFC 1951 compliant. That is, any valid DEFLATE decompressor will
// continue to be able to decompress this output.
HuffmanOnly = -2
)
```
func NewReader
--------------
```
func NewReader(r io.Reader) io.ReadCloser
```
NewReader returns a new ReadCloser that can be used to read the uncompressed version of r. If r does not also implement io.ByteReader, the decompressor may read more data than necessary from r. The reader returns io.EOF after the final block in the DEFLATE stream has been encountered. Any trailing data after the final block is ignored.
The ReadCloser returned by NewReader also implements Resetter.
func NewReaderDict
------------------
```
func NewReaderDict(r io.Reader, dict []byte) io.ReadCloser
```
NewReaderDict is like NewReader but initializes the reader with a preset dictionary. The returned Reader behaves as if the uncompressed data stream started with the given dictionary, which has already been read. NewReaderDict is typically used to read data compressed by NewWriterDict.
The ReadCloser returned by NewReader also implements Resetter.
type CorruptInputError
----------------------
A CorruptInputError reports the presence of corrupt input at a given offset.
```
type CorruptInputError int64
```
### func (CorruptInputError) Error
```
func (e CorruptInputError) Error() string
```
type InternalError
------------------
An InternalError reports an error in the flate code itself.
```
type InternalError string
```
### func (InternalError) Error
```
func (e InternalError) Error() string
```
type ReadError
--------------
A ReadError reports an error encountered while reading input.
Deprecated: No longer returned.
```
type ReadError struct {
Offset int64 // byte offset where error occurred
Err error // error returned by underlying Read
}
```
### func (\*ReadError) Error
```
func (e *ReadError) Error() string
```
type Reader
-----------
The actual read interface needed by NewReader. If the passed in io.Reader does not also have ReadByte, the NewReader will introduce its own buffering.
```
type Reader interface {
io.Reader
io.ByteReader
}
```
type Resetter 1.4
-----------------
Resetter resets a ReadCloser returned by NewReader or NewReaderDict to switch to a new underlying Reader. This permits reusing a ReadCloser instead of allocating a new one.
```
type Resetter interface {
// Reset discards any buffered data and resets the Resetter as if it was
// newly initialized with the given reader.
Reset(r io.Reader, dict []byte) error
}
```
type WriteError
---------------
A WriteError reports an error encountered while writing output.
Deprecated: No longer returned.
```
type WriteError struct {
Offset int64 // byte offset where error occurred
Err error // error returned by underlying Write
}
```
### func (\*WriteError) Error
```
func (e *WriteError) Error() string
```
type Writer
-----------
A Writer takes data written to it and writes the compressed form of that data to an underlying writer (see NewWriter).
```
type Writer struct {
// contains filtered or unexported fields
}
```
### func NewWriter
```
func NewWriter(w io.Writer, level int) (*Writer, error)
```
NewWriter returns a new Writer compressing data at the given level. Following zlib, levels range from 1 (BestSpeed) to 9 (BestCompression); higher levels typically run slower but compress more. Level 0 (NoCompression) does not attempt any compression; it only adds the necessary DEFLATE framing. Level -1 (DefaultCompression) uses the default compression level. Level -2 (HuffmanOnly) will use Huffman compression only, giving a very fast compression for all types of input, but sacrificing considerable compression efficiency.
If level is in the range [-2, 9] then the error returned will be nil. Otherwise the error returned will be non-nil.
### func NewWriterDict
```
func NewWriterDict(w io.Writer, level int, dict []byte) (*Writer, error)
```
NewWriterDict is like NewWriter but initializes the new Writer with a preset dictionary. The returned Writer behaves as if the dictionary had been written to it without producing any compressed output. The compressed data written to w can only be decompressed by a Reader initialized with the same dictionary.
### func (\*Writer) Close
```
func (w *Writer) Close() error
```
Close flushes and closes the writer.
### func (\*Writer) Flush
```
func (w *Writer) Flush() error
```
Flush flushes any pending data to the underlying writer. It is useful mainly in compressed network protocols, to ensure that a remote reader has enough data to reconstruct a packet. Flush does not return until the data has been written. Calling Flush when there is no pending data still causes the Writer to emit a sync marker of at least 4 bytes. If the underlying writer returns an error, Flush returns that error.
In the terminology of the zlib library, Flush is equivalent to Z\_SYNC\_FLUSH.
### func (\*Writer) Reset 1.2
```
func (w *Writer) Reset(dst io.Writer)
```
Reset discards the writer's state and makes it equivalent to the result of NewWriter or NewWriterDict called with dst and w's level and dictionary.
### func (\*Writer) Write
```
func (w *Writer) Write(data []byte) (n int, err error)
```
Write writes data to w, which will eventually write the compressed form of data to its underlying writer.
go Package gzip Package gzip
=============
* `import "compress/gzip"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package gzip implements reading and writing of gzip format compressed files, as specified in RFC 1952.
#### Example (CompressingReader)
Code:
```
// This is an example of writing a compressing reader.
// This can be useful for an HTTP client body, as shown.
const testdata = "the data to be compressed"
// This HTTP handler is just for testing purposes.
handler := http.HandlerFunc(func(rw http.ResponseWriter, req *http.Request) {
zr, err := gzip.NewReader(req.Body)
if err != nil {
log.Fatal(err)
}
// Just output the data for the example.
if _, err := io.Copy(os.Stdout, zr); err != nil {
log.Fatal(err)
}
})
ts := httptest.NewServer(handler)
defer ts.Close()
// The remainder is the example code.
// The data we want to compress, as an io.Reader
dataReader := strings.NewReader(testdata)
// bodyReader is the body of the HTTP request, as an io.Reader.
// httpWriter is the body of the HTTP request, as an io.Writer.
bodyReader, httpWriter := io.Pipe()
// Make sure that bodyReader is always closed, so that the
// goroutine below will always exit.
defer bodyReader.Close()
// gzipWriter compresses data to httpWriter.
gzipWriter := gzip.NewWriter(httpWriter)
// errch collects any errors from the writing goroutine.
errch := make(chan error, 1)
go func() {
defer close(errch)
sentErr := false
sendErr := func(err error) {
if !sentErr {
errch <- err
sentErr = true
}
}
// Copy our data to gzipWriter, which compresses it to
// gzipWriter, which feeds it to bodyReader.
if _, err := io.Copy(gzipWriter, dataReader); err != nil && err != io.ErrClosedPipe {
sendErr(err)
}
if err := gzipWriter.Close(); err != nil && err != io.ErrClosedPipe {
sendErr(err)
}
if err := httpWriter.Close(); err != nil && err != io.ErrClosedPipe {
sendErr(err)
}
}()
// Send an HTTP request to the test server.
req, err := http.NewRequest("PUT", ts.URL, bodyReader)
if err != nil {
log.Fatal(err)
}
// Note that passing req to http.Client.Do promises that it
// will close the body, in this case bodyReader.
resp, err := ts.Client().Do(req)
if err != nil {
log.Fatal(err)
}
// Check whether there was an error compressing the data.
if err := <-errch; err != nil {
log.Fatal(err)
}
// For this example we don't care about the response.
resp.Body.Close()
```
Output:
```
the data to be compressed
```
#### Example (WriterReader)
Code:
```
var buf bytes.Buffer
zw := gzip.NewWriter(&buf)
// Setting the Header fields is optional.
zw.Name = "a-new-hope.txt"
zw.Comment = "an epic space opera by George Lucas"
zw.ModTime = time.Date(1977, time.May, 25, 0, 0, 0, 0, time.UTC)
_, err := zw.Write([]byte("A long time ago in a galaxy far, far away..."))
if err != nil {
log.Fatal(err)
}
if err := zw.Close(); err != nil {
log.Fatal(err)
}
zr, err := gzip.NewReader(&buf)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Name: %s\nComment: %s\nModTime: %s\n\n", zr.Name, zr.Comment, zr.ModTime.UTC())
if _, err := io.Copy(os.Stdout, zr); err != nil {
log.Fatal(err)
}
if err := zr.Close(); err != nil {
log.Fatal(err)
}
```
Output:
```
Name: a-new-hope.txt
Comment: an epic space opera by George Lucas
ModTime: 1977-05-25 00:00:00 +0000 UTC
A long time ago in a galaxy far, far away...
```
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [type Header](#Header)
* [type Reader](#Reader)
* [func NewReader(r io.Reader) (\*Reader, error)](#NewReader)
* [func (z \*Reader) Close() error](#Reader.Close)
* [func (z \*Reader) Multistream(ok bool)](#Reader.Multistream)
* [func (z \*Reader) Read(p []byte) (n int, err error)](#Reader.Read)
* [func (z \*Reader) Reset(r io.Reader) error](#Reader.Reset)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer) \*Writer](#NewWriter)
* [func NewWriterLevel(w io.Writer, level int) (\*Writer, error)](#NewWriterLevel)
* [func (z \*Writer) Close() error](#Writer.Close)
* [func (z \*Writer) Flush() error](#Writer.Flush)
* [func (z \*Writer) Reset(w io.Writer)](#Writer.Reset)
* [func (z \*Writer) Write(p []byte) (int, error)](#Writer.Write)
### Examples
[Reader.Multistream](#example_Reader_Multistream) [Package (CompressingReader)](#example__compressingReader) [Package (WriterReader)](#example__writerReader) ### Package files
gunzip.go gzip.go
Constants
---------
These constants are copied from the flate package, so that code that imports "compress/gzip" does not also have to import "compress/flate".
```
const (
NoCompression = flate.NoCompression
BestSpeed = flate.BestSpeed
BestCompression = flate.BestCompression
DefaultCompression = flate.DefaultCompression
HuffmanOnly = flate.HuffmanOnly
)
```
Variables
---------
```
var (
// ErrChecksum is returned when reading GZIP data that has an invalid checksum.
ErrChecksum = errors.New("gzip: invalid checksum")
// ErrHeader is returned when reading GZIP data that has an invalid header.
ErrHeader = errors.New("gzip: invalid header")
)
```
type Header
-----------
The gzip file stores a header giving metadata about the compressed file. That header is exposed as the fields of the Writer and Reader structs.
Strings must be UTF-8 encoded and may only contain Unicode code points U+0001 through U+00FF, due to limitations of the GZIP file format.
```
type Header struct {
Comment string // comment
Extra []byte // "extra data"
ModTime time.Time // modification time
Name string // file name
OS byte // operating system type
}
```
type Reader
-----------
A Reader is an io.Reader that can be read to retrieve uncompressed data from a gzip-format compressed file.
In general, a gzip file can be a concatenation of gzip files, each with its own header. Reads from the Reader return the concatenation of the uncompressed data of each. Only the first header is recorded in the Reader fields.
Gzip files store a length and checksum of the uncompressed data. The Reader will return an ErrChecksum when Read reaches the end of the uncompressed data if it does not have the expected length or checksum. Clients should treat data returned by Read as tentative until they receive the io.EOF marking the end of the data.
```
type Reader struct {
Header // valid after NewReader or Reader.Reset
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(r io.Reader) (*Reader, error)
```
NewReader creates a new Reader reading the given reader. If r does not also implement io.ByteReader, the decompressor may read more data than necessary from r.
It is the caller's responsibility to call Close on the Reader when done.
The Reader.Header fields will be valid in the Reader returned.
### func (\*Reader) Close
```
func (z *Reader) Close() error
```
Close closes the Reader. It does not close the underlying io.Reader. In order for the GZIP checksum to be verified, the reader must be fully consumed until the io.EOF.
### func (\*Reader) Multistream 1.4
```
func (z *Reader) Multistream(ok bool)
```
Multistream controls whether the reader supports multistream files.
If enabled (the default), the Reader expects the input to be a sequence of individually gzipped data streams, each with its own header and trailer, ending at EOF. The effect is that the concatenation of a sequence of gzipped files is treated as equivalent to the gzip of the concatenation of the sequence. This is standard behavior for gzip readers.
Calling Multistream(false) disables this behavior; disabling the behavior can be useful when reading file formats that distinguish individual gzip data streams or mix gzip data streams with other data streams. In this mode, when the Reader reaches the end of the data stream, Read returns io.EOF. The underlying reader must implement io.ByteReader in order to be left positioned just after the gzip stream. To start the next stream, call z.Reset(r) followed by z.Multistream(false). If there is no next stream, z.Reset(r) will return io.EOF.
#### Example
Code:
```
var buf bytes.Buffer
zw := gzip.NewWriter(&buf)
var files = []struct {
name string
comment string
modTime time.Time
data string
}{
{"file-1.txt", "file-header-1", time.Date(2006, time.February, 1, 3, 4, 5, 0, time.UTC), "Hello Gophers - 1"},
{"file-2.txt", "file-header-2", time.Date(2007, time.March, 2, 4, 5, 6, 1, time.UTC), "Hello Gophers - 2"},
}
for _, file := range files {
zw.Name = file.name
zw.Comment = file.comment
zw.ModTime = file.modTime
if _, err := zw.Write([]byte(file.data)); err != nil {
log.Fatal(err)
}
if err := zw.Close(); err != nil {
log.Fatal(err)
}
zw.Reset(&buf)
}
zr, err := gzip.NewReader(&buf)
if err != nil {
log.Fatal(err)
}
for {
zr.Multistream(false)
fmt.Printf("Name: %s\nComment: %s\nModTime: %s\n\n", zr.Name, zr.Comment, zr.ModTime.UTC())
if _, err := io.Copy(os.Stdout, zr); err != nil {
log.Fatal(err)
}
fmt.Print("\n\n")
err = zr.Reset(&buf)
if err == io.EOF {
break
}
if err != nil {
log.Fatal(err)
}
}
if err := zr.Close(); err != nil {
log.Fatal(err)
}
```
Output:
```
Name: file-1.txt
Comment: file-header-1
ModTime: 2006-02-01 03:04:05 +0000 UTC
Hello Gophers - 1
Name: file-2.txt
Comment: file-header-2
ModTime: 2007-03-02 04:05:06 +0000 UTC
Hello Gophers - 2
```
### func (\*Reader) Read
```
func (z *Reader) Read(p []byte) (n int, err error)
```
Read implements io.Reader, reading uncompressed bytes from its underlying Reader.
### func (\*Reader) Reset 1.3
```
func (z *Reader) Reset(r io.Reader) error
```
Reset discards the Reader z's state and makes it equivalent to the result of its original state from NewReader, but reading from r instead. This permits reusing a Reader rather than allocating a new one.
type Writer
-----------
A Writer is an io.WriteCloser. Writes to a Writer are compressed and written to w.
```
type Writer struct {
Header // written at first call to Write, Flush, or Close
// contains filtered or unexported fields
}
```
### func NewWriter
```
func NewWriter(w io.Writer) *Writer
```
NewWriter returns a new Writer. Writes to the returned writer are compressed and written to w.
It is the caller's responsibility to call Close on the Writer when done. Writes may be buffered and not flushed until Close.
Callers that wish to set the fields in Writer.Header must do so before the first call to Write, Flush, or Close.
### func NewWriterLevel
```
func NewWriterLevel(w io.Writer, level int) (*Writer, error)
```
NewWriterLevel is like NewWriter but specifies the compression level instead of assuming DefaultCompression.
The compression level can be DefaultCompression, NoCompression, HuffmanOnly or any integer value between BestSpeed and BestCompression inclusive. The error returned will be nil if the level is valid.
### func (\*Writer) Close
```
func (z *Writer) Close() error
```
Close closes the Writer by flushing any unwritten data to the underlying io.Writer and writing the GZIP footer. It does not close the underlying io.Writer.
### func (\*Writer) Flush 1.1
```
func (z *Writer) Flush() error
```
Flush flushes any pending compressed data to the underlying writer.
It is useful mainly in compressed network protocols, to ensure that a remote reader has enough data to reconstruct a packet. Flush does not return until the data has been written. If the underlying writer returns an error, Flush returns that error.
In the terminology of the zlib library, Flush is equivalent to Z\_SYNC\_FLUSH.
### func (\*Writer) Reset 1.2
```
func (z *Writer) Reset(w io.Writer)
```
Reset discards the Writer z's state and makes it equivalent to the result of its original state from NewWriter or NewWriterLevel, but writing to w instead. This permits reusing a Writer rather than allocating a new one.
### func (\*Writer) Write
```
func (z *Writer) Write(p []byte) (int, error)
```
Write writes a compressed form of p to the underlying io.Writer. The compressed bytes are not necessarily flushed until the Writer is closed.
| programming_docs |
go Package lzw Package lzw
============
* `import "compress/lzw"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package lzw implements the Lempel-Ziv-Welch compressed data format, described in T. A. Welch, “A Technique for High-Performance Data Compression”, Computer, 17(6) (June 1984), pp 8-19.
In particular, it implements LZW as used by the GIF and PDF file formats, which means variable-width codes up to 12 bits and the first two non-literal codes are a clear code and an EOF code.
The TIFF file format uses a similar but incompatible version of the LZW algorithm. See the golang.org/x/image/tiff/lzw package for an implementation.
Index
-----
* [func NewReader(r io.Reader, order Order, litWidth int) io.ReadCloser](#NewReader)
* [func NewWriter(w io.Writer, order Order, litWidth int) io.WriteCloser](#NewWriter)
* [type Order](#Order)
* [type Reader](#Reader)
* [func (r \*Reader) Close() error](#Reader.Close)
* [func (r \*Reader) Read(b []byte) (int, error)](#Reader.Read)
* [func (r \*Reader) Reset(src io.Reader, order Order, litWidth int)](#Reader.Reset)
* [type Writer](#Writer)
* [func (w \*Writer) Close() error](#Writer.Close)
* [func (w \*Writer) Reset(dst io.Writer, order Order, litWidth int)](#Writer.Reset)
* [func (w \*Writer) Write(p []byte) (n int, err error)](#Writer.Write)
### Package files
reader.go writer.go
func NewReader
--------------
```
func NewReader(r io.Reader, order Order, litWidth int) io.ReadCloser
```
NewReader creates a new io.ReadCloser. Reads from the returned io.ReadCloser read and decompress data from r. If r does not also implement io.ByteReader, the decompressor may read more data than necessary from r. It is the caller's responsibility to call Close on the ReadCloser when finished reading. The number of bits to use for literal codes, litWidth, must be in the range [2,8] and is typically 8. It must equal the litWidth used during compression.
It is guaranteed that the underlying type of the returned io.ReadCloser is a \*Reader.
func NewWriter
--------------
```
func NewWriter(w io.Writer, order Order, litWidth int) io.WriteCloser
```
NewWriter creates a new io.WriteCloser. Writes to the returned io.WriteCloser are compressed and written to w. It is the caller's responsibility to call Close on the WriteCloser when finished writing. The number of bits to use for literal codes, litWidth, must be in the range [2,8] and is typically 8. Input bytes must be less than 1<<litWidth.
It is guaranteed that the underlying type of the returned io.WriteCloser is a \*Writer.
type Order
----------
Order specifies the bit ordering in an LZW data stream.
```
type Order int
```
```
const (
// LSB means Least Significant Bits first, as used in the GIF file format.
LSB Order = iota
// MSB means Most Significant Bits first, as used in the TIFF and PDF
// file formats.
MSB
)
```
type Reader 1.17
----------------
Reader is an io.Reader which can be used to read compressed data in the LZW format.
```
type Reader struct {
// contains filtered or unexported fields
}
```
### func (\*Reader) Close 1.17
```
func (r *Reader) Close() error
```
Close closes the Reader and returns an error for any future read operation. It does not close the underlying io.Reader.
### func (\*Reader) Read 1.17
```
func (r *Reader) Read(b []byte) (int, error)
```
Read implements io.Reader, reading uncompressed bytes from its underlying Reader.
### func (\*Reader) Reset 1.17
```
func (r *Reader) Reset(src io.Reader, order Order, litWidth int)
```
Reset clears the Reader's state and allows it to be reused again as a new Reader.
type Writer 1.17
----------------
Writer is an LZW compressor. It writes the compressed form of the data to an underlying writer (see NewWriter).
```
type Writer struct {
// contains filtered or unexported fields
}
```
### func (\*Writer) Close 1.17
```
func (w *Writer) Close() error
```
Close closes the Writer, flushing any pending output. It does not close w's underlying writer.
### func (\*Writer) Reset 1.17
```
func (w *Writer) Reset(dst io.Writer, order Order, litWidth int)
```
Reset clears the Writer's state and allows it to be reused again as a new Writer.
### func (\*Writer) Write 1.17
```
func (w *Writer) Write(p []byte) (n int, err error)
```
Write writes a compressed representation of p to w's underlying writer.
go Package zlib Package zlib
=============
* `import "compress/zlib"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package zlib implements reading and writing of zlib format compressed data, as specified in RFC 1950.
The implementation provides filters that uncompress during reading and compress during writing. For example, to write compressed data to a buffer:
```
var b bytes.Buffer
w := zlib.NewWriter(&b)
w.Write([]byte("hello, world\n"))
w.Close()
```
and to read that data back:
```
r, err := zlib.NewReader(&b)
io.Copy(os.Stdout, r)
r.Close()
```
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func NewReader(r io.Reader) (io.ReadCloser, error)](#NewReader)
* [func NewReaderDict(r io.Reader, dict []byte) (io.ReadCloser, error)](#NewReaderDict)
* [type Resetter](#Resetter)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer) \*Writer](#NewWriter)
* [func NewWriterLevel(w io.Writer, level int) (\*Writer, error)](#NewWriterLevel)
* [func NewWriterLevelDict(w io.Writer, level int, dict []byte) (\*Writer, error)](#NewWriterLevelDict)
* [func (z \*Writer) Close() error](#Writer.Close)
* [func (z \*Writer) Flush() error](#Writer.Flush)
* [func (z \*Writer) Reset(w io.Writer)](#Writer.Reset)
* [func (z \*Writer) Write(p []byte) (n int, err error)](#Writer.Write)
### Examples
[NewReader](#example_NewReader) [NewWriter](#example_NewWriter) ### Package files
reader.go writer.go
Constants
---------
These constants are copied from the flate package, so that code that imports "compress/zlib" does not also have to import "compress/flate".
```
const (
NoCompression = flate.NoCompression
BestSpeed = flate.BestSpeed
BestCompression = flate.BestCompression
DefaultCompression = flate.DefaultCompression
HuffmanOnly = flate.HuffmanOnly
)
```
Variables
---------
```
var (
// ErrChecksum is returned when reading ZLIB data that has an invalid checksum.
ErrChecksum = errors.New("zlib: invalid checksum")
// ErrDictionary is returned when reading ZLIB data that has an invalid dictionary.
ErrDictionary = errors.New("zlib: invalid dictionary")
// ErrHeader is returned when reading ZLIB data that has an invalid header.
ErrHeader = errors.New("zlib: invalid header")
)
```
func NewReader
--------------
```
func NewReader(r io.Reader) (io.ReadCloser, error)
```
NewReader creates a new ReadCloser. Reads from the returned ReadCloser read and decompress data from r. If r does not implement io.ByteReader, the decompressor may read more data than necessary from r. It is the caller's responsibility to call Close on the ReadCloser when done.
The ReadCloser returned by NewReader also implements Resetter.
#### Example
Code:
```
buff := []byte{120, 156, 202, 72, 205, 201, 201, 215, 81, 40, 207,
47, 202, 73, 225, 2, 4, 0, 0, 255, 255, 33, 231, 4, 147}
b := bytes.NewReader(buff)
r, err := zlib.NewReader(b)
if err != nil {
panic(err)
}
io.Copy(os.Stdout, r)
```
Output:
```
hello, world
```
func NewReaderDict
------------------
```
func NewReaderDict(r io.Reader, dict []byte) (io.ReadCloser, error)
```
NewReaderDict is like NewReader but uses a preset dictionary. NewReaderDict ignores the dictionary if the compressed data does not refer to it. If the compressed data refers to a different dictionary, NewReaderDict returns ErrDictionary.
The ReadCloser returned by NewReaderDict also implements Resetter.
type Resetter 1.4
-----------------
Resetter resets a ReadCloser returned by NewReader or NewReaderDict to switch to a new underlying Reader. This permits reusing a ReadCloser instead of allocating a new one.
```
type Resetter interface {
// Reset discards any buffered data and resets the Resetter as if it was
// newly initialized with the given reader.
Reset(r io.Reader, dict []byte) error
}
```
type Writer
-----------
A Writer takes data written to it and writes the compressed form of that data to an underlying writer (see NewWriter).
```
type Writer struct {
// contains filtered or unexported fields
}
```
### func NewWriter
```
func NewWriter(w io.Writer) *Writer
```
NewWriter creates a new Writer. Writes to the returned Writer are compressed and written to w.
It is the caller's responsibility to call Close on the Writer when done. Writes may be buffered and not flushed until Close.
#### Example
Code:
```
var b bytes.Buffer
w := zlib.NewWriter(&b)
w.Write([]byte("hello, world\n"))
w.Close()
fmt.Println(b.Bytes())
```
Output:
```
[120 156 202 72 205 201 201 215 81 40 207 47 202 73 225 2 4 0 0 255 255 33 231 4 147]
```
### func NewWriterLevel
```
func NewWriterLevel(w io.Writer, level int) (*Writer, error)
```
NewWriterLevel is like NewWriter but specifies the compression level instead of assuming DefaultCompression.
The compression level can be DefaultCompression, NoCompression, HuffmanOnly or any integer value between BestSpeed and BestCompression inclusive. The error returned will be nil if the level is valid.
### func NewWriterLevelDict
```
func NewWriterLevelDict(w io.Writer, level int, dict []byte) (*Writer, error)
```
NewWriterLevelDict is like NewWriterLevel but specifies a dictionary to compress with.
The dictionary may be nil. If not, its contents should not be modified until the Writer is closed.
### func (\*Writer) Close
```
func (z *Writer) Close() error
```
Close closes the Writer, flushing any unwritten data to the underlying io.Writer, but does not close the underlying io.Writer.
### func (\*Writer) Flush
```
func (z *Writer) Flush() error
```
Flush flushes the Writer to its underlying io.Writer.
### func (\*Writer) Reset 1.2
```
func (z *Writer) Reset(w io.Writer)
```
Reset clears the state of the Writer z such that it is equivalent to its initial state from NewWriterLevel or NewWriterLevelDict, but instead writing to w.
### func (\*Writer) Write
```
func (z *Writer) Write(p []byte) (n int, err error)
```
Write writes a compressed form of p to the underlying io.Writer. The compressed bytes are not necessarily flushed until the Writer is closed or explicitly flushed.
go Package bzip2 Package bzip2
==============
* `import "compress/bzip2"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package bzip2 implements bzip2 decompression.
Index
-----
* [func NewReader(r io.Reader) io.Reader](#NewReader)
* [type StructuralError](#StructuralError)
* [func (s StructuralError) Error() string](#StructuralError.Error)
### Package files
bit\_reader.go bzip2.go huffman.go move\_to\_front.go
func NewReader
--------------
```
func NewReader(r io.Reader) io.Reader
```
NewReader returns an io.Reader which decompresses bzip2 data from r. If r does not also implement io.ByteReader, the decompressor may read more data than necessary from r.
type StructuralError
--------------------
A StructuralError is returned when the bzip2 data is found to be syntactically invalid.
```
type StructuralError string
```
### func (StructuralError) Error
```
func (s StructuralError) Error() string
```
go Package flag Package flag
=============
* `import "flag"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package flag implements command-line flag parsing.
### Usage
Define flags using flag.String(), Bool(), Int(), etc.
This declares an integer flag, -n, stored in the pointer nFlag, with type \*int:
```
import "flag"
var nFlag = flag.Int("n", 1234, "help message for flag n")
```
If you like, you can bind the flag to a variable using the Var() functions.
```
var flagvar int
func init() {
flag.IntVar(&flagvar, "flagname", 1234, "help message for flagname")
}
```
Or you can create custom flags that satisfy the Value interface (with pointer receivers) and couple them to flag parsing by
```
flag.Var(&flagVal, "name", "help message for flagname")
```
For such flags, the default value is just the initial value of the variable.
After all flags are defined, call
```
flag.Parse()
```
to parse the command line into the defined flags.
Flags may then be used directly. If you're using the flags themselves, they are all pointers; if you bind to variables, they're values.
```
fmt.Println("ip has value ", *ip)
fmt.Println("flagvar has value ", flagvar)
```
After parsing, the arguments following the flags are available as the slice flag.Args() or individually as flag.Arg(i). The arguments are indexed from 0 through flag.NArg()-1.
### Command line flag syntax
The following forms are permitted:
```
-flag
--flag // double dashes are also permitted
-flag=x
-flag x // non-boolean flags only
```
One or two dashes may be used; they are equivalent. The last form is not permitted for boolean flags because the meaning of the command
```
cmd -x *
```
where \* is a Unix shell wildcard, will change if there is a file called 0, false, etc. You must use the -flag=false form to turn off a boolean flag.
Flag parsing stops just before the first non-flag argument ("-" is a non-flag argument) or after the terminator "--".
Integer flags accept 1234, 0664, 0x1234 and may be negative. Boolean flags may be:
```
1, 0, t, f, T, F, true, false, TRUE, FALSE, True, False
```
Duration flags accept any input valid for time.ParseDuration.
The default set of command-line flags is controlled by top-level functions. The FlagSet type allows one to define independent sets of flags, such as to implement subcommands in a command-line interface. The methods of FlagSet are analogous to the top-level functions for the command-line flag set.
#### Example
Code:
```
// These examples demonstrate more intricate uses of the flag package.
package flag_test
import (
"errors"
"flag"
"fmt"
"strings"
"time"
)
// Example 1: A single string flag called "species" with default value "gopher".
var species = flag.String("species", "gopher", "the species we are studying")
// Example 2: Two flags sharing a variable, so we can have a shorthand.
// The order of initialization is undefined, so make sure both use the
// same default value. They must be set up with an init function.
var gopherType string
func init() {
const (
defaultGopher = "pocket"
usage = "the variety of gopher"
)
flag.StringVar(&gopherType, "gopher_type", defaultGopher, usage)
flag.StringVar(&gopherType, "g", defaultGopher, usage+" (shorthand)")
}
// Example 3: A user-defined flag type, a slice of durations.
type interval []time.Duration
// String is the method to format the flag's value, part of the flag.Value interface.
// The String method's output will be used in diagnostics.
func (i *interval) String() string {
return fmt.Sprint(*i)
}
// Set is the method to set the flag value, part of the flag.Value interface.
// Set's argument is a string to be parsed to set the flag.
// It's a comma-separated list, so we split it.
func (i *interval) Set(value string) error {
// If we wanted to allow the flag to be set multiple times,
// accumulating values, we would delete this if statement.
// That would permit usages such as
// -deltaT 10s -deltaT 15s
// and other combinations.
if len(*i) > 0 {
return errors.New("interval flag already set")
}
for _, dt := range strings.Split(value, ",") {
duration, err := time.ParseDuration(dt)
if err != nil {
return err
}
*i = append(*i, duration)
}
return nil
}
// Define a flag to accumulate durations. Because it has a special type,
// we need to use the Var function and therefore create the flag during
// init.
var intervalFlag interval
func init() {
// Tie the command-line flag to the intervalFlag variable and
// set a usage message.
flag.Var(&intervalFlag, "deltaT", "comma-separated list of intervals to use between events")
}
func Example() {
// All the interesting pieces are with the variables declared above, but
// to enable the flag package to see the flags defined there, one must
// execute, typically at the start of main (not init!):
// flag.Parse()
// We don't call it here because this code is a function called "Example"
// that is part of the testing suite for the package, which has already
// parsed the flags. When viewed at pkg.go.dev, however, the function is
// renamed to "main" and it could be run as a standalone example.
}
```
Index
-----
* [Variables](#pkg-variables)
* [func Arg(i int) string](#Arg)
* [func Args() []string](#Args)
* [func Bool(name string, value bool, usage string) \*bool](#Bool)
* [func BoolVar(p \*bool, name string, value bool, usage string)](#BoolVar)
* [func Duration(name string, value time.Duration, usage string) \*time.Duration](#Duration)
* [func DurationVar(p \*time.Duration, name string, value time.Duration, usage string)](#DurationVar)
* [func Float64(name string, value float64, usage string) \*float64](#Float64)
* [func Float64Var(p \*float64, name string, value float64, usage string)](#Float64Var)
* [func Func(name, usage string, fn func(string) error)](#Func)
* [func Int(name string, value int, usage string) \*int](#Int)
* [func Int64(name string, value int64, usage string) \*int64](#Int64)
* [func Int64Var(p \*int64, name string, value int64, usage string)](#Int64Var)
* [func IntVar(p \*int, name string, value int, usage string)](#IntVar)
* [func NArg() int](#NArg)
* [func NFlag() int](#NFlag)
* [func Parse()](#Parse)
* [func Parsed() bool](#Parsed)
* [func PrintDefaults()](#PrintDefaults)
* [func Set(name, value string) error](#Set)
* [func String(name string, value string, usage string) \*string](#String)
* [func StringVar(p \*string, name string, value string, usage string)](#StringVar)
* [func TextVar(p encoding.TextUnmarshaler, name string, value encoding.TextMarshaler, usage string)](#TextVar)
* [func Uint(name string, value uint, usage string) \*uint](#Uint)
* [func Uint64(name string, value uint64, usage string) \*uint64](#Uint64)
* [func Uint64Var(p \*uint64, name string, value uint64, usage string)](#Uint64Var)
* [func UintVar(p \*uint, name string, value uint, usage string)](#UintVar)
* [func UnquoteUsage(flag \*Flag) (name string, usage string)](#UnquoteUsage)
* [func Var(value Value, name string, usage string)](#Var)
* [func Visit(fn func(\*Flag))](#Visit)
* [func VisitAll(fn func(\*Flag))](#VisitAll)
* [type ErrorHandling](#ErrorHandling)
* [type Flag](#Flag)
* [func Lookup(name string) \*Flag](#Lookup)
* [type FlagSet](#FlagSet)
* [func NewFlagSet(name string, errorHandling ErrorHandling) \*FlagSet](#NewFlagSet)
* [func (f \*FlagSet) Arg(i int) string](#FlagSet.Arg)
* [func (f \*FlagSet) Args() []string](#FlagSet.Args)
* [func (f \*FlagSet) Bool(name string, value bool, usage string) \*bool](#FlagSet.Bool)
* [func (f \*FlagSet) BoolVar(p \*bool, name string, value bool, usage string)](#FlagSet.BoolVar)
* [func (f \*FlagSet) Duration(name string, value time.Duration, usage string) \*time.Duration](#FlagSet.Duration)
* [func (f \*FlagSet) DurationVar(p \*time.Duration, name string, value time.Duration, usage string)](#FlagSet.DurationVar)
* [func (f \*FlagSet) ErrorHandling() ErrorHandling](#FlagSet.ErrorHandling)
* [func (f \*FlagSet) Float64(name string, value float64, usage string) \*float64](#FlagSet.Float64)
* [func (f \*FlagSet) Float64Var(p \*float64, name string, value float64, usage string)](#FlagSet.Float64Var)
* [func (f \*FlagSet) Func(name, usage string, fn func(string) error)](#FlagSet.Func)
* [func (f \*FlagSet) Init(name string, errorHandling ErrorHandling)](#FlagSet.Init)
* [func (f \*FlagSet) Int(name string, value int, usage string) \*int](#FlagSet.Int)
* [func (f \*FlagSet) Int64(name string, value int64, usage string) \*int64](#FlagSet.Int64)
* [func (f \*FlagSet) Int64Var(p \*int64, name string, value int64, usage string)](#FlagSet.Int64Var)
* [func (f \*FlagSet) IntVar(p \*int, name string, value int, usage string)](#FlagSet.IntVar)
* [func (f \*FlagSet) Lookup(name string) \*Flag](#FlagSet.Lookup)
* [func (f \*FlagSet) NArg() int](#FlagSet.NArg)
* [func (f \*FlagSet) NFlag() int](#FlagSet.NFlag)
* [func (f \*FlagSet) Name() string](#FlagSet.Name)
* [func (f \*FlagSet) Output() io.Writer](#FlagSet.Output)
* [func (f \*FlagSet) Parse(arguments []string) error](#FlagSet.Parse)
* [func (f \*FlagSet) Parsed() bool](#FlagSet.Parsed)
* [func (f \*FlagSet) PrintDefaults()](#FlagSet.PrintDefaults)
* [func (f \*FlagSet) Set(name, value string) error](#FlagSet.Set)
* [func (f \*FlagSet) SetOutput(output io.Writer)](#FlagSet.SetOutput)
* [func (f \*FlagSet) String(name string, value string, usage string) \*string](#FlagSet.String)
* [func (f \*FlagSet) StringVar(p \*string, name string, value string, usage string)](#FlagSet.StringVar)
* [func (f \*FlagSet) TextVar(p encoding.TextUnmarshaler, name string, value encoding.TextMarshaler, usage string)](#FlagSet.TextVar)
* [func (f \*FlagSet) Uint(name string, value uint, usage string) \*uint](#FlagSet.Uint)
* [func (f \*FlagSet) Uint64(name string, value uint64, usage string) \*uint64](#FlagSet.Uint64)
* [func (f \*FlagSet) Uint64Var(p \*uint64, name string, value uint64, usage string)](#FlagSet.Uint64Var)
* [func (f \*FlagSet) UintVar(p \*uint, name string, value uint, usage string)](#FlagSet.UintVar)
* [func (f \*FlagSet) Var(value Value, name string, usage string)](#FlagSet.Var)
* [func (f \*FlagSet) Visit(fn func(\*Flag))](#FlagSet.Visit)
* [func (f \*FlagSet) VisitAll(fn func(\*Flag))](#FlagSet.VisitAll)
* [type Getter](#Getter)
* [type Value](#Value)
### Examples
[Package](#example_) [Func](#example_Func) [TextVar](#example_TextVar) [Value](#example_Value) ### Package files
flag.go
Variables
---------
CommandLine is the default set of command-line flags, parsed from os.Args. The top-level functions such as BoolVar, Arg, and so on are wrappers for the methods of CommandLine.
```
var CommandLine = NewFlagSet(os.Args[0], ExitOnError)
```
ErrHelp is the error returned if the -help or -h flag is invoked but no such flag is defined.
```
var ErrHelp = errors.New("flag: help requested")
```
Usage prints a usage message documenting all defined command-line flags to CommandLine's output, which by default is os.Stderr. It is called when an error occurs while parsing flags. The function is a variable that may be changed to point to a custom function. By default it prints a simple header and calls PrintDefaults; for details about the format of the output and how to control it, see the documentation for PrintDefaults. Custom usage functions may choose to exit the program; by default exiting happens anyway as the command line's error handling strategy is set to ExitOnError.
```
var Usage = func() {
fmt.Fprintf(CommandLine.Output(), "Usage of %s:\n", os.Args[0])
PrintDefaults()
}
```
func Arg
--------
```
func Arg(i int) string
```
Arg returns the i'th command-line argument. Arg(0) is the first remaining argument after flags have been processed. Arg returns an empty string if the requested element does not exist.
func Args
---------
```
func Args() []string
```
Args returns the non-flag command-line arguments.
func Bool
---------
```
func Bool(name string, value bool, usage string) *bool
```
Bool defines a bool flag with specified name, default value, and usage string. The return value is the address of a bool variable that stores the value of the flag.
func BoolVar
------------
```
func BoolVar(p *bool, name string, value bool, usage string)
```
BoolVar defines a bool flag with specified name, default value, and usage string. The argument p points to a bool variable in which to store the value of the flag.
func Duration
-------------
```
func Duration(name string, value time.Duration, usage string) *time.Duration
```
Duration defines a time.Duration flag with specified name, default value, and usage string. The return value is the address of a time.Duration variable that stores the value of the flag. The flag accepts a value acceptable to time.ParseDuration.
func DurationVar
----------------
```
func DurationVar(p *time.Duration, name string, value time.Duration, usage string)
```
DurationVar defines a time.Duration flag with specified name, default value, and usage string. The argument p points to a time.Duration variable in which to store the value of the flag. The flag accepts a value acceptable to time.ParseDuration.
func Float64
------------
```
func Float64(name string, value float64, usage string) *float64
```
Float64 defines a float64 flag with specified name, default value, and usage string. The return value is the address of a float64 variable that stores the value of the flag.
func Float64Var
---------------
```
func Float64Var(p *float64, name string, value float64, usage string)
```
Float64Var defines a float64 flag with specified name, default value, and usage string. The argument p points to a float64 variable in which to store the value of the flag.
func Func 1.16
--------------
```
func Func(name, usage string, fn func(string) error)
```
Func defines a flag with the specified name and usage string. Each time the flag is seen, fn is called with the value of the flag. If fn returns a non-nil error, it will be treated as a flag value parsing error.
#### Example
Code:
```
fs := flag.NewFlagSet("ExampleFunc", flag.ContinueOnError)
fs.SetOutput(os.Stdout)
var ip net.IP
fs.Func("ip", "`IP address` to parse", func(s string) error {
ip = net.ParseIP(s)
if ip == nil {
return errors.New("could not parse IP")
}
return nil
})
fs.Parse([]string{"-ip", "127.0.0.1"})
fmt.Printf("{ip: %v, loopback: %t}\n\n", ip, ip.IsLoopback())
// 256 is not a valid IPv4 component
fs.Parse([]string{"-ip", "256.0.0.1"})
fmt.Printf("{ip: %v, loopback: %t}\n\n", ip, ip.IsLoopback())
```
Output:
```
{ip: 127.0.0.1, loopback: true}
invalid value "256.0.0.1" for flag -ip: could not parse IP
Usage of ExampleFunc:
-ip IP address
IP address to parse
{ip: <nil>, loopback: false}
```
func Int
--------
```
func Int(name string, value int, usage string) *int
```
Int defines an int flag with specified name, default value, and usage string. The return value is the address of an int variable that stores the value of the flag.
func Int64
----------
```
func Int64(name string, value int64, usage string) *int64
```
Int64 defines an int64 flag with specified name, default value, and usage string. The return value is the address of an int64 variable that stores the value of the flag.
func Int64Var
-------------
```
func Int64Var(p *int64, name string, value int64, usage string)
```
Int64Var defines an int64 flag with specified name, default value, and usage string. The argument p points to an int64 variable in which to store the value of the flag.
func IntVar
-----------
```
func IntVar(p *int, name string, value int, usage string)
```
IntVar defines an int flag with specified name, default value, and usage string. The argument p points to an int variable in which to store the value of the flag.
func NArg
---------
```
func NArg() int
```
NArg is the number of arguments remaining after flags have been processed.
func NFlag
----------
```
func NFlag() int
```
NFlag returns the number of command-line flags that have been set.
func Parse
----------
```
func Parse()
```
Parse parses the command-line flags from os.Args[1:]. Must be called after all flags are defined and before flags are accessed by the program.
func Parsed
-----------
```
func Parsed() bool
```
Parsed reports whether the command-line flags have been parsed.
func PrintDefaults
------------------
```
func PrintDefaults()
```
PrintDefaults prints, to standard error unless configured otherwise, a usage message showing the default settings of all defined command-line flags. For an integer valued flag x, the default output has the form
```
-x int
usage-message-for-x (default 7)
```
The usage message will appear on a separate line for anything but a bool flag with a one-byte name. For bool flags, the type is omitted and if the flag name is one byte the usage message appears on the same line. The parenthetical default is omitted if the default is the zero value for the type. The listed type, here int, can be changed by placing a back-quoted name in the flag's usage string; the first such item in the message is taken to be a parameter name to show in the message and the back quotes are stripped from the message when displayed. For instance, given
```
flag.String("I", "", "search `directory` for include files")
```
the output will be
```
-I directory
search directory for include files.
```
To change the destination for flag messages, call CommandLine.SetOutput.
func Set
--------
```
func Set(name, value string) error
```
Set sets the value of the named command-line flag.
func String
-----------
```
func String(name string, value string, usage string) *string
```
String defines a string flag with specified name, default value, and usage string. The return value is the address of a string variable that stores the value of the flag.
func StringVar
--------------
```
func StringVar(p *string, name string, value string, usage string)
```
StringVar defines a string flag with specified name, default value, and usage string. The argument p points to a string variable in which to store the value of the flag.
func TextVar 1.19
-----------------
```
func TextVar(p encoding.TextUnmarshaler, name string, value encoding.TextMarshaler, usage string)
```
TextVar defines a flag with a specified name, default value, and usage string. The argument p must be a pointer to a variable that will hold the value of the flag, and p must implement encoding.TextUnmarshaler. If the flag is used, the flag value will be passed to p's UnmarshalText method. The type of the default value must be the same as the type of p.
#### Example
Code:
```
fs := flag.NewFlagSet("ExampleTextVar", flag.ContinueOnError)
fs.SetOutput(os.Stdout)
var ip net.IP
fs.TextVar(&ip, "ip", net.IPv4(192, 168, 0, 100), "`IP address` to parse")
fs.Parse([]string{"-ip", "127.0.0.1"})
fmt.Printf("{ip: %v}\n\n", ip)
// 256 is not a valid IPv4 component
ip = nil
fs.Parse([]string{"-ip", "256.0.0.1"})
fmt.Printf("{ip: %v}\n\n", ip)
```
Output:
```
{ip: 127.0.0.1}
invalid value "256.0.0.1" for flag -ip: invalid IP address: 256.0.0.1
Usage of ExampleTextVar:
-ip IP address
IP address to parse (default 192.168.0.100)
{ip: <nil>}
```
func Uint
---------
```
func Uint(name string, value uint, usage string) *uint
```
Uint defines a uint flag with specified name, default value, and usage string. The return value is the address of a uint variable that stores the value of the flag.
func Uint64
-----------
```
func Uint64(name string, value uint64, usage string) *uint64
```
Uint64 defines a uint64 flag with specified name, default value, and usage string. The return value is the address of a uint64 variable that stores the value of the flag.
func Uint64Var
--------------
```
func Uint64Var(p *uint64, name string, value uint64, usage string)
```
Uint64Var defines a uint64 flag with specified name, default value, and usage string. The argument p points to a uint64 variable in which to store the value of the flag.
func UintVar
------------
```
func UintVar(p *uint, name string, value uint, usage string)
```
UintVar defines a uint flag with specified name, default value, and usage string. The argument p points to a uint variable in which to store the value of the flag.
func UnquoteUsage 1.5
---------------------
```
func UnquoteUsage(flag *Flag) (name string, usage string)
```
UnquoteUsage extracts a back-quoted name from the usage string for a flag and returns it and the un-quoted usage. Given "a `name` to show" it returns ("name", "a name to show"). If there are no back quotes, the name is an educated guess of the type of the flag's value, or the empty string if the flag is boolean.
func Var
--------
```
func Var(value Value, name string, usage string)
```
Var defines a flag with the specified name and usage string. The type and value of the flag are represented by the first argument, of type Value, which typically holds a user-defined implementation of Value. For instance, the caller could create a flag that turns a comma-separated string into a slice of strings by giving the slice the methods of Value; in particular, Set would decompose the comma-separated string into the slice.
func Visit
----------
```
func Visit(fn func(*Flag))
```
Visit visits the command-line flags in lexicographical order, calling fn for each. It visits only those flags that have been set.
func VisitAll
-------------
```
func VisitAll(fn func(*Flag))
```
VisitAll visits the command-line flags in lexicographical order, calling fn for each. It visits all flags, even those not set.
type ErrorHandling
------------------
ErrorHandling defines how FlagSet.Parse behaves if the parse fails.
```
type ErrorHandling int
```
These constants cause FlagSet.Parse to behave as described if the parse fails.
```
const (
ContinueOnError ErrorHandling = iota // Return a descriptive error.
ExitOnError // Call os.Exit(2) or for -h/-help Exit(0).
PanicOnError // Call panic with a descriptive error.
)
```
type Flag
---------
A Flag represents the state of a flag.
```
type Flag struct {
Name string // name as it appears on command line
Usage string // help message
Value Value // value as set
DefValue string // default value (as text); for usage message
}
```
### func Lookup
```
func Lookup(name string) *Flag
```
Lookup returns the Flag structure of the named command-line flag, returning nil if none exists.
type FlagSet
------------
A FlagSet represents a set of defined flags. The zero value of a FlagSet has no name and has ContinueOnError error handling.
Flag names must be unique within a FlagSet. An attempt to define a flag whose name is already in use will cause a panic.
```
type FlagSet struct {
// Usage is the function called when an error occurs while parsing flags.
// The field is a function (not a method) that may be changed to point to
// a custom error handler. What happens after Usage is called depends
// on the ErrorHandling setting; for the command line, this defaults
// to ExitOnError, which exits the program after calling Usage.
Usage func()
// contains filtered or unexported fields
}
```
### func NewFlagSet
```
func NewFlagSet(name string, errorHandling ErrorHandling) *FlagSet
```
NewFlagSet returns a new, empty flag set with the specified name and error handling property. If the name is not empty, it will be printed in the default usage message and in error messages.
### func (\*FlagSet) Arg
```
func (f *FlagSet) Arg(i int) string
```
Arg returns the i'th argument. Arg(0) is the first remaining argument after flags have been processed. Arg returns an empty string if the requested element does not exist.
### func (\*FlagSet) Args
```
func (f *FlagSet) Args() []string
```
Args returns the non-flag arguments.
### func (\*FlagSet) Bool
```
func (f *FlagSet) Bool(name string, value bool, usage string) *bool
```
Bool defines a bool flag with specified name, default value, and usage string. The return value is the address of a bool variable that stores the value of the flag.
### func (\*FlagSet) BoolVar
```
func (f *FlagSet) BoolVar(p *bool, name string, value bool, usage string)
```
BoolVar defines a bool flag with specified name, default value, and usage string. The argument p points to a bool variable in which to store the value of the flag.
### func (\*FlagSet) Duration
```
func (f *FlagSet) Duration(name string, value time.Duration, usage string) *time.Duration
```
Duration defines a time.Duration flag with specified name, default value, and usage string. The return value is the address of a time.Duration variable that stores the value of the flag. The flag accepts a value acceptable to time.ParseDuration.
### func (\*FlagSet) DurationVar
```
func (f *FlagSet) DurationVar(p *time.Duration, name string, value time.Duration, usage string)
```
DurationVar defines a time.Duration flag with specified name, default value, and usage string. The argument p points to a time.Duration variable in which to store the value of the flag. The flag accepts a value acceptable to time.ParseDuration.
### func (\*FlagSet) ErrorHandling 1.10
```
func (f *FlagSet) ErrorHandling() ErrorHandling
```
ErrorHandling returns the error handling behavior of the flag set.
### func (\*FlagSet) Float64
```
func (f *FlagSet) Float64(name string, value float64, usage string) *float64
```
Float64 defines a float64 flag with specified name, default value, and usage string. The return value is the address of a float64 variable that stores the value of the flag.
### func (\*FlagSet) Float64Var
```
func (f *FlagSet) Float64Var(p *float64, name string, value float64, usage string)
```
Float64Var defines a float64 flag with specified name, default value, and usage string. The argument p points to a float64 variable in which to store the value of the flag.
### func (\*FlagSet) Func 1.16
```
func (f *FlagSet) Func(name, usage string, fn func(string) error)
```
Func defines a flag with the specified name and usage string. Each time the flag is seen, fn is called with the value of the flag. If fn returns a non-nil error, it will be treated as a flag value parsing error.
### func (\*FlagSet) Init
```
func (f *FlagSet) Init(name string, errorHandling ErrorHandling)
```
Init sets the name and error handling property for a flag set. By default, the zero FlagSet uses an empty name and the ContinueOnError error handling policy.
### func (\*FlagSet) Int
```
func (f *FlagSet) Int(name string, value int, usage string) *int
```
Int defines an int flag with specified name, default value, and usage string. The return value is the address of an int variable that stores the value of the flag.
### func (\*FlagSet) Int64
```
func (f *FlagSet) Int64(name string, value int64, usage string) *int64
```
Int64 defines an int64 flag with specified name, default value, and usage string. The return value is the address of an int64 variable that stores the value of the flag.
### func (\*FlagSet) Int64Var
```
func (f *FlagSet) Int64Var(p *int64, name string, value int64, usage string)
```
Int64Var defines an int64 flag with specified name, default value, and usage string. The argument p points to an int64 variable in which to store the value of the flag.
### func (\*FlagSet) IntVar
```
func (f *FlagSet) IntVar(p *int, name string, value int, usage string)
```
IntVar defines an int flag with specified name, default value, and usage string. The argument p points to an int variable in which to store the value of the flag.
### func (\*FlagSet) Lookup
```
func (f *FlagSet) Lookup(name string) *Flag
```
Lookup returns the Flag structure of the named flag, returning nil if none exists.
### func (\*FlagSet) NArg
```
func (f *FlagSet) NArg() int
```
NArg is the number of arguments remaining after flags have been processed.
### func (\*FlagSet) NFlag
```
func (f *FlagSet) NFlag() int
```
NFlag returns the number of flags that have been set.
### func (\*FlagSet) Name 1.10
```
func (f *FlagSet) Name() string
```
Name returns the name of the flag set.
### func (\*FlagSet) Output 1.10
```
func (f *FlagSet) Output() io.Writer
```
Output returns the destination for usage and error messages. os.Stderr is returned if output was not set or was set to nil.
### func (\*FlagSet) Parse
```
func (f *FlagSet) Parse(arguments []string) error
```
Parse parses flag definitions from the argument list, which should not include the command name. Must be called after all flags in the FlagSet are defined and before flags are accessed by the program. The return value will be ErrHelp if -help or -h were set but not defined.
### func (\*FlagSet) Parsed
```
func (f *FlagSet) Parsed() bool
```
Parsed reports whether f.Parse has been called.
### func (\*FlagSet) PrintDefaults
```
func (f *FlagSet) PrintDefaults()
```
PrintDefaults prints, to standard error unless configured otherwise, the default values of all defined command-line flags in the set. See the documentation for the global function PrintDefaults for more information.
### func (\*FlagSet) Set
```
func (f *FlagSet) Set(name, value string) error
```
Set sets the value of the named flag.
### func (\*FlagSet) SetOutput
```
func (f *FlagSet) SetOutput(output io.Writer)
```
SetOutput sets the destination for usage and error messages. If output is nil, os.Stderr is used.
### func (\*FlagSet) String
```
func (f *FlagSet) String(name string, value string, usage string) *string
```
String defines a string flag with specified name, default value, and usage string. The return value is the address of a string variable that stores the value of the flag.
### func (\*FlagSet) StringVar
```
func (f *FlagSet) StringVar(p *string, name string, value string, usage string)
```
StringVar defines a string flag with specified name, default value, and usage string. The argument p points to a string variable in which to store the value of the flag.
### func (\*FlagSet) TextVar 1.19
```
func (f *FlagSet) TextVar(p encoding.TextUnmarshaler, name string, value encoding.TextMarshaler, usage string)
```
TextVar defines a flag with a specified name, default value, and usage string. The argument p must be a pointer to a variable that will hold the value of the flag, and p must implement encoding.TextUnmarshaler. If the flag is used, the flag value will be passed to p's UnmarshalText method. The type of the default value must be the same as the type of p.
### func (\*FlagSet) Uint
```
func (f *FlagSet) Uint(name string, value uint, usage string) *uint
```
Uint defines a uint flag with specified name, default value, and usage string. The return value is the address of a uint variable that stores the value of the flag.
### func (\*FlagSet) Uint64
```
func (f *FlagSet) Uint64(name string, value uint64, usage string) *uint64
```
Uint64 defines a uint64 flag with specified name, default value, and usage string. The return value is the address of a uint64 variable that stores the value of the flag.
### func (\*FlagSet) Uint64Var
```
func (f *FlagSet) Uint64Var(p *uint64, name string, value uint64, usage string)
```
Uint64Var defines a uint64 flag with specified name, default value, and usage string. The argument p points to a uint64 variable in which to store the value of the flag.
### func (\*FlagSet) UintVar
```
func (f *FlagSet) UintVar(p *uint, name string, value uint, usage string)
```
UintVar defines a uint flag with specified name, default value, and usage string. The argument p points to a uint variable in which to store the value of the flag.
### func (\*FlagSet) Var
```
func (f *FlagSet) Var(value Value, name string, usage string)
```
Var defines a flag with the specified name and usage string. The type and value of the flag are represented by the first argument, of type Value, which typically holds a user-defined implementation of Value. For instance, the caller could create a flag that turns a comma-separated string into a slice of strings by giving the slice the methods of Value; in particular, Set would decompose the comma-separated string into the slice.
### func (\*FlagSet) Visit
```
func (f *FlagSet) Visit(fn func(*Flag))
```
Visit visits the flags in lexicographical order, calling fn for each. It visits only those flags that have been set.
### func (\*FlagSet) VisitAll
```
func (f *FlagSet) VisitAll(fn func(*Flag))
```
VisitAll visits the flags in lexicographical order, calling fn for each. It visits all flags, even those not set.
type Getter 1.2
---------------
Getter is an interface that allows the contents of a Value to be retrieved. It wraps the Value interface, rather than being part of it, because it appeared after Go 1 and its compatibility rules. All Value types provided by this package satisfy the Getter interface, except the type used by Func.
```
type Getter interface {
Value
Get() any
}
```
type Value
----------
Value is the interface to the dynamic value stored in a flag. (The default value is represented as a string.)
If a Value has an IsBoolFlag() bool method returning true, the command-line parser makes -name equivalent to -name=true rather than using the next command-line argument.
Set is called once, in command line order, for each flag present. The flag package may call the String method with a zero-valued receiver, such as a nil pointer.
```
type Value interface {
String() string
Set(string) error
}
```
#### Example
Code:
```
package flag_test
import (
"flag"
"fmt"
"net/url"
)
type URLValue struct {
URL *url.URL
}
func (v URLValue) String() string {
if v.URL != nil {
return v.URL.String()
}
return ""
}
func (v URLValue) Set(s string) error {
if u, err := url.Parse(s); err != nil {
return err
} else {
*v.URL = *u
}
return nil
}
var u = &url.URL{}
func ExampleValue() {
fs := flag.NewFlagSet("ExampleValue", flag.ExitOnError)
fs.Var(&URLValue{u}, "url", "URL to parse")
fs.Parse([]string{"-url", "https://golang.org/pkg/flag/"})
fmt.Printf(`{scheme: %q, host: %q, path: %q}`, u.Scheme, u.Host, u.Path)
// Output:
// {scheme: "https", host: "golang.org", path: "/pkg/flag/"}
}
```
| programming_docs |
go Package strconv Package strconv
================
* `import "strconv"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package strconv implements conversions to and from string representations of basic data types.
### Numeric Conversions
The most common numeric conversions are Atoi (string to int) and Itoa (int to string).
```
i, err := strconv.Atoi("-42")
s := strconv.Itoa(-42)
```
These assume decimal and the Go int type.
ParseBool, ParseFloat, ParseInt, and ParseUint convert strings to values:
```
b, err := strconv.ParseBool("true")
f, err := strconv.ParseFloat("3.1415", 64)
i, err := strconv.ParseInt("-42", 10, 64)
u, err := strconv.ParseUint("42", 10, 64)
```
The parse functions return the widest type (float64, int64, and uint64), but if the size argument specifies a narrower width the result can be converted to that narrower type without data loss:
```
s := "2147483647" // biggest int32
i64, err := strconv.ParseInt(s, 10, 32)
...
i := int32(i64)
```
FormatBool, FormatFloat, FormatInt, and FormatUint convert values to strings:
```
s := strconv.FormatBool(true)
s := strconv.FormatFloat(3.1415, 'E', -1, 64)
s := strconv.FormatInt(-42, 16)
s := strconv.FormatUint(42, 16)
```
AppendBool, AppendFloat, AppendInt, and AppendUint are similar but append the formatted value to a destination slice.
### String Conversions
Quote and QuoteToASCII convert strings to quoted Go string literals. The latter guarantees that the result is an ASCII string, by escaping any non-ASCII Unicode with \u:
```
q := strconv.Quote("Hello, 世界")
q := strconv.QuoteToASCII("Hello, 世界")
```
QuoteRune and QuoteRuneToASCII are similar but accept runes and return quoted Go rune literals.
Unquote and UnquoteChar unquote Go string and rune literals.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func AppendBool(dst []byte, b bool) []byte](#AppendBool)
* [func AppendFloat(dst []byte, f float64, fmt byte, prec, bitSize int) []byte](#AppendFloat)
* [func AppendInt(dst []byte, i int64, base int) []byte](#AppendInt)
* [func AppendQuote(dst []byte, s string) []byte](#AppendQuote)
* [func AppendQuoteRune(dst []byte, r rune) []byte](#AppendQuoteRune)
* [func AppendQuoteRuneToASCII(dst []byte, r rune) []byte](#AppendQuoteRuneToASCII)
* [func AppendQuoteRuneToGraphic(dst []byte, r rune) []byte](#AppendQuoteRuneToGraphic)
* [func AppendQuoteToASCII(dst []byte, s string) []byte](#AppendQuoteToASCII)
* [func AppendQuoteToGraphic(dst []byte, s string) []byte](#AppendQuoteToGraphic)
* [func AppendUint(dst []byte, i uint64, base int) []byte](#AppendUint)
* [func Atoi(s string) (int, error)](#Atoi)
* [func CanBackquote(s string) bool](#CanBackquote)
* [func FormatBool(b bool) string](#FormatBool)
* [func FormatComplex(c complex128, fmt byte, prec, bitSize int) string](#FormatComplex)
* [func FormatFloat(f float64, fmt byte, prec, bitSize int) string](#FormatFloat)
* [func FormatInt(i int64, base int) string](#FormatInt)
* [func FormatUint(i uint64, base int) string](#FormatUint)
* [func IsGraphic(r rune) bool](#IsGraphic)
* [func IsPrint(r rune) bool](#IsPrint)
* [func Itoa(i int) string](#Itoa)
* [func ParseBool(str string) (bool, error)](#ParseBool)
* [func ParseComplex(s string, bitSize int) (complex128, error)](#ParseComplex)
* [func ParseFloat(s string, bitSize int) (float64, error)](#ParseFloat)
* [func ParseInt(s string, base int, bitSize int) (i int64, err error)](#ParseInt)
* [func ParseUint(s string, base int, bitSize int) (uint64, error)](#ParseUint)
* [func Quote(s string) string](#Quote)
* [func QuoteRune(r rune) string](#QuoteRune)
* [func QuoteRuneToASCII(r rune) string](#QuoteRuneToASCII)
* [func QuoteRuneToGraphic(r rune) string](#QuoteRuneToGraphic)
* [func QuoteToASCII(s string) string](#QuoteToASCII)
* [func QuoteToGraphic(s string) string](#QuoteToGraphic)
* [func QuotedPrefix(s string) (string, error)](#QuotedPrefix)
* [func Unquote(s string) (string, error)](#Unquote)
* [func UnquoteChar(s string, quote byte) (value rune, multibyte bool, tail string, err error)](#UnquoteChar)
* [type NumError](#NumError)
* [func (e \*NumError) Error() string](#NumError.Error)
* [func (e \*NumError) Unwrap() error](#NumError.Unwrap)
### Examples
[AppendBool](#example_AppendBool) [AppendFloat](#example_AppendFloat) [AppendInt](#example_AppendInt) [AppendQuote](#example_AppendQuote) [AppendQuoteRune](#example_AppendQuoteRune) [AppendQuoteRuneToASCII](#example_AppendQuoteRuneToASCII) [AppendQuoteToASCII](#example_AppendQuoteToASCII) [AppendUint](#example_AppendUint) [Atoi](#example_Atoi) [CanBackquote](#example_CanBackquote) [FormatBool](#example_FormatBool) [FormatFloat](#example_FormatFloat) [FormatInt](#example_FormatInt) [FormatUint](#example_FormatUint) [IsGraphic](#example_IsGraphic) [IsPrint](#example_IsPrint) [Itoa](#example_Itoa) [NumError](#example_NumError) [ParseBool](#example_ParseBool) [ParseFloat](#example_ParseFloat) [ParseInt](#example_ParseInt) [ParseUint](#example_ParseUint) [Quote](#example_Quote) [QuoteRune](#example_QuoteRune) [QuoteRuneToASCII](#example_QuoteRuneToASCII) [QuoteRuneToGraphic](#example_QuoteRuneToGraphic) [QuoteToASCII](#example_QuoteToASCII) [QuoteToGraphic](#example_QuoteToGraphic) [Unquote](#example_Unquote) [UnquoteChar](#example_UnquoteChar) ### Package files
atob.go atoc.go atof.go atoi.go bytealg.go ctoa.go decimal.go doc.go eisel\_lemire.go ftoa.go ftoaryu.go isprint.go itoa.go quote.go
Constants
---------
IntSize is the size in bits of an int or uint value.
```
const IntSize = intSize
```
Variables
---------
ErrRange indicates that a value is out of range for the target type.
```
var ErrRange = errors.New("value out of range")
```
ErrSyntax indicates that a value does not have the right syntax for the target type.
```
var ErrSyntax = errors.New("invalid syntax")
```
func AppendBool
---------------
```
func AppendBool(dst []byte, b bool) []byte
```
AppendBool appends "true" or "false", according to the value of b, to dst and returns the extended buffer.
#### Example
Code:
```
b := []byte("bool:")
b = strconv.AppendBool(b, true)
fmt.Println(string(b))
```
Output:
```
bool:true
```
func AppendFloat
----------------
```
func AppendFloat(dst []byte, f float64, fmt byte, prec, bitSize int) []byte
```
AppendFloat appends the string form of the floating-point number f, as generated by FormatFloat, to dst and returns the extended buffer.
#### Example
Code:
```
b32 := []byte("float32:")
b32 = strconv.AppendFloat(b32, 3.1415926535, 'E', -1, 32)
fmt.Println(string(b32))
b64 := []byte("float64:")
b64 = strconv.AppendFloat(b64, 3.1415926535, 'E', -1, 64)
fmt.Println(string(b64))
```
Output:
```
float32:3.1415927E+00
float64:3.1415926535E+00
```
func AppendInt
--------------
```
func AppendInt(dst []byte, i int64, base int) []byte
```
AppendInt appends the string form of the integer i, as generated by FormatInt, to dst and returns the extended buffer.
#### Example
Code:
```
b10 := []byte("int (base 10):")
b10 = strconv.AppendInt(b10, -42, 10)
fmt.Println(string(b10))
b16 := []byte("int (base 16):")
b16 = strconv.AppendInt(b16, -42, 16)
fmt.Println(string(b16))
```
Output:
```
int (base 10):-42
int (base 16):-2a
```
func AppendQuote
----------------
```
func AppendQuote(dst []byte, s string) []byte
```
AppendQuote appends a double-quoted Go string literal representing s, as generated by Quote, to dst and returns the extended buffer.
#### Example
Code:
```
b := []byte("quote:")
b = strconv.AppendQuote(b, `"Fran & Freddie's Diner"`)
fmt.Println(string(b))
```
Output:
```
quote:"\"Fran & Freddie's Diner\""
```
func AppendQuoteRune
--------------------
```
func AppendQuoteRune(dst []byte, r rune) []byte
```
AppendQuoteRune appends a single-quoted Go character literal representing the rune, as generated by QuoteRune, to dst and returns the extended buffer.
#### Example
Code:
```
b := []byte("rune:")
b = strconv.AppendQuoteRune(b, '☺')
fmt.Println(string(b))
```
Output:
```
rune:'☺'
```
func AppendQuoteRuneToASCII
---------------------------
```
func AppendQuoteRuneToASCII(dst []byte, r rune) []byte
```
AppendQuoteRuneToASCII appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToASCII, to dst and returns the extended buffer.
#### Example
Code:
```
b := []byte("rune (ascii):")
b = strconv.AppendQuoteRuneToASCII(b, '☺')
fmt.Println(string(b))
```
Output:
```
rune (ascii):'\u263a'
```
func AppendQuoteRuneToGraphic 1.6
---------------------------------
```
func AppendQuoteRuneToGraphic(dst []byte, r rune) []byte
```
AppendQuoteRuneToGraphic appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToGraphic, to dst and returns the extended buffer.
func AppendQuoteToASCII
-----------------------
```
func AppendQuoteToASCII(dst []byte, s string) []byte
```
AppendQuoteToASCII appends a double-quoted Go string literal representing s, as generated by QuoteToASCII, to dst and returns the extended buffer.
#### Example
Code:
```
b := []byte("quote (ascii):")
b = strconv.AppendQuoteToASCII(b, `"Fran & Freddie's Diner"`)
fmt.Println(string(b))
```
Output:
```
quote (ascii):"\"Fran & Freddie's Diner\""
```
func AppendQuoteToGraphic 1.6
-----------------------------
```
func AppendQuoteToGraphic(dst []byte, s string) []byte
```
AppendQuoteToGraphic appends a double-quoted Go string literal representing s, as generated by QuoteToGraphic, to dst and returns the extended buffer.
func AppendUint
---------------
```
func AppendUint(dst []byte, i uint64, base int) []byte
```
AppendUint appends the string form of the unsigned integer i, as generated by FormatUint, to dst and returns the extended buffer.
#### Example
Code:
```
b10 := []byte("uint (base 10):")
b10 = strconv.AppendUint(b10, 42, 10)
fmt.Println(string(b10))
b16 := []byte("uint (base 16):")
b16 = strconv.AppendUint(b16, 42, 16)
fmt.Println(string(b16))
```
Output:
```
uint (base 10):42
uint (base 16):2a
```
func Atoi
---------
```
func Atoi(s string) (int, error)
```
Atoi is equivalent to ParseInt(s, 10, 0), converted to type int.
#### Example
Code:
```
v := "10"
if s, err := strconv.Atoi(v); err == nil {
fmt.Printf("%T, %v", s, s)
}
```
Output:
```
int, 10
```
func CanBackquote
-----------------
```
func CanBackquote(s string) bool
```
CanBackquote reports whether the string s can be represented unchanged as a single-line backquoted string without control characters other than tab.
#### Example
Code:
```
fmt.Println(strconv.CanBackquote("Fran & Freddie's Diner ☺"))
fmt.Println(strconv.CanBackquote("`can't backquote this`"))
```
Output:
```
true
false
```
func FormatBool
---------------
```
func FormatBool(b bool) string
```
FormatBool returns "true" or "false" according to the value of b.
#### Example
Code:
```
v := true
s := strconv.FormatBool(v)
fmt.Printf("%T, %v\n", s, s)
```
Output:
```
string, true
```
func FormatComplex 1.15
-----------------------
```
func FormatComplex(c complex128, fmt byte, prec, bitSize int) string
```
FormatComplex converts the complex number c to a string of the form (a+bi) where a and b are the real and imaginary parts, formatted according to the format fmt and precision prec.
The format fmt and precision prec have the same meaning as in FormatFloat. It rounds the result assuming that the original was obtained from a complex value of bitSize bits, which must be 64 for complex64 and 128 for complex128.
func FormatFloat
----------------
```
func FormatFloat(f float64, fmt byte, prec, bitSize int) string
```
FormatFloat converts the floating-point number f to a string, according to the format fmt and precision prec. It rounds the result assuming that the original was obtained from a floating-point value of bitSize bits (32 for float32, 64 for float64).
The format fmt is one of 'b' (-ddddp±ddd, a binary exponent), 'e' (-d.dddde±dd, a decimal exponent), 'E' (-d.ddddE±dd, a decimal exponent), 'f' (-ddd.dddd, no exponent), 'g' ('e' for large exponents, 'f' otherwise), 'G' ('E' for large exponents, 'f' otherwise), 'x' (-0xd.ddddp±ddd, a hexadecimal fraction and binary exponent), or 'X' (-0Xd.ddddP±ddd, a hexadecimal fraction and binary exponent).
The precision prec controls the number of digits (excluding the exponent) printed by the 'e', 'E', 'f', 'g', 'G', 'x', and 'X' formats. For 'e', 'E', 'f', 'x', and 'X', it is the number of digits after the decimal point. For 'g' and 'G' it is the maximum number of significant digits (trailing zeros are removed). The special precision -1 uses the smallest number of digits necessary such that ParseFloat will return f exactly.
#### Example
Code:
```
v := 3.1415926535
s32 := strconv.FormatFloat(v, 'E', -1, 32)
fmt.Printf("%T, %v\n", s32, s32)
s64 := strconv.FormatFloat(v, 'E', -1, 64)
fmt.Printf("%T, %v\n", s64, s64)
```
Output:
```
string, 3.1415927E+00
string, 3.1415926535E+00
```
func FormatInt
--------------
```
func FormatInt(i int64, base int) string
```
FormatInt returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
#### Example
Code:
```
v := int64(-42)
s10 := strconv.FormatInt(v, 10)
fmt.Printf("%T, %v\n", s10, s10)
s16 := strconv.FormatInt(v, 16)
fmt.Printf("%T, %v\n", s16, s16)
```
Output:
```
string, -42
string, -2a
```
func FormatUint
---------------
```
func FormatUint(i uint64, base int) string
```
FormatUint returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
#### Example
Code:
```
v := uint64(42)
s10 := strconv.FormatUint(v, 10)
fmt.Printf("%T, %v\n", s10, s10)
s16 := strconv.FormatUint(v, 16)
fmt.Printf("%T, %v\n", s16, s16)
```
Output:
```
string, 42
string, 2a
```
func IsGraphic 1.6
------------------
```
func IsGraphic(r rune) bool
```
IsGraphic reports whether the rune is defined as a Graphic by Unicode. Such characters include letters, marks, numbers, punctuation, symbols, and spaces, from categories L, M, N, P, S, and Zs.
#### Example
Code:
```
shamrock := strconv.IsGraphic('☘')
fmt.Println(shamrock)
a := strconv.IsGraphic('a')
fmt.Println(a)
bel := strconv.IsGraphic('\007')
fmt.Println(bel)
```
Output:
```
true
true
false
```
func IsPrint
------------
```
func IsPrint(r rune) bool
```
IsPrint reports whether the rune is defined as printable by Go, with the same definition as unicode.IsPrint: letters, numbers, punctuation, symbols and ASCII space.
#### Example
Code:
```
c := strconv.IsPrint('\u263a')
fmt.Println(c)
bel := strconv.IsPrint('\007')
fmt.Println(bel)
```
Output:
```
true
false
```
func Itoa
---------
```
func Itoa(i int) string
```
Itoa is equivalent to FormatInt(int64(i), 10).
#### Example
Code:
```
i := 10
s := strconv.Itoa(i)
fmt.Printf("%T, %v\n", s, s)
```
Output:
```
string, 10
```
func ParseBool
--------------
```
func ParseBool(str string) (bool, error)
```
ParseBool returns the boolean value represented by the string. It accepts 1, t, T, TRUE, true, True, 0, f, F, FALSE, false, False. Any other value returns an error.
#### Example
Code:
```
v := "true"
if s, err := strconv.ParseBool(v); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
```
Output:
```
bool, true
```
func ParseComplex 1.15
----------------------
```
func ParseComplex(s string, bitSize int) (complex128, error)
```
ParseComplex converts the string s to a complex number with the precision specified by bitSize: 64 for complex64, or 128 for complex128. When bitSize=64, the result still has type complex128, but it will be convertible to complex64 without changing its value.
The number represented by s must be of the form N, Ni, or N±Ni, where N stands for a floating-point number as recognized by ParseFloat, and i is the imaginary component. If the second N is unsigned, a + sign is required between the two components as indicated by the ±. If the second N is NaN, only a + sign is accepted. The form may be parenthesized and cannot contain any spaces. The resulting complex number consists of the two components converted by ParseFloat.
The errors that ParseComplex returns have concrete type \*NumError and include err.Num = s.
If s is not syntactically well-formed, ParseComplex returns err.Err = ErrSyntax.
If s is syntactically well-formed but either component is more than 1/2 ULP away from the largest floating point number of the given component's size, ParseComplex returns err.Err = ErrRange and c = ±Inf for the respective component.
func ParseFloat
---------------
```
func ParseFloat(s string, bitSize int) (float64, error)
```
ParseFloat converts the string s to a floating-point number with the precision specified by bitSize: 32 for float32, or 64 for float64. When bitSize=32, the result still has type float64, but it will be convertible to float32 without changing its value.
ParseFloat accepts decimal and hexadecimal floating-point numbers as defined by the Go syntax for [floating-point literals](https://go.dev/ref/spec#Floating-point_literals). If s is well-formed and near a valid floating-point number, ParseFloat returns the nearest floating-point number rounded using IEEE754 unbiased rounding. (Parsing a hexadecimal floating-point value only rounds when there are more bits in the hexadecimal representation than will fit in the mantissa.)
The errors that ParseFloat returns have concrete type \*NumError and include err.Num = s.
If s is not syntactically well-formed, ParseFloat returns err.Err = ErrSyntax.
If s is syntactically well-formed but is more than 1/2 ULP away from the largest floating point number of the given size, ParseFloat returns f = ±Inf, err.Err = ErrRange.
ParseFloat recognizes the string "NaN", and the (possibly signed) strings "Inf" and "Infinity" as their respective special floating point values. It ignores case when matching.
#### Example
Code:
```
v := "3.1415926535"
if s, err := strconv.ParseFloat(v, 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat(v, 64); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("NaN", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
// ParseFloat is case insensitive
if s, err := strconv.ParseFloat("nan", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("inf", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("+Inf", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("-Inf", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("-0", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseFloat("+0", 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
```
Output:
```
float64, 3.1415927410125732
float64, 3.1415926535
float64, NaN
float64, NaN
float64, +Inf
float64, +Inf
float64, -Inf
float64, -0
float64, 0
```
func ParseInt
-------------
```
func ParseInt(s string, base int, bitSize int) (i int64, err error)
```
ParseInt interprets a string s in the given base (0, 2 to 36) and bit size (0 to 64) and returns the corresponding value i.
The string may begin with a leading sign: "+" or "-".
If the base argument is 0, the true base is implied by the string's prefix following the sign (if present): 2 for "0b", 8 for "0" or "0o", 16 for "0x", and 10 otherwise. Also, for argument base 0 only, underscore characters are permitted as defined by the Go syntax for [integer literals](https://go.dev/ref/spec#Integer_literals).
The bitSize argument specifies the integer type that the result must fit into. Bit sizes 0, 8, 16, 32, and 64 correspond to int, int8, int16, int32, and int64. If bitSize is below 0 or above 64, an error is returned.
The errors that ParseInt returns have concrete type \*NumError and include err.Num = s. If s is empty or contains invalid digits, err.Err = ErrSyntax and the returned value is 0; if the value corresponding to s cannot be represented by a signed integer of the given size, err.Err = ErrRange and the returned value is the maximum magnitude integer of the appropriate bitSize and sign.
#### Example
Code:
```
v32 := "-354634382"
if s, err := strconv.ParseInt(v32, 10, 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseInt(v32, 16, 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
v64 := "-3546343826724305832"
if s, err := strconv.ParseInt(v64, 10, 64); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseInt(v64, 16, 64); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
```
Output:
```
int64, -354634382
int64, -3546343826724305832
```
func ParseUint
--------------
```
func ParseUint(s string, base int, bitSize int) (uint64, error)
```
ParseUint is like ParseInt but for unsigned numbers.
A sign prefix is not permitted.
#### Example
Code:
```
v := "42"
if s, err := strconv.ParseUint(v, 10, 32); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
if s, err := strconv.ParseUint(v, 10, 64); err == nil {
fmt.Printf("%T, %v\n", s, s)
}
```
Output:
```
uint64, 42
uint64, 42
```
func Quote
----------
```
func Quote(s string) string
```
Quote returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint.
#### Example
Code:
```
// This string literal contains a tab character.
s := strconv.Quote(`"Fran & Freddie's Diner ☺"`)
fmt.Println(s)
```
Output:
```
"\"Fran & Freddie's Diner\t☺\""
```
func QuoteRune
--------------
```
func QuoteRune(r rune) string
```
QuoteRune returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint. If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
#### Example
Code:
```
s := strconv.QuoteRune('☺')
fmt.Println(s)
```
Output:
```
'☺'
```
func QuoteRuneToASCII
---------------------
```
func QuoteRuneToASCII(r rune) string
```
QuoteRuneToASCII returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint. If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
#### Example
Code:
```
s := strconv.QuoteRuneToASCII('☺')
fmt.Println(s)
```
Output:
```
'\u263a'
```
func QuoteRuneToGraphic 1.6
---------------------------
```
func QuoteRuneToGraphic(r rune) string
```
QuoteRuneToGraphic returns a single-quoted Go character literal representing the rune. If the rune is not a Unicode graphic character, as defined by IsGraphic, the returned string will use a Go escape sequence (\t, \n, \xFF, \u0100). If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
#### Example
Code:
```
s := strconv.QuoteRuneToGraphic('☺')
fmt.Println(s)
s = strconv.QuoteRuneToGraphic('\u263a')
fmt.Println(s)
s = strconv.QuoteRuneToGraphic('\u000a')
fmt.Println(s)
s = strconv.QuoteRuneToGraphic(' ') // tab character
fmt.Println(s)
```
Output:
```
'☺'
'☺'
'\n'
'\t'
```
func QuoteToASCII
-----------------
```
func QuoteToASCII(s string) string
```
QuoteToASCII returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint.
#### Example
Code:
```
// This string literal contains a tab character.
s := strconv.QuoteToASCII(`"Fran & Freddie's Diner ☺"`)
fmt.Println(s)
```
Output:
```
"\"Fran & Freddie's Diner\t\u263a\""
```
func QuoteToGraphic 1.6
-----------------------
```
func QuoteToGraphic(s string) string
```
QuoteToGraphic returns a double-quoted Go string literal representing s. The returned string leaves Unicode graphic characters, as defined by IsGraphic, unchanged and uses Go escape sequences (\t, \n, \xFF, \u0100) for non-graphic characters.
#### Example
Code:
```
s := strconv.QuoteToGraphic("☺")
fmt.Println(s)
// This string literal contains a tab character.
s = strconv.QuoteToGraphic("This is a \u263a \u000a")
fmt.Println(s)
s = strconv.QuoteToGraphic(`" This is a ☺ \n "`)
fmt.Println(s)
```
Output:
```
"☺"
"This is a ☺\t\n"
"\" This is a ☺ \\n \""
```
func QuotedPrefix 1.17
----------------------
```
func QuotedPrefix(s string) (string, error)
```
QuotedPrefix returns the quoted string (as understood by Unquote) at the prefix of s. If s does not start with a valid quoted string, QuotedPrefix returns an error.
func Unquote
------------
```
func Unquote(s string) (string, error)
```
Unquote interprets s as a single-quoted, double-quoted, or backquoted Go string literal, returning the string value that s quotes. (If s is single-quoted, it would be a Go character literal; Unquote returns the corresponding one-character string.)
#### Example
Code:
```
s, err := strconv.Unquote("You can't unquote a string without quotes")
fmt.Printf("%q, %v\n", s, err)
s, err = strconv.Unquote("\"The string must be either double-quoted\"")
fmt.Printf("%q, %v\n", s, err)
s, err = strconv.Unquote("`or backquoted.`")
fmt.Printf("%q, %v\n", s, err)
s, err = strconv.Unquote("'\u263a'") // single character only allowed in single quotes
fmt.Printf("%q, %v\n", s, err)
s, err = strconv.Unquote("'\u2639\u2639'")
fmt.Printf("%q, %v\n", s, err)
```
Output:
```
"", invalid syntax
"The string must be either double-quoted", <nil>
"or backquoted.", <nil>
"☺", <nil>
"", invalid syntax
```
func UnquoteChar
----------------
```
func UnquoteChar(s string, quote byte) (value rune, multibyte bool, tail string, err error)
```
UnquoteChar decodes the first character or byte in the escaped string or character literal represented by the string s. It returns four values:
1. value, the decoded Unicode code point or byte value;
2. multibyte, a boolean indicating whether the decoded character requires a multibyte UTF-8 representation;
3. tail, the remainder of the string after the character; and
4. an error that will be nil if the character is syntactically valid.
The second argument, quote, specifies the type of literal being parsed and therefore which escaped quote character is permitted. If set to a single quote, it permits the sequence \' and disallows unescaped '. If set to a double quote, it permits \" and disallows unescaped ". If set to zero, it does not permit either escape and allows both quote characters to appear unescaped.
#### Example
Code:
```
v, mb, t, err := strconv.UnquoteChar(`\"Fran & Freddie's Diner\"`, '"')
if err != nil {
log.Fatal(err)
}
fmt.Println("value:", string(v))
fmt.Println("multibyte:", mb)
fmt.Println("tail:", t)
```
Output:
```
value: "
multibyte: false
tail: Fran & Freddie's Diner\"
```
type NumError
-------------
A NumError records a failed conversion.
```
type NumError struct {
Func string // the failing function (ParseBool, ParseInt, ParseUint, ParseFloat, ParseComplex)
Num string // the input
Err error // the reason the conversion failed (e.g. ErrRange, ErrSyntax, etc.)
}
```
#### Example
Code:
```
str := "Not a number"
if _, err := strconv.ParseFloat(str, 64); err != nil {
e := err.(*strconv.NumError)
fmt.Println("Func:", e.Func)
fmt.Println("Num:", e.Num)
fmt.Println("Err:", e.Err)
fmt.Println(err)
}
```
Output:
```
Func: ParseFloat
Num: Not a number
Err: invalid syntax
strconv.ParseFloat: parsing "Not a number": invalid syntax
```
### func (\*NumError) Error
```
func (e *NumError) Error() string
```
### func (\*NumError) Unwrap 1.14
```
func (e *NumError) Unwrap() error
```
| programming_docs |
go Package strings Package strings
================
* `import "strings"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package strings implements simple functions to manipulate UTF-8 encoded strings.
For information about UTF-8 strings in Go, see <https://blog.golang.org/strings>.
Index
-----
* [func Clone(s string) string](#Clone)
* [func Compare(a, b string) int](#Compare)
* [func Contains(s, substr string) bool](#Contains)
* [func ContainsAny(s, chars string) bool](#ContainsAny)
* [func ContainsRune(s string, r rune) bool](#ContainsRune)
* [func Count(s, substr string) int](#Count)
* [func Cut(s, sep string) (before, after string, found bool)](#Cut)
* [func CutPrefix(s, prefix string) (after string, found bool)](#CutPrefix)
* [func CutSuffix(s, suffix string) (before string, found bool)](#CutSuffix)
* [func EqualFold(s, t string) bool](#EqualFold)
* [func Fields(s string) []string](#Fields)
* [func FieldsFunc(s string, f func(rune) bool) []string](#FieldsFunc)
* [func HasPrefix(s, prefix string) bool](#HasPrefix)
* [func HasSuffix(s, suffix string) bool](#HasSuffix)
* [func Index(s, substr string) int](#Index)
* [func IndexAny(s, chars string) int](#IndexAny)
* [func IndexByte(s string, c byte) int](#IndexByte)
* [func IndexFunc(s string, f func(rune) bool) int](#IndexFunc)
* [func IndexRune(s string, r rune) int](#IndexRune)
* [func Join(elems []string, sep string) string](#Join)
* [func LastIndex(s, substr string) int](#LastIndex)
* [func LastIndexAny(s, chars string) int](#LastIndexAny)
* [func LastIndexByte(s string, c byte) int](#LastIndexByte)
* [func LastIndexFunc(s string, f func(rune) bool) int](#LastIndexFunc)
* [func Map(mapping func(rune) rune, s string) string](#Map)
* [func Repeat(s string, count int) string](#Repeat)
* [func Replace(s, old, new string, n int) string](#Replace)
* [func ReplaceAll(s, old, new string) string](#ReplaceAll)
* [func Split(s, sep string) []string](#Split)
* [func SplitAfter(s, sep string) []string](#SplitAfter)
* [func SplitAfterN(s, sep string, n int) []string](#SplitAfterN)
* [func SplitN(s, sep string, n int) []string](#SplitN)
* [func Title(s string) string](#Title)
* [func ToLower(s string) string](#ToLower)
* [func ToLowerSpecial(c unicode.SpecialCase, s string) string](#ToLowerSpecial)
* [func ToTitle(s string) string](#ToTitle)
* [func ToTitleSpecial(c unicode.SpecialCase, s string) string](#ToTitleSpecial)
* [func ToUpper(s string) string](#ToUpper)
* [func ToUpperSpecial(c unicode.SpecialCase, s string) string](#ToUpperSpecial)
* [func ToValidUTF8(s, replacement string) string](#ToValidUTF8)
* [func Trim(s, cutset string) string](#Trim)
* [func TrimFunc(s string, f func(rune) bool) string](#TrimFunc)
* [func TrimLeft(s, cutset string) string](#TrimLeft)
* [func TrimLeftFunc(s string, f func(rune) bool) string](#TrimLeftFunc)
* [func TrimPrefix(s, prefix string) string](#TrimPrefix)
* [func TrimRight(s, cutset string) string](#TrimRight)
* [func TrimRightFunc(s string, f func(rune) bool) string](#TrimRightFunc)
* [func TrimSpace(s string) string](#TrimSpace)
* [func TrimSuffix(s, suffix string) string](#TrimSuffix)
* [type Builder](#Builder)
* [func (b \*Builder) Cap() int](#Builder.Cap)
* [func (b \*Builder) Grow(n int)](#Builder.Grow)
* [func (b \*Builder) Len() int](#Builder.Len)
* [func (b \*Builder) Reset()](#Builder.Reset)
* [func (b \*Builder) String() string](#Builder.String)
* [func (b \*Builder) Write(p []byte) (int, error)](#Builder.Write)
* [func (b \*Builder) WriteByte(c byte) error](#Builder.WriteByte)
* [func (b \*Builder) WriteRune(r rune) (int, error)](#Builder.WriteRune)
* [func (b \*Builder) WriteString(s string) (int, error)](#Builder.WriteString)
* [type Reader](#Reader)
* [func NewReader(s string) \*Reader](#NewReader)
* [func (r \*Reader) Len() int](#Reader.Len)
* [func (r \*Reader) Read(b []byte) (n int, err error)](#Reader.Read)
* [func (r \*Reader) ReadAt(b []byte, off int64) (n int, err error)](#Reader.ReadAt)
* [func (r \*Reader) ReadByte() (byte, error)](#Reader.ReadByte)
* [func (r \*Reader) ReadRune() (ch rune, size int, err error)](#Reader.ReadRune)
* [func (r \*Reader) Reset(s string)](#Reader.Reset)
* [func (r \*Reader) Seek(offset int64, whence int) (int64, error)](#Reader.Seek)
* [func (r \*Reader) Size() int64](#Reader.Size)
* [func (r \*Reader) UnreadByte() error](#Reader.UnreadByte)
* [func (r \*Reader) UnreadRune() error](#Reader.UnreadRune)
* [func (r \*Reader) WriteTo(w io.Writer) (n int64, err error)](#Reader.WriteTo)
* [type Replacer](#Replacer)
* [func NewReplacer(oldnew ...string) \*Replacer](#NewReplacer)
* [func (r \*Replacer) Replace(s string) string](#Replacer.Replace)
* [func (r \*Replacer) WriteString(w io.Writer, s string) (n int, err error)](#Replacer.WriteString)
### Examples
[Builder](#example_Builder) [Compare](#example_Compare) [Contains](#example_Contains) [ContainsAny](#example_ContainsAny) [ContainsRune](#example_ContainsRune) [Count](#example_Count) [Cut](#example_Cut) [EqualFold](#example_EqualFold) [Fields](#example_Fields) [FieldsFunc](#example_FieldsFunc) [HasPrefix](#example_HasPrefix) [HasSuffix](#example_HasSuffix) [Index](#example_Index) [IndexAny](#example_IndexAny) [IndexByte](#example_IndexByte) [IndexFunc](#example_IndexFunc) [IndexRune](#example_IndexRune) [Join](#example_Join) [LastIndex](#example_LastIndex) [LastIndexAny](#example_LastIndexAny) [LastIndexByte](#example_LastIndexByte) [LastIndexFunc](#example_LastIndexFunc) [Map](#example_Map) [NewReplacer](#example_NewReplacer) [Repeat](#example_Repeat) [Replace](#example_Replace) [ReplaceAll](#example_ReplaceAll) [Split](#example_Split) [SplitAfter](#example_SplitAfter) [SplitAfterN](#example_SplitAfterN) [SplitN](#example_SplitN) [Title](#example_Title) [ToLower](#example_ToLower) [ToLowerSpecial](#example_ToLowerSpecial) [ToTitle](#example_ToTitle) [ToTitleSpecial](#example_ToTitleSpecial) [ToUpper](#example_ToUpper) [ToUpperSpecial](#example_ToUpperSpecial) [Trim](#example_Trim) [TrimFunc](#example_TrimFunc) [TrimLeft](#example_TrimLeft) [TrimLeftFunc](#example_TrimLeftFunc) [TrimPrefix](#example_TrimPrefix) [TrimRight](#example_TrimRight) [TrimRightFunc](#example_TrimRightFunc) [TrimSpace](#example_TrimSpace) [TrimSuffix](#example_TrimSuffix) ### Package files
builder.go clone.go compare.go reader.go replace.go search.go strings.go
func Clone 1.18
---------------
```
func Clone(s string) string
```
Clone returns a fresh copy of s. It guarantees to make a copy of s into a new allocation, which can be important when retaining only a small substring of a much larger string. Using Clone can help such programs use less memory. Of course, since using Clone makes a copy, overuse of Clone can make programs use more memory. Clone should typically be used only rarely, and only when profiling indicates that it is needed. For strings of length zero the string "" will be returned and no allocation is made.
func Compare 1.5
----------------
```
func Compare(a, b string) int
```
Compare returns an integer comparing two strings lexicographically. The result will be 0 if a == b, -1 if a < b, and +1 if a > b.
Compare is included only for symmetry with package bytes. It is usually clearer and always faster to use the built-in string comparison operators ==, <, >, and so on.
#### Example
Code:
```
fmt.Println(strings.Compare("a", "b"))
fmt.Println(strings.Compare("a", "a"))
fmt.Println(strings.Compare("b", "a"))
```
Output:
```
-1
0
1
```
func Contains
-------------
```
func Contains(s, substr string) bool
```
Contains reports whether substr is within s.
#### Example
Code:
```
fmt.Println(strings.Contains("seafood", "foo"))
fmt.Println(strings.Contains("seafood", "bar"))
fmt.Println(strings.Contains("seafood", ""))
fmt.Println(strings.Contains("", ""))
```
Output:
```
true
false
true
true
```
func ContainsAny
----------------
```
func ContainsAny(s, chars string) bool
```
ContainsAny reports whether any Unicode code points in chars are within s.
#### Example
Code:
```
fmt.Println(strings.ContainsAny("team", "i"))
fmt.Println(strings.ContainsAny("fail", "ui"))
fmt.Println(strings.ContainsAny("ure", "ui"))
fmt.Println(strings.ContainsAny("failure", "ui"))
fmt.Println(strings.ContainsAny("foo", ""))
fmt.Println(strings.ContainsAny("", ""))
```
Output:
```
false
true
true
true
false
false
```
func ContainsRune
-----------------
```
func ContainsRune(s string, r rune) bool
```
ContainsRune reports whether the Unicode code point r is within s.
#### Example
Code:
```
// Finds whether a string contains a particular Unicode code point.
// The code point for the lowercase letter "a", for example, is 97.
fmt.Println(strings.ContainsRune("aardvark", 97))
fmt.Println(strings.ContainsRune("timeout", 97))
```
Output:
```
true
false
```
func Count
----------
```
func Count(s, substr string) int
```
Count counts the number of non-overlapping instances of substr in s. If substr is an empty string, Count returns 1 + the number of Unicode code points in s.
#### Example
Code:
```
fmt.Println(strings.Count("cheese", "e"))
fmt.Println(strings.Count("five", "")) // before & after each rune
```
Output:
```
3
5
```
func Cut 1.18
-------------
```
func Cut(s, sep string) (before, after string, found bool)
```
Cut slices s around the first instance of sep, returning the text before and after sep. The found result reports whether sep appears in s. If sep does not appear in s, cut returns s, "", false.
#### Example
Code:
```
show := func(s, sep string) {
before, after, found := strings.Cut(s, sep)
fmt.Printf("Cut(%q, %q) = %q, %q, %v\n", s, sep, before, after, found)
}
show("Gopher", "Go")
show("Gopher", "ph")
show("Gopher", "er")
show("Gopher", "Badger")
```
Output:
```
Cut("Gopher", "Go") = "", "pher", true
Cut("Gopher", "ph") = "Go", "er", true
Cut("Gopher", "er") = "Goph", "", true
Cut("Gopher", "Badger") = "Gopher", "", false
```
func CutPrefix 1.20
-------------------
```
func CutPrefix(s, prefix string) (after string, found bool)
```
CutPrefix returns s without the provided leading prefix string and reports whether it found the prefix. If s doesn't start with prefix, CutPrefix returns s, false. If prefix is the empty string, CutPrefix returns s, true.
func CutSuffix 1.20
-------------------
```
func CutSuffix(s, suffix string) (before string, found bool)
```
CutSuffix returns s without the provided ending suffix string and reports whether it found the suffix. If s doesn't end with suffix, CutSuffix returns s, false. If suffix is the empty string, CutSuffix returns s, true.
func EqualFold
--------------
```
func EqualFold(s, t string) bool
```
EqualFold reports whether s and t, interpreted as UTF-8 strings, are equal under simple Unicode case-folding, which is a more general form of case-insensitivity.
#### Example
Code:
```
fmt.Println(strings.EqualFold("Go", "go"))
fmt.Println(strings.EqualFold("AB", "ab")) // true because comparison uses simple case-folding
fmt.Println(strings.EqualFold("ß", "ss")) // false because comparison does not use full case-folding
```
Output:
```
true
true
false
```
func Fields
-----------
```
func Fields(s string) []string
```
Fields splits the string s around each instance of one or more consecutive white space characters, as defined by unicode.IsSpace, returning a slice of substrings of s or an empty slice if s contains only white space.
#### Example
Code:
```
fmt.Printf("Fields are: %q", strings.Fields(" foo bar baz "))
```
Output:
```
Fields are: ["foo" "bar" "baz"]
```
func FieldsFunc
---------------
```
func FieldsFunc(s string, f func(rune) bool) []string
```
FieldsFunc splits the string s at each run of Unicode code points c satisfying f(c) and returns an array of slices of s. If all code points in s satisfy f(c) or the string is empty, an empty slice is returned.
FieldsFunc makes no guarantees about the order in which it calls f(c) and assumes that f always returns the same value for a given c.
#### Example
Code:
```
f := func(c rune) bool {
return !unicode.IsLetter(c) && !unicode.IsNumber(c)
}
fmt.Printf("Fields are: %q", strings.FieldsFunc(" foo1;bar2,baz3...", f))
```
Output:
```
Fields are: ["foo1" "bar2" "baz3"]
```
func HasPrefix
--------------
```
func HasPrefix(s, prefix string) bool
```
HasPrefix tests whether the string s begins with prefix.
#### Example
Code:
```
fmt.Println(strings.HasPrefix("Gopher", "Go"))
fmt.Println(strings.HasPrefix("Gopher", "C"))
fmt.Println(strings.HasPrefix("Gopher", ""))
```
Output:
```
true
false
true
```
func HasSuffix
--------------
```
func HasSuffix(s, suffix string) bool
```
HasSuffix tests whether the string s ends with suffix.
#### Example
Code:
```
fmt.Println(strings.HasSuffix("Amigo", "go"))
fmt.Println(strings.HasSuffix("Amigo", "O"))
fmt.Println(strings.HasSuffix("Amigo", "Ami"))
fmt.Println(strings.HasSuffix("Amigo", ""))
```
Output:
```
true
false
false
true
```
func Index
----------
```
func Index(s, substr string) int
```
Index returns the index of the first instance of substr in s, or -1 if substr is not present in s.
#### Example
Code:
```
fmt.Println(strings.Index("chicken", "ken"))
fmt.Println(strings.Index("chicken", "dmr"))
```
Output:
```
4
-1
```
func IndexAny
-------------
```
func IndexAny(s, chars string) int
```
IndexAny returns the index of the first instance of any Unicode code point from chars in s, or -1 if no Unicode code point from chars is present in s.
#### Example
Code:
```
fmt.Println(strings.IndexAny("chicken", "aeiouy"))
fmt.Println(strings.IndexAny("crwth", "aeiouy"))
```
Output:
```
2
-1
```
func IndexByte 1.2
------------------
```
func IndexByte(s string, c byte) int
```
IndexByte returns the index of the first instance of c in s, or -1 if c is not present in s.
#### Example
Code:
```
fmt.Println(strings.IndexByte("golang", 'g'))
fmt.Println(strings.IndexByte("gophers", 'h'))
fmt.Println(strings.IndexByte("golang", 'x'))
```
Output:
```
0
3
-1
```
func IndexFunc
--------------
```
func IndexFunc(s string, f func(rune) bool) int
```
IndexFunc returns the index into s of the first Unicode code point satisfying f(c), or -1 if none do.
#### Example
Code:
```
f := func(c rune) bool {
return unicode.Is(unicode.Han, c)
}
fmt.Println(strings.IndexFunc("Hello, 世界", f))
fmt.Println(strings.IndexFunc("Hello, world", f))
```
Output:
```
7
-1
```
func IndexRune
--------------
```
func IndexRune(s string, r rune) int
```
IndexRune returns the index of the first instance of the Unicode code point r, or -1 if rune is not present in s. If r is utf8.RuneError, it returns the first instance of any invalid UTF-8 byte sequence.
#### Example
Code:
```
fmt.Println(strings.IndexRune("chicken", 'k'))
fmt.Println(strings.IndexRune("chicken", 'd'))
```
Output:
```
4
-1
```
func Join
---------
```
func Join(elems []string, sep string) string
```
Join concatenates the elements of its first argument to create a single string. The separator string sep is placed between elements in the resulting string.
#### Example
Code:
```
s := []string{"foo", "bar", "baz"}
fmt.Println(strings.Join(s, ", "))
```
Output:
```
foo, bar, baz
```
func LastIndex
--------------
```
func LastIndex(s, substr string) int
```
LastIndex returns the index of the last instance of substr in s, or -1 if substr is not present in s.
#### Example
Code:
```
fmt.Println(strings.Index("go gopher", "go"))
fmt.Println(strings.LastIndex("go gopher", "go"))
fmt.Println(strings.LastIndex("go gopher", "rodent"))
```
Output:
```
0
3
-1
```
func LastIndexAny
-----------------
```
func LastIndexAny(s, chars string) int
```
LastIndexAny returns the index of the last instance of any Unicode code point from chars in s, or -1 if no Unicode code point from chars is present in s.
#### Example
Code:
```
fmt.Println(strings.LastIndexAny("go gopher", "go"))
fmt.Println(strings.LastIndexAny("go gopher", "rodent"))
fmt.Println(strings.LastIndexAny("go gopher", "fail"))
```
Output:
```
4
8
-1
```
func LastIndexByte 1.5
----------------------
```
func LastIndexByte(s string, c byte) int
```
LastIndexByte returns the index of the last instance of c in s, or -1 if c is not present in s.
#### Example
Code:
```
fmt.Println(strings.LastIndexByte("Hello, world", 'l'))
fmt.Println(strings.LastIndexByte("Hello, world", 'o'))
fmt.Println(strings.LastIndexByte("Hello, world", 'x'))
```
Output:
```
10
8
-1
```
func LastIndexFunc
------------------
```
func LastIndexFunc(s string, f func(rune) bool) int
```
LastIndexFunc returns the index into s of the last Unicode code point satisfying f(c), or -1 if none do.
#### Example
Code:
```
fmt.Println(strings.LastIndexFunc("go 123", unicode.IsNumber))
fmt.Println(strings.LastIndexFunc("123 go", unicode.IsNumber))
fmt.Println(strings.LastIndexFunc("go", unicode.IsNumber))
```
Output:
```
5
2
-1
```
func Map
--------
```
func Map(mapping func(rune) rune, s string) string
```
Map returns a copy of the string s with all its characters modified according to the mapping function. If mapping returns a negative value, the character is dropped from the string with no replacement.
#### Example
Code:
```
rot13 := func(r rune) rune {
switch {
case r >= 'A' && r <= 'Z':
return 'A' + (r-'A'+13)%26
case r >= 'a' && r <= 'z':
return 'a' + (r-'a'+13)%26
}
return r
}
fmt.Println(strings.Map(rot13, "'Twas brillig and the slithy gopher..."))
```
Output:
```
'Gjnf oevyyvt naq gur fyvgul tbcure...
```
func Repeat
-----------
```
func Repeat(s string, count int) string
```
Repeat returns a new string consisting of count copies of the string s.
It panics if count is negative or if the result of (len(s) \* count) overflows.
#### Example
Code:
```
fmt.Println("ba" + strings.Repeat("na", 2))
```
Output:
```
banana
```
func Replace
------------
```
func Replace(s, old, new string, n int) string
```
Replace returns a copy of the string s with the first n non-overlapping instances of old replaced by new. If old is empty, it matches at the beginning of the string and after each UTF-8 sequence, yielding up to k+1 replacements for a k-rune string. If n < 0, there is no limit on the number of replacements.
#### Example
Code:
```
fmt.Println(strings.Replace("oink oink oink", "k", "ky", 2))
fmt.Println(strings.Replace("oink oink oink", "oink", "moo", -1))
```
Output:
```
oinky oinky oink
moo moo moo
```
func ReplaceAll 1.12
--------------------
```
func ReplaceAll(s, old, new string) string
```
ReplaceAll returns a copy of the string s with all non-overlapping instances of old replaced by new. If old is empty, it matches at the beginning of the string and after each UTF-8 sequence, yielding up to k+1 replacements for a k-rune string.
#### Example
Code:
```
fmt.Println(strings.ReplaceAll("oink oink oink", "oink", "moo"))
```
Output:
```
moo moo moo
```
func Split
----------
```
func Split(s, sep string) []string
```
Split slices s into all substrings separated by sep and returns a slice of the substrings between those separators.
If s does not contain sep and sep is not empty, Split returns a slice of length 1 whose only element is s.
If sep is empty, Split splits after each UTF-8 sequence. If both s and sep are empty, Split returns an empty slice.
It is equivalent to SplitN with a count of -1.
To split around the first instance of a separator, see Cut.
#### Example
Code:
```
fmt.Printf("%q\n", strings.Split("a,b,c", ","))
fmt.Printf("%q\n", strings.Split("a man a plan a canal panama", "a "))
fmt.Printf("%q\n", strings.Split(" xyz ", ""))
fmt.Printf("%q\n", strings.Split("", "Bernardo O'Higgins"))
```
Output:
```
["a" "b" "c"]
["" "man " "plan " "canal panama"]
[" " "x" "y" "z" " "]
[""]
```
func SplitAfter
---------------
```
func SplitAfter(s, sep string) []string
```
SplitAfter slices s into all substrings after each instance of sep and returns a slice of those substrings.
If s does not contain sep and sep is not empty, SplitAfter returns a slice of length 1 whose only element is s.
If sep is empty, SplitAfter splits after each UTF-8 sequence. If both s and sep are empty, SplitAfter returns an empty slice.
It is equivalent to SplitAfterN with a count of -1.
#### Example
Code:
```
fmt.Printf("%q\n", strings.SplitAfter("a,b,c", ","))
```
Output:
```
["a," "b," "c"]
```
func SplitAfterN
----------------
```
func SplitAfterN(s, sep string, n int) []string
```
SplitAfterN slices s into substrings after each instance of sep and returns a slice of those substrings.
The count determines the number of substrings to return:
```
n > 0: at most n substrings; the last substring will be the unsplit remainder.
n == 0: the result is nil (zero substrings)
n < 0: all substrings
```
Edge cases for s and sep (for example, empty strings) are handled as described in the documentation for SplitAfter.
#### Example
Code:
```
fmt.Printf("%q\n", strings.SplitAfterN("a,b,c", ",", 2))
```
Output:
```
["a," "b,c"]
```
func SplitN
-----------
```
func SplitN(s, sep string, n int) []string
```
SplitN slices s into substrings separated by sep and returns a slice of the substrings between those separators.
The count determines the number of substrings to return:
```
n > 0: at most n substrings; the last substring will be the unsplit remainder.
n == 0: the result is nil (zero substrings)
n < 0: all substrings
```
Edge cases for s and sep (for example, empty strings) are handled as described in the documentation for Split.
To split around the first instance of a separator, see Cut.
#### Example
Code:
```
fmt.Printf("%q\n", strings.SplitN("a,b,c", ",", 2))
z := strings.SplitN("a,b,c", ",", 0)
fmt.Printf("%q (nil = %v)\n", z, z == nil)
```
Output:
```
["a" "b,c"]
[] (nil = true)
```
func Title
----------
```
func Title(s string) string
```
Title returns a copy of the string s with all Unicode letters that begin words mapped to their Unicode title case.
Deprecated: The rule Title uses for word boundaries does not handle Unicode punctuation properly. Use golang.org/x/text/cases instead.
#### Example
Code:
```
// Compare this example to the ToTitle example.
fmt.Println(strings.Title("her royal highness"))
fmt.Println(strings.Title("loud noises"))
fmt.Println(strings.Title("хлеб"))
```
Output:
```
Her Royal Highness
Loud Noises
Хлеб
```
func ToLower
------------
```
func ToLower(s string) string
```
ToLower returns s with all Unicode letters mapped to their lower case.
#### Example
Code:
```
fmt.Println(strings.ToLower("Gopher"))
```
Output:
```
gopher
```
func ToLowerSpecial
-------------------
```
func ToLowerSpecial(c unicode.SpecialCase, s string) string
```
ToLowerSpecial returns a copy of the string s with all Unicode letters mapped to their lower case using the case mapping specified by c.
#### Example
Code:
```
fmt.Println(strings.ToLowerSpecial(unicode.TurkishCase, "Önnek İş"))
```
Output:
```
önnek iş
```
func ToTitle
------------
```
func ToTitle(s string) string
```
ToTitle returns a copy of the string s with all Unicode letters mapped to their Unicode title case.
#### Example
Code:
```
// Compare this example to the Title example.
fmt.Println(strings.ToTitle("her royal highness"))
fmt.Println(strings.ToTitle("loud noises"))
fmt.Println(strings.ToTitle("хлеб"))
```
Output:
```
HER ROYAL HIGHNESS
LOUD NOISES
ХЛЕБ
```
func ToTitleSpecial
-------------------
```
func ToTitleSpecial(c unicode.SpecialCase, s string) string
```
ToTitleSpecial returns a copy of the string s with all Unicode letters mapped to their Unicode title case, giving priority to the special casing rules.
#### Example
Code:
```
fmt.Println(strings.ToTitleSpecial(unicode.TurkishCase, "dünyanın ilk borsa yapısı Aizonai kabul edilir"))
```
Output:
```
DÜNYANIN İLK BORSA YAPISI AİZONAİ KABUL EDİLİR
```
func ToUpper
------------
```
func ToUpper(s string) string
```
ToUpper returns s with all Unicode letters mapped to their upper case.
#### Example
Code:
```
fmt.Println(strings.ToUpper("Gopher"))
```
Output:
```
GOPHER
```
func ToUpperSpecial
-------------------
```
func ToUpperSpecial(c unicode.SpecialCase, s string) string
```
ToUpperSpecial returns a copy of the string s with all Unicode letters mapped to their upper case using the case mapping specified by c.
#### Example
Code:
```
fmt.Println(strings.ToUpperSpecial(unicode.TurkishCase, "örnek iş"))
```
Output:
```
ÖRNEK İŞ
```
func ToValidUTF8 1.13
---------------------
```
func ToValidUTF8(s, replacement string) string
```
ToValidUTF8 returns a copy of the string s with each run of invalid UTF-8 byte sequences replaced by the replacement string, which may be empty.
func Trim
---------
```
func Trim(s, cutset string) string
```
Trim returns a slice of the string s with all leading and trailing Unicode code points contained in cutset removed.
#### Example
Code:
```
fmt.Print(strings.Trim("¡¡¡Hello, Gophers!!!", "!¡"))
```
Output:
```
Hello, Gophers
```
func TrimFunc
-------------
```
func TrimFunc(s string, f func(rune) bool) string
```
TrimFunc returns a slice of the string s with all leading and trailing Unicode code points c satisfying f(c) removed.
#### Example
Code:
```
fmt.Print(strings.TrimFunc("¡¡¡Hello, Gophers!!!", func(r rune) bool {
return !unicode.IsLetter(r) && !unicode.IsNumber(r)
}))
```
Output:
```
Hello, Gophers
```
func TrimLeft
-------------
```
func TrimLeft(s, cutset string) string
```
TrimLeft returns a slice of the string s with all leading Unicode code points contained in cutset removed.
To remove a prefix, use TrimPrefix instead.
#### Example
Code:
```
fmt.Print(strings.TrimLeft("¡¡¡Hello, Gophers!!!", "!¡"))
```
Output:
```
Hello, Gophers!!!
```
func TrimLeftFunc
-----------------
```
func TrimLeftFunc(s string, f func(rune) bool) string
```
TrimLeftFunc returns a slice of the string s with all leading Unicode code points c satisfying f(c) removed.
#### Example
Code:
```
fmt.Print(strings.TrimLeftFunc("¡¡¡Hello, Gophers!!!", func(r rune) bool {
return !unicode.IsLetter(r) && !unicode.IsNumber(r)
}))
```
Output:
```
Hello, Gophers!!!
```
func TrimPrefix 1.1
-------------------
```
func TrimPrefix(s, prefix string) string
```
TrimPrefix returns s without the provided leading prefix string. If s doesn't start with prefix, s is returned unchanged.
#### Example
Code:
```
var s = "¡¡¡Hello, Gophers!!!"
s = strings.TrimPrefix(s, "¡¡¡Hello, ")
s = strings.TrimPrefix(s, "¡¡¡Howdy, ")
fmt.Print(s)
```
Output:
```
Gophers!!!
```
func TrimRight
--------------
```
func TrimRight(s, cutset string) string
```
TrimRight returns a slice of the string s, with all trailing Unicode code points contained in cutset removed.
To remove a suffix, use TrimSuffix instead.
#### Example
Code:
```
fmt.Print(strings.TrimRight("¡¡¡Hello, Gophers!!!", "!¡"))
```
Output:
```
¡¡¡Hello, Gophers
```
func TrimRightFunc
------------------
```
func TrimRightFunc(s string, f func(rune) bool) string
```
TrimRightFunc returns a slice of the string s with all trailing Unicode code points c satisfying f(c) removed.
#### Example
Code:
```
fmt.Print(strings.TrimRightFunc("¡¡¡Hello, Gophers!!!", func(r rune) bool {
return !unicode.IsLetter(r) && !unicode.IsNumber(r)
}))
```
Output:
```
¡¡¡Hello, Gophers
```
func TrimSpace
--------------
```
func TrimSpace(s string) string
```
TrimSpace returns a slice of the string s, with all leading and trailing white space removed, as defined by Unicode.
#### Example
Code:
```
fmt.Println(strings.TrimSpace(" \t\n Hello, Gophers \n\t\r\n"))
```
Output:
```
Hello, Gophers
```
func TrimSuffix 1.1
-------------------
```
func TrimSuffix(s, suffix string) string
```
TrimSuffix returns s without the provided trailing suffix string. If s doesn't end with suffix, s is returned unchanged.
#### Example
Code:
```
var s = "¡¡¡Hello, Gophers!!!"
s = strings.TrimSuffix(s, ", Gophers!!!")
s = strings.TrimSuffix(s, ", Marmots!!!")
fmt.Print(s)
```
Output:
```
¡¡¡Hello
```
type Builder 1.10
-----------------
A Builder is used to efficiently build a string using Write methods. It minimizes memory copying. The zero value is ready to use. Do not copy a non-zero Builder.
```
type Builder struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
var b strings.Builder
for i := 3; i >= 1; i-- {
fmt.Fprintf(&b, "%d...", i)
}
b.WriteString("ignition")
fmt.Println(b.String())
```
Output:
```
3...2...1...ignition
```
### func (\*Builder) Cap 1.12
```
func (b *Builder) Cap() int
```
Cap returns the capacity of the builder's underlying byte slice. It is the total space allocated for the string being built and includes any bytes already written.
### func (\*Builder) Grow 1.10
```
func (b *Builder) Grow(n int)
```
Grow grows b's capacity, if necessary, to guarantee space for another n bytes. After Grow(n), at least n bytes can be written to b without another allocation. If n is negative, Grow panics.
### func (\*Builder) Len 1.10
```
func (b *Builder) Len() int
```
Len returns the number of accumulated bytes; b.Len() == len(b.String()).
### func (\*Builder) Reset 1.10
```
func (b *Builder) Reset()
```
Reset resets the Builder to be empty.
### func (\*Builder) String 1.10
```
func (b *Builder) String() string
```
String returns the accumulated string.
### func (\*Builder) Write 1.10
```
func (b *Builder) Write(p []byte) (int, error)
```
Write appends the contents of p to b's buffer. Write always returns len(p), nil.
### func (\*Builder) WriteByte 1.10
```
func (b *Builder) WriteByte(c byte) error
```
WriteByte appends the byte c to b's buffer. The returned error is always nil.
### func (\*Builder) WriteRune 1.10
```
func (b *Builder) WriteRune(r rune) (int, error)
```
WriteRune appends the UTF-8 encoding of Unicode code point r to b's buffer. It returns the length of r and a nil error.
### func (\*Builder) WriteString 1.10
```
func (b *Builder) WriteString(s string) (int, error)
```
WriteString appends the contents of s to b's buffer. It returns the length of s and a nil error.
type Reader
-----------
A Reader implements the io.Reader, io.ReaderAt, io.ByteReader, io.ByteScanner, io.RuneReader, io.RuneScanner, io.Seeker, and io.WriterTo interfaces by reading from a string. The zero value for Reader operates like a Reader of an empty string.
```
type Reader struct {
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(s string) *Reader
```
NewReader returns a new Reader reading from s. It is similar to bytes.NewBufferString but more efficient and read-only.
### func (\*Reader) Len
```
func (r *Reader) Len() int
```
Len returns the number of bytes of the unread portion of the string.
### func (\*Reader) Read
```
func (r *Reader) Read(b []byte) (n int, err error)
```
Read implements the io.Reader interface.
### func (\*Reader) ReadAt
```
func (r *Reader) ReadAt(b []byte, off int64) (n int, err error)
```
ReadAt implements the io.ReaderAt interface.
### func (\*Reader) ReadByte
```
func (r *Reader) ReadByte() (byte, error)
```
ReadByte implements the io.ByteReader interface.
### func (\*Reader) ReadRune
```
func (r *Reader) ReadRune() (ch rune, size int, err error)
```
ReadRune implements the io.RuneReader interface.
### func (\*Reader) Reset 1.7
```
func (r *Reader) Reset(s string)
```
Reset resets the Reader to be reading from s.
### func (\*Reader) Seek
```
func (r *Reader) Seek(offset int64, whence int) (int64, error)
```
Seek implements the io.Seeker interface.
### func (\*Reader) Size 1.5
```
func (r *Reader) Size() int64
```
Size returns the original length of the underlying string. Size is the number of bytes available for reading via ReadAt. The returned value is always the same and is not affected by calls to any other method.
### func (\*Reader) UnreadByte
```
func (r *Reader) UnreadByte() error
```
UnreadByte implements the io.ByteScanner interface.
### func (\*Reader) UnreadRune
```
func (r *Reader) UnreadRune() error
```
UnreadRune implements the io.RuneScanner interface.
### func (\*Reader) WriteTo 1.1
```
func (r *Reader) WriteTo(w io.Writer) (n int64, err error)
```
WriteTo implements the io.WriterTo interface.
type Replacer
-------------
Replacer replaces a list of strings with replacements. It is safe for concurrent use by multiple goroutines.
```
type Replacer struct {
// contains filtered or unexported fields
}
```
### func NewReplacer
```
func NewReplacer(oldnew ...string) *Replacer
```
NewReplacer returns a new Replacer from a list of old, new string pairs. Replacements are performed in the order they appear in the target string, without overlapping matches. The old string comparisons are done in argument order.
NewReplacer panics if given an odd number of arguments.
#### Example
Code:
```
r := strings.NewReplacer("<", "<", ">", ">")
fmt.Println(r.Replace("This is <b>HTML</b>!"))
```
Output:
```
This is <b>HTML</b>!
```
### func (\*Replacer) Replace
```
func (r *Replacer) Replace(s string) string
```
Replace returns a copy of s with all replacements performed.
### func (\*Replacer) WriteString
```
func (r *Replacer) WriteString(w io.Writer, s string) (n int, err error)
```
WriteString writes s to w with all replacements performed.
| programming_docs |
go Package net Package net
============
* `import "net"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package net provides a portable interface for network I/O, including TCP/IP, UDP, domain name resolution, and Unix domain sockets.
Although the package provides access to low-level networking primitives, most clients will need only the basic interface provided by the Dial, Listen, and Accept functions and the associated Conn and Listener interfaces. The crypto/tls package uses the same interfaces and similar Dial and Listen functions.
The Dial function connects to a server:
```
conn, err := net.Dial("tcp", "golang.org:80")
if err != nil {
// handle error
}
fmt.Fprintf(conn, "GET / HTTP/1.0\r\n\r\n")
status, err := bufio.NewReader(conn).ReadString('\n')
// ...
```
The Listen function creates servers:
```
ln, err := net.Listen("tcp", ":8080")
if err != nil {
// handle error
}
for {
conn, err := ln.Accept()
if err != nil {
// handle error
}
go handleConnection(conn)
}
```
### Name Resolution
The method for resolving domain names, whether indirectly with functions like Dial or directly with functions like LookupHost and LookupAddr, varies by operating system.
On Unix systems, the resolver has two options for resolving names. It can use a pure Go resolver that sends DNS requests directly to the servers listed in /etc/resolv.conf, or it can use a cgo-based resolver that calls C library routines such as getaddrinfo and getnameinfo.
By default the pure Go resolver is used, because a blocked DNS request consumes only a goroutine, while a blocked C call consumes an operating system thread. When cgo is available, the cgo-based resolver is used instead under a variety of conditions: on systems that do not let programs make direct DNS requests (OS X), when the LOCALDOMAIN environment variable is present (even if empty), when the RES\_OPTIONS or HOSTALIASES environment variable is non-empty, when the ASR\_CONFIG environment variable is non-empty (OpenBSD only), when /etc/resolv.conf or /etc/nsswitch.conf specify the use of features that the Go resolver does not implement, and when the name being looked up ends in .local or is an mDNS name.
The resolver decision can be overridden by setting the netdns value of the GODEBUG environment variable (see package runtime) to go or cgo, as in:
```
export GODEBUG=netdns=go # force pure Go resolver
export GODEBUG=netdns=cgo # force native resolver (cgo, win32)
```
The decision can also be forced while building the Go source tree by setting the netgo or netcgo build tag.
A numeric netdns setting, as in GODEBUG=netdns=1, causes the resolver to print debugging information about its decisions. To force a particular resolver while also printing debugging information, join the two settings by a plus sign, as in GODEBUG=netdns=go+1.
On Plan 9, the resolver always accesses /net/cs and /net/dns.
On Windows, in Go 1.18.x and earlier, the resolver always used C library functions, such as GetAddrInfo and DnsQuery.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func JoinHostPort(host, port string) string](#JoinHostPort)
* [func LookupAddr(addr string) (names []string, err error)](#LookupAddr)
* [func LookupCNAME(host string) (cname string, err error)](#LookupCNAME)
* [func LookupHost(host string) (addrs []string, err error)](#LookupHost)
* [func LookupPort(network, service string) (port int, err error)](#LookupPort)
* [func LookupTXT(name string) ([]string, error)](#LookupTXT)
* [func ParseCIDR(s string) (IP, \*IPNet, error)](#ParseCIDR)
* [func Pipe() (Conn, Conn)](#Pipe)
* [func SplitHostPort(hostport string) (host, port string, err error)](#SplitHostPort)
* [type Addr](#Addr)
* [func InterfaceAddrs() ([]Addr, error)](#InterfaceAddrs)
* [type AddrError](#AddrError)
* [func (e \*AddrError) Error() string](#AddrError.Error)
* [func (e \*AddrError) Temporary() bool](#AddrError.Temporary)
* [func (e \*AddrError) Timeout() bool](#AddrError.Timeout)
* [type Buffers](#Buffers)
* [func (v \*Buffers) Read(p []byte) (n int, err error)](#Buffers.Read)
* [func (v \*Buffers) WriteTo(w io.Writer) (n int64, err error)](#Buffers.WriteTo)
* [type Conn](#Conn)
* [func Dial(network, address string) (Conn, error)](#Dial)
* [func DialTimeout(network, address string, timeout time.Duration) (Conn, error)](#DialTimeout)
* [func FileConn(f \*os.File) (c Conn, err error)](#FileConn)
* [type DNSConfigError](#DNSConfigError)
* [func (e \*DNSConfigError) Error() string](#DNSConfigError.Error)
* [func (e \*DNSConfigError) Temporary() bool](#DNSConfigError.Temporary)
* [func (e \*DNSConfigError) Timeout() bool](#DNSConfigError.Timeout)
* [func (e \*DNSConfigError) Unwrap() error](#DNSConfigError.Unwrap)
* [type DNSError](#DNSError)
* [func (e \*DNSError) Error() string](#DNSError.Error)
* [func (e \*DNSError) Temporary() bool](#DNSError.Temporary)
* [func (e \*DNSError) Timeout() bool](#DNSError.Timeout)
* [type Dialer](#Dialer)
* [func (d \*Dialer) Dial(network, address string) (Conn, error)](#Dialer.Dial)
* [func (d \*Dialer) DialContext(ctx context.Context, network, address string) (Conn, error)](#Dialer.DialContext)
* [type Error](#Error)
* [type Flags](#Flags)
* [func (f Flags) String() string](#Flags.String)
* [type HardwareAddr](#HardwareAddr)
* [func ParseMAC(s string) (hw HardwareAddr, err error)](#ParseMAC)
* [func (a HardwareAddr) String() string](#HardwareAddr.String)
* [type IP](#IP)
* [func IPv4(a, b, c, d byte) IP](#IPv4)
* [func LookupIP(host string) ([]IP, error)](#LookupIP)
* [func ParseIP(s string) IP](#ParseIP)
* [func (ip IP) DefaultMask() IPMask](#IP.DefaultMask)
* [func (ip IP) Equal(x IP) bool](#IP.Equal)
* [func (ip IP) IsGlobalUnicast() bool](#IP.IsGlobalUnicast)
* [func (ip IP) IsInterfaceLocalMulticast() bool](#IP.IsInterfaceLocalMulticast)
* [func (ip IP) IsLinkLocalMulticast() bool](#IP.IsLinkLocalMulticast)
* [func (ip IP) IsLinkLocalUnicast() bool](#IP.IsLinkLocalUnicast)
* [func (ip IP) IsLoopback() bool](#IP.IsLoopback)
* [func (ip IP) IsMulticast() bool](#IP.IsMulticast)
* [func (ip IP) IsPrivate() bool](#IP.IsPrivate)
* [func (ip IP) IsUnspecified() bool](#IP.IsUnspecified)
* [func (ip IP) MarshalText() ([]byte, error)](#IP.MarshalText)
* [func (ip IP) Mask(mask IPMask) IP](#IP.Mask)
* [func (ip IP) String() string](#IP.String)
* [func (ip IP) To16() IP](#IP.To16)
* [func (ip IP) To4() IP](#IP.To4)
* [func (ip \*IP) UnmarshalText(text []byte) error](#IP.UnmarshalText)
* [type IPAddr](#IPAddr)
* [func ResolveIPAddr(network, address string) (\*IPAddr, error)](#ResolveIPAddr)
* [func (a \*IPAddr) Network() string](#IPAddr.Network)
* [func (a \*IPAddr) String() string](#IPAddr.String)
* [type IPConn](#IPConn)
* [func DialIP(network string, laddr, raddr \*IPAddr) (\*IPConn, error)](#DialIP)
* [func ListenIP(network string, laddr \*IPAddr) (\*IPConn, error)](#ListenIP)
* [func (c \*IPConn) Close() error](#IPConn.Close)
* [func (c \*IPConn) File() (f \*os.File, err error)](#IPConn.File)
* [func (c \*IPConn) LocalAddr() Addr](#IPConn.LocalAddr)
* [func (c \*IPConn) Read(b []byte) (int, error)](#IPConn.Read)
* [func (c \*IPConn) ReadFrom(b []byte) (int, Addr, error)](#IPConn.ReadFrom)
* [func (c \*IPConn) ReadFromIP(b []byte) (int, \*IPAddr, error)](#IPConn.ReadFromIP)
* [func (c \*IPConn) ReadMsgIP(b, oob []byte) (n, oobn, flags int, addr \*IPAddr, err error)](#IPConn.ReadMsgIP)
* [func (c \*IPConn) RemoteAddr() Addr](#IPConn.RemoteAddr)
* [func (c \*IPConn) SetDeadline(t time.Time) error](#IPConn.SetDeadline)
* [func (c \*IPConn) SetReadBuffer(bytes int) error](#IPConn.SetReadBuffer)
* [func (c \*IPConn) SetReadDeadline(t time.Time) error](#IPConn.SetReadDeadline)
* [func (c \*IPConn) SetWriteBuffer(bytes int) error](#IPConn.SetWriteBuffer)
* [func (c \*IPConn) SetWriteDeadline(t time.Time) error](#IPConn.SetWriteDeadline)
* [func (c \*IPConn) SyscallConn() (syscall.RawConn, error)](#IPConn.SyscallConn)
* [func (c \*IPConn) Write(b []byte) (int, error)](#IPConn.Write)
* [func (c \*IPConn) WriteMsgIP(b, oob []byte, addr \*IPAddr) (n, oobn int, err error)](#IPConn.WriteMsgIP)
* [func (c \*IPConn) WriteTo(b []byte, addr Addr) (int, error)](#IPConn.WriteTo)
* [func (c \*IPConn) WriteToIP(b []byte, addr \*IPAddr) (int, error)](#IPConn.WriteToIP)
* [type IPMask](#IPMask)
* [func CIDRMask(ones, bits int) IPMask](#CIDRMask)
* [func IPv4Mask(a, b, c, d byte) IPMask](#IPv4Mask)
* [func (m IPMask) Size() (ones, bits int)](#IPMask.Size)
* [func (m IPMask) String() string](#IPMask.String)
* [type IPNet](#IPNet)
* [func (n \*IPNet) Contains(ip IP) bool](#IPNet.Contains)
* [func (n \*IPNet) Network() string](#IPNet.Network)
* [func (n \*IPNet) String() string](#IPNet.String)
* [type Interface](#Interface)
* [func InterfaceByIndex(index int) (\*Interface, error)](#InterfaceByIndex)
* [func InterfaceByName(name string) (\*Interface, error)](#InterfaceByName)
* [func Interfaces() ([]Interface, error)](#Interfaces)
* [func (ifi \*Interface) Addrs() ([]Addr, error)](#Interface.Addrs)
* [func (ifi \*Interface) MulticastAddrs() ([]Addr, error)](#Interface.MulticastAddrs)
* [type InvalidAddrError](#InvalidAddrError)
* [func (e InvalidAddrError) Error() string](#InvalidAddrError.Error)
* [func (e InvalidAddrError) Temporary() bool](#InvalidAddrError.Temporary)
* [func (e InvalidAddrError) Timeout() bool](#InvalidAddrError.Timeout)
* [type ListenConfig](#ListenConfig)
* [func (lc \*ListenConfig) Listen(ctx context.Context, network, address string) (Listener, error)](#ListenConfig.Listen)
* [func (lc \*ListenConfig) ListenPacket(ctx context.Context, network, address string) (PacketConn, error)](#ListenConfig.ListenPacket)
* [type Listener](#Listener)
* [func FileListener(f \*os.File) (ln Listener, err error)](#FileListener)
* [func Listen(network, address string) (Listener, error)](#Listen)
* [type MX](#MX)
* [func LookupMX(name string) ([]\*MX, error)](#LookupMX)
* [type NS](#NS)
* [func LookupNS(name string) ([]\*NS, error)](#LookupNS)
* [type OpError](#OpError)
* [func (e \*OpError) Error() string](#OpError.Error)
* [func (e \*OpError) Temporary() bool](#OpError.Temporary)
* [func (e \*OpError) Timeout() bool](#OpError.Timeout)
* [func (e \*OpError) Unwrap() error](#OpError.Unwrap)
* [type PacketConn](#PacketConn)
* [func FilePacketConn(f \*os.File) (c PacketConn, err error)](#FilePacketConn)
* [func ListenPacket(network, address string) (PacketConn, error)](#ListenPacket)
* [type ParseError](#ParseError)
* [func (e \*ParseError) Error() string](#ParseError.Error)
* [func (e \*ParseError) Temporary() bool](#ParseError.Temporary)
* [func (e \*ParseError) Timeout() bool](#ParseError.Timeout)
* [type Resolver](#Resolver)
* [func (r \*Resolver) LookupAddr(ctx context.Context, addr string) ([]string, error)](#Resolver.LookupAddr)
* [func (r \*Resolver) LookupCNAME(ctx context.Context, host string) (string, error)](#Resolver.LookupCNAME)
* [func (r \*Resolver) LookupHost(ctx context.Context, host string) (addrs []string, err error)](#Resolver.LookupHost)
* [func (r \*Resolver) LookupIP(ctx context.Context, network, host string) ([]IP, error)](#Resolver.LookupIP)
* [func (r \*Resolver) LookupIPAddr(ctx context.Context, host string) ([]IPAddr, error)](#Resolver.LookupIPAddr)
* [func (r \*Resolver) LookupMX(ctx context.Context, name string) ([]\*MX, error)](#Resolver.LookupMX)
* [func (r \*Resolver) LookupNS(ctx context.Context, name string) ([]\*NS, error)](#Resolver.LookupNS)
* [func (r \*Resolver) LookupNetIP(ctx context.Context, network, host string) ([]netip.Addr, error)](#Resolver.LookupNetIP)
* [func (r \*Resolver) LookupPort(ctx context.Context, network, service string) (port int, err error)](#Resolver.LookupPort)
* [func (r \*Resolver) LookupSRV(ctx context.Context, service, proto, name string) (string, []\*SRV, error)](#Resolver.LookupSRV)
* [func (r \*Resolver) LookupTXT(ctx context.Context, name string) ([]string, error)](#Resolver.LookupTXT)
* [type SRV](#SRV)
* [func LookupSRV(service, proto, name string) (cname string, addrs []\*SRV, err error)](#LookupSRV)
* [type TCPAddr](#TCPAddr)
* [func ResolveTCPAddr(network, address string) (\*TCPAddr, error)](#ResolveTCPAddr)
* [func TCPAddrFromAddrPort(addr netip.AddrPort) \*TCPAddr](#TCPAddrFromAddrPort)
* [func (a \*TCPAddr) AddrPort() netip.AddrPort](#TCPAddr.AddrPort)
* [func (a \*TCPAddr) Network() string](#TCPAddr.Network)
* [func (a \*TCPAddr) String() string](#TCPAddr.String)
* [type TCPConn](#TCPConn)
* [func DialTCP(network string, laddr, raddr \*TCPAddr) (\*TCPConn, error)](#DialTCP)
* [func (c \*TCPConn) Close() error](#TCPConn.Close)
* [func (c \*TCPConn) CloseRead() error](#TCPConn.CloseRead)
* [func (c \*TCPConn) CloseWrite() error](#TCPConn.CloseWrite)
* [func (c \*TCPConn) File() (f \*os.File, err error)](#TCPConn.File)
* [func (c \*TCPConn) LocalAddr() Addr](#TCPConn.LocalAddr)
* [func (c \*TCPConn) Read(b []byte) (int, error)](#TCPConn.Read)
* [func (c \*TCPConn) ReadFrom(r io.Reader) (int64, error)](#TCPConn.ReadFrom)
* [func (c \*TCPConn) RemoteAddr() Addr](#TCPConn.RemoteAddr)
* [func (c \*TCPConn) SetDeadline(t time.Time) error](#TCPConn.SetDeadline)
* [func (c \*TCPConn) SetKeepAlive(keepalive bool) error](#TCPConn.SetKeepAlive)
* [func (c \*TCPConn) SetKeepAlivePeriod(d time.Duration) error](#TCPConn.SetKeepAlivePeriod)
* [func (c \*TCPConn) SetLinger(sec int) error](#TCPConn.SetLinger)
* [func (c \*TCPConn) SetNoDelay(noDelay bool) error](#TCPConn.SetNoDelay)
* [func (c \*TCPConn) SetReadBuffer(bytes int) error](#TCPConn.SetReadBuffer)
* [func (c \*TCPConn) SetReadDeadline(t time.Time) error](#TCPConn.SetReadDeadline)
* [func (c \*TCPConn) SetWriteBuffer(bytes int) error](#TCPConn.SetWriteBuffer)
* [func (c \*TCPConn) SetWriteDeadline(t time.Time) error](#TCPConn.SetWriteDeadline)
* [func (c \*TCPConn) SyscallConn() (syscall.RawConn, error)](#TCPConn.SyscallConn)
* [func (c \*TCPConn) Write(b []byte) (int, error)](#TCPConn.Write)
* [type TCPListener](#TCPListener)
* [func ListenTCP(network string, laddr \*TCPAddr) (\*TCPListener, error)](#ListenTCP)
* [func (l \*TCPListener) Accept() (Conn, error)](#TCPListener.Accept)
* [func (l \*TCPListener) AcceptTCP() (\*TCPConn, error)](#TCPListener.AcceptTCP)
* [func (l \*TCPListener) Addr() Addr](#TCPListener.Addr)
* [func (l \*TCPListener) Close() error](#TCPListener.Close)
* [func (l \*TCPListener) File() (f \*os.File, err error)](#TCPListener.File)
* [func (l \*TCPListener) SetDeadline(t time.Time) error](#TCPListener.SetDeadline)
* [func (l \*TCPListener) SyscallConn() (syscall.RawConn, error)](#TCPListener.SyscallConn)
* [type UDPAddr](#UDPAddr)
* [func ResolveUDPAddr(network, address string) (\*UDPAddr, error)](#ResolveUDPAddr)
* [func UDPAddrFromAddrPort(addr netip.AddrPort) \*UDPAddr](#UDPAddrFromAddrPort)
* [func (a \*UDPAddr) AddrPort() netip.AddrPort](#UDPAddr.AddrPort)
* [func (a \*UDPAddr) Network() string](#UDPAddr.Network)
* [func (a \*UDPAddr) String() string](#UDPAddr.String)
* [type UDPConn](#UDPConn)
* [func DialUDP(network string, laddr, raddr \*UDPAddr) (\*UDPConn, error)](#DialUDP)
* [func ListenMulticastUDP(network string, ifi \*Interface, gaddr \*UDPAddr) (\*UDPConn, error)](#ListenMulticastUDP)
* [func ListenUDP(network string, laddr \*UDPAddr) (\*UDPConn, error)](#ListenUDP)
* [func (c \*UDPConn) Close() error](#UDPConn.Close)
* [func (c \*UDPConn) File() (f \*os.File, err error)](#UDPConn.File)
* [func (c \*UDPConn) LocalAddr() Addr](#UDPConn.LocalAddr)
* [func (c \*UDPConn) Read(b []byte) (int, error)](#UDPConn.Read)
* [func (c \*UDPConn) ReadFrom(b []byte) (int, Addr, error)](#UDPConn.ReadFrom)
* [func (c \*UDPConn) ReadFromUDP(b []byte) (n int, addr \*UDPAddr, err error)](#UDPConn.ReadFromUDP)
* [func (c \*UDPConn) ReadFromUDPAddrPort(b []byte) (n int, addr netip.AddrPort, err error)](#UDPConn.ReadFromUDPAddrPort)
* [func (c \*UDPConn) ReadMsgUDP(b, oob []byte) (n, oobn, flags int, addr \*UDPAddr, err error)](#UDPConn.ReadMsgUDP)
* [func (c \*UDPConn) ReadMsgUDPAddrPort(b, oob []byte) (n, oobn, flags int, addr netip.AddrPort, err error)](#UDPConn.ReadMsgUDPAddrPort)
* [func (c \*UDPConn) RemoteAddr() Addr](#UDPConn.RemoteAddr)
* [func (c \*UDPConn) SetDeadline(t time.Time) error](#UDPConn.SetDeadline)
* [func (c \*UDPConn) SetReadBuffer(bytes int) error](#UDPConn.SetReadBuffer)
* [func (c \*UDPConn) SetReadDeadline(t time.Time) error](#UDPConn.SetReadDeadline)
* [func (c \*UDPConn) SetWriteBuffer(bytes int) error](#UDPConn.SetWriteBuffer)
* [func (c \*UDPConn) SetWriteDeadline(t time.Time) error](#UDPConn.SetWriteDeadline)
* [func (c \*UDPConn) SyscallConn() (syscall.RawConn, error)](#UDPConn.SyscallConn)
* [func (c \*UDPConn) Write(b []byte) (int, error)](#UDPConn.Write)
* [func (c \*UDPConn) WriteMsgUDP(b, oob []byte, addr \*UDPAddr) (n, oobn int, err error)](#UDPConn.WriteMsgUDP)
* [func (c \*UDPConn) WriteMsgUDPAddrPort(b, oob []byte, addr netip.AddrPort) (n, oobn int, err error)](#UDPConn.WriteMsgUDPAddrPort)
* [func (c \*UDPConn) WriteTo(b []byte, addr Addr) (int, error)](#UDPConn.WriteTo)
* [func (c \*UDPConn) WriteToUDP(b []byte, addr \*UDPAddr) (int, error)](#UDPConn.WriteToUDP)
* [func (c \*UDPConn) WriteToUDPAddrPort(b []byte, addr netip.AddrPort) (int, error)](#UDPConn.WriteToUDPAddrPort)
* [type UnixAddr](#UnixAddr)
* [func ResolveUnixAddr(network, address string) (\*UnixAddr, error)](#ResolveUnixAddr)
* [func (a \*UnixAddr) Network() string](#UnixAddr.Network)
* [func (a \*UnixAddr) String() string](#UnixAddr.String)
* [type UnixConn](#UnixConn)
* [func DialUnix(network string, laddr, raddr \*UnixAddr) (\*UnixConn, error)](#DialUnix)
* [func ListenUnixgram(network string, laddr \*UnixAddr) (\*UnixConn, error)](#ListenUnixgram)
* [func (c \*UnixConn) Close() error](#UnixConn.Close)
* [func (c \*UnixConn) CloseRead() error](#UnixConn.CloseRead)
* [func (c \*UnixConn) CloseWrite() error](#UnixConn.CloseWrite)
* [func (c \*UnixConn) File() (f \*os.File, err error)](#UnixConn.File)
* [func (c \*UnixConn) LocalAddr() Addr](#UnixConn.LocalAddr)
* [func (c \*UnixConn) Read(b []byte) (int, error)](#UnixConn.Read)
* [func (c \*UnixConn) ReadFrom(b []byte) (int, Addr, error)](#UnixConn.ReadFrom)
* [func (c \*UnixConn) ReadFromUnix(b []byte) (int, \*UnixAddr, error)](#UnixConn.ReadFromUnix)
* [func (c \*UnixConn) ReadMsgUnix(b, oob []byte) (n, oobn, flags int, addr \*UnixAddr, err error)](#UnixConn.ReadMsgUnix)
* [func (c \*UnixConn) RemoteAddr() Addr](#UnixConn.RemoteAddr)
* [func (c \*UnixConn) SetDeadline(t time.Time) error](#UnixConn.SetDeadline)
* [func (c \*UnixConn) SetReadBuffer(bytes int) error](#UnixConn.SetReadBuffer)
* [func (c \*UnixConn) SetReadDeadline(t time.Time) error](#UnixConn.SetReadDeadline)
* [func (c \*UnixConn) SetWriteBuffer(bytes int) error](#UnixConn.SetWriteBuffer)
* [func (c \*UnixConn) SetWriteDeadline(t time.Time) error](#UnixConn.SetWriteDeadline)
* [func (c \*UnixConn) SyscallConn() (syscall.RawConn, error)](#UnixConn.SyscallConn)
* [func (c \*UnixConn) Write(b []byte) (int, error)](#UnixConn.Write)
* [func (c \*UnixConn) WriteMsgUnix(b, oob []byte, addr \*UnixAddr) (n, oobn int, err error)](#UnixConn.WriteMsgUnix)
* [func (c \*UnixConn) WriteTo(b []byte, addr Addr) (int, error)](#UnixConn.WriteTo)
* [func (c \*UnixConn) WriteToUnix(b []byte, addr \*UnixAddr) (int, error)](#UnixConn.WriteToUnix)
* [type UnixListener](#UnixListener)
* [func ListenUnix(network string, laddr \*UnixAddr) (\*UnixListener, error)](#ListenUnix)
* [func (l \*UnixListener) Accept() (Conn, error)](#UnixListener.Accept)
* [func (l \*UnixListener) AcceptUnix() (\*UnixConn, error)](#UnixListener.AcceptUnix)
* [func (l \*UnixListener) Addr() Addr](#UnixListener.Addr)
* [func (l \*UnixListener) Close() error](#UnixListener.Close)
* [func (l \*UnixListener) File() (f \*os.File, err error)](#UnixListener.File)
* [func (l \*UnixListener) SetDeadline(t time.Time) error](#UnixListener.SetDeadline)
* [func (l \*UnixListener) SetUnlinkOnClose(unlink bool)](#UnixListener.SetUnlinkOnClose)
* [func (l \*UnixListener) SyscallConn() (syscall.RawConn, error)](#UnixListener.SyscallConn)
* [type UnknownNetworkError](#UnknownNetworkError)
* [func (e UnknownNetworkError) Error() string](#UnknownNetworkError.Error)
* [func (e UnknownNetworkError) Temporary() bool](#UnknownNetworkError.Temporary)
* [func (e UnknownNetworkError) Timeout() bool](#UnknownNetworkError.Timeout)
* [Bugs](#pkg-note-BUG)
### Examples
[CIDRMask](#example_CIDRMask) [Dialer](#example_Dialer) [Dialer (Unix)](#example_Dialer_unix) [IP.DefaultMask](#example_IP_DefaultMask) [IP.Equal](#example_IP_Equal) [IP.IsGlobalUnicast](#example_IP_IsGlobalUnicast) [IP.IsInterfaceLocalMulticast](#example_IP_IsInterfaceLocalMulticast) [IP.IsLinkLocalMulticast](#example_IP_IsLinkLocalMulticast) [IP.IsLinkLocalUnicast](#example_IP_IsLinkLocalUnicast) [IP.IsLoopback](#example_IP_IsLoopback) [IP.IsMulticast](#example_IP_IsMulticast) [IP.IsPrivate](#example_IP_IsPrivate) [IP.IsUnspecified](#example_IP_IsUnspecified) [IP.Mask](#example_IP_Mask) [IP.String](#example_IP_String) [IP.To16](#example_IP_To16) [IP (To4)](#example_IP_to4) [IPv4](#example_IPv4) [IPv4Mask](#example_IPv4Mask) [Listener](#example_Listener) [ParseCIDR](#example_ParseCIDR) [ParseIP](#example_ParseIP) [UDPConn.WriteTo](#example_UDPConn_WriteTo) ### Package files
addrselect.go cgo\_linux.go cgo\_resnew.go cgo\_socknew.go cgo\_unix.go cgo\_unix\_cgo.go cgo\_unix\_cgo\_res.go conf.go dial.go dnsclient.go dnsclient\_unix.go dnsconfig.go dnsconfig\_unix.go error\_posix.go error\_unix.go fd\_posix.go fd\_unix.go file.go file\_unix.go hook.go hook\_unix.go hosts.go interface.go interface\_linux.go ip.go iprawsock.go iprawsock\_posix.go ipsock.go ipsock\_posix.go lookup.go lookup\_unix.go mac.go net.go nss.go parse.go pipe.go port.go port\_unix.go rawconn.go sendfile\_linux.go sock\_cloexec.go sock\_linux.go sock\_posix.go sockaddr\_posix.go sockopt\_linux.go sockopt\_posix.go sockoptip\_linux.go sockoptip\_posix.go splice\_linux.go tcpsock.go tcpsock\_posix.go tcpsockopt\_posix.go tcpsockopt\_unix.go udpsock.go udpsock\_posix.go unixsock.go unixsock\_posix.go unixsock\_readmsg\_cmsg\_cloexec.go writev\_unix.go
Constants
---------
IP address lengths (bytes).
```
const (
IPv4len = 4
IPv6len = 16
)
```
Variables
---------
Well-known IPv4 addresses
```
var (
IPv4bcast = IPv4(255, 255, 255, 255) // limited broadcast
IPv4allsys = IPv4(224, 0, 0, 1) // all systems
IPv4allrouter = IPv4(224, 0, 0, 2) // all routers
IPv4zero = IPv4(0, 0, 0, 0) // all zeros
)
```
Well-known IPv6 addresses
```
var (
IPv6zero = IP{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
IPv6unspecified = IP{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
IPv6loopback = IP{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1}
IPv6interfacelocalallnodes = IP{0xff, 0x01, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0x01}
IPv6linklocalallnodes = IP{0xff, 0x02, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0x01}
IPv6linklocalallrouters = IP{0xff, 0x02, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0x02}
)
```
DefaultResolver is the resolver used by the package-level Lookup functions and by Dialers without a specified Resolver.
```
var DefaultResolver = &Resolver{}
```
ErrClosed is the error returned by an I/O call on a network connection that has already been closed, or that is closed by another goroutine before the I/O is completed. This may be wrapped in another error, and should normally be tested using errors.Is(err, net.ErrClosed).
```
var ErrClosed error = errClosed
```
Various errors contained in OpError.
```
var (
ErrWriteToConnected = errors.New("use of WriteTo with pre-connected connection")
)
```
func JoinHostPort
-----------------
```
func JoinHostPort(host, port string) string
```
JoinHostPort combines host and port into a network address of the form "host:port". If host contains a colon, as found in literal IPv6 addresses, then JoinHostPort returns "[host]:port".
See func Dial for a description of the host and port parameters.
func LookupAddr
---------------
```
func LookupAddr(addr string) (names []string, err error)
```
LookupAddr performs a reverse lookup for the given address, returning a list of names mapping to that address.
The returned names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
When using the host C library resolver, at most one result will be returned. To bypass the host resolver, use a custom Resolver.
LookupAddr uses context.Background internally; to specify the context, use Resolver.LookupAddr.
func LookupCNAME
----------------
```
func LookupCNAME(host string) (cname string, err error)
```
LookupCNAME returns the canonical name for the given host. Callers that do not care about the canonical name can call LookupHost or LookupIP directly; both take care of resolving the canonical name as part of the lookup.
A canonical name is the final name after following zero or more CNAME records. LookupCNAME does not return an error if host does not contain DNS "CNAME" records, as long as host resolves to address records.
The returned canonical name is validated to be a properly formatted presentation-format domain name.
LookupCNAME uses context.Background internally; to specify the context, use Resolver.LookupCNAME.
func LookupHost
---------------
```
func LookupHost(host string) (addrs []string, err error)
```
LookupHost looks up the given host using the local resolver. It returns a slice of that host's addresses.
LookupHost uses context.Background internally; to specify the context, use Resolver.LookupHost.
func LookupPort
---------------
```
func LookupPort(network, service string) (port int, err error)
```
LookupPort looks up the port for the given network and service.
LookupPort uses context.Background internally; to specify the context, use Resolver.LookupPort.
func LookupTXT
--------------
```
func LookupTXT(name string) ([]string, error)
```
LookupTXT returns the DNS TXT records for the given domain name.
LookupTXT uses context.Background internally; to specify the context, use Resolver.LookupTXT.
func ParseCIDR
--------------
```
func ParseCIDR(s string) (IP, *IPNet, error)
```
ParseCIDR parses s as a CIDR notation IP address and prefix length, like "192.0.2.0/24" or "2001:db8::/32", as defined in RFC 4632 and RFC 4291.
It returns the IP address and the network implied by the IP and prefix length. For example, ParseCIDR("192.0.2.1/24") returns the IP address 192.0.2.1 and the network 192.0.2.0/24.
#### Example
Code:
```
ipv4Addr, ipv4Net, err := net.ParseCIDR("192.0.2.1/24")
if err != nil {
log.Fatal(err)
}
fmt.Println(ipv4Addr)
fmt.Println(ipv4Net)
ipv6Addr, ipv6Net, err := net.ParseCIDR("2001:db8:a0b:12f0::1/32")
if err != nil {
log.Fatal(err)
}
fmt.Println(ipv6Addr)
fmt.Println(ipv6Net)
```
Output:
```
192.0.2.1
192.0.2.0/24
2001:db8:a0b:12f0::1
2001:db8::/32
```
func Pipe
---------
```
func Pipe() (Conn, Conn)
```
Pipe creates a synchronous, in-memory, full duplex network connection; both ends implement the Conn interface. Reads on one end are matched with writes on the other, copying data directly between the two; there is no internal buffering.
func SplitHostPort
------------------
```
func SplitHostPort(hostport string) (host, port string, err error)
```
SplitHostPort splits a network address of the form "host:port", "host%zone:port", "[host]:port" or "[host%zone]:port" into host or host%zone and port.
A literal IPv6 address in hostport must be enclosed in square brackets, as in "[::1]:80", "[::1%lo0]:80".
See func Dial for a description of the hostport parameter, and host and port results.
type Addr
---------
Addr represents a network end point address.
The two methods Network and String conventionally return strings that can be passed as the arguments to Dial, but the exact form and meaning of the strings is up to the implementation.
```
type Addr interface {
Network() string // name of the network (for example, "tcp", "udp")
String() string // string form of address (for example, "192.0.2.1:25", "[2001:db8::1]:80")
}
```
### func InterfaceAddrs
```
func InterfaceAddrs() ([]Addr, error)
```
InterfaceAddrs returns a list of the system's unicast interface addresses.
The returned list does not identify the associated interface; use Interfaces and Interface.Addrs for more detail.
type AddrError
--------------
```
type AddrError struct {
Err string
Addr string
}
```
### func (\*AddrError) Error
```
func (e *AddrError) Error() string
```
### func (\*AddrError) Temporary
```
func (e *AddrError) Temporary() bool
```
### func (\*AddrError) Timeout
```
func (e *AddrError) Timeout() bool
```
type Buffers 1.8
----------------
Buffers contains zero or more runs of bytes to write.
On certain machines, for certain types of connections, this is optimized into an OS-specific batch write operation (such as "writev").
```
type Buffers [][]byte
```
### func (\*Buffers) Read 1.8
```
func (v *Buffers) Read(p []byte) (n int, err error)
```
Read from the buffers.
Read implements io.Reader for Buffers.
Read modifies the slice v as well as v[i] for 0 <= i < len(v), but does not modify v[i][j] for any i, j.
### func (\*Buffers) WriteTo 1.8
```
func (v *Buffers) WriteTo(w io.Writer) (n int64, err error)
```
WriteTo writes contents of the buffers to w.
WriteTo implements io.WriterTo for Buffers.
WriteTo modifies the slice v as well as v[i] for 0 <= i < len(v), but does not modify v[i][j] for any i, j.
type Conn
---------
Conn is a generic stream-oriented network connection.
Multiple goroutines may invoke methods on a Conn simultaneously.
```
type Conn interface {
// Read reads data from the connection.
// Read can be made to time out and return an error after a fixed
// time limit; see SetDeadline and SetReadDeadline.
Read(b []byte) (n int, err error)
// Write writes data to the connection.
// Write can be made to time out and return an error after a fixed
// time limit; see SetDeadline and SetWriteDeadline.
Write(b []byte) (n int, err error)
// Close closes the connection.
// Any blocked Read or Write operations will be unblocked and return errors.
Close() error
// LocalAddr returns the local network address, if known.
LocalAddr() Addr
// RemoteAddr returns the remote network address, if known.
RemoteAddr() Addr
// SetDeadline sets the read and write deadlines associated
// with the connection. It is equivalent to calling both
// SetReadDeadline and SetWriteDeadline.
//
// A deadline is an absolute time after which I/O operations
// fail instead of blocking. The deadline applies to all future
// and pending I/O, not just the immediately following call to
// Read or Write. After a deadline has been exceeded, the
// connection can be refreshed by setting a deadline in the future.
//
// If the deadline is exceeded a call to Read or Write or to other
// I/O methods will return an error that wraps os.ErrDeadlineExceeded.
// This can be tested using errors.Is(err, os.ErrDeadlineExceeded).
// The error's Timeout method will return true, but note that there
// are other possible errors for which the Timeout method will
// return true even if the deadline has not been exceeded.
//
// An idle timeout can be implemented by repeatedly extending
// the deadline after successful Read or Write calls.
//
// A zero value for t means I/O operations will not time out.
SetDeadline(t time.Time) error
// SetReadDeadline sets the deadline for future Read calls
// and any currently-blocked Read call.
// A zero value for t means Read will not time out.
SetReadDeadline(t time.Time) error
// SetWriteDeadline sets the deadline for future Write calls
// and any currently-blocked Write call.
// Even if write times out, it may return n > 0, indicating that
// some of the data was successfully written.
// A zero value for t means Write will not time out.
SetWriteDeadline(t time.Time) error
}
```
### func Dial
```
func Dial(network, address string) (Conn, error)
```
Dial connects to the address on the named network.
Known networks are "tcp", "tcp4" (IPv4-only), "tcp6" (IPv6-only), "udp", "udp4" (IPv4-only), "udp6" (IPv6-only), "ip", "ip4" (IPv4-only), "ip6" (IPv6-only), "unix", "unixgram" and "unixpacket".
For TCP and UDP networks, the address has the form "host:port". The host must be a literal IP address, or a host name that can be resolved to IP addresses. The port must be a literal port number or a service name. If the host is a literal IPv6 address it must be enclosed in square brackets, as in "[2001:db8::1]:80" or "[fe80::1%zone]:80". The zone specifies the scope of the literal IPv6 address as defined in RFC 4007. The functions JoinHostPort and SplitHostPort manipulate a pair of host and port in this form. When using TCP, and the host resolves to multiple IP addresses, Dial will try each IP address in order until one succeeds.
Examples:
```
Dial("tcp", "golang.org:http")
Dial("tcp", "192.0.2.1:http")
Dial("tcp", "198.51.100.1:80")
Dial("udp", "[2001:db8::1]:domain")
Dial("udp", "[fe80::1%lo0]:53")
Dial("tcp", ":80")
```
For IP networks, the network must be "ip", "ip4" or "ip6" followed by a colon and a literal protocol number or a protocol name, and the address has the form "host". The host must be a literal IP address or a literal IPv6 address with zone. It depends on each operating system how the operating system behaves with a non-well known protocol number such as "0" or "255".
Examples:
```
Dial("ip4:1", "192.0.2.1")
Dial("ip6:ipv6-icmp", "2001:db8::1")
Dial("ip6:58", "fe80::1%lo0")
```
For TCP, UDP and IP networks, if the host is empty or a literal unspecified IP address, as in ":80", "0.0.0.0:80" or "[::]:80" for TCP and UDP, "", "0.0.0.0" or "::" for IP, the local system is assumed.
For Unix networks, the address must be a file system path.
### func DialTimeout
```
func DialTimeout(network, address string, timeout time.Duration) (Conn, error)
```
DialTimeout acts like Dial but takes a timeout.
The timeout includes name resolution, if required. When using TCP, and the host in the address parameter resolves to multiple IP addresses, the timeout is spread over each consecutive dial, such that each is given an appropriate fraction of the time to connect.
See func Dial for a description of the network and address parameters.
### func FileConn
```
func FileConn(f *os.File) (c Conn, err error)
```
FileConn returns a copy of the network connection corresponding to the open file f. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
type DNSConfigError
-------------------
DNSConfigError represents an error reading the machine's DNS configuration. (No longer used; kept for compatibility.)
```
type DNSConfigError struct {
Err error
}
```
### func (\*DNSConfigError) Error
```
func (e *DNSConfigError) Error() string
```
### func (\*DNSConfigError) Temporary
```
func (e *DNSConfigError) Temporary() bool
```
### func (\*DNSConfigError) Timeout
```
func (e *DNSConfigError) Timeout() bool
```
### func (\*DNSConfigError) Unwrap 1.13
```
func (e *DNSConfigError) Unwrap() error
```
type DNSError
-------------
DNSError represents a DNS lookup error.
```
type DNSError struct {
Err string // description of the error
Name string // name looked for
Server string // server used
IsTimeout bool // if true, timed out; not all timeouts set this
IsTemporary bool // if true, error is temporary; not all errors set this; added in Go 1.6
IsNotFound bool // if true, host could not be found; added in Go 1.13
}
```
### func (\*DNSError) Error
```
func (e *DNSError) Error() string
```
### func (\*DNSError) Temporary
```
func (e *DNSError) Temporary() bool
```
Temporary reports whether the DNS error is known to be temporary. This is not always known; a DNS lookup may fail due to a temporary error and return a DNSError for which Temporary returns false.
### func (\*DNSError) Timeout
```
func (e *DNSError) Timeout() bool
```
Timeout reports whether the DNS lookup is known to have timed out. This is not always known; a DNS lookup may fail due to a timeout and return a DNSError for which Timeout returns false.
type Dialer 1.1
---------------
A Dialer contains options for connecting to an address.
The zero value for each field is equivalent to dialing without that option. Dialing with the zero value of Dialer is therefore equivalent to just calling the Dial function.
It is safe to call Dialer's methods concurrently.
```
type Dialer struct {
// Timeout is the maximum amount of time a dial will wait for
// a connect to complete. If Deadline is also set, it may fail
// earlier.
//
// The default is no timeout.
//
// When using TCP and dialing a host name with multiple IP
// addresses, the timeout may be divided between them.
//
// With or without a timeout, the operating system may impose
// its own earlier timeout. For instance, TCP timeouts are
// often around 3 minutes.
Timeout time.Duration
// Deadline is the absolute point in time after which dials
// will fail. If Timeout is set, it may fail earlier.
// Zero means no deadline, or dependent on the operating system
// as with the Timeout option.
Deadline time.Time
// LocalAddr is the local address to use when dialing an
// address. The address must be of a compatible type for the
// network being dialed.
// If nil, a local address is automatically chosen.
LocalAddr Addr
// DualStack previously enabled RFC 6555 Fast Fallback
// support, also known as "Happy Eyeballs", in which IPv4 is
// tried soon if IPv6 appears to be misconfigured and
// hanging.
//
// Deprecated: Fast Fallback is enabled by default. To
// disable, set FallbackDelay to a negative value.
DualStack bool // Go 1.2
// FallbackDelay specifies the length of time to wait before
// spawning a RFC 6555 Fast Fallback connection. That is, this
// is the amount of time to wait for IPv6 to succeed before
// assuming that IPv6 is misconfigured and falling back to
// IPv4.
//
// If zero, a default delay of 300ms is used.
// A negative value disables Fast Fallback support.
FallbackDelay time.Duration // Go 1.5
// KeepAlive specifies the interval between keep-alive
// probes for an active network connection.
// If zero, keep-alive probes are sent with a default value
// (currently 15 seconds), if supported by the protocol and operating
// system. Network protocols or operating systems that do
// not support keep-alives ignore this field.
// If negative, keep-alive probes are disabled.
KeepAlive time.Duration // Go 1.3
// Resolver optionally specifies an alternate resolver to use.
Resolver *Resolver // Go 1.8
// Cancel is an optional channel whose closure indicates that
// the dial should be canceled. Not all types of dials support
// cancellation.
//
// Deprecated: Use DialContext instead.
Cancel <-chan struct{} // Go 1.6
// If Control is not nil, it is called after creating the network
// connection but before actually dialing.
//
// Network and address parameters passed to Control method are not
// necessarily the ones passed to Dial. For example, passing "tcp" to Dial
// will cause the Control function to be called with "tcp4" or "tcp6".
//
// Control is ignored if ControlContext is not nil.
Control func(network, address string, c syscall.RawConn) error // Go 1.11
// If ControlContext is not nil, it is called after creating the network
// connection but before actually dialing.
//
// Network and address parameters passed to Control method are not
// necessarily the ones passed to Dial. For example, passing "tcp" to Dial
// will cause the Control function to be called with "tcp4" or "tcp6".
//
// If ControlContext is not nil, Control is ignored.
ControlContext func(ctx context.Context, network, address string, c syscall.RawConn) error // Go 1.20
}
```
#### Example
Code:
```
var d net.Dialer
ctx, cancel := context.WithTimeout(context.Background(), time.Minute)
defer cancel()
conn, err := d.DialContext(ctx, "tcp", "localhost:12345")
if err != nil {
log.Fatalf("Failed to dial: %v", err)
}
defer conn.Close()
if _, err := conn.Write([]byte("Hello, World!")); err != nil {
log.Fatal(err)
}
```
#### Example (Unix)
Code:
```
// DialUnix does not take a context.Context parameter. This example shows
// how to dial a Unix socket with a Context. Note that the Context only
// applies to the dial operation; it does not apply to the connection once
// it has been established.
var d net.Dialer
ctx, cancel := context.WithTimeout(context.Background(), time.Minute)
defer cancel()
d.LocalAddr = nil // if you have a local addr, add it here
raddr := net.UnixAddr{Name: "/path/to/unix.sock", Net: "unix"}
conn, err := d.DialContext(ctx, "unix", raddr.String())
if err != nil {
log.Fatalf("Failed to dial: %v", err)
}
defer conn.Close()
if _, err := conn.Write([]byte("Hello, socket!")); err != nil {
log.Fatal(err)
}
```
### func (\*Dialer) Dial 1.1
```
func (d *Dialer) Dial(network, address string) (Conn, error)
```
Dial connects to the address on the named network.
See func Dial for a description of the network and address parameters.
Dial uses context.Background internally; to specify the context, use DialContext.
### func (\*Dialer) DialContext 1.7
```
func (d *Dialer) DialContext(ctx context.Context, network, address string) (Conn, error)
```
DialContext connects to the address on the named network using the provided context.
The provided Context must be non-nil. If the context expires before the connection is complete, an error is returned. Once successfully connected, any expiration of the context will not affect the connection.
When using TCP, and the host in the address parameter resolves to multiple network addresses, any dial timeout (from d.Timeout or ctx) is spread over each consecutive dial, such that each is given an appropriate fraction of the time to connect. For example, if a host has 4 IP addresses and the timeout is 1 minute, the connect to each single address will be given 15 seconds to complete before trying the next one.
See func Dial for a description of the network and address parameters.
type Error
----------
An Error represents a network error.
```
type Error interface {
error
Timeout() bool // Is the error a timeout?
// Deprecated: Temporary errors are not well-defined.
// Most "temporary" errors are timeouts, and the few exceptions are surprising.
// Do not use this method.
Temporary() bool
}
```
type Flags
----------
```
type Flags uint
```
```
const (
FlagUp Flags = 1 << iota // interface is administratively up
FlagBroadcast // interface supports broadcast access capability
FlagLoopback // interface is a loopback interface
FlagPointToPoint // interface belongs to a point-to-point link
FlagMulticast // interface supports multicast access capability
FlagRunning // interface is in running state
)
```
### func (Flags) String
```
func (f Flags) String() string
```
type HardwareAddr
-----------------
A HardwareAddr represents a physical hardware address.
```
type HardwareAddr []byte
```
### func ParseMAC
```
func ParseMAC(s string) (hw HardwareAddr, err error)
```
ParseMAC parses s as an IEEE 802 MAC-48, EUI-48, EUI-64, or a 20-octet IP over InfiniBand link-layer address using one of the following formats:
```
00:00:5e:00:53:01
02:00:5e:10:00:00:00:01
00:00:00:00:fe:80:00:00:00:00:00:00:02:00:5e:10:00:00:00:01
00-00-5e-00-53-01
02-00-5e-10-00-00-00-01
00-00-00-00-fe-80-00-00-00-00-00-00-02-00-5e-10-00-00-00-01
0000.5e00.5301
0200.5e10.0000.0001
0000.0000.fe80.0000.0000.0000.0200.5e10.0000.0001
```
### func (HardwareAddr) String
```
func (a HardwareAddr) String() string
```
type IP
-------
An IP is a single IP address, a slice of bytes. Functions in this package accept either 4-byte (IPv4) or 16-byte (IPv6) slices as input.
Note that in this documentation, referring to an IP address as an IPv4 address or an IPv6 address is a semantic property of the address, not just the length of the byte slice: a 16-byte slice can still be an IPv4 address.
```
type IP []byte
```
#### Example (To4)
Code:
```
ipv6 := net.IP{0xfc, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
ipv4 := net.IPv4(10, 255, 0, 0)
fmt.Println(ipv6.To4())
fmt.Println(ipv4.To4())
```
Output:
```
<nil>
10.255.0.0
```
### func IPv4
```
func IPv4(a, b, c, d byte) IP
```
IPv4 returns the IP address (in 16-byte form) of the IPv4 address a.b.c.d.
#### Example
Code:
```
fmt.Println(net.IPv4(8, 8, 8, 8))
```
Output:
```
8.8.8.8
```
### func LookupIP
```
func LookupIP(host string) ([]IP, error)
```
LookupIP looks up host using the local resolver. It returns a slice of that host's IPv4 and IPv6 addresses.
### func ParseIP
```
func ParseIP(s string) IP
```
ParseIP parses s as an IP address, returning the result. The string s can be in IPv4 dotted decimal ("192.0.2.1"), IPv6 ("2001:db8::68"), or IPv4-mapped IPv6 ("::ffff:192.0.2.1") form. If s is not a valid textual representation of an IP address, ParseIP returns nil.
#### Example
Code:
```
fmt.Println(net.ParseIP("192.0.2.1"))
fmt.Println(net.ParseIP("2001:db8::68"))
fmt.Println(net.ParseIP("192.0.2"))
```
Output:
```
192.0.2.1
2001:db8::68
<nil>
```
### func (IP) DefaultMask
```
func (ip IP) DefaultMask() IPMask
```
DefaultMask returns the default IP mask for the IP address ip. Only IPv4 addresses have default masks; DefaultMask returns nil if ip is not a valid IPv4 address.
#### Example
Code:
```
ip := net.ParseIP("192.0.2.1")
fmt.Println(ip.DefaultMask())
```
Output:
```
ffffff00
```
### func (IP) Equal
```
func (ip IP) Equal(x IP) bool
```
Equal reports whether ip and x are the same IP address. An IPv4 address and that same address in IPv6 form are considered to be equal.
#### Example
Code:
```
ipv4DNS := net.ParseIP("8.8.8.8")
ipv4Lo := net.ParseIP("127.0.0.1")
ipv6DNS := net.ParseIP("0:0:0:0:0:FFFF:0808:0808")
fmt.Println(ipv4DNS.Equal(ipv4DNS))
fmt.Println(ipv4DNS.Equal(ipv4Lo))
fmt.Println(ipv4DNS.Equal(ipv6DNS))
```
Output:
```
true
false
true
```
### func (IP) IsGlobalUnicast
```
func (ip IP) IsGlobalUnicast() bool
```
IsGlobalUnicast reports whether ip is a global unicast address.
The identification of global unicast addresses uses address type identification as defined in RFC 1122, RFC 4632 and RFC 4291 with the exception of IPv4 directed broadcast addresses. It returns true even if ip is in IPv4 private address space or local IPv6 unicast address space.
#### Example
Code:
```
ipv6Global := net.ParseIP("2000::")
ipv6UniqLocal := net.ParseIP("2000::")
ipv6Multi := net.ParseIP("FF00::")
ipv4Private := net.ParseIP("10.255.0.0")
ipv4Public := net.ParseIP("8.8.8.8")
ipv4Broadcast := net.ParseIP("255.255.255.255")
fmt.Println(ipv6Global.IsGlobalUnicast())
fmt.Println(ipv6UniqLocal.IsGlobalUnicast())
fmt.Println(ipv6Multi.IsGlobalUnicast())
fmt.Println(ipv4Private.IsGlobalUnicast())
fmt.Println(ipv4Public.IsGlobalUnicast())
fmt.Println(ipv4Broadcast.IsGlobalUnicast())
```
Output:
```
true
true
false
true
true
false
```
### func (IP) IsInterfaceLocalMulticast
```
func (ip IP) IsInterfaceLocalMulticast() bool
```
IsInterfaceLocalMulticast reports whether ip is an interface-local multicast address.
#### Example
Code:
```
ipv6InterfaceLocalMulti := net.ParseIP("ff01::1")
ipv6Global := net.ParseIP("2000::")
ipv4 := net.ParseIP("255.0.0.0")
fmt.Println(ipv6InterfaceLocalMulti.IsInterfaceLocalMulticast())
fmt.Println(ipv6Global.IsInterfaceLocalMulticast())
fmt.Println(ipv4.IsInterfaceLocalMulticast())
```
Output:
```
true
false
false
```
### func (IP) IsLinkLocalMulticast
```
func (ip IP) IsLinkLocalMulticast() bool
```
IsLinkLocalMulticast reports whether ip is a link-local multicast address.
#### Example
Code:
```
ipv6LinkLocalMulti := net.ParseIP("ff02::2")
ipv6LinkLocalUni := net.ParseIP("fe80::")
ipv4LinkLocalMulti := net.ParseIP("224.0.0.0")
ipv4LinkLocalUni := net.ParseIP("169.254.0.0")
fmt.Println(ipv6LinkLocalMulti.IsLinkLocalMulticast())
fmt.Println(ipv6LinkLocalUni.IsLinkLocalMulticast())
fmt.Println(ipv4LinkLocalMulti.IsLinkLocalMulticast())
fmt.Println(ipv4LinkLocalUni.IsLinkLocalMulticast())
```
Output:
```
true
false
true
false
```
### func (IP) IsLinkLocalUnicast
```
func (ip IP) IsLinkLocalUnicast() bool
```
IsLinkLocalUnicast reports whether ip is a link-local unicast address.
#### Example
Code:
```
ipv6LinkLocalUni := net.ParseIP("fe80::")
ipv6Global := net.ParseIP("2000::")
ipv4LinkLocalUni := net.ParseIP("169.254.0.0")
ipv4LinkLocalMulti := net.ParseIP("224.0.0.0")
fmt.Println(ipv6LinkLocalUni.IsLinkLocalUnicast())
fmt.Println(ipv6Global.IsLinkLocalUnicast())
fmt.Println(ipv4LinkLocalUni.IsLinkLocalUnicast())
fmt.Println(ipv4LinkLocalMulti.IsLinkLocalUnicast())
```
Output:
```
true
false
true
false
```
### func (IP) IsLoopback
```
func (ip IP) IsLoopback() bool
```
IsLoopback reports whether ip is a loopback address.
#### Example
Code:
```
ipv6Lo := net.ParseIP("::1")
ipv6 := net.ParseIP("ff02::1")
ipv4Lo := net.ParseIP("127.0.0.0")
ipv4 := net.ParseIP("128.0.0.0")
fmt.Println(ipv6Lo.IsLoopback())
fmt.Println(ipv6.IsLoopback())
fmt.Println(ipv4Lo.IsLoopback())
fmt.Println(ipv4.IsLoopback())
```
Output:
```
true
false
true
false
```
### func (IP) IsMulticast
```
func (ip IP) IsMulticast() bool
```
IsMulticast reports whether ip is a multicast address.
#### Example
Code:
```
ipv6Multi := net.ParseIP("FF00::")
ipv6LinkLocalMulti := net.ParseIP("ff02::1")
ipv6Lo := net.ParseIP("::1")
ipv4Multi := net.ParseIP("239.0.0.0")
ipv4LinkLocalMulti := net.ParseIP("224.0.0.0")
ipv4Lo := net.ParseIP("127.0.0.0")
fmt.Println(ipv6Multi.IsMulticast())
fmt.Println(ipv6LinkLocalMulti.IsMulticast())
fmt.Println(ipv6Lo.IsMulticast())
fmt.Println(ipv4Multi.IsMulticast())
fmt.Println(ipv4LinkLocalMulti.IsMulticast())
fmt.Println(ipv4Lo.IsMulticast())
```
Output:
```
true
true
false
true
true
false
```
### func (IP) IsPrivate 1.17
```
func (ip IP) IsPrivate() bool
```
IsPrivate reports whether ip is a private address, according to RFC 1918 (IPv4 addresses) and RFC 4193 (IPv6 addresses).
#### Example
Code:
```
ipv6Private := net.ParseIP("fc00::")
ipv6Public := net.ParseIP("fe00::")
ipv4Private := net.ParseIP("10.255.0.0")
ipv4Public := net.ParseIP("11.0.0.0")
fmt.Println(ipv6Private.IsPrivate())
fmt.Println(ipv6Public.IsPrivate())
fmt.Println(ipv4Private.IsPrivate())
fmt.Println(ipv4Public.IsPrivate())
```
Output:
```
true
false
true
false
```
### func (IP) IsUnspecified
```
func (ip IP) IsUnspecified() bool
```
IsUnspecified reports whether ip is an unspecified address, either the IPv4 address "0.0.0.0" or the IPv6 address "::".
#### Example
Code:
```
ipv6Unspecified := net.ParseIP("::")
ipv6Specified := net.ParseIP("fe00::")
ipv4Unspecified := net.ParseIP("0.0.0.0")
ipv4Specified := net.ParseIP("8.8.8.8")
fmt.Println(ipv6Unspecified.IsUnspecified())
fmt.Println(ipv6Specified.IsUnspecified())
fmt.Println(ipv4Unspecified.IsUnspecified())
fmt.Println(ipv4Specified.IsUnspecified())
```
Output:
```
true
false
true
false
```
### func (IP) MarshalText 1.2
```
func (ip IP) MarshalText() ([]byte, error)
```
MarshalText implements the encoding.TextMarshaler interface. The encoding is the same as returned by String, with one exception: When len(ip) is zero, it returns an empty slice.
### func (IP) Mask
```
func (ip IP) Mask(mask IPMask) IP
```
Mask returns the result of masking the IP address ip with mask.
#### Example
Code:
```
ipv4Addr := net.ParseIP("192.0.2.1")
// This mask corresponds to a /24 subnet for IPv4.
ipv4Mask := net.CIDRMask(24, 32)
fmt.Println(ipv4Addr.Mask(ipv4Mask))
ipv6Addr := net.ParseIP("2001:db8:a0b:12f0::1")
// This mask corresponds to a /32 subnet for IPv6.
ipv6Mask := net.CIDRMask(32, 128)
fmt.Println(ipv6Addr.Mask(ipv6Mask))
```
Output:
```
192.0.2.0
2001:db8::
```
### func (IP) String
```
func (ip IP) String() string
```
String returns the string form of the IP address ip. It returns one of 4 forms:
* "<nil>", if ip has length 0
* dotted decimal ("192.0.2.1"), if ip is an IPv4 or IP4-mapped IPv6 address
* IPv6 conforming to RFC 5952 ("2001:db8::1"), if ip is a valid IPv6 address
* the hexadecimal form of ip, without punctuation, if no other cases apply
#### Example
Code:
```
ipv6 := net.IP{0xfc, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
ipv4 := net.IPv4(10, 255, 0, 0)
fmt.Println(ipv6.String())
fmt.Println(ipv4.String())
```
Output:
```
fc00::
10.255.0.0
```
### func (IP) To16
```
func (ip IP) To16() IP
```
To16 converts the IP address ip to a 16-byte representation. If ip is not an IP address (it is the wrong length), To16 returns nil.
#### Example
Code:
```
ipv6 := net.IP{0xfc, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
ipv4 := net.IPv4(10, 255, 0, 0)
fmt.Println(ipv6.To16())
fmt.Println(ipv4.To16())
```
Output:
```
fc00::
10.255.0.0
```
### func (IP) To4
```
func (ip IP) To4() IP
```
To4 converts the IPv4 address ip to a 4-byte representation. If ip is not an IPv4 address, To4 returns nil.
### func (\*IP) UnmarshalText 1.2
```
func (ip *IP) UnmarshalText(text []byte) error
```
UnmarshalText implements the encoding.TextUnmarshaler interface. The IP address is expected in a form accepted by ParseIP.
type IPAddr
-----------
IPAddr represents the address of an IP end point.
```
type IPAddr struct {
IP IP
Zone string // IPv6 scoped addressing zone; added in Go 1.1
}
```
### func ResolveIPAddr
```
func ResolveIPAddr(network, address string) (*IPAddr, error)
```
ResolveIPAddr returns an address of IP end point.
The network must be an IP network name.
If the host in the address parameter is not a literal IP address, ResolveIPAddr resolves the address to an address of IP end point. Otherwise, it parses the address as a literal IP address. The address parameter can use a host name, but this is not recommended, because it will return at most one of the host name's IP addresses.
See func Dial for a description of the network and address parameters.
### func (\*IPAddr) Network
```
func (a *IPAddr) Network() string
```
Network returns the address's network name, "ip".
### func (\*IPAddr) String
```
func (a *IPAddr) String() string
```
type IPConn
-----------
IPConn is the implementation of the Conn and PacketConn interfaces for IP network connections.
```
type IPConn struct {
// contains filtered or unexported fields
}
```
### func DialIP
```
func DialIP(network string, laddr, raddr *IPAddr) (*IPConn, error)
```
DialIP acts like Dial for IP networks.
The network must be an IP network name; see func Dial for details.
If laddr is nil, a local address is automatically chosen. If the IP field of raddr is nil or an unspecified IP address, the local system is assumed.
### func ListenIP
```
func ListenIP(network string, laddr *IPAddr) (*IPConn, error)
```
ListenIP acts like ListenPacket for IP networks.
The network must be an IP network name; see func Dial for details.
If the IP field of laddr is nil or an unspecified IP address, ListenIP listens on all available IP addresses of the local system except multicast IP addresses.
### func (\*IPConn) Close
```
func (c *IPConn) Close() error
```
Close closes the connection.
### func (\*IPConn) File
```
func (c *IPConn) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*IPConn) LocalAddr
```
func (c *IPConn) LocalAddr() Addr
```
LocalAddr returns the local network address. The Addr returned is shared by all invocations of LocalAddr, so do not modify it.
### func (\*IPConn) Read
```
func (c *IPConn) Read(b []byte) (int, error)
```
Read implements the Conn Read method.
### func (\*IPConn) ReadFrom
```
func (c *IPConn) ReadFrom(b []byte) (int, Addr, error)
```
ReadFrom implements the PacketConn ReadFrom method.
### func (\*IPConn) ReadFromIP
```
func (c *IPConn) ReadFromIP(b []byte) (int, *IPAddr, error)
```
ReadFromIP acts like ReadFrom but returns an IPAddr.
### func (\*IPConn) ReadMsgIP 1.1
```
func (c *IPConn) ReadMsgIP(b, oob []byte) (n, oobn, flags int, addr *IPAddr, err error)
```
ReadMsgIP reads a message from c, copying the payload into b and the associated out-of-band data into oob. It returns the number of bytes copied into b, the number of bytes copied into oob, the flags that were set on the message and the source address of the message.
The packages golang.org/x/net/ipv4 and golang.org/x/net/ipv6 can be used to manipulate IP-level socket options in oob.
### func (\*IPConn) RemoteAddr
```
func (c *IPConn) RemoteAddr() Addr
```
RemoteAddr returns the remote network address. The Addr returned is shared by all invocations of RemoteAddr, so do not modify it.
### func (\*IPConn) SetDeadline
```
func (c *IPConn) SetDeadline(t time.Time) error
```
SetDeadline implements the Conn SetDeadline method.
### func (\*IPConn) SetReadBuffer
```
func (c *IPConn) SetReadBuffer(bytes int) error
```
SetReadBuffer sets the size of the operating system's receive buffer associated with the connection.
### func (\*IPConn) SetReadDeadline
```
func (c *IPConn) SetReadDeadline(t time.Time) error
```
SetReadDeadline implements the Conn SetReadDeadline method.
### func (\*IPConn) SetWriteBuffer
```
func (c *IPConn) SetWriteBuffer(bytes int) error
```
SetWriteBuffer sets the size of the operating system's transmit buffer associated with the connection.
### func (\*IPConn) SetWriteDeadline
```
func (c *IPConn) SetWriteDeadline(t time.Time) error
```
SetWriteDeadline implements the Conn SetWriteDeadline method.
### func (\*IPConn) SyscallConn 1.9
```
func (c *IPConn) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
### func (\*IPConn) Write
```
func (c *IPConn) Write(b []byte) (int, error)
```
Write implements the Conn Write method.
### func (\*IPConn) WriteMsgIP 1.1
```
func (c *IPConn) WriteMsgIP(b, oob []byte, addr *IPAddr) (n, oobn int, err error)
```
WriteMsgIP writes a message to addr via c, copying the payload from b and the associated out-of-band data from oob. It returns the number of payload and out-of-band bytes written.
The packages golang.org/x/net/ipv4 and golang.org/x/net/ipv6 can be used to manipulate IP-level socket options in oob.
### func (\*IPConn) WriteTo
```
func (c *IPConn) WriteTo(b []byte, addr Addr) (int, error)
```
WriteTo implements the PacketConn WriteTo method.
### func (\*IPConn) WriteToIP
```
func (c *IPConn) WriteToIP(b []byte, addr *IPAddr) (int, error)
```
WriteToIP acts like WriteTo but takes an IPAddr.
type IPMask
-----------
An IPMask is a bitmask that can be used to manipulate IP addresses for IP addressing and routing.
See type IPNet and func ParseCIDR for details.
```
type IPMask []byte
```
### func CIDRMask
```
func CIDRMask(ones, bits int) IPMask
```
CIDRMask returns an IPMask consisting of 'ones' 1 bits followed by 0s up to a total length of 'bits' bits. For a mask of this form, CIDRMask is the inverse of IPMask.Size.
#### Example
Code:
```
// This mask corresponds to a /31 subnet for IPv4.
fmt.Println(net.CIDRMask(31, 32))
// This mask corresponds to a /64 subnet for IPv6.
fmt.Println(net.CIDRMask(64, 128))
```
Output:
```
fffffffe
ffffffffffffffff0000000000000000
```
### func IPv4Mask
```
func IPv4Mask(a, b, c, d byte) IPMask
```
IPv4Mask returns the IP mask (in 4-byte form) of the IPv4 mask a.b.c.d.
#### Example
Code:
```
fmt.Println(net.IPv4Mask(255, 255, 255, 0))
```
Output:
```
ffffff00
```
### func (IPMask) Size
```
func (m IPMask) Size() (ones, bits int)
```
Size returns the number of leading ones and total bits in the mask. If the mask is not in the canonical form--ones followed by zeros--then Size returns 0, 0.
### func (IPMask) String
```
func (m IPMask) String() string
```
String returns the hexadecimal form of m, with no punctuation.
type IPNet
----------
An IPNet represents an IP network.
```
type IPNet struct {
IP IP // network number
Mask IPMask // network mask
}
```
### func (\*IPNet) Contains
```
func (n *IPNet) Contains(ip IP) bool
```
Contains reports whether the network includes ip.
### func (\*IPNet) Network
```
func (n *IPNet) Network() string
```
Network returns the address's network name, "ip+net".
### func (\*IPNet) String
```
func (n *IPNet) String() string
```
String returns the CIDR notation of n like "192.0.2.0/24" or "2001:db8::/48" as defined in RFC 4632 and RFC 4291. If the mask is not in the canonical form, it returns the string which consists of an IP address, followed by a slash character and a mask expressed as hexadecimal form with no punctuation like "198.51.100.0/c000ff00".
type Interface
--------------
Interface represents a mapping between network interface name and index. It also represents network interface facility information.
```
type Interface struct {
Index int // positive integer that starts at one, zero is never used
MTU int // maximum transmission unit
Name string // e.g., "en0", "lo0", "eth0.100"
HardwareAddr HardwareAddr // IEEE MAC-48, EUI-48 and EUI-64 form
Flags Flags // e.g., FlagUp, FlagLoopback, FlagMulticast
}
```
### func InterfaceByIndex
```
func InterfaceByIndex(index int) (*Interface, error)
```
InterfaceByIndex returns the interface specified by index.
On Solaris, it returns one of the logical network interfaces sharing the logical data link; for more precision use InterfaceByName.
### func InterfaceByName
```
func InterfaceByName(name string) (*Interface, error)
```
InterfaceByName returns the interface specified by name.
### func Interfaces
```
func Interfaces() ([]Interface, error)
```
Interfaces returns a list of the system's network interfaces.
### func (\*Interface) Addrs
```
func (ifi *Interface) Addrs() ([]Addr, error)
```
Addrs returns a list of unicast interface addresses for a specific interface.
### func (\*Interface) MulticastAddrs
```
func (ifi *Interface) MulticastAddrs() ([]Addr, error)
```
MulticastAddrs returns a list of multicast, joined group addresses for a specific interface.
type InvalidAddrError
---------------------
```
type InvalidAddrError string
```
### func (InvalidAddrError) Error
```
func (e InvalidAddrError) Error() string
```
### func (InvalidAddrError) Temporary
```
func (e InvalidAddrError) Temporary() bool
```
### func (InvalidAddrError) Timeout
```
func (e InvalidAddrError) Timeout() bool
```
type ListenConfig 1.11
----------------------
ListenConfig contains options for listening to an address.
```
type ListenConfig struct {
// If Control is not nil, it is called after creating the network
// connection but before binding it to the operating system.
//
// Network and address parameters passed to Control method are not
// necessarily the ones passed to Listen. For example, passing "tcp" to
// Listen will cause the Control function to be called with "tcp4" or "tcp6".
Control func(network, address string, c syscall.RawConn) error
// KeepAlive specifies the keep-alive period for network
// connections accepted by this listener.
// If zero, keep-alives are enabled if supported by the protocol
// and operating system. Network protocols or operating systems
// that do not support keep-alives ignore this field.
// If negative, keep-alives are disabled.
KeepAlive time.Duration // Go 1.13
}
```
### func (\*ListenConfig) Listen 1.11
```
func (lc *ListenConfig) Listen(ctx context.Context, network, address string) (Listener, error)
```
Listen announces on the local network address.
See func Listen for a description of the network and address parameters.
### func (\*ListenConfig) ListenPacket 1.11
```
func (lc *ListenConfig) ListenPacket(ctx context.Context, network, address string) (PacketConn, error)
```
ListenPacket announces on the local network address.
See func ListenPacket for a description of the network and address parameters.
type Listener
-------------
A Listener is a generic network listener for stream-oriented protocols.
Multiple goroutines may invoke methods on a Listener simultaneously.
```
type Listener interface {
// Accept waits for and returns the next connection to the listener.
Accept() (Conn, error)
// Close closes the listener.
// Any blocked Accept operations will be unblocked and return errors.
Close() error
// Addr returns the listener's network address.
Addr() Addr
}
```
#### Example
Code:
```
// Listen on TCP port 2000 on all available unicast and
// anycast IP addresses of the local system.
l, err := net.Listen("tcp", ":2000")
if err != nil {
log.Fatal(err)
}
defer l.Close()
for {
// Wait for a connection.
conn, err := l.Accept()
if err != nil {
log.Fatal(err)
}
// Handle the connection in a new goroutine.
// The loop then returns to accepting, so that
// multiple connections may be served concurrently.
go func(c net.Conn) {
// Echo all incoming data.
io.Copy(c, c)
// Shut down the connection.
c.Close()
}(conn)
}
```
### func FileListener
```
func FileListener(f *os.File) (ln Listener, err error)
```
FileListener returns a copy of the network listener corresponding to the open file f. It is the caller's responsibility to close ln when finished. Closing ln does not affect f, and closing f does not affect ln.
### func Listen
```
func Listen(network, address string) (Listener, error)
```
Listen announces on the local network address.
The network must be "tcp", "tcp4", "tcp6", "unix" or "unixpacket".
For TCP networks, if the host in the address parameter is empty or a literal unspecified IP address, Listen listens on all available unicast and anycast IP addresses of the local system. To only use IPv4, use network "tcp4". The address can use a host name, but this is not recommended, because it will create a listener for at most one of the host's IP addresses. If the port in the address parameter is empty or "0", as in "127.0.0.1:" or "[::1]:0", a port number is automatically chosen. The Addr method of Listener can be used to discover the chosen port.
See func Dial for a description of the network and address parameters.
Listen uses context.Background internally; to specify the context, use ListenConfig.Listen.
type MX
-------
An MX represents a single DNS MX record.
```
type MX struct {
Host string
Pref uint16
}
```
### func LookupMX
```
func LookupMX(name string) ([]*MX, error)
```
LookupMX returns the DNS MX records for the given domain name sorted by preference.
The returned mail server names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
LookupMX uses context.Background internally; to specify the context, use Resolver.LookupMX.
type NS 1.1
-----------
An NS represents a single DNS NS record.
```
type NS struct {
Host string
}
```
### func LookupNS 1.1
```
func LookupNS(name string) ([]*NS, error)
```
LookupNS returns the DNS NS records for the given domain name.
The returned name server names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
LookupNS uses context.Background internally; to specify the context, use Resolver.LookupNS.
type OpError
------------
OpError is the error type usually returned by functions in the net package. It describes the operation, network type, and address of an error.
```
type OpError struct {
// Op is the operation which caused the error, such as
// "read" or "write".
Op string
// Net is the network type on which this error occurred,
// such as "tcp" or "udp6".
Net string
// For operations involving a remote network connection, like
// Dial, Read, or Write, Source is the corresponding local
// network address.
Source Addr // Go 1.5
// Addr is the network address for which this error occurred.
// For local operations, like Listen or SetDeadline, Addr is
// the address of the local endpoint being manipulated.
// For operations involving a remote network connection, like
// Dial, Read, or Write, Addr is the remote address of that
// connection.
Addr Addr
// Err is the error that occurred during the operation.
// The Error method panics if the error is nil.
Err error
}
```
### func (\*OpError) Error
```
func (e *OpError) Error() string
```
### func (\*OpError) Temporary
```
func (e *OpError) Temporary() bool
```
### func (\*OpError) Timeout
```
func (e *OpError) Timeout() bool
```
### func (\*OpError) Unwrap 1.13
```
func (e *OpError) Unwrap() error
```
type PacketConn
---------------
PacketConn is a generic packet-oriented network connection.
Multiple goroutines may invoke methods on a PacketConn simultaneously.
```
type PacketConn interface {
// ReadFrom reads a packet from the connection,
// copying the payload into p. It returns the number of
// bytes copied into p and the return address that
// was on the packet.
// It returns the number of bytes read (0 <= n <= len(p))
// and any error encountered. Callers should always process
// the n > 0 bytes returned before considering the error err.
// ReadFrom can be made to time out and return an error after a
// fixed time limit; see SetDeadline and SetReadDeadline.
ReadFrom(p []byte) (n int, addr Addr, err error)
// WriteTo writes a packet with payload p to addr.
// WriteTo can be made to time out and return an Error after a
// fixed time limit; see SetDeadline and SetWriteDeadline.
// On packet-oriented connections, write timeouts are rare.
WriteTo(p []byte, addr Addr) (n int, err error)
// Close closes the connection.
// Any blocked ReadFrom or WriteTo operations will be unblocked and return errors.
Close() error
// LocalAddr returns the local network address, if known.
LocalAddr() Addr
// SetDeadline sets the read and write deadlines associated
// with the connection. It is equivalent to calling both
// SetReadDeadline and SetWriteDeadline.
//
// A deadline is an absolute time after which I/O operations
// fail instead of blocking. The deadline applies to all future
// and pending I/O, not just the immediately following call to
// Read or Write. After a deadline has been exceeded, the
// connection can be refreshed by setting a deadline in the future.
//
// If the deadline is exceeded a call to Read or Write or to other
// I/O methods will return an error that wraps os.ErrDeadlineExceeded.
// This can be tested using errors.Is(err, os.ErrDeadlineExceeded).
// The error's Timeout method will return true, but note that there
// are other possible errors for which the Timeout method will
// return true even if the deadline has not been exceeded.
//
// An idle timeout can be implemented by repeatedly extending
// the deadline after successful ReadFrom or WriteTo calls.
//
// A zero value for t means I/O operations will not time out.
SetDeadline(t time.Time) error
// SetReadDeadline sets the deadline for future ReadFrom calls
// and any currently-blocked ReadFrom call.
// A zero value for t means ReadFrom will not time out.
SetReadDeadline(t time.Time) error
// SetWriteDeadline sets the deadline for future WriteTo calls
// and any currently-blocked WriteTo call.
// Even if write times out, it may return n > 0, indicating that
// some of the data was successfully written.
// A zero value for t means WriteTo will not time out.
SetWriteDeadline(t time.Time) error
}
```
### func FilePacketConn
```
func FilePacketConn(f *os.File) (c PacketConn, err error)
```
FilePacketConn returns a copy of the packet network connection corresponding to the open file f. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
### func ListenPacket
```
func ListenPacket(network, address string) (PacketConn, error)
```
ListenPacket announces on the local network address.
The network must be "udp", "udp4", "udp6", "unixgram", or an IP transport. The IP transports are "ip", "ip4", or "ip6" followed by a colon and a literal protocol number or a protocol name, as in "ip:1" or "ip:icmp".
For UDP and IP networks, if the host in the address parameter is empty or a literal unspecified IP address, ListenPacket listens on all available IP addresses of the local system except multicast IP addresses. To only use IPv4, use network "udp4" or "ip4:proto". The address can use a host name, but this is not recommended, because it will create a listener for at most one of the host's IP addresses. If the port in the address parameter is empty or "0", as in "127.0.0.1:" or "[::1]:0", a port number is automatically chosen. The LocalAddr method of PacketConn can be used to discover the chosen port.
See func Dial for a description of the network and address parameters.
ListenPacket uses context.Background internally; to specify the context, use ListenConfig.ListenPacket.
type ParseError
---------------
A ParseError is the error type of literal network address parsers.
```
type ParseError struct {
// Type is the type of string that was expected, such as
// "IP address", "CIDR address".
Type string
// Text is the malformed text string.
Text string
}
```
### func (\*ParseError) Error
```
func (e *ParseError) Error() string
```
### func (\*ParseError) Temporary 1.17
```
func (e *ParseError) Temporary() bool
```
### func (\*ParseError) Timeout 1.17
```
func (e *ParseError) Timeout() bool
```
type Resolver 1.8
-----------------
A Resolver looks up names and numbers.
A nil \*Resolver is equivalent to a zero Resolver.
```
type Resolver struct {
// PreferGo controls whether Go's built-in DNS resolver is preferred
// on platforms where it's available. It is equivalent to setting
// GODEBUG=netdns=go, but scoped to just this resolver.
PreferGo bool
// StrictErrors controls the behavior of temporary errors
// (including timeout, socket errors, and SERVFAIL) when using
// Go's built-in resolver. For a query composed of multiple
// sub-queries (such as an A+AAAA address lookup, or walking the
// DNS search list), this option causes such errors to abort the
// whole query instead of returning a partial result. This is
// not enabled by default because it may affect compatibility
// with resolvers that process AAAA queries incorrectly.
StrictErrors bool // Go 1.9
// Dial optionally specifies an alternate dialer for use by
// Go's built-in DNS resolver to make TCP and UDP connections
// to DNS services. The host in the address parameter will
// always be a literal IP address and not a host name, and the
// port in the address parameter will be a literal port number
// and not a service name.
// If the Conn returned is also a PacketConn, sent and received DNS
// messages must adhere to RFC 1035 section 4.2.1, "UDP usage".
// Otherwise, DNS messages transmitted over Conn must adhere
// to RFC 7766 section 5, "Transport Protocol Selection".
// If nil, the default dialer is used.
Dial func(ctx context.Context, network, address string) (Conn, error) // Go 1.9
// contains filtered or unexported fields
}
```
### func (\*Resolver) LookupAddr 1.8
```
func (r *Resolver) LookupAddr(ctx context.Context, addr string) ([]string, error)
```
LookupAddr performs a reverse lookup for the given address, returning a list of names mapping to that address.
The returned names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
### func (\*Resolver) LookupCNAME 1.8
```
func (r *Resolver) LookupCNAME(ctx context.Context, host string) (string, error)
```
LookupCNAME returns the canonical name for the given host. Callers that do not care about the canonical name can call LookupHost or LookupIP directly; both take care of resolving the canonical name as part of the lookup.
A canonical name is the final name after following zero or more CNAME records. LookupCNAME does not return an error if host does not contain DNS "CNAME" records, as long as host resolves to address records.
The returned canonical name is validated to be a properly formatted presentation-format domain name.
### func (\*Resolver) LookupHost 1.8
```
func (r *Resolver) LookupHost(ctx context.Context, host string) (addrs []string, err error)
```
LookupHost looks up the given host using the local resolver. It returns a slice of that host's addresses.
### func (\*Resolver) LookupIP 1.15
```
func (r *Resolver) LookupIP(ctx context.Context, network, host string) ([]IP, error)
```
LookupIP looks up host for the given network using the local resolver. It returns a slice of that host's IP addresses of the type specified by network. network must be one of "ip", "ip4" or "ip6".
### func (\*Resolver) LookupIPAddr 1.8
```
func (r *Resolver) LookupIPAddr(ctx context.Context, host string) ([]IPAddr, error)
```
LookupIPAddr looks up host using the local resolver. It returns a slice of that host's IPv4 and IPv6 addresses.
### func (\*Resolver) LookupMX 1.8
```
func (r *Resolver) LookupMX(ctx context.Context, name string) ([]*MX, error)
```
LookupMX returns the DNS MX records for the given domain name sorted by preference.
The returned mail server names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
### func (\*Resolver) LookupNS 1.8
```
func (r *Resolver) LookupNS(ctx context.Context, name string) ([]*NS, error)
```
LookupNS returns the DNS NS records for the given domain name.
The returned name server names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
### func (\*Resolver) LookupNetIP 1.18
```
func (r *Resolver) LookupNetIP(ctx context.Context, network, host string) ([]netip.Addr, error)
```
LookupNetIP looks up host using the local resolver. It returns a slice of that host's IP addresses of the type specified by network. The network must be one of "ip", "ip4" or "ip6".
### func (\*Resolver) LookupPort 1.8
```
func (r *Resolver) LookupPort(ctx context.Context, network, service string) (port int, err error)
```
LookupPort looks up the port for the given network and service.
### func (\*Resolver) LookupSRV 1.8
```
func (r *Resolver) LookupSRV(ctx context.Context, service, proto, name string) (string, []*SRV, error)
```
LookupSRV tries to resolve an SRV query of the given service, protocol, and domain name. The proto is "tcp" or "udp". The returned records are sorted by priority and randomized by weight within a priority.
LookupSRV constructs the DNS name to look up following RFC 2782. That is, it looks up \_service.\_proto.name. To accommodate services publishing SRV records under non-standard names, if both service and proto are empty strings, LookupSRV looks up name directly.
The returned service names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
### func (\*Resolver) LookupTXT 1.8
```
func (r *Resolver) LookupTXT(ctx context.Context, name string) ([]string, error)
```
LookupTXT returns the DNS TXT records for the given domain name.
type SRV
--------
An SRV represents a single DNS SRV record.
```
type SRV struct {
Target string
Port uint16
Priority uint16
Weight uint16
}
```
### func LookupSRV
```
func LookupSRV(service, proto, name string) (cname string, addrs []*SRV, err error)
```
LookupSRV tries to resolve an SRV query of the given service, protocol, and domain name. The proto is "tcp" or "udp". The returned records are sorted by priority and randomized by weight within a priority.
LookupSRV constructs the DNS name to look up following RFC 2782. That is, it looks up \_service.\_proto.name. To accommodate services publishing SRV records under non-standard names, if both service and proto are empty strings, LookupSRV looks up name directly.
The returned service names are validated to be properly formatted presentation-format domain names. If the response contains invalid names, those records are filtered out and an error will be returned alongside the remaining results, if any.
type TCPAddr
------------
TCPAddr represents the address of a TCP end point.
```
type TCPAddr struct {
IP IP
Port int
Zone string // IPv6 scoped addressing zone; added in Go 1.1
}
```
### func ResolveTCPAddr
```
func ResolveTCPAddr(network, address string) (*TCPAddr, error)
```
ResolveTCPAddr returns an address of TCP end point.
The network must be a TCP network name.
If the host in the address parameter is not a literal IP address or the port is not a literal port number, ResolveTCPAddr resolves the address to an address of TCP end point. Otherwise, it parses the address as a pair of literal IP address and port number. The address parameter can use a host name, but this is not recommended, because it will return at most one of the host name's IP addresses.
See func Dial for a description of the network and address parameters.
### func TCPAddrFromAddrPort 1.18
```
func TCPAddrFromAddrPort(addr netip.AddrPort) *TCPAddr
```
TCPAddrFromAddrPort returns addr as a TCPAddr. If addr.IsValid() is false, then the returned TCPAddr will contain a nil IP field, indicating an address family-agnostic unspecified address.
### func (\*TCPAddr) AddrPort 1.18
```
func (a *TCPAddr) AddrPort() netip.AddrPort
```
AddrPort returns the TCPAddr a as a netip.AddrPort.
If a.Port does not fit in a uint16, it's silently truncated.
If a is nil, a zero value is returned.
### func (\*TCPAddr) Network
```
func (a *TCPAddr) Network() string
```
Network returns the address's network name, "tcp".
### func (\*TCPAddr) String
```
func (a *TCPAddr) String() string
```
type TCPConn
------------
TCPConn is an implementation of the Conn interface for TCP network connections.
```
type TCPConn struct {
// contains filtered or unexported fields
}
```
### func DialTCP
```
func DialTCP(network string, laddr, raddr *TCPAddr) (*TCPConn, error)
```
DialTCP acts like Dial for TCP networks.
The network must be a TCP network name; see func Dial for details.
If laddr is nil, a local address is automatically chosen. If the IP field of raddr is nil or an unspecified IP address, the local system is assumed.
### func (\*TCPConn) Close
```
func (c *TCPConn) Close() error
```
Close closes the connection.
### func (\*TCPConn) CloseRead
```
func (c *TCPConn) CloseRead() error
```
CloseRead shuts down the reading side of the TCP connection. Most callers should just use Close.
### func (\*TCPConn) CloseWrite
```
func (c *TCPConn) CloseWrite() error
```
CloseWrite shuts down the writing side of the TCP connection. Most callers should just use Close.
### func (\*TCPConn) File
```
func (c *TCPConn) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*TCPConn) LocalAddr
```
func (c *TCPConn) LocalAddr() Addr
```
LocalAddr returns the local network address. The Addr returned is shared by all invocations of LocalAddr, so do not modify it.
### func (\*TCPConn) Read
```
func (c *TCPConn) Read(b []byte) (int, error)
```
Read implements the Conn Read method.
### func (\*TCPConn) ReadFrom
```
func (c *TCPConn) ReadFrom(r io.Reader) (int64, error)
```
ReadFrom implements the io.ReaderFrom ReadFrom method.
### func (\*TCPConn) RemoteAddr
```
func (c *TCPConn) RemoteAddr() Addr
```
RemoteAddr returns the remote network address. The Addr returned is shared by all invocations of RemoteAddr, so do not modify it.
### func (\*TCPConn) SetDeadline
```
func (c *TCPConn) SetDeadline(t time.Time) error
```
SetDeadline implements the Conn SetDeadline method.
### func (\*TCPConn) SetKeepAlive
```
func (c *TCPConn) SetKeepAlive(keepalive bool) error
```
SetKeepAlive sets whether the operating system should send keep-alive messages on the connection.
### func (\*TCPConn) SetKeepAlivePeriod 1.2
```
func (c *TCPConn) SetKeepAlivePeriod(d time.Duration) error
```
SetKeepAlivePeriod sets period between keep-alives.
### func (\*TCPConn) SetLinger
```
func (c *TCPConn) SetLinger(sec int) error
```
SetLinger sets the behavior of Close on a connection which still has data waiting to be sent or to be acknowledged.
If sec < 0 (the default), the operating system finishes sending the data in the background.
If sec == 0, the operating system discards any unsent or unacknowledged data.
If sec > 0, the data is sent in the background as with sec < 0. On some operating systems after sec seconds have elapsed any remaining unsent data may be discarded.
### func (\*TCPConn) SetNoDelay
```
func (c *TCPConn) SetNoDelay(noDelay bool) error
```
SetNoDelay controls whether the operating system should delay packet transmission in hopes of sending fewer packets (Nagle's algorithm). The default is true (no delay), meaning that data is sent as soon as possible after a Write.
### func (\*TCPConn) SetReadBuffer
```
func (c *TCPConn) SetReadBuffer(bytes int) error
```
SetReadBuffer sets the size of the operating system's receive buffer associated with the connection.
### func (\*TCPConn) SetReadDeadline
```
func (c *TCPConn) SetReadDeadline(t time.Time) error
```
SetReadDeadline implements the Conn SetReadDeadline method.
### func (\*TCPConn) SetWriteBuffer
```
func (c *TCPConn) SetWriteBuffer(bytes int) error
```
SetWriteBuffer sets the size of the operating system's transmit buffer associated with the connection.
### func (\*TCPConn) SetWriteDeadline
```
func (c *TCPConn) SetWriteDeadline(t time.Time) error
```
SetWriteDeadline implements the Conn SetWriteDeadline method.
### func (\*TCPConn) SyscallConn 1.9
```
func (c *TCPConn) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
### func (\*TCPConn) Write
```
func (c *TCPConn) Write(b []byte) (int, error)
```
Write implements the Conn Write method.
type TCPListener
----------------
TCPListener is a TCP network listener. Clients should typically use variables of type Listener instead of assuming TCP.
```
type TCPListener struct {
// contains filtered or unexported fields
}
```
### func ListenTCP
```
func ListenTCP(network string, laddr *TCPAddr) (*TCPListener, error)
```
ListenTCP acts like Listen for TCP networks.
The network must be a TCP network name; see func Dial for details.
If the IP field of laddr is nil or an unspecified IP address, ListenTCP listens on all available unicast and anycast IP addresses of the local system. If the Port field of laddr is 0, a port number is automatically chosen.
### func (\*TCPListener) Accept
```
func (l *TCPListener) Accept() (Conn, error)
```
Accept implements the Accept method in the Listener interface; it waits for the next call and returns a generic Conn.
### func (\*TCPListener) AcceptTCP
```
func (l *TCPListener) AcceptTCP() (*TCPConn, error)
```
AcceptTCP accepts the next incoming call and returns the new connection.
### func (\*TCPListener) Addr
```
func (l *TCPListener) Addr() Addr
```
Addr returns the listener's network address, a \*TCPAddr. The Addr returned is shared by all invocations of Addr, so do not modify it.
### func (\*TCPListener) Close
```
func (l *TCPListener) Close() error
```
Close stops listening on the TCP address. Already Accepted connections are not closed.
### func (\*TCPListener) File
```
func (l *TCPListener) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing l does not affect f, and closing f does not affect l.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*TCPListener) SetDeadline
```
func (l *TCPListener) SetDeadline(t time.Time) error
```
SetDeadline sets the deadline associated with the listener. A zero time value disables the deadline.
### func (\*TCPListener) SyscallConn 1.10
```
func (l *TCPListener) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
The returned RawConn only supports calling Control. Read and Write return an error.
type UDPAddr
------------
UDPAddr represents the address of a UDP end point.
```
type UDPAddr struct {
IP IP
Port int
Zone string // IPv6 scoped addressing zone; added in Go 1.1
}
```
### func ResolveUDPAddr
```
func ResolveUDPAddr(network, address string) (*UDPAddr, error)
```
ResolveUDPAddr returns an address of UDP end point.
The network must be a UDP network name.
If the host in the address parameter is not a literal IP address or the port is not a literal port number, ResolveUDPAddr resolves the address to an address of UDP end point. Otherwise, it parses the address as a pair of literal IP address and port number. The address parameter can use a host name, but this is not recommended, because it will return at most one of the host name's IP addresses.
See func Dial for a description of the network and address parameters.
### func UDPAddrFromAddrPort 1.18
```
func UDPAddrFromAddrPort(addr netip.AddrPort) *UDPAddr
```
UDPAddrFromAddrPort returns addr as a UDPAddr. If addr.IsValid() is false, then the returned UDPAddr will contain a nil IP field, indicating an address family-agnostic unspecified address.
### func (\*UDPAddr) AddrPort 1.18
```
func (a *UDPAddr) AddrPort() netip.AddrPort
```
AddrPort returns the UDPAddr a as a netip.AddrPort.
If a.Port does not fit in a uint16, it's silently truncated.
If a is nil, a zero value is returned.
### func (\*UDPAddr) Network
```
func (a *UDPAddr) Network() string
```
Network returns the address's network name, "udp".
### func (\*UDPAddr) String
```
func (a *UDPAddr) String() string
```
type UDPConn
------------
UDPConn is the implementation of the Conn and PacketConn interfaces for UDP network connections.
```
type UDPConn struct {
// contains filtered or unexported fields
}
```
### func DialUDP
```
func DialUDP(network string, laddr, raddr *UDPAddr) (*UDPConn, error)
```
DialUDP acts like Dial for UDP networks.
The network must be a UDP network name; see func Dial for details.
If laddr is nil, a local address is automatically chosen. If the IP field of raddr is nil or an unspecified IP address, the local system is assumed.
### func ListenMulticastUDP
```
func ListenMulticastUDP(network string, ifi *Interface, gaddr *UDPAddr) (*UDPConn, error)
```
ListenMulticastUDP acts like ListenPacket for UDP networks but takes a group address on a specific network interface.
The network must be a UDP network name; see func Dial for details.
ListenMulticastUDP listens on all available IP addresses of the local system including the group, multicast IP address. If ifi is nil, ListenMulticastUDP uses the system-assigned multicast interface, although this is not recommended because the assignment depends on platforms and sometimes it might require routing configuration. If the Port field of gaddr is 0, a port number is automatically chosen.
ListenMulticastUDP is just for convenience of simple, small applications. There are golang.org/x/net/ipv4 and golang.org/x/net/ipv6 packages for general purpose uses.
Note that ListenMulticastUDP will set the IP\_MULTICAST\_LOOP socket option to 0 under IPPROTO\_IP, to disable loopback of multicast packets.
### func ListenUDP
```
func ListenUDP(network string, laddr *UDPAddr) (*UDPConn, error)
```
ListenUDP acts like ListenPacket for UDP networks.
The network must be a UDP network name; see func Dial for details.
If the IP field of laddr is nil or an unspecified IP address, ListenUDP listens on all available IP addresses of the local system except multicast IP addresses. If the Port field of laddr is 0, a port number is automatically chosen.
### func (\*UDPConn) Close
```
func (c *UDPConn) Close() error
```
Close closes the connection.
### func (\*UDPConn) File
```
func (c *UDPConn) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*UDPConn) LocalAddr
```
func (c *UDPConn) LocalAddr() Addr
```
LocalAddr returns the local network address. The Addr returned is shared by all invocations of LocalAddr, so do not modify it.
### func (\*UDPConn) Read
```
func (c *UDPConn) Read(b []byte) (int, error)
```
Read implements the Conn Read method.
### func (\*UDPConn) ReadFrom
```
func (c *UDPConn) ReadFrom(b []byte) (int, Addr, error)
```
ReadFrom implements the PacketConn ReadFrom method.
### func (\*UDPConn) ReadFromUDP
```
func (c *UDPConn) ReadFromUDP(b []byte) (n int, addr *UDPAddr, err error)
```
ReadFromUDP acts like ReadFrom but returns a UDPAddr.
### func (\*UDPConn) ReadFromUDPAddrPort 1.18
```
func (c *UDPConn) ReadFromUDPAddrPort(b []byte) (n int, addr netip.AddrPort, err error)
```
ReadFromUDPAddrPort acts like ReadFrom but returns a netip.AddrPort.
If c is bound to an unspecified address, the returned netip.AddrPort's address might be an IPv4-mapped IPv6 address. Use netip.Addr.Unmap to get the address without the IPv6 prefix.
### func (\*UDPConn) ReadMsgUDP 1.1
```
func (c *UDPConn) ReadMsgUDP(b, oob []byte) (n, oobn, flags int, addr *UDPAddr, err error)
```
ReadMsgUDP reads a message from c, copying the payload into b and the associated out-of-band data into oob. It returns the number of bytes copied into b, the number of bytes copied into oob, the flags that were set on the message and the source address of the message.
The packages golang.org/x/net/ipv4 and golang.org/x/net/ipv6 can be used to manipulate IP-level socket options in oob.
### func (\*UDPConn) ReadMsgUDPAddrPort 1.18
```
func (c *UDPConn) ReadMsgUDPAddrPort(b, oob []byte) (n, oobn, flags int, addr netip.AddrPort, err error)
```
ReadMsgUDPAddrPort is like ReadMsgUDP but returns an netip.AddrPort instead of a UDPAddr.
### func (\*UDPConn) RemoteAddr
```
func (c *UDPConn) RemoteAddr() Addr
```
RemoteAddr returns the remote network address. The Addr returned is shared by all invocations of RemoteAddr, so do not modify it.
### func (\*UDPConn) SetDeadline
```
func (c *UDPConn) SetDeadline(t time.Time) error
```
SetDeadline implements the Conn SetDeadline method.
### func (\*UDPConn) SetReadBuffer
```
func (c *UDPConn) SetReadBuffer(bytes int) error
```
SetReadBuffer sets the size of the operating system's receive buffer associated with the connection.
### func (\*UDPConn) SetReadDeadline
```
func (c *UDPConn) SetReadDeadline(t time.Time) error
```
SetReadDeadline implements the Conn SetReadDeadline method.
### func (\*UDPConn) SetWriteBuffer
```
func (c *UDPConn) SetWriteBuffer(bytes int) error
```
SetWriteBuffer sets the size of the operating system's transmit buffer associated with the connection.
### func (\*UDPConn) SetWriteDeadline
```
func (c *UDPConn) SetWriteDeadline(t time.Time) error
```
SetWriteDeadline implements the Conn SetWriteDeadline method.
### func (\*UDPConn) SyscallConn 1.9
```
func (c *UDPConn) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
### func (\*UDPConn) Write
```
func (c *UDPConn) Write(b []byte) (int, error)
```
Write implements the Conn Write method.
### func (\*UDPConn) WriteMsgUDP 1.1
```
func (c *UDPConn) WriteMsgUDP(b, oob []byte, addr *UDPAddr) (n, oobn int, err error)
```
WriteMsgUDP writes a message to addr via c if c isn't connected, or to c's remote address if c is connected (in which case addr must be nil). The payload is copied from b and the associated out-of-band data is copied from oob. It returns the number of payload and out-of-band bytes written.
The packages golang.org/x/net/ipv4 and golang.org/x/net/ipv6 can be used to manipulate IP-level socket options in oob.
### func (\*UDPConn) WriteMsgUDPAddrPort 1.18
```
func (c *UDPConn) WriteMsgUDPAddrPort(b, oob []byte, addr netip.AddrPort) (n, oobn int, err error)
```
WriteMsgUDPAddrPort is like WriteMsgUDP but takes a netip.AddrPort instead of a UDPAddr.
### func (\*UDPConn) WriteTo
```
func (c *UDPConn) WriteTo(b []byte, addr Addr) (int, error)
```
WriteTo implements the PacketConn WriteTo method.
#### Example
Code:
```
// Unlike Dial, ListenPacket creates a connection without any
// association with peers.
conn, err := net.ListenPacket("udp", ":0")
if err != nil {
log.Fatal(err)
}
defer conn.Close()
dst, err := net.ResolveUDPAddr("udp", "192.0.2.1:2000")
if err != nil {
log.Fatal(err)
}
// The connection can write data to the desired address.
_, err = conn.WriteTo([]byte("data"), dst)
if err != nil {
log.Fatal(err)
}
```
### func (\*UDPConn) WriteToUDP
```
func (c *UDPConn) WriteToUDP(b []byte, addr *UDPAddr) (int, error)
```
WriteToUDP acts like WriteTo but takes a UDPAddr.
### func (\*UDPConn) WriteToUDPAddrPort 1.18
```
func (c *UDPConn) WriteToUDPAddrPort(b []byte, addr netip.AddrPort) (int, error)
```
WriteToUDPAddrPort acts like WriteTo but takes a netip.AddrPort.
type UnixAddr
-------------
UnixAddr represents the address of a Unix domain socket end point.
```
type UnixAddr struct {
Name string
Net string
}
```
### func ResolveUnixAddr
```
func ResolveUnixAddr(network, address string) (*UnixAddr, error)
```
ResolveUnixAddr returns an address of Unix domain socket end point.
The network must be a Unix network name.
See func Dial for a description of the network and address parameters.
### func (\*UnixAddr) Network
```
func (a *UnixAddr) Network() string
```
Network returns the address's network name, "unix", "unixgram" or "unixpacket".
### func (\*UnixAddr) String
```
func (a *UnixAddr) String() string
```
type UnixConn
-------------
UnixConn is an implementation of the Conn interface for connections to Unix domain sockets.
```
type UnixConn struct {
// contains filtered or unexported fields
}
```
### func DialUnix
```
func DialUnix(network string, laddr, raddr *UnixAddr) (*UnixConn, error)
```
DialUnix acts like Dial for Unix networks.
The network must be a Unix network name; see func Dial for details.
If laddr is non-nil, it is used as the local address for the connection.
### func ListenUnixgram
```
func ListenUnixgram(network string, laddr *UnixAddr) (*UnixConn, error)
```
ListenUnixgram acts like ListenPacket for Unix networks.
The network must be "unixgram".
### func (\*UnixConn) Close
```
func (c *UnixConn) Close() error
```
Close closes the connection.
### func (\*UnixConn) CloseRead 1.1
```
func (c *UnixConn) CloseRead() error
```
CloseRead shuts down the reading side of the Unix domain connection. Most callers should just use Close.
### func (\*UnixConn) CloseWrite 1.1
```
func (c *UnixConn) CloseWrite() error
```
CloseWrite shuts down the writing side of the Unix domain connection. Most callers should just use Close.
### func (\*UnixConn) File
```
func (c *UnixConn) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing c does not affect f, and closing f does not affect c.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*UnixConn) LocalAddr
```
func (c *UnixConn) LocalAddr() Addr
```
LocalAddr returns the local network address. The Addr returned is shared by all invocations of LocalAddr, so do not modify it.
### func (\*UnixConn) Read
```
func (c *UnixConn) Read(b []byte) (int, error)
```
Read implements the Conn Read method.
### func (\*UnixConn) ReadFrom
```
func (c *UnixConn) ReadFrom(b []byte) (int, Addr, error)
```
ReadFrom implements the PacketConn ReadFrom method.
### func (\*UnixConn) ReadFromUnix
```
func (c *UnixConn) ReadFromUnix(b []byte) (int, *UnixAddr, error)
```
ReadFromUnix acts like ReadFrom but returns a UnixAddr.
### func (\*UnixConn) ReadMsgUnix
```
func (c *UnixConn) ReadMsgUnix(b, oob []byte) (n, oobn, flags int, addr *UnixAddr, err error)
```
ReadMsgUnix reads a message from c, copying the payload into b and the associated out-of-band data into oob. It returns the number of bytes copied into b, the number of bytes copied into oob, the flags that were set on the message and the source address of the message.
Note that if len(b) == 0 and len(oob) > 0, this function will still read (and discard) 1 byte from the connection.
### func (\*UnixConn) RemoteAddr
```
func (c *UnixConn) RemoteAddr() Addr
```
RemoteAddr returns the remote network address. The Addr returned is shared by all invocations of RemoteAddr, so do not modify it.
### func (\*UnixConn) SetDeadline
```
func (c *UnixConn) SetDeadline(t time.Time) error
```
SetDeadline implements the Conn SetDeadline method.
### func (\*UnixConn) SetReadBuffer
```
func (c *UnixConn) SetReadBuffer(bytes int) error
```
SetReadBuffer sets the size of the operating system's receive buffer associated with the connection.
### func (\*UnixConn) SetReadDeadline
```
func (c *UnixConn) SetReadDeadline(t time.Time) error
```
SetReadDeadline implements the Conn SetReadDeadline method.
### func (\*UnixConn) SetWriteBuffer
```
func (c *UnixConn) SetWriteBuffer(bytes int) error
```
SetWriteBuffer sets the size of the operating system's transmit buffer associated with the connection.
### func (\*UnixConn) SetWriteDeadline
```
func (c *UnixConn) SetWriteDeadline(t time.Time) error
```
SetWriteDeadline implements the Conn SetWriteDeadline method.
### func (\*UnixConn) SyscallConn 1.9
```
func (c *UnixConn) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
### func (\*UnixConn) Write
```
func (c *UnixConn) Write(b []byte) (int, error)
```
Write implements the Conn Write method.
### func (\*UnixConn) WriteMsgUnix
```
func (c *UnixConn) WriteMsgUnix(b, oob []byte, addr *UnixAddr) (n, oobn int, err error)
```
WriteMsgUnix writes a message to addr via c, copying the payload from b and the associated out-of-band data from oob. It returns the number of payload and out-of-band bytes written.
Note that if len(b) == 0 and len(oob) > 0, this function will still write 1 byte to the connection.
### func (\*UnixConn) WriteTo
```
func (c *UnixConn) WriteTo(b []byte, addr Addr) (int, error)
```
WriteTo implements the PacketConn WriteTo method.
### func (\*UnixConn) WriteToUnix
```
func (c *UnixConn) WriteToUnix(b []byte, addr *UnixAddr) (int, error)
```
WriteToUnix acts like WriteTo but takes a UnixAddr.
type UnixListener
-----------------
UnixListener is a Unix domain socket listener. Clients should typically use variables of type Listener instead of assuming Unix domain sockets.
```
type UnixListener struct {
// contains filtered or unexported fields
}
```
### func ListenUnix
```
func ListenUnix(network string, laddr *UnixAddr) (*UnixListener, error)
```
ListenUnix acts like Listen for Unix networks.
The network must be "unix" or "unixpacket".
### func (\*UnixListener) Accept
```
func (l *UnixListener) Accept() (Conn, error)
```
Accept implements the Accept method in the Listener interface. Returned connections will be of type \*UnixConn.
### func (\*UnixListener) AcceptUnix
```
func (l *UnixListener) AcceptUnix() (*UnixConn, error)
```
AcceptUnix accepts the next incoming call and returns the new connection.
### func (\*UnixListener) Addr
```
func (l *UnixListener) Addr() Addr
```
Addr returns the listener's network address. The Addr returned is shared by all invocations of Addr, so do not modify it.
### func (\*UnixListener) Close
```
func (l *UnixListener) Close() error
```
Close stops listening on the Unix address. Already accepted connections are not closed.
### func (\*UnixListener) File
```
func (l *UnixListener) File() (f *os.File, err error)
```
File returns a copy of the underlying os.File. It is the caller's responsibility to close f when finished. Closing l does not affect f, and closing f does not affect l.
The returned os.File's file descriptor is different from the connection's. Attempting to change properties of the original using this duplicate may or may not have the desired effect.
### func (\*UnixListener) SetDeadline
```
func (l *UnixListener) SetDeadline(t time.Time) error
```
SetDeadline sets the deadline associated with the listener. A zero time value disables the deadline.
### func (\*UnixListener) SetUnlinkOnClose 1.8
```
func (l *UnixListener) SetUnlinkOnClose(unlink bool)
```
SetUnlinkOnClose sets whether the underlying socket file should be removed from the file system when the listener is closed.
The default behavior is to unlink the socket file only when package net created it. That is, when the listener and the underlying socket file were created by a call to Listen or ListenUnix, then by default closing the listener will remove the socket file. but if the listener was created by a call to FileListener to use an already existing socket file, then by default closing the listener will not remove the socket file.
### func (\*UnixListener) SyscallConn 1.10
```
func (l *UnixListener) SyscallConn() (syscall.RawConn, error)
```
SyscallConn returns a raw network connection. This implements the syscall.Conn interface.
The returned RawConn only supports calling Control. Read and Write return an error.
type UnknownNetworkError
------------------------
```
type UnknownNetworkError string
```
### func (UnknownNetworkError) Error
```
func (e UnknownNetworkError) Error() string
```
### func (UnknownNetworkError) Temporary
```
func (e UnknownNetworkError) Temporary() bool
```
### func (UnknownNetworkError) Timeout
```
func (e UnknownNetworkError) Timeout() bool
```
Bugs
----
* ☞ On JS and Windows, the FileConn, FileListener and FilePacketConn functions are not implemented.
* ☞ On JS, methods and functions related to Interface are not implemented.
* ☞ On AIX, DragonFly BSD, NetBSD, OpenBSD, Plan 9 and Solaris, the MulticastAddrs method of Interface is not implemented.
* ☞ On every POSIX platform, reads from the "ip4" network using the ReadFrom or ReadFromIP method might not return a complete IPv4 packet, including its header, even if there is space available. This can occur even in cases where Read or ReadMsgIP could return a complete packet. For this reason, it is recommended that you do not use these methods if it is important to receive a full packet.
The Go 1 compatibility guidelines make it impossible for us to change the behavior of these methods; use Read or ReadMsgIP instead.
* ☞ On JS and Plan 9, methods and functions related to IPConn are not implemented.
* ☞ On Windows, the File method of IPConn is not implemented.
* ☞ On DragonFly BSD and OpenBSD, listening on the "tcp" and "udp" networks does not listen for both IPv4 and IPv6 connections. This is due to the fact that IPv4 traffic will not be routed to an IPv6 socket - two separate sockets are required if both address families are to be supported. See inet6(4) for details.
* ☞ On Windows, the Write method of syscall.RawConn does not integrate with the runtime's network poller. It cannot wait for the connection to become writeable, and does not respect deadlines. If the user-provided callback returns false, the Write method will fail immediately.
* ☞ On JS and Plan 9, the Control, Read and Write methods of syscall.RawConn are not implemented.
* ☞ On JS and Windows, the File method of TCPConn and TCPListener is not implemented.
* ☞ On Plan 9, the ReadMsgUDP and WriteMsgUDP methods of UDPConn are not implemented.
* ☞ On Windows, the File method of UDPConn is not implemented.
* ☞ On JS, methods and functions related to UDPConn are not implemented.
* ☞ On JS and Plan 9, methods and functions related to UnixConn and UnixListener are not implemented.
* ☞ On Windows, methods and functions related to UnixConn and UnixListener don't work for "unixgram" and "unixpacket".
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [http](http/index) | Package http provides HTTP client and server implementations. |
| [cgi](http/cgi/index) | Package cgi implements CGI (Common Gateway Interface) as specified in RFC 3875. |
| [cookiejar](http/cookiejar/index) | Package cookiejar implements an in-memory RFC 6265-compliant http.CookieJar. |
| [fcgi](http/fcgi/index) | Package fcgi implements the FastCGI protocol. |
| [httptest](http/httptest/index) | Package httptest provides utilities for HTTP testing. |
| [httptrace](http/httptrace/index) | Package httptrace provides mechanisms to trace the events within HTTP client requests. |
| [httputil](http/httputil/index) | Package httputil provides HTTP utility functions, complementing the more common ones in the net/http package. |
| [pprof](http/pprof/index) | Package pprof serves via its HTTP server runtime profiling data in the format expected by the pprof visualization tool. |
| [mail](mail/index) | Package mail implements parsing of mail messages. |
| [netip](netip/index) | Package netip defines an IP address type that's a small value type. |
| [rpc](rpc/index) | Package rpc provides access to the exported methods of an object across a network or other I/O connection. |
| [jsonrpc](rpc/jsonrpc/index) | Package jsonrpc implements a JSON-RPC 1.0 ClientCodec and ServerCodec for the rpc package. |
| [smtp](smtp/index) | Package smtp implements the Simple Mail Transfer Protocol as defined in RFC 5321. |
| [textproto](textproto/index) | Package textproto implements generic support for text-based request/response protocols in the style of HTTP, NNTP, and SMTP. |
| [url](url/index) | Package url parses URLs and implements query escaping. |
| programming_docs |
go Package textproto Package textproto
==================
* `import "net/textproto"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package textproto implements generic support for text-based request/response protocols in the style of HTTP, NNTP, and SMTP.
The package provides:
Error, which represents a numeric error response from a server.
Pipeline, to manage pipelined requests and responses in a client.
Reader, to read numeric response code lines, key: value headers, lines wrapped with leading spaces on continuation lines, and whole text blocks ending with a dot on a line by itself.
Writer, to write dot-encoded text blocks.
Conn, a convenient packaging of Reader, Writer, and Pipeline for use with a single network connection.
Index
-----
* [func CanonicalMIMEHeaderKey(s string) string](#CanonicalMIMEHeaderKey)
* [func TrimBytes(b []byte) []byte](#TrimBytes)
* [func TrimString(s string) string](#TrimString)
* [type Conn](#Conn)
* [func Dial(network, addr string) (\*Conn, error)](#Dial)
* [func NewConn(conn io.ReadWriteCloser) \*Conn](#NewConn)
* [func (c \*Conn) Close() error](#Conn.Close)
* [func (c \*Conn) Cmd(format string, args ...any) (id uint, err error)](#Conn.Cmd)
* [type Error](#Error)
* [func (e \*Error) Error() string](#Error.Error)
* [type MIMEHeader](#MIMEHeader)
* [func (h MIMEHeader) Add(key, value string)](#MIMEHeader.Add)
* [func (h MIMEHeader) Del(key string)](#MIMEHeader.Del)
* [func (h MIMEHeader) Get(key string) string](#MIMEHeader.Get)
* [func (h MIMEHeader) Set(key, value string)](#MIMEHeader.Set)
* [func (h MIMEHeader) Values(key string) []string](#MIMEHeader.Values)
* [type Pipeline](#Pipeline)
* [func (p \*Pipeline) EndRequest(id uint)](#Pipeline.EndRequest)
* [func (p \*Pipeline) EndResponse(id uint)](#Pipeline.EndResponse)
* [func (p \*Pipeline) Next() uint](#Pipeline.Next)
* [func (p \*Pipeline) StartRequest(id uint)](#Pipeline.StartRequest)
* [func (p \*Pipeline) StartResponse(id uint)](#Pipeline.StartResponse)
* [type ProtocolError](#ProtocolError)
* [func (p ProtocolError) Error() string](#ProtocolError.Error)
* [type Reader](#Reader)
* [func NewReader(r \*bufio.Reader) \*Reader](#NewReader)
* [func (r \*Reader) DotReader() io.Reader](#Reader.DotReader)
* [func (r \*Reader) ReadCodeLine(expectCode int) (code int, message string, err error)](#Reader.ReadCodeLine)
* [func (r \*Reader) ReadContinuedLine() (string, error)](#Reader.ReadContinuedLine)
* [func (r \*Reader) ReadContinuedLineBytes() ([]byte, error)](#Reader.ReadContinuedLineBytes)
* [func (r \*Reader) ReadDotBytes() ([]byte, error)](#Reader.ReadDotBytes)
* [func (r \*Reader) ReadDotLines() ([]string, error)](#Reader.ReadDotLines)
* [func (r \*Reader) ReadLine() (string, error)](#Reader.ReadLine)
* [func (r \*Reader) ReadLineBytes() ([]byte, error)](#Reader.ReadLineBytes)
* [func (r \*Reader) ReadMIMEHeader() (MIMEHeader, error)](#Reader.ReadMIMEHeader)
* [func (r \*Reader) ReadResponse(expectCode int) (code int, message string, err error)](#Reader.ReadResponse)
* [type Writer](#Writer)
* [func NewWriter(w \*bufio.Writer) \*Writer](#NewWriter)
* [func (w \*Writer) DotWriter() io.WriteCloser](#Writer.DotWriter)
* [func (w \*Writer) PrintfLine(format string, args ...any) error](#Writer.PrintfLine)
### Package files
header.go pipeline.go reader.go textproto.go writer.go
func CanonicalMIMEHeaderKey
---------------------------
```
func CanonicalMIMEHeaderKey(s string) string
```
CanonicalMIMEHeaderKey returns the canonical format of the MIME header key s. The canonicalization converts the first letter and any letter following a hyphen to upper case; the rest are converted to lowercase. For example, the canonical key for "accept-encoding" is "Accept-Encoding". MIME header keys are assumed to be ASCII only. If s contains a space or invalid header field bytes, it is returned without modifications.
func TrimBytes 1.1
------------------
```
func TrimBytes(b []byte) []byte
```
TrimBytes returns b without leading and trailing ASCII space.
func TrimString 1.1
-------------------
```
func TrimString(s string) string
```
TrimString returns s without leading and trailing ASCII space.
type Conn
---------
A Conn represents a textual network protocol connection. It consists of a Reader and Writer to manage I/O and a Pipeline to sequence concurrent requests on the connection. These embedded types carry methods with them; see the documentation of those types for details.
```
type Conn struct {
Reader
Writer
Pipeline
// contains filtered or unexported fields
}
```
### func Dial
```
func Dial(network, addr string) (*Conn, error)
```
Dial connects to the given address on the given network using net.Dial and then returns a new Conn for the connection.
### func NewConn
```
func NewConn(conn io.ReadWriteCloser) *Conn
```
NewConn returns a new Conn using conn for I/O.
### func (\*Conn) Close
```
func (c *Conn) Close() error
```
Close closes the connection.
### func (\*Conn) Cmd
```
func (c *Conn) Cmd(format string, args ...any) (id uint, err error)
```
Cmd is a convenience method that sends a command after waiting its turn in the pipeline. The command text is the result of formatting format with args and appending \r\n. Cmd returns the id of the command, for use with StartResponse and EndResponse.
For example, a client might run a HELP command that returns a dot-body by using:
```
id, err := c.Cmd("HELP")
if err != nil {
return nil, err
}
c.StartResponse(id)
defer c.EndResponse(id)
if _, _, err = c.ReadCodeLine(110); err != nil {
return nil, err
}
text, err := c.ReadDotBytes()
if err != nil {
return nil, err
}
return c.ReadCodeLine(250)
```
type Error
----------
An Error represents a numeric error response from a server.
```
type Error struct {
Code int
Msg string
}
```
### func (\*Error) Error
```
func (e *Error) Error() string
```
type MIMEHeader
---------------
A MIMEHeader represents a MIME-style header mapping keys to sets of values.
```
type MIMEHeader map[string][]string
```
### func (MIMEHeader) Add
```
func (h MIMEHeader) Add(key, value string)
```
Add adds the key, value pair to the header. It appends to any existing values associated with key.
### func (MIMEHeader) Del
```
func (h MIMEHeader) Del(key string)
```
Del deletes the values associated with key.
### func (MIMEHeader) Get
```
func (h MIMEHeader) Get(key string) string
```
Get gets the first value associated with the given key. It is case insensitive; CanonicalMIMEHeaderKey is used to canonicalize the provided key. If there are no values associated with the key, Get returns "". To use non-canonical keys, access the map directly.
### func (MIMEHeader) Set
```
func (h MIMEHeader) Set(key, value string)
```
Set sets the header entries associated with key to the single element value. It replaces any existing values associated with key.
### func (MIMEHeader) Values 1.14
```
func (h MIMEHeader) Values(key string) []string
```
Values returns all values associated with the given key. It is case insensitive; CanonicalMIMEHeaderKey is used to canonicalize the provided key. To use non-canonical keys, access the map directly. The returned slice is not a copy.
type Pipeline
-------------
A Pipeline manages a pipelined in-order request/response sequence.
To use a Pipeline p to manage multiple clients on a connection, each client should run:
```
id := p.Next() // take a number
p.StartRequest(id) // wait for turn to send request
«send request»
p.EndRequest(id) // notify Pipeline that request is sent
p.StartResponse(id) // wait for turn to read response
«read response»
p.EndResponse(id) // notify Pipeline that response is read
```
A pipelined server can use the same calls to ensure that responses computed in parallel are written in the correct order.
```
type Pipeline struct {
// contains filtered or unexported fields
}
```
### func (\*Pipeline) EndRequest
```
func (p *Pipeline) EndRequest(id uint)
```
EndRequest notifies p that the request with the given id has been sent (or, if this is a server, received).
### func (\*Pipeline) EndResponse
```
func (p *Pipeline) EndResponse(id uint)
```
EndResponse notifies p that the response with the given id has been received (or, if this is a server, sent).
### func (\*Pipeline) Next
```
func (p *Pipeline) Next() uint
```
Next returns the next id for a request/response pair.
### func (\*Pipeline) StartRequest
```
func (p *Pipeline) StartRequest(id uint)
```
StartRequest blocks until it is time to send (or, if this is a server, receive) the request with the given id.
### func (\*Pipeline) StartResponse
```
func (p *Pipeline) StartResponse(id uint)
```
StartResponse blocks until it is time to receive (or, if this is a server, send) the request with the given id.
type ProtocolError
------------------
A ProtocolError describes a protocol violation such as an invalid response or a hung-up connection.
```
type ProtocolError string
```
### func (ProtocolError) Error
```
func (p ProtocolError) Error() string
```
type Reader
-----------
A Reader implements convenience methods for reading requests or responses from a text protocol network connection.
```
type Reader struct {
R *bufio.Reader
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(r *bufio.Reader) *Reader
```
NewReader returns a new Reader reading from r.
To avoid denial of service attacks, the provided bufio.Reader should be reading from an io.LimitReader or similar Reader to bound the size of responses.
### func (\*Reader) DotReader
```
func (r *Reader) DotReader() io.Reader
```
DotReader returns a new Reader that satisfies Reads using the decoded text of a dot-encoded block read from r. The returned Reader is only valid until the next call to a method on r.
Dot encoding is a common framing used for data blocks in text protocols such as SMTP. The data consists of a sequence of lines, each of which ends in "\r\n". The sequence itself ends at a line containing just a dot: ".\r\n". Lines beginning with a dot are escaped with an additional dot to avoid looking like the end of the sequence.
The decoded form returned by the Reader's Read method rewrites the "\r\n" line endings into the simpler "\n", removes leading dot escapes if present, and stops with error io.EOF after consuming (and discarding) the end-of-sequence line.
### func (\*Reader) ReadCodeLine
```
func (r *Reader) ReadCodeLine(expectCode int) (code int, message string, err error)
```
ReadCodeLine reads a response code line of the form
```
code message
```
where code is a three-digit status code and the message extends to the rest of the line. An example of such a line is:
```
220 plan9.bell-labs.com ESMTP
```
If the prefix of the status does not match the digits in expectCode, ReadCodeLine returns with err set to &Error{code, message}. For example, if expectCode is 31, an error will be returned if the status is not in the range [310,319].
If the response is multi-line, ReadCodeLine returns an error.
An expectCode <= 0 disables the check of the status code.
### func (\*Reader) ReadContinuedLine
```
func (r *Reader) ReadContinuedLine() (string, error)
```
ReadContinuedLine reads a possibly continued line from r, eliding the final trailing ASCII white space. Lines after the first are considered continuations if they begin with a space or tab character. In the returned data, continuation lines are separated from the previous line only by a single space: the newline and leading white space are removed.
For example, consider this input:
```
Line 1
continued...
Line 2
```
The first call to ReadContinuedLine will return "Line 1 continued..." and the second will return "Line 2".
Empty lines are never continued.
### func (\*Reader) ReadContinuedLineBytes
```
func (r *Reader) ReadContinuedLineBytes() ([]byte, error)
```
ReadContinuedLineBytes is like ReadContinuedLine but returns a []byte instead of a string.
### func (\*Reader) ReadDotBytes
```
func (r *Reader) ReadDotBytes() ([]byte, error)
```
ReadDotBytes reads a dot-encoding and returns the decoded data.
See the documentation for the DotReader method for details about dot-encoding.
### func (\*Reader) ReadDotLines
```
func (r *Reader) ReadDotLines() ([]string, error)
```
ReadDotLines reads a dot-encoding and returns a slice containing the decoded lines, with the final \r\n or \n elided from each.
See the documentation for the DotReader method for details about dot-encoding.
### func (\*Reader) ReadLine
```
func (r *Reader) ReadLine() (string, error)
```
ReadLine reads a single line from r, eliding the final \n or \r\n from the returned string.
### func (\*Reader) ReadLineBytes
```
func (r *Reader) ReadLineBytes() ([]byte, error)
```
ReadLineBytes is like ReadLine but returns a []byte instead of a string.
### func (\*Reader) ReadMIMEHeader
```
func (r *Reader) ReadMIMEHeader() (MIMEHeader, error)
```
ReadMIMEHeader reads a MIME-style header from r. The header is a sequence of possibly continued Key: Value lines ending in a blank line. The returned map m maps CanonicalMIMEHeaderKey(key) to a sequence of values in the same order encountered in the input.
For example, consider this input:
```
My-Key: Value 1
Long-Key: Even
Longer Value
My-Key: Value 2
```
Given that input, ReadMIMEHeader returns the map:
```
map[string][]string{
"My-Key": {"Value 1", "Value 2"},
"Long-Key": {"Even Longer Value"},
}
```
### func (\*Reader) ReadResponse
```
func (r *Reader) ReadResponse(expectCode int) (code int, message string, err error)
```
ReadResponse reads a multi-line response of the form:
```
code-message line 1
code-message line 2
...
code message line n
```
where code is a three-digit status code. The first line starts with the code and a hyphen. The response is terminated by a line that starts with the same code followed by a space. Each line in message is separated by a newline (\n).
See page 36 of RFC 959 (<https://www.ietf.org/rfc/rfc959.txt>) for details of another form of response accepted:
```
code-message line 1
message line 2
...
code message line n
```
If the prefix of the status does not match the digits in expectCode, ReadResponse returns with err set to &Error{code, message}. For example, if expectCode is 31, an error will be returned if the status is not in the range [310,319].
An expectCode <= 0 disables the check of the status code.
type Writer
-----------
A Writer implements convenience methods for writing requests or responses to a text protocol network connection.
```
type Writer struct {
W *bufio.Writer
// contains filtered or unexported fields
}
```
### func NewWriter
```
func NewWriter(w *bufio.Writer) *Writer
```
NewWriter returns a new Writer writing to w.
### func (\*Writer) DotWriter
```
func (w *Writer) DotWriter() io.WriteCloser
```
DotWriter returns a writer that can be used to write a dot-encoding to w. It takes care of inserting leading dots when necessary, translating line-ending \n into \r\n, and adding the final .\r\n line when the DotWriter is closed. The caller should close the DotWriter before the next call to a method on w.
See the documentation for Reader's DotReader method for details about dot-encoding.
### func (\*Writer) PrintfLine
```
func (w *Writer) PrintfLine(format string, args ...any) error
```
PrintfLine writes the formatted output followed by \r\n.
go Package mail Package mail
=============
* `import "net/mail"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package mail implements parsing of mail messages.
For the most part, this package follows the syntax as specified by RFC 5322 and extended by RFC 6532. Notable divergences:
* Obsolete address formats are not parsed, including addresses with embedded route information.
* The full range of spacing (the CFWS syntax element) is not supported, such as breaking addresses across lines.
* No unicode normalization is performed.
* The special characters ()[]:;@\, are allowed to appear unquoted in names.
Index
-----
* [Variables](#pkg-variables)
* [func ParseDate(date string) (time.Time, error)](#ParseDate)
* [type Address](#Address)
* [func ParseAddress(address string) (\*Address, error)](#ParseAddress)
* [func ParseAddressList(list string) ([]\*Address, error)](#ParseAddressList)
* [func (a \*Address) String() string](#Address.String)
* [type AddressParser](#AddressParser)
* [func (p \*AddressParser) Parse(address string) (\*Address, error)](#AddressParser.Parse)
* [func (p \*AddressParser) ParseList(list string) ([]\*Address, error)](#AddressParser.ParseList)
* [type Header](#Header)
* [func (h Header) AddressList(key string) ([]\*Address, error)](#Header.AddressList)
* [func (h Header) Date() (time.Time, error)](#Header.Date)
* [func (h Header) Get(key string) string](#Header.Get)
* [type Message](#Message)
* [func ReadMessage(r io.Reader) (msg \*Message, err error)](#ReadMessage)
### Examples
[ParseAddress](#example_ParseAddress) [ParseAddressList](#example_ParseAddressList) [ReadMessage](#example_ReadMessage) ### Package files
message.go
Variables
---------
```
var ErrHeaderNotPresent = errors.New("mail: header not in message")
```
func ParseDate 1.8
------------------
```
func ParseDate(date string) (time.Time, error)
```
ParseDate parses an RFC 5322 date string.
type Address
------------
Address represents a single mail address. An address such as "Barry Gibbs <[email protected]>" is represented as Address{Name: "Barry Gibbs", Address: "[email protected]"}.
```
type Address struct {
Name string // Proper name; may be empty.
Address string // user@domain
}
```
### func ParseAddress 1.1
```
func ParseAddress(address string) (*Address, error)
```
ParseAddress parses a single RFC 5322 address, e.g. "Barry Gibbs <[email protected]>"
#### Example
Code:
```
e, err := mail.ParseAddress("Alice <[email protected]>")
if err != nil {
log.Fatal(err)
}
fmt.Println(e.Name, e.Address)
```
Output:
```
Alice [email protected]
```
### func ParseAddressList 1.1
```
func ParseAddressList(list string) ([]*Address, error)
```
ParseAddressList parses the given string as a list of addresses.
#### Example
Code:
```
const list = "Alice <[email protected]>, Bob <[email protected]>, Eve <[email protected]>"
emails, err := mail.ParseAddressList(list)
if err != nil {
log.Fatal(err)
}
for _, v := range emails {
fmt.Println(v.Name, v.Address)
}
```
Output:
```
Alice [email protected]
Bob [email protected]
Eve [email protected]
```
### func (\*Address) String
```
func (a *Address) String() string
```
String formats the address as a valid RFC 5322 address. If the address's name contains non-ASCII characters the name will be rendered according to RFC 2047.
type AddressParser 1.5
----------------------
An AddressParser is an RFC 5322 address parser.
```
type AddressParser struct {
// WordDecoder optionally specifies a decoder for RFC 2047 encoded-words.
WordDecoder *mime.WordDecoder
}
```
### func (\*AddressParser) Parse 1.5
```
func (p *AddressParser) Parse(address string) (*Address, error)
```
Parse parses a single RFC 5322 address of the form "Gogh Fir <[email protected]>" or "[email protected]".
### func (\*AddressParser) ParseList 1.5
```
func (p *AddressParser) ParseList(list string) ([]*Address, error)
```
ParseList parses the given string as a list of comma-separated addresses of the form "Gogh Fir <[email protected]>" or "[email protected]".
type Header
-----------
A Header represents the key-value pairs in a mail message header.
```
type Header map[string][]string
```
### func (Header) AddressList
```
func (h Header) AddressList(key string) ([]*Address, error)
```
AddressList parses the named header field as a list of addresses.
### func (Header) Date
```
func (h Header) Date() (time.Time, error)
```
Date parses the Date header field.
### func (Header) Get
```
func (h Header) Get(key string) string
```
Get gets the first value associated with the given key. It is case insensitive; CanonicalMIMEHeaderKey is used to canonicalize the provided key. If there are no values associated with the key, Get returns "". To access multiple values of a key, or to use non-canonical keys, access the map directly.
type Message
------------
A Message represents a parsed mail message.
```
type Message struct {
Header Header
Body io.Reader
}
```
### func ReadMessage
```
func ReadMessage(r io.Reader) (msg *Message, err error)
```
ReadMessage reads a message from r. The headers are parsed, and the body of the message will be available for reading from msg.Body.
#### Example
Code:
```
msg := `Date: Mon, 23 Jun 2015 11:40:36 -0400
From: Gopher <[email protected]>
To: Another Gopher <[email protected]>
Subject: Gophers at Gophercon
Message body
`
r := strings.NewReader(msg)
m, err := mail.ReadMessage(r)
if err != nil {
log.Fatal(err)
}
header := m.Header
fmt.Println("Date:", header.Get("Date"))
fmt.Println("From:", header.Get("From"))
fmt.Println("To:", header.Get("To"))
fmt.Println("Subject:", header.Get("Subject"))
body, err := io.ReadAll(m.Body)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", body)
```
Output:
```
Date: Mon, 23 Jun 2015 11:40:36 -0400
From: Gopher <[email protected]>
To: Another Gopher <[email protected]>
Subject: Gophers at Gophercon
Message body
```
| programming_docs |
go Package url Package url
============
* `import "net/url"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package url parses URLs and implements query escaping.
Index
-----
* [func JoinPath(base string, elem ...string) (result string, err error)](#JoinPath)
* [func PathEscape(s string) string](#PathEscape)
* [func PathUnescape(s string) (string, error)](#PathUnescape)
* [func QueryEscape(s string) string](#QueryEscape)
* [func QueryUnescape(s string) (string, error)](#QueryUnescape)
* [type Error](#Error)
* [func (e \*Error) Error() string](#Error.Error)
* [func (e \*Error) Temporary() bool](#Error.Temporary)
* [func (e \*Error) Timeout() bool](#Error.Timeout)
* [func (e \*Error) Unwrap() error](#Error.Unwrap)
* [type EscapeError](#EscapeError)
* [func (e EscapeError) Error() string](#EscapeError.Error)
* [type InvalidHostError](#InvalidHostError)
* [func (e InvalidHostError) Error() string](#InvalidHostError.Error)
* [type URL](#URL)
* [func Parse(rawURL string) (\*URL, error)](#Parse)
* [func ParseRequestURI(rawURL string) (\*URL, error)](#ParseRequestURI)
* [func (u \*URL) EscapedFragment() string](#URL.EscapedFragment)
* [func (u \*URL) EscapedPath() string](#URL.EscapedPath)
* [func (u \*URL) Hostname() string](#URL.Hostname)
* [func (u \*URL) IsAbs() bool](#URL.IsAbs)
* [func (u \*URL) JoinPath(elem ...string) \*URL](#URL.JoinPath)
* [func (u \*URL) MarshalBinary() (text []byte, err error)](#URL.MarshalBinary)
* [func (u \*URL) Parse(ref string) (\*URL, error)](#URL.Parse)
* [func (u \*URL) Port() string](#URL.Port)
* [func (u \*URL) Query() Values](#URL.Query)
* [func (u \*URL) Redacted() string](#URL.Redacted)
* [func (u \*URL) RequestURI() string](#URL.RequestURI)
* [func (u \*URL) ResolveReference(ref \*URL) \*URL](#URL.ResolveReference)
* [func (u \*URL) String() string](#URL.String)
* [func (u \*URL) UnmarshalBinary(text []byte) error](#URL.UnmarshalBinary)
* [type Userinfo](#Userinfo)
* [func User(username string) \*Userinfo](#User)
* [func UserPassword(username, password string) \*Userinfo](#UserPassword)
* [func (u \*Userinfo) Password() (string, bool)](#Userinfo.Password)
* [func (u \*Userinfo) String() string](#Userinfo.String)
* [func (u \*Userinfo) Username() string](#Userinfo.Username)
* [type Values](#Values)
* [func ParseQuery(query string) (Values, error)](#ParseQuery)
* [func (v Values) Add(key, value string)](#Values.Add)
* [func (v Values) Del(key string)](#Values.Del)
* [func (v Values) Encode() string](#Values.Encode)
* [func (v Values) Get(key string) string](#Values.Get)
* [func (v Values) Has(key string) bool](#Values.Has)
* [func (v Values) Set(key, value string)](#Values.Set)
### Examples
[ParseQuery](#example_ParseQuery) [PathEscape](#example_PathEscape) [PathUnescape](#example_PathUnescape) [QueryEscape](#example_QueryEscape) [QueryUnescape](#example_QueryUnescape) [URL](#example_URL) [URL.EscapedFragment](#example_URL_EscapedFragment) [URL.EscapedPath](#example_URL_EscapedPath) [URL.Hostname](#example_URL_Hostname) [URL.IsAbs](#example_URL_IsAbs) [URL.MarshalBinary](#example_URL_MarshalBinary) [URL.Parse](#example_URL_Parse) [URL.Port](#example_URL_Port) [URL.Query](#example_URL_Query) [URL.Redacted](#example_URL_Redacted) [URL.RequestURI](#example_URL_RequestURI) [URL.ResolveReference](#example_URL_ResolveReference) [URL.String](#example_URL_String) [URL.UnmarshalBinary](#example_URL_UnmarshalBinary) [URL (Roundtrip)](#example_URL_roundtrip) [Values](#example_Values) [Values.Add](#example_Values_Add) [Values.Del](#example_Values_Del) [Values.Encode](#example_Values_Encode) [Values.Get](#example_Values_Get) [Values.Has](#example_Values_Has) [Values.Set](#example_Values_Set) ### Package files
url.go
func JoinPath 1.19
------------------
```
func JoinPath(base string, elem ...string) (result string, err error)
```
JoinPath returns a URL string with the provided path elements joined to the existing path of base and the resulting path cleaned of any ./ or ../ elements.
func PathEscape 1.8
-------------------
```
func PathEscape(s string) string
```
PathEscape escapes the string so it can be safely placed inside a URL path segment, replacing special characters (including /) with %XX sequences as needed.
#### Example
Code:
```
path := url.PathEscape("my/cool+blog&about,stuff")
fmt.Println(path)
```
Output:
```
my%2Fcool+blog&about%2Cstuff
```
func PathUnescape 1.8
---------------------
```
func PathUnescape(s string) (string, error)
```
PathUnescape does the inverse transformation of PathEscape, converting each 3-byte encoded substring of the form "%AB" into the hex-decoded byte 0xAB. It returns an error if any % is not followed by two hexadecimal digits.
PathUnescape is identical to QueryUnescape except that it does not unescape '+' to ' ' (space).
#### Example
Code:
```
escapedPath := "my%2Fcool+blog&about%2Cstuff"
path, err := url.PathUnescape(escapedPath)
if err != nil {
log.Fatal(err)
}
fmt.Println(path)
```
Output:
```
my/cool+blog&about,stuff
```
func QueryEscape
----------------
```
func QueryEscape(s string) string
```
QueryEscape escapes the string so it can be safely placed inside a URL query.
#### Example
Code:
```
query := url.QueryEscape("my/cool+blog&about,stuff")
fmt.Println(query)
```
Output:
```
my%2Fcool%2Bblog%26about%2Cstuff
```
func QueryUnescape
------------------
```
func QueryUnescape(s string) (string, error)
```
QueryUnescape does the inverse transformation of QueryEscape, converting each 3-byte encoded substring of the form "%AB" into the hex-decoded byte 0xAB. It returns an error if any % is not followed by two hexadecimal digits.
#### Example
Code:
```
escapedQuery := "my%2Fcool%2Bblog%26about%2Cstuff"
query, err := url.QueryUnescape(escapedQuery)
if err != nil {
log.Fatal(err)
}
fmt.Println(query)
```
Output:
```
my/cool+blog&about,stuff
```
type Error
----------
Error reports an error and the operation and URL that caused it.
```
type Error struct {
Op string
URL string
Err error
}
```
### func (\*Error) Error
```
func (e *Error) Error() string
```
### func (\*Error) Temporary 1.6
```
func (e *Error) Temporary() bool
```
### func (\*Error) Timeout 1.6
```
func (e *Error) Timeout() bool
```
### func (\*Error) Unwrap 1.13
```
func (e *Error) Unwrap() error
```
type EscapeError
----------------
```
type EscapeError string
```
### func (EscapeError) Error
```
func (e EscapeError) Error() string
```
type InvalidHostError 1.6
-------------------------
```
type InvalidHostError string
```
### func (InvalidHostError) Error 1.6
```
func (e InvalidHostError) Error() string
```
type URL
--------
A URL represents a parsed URL (technically, a URI reference).
The general form represented is:
```
[scheme:][//[userinfo@]host][/]path[?query][#fragment]
```
URLs that do not start with a slash after the scheme are interpreted as:
```
scheme:opaque[?query][#fragment]
```
Note that the Path field is stored in decoded form: /%47%6f%2f becomes /Go/. A consequence is that it is impossible to tell which slashes in the Path were slashes in the raw URL and which were %2f. This distinction is rarely important, but when it is, the code should use the EscapedPath method, which preserves the original encoding of Path.
The RawPath field is an optional field which is only set when the default encoding of Path is different from the escaped path. See the EscapedPath method for more details.
URL's String method uses the EscapedPath method to obtain the path.
```
type URL struct {
Scheme string
Opaque string // encoded opaque data
User *Userinfo // username and password information
Host string // host or host:port
Path string // path (relative paths may omit leading slash)
RawPath string // encoded path hint (see EscapedPath method); added in Go 1.5
OmitHost bool // do not emit empty host (authority); added in Go 1.19
ForceQuery bool // append a query ('?') even if RawQuery is empty; added in Go 1.7
RawQuery string // encoded query values, without '?'
Fragment string // fragment for references, without '#'
RawFragment string // encoded fragment hint (see EscapedFragment method); added in Go 1.15
}
```
#### Example
Code:
```
u, err := url.Parse("http://bing.com/search?q=dotnet")
if err != nil {
log.Fatal(err)
}
u.Scheme = "https"
u.Host = "google.com"
q := u.Query()
q.Set("q", "golang")
u.RawQuery = q.Encode()
fmt.Println(u)
```
Output:
```
https://google.com/search?q=golang
```
#### Example (Roundtrip)
Code:
```
// Parse + String preserve the original encoding.
u, err := url.Parse("https://example.com/foo%2fbar")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.Path)
fmt.Println(u.RawPath)
fmt.Println(u.String())
```
Output:
```
/foo/bar
/foo%2fbar
https://example.com/foo%2fbar
```
### func Parse
```
func Parse(rawURL string) (*URL, error)
```
Parse parses a raw url into a URL structure.
The url may be relative (a path, without a host) or absolute (starting with a scheme). Trying to parse a hostname and path without a scheme is invalid but may not necessarily return an error, due to parsing ambiguities.
### func ParseRequestURI
```
func ParseRequestURI(rawURL string) (*URL, error)
```
ParseRequestURI parses a raw url into a URL structure. It assumes that url was received in an HTTP request, so the url is interpreted only as an absolute URI or an absolute path. The string url is assumed not to have a #fragment suffix. (Web browsers strip #fragment before sending the URL to a web server.)
### func (\*URL) EscapedFragment 1.15
```
func (u *URL) EscapedFragment() string
```
EscapedFragment returns the escaped form of u.Fragment. In general there are multiple possible escaped forms of any fragment. EscapedFragment returns u.RawFragment when it is a valid escaping of u.Fragment. Otherwise EscapedFragment ignores u.RawFragment and computes an escaped form on its own. The String method uses EscapedFragment to construct its result. In general, code should call EscapedFragment instead of reading u.RawFragment directly.
#### Example
Code:
```
u, err := url.Parse("http://example.com/#x/y%2Fz")
if err != nil {
log.Fatal(err)
}
fmt.Println("Fragment:", u.Fragment)
fmt.Println("RawFragment:", u.RawFragment)
fmt.Println("EscapedFragment:", u.EscapedFragment())
```
Output:
```
Fragment: x/y/z
RawFragment: x/y%2Fz
EscapedFragment: x/y%2Fz
```
### func (\*URL) EscapedPath 1.5
```
func (u *URL) EscapedPath() string
```
EscapedPath returns the escaped form of u.Path. In general there are multiple possible escaped forms of any path. EscapedPath returns u.RawPath when it is a valid escaping of u.Path. Otherwise EscapedPath ignores u.RawPath and computes an escaped form on its own. The String and RequestURI methods use EscapedPath to construct their results. In general, code should call EscapedPath instead of reading u.RawPath directly.
#### Example
Code:
```
u, err := url.Parse("http://example.com/x/y%2Fz")
if err != nil {
log.Fatal(err)
}
fmt.Println("Path:", u.Path)
fmt.Println("RawPath:", u.RawPath)
fmt.Println("EscapedPath:", u.EscapedPath())
```
Output:
```
Path: /x/y/z
RawPath: /x/y%2Fz
EscapedPath: /x/y%2Fz
```
### func (\*URL) Hostname 1.8
```
func (u *URL) Hostname() string
```
Hostname returns u.Host, stripping any valid port number if present.
If the result is enclosed in square brackets, as literal IPv6 addresses are, the square brackets are removed from the result.
#### Example
Code:
```
u, err := url.Parse("https://example.org:8000/path")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.Hostname())
u, err = url.Parse("https://[2001:0db8:85a3:0000:0000:8a2e:0370:7334]:17000")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.Hostname())
```
Output:
```
example.org
2001:0db8:85a3:0000:0000:8a2e:0370:7334
```
### func (\*URL) IsAbs
```
func (u *URL) IsAbs() bool
```
IsAbs reports whether the URL is absolute. Absolute means that it has a non-empty scheme.
#### Example
Code:
```
u := url.URL{Host: "example.com", Path: "foo"}
fmt.Println(u.IsAbs())
u.Scheme = "http"
fmt.Println(u.IsAbs())
```
Output:
```
false
true
```
### func (\*URL) JoinPath 1.19
```
func (u *URL) JoinPath(elem ...string) *URL
```
JoinPath returns a new URL with the provided path elements joined to any existing path and the resulting path cleaned of any ./ or ../ elements. Any sequences of multiple / characters will be reduced to a single /.
### func (\*URL) MarshalBinary 1.8
```
func (u *URL) MarshalBinary() (text []byte, err error)
```
#### Example
Code:
```
u, _ := url.Parse("https://example.org")
b, err := u.MarshalBinary()
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s\n", b)
```
Output:
```
https://example.org
```
### func (\*URL) Parse
```
func (u *URL) Parse(ref string) (*URL, error)
```
Parse parses a URL in the context of the receiver. The provided URL may be relative or absolute. Parse returns nil, err on parse failure, otherwise its return value is the same as ResolveReference.
#### Example
Code:
```
u, err := url.Parse("https://example.org")
if err != nil {
log.Fatal(err)
}
rel, err := u.Parse("/foo")
if err != nil {
log.Fatal(err)
}
fmt.Println(rel)
_, err = u.Parse(":foo")
if _, ok := err.(*url.Error); !ok {
log.Fatal(err)
}
```
Output:
```
https://example.org/foo
```
### func (\*URL) Port 1.8
```
func (u *URL) Port() string
```
Port returns the port part of u.Host, without the leading colon.
If u.Host doesn't contain a valid numeric port, Port returns an empty string.
#### Example
Code:
```
u, err := url.Parse("https://example.org")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.Port())
u, err = url.Parse("https://example.org:8080")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.Port())
```
Output:
```
8080
```
### func (\*URL) Query
```
func (u *URL) Query() Values
```
Query parses RawQuery and returns the corresponding values. It silently discards malformed value pairs. To check errors use ParseQuery.
#### Example
Code:
```
u, err := url.Parse("https://example.org/?a=1&a=2&b=&=3&&&&")
if err != nil {
log.Fatal(err)
}
q := u.Query()
fmt.Println(q["a"])
fmt.Println(q.Get("b"))
fmt.Println(q.Get(""))
```
Output:
```
[1 2]
3
```
### func (\*URL) Redacted 1.15
```
func (u *URL) Redacted() string
```
Redacted is like String but replaces any password with "xxxxx". Only the password in u.URL is redacted.
#### Example
Code:
```
u := &url.URL{
Scheme: "https",
User: url.UserPassword("user", "password"),
Host: "example.com",
Path: "foo/bar",
}
fmt.Println(u.Redacted())
u.User = url.UserPassword("me", "newerPassword")
fmt.Println(u.Redacted())
```
Output:
```
https://user:[email protected]/foo/bar
https://me:[email protected]/foo/bar
```
### func (\*URL) RequestURI
```
func (u *URL) RequestURI() string
```
RequestURI returns the encoded path?query or opaque?query string that would be used in an HTTP request for u.
#### Example
Code:
```
u, err := url.Parse("https://example.org/path?foo=bar")
if err != nil {
log.Fatal(err)
}
fmt.Println(u.RequestURI())
```
Output:
```
/path?foo=bar
```
### func (\*URL) ResolveReference
```
func (u *URL) ResolveReference(ref *URL) *URL
```
ResolveReference resolves a URI reference to an absolute URI from an absolute base URI u, per RFC 3986 Section 5.2. The URI reference may be relative or absolute. ResolveReference always returns a new URL instance, even if the returned URL is identical to either the base or reference. If ref is an absolute URL, then ResolveReference ignores base and returns a copy of ref.
#### Example
Code:
```
u, err := url.Parse("../../..//search?q=dotnet")
if err != nil {
log.Fatal(err)
}
base, err := url.Parse("http://example.com/directory/")
if err != nil {
log.Fatal(err)
}
fmt.Println(base.ResolveReference(u))
```
Output:
```
http://example.com/search?q=dotnet
```
### func (\*URL) String
```
func (u *URL) String() string
```
String reassembles the URL into a valid URL string. The general form of the result is one of:
```
scheme:opaque?query#fragment
scheme://userinfo@host/path?query#fragment
```
If u.Opaque is non-empty, String uses the first form; otherwise it uses the second form. Any non-ASCII characters in host are escaped. To obtain the path, String uses u.EscapedPath().
In the second form, the following rules apply:
* if u.Scheme is empty, scheme: is omitted.
* if u.User is nil, userinfo@ is omitted.
* if u.Host is empty, host/ is omitted.
* if u.Scheme and u.Host are empty and u.User is nil, the entire scheme://userinfo@host/ is omitted.
* if u.Host is non-empty and u.Path begins with a /, the form host/path does not add its own /.
* if u.RawQuery is empty, ?query is omitted.
* if u.Fragment is empty, #fragment is omitted.
#### Example
Code:
```
u := &url.URL{
Scheme: "https",
User: url.UserPassword("me", "pass"),
Host: "example.com",
Path: "foo/bar",
RawQuery: "x=1&y=2",
Fragment: "anchor",
}
fmt.Println(u.String())
u.Opaque = "opaque"
fmt.Println(u.String())
```
Output:
```
https://me:[email protected]/foo/bar?x=1&y=2#anchor
https:opaque?x=1&y=2#anchor
```
### func (\*URL) UnmarshalBinary 1.8
```
func (u *URL) UnmarshalBinary(text []byte) error
```
#### Example
Code:
```
u := &url.URL{}
err := u.UnmarshalBinary([]byte("https://example.org/foo"))
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s\n", u)
```
Output:
```
https://example.org/foo
```
type Userinfo
-------------
The Userinfo type is an immutable encapsulation of username and password details for a URL. An existing Userinfo value is guaranteed to have a username set (potentially empty, as allowed by RFC 2396), and optionally a password.
```
type Userinfo struct {
// contains filtered or unexported fields
}
```
### func User
```
func User(username string) *Userinfo
```
User returns a Userinfo containing the provided username and no password set.
### func UserPassword
```
func UserPassword(username, password string) *Userinfo
```
UserPassword returns a Userinfo containing the provided username and password.
This functionality should only be used with legacy web sites. RFC 2396 warns that interpreting Userinfo this way “is NOT RECOMMENDED, because the passing of authentication information in clear text (such as URI) has proven to be a security risk in almost every case where it has been used.”
### func (\*Userinfo) Password
```
func (u *Userinfo) Password() (string, bool)
```
Password returns the password in case it is set, and whether it is set.
### func (\*Userinfo) String
```
func (u *Userinfo) String() string
```
String returns the encoded userinfo information in the standard form of "username[:password]".
### func (\*Userinfo) Username
```
func (u *Userinfo) Username() string
```
Username returns the username.
type Values
-----------
Values maps a string key to a list of values. It is typically used for query parameters and form values. Unlike in the http.Header map, the keys in a Values map are case-sensitive.
```
type Values map[string][]string
```
#### Example
Code:
```
v := url.Values{}
v.Set("name", "Ava")
v.Add("friend", "Jess")
v.Add("friend", "Sarah")
v.Add("friend", "Zoe")
// v.Encode() == "name=Ava&friend=Jess&friend=Sarah&friend=Zoe"
fmt.Println(v.Get("name"))
fmt.Println(v.Get("friend"))
fmt.Println(v["friend"])
```
Output:
```
Ava
Jess
[Jess Sarah Zoe]
```
### func ParseQuery
```
func ParseQuery(query string) (Values, error)
```
ParseQuery parses the URL-encoded query string and returns a map listing the values specified for each key. ParseQuery always returns a non-nil map containing all the valid query parameters found; err describes the first decoding error encountered, if any.
Query is expected to be a list of key=value settings separated by ampersands. A setting without an equals sign is interpreted as a key set to an empty value. Settings containing a non-URL-encoded semicolon are considered invalid.
#### Example
Code:
```
m, err := url.ParseQuery(`x=1&y=2&y=3`)
if err != nil {
log.Fatal(err)
}
fmt.Println(toJSON(m))
```
Output:
```
{"x":["1"], "y":["2", "3"]}
```
### func (Values) Add
```
func (v Values) Add(key, value string)
```
Add adds the value to key. It appends to any existing values associated with key.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew")
v.Add("cat sounds", "mau")
fmt.Println(v["cat sounds"])
```
Output:
```
[meow mew mau]
```
### func (Values) Del
```
func (v Values) Del(key string)
```
Del deletes the values associated with key.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew")
v.Add("cat sounds", "mau")
fmt.Println(v["cat sounds"])
v.Del("cat sounds")
fmt.Println(v["cat sounds"])
```
Output:
```
[meow mew mau]
[]
```
### func (Values) Encode
```
func (v Values) Encode() string
```
Encode encodes the values into “URL encoded” form ("bar=baz&foo=quux") sorted by key.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew/")
v.Add("cat sounds", "mau$")
fmt.Println(v.Encode())
```
Output:
```
cat+sounds=meow&cat+sounds=mew%2F&cat+sounds=mau%24
```
### func (Values) Get
```
func (v Values) Get(key string) string
```
Get gets the first value associated with the given key. If there are no values associated with the key, Get returns the empty string. To access multiple values, use the map directly.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew")
v.Add("cat sounds", "mau")
fmt.Printf("%q\n", v.Get("cat sounds"))
fmt.Printf("%q\n", v.Get("dog sounds"))
```
Output:
```
"meow"
""
```
### func (Values) Has 1.17
```
func (v Values) Has(key string) bool
```
Has checks whether a given key is set.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew")
v.Add("cat sounds", "mau")
fmt.Println(v.Has("cat sounds"))
fmt.Println(v.Has("dog sounds"))
```
Output:
```
true
false
```
### func (Values) Set
```
func (v Values) Set(key, value string)
```
Set sets the key to value. It replaces any existing values.
#### Example
Code:
```
v := url.Values{}
v.Add("cat sounds", "meow")
v.Add("cat sounds", "mew")
v.Add("cat sounds", "mau")
fmt.Println(v["cat sounds"])
v.Set("cat sounds", "meow")
fmt.Println(v["cat sounds"])
```
Output:
```
[meow mew mau]
[meow]
```
| programming_docs |
go Package http Package http
=============
* `import "net/http"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package http provides HTTP client and server implementations.
Get, Head, Post, and PostForm make HTTP (or HTTPS) requests:
```
resp, err := http.Get("http://example.com/")
...
resp, err := http.Post("http://example.com/upload", "image/jpeg", &buf)
...
resp, err := http.PostForm("http://example.com/form",
url.Values{"key": {"Value"}, "id": {"123"}})
```
The client must close the response body when finished with it:
```
resp, err := http.Get("http://example.com/")
if err != nil {
// handle error
}
defer resp.Body.Close()
body, err := io.ReadAll(resp.Body)
// ...
```
For control over HTTP client headers, redirect policy, and other settings, create a Client:
```
client := &http.Client{
CheckRedirect: redirectPolicyFunc,
}
resp, err := client.Get("http://example.com")
// ...
req, err := http.NewRequest("GET", "http://example.com", nil)
// ...
req.Header.Add("If-None-Match", `W/"wyzzy"`)
resp, err := client.Do(req)
// ...
```
For control over proxies, TLS configuration, keep-alives, compression, and other settings, create a Transport:
```
tr := &http.Transport{
MaxIdleConns: 10,
IdleConnTimeout: 30 * time.Second,
DisableCompression: true,
}
client := &http.Client{Transport: tr}
resp, err := client.Get("https://example.com")
```
Clients and Transports are safe for concurrent use by multiple goroutines and for efficiency should only be created once and re-used.
ListenAndServe starts an HTTP server with a given address and handler. The handler is usually nil, which means to use DefaultServeMux. Handle and HandleFunc add handlers to DefaultServeMux:
```
http.Handle("/foo", fooHandler)
http.HandleFunc("/bar", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, %q", html.EscapeString(r.URL.Path))
})
log.Fatal(http.ListenAndServe(":8080", nil))
```
More control over the server's behavior is available by creating a custom Server:
```
s := &http.Server{
Addr: ":8080",
Handler: myHandler,
ReadTimeout: 10 * time.Second,
WriteTimeout: 10 * time.Second,
MaxHeaderBytes: 1 << 20,
}
log.Fatal(s.ListenAndServe())
```
Starting with Go 1.6, the http package has transparent support for the HTTP/2 protocol when using HTTPS. Programs that must disable HTTP/2 can do so by setting Transport.TLSNextProto (for clients) or Server.TLSNextProto (for servers) to a non-nil, empty map. Alternatively, the following GODEBUG environment variables are currently supported:
```
GODEBUG=http2client=0 # disable HTTP/2 client support
GODEBUG=http2server=0 # disable HTTP/2 server support
GODEBUG=http2debug=1 # enable verbose HTTP/2 debug logs
GODEBUG=http2debug=2 # ... even more verbose, with frame dumps
```
The GODEBUG variables are not covered by Go's API compatibility promise. Please report any issues before disabling HTTP/2 support: <https://golang.org/s/http2bug>
The http package's Transport and Server both automatically enable HTTP/2 support for simple configurations. To enable HTTP/2 for more complex configurations, to use lower-level HTTP/2 features, or to use a newer version of Go's http2 package, import "golang.org/x/net/http2" directly and use its ConfigureTransport and/or ConfigureServer functions. Manually configuring HTTP/2 via the golang.org/x/net/http2 package takes precedence over the net/http package's built-in HTTP/2 support.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func CanonicalHeaderKey(s string) string](#CanonicalHeaderKey)
* [func DetectContentType(data []byte) string](#DetectContentType)
* [func Error(w ResponseWriter, error string, code int)](#Error)
* [func Handle(pattern string, handler Handler)](#Handle)
* [func HandleFunc(pattern string, handler func(ResponseWriter, \*Request))](#HandleFunc)
* [func ListenAndServe(addr string, handler Handler) error](#ListenAndServe)
* [func ListenAndServeTLS(addr, certFile, keyFile string, handler Handler) error](#ListenAndServeTLS)
* [func MaxBytesReader(w ResponseWriter, r io.ReadCloser, n int64) io.ReadCloser](#MaxBytesReader)
* [func NotFound(w ResponseWriter, r \*Request)](#NotFound)
* [func ParseHTTPVersion(vers string) (major, minor int, ok bool)](#ParseHTTPVersion)
* [func ParseTime(text string) (t time.Time, err error)](#ParseTime)
* [func ProxyFromEnvironment(req \*Request) (\*url.URL, error)](#ProxyFromEnvironment)
* [func ProxyURL(fixedURL \*url.URL) func(\*Request) (\*url.URL, error)](#ProxyURL)
* [func Redirect(w ResponseWriter, r \*Request, url string, code int)](#Redirect)
* [func Serve(l net.Listener, handler Handler) error](#Serve)
* [func ServeContent(w ResponseWriter, req \*Request, name string, modtime time.Time, content io.ReadSeeker)](#ServeContent)
* [func ServeFile(w ResponseWriter, r \*Request, name string)](#ServeFile)
* [func ServeTLS(l net.Listener, handler Handler, certFile, keyFile string) error](#ServeTLS)
* [func SetCookie(w ResponseWriter, cookie \*Cookie)](#SetCookie)
* [func StatusText(code int) string](#StatusText)
* [type Client](#Client)
* [func (c \*Client) CloseIdleConnections()](#Client.CloseIdleConnections)
* [func (c \*Client) Do(req \*Request) (\*Response, error)](#Client.Do)
* [func (c \*Client) Get(url string) (resp \*Response, err error)](#Client.Get)
* [func (c \*Client) Head(url string) (resp \*Response, err error)](#Client.Head)
* [func (c \*Client) Post(url, contentType string, body io.Reader) (resp \*Response, err error)](#Client.Post)
* [func (c \*Client) PostForm(url string, data url.Values) (resp \*Response, err error)](#Client.PostForm)
* [type CloseNotifier](#CloseNotifier)
* [type ConnState](#ConnState)
* [func (c ConnState) String() string](#ConnState.String)
* [type Cookie](#Cookie)
* [func (c \*Cookie) String() string](#Cookie.String)
* [func (c \*Cookie) Valid() error](#Cookie.Valid)
* [type CookieJar](#CookieJar)
* [type Dir](#Dir)
* [func (d Dir) Open(name string) (File, error)](#Dir.Open)
* [type File](#File)
* [type FileSystem](#FileSystem)
* [func FS(fsys fs.FS) FileSystem](#FS)
* [type Flusher](#Flusher)
* [type Handler](#Handler)
* [func AllowQuerySemicolons(h Handler) Handler](#AllowQuerySemicolons)
* [func FileServer(root FileSystem) Handler](#FileServer)
* [func MaxBytesHandler(h Handler, n int64) Handler](#MaxBytesHandler)
* [func NotFoundHandler() Handler](#NotFoundHandler)
* [func RedirectHandler(url string, code int) Handler](#RedirectHandler)
* [func StripPrefix(prefix string, h Handler) Handler](#StripPrefix)
* [func TimeoutHandler(h Handler, dt time.Duration, msg string) Handler](#TimeoutHandler)
* [type HandlerFunc](#HandlerFunc)
* [func (f HandlerFunc) ServeHTTP(w ResponseWriter, r \*Request)](#HandlerFunc.ServeHTTP)
* [type Header](#Header)
* [func (h Header) Add(key, value string)](#Header.Add)
* [func (h Header) Clone() Header](#Header.Clone)
* [func (h Header) Del(key string)](#Header.Del)
* [func (h Header) Get(key string) string](#Header.Get)
* [func (h Header) Set(key, value string)](#Header.Set)
* [func (h Header) Values(key string) []string](#Header.Values)
* [func (h Header) Write(w io.Writer) error](#Header.Write)
* [func (h Header) WriteSubset(w io.Writer, exclude map[string]bool) error](#Header.WriteSubset)
* [type Hijacker](#Hijacker)
* [type MaxBytesError](#MaxBytesError)
* [func (e \*MaxBytesError) Error() string](#MaxBytesError.Error)
* [type ProtocolError](#ProtocolError)
* [func (pe \*ProtocolError) Error() string](#ProtocolError.Error)
* [type PushOptions](#PushOptions)
* [type Pusher](#Pusher)
* [type Request](#Request)
* [func NewRequest(method, url string, body io.Reader) (\*Request, error)](#NewRequest)
* [func NewRequestWithContext(ctx context.Context, method, url string, body io.Reader) (\*Request, error)](#NewRequestWithContext)
* [func ReadRequest(b \*bufio.Reader) (\*Request, error)](#ReadRequest)
* [func (r \*Request) AddCookie(c \*Cookie)](#Request.AddCookie)
* [func (r \*Request) BasicAuth() (username, password string, ok bool)](#Request.BasicAuth)
* [func (r \*Request) Clone(ctx context.Context) \*Request](#Request.Clone)
* [func (r \*Request) Context() context.Context](#Request.Context)
* [func (r \*Request) Cookie(name string) (\*Cookie, error)](#Request.Cookie)
* [func (r \*Request) Cookies() []\*Cookie](#Request.Cookies)
* [func (r \*Request) FormFile(key string) (multipart.File, \*multipart.FileHeader, error)](#Request.FormFile)
* [func (r \*Request) FormValue(key string) string](#Request.FormValue)
* [func (r \*Request) MultipartReader() (\*multipart.Reader, error)](#Request.MultipartReader)
* [func (r \*Request) ParseForm() error](#Request.ParseForm)
* [func (r \*Request) ParseMultipartForm(maxMemory int64) error](#Request.ParseMultipartForm)
* [func (r \*Request) PostFormValue(key string) string](#Request.PostFormValue)
* [func (r \*Request) ProtoAtLeast(major, minor int) bool](#Request.ProtoAtLeast)
* [func (r \*Request) Referer() string](#Request.Referer)
* [func (r \*Request) SetBasicAuth(username, password string)](#Request.SetBasicAuth)
* [func (r \*Request) UserAgent() string](#Request.UserAgent)
* [func (r \*Request) WithContext(ctx context.Context) \*Request](#Request.WithContext)
* [func (r \*Request) Write(w io.Writer) error](#Request.Write)
* [func (r \*Request) WriteProxy(w io.Writer) error](#Request.WriteProxy)
* [type Response](#Response)
* [func Get(url string) (resp \*Response, err error)](#Get)
* [func Head(url string) (resp \*Response, err error)](#Head)
* [func Post(url, contentType string, body io.Reader) (resp \*Response, err error)](#Post)
* [func PostForm(url string, data url.Values) (resp \*Response, err error)](#PostForm)
* [func ReadResponse(r \*bufio.Reader, req \*Request) (\*Response, error)](#ReadResponse)
* [func (r \*Response) Cookies() []\*Cookie](#Response.Cookies)
* [func (r \*Response) Location() (\*url.URL, error)](#Response.Location)
* [func (r \*Response) ProtoAtLeast(major, minor int) bool](#Response.ProtoAtLeast)
* [func (r \*Response) Write(w io.Writer) error](#Response.Write)
* [type ResponseController](#ResponseController)
* [func NewResponseController(rw ResponseWriter) \*ResponseController](#NewResponseController)
* [func (c \*ResponseController) Flush() error](#ResponseController.Flush)
* [func (c \*ResponseController) Hijack() (net.Conn, \*bufio.ReadWriter, error)](#ResponseController.Hijack)
* [func (c \*ResponseController) SetReadDeadline(deadline time.Time) error](#ResponseController.SetReadDeadline)
* [func (c \*ResponseController) SetWriteDeadline(deadline time.Time) error](#ResponseController.SetWriteDeadline)
* [type ResponseWriter](#ResponseWriter)
* [type RoundTripper](#RoundTripper)
* [func NewFileTransport(fs FileSystem) RoundTripper](#NewFileTransport)
* [type SameSite](#SameSite)
* [type ServeMux](#ServeMux)
* [func NewServeMux() \*ServeMux](#NewServeMux)
* [func (mux \*ServeMux) Handle(pattern string, handler Handler)](#ServeMux.Handle)
* [func (mux \*ServeMux) HandleFunc(pattern string, handler func(ResponseWriter, \*Request))](#ServeMux.HandleFunc)
* [func (mux \*ServeMux) Handler(r \*Request) (h Handler, pattern string)](#ServeMux.Handler)
* [func (mux \*ServeMux) ServeHTTP(w ResponseWriter, r \*Request)](#ServeMux.ServeHTTP)
* [type Server](#Server)
* [func (srv \*Server) Close() error](#Server.Close)
* [func (srv \*Server) ListenAndServe() error](#Server.ListenAndServe)
* [func (srv \*Server) ListenAndServeTLS(certFile, keyFile string) error](#Server.ListenAndServeTLS)
* [func (srv \*Server) RegisterOnShutdown(f func())](#Server.RegisterOnShutdown)
* [func (srv \*Server) Serve(l net.Listener) error](#Server.Serve)
* [func (srv \*Server) ServeTLS(l net.Listener, certFile, keyFile string) error](#Server.ServeTLS)
* [func (srv \*Server) SetKeepAlivesEnabled(v bool)](#Server.SetKeepAlivesEnabled)
* [func (srv \*Server) Shutdown(ctx context.Context) error](#Server.Shutdown)
* [type Transport](#Transport)
* [func (t \*Transport) CancelRequest(req \*Request)](#Transport.CancelRequest)
* [func (t \*Transport) Clone() \*Transport](#Transport.Clone)
* [func (t \*Transport) CloseIdleConnections()](#Transport.CloseIdleConnections)
* [func (t \*Transport) RegisterProtocol(scheme string, rt RoundTripper)](#Transport.RegisterProtocol)
* [func (t \*Transport) RoundTrip(req \*Request) (\*Response, error)](#Transport.RoundTrip)
### Examples
[FileServer](#example_FileServer) [FileServer (DotFileHiding)](#example_FileServer_dotFileHiding) [FileServer (StripPrefix)](#example_FileServer_stripPrefix) [Get](#example_Get) [Handle](#example_Handle) [HandleFunc](#example_HandleFunc) [Hijacker](#example_Hijacker) [ListenAndServe](#example_ListenAndServe) [ListenAndServeTLS](#example_ListenAndServeTLS) [NotFoundHandler](#example_NotFoundHandler) [ResponseWriter (Trailers)](#example_ResponseWriter_trailers) [ServeMux.Handle](#example_ServeMux_Handle) [Server.Shutdown](#example_Server_Shutdown) [StripPrefix](#example_StripPrefix) ### Package files
client.go clone.go cookie.go doc.go filetransport.go fs.go h2\_bundle.go h2\_error.go header.go http.go jar.go method.go request.go response.go responsecontroller.go roundtrip.go server.go sniff.go socks\_bundle.go status.go transfer.go transport.go transport\_default\_other.go
Constants
---------
Common HTTP methods.
Unless otherwise noted, these are defined in RFC 7231 section 4.3.
```
const (
MethodGet = "GET"
MethodHead = "HEAD"
MethodPost = "POST"
MethodPut = "PUT"
MethodPatch = "PATCH" // RFC 5789
MethodDelete = "DELETE"
MethodConnect = "CONNECT"
MethodOptions = "OPTIONS"
MethodTrace = "TRACE"
)
```
HTTP status codes as registered with IANA. See: <https://www.iana.org/assignments/http-status-codes/http-status-codes.xhtml>
```
const (
StatusContinue = 100 // RFC 9110, 15.2.1
StatusSwitchingProtocols = 101 // RFC 9110, 15.2.2
StatusProcessing = 102 // RFC 2518, 10.1
StatusEarlyHints = 103 // RFC 8297
StatusOK = 200 // RFC 9110, 15.3.1
StatusCreated = 201 // RFC 9110, 15.3.2
StatusAccepted = 202 // RFC 9110, 15.3.3
StatusNonAuthoritativeInfo = 203 // RFC 9110, 15.3.4
StatusNoContent = 204 // RFC 9110, 15.3.5
StatusResetContent = 205 // RFC 9110, 15.3.6
StatusPartialContent = 206 // RFC 9110, 15.3.7
StatusMultiStatus = 207 // RFC 4918, 11.1
StatusAlreadyReported = 208 // RFC 5842, 7.1
StatusIMUsed = 226 // RFC 3229, 10.4.1
StatusMultipleChoices = 300 // RFC 9110, 15.4.1
StatusMovedPermanently = 301 // RFC 9110, 15.4.2
StatusFound = 302 // RFC 9110, 15.4.3
StatusSeeOther = 303 // RFC 9110, 15.4.4
StatusNotModified = 304 // RFC 9110, 15.4.5
StatusUseProxy = 305 // RFC 9110, 15.4.6
StatusTemporaryRedirect = 307 // RFC 9110, 15.4.8
StatusPermanentRedirect = 308 // RFC 9110, 15.4.9
StatusBadRequest = 400 // RFC 9110, 15.5.1
StatusUnauthorized = 401 // RFC 9110, 15.5.2
StatusPaymentRequired = 402 // RFC 9110, 15.5.3
StatusForbidden = 403 // RFC 9110, 15.5.4
StatusNotFound = 404 // RFC 9110, 15.5.5
StatusMethodNotAllowed = 405 // RFC 9110, 15.5.6
StatusNotAcceptable = 406 // RFC 9110, 15.5.7
StatusProxyAuthRequired = 407 // RFC 9110, 15.5.8
StatusRequestTimeout = 408 // RFC 9110, 15.5.9
StatusConflict = 409 // RFC 9110, 15.5.10
StatusGone = 410 // RFC 9110, 15.5.11
StatusLengthRequired = 411 // RFC 9110, 15.5.12
StatusPreconditionFailed = 412 // RFC 9110, 15.5.13
StatusRequestEntityTooLarge = 413 // RFC 9110, 15.5.14
StatusRequestURITooLong = 414 // RFC 9110, 15.5.15
StatusUnsupportedMediaType = 415 // RFC 9110, 15.5.16
StatusRequestedRangeNotSatisfiable = 416 // RFC 9110, 15.5.17
StatusExpectationFailed = 417 // RFC 9110, 15.5.18
StatusTeapot = 418 // RFC 9110, 15.5.19 (Unused)
StatusMisdirectedRequest = 421 // RFC 9110, 15.5.20
StatusUnprocessableEntity = 422 // RFC 9110, 15.5.21
StatusLocked = 423 // RFC 4918, 11.3
StatusFailedDependency = 424 // RFC 4918, 11.4
StatusTooEarly = 425 // RFC 8470, 5.2.
StatusUpgradeRequired = 426 // RFC 9110, 15.5.22
StatusPreconditionRequired = 428 // RFC 6585, 3
StatusTooManyRequests = 429 // RFC 6585, 4
StatusRequestHeaderFieldsTooLarge = 431 // RFC 6585, 5
StatusUnavailableForLegalReasons = 451 // RFC 7725, 3
StatusInternalServerError = 500 // RFC 9110, 15.6.1
StatusNotImplemented = 501 // RFC 9110, 15.6.2
StatusBadGateway = 502 // RFC 9110, 15.6.3
StatusServiceUnavailable = 503 // RFC 9110, 15.6.4
StatusGatewayTimeout = 504 // RFC 9110, 15.6.5
StatusHTTPVersionNotSupported = 505 // RFC 9110, 15.6.6
StatusVariantAlsoNegotiates = 506 // RFC 2295, 8.1
StatusInsufficientStorage = 507 // RFC 4918, 11.5
StatusLoopDetected = 508 // RFC 5842, 7.2
StatusNotExtended = 510 // RFC 2774, 7
StatusNetworkAuthenticationRequired = 511 // RFC 6585, 6
)
```
DefaultMaxHeaderBytes is the maximum permitted size of the headers in an HTTP request. This can be overridden by setting Server.MaxHeaderBytes.
```
const DefaultMaxHeaderBytes = 1 << 20 // 1 MB
```
DefaultMaxIdleConnsPerHost is the default value of Transport's MaxIdleConnsPerHost.
```
const DefaultMaxIdleConnsPerHost = 2
```
TimeFormat is the time format to use when generating times in HTTP headers. It is like time.RFC1123 but hard-codes GMT as the time zone. The time being formatted must be in UTC for Format to generate the correct format.
For parsing this time format, see ParseTime.
```
const TimeFormat = "Mon, 02 Jan 2006 15:04:05 GMT"
```
TrailerPrefix is a magic prefix for ResponseWriter.Header map keys that, if present, signals that the map entry is actually for the response trailers, and not the response headers. The prefix is stripped after the ServeHTTP call finishes and the values are sent in the trailers.
This mechanism is intended only for trailers that are not known prior to the headers being written. If the set of trailers is fixed or known before the header is written, the normal Go trailers mechanism is preferred:
```
https://pkg.go.dev/net/http#ResponseWriter
https://pkg.go.dev/net/http#example-ResponseWriter-Trailers
```
```
const TrailerPrefix = "Trailer:"
```
Variables
---------
```
var (
// ErrNotSupported indicates that a feature is not supported.
//
// It is returned by ResponseController methods to indicate that
// the handler does not support the method, and by the Push method
// of Pusher implementations to indicate that HTTP/2 Push support
// is not available.
ErrNotSupported = &ProtocolError{"feature not supported"}
// Deprecated: ErrUnexpectedTrailer is no longer returned by
// anything in the net/http package. Callers should not
// compare errors against this variable.
ErrUnexpectedTrailer = &ProtocolError{"trailer header without chunked transfer encoding"}
// ErrMissingBoundary is returned by Request.MultipartReader when the
// request's Content-Type does not include a "boundary" parameter.
ErrMissingBoundary = &ProtocolError{"no multipart boundary param in Content-Type"}
// ErrNotMultipart is returned by Request.MultipartReader when the
// request's Content-Type is not multipart/form-data.
ErrNotMultipart = &ProtocolError{"request Content-Type isn't multipart/form-data"}
// Deprecated: ErrHeaderTooLong is no longer returned by
// anything in the net/http package. Callers should not
// compare errors against this variable.
ErrHeaderTooLong = &ProtocolError{"header too long"}
// Deprecated: ErrShortBody is no longer returned by
// anything in the net/http package. Callers should not
// compare errors against this variable.
ErrShortBody = &ProtocolError{"entity body too short"}
// Deprecated: ErrMissingContentLength is no longer returned by
// anything in the net/http package. Callers should not
// compare errors against this variable.
ErrMissingContentLength = &ProtocolError{"missing ContentLength in HEAD response"}
)
```
Errors used by the HTTP server.
```
var (
// ErrBodyNotAllowed is returned by ResponseWriter.Write calls
// when the HTTP method or response code does not permit a
// body.
ErrBodyNotAllowed = errors.New("http: request method or response status code does not allow body")
// ErrHijacked is returned by ResponseWriter.Write calls when
// the underlying connection has been hijacked using the
// Hijacker interface. A zero-byte write on a hijacked
// connection will return ErrHijacked without any other side
// effects.
ErrHijacked = errors.New("http: connection has been hijacked")
// ErrContentLength is returned by ResponseWriter.Write calls
// when a Handler set a Content-Length response header with a
// declared size and then attempted to write more bytes than
// declared.
ErrContentLength = errors.New("http: wrote more than the declared Content-Length")
// Deprecated: ErrWriteAfterFlush is no longer returned by
// anything in the net/http package. Callers should not
// compare errors against this variable.
ErrWriteAfterFlush = errors.New("unused")
)
```
```
var (
// ServerContextKey is a context key. It can be used in HTTP
// handlers with Context.Value to access the server that
// started the handler. The associated value will be of
// type *Server.
ServerContextKey = &contextKey{"http-server"}
// LocalAddrContextKey is a context key. It can be used in
// HTTP handlers with Context.Value to access the local
// address the connection arrived on.
// The associated value will be of type net.Addr.
LocalAddrContextKey = &contextKey{"local-addr"}
)
```
DefaultClient is the default Client and is used by Get, Head, and Post.
```
var DefaultClient = &Client{}
```
DefaultServeMux is the default ServeMux used by Serve.
```
var DefaultServeMux = &defaultServeMux
```
ErrAbortHandler is a sentinel panic value to abort a handler. While any panic from ServeHTTP aborts the response to the client, panicking with ErrAbortHandler also suppresses logging of a stack trace to the server's error log.
```
var ErrAbortHandler = errors.New("net/http: abort Handler")
```
ErrBodyReadAfterClose is returned when reading a Request or Response Body after the body has been closed. This typically happens when the body is read after an HTTP Handler calls WriteHeader or Write on its ResponseWriter.
```
var ErrBodyReadAfterClose = errors.New("http: invalid Read on closed Body")
```
ErrHandlerTimeout is returned on ResponseWriter Write calls in handlers which have timed out.
```
var ErrHandlerTimeout = errors.New("http: Handler timeout")
```
ErrLineTooLong is returned when reading request or response bodies with malformed chunked encoding.
```
var ErrLineTooLong = internal.ErrLineTooLong
```
ErrMissingFile is returned by FormFile when the provided file field name is either not present in the request or not a file field.
```
var ErrMissingFile = errors.New("http: no such file")
```
ErrNoCookie is returned by Request's Cookie method when a cookie is not found.
```
var ErrNoCookie = errors.New("http: named cookie not present")
```
ErrNoLocation is returned by Response's Location method when no Location header is present.
```
var ErrNoLocation = errors.New("http: no Location header in response")
```
ErrServerClosed is returned by the Server's Serve, ServeTLS, ListenAndServe, and ListenAndServeTLS methods after a call to Shutdown or Close.
```
var ErrServerClosed = errors.New("http: Server closed")
```
ErrSkipAltProtocol is a sentinel error value defined by Transport.RegisterProtocol.
```
var ErrSkipAltProtocol = errors.New("net/http: skip alternate protocol")
```
ErrUseLastResponse can be returned by Client.CheckRedirect hooks to control how redirects are processed. If returned, the next request is not sent and the most recent response is returned with its body unclosed.
```
var ErrUseLastResponse = errors.New("net/http: use last response")
```
NoBody is an io.ReadCloser with no bytes. Read always returns EOF and Close always returns nil. It can be used in an outgoing client request to explicitly signal that a request has zero bytes. An alternative, however, is to simply set Request.Body to nil.
```
var NoBody = noBody{}
```
func CanonicalHeaderKey
-----------------------
```
func CanonicalHeaderKey(s string) string
```
CanonicalHeaderKey returns the canonical format of the header key s. The canonicalization converts the first letter and any letter following a hyphen to upper case; the rest are converted to lowercase. For example, the canonical key for "accept-encoding" is "Accept-Encoding". If s contains a space or invalid header field bytes, it is returned without modifications.
func DetectContentType
----------------------
```
func DetectContentType(data []byte) string
```
DetectContentType implements the algorithm described at <https://mimesniff.spec.whatwg.org/> to determine the Content-Type of the given data. It considers at most the first 512 bytes of data. DetectContentType always returns a valid MIME type: if it cannot determine a more specific one, it returns "application/octet-stream".
func Error
----------
```
func Error(w ResponseWriter, error string, code int)
```
Error replies to the request with the specified error message and HTTP code. It does not otherwise end the request; the caller should ensure no further writes are done to w. The error message should be plain text.
func Handle
-----------
```
func Handle(pattern string, handler Handler)
```
Handle registers the handler for the given pattern in the DefaultServeMux. The documentation for ServeMux explains how patterns are matched.
#### Example
Code:
```
package http_test
import (
"fmt"
"log"
"net/http"
"sync"
)
type countHandler struct {
mu sync.Mutex // guards n
n int
}
func (h *countHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
h.mu.Lock()
defer h.mu.Unlock()
h.n++
fmt.Fprintf(w, "count is %d\n", h.n)
}
func ExampleHandle() {
http.Handle("/count", new(countHandler))
log.Fatal(http.ListenAndServe(":8080", nil))
}
```
func HandleFunc
---------------
```
func HandleFunc(pattern string, handler func(ResponseWriter, *Request))
```
HandleFunc registers the handler function for the given pattern in the DefaultServeMux. The documentation for ServeMux explains how patterns are matched.
#### Example
Code:
```
h1 := func(w http.ResponseWriter, _ *http.Request) {
io.WriteString(w, "Hello from a HandleFunc #1!\n")
}
h2 := func(w http.ResponseWriter, _ *http.Request) {
io.WriteString(w, "Hello from a HandleFunc #2!\n")
}
http.HandleFunc("/", h1)
http.HandleFunc("/endpoint", h2)
log.Fatal(http.ListenAndServe(":8080", nil))
```
func ListenAndServe
-------------------
```
func ListenAndServe(addr string, handler Handler) error
```
ListenAndServe listens on the TCP network address addr and then calls Serve with handler to handle requests on incoming connections. Accepted connections are configured to enable TCP keep-alives.
The handler is typically nil, in which case the DefaultServeMux is used.
ListenAndServe always returns a non-nil error.
#### Example
Code:
```
// Hello world, the web server
helloHandler := func(w http.ResponseWriter, req *http.Request) {
io.WriteString(w, "Hello, world!\n")
}
http.HandleFunc("/hello", helloHandler)
log.Fatal(http.ListenAndServe(":8080", nil))
```
func ListenAndServeTLS
----------------------
```
func ListenAndServeTLS(addr, certFile, keyFile string, handler Handler) error
```
ListenAndServeTLS acts identically to ListenAndServe, except that it expects HTTPS connections. Additionally, files containing a certificate and matching private key for the server must be provided. If the certificate is signed by a certificate authority, the certFile should be the concatenation of the server's certificate, any intermediates, and the CA's certificate.
#### Example
Code:
```
http.HandleFunc("/", func(w http.ResponseWriter, req *http.Request) {
io.WriteString(w, "Hello, TLS!\n")
})
// One can use generate_cert.go in crypto/tls to generate cert.pem and key.pem.
log.Printf("About to listen on 8443. Go to https://127.0.0.1:8443/")
err := http.ListenAndServeTLS(":8443", "cert.pem", "key.pem", nil)
log.Fatal(err)
```
func MaxBytesReader
-------------------
```
func MaxBytesReader(w ResponseWriter, r io.ReadCloser, n int64) io.ReadCloser
```
MaxBytesReader is similar to io.LimitReader but is intended for limiting the size of incoming request bodies. In contrast to io.LimitReader, MaxBytesReader's result is a ReadCloser, returns a non-nil error of type \*MaxBytesError for a Read beyond the limit, and closes the underlying reader when its Close method is called.
MaxBytesReader prevents clients from accidentally or maliciously sending a large request and wasting server resources. If possible, it tells the ResponseWriter to close the connection after the limit has been reached.
func NotFound
-------------
```
func NotFound(w ResponseWriter, r *Request)
```
NotFound replies to the request with an HTTP 404 not found error.
func ParseHTTPVersion
---------------------
```
func ParseHTTPVersion(vers string) (major, minor int, ok bool)
```
ParseHTTPVersion parses an HTTP version string according to RFC 7230, section 2.6. "HTTP/1.0" returns (1, 0, true). Note that strings without a minor version, such as "HTTP/2", are not valid.
func ParseTime 1.1
------------------
```
func ParseTime(text string) (t time.Time, err error)
```
ParseTime parses a time header (such as the Date: header), trying each of the three formats allowed by HTTP/1.1: TimeFormat, time.RFC850, and time.ANSIC.
func ProxyFromEnvironment
-------------------------
```
func ProxyFromEnvironment(req *Request) (*url.URL, error)
```
ProxyFromEnvironment returns the URL of the proxy to use for a given request, as indicated by the environment variables HTTP\_PROXY, HTTPS\_PROXY and NO\_PROXY (or the lowercase versions thereof). Requests use the proxy from the environment variable matching their scheme, unless excluded by NO\_PROXY.
The environment values may be either a complete URL or a "host[:port]", in which case the "http" scheme is assumed. The schemes "http", "https", and "socks5" are supported. An error is returned if the value is a different form.
A nil URL and nil error are returned if no proxy is defined in the environment, or a proxy should not be used for the given request, as defined by NO\_PROXY.
As a special case, if req.URL.Host is "localhost" (with or without a port number), then a nil URL and nil error will be returned.
func ProxyURL
-------------
```
func ProxyURL(fixedURL *url.URL) func(*Request) (*url.URL, error)
```
ProxyURL returns a proxy function (for use in a Transport) that always returns the same URL.
func Redirect
-------------
```
func Redirect(w ResponseWriter, r *Request, url string, code int)
```
Redirect replies to the request with a redirect to url, which may be a path relative to the request path.
The provided code should be in the 3xx range and is usually StatusMovedPermanently, StatusFound or StatusSeeOther.
If the Content-Type header has not been set, Redirect sets it to "text/html; charset=utf-8" and writes a small HTML body. Setting the Content-Type header to any value, including nil, disables that behavior.
func Serve
----------
```
func Serve(l net.Listener, handler Handler) error
```
Serve accepts incoming HTTP connections on the listener l, creating a new service goroutine for each. The service goroutines read requests and then call handler to reply to them.
The handler is typically nil, in which case the DefaultServeMux is used.
HTTP/2 support is only enabled if the Listener returns \*tls.Conn connections and they were configured with "h2" in the TLS Config.NextProtos.
Serve always returns a non-nil error.
func ServeContent
-----------------
```
func ServeContent(w ResponseWriter, req *Request, name string, modtime time.Time, content io.ReadSeeker)
```
ServeContent replies to the request using the content in the provided ReadSeeker. The main benefit of ServeContent over io.Copy is that it handles Range requests properly, sets the MIME type, and handles If-Match, If-Unmodified-Since, If-None-Match, If-Modified-Since, and If-Range requests.
If the response's Content-Type header is not set, ServeContent first tries to deduce the type from name's file extension and, if that fails, falls back to reading the first block of the content and passing it to DetectContentType. The name is otherwise unused; in particular it can be empty and is never sent in the response.
If modtime is not the zero time or Unix epoch, ServeContent includes it in a Last-Modified header in the response. If the request includes an If-Modified-Since header, ServeContent uses modtime to decide whether the content needs to be sent at all.
The content's Seek method must work: ServeContent uses a seek to the end of the content to determine its size.
If the caller has set w's ETag header formatted per RFC 7232, section 2.3, ServeContent uses it to handle requests using If-Match, If-None-Match, or If-Range.
Note that \*os.File implements the io.ReadSeeker interface.
func ServeFile
--------------
```
func ServeFile(w ResponseWriter, r *Request, name string)
```
ServeFile replies to the request with the contents of the named file or directory.
If the provided file or directory name is a relative path, it is interpreted relative to the current directory and may ascend to parent directories. If the provided name is constructed from user input, it should be sanitized before calling ServeFile.
As a precaution, ServeFile will reject requests where r.URL.Path contains a ".." path element; this protects against callers who might unsafely use filepath.Join on r.URL.Path without sanitizing it and then use that filepath.Join result as the name argument.
As another special case, ServeFile redirects any request where r.URL.Path ends in "/index.html" to the same path, without the final "index.html". To avoid such redirects either modify the path or use ServeContent.
Outside of those two special cases, ServeFile does not use r.URL.Path for selecting the file or directory to serve; only the file or directory provided in the name argument is used.
func ServeTLS 1.9
-----------------
```
func ServeTLS(l net.Listener, handler Handler, certFile, keyFile string) error
```
ServeTLS accepts incoming HTTPS connections on the listener l, creating a new service goroutine for each. The service goroutines read requests and then call handler to reply to them.
The handler is typically nil, in which case the DefaultServeMux is used.
Additionally, files containing a certificate and matching private key for the server must be provided. If the certificate is signed by a certificate authority, the certFile should be the concatenation of the server's certificate, any intermediates, and the CA's certificate.
ServeTLS always returns a non-nil error.
func SetCookie
--------------
```
func SetCookie(w ResponseWriter, cookie *Cookie)
```
SetCookie adds a Set-Cookie header to the provided ResponseWriter's headers. The provided cookie must have a valid Name. Invalid cookies may be silently dropped.
func StatusText
---------------
```
func StatusText(code int) string
```
StatusText returns a text for the HTTP status code. It returns the empty string if the code is unknown.
type Client
-----------
A Client is an HTTP client. Its zero value (DefaultClient) is a usable client that uses DefaultTransport.
The Client's Transport typically has internal state (cached TCP connections), so Clients should be reused instead of created as needed. Clients are safe for concurrent use by multiple goroutines.
A Client is higher-level than a RoundTripper (such as Transport) and additionally handles HTTP details such as cookies and redirects.
When following redirects, the Client will forward all headers set on the initial Request except:
• when forwarding sensitive headers like "Authorization", "WWW-Authenticate", and "Cookie" to untrusted targets. These headers will be ignored when following a redirect to a domain that is not a subdomain match or exact match of the initial domain. For example, a redirect from "foo.com" to either "foo.com" or "sub.foo.com" will forward the sensitive headers, but a redirect to "bar.com" will not.
• when forwarding the "Cookie" header with a non-nil cookie Jar. Since each redirect may mutate the state of the cookie jar, a redirect may possibly alter a cookie set in the initial request. When forwarding the "Cookie" header, any mutated cookies will be omitted, with the expectation that the Jar will insert those mutated cookies with the updated values (assuming the origin matches). If Jar is nil, the initial cookies are forwarded without change.
```
type Client struct {
// Transport specifies the mechanism by which individual
// HTTP requests are made.
// If nil, DefaultTransport is used.
Transport RoundTripper
// CheckRedirect specifies the policy for handling redirects.
// If CheckRedirect is not nil, the client calls it before
// following an HTTP redirect. The arguments req and via are
// the upcoming request and the requests made already, oldest
// first. If CheckRedirect returns an error, the Client's Get
// method returns both the previous Response (with its Body
// closed) and CheckRedirect's error (wrapped in a url.Error)
// instead of issuing the Request req.
// As a special case, if CheckRedirect returns ErrUseLastResponse,
// then the most recent response is returned with its body
// unclosed, along with a nil error.
//
// If CheckRedirect is nil, the Client uses its default policy,
// which is to stop after 10 consecutive requests.
CheckRedirect func(req *Request, via []*Request) error
// Jar specifies the cookie jar.
//
// The Jar is used to insert relevant cookies into every
// outbound Request and is updated with the cookie values
// of every inbound Response. The Jar is consulted for every
// redirect that the Client follows.
//
// If Jar is nil, cookies are only sent if they are explicitly
// set on the Request.
Jar CookieJar
// Timeout specifies a time limit for requests made by this
// Client. The timeout includes connection time, any
// redirects, and reading the response body. The timer remains
// running after Get, Head, Post, or Do return and will
// interrupt reading of the Response.Body.
//
// A Timeout of zero means no timeout.
//
// The Client cancels requests to the underlying Transport
// as if the Request's Context ended.
//
// For compatibility, the Client will also use the deprecated
// CancelRequest method on Transport if found. New
// RoundTripper implementations should use the Request's Context
// for cancellation instead of implementing CancelRequest.
Timeout time.Duration // Go 1.3
}
```
### func (\*Client) CloseIdleConnections 1.12
```
func (c *Client) CloseIdleConnections()
```
CloseIdleConnections closes any connections on its Transport which were previously connected from previous requests but are now sitting idle in a "keep-alive" state. It does not interrupt any connections currently in use.
If the Client's Transport does not have a CloseIdleConnections method then this method does nothing.
### func (\*Client) Do
```
func (c *Client) Do(req *Request) (*Response, error)
```
Do sends an HTTP request and returns an HTTP response, following policy (such as redirects, cookies, auth) as configured on the client.
An error is returned if caused by client policy (such as CheckRedirect), or failure to speak HTTP (such as a network connectivity problem). A non-2xx status code doesn't cause an error.
If the returned error is nil, the Response will contain a non-nil Body which the user is expected to close. If the Body is not both read to EOF and closed, the Client's underlying RoundTripper (typically Transport) may not be able to re-use a persistent TCP connection to the server for a subsequent "keep-alive" request.
The request Body, if non-nil, will be closed by the underlying Transport, even on errors.
On error, any Response can be ignored. A non-nil Response with a non-nil error only occurs when CheckRedirect fails, and even then the returned Response.Body is already closed.
Generally Get, Post, or PostForm will be used instead of Do.
If the server replies with a redirect, the Client first uses the CheckRedirect function to determine whether the redirect should be followed. If permitted, a 301, 302, or 303 redirect causes subsequent requests to use HTTP method GET (or HEAD if the original request was HEAD), with no body. A 307 or 308 redirect preserves the original HTTP method and body, provided that the Request.GetBody function is defined. The NewRequest function automatically sets GetBody for common standard library body types.
Any returned error will be of type \*url.Error. The url.Error value's Timeout method will report true if the request timed out.
### func (\*Client) Get
```
func (c *Client) Get(url string) (resp *Response, err error)
```
Get issues a GET to the specified URL. If the response is one of the following redirect codes, Get follows the redirect after calling the Client's CheckRedirect function:
```
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
```
An error is returned if the Client's CheckRedirect function fails or if there was an HTTP protocol error. A non-2xx response doesn't cause an error. Any returned error will be of type \*url.Error. The url.Error value's Timeout method will report true if the request timed out.
When err is nil, resp always contains a non-nil resp.Body. Caller should close resp.Body when done reading from it.
To make a request with custom headers, use NewRequest and Client.Do.
To make a request with a specified context.Context, use NewRequestWithContext and Client.Do.
### func (\*Client) Head
```
func (c *Client) Head(url string) (resp *Response, err error)
```
Head issues a HEAD to the specified URL. If the response is one of the following redirect codes, Head follows the redirect after calling the Client's CheckRedirect function:
```
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
```
To make a request with a specified context.Context, use NewRequestWithContext and Client.Do.
### func (\*Client) Post
```
func (c *Client) Post(url, contentType string, body io.Reader) (resp *Response, err error)
```
Post issues a POST to the specified URL.
Caller should close resp.Body when done reading from it.
If the provided body is an io.Closer, it is closed after the request.
To set custom headers, use NewRequest and Client.Do.
To make a request with a specified context.Context, use NewRequestWithContext and Client.Do.
See the Client.Do method documentation for details on how redirects are handled.
### func (\*Client) PostForm
```
func (c *Client) PostForm(url string, data url.Values) (resp *Response, err error)
```
PostForm issues a POST to the specified URL, with data's keys and values URL-encoded as the request body.
The Content-Type header is set to application/x-www-form-urlencoded. To set other headers, use NewRequest and Client.Do.
When err is nil, resp always contains a non-nil resp.Body. Caller should close resp.Body when done reading from it.
See the Client.Do method documentation for details on how redirects are handled.
To make a request with a specified context.Context, use NewRequestWithContext and Client.Do.
type CloseNotifier 1.1
----------------------
The CloseNotifier interface is implemented by ResponseWriters which allow detecting when the underlying connection has gone away.
This mechanism can be used to cancel long operations on the server if the client has disconnected before the response is ready.
Deprecated: the CloseNotifier interface predates Go's context package. New code should use Request.Context instead.
```
type CloseNotifier interface {
// CloseNotify returns a channel that receives at most a
// single value (true) when the client connection has gone
// away.
//
// CloseNotify may wait to notify until Request.Body has been
// fully read.
//
// After the Handler has returned, there is no guarantee
// that the channel receives a value.
//
// If the protocol is HTTP/1.1 and CloseNotify is called while
// processing an idempotent request (such a GET) while
// HTTP/1.1 pipelining is in use, the arrival of a subsequent
// pipelined request may cause a value to be sent on the
// returned channel. In practice HTTP/1.1 pipelining is not
// enabled in browsers and not seen often in the wild. If this
// is a problem, use HTTP/2 or only use CloseNotify on methods
// such as POST.
CloseNotify() <-chan bool
}
```
type ConnState 1.3
------------------
A ConnState represents the state of a client connection to a server. It's used by the optional Server.ConnState hook.
```
type ConnState int
```
```
const (
// StateNew represents a new connection that is expected to
// send a request immediately. Connections begin at this
// state and then transition to either StateActive or
// StateClosed.
StateNew ConnState = iota
// StateActive represents a connection that has read 1 or more
// bytes of a request. The Server.ConnState hook for
// StateActive fires before the request has entered a handler
// and doesn't fire again until the request has been
// handled. After the request is handled, the state
// transitions to StateClosed, StateHijacked, or StateIdle.
// For HTTP/2, StateActive fires on the transition from zero
// to one active request, and only transitions away once all
// active requests are complete. That means that ConnState
// cannot be used to do per-request work; ConnState only notes
// the overall state of the connection.
StateActive
// StateIdle represents a connection that has finished
// handling a request and is in the keep-alive state, waiting
// for a new request. Connections transition from StateIdle
// to either StateActive or StateClosed.
StateIdle
// StateHijacked represents a hijacked connection.
// This is a terminal state. It does not transition to StateClosed.
StateHijacked
// StateClosed represents a closed connection.
// This is a terminal state. Hijacked connections do not
// transition to StateClosed.
StateClosed
)
```
### func (ConnState) String 1.3
```
func (c ConnState) String() string
```
type Cookie
-----------
A Cookie represents an HTTP cookie as sent in the Set-Cookie header of an HTTP response or the Cookie header of an HTTP request.
See <https://tools.ietf.org/html/rfc6265> for details.
```
type Cookie struct {
Name string
Value string
Path string // optional
Domain string // optional
Expires time.Time // optional
RawExpires string // for reading cookies only
// MaxAge=0 means no 'Max-Age' attribute specified.
// MaxAge<0 means delete cookie now, equivalently 'Max-Age: 0'
// MaxAge>0 means Max-Age attribute present and given in seconds
MaxAge int
Secure bool
HttpOnly bool
SameSite SameSite // Go 1.11
Raw string
Unparsed []string // Raw text of unparsed attribute-value pairs
}
```
### func (\*Cookie) String
```
func (c *Cookie) String() string
```
String returns the serialization of the cookie for use in a Cookie header (if only Name and Value are set) or a Set-Cookie response header (if other fields are set). If c is nil or c.Name is invalid, the empty string is returned.
### func (\*Cookie) Valid 1.18
```
func (c *Cookie) Valid() error
```
Valid reports whether the cookie is valid.
type CookieJar
--------------
A CookieJar manages storage and use of cookies in HTTP requests.
Implementations of CookieJar must be safe for concurrent use by multiple goroutines.
The net/http/cookiejar package provides a CookieJar implementation.
```
type CookieJar interface {
// SetCookies handles the receipt of the cookies in a reply for the
// given URL. It may or may not choose to save the cookies, depending
// on the jar's policy and implementation.
SetCookies(u *url.URL, cookies []*Cookie)
// Cookies returns the cookies to send in a request for the given URL.
// It is up to the implementation to honor the standard cookie use
// restrictions such as in RFC 6265.
Cookies(u *url.URL) []*Cookie
}
```
type Dir
--------
A Dir implements FileSystem using the native file system restricted to a specific directory tree.
While the FileSystem.Open method takes '/'-separated paths, a Dir's string value is a filename on the native file system, not a URL, so it is separated by filepath.Separator, which isn't necessarily '/'.
Note that Dir could expose sensitive files and directories. Dir will follow symlinks pointing out of the directory tree, which can be especially dangerous if serving from a directory in which users are able to create arbitrary symlinks. Dir will also allow access to files and directories starting with a period, which could expose sensitive directories like .git or sensitive files like .htpasswd. To exclude files with a leading period, remove the files/directories from the server or create a custom FileSystem implementation.
An empty Dir is treated as ".".
```
type Dir string
```
### func (Dir) Open
```
func (d Dir) Open(name string) (File, error)
```
Open implements FileSystem using os.Open, opening files for reading rooted and relative to the directory d.
type File
---------
A File is returned by a FileSystem's Open method and can be served by the FileServer implementation.
The methods should behave the same as those on an \*os.File.
```
type File interface {
io.Closer
io.Reader
io.Seeker
Readdir(count int) ([]fs.FileInfo, error)
Stat() (fs.FileInfo, error)
}
```
type FileSystem
---------------
A FileSystem implements access to a collection of named files. The elements in a file path are separated by slash ('/', U+002F) characters, regardless of host operating system convention. See the FileServer function to convert a FileSystem to a Handler.
This interface predates the fs.FS interface, which can be used instead: the FS adapter function converts an fs.FS to a FileSystem.
```
type FileSystem interface {
Open(name string) (File, error)
}
```
### func FS 1.16
```
func FS(fsys fs.FS) FileSystem
```
FS converts fsys to a FileSystem implementation, for use with FileServer and NewFileTransport. The files provided by fsys must implement io.Seeker.
type Flusher
------------
The Flusher interface is implemented by ResponseWriters that allow an HTTP handler to flush buffered data to the client.
The default HTTP/1.x and HTTP/2 ResponseWriter implementations support Flusher, but ResponseWriter wrappers may not. Handlers should always test for this ability at runtime.
Note that even for ResponseWriters that support Flush, if the client is connected through an HTTP proxy, the buffered data may not reach the client until the response completes.
```
type Flusher interface {
// Flush sends any buffered data to the client.
Flush()
}
```
type Handler
------------
A Handler responds to an HTTP request.
ServeHTTP should write reply headers and data to the ResponseWriter and then return. Returning signals that the request is finished; it is not valid to use the ResponseWriter or read from the Request.Body after or concurrently with the completion of the ServeHTTP call.
Depending on the HTTP client software, HTTP protocol version, and any intermediaries between the client and the Go server, it may not be possible to read from the Request.Body after writing to the ResponseWriter. Cautious handlers should read the Request.Body first, and then reply.
Except for reading the body, handlers should not modify the provided Request.
If ServeHTTP panics, the server (the caller of ServeHTTP) assumes that the effect of the panic was isolated to the active request. It recovers the panic, logs a stack trace to the server error log, and either closes the network connection or sends an HTTP/2 RST\_STREAM, depending on the HTTP protocol. To abort a handler so the client sees an interrupted response but the server doesn't log an error, panic with the value ErrAbortHandler.
```
type Handler interface {
ServeHTTP(ResponseWriter, *Request)
}
```
### func AllowQuerySemicolons 1.17
```
func AllowQuerySemicolons(h Handler) Handler
```
AllowQuerySemicolons returns a handler that serves requests by converting any unescaped semicolons in the URL query to ampersands, and invoking the handler h.
This restores the pre-Go 1.17 behavior of splitting query parameters on both semicolons and ampersands. (See golang.org/issue/25192). Note that this behavior doesn't match that of many proxies, and the mismatch can lead to security issues.
AllowQuerySemicolons should be invoked before Request.ParseForm is called.
### func FileServer
```
func FileServer(root FileSystem) Handler
```
FileServer returns a handler that serves HTTP requests with the contents of the file system rooted at root.
As a special case, the returned file server redirects any request ending in "/index.html" to the same path, without the final "index.html".
To use the operating system's file system implementation, use http.Dir:
```
http.Handle("/", http.FileServer(http.Dir("/tmp")))
```
To use an fs.FS implementation, use http.FS to convert it:
```
http.Handle("/", http.FileServer(http.FS(fsys)))
```
#### Example
Code:
```
// Simple static webserver:
log.Fatal(http.ListenAndServe(":8080", http.FileServer(http.Dir("/usr/share/doc"))))
```
#### Example (DotFileHiding)
Code:
```
package http_test
import (
"io/fs"
"log"
"net/http"
"strings"
)
// containsDotFile reports whether name contains a path element starting with a period.
// The name is assumed to be a delimited by forward slashes, as guaranteed
// by the http.FileSystem interface.
func containsDotFile(name string) bool {
parts := strings.Split(name, "/")
for _, part := range parts {
if strings.HasPrefix(part, ".") {
return true
}
}
return false
}
// dotFileHidingFile is the http.File use in dotFileHidingFileSystem.
// It is used to wrap the Readdir method of http.File so that we can
// remove files and directories that start with a period from its output.
type dotFileHidingFile struct {
http.File
}
// Readdir is a wrapper around the Readdir method of the embedded File
// that filters out all files that start with a period in their name.
func (f dotFileHidingFile) Readdir(n int) (fis []fs.FileInfo, err error) {
files, err := f.File.Readdir(n)
for _, file := range files { // Filters out the dot files
if !strings.HasPrefix(file.Name(), ".") {
fis = append(fis, file)
}
}
return
}
// dotFileHidingFileSystem is an http.FileSystem that hides
// hidden "dot files" from being served.
type dotFileHidingFileSystem struct {
http.FileSystem
}
// Open is a wrapper around the Open method of the embedded FileSystem
// that serves a 403 permission error when name has a file or directory
// with whose name starts with a period in its path.
func (fsys dotFileHidingFileSystem) Open(name string) (http.File, error) {
if containsDotFile(name) { // If dot file, return 403 response
return nil, fs.ErrPermission
}
file, err := fsys.FileSystem.Open(name)
if err != nil {
return nil, err
}
return dotFileHidingFile{file}, err
}
func ExampleFileServer_dotFileHiding() {
fsys := dotFileHidingFileSystem{http.Dir(".")}
http.Handle("/", http.FileServer(fsys))
log.Fatal(http.ListenAndServe(":8080", nil))
}
```
#### Example (StripPrefix)
Code:
```
// To serve a directory on disk (/tmp) under an alternate URL
// path (/tmpfiles/), use StripPrefix to modify the request
// URL's path before the FileServer sees it:
http.Handle("/tmpfiles/", http.StripPrefix("/tmpfiles/", http.FileServer(http.Dir("/tmp"))))
```
### func MaxBytesHandler 1.18
```
func MaxBytesHandler(h Handler, n int64) Handler
```
MaxBytesHandler returns a Handler that runs h with its ResponseWriter and Request.Body wrapped by a MaxBytesReader.
### func NotFoundHandler
```
func NotFoundHandler() Handler
```
NotFoundHandler returns a simple request handler that replies to each request with a “404 page not found” reply.
#### Example
Code:
```
mux := http.NewServeMux()
// Create sample handler to returns 404
mux.Handle("/resources", http.NotFoundHandler())
// Create sample handler that returns 200
mux.Handle("/resources/people/", newPeopleHandler())
log.Fatal(http.ListenAndServe(":8080", mux))
```
### func RedirectHandler
```
func RedirectHandler(url string, code int) Handler
```
RedirectHandler returns a request handler that redirects each request it receives to the given url using the given status code.
The provided code should be in the 3xx range and is usually StatusMovedPermanently, StatusFound or StatusSeeOther.
### func StripPrefix
```
func StripPrefix(prefix string, h Handler) Handler
```
StripPrefix returns a handler that serves HTTP requests by removing the given prefix from the request URL's Path (and RawPath if set) and invoking the handler h. StripPrefix handles a request for a path that doesn't begin with prefix by replying with an HTTP 404 not found error. The prefix must match exactly: if the prefix in the request contains escaped characters the reply is also an HTTP 404 not found error.
#### Example
Code:
```
// To serve a directory on disk (/tmp) under an alternate URL
// path (/tmpfiles/), use StripPrefix to modify the request
// URL's path before the FileServer sees it:
http.Handle("/tmpfiles/", http.StripPrefix("/tmpfiles/", http.FileServer(http.Dir("/tmp"))))
```
### func TimeoutHandler
```
func TimeoutHandler(h Handler, dt time.Duration, msg string) Handler
```
TimeoutHandler returns a Handler that runs h with the given time limit.
The new Handler calls h.ServeHTTP to handle each request, but if a call runs for longer than its time limit, the handler responds with a 503 Service Unavailable error and the given message in its body. (If msg is empty, a suitable default message will be sent.) After such a timeout, writes by h to its ResponseWriter will return ErrHandlerTimeout.
TimeoutHandler supports the Pusher interface but does not support the Hijacker or Flusher interfaces.
type HandlerFunc
----------------
The HandlerFunc type is an adapter to allow the use of ordinary functions as HTTP handlers. If f is a function with the appropriate signature, HandlerFunc(f) is a Handler that calls f.
```
type HandlerFunc func(ResponseWriter, *Request)
```
### func (HandlerFunc) ServeHTTP
```
func (f HandlerFunc) ServeHTTP(w ResponseWriter, r *Request)
```
ServeHTTP calls f(w, r).
type Header
-----------
A Header represents the key-value pairs in an HTTP header.
The keys should be in canonical form, as returned by CanonicalHeaderKey.
```
type Header map[string][]string
```
### func (Header) Add
```
func (h Header) Add(key, value string)
```
Add adds the key, value pair to the header. It appends to any existing values associated with key. The key is case insensitive; it is canonicalized by CanonicalHeaderKey.
### func (Header) Clone 1.13
```
func (h Header) Clone() Header
```
Clone returns a copy of h or nil if h is nil.
### func (Header) Del
```
func (h Header) Del(key string)
```
Del deletes the values associated with key. The key is case insensitive; it is canonicalized by CanonicalHeaderKey.
### func (Header) Get
```
func (h Header) Get(key string) string
```
Get gets the first value associated with the given key. If there are no values associated with the key, Get returns "". It is case insensitive; textproto.CanonicalMIMEHeaderKey is used to canonicalize the provided key. Get assumes that all keys are stored in canonical form. To use non-canonical keys, access the map directly.
### func (Header) Set
```
func (h Header) Set(key, value string)
```
Set sets the header entries associated with key to the single element value. It replaces any existing values associated with key. The key is case insensitive; it is canonicalized by textproto.CanonicalMIMEHeaderKey. To use non-canonical keys, assign to the map directly.
### func (Header) Values 1.14
```
func (h Header) Values(key string) []string
```
Values returns all values associated with the given key. It is case insensitive; textproto.CanonicalMIMEHeaderKey is used to canonicalize the provided key. To use non-canonical keys, access the map directly. The returned slice is not a copy.
### func (Header) Write
```
func (h Header) Write(w io.Writer) error
```
Write writes a header in wire format.
### func (Header) WriteSubset
```
func (h Header) WriteSubset(w io.Writer, exclude map[string]bool) error
```
WriteSubset writes a header in wire format. If exclude is not nil, keys where exclude[key] == true are not written. Keys are not canonicalized before checking the exclude map.
type Hijacker
-------------
The Hijacker interface is implemented by ResponseWriters that allow an HTTP handler to take over the connection.
The default ResponseWriter for HTTP/1.x connections supports Hijacker, but HTTP/2 connections intentionally do not. ResponseWriter wrappers may also not support Hijacker. Handlers should always test for this ability at runtime.
```
type Hijacker interface {
// Hijack lets the caller take over the connection.
// After a call to Hijack the HTTP server library
// will not do anything else with the connection.
//
// It becomes the caller's responsibility to manage
// and close the connection.
//
// The returned net.Conn may have read or write deadlines
// already set, depending on the configuration of the
// Server. It is the caller's responsibility to set
// or clear those deadlines as needed.
//
// The returned bufio.Reader may contain unprocessed buffered
// data from the client.
//
// After a call to Hijack, the original Request.Body must not
// be used. The original Request's Context remains valid and
// is not canceled until the Request's ServeHTTP method
// returns.
Hijack() (net.Conn, *bufio.ReadWriter, error)
}
```
#### Example
Code:
```
http.HandleFunc("/hijack", func(w http.ResponseWriter, r *http.Request) {
hj, ok := w.(http.Hijacker)
if !ok {
http.Error(w, "webserver doesn't support hijacking", http.StatusInternalServerError)
return
}
conn, bufrw, err := hj.Hijack()
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Don't forget to close the connection:
defer conn.Close()
bufrw.WriteString("Now we're speaking raw TCP. Say hi: ")
bufrw.Flush()
s, err := bufrw.ReadString('\n')
if err != nil {
log.Printf("error reading string: %v", err)
return
}
fmt.Fprintf(bufrw, "You said: %q\nBye.\n", s)
bufrw.Flush()
})
```
type MaxBytesError 1.19
-----------------------
MaxBytesError is returned by MaxBytesReader when its read limit is exceeded.
```
type MaxBytesError struct {
Limit int64
}
```
### func (\*MaxBytesError) Error 1.19
```
func (e *MaxBytesError) Error() string
```
type ProtocolError
------------------
ProtocolError represents an HTTP protocol error.
Deprecated: Not all errors in the http package related to protocol errors are of type ProtocolError.
```
type ProtocolError struct {
ErrorString string
}
```
### func (\*ProtocolError) Error
```
func (pe *ProtocolError) Error() string
```
type PushOptions 1.8
--------------------
PushOptions describes options for Pusher.Push.
```
type PushOptions struct {
// Method specifies the HTTP method for the promised request.
// If set, it must be "GET" or "HEAD". Empty means "GET".
Method string
// Header specifies additional promised request headers. This cannot
// include HTTP/2 pseudo header fields like ":path" and ":scheme",
// which will be added automatically.
Header Header
}
```
type Pusher 1.8
---------------
Pusher is the interface implemented by ResponseWriters that support HTTP/2 server push. For more background, see <https://tools.ietf.org/html/rfc7540#section-8.2>.
```
type Pusher interface {
// Push initiates an HTTP/2 server push. This constructs a synthetic
// request using the given target and options, serializes that request
// into a PUSH_PROMISE frame, then dispatches that request using the
// server's request handler. If opts is nil, default options are used.
//
// The target must either be an absolute path (like "/path") or an absolute
// URL that contains a valid host and the same scheme as the parent request.
// If the target is a path, it will inherit the scheme and host of the
// parent request.
//
// The HTTP/2 spec disallows recursive pushes and cross-authority pushes.
// Push may or may not detect these invalid pushes; however, invalid
// pushes will be detected and canceled by conforming clients.
//
// Handlers that wish to push URL X should call Push before sending any
// data that may trigger a request for URL X. This avoids a race where the
// client issues requests for X before receiving the PUSH_PROMISE for X.
//
// Push will run in a separate goroutine making the order of arrival
// non-deterministic. Any required synchronization needs to be implemented
// by the caller.
//
// Push returns ErrNotSupported if the client has disabled push or if push
// is not supported on the underlying connection.
Push(target string, opts *PushOptions) error
}
```
type Request
------------
A Request represents an HTTP request received by a server or to be sent by a client.
The field semantics differ slightly between client and server usage. In addition to the notes on the fields below, see the documentation for Request.Write and RoundTripper.
```
type Request struct {
// Method specifies the HTTP method (GET, POST, PUT, etc.).
// For client requests, an empty string means GET.
//
// Go's HTTP client does not support sending a request with
// the CONNECT method. See the documentation on Transport for
// details.
Method string
// URL specifies either the URI being requested (for server
// requests) or the URL to access (for client requests).
//
// For server requests, the URL is parsed from the URI
// supplied on the Request-Line as stored in RequestURI. For
// most requests, fields other than Path and RawQuery will be
// empty. (See RFC 7230, Section 5.3)
//
// For client requests, the URL's Host specifies the server to
// connect to, while the Request's Host field optionally
// specifies the Host header value to send in the HTTP
// request.
URL *url.URL
// The protocol version for incoming server requests.
//
// For client requests, these fields are ignored. The HTTP
// client code always uses either HTTP/1.1 or HTTP/2.
// See the docs on Transport for details.
Proto string // "HTTP/1.0"
ProtoMajor int // 1
ProtoMinor int // 0
// Header contains the request header fields either received
// by the server or to be sent by the client.
//
// If a server received a request with header lines,
//
// Host: example.com
// accept-encoding: gzip, deflate
// Accept-Language: en-us
// fOO: Bar
// foo: two
//
// then
//
// Header = map[string][]string{
// "Accept-Encoding": {"gzip, deflate"},
// "Accept-Language": {"en-us"},
// "Foo": {"Bar", "two"},
// }
//
// For incoming requests, the Host header is promoted to the
// Request.Host field and removed from the Header map.
//
// HTTP defines that header names are case-insensitive. The
// request parser implements this by using CanonicalHeaderKey,
// making the first character and any characters following a
// hyphen uppercase and the rest lowercase.
//
// For client requests, certain headers such as Content-Length
// and Connection are automatically written when needed and
// values in Header may be ignored. See the documentation
// for the Request.Write method.
Header Header
// Body is the request's body.
//
// For client requests, a nil body means the request has no
// body, such as a GET request. The HTTP Client's Transport
// is responsible for calling the Close method.
//
// For server requests, the Request Body is always non-nil
// but will return EOF immediately when no body is present.
// The Server will close the request body. The ServeHTTP
// Handler does not need to.
//
// Body must allow Read to be called concurrently with Close.
// In particular, calling Close should unblock a Read waiting
// for input.
Body io.ReadCloser
// GetBody defines an optional func to return a new copy of
// Body. It is used for client requests when a redirect requires
// reading the body more than once. Use of GetBody still
// requires setting Body.
//
// For server requests, it is unused.
GetBody func() (io.ReadCloser, error) // Go 1.8
// ContentLength records the length of the associated content.
// The value -1 indicates that the length is unknown.
// Values >= 0 indicate that the given number of bytes may
// be read from Body.
//
// For client requests, a value of 0 with a non-nil Body is
// also treated as unknown.
ContentLength int64
// TransferEncoding lists the transfer encodings from outermost to
// innermost. An empty list denotes the "identity" encoding.
// TransferEncoding can usually be ignored; chunked encoding is
// automatically added and removed as necessary when sending and
// receiving requests.
TransferEncoding []string
// Close indicates whether to close the connection after
// replying to this request (for servers) or after sending this
// request and reading its response (for clients).
//
// For server requests, the HTTP server handles this automatically
// and this field is not needed by Handlers.
//
// For client requests, setting this field prevents re-use of
// TCP connections between requests to the same hosts, as if
// Transport.DisableKeepAlives were set.
Close bool
// For server requests, Host specifies the host on which the
// URL is sought. For HTTP/1 (per RFC 7230, section 5.4), this
// is either the value of the "Host" header or the host name
// given in the URL itself. For HTTP/2, it is the value of the
// ":authority" pseudo-header field.
// It may be of the form "host:port". For international domain
// names, Host may be in Punycode or Unicode form. Use
// golang.org/x/net/idna to convert it to either format if
// needed.
// To prevent DNS rebinding attacks, server Handlers should
// validate that the Host header has a value for which the
// Handler considers itself authoritative. The included
// ServeMux supports patterns registered to particular host
// names and thus protects its registered Handlers.
//
// For client requests, Host optionally overrides the Host
// header to send. If empty, the Request.Write method uses
// the value of URL.Host. Host may contain an international
// domain name.
Host string
// Form contains the parsed form data, including both the URL
// field's query parameters and the PATCH, POST, or PUT form data.
// This field is only available after ParseForm is called.
// The HTTP client ignores Form and uses Body instead.
Form url.Values
// PostForm contains the parsed form data from PATCH, POST
// or PUT body parameters.
//
// This field is only available after ParseForm is called.
// The HTTP client ignores PostForm and uses Body instead.
PostForm url.Values // Go 1.1
// MultipartForm is the parsed multipart form, including file uploads.
// This field is only available after ParseMultipartForm is called.
// The HTTP client ignores MultipartForm and uses Body instead.
MultipartForm *multipart.Form
// Trailer specifies additional headers that are sent after the request
// body.
//
// For server requests, the Trailer map initially contains only the
// trailer keys, with nil values. (The client declares which trailers it
// will later send.) While the handler is reading from Body, it must
// not reference Trailer. After reading from Body returns EOF, Trailer
// can be read again and will contain non-nil values, if they were sent
// by the client.
//
// For client requests, Trailer must be initialized to a map containing
// the trailer keys to later send. The values may be nil or their final
// values. The ContentLength must be 0 or -1, to send a chunked request.
// After the HTTP request is sent the map values can be updated while
// the request body is read. Once the body returns EOF, the caller must
// not mutate Trailer.
//
// Few HTTP clients, servers, or proxies support HTTP trailers.
Trailer Header
// RemoteAddr allows HTTP servers and other software to record
// the network address that sent the request, usually for
// logging. This field is not filled in by ReadRequest and
// has no defined format. The HTTP server in this package
// sets RemoteAddr to an "IP:port" address before invoking a
// handler.
// This field is ignored by the HTTP client.
RemoteAddr string
// RequestURI is the unmodified request-target of the
// Request-Line (RFC 7230, Section 3.1.1) as sent by the client
// to a server. Usually the URL field should be used instead.
// It is an error to set this field in an HTTP client request.
RequestURI string
// TLS allows HTTP servers and other software to record
// information about the TLS connection on which the request
// was received. This field is not filled in by ReadRequest.
// The HTTP server in this package sets the field for
// TLS-enabled connections before invoking a handler;
// otherwise it leaves the field nil.
// This field is ignored by the HTTP client.
TLS *tls.ConnectionState
// Cancel is an optional channel whose closure indicates that the client
// request should be regarded as canceled. Not all implementations of
// RoundTripper may support Cancel.
//
// For server requests, this field is not applicable.
//
// Deprecated: Set the Request's context with NewRequestWithContext
// instead. If a Request's Cancel field and context are both
// set, it is undefined whether Cancel is respected.
Cancel <-chan struct{} // Go 1.5
// Response is the redirect response which caused this request
// to be created. This field is only populated during client
// redirects.
Response *Response // Go 1.7
// contains filtered or unexported fields
}
```
### func NewRequest
```
func NewRequest(method, url string, body io.Reader) (*Request, error)
```
NewRequest wraps NewRequestWithContext using context.Background.
### func NewRequestWithContext 1.13
```
func NewRequestWithContext(ctx context.Context, method, url string, body io.Reader) (*Request, error)
```
NewRequestWithContext returns a new Request given a method, URL, and optional body.
If the provided body is also an io.Closer, the returned Request.Body is set to body and will be closed by the Client methods Do, Post, and PostForm, and Transport.RoundTrip.
NewRequestWithContext returns a Request suitable for use with Client.Do or Transport.RoundTrip. To create a request for use with testing a Server Handler, either use the NewRequest function in the net/http/httptest package, use ReadRequest, or manually update the Request fields. For an outgoing client request, the context controls the entire lifetime of a request and its response: obtaining a connection, sending the request, and reading the response headers and body. See the Request type's documentation for the difference between inbound and outbound request fields.
If body is of type \*bytes.Buffer, \*bytes.Reader, or \*strings.Reader, the returned request's ContentLength is set to its exact value (instead of -1), GetBody is populated (so 307 and 308 redirects can replay the body), and Body is set to NoBody if the ContentLength is 0.
### func ReadRequest
```
func ReadRequest(b *bufio.Reader) (*Request, error)
```
ReadRequest reads and parses an incoming request from b.
ReadRequest is a low-level function and should only be used for specialized applications; most code should use the Server to read requests and handle them via the Handler interface. ReadRequest only supports HTTP/1.x requests. For HTTP/2, use golang.org/x/net/http2.
### func (\*Request) AddCookie
```
func (r *Request) AddCookie(c *Cookie)
```
AddCookie adds a cookie to the request. Per RFC 6265 section 5.4, AddCookie does not attach more than one Cookie header field. That means all cookies, if any, are written into the same line, separated by semicolon. AddCookie only sanitizes c's name and value, and does not sanitize a Cookie header already present in the request.
### func (\*Request) BasicAuth 1.4
```
func (r *Request) BasicAuth() (username, password string, ok bool)
```
BasicAuth returns the username and password provided in the request's Authorization header, if the request uses HTTP Basic Authentication. See RFC 2617, Section 2.
### func (\*Request) Clone 1.13
```
func (r *Request) Clone(ctx context.Context) *Request
```
Clone returns a deep copy of r with its context changed to ctx. The provided ctx must be non-nil.
For an outgoing client request, the context controls the entire lifetime of a request and its response: obtaining a connection, sending the request, and reading the response headers and body.
### func (\*Request) Context 1.7
```
func (r *Request) Context() context.Context
```
Context returns the request's context. To change the context, use Clone or WithContext.
The returned context is always non-nil; it defaults to the background context.
For outgoing client requests, the context controls cancellation.
For incoming server requests, the context is canceled when the client's connection closes, the request is canceled (with HTTP/2), or when the ServeHTTP method returns.
### func (\*Request) Cookie
```
func (r *Request) Cookie(name string) (*Cookie, error)
```
Cookie returns the named cookie provided in the request or ErrNoCookie if not found. If multiple cookies match the given name, only one cookie will be returned.
### func (\*Request) Cookies
```
func (r *Request) Cookies() []*Cookie
```
Cookies parses and returns the HTTP cookies sent with the request.
### func (\*Request) FormFile
```
func (r *Request) FormFile(key string) (multipart.File, *multipart.FileHeader, error)
```
FormFile returns the first file for the provided form key. FormFile calls ParseMultipartForm and ParseForm if necessary.
### func (\*Request) FormValue
```
func (r *Request) FormValue(key string) string
```
FormValue returns the first value for the named component of the query. POST and PUT body parameters take precedence over URL query string values. FormValue calls ParseMultipartForm and ParseForm if necessary and ignores any errors returned by these functions. If key is not present, FormValue returns the empty string. To access multiple values of the same key, call ParseForm and then inspect Request.Form directly.
### func (\*Request) MultipartReader
```
func (r *Request) MultipartReader() (*multipart.Reader, error)
```
MultipartReader returns a MIME multipart reader if this is a multipart/form-data or a multipart/mixed POST request, else returns nil and an error. Use this function instead of ParseMultipartForm to process the request body as a stream.
### func (\*Request) ParseForm
```
func (r *Request) ParseForm() error
```
ParseForm populates r.Form and r.PostForm.
For all requests, ParseForm parses the raw query from the URL and updates r.Form.
For POST, PUT, and PATCH requests, it also reads the request body, parses it as a form and puts the results into both r.PostForm and r.Form. Request body parameters take precedence over URL query string values in r.Form.
If the request Body's size has not already been limited by MaxBytesReader, the size is capped at 10MB.
For other HTTP methods, or when the Content-Type is not application/x-www-form-urlencoded, the request Body is not read, and r.PostForm is initialized to a non-nil, empty value.
ParseMultipartForm calls ParseForm automatically. ParseForm is idempotent.
### func (\*Request) ParseMultipartForm
```
func (r *Request) ParseMultipartForm(maxMemory int64) error
```
ParseMultipartForm parses a request body as multipart/form-data. The whole request body is parsed and up to a total of maxMemory bytes of its file parts are stored in memory, with the remainder stored on disk in temporary files. ParseMultipartForm calls ParseForm if necessary. If ParseForm returns an error, ParseMultipartForm returns it but also continues parsing the request body. After one call to ParseMultipartForm, subsequent calls have no effect.
### func (\*Request) PostFormValue 1.1
```
func (r *Request) PostFormValue(key string) string
```
PostFormValue returns the first value for the named component of the POST, PATCH, or PUT request body. URL query parameters are ignored. PostFormValue calls ParseMultipartForm and ParseForm if necessary and ignores any errors returned by these functions. If key is not present, PostFormValue returns the empty string.
### func (\*Request) ProtoAtLeast
```
func (r *Request) ProtoAtLeast(major, minor int) bool
```
ProtoAtLeast reports whether the HTTP protocol used in the request is at least major.minor.
### func (\*Request) Referer
```
func (r *Request) Referer() string
```
Referer returns the referring URL, if sent in the request.
Referer is misspelled as in the request itself, a mistake from the earliest days of HTTP. This value can also be fetched from the Header map as Header["Referer"]; the benefit of making it available as a method is that the compiler can diagnose programs that use the alternate (correct English) spelling req.Referrer() but cannot diagnose programs that use Header["Referrer"].
### func (\*Request) SetBasicAuth
```
func (r *Request) SetBasicAuth(username, password string)
```
SetBasicAuth sets the request's Authorization header to use HTTP Basic Authentication with the provided username and password.
With HTTP Basic Authentication the provided username and password are not encrypted. It should generally only be used in an HTTPS request.
The username may not contain a colon. Some protocols may impose additional requirements on pre-escaping the username and password. For instance, when used with OAuth2, both arguments must be URL encoded first with url.QueryEscape.
### func (\*Request) UserAgent
```
func (r *Request) UserAgent() string
```
UserAgent returns the client's User-Agent, if sent in the request.
### func (\*Request) WithContext 1.7
```
func (r *Request) WithContext(ctx context.Context) *Request
```
WithContext returns a shallow copy of r with its context changed to ctx. The provided ctx must be non-nil.
For outgoing client request, the context controls the entire lifetime of a request and its response: obtaining a connection, sending the request, and reading the response headers and body.
To create a new request with a context, use NewRequestWithContext. To make a deep copy of a request with a new context, use Request.Clone.
### func (\*Request) Write
```
func (r *Request) Write(w io.Writer) error
```
Write writes an HTTP/1.1 request, which is the header and body, in wire format. This method consults the following fields of the request:
```
Host
URL
Method (defaults to "GET")
Header
ContentLength
TransferEncoding
Body
```
If Body is present, Content-Length is <= 0 and TransferEncoding hasn't been set to "identity", Write adds "Transfer-Encoding: chunked" to the header. Body is closed after it is sent.
### func (\*Request) WriteProxy
```
func (r *Request) WriteProxy(w io.Writer) error
```
WriteProxy is like Write but writes the request in the form expected by an HTTP proxy. In particular, WriteProxy writes the initial Request-URI line of the request with an absolute URI, per section 5.3 of RFC 7230, including the scheme and host. In either case, WriteProxy also writes a Host header, using either r.Host or r.URL.Host.
type Response
-------------
Response represents the response from an HTTP request.
The Client and Transport return Responses from servers once the response headers have been received. The response body is streamed on demand as the Body field is read.
```
type Response struct {
Status string // e.g. "200 OK"
StatusCode int // e.g. 200
Proto string // e.g. "HTTP/1.0"
ProtoMajor int // e.g. 1
ProtoMinor int // e.g. 0
// Header maps header keys to values. If the response had multiple
// headers with the same key, they may be concatenated, with comma
// delimiters. (RFC 7230, section 3.2.2 requires that multiple headers
// be semantically equivalent to a comma-delimited sequence.) When
// Header values are duplicated by other fields in this struct (e.g.,
// ContentLength, TransferEncoding, Trailer), the field values are
// authoritative.
//
// Keys in the map are canonicalized (see CanonicalHeaderKey).
Header Header
// Body represents the response body.
//
// The response body is streamed on demand as the Body field
// is read. If the network connection fails or the server
// terminates the response, Body.Read calls return an error.
//
// The http Client and Transport guarantee that Body is always
// non-nil, even on responses without a body or responses with
// a zero-length body. It is the caller's responsibility to
// close Body. The default HTTP client's Transport may not
// reuse HTTP/1.x "keep-alive" TCP connections if the Body is
// not read to completion and closed.
//
// The Body is automatically dechunked if the server replied
// with a "chunked" Transfer-Encoding.
//
// As of Go 1.12, the Body will also implement io.Writer
// on a successful "101 Switching Protocols" response,
// as used by WebSockets and HTTP/2's "h2c" mode.
Body io.ReadCloser
// ContentLength records the length of the associated content. The
// value -1 indicates that the length is unknown. Unless Request.Method
// is "HEAD", values >= 0 indicate that the given number of bytes may
// be read from Body.
ContentLength int64
// Contains transfer encodings from outer-most to inner-most. Value is
// nil, means that "identity" encoding is used.
TransferEncoding []string
// Close records whether the header directed that the connection be
// closed after reading Body. The value is advice for clients: neither
// ReadResponse nor Response.Write ever closes a connection.
Close bool
// Uncompressed reports whether the response was sent compressed but
// was decompressed by the http package. When true, reading from
// Body yields the uncompressed content instead of the compressed
// content actually set from the server, ContentLength is set to -1,
// and the "Content-Length" and "Content-Encoding" fields are deleted
// from the responseHeader. To get the original response from
// the server, set Transport.DisableCompression to true.
Uncompressed bool // Go 1.7
// Trailer maps trailer keys to values in the same
// format as Header.
//
// The Trailer initially contains only nil values, one for
// each key specified in the server's "Trailer" header
// value. Those values are not added to Header.
//
// Trailer must not be accessed concurrently with Read calls
// on the Body.
//
// After Body.Read has returned io.EOF, Trailer will contain
// any trailer values sent by the server.
Trailer Header
// Request is the request that was sent to obtain this Response.
// Request's Body is nil (having already been consumed).
// This is only populated for Client requests.
Request *Request
// TLS contains information about the TLS connection on which the
// response was received. It is nil for unencrypted responses.
// The pointer is shared between responses and should not be
// modified.
TLS *tls.ConnectionState // Go 1.3
}
```
### func Get
```
func Get(url string) (resp *Response, err error)
```
Get issues a GET to the specified URL. If the response is one of the following redirect codes, Get follows the redirect, up to a maximum of 10 redirects:
```
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
```
An error is returned if there were too many redirects or if there was an HTTP protocol error. A non-2xx response doesn't cause an error. Any returned error will be of type \*url.Error. The url.Error value's Timeout method will report true if the request timed out.
When err is nil, resp always contains a non-nil resp.Body. Caller should close resp.Body when done reading from it.
Get is a wrapper around DefaultClient.Get.
To make a request with custom headers, use NewRequest and DefaultClient.Do.
To make a request with a specified context.Context, use NewRequestWithContext and DefaultClient.Do.
#### Example
Code:
```
res, err := http.Get("http://www.google.com/robots.txt")
if err != nil {
log.Fatal(err)
}
body, err := io.ReadAll(res.Body)
res.Body.Close()
if res.StatusCode > 299 {
log.Fatalf("Response failed with status code: %d and\nbody: %s\n", res.StatusCode, body)
}
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", body)
```
### func Head
```
func Head(url string) (resp *Response, err error)
```
Head issues a HEAD to the specified URL. If the response is one of the following redirect codes, Head follows the redirect, up to a maximum of 10 redirects:
```
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
```
Head is a wrapper around DefaultClient.Head.
To make a request with a specified context.Context, use NewRequestWithContext and DefaultClient.Do.
### func Post
```
func Post(url, contentType string, body io.Reader) (resp *Response, err error)
```
Post issues a POST to the specified URL.
Caller should close resp.Body when done reading from it.
If the provided body is an io.Closer, it is closed after the request.
Post is a wrapper around DefaultClient.Post.
To set custom headers, use NewRequest and DefaultClient.Do.
See the Client.Do method documentation for details on how redirects are handled.
To make a request with a specified context.Context, use NewRequestWithContext and DefaultClient.Do.
### func PostForm
```
func PostForm(url string, data url.Values) (resp *Response, err error)
```
PostForm issues a POST to the specified URL, with data's keys and values URL-encoded as the request body.
The Content-Type header is set to application/x-www-form-urlencoded. To set other headers, use NewRequest and DefaultClient.Do.
When err is nil, resp always contains a non-nil resp.Body. Caller should close resp.Body when done reading from it.
PostForm is a wrapper around DefaultClient.PostForm.
See the Client.Do method documentation for details on how redirects are handled.
To make a request with a specified context.Context, use NewRequestWithContext and DefaultClient.Do.
### func ReadResponse
```
func ReadResponse(r *bufio.Reader, req *Request) (*Response, error)
```
ReadResponse reads and returns an HTTP response from r. The req parameter optionally specifies the Request that corresponds to this Response. If nil, a GET request is assumed. Clients must call resp.Body.Close when finished reading resp.Body. After that call, clients can inspect resp.Trailer to find key/value pairs included in the response trailer.
### func (\*Response) Cookies
```
func (r *Response) Cookies() []*Cookie
```
Cookies parses and returns the cookies set in the Set-Cookie headers.
### func (\*Response) Location
```
func (r *Response) Location() (*url.URL, error)
```
Location returns the URL of the response's "Location" header, if present. Relative redirects are resolved relative to the Response's Request. ErrNoLocation is returned if no Location header is present.
### func (\*Response) ProtoAtLeast
```
func (r *Response) ProtoAtLeast(major, minor int) bool
```
ProtoAtLeast reports whether the HTTP protocol used in the response is at least major.minor.
### func (\*Response) Write
```
func (r *Response) Write(w io.Writer) error
```
Write writes r to w in the HTTP/1.x server response format, including the status line, headers, body, and optional trailer.
This method consults the following fields of the response r:
```
StatusCode
ProtoMajor
ProtoMinor
Request.Method
TransferEncoding
Trailer
Body
ContentLength
Header, values for non-canonical keys will have unpredictable behavior
```
The Response Body is closed after it is sent.
type ResponseController 1.20
----------------------------
A ResponseController is used by an HTTP handler to control the response.
A ResponseController may not be used after the Handler.ServeHTTP method has returned.
```
type ResponseController struct {
// contains filtered or unexported fields
}
```
### func NewResponseController 1.20
```
func NewResponseController(rw ResponseWriter) *ResponseController
```
NewResponseController creates a ResponseController for a request.
The ResponseWriter should be the original value passed to the Handler.ServeHTTP method, or have an Unwrap method returning the original ResponseWriter.
If the ResponseWriter implements any of the following methods, the ResponseController will call them as appropriate:
```
Flush()
FlushError() error // alternative Flush returning an error
Hijack() (net.Conn, *bufio.ReadWriter, error)
SetReadDeadline(deadline time.Time) error
SetWriteDeadline(deadline time.Time) error
```
If the ResponseWriter does not support a method, ResponseController returns an error matching ErrNotSupported.
### func (\*ResponseController) Flush 1.20
```
func (c *ResponseController) Flush() error
```
Flush flushes buffered data to the client.
### func (\*ResponseController) Hijack 1.20
```
func (c *ResponseController) Hijack() (net.Conn, *bufio.ReadWriter, error)
```
Hijack lets the caller take over the connection. See the Hijacker interface for details.
### func (\*ResponseController) SetReadDeadline 1.20
```
func (c *ResponseController) SetReadDeadline(deadline time.Time) error
```
SetReadDeadline sets the deadline for reading the entire request, including the body. Reads from the request body after the deadline has been exceeded will return an error. A zero value means no deadline.
Setting the read deadline after it has been exceeded will not extend it.
### func (\*ResponseController) SetWriteDeadline 1.20
```
func (c *ResponseController) SetWriteDeadline(deadline time.Time) error
```
SetWriteDeadline sets the deadline for writing the response. Writes to the response body after the deadline has been exceeded will not block, but may succeed if the data has been buffered. A zero value means no deadline.
Setting the write deadline after it has been exceeded will not extend it.
type ResponseWriter
-------------------
A ResponseWriter interface is used by an HTTP handler to construct an HTTP response.
A ResponseWriter may not be used after the Handler.ServeHTTP method has returned.
```
type ResponseWriter interface {
// Header returns the header map that will be sent by
// WriteHeader. The Header map also is the mechanism with which
// Handlers can set HTTP trailers.
//
// Changing the header map after a call to WriteHeader (or
// Write) has no effect unless the HTTP status code was of the
// 1xx class or the modified headers are trailers.
//
// There are two ways to set Trailers. The preferred way is to
// predeclare in the headers which trailers you will later
// send by setting the "Trailer" header to the names of the
// trailer keys which will come later. In this case, those
// keys of the Header map are treated as if they were
// trailers. See the example. The second way, for trailer
// keys not known to the Handler until after the first Write,
// is to prefix the Header map keys with the TrailerPrefix
// constant value. See TrailerPrefix.
//
// To suppress automatic response headers (such as "Date"), set
// their value to nil.
Header() Header
// Write writes the data to the connection as part of an HTTP reply.
//
// If WriteHeader has not yet been called, Write calls
// WriteHeader(http.StatusOK) before writing the data. If the Header
// does not contain a Content-Type line, Write adds a Content-Type set
// to the result of passing the initial 512 bytes of written data to
// DetectContentType. Additionally, if the total size of all written
// data is under a few KB and there are no Flush calls, the
// Content-Length header is added automatically.
//
// Depending on the HTTP protocol version and the client, calling
// Write or WriteHeader may prevent future reads on the
// Request.Body. For HTTP/1.x requests, handlers should read any
// needed request body data before writing the response. Once the
// headers have been flushed (due to either an explicit Flusher.Flush
// call or writing enough data to trigger a flush), the request body
// may be unavailable. For HTTP/2 requests, the Go HTTP server permits
// handlers to continue to read the request body while concurrently
// writing the response. However, such behavior may not be supported
// by all HTTP/2 clients. Handlers should read before writing if
// possible to maximize compatibility.
Write([]byte) (int, error)
// WriteHeader sends an HTTP response header with the provided
// status code.
//
// If WriteHeader is not called explicitly, the first call to Write
// will trigger an implicit WriteHeader(http.StatusOK).
// Thus explicit calls to WriteHeader are mainly used to
// send error codes or 1xx informational responses.
//
// The provided code must be a valid HTTP 1xx-5xx status code.
// Any number of 1xx headers may be written, followed by at most
// one 2xx-5xx header. 1xx headers are sent immediately, but 2xx-5xx
// headers may be buffered. Use the Flusher interface to send
// buffered data. The header map is cleared when 2xx-5xx headers are
// sent, but not with 1xx headers.
//
// The server will automatically send a 100 (Continue) header
// on the first read from the request body if the request has
// an "Expect: 100-continue" header.
WriteHeader(statusCode int)
}
```
#### Example (Trailers)
HTTP Trailers are a set of key/value pairs like headers that come after the HTTP response, instead of before.
Code:
```
mux := http.NewServeMux()
mux.HandleFunc("/sendstrailers", func(w http.ResponseWriter, req *http.Request) {
// Before any call to WriteHeader or Write, declare
// the trailers you will set during the HTTP
// response. These three headers are actually sent in
// the trailer.
w.Header().Set("Trailer", "AtEnd1, AtEnd2")
w.Header().Add("Trailer", "AtEnd3")
w.Header().Set("Content-Type", "text/plain; charset=utf-8") // normal header
w.WriteHeader(http.StatusOK)
w.Header().Set("AtEnd1", "value 1")
io.WriteString(w, "This HTTP response has both headers before this text and trailers at the end.\n")
w.Header().Set("AtEnd2", "value 2")
w.Header().Set("AtEnd3", "value 3") // These will appear as trailers.
})
```
type RoundTripper
-----------------
RoundTripper is an interface representing the ability to execute a single HTTP transaction, obtaining the Response for a given Request.
A RoundTripper must be safe for concurrent use by multiple goroutines.
```
type RoundTripper interface {
// RoundTrip executes a single HTTP transaction, returning
// a Response for the provided Request.
//
// RoundTrip should not attempt to interpret the response. In
// particular, RoundTrip must return err == nil if it obtained
// a response, regardless of the response's HTTP status code.
// A non-nil err should be reserved for failure to obtain a
// response. Similarly, RoundTrip should not attempt to
// handle higher-level protocol details such as redirects,
// authentication, or cookies.
//
// RoundTrip should not modify the request, except for
// consuming and closing the Request's Body. RoundTrip may
// read fields of the request in a separate goroutine. Callers
// should not mutate or reuse the request until the Response's
// Body has been closed.
//
// RoundTrip must always close the body, including on errors,
// but depending on the implementation may do so in a separate
// goroutine even after RoundTrip returns. This means that
// callers wanting to reuse the body for subsequent requests
// must arrange to wait for the Close call before doing so.
//
// The Request's URL and Header fields must be initialized.
RoundTrip(*Request) (*Response, error)
}
```
DefaultTransport is the default implementation of Transport and is used by DefaultClient. It establishes network connections as needed and caches them for reuse by subsequent calls. It uses HTTP proxies as directed by the environment variables HTTP\_PROXY, HTTPS\_PROXY and NO\_PROXY (or the lowercase versions thereof).
```
var DefaultTransport RoundTripper = &Transport{
Proxy: ProxyFromEnvironment,
DialContext: defaultTransportDialContext(&net.Dialer{
Timeout: 30 * time.Second,
KeepAlive: 30 * time.Second,
}),
ForceAttemptHTTP2: true,
MaxIdleConns: 100,
IdleConnTimeout: 90 * time.Second,
TLSHandshakeTimeout: 10 * time.Second,
ExpectContinueTimeout: 1 * time.Second,
}
```
### func NewFileTransport
```
func NewFileTransport(fs FileSystem) RoundTripper
```
NewFileTransport returns a new RoundTripper, serving the provided FileSystem. The returned RoundTripper ignores the URL host in its incoming requests, as well as most other properties of the request.
The typical use case for NewFileTransport is to register the "file" protocol with a Transport, as in:
```
t := &http.Transport{}
t.RegisterProtocol("file", http.NewFileTransport(http.Dir("/")))
c := &http.Client{Transport: t}
res, err := c.Get("file:///etc/passwd")
...
```
type SameSite 1.11
------------------
SameSite allows a server to define a cookie attribute making it impossible for the browser to send this cookie along with cross-site requests. The main goal is to mitigate the risk of cross-origin information leakage, and provide some protection against cross-site request forgery attacks.
See <https://tools.ietf.org/html/draft-ietf-httpbis-cookie-same-site-00> for details.
```
type SameSite int
```
```
const (
SameSiteDefaultMode SameSite = iota + 1
SameSiteLaxMode
SameSiteStrictMode
SameSiteNoneMode
)
```
type ServeMux
-------------
ServeMux is an HTTP request multiplexer. It matches the URL of each incoming request against a list of registered patterns and calls the handler for the pattern that most closely matches the URL.
Patterns name fixed, rooted paths, like "/favicon.ico", or rooted subtrees, like "/images/" (note the trailing slash). Longer patterns take precedence over shorter ones, so that if there are handlers registered for both "/images/" and "/images/thumbnails/", the latter handler will be called for paths beginning "/images/thumbnails/" and the former will receive requests for any other paths in the "/images/" subtree.
Note that since a pattern ending in a slash names a rooted subtree, the pattern "/" matches all paths not matched by other registered patterns, not just the URL with Path == "/".
If a subtree has been registered and a request is received naming the subtree root without its trailing slash, ServeMux redirects that request to the subtree root (adding the trailing slash). This behavior can be overridden with a separate registration for the path without the trailing slash. For example, registering "/images/" causes ServeMux to redirect a request for "/images" to "/images/", unless "/images" has been registered separately.
Patterns may optionally begin with a host name, restricting matches to URLs on that host only. Host-specific patterns take precedence over general patterns, so that a handler might register for the two patterns "/codesearch" and "codesearch.google.com/" without also taking over requests for "<http://www.google.com/>".
ServeMux also takes care of sanitizing the URL request path and the Host header, stripping the port number and redirecting any request containing . or .. elements or repeated slashes to an equivalent, cleaner URL.
```
type ServeMux struct {
// contains filtered or unexported fields
}
```
### func NewServeMux
```
func NewServeMux() *ServeMux
```
NewServeMux allocates and returns a new ServeMux.
### func (\*ServeMux) Handle
```
func (mux *ServeMux) Handle(pattern string, handler Handler)
```
Handle registers the handler for the given pattern. If a handler already exists for pattern, Handle panics.
#### Example
Code:
```
mux := http.NewServeMux()
mux.Handle("/api/", apiHandler{})
mux.HandleFunc("/", func(w http.ResponseWriter, req *http.Request) {
// The "/" pattern matches everything, so we need to check
// that we're at the root here.
if req.URL.Path != "/" {
http.NotFound(w, req)
return
}
fmt.Fprintf(w, "Welcome to the home page!")
})
```
### func (\*ServeMux) HandleFunc
```
func (mux *ServeMux) HandleFunc(pattern string, handler func(ResponseWriter, *Request))
```
HandleFunc registers the handler function for the given pattern.
### func (\*ServeMux) Handler 1.1
```
func (mux *ServeMux) Handler(r *Request) (h Handler, pattern string)
```
Handler returns the handler to use for the given request, consulting r.Method, r.Host, and r.URL.Path. It always returns a non-nil handler. If the path is not in its canonical form, the handler will be an internally-generated handler that redirects to the canonical path. If the host contains a port, it is ignored when matching handlers.
The path and host are used unchanged for CONNECT requests.
Handler also returns the registered pattern that matches the request or, in the case of internally-generated redirects, the pattern that will match after following the redirect.
If there is no registered handler that applies to the request, Handler returns a “page not found” handler and an empty pattern.
### func (\*ServeMux) ServeHTTP
```
func (mux *ServeMux) ServeHTTP(w ResponseWriter, r *Request)
```
ServeHTTP dispatches the request to the handler whose pattern most closely matches the request URL.
type Server
-----------
A Server defines parameters for running an HTTP server. The zero value for Server is a valid configuration.
```
type Server struct {
// Addr optionally specifies the TCP address for the server to listen on,
// in the form "host:port". If empty, ":http" (port 80) is used.
// The service names are defined in RFC 6335 and assigned by IANA.
// See net.Dial for details of the address format.
Addr string
Handler Handler // handler to invoke, http.DefaultServeMux if nil
// DisableGeneralOptionsHandler, if true, passes "OPTIONS *" requests to the Handler,
// otherwise responds with 200 OK and Content-Length: 0.
DisableGeneralOptionsHandler bool // Go 1.20
// TLSConfig optionally provides a TLS configuration for use
// by ServeTLS and ListenAndServeTLS. Note that this value is
// cloned by ServeTLS and ListenAndServeTLS, so it's not
// possible to modify the configuration with methods like
// tls.Config.SetSessionTicketKeys. To use
// SetSessionTicketKeys, use Server.Serve with a TLS Listener
// instead.
TLSConfig *tls.Config
// ReadTimeout is the maximum duration for reading the entire
// request, including the body. A zero or negative value means
// there will be no timeout.
//
// Because ReadTimeout does not let Handlers make per-request
// decisions on each request body's acceptable deadline or
// upload rate, most users will prefer to use
// ReadHeaderTimeout. It is valid to use them both.
ReadTimeout time.Duration
// ReadHeaderTimeout is the amount of time allowed to read
// request headers. The connection's read deadline is reset
// after reading the headers and the Handler can decide what
// is considered too slow for the body. If ReadHeaderTimeout
// is zero, the value of ReadTimeout is used. If both are
// zero, there is no timeout.
ReadHeaderTimeout time.Duration // Go 1.8
// WriteTimeout is the maximum duration before timing out
// writes of the response. It is reset whenever a new
// request's header is read. Like ReadTimeout, it does not
// let Handlers make decisions on a per-request basis.
// A zero or negative value means there will be no timeout.
WriteTimeout time.Duration
// IdleTimeout is the maximum amount of time to wait for the
// next request when keep-alives are enabled. If IdleTimeout
// is zero, the value of ReadTimeout is used. If both are
// zero, there is no timeout.
IdleTimeout time.Duration // Go 1.8
// MaxHeaderBytes controls the maximum number of bytes the
// server will read parsing the request header's keys and
// values, including the request line. It does not limit the
// size of the request body.
// If zero, DefaultMaxHeaderBytes is used.
MaxHeaderBytes int
// TLSNextProto optionally specifies a function to take over
// ownership of the provided TLS connection when an ALPN
// protocol upgrade has occurred. The map key is the protocol
// name negotiated. The Handler argument should be used to
// handle HTTP requests and will initialize the Request's TLS
// and RemoteAddr if not already set. The connection is
// automatically closed when the function returns.
// If TLSNextProto is not nil, HTTP/2 support is not enabled
// automatically.
TLSNextProto map[string]func(*Server, *tls.Conn, Handler) // Go 1.1
// ConnState specifies an optional callback function that is
// called when a client connection changes state. See the
// ConnState type and associated constants for details.
ConnState func(net.Conn, ConnState) // Go 1.3
// ErrorLog specifies an optional logger for errors accepting
// connections, unexpected behavior from handlers, and
// underlying FileSystem errors.
// If nil, logging is done via the log package's standard logger.
ErrorLog *log.Logger // Go 1.3
// BaseContext optionally specifies a function that returns
// the base context for incoming requests on this server.
// The provided Listener is the specific Listener that's
// about to start accepting requests.
// If BaseContext is nil, the default is context.Background().
// If non-nil, it must return a non-nil context.
BaseContext func(net.Listener) context.Context // Go 1.13
// ConnContext optionally specifies a function that modifies
// the context used for a new connection c. The provided ctx
// is derived from the base context and has a ServerContextKey
// value.
ConnContext func(ctx context.Context, c net.Conn) context.Context // Go 1.13
// contains filtered or unexported fields
}
```
### func (\*Server) Close 1.8
```
func (srv *Server) Close() error
```
Close immediately closes all active net.Listeners and any connections in state StateNew, StateActive, or StateIdle. For a graceful shutdown, use Shutdown.
Close does not attempt to close (and does not even know about) any hijacked connections, such as WebSockets.
Close returns any error returned from closing the Server's underlying Listener(s).
### func (\*Server) ListenAndServe
```
func (srv *Server) ListenAndServe() error
```
ListenAndServe listens on the TCP network address srv.Addr and then calls Serve to handle requests on incoming connections. Accepted connections are configured to enable TCP keep-alives.
If srv.Addr is blank, ":http" is used.
ListenAndServe always returns a non-nil error. After Shutdown or Close, the returned error is ErrServerClosed.
### func (\*Server) ListenAndServeTLS
```
func (srv *Server) ListenAndServeTLS(certFile, keyFile string) error
```
ListenAndServeTLS listens on the TCP network address srv.Addr and then calls ServeTLS to handle requests on incoming TLS connections. Accepted connections are configured to enable TCP keep-alives.
Filenames containing a certificate and matching private key for the server must be provided if neither the Server's TLSConfig.Certificates nor TLSConfig.GetCertificate are populated. If the certificate is signed by a certificate authority, the certFile should be the concatenation of the server's certificate, any intermediates, and the CA's certificate.
If srv.Addr is blank, ":https" is used.
ListenAndServeTLS always returns a non-nil error. After Shutdown or Close, the returned error is ErrServerClosed.
### func (\*Server) RegisterOnShutdown 1.9
```
func (srv *Server) RegisterOnShutdown(f func())
```
RegisterOnShutdown registers a function to call on Shutdown. This can be used to gracefully shutdown connections that have undergone ALPN protocol upgrade or that have been hijacked. This function should start protocol-specific graceful shutdown, but should not wait for shutdown to complete.
### func (\*Server) Serve
```
func (srv *Server) Serve(l net.Listener) error
```
Serve accepts incoming connections on the Listener l, creating a new service goroutine for each. The service goroutines read requests and then call srv.Handler to reply to them.
HTTP/2 support is only enabled if the Listener returns \*tls.Conn connections and they were configured with "h2" in the TLS Config.NextProtos.
Serve always returns a non-nil error and closes l. After Shutdown or Close, the returned error is ErrServerClosed.
### func (\*Server) ServeTLS 1.9
```
func (srv *Server) ServeTLS(l net.Listener, certFile, keyFile string) error
```
ServeTLS accepts incoming connections on the Listener l, creating a new service goroutine for each. The service goroutines perform TLS setup and then read requests, calling srv.Handler to reply to them.
Files containing a certificate and matching private key for the server must be provided if neither the Server's TLSConfig.Certificates nor TLSConfig.GetCertificate are populated. If the certificate is signed by a certificate authority, the certFile should be the concatenation of the server's certificate, any intermediates, and the CA's certificate.
ServeTLS always returns a non-nil error. After Shutdown or Close, the returned error is ErrServerClosed.
### func (\*Server) SetKeepAlivesEnabled 1.3
```
func (srv *Server) SetKeepAlivesEnabled(v bool)
```
SetKeepAlivesEnabled controls whether HTTP keep-alives are enabled. By default, keep-alives are always enabled. Only very resource-constrained environments or servers in the process of shutting down should disable them.
### func (\*Server) Shutdown 1.8
```
func (srv *Server) Shutdown(ctx context.Context) error
```
Shutdown gracefully shuts down the server without interrupting any active connections. Shutdown works by first closing all open listeners, then closing all idle connections, and then waiting indefinitely for connections to return to idle and then shut down. If the provided context expires before the shutdown is complete, Shutdown returns the context's error, otherwise it returns any error returned from closing the Server's underlying Listener(s).
When Shutdown is called, Serve, ListenAndServe, and ListenAndServeTLS immediately return ErrServerClosed. Make sure the program doesn't exit and waits instead for Shutdown to return.
Shutdown does not attempt to close nor wait for hijacked connections such as WebSockets. The caller of Shutdown should separately notify such long-lived connections of shutdown and wait for them to close, if desired. See RegisterOnShutdown for a way to register shutdown notification functions.
Once Shutdown has been called on a server, it may not be reused; future calls to methods such as Serve will return ErrServerClosed.
#### Example
Code:
```
var srv http.Server
idleConnsClosed := make(chan struct{})
go func() {
sigint := make(chan os.Signal, 1)
signal.Notify(sigint, os.Interrupt)
<-sigint
// We received an interrupt signal, shut down.
if err := srv.Shutdown(context.Background()); err != nil {
// Error from closing listeners, or context timeout:
log.Printf("HTTP server Shutdown: %v", err)
}
close(idleConnsClosed)
}()
if err := srv.ListenAndServe(); err != http.ErrServerClosed {
// Error starting or closing listener:
log.Fatalf("HTTP server ListenAndServe: %v", err)
}
<-idleConnsClosed
```
type Transport
--------------
Transport is an implementation of RoundTripper that supports HTTP, HTTPS, and HTTP proxies (for either HTTP or HTTPS with CONNECT).
By default, Transport caches connections for future re-use. This may leave many open connections when accessing many hosts. This behavior can be managed using Transport's CloseIdleConnections method and the MaxIdleConnsPerHost and DisableKeepAlives fields.
Transports should be reused instead of created as needed. Transports are safe for concurrent use by multiple goroutines.
A Transport is a low-level primitive for making HTTP and HTTPS requests. For high-level functionality, such as cookies and redirects, see Client.
Transport uses HTTP/1.1 for HTTP URLs and either HTTP/1.1 or HTTP/2 for HTTPS URLs, depending on whether the server supports HTTP/2, and how the Transport is configured. The DefaultTransport supports HTTP/2. To explicitly enable HTTP/2 on a transport, use golang.org/x/net/http2 and call ConfigureTransport. See the package docs for more about HTTP/2.
Responses with status codes in the 1xx range are either handled automatically (100 expect-continue) or ignored. The one exception is HTTP status code 101 (Switching Protocols), which is considered a terminal status and returned by RoundTrip. To see the ignored 1xx responses, use the httptrace trace package's ClientTrace.Got1xxResponse.
Transport only retries a request upon encountering a network error if the request is idempotent and either has no body or has its Request.GetBody defined. HTTP requests are considered idempotent if they have HTTP methods GET, HEAD, OPTIONS, or TRACE; or if their Header map contains an "Idempotency-Key" or "X-Idempotency-Key" entry. If the idempotency key value is a zero-length slice, the request is treated as idempotent but the header is not sent on the wire.
```
type Transport struct {
// Proxy specifies a function to return a proxy for a given
// Request. If the function returns a non-nil error, the
// request is aborted with the provided error.
//
// The proxy type is determined by the URL scheme. "http",
// "https", and "socks5" are supported. If the scheme is empty,
// "http" is assumed.
//
// If Proxy is nil or returns a nil *URL, no proxy is used.
Proxy func(*Request) (*url.URL, error)
// OnProxyConnectResponse is called when the Transport gets an HTTP response from
// a proxy for a CONNECT request. It's called before the check for a 200 OK response.
// If it returns an error, the request fails with that error.
OnProxyConnectResponse func(ctx context.Context, proxyURL *url.URL, connectReq *Request, connectRes *Response) error // Go 1.20
// DialContext specifies the dial function for creating unencrypted TCP connections.
// If DialContext is nil (and the deprecated Dial below is also nil),
// then the transport dials using package net.
//
// DialContext runs concurrently with calls to RoundTrip.
// A RoundTrip call that initiates a dial may end up using
// a connection dialed previously when the earlier connection
// becomes idle before the later DialContext completes.
DialContext func(ctx context.Context, network, addr string) (net.Conn, error) // Go 1.7
// Dial specifies the dial function for creating unencrypted TCP connections.
//
// Dial runs concurrently with calls to RoundTrip.
// A RoundTrip call that initiates a dial may end up using
// a connection dialed previously when the earlier connection
// becomes idle before the later Dial completes.
//
// Deprecated: Use DialContext instead, which allows the transport
// to cancel dials as soon as they are no longer needed.
// If both are set, DialContext takes priority.
Dial func(network, addr string) (net.Conn, error)
// DialTLSContext specifies an optional dial function for creating
// TLS connections for non-proxied HTTPS requests.
//
// If DialTLSContext is nil (and the deprecated DialTLS below is also nil),
// DialContext and TLSClientConfig are used.
//
// If DialTLSContext is set, the Dial and DialContext hooks are not used for HTTPS
// requests and the TLSClientConfig and TLSHandshakeTimeout
// are ignored. The returned net.Conn is assumed to already be
// past the TLS handshake.
DialTLSContext func(ctx context.Context, network, addr string) (net.Conn, error) // Go 1.14
// DialTLS specifies an optional dial function for creating
// TLS connections for non-proxied HTTPS requests.
//
// Deprecated: Use DialTLSContext instead, which allows the transport
// to cancel dials as soon as they are no longer needed.
// If both are set, DialTLSContext takes priority.
DialTLS func(network, addr string) (net.Conn, error) // Go 1.4
// TLSClientConfig specifies the TLS configuration to use with
// tls.Client.
// If nil, the default configuration is used.
// If non-nil, HTTP/2 support may not be enabled by default.
TLSClientConfig *tls.Config
// TLSHandshakeTimeout specifies the maximum amount of time waiting to
// wait for a TLS handshake. Zero means no timeout.
TLSHandshakeTimeout time.Duration // Go 1.3
// DisableKeepAlives, if true, disables HTTP keep-alives and
// will only use the connection to the server for a single
// HTTP request.
//
// This is unrelated to the similarly named TCP keep-alives.
DisableKeepAlives bool
// DisableCompression, if true, prevents the Transport from
// requesting compression with an "Accept-Encoding: gzip"
// request header when the Request contains no existing
// Accept-Encoding value. If the Transport requests gzip on
// its own and gets a gzipped response, it's transparently
// decoded in the Response.Body. However, if the user
// explicitly requested gzip it is not automatically
// uncompressed.
DisableCompression bool
// MaxIdleConns controls the maximum number of idle (keep-alive)
// connections across all hosts. Zero means no limit.
MaxIdleConns int // Go 1.7
// MaxIdleConnsPerHost, if non-zero, controls the maximum idle
// (keep-alive) connections to keep per-host. If zero,
// DefaultMaxIdleConnsPerHost is used.
MaxIdleConnsPerHost int
// MaxConnsPerHost optionally limits the total number of
// connections per host, including connections in the dialing,
// active, and idle states. On limit violation, dials will block.
//
// Zero means no limit.
MaxConnsPerHost int // Go 1.11
// IdleConnTimeout is the maximum amount of time an idle
// (keep-alive) connection will remain idle before closing
// itself.
// Zero means no limit.
IdleConnTimeout time.Duration // Go 1.7
// ResponseHeaderTimeout, if non-zero, specifies the amount of
// time to wait for a server's response headers after fully
// writing the request (including its body, if any). This
// time does not include the time to read the response body.
ResponseHeaderTimeout time.Duration // Go 1.1
// ExpectContinueTimeout, if non-zero, specifies the amount of
// time to wait for a server's first response headers after fully
// writing the request headers if the request has an
// "Expect: 100-continue" header. Zero means no timeout and
// causes the body to be sent immediately, without
// waiting for the server to approve.
// This time does not include the time to send the request header.
ExpectContinueTimeout time.Duration // Go 1.6
// TLSNextProto specifies how the Transport switches to an
// alternate protocol (such as HTTP/2) after a TLS ALPN
// protocol negotiation. If Transport dials an TLS connection
// with a non-empty protocol name and TLSNextProto contains a
// map entry for that key (such as "h2"), then the func is
// called with the request's authority (such as "example.com"
// or "example.com:1234") and the TLS connection. The function
// must return a RoundTripper that then handles the request.
// If TLSNextProto is not nil, HTTP/2 support is not enabled
// automatically.
TLSNextProto map[string]func(authority string, c *tls.Conn) RoundTripper // Go 1.6
// ProxyConnectHeader optionally specifies headers to send to
// proxies during CONNECT requests.
// To set the header dynamically, see GetProxyConnectHeader.
ProxyConnectHeader Header // Go 1.8
// GetProxyConnectHeader optionally specifies a func to return
// headers to send to proxyURL during a CONNECT request to the
// ip:port target.
// If it returns an error, the Transport's RoundTrip fails with
// that error. It can return (nil, nil) to not add headers.
// If GetProxyConnectHeader is non-nil, ProxyConnectHeader is
// ignored.
GetProxyConnectHeader func(ctx context.Context, proxyURL *url.URL, target string) (Header, error) // Go 1.16
// MaxResponseHeaderBytes specifies a limit on how many
// response bytes are allowed in the server's response
// header.
//
// Zero means to use a default limit.
MaxResponseHeaderBytes int64 // Go 1.7
// WriteBufferSize specifies the size of the write buffer used
// when writing to the transport.
// If zero, a default (currently 4KB) is used.
WriteBufferSize int // Go 1.13
// ReadBufferSize specifies the size of the read buffer used
// when reading from the transport.
// If zero, a default (currently 4KB) is used.
ReadBufferSize int // Go 1.13
// ForceAttemptHTTP2 controls whether HTTP/2 is enabled when a non-zero
// Dial, DialTLS, or DialContext func or TLSClientConfig is provided.
// By default, use of any those fields conservatively disables HTTP/2.
// To use a custom dialer or TLS config and still attempt HTTP/2
// upgrades, set this to true.
ForceAttemptHTTP2 bool // Go 1.13
// contains filtered or unexported fields
}
```
### func (\*Transport) CancelRequest 1.1
```
func (t *Transport) CancelRequest(req *Request)
```
CancelRequest cancels an in-flight request by closing its connection. CancelRequest should only be called after RoundTrip has returned.
Deprecated: Use Request.WithContext to create a request with a cancelable context instead. CancelRequest cannot cancel HTTP/2 requests.
### func (\*Transport) Clone 1.13
```
func (t *Transport) Clone() *Transport
```
Clone returns a deep copy of t's exported fields.
### func (\*Transport) CloseIdleConnections
```
func (t *Transport) CloseIdleConnections()
```
CloseIdleConnections closes any connections which were previously connected from previous requests but are now sitting idle in a "keep-alive" state. It does not interrupt any connections currently in use.
### func (\*Transport) RegisterProtocol
```
func (t *Transport) RegisterProtocol(scheme string, rt RoundTripper)
```
RegisterProtocol registers a new protocol with scheme. The Transport will pass requests using the given scheme to rt. It is rt's responsibility to simulate HTTP request semantics.
RegisterProtocol can be used by other packages to provide implementations of protocol schemes like "ftp" or "file".
If rt.RoundTrip returns ErrSkipAltProtocol, the Transport will handle the RoundTrip itself for that one request, as if the protocol were not registered.
### func (\*Transport) RoundTrip
```
func (t *Transport) RoundTrip(req *Request) (*Response, error)
```
RoundTrip implements the RoundTripper interface.
For higher-level HTTP client support (such as handling of cookies and redirects), see Get, Post, and the Client type.
Like the RoundTripper interface, the error types returned by RoundTrip are unspecified.
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [cgi](cgi/index) | Package cgi implements CGI (Common Gateway Interface) as specified in RFC 3875. |
| [cookiejar](cookiejar/index) | Package cookiejar implements an in-memory RFC 6265-compliant http.CookieJar. |
| [fcgi](fcgi/index) | Package fcgi implements the FastCGI protocol. |
| [httptest](httptest/index) | Package httptest provides utilities for HTTP testing. |
| [httptrace](httptrace/index) | Package httptrace provides mechanisms to trace the events within HTTP client requests. |
| [httputil](httputil/index) | Package httputil provides HTTP utility functions, complementing the more common ones in the net/http package. |
| [pprof](pprof/index) | Package pprof serves via its HTTP server runtime profiling data in the format expected by the pprof visualization tool. |
| programming_docs |
go Package httptest Package httptest
=================
* `import "net/http/httptest"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package httptest provides utilities for HTTP testing.
Index
-----
* [Constants](#pkg-constants)
* [func NewRequest(method, target string, body io.Reader) \*http.Request](#NewRequest)
* [type ResponseRecorder](#ResponseRecorder)
* [func NewRecorder() \*ResponseRecorder](#NewRecorder)
* [func (rw \*ResponseRecorder) Flush()](#ResponseRecorder.Flush)
* [func (rw \*ResponseRecorder) Header() http.Header](#ResponseRecorder.Header)
* [func (rw \*ResponseRecorder) Result() \*http.Response](#ResponseRecorder.Result)
* [func (rw \*ResponseRecorder) Write(buf []byte) (int, error)](#ResponseRecorder.Write)
* [func (rw \*ResponseRecorder) WriteHeader(code int)](#ResponseRecorder.WriteHeader)
* [func (rw \*ResponseRecorder) WriteString(str string) (int, error)](#ResponseRecorder.WriteString)
* [type Server](#Server)
* [func NewServer(handler http.Handler) \*Server](#NewServer)
* [func NewTLSServer(handler http.Handler) \*Server](#NewTLSServer)
* [func NewUnstartedServer(handler http.Handler) \*Server](#NewUnstartedServer)
* [func (s \*Server) Certificate() \*x509.Certificate](#Server.Certificate)
* [func (s \*Server) Client() \*http.Client](#Server.Client)
* [func (s \*Server) Close()](#Server.Close)
* [func (s \*Server) CloseClientConnections()](#Server.CloseClientConnections)
* [func (s \*Server) Start()](#Server.Start)
* [func (s \*Server) StartTLS()](#Server.StartTLS)
### Examples
[NewTLSServer](#example_NewTLSServer) [ResponseRecorder](#example_ResponseRecorder) [Server](#example_Server) [Server (HTTP2)](#example_Server_hTTP2) ### Package files
httptest.go recorder.go server.go
Constants
---------
DefaultRemoteAddr is the default remote address to return in RemoteAddr if an explicit DefaultRemoteAddr isn't set on ResponseRecorder.
```
const DefaultRemoteAddr = "1.2.3.4"
```
func NewRequest 1.7
-------------------
```
func NewRequest(method, target string, body io.Reader) *http.Request
```
NewRequest returns a new incoming server Request, suitable for passing to an http.Handler for testing.
The target is the RFC 7230 "request-target": it may be either a path or an absolute URL. If target is an absolute URL, the host name from the URL is used. Otherwise, "example.com" is used.
The TLS field is set to a non-nil dummy value if target has scheme "https".
The Request.Proto is always HTTP/1.1.
An empty method means "GET".
The provided body may be nil. If the body is of type \*bytes.Reader, \*strings.Reader, or \*bytes.Buffer, the Request.ContentLength is set.
NewRequest panics on error for ease of use in testing, where a panic is acceptable.
To generate a client HTTP request instead of a server request, see the NewRequest function in the net/http package.
type ResponseRecorder
---------------------
ResponseRecorder is an implementation of http.ResponseWriter that records its mutations for later inspection in tests.
```
type ResponseRecorder struct {
// Code is the HTTP response code set by WriteHeader.
//
// Note that if a Handler never calls WriteHeader or Write,
// this might end up being 0, rather than the implicit
// http.StatusOK. To get the implicit value, use the Result
// method.
Code int
// HeaderMap contains the headers explicitly set by the Handler.
// It is an internal detail.
//
// Deprecated: HeaderMap exists for historical compatibility
// and should not be used. To access the headers returned by a handler,
// use the Response.Header map as returned by the Result method.
HeaderMap http.Header
// Body is the buffer to which the Handler's Write calls are sent.
// If nil, the Writes are silently discarded.
Body *bytes.Buffer
// Flushed is whether the Handler called Flush.
Flushed bool
// contains filtered or unexported fields
}
```
#### Example
Code:
```
handler := func(w http.ResponseWriter, r *http.Request) {
io.WriteString(w, "<html><body>Hello World!</body></html>")
}
req := httptest.NewRequest("GET", "http://example.com/foo", nil)
w := httptest.NewRecorder()
handler(w, req)
resp := w.Result()
body, _ := io.ReadAll(resp.Body)
fmt.Println(resp.StatusCode)
fmt.Println(resp.Header.Get("Content-Type"))
fmt.Println(string(body))
```
Output:
```
200
text/html; charset=utf-8
<html><body>Hello World!</body></html>
```
### func NewRecorder
```
func NewRecorder() *ResponseRecorder
```
NewRecorder returns an initialized ResponseRecorder.
### func (\*ResponseRecorder) Flush
```
func (rw *ResponseRecorder) Flush()
```
Flush implements http.Flusher. To test whether Flush was called, see rw.Flushed.
### func (\*ResponseRecorder) Header
```
func (rw *ResponseRecorder) Header() http.Header
```
Header implements http.ResponseWriter. It returns the response headers to mutate within a handler. To test the headers that were written after a handler completes, use the Result method and see the returned Response value's Header.
### func (\*ResponseRecorder) Result 1.7
```
func (rw *ResponseRecorder) Result() *http.Response
```
Result returns the response generated by the handler.
The returned Response will have at least its StatusCode, Header, Body, and optionally Trailer populated. More fields may be populated in the future, so callers should not DeepEqual the result in tests.
The Response.Header is a snapshot of the headers at the time of the first write call, or at the time of this call, if the handler never did a write.
The Response.Body is guaranteed to be non-nil and Body.Read call is guaranteed to not return any error other than io.EOF.
Result must only be called after the handler has finished running.
### func (\*ResponseRecorder) Write
```
func (rw *ResponseRecorder) Write(buf []byte) (int, error)
```
Write implements http.ResponseWriter. The data in buf is written to rw.Body, if not nil.
### func (\*ResponseRecorder) WriteHeader
```
func (rw *ResponseRecorder) WriteHeader(code int)
```
WriteHeader implements http.ResponseWriter.
### func (\*ResponseRecorder) WriteString 1.6
```
func (rw *ResponseRecorder) WriteString(str string) (int, error)
```
WriteString implements io.StringWriter. The data in str is written to rw.Body, if not nil.
type Server
-----------
A Server is an HTTP server listening on a system-chosen port on the local loopback interface, for use in end-to-end HTTP tests.
```
type Server struct {
URL string // base URL of form http://ipaddr:port with no trailing slash
Listener net.Listener
// EnableHTTP2 controls whether HTTP/2 is enabled
// on the server. It must be set between calling
// NewUnstartedServer and calling Server.StartTLS.
EnableHTTP2 bool // Go 1.14
// TLS is the optional TLS configuration, populated with a new config
// after TLS is started. If set on an unstarted server before StartTLS
// is called, existing fields are copied into the new config.
TLS *tls.Config
// Config may be changed after calling NewUnstartedServer and
// before Start or StartTLS.
Config *http.Server
// contains filtered or unexported fields
}
```
#### Example
Code:
```
ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, client")
}))
defer ts.Close()
res, err := http.Get(ts.URL)
if err != nil {
log.Fatal(err)
}
greeting, err := io.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", greeting)
```
Output:
```
Hello, client
```
#### Example (HTTP2)
Code:
```
ts := httptest.NewUnstartedServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, %s", r.Proto)
}))
ts.EnableHTTP2 = true
ts.StartTLS()
defer ts.Close()
res, err := ts.Client().Get(ts.URL)
if err != nil {
log.Fatal(err)
}
greeting, err := io.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", greeting)
```
Output:
```
Hello, HTTP/2.0
```
### func NewServer
```
func NewServer(handler http.Handler) *Server
```
NewServer starts and returns a new Server. The caller should call Close when finished, to shut it down.
### func NewTLSServer
```
func NewTLSServer(handler http.Handler) *Server
```
NewTLSServer starts and returns a new Server using TLS. The caller should call Close when finished, to shut it down.
#### Example
Code:
```
ts := httptest.NewTLSServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, client")
}))
defer ts.Close()
client := ts.Client()
res, err := client.Get(ts.URL)
if err != nil {
log.Fatal(err)
}
greeting, err := io.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", greeting)
```
Output:
```
Hello, client
```
### func NewUnstartedServer
```
func NewUnstartedServer(handler http.Handler) *Server
```
NewUnstartedServer returns a new Server but doesn't start it.
After changing its configuration, the caller should call Start or StartTLS.
The caller should call Close when finished, to shut it down.
### func (\*Server) Certificate 1.9
```
func (s *Server) Certificate() *x509.Certificate
```
Certificate returns the certificate used by the server, or nil if the server doesn't use TLS.
### func (\*Server) Client 1.9
```
func (s *Server) Client() *http.Client
```
Client returns an HTTP client configured for making requests to the server. It is configured to trust the server's TLS test certificate and will close its idle connections on Server.Close.
### func (\*Server) Close
```
func (s *Server) Close()
```
Close shuts down the server and blocks until all outstanding requests on this server have completed.
### func (\*Server) CloseClientConnections
```
func (s *Server) CloseClientConnections()
```
CloseClientConnections closes any open HTTP connections to the test Server.
### func (\*Server) Start
```
func (s *Server) Start()
```
Start starts a server from NewUnstartedServer.
### func (\*Server) StartTLS
```
func (s *Server) StartTLS()
```
StartTLS starts TLS on a server from NewUnstartedServer.
go Package httputil Package httputil
=================
* `import "net/http/httputil"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package httputil provides HTTP utility functions, complementing the more common ones in the net/http package.
Index
-----
* [Variables](#pkg-variables)
* [func DumpRequest(req \*http.Request, body bool) ([]byte, error)](#DumpRequest)
* [func DumpRequestOut(req \*http.Request, body bool) ([]byte, error)](#DumpRequestOut)
* [func DumpResponse(resp \*http.Response, body bool) ([]byte, error)](#DumpResponse)
* [func NewChunkedReader(r io.Reader) io.Reader](#NewChunkedReader)
* [func NewChunkedWriter(w io.Writer) io.WriteCloser](#NewChunkedWriter)
* [type BufferPool](#BufferPool)
* [type ClientConn](#ClientConn)
* [func NewClientConn(c net.Conn, r \*bufio.Reader) \*ClientConn](#NewClientConn)
* [func NewProxyClientConn(c net.Conn, r \*bufio.Reader) \*ClientConn](#NewProxyClientConn)
* [func (cc \*ClientConn) Close() error](#ClientConn.Close)
* [func (cc \*ClientConn) Do(req \*http.Request) (\*http.Response, error)](#ClientConn.Do)
* [func (cc \*ClientConn) Hijack() (c net.Conn, r \*bufio.Reader)](#ClientConn.Hijack)
* [func (cc \*ClientConn) Pending() int](#ClientConn.Pending)
* [func (cc \*ClientConn) Read(req \*http.Request) (resp \*http.Response, err error)](#ClientConn.Read)
* [func (cc \*ClientConn) Write(req \*http.Request) error](#ClientConn.Write)
* [type ProxyRequest](#ProxyRequest)
* [func (r \*ProxyRequest) SetURL(target \*url.URL)](#ProxyRequest.SetURL)
* [func (r \*ProxyRequest) SetXForwarded()](#ProxyRequest.SetXForwarded)
* [type ReverseProxy](#ReverseProxy)
* [func NewSingleHostReverseProxy(target \*url.URL) \*ReverseProxy](#NewSingleHostReverseProxy)
* [func (p \*ReverseProxy) ServeHTTP(rw http.ResponseWriter, req \*http.Request)](#ReverseProxy.ServeHTTP)
* [type ServerConn](#ServerConn)
* [func NewServerConn(c net.Conn, r \*bufio.Reader) \*ServerConn](#NewServerConn)
* [func (sc \*ServerConn) Close() error](#ServerConn.Close)
* [func (sc \*ServerConn) Hijack() (net.Conn, \*bufio.Reader)](#ServerConn.Hijack)
* [func (sc \*ServerConn) Pending() int](#ServerConn.Pending)
* [func (sc \*ServerConn) Read() (\*http.Request, error)](#ServerConn.Read)
* [func (sc \*ServerConn) Write(req \*http.Request, resp \*http.Response) error](#ServerConn.Write)
### Examples
[DumpRequest](#example_DumpRequest) [DumpRequestOut](#example_DumpRequestOut) [DumpResponse](#example_DumpResponse) [ReverseProxy](#example_ReverseProxy) ### Package files
dump.go httputil.go persist.go reverseproxy.go
Variables
---------
```
var (
// Deprecated: No longer used.
ErrPersistEOF = &http.ProtocolError{ErrorString: "persistent connection closed"}
// Deprecated: No longer used.
ErrClosed = &http.ProtocolError{ErrorString: "connection closed by user"}
// Deprecated: No longer used.
ErrPipeline = &http.ProtocolError{ErrorString: "pipeline error"}
)
```
ErrLineTooLong is returned when reading malformed chunked data with lines that are too long.
```
var ErrLineTooLong = internal.ErrLineTooLong
```
func DumpRequest
----------------
```
func DumpRequest(req *http.Request, body bool) ([]byte, error)
```
DumpRequest returns the given request in its HTTP/1.x wire representation. It should only be used by servers to debug client requests. The returned representation is an approximation only; some details of the initial request are lost while parsing it into an http.Request. In particular, the order and case of header field names are lost. The order of values in multi-valued headers is kept intact. HTTP/2 requests are dumped in HTTP/1.x form, not in their original binary representations.
If body is true, DumpRequest also returns the body. To do so, it consumes req.Body and then replaces it with a new io.ReadCloser that yields the same bytes. If DumpRequest returns an error, the state of req is undefined.
The documentation for http.Request.Write details which fields of req are included in the dump.
#### Example
Code:
```
ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
dump, err := httputil.DumpRequest(r, true)
if err != nil {
http.Error(w, fmt.Sprint(err), http.StatusInternalServerError)
return
}
fmt.Fprintf(w, "%q", dump)
}))
defer ts.Close()
const body = "Go is a general-purpose language designed with systems programming in mind."
req, err := http.NewRequest("POST", ts.URL, strings.NewReader(body))
if err != nil {
log.Fatal(err)
}
req.Host = "www.example.org"
resp, err := http.DefaultClient.Do(req)
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
b, err := io.ReadAll(resp.Body)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", b)
```
Output:
```
"POST / HTTP/1.1\r\nHost: www.example.org\r\nAccept-Encoding: gzip\r\nContent-Length: 75\r\nUser-Agent: Go-http-client/1.1\r\n\r\nGo is a general-purpose language designed with systems programming in mind."
```
func DumpRequestOut
-------------------
```
func DumpRequestOut(req *http.Request, body bool) ([]byte, error)
```
DumpRequestOut is like DumpRequest but for outgoing client requests. It includes any headers that the standard http.Transport adds, such as User-Agent.
#### Example
Code:
```
const body = "Go is a general-purpose language designed with systems programming in mind."
req, err := http.NewRequest("PUT", "http://www.example.org", strings.NewReader(body))
if err != nil {
log.Fatal(err)
}
dump, err := httputil.DumpRequestOut(req, true)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%q", dump)
```
Output:
```
"PUT / HTTP/1.1\r\nHost: www.example.org\r\nUser-Agent: Go-http-client/1.1\r\nContent-Length: 75\r\nAccept-Encoding: gzip\r\n\r\nGo is a general-purpose language designed with systems programming in mind."
```
func DumpResponse
-----------------
```
func DumpResponse(resp *http.Response, body bool) ([]byte, error)
```
DumpResponse is like DumpRequest but dumps a response.
#### Example
Code:
```
const body = "Go is a general-purpose language designed with systems programming in mind."
ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Date", "Wed, 19 Jul 1972 19:00:00 GMT")
fmt.Fprintln(w, body)
}))
defer ts.Close()
resp, err := http.Get(ts.URL)
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
dump, err := httputil.DumpResponse(resp, true)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%q", dump)
```
Output:
```
"HTTP/1.1 200 OK\r\nContent-Length: 76\r\nContent-Type: text/plain; charset=utf-8\r\nDate: Wed, 19 Jul 1972 19:00:00 GMT\r\n\r\nGo is a general-purpose language designed with systems programming in mind.\n"
```
func NewChunkedReader
---------------------
```
func NewChunkedReader(r io.Reader) io.Reader
```
NewChunkedReader returns a new chunkedReader that translates the data read from r out of HTTP "chunked" format before returning it. The chunkedReader returns io.EOF when the final 0-length chunk is read.
NewChunkedReader is not needed by normal applications. The http package automatically decodes chunking when reading response bodies.
func NewChunkedWriter
---------------------
```
func NewChunkedWriter(w io.Writer) io.WriteCloser
```
NewChunkedWriter returns a new chunkedWriter that translates writes into HTTP "chunked" format before writing them to w. Closing the returned chunkedWriter sends the final 0-length chunk that marks the end of the stream but does not send the final CRLF that appears after trailers; trailers and the last CRLF must be written separately.
NewChunkedWriter is not needed by normal applications. The http package adds chunking automatically if handlers don't set a Content-Length header. Using NewChunkedWriter inside a handler would result in double chunking or chunking with a Content-Length length, both of which are wrong.
type BufferPool 1.6
-------------------
A BufferPool is an interface for getting and returning temporary byte slices for use by io.CopyBuffer.
```
type BufferPool interface {
Get() []byte
Put([]byte)
}
```
type ClientConn
---------------
ClientConn is an artifact of Go's early HTTP implementation. It is low-level, old, and unused by Go's current HTTP stack. We should have deleted it before Go 1.
Deprecated: Use Client or Transport in package net/http instead.
```
type ClientConn struct {
// contains filtered or unexported fields
}
```
### func NewClientConn
```
func NewClientConn(c net.Conn, r *bufio.Reader) *ClientConn
```
NewClientConn is an artifact of Go's early HTTP implementation. It is low-level, old, and unused by Go's current HTTP stack. We should have deleted it before Go 1.
Deprecated: Use the Client or Transport in package net/http instead.
### func NewProxyClientConn
```
func NewProxyClientConn(c net.Conn, r *bufio.Reader) *ClientConn
```
NewProxyClientConn is an artifact of Go's early HTTP implementation. It is low-level, old, and unused by Go's current HTTP stack. We should have deleted it before Go 1.
Deprecated: Use the Client or Transport in package net/http instead.
### func (\*ClientConn) Close
```
func (cc *ClientConn) Close() error
```
Close calls Hijack and then also closes the underlying connection.
### func (\*ClientConn) Do
```
func (cc *ClientConn) Do(req *http.Request) (*http.Response, error)
```
Do is convenience method that writes a request and reads a response.
### func (\*ClientConn) Hijack
```
func (cc *ClientConn) Hijack() (c net.Conn, r *bufio.Reader)
```
Hijack detaches the ClientConn and returns the underlying connection as well as the read-side bufio which may have some left over data. Hijack may be called before the user or Read have signaled the end of the keep-alive logic. The user should not call Hijack while Read or Write is in progress.
### func (\*ClientConn) Pending
```
func (cc *ClientConn) Pending() int
```
Pending returns the number of unanswered requests that have been sent on the connection.
### func (\*ClientConn) Read
```
func (cc *ClientConn) Read(req *http.Request) (resp *http.Response, err error)
```
Read reads the next response from the wire. A valid response might be returned together with an ErrPersistEOF, which means that the remote requested that this be the last request serviced. Read can be called concurrently with Write, but not with another Read.
### func (\*ClientConn) Write
```
func (cc *ClientConn) Write(req *http.Request) error
```
Write writes a request. An ErrPersistEOF error is returned if the connection has been closed in an HTTP keep-alive sense. If req.Close equals true, the keep-alive connection is logically closed after this request and the opposing server is informed. An ErrUnexpectedEOF indicates the remote closed the underlying TCP connection, which is usually considered as graceful close.
type ProxyRequest 1.20
----------------------
A ProxyRequest contains a request to be rewritten by a ReverseProxy.
```
type ProxyRequest struct {
// In is the request received by the proxy.
// The Rewrite function must not modify In.
In *http.Request
// Out is the request which will be sent by the proxy.
// The Rewrite function may modify or replace this request.
// Hop-by-hop headers are removed from this request
// before Rewrite is called.
Out *http.Request
}
```
### func (\*ProxyRequest) SetURL 1.20
```
func (r *ProxyRequest) SetURL(target *url.URL)
```
SetURL routes the outbound request to the scheme, host, and base path provided in target. If the target's path is "/base" and the incoming request was for "/dir", the target request will be for "/base/dir".
SetURL rewrites the outbound Host header to match the target's host. To preserve the inbound request's Host header (the default behavior of NewSingleHostReverseProxy):
```
rewriteFunc := func(r *httputil.ProxyRequest) {
r.SetURL(url)
r.Out.Host = r.In.Host
}
```
### func (\*ProxyRequest) SetXForwarded 1.20
```
func (r *ProxyRequest) SetXForwarded()
```
SetXForwarded sets the X-Forwarded-For, X-Forwarded-Host, and X-Forwarded-Proto headers of the outbound request.
* The X-Forwarded-For header is set to the client IP address.
* The X-Forwarded-Host header is set to the host name requested by the client.
* The X-Forwarded-Proto header is set to "http" or "https", depending on whether the inbound request was made on a TLS-enabled connection.
If the outbound request contains an existing X-Forwarded-For header, SetXForwarded appends the client IP address to it. To append to the inbound request's X-Forwarded-For header (the default behavior of ReverseProxy when using a Director function), copy the header from the inbound request before calling SetXForwarded:
```
rewriteFunc := func(r *httputil.ProxyRequest) {
r.Out.Header["X-Forwarded-For"] = r.In.Header["X-Forwarded-For"]
r.SetXForwarded()
}
```
type ReverseProxy
-----------------
ReverseProxy is an HTTP Handler that takes an incoming request and sends it to another server, proxying the response back to the client.
1xx responses are forwarded to the client if the underlying transport supports ClientTrace.Got1xxResponse.
```
type ReverseProxy struct {
// Rewrite must be a function which modifies
// the request into a new request to be sent
// using Transport. Its response is then copied
// back to the original client unmodified.
// Rewrite must not access the provided ProxyRequest
// or its contents after returning.
//
// The Forwarded, X-Forwarded, X-Forwarded-Host,
// and X-Forwarded-Proto headers are removed from the
// outbound request before Rewrite is called. See also
// the ProxyRequest.SetXForwarded method.
//
// Unparsable query parameters are removed from the
// outbound request before Rewrite is called.
// The Rewrite function may copy the inbound URL's
// RawQuery to the outbound URL to preserve the original
// parameter string. Note that this can lead to security
// issues if the proxy's interpretation of query parameters
// does not match that of the downstream server.
//
// At most one of Rewrite or Director may be set.
Rewrite func(*ProxyRequest) // Go 1.20
// Director is a function which modifies
// the request into a new request to be sent
// using Transport. Its response is then copied
// back to the original client unmodified.
// Director must not access the provided Request
// after returning.
//
// By default, the X-Forwarded-For header is set to the
// value of the client IP address. If an X-Forwarded-For
// header already exists, the client IP is appended to the
// existing values. As a special case, if the header
// exists in the Request.Header map but has a nil value
// (such as when set by the Director func), the X-Forwarded-For
// header is not modified.
//
// To prevent IP spoofing, be sure to delete any pre-existing
// X-Forwarded-For header coming from the client or
// an untrusted proxy.
//
// Hop-by-hop headers are removed from the request after
// Director returns, which can remove headers added by
// Director. Use a Rewrite function instead to ensure
// modifications to the request are preserved.
//
// Unparsable query parameters are removed from the outbound
// request if Request.Form is set after Director returns.
//
// At most one of Rewrite or Director may be set.
Director func(*http.Request)
// The transport used to perform proxy requests.
// If nil, http.DefaultTransport is used.
Transport http.RoundTripper
// FlushInterval specifies the flush interval
// to flush to the client while copying the
// response body.
// If zero, no periodic flushing is done.
// A negative value means to flush immediately
// after each write to the client.
// The FlushInterval is ignored when ReverseProxy
// recognizes a response as a streaming response, or
// if its ContentLength is -1; for such responses, writes
// are flushed to the client immediately.
FlushInterval time.Duration
// ErrorLog specifies an optional logger for errors
// that occur when attempting to proxy the request.
// If nil, logging is done via the log package's standard logger.
ErrorLog *log.Logger // Go 1.4
// BufferPool optionally specifies a buffer pool to
// get byte slices for use by io.CopyBuffer when
// copying HTTP response bodies.
BufferPool BufferPool // Go 1.6
// ModifyResponse is an optional function that modifies the
// Response from the backend. It is called if the backend
// returns a response at all, with any HTTP status code.
// If the backend is unreachable, the optional ErrorHandler is
// called without any call to ModifyResponse.
//
// If ModifyResponse returns an error, ErrorHandler is called
// with its error value. If ErrorHandler is nil, its default
// implementation is used.
ModifyResponse func(*http.Response) error // Go 1.8
// ErrorHandler is an optional function that handles errors
// reaching the backend or errors from ModifyResponse.
//
// If nil, the default is to log the provided error and return
// a 502 Status Bad Gateway response.
ErrorHandler func(http.ResponseWriter, *http.Request, error) // Go 1.11
}
```
#### Example
Code:
```
backendServer := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "this call was relayed by the reverse proxy")
}))
defer backendServer.Close()
rpURL, err := url.Parse(backendServer.URL)
if err != nil {
log.Fatal(err)
}
frontendProxy := httptest.NewServer(&httputil.ReverseProxy{
Rewrite: func(r *httputil.ProxyRequest) {
r.SetXForwarded()
r.SetURL(rpURL)
},
})
defer frontendProxy.Close()
resp, err := http.Get(frontendProxy.URL)
if err != nil {
log.Fatal(err)
}
b, err := io.ReadAll(resp.Body)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", b)
```
Output:
```
this call was relayed by the reverse proxy
```
### func NewSingleHostReverseProxy
```
func NewSingleHostReverseProxy(target *url.URL) *ReverseProxy
```
NewSingleHostReverseProxy returns a new ReverseProxy that routes URLs to the scheme, host, and base path provided in target. If the target's path is "/base" and the incoming request was for "/dir", the target request will be for /base/dir.
NewSingleHostReverseProxy does not rewrite the Host header.
To customize the ReverseProxy behavior beyond what NewSingleHostReverseProxy provides, use ReverseProxy directly with a Rewrite function. The ProxyRequest SetURL method may be used to route the outbound request. (Note that SetURL, unlike NewSingleHostReverseProxy, rewrites the Host header of the outbound request by default.)
```
proxy := &ReverseProxy{
Rewrite: func(r *ProxyRequest) {
r.SetURL(target)
r.Out.Host = r.In.Host // if desired
}
}
```
### func (\*ReverseProxy) ServeHTTP
```
func (p *ReverseProxy) ServeHTTP(rw http.ResponseWriter, req *http.Request)
```
type ServerConn
---------------
ServerConn is an artifact of Go's early HTTP implementation. It is low-level, old, and unused by Go's current HTTP stack. We should have deleted it before Go 1.
Deprecated: Use the Server in package net/http instead.
```
type ServerConn struct {
// contains filtered or unexported fields
}
```
### func NewServerConn
```
func NewServerConn(c net.Conn, r *bufio.Reader) *ServerConn
```
NewServerConn is an artifact of Go's early HTTP implementation. It is low-level, old, and unused by Go's current HTTP stack. We should have deleted it before Go 1.
Deprecated: Use the Server in package net/http instead.
### func (\*ServerConn) Close
```
func (sc *ServerConn) Close() error
```
Close calls Hijack and then also closes the underlying connection.
### func (\*ServerConn) Hijack
```
func (sc *ServerConn) Hijack() (net.Conn, *bufio.Reader)
```
Hijack detaches the ServerConn and returns the underlying connection as well as the read-side bufio which may have some left over data. Hijack may be called before Read has signaled the end of the keep-alive logic. The user should not call Hijack while Read or Write is in progress.
### func (\*ServerConn) Pending
```
func (sc *ServerConn) Pending() int
```
Pending returns the number of unanswered requests that have been received on the connection.
### func (\*ServerConn) Read
```
func (sc *ServerConn) Read() (*http.Request, error)
```
Read returns the next request on the wire. An ErrPersistEOF is returned if it is gracefully determined that there are no more requests (e.g. after the first request on an HTTP/1.0 connection, or after a Connection:close on a HTTP/1.1 connection).
### func (\*ServerConn) Write
```
func (sc *ServerConn) Write(req *http.Request, resp *http.Response) error
```
Write writes resp in response to req. To close the connection gracefully, set the Response.Close field to true. Write should be considered operational until it returns an error, regardless of any errors returned on the Read side.
| programming_docs |
go Package pprof Package pprof
==============
* `import "net/http/pprof"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package pprof serves via its HTTP server runtime profiling data in the format expected by the pprof visualization tool.
The package is typically only imported for the side effect of registering its HTTP handlers. The handled paths all begin with /debug/pprof/.
To use pprof, link this package into your program:
```
import _ "net/http/pprof"
```
If your application is not already running an http server, you need to start one. Add "net/http" and "log" to your imports and the following code to your main function:
```
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
```
By default, all the profiles listed in runtime/pprof.Profile are available (via [Handler](#Handler)), in addition to the [Cmdline](#Cmdline), [Profile](#Profile), [Symbol](#Symbol), and [Trace](#Trace) profiles defined in this package. If you are not using DefaultServeMux, you will have to register handlers with the mux you are using.
### Usage examples
Use the pprof tool to look at the heap profile:
```
go tool pprof http://localhost:6060/debug/pprof/heap
```
Or to look at a 30-second CPU profile:
```
go tool pprof http://localhost:6060/debug/pprof/profile?seconds=30
```
Or to look at the goroutine blocking profile, after calling runtime.SetBlockProfileRate in your program:
```
go tool pprof http://localhost:6060/debug/pprof/block
```
Or to look at the holders of contended mutexes, after calling runtime.SetMutexProfileFraction in your program:
```
go tool pprof http://localhost:6060/debug/pprof/mutex
```
The package also exports a handler that serves execution trace data for the "go tool trace" command. To collect a 5-second execution trace:
```
curl -o trace.out http://localhost:6060/debug/pprof/trace?seconds=5
go tool trace trace.out
```
To view all available profiles, open http://localhost:6060/debug/pprof/ in your browser.
For a study of the facility in action, visit
```
https://blog.golang.org/2011/06/profiling-go-programs.html
```
Index
-----
* [func Cmdline(w http.ResponseWriter, r \*http.Request)](#Cmdline)
* [func Handler(name string) http.Handler](#Handler)
* [func Index(w http.ResponseWriter, r \*http.Request)](#Index)
* [func Profile(w http.ResponseWriter, r \*http.Request)](#Profile)
* [func Symbol(w http.ResponseWriter, r \*http.Request)](#Symbol)
* [func Trace(w http.ResponseWriter, r \*http.Request)](#Trace)
### Package files
pprof.go
func Cmdline
------------
```
func Cmdline(w http.ResponseWriter, r *http.Request)
```
Cmdline responds with the running program's command line, with arguments separated by NUL bytes. The package initialization registers it as /debug/pprof/cmdline.
func Handler
------------
```
func Handler(name string) http.Handler
```
Handler returns an HTTP handler that serves the named profile. Available profiles can be found in runtime/pprof.Profile.
func Index
----------
```
func Index(w http.ResponseWriter, r *http.Request)
```
Index responds with the pprof-formatted profile named by the request. For example, "/debug/pprof/heap" serves the "heap" profile. Index responds to a request for "/debug/pprof/" with an HTML page listing the available profiles.
func Profile
------------
```
func Profile(w http.ResponseWriter, r *http.Request)
```
Profile responds with the pprof-formatted cpu profile. Profiling lasts for duration specified in seconds GET parameter, or for 30 seconds if not specified. The package initialization registers it as /debug/pprof/profile.
func Symbol
-----------
```
func Symbol(w http.ResponseWriter, r *http.Request)
```
Symbol looks up the program counters listed in the request, responding with a table mapping program counters to function names. The package initialization registers it as /debug/pprof/symbol.
func Trace 1.5
--------------
```
func Trace(w http.ResponseWriter, r *http.Request)
```
Trace responds with the execution trace in binary form. Tracing lasts for duration specified in seconds GET parameter, or for 1 second if not specified. The package initialization registers it as /debug/pprof/trace.
go Package fcgi Package fcgi
=============
* `import "net/http/fcgi"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package fcgi implements the FastCGI protocol.
See <https://fast-cgi.github.io/> for an unofficial mirror of the original documentation.
Currently only the responder role is supported.
Index
-----
* [Variables](#pkg-variables)
* [func ProcessEnv(r \*http.Request) map[string]string](#ProcessEnv)
* [func Serve(l net.Listener, handler http.Handler) error](#Serve)
### Package files
child.go fcgi.go
Variables
---------
ErrConnClosed is returned by Read when a handler attempts to read the body of a request after the connection to the web server has been closed.
```
var ErrConnClosed = errors.New("fcgi: connection to web server closed")
```
ErrRequestAborted is returned by Read when a handler attempts to read the body of a request that has been aborted by the web server.
```
var ErrRequestAborted = errors.New("fcgi: request aborted by web server")
```
func ProcessEnv 1.9
-------------------
```
func ProcessEnv(r *http.Request) map[string]string
```
ProcessEnv returns FastCGI environment variables associated with the request r for which no effort was made to be included in the request itself - the data is hidden in the request's context. As an example, if REMOTE\_USER is set for a request, it will not be found anywhere in r, but it will be included in ProcessEnv's response (via r's context).
func Serve
----------
```
func Serve(l net.Listener, handler http.Handler) error
```
Serve accepts incoming FastCGI connections on the listener l, creating a new goroutine for each. The goroutine reads requests and then calls handler to reply to them. If l is nil, Serve accepts connections from os.Stdin. If handler is nil, http.DefaultServeMux is used.
go Package internal Package internal
=================
* `import "net/http/internal"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package internal contains HTTP internals shared by net/http and net/http/httputil.
Index
-----
* [Variables](#pkg-variables)
* [func NewChunkedReader(r io.Reader) io.Reader](#NewChunkedReader)
* [func NewChunkedWriter(w io.Writer) io.WriteCloser](#NewChunkedWriter)
* [type FlushAfterChunkWriter](#FlushAfterChunkWriter)
### Package files
chunked.go
Variables
---------
```
var ErrLineTooLong = errors.New("header line too long")
```
func NewChunkedReader
---------------------
```
func NewChunkedReader(r io.Reader) io.Reader
```
NewChunkedReader returns a new chunkedReader that translates the data read from r out of HTTP "chunked" format before returning it. The chunkedReader returns io.EOF when the final 0-length chunk is read.
NewChunkedReader is not needed by normal applications. The http package automatically decodes chunking when reading response bodies.
func NewChunkedWriter
---------------------
```
func NewChunkedWriter(w io.Writer) io.WriteCloser
```
NewChunkedWriter returns a new chunkedWriter that translates writes into HTTP "chunked" format before writing them to w. Closing the returned chunkedWriter sends the final 0-length chunk that marks the end of the stream but does not send the final CRLF that appears after trailers; trailers and the last CRLF must be written separately.
NewChunkedWriter is not needed by normal applications. The http package adds chunking automatically if handlers don't set a Content-Length header. Using newChunkedWriter inside a handler would result in double chunking or chunking with a Content-Length length, both of which are wrong.
type FlushAfterChunkWriter
--------------------------
FlushAfterChunkWriter signals from the caller of NewChunkedWriter that each chunk should be followed by a flush. It is used by the http.Transport code to keep the buffering behavior for headers and trailers, but flush out chunks aggressively in the middle for request bodies which may be generated slowly. See Issue 6574.
```
type FlushAfterChunkWriter struct {
*bufio.Writer
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
go Package cgi Package cgi
============
* `import "net/http/cgi"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package cgi implements CGI (Common Gateway Interface) as specified in RFC 3875.
Note that using CGI means starting a new process to handle each request, which is typically less efficient than using a long-running server. This package is intended primarily for compatibility with existing systems.
Index
-----
* [func Request() (\*http.Request, error)](#Request)
* [func RequestFromMap(params map[string]string) (\*http.Request, error)](#RequestFromMap)
* [func Serve(handler http.Handler) error](#Serve)
* [type Handler](#Handler)
* [func (h \*Handler) ServeHTTP(rw http.ResponseWriter, req \*http.Request)](#Handler.ServeHTTP)
### Package files
child.go host.go
func Request
------------
```
func Request() (*http.Request, error)
```
Request returns the HTTP request as represented in the current environment. This assumes the current program is being run by a web server in a CGI environment. The returned Request's Body is populated, if applicable.
func RequestFromMap
-------------------
```
func RequestFromMap(params map[string]string) (*http.Request, error)
```
RequestFromMap creates an http.Request from CGI variables. The returned Request's Body field is not populated.
func Serve
----------
```
func Serve(handler http.Handler) error
```
Serve executes the provided Handler on the currently active CGI request, if any. If there's no current CGI environment an error is returned. The provided handler may be nil to use http.DefaultServeMux.
type Handler
------------
Handler runs an executable in a subprocess with a CGI environment.
```
type Handler struct {
Path string // path to the CGI executable
Root string // root URI prefix of handler or empty for "/"
// Dir specifies the CGI executable's working directory.
// If Dir is empty, the base directory of Path is used.
// If Path has no base directory, the current working
// directory is used.
Dir string
Env []string // extra environment variables to set, if any, as "key=value"
InheritEnv []string // environment variables to inherit from host, as "key"
Logger *log.Logger // optional log for errors or nil to use log.Print
Args []string // optional arguments to pass to child process
Stderr io.Writer // optional stderr for the child process; nil means os.Stderr; added in Go 1.7
// PathLocationHandler specifies the root http Handler that
// should handle internal redirects when the CGI process
// returns a Location header value starting with a "/", as
// specified in RFC 3875 § 6.3.2. This will likely be
// http.DefaultServeMux.
//
// If nil, a CGI response with a local URI path is instead sent
// back to the client and not redirected internally.
PathLocationHandler http.Handler
}
```
### func (\*Handler) ServeHTTP
```
func (h *Handler) ServeHTTP(rw http.ResponseWriter, req *http.Request)
```
go Package cookiejar Package cookiejar
==================
* `import "net/http/cookiejar"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package cookiejar implements an in-memory RFC 6265-compliant http.CookieJar.
Index
-----
* [type Jar](#Jar)
* [func New(o \*Options) (\*Jar, error)](#New)
* [func (j \*Jar) Cookies(u \*url.URL) (cookies []\*http.Cookie)](#Jar.Cookies)
* [func (j \*Jar) SetCookies(u \*url.URL, cookies []\*http.Cookie)](#Jar.SetCookies)
* [type Options](#Options)
* [type PublicSuffixList](#PublicSuffixList)
### Examples
[New](#example_New) ### Package files
jar.go punycode.go
type Jar 1.1
------------
Jar implements the http.CookieJar interface from the net/http package.
```
type Jar struct {
// contains filtered or unexported fields
}
```
### func New 1.1
```
func New(o *Options) (*Jar, error)
```
New returns a new cookie jar. A nil \*Options is equivalent to a zero Options.
#### Example
Code:
```
// Start a server to give us cookies.
ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
if cookie, err := r.Cookie("Flavor"); err != nil {
http.SetCookie(w, &http.Cookie{Name: "Flavor", Value: "Chocolate Chip"})
} else {
cookie.Value = "Oatmeal Raisin"
http.SetCookie(w, cookie)
}
}))
defer ts.Close()
u, err := url.Parse(ts.URL)
if err != nil {
log.Fatal(err)
}
// All users of cookiejar should import "golang.org/x/net/publicsuffix"
jar, err := cookiejar.New(&cookiejar.Options{PublicSuffixList: publicsuffix.List})
if err != nil {
log.Fatal(err)
}
client := &http.Client{
Jar: jar,
}
if _, err = client.Get(u.String()); err != nil {
log.Fatal(err)
}
fmt.Println("After 1st request:")
for _, cookie := range jar.Cookies(u) {
fmt.Printf(" %s: %s\n", cookie.Name, cookie.Value)
}
if _, err = client.Get(u.String()); err != nil {
log.Fatal(err)
}
fmt.Println("After 2nd request:")
for _, cookie := range jar.Cookies(u) {
fmt.Printf(" %s: %s\n", cookie.Name, cookie.Value)
}
```
Output:
```
After 1st request:
Flavor: Chocolate Chip
After 2nd request:
Flavor: Oatmeal Raisin
```
### func (\*Jar) Cookies 1.1
```
func (j *Jar) Cookies(u *url.URL) (cookies []*http.Cookie)
```
Cookies implements the Cookies method of the http.CookieJar interface.
It returns an empty slice if the URL's scheme is not HTTP or HTTPS.
### func (\*Jar) SetCookies 1.1
```
func (j *Jar) SetCookies(u *url.URL, cookies []*http.Cookie)
```
SetCookies implements the SetCookies method of the http.CookieJar interface.
It does nothing if the URL's scheme is not HTTP or HTTPS.
type Options 1.1
----------------
Options are the options for creating a new Jar.
```
type Options struct {
// PublicSuffixList is the public suffix list that determines whether
// an HTTP server can set a cookie for a domain.
//
// A nil value is valid and may be useful for testing but it is not
// secure: it means that the HTTP server for foo.co.uk can set a cookie
// for bar.co.uk.
PublicSuffixList PublicSuffixList
}
```
type PublicSuffixList 1.1
-------------------------
PublicSuffixList provides the public suffix of a domain. For example:
* the public suffix of "example.com" is "com",
* the public suffix of "foo1.foo2.foo3.co.uk" is "co.uk", and
* the public suffix of "bar.pvt.k12.ma.us" is "pvt.k12.ma.us".
Implementations of PublicSuffixList must be safe for concurrent use by multiple goroutines.
An implementation that always returns "" is valid and may be useful for testing but it is not secure: it means that the HTTP server for foo.com can set a cookie for bar.com.
A public suffix list implementation is in the package golang.org/x/net/publicsuffix.
```
type PublicSuffixList interface {
// PublicSuffix returns the public suffix of domain.
//
// TODO: specify which of the caller and callee is responsible for IP
// addresses, for leading and trailing dots, for case sensitivity, and
// for IDN/Punycode.
PublicSuffix(domain string) string
// String returns a description of the source of this public suffix
// list. The description will typically contain something like a time
// stamp or version number.
String() string
}
```
go Package httptrace Package httptrace
==================
* `import "net/http/httptrace"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package httptrace provides mechanisms to trace the events within HTTP client requests.
#### Example
Code:
```
req, _ := http.NewRequest("GET", "http://example.com", nil)
trace := &httptrace.ClientTrace{
GotConn: func(connInfo httptrace.GotConnInfo) {
fmt.Printf("Got Conn: %+v\n", connInfo)
},
DNSDone: func(dnsInfo httptrace.DNSDoneInfo) {
fmt.Printf("DNS Info: %+v\n", dnsInfo)
},
}
req = req.WithContext(httptrace.WithClientTrace(req.Context(), trace))
_, err := http.DefaultTransport.RoundTrip(req)
if err != nil {
log.Fatal(err)
}
```
Index
-----
* [func WithClientTrace(ctx context.Context, trace \*ClientTrace) context.Context](#WithClientTrace)
* [type ClientTrace](#ClientTrace)
* [func ContextClientTrace(ctx context.Context) \*ClientTrace](#ContextClientTrace)
* [type DNSDoneInfo](#DNSDoneInfo)
* [type DNSStartInfo](#DNSStartInfo)
* [type GotConnInfo](#GotConnInfo)
* [type WroteRequestInfo](#WroteRequestInfo)
### Examples
[Package](#example_) ### Package files
trace.go
func WithClientTrace 1.7
------------------------
```
func WithClientTrace(ctx context.Context, trace *ClientTrace) context.Context
```
WithClientTrace returns a new context based on the provided parent ctx. HTTP client requests made with the returned context will use the provided trace hooks, in addition to any previous hooks registered with ctx. Any hooks defined in the provided trace will be called first.
type ClientTrace 1.7
--------------------
ClientTrace is a set of hooks to run at various stages of an outgoing HTTP request. Any particular hook may be nil. Functions may be called concurrently from different goroutines and some may be called after the request has completed or failed.
ClientTrace currently traces a single HTTP request & response during a single round trip and has no hooks that span a series of redirected requests.
See <https://blog.golang.org/http-tracing> for more.
```
type ClientTrace struct {
// GetConn is called before a connection is created or
// retrieved from an idle pool. The hostPort is the
// "host:port" of the target or proxy. GetConn is called even
// if there's already an idle cached connection available.
GetConn func(hostPort string)
// GotConn is called after a successful connection is
// obtained. There is no hook for failure to obtain a
// connection; instead, use the error from
// Transport.RoundTrip.
GotConn func(GotConnInfo)
// PutIdleConn is called when the connection is returned to
// the idle pool. If err is nil, the connection was
// successfully returned to the idle pool. If err is non-nil,
// it describes why not. PutIdleConn is not called if
// connection reuse is disabled via Transport.DisableKeepAlives.
// PutIdleConn is called before the caller's Response.Body.Close
// call returns.
// For HTTP/2, this hook is not currently used.
PutIdleConn func(err error)
// GotFirstResponseByte is called when the first byte of the response
// headers is available.
GotFirstResponseByte func()
// Got100Continue is called if the server replies with a "100
// Continue" response.
Got100Continue func()
// Got1xxResponse is called for each 1xx informational response header
// returned before the final non-1xx response. Got1xxResponse is called
// for "100 Continue" responses, even if Got100Continue is also defined.
// If it returns an error, the client request is aborted with that error value.
Got1xxResponse func(code int, header textproto.MIMEHeader) error // Go 1.11
// DNSStart is called when a DNS lookup begins.
DNSStart func(DNSStartInfo)
// DNSDone is called when a DNS lookup ends.
DNSDone func(DNSDoneInfo)
// ConnectStart is called when a new connection's Dial begins.
// If net.Dialer.DualStack (IPv6 "Happy Eyeballs") support is
// enabled, this may be called multiple times.
ConnectStart func(network, addr string)
// ConnectDone is called when a new connection's Dial
// completes. The provided err indicates whether the
// connection completed successfully.
// If net.Dialer.DualStack ("Happy Eyeballs") support is
// enabled, this may be called multiple times.
ConnectDone func(network, addr string, err error)
// TLSHandshakeStart is called when the TLS handshake is started. When
// connecting to an HTTPS site via an HTTP proxy, the handshake happens
// after the CONNECT request is processed by the proxy.
TLSHandshakeStart func() // Go 1.8
// TLSHandshakeDone is called after the TLS handshake with either the
// successful handshake's connection state, or a non-nil error on handshake
// failure.
TLSHandshakeDone func(tls.ConnectionState, error) // Go 1.8
// WroteHeaderField is called after the Transport has written
// each request header. At the time of this call the values
// might be buffered and not yet written to the network.
WroteHeaderField func(key string, value []string) // Go 1.11
// WroteHeaders is called after the Transport has written
// all request headers.
WroteHeaders func()
// Wait100Continue is called if the Request specified
// "Expect: 100-continue" and the Transport has written the
// request headers but is waiting for "100 Continue" from the
// server before writing the request body.
Wait100Continue func()
// WroteRequest is called with the result of writing the
// request and any body. It may be called multiple times
// in the case of retried requests.
WroteRequest func(WroteRequestInfo)
}
```
### func ContextClientTrace 1.7
```
func ContextClientTrace(ctx context.Context) *ClientTrace
```
ContextClientTrace returns the ClientTrace associated with the provided context. If none, it returns nil.
type DNSDoneInfo 1.7
--------------------
DNSDoneInfo contains information about the results of a DNS lookup.
```
type DNSDoneInfo struct {
// Addrs are the IPv4 and/or IPv6 addresses found in the DNS
// lookup. The contents of the slice should not be mutated.
Addrs []net.IPAddr
// Err is any error that occurred during the DNS lookup.
Err error
// Coalesced is whether the Addrs were shared with another
// caller who was doing the same DNS lookup concurrently.
Coalesced bool
}
```
type DNSStartInfo 1.7
---------------------
DNSStartInfo contains information about a DNS request.
```
type DNSStartInfo struct {
Host string
}
```
type GotConnInfo 1.7
--------------------
GotConnInfo is the argument to the ClientTrace.GotConn function and contains information about the obtained connection.
```
type GotConnInfo struct {
// Conn is the connection that was obtained. It is owned by
// the http.Transport and should not be read, written or
// closed by users of ClientTrace.
Conn net.Conn
// Reused is whether this connection has been previously
// used for another HTTP request.
Reused bool
// WasIdle is whether this connection was obtained from an
// idle pool.
WasIdle bool
// IdleTime reports how long the connection was previously
// idle, if WasIdle is true.
IdleTime time.Duration
}
```
type WroteRequestInfo 1.7
-------------------------
WroteRequestInfo contains information provided to the WroteRequest hook.
```
type WroteRequestInfo struct {
// Err is any error encountered while writing the Request.
Err error
}
```
| programming_docs |
go Package smtp Package smtp
=============
* `import "net/smtp"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package smtp implements the Simple Mail Transfer Protocol as defined in RFC 5321. It also implements the following extensions:
```
8BITMIME RFC 1652
AUTH RFC 2554
STARTTLS RFC 3207
```
Additional extensions may be handled by clients.
The smtp package is frozen and is not accepting new features. Some external packages provide more functionality. See:
```
https://godoc.org/?q=smtp
```
#### Example
Code:
```
// Connect to the remote SMTP server.
c, err := smtp.Dial("mail.example.com:25")
if err != nil {
log.Fatal(err)
}
// Set the sender and recipient first
if err := c.Mail("[email protected]"); err != nil {
log.Fatal(err)
}
if err := c.Rcpt("[email protected]"); err != nil {
log.Fatal(err)
}
// Send the email body.
wc, err := c.Data()
if err != nil {
log.Fatal(err)
}
_, err = fmt.Fprintf(wc, "This is the email body")
if err != nil {
log.Fatal(err)
}
err = wc.Close()
if err != nil {
log.Fatal(err)
}
// Send the QUIT command and close the connection.
err = c.Quit()
if err != nil {
log.Fatal(err)
}
```
Index
-----
* [func SendMail(addr string, a Auth, from string, to []string, msg []byte) error](#SendMail)
* [type Auth](#Auth)
* [func CRAMMD5Auth(username, secret string) Auth](#CRAMMD5Auth)
* [func PlainAuth(identity, username, password, host string) Auth](#PlainAuth)
* [type Client](#Client)
* [func Dial(addr string) (\*Client, error)](#Dial)
* [func NewClient(conn net.Conn, host string) (\*Client, error)](#NewClient)
* [func (c \*Client) Auth(a Auth) error](#Client.Auth)
* [func (c \*Client) Close() error](#Client.Close)
* [func (c \*Client) Data() (io.WriteCloser, error)](#Client.Data)
* [func (c \*Client) Extension(ext string) (bool, string)](#Client.Extension)
* [func (c \*Client) Hello(localName string) error](#Client.Hello)
* [func (c \*Client) Mail(from string) error](#Client.Mail)
* [func (c \*Client) Noop() error](#Client.Noop)
* [func (c \*Client) Quit() error](#Client.Quit)
* [func (c \*Client) Rcpt(to string) error](#Client.Rcpt)
* [func (c \*Client) Reset() error](#Client.Reset)
* [func (c \*Client) StartTLS(config \*tls.Config) error](#Client.StartTLS)
* [func (c \*Client) TLSConnectionState() (state tls.ConnectionState, ok bool)](#Client.TLSConnectionState)
* [func (c \*Client) Verify(addr string) error](#Client.Verify)
* [type ServerInfo](#ServerInfo)
### Examples
[Package](#example_) [PlainAuth](#example_PlainAuth) [SendMail](#example_SendMail) ### Package files
auth.go smtp.go
func SendMail
-------------
```
func SendMail(addr string, a Auth, from string, to []string, msg []byte) error
```
SendMail connects to the server at addr, switches to TLS if possible, authenticates with the optional mechanism a if possible, and then sends an email from address from, to addresses to, with message msg. The addr must include a port, as in "mail.example.com:smtp".
The addresses in the to parameter are the SMTP RCPT addresses.
The msg parameter should be an RFC 822-style email with headers first, a blank line, and then the message body. The lines of msg should be CRLF terminated. The msg headers should usually include fields such as "From", "To", "Subject", and "Cc". Sending "Bcc" messages is accomplished by including an email address in the to parameter but not including it in the msg headers.
The SendMail function and the net/smtp package are low-level mechanisms and provide no support for DKIM signing, MIME attachments (see the mime/multipart package), or other mail functionality. Higher-level packages exist outside of the standard library.
#### Example
Code:
```
// Set up authentication information.
auth := smtp.PlainAuth("", "[email protected]", "password", "mail.example.com")
// Connect to the server, authenticate, set the sender and recipient,
// and send the email all in one step.
to := []string{"[email protected]"}
msg := []byte("To: [email protected]\r\n" +
"Subject: discount Gophers!\r\n" +
"\r\n" +
"This is the email body.\r\n")
err := smtp.SendMail("mail.example.com:25", auth, "[email protected]", to, msg)
if err != nil {
log.Fatal(err)
}
```
type Auth
---------
Auth is implemented by an SMTP authentication mechanism.
```
type Auth interface {
// Start begins an authentication with a server.
// It returns the name of the authentication protocol
// and optionally data to include in the initial AUTH message
// sent to the server.
// If it returns a non-nil error, the SMTP client aborts
// the authentication attempt and closes the connection.
Start(server *ServerInfo) (proto string, toServer []byte, err error)
// Next continues the authentication. The server has just sent
// the fromServer data. If more is true, the server expects a
// response, which Next should return as toServer; otherwise
// Next should return toServer == nil.
// If Next returns a non-nil error, the SMTP client aborts
// the authentication attempt and closes the connection.
Next(fromServer []byte, more bool) (toServer []byte, err error)
}
```
### func CRAMMD5Auth
```
func CRAMMD5Auth(username, secret string) Auth
```
CRAMMD5Auth returns an Auth that implements the CRAM-MD5 authentication mechanism as defined in RFC 2195. The returned Auth uses the given username and secret to authenticate to the server using the challenge-response mechanism.
### func PlainAuth
```
func PlainAuth(identity, username, password, host string) Auth
```
PlainAuth returns an Auth that implements the PLAIN authentication mechanism as defined in RFC 4616. The returned Auth uses the given username and password to authenticate to host and act as identity. Usually identity should be the empty string, to act as username.
PlainAuth will only send the credentials if the connection is using TLS or is connected to localhost. Otherwise authentication will fail with an error, without sending the credentials.
#### Example
Code:
```
// hostname is used by PlainAuth to validate the TLS certificate.
hostname := "mail.example.com"
auth := smtp.PlainAuth("", "[email protected]", "password", hostname)
err := smtp.SendMail(hostname+":25", auth, from, recipients, msg)
if err != nil {
log.Fatal(err)
}
```
type Client
-----------
A Client represents a client connection to an SMTP server.
```
type Client struct {
// Text is the textproto.Conn used by the Client. It is exported to allow for
// clients to add extensions.
Text *textproto.Conn
// contains filtered or unexported fields
}
```
### func Dial
```
func Dial(addr string) (*Client, error)
```
Dial returns a new Client connected to an SMTP server at addr. The addr must include a port, as in "mail.example.com:smtp".
### func NewClient
```
func NewClient(conn net.Conn, host string) (*Client, error)
```
NewClient returns a new Client using an existing connection and host as a server name to be used when authenticating.
### func (\*Client) Auth
```
func (c *Client) Auth(a Auth) error
```
Auth authenticates a client using the provided authentication mechanism. A failed authentication closes the connection. Only servers that advertise the AUTH extension support this function.
### func (\*Client) Close 1.2
```
func (c *Client) Close() error
```
Close closes the connection.
### func (\*Client) Data
```
func (c *Client) Data() (io.WriteCloser, error)
```
Data issues a DATA command to the server and returns a writer that can be used to write the mail headers and body. The caller should close the writer before calling any more methods on c. A call to Data must be preceded by one or more calls to Rcpt.
### func (\*Client) Extension
```
func (c *Client) Extension(ext string) (bool, string)
```
Extension reports whether an extension is support by the server. The extension name is case-insensitive. If the extension is supported, Extension also returns a string that contains any parameters the server specifies for the extension.
### func (\*Client) Hello 1.1
```
func (c *Client) Hello(localName string) error
```
Hello sends a HELO or EHLO to the server as the given host name. Calling this method is only necessary if the client needs control over the host name used. The client will introduce itself as "localhost" automatically otherwise. If Hello is called, it must be called before any of the other methods.
### func (\*Client) Mail
```
func (c *Client) Mail(from string) error
```
Mail issues a MAIL command to the server using the provided email address. If the server supports the 8BITMIME extension, Mail adds the BODY=8BITMIME parameter. If the server supports the SMTPUTF8 extension, Mail adds the SMTPUTF8 parameter. This initiates a mail transaction and is followed by one or more Rcpt calls.
### func (\*Client) Noop 1.10
```
func (c *Client) Noop() error
```
Noop sends the NOOP command to the server. It does nothing but check that the connection to the server is okay.
### func (\*Client) Quit
```
func (c *Client) Quit() error
```
Quit sends the QUIT command and closes the connection to the server.
### func (\*Client) Rcpt
```
func (c *Client) Rcpt(to string) error
```
Rcpt issues a RCPT command to the server using the provided email address. A call to Rcpt must be preceded by a call to Mail and may be followed by a Data call or another Rcpt call.
### func (\*Client) Reset
```
func (c *Client) Reset() error
```
Reset sends the RSET command to the server, aborting the current mail transaction.
### func (\*Client) StartTLS
```
func (c *Client) StartTLS(config *tls.Config) error
```
StartTLS sends the STARTTLS command and encrypts all further communication. Only servers that advertise the STARTTLS extension support this function.
### func (\*Client) TLSConnectionState 1.5
```
func (c *Client) TLSConnectionState() (state tls.ConnectionState, ok bool)
```
TLSConnectionState returns the client's TLS connection state. The return values are their zero values if StartTLS did not succeed.
### func (\*Client) Verify
```
func (c *Client) Verify(addr string) error
```
Verify checks the validity of an email address on the server. If Verify returns nil, the address is valid. A non-nil return does not necessarily indicate an invalid address. Many servers will not verify addresses for security reasons.
type ServerInfo
---------------
ServerInfo records information about an SMTP server.
```
type ServerInfo struct {
Name string // SMTP server name
TLS bool // using TLS, with valid certificate for Name
Auth []string // advertised authentication mechanisms
}
```
go Package netip Package netip
==============
* `import "net/netip"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package netip defines an IP address type that's a small value type. Building on that Addr type, the package also defines AddrPort (an IP address and a port), and Prefix (an IP address and a bit length prefix).
Compared to the net.IP type, this package's Addr type takes less memory, is immutable, and is comparable (supports == and being a map key).
Index
-----
* [type Addr](#Addr)
* [func AddrFrom16(addr [16]byte) Addr](#AddrFrom16)
* [func AddrFrom4(addr [4]byte) Addr](#AddrFrom4)
* [func AddrFromSlice(slice []byte) (ip Addr, ok bool)](#AddrFromSlice)
* [func IPv4Unspecified() Addr](#IPv4Unspecified)
* [func IPv6LinkLocalAllNodes() Addr](#IPv6LinkLocalAllNodes)
* [func IPv6LinkLocalAllRouters() Addr](#IPv6LinkLocalAllRouters)
* [func IPv6Loopback() Addr](#IPv6Loopback)
* [func IPv6Unspecified() Addr](#IPv6Unspecified)
* [func MustParseAddr(s string) Addr](#MustParseAddr)
* [func ParseAddr(s string) (Addr, error)](#ParseAddr)
* [func (ip Addr) AppendTo(b []byte) []byte](#Addr.AppendTo)
* [func (ip Addr) As16() (a16 [16]byte)](#Addr.As16)
* [func (ip Addr) As4() (a4 [4]byte)](#Addr.As4)
* [func (ip Addr) AsSlice() []byte](#Addr.AsSlice)
* [func (ip Addr) BitLen() int](#Addr.BitLen)
* [func (ip Addr) Compare(ip2 Addr) int](#Addr.Compare)
* [func (ip Addr) Is4() bool](#Addr.Is4)
* [func (ip Addr) Is4In6() bool](#Addr.Is4In6)
* [func (ip Addr) Is6() bool](#Addr.Is6)
* [func (ip Addr) IsGlobalUnicast() bool](#Addr.IsGlobalUnicast)
* [func (ip Addr) IsInterfaceLocalMulticast() bool](#Addr.IsInterfaceLocalMulticast)
* [func (ip Addr) IsLinkLocalMulticast() bool](#Addr.IsLinkLocalMulticast)
* [func (ip Addr) IsLinkLocalUnicast() bool](#Addr.IsLinkLocalUnicast)
* [func (ip Addr) IsLoopback() bool](#Addr.IsLoopback)
* [func (ip Addr) IsMulticast() bool](#Addr.IsMulticast)
* [func (ip Addr) IsPrivate() bool](#Addr.IsPrivate)
* [func (ip Addr) IsUnspecified() bool](#Addr.IsUnspecified)
* [func (ip Addr) IsValid() bool](#Addr.IsValid)
* [func (ip Addr) Less(ip2 Addr) bool](#Addr.Less)
* [func (ip Addr) MarshalBinary() ([]byte, error)](#Addr.MarshalBinary)
* [func (ip Addr) MarshalText() ([]byte, error)](#Addr.MarshalText)
* [func (ip Addr) Next() Addr](#Addr.Next)
* [func (ip Addr) Prefix(b int) (Prefix, error)](#Addr.Prefix)
* [func (ip Addr) Prev() Addr](#Addr.Prev)
* [func (ip Addr) String() string](#Addr.String)
* [func (ip Addr) StringExpanded() string](#Addr.StringExpanded)
* [func (ip Addr) Unmap() Addr](#Addr.Unmap)
* [func (ip \*Addr) UnmarshalBinary(b []byte) error](#Addr.UnmarshalBinary)
* [func (ip \*Addr) UnmarshalText(text []byte) error](#Addr.UnmarshalText)
* [func (ip Addr) WithZone(zone string) Addr](#Addr.WithZone)
* [func (ip Addr) Zone() string](#Addr.Zone)
* [type AddrPort](#AddrPort)
* [func AddrPortFrom(ip Addr, port uint16) AddrPort](#AddrPortFrom)
* [func MustParseAddrPort(s string) AddrPort](#MustParseAddrPort)
* [func ParseAddrPort(s string) (AddrPort, error)](#ParseAddrPort)
* [func (p AddrPort) Addr() Addr](#AddrPort.Addr)
* [func (p AddrPort) AppendTo(b []byte) []byte](#AddrPort.AppendTo)
* [func (p AddrPort) IsValid() bool](#AddrPort.IsValid)
* [func (p AddrPort) MarshalBinary() ([]byte, error)](#AddrPort.MarshalBinary)
* [func (p AddrPort) MarshalText() ([]byte, error)](#AddrPort.MarshalText)
* [func (p AddrPort) Port() uint16](#AddrPort.Port)
* [func (p AddrPort) String() string](#AddrPort.String)
* [func (p \*AddrPort) UnmarshalBinary(b []byte) error](#AddrPort.UnmarshalBinary)
* [func (p \*AddrPort) UnmarshalText(text []byte) error](#AddrPort.UnmarshalText)
* [type Prefix](#Prefix)
* [func MustParsePrefix(s string) Prefix](#MustParsePrefix)
* [func ParsePrefix(s string) (Prefix, error)](#ParsePrefix)
* [func PrefixFrom(ip Addr, bits int) Prefix](#PrefixFrom)
* [func (p Prefix) Addr() Addr](#Prefix.Addr)
* [func (p Prefix) AppendTo(b []byte) []byte](#Prefix.AppendTo)
* [func (p Prefix) Bits() int](#Prefix.Bits)
* [func (p Prefix) Contains(ip Addr) bool](#Prefix.Contains)
* [func (p Prefix) IsSingleIP() bool](#Prefix.IsSingleIP)
* [func (p Prefix) IsValid() bool](#Prefix.IsValid)
* [func (p Prefix) MarshalBinary() ([]byte, error)](#Prefix.MarshalBinary)
* [func (p Prefix) MarshalText() ([]byte, error)](#Prefix.MarshalText)
* [func (p Prefix) Masked() Prefix](#Prefix.Masked)
* [func (p Prefix) Overlaps(o Prefix) bool](#Prefix.Overlaps)
* [func (p Prefix) String() string](#Prefix.String)
* [func (p \*Prefix) UnmarshalBinary(b []byte) error](#Prefix.UnmarshalBinary)
* [func (p \*Prefix) UnmarshalText(text []byte) error](#Prefix.UnmarshalText)
### Package files
leaf\_alts.go netip.go uint128.go
type Addr 1.18
--------------
Addr represents an IPv4 or IPv6 address (with or without a scoped addressing zone), similar to net.IP or net.IPAddr.
Unlike net.IP or net.IPAddr, Addr is a comparable value type (it supports == and can be a map key) and is immutable.
The zero Addr is not a valid IP address. Addr{} is distinct from both 0.0.0.0 and ::.
```
type Addr struct {
// contains filtered or unexported fields
}
```
### func AddrFrom16 1.18
```
func AddrFrom16(addr [16]byte) Addr
```
AddrFrom16 returns the IPv6 address given by the bytes in addr. An IPv4-mapped IPv6 address is left as an IPv6 address. (Use Unmap to convert them if needed.)
### func AddrFrom4 1.18
```
func AddrFrom4(addr [4]byte) Addr
```
AddrFrom4 returns the address of the IPv4 address given by the bytes in addr.
### func AddrFromSlice 1.18
```
func AddrFromSlice(slice []byte) (ip Addr, ok bool)
```
AddrFromSlice parses the 4- or 16-byte byte slice as an IPv4 or IPv6 address. Note that a net.IP can be passed directly as the []byte argument. If slice's length is not 4 or 16, AddrFromSlice returns Addr{}, false.
### func IPv4Unspecified 1.18
```
func IPv4Unspecified() Addr
```
IPv4Unspecified returns the IPv4 unspecified address "0.0.0.0".
### func IPv6LinkLocalAllNodes 1.18
```
func IPv6LinkLocalAllNodes() Addr
```
IPv6LinkLocalAllNodes returns the IPv6 link-local all nodes multicast address ff02::1.
### func IPv6LinkLocalAllRouters 1.20
```
func IPv6LinkLocalAllRouters() Addr
```
IPv6LinkLocalAllRouters returns the IPv6 link-local all routers multicast address ff02::2.
### func IPv6Loopback 1.20
```
func IPv6Loopback() Addr
```
IPv6Loopback returns the IPv6 loopback address ::1.
### func IPv6Unspecified 1.18
```
func IPv6Unspecified() Addr
```
IPv6Unspecified returns the IPv6 unspecified address "::".
### func MustParseAddr 1.18
```
func MustParseAddr(s string) Addr
```
MustParseAddr calls ParseAddr(s) and panics on error. It is intended for use in tests with hard-coded strings.
### func ParseAddr 1.18
```
func ParseAddr(s string) (Addr, error)
```
ParseAddr parses s as an IP address, returning the result. The string s can be in dotted decimal ("192.0.2.1"), IPv6 ("2001:db8::68"), or IPv6 with a scoped addressing zone ("fe80::1cc0:3e8c:119f:c2e1%ens18").
### func (Addr) AppendTo 1.18
```
func (ip Addr) AppendTo(b []byte) []byte
```
AppendTo appends a text encoding of ip, as generated by MarshalText, to b and returns the extended buffer.
### func (Addr) As16 1.18
```
func (ip Addr) As16() (a16 [16]byte)
```
As16 returns the IP address in its 16-byte representation. IPv4 addresses are returned as IPv4-mapped IPv6 addresses. IPv6 addresses with zones are returned without their zone (use the Zone method to get it). The ip zero value returns all zeroes.
### func (Addr) As4 1.18
```
func (ip Addr) As4() (a4 [4]byte)
```
As4 returns an IPv4 or IPv4-in-IPv6 address in its 4-byte representation. If ip is the zero Addr or an IPv6 address, As4 panics. Note that 0.0.0.0 is not the zero Addr.
### func (Addr) AsSlice 1.18
```
func (ip Addr) AsSlice() []byte
```
AsSlice returns an IPv4 or IPv6 address in its respective 4-byte or 16-byte representation.
### func (Addr) BitLen 1.18
```
func (ip Addr) BitLen() int
```
BitLen returns the number of bits in the IP address: 128 for IPv6, 32 for IPv4, and 0 for the zero Addr.
Note that IPv4-mapped IPv6 addresses are considered IPv6 addresses and therefore have bit length 128.
### func (Addr) Compare 1.18
```
func (ip Addr) Compare(ip2 Addr) int
```
Compare returns an integer comparing two IPs. The result will be 0 if ip == ip2, -1 if ip < ip2, and +1 if ip > ip2. The definition of "less than" is the same as the Less method.
### func (Addr) Is4 1.18
```
func (ip Addr) Is4() bool
```
Is4 reports whether ip is an IPv4 address.
It returns false for IPv4-mapped IPv6 addresses. See Addr.Unmap.
### func (Addr) Is4In6 1.18
```
func (ip Addr) Is4In6() bool
```
Is4In6 reports whether ip is an IPv4-mapped IPv6 address.
### func (Addr) Is6 1.18
```
func (ip Addr) Is6() bool
```
Is6 reports whether ip is an IPv6 address, including IPv4-mapped IPv6 addresses.
### func (Addr) IsGlobalUnicast 1.18
```
func (ip Addr) IsGlobalUnicast() bool
```
IsGlobalUnicast reports whether ip is a global unicast address.
It returns true for IPv6 addresses which fall outside of the current IANA-allocated 2000::/3 global unicast space, with the exception of the link-local address space. It also returns true even if ip is in the IPv4 private address space or IPv6 unique local address space. It returns false for the zero Addr.
For reference, see RFC 1122, RFC 4291, and RFC 4632.
### func (Addr) IsInterfaceLocalMulticast 1.18
```
func (ip Addr) IsInterfaceLocalMulticast() bool
```
IsInterfaceLocalMulticast reports whether ip is an IPv6 interface-local multicast address.
### func (Addr) IsLinkLocalMulticast 1.18
```
func (ip Addr) IsLinkLocalMulticast() bool
```
IsLinkLocalMulticast reports whether ip is a link-local multicast address.
### func (Addr) IsLinkLocalUnicast 1.18
```
func (ip Addr) IsLinkLocalUnicast() bool
```
IsLinkLocalUnicast reports whether ip is a link-local unicast address.
### func (Addr) IsLoopback 1.18
```
func (ip Addr) IsLoopback() bool
```
IsLoopback reports whether ip is a loopback address.
### func (Addr) IsMulticast 1.18
```
func (ip Addr) IsMulticast() bool
```
IsMulticast reports whether ip is a multicast address.
### func (Addr) IsPrivate 1.18
```
func (ip Addr) IsPrivate() bool
```
IsPrivate reports whether ip is a private address, according to RFC 1918 (IPv4 addresses) and RFC 4193 (IPv6 addresses). That is, it reports whether ip is in 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16, or fc00::/7. This is the same as net.IP.IsPrivate.
### func (Addr) IsUnspecified 1.18
```
func (ip Addr) IsUnspecified() bool
```
IsUnspecified reports whether ip is an unspecified address, either the IPv4 address "0.0.0.0" or the IPv6 address "::".
Note that the zero Addr is not an unspecified address.
### func (Addr) IsValid 1.18
```
func (ip Addr) IsValid() bool
```
IsValid reports whether the Addr is an initialized address (not the zero Addr).
Note that "0.0.0.0" and "::" are both valid values.
### func (Addr) Less 1.18
```
func (ip Addr) Less(ip2 Addr) bool
```
Less reports whether ip sorts before ip2. IP addresses sort first by length, then their address. IPv6 addresses with zones sort just after the same address without a zone.
### func (Addr) MarshalBinary 1.18
```
func (ip Addr) MarshalBinary() ([]byte, error)
```
MarshalBinary implements the encoding.BinaryMarshaler interface. It returns a zero-length slice for the zero Addr, the 4-byte form for an IPv4 address, and the 16-byte form with zone appended for an IPv6 address.
### func (Addr) MarshalText 1.18
```
func (ip Addr) MarshalText() ([]byte, error)
```
MarshalText implements the encoding.TextMarshaler interface, The encoding is the same as returned by String, with one exception: If ip is the zero Addr, the encoding is the empty string.
### func (Addr) Next 1.18
```
func (ip Addr) Next() Addr
```
Next returns the address following ip. If there is none, it returns the zero Addr.
### func (Addr) Prefix 1.18
```
func (ip Addr) Prefix(b int) (Prefix, error)
```
Prefix keeps only the top b bits of IP, producing a Prefix of the specified length. If ip is a zero Addr, Prefix always returns a zero Prefix and a nil error. Otherwise, if bits is less than zero or greater than ip.BitLen(), Prefix returns an error.
### func (Addr) Prev 1.18
```
func (ip Addr) Prev() Addr
```
Prev returns the IP before ip. If there is none, it returns the IP zero value.
### func (Addr) String 1.18
```
func (ip Addr) String() string
```
String returns the string form of the IP address ip. It returns one of 5 forms:
* "invalid IP", if ip is the zero Addr
* IPv4 dotted decimal ("192.0.2.1")
* IPv6 ("2001:db8::1")
* "::ffff:1.2.3.4" (if Is4In6)
* IPv6 with zone ("fe80:db8::1%eth0")
Note that unlike package net's IP.String method, IPv4-mapped IPv6 addresses format with a "::ffff:" prefix before the dotted quad.
### func (Addr) StringExpanded 1.18
```
func (ip Addr) StringExpanded() string
```
StringExpanded is like String but IPv6 addresses are expanded with leading zeroes and no "::" compression. For example, "2001:db8::1" becomes "2001:0db8:0000:0000:0000:0000:0000:0001".
### func (Addr) Unmap 1.18
```
func (ip Addr) Unmap() Addr
```
Unmap returns ip with any IPv4-mapped IPv6 address prefix removed.
That is, if ip is an IPv6 address wrapping an IPv4 address, it returns the wrapped IPv4 address. Otherwise it returns ip unmodified.
### func (\*Addr) UnmarshalBinary 1.18
```
func (ip *Addr) UnmarshalBinary(b []byte) error
```
UnmarshalBinary implements the encoding.BinaryUnmarshaler interface. It expects data in the form generated by MarshalBinary.
### func (\*Addr) UnmarshalText 1.18
```
func (ip *Addr) UnmarshalText(text []byte) error
```
UnmarshalText implements the encoding.TextUnmarshaler interface. The IP address is expected in a form accepted by ParseAddr.
If text is empty, UnmarshalText sets \*ip to the zero Addr and returns no error.
### func (Addr) WithZone 1.18
```
func (ip Addr) WithZone(zone string) Addr
```
WithZone returns an IP that's the same as ip but with the provided zone. If zone is empty, the zone is removed. If ip is an IPv4 address, WithZone is a no-op and returns ip unchanged.
### func (Addr) Zone 1.18
```
func (ip Addr) Zone() string
```
Zone returns ip's IPv6 scoped addressing zone, if any.
type AddrPort 1.18
------------------
AddrPort is an IP and a port number.
```
type AddrPort struct {
// contains filtered or unexported fields
}
```
### func AddrPortFrom 1.18
```
func AddrPortFrom(ip Addr, port uint16) AddrPort
```
AddrPortFrom returns an AddrPort with the provided IP and port. It does not allocate.
### func MustParseAddrPort 1.18
```
func MustParseAddrPort(s string) AddrPort
```
MustParseAddrPort calls ParseAddrPort(s) and panics on error. It is intended for use in tests with hard-coded strings.
### func ParseAddrPort 1.18
```
func ParseAddrPort(s string) (AddrPort, error)
```
ParseAddrPort parses s as an AddrPort.
It doesn't do any name resolution: both the address and the port must be numeric.
### func (AddrPort) Addr 1.18
```
func (p AddrPort) Addr() Addr
```
Addr returns p's IP address.
### func (AddrPort) AppendTo 1.18
```
func (p AddrPort) AppendTo(b []byte) []byte
```
AppendTo appends a text encoding of p, as generated by MarshalText, to b and returns the extended buffer.
### func (AddrPort) IsValid 1.18
```
func (p AddrPort) IsValid() bool
```
IsValid reports whether p.Addr() is valid. All ports are valid, including zero.
### func (AddrPort) MarshalBinary 1.18
```
func (p AddrPort) MarshalBinary() ([]byte, error)
```
MarshalBinary implements the encoding.BinaryMarshaler interface. It returns Addr.MarshalBinary with an additional two bytes appended containing the port in little-endian.
### func (AddrPort) MarshalText 1.18
```
func (p AddrPort) MarshalText() ([]byte, error)
```
MarshalText implements the encoding.TextMarshaler interface. The encoding is the same as returned by String, with one exception: if p.Addr() is the zero Addr, the encoding is the empty string.
### func (AddrPort) Port 1.18
```
func (p AddrPort) Port() uint16
```
Port returns p's port.
### func (AddrPort) String 1.18
```
func (p AddrPort) String() string
```
### func (\*AddrPort) UnmarshalBinary 1.18
```
func (p *AddrPort) UnmarshalBinary(b []byte) error
```
UnmarshalBinary implements the encoding.BinaryUnmarshaler interface. It expects data in the form generated by MarshalBinary.
### func (\*AddrPort) UnmarshalText 1.18
```
func (p *AddrPort) UnmarshalText(text []byte) error
```
UnmarshalText implements the encoding.TextUnmarshaler interface. The AddrPort is expected in a form generated by MarshalText or accepted by ParseAddrPort.
type Prefix 1.18
----------------
Prefix is an IP address prefix (CIDR) representing an IP network.
The first Bits() of Addr() are specified. The remaining bits match any address. The range of Bits() is [0,32] for IPv4 or [0,128] for IPv6.
```
type Prefix struct {
// contains filtered or unexported fields
}
```
### func MustParsePrefix 1.18
```
func MustParsePrefix(s string) Prefix
```
MustParsePrefix calls ParsePrefix(s) and panics on error. It is intended for use in tests with hard-coded strings.
### func ParsePrefix 1.18
```
func ParsePrefix(s string) (Prefix, error)
```
ParsePrefix parses s as an IP address prefix. The string can be in the form "192.168.1.0/24" or "2001:db8::/32", the CIDR notation defined in RFC 4632 and RFC 4291. IPv6 zones are not permitted in prefixes, and an error will be returned if a zone is present.
Note that masked address bits are not zeroed. Use Masked for that.
### func PrefixFrom 1.18
```
func PrefixFrom(ip Addr, bits int) Prefix
```
PrefixFrom returns a Prefix with the provided IP address and bit prefix length.
It does not allocate. Unlike Addr.Prefix, PrefixFrom does not mask off the host bits of ip.
If bits is less than zero or greater than ip.BitLen, Prefix.Bits will return an invalid value -1.
### func (Prefix) Addr 1.18
```
func (p Prefix) Addr() Addr
```
Addr returns p's IP address.
### func (Prefix) AppendTo 1.18
```
func (p Prefix) AppendTo(b []byte) []byte
```
AppendTo appends a text encoding of p, as generated by MarshalText, to b and returns the extended buffer.
### func (Prefix) Bits 1.18
```
func (p Prefix) Bits() int
```
Bits returns p's prefix length.
It reports -1 if invalid.
### func (Prefix) Contains 1.18
```
func (p Prefix) Contains(ip Addr) bool
```
Contains reports whether the network p includes ip.
An IPv4 address will not match an IPv6 prefix. An IPv4-mapped IPv6 address will not match an IPv4 prefix. A zero-value IP will not match any prefix. If ip has an IPv6 zone, Contains returns false, because Prefixes strip zones.
### func (Prefix) IsSingleIP 1.18
```
func (p Prefix) IsSingleIP() bool
```
IsSingleIP reports whether p contains exactly one IP.
### func (Prefix) IsValid 1.18
```
func (p Prefix) IsValid() bool
```
IsValid reports whether p.Bits() has a valid range for p.Addr(). If p.Addr() is the zero Addr, IsValid returns false. Note that if p is the zero Prefix, then p.IsValid() == false.
### func (Prefix) MarshalBinary 1.18
```
func (p Prefix) MarshalBinary() ([]byte, error)
```
MarshalBinary implements the encoding.BinaryMarshaler interface. It returns Addr.MarshalBinary with an additional byte appended containing the prefix bits.
### func (Prefix) MarshalText 1.18
```
func (p Prefix) MarshalText() ([]byte, error)
```
MarshalText implements the encoding.TextMarshaler interface, The encoding is the same as returned by String, with one exception: If p is the zero value, the encoding is the empty string.
### func (Prefix) Masked 1.18
```
func (p Prefix) Masked() Prefix
```
Masked returns p in its canonical form, with all but the high p.Bits() bits of p.Addr() masked off.
If p is zero or otherwise invalid, Masked returns the zero Prefix.
### func (Prefix) Overlaps 1.18
```
func (p Prefix) Overlaps(o Prefix) bool
```
Overlaps reports whether p and o contain any IP addresses in common.
If p and o are of different address families or either have a zero IP, it reports false. Like the Contains method, a prefix with an IPv4-mapped IPv6 address is still treated as an IPv6 mask.
### func (Prefix) String 1.18
```
func (p Prefix) String() string
```
String returns the CIDR notation of p: "<ip>/<bits>".
### func (\*Prefix) UnmarshalBinary 1.18
```
func (p *Prefix) UnmarshalBinary(b []byte) error
```
UnmarshalBinary implements the encoding.BinaryUnmarshaler interface. It expects data in the form generated by MarshalBinary.
### func (\*Prefix) UnmarshalText 1.18
```
func (p *Prefix) UnmarshalText(text []byte) error
```
UnmarshalText implements the encoding.TextUnmarshaler interface. The IP address is expected in a form accepted by ParsePrefix or generated by MarshalText.
| programming_docs |
go Package rpc Package rpc
============
* `import "net/rpc"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package rpc provides access to the exported methods of an object across a network or other I/O connection. A server registers an object, making it visible as a service with the name of the type of the object. After registration, exported methods of the object will be accessible remotely. A server may register multiple objects (services) of different types but it is an error to register multiple objects of the same type.
Only methods that satisfy these criteria will be made available for remote access; other methods will be ignored:
* the method's type is exported.
* the method is exported.
* the method has two arguments, both exported (or builtin) types.
* the method's second argument is a pointer.
* the method has return type error.
In effect, the method must look schematically like
```
func (t *T) MethodName(argType T1, replyType *T2) error
```
where T1 and T2 can be marshaled by encoding/gob. These requirements apply even if a different codec is used. (In the future, these requirements may soften for custom codecs.)
The method's first argument represents the arguments provided by the caller; the second argument represents the result parameters to be returned to the caller. The method's return value, if non-nil, is passed back as a string that the client sees as if created by errors.New. If an error is returned, the reply parameter will not be sent back to the client.
The server may handle requests on a single connection by calling ServeConn. More typically it will create a network listener and call Accept or, for an HTTP listener, HandleHTTP and http.Serve.
A client wishing to use the service establishes a connection and then invokes NewClient on the connection. The convenience function Dial (DialHTTP) performs both steps for a raw network connection (an HTTP connection). The resulting Client object has two methods, Call and Go, that specify the service and method to call, a pointer containing the arguments, and a pointer to receive the result parameters.
The Call method waits for the remote call to complete while the Go method launches the call asynchronously and signals completion using the Call structure's Done channel.
Unless an explicit codec is set up, package encoding/gob is used to transport the data.
Here is a simple example. A server wishes to export an object of type Arith:
```
package server
import "errors"
type Args struct {
A, B int
}
type Quotient struct {
Quo, Rem int
}
type Arith int
func (t *Arith) Multiply(args *Args, reply *int) error {
*reply = args.A * args.B
return nil
}
func (t *Arith) Divide(args *Args, quo *Quotient) error {
if args.B == 0 {
return errors.New("divide by zero")
}
quo.Quo = args.A / args.B
quo.Rem = args.A % args.B
return nil
}
```
The server calls (for HTTP service):
```
arith := new(Arith)
rpc.Register(arith)
rpc.HandleHTTP()
l, e := net.Listen("tcp", ":1234")
if e != nil {
log.Fatal("listen error:", e)
}
go http.Serve(l, nil)
```
At this point, clients can see a service "Arith" with methods "Arith.Multiply" and "Arith.Divide". To invoke one, a client first dials the server:
```
client, err := rpc.DialHTTP("tcp", serverAddress + ":1234")
if err != nil {
log.Fatal("dialing:", err)
}
```
Then it can make a remote call:
```
// Synchronous call
args := &server.Args{7,8}
var reply int
err = client.Call("Arith.Multiply", args, &reply)
if err != nil {
log.Fatal("arith error:", err)
}
fmt.Printf("Arith: %d*%d=%d", args.A, args.B, reply)
```
or
```
// Asynchronous call
quotient := new(Quotient)
divCall := client.Go("Arith.Divide", args, quotient, nil)
replyCall := <-divCall.Done // will be equal to divCall
// check errors, print, etc.
```
A server implementation will often provide a simple, type-safe wrapper for the client.
The net/rpc package is frozen and is not accepting new features.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func Accept(lis net.Listener)](#Accept)
* [func HandleHTTP()](#HandleHTTP)
* [func Register(rcvr any) error](#Register)
* [func RegisterName(name string, rcvr any) error](#RegisterName)
* [func ServeCodec(codec ServerCodec)](#ServeCodec)
* [func ServeConn(conn io.ReadWriteCloser)](#ServeConn)
* [func ServeRequest(codec ServerCodec) error](#ServeRequest)
* [type Call](#Call)
* [type Client](#Client)
* [func Dial(network, address string) (\*Client, error)](#Dial)
* [func DialHTTP(network, address string) (\*Client, error)](#DialHTTP)
* [func DialHTTPPath(network, address, path string) (\*Client, error)](#DialHTTPPath)
* [func NewClient(conn io.ReadWriteCloser) \*Client](#NewClient)
* [func NewClientWithCodec(codec ClientCodec) \*Client](#NewClientWithCodec)
* [func (client \*Client) Call(serviceMethod string, args any, reply any) error](#Client.Call)
* [func (client \*Client) Close() error](#Client.Close)
* [func (client \*Client) Go(serviceMethod string, args any, reply any, done chan \*Call) \*Call](#Client.Go)
* [type ClientCodec](#ClientCodec)
* [type Request](#Request)
* [type Response](#Response)
* [type Server](#Server)
* [func NewServer() \*Server](#NewServer)
* [func (server \*Server) Accept(lis net.Listener)](#Server.Accept)
* [func (server \*Server) HandleHTTP(rpcPath, debugPath string)](#Server.HandleHTTP)
* [func (server \*Server) Register(rcvr any) error](#Server.Register)
* [func (server \*Server) RegisterName(name string, rcvr any) error](#Server.RegisterName)
* [func (server \*Server) ServeCodec(codec ServerCodec)](#Server.ServeCodec)
* [func (server \*Server) ServeConn(conn io.ReadWriteCloser)](#Server.ServeConn)
* [func (server \*Server) ServeHTTP(w http.ResponseWriter, req \*http.Request)](#Server.ServeHTTP)
* [func (server \*Server) ServeRequest(codec ServerCodec) error](#Server.ServeRequest)
* [type ServerCodec](#ServerCodec)
* [type ServerError](#ServerError)
* [func (e ServerError) Error() string](#ServerError.Error)
### Package files
client.go debug.go server.go
Constants
---------
```
const (
// Defaults used by HandleHTTP
DefaultRPCPath = "/_goRPC_"
DefaultDebugPath = "/debug/rpc"
)
```
Variables
---------
DefaultServer is the default instance of \*Server.
```
var DefaultServer = NewServer()
```
```
var ErrShutdown = errors.New("connection is shut down")
```
func Accept
-----------
```
func Accept(lis net.Listener)
```
Accept accepts connections on the listener and serves requests to DefaultServer for each incoming connection. Accept blocks; the caller typically invokes it in a go statement.
func HandleHTTP
---------------
```
func HandleHTTP()
```
HandleHTTP registers an HTTP handler for RPC messages to DefaultServer on DefaultRPCPath and a debugging handler on DefaultDebugPath. It is still necessary to invoke http.Serve(), typically in a go statement.
func Register
-------------
```
func Register(rcvr any) error
```
Register publishes the receiver's methods in the DefaultServer.
func RegisterName
-----------------
```
func RegisterName(name string, rcvr any) error
```
RegisterName is like Register but uses the provided name for the type instead of the receiver's concrete type.
func ServeCodec
---------------
```
func ServeCodec(codec ServerCodec)
```
ServeCodec is like ServeConn but uses the specified codec to decode requests and encode responses.
func ServeConn
--------------
```
func ServeConn(conn io.ReadWriteCloser)
```
ServeConn runs the DefaultServer on a single connection. ServeConn blocks, serving the connection until the client hangs up. The caller typically invokes ServeConn in a go statement. ServeConn uses the gob wire format (see package gob) on the connection. To use an alternate codec, use ServeCodec. See NewClient's comment for information about concurrent access.
func ServeRequest
-----------------
```
func ServeRequest(codec ServerCodec) error
```
ServeRequest is like ServeCodec but synchronously serves a single request. It does not close the codec upon completion.
type Call
---------
Call represents an active RPC.
```
type Call struct {
ServiceMethod string // The name of the service and method to call.
Args any // The argument to the function (*struct).
Reply any // The reply from the function (*struct).
Error error // After completion, the error status.
Done chan *Call // Receives *Call when Go is complete.
}
```
type Client
-----------
Client represents an RPC Client. There may be multiple outstanding Calls associated with a single Client, and a Client may be used by multiple goroutines simultaneously.
```
type Client struct {
// contains filtered or unexported fields
}
```
### func Dial
```
func Dial(network, address string) (*Client, error)
```
Dial connects to an RPC server at the specified network address.
### func DialHTTP
```
func DialHTTP(network, address string) (*Client, error)
```
DialHTTP connects to an HTTP RPC server at the specified network address listening on the default HTTP RPC path.
### func DialHTTPPath
```
func DialHTTPPath(network, address, path string) (*Client, error)
```
DialHTTPPath connects to an HTTP RPC server at the specified network address and path.
### func NewClient
```
func NewClient(conn io.ReadWriteCloser) *Client
```
NewClient returns a new Client to handle requests to the set of services at the other end of the connection. It adds a buffer to the write side of the connection so the header and payload are sent as a unit.
The read and write halves of the connection are serialized independently, so no interlocking is required. However each half may be accessed concurrently so the implementation of conn should protect against concurrent reads or concurrent writes.
### func NewClientWithCodec
```
func NewClientWithCodec(codec ClientCodec) *Client
```
NewClientWithCodec is like NewClient but uses the specified codec to encode requests and decode responses.
### func (\*Client) Call
```
func (client *Client) Call(serviceMethod string, args any, reply any) error
```
Call invokes the named function, waits for it to complete, and returns its error status.
### func (\*Client) Close
```
func (client *Client) Close() error
```
Close calls the underlying codec's Close method. If the connection is already shutting down, ErrShutdown is returned.
### func (\*Client) Go
```
func (client *Client) Go(serviceMethod string, args any, reply any, done chan *Call) *Call
```
Go invokes the function asynchronously. It returns the Call structure representing the invocation. The done channel will signal when the call is complete by returning the same Call object. If done is nil, Go will allocate a new channel. If non-nil, done must be buffered or Go will deliberately crash.
type ClientCodec
----------------
A ClientCodec implements writing of RPC requests and reading of RPC responses for the client side of an RPC session. The client calls WriteRequest to write a request to the connection and calls ReadResponseHeader and ReadResponseBody in pairs to read responses. The client calls Close when finished with the connection. ReadResponseBody may be called with a nil argument to force the body of the response to be read and then discarded. See NewClient's comment for information about concurrent access.
```
type ClientCodec interface {
WriteRequest(*Request, any) error
ReadResponseHeader(*Response) error
ReadResponseBody(any) error
Close() error
}
```
type Request
------------
Request is a header written before every RPC call. It is used internally but documented here as an aid to debugging, such as when analyzing network traffic.
```
type Request struct {
ServiceMethod string // format: "Service.Method"
Seq uint64 // sequence number chosen by client
// contains filtered or unexported fields
}
```
type Response
-------------
Response is a header written before every RPC return. It is used internally but documented here as an aid to debugging, such as when analyzing network traffic.
```
type Response struct {
ServiceMethod string // echoes that of the Request
Seq uint64 // echoes that of the request
Error string // error, if any.
// contains filtered or unexported fields
}
```
type Server
-----------
Server represents an RPC Server.
```
type Server struct {
// contains filtered or unexported fields
}
```
### func NewServer
```
func NewServer() *Server
```
NewServer returns a new Server.
### func (\*Server) Accept
```
func (server *Server) Accept(lis net.Listener)
```
Accept accepts connections on the listener and serves requests for each incoming connection. Accept blocks until the listener returns a non-nil error. The caller typically invokes Accept in a go statement.
### func (\*Server) HandleHTTP
```
func (server *Server) HandleHTTP(rpcPath, debugPath string)
```
HandleHTTP registers an HTTP handler for RPC messages on rpcPath, and a debugging handler on debugPath. It is still necessary to invoke http.Serve(), typically in a go statement.
### func (\*Server) Register
```
func (server *Server) Register(rcvr any) error
```
Register publishes in the server the set of methods of the receiver value that satisfy the following conditions:
* exported method of exported type
* two arguments, both of exported type
* the second argument is a pointer
* one return value, of type error
It returns an error if the receiver is not an exported type or has no suitable methods. It also logs the error using package log. The client accesses each method using a string of the form "Type.Method", where Type is the receiver's concrete type.
### func (\*Server) RegisterName
```
func (server *Server) RegisterName(name string, rcvr any) error
```
RegisterName is like Register but uses the provided name for the type instead of the receiver's concrete type.
### func (\*Server) ServeCodec
```
func (server *Server) ServeCodec(codec ServerCodec)
```
ServeCodec is like ServeConn but uses the specified codec to decode requests and encode responses.
### func (\*Server) ServeConn
```
func (server *Server) ServeConn(conn io.ReadWriteCloser)
```
ServeConn runs the server on a single connection. ServeConn blocks, serving the connection until the client hangs up. The caller typically invokes ServeConn in a go statement. ServeConn uses the gob wire format (see package gob) on the connection. To use an alternate codec, use ServeCodec. See NewClient's comment for information about concurrent access.
### func (\*Server) ServeHTTP
```
func (server *Server) ServeHTTP(w http.ResponseWriter, req *http.Request)
```
ServeHTTP implements an http.Handler that answers RPC requests.
### func (\*Server) ServeRequest
```
func (server *Server) ServeRequest(codec ServerCodec) error
```
ServeRequest is like ServeCodec but synchronously serves a single request. It does not close the codec upon completion.
type ServerCodec
----------------
A ServerCodec implements reading of RPC requests and writing of RPC responses for the server side of an RPC session. The server calls ReadRequestHeader and ReadRequestBody in pairs to read requests from the connection, and it calls WriteResponse to write a response back. The server calls Close when finished with the connection. ReadRequestBody may be called with a nil argument to force the body of the request to be read and discarded. See NewClient's comment for information about concurrent access.
```
type ServerCodec interface {
ReadRequestHeader(*Request) error
ReadRequestBody(any) error
WriteResponse(*Response, any) error
// Close can be called multiple times and must be idempotent.
Close() error
}
```
type ServerError
----------------
ServerError represents an error that has been returned from the remote side of the RPC connection.
```
type ServerError string
```
### func (ServerError) Error
```
func (e ServerError) Error() string
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [jsonrpc](jsonrpc/index) | Package jsonrpc implements a JSON-RPC 1.0 ClientCodec and ServerCodec for the rpc package. |
go Package jsonrpc Package jsonrpc
================
* `import "net/rpc/jsonrpc"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package jsonrpc implements a JSON-RPC 1.0 ClientCodec and ServerCodec for the rpc package. For JSON-RPC 2.0 support, see <https://godoc.org/?q=json-rpc+2.0>
Index
-----
* [func Dial(network, address string) (\*rpc.Client, error)](#Dial)
* [func NewClient(conn io.ReadWriteCloser) \*rpc.Client](#NewClient)
* [func NewClientCodec(conn io.ReadWriteCloser) rpc.ClientCodec](#NewClientCodec)
* [func NewServerCodec(conn io.ReadWriteCloser) rpc.ServerCodec](#NewServerCodec)
* [func ServeConn(conn io.ReadWriteCloser)](#ServeConn)
### Package files
client.go server.go
func Dial
---------
```
func Dial(network, address string) (*rpc.Client, error)
```
Dial connects to a JSON-RPC server at the specified network address.
func NewClient
--------------
```
func NewClient(conn io.ReadWriteCloser) *rpc.Client
```
NewClient returns a new rpc.Client to handle requests to the set of services at the other end of the connection.
func NewClientCodec
-------------------
```
func NewClientCodec(conn io.ReadWriteCloser) rpc.ClientCodec
```
NewClientCodec returns a new rpc.ClientCodec using JSON-RPC on conn.
func NewServerCodec
-------------------
```
func NewServerCodec(conn io.ReadWriteCloser) rpc.ServerCodec
```
NewServerCodec returns a new rpc.ServerCodec using JSON-RPC on conn.
func ServeConn
--------------
```
func ServeConn(conn io.ReadWriteCloser)
```
ServeConn runs the JSON-RPC server on a single connection. ServeConn blocks, serving the connection until the client hangs up. The caller typically invokes ServeConn in a go statement.
go Package token Package token
==============
* `import "go/token"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package token defines constants representing the lexical tokens of the Go programming language and basic operations on tokens (printing, predicates).
#### Example (RetrievePositionInfo)
Code:
```
fset := token.NewFileSet()
const src = `package main
import "fmt"
import "go/token"
type p = token.Pos
const bad = token.NoPos
func ok(pos p) bool {
return pos != bad
}
/*line :7:9*/func main() {
fmt.Println(ok(bad) == bad.IsValid())
}
`
f, err := parser.ParseFile(fset, "main.go", src, 0)
if err != nil {
fmt.Println(err)
return
}
// Print the location and kind of each declaration in f.
for _, decl := range f.Decls {
// Get the filename, line, and column back via the file set.
// We get both the relative and absolute position.
// The relative position is relative to the last line directive.
// The absolute position is the exact position in the source.
pos := decl.Pos()
relPosition := fset.Position(pos)
absPosition := fset.PositionFor(pos, false)
// Either a FuncDecl or GenDecl, since we exit on error.
kind := "func"
if gen, ok := decl.(*ast.GenDecl); ok {
kind = gen.Tok.String()
}
// If the relative and absolute positions differ, show both.
fmtPosition := relPosition.String()
if relPosition != absPosition {
fmtPosition += "[" + absPosition.String() + "]"
}
fmt.Printf("%s: %s\n", fmtPosition, kind)
}
```
Output:
```
main.go:3:1: import
main.go:5:1: import
main.go:1:5[main.go:8:1]: type
main.go:3:1[main.go:10:1]: const
fake.go:42:11[main.go:13:1]: func
fake.go:7:9[main.go:17:14]: func
```
Index
-----
* [Constants](#pkg-constants)
* [func IsExported(name string) bool](#IsExported)
* [func IsIdentifier(name string) bool](#IsIdentifier)
* [func IsKeyword(name string) bool](#IsKeyword)
* [type File](#File)
* [func (f \*File) AddLine(offset int)](#File.AddLine)
* [func (f \*File) AddLineColumnInfo(offset int, filename string, line, column int)](#File.AddLineColumnInfo)
* [func (f \*File) AddLineInfo(offset int, filename string, line int)](#File.AddLineInfo)
* [func (f \*File) Base() int](#File.Base)
* [func (f \*File) Line(p Pos) int](#File.Line)
* [func (f \*File) LineCount() int](#File.LineCount)
* [func (f \*File) LineStart(line int) Pos](#File.LineStart)
* [func (f \*File) MergeLine(line int)](#File.MergeLine)
* [func (f \*File) Name() string](#File.Name)
* [func (f \*File) Offset(p Pos) int](#File.Offset)
* [func (f \*File) Pos(offset int) Pos](#File.Pos)
* [func (f \*File) Position(p Pos) (pos Position)](#File.Position)
* [func (f \*File) PositionFor(p Pos, adjusted bool) (pos Position)](#File.PositionFor)
* [func (f \*File) SetLines(lines []int) bool](#File.SetLines)
* [func (f \*File) SetLinesForContent(content []byte)](#File.SetLinesForContent)
* [func (f \*File) Size() int](#File.Size)
* [type FileSet](#FileSet)
* [func NewFileSet() \*FileSet](#NewFileSet)
* [func (s \*FileSet) AddFile(filename string, base, size int) \*File](#FileSet.AddFile)
* [func (s \*FileSet) Base() int](#FileSet.Base)
* [func (s \*FileSet) File(p Pos) (f \*File)](#FileSet.File)
* [func (s \*FileSet) Iterate(f func(\*File) bool)](#FileSet.Iterate)
* [func (s \*FileSet) Position(p Pos) (pos Position)](#FileSet.Position)
* [func (s \*FileSet) PositionFor(p Pos, adjusted bool) (pos Position)](#FileSet.PositionFor)
* [func (s \*FileSet) Read(decode func(any) error) error](#FileSet.Read)
* [func (s \*FileSet) RemoveFile(file \*File)](#FileSet.RemoveFile)
* [func (s \*FileSet) Write(encode func(any) error) error](#FileSet.Write)
* [type Pos](#Pos)
* [func (p Pos) IsValid() bool](#Pos.IsValid)
* [type Position](#Position)
* [func (pos \*Position) IsValid() bool](#Position.IsValid)
* [func (pos Position) String() string](#Position.String)
* [type Token](#Token)
* [func Lookup(ident string) Token](#Lookup)
* [func (tok Token) IsKeyword() bool](#Token.IsKeyword)
* [func (tok Token) IsLiteral() bool](#Token.IsLiteral)
* [func (tok Token) IsOperator() bool](#Token.IsOperator)
* [func (op Token) Precedence() int](#Token.Precedence)
* [func (tok Token) String() string](#Token.String)
### Examples
[Package (RetrievePositionInfo)](#example__retrievePositionInfo) ### Package files
position.go serialize.go token.go
Constants
---------
A set of constants for precedence-based expression parsing. Non-operators have lowest precedence, followed by operators starting with precedence 1 up to unary operators. The highest precedence serves as "catch-all" precedence for selector, indexing, and other operator and delimiter tokens.
```
const (
LowestPrec = 0 // non-operators
UnaryPrec = 6
HighestPrec = 7
)
```
func IsExported 1.13
--------------------
```
func IsExported(name string) bool
```
IsExported reports whether name starts with an upper-case letter.
func IsIdentifier 1.13
----------------------
```
func IsIdentifier(name string) bool
```
IsIdentifier reports whether name is a Go identifier, that is, a non-empty string made up of letters, digits, and underscores, where the first character is not a digit. Keywords are not identifiers.
func IsKeyword 1.13
-------------------
```
func IsKeyword(name string) bool
```
IsKeyword reports whether name is a Go keyword, such as "func" or "return".
type File
---------
A File is a handle for a file belonging to a FileSet. A File has a name, size, and line offset table.
```
type File struct {
// contains filtered or unexported fields
}
```
### func (\*File) AddLine
```
func (f *File) AddLine(offset int)
```
AddLine adds the line offset for a new line. The line offset must be larger than the offset for the previous line and smaller than the file size; otherwise the line offset is ignored.
### func (\*File) AddLineColumnInfo 1.11
```
func (f *File) AddLineColumnInfo(offset int, filename string, line, column int)
```
AddLineColumnInfo adds alternative file, line, and column number information for a given file offset. The offset must be larger than the offset for the previously added alternative line info and smaller than the file size; otherwise the information is ignored.
AddLineColumnInfo is typically used to register alternative position information for line directives such as //line filename:line:column.
### func (\*File) AddLineInfo
```
func (f *File) AddLineInfo(offset int, filename string, line int)
```
AddLineInfo is like AddLineColumnInfo with a column = 1 argument. It is here for backward-compatibility for code prior to Go 1.11.
### func (\*File) Base
```
func (f *File) Base() int
```
Base returns the base offset of file f as registered with AddFile.
### func (\*File) Line
```
func (f *File) Line(p Pos) int
```
Line returns the line number for the given file position p; p must be a Pos value in that file or NoPos.
### func (\*File) LineCount
```
func (f *File) LineCount() int
```
LineCount returns the number of lines in file f.
### func (\*File) LineStart 1.12
```
func (f *File) LineStart(line int) Pos
```
LineStart returns the Pos value of the start of the specified line. It ignores any alternative positions set using AddLineColumnInfo. LineStart panics if the 1-based line number is invalid.
### func (\*File) MergeLine 1.2
```
func (f *File) MergeLine(line int)
```
MergeLine merges a line with the following line. It is akin to replacing the newline character at the end of the line with a space (to not change the remaining offsets). To obtain the line number, consult e.g. Position.Line. MergeLine will panic if given an invalid line number.
### func (\*File) Name
```
func (f *File) Name() string
```
Name returns the file name of file f as registered with AddFile.
### func (\*File) Offset
```
func (f *File) Offset(p Pos) int
```
Offset returns the offset for the given file position p; p must be a valid Pos value in that file. f.Offset(f.Pos(offset)) == offset.
### func (\*File) Pos
```
func (f *File) Pos(offset int) Pos
```
Pos returns the Pos value for the given file offset; the offset must be <= f.Size(). f.Pos(f.Offset(p)) == p.
### func (\*File) Position
```
func (f *File) Position(p Pos) (pos Position)
```
Position returns the Position value for the given file position p. Calling f.Position(p) is equivalent to calling f.PositionFor(p, true).
### func (\*File) PositionFor 1.4
```
func (f *File) PositionFor(p Pos, adjusted bool) (pos Position)
```
PositionFor returns the Position value for the given file position p. If adjusted is set, the position may be adjusted by position-altering //line comments; otherwise those comments are ignored. p must be a Pos value in f or NoPos.
### func (\*File) SetLines
```
func (f *File) SetLines(lines []int) bool
```
SetLines sets the line offsets for a file and reports whether it succeeded. The line offsets are the offsets of the first character of each line; for instance for the content "ab\nc\n" the line offsets are {0, 3}. An empty file has an empty line offset table. Each line offset must be larger than the offset for the previous line and smaller than the file size; otherwise SetLines fails and returns false. Callers must not mutate the provided slice after SetLines returns.
### func (\*File) SetLinesForContent
```
func (f *File) SetLinesForContent(content []byte)
```
SetLinesForContent sets the line offsets for the given file content. It ignores position-altering //line comments.
### func (\*File) Size
```
func (f *File) Size() int
```
Size returns the size of file f as registered with AddFile.
type FileSet
------------
A FileSet represents a set of source files. Methods of file sets are synchronized; multiple goroutines may invoke them concurrently.
The byte offsets for each file in a file set are mapped into distinct (integer) intervals, one interval [base, base+size] per file. Base represents the first byte in the file, and size is the corresponding file size. A Pos value is a value in such an interval. By determining the interval a Pos value belongs to, the file, its file base, and thus the byte offset (position) the Pos value is representing can be computed.
When adding a new file, a file base must be provided. That can be any integer value that is past the end of any interval of any file already in the file set. For convenience, FileSet.Base provides such a value, which is simply the end of the Pos interval of the most recently added file, plus one. Unless there is a need to extend an interval later, using the FileSet.Base should be used as argument for FileSet.AddFile.
A File may be removed from a FileSet when it is no longer needed. This may reduce memory usage in a long-running application.
```
type FileSet struct {
// contains filtered or unexported fields
}
```
### func NewFileSet
```
func NewFileSet() *FileSet
```
NewFileSet creates a new file set.
### func (\*FileSet) AddFile
```
func (s *FileSet) AddFile(filename string, base, size int) *File
```
AddFile adds a new file with a given filename, base offset, and file size to the file set s and returns the file. Multiple files may have the same name. The base offset must not be smaller than the FileSet's Base(), and size must not be negative. As a special case, if a negative base is provided, the current value of the FileSet's Base() is used instead.
Adding the file will set the file set's Base() value to base + size + 1 as the minimum base value for the next file. The following relationship exists between a Pos value p for a given file offset offs:
```
int(p) = base + offs
```
with offs in the range [0, size] and thus p in the range [base, base+size]. For convenience, File.Pos may be used to create file-specific position values from a file offset.
### func (\*FileSet) Base
```
func (s *FileSet) Base() int
```
Base returns the minimum base offset that must be provided to AddFile when adding the next file.
### func (\*FileSet) File
```
func (s *FileSet) File(p Pos) (f *File)
```
File returns the file that contains the position p. If no such file is found (for instance for p == NoPos), the result is nil.
### func (\*FileSet) Iterate
```
func (s *FileSet) Iterate(f func(*File) bool)
```
Iterate calls f for the files in the file set in the order they were added until f returns false.
### func (\*FileSet) Position
```
func (s *FileSet) Position(p Pos) (pos Position)
```
Position converts a Pos p in the fileset into a Position value. Calling s.Position(p) is equivalent to calling s.PositionFor(p, true).
### func (\*FileSet) PositionFor 1.4
```
func (s *FileSet) PositionFor(p Pos, adjusted bool) (pos Position)
```
PositionFor converts a Pos p in the fileset into a Position value. If adjusted is set, the position may be adjusted by position-altering //line comments; otherwise those comments are ignored. p must be a Pos value in s or NoPos.
### func (\*FileSet) Read
```
func (s *FileSet) Read(decode func(any) error) error
```
Read calls decode to deserialize a file set into s; s must not be nil.
### func (\*FileSet) RemoveFile 1.20
```
func (s *FileSet) RemoveFile(file *File)
```
RemoveFile removes a file from the FileSet so that subsequent queries for its Pos interval yield a negative result. This reduces the memory usage of a long-lived FileSet that encounters an unbounded stream of files.
Removing a file that does not belong to the set has no effect.
### func (\*FileSet) Write
```
func (s *FileSet) Write(encode func(any) error) error
```
Write calls encode to serialize the file set s.
type Pos
--------
Pos is a compact encoding of a source position within a file set. It can be converted into a Position for a more convenient, but much larger, representation.
The Pos value for a given file is a number in the range [base, base+size], where base and size are specified when a file is added to the file set. The difference between a Pos value and the corresponding file base corresponds to the byte offset of that position (represented by the Pos value) from the beginning of the file. Thus, the file base offset is the Pos value representing the first byte in the file.
To create the Pos value for a specific source offset (measured in bytes), first add the respective file to the current file set using FileSet.AddFile and then call File.Pos(offset) for that file. Given a Pos value p for a specific file set fset, the corresponding Position value is obtained by calling fset.Position(p).
Pos values can be compared directly with the usual comparison operators: If two Pos values p and q are in the same file, comparing p and q is equivalent to comparing the respective source file offsets. If p and q are in different files, p < q is true if the file implied by p was added to the respective file set before the file implied by q.
```
type Pos int
```
The zero value for Pos is NoPos; there is no file and line information associated with it, and NoPos.IsValid() is false. NoPos is always smaller than any other Pos value. The corresponding Position value for NoPos is the zero value for Position.
```
const NoPos Pos = 0
```
### func (Pos) IsValid
```
func (p Pos) IsValid() bool
```
IsValid reports whether the position is valid.
type Position
-------------
Position describes an arbitrary source position including the file, line, and column location. A Position is valid if the line number is > 0.
```
type Position struct {
Filename string // filename, if any
Offset int // offset, starting at 0
Line int // line number, starting at 1
Column int // column number, starting at 1 (byte count)
}
```
### func (\*Position) IsValid
```
func (pos *Position) IsValid() bool
```
IsValid reports whether the position is valid.
### func (Position) String
```
func (pos Position) String() string
```
String returns a string in one of several forms:
```
file:line:column valid position with file name
file:line valid position with file name but no column (column == 0)
line:column valid position without file name
line valid position without file name and no column (column == 0)
file invalid position with file name
- invalid position without file name
```
type Token
----------
Token is the set of lexical tokens of the Go programming language.
```
type Token int
```
The list of tokens.
```
const (
// Special tokens
ILLEGAL Token = iota
EOF
COMMENT
// Identifiers and basic type literals
// (these tokens stand for classes of literals)
IDENT // main
INT // 12345
FLOAT // 123.45
IMAG // 123.45i
CHAR // 'a'
STRING // "abc"
// Operators and delimiters
ADD // +
SUB // -
MUL // *
QUO // /
REM // %
AND // &
OR // |
XOR // ^
SHL // <<
SHR // >>
AND_NOT // &^
ADD_ASSIGN // +=
SUB_ASSIGN // -=
MUL_ASSIGN // *=
QUO_ASSIGN // /=
REM_ASSIGN // %=
AND_ASSIGN // &=
OR_ASSIGN // |=
XOR_ASSIGN // ^=
SHL_ASSIGN // <<=
SHR_ASSIGN // >>=
AND_NOT_ASSIGN // &^=
LAND // &&
LOR // ||
ARROW // <-
INC // ++
DEC // --
EQL // ==
LSS // <
GTR // >
ASSIGN // =
NOT // !
NEQ // !=
LEQ // <=
GEQ // >=
DEFINE // :=
ELLIPSIS // ...
LPAREN // (
LBRACK // [
LBRACE // {
COMMA // ,
PERIOD // .
RPAREN // )
RBRACK // ]
RBRACE // }
SEMICOLON // ;
COLON // :
// Keywords
BREAK
CASE
CHAN
CONST
CONTINUE
DEFAULT
DEFER
ELSE
FALLTHROUGH
FOR
FUNC
GO
GOTO
IF
IMPORT
INTERFACE
MAP
PACKAGE
RANGE
RETURN
SELECT
STRUCT
SWITCH
TYPE
VAR
// additional tokens, handled in an ad-hoc manner
TILDE
)
```
### func Lookup
```
func Lookup(ident string) Token
```
Lookup maps an identifier to its keyword token or IDENT (if not a keyword).
### func (Token) IsKeyword
```
func (tok Token) IsKeyword() bool
```
IsKeyword returns true for tokens corresponding to keywords; it returns false otherwise.
### func (Token) IsLiteral
```
func (tok Token) IsLiteral() bool
```
IsLiteral returns true for tokens corresponding to identifiers and basic type literals; it returns false otherwise.
### func (Token) IsOperator
```
func (tok Token) IsOperator() bool
```
IsOperator returns true for tokens corresponding to operators and delimiters; it returns false otherwise.
### func (Token) Precedence
```
func (op Token) Precedence() int
```
Precedence returns the operator precedence of the binary operator op. If op is not a binary operator, the result is LowestPrecedence.
### func (Token) String
```
func (tok Token) String() string
```
String returns the string corresponding to the token tok. For operators, delimiters, and keywords the string is the actual token character sequence (e.g., for the token ADD, the string is "+"). For all other tokens the string corresponds to the token constant name (e.g. for the token IDENT, the string is "IDENT").
| programming_docs |
go Package types Package types
==============
* `import "go/types"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package types declares the data types and implements the algorithms for type-checking of Go packages. Use Config.Check to invoke the type checker for a package. Alternatively, create a new type checker with NewChecker and invoke it incrementally by calling Checker.Files.
Type-checking consists of several interdependent phases:
Name resolution maps each identifier (ast.Ident) in the program to the language object (Object) it denotes. Use Info.{Defs,Uses,Implicits} for the results of name resolution.
Constant folding computes the exact constant value (constant.Value) for every expression (ast.Expr) that is a compile-time constant. Use Info.Types[expr].Value for the results of constant folding.
Type inference computes the type (Type) of every expression (ast.Expr) and checks for compliance with the language specification. Use Info.Types[expr].Type for the results of type inference.
For a tutorial, see <https://golang.org/s/types-tutorial>.
Index
-----
* [Variables](#pkg-variables)
* [func AssertableTo(V \*Interface, T Type) bool](#AssertableTo)
* [func AssignableTo(V, T Type) bool](#AssignableTo)
* [func CheckExpr(fset \*token.FileSet, pkg \*Package, pos token.Pos, expr ast.Expr, info \*Info) (err error)](#CheckExpr)
* [func Comparable(T Type) bool](#Comparable)
* [func ConvertibleTo(V, T Type) bool](#ConvertibleTo)
* [func DefPredeclaredTestFuncs()](#DefPredeclaredTestFuncs)
* [func ExprString(x ast.Expr) string](#ExprString)
* [func Id(pkg \*Package, name string) string](#Id)
* [func Identical(x, y Type) bool](#Identical)
* [func IdenticalIgnoreTags(x, y Type) bool](#IdenticalIgnoreTags)
* [func Implements(V Type, T \*Interface) bool](#Implements)
* [func IsInterface(t Type) bool](#IsInterface)
* [func ObjectString(obj Object, qf Qualifier) string](#ObjectString)
* [func Satisfies(V Type, T \*Interface) bool](#Satisfies)
* [func SelectionString(s \*Selection, qf Qualifier) string](#SelectionString)
* [func TypeString(typ Type, qf Qualifier) string](#TypeString)
* [func WriteExpr(buf \*bytes.Buffer, x ast.Expr)](#WriteExpr)
* [func WriteSignature(buf \*bytes.Buffer, sig \*Signature, qf Qualifier)](#WriteSignature)
* [func WriteType(buf \*bytes.Buffer, typ Type, qf Qualifier)](#WriteType)
* [type ArgumentError](#ArgumentError)
* [func (e \*ArgumentError) Error() string](#ArgumentError.Error)
* [func (e \*ArgumentError) Unwrap() error](#ArgumentError.Unwrap)
* [type Array](#Array)
* [func NewArray(elem Type, len int64) \*Array](#NewArray)
* [func (a \*Array) Elem() Type](#Array.Elem)
* [func (a \*Array) Len() int64](#Array.Len)
* [func (t \*Array) String() string](#Array.String)
* [func (t \*Array) Underlying() Type](#Array.Underlying)
* [type Basic](#Basic)
* [func (b \*Basic) Info() BasicInfo](#Basic.Info)
* [func (b \*Basic) Kind() BasicKind](#Basic.Kind)
* [func (b \*Basic) Name() string](#Basic.Name)
* [func (t \*Basic) String() string](#Basic.String)
* [func (t \*Basic) Underlying() Type](#Basic.Underlying)
* [type BasicInfo](#BasicInfo)
* [type BasicKind](#BasicKind)
* [type Builtin](#Builtin)
* [func (obj \*Builtin) Exported() bool](#Builtin.Exported)
* [func (obj \*Builtin) Id() string](#Builtin.Id)
* [func (obj \*Builtin) Name() string](#Builtin.Name)
* [func (obj \*Builtin) Parent() \*Scope](#Builtin.Parent)
* [func (obj \*Builtin) Pkg() \*Package](#Builtin.Pkg)
* [func (obj \*Builtin) Pos() token.Pos](#Builtin.Pos)
* [func (obj \*Builtin) String() string](#Builtin.String)
* [func (obj \*Builtin) Type() Type](#Builtin.Type)
* [type Chan](#Chan)
* [func NewChan(dir ChanDir, elem Type) \*Chan](#NewChan)
* [func (c \*Chan) Dir() ChanDir](#Chan.Dir)
* [func (c \*Chan) Elem() Type](#Chan.Elem)
* [func (t \*Chan) String() string](#Chan.String)
* [func (t \*Chan) Underlying() Type](#Chan.Underlying)
* [type ChanDir](#ChanDir)
* [type Checker](#Checker)
* [func NewChecker(conf \*Config, fset \*token.FileSet, pkg \*Package, info \*Info) \*Checker](#NewChecker)
* [func (check \*Checker) Files(files []\*ast.File) error](#Checker.Files)
* [type Config](#Config)
* [func (conf \*Config) Check(path string, fset \*token.FileSet, files []\*ast.File, info \*Info) (\*Package, error)](#Config.Check)
* [type Const](#Const)
* [func NewConst(pos token.Pos, pkg \*Package, name string, typ Type, val constant.Value) \*Const](#NewConst)
* [func (obj \*Const) Exported() bool](#Const.Exported)
* [func (obj \*Const) Id() string](#Const.Id)
* [func (obj \*Const) Name() string](#Const.Name)
* [func (obj \*Const) Parent() \*Scope](#Const.Parent)
* [func (obj \*Const) Pkg() \*Package](#Const.Pkg)
* [func (obj \*Const) Pos() token.Pos](#Const.Pos)
* [func (obj \*Const) String() string](#Const.String)
* [func (obj \*Const) Type() Type](#Const.Type)
* [func (obj \*Const) Val() constant.Value](#Const.Val)
* [type Context](#Context)
* [func NewContext() \*Context](#NewContext)
* [type Error](#Error)
* [func (err Error) Error() string](#Error.Error)
* [type Func](#Func)
* [func MissingMethod(V Type, T \*Interface, static bool) (method \*Func, wrongType bool)](#MissingMethod)
* [func NewFunc(pos token.Pos, pkg \*Package, name string, sig \*Signature) \*Func](#NewFunc)
* [func (obj \*Func) Exported() bool](#Func.Exported)
* [func (obj \*Func) FullName() string](#Func.FullName)
* [func (obj \*Func) Id() string](#Func.Id)
* [func (obj \*Func) Name() string](#Func.Name)
* [func (obj \*Func) Origin() \*Func](#Func.Origin)
* [func (obj \*Func) Parent() \*Scope](#Func.Parent)
* [func (obj \*Func) Pkg() \*Package](#Func.Pkg)
* [func (obj \*Func) Pos() token.Pos](#Func.Pos)
* [func (obj \*Func) Scope() \*Scope](#Func.Scope)
* [func (obj \*Func) String() string](#Func.String)
* [func (obj \*Func) Type() Type](#Func.Type)
* [type ImportMode](#ImportMode)
* [type Importer](#Importer)
* [type ImporterFrom](#ImporterFrom)
* [type Info](#Info)
* [func (info \*Info) ObjectOf(id \*ast.Ident) Object](#Info.ObjectOf)
* [func (info \*Info) TypeOf(e ast.Expr) Type](#Info.TypeOf)
* [type Initializer](#Initializer)
* [func (init \*Initializer) String() string](#Initializer.String)
* [type Instance](#Instance)
* [type Interface](#Interface)
* [func NewInterface(methods []\*Func, embeddeds []\*Named) \*Interface](#NewInterface)
* [func NewInterfaceType(methods []\*Func, embeddeds []Type) \*Interface](#NewInterfaceType)
* [func (t \*Interface) Complete() \*Interface](#Interface.Complete)
* [func (t \*Interface) Embedded(i int) \*Named](#Interface.Embedded)
* [func (t \*Interface) EmbeddedType(i int) Type](#Interface.EmbeddedType)
* [func (t \*Interface) Empty() bool](#Interface.Empty)
* [func (t \*Interface) ExplicitMethod(i int) \*Func](#Interface.ExplicitMethod)
* [func (t \*Interface) IsComparable() bool](#Interface.IsComparable)
* [func (t \*Interface) IsImplicit() bool](#Interface.IsImplicit)
* [func (t \*Interface) IsMethodSet() bool](#Interface.IsMethodSet)
* [func (t \*Interface) MarkImplicit()](#Interface.MarkImplicit)
* [func (t \*Interface) Method(i int) \*Func](#Interface.Method)
* [func (t \*Interface) NumEmbeddeds() int](#Interface.NumEmbeddeds)
* [func (t \*Interface) NumExplicitMethods() int](#Interface.NumExplicitMethods)
* [func (t \*Interface) NumMethods() int](#Interface.NumMethods)
* [func (t \*Interface) String() string](#Interface.String)
* [func (t \*Interface) Underlying() Type](#Interface.Underlying)
* [type Label](#Label)
* [func NewLabel(pos token.Pos, pkg \*Package, name string) \*Label](#NewLabel)
* [func (obj \*Label) Exported() bool](#Label.Exported)
* [func (obj \*Label) Id() string](#Label.Id)
* [func (obj \*Label) Name() string](#Label.Name)
* [func (obj \*Label) Parent() \*Scope](#Label.Parent)
* [func (obj \*Label) Pkg() \*Package](#Label.Pkg)
* [func (obj \*Label) Pos() token.Pos](#Label.Pos)
* [func (obj \*Label) String() string](#Label.String)
* [func (obj \*Label) Type() Type](#Label.Type)
* [type Map](#Map)
* [func NewMap(key, elem Type) \*Map](#NewMap)
* [func (m \*Map) Elem() Type](#Map.Elem)
* [func (m \*Map) Key() Type](#Map.Key)
* [func (t \*Map) String() string](#Map.String)
* [func (t \*Map) Underlying() Type](#Map.Underlying)
* [type MethodSet](#MethodSet)
* [func NewMethodSet(T Type) \*MethodSet](#NewMethodSet)
* [func (s \*MethodSet) At(i int) \*Selection](#MethodSet.At)
* [func (s \*MethodSet) Len() int](#MethodSet.Len)
* [func (s \*MethodSet) Lookup(pkg \*Package, name string) \*Selection](#MethodSet.Lookup)
* [func (s \*MethodSet) String() string](#MethodSet.String)
* [type Named](#Named)
* [func NewNamed(obj \*TypeName, underlying Type, methods []\*Func) \*Named](#NewNamed)
* [func (t \*Named) AddMethod(m \*Func)](#Named.AddMethod)
* [func (t \*Named) Method(i int) \*Func](#Named.Method)
* [func (t \*Named) NumMethods() int](#Named.NumMethods)
* [func (t \*Named) Obj() \*TypeName](#Named.Obj)
* [func (t \*Named) Origin() \*Named](#Named.Origin)
* [func (t \*Named) SetTypeParams(tparams []\*TypeParam)](#Named.SetTypeParams)
* [func (t \*Named) SetUnderlying(underlying Type)](#Named.SetUnderlying)
* [func (t \*Named) String() string](#Named.String)
* [func (t \*Named) TypeArgs() \*TypeList](#Named.TypeArgs)
* [func (t \*Named) TypeParams() \*TypeParamList](#Named.TypeParams)
* [func (t \*Named) Underlying() Type](#Named.Underlying)
* [type Nil](#Nil)
* [func (obj \*Nil) Exported() bool](#Nil.Exported)
* [func (obj \*Nil) Id() string](#Nil.Id)
* [func (obj \*Nil) Name() string](#Nil.Name)
* [func (obj \*Nil) Parent() \*Scope](#Nil.Parent)
* [func (obj \*Nil) Pkg() \*Package](#Nil.Pkg)
* [func (obj \*Nil) Pos() token.Pos](#Nil.Pos)
* [func (obj \*Nil) String() string](#Nil.String)
* [func (obj \*Nil) Type() Type](#Nil.Type)
* [type Object](#Object)
* [func LookupFieldOrMethod(T Type, addressable bool, pkg \*Package, name string) (obj Object, index []int, indirect bool)](#LookupFieldOrMethod)
* [type Package](#Package)
* [func NewPackage(path, name string) \*Package](#NewPackage)
* [func (pkg \*Package) Complete() bool](#Package.Complete)
* [func (pkg \*Package) Imports() []\*Package](#Package.Imports)
* [func (pkg \*Package) MarkComplete()](#Package.MarkComplete)
* [func (pkg \*Package) Name() string](#Package.Name)
* [func (pkg \*Package) Path() string](#Package.Path)
* [func (pkg \*Package) Scope() \*Scope](#Package.Scope)
* [func (pkg \*Package) SetImports(list []\*Package)](#Package.SetImports)
* [func (pkg \*Package) SetName(name string)](#Package.SetName)
* [func (pkg \*Package) String() string](#Package.String)
* [type PkgName](#PkgName)
* [func NewPkgName(pos token.Pos, pkg \*Package, name string, imported \*Package) \*PkgName](#NewPkgName)
* [func (obj \*PkgName) Exported() bool](#PkgName.Exported)
* [func (obj \*PkgName) Id() string](#PkgName.Id)
* [func (obj \*PkgName) Imported() \*Package](#PkgName.Imported)
* [func (obj \*PkgName) Name() string](#PkgName.Name)
* [func (obj \*PkgName) Parent() \*Scope](#PkgName.Parent)
* [func (obj \*PkgName) Pkg() \*Package](#PkgName.Pkg)
* [func (obj \*PkgName) Pos() token.Pos](#PkgName.Pos)
* [func (obj \*PkgName) String() string](#PkgName.String)
* [func (obj \*PkgName) Type() Type](#PkgName.Type)
* [type Pointer](#Pointer)
* [func NewPointer(elem Type) \*Pointer](#NewPointer)
* [func (p \*Pointer) Elem() Type](#Pointer.Elem)
* [func (t \*Pointer) String() string](#Pointer.String)
* [func (t \*Pointer) Underlying() Type](#Pointer.Underlying)
* [type Qualifier](#Qualifier)
* [func RelativeTo(pkg \*Package) Qualifier](#RelativeTo)
* [type Scope](#Scope)
* [func NewScope(parent \*Scope, pos, end token.Pos, comment string) \*Scope](#NewScope)
* [func (s \*Scope) Child(i int) \*Scope](#Scope.Child)
* [func (s \*Scope) Contains(pos token.Pos) bool](#Scope.Contains)
* [func (s \*Scope) End() token.Pos](#Scope.End)
* [func (s \*Scope) Innermost(pos token.Pos) \*Scope](#Scope.Innermost)
* [func (s \*Scope) Insert(obj Object) Object](#Scope.Insert)
* [func (s \*Scope) Len() int](#Scope.Len)
* [func (s \*Scope) Lookup(name string) Object](#Scope.Lookup)
* [func (s \*Scope) LookupParent(name string, pos token.Pos) (\*Scope, Object)](#Scope.LookupParent)
* [func (s \*Scope) Names() []string](#Scope.Names)
* [func (s \*Scope) NumChildren() int](#Scope.NumChildren)
* [func (s \*Scope) Parent() \*Scope](#Scope.Parent)
* [func (s \*Scope) Pos() token.Pos](#Scope.Pos)
* [func (s \*Scope) String() string](#Scope.String)
* [func (s \*Scope) WriteTo(w io.Writer, n int, recurse bool)](#Scope.WriteTo)
* [type Selection](#Selection)
* [func (s \*Selection) Index() []int](#Selection.Index)
* [func (s \*Selection) Indirect() bool](#Selection.Indirect)
* [func (s \*Selection) Kind() SelectionKind](#Selection.Kind)
* [func (s \*Selection) Obj() Object](#Selection.Obj)
* [func (s \*Selection) Recv() Type](#Selection.Recv)
* [func (s \*Selection) String() string](#Selection.String)
* [func (s \*Selection) Type() Type](#Selection.Type)
* [type SelectionKind](#SelectionKind)
* [type Signature](#Signature)
* [func NewSignature(recv \*Var, params, results \*Tuple, variadic bool) \*Signature](#NewSignature)
* [func NewSignatureType(recv \*Var, recvTypeParams, typeParams []\*TypeParam, params, results \*Tuple, variadic bool) \*Signature](#NewSignatureType)
* [func (s \*Signature) Params() \*Tuple](#Signature.Params)
* [func (s \*Signature) Recv() \*Var](#Signature.Recv)
* [func (s \*Signature) RecvTypeParams() \*TypeParamList](#Signature.RecvTypeParams)
* [func (s \*Signature) Results() \*Tuple](#Signature.Results)
* [func (t \*Signature) String() string](#Signature.String)
* [func (s \*Signature) TypeParams() \*TypeParamList](#Signature.TypeParams)
* [func (t \*Signature) Underlying() Type](#Signature.Underlying)
* [func (s \*Signature) Variadic() bool](#Signature.Variadic)
* [type Sizes](#Sizes)
* [func SizesFor(compiler, arch string) Sizes](#SizesFor)
* [type Slice](#Slice)
* [func NewSlice(elem Type) \*Slice](#NewSlice)
* [func (s \*Slice) Elem() Type](#Slice.Elem)
* [func (t \*Slice) String() string](#Slice.String)
* [func (t \*Slice) Underlying() Type](#Slice.Underlying)
* [type StdSizes](#StdSizes)
* [func (s \*StdSizes) Alignof(T Type) int64](#StdSizes.Alignof)
* [func (s \*StdSizes) Offsetsof(fields []\*Var) []int64](#StdSizes.Offsetsof)
* [func (s \*StdSizes) Sizeof(T Type) int64](#StdSizes.Sizeof)
* [type Struct](#Struct)
* [func NewStruct(fields []\*Var, tags []string) \*Struct](#NewStruct)
* [func (s \*Struct) Field(i int) \*Var](#Struct.Field)
* [func (s \*Struct) NumFields() int](#Struct.NumFields)
* [func (t \*Struct) String() string](#Struct.String)
* [func (s \*Struct) Tag(i int) string](#Struct.Tag)
* [func (t \*Struct) Underlying() Type](#Struct.Underlying)
* [type Term](#Term)
* [func NewTerm(tilde bool, typ Type) \*Term](#NewTerm)
* [func (t \*Term) String() string](#Term.String)
* [func (t \*Term) Tilde() bool](#Term.Tilde)
* [func (t \*Term) Type() Type](#Term.Type)
* [type Tuple](#Tuple)
* [func NewTuple(x ...\*Var) \*Tuple](#NewTuple)
* [func (t \*Tuple) At(i int) \*Var](#Tuple.At)
* [func (t \*Tuple) Len() int](#Tuple.Len)
* [func (t \*Tuple) String() string](#Tuple.String)
* [func (t \*Tuple) Underlying() Type](#Tuple.Underlying)
* [type Type](#Type)
* [func Default(t Type) Type](#Default)
* [func Instantiate(ctxt \*Context, orig Type, targs []Type, validate bool) (Type, error)](#Instantiate)
* [type TypeAndValue](#TypeAndValue)
* [func Eval(fset \*token.FileSet, pkg \*Package, pos token.Pos, expr string) (\_ TypeAndValue, err error)](#Eval)
* [func (tv TypeAndValue) Addressable() bool](#TypeAndValue.Addressable)
* [func (tv TypeAndValue) Assignable() bool](#TypeAndValue.Assignable)
* [func (tv TypeAndValue) HasOk() bool](#TypeAndValue.HasOk)
* [func (tv TypeAndValue) IsBuiltin() bool](#TypeAndValue.IsBuiltin)
* [func (tv TypeAndValue) IsNil() bool](#TypeAndValue.IsNil)
* [func (tv TypeAndValue) IsType() bool](#TypeAndValue.IsType)
* [func (tv TypeAndValue) IsValue() bool](#TypeAndValue.IsValue)
* [func (tv TypeAndValue) IsVoid() bool](#TypeAndValue.IsVoid)
* [type TypeList](#TypeList)
* [func (l \*TypeList) At(i int) Type](#TypeList.At)
* [func (l \*TypeList) Len() int](#TypeList.Len)
* [type TypeName](#TypeName)
* [func NewTypeName(pos token.Pos, pkg \*Package, name string, typ Type) \*TypeName](#NewTypeName)
* [func (obj \*TypeName) Exported() bool](#TypeName.Exported)
* [func (obj \*TypeName) Id() string](#TypeName.Id)
* [func (obj \*TypeName) IsAlias() bool](#TypeName.IsAlias)
* [func (obj \*TypeName) Name() string](#TypeName.Name)
* [func (obj \*TypeName) Parent() \*Scope](#TypeName.Parent)
* [func (obj \*TypeName) Pkg() \*Package](#TypeName.Pkg)
* [func (obj \*TypeName) Pos() token.Pos](#TypeName.Pos)
* [func (obj \*TypeName) String() string](#TypeName.String)
* [func (obj \*TypeName) Type() Type](#TypeName.Type)
* [type TypeParam](#TypeParam)
* [func NewTypeParam(obj \*TypeName, constraint Type) \*TypeParam](#NewTypeParam)
* [func (t \*TypeParam) Constraint() Type](#TypeParam.Constraint)
* [func (t \*TypeParam) Index() int](#TypeParam.Index)
* [func (t \*TypeParam) Obj() \*TypeName](#TypeParam.Obj)
* [func (t \*TypeParam) SetConstraint(bound Type)](#TypeParam.SetConstraint)
* [func (t \*TypeParam) String() string](#TypeParam.String)
* [func (t \*TypeParam) Underlying() Type](#TypeParam.Underlying)
* [type TypeParamList](#TypeParamList)
* [func (l \*TypeParamList) At(i int) \*TypeParam](#TypeParamList.At)
* [func (l \*TypeParamList) Len() int](#TypeParamList.Len)
* [type Union](#Union)
* [func NewUnion(terms []\*Term) \*Union](#NewUnion)
* [func (u \*Union) Len() int](#Union.Len)
* [func (u \*Union) String() string](#Union.String)
* [func (u \*Union) Term(i int) \*Term](#Union.Term)
* [func (u \*Union) Underlying() Type](#Union.Underlying)
* [type Var](#Var)
* [func NewField(pos token.Pos, pkg \*Package, name string, typ Type, embedded bool) \*Var](#NewField)
* [func NewParam(pos token.Pos, pkg \*Package, name string, typ Type) \*Var](#NewParam)
* [func NewVar(pos token.Pos, pkg \*Package, name string, typ Type) \*Var](#NewVar)
* [func (obj \*Var) Anonymous() bool](#Var.Anonymous)
* [func (obj \*Var) Embedded() bool](#Var.Embedded)
* [func (obj \*Var) Exported() bool](#Var.Exported)
* [func (obj \*Var) Id() string](#Var.Id)
* [func (obj \*Var) IsField() bool](#Var.IsField)
* [func (obj \*Var) Name() string](#Var.Name)
* [func (obj \*Var) Origin() \*Var](#Var.Origin)
* [func (obj \*Var) Parent() \*Scope](#Var.Parent)
* [func (obj \*Var) Pkg() \*Package](#Var.Pkg)
* [func (obj \*Var) Pos() token.Pos](#Var.Pos)
* [func (obj \*Var) String() string](#Var.String)
* [func (obj \*Var) Type() Type](#Var.Type)
### Examples
[Info](#example_Info) [MethodSet](#example_MethodSet) [Scope](#example_Scope) ### Package files
api.go array.go assignments.go basic.go builtins.go call.go chan.go check.go context.go conversions.go decl.go errors.go eval.go expr.go exprstring.go gccgosizes.go index.go infer.go initorder.go instantiate.go interface.go labels.go lookup.go map.go methodset.go mono.go named.go object.go objset.go operand.go package.go pointer.go predicates.go resolver.go return.go scope.go selection.go signature.go sizes.go slice.go stmt.go struct.go subst.go termlist.go tuple.go type.go typelists.go typeparam.go typeset.go typestring.go typeterm.go typexpr.go unify.go union.go universe.go validtype.go version.go
Variables
---------
Typ contains the predeclared \*Basic types indexed by their corresponding BasicKind.
The \*Basic type for Typ[Byte] will have the name "uint8". Use Universe.Lookup("byte").Type() to obtain the specific alias basic type named "byte" (and analogous for "rune").
```
var Typ = []*Basic{
Invalid: {Invalid, 0, "invalid type"},
Bool: {Bool, IsBoolean, "bool"},
Int: {Int, IsInteger, "int"},
Int8: {Int8, IsInteger, "int8"},
Int16: {Int16, IsInteger, "int16"},
Int32: {Int32, IsInteger, "int32"},
Int64: {Int64, IsInteger, "int64"},
Uint: {Uint, IsInteger | IsUnsigned, "uint"},
Uint8: {Uint8, IsInteger | IsUnsigned, "uint8"},
Uint16: {Uint16, IsInteger | IsUnsigned, "uint16"},
Uint32: {Uint32, IsInteger | IsUnsigned, "uint32"},
Uint64: {Uint64, IsInteger | IsUnsigned, "uint64"},
Uintptr: {Uintptr, IsInteger | IsUnsigned, "uintptr"},
Float32: {Float32, IsFloat, "float32"},
Float64: {Float64, IsFloat, "float64"},
Complex64: {Complex64, IsComplex, "complex64"},
Complex128: {Complex128, IsComplex, "complex128"},
String: {String, IsString, "string"},
UnsafePointer: {UnsafePointer, 0, "Pointer"},
UntypedBool: {UntypedBool, IsBoolean | IsUntyped, "untyped bool"},
UntypedInt: {UntypedInt, IsInteger | IsUntyped, "untyped int"},
UntypedRune: {UntypedRune, IsInteger | IsUntyped, "untyped rune"},
UntypedFloat: {UntypedFloat, IsFloat | IsUntyped, "untyped float"},
UntypedComplex: {UntypedComplex, IsComplex | IsUntyped, "untyped complex"},
UntypedString: {UntypedString, IsString | IsUntyped, "untyped string"},
UntypedNil: {UntypedNil, IsUntyped, "untyped nil"},
}
```
func AssertableTo 1.5
---------------------
```
func AssertableTo(V *Interface, T Type) bool
```
AssertableTo reports whether a value of type V can be asserted to have type T.
The behavior of AssertableTo is unspecified in three cases:
* if T is Typ[Invalid]
* if V is a generalized interface; i.e., an interface that may only be used as a type constraint in Go code
* if T is an uninstantiated generic type
func AssignableTo 1.5
---------------------
```
func AssignableTo(V, T Type) bool
```
AssignableTo reports whether a value of type V is assignable to a variable of type T.
The behavior of AssignableTo is unspecified if V or T is Typ[Invalid] or an uninstantiated generic type.
func CheckExpr 1.13
-------------------
```
func CheckExpr(fset *token.FileSet, pkg *Package, pos token.Pos, expr ast.Expr, info *Info) (err error)
```
CheckExpr type checks the expression expr as if it had appeared at position pos of package pkg. Type information about the expression is recorded in info. The expression may be an identifier denoting an uninstantiated generic function or type.
If pkg == nil, the Universe scope is used and the provided position pos is ignored. If pkg != nil, and pos is invalid, the package scope is used. Otherwise, pos must belong to the package.
An error is returned if pos is not within the package or if the node cannot be type-checked.
Note: Eval and CheckExpr should not be used instead of running Check to compute types and values, but in addition to Check, as these functions ignore the context in which an expression is used (e.g., an assignment). Thus, top-level untyped constants will return an untyped type rather then the respective context-specific type.
func Comparable 1.5
-------------------
```
func Comparable(T Type) bool
```
Comparable reports whether values of type T are comparable.
func ConvertibleTo 1.5
----------------------
```
func ConvertibleTo(V, T Type) bool
```
ConvertibleTo reports whether a value of type V is convertible to a value of type T.
The behavior of ConvertibleTo is unspecified if V or T is Typ[Invalid] or an uninstantiated generic type.
func DefPredeclaredTestFuncs 1.5
--------------------------------
```
func DefPredeclaredTestFuncs()
```
DefPredeclaredTestFuncs defines the assert and trace built-ins. These built-ins are intended for debugging and testing of this package only.
func ExprString 1.5
-------------------
```
func ExprString(x ast.Expr) string
```
ExprString returns the (possibly shortened) string representation for x. Shortened representations are suitable for user interfaces but may not necessarily follow Go syntax.
func Id 1.5
-----------
```
func Id(pkg *Package, name string) string
```
Id returns name if it is exported, otherwise it returns the name qualified with the package path.
func Identical 1.5
------------------
```
func Identical(x, y Type) bool
```
Identical reports whether x and y are identical types. Receivers of Signature types are ignored.
func IdenticalIgnoreTags 1.8
----------------------------
```
func IdenticalIgnoreTags(x, y Type) bool
```
IdenticalIgnoreTags reports whether x and y are identical types if tags are ignored. Receivers of Signature types are ignored.
func Implements 1.5
-------------------
```
func Implements(V Type, T *Interface) bool
```
Implements reports whether type V implements interface T.
The behavior of Implements is unspecified if V is Typ[Invalid] or an uninstantiated generic type.
func IsInterface 1.5
--------------------
```
func IsInterface(t Type) bool
```
IsInterface reports whether t is an interface type.
func ObjectString 1.5
---------------------
```
func ObjectString(obj Object, qf Qualifier) string
```
ObjectString returns the string form of obj. The Qualifier controls the printing of package-level objects, and may be nil.
func Satisfies 1.20
-------------------
```
func Satisfies(V Type, T *Interface) bool
```
Satisfies reports whether type V satisfies the constraint T.
The behavior of Satisfies is unspecified if V is Typ[Invalid] or an uninstantiated generic type.
func SelectionString 1.5
------------------------
```
func SelectionString(s *Selection, qf Qualifier) string
```
SelectionString returns the string form of s. The Qualifier controls the printing of package-level objects, and may be nil.
Examples:
```
"field (T) f int"
"method (T) f(X) Y"
"method expr (T) f(X) Y"
```
func TypeString 1.5
-------------------
```
func TypeString(typ Type, qf Qualifier) string
```
TypeString returns the string representation of typ. The Qualifier controls the printing of package-level objects, and may be nil.
func WriteExpr 1.5
------------------
```
func WriteExpr(buf *bytes.Buffer, x ast.Expr)
```
WriteExpr writes the (possibly shortened) string representation for x to buf. Shortened representations are suitable for user interfaces but may not necessarily follow Go syntax.
func WriteSignature 1.5
-----------------------
```
func WriteSignature(buf *bytes.Buffer, sig *Signature, qf Qualifier)
```
WriteSignature writes the representation of the signature sig to buf, without a leading "func" keyword. The Qualifier controls the printing of package-level objects, and may be nil.
func WriteType 1.5
------------------
```
func WriteType(buf *bytes.Buffer, typ Type, qf Qualifier)
```
WriteType writes the string representation of typ to buf. The Qualifier controls the printing of package-level objects, and may be nil.
type ArgumentError 1.18
-----------------------
An ArgumentError holds an error associated with an argument index.
```
type ArgumentError struct {
Index int
Err error
}
```
### func (\*ArgumentError) Error 1.18
```
func (e *ArgumentError) Error() string
```
### func (\*ArgumentError) Unwrap 1.18
```
func (e *ArgumentError) Unwrap() error
```
type Array 1.5
--------------
An Array represents an array type.
```
type Array struct {
// contains filtered or unexported fields
}
```
### func NewArray 1.5
```
func NewArray(elem Type, len int64) *Array
```
NewArray returns a new array type for the given element type and length. A negative length indicates an unknown length.
### func (\*Array) Elem 1.5
```
func (a *Array) Elem() Type
```
Elem returns element type of array a.
### func (\*Array) Len 1.5
```
func (a *Array) Len() int64
```
Len returns the length of array a. A negative result indicates an unknown length.
### func (\*Array) String 1.5
```
func (t *Array) String() string
```
### func (\*Array) Underlying 1.5
```
func (t *Array) Underlying() Type
```
type Basic 1.5
--------------
A Basic represents a basic type.
```
type Basic struct {
// contains filtered or unexported fields
}
```
### func (\*Basic) Info 1.5
```
func (b *Basic) Info() BasicInfo
```
Info returns information about properties of basic type b.
### func (\*Basic) Kind 1.5
```
func (b *Basic) Kind() BasicKind
```
Kind returns the kind of basic type b.
### func (\*Basic) Name 1.5
```
func (b *Basic) Name() string
```
Name returns the name of basic type b.
### func (\*Basic) String 1.5
```
func (t *Basic) String() string
```
### func (\*Basic) Underlying 1.5
```
func (t *Basic) Underlying() Type
```
type BasicInfo 1.5
------------------
BasicInfo is a set of flags describing properties of a basic type.
```
type BasicInfo int
```
Properties of basic types.
```
const (
IsBoolean BasicInfo = 1 << iota
IsInteger
IsUnsigned
IsFloat
IsComplex
IsString
IsUntyped
IsOrdered = IsInteger | IsFloat | IsString
IsNumeric = IsInteger | IsFloat | IsComplex
IsConstType = IsBoolean | IsNumeric | IsString
)
```
type BasicKind 1.5
------------------
BasicKind describes the kind of basic type.
```
type BasicKind int
```
```
const (
Invalid BasicKind = iota // type is invalid
// predeclared types
Bool
Int
Int8
Int16
Int32
Int64
Uint
Uint8
Uint16
Uint32
Uint64
Uintptr
Float32
Float64
Complex64
Complex128
String
UnsafePointer
// types for untyped values
UntypedBool
UntypedInt
UntypedRune
UntypedFloat
UntypedComplex
UntypedString
UntypedNil
// aliases
Byte = Uint8
Rune = Int32
)
```
type Builtin 1.5
----------------
A Builtin represents a built-in function. Builtins don't have a valid type.
```
type Builtin struct {
// contains filtered or unexported fields
}
```
### func (\*Builtin) Exported 1.5
```
func (obj *Builtin) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Builtin) Id 1.5
```
func (obj *Builtin) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Builtin) Name 1.5
```
func (obj *Builtin) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Builtin) Parent 1.5
```
func (obj *Builtin) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Builtin) Pkg 1.5
```
func (obj *Builtin) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Builtin) Pos 1.5
```
func (obj *Builtin) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Builtin) String 1.5
```
func (obj *Builtin) String() string
```
### func (\*Builtin) Type 1.5
```
func (obj *Builtin) Type() Type
```
Type returns the object's type.
type Chan 1.5
-------------
A Chan represents a channel type.
```
type Chan struct {
// contains filtered or unexported fields
}
```
### func NewChan 1.5
```
func NewChan(dir ChanDir, elem Type) *Chan
```
NewChan returns a new channel type for the given direction and element type.
### func (\*Chan) Dir 1.5
```
func (c *Chan) Dir() ChanDir
```
Dir returns the direction of channel c.
### func (\*Chan) Elem 1.5
```
func (c *Chan) Elem() Type
```
Elem returns the element type of channel c.
### func (\*Chan) String 1.5
```
func (t *Chan) String() string
```
### func (\*Chan) Underlying 1.5
```
func (t *Chan) Underlying() Type
```
type ChanDir 1.5
----------------
A ChanDir value indicates a channel direction.
```
type ChanDir int
```
The direction of a channel is indicated by one of these constants.
```
const (
SendRecv ChanDir = iota
SendOnly
RecvOnly
)
```
type Checker 1.5
----------------
A Checker maintains the state of the type checker. It must be created with NewChecker.
```
type Checker struct {
*Info
// contains filtered or unexported fields
}
```
### func NewChecker 1.5
```
func NewChecker(conf *Config, fset *token.FileSet, pkg *Package, info *Info) *Checker
```
NewChecker returns a new Checker instance for a given package. Package files may be added incrementally via checker.Files.
### func (\*Checker) Files 1.5
```
func (check *Checker) Files(files []*ast.File) error
```
Files checks the provided files as part of the checker's package.
type Config 1.5
---------------
A Config specifies the configuration for type checking. The zero value for Config is a ready-to-use default configuration.
```
type Config struct {
// Context is the context used for resolving global identifiers. If nil, the
// type checker will initialize this field with a newly created context.
Context *Context // Go 1.18
// GoVersion describes the accepted Go language version. The string
// must follow the format "go%d.%d" (e.g. "go1.12") or it must be
// empty; an empty string indicates the latest language version.
// If the format is invalid, invoking the type checker will cause a
// panic.
GoVersion string // Go 1.18
// If IgnoreFuncBodies is set, function bodies are not
// type-checked.
IgnoreFuncBodies bool
// If FakeImportC is set, `import "C"` (for packages requiring Cgo)
// declares an empty "C" package and errors are omitted for qualified
// identifiers referring to package C (which won't find an object).
// This feature is intended for the standard library cmd/api tool.
//
// Caution: Effects may be unpredictable due to follow-on errors.
// Do not use casually!
FakeImportC bool
// If Error != nil, it is called with each error found
// during type checking; err has dynamic type Error.
// Secondary errors (for instance, to enumerate all types
// involved in an invalid recursive type declaration) have
// error strings that start with a '\t' character.
// If Error == nil, type-checking stops with the first
// error found.
Error func(err error)
// An importer is used to import packages referred to from
// import declarations.
// If the installed importer implements ImporterFrom, the type
// checker calls ImportFrom instead of Import.
// The type checker reports an error if an importer is needed
// but none was installed.
Importer Importer
// If Sizes != nil, it provides the sizing functions for package unsafe.
// Otherwise SizesFor("gc", "amd64") is used instead.
Sizes Sizes
// If DisableUnusedImportCheck is set, packages are not checked
// for unused imports.
DisableUnusedImportCheck bool
// contains filtered or unexported fields
}
```
### func (\*Config) Check 1.5
```
func (conf *Config) Check(path string, fset *token.FileSet, files []*ast.File, info *Info) (*Package, error)
```
Check type-checks a package and returns the resulting package object and the first error if any. Additionally, if info != nil, Check populates each of the non-nil maps in the Info struct.
The package is marked as complete if no errors occurred, otherwise it is incomplete. See Config.Error for controlling behavior in the presence of errors.
The package is specified by a list of \*ast.Files and corresponding file set, and the package path the package is identified with. The clean path must not be empty or dot (".").
type Const 1.5
--------------
A Const represents a declared constant.
```
type Const struct {
// contains filtered or unexported fields
}
```
### func NewConst 1.5
```
func NewConst(pos token.Pos, pkg *Package, name string, typ Type, val constant.Value) *Const
```
NewConst returns a new constant with value val. The remaining arguments set the attributes found with all Objects.
### func (\*Const) Exported 1.5
```
func (obj *Const) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Const) Id 1.5
```
func (obj *Const) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Const) Name 1.5
```
func (obj *Const) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Const) Parent 1.5
```
func (obj *Const) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Const) Pkg 1.5
```
func (obj *Const) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Const) Pos 1.5
```
func (obj *Const) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Const) String 1.5
```
func (obj *Const) String() string
```
### func (\*Const) Type 1.5
```
func (obj *Const) Type() Type
```
Type returns the object's type.
### func (\*Const) Val 1.5
```
func (obj *Const) Val() constant.Value
```
Val returns the constant's value.
type Context 1.18
-----------------
A Context is an opaque type checking context. It may be used to share identical type instances across type-checked packages or calls to Instantiate. Contexts are safe for concurrent use.
The use of a shared context does not guarantee that identical instances are deduplicated in all cases.
```
type Context struct {
// contains filtered or unexported fields
}
```
### func NewContext 1.18
```
func NewContext() *Context
```
NewContext creates a new Context.
type Error 1.5
--------------
An Error describes a type-checking error; it implements the error interface. A "soft" error is an error that still permits a valid interpretation of a package (such as "unused variable"); "hard" errors may lead to unpredictable behavior if ignored.
```
type Error struct {
Fset *token.FileSet // file set for interpretation of Pos
Pos token.Pos // error position
Msg string // error message
Soft bool // if set, error is "soft"
// contains filtered or unexported fields
}
```
### func (Error) Error 1.5
```
func (err Error) Error() string
```
Error returns an error string formatted as follows: filename:line:column: message
type Func 1.5
-------------
A Func represents a declared function, concrete method, or abstract (interface) method. Its Type() is always a \*Signature. An abstract method may belong to many interfaces due to embedding.
```
type Func struct {
// contains filtered or unexported fields
}
```
### func MissingMethod 1.5
```
func MissingMethod(V Type, T *Interface, static bool) (method *Func, wrongType bool)
```
MissingMethod returns (nil, false) if V implements T, otherwise it returns a missing method required by T and whether it is missing or just has the wrong type.
For non-interface types V, or if static is set, V implements T if all methods of T are present in V. Otherwise (V is an interface and static is not set), MissingMethod only checks that methods of T which are also present in V have matching types (e.g., for a type assertion x.(T) where x is of interface type V).
### func NewFunc 1.5
```
func NewFunc(pos token.Pos, pkg *Package, name string, sig *Signature) *Func
```
NewFunc returns a new function with the given signature, representing the function's type.
### func (\*Func) Exported 1.5
```
func (obj *Func) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Func) FullName 1.5
```
func (obj *Func) FullName() string
```
FullName returns the package- or receiver-type-qualified name of function or method obj.
### func (\*Func) Id 1.5
```
func (obj *Func) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Func) Name 1.5
```
func (obj *Func) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Func) Origin 1.19
```
func (obj *Func) Origin() *Func
```
Origin returns the canonical Func for its receiver, i.e. the Func object recorded in Info.Defs.
For synthetic functions created during instantiation (such as methods on an instantiated Named type or interface methods that depend on type arguments), this will be the corresponding Func on the generic (uninstantiated) type. For all other Funcs Origin returns the receiver.
### func (\*Func) Parent 1.5
```
func (obj *Func) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Func) Pkg 1.5
```
func (obj *Func) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Func) Pos 1.5
```
func (obj *Func) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Func) Scope 1.5
```
func (obj *Func) Scope() *Scope
```
Scope returns the scope of the function's body block. The result is nil for imported or instantiated functions and methods (but there is also no mechanism to get to an instantiated function).
### func (\*Func) String 1.5
```
func (obj *Func) String() string
```
### func (\*Func) Type 1.5
```
func (obj *Func) Type() Type
```
Type returns the object's type.
type ImportMode 1.6
-------------------
ImportMode is reserved for future use.
```
type ImportMode int
```
type Importer 1.5
-----------------
An Importer resolves import paths to Packages.
CAUTION: This interface does not support the import of locally vendored packages. See <https://golang.org/s/go15vendor>. If possible, external implementations should implement ImporterFrom.
```
type Importer interface {
// Import returns the imported package for the given import path.
// The semantics is like for ImporterFrom.ImportFrom except that
// dir and mode are ignored (since they are not present).
Import(path string) (*Package, error)
}
```
type ImporterFrom 1.6
---------------------
An ImporterFrom resolves import paths to packages; it supports vendoring per <https://golang.org/s/go15vendor>. Use go/importer to obtain an ImporterFrom implementation.
```
type ImporterFrom interface {
// Importer is present for backward-compatibility. Calling
// Import(path) is the same as calling ImportFrom(path, "", 0);
// i.e., locally vendored packages may not be found.
// The types package does not call Import if an ImporterFrom
// is present.
Importer
// ImportFrom returns the imported package for the given import
// path when imported by a package file located in dir.
// If the import failed, besides returning an error, ImportFrom
// is encouraged to cache and return a package anyway, if one
// was created. This will reduce package inconsistencies and
// follow-on type checker errors due to the missing package.
// The mode value must be 0; it is reserved for future use.
// Two calls to ImportFrom with the same path and dir must
// return the same package.
ImportFrom(path, dir string, mode ImportMode) (*Package, error)
}
```
type Info 1.5
-------------
Info holds result type information for a type-checked package. Only the information for which a map is provided is collected. If the package has type errors, the collected information may be incomplete.
```
type Info struct {
// Types maps expressions to their types, and for constant
// expressions, also their values. Invalid expressions are
// omitted.
//
// For (possibly parenthesized) identifiers denoting built-in
// functions, the recorded signatures are call-site specific:
// if the call result is not a constant, the recorded type is
// an argument-specific signature. Otherwise, the recorded type
// is invalid.
//
// The Types map does not record the type of every identifier,
// only those that appear where an arbitrary expression is
// permitted. For instance, the identifier f in a selector
// expression x.f is found only in the Selections map, the
// identifier z in a variable declaration 'var z int' is found
// only in the Defs map, and identifiers denoting packages in
// qualified identifiers are collected in the Uses map.
Types map[ast.Expr]TypeAndValue
// Instances maps identifiers denoting generic types or functions to their
// type arguments and instantiated type.
//
// For example, Instances will map the identifier for 'T' in the type
// instantiation T[int, string] to the type arguments [int, string] and
// resulting instantiated *Named type. Given a generic function
// func F[A any](A), Instances will map the identifier for 'F' in the call
// expression F(int(1)) to the inferred type arguments [int], and resulting
// instantiated *Signature.
//
// Invariant: Instantiating Uses[id].Type() with Instances[id].TypeArgs
// results in an equivalent of Instances[id].Type.
Instances map[*ast.Ident]Instance // Go 1.18
// Defs maps identifiers to the objects they define (including
// package names, dots "." of dot-imports, and blank "_" identifiers).
// For identifiers that do not denote objects (e.g., the package name
// in package clauses, or symbolic variables t in t := x.(type) of
// type switch headers), the corresponding objects are nil.
//
// For an embedded field, Defs returns the field *Var it defines.
//
// Invariant: Defs[id] == nil || Defs[id].Pos() == id.Pos()
Defs map[*ast.Ident]Object
// Uses maps identifiers to the objects they denote.
//
// For an embedded field, Uses returns the *TypeName it denotes.
//
// Invariant: Uses[id].Pos() != id.Pos()
Uses map[*ast.Ident]Object
// Implicits maps nodes to their implicitly declared objects, if any.
// The following node and object types may appear:
//
// node declared object
//
// *ast.ImportSpec *PkgName for imports without renames
// *ast.CaseClause type-specific *Var for each type switch case clause (incl. default)
// *ast.Field anonymous parameter *Var (incl. unnamed results)
//
Implicits map[ast.Node]Object
// Selections maps selector expressions (excluding qualified identifiers)
// to their corresponding selections.
Selections map[*ast.SelectorExpr]*Selection
// Scopes maps ast.Nodes to the scopes they define. Package scopes are not
// associated with a specific node but with all files belonging to a package.
// Thus, the package scope can be found in the type-checked Package object.
// Scopes nest, with the Universe scope being the outermost scope, enclosing
// the package scope, which contains (one or more) files scopes, which enclose
// function scopes which in turn enclose statement and function literal scopes.
// Note that even though package-level functions are declared in the package
// scope, the function scopes are embedded in the file scope of the file
// containing the function declaration.
//
// The following node types may appear in Scopes:
//
// *ast.File
// *ast.FuncType
// *ast.TypeSpec
// *ast.BlockStmt
// *ast.IfStmt
// *ast.SwitchStmt
// *ast.TypeSwitchStmt
// *ast.CaseClause
// *ast.CommClause
// *ast.ForStmt
// *ast.RangeStmt
//
Scopes map[ast.Node]*Scope
// InitOrder is the list of package-level initializers in the order in which
// they must be executed. Initializers referring to variables related by an
// initialization dependency appear in topological order, the others appear
// in source order. Variables without an initialization expression do not
// appear in this list.
InitOrder []*Initializer
}
```
#### Example
ExampleInfo prints various facts recorded by the type checker in a types.Info struct: definitions of and references to each named object, and the type, value, and mode of every expression in the package.
Code:
```
// Parse a single source file.
const input = `
package fib
type S string
var a, b, c = len(b), S(c), "hello"
func fib(x int) int {
if x < 2 {
return x
}
return fib(x-1) - fib(x-2)
}`
fset := token.NewFileSet()
f := mustParse(fset, "fib.go", input)
// Type-check the package.
// We create an empty map for each kind of input
// we're interested in, and Check populates them.
info := types.Info{
Types: make(map[ast.Expr]types.TypeAndValue),
Defs: make(map[*ast.Ident]types.Object),
Uses: make(map[*ast.Ident]types.Object),
}
var conf types.Config
pkg, err := conf.Check("fib", fset, []*ast.File{f}, &info)
if err != nil {
log.Fatal(err)
}
// Print package-level variables in initialization order.
fmt.Printf("InitOrder: %v\n\n", info.InitOrder)
// For each named object, print the line and
// column of its definition and each of its uses.
fmt.Println("Defs and Uses of each named object:")
usesByObj := make(map[types.Object][]string)
for id, obj := range info.Uses {
posn := fset.Position(id.Pos())
lineCol := fmt.Sprintf("%d:%d", posn.Line, posn.Column)
usesByObj[obj] = append(usesByObj[obj], lineCol)
}
var items []string
for obj, uses := range usesByObj {
sort.Strings(uses)
item := fmt.Sprintf("%s:\n defined at %s\n used at %s",
types.ObjectString(obj, types.RelativeTo(pkg)),
fset.Position(obj.Pos()),
strings.Join(uses, ", "))
items = append(items, item)
}
sort.Strings(items) // sort by line:col, in effect
fmt.Println(strings.Join(items, "\n"))
fmt.Println()
fmt.Println("Types and Values of each expression:")
items = nil
for expr, tv := range info.Types {
var buf strings.Builder
posn := fset.Position(expr.Pos())
tvstr := tv.Type.String()
if tv.Value != nil {
tvstr += " = " + tv.Value.String()
}
// line:col | expr | mode : type = value
fmt.Fprintf(&buf, "%2d:%2d | %-19s | %-7s : %s",
posn.Line, posn.Column, exprString(fset, expr),
mode(tv), tvstr)
items = append(items, buf.String())
}
sort.Strings(items)
fmt.Println(strings.Join(items, "\n"))
```
Output:
```
InitOrder: [c = "hello" b = S(c) a = len(b)]
Defs and Uses of each named object:
builtin len:
defined at -
used at 6:15
func fib(x int) int:
defined at fib.go:8:6
used at 12:20, 12:9
type S string:
defined at fib.go:4:6
used at 6:23
type int:
defined at -
used at 8:12, 8:17
type string:
defined at -
used at 4:8
var b S:
defined at fib.go:6:8
used at 6:19
var c string:
defined at fib.go:6:11
used at 6:25
var x int:
defined at fib.go:8:10
used at 10:10, 12:13, 12:24, 9:5
Types and Values of each expression:
4: 8 | string | type : string
6:15 | len | builtin : func(fib.S) int
6:15 | len(b) | value : int
6:19 | b | var : fib.S
6:23 | S | type : fib.S
6:23 | S(c) | value : fib.S
6:25 | c | var : string
6:29 | "hello" | value : string = "hello"
8:12 | int | type : int
8:17 | int | type : int
9: 5 | x | var : int
9: 5 | x < 2 | value : untyped bool
9: 9 | 2 | value : int = 2
10:10 | x | var : int
12: 9 | fib | value : func(x int) int
12: 9 | fib(x - 1) | value : int
12: 9 | fib(x-1) - fib(x-2) | value : int
12:13 | x | var : int
12:13 | x - 1 | value : int
12:15 | 1 | value : int = 1
12:20 | fib | value : func(x int) int
12:20 | fib(x - 2) | value : int
12:24 | x | var : int
12:24 | x - 2 | value : int
12:26 | 2 | value : int = 2
```
### func (\*Info) ObjectOf 1.5
```
func (info *Info) ObjectOf(id *ast.Ident) Object
```
ObjectOf returns the object denoted by the specified id, or nil if not found.
If id is an embedded struct field, ObjectOf returns the field (\*Var) it defines, not the type (\*TypeName) it uses.
Precondition: the Uses and Defs maps are populated.
### func (\*Info) TypeOf 1.5
```
func (info *Info) TypeOf(e ast.Expr) Type
```
TypeOf returns the type of expression e, or nil if not found. Precondition: the Types, Uses and Defs maps are populated.
type Initializer 1.5
--------------------
An Initializer describes a package-level variable, or a list of variables in case of a multi-valued initialization expression, and the corresponding initialization expression.
```
type Initializer struct {
Lhs []*Var // var Lhs = Rhs
Rhs ast.Expr
}
```
### func (\*Initializer) String 1.5
```
func (init *Initializer) String() string
```
type Instance 1.18
------------------
Instance reports the type arguments and instantiated type for type and function instantiations. For type instantiations, Type will be of dynamic type \*Named. For function instantiations, Type will be of dynamic type \*Signature.
```
type Instance struct {
TypeArgs *TypeList
Type Type
}
```
type Interface 1.5
------------------
An Interface represents an interface type.
```
type Interface struct {
// contains filtered or unexported fields
}
```
### func NewInterface 1.5
```
func NewInterface(methods []*Func, embeddeds []*Named) *Interface
```
NewInterface returns a new interface for the given methods and embedded types. NewInterface takes ownership of the provided methods and may modify their types by setting missing receivers.
Deprecated: Use NewInterfaceType instead which allows arbitrary embedded types.
### func NewInterfaceType 1.11
```
func NewInterfaceType(methods []*Func, embeddeds []Type) *Interface
```
NewInterfaceType returns a new interface for the given methods and embedded types. NewInterfaceType takes ownership of the provided methods and may modify their types by setting missing receivers.
To avoid race conditions, the interface's type set should be computed before concurrent use of the interface, by explicitly calling Complete.
### func (\*Interface) Complete 1.5
```
func (t *Interface) Complete() *Interface
```
Complete computes the interface's type set. It must be called by users of NewInterfaceType and NewInterface after the interface's embedded types are fully defined and before using the interface type in any way other than to form other types. The interface must not contain duplicate methods or a panic occurs. Complete returns the receiver.
Interface types that have been completed are safe for concurrent use.
### func (\*Interface) Embedded 1.5
```
func (t *Interface) Embedded(i int) *Named
```
Embedded returns the i'th embedded defined (\*Named) type of interface t for 0 <= i < t.NumEmbeddeds(). The result is nil if the i'th embedded type is not a defined type.
Deprecated: Use EmbeddedType which is not restricted to defined (\*Named) types.
### func (\*Interface) EmbeddedType 1.11
```
func (t *Interface) EmbeddedType(i int) Type
```
EmbeddedType returns the i'th embedded type of interface t for 0 <= i < t.NumEmbeddeds().
### func (\*Interface) Empty 1.5
```
func (t *Interface) Empty() bool
```
Empty reports whether t is the empty interface.
### func (\*Interface) ExplicitMethod 1.5
```
func (t *Interface) ExplicitMethod(i int) *Func
```
ExplicitMethod returns the i'th explicitly declared method of interface t for 0 <= i < t.NumExplicitMethods(). The methods are ordered by their unique Id.
### func (\*Interface) IsComparable 1.18
```
func (t *Interface) IsComparable() bool
```
IsComparable reports whether each type in interface t's type set is comparable.
### func (\*Interface) IsImplicit 1.18
```
func (t *Interface) IsImplicit() bool
```
IsImplicit reports whether the interface t is a wrapper for a type set literal.
### func (\*Interface) IsMethodSet 1.18
```
func (t *Interface) IsMethodSet() bool
```
IsMethodSet reports whether the interface t is fully described by its method set.
### func (\*Interface) MarkImplicit 1.18
```
func (t *Interface) MarkImplicit()
```
MarkImplicit marks the interface t as implicit, meaning this interface corresponds to a constraint literal such as ~T or A|B without explicit interface embedding. MarkImplicit should be called before any concurrent use of implicit interfaces.
### func (\*Interface) Method 1.5
```
func (t *Interface) Method(i int) *Func
```
Method returns the i'th method of interface t for 0 <= i < t.NumMethods(). The methods are ordered by their unique Id.
### func (\*Interface) NumEmbeddeds 1.5
```
func (t *Interface) NumEmbeddeds() int
```
NumEmbeddeds returns the number of embedded types in interface t.
### func (\*Interface) NumExplicitMethods 1.5
```
func (t *Interface) NumExplicitMethods() int
```
NumExplicitMethods returns the number of explicitly declared methods of interface t.
### func (\*Interface) NumMethods 1.5
```
func (t *Interface) NumMethods() int
```
NumMethods returns the total number of methods of interface t.
### func (\*Interface) String 1.5
```
func (t *Interface) String() string
```
### func (\*Interface) Underlying 1.5
```
func (t *Interface) Underlying() Type
```
type Label 1.5
--------------
A Label represents a declared label. Labels don't have a type.
```
type Label struct {
// contains filtered or unexported fields
}
```
### func NewLabel 1.5
```
func NewLabel(pos token.Pos, pkg *Package, name string) *Label
```
NewLabel returns a new label.
### func (\*Label) Exported 1.5
```
func (obj *Label) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Label) Id 1.5
```
func (obj *Label) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Label) Name 1.5
```
func (obj *Label) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Label) Parent 1.5
```
func (obj *Label) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Label) Pkg 1.5
```
func (obj *Label) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Label) Pos 1.5
```
func (obj *Label) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Label) String 1.5
```
func (obj *Label) String() string
```
### func (\*Label) Type 1.5
```
func (obj *Label) Type() Type
```
Type returns the object's type.
type Map 1.5
------------
A Map represents a map type.
```
type Map struct {
// contains filtered or unexported fields
}
```
### func NewMap 1.5
```
func NewMap(key, elem Type) *Map
```
NewMap returns a new map for the given key and element types.
### func (\*Map) Elem 1.5
```
func (m *Map) Elem() Type
```
Elem returns the element type of map m.
### func (\*Map) Key 1.5
```
func (m *Map) Key() Type
```
Key returns the key type of map m.
### func (\*Map) String 1.5
```
func (t *Map) String() string
```
### func (\*Map) Underlying 1.5
```
func (t *Map) Underlying() Type
```
type MethodSet 1.5
------------------
A MethodSet is an ordered set of concrete or abstract (interface) methods; a method is a MethodVal selection, and they are ordered by ascending m.Obj().Id(). The zero value for a MethodSet is a ready-to-use empty method set.
```
type MethodSet struct {
// contains filtered or unexported fields
}
```
#### Example
ExampleMethodSet prints the method sets of various types.
Code:
```
// Parse a single source file.
const input = `
package temperature
import "fmt"
type Celsius float64
func (c Celsius) String() string { return fmt.Sprintf("%g°C", c) }
func (c *Celsius) SetF(f float64) { *c = Celsius(f - 32 / 9 * 5) }
type S struct { I; m int }
type I interface { m() byte }
`
fset := token.NewFileSet()
f, err := parser.ParseFile(fset, "celsius.go", input, 0)
if err != nil {
log.Fatal(err)
}
// Type-check a package consisting of this file.
// Type information for the imported packages
// comes from $GOROOT/pkg/$GOOS_$GOOARCH/fmt.a.
conf := types.Config{Importer: importer.Default()}
pkg, err := conf.Check("temperature", fset, []*ast.File{f}, nil)
if err != nil {
log.Fatal(err)
}
// Print the method sets of Celsius and *Celsius.
celsius := pkg.Scope().Lookup("Celsius").Type()
for _, t := range []types.Type{celsius, types.NewPointer(celsius)} {
fmt.Printf("Method set of %s:\n", t)
mset := types.NewMethodSet(t)
for i := 0; i < mset.Len(); i++ {
fmt.Println(mset.At(i))
}
fmt.Println()
}
// Print the method set of S.
styp := pkg.Scope().Lookup("S").Type()
fmt.Printf("Method set of %s:\n", styp)
fmt.Println(types.NewMethodSet(styp))
```
Output:
```
Method set of temperature.Celsius:
method (temperature.Celsius) String() string
Method set of *temperature.Celsius:
method (*temperature.Celsius) SetF(f float64)
method (*temperature.Celsius) String() string
Method set of temperature.S:
MethodSet {}
```
### func NewMethodSet 1.5
```
func NewMethodSet(T Type) *MethodSet
```
NewMethodSet returns the method set for the given type T. It always returns a non-nil method set, even if it is empty.
### func (\*MethodSet) At 1.5
```
func (s *MethodSet) At(i int) *Selection
```
At returns the i'th method in s for 0 <= i < s.Len().
### func (\*MethodSet) Len 1.5
```
func (s *MethodSet) Len() int
```
Len returns the number of methods in s.
### func (\*MethodSet) Lookup 1.5
```
func (s *MethodSet) Lookup(pkg *Package, name string) *Selection
```
Lookup returns the method with matching package and name, or nil if not found.
### func (\*MethodSet) String 1.5
```
func (s *MethodSet) String() string
```
type Named 1.5
--------------
A Named represents a named (defined) type.
```
type Named struct {
// contains filtered or unexported fields
}
```
### func NewNamed 1.5
```
func NewNamed(obj *TypeName, underlying Type, methods []*Func) *Named
```
NewNamed returns a new named type for the given type name, underlying type, and associated methods. If the given type name obj doesn't have a type yet, its type is set to the returned named type. The underlying type must not be a \*Named.
### func (\*Named) AddMethod 1.5
```
func (t *Named) AddMethod(m *Func)
```
AddMethod adds method m unless it is already in the method list. t must not have type arguments.
### func (\*Named) Method 1.5
```
func (t *Named) Method(i int) *Func
```
Method returns the i'th method of named type t for 0 <= i < t.NumMethods().
For an ordinary or instantiated type t, the receiver base type of this method is the named type t. For an uninstantiated generic type t, each method receiver is instantiated with its receiver type parameters.
### func (\*Named) NumMethods 1.5
```
func (t *Named) NumMethods() int
```
NumMethods returns the number of explicit methods defined for t.
### func (\*Named) Obj 1.5
```
func (t *Named) Obj() *TypeName
```
Obj returns the type name for the declaration defining the named type t. For instantiated types, this is same as the type name of the origin type.
### func (\*Named) Origin 1.18
```
func (t *Named) Origin() *Named
```
Origin returns the generic type from which the named type t is instantiated. If t is not an instantiated type, the result is t.
### func (\*Named) SetTypeParams 1.18
```
func (t *Named) SetTypeParams(tparams []*TypeParam)
```
SetTypeParams sets the type parameters of the named type t. t must not have type arguments.
### func (\*Named) SetUnderlying 1.5
```
func (t *Named) SetUnderlying(underlying Type)
```
SetUnderlying sets the underlying type and marks t as complete. t must not have type arguments.
### func (\*Named) String 1.5
```
func (t *Named) String() string
```
### func (\*Named) TypeArgs 1.18
```
func (t *Named) TypeArgs() *TypeList
```
TypeArgs returns the type arguments used to instantiate the named type t.
### func (\*Named) TypeParams 1.18
```
func (t *Named) TypeParams() *TypeParamList
```
TypeParams returns the type parameters of the named type t, or nil. The result is non-nil for an (originally) generic type even if it is instantiated.
### func (\*Named) Underlying 1.5
```
func (t *Named) Underlying() Type
```
type Nil 1.5
------------
Nil represents the predeclared value nil.
```
type Nil struct {
// contains filtered or unexported fields
}
```
### func (\*Nil) Exported 1.5
```
func (obj *Nil) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Nil) Id 1.5
```
func (obj *Nil) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Nil) Name 1.5
```
func (obj *Nil) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Nil) Parent 1.5
```
func (obj *Nil) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Nil) Pkg 1.5
```
func (obj *Nil) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Nil) Pos 1.5
```
func (obj *Nil) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Nil) String 1.5
```
func (obj *Nil) String() string
```
### func (\*Nil) Type 1.5
```
func (obj *Nil) Type() Type
```
Type returns the object's type.
type Object 1.5
---------------
An Object describes a named language entity such as a package, constant, type, variable, function (incl. methods), or label. All objects implement the Object interface.
```
type Object interface {
Parent() *Scope // scope in which this object is declared; nil for methods and struct fields
Pos() token.Pos // position of object identifier in declaration
Pkg() *Package // package to which this object belongs; nil for labels and objects in the Universe scope
Name() string // package local object name
Type() Type // object type
Exported() bool // reports whether the name starts with a capital letter
Id() string // object name if exported, qualified name if not exported (see func Id)
// String returns a human-readable string of the object.
String() string
// contains filtered or unexported methods
}
```
### func LookupFieldOrMethod 1.5
```
func LookupFieldOrMethod(T Type, addressable bool, pkg *Package, name string) (obj Object, index []int, indirect bool)
```
LookupFieldOrMethod looks up a field or method with given package and name in T and returns the corresponding \*Var or \*Func, an index sequence, and a bool indicating if there were any pointer indirections on the path to the field or method. If addressable is set, T is the type of an addressable variable (only matters for method lookups). T must not be nil.
The last index entry is the field or method index in the (possibly embedded) type where the entry was found, either:
1. the list of declared methods of a named type; or
2. the list of all methods (method set) of an interface type; or
3. the list of fields of a struct type.
The earlier index entries are the indices of the embedded struct fields traversed to get to the found entry, starting at depth 0.
If no entry is found, a nil object is returned. In this case, the returned index and indirect values have the following meaning:
* If index != nil, the index sequence points to an ambiguous entry (the same name appeared more than once at the same embedding level).
* If indirect is set, a method with a pointer receiver type was found but there was no pointer on the path from the actual receiver type to the method's formal receiver base type, nor was the receiver addressable.
type Package 1.5
----------------
A Package describes a Go package.
```
type Package struct {
// contains filtered or unexported fields
}
```
The Unsafe package is the package returned by an importer for the import path "unsafe".
```
var Unsafe *Package
```
### func NewPackage 1.5
```
func NewPackage(path, name string) *Package
```
NewPackage returns a new Package for the given package path and name. The package is not complete and contains no explicit imports.
### func (\*Package) Complete 1.5
```
func (pkg *Package) Complete() bool
```
A package is complete if its scope contains (at least) all exported objects; otherwise it is incomplete.
### func (\*Package) Imports 1.5
```
func (pkg *Package) Imports() []*Package
```
Imports returns the list of packages directly imported by pkg; the list is in source order.
If pkg was loaded from export data, Imports includes packages that provide package-level objects referenced by pkg. This may be more or less than the set of packages directly imported by pkg's source code.
If pkg uses cgo and the FakeImportC configuration option was enabled, the imports list may contain a fake "C" package.
### func (\*Package) MarkComplete 1.5
```
func (pkg *Package) MarkComplete()
```
MarkComplete marks a package as complete.
### func (\*Package) Name 1.5
```
func (pkg *Package) Name() string
```
Name returns the package name.
### func (\*Package) Path 1.5
```
func (pkg *Package) Path() string
```
Path returns the package path.
### func (\*Package) Scope 1.5
```
func (pkg *Package) Scope() *Scope
```
Scope returns the (complete or incomplete) package scope holding the objects declared at package level (TypeNames, Consts, Vars, and Funcs). For a nil pkg receiver, Scope returns the Universe scope.
### func (\*Package) SetImports 1.5
```
func (pkg *Package) SetImports(list []*Package)
```
SetImports sets the list of explicitly imported packages to list. It is the caller's responsibility to make sure list elements are unique.
### func (\*Package) SetName 1.6
```
func (pkg *Package) SetName(name string)
```
SetName sets the package name.
### func (\*Package) String 1.5
```
func (pkg *Package) String() string
```
type PkgName 1.5
----------------
A PkgName represents an imported Go package. PkgNames don't have a type.
```
type PkgName struct {
// contains filtered or unexported fields
}
```
### func NewPkgName 1.5
```
func NewPkgName(pos token.Pos, pkg *Package, name string, imported *Package) *PkgName
```
NewPkgName returns a new PkgName object representing an imported package. The remaining arguments set the attributes found with all Objects.
### func (\*PkgName) Exported 1.5
```
func (obj *PkgName) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*PkgName) Id 1.5
```
func (obj *PkgName) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*PkgName) Imported 1.5
```
func (obj *PkgName) Imported() *Package
```
Imported returns the package that was imported. It is distinct from Pkg(), which is the package containing the import statement.
### func (\*PkgName) Name 1.5
```
func (obj *PkgName) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*PkgName) Parent 1.5
```
func (obj *PkgName) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*PkgName) Pkg 1.5
```
func (obj *PkgName) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*PkgName) Pos 1.5
```
func (obj *PkgName) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*PkgName) String 1.5
```
func (obj *PkgName) String() string
```
### func (\*PkgName) Type 1.5
```
func (obj *PkgName) Type() Type
```
Type returns the object's type.
type Pointer 1.5
----------------
A Pointer represents a pointer type.
```
type Pointer struct {
// contains filtered or unexported fields
}
```
### func NewPointer 1.5
```
func NewPointer(elem Type) *Pointer
```
NewPointer returns a new pointer type for the given element (base) type.
### func (\*Pointer) Elem 1.5
```
func (p *Pointer) Elem() Type
```
Elem returns the element type for the given pointer p.
### func (\*Pointer) String 1.5
```
func (t *Pointer) String() string
```
### func (\*Pointer) Underlying 1.5
```
func (t *Pointer) Underlying() Type
```
type Qualifier 1.5
------------------
A Qualifier controls how named package-level objects are printed in calls to TypeString, ObjectString, and SelectionString.
These three formatting routines call the Qualifier for each package-level object O, and if the Qualifier returns a non-empty string p, the object is printed in the form p.O. If it returns an empty string, only the object name O is printed.
Using a nil Qualifier is equivalent to using (\*Package).Path: the object is qualified by the import path, e.g., "encoding/json.Marshal".
```
type Qualifier func(*Package) string
```
### func RelativeTo 1.5
```
func RelativeTo(pkg *Package) Qualifier
```
RelativeTo returns a Qualifier that fully qualifies members of all packages other than pkg.
type Scope 1.5
--------------
A Scope maintains a set of objects and links to its containing (parent) and contained (children) scopes. Objects may be inserted and looked up by name. The zero value for Scope is a ready-to-use empty scope.
```
type Scope struct {
// contains filtered or unexported fields
}
```
The Universe scope contains all predeclared objects of Go. It is the outermost scope of any chain of nested scopes.
```
var Universe *Scope
```
#### Example
ExampleScope prints the tree of Scopes of a package created from a set of parsed files.
Code:
```
// Parse the source files for a package.
fset := token.NewFileSet()
var files []*ast.File
for _, file := range []struct{ name, input string }{
{"main.go", `
package main
import "fmt"
func main() {
freezing := FToC(-18)
fmt.Println(freezing, Boiling) }
`},
{"celsius.go", `
package main
import "fmt"
type Celsius float64
func (c Celsius) String() string { return fmt.Sprintf("%g°C", c) }
func FToC(f float64) Celsius { return Celsius(f - 32 / 9 * 5) }
const Boiling Celsius = 100
func Unused() { {}; {{ var x int; _ = x }} } // make sure empty block scopes get printed
`},
} {
files = append(files, mustParse(fset, file.name, file.input))
}
// Type-check a package consisting of these files.
// Type information for the imported "fmt" package
// comes from $GOROOT/pkg/$GOOS_$GOOARCH/fmt.a.
conf := types.Config{Importer: importer.Default()}
pkg, err := conf.Check("temperature", fset, files, nil)
if err != nil {
log.Fatal(err)
}
// Print the tree of scopes.
// For determinism, we redact addresses.
var buf strings.Builder
pkg.Scope().WriteTo(&buf, 0, true)
rx := regexp.MustCompile(` 0x[a-fA-F\d]*`)
fmt.Println(rx.ReplaceAllString(buf.String(), ""))
```
Output:
```
package "temperature" scope {
. const temperature.Boiling temperature.Celsius
. type temperature.Celsius float64
. func temperature.FToC(f float64) temperature.Celsius
. func temperature.Unused()
. func temperature.main()
. main.go scope {
. . package fmt
. . function scope {
. . . var freezing temperature.Celsius
. . }
. }
. celsius.go scope {
. . package fmt
. . function scope {
. . . var c temperature.Celsius
. . }
. . function scope {
. . . var f float64
. . }
. . function scope {
. . . block scope {
. . . }
. . . block scope {
. . . . block scope {
. . . . . var x int
. . . . }
. . . }
. . }
. }
}
```
### func NewScope 1.5
```
func NewScope(parent *Scope, pos, end token.Pos, comment string) *Scope
```
NewScope returns a new, empty scope contained in the given parent scope, if any. The comment is for debugging only.
### func (\*Scope) Child 1.5
```
func (s *Scope) Child(i int) *Scope
```
Child returns the i'th child scope for 0 <= i < NumChildren().
### func (\*Scope) Contains 1.5
```
func (s *Scope) Contains(pos token.Pos) bool
```
Contains reports whether pos is within the scope's extent. The result is guaranteed to be valid only if the type-checked AST has complete position information.
### func (\*Scope) End 1.5
```
func (s *Scope) End() token.Pos
```
### func (\*Scope) Innermost 1.5
```
func (s *Scope) Innermost(pos token.Pos) *Scope
```
Innermost returns the innermost (child) scope containing pos. If pos is not within any scope, the result is nil. The result is also nil for the Universe scope. The result is guaranteed to be valid only if the type-checked AST has complete position information.
### func (\*Scope) Insert 1.5
```
func (s *Scope) Insert(obj Object) Object
```
Insert attempts to insert an object obj into scope s. If s already contains an alternative object alt with the same name, Insert leaves s unchanged and returns alt. Otherwise it inserts obj, sets the object's parent scope if not already set, and returns nil.
### func (\*Scope) Len 1.5
```
func (s *Scope) Len() int
```
Len returns the number of scope elements.
### func (\*Scope) Lookup 1.5
```
func (s *Scope) Lookup(name string) Object
```
Lookup returns the object in scope s with the given name if such an object exists; otherwise the result is nil.
### func (\*Scope) LookupParent 1.5
```
func (s *Scope) LookupParent(name string, pos token.Pos) (*Scope, Object)
```
LookupParent follows the parent chain of scopes starting with s until it finds a scope where Lookup(name) returns a non-nil object, and then returns that scope and object. If a valid position pos is provided, only objects that were declared at or before pos are considered. If no such scope and object exists, the result is (nil, nil).
Note that obj.Parent() may be different from the returned scope if the object was inserted into the scope and already had a parent at that time (see Insert). This can only happen for dot-imported objects whose scope is the scope of the package that exported them.
### func (\*Scope) Names 1.5
```
func (s *Scope) Names() []string
```
Names returns the scope's element names in sorted order.
### func (\*Scope) NumChildren 1.5
```
func (s *Scope) NumChildren() int
```
NumChildren returns the number of scopes nested in s.
### func (\*Scope) Parent 1.5
```
func (s *Scope) Parent() *Scope
```
Parent returns the scope's containing (parent) scope.
### func (\*Scope) Pos 1.5
```
func (s *Scope) Pos() token.Pos
```
Pos and End describe the scope's source code extent [pos, end). The results are guaranteed to be valid only if the type-checked AST has complete position information. The extent is undefined for Universe and package scopes.
### func (\*Scope) String 1.5
```
func (s *Scope) String() string
```
String returns a string representation of the scope, for debugging.
### func (\*Scope) WriteTo 1.5
```
func (s *Scope) WriteTo(w io.Writer, n int, recurse bool)
```
WriteTo writes a string representation of the scope to w, with the scope elements sorted by name. The level of indentation is controlled by n >= 0, with n == 0 for no indentation. If recurse is set, it also writes nested (children) scopes.
type Selection 1.5
------------------
A Selection describes a selector expression x.f. For the declarations:
```
type T struct{ x int; E }
type E struct{}
func (e E) m() {}
var p *T
```
the following relations exist:
```
Selector Kind Recv Obj Type Index Indirect
p.x FieldVal T x int {0} true
p.m MethodVal *T m func() {1, 0} true
T.m MethodExpr T m func(T) {1, 0} false
```
```
type Selection struct {
// contains filtered or unexported fields
}
```
### func (\*Selection) Index 1.5
```
func (s *Selection) Index() []int
```
Index describes the path from x to f in x.f. The last index entry is the field or method index of the type declaring f; either:
1. the list of declared methods of a named type; or
2. the list of methods of an interface type; or
3. the list of fields of a struct type.
The earlier index entries are the indices of the embedded fields implicitly traversed to get from (the type of) x to f, starting at embedding depth 0.
### func (\*Selection) Indirect 1.5
```
func (s *Selection) Indirect() bool
```
Indirect reports whether any pointer indirection was required to get from x to f in x.f.
### func (\*Selection) Kind 1.5
```
func (s *Selection) Kind() SelectionKind
```
Kind returns the selection kind.
### func (\*Selection) Obj 1.5
```
func (s *Selection) Obj() Object
```
Obj returns the object denoted by x.f; a \*Var for a field selection, and a \*Func in all other cases.
### func (\*Selection) Recv 1.5
```
func (s *Selection) Recv() Type
```
Recv returns the type of x in x.f.
### func (\*Selection) String 1.5
```
func (s *Selection) String() string
```
### func (\*Selection) Type 1.5
```
func (s *Selection) Type() Type
```
Type returns the type of x.f, which may be different from the type of f. See Selection for more information.
type SelectionKind 1.5
----------------------
SelectionKind describes the kind of a selector expression x.f (excluding qualified identifiers).
```
type SelectionKind int
```
```
const (
FieldVal SelectionKind = iota // x.f is a struct field selector
MethodVal // x.f is a method selector
MethodExpr // x.f is a method expression
)
```
type Signature 1.5
------------------
A Signature represents a (non-builtin) function or method type. The receiver is ignored when comparing signatures for identity.
```
type Signature struct {
// contains filtered or unexported fields
}
```
### func NewSignature 1.5
```
func NewSignature(recv *Var, params, results *Tuple, variadic bool) *Signature
```
NewSignature returns a new function type for the given receiver, parameters, and results, either of which may be nil. If variadic is set, the function is variadic, it must have at least one parameter, and the last parameter must be of unnamed slice type.
Deprecated: Use NewSignatureType instead which allows for type parameters.
### func NewSignatureType 1.18
```
func NewSignatureType(recv *Var, recvTypeParams, typeParams []*TypeParam, params, results *Tuple, variadic bool) *Signature
```
NewSignatureType creates a new function type for the given receiver, receiver type parameters, type parameters, parameters, and results. If variadic is set, params must hold at least one parameter and the last parameter's core type must be of unnamed slice or bytestring type. If recv is non-nil, typeParams must be empty. If recvTypeParams is non-empty, recv must be non-nil.
### func (\*Signature) Params 1.5
```
func (s *Signature) Params() *Tuple
```
Params returns the parameters of signature s, or nil.
### func (\*Signature) Recv 1.5
```
func (s *Signature) Recv() *Var
```
Recv returns the receiver of signature s (if a method), or nil if a function. It is ignored when comparing signatures for identity.
For an abstract method, Recv returns the enclosing interface either as a \*Named or an \*Interface. Due to embedding, an interface may contain methods whose receiver type is a different interface.
### func (\*Signature) RecvTypeParams 1.18
```
func (s *Signature) RecvTypeParams() *TypeParamList
```
RecvTypeParams returns the receiver type parameters of signature s, or nil.
### func (\*Signature) Results 1.5
```
func (s *Signature) Results() *Tuple
```
Results returns the results of signature s, or nil.
### func (\*Signature) String 1.5
```
func (t *Signature) String() string
```
### func (\*Signature) TypeParams 1.18
```
func (s *Signature) TypeParams() *TypeParamList
```
TypeParams returns the type parameters of signature s, or nil.
### func (\*Signature) Underlying 1.5
```
func (t *Signature) Underlying() Type
```
### func (\*Signature) Variadic 1.5
```
func (s *Signature) Variadic() bool
```
Variadic reports whether the signature s is variadic.
type Sizes 1.5
--------------
Sizes defines the sizing functions for package unsafe.
```
type Sizes interface {
// Alignof returns the alignment of a variable of type T.
// Alignof must implement the alignment guarantees required by the spec.
Alignof(T Type) int64
// Offsetsof returns the offsets of the given struct fields, in bytes.
// Offsetsof must implement the offset guarantees required by the spec.
Offsetsof(fields []*Var) []int64
// Sizeof returns the size of a variable of type T.
// Sizeof must implement the size guarantees required by the spec.
Sizeof(T Type) int64
}
```
### func SizesFor 1.9
```
func SizesFor(compiler, arch string) Sizes
```
SizesFor returns the Sizes used by a compiler for an architecture. The result is nil if a compiler/architecture pair is not known.
Supported architectures for compiler "gc": "386", "amd64", "amd64p32", "arm", "arm64", "loong64", "mips", "mipsle", "mips64", "mips64le", "ppc64", "ppc64le", "riscv64", "s390x", "sparc64", "wasm".
type Slice 1.5
--------------
A Slice represents a slice type.
```
type Slice struct {
// contains filtered or unexported fields
}
```
### func NewSlice 1.5
```
func NewSlice(elem Type) *Slice
```
NewSlice returns a new slice type for the given element type.
### func (\*Slice) Elem 1.5
```
func (s *Slice) Elem() Type
```
Elem returns the element type of slice s.
### func (\*Slice) String 1.5
```
func (t *Slice) String() string
```
### func (\*Slice) Underlying 1.5
```
func (t *Slice) Underlying() Type
```
type StdSizes 1.5
-----------------
StdSizes is a convenience type for creating commonly used Sizes. It makes the following simplifying assumptions:
* The size of explicitly sized basic types (int16, etc.) is the specified size.
* The size of strings and interfaces is 2\*WordSize.
* The size of slices is 3\*WordSize.
* The size of an array of n elements corresponds to the size of a struct of n consecutive fields of the array's element type.
* The size of a struct is the offset of the last field plus that field's size. As with all element types, if the struct is used in an array its size must first be aligned to a multiple of the struct's alignment.
* All other types have size WordSize.
* Arrays and structs are aligned per spec definition; all other types are naturally aligned with a maximum alignment MaxAlign.
\*StdSizes implements Sizes.
```
type StdSizes struct {
WordSize int64 // word size in bytes - must be >= 4 (32bits)
MaxAlign int64 // maximum alignment in bytes - must be >= 1
}
```
### func (\*StdSizes) Alignof 1.5
```
func (s *StdSizes) Alignof(T Type) int64
```
### func (\*StdSizes) Offsetsof 1.5
```
func (s *StdSizes) Offsetsof(fields []*Var) []int64
```
### func (\*StdSizes) Sizeof 1.5
```
func (s *StdSizes) Sizeof(T Type) int64
```
type Struct 1.5
---------------
A Struct represents a struct type.
```
type Struct struct {
// contains filtered or unexported fields
}
```
### func NewStruct 1.5
```
func NewStruct(fields []*Var, tags []string) *Struct
```
NewStruct returns a new struct with the given fields and corresponding field tags. If a field with index i has a tag, tags[i] must be that tag, but len(tags) may be only as long as required to hold the tag with the largest index i. Consequently, if no field has a tag, tags may be nil.
### func (\*Struct) Field 1.5
```
func (s *Struct) Field(i int) *Var
```
Field returns the i'th field for 0 <= i < NumFields().
### func (\*Struct) NumFields 1.5
```
func (s *Struct) NumFields() int
```
NumFields returns the number of fields in the struct (including blank and embedded fields).
### func (\*Struct) String 1.5
```
func (t *Struct) String() string
```
### func (\*Struct) Tag 1.5
```
func (s *Struct) Tag(i int) string
```
Tag returns the i'th field tag for 0 <= i < NumFields().
### func (\*Struct) Underlying 1.5
```
func (t *Struct) Underlying() Type
```
type Term 1.18
--------------
A Term represents a term in a Union.
```
type Term term
```
### func NewTerm 1.18
```
func NewTerm(tilde bool, typ Type) *Term
```
NewTerm returns a new union term.
### func (\*Term) String 1.18
```
func (t *Term) String() string
```
### func (\*Term) Tilde 1.18
```
func (t *Term) Tilde() bool
```
### func (\*Term) Type 1.18
```
func (t *Term) Type() Type
```
type Tuple 1.5
--------------
A Tuple represents an ordered list of variables; a nil \*Tuple is a valid (empty) tuple. Tuples are used as components of signatures and to represent the type of multiple assignments; they are not first class types of Go.
```
type Tuple struct {
// contains filtered or unexported fields
}
```
### func NewTuple 1.5
```
func NewTuple(x ...*Var) *Tuple
```
NewTuple returns a new tuple for the given variables.
### func (\*Tuple) At 1.5
```
func (t *Tuple) At(i int) *Var
```
At returns the i'th variable of tuple t.
### func (\*Tuple) Len 1.5
```
func (t *Tuple) Len() int
```
Len returns the number variables of tuple t.
### func (\*Tuple) String 1.5
```
func (t *Tuple) String() string
```
### func (\*Tuple) Underlying 1.5
```
func (t *Tuple) Underlying() Type
```
type Type 1.5
-------------
A Type represents a type of Go. All types implement the Type interface.
```
type Type interface {
// Underlying returns the underlying type of a type.
Underlying() Type
// String returns a string representation of a type.
String() string
}
```
### func Default 1.8
```
func Default(t Type) Type
```
Default returns the default "typed" type for an "untyped" type; it returns the incoming type for all other types. The default type for untyped nil is untyped nil.
### func Instantiate 1.18
```
func Instantiate(ctxt *Context, orig Type, targs []Type, validate bool) (Type, error)
```
Instantiate instantiates the type orig with the given type arguments targs. orig must be a \*Named or a \*Signature type. If there is no error, the resulting Type is an instantiated type of the same kind (either a \*Named or a \*Signature). Methods attached to a \*Named type are also instantiated, and associated with a new \*Func that has the same position as the original method, but nil function scope.
If ctxt is non-nil, it may be used to de-duplicate the instance against previous instances with the same identity. As a special case, generic \*Signature origin types are only considered identical if they are pointer equivalent, so that instantiating distinct (but possibly identical) signatures will yield different instances. The use of a shared context does not guarantee that identical instances are deduplicated in all cases.
If validate is set, Instantiate verifies that the number of type arguments and parameters match, and that the type arguments satisfy their corresponding type constraints. If verification fails, the resulting error may wrap an \*ArgumentError indicating which type argument did not satisfy its corresponding type parameter constraint, and why.
If validate is not set, Instantiate does not verify the type argument count or whether the type arguments satisfy their constraints. Instantiate is guaranteed to not return an error, but may panic. Specifically, for \*Signature types, Instantiate will panic immediately if the type argument count is incorrect; for \*Named types, a panic may occur later inside the \*Named API.
type TypeAndValue 1.5
---------------------
TypeAndValue reports the type and value (for constants) of the corresponding expression.
```
type TypeAndValue struct {
Type Type
Value constant.Value
// contains filtered or unexported fields
}
```
### func Eval 1.5
```
func Eval(fset *token.FileSet, pkg *Package, pos token.Pos, expr string) (_ TypeAndValue, err error)
```
Eval returns the type and, if constant, the value for the expression expr, evaluated at position pos of package pkg, which must have been derived from type-checking an AST with complete position information relative to the provided file set.
The meaning of the parameters fset, pkg, and pos is the same as in CheckExpr. An error is returned if expr cannot be parsed successfully, or the resulting expr AST cannot be type-checked.
### func (TypeAndValue) Addressable 1.5
```
func (tv TypeAndValue) Addressable() bool
```
Addressable reports whether the corresponding expression is addressable (<https://golang.org/ref/spec#Address_operators>).
### func (TypeAndValue) Assignable 1.5
```
func (tv TypeAndValue) Assignable() bool
```
Assignable reports whether the corresponding expression is assignable to (provided a value of the right type).
### func (TypeAndValue) HasOk 1.5
```
func (tv TypeAndValue) HasOk() bool
```
HasOk reports whether the corresponding expression may be used on the rhs of a comma-ok assignment.
### func (TypeAndValue) IsBuiltin 1.5
```
func (tv TypeAndValue) IsBuiltin() bool
```
IsBuiltin reports whether the corresponding expression denotes a (possibly parenthesized) built-in function.
### func (TypeAndValue) IsNil 1.5
```
func (tv TypeAndValue) IsNil() bool
```
IsNil reports whether the corresponding expression denotes the predeclared value nil.
### func (TypeAndValue) IsType 1.5
```
func (tv TypeAndValue) IsType() bool
```
IsType reports whether the corresponding expression specifies a type.
### func (TypeAndValue) IsValue 1.5
```
func (tv TypeAndValue) IsValue() bool
```
IsValue reports whether the corresponding expression is a value. Builtins are not considered values. Constant values have a non- nil Value.
### func (TypeAndValue) IsVoid 1.5
```
func (tv TypeAndValue) IsVoid() bool
```
IsVoid reports whether the corresponding expression is a function call without results.
type TypeList 1.18
------------------
TypeList holds a list of types.
```
type TypeList struct {
// contains filtered or unexported fields
}
```
### func (\*TypeList) At 1.18
```
func (l *TypeList) At(i int) Type
```
At returns the i'th type in the list.
### func (\*TypeList) Len 1.18
```
func (l *TypeList) Len() int
```
Len returns the number of types in the list. It is safe to call on a nil receiver.
type TypeName 1.5
-----------------
A TypeName represents a name for a (defined or alias) type.
```
type TypeName struct {
// contains filtered or unexported fields
}
```
### func NewTypeName 1.5
```
func NewTypeName(pos token.Pos, pkg *Package, name string, typ Type) *TypeName
```
NewTypeName returns a new type name denoting the given typ. The remaining arguments set the attributes found with all Objects.
The typ argument may be a defined (Named) type or an alias type. It may also be nil such that the returned TypeName can be used as argument for NewNamed, which will set the TypeName's type as a side- effect.
### func (\*TypeName) Exported 1.5
```
func (obj *TypeName) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*TypeName) Id 1.5
```
func (obj *TypeName) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*TypeName) IsAlias 1.9
```
func (obj *TypeName) IsAlias() bool
```
IsAlias reports whether obj is an alias name for a type.
### func (\*TypeName) Name 1.5
```
func (obj *TypeName) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*TypeName) Parent 1.5
```
func (obj *TypeName) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*TypeName) Pkg 1.5
```
func (obj *TypeName) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*TypeName) Pos 1.5
```
func (obj *TypeName) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*TypeName) String 1.5
```
func (obj *TypeName) String() string
```
### func (\*TypeName) Type 1.5
```
func (obj *TypeName) Type() Type
```
Type returns the object's type.
type TypeParam 1.18
-------------------
A TypeParam represents a type parameter type.
```
type TypeParam struct {
// contains filtered or unexported fields
}
```
### func NewTypeParam 1.18
```
func NewTypeParam(obj *TypeName, constraint Type) *TypeParam
```
NewTypeParam returns a new TypeParam. Type parameters may be set on a Named or Signature type by calling SetTypeParams. Setting a type parameter on more than one type will result in a panic.
The constraint argument can be nil, and set later via SetConstraint. If the constraint is non-nil, it must be fully defined.
### func (\*TypeParam) Constraint 1.18
```
func (t *TypeParam) Constraint() Type
```
Constraint returns the type constraint specified for t.
### func (\*TypeParam) Index 1.18
```
func (t *TypeParam) Index() int
```
Index returns the index of the type param within its param list, or -1 if the type parameter has not yet been bound to a type.
### func (\*TypeParam) Obj 1.18
```
func (t *TypeParam) Obj() *TypeName
```
Obj returns the type name for t.
### func (\*TypeParam) SetConstraint 1.18
```
func (t *TypeParam) SetConstraint(bound Type)
```
SetConstraint sets the type constraint for t.
It must be called by users of NewTypeParam after the bound's underlying is fully defined, and before using the type parameter in any way other than to form other types. Once SetConstraint returns the receiver, t is safe for concurrent use.
### func (\*TypeParam) String 1.18
```
func (t *TypeParam) String() string
```
### func (\*TypeParam) Underlying 1.18
```
func (t *TypeParam) Underlying() Type
```
type TypeParamList 1.18
-----------------------
TypeParamList holds a list of type parameters.
```
type TypeParamList struct {
// contains filtered or unexported fields
}
```
### func (\*TypeParamList) At 1.18
```
func (l *TypeParamList) At(i int) *TypeParam
```
At returns the i'th type parameter in the list.
### func (\*TypeParamList) Len 1.18
```
func (l *TypeParamList) Len() int
```
Len returns the number of type parameters in the list. It is safe to call on a nil receiver.
type Union 1.18
---------------
A Union represents a union of terms embedded in an interface.
```
type Union struct {
// contains filtered or unexported fields
}
```
### func NewUnion 1.18
```
func NewUnion(terms []*Term) *Union
```
NewUnion returns a new Union type with the given terms. It is an error to create an empty union; they are syntactically not possible.
### func (\*Union) Len 1.18
```
func (u *Union) Len() int
```
### func (\*Union) String 1.18
```
func (u *Union) String() string
```
### func (\*Union) Term 1.18
```
func (u *Union) Term(i int) *Term
```
### func (\*Union) Underlying 1.18
```
func (u *Union) Underlying() Type
```
type Var 1.5
------------
A Variable represents a declared variable (including function parameters and results, and struct fields).
```
type Var struct {
// contains filtered or unexported fields
}
```
### func NewField 1.5
```
func NewField(pos token.Pos, pkg *Package, name string, typ Type, embedded bool) *Var
```
NewField returns a new variable representing a struct field. For embedded fields, the name is the unqualified type name under which the field is accessible.
### func NewParam 1.5
```
func NewParam(pos token.Pos, pkg *Package, name string, typ Type) *Var
```
NewParam returns a new variable representing a function parameter.
### func NewVar 1.5
```
func NewVar(pos token.Pos, pkg *Package, name string, typ Type) *Var
```
NewVar returns a new variable. The arguments set the attributes found with all Objects.
### func (\*Var) Anonymous 1.5
```
func (obj *Var) Anonymous() bool
```
Anonymous reports whether the variable is an embedded field. Same as Embedded; only present for backward-compatibility.
### func (\*Var) Embedded 1.11
```
func (obj *Var) Embedded() bool
```
Embedded reports whether the variable is an embedded field.
### func (\*Var) Exported 1.5
```
func (obj *Var) Exported() bool
```
Exported reports whether the object is exported (starts with a capital letter). It doesn't take into account whether the object is in a local (function) scope or not.
### func (\*Var) Id 1.5
```
func (obj *Var) Id() string
```
Id is a wrapper for Id(obj.Pkg(), obj.Name()).
### func (\*Var) IsField 1.5
```
func (obj *Var) IsField() bool
```
IsField reports whether the variable is a struct field.
### func (\*Var) Name 1.5
```
func (obj *Var) Name() string
```
Name returns the object's (package-local, unqualified) name.
### func (\*Var) Origin 1.19
```
func (obj *Var) Origin() *Var
```
Origin returns the canonical Var for its receiver, i.e. the Var object recorded in Info.Defs.
For synthetic Vars created during instantiation (such as struct fields or function parameters that depend on type arguments), this will be the corresponding Var on the generic (uninstantiated) type. For all other Vars Origin returns the receiver.
### func (\*Var) Parent 1.5
```
func (obj *Var) Parent() *Scope
```
Parent returns the scope in which the object is declared. The result is nil for methods and struct fields.
### func (\*Var) Pkg 1.5
```
func (obj *Var) Pkg() *Package
```
Pkg returns the package to which the object belongs. The result is nil for labels and objects in the Universe scope.
### func (\*Var) Pos 1.5
```
func (obj *Var) Pos() token.Pos
```
Pos returns the declaration position of the object's identifier.
### func (\*Var) String 1.5
```
func (obj *Var) String() string
```
### func (\*Var) Type 1.5
```
func (obj *Var) Type() Type
```
Type returns the object's type.
| programming_docs |
go Package importer Package importer
=================
* `import "go/importer"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package importer provides access to export data importers.
Index
-----
* [func Default() types.Importer](#Default)
* [func For(compiler string, lookup Lookup) types.Importer](#For)
* [func ForCompiler(fset \*token.FileSet, compiler string, lookup Lookup) types.Importer](#ForCompiler)
* [type Lookup](#Lookup)
### Package files
importer.go
func Default 1.5
----------------
```
func Default() types.Importer
```
Default returns an Importer for the compiler that built the running binary. If available, the result implements types.ImporterFrom.
func For 1.5
------------
```
func For(compiler string, lookup Lookup) types.Importer
```
For calls ForCompiler with a new FileSet.
Deprecated: Use ForCompiler, which populates a FileSet with the positions of objects created by the importer.
func ForCompiler 1.12
---------------------
```
func ForCompiler(fset *token.FileSet, compiler string, lookup Lookup) types.Importer
```
ForCompiler returns an Importer for importing from installed packages for the compilers "gc" and "gccgo", or for importing directly from the source if the compiler argument is "source". In this latter case, importing may fail under circumstances where the exported API is not entirely defined in pure Go source code (if the package API depends on cgo-defined entities, the type checker won't have access to those).
The lookup function is called each time the resulting importer needs to resolve an import path. In this mode the importer can only be invoked with canonical import paths (not relative or absolute ones); it is assumed that the translation to canonical import paths is being done by the client of the importer.
A lookup function must be provided for correct module-aware operation. Deprecated: If lookup is nil, for backwards-compatibility, the importer will attempt to resolve imports in the $GOPATH workspace.
type Lookup 1.5
---------------
A Lookup function returns a reader to access package data for a given import path, or an error if no matching package is found.
```
type Lookup func(path string) (io.ReadCloser, error)
```
go Package printer Package printer
================
* `import "go/printer"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package printer implements printing of AST nodes.
Index
-----
* [func Fprint(output io.Writer, fset \*token.FileSet, node any) error](#Fprint)
* [type CommentedNode](#CommentedNode)
* [type Config](#Config)
* [func (cfg \*Config) Fprint(output io.Writer, fset \*token.FileSet, node any) error](#Config.Fprint)
* [type Mode](#Mode)
### Examples
[Fprint](#example_Fprint) ### Package files
comment.go gobuild.go nodes.go printer.go
func Fprint
-----------
```
func Fprint(output io.Writer, fset *token.FileSet, node any) error
```
Fprint "pretty-prints" an AST node to output. It calls Config.Fprint with default settings. Note that gofmt uses tabs for indentation but spaces for alignment; use format.Node (package go/format) for output that matches gofmt.
#### Example
Code:
```
package printer_test
import (
"bytes"
"fmt"
"go/ast"
"go/parser"
"go/printer"
"go/token"
"strings"
)
func parseFunc(filename, functionname string) (fun *ast.FuncDecl, fset *token.FileSet) {
fset = token.NewFileSet()
if file, err := parser.ParseFile(fset, filename, nil, 0); err == nil {
for _, d := range file.Decls {
if f, ok := d.(*ast.FuncDecl); ok && f.Name.Name == functionname {
fun = f
return
}
}
}
panic("function not found")
}
func printSelf() {
// Parse source file and extract the AST without comments for
// this function, with position information referring to the
// file set fset.
funcAST, fset := parseFunc("example_test.go", "printSelf")
// Print the function body into buffer buf.
// The file set is provided to the printer so that it knows
// about the original source formatting and can add additional
// line breaks where they were present in the source.
var buf bytes.Buffer
printer.Fprint(&buf, fset, funcAST.Body)
// Remove braces {} enclosing the function body, unindent,
// and trim leading and trailing white space.
s := buf.String()
s = s[1 : len(s)-1]
s = strings.TrimSpace(strings.ReplaceAll(s, "\n\t", "\n"))
// Print the cleaned-up body text to stdout.
fmt.Println(s)
}
func ExampleFprint() {
printSelf()
// Output:
// funcAST, fset := parseFunc("example_test.go", "printSelf")
//
// var buf bytes.Buffer
// printer.Fprint(&buf, fset, funcAST.Body)
//
// s := buf.String()
// s = s[1 : len(s)-1]
// s = strings.TrimSpace(strings.ReplaceAll(s, "\n\t", "\n"))
//
// fmt.Println(s)
}
```
type CommentedNode
------------------
A CommentedNode bundles an AST node and corresponding comments. It may be provided as argument to any of the Fprint functions.
```
type CommentedNode struct {
Node any // *ast.File, or ast.Expr, ast.Decl, ast.Spec, or ast.Stmt
Comments []*ast.CommentGroup
}
```
type Config
-----------
A Config node controls the output of Fprint.
```
type Config struct {
Mode Mode // default: 0
Tabwidth int // default: 8
Indent int // default: 0 (all code is indented at least by this much); added in Go 1.1
}
```
### func (\*Config) Fprint
```
func (cfg *Config) Fprint(output io.Writer, fset *token.FileSet, node any) error
```
Fprint "pretty-prints" an AST node to output for a given configuration cfg. Position information is interpreted relative to the file set fset. The node type must be \*ast.File, \*CommentedNode, []ast.Decl, []ast.Stmt, or assignment-compatible to ast.Expr, ast.Decl, ast.Spec, or ast.Stmt.
type Mode
---------
A Mode value is a set of flags (or 0). They control printing.
```
type Mode uint
```
```
const (
RawFormat Mode = 1 << iota // do not use a tabwriter; if set, UseSpaces is ignored
TabIndent // use tabs for indentation independent of UseSpaces
UseSpaces // use spaces instead of tabs for alignment
SourcePos // emit //line directives to preserve original source positions
)
```
go Package parser Package parser
===============
* `import "go/parser"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package parser implements a parser for Go source files. Input may be provided in a variety of forms (see the various Parse\* functions); the output is an abstract syntax tree (AST) representing the Go source. The parser is invoked through one of the Parse\* functions.
The parser accepts a larger language than is syntactically permitted by the Go spec, for simplicity, and for improved robustness in the presence of syntax errors. For instance, in method declarations, the receiver is treated like an ordinary parameter list and thus may contain multiple entries where the spec permits exactly one. Consequently, the corresponding field in the AST (ast.FuncDecl.Recv) field is not restricted to one entry.
Index
-----
* [func ParseDir(fset \*token.FileSet, path string, filter func(fs.FileInfo) bool, mode Mode) (pkgs map[string]\*ast.Package, first error)](#ParseDir)
* [func ParseExpr(x string) (ast.Expr, error)](#ParseExpr)
* [func ParseExprFrom(fset \*token.FileSet, filename string, src any, mode Mode) (expr ast.Expr, err error)](#ParseExprFrom)
* [func ParseFile(fset \*token.FileSet, filename string, src any, mode Mode) (f \*ast.File, err error)](#ParseFile)
* [type Mode](#Mode)
### Examples
[ParseFile](#example_ParseFile) ### Package files
interface.go parser.go resolver.go
func ParseDir
-------------
```
func ParseDir(fset *token.FileSet, path string, filter func(fs.FileInfo) bool, mode Mode) (pkgs map[string]*ast.Package, first error)
```
ParseDir calls ParseFile for all files with names ending in ".go" in the directory specified by path and returns a map of package name -> package AST with all the packages found.
If filter != nil, only the files with fs.FileInfo entries passing through the filter (and ending in ".go") are considered. The mode bits are passed to ParseFile unchanged. Position information is recorded in fset, which must not be nil.
If the directory couldn't be read, a nil map and the respective error are returned. If a parse error occurred, a non-nil but incomplete map and the first error encountered are returned.
func ParseExpr
--------------
```
func ParseExpr(x string) (ast.Expr, error)
```
ParseExpr is a convenience function for obtaining the AST of an expression x. The position information recorded in the AST is undefined. The filename used in error messages is the empty string.
If syntax errors were found, the result is a partial AST (with ast.Bad\* nodes representing the fragments of erroneous source code). Multiple errors are returned via a scanner.ErrorList which is sorted by source position.
func ParseExprFrom 1.5
----------------------
```
func ParseExprFrom(fset *token.FileSet, filename string, src any, mode Mode) (expr ast.Expr, err error)
```
ParseExprFrom is a convenience function for parsing an expression. The arguments have the same meaning as for ParseFile, but the source must be a valid Go (type or value) expression. Specifically, fset must not be nil.
If the source couldn't be read, the returned AST is nil and the error indicates the specific failure. If the source was read but syntax errors were found, the result is a partial AST (with ast.Bad\* nodes representing the fragments of erroneous source code). Multiple errors are returned via a scanner.ErrorList which is sorted by source position.
func ParseFile
--------------
```
func ParseFile(fset *token.FileSet, filename string, src any, mode Mode) (f *ast.File, err error)
```
ParseFile parses the source code of a single Go source file and returns the corresponding ast.File node. The source code may be provided via the filename of the source file, or via the src parameter.
If src != nil, ParseFile parses the source from src and the filename is only used when recording position information. The type of the argument for the src parameter must be string, []byte, or io.Reader. If src == nil, ParseFile parses the file specified by filename.
The mode parameter controls the amount of source text parsed and other optional parser functionality. If the SkipObjectResolution mode bit is set, the object resolution phase of parsing will be skipped, causing File.Scope, File.Unresolved, and all Ident.Obj fields to be nil.
Position information is recorded in the file set fset, which must not be nil.
If the source couldn't be read, the returned AST is nil and the error indicates the specific failure. If the source was read but syntax errors were found, the result is a partial AST (with ast.Bad\* nodes representing the fragments of erroneous source code). Multiple errors are returned via a scanner.ErrorList which is sorted by source position.
#### Example
Code:
```
fset := token.NewFileSet() // positions are relative to fset
src := `package foo
import (
"fmt"
"time"
)
func bar() {
fmt.Println(time.Now())
}`
// Parse src but stop after processing the imports.
f, err := parser.ParseFile(fset, "", src, parser.ImportsOnly)
if err != nil {
fmt.Println(err)
return
}
// Print the imports from the file's AST.
for _, s := range f.Imports {
fmt.Println(s.Path.Value)
}
```
Output:
```
"fmt"
"time"
```
type Mode
---------
A Mode value is a set of flags (or 0). They control the amount of source code parsed and other optional parser functionality.
```
type Mode uint
```
```
const (
PackageClauseOnly Mode = 1 << iota // stop parsing after package clause
ImportsOnly // stop parsing after import declarations
ParseComments // parse comments and add them to AST
Trace // print a trace of parsed productions
DeclarationErrors // report declaration errors
SpuriousErrors // same as AllErrors, for backward-compatibility
SkipObjectResolution // don't resolve identifiers to objects - see ParseFile
AllErrors = SpuriousErrors // report all errors (not just the first 10 on different lines)
)
```
go Package constant Package constant
=================
* `import "go/constant"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package constant implements Values representing untyped Go constants and their corresponding operations.
A special Unknown value may be used when a value is unknown due to an error. Operations on unknown values produce unknown values unless specified otherwise.
#### Example (ComplexNumbers)
Code:
```
// Create the complex number 2.3 + 5i.
ar := constant.MakeFloat64(2.3)
ai := constant.MakeImag(constant.MakeInt64(5))
a := constant.BinaryOp(ar, token.ADD, ai)
// Compute (2.3 + 5i) * 11.
b := constant.MakeUint64(11)
c := constant.BinaryOp(a, token.MUL, b)
// Convert c into a complex128.
Ar, exact := constant.Float64Val(constant.Real(c))
if !exact {
fmt.Printf("Could not represent real part %s exactly as float64\n", constant.Real(c))
}
Ai, exact := constant.Float64Val(constant.Imag(c))
if !exact {
fmt.Printf("Could not represent imaginary part %s as exactly as float64\n", constant.Imag(c))
}
C := complex(Ar, Ai)
fmt.Println("literal", 25.3+55i)
fmt.Println("go/constant", c)
fmt.Println("complex128", C)
```
Output:
```
Could not represent real part 25.3 exactly as float64
literal (25.3+55i)
go/constant (25.3 + 55i)
complex128 (25.299999999999997+55i)
```
Index
-----
* [func BitLen(x Value) int](#BitLen)
* [func BoolVal(x Value) bool](#BoolVal)
* [func Bytes(x Value) []byte](#Bytes)
* [func Compare(x\_ Value, op token.Token, y\_ Value) bool](#Compare)
* [func Float32Val(x Value) (float32, bool)](#Float32Val)
* [func Float64Val(x Value) (float64, bool)](#Float64Val)
* [func Int64Val(x Value) (int64, bool)](#Int64Val)
* [func Sign(x Value) int](#Sign)
* [func StringVal(x Value) string](#StringVal)
* [func Uint64Val(x Value) (uint64, bool)](#Uint64Val)
* [func Val(x Value) any](#Val)
* [type Kind](#Kind)
* [func (i Kind) String() string](#Kind.String)
* [type Value](#Value)
* [func BinaryOp(x\_ Value, op token.Token, y\_ Value) Value](#BinaryOp)
* [func Denom(x Value) Value](#Denom)
* [func Imag(x Value) Value](#Imag)
* [func Make(x any) Value](#Make)
* [func MakeBool(b bool) Value](#MakeBool)
* [func MakeFloat64(x float64) Value](#MakeFloat64)
* [func MakeFromBytes(bytes []byte) Value](#MakeFromBytes)
* [func MakeFromLiteral(lit string, tok token.Token, zero uint) Value](#MakeFromLiteral)
* [func MakeImag(x Value) Value](#MakeImag)
* [func MakeInt64(x int64) Value](#MakeInt64)
* [func MakeString(s string) Value](#MakeString)
* [func MakeUint64(x uint64) Value](#MakeUint64)
* [func MakeUnknown() Value](#MakeUnknown)
* [func Num(x Value) Value](#Num)
* [func Real(x Value) Value](#Real)
* [func Shift(x Value, op token.Token, s uint) Value](#Shift)
* [func ToComplex(x Value) Value](#ToComplex)
* [func ToFloat(x Value) Value](#ToFloat)
* [func ToInt(x Value) Value](#ToInt)
* [func UnaryOp(op token.Token, y Value, prec uint) Value](#UnaryOp)
### Examples
[BinaryOp](#example_BinaryOp) [Compare](#example_Compare) [Sign](#example_Sign) [UnaryOp](#example_UnaryOp) [Val](#example_Val) [Package (ComplexNumbers)](#example__complexNumbers) ### Package files
kind\_string.go value.go
func BitLen 1.5
---------------
```
func BitLen(x Value) int
```
BitLen returns the number of bits required to represent the absolute value x in binary representation; x must be an Int or an Unknown. If x is Unknown, the result is 0.
func BoolVal 1.5
----------------
```
func BoolVal(x Value) bool
```
BoolVal returns the Go boolean value of x, which must be a Bool or an Unknown. If x is Unknown, the result is false.
func Bytes 1.5
--------------
```
func Bytes(x Value) []byte
```
Bytes returns the bytes for the absolute value of x in little- endian binary representation; x must be an Int.
func Compare 1.5
----------------
```
func Compare(x_ Value, op token.Token, y_ Value) bool
```
Compare returns the result of the comparison x op y. The comparison must be defined for the operands. If one of the operands is Unknown, the result is false.
#### Example
Code:
```
vs := []constant.Value{
constant.MakeString("Z"),
constant.MakeString("bacon"),
constant.MakeString("go"),
constant.MakeString("Frame"),
constant.MakeString("defer"),
constant.MakeFromLiteral(`"a"`, token.STRING, 0),
}
sort.Slice(vs, func(i, j int) bool {
// Equivalent to vs[i] <= vs[j].
return constant.Compare(vs[i], token.LEQ, vs[j])
})
for _, v := range vs {
fmt.Println(constant.StringVal(v))
}
```
Output:
```
Frame
Z
a
bacon
defer
go
```
func Float32Val 1.5
-------------------
```
func Float32Val(x Value) (float32, bool)
```
Float32Val is like Float64Val but for float32 instead of float64.
func Float64Val 1.5
-------------------
```
func Float64Val(x Value) (float64, bool)
```
Float64Val returns the nearest Go float64 value of x and whether the result is exact; x must be numeric or an Unknown, but not Complex. For values too small (too close to 0) to represent as float64, Float64Val silently underflows to 0. The result sign always matches the sign of x, even for 0. If x is Unknown, the result is (0, false).
func Int64Val 1.5
-----------------
```
func Int64Val(x Value) (int64, bool)
```
Int64Val returns the Go int64 value of x and whether the result is exact; x must be an Int or an Unknown. If the result is not exact, its value is undefined. If x is Unknown, the result is (0, false).
func Sign 1.5
-------------
```
func Sign(x Value) int
```
Sign returns -1, 0, or 1 depending on whether x < 0, x == 0, or x > 0; x must be numeric or Unknown. For complex values x, the sign is 0 if x == 0, otherwise it is != 0. If x is Unknown, the result is 1.
#### Example
Code:
```
zero := constant.MakeInt64(0)
one := constant.MakeInt64(1)
negOne := constant.MakeInt64(-1)
mkComplex := func(a, b constant.Value) constant.Value {
b = constant.MakeImag(b)
return constant.BinaryOp(a, token.ADD, b)
}
vs := []constant.Value{
negOne,
mkComplex(zero, negOne),
mkComplex(one, negOne),
mkComplex(negOne, one),
mkComplex(negOne, negOne),
zero,
mkComplex(zero, zero),
one,
mkComplex(zero, one),
mkComplex(one, one),
}
for _, v := range vs {
fmt.Printf("% d %s\n", constant.Sign(v), v)
}
```
Output:
```
-1 -1
-1 (0 + -1i)
-1 (1 + -1i)
-1 (-1 + 1i)
-1 (-1 + -1i)
0 0
0 (0 + 0i)
1 1
1 (0 + 1i)
1 (1 + 1i)
```
func StringVal 1.5
------------------
```
func StringVal(x Value) string
```
StringVal returns the Go string value of x, which must be a String or an Unknown. If x is Unknown, the result is "".
func Uint64Val 1.5
------------------
```
func Uint64Val(x Value) (uint64, bool)
```
Uint64Val returns the Go uint64 value of x and whether the result is exact; x must be an Int or an Unknown. If the result is not exact, its value is undefined. If x is Unknown, the result is (0, false).
func Val 1.13
-------------
```
func Val(x Value) any
```
Val returns the underlying value for a given constant. Since it returns an interface, it is up to the caller to type assert the result to the expected type. The possible dynamic return types are:
```
x Kind type of result
-----------------------------------------
Bool bool
String string
Int int64 or *big.Int
Float *big.Float or *big.Rat
everything else nil
```
#### Example
Code:
```
maxint := constant.MakeInt64(math.MaxInt64)
fmt.Printf("%v\n", constant.Val(maxint))
e := constant.MakeFloat64(math.E)
fmt.Printf("%v\n", constant.Val(e))
b := constant.MakeBool(true)
fmt.Printf("%v\n", constant.Val(b))
b = constant.Make(false)
fmt.Printf("%v\n", constant.Val(b))
```
Output:
```
9223372036854775807
6121026514868073/2251799813685248
true
false
```
type Kind 1.5
-------------
Kind specifies the kind of value represented by a Value.
```
type Kind int
```
```
const (
// unknown values
Unknown Kind = iota
// non-numeric values
Bool
String
// numeric values
Int
Float
Complex
)
```
### func (Kind) String 1.18
```
func (i Kind) String() string
```
type Value 1.5
--------------
A Value represents the value of a Go constant.
```
type Value interface {
// Kind returns the value kind.
Kind() Kind
// String returns a short, quoted (human-readable) form of the value.
// For numeric values, the result may be an approximation;
// for String values the result may be a shortened string.
// Use ExactString for a string representing a value exactly.
String() string
// ExactString returns an exact, quoted (human-readable) form of the value.
// If the Value is of Kind String, use StringVal to obtain the unquoted string.
ExactString() string
// contains filtered or unexported methods
}
```
### func BinaryOp 1.5
```
func BinaryOp(x_ Value, op token.Token, y_ Value) Value
```
BinaryOp returns the result of the binary expression x op y. The operation must be defined for the operands. If one of the operands is Unknown, the result is Unknown. BinaryOp doesn't handle comparisons or shifts; use Compare or Shift instead.
To force integer division of Int operands, use op == token.QUO\_ASSIGN instead of token.QUO; the result is guaranteed to be Int in this case. Division by zero leads to a run-time panic.
#### Example
Code:
```
// 11 / 0.5
a := constant.MakeUint64(11)
b := constant.MakeFloat64(0.5)
c := constant.BinaryOp(a, token.QUO, b)
fmt.Println(c)
```
Output:
```
22
```
### func Denom 1.5
```
func Denom(x Value) Value
```
Denom returns the denominator of x; x must be Int, Float, or Unknown. If x is Unknown, or if it is too large or small to represent as a fraction, the result is Unknown. Otherwise the result is an Int >= 1.
### func Imag 1.5
```
func Imag(x Value) Value
```
Imag returns the imaginary part of x, which must be a numeric or unknown value. If x is Unknown, the result is Unknown.
### func Make 1.13
```
func Make(x any) Value
```
Make returns the Value for x.
```
type of x result Kind
----------------------------
bool Bool
string String
int64 Int
*big.Int Int
*big.Float Float
*big.Rat Float
anything else Unknown
```
### func MakeBool 1.5
```
func MakeBool(b bool) Value
```
MakeBool returns the Bool value for b.
### func MakeFloat64 1.5
```
func MakeFloat64(x float64) Value
```
MakeFloat64 returns the Float value for x. If x is -0.0, the result is 0.0. If x is not finite, the result is an Unknown.
### func MakeFromBytes 1.5
```
func MakeFromBytes(bytes []byte) Value
```
MakeFromBytes returns the Int value given the bytes of its little-endian binary representation. An empty byte slice argument represents 0.
### func MakeFromLiteral 1.5
```
func MakeFromLiteral(lit string, tok token.Token, zero uint) Value
```
MakeFromLiteral returns the corresponding integer, floating-point, imaginary, character, or string value for a Go literal string. The tok value must be one of token.INT, token.FLOAT, token.IMAG, token.CHAR, or token.STRING. The final argument must be zero. If the literal string syntax is invalid, the result is an Unknown.
### func MakeImag 1.5
```
func MakeImag(x Value) Value
```
MakeImag returns the Complex value x\*i; x must be Int, Float, or Unknown. If x is Unknown, the result is Unknown.
### func MakeInt64 1.5
```
func MakeInt64(x int64) Value
```
MakeInt64 returns the Int value for x.
### func MakeString 1.5
```
func MakeString(s string) Value
```
MakeString returns the String value for s.
### func MakeUint64 1.5
```
func MakeUint64(x uint64) Value
```
MakeUint64 returns the Int value for x.
### func MakeUnknown 1.5
```
func MakeUnknown() Value
```
MakeUnknown returns the Unknown value.
### func Num 1.5
```
func Num(x Value) Value
```
Num returns the numerator of x; x must be Int, Float, or Unknown. If x is Unknown, or if it is too large or small to represent as a fraction, the result is Unknown. Otherwise the result is an Int with the same sign as x.
### func Real 1.5
```
func Real(x Value) Value
```
Real returns the real part of x, which must be a numeric or unknown value. If x is Unknown, the result is Unknown.
### func Shift 1.5
```
func Shift(x Value, op token.Token, s uint) Value
```
Shift returns the result of the shift expression x op s with op == token.SHL or token.SHR (<< or >>). x must be an Int or an Unknown. If x is Unknown, the result is x.
### func ToComplex 1.6
```
func ToComplex(x Value) Value
```
ToComplex converts x to a Complex value if x is representable as a Complex. Otherwise it returns an Unknown.
### func ToFloat 1.6
```
func ToFloat(x Value) Value
```
ToFloat converts x to a Float value if x is representable as a Float. Otherwise it returns an Unknown.
### func ToInt 1.6
```
func ToInt(x Value) Value
```
ToInt converts x to an Int value if x is representable as an Int. Otherwise it returns an Unknown.
### func UnaryOp 1.5
```
func UnaryOp(op token.Token, y Value, prec uint) Value
```
UnaryOp returns the result of the unary expression op y. The operation must be defined for the operand. If prec > 0 it specifies the ^ (xor) result size in bits. If y is Unknown, the result is Unknown.
#### Example
Code:
```
vs := []constant.Value{
constant.MakeBool(true),
constant.MakeFloat64(2.7),
constant.MakeUint64(42),
}
for i, v := range vs {
switch v.Kind() {
case constant.Bool:
vs[i] = constant.UnaryOp(token.NOT, v, 0)
case constant.Float:
vs[i] = constant.UnaryOp(token.SUB, v, 0)
case constant.Int:
// Use 16-bit precision.
// This would be equivalent to ^uint16(v).
vs[i] = constant.UnaryOp(token.XOR, v, 16)
}
}
for _, v := range vs {
fmt.Println(v)
}
```
Output:
```
false
-2.7
65493
```
| programming_docs |
go Package format Package format
===============
* `import "go/format"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package format implements standard formatting of Go source.
Note that formatting of Go source code changes over time, so tools relying on consistent formatting should execute a specific version of the gofmt binary instead of using this package. That way, the formatting will be stable, and the tools won't need to be recompiled each time gofmt changes.
For example, pre-submit checks that use this package directly would behave differently depending on what Go version each developer uses, causing the check to be inherently fragile.
Index
-----
* [func Node(dst io.Writer, fset \*token.FileSet, node any) error](#Node)
* [func Source(src []byte) ([]byte, error)](#Source)
### Examples
[Node](#example_Node) ### Package files
format.go internal.go
func Node 1.1
-------------
```
func Node(dst io.Writer, fset *token.FileSet, node any) error
```
Node formats node in canonical gofmt style and writes the result to dst.
The node type must be \*ast.File, \*printer.CommentedNode, []ast.Decl, []ast.Stmt, or assignment-compatible to ast.Expr, ast.Decl, ast.Spec, or ast.Stmt. Node does not modify node. Imports are not sorted for nodes representing partial source files (for instance, if the node is not an \*ast.File or a \*printer.CommentedNode not wrapping an \*ast.File).
The function may return early (before the entire result is written) and return a formatting error, for instance due to an incorrect AST.
#### Example
Code:
```
const expr = "(6+2*3)/4"
// parser.ParseExpr parses the argument and returns the
// corresponding ast.Node.
node, err := parser.ParseExpr(expr)
if err != nil {
log.Fatal(err)
}
// Create a FileSet for node. Since the node does not come
// from a real source file, fset will be empty.
fset := token.NewFileSet()
var buf bytes.Buffer
err = format.Node(&buf, fset, node)
if err != nil {
log.Fatal(err)
}
fmt.Println(buf.String())
```
Output:
```
(6 + 2*3) / 4
```
func Source 1.1
---------------
```
func Source(src []byte) ([]byte, error)
```
Source formats src in canonical gofmt style and returns the result or an (I/O or syntax) error. src is expected to be a syntactically correct Go source file, or a list of Go declarations or statements.
If src is a partial source file, the leading and trailing space of src is applied to the result (such that it has the same leading and trailing space as src), and the result is indented by the same amount as the first line of src containing code. Imports are not sorted for partial source files.
go Package ast Package ast
============
* `import "go/ast"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package ast declares the types used to represent syntax trees for Go packages.
Index
-----
* [func FileExports(src \*File) bool](#FileExports)
* [func FilterDecl(decl Decl, f Filter) bool](#FilterDecl)
* [func FilterFile(src \*File, f Filter) bool](#FilterFile)
* [func FilterPackage(pkg \*Package, f Filter) bool](#FilterPackage)
* [func Fprint(w io.Writer, fset \*token.FileSet, x any, f FieldFilter) error](#Fprint)
* [func Inspect(node Node, f func(Node) bool)](#Inspect)
* [func IsExported(name string) bool](#IsExported)
* [func NotNilFilter(\_ string, v reflect.Value) bool](#NotNilFilter)
* [func PackageExports(pkg \*Package) bool](#PackageExports)
* [func Print(fset \*token.FileSet, x any) error](#Print)
* [func SortImports(fset \*token.FileSet, f \*File)](#SortImports)
* [func Walk(v Visitor, node Node)](#Walk)
* [type ArrayType](#ArrayType)
* [func (x \*ArrayType) End() token.Pos](#ArrayType.End)
* [func (x \*ArrayType) Pos() token.Pos](#ArrayType.Pos)
* [type AssignStmt](#AssignStmt)
* [func (s \*AssignStmt) End() token.Pos](#AssignStmt.End)
* [func (s \*AssignStmt) Pos() token.Pos](#AssignStmt.Pos)
* [type BadDecl](#BadDecl)
* [func (d \*BadDecl) End() token.Pos](#BadDecl.End)
* [func (d \*BadDecl) Pos() token.Pos](#BadDecl.Pos)
* [type BadExpr](#BadExpr)
* [func (x \*BadExpr) End() token.Pos](#BadExpr.End)
* [func (x \*BadExpr) Pos() token.Pos](#BadExpr.Pos)
* [type BadStmt](#BadStmt)
* [func (s \*BadStmt) End() token.Pos](#BadStmt.End)
* [func (s \*BadStmt) Pos() token.Pos](#BadStmt.Pos)
* [type BasicLit](#BasicLit)
* [func (x \*BasicLit) End() token.Pos](#BasicLit.End)
* [func (x \*BasicLit) Pos() token.Pos](#BasicLit.Pos)
* [type BinaryExpr](#BinaryExpr)
* [func (x \*BinaryExpr) End() token.Pos](#BinaryExpr.End)
* [func (x \*BinaryExpr) Pos() token.Pos](#BinaryExpr.Pos)
* [type BlockStmt](#BlockStmt)
* [func (s \*BlockStmt) End() token.Pos](#BlockStmt.End)
* [func (s \*BlockStmt) Pos() token.Pos](#BlockStmt.Pos)
* [type BranchStmt](#BranchStmt)
* [func (s \*BranchStmt) End() token.Pos](#BranchStmt.End)
* [func (s \*BranchStmt) Pos() token.Pos](#BranchStmt.Pos)
* [type CallExpr](#CallExpr)
* [func (x \*CallExpr) End() token.Pos](#CallExpr.End)
* [func (x \*CallExpr) Pos() token.Pos](#CallExpr.Pos)
* [type CaseClause](#CaseClause)
* [func (s \*CaseClause) End() token.Pos](#CaseClause.End)
* [func (s \*CaseClause) Pos() token.Pos](#CaseClause.Pos)
* [type ChanDir](#ChanDir)
* [type ChanType](#ChanType)
* [func (x \*ChanType) End() token.Pos](#ChanType.End)
* [func (x \*ChanType) Pos() token.Pos](#ChanType.Pos)
* [type CommClause](#CommClause)
* [func (s \*CommClause) End() token.Pos](#CommClause.End)
* [func (s \*CommClause) Pos() token.Pos](#CommClause.Pos)
* [type Comment](#Comment)
* [func (c \*Comment) End() token.Pos](#Comment.End)
* [func (c \*Comment) Pos() token.Pos](#Comment.Pos)
* [type CommentGroup](#CommentGroup)
* [func (g \*CommentGroup) End() token.Pos](#CommentGroup.End)
* [func (g \*CommentGroup) Pos() token.Pos](#CommentGroup.Pos)
* [func (g \*CommentGroup) Text() string](#CommentGroup.Text)
* [type CommentMap](#CommentMap)
* [func NewCommentMap(fset \*token.FileSet, node Node, comments []\*CommentGroup) CommentMap](#NewCommentMap)
* [func (cmap CommentMap) Comments() []\*CommentGroup](#CommentMap.Comments)
* [func (cmap CommentMap) Filter(node Node) CommentMap](#CommentMap.Filter)
* [func (cmap CommentMap) String() string](#CommentMap.String)
* [func (cmap CommentMap) Update(old, new Node) Node](#CommentMap.Update)
* [type CompositeLit](#CompositeLit)
* [func (x \*CompositeLit) End() token.Pos](#CompositeLit.End)
* [func (x \*CompositeLit) Pos() token.Pos](#CompositeLit.Pos)
* [type Decl](#Decl)
* [type DeclStmt](#DeclStmt)
* [func (s \*DeclStmt) End() token.Pos](#DeclStmt.End)
* [func (s \*DeclStmt) Pos() token.Pos](#DeclStmt.Pos)
* [type DeferStmt](#DeferStmt)
* [func (s \*DeferStmt) End() token.Pos](#DeferStmt.End)
* [func (s \*DeferStmt) Pos() token.Pos](#DeferStmt.Pos)
* [type Ellipsis](#Ellipsis)
* [func (x \*Ellipsis) End() token.Pos](#Ellipsis.End)
* [func (x \*Ellipsis) Pos() token.Pos](#Ellipsis.Pos)
* [type EmptyStmt](#EmptyStmt)
* [func (s \*EmptyStmt) End() token.Pos](#EmptyStmt.End)
* [func (s \*EmptyStmt) Pos() token.Pos](#EmptyStmt.Pos)
* [type Expr](#Expr)
* [type ExprStmt](#ExprStmt)
* [func (s \*ExprStmt) End() token.Pos](#ExprStmt.End)
* [func (s \*ExprStmt) Pos() token.Pos](#ExprStmt.Pos)
* [type Field](#Field)
* [func (f \*Field) End() token.Pos](#Field.End)
* [func (f \*Field) Pos() token.Pos](#Field.Pos)
* [type FieldFilter](#FieldFilter)
* [type FieldList](#FieldList)
* [func (f \*FieldList) End() token.Pos](#FieldList.End)
* [func (f \*FieldList) NumFields() int](#FieldList.NumFields)
* [func (f \*FieldList) Pos() token.Pos](#FieldList.Pos)
* [type File](#File)
* [func MergePackageFiles(pkg \*Package, mode MergeMode) \*File](#MergePackageFiles)
* [func (f \*File) End() token.Pos](#File.End)
* [func (f \*File) Pos() token.Pos](#File.Pos)
* [type Filter](#Filter)
* [type ForStmt](#ForStmt)
* [func (s \*ForStmt) End() token.Pos](#ForStmt.End)
* [func (s \*ForStmt) Pos() token.Pos](#ForStmt.Pos)
* [type FuncDecl](#FuncDecl)
* [func (d \*FuncDecl) End() token.Pos](#FuncDecl.End)
* [func (d \*FuncDecl) Pos() token.Pos](#FuncDecl.Pos)
* [type FuncLit](#FuncLit)
* [func (x \*FuncLit) End() token.Pos](#FuncLit.End)
* [func (x \*FuncLit) Pos() token.Pos](#FuncLit.Pos)
* [type FuncType](#FuncType)
* [func (x \*FuncType) End() token.Pos](#FuncType.End)
* [func (x \*FuncType) Pos() token.Pos](#FuncType.Pos)
* [type GenDecl](#GenDecl)
* [func (d \*GenDecl) End() token.Pos](#GenDecl.End)
* [func (d \*GenDecl) Pos() token.Pos](#GenDecl.Pos)
* [type GoStmt](#GoStmt)
* [func (s \*GoStmt) End() token.Pos](#GoStmt.End)
* [func (s \*GoStmt) Pos() token.Pos](#GoStmt.Pos)
* [type Ident](#Ident)
* [func NewIdent(name string) \*Ident](#NewIdent)
* [func (x \*Ident) End() token.Pos](#Ident.End)
* [func (id \*Ident) IsExported() bool](#Ident.IsExported)
* [func (x \*Ident) Pos() token.Pos](#Ident.Pos)
* [func (id \*Ident) String() string](#Ident.String)
* [type IfStmt](#IfStmt)
* [func (s \*IfStmt) End() token.Pos](#IfStmt.End)
* [func (s \*IfStmt) Pos() token.Pos](#IfStmt.Pos)
* [type ImportSpec](#ImportSpec)
* [func (s \*ImportSpec) End() token.Pos](#ImportSpec.End)
* [func (s \*ImportSpec) Pos() token.Pos](#ImportSpec.Pos)
* [type Importer](#Importer)
* [type IncDecStmt](#IncDecStmt)
* [func (s \*IncDecStmt) End() token.Pos](#IncDecStmt.End)
* [func (s \*IncDecStmt) Pos() token.Pos](#IncDecStmt.Pos)
* [type IndexExpr](#IndexExpr)
* [func (x \*IndexExpr) End() token.Pos](#IndexExpr.End)
* [func (x \*IndexExpr) Pos() token.Pos](#IndexExpr.Pos)
* [type IndexListExpr](#IndexListExpr)
* [func (x \*IndexListExpr) End() token.Pos](#IndexListExpr.End)
* [func (x \*IndexListExpr) Pos() token.Pos](#IndexListExpr.Pos)
* [type InterfaceType](#InterfaceType)
* [func (x \*InterfaceType) End() token.Pos](#InterfaceType.End)
* [func (x \*InterfaceType) Pos() token.Pos](#InterfaceType.Pos)
* [type KeyValueExpr](#KeyValueExpr)
* [func (x \*KeyValueExpr) End() token.Pos](#KeyValueExpr.End)
* [func (x \*KeyValueExpr) Pos() token.Pos](#KeyValueExpr.Pos)
* [type LabeledStmt](#LabeledStmt)
* [func (s \*LabeledStmt) End() token.Pos](#LabeledStmt.End)
* [func (s \*LabeledStmt) Pos() token.Pos](#LabeledStmt.Pos)
* [type MapType](#MapType)
* [func (x \*MapType) End() token.Pos](#MapType.End)
* [func (x \*MapType) Pos() token.Pos](#MapType.Pos)
* [type MergeMode](#MergeMode)
* [type Node](#Node)
* [type ObjKind](#ObjKind)
* [func (kind ObjKind) String() string](#ObjKind.String)
* [type Object](#Object)
* [func NewObj(kind ObjKind, name string) \*Object](#NewObj)
* [func (obj \*Object) Pos() token.Pos](#Object.Pos)
* [type Package](#Package)
* [func NewPackage(fset \*token.FileSet, files map[string]\*File, importer Importer, universe \*Scope) (\*Package, error)](#NewPackage)
* [func (p \*Package) End() token.Pos](#Package.End)
* [func (p \*Package) Pos() token.Pos](#Package.Pos)
* [type ParenExpr](#ParenExpr)
* [func (x \*ParenExpr) End() token.Pos](#ParenExpr.End)
* [func (x \*ParenExpr) Pos() token.Pos](#ParenExpr.Pos)
* [type RangeStmt](#RangeStmt)
* [func (s \*RangeStmt) End() token.Pos](#RangeStmt.End)
* [func (s \*RangeStmt) Pos() token.Pos](#RangeStmt.Pos)
* [type ReturnStmt](#ReturnStmt)
* [func (s \*ReturnStmt) End() token.Pos](#ReturnStmt.End)
* [func (s \*ReturnStmt) Pos() token.Pos](#ReturnStmt.Pos)
* [type Scope](#Scope)
* [func NewScope(outer \*Scope) \*Scope](#NewScope)
* [func (s \*Scope) Insert(obj \*Object) (alt \*Object)](#Scope.Insert)
* [func (s \*Scope) Lookup(name string) \*Object](#Scope.Lookup)
* [func (s \*Scope) String() string](#Scope.String)
* [type SelectStmt](#SelectStmt)
* [func (s \*SelectStmt) End() token.Pos](#SelectStmt.End)
* [func (s \*SelectStmt) Pos() token.Pos](#SelectStmt.Pos)
* [type SelectorExpr](#SelectorExpr)
* [func (x \*SelectorExpr) End() token.Pos](#SelectorExpr.End)
* [func (x \*SelectorExpr) Pos() token.Pos](#SelectorExpr.Pos)
* [type SendStmt](#SendStmt)
* [func (s \*SendStmt) End() token.Pos](#SendStmt.End)
* [func (s \*SendStmt) Pos() token.Pos](#SendStmt.Pos)
* [type SliceExpr](#SliceExpr)
* [func (x \*SliceExpr) End() token.Pos](#SliceExpr.End)
* [func (x \*SliceExpr) Pos() token.Pos](#SliceExpr.Pos)
* [type Spec](#Spec)
* [type StarExpr](#StarExpr)
* [func (x \*StarExpr) End() token.Pos](#StarExpr.End)
* [func (x \*StarExpr) Pos() token.Pos](#StarExpr.Pos)
* [type Stmt](#Stmt)
* [type StructType](#StructType)
* [func (x \*StructType) End() token.Pos](#StructType.End)
* [func (x \*StructType) Pos() token.Pos](#StructType.Pos)
* [type SwitchStmt](#SwitchStmt)
* [func (s \*SwitchStmt) End() token.Pos](#SwitchStmt.End)
* [func (s \*SwitchStmt) Pos() token.Pos](#SwitchStmt.Pos)
* [type TypeAssertExpr](#TypeAssertExpr)
* [func (x \*TypeAssertExpr) End() token.Pos](#TypeAssertExpr.End)
* [func (x \*TypeAssertExpr) Pos() token.Pos](#TypeAssertExpr.Pos)
* [type TypeSpec](#TypeSpec)
* [func (s \*TypeSpec) End() token.Pos](#TypeSpec.End)
* [func (s \*TypeSpec) Pos() token.Pos](#TypeSpec.Pos)
* [type TypeSwitchStmt](#TypeSwitchStmt)
* [func (s \*TypeSwitchStmt) End() token.Pos](#TypeSwitchStmt.End)
* [func (s \*TypeSwitchStmt) Pos() token.Pos](#TypeSwitchStmt.Pos)
* [type UnaryExpr](#UnaryExpr)
* [func (x \*UnaryExpr) End() token.Pos](#UnaryExpr.End)
* [func (x \*UnaryExpr) Pos() token.Pos](#UnaryExpr.Pos)
* [type ValueSpec](#ValueSpec)
* [func (s \*ValueSpec) End() token.Pos](#ValueSpec.End)
* [func (s \*ValueSpec) Pos() token.Pos](#ValueSpec.Pos)
* [type Visitor](#Visitor)
### Examples
[CommentMap](#example_CommentMap) [Inspect](#example_Inspect) [Print](#example_Print) ### Package files
ast.go commentmap.go filter.go import.go print.go resolve.go scope.go walk.go
func FileExports
----------------
```
func FileExports(src *File) bool
```
FileExports trims the AST for a Go source file in place such that only exported nodes remain: all top-level identifiers which are not exported and their associated information (such as type, initial value, or function body) are removed. Non-exported fields and methods of exported types are stripped. The File.Comments list is not changed.
FileExports reports whether there are exported declarations.
func FilterDecl
---------------
```
func FilterDecl(decl Decl, f Filter) bool
```
FilterDecl trims the AST for a Go declaration in place by removing all names (including struct field and interface method names, but not from parameter lists) that don't pass through the filter f.
FilterDecl reports whether there are any declared names left after filtering.
func FilterFile
---------------
```
func FilterFile(src *File, f Filter) bool
```
FilterFile trims the AST for a Go file in place by removing all names from top-level declarations (including struct field and interface method names, but not from parameter lists) that don't pass through the filter f. If the declaration is empty afterwards, the declaration is removed from the AST. Import declarations are always removed. The File.Comments list is not changed.
FilterFile reports whether there are any top-level declarations left after filtering.
func FilterPackage
------------------
```
func FilterPackage(pkg *Package, f Filter) bool
```
FilterPackage trims the AST for a Go package in place by removing all names from top-level declarations (including struct field and interface method names, but not from parameter lists) that don't pass through the filter f. If the declaration is empty afterwards, the declaration is removed from the AST. The pkg.Files list is not changed, so that file names and top-level package comments don't get lost.
FilterPackage reports whether there are any top-level declarations left after filtering.
func Fprint
-----------
```
func Fprint(w io.Writer, fset *token.FileSet, x any, f FieldFilter) error
```
Fprint prints the (sub-)tree starting at AST node x to w. If fset != nil, position information is interpreted relative to that file set. Otherwise positions are printed as integer values (file set specific offsets).
A non-nil FieldFilter f may be provided to control the output: struct fields for which f(fieldname, fieldvalue) is true are printed; all others are filtered from the output. Unexported struct fields are never printed.
func Inspect
------------
```
func Inspect(node Node, f func(Node) bool)
```
Inspect traverses an AST in depth-first order: It starts by calling f(node); node must not be nil. If f returns true, Inspect invokes f recursively for each of the non-nil children of node, followed by a call of f(nil).
#### Example
This example demonstrates how to inspect the AST of a Go program.
Code:
```
// src is the input for which we want to inspect the AST.
src := `
package p
const c = 1.0
var X = f(3.14)*2 + c
`
// Create the AST by parsing src.
fset := token.NewFileSet() // positions are relative to fset
f, err := parser.ParseFile(fset, "src.go", src, 0)
if err != nil {
panic(err)
}
// Inspect the AST and print all identifiers and literals.
ast.Inspect(f, func(n ast.Node) bool {
var s string
switch x := n.(type) {
case *ast.BasicLit:
s = x.Value
case *ast.Ident:
s = x.Name
}
if s != "" {
fmt.Printf("%s:\t%s\n", fset.Position(n.Pos()), s)
}
return true
})
```
Output:
```
src.go:2:9: p
src.go:3:7: c
src.go:3:11: 1.0
src.go:4:5: X
src.go:4:9: f
src.go:4:11: 3.14
src.go:4:17: 2
src.go:4:21: c
```
func IsExported
---------------
```
func IsExported(name string) bool
```
IsExported reports whether name starts with an upper-case letter.
func NotNilFilter
-----------------
```
func NotNilFilter(_ string, v reflect.Value) bool
```
NotNilFilter returns true for field values that are not nil; it returns false otherwise.
func PackageExports
-------------------
```
func PackageExports(pkg *Package) bool
```
PackageExports trims the AST for a Go package in place such that only exported nodes remain. The pkg.Files list is not changed, so that file names and top-level package comments don't get lost.
PackageExports reports whether there are exported declarations; it returns false otherwise.
func Print
----------
```
func Print(fset *token.FileSet, x any) error
```
Print prints x to standard output, skipping nil fields. Print(fset, x) is the same as Fprint(os.Stdout, fset, x, NotNilFilter).
#### Example
This example shows what an AST looks like when printed for debugging.
Code:
```
// src is the input for which we want to print the AST.
src := `
package main
func main() {
println("Hello, World!")
}
`
// Create the AST by parsing src.
fset := token.NewFileSet() // positions are relative to fset
f, err := parser.ParseFile(fset, "", src, 0)
if err != nil {
panic(err)
}
// Print the AST.
ast.Print(fset, f)
```
Output:
```
0 *ast.File {
1 . Package: 2:1
2 . Name: *ast.Ident {
3 . . NamePos: 2:9
4 . . Name: "main"
5 . }
6 . Decls: []ast.Decl (len = 1) {
7 . . 0: *ast.FuncDecl {
8 . . . Name: *ast.Ident {
9 . . . . NamePos: 3:6
10 . . . . Name: "main"
11 . . . . Obj: *ast.Object {
12 . . . . . Kind: func
13 . . . . . Name: "main"
14 . . . . . Decl: *(obj @ 7)
15 . . . . }
16 . . . }
17 . . . Type: *ast.FuncType {
18 . . . . Func: 3:1
19 . . . . Params: *ast.FieldList {
20 . . . . . Opening: 3:10
21 . . . . . Closing: 3:11
22 . . . . }
23 . . . }
24 . . . Body: *ast.BlockStmt {
25 . . . . Lbrace: 3:13
26 . . . . List: []ast.Stmt (len = 1) {
27 . . . . . 0: *ast.ExprStmt {
28 . . . . . . X: *ast.CallExpr {
29 . . . . . . . Fun: *ast.Ident {
30 . . . . . . . . NamePos: 4:2
31 . . . . . . . . Name: "println"
32 . . . . . . . }
33 . . . . . . . Lparen: 4:9
34 . . . . . . . Args: []ast.Expr (len = 1) {
35 . . . . . . . . 0: *ast.BasicLit {
36 . . . . . . . . . ValuePos: 4:10
37 . . . . . . . . . Kind: STRING
38 . . . . . . . . . Value: "\"Hello, World!\""
39 . . . . . . . . }
40 . . . . . . . }
41 . . . . . . . Ellipsis: -
42 . . . . . . . Rparen: 4:25
43 . . . . . . }
44 . . . . . }
45 . . . . }
46 . . . . Rbrace: 5:1
47 . . . }
48 . . }
49 . }
50 . FileStart: 1:1
51 . FileEnd: 5:3
52 . Scope: *ast.Scope {
53 . . Objects: map[string]*ast.Object (len = 1) {
54 . . . "main": *(obj @ 11)
55 . . }
56 . }
57 . Unresolved: []*ast.Ident (len = 1) {
58 . . 0: *(obj @ 29)
59 . }
60 }
```
func SortImports
----------------
```
func SortImports(fset *token.FileSet, f *File)
```
SortImports sorts runs of consecutive import lines in import blocks in f. It also removes duplicate imports when it is possible to do so without data loss.
func Walk
---------
```
func Walk(v Visitor, node Node)
```
Walk traverses an AST in depth-first order: It starts by calling v.Visit(node); node must not be nil. If the visitor w returned by v.Visit(node) is not nil, Walk is invoked recursively with visitor w for each of the non-nil children of node, followed by a call of w.Visit(nil).
type ArrayType
--------------
An ArrayType node represents an array or slice type.
```
type ArrayType struct {
Lbrack token.Pos // position of "["
Len Expr // Ellipsis node for [...]T array types, nil for slice types
Elt Expr // element type
}
```
### func (\*ArrayType) End
```
func (x *ArrayType) End() token.Pos
```
### func (\*ArrayType) Pos
```
func (x *ArrayType) Pos() token.Pos
```
type AssignStmt
---------------
An AssignStmt node represents an assignment or a short variable declaration.
```
type AssignStmt struct {
Lhs []Expr
TokPos token.Pos // position of Tok
Tok token.Token // assignment token, DEFINE
Rhs []Expr
}
```
### func (\*AssignStmt) End
```
func (s *AssignStmt) End() token.Pos
```
### func (\*AssignStmt) Pos
```
func (s *AssignStmt) Pos() token.Pos
```
type BadDecl
------------
A BadDecl node is a placeholder for a declaration containing syntax errors for which a correct declaration node cannot be created.
```
type BadDecl struct {
From, To token.Pos // position range of bad declaration
}
```
### func (\*BadDecl) End
```
func (d *BadDecl) End() token.Pos
```
### func (\*BadDecl) Pos
```
func (d *BadDecl) Pos() token.Pos
```
type BadExpr
------------
A BadExpr node is a placeholder for an expression containing syntax errors for which a correct expression node cannot be created.
```
type BadExpr struct {
From, To token.Pos // position range of bad expression
}
```
### func (\*BadExpr) End
```
func (x *BadExpr) End() token.Pos
```
### func (\*BadExpr) Pos
```
func (x *BadExpr) Pos() token.Pos
```
type BadStmt
------------
A BadStmt node is a placeholder for statements containing syntax errors for which no correct statement nodes can be created.
```
type BadStmt struct {
From, To token.Pos // position range of bad statement
}
```
### func (\*BadStmt) End
```
func (s *BadStmt) End() token.Pos
```
### func (\*BadStmt) Pos
```
func (s *BadStmt) Pos() token.Pos
```
type BasicLit
-------------
A BasicLit node represents a literal of basic type.
```
type BasicLit struct {
ValuePos token.Pos // literal position
Kind token.Token // token.INT, token.FLOAT, token.IMAG, token.CHAR, or token.STRING
Value string // literal string; e.g. 42, 0x7f, 3.14, 1e-9, 2.4i, 'a', '\x7f', "foo" or `\m\n\o`
}
```
### func (\*BasicLit) End
```
func (x *BasicLit) End() token.Pos
```
### func (\*BasicLit) Pos
```
func (x *BasicLit) Pos() token.Pos
```
type BinaryExpr
---------------
A BinaryExpr node represents a binary expression.
```
type BinaryExpr struct {
X Expr // left operand
OpPos token.Pos // position of Op
Op token.Token // operator
Y Expr // right operand
}
```
### func (\*BinaryExpr) End
```
func (x *BinaryExpr) End() token.Pos
```
### func (\*BinaryExpr) Pos
```
func (x *BinaryExpr) Pos() token.Pos
```
type BlockStmt
--------------
A BlockStmt node represents a braced statement list.
```
type BlockStmt struct {
Lbrace token.Pos // position of "{"
List []Stmt
Rbrace token.Pos // position of "}", if any (may be absent due to syntax error)
}
```
### func (\*BlockStmt) End
```
func (s *BlockStmt) End() token.Pos
```
### func (\*BlockStmt) Pos
```
func (s *BlockStmt) Pos() token.Pos
```
type BranchStmt
---------------
A BranchStmt node represents a break, continue, goto, or fallthrough statement.
```
type BranchStmt struct {
TokPos token.Pos // position of Tok
Tok token.Token // keyword token (BREAK, CONTINUE, GOTO, FALLTHROUGH)
Label *Ident // label name; or nil
}
```
### func (\*BranchStmt) End
```
func (s *BranchStmt) End() token.Pos
```
### func (\*BranchStmt) Pos
```
func (s *BranchStmt) Pos() token.Pos
```
type CallExpr
-------------
A CallExpr node represents an expression followed by an argument list.
```
type CallExpr struct {
Fun Expr // function expression
Lparen token.Pos // position of "("
Args []Expr // function arguments; or nil
Ellipsis token.Pos // position of "..." (token.NoPos if there is no "...")
Rparen token.Pos // position of ")"
}
```
### func (\*CallExpr) End
```
func (x *CallExpr) End() token.Pos
```
### func (\*CallExpr) Pos
```
func (x *CallExpr) Pos() token.Pos
```
type CaseClause
---------------
A CaseClause represents a case of an expression or type switch statement.
```
type CaseClause struct {
Case token.Pos // position of "case" or "default" keyword
List []Expr // list of expressions or types; nil means default case
Colon token.Pos // position of ":"
Body []Stmt // statement list; or nil
}
```
### func (\*CaseClause) End
```
func (s *CaseClause) End() token.Pos
```
### func (\*CaseClause) Pos
```
func (s *CaseClause) Pos() token.Pos
```
type ChanDir
------------
The direction of a channel type is indicated by a bit mask including one or both of the following constants.
```
type ChanDir int
```
```
const (
SEND ChanDir = 1 << iota
RECV
)
```
type ChanType
-------------
A ChanType node represents a channel type.
```
type ChanType struct {
Begin token.Pos // position of "chan" keyword or "<-" (whichever comes first)
Arrow token.Pos // position of "<-" (token.NoPos if there is no "<-"); added in Go 1.1
Dir ChanDir // channel direction
Value Expr // value type
}
```
### func (\*ChanType) End
```
func (x *ChanType) End() token.Pos
```
### func (\*ChanType) Pos
```
func (x *ChanType) Pos() token.Pos
```
type CommClause
---------------
A CommClause node represents a case of a select statement.
```
type CommClause struct {
Case token.Pos // position of "case" or "default" keyword
Comm Stmt // send or receive statement; nil means default case
Colon token.Pos // position of ":"
Body []Stmt // statement list; or nil
}
```
### func (\*CommClause) End
```
func (s *CommClause) End() token.Pos
```
### func (\*CommClause) Pos
```
func (s *CommClause) Pos() token.Pos
```
type Comment
------------
A Comment node represents a single //-style or /\*-style comment.
The Text field contains the comment text without carriage returns (\r) that may have been present in the source. Because a comment's end position is computed using len(Text), the position reported by End() does not match the true source end position for comments containing carriage returns.
```
type Comment struct {
Slash token.Pos // position of "/" starting the comment
Text string // comment text (excluding '\n' for //-style comments)
}
```
### func (\*Comment) End
```
func (c *Comment) End() token.Pos
```
### func (\*Comment) Pos
```
func (c *Comment) Pos() token.Pos
```
type CommentGroup
-----------------
A CommentGroup represents a sequence of comments with no other tokens and no empty lines between.
```
type CommentGroup struct {
List []*Comment // len(List) > 0
}
```
### func (\*CommentGroup) End
```
func (g *CommentGroup) End() token.Pos
```
### func (\*CommentGroup) Pos
```
func (g *CommentGroup) Pos() token.Pos
```
### func (\*CommentGroup) Text
```
func (g *CommentGroup) Text() string
```
Text returns the text of the comment. Comment markers (//, /\*, and \*/), the first space of a line comment, and leading and trailing empty lines are removed. Comment directives like "//line" and "//go:noinline" are also removed. Multiple empty lines are reduced to one, and trailing space on lines is trimmed. Unless the result is empty, it is newline-terminated.
type CommentMap 1.1
-------------------
A CommentMap maps an AST node to a list of comment groups associated with it. See NewCommentMap for a description of the association.
```
type CommentMap map[Node][]*CommentGroup
```
#### Example
This example illustrates how to remove a variable declaration in a Go program while maintaining correct comment association using an ast.CommentMap.
Code:
```
// src is the input for which we create the AST that we
// are going to manipulate.
src := `
// This is the package comment.
package main
// This comment is associated with the hello constant.
const hello = "Hello, World!" // line comment 1
// This comment is associated with the foo variable.
var foo = hello // line comment 2
// This comment is associated with the main function.
func main() {
fmt.Println(hello) // line comment 3
}
`
// Create the AST by parsing src.
fset := token.NewFileSet() // positions are relative to fset
f, err := parser.ParseFile(fset, "src.go", src, parser.ParseComments)
if err != nil {
panic(err)
}
// Create an ast.CommentMap from the ast.File's comments.
// This helps keeping the association between comments
// and AST nodes.
cmap := ast.NewCommentMap(fset, f, f.Comments)
// Remove the first variable declaration from the list of declarations.
for i, decl := range f.Decls {
if gen, ok := decl.(*ast.GenDecl); ok && gen.Tok == token.VAR {
copy(f.Decls[i:], f.Decls[i+1:])
f.Decls = f.Decls[:len(f.Decls)-1]
break
}
}
// Use the comment map to filter comments that don't belong anymore
// (the comments associated with the variable declaration), and create
// the new comments list.
f.Comments = cmap.Filter(f).Comments()
// Print the modified AST.
var buf strings.Builder
if err := format.Node(&buf, fset, f); err != nil {
panic(err)
}
fmt.Printf("%s", buf.String())
```
Output:
```
// This is the package comment.
package main
// This comment is associated with the hello constant.
const hello = "Hello, World!" // line comment 1
// This comment is associated with the main function.
func main() {
fmt.Println(hello) // line comment 3
}
```
### func NewCommentMap 1.1
```
func NewCommentMap(fset *token.FileSet, node Node, comments []*CommentGroup) CommentMap
```
NewCommentMap creates a new comment map by associating comment groups of the comments list with the nodes of the AST specified by node.
A comment group g is associated with a node n if:
* g starts on the same line as n ends
* g starts on the line immediately following n, and there is at least one empty line after g and before the next node
* g starts before n and is not associated to the node before n via the previous rules
NewCommentMap tries to associate a comment group to the "largest" node possible: For instance, if the comment is a line comment trailing an assignment, the comment is associated with the entire assignment rather than just the last operand in the assignment.
### func (CommentMap) Comments 1.1
```
func (cmap CommentMap) Comments() []*CommentGroup
```
Comments returns the list of comment groups in the comment map. The result is sorted in source order.
### func (CommentMap) Filter 1.1
```
func (cmap CommentMap) Filter(node Node) CommentMap
```
Filter returns a new comment map consisting of only those entries of cmap for which a corresponding node exists in the AST specified by node.
### func (CommentMap) String 1.1
```
func (cmap CommentMap) String() string
```
### func (CommentMap) Update 1.1
```
func (cmap CommentMap) Update(old, new Node) Node
```
Update replaces an old node in the comment map with the new node and returns the new node. Comments that were associated with the old node are associated with the new node.
type CompositeLit
-----------------
A CompositeLit node represents a composite literal.
```
type CompositeLit struct {
Type Expr // literal type; or nil
Lbrace token.Pos // position of "{"
Elts []Expr // list of composite elements; or nil
Rbrace token.Pos // position of "}"
Incomplete bool // true if (source) expressions are missing in the Elts list; added in Go 1.11
}
```
### func (\*CompositeLit) End
```
func (x *CompositeLit) End() token.Pos
```
### func (\*CompositeLit) Pos
```
func (x *CompositeLit) Pos() token.Pos
```
type Decl
---------
All declaration nodes implement the Decl interface.
```
type Decl interface {
Node
// contains filtered or unexported methods
}
```
type DeclStmt
-------------
A DeclStmt node represents a declaration in a statement list.
```
type DeclStmt struct {
Decl Decl // *GenDecl with CONST, TYPE, or VAR token
}
```
### func (\*DeclStmt) End
```
func (s *DeclStmt) End() token.Pos
```
### func (\*DeclStmt) Pos
```
func (s *DeclStmt) Pos() token.Pos
```
type DeferStmt
--------------
A DeferStmt node represents a defer statement.
```
type DeferStmt struct {
Defer token.Pos // position of "defer" keyword
Call *CallExpr
}
```
### func (\*DeferStmt) End
```
func (s *DeferStmt) End() token.Pos
```
### func (\*DeferStmt) Pos
```
func (s *DeferStmt) Pos() token.Pos
```
type Ellipsis
-------------
An Ellipsis node stands for the "..." type in a parameter list or the "..." length in an array type.
```
type Ellipsis struct {
Ellipsis token.Pos // position of "..."
Elt Expr // ellipsis element type (parameter lists only); or nil
}
```
### func (\*Ellipsis) End
```
func (x *Ellipsis) End() token.Pos
```
### func (\*Ellipsis) Pos
```
func (x *Ellipsis) Pos() token.Pos
```
type EmptyStmt
--------------
An EmptyStmt node represents an empty statement. The "position" of the empty statement is the position of the immediately following (explicit or implicit) semicolon.
```
type EmptyStmt struct {
Semicolon token.Pos // position of following ";"
Implicit bool // if set, ";" was omitted in the source; added in Go 1.5
}
```
### func (\*EmptyStmt) End
```
func (s *EmptyStmt) End() token.Pos
```
### func (\*EmptyStmt) Pos
```
func (s *EmptyStmt) Pos() token.Pos
```
type Expr
---------
All expression nodes implement the Expr interface.
```
type Expr interface {
Node
// contains filtered or unexported methods
}
```
type ExprStmt
-------------
An ExprStmt node represents a (stand-alone) expression in a statement list.
```
type ExprStmt struct {
X Expr // expression
}
```
### func (\*ExprStmt) End
```
func (s *ExprStmt) End() token.Pos
```
### func (\*ExprStmt) Pos
```
func (s *ExprStmt) Pos() token.Pos
```
type Field
----------
A Field represents a Field declaration list in a struct type, a method list in an interface type, or a parameter/result declaration in a signature. Field.Names is nil for unnamed parameters (parameter lists which only contain types) and embedded struct fields. In the latter case, the field name is the type name.
```
type Field struct {
Doc *CommentGroup // associated documentation; or nil
Names []*Ident // field/method/(type) parameter names; or nil
Type Expr // field/method/parameter type; or nil
Tag *BasicLit // field tag; or nil
Comment *CommentGroup // line comments; or nil
}
```
### func (\*Field) End
```
func (f *Field) End() token.Pos
```
### func (\*Field) Pos
```
func (f *Field) Pos() token.Pos
```
type FieldFilter
----------------
A FieldFilter may be provided to Fprint to control the output.
```
type FieldFilter func(name string, value reflect.Value) bool
```
type FieldList
--------------
A FieldList represents a list of Fields, enclosed by parentheses, curly braces, or square brackets.
```
type FieldList struct {
Opening token.Pos // position of opening parenthesis/brace/bracket, if any
List []*Field // field list; or nil
Closing token.Pos // position of closing parenthesis/brace/bracket, if any
}
```
### func (\*FieldList) End
```
func (f *FieldList) End() token.Pos
```
### func (\*FieldList) NumFields
```
func (f *FieldList) NumFields() int
```
NumFields returns the number of parameters or struct fields represented by a FieldList.
### func (\*FieldList) Pos
```
func (f *FieldList) Pos() token.Pos
```
type File
---------
A File node represents a Go source file.
The Comments list contains all comments in the source file in order of appearance, including the comments that are pointed to from other nodes via Doc and Comment fields.
For correct printing of source code containing comments (using packages go/format and go/printer), special care must be taken to update comments when a File's syntax tree is modified: For printing, comments are interspersed between tokens based on their position. If syntax tree nodes are removed or moved, relevant comments in their vicinity must also be removed (from the File.Comments list) or moved accordingly (by updating their positions). A CommentMap may be used to facilitate some of these operations.
Whether and how a comment is associated with a node depends on the interpretation of the syntax tree by the manipulating program: Except for Doc and Comment comments directly associated with nodes, the remaining comments are "free-floating" (see also issues #18593, #20744).
```
type File struct {
Doc *CommentGroup // associated documentation; or nil
Package token.Pos // position of "package" keyword
Name *Ident // package name
Decls []Decl // top-level declarations; or nil
FileStart, FileEnd token.Pos // start and end of entire file; added in Go 1.20
Scope *Scope // package scope (this file only)
Imports []*ImportSpec // imports in this file
Unresolved []*Ident // unresolved identifiers in this file
Comments []*CommentGroup // list of all comments in the source file
}
```
### func MergePackageFiles
```
func MergePackageFiles(pkg *Package, mode MergeMode) *File
```
MergePackageFiles creates a file AST by merging the ASTs of the files belonging to a package. The mode flags control merging behavior.
### func (\*File) End
```
func (f *File) End() token.Pos
```
End returns the end of the last declaration in the file. (Use FileEnd for the end of the entire file.)
### func (\*File) Pos
```
func (f *File) Pos() token.Pos
```
Pos returns the position of the package declaration. (Use FileStart for the start of the entire file.)
type Filter
-----------
```
type Filter func(string) bool
```
type ForStmt
------------
A ForStmt represents a for statement.
```
type ForStmt struct {
For token.Pos // position of "for" keyword
Init Stmt // initialization statement; or nil
Cond Expr // condition; or nil
Post Stmt // post iteration statement; or nil
Body *BlockStmt
}
```
### func (\*ForStmt) End
```
func (s *ForStmt) End() token.Pos
```
### func (\*ForStmt) Pos
```
func (s *ForStmt) Pos() token.Pos
```
type FuncDecl
-------------
A FuncDecl node represents a function declaration.
```
type FuncDecl struct {
Doc *CommentGroup // associated documentation; or nil
Recv *FieldList // receiver (methods); or nil (functions)
Name *Ident // function/method name
Type *FuncType // function signature: type and value parameters, results, and position of "func" keyword
Body *BlockStmt // function body; or nil for external (non-Go) function
}
```
### func (\*FuncDecl) End
```
func (d *FuncDecl) End() token.Pos
```
### func (\*FuncDecl) Pos
```
func (d *FuncDecl) Pos() token.Pos
```
type FuncLit
------------
A FuncLit node represents a function literal.
```
type FuncLit struct {
Type *FuncType // function type
Body *BlockStmt // function body
}
```
### func (\*FuncLit) End
```
func (x *FuncLit) End() token.Pos
```
### func (\*FuncLit) Pos
```
func (x *FuncLit) Pos() token.Pos
```
type FuncType
-------------
A FuncType node represents a function type.
```
type FuncType struct {
Func token.Pos // position of "func" keyword (token.NoPos if there is no "func")
TypeParams *FieldList // type parameters; or nil; added in Go 1.18
Params *FieldList // (incoming) parameters; non-nil
Results *FieldList // (outgoing) results; or nil
}
```
### func (\*FuncType) End
```
func (x *FuncType) End() token.Pos
```
### func (\*FuncType) Pos
```
func (x *FuncType) Pos() token.Pos
```
type GenDecl
------------
A GenDecl node (generic declaration node) represents an import, constant, type or variable declaration. A valid Lparen position (Lparen.IsValid()) indicates a parenthesized declaration.
Relationship between Tok value and Specs element type:
```
token.IMPORT *ImportSpec
token.CONST *ValueSpec
token.TYPE *TypeSpec
token.VAR *ValueSpec
```
```
type GenDecl struct {
Doc *CommentGroup // associated documentation; or nil
TokPos token.Pos // position of Tok
Tok token.Token // IMPORT, CONST, TYPE, or VAR
Lparen token.Pos // position of '(', if any
Specs []Spec
Rparen token.Pos // position of ')', if any
}
```
### func (\*GenDecl) End
```
func (d *GenDecl) End() token.Pos
```
### func (\*GenDecl) Pos
```
func (d *GenDecl) Pos() token.Pos
```
type GoStmt
-----------
A GoStmt node represents a go statement.
```
type GoStmt struct {
Go token.Pos // position of "go" keyword
Call *CallExpr
}
```
### func (\*GoStmt) End
```
func (s *GoStmt) End() token.Pos
```
### func (\*GoStmt) Pos
```
func (s *GoStmt) Pos() token.Pos
```
type Ident
----------
An Ident node represents an identifier.
```
type Ident struct {
NamePos token.Pos // identifier position
Name string // identifier name
Obj *Object // denoted object; or nil
}
```
### func NewIdent
```
func NewIdent(name string) *Ident
```
NewIdent creates a new Ident without position. Useful for ASTs generated by code other than the Go parser.
### func (\*Ident) End
```
func (x *Ident) End() token.Pos
```
### func (\*Ident) IsExported
```
func (id *Ident) IsExported() bool
```
IsExported reports whether id starts with an upper-case letter.
### func (\*Ident) Pos
```
func (x *Ident) Pos() token.Pos
```
### func (\*Ident) String
```
func (id *Ident) String() string
```
type IfStmt
-----------
An IfStmt node represents an if statement.
```
type IfStmt struct {
If token.Pos // position of "if" keyword
Init Stmt // initialization statement; or nil
Cond Expr // condition
Body *BlockStmt
Else Stmt // else branch; or nil
}
```
### func (\*IfStmt) End
```
func (s *IfStmt) End() token.Pos
```
### func (\*IfStmt) Pos
```
func (s *IfStmt) Pos() token.Pos
```
type ImportSpec
---------------
An ImportSpec node represents a single package import.
```
type ImportSpec struct {
Doc *CommentGroup // associated documentation; or nil
Name *Ident // local package name (including "."); or nil
Path *BasicLit // import path
Comment *CommentGroup // line comments; or nil
EndPos token.Pos // end of spec (overrides Path.Pos if nonzero)
}
```
### func (\*ImportSpec) End
```
func (s *ImportSpec) End() token.Pos
```
### func (\*ImportSpec) Pos
```
func (s *ImportSpec) Pos() token.Pos
```
type Importer
-------------
An Importer resolves import paths to package Objects. The imports map records the packages already imported, indexed by package id (canonical import path). An Importer must determine the canonical import path and check the map to see if it is already present in the imports map. If so, the Importer can return the map entry. Otherwise, the Importer should load the package data for the given path into a new \*Object (pkg), record pkg in the imports map, and then return pkg.
```
type Importer func(imports map[string]*Object, path string) (pkg *Object, err error)
```
type IncDecStmt
---------------
An IncDecStmt node represents an increment or decrement statement.
```
type IncDecStmt struct {
X Expr
TokPos token.Pos // position of Tok
Tok token.Token // INC or DEC
}
```
### func (\*IncDecStmt) End
```
func (s *IncDecStmt) End() token.Pos
```
### func (\*IncDecStmt) Pos
```
func (s *IncDecStmt) Pos() token.Pos
```
type IndexExpr
--------------
An IndexExpr node represents an expression followed by an index.
```
type IndexExpr struct {
X Expr // expression
Lbrack token.Pos // position of "["
Index Expr // index expression
Rbrack token.Pos // position of "]"
}
```
### func (\*IndexExpr) End
```
func (x *IndexExpr) End() token.Pos
```
### func (\*IndexExpr) Pos
```
func (x *IndexExpr) Pos() token.Pos
```
type IndexListExpr 1.18
-----------------------
An IndexListExpr node represents an expression followed by multiple indices.
```
type IndexListExpr struct {
X Expr // expression
Lbrack token.Pos // position of "["
Indices []Expr // index expressions
Rbrack token.Pos // position of "]"
}
```
### func (\*IndexListExpr) End 1.18
```
func (x *IndexListExpr) End() token.Pos
```
### func (\*IndexListExpr) Pos 1.18
```
func (x *IndexListExpr) Pos() token.Pos
```
type InterfaceType
------------------
An InterfaceType node represents an interface type.
```
type InterfaceType struct {
Interface token.Pos // position of "interface" keyword
Methods *FieldList // list of embedded interfaces, methods, or types
Incomplete bool // true if (source) methods or types are missing in the Methods list
}
```
### func (\*InterfaceType) End
```
func (x *InterfaceType) End() token.Pos
```
### func (\*InterfaceType) Pos
```
func (x *InterfaceType) Pos() token.Pos
```
type KeyValueExpr
-----------------
A KeyValueExpr node represents (key : value) pairs in composite literals.
```
type KeyValueExpr struct {
Key Expr
Colon token.Pos // position of ":"
Value Expr
}
```
### func (\*KeyValueExpr) End
```
func (x *KeyValueExpr) End() token.Pos
```
### func (\*KeyValueExpr) Pos
```
func (x *KeyValueExpr) Pos() token.Pos
```
type LabeledStmt
----------------
A LabeledStmt node represents a labeled statement.
```
type LabeledStmt struct {
Label *Ident
Colon token.Pos // position of ":"
Stmt Stmt
}
```
### func (\*LabeledStmt) End
```
func (s *LabeledStmt) End() token.Pos
```
### func (\*LabeledStmt) Pos
```
func (s *LabeledStmt) Pos() token.Pos
```
type MapType
------------
A MapType node represents a map type.
```
type MapType struct {
Map token.Pos // position of "map" keyword
Key Expr
Value Expr
}
```
### func (\*MapType) End
```
func (x *MapType) End() token.Pos
```
### func (\*MapType) Pos
```
func (x *MapType) Pos() token.Pos
```
type MergeMode
--------------
The MergeMode flags control the behavior of MergePackageFiles.
```
type MergeMode uint
```
```
const (
// If set, duplicate function declarations are excluded.
FilterFuncDuplicates MergeMode = 1 << iota
// If set, comments that are not associated with a specific
// AST node (as Doc or Comment) are excluded.
FilterUnassociatedComments
// If set, duplicate import declarations are excluded.
FilterImportDuplicates
)
```
type Node
---------
All node types implement the Node interface.
```
type Node interface {
Pos() token.Pos // position of first character belonging to the node
End() token.Pos // position of first character immediately after the node
}
```
type ObjKind
------------
ObjKind describes what an object represents.
```
type ObjKind int
```
The list of possible Object kinds.
```
const (
Bad ObjKind = iota // for error handling
Pkg // package
Con // constant
Typ // type
Var // variable
Fun // function or method
Lbl // label
)
```
### func (ObjKind) String
```
func (kind ObjKind) String() string
```
type Object
-----------
An Object describes a named language entity such as a package, constant, type, variable, function (incl. methods), or label.
The Data fields contains object-specific data:
```
Kind Data type Data value
Pkg *Scope package scope
Con int iota for the respective declaration
```
```
type Object struct {
Kind ObjKind
Name string // declared name
Decl any // corresponding Field, XxxSpec, FuncDecl, LabeledStmt, AssignStmt, Scope; or nil
Data any // object-specific data; or nil
Type any // placeholder for type information; may be nil
}
```
### func NewObj
```
func NewObj(kind ObjKind, name string) *Object
```
NewObj creates a new object of a given kind and name.
### func (\*Object) Pos
```
func (obj *Object) Pos() token.Pos
```
Pos computes the source position of the declaration of an object name. The result may be an invalid position if it cannot be computed (obj.Decl may be nil or not correct).
type Package
------------
A Package node represents a set of source files collectively building a Go package.
```
type Package struct {
Name string // package name
Scope *Scope // package scope across all files
Imports map[string]*Object // map of package id -> package object
Files map[string]*File // Go source files by filename
}
```
### func NewPackage
```
func NewPackage(fset *token.FileSet, files map[string]*File, importer Importer, universe *Scope) (*Package, error)
```
NewPackage creates a new Package node from a set of File nodes. It resolves unresolved identifiers across files and updates each file's Unresolved list accordingly. If a non-nil importer and universe scope are provided, they are used to resolve identifiers not declared in any of the package files. Any remaining unresolved identifiers are reported as undeclared. If the files belong to different packages, one package name is selected and files with different package names are reported and then ignored. The result is a package node and a scanner.ErrorList if there were errors.
### func (\*Package) End
```
func (p *Package) End() token.Pos
```
### func (\*Package) Pos
```
func (p *Package) Pos() token.Pos
```
type ParenExpr
--------------
A ParenExpr node represents a parenthesized expression.
```
type ParenExpr struct {
Lparen token.Pos // position of "("
X Expr // parenthesized expression
Rparen token.Pos // position of ")"
}
```
### func (\*ParenExpr) End
```
func (x *ParenExpr) End() token.Pos
```
### func (\*ParenExpr) Pos
```
func (x *ParenExpr) Pos() token.Pos
```
type RangeStmt
--------------
A RangeStmt represents a for statement with a range clause.
```
type RangeStmt struct {
For token.Pos // position of "for" keyword
Key, Value Expr // Key, Value may be nil
TokPos token.Pos // position of Tok; invalid if Key == nil
Tok token.Token // ILLEGAL if Key == nil, ASSIGN, DEFINE
Range token.Pos // position of "range" keyword; added in Go 1.20
X Expr // value to range over
Body *BlockStmt
}
```
### func (\*RangeStmt) End
```
func (s *RangeStmt) End() token.Pos
```
### func (\*RangeStmt) Pos
```
func (s *RangeStmt) Pos() token.Pos
```
type ReturnStmt
---------------
A ReturnStmt node represents a return statement.
```
type ReturnStmt struct {
Return token.Pos // position of "return" keyword
Results []Expr // result expressions; or nil
}
```
### func (\*ReturnStmt) End
```
func (s *ReturnStmt) End() token.Pos
```
### func (\*ReturnStmt) Pos
```
func (s *ReturnStmt) Pos() token.Pos
```
type Scope
----------
A Scope maintains the set of named language entities declared in the scope and a link to the immediately surrounding (outer) scope.
```
type Scope struct {
Outer *Scope
Objects map[string]*Object
}
```
### func NewScope
```
func NewScope(outer *Scope) *Scope
```
NewScope creates a new scope nested in the outer scope.
### func (\*Scope) Insert
```
func (s *Scope) Insert(obj *Object) (alt *Object)
```
Insert attempts to insert a named object obj into the scope s. If the scope already contains an object alt with the same name, Insert leaves the scope unchanged and returns alt. Otherwise it inserts obj and returns nil.
### func (\*Scope) Lookup
```
func (s *Scope) Lookup(name string) *Object
```
Lookup returns the object with the given name if it is found in scope s, otherwise it returns nil. Outer scopes are ignored.
### func (\*Scope) String
```
func (s *Scope) String() string
```
Debugging support
type SelectStmt
---------------
A SelectStmt node represents a select statement.
```
type SelectStmt struct {
Select token.Pos // position of "select" keyword
Body *BlockStmt // CommClauses only
}
```
### func (\*SelectStmt) End
```
func (s *SelectStmt) End() token.Pos
```
### func (\*SelectStmt) Pos
```
func (s *SelectStmt) Pos() token.Pos
```
type SelectorExpr
-----------------
A SelectorExpr node represents an expression followed by a selector.
```
type SelectorExpr struct {
X Expr // expression
Sel *Ident // field selector
}
```
### func (\*SelectorExpr) End
```
func (x *SelectorExpr) End() token.Pos
```
### func (\*SelectorExpr) Pos
```
func (x *SelectorExpr) Pos() token.Pos
```
type SendStmt
-------------
A SendStmt node represents a send statement.
```
type SendStmt struct {
Chan Expr
Arrow token.Pos // position of "<-"
Value Expr
}
```
### func (\*SendStmt) End
```
func (s *SendStmt) End() token.Pos
```
### func (\*SendStmt) Pos
```
func (s *SendStmt) Pos() token.Pos
```
type SliceExpr
--------------
A SliceExpr node represents an expression followed by slice indices.
```
type SliceExpr struct {
X Expr // expression
Lbrack token.Pos // position of "["
Low Expr // begin of slice range; or nil
High Expr // end of slice range; or nil
Max Expr // maximum capacity of slice; or nil; added in Go 1.2
Slice3 bool // true if 3-index slice (2 colons present); added in Go 1.2
Rbrack token.Pos // position of "]"
}
```
### func (\*SliceExpr) End
```
func (x *SliceExpr) End() token.Pos
```
### func (\*SliceExpr) Pos
```
func (x *SliceExpr) Pos() token.Pos
```
type Spec
---------
The Spec type stands for any of \*ImportSpec, \*ValueSpec, and \*TypeSpec.
```
type Spec interface {
Node
// contains filtered or unexported methods
}
```
type StarExpr
-------------
A StarExpr node represents an expression of the form "\*" Expression. Semantically it could be a unary "\*" expression, or a pointer type.
```
type StarExpr struct {
Star token.Pos // position of "*"
X Expr // operand
}
```
### func (\*StarExpr) End
```
func (x *StarExpr) End() token.Pos
```
### func (\*StarExpr) Pos
```
func (x *StarExpr) Pos() token.Pos
```
type Stmt
---------
All statement nodes implement the Stmt interface.
```
type Stmt interface {
Node
// contains filtered or unexported methods
}
```
type StructType
---------------
A StructType node represents a struct type.
```
type StructType struct {
Struct token.Pos // position of "struct" keyword
Fields *FieldList // list of field declarations
Incomplete bool // true if (source) fields are missing in the Fields list
}
```
### func (\*StructType) End
```
func (x *StructType) End() token.Pos
```
### func (\*StructType) Pos
```
func (x *StructType) Pos() token.Pos
```
type SwitchStmt
---------------
A SwitchStmt node represents an expression switch statement.
```
type SwitchStmt struct {
Switch token.Pos // position of "switch" keyword
Init Stmt // initialization statement; or nil
Tag Expr // tag expression; or nil
Body *BlockStmt // CaseClauses only
}
```
### func (\*SwitchStmt) End
```
func (s *SwitchStmt) End() token.Pos
```
### func (\*SwitchStmt) Pos
```
func (s *SwitchStmt) Pos() token.Pos
```
type TypeAssertExpr
-------------------
A TypeAssertExpr node represents an expression followed by a type assertion.
```
type TypeAssertExpr struct {
X Expr // expression
Lparen token.Pos // position of "("; added in Go 1.2
Type Expr // asserted type; nil means type switch X.(type)
Rparen token.Pos // position of ")"; added in Go 1.2
}
```
### func (\*TypeAssertExpr) End
```
func (x *TypeAssertExpr) End() token.Pos
```
### func (\*TypeAssertExpr) Pos
```
func (x *TypeAssertExpr) Pos() token.Pos
```
type TypeSpec
-------------
A TypeSpec node represents a type declaration (TypeSpec production).
```
type TypeSpec struct {
Doc *CommentGroup // associated documentation; or nil
Name *Ident // type name
TypeParams *FieldList // type parameters; or nil; added in Go 1.18
Assign token.Pos // position of '=', if any; added in Go 1.9
Type Expr // *Ident, *ParenExpr, *SelectorExpr, *StarExpr, or any of the *XxxTypes
Comment *CommentGroup // line comments; or nil
}
```
### func (\*TypeSpec) End
```
func (s *TypeSpec) End() token.Pos
```
### func (\*TypeSpec) Pos
```
func (s *TypeSpec) Pos() token.Pos
```
type TypeSwitchStmt
-------------------
A TypeSwitchStmt node represents a type switch statement.
```
type TypeSwitchStmt struct {
Switch token.Pos // position of "switch" keyword
Init Stmt // initialization statement; or nil
Assign Stmt // x := y.(type) or y.(type)
Body *BlockStmt // CaseClauses only
}
```
### func (\*TypeSwitchStmt) End
```
func (s *TypeSwitchStmt) End() token.Pos
```
### func (\*TypeSwitchStmt) Pos
```
func (s *TypeSwitchStmt) Pos() token.Pos
```
type UnaryExpr
--------------
A UnaryExpr node represents a unary expression. Unary "\*" expressions are represented via StarExpr nodes.
```
type UnaryExpr struct {
OpPos token.Pos // position of Op
Op token.Token // operator
X Expr // operand
}
```
### func (\*UnaryExpr) End
```
func (x *UnaryExpr) End() token.Pos
```
### func (\*UnaryExpr) Pos
```
func (x *UnaryExpr) Pos() token.Pos
```
type ValueSpec
--------------
A ValueSpec node represents a constant or variable declaration (ConstSpec or VarSpec production).
```
type ValueSpec struct {
Doc *CommentGroup // associated documentation; or nil
Names []*Ident // value names (len(Names) > 0)
Type Expr // value type; or nil
Values []Expr // initial values; or nil
Comment *CommentGroup // line comments; or nil
}
```
### func (\*ValueSpec) End
```
func (s *ValueSpec) End() token.Pos
```
### func (\*ValueSpec) Pos
```
func (s *ValueSpec) Pos() token.Pos
```
type Visitor
------------
A Visitor's Visit method is invoked for each node encountered by Walk. If the result visitor w is not nil, Walk visits each of the children of node with the visitor w, followed by a call of w.Visit(nil).
```
type Visitor interface {
Visit(node Node) (w Visitor)
}
```
| programming_docs |
go Package doc Package doc
============
* `import "go/doc"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package doc extracts source code documentation from a Go AST.
Index
-----
* [Variables](#pkg-variables)
* [func IsPredeclared(s string) bool](#IsPredeclared)
* [func Synopsis(text string) string](#Synopsis)
* [func ToHTML(w io.Writer, text string, words map[string]string)](#ToHTML)
* [func ToText(w io.Writer, text string, prefix, codePrefix string, width int)](#ToText)
* [type Example](#Example)
* [func Examples(testFiles ...\*ast.File) []\*Example](#Examples)
* [type Filter](#Filter)
* [type Func](#Func)
* [type Mode](#Mode)
* [type Note](#Note)
* [type Package](#Package)
* [func New(pkg \*ast.Package, importPath string, mode Mode) \*Package](#New)
* [func NewFromFiles(fset \*token.FileSet, files []\*ast.File, importPath string, opts ...any) (\*Package, error)](#NewFromFiles)
* [func (p \*Package) Filter(f Filter)](#Package.Filter)
* [func (p \*Package) HTML(text string) []byte](#Package.HTML)
* [func (p \*Package) Markdown(text string) []byte](#Package.Markdown)
* [func (p \*Package) Parser() \*comment.Parser](#Package.Parser)
* [func (p \*Package) Printer() \*comment.Printer](#Package.Printer)
* [func (p \*Package) Synopsis(text string) string](#Package.Synopsis)
* [func (p \*Package) Text(text string) []byte](#Package.Text)
* [type Type](#Type)
* [type Value](#Value)
### Examples
[NewFromFiles](#example_NewFromFiles) ### Package files
comment.go doc.go example.go exports.go filter.go reader.go synopsis.go
Variables
---------
IllegalPrefixes is a list of lower-case prefixes that identify a comment as not being a doc comment. This helps to avoid misinterpreting the common mistake of a copyright notice immediately before a package statement as being a doc comment.
```
var IllegalPrefixes = []string{
"copyright",
"all rights",
"author",
}
```
func IsPredeclared 1.8
----------------------
```
func IsPredeclared(s string) bool
```
IsPredeclared reports whether s is a predeclared identifier.
func Synopsis
-------------
```
func Synopsis(text string) string
```
Synopsis returns a cleaned version of the first sentence in text.
Deprecated: New programs should use [Package.Synopsis](#Package.Synopsis) instead, which handles links in text properly.
func ToHTML
-----------
```
func ToHTML(w io.Writer, text string, words map[string]string)
```
ToHTML converts comment text to formatted HTML.
Deprecated: ToHTML cannot identify documentation links in the doc comment, because they depend on knowing what package the text came from, which is not included in this API.
Given the \*[doc.Package](#Package) p where text was found, ToHTML(w, text, nil) can be replaced by:
```
w.Write(p.HTML(text))
```
which is in turn shorthand for:
```
w.Write(p.Printer().HTML(p.Parser().Parse(text)))
```
If words may be non-nil, the longer replacement is:
```
parser := p.Parser()
parser.Words = words
w.Write(p.Printer().HTML(parser.Parse(d)))
```
func ToText
-----------
```
func ToText(w io.Writer, text string, prefix, codePrefix string, width int)
```
ToText converts comment text to formatted text.
Deprecated: ToText cannot identify documentation links in the doc comment, because they depend on knowing what package the text came from, which is not included in this API.
Given the \*[doc.Package](#Package) p where text was found, ToText(w, text, "", "\t", 80) can be replaced by:
```
w.Write(p.Text(text))
```
In the general case, ToText(w, text, prefix, codePrefix, width) can be replaced by:
```
d := p.Parser().Parse(text)
pr := p.Printer()
pr.TextPrefix = prefix
pr.TextCodePrefix = codePrefix
pr.TextWidth = width
w.Write(pr.Text(d))
```
See the documentation for [Package.Text](#Package.Text) and comment.Printer.Text for more details.
type Example
------------
An Example represents an example function found in a test source file.
```
type Example struct {
Name string // name of the item being exemplified (including optional suffix)
Suffix string // example suffix, without leading '_' (only populated by NewFromFiles); added in Go 1.14
Doc string // example function doc string
Code ast.Node
Play *ast.File // a whole program version of the example; added in Go 1.1
Comments []*ast.CommentGroup
Output string // expected output
Unordered bool // Go 1.7
EmptyOutput bool // expect empty output; added in Go 1.1
Order int // original source code order; added in Go 1.1
}
```
### func Examples
```
func Examples(testFiles ...*ast.File) []*Example
```
Examples returns the examples found in testFiles, sorted by Name field. The Order fields record the order in which the examples were encountered. The Suffix field is not populated when Examples is called directly, it is only populated by NewFromFiles for examples it finds in \_test.go files.
Playable Examples must be in a package whose name ends in "\_test". An Example is "playable" (the Play field is non-nil) in either of these circumstances:
* The example function is self-contained: the function references only identifiers from other packages (or predeclared identifiers, such as "int") and the test file does not include a dot import.
* The entire test file is the example: the file contains exactly one example function, zero test, fuzz test, or benchmark function, and at least one top-level function, type, variable, or constant declaration other than the example function.
type Filter
-----------
```
type Filter func(string) bool
```
type Func
---------
Func is the documentation for a func declaration.
```
type Func struct {
Doc string
Name string
Decl *ast.FuncDecl
// methods
// (for functions, these fields have the respective zero value)
Recv string // actual receiver "T" or "*T" possibly followed by type parameters [P1, ..., Pn]
Orig string // original receiver "T" or "*T"
Level int // embedding level; 0 means not embedded
// Examples is a sorted list of examples associated with this
// function or method. Examples are extracted from _test.go files
// provided to NewFromFiles.
Examples []*Example // Go 1.14
}
```
type Mode
---------
Mode values control the operation of New and NewFromFiles.
```
type Mode int
```
```
const (
// AllDecls says to extract documentation for all package-level
// declarations, not just exported ones.
AllDecls Mode = 1 << iota
// AllMethods says to show all embedded methods, not just the ones of
// invisible (unexported) anonymous fields.
AllMethods
// PreserveAST says to leave the AST unmodified. Originally, pieces of
// the AST such as function bodies were nil-ed out to save memory in
// godoc, but not all programs want that behavior.
PreserveAST
)
```
type Note 1.1
-------------
A Note represents a marked comment starting with "MARKER(uid): note body". Any note with a marker of 2 or more upper case [A-Z] letters and a uid of at least one character is recognized. The ":" following the uid is optional. Notes are collected in the Package.Notes map indexed by the notes marker.
```
type Note struct {
Pos, End token.Pos // position range of the comment containing the marker
UID string // uid found with the marker
Body string // note body text
}
```
type Package
------------
Package is the documentation for an entire package.
```
type Package struct {
Doc string
Name string
ImportPath string
Imports []string
Filenames []string
Notes map[string][]*Note // Go 1.1
// Deprecated: For backward compatibility Bugs is still populated,
// but all new code should use Notes instead.
Bugs []string
// declarations
Consts []*Value
Types []*Type
Vars []*Value
Funcs []*Func
// Examples is a sorted list of examples associated with
// the package. Examples are extracted from _test.go files
// provided to NewFromFiles.
Examples []*Example // Go 1.14
// contains filtered or unexported fields
}
```
### func New
```
func New(pkg *ast.Package, importPath string, mode Mode) *Package
```
New computes the package documentation for the given package AST. New takes ownership of the AST pkg and may edit or overwrite it. To have the Examples fields populated, use NewFromFiles and include the package's \_test.go files.
### func NewFromFiles 1.14
```
func NewFromFiles(fset *token.FileSet, files []*ast.File, importPath string, opts ...any) (*Package, error)
```
NewFromFiles computes documentation for a package.
The package is specified by a list of \*ast.Files and corresponding file set, which must not be nil. NewFromFiles uses all provided files when computing documentation, so it is the caller's responsibility to provide only the files that match the desired build context. "go/build".Context.MatchFile can be used for determining whether a file matches a build context with the desired GOOS and GOARCH values, and other build constraints. The import path of the package is specified by importPath.
Examples found in \_test.go files are associated with the corresponding type, function, method, or the package, based on their name. If the example has a suffix in its name, it is set in the Example.Suffix field. Examples with malformed names are skipped.
Optionally, a single extra argument of type Mode can be provided to control low-level aspects of the documentation extraction behavior.
NewFromFiles takes ownership of the AST files and may edit them, unless the PreserveAST Mode bit is on.
#### Example
This example illustrates how to use NewFromFiles to compute package documentation with examples.
Code:
```
// src and test are two source files that make up
// a package whose documentation will be computed.
const src = `
// This is the package comment.
package p
import "fmt"
// This comment is associated with the Greet function.
func Greet(who string) {
fmt.Printf("Hello, %s!\n", who)
}
`
const test = `
package p_test
// This comment is associated with the ExampleGreet_world example.
func ExampleGreet_world() {
Greet("world")
}
`
// Create the AST by parsing src and test.
fset := token.NewFileSet()
files := []*ast.File{
mustParse(fset, "src.go", src),
mustParse(fset, "src_test.go", test),
}
// Compute package documentation with examples.
p, err := doc.NewFromFiles(fset, files, "example.com/p")
if err != nil {
panic(err)
}
fmt.Printf("package %s - %s", p.Name, p.Doc)
fmt.Printf("func %s - %s", p.Funcs[0].Name, p.Funcs[0].Doc)
fmt.Printf(" ⤷ example with suffix %q - %s", p.Funcs[0].Examples[0].Suffix, p.Funcs[0].Examples[0].Doc)
```
Output:
```
package p - This is the package comment.
func Greet - This comment is associated with the Greet function.
⤷ example with suffix "world" - This comment is associated with the ExampleGreet_world example.
```
### func (\*Package) Filter
```
func (p *Package) Filter(f Filter)
```
Filter eliminates documentation for names that don't pass through the filter f. TODO(gri): Recognize "Type.Method" as a name.
### func (\*Package) HTML 1.19
```
func (p *Package) HTML(text string) []byte
```
HTML returns formatted HTML for the doc comment text.
To customize details of the HTML, use [Package.Printer](#Package.Printer) to obtain a comment.Printer, and configure it before calling its HTML method.
### func (\*Package) Markdown 1.19
```
func (p *Package) Markdown(text string) []byte
```
Markdown returns formatted Markdown for the doc comment text.
To customize details of the Markdown, use [Package.Printer](#Package.Printer) to obtain a comment.Printer, and configure it before calling its Markdown method.
### func (\*Package) Parser 1.19
```
func (p *Package) Parser() *comment.Parser
```
Parser returns a doc comment parser configured for parsing doc comments from package p. Each call returns a new parser, so that the caller may customize it before use.
### func (\*Package) Printer 1.19
```
func (p *Package) Printer() *comment.Printer
```
Printer returns a doc comment printer configured for printing doc comments from package p. Each call returns a new printer, so that the caller may customize it before use.
### func (\*Package) Synopsis 1.19
```
func (p *Package) Synopsis(text string) string
```
Synopsis returns a cleaned version of the first sentence in text. That sentence ends after the first period followed by space and not preceded by exactly one uppercase letter, or at the first paragraph break. The result string has no \n, \r, or \t characters and uses only single spaces between words. If text starts with any of the IllegalPrefixes, the result is the empty string.
### func (\*Package) Text 1.19
```
func (p *Package) Text(text string) []byte
```
Text returns formatted text for the doc comment text, wrapped to 80 Unicode code points and using tabs for code block indentation.
To customize details of the formatting, use [Package.Printer](#Package.Printer) to obtain a comment.Printer, and configure it before calling its Text method.
type Type
---------
Type is the documentation for a type declaration.
```
type Type struct {
Doc string
Name string
Decl *ast.GenDecl
// associated declarations
Consts []*Value // sorted list of constants of (mostly) this type
Vars []*Value // sorted list of variables of (mostly) this type
Funcs []*Func // sorted list of functions returning this type
Methods []*Func // sorted list of methods (including embedded ones) of this type
// Examples is a sorted list of examples associated with
// this type. Examples are extracted from _test.go files
// provided to NewFromFiles.
Examples []*Example // Go 1.14
}
```
type Value
----------
Value is the documentation for a (possibly grouped) var or const declaration.
```
type Value struct {
Doc string
Names []string // var or const names in declaration order
Decl *ast.GenDecl
// contains filtered or unexported fields
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [comment](comment/index) | Package comment implements parsing and reformatting of Go doc comments, (documentation comments), which are comments that immediately precede a top-level declaration of a package, const, func, type, or var. |
go Package comment Package comment
================
* `import "go/doc/comment"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package comment implements parsing and reformatting of Go doc comments, (documentation comments), which are comments that immediately precede a top-level declaration of a package, const, func, type, or var.
Go doc comment syntax is a simplified subset of Markdown that supports links, headings, paragraphs, lists (without nesting), and preformatted text blocks. The details of the syntax are documented at <https://go.dev/doc/comment>.
To parse the text associated with a doc comment (after removing comment markers), use a [Parser](#Parser):
```
var p comment.Parser
doc := p.Parse(text)
```
The result is a [\*Doc](#Doc). To reformat it as a doc comment, HTML, Markdown, or plain text, use a [Printer](#Printer):
```
var pr comment.Printer
os.Stdout.Write(pr.Text(doc))
```
The [Parser](#Parser) and [Printer](#Printer) types are structs whose fields can be modified to customize the operations. For details, see the documentation for those types.
Use cases that need additional control over reformatting can implement their own logic by inspecting the parsed syntax itself. See the documentation for [Doc](#Doc), [Block](#Block), [Text](#Text) for an overview and links to additional types.
Index
-----
* [func DefaultLookupPackage(name string) (importPath string, ok bool)](#DefaultLookupPackage)
* [type Block](#Block)
* [type Code](#Code)
* [type Doc](#Doc)
* [type DocLink](#DocLink)
* [func (l \*DocLink) DefaultURL(baseURL string) string](#DocLink.DefaultURL)
* [type Heading](#Heading)
* [func (h \*Heading) DefaultID() string](#Heading.DefaultID)
* [type Italic](#Italic)
* [type Link](#Link)
* [type LinkDef](#LinkDef)
* [type List](#List)
* [func (l \*List) BlankBefore() bool](#List.BlankBefore)
* [func (l \*List) BlankBetween() bool](#List.BlankBetween)
* [type ListItem](#ListItem)
* [type Paragraph](#Paragraph)
* [type Parser](#Parser)
* [func (p \*Parser) Parse(text string) \*Doc](#Parser.Parse)
* [type Plain](#Plain)
* [type Printer](#Printer)
* [func (p \*Printer) Comment(d \*Doc) []byte](#Printer.Comment)
* [func (p \*Printer) HTML(d \*Doc) []byte](#Printer.HTML)
* [func (p \*Printer) Markdown(d \*Doc) []byte](#Printer.Markdown)
* [func (p \*Printer) Text(d \*Doc) []byte](#Printer.Text)
* [type Text](#Text)
### Package files
doc.go html.go markdown.go parse.go print.go std.go text.go
func DefaultLookupPackage 1.19
------------------------------
```
func DefaultLookupPackage(name string) (importPath string, ok bool)
```
DefaultLookupPackage is the default package lookup function, used when [Parser](#Parser).LookupPackage is nil. It recognizes names of the packages from the standard library with single-element import paths, such as math, which would otherwise be impossible to name.
Note that the go/doc package provides a more sophisticated lookup based on the imports used in the current package.
type Block 1.19
---------------
A Block is block-level content in a doc comment, one of [\*Code](#Code), [\*Heading](#Heading), [\*List](#List), or [\*Paragraph](#Paragraph).
```
type Block interface {
// contains filtered or unexported methods
}
```
type Code 1.19
--------------
A Code is a preformatted code block.
```
type Code struct {
// Text is the preformatted text, ending with a newline character.
// It may be multiple lines, each of which ends with a newline character.
// It is never empty, nor does it start or end with a blank line.
Text string
}
```
type Doc 1.19
-------------
A Doc is a parsed Go doc comment.
```
type Doc struct {
// Content is the sequence of content blocks in the comment.
Content []Block
// Links is the link definitions in the comment.
Links []*LinkDef
}
```
type DocLink 1.19
-----------------
A DocLink is a link to documentation for a Go package or symbol.
```
type DocLink struct {
Text []Text // text of link
// ImportPath, Recv, and Name identify the Go package or symbol
// that is the link target. The potential combinations of
// non-empty fields are:
// - ImportPath: a link to another package
// - ImportPath, Name: a link to a const, func, type, or var in another package
// - ImportPath, Recv, Name: a link to a method in another package
// - Name: a link to a const, func, type, or var in this package
// - Recv, Name: a link to a method in this package
ImportPath string // import path
Recv string // receiver type, without any pointer star, for methods
Name string // const, func, type, var, or method name
}
```
### func (\*DocLink) DefaultURL 1.19
```
func (l *DocLink) DefaultURL(baseURL string) string
```
DefaultURL constructs and returns the documentation URL for l, using baseURL as a prefix for links to other packages.
The possible forms returned by DefaultURL are:
* baseURL/ImportPath, for a link to another package
* baseURL/ImportPath#Name, for a link to a const, func, type, or var in another package
* baseURL/ImportPath#Recv.Name, for a link to a method in another package
* #Name, for a link to a const, func, type, or var in this package
* #Recv.Name, for a link to a method in this package
If baseURL ends in a trailing slash, then DefaultURL inserts a slash between ImportPath and # in the anchored forms. For example, here are some baseURL values and URLs they can generate:
```
"/pkg/" → "/pkg/math/#Sqrt"
"/pkg" → "/pkg/math#Sqrt"
"/" → "/math/#Sqrt"
"" → "/math#Sqrt"
```
type Heading 1.19
-----------------
A Heading is a doc comment heading.
```
type Heading struct {
Text []Text // the heading text
}
```
### func (\*Heading) DefaultID 1.19
```
func (h *Heading) DefaultID() string
```
DefaultID returns the default anchor ID for the heading h.
The default anchor ID is constructed by converting every rune that is not alphanumeric ASCII to an underscore and then adding the prefix “hdr-”. For example, if the heading text is “Go Doc Comments”, the default ID is “hdr-Go\_Doc\_Comments”.
type Italic 1.19
----------------
An Italic is a string rendered as italicized text.
```
type Italic string
```
type Link 1.19
--------------
A Link is a link to a specific URL.
```
type Link struct {
Auto bool // is this an automatic (implicit) link of a literal URL?
Text []Text // text of link
URL string // target URL of link
}
```
type LinkDef 1.19
-----------------
A LinkDef is a single link definition.
```
type LinkDef struct {
Text string // the link text
URL string // the link URL
Used bool // whether the comment uses the definition
}
```
type List 1.19
--------------
A List is a numbered or bullet list. Lists are always non-empty: len(Items) > 0. In a numbered list, every Items[i].Number is a non-empty string. In a bullet list, every Items[i].Number is an empty string.
```
type List struct {
// Items is the list items.
Items []*ListItem
// ForceBlankBefore indicates that the list must be
// preceded by a blank line when reformatting the comment,
// overriding the usual conditions. See the BlankBefore method.
//
// The comment parser sets ForceBlankBefore for any list
// that is preceded by a blank line, to make sure
// the blank line is preserved when printing.
ForceBlankBefore bool
// ForceBlankBetween indicates that list items must be
// separated by blank lines when reformatting the comment,
// overriding the usual conditions. See the BlankBetween method.
//
// The comment parser sets ForceBlankBetween for any list
// that has a blank line between any two of its items, to make sure
// the blank lines are preserved when printing.
ForceBlankBetween bool
}
```
### func (\*List) BlankBefore 1.19
```
func (l *List) BlankBefore() bool
```
BlankBefore reports whether a reformatting of the comment should include a blank line before the list. The default rule is the same as for [BlankBetween]: if the list item content contains any blank lines (meaning at least one item has multiple paragraphs) then the list itself must be preceded by a blank line. A preceding blank line can be forced by setting [List](#List).ForceBlankBefore.
### func (\*List) BlankBetween 1.19
```
func (l *List) BlankBetween() bool
```
BlankBetween reports whether a reformatting of the comment should include a blank line between each pair of list items. The default rule is that if the list item content contains any blank lines (meaning at least one item has multiple paragraphs) then list items must themselves be separated by blank lines. Blank line separators can be forced by setting [List](#List).ForceBlankBetween.
type ListItem 1.19
------------------
A ListItem is a single item in a numbered or bullet list.
```
type ListItem struct {
// Number is a decimal string in a numbered list
// or an empty string in a bullet list.
Number string // "1", "2", ...; "" for bullet list
// Content is the list content.
// Currently, restrictions in the parser and printer
// require every element of Content to be a *Paragraph.
Content []Block // Content of this item.
}
```
type Paragraph 1.19
-------------------
A Paragraph is a paragraph of text.
```
type Paragraph struct {
Text []Text
}
```
type Parser 1.19
----------------
A Parser is a doc comment parser. The fields in the struct can be filled in before calling Parse in order to customize the details of the parsing process.
```
type Parser struct {
// Words is a map of Go identifier words that
// should be italicized and potentially linked.
// If Words[w] is the empty string, then the word w
// is only italicized. Otherwise it is linked, using
// Words[w] as the link target.
// Words corresponds to the [go/doc.ToHTML] words parameter.
Words map[string]string
// LookupPackage resolves a package name to an import path.
//
// If LookupPackage(name) returns ok == true, then [name]
// (or [name.Sym] or [name.Sym.Method])
// is considered a documentation link to importPath's package docs.
// It is valid to return "", true, in which case name is considered
// to refer to the current package.
//
// If LookupPackage(name) returns ok == false,
// then [name] (or [name.Sym] or [name.Sym.Method])
// will not be considered a documentation link,
// except in the case where name is the full (but single-element) import path
// of a package in the standard library, such as in [math] or [io.Reader].
// LookupPackage is still called for such names,
// in order to permit references to imports of other packages
// with the same package names.
//
// Setting LookupPackage to nil is equivalent to setting it to
// a function that always returns "", false.
LookupPackage func(name string) (importPath string, ok bool)
// LookupSym reports whether a symbol name or method name
// exists in the current package.
//
// If LookupSym("", "Name") returns true, then [Name]
// is considered a documentation link for a const, func, type, or var.
//
// Similarly, if LookupSym("Recv", "Name") returns true,
// then [Recv.Name] is considered a documentation link for
// type Recv's method Name.
//
// Setting LookupSym to nil is equivalent to setting it to a function
// that always returns false.
LookupSym func(recv, name string) (ok bool)
}
```
### func (\*Parser) Parse 1.19
```
func (p *Parser) Parse(text string) *Doc
```
Parse parses the doc comment text and returns the \*Doc form. Comment markers (/\* // and \*/) in the text must have already been removed.
type Plain 1.19
---------------
A Plain is a string rendered as plain text (not italicized).
```
type Plain string
```
type Printer 1.19
-----------------
A Printer is a doc comment printer. The fields in the struct can be filled in before calling any of the printing methods in order to customize the details of the printing process.
```
type Printer struct {
// HeadingLevel is the nesting level used for
// HTML and Markdown headings.
// If HeadingLevel is zero, it defaults to level 3,
// meaning to use <h3> and ###.
HeadingLevel int
// HeadingID is a function that computes the heading ID
// (anchor tag) to use for the heading h when generating
// HTML and Markdown. If HeadingID returns an empty string,
// then the heading ID is omitted.
// If HeadingID is nil, h.DefaultID is used.
HeadingID func(h *Heading) string
// DocLinkURL is a function that computes the URL for the given DocLink.
// If DocLinkURL is nil, then link.DefaultURL(p.DocLinkBaseURL) is used.
DocLinkURL func(link *DocLink) string
// DocLinkBaseURL is used when DocLinkURL is nil,
// passed to [DocLink.DefaultURL] to construct a DocLink's URL.
// See that method's documentation for details.
DocLinkBaseURL string
// TextPrefix is a prefix to print at the start of every line
// when generating text output using the Text method.
TextPrefix string
// TextCodePrefix is the prefix to print at the start of each
// preformatted (code block) line when generating text output,
// instead of (not in addition to) TextPrefix.
// If TextCodePrefix is the empty string, it defaults to TextPrefix+"\t".
TextCodePrefix string
// TextWidth is the maximum width text line to generate,
// measured in Unicode code points,
// excluding TextPrefix and the newline character.
// If TextWidth is zero, it defaults to 80 minus the number of code points in TextPrefix.
// If TextWidth is negative, there is no limit.
TextWidth int
}
```
### func (\*Printer) Comment 1.19
```
func (p *Printer) Comment(d *Doc) []byte
```
Comment returns the standard Go formatting of the Doc, without any comment markers.
### func (\*Printer) HTML 1.19
```
func (p *Printer) HTML(d *Doc) []byte
```
HTML returns an HTML formatting of the Doc. See the [Printer](#Printer) documentation for ways to customize the HTML output.
### func (\*Printer) Markdown 1.19
```
func (p *Printer) Markdown(d *Doc) []byte
```
Markdown returns a Markdown formatting of the Doc. See the [Printer](#Printer) documentation for ways to customize the Markdown output.
### func (\*Printer) Text 1.19
```
func (p *Printer) Text(d *Doc) []byte
```
Text returns a textual formatting of the Doc. See the [Printer](#Printer) documentation for ways to customize the text output.
type Text 1.19
--------------
A Text is text-level content in a doc comment, one of [Plain](#Plain), [Italic](#Italic), [\*Link](#Link), or [\*DocLink](#DocLink).
```
type Text interface {
// contains filtered or unexported methods
}
```
| programming_docs |
go Package build Package build
==============
* `import "go/build"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package build gathers information about Go packages.
### Go Path
The Go path is a list of directory trees containing Go source code. It is consulted to resolve imports that cannot be found in the standard Go tree. The default path is the value of the GOPATH environment variable, interpreted as a path list appropriate to the operating system (on Unix, the variable is a colon-separated string; on Windows, a semicolon-separated string; on Plan 9, a list).
Each directory listed in the Go path must have a prescribed structure:
The src/ directory holds source code. The path below 'src' determines the import path or executable name.
The pkg/ directory holds installed package objects. As in the Go tree, each target operating system and architecture pair has its own subdirectory of pkg (pkg/GOOS\_GOARCH).
If DIR is a directory listed in the Go path, a package with source in DIR/src/foo/bar can be imported as "foo/bar" and has its compiled form installed to "DIR/pkg/GOOS\_GOARCH/foo/bar.a" (or, for gccgo, "DIR/pkg/gccgo/foo/libbar.a").
The bin/ directory holds compiled commands. Each command is named for its source directory, but only using the final element, not the entire path. That is, the command with source in DIR/src/foo/quux is installed into DIR/bin/quux, not DIR/bin/foo/quux. The foo/ is stripped so that you can add DIR/bin to your PATH to get at the installed commands.
Here's an example directory layout:
```
GOPATH=/home/user/gocode
/home/user/gocode/
src/
foo/
bar/ (go code in package bar)
x.go
quux/ (go code in package main)
y.go
bin/
quux (installed command)
pkg/
linux_amd64/
foo/
bar.a (installed package object)
```
### Build Constraints
A build constraint, also known as a build tag, is a condition under which a file should be included in the package. Build constraints are given by a line comment that begins
```
//go:build
```
Build constraints may also be part of a file's name (for example, source\_windows.go will only be included if the target operating system is windows).
See 'go help buildconstraint' (<https://golang.org/cmd/go/#hdr-Build_constraints>) for details.
### Binary-Only Packages
In Go 1.12 and earlier, it was possible to distribute packages in binary form without including the source code used for compiling the package. The package was distributed with a source file not excluded by build constraints and containing a "//go:binary-only-package" comment. Like a build constraint, this comment appeared at the top of a file, preceded only by blank lines and other line comments and with a blank line following the comment, to separate it from the package documentation. Unlike build constraints, this comment is only recognized in non-test Go source files.
The minimal source code for a binary-only package was therefore:
```
//go:binary-only-package
package mypkg
```
The source code could include additional Go code. That code was never compiled but would be processed by tools like godoc and might be useful as end-user documentation.
"go build" and other commands no longer support binary-only-packages. Import and ImportDir will still set the BinaryOnly flag in packages containing these comments for use in tools and error messages.
Index
-----
* [Variables](#pkg-variables)
* [func ArchChar(goarch string) (string, error)](#ArchChar)
* [func IsLocalImport(path string) bool](#IsLocalImport)
* [type Context](#Context)
* [func (ctxt \*Context) Import(path string, srcDir string, mode ImportMode) (\*Package, error)](#Context.Import)
* [func (ctxt \*Context) ImportDir(dir string, mode ImportMode) (\*Package, error)](#Context.ImportDir)
* [func (ctxt \*Context) MatchFile(dir, name string) (match bool, err error)](#Context.MatchFile)
* [func (ctxt \*Context) SrcDirs() []string](#Context.SrcDirs)
* [type ImportMode](#ImportMode)
* [type MultiplePackageError](#MultiplePackageError)
* [func (e \*MultiplePackageError) Error() string](#MultiplePackageError.Error)
* [type NoGoError](#NoGoError)
* [func (e \*NoGoError) Error() string](#NoGoError.Error)
* [type Package](#Package)
* [func Import(path, srcDir string, mode ImportMode) (\*Package, error)](#Import)
* [func ImportDir(dir string, mode ImportMode) (\*Package, error)](#ImportDir)
* [func (p \*Package) IsCommand() bool](#Package.IsCommand)
### Package files
build.go doc.go gc.go read.go syslist.go zcgo.go
Variables
---------
ToolDir is the directory containing build tools.
```
var ToolDir = getToolDir()
```
func ArchChar
-------------
```
func ArchChar(goarch string) (string, error)
```
ArchChar returns "?" and an error. In earlier versions of Go, the returned string was used to derive the compiler and linker tool names, the default object file suffix, and the default linker output name. As of Go 1.5, those strings no longer vary by architecture; they are compile, link, .o, and a.out, respectively.
func IsLocalImport
------------------
```
func IsLocalImport(path string) bool
```
IsLocalImport reports whether the import path is a local import path, like ".", "..", "./foo", or "../foo".
type Context
------------
A Context specifies the supporting context for a build.
```
type Context struct {
GOARCH string // target architecture
GOOS string // target operating system
GOROOT string // Go root
GOPATH string // Go paths
// Dir is the caller's working directory, or the empty string to use
// the current directory of the running process. In module mode, this is used
// to locate the main module.
//
// If Dir is non-empty, directories passed to Import and ImportDir must
// be absolute.
Dir string // Go 1.14
CgoEnabled bool // whether cgo files are included
UseAllFiles bool // use files regardless of go:build lines, file names
Compiler string // compiler to assume when computing target paths
// The build, tool, and release tags specify build constraints
// that should be considered satisfied when processing go:build lines.
// Clients creating a new context may customize BuildTags, which
// defaults to empty, but it is usually an error to customize ToolTags or ReleaseTags.
// ToolTags defaults to build tags appropriate to the current Go toolchain configuration.
// ReleaseTags defaults to the list of Go releases the current release is compatible with.
// BuildTags is not set for the Default build Context.
// In addition to the BuildTags, ToolTags, and ReleaseTags, build constraints
// consider the values of GOARCH and GOOS as satisfied tags.
// The last element in ReleaseTags is assumed to be the current release.
BuildTags []string
ToolTags []string // Go 1.17
ReleaseTags []string // Go 1.1
// The install suffix specifies a suffix to use in the name of the installation
// directory. By default it is empty, but custom builds that need to keep
// their outputs separate can set InstallSuffix to do so. For example, when
// using the race detector, the go command uses InstallSuffix = "race", so
// that on a Linux/386 system, packages are written to a directory named
// "linux_386_race" instead of the usual "linux_386".
InstallSuffix string // Go 1.1
// JoinPath joins the sequence of path fragments into a single path.
// If JoinPath is nil, Import uses filepath.Join.
JoinPath func(elem ...string) string
// SplitPathList splits the path list into a slice of individual paths.
// If SplitPathList is nil, Import uses filepath.SplitList.
SplitPathList func(list string) []string
// IsAbsPath reports whether path is an absolute path.
// If IsAbsPath is nil, Import uses filepath.IsAbs.
IsAbsPath func(path string) bool
// IsDir reports whether the path names a directory.
// If IsDir is nil, Import calls os.Stat and uses the result's IsDir method.
IsDir func(path string) bool
// HasSubdir reports whether dir is lexically a subdirectory of
// root, perhaps multiple levels below. It does not try to check
// whether dir exists.
// If so, HasSubdir sets rel to a slash-separated path that
// can be joined to root to produce a path equivalent to dir.
// If HasSubdir is nil, Import uses an implementation built on
// filepath.EvalSymlinks.
HasSubdir func(root, dir string) (rel string, ok bool)
// ReadDir returns a slice of fs.FileInfo, sorted by Name,
// describing the content of the named directory.
// If ReadDir is nil, Import uses os.ReadDir.
ReadDir func(dir string) ([]fs.FileInfo, error)
// OpenFile opens a file (not a directory) for reading.
// If OpenFile is nil, Import uses os.Open.
OpenFile func(path string) (io.ReadCloser, error)
}
```
Default is the default Context for builds. It uses the GOARCH, GOOS, GOROOT, and GOPATH environment variables if set, or else the compiled code's GOARCH, GOOS, and GOROOT.
```
var Default Context = defaultContext()
```
### func (\*Context) Import
```
func (ctxt *Context) Import(path string, srcDir string, mode ImportMode) (*Package, error)
```
Import returns details about the Go package named by the import path, interpreting local import paths relative to the srcDir directory. If the path is a local import path naming a package that can be imported using a standard import path, the returned package will set p.ImportPath to that path.
In the directory containing the package, .go, .c, .h, and .s files are considered part of the package except for:
* .go files in package documentation
* files starting with \_ or . (likely editor temporary files)
* files with build constraints not satisfied by the context
If an error occurs, Import returns a non-nil error and a non-nil \*Package containing partial information.
### func (\*Context) ImportDir
```
func (ctxt *Context) ImportDir(dir string, mode ImportMode) (*Package, error)
```
ImportDir is like Import but processes the Go package found in the named directory.
### func (\*Context) MatchFile 1.2
```
func (ctxt *Context) MatchFile(dir, name string) (match bool, err error)
```
MatchFile reports whether the file with the given name in the given directory matches the context and would be included in a Package created by ImportDir of that directory.
MatchFile considers the name of the file and may use ctxt.OpenFile to read some or all of the file's content.
### func (\*Context) SrcDirs
```
func (ctxt *Context) SrcDirs() []string
```
SrcDirs returns a list of package source root directories. It draws from the current Go root and Go path but omits directories that do not exist.
type ImportMode
---------------
An ImportMode controls the behavior of the Import method.
```
type ImportMode uint
```
```
const (
// If FindOnly is set, Import stops after locating the directory
// that should contain the sources for a package. It does not
// read any files in the directory.
FindOnly ImportMode = 1 << iota
// If AllowBinary is set, Import can be satisfied by a compiled
// package object without corresponding sources.
//
// Deprecated:
// The supported way to create a compiled-only package is to
// write source code containing a //go:binary-only-package comment at
// the top of the file. Such a package will be recognized
// regardless of this flag setting (because it has source code)
// and will have BinaryOnly set to true in the returned Package.
AllowBinary
// If ImportComment is set, parse import comments on package statements.
// Import returns an error if it finds a comment it cannot understand
// or finds conflicting comments in multiple source files.
// See golang.org/s/go14customimport for more information.
ImportComment
// By default, Import searches vendor directories
// that apply in the given source directory before searching
// the GOROOT and GOPATH roots.
// If an Import finds and returns a package using a vendor
// directory, the resulting ImportPath is the complete path
// to the package, including the path elements leading up
// to and including "vendor".
// For example, if Import("y", "x/subdir", 0) finds
// "x/vendor/y", the returned package's ImportPath is "x/vendor/y",
// not plain "y".
// See golang.org/s/go15vendor for more information.
//
// Setting IgnoreVendor ignores vendor directories.
//
// In contrast to the package's ImportPath,
// the returned package's Imports, TestImports, and XTestImports
// are always the exact import paths from the source files:
// Import makes no attempt to resolve or check those paths.
IgnoreVendor
)
```
type MultiplePackageError 1.4
-----------------------------
MultiplePackageError describes a directory containing multiple buildable Go source files for multiple packages.
```
type MultiplePackageError struct {
Dir string // directory containing files
Packages []string // package names found
Files []string // corresponding files: Files[i] declares package Packages[i]
}
```
### func (\*MultiplePackageError) Error 1.4
```
func (e *MultiplePackageError) Error() string
```
type NoGoError
--------------
NoGoError is the error used by Import to describe a directory containing no buildable Go source files. (It may still contain test files, files hidden by build tags, and so on.)
```
type NoGoError struct {
Dir string
}
```
### func (\*NoGoError) Error
```
func (e *NoGoError) Error() string
```
type Package
------------
A Package describes the Go package found in a directory.
```
type Package struct {
Dir string // directory containing package sources
Name string // package name
ImportComment string // path in import comment on package statement; added in Go 1.4
Doc string // documentation synopsis
ImportPath string // import path of package ("" if unknown)
Root string // root of Go tree where this package lives
SrcRoot string // package source root directory ("" if unknown)
PkgRoot string // package install root directory ("" if unknown)
PkgTargetRoot string // architecture dependent install root directory ("" if unknown); added in Go 1.5
BinDir string // command install directory ("" if unknown)
Goroot bool // package found in Go root
PkgObj string // installed .a file
AllTags []string // tags that can influence file selection in this directory; added in Go 1.2
ConflictDir string // this directory shadows Dir in $GOPATH; added in Go 1.2
BinaryOnly bool // cannot be rebuilt from source (has //go:binary-only-package comment); added in Go 1.7
// Source files
GoFiles []string // .go source files (excluding CgoFiles, TestGoFiles, XTestGoFiles)
CgoFiles []string // .go source files that import "C"
IgnoredGoFiles []string // .go source files ignored for this build (including ignored _test.go files); added in Go 1.1
InvalidGoFiles []string // .go source files with detected problems (parse error, wrong package name, and so on); added in Go 1.6
IgnoredOtherFiles []string // non-.go source files ignored for this build; added in Go 1.16
CFiles []string // .c source files
CXXFiles []string // .cc, .cpp and .cxx source files; added in Go 1.2
MFiles []string // .m (Objective-C) source files; added in Go 1.3
HFiles []string // .h, .hh, .hpp and .hxx source files
FFiles []string // .f, .F, .for and .f90 Fortran source files; added in Go 1.7
SFiles []string // .s source files
SwigFiles []string // .swig files; added in Go 1.1
SwigCXXFiles []string // .swigcxx files; added in Go 1.1
SysoFiles []string // .syso system object files to add to archive
// Cgo directives
CgoCFLAGS []string // Cgo CFLAGS directives
CgoCPPFLAGS []string // Cgo CPPFLAGS directives; added in Go 1.2
CgoCXXFLAGS []string // Cgo CXXFLAGS directives; added in Go 1.2
CgoFFLAGS []string // Cgo FFLAGS directives; added in Go 1.7
CgoLDFLAGS []string // Cgo LDFLAGS directives
CgoPkgConfig []string // Cgo pkg-config directives
// Test information
TestGoFiles []string // _test.go files in package
XTestGoFiles []string // _test.go files outside package
// Dependency information
Imports []string // import paths from GoFiles, CgoFiles
ImportPos map[string][]token.Position // line information for Imports
TestImports []string // import paths from TestGoFiles
TestImportPos map[string][]token.Position // line information for TestImports
XTestImports []string // import paths from XTestGoFiles
XTestImportPos map[string][]token.Position // line information for XTestImports
// //go:embed patterns found in Go source files
// For example, if a source file says
// //go:embed a* b.c
// then the list will contain those two strings as separate entries.
// (See package embed for more details about //go:embed.)
EmbedPatterns []string // patterns from GoFiles, CgoFiles; added in Go 1.16
EmbedPatternPos map[string][]token.Position // line information for EmbedPatterns; added in Go 1.16
TestEmbedPatterns []string // patterns from TestGoFiles; added in Go 1.16
TestEmbedPatternPos map[string][]token.Position // line information for TestEmbedPatterns; added in Go 1.16
XTestEmbedPatterns []string // patterns from XTestGoFiles; added in Go 1.16
XTestEmbedPatternPos map[string][]token.Position // line information for XTestEmbedPatternPos; added in Go 1.16
}
```
### func Import
```
func Import(path, srcDir string, mode ImportMode) (*Package, error)
```
Import is shorthand for Default.Import.
### func ImportDir
```
func ImportDir(dir string, mode ImportMode) (*Package, error)
```
ImportDir is shorthand for Default.ImportDir.
### func (\*Package) IsCommand
```
func (p *Package) IsCommand() bool
```
IsCommand reports whether the package is considered a command to be installed (not just a library). Packages named "main" are treated as commands.
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [constraint](constraint/index) | Package constraint implements parsing and evaluation of build constraint lines. |
go Package constraint Package constraint
===================
* `import "go/build/constraint"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package constraint implements parsing and evaluation of build constraint lines. See <https://golang.org/cmd/go/#hdr-Build_constraints> for documentation about build constraints themselves.
This package parses both the original “// +build” syntax and the “//go:build” syntax that was added in Go 1.17. See <https://golang.org/design/draft-gobuild> for details about the “//go:build” syntax.
Index
-----
* [func IsGoBuild(line string) bool](#IsGoBuild)
* [func IsPlusBuild(line string) bool](#IsPlusBuild)
* [func PlusBuildLines(x Expr) ([]string, error)](#PlusBuildLines)
* [type AndExpr](#AndExpr)
* [func (x \*AndExpr) Eval(ok func(tag string) bool) bool](#AndExpr.Eval)
* [func (x \*AndExpr) String() string](#AndExpr.String)
* [type Expr](#Expr)
* [func Parse(line string) (Expr, error)](#Parse)
* [type NotExpr](#NotExpr)
* [func (x \*NotExpr) Eval(ok func(tag string) bool) bool](#NotExpr.Eval)
* [func (x \*NotExpr) String() string](#NotExpr.String)
* [type OrExpr](#OrExpr)
* [func (x \*OrExpr) Eval(ok func(tag string) bool) bool](#OrExpr.Eval)
* [func (x \*OrExpr) String() string](#OrExpr.String)
* [type SyntaxError](#SyntaxError)
* [func (e \*SyntaxError) Error() string](#SyntaxError.Error)
* [type TagExpr](#TagExpr)
* [func (x \*TagExpr) Eval(ok func(tag string) bool) bool](#TagExpr.Eval)
* [func (x \*TagExpr) String() string](#TagExpr.String)
### Package files
expr.go
func IsGoBuild 1.16
-------------------
```
func IsGoBuild(line string) bool
```
IsGoBuild reports whether the line of text is a “//go:build” constraint. It only checks the prefix of the text, not that the expression itself parses.
func IsPlusBuild 1.16
---------------------
```
func IsPlusBuild(line string) bool
```
IsPlusBuild reports whether the line of text is a “// +build” constraint. It only checks the prefix of the text, not that the expression itself parses.
func PlusBuildLines 1.16
------------------------
```
func PlusBuildLines(x Expr) ([]string, error)
```
PlusBuildLines returns a sequence of “// +build” lines that evaluate to the build expression x. If the expression is too complex to convert directly to “// +build” lines, PlusBuildLines returns an error.
type AndExpr 1.16
-----------------
An AndExpr represents the expression X && Y.
```
type AndExpr struct {
X, Y Expr
}
```
### func (\*AndExpr) Eval 1.16
```
func (x *AndExpr) Eval(ok func(tag string) bool) bool
```
### func (\*AndExpr) String 1.16
```
func (x *AndExpr) String() string
```
type Expr 1.16
--------------
An Expr is a build tag constraint expression. The underlying concrete type is \*AndExpr, \*OrExpr, \*NotExpr, or \*TagExpr.
```
type Expr interface {
// String returns the string form of the expression,
// using the boolean syntax used in //go:build lines.
String() string
// Eval reports whether the expression evaluates to true.
// It calls ok(tag) as needed to find out whether a given build tag
// is satisfied by the current build configuration.
Eval(ok func(tag string) bool) bool
// contains filtered or unexported methods
}
```
### func Parse 1.16
```
func Parse(line string) (Expr, error)
```
Parse parses a single build constraint line of the form “//go:build ...” or “// +build ...” and returns the corresponding boolean expression.
type NotExpr 1.16
-----------------
A NotExpr represents the expression !X (the negation of X).
```
type NotExpr struct {
X Expr
}
```
### func (\*NotExpr) Eval 1.16
```
func (x *NotExpr) Eval(ok func(tag string) bool) bool
```
### func (\*NotExpr) String 1.16
```
func (x *NotExpr) String() string
```
type OrExpr 1.16
----------------
An OrExpr represents the expression X || Y.
```
type OrExpr struct {
X, Y Expr
}
```
### func (\*OrExpr) Eval 1.16
```
func (x *OrExpr) Eval(ok func(tag string) bool) bool
```
### func (\*OrExpr) String 1.16
```
func (x *OrExpr) String() string
```
type SyntaxError 1.16
---------------------
A SyntaxError reports a syntax error in a parsed build expression.
```
type SyntaxError struct {
Offset int // byte offset in input where error was detected
Err string // description of error
}
```
### func (\*SyntaxError) Error 1.16
```
func (e *SyntaxError) Error() string
```
type TagExpr 1.16
-----------------
A TagExpr is an Expr for the single tag Tag.
```
type TagExpr struct {
Tag string // for example, “linux” or “cgo”
}
```
### func (\*TagExpr) Eval 1.16
```
func (x *TagExpr) Eval(ok func(tag string) bool) bool
```
### func (\*TagExpr) String 1.16
```
func (x *TagExpr) String() string
```
| programming_docs |
go Package scanner Package scanner
================
* `import "go/scanner"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package scanner implements a scanner for Go source text. It takes a []byte as source which can then be tokenized through repeated calls to the Scan method.
Index
-----
* [func PrintError(w io.Writer, err error)](#PrintError)
* [type Error](#Error)
* [func (e Error) Error() string](#Error.Error)
* [type ErrorHandler](#ErrorHandler)
* [type ErrorList](#ErrorList)
* [func (p \*ErrorList) Add(pos token.Position, msg string)](#ErrorList.Add)
* [func (p ErrorList) Err() error](#ErrorList.Err)
* [func (p ErrorList) Error() string](#ErrorList.Error)
* [func (p ErrorList) Len() int](#ErrorList.Len)
* [func (p ErrorList) Less(i, j int) bool](#ErrorList.Less)
* [func (p \*ErrorList) RemoveMultiples()](#ErrorList.RemoveMultiples)
* [func (p \*ErrorList) Reset()](#ErrorList.Reset)
* [func (p ErrorList) Sort()](#ErrorList.Sort)
* [func (p ErrorList) Swap(i, j int)](#ErrorList.Swap)
* [type Mode](#Mode)
* [type Scanner](#Scanner)
* [func (s \*Scanner) Init(file \*token.File, src []byte, err ErrorHandler, mode Mode)](#Scanner.Init)
* [func (s \*Scanner) Scan() (pos token.Pos, tok token.Token, lit string)](#Scanner.Scan)
### Examples
[Scanner.Scan](#example_Scanner_Scan) ### Package files
errors.go scanner.go
func PrintError
---------------
```
func PrintError(w io.Writer, err error)
```
PrintError is a utility function that prints a list of errors to w, one error per line, if the err parameter is an ErrorList. Otherwise it prints the err string.
type Error
----------
In an ErrorList, an error is represented by an \*Error. The position Pos, if valid, points to the beginning of the offending token, and the error condition is described by Msg.
```
type Error struct {
Pos token.Position
Msg string
}
```
### func (Error) Error
```
func (e Error) Error() string
```
Error implements the error interface.
type ErrorHandler
-----------------
An ErrorHandler may be provided to Scanner.Init. If a syntax error is encountered and a handler was installed, the handler is called with a position and an error message. The position points to the beginning of the offending token.
```
type ErrorHandler func(pos token.Position, msg string)
```
type ErrorList
--------------
ErrorList is a list of \*Errors. The zero value for an ErrorList is an empty ErrorList ready to use.
```
type ErrorList []*Error
```
### func (\*ErrorList) Add
```
func (p *ErrorList) Add(pos token.Position, msg string)
```
Add adds an Error with given position and error message to an ErrorList.
### func (ErrorList) Err
```
func (p ErrorList) Err() error
```
Err returns an error equivalent to this error list. If the list is empty, Err returns nil.
### func (ErrorList) Error
```
func (p ErrorList) Error() string
```
An ErrorList implements the error interface.
### func (ErrorList) Len
```
func (p ErrorList) Len() int
```
ErrorList implements the sort Interface.
### func (ErrorList) Less
```
func (p ErrorList) Less(i, j int) bool
```
### func (\*ErrorList) RemoveMultiples
```
func (p *ErrorList) RemoveMultiples()
```
RemoveMultiples sorts an ErrorList and removes all but the first error per line.
### func (\*ErrorList) Reset
```
func (p *ErrorList) Reset()
```
Reset resets an ErrorList to no errors.
### func (ErrorList) Sort
```
func (p ErrorList) Sort()
```
Sort sorts an ErrorList. \*Error entries are sorted by position, other errors are sorted by error message, and before any \*Error entry.
### func (ErrorList) Swap
```
func (p ErrorList) Swap(i, j int)
```
type Mode
---------
A mode value is a set of flags (or 0). They control scanner behavior.
```
type Mode uint
```
```
const (
ScanComments Mode = 1 << iota // return comments as COMMENT tokens
)
```
type Scanner
------------
A Scanner holds the scanner's internal state while processing a given text. It can be allocated as part of another data structure but must be initialized via Init before use.
```
type Scanner struct {
// public state - ok to modify
ErrorCount int // number of errors encountered
// contains filtered or unexported fields
}
```
### func (\*Scanner) Init
```
func (s *Scanner) Init(file *token.File, src []byte, err ErrorHandler, mode Mode)
```
Init prepares the scanner s to tokenize the text src by setting the scanner at the beginning of src. The scanner uses the file set file for position information and it adds line information for each line. It is ok to re-use the same file when re-scanning the same file as line information which is already present is ignored. Init causes a panic if the file size does not match the src size.
Calls to Scan will invoke the error handler err if they encounter a syntax error and err is not nil. Also, for each error encountered, the Scanner field ErrorCount is incremented by one. The mode parameter determines how comments are handled.
Note that Init may call err if there is an error in the first character of the file.
### func (\*Scanner) Scan
```
func (s *Scanner) Scan() (pos token.Pos, tok token.Token, lit string)
```
Scan scans the next token and returns the token position, the token, and its literal string if applicable. The source end is indicated by token.EOF.
If the returned token is a literal (token.IDENT, token.INT, token.FLOAT, token.IMAG, token.CHAR, token.STRING) or token.COMMENT, the literal string has the corresponding value.
If the returned token is a keyword, the literal string is the keyword.
If the returned token is token.SEMICOLON, the corresponding literal string is ";" if the semicolon was present in the source, and "\n" if the semicolon was inserted because of a newline or at EOF.
If the returned token is token.ILLEGAL, the literal string is the offending character.
In all other cases, Scan returns an empty literal string.
For more tolerant parsing, Scan will return a valid token if possible even if a syntax error was encountered. Thus, even if the resulting token sequence contains no illegal tokens, a client may not assume that no error occurred. Instead it must check the scanner's ErrorCount or the number of calls of the error handler, if there was one installed.
Scan adds line information to the file added to the file set with Init. Token positions are relative to that file and thus relative to the file set.
#### Example
Code:
```
// src is the input that we want to tokenize.
src := []byte("cos(x) + 1i*sin(x) // Euler")
// Initialize the scanner.
var s scanner.Scanner
fset := token.NewFileSet() // positions are relative to fset
file := fset.AddFile("", fset.Base(), len(src)) // register input "file"
s.Init(file, src, nil /* no error handler */, scanner.ScanComments)
// Repeated calls to Scan yield the token sequence found in the input.
for {
pos, tok, lit := s.Scan()
if tok == token.EOF {
break
}
fmt.Printf("%s\t%s\t%q\n", fset.Position(pos), tok, lit)
}
```
Output:
```
1:1 IDENT "cos"
1:4 ( ""
1:5 IDENT "x"
1:6 ) ""
1:8 + ""
1:10 IMAG "1i"
1:12 * ""
1:13 IDENT "sin"
1:16 ( ""
1:17 IDENT "x"
1:18 ) ""
1:20 COMMENT "// Euler"
1:28 ; "\n"
```
go Package context Package context
================
* `import "context"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package context defines the Context type, which carries deadlines, cancellation signals, and other request-scoped values across API boundaries and between processes.
Incoming requests to a server should create a Context, and outgoing calls to servers should accept a Context. The chain of function calls between them must propagate the Context, optionally replacing it with a derived Context created using WithCancel, WithDeadline, WithTimeout, or WithValue. When a Context is canceled, all Contexts derived from it are also canceled.
The WithCancel, WithDeadline, and WithTimeout functions take a Context (the parent) and return a derived Context (the child) and a CancelFunc. Calling the CancelFunc cancels the child and its children, removes the parent's reference to the child, and stops any associated timers. Failing to call the CancelFunc leaks the child and its children until the parent is canceled or the timer fires. The go vet tool checks that CancelFuncs are used on all control-flow paths.
The WithCancelCause function returns a CancelCauseFunc, which takes an error and records it as the cancellation cause. Calling Cause on the canceled context or any of its children retrieves the cause. If no cause is specified, Cause(ctx) returns the same value as ctx.Err().
Programs that use Contexts should follow these rules to keep interfaces consistent across packages and enable static analysis tools to check context propagation:
Do not store Contexts inside a struct type; instead, pass a Context explicitly to each function that needs it. The Context should be the first parameter, typically named ctx:
```
func DoSomething(ctx context.Context, arg Arg) error {
// ... use ctx ...
}
```
Do not pass a nil Context, even if a function permits it. Pass context.TODO if you are unsure about which Context to use.
Use context Values only for request-scoped data that transits processes and APIs, not for passing optional parameters to functions.
The same Context may be passed to functions running in different goroutines; Contexts are safe for simultaneous use by multiple goroutines.
See <https://blog.golang.org/context> for example code for a server that uses Contexts.
Index
-----
* [Variables](#pkg-variables)
* [func Cause(c Context) error](#Cause)
* [func WithCancel(parent Context) (ctx Context, cancel CancelFunc)](#WithCancel)
* [func WithCancelCause(parent Context) (ctx Context, cancel CancelCauseFunc)](#WithCancelCause)
* [func WithDeadline(parent Context, d time.Time) (Context, CancelFunc)](#WithDeadline)
* [func WithTimeout(parent Context, timeout time.Duration) (Context, CancelFunc)](#WithTimeout)
* [type CancelCauseFunc](#CancelCauseFunc)
* [type CancelFunc](#CancelFunc)
* [type Context](#Context)
* [func Background() Context](#Background)
* [func TODO() Context](#TODO)
* [func WithValue(parent Context, key, val any) Context](#WithValue)
### Examples
[WithCancel](#example_WithCancel) [WithDeadline](#example_WithDeadline) [WithTimeout](#example_WithTimeout) [WithValue](#example_WithValue) ### Package files
context.go
Variables
---------
Canceled is the error returned by Context.Err when the context is canceled.
```
var Canceled = errors.New("context canceled")
```
DeadlineExceeded is the error returned by Context.Err when the context's deadline passes.
```
var DeadlineExceeded error = deadlineExceededError{}
```
func Cause 1.20
---------------
```
func Cause(c Context) error
```
Cause returns a non-nil error explaining why c was canceled. The first cancellation of c or one of its parents sets the cause. If that cancellation happened via a call to CancelCauseFunc(err), then Cause returns err. Otherwise Cause(c) returns the same value as c.Err(). Cause returns nil if c has not been canceled yet.
func WithCancel 1.7
-------------------
```
func WithCancel(parent Context) (ctx Context, cancel CancelFunc)
```
WithCancel returns a copy of parent with a new Done channel. The returned context's Done channel is closed when the returned cancel function is called or when the parent context's Done channel is closed, whichever happens first.
Canceling this context releases resources associated with it, so code should call cancel as soon as the operations running in this Context complete.
#### Example
This example demonstrates the use of a cancelable context to prevent a goroutine leak. By the end of the example function, the goroutine started by gen will return without leaking.
Code:
```
// gen generates integers in a separate goroutine and
// sends them to the returned channel.
// The callers of gen need to cancel the context once
// they are done consuming generated integers not to leak
// the internal goroutine started by gen.
gen := func(ctx context.Context) <-chan int {
dst := make(chan int)
n := 1
go func() {
for {
select {
case <-ctx.Done():
return // returning not to leak the goroutine
case dst <- n:
n++
}
}
}()
return dst
}
ctx, cancel := context.WithCancel(context.Background())
defer cancel() // cancel when we are finished consuming integers
for n := range gen(ctx) {
fmt.Println(n)
if n == 5 {
break
}
}
```
Output:
```
1
2
3
4
5
```
func WithCancelCause 1.20
-------------------------
```
func WithCancelCause(parent Context) (ctx Context, cancel CancelCauseFunc)
```
WithCancelCause behaves like WithCancel but returns a CancelCauseFunc instead of a CancelFunc. Calling cancel with a non-nil error (the "cause") records that error in ctx; it can then be retrieved using Cause(ctx). Calling cancel with nil sets the cause to Canceled.
Example use:
```
ctx, cancel := context.WithCancelCause(parent)
cancel(myError)
ctx.Err() // returns context.Canceled
context.Cause(ctx) // returns myError
```
func WithDeadline 1.7
---------------------
```
func WithDeadline(parent Context, d time.Time) (Context, CancelFunc)
```
WithDeadline returns a copy of the parent context with the deadline adjusted to be no later than d. If the parent's deadline is already earlier than d, WithDeadline(parent, d) is semantically equivalent to parent. The returned context's Done channel is closed when the deadline expires, when the returned cancel function is called, or when the parent context's Done channel is closed, whichever happens first.
Canceling this context releases resources associated with it, so code should call cancel as soon as the operations running in this Context complete.
#### Example
This example passes a context with an arbitrary deadline to tell a blocking function that it should abandon its work as soon as it gets to it.
Code:
```
d := time.Now().Add(shortDuration)
ctx, cancel := context.WithDeadline(context.Background(), d)
// Even though ctx will be expired, it is good practice to call its
// cancellation function in any case. Failure to do so may keep the
// context and its parent alive longer than necessary.
defer cancel()
select {
case <-time.After(1 * time.Second):
fmt.Println("overslept")
case <-ctx.Done():
fmt.Println(ctx.Err())
}
```
Output:
```
context deadline exceeded
```
func WithTimeout 1.7
--------------------
```
func WithTimeout(parent Context, timeout time.Duration) (Context, CancelFunc)
```
WithTimeout returns WithDeadline(parent, time.Now().Add(timeout)).
Canceling this context releases resources associated with it, so code should call cancel as soon as the operations running in this Context complete:
```
func slowOperationWithTimeout(ctx context.Context) (Result, error) {
ctx, cancel := context.WithTimeout(ctx, 100*time.Millisecond)
defer cancel() // releases resources if slowOperation completes before timeout elapses
return slowOperation(ctx)
}
```
#### Example
This example passes a context with a timeout to tell a blocking function that it should abandon its work after the timeout elapses.
Code:
```
// Pass a context with a timeout to tell a blocking function that it
// should abandon its work after the timeout elapses.
ctx, cancel := context.WithTimeout(context.Background(), shortDuration)
defer cancel()
select {
case <-time.After(1 * time.Second):
fmt.Println("overslept")
case <-ctx.Done():
fmt.Println(ctx.Err()) // prints "context deadline exceeded"
}
```
Output:
```
context deadline exceeded
```
type CancelCauseFunc 1.20
-------------------------
A CancelCauseFunc behaves like a CancelFunc but additionally sets the cancellation cause. This cause can be retrieved by calling Cause on the canceled Context or on any of its derived Contexts.
If the context has already been canceled, CancelCauseFunc does not set the cause. For example, if childContext is derived from parentContext:
* if parentContext is canceled with cause1 before childContext is canceled with cause2, then Cause(parentContext) == Cause(childContext) == cause1
* if childContext is canceled with cause2 before parentContext is canceled with cause1, then Cause(parentContext) == cause1 and Cause(childContext) == cause2
```
type CancelCauseFunc func(cause error)
```
type CancelFunc 1.7
-------------------
A CancelFunc tells an operation to abandon its work. A CancelFunc does not wait for the work to stop. A CancelFunc may be called by multiple goroutines simultaneously. After the first call, subsequent calls to a CancelFunc do nothing.
```
type CancelFunc func()
```
type Context 1.7
----------------
A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Context's methods may be called by multiple goroutines simultaneously.
```
type Context interface {
// Deadline returns the time when work done on behalf of this context
// should be canceled. Deadline returns ok==false when no deadline is
// set. Successive calls to Deadline return the same results.
Deadline() (deadline time.Time, ok bool)
// Done returns a channel that's closed when work done on behalf of this
// context should be canceled. Done may return nil if this context can
// never be canceled. Successive calls to Done return the same value.
// The close of the Done channel may happen asynchronously,
// after the cancel function returns.
//
// WithCancel arranges for Done to be closed when cancel is called;
// WithDeadline arranges for Done to be closed when the deadline
// expires; WithTimeout arranges for Done to be closed when the timeout
// elapses.
//
// Done is provided for use in select statements:
//
// // Stream generates values with DoSomething and sends them to out
// // until DoSomething returns an error or ctx.Done is closed.
// func Stream(ctx context.Context, out chan<- Value) error {
// for {
// v, err := DoSomething(ctx)
// if err != nil {
// return err
// }
// select {
// case <-ctx.Done():
// return ctx.Err()
// case out <- v:
// }
// }
// }
//
// See https://blog.golang.org/pipelines for more examples of how to use
// a Done channel for cancellation.
Done() <-chan struct{}
// If Done is not yet closed, Err returns nil.
// If Done is closed, Err returns a non-nil error explaining why:
// Canceled if the context was canceled
// or DeadlineExceeded if the context's deadline passed.
// After Err returns a non-nil error, successive calls to Err return the same error.
Err() error
// Value returns the value associated with this context for key, or nil
// if no value is associated with key. Successive calls to Value with
// the same key returns the same result.
//
// Use context values only for request-scoped data that transits
// processes and API boundaries, not for passing optional parameters to
// functions.
//
// A key identifies a specific value in a Context. Functions that wish
// to store values in Context typically allocate a key in a global
// variable then use that key as the argument to context.WithValue and
// Context.Value. A key can be any type that supports equality;
// packages should define keys as an unexported type to avoid
// collisions.
//
// Packages that define a Context key should provide type-safe accessors
// for the values stored using that key:
//
// // Package user defines a User type that's stored in Contexts.
// package user
//
// import "context"
//
// // User is the type of value stored in the Contexts.
// type User struct {...}
//
// // key is an unexported type for keys defined in this package.
// // This prevents collisions with keys defined in other packages.
// type key int
//
// // userKey is the key for user.User values in Contexts. It is
// // unexported; clients use user.NewContext and user.FromContext
// // instead of using this key directly.
// var userKey key
//
// // NewContext returns a new Context that carries value u.
// func NewContext(ctx context.Context, u *User) context.Context {
// return context.WithValue(ctx, userKey, u)
// }
//
// // FromContext returns the User value stored in ctx, if any.
// func FromContext(ctx context.Context) (*User, bool) {
// u, ok := ctx.Value(userKey).(*User)
// return u, ok
// }
Value(key any) any
}
```
### func Background 1.7
```
func Background() Context
```
Background returns a non-nil, empty Context. It is never canceled, has no values, and has no deadline. It is typically used by the main function, initialization, and tests, and as the top-level Context for incoming requests.
### func TODO 1.7
```
func TODO() Context
```
TODO returns a non-nil, empty Context. Code should use context.TODO when it's unclear which Context to use or it is not yet available (because the surrounding function has not yet been extended to accept a Context parameter).
### func WithValue 1.7
```
func WithValue(parent Context, key, val any) Context
```
WithValue returns a copy of parent in which the value associated with key is val.
Use context Values only for request-scoped data that transits processes and APIs, not for passing optional parameters to functions.
The provided key must be comparable and should not be of type string or any other built-in type to avoid collisions between packages using context. Users of WithValue should define their own types for keys. To avoid allocating when assigning to an interface{}, context keys often have concrete type struct{}. Alternatively, exported context key variables' static type should be a pointer or interface.
#### Example
This example demonstrates how a value can be passed to the context and also how to retrieve it if it exists.
Code:
```
type favContextKey string
f := func(ctx context.Context, k favContextKey) {
if v := ctx.Value(k); v != nil {
fmt.Println("found value:", v)
return
}
fmt.Println("key not found:", k)
}
k := favContextKey("language")
ctx := context.WithValue(context.Background(), k, "Go")
f(ctx, k)
f(ctx, favContextKey("color"))
```
Output:
```
found value: Go
key not found: color
```
| programming_docs |
go Package regexp Package regexp
===============
* `import "regexp"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package regexp implements regular expression search.
The syntax of the regular expressions accepted is the same general syntax used by Perl, Python, and other languages. More precisely, it is the syntax accepted by RE2 and described at <https://golang.org/s/re2syntax>, except for \C. For an overview of the syntax, run
```
go doc regexp/syntax
```
The regexp implementation provided by this package is guaranteed to run in time linear in the size of the input. (This is a property not guaranteed by most open source implementations of regular expressions.) For more information about this property, see
```
https://swtch.com/~rsc/regexp/regexp1.html
```
or any book about automata theory.
All characters are UTF-8-encoded code points. Following utf8.DecodeRune, each byte of an invalid UTF-8 sequence is treated as if it encoded utf8.RuneError (U+FFFD).
There are 16 methods of Regexp that match a regular expression and identify the matched text. Their names are matched by this regular expression:
```
Find(All)?(String)?(Submatch)?(Index)?
```
If 'All' is present, the routine matches successive non-overlapping matches of the entire expression. Empty matches abutting a preceding match are ignored. The return value is a slice containing the successive return values of the corresponding non-'All' routine. These routines take an extra integer argument, n. If n >= 0, the function returns at most n matches/submatches; otherwise, it returns all of them.
If 'String' is present, the argument is a string; otherwise it is a slice of bytes; return values are adjusted as appropriate.
If 'Submatch' is present, the return value is a slice identifying the successive submatches of the expression. Submatches are matches of parenthesized subexpressions (also known as capturing groups) within the regular expression, numbered from left to right in order of opening parenthesis. Submatch 0 is the match of the entire expression, submatch 1 is the match of the first parenthesized subexpression, and so on.
If 'Index' is present, matches and submatches are identified by byte index pairs within the input string: result[2\*n:2\*n+2] identifies the indexes of the nth submatch. The pair for n==0 identifies the match of the entire expression. If 'Index' is not present, the match is identified by the text of the match/submatch. If an index is negative or text is nil, it means that subexpression did not match any string in the input. For 'String' versions an empty string means either no match or an empty match.
There is also a subset of the methods that can be applied to text read from a RuneReader:
```
MatchReader, FindReaderIndex, FindReaderSubmatchIndex
```
This set may grow. Note that regular expression matches may need to examine text beyond the text returned by a match, so the methods that match text from a RuneReader may read arbitrarily far into the input before returning.
(There are a few other methods that do not match this pattern.)
#### Example
Code:
```
// Compile the expression once, usually at init time.
// Use raw strings to avoid having to quote the backslashes.
var validID = regexp.MustCompile(`^[a-z]+\[[0-9]+\]$`)
fmt.Println(validID.MatchString("adam[23]"))
fmt.Println(validID.MatchString("eve[7]"))
fmt.Println(validID.MatchString("Job[48]"))
fmt.Println(validID.MatchString("snakey"))
```
Output:
```
true
true
false
false
```
Index
-----
* [func Match(pattern string, b []byte) (matched bool, err error)](#Match)
* [func MatchReader(pattern string, r io.RuneReader) (matched bool, err error)](#MatchReader)
* [func MatchString(pattern string, s string) (matched bool, err error)](#MatchString)
* [func QuoteMeta(s string) string](#QuoteMeta)
* [type Regexp](#Regexp)
* [func Compile(expr string) (\*Regexp, error)](#Compile)
* [func CompilePOSIX(expr string) (\*Regexp, error)](#CompilePOSIX)
* [func MustCompile(str string) \*Regexp](#MustCompile)
* [func MustCompilePOSIX(str string) \*Regexp](#MustCompilePOSIX)
* [func (re \*Regexp) Copy() \*Regexp](#Regexp.Copy)
* [func (re \*Regexp) Expand(dst []byte, template []byte, src []byte, match []int) []byte](#Regexp.Expand)
* [func (re \*Regexp) ExpandString(dst []byte, template string, src string, match []int) []byte](#Regexp.ExpandString)
* [func (re \*Regexp) Find(b []byte) []byte](#Regexp.Find)
* [func (re \*Regexp) FindAll(b []byte, n int) [][]byte](#Regexp.FindAll)
* [func (re \*Regexp) FindAllIndex(b []byte, n int) [][]int](#Regexp.FindAllIndex)
* [func (re \*Regexp) FindAllString(s string, n int) []string](#Regexp.FindAllString)
* [func (re \*Regexp) FindAllStringIndex(s string, n int) [][]int](#Regexp.FindAllStringIndex)
* [func (re \*Regexp) FindAllStringSubmatch(s string, n int) [][]string](#Regexp.FindAllStringSubmatch)
* [func (re \*Regexp) FindAllStringSubmatchIndex(s string, n int) [][]int](#Regexp.FindAllStringSubmatchIndex)
* [func (re \*Regexp) FindAllSubmatch(b []byte, n int) [][][]byte](#Regexp.FindAllSubmatch)
* [func (re \*Regexp) FindAllSubmatchIndex(b []byte, n int) [][]int](#Regexp.FindAllSubmatchIndex)
* [func (re \*Regexp) FindIndex(b []byte) (loc []int)](#Regexp.FindIndex)
* [func (re \*Regexp) FindReaderIndex(r io.RuneReader) (loc []int)](#Regexp.FindReaderIndex)
* [func (re \*Regexp) FindReaderSubmatchIndex(r io.RuneReader) []int](#Regexp.FindReaderSubmatchIndex)
* [func (re \*Regexp) FindString(s string) string](#Regexp.FindString)
* [func (re \*Regexp) FindStringIndex(s string) (loc []int)](#Regexp.FindStringIndex)
* [func (re \*Regexp) FindStringSubmatch(s string) []string](#Regexp.FindStringSubmatch)
* [func (re \*Regexp) FindStringSubmatchIndex(s string) []int](#Regexp.FindStringSubmatchIndex)
* [func (re \*Regexp) FindSubmatch(b []byte) [][]byte](#Regexp.FindSubmatch)
* [func (re \*Regexp) FindSubmatchIndex(b []byte) []int](#Regexp.FindSubmatchIndex)
* [func (re \*Regexp) LiteralPrefix() (prefix string, complete bool)](#Regexp.LiteralPrefix)
* [func (re \*Regexp) Longest()](#Regexp.Longest)
* [func (re \*Regexp) Match(b []byte) bool](#Regexp.Match)
* [func (re \*Regexp) MatchReader(r io.RuneReader) bool](#Regexp.MatchReader)
* [func (re \*Regexp) MatchString(s string) bool](#Regexp.MatchString)
* [func (re \*Regexp) NumSubexp() int](#Regexp.NumSubexp)
* [func (re \*Regexp) ReplaceAll(src, repl []byte) []byte](#Regexp.ReplaceAll)
* [func (re \*Regexp) ReplaceAllFunc(src []byte, repl func([]byte) []byte) []byte](#Regexp.ReplaceAllFunc)
* [func (re \*Regexp) ReplaceAllLiteral(src, repl []byte) []byte](#Regexp.ReplaceAllLiteral)
* [func (re \*Regexp) ReplaceAllLiteralString(src, repl string) string](#Regexp.ReplaceAllLiteralString)
* [func (re \*Regexp) ReplaceAllString(src, repl string) string](#Regexp.ReplaceAllString)
* [func (re \*Regexp) ReplaceAllStringFunc(src string, repl func(string) string) string](#Regexp.ReplaceAllStringFunc)
* [func (re \*Regexp) Split(s string, n int) []string](#Regexp.Split)
* [func (re \*Regexp) String() string](#Regexp.String)
* [func (re \*Regexp) SubexpIndex(name string) int](#Regexp.SubexpIndex)
* [func (re \*Regexp) SubexpNames() []string](#Regexp.SubexpNames)
### Examples
[Package](#example_) [Match](#example_Match) [MatchString](#example_MatchString) [QuoteMeta](#example_QuoteMeta) [Regexp.Expand](#example_Regexp_Expand) [Regexp.ExpandString](#example_Regexp_ExpandString) [Regexp.Find](#example_Regexp_Find) [Regexp.FindAll](#example_Regexp_FindAll) [Regexp.FindAllIndex](#example_Regexp_FindAllIndex) [Regexp.FindAllString](#example_Regexp_FindAllString) [Regexp.FindAllStringSubmatch](#example_Regexp_FindAllStringSubmatch) [Regexp.FindAllStringSubmatchIndex](#example_Regexp_FindAllStringSubmatchIndex) [Regexp.FindAllSubmatch](#example_Regexp_FindAllSubmatch) [Regexp.FindAllSubmatchIndex](#example_Regexp_FindAllSubmatchIndex) [Regexp.FindIndex](#example_Regexp_FindIndex) [Regexp.FindString](#example_Regexp_FindString) [Regexp.FindStringIndex](#example_Regexp_FindStringIndex) [Regexp.FindStringSubmatch](#example_Regexp_FindStringSubmatch) [Regexp.FindSubmatch](#example_Regexp_FindSubmatch) [Regexp.FindSubmatchIndex](#example_Regexp_FindSubmatchIndex) [Regexp.Longest](#example_Regexp_Longest) [Regexp.Match](#example_Regexp_Match) [Regexp.MatchString](#example_Regexp_MatchString) [Regexp.NumSubexp](#example_Regexp_NumSubexp) [Regexp.ReplaceAll](#example_Regexp_ReplaceAll) [Regexp.ReplaceAllLiteralString](#example_Regexp_ReplaceAllLiteralString) [Regexp.ReplaceAllString](#example_Regexp_ReplaceAllString) [Regexp.ReplaceAllStringFunc](#example_Regexp_ReplaceAllStringFunc) [Regexp.Split](#example_Regexp_Split) [Regexp.SubexpIndex](#example_Regexp_SubexpIndex) [Regexp.SubexpNames](#example_Regexp_SubexpNames) ### Package files
backtrack.go exec.go onepass.go regexp.go
func Match
----------
```
func Match(pattern string, b []byte) (matched bool, err error)
```
Match reports whether the byte slice b contains any match of the regular expression pattern. More complicated queries need to use Compile and the full Regexp interface.
#### Example
Code:
```
matched, err := regexp.Match(`foo.*`, []byte(`seafood`))
fmt.Println(matched, err)
matched, err = regexp.Match(`bar.*`, []byte(`seafood`))
fmt.Println(matched, err)
matched, err = regexp.Match(`a(b`, []byte(`seafood`))
fmt.Println(matched, err)
```
Output:
```
true <nil>
false <nil>
false error parsing regexp: missing closing ): `a(b`
```
func MatchReader
----------------
```
func MatchReader(pattern string, r io.RuneReader) (matched bool, err error)
```
MatchReader reports whether the text returned by the RuneReader contains any match of the regular expression pattern. More complicated queries need to use Compile and the full Regexp interface.
func MatchString
----------------
```
func MatchString(pattern string, s string) (matched bool, err error)
```
MatchString reports whether the string s contains any match of the regular expression pattern. More complicated queries need to use Compile and the full Regexp interface.
#### Example
Code:
```
matched, err := regexp.MatchString(`foo.*`, "seafood")
fmt.Println(matched, err)
matched, err = regexp.MatchString(`bar.*`, "seafood")
fmt.Println(matched, err)
matched, err = regexp.MatchString(`a(b`, "seafood")
fmt.Println(matched, err)
```
Output:
```
true <nil>
false <nil>
false error parsing regexp: missing closing ): `a(b`
```
func QuoteMeta
--------------
```
func QuoteMeta(s string) string
```
QuoteMeta returns a string that escapes all regular expression metacharacters inside the argument text; the returned string is a regular expression matching the literal text.
#### Example
Code:
```
fmt.Println(regexp.QuoteMeta(`Escaping symbols like: .+*?()|[]{}^$`))
```
Output:
```
Escaping symbols like: \.\+\*\?\(\)\|\[\]\{\}\^\$
```
type Regexp
-----------
Regexp is the representation of a compiled regular expression. A Regexp is safe for concurrent use by multiple goroutines, except for configuration methods, such as Longest.
```
type Regexp struct {
// contains filtered or unexported fields
}
```
### func Compile
```
func Compile(expr string) (*Regexp, error)
```
Compile parses a regular expression and returns, if successful, a Regexp object that can be used to match against text.
When matching against text, the regexp returns a match that begins as early as possible in the input (leftmost), and among those it chooses the one that a backtracking search would have found first. This so-called leftmost-first matching is the same semantics that Perl, Python, and other implementations use, although this package implements it without the expense of backtracking. For POSIX leftmost-longest matching, see CompilePOSIX.
### func CompilePOSIX
```
func CompilePOSIX(expr string) (*Regexp, error)
```
CompilePOSIX is like Compile but restricts the regular expression to POSIX ERE (egrep) syntax and changes the match semantics to leftmost-longest.
That is, when matching against text, the regexp returns a match that begins as early as possible in the input (leftmost), and among those it chooses a match that is as long as possible. This so-called leftmost-longest matching is the same semantics that early regular expression implementations used and that POSIX specifies.
However, there can be multiple leftmost-longest matches, with different submatch choices, and here this package diverges from POSIX. Among the possible leftmost-longest matches, this package chooses the one that a backtracking search would have found first, while POSIX specifies that the match be chosen to maximize the length of the first subexpression, then the second, and so on from left to right. The POSIX rule is computationally prohibitive and not even well-defined. See <https://swtch.com/~rsc/regexp/regexp2.html#posix> for details.
### func MustCompile
```
func MustCompile(str string) *Regexp
```
MustCompile is like Compile but panics if the expression cannot be parsed. It simplifies safe initialization of global variables holding compiled regular expressions.
### func MustCompilePOSIX
```
func MustCompilePOSIX(str string) *Regexp
```
MustCompilePOSIX is like CompilePOSIX but panics if the expression cannot be parsed. It simplifies safe initialization of global variables holding compiled regular expressions.
### func (\*Regexp) Copy 1.6
```
func (re *Regexp) Copy() *Regexp
```
Copy returns a new Regexp object copied from re. Calling Longest on one copy does not affect another.
Deprecated: In earlier releases, when using a Regexp in multiple goroutines, giving each goroutine its own copy helped to avoid lock contention. As of Go 1.12, using Copy is no longer necessary to avoid lock contention. Copy may still be appropriate if the reason for its use is to make two copies with different Longest settings.
### func (\*Regexp) Expand
```
func (re *Regexp) Expand(dst []byte, template []byte, src []byte, match []int) []byte
```
Expand appends template to dst and returns the result; during the append, Expand replaces variables in the template with corresponding matches drawn from src. The match slice should have been returned by FindSubmatchIndex.
In the template, a variable is denoted by a substring of the form $name or ${name}, where name is a non-empty sequence of letters, digits, and underscores. A purely numeric name like $1 refers to the submatch with the corresponding index; other names refer to capturing parentheses named with the (?P<name>...) syntax. A reference to an out of range or unmatched index or a name that is not present in the regular expression is replaced with an empty slice.
In the $name form, name is taken to be as long as possible: $1x is equivalent to ${1x}, not ${1}x, and, $10 is equivalent to ${10}, not ${1}0.
To insert a literal $ in the output, use $$ in the template.
#### Example
Code:
```
content := []byte(`
# comment line
option1: value1
option2: value2
# another comment line
option3: value3
`)
// Regex pattern captures "key: value" pair from the content.
pattern := regexp.MustCompile(`(?m)(?P<key>\w+):\s+(?P<value>\w+)$`)
// Template to convert "key: value" to "key=value" by
// referencing the values captured by the regex pattern.
template := []byte("$key=$value\n")
result := []byte{}
// For each match of the regex in the content.
for _, submatches := range pattern.FindAllSubmatchIndex(content, -1) {
// Apply the captured submatches to the template and append the output
// to the result.
result = pattern.Expand(result, template, content, submatches)
}
fmt.Println(string(result))
```
Output:
```
option1=value1
option2=value2
option3=value3
```
### func (\*Regexp) ExpandString
```
func (re *Regexp) ExpandString(dst []byte, template string, src string, match []int) []byte
```
ExpandString is like Expand but the template and source are strings. It appends to and returns a byte slice in order to give the calling code control over allocation.
#### Example
Code:
```
content := `
# comment line
option1: value1
option2: value2
# another comment line
option3: value3
`
// Regex pattern captures "key: value" pair from the content.
pattern := regexp.MustCompile(`(?m)(?P<key>\w+):\s+(?P<value>\w+)$`)
// Template to convert "key: value" to "key=value" by
// referencing the values captured by the regex pattern.
template := "$key=$value\n"
result := []byte{}
// For each match of the regex in the content.
for _, submatches := range pattern.FindAllStringSubmatchIndex(content, -1) {
// Apply the captured submatches to the template and append the output
// to the result.
result = pattern.ExpandString(result, template, content, submatches)
}
fmt.Println(string(result))
```
Output:
```
option1=value1
option2=value2
option3=value3
```
### func (\*Regexp) Find
```
func (re *Regexp) Find(b []byte) []byte
```
Find returns a slice holding the text of the leftmost match in b of the regular expression. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`foo.?`)
fmt.Printf("%q\n", re.Find([]byte(`seafood fool`)))
```
Output:
```
"food"
```
### func (\*Regexp) FindAll
```
func (re *Regexp) FindAll(b []byte, n int) [][]byte
```
FindAll is the 'All' version of Find; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`foo.?`)
fmt.Printf("%q\n", re.FindAll([]byte(`seafood fool`), -1))
```
Output:
```
["food" "fool"]
```
### func (\*Regexp) FindAllIndex
```
func (re *Regexp) FindAllIndex(b []byte, n int) [][]int
```
FindAllIndex is the 'All' version of FindIndex; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
content := []byte("London")
re := regexp.MustCompile(`o.`)
fmt.Println(re.FindAllIndex(content, 1))
fmt.Println(re.FindAllIndex(content, -1))
```
Output:
```
[[1 3]]
[[1 3] [4 6]]
```
### func (\*Regexp) FindAllString
```
func (re *Regexp) FindAllString(s string, n int) []string
```
FindAllString is the 'All' version of FindString; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`a.`)
fmt.Println(re.FindAllString("paranormal", -1))
fmt.Println(re.FindAllString("paranormal", 2))
fmt.Println(re.FindAllString("graal", -1))
fmt.Println(re.FindAllString("none", -1))
```
Output:
```
[ar an al]
[ar an]
[aa]
[]
```
### func (\*Regexp) FindAllStringIndex
```
func (re *Regexp) FindAllStringIndex(s string, n int) [][]int
```
FindAllStringIndex is the 'All' version of FindStringIndex; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
### func (\*Regexp) FindAllStringSubmatch
```
func (re *Regexp) FindAllStringSubmatch(s string, n int) [][]string
```
FindAllStringSubmatch is the 'All' version of FindStringSubmatch; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
fmt.Printf("%q\n", re.FindAllStringSubmatch("-ab-", -1))
fmt.Printf("%q\n", re.FindAllStringSubmatch("-axxb-", -1))
fmt.Printf("%q\n", re.FindAllStringSubmatch("-ab-axb-", -1))
fmt.Printf("%q\n", re.FindAllStringSubmatch("-axxb-ab-", -1))
```
Output:
```
[["ab" ""]]
[["axxb" "xx"]]
[["ab" ""] ["axb" "x"]]
[["axxb" "xx"] ["ab" ""]]
```
### func (\*Regexp) FindAllStringSubmatchIndex
```
func (re *Regexp) FindAllStringSubmatchIndex(s string, n int) [][]int
```
FindAllStringSubmatchIndex is the 'All' version of FindStringSubmatchIndex; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
// Indices:
// 01234567 012345678
// -ab-axb- -axxb-ab-
fmt.Println(re.FindAllStringSubmatchIndex("-ab-", -1))
fmt.Println(re.FindAllStringSubmatchIndex("-axxb-", -1))
fmt.Println(re.FindAllStringSubmatchIndex("-ab-axb-", -1))
fmt.Println(re.FindAllStringSubmatchIndex("-axxb-ab-", -1))
fmt.Println(re.FindAllStringSubmatchIndex("-foo-", -1))
```
Output:
```
[[1 3 2 2]]
[[1 5 2 4]]
[[1 3 2 2] [4 7 5 6]]
[[1 5 2 4] [6 8 7 7]]
[]
```
### func (\*Regexp) FindAllSubmatch
```
func (re *Regexp) FindAllSubmatch(b []byte, n int) [][][]byte
```
FindAllSubmatch is the 'All' version of FindSubmatch; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`foo(.?)`)
fmt.Printf("%q\n", re.FindAllSubmatch([]byte(`seafood fool`), -1))
```
Output:
```
[["food" "d"] ["fool" "l"]]
```
### func (\*Regexp) FindAllSubmatchIndex
```
func (re *Regexp) FindAllSubmatchIndex(b []byte, n int) [][]int
```
FindAllSubmatchIndex is the 'All' version of FindSubmatchIndex; it returns a slice of all successive matches of the expression, as defined by the 'All' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
content := []byte(`
# comment line
option1: value1
option2: value2
`)
// Regex pattern captures "key: value" pair from the content.
pattern := regexp.MustCompile(`(?m)(?P<key>\w+):\s+(?P<value>\w+)$`)
allIndexes := pattern.FindAllSubmatchIndex(content, -1)
for _, loc := range allIndexes {
fmt.Println(loc)
fmt.Println(string(content[loc[0]:loc[1]]))
fmt.Println(string(content[loc[2]:loc[3]]))
fmt.Println(string(content[loc[4]:loc[5]]))
}
```
Output:
```
[18 33 18 25 27 33]
option1: value1
option1
value1
[35 50 35 42 44 50]
option2: value2
option2
value2
```
### func (\*Regexp) FindIndex
```
func (re *Regexp) FindIndex(b []byte) (loc []int)
```
FindIndex returns a two-element slice of integers defining the location of the leftmost match in b of the regular expression. The match itself is at b[loc[0]:loc[1]]. A return value of nil indicates no match.
#### Example
Code:
```
content := []byte(`
# comment line
option1: value1
option2: value2
`)
// Regex pattern captures "key: value" pair from the content.
pattern := regexp.MustCompile(`(?m)(?P<key>\w+):\s+(?P<value>\w+)$`)
loc := pattern.FindIndex(content)
fmt.Println(loc)
fmt.Println(string(content[loc[0]:loc[1]]))
```
Output:
```
[18 33]
option1: value1
```
### func (\*Regexp) FindReaderIndex
```
func (re *Regexp) FindReaderIndex(r io.RuneReader) (loc []int)
```
FindReaderIndex returns a two-element slice of integers defining the location of the leftmost match of the regular expression in text read from the RuneReader. The match text was found in the input stream at byte offset loc[0] through loc[1]-1. A return value of nil indicates no match.
### func (\*Regexp) FindReaderSubmatchIndex
```
func (re *Regexp) FindReaderSubmatchIndex(r io.RuneReader) []int
```
FindReaderSubmatchIndex returns a slice holding the index pairs identifying the leftmost match of the regular expression of text read by the RuneReader, and the matches, if any, of its subexpressions, as defined by the 'Submatch' and 'Index' descriptions in the package comment. A return value of nil indicates no match.
### func (\*Regexp) FindString
```
func (re *Regexp) FindString(s string) string
```
FindString returns a string holding the text of the leftmost match in s of the regular expression. If there is no match, the return value is an empty string, but it will also be empty if the regular expression successfully matches an empty string. Use FindStringIndex or FindStringSubmatch if it is necessary to distinguish these cases.
#### Example
Code:
```
re := regexp.MustCompile(`foo.?`)
fmt.Printf("%q\n", re.FindString("seafood fool"))
fmt.Printf("%q\n", re.FindString("meat"))
```
Output:
```
"food"
""
```
### func (\*Regexp) FindStringIndex
```
func (re *Regexp) FindStringIndex(s string) (loc []int)
```
FindStringIndex returns a two-element slice of integers defining the location of the leftmost match in s of the regular expression. The match itself is at s[loc[0]:loc[1]]. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`ab?`)
fmt.Println(re.FindStringIndex("tablett"))
fmt.Println(re.FindStringIndex("foo") == nil)
```
Output:
```
[1 3]
true
```
### func (\*Regexp) FindStringSubmatch
```
func (re *Regexp) FindStringSubmatch(s string) []string
```
FindStringSubmatch returns a slice of strings holding the text of the leftmost match of the regular expression in s and the matches, if any, of its subexpressions, as defined by the 'Submatch' description in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b(y|z)c`)
fmt.Printf("%q\n", re.FindStringSubmatch("-axxxbyc-"))
fmt.Printf("%q\n", re.FindStringSubmatch("-abzc-"))
```
Output:
```
["axxxbyc" "xxx" "y"]
["abzc" "" "z"]
```
### func (\*Regexp) FindStringSubmatchIndex
```
func (re *Regexp) FindStringSubmatchIndex(s string) []int
```
FindStringSubmatchIndex returns a slice holding the index pairs identifying the leftmost match of the regular expression in s and the matches, if any, of its subexpressions, as defined by the 'Submatch' and 'Index' descriptions in the package comment. A return value of nil indicates no match.
### func (\*Regexp) FindSubmatch
```
func (re *Regexp) FindSubmatch(b []byte) [][]byte
```
FindSubmatch returns a slice of slices holding the text of the leftmost match of the regular expression in b and the matches, if any, of its subexpressions, as defined by the 'Submatch' descriptions in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`foo(.?)`)
fmt.Printf("%q\n", re.FindSubmatch([]byte(`seafood fool`)))
```
Output:
```
["food" "d"]
```
### func (\*Regexp) FindSubmatchIndex
```
func (re *Regexp) FindSubmatchIndex(b []byte) []int
```
FindSubmatchIndex returns a slice holding the index pairs identifying the leftmost match of the regular expression in b and the matches, if any, of its subexpressions, as defined by the 'Submatch' and 'Index' descriptions in the package comment. A return value of nil indicates no match.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
// Indices:
// 01234567 012345678
// -ab-axb- -axxb-ab-
fmt.Println(re.FindSubmatchIndex([]byte("-ab-")))
fmt.Println(re.FindSubmatchIndex([]byte("-axxb-")))
fmt.Println(re.FindSubmatchIndex([]byte("-ab-axb-")))
fmt.Println(re.FindSubmatchIndex([]byte("-axxb-ab-")))
fmt.Println(re.FindSubmatchIndex([]byte("-foo-")))
```
Output:
```
[1 3 2 2]
[1 5 2 4]
[1 3 2 2]
[1 5 2 4]
[]
```
### func (\*Regexp) LiteralPrefix
```
func (re *Regexp) LiteralPrefix() (prefix string, complete bool)
```
LiteralPrefix returns a literal string that must begin any match of the regular expression re. It returns the boolean true if the literal string comprises the entire regular expression.
### func (\*Regexp) Longest 1.1
```
func (re *Regexp) Longest()
```
Longest makes future searches prefer the leftmost-longest match. That is, when matching against text, the regexp returns a match that begins as early as possible in the input (leftmost), and among those it chooses a match that is as long as possible. This method modifies the Regexp and may not be called concurrently with any other methods.
#### Example
Code:
```
re := regexp.MustCompile(`a(|b)`)
fmt.Println(re.FindString("ab"))
re.Longest()
fmt.Println(re.FindString("ab"))
```
Output:
```
a
ab
```
### func (\*Regexp) Match
```
func (re *Regexp) Match(b []byte) bool
```
Match reports whether the byte slice b contains any match of the regular expression re.
#### Example
Code:
```
re := regexp.MustCompile(`foo.?`)
fmt.Println(re.Match([]byte(`seafood fool`)))
fmt.Println(re.Match([]byte(`something else`)))
```
Output:
```
true
false
```
### func (\*Regexp) MatchReader
```
func (re *Regexp) MatchReader(r io.RuneReader) bool
```
MatchReader reports whether the text returned by the RuneReader contains any match of the regular expression re.
### func (\*Regexp) MatchString
```
func (re *Regexp) MatchString(s string) bool
```
MatchString reports whether the string s contains any match of the regular expression re.
#### Example
Code:
```
re := regexp.MustCompile(`(gopher){2}`)
fmt.Println(re.MatchString("gopher"))
fmt.Println(re.MatchString("gophergopher"))
fmt.Println(re.MatchString("gophergophergopher"))
```
Output:
```
false
true
true
```
### func (\*Regexp) NumSubexp
```
func (re *Regexp) NumSubexp() int
```
NumSubexp returns the number of parenthesized subexpressions in this Regexp.
#### Example
Code:
```
re0 := regexp.MustCompile(`a.`)
fmt.Printf("%d\n", re0.NumSubexp())
re := regexp.MustCompile(`(.*)((a)b)(.*)a`)
fmt.Println(re.NumSubexp())
```
Output:
```
0
4
```
### func (\*Regexp) ReplaceAll
```
func (re *Regexp) ReplaceAll(src, repl []byte) []byte
```
ReplaceAll returns a copy of src, replacing matches of the Regexp with the replacement text repl. Inside repl, $ signs are interpreted as in Expand, so for instance $1 represents the text of the first submatch.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
fmt.Printf("%s\n", re.ReplaceAll([]byte("-ab-axxb-"), []byte("T")))
fmt.Printf("%s\n", re.ReplaceAll([]byte("-ab-axxb-"), []byte("$1")))
fmt.Printf("%s\n", re.ReplaceAll([]byte("-ab-axxb-"), []byte("$1W")))
fmt.Printf("%s\n", re.ReplaceAll([]byte("-ab-axxb-"), []byte("${1}W")))
```
Output:
```
-T-T-
--xx-
---
-W-xxW-
```
### func (\*Regexp) ReplaceAllFunc
```
func (re *Regexp) ReplaceAllFunc(src []byte, repl func([]byte) []byte) []byte
```
ReplaceAllFunc returns a copy of src in which all matches of the Regexp have been replaced by the return value of function repl applied to the matched byte slice. The replacement returned by repl is substituted directly, without using Expand.
### func (\*Regexp) ReplaceAllLiteral
```
func (re *Regexp) ReplaceAllLiteral(src, repl []byte) []byte
```
ReplaceAllLiteral returns a copy of src, replacing matches of the Regexp with the replacement bytes repl. The replacement repl is substituted directly, without using Expand.
### func (\*Regexp) ReplaceAllLiteralString
```
func (re *Regexp) ReplaceAllLiteralString(src, repl string) string
```
ReplaceAllLiteralString returns a copy of src, replacing matches of the Regexp with the replacement string repl. The replacement repl is substituted directly, without using Expand.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
fmt.Println(re.ReplaceAllLiteralString("-ab-axxb-", "T"))
fmt.Println(re.ReplaceAllLiteralString("-ab-axxb-", "$1"))
fmt.Println(re.ReplaceAllLiteralString("-ab-axxb-", "${1}"))
```
Output:
```
-T-T-
-$1-$1-
-${1}-${1}-
```
### func (\*Regexp) ReplaceAllString
```
func (re *Regexp) ReplaceAllString(src, repl string) string
```
ReplaceAllString returns a copy of src, replacing matches of the Regexp with the replacement string repl. Inside repl, $ signs are interpreted as in Expand, so for instance $1 represents the text of the first submatch.
#### Example
Code:
```
re := regexp.MustCompile(`a(x*)b`)
fmt.Println(re.ReplaceAllString("-ab-axxb-", "T"))
fmt.Println(re.ReplaceAllString("-ab-axxb-", "$1"))
fmt.Println(re.ReplaceAllString("-ab-axxb-", "$1W"))
fmt.Println(re.ReplaceAllString("-ab-axxb-", "${1}W"))
```
Output:
```
-T-T-
--xx-
---
-W-xxW-
```
### func (\*Regexp) ReplaceAllStringFunc
```
func (re *Regexp) ReplaceAllStringFunc(src string, repl func(string) string) string
```
ReplaceAllStringFunc returns a copy of src in which all matches of the Regexp have been replaced by the return value of function repl applied to the matched substring. The replacement returned by repl is substituted directly, without using Expand.
#### Example
Code:
```
re := regexp.MustCompile(`[^aeiou]`)
fmt.Println(re.ReplaceAllStringFunc("seafood fool", strings.ToUpper))
```
Output:
```
SeaFooD FooL
```
### func (\*Regexp) Split 1.1
```
func (re *Regexp) Split(s string, n int) []string
```
Split slices s into substrings separated by the expression and returns a slice of the substrings between those expression matches.
The slice returned by this method consists of all the substrings of s not contained in the slice returned by FindAllString. When called on an expression that contains no metacharacters, it is equivalent to strings.SplitN.
Example:
```
s := regexp.MustCompile("a*").Split("abaabaccadaaae", 5)
// s: ["", "b", "b", "c", "cadaaae"]
```
The count determines the number of substrings to return:
```
n > 0: at most n substrings; the last substring will be the unsplit remainder.
n == 0: the result is nil (zero substrings)
n < 0: all substrings
```
#### Example
Code:
```
a := regexp.MustCompile(`a`)
fmt.Println(a.Split("banana", -1))
fmt.Println(a.Split("banana", 0))
fmt.Println(a.Split("banana", 1))
fmt.Println(a.Split("banana", 2))
zp := regexp.MustCompile(`z+`)
fmt.Println(zp.Split("pizza", -1))
fmt.Println(zp.Split("pizza", 0))
fmt.Println(zp.Split("pizza", 1))
fmt.Println(zp.Split("pizza", 2))
```
Output:
```
[b n n ]
[]
[banana]
[b nana]
[pi a]
[]
[pizza]
[pi a]
```
### func (\*Regexp) String
```
func (re *Regexp) String() string
```
String returns the source text used to compile the regular expression.
### func (\*Regexp) SubexpIndex 1.15
```
func (re *Regexp) SubexpIndex(name string) int
```
SubexpIndex returns the index of the first subexpression with the given name, or -1 if there is no subexpression with that name.
Note that multiple subexpressions can be written using the same name, as in (?P<bob>a+)(?P<bob>b+), which declares two subexpressions named "bob". In this case, SubexpIndex returns the index of the leftmost such subexpression in the regular expression.
#### Example
Code:
```
re := regexp.MustCompile(`(?P<first>[a-zA-Z]+) (?P<last>[a-zA-Z]+)`)
fmt.Println(re.MatchString("Alan Turing"))
matches := re.FindStringSubmatch("Alan Turing")
lastIndex := re.SubexpIndex("last")
fmt.Printf("last => %d\n", lastIndex)
fmt.Println(matches[lastIndex])
```
Output:
```
true
last => 2
Turing
```
### func (\*Regexp) SubexpNames
```
func (re *Regexp) SubexpNames() []string
```
SubexpNames returns the names of the parenthesized subexpressions in this Regexp. The name for the first sub-expression is names[1], so that if m is a match slice, the name for m[i] is SubexpNames()[i]. Since the Regexp as a whole cannot be named, names[0] is always the empty string. The slice should not be modified.
#### Example
Code:
```
re := regexp.MustCompile(`(?P<first>[a-zA-Z]+) (?P<last>[a-zA-Z]+)`)
fmt.Println(re.MatchString("Alan Turing"))
fmt.Printf("%q\n", re.SubexpNames())
reversed := fmt.Sprintf("${%s} ${%s}", re.SubexpNames()[2], re.SubexpNames()[1])
fmt.Println(reversed)
fmt.Println(re.ReplaceAllString("Alan Turing", reversed))
```
Output:
```
true
["" "first" "last"]
${last} ${first}
Turing Alan
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [syntax](syntax/index) | Package syntax parses regular expressions into parse trees and compiles parse trees into programs. |
| programming_docs |
go Package syntax Package syntax
===============
* `import "regexp/syntax"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package syntax parses regular expressions into parse trees and compiles parse trees into programs. Most clients of regular expressions will use the facilities of package regexp (such as Compile and Match) instead of this package.
### Syntax
The regular expression syntax understood by this package when parsing with the Perl flag is as follows. Parts of the syntax can be disabled by passing alternate flags to Parse.
Single characters:
```
. any character, possibly including newline (flag s=true)
[xyz] character class
[^xyz] negated character class
\d Perl character class
\D negated Perl character class
[[:alpha:]] ASCII character class
[[:^alpha:]] negated ASCII character class
\pN Unicode character class (one-letter name)
\p{Greek} Unicode character class
\PN negated Unicode character class (one-letter name)
\P{Greek} negated Unicode character class
```
Composites:
```
xy x followed by y
x|y x or y (prefer x)
```
Repetitions:
```
x* zero or more x, prefer more
x+ one or more x, prefer more
x? zero or one x, prefer one
x{n,m} n or n+1 or ... or m x, prefer more
x{n,} n or more x, prefer more
x{n} exactly n x
x*? zero or more x, prefer fewer
x+? one or more x, prefer fewer
x?? zero or one x, prefer zero
x{n,m}? n or n+1 or ... or m x, prefer fewer
x{n,}? n or more x, prefer fewer
x{n}? exactly n x
```
Implementation restriction: The counting forms x{n,m}, x{n,}, and x{n} reject forms that create a minimum or maximum repetition count above 1000. Unlimited repetitions are not subject to this restriction.
Grouping:
```
(re) numbered capturing group (submatch)
(?P<name>re) named & numbered capturing group (submatch)
(?:re) non-capturing group
(?flags) set flags within current group; non-capturing
(?flags:re) set flags during re; non-capturing
Flag syntax is xyz (set) or -xyz (clear) or xy-z (set xy, clear z). The flags are:
i case-insensitive (default false)
m multi-line mode: ^ and $ match begin/end line in addition to begin/end text (default false)
s let . match \n (default false)
U ungreedy: swap meaning of x* and x*?, x+ and x+?, etc (default false)
```
Empty strings:
```
^ at beginning of text or line (flag m=true)
$ at end of text (like \z not \Z) or line (flag m=true)
\A at beginning of text
\b at ASCII word boundary (\w on one side and \W, \A, or \z on the other)
\B not at ASCII word boundary
\z at end of text
```
Escape sequences:
```
\a bell (== \007)
\f form feed (== \014)
\t horizontal tab (== \011)
\n newline (== \012)
\r carriage return (== \015)
\v vertical tab character (== \013)
\* literal *, for any punctuation character *
\123 octal character code (up to three digits)
\x7F hex character code (exactly two digits)
\x{10FFFF} hex character code
\Q...\E literal text ... even if ... has punctuation
```
Character class elements:
```
x single character
A-Z character range (inclusive)
\d Perl character class
[:foo:] ASCII character class foo
\p{Foo} Unicode character class Foo
\pF Unicode character class F (one-letter name)
```
Named character classes as character class elements:
```
[\d] digits (== \d)
[^\d] not digits (== \D)
[\D] not digits (== \D)
[^\D] not not digits (== \d)
[[:name:]] named ASCII class inside character class (== [:name:])
[^[:name:]] named ASCII class inside negated character class (== [:^name:])
[\p{Name}] named Unicode property inside character class (== \p{Name})
[^\p{Name}] named Unicode property inside negated character class (== \P{Name})
```
Perl character classes (all ASCII-only):
```
\d digits (== [0-9])
\D not digits (== [^0-9])
\s whitespace (== [\t\n\f\r ])
\S not whitespace (== [^\t\n\f\r ])
\w word characters (== [0-9A-Za-z_])
\W not word characters (== [^0-9A-Za-z_])
```
ASCII character classes:
```
[[:alnum:]] alphanumeric (== [0-9A-Za-z])
[[:alpha:]] alphabetic (== [A-Za-z])
[[:ascii:]] ASCII (== [\x00-\x7F])
[[:blank:]] blank (== [\t ])
[[:cntrl:]] control (== [\x00-\x1F\x7F])
[[:digit:]] digits (== [0-9])
[[:graph:]] graphical (== [!-~] == [A-Za-z0-9!"#$%&'()*+,\-./:;<=>?@[\\\]^_`{|}~])
[[:lower:]] lower case (== [a-z])
[[:print:]] printable (== [ -~] == [ [:graph:]])
[[:punct:]] punctuation (== [!-/:-@[-`{-~])
[[:space:]] whitespace (== [\t\n\v\f\r ])
[[:upper:]] upper case (== [A-Z])
[[:word:]] word characters (== [0-9A-Za-z_])
[[:xdigit:]] hex digit (== [0-9A-Fa-f])
```
Unicode character classes are those in unicode.Categories and unicode.Scripts.
Index
-----
* [func IsWordChar(r rune) bool](#IsWordChar)
* [type EmptyOp](#EmptyOp)
* [func EmptyOpContext(r1, r2 rune) EmptyOp](#EmptyOpContext)
* [type Error](#Error)
* [func (e \*Error) Error() string](#Error.Error)
* [type ErrorCode](#ErrorCode)
* [func (e ErrorCode) String() string](#ErrorCode.String)
* [type Flags](#Flags)
* [type Inst](#Inst)
* [func (i \*Inst) MatchEmptyWidth(before rune, after rune) bool](#Inst.MatchEmptyWidth)
* [func (i \*Inst) MatchRune(r rune) bool](#Inst.MatchRune)
* [func (i \*Inst) MatchRunePos(r rune) int](#Inst.MatchRunePos)
* [func (i \*Inst) String() string](#Inst.String)
* [type InstOp](#InstOp)
* [func (i InstOp) String() string](#InstOp.String)
* [type Op](#Op)
* [func (i Op) String() string](#Op.String)
* [type Prog](#Prog)
* [func Compile(re \*Regexp) (\*Prog, error)](#Compile)
* [func (p \*Prog) Prefix() (prefix string, complete bool)](#Prog.Prefix)
* [func (p \*Prog) StartCond() EmptyOp](#Prog.StartCond)
* [func (p \*Prog) String() string](#Prog.String)
* [type Regexp](#Regexp)
* [func Parse(s string, flags Flags) (\*Regexp, error)](#Parse)
* [func (re \*Regexp) CapNames() []string](#Regexp.CapNames)
* [func (x \*Regexp) Equal(y \*Regexp) bool](#Regexp.Equal)
* [func (re \*Regexp) MaxCap() int](#Regexp.MaxCap)
* [func (re \*Regexp) Simplify() \*Regexp](#Regexp.Simplify)
* [func (re \*Regexp) String() string](#Regexp.String)
### Package files
compile.go doc.go op\_string.go parse.go perl\_groups.go prog.go regexp.go simplify.go
func IsWordChar
---------------
```
func IsWordChar(r rune) bool
```
IsWordChar reports whether r is considered a “word character” during the evaluation of the \b and \B zero-width assertions. These assertions are ASCII-only: the word characters are [A-Za-z0-9\_].
type EmptyOp
------------
An EmptyOp specifies a kind or mixture of zero-width assertions.
```
type EmptyOp uint8
```
```
const (
EmptyBeginLine EmptyOp = 1 << iota
EmptyEndLine
EmptyBeginText
EmptyEndText
EmptyWordBoundary
EmptyNoWordBoundary
)
```
### func EmptyOpContext
```
func EmptyOpContext(r1, r2 rune) EmptyOp
```
EmptyOpContext returns the zero-width assertions satisfied at the position between the runes r1 and r2. Passing r1 == -1 indicates that the position is at the beginning of the text. Passing r2 == -1 indicates that the position is at the end of the text.
type Error
----------
An Error describes a failure to parse a regular expression and gives the offending expression.
```
type Error struct {
Code ErrorCode
Expr string
}
```
### func (\*Error) Error
```
func (e *Error) Error() string
```
type ErrorCode
--------------
An ErrorCode describes a failure to parse a regular expression.
```
type ErrorCode string
```
```
const (
// Unexpected error
ErrInternalError ErrorCode = "regexp/syntax: internal error"
// Parse errors
ErrInvalidCharClass ErrorCode = "invalid character class"
ErrInvalidCharRange ErrorCode = "invalid character class range"
ErrInvalidEscape ErrorCode = "invalid escape sequence"
ErrInvalidNamedCapture ErrorCode = "invalid named capture"
ErrInvalidPerlOp ErrorCode = "invalid or unsupported Perl syntax"
ErrInvalidRepeatOp ErrorCode = "invalid nested repetition operator"
ErrInvalidRepeatSize ErrorCode = "invalid repeat count"
ErrInvalidUTF8 ErrorCode = "invalid UTF-8"
ErrMissingBracket ErrorCode = "missing closing ]"
ErrMissingParen ErrorCode = "missing closing )"
ErrMissingRepeatArgument ErrorCode = "missing argument to repetition operator"
ErrTrailingBackslash ErrorCode = "trailing backslash at end of expression"
ErrUnexpectedParen ErrorCode = "unexpected )"
ErrNestingDepth ErrorCode = "expression nests too deeply"
ErrLarge ErrorCode = "expression too large"
)
```
### func (ErrorCode) String
```
func (e ErrorCode) String() string
```
type Flags
----------
Flags control the behavior of the parser and record information about regexp context.
```
type Flags uint16
```
```
const (
FoldCase Flags = 1 << iota // case-insensitive match
Literal // treat pattern as literal string
ClassNL // allow character classes like [^a-z] and [[:space:]] to match newline
DotNL // allow . to match newline
OneLine // treat ^ and $ as only matching at beginning and end of text
NonGreedy // make repetition operators default to non-greedy
PerlX // allow Perl extensions
UnicodeGroups // allow \p{Han}, \P{Han} for Unicode group and negation
WasDollar // regexp OpEndText was $, not \z
Simple // regexp contains no counted repetition
MatchNL = ClassNL | DotNL
Perl = ClassNL | OneLine | PerlX | UnicodeGroups // as close to Perl as possible
POSIX Flags = 0 // POSIX syntax
)
```
type Inst
---------
An Inst is a single instruction in a regular expression program.
```
type Inst struct {
Op InstOp
Out uint32 // all but InstMatch, InstFail
Arg uint32 // InstAlt, InstAltMatch, InstCapture, InstEmptyWidth
Rune []rune
}
```
### func (\*Inst) MatchEmptyWidth
```
func (i *Inst) MatchEmptyWidth(before rune, after rune) bool
```
MatchEmptyWidth reports whether the instruction matches an empty string between the runes before and after. It should only be called when i.Op == InstEmptyWidth.
### func (\*Inst) MatchRune
```
func (i *Inst) MatchRune(r rune) bool
```
MatchRune reports whether the instruction matches (and consumes) r. It should only be called when i.Op == InstRune.
### func (\*Inst) MatchRunePos 1.3
```
func (i *Inst) MatchRunePos(r rune) int
```
MatchRunePos checks whether the instruction matches (and consumes) r. If so, MatchRunePos returns the index of the matching rune pair (or, when len(i.Rune) == 1, rune singleton). If not, MatchRunePos returns -1. MatchRunePos should only be called when i.Op == InstRune.
### func (\*Inst) String
```
func (i *Inst) String() string
```
type InstOp
-----------
An InstOp is an instruction opcode.
```
type InstOp uint8
```
```
const (
InstAlt InstOp = iota
InstAltMatch
InstCapture
InstEmptyWidth
InstMatch
InstFail
InstNop
InstRune
InstRune1
InstRuneAny
InstRuneAnyNotNL
)
```
### func (InstOp) String 1.3
```
func (i InstOp) String() string
```
type Op
-------
An Op is a single regular expression operator.
```
type Op uint8
```
```
const (
OpNoMatch Op = 1 + iota // matches no strings
OpEmptyMatch // matches empty string
OpLiteral // matches Runes sequence
OpCharClass // matches Runes interpreted as range pair list
OpAnyCharNotNL // matches any character except newline
OpAnyChar // matches any character
OpBeginLine // matches empty string at beginning of line
OpEndLine // matches empty string at end of line
OpBeginText // matches empty string at beginning of text
OpEndText // matches empty string at end of text
OpWordBoundary // matches word boundary `\b`
OpNoWordBoundary // matches word non-boundary `\B`
OpCapture // capturing subexpression with index Cap, optional name Name
OpStar // matches Sub[0] zero or more times
OpPlus // matches Sub[0] one or more times
OpQuest // matches Sub[0] zero or one times
OpRepeat // matches Sub[0] at least Min times, at most Max (Max == -1 is no limit)
OpConcat // matches concatenation of Subs
OpAlternate // matches alternation of Subs
)
```
### func (Op) String 1.11
```
func (i Op) String() string
```
type Prog
---------
A Prog is a compiled regular expression program.
```
type Prog struct {
Inst []Inst
Start int // index of start instruction
NumCap int // number of InstCapture insts in re
}
```
### func Compile
```
func Compile(re *Regexp) (*Prog, error)
```
Compile compiles the regexp into a program to be executed. The regexp should have been simplified already (returned from re.Simplify).
### func (\*Prog) Prefix
```
func (p *Prog) Prefix() (prefix string, complete bool)
```
Prefix returns a literal string that all matches for the regexp must start with. Complete is true if the prefix is the entire match.
### func (\*Prog) StartCond
```
func (p *Prog) StartCond() EmptyOp
```
StartCond returns the leading empty-width conditions that must be true in any match. It returns ^EmptyOp(0) if no matches are possible.
### func (\*Prog) String
```
func (p *Prog) String() string
```
type Regexp
-----------
A Regexp is a node in a regular expression syntax tree.
```
type Regexp struct {
Op Op // operator
Flags Flags
Sub []*Regexp // subexpressions, if any
Sub0 [1]*Regexp // storage for short Sub
Rune []rune // matched runes, for OpLiteral, OpCharClass
Rune0 [2]rune // storage for short Rune
Min, Max int // min, max for OpRepeat
Cap int // capturing index, for OpCapture
Name string // capturing name, for OpCapture
}
```
### func Parse
```
func Parse(s string, flags Flags) (*Regexp, error)
```
Parse parses a regular expression string s, controlled by the specified Flags, and returns a regular expression parse tree. The syntax is described in the top-level comment.
### func (\*Regexp) CapNames
```
func (re *Regexp) CapNames() []string
```
CapNames walks the regexp to find the names of capturing groups.
### func (\*Regexp) Equal
```
func (x *Regexp) Equal(y *Regexp) bool
```
Equal reports whether x and y have identical structure.
### func (\*Regexp) MaxCap
```
func (re *Regexp) MaxCap() int
```
MaxCap walks the regexp to find the maximum capture index.
### func (\*Regexp) Simplify
```
func (re *Regexp) Simplify() *Regexp
```
Simplify returns a regexp equivalent to re but without counted repetitions and with various other simplifications, such as rewriting /(?:a+)+/ to /a+/. The resulting regexp will execute correctly but its string representation will not produce the same parse tree, because capturing parentheses may have been duplicated or removed. For example, the simplified form for /(x){1,2}/ is /(x)(x)?/ but both parentheses capture as $1. The returned regexp may share structure with or be the original.
### func (\*Regexp) String
```
func (re *Regexp) String() string
```
go Package embed Package embed
==============
* `import "embed"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package embed provides access to files embedded in the running Go program.
Go source files that import "embed" can use the //go:embed directive to initialize a variable of type string, []byte, or FS with the contents of files read from the package directory or subdirectories at compile time.
For example, here are three ways to embed a file named hello.txt and then print its contents at run time.
Embedding one file into a string:
```
import _ "embed"
//go:embed hello.txt
var s string
print(s)
```
Embedding one file into a slice of bytes:
```
import _ "embed"
//go:embed hello.txt
var b []byte
print(string(b))
```
Embedded one or more files into a file system:
```
import "embed"
//go:embed hello.txt
var f embed.FS
data, _ := f.ReadFile("hello.txt")
print(string(data))
```
### Directives
A //go:embed directive above a variable declaration specifies which files to embed, using one or more path.Match patterns.
The directive must immediately precede a line containing the declaration of a single variable. Only blank lines and ‘//’ line comments are permitted between the directive and the declaration.
The type of the variable must be a string type, or a slice of a byte type, or FS (or an alias of FS).
For example:
```
package server
import "embed"
// content holds our static web server content.
//go:embed image/* template/*
//go:embed html/index.html
var content embed.FS
```
The Go build system will recognize the directives and arrange for the declared variable (in the example above, content) to be populated with the matching files from the file system.
The //go:embed directive accepts multiple space-separated patterns for brevity, but it can also be repeated, to avoid very long lines when there are many patterns. The patterns are interpreted relative to the package directory containing the source file. The path separator is a forward slash, even on Windows systems. Patterns may not contain ‘.’ or ‘..’ or empty path elements, nor may they begin or end with a slash. To match everything in the current directory, use ‘\*’ instead of ‘.’. To allow for naming files with spaces in their names, patterns can be written as Go double-quoted or back-quoted string literals.
If a pattern names a directory, all files in the subtree rooted at that directory are embedded (recursively), except that files with names beginning with ‘.’ or ‘\_’ are excluded. So the variable in the above example is almost equivalent to:
```
// content is our static web server content.
//go:embed image template html/index.html
var content embed.FS
```
The difference is that ‘image/\*’ embeds ‘image/.tempfile’ while ‘image’ does not. Neither embeds ‘image/dir/.tempfile’.
If a pattern begins with the prefix ‘all:’, then the rule for walking directories is changed to include those files beginning with ‘.’ or ‘\_’. For example, ‘all:image’ embeds both ‘image/.tempfile’ and ‘image/dir/.tempfile’.
The //go:embed directive can be used with both exported and unexported variables, depending on whether the package wants to make the data available to other packages. It can only be used with variables at package scope, not with local variables.
Patterns must not match files outside the package's module, such as ‘.git/\*’ or symbolic links. Patterns must not match files whose names include the special punctuation characters " \* < > ? ` ' | / \ and :. Matches for empty directories are ignored. After that, each pattern in a //go:embed line must match at least one file or non-empty directory.
If any patterns are invalid or have invalid matches, the build will fail.
### Strings and Bytes
The //go:embed line for a variable of type string or []byte can have only a single pattern, and that pattern can match only a single file. The string or []byte is initialized with the contents of that file.
The //go:embed directive requires importing "embed", even when using a string or []byte. In source files that don't refer to embed.FS, use a blank import (import \_ "embed").
### File Systems
For embedding a single file, a variable of type string or []byte is often best. The FS type enables embedding a tree of files, such as a directory of static web server content, as in the example above.
FS implements the io/fs package's FS interface, so it can be used with any package that understands file systems, including net/http, text/template, and html/template.
For example, given the content variable in the example above, we can write:
```
http.Handle("/static/", http.StripPrefix("/static/", http.FileServer(http.FS(content))))
template.ParseFS(content, "*.tmpl")
```
### Tools
To support tools that analyze Go packages, the patterns found in //go:embed lines are available in “go list” output. See the EmbedPatterns, TestEmbedPatterns, and XTestEmbedPatterns fields in the “go help list” output.
#### Example
Code:
```
package embed_test
import (
"embed"
"log"
"net/http"
)
//go:embed internal/embedtest/testdata/*.txt
var content embed.FS
func Example() {
mutex := http.NewServeMux()
mutex.Handle("/", http.FileServer(http.FS(content)))
err := http.ListenAndServe(":8080", mutex)
if err != nil {
log.Fatal(err)
}
}
```
Index
-----
* [type FS](#FS)
* [func (f FS) Open(name string) (fs.File, error)](#FS.Open)
* [func (f FS) ReadDir(name string) ([]fs.DirEntry, error)](#FS.ReadDir)
* [func (f FS) ReadFile(name string) ([]byte, error)](#FS.ReadFile)
### Examples
[Package](#example_) ### Package files
embed.go
type FS 1.16
------------
An FS is a read-only collection of files, usually initialized with a //go:embed directive. When declared without a //go:embed directive, an FS is an empty file system.
An FS is a read-only value, so it is safe to use from multiple goroutines simultaneously and also safe to assign values of type FS to each other.
FS implements fs.FS, so it can be used with any package that understands file system interfaces, including net/http, text/template, and html/template.
See the package documentation for more details about initializing an FS.
```
type FS struct {
// contains filtered or unexported fields
}
```
### func (FS) Open 1.16
```
func (f FS) Open(name string) (fs.File, error)
```
Open opens the named file for reading and returns it as an fs.File.
The returned file implements io.Seeker when the file is not a directory.
### func (FS) ReadDir 1.16
```
func (f FS) ReadDir(name string) ([]fs.DirEntry, error)
```
ReadDir reads and returns the entire named directory.
### func (FS) ReadFile 1.16
```
func (f FS) ReadFile(name string) ([]byte, error)
```
ReadFile reads and returns the content of the named file.
| programming_docs |
go Package zip Package zip
============
* `import "archive/zip"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package zip provides support for reading and writing ZIP archives.
See the [ZIP specification](https://www.pkware.com/appnote) for details.
This package does not support disk spanning.
A note about ZIP64:
To be backwards compatible the FileHeader has both 32 and 64 bit Size fields. The 64 bit fields will always contain the correct value and for normal archives both fields will be the same. For files requiring the ZIP64 format the 32 bit fields will be 0xffffffff and the 64 bit fields must be used instead.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func RegisterCompressor(method uint16, comp Compressor)](#RegisterCompressor)
* [func RegisterDecompressor(method uint16, dcomp Decompressor)](#RegisterDecompressor)
* [type Compressor](#Compressor)
* [type Decompressor](#Decompressor)
* [type File](#File)
* [func (f \*File) DataOffset() (offset int64, err error)](#File.DataOffset)
* [func (f \*File) Open() (io.ReadCloser, error)](#File.Open)
* [func (f \*File) OpenRaw() (io.Reader, error)](#File.OpenRaw)
* [type FileHeader](#FileHeader)
* [func FileInfoHeader(fi fs.FileInfo) (\*FileHeader, error)](#FileInfoHeader)
* [func (h \*FileHeader) FileInfo() fs.FileInfo](#FileHeader.FileInfo)
* [func (h \*FileHeader) ModTime() time.Time](#FileHeader.ModTime)
* [func (h \*FileHeader) Mode() (mode fs.FileMode)](#FileHeader.Mode)
* [func (h \*FileHeader) SetModTime(t time.Time)](#FileHeader.SetModTime)
* [func (h \*FileHeader) SetMode(mode fs.FileMode)](#FileHeader.SetMode)
* [type ReadCloser](#ReadCloser)
* [func OpenReader(name string) (\*ReadCloser, error)](#OpenReader)
* [func (rc \*ReadCloser) Close() error](#ReadCloser.Close)
* [type Reader](#Reader)
* [func NewReader(r io.ReaderAt, size int64) (\*Reader, error)](#NewReader)
* [func (r \*Reader) Open(name string) (fs.File, error)](#Reader.Open)
* [func (z \*Reader) RegisterDecompressor(method uint16, dcomp Decompressor)](#Reader.RegisterDecompressor)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer) \*Writer](#NewWriter)
* [func (w \*Writer) Close() error](#Writer.Close)
* [func (w \*Writer) Copy(f \*File) error](#Writer.Copy)
* [func (w \*Writer) Create(name string) (io.Writer, error)](#Writer.Create)
* [func (w \*Writer) CreateHeader(fh \*FileHeader) (io.Writer, error)](#Writer.CreateHeader)
* [func (w \*Writer) CreateRaw(fh \*FileHeader) (io.Writer, error)](#Writer.CreateRaw)
* [func (w \*Writer) Flush() error](#Writer.Flush)
* [func (w \*Writer) RegisterCompressor(method uint16, comp Compressor)](#Writer.RegisterCompressor)
* [func (w \*Writer) SetComment(comment string) error](#Writer.SetComment)
* [func (w \*Writer) SetOffset(n int64)](#Writer.SetOffset)
### Examples
[Reader](#example_Reader) [Writer](#example_Writer) [Writer.RegisterCompressor](#example_Writer_RegisterCompressor) ### Package files
reader.go register.go struct.go writer.go
Constants
---------
Compression methods.
```
const (
Store uint16 = 0 // no compression
Deflate uint16 = 8 // DEFLATE compressed
)
```
Variables
---------
```
var (
ErrFormat = errors.New("zip: not a valid zip file")
ErrAlgorithm = errors.New("zip: unsupported compression algorithm")
ErrChecksum = errors.New("zip: checksum error")
ErrInsecurePath = errors.New("zip: insecure file path")
)
```
func RegisterCompressor 1.2
---------------------------
```
func RegisterCompressor(method uint16, comp Compressor)
```
RegisterCompressor registers custom compressors for a specified method ID. The common methods Store and Deflate are built in.
func RegisterDecompressor 1.2
-----------------------------
```
func RegisterDecompressor(method uint16, dcomp Decompressor)
```
RegisterDecompressor allows custom decompressors for a specified method ID. The common methods Store and Deflate are built in.
type Compressor 1.2
-------------------
A Compressor returns a new compressing writer, writing to w. The WriteCloser's Close method must be used to flush pending data to w. The Compressor itself must be safe to invoke from multiple goroutines simultaneously, but each returned writer will be used only by one goroutine at a time.
```
type Compressor func(w io.Writer) (io.WriteCloser, error)
```
type Decompressor 1.2
---------------------
A Decompressor returns a new decompressing reader, reading from r. The ReadCloser's Close method must be used to release associated resources. The Decompressor itself must be safe to invoke from multiple goroutines simultaneously, but each returned reader will be used only by one goroutine at a time.
```
type Decompressor func(r io.Reader) io.ReadCloser
```
type File
---------
A File is a single file in a ZIP archive. The file information is in the embedded FileHeader. The file content can be accessed by calling Open.
```
type File struct {
FileHeader
// contains filtered or unexported fields
}
```
### func (\*File) DataOffset 1.2
```
func (f *File) DataOffset() (offset int64, err error)
```
DataOffset returns the offset of the file's possibly-compressed data, relative to the beginning of the zip file.
Most callers should instead use Open, which transparently decompresses data and verifies checksums.
### func (\*File) Open
```
func (f *File) Open() (io.ReadCloser, error)
```
Open returns a ReadCloser that provides access to the File's contents. Multiple files may be read concurrently.
### func (\*File) OpenRaw 1.17
```
func (f *File) OpenRaw() (io.Reader, error)
```
OpenRaw returns a Reader that provides access to the File's contents without decompression.
type FileHeader
---------------
FileHeader describes a file within a ZIP file. See the [ZIP specification](https://www.pkware.com/appnote) for details.
```
type FileHeader struct {
// Name is the name of the file.
//
// It must be a relative path, not start with a drive letter (such as "C:"),
// and must use forward slashes instead of back slashes. A trailing slash
// indicates that this file is a directory and should have no data.
Name string
// Comment is any arbitrary user-defined string shorter than 64KiB.
Comment string
// NonUTF8 indicates that Name and Comment are not encoded in UTF-8.
//
// By specification, the only other encoding permitted should be CP-437,
// but historically many ZIP readers interpret Name and Comment as whatever
// the system's local character encoding happens to be.
//
// This flag should only be set if the user intends to encode a non-portable
// ZIP file for a specific localized region. Otherwise, the Writer
// automatically sets the ZIP format's UTF-8 flag for valid UTF-8 strings.
NonUTF8 bool // Go 1.10
CreatorVersion uint16
ReaderVersion uint16
Flags uint16
// Method is the compression method. If zero, Store is used.
Method uint16
// Modified is the modified time of the file.
//
// When reading, an extended timestamp is preferred over the legacy MS-DOS
// date field, and the offset between the times is used as the timezone.
// If only the MS-DOS date is present, the timezone is assumed to be UTC.
//
// When writing, an extended timestamp (which is timezone-agnostic) is
// always emitted. The legacy MS-DOS date field is encoded according to the
// location of the Modified time.
Modified time.Time // Go 1.10
// ModifiedTime is an MS-DOS-encoded time.
//
// Deprecated: Use Modified instead.
ModifiedTime uint16
// ModifiedDate is an MS-DOS-encoded date.
//
// Deprecated: Use Modified instead.
ModifiedDate uint16
// CRC32 is the CRC32 checksum of the file content.
CRC32 uint32
// CompressedSize is the compressed size of the file in bytes.
// If either the uncompressed or compressed size of the file
// does not fit in 32 bits, CompressedSize is set to ^uint32(0).
//
// Deprecated: Use CompressedSize64 instead.
CompressedSize uint32
// UncompressedSize is the compressed size of the file in bytes.
// If either the uncompressed or compressed size of the file
// does not fit in 32 bits, CompressedSize is set to ^uint32(0).
//
// Deprecated: Use UncompressedSize64 instead.
UncompressedSize uint32
// CompressedSize64 is the compressed size of the file in bytes.
CompressedSize64 uint64 // Go 1.1
// UncompressedSize64 is the uncompressed size of the file in bytes.
UncompressedSize64 uint64 // Go 1.1
Extra []byte
ExternalAttrs uint32 // Meaning depends on CreatorVersion
}
```
### func FileInfoHeader
```
func FileInfoHeader(fi fs.FileInfo) (*FileHeader, error)
```
FileInfoHeader creates a partially-populated FileHeader from an fs.FileInfo. Because fs.FileInfo's Name method returns only the base name of the file it describes, it may be necessary to modify the Name field of the returned header to provide the full path name of the file. If compression is desired, callers should set the FileHeader.Method field; it is unset by default.
### func (\*FileHeader) FileInfo
```
func (h *FileHeader) FileInfo() fs.FileInfo
```
FileInfo returns an fs.FileInfo for the FileHeader.
### func (\*FileHeader) ModTime
```
func (h *FileHeader) ModTime() time.Time
```
ModTime returns the modification time in UTC using the legacy ModifiedDate and ModifiedTime fields.
Deprecated: Use Modified instead.
### func (\*FileHeader) Mode
```
func (h *FileHeader) Mode() (mode fs.FileMode)
```
Mode returns the permission and mode bits for the FileHeader.
### func (\*FileHeader) SetModTime
```
func (h *FileHeader) SetModTime(t time.Time)
```
SetModTime sets the Modified, ModifiedTime, and ModifiedDate fields to the given time in UTC.
Deprecated: Use Modified instead.
### func (\*FileHeader) SetMode
```
func (h *FileHeader) SetMode(mode fs.FileMode)
```
SetMode changes the permission and mode bits for the FileHeader.
type ReadCloser
---------------
A ReadCloser is a Reader that must be closed when no longer needed.
```
type ReadCloser struct {
Reader
// contains filtered or unexported fields
}
```
### func OpenReader
```
func OpenReader(name string) (*ReadCloser, error)
```
OpenReader will open the Zip file specified by name and return a ReadCloser.
### func (\*ReadCloser) Close
```
func (rc *ReadCloser) Close() error
```
Close closes the Zip file, rendering it unusable for I/O.
type Reader
-----------
A Reader serves content from a ZIP archive.
```
type Reader struct {
File []*File
Comment string
// contains filtered or unexported fields
}
```
#### Example
Code:
```
// Open a zip archive for reading.
r, err := zip.OpenReader("testdata/readme.zip")
if err != nil {
log.Fatal(err)
}
defer r.Close()
// Iterate through the files in the archive,
// printing some of their contents.
for _, f := range r.File {
fmt.Printf("Contents of %s:\n", f.Name)
rc, err := f.Open()
if err != nil {
log.Fatal(err)
}
_, err = io.CopyN(os.Stdout, rc, 68)
if err != nil {
log.Fatal(err)
}
rc.Close()
fmt.Println()
}
```
Output:
```
Contents of README:
This is the source code repository for the Go programming language.
```
### func NewReader
```
func NewReader(r io.ReaderAt, size int64) (*Reader, error)
```
NewReader returns a new Reader reading from r, which is assumed to have the given size in bytes.
If any file inside the archive uses a non-local name (as defined by filepath.IsLocal) or a name containing backslashes and the GODEBUG environment variable contains `zipinsecurepath=0`, NewReader returns the reader with an ErrInsecurePath error. A future version of Go may introduce this behavior by default. Programs that want to accept non-local names can ignore the ErrInsecurePath error and use the returned reader.
### func (\*Reader) Open 1.16
```
func (r *Reader) Open(name string) (fs.File, error)
```
Open opens the named file in the ZIP archive, using the semantics of fs.FS.Open: paths are always slash separated, with no leading / or ../ elements.
### func (\*Reader) RegisterDecompressor 1.6
```
func (z *Reader) RegisterDecompressor(method uint16, dcomp Decompressor)
```
RegisterDecompressor registers or overrides a custom decompressor for a specific method ID. If a decompressor for a given method is not found, Reader will default to looking up the decompressor at the package level.
type Writer
-----------
Writer implements a zip file writer.
```
type Writer struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
// Create a buffer to write our archive to.
buf := new(bytes.Buffer)
// Create a new zip archive.
w := zip.NewWriter(buf)
// Add some files to the archive.
var files = []struct {
Name, Body string
}{
{"readme.txt", "This archive contains some text files."},
{"gopher.txt", "Gopher names:\nGeorge\nGeoffrey\nGonzo"},
{"todo.txt", "Get animal handling licence.\nWrite more examples."},
}
for _, file := range files {
f, err := w.Create(file.Name)
if err != nil {
log.Fatal(err)
}
_, err = f.Write([]byte(file.Body))
if err != nil {
log.Fatal(err)
}
}
// Make sure to check the error on Close.
err := w.Close()
if err != nil {
log.Fatal(err)
}
```
### func NewWriter
```
func NewWriter(w io.Writer) *Writer
```
NewWriter returns a new Writer writing a zip file to w.
### func (\*Writer) Close
```
func (w *Writer) Close() error
```
Close finishes writing the zip file by writing the central directory. It does not close the underlying writer.
### func (\*Writer) Copy 1.17
```
func (w *Writer) Copy(f *File) error
```
Copy copies the file f (obtained from a Reader) into w. It copies the raw form directly bypassing decompression, compression, and validation.
### func (\*Writer) Create
```
func (w *Writer) Create(name string) (io.Writer, error)
```
Create adds a file to the zip file using the provided name. It returns a Writer to which the file contents should be written. The file contents will be compressed using the Deflate method. The name must be a relative path: it must not start with a drive letter (e.g. C:) or leading slash, and only forward slashes are allowed. To create a directory instead of a file, add a trailing slash to the name. The file's contents must be written to the io.Writer before the next call to Create, CreateHeader, or Close.
### func (\*Writer) CreateHeader
```
func (w *Writer) CreateHeader(fh *FileHeader) (io.Writer, error)
```
CreateHeader adds a file to the zip archive using the provided FileHeader for the file metadata. Writer takes ownership of fh and may mutate its fields. The caller must not modify fh after calling CreateHeader.
This returns a Writer to which the file contents should be written. The file's contents must be written to the io.Writer before the next call to Create, CreateHeader, CreateRaw, or Close.
### func (\*Writer) CreateRaw 1.17
```
func (w *Writer) CreateRaw(fh *FileHeader) (io.Writer, error)
```
CreateRaw adds a file to the zip archive using the provided FileHeader and returns a Writer to which the file contents should be written. The file's contents must be written to the io.Writer before the next call to Create, CreateHeader, CreateRaw, or Close.
In contrast to CreateHeader, the bytes passed to Writer are not compressed.
### func (\*Writer) Flush 1.4
```
func (w *Writer) Flush() error
```
Flush flushes any buffered data to the underlying writer. Calling Flush is not normally necessary; calling Close is sufficient.
### func (\*Writer) RegisterCompressor 1.6
```
func (w *Writer) RegisterCompressor(method uint16, comp Compressor)
```
RegisterCompressor registers or overrides a custom compressor for a specific method ID. If a compressor for a given method is not found, Writer will default to looking up the compressor at the package level.
#### Example
Code:
```
// Override the default Deflate compressor with a higher compression level.
// Create a buffer to write our archive to.
buf := new(bytes.Buffer)
// Create a new zip archive.
w := zip.NewWriter(buf)
// Register a custom Deflate compressor.
w.RegisterCompressor(zip.Deflate, func(out io.Writer) (io.WriteCloser, error) {
return flate.NewWriter(out, flate.BestCompression)
})
// Proceed to add files to w.
```
### func (\*Writer) SetComment 1.10
```
func (w *Writer) SetComment(comment string) error
```
SetComment sets the end-of-central-directory comment field. It can only be called before Close.
### func (\*Writer) SetOffset 1.5
```
func (w *Writer) SetOffset(n int64)
```
SetOffset sets the offset of the beginning of the zip data within the underlying writer. It should be used when the zip data is appended to an existing file, such as a binary executable. It must be called before any data is written.
go Package tar Package tar
============
* `import "archive/tar"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package tar implements access to tar archives.
Tape archives (tar) are a file format for storing a sequence of files that can be read and written in a streaming manner. This package aims to cover most variations of the format, including those produced by GNU and BSD tar tools.
#### Example (Minimal)
Code:
```
// Create and add some files to the archive.
var buf bytes.Buffer
tw := tar.NewWriter(&buf)
var files = []struct {
Name, Body string
}{
{"readme.txt", "This archive contains some text files."},
{"gopher.txt", "Gopher names:\nGeorge\nGeoffrey\nGonzo"},
{"todo.txt", "Get animal handling license."},
}
for _, file := range files {
hdr := &tar.Header{
Name: file.Name,
Mode: 0600,
Size: int64(len(file.Body)),
}
if err := tw.WriteHeader(hdr); err != nil {
log.Fatal(err)
}
if _, err := tw.Write([]byte(file.Body)); err != nil {
log.Fatal(err)
}
}
if err := tw.Close(); err != nil {
log.Fatal(err)
}
// Open and iterate through the files in the archive.
tr := tar.NewReader(&buf)
for {
hdr, err := tr.Next()
if err == io.EOF {
break // End of archive
}
if err != nil {
log.Fatal(err)
}
fmt.Printf("Contents of %s:\n", hdr.Name)
if _, err := io.Copy(os.Stdout, tr); err != nil {
log.Fatal(err)
}
fmt.Println()
}
```
Output:
```
Contents of readme.txt:
This archive contains some text files.
Contents of gopher.txt:
Gopher names:
George
Geoffrey
Gonzo
Contents of todo.txt:
Get animal handling license.
```
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [type Format](#Format)
* [func (f Format) String() string](#Format.String)
* [type Header](#Header)
* [func FileInfoHeader(fi fs.FileInfo, link string) (\*Header, error)](#FileInfoHeader)
* [func (h \*Header) FileInfo() fs.FileInfo](#Header.FileInfo)
* [type Reader](#Reader)
* [func NewReader(r io.Reader) \*Reader](#NewReader)
* [func (tr \*Reader) Next() (\*Header, error)](#Reader.Next)
* [func (tr \*Reader) Read(b []byte) (int, error)](#Reader.Read)
* [type Writer](#Writer)
* [func NewWriter(w io.Writer) \*Writer](#NewWriter)
* [func (tw \*Writer) Close() error](#Writer.Close)
* [func (tw \*Writer) Flush() error](#Writer.Flush)
* [func (tw \*Writer) Write(b []byte) (int, error)](#Writer.Write)
* [func (tw \*Writer) WriteHeader(hdr \*Header) error](#Writer.WriteHeader)
### Examples
[Package (Minimal)](#example__minimal) ### Package files
common.go format.go reader.go stat\_actime1.go stat\_unix.go strconv.go writer.go
Constants
---------
Type flags for Header.Typeflag.
```
const (
// Type '0' indicates a regular file.
TypeReg = '0'
// Deprecated: Use TypeReg instead.
TypeRegA = '\x00'
// Type '1' to '6' are header-only flags and may not have a data body.
TypeLink = '1' // Hard link
TypeSymlink = '2' // Symbolic link
TypeChar = '3' // Character device node
TypeBlock = '4' // Block device node
TypeDir = '5' // Directory
TypeFifo = '6' // FIFO node
// Type '7' is reserved.
TypeCont = '7'
// Type 'x' is used by the PAX format to store key-value records that
// are only relevant to the next file.
// This package transparently handles these types.
TypeXHeader = 'x'
// Type 'g' is used by the PAX format to store key-value records that
// are relevant to all subsequent files.
// This package only supports parsing and composing such headers,
// but does not currently support persisting the global state across files.
TypeXGlobalHeader = 'g'
// Type 'S' indicates a sparse file in the GNU format.
TypeGNUSparse = 'S'
// Types 'L' and 'K' are used by the GNU format for a meta file
// used to store the path or link name for the next file.
// This package transparently handles these types.
TypeGNULongName = 'L'
TypeGNULongLink = 'K'
)
```
Variables
---------
```
var (
ErrHeader = errors.New("archive/tar: invalid tar header")
ErrWriteTooLong = errors.New("archive/tar: write too long")
ErrFieldTooLong = errors.New("archive/tar: header field too long")
ErrWriteAfterClose = errors.New("archive/tar: write after close")
ErrInsecurePath = errors.New("archive/tar: insecure file path")
)
```
type Format 1.10
----------------
Format represents the tar archive format.
The original tar format was introduced in Unix V7. Since then, there have been multiple competing formats attempting to standardize or extend the V7 format to overcome its limitations. The most common formats are the USTAR, PAX, and GNU formats, each with their own advantages and limitations.
The following table captures the capabilities of each format:
```
| USTAR | PAX | GNU
------------------+--------+-----------+----------
Name | 256B | unlimited | unlimited
Linkname | 100B | unlimited | unlimited
Size | uint33 | unlimited | uint89
Mode | uint21 | uint21 | uint57
Uid/Gid | uint21 | unlimited | uint57
Uname/Gname | 32B | unlimited | 32B
ModTime | uint33 | unlimited | int89
AccessTime | n/a | unlimited | int89
ChangeTime | n/a | unlimited | int89
Devmajor/Devminor | uint21 | uint21 | uint57
------------------+--------+-----------+----------
string encoding | ASCII | UTF-8 | binary
sub-second times | no | yes | no
sparse files | no | yes | yes
```
The table's upper portion shows the Header fields, where each format reports the maximum number of bytes allowed for each string field and the integer type used to store each numeric field (where timestamps are stored as the number of seconds since the Unix epoch).
The table's lower portion shows specialized features of each format, such as supported string encodings, support for sub-second timestamps, or support for sparse files.
The Writer currently provides no support for sparse files.
```
type Format int
```
Constants to identify various tar formats.
```
const (
// FormatUnknown indicates that the format is unknown.
FormatUnknown Format
// FormatUSTAR represents the USTAR header format defined in POSIX.1-1988.
//
// While this format is compatible with most tar readers,
// the format has several limitations making it unsuitable for some usages.
// Most notably, it cannot support sparse files, files larger than 8GiB,
// filenames larger than 256 characters, and non-ASCII filenames.
//
// Reference:
// http://pubs.opengroup.org/onlinepubs/9699919799/utilities/pax.html#tag_20_92_13_06
FormatUSTAR
// FormatPAX represents the PAX header format defined in POSIX.1-2001.
//
// PAX extends USTAR by writing a special file with Typeflag TypeXHeader
// preceding the original header. This file contains a set of key-value
// records, which are used to overcome USTAR's shortcomings, in addition to
// providing the ability to have sub-second resolution for timestamps.
//
// Some newer formats add their own extensions to PAX by defining their
// own keys and assigning certain semantic meaning to the associated values.
// For example, sparse file support in PAX is implemented using keys
// defined by the GNU manual (e.g., "GNU.sparse.map").
//
// Reference:
// http://pubs.opengroup.org/onlinepubs/009695399/utilities/pax.html
FormatPAX
// FormatGNU represents the GNU header format.
//
// The GNU header format is older than the USTAR and PAX standards and
// is not compatible with them. The GNU format supports
// arbitrary file sizes, filenames of arbitrary encoding and length,
// sparse files, and other features.
//
// It is recommended that PAX be chosen over GNU unless the target
// application can only parse GNU formatted archives.
//
// Reference:
// https://www.gnu.org/software/tar/manual/html_node/Standard.html
FormatGNU
)
```
### func (Format) String 1.10
```
func (f Format) String() string
```
type Header
-----------
A Header represents a single header in a tar archive. Some fields may not be populated.
For forward compatibility, users that retrieve a Header from Reader.Next, mutate it in some ways, and then pass it back to Writer.WriteHeader should do so by creating a new Header and copying the fields that they are interested in preserving.
```
type Header struct {
// Typeflag is the type of header entry.
// The zero value is automatically promoted to either TypeReg or TypeDir
// depending on the presence of a trailing slash in Name.
Typeflag byte
Name string // Name of file entry
Linkname string // Target name of link (valid for TypeLink or TypeSymlink)
Size int64 // Logical file size in bytes
Mode int64 // Permission and mode bits
Uid int // User ID of owner
Gid int // Group ID of owner
Uname string // User name of owner
Gname string // Group name of owner
// If the Format is unspecified, then Writer.WriteHeader rounds ModTime
// to the nearest second and ignores the AccessTime and ChangeTime fields.
//
// To use AccessTime or ChangeTime, specify the Format as PAX or GNU.
// To use sub-second resolution, specify the Format as PAX.
ModTime time.Time // Modification time
AccessTime time.Time // Access time (requires either PAX or GNU support)
ChangeTime time.Time // Change time (requires either PAX or GNU support)
Devmajor int64 // Major device number (valid for TypeChar or TypeBlock)
Devminor int64 // Minor device number (valid for TypeChar or TypeBlock)
// Xattrs stores extended attributes as PAX records under the
// "SCHILY.xattr." namespace.
//
// The following are semantically equivalent:
// h.Xattrs[key] = value
// h.PAXRecords["SCHILY.xattr."+key] = value
//
// When Writer.WriteHeader is called, the contents of Xattrs will take
// precedence over those in PAXRecords.
//
// Deprecated: Use PAXRecords instead.
Xattrs map[string]string // Go 1.3
// PAXRecords is a map of PAX extended header records.
//
// User-defined records should have keys of the following form:
// VENDOR.keyword
// Where VENDOR is some namespace in all uppercase, and keyword may
// not contain the '=' character (e.g., "GOLANG.pkg.version").
// The key and value should be non-empty UTF-8 strings.
//
// When Writer.WriteHeader is called, PAX records derived from the
// other fields in Header take precedence over PAXRecords.
PAXRecords map[string]string // Go 1.10
// Format specifies the format of the tar header.
//
// This is set by Reader.Next as a best-effort guess at the format.
// Since the Reader liberally reads some non-compliant files,
// it is possible for this to be FormatUnknown.
//
// If the format is unspecified when Writer.WriteHeader is called,
// then it uses the first format (in the order of USTAR, PAX, GNU)
// capable of encoding this Header (see Format).
Format Format // Go 1.10
}
```
### func FileInfoHeader 1.1
```
func FileInfoHeader(fi fs.FileInfo, link string) (*Header, error)
```
FileInfoHeader creates a partially-populated Header from fi. If fi describes a symlink, FileInfoHeader records link as the link target. If fi describes a directory, a slash is appended to the name.
Since fs.FileInfo's Name method only returns the base name of the file it describes, it may be necessary to modify Header.Name to provide the full path name of the file.
### func (\*Header) FileInfo 1.1
```
func (h *Header) FileInfo() fs.FileInfo
```
FileInfo returns an fs.FileInfo for the Header.
type Reader
-----------
Reader provides sequential access to the contents of a tar archive. Reader.Next advances to the next file in the archive (including the first), and then Reader can be treated as an io.Reader to access the file's data.
```
type Reader struct {
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(r io.Reader) *Reader
```
NewReader creates a new Reader reading from r.
### func (\*Reader) Next
```
func (tr *Reader) Next() (*Header, error)
```
Next advances to the next entry in the tar archive. The Header.Size determines how many bytes can be read for the next file. Any remaining data in the current file is automatically discarded. At the end of the archive, Next returns the error io.EOF.
If Next encounters a non-local name (as defined by filepath.IsLocal) and the GODEBUG environment variable contains `tarinsecurepath=0`, Next returns the header with an ErrInsecurePath error. A future version of Go may introduce this behavior by default. Programs that want to accept non-local names can ignore the ErrInsecurePath error and use the returned header.
### func (\*Reader) Read
```
func (tr *Reader) Read(b []byte) (int, error)
```
Read reads from the current file in the tar archive. It returns (0, io.EOF) when it reaches the end of that file, until Next is called to advance to the next file.
If the current file is sparse, then the regions marked as a hole are read back as NUL-bytes.
Calling Read on special types like TypeLink, TypeSymlink, TypeChar, TypeBlock, TypeDir, and TypeFifo returns (0, io.EOF) regardless of what the Header.Size claims.
type Writer
-----------
Writer provides sequential writing of a tar archive. Write.WriteHeader begins a new file with the provided Header, and then Writer can be treated as an io.Writer to supply that file's data.
```
type Writer struct {
// contains filtered or unexported fields
}
```
### func NewWriter
```
func NewWriter(w io.Writer) *Writer
```
NewWriter creates a new Writer writing to w.
### func (\*Writer) Close
```
func (tw *Writer) Close() error
```
Close closes the tar archive by flushing the padding, and writing the footer. If the current file (from a prior call to WriteHeader) is not fully written, then this returns an error.
### func (\*Writer) Flush
```
func (tw *Writer) Flush() error
```
Flush finishes writing the current file's block padding. The current file must be fully written before Flush can be called.
This is unnecessary as the next call to WriteHeader or Close will implicitly flush out the file's padding.
### func (\*Writer) Write
```
func (tw *Writer) Write(b []byte) (int, error)
```
Write writes to the current file in the tar archive. Write returns the error ErrWriteTooLong if more than Header.Size bytes are written after WriteHeader.
Calling Write on special types like TypeLink, TypeSymlink, TypeChar, TypeBlock, TypeDir, and TypeFifo returns (0, ErrWriteTooLong) regardless of what the Header.Size claims.
### func (\*Writer) WriteHeader
```
func (tw *Writer) WriteHeader(hdr *Header) error
```
WriteHeader writes hdr and prepares to accept the file's contents. The Header.Size determines how many bytes can be written for the next file. If the current file is not fully written, then this returns an error. This implicitly flushes any padding necessary before writing the header.
| programming_docs |
go Package bytes Package bytes
==============
* `import "bytes"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package bytes implements functions for the manipulation of byte slices. It is analogous to the facilities of the strings package.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func Clone(b []byte) []byte](#Clone)
* [func Compare(a, b []byte) int](#Compare)
* [func Contains(b, subslice []byte) bool](#Contains)
* [func ContainsAny(b []byte, chars string) bool](#ContainsAny)
* [func ContainsRune(b []byte, r rune) bool](#ContainsRune)
* [func Count(s, sep []byte) int](#Count)
* [func Cut(s, sep []byte) (before, after []byte, found bool)](#Cut)
* [func CutPrefix(s, prefix []byte) (after []byte, found bool)](#CutPrefix)
* [func CutSuffix(s, suffix []byte) (before []byte, found bool)](#CutSuffix)
* [func Equal(a, b []byte) bool](#Equal)
* [func EqualFold(s, t []byte) bool](#EqualFold)
* [func Fields(s []byte) [][]byte](#Fields)
* [func FieldsFunc(s []byte, f func(rune) bool) [][]byte](#FieldsFunc)
* [func HasPrefix(s, prefix []byte) bool](#HasPrefix)
* [func HasSuffix(s, suffix []byte) bool](#HasSuffix)
* [func Index(s, sep []byte) int](#Index)
* [func IndexAny(s []byte, chars string) int](#IndexAny)
* [func IndexByte(b []byte, c byte) int](#IndexByte)
* [func IndexFunc(s []byte, f func(r rune) bool) int](#IndexFunc)
* [func IndexRune(s []byte, r rune) int](#IndexRune)
* [func Join(s [][]byte, sep []byte) []byte](#Join)
* [func LastIndex(s, sep []byte) int](#LastIndex)
* [func LastIndexAny(s []byte, chars string) int](#LastIndexAny)
* [func LastIndexByte(s []byte, c byte) int](#LastIndexByte)
* [func LastIndexFunc(s []byte, f func(r rune) bool) int](#LastIndexFunc)
* [func Map(mapping func(r rune) rune, s []byte) []byte](#Map)
* [func Repeat(b []byte, count int) []byte](#Repeat)
* [func Replace(s, old, new []byte, n int) []byte](#Replace)
* [func ReplaceAll(s, old, new []byte) []byte](#ReplaceAll)
* [func Runes(s []byte) []rune](#Runes)
* [func Split(s, sep []byte) [][]byte](#Split)
* [func SplitAfter(s, sep []byte) [][]byte](#SplitAfter)
* [func SplitAfterN(s, sep []byte, n int) [][]byte](#SplitAfterN)
* [func SplitN(s, sep []byte, n int) [][]byte](#SplitN)
* [func Title(s []byte) []byte](#Title)
* [func ToLower(s []byte) []byte](#ToLower)
* [func ToLowerSpecial(c unicode.SpecialCase, s []byte) []byte](#ToLowerSpecial)
* [func ToTitle(s []byte) []byte](#ToTitle)
* [func ToTitleSpecial(c unicode.SpecialCase, s []byte) []byte](#ToTitleSpecial)
* [func ToUpper(s []byte) []byte](#ToUpper)
* [func ToUpperSpecial(c unicode.SpecialCase, s []byte) []byte](#ToUpperSpecial)
* [func ToValidUTF8(s, replacement []byte) []byte](#ToValidUTF8)
* [func Trim(s []byte, cutset string) []byte](#Trim)
* [func TrimFunc(s []byte, f func(r rune) bool) []byte](#TrimFunc)
* [func TrimLeft(s []byte, cutset string) []byte](#TrimLeft)
* [func TrimLeftFunc(s []byte, f func(r rune) bool) []byte](#TrimLeftFunc)
* [func TrimPrefix(s, prefix []byte) []byte](#TrimPrefix)
* [func TrimRight(s []byte, cutset string) []byte](#TrimRight)
* [func TrimRightFunc(s []byte, f func(r rune) bool) []byte](#TrimRightFunc)
* [func TrimSpace(s []byte) []byte](#TrimSpace)
* [func TrimSuffix(s, suffix []byte) []byte](#TrimSuffix)
* [type Buffer](#Buffer)
* [func NewBuffer(buf []byte) \*Buffer](#NewBuffer)
* [func NewBufferString(s string) \*Buffer](#NewBufferString)
* [func (b \*Buffer) Bytes() []byte](#Buffer.Bytes)
* [func (b \*Buffer) Cap() int](#Buffer.Cap)
* [func (b \*Buffer) Grow(n int)](#Buffer.Grow)
* [func (b \*Buffer) Len() int](#Buffer.Len)
* [func (b \*Buffer) Next(n int) []byte](#Buffer.Next)
* [func (b \*Buffer) Read(p []byte) (n int, err error)](#Buffer.Read)
* [func (b \*Buffer) ReadByte() (byte, error)](#Buffer.ReadByte)
* [func (b \*Buffer) ReadBytes(delim byte) (line []byte, err error)](#Buffer.ReadBytes)
* [func (b \*Buffer) ReadFrom(r io.Reader) (n int64, err error)](#Buffer.ReadFrom)
* [func (b \*Buffer) ReadRune() (r rune, size int, err error)](#Buffer.ReadRune)
* [func (b \*Buffer) ReadString(delim byte) (line string, err error)](#Buffer.ReadString)
* [func (b \*Buffer) Reset()](#Buffer.Reset)
* [func (b \*Buffer) String() string](#Buffer.String)
* [func (b \*Buffer) Truncate(n int)](#Buffer.Truncate)
* [func (b \*Buffer) UnreadByte() error](#Buffer.UnreadByte)
* [func (b \*Buffer) UnreadRune() error](#Buffer.UnreadRune)
* [func (b \*Buffer) Write(p []byte) (n int, err error)](#Buffer.Write)
* [func (b \*Buffer) WriteByte(c byte) error](#Buffer.WriteByte)
* [func (b \*Buffer) WriteRune(r rune) (n int, err error)](#Buffer.WriteRune)
* [func (b \*Buffer) WriteString(s string) (n int, err error)](#Buffer.WriteString)
* [func (b \*Buffer) WriteTo(w io.Writer) (n int64, err error)](#Buffer.WriteTo)
* [type Reader](#Reader)
* [func NewReader(b []byte) \*Reader](#NewReader)
* [func (r \*Reader) Len() int](#Reader.Len)
* [func (r \*Reader) Read(b []byte) (n int, err error)](#Reader.Read)
* [func (r \*Reader) ReadAt(b []byte, off int64) (n int, err error)](#Reader.ReadAt)
* [func (r \*Reader) ReadByte() (byte, error)](#Reader.ReadByte)
* [func (r \*Reader) ReadRune() (ch rune, size int, err error)](#Reader.ReadRune)
* [func (r \*Reader) Reset(b []byte)](#Reader.Reset)
* [func (r \*Reader) Seek(offset int64, whence int) (int64, error)](#Reader.Seek)
* [func (r \*Reader) Size() int64](#Reader.Size)
* [func (r \*Reader) UnreadByte() error](#Reader.UnreadByte)
* [func (r \*Reader) UnreadRune() error](#Reader.UnreadRune)
* [func (r \*Reader) WriteTo(w io.Writer) (n int64, err error)](#Reader.WriteTo)
### Examples
[Buffer](#example_Buffer) [Buffer.Bytes](#example_Buffer_Bytes) [Buffer.Cap](#example_Buffer_Cap) [Buffer.Grow](#example_Buffer_Grow) [Buffer.Len](#example_Buffer_Len) [Buffer.Next](#example_Buffer_Next) [Buffer.Read](#example_Buffer_Read) [Buffer.ReadByte](#example_Buffer_ReadByte) [Buffer (Reader)](#example_Buffer_reader) [Compare](#example_Compare) [Compare (Search)](#example_Compare_search) [Contains](#example_Contains) [ContainsAny](#example_ContainsAny) [ContainsRune](#example_ContainsRune) [Count](#example_Count) [Cut](#example_Cut) [Equal](#example_Equal) [EqualFold](#example_EqualFold) [Fields](#example_Fields) [FieldsFunc](#example_FieldsFunc) [HasPrefix](#example_HasPrefix) [HasSuffix](#example_HasSuffix) [Index](#example_Index) [IndexAny](#example_IndexAny) [IndexByte](#example_IndexByte) [IndexFunc](#example_IndexFunc) [IndexRune](#example_IndexRune) [Join](#example_Join) [LastIndex](#example_LastIndex) [LastIndexAny](#example_LastIndexAny) [LastIndexByte](#example_LastIndexByte) [LastIndexFunc](#example_LastIndexFunc) [Reader.Len](#example_Reader_Len) [Repeat](#example_Repeat) [Replace](#example_Replace) [ReplaceAll](#example_ReplaceAll) [Runes](#example_Runes) [Split](#example_Split) [SplitAfter](#example_SplitAfter) [SplitAfterN](#example_SplitAfterN) [SplitN](#example_SplitN) [Title](#example_Title) [ToLower](#example_ToLower) [ToLowerSpecial](#example_ToLowerSpecial) [ToTitle](#example_ToTitle) [ToTitleSpecial](#example_ToTitleSpecial) [ToUpper](#example_ToUpper) [ToUpperSpecial](#example_ToUpperSpecial) [Trim](#example_Trim) [TrimFunc](#example_TrimFunc) [TrimLeft](#example_TrimLeft) [TrimLeftFunc](#example_TrimLeftFunc) [TrimPrefix](#example_TrimPrefix) [TrimRight](#example_TrimRight) [TrimRightFunc](#example_TrimRightFunc) [TrimSpace](#example_TrimSpace) [TrimSuffix](#example_TrimSuffix) ### Package files
buffer.go bytes.go reader.go
Constants
---------
MinRead is the minimum slice size passed to a Read call by Buffer.ReadFrom. As long as the Buffer has at least MinRead bytes beyond what is required to hold the contents of r, ReadFrom will not grow the underlying buffer.
```
const MinRead = 512
```
Variables
---------
ErrTooLarge is passed to panic if memory cannot be allocated to store data in a buffer.
```
var ErrTooLarge = errors.New("bytes.Buffer: too large")
```
func Clone 1.20
---------------
```
func Clone(b []byte) []byte
```
Clone returns a copy of b[:len(b)]. The result may have additional unused capacity. Clone(nil) returns nil.
func Compare
------------
```
func Compare(a, b []byte) int
```
Compare returns an integer comparing two byte slices lexicographically. The result will be 0 if a == b, -1 if a < b, and +1 if a > b. A nil argument is equivalent to an empty slice.
#### Example
Code:
```
// Interpret Compare's result by comparing it to zero.
var a, b []byte
if bytes.Compare(a, b) < 0 {
// a less b
}
if bytes.Compare(a, b) <= 0 {
// a less or equal b
}
if bytes.Compare(a, b) > 0 {
// a greater b
}
if bytes.Compare(a, b) >= 0 {
// a greater or equal b
}
// Prefer Equal to Compare for equality comparisons.
if bytes.Equal(a, b) {
// a equal b
}
if !bytes.Equal(a, b) {
// a not equal b
}
```
#### Example (Search)
Code:
```
// Binary search to find a matching byte slice.
var needle []byte
var haystack [][]byte // Assume sorted
i := sort.Search(len(haystack), func(i int) bool {
// Return haystack[i] >= needle.
return bytes.Compare(haystack[i], needle) >= 0
})
if i < len(haystack) && bytes.Equal(haystack[i], needle) {
// Found it!
}
```
func Contains
-------------
```
func Contains(b, subslice []byte) bool
```
Contains reports whether subslice is within b.
#### Example
Code:
```
fmt.Println(bytes.Contains([]byte("seafood"), []byte("foo")))
fmt.Println(bytes.Contains([]byte("seafood"), []byte("bar")))
fmt.Println(bytes.Contains([]byte("seafood"), []byte("")))
fmt.Println(bytes.Contains([]byte(""), []byte("")))
```
Output:
```
true
false
true
true
```
func ContainsAny 1.7
--------------------
```
func ContainsAny(b []byte, chars string) bool
```
ContainsAny reports whether any of the UTF-8-encoded code points in chars are within b.
#### Example
Code:
```
fmt.Println(bytes.ContainsAny([]byte("I like seafood."), "fÄo!"))
fmt.Println(bytes.ContainsAny([]byte("I like seafood."), "去是伟大的."))
fmt.Println(bytes.ContainsAny([]byte("I like seafood."), ""))
fmt.Println(bytes.ContainsAny([]byte(""), ""))
```
Output:
```
true
true
false
false
```
func ContainsRune 1.7
---------------------
```
func ContainsRune(b []byte, r rune) bool
```
ContainsRune reports whether the rune is contained in the UTF-8-encoded byte slice b.
#### Example
Code:
```
fmt.Println(bytes.ContainsRune([]byte("I like seafood."), 'f'))
fmt.Println(bytes.ContainsRune([]byte("I like seafood."), 'ö'))
fmt.Println(bytes.ContainsRune([]byte("去是伟大的!"), '大'))
fmt.Println(bytes.ContainsRune([]byte("去是伟大的!"), '!'))
fmt.Println(bytes.ContainsRune([]byte(""), '@'))
```
Output:
```
true
false
true
true
false
```
func Count
----------
```
func Count(s, sep []byte) int
```
Count counts the number of non-overlapping instances of sep in s. If sep is an empty slice, Count returns 1 + the number of UTF-8-encoded code points in s.
#### Example
Code:
```
fmt.Println(bytes.Count([]byte("cheese"), []byte("e")))
fmt.Println(bytes.Count([]byte("five"), []byte(""))) // before & after each rune
```
Output:
```
3
5
```
func Cut 1.18
-------------
```
func Cut(s, sep []byte) (before, after []byte, found bool)
```
Cut slices s around the first instance of sep, returning the text before and after sep. The found result reports whether sep appears in s. If sep does not appear in s, cut returns s, nil, false.
Cut returns slices of the original slice s, not copies.
#### Example
Code:
```
show := func(s, sep string) {
before, after, found := bytes.Cut([]byte(s), []byte(sep))
fmt.Printf("Cut(%q, %q) = %q, %q, %v\n", s, sep, before, after, found)
}
show("Gopher", "Go")
show("Gopher", "ph")
show("Gopher", "er")
show("Gopher", "Badger")
```
Output:
```
Cut("Gopher", "Go") = "", "pher", true
Cut("Gopher", "ph") = "Go", "er", true
Cut("Gopher", "er") = "Goph", "", true
Cut("Gopher", "Badger") = "Gopher", "", false
```
func CutPrefix 1.20
-------------------
```
func CutPrefix(s, prefix []byte) (after []byte, found bool)
```
CutPrefix returns s without the provided leading prefix byte slice and reports whether it found the prefix. If s doesn't start with prefix, CutPrefix returns s, false. If prefix is the empty byte slice, CutPrefix returns s, true.
CutPrefix returns slices of the original slice s, not copies.
func CutSuffix 1.20
-------------------
```
func CutSuffix(s, suffix []byte) (before []byte, found bool)
```
CutSuffix returns s without the provided ending suffix byte slice and reports whether it found the suffix. If s doesn't end with suffix, CutSuffix returns s, false. If suffix is the empty byte slice, CutSuffix returns s, true.
CutSuffix returns slices of the original slice s, not copies.
func Equal
----------
```
func Equal(a, b []byte) bool
```
Equal reports whether a and b are the same length and contain the same bytes. A nil argument is equivalent to an empty slice.
#### Example
Code:
```
fmt.Println(bytes.Equal([]byte("Go"), []byte("Go")))
fmt.Println(bytes.Equal([]byte("Go"), []byte("C++")))
```
Output:
```
true
false
```
func EqualFold
--------------
```
func EqualFold(s, t []byte) bool
```
EqualFold reports whether s and t, interpreted as UTF-8 strings, are equal under simple Unicode case-folding, which is a more general form of case-insensitivity.
#### Example
Code:
```
fmt.Println(bytes.EqualFold([]byte("Go"), []byte("go")))
```
Output:
```
true
```
func Fields
-----------
```
func Fields(s []byte) [][]byte
```
Fields interprets s as a sequence of UTF-8-encoded code points. It splits the slice s around each instance of one or more consecutive white space characters, as defined by unicode.IsSpace, returning a slice of subslices of s or an empty slice if s contains only white space.
#### Example
Code:
```
fmt.Printf("Fields are: %q", bytes.Fields([]byte(" foo bar baz ")))
```
Output:
```
Fields are: ["foo" "bar" "baz"]
```
func FieldsFunc
---------------
```
func FieldsFunc(s []byte, f func(rune) bool) [][]byte
```
FieldsFunc interprets s as a sequence of UTF-8-encoded code points. It splits the slice s at each run of code points c satisfying f(c) and returns a slice of subslices of s. If all code points in s satisfy f(c), or len(s) == 0, an empty slice is returned.
FieldsFunc makes no guarantees about the order in which it calls f(c) and assumes that f always returns the same value for a given c.
#### Example
Code:
```
f := func(c rune) bool {
return !unicode.IsLetter(c) && !unicode.IsNumber(c)
}
fmt.Printf("Fields are: %q", bytes.FieldsFunc([]byte(" foo1;bar2,baz3..."), f))
```
Output:
```
Fields are: ["foo1" "bar2" "baz3"]
```
func HasPrefix
--------------
```
func HasPrefix(s, prefix []byte) bool
```
HasPrefix tests whether the byte slice s begins with prefix.
#### Example
Code:
```
fmt.Println(bytes.HasPrefix([]byte("Gopher"), []byte("Go")))
fmt.Println(bytes.HasPrefix([]byte("Gopher"), []byte("C")))
fmt.Println(bytes.HasPrefix([]byte("Gopher"), []byte("")))
```
Output:
```
true
false
true
```
func HasSuffix
--------------
```
func HasSuffix(s, suffix []byte) bool
```
HasSuffix tests whether the byte slice s ends with suffix.
#### Example
Code:
```
fmt.Println(bytes.HasSuffix([]byte("Amigo"), []byte("go")))
fmt.Println(bytes.HasSuffix([]byte("Amigo"), []byte("O")))
fmt.Println(bytes.HasSuffix([]byte("Amigo"), []byte("Ami")))
fmt.Println(bytes.HasSuffix([]byte("Amigo"), []byte("")))
```
Output:
```
true
false
false
true
```
func Index
----------
```
func Index(s, sep []byte) int
```
Index returns the index of the first instance of sep in s, or -1 if sep is not present in s.
#### Example
Code:
```
fmt.Println(bytes.Index([]byte("chicken"), []byte("ken")))
fmt.Println(bytes.Index([]byte("chicken"), []byte("dmr")))
```
Output:
```
4
-1
```
func IndexAny
-------------
```
func IndexAny(s []byte, chars string) int
```
IndexAny interprets s as a sequence of UTF-8-encoded Unicode code points. It returns the byte index of the first occurrence in s of any of the Unicode code points in chars. It returns -1 if chars is empty or if there is no code point in common.
#### Example
Code:
```
fmt.Println(bytes.IndexAny([]byte("chicken"), "aeiouy"))
fmt.Println(bytes.IndexAny([]byte("crwth"), "aeiouy"))
```
Output:
```
2
-1
```
func IndexByte
--------------
```
func IndexByte(b []byte, c byte) int
```
IndexByte returns the index of the first instance of c in b, or -1 if c is not present in b.
#### Example
Code:
```
fmt.Println(bytes.IndexByte([]byte("chicken"), byte('k')))
fmt.Println(bytes.IndexByte([]byte("chicken"), byte('g')))
```
Output:
```
4
-1
```
func IndexFunc
--------------
```
func IndexFunc(s []byte, f func(r rune) bool) int
```
IndexFunc interprets s as a sequence of UTF-8-encoded code points. It returns the byte index in s of the first Unicode code point satisfying f(c), or -1 if none do.
#### Example
Code:
```
f := func(c rune) bool {
return unicode.Is(unicode.Han, c)
}
fmt.Println(bytes.IndexFunc([]byte("Hello, 世界"), f))
fmt.Println(bytes.IndexFunc([]byte("Hello, world"), f))
```
Output:
```
7
-1
```
func IndexRune
--------------
```
func IndexRune(s []byte, r rune) int
```
IndexRune interprets s as a sequence of UTF-8-encoded code points. It returns the byte index of the first occurrence in s of the given rune. It returns -1 if rune is not present in s. If r is utf8.RuneError, it returns the first instance of any invalid UTF-8 byte sequence.
#### Example
Code:
```
fmt.Println(bytes.IndexRune([]byte("chicken"), 'k'))
fmt.Println(bytes.IndexRune([]byte("chicken"), 'd'))
```
Output:
```
4
-1
```
func Join
---------
```
func Join(s [][]byte, sep []byte) []byte
```
Join concatenates the elements of s to create a new byte slice. The separator sep is placed between elements in the resulting slice.
#### Example
Code:
```
s := [][]byte{[]byte("foo"), []byte("bar"), []byte("baz")}
fmt.Printf("%s", bytes.Join(s, []byte(", ")))
```
Output:
```
foo, bar, baz
```
func LastIndex
--------------
```
func LastIndex(s, sep []byte) int
```
LastIndex returns the index of the last instance of sep in s, or -1 if sep is not present in s.
#### Example
Code:
```
fmt.Println(bytes.Index([]byte("go gopher"), []byte("go")))
fmt.Println(bytes.LastIndex([]byte("go gopher"), []byte("go")))
fmt.Println(bytes.LastIndex([]byte("go gopher"), []byte("rodent")))
```
Output:
```
0
3
-1
```
func LastIndexAny
-----------------
```
func LastIndexAny(s []byte, chars string) int
```
LastIndexAny interprets s as a sequence of UTF-8-encoded Unicode code points. It returns the byte index of the last occurrence in s of any of the Unicode code points in chars. It returns -1 if chars is empty or if there is no code point in common.
#### Example
Code:
```
fmt.Println(bytes.LastIndexAny([]byte("go gopher"), "MüQp"))
fmt.Println(bytes.LastIndexAny([]byte("go 地鼠"), "地大"))
fmt.Println(bytes.LastIndexAny([]byte("go gopher"), "z,!."))
```
Output:
```
5
3
-1
```
func LastIndexByte 1.5
----------------------
```
func LastIndexByte(s []byte, c byte) int
```
LastIndexByte returns the index of the last instance of c in s, or -1 if c is not present in s.
#### Example
Code:
```
fmt.Println(bytes.LastIndexByte([]byte("go gopher"), byte('g')))
fmt.Println(bytes.LastIndexByte([]byte("go gopher"), byte('r')))
fmt.Println(bytes.LastIndexByte([]byte("go gopher"), byte('z')))
```
Output:
```
3
8
-1
```
func LastIndexFunc
------------------
```
func LastIndexFunc(s []byte, f func(r rune) bool) int
```
LastIndexFunc interprets s as a sequence of UTF-8-encoded code points. It returns the byte index in s of the last Unicode code point satisfying f(c), or -1 if none do.
#### Example
Code:
```
fmt.Println(bytes.LastIndexFunc([]byte("go gopher!"), unicode.IsLetter))
fmt.Println(bytes.LastIndexFunc([]byte("go gopher!"), unicode.IsPunct))
fmt.Println(bytes.LastIndexFunc([]byte("go gopher!"), unicode.IsNumber))
```
Output:
```
8
9
-1
```
func Map
--------
```
func Map(mapping func(r rune) rune, s []byte) []byte
```
Map returns a copy of the byte slice s with all its characters modified according to the mapping function. If mapping returns a negative value, the character is dropped from the byte slice with no replacement. The characters in s and the output are interpreted as UTF-8-encoded code points.
func Repeat
-----------
```
func Repeat(b []byte, count int) []byte
```
Repeat returns a new byte slice consisting of count copies of b.
It panics if count is negative or if the result of (len(b) \* count) overflows.
#### Example
Code:
```
fmt.Printf("ba%s", bytes.Repeat([]byte("na"), 2))
```
Output:
```
banana
```
func Replace
------------
```
func Replace(s, old, new []byte, n int) []byte
```
Replace returns a copy of the slice s with the first n non-overlapping instances of old replaced by new. If old is empty, it matches at the beginning of the slice and after each UTF-8 sequence, yielding up to k+1 replacements for a k-rune slice. If n < 0, there is no limit on the number of replacements.
#### Example
Code:
```
fmt.Printf("%s\n", bytes.Replace([]byte("oink oink oink"), []byte("k"), []byte("ky"), 2))
fmt.Printf("%s\n", bytes.Replace([]byte("oink oink oink"), []byte("oink"), []byte("moo"), -1))
```
Output:
```
oinky oinky oink
moo moo moo
```
func ReplaceAll 1.12
--------------------
```
func ReplaceAll(s, old, new []byte) []byte
```
ReplaceAll returns a copy of the slice s with all non-overlapping instances of old replaced by new. If old is empty, it matches at the beginning of the slice and after each UTF-8 sequence, yielding up to k+1 replacements for a k-rune slice.
#### Example
Code:
```
fmt.Printf("%s\n", bytes.ReplaceAll([]byte("oink oink oink"), []byte("oink"), []byte("moo")))
```
Output:
```
moo moo moo
```
func Runes
----------
```
func Runes(s []byte) []rune
```
Runes interprets s as a sequence of UTF-8-encoded code points. It returns a slice of runes (Unicode code points) equivalent to s.
#### Example
Code:
```
rs := bytes.Runes([]byte("go gopher"))
for _, r := range rs {
fmt.Printf("%#U\n", r)
}
```
Output:
```
U+0067 'g'
U+006F 'o'
U+0020 ' '
U+0067 'g'
U+006F 'o'
U+0070 'p'
U+0068 'h'
U+0065 'e'
U+0072 'r'
```
func Split
----------
```
func Split(s, sep []byte) [][]byte
```
Split slices s into all subslices separated by sep and returns a slice of the subslices between those separators. If sep is empty, Split splits after each UTF-8 sequence. It is equivalent to SplitN with a count of -1.
To split around the first instance of a separator, see Cut.
#### Example
Code:
```
fmt.Printf("%q\n", bytes.Split([]byte("a,b,c"), []byte(",")))
fmt.Printf("%q\n", bytes.Split([]byte("a man a plan a canal panama"), []byte("a ")))
fmt.Printf("%q\n", bytes.Split([]byte(" xyz "), []byte("")))
fmt.Printf("%q\n", bytes.Split([]byte(""), []byte("Bernardo O'Higgins")))
```
Output:
```
["a" "b" "c"]
["" "man " "plan " "canal panama"]
[" " "x" "y" "z" " "]
[""]
```
func SplitAfter
---------------
```
func SplitAfter(s, sep []byte) [][]byte
```
SplitAfter slices s into all subslices after each instance of sep and returns a slice of those subslices. If sep is empty, SplitAfter splits after each UTF-8 sequence. It is equivalent to SplitAfterN with a count of -1.
#### Example
Code:
```
fmt.Printf("%q\n", bytes.SplitAfter([]byte("a,b,c"), []byte(",")))
```
Output:
```
["a," "b," "c"]
```
func SplitAfterN
----------------
```
func SplitAfterN(s, sep []byte, n int) [][]byte
```
SplitAfterN slices s into subslices after each instance of sep and returns a slice of those subslices. If sep is empty, SplitAfterN splits after each UTF-8 sequence. The count determines the number of subslices to return:
```
n > 0: at most n subslices; the last subslice will be the unsplit remainder.
n == 0: the result is nil (zero subslices)
n < 0: all subslices
```
#### Example
Code:
```
fmt.Printf("%q\n", bytes.SplitAfterN([]byte("a,b,c"), []byte(","), 2))
```
Output:
```
["a," "b,c"]
```
func SplitN
-----------
```
func SplitN(s, sep []byte, n int) [][]byte
```
SplitN slices s into subslices separated by sep and returns a slice of the subslices between those separators. If sep is empty, SplitN splits after each UTF-8 sequence. The count determines the number of subslices to return:
```
n > 0: at most n subslices; the last subslice will be the unsplit remainder.
n == 0: the result is nil (zero subslices)
n < 0: all subslices
```
To split around the first instance of a separator, see Cut.
#### Example
Code:
```
fmt.Printf("%q\n", bytes.SplitN([]byte("a,b,c"), []byte(","), 2))
z := bytes.SplitN([]byte("a,b,c"), []byte(","), 0)
fmt.Printf("%q (nil = %v)\n", z, z == nil)
```
Output:
```
["a" "b,c"]
[] (nil = true)
```
func Title
----------
```
func Title(s []byte) []byte
```
Title treats s as UTF-8-encoded bytes and returns a copy with all Unicode letters that begin words mapped to their title case.
Deprecated: The rule Title uses for word boundaries does not handle Unicode punctuation properly. Use golang.org/x/text/cases instead.
#### Example
Code:
```
fmt.Printf("%s", bytes.Title([]byte("her royal highness")))
```
Output:
```
Her Royal Highness
```
func ToLower
------------
```
func ToLower(s []byte) []byte
```
ToLower returns a copy of the byte slice s with all Unicode letters mapped to their lower case.
#### Example
Code:
```
fmt.Printf("%s", bytes.ToLower([]byte("Gopher")))
```
Output:
```
gopher
```
func ToLowerSpecial
-------------------
```
func ToLowerSpecial(c unicode.SpecialCase, s []byte) []byte
```
ToLowerSpecial treats s as UTF-8-encoded bytes and returns a copy with all the Unicode letters mapped to their lower case, giving priority to the special casing rules.
#### Example
Code:
```
str := []byte("AHOJ VÝVOJÁRİ GOLANG")
totitle := bytes.ToLowerSpecial(unicode.AzeriCase, str)
fmt.Println("Original : " + string(str))
fmt.Println("ToLower : " + string(totitle))
```
Output:
```
Original : AHOJ VÝVOJÁRİ GOLANG
ToLower : ahoj vývojári golang
```
func ToTitle
------------
```
func ToTitle(s []byte) []byte
```
ToTitle treats s as UTF-8-encoded bytes and returns a copy with all the Unicode letters mapped to their title case.
#### Example
Code:
```
fmt.Printf("%s\n", bytes.ToTitle([]byte("loud noises")))
fmt.Printf("%s\n", bytes.ToTitle([]byte("хлеб")))
```
Output:
```
LOUD NOISES
ХЛЕБ
```
func ToTitleSpecial
-------------------
```
func ToTitleSpecial(c unicode.SpecialCase, s []byte) []byte
```
ToTitleSpecial treats s as UTF-8-encoded bytes and returns a copy with all the Unicode letters mapped to their title case, giving priority to the special casing rules.
#### Example
Code:
```
str := []byte("ahoj vývojári golang")
totitle := bytes.ToTitleSpecial(unicode.AzeriCase, str)
fmt.Println("Original : " + string(str))
fmt.Println("ToTitle : " + string(totitle))
```
Output:
```
Original : ahoj vývojári golang
ToTitle : AHOJ VÝVOJÁRİ GOLANG
```
func ToUpper
------------
```
func ToUpper(s []byte) []byte
```
ToUpper returns a copy of the byte slice s with all Unicode letters mapped to their upper case.
#### Example
Code:
```
fmt.Printf("%s", bytes.ToUpper([]byte("Gopher")))
```
Output:
```
GOPHER
```
func ToUpperSpecial
-------------------
```
func ToUpperSpecial(c unicode.SpecialCase, s []byte) []byte
```
ToUpperSpecial treats s as UTF-8-encoded bytes and returns a copy with all the Unicode letters mapped to their upper case, giving priority to the special casing rules.
#### Example
Code:
```
str := []byte("ahoj vývojári golang")
totitle := bytes.ToUpperSpecial(unicode.AzeriCase, str)
fmt.Println("Original : " + string(str))
fmt.Println("ToUpper : " + string(totitle))
```
Output:
```
Original : ahoj vývojári golang
ToUpper : AHOJ VÝVOJÁRİ GOLANG
```
func ToValidUTF8 1.13
---------------------
```
func ToValidUTF8(s, replacement []byte) []byte
```
ToValidUTF8 treats s as UTF-8-encoded bytes and returns a copy with each run of bytes representing invalid UTF-8 replaced with the bytes in replacement, which may be empty.
func Trim
---------
```
func Trim(s []byte, cutset string) []byte
```
Trim returns a subslice of s by slicing off all leading and trailing UTF-8-encoded code points contained in cutset.
#### Example
Code:
```
fmt.Printf("[%q]", bytes.Trim([]byte(" !!! Achtung! Achtung! !!! "), "! "))
```
Output:
```
["Achtung! Achtung"]
```
func TrimFunc
-------------
```
func TrimFunc(s []byte, f func(r rune) bool) []byte
```
TrimFunc returns a subslice of s by slicing off all leading and trailing UTF-8-encoded code points c that satisfy f(c).
#### Example
Code:
```
fmt.Println(string(bytes.TrimFunc([]byte("go-gopher!"), unicode.IsLetter)))
fmt.Println(string(bytes.TrimFunc([]byte("\"go-gopher!\""), unicode.IsLetter)))
fmt.Println(string(bytes.TrimFunc([]byte("go-gopher!"), unicode.IsPunct)))
fmt.Println(string(bytes.TrimFunc([]byte("1234go-gopher!567"), unicode.IsNumber)))
```
Output:
```
-gopher!
"go-gopher!"
go-gopher
go-gopher!
```
func TrimLeft
-------------
```
func TrimLeft(s []byte, cutset string) []byte
```
TrimLeft returns a subslice of s by slicing off all leading UTF-8-encoded code points contained in cutset.
#### Example
Code:
```
fmt.Print(string(bytes.TrimLeft([]byte("453gopher8257"), "0123456789")))
```
Output:
```
gopher8257
```
func TrimLeftFunc
-----------------
```
func TrimLeftFunc(s []byte, f func(r rune) bool) []byte
```
TrimLeftFunc treats s as UTF-8-encoded bytes and returns a subslice of s by slicing off all leading UTF-8-encoded code points c that satisfy f(c).
#### Example
Code:
```
fmt.Println(string(bytes.TrimLeftFunc([]byte("go-gopher"), unicode.IsLetter)))
fmt.Println(string(bytes.TrimLeftFunc([]byte("go-gopher!"), unicode.IsPunct)))
fmt.Println(string(bytes.TrimLeftFunc([]byte("1234go-gopher!567"), unicode.IsNumber)))
```
Output:
```
-gopher
go-gopher!
go-gopher!567
```
func TrimPrefix 1.1
-------------------
```
func TrimPrefix(s, prefix []byte) []byte
```
TrimPrefix returns s without the provided leading prefix string. If s doesn't start with prefix, s is returned unchanged.
#### Example
Code:
```
var b = []byte("Goodbye,, world!")
b = bytes.TrimPrefix(b, []byte("Goodbye,"))
b = bytes.TrimPrefix(b, []byte("See ya,"))
fmt.Printf("Hello%s", b)
```
Output:
```
Hello, world!
```
func TrimRight
--------------
```
func TrimRight(s []byte, cutset string) []byte
```
TrimRight returns a subslice of s by slicing off all trailing UTF-8-encoded code points that are contained in cutset.
#### Example
Code:
```
fmt.Print(string(bytes.TrimRight([]byte("453gopher8257"), "0123456789")))
```
Output:
```
453gopher
```
func TrimRightFunc
------------------
```
func TrimRightFunc(s []byte, f func(r rune) bool) []byte
```
TrimRightFunc returns a subslice of s by slicing off all trailing UTF-8-encoded code points c that satisfy f(c).
#### Example
Code:
```
fmt.Println(string(bytes.TrimRightFunc([]byte("go-gopher"), unicode.IsLetter)))
fmt.Println(string(bytes.TrimRightFunc([]byte("go-gopher!"), unicode.IsPunct)))
fmt.Println(string(bytes.TrimRightFunc([]byte("1234go-gopher!567"), unicode.IsNumber)))
```
Output:
```
go-
go-gopher
1234go-gopher!
```
func TrimSpace
--------------
```
func TrimSpace(s []byte) []byte
```
TrimSpace returns a subslice of s by slicing off all leading and trailing white space, as defined by Unicode.
#### Example
Code:
```
fmt.Printf("%s", bytes.TrimSpace([]byte(" \t\n a lone gopher \n\t\r\n")))
```
Output:
```
a lone gopher
```
func TrimSuffix 1.1
-------------------
```
func TrimSuffix(s, suffix []byte) []byte
```
TrimSuffix returns s without the provided trailing suffix string. If s doesn't end with suffix, s is returned unchanged.
#### Example
Code:
```
var b = []byte("Hello, goodbye, etc!")
b = bytes.TrimSuffix(b, []byte("goodbye, etc!"))
b = bytes.TrimSuffix(b, []byte("gopher"))
b = append(b, bytes.TrimSuffix([]byte("world!"), []byte("x!"))...)
os.Stdout.Write(b)
```
Output:
```
Hello, world!
```
type Buffer
-----------
A Buffer is a variable-sized buffer of bytes with Read and Write methods. The zero value for Buffer is an empty buffer ready to use.
```
type Buffer struct {
// contains filtered or unexported fields
}
```
#### Example
Code:
```
var b bytes.Buffer // A Buffer needs no initialization.
b.Write([]byte("Hello "))
fmt.Fprintf(&b, "world!")
b.WriteTo(os.Stdout)
```
Output:
```
Hello world!
```
#### Example (Reader)
Code:
```
// A Buffer can turn a string or a []byte into an io.Reader.
buf := bytes.NewBufferString("R29waGVycyBydWxlIQ==")
dec := base64.NewDecoder(base64.StdEncoding, buf)
io.Copy(os.Stdout, dec)
```
Output:
```
Gophers rule!
```
### func NewBuffer
```
func NewBuffer(buf []byte) *Buffer
```
NewBuffer creates and initializes a new Buffer using buf as its initial contents. The new Buffer takes ownership of buf, and the caller should not use buf after this call. NewBuffer is intended to prepare a Buffer to read existing data. It can also be used to set the initial size of the internal buffer for writing. To do that, buf should have the desired capacity but a length of zero.
In most cases, new(Buffer) (or just declaring a Buffer variable) is sufficient to initialize a Buffer.
### func NewBufferString
```
func NewBufferString(s string) *Buffer
```
NewBufferString creates and initializes a new Buffer using string s as its initial contents. It is intended to prepare a buffer to read an existing string.
In most cases, new(Buffer) (or just declaring a Buffer variable) is sufficient to initialize a Buffer.
### func (\*Buffer) Bytes
```
func (b *Buffer) Bytes() []byte
```
Bytes returns a slice of length b.Len() holding the unread portion of the buffer. The slice is valid for use only until the next buffer modification (that is, only until the next call to a method like Read, Write, Reset, or Truncate). The slice aliases the buffer content at least until the next buffer modification, so immediate changes to the slice will affect the result of future reads.
#### Example
Code:
```
buf := bytes.Buffer{}
buf.Write([]byte{'h', 'e', 'l', 'l', 'o', ' ', 'w', 'o', 'r', 'l', 'd'})
os.Stdout.Write(buf.Bytes())
```
Output:
```
hello world
```
### func (\*Buffer) Cap 1.5
```
func (b *Buffer) Cap() int
```
Cap returns the capacity of the buffer's underlying byte slice, that is, the total space allocated for the buffer's data.
#### Example
Code:
```
buf1 := bytes.NewBuffer(make([]byte, 10))
buf2 := bytes.NewBuffer(make([]byte, 0, 10))
fmt.Println(buf1.Cap())
fmt.Println(buf2.Cap())
```
Output:
```
10
10
```
### func (\*Buffer) Grow 1.1
```
func (b *Buffer) Grow(n int)
```
Grow grows the buffer's capacity, if necessary, to guarantee space for another n bytes. After Grow(n), at least n bytes can be written to the buffer without another allocation. If n is negative, Grow will panic. If the buffer can't grow it will panic with ErrTooLarge.
#### Example
Code:
```
var b bytes.Buffer
b.Grow(64)
bb := b.Bytes()
b.Write([]byte("64 bytes or fewer"))
fmt.Printf("%q", bb[:b.Len()])
```
Output:
```
"64 bytes or fewer"
```
### func (\*Buffer) Len
```
func (b *Buffer) Len() int
```
Len returns the number of bytes of the unread portion of the buffer; b.Len() == len(b.Bytes()).
#### Example
Code:
```
var b bytes.Buffer
b.Grow(64)
b.Write([]byte("abcde"))
fmt.Printf("%d", b.Len())
```
Output:
```
5
```
### func (\*Buffer) Next
```
func (b *Buffer) Next(n int) []byte
```
Next returns a slice containing the next n bytes from the buffer, advancing the buffer as if the bytes had been returned by Read. If there are fewer than n bytes in the buffer, Next returns the entire buffer. The slice is only valid until the next call to a read or write method.
#### Example
Code:
```
var b bytes.Buffer
b.Grow(64)
b.Write([]byte("abcde"))
fmt.Printf("%s\n", string(b.Next(2)))
fmt.Printf("%s\n", string(b.Next(2)))
fmt.Printf("%s", string(b.Next(2)))
```
Output:
```
ab
cd
e
```
### func (\*Buffer) Read
```
func (b *Buffer) Read(p []byte) (n int, err error)
```
Read reads the next len(p) bytes from the buffer or until the buffer is drained. The return value n is the number of bytes read. If the buffer has no data to return, err is io.EOF (unless len(p) is zero); otherwise it is nil.
#### Example
Code:
```
var b bytes.Buffer
b.Grow(64)
b.Write([]byte("abcde"))
rdbuf := make([]byte, 1)
n, err := b.Read(rdbuf)
if err != nil {
panic(err)
}
fmt.Println(n)
fmt.Println(b.String())
fmt.Println(string(rdbuf))
// Output
// 1
// bcde
// a
```
### func (\*Buffer) ReadByte
```
func (b *Buffer) ReadByte() (byte, error)
```
ReadByte reads and returns the next byte from the buffer. If no byte is available, it returns error io.EOF.
#### Example
Code:
```
var b bytes.Buffer
b.Grow(64)
b.Write([]byte("abcde"))
c, err := b.ReadByte()
if err != nil {
panic(err)
}
fmt.Println(c)
fmt.Println(b.String())
// Output
// 97
// bcde
```
### func (\*Buffer) ReadBytes
```
func (b *Buffer) ReadBytes(delim byte) (line []byte, err error)
```
ReadBytes reads until the first occurrence of delim in the input, returning a slice containing the data up to and including the delimiter. If ReadBytes encounters an error before finding a delimiter, it returns the data read before the error and the error itself (often io.EOF). ReadBytes returns err != nil if and only if the returned data does not end in delim.
### func (\*Buffer) ReadFrom
```
func (b *Buffer) ReadFrom(r io.Reader) (n int64, err error)
```
ReadFrom reads data from r until EOF and appends it to the buffer, growing the buffer as needed. The return value n is the number of bytes read. Any error except io.EOF encountered during the read is also returned. If the buffer becomes too large, ReadFrom will panic with ErrTooLarge.
### func (\*Buffer) ReadRune
```
func (b *Buffer) ReadRune() (r rune, size int, err error)
```
ReadRune reads and returns the next UTF-8-encoded Unicode code point from the buffer. If no bytes are available, the error returned is io.EOF. If the bytes are an erroneous UTF-8 encoding, it consumes one byte and returns U+FFFD, 1.
### func (\*Buffer) ReadString
```
func (b *Buffer) ReadString(delim byte) (line string, err error)
```
ReadString reads until the first occurrence of delim in the input, returning a string containing the data up to and including the delimiter. If ReadString encounters an error before finding a delimiter, it returns the data read before the error and the error itself (often io.EOF). ReadString returns err != nil if and only if the returned data does not end in delim.
### func (\*Buffer) Reset
```
func (b *Buffer) Reset()
```
Reset resets the buffer to be empty, but it retains the underlying storage for use by future writes. Reset is the same as Truncate(0).
### func (\*Buffer) String
```
func (b *Buffer) String() string
```
String returns the contents of the unread portion of the buffer as a string. If the Buffer is a nil pointer, it returns "<nil>".
To build strings more efficiently, see the strings.Builder type.
### func (\*Buffer) Truncate
```
func (b *Buffer) Truncate(n int)
```
Truncate discards all but the first n unread bytes from the buffer but continues to use the same allocated storage. It panics if n is negative or greater than the length of the buffer.
### func (\*Buffer) UnreadByte
```
func (b *Buffer) UnreadByte() error
```
UnreadByte unreads the last byte returned by the most recent successful read operation that read at least one byte. If a write has happened since the last read, if the last read returned an error, or if the read read zero bytes, UnreadByte returns an error.
### func (\*Buffer) UnreadRune
```
func (b *Buffer) UnreadRune() error
```
UnreadRune unreads the last rune returned by ReadRune. If the most recent read or write operation on the buffer was not a successful ReadRune, UnreadRune returns an error. (In this regard it is stricter than UnreadByte, which will unread the last byte from any read operation.)
### func (\*Buffer) Write
```
func (b *Buffer) Write(p []byte) (n int, err error)
```
Write appends the contents of p to the buffer, growing the buffer as needed. The return value n is the length of p; err is always nil. If the buffer becomes too large, Write will panic with ErrTooLarge.
### func (\*Buffer) WriteByte
```
func (b *Buffer) WriteByte(c byte) error
```
WriteByte appends the byte c to the buffer, growing the buffer as needed. The returned error is always nil, but is included to match bufio.Writer's WriteByte. If the buffer becomes too large, WriteByte will panic with ErrTooLarge.
### func (\*Buffer) WriteRune
```
func (b *Buffer) WriteRune(r rune) (n int, err error)
```
WriteRune appends the UTF-8 encoding of Unicode code point r to the buffer, returning its length and an error, which is always nil but is included to match bufio.Writer's WriteRune. The buffer is grown as needed; if it becomes too large, WriteRune will panic with ErrTooLarge.
### func (\*Buffer) WriteString
```
func (b *Buffer) WriteString(s string) (n int, err error)
```
WriteString appends the contents of s to the buffer, growing the buffer as needed. The return value n is the length of s; err is always nil. If the buffer becomes too large, WriteString will panic with ErrTooLarge.
### func (\*Buffer) WriteTo
```
func (b *Buffer) WriteTo(w io.Writer) (n int64, err error)
```
WriteTo writes data to w until the buffer is drained or an error occurs. The return value n is the number of bytes written; it always fits into an int, but it is int64 to match the io.WriterTo interface. Any error encountered during the write is also returned.
type Reader
-----------
A Reader implements the io.Reader, io.ReaderAt, io.WriterTo, io.Seeker, io.ByteScanner, and io.RuneScanner interfaces by reading from a byte slice. Unlike a Buffer, a Reader is read-only and supports seeking. The zero value for Reader operates like a Reader of an empty slice.
```
type Reader struct {
// contains filtered or unexported fields
}
```
### func NewReader
```
func NewReader(b []byte) *Reader
```
NewReader returns a new Reader reading from b.
### func (\*Reader) Len
```
func (r *Reader) Len() int
```
Len returns the number of bytes of the unread portion of the slice.
#### Example
Code:
```
fmt.Println(bytes.NewReader([]byte("Hi!")).Len())
fmt.Println(bytes.NewReader([]byte("こんにちは!")).Len())
```
Output:
```
3
16
```
### func (\*Reader) Read
```
func (r *Reader) Read(b []byte) (n int, err error)
```
Read implements the io.Reader interface.
### func (\*Reader) ReadAt
```
func (r *Reader) ReadAt(b []byte, off int64) (n int, err error)
```
ReadAt implements the io.ReaderAt interface.
### func (\*Reader) ReadByte
```
func (r *Reader) ReadByte() (byte, error)
```
ReadByte implements the io.ByteReader interface.
### func (\*Reader) ReadRune
```
func (r *Reader) ReadRune() (ch rune, size int, err error)
```
ReadRune implements the io.RuneReader interface.
### func (\*Reader) Reset 1.7
```
func (r *Reader) Reset(b []byte)
```
Reset resets the Reader to be reading from b.
### func (\*Reader) Seek
```
func (r *Reader) Seek(offset int64, whence int) (int64, error)
```
Seek implements the io.Seeker interface.
### func (\*Reader) Size 1.5
```
func (r *Reader) Size() int64
```
Size returns the original length of the underlying byte slice. Size is the number of bytes available for reading via ReadAt. The result is unaffected by any method calls except Reset.
### func (\*Reader) UnreadByte
```
func (r *Reader) UnreadByte() error
```
UnreadByte complements ReadByte in implementing the io.ByteScanner interface.
### func (\*Reader) UnreadRune
```
func (r *Reader) UnreadRune() error
```
UnreadRune complements ReadRune in implementing the io.RuneScanner interface.
### func (\*Reader) WriteTo 1.1
```
func (r *Reader) WriteTo(w io.Writer) (n int64, err error)
```
WriteTo implements the io.WriterTo interface.
| programming_docs |
go Package sort Package sort
=============
* `import "sort"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package sort provides primitives for sorting slices and user-defined collections.
#### Example
Code:
```
package sort_test
import (
"fmt"
"sort"
)
type Person struct {
Name string
Age int
}
func (p Person) String() string {
return fmt.Sprintf("%s: %d", p.Name, p.Age)
}
// ByAge implements sort.Interface for []Person based on
// the Age field.
type ByAge []Person
func (a ByAge) Len() int { return len(a) }
func (a ByAge) Swap(i, j int) { a[i], a[j] = a[j], a[i] }
func (a ByAge) Less(i, j int) bool { return a[i].Age < a[j].Age }
func Example() {
people := []Person{
{"Bob", 31},
{"John", 42},
{"Michael", 17},
{"Jenny", 26},
}
fmt.Println(people)
// There are two ways to sort a slice. First, one can define
// a set of methods for the slice type, as with ByAge, and
// call sort.Sort. In this first example we use that technique.
sort.Sort(ByAge(people))
fmt.Println(people)
// The other way is to use sort.Slice with a custom Less
// function, which can be provided as a closure. In this
// case no methods are needed. (And if they exist, they
// are ignored.) Here we re-sort in reverse order: compare
// the closure with ByAge.Less.
sort.Slice(people, func(i, j int) bool {
return people[i].Age > people[j].Age
})
fmt.Println(people)
// Output:
// [Bob: 31 John: 42 Michael: 17 Jenny: 26]
// [Michael: 17 Jenny: 26 Bob: 31 John: 42]
// [John: 42 Bob: 31 Jenny: 26 Michael: 17]
}
```
#### Example (SortKeys)
ExampleSortKeys demonstrates a technique for sorting a struct type using programmable sort criteria.
Code:
```
package sort_test
import (
"fmt"
"sort"
)
// A couple of type definitions to make the units clear.
type earthMass float64
type au float64
// A Planet defines the properties of a solar system object.
type Planet struct {
name string
mass earthMass
distance au
}
// By is the type of a "less" function that defines the ordering of its Planet arguments.
type By func(p1, p2 *Planet) bool
// Sort is a method on the function type, By, that sorts the argument slice according to the function.
func (by By) Sort(planets []Planet) {
ps := &planetSorter{
planets: planets,
by: by, // The Sort method's receiver is the function (closure) that defines the sort order.
}
sort.Sort(ps)
}
// planetSorter joins a By function and a slice of Planets to be sorted.
type planetSorter struct {
planets []Planet
by func(p1, p2 *Planet) bool // Closure used in the Less method.
}
// Len is part of sort.Interface.
func (s *planetSorter) Len() int {
return len(s.planets)
}
// Swap is part of sort.Interface.
func (s *planetSorter) Swap(i, j int) {
s.planets[i], s.planets[j] = s.planets[j], s.planets[i]
}
// Less is part of sort.Interface. It is implemented by calling the "by" closure in the sorter.
func (s *planetSorter) Less(i, j int) bool {
return s.by(&s.planets[i], &s.planets[j])
}
var planets = []Planet{
{"Mercury", 0.055, 0.4},
{"Venus", 0.815, 0.7},
{"Earth", 1.0, 1.0},
{"Mars", 0.107, 1.5},
}
// ExampleSortKeys demonstrates a technique for sorting a struct type using programmable sort criteria.
func Example_sortKeys() {
// Closures that order the Planet structure.
name := func(p1, p2 *Planet) bool {
return p1.name < p2.name
}
mass := func(p1, p2 *Planet) bool {
return p1.mass < p2.mass
}
distance := func(p1, p2 *Planet) bool {
return p1.distance < p2.distance
}
decreasingDistance := func(p1, p2 *Planet) bool {
return distance(p2, p1)
}
// Sort the planets by the various criteria.
By(name).Sort(planets)
fmt.Println("By name:", planets)
By(mass).Sort(planets)
fmt.Println("By mass:", planets)
By(distance).Sort(planets)
fmt.Println("By distance:", planets)
By(decreasingDistance).Sort(planets)
fmt.Println("By decreasing distance:", planets)
// Output: By name: [{Earth 1 1} {Mars 0.107 1.5} {Mercury 0.055 0.4} {Venus 0.815 0.7}]
// By mass: [{Mercury 0.055 0.4} {Mars 0.107 1.5} {Venus 0.815 0.7} {Earth 1 1}]
// By distance: [{Mercury 0.055 0.4} {Venus 0.815 0.7} {Earth 1 1} {Mars 0.107 1.5}]
// By decreasing distance: [{Mars 0.107 1.5} {Earth 1 1} {Venus 0.815 0.7} {Mercury 0.055 0.4}]
}
```
#### Example (SortMultiKeys)
ExampleMultiKeys demonstrates a technique for sorting a struct type using different sets of multiple fields in the comparison. We chain together "Less" functions, each of which compares a single field.
Code:
```
package sort_test
import (
"fmt"
"sort"
)
// A Change is a record of source code changes, recording user, language, and delta size.
type Change struct {
user string
language string
lines int
}
type lessFunc func(p1, p2 *Change) bool
// multiSorter implements the Sort interface, sorting the changes within.
type multiSorter struct {
changes []Change
less []lessFunc
}
// Sort sorts the argument slice according to the less functions passed to OrderedBy.
func (ms *multiSorter) Sort(changes []Change) {
ms.changes = changes
sort.Sort(ms)
}
// OrderedBy returns a Sorter that sorts using the less functions, in order.
// Call its Sort method to sort the data.
func OrderedBy(less ...lessFunc) *multiSorter {
return &multiSorter{
less: less,
}
}
// Len is part of sort.Interface.
func (ms *multiSorter) Len() int {
return len(ms.changes)
}
// Swap is part of sort.Interface.
func (ms *multiSorter) Swap(i, j int) {
ms.changes[i], ms.changes[j] = ms.changes[j], ms.changes[i]
}
// Less is part of sort.Interface. It is implemented by looping along the
// less functions until it finds a comparison that discriminates between
// the two items (one is less than the other). Note that it can call the
// less functions twice per call. We could change the functions to return
// -1, 0, 1 and reduce the number of calls for greater efficiency: an
// exercise for the reader.
func (ms *multiSorter) Less(i, j int) bool {
p, q := &ms.changes[i], &ms.changes[j]
// Try all but the last comparison.
var k int
for k = 0; k < len(ms.less)-1; k++ {
less := ms.less[k]
switch {
case less(p, q):
// p < q, so we have a decision.
return true
case less(q, p):
// p > q, so we have a decision.
return false
}
// p == q; try the next comparison.
}
// All comparisons to here said "equal", so just return whatever
// the final comparison reports.
return ms.less[k](p, q)
}
var changes = []Change{
{"gri", "Go", 100},
{"ken", "C", 150},
{"glenda", "Go", 200},
{"rsc", "Go", 200},
{"r", "Go", 100},
{"ken", "Go", 200},
{"dmr", "C", 100},
{"r", "C", 150},
{"gri", "Smalltalk", 80},
}
// ExampleMultiKeys demonstrates a technique for sorting a struct type using different
// sets of multiple fields in the comparison. We chain together "Less" functions, each of
// which compares a single field.
func Example_sortMultiKeys() {
// Closures that order the Change structure.
user := func(c1, c2 *Change) bool {
return c1.user < c2.user
}
language := func(c1, c2 *Change) bool {
return c1.language < c2.language
}
increasingLines := func(c1, c2 *Change) bool {
return c1.lines < c2.lines
}
decreasingLines := func(c1, c2 *Change) bool {
return c1.lines > c2.lines // Note: > orders downwards.
}
// Simple use: Sort by user.
OrderedBy(user).Sort(changes)
fmt.Println("By user:", changes)
// More examples.
OrderedBy(user, increasingLines).Sort(changes)
fmt.Println("By user,<lines:", changes)
OrderedBy(user, decreasingLines).Sort(changes)
fmt.Println("By user,>lines:", changes)
OrderedBy(language, increasingLines).Sort(changes)
fmt.Println("By language,<lines:", changes)
OrderedBy(language, increasingLines, user).Sort(changes)
fmt.Println("By language,<lines,user:", changes)
// Output:
// By user: [{dmr C 100} {glenda Go 200} {gri Go 100} {gri Smalltalk 80} {ken C 150} {ken Go 200} {r Go 100} {r C 150} {rsc Go 200}]
// By user,<lines: [{dmr C 100} {glenda Go 200} {gri Smalltalk 80} {gri Go 100} {ken C 150} {ken Go 200} {r Go 100} {r C 150} {rsc Go 200}]
// By user,>lines: [{dmr C 100} {glenda Go 200} {gri Go 100} {gri Smalltalk 80} {ken Go 200} {ken C 150} {r C 150} {r Go 100} {rsc Go 200}]
// By language,<lines: [{dmr C 100} {ken C 150} {r C 150} {gri Go 100} {r Go 100} {glenda Go 200} {ken Go 200} {rsc Go 200} {gri Smalltalk 80}]
// By language,<lines,user: [{dmr C 100} {ken C 150} {r C 150} {gri Go 100} {r Go 100} {glenda Go 200} {ken Go 200} {rsc Go 200} {gri Smalltalk 80}]
}
```
#### Example (SortWrapper)
Code:
```
package sort_test
import (
"fmt"
"sort"
)
type Grams int
func (g Grams) String() string { return fmt.Sprintf("%dg", int(g)) }
type Organ struct {
Name string
Weight Grams
}
type Organs []*Organ
func (s Organs) Len() int { return len(s) }
func (s Organs) Swap(i, j int) { s[i], s[j] = s[j], s[i] }
// ByName implements sort.Interface by providing Less and using the Len and
// Swap methods of the embedded Organs value.
type ByName struct{ Organs }
func (s ByName) Less(i, j int) bool { return s.Organs[i].Name < s.Organs[j].Name }
// ByWeight implements sort.Interface by providing Less and using the Len and
// Swap methods of the embedded Organs value.
type ByWeight struct{ Organs }
func (s ByWeight) Less(i, j int) bool { return s.Organs[i].Weight < s.Organs[j].Weight }
func Example_sortWrapper() {
s := []*Organ{
{"brain", 1340},
{"heart", 290},
{"liver", 1494},
{"pancreas", 131},
{"prostate", 62},
{"spleen", 162},
}
sort.Sort(ByWeight{s})
fmt.Println("Organs by weight:")
printOrgans(s)
sort.Sort(ByName{s})
fmt.Println("Organs by name:")
printOrgans(s)
// Output:
// Organs by weight:
// prostate (62g)
// pancreas (131g)
// spleen (162g)
// heart (290g)
// brain (1340g)
// liver (1494g)
// Organs by name:
// brain (1340g)
// heart (290g)
// liver (1494g)
// pancreas (131g)
// prostate (62g)
// spleen (162g)
}
func printOrgans(s []*Organ) {
for _, o := range s {
fmt.Printf("%-8s (%v)\n", o.Name, o.Weight)
}
}
```
Index
-----
* [func Find(n int, cmp func(int) int) (i int, found bool)](#Find)
* [func Float64s(x []float64)](#Float64s)
* [func Float64sAreSorted(x []float64) bool](#Float64sAreSorted)
* [func Ints(x []int)](#Ints)
* [func IntsAreSorted(x []int) bool](#IntsAreSorted)
* [func IsSorted(data Interface) bool](#IsSorted)
* [func Search(n int, f func(int) bool) int](#Search)
* [func SearchFloat64s(a []float64, x float64) int](#SearchFloat64s)
* [func SearchInts(a []int, x int) int](#SearchInts)
* [func SearchStrings(a []string, x string) int](#SearchStrings)
* [func Slice(x any, less func(i, j int) bool)](#Slice)
* [func SliceIsSorted(x any, less func(i, j int) bool) bool](#SliceIsSorted)
* [func SliceStable(x any, less func(i, j int) bool)](#SliceStable)
* [func Sort(data Interface)](#Sort)
* [func Stable(data Interface)](#Stable)
* [func Strings(x []string)](#Strings)
* [func StringsAreSorted(x []string) bool](#StringsAreSorted)
* [type Float64Slice](#Float64Slice)
* [func (x Float64Slice) Len() int](#Float64Slice.Len)
* [func (x Float64Slice) Less(i, j int) bool](#Float64Slice.Less)
* [func (p Float64Slice) Search(x float64) int](#Float64Slice.Search)
* [func (x Float64Slice) Sort()](#Float64Slice.Sort)
* [func (x Float64Slice) Swap(i, j int)](#Float64Slice.Swap)
* [type IntSlice](#IntSlice)
* [func (x IntSlice) Len() int](#IntSlice.Len)
* [func (x IntSlice) Less(i, j int) bool](#IntSlice.Less)
* [func (p IntSlice) Search(x int) int](#IntSlice.Search)
* [func (x IntSlice) Sort()](#IntSlice.Sort)
* [func (x IntSlice) Swap(i, j int)](#IntSlice.Swap)
* [type Interface](#Interface)
* [func Reverse(data Interface) Interface](#Reverse)
* [type StringSlice](#StringSlice)
* [func (x StringSlice) Len() int](#StringSlice.Len)
* [func (x StringSlice) Less(i, j int) bool](#StringSlice.Less)
* [func (p StringSlice) Search(x string) int](#StringSlice.Search)
* [func (x StringSlice) Sort()](#StringSlice.Sort)
* [func (x StringSlice) Swap(i, j int)](#StringSlice.Swap)
### Examples
[Package](#example_) [Float64s](#example_Float64s) [Float64sAreSorted](#example_Float64sAreSorted) [Ints](#example_Ints) [IntsAreSorted](#example_IntsAreSorted) [Reverse](#example_Reverse) [Search](#example_Search) [SearchFloat64s](#example_SearchFloat64s) [SearchInts](#example_SearchInts) [Search (DescendingOrder)](#example_Search_descendingOrder) [Slice](#example_Slice) [SliceStable](#example_SliceStable) [Strings](#example_Strings) [Package (SortKeys)](#example__sortKeys) [Package (SortMultiKeys)](#example__sortMultiKeys) [Package (SortWrapper)](#example__sortWrapper) ### Package files
search.go slice.go sort.go zsortfunc.go zsortinterface.go
func Find 1.19
--------------
```
func Find(n int, cmp func(int) int) (i int, found bool)
```
Find uses binary search to find and return the smallest index i in [0, n) at which cmp(i) <= 0. If there is no such index i, Find returns i = n. The found result is true if i < n and cmp(i) == 0. Find calls cmp(i) only for i in the range [0, n).
To permit binary search, Find requires that cmp(i) > 0 for a leading prefix of the range, cmp(i) == 0 in the middle, and cmp(i) < 0 for the final suffix of the range. (Each subrange could be empty.) The usual way to establish this condition is to interpret cmp(i) as a comparison of a desired target value t against entry i in an underlying indexed data structure x, returning <0, 0, and >0 when t < x[i], t == x[i], and t > x[i], respectively.
For example, to look for a particular string in a sorted, random-access list of strings:
```
i, found := sort.Find(x.Len(), func(i int) int {
return strings.Compare(target, x.At(i))
})
if found {
fmt.Printf("found %s at entry %d\n", target, i)
} else {
fmt.Printf("%s not found, would insert at %d", target, i)
}
```
func Float64s
-------------
```
func Float64s(x []float64)
```
Float64s sorts a slice of float64s in increasing order. Not-a-number (NaN) values are ordered before other values.
#### Example
Code:
```
s := []float64{5.2, -1.3, 0.7, -3.8, 2.6} // unsorted
sort.Float64s(s)
fmt.Println(s)
s = []float64{math.Inf(1), math.NaN(), math.Inf(-1), 0.0} // unsorted
sort.Float64s(s)
fmt.Println(s)
```
Output:
```
[-3.8 -1.3 0.7 2.6 5.2]
[NaN -Inf 0 +Inf]
```
func Float64sAreSorted
----------------------
```
func Float64sAreSorted(x []float64) bool
```
Float64sAreSorted reports whether the slice x is sorted in increasing order, with not-a-number (NaN) values before any other values.
#### Example
Code:
```
s := []float64{0.7, 1.3, 2.6, 3.8, 5.2} // sorted ascending
fmt.Println(sort.Float64sAreSorted(s))
s = []float64{5.2, 3.8, 2.6, 1.3, 0.7} // sorted descending
fmt.Println(sort.Float64sAreSorted(s))
s = []float64{5.2, 1.3, 0.7, 3.8, 2.6} // unsorted
fmt.Println(sort.Float64sAreSorted(s))
```
Output:
```
true
false
false
```
func Ints
---------
```
func Ints(x []int)
```
Ints sorts a slice of ints in increasing order.
#### Example
Code:
```
s := []int{5, 2, 6, 3, 1, 4} // unsorted
sort.Ints(s)
fmt.Println(s)
```
Output:
```
[1 2 3 4 5 6]
```
func IntsAreSorted
------------------
```
func IntsAreSorted(x []int) bool
```
IntsAreSorted reports whether the slice x is sorted in increasing order.
#### Example
Code:
```
s := []int{1, 2, 3, 4, 5, 6} // sorted ascending
fmt.Println(sort.IntsAreSorted(s))
s = []int{6, 5, 4, 3, 2, 1} // sorted descending
fmt.Println(sort.IntsAreSorted(s))
s = []int{3, 2, 4, 1, 5} // unsorted
fmt.Println(sort.IntsAreSorted(s))
```
Output:
```
true
false
false
```
func IsSorted
-------------
```
func IsSorted(data Interface) bool
```
IsSorted reports whether data is sorted.
func Search
-----------
```
func Search(n int, f func(int) bool) int
```
Search uses binary search to find and return the smallest index i in [0, n) at which f(i) is true, assuming that on the range [0, n), f(i) == true implies f(i+1) == true. That is, Search requires that f is false for some (possibly empty) prefix of the input range [0, n) and then true for the (possibly empty) remainder; Search returns the first true index. If there is no such index, Search returns n. (Note that the "not found" return value is not -1 as in, for instance, strings.Index.) Search calls f(i) only for i in the range [0, n).
A common use of Search is to find the index i for a value x in a sorted, indexable data structure such as an array or slice. In this case, the argument f, typically a closure, captures the value to be searched for, and how the data structure is indexed and ordered.
For instance, given a slice data sorted in ascending order, the call Search(len(data), func(i int) bool { return data[i] >= 23 }) returns the smallest index i such that data[i] >= 23. If the caller wants to find whether 23 is in the slice, it must test data[i] == 23 separately.
Searching data sorted in descending order would use the <= operator instead of the >= operator.
To complete the example above, the following code tries to find the value x in an integer slice data sorted in ascending order:
```
x := 23
i := sort.Search(len(data), func(i int) bool { return data[i] >= x })
if i < len(data) && data[i] == x {
// x is present at data[i]
} else {
// x is not present in data,
// but i is the index where it would be inserted.
}
```
As a more whimsical example, this program guesses your number:
```
func GuessingGame() {
var s string
fmt.Printf("Pick an integer from 0 to 100.\n")
answer := sort.Search(100, func(i int) bool {
fmt.Printf("Is your number <= %d? ", i)
fmt.Scanf("%s", &s)
return s != "" && s[0] == 'y'
})
fmt.Printf("Your number is %d.\n", answer)
}
```
#### Example
This example demonstrates searching a list sorted in ascending order.
Code:
```
a := []int{1, 3, 6, 10, 15, 21, 28, 36, 45, 55}
x := 6
i := sort.Search(len(a), func(i int) bool { return a[i] >= x })
if i < len(a) && a[i] == x {
fmt.Printf("found %d at index %d in %v\n", x, i, a)
} else {
fmt.Printf("%d not found in %v\n", x, a)
}
```
Output:
```
found 6 at index 2 in [1 3 6 10 15 21 28 36 45 55]
```
#### Example (DescendingOrder)
This example demonstrates searching a list sorted in descending order. The approach is the same as searching a list in ascending order, but with the condition inverted.
Code:
```
a := []int{55, 45, 36, 28, 21, 15, 10, 6, 3, 1}
x := 6
i := sort.Search(len(a), func(i int) bool { return a[i] <= x })
if i < len(a) && a[i] == x {
fmt.Printf("found %d at index %d in %v\n", x, i, a)
} else {
fmt.Printf("%d not found in %v\n", x, a)
}
```
Output:
```
found 6 at index 7 in [55 45 36 28 21 15 10 6 3 1]
```
func SearchFloat64s
-------------------
```
func SearchFloat64s(a []float64, x float64) int
```
SearchFloat64s searches for x in a sorted slice of float64s and returns the index as specified by Search. The return value is the index to insert x if x is not present (it could be len(a)). The slice must be sorted in ascending order.
#### Example
This example demonstrates searching for float64 in a list sorted in ascending order.
Code:
```
a := []float64{1.0, 2.0, 3.3, 4.6, 6.1, 7.2, 8.0}
x := 2.0
i := sort.SearchFloat64s(a, x)
fmt.Printf("found %g at index %d in %v\n", x, i, a)
x = 0.5
i = sort.SearchFloat64s(a, x)
fmt.Printf("%g not found, can be inserted at index %d in %v\n", x, i, a)
```
Output:
```
found 2 at index 1 in [1 2 3.3 4.6 6.1 7.2 8]
0.5 not found, can be inserted at index 0 in [1 2 3.3 4.6 6.1 7.2 8]
```
func SearchInts
---------------
```
func SearchInts(a []int, x int) int
```
SearchInts searches for x in a sorted slice of ints and returns the index as specified by Search. The return value is the index to insert x if x is not present (it could be len(a)). The slice must be sorted in ascending order.
#### Example
This example demonstrates searching for int in a list sorted in ascending order.
Code:
```
a := []int{1, 2, 3, 4, 6, 7, 8}
x := 2
i := sort.SearchInts(a, x)
fmt.Printf("found %d at index %d in %v\n", x, i, a)
x = 5
i = sort.SearchInts(a, x)
fmt.Printf("%d not found, can be inserted at index %d in %v\n", x, i, a)
```
Output:
```
found 2 at index 1 in [1 2 3 4 6 7 8]
5 not found, can be inserted at index 4 in [1 2 3 4 6 7 8]
```
func SearchStrings
------------------
```
func SearchStrings(a []string, x string) int
```
SearchStrings searches for x in a sorted slice of strings and returns the index as specified by Search. The return value is the index to insert x if x is not present (it could be len(a)). The slice must be sorted in ascending order.
func Slice 1.8
--------------
```
func Slice(x any, less func(i, j int) bool)
```
Slice sorts the slice x given the provided less function. It panics if x is not a slice.
The sort is not guaranteed to be stable: equal elements may be reversed from their original order. For a stable sort, use SliceStable.
The less function must satisfy the same requirements as the Interface type's Less method.
#### Example
Code:
```
people := []struct {
Name string
Age int
}{
{"Gopher", 7},
{"Alice", 55},
{"Vera", 24},
{"Bob", 75},
}
sort.Slice(people, func(i, j int) bool { return people[i].Name < people[j].Name })
fmt.Println("By name:", people)
sort.Slice(people, func(i, j int) bool { return people[i].Age < people[j].Age })
fmt.Println("By age:", people)
```
Output:
```
By name: [{Alice 55} {Bob 75} {Gopher 7} {Vera 24}]
By age: [{Gopher 7} {Vera 24} {Alice 55} {Bob 75}]
```
func SliceIsSorted 1.8
----------------------
```
func SliceIsSorted(x any, less func(i, j int) bool) bool
```
SliceIsSorted reports whether the slice x is sorted according to the provided less function. It panics if x is not a slice.
func SliceStable 1.8
--------------------
```
func SliceStable(x any, less func(i, j int) bool)
```
SliceStable sorts the slice x using the provided less function, keeping equal elements in their original order. It panics if x is not a slice.
The less function must satisfy the same requirements as the Interface type's Less method.
#### Example
Code:
```
people := []struct {
Name string
Age int
}{
{"Alice", 25},
{"Elizabeth", 75},
{"Alice", 75},
{"Bob", 75},
{"Alice", 75},
{"Bob", 25},
{"Colin", 25},
{"Elizabeth", 25},
}
// Sort by name, preserving original order
sort.SliceStable(people, func(i, j int) bool { return people[i].Name < people[j].Name })
fmt.Println("By name:", people)
// Sort by age preserving name order
sort.SliceStable(people, func(i, j int) bool { return people[i].Age < people[j].Age })
fmt.Println("By age,name:", people)
```
Output:
```
By name: [{Alice 25} {Alice 75} {Alice 75} {Bob 75} {Bob 25} {Colin 25} {Elizabeth 75} {Elizabeth 25}]
By age,name: [{Alice 25} {Bob 25} {Colin 25} {Elizabeth 25} {Alice 75} {Alice 75} {Bob 75} {Elizabeth 75}]
```
func Sort
---------
```
func Sort(data Interface)
```
Sort sorts data in ascending order as determined by the Less method. It makes one call to data.Len to determine n and O(n\*log(n)) calls to data.Less and data.Swap. The sort is not guaranteed to be stable.
func Stable 1.2
---------------
```
func Stable(data Interface)
```
Stable sorts data in ascending order as determined by the Less method, while keeping the original order of equal elements.
It makes one call to data.Len to determine n, O(n\*log(n)) calls to data.Less and O(n\*log(n)\*log(n)) calls to data.Swap.
func Strings
------------
```
func Strings(x []string)
```
Strings sorts a slice of strings in increasing order.
#### Example
Code:
```
s := []string{"Go", "Bravo", "Gopher", "Alpha", "Grin", "Delta"}
sort.Strings(s)
fmt.Println(s)
```
Output:
```
[Alpha Bravo Delta Go Gopher Grin]
```
func StringsAreSorted
---------------------
```
func StringsAreSorted(x []string) bool
```
StringsAreSorted reports whether the slice x is sorted in increasing order.
type Float64Slice
-----------------
Float64Slice implements Interface for a []float64, sorting in increasing order, with not-a-number (NaN) values ordered before other values.
```
type Float64Slice []float64
```
### func (Float64Slice) Len
```
func (x Float64Slice) Len() int
```
### func (Float64Slice) Less
```
func (x Float64Slice) Less(i, j int) bool
```
Less reports whether x[i] should be ordered before x[j], as required by the sort Interface. Note that floating-point comparison by itself is not a transitive relation: it does not report a consistent ordering for not-a-number (NaN) values. This implementation of Less places NaN values before any others, by using:
```
x[i] < x[j] || (math.IsNaN(x[i]) && !math.IsNaN(x[j]))
```
### func (Float64Slice) Search
```
func (p Float64Slice) Search(x float64) int
```
Search returns the result of applying SearchFloat64s to the receiver and x.
### func (Float64Slice) Sort
```
func (x Float64Slice) Sort()
```
Sort is a convenience method: x.Sort() calls Sort(x).
### func (Float64Slice) Swap
```
func (x Float64Slice) Swap(i, j int)
```
type IntSlice
-------------
IntSlice attaches the methods of Interface to []int, sorting in increasing order.
```
type IntSlice []int
```
### func (IntSlice) Len
```
func (x IntSlice) Len() int
```
### func (IntSlice) Less
```
func (x IntSlice) Less(i, j int) bool
```
### func (IntSlice) Search
```
func (p IntSlice) Search(x int) int
```
Search returns the result of applying SearchInts to the receiver and x.
### func (IntSlice) Sort
```
func (x IntSlice) Sort()
```
Sort is a convenience method: x.Sort() calls Sort(x).
### func (IntSlice) Swap
```
func (x IntSlice) Swap(i, j int)
```
type Interface
--------------
An implementation of Interface can be sorted by the routines in this package. The methods refer to elements of the underlying collection by integer index.
```
type Interface interface {
// Len is the number of elements in the collection.
Len() int
// Less reports whether the element with index i
// must sort before the element with index j.
//
// If both Less(i, j) and Less(j, i) are false,
// then the elements at index i and j are considered equal.
// Sort may place equal elements in any order in the final result,
// while Stable preserves the original input order of equal elements.
//
// Less must describe a transitive ordering:
// - if both Less(i, j) and Less(j, k) are true, then Less(i, k) must be true as well.
// - if both Less(i, j) and Less(j, k) are false, then Less(i, k) must be false as well.
//
// Note that floating-point comparison (the < operator on float32 or float64 values)
// is not a transitive ordering when not-a-number (NaN) values are involved.
// See Float64Slice.Less for a correct implementation for floating-point values.
Less(i, j int) bool
// Swap swaps the elements with indexes i and j.
Swap(i, j int)
}
```
### func Reverse 1.1
```
func Reverse(data Interface) Interface
```
Reverse returns the reverse order for data.
#### Example
Code:
```
s := []int{5, 2, 6, 3, 1, 4} // unsorted
sort.Sort(sort.Reverse(sort.IntSlice(s)))
fmt.Println(s)
```
Output:
```
[6 5 4 3 2 1]
```
type StringSlice
----------------
StringSlice attaches the methods of Interface to []string, sorting in increasing order.
```
type StringSlice []string
```
### func (StringSlice) Len
```
func (x StringSlice) Len() int
```
### func (StringSlice) Less
```
func (x StringSlice) Less(i, j int) bool
```
### func (StringSlice) Search
```
func (p StringSlice) Search(x string) int
```
Search returns the result of applying SearchStrings to the receiver and x.
### func (StringSlice) Sort
```
func (x StringSlice) Sort()
```
Sort is a convenience method: x.Sort() calls Sort(x).
### func (StringSlice) Swap
```
func (x StringSlice) Swap(i, j int)
```
| programming_docs |
go Package unicode Package unicode
================
* `import "unicode"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package unicode provides data and functions to test some properties of Unicode code points.
#### Example (Is)
Functions starting with "Is" can be used to inspect which table of range a rune belongs to. Note that runes may fit into more than one range.
Code:
```
// constant with mixed type runes
const mixed = "\b5Ὂg̀9! ℃ᾭG"
for _, c := range mixed {
fmt.Printf("For %q:\n", c)
if unicode.IsControl(c) {
fmt.Println("\tis control rune")
}
if unicode.IsDigit(c) {
fmt.Println("\tis digit rune")
}
if unicode.IsGraphic(c) {
fmt.Println("\tis graphic rune")
}
if unicode.IsLetter(c) {
fmt.Println("\tis letter rune")
}
if unicode.IsLower(c) {
fmt.Println("\tis lower case rune")
}
if unicode.IsMark(c) {
fmt.Println("\tis mark rune")
}
if unicode.IsNumber(c) {
fmt.Println("\tis number rune")
}
if unicode.IsPrint(c) {
fmt.Println("\tis printable rune")
}
if !unicode.IsPrint(c) {
fmt.Println("\tis not printable rune")
}
if unicode.IsPunct(c) {
fmt.Println("\tis punct rune")
}
if unicode.IsSpace(c) {
fmt.Println("\tis space rune")
}
if unicode.IsSymbol(c) {
fmt.Println("\tis symbol rune")
}
if unicode.IsTitle(c) {
fmt.Println("\tis title case rune")
}
if unicode.IsUpper(c) {
fmt.Println("\tis upper case rune")
}
}
```
Output:
```
For '\b':
is control rune
is not printable rune
For '5':
is digit rune
is graphic rune
is number rune
is printable rune
For 'Ὂ':
is graphic rune
is letter rune
is printable rune
is upper case rune
For 'g':
is graphic rune
is letter rune
is lower case rune
is printable rune
For '̀':
is graphic rune
is mark rune
is printable rune
For '9':
is digit rune
is graphic rune
is number rune
is printable rune
For '!':
is graphic rune
is printable rune
is punct rune
For ' ':
is graphic rune
is printable rune
is space rune
For '℃':
is graphic rune
is printable rune
is symbol rune
For 'ᾭ':
is graphic rune
is letter rune
is printable rune
is title case rune
For 'G':
is graphic rune
is letter rune
is printable rune
is upper case rune
```
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func In(r rune, ranges ...\*RangeTable) bool](#In)
* [func Is(rangeTab \*RangeTable, r rune) bool](#Is)
* [func IsControl(r rune) bool](#IsControl)
* [func IsDigit(r rune) bool](#IsDigit)
* [func IsGraphic(r rune) bool](#IsGraphic)
* [func IsLetter(r rune) bool](#IsLetter)
* [func IsLower(r rune) bool](#IsLower)
* [func IsMark(r rune) bool](#IsMark)
* [func IsNumber(r rune) bool](#IsNumber)
* [func IsOneOf(ranges []\*RangeTable, r rune) bool](#IsOneOf)
* [func IsPrint(r rune) bool](#IsPrint)
* [func IsPunct(r rune) bool](#IsPunct)
* [func IsSpace(r rune) bool](#IsSpace)
* [func IsSymbol(r rune) bool](#IsSymbol)
* [func IsTitle(r rune) bool](#IsTitle)
* [func IsUpper(r rune) bool](#IsUpper)
* [func SimpleFold(r rune) rune](#SimpleFold)
* [func To(\_case int, r rune) rune](#To)
* [func ToLower(r rune) rune](#ToLower)
* [func ToTitle(r rune) rune](#ToTitle)
* [func ToUpper(r rune) rune](#ToUpper)
* [type CaseRange](#CaseRange)
* [type Range16](#Range16)
* [type Range32](#Range32)
* [type RangeTable](#RangeTable)
* [type SpecialCase](#SpecialCase)
* [func (special SpecialCase) ToLower(r rune) rune](#SpecialCase.ToLower)
* [func (special SpecialCase) ToTitle(r rune) rune](#SpecialCase.ToTitle)
* [func (special SpecialCase) ToUpper(r rune) rune](#SpecialCase.ToUpper)
* [Bugs](#pkg-note-BUG)
### Examples
[IsDigit](#example_IsDigit) [IsLetter](#example_IsLetter) [IsLower](#example_IsLower) [IsNumber](#example_IsNumber) [IsSpace](#example_IsSpace) [IsTitle](#example_IsTitle) [IsUpper](#example_IsUpper) [SimpleFold](#example_SimpleFold) [SpecialCase](#example_SpecialCase) [To](#example_To) [ToLower](#example_ToLower) [ToTitle](#example_ToTitle) [ToUpper](#example_ToUpper) [Package (Is)](#example__is) ### Package files
casetables.go digit.go graphic.go letter.go tables.go
Constants
---------
```
const (
MaxRune = '\U0010FFFF' // Maximum valid Unicode code point.
ReplacementChar = '\uFFFD' // Represents invalid code points.
MaxASCII = '\u007F' // maximum ASCII value.
MaxLatin1 = '\u00FF' // maximum Latin-1 value.
)
```
Indices into the Delta arrays inside CaseRanges for case mapping.
```
const (
UpperCase = iota
LowerCase
TitleCase
MaxCase
)
```
If the Delta field of a CaseRange is UpperLower, it means this CaseRange represents a sequence of the form (say) Upper Lower Upper Lower.
```
const (
UpperLower = MaxRune + 1 // (Cannot be a valid delta.)
)
```
Version is the Unicode edition from which the tables are derived.
```
const Version = "13.0.0"
```
Variables
---------
These variables have type \*RangeTable.
```
var (
Cc = _Cc // Cc is the set of Unicode characters in category Cc (Other, control).
Cf = _Cf // Cf is the set of Unicode characters in category Cf (Other, format).
Co = _Co // Co is the set of Unicode characters in category Co (Other, private use).
Cs = _Cs // Cs is the set of Unicode characters in category Cs (Other, surrogate).
Digit = _Nd // Digit is the set of Unicode characters with the "decimal digit" property.
Nd = _Nd // Nd is the set of Unicode characters in category Nd (Number, decimal digit).
Letter = _L // Letter/L is the set of Unicode letters, category L.
L = _L
Lm = _Lm // Lm is the set of Unicode characters in category Lm (Letter, modifier).
Lo = _Lo // Lo is the set of Unicode characters in category Lo (Letter, other).
Lower = _Ll // Lower is the set of Unicode lower case letters.
Ll = _Ll // Ll is the set of Unicode characters in category Ll (Letter, lowercase).
Mark = _M // Mark/M is the set of Unicode mark characters, category M.
M = _M
Mc = _Mc // Mc is the set of Unicode characters in category Mc (Mark, spacing combining).
Me = _Me // Me is the set of Unicode characters in category Me (Mark, enclosing).
Mn = _Mn // Mn is the set of Unicode characters in category Mn (Mark, nonspacing).
Nl = _Nl // Nl is the set of Unicode characters in category Nl (Number, letter).
No = _No // No is the set of Unicode characters in category No (Number, other).
Number = _N // Number/N is the set of Unicode number characters, category N.
N = _N
Other = _C // Other/C is the set of Unicode control and special characters, category C.
C = _C
Pc = _Pc // Pc is the set of Unicode characters in category Pc (Punctuation, connector).
Pd = _Pd // Pd is the set of Unicode characters in category Pd (Punctuation, dash).
Pe = _Pe // Pe is the set of Unicode characters in category Pe (Punctuation, close).
Pf = _Pf // Pf is the set of Unicode characters in category Pf (Punctuation, final quote).
Pi = _Pi // Pi is the set of Unicode characters in category Pi (Punctuation, initial quote).
Po = _Po // Po is the set of Unicode characters in category Po (Punctuation, other).
Ps = _Ps // Ps is the set of Unicode characters in category Ps (Punctuation, open).
Punct = _P // Punct/P is the set of Unicode punctuation characters, category P.
P = _P
Sc = _Sc // Sc is the set of Unicode characters in category Sc (Symbol, currency).
Sk = _Sk // Sk is the set of Unicode characters in category Sk (Symbol, modifier).
Sm = _Sm // Sm is the set of Unicode characters in category Sm (Symbol, math).
So = _So // So is the set of Unicode characters in category So (Symbol, other).
Space = _Z // Space/Z is the set of Unicode space characters, category Z.
Z = _Z
Symbol = _S // Symbol/S is the set of Unicode symbol characters, category S.
S = _S
Title = _Lt // Title is the set of Unicode title case letters.
Lt = _Lt // Lt is the set of Unicode characters in category Lt (Letter, titlecase).
Upper = _Lu // Upper is the set of Unicode upper case letters.
Lu = _Lu // Lu is the set of Unicode characters in category Lu (Letter, uppercase).
Zl = _Zl // Zl is the set of Unicode characters in category Zl (Separator, line).
Zp = _Zp // Zp is the set of Unicode characters in category Zp (Separator, paragraph).
Zs = _Zs // Zs is the set of Unicode characters in category Zs (Separator, space).
)
```
These variables have type \*RangeTable.
```
var (
Adlam = _Adlam // Adlam is the set of Unicode characters in script Adlam.
Ahom = _Ahom // Ahom is the set of Unicode characters in script Ahom.
Anatolian_Hieroglyphs = _Anatolian_Hieroglyphs // Anatolian_Hieroglyphs is the set of Unicode characters in script Anatolian_Hieroglyphs.
Arabic = _Arabic // Arabic is the set of Unicode characters in script Arabic.
Armenian = _Armenian // Armenian is the set of Unicode characters in script Armenian.
Avestan = _Avestan // Avestan is the set of Unicode characters in script Avestan.
Balinese = _Balinese // Balinese is the set of Unicode characters in script Balinese.
Bamum = _Bamum // Bamum is the set of Unicode characters in script Bamum.
Bassa_Vah = _Bassa_Vah // Bassa_Vah is the set of Unicode characters in script Bassa_Vah.
Batak = _Batak // Batak is the set of Unicode characters in script Batak.
Bengali = _Bengali // Bengali is the set of Unicode characters in script Bengali.
Bhaiksuki = _Bhaiksuki // Bhaiksuki is the set of Unicode characters in script Bhaiksuki.
Bopomofo = _Bopomofo // Bopomofo is the set of Unicode characters in script Bopomofo.
Brahmi = _Brahmi // Brahmi is the set of Unicode characters in script Brahmi.
Braille = _Braille // Braille is the set of Unicode characters in script Braille.
Buginese = _Buginese // Buginese is the set of Unicode characters in script Buginese.
Buhid = _Buhid // Buhid is the set of Unicode characters in script Buhid.
Canadian_Aboriginal = _Canadian_Aboriginal // Canadian_Aboriginal is the set of Unicode characters in script Canadian_Aboriginal.
Carian = _Carian // Carian is the set of Unicode characters in script Carian.
Caucasian_Albanian = _Caucasian_Albanian // Caucasian_Albanian is the set of Unicode characters in script Caucasian_Albanian.
Chakma = _Chakma // Chakma is the set of Unicode characters in script Chakma.
Cham = _Cham // Cham is the set of Unicode characters in script Cham.
Cherokee = _Cherokee // Cherokee is the set of Unicode characters in script Cherokee.
Chorasmian = _Chorasmian // Chorasmian is the set of Unicode characters in script Chorasmian.
Common = _Common // Common is the set of Unicode characters in script Common.
Coptic = _Coptic // Coptic is the set of Unicode characters in script Coptic.
Cuneiform = _Cuneiform // Cuneiform is the set of Unicode characters in script Cuneiform.
Cypriot = _Cypriot // Cypriot is the set of Unicode characters in script Cypriot.
Cyrillic = _Cyrillic // Cyrillic is the set of Unicode characters in script Cyrillic.
Deseret = _Deseret // Deseret is the set of Unicode characters in script Deseret.
Devanagari = _Devanagari // Devanagari is the set of Unicode characters in script Devanagari.
Dives_Akuru = _Dives_Akuru // Dives_Akuru is the set of Unicode characters in script Dives_Akuru.
Dogra = _Dogra // Dogra is the set of Unicode characters in script Dogra.
Duployan = _Duployan // Duployan is the set of Unicode characters in script Duployan.
Egyptian_Hieroglyphs = _Egyptian_Hieroglyphs // Egyptian_Hieroglyphs is the set of Unicode characters in script Egyptian_Hieroglyphs.
Elbasan = _Elbasan // Elbasan is the set of Unicode characters in script Elbasan.
Elymaic = _Elymaic // Elymaic is the set of Unicode characters in script Elymaic.
Ethiopic = _Ethiopic // Ethiopic is the set of Unicode characters in script Ethiopic.
Georgian = _Georgian // Georgian is the set of Unicode characters in script Georgian.
Glagolitic = _Glagolitic // Glagolitic is the set of Unicode characters in script Glagolitic.
Gothic = _Gothic // Gothic is the set of Unicode characters in script Gothic.
Grantha = _Grantha // Grantha is the set of Unicode characters in script Grantha.
Greek = _Greek // Greek is the set of Unicode characters in script Greek.
Gujarati = _Gujarati // Gujarati is the set of Unicode characters in script Gujarati.
Gunjala_Gondi = _Gunjala_Gondi // Gunjala_Gondi is the set of Unicode characters in script Gunjala_Gondi.
Gurmukhi = _Gurmukhi // Gurmukhi is the set of Unicode characters in script Gurmukhi.
Han = _Han // Han is the set of Unicode characters in script Han.
Hangul = _Hangul // Hangul is the set of Unicode characters in script Hangul.
Hanifi_Rohingya = _Hanifi_Rohingya // Hanifi_Rohingya is the set of Unicode characters in script Hanifi_Rohingya.
Hanunoo = _Hanunoo // Hanunoo is the set of Unicode characters in script Hanunoo.
Hatran = _Hatran // Hatran is the set of Unicode characters in script Hatran.
Hebrew = _Hebrew // Hebrew is the set of Unicode characters in script Hebrew.
Hiragana = _Hiragana // Hiragana is the set of Unicode characters in script Hiragana.
Imperial_Aramaic = _Imperial_Aramaic // Imperial_Aramaic is the set of Unicode characters in script Imperial_Aramaic.
Inherited = _Inherited // Inherited is the set of Unicode characters in script Inherited.
Inscriptional_Pahlavi = _Inscriptional_Pahlavi // Inscriptional_Pahlavi is the set of Unicode characters in script Inscriptional_Pahlavi.
Inscriptional_Parthian = _Inscriptional_Parthian // Inscriptional_Parthian is the set of Unicode characters in script Inscriptional_Parthian.
Javanese = _Javanese // Javanese is the set of Unicode characters in script Javanese.
Kaithi = _Kaithi // Kaithi is the set of Unicode characters in script Kaithi.
Kannada = _Kannada // Kannada is the set of Unicode characters in script Kannada.
Katakana = _Katakana // Katakana is the set of Unicode characters in script Katakana.
Kayah_Li = _Kayah_Li // Kayah_Li is the set of Unicode characters in script Kayah_Li.
Kharoshthi = _Kharoshthi // Kharoshthi is the set of Unicode characters in script Kharoshthi.
Khitan_Small_Script = _Khitan_Small_Script // Khitan_Small_Script is the set of Unicode characters in script Khitan_Small_Script.
Khmer = _Khmer // Khmer is the set of Unicode characters in script Khmer.
Khojki = _Khojki // Khojki is the set of Unicode characters in script Khojki.
Khudawadi = _Khudawadi // Khudawadi is the set of Unicode characters in script Khudawadi.
Lao = _Lao // Lao is the set of Unicode characters in script Lao.
Latin = _Latin // Latin is the set of Unicode characters in script Latin.
Lepcha = _Lepcha // Lepcha is the set of Unicode characters in script Lepcha.
Limbu = _Limbu // Limbu is the set of Unicode characters in script Limbu.
Linear_A = _Linear_A // Linear_A is the set of Unicode characters in script Linear_A.
Linear_B = _Linear_B // Linear_B is the set of Unicode characters in script Linear_B.
Lisu = _Lisu // Lisu is the set of Unicode characters in script Lisu.
Lycian = _Lycian // Lycian is the set of Unicode characters in script Lycian.
Lydian = _Lydian // Lydian is the set of Unicode characters in script Lydian.
Mahajani = _Mahajani // Mahajani is the set of Unicode characters in script Mahajani.
Makasar = _Makasar // Makasar is the set of Unicode characters in script Makasar.
Malayalam = _Malayalam // Malayalam is the set of Unicode characters in script Malayalam.
Mandaic = _Mandaic // Mandaic is the set of Unicode characters in script Mandaic.
Manichaean = _Manichaean // Manichaean is the set of Unicode characters in script Manichaean.
Marchen = _Marchen // Marchen is the set of Unicode characters in script Marchen.
Masaram_Gondi = _Masaram_Gondi // Masaram_Gondi is the set of Unicode characters in script Masaram_Gondi.
Medefaidrin = _Medefaidrin // Medefaidrin is the set of Unicode characters in script Medefaidrin.
Meetei_Mayek = _Meetei_Mayek // Meetei_Mayek is the set of Unicode characters in script Meetei_Mayek.
Mende_Kikakui = _Mende_Kikakui // Mende_Kikakui is the set of Unicode characters in script Mende_Kikakui.
Meroitic_Cursive = _Meroitic_Cursive // Meroitic_Cursive is the set of Unicode characters in script Meroitic_Cursive.
Meroitic_Hieroglyphs = _Meroitic_Hieroglyphs // Meroitic_Hieroglyphs is the set of Unicode characters in script Meroitic_Hieroglyphs.
Miao = _Miao // Miao is the set of Unicode characters in script Miao.
Modi = _Modi // Modi is the set of Unicode characters in script Modi.
Mongolian = _Mongolian // Mongolian is the set of Unicode characters in script Mongolian.
Mro = _Mro // Mro is the set of Unicode characters in script Mro.
Multani = _Multani // Multani is the set of Unicode characters in script Multani.
Myanmar = _Myanmar // Myanmar is the set of Unicode characters in script Myanmar.
Nabataean = _Nabataean // Nabataean is the set of Unicode characters in script Nabataean.
Nandinagari = _Nandinagari // Nandinagari is the set of Unicode characters in script Nandinagari.
New_Tai_Lue = _New_Tai_Lue // New_Tai_Lue is the set of Unicode characters in script New_Tai_Lue.
Newa = _Newa // Newa is the set of Unicode characters in script Newa.
Nko = _Nko // Nko is the set of Unicode characters in script Nko.
Nushu = _Nushu // Nushu is the set of Unicode characters in script Nushu.
Nyiakeng_Puachue_Hmong = _Nyiakeng_Puachue_Hmong // Nyiakeng_Puachue_Hmong is the set of Unicode characters in script Nyiakeng_Puachue_Hmong.
Ogham = _Ogham // Ogham is the set of Unicode characters in script Ogham.
Ol_Chiki = _Ol_Chiki // Ol_Chiki is the set of Unicode characters in script Ol_Chiki.
Old_Hungarian = _Old_Hungarian // Old_Hungarian is the set of Unicode characters in script Old_Hungarian.
Old_Italic = _Old_Italic // Old_Italic is the set of Unicode characters in script Old_Italic.
Old_North_Arabian = _Old_North_Arabian // Old_North_Arabian is the set of Unicode characters in script Old_North_Arabian.
Old_Permic = _Old_Permic // Old_Permic is the set of Unicode characters in script Old_Permic.
Old_Persian = _Old_Persian // Old_Persian is the set of Unicode characters in script Old_Persian.
Old_Sogdian = _Old_Sogdian // Old_Sogdian is the set of Unicode characters in script Old_Sogdian.
Old_South_Arabian = _Old_South_Arabian // Old_South_Arabian is the set of Unicode characters in script Old_South_Arabian.
Old_Turkic = _Old_Turkic // Old_Turkic is the set of Unicode characters in script Old_Turkic.
Oriya = _Oriya // Oriya is the set of Unicode characters in script Oriya.
Osage = _Osage // Osage is the set of Unicode characters in script Osage.
Osmanya = _Osmanya // Osmanya is the set of Unicode characters in script Osmanya.
Pahawh_Hmong = _Pahawh_Hmong // Pahawh_Hmong is the set of Unicode characters in script Pahawh_Hmong.
Palmyrene = _Palmyrene // Palmyrene is the set of Unicode characters in script Palmyrene.
Pau_Cin_Hau = _Pau_Cin_Hau // Pau_Cin_Hau is the set of Unicode characters in script Pau_Cin_Hau.
Phags_Pa = _Phags_Pa // Phags_Pa is the set of Unicode characters in script Phags_Pa.
Phoenician = _Phoenician // Phoenician is the set of Unicode characters in script Phoenician.
Psalter_Pahlavi = _Psalter_Pahlavi // Psalter_Pahlavi is the set of Unicode characters in script Psalter_Pahlavi.
Rejang = _Rejang // Rejang is the set of Unicode characters in script Rejang.
Runic = _Runic // Runic is the set of Unicode characters in script Runic.
Samaritan = _Samaritan // Samaritan is the set of Unicode characters in script Samaritan.
Saurashtra = _Saurashtra // Saurashtra is the set of Unicode characters in script Saurashtra.
Sharada = _Sharada // Sharada is the set of Unicode characters in script Sharada.
Shavian = _Shavian // Shavian is the set of Unicode characters in script Shavian.
Siddham = _Siddham // Siddham is the set of Unicode characters in script Siddham.
SignWriting = _SignWriting // SignWriting is the set of Unicode characters in script SignWriting.
Sinhala = _Sinhala // Sinhala is the set of Unicode characters in script Sinhala.
Sogdian = _Sogdian // Sogdian is the set of Unicode characters in script Sogdian.
Sora_Sompeng = _Sora_Sompeng // Sora_Sompeng is the set of Unicode characters in script Sora_Sompeng.
Soyombo = _Soyombo // Soyombo is the set of Unicode characters in script Soyombo.
Sundanese = _Sundanese // Sundanese is the set of Unicode characters in script Sundanese.
Syloti_Nagri = _Syloti_Nagri // Syloti_Nagri is the set of Unicode characters in script Syloti_Nagri.
Syriac = _Syriac // Syriac is the set of Unicode characters in script Syriac.
Tagalog = _Tagalog // Tagalog is the set of Unicode characters in script Tagalog.
Tagbanwa = _Tagbanwa // Tagbanwa is the set of Unicode characters in script Tagbanwa.
Tai_Le = _Tai_Le // Tai_Le is the set of Unicode characters in script Tai_Le.
Tai_Tham = _Tai_Tham // Tai_Tham is the set of Unicode characters in script Tai_Tham.
Tai_Viet = _Tai_Viet // Tai_Viet is the set of Unicode characters in script Tai_Viet.
Takri = _Takri // Takri is the set of Unicode characters in script Takri.
Tamil = _Tamil // Tamil is the set of Unicode characters in script Tamil.
Tangut = _Tangut // Tangut is the set of Unicode characters in script Tangut.
Telugu = _Telugu // Telugu is the set of Unicode characters in script Telugu.
Thaana = _Thaana // Thaana is the set of Unicode characters in script Thaana.
Thai = _Thai // Thai is the set of Unicode characters in script Thai.
Tibetan = _Tibetan // Tibetan is the set of Unicode characters in script Tibetan.
Tifinagh = _Tifinagh // Tifinagh is the set of Unicode characters in script Tifinagh.
Tirhuta = _Tirhuta // Tirhuta is the set of Unicode characters in script Tirhuta.
Ugaritic = _Ugaritic // Ugaritic is the set of Unicode characters in script Ugaritic.
Vai = _Vai // Vai is the set of Unicode characters in script Vai.
Wancho = _Wancho // Wancho is the set of Unicode characters in script Wancho.
Warang_Citi = _Warang_Citi // Warang_Citi is the set of Unicode characters in script Warang_Citi.
Yezidi = _Yezidi // Yezidi is the set of Unicode characters in script Yezidi.
Yi = _Yi // Yi is the set of Unicode characters in script Yi.
Zanabazar_Square = _Zanabazar_Square // Zanabazar_Square is the set of Unicode characters in script Zanabazar_Square.
)
```
These variables have type \*RangeTable.
```
var (
ASCII_Hex_Digit = _ASCII_Hex_Digit // ASCII_Hex_Digit is the set of Unicode characters with property ASCII_Hex_Digit.
Bidi_Control = _Bidi_Control // Bidi_Control is the set of Unicode characters with property Bidi_Control.
Dash = _Dash // Dash is the set of Unicode characters with property Dash.
Deprecated = _Deprecated // Deprecated is the set of Unicode characters with property Deprecated.
Diacritic = _Diacritic // Diacritic is the set of Unicode characters with property Diacritic.
Extender = _Extender // Extender is the set of Unicode characters with property Extender.
Hex_Digit = _Hex_Digit // Hex_Digit is the set of Unicode characters with property Hex_Digit.
Hyphen = _Hyphen // Hyphen is the set of Unicode characters with property Hyphen.
IDS_Binary_Operator = _IDS_Binary_Operator // IDS_Binary_Operator is the set of Unicode characters with property IDS_Binary_Operator.
IDS_Trinary_Operator = _IDS_Trinary_Operator // IDS_Trinary_Operator is the set of Unicode characters with property IDS_Trinary_Operator.
Ideographic = _Ideographic // Ideographic is the set of Unicode characters with property Ideographic.
Join_Control = _Join_Control // Join_Control is the set of Unicode characters with property Join_Control.
Logical_Order_Exception = _Logical_Order_Exception // Logical_Order_Exception is the set of Unicode characters with property Logical_Order_Exception.
Noncharacter_Code_Point = _Noncharacter_Code_Point // Noncharacter_Code_Point is the set of Unicode characters with property Noncharacter_Code_Point.
Other_Alphabetic = _Other_Alphabetic // Other_Alphabetic is the set of Unicode characters with property Other_Alphabetic.
Other_Default_Ignorable_Code_Point = _Other_Default_Ignorable_Code_Point // Other_Default_Ignorable_Code_Point is the set of Unicode characters with property Other_Default_Ignorable_Code_Point.
Other_Grapheme_Extend = _Other_Grapheme_Extend // Other_Grapheme_Extend is the set of Unicode characters with property Other_Grapheme_Extend.
Other_ID_Continue = _Other_ID_Continue // Other_ID_Continue is the set of Unicode characters with property Other_ID_Continue.
Other_ID_Start = _Other_ID_Start // Other_ID_Start is the set of Unicode characters with property Other_ID_Start.
Other_Lowercase = _Other_Lowercase // Other_Lowercase is the set of Unicode characters with property Other_Lowercase.
Other_Math = _Other_Math // Other_Math is the set of Unicode characters with property Other_Math.
Other_Uppercase = _Other_Uppercase // Other_Uppercase is the set of Unicode characters with property Other_Uppercase.
Pattern_Syntax = _Pattern_Syntax // Pattern_Syntax is the set of Unicode characters with property Pattern_Syntax.
Pattern_White_Space = _Pattern_White_Space // Pattern_White_Space is the set of Unicode characters with property Pattern_White_Space.
Prepended_Concatenation_Mark = _Prepended_Concatenation_Mark // Prepended_Concatenation_Mark is the set of Unicode characters with property Prepended_Concatenation_Mark.
Quotation_Mark = _Quotation_Mark // Quotation_Mark is the set of Unicode characters with property Quotation_Mark.
Radical = _Radical // Radical is the set of Unicode characters with property Radical.
Regional_Indicator = _Regional_Indicator // Regional_Indicator is the set of Unicode characters with property Regional_Indicator.
STerm = _Sentence_Terminal // STerm is an alias for Sentence_Terminal.
Sentence_Terminal = _Sentence_Terminal // Sentence_Terminal is the set of Unicode characters with property Sentence_Terminal.
Soft_Dotted = _Soft_Dotted // Soft_Dotted is the set of Unicode characters with property Soft_Dotted.
Terminal_Punctuation = _Terminal_Punctuation // Terminal_Punctuation is the set of Unicode characters with property Terminal_Punctuation.
Unified_Ideograph = _Unified_Ideograph // Unified_Ideograph is the set of Unicode characters with property Unified_Ideograph.
Variation_Selector = _Variation_Selector // Variation_Selector is the set of Unicode characters with property Variation_Selector.
White_Space = _White_Space // White_Space is the set of Unicode characters with property White_Space.
)
```
CaseRanges is the table describing case mappings for all letters with non-self mappings.
```
var CaseRanges = _CaseRanges
```
Categories is the set of Unicode category tables.
```
var Categories = map[string]*RangeTable{
"C": C,
"Cc": Cc,
"Cf": Cf,
"Co": Co,
"Cs": Cs,
"L": L,
"Ll": Ll,
"Lm": Lm,
"Lo": Lo,
"Lt": Lt,
"Lu": Lu,
"M": M,
"Mc": Mc,
"Me": Me,
"Mn": Mn,
"N": N,
"Nd": Nd,
"Nl": Nl,
"No": No,
"P": P,
"Pc": Pc,
"Pd": Pd,
"Pe": Pe,
"Pf": Pf,
"Pi": Pi,
"Po": Po,
"Ps": Ps,
"S": S,
"Sc": Sc,
"Sk": Sk,
"Sm": Sm,
"So": So,
"Z": Z,
"Zl": Zl,
"Zp": Zp,
"Zs": Zs,
}
```
FoldCategory maps a category name to a table of code points outside the category that are equivalent under simple case folding to code points inside the category. If there is no entry for a category name, there are no such points.
```
var FoldCategory = map[string]*RangeTable{
"L": foldL,
"Ll": foldLl,
"Lt": foldLt,
"Lu": foldLu,
"M": foldM,
"Mn": foldMn,
}
```
FoldScript maps a script name to a table of code points outside the script that are equivalent under simple case folding to code points inside the script. If there is no entry for a script name, there are no such points.
```
var FoldScript = map[string]*RangeTable{
"Common": foldCommon,
"Greek": foldGreek,
"Inherited": foldInherited,
}
```
GraphicRanges defines the set of graphic characters according to Unicode.
```
var GraphicRanges = []*RangeTable{
L, M, N, P, S, Zs,
}
```
PrintRanges defines the set of printable characters according to Go. ASCII space, U+0020, is handled separately.
```
var PrintRanges = []*RangeTable{
L, M, N, P, S,
}
```
Properties is the set of Unicode property tables.
```
var Properties = map[string]*RangeTable{
"ASCII_Hex_Digit": ASCII_Hex_Digit,
"Bidi_Control": Bidi_Control,
"Dash": Dash,
"Deprecated": Deprecated,
"Diacritic": Diacritic,
"Extender": Extender,
"Hex_Digit": Hex_Digit,
"Hyphen": Hyphen,
"IDS_Binary_Operator": IDS_Binary_Operator,
"IDS_Trinary_Operator": IDS_Trinary_Operator,
"Ideographic": Ideographic,
"Join_Control": Join_Control,
"Logical_Order_Exception": Logical_Order_Exception,
"Noncharacter_Code_Point": Noncharacter_Code_Point,
"Other_Alphabetic": Other_Alphabetic,
"Other_Default_Ignorable_Code_Point": Other_Default_Ignorable_Code_Point,
"Other_Grapheme_Extend": Other_Grapheme_Extend,
"Other_ID_Continue": Other_ID_Continue,
"Other_ID_Start": Other_ID_Start,
"Other_Lowercase": Other_Lowercase,
"Other_Math": Other_Math,
"Other_Uppercase": Other_Uppercase,
"Pattern_Syntax": Pattern_Syntax,
"Pattern_White_Space": Pattern_White_Space,
"Prepended_Concatenation_Mark": Prepended_Concatenation_Mark,
"Quotation_Mark": Quotation_Mark,
"Radical": Radical,
"Regional_Indicator": Regional_Indicator,
"Sentence_Terminal": Sentence_Terminal,
"STerm": Sentence_Terminal,
"Soft_Dotted": Soft_Dotted,
"Terminal_Punctuation": Terminal_Punctuation,
"Unified_Ideograph": Unified_Ideograph,
"Variation_Selector": Variation_Selector,
"White_Space": White_Space,
}
```
Scripts is the set of Unicode script tables.
```
var Scripts = map[string]*RangeTable{
"Adlam": Adlam,
"Ahom": Ahom,
"Anatolian_Hieroglyphs": Anatolian_Hieroglyphs,
"Arabic": Arabic,
"Armenian": Armenian,
"Avestan": Avestan,
"Balinese": Balinese,
"Bamum": Bamum,
"Bassa_Vah": Bassa_Vah,
"Batak": Batak,
"Bengali": Bengali,
"Bhaiksuki": Bhaiksuki,
"Bopomofo": Bopomofo,
"Brahmi": Brahmi,
"Braille": Braille,
"Buginese": Buginese,
"Buhid": Buhid,
"Canadian_Aboriginal": Canadian_Aboriginal,
"Carian": Carian,
"Caucasian_Albanian": Caucasian_Albanian,
"Chakma": Chakma,
"Cham": Cham,
"Cherokee": Cherokee,
"Chorasmian": Chorasmian,
"Common": Common,
"Coptic": Coptic,
"Cuneiform": Cuneiform,
"Cypriot": Cypriot,
"Cyrillic": Cyrillic,
"Deseret": Deseret,
"Devanagari": Devanagari,
"Dives_Akuru": Dives_Akuru,
"Dogra": Dogra,
"Duployan": Duployan,
"Egyptian_Hieroglyphs": Egyptian_Hieroglyphs,
"Elbasan": Elbasan,
"Elymaic": Elymaic,
"Ethiopic": Ethiopic,
"Georgian": Georgian,
"Glagolitic": Glagolitic,
"Gothic": Gothic,
"Grantha": Grantha,
"Greek": Greek,
"Gujarati": Gujarati,
"Gunjala_Gondi": Gunjala_Gondi,
"Gurmukhi": Gurmukhi,
"Han": Han,
"Hangul": Hangul,
"Hanifi_Rohingya": Hanifi_Rohingya,
"Hanunoo": Hanunoo,
"Hatran": Hatran,
"Hebrew": Hebrew,
"Hiragana": Hiragana,
"Imperial_Aramaic": Imperial_Aramaic,
"Inherited": Inherited,
"Inscriptional_Pahlavi": Inscriptional_Pahlavi,
"Inscriptional_Parthian": Inscriptional_Parthian,
"Javanese": Javanese,
"Kaithi": Kaithi,
"Kannada": Kannada,
"Katakana": Katakana,
"Kayah_Li": Kayah_Li,
"Kharoshthi": Kharoshthi,
"Khitan_Small_Script": Khitan_Small_Script,
"Khmer": Khmer,
"Khojki": Khojki,
"Khudawadi": Khudawadi,
"Lao": Lao,
"Latin": Latin,
"Lepcha": Lepcha,
"Limbu": Limbu,
"Linear_A": Linear_A,
"Linear_B": Linear_B,
"Lisu": Lisu,
"Lycian": Lycian,
"Lydian": Lydian,
"Mahajani": Mahajani,
"Makasar": Makasar,
"Malayalam": Malayalam,
"Mandaic": Mandaic,
"Manichaean": Manichaean,
"Marchen": Marchen,
"Masaram_Gondi": Masaram_Gondi,
"Medefaidrin": Medefaidrin,
"Meetei_Mayek": Meetei_Mayek,
"Mende_Kikakui": Mende_Kikakui,
"Meroitic_Cursive": Meroitic_Cursive,
"Meroitic_Hieroglyphs": Meroitic_Hieroglyphs,
"Miao": Miao,
"Modi": Modi,
"Mongolian": Mongolian,
"Mro": Mro,
"Multani": Multani,
"Myanmar": Myanmar,
"Nabataean": Nabataean,
"Nandinagari": Nandinagari,
"New_Tai_Lue": New_Tai_Lue,
"Newa": Newa,
"Nko": Nko,
"Nushu": Nushu,
"Nyiakeng_Puachue_Hmong": Nyiakeng_Puachue_Hmong,
"Ogham": Ogham,
"Ol_Chiki": Ol_Chiki,
"Old_Hungarian": Old_Hungarian,
"Old_Italic": Old_Italic,
"Old_North_Arabian": Old_North_Arabian,
"Old_Permic": Old_Permic,
"Old_Persian": Old_Persian,
"Old_Sogdian": Old_Sogdian,
"Old_South_Arabian": Old_South_Arabian,
"Old_Turkic": Old_Turkic,
"Oriya": Oriya,
"Osage": Osage,
"Osmanya": Osmanya,
"Pahawh_Hmong": Pahawh_Hmong,
"Palmyrene": Palmyrene,
"Pau_Cin_Hau": Pau_Cin_Hau,
"Phags_Pa": Phags_Pa,
"Phoenician": Phoenician,
"Psalter_Pahlavi": Psalter_Pahlavi,
"Rejang": Rejang,
"Runic": Runic,
"Samaritan": Samaritan,
"Saurashtra": Saurashtra,
"Sharada": Sharada,
"Shavian": Shavian,
"Siddham": Siddham,
"SignWriting": SignWriting,
"Sinhala": Sinhala,
"Sogdian": Sogdian,
"Sora_Sompeng": Sora_Sompeng,
"Soyombo": Soyombo,
"Sundanese": Sundanese,
"Syloti_Nagri": Syloti_Nagri,
"Syriac": Syriac,
"Tagalog": Tagalog,
"Tagbanwa": Tagbanwa,
"Tai_Le": Tai_Le,
"Tai_Tham": Tai_Tham,
"Tai_Viet": Tai_Viet,
"Takri": Takri,
"Tamil": Tamil,
"Tangut": Tangut,
"Telugu": Telugu,
"Thaana": Thaana,
"Thai": Thai,
"Tibetan": Tibetan,
"Tifinagh": Tifinagh,
"Tirhuta": Tirhuta,
"Ugaritic": Ugaritic,
"Vai": Vai,
"Wancho": Wancho,
"Warang_Citi": Warang_Citi,
"Yezidi": Yezidi,
"Yi": Yi,
"Zanabazar_Square": Zanabazar_Square,
}
```
func In 1.2
-----------
```
func In(r rune, ranges ...*RangeTable) bool
```
In reports whether the rune is a member of one of the ranges.
func Is
-------
```
func Is(rangeTab *RangeTable, r rune) bool
```
Is reports whether the rune is in the specified table of ranges.
func IsControl
--------------
```
func IsControl(r rune) bool
```
IsControl reports whether the rune is a control character. The C (Other) Unicode category includes more code points such as surrogates; use Is(C, r) to test for them.
func IsDigit
------------
```
func IsDigit(r rune) bool
```
IsDigit reports whether the rune is a decimal digit.
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsDigit('৩'))
fmt.Printf("%t\n", unicode.IsDigit('A'))
```
Output:
```
true
false
```
func IsGraphic
--------------
```
func IsGraphic(r rune) bool
```
IsGraphic reports whether the rune is defined as a Graphic by Unicode. Such characters include letters, marks, numbers, punctuation, symbols, and spaces, from categories L, M, N, P, S, Zs.
func IsLetter
-------------
```
func IsLetter(r rune) bool
```
IsLetter reports whether the rune is a letter (category L).
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsLetter('A'))
fmt.Printf("%t\n", unicode.IsLetter('7'))
```
Output:
```
true
false
```
func IsLower
------------
```
func IsLower(r rune) bool
```
IsLower reports whether the rune is a lower case letter.
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsLower('a'))
fmt.Printf("%t\n", unicode.IsLower('A'))
```
Output:
```
true
false
```
func IsMark
-----------
```
func IsMark(r rune) bool
```
IsMark reports whether the rune is a mark character (category M).
func IsNumber
-------------
```
func IsNumber(r rune) bool
```
IsNumber reports whether the rune is a number (category N).
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsNumber('Ⅷ'))
fmt.Printf("%t\n", unicode.IsNumber('A'))
```
Output:
```
true
false
```
func IsOneOf
------------
```
func IsOneOf(ranges []*RangeTable, r rune) bool
```
IsOneOf reports whether the rune is a member of one of the ranges. The function "In" provides a nicer signature and should be used in preference to IsOneOf.
func IsPrint
------------
```
func IsPrint(r rune) bool
```
IsPrint reports whether the rune is defined as printable by Go. Such characters include letters, marks, numbers, punctuation, symbols, and the ASCII space character, from categories L, M, N, P, S and the ASCII space character. This categorization is the same as IsGraphic except that the only spacing character is ASCII space, U+0020.
func IsPunct
------------
```
func IsPunct(r rune) bool
```
IsPunct reports whether the rune is a Unicode punctuation character (category P).
func IsSpace
------------
```
func IsSpace(r rune) bool
```
IsSpace reports whether the rune is a space character as defined by Unicode's White Space property; in the Latin-1 space this is
```
'\t', '\n', '\v', '\f', '\r', ' ', U+0085 (NEL), U+00A0 (NBSP).
```
Other definitions of spacing characters are set by category Z and property Pattern\_White\_Space.
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsSpace(' '))
fmt.Printf("%t\n", unicode.IsSpace('\n'))
fmt.Printf("%t\n", unicode.IsSpace('\t'))
fmt.Printf("%t\n", unicode.IsSpace('a'))
```
Output:
```
true
true
true
false
```
func IsSymbol
-------------
```
func IsSymbol(r rune) bool
```
IsSymbol reports whether the rune is a symbolic character.
func IsTitle
------------
```
func IsTitle(r rune) bool
```
IsTitle reports whether the rune is a title case letter.
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsTitle('Dž'))
fmt.Printf("%t\n", unicode.IsTitle('a'))
```
Output:
```
true
false
```
func IsUpper
------------
```
func IsUpper(r rune) bool
```
IsUpper reports whether the rune is an upper case letter.
#### Example
Code:
```
fmt.Printf("%t\n", unicode.IsUpper('A'))
fmt.Printf("%t\n", unicode.IsUpper('a'))
```
Output:
```
true
false
```
func SimpleFold
---------------
```
func SimpleFold(r rune) rune
```
SimpleFold iterates over Unicode code points equivalent under the Unicode-defined simple case folding. Among the code points equivalent to rune (including rune itself), SimpleFold returns the smallest rune > r if one exists, or else the smallest rune >= 0. If r is not a valid Unicode code point, SimpleFold(r) returns r.
For example:
```
SimpleFold('A') = 'a'
SimpleFold('a') = 'A'
SimpleFold('K') = 'k'
SimpleFold('k') = '\u212A' (Kelvin symbol, K)
SimpleFold('\u212A') = 'K'
SimpleFold('1') = '1'
SimpleFold(-2) = -2
```
#### Example
Code:
```
fmt.Printf("%#U\n", unicode.SimpleFold('A')) // 'a'
fmt.Printf("%#U\n", unicode.SimpleFold('a')) // 'A'
fmt.Printf("%#U\n", unicode.SimpleFold('K')) // 'k'
fmt.Printf("%#U\n", unicode.SimpleFold('k')) // '\u212A' (Kelvin symbol, K)
fmt.Printf("%#U\n", unicode.SimpleFold('\u212A')) // 'K'
fmt.Printf("%#U\n", unicode.SimpleFold('1')) // '1'
```
Output:
```
U+0061 'a'
U+0041 'A'
U+006B 'k'
U+212A 'K'
U+004B 'K'
U+0031 '1'
```
func To
-------
```
func To(_case int, r rune) rune
```
To maps the rune to the specified case: UpperCase, LowerCase, or TitleCase.
#### Example
Code:
```
const lcG = 'g'
fmt.Printf("%#U\n", unicode.To(unicode.UpperCase, lcG))
fmt.Printf("%#U\n", unicode.To(unicode.LowerCase, lcG))
fmt.Printf("%#U\n", unicode.To(unicode.TitleCase, lcG))
const ucG = 'G'
fmt.Printf("%#U\n", unicode.To(unicode.UpperCase, ucG))
fmt.Printf("%#U\n", unicode.To(unicode.LowerCase, ucG))
fmt.Printf("%#U\n", unicode.To(unicode.TitleCase, ucG))
```
Output:
```
U+0047 'G'
U+0067 'g'
U+0047 'G'
U+0047 'G'
U+0067 'g'
U+0047 'G'
```
func ToLower
------------
```
func ToLower(r rune) rune
```
ToLower maps the rune to lower case.
#### Example
Code:
```
const ucG = 'G'
fmt.Printf("%#U\n", unicode.ToLower(ucG))
```
Output:
```
U+0067 'g'
```
func ToTitle
------------
```
func ToTitle(r rune) rune
```
ToTitle maps the rune to title case.
#### Example
Code:
```
const ucG = 'g'
fmt.Printf("%#U\n", unicode.ToTitle(ucG))
```
Output:
```
U+0047 'G'
```
func ToUpper
------------
```
func ToUpper(r rune) rune
```
ToUpper maps the rune to upper case.
#### Example
Code:
```
const ucG = 'g'
fmt.Printf("%#U\n", unicode.ToUpper(ucG))
```
Output:
```
U+0047 'G'
```
type CaseRange
--------------
CaseRange represents a range of Unicode code points for simple (one code point to one code point) case conversion. The range runs from Lo to Hi inclusive, with a fixed stride of 1. Deltas are the number to add to the code point to reach the code point for a different case for that character. They may be negative. If zero, it means the character is in the corresponding case. There is a special case representing sequences of alternating corresponding Upper and Lower pairs. It appears with a fixed Delta of
```
{UpperLower, UpperLower, UpperLower}
```
The constant UpperLower has an otherwise impossible delta value.
```
type CaseRange struct {
Lo uint32
Hi uint32
Delta d
}
```
type Range16
------------
Range16 represents of a range of 16-bit Unicode code points. The range runs from Lo to Hi inclusive and has the specified stride.
```
type Range16 struct {
Lo uint16
Hi uint16
Stride uint16
}
```
type Range32
------------
Range32 represents of a range of Unicode code points and is used when one or more of the values will not fit in 16 bits. The range runs from Lo to Hi inclusive and has the specified stride. Lo and Hi must always be >= 1<<16.
```
type Range32 struct {
Lo uint32
Hi uint32
Stride uint32
}
```
type RangeTable
---------------
RangeTable defines a set of Unicode code points by listing the ranges of code points within the set. The ranges are listed in two slices to save space: a slice of 16-bit ranges and a slice of 32-bit ranges. The two slices must be in sorted order and non-overlapping. Also, R32 should contain only values >= 0x10000 (1<<16).
```
type RangeTable struct {
R16 []Range16
R32 []Range32
LatinOffset int // number of entries in R16 with Hi <= MaxLatin1; added in Go 1.1
}
```
type SpecialCase
----------------
SpecialCase represents language-specific case mappings such as Turkish. Methods of SpecialCase customize (by overriding) the standard mappings.
```
type SpecialCase []CaseRange
```
```
var AzeriCase SpecialCase = _TurkishCase
```
```
var TurkishCase SpecialCase = _TurkishCase
```
#### Example
Code:
```
t := unicode.TurkishCase
const lci = 'i'
fmt.Printf("%#U\n", t.ToLower(lci))
fmt.Printf("%#U\n", t.ToTitle(lci))
fmt.Printf("%#U\n", t.ToUpper(lci))
const uci = 'İ'
fmt.Printf("%#U\n", t.ToLower(uci))
fmt.Printf("%#U\n", t.ToTitle(uci))
fmt.Printf("%#U\n", t.ToUpper(uci))
```
Output:
```
U+0069 'i'
U+0130 'İ'
U+0130 'İ'
U+0069 'i'
U+0130 'İ'
U+0130 'İ'
```
### func (SpecialCase) ToLower
```
func (special SpecialCase) ToLower(r rune) rune
```
ToLower maps the rune to lower case giving priority to the special mapping.
### func (SpecialCase) ToTitle
```
func (special SpecialCase) ToTitle(r rune) rune
```
ToTitle maps the rune to title case giving priority to the special mapping.
### func (SpecialCase) ToUpper
```
func (special SpecialCase) ToUpper(r rune) rune
```
ToUpper maps the rune to upper case giving priority to the special mapping.
Bugs
----
* ☞ There is no mechanism for full case folding, that is, for characters that involve multiple runes in the input or output.
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [utf16](utf16/index) | Package utf16 implements encoding and decoding of UTF-16 sequences. |
| [utf8](utf8/index) | Package utf8 implements functions and constants to support text encoded in UTF-8. |
| programming_docs |
go Package utf16 Package utf16
==============
* `import "unicode/utf16"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package utf16 implements encoding and decoding of UTF-16 sequences.
Index
-----
* [func AppendRune(a []uint16, r rune) []uint16](#AppendRune)
* [func Decode(s []uint16) []rune](#Decode)
* [func DecodeRune(r1, r2 rune) rune](#DecodeRune)
* [func Encode(s []rune) []uint16](#Encode)
* [func EncodeRune(r rune) (r1, r2 rune)](#EncodeRune)
* [func IsSurrogate(r rune) bool](#IsSurrogate)
### Package files
utf16.go
func AppendRune 1.20
--------------------
```
func AppendRune(a []uint16, r rune) []uint16
```
AppendRune appends the UTF-16 encoding of the Unicode code point r to the end of p and returns the extended buffer. If the rune is not a valid Unicode code point, it appends the encoding of U+FFFD.
func Decode
-----------
```
func Decode(s []uint16) []rune
```
Decode returns the Unicode code point sequence represented by the UTF-16 encoding s.
func DecodeRune
---------------
```
func DecodeRune(r1, r2 rune) rune
```
DecodeRune returns the UTF-16 decoding of a surrogate pair. If the pair is not a valid UTF-16 surrogate pair, DecodeRune returns the Unicode replacement code point U+FFFD.
func Encode
-----------
```
func Encode(s []rune) []uint16
```
Encode returns the UTF-16 encoding of the Unicode code point sequence s.
func EncodeRune
---------------
```
func EncodeRune(r rune) (r1, r2 rune)
```
EncodeRune returns the UTF-16 surrogate pair r1, r2 for the given rune. If the rune is not a valid Unicode code point or does not need encoding, EncodeRune returns U+FFFD, U+FFFD.
func IsSurrogate
----------------
```
func IsSurrogate(r rune) bool
```
IsSurrogate reports whether the specified Unicode code point can appear in a surrogate pair.
go Package utf8 Package utf8
=============
* `import "unicode/utf8"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package utf8 implements functions and constants to support text encoded in UTF-8. It includes functions to translate between runes and UTF-8 byte sequences. See <https://en.wikipedia.org/wiki/UTF-8>
Index
-----
* [Constants](#pkg-constants)
* [func AppendRune(p []byte, r rune) []byte](#AppendRune)
* [func DecodeLastRune(p []byte) (r rune, size int)](#DecodeLastRune)
* [func DecodeLastRuneInString(s string) (r rune, size int)](#DecodeLastRuneInString)
* [func DecodeRune(p []byte) (r rune, size int)](#DecodeRune)
* [func DecodeRuneInString(s string) (r rune, size int)](#DecodeRuneInString)
* [func EncodeRune(p []byte, r rune) int](#EncodeRune)
* [func FullRune(p []byte) bool](#FullRune)
* [func FullRuneInString(s string) bool](#FullRuneInString)
* [func RuneCount(p []byte) int](#RuneCount)
* [func RuneCountInString(s string) (n int)](#RuneCountInString)
* [func RuneLen(r rune) int](#RuneLen)
* [func RuneStart(b byte) bool](#RuneStart)
* [func Valid(p []byte) bool](#Valid)
* [func ValidRune(r rune) bool](#ValidRune)
* [func ValidString(s string) bool](#ValidString)
### Examples
[AppendRune](#example_AppendRune) [DecodeLastRune](#example_DecodeLastRune) [DecodeLastRuneInString](#example_DecodeLastRuneInString) [DecodeRune](#example_DecodeRune) [DecodeRuneInString](#example_DecodeRuneInString) [EncodeRune](#example_EncodeRune) [EncodeRune (OutOfRange)](#example_EncodeRune_outOfRange) [FullRune](#example_FullRune) [FullRuneInString](#example_FullRuneInString) [RuneCount](#example_RuneCount) [RuneCountInString](#example_RuneCountInString) [RuneLen](#example_RuneLen) [RuneStart](#example_RuneStart) [Valid](#example_Valid) [ValidRune](#example_ValidRune) [ValidString](#example_ValidString) ### Package files
utf8.go
Constants
---------
Numbers fundamental to the encoding.
```
const (
RuneError = '\uFFFD' // the "error" Rune or "Unicode replacement character"
RuneSelf = 0x80 // characters below RuneSelf are represented as themselves in a single byte.
MaxRune = '\U0010FFFF' // Maximum valid Unicode code point.
UTFMax = 4 // maximum number of bytes of a UTF-8 encoded Unicode character.
)
```
func AppendRune 1.18
--------------------
```
func AppendRune(p []byte, r rune) []byte
```
AppendRune appends the UTF-8 encoding of r to the end of p and returns the extended buffer. If the rune is out of range, it appends the encoding of RuneError.
#### Example
Code:
```
buf1 := utf8.AppendRune(nil, 0x10000)
buf2 := utf8.AppendRune([]byte("init"), 0x10000)
fmt.Println(string(buf1))
fmt.Println(string(buf2))
```
Output:
```
𐀀
init𐀀
```
func DecodeLastRune
-------------------
```
func DecodeLastRune(p []byte) (r rune, size int)
```
DecodeLastRune unpacks the last UTF-8 encoding in p and returns the rune and its width in bytes. If p is empty it returns (RuneError, 0). Otherwise, if the encoding is invalid, it returns (RuneError, 1). Both are impossible results for correct, non-empty UTF-8.
An encoding is invalid if it is incorrect UTF-8, encodes a rune that is out of range, or is not the shortest possible UTF-8 encoding for the value. No other validation is performed.
#### Example
Code:
```
b := []byte("Hello, 世界")
for len(b) > 0 {
r, size := utf8.DecodeLastRune(b)
fmt.Printf("%c %v\n", r, size)
b = b[:len(b)-size]
}
```
Output:
```
界 3
世 3
1
, 1
o 1
l 1
l 1
e 1
H 1
```
func DecodeLastRuneInString
---------------------------
```
func DecodeLastRuneInString(s string) (r rune, size int)
```
DecodeLastRuneInString is like DecodeLastRune but its input is a string. If s is empty it returns (RuneError, 0). Otherwise, if the encoding is invalid, it returns (RuneError, 1). Both are impossible results for correct, non-empty UTF-8.
An encoding is invalid if it is incorrect UTF-8, encodes a rune that is out of range, or is not the shortest possible UTF-8 encoding for the value. No other validation is performed.
#### Example
Code:
```
str := "Hello, 世界"
for len(str) > 0 {
r, size := utf8.DecodeLastRuneInString(str)
fmt.Printf("%c %v\n", r, size)
str = str[:len(str)-size]
}
```
Output:
```
界 3
世 3
1
, 1
o 1
l 1
l 1
e 1
H 1
```
func DecodeRune
---------------
```
func DecodeRune(p []byte) (r rune, size int)
```
DecodeRune unpacks the first UTF-8 encoding in p and returns the rune and its width in bytes. If p is empty it returns (RuneError, 0). Otherwise, if the encoding is invalid, it returns (RuneError, 1). Both are impossible results for correct, non-empty UTF-8.
An encoding is invalid if it is incorrect UTF-8, encodes a rune that is out of range, or is not the shortest possible UTF-8 encoding for the value. No other validation is performed.
#### Example
Code:
```
b := []byte("Hello, 世界")
for len(b) > 0 {
r, size := utf8.DecodeRune(b)
fmt.Printf("%c %v\n", r, size)
b = b[size:]
}
```
Output:
```
H 1
e 1
l 1
l 1
o 1
, 1
1
世 3
界 3
```
func DecodeRuneInString
-----------------------
```
func DecodeRuneInString(s string) (r rune, size int)
```
DecodeRuneInString is like DecodeRune but its input is a string. If s is empty it returns (RuneError, 0). Otherwise, if the encoding is invalid, it returns (RuneError, 1). Both are impossible results for correct, non-empty UTF-8.
An encoding is invalid if it is incorrect UTF-8, encodes a rune that is out of range, or is not the shortest possible UTF-8 encoding for the value. No other validation is performed.
#### Example
Code:
```
str := "Hello, 世界"
for len(str) > 0 {
r, size := utf8.DecodeRuneInString(str)
fmt.Printf("%c %v\n", r, size)
str = str[size:]
}
```
Output:
```
H 1
e 1
l 1
l 1
o 1
, 1
1
世 3
界 3
```
func EncodeRune
---------------
```
func EncodeRune(p []byte, r rune) int
```
EncodeRune writes into p (which must be large enough) the UTF-8 encoding of the rune. If the rune is out of range, it writes the encoding of RuneError. It returns the number of bytes written.
#### Example
Code:
```
r := '世'
buf := make([]byte, 3)
n := utf8.EncodeRune(buf, r)
fmt.Println(buf)
fmt.Println(n)
```
Output:
```
[228 184 150]
3
```
#### Example (OutOfRange)
Code:
```
runes := []rune{
// Less than 0, out of range.
-1,
// Greater than 0x10FFFF, out of range.
0x110000,
// The Unicode replacement character.
utf8.RuneError,
}
for i, c := range runes {
buf := make([]byte, 3)
size := utf8.EncodeRune(buf, c)
fmt.Printf("%d: %d %[2]s %d\n", i, buf, size)
}
```
Output:
```
0: [239 191 189] � 3
1: [239 191 189] � 3
2: [239 191 189] � 3
```
func FullRune
-------------
```
func FullRune(p []byte) bool
```
FullRune reports whether the bytes in p begin with a full UTF-8 encoding of a rune. An invalid encoding is considered a full Rune since it will convert as a width-1 error rune.
#### Example
Code:
```
buf := []byte{228, 184, 150} // 世
fmt.Println(utf8.FullRune(buf))
fmt.Println(utf8.FullRune(buf[:2]))
```
Output:
```
true
false
```
func FullRuneInString
---------------------
```
func FullRuneInString(s string) bool
```
FullRuneInString is like FullRune but its input is a string.
#### Example
Code:
```
str := "世"
fmt.Println(utf8.FullRuneInString(str))
fmt.Println(utf8.FullRuneInString(str[:2]))
```
Output:
```
true
false
```
func RuneCount
--------------
```
func RuneCount(p []byte) int
```
RuneCount returns the number of runes in p. Erroneous and short encodings are treated as single runes of width 1 byte.
#### Example
Code:
```
buf := []byte("Hello, 世界")
fmt.Println("bytes =", len(buf))
fmt.Println("runes =", utf8.RuneCount(buf))
```
Output:
```
bytes = 13
runes = 9
```
func RuneCountInString
----------------------
```
func RuneCountInString(s string) (n int)
```
RuneCountInString is like RuneCount but its input is a string.
#### Example
Code:
```
str := "Hello, 世界"
fmt.Println("bytes =", len(str))
fmt.Println("runes =", utf8.RuneCountInString(str))
```
Output:
```
bytes = 13
runes = 9
```
func RuneLen
------------
```
func RuneLen(r rune) int
```
RuneLen returns the number of bytes required to encode the rune. It returns -1 if the rune is not a valid value to encode in UTF-8.
#### Example
Code:
```
fmt.Println(utf8.RuneLen('a'))
fmt.Println(utf8.RuneLen('界'))
```
Output:
```
1
3
```
func RuneStart
--------------
```
func RuneStart(b byte) bool
```
RuneStart reports whether the byte could be the first byte of an encoded, possibly invalid rune. Second and subsequent bytes always have the top two bits set to 10.
#### Example
Code:
```
buf := []byte("a界")
fmt.Println(utf8.RuneStart(buf[0]))
fmt.Println(utf8.RuneStart(buf[1]))
fmt.Println(utf8.RuneStart(buf[2]))
```
Output:
```
true
true
false
```
func Valid
----------
```
func Valid(p []byte) bool
```
Valid reports whether p consists entirely of valid UTF-8-encoded runes.
#### Example
Code:
```
valid := []byte("Hello, 世界")
invalid := []byte{0xff, 0xfe, 0xfd}
fmt.Println(utf8.Valid(valid))
fmt.Println(utf8.Valid(invalid))
```
Output:
```
true
false
```
func ValidRune 1.1
------------------
```
func ValidRune(r rune) bool
```
ValidRune reports whether r can be legally encoded as UTF-8. Code points that are out of range or a surrogate half are illegal.
#### Example
Code:
```
valid := 'a'
invalid := rune(0xfffffff)
fmt.Println(utf8.ValidRune(valid))
fmt.Println(utf8.ValidRune(invalid))
```
Output:
```
true
false
```
func ValidString
----------------
```
func ValidString(s string) bool
```
ValidString reports whether s consists entirely of valid UTF-8-encoded runes.
#### Example
Code:
```
valid := "Hello, 世界"
invalid := string([]byte{0xff, 0xfe, 0xfd})
fmt.Println(utf8.ValidString(valid))
fmt.Println(utf8.ValidString(invalid))
```
Output:
```
true
false
```
go Package hash Package hash
=============
* `import "hash"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package hash provides interfaces for hash functions.
#### Example (BinaryMarshaler)
Code:
```
const (
input1 = "The tunneling gopher digs downwards, "
input2 = "unaware of what he will find."
)
first := sha256.New()
first.Write([]byte(input1))
marshaler, ok := first.(encoding.BinaryMarshaler)
if !ok {
log.Fatal("first does not implement encoding.BinaryMarshaler")
}
state, err := marshaler.MarshalBinary()
if err != nil {
log.Fatal("unable to marshal hash:", err)
}
second := sha256.New()
unmarshaler, ok := second.(encoding.BinaryUnmarshaler)
if !ok {
log.Fatal("second does not implement encoding.BinaryUnmarshaler")
}
if err := unmarshaler.UnmarshalBinary(state); err != nil {
log.Fatal("unable to unmarshal hash:", err)
}
first.Write([]byte(input2))
second.Write([]byte(input2))
fmt.Printf("%x\n", first.Sum(nil))
fmt.Println(bytes.Equal(first.Sum(nil), second.Sum(nil)))
```
Output:
```
57d51a066f3a39942649cd9a76c77e97ceab246756ff3888659e6aa5a07f4a52
true
```
Index
-----
* [type Hash](#Hash)
* [type Hash32](#Hash32)
* [type Hash64](#Hash64)
### Examples
[Package (BinaryMarshaler)](#example__binaryMarshaler) ### Package files
hash.go
type Hash
---------
Hash is the common interface implemented by all hash functions.
Hash implementations in the standard library (e.g. hash/crc32 and crypto/sha256) implement the encoding.BinaryMarshaler and encoding.BinaryUnmarshaler interfaces. Marshaling a hash implementation allows its internal state to be saved and used for additional processing later, without having to re-write the data previously written to the hash. The hash state may contain portions of the input in its original form, which users are expected to handle for any possible security implications.
Compatibility: Any future changes to hash or crypto packages will endeavor to maintain compatibility with state encoded using previous versions. That is, any released versions of the packages should be able to decode data written with any previously released version, subject to issues such as security fixes. See the Go compatibility document for background: <https://golang.org/doc/go1compat>
```
type Hash interface {
// Write (via the embedded io.Writer interface) adds more data to the running hash.
// It never returns an error.
io.Writer
// Sum appends the current hash to b and returns the resulting slice.
// It does not change the underlying hash state.
Sum(b []byte) []byte
// Reset resets the Hash to its initial state.
Reset()
// Size returns the number of bytes Sum will return.
Size() int
// BlockSize returns the hash's underlying block size.
// The Write method must be able to accept any amount
// of data, but it may operate more efficiently if all writes
// are a multiple of the block size.
BlockSize() int
}
```
type Hash32
-----------
Hash32 is the common interface implemented by all 32-bit hash functions.
```
type Hash32 interface {
Hash
Sum32() uint32
}
```
type Hash64
-----------
Hash64 is the common interface implemented by all 64-bit hash functions.
```
type Hash64 interface {
Hash
Sum64() uint64
}
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [adler32](adler32/index) | Package adler32 implements the Adler-32 checksum. |
| [crc32](crc32/index) | Package crc32 implements the 32-bit cyclic redundancy check, or CRC-32, checksum. |
| [crc64](crc64/index) | Package crc64 implements the 64-bit cyclic redundancy check, or CRC-64, checksum. |
| [fnv](fnv/index) | Package fnv implements FNV-1 and FNV-1a, non-cryptographic hash functions created by Glenn Fowler, Landon Curt Noll, and Phong Vo. |
| [maphash](maphash/index) | Package maphash provides hash functions on byte sequences. |
go Package maphash Package maphash
================
* `import "hash/maphash"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package maphash provides hash functions on byte sequences. These hash functions are intended to be used to implement hash tables or other data structures that need to map arbitrary strings or byte sequences to a uniform distribution on unsigned 64-bit integers. Each different instance of a hash table or data structure should use its own Seed.
The hash functions are not cryptographically secure. (See crypto/sha256 and crypto/sha512 for cryptographic use.)
#### Example
Code:
```
// The zero Hash value is valid and ready to use; setting an
// initial seed is not necessary.
var h maphash.Hash
// Add a string to the hash, and print the current hash value.
h.WriteString("hello, ")
fmt.Printf("%#x\n", h.Sum64())
// Append additional data (in the form of a byte array).
h.Write([]byte{'w', 'o', 'r', 'l', 'd'})
fmt.Printf("%#x\n", h.Sum64())
// Reset discards all data previously added to the Hash, without
// changing its seed.
h.Reset()
// Use SetSeed to create a new Hash h2 which will behave
// identically to h.
var h2 maphash.Hash
h2.SetSeed(h.Seed())
h.WriteString("same")
h2.WriteString("same")
fmt.Printf("%#x == %#x\n", h.Sum64(), h2.Sum64())
```
Index
-----
* [func Bytes(seed Seed, b []byte) uint64](#Bytes)
* [func String(seed Seed, s string) uint64](#String)
* [type Hash](#Hash)
* [func (h \*Hash) BlockSize() int](#Hash.BlockSize)
* [func (h \*Hash) Reset()](#Hash.Reset)
* [func (h \*Hash) Seed() Seed](#Hash.Seed)
* [func (h \*Hash) SetSeed(seed Seed)](#Hash.SetSeed)
* [func (h \*Hash) Size() int](#Hash.Size)
* [func (h \*Hash) Sum(b []byte) []byte](#Hash.Sum)
* [func (h \*Hash) Sum64() uint64](#Hash.Sum64)
* [func (h \*Hash) Write(b []byte) (int, error)](#Hash.Write)
* [func (h \*Hash) WriteByte(b byte) error](#Hash.WriteByte)
* [func (h \*Hash) WriteString(s string) (int, error)](#Hash.WriteString)
* [type Seed](#Seed)
* [func MakeSeed() Seed](#MakeSeed)
### Examples
[Package](#example_) ### Package files
maphash.go
func Bytes 1.19
---------------
```
func Bytes(seed Seed, b []byte) uint64
```
Bytes returns the hash of b with the given seed.
Bytes is equivalent to, but more convenient and efficient than:
```
var h Hash
h.SetSeed(seed)
h.Write(b)
return h.Sum64()
```
func String 1.19
----------------
```
func String(seed Seed, s string) uint64
```
String returns the hash of s with the given seed.
String is equivalent to, but more convenient and efficient than:
```
var h Hash
h.SetSeed(seed)
h.WriteString(s)
return h.Sum64()
```
type Hash 1.14
--------------
A Hash computes a seeded hash of a byte sequence.
The zero Hash is a valid Hash ready to use. A zero Hash chooses a random seed for itself during the first call to a Reset, Write, Seed, or Sum64 method. For control over the seed, use SetSeed.
The computed hash values depend only on the initial seed and the sequence of bytes provided to the Hash object, not on the way in which the bytes are provided. For example, the three sequences
```
h.Write([]byte{'f','o','o'})
h.WriteByte('f'); h.WriteByte('o'); h.WriteByte('o')
h.WriteString("foo")
```
all have the same effect.
Hashes are intended to be collision-resistant, even for situations where an adversary controls the byte sequences being hashed.
A Hash is not safe for concurrent use by multiple goroutines, but a Seed is. If multiple goroutines must compute the same seeded hash, each can declare its own Hash and call SetSeed with a common Seed.
```
type Hash struct {
// contains filtered or unexported fields
}
```
### func (\*Hash) BlockSize 1.14
```
func (h *Hash) BlockSize() int
```
BlockSize returns h's block size.
### func (\*Hash) Reset 1.14
```
func (h *Hash) Reset()
```
Reset discards all bytes added to h. (The seed remains the same.)
### func (\*Hash) Seed 1.14
```
func (h *Hash) Seed() Seed
```
Seed returns h's seed value.
### func (\*Hash) SetSeed 1.14
```
func (h *Hash) SetSeed(seed Seed)
```
SetSeed sets h to use seed, which must have been returned by MakeSeed or by another Hash's Seed method. Two Hash objects with the same seed behave identically. Two Hash objects with different seeds will very likely behave differently. Any bytes added to h before this call will be discarded.
### func (\*Hash) Size 1.14
```
func (h *Hash) Size() int
```
Size returns h's hash value size, 8 bytes.
### func (\*Hash) Sum 1.14
```
func (h *Hash) Sum(b []byte) []byte
```
Sum appends the hash's current 64-bit value to b. It exists for implementing hash.Hash. For direct calls, it is more efficient to use Sum64.
### func (\*Hash) Sum64 1.14
```
func (h *Hash) Sum64() uint64
```
Sum64 returns h's current 64-bit value, which depends on h's seed and the sequence of bytes added to h since the last call to Reset or SetSeed.
All bits of the Sum64 result are close to uniformly and independently distributed, so it can be safely reduced by using bit masking, shifting, or modular arithmetic.
### func (\*Hash) Write 1.14
```
func (h *Hash) Write(b []byte) (int, error)
```
Write adds b to the sequence of bytes hashed by h. It always writes all of b and never fails; the count and error result are for implementing io.Writer.
### func (\*Hash) WriteByte 1.14
```
func (h *Hash) WriteByte(b byte) error
```
WriteByte adds b to the sequence of bytes hashed by h. It never fails; the error result is for implementing io.ByteWriter.
### func (\*Hash) WriteString 1.14
```
func (h *Hash) WriteString(s string) (int, error)
```
WriteString adds the bytes of s to the sequence of bytes hashed by h. It always writes all of s and never fails; the count and error result are for implementing io.StringWriter.
type Seed 1.14
--------------
A Seed is a random value that selects the specific hash function computed by a Hash. If two Hashes use the same Seeds, they will compute the same hash values for any given input. If two Hashes use different Seeds, they are very likely to compute distinct hash values for any given input.
A Seed must be initialized by calling MakeSeed. The zero seed is uninitialized and not valid for use with Hash's SetSeed method.
Each Seed value is local to a single process and cannot be serialized or otherwise recreated in a different process.
```
type Seed struct {
// contains filtered or unexported fields
}
```
### func MakeSeed 1.14
```
func MakeSeed() Seed
```
MakeSeed returns a new random seed.
| programming_docs |
go Package adler32 Package adler32
================
* `import "hash/adler32"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package adler32 implements the Adler-32 checksum.
It is defined in RFC 1950:
```
Adler-32 is composed of two sums accumulated per byte: s1 is
the sum of all bytes, s2 is the sum of all s1 values. Both sums
are done modulo 65521. s1 is initialized to 1, s2 to zero. The
Adler-32 checksum is stored as s2*65536 + s1 in most-
significant-byte first (network) order.
```
Index
-----
* [Constants](#pkg-constants)
* [func Checksum(data []byte) uint32](#Checksum)
* [func New() hash.Hash32](#New)
### Package files
adler32.go
Constants
---------
The size of an Adler-32 checksum in bytes.
```
const Size = 4
```
func Checksum
-------------
```
func Checksum(data []byte) uint32
```
Checksum returns the Adler-32 checksum of data.
func New
--------
```
func New() hash.Hash32
```
New returns a new hash.Hash32 computing the Adler-32 checksum. Its Sum method will lay the value out in big-endian byte order. The returned Hash32 also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
go Package crc32 Package crc32
==============
* `import "hash/crc32"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package crc32 implements the 32-bit cyclic redundancy check, or CRC-32, checksum. See <https://en.wikipedia.org/wiki/Cyclic_redundancy_check> for information.
Polynomials are represented in LSB-first form also known as reversed representation.
See <https://en.wikipedia.org/wiki/Mathematics_of_cyclic_redundancy_checks#Reversed_representations_and_reciprocal_polynomials> for information.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func Checksum(data []byte, tab \*Table) uint32](#Checksum)
* [func ChecksumIEEE(data []byte) uint32](#ChecksumIEEE)
* [func New(tab \*Table) hash.Hash32](#New)
* [func NewIEEE() hash.Hash32](#NewIEEE)
* [func Update(crc uint32, tab \*Table, p []byte) uint32](#Update)
* [type Table](#Table)
* [func MakeTable(poly uint32) \*Table](#MakeTable)
### Examples
[MakeTable](#example_MakeTable) ### Package files
crc32.go crc32\_amd64.go crc32\_generic.go
Constants
---------
Predefined polynomials.
```
const (
// IEEE is by far and away the most common CRC-32 polynomial.
// Used by ethernet (IEEE 802.3), v.42, fddi, gzip, zip, png, ...
IEEE = 0xedb88320
// Castagnoli's polynomial, used in iSCSI.
// Has better error detection characteristics than IEEE.
// https://dx.doi.org/10.1109/26.231911
Castagnoli = 0x82f63b78
// Koopman's polynomial.
// Also has better error detection characteristics than IEEE.
// https://dx.doi.org/10.1109/DSN.2002.1028931
Koopman = 0xeb31d82e
)
```
The size of a CRC-32 checksum in bytes.
```
const Size = 4
```
Variables
---------
IEEETable is the table for the IEEE polynomial.
```
var IEEETable = simpleMakeTable(IEEE)
```
func Checksum
-------------
```
func Checksum(data []byte, tab *Table) uint32
```
Checksum returns the CRC-32 checksum of data using the polynomial represented by the Table.
func ChecksumIEEE
-----------------
```
func ChecksumIEEE(data []byte) uint32
```
ChecksumIEEE returns the CRC-32 checksum of data using the IEEE polynomial.
func New
--------
```
func New(tab *Table) hash.Hash32
```
New creates a new hash.Hash32 computing the CRC-32 checksum using the polynomial represented by the Table. Its Sum method will lay the value out in big-endian byte order. The returned Hash32 also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
func NewIEEE
------------
```
func NewIEEE() hash.Hash32
```
NewIEEE creates a new hash.Hash32 computing the CRC-32 checksum using the IEEE polynomial. Its Sum method will lay the value out in big-endian byte order. The returned Hash32 also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
func Update
-----------
```
func Update(crc uint32, tab *Table, p []byte) uint32
```
Update returns the result of adding the bytes in p to the crc.
type Table
----------
Table is a 256-word table representing the polynomial for efficient processing.
```
type Table [256]uint32
```
### func MakeTable
```
func MakeTable(poly uint32) *Table
```
MakeTable returns a Table constructed from the specified polynomial. The contents of this Table must not be modified.
#### Example
Code:
```
// In this package, the CRC polynomial is represented in reversed notation,
// or LSB-first representation.
//
// LSB-first representation is a hexadecimal number with n bits, in which the
// most significant bit represents the coefficient of x⁰ and the least significant
// bit represents the coefficient of xⁿ⁻¹ (the coefficient for xⁿ is implicit).
//
// For example, CRC32-Q, as defined by the following polynomial,
// x³²+ x³¹+ x²⁴+ x²²+ x¹⁶+ x¹⁴+ x⁸+ x⁷+ x⁵+ x³+ x¹+ x⁰
// has the reversed notation 0b11010101100000101000001010000001, so the value
// that should be passed to MakeTable is 0xD5828281.
crc32q := crc32.MakeTable(0xD5828281)
fmt.Printf("%08x\n", crc32.Checksum([]byte("Hello world"), crc32q))
```
Output:
```
2964d064
```
go Package fnv Package fnv
============
* `import "hash/fnv"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package fnv implements FNV-1 and FNV-1a, non-cryptographic hash functions created by Glenn Fowler, Landon Curt Noll, and Phong Vo. See <https://en.wikipedia.org/wiki/Fowler-Noll-Vo_hash_function>.
All the hash.Hash implementations returned by this package also implement encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
Index
-----
* [func New128() hash.Hash](#New128)
* [func New128a() hash.Hash](#New128a)
* [func New32() hash.Hash32](#New32)
* [func New32a() hash.Hash32](#New32a)
* [func New64() hash.Hash64](#New64)
* [func New64a() hash.Hash64](#New64a)
### Package files
fnv.go
func New128 1.9
---------------
```
func New128() hash.Hash
```
New128 returns a new 128-bit FNV-1 hash.Hash. Its Sum method will lay the value out in big-endian byte order.
func New128a 1.9
----------------
```
func New128a() hash.Hash
```
New128a returns a new 128-bit FNV-1a hash.Hash. Its Sum method will lay the value out in big-endian byte order.
func New32
----------
```
func New32() hash.Hash32
```
New32 returns a new 32-bit FNV-1 hash.Hash. Its Sum method will lay the value out in big-endian byte order.
func New32a
-----------
```
func New32a() hash.Hash32
```
New32a returns a new 32-bit FNV-1a hash.Hash. Its Sum method will lay the value out in big-endian byte order.
func New64
----------
```
func New64() hash.Hash64
```
New64 returns a new 64-bit FNV-1 hash.Hash. Its Sum method will lay the value out in big-endian byte order.
func New64a
-----------
```
func New64a() hash.Hash64
```
New64a returns a new 64-bit FNV-1a hash.Hash. Its Sum method will lay the value out in big-endian byte order.
go Package crc64 Package crc64
==============
* `import "hash/crc64"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
Overview
--------
Package crc64 implements the 64-bit cyclic redundancy check, or CRC-64, checksum. See <https://en.wikipedia.org/wiki/Cyclic_redundancy_check> for information.
Index
-----
* [Constants](#pkg-constants)
* [func Checksum(data []byte, tab \*Table) uint64](#Checksum)
* [func New(tab \*Table) hash.Hash64](#New)
* [func Update(crc uint64, tab \*Table, p []byte) uint64](#Update)
* [type Table](#Table)
* [func MakeTable(poly uint64) \*Table](#MakeTable)
### Package files
crc64.go
Constants
---------
Predefined polynomials.
```
const (
// The ISO polynomial, defined in ISO 3309 and used in HDLC.
ISO = 0xD800000000000000
// The ECMA polynomial, defined in ECMA 182.
ECMA = 0xC96C5795D7870F42
)
```
The size of a CRC-64 checksum in bytes.
```
const Size = 8
```
func Checksum
-------------
```
func Checksum(data []byte, tab *Table) uint64
```
Checksum returns the CRC-64 checksum of data using the polynomial represented by the Table.
func New
--------
```
func New(tab *Table) hash.Hash64
```
New creates a new hash.Hash64 computing the CRC-64 checksum using the polynomial represented by the Table. Its Sum method will lay the value out in big-endian byte order. The returned Hash64 also implements encoding.BinaryMarshaler and encoding.BinaryUnmarshaler to marshal and unmarshal the internal state of the hash.
func Update
-----------
```
func Update(crc uint64, tab *Table, p []byte) uint64
```
Update returns the result of adding the bytes in p to the crc.
type Table
----------
Table is a 256-word table representing the polynomial for efficient processing.
```
type Table [256]uint64
```
### func MakeTable
```
func MakeTable(poly uint64) *Table
```
MakeTable returns a Table constructed from the specified polynomial. The contents of this Table must not be modified.
go Package fmt Package fmt
============
* `import "fmt"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package fmt implements formatted I/O with functions analogous to C's printf and scanf. The format 'verbs' are derived from C's but are simpler.
### Printing
The verbs:
General:
```
%v the value in a default format
when printing structs, the plus flag (%+v) adds field names
%#v a Go-syntax representation of the value
%T a Go-syntax representation of the type of the value
%% a literal percent sign; consumes no value
```
Boolean:
```
%t the word true or false
```
Integer:
```
%b base 2
%c the character represented by the corresponding Unicode code point
%d base 10
%o base 8
%O base 8 with 0o prefix
%q a single-quoted character literal safely escaped with Go syntax.
%x base 16, with lower-case letters for a-f
%X base 16, with upper-case letters for A-F
%U Unicode format: U+1234; same as "U+%04X"
```
Floating-point and complex constituents:
```
%b decimalless scientific notation with exponent a power of two,
in the manner of strconv.FormatFloat with the 'b' format,
e.g. -123456p-78
%e scientific notation, e.g. -1.234456e+78
%E scientific notation, e.g. -1.234456E+78
%f decimal point but no exponent, e.g. 123.456
%F synonym for %f
%g %e for large exponents, %f otherwise. Precision is discussed below.
%G %E for large exponents, %F otherwise
%x hexadecimal notation (with decimal power of two exponent), e.g. -0x1.23abcp+20
%X upper-case hexadecimal notation, e.g. -0X1.23ABCP+20
```
String and slice of bytes (treated equivalently with these verbs):
```
%s the uninterpreted bytes of the string or slice
%q a double-quoted string safely escaped with Go syntax
%x base 16, lower-case, two characters per byte
%X base 16, upper-case, two characters per byte
```
Slice:
```
%p address of 0th element in base 16 notation, with leading 0x
```
Pointer:
```
%p base 16 notation, with leading 0x
The %b, %d, %o, %x and %X verbs also work with pointers,
formatting the value exactly as if it were an integer.
```
The default format for %v is:
```
bool: %t
int, int8 etc.: %d
uint, uint8 etc.: %d, %#x if printed with %#v
float32, complex64, etc: %g
string: %s
chan: %p
pointer: %p
```
For compound objects, the elements are printed using these rules, recursively, laid out like this:
```
struct: {field0 field1 ...}
array, slice: [elem0 elem1 ...]
maps: map[key1:value1 key2:value2 ...]
pointer to above: &{}, &[], &map[]
```
Width is specified by an optional decimal number immediately preceding the verb. If absent, the width is whatever is necessary to represent the value. Precision is specified after the (optional) width by a period followed by a decimal number. If no period is present, a default precision is used. A period with no following number specifies a precision of zero. Examples:
```
%f default width, default precision
%9f width 9, default precision
%.2f default width, precision 2
%9.2f width 9, precision 2
%9.f width 9, precision 0
```
Width and precision are measured in units of Unicode code points, that is, runes. (This differs from C's printf where the units are always measured in bytes.) Either or both of the flags may be replaced with the character '\*', causing their values to be obtained from the next operand (preceding the one to format), which must be of type int.
For most values, width is the minimum number of runes to output, padding the formatted form with spaces if necessary.
For strings, byte slices and byte arrays, however, precision limits the length of the input to be formatted (not the size of the output), truncating if necessary. Normally it is measured in runes, but for these types when formatted with the %x or %X format it is measured in bytes.
For floating-point values, width sets the minimum width of the field and precision sets the number of places after the decimal, if appropriate, except that for %g/%G precision sets the maximum number of significant digits (trailing zeros are removed). For example, given 12.345 the format %6.3f prints 12.345 while %.3g prints 12.3. The default precision for %e, %f and %#g is 6; for %g it is the smallest number of digits necessary to identify the value uniquely.
For complex numbers, the width and precision apply to the two components independently and the result is parenthesized, so %f applied to 1.2+3.4i produces (1.200000+3.400000i).
When formatting a single integer code point or a rune string (type []rune) with %q, invalid Unicode code points are changed to the Unicode replacement character, U+FFFD, as in strconv.QuoteRune.
Other flags:
```
'+' always print a sign for numeric values;
guarantee ASCII-only output for %q (%+q)
'-' pad with spaces on the right rather than the left (left-justify the field)
'#' alternate format: add leading 0b for binary (%#b), 0 for octal (%#o),
0x or 0X for hex (%#x or %#X); suppress 0x for %p (%#p);
for %q, print a raw (backquoted) string if strconv.CanBackquote
returns true;
always print a decimal point for %e, %E, %f, %F, %g and %G;
do not remove trailing zeros for %g and %G;
write e.g. U+0078 'x' if the character is printable for %U (%#U).
' ' (space) leave a space for elided sign in numbers (% d);
put spaces between bytes printing strings or slices in hex (% x, % X)
'0' pad with leading zeros rather than spaces;
for numbers, this moves the padding after the sign;
ignored for strings, byte slices and byte arrays
```
Flags are ignored by verbs that do not expect them. For example there is no alternate decimal format, so %#d and %d behave identically.
For each Printf-like function, there is also a Print function that takes no format and is equivalent to saying %v for every operand. Another variant Println inserts blanks between operands and appends a newline.
Regardless of the verb, if an operand is an interface value, the internal concrete value is used, not the interface itself. Thus:
```
var i interface{} = 23
fmt.Printf("%v\n", i)
```
will print 23.
Except when printed using the verbs %T and %p, special formatting considerations apply for operands that implement certain interfaces. In order of application:
1. If the operand is a reflect.Value, the operand is replaced by the concrete value that it holds, and printing continues with the next rule.
2. If an operand implements the Formatter interface, it will be invoked. In this case the interpretation of verbs and flags is controlled by that implementation.
3. If the %v verb is used with the # flag (%#v) and the operand implements the GoStringer interface, that will be invoked.
If the format (which is implicitly %v for Println etc.) is valid for a string (%s %q %v %x %X), the following two rules apply:
4. If an operand implements the error interface, the Error method will be invoked to convert the object to a string, which will then be formatted as required by the verb (if any).
5. If an operand implements method String() string, that method will be invoked to convert the object to a string, which will then be formatted as required by the verb (if any).
For compound operands such as slices and structs, the format applies to the elements of each operand, recursively, not to the operand as a whole. Thus %q will quote each element of a slice of strings, and %6.2f will control formatting for each element of a floating-point array.
However, when printing a byte slice with a string-like verb (%s %q %x %X), it is treated identically to a string, as a single item.
To avoid recursion in cases such as
```
type X string
func (x X) String() string { return Sprintf("<%s>", x) }
```
convert the value before recurring:
```
func (x X) String() string { return Sprintf("<%s>", string(x)) }
```
Infinite recursion can also be triggered by self-referential data structures, such as a slice that contains itself as an element, if that type has a String method. Such pathologies are rare, however, and the package does not protect against them.
When printing a struct, fmt cannot and therefore does not invoke formatting methods such as Error or String on unexported fields.
### Explicit argument indexes
In Printf, Sprintf, and Fprintf, the default behavior is for each formatting verb to format successive arguments passed in the call. However, the notation [n] immediately before the verb indicates that the nth one-indexed argument is to be formatted instead. The same notation before a '\*' for a width or precision selects the argument index holding the value. After processing a bracketed expression [n], subsequent verbs will use arguments n+1, n+2, etc. unless otherwise directed.
For example,
```
fmt.Sprintf("%[2]d %[1]d\n", 11, 22)
```
will yield "22 11", while
```
fmt.Sprintf("%[3]*.[2]*[1]f", 12.0, 2, 6)
```
equivalent to
```
fmt.Sprintf("%6.2f", 12.0)
```
will yield " 12.00". Because an explicit index affects subsequent verbs, this notation can be used to print the same values multiple times by resetting the index for the first argument to be repeated:
```
fmt.Sprintf("%d %d %#[1]x %#x", 16, 17)
```
will yield "16 17 0x10 0x11".
### Format errors
If an invalid argument is given for a verb, such as providing a string to %d, the generated string will contain a description of the problem, as in these examples:
```
Wrong type or unknown verb: %!verb(type=value)
Printf("%d", "hi"): %!d(string=hi)
Too many arguments: %!(EXTRA type=value)
Printf("hi", "guys"): hi%!(EXTRA string=guys)
Too few arguments: %!verb(MISSING)
Printf("hi%d"): hi%!d(MISSING)
Non-int for width or precision: %!(BADWIDTH) or %!(BADPREC)
Printf("%*s", 4.5, "hi"): %!(BADWIDTH)hi
Printf("%.*s", 4.5, "hi"): %!(BADPREC)hi
Invalid or invalid use of argument index: %!(BADINDEX)
Printf("%*[2]d", 7): %!d(BADINDEX)
Printf("%.[2]d", 7): %!d(BADINDEX)
```
All errors begin with the string "%!" followed sometimes by a single character (the verb) and end with a parenthesized description.
If an Error or String method triggers a panic when called by a print routine, the fmt package reformats the error message from the panic, decorating it with an indication that it came through the fmt package. For example, if a String method calls panic("bad"), the resulting formatted message will look like
```
%!s(PANIC=bad)
```
The %!s just shows the print verb in use when the failure occurred. If the panic is caused by a nil receiver to an Error or String method, however, the output is the undecorated string, "<nil>".
### Scanning
An analogous set of functions scans formatted text to yield values. Scan, Scanf and Scanln read from os.Stdin; Fscan, Fscanf and Fscanln read from a specified io.Reader; Sscan, Sscanf and Sscanln read from an argument string.
Scan, Fscan, Sscan treat newlines in the input as spaces.
Scanln, Fscanln and Sscanln stop scanning at a newline and require that the items be followed by a newline or EOF.
Scanf, Fscanf, and Sscanf parse the arguments according to a format string, analogous to that of Printf. In the text that follows, 'space' means any Unicode whitespace character except newline.
In the format string, a verb introduced by the % character consumes and parses input; these verbs are described in more detail below. A character other than %, space, or newline in the format consumes exactly that input character, which must be present. A newline with zero or more spaces before it in the format string consumes zero or more spaces in the input followed by a single newline or the end of the input. A space following a newline in the format string consumes zero or more spaces in the input. Otherwise, any run of one or more spaces in the format string consumes as many spaces as possible in the input. Unless the run of spaces in the format string appears adjacent to a newline, the run must consume at least one space from the input or find the end of the input.
The handling of spaces and newlines differs from that of C's scanf family: in C, newlines are treated as any other space, and it is never an error when a run of spaces in the format string finds no spaces to consume in the input.
The verbs behave analogously to those of Printf. For example, %x will scan an integer as a hexadecimal number, and %v will scan the default representation format for the value. The Printf verbs %p and %T and the flags # and + are not implemented. For floating-point and complex values, all valid formatting verbs (%b %e %E %f %F %g %G %x %X and %v) are equivalent and accept both decimal and hexadecimal notation (for example: "2.3e+7", "0x4.5p-8") and digit-separating underscores (for example: "3.14159\_26535\_89793").
Input processed by verbs is implicitly space-delimited: the implementation of every verb except %c starts by discarding leading spaces from the remaining input, and the %s verb (and %v reading into a string) stops consuming input at the first space or newline character.
The familiar base-setting prefixes 0b (binary), 0o and 0 (octal), and 0x (hexadecimal) are accepted when scanning integers without a format or with the %v verb, as are digit-separating underscores.
Width is interpreted in the input text but there is no syntax for scanning with a precision (no %5.2f, just %5f). If width is provided, it applies after leading spaces are trimmed and specifies the maximum number of runes to read to satisfy the verb. For example,
```
Sscanf(" 1234567 ", "%5s%d", &s, &i)
```
will set s to "12345" and i to 67 while
```
Sscanf(" 12 34 567 ", "%5s%d", &s, &i)
```
will set s to "12" and i to 34.
In all the scanning functions, a carriage return followed immediately by a newline is treated as a plain newline (\r\n means the same as \n).
In all the scanning functions, if an operand implements method Scan (that is, it implements the Scanner interface) that method will be used to scan the text for that operand. Also, if the number of arguments scanned is less than the number of arguments provided, an error is returned.
All arguments to be scanned must be either pointers to basic types or implementations of the Scanner interface.
Like Scanf and Fscanf, Sscanf need not consume its entire input. There is no way to recover how much of the input string Sscanf used.
Note: Fscan etc. can read one character (rune) past the input they return, which means that a loop calling a scan routine may skip some of the input. This is usually a problem only when there is no space between input values. If the reader provided to Fscan implements ReadRune, that method will be used to read characters. If the reader also implements UnreadRune, that method will be used to save the character and successive calls will not lose data. To attach ReadRune and UnreadRune methods to a reader without that capability, use bufio.NewReader.
#### Example (Formats)
These examples demonstrate the basics of printing using a format string. Printf, Sprintf, and Fprintf all take a format string that specifies how to format the subsequent arguments. For example, %d (we call that a 'verb') says to print the corresponding argument, which must be an integer (or something containing an integer, such as a slice of ints) in decimal. The verb %v ('v' for 'value') always formats the argument in its default form, just how Print or Println would show it. The special verb %T ('T' for 'Type') prints the type of the argument rather than its value. The examples are not exhaustive; see the package comment for all the details.
Code:
```
// A basic set of examples showing that %v is the default format, in this
// case decimal for integers, which can be explicitly requested with %d;
// the output is just what Println generates.
integer := 23
// Each of these prints "23" (without the quotes).
fmt.Println(integer)
fmt.Printf("%v\n", integer)
fmt.Printf("%d\n", integer)
// The special verb %T shows the type of an item rather than its value.
fmt.Printf("%T %T\n", integer, &integer)
// Result: int *int
// Println(x) is the same as Printf("%v\n", x) so we will use only Printf
// in the following examples. Each one demonstrates how to format values of
// a particular type, such as integers or strings. We start each format
// string with %v to show the default output and follow that with one or
// more custom formats.
// Booleans print as "true" or "false" with %v or %t.
truth := true
fmt.Printf("%v %t\n", truth, truth)
// Result: true true
// Integers print as decimals with %v and %d,
// or in hex with %x, octal with %o, or binary with %b.
answer := 42
fmt.Printf("%v %d %x %o %b\n", answer, answer, answer, answer, answer)
// Result: 42 42 2a 52 101010
// Floats have multiple formats: %v and %g print a compact representation,
// while %f prints a decimal point and %e uses exponential notation. The
// format %6.2f used here shows how to set the width and precision to
// control the appearance of a floating-point value. In this instance, 6 is
// the total width of the printed text for the value (note the extra spaces
// in the output) and 2 is the number of decimal places to show.
pi := math.Pi
fmt.Printf("%v %g %.2f (%6.2f) %e\n", pi, pi, pi, pi, pi)
// Result: 3.141592653589793 3.141592653589793 3.14 ( 3.14) 3.141593e+00
// Complex numbers format as parenthesized pairs of floats, with an 'i'
// after the imaginary part.
point := 110.7 + 22.5i
fmt.Printf("%v %g %.2f %.2e\n", point, point, point, point)
// Result: (110.7+22.5i) (110.7+22.5i) (110.70+22.50i) (1.11e+02+2.25e+01i)
// Runes are integers but when printed with %c show the character with that
// Unicode value. The %q verb shows them as quoted characters, %U as a
// hex Unicode code point, and %#U as both a code point and a quoted
// printable form if the rune is printable.
smile := '😀'
fmt.Printf("%v %d %c %q %U %#U\n", smile, smile, smile, smile, smile, smile)
// Result: 128512 128512 😀 '😀' U+1F600 U+1F600 '😀'
// Strings are formatted with %v and %s as-is, with %q as quoted strings,
// and %#q as backquoted strings.
placeholders := `foo "bar"`
fmt.Printf("%v %s %q %#q\n", placeholders, placeholders, placeholders, placeholders)
// Result: foo "bar" foo "bar" "foo \"bar\"" `foo "bar"`
// Maps formatted with %v show keys and values in their default formats.
// The %#v form (the # is called a "flag" in this context) shows the map in
// the Go source format. Maps are printed in a consistent order, sorted
// by the values of the keys.
isLegume := map[string]bool{
"peanut": true,
"dachshund": false,
}
fmt.Printf("%v %#v\n", isLegume, isLegume)
// Result: map[dachshund:false peanut:true] map[string]bool{"dachshund":false, "peanut":true}
// Structs formatted with %v show field values in their default formats.
// The %+v form shows the fields by name, while %#v formats the struct in
// Go source format.
person := struct {
Name string
Age int
}{"Kim", 22}
fmt.Printf("%v %+v %#v\n", person, person, person)
// Result: {Kim 22} {Name:Kim Age:22} struct { Name string; Age int }{Name:"Kim", Age:22}
// The default format for a pointer shows the underlying value preceded by
// an ampersand. The %p verb prints the pointer value in hex. We use a
// typed nil for the argument to %p here because the value of any non-nil
// pointer would change from run to run; run the commented-out Printf
// call yourself to see.
pointer := &person
fmt.Printf("%v %p\n", pointer, (*int)(nil))
// Result: &{Kim 22} 0x0
// fmt.Printf("%v %p\n", pointer, pointer)
// Result: &{Kim 22} 0x010203 // See comment above.
// Arrays and slices are formatted by applying the format to each element.
greats := [5]string{"Kitano", "Kobayashi", "Kurosawa", "Miyazaki", "Ozu"}
fmt.Printf("%v %q\n", greats, greats)
// Result: [Kitano Kobayashi Kurosawa Miyazaki Ozu] ["Kitano" "Kobayashi" "Kurosawa" "Miyazaki" "Ozu"]
kGreats := greats[:3]
fmt.Printf("%v %q %#v\n", kGreats, kGreats, kGreats)
// Result: [Kitano Kobayashi Kurosawa] ["Kitano" "Kobayashi" "Kurosawa"] []string{"Kitano", "Kobayashi", "Kurosawa"}
// Byte slices are special. Integer verbs like %d print the elements in
// that format. The %s and %q forms treat the slice like a string. The %x
// verb has a special form with the space flag that puts a space between
// the bytes.
cmd := []byte("a⌘")
fmt.Printf("%v %d %s %q %x % x\n", cmd, cmd, cmd, cmd, cmd, cmd)
// Result: [97 226 140 152] [97 226 140 152] a⌘ "a⌘" 61e28c98 61 e2 8c 98
// Types that implement Stringer are printed the same as strings. Because
// Stringers return a string, we can print them using a string-specific
// verb such as %q.
now := time.Unix(123456789, 0).UTC() // time.Time implements fmt.Stringer.
fmt.Printf("%v %q\n", now, now)
// Result: 1973-11-29 21:33:09 +0000 UTC "1973-11-29 21:33:09 +0000 UTC"
```
Output:
```
23
23
23
int *int
true true
42 42 2a 52 101010
3.141592653589793 3.141592653589793 3.14 ( 3.14) 3.141593e+00
(110.7+22.5i) (110.7+22.5i) (110.70+22.50i) (1.11e+02+2.25e+01i)
128512 128512 😀 '😀' U+1F600 U+1F600 '😀'
foo "bar" foo "bar" "foo \"bar\"" `foo "bar"`
map[dachshund:false peanut:true] map[string]bool{"dachshund":false, "peanut":true}
{Kim 22} {Name:Kim Age:22} struct { Name string; Age int }{Name:"Kim", Age:22}
&{Kim 22} 0x0
[Kitano Kobayashi Kurosawa Miyazaki Ozu] ["Kitano" "Kobayashi" "Kurosawa" "Miyazaki" "Ozu"]
[Kitano Kobayashi Kurosawa] ["Kitano" "Kobayashi" "Kurosawa"] []string{"Kitano", "Kobayashi", "Kurosawa"}
[97 226 140 152] [97 226 140 152] a⌘ "a⌘" 61e28c98 61 e2 8c 98
1973-11-29 21:33:09 +0000 UTC "1973-11-29 21:33:09 +0000 UTC"
```
#### Example (Printers)
Print, Println, and Printf lay out their arguments differently. In this example we can compare their behaviors. Println always adds blanks between the items it prints, while Print adds blanks only between non-string arguments and Printf does exactly what it is told. Sprint, Sprintln, Sprintf, Fprint, Fprintln, and Fprintf behave the same as their corresponding Print, Println, and Printf functions shown here.
Code:
```
a, b := 3.0, 4.0
h := math.Hypot(a, b)
// Print inserts blanks between arguments when neither is a string.
// It does not add a newline to the output, so we add one explicitly.
fmt.Print("The vector (", a, b, ") has length ", h, ".\n")
// Println always inserts spaces between its arguments,
// so it cannot be used to produce the same output as Print in this case;
// its output has extra spaces.
// Also, Println always adds a newline to the output.
fmt.Println("The vector (", a, b, ") has length", h, ".")
// Printf provides complete control but is more complex to use.
// It does not add a newline to the output, so we add one explicitly
// at the end of the format specifier string.
fmt.Printf("The vector (%g %g) has length %g.\n", a, b, h)
```
Output:
```
The vector (3 4) has length 5.
The vector ( 3 4 ) has length 5 .
The vector (3 4) has length 5.
```
Index
-----
* [func Append(b []byte, a ...any) []byte](#Append)
* [func Appendf(b []byte, format string, a ...any) []byte](#Appendf)
* [func Appendln(b []byte, a ...any) []byte](#Appendln)
* [func Errorf(format string, a ...any) error](#Errorf)
* [func FormatString(state State, verb rune) string](#FormatString)
* [func Fprint(w io.Writer, a ...any) (n int, err error)](#Fprint)
* [func Fprintf(w io.Writer, format string, a ...any) (n int, err error)](#Fprintf)
* [func Fprintln(w io.Writer, a ...any) (n int, err error)](#Fprintln)
* [func Fscan(r io.Reader, a ...any) (n int, err error)](#Fscan)
* [func Fscanf(r io.Reader, format string, a ...any) (n int, err error)](#Fscanf)
* [func Fscanln(r io.Reader, a ...any) (n int, err error)](#Fscanln)
* [func Print(a ...any) (n int, err error)](#Print)
* [func Printf(format string, a ...any) (n int, err error)](#Printf)
* [func Println(a ...any) (n int, err error)](#Println)
* [func Scan(a ...any) (n int, err error)](#Scan)
* [func Scanf(format string, a ...any) (n int, err error)](#Scanf)
* [func Scanln(a ...any) (n int, err error)](#Scanln)
* [func Sprint(a ...any) string](#Sprint)
* [func Sprintf(format string, a ...any) string](#Sprintf)
* [func Sprintln(a ...any) string](#Sprintln)
* [func Sscan(str string, a ...any) (n int, err error)](#Sscan)
* [func Sscanf(str string, format string, a ...any) (n int, err error)](#Sscanf)
* [func Sscanln(str string, a ...any) (n int, err error)](#Sscanln)
* [type Formatter](#Formatter)
* [type GoStringer](#GoStringer)
* [type ScanState](#ScanState)
* [type Scanner](#Scanner)
* [type State](#State)
* [type Stringer](#Stringer)
### Examples
[Errorf](#example_Errorf) [Fprint](#example_Fprint) [Fprintf](#example_Fprintf) [Fprintln](#example_Fprintln) [Fscanf](#example_Fscanf) [Fscanln](#example_Fscanln) [GoStringer](#example_GoStringer) [Print](#example_Print) [Printf](#example_Printf) [Println](#example_Println) [Sprint](#example_Sprint) [Sprintf](#example_Sprintf) [Sprintln](#example_Sprintln) [Sscanf](#example_Sscanf) [Stringer](#example_Stringer) [Package (Formats)](#example__formats) [Package (Printers)](#example__printers) ### Package files
doc.go errors.go format.go print.go scan.go
func Append 1.19
----------------
```
func Append(b []byte, a ...any) []byte
```
Append formats using the default formats for its operands, appends the result to the byte slice, and returns the updated slice.
func Appendf 1.19
-----------------
```
func Appendf(b []byte, format string, a ...any) []byte
```
Appendf formats according to a format specifier, appends the result to the byte slice, and returns the updated slice.
func Appendln 1.19
------------------
```
func Appendln(b []byte, a ...any) []byte
```
Appendln formats using the default formats for its operands, appends the result to the byte slice, and returns the updated slice. Spaces are always added between operands and a newline is appended.
func Errorf
-----------
```
func Errorf(format string, a ...any) error
```
Errorf formats according to a format specifier and returns the string as a value that satisfies error.
If the format specifier includes a %w verb with an error operand, the returned error will implement an Unwrap method returning the operand. If there is more than one %w verb, the returned error will implement an Unwrap method returning a []error containing all the %w operands in the order they appear in the arguments. It is invalid to supply the %w verb with an operand that does not implement the error interface. The %w verb is otherwise a synonym for %v.
#### Example
The Errorf function lets us use formatting features to create descriptive error messages.
Code:
```
const name, id = "bueller", 17
err := fmt.Errorf("user %q (id %d) not found", name, id)
fmt.Println(err.Error())
```
Output:
```
user "bueller" (id 17) not found
```
func FormatString 1.20
----------------------
```
func FormatString(state State, verb rune) string
```
FormatString returns a string representing the fully qualified formatting directive captured by the State, followed by the argument verb. (State does not itself contain the verb.) The result has a leading percent sign followed by any flags, the width, and the precision. Missing flags, width, and precision are omitted. This function allows a Formatter to reconstruct the original directive triggering the call to Format.
func Fprint
-----------
```
func Fprint(w io.Writer, a ...any) (n int, err error)
```
Fprint formats using the default formats for its operands and writes to w. Spaces are added between operands when neither is a string. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
n, err := fmt.Fprint(os.Stdout, name, " is ", age, " years old.\n")
// The n and err return values from Fprint are
// those returned by the underlying io.Writer.
if err != nil {
fmt.Fprintf(os.Stderr, "Fprint: %v\n", err)
}
fmt.Print(n, " bytes written.\n")
```
Output:
```
Kim is 22 years old.
21 bytes written.
```
func Fprintf
------------
```
func Fprintf(w io.Writer, format string, a ...any) (n int, err error)
```
Fprintf formats according to a format specifier and writes to w. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
n, err := fmt.Fprintf(os.Stdout, "%s is %d years old.\n", name, age)
// The n and err return values from Fprintf are
// those returned by the underlying io.Writer.
if err != nil {
fmt.Fprintf(os.Stderr, "Fprintf: %v\n", err)
}
fmt.Printf("%d bytes written.\n", n)
```
Output:
```
Kim is 22 years old.
21 bytes written.
```
func Fprintln
-------------
```
func Fprintln(w io.Writer, a ...any) (n int, err error)
```
Fprintln formats using the default formats for its operands and writes to w. Spaces are always added between operands and a newline is appended. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
n, err := fmt.Fprintln(os.Stdout, name, "is", age, "years old.")
// The n and err return values from Fprintln are
// those returned by the underlying io.Writer.
if err != nil {
fmt.Fprintf(os.Stderr, "Fprintln: %v\n", err)
}
fmt.Println(n, "bytes written.")
```
Output:
```
Kim is 22 years old.
21 bytes written.
```
func Fscan
----------
```
func Fscan(r io.Reader, a ...any) (n int, err error)
```
Fscan scans text read from r, storing successive space-separated values into successive arguments. Newlines count as space. It returns the number of items successfully scanned. If that is less than the number of arguments, err will report why.
func Fscanf
-----------
```
func Fscanf(r io.Reader, format string, a ...any) (n int, err error)
```
Fscanf scans text read from r, storing successive space-separated values into successive arguments as determined by the format. It returns the number of items successfully parsed. Newlines in the input must match newlines in the format.
#### Example
Code:
```
var (
i int
b bool
s string
)
r := strings.NewReader("5 true gophers")
n, err := fmt.Fscanf(r, "%d %t %s", &i, &b, &s)
if err != nil {
fmt.Fprintf(os.Stderr, "Fscanf: %v\n", err)
}
fmt.Println(i, b, s)
fmt.Println(n)
```
Output:
```
5 true gophers
3
```
func Fscanln
------------
```
func Fscanln(r io.Reader, a ...any) (n int, err error)
```
Fscanln is similar to Fscan, but stops scanning at a newline and after the final item there must be a newline or EOF.
#### Example
Code:
```
s := `dmr 1771 1.61803398875
ken 271828 3.14159`
r := strings.NewReader(s)
var a string
var b int
var c float64
for {
n, err := fmt.Fscanln(r, &a, &b, &c)
if err == io.EOF {
break
}
if err != nil {
panic(err)
}
fmt.Printf("%d: %s, %d, %f\n", n, a, b, c)
}
```
Output:
```
3: dmr, 1771, 1.618034
3: ken, 271828, 3.141590
```
func Print
----------
```
func Print(a ...any) (n int, err error)
```
Print formats using the default formats for its operands and writes to standard output. Spaces are added between operands when neither is a string. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
fmt.Print(name, " is ", age, " years old.\n")
// It is conventional not to worry about any
// error returned by Print.
```
Output:
```
Kim is 22 years old.
```
func Printf
-----------
```
func Printf(format string, a ...any) (n int, err error)
```
Printf formats according to a format specifier and writes to standard output. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
fmt.Printf("%s is %d years old.\n", name, age)
// It is conventional not to worry about any
// error returned by Printf.
```
Output:
```
Kim is 22 years old.
```
func Println
------------
```
func Println(a ...any) (n int, err error)
```
Println formats using the default formats for its operands and writes to standard output. Spaces are always added between operands and a newline is appended. It returns the number of bytes written and any write error encountered.
#### Example
Code:
```
const name, age = "Kim", 22
fmt.Println(name, "is", age, "years old.")
// It is conventional not to worry about any
// error returned by Println.
```
Output:
```
Kim is 22 years old.
```
func Scan
---------
```
func Scan(a ...any) (n int, err error)
```
Scan scans text read from standard input, storing successive space-separated values into successive arguments. Newlines count as space. It returns the number of items successfully scanned. If that is less than the number of arguments, err will report why.
func Scanf
----------
```
func Scanf(format string, a ...any) (n int, err error)
```
Scanf scans text read from standard input, storing successive space-separated values into successive arguments as determined by the format. It returns the number of items successfully scanned. If that is less than the number of arguments, err will report why. Newlines in the input must match newlines in the format. The one exception: the verb %c always scans the next rune in the input, even if it is a space (or tab etc.) or newline.
func Scanln
-----------
```
func Scanln(a ...any) (n int, err error)
```
Scanln is similar to Scan, but stops scanning at a newline and after the final item there must be a newline or EOF.
func Sprint
-----------
```
func Sprint(a ...any) string
```
Sprint formats using the default formats for its operands and returns the resulting string. Spaces are added between operands when neither is a string.
#### Example
Code:
```
const name, age = "Kim", 22
s := fmt.Sprint(name, " is ", age, " years old.\n")
io.WriteString(os.Stdout, s) // Ignoring error for simplicity.
```
Output:
```
Kim is 22 years old.
```
func Sprintf
------------
```
func Sprintf(format string, a ...any) string
```
Sprintf formats according to a format specifier and returns the resulting string.
#### Example
Code:
```
const name, age = "Kim", 22
s := fmt.Sprintf("%s is %d years old.\n", name, age)
io.WriteString(os.Stdout, s) // Ignoring error for simplicity.
```
Output:
```
Kim is 22 years old.
```
func Sprintln
-------------
```
func Sprintln(a ...any) string
```
Sprintln formats using the default formats for its operands and returns the resulting string. Spaces are always added between operands and a newline is appended.
#### Example
Code:
```
const name, age = "Kim", 22
s := fmt.Sprintln(name, "is", age, "years old.")
io.WriteString(os.Stdout, s) // Ignoring error for simplicity.
```
Output:
```
Kim is 22 years old.
```
func Sscan
----------
```
func Sscan(str string, a ...any) (n int, err error)
```
Sscan scans the argument string, storing successive space-separated values into successive arguments. Newlines count as space. It returns the number of items successfully scanned. If that is less than the number of arguments, err will report why.
func Sscanf
-----------
```
func Sscanf(str string, format string, a ...any) (n int, err error)
```
Sscanf scans the argument string, storing successive space-separated values into successive arguments as determined by the format. It returns the number of items successfully parsed. Newlines in the input must match newlines in the format.
#### Example
Code:
```
var name string
var age int
n, err := fmt.Sscanf("Kim is 22 years old", "%s is %d years old", &name, &age)
if err != nil {
panic(err)
}
fmt.Printf("%d: %s, %d\n", n, name, age)
```
Output:
```
2: Kim, 22
```
func Sscanln
------------
```
func Sscanln(str string, a ...any) (n int, err error)
```
Sscanln is similar to Sscan, but stops scanning at a newline and after the final item there must be a newline or EOF.
type Formatter
--------------
Formatter is implemented by any value that has a Format method. The implementation controls how State and rune are interpreted, and may call Sprint(f) or Fprint(f) etc. to generate its output.
```
type Formatter interface {
Format(f State, verb rune)
}
```
type GoStringer
---------------
GoStringer is implemented by any value that has a GoString method, which defines the Go syntax for that value. The GoString method is used to print values passed as an operand to a %#v format.
```
type GoStringer interface {
GoString() string
}
```
#### Example
Code:
```
package fmt_test
import (
"fmt"
)
// Address has a City, State and a Country.
type Address struct {
City string
State string
Country string
}
// Person has a Name, Age and Address.
type Person struct {
Name string
Age uint
Addr *Address
}
// GoString makes Person satisfy the GoStringer interface.
// The return value is valid Go code that can be used to reproduce the Person struct.
func (p Person) GoString() string {
if p.Addr != nil {
return fmt.Sprintf("Person{Name: %q, Age: %d, Addr: &Address{City: %q, State: %q, Country: %q}}", p.Name, int(p.Age), p.Addr.City, p.Addr.State, p.Addr.Country)
}
return fmt.Sprintf("Person{Name: %q, Age: %d}", p.Name, int(p.Age))
}
func ExampleGoStringer() {
p1 := Person{
Name: "Warren",
Age: 31,
Addr: &Address{
City: "Denver",
State: "CO",
Country: "U.S.A.",
},
}
// If GoString() wasn't implemented, the output of `fmt.Printf("%#v", p1)` would be similar to
// Person{Name:"Warren", Age:0x1f, Addr:(*main.Address)(0x10448240)}
fmt.Printf("%#v\n", p1)
p2 := Person{
Name: "Theia",
Age: 4,
}
// If GoString() wasn't implemented, the output of `fmt.Printf("%#v", p2)` would be similar to
// Person{Name:"Theia", Age:0x4, Addr:(*main.Address)(nil)}
fmt.Printf("%#v\n", p2)
// Output:
// Person{Name: "Warren", Age: 31, Addr: &Address{City: "Denver", State: "CO", Country: "U.S.A."}}
// Person{Name: "Theia", Age: 4}
}
```
type ScanState
--------------
ScanState represents the scanner state passed to custom scanners. Scanners may do rune-at-a-time scanning or ask the ScanState to discover the next space-delimited token.
```
type ScanState interface {
// ReadRune reads the next rune (Unicode code point) from the input.
// If invoked during Scanln, Fscanln, or Sscanln, ReadRune() will
// return EOF after returning the first '\n' or when reading beyond
// the specified width.
ReadRune() (r rune, size int, err error)
// UnreadRune causes the next call to ReadRune to return the same rune.
UnreadRune() error
// SkipSpace skips space in the input. Newlines are treated appropriately
// for the operation being performed; see the package documentation
// for more information.
SkipSpace()
// Token skips space in the input if skipSpace is true, then returns the
// run of Unicode code points c satisfying f(c). If f is nil,
// !unicode.IsSpace(c) is used; that is, the token will hold non-space
// characters. Newlines are treated appropriately for the operation being
// performed; see the package documentation for more information.
// The returned slice points to shared data that may be overwritten
// by the next call to Token, a call to a Scan function using the ScanState
// as input, or when the calling Scan method returns.
Token(skipSpace bool, f func(rune) bool) (token []byte, err error)
// Width returns the value of the width option and whether it has been set.
// The unit is Unicode code points.
Width() (wid int, ok bool)
// Because ReadRune is implemented by the interface, Read should never be
// called by the scanning routines and a valid implementation of
// ScanState may choose always to return an error from Read.
Read(buf []byte) (n int, err error)
}
```
type Scanner
------------
Scanner is implemented by any value that has a Scan method, which scans the input for the representation of a value and stores the result in the receiver, which must be a pointer to be useful. The Scan method is called for any argument to Scan, Scanf, or Scanln that implements it.
```
type Scanner interface {
Scan(state ScanState, verb rune) error
}
```
type State
----------
State represents the printer state passed to custom formatters. It provides access to the io.Writer interface plus information about the flags and options for the operand's format specifier.
```
type State interface {
// Write is the function to call to emit formatted output to be printed.
Write(b []byte) (n int, err error)
// Width returns the value of the width option and whether it has been set.
Width() (wid int, ok bool)
// Precision returns the value of the precision option and whether it has been set.
Precision() (prec int, ok bool)
// Flag reports whether the flag c, a character, has been set.
Flag(c int) bool
}
```
type Stringer
-------------
Stringer is implemented by any value that has a String method, which defines the “native” format for that value. The String method is used to print values passed as an operand to any format that accepts a string or to an unformatted printer such as Print.
```
type Stringer interface {
String() string
}
```
#### Example
Code:
```
package fmt_test
import (
"fmt"
)
// Animal has a Name and an Age to represent an animal.
type Animal struct {
Name string
Age uint
}
// String makes Animal satisfy the Stringer interface.
func (a Animal) String() string {
return fmt.Sprintf("%v (%d)", a.Name, a.Age)
}
func ExampleStringer() {
a := Animal{
Name: "Gopher",
Age: 2,
}
fmt.Println(a)
// Output: Gopher (2)
}
```
| programming_docs |
go Package path Package path
=============
* `import "path"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
* [Subdirectories](#pkg-subdirectories)
Overview
--------
Package path implements utility routines for manipulating slash-separated paths.
The path package should only be used for paths separated by forward slashes, such as the paths in URLs. This package does not deal with Windows paths with drive letters or backslashes; to manipulate operating system paths, use the path/filepath package.
Index
-----
* [Variables](#pkg-variables)
* [func Base(path string) string](#Base)
* [func Clean(path string) string](#Clean)
* [func Dir(path string) string](#Dir)
* [func Ext(path string) string](#Ext)
* [func IsAbs(path string) bool](#IsAbs)
* [func Join(elem ...string) string](#Join)
* [func Match(pattern, name string) (matched bool, err error)](#Match)
* [func Split(path string) (dir, file string)](#Split)
### Examples
[Base](#example_Base) [Clean](#example_Clean) [Dir](#example_Dir) [Ext](#example_Ext) [IsAbs](#example_IsAbs) [Join](#example_Join) [Match](#example_Match) [Split](#example_Split) ### Package files
match.go path.go
Variables
---------
ErrBadPattern indicates a pattern was malformed.
```
var ErrBadPattern = errors.New("syntax error in pattern")
```
func Base
---------
```
func Base(path string) string
```
Base returns the last element of path. Trailing slashes are removed before extracting the last element. If the path is empty, Base returns ".". If the path consists entirely of slashes, Base returns "/".
#### Example
Code:
```
fmt.Println(path.Base("/a/b"))
fmt.Println(path.Base("/"))
fmt.Println(path.Base(""))
```
Output:
```
b
/
.
```
func Clean
----------
```
func Clean(path string) string
```
Clean returns the shortest path name equivalent to path by purely lexical processing. It applies the following rules iteratively until no further processing can be done:
1. Replace multiple slashes with a single slash.
2. Eliminate each . path name element (the current directory).
3. Eliminate each inner .. path name element (the parent directory) along with the non-.. element that precedes it.
4. Eliminate .. elements that begin a rooted path: that is, replace "/.." by "/" at the beginning of a path.
The returned path ends in a slash only if it is the root "/".
If the result of this process is an empty string, Clean returns the string ".".
See also Rob Pike, “Lexical File Names in Plan 9 or Getting Dot-Dot Right,” <https://9p.io/sys/doc/lexnames.html>
#### Example
Code:
```
paths := []string{
"a/c",
"a//c",
"a/c/.",
"a/c/b/..",
"/../a/c",
"/../a/b/../././/c",
"",
}
for _, p := range paths {
fmt.Printf("Clean(%q) = %q\n", p, path.Clean(p))
}
```
Output:
```
Clean("a/c") = "a/c"
Clean("a//c") = "a/c"
Clean("a/c/.") = "a/c"
Clean("a/c/b/..") = "a/c"
Clean("/../a/c") = "/a/c"
Clean("/../a/b/../././/c") = "/a/c"
Clean("") = "."
```
func Dir
--------
```
func Dir(path string) string
```
Dir returns all but the last element of path, typically the path's directory. After dropping the final element using Split, the path is Cleaned and trailing slashes are removed. If the path is empty, Dir returns ".". If the path consists entirely of slashes followed by non-slash bytes, Dir returns a single slash. In any other case, the returned path does not end in a slash.
#### Example
Code:
```
fmt.Println(path.Dir("/a/b/c"))
fmt.Println(path.Dir("a/b/c"))
fmt.Println(path.Dir("/a/"))
fmt.Println(path.Dir("a/"))
fmt.Println(path.Dir("/"))
fmt.Println(path.Dir(""))
```
Output:
```
/a/b
a/b
/a
a
/
.
```
func Ext
--------
```
func Ext(path string) string
```
Ext returns the file name extension used by path. The extension is the suffix beginning at the final dot in the final slash-separated element of path; it is empty if there is no dot.
#### Example
Code:
```
fmt.Println(path.Ext("/a/b/c/bar.css"))
fmt.Println(path.Ext("/"))
fmt.Println(path.Ext(""))
```
Output:
```
.css
```
func IsAbs
----------
```
func IsAbs(path string) bool
```
IsAbs reports whether the path is absolute.
#### Example
Code:
```
fmt.Println(path.IsAbs("/dev/null"))
```
Output:
```
true
```
func Join
---------
```
func Join(elem ...string) string
```
Join joins any number of path elements into a single path, separating them with slashes. Empty elements are ignored. The result is Cleaned. However, if the argument list is empty or all its elements are empty, Join returns an empty string.
#### Example
Code:
```
fmt.Println(path.Join("a", "b", "c"))
fmt.Println(path.Join("a", "b/c"))
fmt.Println(path.Join("a/b", "c"))
fmt.Println(path.Join("a/b", "../../../xyz"))
fmt.Println(path.Join("", ""))
fmt.Println(path.Join("a", ""))
fmt.Println(path.Join("", "a"))
```
Output:
```
a/b/c
a/b/c
a/b/c
../xyz
a
a
```
func Match
----------
```
func Match(pattern, name string) (matched bool, err error)
```
Match reports whether name matches the shell pattern. The pattern syntax is:
```
pattern:
{ term }
term:
'*' matches any sequence of non-/ characters
'?' matches any single non-/ character
'[' [ '^' ] { character-range } ']'
character class (must be non-empty)
c matches character c (c != '*', '?', '\\', '[')
'\\' c matches character c
character-range:
c matches character c (c != '\\', '-', ']')
'\\' c matches character c
lo '-' hi matches character c for lo <= c <= hi
```
Match requires pattern to match all of name, not just a substring. The only possible returned error is ErrBadPattern, when pattern is malformed.
#### Example
Code:
```
fmt.Println(path.Match("abc", "abc"))
fmt.Println(path.Match("a*", "abc"))
fmt.Println(path.Match("a*/b", "a/c/b"))
```
Output:
```
true <nil>
true <nil>
false <nil>
```
func Split
----------
```
func Split(path string) (dir, file string)
```
Split splits path immediately following the final slash, separating it into a directory and file name component. If there is no slash in path, Split returns an empty dir and file set to path. The returned values have the property that path = dir+file.
#### Example
Code:
```
split := func(s string) {
dir, file := path.Split(s)
fmt.Printf("path.Split(%q) = dir: %q, file: %q\n", s, dir, file)
}
split("static/myfile.css")
split("myfile.css")
split("")
```
Output:
```
path.Split("static/myfile.css") = dir: "static/", file: "myfile.css"
path.Split("myfile.css") = dir: "", file: "myfile.css"
path.Split("") = dir: "", file: ""
```
Subdirectories
--------------
| Name | Synopsis |
| --- | --- |
| [..](../index) |
| [filepath](filepath/index) | Package filepath implements utility routines for manipulating filename paths in a way compatible with the target operating system-defined file paths. |
go Package filepath Package filepath
=================
* `import "path/filepath"`
* [Overview](#pkg-overview)
* [Index](#pkg-index)
* [Examples](#pkg-examples)
Overview
--------
Package filepath implements utility routines for manipulating filename paths in a way compatible with the target operating system-defined file paths.
The filepath package uses either forward slashes or backslashes, depending on the operating system. To process paths such as URLs that always use forward slashes regardless of the operating system, see the path package.
Index
-----
* [Constants](#pkg-constants)
* [Variables](#pkg-variables)
* [func Abs(path string) (string, error)](#Abs)
* [func Base(path string) string](#Base)
* [func Clean(path string) string](#Clean)
* [func Dir(path string) string](#Dir)
* [func EvalSymlinks(path string) (string, error)](#EvalSymlinks)
* [func Ext(path string) string](#Ext)
* [func FromSlash(path string) string](#FromSlash)
* [func Glob(pattern string) (matches []string, err error)](#Glob)
* [func HasPrefix(p, prefix string) bool](#HasPrefix)
* [func IsAbs(path string) bool](#IsAbs)
* [func IsLocal(path string) bool](#IsLocal)
* [func Join(elem ...string) string](#Join)
* [func Match(pattern, name string) (matched bool, err error)](#Match)
* [func Rel(basepath, targpath string) (string, error)](#Rel)
* [func Split(path string) (dir, file string)](#Split)
* [func SplitList(path string) []string](#SplitList)
* [func ToSlash(path string) string](#ToSlash)
* [func VolumeName(path string) string](#VolumeName)
* [func Walk(root string, fn WalkFunc) error](#Walk)
* [func WalkDir(root string, fn fs.WalkDirFunc) error](#WalkDir)
* [type WalkFunc](#WalkFunc)
### Examples
[Base](#example_Base) [Dir](#example_Dir) [Ext](#example_Ext) [IsAbs](#example_IsAbs) [Join](#example_Join) [Match](#example_Match) [Rel](#example_Rel) [Split](#example_Split) [SplitList](#example_SplitList) [Walk](#example_Walk) ### Package files
match.go path.go path\_unix.go symlink.go symlink\_unix.go
Constants
---------
```
const (
Separator = os.PathSeparator
ListSeparator = os.PathListSeparator
)
```
Variables
---------
ErrBadPattern indicates a pattern was malformed.
```
var ErrBadPattern = errors.New("syntax error in pattern")
```
SkipAll is used as a return value from WalkFuncs to indicate that all remaining files and directories are to be skipped. It is not returned as an error by any function.
```
var SkipAll error = fs.SkipAll
```
SkipDir is used as a return value from WalkFuncs to indicate that the directory named in the call is to be skipped. It is not returned as an error by any function.
```
var SkipDir error = fs.SkipDir
```
func Abs
--------
```
func Abs(path string) (string, error)
```
Abs returns an absolute representation of path. If the path is not absolute it will be joined with the current working directory to turn it into an absolute path. The absolute path name for a given file is not guaranteed to be unique. Abs calls Clean on the result.
func Base
---------
```
func Base(path string) string
```
Base returns the last element of path. Trailing path separators are removed before extracting the last element. If the path is empty, Base returns ".". If the path consists entirely of separators, Base returns a single separator.
#### Example
Code:
```
fmt.Println("On Unix:")
fmt.Println(filepath.Base("/foo/bar/baz.js"))
fmt.Println(filepath.Base("/foo/bar/baz"))
fmt.Println(filepath.Base("/foo/bar/baz/"))
fmt.Println(filepath.Base("dev.txt"))
fmt.Println(filepath.Base("../todo.txt"))
fmt.Println(filepath.Base(".."))
fmt.Println(filepath.Base("."))
fmt.Println(filepath.Base("/"))
fmt.Println(filepath.Base(""))
```
Output:
```
On Unix:
baz.js
baz
baz
dev.txt
todo.txt
..
.
/
.
```
func Clean
----------
```
func Clean(path string) string
```
Clean returns the shortest path name equivalent to path by purely lexical processing. It applies the following rules iteratively until no further processing can be done:
1. Replace multiple Separator elements with a single one.
2. Eliminate each . path name element (the current directory).
3. Eliminate each inner .. path name element (the parent directory) along with the non-.. element that precedes it.
4. Eliminate .. elements that begin a rooted path: that is, replace "/.." by "/" at the beginning of a path, assuming Separator is '/'.
The returned path ends in a slash only if it represents a root directory, such as "/" on Unix or `C:\` on Windows.
Finally, any occurrences of slash are replaced by Separator.
If the result of this process is an empty string, Clean returns the string ".".
See also Rob Pike, “Lexical File Names in Plan 9 or Getting Dot-Dot Right,” <https://9p.io/sys/doc/lexnames.html>
func Dir
--------
```
func Dir(path string) string
```
Dir returns all but the last element of path, typically the path's directory. After dropping the final element, Dir calls Clean on the path and trailing slashes are removed. If the path is empty, Dir returns ".". If the path consists entirely of separators, Dir returns a single separator. The returned path does not end in a separator unless it is the root directory.
#### Example
Code:
```
fmt.Println("On Unix:")
fmt.Println(filepath.Dir("/foo/bar/baz.js"))
fmt.Println(filepath.Dir("/foo/bar/baz"))
fmt.Println(filepath.Dir("/foo/bar/baz/"))
fmt.Println(filepath.Dir("/dirty//path///"))
fmt.Println(filepath.Dir("dev.txt"))
fmt.Println(filepath.Dir("../todo.txt"))
fmt.Println(filepath.Dir(".."))
fmt.Println(filepath.Dir("."))
fmt.Println(filepath.Dir("/"))
fmt.Println(filepath.Dir(""))
```
Output:
```
On Unix:
/foo/bar
/foo/bar
/foo/bar/baz
/dirty/path
.
..
.
.
/
.
```
func EvalSymlinks
-----------------
```
func EvalSymlinks(path string) (string, error)
```
EvalSymlinks returns the path name after the evaluation of any symbolic links. If path is relative the result will be relative to the current directory, unless one of the components is an absolute symbolic link. EvalSymlinks calls Clean on the result.
func Ext
--------
```
func Ext(path string) string
```
Ext returns the file name extension used by path. The extension is the suffix beginning at the final dot in the final element of path; it is empty if there is no dot.
#### Example
Code:
```
fmt.Printf("No dots: %q\n", filepath.Ext("index"))
fmt.Printf("One dot: %q\n", filepath.Ext("index.js"))
fmt.Printf("Two dots: %q\n", filepath.Ext("main.test.js"))
```
Output:
```
No dots: ""
One dot: ".js"
Two dots: ".js"
```
func FromSlash
--------------
```
func FromSlash(path string) string
```
FromSlash returns the result of replacing each slash ('/') character in path with a separator character. Multiple slashes are replaced by multiple separators.
func Glob
---------
```
func Glob(pattern string) (matches []string, err error)
```
Glob returns the names of all files matching pattern or nil if there is no matching file. The syntax of patterns is the same as in Match. The pattern may describe hierarchical names such as /usr/\*/bin/ed (assuming the Separator is '/').
Glob ignores file system errors such as I/O errors reading directories. The only possible returned error is ErrBadPattern, when pattern is malformed.
func HasPrefix
--------------
```
func HasPrefix(p, prefix string) bool
```
HasPrefix exists for historical compatibility and should not be used.
Deprecated: HasPrefix does not respect path boundaries and does not ignore case when required.
func IsAbs
----------
```
func IsAbs(path string) bool
```
IsAbs reports whether the path is absolute.
#### Example
Code:
```
fmt.Println("On Unix:")
fmt.Println(filepath.IsAbs("/home/gopher"))
fmt.Println(filepath.IsAbs(".bashrc"))
fmt.Println(filepath.IsAbs(".."))
fmt.Println(filepath.IsAbs("."))
fmt.Println(filepath.IsAbs("/"))
fmt.Println(filepath.IsAbs(""))
```
Output:
```
On Unix:
true
false
false
false
true
false
```
func IsLocal 1.20
-----------------
```
func IsLocal(path string) bool
```
IsLocal reports whether path, using lexical analysis only, has all of these properties:
* is within the subtree rooted at the directory in which path is evaluated
* is not an absolute path
* is not empty
* on Windows, is not a reserved name such as "NUL"
If IsLocal(path) returns true, then Join(base, path) will always produce a path contained within base and Clean(path) will always produce an unrooted path with no ".." path elements.
IsLocal is a purely lexical operation. In particular, it does not account for the effect of any symbolic links that may exist in the filesystem.
func Join
---------
```
func Join(elem ...string) string
```
Join joins any number of path elements into a single path, separating them with an OS specific Separator. Empty elements are ignored. The result is Cleaned. However, if the argument list is empty or all its elements are empty, Join returns an empty string. On Windows, the result will only be a UNC path if the first non-empty element is a UNC path.
#### Example
Code:
```
fmt.Println("On Unix:")
fmt.Println(filepath.Join("a", "b", "c"))
fmt.Println(filepath.Join("a", "b/c"))
fmt.Println(filepath.Join("a/b", "c"))
fmt.Println(filepath.Join("a/b", "/c"))
fmt.Println(filepath.Join("a/b", "../../../xyz"))
```
Output:
```
On Unix:
a/b/c
a/b/c
a/b/c
a/b/c
../xyz
```
func Match
----------
```
func Match(pattern, name string) (matched bool, err error)
```
Match reports whether name matches the shell file name pattern. The pattern syntax is:
```
pattern:
{ term }
term:
'*' matches any sequence of non-Separator characters
'?' matches any single non-Separator character
'[' [ '^' ] { character-range } ']'
character class (must be non-empty)
c matches character c (c != '*', '?', '\\', '[')
'\\' c matches character c
character-range:
c matches character c (c != '\\', '-', ']')
'\\' c matches character c
lo '-' hi matches character c for lo <= c <= hi
```
Match requires pattern to match all of name, not just a substring. The only possible returned error is ErrBadPattern, when pattern is malformed.
On Windows, escaping is disabled. Instead, '\\' is treated as path separator.
#### Example
Code:
```
fmt.Println("On Unix:")
fmt.Println(filepath.Match("/home/catch/*", "/home/catch/foo"))
fmt.Println(filepath.Match("/home/catch/*", "/home/catch/foo/bar"))
fmt.Println(filepath.Match("/home/?opher", "/home/gopher"))
fmt.Println(filepath.Match("/home/\\*", "/home/*"))
```
Output:
```
On Unix:
true <nil>
false <nil>
true <nil>
true <nil>
```
func Rel
--------
```
func Rel(basepath, targpath string) (string, error)
```
Rel returns a relative path that is lexically equivalent to targpath when joined to basepath with an intervening separator. That is, Join(basepath, Rel(basepath, targpath)) is equivalent to targpath itself. On success, the returned path will always be relative to basepath, even if basepath and targpath share no elements. An error is returned if targpath can't be made relative to basepath or if knowing the current working directory would be necessary to compute it. Rel calls Clean on the result.
#### Example
Code:
```
paths := []string{
"/a/b/c",
"/b/c",
"./b/c",
}
base := "/a"
fmt.Println("On Unix:")
for _, p := range paths {
rel, err := filepath.Rel(base, p)
fmt.Printf("%q: %q %v\n", p, rel, err)
}
```
Output:
```
On Unix:
"/a/b/c": "b/c" <nil>
"/b/c": "../b/c" <nil>
"./b/c": "" Rel: can't make ./b/c relative to /a
```
func Split
----------
```
func Split(path string) (dir, file string)
```
Split splits path immediately following the final Separator, separating it into a directory and file name component. If there is no Separator in path, Split returns an empty dir and file set to path. The returned values have the property that path = dir+file.
#### Example
Code:
```
paths := []string{
"/home/arnie/amelia.jpg",
"/mnt/photos/",
"rabbit.jpg",
"/usr/local//go",
}
fmt.Println("On Unix:")
for _, p := range paths {
dir, file := filepath.Split(p)
fmt.Printf("input: %q\n\tdir: %q\n\tfile: %q\n", p, dir, file)
}
```
Output:
```
On Unix:
input: "/home/arnie/amelia.jpg"
dir: "/home/arnie/"
file: "amelia.jpg"
input: "/mnt/photos/"
dir: "/mnt/photos/"
file: ""
input: "rabbit.jpg"
dir: ""
file: "rabbit.jpg"
input: "/usr/local//go"
dir: "/usr/local//"
file: "go"
```
func SplitList
--------------
```
func SplitList(path string) []string
```
SplitList splits a list of paths joined by the OS-specific ListSeparator, usually found in PATH or GOPATH environment variables. Unlike strings.Split, SplitList returns an empty slice when passed an empty string.
#### Example
Code:
```
fmt.Println("On Unix:", filepath.SplitList("/a/b/c:/usr/bin"))
```
Output:
```
On Unix: [/a/b/c /usr/bin]
```
func ToSlash
------------
```
func ToSlash(path string) string
```
ToSlash returns the result of replacing each separator character in path with a slash ('/') character. Multiple separators are replaced by multiple slashes.
func VolumeName
---------------
```
func VolumeName(path string) string
```
VolumeName returns leading volume name. Given "C:\foo\bar" it returns "C:" on Windows. Given "\\host\share\foo" it returns "\\host\share". On other platforms it returns "".
func Walk
---------
```
func Walk(root string, fn WalkFunc) error
```
Walk walks the file tree rooted at root, calling fn for each file or directory in the tree, including root.
All errors that arise visiting files and directories are filtered by fn: see the WalkFunc documentation for details.
The files are walked in lexical order, which makes the output deterministic but requires Walk to read an entire directory into memory before proceeding to walk that directory.
Walk does not follow symbolic links.
Walk is less efficient than WalkDir, introduced in Go 1.16, which avoids calling os.Lstat on every visited file or directory.
#### Example
Code:
```
package filepath_test
import (
"fmt"
"io/fs"
"os"
"path/filepath"
)
func prepareTestDirTree(tree string) (string, error) {
tmpDir, err := os.MkdirTemp("", "")
if err != nil {
return "", fmt.Errorf("error creating temp directory: %v\n", err)
}
err = os.MkdirAll(filepath.Join(tmpDir, tree), 0755)
if err != nil {
os.RemoveAll(tmpDir)
return "", err
}
return tmpDir, nil
}
func ExampleWalk() {
tmpDir, err := prepareTestDirTree("dir/to/walk/skip")
if err != nil {
fmt.Printf("unable to create test dir tree: %v\n", err)
return
}
defer os.RemoveAll(tmpDir)
os.Chdir(tmpDir)
subDirToSkip := "skip"
fmt.Println("On Unix:")
err = filepath.Walk(".", func(path string, info fs.FileInfo, err error) error {
if err != nil {
fmt.Printf("prevent panic by handling failure accessing a path %q: %v\n", path, err)
return err
}
if info.IsDir() && info.Name() == subDirToSkip {
fmt.Printf("skipping a dir without errors: %+v \n", info.Name())
return filepath.SkipDir
}
fmt.Printf("visited file or dir: %q\n", path)
return nil
})
if err != nil {
fmt.Printf("error walking the path %q: %v\n", tmpDir, err)
return
}
// Output:
// On Unix:
// visited file or dir: "."
// visited file or dir: "dir"
// visited file or dir: "dir/to"
// visited file or dir: "dir/to/walk"
// skipping a dir without errors: skip
}
```
func WalkDir 1.16
-----------------
```
func WalkDir(root string, fn fs.WalkDirFunc) error
```
WalkDir walks the file tree rooted at root, calling fn for each file or directory in the tree, including root.
All errors that arise visiting files and directories are filtered by fn: see the fs.WalkDirFunc documentation for details.
The files are walked in lexical order, which makes the output deterministic but requires WalkDir to read an entire directory into memory before proceeding to walk that directory.
WalkDir does not follow symbolic links.
WalkDir calls fn with paths that use the separator character appropriate for the operating system. This is unlike io/fs.WalkDir, which always uses slash separated paths.
type WalkFunc
-------------
WalkFunc is the type of the function called by Walk to visit each file or directory.
The path argument contains the argument to Walk as a prefix. That is, if Walk is called with root argument "dir" and finds a file named "a" in that directory, the walk function will be called with argument "dir/a".
The directory and file are joined with Join, which may clean the directory name: if Walk is called with the root argument "x/../dir" and finds a file named "a" in that directory, the walk function will be called with argument "dir/a", not "x/../dir/a".
The info argument is the fs.FileInfo for the named path.
The error result returned by the function controls how Walk continues. If the function returns the special value SkipDir, Walk skips the current directory (path if info.IsDir() is true, otherwise path's parent directory). If the function returns the special value SkipAll, Walk skips all remaining files and directories. Otherwise, if the function returns a non-nil error, Walk stops entirely and returns that error.
The err argument reports an error related to path, signaling that Walk will not walk into that directory. The function can decide how to handle that error; as described earlier, returning the error will cause Walk to stop walking the entire tree.
Walk calls the function with a non-nil err argument in two cases.
First, if an os.Lstat on the root directory or any directory or file in the tree fails, Walk calls the function with path set to that directory or file's path, info set to nil, and err set to the error from os.Lstat.
Second, if a directory's Readdirnames method fails, Walk calls the function with path set to the directory's path, info, set to an fs.FileInfo describing the directory, and err set to the error from Readdirnames.
```
type WalkFunc func(path string, info fs.FileInfo, err error) error
```
| programming_docs |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.