code
stringlengths 2.5k
150k
| kind
stringclasses 1
value |
---|---|
pytorch ZeroPad2d ZeroPad2d
=========
`class torch.nn.ZeroPad2d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ZeroPad2d)
Pads the input tensor boundaries with zero.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 4-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} , padding\_top\text{padding\\_top} , padding\_bottom\text{padding\\_bottom} )
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) where
Hout=Hin+padding\_top+padding\_bottomH\_{out} = H\_{in} + \text{padding\\_top} + \text{padding\\_bottom}
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ZeroPad2d(2)
>>> input = torch.randn(1, 1, 3, 3)
>>> input
tensor([[[[-0.1678, -0.4418, 1.9466],
[ 0.9604, -0.4219, -0.5241],
[-0.9162, -0.5436, -0.6446]]]])
>>> m(input)
tensor([[[[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000],
[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000],
[ 0.0000, 0.0000, -0.1678, -0.4418, 1.9466, 0.0000, 0.0000],
[ 0.0000, 0.0000, 0.9604, -0.4219, -0.5241, 0.0000, 0.0000],
[ 0.0000, 0.0000, -0.9162, -0.5436, -0.6446, 0.0000, 0.0000],
[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000],
[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000, 0.0000]]]])
>>> # using different paddings for different sides
>>> m = nn.ZeroPad2d((1, 1, 2, 0))
>>> m(input)
tensor([[[[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000],
[ 0.0000, 0.0000, 0.0000, 0.0000, 0.0000],
[ 0.0000, -0.1678, -0.4418, 1.9466, 0.0000],
[ 0.0000, 0.9604, -0.4219, -0.5241, 0.0000],
[ 0.0000, -0.9162, -0.5436, -0.6446, 0.0000]]]])
```
pytorch RReLU RReLU
=====
`class torch.nn.RReLU(lower=0.125, upper=0.3333333333333333, inplace=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#RReLU)
Applies the randomized leaky rectified liner unit function, element-wise, as described in the paper:
[Empirical Evaluation of Rectified Activations in Convolutional Network](https://arxiv.org/abs/1505.00853).
The function is defined as:
RReLU(x)={xif x≥0ax otherwise \text{RReLU}(x) = \begin{cases} x & \text{if } x \geq 0 \\ ax & \text{ otherwise } \end{cases}
where aa is randomly sampled from uniform distribution U(lower,upper)\mathcal{U}(\text{lower}, \text{upper}) .
See: <https://arxiv.org/pdf/1505.00853.pdf>
Parameters
* **lower** – lower bound of the uniform distribution. Default: 18\frac{1}{8}
* **upper** – upper bound of the uniform distribution. Default: 13\frac{1}{3}
* **inplace** – can optionally do the operation in-place. Default: `False`
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.RReLU(0.1, 0.3)
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.movedim torch.movedim
=============
`torch.movedim(input, source, destination) → Tensor`
Moves the dimension(s) of `input` at the position(s) in `source` to the position(s) in `destination`.
Other dimensions of `input` that are not explicitly moved remain in their original order and appear at the positions not specified in `destination`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **source** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – Original positions of the dims to move. These must be unique.
* **destination** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – Destination positions for each of the original dims. These must also be unique.
Examples:
```
>>> t = torch.randn(3,2,1)
>>> t
tensor([[[-0.3362],
[-0.8437]],
[[-0.9627],
[ 0.1727]],
[[ 0.5173],
[-0.1398]]])
>>> torch.movedim(t, 1, 0).shape
torch.Size([2, 3, 1])
>>> torch.movedim(t, 1, 0)
tensor([[[-0.3362],
[-0.9627],
[ 0.5173]],
[[-0.8437],
[ 0.1727],
[-0.1398]]])
>>> torch.movedim(t, (1, 2), (0, 1)).shape
torch.Size([2, 1, 3])
>>> torch.movedim(t, (1, 2), (0, 1))
tensor([[[-0.3362, -0.9627, 0.5173]],
[[-0.8437, 0.1727, -0.1398]]])
```
pytorch torch.bmm torch.bmm
=========
`torch.bmm(input, mat2, *, deterministic=False, out=None) → Tensor`
Performs a batch matrix-matrix product of matrices stored in `input` and `mat2`.
`input` and `mat2` must be 3-D tensors each containing the same number of matrices.
If `input` is a (b×n×m)(b \times n \times m) tensor, `mat2` is a (b×m×p)(b \times m \times p) tensor, `out` will be a (b×n×p)(b \times n \times p) tensor.
outi=inputi@mat2i\text{out}\_i = \text{input}\_i \mathbin{@} \text{mat2}\_i
This operator supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
Note
This function does not [broadcast](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics). For broadcasting matrix products, see [`torch.matmul()`](torch.matmul#torch.matmul "torch.matmul").
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first batch of matrices to be multiplied
* **mat2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second batch of matrices to be multiplied
Keyword Arguments
* **deterministic** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – flag to choose between a faster non-deterministic calculation, or a slower deterministic calculation. This argument is only available for sparse-dense CUDA bmm. Default: `False`
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> input = torch.randn(10, 3, 4)
>>> mat2 = torch.randn(10, 4, 5)
>>> res = torch.bmm(input, mat2)
>>> res.size()
torch.Size([10, 3, 5])
```
pytorch torch.minimum torch.minimum
=============
`torch.minimum(input, other, *, out=None) → Tensor`
Computes the element-wise minimum of `input` and `other`.
Note
If one of the elements being compared is a NaN, then that element is returned. [`minimum()`](#torch.minimum "torch.minimum") is not supported for tensors with complex dtypes.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor((1, 2, -1))
>>> b = torch.tensor((3, 0, 4))
>>> torch.minimum(a, b)
tensor([1, 0, -1])
```
pytorch torch.linspace torch.linspace
==============
`torch.linspace(start, end, steps, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Creates a one-dimensional tensor of size `steps` whose values are evenly spaced from `start` to `end`, inclusive. That is, the value are:
(start,start+end−startsteps−1,…,start+(steps−2)∗end−startsteps−1,end)(\text{start}, \text{start} + \frac{\text{end} - \text{start}}{\text{steps} - 1}, \ldots, \text{start} + (\text{steps} - 2) \* \frac{\text{end} - \text{start}}{\text{steps} - 1}, \text{end})
Warning
Not providing a value for `steps` is deprecated. For backwards compatibility, not providing a value for `steps` will create a tensor with 100 elements. Note that this behavior is not reflected in the documented function signature and should not be relied on. In a future PyTorch release, failing to provide a value for `steps` will throw a runtime error.
Parameters
* **start** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the starting value for the set of points
* **end** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the ending value for the set of points
* **steps** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – size of the constructed tensor
Keyword Arguments
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Example:
```
>>> torch.linspace(3, 10, steps=5)
tensor([ 3.0000, 4.7500, 6.5000, 8.2500, 10.0000])
>>> torch.linspace(-10, 10, steps=5)
tensor([-10., -5., 0., 5., 10.])
>>> torch.linspace(start=-10, end=10, steps=5)
tensor([-10., -5., 0., 5., 10.])
>>> torch.linspace(start=-10, end=10, steps=1)
tensor([-10.])
```
pytorch torch.load torch.load
==========
`torch.load(f, map_location=None, pickle_module=<module 'pickle' from '/home/matti/miniconda3/lib/python3.7/pickle.py'>, **pickle_load_args)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/serialization.html#load)
Loads an object saved with [`torch.save()`](torch.save#torch.save "torch.save") from a file.
[`torch.load()`](#torch.load "torch.load") uses Python’s unpickling facilities but treats storages, which underlie tensors, specially. They are first deserialized on the CPU and are then moved to the device they were saved from. If this fails (e.g. because the run time system doesn’t have certain devices), an exception is raised. However, storages can be dynamically remapped to an alternative set of devices using the `map_location` argument.
If `map_location` is a callable, it will be called once for each serialized storage with two arguments: storage and location. The storage argument will be the initial deserialization of the storage, residing on the CPU. Each serialized storage has a location tag associated with it which identifies the device it was saved from, and this tag is the second argument passed to `map_location`. The builtin location tags are `'cpu'` for CPU tensors and `'cuda:device_id'` (e.g. `'cuda:2'`) for CUDA tensors. `map_location` should return either `None` or a storage. If `map_location` returns a storage, it will be used as the final deserialized object, already moved to the right device. Otherwise, [`torch.load()`](#torch.load "torch.load") will fall back to the default behavior, as if `map_location` wasn’t specified.
If `map_location` is a [`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device") object or a string containing a device tag, it indicates the location where all tensors should be loaded.
Otherwise, if `map_location` is a dict, it will be used to remap location tags appearing in the file (keys), to ones that specify where to put the storages (values).
User extensions can register their own location tags and tagging and deserialization methods using `torch.serialization.register_package()`.
Parameters
* **f** – a file-like object (has to implement `read()`, `readline()`, `tell()`, and `seek()`), or a string or os.PathLike object containing a file name
* **map\_location** – a function, [`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), string or a dict specifying how to remap storage locations
* **pickle\_module** – module used for unpickling metadata and objects (has to match the `pickle_module` used to serialize file)
* **pickle\_load\_args** – (Python 3 only) optional keyword arguments passed over to `pickle_module.load()` and `pickle_module.Unpickler()`, e.g., `errors=...`.
Warning
[`torch.load()`](#torch.load "torch.load") uses `pickle` module implicitly, which is known to be insecure. It is possible to construct malicious pickle data which will execute arbitrary code during unpickling. Never load data that could have come from an untrusted source, or that could have been tampered with. **Only load data you trust**.
Note
When you call [`torch.load()`](#torch.load "torch.load") on a file which contains GPU tensors, those tensors will be loaded to GPU by default. You can call `torch.load(.., map_location='cpu')` and then `load_state_dict()` to avoid GPU RAM surge when loading a model checkpoint.
Note
By default, we decode byte strings as `utf-8`. This is to avoid a common error case `UnicodeDecodeError: 'ascii' codec can't decode byte 0x...` when loading files saved by Python 2 in Python 3. If this default is incorrect, you may use an extra `encoding` keyword argument to specify how these objects should be loaded, e.g., `encoding='latin1'` decodes them to strings using `latin1` encoding, and `encoding='bytes'` keeps them as byte arrays which can be decoded later with `byte_array.decode(...)`.
#### Example
```
>>> torch.load('tensors.pt')
# Load all tensors onto the CPU
>>> torch.load('tensors.pt', map_location=torch.device('cpu'))
# Load all tensors onto the CPU, using a function
>>> torch.load('tensors.pt', map_location=lambda storage, loc: storage)
# Load all tensors onto GPU 1
>>> torch.load('tensors.pt', map_location=lambda storage, loc: storage.cuda(1))
# Map tensors from GPU 1 to GPU 0
>>> torch.load('tensors.pt', map_location={'cuda:1':'cuda:0'})
# Load tensor from io.BytesIO object
>>> with open('tensor.pt', 'rb') as f:
... buffer = io.BytesIO(f.read())
>>> torch.load(buffer)
# Load a module with 'ascii' encoding for unpickling
>>> torch.load('module.pt', encoding='ascii')
```
pytorch torch.dstack torch.dstack
============
`torch.dstack(tensors, *, out=None) → Tensor`
Stack tensors in sequence depthwise (along third axis).
This is equivalent to concatenation along the third axis after 1-D and 2-D tensors have been reshaped by [`torch.atleast_3d()`](torch.atleast_3d#torch.atleast_3d "torch.atleast_3d").
Parameters
**tensors** (*sequence of Tensors*) – sequence of tensors to concatenate
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example::
```
>>> a = torch.tensor([1, 2, 3])
>>> b = torch.tensor([4, 5, 6])
>>> torch.dstack((a,b))
tensor([[[1, 4],
[2, 5],
[3, 6]]])
>>> a = torch.tensor([[1],[2],[3]])
>>> b = torch.tensor([[4],[5],[6]])
>>> torch.dstack((a,b))
tensor([[[1, 4]],
[[2, 5]],
[[3, 6]]])
```
pytorch torch.slogdet torch.slogdet
=============
`torch.slogdet(input) -> (Tensor, Tensor)`
Calculates the sign and log absolute value of the determinant(s) of a square matrix or batches of square matrices.
Note
[`torch.slogdet()`](#torch.slogdet "torch.slogdet") is deprecated. Please use [`torch.linalg.slogdet()`](../linalg#torch.linalg.slogdet "torch.linalg.slogdet") instead.
Note
If `input` has zero determinant, this returns `(0, -inf)`.
Note
Backward through [`slogdet()`](#torch.slogdet "torch.slogdet") internally uses SVD results when `input` is not invertible. In this case, double backward through [`slogdet()`](#torch.slogdet "torch.slogdet") will be unstable in when `input` doesn’t have distinct singular values. See [`svd()`](torch.svd#torch.svd "torch.svd") for details.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor of size `(*, n, n)` where `*` is zero or more batch dimensions.
Returns
A namedtuple (sign, logabsdet) containing the sign of the determinant, and the log value of the absolute determinant.
Example:
```
>>> A = torch.randn(3, 3)
>>> A
tensor([[ 0.0032, -0.2239, -1.1219],
[-0.6690, 0.1161, 0.4053],
[-1.6218, -0.9273, -0.0082]])
>>> torch.det(A)
tensor(-0.7576)
>>> torch.logdet(A)
tensor(nan)
>>> torch.slogdet(A)
torch.return_types.slogdet(sign=tensor(-1.), logabsdet=tensor(-0.2776))
```
pytorch EmbeddingBag EmbeddingBag
============
`class torch.nn.EmbeddingBag(num_embeddings, embedding_dim, max_norm=None, norm_type=2.0, scale_grad_by_freq=False, mode='mean', sparse=False, _weight=None, include_last_offset=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/sparse.html#EmbeddingBag)
Computes sums or means of ‘bags’ of embeddings, without instantiating the intermediate embeddings.
For bags of constant length and no `per_sample_weights` and 2D inputs, this class
* with `mode="sum"` is equivalent to [`Embedding`](torch.nn.embedding#torch.nn.Embedding "torch.nn.Embedding") followed by `torch.sum(dim=1)`,
* with `mode="mean"` is equivalent to [`Embedding`](torch.nn.embedding#torch.nn.Embedding "torch.nn.Embedding") followed by `torch.mean(dim=1)`,
* with `mode="max"` is equivalent to [`Embedding`](torch.nn.embedding#torch.nn.Embedding "torch.nn.Embedding") followed by `torch.max(dim=1)`.
However, [`EmbeddingBag`](#torch.nn.EmbeddingBag "torch.nn.EmbeddingBag") is much more time and memory efficient than using a chain of these operations.
EmbeddingBag also supports per-sample weights as an argument to the forward pass. This scales the output of the Embedding before performing a weighted reduction as specified by `mode`. If `per_sample_weights`` is passed, the only supported `mode` is `"sum"`, which computes a weighted sum according to `per_sample_weights`.
Parameters
* **num\_embeddings** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – size of the dictionary of embeddings
* **embedding\_dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the size of each embedding vector
* **max\_norm** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – If given, each embedding vector with norm larger than `max_norm` is renormalized to have norm `max_norm`.
* **norm\_type** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – The p of the p-norm to compute for the `max_norm` option. Default `2`.
* **scale\_grad\_by\_freq** (*boolean**,* *optional*) – if given, this will scale gradients by the inverse of frequency of the words in the mini-batch. Default `False`. Note: this option is not supported when `mode="max"`.
* **mode** (*string**,* *optional*) – `"sum"`, `"mean"` or `"max"`. Specifies the way to reduce the bag. `"sum"` computes the weighted sum, taking `per_sample_weights` into consideration. `"mean"` computes the average of the values in the bag, `"max"` computes the max value over each bag. Default: `"mean"`
* **sparse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True`, gradient w.r.t. `weight` matrix will be a sparse tensor. See Notes for more details regarding sparse gradients. Note: this option is not supported when `mode="max"`.
* **include\_last\_offset** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True`, `offsets` has one additional element, where the last element is equivalent to the size of `indices`. This matches the CSR format.
Variables
**~EmbeddingBag.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape `(num_embeddings, embedding_dim)` initialized from N(0,1)\mathcal{N}(0, 1) .
`Inputs: input (IntTensor or LongTensor), offsets (IntTensor or LongTensor, optional), and`
`per_index_weights` (Tensor, optional)
* `input` and `offsets` have to be of the same type, either int or long
* If `input` is 2D of shape `(B, N)`,
it will be treated as `B` bags (sequences) each of fixed length `N`, and this will return `B` values aggregated in a way depending on the `mode`. `offsets` is ignored and required to be `None` in this case.
* If `input` is 1D of shape `(N)`,
it will be treated as a concatenation of multiple bags (sequences). `offsets` is required to be a 1D tensor containing the starting index positions of each bag in `input`. Therefore, for `offsets` of shape `(B)`, `input` will be viewed as having `B` bags. Empty bags (i.e., having 0-length) will have returned vectors filled by zeros.
per\_sample\_weights (Tensor, optional): a tensor of float / double weights, or None
to indicate all weights should be taken to be `1`. If specified, `per_sample_weights` must have exactly the same shape as input and is treated as having the same `offsets`, if those are not `None`. Only supported for `mode='sum'`.
Output shape: `(B, embedding_dim)`
Examples:
```
>>> # an Embedding module containing 10 tensors of size 3
>>> embedding_sum = nn.EmbeddingBag(10, 3, mode='sum')
>>> # a batch of 2 samples of 4 indices each
>>> input = torch.LongTensor([1,2,4,5,4,3,2,9])
>>> offsets = torch.LongTensor([0,4])
>>> embedding_sum(input, offsets)
tensor([[-0.8861, -5.4350, -0.0523],
[ 1.1306, -2.5798, -1.0044]])
```
`classmethod from_pretrained(embeddings, freeze=True, max_norm=None, norm_type=2.0, scale_grad_by_freq=False, mode='mean', sparse=False, include_last_offset=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/sparse.html#EmbeddingBag.from_pretrained)
Creates EmbeddingBag instance from given 2-dimensional FloatTensor.
Parameters
* **embeddings** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – FloatTensor containing weights for the EmbeddingBag. First dimension is being passed to EmbeddingBag as ‘num\_embeddings’, second as ‘embedding\_dim’.
* **freeze** (*boolean**,* *optional*) – If `True`, the tensor does not get updated in the learning process. Equivalent to `embeddingbag.weight.requires_grad = False`. Default: `True`
* **max\_norm** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – See module initialization documentation. Default: `None`
* **norm\_type** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – See module initialization documentation. Default `2`.
* **scale\_grad\_by\_freq** (*boolean**,* *optional*) – See module initialization documentation. Default `False`.
* **mode** (*string**,* *optional*) – See module initialization documentation. Default: `"mean"`
* **sparse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – See module initialization documentation. Default: `False`.
* **include\_last\_offset** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – See module initialization documentation. Default: `False`.
Examples:
```
>>> # FloatTensor containing pretrained weights
>>> weight = torch.FloatTensor([[1, 2.3, 3], [4, 5.1, 6.3]])
>>> embeddingbag = nn.EmbeddingBag.from_pretrained(weight)
>>> # Get embeddings for index 1
>>> input = torch.LongTensor([[1, 0]])
>>> embeddingbag(input)
tensor([[ 2.5000, 3.7000, 4.6500]])
```
| programming_docs |
pytorch torch.mvlgamma torch.mvlgamma
==============
`torch.mvlgamma(input, p) → Tensor`
Computes the [multivariate log-gamma function](https://en.wikipedia.org/wiki/Multivariate_gamma_function)) with dimension pp element-wise, given by
log(Γp(a))=C+∑i=1plog(Γ(a−i−12))\log(\Gamma\_{p}(a)) = C + \displaystyle \sum\_{i=1}^{p} \log\left(\Gamma\left(a - \frac{i - 1}{2}\right)\right)
where C=log(π)×p(p−1)4C = \log(\pi) \times \frac{p (p - 1)}{4} and Γ(⋅)\Gamma(\cdot) is the Gamma function.
All elements must be greater than p−12\frac{p - 1}{2} , otherwise an error would be thrown.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compute the multivariate log-gamma function
* **p** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the number of dimensions
Example:
```
>>> a = torch.empty(2, 3).uniform_(1, 2)
>>> a
tensor([[1.6835, 1.8474, 1.1929],
[1.0475, 1.7162, 1.4180]])
>>> torch.mvlgamma(a, 2)
tensor([[0.3928, 0.4007, 0.7586],
[1.0311, 0.3901, 0.5049]])
```
pytorch torch.jit.ignore torch.jit.ignore
================
`torch.jit.ignore(drop=False, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/_jit_internal.html#ignore)
This decorator indicates to the compiler that a function or method should be ignored and left as a Python function. This allows you to leave code in your model that is not yet TorchScript compatible. If called from TorchScript, ignored functions will dispatch the call to the Python interpreter. Models with ignored functions cannot be exported; use [`@torch.jit.unused`](torch.jit.unused#torch.jit.unused "torch.jit.unused") instead.
Example (using `@torch.jit.ignore` on a method):
```
import torch
import torch.nn as nn
class MyModule(nn.Module):
@torch.jit.ignore
def debugger(self, x):
import pdb
pdb.set_trace()
def forward(self, x):
x += 10
# The compiler would normally try to compile `debugger`,
# but since it is `@ignore`d, it will be left as a call
# to Python
self.debugger(x)
return x
m = torch.jit.script(MyModule())
# Error! The call `debugger` cannot be saved since it calls into Python
m.save("m.pt")
```
Example (using `@torch.jit.ignore(drop=True)` on a method):
```
import torch
import torch.nn as nn
class MyModule(nn.Module):
@torch.jit.ignore(drop=True)
def training_method(self, x):
import pdb
pdb.set_trace()
def forward(self, x):
if self.training:
self.training_method(x)
return x
m = torch.jit.script(MyModule())
# This is OK since `training_method` is not saved, the call is replaced
# with a `raise`.
m.save("m.pt")
```
pytorch AlphaDropout AlphaDropout
============
`class torch.nn.AlphaDropout(p=0.5, inplace=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/dropout.html#AlphaDropout)
Applies Alpha Dropout over the input.
Alpha Dropout is a type of Dropout that maintains the self-normalizing property. For an input with zero mean and unit standard deviation, the output of Alpha Dropout maintains the original mean and standard deviation of the input. Alpha Dropout goes hand-in-hand with SELU activation function, which ensures that the outputs have zero mean and unit standard deviation.
During training, it randomly masks some of the elements of the input tensor with probability *p* using samples from a bernoulli distribution. The elements to masked are randomized on every forward call, and scaled and shifted to maintain zero mean and unit standard deviation.
During evaluation the module simply computes an identity function.
More details can be found in the paper [Self-Normalizing Neural Networks](https://arxiv.org/abs/1706.02515) .
Parameters
* **p** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – probability of an element to be dropped. Default: 0.5
* **inplace** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If set to `True`, will do this operation in-place
Shape:
* Input: (∗)(\*) . Input can be of any shape
* Output: (∗)(\*) . Output is of the same shape as input
Examples:
```
>>> m = nn.AlphaDropout(p=0.2)
>>> input = torch.randn(20, 16)
>>> output = m(input)
```
pytorch Hardtanh Hardtanh
========
`class torch.nn.Hardtanh(min_val=-1.0, max_val=1.0, inplace=False, min_value=None, max_value=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Hardtanh)
Applies the HardTanh function element-wise
HardTanh is defined as:
HardTanh(x)={1 if x>1−1 if x<−1x otherwise \text{HardTanh}(x) = \begin{cases} 1 & \text{ if } x > 1 \\ -1 & \text{ if } x < -1 \\ x & \text{ otherwise } \\ \end{cases}
The range of the linear region [−1,1][-1, 1] can be adjusted using `min_val` and `max_val`.
Parameters
* **min\_val** – minimum value of the linear region range. Default: -1
* **max\_val** – maximum value of the linear region range. Default: 1
* **inplace** – can optionally do the operation in-place. Default: `False`
Keyword arguments `min_value` and `max_value` have been deprecated in favor of `min_val` and `max_val`.
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.Hardtanh(-2, 2)
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch Tanhshrink Tanhshrink
==========
`class torch.nn.Tanhshrink` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Tanhshrink)
Applies the element-wise function:
Tanhshrink(x)=x−tanh(x)\text{Tanhshrink}(x) = x - \tanh(x)
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.Tanhshrink()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch RandomStructured RandomStructured
================
`class torch.nn.utils.prune.RandomStructured(amount, dim=-1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#RandomStructured)
Prune entire (currently unpruned) channels in a tensor at random.
Parameters
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – index of the dim along which we define channels to prune. Default: -1.
`classmethod apply(module, name, amount, dim=-1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#RandomStructured.apply)
Adds the forward pre-hook that enables pruning on the fly and the reparametrization of a tensor in terms of the original tensor and the pruning mask.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – index of the dim along which we define channels to prune. Default: -1.
`apply_mask(module)`
Simply handles the multiplication between the parameter being pruned and the generated mask. Fetches the mask and the original tensor from the module and returns the pruned version of the tensor.
Parameters
**module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
Returns
pruned version of the input tensor
Return type
pruned\_tensor ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
`compute_mask(t, default_mask)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#RandomStructured.compute_mask)
Computes and returns a mask for the input tensor `t`. Starting from a base `default_mask` (which should be a mask of ones if the tensor has not been pruned yet), generate a random mask to apply on top of the `default_mask` by randomly zeroing out channels along the specified dim of the tensor.
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor representing the parameter to prune
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Base mask from previous pruning iterations, that need to be respected after the new mask is applied. Same dims as `t`.
Returns
mask to apply to `t`, of same dims as `t`
Return type
mask ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
Raises
[**IndexError**](https://docs.python.org/3/library/exceptions.html#IndexError "(in Python v3.9)") – if `self.dim >= len(t.shape)`
`prune(t, default_mask=None, importance_scores=None)`
Computes and returns a pruned version of input tensor `t` according to the pruning rule specified in [`compute_mask()`](#torch.nn.utils.prune.RandomStructured.compute_mask "torch.nn.utils.prune.RandomStructured.compute_mask").
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor to prune (of same dimensions as `default_mask`).
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as `t`) used to compute mask for pruning `t`. The values in this tensor indicate the importance of the corresponding elements in the `t` that is being pruned. If unspecified or None, the tensor `t` will be used in its place.
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – mask from previous pruning iteration, if any. To be considered when determining what portion of the tensor that pruning should act on. If None, default to a mask of ones.
Returns
pruned version of tensor `t`.
`remove(module)`
Removes the pruning reparameterization from a module. The pruned parameter named `name` remains permanently pruned, and the parameter named `name+'_orig'` is removed from the parameter list. Similarly, the buffer named `name+'_mask'` is removed from the buffers.
Note
Pruning itself is NOT undone or reversed!
pytorch torch.scatter_add torch.scatter\_add
==================
`torch.scatter_add(input, dim, index, src) → Tensor`
Out-of-place version of [`torch.Tensor.scatter_add_()`](../tensors#torch.Tensor.scatter_add_ "torch.Tensor.scatter_add_")
pytorch GaussianNLLLoss GaussianNLLLoss
===============
`class torch.nn.GaussianNLLLoss(*, full=False, eps=1e-06, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#GaussianNLLLoss)
Gaussian negative log likelihood loss.
The targets are treated as samples from Gaussian distributions with expectations and variances predicted by the neural network. For a D-dimensional `target` tensor modelled as having heteroscedastic Gaussian distributions with a D-dimensional tensor of expectations `input` and a D-dimensional tensor of positive variances `var` the loss is:
loss=12∑i=1D(log(max(var[i], eps))+(input[i]−target[i])2max(var[i], eps))+const.\text{loss} = \frac{1}{2}\sum\_{i=1}^D \left(\log\left(\text{max}\left(\text{var}[i], \ \text{eps}\right)\right) + \frac{\left(\text{input}[i] - \text{target}[i]\right)^2} {\text{max}\left(\text{var}[i], \ \text{eps}\right)}\right) + \text{const.}
where `eps` is used for stability. By default, the constant term of the loss function is omitted unless `full` is `True`. If `var` is a scalar (implying `target` tensor has homoscedastic Gaussian distributions) it is broadcasted to be the same size as the input.
Parameters
* **full** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – include the constant term in the loss calculation. Default: `False`.
* **eps** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – value used to clamp `var` (see note below), for stability. Default: 1e-6.
* **reduction** (*string**,* *optional*) – specifies the reduction to apply to the output:`'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the output is the average of all batch member losses, `'sum'`: the output is the sum of all batch member losses. Default: `'mean'`.
Shape:
* Input: (N,∗)(N, \*) where ∗\* means any number of additional dimensions
* Target: (N,∗)(N, \*) , same shape as the input
* Var: (N,1)(N, 1) or (N,∗)(N, \*) , same shape as the input
* Output: scalar if `reduction` is `'mean'` (default) or `'sum'`. If `reduction` is `'none'`, then (N)(N)
Examples:
```
>>> loss = nn.GaussianNLLLoss()
>>> input = torch.randn(5, 2, requires_grad=True)
>>> target = torch.randn(5, 2)
>>> var = torch.ones(5, 2, requires_grad=True) #heteroscedastic
>>> output = loss(input, target, var)
>>> output.backward()
>>> loss = nn.GaussianNLLLoss()
>>> input = torch.randn(5, 2, requires_grad=True)
>>> target = torch.randn(5, 2)
>>> var = torch.ones(5, 1, requires_grad=True) #homoscedastic
>>> output = loss(input, target, var)
>>> output.backward()
```
Note
The clamping of `var` is ignored with respect to autograd, and so the gradients are unaffected by it.
Reference:
Nix, D. A. and Weigend, A. S., “Estimating the mean and variance of the target probability distribution”, Proceedings of 1994 IEEE International Conference on Neural Networks (ICNN’94), Orlando, FL, USA, 1994, pp. 55-60 vol.1, doi: 10.1109/ICNN.1994.374138.
pytorch torch.tril_indices torch.tril\_indices
===================
`torch.tril_indices(row, col, offset=0, *, dtype=torch.long, device='cpu', layout=torch.strided) → Tensor`
Returns the indices of the lower triangular part of a `row`-by- `col` matrix in a 2-by-N Tensor, where the first row contains row coordinates of all indices and the second row contains column coordinates. Indices are ordered based on rows and then columns.
The lower triangular part of the matrix is defined as the elements on and below the diagonal.
The argument `offset` controls which diagonal to consider. If `offset` = 0, all elements on and below the main diagonal are retained. A positive value includes just as many diagonals above the main diagonal, and similarly a negative value excludes just as many diagonals below the main diagonal. The main diagonal are the set of indices {(i,i)}\lbrace (i, i) \rbrace for i∈[0,min{d1,d2}−1]i \in [0, \min\{d\_{1}, d\_{2}\} - 1] where d1,d2d\_{1}, d\_{2} are the dimensions of the matrix.
Note
When running on CUDA, `row * col` must be less than 2592^{59} to prevent overflow during calculation.
Parameters
* **row** (`int`) – number of rows in the 2-D matrix.
* **col** (`int`) – number of columns in the 2-D matrix.
* **offset** (`int`) – diagonal offset from the main diagonal. Default: if not provided, 0.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, `torch.long`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – currently only support `torch.strided`.
Example::
```
>>> a = torch.tril_indices(3, 3)
>>> a
tensor([[0, 1, 1, 2, 2, 2],
[0, 0, 1, 0, 1, 2]])
```
```
>>> a = torch.tril_indices(4, 3, -1)
>>> a
tensor([[1, 2, 2, 3, 3, 3],
[0, 0, 1, 0, 1, 2]])
```
```
>>> a = torch.tril_indices(4, 3, 1)
>>> a
tensor([[0, 0, 1, 1, 1, 2, 2, 2, 3, 3, 3],
[0, 1, 0, 1, 2, 0, 1, 2, 0, 1, 2]])
```
pytorch torch.square torch.square
============
`torch.square(input, *, out=None) → Tensor`
Returns a new tensor with the square of the elements of `input`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([-2.0755, 1.0226, 0.0831, 0.4806])
>>> torch.square(a)
tensor([ 4.3077, 1.0457, 0.0069, 0.2310])
```
pytorch torch.median torch.median
============
`torch.median(input) → Tensor`
Returns the median of the values in `input`.
Note
The median is not unique for `input` tensors with an even number of elements. In this case the lower of the two medians is returned. To compute the mean of both medians, use [`torch.quantile()`](torch.quantile#torch.quantile "torch.quantile") with `q=0.5` instead.
Warning
This function produces deterministic (sub)gradients unlike `median(dim=0)`
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[ 1.5219, -1.5212, 0.2202]])
>>> torch.median(a)
tensor(0.2202)
```
`torch.median(input, dim=-1, keepdim=False, *, out=None) -> (Tensor, LongTensor)`
Returns a namedtuple `(values, indices)` where `values` contains the median of each row of `input` in the dimension `dim`, and `indices` contains the index of the median values found in the dimension `dim`.
By default, `dim` is the last dimension of the `input` tensor.
If `keepdim` is `True`, the output tensors are of the same size as `input` except in the dimension `dim` where they are of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the outputs tensor having 1 fewer dimension than `input`.
Note
The median is not unique for `input` tensors with an even number of elements in the dimension `dim`. In this case the lower of the two medians is returned. To compute the mean of both medians in `input`, use [`torch.quantile()`](torch.quantile#torch.quantile "torch.quantile") with `q=0.5` instead.
Warning
`indices` does not necessarily contain the first occurrence of each median value found, unless it is unique. The exact implementation details are device-specific. Do not expect the same result when run on CPU and GPU in general. For the same reason do not expect the gradients to be deterministic.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**out** (*(*[Tensor](../tensors#torch.Tensor "torch.Tensor")*,* [Tensor](../tensors#torch.Tensor "torch.Tensor")*)**,* *optional*) – The first tensor will be populated with the median values and the second tensor, which must have dtype long, with their indices in the dimension `dim` of `input`.
Example:
```
>>> a = torch.randn(4, 5)
>>> a
tensor([[ 0.2505, -0.3982, -0.9948, 0.3518, -1.3131],
[ 0.3180, -0.6993, 1.0436, 0.0438, 0.2270],
[-0.2751, 0.7303, 0.2192, 0.3321, 0.2488],
[ 1.0778, -1.9510, 0.7048, 0.4742, -0.7125]])
>>> torch.median(a, 1)
torch.return_types.median(values=tensor([-0.3982, 0.2270, 0.2488, 0.4742]), indices=tensor([1, 4, 4, 3]))
```
| programming_docs |
pytorch Transformer Transformer
===========
`class torch.nn.Transformer(d_model=512, nhead=8, num_encoder_layers=6, num_decoder_layers=6, dim_feedforward=2048, dropout=0.1, activation='relu', custom_encoder=None, custom_decoder=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#Transformer)
A transformer model. User is able to modify the attributes as needed. The architecture is based on the paper “Attention Is All You Need”. Ashish Vaswani, Noam Shazeer, Niki Parmar, Jakob Uszkoreit, Llion Jones, Aidan N Gomez, Lukasz Kaiser, and Illia Polosukhin. 2017. Attention is all you need. In Advances in Neural Information Processing Systems, pages 6000-6010. Users can build the BERT(<https://arxiv.org/abs/1810.04805>) model with corresponding parameters.
Parameters
* **d\_model** – the number of expected features in the encoder/decoder inputs (default=512).
* **nhead** – the number of heads in the multiheadattention models (default=8).
* **num\_encoder\_layers** – the number of sub-encoder-layers in the encoder (default=6).
* **num\_decoder\_layers** – the number of sub-decoder-layers in the decoder (default=6).
* **dim\_feedforward** – the dimension of the feedforward network model (default=2048).
* **dropout** – the dropout value (default=0.1).
* **activation** – the activation function of encoder/decoder intermediate layer, relu or gelu (default=relu).
* **custom\_encoder** – custom encoder (default=None).
* **custom\_decoder** – custom decoder (default=None).
Examples::
```
>>> transformer_model = nn.Transformer(nhead=16, num_encoder_layers=12)
>>> src = torch.rand((10, 32, 512))
>>> tgt = torch.rand((20, 32, 512))
>>> out = transformer_model(src, tgt)
```
Note: A full example to apply nn.Transformer module for the word language model is available in <https://github.com/pytorch/examples/tree/master/word_language_model>
`forward(src, tgt, src_mask=None, tgt_mask=None, memory_mask=None, src_key_padding_mask=None, tgt_key_padding_mask=None, memory_key_padding_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#Transformer.forward)
Take in and process masked source/target sequences.
Parameters
* **src** – the sequence to the encoder (required).
* **tgt** – the sequence to the decoder (required).
* **src\_mask** – the additive mask for the src sequence (optional).
* **tgt\_mask** – the additive mask for the tgt sequence (optional).
* **memory\_mask** – the additive mask for the encoder output (optional).
* **src\_key\_padding\_mask** – the ByteTensor mask for src keys per batch (optional).
* **tgt\_key\_padding\_mask** – the ByteTensor mask for tgt keys per batch (optional).
* **memory\_key\_padding\_mask** – the ByteTensor mask for memory keys per batch (optional).
Shape:
* src: (S,N,E)(S, N, E) .
* tgt: (T,N,E)(T, N, E) .
* src\_mask: (S,S)(S, S) .
* tgt\_mask: (T,T)(T, T) .
* memory\_mask: (T,S)(T, S) .
* src\_key\_padding\_mask: (N,S)(N, S) .
* tgt\_key\_padding\_mask: (N,T)(N, T) .
* memory\_key\_padding\_mask: (N,S)(N, S) .
Note: [src/tgt/memory]\_mask ensures that position i is allowed to attend the unmasked positions. If a ByteTensor is provided, the non-zero positions are not allowed to attend while the zero positions will be unchanged. If a BoolTensor is provided, positions with `True` are not allowed to attend while `False` values will be unchanged. If a FloatTensor is provided, it will be added to the attention weight. [src/tgt/memory]\_key\_padding\_mask provides specified elements in the key to be ignored by the attention. If a ByteTensor is provided, the non-zero positions will be ignored while the zero positions will be unchanged. If a BoolTensor is provided, the positions with the value of `True` will be ignored while the position with the value of `False` will be unchanged.
* output: (T,N,E)(T, N, E) .
Note: Due to the multi-head attention architecture in the transformer model, the output sequence length of a transformer is same as the input sequence (i.e. target) length of the decode.
where S is the source sequence length, T is the target sequence length, N is the batch size, E is the feature number
#### Examples
```
>>> output = transformer_model(src, tgt, src_mask=src_mask, tgt_mask=tgt_mask)
```
`generate_square_subsequent_mask(sz)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#Transformer.generate_square_subsequent_mask)
Generate a square mask for the sequence. The masked positions are filled with float(‘-inf’). Unmasked positions are filled with float(0.0).
pytorch torch.rad2deg torch.rad2deg
=============
`torch.rad2deg(input, *, out=None) → Tensor`
Returns a new tensor with each of the elements of `input` converted from angles in radians to degrees.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([[3.142, -3.142], [6.283, -6.283], [1.570, -1.570]])
>>> torch.rad2deg(a)
tensor([[ 180.0233, -180.0233],
[ 359.9894, -359.9894],
[ 89.9544, -89.9544]])
```
pytorch torch.hamming_window torch.hamming\_window
=====================
`torch.hamming_window(window_length, periodic=True, alpha=0.54, beta=0.46, *, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Hamming window function.
w[n]=α−βcos(2πnN−1),w[n] = \alpha - \beta\ \cos \left( \frac{2 \pi n}{N - 1} \right),
where NN is the full window size.
The input `window_length` is a positive integer controlling the returned window size. `periodic` flag determines whether the returned window trims off the last duplicate value from the symmetric window and is ready to be used as a periodic window with functions like [`torch.stft()`](torch.stft#torch.stft "torch.stft"). Therefore, if `periodic` is true, the NN in above formula is in fact window\_length+1\text{window\\_length} + 1 . Also, we always have `torch.hamming_window(L, periodic=True)` equal to `torch.hamming_window(L + 1, periodic=False)[:-1])`.
Note
If `window_length` =1=1 , the returned window contains a single value 1.
Note
This is a generalized version of [`torch.hann_window()`](torch.hann_window#torch.hann_window "torch.hann_window").
Parameters
* **window\_length** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the size of returned window
* **periodic** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If True, returns a window to be used as periodic function. If False, return a symmetric window.
* **alpha** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – The coefficient α\alpha in the equation above
* **beta** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – The coefficient β\beta in the equation above
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). Only floating point types are supported.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned window tensor. Only `torch.strided` (dense layout) is supported.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Returns
A 1-D tensor of size (window\_length,)(\text{window\\_length},) containing the window
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
pytorch LPPool2d LPPool2d
========
`class torch.nn.LPPool2d(norm_type, kernel_size, stride=None, ceil_mode=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#LPPool2d)
Applies a 2D power-average pooling over an input signal composed of several input planes.
On each window, the function computed is:
f(X)=∑x∈Xxppf(X) = \sqrt[p]{\sum\_{x \in X} x^{p}}
* At p = ∞\infty , one gets Max Pooling
* At p = 1, one gets Sum Pooling (which is proportional to average pooling)
The parameters `kernel_size`, `stride` can either be:
* a single `int` – in which case the same value is used for the height and width dimension
* a `tuple` of two ints – in which case, the first `int` is used for the height dimension, and the second `int` for the width dimension
Note
If the sum to the power of `p` is zero, the gradient of this function is not defined. This implementation will set the gradient to zero in this case.
Parameters
* **kernel\_size** – the size of the window
* **stride** – the stride of the window. Default value is `kernel_size`
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) , where
Hout=⌊Hin−kernel\_size[0]stride[0]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} - \text{kernel\\_size}[0]}{\text{stride}[0]} + 1\right\rfloor
Wout=⌊Win−kernel\_size[1]stride[1]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} - \text{kernel\\_size}[1]}{\text{stride}[1]} + 1\right\rfloor
Examples:
```
>>> # power-2 pool of square window of size=3, stride=2
>>> m = nn.LPPool2d(2, 3, stride=2)
>>> # pool of non-square window of power 1.2
>>> m = nn.LPPool2d(1.2, (3, 2), stride=(2, 1))
>>> input = torch.randn(20, 16, 50, 32)
>>> output = m(input)
```
pytorch torch.logical_xor torch.logical\_xor
==================
`torch.logical_xor(input, other, *, out=None) → Tensor`
Computes the element-wise logical XOR of the given input tensors. Zeros are treated as `False` and nonzeros are treated as `True`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compute XOR with
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.logical_xor(torch.tensor([True, False, True]), torch.tensor([True, False, False]))
tensor([False, False, True])
>>> a = torch.tensor([0, 1, 10, 0], dtype=torch.int8)
>>> b = torch.tensor([4, 0, 1, 0], dtype=torch.int8)
>>> torch.logical_xor(a, b)
tensor([ True, True, False, False])
>>> torch.logical_xor(a.double(), b.double())
tensor([ True, True, False, False])
>>> torch.logical_xor(a.double(), b)
tensor([ True, True, False, False])
>>> torch.logical_xor(a, b, out=torch.empty(4, dtype=torch.bool))
tensor([ True, True, False, False])
```
pytorch torch.log2 torch.log2
==========
`torch.log2(input, *, out=None) → Tensor`
Returns a new tensor with the logarithm to the base 2 of the elements of `input`.
yi=log2(xi)y\_{i} = \log\_{2} (x\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.rand(5)
>>> a
tensor([ 0.8419, 0.8003, 0.9971, 0.5287, 0.0490])
>>> torch.log2(a)
tensor([-0.2483, -0.3213, -0.0042, -0.9196, -4.3504])
```
pytorch TripletMarginWithDistanceLoss TripletMarginWithDistanceLoss
=============================
`class torch.nn.TripletMarginWithDistanceLoss(*, distance_function=None, margin=1.0, swap=False, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#TripletMarginWithDistanceLoss)
Creates a criterion that measures the triplet loss given input tensors aa , pp , and nn (representing anchor, positive, and negative examples, respectively), and a nonnegative, real-valued function (“distance function”) used to compute the relationship between the anchor and positive example (“positive distance”) and the anchor and negative example (“negative distance”).
The unreduced loss (i.e., with `reduction` set to `'none'`) can be described as:
ℓ(a,p,n)=L={l1,…,lN}⊤,li=max{d(ai,pi)−d(ai,ni)+margin,0}\ell(a, p, n) = L = \{l\_1,\dots,l\_N\}^\top, \quad l\_i = \max \{d(a\_i, p\_i) - d(a\_i, n\_i) + {\rm margin}, 0\}
where NN is the batch size; dd is a nonnegative, real-valued function quantifying the closeness of two tensors, referred to as the `distance_function`; and marginmargin is a nonnegative margin representing the minimum difference between the positive and negative distances that is required for the loss to be 0. The input tensors have NN elements each and can be of any shape that the distance function can handle.
If `reduction` is not `'none'` (default `'mean'`), then:
ℓ(x,y)={mean(L),if reduction=‘mean’;sum(L),if reduction=‘sum’.\ell(x, y) = \begin{cases} \operatorname{mean}(L), & \text{if reduction} = \text{`mean';}\\ \operatorname{sum}(L), & \text{if reduction} = \text{`sum'.} \end{cases}
See also [`TripletMarginLoss`](torch.nn.tripletmarginloss#torch.nn.TripletMarginLoss "torch.nn.TripletMarginLoss"), which computes the triplet loss for input tensors using the lpl\_p distance as the distance function.
Parameters
* **distance\_function** (*callable**,* *optional*) – A nonnegative, real-valued function that quantifies the closeness of two tensors. If not specified, `nn.PairwiseDistance` will be used. Default: `None`
* **margin** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – A nonnegative margin representing the minimum difference between the positive and negative distances required for the loss to be 0. Larger margins penalize cases where the negative examples are not distant enough from the anchors, relative to the positives. Default: 11 .
* **swap** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Whether to use the distance swap described in the paper `Learning shallow convolutional feature descriptors with triplet losses` by V. Balntas, E. Riba et al. If True, and if the positive example is closer to the negative example than the anchor is, swaps the positive example and the anchor in the loss computation. Default: `False`.
* **reduction** (*string**,* *optional*) – Specifies the (optional) reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Default: `'mean'`
Shape:
* Input: (N,∗)(N, \*) where ∗\* represents any number of additional dimensions as supported by the distance function.
* Output: A Tensor of shape (N)(N) if `reduction` is `'none'`, or a scalar otherwise.
Examples:
```
>>> # Initialize embeddings
>>> embedding = nn.Embedding(1000, 128)
>>> anchor_ids = torch.randint(0, 1000, (1,))
>>> positive_ids = torch.randint(0, 1000, (1,))
>>> negative_ids = torch.randint(0, 1000, (1,))
>>> anchor = embedding(anchor_ids)
>>> positive = embedding(positive_ids)
>>> negative = embedding(negative_ids)
>>>
>>> # Built-in Distance Function
>>> triplet_loss = \
>>> nn.TripletMarginWithDistanceLoss(distance_function=nn.PairwiseDistance())
>>> output = triplet_loss(anchor, positive, negative)
>>> output.backward()
>>>
>>> # Custom Distance Function
>>> def l_infinity(x1, x2):
>>> return torch.max(torch.abs(x1 - x2), dim=1).values
>>>
>>> triplet_loss = \
>>> nn.TripletMarginWithDistanceLoss(distance_function=l_infinity, margin=1.5)
>>> output = triplet_loss(anchor, positive, negative)
>>> output.backward()
>>>
>>> # Custom Distance Function (Lambda)
>>> triplet_loss = \
>>> nn.TripletMarginWithDistanceLoss(
>>> distance_function=lambda x, y: 1.0 - F.cosine_similarity(x, y))
>>> output = triplet_loss(anchor, positive, negative)
>>> output.backward()
```
Reference:
V. Balntas, et al.: Learning shallow convolutional feature descriptors with triplet losses: <http://www.bmva.org/bmvc/2016/papers/paper119/index.html>
pytorch BatchNorm1d BatchNorm1d
===========
`class torch.nn.BatchNorm1d(num_features, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/batchnorm.html#BatchNorm1d)
Applies Batch Normalization over a 2D or 3D input (a mini-batch of 1D inputs with optional additional channel dimension) as described in the paper [Batch Normalization: Accelerating Deep Network Training by Reducing Internal Covariate Shift](https://arxiv.org/abs/1502.03167) .
y=x−E[x]Var[x]+ϵ∗γ+βy = \frac{x - \mathrm{E}[x]}{\sqrt{\mathrm{Var}[x] + \epsilon}} \* \gamma + \beta
The mean and standard-deviation are calculated per-dimension over the mini-batches and γ\gamma and β\beta are learnable parameter vectors of size `C` (where `C` is the input size). By default, the elements of γ\gamma are set to 1 and the elements of β\beta are set to 0. The standard-deviation is calculated via the biased estimator, equivalent to `torch.var(input, unbiased=False)`.
Also by default, during training this layer keeps running estimates of its computed mean and variance, which are then used for normalization during evaluation. The running estimates are kept with a default `momentum` of 0.1.
If `track_running_stats` is set to `False`, this layer then does not keep running estimates, and batch statistics are instead used during evaluation time as well.
Note
This `momentum` argument is different from one used in optimizer classes and the conventional notion of momentum. Mathematically, the update rule for running statistics here is x^new=(1−momentum)×x^+momentum×xt\hat{x}\_\text{new} = (1 - \text{momentum}) \times \hat{x} + \text{momentum} \times x\_t , where x^\hat{x} is the estimated statistic and xtx\_t is the new observed value.
Because the Batch Normalization is done over the `C` dimension, computing statistics on `(N, L)` slices, it’s common terminology to call this Temporal Batch Normalization.
Parameters
* **num\_features** – CC from an expected input of size (N,C,L)(N, C, L) or LL from input of size (N,L)(N, L)
* **eps** – a value added to the denominator for numerical stability. Default: 1e-5
* **momentum** – the value used for the running\_mean and running\_var computation. Can be set to `None` for cumulative moving average (i.e. simple average). Default: 0.1
* **affine** – a boolean value that when set to `True`, this module has learnable affine parameters. Default: `True`
* **track\_running\_stats** – a boolean value that when set to `True`, this module tracks the running mean and variance, and when set to `False`, this module does not track such statistics, and initializes statistics buffers `running_mean` and `running_var` as `None`. When these buffers are `None`, this module always uses batch statistics. in both training and eval modes. Default: `True`
Shape:
* Input: (N,C)(N, C) or (N,C,L)(N, C, L)
* Output: (N,C)(N, C) or (N,C,L)(N, C, L) (same shape as input)
Examples:
```
>>> # With Learnable Parameters
>>> m = nn.BatchNorm1d(100)
>>> # Without Learnable Parameters
>>> m = nn.BatchNorm1d(100, affine=False)
>>> input = torch.randn(20, 100)
>>> output = m(input)
```
| programming_docs |
pytorch TransformerDecoder TransformerDecoder
==================
`class torch.nn.TransformerDecoder(decoder_layer, num_layers, norm=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerDecoder)
TransformerDecoder is a stack of N decoder layers
Parameters
* **decoder\_layer** – an instance of the TransformerDecoderLayer() class (required).
* **num\_layers** – the number of sub-decoder-layers in the decoder (required).
* **norm** – the layer normalization component (optional).
Examples::
```
>>> decoder_layer = nn.TransformerDecoderLayer(d_model=512, nhead=8)
>>> transformer_decoder = nn.TransformerDecoder(decoder_layer, num_layers=6)
>>> memory = torch.rand(10, 32, 512)
>>> tgt = torch.rand(20, 32, 512)
>>> out = transformer_decoder(tgt, memory)
```
`forward(tgt, memory, tgt_mask=None, memory_mask=None, tgt_key_padding_mask=None, memory_key_padding_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerDecoder.forward)
Pass the inputs (and mask) through the decoder layer in turn.
Parameters
* **tgt** – the sequence to the decoder (required).
* **memory** – the sequence from the last layer of the encoder (required).
* **tgt\_mask** – the mask for the tgt sequence (optional).
* **memory\_mask** – the mask for the memory sequence (optional).
* **tgt\_key\_padding\_mask** – the mask for the tgt keys per batch (optional).
* **memory\_key\_padding\_mask** – the mask for the memory keys per batch (optional).
Shape:
see the docs in Transformer class.
pytorch torch.multiply torch.multiply
==============
`torch.multiply(input, other, *, out=None)`
Alias for [`torch.mul()`](torch.mul#torch.mul "torch.mul").
pytorch torch.argmax torch.argmax
============
`torch.argmax(input) → LongTensor`
Returns the indices of the maximum value of all elements in the `input` tensor.
This is the second value returned by [`torch.max()`](torch.max#torch.max "torch.max"). See its documentation for the exact semantics of this method.
Note
If there are multiple minimal values then the indices of the first minimal value are returned.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[ 1.3398, 0.2663, -0.2686, 0.2450],
[-0.7401, -0.8805, -0.3402, -1.1936],
[ 0.4907, -1.3948, -1.0691, -0.3132],
[-1.6092, 0.5419, -0.2993, 0.3195]])
>>> torch.argmax(a)
tensor(0)
```
`torch.argmax(input, dim, keepdim=False) → LongTensor`
Returns the indices of the maximum values of a tensor across a dimension.
This is the second value returned by [`torch.max()`](torch.max#torch.max "torch.max"). See its documentation for the exact semantics of this method.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce. If `None`, the argmax of the flattened input is returned.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not. Ignored if `dim=None`.
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[ 1.3398, 0.2663, -0.2686, 0.2450],
[-0.7401, -0.8805, -0.3402, -1.1936],
[ 0.4907, -1.3948, -1.0691, -0.3132],
[-1.6092, 0.5419, -0.2993, 0.3195]])
>>> torch.argmax(a, dim=1)
tensor([ 0, 2, 0, 1])
```
pytorch torch.prod torch.prod
==========
`torch.prod(input, *, dtype=None) → Tensor`
Returns the product of all elements in the `input` tensor.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. If specified, the input tensor is casted to `dtype` before the operation is performed. This is useful for preventing data type overflows. Default: None.
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[-0.8020, 0.5428, -1.5854]])
>>> torch.prod(a)
tensor(0.6902)
```
`torch.prod(input, dim, keepdim=False, *, dtype=None) → Tensor`
Returns the product of each row of the `input` tensor in the given dimension `dim`.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 fewer dimension than `input`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. If specified, the input tensor is casted to `dtype` before the operation is performed. This is useful for preventing data type overflows. Default: None.
Example:
```
>>> a = torch.randn(4, 2)
>>> a
tensor([[ 0.5261, -0.3837],
[ 1.1857, -0.2498],
[-1.1646, 0.0705],
[ 1.1131, -1.0629]])
>>> torch.prod(a, 1)
tensor([-0.2018, -0.2962, -0.0821, -1.1831])
```
pytorch torch.less torch.less
==========
`torch.less(input, other, *, out=None) → Tensor`
Alias for [`torch.lt()`](torch.lt#torch.lt "torch.lt").
pytorch torch.quantize_per_tensor torch.quantize\_per\_tensor
===========================
`torch.quantize_per_tensor(input, scale, zero_point, dtype) → Tensor`
Converts a float tensor to a quantized tensor with given scale and zero point.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – float tensor to quantize
* **scale** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – scale to apply in quantization formula
* **zero\_point** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – offset in integer value that maps to float zero
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype")) – the desired data type of returned tensor. Has to be one of the quantized dtypes: `torch.quint8`, `torch.qint8`, `torch.qint32`
Returns
A newly quantized tensor
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> torch.quantize_per_tensor(torch.tensor([-1.0, 0.0, 1.0, 2.0]), 0.1, 10, torch.quint8)
tensor([-1., 0., 1., 2.], size=(4,), dtype=torch.quint8,
quantization_scheme=torch.per_tensor_affine, scale=0.1, zero_point=10)
>>> torch.quantize_per_tensor(torch.tensor([-1.0, 0.0, 1.0, 2.0]), 0.1, 10, torch.quint8).int_repr()
tensor([ 0, 10, 20, 30], dtype=torch.uint8)
```
pytorch MaxUnpool1d MaxUnpool1d
===========
`class torch.nn.MaxUnpool1d(kernel_size, stride=None, padding=0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#MaxUnpool1d)
Computes a partial inverse of [`MaxPool1d`](torch.nn.maxpool1d#torch.nn.MaxPool1d "torch.nn.MaxPool1d").
[`MaxPool1d`](torch.nn.maxpool1d#torch.nn.MaxPool1d "torch.nn.MaxPool1d") is not fully invertible, since the non-maximal values are lost.
[`MaxUnpool1d`](#torch.nn.MaxUnpool1d "torch.nn.MaxUnpool1d") takes in as input the output of [`MaxPool1d`](torch.nn.maxpool1d#torch.nn.MaxPool1d "torch.nn.MaxPool1d") including the indices of the maximal values and computes a partial inverse in which all non-maximal values are set to zero.
Note
[`MaxPool1d`](torch.nn.maxpool1d#torch.nn.MaxPool1d "torch.nn.MaxPool1d") can map several input sizes to the same output sizes. Hence, the inversion process can get ambiguous. To accommodate this, you can provide the needed output size as an additional argument `output_size` in the forward call. See the Inputs and Example below.
Parameters
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the max pooling window.
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Stride of the max pooling window. It is set to `kernel_size` by default.
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Padding that was added to the input
Inputs:
* `input`: the input Tensor to invert
* `indices`: the indices given out by [`MaxPool1d`](torch.nn.maxpool1d#torch.nn.MaxPool1d "torch.nn.MaxPool1d")
* `output_size` (optional): the targeted output size
Shape:
* Input: (N,C,Hin)(N, C, H\_{in})
* Output: (N,C,Hout)(N, C, H\_{out}) , where
Hout=(Hin−1)×stride[0]−2×padding[0]+kernel\_size[0]H\_{out} = (H\_{in} - 1) \times \text{stride}[0] - 2 \times \text{padding}[0] + \text{kernel\\_size}[0]
or as given by `output_size` in the call operator
Example:
```
>>> pool = nn.MaxPool1d(2, stride=2, return_indices=True)
>>> unpool = nn.MaxUnpool1d(2, stride=2)
>>> input = torch.tensor([[[1., 2, 3, 4, 5, 6, 7, 8]]])
>>> output, indices = pool(input)
>>> unpool(output, indices)
tensor([[[ 0., 2., 0., 4., 0., 6., 0., 8.]]])
>>> # Example showcasing the use of output_size
>>> input = torch.tensor([[[1., 2, 3, 4, 5, 6, 7, 8, 9]]])
>>> output, indices = pool(input)
>>> unpool(output, indices, output_size=input.size())
tensor([[[ 0., 2., 0., 4., 0., 6., 0., 8., 0.]]])
>>> unpool(output, indices)
tensor([[[ 0., 2., 0., 4., 0., 6., 0., 8.]]])
```
pytorch torch.lu_solve torch.lu\_solve
===============
`torch.lu_solve(b, LU_data, LU_pivots, *, out=None) → Tensor`
Returns the LU solve of the linear system Ax=bAx = b using the partially pivoted LU factorization of A from [`torch.lu()`](torch.lu#torch.lu "torch.lu").
This function supports `float`, `double`, `cfloat` and `cdouble` dtypes for `input`.
Parameters
* **b** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the RHS tensor of size (∗,m,k)(\*, m, k) , where ∗\* is zero or more batch dimensions.
* **LU\_data** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the pivoted LU factorization of A from [`torch.lu()`](torch.lu#torch.lu "torch.lu") of size (∗,m,m)(\*, m, m) , where ∗\* is zero or more batch dimensions.
* **LU\_pivots** (*IntTensor*) – the pivots of the LU factorization from [`torch.lu()`](torch.lu#torch.lu "torch.lu") of size (∗,m)(\*, m) , where ∗\* is zero or more batch dimensions. The batch dimensions of `LU_pivots` must be equal to the batch dimensions of `LU_data`.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> A = torch.randn(2, 3, 3)
>>> b = torch.randn(2, 3, 1)
>>> A_LU = torch.lu(A)
>>> x = torch.lu_solve(b, *A_LU)
>>> torch.norm(torch.bmm(A, x) - b)
tensor(1.00000e-07 *
2.8312)
```
pytorch torch.ge torch.ge
========
`torch.ge(input, other, *, out=None) → Tensor`
Computes input≥other\text{input} \geq \text{other} element-wise.
The second argument can be a number or a tensor whose shape is [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with the first argument.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compare
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the tensor or value to compare
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Returns
A boolean tensor that is True where `input` is greater than or equal to `other` and False elsewhere
Example:
```
>>> torch.ge(torch.tensor([[1, 2], [3, 4]]), torch.tensor([[1, 1], [4, 4]]))
tensor([[True, True], [False, True]])
```
pytorch torch.take torch.take
==========
`torch.take(input, index) → Tensor`
Returns a new tensor with the elements of `input` at the given indices. The input tensor is treated as if it were viewed as a 1-D tensor. The result takes the same shape as the indices.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **indices** (*LongTensor*) – the indices into tensor
Example:
```
>>> src = torch.tensor([[4, 3, 5],
... [6, 7, 8]])
>>> torch.take(src, torch.tensor([0, 2, 5]))
tensor([ 4, 5, 8])
```
pytorch MultiMarginLoss MultiMarginLoss
===============
`class torch.nn.MultiMarginLoss(p=1, margin=1.0, weight=None, size_average=None, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#MultiMarginLoss)
Creates a criterion that optimizes a multi-class classification hinge loss (margin-based loss) between input xx (a 2D mini-batch `Tensor`) and output yy (which is a 1D tensor of target class indices, 0≤y≤x.size(1)−10 \leq y \leq \text{x.size}(1)-1 ):
For each mini-batch sample, the loss in terms of the 1D input xx and scalar output yy is:
loss(x,y)=∑imax(0,margin−x[y]+x[i]))px.size(0)\text{loss}(x, y) = \frac{\sum\_i \max(0, \text{margin} - x[y] + x[i]))^p}{\text{x.size}(0)}
where x∈{0,⋯,x.size(0)−1}x \in \left\{0, \; \cdots , \; \text{x.size}(0) - 1\right\} and i≠yi \neq y .
Optionally, you can give non-equal weighting on the classes by passing a 1D `weight` tensor into the constructor.
The loss function then becomes:
loss(x,y)=∑imax(0,w[y]∗(margin−x[y]+x[i]))p)x.size(0)\text{loss}(x, y) = \frac{\sum\_i \max(0, w[y] \* (\text{margin} - x[y] + x[i]))^p)}{\text{x.size}(0)}
Parameters
* **p** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Has a default value of 11 . 11 and 22 are the only supported values.
* **margin** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Has a default value of 11 .
* **weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – a manual rescaling weight given to each class. If given, it has to be a Tensor of size `C`. Otherwise, it is treated as if having all ones.
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
pytorch torch.erfc torch.erfc
==========
`torch.erfc(input, *, out=None) → Tensor`
Computes the complementary error function of each element of `input`. The complementary error function is defined as follows:
erfc(x)=1−2π∫0xe−t2dt\mathrm{erfc}(x) = 1 - \frac{2}{\sqrt{\pi}} \int\_{0}^{x} e^{-t^2} dt
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.erfc(torch.tensor([0, -1., 10.]))
tensor([ 1.0000, 1.8427, 0.0000])
```
pytorch torch.nn.modules.module.register_module_backward_hook torch.nn.modules.module.register\_module\_backward\_hook
========================================================
`torch.nn.modules.module.register_module_backward_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#register_module_backward_hook)
Registers a backward hook common to all the modules.
This function is deprecated in favor of `nn.module.register_module_full_backward_hook()` and the behavior of this function will change in future versions.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
pytorch torch.split torch.split
===========
`torch.split(tensor, split_size_or_sections, dim=0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#split)
Splits the tensor into chunks. Each chunk is a view of the original tensor.
If `split_size_or_sections` is an integer type, then [`tensor`](torch.tensor#torch.tensor "torch.tensor") will be split into equally sized chunks (if possible). Last chunk will be smaller if the tensor size along the given dimension `dim` is not divisible by `split_size`.
If `split_size_or_sections` is a list, then [`tensor`](torch.tensor#torch.tensor "torch.tensor") will be split into `len(split_size_or_sections)` chunks with sizes in `dim` according to `split_size_or_sections`.
Parameters
* **tensor** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor to split.
* **split\_size\_or\_sections** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*) or* *(*[list](https://docs.python.org/3/library/stdtypes.html#list "(in Python v3.9)")*(*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*)*) – size of a single chunk or list of sizes for each chunk
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – dimension along which to split the tensor.
Example::
```
>>> a = torch.arange(10).reshape(5,2)
>>> a
tensor([[0, 1],
[2, 3],
[4, 5],
[6, 7],
[8, 9]])
>>> torch.split(a, 2)
(tensor([[0, 1],
[2, 3]]),
tensor([[4, 5],
[6, 7]]),
tensor([[8, 9]]))
>>> torch.split(a, [1,4])
(tensor([[0, 1]]),
tensor([[2, 3],
[4, 5],
[6, 7],
[8, 9]]))
```
pytorch torch.angle torch.angle
===========
`torch.angle(input, *, out=None) → Tensor`
Computes the element-wise angle (in radians) of the given `input` tensor.
outi=angle(inputi)\text{out}\_{i} = angle(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Note
Starting in PyTorch 1.8, angle returns pi for negative real numbers, zero for non-negative real numbers, and propagates NaNs. Previously the function would return zero for all real numbers and not propagate floating-point NaNs.
Example:
```
>>> torch.angle(torch.tensor([-1 + 1j, -2 + 2j, 3 - 3j]))*180/3.14159
tensor([ 135., 135, -45])
```
pytorch torch.nn.utils.remove_weight_norm torch.nn.utils.remove\_weight\_norm
===================================
`torch.nn.utils.remove_weight_norm(module, name='weight')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/weight_norm.html#remove_weight_norm)
Removes the weight normalization reparameterization from a module.
Parameters
* **module** ([Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – containing module
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")*,* *optional*) – name of weight parameter
#### Example
```
>>> m = weight_norm(nn.Linear(20, 40))
>>> remove_weight_norm(m)
```
| programming_docs |
pytorch torch.matrix_power torch.matrix\_power
===================
`torch.matrix_power(input, n) → Tensor`
Returns the matrix raised to the power `n` for square matrices. For batch of matrices, each individual matrix is raised to the power `n`.
If `n` is negative, then the inverse of the matrix (if invertible) is raised to the power `n`. For a batch of matrices, the batched inverse (if invertible) is raised to the power `n`. If `n` is 0, then an identity matrix is returned.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the power to raise the matrix to
Example:
```
>>> a = torch.randn(2, 2, 2)
>>> a
tensor([[[-1.9975, -1.9610],
[ 0.9592, -2.3364]],
[[-1.2534, -1.3429],
[ 0.4153, -1.4664]]])
>>> torch.matrix_power(a, 3)
tensor([[[ 3.9392, -23.9916],
[ 11.7357, -0.2070]],
[[ 0.2468, -6.7168],
[ 2.0774, -0.8187]]])
```
pytorch torch.randint torch.randint
=============
`torch.randint(low=0, high, size, *, generator=None, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Returns a tensor filled with random integers generated uniformly between `low` (inclusive) and `high` (exclusive).
The shape of the tensor is defined by the variable argument `size`.
Note
With the global dtype default (`torch.float32`), this function returns a tensor with dtype `torch.int64`.
Parameters
* **low** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Lowest integer to be drawn from the distribution. Default: 0.
* **high** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – One above the highest integer to be drawn from the distribution.
* **size** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – a tuple defining the shape of the output tensor.
Keyword Arguments
* **generator** ([`torch.Generator`](torch.generator#torch.Generator "torch.Generator"), optional) – a pseudorandom number generator for sampling
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Example:
```
>>> torch.randint(3, 5, (3,))
tensor([4, 3, 4])
>>> torch.randint(10, (2, 2))
tensor([[0, 2],
[5, 5]])
>>> torch.randint(3, 10, (2, 2))
tensor([[4, 5],
[6, 7]])
```
pytorch Softmin Softmin
=======
`class torch.nn.Softmin(dim=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Softmin)
Applies the Softmin function to an n-dimensional input Tensor rescaling them so that the elements of the n-dimensional output Tensor lie in the range `[0, 1]` and sum to 1.
Softmin is defined as:
Softmin(xi)=exp(−xi)∑jexp(−xj)\text{Softmin}(x\_{i}) = \frac{\exp(-x\_i)}{\sum\_j \exp(-x\_j)}
Shape:
* Input: (∗)(\*) where `*` means, any number of additional dimensions
* Output: (∗)(\*) , same shape as the input
Parameters
**dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – A dimension along which Softmin will be computed (so every slice along dim will sum to 1).
Returns
a Tensor of the same dimension and shape as the input, with values in the range [0, 1]
Examples:
```
>>> m = nn.Softmin()
>>> input = torch.randn(2, 3)
>>> output = m(input)
```
pytorch NLLLoss NLLLoss
=======
`class torch.nn.NLLLoss(weight=None, size_average=None, ignore_index=-100, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#NLLLoss)
The negative log likelihood loss. It is useful to train a classification problem with `C` classes.
If provided, the optional argument `weight` should be a 1D Tensor assigning weight to each of the classes. This is particularly useful when you have an unbalanced training set.
The `input` given through a forward call is expected to contain log-probabilities of each class. `input` has to be a Tensor of size either (minibatch,C)(minibatch, C) or (minibatch,C,d1,d2,...,dK)(minibatch, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 for the `K`-dimensional case (described later).
Obtaining log-probabilities in a neural network is easily achieved by adding a `LogSoftmax` layer in the last layer of your network. You may use `CrossEntropyLoss` instead, if you prefer not to add an extra layer.
The `target` that this loss expects should be a class index in the range [0,C−1][0, C-1] where `C = number of classes`; if `ignore_index` is specified, this loss also accepts this class index (this index may not necessarily be in the class range).
The unreduced (i.e. with `reduction` set to `'none'`) loss can be described as:
ℓ(x,y)=L={l1,…,lN}⊤,ln=−wynxn,yn,wc=weight[c]⋅1{c≠ignore\_index},\ell(x, y) = L = \{l\_1,\dots,l\_N\}^\top, \quad l\_n = - w\_{y\_n} x\_{n,y\_n}, \quad w\_{c} = \text{weight}[c] \cdot \mathbb{1}\{c \not= \text{ignore\\_index}\},
where xx is the input, yy is the target, ww is the weight, and NN is the batch size. If `reduction` is not `'none'` (default `'mean'`), then
ℓ(x,y)={∑n=1N1∑n=1Nwynln,if reduction=‘mean’;∑n=1Nln,if reduction=‘sum’.\ell(x, y) = \begin{cases} \sum\_{n=1}^N \frac{1}{\sum\_{n=1}^N w\_{y\_n}} l\_n, & \text{if reduction} = \text{`mean';}\\ \sum\_{n=1}^N l\_n, & \text{if reduction} = \text{`sum'.} \end{cases}
Can also be used for higher dimension inputs, such as 2D images, by providing an input of size (minibatch,C,d1,d2,...,dK)(minibatch, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 , where KK is the number of dimensions, and a target of appropriate shape (see below). In the case of images, it computes NLL loss per-pixel.
Parameters
* **weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – a manual rescaling weight given to each class. If given, it has to be a Tensor of size `C`. Otherwise, it is treated as if having all ones.
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **ignore\_index** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Specifies a target value that is ignored and does not contribute to the input gradient. When `size_average` is `True`, the loss is averaged over non-ignored targets.
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the weighted mean of the output is taken, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Shape:
* Input: (N,C)(N, C) where `C = number of classes`, or (N,C,d1,d2,...,dK)(N, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of `K`-dimensional loss.
* Target: (N)(N) where each value is 0≤targets[i]≤C−10 \leq \text{targets}[i] \leq C-1 , or (N,d1,d2,...,dK)(N, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of K-dimensional loss.
* Output: scalar. If `reduction` is `'none'`, then the same size as the target: (N)(N) , or (N,d1,d2,...,dK)(N, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of K-dimensional loss.
Examples:
```
>>> m = nn.LogSoftmax(dim=1)
>>> loss = nn.NLLLoss()
>>> # input is of size N x C = 3 x 5
>>> input = torch.randn(3, 5, requires_grad=True)
>>> # each element in target has to have 0 <= value < C
>>> target = torch.tensor([1, 0, 4])
>>> output = loss(m(input), target)
>>> output.backward()
>>>
>>>
>>> # 2D loss example (used, for example, with image inputs)
>>> N, C = 5, 4
>>> loss = nn.NLLLoss()
>>> # input is of size N x C x height x width
>>> data = torch.randn(N, 16, 10, 10)
>>> conv = nn.Conv2d(16, C, (3, 3))
>>> m = nn.LogSoftmax(dim=1)
>>> # each element in target has to have 0 <= value < C
>>> target = torch.empty(N, 8, 8, dtype=torch.long).random_(0, C)
>>> output = loss(m(conv(data)), target)
>>> output.backward()
```
pytorch torch.signbit torch.signbit
=============
`torch.signbit(input, *, out=None) → Tensor`
Tests if each element of `input` has its sign bit set (is less than zero) or not.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([0.7, -1.2, 0., 2.3])
>>> torch.signbit(a)
tensor([ False, True, False, False])
```
pytorch torch.rand torch.rand
==========
`torch.rand(*size, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Returns a tensor filled with random numbers from a uniform distribution on the interval [0,1)[0, 1)
The shape of the tensor is defined by the variable argument `size`.
Parameters
**size** (*int...*) – a sequence of integers defining the shape of the output tensor. Can be a variable number of arguments or a collection like a list or tuple.
Keyword Arguments
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Example:
```
>>> torch.rand(4)
tensor([ 0.5204, 0.2503, 0.3525, 0.5673])
>>> torch.rand(2, 3)
tensor([[ 0.8237, 0.5781, 0.6879],
[ 0.3816, 0.7249, 0.0998]])
```
pytorch torch.can_cast torch.can\_cast
===============
`torch.can_cast(from, to) → bool`
Determines if a type conversion is allowed under PyTorch casting rules described in the type promotion [documentation](../tensor_attributes#type-promotion-doc).
Parameters
* **from** (*dpython:type*) – The original [`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype").
* **to** (*dpython:type*) – The target [`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype").
Example:
```
>>> torch.can_cast(torch.double, torch.float)
True
>>> torch.can_cast(torch.float, torch.int)
False
```
pytorch torch.fmin torch.fmin
==========
`torch.fmin(input, other, *, out=None) → Tensor`
Computes the element-wise minimum of `input` and `other`.
This is like [`torch.minimum()`](torch.minimum#torch.minimum "torch.minimum") except it handles NaNs differently: if exactly one of the two elements being compared is a NaN then the non-NaN element is taken as the minimum. Only if both elements are NaN is NaN propagated.
This function is a wrapper around C++’s `std::fmin` and is similar to NumPy’s `fmin` function.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), [type promotion](../tensor_attributes#type-promotion-doc), and integer and floating-point inputs.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([2.2, float('nan'), 2.1, float('nan')])
>>> b = torch.tensor([-9.3, 0.1, float('nan'), float('nan')])
>>> torch.fmin(a, b)
tensor([-9.3000, 0.1000, 2.1000, nan])
```
pytorch torch.cos torch.cos
=========
`torch.cos(input, *, out=None) → Tensor`
Returns a new tensor with the cosine of the elements of `input`.
outi=cos(inputi)\text{out}\_{i} = \cos(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 1.4309, 1.2706, -0.8562, 0.9796])
>>> torch.cos(a)
tensor([ 0.1395, 0.2957, 0.6553, 0.5574])
```
pytorch Flatten Flatten
=======
`class torch.nn.Flatten(start_dim=1, end_dim=-1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/flatten.html#Flatten)
Flattens a contiguous range of dims into a tensor. For use with `Sequential`.
Shape:
* Input: (N,∗dims)(N, \*dims)
* Output: (N,∏∗dims)(N, \prod \*dims) (for the default case).
Parameters
* **start\_dim** – first dim to flatten (default = 1).
* **end\_dim** – last dim to flatten (default = -1).
Examples::
```
>>> input = torch.randn(32, 1, 5, 5)
>>> m = nn.Sequential(
>>> nn.Conv2d(1, 32, 5, 1, 1),
>>> nn.Flatten()
>>> )
>>> output = m(input)
>>> output.size()
torch.Size([32, 288])
```
`add_module(name, module)`
Adds a child module to the current module.
The module can be accessed as an attribute using the given name.
Parameters
* **name** (*string*) – name of the child module. The child module can be accessed from this module using the given name
* **module** ([Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – child module to be added to the module.
`apply(fn)`
Applies `fn` recursively to every submodule (as returned by `.children()`) as well as self. Typical use includes initializing the parameters of a model (see also [torch.nn.init](../nn.init#nn-init-doc)).
Parameters
**fn** ([`Module`](torch.nn.module#torch.nn.Module "torch.nn.Module") -> None) – function to be applied to each submodule
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Example:
```
>>> @torch.no_grad()
>>> def init_weights(m):
>>> print(m)
>>> if type(m) == nn.Linear:
>>> m.weight.fill_(1.0)
>>> print(m.weight)
>>> net = nn.Sequential(nn.Linear(2, 2), nn.Linear(2, 2))
>>> net.apply(init_weights)
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
```
`bfloat16()`
Casts all floating point parameters and buffers to `bfloat16` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`buffers(recurse=True)`
Returns an iterator over module buffers.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*torch.Tensor* – module buffer
Example:
```
>>> for buf in model.buffers():
>>> print(type(buf), buf.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`children()`
Returns an iterator over immediate children modules.
Yields
*Module* – a child module
`cpu()`
Moves all model parameters and buffers to the CPU.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`cuda(device=None)`
Moves all model parameters and buffers to the GPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on GPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`double()`
Casts all floating point parameters and buffers to `double` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`eval()`
Sets the module in evaluation mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
This is equivalent with [`self.train(False)`](torch.nn.module#torch.nn.Module.train "torch.nn.Module.train").
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`float()`
Casts all floating point parameters and buffers to float datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`half()`
Casts all floating point parameters and buffers to `half` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`load_state_dict(state_dict, strict=True)`
Copies parameters and buffers from [`state_dict`](#torch.nn.Flatten.state_dict "torch.nn.Flatten.state_dict") into this module and its descendants. If `strict` is `True`, then the keys of [`state_dict`](#torch.nn.Flatten.state_dict "torch.nn.Flatten.state_dict") must exactly match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function.
Parameters
* **state\_dict** ([dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")) – a dict containing parameters and persistent buffers.
* **strict** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to strictly enforce that the keys in [`state_dict`](#torch.nn.Flatten.state_dict "torch.nn.Flatten.state_dict") match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function. Default: `True`
Returns
* **missing\_keys** is a list of str containing the missing keys
* **unexpected\_keys** is a list of str containing the unexpected keys
Return type
`NamedTuple` with `missing_keys` and `unexpected_keys` fields
`modules()`
Returns an iterator over all modules in the network.
Yields
*Module* – a module in the network
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.modules()):
print(idx, '->', m)
0 -> Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
1 -> Linear(in_features=2, out_features=2, bias=True)
```
`named_buffers(prefix='', recurse=True)`
Returns an iterator over module buffers, yielding both the name of the buffer as well as the buffer itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all buffer names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*(string, torch.Tensor)* – Tuple containing the name and buffer
Example:
```
>>> for name, buf in self.named_buffers():
>>> if name in ['running_var']:
>>> print(buf.size())
```
`named_children()`
Returns an iterator over immediate children modules, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple containing a name and child module
Example:
```
>>> for name, module in model.named_children():
>>> if name in ['conv4', 'conv5']:
>>> print(module)
```
`named_modules(memo=None, prefix='')`
Returns an iterator over all modules in the network, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple of name and module
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.named_modules()):
print(idx, '->', m)
0 -> ('', Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
))
1 -> ('0', Linear(in_features=2, out_features=2, bias=True))
```
`named_parameters(prefix='', recurse=True)`
Returns an iterator over module parameters, yielding both the name of the parameter as well as the parameter itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all parameter names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*(string, Parameter)* – Tuple containing the name and parameter
Example:
```
>>> for name, param in self.named_parameters():
>>> if name in ['bias']:
>>> print(param.size())
```
`parameters(recurse=True)`
Returns an iterator over module parameters.
This is typically passed to an optimizer.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*Parameter* – module parameter
Example:
```
>>> for param in model.parameters():
>>> print(type(param), param.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`register_backward_hook(hook)`
Registers a backward hook on the module.
This function is deprecated in favor of `nn.Module.register_full_backward_hook()` and the behavior of this function will change in future versions.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_buffer(name, tensor, persistent=True)`
Adds a buffer to the module.
This is typically used to register a buffer that should not to be considered a model parameter. For example, BatchNorm’s `running_mean` is not a parameter, but is part of the module’s state. Buffers, by default, are persistent and will be saved alongside parameters. This behavior can be changed by setting `persistent` to `False`. The only difference between a persistent buffer and a non-persistent buffer is that the latter will not be a part of this module’s [`state_dict`](#torch.nn.Flatten.state_dict "torch.nn.Flatten.state_dict").
Buffers can be accessed as attributes using given names.
Parameters
* **name** (*string*) – name of the buffer. The buffer can be accessed from this module using the given name
* **tensor** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – buffer to be registered.
* **persistent** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the buffer is part of this module’s [`state_dict`](#torch.nn.Flatten.state_dict "torch.nn.Flatten.state_dict").
Example:
```
>>> self.register_buffer('running_mean', torch.zeros(num_features))
```
`register_forward_hook(hook)`
Registers a forward hook on the module.
The hook will be called every time after `forward()` has computed an output. It should have the following signature:
```
hook(module, input, output) -> None or modified output
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the output. It can modify the input inplace but it will not have effect on forward since this is called after `forward()` is called.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_forward_pre_hook(hook)`
Registers a forward pre-hook on the module.
The hook will be called every time before `forward()` is invoked. It should have the following signature:
```
hook(module, input) -> None or modified input
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the input. User can either return a tuple or a single modified value in the hook. We will wrap the value into a tuple if a single value is returned(unless that value is already a tuple).
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_full_backward_hook(hook)`
Registers a backward hook on the module.
The hook will be called every time the gradients with respect to module inputs are computed. The hook should have the following signature:
```
hook(module, grad_input, grad_output) -> tuple(Tensor) or None
```
The `grad_input` and `grad_output` are tuples that contain the gradients with respect to the inputs and outputs respectively. The hook should not modify its arguments, but it can optionally return a new gradient with respect to the input that will be used in place of `grad_input` in subsequent computations. `grad_input` will only correspond to the inputs given as positional arguments and all kwarg arguments are ignored. Entries in `grad_input` and `grad_output` will be `None` for all non-Tensor arguments.
Warning
Modifying inputs or outputs inplace is not allowed when using backward hooks and will raise an error.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_parameter(name, param)`
Adds a parameter to the module.
The parameter can be accessed as an attribute using given name.
Parameters
* **name** (*string*) – name of the parameter. The parameter can be accessed from this module using the given name
* **param** ([Parameter](torch.nn.parameter.parameter#torch.nn.parameter.Parameter "torch.nn.parameter.Parameter")) – parameter to be added to the module.
`requires_grad_(requires_grad=True)`
Change if autograd should record operations on parameters in this module.
This method sets the parameters’ `requires_grad` attributes in-place.
This method is helpful for freezing part of the module for finetuning or training parts of a model individually (e.g., GAN training).
Parameters
**requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether autograd should record operations on parameters in this module. Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`state_dict(destination=None, prefix='', keep_vars=False)`
Returns a dictionary containing a whole state of the module.
Both parameters and persistent buffers (e.g. running averages) are included. Keys are corresponding parameter and buffer names.
Returns
a dictionary containing a whole state of the module
Return type
[dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")
Example:
```
>>> module.state_dict().keys()
['bias', 'weight']
```
`to(*args, **kwargs)`
Moves and/or casts the parameters and buffers.
This can be called as
`to(device=None, dtype=None, non_blocking=False)`
`to(dtype, non_blocking=False)`
`to(tensor, non_blocking=False)`
`to(memory_format=torch.channels_last)`
Its signature is similar to [`torch.Tensor.to()`](../tensors#torch.Tensor.to "torch.Tensor.to"), but only accepts floating point or complex `dtype`s. In addition, this method will
only cast the floating point or complex parameters and buffers to :attr:`dtype` (if given). The integral parameters and buffers will be moved `device`, if that is given, but with dtypes unchanged. When `non_blocking` is set, it tries to convert/move asynchronously with respect to the host if possible, e.g., moving CPU Tensors with pinned memory to CUDA devices.
See below for examples.
Note
This method modifies the module in-place.
Parameters
* **device** (`torch.device`) – the desired device of the parameters and buffers in this module
* **dtype** (`torch.dtype`) – the desired floating point or complex dtype of the parameters and buffers in this module
* **tensor** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Tensor whose dtype and device are the desired dtype and device for all parameters and buffers in this module
* **memory\_format** (`torch.memory_format`) – the desired memory format for 4D parameters and buffers in this module (keyword only argument)
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Examples:
```
>>> linear = nn.Linear(2, 2)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]])
>>> linear.to(torch.double)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]], dtype=torch.float64)
>>> gpu1 = torch.device("cuda:1")
>>> linear.to(gpu1, dtype=torch.half, non_blocking=True)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16, device='cuda:1')
>>> cpu = torch.device("cpu")
>>> linear.to(cpu)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16)
>>> linear = nn.Linear(2, 2, bias=None).to(torch.cdouble)
>>> linear.weight
Parameter containing:
tensor([[ 0.3741+0.j, 0.2382+0.j],
[ 0.5593+0.j, -0.4443+0.j]], dtype=torch.complex128)
>>> linear(torch.ones(3, 2, dtype=torch.cdouble))
tensor([[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j]], dtype=torch.complex128)
```
`train(mode=True)`
Sets the module in training mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
Parameters
**mode** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to set training mode (`True`) or evaluation mode (`False`). Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`type(dst_type)`
Casts all parameters and buffers to `dst_type`.
Parameters
**dst\_type** ([type](https://docs.python.org/3/library/functions.html#type "(in Python v3.9)") *or* *string*) – the desired type
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`xpu(device=None)`
Moves all model parameters and buffers to the XPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on XPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`zero_grad(set_to_none=False)`
Sets gradients of all model parameters to zero. See similar function under [`torch.optim.Optimizer`](../optim#torch.optim.Optimizer "torch.optim.Optimizer") for more context.
Parameters
**set\_to\_none** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – instead of setting to zero, set the grads to None. See [`torch.optim.Optimizer.zero_grad()`](../optim#torch.optim.Optimizer.zero_grad "torch.optim.Optimizer.zero_grad") for details.
| programming_docs |
pytorch torch.nn.utils.prune.global_unstructured torch.nn.utils.prune.global\_unstructured
=========================================
`torch.nn.utils.prune.global_unstructured(parameters, pruning_method, importance_scores=None, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#global_unstructured)
Globally prunes tensors corresponding to all parameters in `parameters` by applying the specified `pruning_method`. Modifies modules in place by: 1) adding a named buffer called `name+'_mask'` corresponding to the binary mask applied to the parameter `name` by the pruning method. 2) replacing the parameter `name` by its pruned version, while the original (unpruned) parameter is stored in a new parameter named `name+'_orig'`.
Parameters
* **parameters** (*Iterable of* *(**module**,* *name**)* *tuples*) – parameters of the model to prune in a global fashion, i.e. by aggregating all weights prior to deciding which ones to prune. module must be of type `nn.Module`, and name must be a string.
* **pruning\_method** (*function*) – a valid pruning function from this module, or a custom one implemented by the user that satisfies the implementation guidelines and has `PRUNING_TYPE='unstructured'`.
* **importance\_scores** ([dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")) – a dictionary mapping (module, name) tuples to the corresponding parameter’s importance scores tensor. The tensor should be the same shape as the parameter, and is used for computing mask for pruning. If unspecified or None, the parameter will be used in place of its importance scores.
* **kwargs** – other keyword arguments such as: amount (int or float): quantity of parameters to prune across the specified parameters. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
Raises
[**TypeError**](https://docs.python.org/3/library/exceptions.html#TypeError "(in Python v3.9)") – if `PRUNING_TYPE != 'unstructured'`
Note
Since global structured pruning doesn’t make much sense unless the norm is normalized by the size of the parameter, we now limit the scope of global pruning to unstructured methods.
#### Examples
```
>>> net = nn.Sequential(OrderedDict([
('first', nn.Linear(10, 4)),
('second', nn.Linear(4, 1)),
]))
>>> parameters_to_prune = (
(net.first, 'weight'),
(net.second, 'weight'),
)
>>> prune.global_unstructured(
parameters_to_prune,
pruning_method=prune.L1Unstructured,
amount=10,
)
>>> print(sum(torch.nn.utils.parameters_to_vector(net.buffers()) == 0))
tensor(10, dtype=torch.uint8)
```
pytorch torch.inner torch.inner
===========
`torch.inner(input, other, *, out=None) → Tensor`
Computes the dot product for 1D tensors. For higher dimensions, sums the product of elements from `input` and `other` along their last dimension.
Note
If either `input` or `other` is a scalar, the result is equivalent to `torch.mul(input, other)`.
If both `input` and `other` are non-scalars, the size of their last dimension must match and the result is equivalent to `torch.tensordot(input, other, dims=([-1], [-1]))`
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – First input tensor
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – Second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – Optional output tensor to write result into. The output shape is `input.shape[:-1] + other.shape[:-1]`.
Example:
```
# Dot product
>>> torch.inner(torch.tensor([1, 2, 3]), torch.tensor([0, 2, 1]))
tensor(7)
# Multidimensional input tensors
>>> a = torch.randn(2, 3)
>>> a
tensor([[0.8173, 1.0874, 1.1784],
[0.3279, 0.1234, 2.7894]])
>>> b = torch.randn(2, 4, 3)
>>> b
tensor([[[-0.4682, -0.7159, 0.1506],
[ 0.4034, -0.3657, 1.0387],
[ 0.9892, -0.6684, 0.1774],
[ 0.9482, 1.3261, 0.3917]],
[[ 0.4537, 0.7493, 1.1724],
[ 0.2291, 0.5749, -0.2267],
[-0.7920, 0.3607, -0.3701],
[ 1.3666, -0.5850, -1.7242]]])
>>> torch.inner(a, b)
tensor([[[-0.9837, 1.1560, 0.2907, 2.6785],
[ 2.5671, 0.5452, -0.6912, -1.5509]],
[[ 0.1782, 2.9843, 0.7366, 1.5672],
[ 3.5115, -0.4864, -1.2476, -4.4337]]])
# Scalar input
>>> torch.inner(a, torch.tensor(2))
tensor([[1.6347, 2.1748, 2.3567],
[0.6558, 0.2469, 5.5787]])
```
pytorch AdaptiveAvgPool3d AdaptiveAvgPool3d
=================
`class torch.nn.AdaptiveAvgPool3d(output_size)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveAvgPool3d)
Applies a 3D adaptive average pooling over an input signal composed of several input planes.
The output is of size D x H x W, for any input size. The number of output features is equal to the number of input planes.
Parameters
**output\_size** – the target output size of the form D x H x W. Can be a tuple (D, H, W) or a single number D for a cube D x D x D. D, H and W can be either a `int`, or `None` which means the size will be the same as that of the input.
#### Examples
```
>>> # target output size of 5x7x9
>>> m = nn.AdaptiveAvgPool3d((5,7,9))
>>> input = torch.randn(1, 64, 8, 9, 10)
>>> output = m(input)
>>> # target output size of 7x7x7 (cube)
>>> m = nn.AdaptiveAvgPool3d(7)
>>> input = torch.randn(1, 64, 10, 9, 8)
>>> output = m(input)
>>> # target output size of 7x9x8
>>> m = nn.AdaptiveAvgPool3d((7, None, None))
>>> input = torch.randn(1, 64, 10, 9, 8)
>>> output = m(input)
```
pytorch torch.istft torch.istft
===========
`torch.istft(input, n_fft, hop_length=None, win_length=None, window=None, center=True, normalized=False, onesided=None, length=None, return_complex=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#istft)
Inverse short time Fourier Transform. This is expected to be the inverse of [`stft()`](torch.stft#torch.stft "torch.stft"). It has the same parameters (+ additional optional parameter of `length`) and it should return the least squares estimation of the original signal. The algorithm will check using the NOLA condition ( nonzero overlap).
Important consideration in the parameters `window` and `center` so that the envelop created by the summation of all the windows is never zero at certain point in time. Specifically, ∑t=−∞∞∣w∣2[n−t×hop\_length]=0\sum\_{t=-\infty}^{\infty} |w|^2[n-t\times hop\\_length] \cancel{=} 0 .
Since [`stft()`](torch.stft#torch.stft "torch.stft") discards elements at the end of the signal if they do not fit in a frame, `istft` may return a shorter signal than the original signal (can occur if `center` is False since the signal isn’t padded).
If `center` is `True`, then there will be padding e.g. `'constant'`, `'reflect'`, etc. Left padding can be trimmed off exactly because they can be calculated but right padding cannot be calculated without additional information.
Example: Suppose the last window is: `[17, 18, 0, 0, 0]` vs `[18, 0, 0, 0, 0]`
The `n_fft`, `hop_length`, `win_length` are all the same which prevents the calculation of right padding. These additional values could be zeros or a reflection of the signal so providing `length` could be useful. If `length` is `None` then padding will be aggressively removed (some loss of signal).
[1] D. W. Griffin and J. S. Lim, “Signal estimation from modified short-time Fourier transform,” IEEE Trans. ASSP, vol.32, no.2, pp.236-243, Apr. 1984.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) –
The input tensor. Expected to be output of [`stft()`](torch.stft#torch.stft "torch.stft"), can either be complex (`channel`, `fft_size`, `n_frame`), or real (`channel`, `fft_size`, `n_frame`, 2) where the `channel` dimension is optional.
Deprecated since version 1.8.0: Real input is deprecated, use complex inputs as returned by `stft(..., return_complex=True)` instead.
* **n\_fft** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Size of Fourier transform
* **hop\_length** (*Optional**[*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*]*) – The distance between neighboring sliding window frames. (Default: `n_fft // 4`)
* **win\_length** (*Optional**[*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*]*) – The size of window frame and STFT filter. (Default: `n_fft`)
* **window** (*Optional**[*[torch.Tensor](../tensors#torch.Tensor "torch.Tensor")*]*) – The optional window function. (Default: `torch.ones(win_length)`)
* **center** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – Whether `input` was padded on both sides so that the tt -th frame is centered at time t×hop\_lengtht \times \text{hop\\_length} . (Default: `True`)
* **normalized** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – Whether the STFT was normalized. (Default: `False`)
* **onesided** (*Optional**[*[bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*]*) – Whether the STFT was onesided. (Default: `True` if `n_fft != fft_size` in the input size)
* **length** (*Optional**[*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*]*) – The amount to trim the signal by (i.e. the original signal length). (Default: whole signal)
* **return\_complex** (*Optional**[*[bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*]*) – Whether the output should be complex, or if the input should be assumed to derive from a real signal and window. Note that this is incompatible with `onesided=True`. (Default: `False`)
Returns
Least squares estimation of the original signal of size (…, signal\_length)
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
pytorch torch.masked_select torch.masked\_select
====================
`torch.masked_select(input, mask, *, out=None) → Tensor`
Returns a new 1-D tensor which indexes the `input` tensor according to the boolean mask `mask` which is a `BoolTensor`.
The shapes of the `mask` tensor and the `input` tensor don’t need to match, but they must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
Note
The returned tensor does **not** use the same storage as the original tensor
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **mask** (*BoolTensor*) – the tensor containing the binary mask to index with
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> x = torch.randn(3, 4)
>>> x
tensor([[ 0.3552, -2.3825, -0.8297, 0.3477],
[-1.2035, 1.2252, 0.5002, 0.6248],
[ 0.1307, -2.0608, 0.1244, 2.0139]])
>>> mask = x.ge(0.5)
>>> mask
tensor([[False, False, False, False],
[False, True, True, True],
[False, False, False, True]])
>>> torch.masked_select(x, mask)
tensor([ 1.2252, 0.5002, 0.6248, 2.0139])
```
pytorch ConvTranspose2d ConvTranspose2d
===============
`class torch.nn.ConvTranspose2d(in_channels, out_channels, kernel_size, stride=1, padding=0, output_padding=0, groups=1, bias=True, dilation=1, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#ConvTranspose2d)
Applies a 2D transposed convolution operator over an input image composed of several input planes.
This module can be seen as the gradient of Conv2d with respect to its input. It is also known as a fractionally-strided convolution or a deconvolution (although it is not an actual deconvolution operation).
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation.
* `padding` controls the amount of implicit zero padding on both sides for `dilation * (kernel_size - 1) - padding` number of points. See note below for details.
* `output_padding` controls the additional size added to one side of the output shape. See note below for details.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
The parameters `kernel_size`, `stride`, `padding`, `output_padding` can either be:
* a single `int` – in which case the same value is used for the height and width dimensions
* a `tuple` of two ints – in which case, the first `int` is used for the height dimension, and the second `int` for the width dimension
Note
The `padding` argument effectively adds `dilation * (kernel_size - 1) - padding` amount of zero padding to both sizes of the input. This is set so that when a [`Conv2d`](torch.nn.conv2d#torch.nn.Conv2d "torch.nn.Conv2d") and a [`ConvTranspose2d`](#torch.nn.ConvTranspose2d "torch.nn.ConvTranspose2d") are initialized with same parameters, they are inverses of each other in regard to the input and output shapes. However, when `stride > 1`, [`Conv2d`](torch.nn.conv2d#torch.nn.Conv2d "torch.nn.Conv2d") maps multiple input shapes to the same output shape. `output_padding` is provided to resolve this ambiguity by effectively increasing the calculated output shape on one side. Note that `output_padding` is only used to find output shape, but does not actually add zero-padding to output.
Note
In some circumstances when given tensors on a CUDA device and using CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic = True`. See [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for more information.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – `dilation * (kernel_size - 1) - padding` zero-padding will be added to both sides of each dimension in the input. Default: 0
* **output\_padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Additional size added to one side of each dimension in the output shape. Default: 0
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
Shape:
* Input: (N,Cin,Hin,Win)(N, C\_{in}, H\_{in}, W\_{in})
* Output: (N,Cout,Hout,Wout)(N, C\_{out}, H\_{out}, W\_{out}) where
Hout=(Hin−1)×stride[0]−2×padding[0]+dilation[0]×(kernel\_size[0]−1)+output\_padding[0]+1H\_{out} = (H\_{in} - 1) \times \text{stride}[0] - 2 \times \text{padding}[0] + \text{dilation}[0] \times (\text{kernel\\_size}[0] - 1) + \text{output\\_padding}[0] + 1
Wout=(Win−1)×stride[1]−2×padding[1]+dilation[1]×(kernel\_size[1]−1)+output\_padding[1]+1W\_{out} = (W\_{in} - 1) \times \text{stride}[1] - 2 \times \text{padding}[1] + \text{dilation}[1] \times (\text{kernel\\_size}[1] - 1) + \text{output\\_padding}[1] + 1
Variables
* **~ConvTranspose2d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (in\_channels,out\_channelsgroups,(\text{in\\_channels}, \frac{\text{out\\_channels}}{\text{groups}}, kernel\_size[0],kernel\_size[1])\text{kernel\\_size[0]}, \text{kernel\\_size[1]}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗∏i=01kernel\_size[i]k = \frac{groups}{C\_\text{out} \* \prod\_{i=0}^{1}\text{kernel\\_size}[i]}
* **~ConvTranspose2d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels) If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗∏i=01kernel\_size[i]k = \frac{groups}{C\_\text{out} \* \prod\_{i=0}^{1}\text{kernel\\_size}[i]}
Examples:
```
>>> # With square kernels and equal stride
>>> m = nn.ConvTranspose2d(16, 33, 3, stride=2)
>>> # non-square kernels and unequal stride and with padding
>>> m = nn.ConvTranspose2d(16, 33, (3, 5), stride=(2, 1), padding=(4, 2))
>>> input = torch.randn(20, 16, 50, 100)
>>> output = m(input)
>>> # exact output size can be also specified as an argument
>>> input = torch.randn(1, 16, 12, 12)
>>> downsample = nn.Conv2d(16, 16, 3, stride=2, padding=1)
>>> upsample = nn.ConvTranspose2d(16, 16, 3, stride=2, padding=1)
>>> h = downsample(input)
>>> h.size()
torch.Size([1, 16, 6, 6])
>>> output = upsample(h, output_size=input.size())
>>> output.size()
torch.Size([1, 16, 12, 12])
```
pytorch Conv1d Conv1d
======
`class torch.nn.Conv1d(in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#Conv1d)
Applies a 1D convolution over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,Cin,L)(N, C\_{\text{in}}, L) and output (N,Cout,Lout)(N, C\_{\text{out}}, L\_{\text{out}}) can be precisely described as:
out(Ni,Coutj)=bias(Coutj)+∑k=0Cin−1weight(Coutj,k)⋆input(Ni,k)\text{out}(N\_i, C\_{\text{out}\_j}) = \text{bias}(C\_{\text{out}\_j}) + \sum\_{k = 0}^{C\_{in} - 1} \text{weight}(C\_{\text{out}\_j}, k) \star \text{input}(N\_i, k)
where ⋆\star is the valid [cross-correlation](https://en.wikipedia.org/wiki/Cross-correlation) operator, NN is a batch size, CC denotes a number of channels, LL is a length of signal sequence.
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation, a single number or a one-element tuple.
* `padding` controls the amount of implicit padding on both sides for `padding` number of points.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
Note
When `groups == in_channels` and `out_channels == K * in_channels`, where `K` is a positive integer, this operation is also known as a “depthwise convolution”.
In other words, for an input of size (N,Cin,Lin)(N, C\_{in}, L\_{in}) , a depthwise convolution with a depthwise multiplier `K` can be performed with the arguments (Cin=Cin,Cout=Cin×K,...,groups=Cin)(C\_\text{in}=C\_\text{in}, C\_\text{out}=C\_\text{in} \times \text{K}, ..., \text{groups}=C\_\text{in}) .
Note
In some circumstances when given tensors on a CUDA device and using CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic = True`. See [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for more information.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Zero-padding added to both sides of the input. Default: 0
* **padding\_mode** (*string**,* *optional*) – `'zeros'`, `'reflect'`, `'replicate'` or `'circular'`. Default: `'zeros'`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
Shape:
* Input: (N,Cin,Lin)(N, C\_{in}, L\_{in})
* Output: (N,Cout,Lout)(N, C\_{out}, L\_{out}) where
Lout=⌊Lin+2×padding−dilation×(kernel\_size−1)−1stride+1⌋L\_{out} = \left\lfloor\frac{L\_{in} + 2 \times \text{padding} - \text{dilation} \times (\text{kernel\\_size} - 1) - 1}{\text{stride}} + 1\right\rfloor
Variables
* **~Conv1d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (out\_channels,in\_channelsgroups,kernel\_size)(\text{out\\_channels}, \frac{\text{in\\_channels}}{\text{groups}}, \text{kernel\\_size}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗kernel\_sizek = \frac{groups}{C\_\text{in} \* \text{kernel\\_size}}
* **~Conv1d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels). If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗kernel\_sizek = \frac{groups}{C\_\text{in} \* \text{kernel\\_size}}
Examples:
```
>>> m = nn.Conv1d(16, 33, 3, stride=2)
>>> input = torch.randn(20, 16, 50)
>>> output = m(input)
```
| programming_docs |
pytorch torch.nn.utils.prune.random_structured torch.nn.utils.prune.random\_structured
=======================================
`torch.nn.utils.prune.random_structured(module, name, amount, dim)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#random_structured)
Prunes tensor corresponding to parameter called `name` in `module` by removing the specified `amount` of (currently unpruned) channels along the specified `dim` selected at random. Modifies module in place (and also return the modified module) by: 1) adding a named buffer called `name+'_mask'` corresponding to the binary mask applied to the parameter `name` by the pruning method. 2) replacing the parameter `name` by its pruned version, while the original (unpruned) parameter is stored in a new parameter named `name+'_orig'`.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – index of the dim along which we define channels to prune.
Returns
modified (i.e. pruned) version of the input module
Return type
module ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module"))
#### Examples
```
>>> m = prune.random_structured(
nn.Linear(5, 3), 'weight', amount=3, dim=1
)
>>> columns_pruned = int(sum(torch.sum(m.weight, dim=0) == 0))
>>> print(columns_pruned)
3
```
pytorch torch.eye torch.eye
=========
`torch.eye(n, m=None, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Returns a 2-D tensor with ones on the diagonal and zeros elsewhere.
Parameters
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the number of rows
* **m** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the number of columns with default being `n`
Keyword Arguments
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Returns
A 2-D tensor with ones on the diagonal and zeros elsewhere
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> torch.eye(3)
tensor([[ 1., 0., 0.],
[ 0., 1., 0.],
[ 0., 0., 1.]])
```
pytorch torch.acos torch.acos
==========
`torch.acos(input, *, out=None) → Tensor`
Computes the inverse cosine of each element in `input`.
outi=cos−1(inputi)\text{out}\_{i} = \cos^{-1}(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.3348, -0.5889, 0.2005, -0.1584])
>>> torch.acos(a)
tensor([ 1.2294, 2.2004, 1.3690, 1.7298])
```
pytorch AdaptiveMaxPool2d AdaptiveMaxPool2d
=================
`class torch.nn.AdaptiveMaxPool2d(output_size, return_indices=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveMaxPool2d)
Applies a 2D adaptive max pooling over an input signal composed of several input planes.
The output is of size H x W, for any input size. The number of output features is equal to the number of input planes.
Parameters
* **output\_size** – the target output size of the image of the form H x W. Can be a tuple (H, W) or a single H for a square image H x H. H and W can be either a `int`, or `None` which means the size will be the same as that of the input.
* **return\_indices** – if `True`, will return the indices along with the outputs. Useful to pass to nn.MaxUnpool2d. Default: `False`
#### Examples
```
>>> # target output size of 5x7
>>> m = nn.AdaptiveMaxPool2d((5,7))
>>> input = torch.randn(1, 64, 8, 9)
>>> output = m(input)
>>> # target output size of 7x7 (square)
>>> m = nn.AdaptiveMaxPool2d(7)
>>> input = torch.randn(1, 64, 10, 9)
>>> output = m(input)
>>> # target output size of 10x7
>>> m = nn.AdaptiveMaxPool2d((None, 7))
>>> input = torch.randn(1, 64, 10, 9)
>>> output = m(input)
```
pytorch torch.is_tensor torch.is\_tensor
================
`torch.is_tensor(obj)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#is_tensor)
Returns True if `obj` is a PyTorch tensor.
Note that this function is simply doing `isinstance(obj, Tensor)`. Using that `isinstance` check is better for typechecking with mypy, and more explicit - so it’s recommended to use that instead of `is_tensor`.
Parameters
**obj** (*Object*) – Object to test
pytorch torch.polygamma torch.polygamma
===============
`torch.polygamma(n, input, *, out=None) → Tensor`
Computes the nthn^{th} derivative of the digamma function on `input`. n≥0n \geq 0 is called the order of the polygamma function.
ψ(n)(x)=d(n)dx(n)ψ(x)\psi^{(n)}(x) = \frac{d^{(n)}}{dx^{(n)}} \psi(x)
Note
This function is implemented only for nonnegative integers n≥0n \geq 0 .
Parameters
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the order of the polygamma function
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example::
```
>>> a = torch.tensor([1, 0.5])
>>> torch.polygamma(1, a)
tensor([1.64493, 4.9348])
>>> torch.polygamma(2, a)
tensor([ -2.4041, -16.8288])
>>> torch.polygamma(3, a)
tensor([ 6.4939, 97.4091])
>>> torch.polygamma(4, a)
tensor([ -24.8863, -771.4742])
```
pytorch torch.round torch.round
===========
`torch.round(input, *, out=None) → Tensor`
Returns a new tensor with each of the elements of `input` rounded to the closest integer.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.9920, 0.6077, 0.9734, -1.0362])
>>> torch.round(a)
tensor([ 1., 1., 1., -1.])
```
pytorch torch.logaddexp2 torch.logaddexp2
================
`torch.logaddexp2(input, other, *, out=None) → Tensor`
Logarithm of the sum of exponentiations of the inputs in base-2.
Calculates pointwise log2(2x+2y)\log\_2\left(2^x + 2^y\right) . See [`torch.logaddexp()`](torch.logaddexp#torch.logaddexp "torch.logaddexp") for more details.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
pytorch torch.triangular_solve torch.triangular\_solve
=======================
`torch.triangular_solve(input, A, upper=True, transpose=False, unitriangular=False) -> (Tensor, Tensor)`
Solves a system of equations with a triangular coefficient matrix AA and multiple right-hand sides bb .
In particular, solves AX=bAX = b and assumes AA is upper-triangular with the default keyword arguments.
`torch.triangular_solve(b, A)` can take in 2D inputs `b, A` or inputs that are batches of 2D matrices. If the inputs are batches, then returns batched outputs `X`
Supports real-valued and complex-valued inputs.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – multiple right-hand sides of size (∗,m,k)(\*, m, k) where ∗\* is zero of more batch dimensions (bb )
* **A** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input triangular coefficient matrix of size (∗,m,m)(\*, m, m) where ∗\* is zero or more batch dimensions
* **upper** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to solve the upper-triangular system of equations (default) or the lower-triangular system of equations. Default: `True`.
* **transpose** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether AA should be transposed before being sent into the solver. Default: `False`.
* **unitriangular** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether AA is unit triangular. If True, the diagonal elements of AA are assumed to be 1 and not referenced from AA . Default: `False`.
Returns
A namedtuple `(solution, cloned_coefficient)` where `cloned_coefficient` is a clone of AA and `solution` is the solution XX to AX=bAX = b (or whatever variant of the system of equations, depending on the keyword arguments.)
Examples:
```
>>> A = torch.randn(2, 2).triu()
>>> A
tensor([[ 1.1527, -1.0753],
[ 0.0000, 0.7986]])
>>> b = torch.randn(2, 3)
>>> b
tensor([[-0.0210, 2.3513, -1.5492],
[ 1.5429, 0.7403, -1.0243]])
>>> torch.triangular_solve(b, A)
torch.return_types.triangular_solve(
solution=tensor([[ 1.7841, 2.9046, -2.5405],
[ 1.9320, 0.9270, -1.2826]]),
cloned_coefficient=tensor([[ 1.1527, -1.0753],
[ 0.0000, 0.7986]]))
```
pytorch Softsign Softsign
========
`class torch.nn.Softsign` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Softsign)
Applies the element-wise function:
SoftSign(x)=x1+∣x∣\text{SoftSign}(x) = \frac{x}{ 1 + |x|}
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.Softsign()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.cross torch.cross
===========
`torch.cross(input, other, dim=None, *, out=None) → Tensor`
Returns the cross product of vectors in dimension `dim` of `input` and `other`.
`input` and `other` must have the same size, and the size of their `dim` dimension should be 3.
If `dim` is not given, it defaults to the first dimension found with the size 3. Note that this might be unexpected.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the dimension to take the cross-product in.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4, 3)
>>> a
tensor([[-0.3956, 1.1455, 1.6895],
[-0.5849, 1.3672, 0.3599],
[-1.1626, 0.7180, -0.0521],
[-0.1339, 0.9902, -2.0225]])
>>> b = torch.randn(4, 3)
>>> b
tensor([[-0.0257, -1.4725, -1.2251],
[-1.1479, -0.7005, -1.9757],
[-1.3904, 0.3726, -1.1836],
[-0.9688, -0.7153, 0.2159]])
>>> torch.cross(a, b, dim=1)
tensor([[ 1.0844, -0.5281, 0.6120],
[-2.4490, -1.5687, 1.9792],
[-0.8304, -1.3037, 0.5650],
[-1.2329, 1.9883, 1.0551]])
>>> torch.cross(a, b)
tensor([[ 1.0844, -0.5281, 0.6120],
[-2.4490, -1.5687, 1.9792],
[-0.8304, -1.3037, 0.5650],
[-1.2329, 1.9883, 1.0551]])
```
pytorch LocalResponseNorm LocalResponseNorm
=================
`class torch.nn.LocalResponseNorm(size, alpha=0.0001, beta=0.75, k=1.0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/normalization.html#LocalResponseNorm)
Applies local response normalization over an input signal composed of several input planes, where channels occupy the second dimension. Applies normalization across channels.
bc=ac(k+αn∑c′=max(0,c−n/2)min(N−1,c+n/2)ac′2)−βb\_{c} = a\_{c}\left(k + \frac{\alpha}{n} \sum\_{c'=\max(0, c-n/2)}^{\min(N-1,c+n/2)}a\_{c'}^2\right)^{-\beta}
Parameters
* **size** – amount of neighbouring channels used for normalization
* **alpha** – multiplicative factor. Default: 0.0001
* **beta** – exponent. Default: 0.75
* **k** – additive factor. Default: 1
Shape:
* Input: (N,C,∗)(N, C, \*)
* Output: (N,C,∗)(N, C, \*) (same shape as input)
Examples:
```
>>> lrn = nn.LocalResponseNorm(2)
>>> signal_2d = torch.randn(32, 5, 24, 24)
>>> signal_4d = torch.randn(16, 5, 7, 7, 7, 7)
>>> output_2d = lrn(signal_2d)
>>> output_4d = lrn(signal_4d)
```
pytorch Unflatten Unflatten
=========
`class torch.nn.Unflatten(dim, unflattened_size)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/flatten.html#Unflatten)
Unflattens a tensor dim expanding it to a desired shape. For use with `Sequential`.
* `dim` specifies the dimension of the input tensor to be unflattened, and it can be either `int` or `str` when `Tensor` or `NamedTensor` is used, respectively.
* `unflattened_size` is the new shape of the unflattened dimension of the tensor and it can be a `tuple` of ints or a `list` of ints or `torch.Size` for `Tensor` input; a `NamedShape` (tuple of `(name, size)` tuples) for `NamedTensor` input.
Shape:
* Input: (N,∗dims)(N, \*dims)
* Output: (N,Cout,Hout,Wout)(N, C\_{\text{out}}, H\_{\text{out}}, W\_{\text{out}})
Parameters
* **dim** (*Union**[*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")*]*) – Dimension to be unflattened
* **unflattened\_size** (*Union**[**torch.Size**,* *Tuple**,* *List**,* *NamedShape**]*) – New shape of the unflattened dimension
#### Examples
```
>>> input = torch.randn(2, 50)
>>> # With tuple of ints
>>> m = nn.Sequential(
>>> nn.Linear(50, 50),
>>> nn.Unflatten(1, (2, 5, 5))
>>> )
>>> output = m(input)
>>> output.size()
torch.Size([2, 2, 5, 5])
>>> # With torch.Size
>>> m = nn.Sequential(
>>> nn.Linear(50, 50),
>>> nn.Unflatten(1, torch.Size([2, 5, 5]))
>>> )
>>> output = m(input)
>>> output.size()
torch.Size([2, 2, 5, 5])
>>> # With namedshape (tuple of tuples)
>>> input = torch.randn(2, 50, names=('N', 'features'))
>>> unflatten = nn.Unflatten('features', (('C', 2), ('H', 5), ('W', 5)))
>>> output = unflatten(input)
>>> output.size()
torch.Size([2, 2, 5, 5])
```
`add_module(name, module)`
Adds a child module to the current module.
The module can be accessed as an attribute using the given name.
Parameters
* **name** (*string*) – name of the child module. The child module can be accessed from this module using the given name
* **module** ([Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – child module to be added to the module.
`apply(fn)`
Applies `fn` recursively to every submodule (as returned by `.children()`) as well as self. Typical use includes initializing the parameters of a model (see also [torch.nn.init](../nn.init#nn-init-doc)).
Parameters
**fn** ([`Module`](torch.nn.module#torch.nn.Module "torch.nn.Module") -> None) – function to be applied to each submodule
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Example:
```
>>> @torch.no_grad()
>>> def init_weights(m):
>>> print(m)
>>> if type(m) == nn.Linear:
>>> m.weight.fill_(1.0)
>>> print(m.weight)
>>> net = nn.Sequential(nn.Linear(2, 2), nn.Linear(2, 2))
>>> net.apply(init_weights)
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
```
`bfloat16()`
Casts all floating point parameters and buffers to `bfloat16` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`buffers(recurse=True)`
Returns an iterator over module buffers.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*torch.Tensor* – module buffer
Example:
```
>>> for buf in model.buffers():
>>> print(type(buf), buf.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`children()`
Returns an iterator over immediate children modules.
Yields
*Module* – a child module
`cpu()`
Moves all model parameters and buffers to the CPU.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`cuda(device=None)`
Moves all model parameters and buffers to the GPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on GPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`double()`
Casts all floating point parameters and buffers to `double` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`eval()`
Sets the module in evaluation mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
This is equivalent with [`self.train(False)`](torch.nn.module#torch.nn.Module.train "torch.nn.Module.train").
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`float()`
Casts all floating point parameters and buffers to float datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`half()`
Casts all floating point parameters and buffers to `half` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`load_state_dict(state_dict, strict=True)`
Copies parameters and buffers from [`state_dict`](#torch.nn.Unflatten.state_dict "torch.nn.Unflatten.state_dict") into this module and its descendants. If `strict` is `True`, then the keys of [`state_dict`](#torch.nn.Unflatten.state_dict "torch.nn.Unflatten.state_dict") must exactly match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function.
Parameters
* **state\_dict** ([dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")) – a dict containing parameters and persistent buffers.
* **strict** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to strictly enforce that the keys in [`state_dict`](#torch.nn.Unflatten.state_dict "torch.nn.Unflatten.state_dict") match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function. Default: `True`
Returns
* **missing\_keys** is a list of str containing the missing keys
* **unexpected\_keys** is a list of str containing the unexpected keys
Return type
`NamedTuple` with `missing_keys` and `unexpected_keys` fields
`modules()`
Returns an iterator over all modules in the network.
Yields
*Module* – a module in the network
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.modules()):
print(idx, '->', m)
0 -> Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
1 -> Linear(in_features=2, out_features=2, bias=True)
```
`named_buffers(prefix='', recurse=True)`
Returns an iterator over module buffers, yielding both the name of the buffer as well as the buffer itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all buffer names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*(string, torch.Tensor)* – Tuple containing the name and buffer
Example:
```
>>> for name, buf in self.named_buffers():
>>> if name in ['running_var']:
>>> print(buf.size())
```
`named_children()`
Returns an iterator over immediate children modules, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple containing a name and child module
Example:
```
>>> for name, module in model.named_children():
>>> if name in ['conv4', 'conv5']:
>>> print(module)
```
`named_modules(memo=None, prefix='')`
Returns an iterator over all modules in the network, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple of name and module
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.named_modules()):
print(idx, '->', m)
0 -> ('', Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
))
1 -> ('0', Linear(in_features=2, out_features=2, bias=True))
```
`named_parameters(prefix='', recurse=True)`
Returns an iterator over module parameters, yielding both the name of the parameter as well as the parameter itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all parameter names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*(string, Parameter)* – Tuple containing the name and parameter
Example:
```
>>> for name, param in self.named_parameters():
>>> if name in ['bias']:
>>> print(param.size())
```
`parameters(recurse=True)`
Returns an iterator over module parameters.
This is typically passed to an optimizer.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*Parameter* – module parameter
Example:
```
>>> for param in model.parameters():
>>> print(type(param), param.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`register_backward_hook(hook)`
Registers a backward hook on the module.
This function is deprecated in favor of `nn.Module.register_full_backward_hook()` and the behavior of this function will change in future versions.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_buffer(name, tensor, persistent=True)`
Adds a buffer to the module.
This is typically used to register a buffer that should not to be considered a model parameter. For example, BatchNorm’s `running_mean` is not a parameter, but is part of the module’s state. Buffers, by default, are persistent and will be saved alongside parameters. This behavior can be changed by setting `persistent` to `False`. The only difference between a persistent buffer and a non-persistent buffer is that the latter will not be a part of this module’s [`state_dict`](#torch.nn.Unflatten.state_dict "torch.nn.Unflatten.state_dict").
Buffers can be accessed as attributes using given names.
Parameters
* **name** (*string*) – name of the buffer. The buffer can be accessed from this module using the given name
* **tensor** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – buffer to be registered.
* **persistent** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the buffer is part of this module’s [`state_dict`](#torch.nn.Unflatten.state_dict "torch.nn.Unflatten.state_dict").
Example:
```
>>> self.register_buffer('running_mean', torch.zeros(num_features))
```
`register_forward_hook(hook)`
Registers a forward hook on the module.
The hook will be called every time after `forward()` has computed an output. It should have the following signature:
```
hook(module, input, output) -> None or modified output
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the output. It can modify the input inplace but it will not have effect on forward since this is called after `forward()` is called.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_forward_pre_hook(hook)`
Registers a forward pre-hook on the module.
The hook will be called every time before `forward()` is invoked. It should have the following signature:
```
hook(module, input) -> None or modified input
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the input. User can either return a tuple or a single modified value in the hook. We will wrap the value into a tuple if a single value is returned(unless that value is already a tuple).
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_full_backward_hook(hook)`
Registers a backward hook on the module.
The hook will be called every time the gradients with respect to module inputs are computed. The hook should have the following signature:
```
hook(module, grad_input, grad_output) -> tuple(Tensor) or None
```
The `grad_input` and `grad_output` are tuples that contain the gradients with respect to the inputs and outputs respectively. The hook should not modify its arguments, but it can optionally return a new gradient with respect to the input that will be used in place of `grad_input` in subsequent computations. `grad_input` will only correspond to the inputs given as positional arguments and all kwarg arguments are ignored. Entries in `grad_input` and `grad_output` will be `None` for all non-Tensor arguments.
Warning
Modifying inputs or outputs inplace is not allowed when using backward hooks and will raise an error.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_parameter(name, param)`
Adds a parameter to the module.
The parameter can be accessed as an attribute using given name.
Parameters
* **name** (*string*) – name of the parameter. The parameter can be accessed from this module using the given name
* **param** ([Parameter](torch.nn.parameter.parameter#torch.nn.parameter.Parameter "torch.nn.parameter.Parameter")) – parameter to be added to the module.
`requires_grad_(requires_grad=True)`
Change if autograd should record operations on parameters in this module.
This method sets the parameters’ `requires_grad` attributes in-place.
This method is helpful for freezing part of the module for finetuning or training parts of a model individually (e.g., GAN training).
Parameters
**requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether autograd should record operations on parameters in this module. Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`state_dict(destination=None, prefix='', keep_vars=False)`
Returns a dictionary containing a whole state of the module.
Both parameters and persistent buffers (e.g. running averages) are included. Keys are corresponding parameter and buffer names.
Returns
a dictionary containing a whole state of the module
Return type
[dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")
Example:
```
>>> module.state_dict().keys()
['bias', 'weight']
```
`to(*args, **kwargs)`
Moves and/or casts the parameters and buffers.
This can be called as
`to(device=None, dtype=None, non_blocking=False)`
`to(dtype, non_blocking=False)`
`to(tensor, non_blocking=False)`
`to(memory_format=torch.channels_last)`
Its signature is similar to [`torch.Tensor.to()`](../tensors#torch.Tensor.to "torch.Tensor.to"), but only accepts floating point or complex `dtype`s. In addition, this method will
only cast the floating point or complex parameters and buffers to :attr:`dtype` (if given). The integral parameters and buffers will be moved `device`, if that is given, but with dtypes unchanged. When `non_blocking` is set, it tries to convert/move asynchronously with respect to the host if possible, e.g., moving CPU Tensors with pinned memory to CUDA devices.
See below for examples.
Note
This method modifies the module in-place.
Parameters
* **device** (`torch.device`) – the desired device of the parameters and buffers in this module
* **dtype** (`torch.dtype`) – the desired floating point or complex dtype of the parameters and buffers in this module
* **tensor** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Tensor whose dtype and device are the desired dtype and device for all parameters and buffers in this module
* **memory\_format** (`torch.memory_format`) – the desired memory format for 4D parameters and buffers in this module (keyword only argument)
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Examples:
```
>>> linear = nn.Linear(2, 2)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]])
>>> linear.to(torch.double)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]], dtype=torch.float64)
>>> gpu1 = torch.device("cuda:1")
>>> linear.to(gpu1, dtype=torch.half, non_blocking=True)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16, device='cuda:1')
>>> cpu = torch.device("cpu")
>>> linear.to(cpu)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16)
>>> linear = nn.Linear(2, 2, bias=None).to(torch.cdouble)
>>> linear.weight
Parameter containing:
tensor([[ 0.3741+0.j, 0.2382+0.j],
[ 0.5593+0.j, -0.4443+0.j]], dtype=torch.complex128)
>>> linear(torch.ones(3, 2, dtype=torch.cdouble))
tensor([[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j]], dtype=torch.complex128)
```
`train(mode=True)`
Sets the module in training mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
Parameters
**mode** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to set training mode (`True`) or evaluation mode (`False`). Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`type(dst_type)`
Casts all parameters and buffers to `dst_type`.
Parameters
**dst\_type** ([type](https://docs.python.org/3/library/functions.html#type "(in Python v3.9)") *or* *string*) – the desired type
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`xpu(device=None)`
Moves all model parameters and buffers to the XPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on XPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`zero_grad(set_to_none=False)`
Sets gradients of all model parameters to zero. See similar function under [`torch.optim.Optimizer`](../optim#torch.optim.Optimizer "torch.optim.Optimizer") for more context.
Parameters
**set\_to\_none** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – instead of setting to zero, set the grads to None. See [`torch.optim.Optimizer.zero_grad()`](../optim#torch.optim.Optimizer.zero_grad "torch.optim.Optimizer.zero_grad") for details.
| programming_docs |
pytorch torch.arccosh torch.arccosh
=============
`torch.arccosh(input, *, out=None) → Tensor`
Alias for [`torch.acosh()`](torch.acosh#torch.acosh "torch.acosh").
pytorch torch.topk torch.topk
==========
`torch.topk(input, k, dim=None, largest=True, sorted=True, *, out=None) -> (Tensor, LongTensor)`
Returns the `k` largest elements of the given `input` tensor along a given dimension.
If `dim` is not given, the last dimension of the `input` is chosen.
If `largest` is `False` then the `k` smallest elements are returned.
A namedtuple of `(values, indices)` is returned, where the `indices` are the indices of the elements in the original `input` tensor.
The boolean option `sorted` if `True`, will make sure that the returned `k` elements are themselves sorted
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **k** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the k in “top-k”
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the dimension to sort along
* **largest** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – controls whether to return largest or smallest elements
* **sorted** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – controls whether to return the elements in sorted order
Keyword Arguments
**out** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the output tuple of (Tensor, LongTensor) that can be optionally given to be used as output buffers
Example:
```
>>> x = torch.arange(1., 6.)
>>> x
tensor([ 1., 2., 3., 4., 5.])
>>> torch.topk(x, 3)
torch.return_types.topk(values=tensor([5., 4., 3.]), indices=tensor([4, 3, 2]))
```
pytorch torch.clip torch.clip
==========
`torch.clip(input, min, max, *, out=None) → Tensor`
Alias for [`torch.clamp()`](torch.clamp#torch.clamp "torch.clamp").
pytorch torch.kaiser_window torch.kaiser\_window
====================
`torch.kaiser_window(window_length, periodic=True, beta=12.0, *, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Computes the Kaiser window with window length `window_length` and shape parameter `beta`.
Let I\_0 be the zeroth order modified Bessel function of the first kind (see [`torch.i0()`](torch.i0#torch.i0 "torch.i0")) and `N = L - 1` if `periodic` is False and `L` if `periodic` is True, where `L` is the `window_length`. This function computes:
outi=I0(β1−(i−N/2N/2)2)/I0(β)out\_i = I\_0 \left( \beta \sqrt{1 - \left( {\frac{i - N/2}{N/2}} \right) ^2 } \right) / I\_0( \beta )
Calling `torch.kaiser_window(L, B, periodic=True)` is equivalent to calling `torch.kaiser_window(L + 1, B, periodic=False)[:-1])`. The `periodic` argument is intended as a helpful shorthand to produce a periodic window as input to functions like [`torch.stft()`](torch.stft#torch.stft "torch.stft").
Note
If `window_length` is one, then the returned window is a single element tensor containing a one.
Parameters
* **window\_length** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – length of the window.
* **periodic** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If True, returns a periodic window suitable for use in spectral analysis. If False, returns a symmetric window suitable for use in filter design.
* **beta** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – shape parameter for the window.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned window tensor. Only `torch.strided` (dense layout) is supported.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
pytorch torch.nn.utils.prune.ln_structured torch.nn.utils.prune.ln\_structured
===================================
`torch.nn.utils.prune.ln_structured(module, name, amount, n, dim, importance_scores=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#ln_structured)
Prunes tensor corresponding to parameter called `name` in `module` by removing the specified `amount` of (currently unpruned) channels along the specified `dim` with the lowest L``n``-norm. Modifies module in place (and also return the modified module) by: 1) adding a named buffer called `name+'_mask'` corresponding to the binary mask applied to the parameter `name` by the pruning method. 2) replacing the parameter `name` by its pruned version, while the original (unpruned) parameter is stored in a new parameter named `name+'_orig'`.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *inf**,* *-inf**,* *'fro'**,* *'nuc'*) – See documentation of valid entries for argument `p` in [`torch.norm()`](torch.norm#torch.norm "torch.norm").
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – index of the dim along which we define channels to prune.
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as module parameter) used to compute mask for pruning. The values in this tensor indicate the importance of the corresponding elements in the parameter being pruned. If unspecified or None, the module parameter will be used in its place.
Returns
modified (i.e. pruned) version of the input module
Return type
module ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module"))
#### Examples
```
>>> m = prune.ln_structured(
nn.Conv2d(5, 3, 2), 'weight', amount=0.3, dim=1, n=float('-inf')
)
```
pytorch torch.poisson torch.poisson
=============
`torch.poisson(input, generator=None) → Tensor`
Returns a tensor of the same size as `input` with each element sampled from a Poisson distribution with rate parameter given by the corresponding element in `input` i.e.,
outi∼Poisson(inputi)\text{out}\_i \sim \text{Poisson}(\text{input}\_i)
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor containing the rates of the Poisson distribution
Keyword Arguments
**generator** ([`torch.Generator`](torch.generator#torch.Generator "torch.Generator"), optional) – a pseudorandom number generator for sampling
Example:
```
>>> rates = torch.rand(4, 4) * 5 # rate parameter between 0 and 5
>>> torch.poisson(rates)
tensor([[9., 1., 3., 5.],
[8., 6., 6., 0.],
[0., 4., 5., 3.],
[2., 1., 4., 2.]])
```
pytorch Conv3d Conv3d
======
`class torch.nn.Conv3d(in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#Conv3d)
Applies a 3D convolution over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,Cin,D,H,W)(N, C\_{in}, D, H, W) and output (N,Cout,Dout,Hout,Wout)(N, C\_{out}, D\_{out}, H\_{out}, W\_{out}) can be precisely described as:
out(Ni,Coutj)=bias(Coutj)+∑k=0Cin−1weight(Coutj,k)⋆input(Ni,k)out(N\_i, C\_{out\_j}) = bias(C\_{out\_j}) + \sum\_{k = 0}^{C\_{in} - 1} weight(C\_{out\_j}, k) \star input(N\_i, k)
where ⋆\star is the valid 3D [cross-correlation](https://en.wikipedia.org/wiki/Cross-correlation) operator
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation.
* `padding` controls the amount of implicit padding on both sides for `padding` number of points for each dimension.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
The parameters `kernel_size`, `stride`, `padding`, `dilation` can either be:
* a single `int` – in which case the same value is used for the depth, height and width dimension
* a `tuple` of three ints – in which case, the first `int` is used for the depth dimension, the second `int` for the height dimension and the third `int` for the width dimension
Note
When `groups == in_channels` and `out_channels == K * in_channels`, where `K` is a positive integer, this operation is also known as a “depthwise convolution”.
In other words, for an input of size (N,Cin,Lin)(N, C\_{in}, L\_{in}) , a depthwise convolution with a depthwise multiplier `K` can be performed with the arguments (Cin=Cin,Cout=Cin×K,...,groups=Cin)(C\_\text{in}=C\_\text{in}, C\_\text{out}=C\_\text{in} \times \text{K}, ..., \text{groups}=C\_\text{in}) .
Note
In some circumstances when given tensors on a CUDA device and using CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic = True`. See [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for more information.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Zero-padding added to all three sides of the input. Default: 0
* **padding\_mode** (*string**,* *optional*) – `'zeros'`, `'reflect'`, `'replicate'` or `'circular'`. Default: `'zeros'`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
Shape:
* Input: (N,Cin,Din,Hin,Win)(N, C\_{in}, D\_{in}, H\_{in}, W\_{in})
* Output: (N,Cout,Dout,Hout,Wout)(N, C\_{out}, D\_{out}, H\_{out}, W\_{out}) where
Dout=⌊Din+2×padding[0]−dilation[0]×(kernel\_size[0]−1)−1stride[0]+1⌋D\_{out} = \left\lfloor\frac{D\_{in} + 2 \times \text{padding}[0] - \text{dilation}[0] \times (\text{kernel\\_size}[0] - 1) - 1}{\text{stride}[0]} + 1\right\rfloor
Hout=⌊Hin+2×padding[1]−dilation[1]×(kernel\_size[1]−1)−1stride[1]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \times \text{padding}[1] - \text{dilation}[1] \times (\text{kernel\\_size}[1] - 1) - 1}{\text{stride}[1]} + 1\right\rfloor
Wout=⌊Win+2×padding[2]−dilation[2]×(kernel\_size[2]−1)−1stride[2]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \times \text{padding}[2] - \text{dilation}[2] \times (\text{kernel\\_size}[2] - 1) - 1}{\text{stride}[2]} + 1\right\rfloor
Variables
* **~Conv3d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (out\_channels,in\_channelsgroups,(\text{out\\_channels}, \frac{\text{in\\_channels}}{\text{groups}}, kernel\_size[0],kernel\_size[1],kernel\_size[2])\text{kernel\\_size[0]}, \text{kernel\\_size[1]}, \text{kernel\\_size[2]}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗∏i=02kernel\_size[i]k = \frac{groups}{C\_\text{in} \* \prod\_{i=0}^{2}\text{kernel\\_size}[i]}
* **~Conv3d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels). If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗∏i=02kernel\_size[i]k = \frac{groups}{C\_\text{in} \* \prod\_{i=0}^{2}\text{kernel\\_size}[i]}
Examples:
```
>>> # With square kernels and equal stride
>>> m = nn.Conv3d(16, 33, 3, stride=2)
>>> # non-square kernels and unequal stride and with padding
>>> m = nn.Conv3d(16, 33, (3, 5, 2), stride=(2, 1, 1), padding=(4, 2, 0))
>>> input = torch.randn(20, 16, 10, 50, 100)
>>> output = m(input)
```
pytorch torch.pinverse torch.pinverse
==============
`torch.pinverse(input, rcond=1e-15) → Tensor`
Calculates the pseudo-inverse (also known as the Moore-Penrose inverse) of a 2D tensor. Please look at [Moore-Penrose inverse](https://en.wikipedia.org/wiki/Moore%E2%80%93Penrose_inverse) for more details
Note
[`torch.pinverse()`](#torch.pinverse "torch.pinverse") is deprecated. Please use [`torch.linalg.pinv()`](../linalg#torch.linalg.pinv "torch.linalg.pinv") instead which includes new parameters `hermitian` and `out`.
Note
This method is implemented using the Singular Value Decomposition.
Note
The pseudo-inverse is not necessarily a continuous function in the elements of the matrix [[1]](https://epubs.siam.org/doi/10.1137/0117004). Therefore, derivatives are not always existent, and exist for a constant rank only [[2]](https://www.jstor.org/stable/2156365). However, this method is backprop-able due to the implementation by using SVD results, and could be unstable. Double-backward will also be unstable due to the usage of SVD internally. See [`svd()`](torch.svd#torch.svd "torch.svd") for more details.
Note
Supports real and complex inputs. Batched version for complex inputs is only supported on the CPU.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The input tensor of size (∗,m,n)(\*, m, n) where ∗\* is zero or more batch dimensions.
* **rcond** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – A floating point value to determine the cutoff for small singular values. Default: `1e-15`.
Returns
The pseudo-inverse of `input` of dimensions (∗,n,m)(\*, n, m)
Example:
```
>>> input = torch.randn(3, 5)
>>> input
tensor([[ 0.5495, 0.0979, -1.4092, -0.1128, 0.4132],
[-1.1143, -0.3662, 0.3042, 1.6374, -0.9294],
[-0.3269, -0.5745, -0.0382, -0.5922, -0.6759]])
>>> torch.pinverse(input)
tensor([[ 0.0600, -0.1933, -0.2090],
[-0.0903, -0.0817, -0.4752],
[-0.7124, -0.1631, -0.2272],
[ 0.1356, 0.3933, -0.5023],
[-0.0308, -0.1725, -0.5216]])
>>> # Batched pinverse example
>>> a = torch.randn(2,6,3)
>>> b = torch.pinverse(a)
>>> torch.matmul(b, a)
tensor([[[ 1.0000e+00, 1.6391e-07, -1.1548e-07],
[ 8.3121e-08, 1.0000e+00, -2.7567e-07],
[ 3.5390e-08, 1.4901e-08, 1.0000e+00]],
[[ 1.0000e+00, -8.9407e-08, 2.9802e-08],
[-2.2352e-07, 1.0000e+00, 1.1921e-07],
[ 0.0000e+00, 8.9407e-08, 1.0000e+00]]])
```
pytorch LazyModuleMixin LazyModuleMixin
===============
`class torch.nn.modules.lazy.LazyModuleMixin(*args, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/lazy.html#LazyModuleMixin)
A mixin for modules that lazily initialize parameters, also known as “lazy modules.”
Modules that lazily initialize parameters, or “lazy modules”, derive the shapes of their parameters from the first input(s) to their forward method. Until that first forward they contain `torch.nn.UninitializedParameter`s that should not be accessed
or used, and afterward they contain regular :class:`torch.nn.Parameter`s.
Lazy modules are convenient since they don't require computing some
module arguments, like the `in_features` argument of a typical [`torch.nn.Linear`](torch.nn.linear#torch.nn.Linear "torch.nn.Linear").
After construction, networks with lazy modules should first be converted to the desired dtype and placed on the desired device. The lazy modules should then be initialized with one or more “dry runs”. These “dry runs” send inputs of the correct size, dtype, and device through the network and to each one of its lazy modules. After this the network can be used as usual.
```
>>> class LazyMLP(torch.nn.Module):
... def __init__(self):
... super().__init__()
... self.fc1 = torch.nn.LazyLinear(10)
... self.relu1 = torch.nn.ReLU()
... self.fc2 = torch.nn.LazyLinear(1)
... self.relu2 = torch.nn.ReLU()
...
... def forward(self, input):
... x = self.relu1(self.fc1(input))
... y = self.relu2(self.fc2(x))
... return y
>>> # constructs a network with lazy modules
>>> lazy_mlp = LazyMLP()
>>> # transforms the network's device and dtype
>>> # NOTE: these transforms can and should be applied after construction and before any 'dry runs'
>>> lazy_mlp = mlp.cuda().double()
>>> lazy_mlp
LazyMLP(
(fc1): LazyLinear(in_features=0, out_features=10, bias=True)
(relu1): ReLU()
(fc2): LazyLinear(in_features=0, out_features=1, bias=True)
(relu2): ReLU()
)
>>> # performs a dry run to initialize the network's lazy modules
>>> lazy_mlp(torch.ones(10,10).cuda())
>>> # after initialization, LazyLinear modules become regular Linear modules
>>> lazy_mlp
LazyMLP(
(fc1): Linear(in_features=10, out_features=10, bias=True)
(relu1): ReLU()
(fc2): Linear(in_features=10, out_features=1, bias=True)
(relu2): ReLU()
)
>>> # attaches an optimizer, since parameters can now be used as usual
>>> optim = torch.optim.SGD(mlp.parameters(), lr=0.01)
```
A final caveat when using lazy modules is that the order of initialization of a network’s parameters may change, since the lazy modules are always initialized after other modules. This can cause the parameters of a network using lazy modules to be initialized differently than the parameters of a network without lazy modules. For example, if the LazyMLP class defined above had a [`torch.nn.LazyLinear`](torch.nn.lazylinear#torch.nn.LazyLinear "torch.nn.LazyLinear") module first and then a regular [`torch.nn.Linear`](torch.nn.linear#torch.nn.Linear "torch.nn.Linear") second, the second module would be initialized on construction and the first module would be initialized during the first dry run.
Lazy modules can be serialized with a state dict like other modules. For example:
```
>>> lazy_mlp = LazyMLP()
>>> # The state dict shows the uninitialized parameters
>>> lazy_mlp.state_dict()
OrderedDict([('fc1.weight', Uninitialized parameter),
('fc1.bias',
tensor([-1.8832e+25, 4.5636e-41, -1.8832e+25, 4.5636e-41, -6.1598e-30,
4.5637e-41, -1.8788e+22, 4.5636e-41, -2.0042e-31, 4.5637e-41])),
('fc2.weight', Uninitialized parameter),
('fc2.bias', tensor([0.0019]))])
```
Lazy modules can also load regular `torch.nn.Parameter` s, which replace their `torch.nn.UninitializedParameter` s:
```
>>> full_mlp = LazyMLP()
>>> # Dry run to initialize another module
>>> full_mlp.forward(torch.ones(10, 1))
>>> # Load an initialized state into a lazy module
>>> lazy_mlp.load_state_dict(full_mlp.state_dict())
>>> # The state dict now holds valid values
>>> lazy_mlp.state_dict()
OrderedDict([('fc1.weight',
tensor([[-0.3837],
[ 0.0907],
[ 0.6708],
[-0.5223],
[-0.9028],
[ 0.2851],
[-0.4537],
[ 0.6813],
[ 0.5766],
[-0.8678]])),
('fc1.bias',
tensor([-1.8832e+25, 4.5636e-41, -1.8832e+25, 4.5636e-41, -6.1598e-30,
4.5637e-41, -1.8788e+22, 4.5636e-41, -2.0042e-31, 4.5637e-41])),
('fc2.weight',
tensor([[ 0.1320, 0.2938, 0.0679, 0.2793, 0.1088, -0.1795, -0.2301, 0.2807,
0.2479, 0.1091]])),
('fc2.bias', tensor([0.0019]))])
```
Note, however, that lazy modules cannot validate that the shape of parameters they load is correct.
`has_uninitialized_params()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/lazy.html#LazyModuleMixin.has_uninitialized_params)
Check if a module has parameters that are not initialized
`initialize_parameters(*args, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/lazy.html#LazyModuleMixin.initialize_parameters)
Initialize parameters according to the input batch properties. This adds an interface to isolate parameter initialization from the forward pass when doing parameter shape inference.
| programming_docs |
pytorch torch.compiled_with_cxx11_abi torch.compiled\_with\_cxx11\_abi
================================
`torch.compiled_with_cxx11_abi()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#compiled_with_cxx11_abi)
Returns whether PyTorch was built with \_GLIBCXX\_USE\_CXX11\_ABI=1
pytorch torch.zeros_like torch.zeros\_like
=================
`torch.zeros_like(input, *, dtype=None, layout=None, device=None, requires_grad=False, memory_format=torch.preserve_format) → Tensor`
Returns a tensor filled with the scalar value `0`, with the same size as `input`. `torch.zeros_like(input)` is equivalent to `torch.zeros(input.size(), dtype=input.dtype, layout=input.layout, device=input.device)`.
Warning
As of 0.4, this function does not support an `out` keyword. As an alternative, the old `torch.zeros_like(input, out=output)` is equivalent to `torch.zeros(input.size(), out=output)`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the size of `input` will determine size of the output tensor.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned Tensor. Default: if `None`, defaults to the dtype of `input`.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned tensor. Default: if `None`, defaults to the layout of `input`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, defaults to the device of `input`.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
* **memory\_format** ([`torch.memory_format`](../tensor_attributes#torch.torch.memory_format "torch.torch.memory_format"), optional) – the desired memory format of returned Tensor. Default: `torch.preserve_format`.
Example:
```
>>> input = torch.empty(2, 3)
>>> torch.zeros_like(input)
tensor([[ 0., 0., 0.],
[ 0., 0., 0.]])
```
pytorch TransformerDecoderLayer TransformerDecoderLayer
=======================
`class torch.nn.TransformerDecoderLayer(d_model, nhead, dim_feedforward=2048, dropout=0.1, activation='relu')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerDecoderLayer)
TransformerDecoderLayer is made up of self-attn, multi-head-attn and feedforward network. This standard decoder layer is based on the paper “Attention Is All You Need”. Ashish Vaswani, Noam Shazeer, Niki Parmar, Jakob Uszkoreit, Llion Jones, Aidan N Gomez, Lukasz Kaiser, and Illia Polosukhin. 2017. Attention is all you need. In Advances in Neural Information Processing Systems, pages 6000-6010. Users may modify or implement in a different way during application.
Parameters
* **d\_model** – the number of expected features in the input (required).
* **nhead** – the number of heads in the multiheadattention models (required).
* **dim\_feedforward** – the dimension of the feedforward network model (default=2048).
* **dropout** – the dropout value (default=0.1).
* **activation** – the activation function of intermediate layer, relu or gelu (default=relu).
Examples::
```
>>> decoder_layer = nn.TransformerDecoderLayer(d_model=512, nhead=8)
>>> memory = torch.rand(10, 32, 512)
>>> tgt = torch.rand(20, 32, 512)
>>> out = decoder_layer(tgt, memory)
```
`forward(tgt, memory, tgt_mask=None, memory_mask=None, tgt_key_padding_mask=None, memory_key_padding_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerDecoderLayer.forward)
Pass the inputs (and mask) through the decoder layer.
Parameters
* **tgt** – the sequence to the decoder layer (required).
* **memory** – the sequence from the last layer of the encoder (required).
* **tgt\_mask** – the mask for the tgt sequence (optional).
* **memory\_mask** – the mask for the memory sequence (optional).
* **tgt\_key\_padding\_mask** – the mask for the tgt keys per batch (optional).
* **memory\_key\_padding\_mask** – the mask for the memory keys per batch (optional).
Shape:
see the docs in Transformer class.
pytorch torch.svd_lowrank torch.svd\_lowrank
==================
`torch.svd_lowrank(A, q=6, niter=2, M=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/_lowrank.html#svd_lowrank)
Return the singular value decomposition `(U, S, V)` of a matrix, batches of matrices, or a sparse matrix AA such that A≈Udiag(S)VTA \approx U diag(S) V^T . In case MM is given, then SVD is computed for the matrix A−MA - M .
Note
The implementation is based on the Algorithm 5.1 from Halko et al, 2009.
Note
To obtain repeatable results, reset the seed for the pseudorandom number generator
Note
The input is assumed to be a low-rank matrix.
Note
In general, use the full-rank SVD implementation `torch.svd` for dense matrices due to its 10-fold higher performance characteristics. The low-rank SVD will be useful for huge sparse matrices that `torch.svd` cannot handle.
Args::
A (Tensor): the input tensor of size (∗,m,n)(\*, m, n)
q (int, optional): a slightly overestimated rank of A.
niter (int, optional): the number of subspace iterations to
conduct; niter must be a nonnegative integer, and defaults to 2
M (Tensor, optional): the input tensor’s mean of size
(∗,1,n)(\*, 1, n) .
References::
* Nathan Halko, Per-Gunnar Martinsson, and Joel Tropp, Finding structure with randomness: probabilistic algorithms for constructing approximate matrix decompositions, arXiv:0909.4061 [math.NA; math.PR], 2009 (available at [arXiv](https://arxiv.org/abs/0909.4061)).
pytorch AdaptiveAvgPool1d AdaptiveAvgPool1d
=================
`class torch.nn.AdaptiveAvgPool1d(output_size)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveAvgPool1d)
Applies a 1D adaptive average pooling over an input signal composed of several input planes.
The output size is H, for any input size. The number of output features is equal to the number of input planes.
Parameters
**output\_size** – the target output size H
#### Examples
```
>>> # target output size of 5
>>> m = nn.AdaptiveAvgPool1d(5)
>>> input = torch.randn(1, 64, 8)
>>> output = m(input)
```
pytorch torch.logcumsumexp torch.logcumsumexp
==================
`torch.logcumsumexp(input, dim, *, out=None) → Tensor`
Returns the logarithm of the cumulative summation of the exponentiation of elements of `input` in the dimension `dim`.
For summation index jj given by `dim` and other indices ii , the result is
logcumsumexp(x)ij=log∑j=0iexp(xij)\text{logcumsumexp}(x)\_{ij} = \log \sum\limits\_{j=0}^{i} \exp(x\_{ij}) Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to do the operation over
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example::
```
>>> a = torch.randn(10)
>>> torch.logcumsumexp(a, dim=0)
tensor([-0.42296738, -0.04462666, 0.86278635, 0.94622083, 1.05277811,
1.39202815, 1.83525007, 1.84492621, 2.06084887, 2.06844475]))
```
pytorch torch.float_power torch.float\_power
==================
`torch.float_power(input, exponent, *, out=None) → Tensor`
Raises `input` to the power of `exponent`, elementwise, in double precision. If neither input is complex returns a `torch.float64` tensor, and if one or more inputs is complex returns a `torch.complex128` tensor.
Note
This function always computes in double precision, unlike [`torch.pow()`](torch.pow#torch.pow "torch.pow"), which implements more typical [type promotion](../tensor_attributes#type-promotion-doc). This is useful when the computation needs to be performed in a wider or more precise dtype, or the results of the computation may contain fractional values not representable in the input dtypes, like when an integer base is raised to a negative integer exponent.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Number*) – the base value(s)
* **exponent** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Number*) – the exponent value(s)
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randint(10, (4,))
>>> a
tensor([6, 4, 7, 1])
>>> torch.float_power(a, 2)
tensor([36., 16., 49., 1.], dtype=torch.float64)
>>> a = torch.arange(1, 5)
>>> a
tensor([ 1, 2, 3, 4])
>>> exp = torch.tensor([2, -3, 4, -5])
>>> exp
tensor([ 2, -3, 4, -5])
>>> torch.float_power(a, exp)
tensor([1.0000e+00, 1.2500e-01, 8.1000e+01, 9.7656e-04], dtype=torch.float64)
```
pytorch torch.quantize_per_channel torch.quantize\_per\_channel
============================
`torch.quantize_per_channel(input, scales, zero_points, axis, dtype) → Tensor`
Converts a float tensor to a per-channel quantized tensor with given scales and zero points.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – float tensor to quantize
* **scales** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – float 1D tensor of scales to use, size should match `input.size(axis)`
* **zero\_points** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – integer 1D tensor of offset to use, size should match `input.size(axis)`
* **axis** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – dimension on which apply per-channel quantization
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype")) – the desired data type of returned tensor. Has to be one of the quantized dtypes: `torch.quint8`, `torch.qint8`, `torch.qint32`
Returns
A newly quantized tensor
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> x = torch.tensor([[-1.0, 0.0], [1.0, 2.0]])
>>> torch.quantize_per_channel(x, torch.tensor([0.1, 0.01]), torch.tensor([10, 0]), 0, torch.quint8)
tensor([[-1., 0.],
[ 1., 2.]], size=(2, 2), dtype=torch.quint8,
quantization_scheme=torch.per_channel_affine,
scale=tensor([0.1000, 0.0100], dtype=torch.float64),
zero_point=tensor([10, 0]), axis=0)
>>> torch.quantize_per_channel(x, torch.tensor([0.1, 0.01]), torch.tensor([10, 0]), 0, torch.quint8).int_repr()
tensor([[ 0, 10],
[100, 200]], dtype=torch.uint8)
```
pytorch Module Module
======
`class torch.nn.Module` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module)
Base class for all neural network modules.
Your models should also subclass this class.
Modules can also contain other Modules, allowing to nest them in a tree structure. You can assign the submodules as regular attributes:
```
import torch.nn as nn
import torch.nn.functional as F
class Model(nn.Module):
def __init__(self):
super(Model, self).__init__()
self.conv1 = nn.Conv2d(1, 20, 5)
self.conv2 = nn.Conv2d(20, 20, 5)
def forward(self, x):
x = F.relu(self.conv1(x))
return F.relu(self.conv2(x))
```
Submodules assigned in this way will be registered, and will have their parameters converted too when you call [`to()`](#torch.nn.Module.to "torch.nn.Module.to"), etc.
Variables
**training** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – Boolean represents whether this module is in training or evaluation mode.
`add_module(name, module)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.add_module)
Adds a child module to the current module.
The module can be accessed as an attribute using the given name.
Parameters
* **name** (*string*) – name of the child module. The child module can be accessed from this module using the given name
* **module** ([Module](#torch.nn.Module "torch.nn.Module")) – child module to be added to the module.
`apply(fn)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.apply)
Applies `fn` recursively to every submodule (as returned by `.children()`) as well as self. Typical use includes initializing the parameters of a model (see also [torch.nn.init](../nn.init#nn-init-doc)).
Parameters
**fn** ([`Module`](#torch.nn.Module "torch.nn.Module") -> None) – function to be applied to each submodule
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
Example:
```
>>> @torch.no_grad()
>>> def init_weights(m):
>>> print(m)
>>> if type(m) == nn.Linear:
>>> m.weight.fill_(1.0)
>>> print(m.weight)
>>> net = nn.Sequential(nn.Linear(2, 2), nn.Linear(2, 2))
>>> net.apply(init_weights)
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
```
`bfloat16()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.bfloat16)
Casts all floating point parameters and buffers to `bfloat16` datatype.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`buffers(recurse=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.buffers)
Returns an iterator over module buffers.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*torch.Tensor* – module buffer
Example:
```
>>> for buf in model.buffers():
>>> print(type(buf), buf.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`children()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.children)
Returns an iterator over immediate children modules.
Yields
*Module* – a child module
`cpu()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.cpu)
Moves all model parameters and buffers to the CPU.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`cuda(device=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.cuda)
Moves all model parameters and buffers to the GPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on GPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`double()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.double)
Casts all floating point parameters and buffers to `double` datatype.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`dump_patches: bool = False`
This allows better BC support for [`load_state_dict()`](#torch.nn.Module.load_state_dict "torch.nn.Module.load_state_dict"). In [`state_dict()`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict"), the version number will be saved as in the attribute `_metadata` of the returned state dict, and thus pickled. `_metadata` is a dictionary with keys that follow the naming convention of state dict. See `_load_from_state_dict` on how to use this information in loading.
If new parameters/buffers are added/removed from a module, this number shall be bumped, and the module’s `_load_from_state_dict` method can compare the version number and do appropriate changes if the state dict is from before the change.
`eval()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.eval)
Sets the module in evaluation mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
This is equivalent with [`self.train(False)`](#torch.nn.Module.train "torch.nn.Module.train").
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`extra_repr()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.extra_repr)
Set the extra representation of the module
To print customized extra information, you should re-implement this method in your own modules. Both single-line and multi-line strings are acceptable.
`float()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.float)
Casts all floating point parameters and buffers to float datatype.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`forward(*input)`
Defines the computation performed at every call.
Should be overridden by all subclasses.
Note
Although the recipe for forward pass needs to be defined within this function, one should call the [`Module`](#torch.nn.Module "torch.nn.Module") instance afterwards instead of this since the former takes care of running the registered hooks while the latter silently ignores them.
`half()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.half)
Casts all floating point parameters and buffers to `half` datatype.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`load_state_dict(state_dict, strict=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.load_state_dict)
Copies parameters and buffers from [`state_dict`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict") into this module and its descendants. If `strict` is `True`, then the keys of [`state_dict`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict") must exactly match the keys returned by this module’s [`state_dict()`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function.
Parameters
* **state\_dict** ([dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")) – a dict containing parameters and persistent buffers.
* **strict** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to strictly enforce that the keys in [`state_dict`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict") match the keys returned by this module’s [`state_dict()`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function. Default: `True`
Returns
* **missing\_keys** is a list of str containing the missing keys
* **unexpected\_keys** is a list of str containing the unexpected keys
Return type
`NamedTuple` with `missing_keys` and `unexpected_keys` fields
`modules()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.modules)
Returns an iterator over all modules in the network.
Yields
*Module* – a module in the network
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.modules()):
print(idx, '->', m)
0 -> Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
1 -> Linear(in_features=2, out_features=2, bias=True)
```
`named_buffers(prefix='', recurse=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.named_buffers)
Returns an iterator over module buffers, yielding both the name of the buffer as well as the buffer itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all buffer names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*(string, torch.Tensor)* – Tuple containing the name and buffer
Example:
```
>>> for name, buf in self.named_buffers():
>>> if name in ['running_var']:
>>> print(buf.size())
```
`named_children()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.named_children)
Returns an iterator over immediate children modules, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple containing a name and child module
Example:
```
>>> for name, module in model.named_children():
>>> if name in ['conv4', 'conv5']:
>>> print(module)
```
`named_modules(memo=None, prefix='')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.named_modules)
Returns an iterator over all modules in the network, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple of name and module
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.named_modules()):
print(idx, '->', m)
0 -> ('', Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
))
1 -> ('0', Linear(in_features=2, out_features=2, bias=True))
```
`named_parameters(prefix='', recurse=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.named_parameters)
Returns an iterator over module parameters, yielding both the name of the parameter as well as the parameter itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all parameter names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*(string, Parameter)* – Tuple containing the name and parameter
Example:
```
>>> for name, param in self.named_parameters():
>>> if name in ['bias']:
>>> print(param.size())
```
`parameters(recurse=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.parameters)
Returns an iterator over module parameters.
This is typically passed to an optimizer.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*Parameter* – module parameter
Example:
```
>>> for param in model.parameters():
>>> print(type(param), param.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`register_backward_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_backward_hook)
Registers a backward hook on the module.
This function is deprecated in favor of `nn.Module.register_full_backward_hook()` and the behavior of this function will change in future versions.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_buffer(name, tensor, persistent=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_buffer)
Adds a buffer to the module.
This is typically used to register a buffer that should not to be considered a model parameter. For example, BatchNorm’s `running_mean` is not a parameter, but is part of the module’s state. Buffers, by default, are persistent and will be saved alongside parameters. This behavior can be changed by setting `persistent` to `False`. The only difference between a persistent buffer and a non-persistent buffer is that the latter will not be a part of this module’s [`state_dict`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict").
Buffers can be accessed as attributes using given names.
Parameters
* **name** (*string*) – name of the buffer. The buffer can be accessed from this module using the given name
* **tensor** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – buffer to be registered.
* **persistent** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the buffer is part of this module’s [`state_dict`](#torch.nn.Module.state_dict "torch.nn.Module.state_dict").
Example:
```
>>> self.register_buffer('running_mean', torch.zeros(num_features))
```
`register_forward_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_forward_hook)
Registers a forward hook on the module.
The hook will be called every time after [`forward()`](#torch.nn.Module.forward "torch.nn.Module.forward") has computed an output. It should have the following signature:
```
hook(module, input, output) -> None or modified output
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the output. It can modify the input inplace but it will not have effect on forward since this is called after [`forward()`](#torch.nn.Module.forward "torch.nn.Module.forward") is called.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_forward_pre_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_forward_pre_hook)
Registers a forward pre-hook on the module.
The hook will be called every time before [`forward()`](#torch.nn.Module.forward "torch.nn.Module.forward") is invoked. It should have the following signature:
```
hook(module, input) -> None or modified input
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the input. User can either return a tuple or a single modified value in the hook. We will wrap the value into a tuple if a single value is returned(unless that value is already a tuple).
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_full_backward_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_full_backward_hook)
Registers a backward hook on the module.
The hook will be called every time the gradients with respect to module inputs are computed. The hook should have the following signature:
```
hook(module, grad_input, grad_output) -> tuple(Tensor) or None
```
The `grad_input` and `grad_output` are tuples that contain the gradients with respect to the inputs and outputs respectively. The hook should not modify its arguments, but it can optionally return a new gradient with respect to the input that will be used in place of `grad_input` in subsequent computations. `grad_input` will only correspond to the inputs given as positional arguments and all kwarg arguments are ignored. Entries in `grad_input` and `grad_output` will be `None` for all non-Tensor arguments.
Warning
Modifying inputs or outputs inplace is not allowed when using backward hooks and will raise an error.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_parameter(name, param)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.register_parameter)
Adds a parameter to the module.
The parameter can be accessed as an attribute using given name.
Parameters
* **name** (*string*) – name of the parameter. The parameter can be accessed from this module using the given name
* **param** ([Parameter](torch.nn.parameter.parameter#torch.nn.parameter.Parameter "torch.nn.parameter.Parameter")) – parameter to be added to the module.
`requires_grad_(requires_grad=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.requires_grad_)
Change if autograd should record operations on parameters in this module.
This method sets the parameters’ `requires_grad` attributes in-place.
This method is helpful for freezing part of the module for finetuning or training parts of a model individually (e.g., GAN training).
Parameters
**requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether autograd should record operations on parameters in this module. Default: `True`.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`state_dict(destination=None, prefix='', keep_vars=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.state_dict)
Returns a dictionary containing a whole state of the module.
Both parameters and persistent buffers (e.g. running averages) are included. Keys are corresponding parameter and buffer names.
Returns
a dictionary containing a whole state of the module
Return type
[dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")
Example:
```
>>> module.state_dict().keys()
['bias', 'weight']
```
`to(*args, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.to)
Moves and/or casts the parameters and buffers.
This can be called as
`to(device=None, dtype=None, non_blocking=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.to)
`to(dtype, non_blocking=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.to)
`to(tensor, non_blocking=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.to)
`to(memory_format=torch.channels_last)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.to)
Its signature is similar to [`torch.Tensor.to()`](../tensors#torch.Tensor.to "torch.Tensor.to"), but only accepts floating point or complex `dtype`s. In addition, this method will
only cast the floating point or complex parameters and buffers to :attr:`dtype` (if given). The integral parameters and buffers will be moved `device`, if that is given, but with dtypes unchanged. When `non_blocking` is set, it tries to convert/move asynchronously with respect to the host if possible, e.g., moving CPU Tensors with pinned memory to CUDA devices.
See below for examples.
Note
This method modifies the module in-place.
Parameters
* **device** (`torch.device`) – the desired device of the parameters and buffers in this module
* **dtype** (`torch.dtype`) – the desired floating point or complex dtype of the parameters and buffers in this module
* **tensor** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Tensor whose dtype and device are the desired dtype and device for all parameters and buffers in this module
* **memory\_format** (`torch.memory_format`) – the desired memory format for 4D parameters and buffers in this module (keyword only argument)
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
Examples:
```
>>> linear = nn.Linear(2, 2)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]])
>>> linear.to(torch.double)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]], dtype=torch.float64)
>>> gpu1 = torch.device("cuda:1")
>>> linear.to(gpu1, dtype=torch.half, non_blocking=True)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16, device='cuda:1')
>>> cpu = torch.device("cpu")
>>> linear.to(cpu)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16)
>>> linear = nn.Linear(2, 2, bias=None).to(torch.cdouble)
>>> linear.weight
Parameter containing:
tensor([[ 0.3741+0.j, 0.2382+0.j],
[ 0.5593+0.j, -0.4443+0.j]], dtype=torch.complex128)
>>> linear(torch.ones(3, 2, dtype=torch.cdouble))
tensor([[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j]], dtype=torch.complex128)
```
`train(mode=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.train)
Sets the module in training mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. [`Dropout`](torch.nn.dropout#torch.nn.Dropout "torch.nn.Dropout"), `BatchNorm`, etc.
Parameters
**mode** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to set training mode (`True`) or evaluation mode (`False`). Default: `True`.
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`type(dst_type)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.type)
Casts all parameters and buffers to `dst_type`.
Parameters
**dst\_type** ([type](https://docs.python.org/3/library/functions.html#type "(in Python v3.9)") *or* *string*) – the desired type
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`xpu(device=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.xpu)
Moves all model parameters and buffers to the XPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on XPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](#torch.nn.Module "torch.nn.Module")
`zero_grad(set_to_none=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#Module.zero_grad)
Sets gradients of all model parameters to zero. See similar function under [`torch.optim.Optimizer`](../optim#torch.optim.Optimizer "torch.optim.Optimizer") for more context.
Parameters
**set\_to\_none** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – instead of setting to zero, set the grads to None. See [`torch.optim.Optimizer.zero_grad()`](../optim#torch.optim.Optimizer.zero_grad "torch.optim.Optimizer.zero_grad") for details.
| programming_docs |
pytorch torch.reciprocal torch.reciprocal
================
`torch.reciprocal(input, *, out=None) → Tensor`
Returns a new tensor with the reciprocal of the elements of `input`
Note
Unlike NumPy’s reciprocal, torch.reciprocal supports integral inputs. Integral inputs to reciprocal are automatically [promoted](../tensor_attributes#type-promotion-doc) to the default scalar type.
outi=1inputi\text{out}\_{i} = \frac{1}{\text{input}\_{i}}
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([-0.4595, -2.1219, -1.4314, 0.7298])
>>> torch.reciprocal(a)
tensor([-2.1763, -0.4713, -0.6986, 1.3702])
```
pytorch torch.lcm torch.lcm
=========
`torch.lcm(input, other, *, out=None) → Tensor`
Computes the element-wise least common multiple (LCM) of `input` and `other`.
Both `input` and `other` must have integer types.
Note
This defines lcm(0,0)=0lcm(0, 0) = 0 and lcm(0,a)=0lcm(0, a) = 0 .
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([5, 10, 15])
>>> b = torch.tensor([3, 4, 5])
>>> torch.lcm(a, b)
tensor([15, 20, 15])
>>> c = torch.tensor([3])
>>> torch.lcm(a, c)
tensor([15, 30, 15])
```
pytorch torch.asinh torch.asinh
===========
`torch.asinh(input, *, out=None) → Tensor`
Returns a new tensor with the inverse hyperbolic sine of the elements of `input`.
outi=sinh−1(inputi)\text{out}\_{i} = \sinh^{-1}(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.1606, -1.4267, -1.0899, -1.0250 ])
>>> torch.asinh(a)
tensor([ 0.1599, -1.1534, -0.9435, -0.8990 ])
```
pytorch ReflectionPad1d ReflectionPad1d
===============
`class torch.nn.ReflectionPad1d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ReflectionPad1d)
Pads the input tensor using the reflection of the input boundary.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 2-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} )
Shape:
* Input: (N,C,Win)(N, C, W\_{in})
* Output: (N,C,Wout)(N, C, W\_{out}) where
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ReflectionPad1d(2)
>>> input = torch.arange(8, dtype=torch.float).reshape(1, 2, 4)
>>> input
tensor([[[0., 1., 2., 3.],
[4., 5., 6., 7.]]])
>>> m(input)
tensor([[[2., 1., 0., 1., 2., 3., 2., 1.],
[6., 5., 4., 5., 6., 7., 6., 5.]]])
>>> # using different paddings for different sides
>>> m = nn.ReflectionPad1d((3, 1))
>>> m(input)
tensor([[[3., 2., 1., 0., 1., 2., 3., 2.],
[7., 6., 5., 4., 5., 6., 7., 6.]]])
```
pytorch AvgPool3d AvgPool3d
=========
`class torch.nn.AvgPool3d(kernel_size, stride=None, padding=0, ceil_mode=False, count_include_pad=True, divisor_override=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AvgPool3d)
Applies a 3D average pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,D,H,W)(N, C, D, H, W) , output (N,C,Dout,Hout,Wout)(N, C, D\_{out}, H\_{out}, W\_{out}) and `kernel_size` (kD,kH,kW)(kD, kH, kW) can be precisely described as:
out(Ni,Cj,d,h,w)=∑k=0kD−1∑m=0kH−1∑n=0kW−1input(Ni,Cj,stride[0]×d+k,stride[1]×h+m,stride[2]×w+n)kD×kH×kW\begin{aligned} \text{out}(N\_i, C\_j, d, h, w) ={} & \sum\_{k=0}^{kD-1} \sum\_{m=0}^{kH-1} \sum\_{n=0}^{kW-1} \\ & \frac{\text{input}(N\_i, C\_j, \text{stride}[0] \times d + k, \text{stride}[1] \times h + m, \text{stride}[2] \times w + n)} {kD \times kH \times kW} \end{aligned}
If `padding` is non-zero, then the input is implicitly zero-padded on all three sides for `padding` number of points.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
The parameters `kernel_size`, `stride` can either be:
* a single `int` – in which case the same value is used for the depth, height and width dimension
* a `tuple` of three ints – in which case, the first `int` is used for the depth dimension, the second `int` for the height dimension and the third `int` for the width dimension
Parameters
* **kernel\_size** – the size of the window
* **stride** – the stride of the window. Default value is `kernel_size`
* **padding** – implicit zero padding to be added on all three sides
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
* **count\_include\_pad** – when True, will include the zero-padding in the averaging calculation
* **divisor\_override** – if specified, it will be used as divisor, otherwise `kernel_size` will be used
Shape:
* Input: (N,C,Din,Hin,Win)(N, C, D\_{in}, H\_{in}, W\_{in})
* Output: (N,C,Dout,Hout,Wout)(N, C, D\_{out}, H\_{out}, W\_{out}) , where
Dout=⌊Din+2×padding[0]−kernel\_size[0]stride[0]+1⌋D\_{out} = \left\lfloor\frac{D\_{in} + 2 \times \text{padding}[0] - \text{kernel\\_size}[0]}{\text{stride}[0]} + 1\right\rfloor
Hout=⌊Hin+2×padding[1]−kernel\_size[1]stride[1]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \times \text{padding}[1] - \text{kernel\\_size}[1]}{\text{stride}[1]} + 1\right\rfloor
Wout=⌊Win+2×padding[2]−kernel\_size[2]stride[2]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \times \text{padding}[2] - \text{kernel\\_size}[2]}{\text{stride}[2]} + 1\right\rfloor
Examples:
```
>>> # pool of square window of size=3, stride=2
>>> m = nn.AvgPool3d(3, stride=2)
>>> # pool of non-square window
>>> m = nn.AvgPool3d((3, 2, 2), stride=(2, 1, 2))
>>> input = torch.randn(20, 16, 50,44, 31)
>>> output = m(input)
```
pytorch torch.conj torch.conj
==========
`torch.conj(input, *, out=None) → Tensor`
Computes the element-wise conjugate of the given `input` tensor. If :attr`input` has a non-complex dtype, this function just returns `input`.
Warning
In the future, [`torch.conj()`](#torch.conj "torch.conj") may return a non-writeable view for an `input` of non-complex dtype. It’s recommended that programs not modify the tensor returned by [`torch.conj()`](#torch.conj "torch.conj") when `input` is of non-complex dtype to be compatible with this change.
outi=conj(inputi)\text{out}\_{i} = conj(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.conj(torch.tensor([-1 + 1j, -2 + 2j, 3 - 3j]))
tensor([-1 - 1j, -2 - 2j, 3 + 3j])
```
pytorch torch.trunc torch.trunc
===========
`torch.trunc(input, *, out=None) → Tensor`
Returns a new tensor with the truncated integer values of the elements of `input`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 3.4742, 0.5466, -0.8008, -0.9079])
>>> torch.trunc(a)
tensor([ 3., 0., -0., -0.])
```
pytorch torch.nn.utils.prune.remove torch.nn.utils.prune.remove
===========================
`torch.nn.utils.prune.remove(module, name)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#remove)
Removes the pruning reparameterization from a module and the pruning method from the forward hook. The pruned parameter named `name` remains permanently pruned, and the parameter named `name+'_orig'` is removed from the parameter list. Similarly, the buffer named `name+'_mask'` is removed from the buffers.
Note
Pruning itself is NOT undone or reversed!
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
#### Examples
```
>>> m = random_unstructured(nn.Linear(5, 7), name='weight', amount=0.2)
>>> m = remove(m, name='weight')
```
pytorch torch.any torch.any
=========
`torch.any(input) → Tensor`
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Tests if any element in `input` evaluates to `True`.
Note
This function matches the behaviour of NumPy in returning output of dtype `bool` for all supported dtypes except `uint8`. For `uint8` the dtype of output is `uint8` itself.
Example:
```
>>> a = torch.rand(1, 2).bool()
>>> a
tensor([[False, True]], dtype=torch.bool)
>>> torch.any(a)
tensor(True, dtype=torch.bool)
>>> a = torch.arange(0, 3)
>>> a
tensor([0, 1, 2])
>>> torch.any(a)
tensor(True)
```
`torch.any(input, dim, keepdim=False, *, out=None) → Tensor`
For each row of `input` in the given dimension `dim`, returns `True` if any element in the row evaluate to `True` and `False` otherwise.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 fewer dimension than `input`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4, 2) < 0
>>> a
tensor([[ True, True],
[False, True],
[ True, True],
[False, False]])
>>> torch.any(a, 1)
tensor([ True, True, True, False])
>>> torch.any(a, 0)
tensor([True, True])
```
pytorch ParameterList ParameterList
=============
`class torch.nn.ParameterList(parameters=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ParameterList)
Holds parameters in a list.
[`ParameterList`](#torch.nn.ParameterList "torch.nn.ParameterList") can be indexed like a regular Python list, but parameters it contains are properly registered, and will be visible by all [`Module`](torch.nn.module#torch.nn.Module "torch.nn.Module") methods.
Parameters
**parameters** (*iterable**,* *optional*) – an iterable of `Parameter` to add
Example:
```
class MyModule(nn.Module):
def __init__(self):
super(MyModule, self).__init__()
self.params = nn.ParameterList([nn.Parameter(torch.randn(10, 10)) for i in range(10)])
def forward(self, x):
# ParameterList can act as an iterable, or be indexed using ints
for i, p in enumerate(self.params):
x = self.params[i // 2].mm(x) + p.mm(x)
return x
```
`append(parameter)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ParameterList.append)
Appends a given parameter at the end of the list.
Parameters
**parameter** (*nn.Parameter*) – parameter to append
`extend(parameters)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ParameterList.extend)
Appends parameters from a Python iterable to the end of the list.
Parameters
**parameters** (*iterable*) – iterable of parameters to append
pytorch torch.frac torch.frac
==========
`torch.frac(input, *, out=None) → Tensor`
Computes the fractional portion of each element in `input`.
outi=inputi−⌊∣inputi∣⌋∗sgn(inputi)\text{out}\_{i} = \text{input}\_{i} - \left\lfloor |\text{input}\_{i}| \right\rfloor \* \operatorname{sgn}(\text{input}\_{i})
Example:
```
>>> torch.frac(torch.tensor([1, 2.5, -3.2]))
tensor([ 0.0000, 0.5000, -0.2000])
```
pytorch torch.sgn torch.sgn
=========
`torch.sgn(input, *, out=None) → Tensor`
For complex tensors, this function returns a new tensor whose elemants have the same angle as that of the elements of `input` and absolute value 1. For a non-complex tensor, this function returns the signs of the elements of `input` (see [`torch.sign()`](torch.sign#torch.sign "torch.sign")).
outi=0\text{out}\_{i} = 0 , if ∣inputi∣==0|{\text{{input}}\_i}| == 0 outi=inputi∣inputi∣\text{out}\_{i} = \frac{{\text{{input}}\_i}}{|{\text{{input}}\_i}|} , otherwise
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> x=torch.tensor([3+4j, 7-24j, 0, 1+2j])
>>> x.sgn()
tensor([0.6000+0.8000j, 0.2800-0.9600j, 0.0000+0.0000j, 0.4472+0.8944j])
```
pytorch torch.greater torch.greater
=============
`torch.greater(input, other, *, out=None) → Tensor`
Alias for [`torch.gt()`](torch.gt#torch.gt "torch.gt").
pytorch torch.histc torch.histc
===========
`torch.histc(input, bins=100, min=0, max=0, *, out=None) → Tensor`
Computes the histogram of a tensor.
The elements are sorted into equal width bins between [`min`](torch.min#torch.min "torch.min") and [`max`](torch.max#torch.max "torch.max"). If [`min`](torch.min#torch.min "torch.min") and [`max`](torch.max#torch.max "torch.max") are both zero, the minimum and maximum values of the data are used.
Elements lower than min and higher than max are ignored.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **bins** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – number of histogram bins
* **min** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – lower end of the range (inclusive)
* **max** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – upper end of the range (inclusive)
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Returns
Histogram represented as a tensor
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> torch.histc(torch.tensor([1., 2, 1]), bins=4, min=0, max=3)
tensor([ 0., 2., 1., 0.])
```
pytorch torch.cholesky_solve torch.cholesky\_solve
=====================
`torch.cholesky_solve(input, input2, upper=False, *, out=None) → Tensor`
Solves a linear system of equations with a positive semidefinite matrix to be inverted given its Cholesky factor matrix uu .
If `upper` is `False`, uu is and lower triangular and `c` is returned such that:
c=(uuT)−1bc = (u u^T)^{{-1}} b
If `upper` is `True` or not provided, uu is upper triangular and `c` is returned such that:
c=(uTu)−1bc = (u^T u)^{{-1}} b
`torch.cholesky_solve(b, u)` can take in 2D inputs `b, u` or inputs that are batches of 2D matrices. If the inputs are batches, then returns batched outputs `c`
Supports real-valued and complex-valued inputs. For the complex-valued inputs the transpose operator above is the conjugate transpose.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – input matrix bb of size (∗,m,k)(\*, m, k) , where ∗\* is zero or more batch dimensions
* **input2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – input matrix uu of size (∗,m,m)(\*, m, m) , where ∗\* is zero of more batch dimensions composed of upper or lower triangular Cholesky factor
* **upper** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to consider the Cholesky factor as a lower or upper triangular matrix. Default: `False`.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor for `c`
Example:
```
>>> a = torch.randn(3, 3)
>>> a = torch.mm(a, a.t()) # make symmetric positive definite
>>> u = torch.cholesky(a)
>>> a
tensor([[ 0.7747, -1.9549, 1.3086],
[-1.9549, 6.7546, -5.4114],
[ 1.3086, -5.4114, 4.8733]])
>>> b = torch.randn(3, 2)
>>> b
tensor([[-0.6355, 0.9891],
[ 0.1974, 1.4706],
[-0.4115, -0.6225]])
>>> torch.cholesky_solve(b, u)
tensor([[ -8.1625, 19.6097],
[ -5.8398, 14.2387],
[ -4.3771, 10.4173]])
>>> torch.mm(a.inverse(), b)
tensor([[ -8.1626, 19.6097],
[ -5.8398, 14.2387],
[ -4.3771, 10.4173]])
```
pytorch torch.ravel torch.ravel
===========
`torch.ravel(input) → Tensor`
Return a contiguous flattened tensor. A copy is made only if needed.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example:
```
>>> t = torch.tensor([[[1, 2],
... [3, 4]],
... [[5, 6],
... [7, 8]]])
>>> torch.ravel(t)
tensor([1, 2, 3, 4, 5, 6, 7, 8])
```
pytorch torch.symeig torch.symeig
============
`torch.symeig(input, eigenvectors=False, upper=True, *, out=None) -> (Tensor, Tensor)`
This function returns eigenvalues and eigenvectors of a real symmetric matrix `input` or a batch of real symmetric matrices, represented by a namedtuple (eigenvalues, eigenvectors).
This function calculates all eigenvalues (and vectors) of `input` such that input=Vdiag(e)VT\text{input} = V \text{diag}(e) V^T .
The boolean argument `eigenvectors` defines computation of both eigenvectors and eigenvalues or eigenvalues only.
If it is `False`, only eigenvalues are computed. If it is `True`, both eigenvalues and eigenvectors are computed.
Since the input matrix `input` is supposed to be symmetric, only the upper triangular portion is used by default.
If `upper` is `False`, then lower triangular portion is used.
Note
The eigenvalues are returned in ascending order. If `input` is a batch of matrices, then the eigenvalues of each matrix in the batch is returned in ascending order.
Note
Irrespective of the original strides, the returned matrix `V` will be transposed, i.e. with strides `V.contiguous().transpose(-1, -2).stride()`.
Warning
Extra care needs to be taken when backward through outputs. Such operation is only stable when all eigenvalues are distinct and becomes less stable the smaller mini≠j∣λi−λj∣\min\_{i \neq j} |\lambda\_i - \lambda\_j| is.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor of size (∗,n,n)(\*, n, n) where `*` is zero or more batch dimensions consisting of symmetric matrices.
* **eigenvectors** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – controls whether eigenvectors have to be computed
* **upper** (*boolean**,* *optional*) – controls whether to consider upper-triangular or lower-triangular region
Keyword Arguments
**out** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the output tuple of (Tensor, Tensor)
Returns
A namedtuple (eigenvalues, eigenvectors) containing
* **eigenvalues** (*Tensor*): Shape (∗,m)(\*, m) . The eigenvalues in ascending order.
* **eigenvectors** (*Tensor*): Shape (∗,m,m)(\*, m, m) . If `eigenvectors=False`, it’s an empty tensor. Otherwise, this tensor contains the orthonormal eigenvectors of the `input`.
Return type
([Tensor](../tensors#torch.Tensor "torch.Tensor"), [Tensor](../tensors#torch.Tensor "torch.Tensor"))
Examples:
```
>>> a = torch.randn(5, 5)
>>> a = a + a.t() # To make a symmetric
>>> a
tensor([[-5.7827, 4.4559, -0.2344, -1.7123, -1.8330],
[ 4.4559, 1.4250, -2.8636, -3.2100, -0.1798],
[-0.2344, -2.8636, 1.7112, -5.5785, 7.1988],
[-1.7123, -3.2100, -5.5785, -2.6227, 3.1036],
[-1.8330, -0.1798, 7.1988, 3.1036, -5.1453]])
>>> e, v = torch.symeig(a, eigenvectors=True)
>>> e
tensor([-13.7012, -7.7497, -2.3163, 5.2477, 8.1050])
>>> v
tensor([[ 0.1643, 0.9034, -0.0291, 0.3508, 0.1817],
[-0.2417, -0.3071, -0.5081, 0.6534, 0.4026],
[-0.5176, 0.1223, -0.0220, 0.3295, -0.7798],
[-0.4850, 0.2695, -0.5773, -0.5840, 0.1337],
[ 0.6415, -0.0447, -0.6381, -0.0193, -0.4230]])
>>> a_big = torch.randn(5, 2, 2)
>>> a_big = a_big + a_big.transpose(-2, -1) # To make a_big symmetric
>>> e, v = a_big.symeig(eigenvectors=True)
>>> torch.allclose(torch.matmul(v, torch.matmul(e.diag_embed(), v.transpose(-2, -1))), a_big)
True
```
| programming_docs |
pytorch torch.set_num_interop_threads torch.set\_num\_interop\_threads
================================
`torch.set_num_interop_threads(int)`
Sets the number of threads used for interop parallelism (e.g. in JIT interpreter) on CPU.
Warning
Can only be called once and before any inter-op parallel work is started (e.g. JIT execution).
pytorch torch.set_printoptions torch.set\_printoptions
=======================
`torch.set_printoptions(precision=None, threshold=None, edgeitems=None, linewidth=None, profile=None, sci_mode=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/_tensor_str.html#set_printoptions)
Set options for printing. Items shamelessly taken from NumPy
Parameters
* **precision** – Number of digits of precision for floating point output (default = 4).
* **threshold** – Total number of array elements which trigger summarization rather than full `repr` (default = 1000).
* **edgeitems** – Number of array items in summary at beginning and end of each dimension (default = 3).
* **linewidth** – The number of characters per line for the purpose of inserting line breaks (default = 80). Thresholded matrices will ignore this parameter.
* **profile** – Sane defaults for pretty printing. Can override with any of the above options. (any one of `default`, `short`, `full`)
* **sci\_mode** – Enable (True) or disable (False) scientific notation. If None (default) is specified, the value is defined by `torch._tensor_str._Formatter`. This value is automatically chosen by the framework.
pytorch torch.mul torch.mul
=========
`torch.mul(input, other, *, out=None)`
Multiplies each element of the input `input` with the scalar `other` and returns a new resulting tensor.
outi=other×inputi\text{out}\_i = \text{other} \times \text{input}\_i
If `input` is of type `FloatTensor` or `DoubleTensor`, `other` should be a real number, otherwise it should be an integer
Parameters
* **{input}** –
* **other** (*Number*) – the number to be multiplied to each element of `input`
Keyword Arguments
**{out}** –
Example:
```
>>> a = torch.randn(3)
>>> a
tensor([ 0.2015, -0.4255, 2.6087])
>>> torch.mul(a, 100)
tensor([ 20.1494, -42.5491, 260.8663])
```
`torch.mul(input, other, *, out=None)`
Each element of the tensor `input` is multiplied by the corresponding element of the Tensor `other`. The resulting tensor is returned.
The shapes of `input` and `other` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
outi=inputi×otheri\text{out}\_i = \text{input}\_i \times \text{other}\_i
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first multiplicand tensor
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second multiplicand tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4, 1)
>>> a
tensor([[ 1.1207],
[-0.3137],
[ 0.0700],
[ 0.8378]])
>>> b = torch.randn(1, 4)
>>> b
tensor([[ 0.5146, 0.1216, -0.5244, 2.2382]])
>>> torch.mul(a, b)
tensor([[ 0.5767, 0.1363, -0.5877, 2.5083],
[-0.1614, -0.0382, 0.1645, -0.7021],
[ 0.0360, 0.0085, -0.0367, 0.1567],
[ 0.4312, 0.1019, -0.4394, 1.8753]])
```
pytorch torch.repeat_interleave torch.repeat\_interleave
========================
`torch.repeat_interleave(input, repeats, dim=None) → Tensor`
Repeat elements of a tensor.
Warning
This is different from [`torch.Tensor.repeat()`](../tensors#torch.Tensor.repeat "torch.Tensor.repeat") but similar to `numpy.repeat`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **repeats** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* [int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – The number of repetitions for each element. repeats is broadcasted to fit the shape of the given axis.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – The dimension along which to repeat values. By default, use the flattened input array, and return a flat output array.
Returns
Repeated tensor which has the same shape as input, except along the given axis.
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> x = torch.tensor([1, 2, 3])
>>> x.repeat_interleave(2)
tensor([1, 1, 2, 2, 3, 3])
>>> y = torch.tensor([[1, 2], [3, 4]])
>>> torch.repeat_interleave(y, 2)
tensor([1, 1, 2, 2, 3, 3, 4, 4])
>>> torch.repeat_interleave(y, 3, dim=1)
tensor([[1, 1, 1, 2, 2, 2],
[3, 3, 3, 4, 4, 4]])
>>> torch.repeat_interleave(y, torch.tensor([1, 2]), dim=0)
tensor([[1, 2],
[3, 4],
[3, 4]])
```
`torch.repeat_interleave(repeats) → Tensor`
If the `repeats` is `tensor([n1, n2, n3, …])`, then the output will be `tensor([0, 0, …, 1, 1, …, 2, 2, …, …])` where `0` appears `n1` times, `1` appears `n2` times, `2` appears `n3` times, etc.
pytorch Embedding Embedding
=========
`class torch.nn.Embedding(num_embeddings, embedding_dim, padding_idx=None, max_norm=None, norm_type=2.0, scale_grad_by_freq=False, sparse=False, _weight=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/sparse.html#Embedding)
A simple lookup table that stores embeddings of a fixed dictionary and size.
This module is often used to store word embeddings and retrieve them using indices. The input to the module is a list of indices, and the output is the corresponding word embeddings.
Parameters
* **num\_embeddings** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – size of the dictionary of embeddings
* **embedding\_dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the size of each embedding vector
* **padding\_idx** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – If given, pads the output with the embedding vector at `padding_idx` (initialized to zeros) whenever it encounters the index.
* **max\_norm** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – If given, each embedding vector with norm larger than `max_norm` is renormalized to have norm `max_norm`.
* **norm\_type** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – The p of the p-norm to compute for the `max_norm` option. Default `2`.
* **scale\_grad\_by\_freq** (*boolean**,* *optional*) – If given, this will scale gradients by the inverse of frequency of the words in the mini-batch. Default `False`.
* **sparse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, gradient w.r.t. `weight` matrix will be a sparse tensor. See Notes for more details regarding sparse gradients.
Variables
**~Embedding.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (num\_embeddings, embedding\_dim) initialized from N(0,1)\mathcal{N}(0, 1)
Shape:
* Input: (∗)(\*) , IntTensor or LongTensor of arbitrary shape containing the indices to extract
* Output: (∗,H)(\*, H) , where `*` is the input shape and H=embedding\_dimH=\text{embedding\\_dim}
Note
Keep in mind that only a limited number of optimizers support sparse gradients: currently it’s `optim.SGD` (`CUDA` and `CPU`), `optim.SparseAdam` (`CUDA` and `CPU`) and `optim.Adagrad` (`CPU`)
Note
With `padding_idx` set, the embedding vector at `padding_idx` is initialized to all zeros. However, note that this vector can be modified afterwards, e.g., using a customized initialization method, and thus changing the vector used to pad the output. The gradient for this vector from [`Embedding`](#torch.nn.Embedding "torch.nn.Embedding") is always zero.
Note
When `max_norm` is not `None`, [`Embedding`](#torch.nn.Embedding "torch.nn.Embedding")’s forward method will modify the `weight` tensor in-place. Since tensors needed for gradient computations cannot be modified in-place, performing a differentiable operation on `Embedding.weight` before calling [`Embedding`](#torch.nn.Embedding "torch.nn.Embedding")’s forward method requires cloning `Embedding.weight` when `max_norm` is not `None`. For example:
```
n, d, m = 3, 5, 7
embedding = nn.Embedding(n, d, max_norm=True)
W = torch.randn((m, d), requires_grad=True)
idx = torch.tensor([1, 2])
a = embedding.weight.clone() @ W.t() # weight must be cloned for this to be differentiable
b = embedding(idx) @ W.t() # modifies weight in-place
out = (a.unsqueeze(0) + b.unsqueeze(1))
loss = out.sigmoid().prod()
loss.backward()
```
Examples:
```
>>> # an Embedding module containing 10 tensors of size 3
>>> embedding = nn.Embedding(10, 3)
>>> # a batch of 2 samples of 4 indices each
>>> input = torch.LongTensor([[1,2,4,5],[4,3,2,9]])
>>> embedding(input)
tensor([[[-0.0251, -1.6902, 0.7172],
[-0.6431, 0.0748, 0.6969],
[ 1.4970, 1.3448, -0.9685],
[-0.3677, -2.7265, -0.1685]],
[[ 1.4970, 1.3448, -0.9685],
[ 0.4362, -0.4004, 0.9400],
[-0.6431, 0.0748, 0.6969],
[ 0.9124, -2.3616, 1.1151]]])
>>> # example with padding_idx
>>> embedding = nn.Embedding(10, 3, padding_idx=0)
>>> input = torch.LongTensor([[0,2,0,5]])
>>> embedding(input)
tensor([[[ 0.0000, 0.0000, 0.0000],
[ 0.1535, -2.0309, 0.9315],
[ 0.0000, 0.0000, 0.0000],
[-0.1655, 0.9897, 0.0635]]])
```
`classmethod from_pretrained(embeddings, freeze=True, padding_idx=None, max_norm=None, norm_type=2.0, scale_grad_by_freq=False, sparse=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/sparse.html#Embedding.from_pretrained)
Creates Embedding instance from given 2-dimensional FloatTensor.
Parameters
* **embeddings** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – FloatTensor containing weights for the Embedding. First dimension is being passed to Embedding as `num_embeddings`, second as `embedding_dim`.
* **freeze** (*boolean**,* *optional*) – If `True`, the tensor does not get updated in the learning process. Equivalent to `embedding.weight.requires_grad = False`. Default: `True`
* **padding\_idx** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – See module initialization documentation.
* **max\_norm** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – See module initialization documentation.
* **norm\_type** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – See module initialization documentation. Default `2`.
* **scale\_grad\_by\_freq** (*boolean**,* *optional*) – See module initialization documentation. Default `False`.
* **sparse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – See module initialization documentation.
Examples:
```
>>> # FloatTensor containing pretrained weights
>>> weight = torch.FloatTensor([[1, 2.3, 3], [4, 5.1, 6.3]])
>>> embedding = nn.Embedding.from_pretrained(weight)
>>> # Get embeddings for index 1
>>> input = torch.LongTensor([1])
>>> embedding(input)
tensor([[ 4.0000, 5.1000, 6.3000]])
```
pytorch torch.jit.script_if_tracing torch.jit.script\_if\_tracing
=============================
`torch.jit.script_if_tracing(fn)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/jit.html#script_if_tracing)
Compiles `fn` when it is first called during tracing. `torch.jit.script` has a non-negligible start up time when it is first called due to lazy-initializations of many compiler builtins. Therefore you should not use it in library code. However, you may want to have parts of your library work in tracing even if they use control flow. In these cases, you should use `@torch.jit.script_if_tracing` to substitute for `torch.jit.script`.
Parameters
**fn** – A function to compile.
Returns
If called during tracing, a [`ScriptFunction`](torch.jit.scriptfunction#torch.jit.ScriptFunction "torch.jit.ScriptFunction") created by `torch.jit.script` is returned. Otherwise, the original function `fn` is returned.
pytorch ReplicationPad1d ReplicationPad1d
================
`class torch.nn.ReplicationPad1d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ReplicationPad1d)
Pads the input tensor using replication of the input boundary.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 2-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} )
Shape:
* Input: (N,C,Win)(N, C, W\_{in})
* Output: (N,C,Wout)(N, C, W\_{out}) where
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ReplicationPad1d(2)
>>> input = torch.arange(8, dtype=torch.float).reshape(1, 2, 4)
>>> input
tensor([[[0., 1., 2., 3.],
[4., 5., 6., 7.]]])
>>> m(input)
tensor([[[0., 0., 0., 1., 2., 3., 3., 3.],
[4., 4., 4., 5., 6., 7., 7., 7.]]])
>>> # using different paddings for different sides
>>> m = nn.ReplicationPad1d((3, 1))
>>> m(input)
tensor([[[0., 0., 0., 0., 1., 2., 3., 3.],
[4., 4., 4., 4., 5., 6., 7., 7.]]])
```
pytorch torch.remainder torch.remainder
===============
`torch.remainder(input, other, *, out=None) → Tensor`
Computes the element-wise remainder of division.
The dividend and divisor may contain both for integer and floating point numbers. The remainder has the same sign as the divisor `other`.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), [type promotion](../tensor_attributes#type-promotion-doc), and integer and float inputs.
Note
Complex inputs are not supported. In some cases, it is not mathematically possible to satisfy the definition of a modulo operation with complex numbers. See [`torch.fmod()`](torch.fmod#torch.fmod "torch.fmod") for how division by zero is handled.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the dividend
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Scalar*) – the divisor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.remainder(torch.tensor([-3., -2, -1, 1, 2, 3]), 2)
tensor([ 1., 0., 1., 1., 0., 1.])
>>> torch.remainder(torch.tensor([1, 2, 3, 4, 5]), 1.5)
tensor([ 1.0000, 0.5000, 0.0000, 1.0000, 0.5000])
```
See also
[`torch.fmod()`](torch.fmod#torch.fmod "torch.fmod"), which computes the element-wise remainder of division equivalently to the C library function `fmod()`.
pytorch MaxPool2d MaxPool2d
=========
`class torch.nn.MaxPool2d(kernel_size, stride=None, padding=0, dilation=1, return_indices=False, ceil_mode=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#MaxPool2d)
Applies a 2D max pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,H,W)(N, C, H, W) , output (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) and `kernel_size` (kH,kW)(kH, kW) can be precisely described as:
out(Ni,Cj,h,w)=maxm=0,…,kH−1maxn=0,…,kW−1input(Ni,Cj,stride[0]×h+m,stride[1]×w+n)\begin{aligned} out(N\_i, C\_j, h, w) ={} & \max\_{m=0, \ldots, kH-1} \max\_{n=0, \ldots, kW-1} \\ & \text{input}(N\_i, C\_j, \text{stride[0]} \times h + m, \text{stride[1]} \times w + n) \end{aligned}
If `padding` is non-zero, then the input is implicitly zero-padded on both sides for `padding` number of points. `dilation` controls the spacing between the kernel points. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
The parameters `kernel_size`, `stride`, `padding`, `dilation` can either be:
* a single `int` – in which case the same value is used for the height and width dimension
* a `tuple` of two ints – in which case, the first `int` is used for the height dimension, and the second `int` for the width dimension
Parameters
* **kernel\_size** – the size of the window to take a max over
* **stride** – the stride of the window. Default value is `kernel_size`
* **padding** – implicit zero padding to be added on both sides
* **dilation** – a parameter that controls the stride of elements in the window
* **return\_indices** – if `True`, will return the max indices along with the outputs. Useful for [`torch.nn.MaxUnpool2d`](torch.nn.maxunpool2d#torch.nn.MaxUnpool2d "torch.nn.MaxUnpool2d") later
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) , where
Hout=⌊Hin+2∗padding[0]−dilation[0]×(kernel\_size[0]−1)−1stride[0]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \* \text{padding[0]} - \text{dilation[0]} \times (\text{kernel\\_size[0]} - 1) - 1}{\text{stride[0]}} + 1\right\rfloor
Wout=⌊Win+2∗padding[1]−dilation[1]×(kernel\_size[1]−1)−1stride[1]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \* \text{padding[1]} - \text{dilation[1]} \times (\text{kernel\\_size[1]} - 1) - 1}{\text{stride[1]}} + 1\right\rfloor
Examples:
```
>>> # pool of square window of size=3, stride=2
>>> m = nn.MaxPool2d(3, stride=2)
>>> # pool of non-square window
>>> m = nn.MaxPool2d((3, 2), stride=(2, 1))
>>> input = torch.randn(20, 16, 50, 32)
>>> output = m(input)
```
pytorch torch.copysign torch.copysign
==============
`torch.copysign(input, other, *, out=None) → Tensor`
Create a new floating-point tensor with the magnitude of `input` and the sign of `other`, elementwise.
outi={−∣inputi∣ifotheri≤−0.0∣inputi∣ifotheri≥0.0\text{out}\_{i} = \begin{cases} -|\text{input}\_{i}| & \text{if} \text{other}\_{i} \leq -0.0 \\ |\text{input}\_{i}| & \text{if} \text{other}\_{i} \geq 0.0 \\ \end{cases}
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), and integer and float inputs.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – magnitudes.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Number*) – contains value(s) whose signbit(s) are applied to the magnitudes in `input`.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(5)
>>> a
tensor([-1.2557, -0.0026, -0.5387, 0.4740, -0.9244])
>>> torch.copysign(a, 1)
tensor([1.2557, 0.0026, 0.5387, 0.4740, 0.9244])
>>> a = torch.randn(4, 4)
>>> a
tensor([[ 0.7079, 0.2778, -1.0249, 0.5719],
[-0.0059, -0.2600, -0.4475, -1.3948],
[ 0.3667, -0.9567, -2.5757, -0.1751],
[ 0.2046, -0.0742, 0.2998, -0.1054]])
>>> b = torch.randn(4)
tensor([ 0.2373, 0.3120, 0.3190, -1.1128])
>>> torch.copysign(a, b)
tensor([[ 0.7079, 0.2778, 1.0249, -0.5719],
[ 0.0059, 0.2600, 0.4475, -1.3948],
[ 0.3667, 0.9567, 2.5757, -0.1751],
[ 0.2046, 0.0742, 0.2998, -0.1054]])
```
| programming_docs |
pytorch torch.scatter torch.scatter
=============
`torch.scatter(input, dim, index, src) → Tensor`
Out-of-place version of [`torch.Tensor.scatter_()`](../tensors#torch.Tensor.scatter_ "torch.Tensor.scatter_")
pytorch torch.lobpcg torch.lobpcg
============
`torch.lobpcg(A, k=None, B=None, X=None, n=None, iK=None, niter=None, tol=None, largest=None, method=None, tracker=None, ortho_iparams=None, ortho_fparams=None, ortho_bparams=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/_lobpcg.html#lobpcg)
Find the k largest (or smallest) eigenvalues and the corresponding eigenvectors of a symmetric positive defined generalized eigenvalue problem using matrix-free LOBPCG methods.
This function is a front-end to the following LOBPCG algorithms selectable via `method` argument:
`method=”basic”` - the LOBPCG method introduced by Andrew Knyazev, see [Knyazev2001]. A less robust method, may fail when Cholesky is applied to singular input.
`method=”ortho”` - the LOBPCG method with orthogonal basis selection [StathopoulosEtal2002]. A robust method.
Supported inputs are dense, sparse, and batches of dense matrices.
Note
In general, the basic method spends least time per iteration. However, the robust methods converge much faster and are more stable. So, the usage of the basic method is generally not recommended but there exist cases where the usage of the basic method may be preferred.
Warning
The backward method does not support sparse and complex inputs. It works only when `B` is not provided (i.e. `B == None`). We are actively working on extensions, and the details of the algorithms are going to be published promptly.
Warning
While it is assumed that `A` is symmetric, `A.grad` is not. To make sure that `A.grad` is symmetric, so that `A - t * A.grad` is symmetric in first-order optimization routines, prior to running `lobpcg` we do the following symmetrization map: `A -> (A + A.t()) / 2`. The map is performed only when the `A` requires gradients.
Parameters
* **A** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor of size (∗,m,m)(\*, m, m)
* **B** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the input tensor of size (∗,m,m)(\*, m, m) . When not specified, `B` is interpereted as identity matrix.
* **X** (*tensor**,* *optional*) – the input tensor of size (∗,m,n)(\*, m, n) where `k <= n <= m`. When specified, it is used as initial approximation of eigenvectors. X must be a dense tensor.
* **iK** (*tensor**,* *optional*) – the input tensor of size (∗,m,m)(\*, m, m) . When specified, it will be used as preconditioner.
* **k** (*integer**,* *optional*) – the number of requested eigenpairs. Default is the number of XX columns (when specified) or `1`.
* **n** (*integer**,* *optional*) – if XX is not specified then `n` specifies the size of the generated random approximation of eigenvectors. Default value for `n` is `k`. If XX is specified, the value of `n` (when specified) must be the number of XX columns.
* **tol** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – residual tolerance for stopping criterion. Default is `feps ** 0.5` where `feps` is smallest non-zero floating-point number of the given input tensor `A` data type.
* **largest** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – when True, solve the eigenproblem for the largest eigenvalues. Otherwise, solve the eigenproblem for smallest eigenvalues. Default is `True`.
* **method** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")*,* *optional*) – select LOBPCG method. See the description of the function above. Default is “ortho”.
* **niter** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – maximum number of iterations. When reached, the iteration process is hard-stopped and the current approximation of eigenpairs is returned. For infinite iteration but until convergence criteria is met, use `-1`.
* **tracker** (*callable**,* *optional*) –
a function for tracing the iteration process. When specified, it is called at each iteration step with LOBPCG instance as an argument. The LOBPCG instance holds the full state of the iteration process in the following attributes:
`iparams`, `fparams`, `bparams` - dictionaries of integer, float, and boolean valued input parameters, respectively
`ivars`, `fvars`, `bvars`, `tvars` - dictionaries of integer, float, boolean, and Tensor valued iteration variables, respectively.
`A`, `B`, `iK` - input Tensor arguments.
`E`, `X`, `S`, `R` - iteration Tensor variables.
For instance:
`ivars[“istep”]` - the current iteration step `X` - the current approximation of eigenvectors `E` - the current approximation of eigenvalues `R` - the current residual `ivars[“converged_count”]` - the current number of converged eigenpairs `tvars[“rerr”]` - the current state of convergence criteria
Note that when `tracker` stores Tensor objects from the LOBPCG instance, it must make copies of these.
If `tracker` sets `bvars[“force_stop”] = True`, the iteration process will be hard-stopped.
* **ortho\_fparams, ortho\_bparams** (*ortho\_iparams**,*) – various parameters to LOBPCG algorithm when using `method=”ortho”`.
Returns
tensor of eigenvalues of size (∗,k)(\*, k)
X (Tensor): tensor of eigenvectors of size (∗,m,k)(\*, m, k)
Return type
E ([Tensor](../tensors#torch.Tensor "torch.Tensor"))
#### References
[Knyazev2001] Andrew V. Knyazev. (2001) Toward the Optimal Preconditioned Eigensolver: Locally Optimal Block Preconditioned Conjugate Gradient Method. SIAM J. Sci. Comput., 23(2), 517-541. (25 pages) <https://epubs.siam.org/doi/abs/10.1137/S1064827500366124>
[StathopoulosEtal2002] Andreas Stathopoulos and Kesheng Wu. (2002) A Block Orthogonalization Procedure with Constant Synchronization Requirements. SIAM J. Sci. Comput., 23(6), 2165-2182. (18 pages) <https://epubs.siam.org/doi/10.1137/S1064827500370883>
[DuerschEtal2018] Jed A. Duersch, Meiyue Shao, Chao Yang, Ming Gu. (2018) A Robust and Efficient Implementation of LOBPCG. SIAM J. Sci. Comput., 40(5), C655-C676. (22 pages) <https://epubs.siam.org/doi/abs/10.1137/17M1129830>
pytorch FractionalMaxPool2d FractionalMaxPool2d
===================
`class torch.nn.FractionalMaxPool2d(kernel_size, output_size=None, output_ratio=None, return_indices=False, _random_samples=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#FractionalMaxPool2d)
Applies a 2D fractional max pooling over an input signal composed of several input planes.
Fractional MaxPooling is described in detail in the paper [Fractional MaxPooling](https://arxiv.org/abs/1412.6071) by Ben Graham
The max-pooling operation is applied in kH×kWkH \times kW regions by a stochastic step size determined by the target output size. The number of output features is equal to the number of input planes.
Parameters
* **kernel\_size** – the size of the window to take a max over. Can be a single number k (for a square kernel of k x k) or a tuple `(kh, kw)`
* **output\_size** – the target output size of the image of the form `oH x oW`. Can be a tuple `(oH, oW)` or a single number oH for a square image `oH x oH`
* **output\_ratio** – If one wants to have an output size as a ratio of the input size, this option can be given. This has to be a number or tuple in the range (0, 1)
* **return\_indices** – if `True`, will return the indices along with the outputs. Useful to pass to `nn.MaxUnpool2d()`. Default: `False`
#### Examples
```
>>> # pool of square window of size=3, and target output size 13x12
>>> m = nn.FractionalMaxPool2d(3, output_size=(13, 12))
>>> # pool of square window and target output size being half of input image size
>>> m = nn.FractionalMaxPool2d(3, output_ratio=(0.5, 0.5))
>>> input = torch.randn(20, 16, 50, 32)
>>> output = m(input)
```
pytorch torch.absolute torch.absolute
==============
`torch.absolute(input, *, out=None) → Tensor`
Alias for [`torch.abs()`](torch.abs#torch.abs "torch.abs")
pytorch Hardswish Hardswish
=========
`class torch.nn.Hardswish(inplace=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Hardswish)
Applies the hardswish function, element-wise, as described in the paper:
[Searching for MobileNetV3](https://arxiv.org/abs/1905.02244).
Hardswish(x)={0if x≤−3,xif x≥+3,x⋅(x+3)/6otherwise\text{Hardswish}(x) = \begin{cases} 0 & \text{if~} x \le -3, \\ x & \text{if~} x \ge +3, \\ x \cdot (x + 3) /6 & \text{otherwise} \end{cases}
Parameters
**inplace** – can optionally do the operation in-place. Default: `False`
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.Hardswish()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.orgqr torch.orgqr
===========
`torch.orgqr(input, input2) → Tensor`
Computes the orthogonal matrix `Q` of a QR factorization, from the `(input, input2)` tuple returned by [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf").
This directly calls the underlying LAPACK function `?orgqr`. See [LAPACK documentation for orgqr](https://software.intel.com/en-us/mkl-developer-reference-c-orgqr) for further details.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the `a` from [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf").
* **input2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the `tau` from [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf").
pytorch torch.diff torch.diff
==========
`torch.diff(input, n=1, dim=-1, prepend=None, append=None) → Tensor`
Computes the n-th forward difference along the given dimension.
The first-order differences are given by `out[i] = input[i + 1] - input[i]`. Higher-order differences are calculated by using [`torch.diff()`](#torch.diff "torch.diff") recursively.
Note
Only `n = 1` is currently supported
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compute the differences on
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the number of times to recursively compute the difference
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the dimension to compute the difference along. Default is the last dimension.
* **append** (*prepend**,*) – values to prepend or append to `input` along `dim` before computing the difference. Their dimensions must be equivalent to that of input, and their shapes must match input’s shape except on `dim`.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([1, 3, 2])
>>> torch.diff(a)
tensor([ 2, -1])
>>> b = torch.tensor([4, 5])
>>> torch.diff(a, append=b)
tensor([ 2, -1, 2, 1])
>>> c = torch.tensor([[1, 2, 3], [3, 4, 5]])
>>> torch.diff(c, dim=0)
tensor([[2, 2, 2]])
>>> torch.diff(c, dim=1)
tensor([[1, 1],
[1, 1]])
```
pytorch LayerNorm LayerNorm
=========
`class torch.nn.LayerNorm(normalized_shape, eps=1e-05, elementwise_affine=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/normalization.html#LayerNorm)
Applies Layer Normalization over a mini-batch of inputs as described in the paper [Layer Normalization](https://arxiv.org/abs/1607.06450)
y=x−E[x]Var[x]+ϵ∗γ+βy = \frac{x - \mathrm{E}[x]}{ \sqrt{\mathrm{Var}[x] + \epsilon}} \* \gamma + \beta
The mean and standard-deviation are calculated separately over the last certain number dimensions which have to be of the shape specified by `normalized_shape`. γ\gamma and β\beta are learnable affine transform parameters of `normalized_shape` if `elementwise_affine` is `True`. The standard-deviation is calculated via the biased estimator, equivalent to `torch.var(input, unbiased=False)`.
Note
Unlike Batch Normalization and Instance Normalization, which applies scalar scale and bias for each entire channel/plane with the `affine` option, Layer Normalization applies per-element scale and bias with `elementwise_affine`.
This layer uses statistics computed from input data in both training and evaluation modes.
Parameters
* **normalized\_shape** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [list](https://docs.python.org/3/library/stdtypes.html#list "(in Python v3.9)") *or* *torch.Size*) –
input shape from an expected input of size
[∗×normalized\_shape[0]×normalized\_shape[1]×…×normalized\_shape[−1]][\* \times \text{normalized\\_shape}[0] \times \text{normalized\\_shape}[1] \times \ldots \times \text{normalized\\_shape}[-1]]
If a single integer is used, it is treated as a singleton list, and this module will normalize over the last dimension which is expected to be of that specific size.
* **eps** – a value added to the denominator for numerical stability. Default: 1e-5
* **elementwise\_affine** – a boolean value that when set to `True`, this module has learnable per-element affine parameters initialized to ones (for weights) and zeros (for biases). Default: `True`.
Shape:
* Input: (N,∗)(N, \*)
* Output: (N,∗)(N, \*) (same shape as input)
Examples:
```
>>> input = torch.randn(20, 5, 10, 10)
>>> # With Learnable Parameters
>>> m = nn.LayerNorm(input.size()[1:])
>>> # Without Learnable Parameters
>>> m = nn.LayerNorm(input.size()[1:], elementwise_affine=False)
>>> # Normalize over last two dimensions
>>> m = nn.LayerNorm([10, 10])
>>> # Normalize over last dimension of size 10
>>> m = nn.LayerNorm(10)
>>> # Activating the module
>>> output = m(input)
```
pytorch torch.jit.save torch.jit.save
==============
`torch.jit.save(m, f, _extra_files=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/jit/_serialization.html#save)
Save an offline version of this module for use in a separate process. The saved module serializes all of the methods, submodules, parameters, and attributes of this module. It can be loaded into the C++ API using `torch::jit::load(filename)` or into the Python API with [`torch.jit.load`](torch.jit.load#torch.jit.load "torch.jit.load").
To be able to save a module, it must not make any calls to native Python functions. This means that all submodules must be subclasses of [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") as well.
Danger
All modules, no matter their device, are always loaded onto the CPU during loading. This is different from [`torch.load()`](torch.load#torch.load "torch.load")’s semantics and may change in the future.
Parameters
* **m** – A [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") to save.
* **f** – A file-like object (has to implement write and flush) or a string containing a file name.
* **\_extra\_files** – Map from filename to contents which will be stored as part of `f`.
Note
torch.jit.save attempts to preserve the behavior of some operators across versions. For example, dividing two integer tensors in PyTorch 1.5 performed floor division, and if the module containing that code is saved in PyTorch 1.5 and loaded in PyTorch 1.6 its division behavior will be preserved. The same module saved in PyTorch 1.6 will fail to load in PyTorch 1.5, however, since the behavior of division changed in 1.6, and 1.5 does not know how to replicate the 1.6 behavior.
Example:
```
import torch
import io
class MyModule(torch.nn.Module):
def forward(self, x):
return x + 10
m = torch.jit.script(MyModule())
# Save to file
torch.jit.save(m, 'scriptmodule.pt')
# This line is equivalent to the previous
m.save("scriptmodule.pt")
# Save to io.BytesIO buffer
buffer = io.BytesIO()
torch.jit.save(m, buffer)
# Save with extra files
extra_files = {'foo.txt': b'bar'}
torch.jit.save(m, 'scriptmodule.pt', _extra_files=extra_files)
```
pytorch ReplicationPad3d ReplicationPad3d
================
`class torch.nn.ReplicationPad3d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ReplicationPad3d)
Pads the input tensor using replication of the input boundary.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 6-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} , padding\_top\text{padding\\_top} , padding\_bottom\text{padding\\_bottom} , padding\_front\text{padding\\_front} , padding\_back\text{padding\\_back} )
Shape:
* Input: (N,C,Din,Hin,Win)(N, C, D\_{in}, H\_{in}, W\_{in})
* Output: (N,C,Dout,Hout,Wout)(N, C, D\_{out}, H\_{out}, W\_{out}) where
Dout=Din+padding\_front+padding\_backD\_{out} = D\_{in} + \text{padding\\_front} + \text{padding\\_back}
Hout=Hin+padding\_top+padding\_bottomH\_{out} = H\_{in} + \text{padding\\_top} + \text{padding\\_bottom}
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ReplicationPad3d(3)
>>> input = torch.randn(16, 3, 8, 320, 480)
>>> output = m(input)
>>> # using different paddings for different sides
>>> m = nn.ReplicationPad3d((3, 3, 6, 6, 1, 1))
>>> output = m(input)
```
pytorch torch.deg2rad torch.deg2rad
=============
`torch.deg2rad(input, *, out=None) → Tensor`
Returns a new tensor with each of the elements of `input` converted from angles in degrees to radians.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([[180.0, -180.0], [360.0, -360.0], [90.0, -90.0]])
>>> torch.deg2rad(a)
tensor([[ 3.1416, -3.1416],
[ 6.2832, -6.2832],
[ 1.5708, -1.5708]])
```
pytorch torch.fliplr torch.fliplr
============
`torch.fliplr(input) → Tensor`
Flip tensor in the left/right direction, returning a new tensor.
Flip the entries in each row in the left/right direction. Columns are preserved, but appear in a different order than before.
Note
Requires the tensor to be at least 2-D.
Note
`torch.fliplr` makes a copy of `input`’s data. This is different from NumPy’s `np.fliplr`, which returns a view in constant time. Since copying a tensor’s data is more work than viewing that data, `torch.fliplr` is expected to be slower than `np.fliplr`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – Must be at least 2-dimensional.
Example:
```
>>> x = torch.arange(4).view(2, 2)
>>> x
tensor([[0, 1],
[2, 3]])
>>> torch.fliplr(x)
tensor([[1, 0],
[3, 2]])
```
pytorch torch.lu_unpack torch.lu\_unpack
================
`torch.lu_unpack(LU_data, LU_pivots, unpack_data=True, unpack_pivots=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#lu_unpack)
Unpacks the data and pivots from a LU factorization of a tensor.
Returns a tuple of tensors as `(the pivots, the L tensor, the U tensor)`.
Parameters
* **LU\_data** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the packed LU factorization data
* **LU\_pivots** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the packed LU factorization pivots
* **unpack\_data** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – flag indicating if the data should be unpacked
* **unpack\_pivots** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – flag indicating if the pivots should be unpacked
Examples:
```
>>> A = torch.randn(2, 3, 3)
>>> A_LU, pivots = A.lu()
>>> P, A_L, A_U = torch.lu_unpack(A_LU, pivots)
>>>
>>> # can recover A from factorization
>>> A_ = torch.bmm(P, torch.bmm(A_L, A_U))
>>> # LU factorization of a rectangular matrix:
>>> A = torch.randn(2, 3, 2)
>>> A_LU, pivots = A.lu()
>>> P, A_L, A_U = torch.lu_unpack(A_LU, pivots)
>>> P
tensor([[[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]],
[[0., 0., 1.],
[0., 1., 0.],
[1., 0., 0.]]])
>>> A_L
tensor([[[ 1.0000, 0.0000],
[ 0.4763, 1.0000],
[ 0.3683, 0.1135]],
[[ 1.0000, 0.0000],
[ 0.2957, 1.0000],
[-0.9668, -0.3335]]])
>>> A_U
tensor([[[ 2.1962, 1.0881],
[ 0.0000, -0.8681]],
[[-1.0947, 0.3736],
[ 0.0000, 0.5718]]])
>>> A_ = torch.bmm(P, torch.bmm(A_L, A_U))
>>> torch.norm(A_ - A)
tensor(2.9802e-08)
```
| programming_docs |
pytorch torch.all torch.all
=========
`torch.all(input) → Tensor`
Tests if all elements in `input` evaluate to `True`.
Note
This function matches the behaviour of NumPy in returning output of dtype `bool` for all supported dtypes except `uint8`. For `uint8` the dtype of output is `uint8` itself.
Example:
```
>>> a = torch.rand(1, 2).bool()
>>> a
tensor([[False, True]], dtype=torch.bool)
>>> torch.all(a)
tensor(False, dtype=torch.bool)
>>> a = torch.arange(0, 3)
>>> a
tensor([0, 1, 2])
>>> torch.all(a)
tensor(False)
```
`torch.all(input, dim, keepdim=False, *, out=None) → Tensor`
For each row of `input` in the given dimension `dim`, returns `True` if all elements in the row evaluate to `True` and `False` otherwise.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 fewer dimension than `input`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.rand(4, 2).bool()
>>> a
tensor([[True, True],
[True, False],
[True, True],
[True, True]], dtype=torch.bool)
>>> torch.all(a, dim=1)
tensor([ True, False, True, True], dtype=torch.bool)
>>> torch.all(a, dim=0)
tensor([ True, False], dtype=torch.bool)
```
pytorch torch.numel torch.numel
===========
`torch.numel(input) → int`
Returns the total number of elements in the `input` tensor.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example:
```
>>> a = torch.randn(1, 2, 3, 4, 5)
>>> torch.numel(a)
120
>>> a = torch.zeros(4,4)
>>> torch.numel(a)
16
```
pytorch torch.sinc torch.sinc
==========
`torch.sinc(input, *, out=None) → Tensor`
Computes the normalized sinc of `input.`
outi={1,if inputi=0sin(πinputi)/(πinputi),otherwise\text{out}\_{i} = \begin{cases} 1, & \text{if}\ \text{input}\_{i}=0 \\ \sin(\pi \text{input}\_{i}) / (\pi \text{input}\_{i}), & \text{otherwise} \end{cases}
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.2252, -0.2948, 1.0267, -1.1566])
>>> torch.sinc(a)
tensor([ 0.9186, 0.8631, -0.0259, -0.1300])
```
pytorch torch.randn_like torch.randn\_like
=================
`torch.randn_like(input, *, dtype=None, layout=None, device=None, requires_grad=False, memory_format=torch.preserve_format) → Tensor`
Returns a tensor with the same size as `input` that is filled with random numbers from a normal distribution with mean 0 and variance 1. `torch.randn_like(input)` is equivalent to `torch.randn(input.size(), dtype=input.dtype, layout=input.layout, device=input.device)`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the size of `input` will determine size of the output tensor.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned Tensor. Default: if `None`, defaults to the dtype of `input`.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned tensor. Default: if `None`, defaults to the layout of `input`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, defaults to the device of `input`.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
* **memory\_format** ([`torch.memory_format`](../tensor_attributes#torch.torch.memory_format "torch.torch.memory_format"), optional) – the desired memory format of returned Tensor. Default: `torch.preserve_format`.
pytorch torch.is_storage torch.is\_storage
=================
`torch.is_storage(obj)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#is_storage)
Returns True if `obj` is a PyTorch storage object.
Parameters
**obj** (*Object*) – Object to test
pytorch PixelUnshuffle PixelUnshuffle
==============
`class torch.nn.PixelUnshuffle(downscale_factor)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pixelshuffle.html#PixelUnshuffle)
Reverses the [`PixelShuffle`](torch.nn.pixelshuffle#torch.nn.PixelShuffle "torch.nn.PixelShuffle") operation by rearranging elements in a tensor of shape (∗,C,H×r,W×r)(\*, C, H \times r, W \times r) to a tensor of shape (∗,C×r2,H,W)(\*, C \times r^2, H, W) , where r is a downscale factor.
See the paper: [Real-Time Single Image and Video Super-Resolution Using an Efficient Sub-Pixel Convolutional Neural Network](https://arxiv.org/abs/1609.05158) by Shi et. al (2016) for more details.
Parameters
**downscale\_factor** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – factor to decrease spatial resolution by
Shape:
* Input: (∗,Cin,Hin,Win)(\*, C\_{in}, H\_{in}, W\_{in}) , where \* is zero or more batch dimensions
* Output: (∗,Cout,Hout,Wout)(\*, C\_{out}, H\_{out}, W\_{out}) , where
Cout=Cin×downscale\_factor2C\_{out} = C\_{in} \times \text{downscale\\_factor}^2
Hout=Hin÷downscale\_factorH\_{out} = H\_{in} \div \text{downscale\\_factor}
Wout=Win÷downscale\_factorW\_{out} = W\_{in} \div \text{downscale\\_factor}
Examples:
```
>>> pixel_unshuffle = nn.PixelUnshuffle(3)
>>> input = torch.randn(1, 1, 12, 12)
>>> output = pixel_unshuffle(input)
>>> print(output.size())
torch.Size([1, 9, 4, 4])
```
pytorch torch.addmm torch.addmm
===========
`torch.addmm(input, mat1, mat2, *, beta=1, alpha=1, out=None) → Tensor`
Performs a matrix multiplication of the matrices `mat1` and `mat2`. The matrix `input` is added to the final result.
If `mat1` is a (n×m)(n \times m) tensor, `mat2` is a (m×p)(m \times p) tensor, then `input` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with a (n×p)(n \times p) tensor and `out` will be a (n×p)(n \times p) tensor.
`alpha` and `beta` are scaling factors on matrix-vector product between `mat1` and `mat2` and the added matrix `input` respectively.
out=β input+α(mat1i@mat2i)\text{out} = \beta\ \text{input} + \alpha\ (\text{mat1}\_i \mathbin{@} \text{mat2}\_i)
If `beta` is 0, then `input` will be ignored, and `nan` and `inf` in it will not be propagated.
For inputs of type `FloatTensor` or `DoubleTensor`, arguments `beta` and `alpha` must be real numbers, otherwise they should be integers.
This operator supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – matrix to be added
* **mat1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first matrix to be matrix multiplied
* **mat2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second matrix to be matrix multiplied
Keyword Arguments
* **beta** (*Number**,* *optional*) – multiplier for `input` (β\beta )
* **alpha** (*Number**,* *optional*) – multiplier for mat1@mat2mat1 @ mat2 (α\alpha )
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> M = torch.randn(2, 3)
>>> mat1 = torch.randn(2, 3)
>>> mat2 = torch.randn(3, 3)
>>> torch.addmm(M, mat1, mat2)
tensor([[-4.8716, 1.4671, -1.3746],
[ 0.7573, -3.9555, -2.8681]])
```
pytorch torch.kron torch.kron
==========
`torch.kron(input, other, *, out=None) → Tensor`
Computes the Kronecker product, denoted by ⊗\otimes , of `input` and `other`.
If `input` is a (a0×a1×⋯×an)(a\_0 \times a\_1 \times \dots \times a\_n) tensor and `other` is a (b0×b1×⋯×bn)(b\_0 \times b\_1 \times \dots \times b\_n) tensor, the result will be a (a0∗b0×a1∗b1×⋯×an∗bn)(a\_0\*b\_0 \times a\_1\*b\_1 \times \dots \times a\_n\*b\_n) tensor with the following entries:
(input⊗other)k0,k1,…,kn=inputi0,i1,…,in∗otherj0,j1,…,jn,(\text{input} \otimes \text{other})\_{k\_0, k\_1, \dots, k\_n} = \text{input}\_{i\_0, i\_1, \dots, i\_n} \* \text{other}\_{j\_0, j\_1, \dots, j\_n},
where kt=it∗bt+jtk\_t = i\_t \* b\_t + j\_t for 0≤t≤n0 \leq t \leq n . If one tensor has fewer dimensions than the other it is unsqueezed until it has the same number of dimensions.
Supports real-valued and complex-valued inputs.
Note
This function generalizes the typical definition of the Kronecker product for two matrices to two tensors, as described above. When `input` is a (m×n)(m \times n) matrix and `other` is a (p×q)(p \times q) matrix, the result will be a (p∗m×q∗n)(p\*m \times q\*n) block matrix:
A⊗B=[a11B⋯a1nB⋮⋱⋮am1B⋯amnB]\mathbf{A} \otimes \mathbf{B}=\begin{bmatrix} a\_{11} \mathbf{B} & \cdots & a\_{1 n} \mathbf{B} \\ \vdots & \ddots & \vdots \\ a\_{m 1} \mathbf{B} & \cdots & a\_{m n} \mathbf{B} \end{bmatrix}
where `input` is A\mathbf{A} and `other` is B\mathbf{B} .
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) –
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) –
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – The output tensor. Ignored if `None`. Default: `None`
Examples:
```
>>> mat1 = torch.eye(2)
>>> mat2 = torch.ones(2, 2)
>>> torch.kron(mat1, mat2)
tensor([[1., 1., 0., 0.],
[1., 1., 0., 0.],
[0., 0., 1., 1.],
[0., 0., 1., 1.]])
>>> mat1 = torch.eye(2)
>>> mat2 = torch.arange(1, 5).reshape(2, 2)
>>> torch.kron(mat1, mat2)
tensor([[1., 2., 0., 0.],
[3., 4., 0., 0.],
[0., 0., 1., 2.],
[0., 0., 3., 4.]])
```
pytorch torch.negative torch.negative
==============
`torch.negative(input, *, out=None) → Tensor`
Alias for [`torch.neg()`](torch.neg#torch.neg "torch.neg")
pytorch torch.fmax torch.fmax
==========
`torch.fmax(input, other, *, out=None) → Tensor`
Computes the element-wise maximum of `input` and `other`.
This is like [`torch.maximum()`](torch.maximum#torch.maximum "torch.maximum") except it handles NaNs differently: if exactly one of the two elements being compared is a NaN then the non-NaN element is taken as the maximum. Only if both elements are NaN is NaN propagated.
This function is a wrapper around C++’s `std::fmax` and is similar to NumPy’s `fmax` function.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), [type promotion](../tensor_attributes#type-promotion-doc), and integer and floating-point inputs.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([9.7, float('nan'), 3.1, float('nan')])
>>> b = torch.tensor([-2.2, 0.5, float('nan'), float('nan')])
>>> torch.fmax(a, b)
tensor([9.7000, 0.5000, 3.1000, nan])
```
pytorch torch.addcmul torch.addcmul
=============
`torch.addcmul(input, tensor1, tensor2, *, value=1, out=None) → Tensor`
Performs the element-wise multiplication of `tensor1` by `tensor2`, multiply the result by the scalar `value` and add it to `input`.
outi=inputi+value×tensor1i×tensor2i\text{out}\_i = \text{input}\_i + \text{value} \times \text{tensor1}\_i \times \text{tensor2}\_i
The shapes of [`tensor`](torch.tensor#torch.tensor "torch.tensor"), `tensor1`, and `tensor2` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
For inputs of type `FloatTensor` or `DoubleTensor`, `value` must be a real number, otherwise an integer.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be added
* **tensor1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be multiplied
* **tensor2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be multiplied
Keyword Arguments
* **value** (*Number**,* *optional*) – multiplier for tensor1.∗tensor2tensor1 .\* tensor2
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> t = torch.randn(1, 3)
>>> t1 = torch.randn(3, 1)
>>> t2 = torch.randn(1, 3)
>>> torch.addcmul(t, t1, t2, value=0.1)
tensor([[-0.8635, -0.6391, 1.6174],
[-0.7617, -0.5879, 1.7388],
[-0.8353, -0.6249, 1.6511]])
```
pytorch torch.fake_quantize_per_channel_affine torch.fake\_quantize\_per\_channel\_affine
==========================================
`torch.fake_quantize_per_channel_affine(input, scale, zero_point, quant_min, quant_max) → Tensor`
Returns a new tensor with the data in `input` fake quantized per channel using `scale`, `zero_point`, `quant_min` and `quant_max`, across the channel specified by `axis`.
output=min(quant\_max,max(quant\_min,std::nearby\_int(input/scale)+zero\_point))\text{output} = min( \text{quant\\_max}, max( \text{quant\\_min}, \text{std::nearby\\_int}(\text{input} / \text{scale}) + \text{zero\\_point} ) )
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input value(s), in `torch.float32`.
* **scale** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – quantization scale, per channel
* **zero\_point** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – quantization zero\_point, per channel
* **axis** (*int32*) – channel axis
* **quant\_min** (*int64*) – lower bound of the quantized domain
* **quant\_max** (*int64*) – upper bound of the quantized domain
Returns
A newly fake\_quantized per channel tensor
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> x = torch.randn(2, 2, 2)
>>> x
tensor([[[-0.2525, -0.0466],
[ 0.3491, -0.2168]],
[[-0.5906, 1.6258],
[ 0.6444, -0.0542]]])
>>> scales = (torch.randn(2) + 1) * 0.05
>>> scales
tensor([0.0475, 0.0486])
>>> zero_points = torch.zeros(2).to(torch.long)
>>> zero_points
tensor([0, 0])
>>> torch.fake_quantize_per_channel_affine(x, scales, zero_points, 1, 0, 255)
tensor([[[0.0000, 0.0000],
[0.3405, 0.0000]],
[[0.0000, 1.6134],
[0.6323, 0.0000]]])
```
pytorch Sequential Sequential
==========
`class torch.nn.Sequential(*args)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#Sequential)
A sequential container. Modules will be added to it in the order they are passed in the constructor. Alternatively, an ordered dict of modules can also be passed in.
To make it easier to understand, here is a small example:
```
# Example of using Sequential
model = nn.Sequential(
nn.Conv2d(1,20,5),
nn.ReLU(),
nn.Conv2d(20,64,5),
nn.ReLU()
)
# Example of using Sequential with OrderedDict
model = nn.Sequential(OrderedDict([
('conv1', nn.Conv2d(1,20,5)),
('relu1', nn.ReLU()),
('conv2', nn.Conv2d(20,64,5)),
('relu2', nn.ReLU())
]))
```
pytorch LnStructured LnStructured
============
`class torch.nn.utils.prune.LnStructured(amount, n, dim=-1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#LnStructured)
Prune entire (currently unpruned) channels in a tensor based on their Ln-norm.
Parameters
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of channels to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *inf**,* *-inf**,* *'fro'**,* *'nuc'*) – See documentation of valid entries for argument `p` in [`torch.norm()`](torch.norm#torch.norm "torch.norm").
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – index of the dim along which we define channels to prune. Default: -1.
`classmethod apply(module, name, amount, n, dim, importance_scores=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#LnStructured.apply)
Adds the forward pre-hook that enables pruning on the fly and the reparametrization of a tensor in terms of the original tensor and the pruning mask.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **n** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *inf**,* *-inf**,* *'fro'**,* *'nuc'*) – See documentation of valid entries for argument `p` in [`torch.norm()`](torch.norm#torch.norm "torch.norm").
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – index of the dim along which we define channels to prune.
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as module parameter) used to compute mask for pruning. The values in this tensor indicate the importance of the corresponding elements in the parameter being pruned. If unspecified or None, the module parameter will be used in its place.
`apply_mask(module)`
Simply handles the multiplication between the parameter being pruned and the generated mask. Fetches the mask and the original tensor from the module and returns the pruned version of the tensor.
Parameters
**module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
Returns
pruned version of the input tensor
Return type
pruned\_tensor ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
`compute_mask(t, default_mask)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#LnStructured.compute_mask)
Computes and returns a mask for the input tensor `t`. Starting from a base `default_mask` (which should be a mask of ones if the tensor has not been pruned yet), generate a mask to apply on top of the `default_mask` by zeroing out the channels along the specified dim with the lowest Ln-norm.
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor representing the parameter to prune
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Base mask from previous pruning iterations, that need to be respected after the new mask is applied. Same dims as `t`.
Returns
mask to apply to `t`, of same dims as `t`
Return type
mask ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
Raises
[**IndexError**](https://docs.python.org/3/library/exceptions.html#IndexError "(in Python v3.9)") – if `self.dim >= len(t.shape)`
`prune(t, default_mask=None, importance_scores=None)`
Computes and returns a pruned version of input tensor `t` according to the pruning rule specified in [`compute_mask()`](#torch.nn.utils.prune.LnStructured.compute_mask "torch.nn.utils.prune.LnStructured.compute_mask").
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor to prune (of same dimensions as `default_mask`).
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as `t`) used to compute mask for pruning `t`. The values in this tensor indicate the importance of the corresponding elements in the `t` that is being pruned. If unspecified or None, the tensor `t` will be used in its place.
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – mask from previous pruning iteration, if any. To be considered when determining what portion of the tensor that pruning should act on. If None, default to a mask of ones.
Returns
pruned version of tensor `t`.
`remove(module)`
Removes the pruning reparameterization from a module. The pruned parameter named `name` remains permanently pruned, and the parameter named `name+'_orig'` is removed from the parameter list. Similarly, the buffer named `name+'_mask'` is removed from the buffers.
Note
Pruning itself is NOT undone or reversed!
| programming_docs |
pytorch Softmax Softmax
=======
`class torch.nn.Softmax(dim=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Softmax)
Applies the Softmax function to an n-dimensional input Tensor rescaling them so that the elements of the n-dimensional output Tensor lie in the range [0,1] and sum to 1.
Softmax is defined as:
Softmax(xi)=exp(xi)∑jexp(xj)\text{Softmax}(x\_{i}) = \frac{\exp(x\_i)}{\sum\_j \exp(x\_j)}
When the input Tensor is a sparse tensor then the unspecifed values are treated as `-inf`.
Shape:
* Input: (∗)(\*) where `*` means, any number of additional dimensions
* Output: (∗)(\*) , same shape as the input
Returns
a Tensor of the same dimension and shape as the input with values in the range [0, 1]
Parameters
**dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – A dimension along which Softmax will be computed (so every slice along dim will sum to 1).
Note
This module doesn’t work directly with NLLLoss, which expects the Log to be computed between the Softmax and itself. Use `LogSoftmax` instead (it’s faster and has better numerical properties).
Examples:
```
>>> m = nn.Softmax(dim=1)
>>> input = torch.randn(2, 3)
>>> output = m(input)
```
pytorch torch.view_as_complex torch.view\_as\_complex
=======================
`torch.view_as_complex(input) → Tensor`
Returns a view of `input` as a complex tensor. For an input complex tensor of `size` m1,m2,…,mi,2m1, m2, \dots, mi, 2 , this function returns a new complex tensor of `size` m1,m2,…,mim1, m2, \dots, mi where the last dimension of the input tensor is expected to represent the real and imaginary components of complex numbers.
Warning
[`view_as_complex()`](#torch.view_as_complex "torch.view_as_complex") is only supported for tensors with [`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype") `torch.float64` and `torch.float32`. The input is expected to have the last dimension of `size` 2. In addition, the tensor must have a `stride` of 1 for its last dimension. The strides of all other dimensions must be even numbers.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example::
```
>>> x=torch.randn(4, 2)
>>> x
tensor([[ 1.6116, -0.5772],
[-1.4606, -0.9120],
[ 0.0786, -1.7497],
[-0.6561, -1.6623]])
>>> torch.view_as_complex(x)
tensor([(1.6116-0.5772j), (-1.4606-0.9120j), (0.0786-1.7497j), (-0.6561-1.6623j)])
```
pytorch torch.bitwise_not torch.bitwise\_not
==================
`torch.bitwise_not(input, *, out=None) → Tensor`
Computes the bitwise NOT of the given input tensor. The input tensor must be of integral or Boolean types. For bool tensors, it computes the logical NOT.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
#### Example
```
>>> torch.bitwise_not(torch.tensor([-1, -2, 3], dtype=torch.int8))
tensor([ 0, 1, -4], dtype=torch.int8)
```
pytorch RNNBase RNNBase
=======
`class torch.nn.RNNBase(mode, input_size, hidden_size, num_layers=1, bias=True, batch_first=False, dropout=0.0, bidirectional=False, proj_size=0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/rnn.html#RNNBase)
`flatten_parameters()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/rnn.html#RNNBase.flatten_parameters)
Resets parameter data pointer so that they can use faster code paths.
Right now, this works only if the module is on the GPU and cuDNN is enabled. Otherwise, it’s a no-op.
pytorch MSELoss MSELoss
=======
`class torch.nn.MSELoss(size_average=None, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#MSELoss)
Creates a criterion that measures the mean squared error (squared L2 norm) between each element in the input xx and target yy .
The unreduced (i.e. with `reduction` set to `'none'`) loss can be described as:
ℓ(x,y)=L={l1,…,lN}⊤,ln=(xn−yn)2,\ell(x, y) = L = \{l\_1,\dots,l\_N\}^\top, \quad l\_n = \left( x\_n - y\_n \right)^2,
where NN is the batch size. If `reduction` is not `'none'` (default `'mean'`), then:
ℓ(x,y)={mean(L),if reduction=‘mean’;sum(L),if reduction=‘sum’.\ell(x, y) = \begin{cases} \operatorname{mean}(L), & \text{if reduction} = \text{`mean';}\\ \operatorname{sum}(L), & \text{if reduction} = \text{`sum'.} \end{cases}
xx and yy are tensors of arbitrary shapes with a total of nn elements each.
The mean operation still operates over all the elements, and divides by nn .
The division by nn can be avoided if one sets `reduction = 'sum'`.
Parameters
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Shape:
* Input: (N,∗)(N, \*) where ∗\* means, any number of additional dimensions
* Target: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> loss = nn.MSELoss()
>>> input = torch.randn(3, 5, requires_grad=True)
>>> target = torch.randn(3, 5)
>>> output = loss(input, target)
>>> output.backward()
```
pytorch GroupNorm GroupNorm
=========
`class torch.nn.GroupNorm(num_groups, num_channels, eps=1e-05, affine=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/normalization.html#GroupNorm)
Applies Group Normalization over a mini-batch of inputs as described in the paper [Group Normalization](https://arxiv.org/abs/1803.08494)
y=x−E[x]Var[x]+ϵ∗γ+βy = \frac{x - \mathrm{E}[x]}{ \sqrt{\mathrm{Var}[x] + \epsilon}} \* \gamma + \beta
The input channels are separated into `num_groups` groups, each containing `num_channels / num_groups` channels. The mean and standard-deviation are calculated separately over the each group. γ\gamma and β\beta are learnable per-channel affine transform parameter vectors of size `num_channels` if `affine` is `True`. The standard-deviation is calculated via the biased estimator, equivalent to `torch.var(input, unbiased=False)`.
This layer uses statistics computed from input data in both training and evaluation modes.
Parameters
* **num\_groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – number of groups to separate the channels into
* **num\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – number of channels expected in input
* **eps** – a value added to the denominator for numerical stability. Default: 1e-5
* **affine** – a boolean value that when set to `True`, this module has learnable per-channel affine parameters initialized to ones (for weights) and zeros (for biases). Default: `True`.
Shape:
* Input: (N,C,∗)(N, C, \*) where C=num\_channelsC=\text{num\\_channels}
* Output: (N,C,∗)(N, C, \*) (same shape as input)
Examples:
```
>>> input = torch.randn(20, 6, 10, 10)
>>> # Separate 6 channels into 3 groups
>>> m = nn.GroupNorm(3, 6)
>>> # Separate 6 channels into 6 groups (equivalent with InstanceNorm)
>>> m = nn.GroupNorm(6, 6)
>>> # Put all 6 channels into a single group (equivalent with LayerNorm)
>>> m = nn.GroupNorm(1, 6)
>>> # Activating the module
>>> output = m(input)
```
pytorch SmoothL1Loss SmoothL1Loss
============
`class torch.nn.SmoothL1Loss(size_average=None, reduce=None, reduction='mean', beta=1.0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#SmoothL1Loss)
Creates a criterion that uses a squared term if the absolute element-wise error falls below beta and an L1 term otherwise. It is less sensitive to outliers than the [`torch.nn.MSELoss`](torch.nn.mseloss#torch.nn.MSELoss "torch.nn.MSELoss") and in some cases prevents exploding gradients (e.g. see `Fast R-CNN` paper by Ross Girshick). Omitting a scaling factor of `beta`, this loss is also known as the Huber loss:
loss(x,y)=1n∑izi\text{loss}(x, y) = \frac{1}{n} \sum\_{i} z\_{i}
where ziz\_{i} is given by:
zi={0.5(xi−yi)2/beta,if ∣xi−yi∣<beta∣xi−yi∣−0.5∗beta,otherwise z\_{i} = \begin{cases} 0.5 (x\_i - y\_i)^2 / beta, & \text{if } |x\_i - y\_i| < beta \\ |x\_i - y\_i| - 0.5 \* beta, & \text{otherwise } \end{cases}
xx and yy arbitrary shapes with a total of nn elements each the sum operation still operates over all the elements, and divides by nn .
`beta` is an optional parameter that defaults to 1.
Note: When `beta` is set to 0, this is equivalent to [`L1Loss`](torch.nn.l1loss#torch.nn.L1Loss "torch.nn.L1Loss"). Passing a negative value in for `beta` will result in an exception.
The division by nn can be avoided if sets `reduction = 'sum'`.
Parameters
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
* **beta** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Specifies the threshold at which to change between L1 and L2 loss. This value defaults to 1.0.
Shape:
* Input: (N,∗)(N, \*) where ∗\* means, any number of additional dimensions
* Target: (N,∗)(N, \*) , same shape as the input
* Output: scalar. If `reduction` is `'none'`, then (N,∗)(N, \*) , same shape as the input
pytorch TransformerEncoderLayer TransformerEncoderLayer
=======================
`class torch.nn.TransformerEncoderLayer(d_model, nhead, dim_feedforward=2048, dropout=0.1, activation='relu')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerEncoderLayer)
TransformerEncoderLayer is made up of self-attn and feedforward network. This standard encoder layer is based on the paper “Attention Is All You Need”. Ashish Vaswani, Noam Shazeer, Niki Parmar, Jakob Uszkoreit, Llion Jones, Aidan N Gomez, Lukasz Kaiser, and Illia Polosukhin. 2017. Attention is all you need. In Advances in Neural Information Processing Systems, pages 6000-6010. Users may modify or implement in a different way during application.
Parameters
* **d\_model** – the number of expected features in the input (required).
* **nhead** – the number of heads in the multiheadattention models (required).
* **dim\_feedforward** – the dimension of the feedforward network model (default=2048).
* **dropout** – the dropout value (default=0.1).
* **activation** – the activation function of intermediate layer, relu or gelu (default=relu).
Examples::
```
>>> encoder_layer = nn.TransformerEncoderLayer(d_model=512, nhead=8)
>>> src = torch.rand(10, 32, 512)
>>> out = encoder_layer(src)
```
`forward(src, src_mask=None, src_key_padding_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerEncoderLayer.forward)
Pass the input through the encoder layer.
Parameters
* **src** – the sequence to the encoder layer (required).
* **src\_mask** – the mask for the src sequence (optional).
* **src\_key\_padding\_mask** – the mask for the src keys per batch (optional).
Shape:
see the docs in Transformer class.
pytorch AvgPool1d AvgPool1d
=========
`class torch.nn.AvgPool1d(kernel_size, stride=None, padding=0, ceil_mode=False, count_include_pad=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AvgPool1d)
Applies a 1D average pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,L)(N, C, L) , output (N,C,Lout)(N, C, L\_{out}) and `kernel_size` kk can be precisely described as:
out(Ni,Cj,l)=1k∑m=0k−1input(Ni,Cj,stride×l+m)\text{out}(N\_i, C\_j, l) = \frac{1}{k} \sum\_{m=0}^{k-1} \text{input}(N\_i, C\_j, \text{stride} \times l + m)
If `padding` is non-zero, then the input is implicitly zero-padded on both sides for `padding` number of points.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
The parameters `kernel_size`, `stride`, `padding` can each be an `int` or a one-element tuple.
Parameters
* **kernel\_size** – the size of the window
* **stride** – the stride of the window. Default value is `kernel_size`
* **padding** – implicit zero padding to be added on both sides
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
* **count\_include\_pad** – when True, will include the zero-padding in the averaging calculation
Shape:
* Input: (N,C,Lin)(N, C, L\_{in})
* Output: (N,C,Lout)(N, C, L\_{out}) , where
Lout=⌊Lin+2×padding−kernel\_sizestride+1⌋L\_{out} = \left\lfloor \frac{L\_{in} + 2 \times \text{padding} - \text{kernel\\_size}}{\text{stride}} + 1\right\rfloor
Examples:
```
>>> # pool with window of size=3, stride=2
>>> m = nn.AvgPool1d(3, stride=2)
>>> m(torch.tensor([[[1.,2,3,4,5,6,7]]]))
tensor([[[ 2., 4., 6.]]])
```
pytorch torch.nn.utils.rnn.pack_sequence torch.nn.utils.rnn.pack\_sequence
=================================
`torch.nn.utils.rnn.pack_sequence(sequences, enforce_sorted=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/rnn.html#pack_sequence)
Packs a list of variable length Tensors
`sequences` should be a list of Tensors of size `L x *`, where `L` is the length of a sequence and `*` is any number of trailing dimensions, including zero.
For unsorted sequences, use `enforce_sorted = False`. If `enforce_sorted` is `True`, the sequences should be sorted in the order of decreasing length. `enforce_sorted = True` is only necessary for ONNX export.
#### Example
```
>>> from torch.nn.utils.rnn import pack_sequence
>>> a = torch.tensor([1,2,3])
>>> b = torch.tensor([4,5])
>>> c = torch.tensor([6])
>>> pack_sequence([a, b, c])
PackedSequence(data=tensor([ 1, 4, 6, 2, 5, 3]), batch_sizes=tensor([ 3, 2, 1]))
```
Parameters
* **sequences** ([list](https://docs.python.org/3/library/stdtypes.html#list "(in Python v3.9)")*[*[Tensor](../tensors#torch.Tensor "torch.Tensor")*]*) – A list of sequences of decreasing length.
* **enforce\_sorted** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True`, checks that the input contains sequences sorted by length in a decreasing order. If `False`, this condition is not checked. Default: `True`.
Returns
a [`PackedSequence`](torch.nn.utils.rnn.packedsequence#torch.nn.utils.rnn.PackedSequence "torch.nn.utils.rnn.PackedSequence") object
pytorch torch.as_strided torch.as\_strided
=================
`torch.as_strided(input, size, stride, storage_offset=0) → Tensor`
Create a view of an existing `torch.Tensor` `input` with specified `size`, `stride` and `storage_offset`.
Warning
More than one element of a created tensor may refer to a single memory location. As a result, in-place operations (especially ones that are vectorized) may result in incorrect behavior. If you need to write to the tensors, please clone them first.
Many PyTorch functions, which return a view of a tensor, are internally implemented with this function. Those functions, like [`torch.Tensor.expand()`](../tensors#torch.Tensor.expand "torch.Tensor.expand"), are easier to read and are therefore more advisable to use.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **size** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)") *or* *ints*) – the shape of the output tensor
* **stride** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)") *or* *ints*) – the stride of the output tensor
* **storage\_offset** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the offset in the underlying storage of the output tensor
Example:
```
>>> x = torch.randn(3, 3)
>>> x
tensor([[ 0.9039, 0.6291, 1.0795],
[ 0.1586, 2.1939, -0.4900],
[-0.1909, -0.7503, 1.9355]])
>>> t = torch.as_strided(x, (2, 2), (1, 2))
>>> t
tensor([[0.9039, 1.0795],
[0.6291, 0.1586]])
>>> t = torch.as_strided(x, (2, 2), (1, 2), 1)
tensor([[0.6291, 0.1586],
[1.0795, 2.1939]])
```
pytorch CELU CELU
====
`class torch.nn.CELU(alpha=1.0, inplace=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#CELU)
Applies the element-wise function:
CELU(x)=max(0,x)+min(0,α∗(exp(x/α)−1))\text{CELU}(x) = \max(0,x) + \min(0, \alpha \* (\exp(x/\alpha) - 1))
More details can be found in the paper [Continuously Differentiable Exponential Linear Units](https://arxiv.org/abs/1704.07483) .
Parameters
* **alpha** – the α\alpha value for the CELU formulation. Default: 1.0
* **inplace** – can optionally do the operation in-place. Default: `False`
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.CELU()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch AdaptiveMaxPool1d AdaptiveMaxPool1d
=================
`class torch.nn.AdaptiveMaxPool1d(output_size, return_indices=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveMaxPool1d)
Applies a 1D adaptive max pooling over an input signal composed of several input planes.
The output size is H, for any input size. The number of output features is equal to the number of input planes.
Parameters
* **output\_size** – the target output size H
* **return\_indices** – if `True`, will return the indices along with the outputs. Useful to pass to nn.MaxUnpool1d. Default: `False`
#### Examples
```
>>> # target output size of 5
>>> m = nn.AdaptiveMaxPool1d(5)
>>> input = torch.randn(1, 64, 8)
>>> output = m(input)
```
| programming_docs |
pytorch MarginRankingLoss MarginRankingLoss
=================
`class torch.nn.MarginRankingLoss(margin=0.0, size_average=None, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#MarginRankingLoss)
Creates a criterion that measures the loss given inputs x1x1 , x2x2 , two 1D mini-batch `Tensors`, and a label 1D mini-batch tensor yy (containing 1 or -1).
If y=1y = 1 then it assumed the first input should be ranked higher (have a larger value) than the second input, and vice-versa for y=−1y = -1 .
The loss function for each pair of samples in the mini-batch is:
loss(x1,x2,y)=max(0,−y∗(x1−x2)+margin)\text{loss}(x1, x2, y) = \max(0, -y \* (x1 - x2) + \text{margin})
Parameters
* **margin** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Has a default value of 00 .
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Shape:
* Input1: (N)(N) where `N` is the batch size.
* Input2: (N)(N) , same shape as the Input1.
* Target: (N)(N) , same shape as the inputs.
* Output: scalar. If `reduction` is `'none'`, then (N)(N) .
Examples:
```
>>> loss = nn.MarginRankingLoss()
>>> input1 = torch.randn(3, requires_grad=True)
>>> input2 = torch.randn(3, requires_grad=True)
>>> target = torch.randn(3).sign()
>>> output = loss(input1, input2, target)
>>> output.backward()
```
pytorch ScriptModule ScriptModule
============
`class torch.jit.ScriptModule` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/jit/_script.html#ScriptModule)
A wrapper around C++ `torch::jit::Module`. `ScriptModule`s contain methods, attributes, parameters, and constants. These can be accessed the same as on a normal `nn.Module`.
`add_module(name, module)`
Adds a child module to the current module.
The module can be accessed as an attribute using the given name.
Parameters
* **name** (*string*) – name of the child module. The child module can be accessed from this module using the given name
* **module** ([Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – child module to be added to the module.
`apply(fn)`
Applies `fn` recursively to every submodule (as returned by `.children()`) as well as self. Typical use includes initializing the parameters of a model (see also [torch.nn.init](../nn.init#nn-init-doc)).
Parameters
**fn** (`Module` -> None) – function to be applied to each submodule
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Example:
```
>>> @torch.no_grad()
>>> def init_weights(m):
>>> print(m)
>>> if type(m) == nn.Linear:
>>> m.weight.fill_(1.0)
>>> print(m.weight)
>>> net = nn.Sequential(nn.Linear(2, 2), nn.Linear(2, 2))
>>> net.apply(init_weights)
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Linear(in_features=2, out_features=2, bias=True)
Parameter containing:
tensor([[ 1., 1.],
[ 1., 1.]])
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
```
`bfloat16()`
Casts all floating point parameters and buffers to `bfloat16` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`buffers(recurse=True)`
Returns an iterator over module buffers.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*torch.Tensor* – module buffer
Example:
```
>>> for buf in model.buffers():
>>> print(type(buf), buf.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`children()`
Returns an iterator over immediate children modules.
Yields
*Module* – a child module
`property code`
Returns a pretty-printed representation (as valid Python syntax) of the internal graph for the `forward` method. See [Inspecting Code](../jit#inspecting-code) for details.
`property code_with_constants`
Returns a tuple of:
[0] a pretty-printed representation (as valid Python syntax) of the internal graph for the `forward` method. See `code`. [1] a ConstMap following the CONSTANT.cN format of the output in [0]. The indices in the [0] output are keys to the underlying constant’s values.
See [Inspecting Code](../jit#inspecting-code) for details.
`cpu()`
Moves all model parameters and buffers to the CPU.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`cuda(device=None)`
Moves all model parameters and buffers to the GPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on GPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`double()`
Casts all floating point parameters and buffers to `double` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`eval()`
Sets the module in evaluation mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. `Dropout`, `BatchNorm`, etc.
This is equivalent with [`self.train(False)`](torch.nn.module#torch.nn.Module.train "torch.nn.Module.train").
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`extra_repr()`
Set the extra representation of the module
To print customized extra information, you should re-implement this method in your own modules. Both single-line and multi-line strings are acceptable.
`float()`
Casts all floating point parameters and buffers to float datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`property graph`
Returns a string representation of the internal graph for the `forward` method. See [Interpreting Graphs](../jit#interpreting-graphs) for details.
`half()`
Casts all floating point parameters and buffers to `half` datatype.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`property inlined_graph`
Returns a string representation of the internal graph for the `forward` method. This graph will be preprocessed to inline all function and method calls. See [Interpreting Graphs](../jit#interpreting-graphs) for details.
`load_state_dict(state_dict, strict=True)`
Copies parameters and buffers from [`state_dict`](#torch.jit.ScriptModule.state_dict "torch.jit.ScriptModule.state_dict") into this module and its descendants. If `strict` is `True`, then the keys of [`state_dict`](#torch.jit.ScriptModule.state_dict "torch.jit.ScriptModule.state_dict") must exactly match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function.
Parameters
* **state\_dict** ([dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")) – a dict containing parameters and persistent buffers.
* **strict** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – whether to strictly enforce that the keys in [`state_dict`](#torch.jit.ScriptModule.state_dict "torch.jit.ScriptModule.state_dict") match the keys returned by this module’s [`state_dict()`](torch.nn.module#torch.nn.Module.state_dict "torch.nn.Module.state_dict") function. Default: `True`
Returns
* **missing\_keys** is a list of str containing the missing keys
* **unexpected\_keys** is a list of str containing the unexpected keys
Return type
`NamedTuple` with `missing_keys` and `unexpected_keys` fields
`modules()`
Returns an iterator over all modules in the network.
Yields
*Module* – a module in the network
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.modules()):
print(idx, '->', m)
0 -> Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
)
1 -> Linear(in_features=2, out_features=2, bias=True)
```
`named_buffers(prefix='', recurse=True)`
Returns an iterator over module buffers, yielding both the name of the buffer as well as the buffer itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all buffer names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields buffers of this module and all submodules. Otherwise, yields only buffers that are direct members of this module.
Yields
*(string, torch.Tensor)* – Tuple containing the name and buffer
Example:
```
>>> for name, buf in self.named_buffers():
>>> if name in ['running_var']:
>>> print(buf.size())
```
`named_children()`
Returns an iterator over immediate children modules, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple containing a name and child module
Example:
```
>>> for name, module in model.named_children():
>>> if name in ['conv4', 'conv5']:
>>> print(module)
```
`named_modules(memo=None, prefix='')`
Returns an iterator over all modules in the network, yielding both the name of the module as well as the module itself.
Yields
*(string, Module)* – Tuple of name and module
Note
Duplicate modules are returned only once. In the following example, `l` will be returned only once.
Example:
```
>>> l = nn.Linear(2, 2)
>>> net = nn.Sequential(l, l)
>>> for idx, m in enumerate(net.named_modules()):
print(idx, '->', m)
0 -> ('', Sequential(
(0): Linear(in_features=2, out_features=2, bias=True)
(1): Linear(in_features=2, out_features=2, bias=True)
))
1 -> ('0', Linear(in_features=2, out_features=2, bias=True))
```
`named_parameters(prefix='', recurse=True)`
Returns an iterator over module parameters, yielding both the name of the parameter as well as the parameter itself.
Parameters
* **prefix** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – prefix to prepend to all parameter names.
* **recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*(string, Parameter)* – Tuple containing the name and parameter
Example:
```
>>> for name, param in self.named_parameters():
>>> if name in ['bias']:
>>> print(param.size())
```
`parameters(recurse=True)`
Returns an iterator over module parameters.
This is typically passed to an optimizer.
Parameters
**recurse** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – if True, then yields parameters of this module and all submodules. Otherwise, yields only parameters that are direct members of this module.
Yields
*Parameter* – module parameter
Example:
```
>>> for param in model.parameters():
>>> print(type(param), param.size())
<class 'torch.Tensor'> (20L,)
<class 'torch.Tensor'> (20L, 1L, 5L, 5L)
```
`register_backward_hook(hook)`
Registers a backward hook on the module.
This function is deprecated in favor of `nn.Module.register_full_backward_hook()` and the behavior of this function will change in future versions.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_buffer(name, tensor, persistent=True)`
Adds a buffer to the module.
This is typically used to register a buffer that should not to be considered a model parameter. For example, BatchNorm’s `running_mean` is not a parameter, but is part of the module’s state. Buffers, by default, are persistent and will be saved alongside parameters. This behavior can be changed by setting `persistent` to `False`. The only difference between a persistent buffer and a non-persistent buffer is that the latter will not be a part of this module’s [`state_dict`](#torch.jit.ScriptModule.state_dict "torch.jit.ScriptModule.state_dict").
Buffers can be accessed as attributes using given names.
Parameters
* **name** (*string*) – name of the buffer. The buffer can be accessed from this module using the given name
* **tensor** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – buffer to be registered.
* **persistent** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the buffer is part of this module’s [`state_dict`](#torch.jit.ScriptModule.state_dict "torch.jit.ScriptModule.state_dict").
Example:
```
>>> self.register_buffer('running_mean', torch.zeros(num_features))
```
`register_forward_hook(hook)`
Registers a forward hook on the module.
The hook will be called every time after `forward()` has computed an output. It should have the following signature:
```
hook(module, input, output) -> None or modified output
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the output. It can modify the input inplace but it will not have effect on forward since this is called after `forward()` is called.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_forward_pre_hook(hook)`
Registers a forward pre-hook on the module.
The hook will be called every time before `forward()` is invoked. It should have the following signature:
```
hook(module, input) -> None or modified input
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the input. User can either return a tuple or a single modified value in the hook. We will wrap the value into a tuple if a single value is returned(unless that value is already a tuple).
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_full_backward_hook(hook)`
Registers a backward hook on the module.
The hook will be called every time the gradients with respect to module inputs are computed. The hook should have the following signature:
```
hook(module, grad_input, grad_output) -> tuple(Tensor) or None
```
The `grad_input` and `grad_output` are tuples that contain the gradients with respect to the inputs and outputs respectively. The hook should not modify its arguments, but it can optionally return a new gradient with respect to the input that will be used in place of `grad_input` in subsequent computations. `grad_input` will only correspond to the inputs given as positional arguments and all kwarg arguments are ignored. Entries in `grad_input` and `grad_output` will be `None` for all non-Tensor arguments.
Warning
Modifying inputs or outputs inplace is not allowed when using backward hooks and will raise an error.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
`register_parameter(name, param)`
Adds a parameter to the module.
The parameter can be accessed as an attribute using given name.
Parameters
* **name** (*string*) – name of the parameter. The parameter can be accessed from this module using the given name
* **param** ([Parameter](torch.nn.parameter.parameter#torch.nn.parameter.Parameter "torch.nn.parameter.Parameter")) – parameter to be added to the module.
`requires_grad_(requires_grad=True)`
Change if autograd should record operations on parameters in this module.
This method sets the parameters’ `requires_grad` attributes in-place.
This method is helpful for freezing part of the module for finetuning or training parts of a model individually (e.g., GAN training).
Parameters
**requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether autograd should record operations on parameters in this module. Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`save(f, _extra_files={})`
See [`torch.jit.save`](torch.jit.save#torch.jit.save "torch.jit.save") for details.
`state_dict(destination=None, prefix='', keep_vars=False)`
Returns a dictionary containing a whole state of the module.
Both parameters and persistent buffers (e.g. running averages) are included. Keys are corresponding parameter and buffer names.
Returns
a dictionary containing a whole state of the module
Return type
[dict](https://docs.python.org/3/library/stdtypes.html#dict "(in Python v3.9)")
Example:
```
>>> module.state_dict().keys()
['bias', 'weight']
```
`to(*args, **kwargs)`
Moves and/or casts the parameters and buffers.
This can be called as
`to(device=None, dtype=None, non_blocking=False)`
`to(dtype, non_blocking=False)`
`to(tensor, non_blocking=False)`
`to(memory_format=torch.channels_last)`
Its signature is similar to [`torch.Tensor.to()`](../tensors#torch.Tensor.to "torch.Tensor.to"), but only accepts floating point or complex `dtype`s. In addition, this method will
only cast the floating point or complex parameters and buffers to :attr:`dtype` (if given). The integral parameters and buffers will be moved `device`, if that is given, but with dtypes unchanged. When `non_blocking` is set, it tries to convert/move asynchronously with respect to the host if possible, e.g., moving CPU Tensors with pinned memory to CUDA devices.
See below for examples.
Note
This method modifies the module in-place.
Parameters
* **device** (`torch.device`) – the desired device of the parameters and buffers in this module
* **dtype** (`torch.dtype`) – the desired floating point or complex dtype of the parameters and buffers in this module
* **tensor** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Tensor whose dtype and device are the desired dtype and device for all parameters and buffers in this module
* **memory\_format** (`torch.memory_format`) – the desired memory format for 4D parameters and buffers in this module (keyword only argument)
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
Examples:
```
>>> linear = nn.Linear(2, 2)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]])
>>> linear.to(torch.double)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1913, -0.3420],
[-0.5113, -0.2325]], dtype=torch.float64)
>>> gpu1 = torch.device("cuda:1")
>>> linear.to(gpu1, dtype=torch.half, non_blocking=True)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16, device='cuda:1')
>>> cpu = torch.device("cpu")
>>> linear.to(cpu)
Linear(in_features=2, out_features=2, bias=True)
>>> linear.weight
Parameter containing:
tensor([[ 0.1914, -0.3420],
[-0.5112, -0.2324]], dtype=torch.float16)
>>> linear = nn.Linear(2, 2, bias=None).to(torch.cdouble)
>>> linear.weight
Parameter containing:
tensor([[ 0.3741+0.j, 0.2382+0.j],
[ 0.5593+0.j, -0.4443+0.j]], dtype=torch.complex128)
>>> linear(torch.ones(3, 2, dtype=torch.cdouble))
tensor([[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j],
[0.6122+0.j, 0.1150+0.j]], dtype=torch.complex128)
```
`train(mode=True)`
Sets the module in training mode.
This has any effect only on certain modules. See documentations of particular modules for details of their behaviors in training/evaluation mode, if they are affected, e.g. `Dropout`, `BatchNorm`, etc.
Parameters
**mode** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to set training mode (`True`) or evaluation mode (`False`). Default: `True`.
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`type(dst_type)`
Casts all parameters and buffers to `dst_type`.
Parameters
**dst\_type** ([type](https://docs.python.org/3/library/functions.html#type "(in Python v3.9)") *or* *string*) – the desired type
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`xpu(device=None)`
Moves all model parameters and buffers to the XPU.
This also makes associated parameters and buffers different objects. So it should be called before constructing optimizer if the module will live on XPU while being optimized.
Parameters
**device** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – if specified, all parameters will be copied to that device
Returns
self
Return type
[Module](torch.nn.module#torch.nn.Module "torch.nn.Module")
`zero_grad(set_to_none=False)`
Sets gradients of all model parameters to zero. See similar function under [`torch.optim.Optimizer`](../optim#torch.optim.Optimizer "torch.optim.Optimizer") for more context.
Parameters
**set\_to\_none** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – instead of setting to zero, set the grads to None. See [`torch.optim.Optimizer.zero_grad()`](../optim#torch.optim.Optimizer.zero_grad "torch.optim.Optimizer.zero_grad") for details.
| programming_docs |
pytorch torch.div torch.div
=========
`torch.div(input, other, *, rounding_mode=None, out=None) → Tensor`
Divides each element of the input `input` by the corresponding element of `other`.
outi=inputiotheri\text{out}\_i = \frac{\text{input}\_i}{\text{other}\_i}
Note
By default, this performs a “true” division like Python 3. See the `rounding_mode` argument for floor division.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), [type promotion](../tensor_attributes#type-promotion-doc), and integer, float, and complex inputs. Always promotes integer types to the default scalar type.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the dividend
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Number*) – the divisor
Keyword Arguments
* **rounding\_mode** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")*,* *optional*) –
Type of rounding applied to the result:
+ None - default behavior. Performs no rounding and, if both `input` and `other` are integer types, promotes the inputs to the default scalar type. Equivalent to true division in Python (the `/` operator) and NumPy’s `np.true_divide`.
+ `"trunc"` - rounds the results of the division towards zero. Equivalent to C-style integer division.
+ `"floor"` - rounds the results of the division down. Equivalent to floor division in Python (the `//` operator) and NumPy’s `np.floor_divide`.
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Examples:
```
>>> x = torch.tensor([ 0.3810, 1.2774, -0.2972, -0.3719, 0.4637])
>>> torch.div(x, 0.5)
tensor([ 0.7620, 2.5548, -0.5944, -0.7438, 0.9274])
>>> a = torch.tensor([[-0.3711, -1.9353, -0.4605, -0.2917],
... [ 0.1815, -1.0111, 0.9805, -1.5923],
... [ 0.1062, 1.4581, 0.7759, -1.2344],
... [-0.1830, -0.0313, 1.1908, -1.4757]])
>>> b = torch.tensor([ 0.8032, 0.2930, -0.8113, -0.2308])
>>> torch.div(a, b)
tensor([[-0.4620, -6.6051, 0.5676, 1.2639],
[ 0.2260, -3.4509, -1.2086, 6.8990],
[ 0.1322, 4.9764, -0.9564, 5.3484],
[-0.2278, -0.1068, -1.4678, 6.3938]])
>>> torch.div(a, b, rounding_mode='trunc')
tensor([[-0., -6., 0., 1.],
[ 0., -3., -1., 6.],
[ 0., 4., -0., 5.],
[-0., -0., -1., 6.]])
>>> torch.div(a, b, rounding_mode='floor')
tensor([[-1., -7., 0., 1.],
[ 0., -4., -2., 6.],
[ 0., 4., -1., 5.],
[-1., -1., -2., 6.]])
```
pytorch ReLU6 ReLU6
=====
`class torch.nn.ReLU6(inplace=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#ReLU6)
Applies the element-wise function:
ReLU6(x)=min(max(0,x),6)\text{ReLU6}(x) = \min(\max(0,x), 6)
Parameters
**inplace** – can optionally do the operation in-place. Default: `False`
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.ReLU6()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.ones_like torch.ones\_like
================
`torch.ones_like(input, *, dtype=None, layout=None, device=None, requires_grad=False, memory_format=torch.preserve_format) → Tensor`
Returns a tensor filled with the scalar value `1`, with the same size as `input`. `torch.ones_like(input)` is equivalent to `torch.ones(input.size(), dtype=input.dtype, layout=input.layout, device=input.device)`.
Warning
As of 0.4, this function does not support an `out` keyword. As an alternative, the old `torch.ones_like(input, out=output)` is equivalent to `torch.ones(input.size(), out=output)`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the size of `input` will determine size of the output tensor.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned Tensor. Default: if `None`, defaults to the dtype of `input`.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned tensor. Default: if `None`, defaults to the layout of `input`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, defaults to the device of `input`.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
* **memory\_format** ([`torch.memory_format`](../tensor_attributes#torch.torch.memory_format "torch.torch.memory_format"), optional) – the desired memory format of returned Tensor. Default: `torch.preserve_format`.
Example:
```
>>> input = torch.empty(2, 3)
>>> torch.ones_like(input)
tensor([[ 1., 1., 1.],
[ 1., 1., 1.]])
```
pytorch ConvTranspose1d ConvTranspose1d
===============
`class torch.nn.ConvTranspose1d(in_channels, out_channels, kernel_size, stride=1, padding=0, output_padding=0, groups=1, bias=True, dilation=1, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#ConvTranspose1d)
Applies a 1D transposed convolution operator over an input image composed of several input planes.
This module can be seen as the gradient of Conv1d with respect to its input. It is also known as a fractionally-strided convolution or a deconvolution (although it is not an actual deconvolution operation).
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation.
* `padding` controls the amount of implicit zero padding on both sides for `dilation * (kernel_size - 1) - padding` number of points. See note below for details.
* `output_padding` controls the additional size added to one side of the output shape. See note below for details.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
Note
The `padding` argument effectively adds `dilation * (kernel_size - 1) - padding` amount of zero padding to both sizes of the input. This is set so that when a [`Conv1d`](torch.nn.conv1d#torch.nn.Conv1d "torch.nn.Conv1d") and a [`ConvTranspose1d`](#torch.nn.ConvTranspose1d "torch.nn.ConvTranspose1d") are initialized with same parameters, they are inverses of each other in regard to the input and output shapes. However, when `stride > 1`, [`Conv1d`](torch.nn.conv1d#torch.nn.Conv1d "torch.nn.Conv1d") maps multiple input shapes to the same output shape. `output_padding` is provided to resolve this ambiguity by effectively increasing the calculated output shape on one side. Note that `output_padding` is only used to find output shape, but does not actually add zero-padding to output.
Note
In some circumstances when using the CUDA backend with CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic =
True`. Please see the notes on [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for background.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – `dilation * (kernel_size - 1) - padding` zero-padding will be added to both sides of the input. Default: 0
* **output\_padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Additional size added to one side of the output shape. Default: 0
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
Shape:
* Input: (N,Cin,Lin)(N, C\_{in}, L\_{in})
* Output: (N,Cout,Lout)(N, C\_{out}, L\_{out}) where
Lout=(Lin−1)×stride−2×padding+dilation×(kernel\_size−1)+output\_padding+1L\_{out} = (L\_{in} - 1) \times \text{stride} - 2 \times \text{padding} + \text{dilation} \times (\text{kernel\\_size} - 1) + \text{output\\_padding} + 1
Variables
* **~ConvTranspose1d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (in\_channels,out\_channelsgroups,(\text{in\\_channels}, \frac{\text{out\\_channels}}{\text{groups}}, kernel\_size)\text{kernel\\_size}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗kernel\_sizek = \frac{groups}{C\_\text{out} \* \text{kernel\\_size}}
* **~ConvTranspose1d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels). If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗kernel\_sizek = \frac{groups}{C\_\text{out} \* \text{kernel\\_size}}
pytorch Identity Identity
========
`class torch.nn.Identity(*args, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/linear.html#Identity)
A placeholder identity operator that is argument-insensitive.
Parameters
* **args** – any argument (unused)
* **kwargs** – any keyword argument (unused)
Examples:
```
>>> m = nn.Identity(54, unused_argument1=0.1, unused_argument2=False)
>>> input = torch.randn(128, 20)
>>> output = m(input)
>>> print(output.size())
torch.Size([128, 20])
```
pytorch Conv2d Conv2d
======
`class torch.nn.Conv2d(in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#Conv2d)
Applies a 2D convolution over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,Cin,H,W)(N, C\_{\text{in}}, H, W) and output (N,Cout,Hout,Wout)(N, C\_{\text{out}}, H\_{\text{out}}, W\_{\text{out}}) can be precisely described as:
out(Ni,Coutj)=bias(Coutj)+∑k=0Cin−1weight(Coutj,k)⋆input(Ni,k)\text{out}(N\_i, C\_{\text{out}\_j}) = \text{bias}(C\_{\text{out}\_j}) + \sum\_{k = 0}^{C\_{\text{in}} - 1} \text{weight}(C\_{\text{out}\_j}, k) \star \text{input}(N\_i, k)
where ⋆\star is the valid 2D [cross-correlation](https://en.wikipedia.org/wiki/Cross-correlation) operator, NN is a batch size, CC denotes a number of channels, HH is a height of input planes in pixels, and WW is width in pixels.
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation, a single number or a tuple.
* `padding` controls the amount of implicit padding on both sides for `padding` number of points for each dimension.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
The parameters `kernel_size`, `stride`, `padding`, `dilation` can either be:
* a single `int` – in which case the same value is used for the height and width dimension
* a `tuple` of two ints – in which case, the first `int` is used for the height dimension, and the second `int` for the width dimension
Note
When `groups == in_channels` and `out_channels == K * in_channels`, where `K` is a positive integer, this operation is also known as a “depthwise convolution”.
In other words, for an input of size (N,Cin,Lin)(N, C\_{in}, L\_{in}) , a depthwise convolution with a depthwise multiplier `K` can be performed with the arguments (Cin=Cin,Cout=Cin×K,...,groups=Cin)(C\_\text{in}=C\_\text{in}, C\_\text{out}=C\_\text{in} \times \text{K}, ..., \text{groups}=C\_\text{in}) .
Note
In some circumstances when given tensors on a CUDA device and using CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic = True`. See [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for more information.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Zero-padding added to both sides of the input. Default: 0
* **padding\_mode** (*string**,* *optional*) – `'zeros'`, `'reflect'`, `'replicate'` or `'circular'`. Default: `'zeros'`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
Shape:
* Input: (N,Cin,Hin,Win)(N, C\_{in}, H\_{in}, W\_{in})
* Output: (N,Cout,Hout,Wout)(N, C\_{out}, H\_{out}, W\_{out}) where
Hout=⌊Hin+2×padding[0]−dilation[0]×(kernel\_size[0]−1)−1stride[0]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \times \text{padding}[0] - \text{dilation}[0] \times (\text{kernel\\_size}[0] - 1) - 1}{\text{stride}[0]} + 1\right\rfloor
Wout=⌊Win+2×padding[1]−dilation[1]×(kernel\_size[1]−1)−1stride[1]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \times \text{padding}[1] - \text{dilation}[1] \times (\text{kernel\\_size}[1] - 1) - 1}{\text{stride}[1]} + 1\right\rfloor
Variables
* **~Conv2d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (out\_channels,in\_channelsgroups,(\text{out\\_channels}, \frac{\text{in\\_channels}}{\text{groups}}, kernel\_size[0],kernel\_size[1])\text{kernel\\_size[0]}, \text{kernel\\_size[1]}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗∏i=01kernel\_size[i]k = \frac{groups}{C\_\text{in} \* \prod\_{i=0}^{1}\text{kernel\\_size}[i]}
* **~Conv2d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels). If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCin∗∏i=01kernel\_size[i]k = \frac{groups}{C\_\text{in} \* \prod\_{i=0}^{1}\text{kernel\\_size}[i]}
#### Examples
```
>>> # With square kernels and equal stride
>>> m = nn.Conv2d(16, 33, 3, stride=2)
>>> # non-square kernels and unequal stride and with padding
>>> m = nn.Conv2d(16, 33, (3, 5), stride=(2, 1), padding=(4, 2))
>>> # non-square kernels and unequal stride and with padding and dilation
>>> m = nn.Conv2d(16, 33, (3, 5), stride=(2, 1), padding=(4, 2), dilation=(3, 1))
>>> input = torch.randn(20, 16, 50, 100)
>>> output = m(input)
```
pytorch torch.sum torch.sum
=========
`torch.sum(input, *, dtype=None) → Tensor`
Returns the sum of all elements in the `input` tensor.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. If specified, the input tensor is casted to `dtype` before the operation is performed. This is useful for preventing data type overflows. Default: None.
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[ 0.1133, -0.9567, 0.2958]])
>>> torch.sum(a)
tensor(-0.5475)
```
`torch.sum(input, dim, keepdim=False, *, dtype=None) → Tensor`
Returns the sum of each row of the `input` tensor in the given dimension `dim`. If `dim` is a list of dimensions, reduce over all of them.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension(s) `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 (or `len(dim)`) fewer dimension(s).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – the dimension or dimensions to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. If specified, the input tensor is casted to `dtype` before the operation is performed. This is useful for preventing data type overflows. Default: None.
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[ 0.0569, -0.2475, 0.0737, -0.3429],
[-0.2993, 0.9138, 0.9337, -1.6864],
[ 0.1132, 0.7892, -0.1003, 0.5688],
[ 0.3637, -0.9906, -0.4752, -1.5197]])
>>> torch.sum(a, 1)
tensor([-0.4598, -0.1381, 1.3708, -2.6217])
>>> b = torch.arange(4 * 5 * 6).view(4, 5, 6)
>>> torch.sum(b, (2, 1))
tensor([ 435., 1335., 2235., 3135.])
```
| programming_docs |
pytorch TripletMarginLoss TripletMarginLoss
=================
`class torch.nn.TripletMarginLoss(margin=1.0, p=2.0, eps=1e-06, swap=False, size_average=None, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#TripletMarginLoss)
Creates a criterion that measures the triplet loss given an input tensors x1x1 , x2x2 , x3x3 and a margin with a value greater than 00 . This is used for measuring a relative similarity between samples. A triplet is composed by `a`, `p` and `n` (i.e., `anchor`, `positive examples` and `negative examples` respectively). The shapes of all input tensors should be (N,D)(N, D) .
The distance swap is described in detail in the paper [Learning shallow convolutional feature descriptors with triplet losses](http://www.bmva.org/bmvc/2016/papers/paper119/index.html) by V. Balntas, E. Riba et al.
The loss function for each sample in the mini-batch is:
L(a,p,n)=max{d(ai,pi)−d(ai,ni)+margin,0}L(a, p, n) = \max \{d(a\_i, p\_i) - d(a\_i, n\_i) + {\rm margin}, 0\}
where
d(xi,yi)=∥xi−yi∥pd(x\_i, y\_i) = \left\lVert {\bf x}\_i - {\bf y}\_i \right\rVert\_p
See also [`TripletMarginWithDistanceLoss`](torch.nn.tripletmarginwithdistanceloss#torch.nn.TripletMarginWithDistanceLoss "torch.nn.TripletMarginWithDistanceLoss"), which computes the triplet margin loss for input tensors using a custom distance function.
Parameters
* **margin** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Default: 11 .
* **p** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – The norm degree for pairwise distance. Default: 22 .
* **swap** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – The distance swap is described in detail in the paper `Learning shallow convolutional feature descriptors with triplet losses` by V. Balntas, E. Riba et al. Default: `False`.
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Shape:
* Input: (N,D)(N, D) where DD is the vector dimension.
* `Output: A Tensor of shape (N)(N) if reduction is 'none', or a scalar`
otherwise.
```
>>> triplet_loss = nn.TripletMarginLoss(margin=1.0, p=2)
>>> anchor = torch.randn(100, 128, requires_grad=True)
>>> positive = torch.randn(100, 128, requires_grad=True)
>>> negative = torch.randn(100, 128, requires_grad=True)
>>> output = triplet_loss(anchor, positive, negative)
>>> output.backward()
```
pytorch torch.cartesian_prod torch.cartesian\_prod
=====================
`torch.cartesian_prod(*tensors)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#cartesian_prod)
Do cartesian product of the given sequence of tensors. The behavior is similar to python’s `itertools.product`.
Parameters
**\*tensors** – any number of 1 dimensional tensors.
Returns
A tensor equivalent to converting all the input tensors into lists,
do `itertools.product` on these lists, and finally convert the resulting list into tensor.
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> a = [1, 2, 3]
>>> b = [4, 5]
>>> list(itertools.product(a, b))
[(1, 4), (1, 5), (2, 4), (2, 5), (3, 4), (3, 5)]
>>> tensor_a = torch.tensor(a)
>>> tensor_b = torch.tensor(b)
>>> torch.cartesian_prod(tensor_a, tensor_b)
tensor([[1, 4],
[1, 5],
[2, 4],
[2, 5],
[3, 4],
[3, 5]])
```
pytorch PairwiseDistance PairwiseDistance
================
`class torch.nn.PairwiseDistance(p=2.0, eps=1e-06, keepdim=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/distance.html#PairwiseDistance)
Computes the batchwise pairwise distance between vectors v1v\_1 , v2v\_2 using the p-norm:
∥x∥p=(∑i=1n∣xi∣p)1/p.\Vert x \Vert \_p = \left( \sum\_{i=1}^n \vert x\_i \vert ^ p \right) ^ {1/p}.
Parameters
* **p** (*real*) – the norm degree. Default: 2
* **eps** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Small value to avoid division by zero. Default: 1e-6
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Determines whether or not to keep the vector dimension. Default: False
Shape:
* Input1: (N,D)(N, D) where `D = vector dimension`
* Input2: (N,D)(N, D) , same shape as the Input1
* Output: (N)(N) . If `keepdim` is `True`, then (N,1)(N, 1) .
Examples::
```
>>> pdist = nn.PairwiseDistance(p=2)
>>> input1 = torch.randn(100, 128)
>>> input2 = torch.randn(100, 128)
>>> output = pdist(input1, input2)
```
pytorch torch.save torch.save
==========
`torch.save(obj, f, pickle_module=<module 'pickle' from '/home/matti/miniconda3/lib/python3.7/pickle.py'>, pickle_protocol=2, _use_new_zipfile_serialization=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/serialization.html#save)
Saves an object to a disk file.
See also: `saving-loading-tensors`
Parameters
* **obj** – saved object
* **f** – a file-like object (has to implement write and flush) or a string or os.PathLike object containing a file name
* **pickle\_module** – module used for pickling metadata and objects
* **pickle\_protocol** – can be specified to override the default protocol
Note
A common PyTorch convention is to save tensors using .pt file extension.
Note
PyTorch preserves storage sharing across serialization. See `preserve-storage-sharing` for more details.
Note
The 1.6 release of PyTorch switched `torch.save` to use a new zipfile-based file format. `torch.load` still retains the ability to load files in the old format. If for any reason you want `torch.save` to use the old format, pass the kwarg `_use_new_zipfile_serialization=False`.
#### Example
```
>>> # Save to file
>>> x = torch.tensor([0, 1, 2, 3, 4])
>>> torch.save(x, 'tensor.pt')
>>> # Save to io.BytesIO buffer
>>> buffer = io.BytesIO()
>>> torch.save(x, buffer)
```
pytorch enable_grad enable\_grad
============
`class torch.enable_grad` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/autograd/grad_mode.html#enable_grad)
Context-manager that enables gradient calculation.
Enables gradient calculation, if it has been disabled via [`no_grad`](torch.no_grad#torch.no_grad "torch.no_grad") or [`set_grad_enabled`](torch.set_grad_enabled#torch.set_grad_enabled "torch.set_grad_enabled").
This context manager is thread local; it will not affect computation in other threads.
Also functions as a decorator. (Make sure to instantiate with parenthesis.)
Example:
```
>>> x = torch.tensor([1], requires_grad=True)
>>> with torch.no_grad():
... with torch.enable_grad():
... y = x * 2
>>> y.requires_grad
True
>>> y.backward()
>>> x.grad
>>> @torch.enable_grad()
... def doubler(x):
... return x * 2
>>> with torch.no_grad():
... z = doubler(x)
>>> z.requires_grad
True
```
pytorch torch.var_mean torch.var\_mean
===============
`torch.var_mean(input, unbiased=True) -> (Tensor, Tensor)`
Returns the variance and mean of all elements in the `input` tensor.
If `unbiased` is `False`, then the variance will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[0.0146, 0.4258, 0.2211]])
>>> torch.var_mean(a)
(tensor(0.0423), tensor(0.2205))
```
`torch.var_mean(input, dim, keepdim=False, unbiased=True) -> (Tensor, Tensor)`
Returns the variance and mean of each row of the `input` tensor in the given dimension `dim`.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension(s) `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 (or `len(dim)`) fewer dimension(s).
If `unbiased` is `False`, then the variance will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – the dimension or dimensions to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[-1.5650, 2.0415, -0.1024, -0.5790],
[ 0.2325, -2.6145, -1.6428, -0.3537],
[-0.2159, -1.1069, 1.2882, -1.3265],
[-0.6706, -1.5893, 0.6827, 1.6727]])
>>> torch.var_mean(a, 1)
(tensor([2.3174, 1.6403, 1.4092, 2.0791]), tensor([-0.0512, -1.0946, -0.3403, 0.0239]))
```
pytorch torch.from_numpy torch.from\_numpy
=================
`torch.from_numpy(ndarray) → Tensor`
Creates a [`Tensor`](../tensors#torch.Tensor "torch.Tensor") from a [`numpy.ndarray`](https://numpy.org/doc/stable/reference/generated/numpy.ndarray.html#numpy.ndarray "(in NumPy v1.20)").
The returned tensor and `ndarray` share the same memory. Modifications to the tensor will be reflected in the `ndarray` and vice versa. The returned tensor is not resizable.
It currently accepts `ndarray` with dtypes of `numpy.float64`, `numpy.float32`, `numpy.float16`, `numpy.complex64`, `numpy.complex128`, `numpy.int64`, `numpy.int32`, `numpy.int16`, `numpy.int8`, `numpy.uint8`, and `numpy.bool`.
Example:
```
>>> a = numpy.array([1, 2, 3])
>>> t = torch.from_numpy(a)
>>> t
tensor([ 1, 2, 3])
>>> t[0] = -1
>>> a
array([-1, 2, 3])
```
pytorch torch.einsum torch.einsum
============
`torch.einsum(equation, *operands) → Tensor` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#einsum)
Sums the product of the elements of the input `operands` along dimensions specified using a notation based on the Einstein summation convention.
Einsum allows computing many common multi-dimensional linear algebraic array operations by representing them in a short-hand format based on the Einstein summation convention, given by `equation`. The details of this format are described below, but the general idea is to label every dimension of the input `operands` with some subscript and define which subscripts are part of the output. The output is then computed by summing the product of the elements of the `operands` along the dimensions whose subscripts are not part of the output. For example, matrix multiplication can be computed using einsum as `torch.einsum(“ij,jk->ik”, A, B)`. Here, j is the summation subscript and i and k the output subscripts (see section below for more details on why).
Equation:
The `equation` string specifies the subscripts (lower case letters `[‘a’, ‘z’]`) for each dimension of the input `operands` in the same order as the dimensions, separating subcripts for each operand by a comma (‘,’), e.g. `‘ij,jk’` specify subscripts for two 2D operands. The dimensions labeled with the same subscript must be broadcastable, that is, their size must either match or be `1`. The exception is if a subscript is repeated for the same input operand, in which case the dimensions labeled with this subscript for this operand must match in size and the operand will be replaced by its diagonal along these dimensions. The subscripts that appear exactly once in the `equation` will be part of the output, sorted in increasing alphabetical order. The output is computed by multiplying the input `operands` element-wise, with their dimensions aligned based on the subscripts, and then summing out the dimensions whose subscripts are not part of the output.
Optionally, the output subscripts can be explicitly defined by adding an arrow (‘->’) at the end of the equation followed by the subscripts for the output. For instance, the following equation computes the transpose of a matrix multiplication: ‘ij,jk->ki’. The output subscripts must appear at least once for some input operand and at most once for the output.
Ellipsis (‘…’) can be used in place of subscripts to broadcast the dimensions covered by the ellipsis. Each input operand may contain at most one ellipsis which will cover the dimensions not covered by subscripts, e.g. for an input operand with 5 dimensions, the ellipsis in the equation `‘ab…c’` cover the third and fourth dimensions. The ellipsis does not need to cover the same number of dimensions across the `operands` but the ‘shape’ of the ellipsis (the size of the dimensions covered by them) must broadcast together. If the output is not explicitly defined with the arrow (‘->’) notation, the ellipsis will come first in the output (left-most dimensions), before the subscript labels that appear exactly once for the input operands. e.g. the following equation implements batch matrix multiplication `‘…ij,…jk’`.
A few final notes: the equation may contain whitespaces between the different elements (subscripts, ellipsis, arrow and comma) but something like `‘…’` is not valid. An empty string `‘’` is valid for scalar operands.
Note
`torch.einsum` handles ellipsis (‘…’) differently from NumPy in that it allows dimensions covered by the ellipsis to be summed over, that is, ellipsis are not required to be part of the output.
Note
This function does not optimize the given expression, so a different formula for the same computation may run faster or consume less memory. Projects like opt\_einsum (<https://optimized-einsum.readthedocs.io/en/stable/>) can optimize the formula for you.
Parameters
* **equation** (*string*) – The subscripts for the Einstein summation.
* **operands** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The operands to compute the Einstein sum of.
Examples:
```
# trace
>>> torch.einsum('ii', torch.randn(4, 4))
tensor(-1.2104)
# diagonal
>>> torch.einsum('ii->i', torch.randn(4, 4))
tensor([-0.1034, 0.7952, -0.2433, 0.4545])
# outer product
>>> x = torch.randn(5)
>>> y = torch.randn(4)
>>> torch.einsum('i,j->ij', x, y)
tensor([[ 0.1156, -0.2897, -0.3918, 0.4963],
[-0.3744, 0.9381, 1.2685, -1.6070],
[ 0.7208, -1.8058, -2.4419, 3.0936],
[ 0.1713, -0.4291, -0.5802, 0.7350],
[ 0.5704, -1.4290, -1.9323, 2.4480]])
# batch matrix multiplication
>>> As = torch.randn(3,2,5)
>>> Bs = torch.randn(3,5,4)
>>> torch.einsum('bij,bjk->bik', As, Bs)
tensor([[[-1.0564, -1.5904, 3.2023, 3.1271],
[-1.6706, -0.8097, -0.8025, -2.1183]],
[[ 4.2239, 0.3107, -0.5756, -0.2354],
[-1.4558, -0.3460, 1.5087, -0.8530]],
[[ 2.8153, 1.8787, -4.3839, -1.2112],
[ 0.3728, -2.1131, 0.0921, 0.8305]]])
# batch permute
>>> A = torch.randn(2, 3, 4, 5)
>>> torch.einsum('...ij->...ji', A).shape
torch.Size([2, 3, 5, 4])
# equivalent to torch.nn.functional.bilinear
>>> A = torch.randn(3,5,4)
>>> l = torch.randn(2,5)
>>> r = torch.randn(2,4)
>>> torch.einsum('bn,anm,bm->ba', l, A, r)
tensor([[-0.3430, -5.2405, 0.4494],
[ 0.3311, 5.5201, -3.0356]])
```
pytorch torch.nonzero torch.nonzero
=============
`torch.nonzero(input, *, out=None, as_tuple=False) → LongTensor or tuple of LongTensors`
Note
[`torch.nonzero(..., as_tuple=False)`](#torch.nonzero "torch.nonzero") (default) returns a 2-D tensor where each row is the index for a nonzero value.
[`torch.nonzero(..., as_tuple=True)`](#torch.nonzero "torch.nonzero") returns a tuple of 1-D index tensors, allowing for advanced indexing, so `x[x.nonzero(as_tuple=True)]` gives all nonzero values of tensor `x`. Of the returned tuple, each index tensor contains nonzero indices for a certain dimension.
See below for more details on the two behaviors.
When `input` is on CUDA, [`torch.nonzero()`](#torch.nonzero "torch.nonzero") causes host-device synchronization.
**When** `as_tuple` **is ``False`` (default)**:
Returns a tensor containing the indices of all non-zero elements of `input`. Each row in the result contains the indices of a non-zero element in `input`. The result is sorted lexicographically, with the last index changing the fastest (C-style).
If `input` has nn dimensions, then the resulting indices tensor `out` is of size (z×n)(z \times n) , where zz is the total number of non-zero elements in the `input` tensor.
**When** `as_tuple` **is ``True``**:
Returns a tuple of 1-D tensors, one for each dimension in `input`, each containing the indices (in that dimension) of all non-zero elements of `input` .
If `input` has nn dimensions, then the resulting tuple contains nn tensors of size zz , where zz is the total number of non-zero elements in the `input` tensor.
As a special case, when `input` has zero dimensions and a nonzero scalar value, it is treated as a one-dimensional tensor with one element.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** (*LongTensor**,* *optional*) – the output tensor containing indices
Returns
If `as_tuple` is `False`, the output tensor containing indices. If `as_tuple` is `True`, one 1-D tensor for each dimension, containing the indices of each nonzero element along that dimension.
Return type
LongTensor or tuple of LongTensor
Example:
```
>>> torch.nonzero(torch.tensor([1, 1, 1, 0, 1]))
tensor([[ 0],
[ 1],
[ 2],
[ 4]])
>>> torch.nonzero(torch.tensor([[0.6, 0.0, 0.0, 0.0],
... [0.0, 0.4, 0.0, 0.0],
... [0.0, 0.0, 1.2, 0.0],
... [0.0, 0.0, 0.0,-0.4]]))
tensor([[ 0, 0],
[ 1, 1],
[ 2, 2],
[ 3, 3]])
>>> torch.nonzero(torch.tensor([1, 1, 1, 0, 1]), as_tuple=True)
(tensor([0, 1, 2, 4]),)
>>> torch.nonzero(torch.tensor([[0.6, 0.0, 0.0, 0.0],
... [0.0, 0.4, 0.0, 0.0],
... [0.0, 0.0, 1.2, 0.0],
... [0.0, 0.0, 0.0,-0.4]]), as_tuple=True)
(tensor([0, 1, 2, 3]), tensor([0, 1, 2, 3]))
>>> torch.nonzero(torch.tensor(5), as_tuple=True)
(tensor([0]),)
```
pytorch torch.var torch.var
=========
`torch.var(input, unbiased=True) → Tensor`
Returns the variance of all elements in the `input` tensor.
If `unbiased` is `False`, then the variance will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[-0.3425, -1.2636, -0.4864]])
>>> torch.var(a)
tensor(0.2455)
```
`torch.var(input, dim, unbiased=True, keepdim=False, *, out=None) → Tensor`
Returns the variance of each row of the `input` tensor in the given dimension `dim`.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension(s) `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 (or `len(dim)`) fewer dimension(s).
If `unbiased` is `False`, then the variance will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – the dimension or dimensions to reduce.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[-0.3567, 1.7385, -1.3042, 0.7423],
[ 1.3436, -0.1015, -0.9834, -0.8438],
[ 0.6056, 0.1089, -0.3112, -1.4085],
[-0.7700, 0.6074, -0.1469, 0.7777]])
>>> torch.var(a, 1)
tensor([ 1.7444, 1.1363, 0.7356, 0.5112])
```
| programming_docs |
pytorch torch.eig torch.eig
=========
`torch.eig(input, eigenvectors=False, *, out=None) -> (Tensor, Tensor)`
Computes the eigenvalues and eigenvectors of a real square matrix.
Note
Since eigenvalues and eigenvectors might be complex, backward pass is supported only if eigenvalues and eigenvectors are all real valued.
When `input` is on CUDA, [`torch.eig()`](#torch.eig "torch.eig") causes host-device synchronization.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the square matrix of shape (n×n)(n \times n) for which the eigenvalues and eigenvectors will be computed
* **eigenvectors** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – `True` to compute both eigenvalues and eigenvectors; otherwise, only eigenvalues will be computed
Keyword Arguments
**out** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the output tensors
Returns
A namedtuple (eigenvalues, eigenvectors) containing
* **eigenvalues** (*Tensor*): Shape (n×2)(n \times 2) . Each row is an eigenvalue of `input`, where the first element is the real part and the second element is the imaginary part. The eigenvalues are not necessarily ordered.
* **eigenvectors** (*Tensor*): If `eigenvectors=False`, it’s an empty tensor. Otherwise, this tensor of shape (n×n)(n \times n) can be used to compute normalized (unit length) eigenvectors of corresponding eigenvalues as follows. If the corresponding `eigenvalues[j]` is a real number, column `eigenvectors[:, j]` is the eigenvector corresponding to `eigenvalues[j]`. If the corresponding `eigenvalues[j]` and `eigenvalues[j + 1]` form a complex conjugate pair, then the true eigenvectors can be computed as true eigenvector[j]=eigenvectors[:,j]+i×eigenvectors[:,j+1]\text{true eigenvector}[j] = eigenvectors[:, j] + i \times eigenvectors[:, j + 1] , true eigenvector[j+1]=eigenvectors[:,j]−i×eigenvectors[:,j+1]\text{true eigenvector}[j + 1] = eigenvectors[:, j] - i \times eigenvectors[:, j + 1] .
Return type
([Tensor](../tensors#torch.Tensor "torch.Tensor"), [Tensor](../tensors#torch.Tensor "torch.Tensor"))
Example:
```
Trivial example with a diagonal matrix. By default, only eigenvalues are computed:
>>> a = torch.diag(torch.tensor([1, 2, 3], dtype=torch.double))
>>> e, v = torch.eig(a)
>>> e
tensor([[1., 0.],
[2., 0.],
[3., 0.]], dtype=torch.float64)
>>> v
tensor([], dtype=torch.float64)
Compute also the eigenvectors:
>>> e, v = torch.eig(a, eigenvectors=True)
>>> e
tensor([[1., 0.],
[2., 0.],
[3., 0.]], dtype=torch.float64)
>>> v
tensor([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]], dtype=torch.float64)
```
pytorch torch.sinh torch.sinh
==========
`torch.sinh(input, *, out=None) → Tensor`
Returns a new tensor with the hyperbolic sine of the elements of `input`.
outi=sinh(inputi)\text{out}\_{i} = \sinh(\text{input}\_{i})
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.5380, -0.8632, -0.1265, 0.9399])
>>> torch.sinh(a)
tensor([ 0.5644, -0.9744, -0.1268, 1.0845])
```
Note
When `input` is on the CPU, the implementation of torch.sinh may use the Sleef library, which rounds very large results to infinity or negative infinity. See [here](https://sleef.org/purec.xhtml) for details.
pytorch torch.exp torch.exp
=========
`torch.exp(input, *, out=None) → Tensor`
Returns a new tensor with the exponential of the elements of the input tensor `input`.
yi=exiy\_{i} = e^{x\_{i}}
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.exp(torch.tensor([0, math.log(2.)]))
tensor([ 1., 2.])
```
pytorch AdaptiveAvgPool2d AdaptiveAvgPool2d
=================
`class torch.nn.AdaptiveAvgPool2d(output_size)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveAvgPool2d)
Applies a 2D adaptive average pooling over an input signal composed of several input planes.
The output is of size H x W, for any input size. The number of output features is equal to the number of input planes.
Parameters
**output\_size** – the target output size of the image of the form H x W. Can be a tuple (H, W) or a single H for a square image H x H. H and W can be either a `int`, or `None` which means the size will be the same as that of the input.
#### Examples
```
>>> # target output size of 5x7
>>> m = nn.AdaptiveAvgPool2d((5,7))
>>> input = torch.randn(1, 64, 8, 9)
>>> output = m(input)
>>> # target output size of 7x7 (square)
>>> m = nn.AdaptiveAvgPool2d(7)
>>> input = torch.randn(1, 64, 10, 9)
>>> output = m(input)
>>> # target output size of 10x7
>>> m = nn.AdaptiveAvgPool2d((None, 7))
>>> input = torch.randn(1, 64, 10, 9)
>>> output = m(input)
```
pytorch torch.pow torch.pow
=========
`torch.pow(input, exponent, *, out=None) → Tensor`
Takes the power of each element in `input` with `exponent` and returns a tensor with the result.
`exponent` can be either a single `float` number or a `Tensor` with the same number of elements as `input`.
When `exponent` is a scalar value, the operation applied is:
outi=xiexponent\text{out}\_i = x\_i ^ \text{exponent}
When `exponent` is a tensor, the operation applied is:
outi=xiexponenti\text{out}\_i = x\_i ^ {\text{exponent}\_i}
When `exponent` is a tensor, the shapes of `input` and `exponent` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **exponent** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* *tensor*) – the exponent value
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.4331, 1.2475, 0.6834, -0.2791])
>>> torch.pow(a, 2)
tensor([ 0.1875, 1.5561, 0.4670, 0.0779])
>>> exp = torch.arange(1., 5.)
>>> a = torch.arange(1., 5.)
>>> a
tensor([ 1., 2., 3., 4.])
>>> exp
tensor([ 1., 2., 3., 4.])
>>> torch.pow(a, exp)
tensor([ 1., 4., 27., 256.])
```
`torch.pow(self, exponent, *, out=None) → Tensor`
`self` is a scalar `float` value, and `exponent` is a tensor. The returned tensor `out` is of the same shape as `exponent`
The operation applied is:
outi=selfexponenti\text{out}\_i = \text{self} ^ {\text{exponent}\_i}
Parameters
* **self** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the scalar base value for the power operation
* **exponent** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the exponent tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> exp = torch.arange(1., 5.)
>>> base = 2
>>> torch.pow(base, exp)
tensor([ 2., 4., 8., 16.])
```
pytorch torch.isreal torch.isreal
============
`torch.isreal(input) → Tensor`
Returns a new tensor with boolean elements representing if each element of `input` is real-valued or not. All real-valued types are considered real. Complex values are considered real when their imaginary part is 0.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Returns
A boolean tensor that is True where `input` is real and False elsewhere
Example:
```
>>> torch.isreal(torch.tensor([1, 1+1j, 2+0j]))
tensor([True, False, True])
```
pytorch torch.empty_strided torch.empty\_strided
====================
`torch.empty_strided(size, stride, *, dtype=None, layout=None, device=None, requires_grad=False, pin_memory=False) → Tensor`
Returns a tensor filled with uninitialized data. The shape and strides of the tensor is defined by the variable argument `size` and `stride` respectively. `torch.empty_strided(size, stride)` is equivalent to `torch.empty(size).as_strided(size, stride)`.
Warning
More than one element of the created tensor may refer to a single memory location. As a result, in-place operations (especially ones that are vectorized) may result in incorrect behavior. If you need to write to the tensors, please clone them first.
Parameters
* **size** (*tuple of python:ints*) – the shape of the output tensor
* **stride** (*tuple of python:ints*) – the strides of the output tensor
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")).
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
* **pin\_memory** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If set, returned tensor would be allocated in the pinned memory. Works only for CPU tensors. Default: `False`.
Example:
```
>>> a = torch.empty_strided((2, 3), (1, 2))
>>> a
tensor([[8.9683e-44, 4.4842e-44, 5.1239e+07],
[0.0000e+00, 0.0000e+00, 3.0705e-41]])
>>> a.stride()
(1, 2)
>>> a.size()
torch.Size([2, 3])
```
pytorch CrossEntropyLoss CrossEntropyLoss
================
`class torch.nn.CrossEntropyLoss(weight=None, size_average=None, ignore_index=-100, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#CrossEntropyLoss)
This criterion combines [`LogSoftmax`](torch.nn.logsoftmax#torch.nn.LogSoftmax "torch.nn.LogSoftmax") and [`NLLLoss`](torch.nn.nllloss#torch.nn.NLLLoss "torch.nn.NLLLoss") in one single class.
It is useful when training a classification problem with `C` classes. If provided, the optional argument `weight` should be a 1D `Tensor` assigning weight to each of the classes. This is particularly useful when you have an unbalanced training set.
The `input` is expected to contain raw, unnormalized scores for each class.
`input` has to be a Tensor of size either (minibatch,C)(minibatch, C) or (minibatch,C,d1,d2,...,dK)(minibatch, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 for the `K`-dimensional case (described later).
This criterion expects a class index in the range [0,C−1][0, C-1] as the `target` for each value of a 1D tensor of size `minibatch`; if `ignore_index` is specified, this criterion also accepts this class index (this index may not necessarily be in the class range).
The loss can be described as:
loss(x,class)=−log(exp(x[class])∑jexp(x[j]))=−x[class]+log(∑jexp(x[j]))\text{loss}(x, class) = -\log\left(\frac{\exp(x[class])}{\sum\_j \exp(x[j])}\right) = -x[class] + \log\left(\sum\_j \exp(x[j])\right)
or in the case of the `weight` argument being specified:
loss(x,class)=weight[class](−x[class]+log(∑jexp(x[j])))\text{loss}(x, class) = weight[class] \left(-x[class] + \log\left(\sum\_j \exp(x[j])\right)\right)
The losses are averaged across observations for each minibatch. If the `weight` argument is specified then this is a weighted average:
loss=∑i=1Nloss(i,class[i])∑i=1Nweight[class[i]]\text{loss} = \frac{\sum^{N}\_{i=1} loss(i, class[i])}{\sum^{N}\_{i=1} weight[class[i]]}
Can also be used for higher dimension inputs, such as 2D images, by providing an input of size (minibatch,C,d1,d2,...,dK)(minibatch, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 , where KK is the number of dimensions, and a target of appropriate shape (see below).
Parameters
* **weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – a manual rescaling weight given to each class. If given, has to be a Tensor of size `C`
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **ignore\_index** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Specifies a target value that is ignored and does not contribute to the input gradient. When `size_average` is `True`, the loss is averaged over non-ignored targets.
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the weighted mean of the output is taken, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Shape:
* Input: (N,C)(N, C) where `C = number of classes`, or (N,C,d1,d2,...,dK)(N, C, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of `K`-dimensional loss.
* Target: (N)(N) where each value is 0≤targets[i]≤C−10 \leq \text{targets}[i] \leq C-1 , or (N,d1,d2,...,dK)(N, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of K-dimensional loss.
* Output: scalar. If `reduction` is `'none'`, then the same size as the target: (N)(N) , or (N,d1,d2,...,dK)(N, d\_1, d\_2, ..., d\_K) with K≥1K \geq 1 in the case of K-dimensional loss.
Examples:
```
>>> loss = nn.CrossEntropyLoss()
>>> input = torch.randn(3, 5, requires_grad=True)
>>> target = torch.empty(3, dtype=torch.long).random_(5)
>>> output = loss(input, target)
>>> output.backward()
```
pytorch torch.bernoulli torch.bernoulli
===============
`torch.bernoulli(input, *, generator=None, out=None) → Tensor`
Draws binary random numbers (0 or 1) from a Bernoulli distribution.
The `input` tensor should be a tensor containing probabilities to be used for drawing the binary random number. Hence, all values in `input` have to be in the range: 0≤inputi≤10 \leq \text{input}\_i \leq 1 .
The ith\text{i}^{th} element of the output tensor will draw a value 11 according to the ith\text{i}^{th} probability value given in `input`.
outi∼Bernoulli(p=inputi)\text{out}\_{i} \sim \mathrm{Bernoulli}(p = \text{input}\_{i})
The returned `out` tensor only has values 0 or 1 and is of the same shape as `input`.
`out` can have integral `dtype`, but `input` must have floating point `dtype`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor of probability values for the Bernoulli distribution
Keyword Arguments
* **generator** ([`torch.Generator`](torch.generator#torch.Generator "torch.Generator"), optional) – a pseudorandom number generator for sampling
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.empty(3, 3).uniform_(0, 1) # generate a uniform random matrix with range [0, 1]
>>> a
tensor([[ 0.1737, 0.0950, 0.3609],
[ 0.7148, 0.0289, 0.2676],
[ 0.9456, 0.8937, 0.7202]])
>>> torch.bernoulli(a)
tensor([[ 1., 0., 0.],
[ 0., 0., 0.],
[ 1., 1., 1.]])
>>> a = torch.ones(3, 3) # probability of drawing "1" is 1
>>> torch.bernoulli(a)
tensor([[ 1., 1., 1.],
[ 1., 1., 1.],
[ 1., 1., 1.]])
>>> a = torch.zeros(3, 3) # probability of drawing "1" is 0
>>> torch.bernoulli(a)
tensor([[ 0., 0., 0.],
[ 0., 0., 0.],
[ 0., 0., 0.]])
```
pytorch torch.logaddexp torch.logaddexp
===============
`torch.logaddexp(input, other, *, out=None) → Tensor`
Logarithm of the sum of exponentiations of the inputs.
Calculates pointwise log(ex+ey)\log\left(e^x + e^y\right) . This function is useful in statistics where the calculated probabilities of events may be so small as to exceed the range of normal floating point numbers. In such cases the logarithm of the calculated probability is stored. This function allows adding probabilities stored in such a fashion.
This op should be disambiguated with [`torch.logsumexp()`](torch.logsumexp#torch.logsumexp "torch.logsumexp") which performs a reduction on a single tensor.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.logaddexp(torch.tensor([-1.0]), torch.tensor([-1.0, -2, -3]))
tensor([-0.3069, -0.6867, -0.8731])
>>> torch.logaddexp(torch.tensor([-100.0, -200, -300]), torch.tensor([-1.0, -2, -3]))
tensor([-1., -2., -3.])
>>> torch.logaddexp(torch.tensor([1.0, 2000, 30000]), torch.tensor([-1.0, -2, -3]))
tensor([1.1269e+00, 2.0000e+03, 3.0000e+04])
```
pytorch torch.log1p torch.log1p
===========
`torch.log1p(input, *, out=None) → Tensor`
Returns a new tensor with the natural logarithm of (1 + `input`).
yi=loge(xi+1)y\_i = \log\_{e} (x\_i + 1)
Note
This function is more accurate than [`torch.log()`](torch.log#torch.log "torch.log") for small values of `input`
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(5)
>>> a
tensor([-1.0090, -0.9923, 1.0249, -0.5372, 0.2492])
>>> torch.log1p(a)
tensor([ nan, -4.8653, 0.7055, -0.7705, 0.2225])
```
pytorch MultiheadAttention MultiheadAttention
==================
`class torch.nn.MultiheadAttention(embed_dim, num_heads, dropout=0.0, bias=True, add_bias_kv=False, add_zero_attn=False, kdim=None, vdim=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#MultiheadAttention)
Allows the model to jointly attend to information from different representation subspaces. See [Attention Is All You Need](https://arxiv.org/abs/1706.03762)
MultiHead(Q,K,V)=Concat(head1,…,headh)WO\text{MultiHead}(Q, K, V) = \text{Concat}(head\_1,\dots,head\_h)W^O
where headi=Attention(QWiQ,KWiK,VWiV)head\_i = \text{Attention}(QW\_i^Q, KW\_i^K, VW\_i^V) .
Parameters
* **embed\_dim** – total dimension of the model.
* **num\_heads** – parallel attention heads.
* **dropout** – a Dropout layer on attn\_output\_weights. Default: 0.0.
* **bias** – add bias as module parameter. Default: True.
* **add\_bias\_kv** – add bias to the key and value sequences at dim=0.
* **add\_zero\_attn** – add a new batch of zeros to the key and value sequences at dim=1.
* **kdim** – total number of features in key. Default: None.
* **vdim** – total number of features in value. Default: None.
Note that if `kdim` and `vdim` are None, they will be set to `embed_dim` such that query, key, and value have the same number of features.
Examples:
```
>>> multihead_attn = nn.MultiheadAttention(embed_dim, num_heads)
>>> attn_output, attn_output_weights = multihead_attn(query, key, value)
```
`forward(query, key, value, key_padding_mask=None, need_weights=True, attn_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#MultiheadAttention.forward)
Parameters
* **key, value** (*query**,*) – map a query and a set of key-value pairs to an output. See “Attention Is All You Need” for more details.
* **key\_padding\_mask** – if provided, specified padding elements in the key will be ignored by the attention. When given a binary mask and a value is True, the corresponding value on the attention layer will be ignored. When given a byte mask and a value is non-zero, the corresponding value on the attention layer will be ignored
* **need\_weights** – output attn\_output\_weights.
* **attn\_mask** – 2D or 3D mask that prevents attention to certain positions. A 2D mask will be broadcasted for all the batches while a 3D mask allows to specify a different mask for the entries of each batch.
Shapes for inputs:
* query: (L,N,E)(L, N, E) where L is the target sequence length, N is the batch size, E is the embedding dimension.
* key: (S,N,E)(S, N, E) , where S is the source sequence length, N is the batch size, E is the embedding dimension.
* value: (S,N,E)(S, N, E) where S is the source sequence length, N is the batch size, E is the embedding dimension.
* key\_padding\_mask: (N,S)(N, S) where N is the batch size, S is the source sequence length. If a ByteTensor is provided, the non-zero positions will be ignored while the position with the zero positions will be unchanged. If a BoolTensor is provided, the positions with the value of `True` will be ignored while the position with the value of `False` will be unchanged.
* attn\_mask: if a 2D mask: (L,S)(L, S) where L is the target sequence length, S is the source sequence length.
If a 3D mask: (N⋅num\_heads,L,S)(N\cdot\text{num\\_heads}, L, S) where N is the batch size, L is the target sequence length, S is the source sequence length. `attn_mask` ensure that position i is allowed to attend the unmasked positions. If a ByteTensor is provided, the non-zero positions are not allowed to attend while the zero positions will be unchanged. If a BoolTensor is provided, positions with `True` is not allowed to attend while `False` values will be unchanged. If a FloatTensor is provided, it will be added to the attention weight.
Shapes for outputs:
* attn\_output: (L,N,E)(L, N, E) where L is the target sequence length, N is the batch size, E is the embedding dimension.
* attn\_output\_weights: (N,L,S)(N, L, S) where N is the batch size, L is the target sequence length, S is the source sequence length.
| programming_docs |
pytorch torch.chain_matmul torch.chain\_matmul
===================
`torch.chain_matmul(*matrices)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#chain_matmul)
Returns the matrix product of the NN 2-D tensors. This product is efficiently computed using the matrix chain order algorithm which selects the order in which incurs the lowest cost in terms of arithmetic operations ([[CLRS]](https://mitpress.mit.edu/books/introduction-algorithms-third-edition)). Note that since this is a function to compute the product, NN needs to be greater than or equal to 2; if equal to 2 then a trivial matrix-matrix product is returned. If NN is 1, then this is a no-op - the original matrix is returned as is.
Parameters
**matrices** (*Tensors...*) – a sequence of 2 or more 2-D tensors whose product is to be determined.
Returns
if the ithi^{th} tensor was of dimensions pi×pi+1p\_{i} \times p\_{i + 1} , then the product would be of dimensions p1×pN+1p\_{1} \times p\_{N + 1} .
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> a = torch.randn(3, 4)
>>> b = torch.randn(4, 5)
>>> c = torch.randn(5, 6)
>>> d = torch.randn(6, 7)
>>> torch.chain_matmul(a, b, c, d)
tensor([[ -2.3375, -3.9790, -4.1119, -6.6577, 9.5609, -11.5095, -3.2614],
[ 21.4038, 3.3378, -8.4982, -5.2457, -10.2561, -2.4684, 2.7163],
[ -0.9647, -5.8917, -2.3213, -5.2284, 12.8615, -12.2816, -2.5095]])
```
pytorch torch.reshape torch.reshape
=============
`torch.reshape(input, shape) → Tensor`
Returns a tensor with the same data and number of elements as `input`, but with the specified shape. When possible, the returned tensor will be a view of `input`. Otherwise, it will be a copy. Contiguous inputs and inputs with compatible strides can be reshaped without copying, but you should not depend on the copying vs. viewing behavior.
See [`torch.Tensor.view()`](../tensors#torch.Tensor.view "torch.Tensor.view") on when it is possible to return a view.
A single dimension may be -1, in which case it’s inferred from the remaining dimensions and the number of elements in `input`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be reshaped
* **shape** (*tuple of python:ints*) – the new shape
Example:
```
>>> a = torch.arange(4.)
>>> torch.reshape(a, (2, 2))
tensor([[ 0., 1.],
[ 2., 3.]])
>>> b = torch.tensor([[0, 1], [2, 3]])
>>> torch.reshape(b, (-1,))
tensor([ 0, 1, 2, 3])
```
pytorch torch.jit.wait torch.jit.wait
==============
`torch.jit.wait(future)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/jit/_async.html#wait)
Forces completion of a `torch.jit.Future[T]` asynchronous task, returning the result of the task. See [`fork()`](torch.jit.fork#torch.jit.fork "torch.jit.fork") for docs and examples. :param func: an asynchronous task reference, created through `torch.jit.fork` :type func: torch.jit.Future[T]
Returns
the return value of the the completed task
Return type
`T`
pytorch torch.greater_equal torch.greater\_equal
====================
`torch.greater_equal(input, other, *, out=None) → Tensor`
Alias for [`torch.ge()`](torch.ge#torch.ge "torch.ge").
pytorch torch.cummax torch.cummax
============
`torch.cummax(input, dim, *, out=None) -> (Tensor, LongTensor)`
Returns a namedtuple `(values, indices)` where `values` is the cumulative maximum of elements of `input` in the dimension `dim`. And `indices` is the index location of each maximum value found in the dimension `dim`.
yi=max(x1,x2,x3,…,xi)y\_i = max(x\_1, x\_2, x\_3, \dots, x\_i)
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to do the operation over
Keyword Arguments
**out** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the result tuple of two output tensors (values, indices)
Example:
```
>>> a = torch.randn(10)
>>> a
tensor([-0.3449, -1.5447, 0.0685, -1.5104, -1.1706, 0.2259, 1.4696, -1.3284,
1.9946, -0.8209])
>>> torch.cummax(a, dim=0)
torch.return_types.cummax(
values=tensor([-0.3449, -0.3449, 0.0685, 0.0685, 0.0685, 0.2259, 1.4696, 1.4696,
1.9946, 1.9946]),
indices=tensor([0, 0, 2, 2, 2, 5, 6, 6, 8, 8]))
```
pytorch torch.nan_to_num torch.nan\_to\_num
==================
`torch.nan_to_num(input, nan=0.0, posinf=None, neginf=None, *, out=None) → Tensor`
Replaces `NaN`, positive infinity, and negative infinity values in `input` with the values specified by `nan`, `posinf`, and `neginf`, respectively. By default, `NaN`s are replaced with zero, positive infinity is replaced with the
greatest finite value representable by :attr:`input`’s dtype, and negative infinity is replaced with the least finite value representable by `input`’s dtype.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **nan** (*Number**,* *optional*) – the value to replace `NaN`s with. Default is zero.
* **posinf** (*Number**,* *optional*) – if a Number, the value to replace positive infinity values with. If None, positive infinity values are replaced with the greatest finite value representable by `input`’s dtype. Default is None.
* **neginf** (*Number**,* *optional*) – if a Number, the value to replace negative infinity values with. If None, negative infinity values are replaced with the lowest finite value representable by `input`’s dtype. Default is None.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> x = torch.tensor([float('nan'), float('inf'), -float('inf'), 3.14])
>>> torch.nan_to_num(x)
tensor([ 0.0000e+00, 3.4028e+38, -3.4028e+38, 3.1400e+00])
>>> torch.nan_to_num(x, nan=2.0)
tensor([ 2.0000e+00, 3.4028e+38, -3.4028e+38, 3.1400e+00])
>>> torch.nan_to_num(x, nan=2.0, posinf=1.0)
tensor([ 2.0000e+00, 1.0000e+00, -3.4028e+38, 3.1400e+00])
```
pytorch torch.nn.utils.rnn.pad_sequence torch.nn.utils.rnn.pad\_sequence
================================
`torch.nn.utils.rnn.pad_sequence(sequences, batch_first=False, padding_value=0.0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/rnn.html#pad_sequence)
Pad a list of variable length Tensors with `padding_value`
`pad_sequence` stacks a list of Tensors along a new dimension, and pads them to equal length. For example, if the input is list of sequences with size `L x *` and if batch\_first is False, and `T x B x *` otherwise.
`B` is batch size. It is equal to the number of elements in `sequences`. `T` is length of the longest sequence. `L` is length of the sequence. `*` is any number of trailing dimensions, including none.
#### Example
```
>>> from torch.nn.utils.rnn import pad_sequence
>>> a = torch.ones(25, 300)
>>> b = torch.ones(22, 300)
>>> c = torch.ones(15, 300)
>>> pad_sequence([a, b, c]).size()
torch.Size([25, 3, 300])
```
Note
This function returns a Tensor of size `T x B x *` or `B x T x *` where `T` is the length of the longest sequence. This function assumes trailing dimensions and type of all the Tensors in sequences are same.
Parameters
* **sequences** ([list](https://docs.python.org/3/library/stdtypes.html#list "(in Python v3.9)")*[*[Tensor](../tensors#torch.Tensor "torch.Tensor")*]*) – list of variable length sequences.
* **batch\_first** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – output will be in `B x T x *` if True, or in `T x B x *` otherwise
* **padding\_value** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – value for padded elements. Default: 0.
Returns
Tensor of size `T x B x *` if `batch_first` is `False`. Tensor of size `B x T x *` otherwise
pytorch torch.addcdiv torch.addcdiv
=============
`torch.addcdiv(input, tensor1, tensor2, *, value=1, out=None) → Tensor`
Performs the element-wise division of `tensor1` by `tensor2`, multiply the result by the scalar `value` and add it to `input`.
Warning
Integer division with addcdiv is no longer supported, and in a future release addcdiv will perform a true division of tensor1 and tensor2. The historic addcdiv behavior can be implemented as (input + value \* torch.trunc(tensor1 / tensor2)).to(input.dtype) for integer inputs and as (input + value \* tensor1 / tensor2) for float inputs. The future addcdiv behavior is just the latter implementation: (input + value \* tensor1 / tensor2), for all dtypes.
outi=inputi+value×tensor1itensor2i\text{out}\_i = \text{input}\_i + \text{value} \times \frac{\text{tensor1}\_i}{\text{tensor2}\_i}
The shapes of `input`, `tensor1`, and `tensor2` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
For inputs of type `FloatTensor` or `DoubleTensor`, `value` must be a real number, otherwise an integer.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be added
* **tensor1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the numerator tensor
* **tensor2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the denominator tensor
Keyword Arguments
* **value** (*Number**,* *optional*) – multiplier for tensor1/tensor2\text{tensor1} / \text{tensor2}
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> t = torch.randn(1, 3)
>>> t1 = torch.randn(3, 1)
>>> t2 = torch.randn(1, 3)
>>> torch.addcdiv(t, t1, t2, value=0.1)
tensor([[-0.2312, -3.6496, 0.1312],
[-1.0428, 3.4292, -0.1030],
[-0.5369, -0.9829, 0.0430]])
```
pytorch torch.gcd torch.gcd
=========
`torch.gcd(input, other, *, out=None) → Tensor`
Computes the element-wise greatest common divisor (GCD) of `input` and `other`.
Both `input` and `other` must have integer types.
Note
This defines gcd(0,0)=0gcd(0, 0) = 0 .
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.tensor([5, 10, 15])
>>> b = torch.tensor([3, 4, 5])
>>> torch.gcd(a, b)
tensor([1, 2, 5])
>>> c = torch.tensor([3])
>>> torch.gcd(a, c)
tensor([1, 1, 3])
```
pytorch torch.bitwise_xor torch.bitwise\_xor
==================
`torch.bitwise_xor(input, other, *, out=None) → Tensor`
Computes the bitwise XOR of `input` and `other`. The input tensor must be of integral or Boolean types. For bool tensors, it computes the logical XOR.
Parameters
* **input** – the first input tensor
* **other** – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
#### Example
```
>>> torch.bitwise_xor(torch.tensor([-1, -2, 3], dtype=torch.int8), torch.tensor([1, 0, 3], dtype=torch.int8))
tensor([-2, -2, 0], dtype=torch.int8)
>>> torch.bitwise_xor(torch.tensor([True, True, False]), torch.tensor([False, True, False]))
tensor([ True, False, False])
```
pytorch torch.real torch.real
==========
`torch.real(input) → Tensor`
Returns a new tensor containing real values of the `self` tensor. The returned tensor and `self` share the same underlying storage.
Warning
[`real()`](#torch.real "torch.real") is only supported for tensors with complex dtypes.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example::
```
>>> x=torch.randn(4, dtype=torch.cfloat)
>>> x
tensor([(0.3100+0.3553j), (-0.5445-0.7896j), (-1.6492-0.0633j), (-0.0638-0.8119j)])
>>> x.real
tensor([ 0.3100, -0.5445, -1.6492, -0.0638])
```
pytorch torch.get_default_dtype torch.get\_default\_dtype
=========================
`torch.get_default_dtype() → torch.dtype`
Get the current default floating point [`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype").
Example:
```
>>> torch.get_default_dtype() # initial default for floating point is torch.float32
torch.float32
>>> torch.set_default_dtype(torch.float64)
>>> torch.get_default_dtype() # default is now changed to torch.float64
torch.float64
>>> torch.set_default_tensor_type(torch.FloatTensor) # setting tensor type also affects this
>>> torch.get_default_dtype() # changed to torch.float32, the dtype for torch.FloatTensor
torch.float32
```
pytorch torch.isinf torch.isinf
===========
`torch.isinf(input) → Tensor`
Tests if each element of `input` is infinite (positive or negative infinity) or not.
Note
Complex values are infinite when their real or imaginary part is infinite.
Args:
{input}
Returns:
A boolean tensor that is True where `input` is infinite and False elsewhere
Example:
```
>>> torch.isinf(torch.tensor([1, float('inf'), 2, float('-inf'), float('nan')]))
tensor([False, True, False, True, False])
```
pytorch torch.lerp torch.lerp
==========
`torch.lerp(input, end, weight, *, out=None)`
Does a linear interpolation of two tensors `start` (given by `input`) and `end` based on a scalar or tensor `weight` and returns the resulting `out` tensor.
outi=starti+weighti×(endi−starti)\text{out}\_i = \text{start}\_i + \text{weight}\_i \times (\text{end}\_i - \text{start}\_i)
The shapes of `start` and `end` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics). If `weight` is a tensor, then the shapes of `weight`, `start`, and `end` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor with the starting points
* **end** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor with the ending points
* **weight** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* *tensor*) – the weight for the interpolation formula
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> start = torch.arange(1., 5.)
>>> end = torch.empty(4).fill_(10)
>>> start
tensor([ 1., 2., 3., 4.])
>>> end
tensor([ 10., 10., 10., 10.])
>>> torch.lerp(start, end, 0.5)
tensor([ 5.5000, 6.0000, 6.5000, 7.0000])
>>> torch.lerp(start, end, torch.full_like(start, 0.5))
tensor([ 5.5000, 6.0000, 6.5000, 7.0000])
```
pytorch torch.arctan torch.arctan
============
`torch.arctan(input, *, out=None) → Tensor`
Alias for [`torch.atan()`](torch.atan#torch.atan "torch.atan").
pytorch torch.trapz torch.trapz
===========
`torch.trapz(y, x, *, dim=-1) → Tensor`
Estimate ∫ydx\int y\,dx along `dim`, using the trapezoid rule.
Parameters
* **y** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The values of the function to integrate
* **x** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The points at which the function `y` is sampled. If `x` is not in ascending order, intervals on which it is decreasing contribute negatively to the estimated integral (i.e., the convention ∫abf=−∫baf\int\_a^b f = -\int\_b^a f is followed).
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – The dimension along which to integrate. By default, use the last dimension.
Returns
A Tensor with the same shape as the input, except with `dim` removed. Each element of the returned tensor represents the estimated integral ∫ydx\int y\,dx along `dim`.
Example:
```
>>> y = torch.randn((2, 3))
>>> y
tensor([[-2.1156, 0.6857, -0.2700],
[-1.2145, 0.5540, 2.0431]])
>>> x = torch.tensor([[1, 3, 4], [1, 2, 3]])
>>> torch.trapz(y, x)
tensor([-1.2220, 0.9683])
```
`torch.trapz(y, *, dx=1, dim=-1) → Tensor`
As above, but the sample points are spaced uniformly at a distance of `dx`.
Parameters
**y** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The values of the function to integrate
Keyword Arguments
* **dx** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – The distance between points at which `y` is sampled.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – The dimension along which to integrate. By default, use the last dimension.
Returns
A Tensor with the same shape as the input, except with `dim` removed. Each element of the returned tensor represents the estimated integral ∫ydx\int y\,dx along `dim`.
pytorch torch.arange torch.arange
============
`torch.arange(start=0, end, step=1, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Returns a 1-D tensor of size ⌈end−startstep⌉\left\lceil \frac{\text{end} - \text{start}}{\text{step}} \right\rceil with values from the interval `[start, end)` taken with common difference `step` beginning from `start`.
Note that non-integer `step` is subject to floating point rounding errors when comparing against `end`; to avoid inconsistency, we advise adding a small epsilon to `end` in such cases.
outi+1=outi+step\text{out}\_{{i+1}} = \text{out}\_{i} + \text{step}
Parameters
* **start** (*Number*) – the starting value for the set of points. Default: `0`.
* **end** (*Number*) – the ending value for the set of points
* **step** (*Number*) – the gap between each pair of adjacent points. Default: `1`.
Keyword Arguments
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). If `dtype` is not given, infer the data type from the other input arguments. If any of `start`, `end`, or `stop` are floating-point, the `dtype` is inferred to be the default dtype, see [`get_default_dtype()`](torch.get_default_dtype#torch.get_default_dtype "torch.get_default_dtype"). Otherwise, the `dtype` is inferred to be `torch.int64`.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned Tensor. Default: `torch.strided`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Example:
```
>>> torch.arange(5)
tensor([ 0, 1, 2, 3, 4])
>>> torch.arange(1, 4)
tensor([ 1, 2, 3])
>>> torch.arange(1, 2.5, 0.5)
tensor([ 1.0000, 1.5000, 2.0000])
```
pytorch torch.arcsin torch.arcsin
============
`torch.arcsin(input, *, out=None) → Tensor`
Alias for [`torch.asin()`](torch.asin#torch.asin "torch.asin").
pytorch AdaptiveMaxPool3d AdaptiveMaxPool3d
=================
`class torch.nn.AdaptiveMaxPool3d(output_size, return_indices=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AdaptiveMaxPool3d)
Applies a 3D adaptive max pooling over an input signal composed of several input planes.
The output is of size D x H x W, for any input size. The number of output features is equal to the number of input planes.
Parameters
* **output\_size** – the target output size of the image of the form D x H x W. Can be a tuple (D, H, W) or a single D for a cube D x D x D. D, H and W can be either a `int`, or `None` which means the size will be the same as that of the input.
* **return\_indices** – if `True`, will return the indices along with the outputs. Useful to pass to nn.MaxUnpool3d. Default: `False`
#### Examples
```
>>> # target output size of 5x7x9
>>> m = nn.AdaptiveMaxPool3d((5,7,9))
>>> input = torch.randn(1, 64, 8, 9, 10)
>>> output = m(input)
>>> # target output size of 7x7x7 (cube)
>>> m = nn.AdaptiveMaxPool3d(7)
>>> input = torch.randn(1, 64, 10, 9, 8)
>>> output = m(input)
>>> # target output size of 7x9x8
>>> m = nn.AdaptiveMaxPool3d((7, None, None))
>>> input = torch.randn(1, 64, 10, 9, 8)
>>> output = m(input)
```
| programming_docs |
pytorch PReLU PReLU
=====
`class torch.nn.PReLU(num_parameters=1, init=0.25)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#PReLU)
Applies the element-wise function:
PReLU(x)=max(0,x)+a∗min(0,x)\text{PReLU}(x) = \max(0,x) + a \* \min(0,x)
or
PReLU(x)={x, if x≥0ax, otherwise \text{PReLU}(x) = \begin{cases} x, & \text{ if } x \geq 0 \\ ax, & \text{ otherwise } \end{cases}
Here aa is a learnable parameter. When called without arguments, `nn.PReLU()` uses a single parameter aa across all input channels. If called with `nn.PReLU(nChannels)`, a separate aa is used for each input channel.
Note
weight decay should not be used when learning aa for good performance.
Note
Channel dim is the 2nd dim of input. When input has dims < 2, then there is no channel dim and the number of channels = 1.
Parameters
* **num\_parameters** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – number of aa to learn. Although it takes an int as input, there is only two values are legitimate: 1, or the number of channels at input. Default: 1
* **init** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the initial value of aa . Default: 0.25
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Variables
**~PReLU.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of shape (`num_parameters`).
Examples:
```
>>> m = nn.PReLU()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.swapaxes torch.swapaxes
==============
`torch.swapaxes(input, axis0, axis1) → Tensor`
Alias for [`torch.transpose()`](torch.transpose#torch.transpose "torch.transpose").
This function is equivalent to NumPy’s swapaxes function.
Examples:
```
>>> x = torch.tensor([[[0,1],[2,3]],[[4,5],[6,7]]])
>>> x
tensor([[[0, 1],
[2, 3]],
[[4, 5],
[6, 7]]])
>>> torch.swapaxes(x, 0, 1)
tensor([[[0, 1],
[4, 5]],
[[2, 3],
[6, 7]]])
>>> torch.swapaxes(x, 0, 2)
tensor([[[0, 4],
[2, 6]],
[[1, 5],
[3, 7]]])
```
pytorch torch.ceil torch.ceil
==========
`torch.ceil(input, *, out=None) → Tensor`
Returns a new tensor with the ceil of the elements of `input`, the smallest integer greater than or equal to each element.
outi=⌈inputi⌉=⌊inputi⌋+1\text{out}\_{i} = \left\lceil \text{input}\_{i} \right\rceil = \left\lfloor \text{input}\_{i} \right\rfloor + 1
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([-0.6341, -1.4208, -1.0900, 0.5826])
>>> torch.ceil(a)
tensor([-0., -1., -1., 1.])
```
pytorch ConvTranspose3d ConvTranspose3d
===============
`class torch.nn.ConvTranspose3d(in_channels, out_channels, kernel_size, stride=1, padding=0, output_padding=0, groups=1, bias=True, dilation=1, padding_mode='zeros')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/conv.html#ConvTranspose3d)
Applies a 3D transposed convolution operator over an input image composed of several input planes. The transposed convolution operator multiplies each input value element-wise by a learnable kernel, and sums over the outputs from all input feature planes.
This module can be seen as the gradient of Conv3d with respect to its input. It is also known as a fractionally-strided convolution or a deconvolution (although it is not an actual deconvolution operation).
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
* `stride` controls the stride for the cross-correlation.
* `padding` controls the amount of implicit zero padding on both sides for `dilation * (kernel_size - 1) - padding` number of points. See note below for details.
* `output_padding` controls the additional size added to one side of the output shape. See note below for details.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
* `groups` controls the connections between inputs and outputs. `in_channels` and `out_channels` must both be divisible by `groups`. For example,
+ At groups=1, all inputs are convolved to all outputs.
+ At groups=2, the operation becomes equivalent to having two conv layers side by side, each seeing half the input channels and producing half the output channels, and both subsequently concatenated.
+ At groups= `in_channels`, each input channel is convolved with its own set of filters (of size out\_channelsin\_channels\frac{\text{out\\_channels}}{\text{in\\_channels}} ).
The parameters `kernel_size`, `stride`, `padding`, `output_padding` can either be:
* a single `int` – in which case the same value is used for the depth, height and width dimensions
* a `tuple` of three ints – in which case, the first `int` is used for the depth dimension, the second `int` for the height dimension and the third `int` for the width dimension
Note
The `padding` argument effectively adds `dilation * (kernel_size - 1) - padding` amount of zero padding to both sizes of the input. This is set so that when a [`Conv3d`](torch.nn.conv3d#torch.nn.Conv3d "torch.nn.Conv3d") and a [`ConvTranspose3d`](#torch.nn.ConvTranspose3d "torch.nn.ConvTranspose3d") are initialized with same parameters, they are inverses of each other in regard to the input and output shapes. However, when `stride > 1`, [`Conv3d`](torch.nn.conv3d#torch.nn.Conv3d "torch.nn.Conv3d") maps multiple input shapes to the same output shape. `output_padding` is provided to resolve this ambiguity by effectively increasing the calculated output shape on one side. Note that `output_padding` is only used to find output shape, but does not actually add zero-padding to output.
Note
In some circumstances when given tensors on a CUDA device and using CuDNN, this operator may select a nondeterministic algorithm to increase performance. If this is undesirable, you can try to make the operation deterministic (potentially at a performance cost) by setting `torch.backends.cudnn.deterministic = True`. See [Reproducibility](https://pytorch.org/docs/1.8.0/notes/randomness.html) for more information.
Parameters
* **in\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels in the input image
* **out\_channels** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – Number of channels produced by the convolution
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – Size of the convolving kernel
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Stride of the convolution. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – `dilation * (kernel_size - 1) - padding` zero-padding will be added to both sides of each dimension in the input. Default: 0
* **output\_padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Additional size added to one side of each dimension in the output shape. Default: 0
* **groups** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – Number of blocked connections from input channels to output channels. Default: 1
* **bias** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If `True`, adds a learnable bias to the output. Default: `True`
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – Spacing between kernel elements. Default: 1
Shape:
* Input: (N,Cin,Din,Hin,Win)(N, C\_{in}, D\_{in}, H\_{in}, W\_{in})
* Output: (N,Cout,Dout,Hout,Wout)(N, C\_{out}, D\_{out}, H\_{out}, W\_{out}) where
Dout=(Din−1)×stride[0]−2×padding[0]+dilation[0]×(kernel\_size[0]−1)+output\_padding[0]+1D\_{out} = (D\_{in} - 1) \times \text{stride}[0] - 2 \times \text{padding}[0] + \text{dilation}[0] \times (\text{kernel\\_size}[0] - 1) + \text{output\\_padding}[0] + 1
Hout=(Hin−1)×stride[1]−2×padding[1]+dilation[1]×(kernel\_size[1]−1)+output\_padding[1]+1H\_{out} = (H\_{in} - 1) \times \text{stride}[1] - 2 \times \text{padding}[1] + \text{dilation}[1] \times (\text{kernel\\_size}[1] - 1) + \text{output\\_padding}[1] + 1
Wout=(Win−1)×stride[2]−2×padding[2]+dilation[2]×(kernel\_size[2]−1)+output\_padding[2]+1W\_{out} = (W\_{in} - 1) \times \text{stride}[2] - 2 \times \text{padding}[2] + \text{dilation}[2] \times (\text{kernel\\_size}[2] - 1) + \text{output\\_padding}[2] + 1
Variables
* **~ConvTranspose3d.weight** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable weights of the module of shape (in\_channels,out\_channelsgroups,(\text{in\\_channels}, \frac{\text{out\\_channels}}{\text{groups}}, kernel\_size[0],kernel\_size[1],kernel\_size[2])\text{kernel\\_size[0]}, \text{kernel\\_size[1]}, \text{kernel\\_size[2]}) . The values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗∏i=02kernel\_size[i]k = \frac{groups}{C\_\text{out} \* \prod\_{i=0}^{2}\text{kernel\\_size}[i]}
* **~ConvTranspose3d.bias** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the learnable bias of the module of shape (out\_channels) If `bias` is `True`, then the values of these weights are sampled from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=groupsCout∗∏i=02kernel\_size[i]k = \frac{groups}{C\_\text{out} \* \prod\_{i=0}^{2}\text{kernel\\_size}[i]}
Examples:
```
>>> # With square kernels and equal stride
>>> m = nn.ConvTranspose3d(16, 33, 3, stride=2)
>>> # non-square kernels and unequal stride and with padding
>>> m = nn.ConvTranspose3d(16, 33, (3, 5, 2), stride=(2, 1, 1), padding=(0, 4, 2))
>>> input = torch.randn(20, 16, 10, 50, 100)
>>> output = m(input)
```
pytorch torch.cholesky torch.cholesky
==============
`torch.cholesky(input, upper=False, *, out=None) → Tensor`
Computes the Cholesky decomposition of a symmetric positive-definite matrix AA or for batches of symmetric positive-definite matrices.
If `upper` is `True`, the returned matrix `U` is upper-triangular, and the decomposition has the form:
A=UTUA = U^TU
If `upper` is `False`, the returned matrix `L` is lower-triangular, and the decomposition has the form:
A=LLTA = LL^T
If `upper` is `True`, and AA is a batch of symmetric positive-definite matrices, then the returned tensor will be composed of upper-triangular Cholesky factors of each of the individual matrices. Similarly, when `upper` is `False`, the returned tensor will be composed of lower-triangular Cholesky factors of each of the individual matrices.
Note
[`torch.linalg.cholesky()`](../linalg#torch.linalg.cholesky "torch.linalg.cholesky") should be used over `torch.cholesky` when possible. Note however that [`torch.linalg.cholesky()`](../linalg#torch.linalg.cholesky "torch.linalg.cholesky") does not yet support the `upper` parameter and instead always returns the lower triangular matrix.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor AA of size (∗,n,n)(\*, n, n) where `*` is zero or more batch dimensions consisting of symmetric positive-definite matrices.
* **upper** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – flag that indicates whether to return a upper or lower triangular matrix. Default: `False`
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output matrix
Example:
```
>>> a = torch.randn(3, 3)
>>> a = torch.mm(a, a.t()) # make symmetric positive-definite
>>> l = torch.cholesky(a)
>>> a
tensor([[ 2.4112, -0.7486, 1.4551],
[-0.7486, 1.3544, 0.1294],
[ 1.4551, 0.1294, 1.6724]])
>>> l
tensor([[ 1.5528, 0.0000, 0.0000],
[-0.4821, 1.0592, 0.0000],
[ 0.9371, 0.5487, 0.7023]])
>>> torch.mm(l, l.t())
tensor([[ 2.4112, -0.7486, 1.4551],
[-0.7486, 1.3544, 0.1294],
[ 1.4551, 0.1294, 1.6724]])
>>> a = torch.randn(3, 2, 2)
>>> a = torch.matmul(a, a.transpose(-1, -2)) + 1e-03 # make symmetric positive-definite
>>> l = torch.cholesky(a)
>>> z = torch.matmul(l, l.transpose(-1, -2))
>>> torch.max(torch.abs(z - a)) # Max non-zero
tensor(2.3842e-07)
```
pytorch torch.polar torch.polar
===========
`torch.polar(abs, angle, *, out=None) → Tensor`
Constructs a complex tensor whose elements are Cartesian coordinates corresponding to the polar coordinates with absolute value [`abs`](torch.abs#torch.abs "torch.abs") and angle [`angle`](torch.angle#torch.angle "torch.angle").
out=abs⋅cos(angle)+abs⋅sin(angle)⋅j\text{out} = \text{abs} \cdot \cos(\text{angle}) + \text{abs} \cdot \sin(\text{angle}) \cdot j
Parameters
* **abs** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The absolute value the complex tensor. Must be float or double.
* **angle** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The angle of the complex tensor. Must be same dtype as [`abs`](torch.abs#torch.abs "torch.abs").
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – If the inputs are `torch.float32`, must be `torch.complex64`. If the inputs are `torch.float64`, must be `torch.complex128`.
Example::
```
>>> import numpy as np
>>> abs = torch.tensor([1, 2], dtype=torch.float64)
>>> angle = torch.tensor([np.pi / 2, 5 * np.pi / 4], dtype=torch.float64)
>>> z = torch.polar(abs, angle)
>>> z
tensor([(0.0000+1.0000j), (-1.4142-1.4142j)], dtype=torch.complex128)
```
pytorch torch.nn.utils.prune.random_unstructured torch.nn.utils.prune.random\_unstructured
=========================================
`torch.nn.utils.prune.random_unstructured(module, name, amount)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#random_unstructured)
Prunes tensor corresponding to parameter called `name` in `module` by removing the specified `amount` of (currently unpruned) units selected at random. Modifies module in place (and also return the modified module) by: 1) adding a named buffer called `name+'_mask'` corresponding to the binary mask applied to the parameter `name` by the pruning method. 2) replacing the parameter `name` by its pruned version, while the original (unpruned) parameter is stored in a new parameter named `name+'_orig'`.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
Returns
modified (i.e. pruned) version of the input module
Return type
module ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module"))
#### Examples
```
>>> m = prune.random_unstructured(nn.Linear(2, 3), 'weight', amount=1)
>>> torch.sum(m.weight_mask == 0)
tensor(1)
```
pytorch BasePruningMethod BasePruningMethod
=================
`class torch.nn.utils.prune.BasePruningMethod` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod)
Abstract base class for creation of new pruning techniques.
Provides a skeleton for customization requiring the overriding of methods such as [`compute_mask()`](#torch.nn.utils.prune.BasePruningMethod.compute_mask "torch.nn.utils.prune.BasePruningMethod.compute_mask") and [`apply()`](#torch.nn.utils.prune.BasePruningMethod.apply "torch.nn.utils.prune.BasePruningMethod.apply").
`classmethod apply(module, name, *args, importance_scores=None, **kwargs)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod.apply)
Adds the forward pre-hook that enables pruning on the fly and the reparametrization of a tensor in terms of the original tensor and the pruning mask.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **args** – arguments passed on to a subclass of [`BasePruningMethod`](#torch.nn.utils.prune.BasePruningMethod "torch.nn.utils.prune.BasePruningMethod")
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as module parameter) used to compute mask for pruning. The values in this tensor indicate the importance of the corresponding elements in the parameter being pruned. If unspecified or None, the parameter will be used in its place.
* **kwargs** – keyword arguments passed on to a subclass of a [`BasePruningMethod`](#torch.nn.utils.prune.BasePruningMethod "torch.nn.utils.prune.BasePruningMethod")
`apply_mask(module)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod.apply_mask)
Simply handles the multiplication between the parameter being pruned and the generated mask. Fetches the mask and the original tensor from the module and returns the pruned version of the tensor.
Parameters
**module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
Returns
pruned version of the input tensor
Return type
pruned\_tensor ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
`abstract compute_mask(t, default_mask)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod.compute_mask)
Computes and returns a mask for the input tensor `t`. Starting from a base `default_mask` (which should be a mask of ones if the tensor has not been pruned yet), generate a random mask to apply on top of the `default_mask` according to the specific pruning method recipe.
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor representing the importance scores of the
* **to prune.** (*parameter*) –
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – Base mask from previous pruning
* **that need to be respected after the new mask is** (*iterations**,*) –
* **Same dims as t.** (*applied.*) –
Returns
mask to apply to `t`, of same dims as `t`
Return type
mask ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor"))
`prune(t, default_mask=None, importance_scores=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod.prune)
Computes and returns a pruned version of input tensor `t` according to the pruning rule specified in [`compute_mask()`](#torch.nn.utils.prune.BasePruningMethod.compute_mask "torch.nn.utils.prune.BasePruningMethod.compute_mask").
Parameters
* **t** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor to prune (of same dimensions as `default_mask`).
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as `t`) used to compute mask for pruning `t`. The values in this tensor indicate the importance of the corresponding elements in the `t` that is being pruned. If unspecified or None, the tensor `t` will be used in its place.
* **default\_mask** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – mask from previous pruning iteration, if any. To be considered when determining what portion of the tensor that pruning should act on. If None, default to a mask of ones.
Returns
pruned version of tensor `t`.
`remove(module)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#BasePruningMethod.remove)
Removes the pruning reparameterization from a module. The pruned parameter named `name` remains permanently pruned, and the parameter named `name+'_orig'` is removed from the parameter list. Similarly, the buffer named `name+'_mask'` is removed from the buffers.
Note
Pruning itself is NOT undone or reversed!
| programming_docs |
pytorch torch.complex torch.complex
=============
`torch.complex(real, imag, *, out=None) → Tensor`
Constructs a complex tensor with its real part equal to [`real`](torch.real#torch.real "torch.real") and its imaginary part equal to [`imag`](torch.imag#torch.imag "torch.imag").
Parameters
* **real** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The real part of the complex tensor. Must be float or double.
* **imag** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – The imaginary part of the complex tensor. Must be same dtype as [`real`](torch.real#torch.real "torch.real").
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – If the inputs are `torch.float32`, must be `torch.complex64`. If the inputs are `torch.float64`, must be `torch.complex128`.
Example::
```
>>> real = torch.tensor([1, 2], dtype=torch.float32)
>>> imag = torch.tensor([3, 4], dtype=torch.float32)
>>> z = torch.complex(real, imag)
>>> z
tensor([(1.+3.j), (2.+4.j)])
>>> z.dtype
torch.complex64
```
pytorch Hardshrink Hardshrink
==========
`class torch.nn.Hardshrink(lambd=0.5)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Hardshrink)
Applies the hard shrinkage function element-wise:
HardShrink(x)={x, if x>λx, if x<−λ0, otherwise \text{HardShrink}(x) = \begin{cases} x, & \text{ if } x > \lambda \\ x, & \text{ if } x < -\lambda \\ 0, & \text{ otherwise } \end{cases}
Parameters
**lambd** – the λ\lambda value for the Hardshrink formulation. Default: 0.5
Shape:
* Input: (N,∗)(N, \*) where `*` means, any number of additional dimensions
* Output: (N,∗)(N, \*) , same shape as the input
Examples:
```
>>> m = nn.Hardshrink()
>>> input = torch.randn(2)
>>> output = m(input)
```
pytorch torch.combinations torch.combinations
==================
`torch.combinations(input, r=2, with_replacement=False) → seq`
Compute combinations of length rr of the given tensor. The behavior is similar to python’s `itertools.combinations` when `with_replacement` is set to `False`, and `itertools.combinations_with_replacement` when `with_replacement` is set to `True`.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – 1D vector.
* **r** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – number of elements to combine
* **with\_replacement** (*boolean**,* *optional*) – whether to allow duplication in combination
Returns
A tensor equivalent to converting all the input tensors into lists, do `itertools.combinations` or `itertools.combinations_with_replacement` on these lists, and finally convert the resulting list into tensor.
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> a = [1, 2, 3]
>>> list(itertools.combinations(a, r=2))
[(1, 2), (1, 3), (2, 3)]
>>> list(itertools.combinations(a, r=3))
[(1, 2, 3)]
>>> list(itertools.combinations_with_replacement(a, r=2))
[(1, 1), (1, 2), (1, 3), (2, 2), (2, 3), (3, 3)]
>>> tensor_a = torch.tensor(a)
>>> torch.combinations(tensor_a)
tensor([[1, 2],
[1, 3],
[2, 3]])
>>> torch.combinations(tensor_a, r=3)
tensor([[1, 2, 3]])
>>> torch.combinations(tensor_a, with_replacement=True)
tensor([[1, 1],
[1, 2],
[1, 3],
[2, 2],
[2, 3],
[3, 3]])
```
pytorch torch.fmod torch.fmod
==========
`torch.fmod(input, other, *, out=None) → Tensor`
Computes the element-wise remainder of division.
The dividend and divisor may contain both for integer and floating point numbers. The remainder has the same sign as the dividend `input`.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics), [type promotion](../tensor_attributes#type-promotion-doc), and integer and float inputs.
Note
When the divisor is zero, returns `NaN` for floating point dtypes on both CPU and GPU; raises `RuntimeError` for integer division by zero on CPU; Integer division by zero on GPU may return any value.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the dividend
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Scalar*) – the divisor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.fmod(torch.tensor([-3., -2, -1, 1, 2, 3]), 2)
tensor([-1., -0., -1., 1., 0., 1.])
>>> torch.fmod(torch.tensor([1, 2, 3, 4, 5]), 1.5)
tensor([1.0000, 0.5000, 0.0000, 1.0000, 0.5000])
```
pytorch Unfold Unfold
======
`class torch.nn.Unfold(kernel_size, dilation=1, padding=0, stride=1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/fold.html#Unfold)
Extracts sliding local blocks from a batched input tensor.
Consider a batched `input` tensor of shape (N,C,∗)(N, C, \*) , where NN is the batch dimension, CC is the channel dimension, and ∗\* represent arbitrary spatial dimensions. This operation flattens each sliding `kernel_size`-sized block within the spatial dimensions of `input` into a column (i.e., last dimension) of a 3-D `output` tensor of shape (N,C×∏(kernel\_size),L)(N, C \times \prod(\text{kernel\\_size}), L) , where C×∏(kernel\_size)C \times \prod(\text{kernel\\_size}) is the total number of values within each block (a block has ∏(kernel\_size)\prod(\text{kernel\\_size}) spatial locations each containing a CC -channeled vector), and LL is the total number of such blocks:
L=∏d⌊spatial\_size[d]+2×padding[d]−dilation[d]×(kernel\_size[d]−1)−1stride[d]+1⌋,L = \prod\_d \left\lfloor\frac{\text{spatial\\_size}[d] + 2 \times \text{padding}[d] % - \text{dilation}[d] \times (\text{kernel\\_size}[d] - 1) - 1}{\text{stride}[d]} + 1\right\rfloor,
where spatial\_size\text{spatial\\_size} is formed by the spatial dimensions of `input` (∗\* above), and dd is over all spatial dimensions.
Therefore, indexing `output` at the last dimension (column dimension) gives all values within a certain block.
The `padding`, `stride` and `dilation` arguments specify how the sliding blocks are retrieved.
* `stride` controls the stride for the sliding blocks.
* `padding` controls the amount of implicit zero-paddings on both sides for `padding` number of points for each dimension before reshaping.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
Parameters
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the sliding blocks
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the stride of the sliding blocks in the input spatial dimensions. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – implicit zero padding to be added on both sides of input. Default: 0
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – a parameter that controls the stride of elements within the neighborhood. Default: 1
* If `kernel_size`, `dilation`, `padding` or `stride` is an int or a tuple of length 1, their values will be replicated across all spatial dimensions.
* For the case of two input spatial dimensions this operation is sometimes called `im2col`.
Note
[`Fold`](torch.nn.fold#torch.nn.Fold "torch.nn.Fold") calculates each combined value in the resulting large tensor by summing all values from all containing blocks. [`Unfold`](#torch.nn.Unfold "torch.nn.Unfold") extracts the values in the local blocks by copying from the large tensor. So, if the blocks overlap, they are not inverses of each other.
In general, folding and unfolding operations are related as follows. Consider [`Fold`](torch.nn.fold#torch.nn.Fold "torch.nn.Fold") and [`Unfold`](#torch.nn.Unfold "torch.nn.Unfold") instances created with the same parameters:
```
>>> fold_params = dict(kernel_size=..., dilation=..., padding=..., stride=...)
>>> fold = nn.Fold(output_size=..., **fold_params)
>>> unfold = nn.Unfold(**fold_params)
```
Then for any (supported) `input` tensor the following equality holds:
```
fold(unfold(input)) == divisor * input
```
where `divisor` is a tensor that depends only on the shape and dtype of the `input`:
```
>>> input_ones = torch.ones(input.shape, dtype=input.dtype)
>>> divisor = fold(unfold(input_ones))
```
When the `divisor` tensor contains no zero elements, then `fold` and `unfold` operations are inverses of each other (up to constant divisor).
Warning
Currently, only 4-D input tensors (batched image-like tensors) are supported.
Shape:
* Input: (N,C,∗)(N, C, \*)
* Output: (N,C×∏(kernel\_size),L)(N, C \times \prod(\text{kernel\\_size}), L) as described above
Examples:
```
>>> unfold = nn.Unfold(kernel_size=(2, 3))
>>> input = torch.randn(2, 5, 3, 4)
>>> output = unfold(input)
>>> # each patch contains 30 values (2x3=6 vectors, each of 5 channels)
>>> # 4 blocks (2x3 kernels) in total in the 3x4 input
>>> output.size()
torch.Size([2, 30, 4])
>>> # Convolution is equivalent with Unfold + Matrix Multiplication + Fold (or view to output shape)
>>> inp = torch.randn(1, 3, 10, 12)
>>> w = torch.randn(2, 3, 4, 5)
>>> inp_unf = torch.nn.functional.unfold(inp, (4, 5))
>>> out_unf = inp_unf.transpose(1, 2).matmul(w.view(w.size(0), -1).t()).transpose(1, 2)
>>> out = torch.nn.functional.fold(out_unf, (7, 8), (1, 1))
>>> # or equivalently (and avoiding a copy),
>>> # out = out_unf.view(1, 2, 7, 8)
>>> (torch.nn.functional.conv2d(inp, w) - out).abs().max()
tensor(1.9073e-06)
```
pytorch LogSoftmax LogSoftmax
==========
`class torch.nn.LogSoftmax(dim=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#LogSoftmax)
Applies the log(Softmax(x))\log(\text{Softmax}(x)) function to an n-dimensional input Tensor. The LogSoftmax formulation can be simplified as:
LogSoftmax(xi)=log(exp(xi)∑jexp(xj))\text{LogSoftmax}(x\_{i}) = \log\left(\frac{\exp(x\_i) }{ \sum\_j \exp(x\_j)} \right)
Shape:
* Input: (∗)(\*) where `*` means, any number of additional dimensions
* Output: (∗)(\*) , same shape as the input
Parameters
**dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – A dimension along which LogSoftmax will be computed.
Returns
a Tensor of the same dimension and shape as the input with values in the range [-inf, 0)
Examples:
```
>>> m = nn.LogSoftmax()
>>> input = torch.randn(2, 3)
>>> output = m(input)
```
pytorch MaxPool1d MaxPool1d
=========
`class torch.nn.MaxPool1d(kernel_size, stride=None, padding=0, dilation=1, return_indices=False, ceil_mode=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#MaxPool1d)
Applies a 1D max pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,L)(N, C, L) and output (N,C,Lout)(N, C, L\_{out}) can be precisely described as:
out(Ni,Cj,k)=maxm=0,…,kernel\_size−1input(Ni,Cj,stride×k+m)out(N\_i, C\_j, k) = \max\_{m=0, \ldots, \text{kernel\\_size} - 1} input(N\_i, C\_j, stride \times k + m)
If `padding` is non-zero, then the input is implicitly padded with negative infinity on both sides for `padding` number of points. `dilation` is the stride between the elements within the sliding window. This [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of the pooling parameters.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
Parameters
* **kernel\_size** – The size of the sliding window, must be > 0.
* **stride** – The stride of the sliding window, must be > 0. Default value is `kernel_size`.
* **padding** – Implicit negative infinity padding to be added on both sides, must be >= 0 and <= kernel\_size / 2.
* **dilation** – The stride between elements within a sliding window, must be > 0.
* **return\_indices** – If `True`, will return the argmax along with the max values. Useful for [`torch.nn.MaxUnpool1d`](torch.nn.maxunpool1d#torch.nn.MaxUnpool1d "torch.nn.MaxUnpool1d") later
* **ceil\_mode** – If `True`, will use `ceil` instead of `floor` to compute the output shape. This ensures that every element in the input tensor is covered by a sliding window.
Shape:
* Input: (N,C,Lin)(N, C, L\_{in})
* Output: (N,C,Lout)(N, C, L\_{out}) , where
Lout=⌊Lin+2×padding−dilation×(kernel\_size−1)−1stride+1⌋L\_{out} = \left\lfloor \frac{L\_{in} + 2 \times \text{padding} - \text{dilation} \times (\text{kernel\\_size} - 1) - 1}{\text{stride}} + 1\right\rfloor
Examples:
```
>>> # pool of size=3, stride=2
>>> m = nn.MaxPool1d(3, stride=2)
>>> input = torch.randn(20, 16, 50)
>>> output = m(input)
```
pytorch torch.xlogy torch.xlogy
===========
`torch.xlogy(input, other, *, out=None) → Tensor`
Computes `input * log(other)` with the following cases.
outi={NaNif otheri=NaN0if inputi=0.0inputi∗log(otheri)otherwise\text{out}\_{i} = \begin{cases} \text{NaN} & \text{if } \text{other}\_{i} = \text{NaN} \\ 0 & \text{if } \text{input}\_{i} = 0.0 \\ \text{input}\_{i} \* \log{(\text{other}\_{i})} & \text{otherwise} \end{cases}
Similar to SciPy’s `scipy.special.xlogy`.
Parameters
* **input** (*Number* *or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) –
* **other** (*Number* *or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) –
Note
At least one of `input` or `other` must be a tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> x = torch.zeros(5,)
>>> y = torch.tensor([-1, 0, 1, float('inf'), float('nan')])
>>> torch.xlogy(x, y)
tensor([0., 0., 0., 0., nan])
>>> x = torch.tensor([1, 2, 3])
>>> y = torch.tensor([3, 2, 1])
>>> torch.xlogy(x, y)
tensor([1.0986, 1.3863, 0.0000])
>>> torch.xlogy(x, 4)
tensor([1.3863, 2.7726, 4.1589])
>>> torch.xlogy(2, y)
tensor([2.1972, 1.3863, 0.0000])
```
pytorch ReplicationPad2d ReplicationPad2d
================
`class torch.nn.ReplicationPad2d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ReplicationPad2d)
Pads the input tensor using replication of the input boundary.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 4-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} , padding\_top\text{padding\\_top} , padding\_bottom\text{padding\\_bottom} )
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) where
Hout=Hin+padding\_top+padding\_bottomH\_{out} = H\_{in} + \text{padding\\_top} + \text{padding\\_bottom}
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ReplicationPad2d(2)
>>> input = torch.arange(9, dtype=torch.float).reshape(1, 1, 3, 3)
>>> input
tensor([[[[0., 1., 2.],
[3., 4., 5.],
[6., 7., 8.]]]])
>>> m(input)
tensor([[[[0., 0., 0., 1., 2., 2., 2.],
[0., 0., 0., 1., 2., 2., 2.],
[0., 0., 0., 1., 2., 2., 2.],
[3., 3., 3., 4., 5., 5., 5.],
[6., 6., 6., 7., 8., 8., 8.],
[6., 6., 6., 7., 8., 8., 8.],
[6., 6., 6., 7., 8., 8., 8.]]]])
>>> # using different paddings for different sides
>>> m = nn.ReplicationPad2d((1, 1, 2, 0))
>>> m(input)
tensor([[[[0., 0., 1., 2., 2.],
[0., 0., 1., 2., 2.],
[0., 0., 1., 2., 2.],
[3., 3., 4., 5., 5.],
[6., 6., 7., 8., 8.]]]])
```
pytorch TransformerEncoder TransformerEncoder
==================
`class torch.nn.TransformerEncoder(encoder_layer, num_layers, norm=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerEncoder)
TransformerEncoder is a stack of N encoder layers
Parameters
* **encoder\_layer** – an instance of the TransformerEncoderLayer() class (required).
* **num\_layers** – the number of sub-encoder-layers in the encoder (required).
* **norm** – the layer normalization component (optional).
Examples::
```
>>> encoder_layer = nn.TransformerEncoderLayer(d_model=512, nhead=8)
>>> transformer_encoder = nn.TransformerEncoder(encoder_layer, num_layers=6)
>>> src = torch.rand(10, 32, 512)
>>> out = transformer_encoder(src)
```
`forward(src, mask=None, src_key_padding_mask=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/transformer.html#TransformerEncoder.forward)
Pass the input through the encoder layers in turn.
Parameters
* **src** – the sequence to the encoder (required).
* **mask** – the mask for the src sequence (optional).
* **src\_key\_padding\_mask** – the mask for the src keys per batch (optional).
Shape:
see the docs in Transformer class.
pytorch torch.sigmoid torch.sigmoid
=============
`torch.sigmoid(input, *, out=None) → Tensor`
Returns a new tensor with the sigmoid of the elements of `input`.
outi=11+e−inputi\text{out}\_{i} = \frac{1}{1 + e^{-\text{input}\_{i}}}
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.9213, 1.0887, -0.8858, -1.7683])
>>> torch.sigmoid(a)
tensor([ 0.7153, 0.7481, 0.2920, 0.1458])
```
pytorch ModuleList ModuleList
==========
`class torch.nn.ModuleList(modules=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ModuleList)
Holds submodules in a list.
[`ModuleList`](#torch.nn.ModuleList "torch.nn.ModuleList") can be indexed like a regular Python list, but modules it contains are properly registered, and will be visible by all [`Module`](torch.nn.module#torch.nn.Module "torch.nn.Module") methods.
Parameters
**modules** (*iterable**,* *optional*) – an iterable of modules to add
Example:
```
class MyModule(nn.Module):
def __init__(self):
super(MyModule, self).__init__()
self.linears = nn.ModuleList([nn.Linear(10, 10) for i in range(10)])
def forward(self, x):
# ModuleList can act as an iterable, or be indexed using ints
for i, l in enumerate(self.linears):
x = self.linears[i // 2](x) + l(x)
return x
```
`append(module)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ModuleList.append)
Appends a given module to the end of the list.
Parameters
**module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module to append
`extend(modules)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ModuleList.extend)
Appends modules from a Python iterable to the end of the list.
Parameters
**modules** (*iterable*) – iterable of modules to append
`insert(index, module)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/container.html#ModuleList.insert)
Insert a given module before a given index in the list.
Parameters
* **index** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – index to insert.
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module to insert
| programming_docs |
pytorch torch.empty_like torch.empty\_like
=================
`torch.empty_like(input, *, dtype=None, layout=None, device=None, requires_grad=False, memory_format=torch.preserve_format) → Tensor`
Returns an uninitialized tensor with the same size as `input`. `torch.empty_like(input)` is equivalent to `torch.empty(input.size(), dtype=input.dtype, layout=input.layout, device=input.device)`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the size of `input` will determine size of the output tensor.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned Tensor. Default: if `None`, defaults to the dtype of `input`.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned tensor. Default: if `None`, defaults to the layout of `input`.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, defaults to the device of `input`.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
* **memory\_format** ([`torch.memory_format`](../tensor_attributes#torch.torch.memory_format "torch.torch.memory_format"), optional) – the desired memory format of returned Tensor. Default: `torch.preserve_format`.
Example:
```
>>> torch.empty((2,3), dtype=torch.int64)
tensor([[ 9.4064e+13, 2.8000e+01, 9.3493e+13],
[ 7.5751e+18, 7.1428e+18, 7.5955e+18]])
```
pytorch torch.arctanh torch.arctanh
=============
`torch.arctanh(input, *, out=None) → Tensor`
Alias for [`torch.atanh()`](torch.atanh#torch.atanh "torch.atanh").
pytorch torch.cummin torch.cummin
============
`torch.cummin(input, dim, *, out=None) -> (Tensor, LongTensor)`
Returns a namedtuple `(values, indices)` where `values` is the cumulative minimum of elements of `input` in the dimension `dim`. And `indices` is the index location of each maximum value found in the dimension `dim`.
yi=min(x1,x2,x3,…,xi)y\_i = min(x\_1, x\_2, x\_3, \dots, x\_i)
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to do the operation over
Keyword Arguments
**out** ([tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – the result tuple of two output tensors (values, indices)
Example:
```
>>> a = torch.randn(10)
>>> a
tensor([-0.2284, -0.6628, 0.0975, 0.2680, -1.3298, -0.4220, -0.3885, 1.1762,
0.9165, 1.6684])
>>> torch.cummin(a, dim=0)
torch.return_types.cummin(
values=tensor([-0.2284, -0.6628, -0.6628, -0.6628, -1.3298, -1.3298, -1.3298, -1.3298,
-1.3298, -1.3298]),
indices=tensor([0, 1, 1, 1, 4, 4, 4, 4, 4, 4]))
```
pytorch torch.where torch.where
===========
`torch.where(condition, x, y) → Tensor`
Return a tensor of elements selected from either `x` or `y`, depending on `condition`.
The operation is defined as:
outi={xiif conditioniyiotherwise\text{out}\_i = \begin{cases} \text{x}\_i & \text{if } \text{condition}\_i \\ \text{y}\_i & \text{otherwise} \\ \end{cases}
Note
The tensors `condition`, `x`, `y` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
Note
Currently valid scalar and tensor combination are 1. Scalar of floating dtype and torch.double 2. Scalar of integral dtype and torch.long 3. Scalar of complex dtype and torch.complex128
Parameters
* **condition** (*BoolTensor*) – When True (nonzero), yield x, otherwise yield y
* **x** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Scalar*) – value (if :attr:x is a scalar) or values selected at indices where `condition` is `True`
* **y** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Scalar*) – value (if :attr:x is a scalar) or values selected at indices where `condition` is `False`
Returns
A tensor of shape equal to the broadcasted shape of `condition`, `x`, `y`
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
Example:
```
>>> x = torch.randn(3, 2)
>>> y = torch.ones(3, 2)
>>> x
tensor([[-0.4620, 0.3139],
[ 0.3898, -0.7197],
[ 0.0478, -0.1657]])
>>> torch.where(x > 0, x, y)
tensor([[ 1.0000, 0.3139],
[ 0.3898, 1.0000],
[ 0.0478, 1.0000]])
>>> x = torch.randn(2, 2, dtype=torch.double)
>>> x
tensor([[ 1.0779, 0.0383],
[-0.8785, -1.1089]], dtype=torch.float64)
>>> torch.where(x > 0, x, 0.)
tensor([[1.0779, 0.0383],
[0.0000, 0.0000]], dtype=torch.float64)
```
`torch.where(condition) → tuple of LongTensor`
`torch.where(condition)` is identical to `torch.nonzero(condition, as_tuple=True)`.
Note
See also [`torch.nonzero()`](torch.nonzero#torch.nonzero "torch.nonzero").
pytorch torch.addbmm torch.addbmm
============
`torch.addbmm(input, batch1, batch2, *, beta=1, alpha=1, out=None) → Tensor`
Performs a batch matrix-matrix product of matrices stored in `batch1` and `batch2`, with a reduced add step (all matrix multiplications get accumulated along the first dimension). `input` is added to the final result.
`batch1` and `batch2` must be 3-D tensors each containing the same number of matrices.
If `batch1` is a (b×n×m)(b \times n \times m) tensor, `batch2` is a (b×m×p)(b \times m \times p) tensor, `input` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with a (n×p)(n \times p) tensor and `out` will be a (n×p)(n \times p) tensor.
out=β input+α(∑i=0b−1batch1i@batch2i)out = \beta\ \text{input} + \alpha\ (\sum\_{i=0}^{b-1} \text{batch1}\_i \mathbin{@} \text{batch2}\_i)
If `beta` is 0, then `input` will be ignored, and `nan` and `inf` in it will not be propagated.
For inputs of type `FloatTensor` or `DoubleTensor`, arguments `beta` and `alpha` must be real numbers, otherwise they should be integers.
This operator supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
Parameters
* **batch1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first batch of matrices to be multiplied
* **batch2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second batch of matrices to be multiplied
Keyword Arguments
* **beta** (*Number**,* *optional*) – multiplier for `input` (β\beta )
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – matrix to be added
* **alpha** (*Number**,* *optional*) – multiplier for `batch1 @ batch2` (α\alpha )
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> M = torch.randn(3, 5)
>>> batch1 = torch.randn(10, 3, 4)
>>> batch2 = torch.randn(10, 4, 5)
>>> torch.addbmm(M, batch1, batch2)
tensor([[ 6.6311, 0.0503, 6.9768, -12.0362, -2.1653],
[ -4.8185, -1.4255, -6.6760, 8.9453, 2.5743],
[ -3.8202, 4.3691, 1.0943, -1.1109, 5.4730]])
```
pytorch torch.cdist torch.cdist
===========
`torch.cdist(x1, x2, p=2.0, compute_mode='use_mm_for_euclid_dist_if_necessary')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#cdist)
Computes batched the p-norm distance between each pair of the two collections of row vectors.
Parameters
* **x1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – input tensor of shape B×P×MB \times P \times M .
* **x2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – input tensor of shape B×R×MB \times R \times M .
* **p** – p value for the p-norm distance to calculate between each vector pair ∈[0,∞]\in [0, \infty] .
* **compute\_mode** – ‘use\_mm\_for\_euclid\_dist\_if\_necessary’ - will use matrix multiplication approach to calculate euclidean distance (p = 2) if P > 25 or R > 25 ‘use\_mm\_for\_euclid\_dist’ - will always use matrix multiplication approach to calculate euclidean distance (p = 2) ‘donot\_use\_mm\_for\_euclid\_dist’ - will never use matrix multiplication approach to calculate euclidean distance (p = 2) Default: use\_mm\_for\_euclid\_dist\_if\_necessary.
If x1 has shape B×P×MB \times P \times M and x2 has shape B×R×MB \times R \times M then the output will have shape B×P×RB \times P \times R .
This function is equivalent to `scipy.spatial.distance.cdist(input,’minkowski’, p=p)` if p∈(0,∞)p \in (0, \infty) . When p=0p = 0 it is equivalent to `scipy.spatial.distance.cdist(input, ‘hamming’) * M`. When p=∞p = \infty , the closest scipy function is `scipy.spatial.distance.cdist(xn, lambda x, y: np.abs(x - y).max())`.
#### Example
```
>>> a = torch.tensor([[0.9041, 0.0196], [-0.3108, -2.4423], [-0.4821, 1.059]])
>>> a
tensor([[ 0.9041, 0.0196],
[-0.3108, -2.4423],
[-0.4821, 1.0590]])
>>> b = torch.tensor([[-2.1763, -0.4713], [-0.6986, 1.3702]])
>>> b
tensor([[-2.1763, -0.4713],
[-0.6986, 1.3702]])
>>> torch.cdist(a, b, p=2)
tensor([[3.1193, 2.0959],
[2.7138, 3.8322],
[2.2830, 0.3791]])
```
pytorch torch.moveaxis torch.moveaxis
==============
`torch.moveaxis(input, source, destination) → Tensor`
Alias for [`torch.movedim()`](torch.movedim#torch.movedim "torch.movedim").
This function is equivalent to NumPy’s moveaxis function.
Examples:
```
>>> t = torch.randn(3,2,1)
>>> t
tensor([[[-0.3362],
[-0.8437]],
[[-0.9627],
[ 0.1727]],
[[ 0.5173],
[-0.1398]]])
>>> torch.moveaxis(t, 1, 0).shape
torch.Size([2, 3, 1])
>>> torch.moveaxis(t, 1, 0)
tensor([[[-0.3362],
[-0.9627],
[ 0.5173]],
[[-0.8437],
[ 0.1727],
[-0.1398]]])
>>> torch.moveaxis(t, (1, 2), (0, 1)).shape
torch.Size([2, 1, 3])
>>> torch.moveaxis(t, (1, 2), (0, 1))
tensor([[[-0.3362, -0.9627, 0.5173]],
[[-0.8437, 0.1727, -0.1398]]])
```
pytorch ReflectionPad2d ReflectionPad2d
===============
`class torch.nn.ReflectionPad2d(padding)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/padding.html#ReflectionPad2d)
Pads the input tensor using the reflection of the input boundary.
For `N`-dimensional padding, use [`torch.nn.functional.pad()`](../nn.functional#torch.nn.functional.pad "torch.nn.functional.pad").
Parameters
**padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the padding. If is `int`, uses the same padding in all boundaries. If a 4-`tuple`, uses (padding\_left\text{padding\\_left} , padding\_right\text{padding\\_right} , padding\_top\text{padding\\_top} , padding\_bottom\text{padding\\_bottom} )
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) where
Hout=Hin+padding\_top+padding\_bottomH\_{out} = H\_{in} + \text{padding\\_top} + \text{padding\\_bottom}
Wout=Win+padding\_left+padding\_rightW\_{out} = W\_{in} + \text{padding\\_left} + \text{padding\\_right}
Examples:
```
>>> m = nn.ReflectionPad2d(2)
>>> input = torch.arange(9, dtype=torch.float).reshape(1, 1, 3, 3)
>>> input
tensor([[[[0., 1., 2.],
[3., 4., 5.],
[6., 7., 8.]]]])
>>> m(input)
tensor([[[[8., 7., 6., 7., 8., 7., 6.],
[5., 4., 3., 4., 5., 4., 3.],
[2., 1., 0., 1., 2., 1., 0.],
[5., 4., 3., 4., 5., 4., 3.],
[8., 7., 6., 7., 8., 7., 6.],
[5., 4., 3., 4., 5., 4., 3.],
[2., 1., 0., 1., 2., 1., 0.]]]])
>>> # using different paddings for different sides
>>> m = nn.ReflectionPad2d((1, 1, 2, 0))
>>> m(input)
tensor([[[[7., 6., 7., 8., 7.],
[4., 3., 4., 5., 4.],
[1., 0., 1., 2., 1.],
[4., 3., 4., 5., 4.],
[7., 6., 7., 8., 7.]]]])
```
pytorch torch.bitwise_and torch.bitwise\_and
==================
`torch.bitwise_and(input, other, *, out=None) → Tensor`
Computes the bitwise AND of `input` and `other`. The input tensor must be of integral or Boolean types. For bool tensors, it computes the logical AND.
Parameters
* **input** – the first input tensor
* **other** – the second input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
#### Example
```
>>> torch.bitwise_and(torch.tensor([-1, -2, 3], dtype=torch.int8), torch.tensor([1, 0, 3], dtype=torch.int8))
tensor([1, 0, 3], dtype=torch.int8)
>>> torch.bitwise_and(torch.tensor([True, True, False]), torch.tensor([False, True, False]))
tensor([ False, True, False])
```
pytorch torch.hann_window torch.hann\_window
==================
`torch.hann_window(window_length, periodic=True, *, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor`
Hann window function.
w[n]=12[1−cos(2πnN−1)]=sin2(πnN−1),w[n] = \frac{1}{2}\ \left[1 - \cos \left( \frac{2 \pi n}{N - 1} \right)\right] = \sin^2 \left( \frac{\pi n}{N - 1} \right),
where NN is the full window size.
The input `window_length` is a positive integer controlling the returned window size. `periodic` flag determines whether the returned window trims off the last duplicate value from the symmetric window and is ready to be used as a periodic window with functions like [`torch.stft()`](torch.stft#torch.stft "torch.stft"). Therefore, if `periodic` is true, the NN in above formula is in fact window\_length+1\text{window\\_length} + 1 . Also, we always have `torch.hann_window(L, periodic=True)` equal to `torch.hann_window(L + 1, periodic=False)[:-1])`.
Note
If `window_length` =1=1 , the returned window contains a single value 1.
Parameters
* **window\_length** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the size of returned window
* **periodic** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If True, returns a window to be used as periodic function. If False, return a symmetric window.
Keyword Arguments
* **dtype** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype"), optional) – the desired data type of returned tensor. Default: if `None`, uses a global default (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). Only floating point types are supported.
* **layout** ([`torch.layout`](../tensor_attributes#torch.torch.layout "torch.torch.layout"), optional) – the desired layout of returned window tensor. Only `torch.strided` (dense layout) is supported.
* **device** ([`torch.device`](../tensor_attributes#torch.torch.device "torch.torch.device"), optional) – the desired device of returned tensor. Default: if `None`, uses the current device for the default tensor type (see [`torch.set_default_tensor_type()`](torch.set_default_tensor_type#torch.set_default_tensor_type "torch.set_default_tensor_type")). `device` will be the CPU for CPU tensor types and the current CUDA device for CUDA tensor types.
* **requires\_grad** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – If autograd should record operations on the returned tensor. Default: `False`.
Returns
A 1-D tensor of size (window\_length,)(\text{window\\_length},) containing the window
Return type
[Tensor](../tensors#torch.Tensor "torch.Tensor")
pytorch torch.igamma torch.igamma
============
`torch.igamma(input, other, *, out=None) → Tensor`
Computes the regularized lower incomplete gamma function:
outi=1Γ(inputi)∫0otheritinputi−1e−tdt\text{out}\_{i} = \frac{1}{\Gamma(\text{input}\_i)} \int\_0^{\text{other}\_i} t^{\text{input}\_i-1} e^{-t} dt
where both inputi\text{input}\_i and otheri\text{other}\_i are weakly positive and at least one is strictly positive. If both are zero or either is negative then outi=nan\text{out}\_i=\text{nan} . Γ(⋅)\Gamma(\cdot) in the equation above is the gamma function,
Γ(inputi)=∫0∞t(inputi−1)e−tdt.\Gamma(\text{input}\_i) = \int\_0^\infty t^{(\text{input}\_i-1)} e^{-t} dt.
See [`torch.igammac()`](torch.igammac#torch.igammac "torch.igammac") and [`torch.lgamma()`](torch.lgamma#torch.lgamma "torch.lgamma") for related functions.
Supports [broadcasting to a common shape](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) and float inputs.
Note
The backward pass with respect to `input` is not yet supported. Please open an issue on PyTorch’s Github to request it.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first non-negative input tensor
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second non-negative input tensor
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a1 = torch.tensor([4.0])
>>> a2 = torch.tensor([3.0, 4.0, 5.0])
>>> a = torch.igammac(a1, a2)
tensor([0.3528, 0.5665, 0.7350])
tensor([0.3528, 0.5665, 0.7350])
>>> b = torch.igamma(a1, a2) + torch.igammac(a1, a2)
tensor([1., 1., 1.])
```
pytorch torch.use_deterministic_algorithms torch.use\_deterministic\_algorithms
====================================
`torch.use_deterministic_algorithms(d)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#use_deterministic_algorithms)
Sets whether PyTorch operations must use “deterministic” algorithms. That is, algorithms which, given the same input, and when run on the same software and hardware, always produce the same output. When True, operations will use deterministic algorithms when available, and if only nondeterministic algorithms are available they will throw a :class:RuntimeError when called.
Warning
This feature is in beta, and its design and implementation may change in the future.
The following normally-nondeterministic operations will act deterministically when `d=True`:
* [`torch.nn.Conv1d`](torch.nn.conv1d#torch.nn.Conv1d "torch.nn.Conv1d") when called on CUDA tensor
* [`torch.nn.Conv2d`](torch.nn.conv2d#torch.nn.Conv2d "torch.nn.Conv2d") when called on CUDA tensor
* [`torch.nn.Conv3d`](torch.nn.conv3d#torch.nn.Conv3d "torch.nn.Conv3d") when called on CUDA tensor
* [`torch.nn.ConvTranspose1d`](torch.nn.convtranspose1d#torch.nn.ConvTranspose1d "torch.nn.ConvTranspose1d") when called on CUDA tensor
* [`torch.nn.ConvTranspose2d`](torch.nn.convtranspose2d#torch.nn.ConvTranspose2d "torch.nn.ConvTranspose2d") when called on CUDA tensor
* [`torch.nn.ConvTranspose3d`](torch.nn.convtranspose3d#torch.nn.ConvTranspose3d "torch.nn.ConvTranspose3d") when called on CUDA tensor
* [`torch.bmm()`](torch.bmm#torch.bmm "torch.bmm") when called on sparse-dense CUDA tensors
* `torch.__getitem__()` backward when `self` is a CPU tensor and `indices` is a list of tensors
* `torch.index_put()` with `accumulate=True` when called on a CPU tensor
The following normally-nondeterministic operations will throw a [`RuntimeError`](https://docs.python.org/3/library/exceptions.html#RuntimeError "(in Python v3.9)") when `d=True`:
* [`torch.nn.AvgPool3d`](torch.nn.avgpool3d#torch.nn.AvgPool3d "torch.nn.AvgPool3d") when called on a CUDA tensor that requires grad
* [`torch.nn.AdaptiveAvgPool2d`](torch.nn.adaptiveavgpool2d#torch.nn.AdaptiveAvgPool2d "torch.nn.AdaptiveAvgPool2d") when called on a CUDA tensor that requires grad
* [`torch.nn.AdaptiveAvgPool3d`](torch.nn.adaptiveavgpool3d#torch.nn.AdaptiveAvgPool3d "torch.nn.AdaptiveAvgPool3d") when called on a CUDA tensor that requires grad
* [`torch.nn.MaxPool3d`](torch.nn.maxpool3d#torch.nn.MaxPool3d "torch.nn.MaxPool3d") when called on a CUDA tensor that requires grad
* [`torch.nn.AdaptiveMaxPool2d`](torch.nn.adaptivemaxpool2d#torch.nn.AdaptiveMaxPool2d "torch.nn.AdaptiveMaxPool2d") when called on a CUDA tensor that requires grad
* [`torch.nn.FractionalMaxPool2d`](torch.nn.fractionalmaxpool2d#torch.nn.FractionalMaxPool2d "torch.nn.FractionalMaxPool2d") when called on a CUDA tensor that requires grad
* `torch.nn.FractionalMaxPool3d` when called on a CUDA tensor that requires grad
* [`torch.nn.functional.interpolate()`](../nn.functional#torch.nn.functional.interpolate "torch.nn.functional.interpolate") when called on a CUDA tensor that requires grad and one of the following modes is used:
+ `linear`
+ `bilinear`
+ `bicubic`
+ `trilinear`
* [`torch.nn.ReflectionPad1d`](torch.nn.reflectionpad1d#torch.nn.ReflectionPad1d "torch.nn.ReflectionPad1d") when called on a CUDA tensor that requires grad
* [`torch.nn.ReflectionPad2d`](torch.nn.reflectionpad2d#torch.nn.ReflectionPad2d "torch.nn.ReflectionPad2d") when called on a CUDA tensor that requires grad
* [`torch.nn.ReplicationPad1d`](torch.nn.replicationpad1d#torch.nn.ReplicationPad1d "torch.nn.ReplicationPad1d") when called on a CUDA tensor that requires grad
* [`torch.nn.ReplicationPad2d`](torch.nn.replicationpad2d#torch.nn.ReplicationPad2d "torch.nn.ReplicationPad2d") when called on a CUDA tensor that requires grad
* [`torch.nn.ReplicationPad3d`](torch.nn.replicationpad3d#torch.nn.ReplicationPad3d "torch.nn.ReplicationPad3d") when called on a CUDA tensor that requires grad
* [`torch.nn.NLLLoss`](torch.nn.nllloss#torch.nn.NLLLoss "torch.nn.NLLLoss") when called on a CUDA tensor that requires grad
* [`torch.nn.CTCLoss`](torch.nn.ctcloss#torch.nn.CTCLoss "torch.nn.CTCLoss") when called on a CUDA tensor that requires grad
* [`torch.nn.EmbeddingBag`](torch.nn.embeddingbag#torch.nn.EmbeddingBag "torch.nn.EmbeddingBag") when called on a CUDA tensor that requires grad
* `torch.scatter_add_()` when called on a CUDA tensor
* `torch.index_add_()` when called on a CUDA tensor
* `torch.index_copy()`
* [`torch.index_select()`](torch.index_select#torch.index_select "torch.index_select") when called on a CUDA tensor that requires grad
* [`torch.repeat_interleave()`](torch.repeat_interleave#torch.repeat_interleave "torch.repeat_interleave") when called on a CUDA tensor that requires grad
* [`torch.histc()`](torch.histc#torch.histc "torch.histc") when called on a CUDA tensor
* [`torch.bincount()`](torch.bincount#torch.bincount "torch.bincount") when called on a CUDA tensor
* [`torch.kthvalue()`](torch.kthvalue#torch.kthvalue "torch.kthvalue") with called on a CUDA tensor
* [`torch.median()`](torch.median#torch.median "torch.median") with indices output when called on a CUDA tensor
A handful of CUDA operations are nondeterministic if the CUDA version is 10.2 or greater, unless the environment variable `CUBLAS_WORKSPACE_CONFIG=:4096:8` or `CUBLAS_WORKSPACE_CONFIG=:16:8` is set. See the CUDA documentation for more details: <https://docs.nvidia.com/cuda/cublas/index.html#cublasApi_reproducibility> If one of these environment variable configurations is not set, a [`RuntimeError`](https://docs.python.org/3/library/exceptions.html#RuntimeError "(in Python v3.9)") will be raised from these operations when called with CUDA tensors:
* [`torch.mm()`](torch.mm#torch.mm "torch.mm")
* [`torch.mv()`](torch.mv#torch.mv "torch.mv")
* [`torch.bmm()`](torch.bmm#torch.bmm "torch.bmm")
Note that deterministic operations tend to have worse performance than non-deterministic operations.
Parameters
**d** ([`bool`](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – If True, force operations to be deterministic. If False, allow non-deterministic operations.
| programming_docs |
pytorch torch.gt torch.gt
========
`torch.gt(input, other, *, out=None) → Tensor`
Computes input>other\text{input} > \text{other} element-wise.
The second argument can be a number or a tensor whose shape is [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with the first argument.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compare
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the tensor or value to compare
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Returns
A boolean tensor that is True where `input` is greater than `other` and False elsewhere
Example:
```
>>> torch.gt(torch.tensor([[1, 2], [3, 4]]), torch.tensor([[1, 1], [4, 4]]))
tensor([[False, True], [False, False]])
```
pytorch Softmax2d Softmax2d
=========
`class torch.nn.Softmax2d` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/activation.html#Softmax2d)
Applies SoftMax over features to each spatial location.
When given an image of `Channels x Height x Width`, it will apply `Softmax` to each location (Channels,hi,wj)(Channels, h\_i, w\_j)
Shape:
* Input: (N,C,H,W)(N, C, H, W)
* Output: (N,C,H,W)(N, C, H, W) (same shape as input)
Returns
a Tensor of the same dimension and shape as the input with values in the range [0, 1]
Examples:
```
>>> m = nn.Softmax2d()
>>> # you softmax over the 2nd dimension
>>> input = torch.randn(2, 3, 12, 13)
>>> output = m(input)
```
pytorch torch.nn.utils.clip_grad_value_ torch.nn.utils.clip\_grad\_value\_
==================================
`torch.nn.utils.clip_grad_value_(parameters, clip_value)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/clip_grad.html#clip_grad_value_)
Clips gradient of an iterable of parameters at specified value.
Gradients are modified in-place.
Parameters
* **parameters** (*Iterable**[*[Tensor](../tensors#torch.Tensor "torch.Tensor")*] or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) – an iterable of Tensors or a single Tensor that will have gradients normalized
* **clip\_value** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* [int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – maximum allowed value of the gradients. The gradients are clipped in the range [-clip\_value,clip\_value]\left[\text{-clip\\_value}, \text{clip\\_value}\right]
pytorch torch.nn.utils.clip_grad_norm_ torch.nn.utils.clip\_grad\_norm\_
=================================
`torch.nn.utils.clip_grad_norm_(parameters, max_norm, norm_type=2.0)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/clip_grad.html#clip_grad_norm_)
Clips gradient norm of an iterable of parameters.
The norm is computed over all gradients together, as if they were concatenated into a single vector. Gradients are modified in-place.
Parameters
* **parameters** (*Iterable**[*[Tensor](../tensors#torch.Tensor "torch.Tensor")*] or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) – an iterable of Tensors or a single Tensor that will have gradients normalized
* **max\_norm** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* [int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – max norm of the gradients
* **norm\_type** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* [int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – type of the used p-norm. Can be `'inf'` for infinity norm.
Returns
Total norm of the parameters (viewed as a single vector).
pytorch torch.erfinv torch.erfinv
============
`torch.erfinv(input, *, out=None) → Tensor`
Computes the inverse error function of each element of `input`. The inverse error function is defined in the range (−1,1)(-1, 1) as:
erfinv(erf(x))=x\mathrm{erfinv}(\mathrm{erf}(x)) = x
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.erfinv(torch.tensor([0, 0.5, -1.]))
tensor([ 0.0000, 0.4769, -inf])
```
pytorch torch.ne torch.ne
========
`torch.ne(input, other, *, out=None) → Tensor`
Computes input≠other\text{input} \neq \text{other} element-wise.
The second argument can be a number or a tensor whose shape is [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with the first argument.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compare
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – the tensor or value to compare
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Returns
A boolean tensor that is True where `input` is not equal to `other` and False elsewhere
Example:
```
>>> torch.ne(torch.tensor([[1, 2], [3, 4]]), torch.tensor([[1, 1], [4, 4]]))
tensor([[False, True], [True, False]])
```
pytorch torch.ormqr torch.ormqr
===========
`torch.ormqr(input, input2, input3, left=True, transpose=False) → Tensor`
Multiplies `mat` (given by `input3`) by the orthogonal `Q` matrix of the QR factorization formed by [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf") that is represented by `(a, tau)` (given by (`input`, `input2`)).
This directly calls the underlying LAPACK function `?ormqr`. See [LAPACK documentation for ormqr](https://software.intel.com/en-us/mkl-developer-reference-c-ormqr) for further details.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the `a` from [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf").
* **input2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the `tau` from [`torch.geqrf()`](torch.geqrf#torch.geqrf "torch.geqrf").
* **input3** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the matrix to be multiplied.
pytorch torch.neg torch.neg
=========
`torch.neg(input, *, out=None) → Tensor`
Returns a new tensor with the negative of the elements of `input`.
out=−1×input\text{out} = -1 \times \text{input}
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(5)
>>> a
tensor([ 0.0090, -0.2262, -0.0682, -0.2866, 0.3940])
>>> torch.neg(a)
tensor([-0.0090, 0.2262, 0.0682, 0.2866, -0.3940])
```
pytorch torch.nn.modules.module.register_module_forward_pre_hook torch.nn.modules.module.register\_module\_forward\_pre\_hook
============================================================
`torch.nn.modules.module.register_module_forward_pre_hook(hook)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/module.html#register_module_forward_pre_hook)
Registers a forward pre-hook common to all modules.
Warning
This adds global state to the `nn.module` module and it is only intended for debugging/profiling purposes.
The hook will be called every time before `forward()` is invoked. It should have the following signature:
```
hook(module, input) -> None or modified input
```
The input contains only the positional arguments given to the module. Keyword arguments won’t be passed to the hooks and only to the `forward`. The hook can modify the input. User can either return a tuple or a single modified value in the hook. We will wrap the value into a tuple if a single value is returned(unless that value is already a tuple).
This hook has precedence over the specific module hooks registered with `register_forward_pre_hook`.
Returns
a handle that can be used to remove the added hook by calling `handle.remove()`
Return type
`torch.utils.hooks.RemovableHandle`
pytorch torch.det torch.det
=========
`torch.det(input) → Tensor`
Calculates determinant of a square matrix or batches of square matrices.
Note
[`torch.det()`](#torch.det "torch.det") is deprecated. Please use [`torch.linalg.det()`](../linalg#torch.linalg.det "torch.linalg.det") instead.
Note
Backward through detdet internally uses SVD results when `input` is not invertible. In this case, double backward through detdet will be unstable when `input` doesn’t have distinct singular values. See torch.svd~torch.svd for details.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor of size `(*, n, n)` where `*` is zero or more batch dimensions.
Example:
```
>>> A = torch.randn(3, 3)
>>> torch.det(A)
tensor(3.7641)
>>> A = torch.randn(3, 2, 2)
>>> A
tensor([[[ 0.9254, -0.6213],
[-0.5787, 1.6843]],
[[ 0.3242, -0.9665],
[ 0.4539, -0.0887]],
[[ 1.1336, -0.4025],
[-0.7089, 0.9032]]])
>>> A.det()
tensor([1.1990, 0.4099, 0.7386])
```
pytorch torch.set_rng_state torch.set\_rng\_state
=====================
`torch.set_rng_state(new_state)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/random.html#set_rng_state)
Sets the random number generator state.
Parameters
**new\_state** (*torch.ByteTensor*) – The desired state
pytorch torch.nn.utils.rnn.pack_padded_sequence torch.nn.utils.rnn.pack\_padded\_sequence
=========================================
`torch.nn.utils.rnn.pack_padded_sequence(input, lengths, batch_first=False, enforce_sorted=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/rnn.html#pack_padded_sequence)
Packs a Tensor containing padded sequences of variable length.
`input` can be of size `T x B x *` where `T` is the length of the longest sequence (equal to `lengths[0]`), `B` is the batch size, and `*` is any number of dimensions (including 0). If `batch_first` is `True`, `B x T x *` `input` is expected.
For unsorted sequences, use `enforce_sorted = False`. If `enforce_sorted` is `True`, the sequences should be sorted by length in a decreasing order, i.e. `input[:,0]` should be the longest sequence, and `input[:,B-1]` the shortest one. `enforce_sorted = True` is only necessary for ONNX export.
Note
This function accepts any input that has at least two dimensions. You can apply it to pack the labels, and use the output of the RNN with them to compute the loss directly. A Tensor can be retrieved from a [`PackedSequence`](torch.nn.utils.rnn.packedsequence#torch.nn.utils.rnn.PackedSequence "torch.nn.utils.rnn.PackedSequence") object by accessing its `.data` attribute.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – padded batch of variable length sequences.
* **lengths** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* [list](https://docs.python.org/3/library/stdtypes.html#list "(in Python v3.9)")*(*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*)*) – list of sequence lengths of each batch element (must be on the CPU if provided as a tensor).
* **batch\_first** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True`, the input is expected in `B x T x *` format.
* **enforce\_sorted** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True`, the input is expected to contain sequences sorted by length in a decreasing order. If `False`, the input will get sorted unconditionally. Default: `True`.
Returns
a [`PackedSequence`](torch.nn.utils.rnn.packedsequence#torch.nn.utils.rnn.PackedSequence "torch.nn.utils.rnn.PackedSequence") object
pytorch torch.le torch.le
========
`torch.le(input, other, *, out=None) → Tensor`
Computes input≤other\text{input} \leq \text{other} element-wise.
The second argument can be a number or a tensor whose shape is [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with the first argument.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to compare
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor") *or* *Scalar*) – the tensor or value to compare
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Returns
A boolean tensor that is True where `input` is less than or equal to `other` and False elsewhere
Example:
```
>>> torch.le(torch.tensor([[1, 2], [3, 4]]), torch.tensor([[1, 1], [4, 4]]))
tensor([[True, False], [True, True]])
```
pytorch torch.baddbmm torch.baddbmm
=============
`torch.baddbmm(input, batch1, batch2, *, beta=1, alpha=1, out=None) → Tensor`
Performs a batch matrix-matrix product of matrices in `batch1` and `batch2`. `input` is added to the final result.
`batch1` and `batch2` must be 3-D tensors each containing the same number of matrices.
If `batch1` is a (b×n×m)(b \times n \times m) tensor, `batch2` is a (b×m×p)(b \times m \times p) tensor, then `input` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with a (b×n×p)(b \times n \times p) tensor and `out` will be a (b×n×p)(b \times n \times p) tensor. Both `alpha` and `beta` mean the same as the scaling factors used in [`torch.addbmm()`](torch.addbmm#torch.addbmm "torch.addbmm").
outi=βinputi+α(batch1i@batch2i)\text{out}\_i = \beta\ \text{input}\_i + \alpha\ (\text{batch1}\_i \mathbin{@} \text{batch2}\_i)
If `beta` is 0, then `input` will be ignored, and `nan` and `inf` in it will not be propagated.
For inputs of type `FloatTensor` or `DoubleTensor`, arguments `beta` and `alpha` must be real numbers, otherwise they should be integers.
This operator supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the tensor to be added
* **batch1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first batch of matrices to be multiplied
* **batch2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second batch of matrices to be multiplied
Keyword Arguments
* **beta** (*Number**,* *optional*) – multiplier for `input` (β\beta )
* **alpha** (*Number**,* *optional*) – multiplier for batch1@batch2\text{batch1} \mathbin{@} \text{batch2} (α\alpha )
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> M = torch.randn(10, 3, 5)
>>> batch1 = torch.randn(10, 3, 4)
>>> batch2 = torch.randn(10, 4, 5)
>>> torch.baddbmm(M, batch1, batch2).size()
torch.Size([10, 3, 5])
```
pytorch torch.get_rng_state torch.get\_rng\_state
=====================
`torch.get_rng_state()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/random.html#get_rng_state)
Returns the random number generator state as a `torch.ByteTensor`.
pytorch AvgPool2d AvgPool2d
=========
`class torch.nn.AvgPool2d(kernel_size, stride=None, padding=0, ceil_mode=False, count_include_pad=True, divisor_override=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#AvgPool2d)
Applies a 2D average pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,H,W)(N, C, H, W) , output (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) and `kernel_size` (kH,kW)(kH, kW) can be precisely described as:
out(Ni,Cj,h,w)=1kH∗kW∑m=0kH−1∑n=0kW−1input(Ni,Cj,stride[0]×h+m,stride[1]×w+n)out(N\_i, C\_j, h, w) = \frac{1}{kH \* kW} \sum\_{m=0}^{kH-1} \sum\_{n=0}^{kW-1} input(N\_i, C\_j, stride[0] \times h + m, stride[1] \times w + n)
If `padding` is non-zero, then the input is implicitly zero-padded on both sides for `padding` number of points.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
The parameters `kernel_size`, `stride`, `padding` can either be:
* a single `int` – in which case the same value is used for the height and width dimension
* a `tuple` of two ints – in which case, the first `int` is used for the height dimension, and the second `int` for the width dimension
Parameters
* **kernel\_size** – the size of the window
* **stride** – the stride of the window. Default value is `kernel_size`
* **padding** – implicit zero padding to be added on both sides
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
* **count\_include\_pad** – when True, will include the zero-padding in the averaging calculation
* **divisor\_override** – if specified, it will be used as divisor, otherwise `kernel_size` will be used
Shape:
* Input: (N,C,Hin,Win)(N, C, H\_{in}, W\_{in})
* Output: (N,C,Hout,Wout)(N, C, H\_{out}, W\_{out}) , where
Hout=⌊Hin+2×padding[0]−kernel\_size[0]stride[0]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \times \text{padding}[0] - \text{kernel\\_size}[0]}{\text{stride}[0]} + 1\right\rfloor
Wout=⌊Win+2×padding[1]−kernel\_size[1]stride[1]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \times \text{padding}[1] - \text{kernel\\_size}[1]}{\text{stride}[1]} + 1\right\rfloor
Examples:
```
>>> # pool of square window of size=3, stride=2
>>> m = nn.AvgPool2d(3, stride=2)
>>> # pool of non-square window
>>> m = nn.AvgPool2d((3, 2), stride=(2, 1))
>>> input = torch.randn(20, 16, 50, 32)
>>> output = m(input)
```
pytorch Linear Linear
======
`class torch.nn.Linear(in_features, out_features, bias=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/linear.html#Linear)
Applies a linear transformation to the incoming data: y=xAT+by = xA^T + b
This module supports [TensorFloat32](https://pytorch.org/docs/1.8.0/notes/cuda.html#tf32-on-ampere).
Parameters
* **in\_features** – size of each input sample
* **out\_features** – size of each output sample
* **bias** – If set to `False`, the layer will not learn an additive bias. Default: `True`
Shape:
* Input: (N,∗,Hin)(N, \*, H\_{in}) where ∗\* means any number of additional dimensions and Hin=in\_featuresH\_{in} = \text{in\\_features}
* Output: (N,∗,Hout)(N, \*, H\_{out}) where all but the last dimension are the same shape as the input and Hout=out\_featuresH\_{out} = \text{out\\_features} .
Variables
* **~Linear.weight** – the learnable weights of the module of shape (out\_features,in\_features)(\text{out\\_features}, \text{in\\_features}) . The values are initialized from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) , where k=1in\_featuresk = \frac{1}{\text{in\\_features}}
* **~Linear.bias** – the learnable bias of the module of shape (out\_features)(\text{out\\_features}) . If `bias` is `True`, the values are initialized from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=1in\_featuresk = \frac{1}{\text{in\\_features}}
Examples:
```
>>> m = nn.Linear(20, 30)
>>> input = torch.randn(128, 20)
>>> output = m(input)
>>> print(output.size())
torch.Size([128, 30])
```
pytorch Bilinear Bilinear
========
`class torch.nn.Bilinear(in1_features, in2_features, out_features, bias=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/linear.html#Bilinear)
Applies a bilinear transformation to the incoming data: y=x1TAx2+by = x\_1^T A x\_2 + b
Parameters
* **in1\_features** – size of each first input sample
* **in2\_features** – size of each second input sample
* **out\_features** – size of each output sample
* **bias** – If set to False, the layer will not learn an additive bias. Default: `True`
Shape:
* Input1: (N,∗,Hin1)(N, \*, H\_{in1}) where Hin1=in1\_featuresH\_{in1}=\text{in1\\_features} and ∗\* means any number of additional dimensions. All but the last dimension of the inputs should be the same.
* Input2: (N,∗,Hin2)(N, \*, H\_{in2}) where Hin2=in2\_featuresH\_{in2}=\text{in2\\_features} .
* Output: (N,∗,Hout)(N, \*, H\_{out}) where Hout=out\_featuresH\_{out}=\text{out\\_features} and all but the last dimension are the same shape as the input.
Variables
* **~Bilinear.weight** – the learnable weights of the module of shape (out\_features,in1\_features,in2\_features)(\text{out\\_features}, \text{in1\\_features}, \text{in2\\_features}) . The values are initialized from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) , where k=1in1\_featuresk = \frac{1}{\text{in1\\_features}}
* **~Bilinear.bias** – the learnable bias of the module of shape (out\_features)(\text{out\\_features}) . If `bias` is `True`, the values are initialized from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) , where k=1in1\_featuresk = \frac{1}{\text{in1\\_features}}
Examples:
```
>>> m = nn.Bilinear(20, 30, 40)
>>> input1 = torch.randn(128, 20)
>>> input2 = torch.randn(128, 30)
>>> output = m(input1, input2)
>>> print(output.size())
torch.Size([128, 40])
```
| programming_docs |
pytorch PoissonNLLLoss PoissonNLLLoss
==============
`class torch.nn.PoissonNLLLoss(log_input=True, full=False, size_average=None, eps=1e-08, reduce=None, reduction='mean')` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/loss.html#PoissonNLLLoss)
Negative log likelihood loss with Poisson distribution of target.
The loss can be described as:
target∼Poisson(input)loss(input,target)=input−target∗log(input)+log(target!)\text{target} \sim \mathrm{Poisson}(\text{input}) \text{loss}(\text{input}, \text{target}) = \text{input} - \text{target} \* \log(\text{input}) + \log(\text{target!})
The last term can be omitted or approximated with Stirling formula. The approximation is used for target values more than 1. For targets less or equal to 1 zeros are added to the loss.
Parameters
* **log\_input** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – if `True` the loss is computed as exp(input)−target∗input\exp(\text{input}) - \text{target}\*\text{input} , if `False` the loss is input−target∗log(input+eps)\text{input} - \text{target}\*\log(\text{input}+\text{eps}) .
* **full** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) –
whether to compute full loss, i. e. to add the Stirling approximation term
target∗log(target)−target+0.5∗log(2πtarget).\text{target}\*\log(\text{target}) - \text{target} + 0.5 \* \log(2\pi\text{target}).
* **size\_average** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged over each loss element in the batch. Note that for some losses, there are multiple elements per sample. If the field `size_average` is set to `False`, the losses are instead summed for each minibatch. Ignored when `reduce` is `False`. Default: `True`
* **eps** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – Small value to avoid evaluation of log(0)\log(0) when `log_input = False`. Default: 1e-8
* **reduce** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")*,* *optional*) – Deprecated (see `reduction`). By default, the losses are averaged or summed over observations for each minibatch depending on `size_average`. When `reduce` is `False`, returns a loss per batch element instead and ignores `size_average`. Default: `True`
* **reduction** (*string**,* *optional*) – Specifies the reduction to apply to the output: `'none'` | `'mean'` | `'sum'`. `'none'`: no reduction will be applied, `'mean'`: the sum of the output will be divided by the number of elements in the output, `'sum'`: the output will be summed. Note: `size_average` and `reduce` are in the process of being deprecated, and in the meantime, specifying either of those two args will override `reduction`. Default: `'mean'`
Examples:
```
>>> loss = nn.PoissonNLLLoss()
>>> log_input = torch.randn(5, 2, requires_grad=True)
>>> target = torch.randn(5, 2)
>>> output = loss(log_input, target)
>>> output.backward()
```
Shape:
* Input: (N,∗)(N, \*) where ∗\* means, any number of additional dimensions
* Target: (N,∗)(N, \*) , same shape as the input
* Output: scalar by default. If `reduction` is `'none'`, then (N,∗)(N, \*) , the same shape as the input
pytorch torch.std_mean torch.std\_mean
===============
`torch.std_mean(input, unbiased=True) -> (Tensor, Tensor)`
Returns the standard-deviation and mean of all elements in the `input` tensor.
If `unbiased` is `False`, then the standard-deviation will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[0.3364, 0.3591, 0.9462]])
>>> torch.std_mean(a)
(tensor(0.3457), tensor(0.5472))
```
`torch.std_mean(input, dim, unbiased=True, keepdim=False) -> (Tensor, Tensor)`
Returns the standard-deviation and mean of each row of the `input` tensor in the dimension `dim`. If `dim` is a list of dimensions, reduce over all of them.
If `keepdim` is `True`, the output tensor is of the same size as `input` except in the dimension(s) `dim` where it is of size 1. Otherwise, `dim` is squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")), resulting in the output tensor having 1 (or `len(dim)`) fewer dimension(s).
If `unbiased` is `False`, then the standard-deviation will be calculated via the biased estimator. Otherwise, Bessel’s correction will be used.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *tuple of python:ints*) – the dimension or dimensions to reduce.
* **unbiased** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether to use the unbiased estimation or not
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Example:
```
>>> a = torch.randn(4, 4)
>>> a
tensor([[ 0.5648, -0.5984, -1.2676, -1.4471],
[ 0.9267, 1.0612, 1.1050, -0.6014],
[ 0.0154, 1.9301, 0.0125, -1.0904],
[-1.9711, -0.7748, -1.3840, 0.5067]])
>>> torch.std_mean(a, 1)
(tensor([0.9110, 0.8197, 1.2552, 1.0608]), tensor([-0.6871, 0.6229, 0.2169, -0.9058]))
```
pytorch torch.erf torch.erf
=========
`torch.erf(input, *, out=None) → Tensor`
Computes the error function of each element. The error function is defined as follows:
erf(x)=2π∫0xe−t2dt\mathrm{erf}(x) = \frac{2}{\sqrt{\pi}} \int\_{0}^{x} e^{-t^2} dt
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> torch.erf(torch.tensor([0, -1., 10.]))
tensor([ 0.0000, -0.8427, 1.0000])
```
pytorch torch.jit.script torch.jit.script
================
`torch.jit.script(obj, optimize=None, _frames_up=0, _rcb=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/jit/_script.html#script)
Scripting a function or `nn.Module` will inspect the source code, compile it as TorchScript code using the TorchScript compiler, and return a [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") or [`ScriptFunction`](torch.jit.scriptfunction#torch.jit.ScriptFunction "torch.jit.ScriptFunction"). TorchScript itself is a subset of the Python language, so not all features in Python work, but we provide enough functionality to compute on tensors and do control-dependent operations. For a complete guide, see the [TorchScript Language Reference](../jit_language_reference#language-reference).
`torch.jit.script` can be used as a function for modules and functions, and as a decorator `@torch.jit.script` for [TorchScript Classes](../jit_language_reference#id2) and functions.
Parameters
**obj** (callable, class, or `nn.Module`) – The `nn.Module`, function, or class type to compile.
Returns
If `obj` is `nn.Module`, `script` returns a [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") object. The returned [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") will have the same set of sub-modules and parameters as the original `nn.Module`. If `obj` is a standalone function, a [`ScriptFunction`](torch.jit.scriptfunction#torch.jit.ScriptFunction "torch.jit.ScriptFunction") will be returned.
**Scripting a function**
The `@torch.jit.script` decorator will construct a [`ScriptFunction`](torch.jit.scriptfunction#torch.jit.ScriptFunction "torch.jit.ScriptFunction") by compiling the body of the function.
Example (scripting a function):
```
import torch
@torch.jit.script
def foo(x, y):
if x.max() > y.max():
r = x
else:
r = y
return r
print(type(foo)) # torch.jit.ScriptFuncion
# See the compiled graph as Python code
print(foo.code)
# Call the function using the TorchScript interpreter
foo(torch.ones(2, 2), torch.ones(2, 2))
```
**Scripting an nn.Module**
Scripting an `nn.Module` by default will compile the `forward` method and recursively compile any methods, submodules, and functions called by `forward`. If a `nn.Module` only uses features supported in TorchScript, no changes to the original module code should be necessary. `script` will construct [`ScriptModule`](torch.jit.scriptmodule#torch.jit.ScriptModule "torch.jit.ScriptModule") that has copies of the attributes, parameters, and methods of the original module.
Example (scripting a simple module with a Parameter):
```
import torch
class MyModule(torch.nn.Module):
def __init__(self, N, M):
super(MyModule, self).__init__()
# This parameter will be copied to the new ScriptModule
self.weight = torch.nn.Parameter(torch.rand(N, M))
# When this submodule is used, it will be compiled
self.linear = torch.nn.Linear(N, M)
def forward(self, input):
output = self.weight.mv(input)
# This calls the `forward` method of the `nn.Linear` module, which will
# cause the `self.linear` submodule to be compiled to a `ScriptModule` here
output = self.linear(output)
return output
scripted_module = torch.jit.script(MyModule(2, 3))
```
Example (scripting a module with traced submodules):
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class MyModule(nn.Module):
def __init__(self):
super(MyModule, self).__init__()
# torch.jit.trace produces a ScriptModule's conv1 and conv2
self.conv1 = torch.jit.trace(nn.Conv2d(1, 20, 5), torch.rand(1, 1, 16, 16))
self.conv2 = torch.jit.trace(nn.Conv2d(20, 20, 5), torch.rand(1, 20, 16, 16))
def forward(self, input):
input = F.relu(self.conv1(input))
input = F.relu(self.conv2(input))
return input
scripted_module = torch.jit.script(MyModule())
```
To compile a method other than `forward` (and recursively compile anything it calls), add the [`@torch.jit.export`](../jit#torch.jit.export "torch.jit.export") decorator to the method. To opt out of compilation use [`@torch.jit.ignore`](torch.jit.ignore#torch.jit.ignore "torch.jit.ignore") or [`@torch.jit.unused`](torch.jit.unused#torch.jit.unused "torch.jit.unused").
Example (an exported and ignored method in a module):
```
import torch
import torch.nn as nn
class MyModule(nn.Module):
def __init__(self):
super(MyModule, self).__init__()
@torch.jit.export
def some_entry_point(self, input):
return input + 10
@torch.jit.ignore
def python_only_fn(self, input):
# This function won't be compiled, so any
# Python APIs can be used
import pdb
pdb.set_trace()
def forward(self, input):
if self.training:
self.python_only_fn(input)
return input * 99
scripted_module = torch.jit.script(MyModule())
print(scripted_module.some_entry_point(torch.randn(2, 2)))
print(scripted_module(torch.randn(2, 2)))
```
pytorch torch.tensordot torch.tensordot
===============
`torch.tensordot(a, b, dims=2, out=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/functional.html#tensordot)
Returns a contraction of a and b over multiple dimensions.
[`tensordot`](#torch.tensordot "torch.tensordot") implements a generalized matrix product.
Parameters
* **a** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – Left tensor to contract
* **b** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – Right tensor to contract
* **dims** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* *Tuple**[**List**[*[int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*]**]* *containing two lists*) – number of dimensions to contract or explicit lists of dimensions for `a` and `b` respectively
When called with a non-negative integer argument `dims` = dd , and the number of dimensions of `a` and `b` is mm and nn , respectively, [`tensordot()`](#torch.tensordot "torch.tensordot") computes
ri0,...,im−d,id,...,in=∑k0,...,kd−1ai0,...,im−d,k0,...,kd−1×bk0,...,kd−1,id,...,in.r\_{i\_0,...,i\_{m-d}, i\_d,...,i\_n} = \sum\_{k\_0,...,k\_{d-1}} a\_{i\_0,...,i\_{m-d},k\_0,...,k\_{d-1}} \times b\_{k\_0,...,k\_{d-1}, i\_d,...,i\_n}.
When called with `dims` of the list form, the given dimensions will be contracted in place of the last dd of `a` and the first dd of bb . The sizes in these dimensions must match, but [`tensordot()`](#torch.tensordot "torch.tensordot") will deal with broadcasted dimensions.
Examples:
```
>>> a = torch.arange(60.).reshape(3, 4, 5)
>>> b = torch.arange(24.).reshape(4, 3, 2)
>>> torch.tensordot(a, b, dims=([1, 0], [0, 1]))
tensor([[4400., 4730.],
[4532., 4874.],
[4664., 5018.],
[4796., 5162.],
[4928., 5306.]])
>>> a = torch.randn(3, 4, 5, device='cuda')
>>> b = torch.randn(4, 5, 6, device='cuda')
>>> c = torch.tensordot(a, b, dims=2).cpu()
tensor([[ 8.3504, -2.5436, 6.2922, 2.7556, -1.0732, 3.2741],
[ 3.3161, 0.0704, 5.0187, -0.4079, -4.3126, 4.8744],
[ 0.8223, 3.9445, 3.2168, -0.2400, 3.4117, 1.7780]])
>>> a = torch.randn(3, 5, 4, 6)
>>> b = torch.randn(6, 4, 5, 3)
>>> torch.tensordot(a, b, dims=([2, 1, 3], [1, 2, 0]))
tensor([[ 7.7193, -2.4867, -10.3204],
[ 1.5513, -14.4737, -6.5113],
[ -0.2850, 4.2573, -3.5997]])
```
pytorch Fold Fold
====
`class torch.nn.Fold(output_size, kernel_size, dilation=1, padding=0, stride=1)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/fold.html#Fold)
Combines an array of sliding local blocks into a large containing tensor.
Consider a batched `input` tensor containing sliding local blocks, e.g., patches of images, of shape (N,C×∏(kernel\_size),L)(N, C \times \prod(\text{kernel\\_size}), L) , where NN is batch dimension, C×∏(kernel\_size)C \times \prod(\text{kernel\\_size}) is the number of values within a block (a block has ∏(kernel\_size)\prod(\text{kernel\\_size}) spatial locations each containing a CC -channeled vector), and LL is the total number of blocks. (This is exactly the same specification as the output shape of [`Unfold`](torch.nn.unfold#torch.nn.Unfold "torch.nn.Unfold").) This operation combines these local blocks into the large `output` tensor of shape (N,C,output\_size[0],output\_size[1],…)(N, C, \text{output\\_size}[0], \text{output\\_size}[1], \dots) by summing the overlapping values. Similar to [`Unfold`](torch.nn.unfold#torch.nn.Unfold "torch.nn.Unfold"), the arguments must satisfy
L=∏d⌊output\_size[d]+2×padding[d]−dilation[d]×(kernel\_size[d]−1)−1stride[d]+1⌋,L = \prod\_d \left\lfloor\frac{\text{output\\_size}[d] + 2 \times \text{padding}[d] % - \text{dilation}[d] \times (\text{kernel\\_size}[d] - 1) - 1}{\text{stride}[d]} + 1\right\rfloor,
where dd is over all spatial dimensions.
* `output_size` describes the spatial shape of the large containing tensor of the sliding local blocks. It is useful to resolve the ambiguity when multiple input shapes map to same number of sliding blocks, e.g., with `stride > 0`.
The `padding`, `stride` and `dilation` arguments specify how the sliding blocks are retrieved.
* `stride` controls the stride for the sliding blocks.
* `padding` controls the amount of implicit zero-paddings on both sides for `padding` number of points for each dimension before reshaping.
* `dilation` controls the spacing between the kernel points; also known as the à trous algorithm. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
Parameters
* **output\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the shape of the spatial dimensions of the output (i.e., `output.sizes()[2:]`)
* **kernel\_size** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the size of the sliding blocks
* **stride** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")) – the stride of the sliding blocks in the input spatial dimensions. Default: 1
* **padding** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – implicit zero padding to be added on both sides of input. Default: 0
* **dilation** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [tuple](https://docs.python.org/3/library/stdtypes.html#tuple "(in Python v3.9)")*,* *optional*) – a parameter that controls the stride of elements within the neighborhood. Default: 1
* If `output_size`, `kernel_size`, `dilation`, `padding` or `stride` is an int or a tuple of length 1 then their values will be replicated across all spatial dimensions.
* For the case of two output spatial dimensions this operation is sometimes called `col2im`.
Note
[`Fold`](#torch.nn.Fold "torch.nn.Fold") calculates each combined value in the resulting large tensor by summing all values from all containing blocks. [`Unfold`](torch.nn.unfold#torch.nn.Unfold "torch.nn.Unfold") extracts the values in the local blocks by copying from the large tensor. So, if the blocks overlap, they are not inverses of each other.
In general, folding and unfolding operations are related as follows. Consider [`Fold`](#torch.nn.Fold "torch.nn.Fold") and [`Unfold`](torch.nn.unfold#torch.nn.Unfold "torch.nn.Unfold") instances created with the same parameters:
```
>>> fold_params = dict(kernel_size=..., dilation=..., padding=..., stride=...)
>>> fold = nn.Fold(output_size=..., **fold_params)
>>> unfold = nn.Unfold(**fold_params)
```
Then for any (supported) `input` tensor the following equality holds:
```
fold(unfold(input)) == divisor * input
```
where `divisor` is a tensor that depends only on the shape and dtype of the `input`:
```
>>> input_ones = torch.ones(input.shape, dtype=input.dtype)
>>> divisor = fold(unfold(input_ones))
```
When the `divisor` tensor contains no zero elements, then `fold` and `unfold` operations are inverses of each other (up to constant divisor).
Warning
Currently, only 4-D output tensors (batched image-like tensors) are supported.
Shape:
* Input: (N,C×∏(kernel\_size),L)(N, C \times \prod(\text{kernel\\_size}), L)
* Output: (N,C,output\_size[0],output\_size[1],…)(N, C, \text{output\\_size}[0], \text{output\\_size}[1], \dots) as described above
Examples:
```
>>> fold = nn.Fold(output_size=(4, 5), kernel_size=(2, 2))
>>> input = torch.randn(1, 3 * 2 * 2, 12)
>>> output = fold(input)
>>> output.size()
torch.Size([1, 3, 4, 5])
```
pytorch torch.set_default_dtype torch.set\_default\_dtype
=========================
`torch.set_default_dtype(d)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#set_default_dtype)
Sets the default floating point dtype to `d`. This dtype is:
1. The inferred dtype for python floats in [`torch.tensor()`](torch.tensor#torch.tensor "torch.tensor").
2. Used to infer dtype for python complex numbers. The default complex dtype is set to `torch.complex128` if default floating point dtype is `torch.float64`, otherwise it’s set to `torch.complex64`
The default floating point dtype is initially `torch.float32`.
Parameters
**d** ([`torch.dtype`](../tensor_attributes#torch.torch.dtype "torch.torch.dtype")) – the floating point dtype to make the default
#### Example
```
>>> # initial default for floating point is torch.float32
>>> torch.tensor([1.2, 3]).dtype
torch.float32
>>> # initial default for floating point is torch.complex64
>>> torch.tensor([1.2, 3j]).dtype
torch.complex64
>>> torch.set_default_dtype(torch.float64)
>>> torch.tensor([1.2, 3]).dtype # a new floating point tensor
torch.float64
>>> torch.tensor([1.2, 3j]).dtype # a new complex tensor
torch.complex128
```
| programming_docs |
pytorch torch.view_as_real torch.view\_as\_real
====================
`torch.view_as_real(input) → Tensor`
Returns a view of `input` as a real tensor. For an input complex tensor of `size` m1,m2,…,mim1, m2, \dots, mi , this function returns a new real tensor of size m1,m2,…,mi,2m1, m2, \dots, mi, 2 , where the last dimension of size 2 represents the real and imaginary components of complex numbers.
Warning
[`view_as_real()`](#torch.view_as_real "torch.view_as_real") is only supported for tensors with `complex dtypes`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
Example::
```
>>> x=torch.randn(4, dtype=torch.cfloat)
>>> x
tensor([(0.4737-0.3839j), (-0.2098-0.6699j), (0.3470-0.9451j), (-0.5174-1.3136j)])
>>> torch.view_as_real(x)
tensor([[ 0.4737, -0.3839],
[-0.2098, -0.6699],
[ 0.3470, -0.9451],
[-0.5174, -1.3136]])
```
pytorch torch.are_deterministic_algorithms_enabled torch.are\_deterministic\_algorithms\_enabled
=============================================
`torch.are_deterministic_algorithms_enabled()` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch.html#are_deterministic_algorithms_enabled)
Returns True if the global deterministic flag is turned on. Refer to [`torch.use_deterministic_algorithms()`](torch.use_deterministic_algorithms#torch.use_deterministic_algorithms "torch.use_deterministic_algorithms") documentation for more details.
pytorch torch.addr torch.addr
==========
`torch.addr(input, vec1, vec2, *, beta=1, alpha=1, out=None) → Tensor`
Performs the outer-product of vectors `vec1` and `vec2` and adds it to the matrix `input`.
Optional values `beta` and `alpha` are scaling factors on the outer product between `vec1` and `vec2` and the added matrix `input` respectively.
out=β input+α(vec1⊗vec2)\text{out} = \beta\ \text{input} + \alpha\ (\text{vec1} \otimes \text{vec2})
If `beta` is 0, then `input` will be ignored, and `nan` and `inf` in it will not be propagated.
If `vec1` is a vector of size `n` and `vec2` is a vector of size `m`, then `input` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics) with a matrix of size (n×m)(n \times m) and `out` will be a matrix of size (n×m)(n \times m) .
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – matrix to be added
* **vec1** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first vector of the outer product
* **vec2** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second vector of the outer product
Keyword Arguments
* **beta** (*Number**,* *optional*) – multiplier for `input` (β\beta )
* **alpha** (*Number**,* *optional*) – multiplier for vec1⊗vec2\text{vec1} \otimes \text{vec2} (α\alpha )
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> vec1 = torch.arange(1., 4.)
>>> vec2 = torch.arange(1., 3.)
>>> M = torch.zeros(3, 2)
>>> torch.addr(M, vec1, vec2)
tensor([[ 1., 2.],
[ 2., 4.],
[ 3., 6.]])
```
pytorch torch.mv torch.mv
========
`torch.mv(input, vec, *, out=None) → Tensor`
Performs a matrix-vector product of the matrix `input` and the vector `vec`.
If `input` is a (n×m)(n \times m) tensor, `vec` is a 1-D tensor of size mm , `out` will be 1-D of size nn .
Note
This function does not [broadcast](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – matrix to be multiplied
* **vec** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – vector to be multiplied
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> mat = torch.randn(2, 3)
>>> vec = torch.randn(3)
>>> torch.mv(mat, vec)
tensor([ 1.0404, -0.6361])
```
pytorch torch.is_floating_point torch.is\_floating\_point
=========================
`torch.is_floating_point(input) -> (bool)`
Returns True if the data type of `input` is a floating point data type i.e., one of `torch.float64`, `torch.float32`, `torch.float16`, and `torch.bfloat16`.
Parameters
**input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
pytorch MaxPool3d MaxPool3d
=========
`class torch.nn.MaxPool3d(kernel_size, stride=None, padding=0, dilation=1, return_indices=False, ceil_mode=False)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/pooling.html#MaxPool3d)
Applies a 3D max pooling over an input signal composed of several input planes.
In the simplest case, the output value of the layer with input size (N,C,D,H,W)(N, C, D, H, W) , output (N,C,Dout,Hout,Wout)(N, C, D\_{out}, H\_{out}, W\_{out}) and `kernel_size` (kD,kH,kW)(kD, kH, kW) can be precisely described as:
out(Ni,Cj,d,h,w)=maxk=0,…,kD−1maxm=0,…,kH−1maxn=0,…,kW−1input(Ni,Cj,stride[0]×d+k,stride[1]×h+m,stride[2]×w+n)\begin{aligned} \text{out}(N\_i, C\_j, d, h, w) ={} & \max\_{k=0, \ldots, kD-1} \max\_{m=0, \ldots, kH-1} \max\_{n=0, \ldots, kW-1} \\ & \text{input}(N\_i, C\_j, \text{stride[0]} \times d + k, \text{stride[1]} \times h + m, \text{stride[2]} \times w + n) \end{aligned}
If `padding` is non-zero, then the input is implicitly zero-padded on both sides for `padding` number of points. `dilation` controls the spacing between the kernel points. It is harder to describe, but this [link](https://github.com/vdumoulin/conv_arithmetic/blob/master/README.md) has a nice visualization of what `dilation` does.
Note
When ceil\_mode=True, sliding windows are allowed to go off-bounds if they start within the left padding or the input. Sliding windows that would start in the right padded region are ignored.
The parameters `kernel_size`, `stride`, `padding`, `dilation` can either be:
* a single `int` – in which case the same value is used for the depth, height and width dimension
* a `tuple` of three ints – in which case, the first `int` is used for the depth dimension, the second `int` for the height dimension and the third `int` for the width dimension
Parameters
* **kernel\_size** – the size of the window to take a max over
* **stride** – the stride of the window. Default value is `kernel_size`
* **padding** – implicit zero padding to be added on all three sides
* **dilation** – a parameter that controls the stride of elements in the window
* **return\_indices** – if `True`, will return the max indices along with the outputs. Useful for [`torch.nn.MaxUnpool3d`](torch.nn.maxunpool3d#torch.nn.MaxUnpool3d "torch.nn.MaxUnpool3d") later
* **ceil\_mode** – when True, will use `ceil` instead of `floor` to compute the output shape
Shape:
* Input: (N,C,Din,Hin,Win)(N, C, D\_{in}, H\_{in}, W\_{in})
* Output: (N,C,Dout,Hout,Wout)(N, C, D\_{out}, H\_{out}, W\_{out}) , where
Dout=⌊Din+2×padding[0]−dilation[0]×(kernel\_size[0]−1)−1stride[0]+1⌋D\_{out} = \left\lfloor\frac{D\_{in} + 2 \times \text{padding}[0] - \text{dilation}[0] \times (\text{kernel\\_size}[0] - 1) - 1}{\text{stride}[0]} + 1\right\rfloor
Hout=⌊Hin+2×padding[1]−dilation[1]×(kernel\_size[1]−1)−1stride[1]+1⌋H\_{out} = \left\lfloor\frac{H\_{in} + 2 \times \text{padding}[1] - \text{dilation}[1] \times (\text{kernel\\_size}[1] - 1) - 1}{\text{stride}[1]} + 1\right\rfloor
Wout=⌊Win+2×padding[2]−dilation[2]×(kernel\_size[2]−1)−1stride[2]+1⌋W\_{out} = \left\lfloor\frac{W\_{in} + 2 \times \text{padding}[2] - \text{dilation}[2] \times (\text{kernel\\_size}[2] - 1) - 1}{\text{stride}[2]} + 1\right\rfloor
Examples:
```
>>> # pool of square window of size=3, stride=2
>>> m = nn.MaxPool3d(3, stride=2)
>>> # pool of non-square window
>>> m = nn.MaxPool3d((3, 2, 2), stride=(2, 1, 2))
>>> input = torch.randn(20, 16, 50,44, 31)
>>> output = m(input)
```
pytorch torch.nn.utils.prune.l1_unstructured torch.nn.utils.prune.l1\_unstructured
=====================================
`torch.nn.utils.prune.l1_unstructured(module, name, amount, importance_scores=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/prune.html#l1_unstructured)
Prunes tensor corresponding to parameter called `name` in `module` by removing the specified `amount` of (currently unpruned) units with the lowest L1-norm. Modifies module in place (and also return the modified module) by: 1) adding a named buffer called `name+'_mask'` corresponding to the binary mask applied to the parameter `name` by the pruning method. 2) replacing the parameter `name` by its pruned version, while the original (unpruned) parameter is stored in a new parameter named `name+'_orig'`.
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – module containing the tensor to prune
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")) – parameter name within `module` on which pruning will act.
* **amount** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)") *or* [float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")) – quantity of parameters to prune. If `float`, should be between 0.0 and 1.0 and represent the fraction of parameters to prune. If `int`, it represents the absolute number of parameters to prune.
* **importance\_scores** ([torch.Tensor](../tensors#torch.Tensor "torch.Tensor")) – tensor of importance scores (of same shape as module parameter) used to compute mask for pruning. The values in this tensor indicate the importance of the corresponding elements in the parameter being pruned. If unspecified or None, the module parameter will be used in its place.
Returns
modified (i.e. pruned) version of the input module
Return type
module ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module"))
#### Examples
```
>>> m = prune.l1_unstructured(nn.Linear(2, 3), 'weight', amount=0.2)
>>> m.state_dict().keys()
odict_keys(['bias', 'weight_orig', 'weight_mask'])
```
pytorch torch.nn.utils.spectral_norm torch.nn.utils.spectral\_norm
=============================
`torch.nn.utils.spectral_norm(module, name='weight', n_power_iterations=1, eps=1e-12, dim=None)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/utils/spectral_norm.html#spectral_norm)
Applies spectral normalization to a parameter in the given module.
WSN=Wσ(W),σ(W)=maxh:h≠0∥Wh∥2∥h∥2\mathbf{W}\_{SN} = \dfrac{\mathbf{W}}{\sigma(\mathbf{W})}, \sigma(\mathbf{W}) = \max\_{\mathbf{h}: \mathbf{h} \ne 0} \dfrac{\|\mathbf{W} \mathbf{h}\|\_2}{\|\mathbf{h}\|\_2}
Spectral normalization stabilizes the training of discriminators (critics) in Generative Adversarial Networks (GANs) by rescaling the weight tensor with spectral norm σ\sigma of the weight matrix calculated using power iteration method. If the dimension of the weight tensor is greater than 2, it is reshaped to 2D in power iteration method to get spectral norm. This is implemented via a hook that calculates spectral norm and rescales weight before every `forward()` call.
See [Spectral Normalization for Generative Adversarial Networks](https://arxiv.org/abs/1802.05957) .
Parameters
* **module** ([nn.Module](torch.nn.module#torch.nn.Module "torch.nn.Module")) – containing module
* **name** ([str](https://docs.python.org/3/library/stdtypes.html#str "(in Python v3.9)")*,* *optional*) – name of weight parameter
* **n\_power\_iterations** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – number of power iterations to calculate spectral norm
* **eps** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)")*,* *optional*) – epsilon for numerical stability in calculating norms
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – dimension corresponding to number of outputs, the default is `0`, except for modules that are instances of ConvTranspose{1,2,3}d, when it is `1`
Returns
The original module with the spectral norm hook
Example:
```
>>> m = spectral_norm(nn.Linear(20, 40))
>>> m
Linear(in_features=20, out_features=40, bias=True)
>>> m.weight_u.size()
torch.Size([40])
```
pytorch GRUCell GRUCell
=======
`class torch.nn.GRUCell(input_size, hidden_size, bias=True)` [[source]](https://pytorch.org/docs/1.8.0/_modules/torch/nn/modules/rnn.html#GRUCell)
A gated recurrent unit (GRU) cell
r=σ(Wirx+bir+Whrh+bhr)z=σ(Wizx+biz+Whzh+bhz)n=tanh(Winx+bin+r∗(Whnh+bhn))h′=(1−z)∗n+z∗h\begin{array}{ll} r = \sigma(W\_{ir} x + b\_{ir} + W\_{hr} h + b\_{hr}) \\ z = \sigma(W\_{iz} x + b\_{iz} + W\_{hz} h + b\_{hz}) \\ n = \tanh(W\_{in} x + b\_{in} + r \* (W\_{hn} h + b\_{hn})) \\ h' = (1 - z) \* n + z \* h \end{array}
where σ\sigma is the sigmoid function, and ∗\* is the Hadamard product.
Parameters
* **input\_size** – The number of expected features in the input `x`
* **hidden\_size** – The number of features in the hidden state `h`
* **bias** – If `False`, then the layer does not use bias weights `b_ih` and `b_hh`. Default: `True`
Inputs: input, hidden
* **input** of shape `(batch, input_size)`: tensor containing input features
* **hidden** of shape `(batch, hidden_size)`: tensor containing the initial hidden state for each element in the batch. Defaults to zero if not provided.
Outputs: h’
* **h’** of shape `(batch, hidden_size)`: tensor containing the next hidden state for each element in the batch
Shape:
* Input1: (N,Hin)(N, H\_{in}) tensor containing input features where HinH\_{in} = `input_size`
* Input2: (N,Hout)(N, H\_{out}) tensor containing the initial hidden state for each element in the batch where HoutH\_{out} = `hidden_size` Defaults to zero if not provided.
* Output: (N,Hout)(N, H\_{out}) tensor containing the next hidden state for each element in the batch
Variables
* **~GRUCell.weight\_ih** – the learnable input-hidden weights, of shape `(3*hidden_size, input_size)`
* **~GRUCell.weight\_hh** – the learnable hidden-hidden weights, of shape `(3*hidden_size, hidden_size)`
* **~GRUCell.bias\_ih** – the learnable input-hidden bias, of shape `(3*hidden_size)`
* **~GRUCell.bias\_hh** – the learnable hidden-hidden bias, of shape `(3*hidden_size)`
Note
All the weights and biases are initialized from U(−k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k}) where k=1hidden\_sizek = \frac{1}{\text{hidden\\_size}}
Examples:
```
>>> rnn = nn.GRUCell(10, 20)
>>> input = torch.randn(6, 3, 10)
>>> hx = torch.randn(3, 20)
>>> output = []
>>> for i in range(6):
hx = rnn(input[i], hx)
output.append(hx)
```
pytorch torch.quantile torch.quantile
==============
`torch.quantile(input, q) → Tensor`
Returns the q-th quantiles of all elements in the `input` tensor, doing a linear interpolation when the q-th quantile lies between two data points.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **q** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) – a scalar or 1D tensor of quantile values in the range [0, 1]
Example:
```
>>> a = torch.randn(1, 3)
>>> a
tensor([[ 0.0700, -0.5446, 0.9214]])
>>> q = torch.tensor([0, 0.5, 1])
>>> torch.quantile(a, q)
tensor([-0.5446, 0.0700, 0.9214])
```
`torch.quantile(input, q, dim=None, keepdim=False, *, out=None) → Tensor`
Returns the q-th quantiles of each row of the `input` tensor along the dimension `dim`, doing a linear interpolation when the q-th quantile lies between two data points. By default, `dim` is `None` resulting in the `input` tensor being flattened before computation.
If `keepdim` is `True`, the output dimensions are of the same size as `input` except in the dimensions being reduced (`dim` or all if `dim` is `None`) where they have size 1. Otherwise, the dimensions being reduced are squeezed (see [`torch.squeeze()`](torch.squeeze#torch.squeeze "torch.squeeze")). If `q` is a 1D tensor, an extra dimension is prepended to the output tensor with the same size as `q` which represents the quantiles.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **q** ([float](https://docs.python.org/3/library/functions.html#float "(in Python v3.9)") *or* [Tensor](../tensors#torch.Tensor "torch.Tensor")) – a scalar or 1D tensor of quantile values in the range [0, 1]
* **dim** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")) – the dimension to reduce.
* **keepdim** ([bool](https://docs.python.org/3/library/functions.html#bool "(in Python v3.9)")) – whether the output tensor has `dim` retained or not.
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(2, 3)
>>> a
tensor([[ 0.0795, -1.2117, 0.9765],
[ 1.1707, 0.6706, 0.4884]])
>>> q = torch.tensor([0.25, 0.5, 0.75])
>>> torch.quantile(a, q, dim=1, keepdim=True)
tensor([[[-0.5661],
[ 0.5795]],
[[ 0.0795],
[ 0.6706]],
[[ 0.5280],
[ 0.9206]]])
>>> torch.quantile(a, q, dim=1, keepdim=True).shape
torch.Size([3, 2, 1])
```
pytorch torch.subtract torch.subtract
==============
`torch.subtract(input, other, *, alpha=1, out=None) → Tensor`
Alias for [`torch.sub()`](torch.sub#torch.sub "torch.sub").
pytorch torch.diag torch.diag
==========
`torch.diag(input, diagonal=0, *, out=None) → Tensor`
* If `input` is a vector (1-D tensor), then returns a 2-D square tensor with the elements of `input` as the diagonal.
* If `input` is a matrix (2-D tensor), then returns a 1-D tensor with the diagonal elements of `input`.
The argument [`diagonal`](torch.diagonal#torch.diagonal "torch.diagonal") controls which diagonal to consider:
* If [`diagonal`](torch.diagonal#torch.diagonal "torch.diagonal") = 0, it is the main diagonal.
* If [`diagonal`](torch.diagonal#torch.diagonal "torch.diagonal") > 0, it is above the main diagonal.
* If [`diagonal`](torch.diagonal#torch.diagonal "torch.diagonal") < 0, it is below the main diagonal.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **diagonal** ([int](https://docs.python.org/3/library/functions.html#int "(in Python v3.9)")*,* *optional*) – the diagonal to consider
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
See also
[`torch.diagonal()`](torch.diagonal#torch.diagonal "torch.diagonal") always returns the diagonal of its input.
[`torch.diagflat()`](torch.diagflat#torch.diagflat "torch.diagflat") always constructs a tensor with diagonal elements specified by the input.
Examples:
Get the square matrix where the input vector is the diagonal:
```
>>> a = torch.randn(3)
>>> a
tensor([ 0.5950,-0.0872, 2.3298])
>>> torch.diag(a)
tensor([[ 0.5950, 0.0000, 0.0000],
[ 0.0000,-0.0872, 0.0000],
[ 0.0000, 0.0000, 2.3298]])
>>> torch.diag(a, 1)
tensor([[ 0.0000, 0.5950, 0.0000, 0.0000],
[ 0.0000, 0.0000,-0.0872, 0.0000],
[ 0.0000, 0.0000, 0.0000, 2.3298],
[ 0.0000, 0.0000, 0.0000, 0.0000]])
```
Get the k-th diagonal of a given matrix:
```
>>> a = torch.randn(3, 3)
>>> a
tensor([[-0.4264, 0.0255,-0.1064],
[ 0.8795,-0.2429, 0.1374],
[ 0.1029,-0.6482,-1.6300]])
>>> torch.diag(a, 0)
tensor([-0.4264,-0.2429,-1.6300])
>>> torch.diag(a, 1)
tensor([ 0.0255, 0.1374])
```
pytorch torch.add torch.add
=========
`torch.add(input, other, *, out=None)`
Adds the scalar `other` to each element of the input `input` and returns a new resulting tensor.
out=input+other\text{out} = \text{input} + \text{other}
If `input` is of type FloatTensor or DoubleTensor, `other` must be a real number, otherwise it should be an integer.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the input tensor.
* **value** (*Number*) – the number to be added to each element of `input`
Keyword Arguments
**out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([ 0.0202, 1.0985, 1.3506, -0.6056])
>>> torch.add(a, 20)
tensor([ 20.0202, 21.0985, 21.3506, 19.3944])
```
`torch.add(input, other, *, alpha=1, out=None)`
Each element of the tensor `other` is multiplied by the scalar `alpha` and added to each element of the tensor `input`. The resulting tensor is returned.
The shapes of `input` and `other` must be [broadcastable](https://pytorch.org/docs/1.8.0/notes/broadcasting.html#broadcasting-semantics).
out=input+alpha×other\text{out} = \text{input} + \text{alpha} \times \text{other}
If `other` is of type FloatTensor or DoubleTensor, `alpha` must be a real number, otherwise it should be an integer.
Parameters
* **input** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the first input tensor
* **other** ([Tensor](../tensors#torch.Tensor "torch.Tensor")) – the second input tensor
Keyword Arguments
* **alpha** (*Number*) – the scalar multiplier for `other`
* **out** ([Tensor](../tensors#torch.Tensor "torch.Tensor")*,* *optional*) – the output tensor.
Example:
```
>>> a = torch.randn(4)
>>> a
tensor([-0.9732, -0.3497, 0.6245, 0.4022])
>>> b = torch.randn(4, 1)
>>> b
tensor([[ 0.3743],
[-1.7724],
[-0.5811],
[-0.8017]])
>>> torch.add(a, b, alpha=10)
tensor([[ 2.7695, 3.3930, 4.3672, 4.1450],
[-18.6971, -18.0736, -17.0994, -17.3216],
[ -6.7845, -6.1610, -5.1868, -5.4090],
[ -8.9902, -8.3667, -7.3925, -7.6147]])
```
| programming_docs |
vueuse Functions Functions
=========
| | |
| --- | --- |
| Core | |
| Add-ons | |
| Sort by | |
| Filters | Has ComponentHas Directive |
### State
[createGlobalState](shared/createglobalstate/index)-keep states in the global scope to be reusable across Vue instances
[createInjectionState](shared/createinjectionstate/index)-create global state that can be injected into components
[createSharedComposable](shared/createsharedcomposable/index)-make a composable function usable with multiple Vue instances
[useAsyncState](core/useasyncstate/index)-reactive async state
[useDebouncedRefHistory](core/usedebouncedrefhistory/index)-shorthand for `useRefHistory` with debounced filter
[useLastChanged](shared/uselastchanged/index)-records the timestamp of the last change
[useLocalStorage](core/uselocalstorage/index)-reactive [LocalStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage)
[useManualRefHistory](core/usemanualrefhistory/index)-manually track the change history of a ref when the using calls `commit()`
[useRefHistory](core/userefhistory/index)-track the change history of a ref
[useSessionStorage](core/usesessionstorage/index)-reactive [SessionStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/sessionStorage)
[useStorage](core/usestorage/index)-reactive [LocalStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage)/[SessionStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/sessionStorage)
[useStorageAsync](core/usestorageasync/index)-reactive Storage in with async support
[useThrottledRefHistory](core/usethrottledrefhistory/index)-shorthand for `useRefHistory` with throttled filter
### Elements
[useActiveElement](core/useactiveelement/index)-reactive `document.activeElement`
[useDocumentVisibility](core/usedocumentvisibility/index)-reactively track [`document.visibilityState`](https://developer.mozilla.org/en-US/docs/Web/API/Document/visibilityState)
[useDraggable](core/usedraggable/index)-make elements draggable
[useDropZone](core/usedropzone/index)-create an zone where files can be dropped
[useElementBounding](core/useelementbounding/index)-reactive [bounding box](https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect) of an HTML element
[useElementSize](core/useelementsize/index)-reactive size of an HTML element
[useElementVisibility](core/useelementvisibility/index)-tracks the visibility of an element within the viewport
[useIntersectionObserver](core/useintersectionobserver/index)-detects that a target element's visibility
[useMouseInElement](core/usemouseinelement/index)-reactive mouse position related to an element
[useMutationObserver](core/usemutationobserver/index)-watch for changes being made to the DOM tree
[useResizeObserver](core/useresizeobserver/index)-reports changes to the dimensions of an Element's content or the border-box
[useWindowFocus](core/usewindowfocus/index)-reactively track window focus with `window.onfocus` and `window.onblur` events
[useWindowScroll](core/usewindowscroll/index)-reactive window scroll
[useWindowSize](core/usewindowsize/index)-reactive window size
### Browser
[useBluetooth](core/usebluetooth/index)-reactive [Web Bluetooth API](https://developer.mozilla.org/en-US/docs/Web/API/Web_Bluetooth_API)
[useBreakpoints](core/usebreakpoints/index)-reactive viewport breakpoints
[useBroadcastChannel](core/usebroadcastchannel/index)-reactive [BroadcastChannel API](https://developer.mozilla.org/en-US/docs/Web/API/BroadcastChannel)
[useBrowserLocation](core/usebrowserlocation/index)-reactive browser location
[useClipboard](core/useclipboard/index)-reactive [Clipboard API](https://developer.mozilla.org/en-US/docs/Web/API/Clipboard_API)
[useColorMode](core/usecolormode/index)-reactive color mode (dark / light / customs) with auto data persistence
[useCssVar](core/usecssvar/index)-manipulate CSS variables
[useDark](core/usedark/index)-reactive dark mode with auto data persistence
[useEventListener](core/useeventlistener/index)-use EventListener with ease
[useEyeDropper](core/useeyedropper/index)-reactive [EyeDropper API](https://developer.mozilla.org/en-US/docs/Web/API/EyeDropper_API)
[useFavicon](core/usefavicon/index)-reactive favicon
[useFileDialog](core/usefiledialog/index)-open file dialog with ease
[useFileSystemAccess](core/usefilesystemaccess/index)-create and read and write local files with [FileSystemAccessAPI](https://developer.mozilla.org/en-US/docs/Web/API/File_System_Access_API)
[useFullscreen](core/usefullscreen/index)-reactive [Fullscreen API](https://developer.mozilla.org/en-US/docs/Web/API/Fullscreen_API)
[useGamepad](core/usegamepad/index)-provides reactive bindings for the [Gamepad API](https://developer.mozilla.org/en-US/docs/Web/API/Gamepad_API)
[useImage](core/useimage/index)-reactive load an image in the browser
[useMediaControls](core/usemediacontrols/index)-reactive media controls for both `audio` and `video` elements
[useMediaQuery](core/usemediaquery/index)-reactive [Media Query](https://developer.mozilla.org/en-US/docs/Web/CSS/Media_Queries/Testing_media_queries)
[useMemory](core/usememory/index)-reactive Memory Info
[useObjectUrl](core/useobjecturl/index)-reactive URL representing an object
[usePermission](core/usepermission/index)-reactive [Permissions API](https://developer.mozilla.org/en-US/docs/Web/API/Permissions_API)
[usePreferredColorScheme](core/usepreferredcolorscheme/index)-reactive [prefers-color-scheme](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-color-scheme) media query
[usePreferredContrast](core/usepreferredcontrast/index)-reactive [prefers-contrast](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-contrast) media query
[usePreferredDark](core/usepreferreddark/index)-reactive dark theme preference
[usePreferredLanguages](core/usepreferredlanguages/index)-reactive [Navigator Languages](https://developer.mozilla.org/en-US/docs/Web/API/NavigatorLanguage/languages)
[usePreferredReducedMotion](core/usepreferredreducedmotion/index)-reactive [prefers-reduced-motion](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-reduced-motion) media query
[useScreenOrientation](core/usescreenorientation/index)-reactive [Screen Orientation API](https://developer.mozilla.org/en-US/docs/Web/API/Screen_Orientation_API)
[useScreenSafeArea](core/usescreensafearea/index)-reactive `env(safe-area-inset-*)`
[useScriptTag](core/usescripttag/index)-script tag injecting
[useShare](core/useshare/index)-reactive [Web Share API](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/share)
[useStyleTag](core/usestyletag/index)-inject reactive `style` element in head
[useTextareaAutosize](core/usetextareaautosize/index)-automatically update the height of a textarea depending on the content
[useTextDirection](core/usetextdirection/index)-reactive [dir](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/dir) of the element's text
[useTitle](core/usetitle/index)-reactive document title
[useUrlSearchParams](core/useurlsearchparams/index)-reactive [URLSearchParams](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams)
[useVibrate](core/usevibrate/index)-reactive [Vibration API](https://developer.mozilla.org/en-US/docs/Web/API/Vibration_API)
[useWakeLock](core/usewakelock/index)-reactive [Screen Wake Lock API](https://developer.mozilla.org/en-US/docs/Web/API/Screen_Wake_Lock_API)
[useWebNotification](core/usewebnotification/index)-reactive [Notification](https://developer.mozilla.org/en-US/docs/Web/API/notification)
[useWebWorker](core/usewebworker/index)-simple [Web Workers](https://developer.mozilla.org/en-US/docs/Web/API/Web_Workers_API/Using_web_workers) registration and communication
[useWebWorkerFn](core/usewebworkerfn/index)-run expensive functions without blocking the UI
### Sensors
[onClickOutside](core/onclickoutside/index)-listen for clicks outside of an element
[onKeyStroke](core/onkeystroke/index)-listen for keyboard key being stroked
[onLongPress](core/onlongpress/index)-listen for a long press on an element
[onStartTyping](core/onstarttyping/index)-fires when users start typing on non-editable elements
[useBattery](core/usebattery/index)-reactive [Battery Status API](https://developer.mozilla.org/en-US/docs/Web/API/Battery_Status_API)
[useDeviceMotion](core/usedevicemotion/index)-reactive [DeviceMotionEvent](https://developer.mozilla.org/en-US/docs/Web/API/DeviceMotionEvent)
[useDeviceOrientation](core/usedeviceorientation/index)-reactive [DeviceOrientationEvent](https://developer.mozilla.org/en-US/docs/Web/API/DeviceOrientationEvent)
[useDevicePixelRatio](core/usedevicepixelratio/index)-reactively track [`window.devicePixelRatio`](https://developer.mozilla.org/ru/docs/Web/API/Window/devicePixelRatio)
[useDevicesList](core/usedeviceslist/index)-reactive [enumerateDevices](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/enumerateDevices) listing available input/output devices
[useDisplayMedia](core/usedisplaymedia/index)-reactive [`mediaDevices.getDisplayMedia`](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/getDisplayMedia) streaming
[useElementByPoint](core/useelementbypoint/index)-reactive element by point
[useElementHover](core/useelementhover/index)-reactive element's hover state
[useFocus](core/usefocus/index)-reactive utility to track or set the focus state of a DOM element
[useFocusWithin](core/usefocuswithin/index)-reactive utility to track if an element or one of its decendants has focus
[useFps](core/usefps/index)-reactive FPS (frames per second)
[useGeolocation](core/usegeolocation/index)-reactive [Geolocation API](https://developer.mozilla.org/en-US/docs/Web/API/Geolocation_API)
[useIdle](core/useidle/index)-tracks whether the user is being inactive
[useInfiniteScroll](core/useinfinitescroll/index)-infinite scrolling of the element
[useKeyModifier](core/usekeymodifier/index)-reactive [Modifier State](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/getModifierState)
[useMagicKeys](core/usemagickeys/index)-reactive keys pressed state
[useMouse](core/usemouse/index)-reactive mouse position
[useMousePressed](core/usemousepressed/index)-reactive mouse pressing state
[useNavigatorLanguage](core/usenavigatorlanguage/index)-reactive [navigator.language](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/language)
[useNetwork](core/usenetwork/index)-reactive [Network status](https://developer.mozilla.org/en-US/docs/Web/API/Network_Information_API)
[useOnline](core/useonline/index)-reactive online state
[usePageLeave](core/usepageleave/index)-reactive state to show whether the mouse leaves the page
[useParallax](core/useparallax/index)-create parallax effect easily
[usePointer](core/usepointer/index)-reactive [pointer state](https://developer.mozilla.org/en-US/docs/Web/API/Pointer_events)
[usePointerSwipe](core/usepointerswipe/index)-reactive swipe detection based on [PointerEvents](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent)
[useScroll](core/usescroll/index)-reactive scroll position and state
[useScrollLock](core/usescrolllock/index)-lock scrolling of the element
[useSpeechRecognition](core/usespeechrecognition/index)-reactive [SpeechRecognition](https://developer.mozilla.org/en-US/docs/Web/API/SpeechRecognition)
[useSpeechSynthesis](core/usespeechsynthesis/index)-reactive [SpeechSynthesis](https://developer.mozilla.org/en-US/docs/Web/API/SpeechSynthesis)
[useSwipe](core/useswipe/index)-reactive swipe detection based on [`TouchEvents`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent)
[useTextSelection](core/usetextselection/index)-reactively track user text selection based on [`Window.getSelection`](https://developer.mozilla.org/en-US/docs/Web/API/Window/getSelection)
[useUserMedia](core/useusermedia/index)-reactive [`mediaDevices.getUserMedia`](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/getUserMedia) streaming
### Network
[useEventSource](core/useeventsource/index)-an [EventSource](https://developer.mozilla.org/en-US/docs/Web/API/EventSource) or [Server-Sent-Events](https://developer.mozilla.org/en-US/docs/Web/API/Server-sent_events) instance opens a persistent connection to an HTTP server
[useFetch](core/usefetch/index)-reactive [Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) provides the ability to abort requests
[useWebSocket](core/usewebsocket/index)-reactive [WebSocket](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket/WebSocket) client
### Animation
[useInterval](shared/useinterval/index)-reactive counter increases on every interval
[useIntervalFn](shared/useintervalfn/index)-wrapper for `setInterval` with controls
[useNow](core/usenow/index)-reactive current Date instance
[useRafFn](core/useraffn/index)-call function on every `requestAnimationFrame`
[useTimeout](shared/usetimeout/index)-update value after a given time with controls
[useTimeoutFn](shared/usetimeoutfn/index)-wrapper for `setTimeout` with controls
[useTimestamp](core/usetimestamp/index)-reactive current timestamp
[useTransition](core/usetransition/index)-transition between values
### Component
[computedInject](core/computedinject/index)-combine computed and inject
[templateRef](core/templateref/index)-shorthand for binding ref to template element
[tryOnBeforeMount](shared/tryonbeforemount/index)-safe `onBeforeMount`
[tryOnBeforeUnmount](shared/tryonbeforeunmount/index)-safe `onBeforeUnmount`
[tryOnMounted](shared/tryonmounted/index)-safe `onMounted`
[tryOnScopeDispose](shared/tryonscopedispose/index)-safe `onScopeDispose`
[tryOnUnmounted](shared/tryonunmounted/index)-safe `onUnmounted`
[unrefElement](core/unrefelement/index)-unref for dom element
[useCurrentElement](core/usecurrentelement/index)-get the DOM element of current component as a ref
[useMounted](core/usemounted/index)-mounted state in ref
[useTemplateRefsList](core/usetemplaterefslist/index)-shorthand for binding refs to template elements and components inside `v-for`
[useVirtualList](core/usevirtuallist/index)-create virtual lists with ease
[useVModel](core/usevmodel/index)-shorthand for v-model binding
[useVModels](core/usevmodels/index)-shorthand for props v-model binding
### Watch
[until](shared/until/index)-promised one-time watch for changes
[watchArray](shared/watcharray/index)-watch for an array with additions and removals
[watchAtMost](shared/watchatmost/index)-`watch` with the number of times triggered
[watchDebounced](shared/watchdebounced/index)-debounced watch
[watchIgnorable](shared/watchignorable/index)-ignorable watch
[watchOnce](shared/watchonce/index)-`watch` that only triggers once
[watchPausable](shared/watchpausable/index)-pausable watch
[watchThrottled](shared/watchthrottled/index)-throttled watch
[watchTriggerable](shared/watchtriggerable/index)-watch that can be triggered manually
[watchWithFilter](shared/watchwithfilter/index)-`watch` with additional EventFilter control
[whenever](shared/whenever/index)-shorthand for watching value to be truthy
### Reactivity
[computedAsync](core/computedasync/index)-computed for async functions
[computedEager](shared/computedeager/index)-eager computed without lazy evaluation
[computedWithControl](shared/computedwithcontrol/index)-explicitly define the dependencies of computed
[extendRef](shared/extendref/index)-add extra attributes to Ref
[reactify](shared/reactify/index)-converts plain functions into reactive functions
[reactifyObject](shared/reactifyobject/index)-apply `reactify` to an object
[reactiveComputed](shared/reactivecomputed/index)-computed reactive object
[reactiveOmit](shared/reactiveomit/index)-reactively omit fields from a reactive object
[reactivePick](shared/reactivepick/index)-reactively pick fields from a reactive object
[refAutoReset](shared/refautoreset/index)-a ref which will be reset to the default value after some time
[refDebounced](shared/refdebounced/index)-debounce execution of a ref value
[refDefault](shared/refdefault/index)-apply default value to a ref
[refThrottled](shared/refthrottled/index)-throttle changing of a ref value
[refWithControl](shared/refwithcontrol/index)-fine-grained controls over ref and its reactivity
[resolveRef](shared/resolveref/index)-normalize value/ref/getter to `ref` or `computed`
[resolveUnref](shared/resolveunref/index)-get the value of value/ref/getter
[syncRef](shared/syncref/index)-two-way refs synchronization
[syncRefs](shared/syncrefs/index)-keep target refs in sync with a source ref
[toReactive](shared/toreactive/index)-converts ref to reactive
[toRefs](shared/torefs/index)-extended [`toRefs`](https://v3.vuejs.org/api/refs-api.html#torefs) that also accepts refs of an object
### Array
[useArrayEvery](shared/usearrayevery/index)-reactive `Array.every`
[useArrayFilter](shared/usearrayfilter/index)-reactive `Array.filter`
[useArrayFind](shared/usearrayfind/index)-reactive `Array.find`
[useArrayFindIndex](shared/usearrayfindindex/index)-reactive `Array.findIndex`
[useArrayJoin](shared/usearrayjoin/index)-reactive `Array.join`
[useArrayMap](shared/usearraymap/index)-reactive `Array.map`
[useArrayReduce](shared/usearrayreduce/index)-reactive `Array.reduce`
[useArraySome](shared/usearraysome/index)-reactive `Array.some`
[useArrayUnique](shared/usearrayunique/index)-reactive unique array
[useSorted](core/usesorted/index)-reactive sort array
### Time
[useDateFormat](shared/usedateformat/index)-get the formatted date according to the string of tokens passed in
[useTimeAgo](core/usetimeago/index)-reactive time ago
### Utilities
[createEventHook](shared/createeventhook/index)-utility for creating event hooks
[createUnrefFn](core/createunreffn/index)-make a plain function accepting ref and raw values as arguments
[get](shared/get/index)-shorthand for accessing `ref.value`
[isDefined](shared/isdefined/index)-non-nullish checking type guard for Ref
[makeDestructurable](shared/makedestructurable/index)-make isomorphic destructurable for object and array at the same time
[set](shared/set/index)-shorthand for `ref.value = x`
[useAsyncQueue](core/useasyncqueue/index)-executes each asynchronous task sequentially and passes the current task result to the next task
[useBase64](core/usebase64/index)-reactive base64 transforming
[useCached](core/usecached/index)-cache a ref with a custom comparator
[useCloned](core/usecloned/index)-reactive clone of a ref
[useConfirmDialog](core/useconfirmdialog/index)-creates event hooks to support modals and confirmation dialog chains
[useCounter](shared/usecounter/index)-basic counter with utility functions
[useCycleList](core/usecyclelist/index)-cycle through a list of items
[useDebounceFn](shared/usedebouncefn/index)-debounce execution of a function
[useEventBus](core/useeventbus/index)-a basic event bus
[useMemoize](core/usememoize/index)-cache results of functions depending on arguments and keep it reactive
[useOffsetPagination](core/useoffsetpagination/index)-reactive offset pagination
[useStepper](core/usestepper/index)-provides helpers for building a multi-step wizard interface
[useSupported](core/usesupported/index)-sSR compatibility `isSupported`
[useThrottleFn](shared/usethrottlefn/index)-throttle execution of a function
[useTimeoutPoll](core/usetimeoutpoll/index)-use timeout to poll something
[useToggle](shared/usetoggle/index)-a boolean switcher with utility functions
[useToNumber](shared/usetonumber/index)-reactively convert a string ref to number
[useToString](shared/usetostring/index)-reactively convert a ref to string
### @Electron
[useIpcRenderer](electron/useipcrenderer/index)-provides [ipcRenderer](https://www.electronjs.org/docs/api/ipc-renderer) and all of its APIs
[useIpcRendererInvoke](electron/useipcrendererinvoke/index)-reactive [ipcRenderer.invoke API](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererinvokechannel-args) result
[useIpcRendererOn](electron/useipcrendereron/index)-use [ipcRenderer.on](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereronchannel-listener) with ease and [ipcRenderer.removeListener](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener) automatically on unmounted
[useZoomFactor](electron/usezoomfactor/index)-reactive [WebFrame](https://www.electronjs.org/docs/api/web-frame#webframe) zoom factor
[useZoomLevel](electron/usezoomlevel/index)-reactive [WebFrame](https://www.electronjs.org/docs/api/web-frame#webframe) zoom level
### @Firebase
[useAuth](firebase/useauth/index)-reactive [Firebase Auth](https://firebase.google.com/docs/auth) binding
[useFirestore](firebase/usefirestore/index)-reactive [Firestore](https://firebase.google.com/docs/firestore) binding
[useRTDB](firebase/usertdb/index)-reactive [Firebase Realtime Database](https://firebase.google.com/docs/database) binding
### @Head
[createHead](https://github.com/vueuse/head#api)-create the head manager instance.
[useHead](https://github.com/vueuse/head#api)-update head meta tags reactively.
### @Integrations
[useAsyncValidator](integrations/useasyncvalidator/index)-wrapper for [`async-validator`](https://github.com/yiminghe/async-validator)
[useAxios](integrations/useaxios/index)-wrapper for [`axios`](https://github.com/axios/axios)
[useChangeCase](integrations/usechangecase/index)-reactive wrapper for [`change-case`](https://github.com/blakeembrey/change-case)
[useCookies](integrations/usecookies/index)-wrapper for [`universal-cookie`](https://www.npmjs.com/package/universal-cookie)
[useDrauu](integrations/usedrauu/index)-reactive instance for [drauu](https://github.com/antfu/drauu)
[useFocusTrap](integrations/usefocustrap/index)-reactive wrapper for [`focus-trap`](https://github.com/focus-trap/focus-trap)
[useFuse](integrations/usefuse/index)-easily implement fuzzy search using a composable with [Fuse.js](https://github.com/krisk/fuse)
[useIDBKeyval](integrations/useidbkeyval/index)-wrapper for [`idb-keyval`](https://www.npmjs.com/package/idb-keyval)
[useJwt](integrations/usejwt/index)-wrapper for [`jwt-decode`](https://github.com/auth0/jwt-decode)
[useNProgress](integrations/usenprogress/index)-reactive wrapper for [`nprogress`](https://github.com/rstacruz/nprogress)
[useQRCode](integrations/useqrcode/index)-wrapper for [`qrcode`](https://github.com/soldair/node-qrcode)
### @Math
[createGenericProjection](math/creategenericprojection/index)-generic version of `createProjection`
[createProjection](math/createprojection/index)-reactive numeric projection from one domain to another
[logicAnd](math/logicand/index)-`AND` condition for refs
[logicNot](math/logicnot/index)-`NOT` condition for ref
[logicOr](math/logicor/index)-`OR` conditions for refs
[useAbs](math/useabs/index)-reactive `Math.abs`
[useAverage](math/useaverage/index)-get the average of an array reactively
[useCeil](math/useceil/index)-reactive `Math.ceil`
[useClamp](math/useclamp/index)-reactively clamp a value between two other values
[useFloor](math/usefloor/index)-reactive `Math.floor`
[useMath](math/usemath/index)-reactive `Math` methods
[useMax](math/usemax/index)-reactive `Math.max`
[useMin](math/usemin/index)-reactive `Math.min`
[usePrecision](math/useprecision/index)-reactively set the precision of a number
[useProjection](math/useprojection/index)-reactive numeric projection from one domain to another
[useRound](math/useround/index)-reactive `Math.round`
[useSum](math/usesum/index)-get the sum of an array reactively
[useToFixed](math/usetofixed/index)-reactive `toFixed`
[useTrunc](math/usetrunc/index)-reactive `Math.trunc`
### @Motion
[useElementStyle](https://motion.vueuse.org/api/use-element-style.html)-sync a reactive object to a target element CSS styling
[useElementTransform](https://motion.vueuse.org/api/use-element-transform.html)-sync a reactive object to a target element CSS transform.
[useMotion](https://motion.vueuse.org/api/use-motion.html)-putting your components in motion.
[useMotionProperties](https://motion.vueuse.org/api/use-motion-properties.html)-access Motion Properties for a target element.
[useMotionVariants](https://motion.vueuse.org/api/use-motion-variants.html)-handle the Variants state and selection.
[useSpring](https://motion.vueuse.org/api/use-spring.html)-spring animations.
### @Router
[useRouteHash](router/useroutehash/index)-shorthand for a reactive `route.hash`
[useRouteParams](router/userouteparams/index)-shorthand for a reactive `route.params`
[useRouteQuery](router/useroutequery/index)-shorthand for a reactive `route.query`
### @RxJS
[from](rxjs/from/index)-/ fromEvent
[toObserver](rxjs/toobserver/index)-sugar function to convert a `ref` into an RxJS [Observer](https://rxjs.dev/guide/observer)
[useObservable](rxjs/useobservable/index)-use an RxJS [`Observable`](https://rxjs.dev/guide/observable)
[useSubject](rxjs/usesubject/index)-bind an RxJS [`Subject`](https://rxjs.dev/guide/subject) to a `ref` and propagate value changes both ways
[useSubscription](rxjs/usesubscription/index)-use an RxJS [`Subscription`](https://rxjs.dev/guide/subscription) without worrying about unsubscribing from it or creating memory leaks
### @SchemaOrg
[createSchemaOrg](https://vue-schema-org.netlify.app/api/core/create-schema-org.html)-create the schema.org manager instance.
[useSchemaOrg](https://vue-schema-org.netlify.app/api/core/use-schema-org.html)-update schema.org reactively.
### @Sound
[useSound](https://github.com/vueuse/sound#examples)-play sound effects reactively.
| programming_docs |
vueuse Export size Export size
===========
generated by [export-size](https://github.com/antfu/export-size)
version: 9.1.0
date: 2022-08-13T04:10:16.215Z
> Please note this is bundle size for each individual APIs (excluding Vue). Since we have a lot shared utilities underneath each function, importing two different functions does NOT necessarily mean the bundle size will be the sum of them (usually smaller). Depends on the bundler and minifier you use, the final result might vary, this list is for reference only.
>
>
`@vueuse/core`
| Function | min+gzipped |
| --- | --- |
| `createFetch` | 3.08 kB |
| [`useDark`](core/usedark/index) | 3.02 kB |
| [`useColorMode`](core/usecolormode/index) | 2.86 kB |
| [`useFetch`](core/usefetch/index) | 2.85 kB |
| [`useMediaControls`](core/usemediacontrols/index) | 2.75 kB |
| [`useLocalStorage`](core/uselocalstorage/index) | 2.19 kB |
| [`useSessionStorage`](core/usesessionstorage/index) | 2.19 kB |
| [`useThrottledRefHistory`](core/usethrottledrefhistory/index) | 2.17 kB |
| [`useStorage`](core/usestorage/index) | 2.16 kB |
| [`useDebouncedRefHistory`](core/usedebouncedrefhistory/index) | 2.12 kB |
| [`useUrlSearchParams`](core/useurlsearchparams/index) | 1.98 kB |
| [`useInfiniteScroll`](core/useinfinitescroll/index) | 1.96 kB |
| [`useParallax`](core/useparallax/index) | 1.96 kB |
| [`useTimeAgo`](core/usetimeago/index) | 1.93 kB |
| [`useRefHistory`](core/userefhistory/index) | 1.91 kB |
| [`useStorageAsync`](core/usestorageasync/index) | 1.83 kB |
| [`useVirtualList`](core/usevirtuallist/index) | 1.76 kB |
| [`useGamepad`](core/usegamepad/index) | 1.73 kB |
| [`useWebSocket`](core/usewebsocket/index) | 1.7 kB |
| [`useScroll`](core/usescroll/index) | 1.68 kB |
| [`useDraggable`](core/usedraggable/index) | 1.68 kB |
| [`useTransition`](core/usetransition/index) | 1.66 kB |
| [`useDevicesList`](core/usedeviceslist/index) | 1.63 kB |
| [`useScreenSafeArea`](core/usescreensafearea/index) | 1.57 kB |
| [`useMagicKeys`](core/usemagickeys/index) | 1.57 kB |
| [`useMouseInElement`](core/usemouseinelement/index) | 1.56 kB |
| [`usePointer`](core/usepointer/index) | 1.55 kB |
| [`useElementBounding`](core/useelementbounding/index) | 1.54 kB |
| [`useSwipe`](core/useswipe/index) | 1.53 kB |
| [`usePointerSwipe`](core/usepointerswipe/index) | 1.52 kB |
| [`useFullscreen`](core/usefullscreen/index) | 1.48 kB |
| [`useWebNotification`](core/usewebnotification/index) | 1.48 kB |
| [`useIdle`](core/useidle/index) | 1.43 kB |
| [`useClipboard`](core/useclipboard/index) | 1.41 kB |
| [`useDevicePixelRatio`](core/usedevicepixelratio/index) | 1.41 kB |
| [`useTitle`](core/usetitle/index) | 1.41 kB |
| [`useBase64`](core/usebase64/index) | 1.39 kB |
| [`useBreakpoints`](core/usebreakpoints/index) | 1.38 kB |
| [`useTextDirection`](core/usetextdirection/index) | 1.38 kB |
| [`useWebWorkerFn`](core/usewebworkerfn/index) | 1.37 kB |
| [`useOnline`](core/useonline/index) | 1.37 kB |
| [`useNetwork`](core/usenetwork/index) | 1.35 kB |
| [`onClickOutside`](core/onclickoutside/index) | 1.34 kB |
| [`useFileSystemAccess`](core/usefilesystemaccess/index) | 1.32 kB |
| [`usePermission`](core/usepermission/index) | 1.3 kB |
| [`useMouse`](core/usemouse/index) | 1.29 kB |
| [`useScriptTag`](core/usescripttag/index) | 1.29 kB |
| [`useSpeechSynthesis`](core/usespeechsynthesis/index) | 1.28 kB |
| [`useWakeLock`](core/usewakelock/index) | 1.27 kB |
| [`useElementSize`](core/useelementsize/index) | 1.27 kB |
| [`useBattery`](core/usebattery/index) | 1.27 kB |
| `onKeyPressed` | 1.27 kB |
| `onKeyUp` | 1.27 kB |
| `onKeyDown` | 1.26 kB |
| [`useOffsetPagination`](core/useoffsetpagination/index) | 1.26 kB |
| [`useSpeechRecognition`](core/usespeechrecognition/index) | 1.25 kB |
| [`useScreenOrientation`](core/usescreenorientation/index) | 1.25 kB |
| [`onStartTyping`](core/onstarttyping/index) | 1.24 kB |
| [`useImage`](core/useimage/index) | 1.24 kB |
| [`useVibrate`](core/usevibrate/index) | 1.23 kB |
| [`useScrollLock`](core/usescrolllock/index) | 1.23 kB |
| [`useTimestamp`](core/usetimestamp/index) | 1.23 kB |
| [`useTextSelection`](core/usetextselection/index) | 1.22 kB |
| [`useElementVisibility`](core/useelementvisibility/index) | 1.22 kB |
| [`useDeviceMotion`](core/usedevicemotion/index) | 1.21 kB |
| [`useFocus`](core/usefocus/index) | 1.21 kB |
| [`useMousePressed`](core/usemousepressed/index) | 1.21 kB |
| [`useEventSource`](core/useeventsource/index) | 1.21 kB |
| [`useNow`](core/usenow/index) | 1.21 kB |
| [`useUserMedia`](core/useusermedia/index) | 1.2 kB |
| [`onLongPress`](core/onlongpress/index) | 1.2 kB |
| [`useDeviceOrientation`](core/usedeviceorientation/index) | 1.2 kB |
| [`useResizeObserver`](core/useresizeobserver/index) | 1.19 kB |
| [`useBluetooth`](core/usebluetooth/index) | 1.18 kB |
| [`useMutationObserver`](core/usemutationobserver/index) | 1.18 kB |
| [`useBrowserLocation`](core/usebrowserlocation/index) | 1.17 kB |
| [`useNavigatorLanguage`](core/usenavigatorlanguage/index) | 1.17 kB |
| [`useDropZone`](core/usedropzone/index) | 1.16 kB |
| [`useWindowSize`](core/usewindowsize/index) | 1.16 kB |
| [`onKeyStroke`](core/onkeystroke/index) | 1.16 kB |
| [`useFocusWithin`](core/usefocuswithin/index) | 1.14 kB |
| [`useMemory`](core/usememory/index) | 1.14 kB |
| [`useIntersectionObserver`](core/useintersectionobserver/index) | 1.14 kB |
| [`useManualRefHistory`](core/usemanualrefhistory/index) | 1.14 kB |
| [`useKeyModifier`](core/usekeymodifier/index) | 1.13 kB |
| [`usePageLeave`](core/usepageleave/index) | 1.11 kB |
| [`usePreferredColorScheme`](core/usepreferredcolorscheme/index) | 1.1 kB |
| [`useGeolocation`](core/usegeolocation/index) | 1.1 kB |
| [`useDisplayMedia`](core/usedisplaymedia/index) | 1.1 kB |
| [`useWindowScroll`](core/usewindowscroll/index) | 1.1 kB |
| [`useVModels`](core/usevmodels/index) | 1.09 kB |
| [`usePreferredReducedMotion`](core/usepreferredreducedmotion/index) | 1.09 kB |
| [`useStyleTag`](core/usestyletag/index) | 1.09 kB |
| [`useActiveElement`](core/useactiveelement/index) | 1.08 kB |
| [`useDocumentVisibility`](core/usedocumentvisibility/index) | 1.07 kB |
| [`useWindowFocus`](core/usewindowfocus/index) | 1.07 kB |
| [`usePreferredLanguages`](core/usepreferredlanguages/index) | 1.07 kB |
| [`useBroadcastChannel`](core/usebroadcastchannel/index) | 1.07 kB |
| [`useVModel`](core/usevmodel/index) | 1.06 kB |
| [`usePreferredDark`](core/usepreferreddark/index) | 1.06 kB |
| [`useElementByPoint`](core/useelementbypoint/index) | 1.05 kB |
| [`useElementHover`](core/useelementhover/index) | 1.05 kB |
| [`useAsyncState`](core/useasyncstate/index) | 1.04 kB |
| [`useFps`](core/usefps/index) | 1.04 kB |
| [`useStepper`](core/usestepper/index) | 1.03 kB |
| [`useMediaQuery`](core/usemediaquery/index) | 1.02 kB |
| [`useShare`](core/useshare/index) | 1.02 kB |
| [`useAsyncQueue`](core/useasyncqueue/index) | 1.02 kB |
| [`useTimeoutPoll`](core/usetimeoutpoll/index) | 1.02 kB |
| [`useMemoize`](core/usememoize/index) | 1 kB |
| [`useEventListener`](core/useeventlistener/index) | 997 B |
| [`useCssVar`](core/usecssvar/index) | 990 B |
| [`useConfirmDialog`](core/useconfirmdialog/index) | 985 B |
| [`useFileDialog`](core/usefiledialog/index) | 978 B |
| `asyncComputed` | 960 B |
| `computedAsync` | 960 B |
| [`useEventBus`](core/useeventbus/index) | 948 B |
| [`useEyeDropper`](core/useeyedropper/index) | 940 B |
| [`useCycleList`](core/usecyclelist/index) | 931 B |
| [`useFavicon`](core/usefavicon/index) | 930 B |
| [`useRafFn`](core/useraffn/index) | 928 B |
| [`useWebWorker`](core/usewebworker/index) | 927 B |
| `mapGamepadToXbox360Controller` | 911 B |
| [`templateRef`](core/templateref/index) | 905 B |
| [`useTextareaAutosize`](core/usetextareaautosize/index) | 889 B |
| [`useObjectUrl`](core/useobjecturl/index) | 884 B |
| `StorageSerializers` | 874 B |
| [`useSupported`](core/usesupported/index) | 823 B |
| [`useCurrentElement`](core/usecurrentelement/index) | 813 B |
| [`computedInject`](core/computedinject/index) | 803 B |
| `DefaultMagicKeysAliasMap` | 793 B |
| [`useTemplateRefsList`](core/usetemplaterefslist/index) | 793 B |
| [`useCached`](core/usecached/index) | 792 B |
| [`unrefElement`](core/unrefelement/index) | 783 B |
| `breakpointsSematic` | 780 B |
| [`useMounted`](core/usemounted/index) | 777 B |
| [`createUnrefFn`](core/createunreffn/index) | 770 B |
| `breakpointsAntDesign` | 769 B |
| `breakpointsBootstrapV5` | 767 B |
| `breakpointsTailwind` | 763 B |
| `breakpointsQuasar` | 762 B |
| `breakpointsVuetify` | 754 B |
| `getSSRHandler` | 745 B |
| `setSSRHandler` | 743 B |
| `defaultNavigator` | 735 B |
| `defaultLocation` | 732 B |
| `defaultDocument` | 731 B |
| `defaultWindow` | 731 B |
| `SwipeDirection` | 724 B |
| `TransitionPresets` | 723 B |
`@vueuse/components`
| Function | min+gzipped |
| --- | --- |
| `VOnClickOutside` | 7.75 kB |
| `vOnClickOutside` | 7.75 kB |
| `vScroll` | 7.53 kB |
| `vOnKeyStroke` | 7.52 kB |
| `vElementSize` | 7.52 kB |
| `vElementVisibility` | 7.51 kB |
| `vElementHover` | 7.5 kB |
| `VOnLongPress` | 7.5 kB |
| `vOnLongPress` | 7.5 kB |
| `vIntersectionObserver` | 7.49 kB |
| `vInfiniteScroll` | 7.48 kB |
| `UseDraggable` | 7.48 kB |
| `UseEyeDropper` | 7.47 kB |
| `UseFullscreen` | 7.47 kB |
| `UseDeviceOrientation` | 7.46 kB |
| `UseDevicesList` | 7.46 kB |
| `UseOnline` | 7.46 kB |
| `UseGeolocation` | 7.46 kB |
| `UseMouseInElement` | 7.46 kB |
| `UseColorMode` | 7.46 kB |
| `UsePreferredDark` | 7.46 kB |
| `UseTimestamp` | 7.46 kB |
| `OnLongPress` | 7.46 kB |
| `UseNetwork` | 7.46 kB |
| `UseBrowserLocation` | 7.46 kB |
| `UseActiveElement` | 7.46 kB |
| `UseMousePressed` | 7.46 kB |
| `OnClickOutside` | 7.45 kB |
| `vScrollLock` | 7.45 kB |
| `UsePreferredLanguages` | 7.45 kB |
| `UseDocumentVisibility` | 7.45 kB |
| `UseElementVisibility` | 7.45 kB |
| `UseTimeAgo` | 7.45 kB |
| `UseWindowSize` | 7.45 kB |
| `UseBattery` | 7.45 kB |
| `UsePointer` | 7.45 kB |
| `UseObjectUrl` | 7.45 kB |
| `UsePageLeave` | 7.45 kB |
| `UseIdle` | 7.44 kB |
| `UseMouse` | 7.44 kB |
| `UseDark` | 7.44 kB |
| `UseWindowFocus` | 7.44 kB |
| `UseVirtualList` | 7.44 kB |
| `UseScreenSafeArea` | 7.42 kB |
| `UseElementBounding` | 7.42 kB |
| `UseNow` | 7.41 kB |
| `UsePreferredReducedMotion` | 7.41 kB |
| `UseDevicePixelRatio` | 7.41 kB |
| `UseElementSize` | 7.41 kB |
| `UseImage` | 7.4 kB |
| `UsePreferredColorScheme` | 7.4 kB |
| `UseDeviceMotion` | 7.4 kB |
| `UseOffsetPagination` | 7.4 kB |
`@vueuse/math`
| Function | min+gzipped |
| --- | --- |
| [`useToFixed`](math/usetofixed/index) | 300 B |
| [`useProjection`](math/useprojection/index) | 278 B |
| [`useMath`](math/usemath/index) | 274 B |
| [`useAverage`](math/useaverage/index) | 273 B |
| [`useSum`](math/usesum/index) | 270 B |
| [`useMin`](math/usemin/index) | 266 B |
| [`createProjection`](math/createprojection/index) | 264 B |
| [`useMax`](math/usemax/index) | 261 B |
| [`useClamp`](math/useclamp/index) | 259 B |
| [`usePrecision`](math/useprecision/index) | 246 B |
| [`createGenericProjection`](math/creategenericprojection/index) | 210 B |
| `and` | 204 B |
| [`logicAnd`](math/logicand/index) | 204 B |
| [`useTrunc`](math/usetrunc/index) | 204 B |
| [`useCeil`](math/useceil/index) | 202 B |
| [`logicOr`](math/logicor/index) | 201 B |
| `or` | 201 B |
| [`useRound`](math/useround/index) | 200 B |
| [`useAbs`](math/useabs/index) | 199 B |
| [`useFloor`](math/usefloor/index) | 199 B |
| [`logicNot`](math/logicnot/index) | 192 B |
| `not` | 192 B |
`@vueuse/nuxt`
| Function | min+gzipped |
| --- | --- |
| `default` | 671 B |
`@vueuse/router`
| Function | min+gzipped |
| --- | --- |
| [`useRouteQuery`](router/useroutequery/index) | 461 B |
| [`useRouteParams`](router/userouteparams/index) | 454 B |
| [`useRouteHash`](router/useroutehash/index) | 185 B |
`@vueuse/integrations`
| Function | min+gzipped |
| --- | --- |
| [`useAxios`](integrations/useaxios/index) | 1.4 kB |
| [`useAsyncValidator`](integrations/useasyncvalidator/index) | 1.2 kB |
| [`useDrauu`](integrations/usedrauu/index) | 957 B |
| `createCookies` | 771 B |
| [`useCookies`](integrations/usecookies/index) | 730 B |
| [`useFocusTrap`](integrations/usefocustrap/index) | 681 B |
| [`useFuse`](integrations/usefuse/index) | 490 B |
| [`useNProgress`](integrations/usenprogress/index) | 462 B |
| [`useChangeCase`](integrations/usechangecase/index) | 447 B |
| [`useJwt`](integrations/usejwt/index) | 359 B |
| [`useQRCode`](integrations/useqrcode/index) | 339 B |
`@vueuse/rxjs`
| Function | min+gzipped |
| --- | --- |
| [`useSubject`](rxjs/usesubject/index) | 310 B |
| [`useObservable`](rxjs/useobservable/index) | 279 B |
| [`useSubscription`](rxjs/usesubscription/index) | 222 B |
| `fromEvent` | 210 B |
| [`from`](rxjs/from/index) | 153 B |
| [`toObserver`](rxjs/toobserver/index) | 88 B |
`@vueuse/firebase`
| Function | min+gzipped |
| --- | --- |
| [`useFirestore`](firebase/usefirestore/index) | 506 B |
| [`useRTDB`](firebase/usertdb/index) | 287 B |
| [`useAuth`](firebase/useauth/index) | 164 B |
`@vueuse/electron`
| Function | min+gzipped |
| --- | --- |
| [`useIpcRenderer`](electron/useipcrenderer/index) | 535 B |
| [`useZoomFactor`](electron/usezoomfactor/index) | 393 B |
| [`useZoomLevel`](electron/usezoomlevel/index) | 358 B |
| [`useIpcRendererOn`](electron/useipcrendereron/index) | 336 B |
| [`useIpcRendererInvoke`](electron/useipcrendererinvoke/index) | 303 B |
`@vueuse/shared`
| Function | min+gzipped |
| --- | --- |
| [`watchTriggerable`](shared/watchtriggerable/index) | 987 B |
| `throttledWatch` | 824 B |
| [`watchThrottled`](shared/watchthrottled/index) | 824 B |
| `debouncedWatch` | 786 B |
| [`watchDebounced`](shared/watchdebounced/index) | 786 B |
| `ignorableWatch` | 763 B |
| [`watchIgnorable`](shared/watchignorable/index) | 763 B |
| [`useDateFormat`](shared/usedateformat/index) | 734 B |
| `pausableWatch` | 714 B |
| [`watchPausable`](shared/watchpausable/index) | 714 B |
| [`until`](shared/until/index) | 653 B |
| [`useInterval`](shared/useinterval/index) | 638 B |
| [`useTimeout`](shared/usetimeout/index) | 621 B |
| [`watchAtMost`](shared/watchatmost/index) | 561 B |
| [`toRefs`](shared/torefs/index) | 525 B |
| `controlledRef` | 523 B |
| [`refWithControl`](shared/refwithcontrol/index) | 516 B |
| [`computedEager`](shared/computedeager/index) | 468 B |
| `eagerComputed` | 468 B |
| `formatDate` | 458 B |
| [`refThrottled`](shared/refthrottled/index) | 454 B |
| `throttledRef` | 454 B |
| `useThrottle` | 454 B |
| [`makeDestructurable`](shared/makedestructurable/index) | 430 B |
| [`watchWithFilter`](shared/watchwithfilter/index) | 421 B |
| `debouncedRef` | 415 B |
| [`refDebounced`](shared/refdebounced/index) | 415 B |
| `useDebounce` | 415 B |
| [`reactiveOmit`](shared/reactiveomit/index) | 407 B |
| [`useThrottleFn`](shared/usethrottlefn/index) | 400 B |
| [`useIntervalFn`](shared/useintervalfn/index) | 394 B |
| [`reactifyObject`](shared/reactifyobject/index) | 374 B |
| [`useDebounceFn`](shared/usedebouncefn/index) | 368 B |
| [`useTimeoutFn`](shared/usetimeoutfn/index) | 347 B |
| [`reactiveComputed`](shared/reactivecomputed/index) | 342 B |
| `normalizeDate` | 332 B |
| [`computedWithControl`](shared/computedwithcontrol/index) | 330 B |
| `controlledComputed` | 330 B |
| [`extendRef`](shared/extendref/index) | 330 B |
| [`watchArray`](shared/watcharray/index) | 330 B |
| [`toReactive`](shared/toreactive/index) | 320 B |
| `autoResetRef` | 315 B |
| [`refAutoReset`](shared/refautoreset/index) | 315 B |
| [`syncRef`](shared/syncref/index) | 315 B |
| `throttleFilter` | 313 B |
| `debounceFilter` | 289 B |
| [`createSharedComposable`](shared/createsharedcomposable/index) | 287 B |
| [`useToggle`](shared/usetoggle/index) | 285 B |
| [`useToNumber`](shared/usetonumber/index) | 278 B |
| [`useCounter`](shared/usecounter/index) | 258 B |
| `createReactiveFn` | 250 B |
| [`reactify`](shared/reactify/index) | 250 B |
| [`useArrayReduce`](shared/usearrayreduce/index) | 246 B |
| `increaseWithUnit` | 239 B |
| [`syncRefs`](shared/syncrefs/index) | 236 B |
| [`createEventHook`](shared/createeventhook/index) | 232 B |
| `pausableFilter` | 224 B |
| [`useArrayFindIndex`](shared/usearrayfindindex/index) | 220 B |
| [`useArrayFind`](shared/usearrayfind/index) | 218 B |
| [`useLastChanged`](shared/uselastchanged/index) | 218 B |
| [`useArrayJoin`](shared/usearrayjoin/index) | 215 B |
| [`useArraySome`](shared/usearraysome/index) | 215 B |
| [`set`](shared/set/index) | 214 B |
| [`useArrayEvery`](shared/usearrayevery/index) | 210 B |
| [`useArrayFilter`](shared/usearrayfilter/index) | 209 B |
| [`useArrayMap`](shared/usearraymap/index) | 208 B |
| `createSingletonPromise` | 203 B |
| [`reactivePick`](shared/reactivepick/index) | 201 B |
| [`createInjectionState`](shared/createinjectionstate/index) | 200 B |
| `__onlyVue3` | 199 B |
| `directiveHooks` | 198 B |
| [`createGlobalState`](shared/createglobalstate/index) | 197 B |
| [`useToString`](shared/usetostring/index) | 195 B |
| `createFilterWrapper` | 193 B |
| `objectPick` | 193 B |
| [`refDefault`](shared/refdefault/index) | 193 B |
| [`tryOnBeforeMount`](shared/tryonbeforemount/index) | 192 B |
| [`tryOnMounted`](shared/tryonmounted/index) | 191 B |
| `promiseTimeout` | 189 B |
| [`resolveRef`](shared/resolveref/index) | 188 B |
| [`watchOnce`](shared/watchonce/index) | 187 B |
| [`tryOnScopeDispose`](shared/tryonscopedispose/index) | 183 B |
| [`get`](shared/get/index) | 179 B |
| `rand` | 177 B |
| [`resolveUnref`](shared/resolveunref/index) | 176 B |
| `isWindow` | 175 B |
| `whenever` | 174 B |
| [`tryOnBeforeUnmount`](shared/tryonbeforeunmount/index) | 173 B |
| [`tryOnUnmounted`](shared/tryonunmounted/index) | 169 B |
| `clamp` | 165 B |
| `containsProp` | 165 B |
| `isObject` | 164 B |
| [`isDefined`](shared/isdefined/index) | 158 B |
| `assert` | 155 B |
| `isBoolean` | 151 B |
| `now` | 151 B |
| `isFunction` | 148 B |
| `bypassFilter` | 147 B |
| `isString` | 146 B |
| `invoke` | 145 B |
| `isNumber` | 145 B |
| `timestamp` | 145 B |
| `noop` | 144 B |
| `isDef` | 143 B |
| `identity` | 138 B |
| `isIOS` | 136 B |
| `isClient` | 133 B |
vueuse watchIgnorable watchIgnorable
==============
| | |
| --- | --- |
| Category | [Watch](functions#category=Watch) |
| Export Size | 763 B |
| Last Changed | 7 months ago |
| Alias | `ignorableWatch` |
Ignorable watch
Usage
-----
Extended `watch` that returns extra `ignoreUpdates(updater)` and `ignorePrevAsyncUpdates()` to ignore particular updates to the source.
```
import { watchIgnorable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref('foo')
const { stop, ignoreUpdates } = watchIgnorable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // logs: Changed to bar!
ignoreUpdates(() => {
source.value = 'foobar'
})
await nextTick() // (nothing happened)
source.value = 'hello'
await nextTick() // logs: Changed to hello!
ignoreUpdates(() => {
source.value = 'ignored'
})
source.value = 'logged'
await nextTick() // logs: Changed to logged!
```
Flush timing
------------
[`watchIgnorable`](shared/watchignorable/index)accepts the same options as `watch` and uses the same defaults. So, by default the composable works using `flush: 'pre'`.
`ignorePrevAsyncUpdates`
-------------------------
This feature is only for async flush `'pre'` and `'post'`. If `flush: 'sync'` is used, `ignorePrevAsyncUpdates()` is a no-op as the watch will trigger immediately after each update to the source. It is still provided for sync flush so the code can be more generic.
```
import { watchIgnorable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref('foo')
const { ignorePrevAsyncUpdates } = watchIgnorable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // logs: Changed to bar!
source.value = 'good'
source.value = 'by'
ignorePrevAsyncUpdates()
await nextTick() // (nothing happened)
source.value = 'prev'
ignorePrevAsyncUpdates()
source.value = 'after'
await nextTick() // logs: Changed to after!
```
Recommended Readings
--------------------
* [Ignorable Watch](https://patak.dev/vue/ignorable-watch.html) - by [@matias-capeletto](https://github.com/matias-capeletto)
Type Declarations
-----------------
Show Type Declarations
```
export type IgnoredUpdater = (updater: () => void) => void
export interface WatchIgnorableReturn {
ignoreUpdates: IgnoredUpdater
ignorePrevAsyncUpdates: () => void
stop: WatchStopHandle
}
export declare function watchIgnorable<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export declare function watchIgnorable<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export declare function watchIgnorable<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export { watchIgnorable as ignorableWatch }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/index.md)
| programming_docs |
vueuse Guidelines Guidelines
==========
Here are the guidelines for VueUse functions. You could also take them as a reference for authoring your own composable functions or apps.
You can also find some reasons for those design decisions and also some tips for writing composable functions with [Anthony Fu](https://github.com/antfu)'s talk about VueUse:
* [Composable Vue](https://antfu.me/posts/composable-vue-vueday-2021) - at VueDay 2021
* [可组合的 Vue](https://antfu.me/posts/composable-vue-vueconf-china-2021) - at VueConf China 2021 (in Chinese)
General
-------
* Import all Vue APIs from `"vue-demi"`
* Use `ref` instead `reactive` whenever possible
* Use options object as arguments whenever possible to be more flexible for future extensions.
* Use `shallowRef` instead of `ref` when wrapping large amounts of data.
* Use `configurableWindow` (etc.) when using global variables like `window` to be flexible when working with multi-windows, testing mocks, and SSR.
* When involved with Web APIs that are not yet implemented by the browser widely, also outputs `isSupported` flag
* When using `watch` or `watchEffect` internally, also make the `immediate` and `flush` options configurable whenever possible
* Use [`tryOnUnmounted`](shared/tryonunmounted/index) to clear the side-effects gracefully
* Avoid using console logs
* When the function is asynchronous, return a PromiseLike
Read also: [Best Practice](guide/best-practice)
ShallowRef
----------
Use `shallowRef` instead of `ref` when wrapping large amounts of data.
```
export function useFetch<T>(url: MaybeRef<string>) {
// use `shallowRef` to prevent deep reactivity
const data = shallowRef<T | undefined>()
const error = shallowRef<Error | undefined>()
fetch(unref(url))
.then(r => r.json())
.then(r => data.value = r)
.catch(e => error.value = e)
/* ... */
}
```
Configurable Globals
--------------------
When using global variables like `window` or `document`, support `configurableWindow` or `configurableDocument` in the options interface to make the function flexible when for scenarios like multi-windows, testing mocks, and SSR.
Learn more about the implementation: [`_configurable.ts`](https://github.com/vueuse/vueuse/blob/main/packages/core/_configurable.ts)
```
import type { ConfigurableWindow } from '../_configurable'
import { defaultWindow } from '../_configurable'
export function useActiveElement<T extends HTMLElement>(
options: ConfigurableWindow = {},
) {
const {
// defaultWindow = isClient ? window : undefined
window = defaultWindow,
} = options
let el: T
// skip when in Node.js environment (SSR)
if (window) {
window.addEventListener('blur', () => {
el = window?.document.activeElement
}, true)
}
/* ... */
}
```
Usage example:
```
// in iframe and bind to the parent window
useActiveElement({ window: window.parent })
```
Watch Options
-------------
When using `watch` or `watchEffect` internally, also make the `immediate` and `flush` options configurable whenever possible. For example [`watchDebounced`](shared/watchdebounced/index)
```
import type { WatchOptions } from 'vue-demi'
// extend the watch options
export interface WatchDebouncedOptions extends WatchOptions {
debounce?: number
}
export function watchDebounced(
source: any,
cb: any,
options: WatchDebouncedOptions = {},
): WatchStopHandle {
return watch(
source,
() => { /* ... */ },
options, // pass watch options
)
}
```
Controls
--------
We use the `controls` option allowing users to use functions with a single return for simple usages, while being able to have more controls and flexibility when needed. Read more: [#362](https://github.com/vueuse/vueuse/pull/362).
#### When to provide a `controls` option
* The function is more commonly used with single `ref` or
* Examples: [`useTimestamp`](core/usetimestamp/index) [`useInterval`](shared/useinterval/index)
```
// common usage
const timestamp = useTimestamp()
// more controls for flexibility
const { timestamp, pause, resume } = useTimestamp({ controls: true })
```
Refer to [`useTimestamp`](core/usetimestamp/index)s source code for the implementation of proper TypeScript support.
#### When **NOT** to provide a `controls` option
* The function is more commonly used with multiple returns
* Examples: [`useRafFn`](core/useraffn/index) [`useRefHistory`](core/userefhistory/index)
```
const { pause, resume } = useRafFn(() => {})
```
`isSupported` Flag
-------------------
When involved with Web APIs that are not yet implemented by the browser widely, also outputs `isSupported` flag.
For example [`useShare`](core/useshare/index)
```
export function useShare(
shareOptions: MaybeRef<ShareOptions> = {},
options: ConfigurableNavigator = {},
) {
const { navigator = defaultNavigator } = options
const isSupported = useSupported(() => navigator && 'canShare' in navigator)
const share = async (overrideOptions) => {
if (isSupported.value) {
/* ...implementation */
}
}
return {
isSupported,
share,
}
}
```
Asynchronous Composables
------------------------
When a composable is asynchronous, like [`useFetch`](core/usefetch/index) it is a good idea to return a PromiseLike object from the composable so the user is able to await the function. This is especially useful in the case of Vue's `<Suspense>` api.
* Use a `ref` to determine when the function should resolve e.g. `isFinished`
* Store the return state in a variable as it must be returned twice, once in the return and once in the promise.
* The return type should be an intersection between the return type and a PromiseLike, e.g. `UseFetchReturn & PromiseLike<UseFetchReturn>`
```
export function useFetch<T>(url: MaybeRef<string>): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>> {
const data = shallowRef<T | undefined>()
const error = shallowRef<Error | undefined>()
const isFinished = ref(false)
fetch(unref(url))
.then(r => r.json())
.then(r => data.value = r)
.catch(e => error.value = e)
.finally(() => isFinished.value = true)
// Store the return state in a variable
const state: UseFetchReturn<T> = {
data,
error,
isFinished,
}
return {
...state,
// Adding `then` to an object allows it to be awaited.
then(onFulfilled, onRejected) {
return new Promise<UseFetchReturn<T>>((resolve, reject) => {
until(isFinished)
.toBeTruthy()
.then(() => resolve(state))
.then(() => reject(state))
}).then(onFulfilled, onRejected)
},
}
}
```
Renderless Components
---------------------
* Use render functions instead of Vue SFC
* Wrap the props in `reactive` to easily pass them as props to the slot
* Prefer to use the functions options as prop types instead of recreating them yourself
* Only wrap the slot in an HTML element if the function needs a target to bind to
```
import { defineComponent, reactive } from 'vue-demi'
import type { MouseOptions } from '@vueuse/core'
import { useMouse } from '@vueuse/core'
export const UseMouse = defineComponent<MouseOptions>({
name: 'UseMouse',
props: ['touch', 'resetOnTouchEnds', 'initialValue'] as unknown as undefined,
setup(props, { slots }) {
const data = reactive(useMouse(props))
return () => {
if (slots.default)
return slots.default(data)
}
},
})
```
Sometimes a function may have multiple parameters, in that case, you maybe need to create a new interface to merge all the interfaces into a single interface for the component props.
```
import type { TimeAgoOptions } from '@vueuse/core'
import { useTimeAgo } from '@vueuse/core'
interface UseTimeAgoComponentOptions extends Omit<TimeAgoOptions<true>, 'controls'> {
time: MaybeRef<Date | number | string>
}
export const UseTimeAgo = defineComponent<UseTimeAgoComponentOptions>({ /* ... */ })
```
vueuse Get Started Get Started
===========
[Learn VueUse with video](https://vueschool.io/courses/vueuse-for-everyone?friend=vueuse)VueUse is a collection of utility functions based on [Composition API](https://v3.vuejs.org/guide/composition-api-introduction.html). We assume you are already familiar with the basic ideas of [Composition API](https://v3.vuejs.org/guide/composition-api-introduction.html) before you continue.
Installation
------------
> 🎩 From v4.0, it works for Vue 2 & 3 **within a single package** by the power of [vue-demi](https://github.com/vueuse/vue-demi)!
>
>
```
npm i @vueuse/core
```
[Add ons](https://vueuse.org/add-ons) | [Nuxt Module](index#nuxt)
> From v6.0, VueUse requires `vue` >= v3.2 or `@vue/composition-api` >= v1.1
>
>
###### Demos
* [Vite + Vue 3](https://github.com/vueuse/vueuse-vite-starter)
* [Nuxt 3 + Vue 3](https://github.com/antfu/vitesse-nuxt3)
* [Webpack + Vue 3](https://github.com/vueuse/vueuse-vue3-example)
* [Nuxt 2 + Vue 2](https://github.com/antfu/vitesse-nuxt-bridge)
* [Vue CLI + Vue 2](https://github.com/vueuse/vueuse-vue2-example)
### CDN
```
<script src="https://unpkg.com/@vueuse/shared"></script>
<script src="https://unpkg.com/@vueuse/core"></script>
```
It will be exposed to global as `window.VueUse`
### Nuxt
From v7.2.0, we shipped a Nuxt module to enable auto importing for Nuxt 3 and Nuxt Bridge.
```
npm i -D @vueuse/nuxt @vueuse/core
```
Nuxt 3
```
// nuxt.config.ts
export default {
modules: [
'@vueuse/nuxt',
],
}
```
Nuxt 2
```
// nuxt.config.js
export default {
buildModules: [
'@vueuse/nuxt',
],
}
```
And then use VueUse function anywhere in your Nuxt app. For example:
```
<script setup lang="ts">
const { x, y } = useMouse()
</script>
<template>
<div>pos: {{x}}, {{y}}</div>
</template>
```
Usage Example
-------------
Simply importing the functions you need from `@vueuse/core`
```
import { useLocalStorage, useMouse, usePreferredDark } from '@vueuse/core'
export default {
setup() {
// tracks mouse position
const { x, y } = useMouse()
// is user prefers dark theme
const isDark = usePreferredDark()
// persist state in localStorage
const store = useLocalStorage(
'my-storage',
{
name: 'Apple',
color: 'red',
},
)
return { x, y, isDark, store }
},
}
```
Refer to [functions list](../functions) for more details.
vueuse Components Components
==========
In v5.0, we introduced a new package, `@vueuse/components` providing renderless component versions of composable functions.
Install
-------
```
$ npm i @vueuse/core @vueuse/components
```
Usage
-----
For example of [`onClickOutside`](../core/onclickoutside/index) instead of binding the component ref for functions to consume:
```
<script setup>
import { ref } from 'vue'
import { onClickOutside } from '@vueuse/core'
const el = ref()
function close () {
/* ... */
}
onClickOutside(el, close)
</script>
<template>
<div ref="el">
Click Outside of Me
</div>
</template>
```
We can now use the renderless component which the binding is done automatically:
```
<script setup>
import { OnClickOutside } from '@vueuse/components'
function close () {
/* ... */
}
</script>
<template>
<OnClickOutside @trigger="close">
<div>
Click Outside of Me
</div>
</OnClickOutside>
</template>
```
Return Value
------------
You can access return values with `v-slot`:
```
<UseMouse v-slot="{ x, y }">
x: {{ x }}
y: {{ y }}
</UseMouse>
```
```
<UseDark v-slot="{ isDark, toggleDark }">
<button @click="toggleDark()">
Is Dark: {{ isDark }}
</button>
</UseDark>
```
Refer to each function's documentation for the detailed usage of component style.
vueuse Configurations Configurations
==============
These show the general configurations for most of the functions in VueUse.
### Event Filters
From v4.0, we provide the Event Filters system to give the flexibility to control when events will get triggered. For example, you can use `throttleFilter` and `debounceFilter` to control the event trigger rate:
```
import { debounceFilter, throttleFilter, useLocalStorage, useMouse } from '@vueuse/core'
// changes will write to localStorage with a throttled 1s
const storage = useLocalStorage('my-key', { foo: 'bar' }, { eventFilter: throttleFilter(1000) })
// mouse position will be updated after mouse idle for 100ms
const { x, y } = useMouse({ eventFilter: debounceFilter(100) })
```
Moreover, you can utilize `pausableFilter` to temporarily pause some events.
```
import { pausableFilter, useDeviceMotion } from '@vueuse/core'
const motionControl = pausableFilter()
const motion = useDeviceMotion({ eventFilter: motionControl.eventFilter })
motionControl.pause()
// motion updates paused
motionControl.resume()
// motion updates resumed
```
### Reactive Timing
VueUse's functions follow Vue's reactivity system defaults for [flush timing](https://vuejs.org/guide/essentials/watchers.html#callback-flush-timing) where possible.
For `watch`-like composables (e.g. `pausableWatch`, `whenever`, [`useStorage`](../core/usestorage/index) [`useRefHistory`](../core/userefhistory/index) the default is `{ flush: 'pre' }`. Which means they will buffer invalidated effects and flush them asynchronously. This avoids unnecessary duplicate invocation when there are multiple state mutations happening in the same "tick".
In the same way as with `watch`, VueUse allows you to configure the timing by passing the `flush` option:
```
const { pause, resume } = pausableWatch(
() => {
// Safely access updated DOM
},
{ flush: 'post' },
)
```
**flush option (default: `'pre'`)**
* `'pre'`: buffers invalidated effects in the same 'tick' and flushes them before rendering
* `'post'`: async like 'pre' but fires after component updates so you can access the updated DOM
* `'sync'`: forces the effect to always trigger synchronously
**Note:** For `computed`-like composables (e.g. [`syncRef`](../shared/syncref/index) `controlledComputed`), when flush timing is configurable, the default is changed to `{ flush: 'sync' }` to align them with the way computed refs works in Vue.
### Configurable Global Dependencies
From v4.0, functions that access the browser APIs will provide an option fields for you to specify the global dependencies (e.g. `window`, `document` and `navigator`). It will use the global instance by default, so for most of the time, you don't need to worry about it. This configure is useful when working with iframes and testing environments.
```
// accessing parent context
const parentMousePos = useMouse({ window: window.parent })
const iframe = document.querySelect('#my-iframe')
// accessing child context
const childMousePos = useMouse({ window: iframe.contentWindow })
```
```
// testing
const mockWindow = { /* ... */ }
const { x, y } = useMouse({ window: mockWindow })
```
vueuse Best Practice Best Practice
=============
### Destructuring
Most of the functions in VueUse return an **object of refs** that you can use [ES6's object destructure](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment) syntax on to take what you need. For example:
```
import { useMouse } from '@vueuse/core'
// "x" and "y" are refs
const { x, y } = useMouse()
console.log(x.value)
const mouse = useMouse()
console.log(mouse.x.value)
```
If you prefer to use them as object properties style, you can unwrap the refs by using `reactive()`. For example:
```
import { reactive } from 'vue'
import { useMouse } from '@vueuse/core'
const mouse = reactive(useMouse())
// "x" and "y" will be auto unwrapped, no `.value` needed
console.log(mouse.x)
```
### Side-effect Clean Up
Similar to Vue's `watch` and `computed` that will be disposed when the component is unmounted, VueUse's functions also clean up the side-effects automatically.
For example, [`useEventListener`](../core/useeventlistener/index)will call `removeEventListener` when the component is unmounted so you don't need to worry about it.
```
// will cleanup automatically
useEventListener('mousemove', () => {})
```
All VueUse functions follow this convention.
To manually dispose the side-effects, some function returns a stop handler just like the `watch` function. For example:
```
const stop = useEventListener('mousemove', () => {})
// ...
// unregister the event listener manually
stop()
```
While not all function return the handler, a more general solution is to use the [`effectScope` API](https://vuejs.org/api/reactivity-advanced.html#effectscope) from Vue.
```
import { effectScope } from 'vue'
const scope = effectScope()
scope.run(() => {
// ...
useEventListener('mousemove', () => {})
onClickOutside(el, () => {})
watch(source, () => {})
})
// all composables called inside `scope.run` will be disposed
scope.stop()
```
You can learn more about effect scope in [this RFC](https://github.com/vuejs/rfcs/blob/master/active-rfcs/0041-reactivity-effect-scope.md).
### Passing Ref as Argument
In Vue, we use the `setup()` function to construct the "connections" between the data and logics. To make it flexible, most of the VueUse function also accpets ref version of the arguments.
Taking [`useTitle`](../core/usetitle/index)as an example:
###### Normal usage
Normally [`useTitle`](../core/usetitle/index)return a ref that reflects to the page's title. When you assign new value to the ref, it automatically updates the title.
```
const isDark = useDark()
const title = useTitle('Set title')
watch(isDark, () => {
title.value = isDark.value ? '🌙 Good evening!' : '☀️ Good morning!'
})
```
###### Connection usage
If you think in "connection", you can instead passing a ref that make it bind to the page's title.
```
const isDark = useDark()
const title = computed(() => isDark.value ? '🌙 Good evening!' : '☀️ Good morning!')
useTitle(title)
```
###### Reactive Getter
Since VueUse 9.0, we introduce a new convention for passing "Reactive Getter" as the argument. Which works great with reactive object and [Reactivity Transform](https://vuejs.org/guide/extras/reactivity-transform.html#reactivity-transform).
```
const isDark = useDark()
useTitle(() => isDark.value ? '🌙 Good evening!' : '☀️ Good morning!')
```
vueuse useRefHistory useRefHistory
=============
| | |
| --- | --- |
| Category | [State](../functions#category=State) |
| Export Size | 1.91 kB |
| Last Changed | 4 months ago |
| Related | [`useDebouncedRefHistory`](usedebouncedrefhistory/index)[`useManualRefHistory`](usemanualrefhistory/index)[`useThrottledRefHistory`](usethrottledrefhistory/index) |
Track the change history of a ref, also provides undo and redo functionality
[Learn useRefHistory with this FREE video lesson from Vue School!](https://vueschool.io/lessons/ref-history-with-vueuse?friend=vueuse)Usage
-----
```
import { ref } from 'vue'
import { useRefHistory } from '@vueuse/core'
const counter = ref(0)
const { history, undo, redo } = useRefHistory(counter)
```
Internally, `watch` is used to trigger a history point when the ref value is modified. This means that history points are triggered asynchronously batching modifications in the same "tick".
```
counter.value += 1
await nextTick()
console.log(history.value)
/* [
{ snapshot: 1, timestamp: 1601912898062 },
{ snapshot: 0, timestamp: 1601912898061 }
] */
```
You can use `undo` to reset the ref value to the last history point.
```
console.log(counter.value) // 1
undo()
console.log(counter.value) // 0
```
### Objects / arrays
When working with objects or arrays, since changing their attributes does not change the reference, it will not trigger the committing. To track attribute changes, you would need to pass `deep: true`. It will create clones for each history record.
```
const state = ref({
foo: 1,
bar: 'bar',
})
const { history, undo, redo } = useRefHistory(state, {
deep: true,
})
state.value.foo = 2
await nextTick()
console.log(history.value)
/* [
{ snapshot: { foo: 2, bar: 'bar' } },
{ snapshot: { foo: 1, bar: 'bar' } }
] */
```
#### Custom Clone Function
[`useRefHistory`](userefhistory/index)only embeds the minimal clone function `x => JSON.parse(JSON.stringify(x))`. To use a full featured or custom clone function, you can set up via the `dump` options.
For example, using [structuredClone](https://developer.mozilla.org/en-US/docs/Web/API/structuredClone):
```
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: structuredClone })
```
Or by using [lodash's `cloneDeep`](https://lodash.com/docs/4.17.15#cloneDeep):
```
import { cloneDeep } from 'lodash-es'
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: cloneDeep })
```
Or a more lightweight [`klona`](https://github.com/lukeed/klona):
```
import { klona } from 'klona'
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: klona })
```
#### Custom Dump and Parse Function
Instead of using the `clone` param, you can pass custom functions to control the serialization and parsing. In case you do not need history values to be objects, this can save an extra clone when undoing. It is also useful in case you want to have the snapshots already stringified to be saved to local storage for example.
```
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, {
dump: JSON.stringify,
parse: JSON.parse,
})
```
### History Capacity
We will keep all the history by default (unlimited) until you explicitly clear them up, you can set the maximal amount of history to be kept by `capacity` options.
```
const refHistory = useRefHistory(target, {
capacity: 15, // limit to 15 history records
})
refHistory.clear() // explicitly clear all the history
```
### History Flush Timing
From [Vue's documentation](https://vuejs.org/guide/essentials/watchers.html#callback-flush-timing): Vue's reactivity system buffers invalidated effects and flush them asynchronously to avoid unnecessary duplicate invocation when there are many state mutations happening in the same "tick".
In the same way as `watch`, you can modify the flush timing using the `flush` option.
```
const refHistory = useRefHistory(target, {
flush: 'sync', // options 'pre' (default), 'post' and 'sync'
})
```
The default is `'pre'`, to align this composable with the default for Vue's watchers. This also helps to avoid common issues, like several history points generated as part of a multi-step update to a ref value that can break invariants of the app state. You can use `commit()` in case you need to create multiple history points in the same "tick"
```
const r = ref(0)
const { history, commit } = useRefHistory(r)
r.value = 1
commit()
r.value = 2
commit()
console.log(history.value)
/* [
{ snapshot: 2 },
{ snapshot: 1 },
{ snapshot: 0 },
] */
```
On the other hand, when using flush `'sync'`, you can use `batch(fn)` to generate a single history point for several sync operations
```
const r = ref({ names: [], version: 1 })
const { history, batch } = useRefHistory(r, { flush: 'sync' })
batch(() => {
r.names.push('Lena')
r.version++
})
console.log(history.value)
/* [
{ snapshot: { names: [ 'Lena' ], version: 2 },
{ snapshot: { names: [], version: 1 },
] */
```
If `{ flush: 'sync', deep: true }` is used, `batch` is also useful when doing a mutable `splice` in an array. `splice` can generate up to three atomic operations that will be pushed to the ref history.
```
const arr = ref([1, 2, 3])
const { history, batch } = useRefHistory(r, { deep: true, flush: 'sync' })
batch(() => {
arr.value.splice(1, 1) // batch ensures only one history point is generated
})
```
Another option is to avoid mutating the original ref value using `arr.value = [...arr.value].splice(1,1)`.
Recommended Readings
--------------------
* [History and Persistence](https://patak.dev/vue/history-and-persistence.html) - by [@matias-capeletto](https://github.com/matias-capeletto)
Type Declarations
-----------------
Show Type Declarations
```
export interface UseRefHistoryOptions<Raw, Serialized = Raw>
extends ConfigurableEventFilter {
/**
* Watch for deep changes, default to false
*
* When set to true, it will also create clones for values store in the history
*
* @default false
*/
deep?: boolean
/**
* The flush option allows for greater control over the timing of a history point, default to 'pre'
*
* Possible values: 'pre', 'post', 'sync'
* It works in the same way as the flush option in watch and watch effect in vue reactivity
*
* @default 'pre'
*/
flush?: "pre" | "post" | "sync"
/**
* Maximum number of history to be kept. Default to unlimited.
*/
capacity?: number
/**
* Clone when taking a snapshot, shortcut for dump: JSON.parse(JSON.stringify(value)).
* Default to false
*
* @default false
*/
clone?: boolean | CloneFn<Raw>
/**
* Serialize data into the history
*/
dump?: (v: Raw) => Serialized
/**
* Deserialize data from the history
*/
parse?: (v: Serialized) => Raw
}
export interface UseRefHistoryReturn<Raw, Serialized>
extends UseManualRefHistoryReturn<Raw, Serialized> {
/**
* A ref representing if the tracking is enabled
*/
isTracking: Ref<boolean>
/**
* Pause change tracking
*/
pause(): void
/**
* Resume change tracking
*
* @param [commit] if true, a history record will be create after resuming
*/
resume(commit?: boolean): void
/**
* A sugar for auto pause and auto resuming within a function scope
*
* @param fn
*/
batch(fn: (cancel: Fn) => void): void
/**
* Clear the data and stop the watch
*/
dispose(): void
}
/**
* Track the change history of a ref, also provides undo and redo functionality.
*
* @see https://vueuse.org/useRefHistory
* @param source
* @param options
*/
export declare function useRefHistory<Raw, Serialized = Raw>(
source: Ref<Raw>,
options?: UseRefHistoryOptions<Raw, Serialized>
): UseRefHistoryReturn<Raw, Serialized>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/index.md)
| programming_docs |
vueuse onClickOutside onClickOutside
==============
| | |
| --- | --- |
| Category | [Sensors](../functions#category=Sensors) |
| Export Size | 1.34 kB |
| Last Changed | 2 weeks ago |
Listen for clicks outside of an element. Useful for modal or dropdown.
Usage
-----
```
<template>
<div ref="target">
Hello world
</div>
<div>
Outside element
</div>
</template>
<script>
import { ref } from 'vue'
import { onClickOutside } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
onClickOutside(target, (event) => console.log(event))
return { target }
}
}
</script>
```
> This function uses [Event.composedPath()](https://developer.mozilla.org/en-US/docs/Web/API/Event/composedPath) which is NOT supported by IE 11, Edge 18 and below. If you are targeting these browsers, we recommend you to include [this code snippet](https://gist.github.com/sibbng/13e83b1dd1b733317ce0130ef07d4efd) on your project.
>
>
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<OnClickOutside @trigger="count++" :options="{ ignore: [/* ... */] }">
<div>
Click Outside of Me
</div>
</OnClickOutside>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vOnClickOutside } from '@vueuse/components'
const modal = ref(false)
function closeModal() {
modal.value = false
}
</script>
<template>
<button @click="modal = true">
Open Modal
</button>
<div v-if="modal" v-on-click-outside="closeModal">
Hello World
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface OnClickOutsideOptions extends ConfigurableWindow {
/**
* List of elements that should not trigger the event.
*/
ignore?: (MaybeElementRef | string)[]
/**
* Use capturing phase for internal event listener.
* @default true
*/
capture?: boolean
/**
* Run handler function if focus moves to an iframe.
* @default false
*/
detectIframe?: boolean
}
export type OnClickOutsideHandler<
T extends {
detectIframe: OnClickOutsideOptions["detectIframe"]
} = {
detectIframe: false
}
> = (
evt: T["detectIframe"] extends true ? PointerEvent | FocusEvent : PointerEvent
) => void
/**
* Listen for clicks outside of an element.
*
* @see https://vueuse.org/onClickOutside
* @param target
* @param handler
* @param options
*/
export declare function onClickOutside<T extends OnClickOutsideOptions>(
target: MaybeElementRef,
handler: OnClickOutsideHandler<{
detectIframe: T["detectIframe"]
}>,
options?: T
): (() => void) | undefined
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/index.md)
vueuse useElementVisibility useElementVisibility
====================
| | |
| --- | --- |
| Category | [Elements](../functions#category=Elements) |
| Export Size | 1.22 kB |
| Last Changed | 4 months ago |
Tracks the visibility of an element within the viewport.
Usage
-----
```
<template>
<div ref="target">
<h1>Hello world</h1>
</div>
</template>
<script>
import { ref } from 'vue'
import { useElementVisibility } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
const targetIsVisible = useElementVisibility(target)
return {
target,
targetIsVisible,
}
}
}
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<UseElementVisibility v-slot="{ isVisible }">
Is Visible: {{ isVisible }}
</UseElementVisibility>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vElementVisibility } from '@vueuse/components'
const target = ref(null)
const isVisible = ref(false)
function onElementVisibility(state) {
isVisible.value = state
}
</script>
<template>
<div v-element-visibility="onElementVisibility">
{{ isVisible ? 'inside' : 'outside' }}
</div>
<!-- with options -->
<div ref="target">
<div v-element-visibility="[onElementVisibility, { scrollTarget: target }]">
{{ isVisible ? 'inside' : 'outside' }}
</div>
</div>
</template>
```
Type Declarations
-----------------
```
export interface UseElementVisibilityOptions extends ConfigurableWindow {
scrollTarget?: MaybeComputedRef<HTMLElement | undefined | null>
}
/**
* Tracks the visibility of an element within the viewport.
*
* @see https://vueuse.org/useElementVisibility
* @param element
* @param options
*/
export declare function useElementVisibility(
element: MaybeComputedElementRef,
{ window, scrollTarget }?: UseElementVisibilityOptions
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/index.md)
vueuse useMediaControls useMediaControls
================
| | |
| --- | --- |
| Category | [Browser](../functions#category=Browser) |
| Export Size | 2.75 kB |
| Last Changed | 6 months ago |
Reactive media controls for both `audio` and `video` elements
Usage
-----
### Basic Usage
```
<script setup lang="ts">
import { onMounted, ref } from 'vue'
import { useMediaControls } from '@vueuse/core'
const video = ref()
const { playing, currentTime, duration, volume } = useMediaControls(video, {
src: 'video.mp4',
})
// Change initial media properties
onMounted(() => {
volume.value = 0.5
currentTime.value = 60
})
</script>
<template>
<video ref="video" />
<button @click="playing = !playing">Play / Pause</button>
<span>{{ currentTime }} / {{ duration }}</span>
</template>
```
### Providing Captions, Subtitles, etc...
You can provide captions, subtitles, etc in the `tracks` options of the [`useMediaControls`](usemediacontrols/index)function. The function will return an array of tracks along with two functions for controlling them, `enableTrack`, `disableTrack`, and `selectedTrack`. Using these you can manage the currently selected track. `selectedTrack` will be `-1` if there is no selected track.
```
<script setup lang="ts">
const { tracks, enableTrack } = useMediaControls(video, {
src: 'video.mp4',
tracks: [
{
default: true,
src: './subtitles.vtt',
kind: 'subtitles',
label: 'English',
srcLang: 'en',
},
]
})
</script>
<template>
<video ref="video" />
<button v-for="track in tracks" :key="track.id" @click="enableTrack(track)">
{{ track.label }}
</button>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Many of the jsdoc definitions here are modified version of the
* documentation from MDN(https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement)
*/
export interface UseMediaSource {
/**
* The source url for the media
*/
src: string
/**
* The media codec type
*/
type?: string
}
export interface UseMediaTextTrackSource {
/**
* Indicates that the track should be enabled unless the user's preferences indicate
* that another track is more appropriate
*/
default?: boolean
/**
* How the text track is meant to be used. If omitted the default kind is subtitles.
*/
kind: TextTrackKind
/**
* A user-readable title of the text track which is used by the browser
* when listing available text tracks.
*/
label: string
/**
* Address of the track (.vtt file). Must be a valid URL. This attribute
* must be specified and its URL value must have the same origin as the document
*/
src: string
/**
* Language of the track text data. It must be a valid BCP 47 language tag.
* If the kind attribute is set to subtitles, then srclang must be defined.
*/
srcLang: string
}
interface UseMediaControlsOptions extends ConfigurableDocument {
/**
* The source for the media, may either be a string, a `UseMediaSource` object, or a list
* of `UseMediaSource` objects.
*/
src?: MaybeComputedRef<string | UseMediaSource | UseMediaSource[]>
/**
* A list of text tracks for the media
*/
tracks?: MaybeComputedRef<UseMediaTextTrackSource[]>
}
export interface UseMediaTextTrack {
/**
* The index of the text track
*/
id: number
/**
* The text track label
*/
label: string
/**
* Language of the track text data. It must be a valid BCP 47 language tag.
* If the kind attribute is set to subtitles, then srclang must be defined.
*/
language: string
/**
* Specifies the display mode of the text track, either `disabled`,
* `hidden`, or `showing`
*/
mode: TextTrackMode
/**
* How the text track is meant to be used. If omitted the default kind is subtitles.
*/
kind: TextTrackKind
/**
* Indicates the track's in-band metadata track dispatch type.
*/
inBandMetadataTrackDispatchType: string
/**
* A list of text track cues
*/
cues: TextTrackCueList | null
/**
* A list of active text track cues
*/
activeCues: TextTrackCueList | null
}
export declare function useMediaControls(
target: MaybeRef<HTMLMediaElement | null | undefined>,
options?: UseMediaControlsOptions
): {
currentTime: Ref<number>
duration: Ref<number>
waiting: Ref<boolean>
seeking: Ref<boolean>
ended: Ref<boolean>
stalled: Ref<boolean>
buffered: Ref<[number, number][]>
playing: Ref<boolean>
rate: Ref<number>
volume: Ref<number>
muted: Ref<boolean>
tracks: Ref<
{
id: number
label: string
language: string
mode: TextTrackMode
kind: TextTrackKind
inBandMetadataTrackDispatchType: string
cues: {
[x: number]: {
endTime: number
id: string
onenter: ((this: TextTrackCue, ev: Event) => any) | null
onexit: ((this: TextTrackCue, ev: Event) => any) | null
pauseOnExit: boolean
startTime: number
readonly track: {
readonly activeCues: any | null
readonly cues: any | null
readonly id: string
readonly inBandMetadataTrackDispatchType: string
readonly kind: TextTrackKind
readonly label: string
readonly language: string
mode: TextTrackMode
oncuechange: ((this: TextTrack, ev: Event) => any) | null
addCue: (cue: TextTrackCue) => void
removeCue: (cue: TextTrackCue) => void
addEventListener: {
<K extends "cuechange">(
type: K,
listener: (this: TextTrack, ev: TextTrackEventMap[K]) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_1 extends "cuechange">(
type: K_1,
listener: (this: TextTrack, ev: TextTrackEventMap[K_1]) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
} | null
addEventListener: {
<K_2 extends keyof TextTrackCueEventMap>(
type: K_2,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_2]
) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_3 extends keyof TextTrackCueEventMap>(
type: K_3,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_3]
) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
}
readonly length: number
getCueById: (id: string) => TextTrackCue | null
[Symbol.iterator]: () => IterableIterator<TextTrackCue>
} | null
activeCues: {
[x: number]: {
endTime: number
id: string
onenter: ((this: TextTrackCue, ev: Event) => any) | null
onexit: ((this: TextTrackCue, ev: Event) => any) | null
pauseOnExit: boolean
startTime: number
readonly track: {
readonly activeCues: any | null
readonly cues: any | null
readonly id: string
readonly inBandMetadataTrackDispatchType: string
readonly kind: TextTrackKind
readonly label: string
readonly language: string
mode: TextTrackMode
oncuechange: ((this: TextTrack, ev: Event) => any) | null
addCue: (cue: TextTrackCue) => void
removeCue: (cue: TextTrackCue) => void
addEventListener: {
<K extends "cuechange">(
type: K,
listener: (this: TextTrack, ev: TextTrackEventMap[K]) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_1 extends "cuechange">(
type: K_1,
listener: (this: TextTrack, ev: TextTrackEventMap[K_1]) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
} | null
addEventListener: {
<K_2 extends keyof TextTrackCueEventMap>(
type: K_2,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_2]
) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_3 extends keyof TextTrackCueEventMap>(
type: K_3,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_3]
) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
}
readonly length: number
getCueById: (id: string) => TextTrackCue | null
[Symbol.iterator]: () => IterableIterator<TextTrackCue>
} | null
}[]
>
selectedTrack: Ref<number>
enableTrack: (
track: number | UseMediaTextTrack,
disableTracks?: boolean
) => void
disableTrack: (track?: number | UseMediaTextTrack) => void
supportsPictureInPicture: boolean | undefined
togglePictureInPicture: () => Promise<unknown>
isPictureInPicture: Ref<boolean>
onSourceError: EventHookOn<Event>
}
export type UseMediaControlsReturn = ReturnType<typeof useMediaControls>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/index.md)
vueuse useElementByPoint useElementByPoint
=================
| | |
| --- | --- |
| Category | [Sensors](../functions#category=Sensors) |
| Export Size | 1.05 kB |
| Last Changed | yesterday |
Reactive element by point.
Usage
-----
```
import { useElementByPoint, useMouse } from '@vueuse/core'
const { x, y } = useMouse({ type: 'client' })
const { element } = useElementByPoint({ x, y })
```
Type Declarations
-----------------
```
export interface UseElementByPointOptions extends ConfigurableDocument {
x: MaybeComputedRef<number>
y: MaybeComputedRef<number>
}
/**
* Reactive element by point.
*
* @see https://vueuse.org/useElementByPoint
* @param options - UseElementByPointOptions
*/
export declare function useElementByPoint(options: UseElementByPointOptions): {
isActive: Ref<boolean>
pause: Fn
resume: Fn
element: Ref<HTMLElement | null>
}
export type UseElementByPointReturn = ReturnType<typeof useElementByPoint>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/index.md)
vueuse useUrlSearchParams useUrlSearchParams
==================
| | |
| --- | --- |
| Category | [Browser](../functions#category=Browser) |
| Export Size | 1.98 kB |
| Last Changed | 4 months ago |
Reactive [URLSearchParams](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams)
Usage
-----
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('history')
console.log(params.foo) // 'bar'
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `?foo=bar&vueuse=awesome`
```
### Hash Mode
When using with hash mode route, specify the `mode` to `hash`
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('hash')
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `#/your/route?foo=bar&vueuse=awesome`
```
### Hash Params
When using with history mode route, but want to use hash as params, specify the `mode` to `hash-params`
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('hash-params')
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `/your/route#foo=bar&vueuse=awesome`
```
Type Declarations
-----------------
```
export type UrlParams = Record<string, string[] | string>
export interface UseUrlSearchParamsOptions<T> extends ConfigurableWindow {
/**
* @default true
*/
removeNullishValues?: boolean
/**
* @default false
*/
removeFalsyValues?: boolean
/**
* @default {}
*/
initialValue?: T
/**
* Write back to `window.history` automatically
*
* @default true
*/
write?: boolean
}
/**
* Reactive URLSearchParams
*
* @see https://vueuse.org/useUrlSearchParams
* @param mode
* @param options
*/
export declare function useUrlSearchParams<
T extends Record<string, any> = UrlParams
>(
mode?: "history" | "hash" | "hash-params",
options?: UseUrlSearchParamsOptions<T>
): T
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/index.md)
| programming_docs |
vueuse useFetch useFetch
========
| | |
| --- | --- |
| Category | [Network](../functions#category=Network) |
| Export Size | 2.85 kB |
| Last Changed | 2 days ago |
Reactive [Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) provides the ability to abort requests, intercept requests before they are fired, automatically refetch requests when the url changes, and create your own [`useFetch`](usefetch/index)with predefined options.
**TIP**When using with Nuxt 3, this functions will **NOT** be auto imported in favor of Nuxt's built-in [`useFetch()`](https://v3.nuxtjs.org/api/composables/use-fetch). Use explicit import if you want to use the function from VueUse.
Usage
-----
### Basic Usage
The [`useFetch`](usefetch/index)function can be used by simply providing a url. The url can be either a string or a `ref`. The `data` object will contain the result of the request, the `error` object will contain any errors, and the `isFetching` object will indicate if the request is loading.
```
import { useFetch } from '@vueuse/core'
const { isFetching, error, data } = useFetch(url)
```
### Asynchronous Usage
[`useFetch`](usefetch/index)can also be awaited just like a normal fetch. Note that whenever a component is asynchronous, whatever component that uses it must wrap the component in a `<Suspense>` tag. You can read more about the suspense api in the [Offical Vue 3 Docs](https://vuejs.org/guide/built-ins/suspense.html)
```
import { useFetch } from '@vueuse/core'
const { isFetching, error, data } = await useFetch(url)
```
### Refetching on URL change
Using a `ref` for the url parameter will allow the [`useFetch`](usefetch/index)function to automatically trigger another request when the url is changed.
```
const url = ref('https://my-api.com/user/1')
const { data } = useFetch(url, { refetch: true })
url.value = 'https://my-api.com/user/2' // Will trigger another request
```
### Prevent request from firing immediately
Setting the `immediate` option to false will prevent the request from firing until the `execute` function is called.
```
const { execute } = useFetch(url, { immediate: false })
execute()
```
### Aborting a request
A request can be aborted by using the `abort` function from the [`useFetch`](usefetch/index)function. The `canAbort` property indicates if the request can be aborted.
```
const { abort, canAbort } = useFetch(url)
setTimeout(() => {
if (canAbort.value)
abort()
}, 100)
```
A request can also be aborted automatically by using `timeout` property. It will call `abort` function when the given timeout is reached.
```
const { data } = useFetch(url, { timeout: 100 })
```
### Intercepting a request
The `beforeFetch` option can intercept a request before it is sent and modify the request options and url.
```
const { data } = useFetch(url, {
async beforeFetch({ url, options, cancel }) {
const myToken = await getMyToken()
if (!myToken)
cancel()
options.headers = {
...options.headers,
Authorization: `Bearer ${myToken}`,
}
return {
options,
}
},
})
```
The `afterFetch` option can intercept the response data before it is updated.
```
const { data } = useFetch(url, {
afterFetch(ctx) {
if (ctx.data.title === 'HxH')
ctx.data.title = 'Hunter x Hunter' // Modifies the response data
return ctx
},
})
```
The `onFetchError` option can intercept the response data and error before it is updated.
```
const { data } = useFetch(url, {
onFetchError(ctx) {
// ctx.data can be null when 5xx response
if (ctx.data === null)
ctx.data = { title: 'Hunter x Hunter' } // Modifies the response data
ctx.error = new Error('Custom Error') // Modifies the error
return ctx
},
})
```
### Setting the request method and return type
The request method and return type can be set by adding the appropriate methods to the end of `useFetch`
```
// Request will be sent with GET method and data will be parsed as JSON
const { data } = useFetch(url).get().json()
// Request will be sent with POST method and data will be parsed as text
const { data } = useFetch(url).post().text()
// Or set the method using the options
// Request will be sent with GET method and data will be parsed as blob
const { data } = useFetch(url, { method: 'GET' }, { refetch: true }).blob()
```
### Creating a Custom Instance
The `createFetch` function will return a useFetch function with whatever pre-configured options that are provided to it. This is useful for interacting with API's throughout an application that uses the same base URL or needs Authorization headers.
```
const useMyFetch = createFetch({
baseUrl: 'https://my-api.com',
options: {
async beforeFetch({ options }) {
const myToken = await getMyToken()
options.headers.Authorization = `Bearer ${myToken}`
return { options }
},
},
fetchOptions: {
mode: 'cors',
},
})
const { isFetching, error, data } = useMyFetch('users')
```
If you want to control the behavior of `beforeFetch`, `afterFetch`, `onFetchError` between the pre-configured instance and newly spawned instance. You can provide a `combination` option to toggle between `overwrite` or `chaining`.
```
const useMyFetch = createFetch({
baseUrl: 'https://my-api.com',
combination: 'overwrite',
options: {
// beforeFetch in pre-configured instance will only run when the newly spawned instance do not pass beforeFetch
async beforeFetch({ options }) {
const myToken = await getMyToken()
options.headers.Authorization = `Bearer ${myToken}`
return { options }
},
},
})
// use useMyFetch beforeFetch
const { isFetching, error, data } = useMyFetch('users')
// use custom beforeFetch
const { isFetching, error, data } = useMyFetch('users', {
async beforeFetch({ url, options, cancel }) {
const myToken = await getMyToken()
if (!myToken)
cancel()
options.headers = {
...options.headers,
Authorization: `Bearer ${myToken}`,
}
return {
options,
}
},
})
```
### Events
The `onFetchResponse` and `onFetchError` will fire on fetch request responses and errors respectively.
```
const { onFetchResponse, onFetchError } = useFetch(url)
onFetchResponse((response) => {
console.log(response.status)
})
onFetchError((error) => {
console.error(error.message)
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseFetchReturn<T> {
/**
* Indicates if the fetch request has finished
*/
isFinished: Ref<boolean>
/**
* The statusCode of the HTTP fetch response
*/
statusCode: Ref<number | null>
/**
* The raw response of the fetch response
*/
response: Ref<Response | null>
/**
* Any fetch errors that may have occurred
*/
error: Ref<any>
/**
* The fetch response body, may either be JSON or text
*/
data: Ref<T | null>
/**
* Indicates if the request is currently being fetched.
*/
isFetching: Ref<boolean>
/**
* Indicates if the fetch request is able to be aborted
*/
canAbort: ComputedRef<boolean>
/**
* Indicates if the fetch request was aborted
*/
aborted: Ref<boolean>
/**
* Abort the fetch request
*/
abort: Fn
/**
* Manually call the fetch
* (default not throwing error)
*/
execute: (throwOnFailed?: boolean) => Promise<any>
/**
* Fires after the fetch request has finished
*/
onFetchResponse: EventHookOn<Response>
/**
* Fires after a fetch request error
*/
onFetchError: EventHookOn
/**
* Fires after a fetch has completed
*/
onFetchFinally: EventHookOn
get(): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
post(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
put(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
delete(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
patch(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
head(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
options(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
json<JSON = any>(): UseFetchReturn<JSON> & PromiseLike<UseFetchReturn<JSON>>
text(): UseFetchReturn<string> & PromiseLike<UseFetchReturn<string>>
blob(): UseFetchReturn<Blob> & PromiseLike<UseFetchReturn<Blob>>
arrayBuffer(): UseFetchReturn<ArrayBuffer> &
PromiseLike<UseFetchReturn<ArrayBuffer>>
formData(): UseFetchReturn<FormData> & PromiseLike<UseFetchReturn<FormData>>
}
type Combination = "overwrite" | "chain"
export interface BeforeFetchContext {
/**
* The computed url of the current request
*/
url: string
/**
* The request options of the current request
*/
options: RequestInit
/**
* Cancels the current request
*/
cancel: Fn
}
export interface AfterFetchContext<T = any> {
response: Response
data: T | null
}
export interface OnFetchErrorContext<T = any, E = any> {
error: E
data: T | null
}
export interface UseFetchOptions {
/**
* Fetch function
*/
fetch?: typeof window.fetch
/**
* Will automatically run fetch when `useFetch` is used
*
* @default true
*/
immediate?: boolean
/**
* Will automatically refetch when:
* - the URL is changed if the URL is a ref
* - the payload is changed if the payload is a ref
*
* @default false
*/
refetch?: MaybeComputedRef<boolean>
/**
* Initial data before the request finished
*
* @default null
*/
initialData?: any
/**
* Timeout for abort request after number of millisecond
* `0` means use browser default
*
* @default 0
*/
timeout?: number
/**
* Will run immediately before the fetch request is dispatched
*/
beforeFetch?: (
ctx: BeforeFetchContext
) =>
| Promise<Partial<BeforeFetchContext> | void>
| Partial<BeforeFetchContext>
| void
/**
* Will run immediately after the fetch request is returned.
* Runs after any 2xx response
*/
afterFetch?: (
ctx: AfterFetchContext
) => Promise<Partial<AfterFetchContext>> | Partial<AfterFetchContext>
/**
* Will run immediately after the fetch request is returned.
* Runs after any 4xx and 5xx response
*/
onFetchError?: (ctx: {
data: any
response: Response | null
error: any
}) => Promise<Partial<OnFetchErrorContext>> | Partial<OnFetchErrorContext>
}
export interface CreateFetchOptions {
/**
* The base URL that will be prefixed to all urls unless urls are absolute
*/
baseUrl?: MaybeComputedRef<string>
/**
* Determine the inherit behavior for beforeFetch, afterFetch, onFetchError
* @default 'chain'
*/
combination?: Combination
/**
* Default Options for the useFetch function
*/
options?: UseFetchOptions
/**
* Options for the fetch request
*/
fetchOptions?: RequestInit
}
export declare function createFetch(
config?: CreateFetchOptions
): typeof useFetch
export declare function useFetch<T>(
url: MaybeComputedRef<string>
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
export declare function useFetch<T>(
url: MaybeComputedRef<string>,
useFetchOptions: UseFetchOptions
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
export declare function useFetch<T>(
url: MaybeComputedRef<string>,
options: RequestInit,
useFetchOptions?: UseFetchOptions
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/index.md)
vueuse useScreenSafeArea useScreenSafeArea
=================
| | |
| --- | --- |
| Category | [Browser](../functions#category=Browser) |
| Export Size | 1.57 kB |
| Last Changed | last year |
Reactive `env(safe-area-inset-*)`
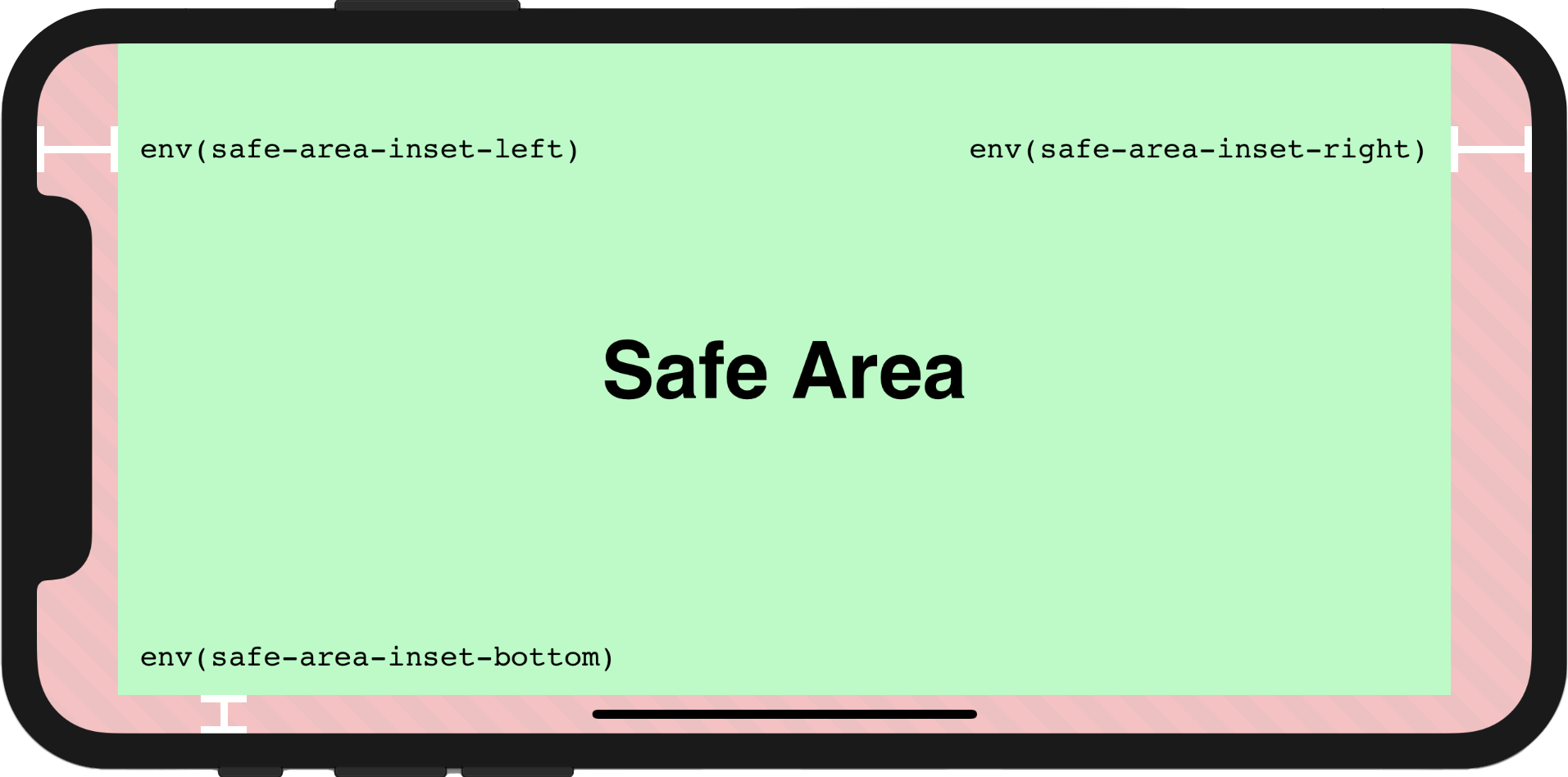
Usage
-----
In order to make the page to be fully rendered in the screen, the additional attribute `viewport-fit=cover` within `viewport` meta tag must be set firstly, the viewport meta tag may look like this:
```
<meta name='viewport' content='initial-scale=1, viewport-fit=cover'>
```
Then we could use [`useScreenSafeArea`](usescreensafearea/index)in the component as shown below:
```
import { useScreenSafeArea } from '@vueuse/core'
const {
top,
right,
bottom,
left,
} = useScreenSafeArea()
```
For further details, you may refer to this documentation: [Designing Websites for iPhone X](https://webkit.org/blog/7929/designing-websites-for-iphone-x/)
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<UseScreenSafeArea top right bottom left>content</UseScreenSafeArea>
```
Type Declarations
-----------------
```
/**
* Reactive `env(safe-area-inset-*)`
*
* @see https://vueuse.org/useScreenSafeArea
*/
export declare function useScreenSafeArea(): {
top: Ref<string>
right: Ref<string>
bottom: Ref<string>
left: Ref<string>
update: () => void
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/index.md)
vueuse useTransition useTransition
=============
| | |
| --- | --- |
| Category | [Animation](../functions#category=Animation) |
| Export Size | 1.66 kB |
| Last Changed | 2 months ago |
Transition between values
Usage
-----
For simple transitions, provide a numeric source value to watch. When changed, the output will transition to the new value. If the source changes while a transition is in progress, a new transition will begin from where the previous one was interrupted.
```
import { ref } from 'vue'
import { TransitionPresets, useTransition } from '@vueuse/core'
const source = ref(0)
const output = useTransition(source, {
duration: 1000,
transition: TransitionPresets.easeInOutCubic,
})
```
To synchronize transitions, use an array of numbers. As an example, here is how we could transition between colors.
```
const source = ref([0, 0, 0])
const output = useTransition(source)
const color = computed(() => {
const [r, g, b] = output.value
return `rgb(${r}, ${g}, ${b})`
})
```
Transition easing can be customized using cubic bezier curves. Transitions defined this way work the same as [CSS easing functions](https://developer.mozilla.org/en-US/docs/Web/CSS/easing-function#easing_functions).
```
useTransition(source, {
transition: [0.75, 0, 0.25, 1],
})
```
The following transitions are available via the `TransitionPresets` constant.
* [`linear`](https://cubic-bezier.com/#0,0,1,1)
* [`easeInSine`](https://cubic-bezier.com/#.12,0,.39,0)
* [`easeOutSine`](https://cubic-bezier.com/#.61,1,.88,1)
* [`easeInOutSine`](https://cubic-bezier.com/#.37,0,.63,1)
* [`easeInQuad`](https://cubic-bezier.com/#.11,0,.5,0)
* [`easeOutQuad`](https://cubic-bezier.com/#.5,1,.89,1)
* [`easeInOutQuad`](https://cubic-bezier.com/#.45,0,.55,1)
* [`easeInCubic`](https://cubic-bezier.com/#.32,0,.67,0)
* [`easeOutCubic`](https://cubic-bezier.com/#.33,1,.68,1)
* [`easeInOutCubic`](https://cubic-bezier.com/#.65,0,.35,1)
* [`easeInQuart`](https://cubic-bezier.com/#.5,0,.75,0)
* [`easeOutQuart`](https://cubic-bezier.com/#.25,1,.5,1)
* [`easeInOutQuart`](https://cubic-bezier.com/#.76,0,.24,1)
* [`easeInQuint`](https://cubic-bezier.com/#.64,0,.78,0)
* [`easeOutQuint`](https://cubic-bezier.com/#.22,1,.36,1)
* [`easeInOutQuint`](https://cubic-bezier.com/#.83,0,.17,1)
* [`easeInExpo`](https://cubic-bezier.com/#.7,0,.84,0)
* [`easeOutExpo`](https://cubic-bezier.com/#.16,1,.3,1)
* [`easeInOutExpo`](https://cubic-bezier.com/#.87,0,.13,1)
* [`easeInCirc`](https://cubic-bezier.com/#.55,0,1,.45)
* [`easeOutCirc`](https://cubic-bezier.com/#0,.55,.45,1)
* [`easeInOutCirc`](https://cubic-bezier.com/#.85,0,.15,1)
* [`easeInBack`](https://cubic-bezier.com/#.36,0,.66,-.56)
* [`easeOutBack`](https://cubic-bezier.com/#.34,1.56,.64,1)
* [`easeInOutBack`](https://cubic-bezier.com/#.68,-.6,.32,1.6)
For more complex transitions, a custom function can be provided.
```
const easeOutElastic = (n) => {
return n === 0
? 0
: n === 1
? 1
: (2 ** (-10 * n)) * Math.sin((n * 10 - 0.75) * ((2 * Math.PI) / 3)) + 1
}
useTransition(source, {
transition: easeOutElastic,
})
```
To control when a transition starts, set a `delay` value. To choreograph behavior around a transition, define `onStarted` or `onFinished` callbacks.
```
useTransition(source, {
delay: 1000,
onStarted() {
// called after the transition starts
},
onFinished() {
// called after the transition ends
},
})
```
To temporarily stop transitioning, define a boolean `disabled` property. Be aware, this is not the same a `duration` of `0`. Disabled transitions track the source value ***synchronously***. They do not respect a `delay`, and do not fire `onStarted` or `onFinished` callbacks.
Type Declarations
-----------------
Show Type Declarations
```
/**
* Cubic bezier points
*/
export type CubicBezierPoints = [number, number, number, number]
/**
* Easing function
*/
export type EasingFunction = (n: number) => number
/**
* Transition options
*/
export interface UseTransitionOptions {
/**
* Milliseconds to wait before starting transition
*/
delay?: MaybeRef<number>
/**
* Disables the transition
*/
disabled?: MaybeRef<boolean>
/**
* Transition duration in milliseconds
*/
duration?: MaybeRef<number>
/**
* Callback to execute after transition finishes
*/
onFinished?: () => void
/**
* Callback to execute after transition starts
*/
onStarted?: () => void
/**
* Easing function or cubic bezier points for calculating transition values
*/
transition?: MaybeRef<EasingFunction | CubicBezierPoints>
}
/**
* Common transitions
*
* @see https://easings.net
*/
export declare const TransitionPresets: Record<
| "easeInSine"
| "easeOutSine"
| "easeInOutSine"
| "easeInQuad"
| "easeOutQuad"
| "easeInOutQuad"
| "easeInCubic"
| "easeOutCubic"
| "easeInOutCubic"
| "easeInQuart"
| "easeOutQuart"
| "easeInOutQuart"
| "easeInQuint"
| "easeOutQuint"
| "easeInOutQuint"
| "easeInExpo"
| "easeOutExpo"
| "easeInOutExpo"
| "easeInCirc"
| "easeOutCirc"
| "easeInOutCirc"
| "easeInBack"
| "easeOutBack"
| "easeInOutBack",
CubicBezierPoints
> & {
linear: EasingFunction
}
export declare function useTransition(
source: Ref<number>,
options?: UseTransitionOptions
): ComputedRef<number>
export declare function useTransition<T extends MaybeRef<number>[]>(
source: [...T],
options?: UseTransitionOptions
): ComputedRef<{
[K in keyof T]: number
}>
export declare function useTransition<T extends Ref<number[]>>(
source: T,
options?: UseTransitionOptions
): ComputedRef<number[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/index.md)
vueuse useDocumentVisibility useDocumentVisibility
=====================
| | |
| --- | --- |
| Category | [Elements](../functions#category=Elements) |
| Export Size | 1.07 kB |
| Last Changed | 6 months ago |
Reactively track [`document.visibilityState`](https://developer.mozilla.org/en-US/docs/Web/API/Document/visibilityState)
Usage
-----
```
import { useDocumentVisibility } from '@vueuse/core'
const visibility = useDocumentVisibility()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../guide/components).
>
>
```
<UseDocumentVisibility v-slot="{ visibility }">
Document Visibility: {{ visibility }}
</UseDocumentVisibility>
```
Type Declarations
-----------------
```
/**
* Reactively track `document.visibilityState`.
*
* @see https://vueuse.org/useDocumentVisibility
* @param options
*/
export declare function useDocumentVisibility({
document,
}?: ConfigurableDocument): Ref<DocumentVisibilityState>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/index.md)
| programming_docs |
vueuse useAsyncQueue useAsyncQueue
=============
| | |
| --- | --- |
| Category | [Utilities](../functions#category=Utilities) |
| Export Size | 1.02 kB |
| Last Changed | last year |
Executes each asynchronous task sequentially and passes the current task result to the next task
Usage
-----
```
import { useAsyncQueue } from '@vueuse/core'
const p1 = () => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(1000)
}, 10)
})
}
const p2 = (result: number) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(1000 + result)
}, 20)
})
}
const { activeIndex, result } = useAsyncQueue([p1, p2])
console.log(activeIndex.value) // current pending task index
console.log(result) // the tasks result
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseAsyncQueueTask<T> = (...args: any[]) => T | Promise<T>
export interface UseAsyncQueueResult<T> {
state: "pending" | "fulfilled" | "rejected"
data: T | null
}
export interface UseAsyncQueueReturn<T> {
activeIndex: Ref<number>
result: T
}
export interface UseAsyncQueueOptions {
/**
* Interrupt tasks when current task fails.
*
* @default true
*/
interrupt?: boolean
/**
* Trigger it when the tasks fails.
*
*/
onError?: () => void
/**
* Trigger it when the tasks ends.
*
*/
onFinished?: () => void
}
/**
* Asynchronous queue task controller.
*
* @see https://vueuse.org/useAsyncQueue
* @param tasks
* @param options
*/
export declare function useAsyncQueue<T1>(
tasks: [UseAsyncQueueTask<T1>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<[UseAsyncQueueResult<T1>]>
export declare function useAsyncQueue<T1, T2>(
tasks: [UseAsyncQueueTask<T1>, UseAsyncQueueTask<T2>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<[UseAsyncQueueResult<T1>, UseAsyncQueueResult<T2>]>
export declare function useAsyncQueue<T1, T2, T3>(
tasks: [UseAsyncQueueTask<T1>, UseAsyncQueueTask<T2>, UseAsyncQueueTask<T3>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[UseAsyncQueueResult<T1>, UseAsyncQueueResult<T2>, UseAsyncQueueResult<T3>]
>
export declare function useAsyncQueue<T1, T2, T3, T4>(
tasks: [
UseAsyncQueueTask<T1>,
UseAsyncQueueTask<T2>,
UseAsyncQueueTask<T3>,
UseAsyncQueueTask<T4>
],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[
UseAsyncQueueResult<T1>,
UseAsyncQueueResult<T2>,
UseAsyncQueueResult<T3>,
UseAsyncQueueResult<T4>
]
>
export declare function useAsyncQueue<T1, T2, T3, T4, T5>(
tasks: [
UseAsyncQueueTask<T1>,
UseAsyncQueueTask<T2>,
UseAsyncQueueTask<T3>,
UseAsyncQueueTask<T4>,
UseAsyncQueueTask<T5>
],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[
UseAsyncQueueResult<T1>,
UseAsyncQueueResult<T2>,
UseAsyncQueueResult<T3>,
UseAsyncQueueResult<T4>,
UseAsyncQueueResult<T5>
]
>
export declare function useAsyncQueue<T>(
tasks: UseAsyncQueueTask<T>[],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<UseAsyncQueueResult<T>[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/index.md)
vueuse useSwipe useSwipe
========
| | |
| --- | --- |
| Category | [Sensors](../functions#category=Sensors) |
| Export Size | 1.53 kB |
| Last Changed | 6 months ago |
Reactive swipe detection based on [`TouchEvents`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent).
Usage
-----
```
<template>
<div ref="el">
Swipe here
</div>
</template>
<script>
setup() {
const el = ref(null)
const { isSwiping, direction } = useSwipe(el)
return { el, isSwiping, direction }
}
</script>
```
Type Declarations
-----------------
Show Type Declarations
```
export declare enum SwipeDirection {
UP = "UP",
RIGHT = "RIGHT",
DOWN = "DOWN",
LEFT = "LEFT",
NONE = "NONE",
}
export interface UseSwipeOptions extends ConfigurableWindow {
/**
* Register events as passive
*
* @default true
*/
passive?: boolean
/**
* @default 50
*/
threshold?: number
/**
* Callback on swipe start
*/
onSwipeStart?: (e: TouchEvent) => void
/**
* Callback on swipe moves
*/
onSwipe?: (e: TouchEvent) => void
/**
* Callback on swipe ends
*/
onSwipeEnd?: (e: TouchEvent, direction: SwipeDirection) => void
}
export interface UseSwipeReturn {
isPassiveEventSupported: boolean
isSwiping: Ref<boolean>
direction: ComputedRef<SwipeDirection | null>
coordsStart: Readonly<Position>
coordsEnd: Readonly<Position>
lengthX: ComputedRef<number>
lengthY: ComputedRef<number>
stop: () => void
}
/**
* Reactive swipe detection.
*
* @see https://vueuse.org/useSwipe
* @param target
* @param options
*/
export declare function useSwipe(
target: MaybeComputedRef<EventTarget | null | undefined>,
options?: UseSwipeOptions
): UseSwipeReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/index.md)
vueuse useStyleTag useStyleTag
===========
| | |
| --- | --- |
| Category | [Browser](../functions#category=Browser) |
| Export Size | 1.09 kB |
| Last Changed | last month |
Inject reactive `style` element in head.
Usage
-----
### Basic usage
Provide a CSS string, then [`useStyleTag`](usestyletag/index)will automatically generate an id and inject it in `<head>`.
```
import { useStyleTag } from '@vueuse/core'
const {
id,
css,
load,
unload,
isLoaded,
} = useStyleTag('.foo { margin-top: 32px; }')
// Later you can modify styles
css.value = '.foo { margin-top: 64px; }'
```
This code will be injected to `<head>`:
```
<style type="text/css" id="vueuse_styletag_1">
.foo { margin-top: 64px; }
</style>
```
### Custom ID
If you need to define your own id, you can pass `id` as first argument.
```
import { useStyleTag } from '@vueuse/core'
useStyleTag('.foo { margin-top: 32px; }', { id: 'custom-id' })
```
```
<!-- injected to <head> -->
<style type="text/css" id="custom-id">
.foo { margin-top: 32px; }
</style>
```
### Media query
You can pass media attributes as last argument within object.
```
useStyleTag('.foo { margin-top: 32px; }', { media: 'print' })
```
```
<!-- injected to <head> -->
<style type="text/css" id="vueuse_styletag_1" media="print">
.foo { margin-top: 32px; }
</style>
```
Type Declarations
-----------------
```
export interface UseStyleTagOptions extends ConfigurableDocument {
/**
* Media query for styles to apply
*/
media?: string
/**
* Load the style immediately
*
* @default true
*/
immediate?: boolean
/**
* Manual controls the timing of loading and unloading
*
* @default false
*/
manual?: boolean
/**
* DOM id of the style tag
*
* @default auto-incremented
*/
id?: string
}
export interface UseStyleTagReturn {
id: string
css: Ref<string>
load: () => void
unload: () => void
isLoaded: Readonly<Ref<boolean>>
}
/**
* Inject <style> element in head.
*
* Overload: Omitted id
*
* @see https://vueuse.org/useStyleTag
* @param css
* @param options
*/
export declare function useStyleTag(
css: MaybeRef<string>,
options?: UseStyleTagOptions
): UseStyleTagReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/index.md)
vueuse useColorMode useColorMode
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 2.86 kB |
| Last Changed | 4 months ago |
| Related | [`useDark`](../usedark/index)[`usePreferredDark`](../usepreferreddark/index)[`useStorage`](../usestorage/index) |
Reactive color mode (dark / light / customs) with auto data persistence.
Basic Usage
-----------
```
import { useColorMode } from '@vueuse/core'
const mode = useColorMode() // Ref<'dark' | 'light'>
```
By default, it will match with users' browser preference using [`usePreferredDark`](../usepreferreddark/index)(a.k.a `auto` mode). When reading the ref, it will by default return the current color mode (`dark`, `light` or your custom modes). The `auto` mode can be included in the returned modes by enabling the `emitAuto` option. When writing to the ref, it will trigger DOM updates and persist the color mode to local storage (or your custom storage). You can pass `auto` to set back to auto mode.
```
mode.value // 'dark' | 'light'
mode.value = 'dark' // change to dark mode and persist
mode.value = 'auto' // change to auto mode
```
Config
------
```
import { useColorMode } from '@vueuse/core'
const mode = useColorMode({
attribute: 'theme',
modes: {
// custom colors
dim: 'dim',
cafe: 'cafe',
},
}) // Ref<'dark' | 'light' | 'dim' | 'cafe'>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseColorMode v-slot="{ mode }">
<button @click="mode = mode === 'dark' ? 'light' : 'dark'">
Mode {{ mode }}
</button>
</UseColorMode>
```
Type Declarations
-----------------
Show Type Declarations
```
export type BasicColorSchema = "light" | "dark" | "auto"
export interface UseColorModeOptions<T extends string = BasicColorSchema>
extends UseStorageOptions<T | BasicColorSchema> {
/**
* CSS Selector for the target element applying to
*
* @default 'html'
*/
selector?: string
/**
* HTML attribute applying the target element
*
* @default 'class'
*/
attribute?: string
/**
* The initial color mode
*
* @default 'auto'
*/
initialValue?: T | BasicColorSchema
/**
* Prefix when adding value to the attribute
*/
modes?: Partial<Record<T | BasicColorSchema, string>>
/**
* A custom handler for handle the updates.
* When specified, the default behavior will be overridden.
*
* @default undefined
*/
onChanged?: (
mode: T | BasicColorSchema,
defaultHandler: (mode: T | BasicColorSchema) => void
) => void
/**
* Custom storage ref
*
* When provided, `useStorage` will be skipped
*/
storageRef?: Ref<T | BasicColorSchema>
/**
* Key to persist the data into localStorage/sessionStorage.
*
* Pass `null` to disable persistence
*
* @default 'vueuse-color-scheme'
*/
storageKey?: string | null
/**
* Storage object, can be localStorage or sessionStorage
*
* @default localStorage
*/
storage?: StorageLike
/**
* Emit `auto` mode from state
*
* When set to `true`, preferred mode won't be translated into `light` or `dark`.
* This is useful when the fact that `auto` mode was selected needs to be known.
*
* @default undefined
*/
emitAuto?: boolean
}
/**
* Reactive color mode with auto data persistence.
*
* @see https://vueuse.org/useColorMode
* @param options
*/
export declare function useColorMode<T extends string = BasicColorSchema>(
options?: UseColorModeOptions<T>
): WritableComputedRef<BasicColorSchema | T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useColorMode/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useColorMode/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useColorMode/index.md)
vueuse useStorage useStorage
==========
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 2.16 kB |
| Last Changed | 2 weeks ago |
| Related | [`useColorMode`](../usecolormode/index)[`useDark`](../usedark/index)[`useLocalStorage`](../uselocalstorage/index)[`useSessionStorage`](../usesessionstorage/index)[`useStorageAsync`](../usestorageasync/index) |
Reactive [LocalStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage)/[SessionStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/sessionStorage)
Usage
-----
**TIP**When using with Nuxt 3, this functions will **NOT** be auto imported in favor of Nitro's built-in [`useStorage()`](https://nitro.unjs.io/guide/introduction/storage). Use explicit import if you want to use the function from VueUse.
```
import { useStorage } from '@vueuse/core'
// bind object
const state = useStorage('my-store', { hello: 'hi', greeting: 'Hello' })
// bind boolean
const flag = useStorage('my-flag', true) // returns Ref<boolean>
// bind number
const count = useStorage('my-count', 0) // returns Ref<number>
// bind string with SessionStorage
const id = useStorage('my-id', 'some-string-id', sessionStorage) // returns Ref<string>
// delete data from storage
state.value = null
```
Merge Defaults
--------------
By default, [`useStorage`](index)will use the value from storage if it presents and ignores the default value. Be aware that when you adding more properties to the default value, the key might be undefined if client's storage does not have that key.
```
localStorage.setItem('my-store', '{"hello": "hello"}')
const state = useStorage('my-store', { hello: 'hi', greeting: 'hello' }, localStorage)
console.log(state.greeting) // undefined, since the value is not presented in storage
```
To solve that, you can enable `mergeDefaults` option.
```
localStorage.setItem('my-store', '{"hello": "nihao"}')
const state = useStorage(
'my-store',
{ hello: 'hi', greeting: 'hello' },
localStorage,
{ mergeDefaults: true } // <--
)
console.log(state.hello) // 'nihao', from storage
console.log(state.greeting) // 'hello', from merged default value
```
When setting it to true, it will perform a **shallow merge** for objects. You can pass a function to perform custom merge (e.g. deep merge), for example:
```
const state = useStorage(
'my-store',
{ hello: 'hi', greeting: 'hello' },
localStorage,
{ mergeDefaults: (storageValue, defaults) => deepMerge(defaults, storageValue) } // <--
)
```
Custom Serialization
--------------------
By default, [`useStorage`](index)will smartly use the corresponding serializer based on the data type of provided default value. For example, `JSON.stringify` / `JSON.parse` will be used for objects, `Number.toString` / `parseFloat` for numbers, etc.
You can also provide your own serialization function to [`useStorage`](index)
```
import { useStorage } from '@vueuse/core'
useStorage(
'key',
{},
undefined,
{
serializer: {
read: (v: any) => v ? JSON.parse(v) : null,
write: (v: any) => JSON.stringify(v),
},
},
)
```
Please note when you provide `null` as the default value, [`useStorage`](index)can't assume the data type from it. In this case, you can provide a custom serializer or reuse the built-in ones explicitly.
```
import { StorageSerializers, useStorage } from '@vueuse/core'
const objectLike = useStorage('key', null, undefined, { serializer: StorageSerializers.object })
objectLike.value = { foo: 'bar' }
```
Type Declarations
-----------------
Show Type Declarations
```
export interface Serializer<T> {
read(raw: string): T
write(value: T): string
}
export interface SerializerAsync<T> {
read(raw: string): Awaitable<T>
write(value: T): Awaitable<string>
}
export declare const StorageSerializers: Record<
"boolean" | "object" | "number" | "any" | "string" | "map" | "set" | "date",
Serializer<any>
>
export interface UseStorageOptions<T>
extends ConfigurableEventFilter,
ConfigurableWindow,
ConfigurableFlush {
/**
* Watch for deep changes
*
* @default true
*/
deep?: boolean
/**
* Listen to storage changes, useful for multiple tabs application
*
* @default true
*/
listenToStorageChanges?: boolean
/**
* Write the default value to the storage when it does not exist
*
* @default true
*/
writeDefaults?: boolean
/**
* Merge the default value with the value read from the storage.
*
* When setting it to true, it will perform a **shallow merge** for objects.
* You can pass a function to perform custom merge (e.g. deep merge), for example:
*
* @default false
*/
mergeDefaults?: boolean | ((storageValue: T, defaults: T) => T)
/**
* Custom data serialization
*/
serializer?: Serializer<T>
/**
* On error callback
*
* Default log error to `console.error`
*/
onError?: (error: unknown) => void
/**
* Use shallow ref as reference
*
* @default false
*/
shallow?: boolean
}
export declare function useStorage(
key: string,
defaults: MaybeComputedRef<string>,
storage?: StorageLike,
options?: UseStorageOptions<string>
): RemovableRef<string>
export declare function useStorage(
key: string,
defaults: MaybeComputedRef<boolean>,
storage?: StorageLike,
options?: UseStorageOptions<boolean>
): RemovableRef<boolean>
export declare function useStorage(
key: string,
defaults: MaybeComputedRef<number>,
storage?: StorageLike,
options?: UseStorageOptions<number>
): RemovableRef<number>
export declare function useStorage<T>(
key: string,
defaults: MaybeComputedRef<T>,
storage?: StorageLike,
options?: UseStorageOptions<T>
): RemovableRef<T>
export declare function useStorage<T = unknown>(
key: string,
defaults: MaybeComputedRef<null>,
storage?: StorageLike,
options?: UseStorageOptions<T>
): RemovableRef<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useStorage/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useStorage/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useStorage/index.md)
vueuse useFetch useFetch
========
| | |
| --- | --- |
| Category | [Network](../../functions#category=Network) |
| Export Size | 2.85 kB |
| Last Changed | 2 days ago |
Reactive [Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) provides the ability to abort requests, intercept requests before they are fired, automatically refetch requests when the url changes, and create your own [`useFetch`](index)with predefined options.
**TIP**When using with Nuxt 3, this functions will **NOT** be auto imported in favor of Nuxt's built-in [`useFetch()`](https://v3.nuxtjs.org/api/composables/use-fetch). Use explicit import if you want to use the function from VueUse.
Usage
-----
### Basic Usage
The [`useFetch`](index)function can be used by simply providing a url. The url can be either a string or a `ref`. The `data` object will contain the result of the request, the `error` object will contain any errors, and the `isFetching` object will indicate if the request is loading.
```
import { useFetch } from '@vueuse/core'
const { isFetching, error, data } = useFetch(url)
```
### Asynchronous Usage
[`useFetch`](index)can also be awaited just like a normal fetch. Note that whenever a component is asynchronous, whatever component that uses it must wrap the component in a `<Suspense>` tag. You can read more about the suspense api in the [Offical Vue 3 Docs](https://vuejs.org/guide/built-ins/suspense.html)
```
import { useFetch } from '@vueuse/core'
const { isFetching, error, data } = await useFetch(url)
```
### Refetching on URL change
Using a `ref` for the url parameter will allow the [`useFetch`](index)function to automatically trigger another request when the url is changed.
```
const url = ref('https://my-api.com/user/1')
const { data } = useFetch(url, { refetch: true })
url.value = 'https://my-api.com/user/2' // Will trigger another request
```
### Prevent request from firing immediately
Setting the `immediate` option to false will prevent the request from firing until the `execute` function is called.
```
const { execute } = useFetch(url, { immediate: false })
execute()
```
### Aborting a request
A request can be aborted by using the `abort` function from the [`useFetch`](index)function. The `canAbort` property indicates if the request can be aborted.
```
const { abort, canAbort } = useFetch(url)
setTimeout(() => {
if (canAbort.value)
abort()
}, 100)
```
A request can also be aborted automatically by using `timeout` property. It will call `abort` function when the given timeout is reached.
```
const { data } = useFetch(url, { timeout: 100 })
```
### Intercepting a request
The `beforeFetch` option can intercept a request before it is sent and modify the request options and url.
```
const { data } = useFetch(url, {
async beforeFetch({ url, options, cancel }) {
const myToken = await getMyToken()
if (!myToken)
cancel()
options.headers = {
...options.headers,
Authorization: `Bearer ${myToken}`,
}
return {
options,
}
},
})
```
The `afterFetch` option can intercept the response data before it is updated.
```
const { data } = useFetch(url, {
afterFetch(ctx) {
if (ctx.data.title === 'HxH')
ctx.data.title = 'Hunter x Hunter' // Modifies the response data
return ctx
},
})
```
The `onFetchError` option can intercept the response data and error before it is updated.
```
const { data } = useFetch(url, {
onFetchError(ctx) {
// ctx.data can be null when 5xx response
if (ctx.data === null)
ctx.data = { title: 'Hunter x Hunter' } // Modifies the response data
ctx.error = new Error('Custom Error') // Modifies the error
return ctx
},
})
```
### Setting the request method and return type
The request method and return type can be set by adding the appropriate methods to the end of `useFetch`
```
// Request will be sent with GET method and data will be parsed as JSON
const { data } = useFetch(url).get().json()
// Request will be sent with POST method and data will be parsed as text
const { data } = useFetch(url).post().text()
// Or set the method using the options
// Request will be sent with GET method and data will be parsed as blob
const { data } = useFetch(url, { method: 'GET' }, { refetch: true }).blob()
```
### Creating a Custom Instance
The `createFetch` function will return a useFetch function with whatever pre-configured options that are provided to it. This is useful for interacting with API's throughout an application that uses the same base URL or needs Authorization headers.
```
const useMyFetch = createFetch({
baseUrl: 'https://my-api.com',
options: {
async beforeFetch({ options }) {
const myToken = await getMyToken()
options.headers.Authorization = `Bearer ${myToken}`
return { options }
},
},
fetchOptions: {
mode: 'cors',
},
})
const { isFetching, error, data } = useMyFetch('users')
```
If you want to control the behavior of `beforeFetch`, `afterFetch`, `onFetchError` between the pre-configured instance and newly spawned instance. You can provide a `combination` option to toggle between `overwrite` or `chaining`.
```
const useMyFetch = createFetch({
baseUrl: 'https://my-api.com',
combination: 'overwrite',
options: {
// beforeFetch in pre-configured instance will only run when the newly spawned instance do not pass beforeFetch
async beforeFetch({ options }) {
const myToken = await getMyToken()
options.headers.Authorization = `Bearer ${myToken}`
return { options }
},
},
})
// use useMyFetch beforeFetch
const { isFetching, error, data } = useMyFetch('users')
// use custom beforeFetch
const { isFetching, error, data } = useMyFetch('users', {
async beforeFetch({ url, options, cancel }) {
const myToken = await getMyToken()
if (!myToken)
cancel()
options.headers = {
...options.headers,
Authorization: `Bearer ${myToken}`,
}
return {
options,
}
},
})
```
### Events
The `onFetchResponse` and `onFetchError` will fire on fetch request responses and errors respectively.
```
const { onFetchResponse, onFetchError } = useFetch(url)
onFetchResponse((response) => {
console.log(response.status)
})
onFetchError((error) => {
console.error(error.message)
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseFetchReturn<T> {
/**
* Indicates if the fetch request has finished
*/
isFinished: Ref<boolean>
/**
* The statusCode of the HTTP fetch response
*/
statusCode: Ref<number | null>
/**
* The raw response of the fetch response
*/
response: Ref<Response | null>
/**
* Any fetch errors that may have occurred
*/
error: Ref<any>
/**
* The fetch response body, may either be JSON or text
*/
data: Ref<T | null>
/**
* Indicates if the request is currently being fetched.
*/
isFetching: Ref<boolean>
/**
* Indicates if the fetch request is able to be aborted
*/
canAbort: ComputedRef<boolean>
/**
* Indicates if the fetch request was aborted
*/
aborted: Ref<boolean>
/**
* Abort the fetch request
*/
abort: Fn
/**
* Manually call the fetch
* (default not throwing error)
*/
execute: (throwOnFailed?: boolean) => Promise<any>
/**
* Fires after the fetch request has finished
*/
onFetchResponse: EventHookOn<Response>
/**
* Fires after a fetch request error
*/
onFetchError: EventHookOn
/**
* Fires after a fetch has completed
*/
onFetchFinally: EventHookOn
get(): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
post(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
put(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
delete(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
patch(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
head(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
options(
payload?: MaybeComputedRef<unknown>,
type?: string
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
json<JSON = any>(): UseFetchReturn<JSON> & PromiseLike<UseFetchReturn<JSON>>
text(): UseFetchReturn<string> & PromiseLike<UseFetchReturn<string>>
blob(): UseFetchReturn<Blob> & PromiseLike<UseFetchReturn<Blob>>
arrayBuffer(): UseFetchReturn<ArrayBuffer> &
PromiseLike<UseFetchReturn<ArrayBuffer>>
formData(): UseFetchReturn<FormData> & PromiseLike<UseFetchReturn<FormData>>
}
type Combination = "overwrite" | "chain"
export interface BeforeFetchContext {
/**
* The computed url of the current request
*/
url: string
/**
* The request options of the current request
*/
options: RequestInit
/**
* Cancels the current request
*/
cancel: Fn
}
export interface AfterFetchContext<T = any> {
response: Response
data: T | null
}
export interface OnFetchErrorContext<T = any, E = any> {
error: E
data: T | null
}
export interface UseFetchOptions {
/**
* Fetch function
*/
fetch?: typeof window.fetch
/**
* Will automatically run fetch when `useFetch` is used
*
* @default true
*/
immediate?: boolean
/**
* Will automatically refetch when:
* - the URL is changed if the URL is a ref
* - the payload is changed if the payload is a ref
*
* @default false
*/
refetch?: MaybeComputedRef<boolean>
/**
* Initial data before the request finished
*
* @default null
*/
initialData?: any
/**
* Timeout for abort request after number of millisecond
* `0` means use browser default
*
* @default 0
*/
timeout?: number
/**
* Will run immediately before the fetch request is dispatched
*/
beforeFetch?: (
ctx: BeforeFetchContext
) =>
| Promise<Partial<BeforeFetchContext> | void>
| Partial<BeforeFetchContext>
| void
/**
* Will run immediately after the fetch request is returned.
* Runs after any 2xx response
*/
afterFetch?: (
ctx: AfterFetchContext
) => Promise<Partial<AfterFetchContext>> | Partial<AfterFetchContext>
/**
* Will run immediately after the fetch request is returned.
* Runs after any 4xx and 5xx response
*/
onFetchError?: (ctx: {
data: any
response: Response | null
error: any
}) => Promise<Partial<OnFetchErrorContext>> | Partial<OnFetchErrorContext>
}
export interface CreateFetchOptions {
/**
* The base URL that will be prefixed to all urls unless urls are absolute
*/
baseUrl?: MaybeComputedRef<string>
/**
* Determine the inherit behavior for beforeFetch, afterFetch, onFetchError
* @default 'chain'
*/
combination?: Combination
/**
* Default Options for the useFetch function
*/
options?: UseFetchOptions
/**
* Options for the fetch request
*/
fetchOptions?: RequestInit
}
export declare function createFetch(
config?: CreateFetchOptions
): typeof useFetch
export declare function useFetch<T>(
url: MaybeComputedRef<string>
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
export declare function useFetch<T>(
url: MaybeComputedRef<string>,
useFetchOptions: UseFetchOptions
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
export declare function useFetch<T>(
url: MaybeComputedRef<string>,
options: RequestInit,
useFetchOptions?: UseFetchOptions
): UseFetchReturn<T> & PromiseLike<UseFetchReturn<T>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFetch/index.md)
| programming_docs |
vueuse useMouse useMouse
========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.29 kB |
| Last Changed | 2 days ago |
Reactive mouse position
Basic Usage
-----------
```
import { useMouse } from '@vueuse/core'
const { x, y, sourceType } = useMouse()
```
Touch is enabled by default. To only detect mouse changes, set `touch` to `false`. The `dragover` event is used to track mouse position while dragging.
```
const { x, y } = useMouse({ touch: false })
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseMouse v-slot="{ x, y }">
x: {{ x }}
y: {{ y }}
</UseMouse>
```
Type Declarations
-----------------
```
export interface UseMouseOptions
extends ConfigurableWindow,
ConfigurableEventFilter {
/**
* Mouse position based by page, client, or relative to previous position
*
* @default 'page'
*/
type?: "page" | "client" | "movement"
/**
* Listen to `touchmove` events
*
* @default true
*/
touch?: boolean
/**
* Reset to initial value when `touchend` event fired
*
* @default false
*/
resetOnTouchEnds?: boolean
/**
* Initial values
*/
initialValue?: Position
}
export type MouseSourceType = "mouse" | "touch" | null
/**
* Reactive mouse position.
*
* @see https://vueuse.org/useMouse
* @param options
*/
export declare function useMouse(options?: UseMouseOptions): {
x: Ref<number>
y: Ref<number>
sourceType: Ref<MouseSourceType>
}
export type UseMouseReturn = ReturnType<typeof useMouse>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouse/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouse/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouse/index.md)
vueuse useTimeAgo useTimeAgo
==========
| | |
| --- | --- |
| Category | [Time](../../functions#category=Time) |
| Export Size | 1.93 kB |
| Last Changed | 2 weeks ago |
Reactive time ago. Automatically update the time ago string when the time changes.
Usage
-----
```
import { useTimeAgo } from '@vueuse/core'
const timeAgo = useTimeAgo(new Date(2021, 0, 1))
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseTimeAgo v-slot="{ timeAgo }" :time="new Date(2021, 0, 1)">
Time Ago: {{ timeAgo }}
</UseTimeAgo>
```
Non-Reactivity Usage
--------------------
In case you don't need the reactivity, you can use the `formatTimeAgo` function to get the formatted string instead of a Ref.
```
import { formatTimeAgo } from '@vueuse/core'
const timeAgo = formatTimeAgo(new Date(2021, 0, 1)) // string
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseTimeAgoFormatter<T = number> = (
value: T,
isPast: boolean
) => string
export type UseTimeAgoUnitNamesDefault =
| "second"
| "minute"
| "hour"
| "day"
| "week"
| "month"
| "year"
export interface UseTimeAgoMessagesBuiltIn {
justNow: string
past: string | UseTimeAgoFormatter<string>
future: string | UseTimeAgoFormatter<string>
invalid: string
}
export type UseTimeAgoMessages<
UnitNames extends string = UseTimeAgoUnitNamesDefault
> = UseTimeAgoMessagesBuiltIn &
Record<UnitNames, string | UseTimeAgoFormatter<number>>
export interface FormatTimeAgoOptions<
UnitNames extends string = UseTimeAgoUnitNamesDefault
> {
/**
* Maximum unit (of diff in milliseconds) to display the full date instead of relative
*
* @default undefined
*/
max?: UnitNames | number
/**
* Formatter for full date
*/
fullDateFormatter?: (date: Date) => string
/**
* Messages for formatting the string
*/
messages?: UseTimeAgoMessages<UnitNames>
/**
* Minimum display time unit (default is minute)
*
* @default false
*/
showSecond?: boolean
/**
* Rounding method to apply.
*
* @default 'round'
*/
rounding?: "round" | "ceil" | "floor" | number
/**
* Custom units
*/
units?: UseTimeAgoUnit<UseTimeAgoUnitNamesDefault>[]
}
export interface UseTimeAgoOptions<
Controls extends boolean,
UnitNames extends string = UseTimeAgoUnitNamesDefault
> extends FormatTimeAgoOptions<UnitNames> {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
/**
* Intervals to update, set 0 to disable auto update
*
* @default 30_000
*/
updateInterval?: number
}
export interface UseTimeAgoUnit<
Unit extends string = UseTimeAgoUnitNamesDefault
> {
max: number
value: number
name: Unit
}
export type UseTimeAgoReturn<Controls extends boolean = false> =
Controls extends true
? {
timeAgo: ComputedRef<string>
} & Pausable
: ComputedRef<string>
/**
* Reactive time ago formatter.
*
* @see https://vueuse.org/useTimeAgo
* @param options
*/
export declare function useTimeAgo<
UnitNames extends string = UseTimeAgoUnitNamesDefault
>(
time: MaybeComputedRef<Date | number | string>,
options?: UseTimeAgoOptions<false, UnitNames>
): UseTimeAgoReturn<false>
export declare function useTimeAgo<
UnitNames extends string = UseTimeAgoUnitNamesDefault
>(
time: MaybeComputedRef<Date | number | string>,
options: UseTimeAgoOptions<true, UnitNames>
): UseTimeAgoReturn<true>
export declare function formatTimeAgo<
UnitNames extends string = UseTimeAgoUnitNamesDefault
>(
from: Date,
options?: FormatTimeAgoOptions<UnitNames>,
now?: Date | number
): string
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeAgo/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeAgo/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeAgo/index.md)
vueuse useFavicon useFavicon
==========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 930 B |
| Last Changed | 4 months ago |
Reactive favicon
Usage
-----
```
import { useFavicon } from '@vueuse/core'
const icon = useFavicon()
icon.value = 'dark.png' // change current icon
```
### Passing a source ref
You can pass a `ref` to it, changes from of the source ref will be reflected to your favicon automatically.
```
import { computed } from 'vue'
import { useFavicon, usePreferredDark } from '@vueuse/core'
const isDark = usePreferredDark()
const favicon = computed(() => isDark.value ? 'dark.png' : 'light.png')
useFavicon(favicon)
```
When a source ref is passed, the return ref will be identical to the source ref
```
const source = ref('icon.png')
const icon = useFavicon(source)
console.log(icon === source) // true
```
Type Declarations
-----------------
```
export interface UseFaviconOptions extends ConfigurableDocument {
baseUrl?: string
rel?: string
}
/**
* Reactive favicon.
*
* @see https://vueuse.org/useFavicon
* @param newIcon
* @param options
*/
export declare function useFavicon(
newIcon: MaybeReadonlyRef<string | null | undefined>,
options?: UseFaviconOptions
): ComputedRef<string | null | undefined>
export declare function useFavicon(
newIcon?: MaybeRef<string | null | undefined>,
options?: UseFaviconOptions
): Ref<string | null | undefined>
export type UseFaviconReturn = ReturnType<typeof useFavicon>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFavicon/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFavicon/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFavicon/index.md)
vueuse useElementSize useElementSize
==============
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.27 kB |
| Last Changed | 4 months ago |
Reactive size of an HTML element. [ResizeObserver MDN](https://developer.mozilla.org/en-US/docs/Web/API/ResizeObserver)
Usage
-----
```
<template>
<div ref="el">
Height: {{ height }}
Width: {{ width }}
</div>
</template>
<script>
import { ref } from 'vue'
import { useElementSize } from '@vueuse/core'
export default {
setup() {
const el = ref(null)
const { width, height } = useElementSize(el)
return {
el,
width,
height,
}
}
})
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseElementSize v-slot="{ width, height }">
Width: {{ width }}
Height: {{ height }}
</UseElementSize>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { vElementSize } from '@vueuse/components'
function onResize({ width, height }: { width: number; height: number }) {
console.log(width, height)
}
</script>
<template>
<textarea v-element-size="onResize" />
<!-- with options -->
<textarea v-element-size="[onResize, {width:100,height:100}, {'box':'content-box'} ]" />
</template>
```
Type Declarations
-----------------
```
export interface ElementSize {
width: number
height: number
}
/**
* Reactive size of an HTML element.
*
* @see https://vueuse.org/useElementSize
* @param target
* @param callback
* @param options
*/
export declare function useElementSize(
target: MaybeComputedElementRef,
initialSize?: ElementSize,
options?: UseResizeObserverOptions
): {
width: Ref<number>
height: Ref<number>
}
export type UseElementSizeReturn = ReturnType<typeof useElementSize>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementSize/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementSize/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementSize/index.md)
vueuse useTimestamp useTimestamp
============
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 1.23 kB |
| Last Changed | 3 months ago |
Reactive current timestamp
Usage
-----
```
import { useTimestamp } from '@vueuse/core'
const timestamp = useTimestamp({ offset: 0 })
```
```
const { timestamp, pause, resume } = useTimestamp({ controls: true })
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseTimestamp v-slot="{ timestamp, pause, resume }">
Current Time: {{ timestamp }}
<button @click="pause()">Pause</button>
<button @click="resume()">Resume</button>
</UseTimestamp>
```
Type Declarations
-----------------
```
export interface UseTimestampOptions<Controls extends boolean> {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
/**
* Offset value adding to the value
*
* @default 0
*/
offset?: number
/**
* Update the timestamp immediately
*
* @default true
*/
immediate?: boolean
/**
* Update interval, or use requestAnimationFrame
*
* @default requestAnimationFrame
*/
interval?: "requestAnimationFrame" | number
/**
* Callback on each update
*/
callback?: (timestamp: number) => void
}
/**
* Reactive current timestamp.
*
* @see https://vueuse.org/useTimestamp
* @param options
*/
export declare function useTimestamp(
options?: UseTimestampOptions<false>
): Ref<number>
export declare function useTimestamp(options: UseTimestampOptions<true>): {
timestamp: Ref<number>
} & Pausable
export type UseTimestampReturn = ReturnType<typeof useTimestamp>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimestamp/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimestamp/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimestamp/index.md)
vueuse useWindowScroll useWindowScroll
===============
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.1 kB |
| Last Changed | 3 weeks ago |
Reactive window scroll
Usage
-----
```
import { useWindowScroll } from '@vueuse/core'
const { x, y } = useWindowScroll()
```
Type Declarations
-----------------
```
/**
* Reactive window scroll.
*
* @see https://vueuse.org/useWindowScroll
* @param options
*/
export declare function useWindowScroll({ window }?: ConfigurableWindow): {
x: Ref<number>
y: Ref<number>
}
export type UseWindowScrollReturn = ReturnType<typeof useWindowScroll>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowScroll/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowScroll/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowScroll/index.md)
vueuse useTimeoutPoll useTimeoutPoll
==============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1.02 kB |
| Last Changed | 6 months ago |
Use timeout to poll something. It's will trigger callback after last task is done.
Usage
-----
```
import { useTimeoutPoll } from '@vueuse/core'
const count = ref(0)
const fetchData = async () => {
await promiseTimeout(1000)
count.value++
}
// Only trigger after last fetch is done
const { isActive, pause, resume } = useTimeoutPoll(fetchData, 1000)
```
Type Declarations
-----------------
```
export declare function useTimeoutPoll(
fn: () => Awaitable<void>,
interval: MaybeComputedRef<number>,
timeoutPollOptions?: UseTimeoutFnOptions
): Pausable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeoutPoll/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeoutPoll/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTimeoutPoll/index.md)
vueuse useDeviceMotion useDeviceMotion
===============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.21 kB |
| Last Changed | last year |
Reactive [DeviceMotionEvent](https://developer.mozilla.org/en-US/docs/Web/API/DeviceMotionEvent). Provide web developers with information about the speed of changes for the device's position and orientation.
Usage
-----
```
import { useDeviceMotion } from '@vueuse/core'
const {
acceleration,
accelerationIncludingGravity,
rotationRate,
interval,
} = useDeviceMotion()
```
| State | Type | Description |
| --- | --- | --- |
| acceleration | `object` | An object giving the acceleration of the device on the three axis X, Y and Z. |
| accelerationIncludingGravity | `object` | An object giving the acceleration of the device on the three axis X, Y and Z with the effect of gravity. |
| rotationRate | `object` | An object giving the rate of change of the device's orientation on the three orientation axis alpha, beta and gamma. |
| interval | `Number` | A number representing the interval of time, in milliseconds, at which data is obtained from the device.. |
You can find [more information about the state on the MDN](https://developer.mozilla.org/en-US/docs/Web/API/DeviceMotionEvent#Properties).
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDeviceMotion v-slot="{ acceleration }">
Acceleration: {{ acceleration }}
</UseDeviceMotion>
```
Type Declarations
-----------------
```
export interface DeviceMotionOptions
extends ConfigurableWindow,
ConfigurableEventFilter {}
/**
* Reactive DeviceMotionEvent.
*
* @see https://vueuse.org/useDeviceMotion
* @param options
*/
export declare function useDeviceMotion(options?: DeviceMotionOptions): {
acceleration: Ref<DeviceMotionEventAcceleration | null>
accelerationIncludingGravity: Ref<DeviceMotionEventAcceleration | null>
rotationRate: Ref<DeviceMotionEventRotationRate | null>
interval: Ref<number>
}
export type UseDeviceMotionReturn = ReturnType<typeof useDeviceMotion>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceMotion/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceMotion/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceMotion/index.md)
vueuse useMousePressed useMousePressed
===============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.21 kB |
| Last Changed | last year |
Reactive mouse pressing state. Triggered by `mousedown` `touchstart` on target element and released by `mouseup` `mouseleave` `touchend` `touchcancel` on window.
Basic Usage
-----------
```
import { useMousePressed } from '@vueuse/core'
const { pressed } = useMousePressed()
```
Touching is enabled by default. To make it only detects mouse changes, set `touch` to `false`
```
const { pressed } = useMousePressed({ touch: false })
```
To only capture `mousedown` and `touchstart` on specific element, you can specify `target` by passing a ref of the element.
```
<template>
<div ref="el">
Only clicking on this element will trigger the update.
</div>
</template>
<script>
import { ref } from 'vue'
import { useMousePressed } from '@vueuse/core'
export default {
setup() {
const el = ref(null)
const { pressed } = useMousePressed({ target: el })
return {
el,
pressed,
}
}
})
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseMousePressed v-slot="{ pressed }">
Is Pressed: {{ pressed }}
</UseMousePressed>
```
Type Declarations
-----------------
```
export interface MousePressedOptions extends ConfigurableWindow {
/**
* Listen to `touchstart` `touchend` events
*
* @default true
*/
touch?: boolean
/**
* Listen to `dragstart` `drop` and `dragend` events
*
* @default true
*/
drag?: boolean
/**
* Initial values
*
* @default false
*/
initialValue?: boolean
/**
* Element target to be capture the click
*/
target?: MaybeElementRef
}
/**
* Reactive mouse position.
*
* @see https://vueuse.org/useMousePressed
* @param options
*/
export declare function useMousePressed(options?: MousePressedOptions): {
pressed: Ref<boolean>
sourceType: Ref<MouseSourceType>
}
export type UseMousePressedReturn = ReturnType<typeof useMousePressed>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMousePressed/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMousePressed/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMousePressed/index.md)
vueuse useStepper useStepper
==========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1.03 kB |
| Last Changed | 6 months ago |
Provides helpers for building a multi-step wizard interface.
Usage
-----
### Steps as array
```
import { useStepper } from '@vueuse/core'
const {
steps,
stepNames,
index,
current,
next,
previous,
isFirst,
isLast,
goTo,
goNext,
goPrevious,
goBackTo,
isNext,
isPrevious,
isCurrent,
isBefore,
isAfter,
} = useStepper([
'billing-address',
'terms',
'payment',
])
// Access the step through `current`
console.log(current.value) // 'billing-address'
```
### Steps as object
```
import { useStepper } from '@vueuse/core'
const {
steps,
stepNames,
index,
current,
next,
previous,
isFirst,
isLast,
goTo,
goNext,
goPrevious,
goBackTo,
isNext,
isPrevious,
isCurrent,
isBefore,
isAfter,
} = useStepper({
'user-information': {
title: 'User information',
},
'billing-address': {
title: 'Billing address',
},
'terms': {
title: 'Terms',
},
'payment': {
title: 'Payment',
},
})
// Access the step object through `current`
console.log(current.value.title) // 'User information'
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseStepperReturn<StepName, Steps, Step> {
/** List of steps. */
steps: Readonly<Ref<Steps>>
/** List of step names. */
stepNames: Readonly<Ref<StepName[]>>
/** Index of the current step. */
index: Ref<number>
/** Current step. */
current: ComputedRef<Step>
/** Next step, or undefined if the current step is the last one. */
next: ComputedRef<StepName | undefined>
/** Previous step, or undefined if the current step is the first one. */
previous: ComputedRef<StepName | undefined>
/** Whether the current step is the first one. */
isFirst: ComputedRef<boolean>
/** Whether the current step is the last one. */
isLast: ComputedRef<boolean>
/** Get the step at the specified index. */
at: (index: number) => Step | undefined
/** Get a step by the specified name. */
get: (step: StepName) => Step | undefined
/** Go to the specified step. */
goTo: (step: StepName) => void
/** Go to the next step. Does nothing if the current step is the last one. */
goToNext: () => void
/** Go to the previous step. Does nothing if the current step is the previous one. */
goToPrevious: () => void
/** Go back to the given step, only if the current step is after. */
goBackTo: (step: StepName) => void
/** Checks whether the given step is the next step. */
isNext: (step: StepName) => boolean
/** Checks whether the given step is the previous step. */
isPrevious: (step: StepName) => boolean
/** Checks whether the given step is the current step. */
isCurrent: (step: StepName) => boolean
/** Checks if the current step is before the given step. */
isBefore: (step: StepName) => boolean
/** Checks if the current step is after the given step. */
isAfter: (step: StepName) => boolean
}
export declare function useStepper<T extends string | number>(
steps: MaybeRef<T[]>,
initialStep?: T
): UseStepperReturn<T, T[], T>
export declare function useStepper<T extends Record<string, any>>(
steps: MaybeRef<T>,
initialStep?: keyof T
): UseStepperReturn<Exclude<keyof T, symbol>, T, T[keyof T]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useStepper/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useStepper/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useStepper/index.md)
| programming_docs |
vueuse useEventListener useEventListener
================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 997 B |
| Last Changed | 2 days ago |
Use EventListener with ease. Register using [addEventListener](https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/addEventListener) on mounted, and [removeEventListener](https://developer.mozilla.org/en-US/docs/Web/API/EventTarget/removeEventListener) automatically on unmounted.
Usage
-----
```
import { useEventListener } from '@vueuse/core'
useEventListener(document, 'visibilitychange', (evt) => {
console.log(evt)
})
```
You can also pass a ref as the event target, [`useEventListener`](index)will unregister the previous event and register the new one when you change the target.
```
import { useEventListener } from '@vueuse/core'
const element = ref<HTMLDivElement>()
useEventListener(element, 'keydown', (e) => {
console.log(e.key)
})
```
```
<template>
<div v-if="cond" ref="element">Div1</div>
<div v-else ref="element">Div2</div>
</template>
```
You can also call the returned to unregister the listener.
```
import { useEventListener } from '@vueuse/core'
const cleanup = useEventListener(document, 'keydown', (e) => {
console.log(e.key)
})
cleanup() // This will unregister the listener.
```
Type Declarations
-----------------
Show Type Declarations
```
interface InferEventTarget<Events> {
addEventListener(event: Events, fn?: any, options?: any): any
removeEventListener(event: Events, fn?: any, options?: any): any
}
export type WindowEventName = keyof WindowEventMap
export type DocumentEventName = keyof DocumentEventMap
export interface GeneralEventListener<E = Event> {
(evt: E): void
}
/**
* Register using addEventListener on mounted, and removeEventListener automatically on unmounted.
*
* Overload 1: Omitted Window target
*
* @see https://vueuse.org/useEventListener
* @param event
* @param listener
* @param options
*/
export declare function useEventListener<E extends keyof WindowEventMap>(
event: Arrayable<E>,
listener: Arrayable<(this: Window, ev: WindowEventMap[E]) => any>,
options?: boolean | AddEventListenerOptions
): Fn
/**
* Register using addEventListener on mounted, and removeEventListener automatically on unmounted.
*
* Overload 2: Explicitly Window target
*
* @see https://vueuse.org/useEventListener
* @param target
* @param event
* @param listener
* @param options
*/
export declare function useEventListener<E extends keyof WindowEventMap>(
target: Window,
event: Arrayable<E>,
listener: Arrayable<(this: Window, ev: WindowEventMap[E]) => any>,
options?: boolean | AddEventListenerOptions
): Fn
/**
* Register using addEventListener on mounted, and removeEventListener automatically on unmounted.
*
* Overload 3: Explicitly Document target
*
* @see https://vueuse.org/useEventListener
* @param target
* @param event
* @param listener
* @param options
*/
export declare function useEventListener<E extends keyof DocumentEventMap>(
target: DocumentOrShadowRoot,
event: Arrayable<E>,
listener: Arrayable<(this: Document, ev: DocumentEventMap[E]) => any>,
options?: boolean | AddEventListenerOptions
): Fn
/**
* Register using addEventListener on mounted, and removeEventListener automatically on unmounted.
*
* Overload 4: Custom event target with event type infer
*
* @see https://vueuse.org/useEventListener
* @param target
* @param event
* @param listener
* @param options
*/
export declare function useEventListener<
Names extends string,
EventType = Event
>(
target: InferEventTarget<Names>,
event: Arrayable<Names>,
listener: Arrayable<GeneralEventListener<EventType>>,
options?: boolean | AddEventListenerOptions
): Fn
/**
* Register using addEventListener on mounted, and removeEventListener automatically on unmounted.
*
* Overload 5: Custom event target fallback
*
* @see https://vueuse.org/useEventListener
* @param target
* @param event
* @param listener
* @param options
*/
export declare function useEventListener<EventType = Event>(
target: MaybeComputedRef<EventTarget | null | undefined>,
event: Arrayable<string>,
listener: Arrayable<GeneralEventListener<EventType>>,
options?: boolean | AddEventListenerOptions
): Fn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventListener/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventListener/index.md)
vueuse useTransition useTransition
=============
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 1.66 kB |
| Last Changed | 2 months ago |
Transition between values
Usage
-----
For simple transitions, provide a numeric source value to watch. When changed, the output will transition to the new value. If the source changes while a transition is in progress, a new transition will begin from where the previous one was interrupted.
```
import { ref } from 'vue'
import { TransitionPresets, useTransition } from '@vueuse/core'
const source = ref(0)
const output = useTransition(source, {
duration: 1000,
transition: TransitionPresets.easeInOutCubic,
})
```
To synchronize transitions, use an array of numbers. As an example, here is how we could transition between colors.
```
const source = ref([0, 0, 0])
const output = useTransition(source)
const color = computed(() => {
const [r, g, b] = output.value
return `rgb(${r}, ${g}, ${b})`
})
```
Transition easing can be customized using cubic bezier curves. Transitions defined this way work the same as [CSS easing functions](https://developer.mozilla.org/en-US/docs/Web/CSS/easing-function#easing_functions).
```
useTransition(source, {
transition: [0.75, 0, 0.25, 1],
})
```
The following transitions are available via the `TransitionPresets` constant.
* [`linear`](https://cubic-bezier.com/#0,0,1,1)
* [`easeInSine`](https://cubic-bezier.com/#.12,0,.39,0)
* [`easeOutSine`](https://cubic-bezier.com/#.61,1,.88,1)
* [`easeInOutSine`](https://cubic-bezier.com/#.37,0,.63,1)
* [`easeInQuad`](https://cubic-bezier.com/#.11,0,.5,0)
* [`easeOutQuad`](https://cubic-bezier.com/#.5,1,.89,1)
* [`easeInOutQuad`](https://cubic-bezier.com/#.45,0,.55,1)
* [`easeInCubic`](https://cubic-bezier.com/#.32,0,.67,0)
* [`easeOutCubic`](https://cubic-bezier.com/#.33,1,.68,1)
* [`easeInOutCubic`](https://cubic-bezier.com/#.65,0,.35,1)
* [`easeInQuart`](https://cubic-bezier.com/#.5,0,.75,0)
* [`easeOutQuart`](https://cubic-bezier.com/#.25,1,.5,1)
* [`easeInOutQuart`](https://cubic-bezier.com/#.76,0,.24,1)
* [`easeInQuint`](https://cubic-bezier.com/#.64,0,.78,0)
* [`easeOutQuint`](https://cubic-bezier.com/#.22,1,.36,1)
* [`easeInOutQuint`](https://cubic-bezier.com/#.83,0,.17,1)
* [`easeInExpo`](https://cubic-bezier.com/#.7,0,.84,0)
* [`easeOutExpo`](https://cubic-bezier.com/#.16,1,.3,1)
* [`easeInOutExpo`](https://cubic-bezier.com/#.87,0,.13,1)
* [`easeInCirc`](https://cubic-bezier.com/#.55,0,1,.45)
* [`easeOutCirc`](https://cubic-bezier.com/#0,.55,.45,1)
* [`easeInOutCirc`](https://cubic-bezier.com/#.85,0,.15,1)
* [`easeInBack`](https://cubic-bezier.com/#.36,0,.66,-.56)
* [`easeOutBack`](https://cubic-bezier.com/#.34,1.56,.64,1)
* [`easeInOutBack`](https://cubic-bezier.com/#.68,-.6,.32,1.6)
For more complex transitions, a custom function can be provided.
```
const easeOutElastic = (n) => {
return n === 0
? 0
: n === 1
? 1
: (2 ** (-10 * n)) * Math.sin((n * 10 - 0.75) * ((2 * Math.PI) / 3)) + 1
}
useTransition(source, {
transition: easeOutElastic,
})
```
To control when a transition starts, set a `delay` value. To choreograph behavior around a transition, define `onStarted` or `onFinished` callbacks.
```
useTransition(source, {
delay: 1000,
onStarted() {
// called after the transition starts
},
onFinished() {
// called after the transition ends
},
})
```
To temporarily stop transitioning, define a boolean `disabled` property. Be aware, this is not the same a `duration` of `0`. Disabled transitions track the source value ***synchronously***. They do not respect a `delay`, and do not fire `onStarted` or `onFinished` callbacks.
Type Declarations
-----------------
Show Type Declarations
```
/**
* Cubic bezier points
*/
export type CubicBezierPoints = [number, number, number, number]
/**
* Easing function
*/
export type EasingFunction = (n: number) => number
/**
* Transition options
*/
export interface UseTransitionOptions {
/**
* Milliseconds to wait before starting transition
*/
delay?: MaybeRef<number>
/**
* Disables the transition
*/
disabled?: MaybeRef<boolean>
/**
* Transition duration in milliseconds
*/
duration?: MaybeRef<number>
/**
* Callback to execute after transition finishes
*/
onFinished?: () => void
/**
* Callback to execute after transition starts
*/
onStarted?: () => void
/**
* Easing function or cubic bezier points for calculating transition values
*/
transition?: MaybeRef<EasingFunction | CubicBezierPoints>
}
/**
* Common transitions
*
* @see https://easings.net
*/
export declare const TransitionPresets: Record<
| "easeInSine"
| "easeOutSine"
| "easeInOutSine"
| "easeInQuad"
| "easeOutQuad"
| "easeInOutQuad"
| "easeInCubic"
| "easeOutCubic"
| "easeInOutCubic"
| "easeInQuart"
| "easeOutQuart"
| "easeInOutQuart"
| "easeInQuint"
| "easeOutQuint"
| "easeInOutQuint"
| "easeInExpo"
| "easeOutExpo"
| "easeInOutExpo"
| "easeInCirc"
| "easeOutCirc"
| "easeInOutCirc"
| "easeInBack"
| "easeOutBack"
| "easeInOutBack",
CubicBezierPoints
> & {
linear: EasingFunction
}
export declare function useTransition(
source: Ref<number>,
options?: UseTransitionOptions
): ComputedRef<number>
export declare function useTransition<T extends MaybeRef<number>[]>(
source: [...T],
options?: UseTransitionOptions
): ComputedRef<{
[K in keyof T]: number
}>
export declare function useTransition<T extends Ref<number[]>>(
source: T,
options?: UseTransitionOptions
): ComputedRef<number[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTransition/index.md)
vueuse useWindowSize useWindowSize
=============
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.16 kB |
| Last Changed | 4 months ago |
Reactive window size
Usage
-----
```
import { useWindowSize } from '@vueuse/core'
const { width, height } = useWindowSize()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseWindowSize v-slot="{ width, height }">
Width: {{ width }}
Height: {{ height }}
</UseWindowSize>
```
Type Declarations
-----------------
```
export interface UseWindowSizeOptions extends ConfigurableWindow {
initialWidth?: number
initialHeight?: number
/**
* Listen to window `orientationchange` event
*
* @default true
*/
listenOrientation?: boolean
/**
* Whether the scrollbar should be included in the width and height
* @default true
*/
includeScrollbar?: boolean
}
/**
* Reactive window size.
*
* @see https://vueuse.org/useWindowSize
* @param options
*/
export declare function useWindowSize(options?: UseWindowSizeOptions): {
width: Ref<number>
height: Ref<number>
}
export type UseWindowSizeReturn = ReturnType<typeof useWindowSize>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowSize/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowSize/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowSize/index.md)
vueuse useOnline useOnline
=========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.37 kB |
| Last Changed | last year |
Reactive online state. A wrapper of [`useNetwork`](../usenetwork/index)
Usage
-----
```
import { useOnline } from '@vueuse/core'
const online = useOnline()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseOnline v-slot="{ isOnline }">
Is Online: {{ isOnline }}
</UseOnline>
```
Type Declarations
-----------------
```
/**
* Reactive online state.
*
* @see https://vueuse.org/useOnline
* @param options
*/
export declare function useOnline(options?: ConfigurableWindow): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useOnline/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useOnline/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useOnline/index.md)
vueuse useElementByPoint useElementByPoint
=================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.05 kB |
| Last Changed | yesterday |
Reactive element by point.
Usage
-----
```
import { useElementByPoint, useMouse } from '@vueuse/core'
const { x, y } = useMouse({ type: 'client' })
const { element } = useElementByPoint({ x, y })
```
Type Declarations
-----------------
```
export interface UseElementByPointOptions extends ConfigurableDocument {
x: MaybeComputedRef<number>
y: MaybeComputedRef<number>
}
/**
* Reactive element by point.
*
* @see https://vueuse.org/useElementByPoint
* @param options - UseElementByPointOptions
*/
export declare function useElementByPoint(options: UseElementByPointOptions): {
isActive: Ref<boolean>
pause: Fn
resume: Fn
element: Ref<HTMLElement | null>
}
export type UseElementByPointReturn = ReturnType<typeof useElementByPoint>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementByPoint/index.md)
vueuse useMediaControls useMediaControls
================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 2.75 kB |
| Last Changed | 6 months ago |
Reactive media controls for both `audio` and `video` elements
Usage
-----
### Basic Usage
```
<script setup lang="ts">
import { onMounted, ref } from 'vue'
import { useMediaControls } from '@vueuse/core'
const video = ref()
const { playing, currentTime, duration, volume } = useMediaControls(video, {
src: 'video.mp4',
})
// Change initial media properties
onMounted(() => {
volume.value = 0.5
currentTime.value = 60
})
</script>
<template>
<video ref="video" />
<button @click="playing = !playing">Play / Pause</button>
<span>{{ currentTime }} / {{ duration }}</span>
</template>
```
### Providing Captions, Subtitles, etc...
You can provide captions, subtitles, etc in the `tracks` options of the [`useMediaControls`](index)function. The function will return an array of tracks along with two functions for controlling them, `enableTrack`, `disableTrack`, and `selectedTrack`. Using these you can manage the currently selected track. `selectedTrack` will be `-1` if there is no selected track.
```
<script setup lang="ts">
const { tracks, enableTrack } = useMediaControls(video, {
src: 'video.mp4',
tracks: [
{
default: true,
src: './subtitles.vtt',
kind: 'subtitles',
label: 'English',
srcLang: 'en',
},
]
})
</script>
<template>
<video ref="video" />
<button v-for="track in tracks" :key="track.id" @click="enableTrack(track)">
{{ track.label }}
</button>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Many of the jsdoc definitions here are modified version of the
* documentation from MDN(https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement)
*/
export interface UseMediaSource {
/**
* The source url for the media
*/
src: string
/**
* The media codec type
*/
type?: string
}
export interface UseMediaTextTrackSource {
/**
* Indicates that the track should be enabled unless the user's preferences indicate
* that another track is more appropriate
*/
default?: boolean
/**
* How the text track is meant to be used. If omitted the default kind is subtitles.
*/
kind: TextTrackKind
/**
* A user-readable title of the text track which is used by the browser
* when listing available text tracks.
*/
label: string
/**
* Address of the track (.vtt file). Must be a valid URL. This attribute
* must be specified and its URL value must have the same origin as the document
*/
src: string
/**
* Language of the track text data. It must be a valid BCP 47 language tag.
* If the kind attribute is set to subtitles, then srclang must be defined.
*/
srcLang: string
}
interface UseMediaControlsOptions extends ConfigurableDocument {
/**
* The source for the media, may either be a string, a `UseMediaSource` object, or a list
* of `UseMediaSource` objects.
*/
src?: MaybeComputedRef<string | UseMediaSource | UseMediaSource[]>
/**
* A list of text tracks for the media
*/
tracks?: MaybeComputedRef<UseMediaTextTrackSource[]>
}
export interface UseMediaTextTrack {
/**
* The index of the text track
*/
id: number
/**
* The text track label
*/
label: string
/**
* Language of the track text data. It must be a valid BCP 47 language tag.
* If the kind attribute is set to subtitles, then srclang must be defined.
*/
language: string
/**
* Specifies the display mode of the text track, either `disabled`,
* `hidden`, or `showing`
*/
mode: TextTrackMode
/**
* How the text track is meant to be used. If omitted the default kind is subtitles.
*/
kind: TextTrackKind
/**
* Indicates the track's in-band metadata track dispatch type.
*/
inBandMetadataTrackDispatchType: string
/**
* A list of text track cues
*/
cues: TextTrackCueList | null
/**
* A list of active text track cues
*/
activeCues: TextTrackCueList | null
}
export declare function useMediaControls(
target: MaybeRef<HTMLMediaElement | null | undefined>,
options?: UseMediaControlsOptions
): {
currentTime: Ref<number>
duration: Ref<number>
waiting: Ref<boolean>
seeking: Ref<boolean>
ended: Ref<boolean>
stalled: Ref<boolean>
buffered: Ref<[number, number][]>
playing: Ref<boolean>
rate: Ref<number>
volume: Ref<number>
muted: Ref<boolean>
tracks: Ref<
{
id: number
label: string
language: string
mode: TextTrackMode
kind: TextTrackKind
inBandMetadataTrackDispatchType: string
cues: {
[x: number]: {
endTime: number
id: string
onenter: ((this: TextTrackCue, ev: Event) => any) | null
onexit: ((this: TextTrackCue, ev: Event) => any) | null
pauseOnExit: boolean
startTime: number
readonly track: {
readonly activeCues: any | null
readonly cues: any | null
readonly id: string
readonly inBandMetadataTrackDispatchType: string
readonly kind: TextTrackKind
readonly label: string
readonly language: string
mode: TextTrackMode
oncuechange: ((this: TextTrack, ev: Event) => any) | null
addCue: (cue: TextTrackCue) => void
removeCue: (cue: TextTrackCue) => void
addEventListener: {
<K extends "cuechange">(
type: K,
listener: (this: TextTrack, ev: TextTrackEventMap[K]) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_1 extends "cuechange">(
type: K_1,
listener: (this: TextTrack, ev: TextTrackEventMap[K_1]) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
} | null
addEventListener: {
<K_2 extends keyof TextTrackCueEventMap>(
type: K_2,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_2]
) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_3 extends keyof TextTrackCueEventMap>(
type: K_3,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_3]
) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
}
readonly length: number
getCueById: (id: string) => TextTrackCue | null
[Symbol.iterator]: () => IterableIterator<TextTrackCue>
} | null
activeCues: {
[x: number]: {
endTime: number
id: string
onenter: ((this: TextTrackCue, ev: Event) => any) | null
onexit: ((this: TextTrackCue, ev: Event) => any) | null
pauseOnExit: boolean
startTime: number
readonly track: {
readonly activeCues: any | null
readonly cues: any | null
readonly id: string
readonly inBandMetadataTrackDispatchType: string
readonly kind: TextTrackKind
readonly label: string
readonly language: string
mode: TextTrackMode
oncuechange: ((this: TextTrack, ev: Event) => any) | null
addCue: (cue: TextTrackCue) => void
removeCue: (cue: TextTrackCue) => void
addEventListener: {
<K extends "cuechange">(
type: K,
listener: (this: TextTrack, ev: TextTrackEventMap[K]) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_1 extends "cuechange">(
type: K_1,
listener: (this: TextTrack, ev: TextTrackEventMap[K_1]) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
} | null
addEventListener: {
<K_2 extends keyof TextTrackCueEventMap>(
type: K_2,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_2]
) => any,
options?: boolean | AddEventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | AddEventListenerOptions | undefined
): void
}
removeEventListener: {
<K_3 extends keyof TextTrackCueEventMap>(
type: K_3,
listener: (
this: TextTrackCue,
ev: TextTrackCueEventMap[K_3]
) => any,
options?: boolean | EventListenerOptions | undefined
): void
(
type: string,
listener: EventListenerOrEventListenerObject,
options?: boolean | EventListenerOptions | undefined
): void
}
dispatchEvent: (event: Event) => boolean
}
readonly length: number
getCueById: (id: string) => TextTrackCue | null
[Symbol.iterator]: () => IterableIterator<TextTrackCue>
} | null
}[]
>
selectedTrack: Ref<number>
enableTrack: (
track: number | UseMediaTextTrack,
disableTracks?: boolean
) => void
disableTrack: (track?: number | UseMediaTextTrack) => void
supportsPictureInPicture: boolean | undefined
togglePictureInPicture: () => Promise<unknown>
isPictureInPicture: Ref<boolean>
onSourceError: EventHookOn<Event>
}
export type UseMediaControlsReturn = ReturnType<typeof useMediaControls>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaControls/index.md)
| programming_docs |
vueuse usePageLeave usePageLeave
============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.11 kB |
| Last Changed | last year |
Reactive state to show whether the mouse leaves the page.
Usage
-----
```
import { usePageLeave } from '@vueuse/core'
const isLeft = usePageLeave()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePageLeave v-slot="{ isLeft }">
Has Left Page: {{ isLeft }}
</UsePageLeave>
```
Type Declarations
-----------------
```
/**
* Reactive state to show whether mouse leaves the page.
*
* @see https://vueuse.org/usePageLeave
* @param options
*/
export declare function usePageLeave(options?: ConfigurableWindow): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePageLeave/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePageLeave/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePageLeave/index.md)
vueuse useNetwork useNetwork
==========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.35 kB |
| Last Changed | 6 months ago |
Reactive [Network status](https://developer.mozilla.org/en-US/docs/Web/API/Network_Information_API). The Network Information API provides information about the system's connection in terms of general connection type (e.g., 'wifi', 'cellular', etc.). This can be used to select high definition content or low definition content based on the user's connection. The entire API consists of the addition of the NetworkInformation interface and a single property to the Navigator interface: Navigator.connection.
Usage
-----
```
import { useNetwork } from '@vueuse/core'
const { isOnline, offlineAt, downlink, downlinkMax, effectiveType, saveData, type } = useNetwork()
console.log(isOnline.value)
```
To use as an object, wrap it with `reactive()`
```
import { reactive } from 'vue'
const network = reactive(useNetwork())
console.log(network.isOnline)
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseNetwork v-slot="{ isOnline, type }">
Is Online: {{ isOnline }}
Type: {{ type }}
<UseNetwork>
```
Type Declarations
-----------------
Show Type Declarations
```
export type NetworkType =
| "bluetooth"
| "cellular"
| "ethernet"
| "none"
| "wifi"
| "wimax"
| "other"
| "unknown"
export type NetworkEffectiveType = "slow-2g" | "2g" | "3g" | "4g" | undefined
export interface NetworkState {
isSupported: Ref<boolean>
/**
* If the user is currently connected.
*/
isOnline: Ref<boolean>
/**
* The time since the user was last connected.
*/
offlineAt: Ref<number | undefined>
/**
* At this time, if the user is offline and reconnects
*/
onlineAt: Ref<number | undefined>
/**
* The download speed in Mbps.
*/
downlink: Ref<number | undefined>
/**
* The max reachable download speed in Mbps.
*/
downlinkMax: Ref<number | undefined>
/**
* The detected effective speed type.
*/
effectiveType: Ref<NetworkEffectiveType | undefined>
/**
* The estimated effective round-trip time of the current connection.
*/
rtt: Ref<number | undefined>
/**
* If the user activated data saver mode.
*/
saveData: Ref<boolean | undefined>
/**
* The detected connection/network type.
*/
type: Ref<NetworkType>
}
/**
* Reactive Network status.
*
* @see https://vueuse.org/useNetwork
* @param options
*/
export declare function useNetwork(
options?: ConfigurableWindow
): Readonly<NetworkState>
export type UseNetworkReturn = ReturnType<typeof useNetwork>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useNetwork/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useNetwork/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useNetwork/index.md)
vueuse useActiveElement useActiveElement
================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.08 kB |
| Last Changed | 2 days ago |
Reactive `document.activeElement`
Usage
-----
```
import { useActiveElement } from '@vueuse/core'
const activeElement = useActiveElement()
watch(activeElement, (el) => {
console.log('focus changed to', el)
})
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseActiveElement v-slot="{ element }">
Active element is {{ element.dataset.id }}
</UseActiveElement>
```
Type Declarations
-----------------
```
export interface UseActiveElementOptions
extends ConfigurableWindow,
ConfigurableDocumentOrShadowRoot {}
/**
* Reactive `document.activeElement`
*
* @see https://vueuse.org/useActiveElement
* @param options
*/
export declare function useActiveElement<T extends HTMLElement>(
options?: UseActiveElementOptions
): ComputedRefWithControl<T | null | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useActiveElement/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useActiveElement/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useActiveElement/index.md)
vueuse useDevicesList useDevicesList
==============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.63 kB |
| Last Changed | 4 months ago |
| Related | [`useUserMedia`](../useusermedia/index) |
Reactive [enumerateDevices](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/enumerateDevices) listing available input/output devices.
Usage
-----
```
import { useDevicesList } from '@vueuse/core'
const {
devices,
videoInputs: cameras,
audioInputs: microphones,
audioOutputs: speakers,
} = useDevicesList()
```
Component
=========
```
<UseDevicesList v-slot="{ videoInputs, audioInputs, audioOutputs }">
Cameras: {{ videoInputs }}
Microphones: {{ audioInputs }}
Speakers: {{ audioOutputs }}
</UseDevicesList>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseDevicesListOptions extends ConfigurableNavigator {
onUpdated?: (devices: MediaDeviceInfo[]) => void
/**
* Request for permissions immediately if it's not granted,
* otherwise label and deviceIds could be empty
*
* @default false
*/
requestPermissions?: boolean
/**
* Request for types of media permissions
*
* @default { audio: true, video: true }
*/
constraints?: MediaStreamConstraints
}
export interface UseDevicesListReturn {
/**
* All devices
*/
devices: Ref<MediaDeviceInfo[]>
videoInputs: ComputedRef<MediaDeviceInfo[]>
audioInputs: ComputedRef<MediaDeviceInfo[]>
audioOutputs: ComputedRef<MediaDeviceInfo[]>
permissionGranted: Ref<boolean>
ensurePermissions: () => Promise<boolean>
isSupported: Ref<boolean>
}
/**
* Reactive `enumerateDevices` listing available input/output devices
*
* @see https://vueuse.org/useDevicesList
* @param options
*/
export declare function useDevicesList(
options?: UseDevicesListOptions
): UseDevicesListReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicesList/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicesList/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicesList/index.md)
vueuse useGamepad useGamepad
==========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.73 kB |
| Last Changed | 6 months ago |
Provides reactive bindings for the [Gamepad API](https://developer.mozilla.org/en-US/docs/Web/API/Gamepad_API).
Usage
-----
> Due to how the Gamepad API works, you must interact with the page using the gamepad before it will be detected.
>
>
```
<script setup>
import { useGamepad } from '@vueuse/core'
const { isSupported, gamepads } = useGamepad()
const gamepad = computed(() => gamepads.find(g => g.mapping === 'standard'))
</script>
<template>
<span>
{{ gamepad.id }}
<span>
</template>
```
### Gamepad Updates
Currently the Gamepad API does not have event support to update the state of the gamepad. To update the gamepad state, `requestAnimationFrame` is used to poll for gamepad changes. You can control this polling by using the `pause` and `resume` functions provided by `useGamepad`
```
const { pause, resume, gamepads } = useGamepad()
pause()
// gamepads object will not update
resume()
// gamepads object will update on user input
```
### Gamepad Connect & Disconnect Events
The `onConnected` and `onDisconnected` events will trigger when a gamepad is connected or disconnected.
```
const { gamepads, onConnected, onDisconnected } = useGamepad()
onConnected((index) => {
console.log(`${gamepads.value[index].id} connected`)
})
onDisconnected((index) => {
console.log(`${index} disconnected`)
})
```
### Vibration
> The Gamepad Haptics API is sparse, so check the [compatibility table](https://developer.mozilla.org/en-US/docs/Web/API/GamepadHapticActuator#browser_compatibility) before using.
>
>
```
const supportsVibration = computed(() => gamepad.hapticActuators.length > 0)
const vibrate = () => {
if (supportsVibration.value) {
const actuator = gamepad.hapticActuators[0]
actuator.playEffect('dual-rumble', {
startDelay: 0,
duration: 1000,
weakMagnitude: 1,
strongMagnitude: 1,
})
}
}
```
### Mappings
To make the Gamepad API easier to use, we provide mappings to map a controller to a controllers button layout.
#### Xbox360 Controller
```
<script setup>
import { mapGamepadToXbox360Controller } from '@vueuse/core'
const controller = mapGamepadToXbox360Controller(gamepad)
</script>
<template>
<span>{{ controller.buttons.a.pressed }}<span>
<span>{{ controller.buttons.b.pressed }}<span>
<span>{{ controller.buttons.x.pressed }}<span>
<span>{{ controller.buttons.y.pressed }}<span>
</template>
```
Currently there are only mappings for the Xbox 360 controller. If you have controller you want to add mappings for, feel free to open a PR for more controller mappings!
Type Declarations
-----------------
Show Type Declarations
```
export interface UseGamepadOptions
extends ConfigurableWindow,
ConfigurableNavigator {}
/**
* Maps a standard standard gamepad to an Xbox 360 Controller.
*/
export declare function mapGamepadToXbox360Controller(
gamepad: Ref<Gamepad | undefined>
): ComputedRef<{
buttons: {
a: GamepadButton
b: GamepadButton
x: GamepadButton
y: GamepadButton
}
bumper: {
left: GamepadButton
right: GamepadButton
}
triggers: {
left: GamepadButton
right: GamepadButton
}
stick: {
left: {
horizontal: number
vertical: number
button: GamepadButton
}
right: {
horizontal: number
vertical: number
button: GamepadButton
}
}
dpad: {
up: GamepadButton
down: GamepadButton
left: GamepadButton
right: GamepadButton
}
back: GamepadButton
start: GamepadButton
} | null>
export declare function useGamepad(options?: UseGamepadOptions): {
isSupported: Ref<boolean>
onConnected: EventHookOn<number>
onDisconnected: EventHookOn<number>
gamepads: Ref<
{
readonly axes: readonly number[]
readonly buttons: readonly {
readonly pressed: boolean
readonly touched: boolean
readonly value: number
}[]
readonly connected: boolean
readonly hapticActuators: readonly {
readonly type: "vibration"
}[]
readonly id: string
readonly index: number
readonly mapping: GamepadMappingType
readonly timestamp: number
}[]
>
pause: Fn
resume: Fn
isActive: Ref<boolean>
}
export type UseGamepadReturn = ReturnType<typeof useGamepad>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useGamepad/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useGamepad/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useGamepad/index.md)
vueuse useParallax useParallax
===========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.96 kB |
| Last Changed | 6 months ago |
Create parallax effect easily. It uses [`useDeviceOrientation`](../usedeviceorientation/index)and fallback to [`useMouse`](../usemouse/index)if orientation is not supported.
Usage
-----
```
<div ref='container'>
</div>
```
```
import { useParallax } from '@vueuse/core'
export default {
setup() {
const container = ref(null)
const { tilt, roll, source } = useParallax(container)
return {
container,
}
},
}
```
Type Declarations
-----------------
```
export interface UseParallaxOptions extends ConfigurableWindow {
deviceOrientationTiltAdjust?: (i: number) => number
deviceOrientationRollAdjust?: (i: number) => number
mouseTiltAdjust?: (i: number) => number
mouseRollAdjust?: (i: number) => number
}
export interface UseParallaxReturn {
/**
* Roll value. Scaled to `-0.5 ~ 0.5`
*/
roll: ComputedRef<number>
/**
* Tilt value. Scaled to `-0.5 ~ 0.5`
*/
tilt: ComputedRef<number>
/**
* Sensor source, can be `mouse` or `deviceOrientation`
*/
source: ComputedRef<"deviceOrientation" | "mouse">
}
/**
* Create parallax effect easily. It uses `useDeviceOrientation` and fallback to `useMouse`
* if orientation is not supported.
*
* @param target
* @param options
*/
export declare function useParallax(
target: MaybeElementRef,
options?: UseParallaxOptions
): UseParallaxReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useParallax/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useParallax/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useParallax/index.md)
vueuse useTemplateRefsList useTemplateRefsList
===================
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 793 B |
| Last Changed | last year |
Shorthand for binding refs to template elements and components inside `v-for`.
> This function only works for Vue 3.x.
>
>
Usage
-----
```
<template>
<div v-for="i of 5" :key="i" :ref="refs.set"></div>
</template>
<script setup lang="ts">
import { onUpdated } from 'vue'
import { useTemplateRefsList } from '@vueuse/core'
const refs = useTemplateRefsList<HTMLDivElement>()
onUpdated(() => {
console.log(refs)
})
</script>
```
Type Declarations
-----------------
```
export type TemplateRefsList<T> = T[] & {
set(el: Object | null): void
}
export declare function useTemplateRefsList<T = Element>(): Readonly<
Ref<Readonly<TemplateRefsList<T>>>
>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTemplateRefsList/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTemplateRefsList/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTemplateRefsList/index.md)
*No recent changes*
vueuse useIdle useIdle
=======
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.43 kB |
| Last Changed | 6 months ago |
Tracks whether the user is being inactive.
Usage
-----
```
import { useIdle } from '@vueuse/core'
const { idle, lastActive } = useIdle(5 * 60 * 1000) // 5 min
console.log(idle.value) // true or false
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseIdle v-slot="{ idle }" :timeout="5 * 60 * 1000">
Is Idle: {{ idle }}
</UseIdle>
```
Type Declarations
-----------------
```
export interface UseIdleOptions
extends ConfigurableWindow,
ConfigurableEventFilter {
/**
* Event names that listen to for detected user activity
*
* @default ['mousemove', 'mousedown', 'resize', 'keydown', 'touchstart', 'wheel']
*/
events?: WindowEventName[]
/**
* Listen for document visibility change
*
* @default true
*/
listenForVisibilityChange?: boolean
/**
* Initial state of the ref idle
*
* @default false
*/
initialState?: boolean
}
export interface UseIdleReturn {
idle: Ref<boolean>
lastActive: Ref<number>
}
/**
* Tracks whether the user is being inactive.
*
* @see https://vueuse.org/useIdle
* @param timeout default to 1 minute
* @param options IdleOptions
*/
export declare function useIdle(
timeout?: number,
options?: UseIdleOptions
): UseIdleReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useIdle/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useIdle/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useIdle/index.md)
vueuse useClipboard useClipboard
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.41 kB |
| Last Changed | 2 months ago |
Reactive [Clipboard API](https://developer.mozilla.org/en-US/docs/Web/API/Clipboard_API). Provides the ability to respond to clipboard commands (cut, copy, and paste) as well as to asynchronously read from and write to the system clipboard. Access to the contents of the clipboard is gated behind the [Permissions API](https://developer.mozilla.org/en-US/docs/Web/API/Permissions_API). Without user permission, reading or altering the clipboard contents is not permitted.
[Learn how to reactively save text to the clipboard with this FREE video lesson from Vue School!](https://vueschool.io/lessons/reactive-browser-wrappers-in-vueuse-useclipboard?friend=vueuse)Usage
-----
```
import { useClipboard } from '@vueuse/core'
const source = ref('Hello')
const { text, copy, copied, isSupported } = useClipboard({ source })
```
```
<button @click='copy()'>
<!-- by default, `copied` will be reset in 1.5s -->
<span v-if='!copied'>Copy</span>
<span v-else>Copied!</span>
</button>
```
Set `legacy: true` to keep the ability to copy if [Clipboard API](https://developer.mozilla.org/en-US/docs/Web/API/Clipboard_API) is not available. It will handle copy with [execCommand](https://developer.mozilla.org/en-US/docs/Web/API/Document/execCommand) as fallback.
Type Declarations
-----------------
Show Type Declarations
```
export interface UseClipboardOptions<Source> extends ConfigurableNavigator {
/**
* Enabled reading for clipboard
*
* @default false
*/
read?: boolean
/**
* Copy source
*/
source?: Source
/**
* Milliseconds to reset state of `copied` ref
*
* @default 1500
*/
copiedDuring?: number
/**
* Whether fallback to document.execCommand('copy') if clipboard is undefined.
*
* @default false
*/
legacy?: boolean
}
export interface UseClipboardReturn<Optional> {
isSupported: Ref<boolean>
text: ComputedRef<string>
copied: ComputedRef<boolean>
copy: Optional extends true
? (text?: string) => Promise<void>
: (text: string) => Promise<void>
}
/**
* Reactive Clipboard API.
*
* @see https://vueuse.org/useClipboard
* @param options
*/
export declare function useClipboard(
options?: UseClipboardOptions<undefined>
): UseClipboardReturn<false>
export declare function useClipboard(
options: UseClipboardOptions<MaybeComputedRef<string>>
): UseClipboardReturn<true>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useClipboard/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useClipboard/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useClipboard/index.md)
| programming_docs |
vueuse useWebWorkerFn useWebWorkerFn
==============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.37 kB |
| Last Changed | 6 months ago |
| Related | [`useWebWorker`](../usewebworker/index) |
Run expensive functions without blocking the UI, using a simple syntax that makes use of Promise. A port of [alewin/useWorker](https://github.com/alewin/useWorker).
Usage
-----
### Basic example
```
import { useWebWorkerFn } from '@vueuse/core'
const { workerFn } = useWebWorkerFn(() => {
// some heavy works to do in web worker
})
```
### With dependencies
```
import { useWebWorkerFn } from '@vueuse/core'
const { workerFn, workerStatus, workerTerminate } = useWebWorkerFn(
dates => dates.sort(dateFns.compareAsc),
{
timeout: 50000,
dependencies: [
'https://cdnjs.cloudflare.com/ajax/libs/date-fns/1.30.1/date_fns.js', // dateFns
],
},
)
```
Web Worker
----------
Before you start using this function, we suggest you read the [Web Worker](https://developer.mozilla.org/en-US/docs/Web/API/Web_Workers_API/Using_web_workers) documentation.
Credit
------
This function is a Vue port of <https://github.com/alewin/useWorker> by Alessio Koci, with the help of [@Donskelle](https://github.com/Donskelle) to migration.
Type Declarations
-----------------
```
export type WebWorkerStatus =
| "PENDING"
| "SUCCESS"
| "RUNNING"
| "ERROR"
| "TIMEOUT_EXPIRED"
export interface UseWebWorkerOptions extends ConfigurableWindow {
/**
* Number of milliseconds before killing the worker
*
* @default undefined
*/
timeout?: number
/**
* An array that contains the external dependencies needed to run the worker
*/
dependencies?: string[]
}
/**
* Run expensive function without blocking the UI, using a simple syntax that makes use of Promise.
*
* @see https://vueuse.org/useWebWorkerFn
* @param fn
* @param options
*/
export declare const useWebWorkerFn: <T extends (...fnArgs: any[]) => any>(
fn: T,
options?: UseWebWorkerOptions
) => {
workerFn: (...fnArgs: Parameters<T>) => Promise<ReturnType<T>>
workerStatus: Ref<WebWorkerStatus>
workerTerminate: (status?: WebWorkerStatus) => void
}
export type UseWebWorkerFnReturn = ReturnType<typeof useWebWorkerFn>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebWorkerFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebWorkerFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebWorkerFn/index.md)
vueuse useGeolocation useGeolocation
==============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.1 kB |
| Last Changed | 3 weeks ago |
Reactive [Geolocation API](https://developer.mozilla.org/en-US/docs/Web/API/Geolocation_API). It allows the user to provide their location to web applications if they so desire. For privacy reasons, the user is asked for permission to report location information.
Usage
-----
```
import { useGeolocation } from '@vueuse/core'
const { coords, locatedAt, error, resume, pause } = useGeolocation()
```
| State | Type | Description |
| --- | --- | --- |
| coords | [`Coordinates`](https://developer.mozilla.org/en-US/docs/Web/API/Coordinates) | information about the position retrieved like the latitude and longitude |
| locatedAt | `Date` | The time of the last geolocation call |
| error | `string` | An error message in case geolocation API fails. |
| resume | `function` | Control function to resume updating geolocation |
| pause | `function` | Control function to pause updating geolocation |
Config
------
[`useGeolocation`](index)function takes [PositionOptions](https://developer.mozilla.org/en-US/docs/Web/API/PositionOptions) object as an optional parameter.
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseGeolocation v-slot="{ coords: { latitude, longitude } }">
Latitude: {{ latitude }}
Longitude: {{ longitude }}
</UseGeolocation>
```
Type Declarations
-----------------
```
export interface UseGeolocationOptions
extends Partial<PositionOptions>,
ConfigurableNavigator {
immediate?: boolean
}
/**
* Reactive Geolocation API.
*
* @see https://vueuse.org/useGeolocation
* @param options
*/
export declare function useGeolocation(options?: UseGeolocationOptions): {
isSupported: Ref<boolean>
coords: Ref<GeolocationCoordinates>
locatedAt: Ref<number | null>
error: Ref<{
readonly code: number
readonly message: string
readonly PERMISSION_DENIED: number
readonly POSITION_UNAVAILABLE: number
readonly TIMEOUT: number
} | null>
resume: () => void
pause: () => void
}
export type UseGeolocationReturn = ReturnType<typeof useGeolocation>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useGeolocation/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useGeolocation/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useGeolocation/index.md)
vueuse computedInject computedInject
==============
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 803 B |
| Last Changed | 4 months ago |
Combine computed and inject
Usage
-----
In Provider Component
```
import type { InjectionKey, Ref } from 'vue-demi'
import { provide, ref } from 'vue-demi'
interface Item {
key: number
value: string
}
export const ArrayKey: InjectionKey<Ref<Item[]>> = Symbol('symbol-key')
const array = ref([{ key: 1, value: '1' }, { key: 2, value: '2' }, { key: 3, value: '3' }])
provide(ArrayKey, array)
```
In Receiver Component
```
import { computedInject } from '@vueuse/core'
import { ArrayKey } from './provider'
const computedArray = computedInject(ArrayKey, (source) => {
const arr = [...source.value]
arr.unshift({ key: 0, value: 'all' })
return arr
})
```
Type Declarations
-----------------
Show Type Declarations
```
export type ComputedInjectGetter<T, K> = (source: T | undefined, ctx?: any) => K
export type ComputedInjectGetterWithDefault<T, K> = (source: T, ctx?: any) => K
export type ComputedInjectSetter<T> = (v: T) => void
export interface WritableComputedInjectOptions<T, K> {
get: ComputedInjectGetter<T, K>
set: ComputedInjectSetter<K>
}
export interface WritableComputedInjectOptionsWithDefault<T, K> {
get: ComputedInjectGetterWithDefault<T, K>
set: ComputedInjectSetter<K>
}
export declare function computedInject<T, K = any>(
key: InjectionKey<T> | string,
getter: ComputedInjectGetter<T, K>
): ComputedRef<K | undefined>
export declare function computedInject<T, K = any>(
key: InjectionKey<T> | string,
options: WritableComputedInjectOptions<T, K>
): ComputedRef<K | undefined>
export declare function computedInject<T, K = any>(
key: InjectionKey<T> | string,
getter: ComputedInjectGetterWithDefault<T, K>,
defaultSource: T,
treatDefaultAsFactory?: false
): ComputedRef<K>
export declare function computedInject<T, K = any>(
key: InjectionKey<T> | string,
options: WritableComputedInjectOptionsWithDefault<T, K>,
defaultSource: T | (() => T),
treatDefaultAsFactory: true
): ComputedRef<K>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/computedInject/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/computedInject/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/computedInject/index.md)
*No recent changes*
vueuse useResizeObserver useResizeObserver
=================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.19 kB |
| Last Changed | 4 months ago |
Reports changes to the dimensions of an Element's content or the border-box
Usage
-----
```
<template>
<div ref="el">
{{text}}
</div>
</template>
<script>
import { ref } from 'vue'
import { useResizeObserver } from '@vueuse/core'
export default {
setup() {
const el = ref(null)
const text = ref('')
useResizeObserver(el, (entries) => {
const entry = entries[0]
const { width, height } = entry.contentRect
text.value = `width: ${width}, height: ${height}`
})
return {
el,
text,
}
}
})
</script>
```
[ResizeObserver MDN](https://developer.mozilla.org/en-US/docs/Web/API/ResizeObserver)
Type Declarations
-----------------
Show Type Declarations
```
export interface ResizeObserverSize {
readonly inlineSize: number
readonly blockSize: number
}
export interface ResizeObserverEntry {
readonly target: Element
readonly contentRect: DOMRectReadOnly
readonly borderBoxSize?: ReadonlyArray<ResizeObserverSize>
readonly contentBoxSize?: ReadonlyArray<ResizeObserverSize>
readonly devicePixelContentBoxSize?: ReadonlyArray<ResizeObserverSize>
}
export type ResizeObserverCallback = (
entries: ReadonlyArray<ResizeObserverEntry>,
observer: ResizeObserver
) => void
export interface UseResizeObserverOptions extends ConfigurableWindow {
/**
* Sets which box model the observer will observe changes to. Possible values
* are `content-box` (the default), `border-box` and `device-pixel-content-box`.
*
* @default 'content-box'
*/
box?: ResizeObserverBoxOptions
}
declare class ResizeObserver {
constructor(callback: ResizeObserverCallback)
disconnect(): void
observe(target: Element, options?: UseResizeObserverOptions): void
unobserve(target: Element): void
}
/**
* Reports changes to the dimensions of an Element's content or the border-box
*
* @see https://vueuse.org/useResizeObserver
* @param target
* @param callback
* @param options
*/
export declare function useResizeObserver(
target: MaybeComputedElementRef,
callback: ResizeObserverCallback,
options?: UseResizeObserverOptions
): {
isSupported: Ref<boolean>
stop: () => void
}
export type UseResizeObserverReturn = ReturnType<typeof useResizeObserver>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useResizeObserver/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useResizeObserver/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useResizeObserver/index.md)
vueuse useCssVar useCssVar
=========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 990 B |
| Last Changed | 6 months ago |
Manipulate CSS variables
Usage
-----
```
import { useCssVar } from '@vueuse/core'
const el = ref(null)
const color = useCssVar('--color', el)
const elv = ref(null)
const key = ref('--color')
const colorVal = useCssVar(key, elv)
const someEl = ref(null)
const color = useCssVar('--color', someEl, { initialValue: '#eee' })
```
Type Declarations
-----------------
```
export interface UseCssVarOptions extends ConfigurableWindow {
initialValue?: string
}
/**
* Manipulate CSS variables.
*
* @see https://vueuse.org/useCssVar
* @param prop
* @param target
* @param initialValue
* @param options
*/
export declare function useCssVar(
prop: MaybeComputedRef<string>,
target?: MaybeElementRef,
{ window, initialValue }?: UseCssVarOptions
): Ref<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useCssVar/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useCssVar/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useCssVar/index.md)
vueuse useMutationObserver useMutationObserver
===================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.18 kB |
| Last Changed | 6 months ago |
Watch for changes being made to the DOM tree. [MutationObserver MDN](https://developer.mozilla.org/en-US/docs/Web/API/MutationObserver)
Usage
-----
```
import { ref } from 'vue'
import { useMutationObserver } from '@vueuse/core'
export default {
setup() {
const el = ref(null)
const messages = ref([])
useMutationObserver(el, (mutations) => {
if (mutations[0])
messages.value.push(mutations[0].attributeName)
}, {
attributes: true,
})
return {
el,
messages,
}
},
}
```
Type Declarations
-----------------
```
export interface UseMutationObserverOptions
extends MutationObserverInit,
ConfigurableWindow {}
/**
* Watch for changes being made to the DOM tree.
*
* @see https://vueuse.org/useMutationObserver
* @see https://developer.mozilla.org/en-US/docs/Web/API/MutationObserver MutationObserver MDN
* @param target
* @param callback
* @param options
*/
export declare function useMutationObserver(
target: MaybeElementRef,
callback: MutationCallback,
options?: UseMutationObserverOptions
): {
isSupported: Ref<boolean>
stop: () => void
}
export type UseMutationObserverReturn = ReturnType<typeof useMutationObserver>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMutationObserver/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMutationObserver/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMutationObserver/index.md)
vueuse useEventBus useEventBus
===========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 948 B |
| Last Changed | 8 months ago |
A basic event bus.
Usage
-----
```
import { useEventBus } from '@vueuse/core'
const bus = useEventBus<string>('news')
const listener = (event: string) => {
console.log(`news: ${event}`)
}
// listen to an event
const unsubscribe = bus.on(listener)
// fire an event
bus.emit('The Tokyo Olympics has begun')
// unregister the listener
unsubscribe()
// or
bus.off(listener)
// clearing all listeners
bus.reset()
```
Listeners registered inside of components `setup` will be unregistered automatically when the component gets unmounted.
TypeScript
----------
Using `EventBusKey` is the key to bind the event type to the key, similar to Vue's [`InjectionKey`](https://antfu.me/notes#typed-provide-and-inject-in-vue) util.
```
// fooKey.ts
import type { EventBusKey } from '@vueuse/core'
export const fooKey: EventBusKey<{ name: foo }> = Symbol('symbol-key')
```
```
import { useEventBus } from '@vueuse/core'
import { fooKey } from './fooKey'
const bus = useEventBus(fooKey)
bus.on((e) => {
// `e` will be `{ name: foo }`
})
```
Type Declarations
-----------------
Show Type Declarations
```
export type EventBusListener<T = unknown, P = any> = (
event: T,
payload?: P
) => void
export type EventBusEvents<T, P = any> = EventBusListener<T, P>[]
export interface EventBusKey<T> extends Symbol {}
export type EventBusIdentifier<T = unknown> = EventBusKey<T> | string | number
export interface UseEventBusReturn<T, P> {
/**
* Subscribe to an event. When calling emit, the listeners will execute.
* @param listener watch listener.
* @returns a stop function to remove the current callback.
*/
on: (listener: EventBusListener<T, P>) => Fn
/**
* Similar to `on`, but only fires once
* @param listener watch listener.
* @returns a stop function to remove the current callback.
*/
once: (listener: EventBusListener<T, P>) => Fn
/**
* Emit an event, the corresponding event listeners will execute.
* @param event data sent.
*/
emit: (event?: T, payload?: P) => void
/**
* Remove the corresponding listener.
* @param listener watch listener.
*/
off: (listener: EventBusListener<T>) => void
/**
* Clear all events
*/
reset: () => void
}
export declare function useEventBus<T = unknown, P = any>(
key: EventBusIdentifier<T>
): UseEventBusReturn<T, P>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventBus/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventBus/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventBus/index.md)
vueuse useKeyModifier useKeyModifier
==============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.13 kB |
| Last Changed | 6 months ago |
Reactive [Modifier State](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/getModifierState). Tracks state of any of the [supported modifiers](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/getModifierState#browser_compatibility) - see Browser Compatibility notes.
Usage
-----
```
import { useKeyModifier } from '@vueuse/core'
const capsLockState = useKeyModifier('CapsLock')
console.log(capsLockState.value)
```
Events
------
You can customize which events will prompt the state to update. By default, these are `mouseup`, `mousedown`, `keyup`, `keydown`. To customize these events:
```
import { useKeyModifier } from '@vueuse/core'
const capsLockState = useKeyModifier('CapsLock', { events: ['mouseup', 'mousedown'] })
console.log(capsLockState) // null
// Caps Lock turned on with key press
console.log(capsLockState) // null
// Mouse button clicked
console.log(capsLockState) // true
```
Initial State
-------------
By default, the returned ref will be `Ref<null>` until the first event is received. You can explicitly pass the initial state to it via:
```
const capsLockState1 = useKeyModifier('CapsLock') // Ref<boolean | null>
const capsLockState2 = useKeyModifier('CapsLock', { initial: false }) // Ref<boolean>
```
Type Declarations
-----------------
```
export type KeyModifier =
| "Alt"
| "AltGraph"
| "CapsLock"
| "Control"
| "Fn"
| "FnLock"
| "Meta"
| "NumLock"
| "ScrollLock"
| "Shift"
| "Symbol"
| "SymbolLock"
export interface UseModifierOptions<Initial> extends ConfigurableDocument {
/**
* Event names that will prompt update to modifier states
*
* @default ['mousedown', 'mouseup', 'keydown', 'keyup']
*/
events?: WindowEventName[]
/**
* Initial value of the returned ref
*
* @default null
*/
initial?: Initial
}
export type UseKeyModifierReturn<Initial> = Ref<
Initial extends boolean ? boolean : boolean | null
>
export declare function useKeyModifier<Initial extends boolean | null>(
modifier: KeyModifier,
options?: UseModifierOptions<Initial>
): UseKeyModifierReturn<Initial>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useKeyModifier/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useKeyModifier/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useKeyModifier/index.md)
vueuse useVModels useVModels
==========
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 1.09 kB |
| Last Changed | 6 months ago |
| Related | [`useVModel`](../usevmodel/index) |
Shorthand for props v-model binding. Think it like `toRefs(props)` but changes will also trigger emit.
Usage
-----
```
import { useVModels } from '@vueuse/core'
export default {
props: {
foo: String,
bar: Number,
},
setup(props, { emit }) {
const { foo, bar } = useVModels(props, emit)
console.log(foo.value) // props.foo
foo.value = 'foo' // emit('update:foo', 'foo')
},
}
```
Type Declarations
-----------------
```
/**
* Shorthand for props v-model binding. Think like `toRefs(props)` but changes will also emit out.
*
* @see https://vueuse.org/useVModels
* @param props
* @param emit
*/
export declare function useVModels<P extends object, Name extends string>(
props: P,
emit?: (name: Name, ...args: any[]) => void,
options?: UseVModelOptions<any>
): ToRefs<P>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useVModels/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useVModels/index.md)
| programming_docs |
vueuse useCloned useCloned
=========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | |
| Last Changed | 4 months ago |
Reactive clone of a ref. By default, it use `JSON.parse(JSON.stringify())` to do the clone.
Usage
-----
```
import { useCloned } from '@vueuse/core'
const original = ref({ key: 'value' })
const { cloned } = useCloned(original)
original.key = 'some new value'
console.log(cloned.value.key) // 'some new value'
```
Manual cloning
--------------
```
import { useCloned } from '@vueuse/core'
const original = ref({ key: 'value' })
const { cloned, sync } = useCloned(original, { manual: true })
original.key = 'manual'
console.log(cloned.value.key) // 'value'
sync()
console.log(cloned.value.key)// 'manual'
```
Custom Clone Function
---------------------
Using [`klona`](https://www.npmjs.com/package/klona) for example:
```
import { useCloned } from '@vueuse/core'
import { klona } from 'klona'
const original = ref({ key: 'value' })
const { cloned, sync } = useCloned(original, { clone: klona })
```
Type Declarations
-----------------
```
export interface UseClonedOptions<T = any> extends WatchOptions {
/**
* Custom clone function.
*
* By default, it use `JSON.parse(JSON.stringify(value))` to clone.
*/
clone?: (source: T) => T
/**
* Manually sync the ref
*
* @default false
*/
manual?: boolean
}
export interface UseClonedReturn<T> {
/**
* Cloned ref
*/
cloned: ComputedRef<T>
/**
* Sync cloned data with source manually
*/
sync: () => void
}
export type CloneFn<F, T = F> = (x: F) => T
export declare function cloneFnJSON<T>(source: T): T
export declare function useCloned<T>(
source: MaybeComputedRef<T>,
options?: UseClonedOptions
): {
cloned: Ref<UnwrapRef<T>>
sync: () => void
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useCloned/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useCloned/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useCloned/index.md)
vueuse createUnrefFn createUnrefFn
=============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 770 B |
| Last Changed | 8 months ago |
| Related | [`reactify`](../../shared/reactify/index) |
Make a plain function accepting ref and raw values as arguments. Returns the same value the unconverted function returns, with proper typing.
**TIP**Make sure you're using the right tool for the job. Using `reactify` might be more pertinent in some cases where you want to evaluate the function on each changes of it's arguments.
Usage
-----
```
import { ref } from 'vue'
import { createUnrefFn } from '@vueuse/core'
const url = ref('https://httpbin.org/post')
const data = ref({ foo: 'bar' })
const post = (url, data) => fetch(url, { data })
const unrefPost = createUnrefFn(post)
post(url, data) /* ❌ Will throw an error because the arguments are refs */
unrefPost(url, data) /* ✔️ Will Work because the arguments will be auto unref */
```
Type Declarations
-----------------
```
export type UnrefFn<T> = T extends (...args: infer A) => infer R
? (
...args: {
[K in keyof A]: MaybeRef<A[K]>
}
) => R
: never
/**
* Make a plain function accepting ref and raw values as arguments.
* Returns the same value the unconverted function returns, with proper typing.
*/
export declare const createUnrefFn: <T extends Function>(fn: T) => UnrefFn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/createUnrefFn/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/createUnrefFn/index.md)
vueuse onLongPress onLongPress
===========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.2 kB |
| Last Changed | 4 months ago |
Listen for a long press on an element.
Function provides modifiers in options
* stop
* once
* prevent
* capture
* self
Usage
-----
```
<script setup lang="ts">
import { ref } from 'vue'
import { onLongPress } from '@vueuse/core'
const htmlRefHook = ref<HTMLElement | null>(null)
const longPressedHook = ref(false)
const onLongPressCallbackHook = (e: PointerEvent) => {
longPressedHook.value = true
}
const resetHook = () => {
longPressedHook.value = false
}
onLongPress(
htmlRefHook,
onLongPressCallbackHook,
{ modifiers: { prevent: true } }
)
</script>
<template>
<p>Long Pressed: {{ longPressedHook }}</p>
<button ref="htmlRefHook" class="ml-2 button small">
Press long
</button>
<button class="ml-2 button small" @click="resetHook">
Reset
</button>
</template>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { OnLongPress } from '@vueuse/components'
const longPressedComponent = ref(false)
const onLongPressCallbackComponent = (e: PointerEvent) => {
longPressedComponent.value = true
}
const resetComponent = () => {
longPressedComponent.value = false
}
</script>
<template>
<p>Long Pressed: {{ longPressedComponent }}</p>
<OnLongPress
as="button"
class="ml-2 button small"
@trigger="onLongPressCallbackComponent"
>
Press long
</OnLongPress>
<button class="ml-2 button small" @click="resetComponent">
Reset
</button>
</template>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vOnLongPress } from '@vueuse/components'
const longPressedDirective = ref(false)
const onLongPressCallbackDirective = (e: PointerEvent) => {
longPressedDirective.value = true
}
const resetDirective = () => {
longPressedDirective.value = false
}
</script>
<template>
<p>Long Pressed: {{ longPressedDirective }}</p>
<button v-on-long-press.prevent="onLongPressCallbackDirective" class="ml-2 button small">
Press long
</button>
<button
v-on-long-press="[onLongPressCallbackDirective, {delay: 1000, modifiers: { stop: true }}]"
class="ml-2button small"
>
Press long (with options)
</button>
<button class="ml-2 button small" @click="resetDirective">
Reset
</button>
</template>
```
Type Declarations
-----------------
```
export interface OnLongPressOptions {
/**
* Time in ms till `longpress` gets called
*
* @default 500
*/
delay?: number
modifiers?: OnLongPressModifiers
}
export interface OnLongPressModifiers {
stop?: boolean
once?: boolean
prevent?: boolean
capture?: boolean
self?: boolean
}
export declare function onLongPress(
target: MaybeElementRef,
handler: (evt: PointerEvent) => void,
options?: OnLongPressOptions
): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/onLongPress/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/onLongPress/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/onLongPress/index.md)
vueuse useTextareaAutosize useTextareaAutosize
===================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 889 B |
| Last Changed | 3 weeks ago |
Automatically update the height of a textarea depending on the content.
Usage
-----
```
<script setup lang="ts">
const { textarea, input } = useTextareaAutosize()
</script>
<template>
<textarea
ref="textarea"
v-model="input"
class="resize-none"
placeholder="What's on your mind?"
/>
</template>
```
Type Declarations
-----------------
```
export interface UseTextareaAutosizeOptions {
/** Textarea element to autosize. */
element?: MaybeRef<HTMLTextAreaElement | undefined>
/** Textarea content. */
input?: MaybeRef<string | undefined>
/** Watch sources that should trigger a textarea resize. */
watch?: WatchSource | Array<WatchSource>
/** Function called when the textarea size changes. */
onResize?: () => void
}
export declare function useTextareaAutosize(
options?: UseTextareaAutosizeOptions
): {
textarea: Ref<HTMLTextAreaElement>
input: Ref<string>
triggerResize: () => void
}
export type UseTextareaAutosizeReturn = ReturnType<typeof useTextareaAutosize>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextareaAutosize/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextareaAutosize/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextareaAutosize/index.md)
vueuse useDevicePixelRatio useDevicePixelRatio
===================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.41 kB |
| Last Changed | 4 months ago |
Reactively track [`window.devicePixelRatio`](https://developer.mozilla.org/ru/docs/Web/API/Window/devicePixelRatio)
> NOTE: there is no event listener for `window.devicePixelRatio` change. So this function uses [`Testing media queries programmatically (window.matchMedia)`](https://developer.mozilla.org/en-US/docs/Web/CSS/Media_Queries/Testing_media_queries) applying the same mechanism as described in [this example](https://developer.mozilla.org/en-US/docs/Web/API/Window/devicePixelRatio#monitoring_screen_resolution_or_zoom_level_changes).
>
>
Usage
-----
```
import { useDevicePixelRatio } from '@vueuse/core'
export default {
setup() {
const { pixelRatio } = useDevicePixelRatio()
return { pixelRatio }
},
}
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDevicePixelRatio v-slot="{ pixelRatio }">
Pixel Ratio: {{ pixelRatio }}
</UseDevicePixelRatio>
```
Type Declarations
-----------------
```
/**
* Reactively track `window.devicePixelRatio`.
*
* @see https://vueuse.org/useDevicePixelRatio
* @param options
*/
export declare function useDevicePixelRatio({ window }?: ConfigurableWindow): {
pixelRatio: Ref<number>
}
export type UseDevicePixelRatioReturn = ReturnType<typeof useDevicePixelRatio>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicePixelRatio/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicePixelRatio/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDevicePixelRatio/index.md)
vueuse useBreakpoints useBreakpoints
==============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.38 kB |
| Last Changed | 4 months ago |
Reactive viewport breakpoints
Usage
-----
```
import { breakpointsTailwind, useBreakpoints } from '@vueuse/core'
const breakpoints = useBreakpoints(breakpointsTailwind)
const smAndLarger = breakpoints.greaterOrEqual('sm') // sm and larger
const largerThanSm = breakpoints.greater('sm') // only larger than sm
const lgAndSmaller = breakpoints.smallerOrEqual('lg') // lg and smaller
const smallerThanLg = breakpoints.smaller('lg') // only smaller than lg
```
```
import { useBreakpoints } from '@vueuse/core'
const breakpoints = useBreakpoints({
tablet: 640,
laptop: 1024,
desktop: 1280,
})
const laptop = breakpoints.between('laptop', 'desktop')
```
Type Declarations
-----------------
Show Type Declarations
```
export * from "./breakpoints"
export type Breakpoints<K extends string = string> = Record<K, number | string>
/**
* Reactively viewport breakpoints
*
* @see https://vueuse.org/useBreakpoints
* @param options
*/
export declare function useBreakpoints<K extends string>(
breakpoints: Breakpoints<K>,
options?: ConfigurableWindow
): {
greater(k: K): Ref<boolean>
greaterOrEqual: (k: K) => Ref<boolean>
smaller(k: K): Ref<boolean>
smallerOrEqual(k: K): Ref<boolean>
between(a: K, b: K): Ref<boolean>
isGreater(k: K): boolean
isGreaterOrEqual(k: K): boolean
isSmaller(k: K): boolean
isSmallerOrEqual(k: K): boolean
isInBetween(a: K, b: K): boolean
} & Record<K, Ref<boolean>>
export type UseBreakpointsReturn<K extends string = string> = {
greater: (k: K) => Ref<boolean>
greaterOrEqual: (k: K) => Ref<boolean>
smaller(k: K): Ref<boolean>
smallerOrEqual: (k: K) => Ref<boolean>
between(a: K, b: K): Ref<boolean>
isGreater(k: K): boolean
isGreaterOrEqual(k: K): boolean
isSmaller(k: K): boolean
isSmallerOrEqual(k: K): boolean
isInBetween(a: K, b: K): boolean
} & Record<K, Ref<boolean>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBreakpoints/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBreakpoints/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBreakpoints/index.md)
vueuse useSupported useSupported
============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 823 B |
| Last Changed | 6 months ago |
SSR compatibility `isSupported`
Usage
-----
```
import { useSupported } from '@vueuse/core'
const isSupported = useSupported(() => navigator && 'getBattery' in navigator)
if (isSupported.value) {
// do something
navigator.getBattery
}
```
Type Declarations
-----------------
```
export declare function useSupported(
callback: () => unknown,
sync?: boolean
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSupported/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSupported/index.md)
vueuse useUserMedia useUserMedia
============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.2 kB |
| Last Changed | 6 months ago |
| Related | [`useDevicesList`](../usedeviceslist/index)[`useDisplayMedia`](../usedisplaymedia/index)[`usePermission`](../usepermission/index) |
Reactive [`mediaDevices.getUserMedia`](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/getUserMedia) streaming.
Usage
-----
```
import { useUserMedia } from '@vueuse/core'
const { stream, start } = useUserMedia()
start()
```
```
const video = document.getElementById('video')
watchEffect(() => {
// preview on a video element
video.srcObject = stream.value
})
```
### Devices
```
import { useDevicesList, useUserMedia } from '@vueuse/core'
const {
videoInputs: cameras,
audioInputs: microphones,
} = useDevicesList({
requestPermissions: true,
})
const currentCamera = computed(() => cameras.value[0]?.deviceId)
const currentMicrophone = computed(() => microphones.value[0]?.deviceId)
const { stream } = useUserMedia({
videoDeviceId: currentCamera,
audioDeviceId: currentMicrophone,
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseUserMediaOptions extends ConfigurableNavigator {
/**
* If the stream is enabled
* @default false
*/
enabled?: MaybeRef<boolean>
/**
* Recreate stream when the input devices id changed
*
* @default true
*/
autoSwitch?: MaybeRef<boolean>
/**
* The device id of video input
*
* When passing with `undefined` the default device will be used.
* Pass `false` or "none" to disabled video input
*
* @default undefined
*/
videoDeviceId?: MaybeRef<string | undefined | false | "none">
/**
* The device id of audi input
*
* When passing with `undefined` the default device will be used.
* Pass `false` or "none" to disabled audi input
*
* @default undefined
*/
audioDeviceId?: MaybeRef<string | undefined | false | "none">
}
/**
* Reactive `mediaDevices.getUserMedia` streaming
*
* @see https://vueuse.org/useUserMedia
* @param options
*/
export declare function useUserMedia(options?: UseUserMediaOptions): {
isSupported: Ref<boolean>
stream: Ref<MediaStream | undefined>
start: () => Promise<MediaStream | undefined>
stop: () => void
restart: () => Promise<MediaStream | undefined>
videoDeviceId: Ref<string | false | undefined>
audioDeviceId: Ref<string | false | undefined>
enabled: Ref<boolean>
autoSwitch: Ref<boolean>
}
export type UseUserMediaReturn = ReturnType<typeof useUserMedia>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useUserMedia/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useUserMedia/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useUserMedia/index.md)
vueuse usePreferredReducedMotion usePreferredReducedMotion
=========================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.09 kB |
| Last Changed | 5 months ago |
Reactive [prefers-reduced-motion](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-reduced-motion) media query.
Usage
-----
```
import { usePreferredReducedMotion } from '@vueuse/core'
const preferredMotion = usePreferredReducedMotion()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePreferredReducedMotion v-slot="{ motion }">
Preferred Color Scheme: {{ motion }}
<UsePreferredReducedMotion>
```
Type Declarations
-----------------
```
export type ReducedMotionType = "reduce" | "no-preference"
/**
* Reactive prefers-reduced-motion media query.
*
* @see https://vueuse.org/usePreferredReducedMotion
* @param [options]
*/
export declare function usePreferredReducedMotion(
options?: ConfigurableWindow
): ComputedRef<ReducedMotionType>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredReducedMotion/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredReducedMotion/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredReducedMotion/index.md)
vueuse useVModel useVModel
=========
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 1.06 kB |
| Last Changed | 4 months ago |
| Related | [`useVModels`](../usevmodels/index) |
Shorthand for v-model binding, props + emit -> ref
Usage
-----
```
import { useVModel } from '@vueuse/core'
export default {
setup(props, { emit }) {
const data = useVModel(props, 'data', emit)
console.log(data.value) // props.data
data.value = 'foo' // emit('update:data', 'foo')
},
}
```
###
`<script setup>`
```
<script lang="ts" setup>
import { useVModel } from '@vueuse/core'
const props = defineProps<{
modelValue: string
}>()
const emit = defineEmits(['update:modelValue'])
const data = useVModel(props, 'modelValue', emit)
</script>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseVModelOptions<T> {
/**
* When passive is set to `true`, it will use `watch` to sync with props and ref.
* Instead of relying on the `v-model` or `.sync` to work.
*
* @default false
*/
passive?: boolean
/**
* When eventName is set, it's value will be used to overwrite the emit event name.
*
* @default undefined
*/
eventName?: string
/**
* Attempting to check for changes of properties in a deeply nested object or array.
* Apply only when `passive` option is set to `true`
*
* @default false
*/
deep?: boolean
/**
* Defining default value for return ref when no value is passed.
*
* @default undefined
*/
defaultValue?: T
/**
* Clone the props.
* Accepts a custom clone function.
* When setting to `true`, it will use `JSON.parse(JSON.stringify(value))` to clone.
*
* @default false
*/
clone?: boolean | CloneFn<T>
}
/**
* Shorthand for v-model binding, props + emit -> ref
*
* @see https://vueuse.org/useVModel
* @param props
* @param key (default 'value' in Vue 2 and 'modelValue' in Vue 3)
* @param emit
*/
export declare function useVModel<
P extends object,
K extends keyof P,
Name extends string
>(
props: P,
key?: K,
emit?: (name: Name, ...args: any[]) => void,
options?: UseVModelOptions<P[K]>
): Ref<UnwrapRef<P[K]>> | WritableComputedRef<P[K]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useVModel/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useVModel/index.md)
| programming_docs |
vueuse useRefHistory useRefHistory
=============
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 1.91 kB |
| Last Changed | 4 months ago |
| Related | [`useDebouncedRefHistory`](../usedebouncedrefhistory/index)[`useManualRefHistory`](../usemanualrefhistory/index)[`useThrottledRefHistory`](../usethrottledrefhistory/index) |
Track the change history of a ref, also provides undo and redo functionality
[Learn useRefHistory with this FREE video lesson from Vue School!](https://vueschool.io/lessons/ref-history-with-vueuse?friend=vueuse)Usage
-----
```
import { ref } from 'vue'
import { useRefHistory } from '@vueuse/core'
const counter = ref(0)
const { history, undo, redo } = useRefHistory(counter)
```
Internally, `watch` is used to trigger a history point when the ref value is modified. This means that history points are triggered asynchronously batching modifications in the same "tick".
```
counter.value += 1
await nextTick()
console.log(history.value)
/* [
{ snapshot: 1, timestamp: 1601912898062 },
{ snapshot: 0, timestamp: 1601912898061 }
] */
```
You can use `undo` to reset the ref value to the last history point.
```
console.log(counter.value) // 1
undo()
console.log(counter.value) // 0
```
### Objects / arrays
When working with objects or arrays, since changing their attributes does not change the reference, it will not trigger the committing. To track attribute changes, you would need to pass `deep: true`. It will create clones for each history record.
```
const state = ref({
foo: 1,
bar: 'bar',
})
const { history, undo, redo } = useRefHistory(state, {
deep: true,
})
state.value.foo = 2
await nextTick()
console.log(history.value)
/* [
{ snapshot: { foo: 2, bar: 'bar' } },
{ snapshot: { foo: 1, bar: 'bar' } }
] */
```
#### Custom Clone Function
[`useRefHistory`](index)only embeds the minimal clone function `x => JSON.parse(JSON.stringify(x))`. To use a full featured or custom clone function, you can set up via the `dump` options.
For example, using [structuredClone](https://developer.mozilla.org/en-US/docs/Web/API/structuredClone):
```
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: structuredClone })
```
Or by using [lodash's `cloneDeep`](https://lodash.com/docs/4.17.15#cloneDeep):
```
import { cloneDeep } from 'lodash-es'
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: cloneDeep })
```
Or a more lightweight [`klona`](https://github.com/lukeed/klona):
```
import { klona } from 'klona'
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, { dump: klona })
```
#### Custom Dump and Parse Function
Instead of using the `clone` param, you can pass custom functions to control the serialization and parsing. In case you do not need history values to be objects, this can save an extra clone when undoing. It is also useful in case you want to have the snapshots already stringified to be saved to local storage for example.
```
import { useRefHistory } from '@vueuse/core'
const refHistory = useRefHistory(target, {
dump: JSON.stringify,
parse: JSON.parse,
})
```
### History Capacity
We will keep all the history by default (unlimited) until you explicitly clear them up, you can set the maximal amount of history to be kept by `capacity` options.
```
const refHistory = useRefHistory(target, {
capacity: 15, // limit to 15 history records
})
refHistory.clear() // explicitly clear all the history
```
### History Flush Timing
From [Vue's documentation](https://vuejs.org/guide/essentials/watchers.html#callback-flush-timing): Vue's reactivity system buffers invalidated effects and flush them asynchronously to avoid unnecessary duplicate invocation when there are many state mutations happening in the same "tick".
In the same way as `watch`, you can modify the flush timing using the `flush` option.
```
const refHistory = useRefHistory(target, {
flush: 'sync', // options 'pre' (default), 'post' and 'sync'
})
```
The default is `'pre'`, to align this composable with the default for Vue's watchers. This also helps to avoid common issues, like several history points generated as part of a multi-step update to a ref value that can break invariants of the app state. You can use `commit()` in case you need to create multiple history points in the same "tick"
```
const r = ref(0)
const { history, commit } = useRefHistory(r)
r.value = 1
commit()
r.value = 2
commit()
console.log(history.value)
/* [
{ snapshot: 2 },
{ snapshot: 1 },
{ snapshot: 0 },
] */
```
On the other hand, when using flush `'sync'`, you can use `batch(fn)` to generate a single history point for several sync operations
```
const r = ref({ names: [], version: 1 })
const { history, batch } = useRefHistory(r, { flush: 'sync' })
batch(() => {
r.names.push('Lena')
r.version++
})
console.log(history.value)
/* [
{ snapshot: { names: [ 'Lena' ], version: 2 },
{ snapshot: { names: [], version: 1 },
] */
```
If `{ flush: 'sync', deep: true }` is used, `batch` is also useful when doing a mutable `splice` in an array. `splice` can generate up to three atomic operations that will be pushed to the ref history.
```
const arr = ref([1, 2, 3])
const { history, batch } = useRefHistory(r, { deep: true, flush: 'sync' })
batch(() => {
arr.value.splice(1, 1) // batch ensures only one history point is generated
})
```
Another option is to avoid mutating the original ref value using `arr.value = [...arr.value].splice(1,1)`.
Recommended Readings
--------------------
* [History and Persistence](https://patak.dev/vue/history-and-persistence.html) - by [@matias-capeletto](https://github.com/matias-capeletto)
Type Declarations
-----------------
Show Type Declarations
```
export interface UseRefHistoryOptions<Raw, Serialized = Raw>
extends ConfigurableEventFilter {
/**
* Watch for deep changes, default to false
*
* When set to true, it will also create clones for values store in the history
*
* @default false
*/
deep?: boolean
/**
* The flush option allows for greater control over the timing of a history point, default to 'pre'
*
* Possible values: 'pre', 'post', 'sync'
* It works in the same way as the flush option in watch and watch effect in vue reactivity
*
* @default 'pre'
*/
flush?: "pre" | "post" | "sync"
/**
* Maximum number of history to be kept. Default to unlimited.
*/
capacity?: number
/**
* Clone when taking a snapshot, shortcut for dump: JSON.parse(JSON.stringify(value)).
* Default to false
*
* @default false
*/
clone?: boolean | CloneFn<Raw>
/**
* Serialize data into the history
*/
dump?: (v: Raw) => Serialized
/**
* Deserialize data from the history
*/
parse?: (v: Serialized) => Raw
}
export interface UseRefHistoryReturn<Raw, Serialized>
extends UseManualRefHistoryReturn<Raw, Serialized> {
/**
* A ref representing if the tracking is enabled
*/
isTracking: Ref<boolean>
/**
* Pause change tracking
*/
pause(): void
/**
* Resume change tracking
*
* @param [commit] if true, a history record will be create after resuming
*/
resume(commit?: boolean): void
/**
* A sugar for auto pause and auto resuming within a function scope
*
* @param fn
*/
batch(fn: (cancel: Fn) => void): void
/**
* Clear the data and stop the watch
*/
dispose(): void
}
/**
* Track the change history of a ref, also provides undo and redo functionality.
*
* @see https://vueuse.org/useRefHistory
* @param source
* @param options
*/
export declare function useRefHistory<Raw, Serialized = Raw>(
source: Ref<Raw>,
options?: UseRefHistoryOptions<Raw, Serialized>
): UseRefHistoryReturn<Raw, Serialized>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useRefHistory/index.md)
vueuse useDebouncedRefHistory useDebouncedRefHistory
======================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 2.12 kB |
| Last Changed | 6 months ago |
| Related | [`useRefHistory`](../userefhistory/index)[`useThrottledRefHistory`](../usethrottledrefhistory/index) |
Shorthand for [`useRefHistory`](../userefhistory/index)with debounced filter.
Usage
-----
This function takes a snapshot of your counter after 1000ms when the value of it starts to change.
```
import { ref } from 'vue'
import { useDebouncedRefHistory } from '@vueuse/core'
const counter = ref(0)
const { history, undo, redo } = useDebouncedRefHistory(counter, { deep: true, debounce: 1000 })
```
Type Declarations
-----------------
```
/**
* Shorthand for [useRefHistory](https://vueuse.org/useRefHistory) with debounce filter.
*
* @see https://vueuse.org/useDebouncedRefHistory
* @param source
* @param options
*/
export declare function useDebouncedRefHistory<Raw, Serialized = Raw>(
source: Ref<Raw>,
options?: Omit<UseRefHistoryOptions<Raw, Serialized>, "eventFilter"> & {
debounce?: MaybeComputedRef<number>
}
): UseRefHistoryReturn<Raw, Serialized>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDebouncedRefHistory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDebouncedRefHistory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDebouncedRefHistory/index.md)
vueuse useLocalStorage useLocalStorage
===============
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 2.19 kB |
| Last Changed | 6 months ago |
| Related | [`useStorage`](../usestorage/index) |
Reactive [LocalStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage).
Usage
-----
Please refer to [`useStorage`](../usestorage/index)
Type Declarations
-----------------
```
export declare function useLocalStorage(
key: string,
initialValue: MaybeComputedRef<string>,
options?: UseStorageOptions<string>
): RemovableRef<string>
export declare function useLocalStorage(
key: string,
initialValue: MaybeComputedRef<boolean>,
options?: UseStorageOptions<boolean>
): RemovableRef<boolean>
export declare function useLocalStorage(
key: string,
initialValue: MaybeComputedRef<number>,
options?: UseStorageOptions<number>
): RemovableRef<number>
export declare function useLocalStorage<T>(
key: string,
initialValue: MaybeComputedRef<T>,
options?: UseStorageOptions<T>
): RemovableRef<T>
export declare function useLocalStorage<T = unknown>(
key: string,
initialValue: MaybeComputedRef<null>,
options?: UseStorageOptions<T>
): RemovableRef<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useLocalStorage/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useLocalStorage/index.md)
vueuse useDraggable useDraggable
============
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.68 kB |
| Last Changed | 3 weeks ago |
Make elements draggable.
Usage
-----
```
<script setup lang="ts">
import { ref } from 'vue'
import { useDraggable } from '@vueuse/core'
const el = ref<HTMLElement | null>(null)
// `style` will be a helper computed for `left: ?px; top: ?px;`
const { x, y, style } = useDraggable(el, {
initialValue: { x: 40, y: 40 },
})
</script>
<template>
<div ref="el" :style="style" style="position: fixed">
Drag me! I am at {{x}}, {{y}}
</div>
</template>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDraggable :initialValue="{ x: 10, y: 10 }" v-slot="{ x, y }">
Drag me! I am at {{x}}, {{y}}
</UseDraggable>
```
For component usage, additional props `storageKey` and `storageType` can be passed to the component and enable the persistence of the element position.
```
<UseDraggable storage-key="vueuse-draggable" storage-type="session">
Refresh the page and I am still in the same position!
</UseDraggable>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseDraggableOptions {
/**
* Only start the dragging when click on the element directly
*
* @default false
*/
exact?: MaybeComputedRef<boolean>
/**
* Prevent events defaults
*
* @default false
*/
preventDefault?: MaybeComputedRef<boolean>
/**
* Prevent events propagation
*
* @default false
*/
stopPropagation?: MaybeComputedRef<boolean>
/**
* Element to attach `pointermove` and `pointerup` events to.
*
* @default window
*/
draggingElement?: MaybeComputedRef<
HTMLElement | SVGElement | Window | Document | null | undefined
>
/**
* Handle that triggers the drag event
*
* @default target
*/
handle?: MaybeComputedRef<HTMLElement | SVGElement | null | undefined>
/**
* Pointer types that listen to.
*
* @default ['mouse', 'touch', 'pen']
*/
pointerTypes?: PointerType[]
/**
* Initial position of the element.
*
* @default { x: 0, y: 0 }
*/
initialValue?: MaybeComputedRef<Position>
/**
* Callback when the dragging starts. Return `false` to prevent dragging.
*/
onStart?: (position: Position, event: PointerEvent) => void | false
/**
* Callback during dragging.
*/
onMove?: (position: Position, event: PointerEvent) => void
/**
* Callback when dragging end.
*/
onEnd?: (position: Position, event: PointerEvent) => void
}
/**
* Make elements draggable.
*
* @see https://vueuse.org/useDraggable
* @param target
* @param options
*/
export declare function useDraggable(
target: MaybeComputedRef<HTMLElement | SVGElement | null | undefined>,
options?: UseDraggableOptions
): {
position: Ref<{
x: number
y: number
}>
isDragging: ComputedRef<boolean>
style: ComputedRef<string>
x: Ref<number>
y: Ref<number>
}
export type UseDraggableReturn = ReturnType<typeof useDraggable>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDraggable/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDraggable/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDraggable/index.md)
vueuse useBluetooth useBluetooth
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.18 kB |
| Last Changed | 3 months ago |
Reactive [Web Bluetooth API](https://developer.mozilla.org/en-US/docs/Web/API/Web_Bluetooth_API). Provides the ability to connect and interact with Bluetooth Low Energy peripherals.
The Web Bluetooth API lets websites discover and communicate with devices over the Bluetooth 4 wireless standard using the Generic Attribute Profile (GATT).
N.B. It is currently partially implemented in Android M, Chrome OS, Mac, and Windows 10. For a full overview of browser compatibility please see [Web Bluetooth API Browser Compatibility](https://developer.mozilla.org/en-US/docs/Web/API/Web_Bluetooth_API#browser_compatibility)
N.B. There are a number of caveats to be aware of with the web bluetooth API specification. Please refer to the [Web Bluetooth W3C Draft Report](https://webbluetoothcg.github.io/web-bluetooth/) for numerous caveats around device detection and connection.
N.B. This API is not available in Web Workers (not exposed via WorkerNavigator).
Usage Default
-------------
```
import { useBluetooth } from '@vueuse/core'
const {
isSupported,
isConnected,
device,
requestDevice,
server,
} = useBluetooth({
acceptAllDevices: true,
})
```
```
<template>
<button @click="requestDevice()">
Request Bluetooth Device
</button>
</template>
```
When the device has paired and is connected, you can then work with the server object as you wish.
Usage Battery Level Example
---------------------------
This sample illustrates the use of the Web Bluetooth API to read battery level and be notified of changes from a nearby Bluetooth Device advertising Battery information with Bluetooth Low Energy.
Here, we use the characteristicvaluechanged event listener to handle reading battery level characteristic value. This event listener will optionally handle upcoming notifications as well.
```
import { pausableWatch, useBluetooth } from '@vueuse/core'
const {
isSupported,
isConnected,
device,
requestDevice,
server,
} = useBluetooth({
acceptAllDevices: true,
optionalServices: [
'battery_service',
],
})
const batteryPercent = ref<undefined | number>()
const isGettingBatteryLevels = ref(false)
const getBatteryLevels = async () => {
isGettingBatteryLevels.value = true
// Get the battery service:
const batteryService = await server.getPrimaryService('battery_service')
// Get the current battery level
const batteryLevelCharacteristic = await batteryService.getCharacteristic(
'battery_level',
)
// Listen to when characteristic value changes on `characteristicvaluechanged` event:
batteryLevelCharacteristic.addEventListener('characteristicvaluechanged', (event) => {
batteryPercent.value = event.target.value.getUint8(0)
})
// Convert received buffer to number:
const batteryLevel = await batteryLevelCharacteristic.readValue()
batteryPercent.value = await batteryLevel.getUint8(0)
}
const { stop } = pausableWatch(isConnected, (newIsConnected) => {
if (!newIsConnected || !server.value || isGettingBatteryLevels.value)
return
// Attempt to get the battery levels of the device:
getBatteryLevels()
// We only want to run this on the initial connection, as we will use a event listener to handle updates:
stop()
})
```
```
<template>
<button @click="requestDevice()">
Request Bluetooth Device
</button>
</template>
```
More samples can be found on [Google Chrome's Web Bluetooth Samples](https://googlechrome.github.io/samples/web-bluetooth/).
Type Declarations
-----------------
Show Type Declarations
```
/// <reference types="@types/web-bluetooth" />
export interface UseBluetoothRequestDeviceOptions {
/**
*
* An array of BluetoothScanFilters. This filter consists of an array
* of BluetoothServiceUUIDs, a name parameter, and a namePrefix parameter.
*
*/
filters?: BluetoothLEScanFilter[] | undefined
/**
*
* An array of BluetoothServiceUUIDs.
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/BluetoothRemoteGATTService/uuid
*
*/
optionalServices?: BluetoothServiceUUID[] | undefined
}
export interface UseBluetoothOptions
extends UseBluetoothRequestDeviceOptions,
ConfigurableNavigator {
/**
*
* A boolean value indicating that the requesting script can accept all Bluetooth
* devices. The default is false.
*
* !! This may result in a bunch of unrelated devices being shown
* in the chooser and energy being wasted as there are no filters.
*
*
* Use it with caution.
*
* @default false
*
*/
acceptAllDevices?: boolean
}
export declare function useBluetooth(
options?: UseBluetoothOptions
): UseBluetoothReturn
export interface UseBluetoothReturn {
isSupported: Ref<boolean>
isConnected: ComputedRef<boolean>
device: Ref<BluetoothDevice | undefined>
requestDevice: () => Promise<void>
server: Ref<BluetoothRemoteGATTServer | undefined>
error: Ref<unknown | null>
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBluetooth/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBluetooth/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBluetooth/index.md)
| programming_docs |
vueuse useBrowserLocation useBrowserLocation
==================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.17 kB |
| Last Changed | last year |
Reactive browser location
> NOTE: If you're using Vue Router, use [`useRoute`](https://router.vuejs.org/guide/advanced/composition-api.html) provided by Vue Router instead.
>
>
Usage
-----
```
import { useBrowserLocation } from '@vueuse/core'
const location = useBrowserLocation()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseBrowserLocation v-slot="{ location }">
Browser Location: {{ location }}
</UseBrowserLocation>
```
Type Declarations
-----------------
```
export interface BrowserLocationState {
trigger: string
state?: any
length?: number
hash?: string
host?: string
hostname?: string
href?: string
origin?: string
pathname?: string
port?: string
protocol?: string
search?: string
}
/**
* Reactive browser location.
*
* @see https://vueuse.org/useBrowserLocation
* @param options
*/
export declare function useBrowserLocation({
window,
}?: ConfigurableWindow): Ref<{
trigger: string
state?: any
length?: number | undefined
hash?: string | undefined
host?: string | undefined
hostname?: string | undefined
href?: string | undefined
origin?: string | undefined
pathname?: string | undefined
port?: string | undefined
protocol?: string | undefined
search?: string | undefined
}>
export type UseBrowserLocationReturn = ReturnType<typeof useBrowserLocation>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBrowserLocation/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBrowserLocation/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBrowserLocation/index.md)
vueuse useUrlSearchParams useUrlSearchParams
==================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.98 kB |
| Last Changed | 4 months ago |
Reactive [URLSearchParams](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams)
Usage
-----
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('history')
console.log(params.foo) // 'bar'
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `?foo=bar&vueuse=awesome`
```
### Hash Mode
When using with hash mode route, specify the `mode` to `hash`
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('hash')
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `#/your/route?foo=bar&vueuse=awesome`
```
### Hash Params
When using with history mode route, but want to use hash as params, specify the `mode` to `hash-params`
```
import { useUrlSearchParams } from '@vueuse/core'
const params = useUrlSearchParams('hash-params')
params.foo = 'bar'
params.vueuse = 'awesome'
// url updated to `/your/route#foo=bar&vueuse=awesome`
```
Type Declarations
-----------------
```
export type UrlParams = Record<string, string[] | string>
export interface UseUrlSearchParamsOptions<T> extends ConfigurableWindow {
/**
* @default true
*/
removeNullishValues?: boolean
/**
* @default false
*/
removeFalsyValues?: boolean
/**
* @default {}
*/
initialValue?: T
/**
* Write back to `window.history` automatically
*
* @default true
*/
write?: boolean
}
/**
* Reactive URLSearchParams
*
* @see https://vueuse.org/useUrlSearchParams
* @param mode
* @param options
*/
export declare function useUrlSearchParams<
T extends Record<string, any> = UrlParams
>(
mode?: "history" | "hash" | "hash-params",
options?: UseUrlSearchParamsOptions<T>
): T
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useUrlSearchParams/index.md)
vueuse usePreferredLanguages usePreferredLanguages
=====================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.07 kB |
| Last Changed | last year |
Reactive [Navigator Languages](https://developer.mozilla.org/en-US/docs/Web/API/NavigatorLanguage/languages). It provides web developers with information about the user's preferred languages. For example, this may be useful to adjust the language of the user interface based on the user's preferred languages in order to provide better experience.
Usage
-----
```
import { usePreferredLanguages } from '@vueuse/core'
const languages = usePreferredLanguages()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePreferredLanguages v-slot="{ languages }">
Preferred Languages: {{ languages }}
</UsePreferredLanguages>
```
Type Declarations
-----------------
```
/**
* Reactive Navigator Languages.
*
* @see https://vueuse.org/usePreferredLanguages
* @param options
*/
export declare function usePreferredLanguages(
options?: ConfigurableWindow
): Ref<readonly string[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredLanguages/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredLanguages/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredLanguages/index.md)
vueuse useAsyncState useAsyncState
=============
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 1.04 kB |
| Last Changed | 2 weeks ago |
Reactive async state. Will not block your setup function and will trigger changes once the promise is ready.
Usage
-----
```
import axios from 'axios'
import { useAsyncState } from '@vueuse/core'
const { state, isReady, isLoading } = useAsyncState(
axios
.get('https://jsonplaceholder.typicode.com/todos/1')
.then(t => t.data),
{ id: null },
)
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseAsyncStateReturn<Data, Shallow extends boolean> {
state: Shallow extends true ? Ref<Data> : Ref<UnwrapRef<Data>>
isReady: Ref<boolean>
isLoading: Ref<boolean>
error: Ref<unknown>
execute: (delay?: number, ...args: any[]) => Promise<Data>
}
export interface UseAsyncStateOptions<Shallow extends boolean, D = any> {
/**
* Delay for executing the promise. In milliseconds.
*
* @default 0
*/
delay?: number
/**
* Execute the promise right after the function is invoked.
* Will apply the delay if any.
*
* When set to false, you will need to execute it manually.
*
* @default true
*/
immediate?: boolean
/**
* Callback when error is caught.
*/
onError?: (e: unknown) => void
/**
* Callback when success is caught.
* @param {D} data
*/
onSuccess?: (data: D) => void
/**
* Sets the state to initialState before executing the promise.
*
* This can be useful when calling the execute function more than once (for
* example, to refresh data). When set to false, the current state remains
* unchanged until the promise resolves.
*
* @default true
*/
resetOnExecute?: boolean
/**
* Use shallowRef.
*
* @default true
*/
shallow?: Shallow
/**
*
* An error is thrown when executing the execute function
*
* @default false
*/
throwError?: boolean
}
/**
* Reactive async state. Will not block your setup function and will trigger changes once
* the promise is ready.
*
* @see https://vueuse.org/useAsyncState
* @param promise The promise / async function to be resolved
* @param initialState The initial state, used until the first evaluation finishes
* @param options
*/
export declare function useAsyncState<Data, Shallow extends boolean = true>(
promise: Promise<Data> | ((...args: any[]) => Promise<Data>),
initialState: Data,
options?: UseAsyncStateOptions<Shallow, Data>
): UseAsyncStateReturn<Data, Shallow>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncState/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncState/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncState/index.md)
vueuse useFileDialog useFileDialog
=============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 978 B |
| Last Changed | 4 months ago |
Open file dialog with ease.
Usage
-----
```
import { useFileDialog } from '@vueuse/core'
const { files, open, reset } = useFileDialog()
```
```
<template>
<button type="button" @click="open">Choose file</button>
</template>
```
Type Declarations
-----------------
```
export interface UseFileDialogOptions extends ConfigurableDocument {
/**
* @default true
*/
multiple?: boolean
/**
* @default '*'
*/
accept?: string
/**
* Select the input source for the capture file.
* @see [HTMLInputElement Capture](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/capture)
*/
capture?: string
}
export interface UseFileDialogReturn {
files: Ref<FileList | null>
open: (localOptions?: Partial<UseFileDialogOptions>) => void
reset: () => void
}
/**
* Open file dialog with ease.
*
* @see https://vueuse.org/useFileDialog
* @param options
*/
export declare function useFileDialog(
options?: UseFileDialogOptions
): UseFileDialogReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileDialog/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileDialog/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileDialog/index.md)
vueuse useBroadcastChannel useBroadcastChannel
===================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.07 kB |
| Last Changed | 6 months ago |
Reactive [BroadcastChannel API](https://developer.mozilla.org/en-US/docs/Web/API/BroadcastChannel).
Closes a broadcast channel automatically component unmounted.
Usage
-----
The BroadcastChannel interface represents a named channel that any browsing context of a given origin can subscribe to. It allows communication between different documents (in different windows, tabs, frames, or iframes) of the same origin.
Messages are broadcasted via a message event fired at all BroadcastChannel objects listening to the channel.
```
import { ref } from 'vue-demi'
import { useBroadcastChannel } from '@vueuse/core'
const {
isSupported,
channel,
post,
close,
error,
isClosed,
} = useBroadcastChannel({ name: 'vueuse-demo-channel' })
const message = ref('')
message.value = 'Hello, VueUse World!'
// Post the message to the broadcast channel:
post(message.value)
// Option to close the channel if you wish:
close()
```
Type Declarations
-----------------
```
export interface UseBroadcastChannelOptions extends ConfigurableWindow {
/**
* The name of the channel.
*/
name: string
}
/**
* Reactive BroadcastChannel
*
* @see https://vueuse.org/useBroadcastChannel
* @see https://developer.mozilla.org/en-US/docs/Web/API/BroadcastChannel
* @param options
*
*/
export declare const useBroadcastChannel: <D, P>(
options: UseBroadcastChannelOptions
) => UseBroadcastChannelReturn<D, P>
export interface UseBroadcastChannelReturn<D, P> {
isSupported: Ref<boolean>
channel: Ref<BroadcastChannel | undefined>
data: Ref<D>
post: (data: P) => void
close: () => void
error: Ref<Event | null>
isClosed: Ref<boolean>
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBroadcastChannel/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBroadcastChannel/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBroadcastChannel/index.md)
vueuse useShare useShare
========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.02 kB |
| Last Changed | 6 months ago |
Reactive [Web Share API](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/share). The Browser provides features that can share content in text or file.
> The `share` method has to be called following a user gesture like a button click. It can’t simply be called on page load for example. That’s in place to help prevent abuse.
>
>
Usage
-----
```
import { useShare } from '@vueuse/core'
const { share, isSupported } = useShare()
function startShare() {
share({
title: 'Hello',
text: 'Hello my friend!',
url: location.href,
})
}
```
### Passing a source ref
You can pass a `ref` to it, changes from the source ref will be reflected to your sharing options.
```
import { ref } from 'vue'
const shareOptions = ref < ShareOptions > ({ text: 'foo' })
const { share, isSupported } = useShare(shareOptions)
shareOptions.value.text = 'bar'
share()
```
Type Declarations
-----------------
```
export interface UseShareOptions {
title?: string
files?: File[]
text?: string
url?: string
}
/**
* Reactive Web Share API.
*
* @see https://vueuse.org/useShare
* @param shareOptions
* @param options
*/
export declare function useShare(
shareOptions?: MaybeComputedRef<UseShareOptions>,
options?: ConfigurableNavigator
): {
isSupported: Ref<boolean>
share: (overrideOptions?: MaybeComputedRef<UseShareOptions>) => Promise<void>
}
export type UseShareReturn = ReturnType<typeof useShare>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useShare/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useShare/demo.client.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useShare/index.md)
vueuse useWakeLock useWakeLock
===========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.27 kB |
| Last Changed | 6 months ago |
Reactive [Screen Wake Lock API](https://developer.mozilla.org/en-US/docs/Web/API/Screen_Wake_Lock_API). Provides a way to prevent devices from dimming or locking the screen when an application needs to keep running.
Usage
-----
```
import { useWakeLock } from '@vueuse/core'
const { isSupported, isActive, request, release } = useWakeLock()
```
If `request` is called, `isActive` will be **true**, and if `release` is called, or other tab is displayed, or the window is minimized,`isActive` will be **false**.
Type Declarations
-----------------
```
type WakeLockType = "screen"
export interface WakeLockSentinel extends EventTarget {
type: WakeLockType
released: boolean
release: () => Promise<void>
}
export type UseWakeLockOptions = ConfigurableNavigator & ConfigurableDocument
/**
* Reactive Screen Wake Lock API.
*
* @see https://vueuse.org/useWakeLock
* @param options
*/
export declare const useWakeLock: (options?: UseWakeLockOptions) => {
isSupported: Ref<boolean>
isActive: Ref<boolean>
request: (type: WakeLockType) => Promise<void>
release: () => Promise<void>
}
export type UseWakeLockReturn = ReturnType<typeof useWakeLock>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWakeLock/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWakeLock/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWakeLock/index.md)
vueuse useConfirmDialog useConfirmDialog
================
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 985 B |
| Last Changed | last year |
Creates event hooks to support modals and confirmation dialog chains.
Functions can be used on the template, and hooks are a handy skeleton for the business logic of modals dialog or other actions that require user confirmation.
Functions and hooks
-------------------
* `reveal()` - triggers `onReveal` hook and sets `revealed.value` to `true`. Returns promise that resolves by `confirm()` or `cancel()`.
* `confirm()` - sets `isRevealed.value` to `false` and triggers `onConfirm` hook.
* `cancel()` - sets `isRevealed.value` to `false` and triggers `onCancel` hook.
Basic Usage
-----------
### Using hooks
```
<script setup>
import { useConfirmDialog } from '@vueuse/core'
const {
isRevealed,
reveal,
confirm,
cancel,
onReveal,
onConfirm,
onCancel,
} = useConfirmDialog()
</script>
<template>
<button @click="reveal">Reveal Modal</button>
<teleport to="body">
<div v-if="isRevealed" class="modal-bg">
<div class="modal">
<h2>Confirm?</h2>
<button @click="confirm">Yes</button>
<button @click="cancel">Cancel</button>
</div>
</div>
</teleport>
</template>
```
### Promise
If you prefer working with promises:
```
<script setup>
import { useConfirmDialog, onClickOutside } from '@vueuse/core'
const {
isRevealed,
reveal,
confirm,
cancel,
} = useConfirmDialog()
const openDialog = async () => {
const { data, isCanceled } = await reveal()
if (!isCanceled) {
console.log(data)
}
}
</script>
<template>
<button @click="openDialog">Show Modal</button>
<teleport to="body">
<div v-if="isRevealed" class="modal-layout">
<div class="modal">
<h2>Confirm?</h2>
<button @click="confirm(true)">Yes</button>
<button @click="confirm(false)">No</button>
</div>
</div>
</teleport>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseConfirmDialogRevealResult<C, D> =
| {
data?: C
isCanceled: false
}
| {
data?: D
isCanceled: true
}
export interface UseConfirmDialogReturn<RevealData, ConfirmData, CancelData> {
/**
* Revealing state
*/
isRevealed: ComputedRef<boolean>
/**
* Opens the dialog.
* Create promise and return it. Triggers `onReveal` hook.
*/
reveal: (
data?: RevealData
) => Promise<UseConfirmDialogRevealResult<ConfirmData, CancelData>>
/**
* Confirms and closes the dialog. Triggers a callback inside `onConfirm` hook.
* Resolves promise from `reveal()` with `data` and `isCanceled` ref with `false` value.
* Can accept any data and to pass it to `onConfirm` hook.
*/
confirm: (data?: ConfirmData) => void
/**
* Cancels and closes the dialog. Triggers a callback inside `onCancel` hook.
* Resolves promise from `reveal()` with `data` and `isCanceled` ref with `true` value.
* Can accept any data and to pass it to `onCancel` hook.
*/
cancel: (data?: CancelData) => void
/**
* Event Hook to be triggered right before dialog creating.
*/
onReveal: EventHookOn<RevealData>
/**
* Event Hook to be called on `confirm()`.
* Gets data object from `confirm` function.
*/
onConfirm: EventHookOn<ConfirmData>
/**
* Event Hook to be called on `cancel()`.
* Gets data object from `cancel` function.
*/
onCancel: EventHookOn<CancelData>
}
/**
* Hooks for creating confirm dialogs. Useful for modal windows, popups and logins.
*
* @see https://vueuse.org/useConfirmDialog/
* @param revealed `boolean` `ref` that handles a modal window
*/
export declare function useConfirmDialog<
RevealData = any,
ConfirmData = any,
CancelData = any
>(
revealed?: Ref<boolean>
): UseConfirmDialogReturn<RevealData, ConfirmData, CancelData>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useConfirmDialog/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useConfirmDialog/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useConfirmDialog/index.md)
*No recent changes*
| programming_docs |
vueuse useSorted useSorted
=========
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | |
| Last Changed | 3 months ago |
reactive sort array
Usage
-----
```
import { useSorted } from '@vueuse/core'
// general sort
const source = [10, 3, 5, 7, 2, 1, 8, 6, 9, 4]
const sorted = useSorted(source)
console.log(sorted.value) // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
console.log(source) // [10, 3, 5, 7, 2, 1, 8, 6, 9, 4]
// object sort
const objArr = [{
name: 'John',
age: 40,
}, {
name: 'Jane',
age: 20,
}, {
name: 'Joe',
age: 30,
}, {
name: 'Jenny',
age: 22,
}]
const objSorted = useSorted(objArr, (a, b) => a.age - b.age)
```
### dirty mode
dirty mode will change the source array.
```
const source = ref([10, 3, 5, 7, 2, 1, 8, 6, 9, 4])
const sorted = useSorted(source, (a, b) => a - b, {
dirty: true,
})
console.log(source)// output: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
```
Type Declarations
-----------------
```
export type UseSortedCompareFn<T = any> = (a: T, b: T) => number
export type UseSortedFn<T = any> = (
arr: T[],
compareFn: UseSortedCompareFn<T>
) => T[]
export interface UseSortedOptions<T = any> {
/**
* sort algorithm
*/
sortFn?: UseSortedFn<T>
/**
* compare function
*/
compareFn?: UseSortedCompareFn<T>
/**
* change the value of the source array
* @default false
*/
dirty?: boolean
}
export declare function useSorted<T = any>(
source: MaybeRef<T[]>,
compareFn?: UseSortedCompareFn<T>
): Ref<T[]>
export declare function useSorted<T = any>(
source: MaybeRef<T[]>,
options?: UseSortedOptions<T>
): Ref<T[]>
export declare function useSorted<T = any>(
source: MaybeRef<T[]>,
compareFn?: UseSortedCompareFn<T>,
options?: Omit<UseSortedOptions<T>, "compareFn">
): Ref<T[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSorted/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useSorted/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSorted/index.md)
vueuse useManualRefHistory useManualRefHistory
===================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 1.14 kB |
| Last Changed | 4 months ago |
| Related | [`useRefHistory`](../userefhistory/index) |
Manually track the change history of a ref when the using calls `commit()`, also provides undo and redo functionality
Usage
-----
```
import { ref } from 'vue'
import { useManualRefHistory } from '@vueuse/core'
const counter = ref(0)
const { history, commit, undo, redo } = useManualRefHistory(counter)
counter.value += 1
commit()
console.log(history.value)
/* [
{ snapshot: 1, timestamp: 1601912898062 },
{ snapshot: 0, timestamp: 1601912898061 }
] */
```
You can use `undo` to reset the ref value to the last history point.
```
console.log(counter.value) // 1
undo()
console.log(counter.value) // 0
```
#### History of mutable objects
If you are going to mutate the source, you need to pass a custom clone function or use `clone` `true` as a param, that is a shortcut for a minimal clone function `x => JSON.parse(JSON.stringify(x))` that will be used in both `dump` and `parse`.
```
import { ref } from 'vue'
import { useManualRefHistory } from '@vueuse/core'
const counter = ref({ foo: 1, bar: 2 })
const { history, commit, undo, redo } = useManualRefHistory(counter, { clone: true })
counter.value.foo += 1
commit()
```
#### Custom Clone Function
To use a full featured or custom clone function, you can set up via the `dump` options.
For example, using [structuredClone](https://developer.mozilla.org/en-US/docs/Web/API/structuredClone):
```
import { useManualRefHistory } from '@vueuse/core'
const refHistory = useManualRefHistory(target, { clone: structuredClone })
```
Or by using [lodash's `cloneDeep`](https://lodash.com/docs/4.17.15#cloneDeep):
```
import { cloneDeep } from 'lodash-es'
import { useManualRefHistory } from '@vueuse/core'
const refHistory = useManualRefHistory(target, { clone: cloneDeep })
```
Or a more lightweight [`klona`](https://github.com/lukeed/klona):
```
import { klona } from 'klona'
import { useManualRefHistory } from '@vueuse/core'
const refHistory = useManualRefHistory(target, { clone: klona })
```
#### Custom Dump and Parse Function
Instead of using the `clone` param, you can pass custom functions to control the serialization and parsing. In case you do not need history values to be objects, this can save an extra clone when undoing. It is also useful in case you want to have the snapshots already stringified to be saved to local storage for example.
```
import { useManualRefHistory } from '@vueuse/core'
const refHistory = useManualRefHistory(target, {
dump: JSON.stringify,
parse: JSON.parse,
})
```
### History Capacity
We will keep all the history by default (unlimited) until you explicitly clear them up, you can set the maximal amount of history to be kept by `capacity` options.
```
const refHistory = useManualRefHistory(target, {
capacity: 15, // limit to 15 history records
})
refHistory.clear() // explicitly clear all the history
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseRefHistoryRecord<T> {
snapshot: T
timestamp: number
}
export interface UseManualRefHistoryOptions<Raw, Serialized = Raw> {
/**
* Maximum number of history to be kept. Default to unlimited.
*/
capacity?: number
/**
* Clone when taking a snapshot, shortcut for dump: JSON.parse(JSON.stringify(value)).
* Default to false
*
* @default false
*/
clone?: boolean | CloneFn<Raw>
/**
* Serialize data into the history
*/
dump?: (v: Raw) => Serialized
/**
* Deserialize data from the history
*/
parse?: (v: Serialized) => Raw
/**
* Deserialize data from the history
*/
setSource?: (source: Ref<Raw>, v: Raw) => void
}
export interface UseManualRefHistoryReturn<Raw, Serialized> {
/**
* Bypassed tracking ref from the argument
*/
source: Ref<Raw>
/**
* An array of history records for undo, newest comes to first
*/
history: Ref<UseRefHistoryRecord<Serialized>[]>
/**
* Last history point, source can be different if paused
*/
last: Ref<UseRefHistoryRecord<Serialized>>
/**
* Same as {@link UseManualRefHistoryReturn.history | history}
*/
undoStack: Ref<UseRefHistoryRecord<Serialized>[]>
/**
* Records array for redo
*/
redoStack: Ref<UseRefHistoryRecord<Serialized>[]>
/**
* A ref representing if undo is possible (non empty undoStack)
*/
canUndo: Ref<boolean>
/**
* A ref representing if redo is possible (non empty redoStack)
*/
canRedo: Ref<boolean>
/**
* Undo changes
*/
undo: () => void
/**
* Redo changes
*/
redo: () => void
/**
* Clear all the history
*/
clear: () => void
/**
* Create new a new history record
*/
commit: () => void
/**
* Reset ref's value with latest history
*/
reset: () => void
}
/**
* Track the change history of a ref, also provides undo and redo functionality.
*
* @see https://vueuse.org/useManualRefHistory
* @param source
* @param options
*/
export declare function useManualRefHistory<Raw, Serialized = Raw>(
source: Ref<Raw>,
options?: UseManualRefHistoryOptions<Raw, Serialized>
): UseManualRefHistoryReturn<Raw, Serialized>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useManualRefHistory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useManualRefHistory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useManualRefHistory/index.md)
vueuse useStorageAsync useStorageAsync
===============
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 1.83 kB |
| Last Changed | 6 months ago |
| Related | [`useStorage`](../usestorage/index) |
Reactive Storage in with async support.
Usage
-----
Please refer to [`useStorage`](../usestorage/index)
Type Declarations
-----------------
Show Type Declarations
```
export interface UseStorageAsyncOptions<T>
extends Omit<UseStorageOptions<T>, "serializer"> {
/**
* Custom data serialization
*/
serializer?: SerializerAsync<T>
}
export declare function useStorageAsync(
key: string,
initialValue: MaybeComputedRef<string>,
storage?: StorageLikeAsync,
options?: UseStorageAsyncOptions<string>
): RemovableRef<string>
export declare function useStorageAsync(
key: string,
initialValue: MaybeComputedRef<boolean>,
storage?: StorageLikeAsync,
options?: UseStorageAsyncOptions<boolean>
): RemovableRef<boolean>
export declare function useStorageAsync(
key: string,
initialValue: MaybeComputedRef<number>,
storage?: StorageLikeAsync,
options?: UseStorageAsyncOptions<number>
): RemovableRef<number>
export declare function useStorageAsync<T>(
key: string,
initialValue: MaybeComputedRef<T>,
storage?: StorageLikeAsync,
options?: UseStorageAsyncOptions<T>
): RemovableRef<T>
export declare function useStorageAsync<T = unknown>(
key: string,
initialValue: MaybeComputedRef<null>,
storage?: StorageLikeAsync,
options?: UseStorageAsyncOptions<T>
): RemovableRef<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useStorageAsync/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useStorageAsync/index.md)
vueuse useMouseInElement useMouseInElement
=================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.56 kB |
| Last Changed | 6 months ago |
Reactive mouse position related to an element
Usage
-----
```
<template>
<div ref="target">
<h1>Hello world</h1>
</div>
</template>
<script>
import { ref } from 'vue'
import { useMouseInElement } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
const { x, y, isOutside } = useMouseInElement(target)
return { x, y, isOutside }
}
}
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseMouseInElement v-slot="{ elementX, elementY, isOutside }">
x: {{ elementX }}
y: {{ elementY }}
Is Outside: {{ isOutside }}
</UseMouseInElement>
```
Type Declarations
-----------------
```
export interface MouseInElementOptions extends UseMouseOptions {
handleOutside?: boolean
}
/**
* Reactive mouse position related to an element.
*
* @see https://vueuse.org/useMouseInElement
* @param target
* @param options
*/
export declare function useMouseInElement(
target?: MaybeElementRef,
options?: MouseInElementOptions
): {
x: Ref<number>
y: Ref<number>
sourceType: Ref<MouseSourceType>
elementX: Ref<number>
elementY: Ref<number>
elementPositionX: Ref<number>
elementPositionY: Ref<number>
elementHeight: Ref<number>
elementWidth: Ref<number>
isOutside: Ref<boolean>
stop: () => void
}
export type UseMouseInElementReturn = ReturnType<typeof useMouseInElement>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouseInElement/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouseInElement/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMouseInElement/index.md)
vueuse useScreenOrientation useScreenOrientation
====================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.25 kB |
| Last Changed | 6 months ago |
Reactive [Screen Orientation API](https://developer.mozilla.org/en-US/docs/Web/API/Screen_Orientation_API). It provides web developers with information about the user's current screen orientation.
Usage
-----
```
import { useScreenOrientation } from '@vueuse/core'
const {
isSupported,
orientation,
angle,
lockOrientation,
unlockOrientation,
} = useScreenOrientation()
```
To lock the orientation, you can pass an [OrientationLockType](https://developer.mozilla.org/en-US/docs/Web/API/ScreenOrientation/type) to the lockOrientation function:
```
import { useScreenOrientation } from '@vueuse/core'
const {
isSupported,
orientation,
angle,
lockOrientation,
unlockOrientation,
} = useScreenOrientation()
lockOrientation('portrait-primary')
```
and then unlock again, with the following:
```
unlockOrientation()
```
Accepted orientation types are one of `"landscape-primary"`, `"landscape-secondary"`, `"portrait-primary"`, `"portrait-secondary"`, `"any"`, `"landscape"`, `"natural"` and `"portrait"`.
[Screen Orientation API MDN](https://developer.mozilla.org/en-US/docs/Web/API/Screen_Orientation_API)
Type Declarations
-----------------
```
/**
* Reactive screen orientation
*
* @see https://vueuse.org/useScreenOrientation
*/
export declare const useScreenOrientation: (options?: ConfigurableWindow) => {
isSupported: Ref<boolean>
orientation: Ref<OrientationType | undefined>
angle: Ref<number>
lockOrientation: (type: OrientationLockType) => Promise<void>
unlockOrientation: () => void
}
export type UseScreenOrientationReturn = ReturnType<typeof useScreenOrientation>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenOrientation/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenOrientation/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenOrientation/index.md)
vueuse useNavigatorLanguage useNavigatorLanguage
====================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.17 kB |
| Last Changed | 4 months ago |
Reactive [navigator.language](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/language).
Usage
-----
```
import { defineComponent, ref } from 'vue'
import { useNavigatorLanguage } from '@vueuse/core'
export default defineComponent({
setup() {
const { language } = useNavigatorLanguage()
watch(language, () => {
// Listen to the value changing
})
return {
language,
}
},
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface NavigatorLanguageState {
isSupported: Ref<boolean>
/**
*
* ISO 639-1 standard Language Code
*
* @info The detected user agent language preference as a language tag
* (which is sometimes referred to as a "locale identifier").
* This consists of a 2-3 letter base language tag that indicates a
* language, optionally followed by additional subtags separated by
* '-'. The most common extra information is the country or region
* variant (like 'en-US' or 'fr-CA').
*
*
* @see https://www.iso.org/iso-639-language-codes.html
* @see https://www.loc.gov/standards/iso639-2/php/code_list.php
*
*/
language: Ref<string | undefined>
}
/**
*
* Reactive useNavigatorLanguage
*
* Detects the currently selected user language and returns a reactive language
* @see https://vueuse.org/useNavigatorLanguage
*
*/
export declare const useNavigatorLanguage: (
options?: ConfigurableWindow
) => Readonly<NavigatorLanguageState>
export type UseNavigatorLanguageReturn = ReturnType<typeof useNavigatorLanguage>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useNavigatorLanguage/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useNavigatorLanguage/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useNavigatorLanguage/index.md)
vueuse useCurrentElement useCurrentElement
=================
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 813 B |
| Last Changed | 5 months ago |
Get the DOM element of current component as a ref.
Usage
-----
```
import { useCurrentElement } from '@vueuse/core'
const el = useCurrentElement() // ComputedRef<Element>
```
Caveats
-------
This functions uses [`$el` under the hood](https://vuejs.org/api/component-instance.html#el).
Value of the ref will be `undefined` until the component is mounted.
* For components with a single root element, it will point to that element.
* For components with text root, it will point to the text node.
* For components with multiple root nodes, it will be the placeholder DOM node that Vue uses to keep track of the component's position in the DOM.
It's recommend to only use this function for components with **a single root element**.
Type Declarations
-----------------
```
export declare function useCurrentElement<
T extends Element = Element
>(): ComputedRefWithControl<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useCurrentElement/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useCurrentElement/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useCurrentElement/index.md)
vueuse onStartTyping onStartTyping
=============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.24 kB |
| Last Changed | last year |
Fires when users start typing on non-editable elements.
Usage
-----
```
<input ref="input" type="text" placeholder="Start typing to focus" />
```
```
import { onStartTyping } from '@vueuse/core'
export default {
setup() {
const input = ref(null)
onStartTyping(() => {
if (!input.value.active)
input.value.focus()
})
return {
input,
}
},
}
```
Type Declarations
-----------------
```
/**
* Fires when users start typing on non-editable elements.
*
* @see https://vueuse.org/onStartTyping
* @param callback
* @param options
*/
export declare function onStartTyping(
callback: (event: KeyboardEvent) => void,
options?: ConfigurableDocument
): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/onStartTyping/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/onStartTyping/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/onStartTyping/index.md)
*No recent changes*
vueuse usePreferredColorScheme usePreferredColorScheme
=======================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.1 kB |
| Last Changed | last year |
Reactive [prefers-color-scheme](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-color-scheme) media query.
Usage
-----
```
import { usePreferredColorScheme } from '@vueuse/core'
const preferredColor = usePreferredColorScheme()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePreferredColorScheme v-slot="{ colorScheme }">
Preferred Color Scheme: {{ colorScheme }}
<UsePreferredColorScheme>
```
Type Declarations
-----------------
```
export type ColorSchemeType = "dark" | "light" | "no-preference"
/**
* Reactive prefers-color-scheme media query.
*
* @see https://vueuse.org/usePreferredColorScheme
* @param [options]
*/
export declare function usePreferredColorScheme(
options?: ConfigurableWindow
): ComputedRef<ColorSchemeType>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredColorScheme/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredColorScheme/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredColorScheme/index.md)
| programming_docs |
vueuse useBase64 useBase64
=========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1.39 kB |
| Last Changed | 6 months ago |
Reactive base64 transforming. Supports plain text, buffer, files, canvas, objects, maps, sets and images.
Usage
-----
```
import { Ref, ref } from 'vue'
import { useBase64 } from '@vueuse/core'
const text = ref('')
const { base64 } = useBase64(text)
```
If you use object, array, map or set you can provide serializer in options. Otherwise, your data will be serialized by default serializer. Objects and arrays will be transformed in string by JSON.stringify. Map and set will be transformed in object and array respectively before stringify.
Type Declarations
-----------------
Show Type Declarations
```
export interface ToDataURLOptions {
/**
* MIME type
*/
type?: string | undefined
/**
* Image quality of jpeg or webp
*/
quality?: any
}
export interface UseBase64ObjectOptions<T> {
serializer: (v: T) => string
}
export interface UseBase64Return {
base64: Ref<string>
promise: Ref<Promise<string>>
execute: () => Promise<string>
}
export declare function useBase64(
target: MaybeComputedRef<string>
): UseBase64Return
export declare function useBase64(
target: MaybeComputedRef<Blob>
): UseBase64Return
export declare function useBase64(
target: MaybeComputedRef<ArrayBuffer>
): UseBase64Return
export declare function useBase64(
target: MaybeComputedRef<HTMLCanvasElement>,
options?: ToDataURLOptions
): UseBase64Return
export declare function useBase64(
target: MaybeComputedRef<HTMLImageElement>,
options?: ToDataURLOptions
): UseBase64Return
export declare function useBase64<T extends Record<string, unknown>>(
target: MaybeComputedRef<T>,
options?: UseBase64ObjectOptions<T>
): UseBase64Return
export declare function useBase64<T extends Map<string, unknown>>(
target: MaybeComputedRef<T>,
options?: UseBase64ObjectOptions<T>
): UseBase64Return
export declare function useBase64<T extends Set<unknown>>(
target: MaybeComputedRef<T>,
options?: UseBase64ObjectOptions<T>
): UseBase64Return
export declare function useBase64<T>(
target: MaybeComputedRef<T[]>,
options?: UseBase64ObjectOptions<T[]>
): UseBase64Return
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBase64/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBase64/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBase64/index.md)
vueuse useDocumentVisibility useDocumentVisibility
=====================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.07 kB |
| Last Changed | 6 months ago |
Reactively track [`document.visibilityState`](https://developer.mozilla.org/en-US/docs/Web/API/Document/visibilityState)
Usage
-----
```
import { useDocumentVisibility } from '@vueuse/core'
const visibility = useDocumentVisibility()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDocumentVisibility v-slot="{ visibility }">
Document Visibility: {{ visibility }}
</UseDocumentVisibility>
```
Type Declarations
-----------------
```
/**
* Reactively track `document.visibilityState`.
*
* @see https://vueuse.org/useDocumentVisibility
* @param options
*/
export declare function useDocumentVisibility({
document,
}?: ConfigurableDocument): Ref<DocumentVisibilityState>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDocumentVisibility/index.md)
vueuse useScriptTag useScriptTag
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.29 kB |
| Last Changed | 5 months ago |
Script tag injecting.
Usage
-----
```
import { useScriptTag } from '@vueuse/core'
useScriptTag(
'https://player.twitch.tv/js/embed/v1.js',
// on script tag loaded.
(el: HTMLScriptElement) => {
// do something
},
)
```
The script will be automatically loaded on the component mounted and removed when the component on unmounting.
Configurations
--------------
Set `manual: true` to have manual control over the timing to load the script.
```
import { useScriptTag } from '@vueuse/core'
const { scriptTag, load, unload } = useScriptTag(
'https://player.twitch.tv/js/embed/v1.js',
() => {
// do something
},
{ manual: true },
)
// manual controls
await load()
await unload()
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseScriptTagOptions extends ConfigurableDocument {
/**
* Load the script immediately
*
* @default true
*/
immediate?: boolean
/**
* Add `async` attribute to the script tag
*
* @default true
*/
async?: boolean
/**
* Script type
*
* @default 'text/javascript'
*/
type?: string
/**
* Manual controls the timing of loading and unloading
*
* @default false
*/
manual?: boolean
crossOrigin?: "anonymous" | "use-credentials"
referrerPolicy?:
| "no-referrer"
| "no-referrer-when-downgrade"
| "origin"
| "origin-when-cross-origin"
| "same-origin"
| "strict-origin"
| "strict-origin-when-cross-origin"
| "unsafe-url"
noModule?: boolean
defer?: boolean
/**
* Add custom attribute to the script tag
*
*/
attrs?: Record<string, string>
}
/**
* Async script tag loading.
*
* @see https://vueuse.org/useScriptTag
* @param src
* @param onLoaded
* @param options
*/
export declare function useScriptTag(
src: MaybeComputedRef<string>,
onLoaded?: (el: HTMLScriptElement) => void,
options?: UseScriptTagOptions
): {
scriptTag: Ref<HTMLScriptElement | null>
load: (waitForScriptLoad?: boolean) => Promise<HTMLScriptElement | boolean>
unload: () => void
}
export type UseScriptTagReturn = ReturnType<typeof useScriptTag>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScriptTag/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScriptTag/index.md)
vueuse useEventSource useEventSource
==============
| | |
| --- | --- |
| Category | [Network](../../functions#category=Network) |
| Export Size | 1.21 kB |
| Last Changed | 6 months ago |
An [EventSource](https://developer.mozilla.org/en-US/docs/Web/API/EventSource) or [Server-Sent-Events](https://developer.mozilla.org/en-US/docs/Web/API/Server-sent_events) instance opens a persistent connection to an HTTP server, which sends events in text/event-stream format.
Usage
-----
```
import { useEventSource } from '@vueuse/core'
const { status, data, error, close } = useEventSource('https://event-source-url')
```
| State | Type | Description |
| --- | --- | --- |
| status | `Ref<string>` | A read-only value representing the state of the connection. Possible values are CONNECTING (0), OPEN (1), or CLOSED (2) |
| data | `Ref<string | null>` | Reference to the latest data received via the EventSource, can be watched to respond to incoming messages |
| eventSource | `Ref<EventSource | null>` | Reference to the current EventSource instance |
| Method | Signature | Description |
| --- | --- | --- |
| close | `() => void` | Closes the EventSource connection gracefully. |
Type Declarations
-----------------
```
export type UseEventSourceOptions = EventSourceInit
/**
* Reactive wrapper for EventSource.
*
* @see https://vueuse.org/useEventSource
* @see https://developer.mozilla.org/en-US/docs/Web/API/EventSource/EventSource EventSource
* @param url
* @param events
* @param options
*/
export declare function useEventSource(
url: string,
events?: Array<string>,
options?: UseEventSourceOptions
): {
eventSource: Ref<EventSource | null>
event: Ref<string | null>
data: Ref<string | null>
status: Ref<"OPEN" | "CONNECTING" | "CLOSED">
error: Ref<Event | null>
close: () => void
}
export type UseEventSourceReturn = ReturnType<typeof useEventSource>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventSource/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useEventSource/index.md)
vueuse useIntersectionObserver useIntersectionObserver
=======================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.14 kB |
| Last Changed | 6 months ago |
Detects that a target element's visibility.
Usage
-----
```
<div ref="target">
<h1>Hello world</h1>
</div>
```
```
import { ref } from 'vue'
import { useIntersectionObserver } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
const targetIsVisible = ref(false)
const { stop } = useIntersectionObserver(
target,
([{ isIntersecting }], observerElement) => {
targetIsVisible.value = isIntersecting
},
)
return {
target,
targetIsVisible,
}
},
}
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vIntersectionObserver } from '@vueuse/components'
const root = ref(null)
const isVisible = ref(false)
function onIntersectionObserver([{ isIntersecting }]) {
isVisible.value = isIntersecting
}
</script>
<template>
<div>
<p>
Scroll me down!
</p>
<div v-intersection-observer="onIntersectionObserver">
<p>Hello world!</p>
</div>
</div>
<!-- with options -->
<div ref="root">
<p>
Scroll me down!
</p>
<div v-intersection-observer="[onIntersectionObserver, { root }]">
<p>Hello world!</p>
</div>
</div>
</template>
```
[IntersectionObserver MDN](https://developer.mozilla.org/en-US/docs/Web/API/IntersectionObserver/IntersectionObserver)
Type Declarations
-----------------
```
export interface UseIntersectionObserverOptions extends ConfigurableWindow {
/**
* The Element or Document whose bounds are used as the bounding box when testing for intersection.
*/
root?: MaybeElementRef
/**
* A string which specifies a set of offsets to add to the root's bounding_box when calculating intersections.
*/
rootMargin?: string
/**
* Either a single number or an array of numbers between 0.0 and 1.
*/
threshold?: number | number[]
}
/**
* Detects that a target element's visibility.
*
* @see https://vueuse.org/useIntersectionObserver
* @param target
* @param callback
* @param options
*/
export declare function useIntersectionObserver(
target: MaybeElementRef,
callback: IntersectionObserverCallback,
options?: UseIntersectionObserverOptions
): {
isSupported: Ref<boolean>
stop: () => void
}
export type UseIntersectionObserverReturn = ReturnType<
typeof useIntersectionObserver
>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useIntersectionObserver/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useIntersectionObserver/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useIntersectionObserver/index.md)
vueuse useMediaQuery useMediaQuery
=============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.02 kB |
| Last Changed | 4 months ago |
Reactive [Media Query](https://developer.mozilla.org/en-US/docs/Web/CSS/Media_Queries/Testing_media_queries). Once you've created a MediaQueryList object, you can check the result of the query or receive notifications when the result changes.
Usage
-----
```
import { useMediaQuery } from '@vueuse/core'
const isLargeScreen = useMediaQuery('(min-width: 1024px)')
const isPreferredDark = useMediaQuery('(prefers-color-scheme: dark)')
```
Type Declarations
-----------------
```
/**
* Reactive Media Query.
*
* @see https://vueuse.org/useMediaQuery
* @param query
* @param options
*/
export declare function useMediaQuery(
query: MaybeComputedRef<string>,
options?: ConfigurableWindow
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaQuery/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaQuery/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMediaQuery/index.md)
vueuse useElementVisibility useElementVisibility
====================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.22 kB |
| Last Changed | 4 months ago |
Tracks the visibility of an element within the viewport.
Usage
-----
```
<template>
<div ref="target">
<h1>Hello world</h1>
</div>
</template>
<script>
import { ref } from 'vue'
import { useElementVisibility } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
const targetIsVisible = useElementVisibility(target)
return {
target,
targetIsVisible,
}
}
}
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseElementVisibility v-slot="{ isVisible }">
Is Visible: {{ isVisible }}
</UseElementVisibility>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vElementVisibility } from '@vueuse/components'
const target = ref(null)
const isVisible = ref(false)
function onElementVisibility(state) {
isVisible.value = state
}
</script>
<template>
<div v-element-visibility="onElementVisibility">
{{ isVisible ? 'inside' : 'outside' }}
</div>
<!-- with options -->
<div ref="target">
<div v-element-visibility="[onElementVisibility, { scrollTarget: target }]">
{{ isVisible ? 'inside' : 'outside' }}
</div>
</div>
</template>
```
Type Declarations
-----------------
```
export interface UseElementVisibilityOptions extends ConfigurableWindow {
scrollTarget?: MaybeComputedRef<HTMLElement | undefined | null>
}
/**
* Tracks the visibility of an element within the viewport.
*
* @see https://vueuse.org/useElementVisibility
* @param element
* @param options
*/
export declare function useElementVisibility(
element: MaybeComputedElementRef,
{ window, scrollTarget }?: UseElementVisibilityOptions
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementVisibility/index.md)
vueuse useInfiniteScroll useInfiniteScroll
=================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.96 kB |
| Last Changed | 6 months ago |
Infinite scrolling of the element.
Usage
-----
```
<script setup lang="ts">
import { ref, toRefs } from 'vue'
import { useInfiniteScroll } from '@vueuse/core'
const el = ref<HTMLElement>(null)
const data = ref([1,2,3,4,5,6])
useInfiniteScroll(
el,
() => {
// load more
data.value.push(...moreData)
},
{ distance: 10 }
)
</script>
<template>
<div ref="el">
<div v-for="item in data">
{{ item }}
</div>
</div>
</template>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vInfiniteScroll } from '@vueuse/components'
const data = ref([1, 2, 3, 4, 5, 6])
function onLoadMore() {
const length = data.value.length + 1
data.value.push(...Array.from({ length: 5 }, (_, i) => length + i))
}
</script>
<template>
<div v-infinite-scroll="onLoadMore">
<div v-for="item in data" :key="item">
{{ item }}
</div>
</div>
<!-- with options -->
<div v-infinite-scroll="[onLoadMore, { 'distance' : 10 }]">
<div v-for="item in data" :key="item">
{{ item }}
</div>
</div>
</template>
```
Type Declarations
-----------------
```
export interface UseInfiniteScrollOptions extends UseScrollOptions {
/**
* The minimum distance between the bottom of the element and the bottom of the viewport
*
* @default 0
*/
distance?: number
/**
* The direction in which to listen the scroll.
*
* @default 'bottom'
*/
direction?: "top" | "bottom" | "left" | "right"
/**
* Whether to preserve the current scroll position when loading more items.
*
* @default false
*/
preserveScrollPosition?: boolean
}
/**
* Reactive infinite scroll.
*
* @see https://vueuse.org/useInfiniteScroll
*/
export declare function useInfiniteScroll(
element: MaybeComputedRef<
HTMLElement | SVGElement | Window | Document | null | undefined
>,
onLoadMore: (
state: UnwrapNestedRefs<ReturnType<typeof useScroll>>
) => void | Promise<void>,
options?: UseInfiniteScrollOptions
): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useInfiniteScroll/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useInfiniteScroll/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useInfiniteScroll/index.md)
vueuse useCached useCached
=========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 792 B |
| Last Changed | last year |
Cache a ref with a custom comparator.
Usage
-----
```
import { useCached } from '@vueuse/core'
interface Data {
value: number
extra: number
}
const source = ref<Data>({ value: 42, extra: 0 })
const cached = useCached(source, (a, b) => a.value === b.value)
source.value = {
value: 42,
extra: 1,
}
console.log(cached.value) // { value: 42, extra: 0 }
source.value = {
value: 43,
extra: 1,
}
console.log(cached.value) // { value: 43, extra: 1 }
```
Type Declarations
-----------------
```
export declare function useCached<T>(
refValue: Ref<T>,
comparator?: (a: T, b: T) => boolean,
watchOptions?: WatchOptions
): Ref<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useCached/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useCached/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useCached/index.md)
vueuse usePreferredContrast usePreferredContrast
====================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | |
| Last Changed | 5 months ago |
Reactive [prefers-contrast](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-contrast) media query.
Usage
-----
```
import { usePreferredContrast } from '@vueuse/core'
const preferredContrast = usePreferredContrast()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePreferredContrast v-slot="{ contrast }">
Preferred Color Scheme: {{ contrast }}
<UsePreferredContrast>
```
Type Declarations
-----------------
```
export type ContrastType = "more" | "less" | "custom" | "no-preference"
/**
* Reactive prefers-contrast media query.
*
* @see https://vueuse.org/usePreferredContrast
* @param [options]
*/
export declare function usePreferredContrast(
options?: ConfigurableWindow
): ComputedRef<ContrastType>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredContrast/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredContrast/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredContrast/index.md)
| programming_docs |
vueuse useWebNotification useWebNotification
==================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.48 kB |
| Last Changed | 6 months ago |
Reactive [Notification](https://developer.mozilla.org/en-US/docs/Web/API/notification)
The Web Notification interface of the Notifications API is used to configure and display desktop notifications to the user.
Usage
-----
```
const {
isSupported,
notification,
show,
close,
onClick,
onShow,
onError,
onClose,
} = useWebNotification({
title: 'Hello, VueUse world!',
dir: 'auto',
lang: 'en',
renotify: true,
tag: 'test',
})
if (isSupported.value)
show()
```
This composable also utilizes the createEventHook utility from '@vueuse/shared`:
```
onClick((evt: Event) => {
// Do something with the notification on:click event...
})
onShow((evt: Event) => {
// Do something with the notification on:show event...
})
onError((evt: Event) => {
// Do something with the notification on:error event...
})
onClose((evt: Event) => {
// Do something with the notification on:close event...
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface WebNotificationOptions {
/**
* The title read-only property of the Notification interface indicates
* the title of the notification
*
* @default ''
*/
title?: string
/**
* The body string of the notification as specified in the constructor's
* options parameter.
*
* @default ''
*/
body?: string
/**
* The text direction of the notification as specified in the constructor's
* options parameter.
*
* @default ''
*/
dir?: "auto" | "ltr" | "rtl"
/**
* The language code of the notification as specified in the constructor's
* options parameter.
*
* @default DOMString
*/
lang?: string
/**
* The ID of the notification(if any) as specified in the constructor's options
* parameter.
*
* @default ''
*/
tag?: string
/**
* The URL of the image used as an icon of the notification as specified
* in the constructor's options parameter.
*
* @default ''
*/
icon?: string
/**
* Specifies whether the user should be notified after a new notification
* replaces an old one.
*
* @default false
*/
renotify?: boolean
/**
* A boolean value indicating that a notification should remain active until the
* user clicks or dismisses it, rather than closing automatically.
*
* @default false
*/
requireInteraction?: boolean
/**
* The silent read-only property of the Notification interface specifies
* whether the notification should be silent, i.e., no sounds or vibrations
* should be issued, regardless of the device settings.
*
* @default false
*/
silent?: boolean
/**
* Specifies a vibration pattern for devices with vibration hardware to emit.
* A vibration pattern, as specified in the Vibration API spec
*
* @see https://w3c.github.io/vibration/
*/
vibrate?: number[]
}
export interface UseWebNotificationOptions
extends WebNotificationOptions,
ConfigurableWindow {}
/**
* Reactive useWebNotification
*
* @see https://vueuse.org/useWebNotification
* @see https://developer.mozilla.org/en-US/docs/Web/API/notification
* @param title
* @param defaultOptions of type WebNotificationOptions
* @param methods of type WebNotificationMethods
*/
export declare const useWebNotification: (
defaultOptions?: UseWebNotificationOptions
) => {
isSupported: Ref<boolean>
notification: Ref<Notification | null>
show: (
overrides?: WebNotificationOptions
) => Promise<Notification | undefined>
close: () => void
onClick: EventHook<any>
onShow: EventHook<any>
onError: EventHook<any>
onClose: EventHook<any>
}
export type UseWebNotificationReturn = ReturnType<typeof useWebNotification>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebNotification/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebNotification/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebNotification/index.md)
vueuse useBattery useBattery
==========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.27 kB |
| Last Changed | 6 months ago |
Reactive [Battery Status API](https://developer.mozilla.org/en-US/docs/Web/API/Battery_Status_API), more often referred to as the Battery API, provides information about the system's battery charge level and lets you be notified by events that are sent when the battery level or charging status change. This can be used to adjust your app's resource usage to reduce battery drain when the battery is low, or to save changes before the battery runs out in order to prevent data loss.
Usage
-----
```
import { useBattery } from '@vueuse/core'
const { charging, chargingTime, dischargingTime, level } = useBattery()
```
| State | Type | Description |
| --- | --- | --- |
| charging | `Boolean` | If the device is currently charging. |
| chargingTime | `Number` | The number of seconds until the device becomes fully charged. |
| dischargingTime | `Number` | The number of seconds before the device becomes fully discharged. |
| level | `Number` | A number between 0 and 1 representing the current charge level. |
Use-cases
---------
Our applications normally are not empathetic to battery level, we can make a few adjustments to our applications that will be more friendly to low battery users.
* Trigger a special "dark-mode" battery saver theme settings.
* Stop auto playing videos in news feeds.
* Disable some background workers that are not critical.
* Limit network calls and reduce CPU/Memory consumption.
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseBattery v-slot="{ charging }">
Is Charging: {{ charging }}
</UseBattery>
```
Type Declarations
-----------------
```
export interface BatteryManager extends EventTarget {
charging: boolean
chargingTime: number
dischargingTime: number
level: number
}
/**
* Reactive Battery Status API.
*
* @see https://vueuse.org/useBattery
* @param options
*/
export declare function useBattery({ navigator }?: ConfigurableNavigator): {
isSupported: Ref<boolean>
charging: Ref<boolean>
chargingTime: Ref<number>
dischargingTime: Ref<number>
level: Ref<number>
}
export type UseBatteryReturn = ReturnType<typeof useBattery>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useBattery/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useBattery/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useBattery/index.md)
vueuse usePointer usePointer
==========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.55 kB |
| Last Changed | 6 months ago |
Reactive [pointer state](https://developer.mozilla.org/en-US/docs/Web/API/Pointer_events).
Basic Usage
-----------
```
import { usePointer } from '@vueuse/core'
const { x, y, pressure, pointerType } = usePointer()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
By default, the component will track the pointer on `window`
```
<UsePointer v-slot="{ x, y }">
x: {{ x }}
y: {{ y }}
</UsePointer>
```
To track local position in the element, set `target="self"`:
```
<UsePointer target="self" v-slot="{ x, y }">
x: {{ x }}
y: {{ y }}
</UsePointer>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UsePointerState extends Position {
pressure: number
pointerId: number
tiltX: number
tiltY: number
width: number
height: number
twist: number
pointerType: PointerType | null
}
export interface UsePointerOptions extends ConfigurableWindow {
/**
* Pointer types that listen to.
*
* @default ['mouse', 'touch', 'pen']
*/
pointerTypes?: PointerType[]
/**
* Initial values
*/
initialValue?: MaybeRef<Partial<UsePointerState>>
/**
* @default window
*/
target?: MaybeRef<EventTarget | null | undefined> | Document | Window
}
/**
* Reactive pointer state.
*
* @see https://vueuse.org/usePointer
* @param options
*/
export declare function usePointer(options?: UsePointerOptions): {
isInside: Ref<boolean>
pressure: Ref<number>
pointerId: Ref<number>
tiltX: Ref<number>
tiltY: Ref<number>
width: Ref<number>
height: Ref<number>
twist: Ref<number>
pointerType: Ref<PointerType | null>
x: Ref<number>
y: Ref<number>
}
export type UsePointerReturn = ReturnType<typeof usePointer>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointer/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointer/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointer/index.md)
vueuse useElementBounding useElementBounding
==================
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.54 kB |
| Last Changed | last week |
Reactive [bounding box](https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect) of an HTML element
Usage
-----
```
<template>
<div ref="el" />
</template>
<script>
import { ref } from 'vue'
import { useElementBounding } from '@vueuse/core'
export default {
setup() {
const el = ref(null)
const { x, y, top, right, bottom, left, width, height } = useElementBounding(el)
return {
el,
/* ... */
}
}
}
</script>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseElementBounding v-slot="{ width, height }">
Width: {{ width }}
Height: {{ height }}
</UseElementBounding>
```
Type Declarations
-----------------
```
export interface UseElementBoundingOptions {
/**
* Reset values to 0 on component unmounted
*
* @default true
*/
reset?: boolean
/**
* Listen to window resize event
*
* @default true
*/
windowResize?: boolean
/**
* Listen to window scroll event
*
* @default true
*/
windowScroll?: boolean
/**
* Immediately call update on component mounted
*
* @default true
*/
immediate?: boolean
}
/**
* Reactive bounding box of an HTML element.
*
* @see https://vueuse.org/useElementBounding
* @param target
*/
export declare function useElementBounding(
target: MaybeComputedElementRef,
options?: UseElementBoundingOptions
): {
height: Ref<number>
bottom: Ref<number>
left: Ref<number>
right: Ref<number>
top: Ref<number>
width: Ref<number>
x: Ref<number>
y: Ref<number>
update: () => void
}
export type UseElementBoundingReturn = ReturnType<typeof useElementBounding>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementBounding/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementBounding/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementBounding/index.md)
vueuse useAsyncQueue useAsyncQueue
=============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1.02 kB |
| Last Changed | last year |
Executes each asynchronous task sequentially and passes the current task result to the next task
Usage
-----
```
import { useAsyncQueue } from '@vueuse/core'
const p1 = () => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(1000)
}, 10)
})
}
const p2 = (result: number) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(1000 + result)
}, 20)
})
}
const { activeIndex, result } = useAsyncQueue([p1, p2])
console.log(activeIndex.value) // current pending task index
console.log(result) // the tasks result
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseAsyncQueueTask<T> = (...args: any[]) => T | Promise<T>
export interface UseAsyncQueueResult<T> {
state: "pending" | "fulfilled" | "rejected"
data: T | null
}
export interface UseAsyncQueueReturn<T> {
activeIndex: Ref<number>
result: T
}
export interface UseAsyncQueueOptions {
/**
* Interrupt tasks when current task fails.
*
* @default true
*/
interrupt?: boolean
/**
* Trigger it when the tasks fails.
*
*/
onError?: () => void
/**
* Trigger it when the tasks ends.
*
*/
onFinished?: () => void
}
/**
* Asynchronous queue task controller.
*
* @see https://vueuse.org/useAsyncQueue
* @param tasks
* @param options
*/
export declare function useAsyncQueue<T1>(
tasks: [UseAsyncQueueTask<T1>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<[UseAsyncQueueResult<T1>]>
export declare function useAsyncQueue<T1, T2>(
tasks: [UseAsyncQueueTask<T1>, UseAsyncQueueTask<T2>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<[UseAsyncQueueResult<T1>, UseAsyncQueueResult<T2>]>
export declare function useAsyncQueue<T1, T2, T3>(
tasks: [UseAsyncQueueTask<T1>, UseAsyncQueueTask<T2>, UseAsyncQueueTask<T3>],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[UseAsyncQueueResult<T1>, UseAsyncQueueResult<T2>, UseAsyncQueueResult<T3>]
>
export declare function useAsyncQueue<T1, T2, T3, T4>(
tasks: [
UseAsyncQueueTask<T1>,
UseAsyncQueueTask<T2>,
UseAsyncQueueTask<T3>,
UseAsyncQueueTask<T4>
],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[
UseAsyncQueueResult<T1>,
UseAsyncQueueResult<T2>,
UseAsyncQueueResult<T3>,
UseAsyncQueueResult<T4>
]
>
export declare function useAsyncQueue<T1, T2, T3, T4, T5>(
tasks: [
UseAsyncQueueTask<T1>,
UseAsyncQueueTask<T2>,
UseAsyncQueueTask<T3>,
UseAsyncQueueTask<T4>,
UseAsyncQueueTask<T5>
],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<
[
UseAsyncQueueResult<T1>,
UseAsyncQueueResult<T2>,
UseAsyncQueueResult<T3>,
UseAsyncQueueResult<T4>,
UseAsyncQueueResult<T5>
]
>
export declare function useAsyncQueue<T>(
tasks: UseAsyncQueueTask<T>[],
options?: UseAsyncQueueOptions
): UseAsyncQueueReturn<UseAsyncQueueResult<T>[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useAsyncQueue/index.md)
vueuse useSpeechSynthesis useSpeechSynthesis
==================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.28 kB |
| Last Changed | 6 months ago |
Reactive [SpeechSynthesis](https://developer.mozilla.org/en-US/docs/Web/API/SpeechSynthesis).
> [Can I use?](https://caniuse.com/mdn-api_speechsynthesis)
>
>
Usage
-----
```
import { useSpeechSynthesis } from '@vueuse/core'
const {
isSupported,
isPlaying,
status,
voiceInfo,
utterance,
error,
toggle,
speak,
} = useSpeechSynthesis()
```
### Options
The following shows the default values of the options, they will be directly passed to [SpeechSynthesis API](https://developer.mozilla.org/en-US/docs/Web/API/SpeechSynthesis).
```
useSpeechSynthesis({
lang: 'en-US',
pitch: 1,
rate: 1,
volume: 1,
})
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseSpeechSynthesisStatus = "init" | "play" | "pause" | "end"
export interface UseSpeechSynthesisOptions extends ConfigurableWindow {
/**
* Language for SpeechSynthesis
*
* @default 'en-US'
*/
lang?: MaybeComputedRef<string>
/**
* Gets and sets the pitch at which the utterance will be spoken at.
*
* @default 1
*/
pitch?: SpeechSynthesisUtterance["pitch"]
/**
* Gets and sets the speed at which the utterance will be spoken at.
*
* @default 1
*/
rate?: SpeechSynthesisUtterance["rate"]
/**
* Gets and sets the voice that will be used to speak the utterance.
*/
voice?: MaybeRef<SpeechSynthesisVoice>
/**
* Gets and sets the volume that the utterance will be spoken at.
*
* @default 1
*/
volume?: SpeechSynthesisUtterance["volume"]
}
/**
* Reactive SpeechSynthesis.
*
* @see https://vueuse.org/useSpeechSynthesis
* @see https://developer.mozilla.org/en-US/docs/Web/API/SpeechSynthesis SpeechSynthesis
* @param options
*/
export declare function useSpeechSynthesis(
text: MaybeComputedRef<string>,
options?: UseSpeechSynthesisOptions
): {
isSupported: Ref<boolean>
isPlaying: Ref<boolean>
status: Ref<UseSpeechSynthesisStatus>
utterance: ComputedRef<SpeechSynthesisUtterance>
error: Ref<SpeechSynthesisErrorEvent | undefined>
toggle: (value?: boolean) => void
speak: () => void
}
export type UseSpeechSynthesisReturn = ReturnType<typeof useSpeechSynthesis>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechSynthesis/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechSynthesis/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechSynthesis/index.md)
vueuse useMagicKeys useMagicKeys
============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.57 kB |
| Last Changed | 2 days ago |
Reactive keys pressed state, with magical keys combination support.
**This function uses [Proxy](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy)** It is **NOT** supported by [IE 11 or below](https://caniuse.com/proxy).
Usage
-----
```
import { useMagicKeys } from '@vueuse/core'
const { shift, space, a /* keys you want to monitor */ } = useMagicKeys()
watch(space, (v) => {
if (v)
console.log('space has been pressed')
})
watchEffect(() => {
if (shift.value && a.value)
console.log('Shift + A have been pressed')
})
```
Check out [all the possible keycodes](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/code/code_values).
### Combinations
You can magically use combinations (shortcuts/hotkeys) by connecting keys with `+` or `_`.
```
import { useMagicKeys } from '@vueuse/core'
const keys = useMagicKeys()
const shiftCtrlA = keys['Shift+Ctrl+A']
watch(shiftCtrlA, (v) => {
if (v)
console.log('Shift + Ctrl + A have been pressed')
})
```
```
import { useMagicKeys } from '@vueuse/core'
const { Ctrl_A_B, space, alt_s /* ... */ } = useMagicKeys()
watch(Ctrl_A_B, (v) => {
if (v)
console.log('Control+A+B have been pressed')
})
```
You can also use `whenever` function to make it shorter
```
import { useMagicKeys, whenever } from '@vueuse/core'
const keys = useMagicKeys()
whenever(keys.shift_space, () => {
console.log('Shift+Space have been pressed')
})
```
### Current Pressed keys
A special property `current` is provided to representing all the keys been pressed currently.
```
import { useMagicKeys } from '@vueuse/core'
const { current } = useMagicKeys()
console.log(current) // Set { 'control', 'a' }
whenever(
() => current.has('a') && !current.has('b'),
() => console.log('A is pressed but not B'),
)
```
### Key Aliasing
```
import { useMagicKeys, whenever } from '@vueuse/core'
const { shift_cool } = useMagicKeys({
aliasMap: {
cool: 'space',
},
})
whenever(shift_cool, () => console.log('Shift + Space have been pressed'))
```
By default, we have some [preconfigured alias for common practices](https://github.com/vueuse/vueuse/blob/main/packages/core/useMagicKeys/aliasMap.ts).
### Conditionally Disable
You might have some `<input />` elements in your apps, and you don't want to trigger the magic keys handling when users focused on those inputs. There is an example of using [`useActiveElement`](../useactiveelement/index)and `and` to do that.
```
import { and, useActiveElement, useMagicKeys, whenever } from '@vueuse/core'
const activeElement = useActiveElement()
const notUsingInput = computed(() =>
activeElement.value?.tagName !== 'INPUT'
&& activeElement.value?.tagName !== 'TEXTAREA',
)
const { tab } = useMagicKeys()
whenever(and(tab, notUsingInput), () => {
console.log('Tab has been pressed outside of inputs!')
})
```
### Custom Event Handler
```
import { useMagicKeys, whenever } from '@vueuse/core'
const { ctrl_s } = useMagicKeys({
passive: false,
onEventFired(e) {
if (e.ctrlKey && e.key === 's' && e.type === 'keydown')
e.preventDefault()
},
})
whenever(ctrl_s, () => console.log('Ctrl+S have been pressed'))
```
> ⚠️ This usage is NOT recommended, please use with caution.
>
>
### Reactive Mode
By default, the values of `useMagicKeys()` are `Ref<boolean>`. If you want to use the object in the template, you can set it to reactive mode.
```
const keys = useMagicKeys({ reactive: true })
```
```
<template>
<div v-if="keys.shift">
You are holding the Shift key!
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseMagicKeysOptions<Reactive extends Boolean> {
/**
* Returns a reactive object instead of an object of refs
*
* @default false
*/
reactive?: Reactive
/**
* Target for listening events
*
* @default window
*/
target?: MaybeComputedRef<EventTarget>
/**
* Alias map for keys, all the keys should be lowercase
* { target: keycode }
*
* @example { ctrl: "control" }
* @default <predefined-map>
*/
aliasMap?: Record<string, string>
/**
* Register passive listener
*
* @default true
*/
passive?: boolean
/**
* Custom event handler for keydown/keyup event.
* Useful when you want to apply custom logic.
*
* When using `e.preventDefault()`, you will need to pass `passive: false` to useMagicKeys().
*/
onEventFired?: (e: KeyboardEvent) => void | boolean
}
export interface MagicKeysInternal {
/**
* A Set of currently pressed keys,
* Stores raw keyCodes.
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/key
*/
current: Set<string>
}
export type UseMagicKeysReturn<Reactive extends Boolean> = Readonly<
Omit<
Reactive extends true
? Record<string, boolean>
: Record<string, ComputedRef<boolean>>,
keyof MagicKeysInternal
> &
MagicKeysInternal
>
/**
* Reactive keys pressed state, with magical keys combination support.
*
* @see https://vueuse.org/useMagicKeys
*/
export declare function useMagicKeys(
options?: UseMagicKeysOptions<false>
): UseMagicKeysReturn<false>
export declare function useMagicKeys(
options: UseMagicKeysOptions<true>
): UseMagicKeysReturn<true>
export { DefaultMagicKeysAliasMap } from "./aliasMap"
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMagicKeys/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMagicKeys/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMagicKeys/index.md)
| programming_docs |
vueuse usePointerSwipe usePointerSwipe
===============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.52 kB |
| Last Changed | 2 months ago |
Reactive swipe detection based on [PointerEvents](https://developer.mozilla.org/en-US/docs/Web/API/PointerEvent).
Usage
-----
```
<script setup>
import { ref } from 'vue'
import { usePointerSwipe } from '@vueuse/core'
const el = ref(null)
const { isSwiping, direction } = usePointerSwipe(el)
</script>
<template>
<div ref="el">
Swipe here
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UsePointerSwipeOptions {
/**
* @default 50
*/
threshold?: number
/**
* Callback on swipe start.
*/
onSwipeStart?: (e: PointerEvent) => void
/**
* Callback on swipe move.
*/
onSwipe?: (e: PointerEvent) => void
/**
* Callback on swipe end.
*/
onSwipeEnd?: (e: PointerEvent, direction: SwipeDirection) => void
/**
* Pointer types to listen to.
*
* @default ['mouse', 'touch', 'pen']
*/
pointerTypes?: PointerType[]
}
export interface UsePointerSwipeReturn {
readonly isSwiping: Ref<boolean>
direction: Readonly<Ref<SwipeDirection | null>>
readonly posStart: Position
readonly posEnd: Position
distanceX: Readonly<Ref<number>>
distanceY: Readonly<Ref<number>>
stop: () => void
}
/**
* Reactive swipe detection based on PointerEvents.
*
* @see https://vueuse.org/usePointerSwipe
* @param target
* @param options
*/
export declare function usePointerSwipe(
target: MaybeComputedRef<HTMLElement | null | undefined>,
options?: UsePointerSwipeOptions
): UsePointerSwipeReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointerSwipe/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointerSwipe/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePointerSwipe/index.md)
vueuse useDeviceOrientation useDeviceOrientation
====================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.2 kB |
| Last Changed | 6 months ago |
Reactive [DeviceOrientationEvent](https://developer.mozilla.org/en-US/docs/Web/API/DeviceOrientationEvent). Provide web developers with information from the physical orientation of the device running the web page.
Usage
-----
```
import { useDeviceOrientation } from '@vueuse/core'
const {
isAbsolute,
alpha,
beta,
gamma,
} = useDeviceOrientation()
```
| State | Type | Description |
| --- | --- | --- |
| isAbsolute | `boolean` | A boolean that indicates whether or not the device is providing orientation data absolutely. |
| alpha | `number` | A number representing the motion of the device around the z axis, express in degrees with values ranging from 0 to 360. |
| beta | `number` | A number representing the motion of the device around the x axis, express in degrees with values ranging from -180 to 180. |
| gamma | `number` | A number representing the motion of the device around the y axis, express in degrees with values ranging from -90 to 90. |
You can find [more information about the state on the MDN](https://developer.mozilla.org/en-US/docs/Web/API/DeviceOrientationEvent#Properties).
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDeviceOrientation v-slot="{ alpha, beta, gamma }">
Alpha: {{ alpha }}
Beta: {{ beta }}
Gamma: {{ gamma }}
</UseDeviceOrientation>
```
Type Declarations
-----------------
```
/**
* Reactive DeviceOrientationEvent.
*
* @see https://vueuse.org/useDeviceOrientation
* @param options
*/
export declare function useDeviceOrientation(options?: ConfigurableWindow): {
isSupported: Ref<boolean>
isAbsolute: Ref<boolean>
alpha: Ref<number | null>
beta: Ref<number | null>
gamma: Ref<number | null>
}
export type UseDeviceOrientationReturn = ReturnType<typeof useDeviceOrientation>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceOrientation/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceOrientation/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDeviceOrientation/index.md)
vueuse useFps useFps
======
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.04 kB |
| Last Changed | 11 months ago |
Reactive FPS (frames per second).
Usage
-----
```
import { useFps } from '@vueuse/core'
const fps = useFps()
```
Type Declarations
-----------------
```
export interface UseFpsOptions {
/**
* Calculate the FPS on every x frames.
* @default 10
*/
every?: number
}
export declare function useFps(options?: UseFpsOptions): Ref<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFps/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFps/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFps/index.md)
vueuse useTitle useTitle
========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.41 kB |
| Last Changed | 3 months ago |
Reactive document title.
**TIP**When using with Nuxt 3, this function will **NOT** be auto imported in favor of Nuxt's built-in `useTitle()`. Use explicit import if you want to use the function from VueUse.
Usage
-----
```
import { useTitle } from '@vueuse/core'
const title = useTitle()
console.log(title.value) // print current title
title.value = 'Hello' // change current title
```
Set initial title immediately:
```
const title = useTitle('New Title')
```
Pass a `ref` and the title will be updated when the source ref changes:
```
import { useTitle } from '@vueuse/core'
const messages = ref(0)
const title = computed(() => {
return !messages.value ? 'No message' : `${messages.value} new messages`
})
useTitle(title) // document title will match with the ref "title"
```
Pass an optional template tag [Vue Meta Title Template](https://vue-meta.nuxtjs.org/guide/metainfo.html) to update the title to be injected into this template:
```
const title = useTitle('New Title', { titleTemplate: '%s | My Awesome Website' })
```
**WARNING**`observe` is incompatible with `titleTemplate`.
Type Declarations
-----------------
```
export type UseTitleOptionsBase =
| {
/**
* Observe `document.title` changes using MutationObserve
* Cannot be used together with `titleTemplate` option.
*
* @default false
*/
observe?: boolean
}
| {
/**
* The template string to parse the title (e.g., '%s | My Website')
* Cannot be used together with `observe` option.
*
* @default '%s'
*/
titleTemplate?: MaybeRef<string> | ((title: string) => string)
}
export type UseTitleOptions = ConfigurableDocument & UseTitleOptionsBase
export declare function useTitle(
newTitle: MaybeReadonlyRef<string | null | undefined>,
options?: UseTitleOptions
): ComputedRef<string | null | undefined>
export declare function useTitle(
newTitle?: MaybeRef<string | null | undefined>,
options?: UseTitleOptions
): Ref<string | null | undefined>
export type UseTitleReturn = ReturnType<typeof useTitle>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTitle/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTitle/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTitle/index.md)
vueuse useDisplayMedia useDisplayMedia
===============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.1 kB |
| Last Changed | 2 days ago |
| Related | [`useUserMedia`](../useusermedia/index) |
Reactive [`mediaDevices.getDisplayMedia`](https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/getDisplayMedia) streaming.
Usage
-----
```
import { useDisplayMedia } from '@vueuse/core'
const { stream, start } = useDisplayMedia()
// start streaming
start()
```
```
const video = document.getElementById('video')
watchEffect(() => {
// preview on a video element
video.srcObject = stream.value
})
```
Type Declarations
-----------------
```
export interface UseDisplayMediaOptions extends ConfigurableNavigator {
/**
* If the stream is enabled
* @default false
*/
enabled?: MaybeRef<boolean>
/**
* If the stream video media constraints
*/
video?: boolean | MediaTrackConstraints | undefined
/**
* If the stream audio media constraints
*/
audio?: boolean | MediaTrackConstraints | undefined
}
/**
* Reactive `mediaDevices.getDisplayMedia` streaming
*
* @see https://vueuse.org/useDisplayMedia
* @param options
*/
export declare function useDisplayMedia(options?: UseDisplayMediaOptions): {
isSupported: Ref<boolean>
stream: Ref<MediaStream | undefined>
start: () => Promise<MediaStream | undefined>
stop: () => void
enabled: Ref<boolean>
}
export type UseDisplayMediaReturn = ReturnType<typeof useDisplayMedia>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDisplayMedia/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDisplayMedia/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDisplayMedia/index.md)
vueuse useScreenSafeArea useScreenSafeArea
=================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.57 kB |
| Last Changed | last year |
Reactive `env(safe-area-inset-*)`
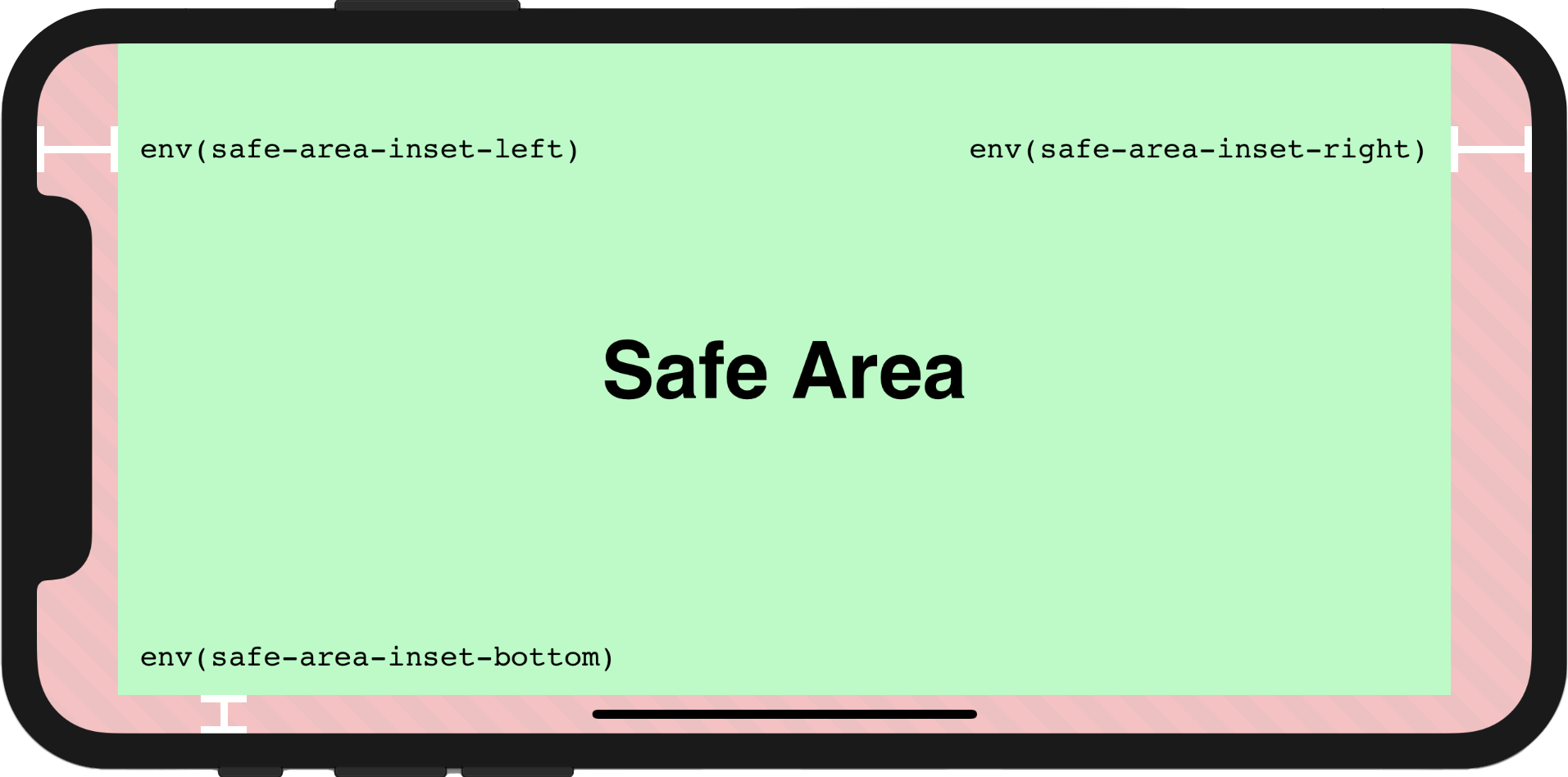
Usage
-----
In order to make the page to be fully rendered in the screen, the additional attribute `viewport-fit=cover` within `viewport` meta tag must be set firstly, the viewport meta tag may look like this:
```
<meta name='viewport' content='initial-scale=1, viewport-fit=cover'>
```
Then we could use [`useScreenSafeArea`](index)in the component as shown below:
```
import { useScreenSafeArea } from '@vueuse/core'
const {
top,
right,
bottom,
left,
} = useScreenSafeArea()
```
For further details, you may refer to this documentation: [Designing Websites for iPhone X](https://webkit.org/blog/7929/designing-websites-for-iphone-x/)
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseScreenSafeArea top right bottom left>content</UseScreenSafeArea>
```
Type Declarations
-----------------
```
/**
* Reactive `env(safe-area-inset-*)`
*
* @see https://vueuse.org/useScreenSafeArea
*/
export declare function useScreenSafeArea(): {
top: Ref<string>
right: Ref<string>
bottom: Ref<string>
left: Ref<string>
update: () => void
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScreenSafeArea/index.md)
vueuse useRafFn useRafFn
========
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 928 B |
| Last Changed | 3 weeks ago |
Call function on every `requestAnimationFrame`. With controls of pausing and resuming.
Usage
-----
```
import { ref } from 'vue'
import { useRafFn } from '@vueuse/core'
const count = ref(0)
const { pause, resume } = useRafFn(() => {
count.value++
console.log(count.value)
})
```
Type Declarations
-----------------
```
export interface UseRafFnCallbackArguments {
/**
* Time elapsed between this and the last frame.
*/
delta: number
/**
* Time elapsed since the creation of the web page. See {@link https://developer.mozilla.org/en-US/docs/Web/API/DOMHighResTimeStamp#the_time_origin Time origin}.
*/
timestamp: DOMHighResTimeStamp
}
export interface UseRafFnOptions extends ConfigurableWindow {
/**
* Start the requestAnimationFrame loop immediately on creation
*
* @default true
*/
immediate?: boolean
}
/**
* Call function on every `requestAnimationFrame`. With controls of pausing and resuming.
*
* @see https://vueuse.org/useRafFn
* @param fn
* @param options
*/
export declare function useRafFn(
fn: (args: UseRafFnCallbackArguments) => void,
options?: UseRafFnOptions
): Pausable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useRafFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useRafFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useRafFn/index.md)
vueuse useEyeDropper useEyeDropper
=============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 940 B |
| Last Changed | 6 months ago |
Reactive [EyeDropper API](https://developer.mozilla.org/en-US/docs/Web/API/EyeDropper_API)
Usage
-----
```
import { useEyeDropper } from '@vueuse/core'
const { isSupported, open, sRGBHex } = useEyeDropper()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseEyeDropper v-slot="{ isSupported, sRGBHex, open }">
<button :disabled="!isSupported" @click="open">
sRGBHex: {{ sRGBHex }}
</button>
</UseEyeDropper>
```
Type Declarations
-----------------
```
export interface EyeDropperOpenOptions {
/**
* @see https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal
*/
signal?: AbortSignal
}
export interface EyeDropper {
new (): EyeDropper
open: (options?: EyeDropperOpenOptions) => Promise<{
sRGBHex: string
}>
[Symbol.toStringTag]: "EyeDropper"
}
export interface UseEyeDropperOptions {
/**
* Initial sRGBHex.
*
* @default ''
*/
initialValue?: string
}
/**
* Reactive [EyeDropper API](https://developer.mozilla.org/en-US/docs/Web/API/EyeDropper_API)
*
* @see https://vueuse.org/useEyeDropper
* @param initialValue string
*/
export declare function useEyeDropper(options?: UseEyeDropperOptions): {
isSupported: Ref<boolean>
sRGBHex: Ref<string>
open: (openOptions?: EyeDropperOpenOptions) => Promise<
| {
sRGBHex: string
}
| undefined
>
}
export type UseEyeDropperReturn = ReturnType<typeof useEyeDropper>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useEyeDropper/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useEyeDropper/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useEyeDropper/index.md)
vueuse useDropZone useDropZone
===========
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.16 kB |
| Last Changed | 6 months ago |
Create an zone where files can be dropped.
Usage
-----
```
<script setup lang="ts">
import { useDropZone } from '@vueuse/core'
const dropZoneRef = ref<HTMLDivElement>()
function onDrop(files: File[] | null) {
// called when files are dropped on zone
}
const { isOverDropZone } = useDropZone(dropZoneRef, onDrop)
</script>
<template>
<div ref="dropZoneRef">
Drop files here
</div>
</template>
```
Type Declarations
-----------------
```
export interface UseDropZoneReturn {
isOverDropZone: Ref<boolean>
}
export declare function useDropZone(
target: MaybeComputedRef<HTMLElement | null | undefined>,
onDrop?: (files: File[] | null) => void
): UseDropZoneReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDropZone/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDropZone/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDropZone/index.md)
vueuse useFullscreen useFullscreen
=============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.48 kB |
| Last Changed | 6 months ago |
Reactive [Fullscreen API](https://developer.mozilla.org/en-US/docs/Web/API/Fullscreen_API). It adds methods to present a specific Element (and its descendants) in full-screen mode, and to exit full-screen mode once it is no longer needed. This makes it possible to present desired content—such as an online game—using the user's entire screen, removing all browser user interface elements and other applications from the screen until full-screen mode is shut off.
Usage
-----
```
import { useFullscreen } from '@vueuse/core'
const { isFullscreen, enter, exit, toggle } = useFullscreen()
```
Fullscreen specified element
```
const el = ref<HTMLElement | null>(null)
const { isFullscreen, enter, exit, toggle } = useFullscreen(el)
```
```
<video ref='el'>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseFullscreen v-slot="{ toggle }">
<video />
<button @click="toggle">
Go Fullscreen
</button>
</UseFullscreen>
```
Type Declarations
-----------------
```
export interface UseFullscreenOptions extends ConfigurableDocument {
/**
* Automatically exit fullscreen when component is unmounted
*
* @default false
*/
autoExit?: boolean
}
/**
* Reactive Fullscreen API.
*
* @see https://vueuse.org/useFullscreen
* @param target
* @param options
*/
export declare function useFullscreen(
target?: MaybeElementRef,
options?: UseFullscreenOptions
): {
isSupported: Ref<boolean>
isFullscreen: Ref<boolean>
enter: () => Promise<void>
exit: () => Promise<void>
toggle: () => Promise<void>
}
export type UseFullscreenReturn = ReturnType<typeof useFullscreen>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFullscreen/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFullscreen/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFullscreen/index.md)
vueuse computedAsync computedAsync
=============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 960 B |
| Last Changed | 2 hours ago |
| Alias | `asyncComputed` |
Computed for async functions
Usage
-----
```
import { ref } from 'vue'
import { computedAsync } from '@vueuse/core'
const name = ref('jack')
const userInfo = computedAsync(
async () => {
return await mockLookUp(name.value)
},
null, // initial state
)
```
### Evaluation State
You will need to pass a ref to track if the async function is evaluating.
```
import { ref } from 'vue'
import { computedAsync } from '@vueuse/core'
const evaluating = ref(false)
const userInfo = computedAsync(
async () => { /* your logic */ },
null,
evaluating,
)
```
### onCancel
When the computed source changed before the previous async function gets resolved, you may want to cancel the previous one. Here is an example showing how to incorporate with the fetch API.
```
const packageName = ref('@vueuse/core')
const downloads = computedAsync(async (onCancel) => {
const abortController = new AbortController()
onCancel(() => abortController.abort())
return await fetch(
`https://api.npmjs.org/downloads/point/last-week/${packageName.value}`,
{ signal: abortController.signal },
)
.then(response => response.ok ? response.json() : { downloads: '—' })
.then(result => result.downloads)
}, 0)
```
### Lazy
By default, `computedAsync` will start resolving immediately on creation, specify `lazy: true` to make it start resolving on the first accessing.
```
import { ref } from 'vue'
import { computedAsync } from '@vueuse/core'
const evaluating = ref(false)
const userInfo = computedAsync(
async () => { /* your logic */ },
null,
{ lazy: true, evaluating },
)
```
Caveats
-------
* Just like Vue's built-in `computed` function, `computedAsync` does dependency tracking and is automatically re-evaluated when dependencies change. Note however that only dependency referenced in the first call stack are considered for this. In other words: **Dependencies that are accessed asynchronously will not trigger re-evaluation of the async computed value.**
* As opposed to Vue's built-in `computed` function, re-evaluation of the async computed value is triggered whenever dependencies are changing, regardless of whether its result is currently being tracked or not.
* The default value of the `shallow` will be changed to `true` in the next major version.
Type Declarations
-----------------
Show Type Declarations
```
/**
* Handle overlapping async evaluations.
*
* @param cancelCallback The provided callback is invoked when a re-evaluation of the computed value is triggered before the previous one finished
*/
export type AsyncComputedOnCancel = (cancelCallback: Fn) => void
export interface AsyncComputedOptions {
/**
* Should value be evaluated lazily
*
* @default false
*/
lazy?: boolean
/**
* Ref passed to receive the updated of async evaluation
*/
evaluating?: Ref<boolean>
/**
* Use shallowRef
*
* The default value will be changed to `true` in the next major version
*
* @default false
*/
shallow?: boolean
/**
* Callback when error is caught.
*/
onError?: (e: unknown) => void
}
/**
* Create an asynchronous computed dependency.
*
* @see https://vueuse.org/computedAsync
* @param evaluationCallback The promise-returning callback which generates the computed value
* @param initialState The initial state, used until the first evaluation finishes
* @param optionsOrRef Additional options or a ref passed to receive the updates of the async evaluation
*/
export declare function computedAsync<T>(
evaluationCallback: (onCancel: AsyncComputedOnCancel) => T | Promise<T>,
initialState?: T,
optionsOrRef?: Ref<boolean> | AsyncComputedOptions
): Ref<T>
export { computedAsync as asyncComputed }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/computedAsync/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/computedAsync/index.md)
| programming_docs |
vueuse useThrottledRefHistory useThrottledRefHistory
======================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 2.17 kB |
| Last Changed | 6 months ago |
| Related | [`useDebouncedRefHistory`](../usedebouncedrefhistory/index)[`useRefHistory`](../userefhistory/index) |
Shorthand for [`useRefHistory`](../userefhistory/index)with throttled filter.
Usage
-----
This function takes the first snapshot right after the counter's value was changed and the second with a delay of 1000ms.
```
import { ref } from 'vue'
import { useThrottledRefHistory } from '@vueuse/core'
const counter = ref(0)
const { history, undo, redo } = useThrottledRefHistory(counter, { deep: true, throttle: 1000 })
```
Type Declarations
-----------------
```
export type UseThrottledRefHistoryOptions<Raw, Serialized = Raw> = Omit<
UseRefHistoryOptions<Raw, Serialized>,
"eventFilter"
> & {
throttle?: MaybeRef<number>
trailing?: boolean
}
export type UseThrottledRefHistoryReturn<
Raw,
Serialized = Raw
> = UseRefHistoryReturn<Raw, Serialized>
/**
* Shorthand for [useRefHistory](https://vueuse.org/useRefHistory) with throttled filter.
*
* @see https://vueuse.org/useThrottledRefHistory
* @param source
* @param options
*/
export declare function useThrottledRefHistory<Raw, Serialized = Raw>(
source: Ref<Raw>,
options?: UseThrottledRefHistoryOptions<Raw, Serialized>
): UseThrottledRefHistoryReturn<Raw, Serialized>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useThrottledRefHistory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useThrottledRefHistory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useThrottledRefHistory/index.md)
*No recent changes*
vueuse templateRef templateRef
===========
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 905 B |
| Last Changed | 4 months ago |
Shorthand for binding ref to template element.
Usage
-----
```
<script lang="ts">
import { templateRef } from '@vueuse/core'
export default {
setup() {
const target = templateRef('target')
// no need to return the `target`, it will bind to the ref magically
},
}
</script>
<template>
<div ref="target" />
</template>
```
### With JSX/TSX
```
import { templateRef } from '@vueuse/core'
export default {
setup() {
const target = templateRef<HTMLElement | null>('target', null)
// use string ref
return () => <div ref="target"></div>
},
}
```
###
`<script setup>`
There is no need for this when using with `<script setup>` since all the variables will be exposed to the template. It will be exactly the same as `ref`.
```
<script setup lang="ts">
import { ref } from 'vue'
const target = ref<HTMLElement | null>(null)
</script>
<template>
<div ref="target" />
</template>
```
Type Declarations
-----------------
```
/**
* Shorthand for binding ref to template element.
*
* @see https://vueuse.org/templateRef
* @param key
* @param initialValue
*/
export declare function templateRef<
T extends HTMLElement | SVGElement | Component | null
>(key: string, initialValue?: T | null): Readonly<Ref<T>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/templateRef/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/templateRef/index.md)
vueuse usePreferredDark usePreferredDark
================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.06 kB |
| Last Changed | last year |
| Related | [`useColorMode`](../usecolormode/index)[`useDark`](../usedark/index) |
Reactive dark theme preference.
Usage
-----
```
import { usePreferredDark } from '@vueuse/core'
const isDark = usePreferredDark()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UsePreferredDark v-slot="{ prefersDark }">
Prefers Dark: {{ prefersDark }}
</UsePreferredDark>
```
Type Declarations
-----------------
```
/**
* Reactive dark theme preference.
*
* @see https://vueuse.org/usePreferredDark
* @param [options]
*/
export declare function usePreferredDark(
options?: ConfigurableWindow
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredDark/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredDark/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePreferredDark/index.md)
vueuse useWindowFocus useWindowFocus
==============
| | |
| --- | --- |
| Category | [Elements](../../functions#category=Elements) |
| Export Size | 1.07 kB |
| Last Changed | last year |
Reactively track window focus with `window.onfocus` and `window.onblur` events.
Usage
-----
```
import { useWindowFocus } from '@vueuse/core'
const focused = useWindowFocus()
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseWindowFocus v-slot="{ focused }">
Document Focus: {{ focused }}
</UseWindowFocus>
```
Type Declarations
-----------------
```
/**
* Reactively track window focus with `window.onfocus` and `window.onblur`.
*
* @see https://vueuse.org/useWindowFocus
* @param options
*/
export declare function useWindowFocus({
window,
}?: ConfigurableWindow): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowFocus/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowFocus/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWindowFocus/index.md)
vueuse onClickOutside onClickOutside
==============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.34 kB |
| Last Changed | 2 weeks ago |
Listen for clicks outside of an element. Useful for modal or dropdown.
Usage
-----
```
<template>
<div ref="target">
Hello world
</div>
<div>
Outside element
</div>
</template>
<script>
import { ref } from 'vue'
import { onClickOutside } from '@vueuse/core'
export default {
setup() {
const target = ref(null)
onClickOutside(target, (event) => console.log(event))
return { target }
}
}
</script>
```
> This function uses [Event.composedPath()](https://developer.mozilla.org/en-US/docs/Web/API/Event/composedPath) which is NOT supported by IE 11, Edge 18 and below. If you are targeting these browsers, we recommend you to include [this code snippet](https://gist.github.com/sibbng/13e83b1dd1b733317ce0130ef07d4efd) on your project.
>
>
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<OnClickOutside @trigger="count++" :options="{ ignore: [/* ... */] }">
<div>
Click Outside of Me
</div>
</OnClickOutside>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vOnClickOutside } from '@vueuse/components'
const modal = ref(false)
function closeModal() {
modal.value = false
}
</script>
<template>
<button @click="modal = true">
Open Modal
</button>
<div v-if="modal" v-on-click-outside="closeModal">
Hello World
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface OnClickOutsideOptions extends ConfigurableWindow {
/**
* List of elements that should not trigger the event.
*/
ignore?: (MaybeElementRef | string)[]
/**
* Use capturing phase for internal event listener.
* @default true
*/
capture?: boolean
/**
* Run handler function if focus moves to an iframe.
* @default false
*/
detectIframe?: boolean
}
export type OnClickOutsideHandler<
T extends {
detectIframe: OnClickOutsideOptions["detectIframe"]
} = {
detectIframe: false
}
> = (
evt: T["detectIframe"] extends true ? PointerEvent | FocusEvent : PointerEvent
) => void
/**
* Listen for clicks outside of an element.
*
* @see https://vueuse.org/onClickOutside
* @param target
* @param handler
* @param options
*/
export declare function onClickOutside<T extends OnClickOutsideOptions>(
target: MaybeElementRef,
handler: OnClickOutsideHandler<{
detectIframe: T["detectIframe"]
}>,
options?: T
): (() => void) | undefined
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/onClickOutside/index.md)
vueuse useOffsetPagination useOffsetPagination
===================
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1.26 kB |
| Last Changed | 5 months ago |
Reactive offset pagination.
Usage
-----
```
import { useOffsetPagination } from '@vueuse/core'
function fetchData({ currentPage, currentPageSize }: { currentPage: number; currentPageSize: number }) {
fetch(currentPage, currentPageSize).then((responseData) => {
data.value = responseData
})
}
const {
currentPage,
currentPageSize,
pageCount,
isFirstPage,
isLastPage,
prev,
next,
} = useOffsetPagination({
total: database.value.length,
page: 1,
pageSize,
onPageChange: fetchData,
onPageSizeChange: fetchData,
})
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseOffsetPagination
v-slot="{
currentPage,
currentPageSize,
next,
prev,
pageCount,
isFirstPage,
isLastPage
}"
:total="database.length"
@page-change="fetchData"
@page-size-change="fetchData"
>
<div class="gap-x-4 gap-y-2 grid-cols-2 inline-grid items-center">
<div opacity="50">
total:
</div>
<div>{{ database.length }}</div>
<div opacity="50">
pageCount:
</div>
<div>{{ pageCount }}</div>
<div opacity="50">
currentPageSize:
</div>
<div>{{ currentPageSize }}</div>
<div opacity="50">
currentPage:
</div>
<div>{{ currentPage }}</div>
<div opacity="50">
isFirstPage:
</div>
<div>{{ isFirstPage }}</div>
<div opacity="50">
isLastPage:
</div>
<div>{{ isLastPage }}</div>
</div>
<div>
<button :disabled="isFirstPage" @click="prev">
prev
</button>
<button :disabled="isLastPage" @click="next">
next
</button>
</div>
</UseOffsetPagination>
```
Component event supported props event callback and event listener.
event listener:
```
<UseOffsetPagination
v-slot="{
currentPage,
currentPageSize,
next,
prev,
pageCount,
isFirstPage,
isLastPage
}"
:total="database.length"
@page-change="fetchData"
@page-size-change="fetchData"
@page-count-change="onPageCountChange"
>
<!-- your code -->
</UseOffsetPagination>
```
or props event callback:
```
<UseOffsetPagination
v-slot="{
currentPage,
currentPageSize,
next,
prev,
pageCount,
isFirstPage,
isLastPage
}"
:total="database.length"
:on-page-change="fetchData"
:on-page-size-change="fetchData"
:on-page-count-change="onPageCountChange"
>
<!-- your code -->
</UseOffsetPagination>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseOffsetPaginationOptions {
/**
* Total number of items.
*/
total?: MaybeRef<number>
/**
* The number of items to display per page.
* @default 10
*/
pageSize?: MaybeRef<number>
/**
* The current page number.
* @default 1
*/
page?: MaybeRef<number>
/**
* Callback when the `page` change.
*/
onPageChange?: (
returnValue: UnwrapNestedRefs<UseOffsetPaginationReturn>
) => unknown
/**
* Callback when the `pageSize` change.
*/
onPageSizeChange?: (
returnValue: UnwrapNestedRefs<UseOffsetPaginationReturn>
) => unknown
/**
* Callback when the `pageCount` change.
*/
onPageCountChange?: (
returnValue: UnwrapNestedRefs<UseOffsetPaginationReturn>
) => unknown
}
export interface UseOffsetPaginationReturn {
currentPage: Ref<number>
currentPageSize: Ref<number>
pageCount: ComputedRef<number>
isFirstPage: ComputedRef<boolean>
isLastPage: ComputedRef<boolean>
prev: () => void
next: () => void
}
export type UseOffsetPaginationInfinityPageReturn = Omit<
UseOffsetPaginationReturn,
"isLastPage"
>
export declare function useOffsetPagination(
options: Omit<UseOffsetPaginationOptions, "total">
): UseOffsetPaginationInfinityPageReturn
export declare function useOffsetPagination(
options: UseOffsetPaginationOptions
): UseOffsetPaginationReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useOffsetPagination/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useOffsetPagination/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useOffsetPagination/index.md)
vueuse usePermission usePermission
=============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.3 kB |
| Last Changed | 6 months ago |
| Related | [`useUserMedia`](../useusermedia/index) |
Reactive [Permissions API](https://developer.mozilla.org/en-US/docs/Web/API/Permissions_API). The Permissions API provides the tools to allow developers to implement a better user experience as far as permissions are concerned.
Usage
-----
```
import { usePermission } from '@vueuse/core'
const microphoneAccess = usePermission('microphone')
```
Type Declarations
-----------------
Show Type Declarations
```
type DescriptorNamePolyfill =
| "accelerometer"
| "accessibility-events"
| "ambient-light-sensor"
| "background-sync"
| "camera"
| "clipboard-read"
| "clipboard-write"
| "gyroscope"
| "magnetometer"
| "microphone"
| "notifications"
| "payment-handler"
| "persistent-storage"
| "push"
| "speaker"
export type GeneralPermissionDescriptor =
| PermissionDescriptor
| {
name: DescriptorNamePolyfill
}
export interface UsePermissionOptions<Controls extends boolean>
extends ConfigurableNavigator {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
}
export type UsePermissionReturn = Readonly<Ref<PermissionState | undefined>>
export interface UsePermissionReturnWithControls {
state: UsePermissionReturn
isSupported: Ref<boolean>
query: () => Promise<PermissionStatus | undefined>
}
/**
* Reactive Permissions API.
*
* @see https://vueuse.org/usePermission
*/
export declare function usePermission(
permissionDesc:
| GeneralPermissionDescriptor
| GeneralPermissionDescriptor["name"],
options?: UsePermissionOptions<false>
): UsePermissionReturn
export declare function usePermission(
permissionDesc:
| GeneralPermissionDescriptor
| GeneralPermissionDescriptor["name"],
options: UsePermissionOptions<true>
): UsePermissionReturnWithControls
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/usePermission/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/usePermission/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/usePermission/index.md)
vueuse useFocusWithin useFocusWithin
==============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.14 kB |
| Last Changed | 4 months ago |
Reactive utility to track if an element or one of its decendants has focus. It is meant to match the behavior of the `:focus-within` CSS pseudo-class. A common use case would be on a form element to see if any of its inputs currently have focus.
Basic Usage
-----------
```
<template>
<form ref="target">
<input type="text" placeholder="First Name">
<input type="text" placeholder="Last Name">
<input type="text" placeholder="Email">
<input type="text" placeholder="Password">
</form>
</template>
<script>
import { useFocusWithin } from '@vueuse/core'
const target = ref();
const { focused } = useFocusWithin(target)
watch(focused, focused => {
if (focused) console.log('Target contains the focused element')
else console.log('Target does NOT contain the focused element')
})
</script>
```
Type Declarations
-----------------
```
export interface UseFocusWithinReturn {
/**
* True if the element or any of its descendants are focused
*/
focused: ComputedRef<boolean>
}
/**
* Track if focus is contained within the target element
*
* @see https://vueuse.org/useFocusWithin
* @param target The target element to track
* @param options Focus within options
*/
export declare function useFocusWithin(
target: MaybeElementRef,
options?: ConfigurableWindow
): UseFocusWithinReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocusWithin/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocusWithin/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocusWithin/index.md)
vueuse useScrollLock useScrollLock
=============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.23 kB |
| Last Changed | 2 days ago |
Lock scrolling of the element.
Usage
-----
```
<script setup lang="ts">
import { useScrollLock } from '@vueuse/core'
const el = ref<HTMLElement | null>(null)
const isLocked = useScrollLock(el)
isLocked.value = true // lock
isLocked.value = false // unlock
</script>
<template>
<div ref="el"></div>
</template>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { vScrollLock } from '@vueuse/components'
const data = ref([1, 2, 3, 4, 5, 6])
const isLocked = ref(false)
const toggleLock = useToggle(isLocked)
</script>
<template>
<div v-scroll-lock="isLocked">
<div v-for="item in data" :key="item">
{{ item }}
</div>
</div>
<button @click="toggleLock()">
Toggle lock state
</button>
</template>
```
Type Declarations
-----------------
```
/**
* Lock scrolling of the element.
*
* @see https://vueuse.org/useScrollLock
* @param element
*/
export declare function useScrollLock(
element: MaybeComputedRef<
HTMLElement | SVGElement | Window | Document | null | undefined
>,
initialState?: boolean
): WritableComputedRef<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScrollLock/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useScrollLock/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScrollLock/index.md)
vueuse useMounted useMounted
==========
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 777 B |
| Last Changed | last year |
Mounted state in ref.
Usage
-----
```
import { useMounted } from '@vueuse/core'
const isMounted = useMounted()
```
Which is essentially a shorthand of:
```
const isMounted = ref(false)
onMounted(() => {
isMounted.value = true
})
```
Type Declarations
-----------------
```
/**
* Mounted state in ref.
*
* @see https://vueuse.org/useMounted
* @param options
*/
export declare function useMounted(): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMounted/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMounted/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMounted/index.md)
| programming_docs |
vueuse unrefElement unrefElement
============
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 783 B |
| Last Changed | 6 months ago |
Unref for dom element.
Usage
-----
```
<template>
<div ref="div"/>
<HelloWorld ref="hello"/>
</template>
<script setup>
import { ref, onMounted } from 'vue'
import { unrefElement } from '@vueuse/core'
const div = ref() // will be bind to the <div> element
const hello = ref() // will be bind to the HelloWorld Component
onMounted(() => {
console.log(unrefElement(div)) // the <div> element
console.log(unrefElement(hello)) // the root element of the HelloWorld Component
})
</script>
```
Type Declarations
-----------------
```
export type VueInstance = ComponentPublicInstance
export type MaybeElementRef<T extends MaybeElement = MaybeElement> = MaybeRef<T>
export type MaybeComputedElementRef<T extends MaybeElement = MaybeElement> =
MaybeComputedRef<T>
export type MaybeElement =
| HTMLElement
| SVGElement
| VueInstance
| undefined
| null
export type UnRefElementReturn<T extends MaybeElement = MaybeElement> =
T extends VueInstance ? Exclude<MaybeElement, VueInstance> : T | undefined
/**
* Get the dom element of a ref of element or Vue component instance
*
* @param elRef
*/
export declare function unrefElement<T extends MaybeElement>(
elRef: MaybeComputedElementRef<T>
): UnRefElementReturn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/unrefElement/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/unrefElement/index.md)
vueuse onKeyStroke onKeyStroke
===========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.16 kB |
| Last Changed | 2 days ago |
Listen for keyboard key being stroked.
Usage
-----
```
import { onKeyStroke } from '@vueuse/core'
onKeyStroke('ArrowDown', (e) => {
e.preventDefault()
})
```
See [this table](https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/key/Key_Values) for all key codes.
### Listen To Multiple Keys
```
import { onKeyStroke } from '@vueuse/core'
onKeyStroke(['s', 'S', 'ArrowDown'], (e) => {
e.preventDefault()
})
// listen to all keys by [true / skip the keyDefine]
onKeyStroke(true, (e) => {
e.preventDefault()
})
onKeyStroke((e) => {
e.preventDefault()
})
```
### Custom Event Target
```
onKeyStroke('A', (e) => {
console.log('Key A pressed on document')
}, { target: document })
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { vOnKeyStroke } from '@vueuse/components'
function onUpdate(e: KeyboardEvent) {
// impl...
}
</script>
<template>
<input v-on-key-stroke:c,v="onUpdate" type="text">
<!-- with options -->
<input v-on-key-stroke:c,v="[onUpdate, { eventName: 'keyup' }]" type="text">
</template>
```
### Custom Keyboard Event
```
onKeyStroke('Shift', (e) => {
console.log('Shift key up')
}, { eventName: 'keyup' })
```
Or
```
onKeyUp('Shift', () => console.log('Shift key up'))
```
Shorthands
----------
* `onKeyDown` - alias for `onKeyStroke(key, handler, {eventName: 'keydown'})`
* `onKeyPressed` - alias for `onKeyStroke(key, handler, {eventName: 'keypress'})`
* `onKeyUp` - alias for `onKeyStroke(key, handler, {eventName: 'keyup'})`
Type Declarations
-----------------
Show Type Declarations
```
export type KeyPredicate = (event: KeyboardEvent) => boolean
export type KeyFilter = true | string | string[] | KeyPredicate
export type KeyStrokeEventName = "keydown" | "keypress" | "keyup"
export interface OnKeyStrokeOptions {
eventName?: KeyStrokeEventName
target?: MaybeComputedRef<EventTarget | null | undefined>
passive?: boolean
}
export declare function onKeyStroke(
key: KeyFilter,
handler: (event: KeyboardEvent) => void,
options?: OnKeyStrokeOptions
): () => void
export declare function onKeyStroke(
handler: (event: KeyboardEvent) => void,
options?: OnKeyStrokeOptions
): () => void
/**
* Listen for keyboard keys being stroked.
*
* @see https://vueuse.org/onKeyStroke
*/
export declare function onKeyStroke(
key: KeyFilter,
handler: (event: KeyboardEvent) => void,
options?: OnKeyStrokeOptions
): () => void
export declare function onKeyStroke(
handler: (event: KeyboardEvent) => void,
options?: OnKeyStrokeOptions
): () => void
/**
* Listen to the keydown event of the given key.
*
* @see https://vueuse.org/onKeyStroke
* @param key
* @param handler
* @param options
*/
export declare function onKeyDown(
key: KeyFilter,
handler: (event: KeyboardEvent) => void,
options?: Omit<OnKeyStrokeOptions, "eventName">
): () => void
/**
* Listen to the keypress event of the given key.
*
* @see https://vueuse.org/onKeyStroke
* @param key
* @param handler
* @param options
*/
export declare function onKeyPressed(
key: KeyFilter,
handler: (event: KeyboardEvent) => void,
options?: Omit<OnKeyStrokeOptions, "eventName">
): () => void
/**
* Listen to the keyup event of the given key.
*
* @see https://vueuse.org/onKeyStroke
* @param key
* @param handler
* @param options
*/
export declare function onKeyUp(
key: KeyFilter,
handler: (event: KeyboardEvent) => void,
options?: Omit<OnKeyStrokeOptions, "eventName">
): () => void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/onKeyStroke/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/onKeyStroke/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/onKeyStroke/index.md)
vueuse useWebSocket useWebSocket
============
| | |
| --- | --- |
| Category | [Network](../../functions#category=Network) |
| Export Size | 1.7 kB |
| Last Changed | 2 months ago |
Reactive [WebSocket](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket/WebSocket) client.
Usage
-----
```
import { useWebSocket } from '@vueuse/core'
const { status, data, send, open, close } = useWebSocket('ws://websocketurl')
```
See the [Type Declarations](#type-declarations) for more options.
### Immediate
Auto-connect (enabled by default).
This will call `open()` automatically for you and you don't need to call it by yourself.
If url is provided as a ref, this also controls whether a connection is re-established when its value is changed (or whether you need to call open() again for the change to take effect).
### Auto-close
Auto-close-connection (enabled by default).
This will call `close()` automatically when the `beforeunload` event is triggered or the associated effect scope is stopped.
### Auto-reconnection
Reconnect on errors automatically (disabled by default).
```
const { status, data, close } = useWebSocket('ws://websocketurl', {
autoReconnect: true,
})
```
Or with more controls over its behavior:
```
const { status, data, close } = useWebSocket('ws://websocketurl', {
autoReconnect: {
retries: 3,
delay: 1000,
onFailed() {
alert('Failed to connect WebSocket after 3 retries')
},
},
})
```
Explicitly calling `close()` won't trigger the auto reconnection.
### Heartbeat
It's common practice to send a small message (heartbeat) for every given time passed to keep the connection active. In this function we provide a convenient helper to do it:
```
const { status, data, close } = useWebSocket('ws://websocketurl', {
heartbeat: true,
})
```
Or with more controls:
```
const { status, data, close } = useWebSocket('ws://websocketurl', {
heartbeat: {
message: 'ping',
interval: 1000,
pongTimeout: 1000,
},
})
```
Type Declarations
-----------------
```
export declare type WebSocketStatus = "OPEN" | "CONNECTING" | "CLOSED"
export interface WebSocketOptions {
onConnected?: (ws: WebSocket) => void
onDisconnected?: (ws: WebSocket, event: CloseEvent) => void
onError?: (ws: WebSocket, event: Event) => void
onMessage?: (ws: WebSocket, event: MessageEvent) => void
/**
* Send heartbeat for every x mileseconds passed
*
* @default false
*/
heartbeat?:
| boolean
| {
/**
* Message for the heartbeat
*
* @default 'ping'
*/
message?: string | ArrayBuffer | Blob
/**
* Interval, in mileseconds
*
* @default 1000
*/
interval?: number
}
/**
* Enabled auto reconnect
*
* @default false
*/
autoReconnect?:
| boolean
| {
/**
* Maximum retry times.
*
* @default -1
*/
retries?: number
/**
* Delay for reconnect, in mileseconds
*
* @default 1000
*/
delay?: number
/**
* On maximum retry times reached.
*/
onFailed?: Fn
}
}
export interface WebSocketResult<T> {
/**
* Reference to the latest data received via the websocket,
* can be watched to respond to incoming messages
*/
data: Ref<T | null>
/**
* The current websocket status, can be only one of:
* 'OPEN', 'CONNECTING', 'CLOSED'
*/
status: Ref<WebSocketStatus>
/**
* Closes the websocket connection gracefully.
*/
close: WebSocket["close"]
/**
* Reopen the websocket connection.
* If there the current one is active, will close it before opening a new one.
*/
open: Fn
/**
* Sends data through the websocket connection.
*
* @param data
* @param useBuffer when the socket is not yet open, store the data into the buffer and sent them one connected. Default to true.
*/
send: (data: string | ArrayBuffer | Blob, useBuffer?: boolean) => boolean
/**
* Reference to the WebSocket instance.
*/
ws: Ref<WebSocket | undefined>
}
/**
* Reactive WebSocket client.
*
* @see {@link /useWebSocket}
* @param url
*/
export declare function useWebSocket<Data = any>(
url: string,
options?: WebSocketOptions
): WebSocketResult<Data>
```
### Sub-protocols
List of one or more subprotocols to use, in this case soap and wamp.
```
import { useWebSocket } from '@vueuse/core'
const { status, data, send, open, close } = useWebSocket('ws://websocketurl', {
protocols: ['soap'], // ['soap', 'wamp']
})
```
Type Declarations
-----------------
Show Type Declarations
```
export type WebSocketStatus = "OPEN" | "CONNECTING" | "CLOSED"
export interface UseWebSocketOptions {
onConnected?: (ws: WebSocket) => void
onDisconnected?: (ws: WebSocket, event: CloseEvent) => void
onError?: (ws: WebSocket, event: Event) => void
onMessage?: (ws: WebSocket, event: MessageEvent) => void
/**
* Send heartbeat for every x milliseconds passed
*
* @default false
*/
heartbeat?:
| boolean
| {
/**
* Message for the heartbeat
*
* @default 'ping'
*/
message?: string | ArrayBuffer | Blob
/**
* Interval, in milliseconds
*
* @default 1000
*/
interval?: number
/**
* Heartbeat response timeout, in milliseconds
*
* @default 1000
*/
pongTimeout?: number
}
/**
* Enabled auto reconnect
*
* @default false
*/
autoReconnect?:
| boolean
| {
/**
* Maximum retry times.
*
* Or you can pass a predicate function (which returns true if you want to retry).
*
* @default -1
*/
retries?: number | (() => boolean)
/**
* Delay for reconnect, in milliseconds
*
* @default 1000
*/
delay?: number
/**
* On maximum retry times reached.
*/
onFailed?: Fn
}
/**
* Automatically open a connection
*
* @default true
*/
immediate?: boolean
/**
* Automatically close a connection
*
* @default true
*/
autoClose?: boolean
/**
* List of one or more sub-protocol strings
*
* @default []
*/
protocols?: string[]
}
export interface UseWebSocketReturn<T> {
/**
* Reference to the latest data received via the websocket,
* can be watched to respond to incoming messages
*/
data: Ref<T | null>
/**
* The current websocket status, can be only one of:
* 'OPEN', 'CONNECTING', 'CLOSED'
*/
status: Ref<WebSocketStatus>
/**
* Closes the websocket connection gracefully.
*/
close: WebSocket["close"]
/**
* Reopen the websocket connection.
* If there the current one is active, will close it before opening a new one.
*/
open: Fn
/**
* Sends data through the websocket connection.
*
* @param data
* @param useBuffer when the socket is not yet open, store the data into the buffer and sent them one connected. Default to true.
*/
send: (data: string | ArrayBuffer | Blob, useBuffer?: boolean) => boolean
/**
* Reference to the WebSocket instance.
*/
ws: Ref<WebSocket | undefined>
}
/**
* Reactive WebSocket client.
*
* @see https://vueuse.org/useWebSocket
* @param url
*/
export declare function useWebSocket<Data = any>(
url: MaybeComputedRef<string | URL>,
options?: UseWebSocketOptions
): UseWebSocketReturn<Data>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebSocket/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebSocket/index.md)
vueuse useFileSystemAccess useFileSystemAccess
===================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.32 kB |
| Last Changed | 6 months ago |
Create and read and write local files with [FileSystemAccessAPI](https://developer.mozilla.org/en-US/docs/Web/API/File_System_Access_API)
Usage
-----
```
import { useFileSystemAccess } from '@vueuse/core'
const { isSupported, data, file, fileName, fileMIME, fileSize, fileLastModified, create, open, save, saveAs, updateData } = useFileSystemAccess()
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* window.showOpenFilePicker parameters
* @see https://developer.mozilla.org/en-US/docs/Web/API/window/showOpenFilePicker#parameters
*/
export interface FileSystemAccessShowOpenFileOptions {
multiple?: boolean
types?: Array<{
description?: string
accept: Record<string, string[]>
}>
excludeAcceptAllOption?: boolean
}
/**
* window.showSaveFilePicker parameters
* @see https://developer.mozilla.org/en-US/docs/Web/API/window/showSaveFilePicker#parameters
*/
export interface FileSystemAccessShowSaveFileOptions {
suggestedName?: string
types?: Array<{
description?: string
accept: Record<string, string[]>
}>
excludeAcceptAllOption?: boolean
}
/**
* FileHandle
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemFileHandle
*/
export interface FileSystemFileHandle {
getFile: () => Promise<File>
createWritable: () => FileSystemWritableFileStream
}
/**
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream
*/
interface FileSystemWritableFileStream extends WritableStream {
/**
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream/write
*/
write: FileSystemWritableFileStreamWrite
/**
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream/seek
*/
seek: (position: number) => Promise<void>
/**
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream/truncate
*/
truncate: (size: number) => Promise<void>
}
/**
* FileStream.write
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream/write
*/
interface FileSystemWritableFileStreamWrite {
(data: string | BufferSource | Blob): Promise<void>
(options: {
type: "write"
position: number
data: string | BufferSource | Blob
}): Promise<void>
(options: { type: "seek"; position: number }): Promise<void>
(options: { type: "truncate"; size: number }): Promise<void>
}
/**
* FileStream.write
* @see https://developer.mozilla.org/en-US/docs/Web/API/FileSystemWritableFileStream/write
*/
export type FileSystemAccessWindow = Window & {
showSaveFilePicker: (
options: FileSystemAccessShowSaveFileOptions
) => Promise<FileSystemFileHandle>
showOpenFilePicker: (
options: FileSystemAccessShowOpenFileOptions
) => Promise<FileSystemFileHandle[]>
}
export type UseFileSystemAccessCommonOptions = Pick<
FileSystemAccessShowOpenFileOptions,
"types" | "excludeAcceptAllOption"
>
export type UseFileSystemAccessShowSaveFileOptions = Pick<
FileSystemAccessShowSaveFileOptions,
"suggestedName"
>
export type UseFileSystemAccessOptions = ConfigurableWindow &
UseFileSystemAccessCommonOptions & {
/**
* file data type
*/
dataType?: MaybeComputedRef<"Text" | "ArrayBuffer" | "Blob">
}
/**
* Create and read and write local files.
* @see https://vueuse.org/useFileSystemAccess
* @param options
*/
export declare function useFileSystemAccess(
options: UseFileSystemAccessOptions & {
dataType: "Text"
}
): UseFileSystemAccessReturn<string>
export declare function useFileSystemAccess(
options: UseFileSystemAccessOptions & {
dataType: "ArrayBuffer"
}
): UseFileSystemAccessReturn<ArrayBuffer>
export declare function useFileSystemAccess(
options: UseFileSystemAccessOptions & {
dataType: "Blob"
}
): UseFileSystemAccessReturn<Blob>
export declare function useFileSystemAccess(
options: UseFileSystemAccessOptions
): UseFileSystemAccessReturn<string | ArrayBuffer | Blob>
export interface UseFileSystemAccessReturn<T = string> {
isSupported: Ref<boolean>
data: Ref<T | undefined>
file: Ref<File | undefined>
fileName: Ref<string>
fileMIME: Ref<string>
fileSize: Ref<number>
fileLastModified: Ref<number>
open: (_options?: UseFileSystemAccessCommonOptions) => Awaitable<void>
create: (_options?: UseFileSystemAccessShowSaveFileOptions) => Awaitable<void>
save: (_options?: UseFileSystemAccessShowSaveFileOptions) => Awaitable<void>
saveAs: (_options?: UseFileSystemAccessShowSaveFileOptions) => Awaitable<void>
updateData: () => Awaitable<void>
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileSystemAccess/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileSystemAccess/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFileSystemAccess/index.md)
vueuse useVibrate useVibrate
==========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.23 kB |
| Last Changed | 6 months ago |
Reactive [Vibration API](https://developer.mozilla.org/en-US/docs/Web/API/Vibration_API)
Most modern mobile devices include vibration hardware, which lets software code provides physical feedback to the user by causing the device to shake.
The Vibration API offers Web apps the ability to access this hardware, if it exists, and does nothing if the device doesn't support it.
Usage
-----
Vibration is described as a pattern of on-off pulses, which may be of varying lengths.
The pattern may consist of either a single integer describing the number of milliseconds to vibrate, or an array of integers describing a pattern of vibrations and pauses.
```
import { useVibrate } from '@vueuse/core'
// This vibrates the device for 300 ms
// then pauses for 100 ms before vibrating the device again for another 300 ms:
const { vibrate, stop, isSupported } = useVibrate({ pattern: [300, 100, 300] })
// Start the vibration, it will automatically stop when the pattern is complete:
vibrate()
// But if you want to stop it, you can:
stop()
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseVibrateOptions extends ConfigurableNavigator {
/**
*
* Vibration Pattern
*
* An array of values describes alternating periods in which the
* device is vibrating and not vibrating. Each value in the array
* is converted to an integer, then interpreted alternately as
* the number of milliseconds the device should vibrate and the
* number of milliseconds it should not be vibrating
*
* @default []
*
*/
pattern?: MaybeComputedRef<number[] | number>
/**
* Interval to run a persistent vibration, in ms
*
* Pass `0` to disable
*
* @default 0
*
*/
interval?: number
}
/**
* Reactive vibrate
*
* @see https://vueuse.org/useVibrate
* @see https://developer.mozilla.org/en-US/docs/Web/API/Vibration_API
* @param options
*/
export declare function useVibrate(options?: UseVibrateOptions): {
isSupported: Ref<boolean>
pattern: MaybeComputedRef<number | number[]>
intervalControls: Pausable | undefined
vibrate: (pattern?: number | number[]) => void
stop: () => void
}
export type UseVibrateReturn = ReturnType<typeof useVibrate>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useVibrate/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useVibrate/index.md)
| programming_docs |
vueuse useObjectUrl useObjectUrl
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 884 B |
| Last Changed | 6 months ago |
Reactive URL representing an object.
Creates an URL for the provided `File`, `Blob`, or `MediaSource` via [URL.createObjectURL()](https://developer.mozilla.org/en-US/docs/Web/API/URL/createObjectURL) and automatically releases the URL via [URL.revokeObjectURL()](https://developer.mozilla.org/en-US/docs/Web/API/URL/revokeObjectURL) when the source changes or the component is unmounted.
Usage
-----
```
<script setup>
import { useObjectUrl } from '@vueuse/core'
import { shallowRef } from 'vue'
const file = shallowRef()
const url = useObjectUrl(file)
const onFileChange = (event) => {
file.value = event.target.files[0]
}
</script>
<template>
<input type="file" @change="onFileChange" />
<a :href="url">Open file</a>
</template>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<template>
<UseObjectUrl v-slot="url" :object="file">
<a :href="url">Open file</a>
</UseObjectUrl>
</template>
```
Type Declarations
-----------------
```
/**
* Reactive URL representing an object.
*
* @see https://vueuse.org/useObjectUrl
* @param object
*/
export declare function useObjectUrl(
object: MaybeRef<Blob | MediaSource | undefined>
): Readonly<Ref<string | undefined>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useObjectUrl/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useObjectUrl/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useObjectUrl/index.md)
vueuse useCycleList useCycleList
============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 931 B |
| Last Changed | 5 months ago |
Cycle through a list of items.
[Learn how to use useCycleList to create an image carousel with this FREE video lesson from Vue School!](https://vueschool.io/lessons/create-an-image-carousel-with-vueuse?friend=vueuse)Usage
-----
```
import { useCycleList } from '@vueuse/core'
const { state, next, prev } = useCycleList([
'Dog',
'Cat',
'Lizard',
'Shark',
'Whale',
'Dolphin',
'Octopus',
'Seal',
])
console.log(state.value) // 'Dog'
prev()
console.log(state.value) // 'Seal'
```
Type Declarations
-----------------
```
export interface UseCycleListOptions<T> {
/**
* The initial value of the state.
* A ref can be provided to reuse.
*/
initialValue?: MaybeRef<T>
/**
* The default index when
*/
fallbackIndex?: number
/**
* Custom function to get the index of the current value.
*/
getIndexOf?: (value: T, list: T[]) => number
}
/**
* Cycle through a list of items
*
* @see https://vueuse.org/useCycleList
*/
export declare function useCycleList<T>(
list: T[],
options?: UseCycleListOptions<T>
): UseCycleListReturn<T>
export interface UseCycleListReturn<T> {
state: Ref<T>
index: Ref<number>
next: (n?: number) => T
prev: (n?: number) => T
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useCycleList/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useCycleList/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useCycleList/index.md)
vueuse useSwipe useSwipe
========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.53 kB |
| Last Changed | 6 months ago |
Reactive swipe detection based on [`TouchEvents`](https://developer.mozilla.org/en-US/docs/Web/API/TouchEvent).
Usage
-----
```
<template>
<div ref="el">
Swipe here
</div>
</template>
<script>
setup() {
const el = ref(null)
const { isSwiping, direction } = useSwipe(el)
return { el, isSwiping, direction }
}
</script>
```
Type Declarations
-----------------
Show Type Declarations
```
export declare enum SwipeDirection {
UP = "UP",
RIGHT = "RIGHT",
DOWN = "DOWN",
LEFT = "LEFT",
NONE = "NONE",
}
export interface UseSwipeOptions extends ConfigurableWindow {
/**
* Register events as passive
*
* @default true
*/
passive?: boolean
/**
* @default 50
*/
threshold?: number
/**
* Callback on swipe start
*/
onSwipeStart?: (e: TouchEvent) => void
/**
* Callback on swipe moves
*/
onSwipe?: (e: TouchEvent) => void
/**
* Callback on swipe ends
*/
onSwipeEnd?: (e: TouchEvent, direction: SwipeDirection) => void
}
export interface UseSwipeReturn {
isPassiveEventSupported: boolean
isSwiping: Ref<boolean>
direction: ComputedRef<SwipeDirection | null>
coordsStart: Readonly<Position>
coordsEnd: Readonly<Position>
lengthX: ComputedRef<number>
lengthY: ComputedRef<number>
stop: () => void
}
/**
* Reactive swipe detection.
*
* @see https://vueuse.org/useSwipe
* @param target
* @param options
*/
export declare function useSwipe(
target: MaybeComputedRef<EventTarget | null | undefined>,
options?: UseSwipeOptions
): UseSwipeReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSwipe/index.md)
vueuse useMemory useMemory
=========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.14 kB |
| Last Changed | 6 months ago |
Reactive Memory Info.
Usage
-----
```
import { useMemory } from '@vueuse/core'
const { isSupported, memory } = useMemory()
```
Type Declarations
-----------------
```
/**
* Performance.memory
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/Performance/memory
*/
export interface MemoryInfo {
/**
* The maximum size of the heap, in bytes, that is available to the context.
*/
readonly jsHeapSizeLimit: number
/**
* The total allocated heap size, in bytes.
*/
readonly totalJSHeapSize: number
/**
* The currently active segment of JS heap, in bytes.
*/
readonly usedJSHeapSize: number
[Symbol.toStringTag]: "MemoryInfo"
}
export interface UseMemoryOptions extends UseIntervalFnOptions {
interval?: number
}
/**
* Reactive Memory Info.
*
* @see https://vueuse.org/useMemory
* @param options
*/
export declare function useMemory(options?: UseMemoryOptions): {
isSupported: Ref<boolean>
memory: Ref<MemoryInfo | undefined>
}
export type UseMemoryReturn = ReturnType<typeof useMemory>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMemory/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useMemory/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMemory/index.md)
vueuse useScroll useScroll
=========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.68 kB |
| Last Changed | 2 months ago |
Reactive scroll position and state.
Usage
-----
```
<script setup lang="ts">
import { useScroll } from '@vueuse/core'
const el = ref<HTMLElement | null>(null)
const { x, y, isScrolling, arrivedState, directions } = useScroll(el)
</script>
<template>
<div ref="el"></div>
</template>
```
### With offsets
```
const { x, y, isScrolling, arrivedState, directions } = useScroll(el, {
offset: { top: 30, bottom: 30, right: 30, left: 30 },
})
```
### Setting scroll position
Set the `x` and `y` values to make the element scroll to that position.
```
<script setup lang="ts">
import { useScroll } from '@vueuse/core'
const el = ref<HTMLElement | null>(null)
const { x, y } = useScroll(el)
</script>
<template>
<div ref="el" />
<button @click="x += 10">
Scroll right 10px
</button>
<button @click="y += 10">
Scroll down 10px
</button>
</template>
```
### Smooth scrolling
Set `behavior: smooth` to enable smooth scrolling. The `behavior` option defaults to `auto`, which means no smooth scrolling. See the `behavior` option on [`window.scrollTo()`](https://developer.mozilla.org/en-US/docs/Web/API/Window/scrollTo) for more information.
```
import { useWindowScroll } from '@vueuse/core'
const el = ref<HTMLElement | null>(null)
const { x, y } = useScroll(el, { behavior: 'smooth' })
// Or as a `ref`:
const smooth = ref(false)
const behavior = computed(() => smooth.value ? 'smooth' : 'auto')
const { x, y } = useScroll(el, { behavior })
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import type { UseScrollReturn } from '@vueuse/core'
import { vScroll } from '@vueuse/components'
const data = ref([1, 2, 3, 4, 5, 6])
function onScroll(state: UseScrollReturn) {
console.log(state) // {x, y, isScrolling, arrivedState, directions}
}
</script>
<template>
<div v-scroll="onScroll">
<div v-for="item in data" :key="item">
{{ item }}
</div>
</div>
<!-- with options -->
<div v-scroll="[onScroll, { 'throttle' : 10 }]">
<div v-for="item in data" :key="item">
{{ item }}
</div>
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseScrollOptions {
/**
* Throttle time for scroll event, it’s disabled by default.
*
* @default 0
*/
throttle?: number
/**
* The check time when scrolling ends.
* This configuration will be setting to (throttle + idle) when the `throttle` is configured.
*
* @default 200
*/
idle?: number
/**
* Offset arrived states by x pixels
*
*/
offset?: {
left?: number
right?: number
top?: number
bottom?: number
}
/**
* Trigger it when scrolling.
*
*/
onScroll?: (e: Event) => void
/**
* Trigger it when scrolling ends.
*
*/
onStop?: (e: Event) => void
/**
* Listener options for scroll event.
*
* @default {capture: false, passive: true}
*/
eventListenerOptions?: boolean | AddEventListenerOptions
/**
* Optionally specify a scroll behavior of `auto` (default, not smooth scrolling) or
* `smooth` (for smooth scrolling) which takes effect when changing the `x` or `y` refs.
*
* @default 'auto'
*/
behavior?: MaybeComputedRef<ScrollBehavior>
}
/**
* Reactive scroll.
*
* @see https://vueuse.org/useScroll
* @param element
* @param options
*/
export declare function useScroll(
element: MaybeComputedRef<
HTMLElement | SVGElement | Window | Document | null | undefined
>,
options?: UseScrollOptions
): {
x: WritableComputedRef<number>
y: WritableComputedRef<number>
isScrolling: Ref<boolean>
arrivedState: {
left: boolean
right: boolean
top: boolean
bottom: boolean
}
directions: {
left: boolean
right: boolean
top: boolean
bottom: boolean
}
}
export type UseScrollReturn = ReturnType<typeof useScroll>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useScroll/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useScroll/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useScroll/index.md)
vueuse useNow useNow
======
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 1.21 kB |
| Last Changed | last year |
Reactive current Date instance.
Usage
-----
```
import { useNow } from '@vueuse/core'
const now = useNow()
```
```
const { now, pause, resume } = useNow({ controls: true })
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseNow v-slot="{ now, pause, resume }">
Now: {{ now }}
<button @click="pause()">Pause</button>
<button @click="resume()">Resume</button>
</UseNow>
```
Type Declarations
-----------------
```
export interface UseNowOptions<Controls extends boolean> {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
/**
* Update interval, or use requestAnimationFrame
*
* @default requestAnimationFrame
*/
interval?: "requestAnimationFrame" | number
}
/**
* Reactive current Date instance.
*
* @see https://vueuse.org/useNow
* @param options
*/
export declare function useNow(options?: UseNowOptions<false>): Ref<Date>
export declare function useNow(options: UseNowOptions<true>): {
now: Ref<Date>
} & Pausable
export type UseNowReturn = ReturnType<typeof useNow>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useNow/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useNow/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useNow/index.md)
vueuse useDark useDark
=======
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 3.02 kB |
| Last Changed | 4 months ago |
| Related | [`useColorMode`](../usecolormode/index)[`usePreferredDark`](../usepreferreddark/index)[`useStorage`](../usestorage/index) |
Reactive dark mode with auto data persistence.
Basic Usage
-----------
```
import { useDark, useToggle } from '@vueuse/core'
const isDark = useDark()
const toggleDark = useToggle(isDark)
```
Behavior
--------
[`useDark`](index)combines with [`usePreferredDark`](../usepreferreddark/index)and [`useStorage`](../usestorage/index) On start up, it reads the value from localStorage/sessionStorage (the key is configurable) to see if there is a user configured color scheme, if not, it will use users' system preferences. When you change the `isDark` ref, it will update the corresponding element's attribute and then store the preference to storage (default key: `vueuse-color-scheme`) for persistence.
> Please note [`useDark`](index)only handles the DOM attribute changes for you to apply proper selector in your CSS. It does NOT handle the actual style, theme or CSS for you.
>
>
Configuration
-------------
By default, it uses [Tailwind CSS favored dark mode](https://tailwindcss.com/docs/dark-mode#toggling-dark-mode-manually), which enables dark mode when class `dark` is applied to the `html` tag, for example:
```
<!--light-->
<html> ... </html>
<!--dark-->
<html class="dark"> ... </html>
```
Still, you can also customize it to make it work with most CSS frameworks.
For example:
```
const isDark = useDark({
selector: 'body',
attribute: 'color-scheme',
valueDark: 'dark',
valueLight: 'light',
})
```
will work like
```
<!--light-->
<html>
<body color-scheme="light"> ... </body>
</html>
<!--dark-->
<html>
<body color-scheme="dark"> ... </body>
</html>
```
If the configuration above still does not fit your needs, you can use the`onChanged` option to take full control over how you handle updates.
```
const isDark = useDark({
onChanged(dark: boolean) {
// update the dom, call the API or something
},
})
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseDark v-slot="{ isDark, toggleDark }">
<button @click="toggleDark()">
Is Dark: {{ isDark }}
</button>
</UseDark>
```
Type Declarations
-----------------
```
export interface UseDarkOptions
extends Omit<UseColorModeOptions<BasicColorSchema>, "modes" | "onChanged"> {
/**
* Value applying to the target element when isDark=true
*
* @default 'dark'
*/
valueDark?: string
/**
* Value applying to the target element when isDark=false
*
* @default ''
*/
valueLight?: string
/**
* A custom handler for handle the updates.
* When specified, the default behavior will be overridden.
*
* @default undefined
*/
onChanged?: (isDark: boolean) => void
}
/**
* Reactive dark mode with auto data persistence.
*
* @see https://vueuse.org/useDark
* @param options
*/
export declare function useDark(
options?: UseDarkOptions
): WritableComputedRef<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useDark/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useDark/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useDark/index.md)
vueuse useStyleTag useStyleTag
===========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.09 kB |
| Last Changed | last month |
Inject reactive `style` element in head.
Usage
-----
### Basic usage
Provide a CSS string, then [`useStyleTag`](index)will automatically generate an id and inject it in `<head>`.
```
import { useStyleTag } from '@vueuse/core'
const {
id,
css,
load,
unload,
isLoaded,
} = useStyleTag('.foo { margin-top: 32px; }')
// Later you can modify styles
css.value = '.foo { margin-top: 64px; }'
```
This code will be injected to `<head>`:
```
<style type="text/css" id="vueuse_styletag_1">
.foo { margin-top: 64px; }
</style>
```
### Custom ID
If you need to define your own id, you can pass `id` as first argument.
```
import { useStyleTag } from '@vueuse/core'
useStyleTag('.foo { margin-top: 32px; }', { id: 'custom-id' })
```
```
<!-- injected to <head> -->
<style type="text/css" id="custom-id">
.foo { margin-top: 32px; }
</style>
```
### Media query
You can pass media attributes as last argument within object.
```
useStyleTag('.foo { margin-top: 32px; }', { media: 'print' })
```
```
<!-- injected to <head> -->
<style type="text/css" id="vueuse_styletag_1" media="print">
.foo { margin-top: 32px; }
</style>
```
Type Declarations
-----------------
```
export interface UseStyleTagOptions extends ConfigurableDocument {
/**
* Media query for styles to apply
*/
media?: string
/**
* Load the style immediately
*
* @default true
*/
immediate?: boolean
/**
* Manual controls the timing of loading and unloading
*
* @default false
*/
manual?: boolean
/**
* DOM id of the style tag
*
* @default auto-incremented
*/
id?: string
}
export interface UseStyleTagReturn {
id: string
css: Ref<string>
load: () => void
unload: () => void
isLoaded: Readonly<Ref<boolean>>
}
/**
* Inject <style> element in head.
*
* Overload: Omitted id
*
* @see https://vueuse.org/useStyleTag
* @param css
* @param options
*/
export declare function useStyleTag(
css: MaybeRef<string>,
options?: UseStyleTagOptions
): UseStyleTagReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useStyleTag/index.md)
vueuse useMemoize useMemoize
==========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 1 kB |
| Last Changed | 2 months ago |
Cache results of functions depending on arguments and keep it reactive. It can also be used for asynchronous functions and will reuse existing promises to avoid fetching the same data at the same time.
**TIP**The results are not cleared automatically. Call `clear()` in case you no longer need the results or use own caching mechanism to avoid memory leaks.
Usage
-----
```
import { useMemoize } from '@vueuse/core'
const getUser = useMemoize(
async (userId: number): Promise<UserData> =>
axios.get(`users/${userId}`).then(({ data }) => data),
)
const user1 = await getUser(1) // Request users/1
const user2 = await getUser(2) // Request users/2
// ...
const user1 = await getUser(1) // Retrieve from cache
// ...
const user1 = await getUser.load(1) // Request users/1
// ...
getUser.delete(1) // Delete cache from user 1
getUser.clear() // Clear full cache
```
Combine with `computed` or `asyncComputed` to achieve reactivity:
```
const user1 = asyncComputed(() => getUser(1))
// ...
await getUser.load(1) // Will also update user1
```
### Resolving cache key
The key for caching is determined by the arguments given to the function and will be serialized by default with `JSON.stringify`. This will allow equal objects to receive the same cache key. In case you want to customize the key you can pass `getKey`
```
const getUser = useMemoize(
async (userId: number, headers: AxiosRequestHeaders): Promise<UserData> =>
axios.get(`users/${userId}`, { headers }).then(({ data }) => data),
{
// Use only userId to get/set cache and ignore headers
getKey: (userId, headers) => userId,
},
)
```
**WARNING**For Vue 2 the key has to be a `string` or `number`
### Customize cache mechanism
By default, the results are cached within a `Map` (normal object for Vue 2). You can implement your own mechanism by passing `cache` as options with following structure:
```
export interface MemoizeCache<Key, Value> {
/**
* Get value for key
*/
get (key: Key): Value | undefined
/**
* Set value for key
*/
set (key: Key, value: Value): void
/**
* Return flag if key exists
*/
has (key: Key): boolean
/**
* Delete value for key
*/
delete (key: Key): void
/**
* Clear cache
*/
clear (): void
}
```
Type Declarations
-----------------
Show Type Declarations
```
type CacheKey = any
/**
* Custom memoize cache handler
*/
export interface UseMemoizeCache<Key, Value> {
/**
* Get value for key
*/
get(key: Key): Value | undefined
/**
* Set value for key
*/
set(key: Key, value: Value): void
/**
* Return flag if key exists
*/
has(key: Key): boolean
/**
* Delete value for key
*/
delete(key: Key): void
/**
* Clear cache
*/
clear(): void
}
/**
* Memoized function
*/
export interface UseMemoizeReturn<Result, Args extends unknown[]> {
/**
* Get result from cache or call memoized function
*/
(...args: Args): Result
/**
* Call memoized function and update cache
*/
load(...args: Args): Result
/**
* Delete cache of given arguments
*/
delete(...args: Args): void
/**
* Clear cache
*/
clear(): void
/**
* Generate cache key for given arguments
*/
generateKey(...args: Args): CacheKey
/**
* Cache container
*/
cache: UseMemoizeCache<CacheKey, Result>
}
export interface UseMemoizeOptions<Result, Args extends unknown[]> {
getKey?: (...args: Args) => string | number
cache?: UseMemoizeCache<CacheKey, Result>
}
/**
* Reactive function result cache based on arguments
*/
export declare function useMemoize<Result, Args extends unknown[]>(
resolver: (...args: Args) => Result,
options?: UseMemoizeOptions<Result, Args>
): UseMemoizeReturn<Result, Args>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useMemoize/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useMemoize/index.md)
| programming_docs |
vueuse useTextDirection useTextDirection
================
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.38 kB |
| Last Changed | 6 months ago |
Reactive [dir](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/dir) of the element's text.
Usage
-----
```
import { useTextDirection } from '@vueuse/core'
const dir = useTextDirection() // Ref<'ltr' | 'rtl' | 'auto'>
```
By default, it returns `rlt` direction when dir `rtl` is applied to the `html` tag, for example:
```
<!--ltr-->
<html> ... </html>
<!--rtl-->
<html dir="rtl"> ... </html>
```
Options
-------
```
import { useTextDirection } from '@vueuse/core'
const mode = useTextDirection({
selector: 'body'
}) // Ref<'ltr' | 'rtl' | 'auto'>
```
Type Declarations
-----------------
```
export type UseTextDirectionValue = "ltr" | "rtl" | "auto"
export interface UseTextDirectionOptions extends ConfigurableDocument {
/**
* CSS Selector for the target element applying to
*
* @default 'html'
*/
selector?: string
/**
* Observe `document.querySelector(selector)` changes using MutationObserve
*
* @default false
*/
observe?: boolean
/**
* Initial value
*
* @default 'ltr'
*/
initialValue?: UseTextDirectionValue
}
/**
* Reactive dir of the element's text.
*
* @see https://vueuse.org/useTextDirection
*/
export declare function useTextDirection(
options?: UseTextDirectionOptions
): WritableComputedRef<UseTextDirectionValue>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextDirection/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextDirection/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextDirection/index.md)
vueuse useElementHover useElementHover
===============
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.05 kB |
| Last Changed | 5 months ago |
Reactive element's hover state.
Usage
-----
```
<script setup>
import { useElementHover } from '@vueuse/core'
const myHoverableElement = ref()
const isHovered = useElementHover(myHoverableElement)
</script>
<template>
<button ref="myHoverableElement">
{{ isHovered }}
</button>
</template>
```
Directive Usage
---------------
> This function also provides a directive version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<script setup lang="ts">
import { ref } from 'vue'
import { vElementHover } from '@vueuse/components'
const isHovered = ref(false)
function onHover(state: boolean) {
isHovered.value = state
}
</script>
<template>
<button v-element-hover="onHover">
{{ isHovered ? 'Thank you!' : 'Hover me' }}
</button>
</template>
```
Type Declarations
-----------------
```
export declare function useElementHover(
el: MaybeComputedRef<EventTarget | null | undefined>
): Ref<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementHover/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementHover/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useElementHover/index.md)
vueuse useSessionStorage useSessionStorage
=================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 2.19 kB |
| Last Changed | 6 months ago |
| Related | [`useStorage`](../usestorage/index) |
Reactive [SessionStorage](https://developer.mozilla.org/en-US/docs/Web/API/Window/sessionStorage).
Usage
-----
Please refer to [`useStorage`](../usestorage/index)
Type Declarations
-----------------
```
export declare function useSessionStorage(
key: string,
initialValue: MaybeComputedRef<string>,
options?: UseStorageOptions<string>
): RemovableRef<string>
export declare function useSessionStorage(
key: string,
initialValue: MaybeComputedRef<boolean>,
options?: UseStorageOptions<boolean>
): RemovableRef<boolean>
export declare function useSessionStorage(
key: string,
initialValue: MaybeComputedRef<number>,
options?: UseStorageOptions<number>
): RemovableRef<number>
export declare function useSessionStorage<T>(
key: string,
initialValue: MaybeComputedRef<T>,
options?: UseStorageOptions<T>
): RemovableRef<T>
export declare function useSessionStorage<T = unknown>(
key: string,
initialValue: MaybeComputedRef<null>,
options?: UseStorageOptions<T>
): RemovableRef<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSessionStorage/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSessionStorage/index.md)
vueuse useFocus useFocus
========
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.21 kB |
| Last Changed | 2 days ago |
Reactive utility to track or set the focus state of a DOM element. State changes to reflect whether the target element is the focused element. Setting reactive value from the outside will trigger `focus` and `blur` events for `true` and `false` values respectively.
Basic Usage
-----------
```
import { useFocus } from '@vueuse/core'
const target = ref()
const { focused } = useFocus(target)
watch(focused, (focused) => {
if (focused)
console.log('input element has been focused')
else console.log('input element has lost focus')
})
```
Setting initial focus
---------------------
To focus the element on its first render one can provide the `initialValue` option as `true`. This will trigger a `focus` event on the target element.
```
import { useFocus } from '@vueuse/core'
const target = ref()
const { focused } = useFocus(target, { initialValue: true })
```
Change focus state
------------------
Changes of the `focused` reactive ref will automatically trigger `focus` and `blur` events for `true` and `false` values respectively. You can utilize this behavior to focus the target element as a result of another action (e.g. when a button click as shown below).
```
<template>
<div>
<button type="button" @click="focused = true">Click me to focus input below</button>
<input ref="input" type="text">
</div>
</template>
<script>
import { ref } from 'vue'
import { useFocus } from '@vueuse/core'
export default {
setup() {
const input = ref()
const { focused } = useFocus(input)
return {
input,
focused,
}
}
})
</script>
```
Type Declarations
-----------------
```
export interface UseFocusOptions extends ConfigurableWindow {
/**
* Initial value. If set true, then focus will be set on the target
*
* @default false
*/
initialValue?: boolean
}
export interface UseFocusReturn {
/**
* If read as true, then the element has focus. If read as false, then the element does not have focus
* If set to true, then the element will be focused. If set to false, the element will be blurred.
*/
focused: Ref<boolean>
}
/**
* Track or set the focus state of a DOM element.
*
* @see https://vueuse.org/useFocus
* @param target The target element for the focus and blur events.
* @param options
*/
export declare function useFocus(
target: MaybeElementRef,
options?: UseFocusOptions
): UseFocusReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocus/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocus/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useFocus/index.md)
vueuse useImage useImage
========
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 1.24 kB |
| Last Changed | 6 months ago |
Reactive load an image in the browser, you can wait the result to display it or show a fallback.
Usage
-----
```
<script setup>
import { useImage } from '@vueuse/core'
const avatarUrl = 'https://place.dog/300/200'
const { isLoading } = useImage({ src: avatarUrl })
</script>
<template>
<span v-if="isLoading">Loading</span>
<img v-else :src="avatarUrl">
</template>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<template>
<UseImage src="https://place.dog/300/200">
<template #loading>
Loading..
</template>
<template #error>
Failed
</template>
</UseImage>
</template>
```
Type Declarations
-----------------
```
export interface UseImageOptions {
/** Address of the resource */
src: string
/** Images to use in different situations, e.g., high-resolution displays, small monitors, etc. */
srcset?: string
/** Image sizes for different page layouts */
sizes?: string
}
/**
* Reactive load an image in the browser, you can wait the result to display it or show a fallback.
*
* @see https://vueuse.org/useImage
* @param options Image attributes, as used in the <img> tag
* @param asyncStateOptions
*/
export declare const useImage: <Shallow extends true>(
options: MaybeComputedRef<UseImageOptions>,
asyncStateOptions?: UseAsyncStateOptions<Shallow, any>
) => UseAsyncStateReturn<HTMLImageElement | undefined, true>
export type UseImageReturn = ReturnType<typeof useImage>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useImage/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useImage/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useImage/index.md)
vueuse useWebWorker useWebWorker
============
| | |
| --- | --- |
| Category | [Browser](../../functions#category=Browser) |
| Export Size | 927 B |
| Last Changed | 2 months ago |
| Related | [`useWebWorkerFn`](../usewebworkerfn/index) |
Simple [Web Workers](https://developer.mozilla.org/en-US/docs/Web/API/Web_Workers_API/Using_web_workers) registration and communication.
Usage
-----
```
import { useWebWorker } from '@vueuse/core'
const { data, post, terminate, worker } = useWebWorker('/path/to/worker.js')
```
| State | Type | Description |
| --- | --- | --- |
| data | `Ref<any>` | Reference to the latest data received via the worker, can be watched to respond to incoming messages |
| worker | `ShallowRef<Worker | undefined>` | Reference to the instance of the WebWorker |
| Method | Signature | Description |
| --- | --- | --- |
| post | `(data: any) => void` | Sends data to the worker thread. |
| terminate | `() => void` | Stops and terminates the worker. |
Type Declarations
-----------------
```
export interface UseWebWorkerReturn<Data = any> {
data: Ref<Data>
post: typeof Worker.prototype["postMessage"]
terminate: () => void
worker: ShallowRef<Worker | undefined>
}
type WorkerFn = (...args: unknown[]) => Worker
/**
* Simple Web Workers registration and communication.
*
* @see https://vueuse.org/useWebWorker
* @param url
* @param workerOptions
* @param options
*/
export declare function useWebWorker<T = any>(
url: string,
workerOptions?: WorkerOptions,
options?: ConfigurableWindow
): UseWebWorkerReturn<T>
/**
* Simple Web Workers registration and communication.
*
* @see https://vueuse.org/useWebWorker
* @param worker
*/
export declare function useWebWorker<T = any>(
worker: Worker | WorkerFn
): UseWebWorkerReturn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebWorker/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useWebWorker/index.md)
vueuse useTextSelection useTextSelection
================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.22 kB |
| Last Changed | 10 months ago |
Reactively track user text selection based on [`Window.getSelection`](https://developer.mozilla.org/en-US/docs/Web/API/Window/getSelection).
Usage
-----
```
<template>
<p>{{state.text}}</p>
</template>
<script setup lang="ts">
import { useTextSelection } from '@vueuse/core'
const state = useTextSelection()
</script>
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Reactively track user text selection based on [`Window.getSelection`](https://developer.mozilla.org/en-US/docs/Web/API/Window/getSelection).
*
* @see https://vueuse.org/useTextSelection
*/
export declare function useTextSelection(options?: ConfigurableWindow): {
text: ComputedRef<string>
rects: ComputedRef<DOMRect[]>
ranges: ComputedRef<Range[]>
selection: Ref<{
readonly anchorNode: Node | null
readonly anchorOffset: number
readonly focusNode: Node | null
readonly focusOffset: number
readonly isCollapsed: boolean
readonly rangeCount: number
readonly type: string
addRange: (range: Range) => void
collapse: (node: Node | null, offset?: number | undefined) => void
collapseToEnd: () => void
collapseToStart: () => void
containsNode: (
node: Node,
allowPartialContainment?: boolean | undefined
) => boolean
deleteFromDocument: () => void
empty: () => void
extend: (node: Node, offset?: number | undefined) => void
getRangeAt: (index: number) => Range
modify: (
alter?: string | undefined,
direction?: string | undefined,
granularity?: string | undefined
) => void
removeAllRanges: () => void
removeRange: (range: Range) => void
selectAllChildren: (node: Node) => void
setBaseAndExtent: (
anchorNode: Node,
anchorOffset: number,
focusNode: Node,
focusOffset: number
) => void
setPosition: (node: Node | null, offset?: number | undefined) => void
toString: () => string
} | null>
}
export type UseTextSelectionReturn = ReturnType<typeof useTextSelection>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextSelection/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextSelection/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useTextSelection/index.md)
vueuse useSpeechRecognition useSpeechRecognition
====================
| | |
| --- | --- |
| Category | [Sensors](../../functions#category=Sensors) |
| Export Size | 1.25 kB |
| Last Changed | 4 months ago |
Reactive [SpeechRecognition](https://developer.mozilla.org/en-US/docs/Web/API/SpeechRecognition).
> [Can I use?](https://caniuse.com/mdn-api_speechrecognition)
>
>
Usage
-----
```
import { useSpeechRecognition } from '@vueuse/core'
const {
isSupported,
isListening,
isFinal,
result,
start,
stop,
} = useSpeechRecognition()
```
### Options
The following shows the default values of the options, they will be directly passed to [SpeechRecognition API](https://developer.mozilla.org/en-US/docs/Web/API/SpeechRecognition).
```
useSpeechRecognition({
lang: 'en-US',
interimResults: true,
continuous: true,
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseSpeechRecognitionOptions extends ConfigurableWindow {
/**
* Controls whether continuous results are returned for each recognition, or only a single result.
*
* @default true
*/
continuous?: boolean
/**
* Controls whether interim results should be returned (true) or not (false.) Interim results are results that are not yet final
*
* @default true
*/
interimResults?: boolean
/**
* Language for SpeechRecognition
*
* @default 'en-US'
*/
lang?: MaybeComputedRef<string>
}
/**
* Reactive SpeechRecognition.
*
* @see https://vueuse.org/useSpeechRecognition
* @see https://developer.mozilla.org/en-US/docs/Web/API/SpeechRecognition SpeechRecognition
* @param options
*/
export declare function useSpeechRecognition(
options?: UseSpeechRecognitionOptions
): {
isSupported: Ref<boolean>
isListening: Ref<boolean>
isFinal: Ref<boolean>
recognition: SpeechRecognition | undefined
result: Ref<string>
error: Ref<SpeechRecognitionErrorEvent | undefined>
toggle: (value?: boolean) => void
start: () => void
stop: () => void
}
export type UseSpeechRecognitionReturn = ReturnType<typeof useSpeechRecognition>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechRecognition/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechRecognition/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useSpeechRecognition/index.md)
vueuse useVirtualList useVirtualList
==============
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 1.76 kB |
| Last Changed | yesterday |
Create virtual lists with ease. Virtual lists (sometimes called [*virtual scrollers*](https://akryum.github.io/vue-virtual-scroller/)) allow you to render a large number of items performantly. They only render the minimum number of DOM nodes necessary to show the items within the `container` element by using the `wrapper` element to emulate the container element's full height.
Usage
-----
### Simple list
```
import { useVirtualList } from '@vueuse/core'
const { list, containerProps, wrapperProps } = useVirtualList(
Array.from(Array(99999).keys()),
{
// Keep `itemHeight` in sync with the item's row.
itemHeight: 22,
},
)
```
### Config
| State | Type | Description |
| --- | --- | --- |
| itemHeight | `number` | ensure that the total height of the `wrapper` element is calculated correctly.\* |
| itemWidth | `number` | ensure that the total width of the `wrapper` element is calculated correctly.\* |
| overscan | `number` | number of pre-rendered DOM nodes. Prevents whitespace between items if you scroll very quickly. |
\* The `itemHeight` or `itemWidth` must be kept in sync with the height of each row rendered. If you are seeing extra whitespace or jitter when scrolling to the bottom of the list, ensure the `itemHeight` or `itemWidth` is the same height as the row.
### Reactive list
```
import { useVirtualList, useToggle } from '@vueuse/core'
import { computed } from 'vue'
const [isEven, toggle] = useToggle()
const allItems = Array.from(Array(99999).keys())
const filteredList = computed(() => allItems.filter(i => isEven.value ? i % 2 === 0 : i % 2 === 1))
const { list, containerProps, wrapperProps } = useVirtualList(
filteredList,
{
itemHeight: 22,
},
)
```
```
<template>
<p>Showing {{ isEven ? 'even' : 'odd' }} items</p>
<button @click="toggle">Toggle Even/Odd</button>
<div v-bind="containerProps" style="height: 300px">
<div v-bind="wrapperProps">
<div v-for="item in list" :key="item.index" style="height: 22px">
Row: {{ item.data }}
</div>
</div>
</div>
</template>
```
### Horizontal list
```
import { useVirtualList } from '@vueuse/core'
const allItems = Array.from(Array(99999).keys())
const { list, containerProps, wrapperProps } = useVirtualList(
allItems,
{
itemWidth: 200,
},
)
```
```
<template>
<div v-bind="containerProps" style="height: 300px">
<div v-bind="wrapperProps">
<div v-for="item in list" :key="item.index" style="width: 200px">
Row: {{ item.data }}
</div>
</div>
</div>
</template>
```
Component Usage
---------------
> This function also provides a renderless component version via the `@vueuse/components` package. [Learn more about the usage](../../guide/components).
>
>
```
<UseVirtualList :list="list" :options="options" height="300px">
<template #="props">
<!-- you can get current item of list here -->
<div style="height: 22px">Row {{ props.data }}</div>
</template>
</UseVirtualList>
```
To scroll to a specific element, the component exposes `scrollTo(index: number) => void`.
Type Declarations
-----------------
Show Type Declarations
```
type UseVirtualListItemSize = number | ((index: number) => number)
export interface UseHorizontalVirtualListOptions
extends UseVirtualListOptionsBase {
/**
* item width, accept a pixel value or a function that returns the height
*
* @default 0
*/
itemWidth: UseVirtualListItemSize
}
export interface UseVerticalVirtualListOptions
extends UseVirtualListOptionsBase {
/**
* item height, accept a pixel value or a function that returns the height
*
* @default 0
*/
itemHeight: UseVirtualListItemSize
}
export interface UseVirtualListOptionsBase {
/**
* the extra buffer items outside of the view area
*
* @default 5
*/
overscan?: number
}
export type UseVirtualListOptions =
| UseHorizontalVirtualListOptions
| UseVerticalVirtualListOptions
export interface UseVirtualListItem<T> {
data: T
index: number
}
export interface UseVirtualListReturn<T> {
list: Ref<UseVirtualListItem<T>[]>
scrollTo: (index: number) => void
containerProps: {
ref: Ref<HTMLElement | null>
onScroll: () => void
style: StyleValue
}
wrapperProps: ComputedRef<{
style:
| {
width: string
height: string
marginTop: string
}
| {
width: string
height: string
marginLeft: string
display: string
}
}>
}
export declare function useVirtualList<T = any>(
list: MaybeRef<T[]>,
options: UseVirtualListOptions
): UseVirtualListReturn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/core/useVirtualList/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/core/useVirtualList/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/core/useVirtualList/index.md)
| programming_docs |
vueuse useThrottleFn useThrottleFn
=============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 400 B |
| Last Changed | 2 days ago |
| Related | [`refDebounced`](../refdebounced/index)[`refThrottled`](../refthrottled/index)[`useDebounceFn`](../usedebouncefn/index) |
Throttle execution of a function. Especially useful for rate limiting execution of handlers on events like resize and scroll.
> Throttle is a spring that throws balls: after a ball flies out it needs some time to shrink back, so it cannot throw any more balls unless it's ready.
>
>
Usage
-----
```
import { useThrottleFn } from '@vueuse/core'
const throttledFn = useThrottleFn(() => {
// do something, it will be called at most 1 time per second
}, 1000)
window.addEventListener('resize', throttledFn)
```
Recommended Reading
-------------------
* [**Debounce vs Throttle**: Definitive Visual Guide](https://redd.one/blog/debounce-vs-throttle)
Type Declarations
-----------------
Show Type Declarations
```
/**
* Throttle execution of a function. Especially useful for rate limiting
* execution of handlers on events like resize and scroll.
*
* @param fn A function to be executed after delay milliseconds. The `this` context and all arguments are passed through, as-is,
* to `callback` when the throttled-function is executed.
* @param ms A zero-or-greater delay in milliseconds. For event callbacks, values around 100 or 250 (or even higher) are most useful.
*
* @param [trailing=false] if true, call fn again after the time is up
*
* @param [leading=true] if true, call fn on the leading edge of the ms timeout
*
* @param [rejectOnCancel=false] if true, reject the last call if it's been cancel
*
* @return A new, throttled, function.
*/
export declare function useThrottleFn<T extends FunctionArgs>(
fn: T,
ms?: MaybeComputedRef<number>,
trailing?: boolean,
leading?: boolean,
rejectOnCancel?: boolean
): PromisifyFn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useThrottleFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useThrottleFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useThrottleFn/index.md)
vueuse tryOnMounted tryOnMounted
============
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 191 B |
| Last Changed | last year |
Safe `onMounted`. Call `onMounted()` if it's inside a component lifecycle, if not, just call the function
Usage
-----
```
import { tryOnMounted } from '@vueuse/core'
tryOnMounted(() => {
})
```
Type Declarations
-----------------
```
/**
* Call onMounted() if it's inside a component lifecycle, if not, just call the function
*
* @param fn
* @param sync if set to false, it will run in the nextTick() of Vue
*/
export declare function tryOnMounted(fn: Fn, sync?: boolean): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnMounted/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnMounted/index.md)
vueuse useLastChanged useLastChanged
==============
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 218 B |
| Last Changed | last year |
Records the timestamp of the last change
Usage
-----
```
import { useLastChanged } from '@vueuse/core'
const a = ref(0)
const lastChanged = useLastChanged(a)
a.value = 1
console.log(lastChanged.value)
```
Type Declarations
-----------------
```
export interface UseLastChangedOptions<
Immediate extends boolean,
InitialValue extends number | null | undefined = undefined
> extends WatchOptions<Immediate> {
initialValue?: InitialValue
}
/**
* Records the timestamp of the last change
*
* @see https://vueuse.org/useLastChanged
*/
export declare function useLastChanged(
source: WatchSource,
options?: UseLastChangedOptions<false>
): Ref<number | null>
export declare function useLastChanged(
source: WatchSource,
options: UseLastChangedOptions<true>
): Ref<number>
export declare function useLastChanged(
source: WatchSource,
options: UseLastChangedOptions<boolean, number>
): Ref<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useLastChanged/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useLastChanged/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useLastChanged/index.md)
*No recent changes*
vueuse reactiveOmit reactiveOmit
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 407 B |
| Last Changed | 6 months ago |
Reactively omit fields from a reactive object.
Usage
-----
```
import { reactiveOmit } from '@vueuse/core'
const obj = reactive({
x: 0,
y: 0,
elementX: 0,
elementY: 0,
})
const picked = reactiveOmit(obj, 'x', 'elementX') // { y: number, elementY: number }
```
### Scenarios
#### Selectively passing props to child
```
<script setup>
import { reactiveOmit } from '@vueuse/core'
const props = defineProps({
value: {
default: 'value',
},
color: {
type: String,
},
font: {
type: String,
}
})
const childProps = reactiveOmit(props, 'value')
</script>
<template>
<div>
<!-- only passes "color" and "font" props to child -->
<ChildComp v-bind="childProps" />
</div>
</template>
```
Type Declarations
-----------------
```
/**
* Reactively omit fields from a reactive object
*
* @see https://vueuse.org/reactiveOmit
*/
export declare function reactiveOmit<T extends object, K extends keyof T>(
obj: T,
...keys: (K | K[])[]
): Omit<T, K>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactiveOmit/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactiveOmit/index.md)
vueuse useArrayFilter useArrayFilter
==============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 209 B |
| Last Changed | 5 months ago |
Reactive `Array.filter`
Usage
-----
### Use with array of multiple refs
```
import { useArrayFilter } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(2)
const item3 = ref(4)
const item4 = ref(6)
const item5 = ref(8)
const list = [item1, item2, item3, item4, item5]
const result = useArrayFilter(list, i => i % 2 === 0)
// result.value: [0, 2, 4, 6, 8]
item2.value = 1
// result.value: [0, 4, 6, 8]
```
### Use with reactive array
```
import { useArrayFilter } from '@vueuse/core'
const list = ref([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
const result = useArrayFilter(list, i => i % 2 === 0)
// result.value: [0, 2, 4, 6, 8]
list.value.shift()
// result.value: [2, 4, 6, 8]
```
Type Declarations
-----------------
```
/**
* Reactive `Array.filter`
*
* @see https://vueuse.org/useArrayFilter
* @param {Array} list - the array was called upon.
* @param fn - a function that is called for every element of the given `list`. Each time `fn` executes, the returned value is added to the new array.
*
* @returns {Array} a shallow copy of a portion of the given array, filtered down to just the elements from the given array that pass the test implemented by the provided function. If no elements pass the test, an empty array will be returned.
*/
export declare function useArrayFilter<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: T[]) => boolean
): ComputedRef<T[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFilter/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFilter/index.md)
vueuse useArrayFind useArrayFind
============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 218 B |
| Last Changed | 5 months ago |
Reactive `Array.find`.
Usage
-----
```
import { useArrayFind } from '@vueuse/core'
const list = [ref(1), ref(-1), ref(2)]
const positive = useArrayFind(list, val => val > 0)
// positive.value: 1
```
### Use with reactive array
```
import { useArrayFind } from '@vueuse/core'
const list = reactive([-1, -2])
const positive = useArrayFind(list, val => val > 0)
// positive.value: undefined
list.push(1)
// positive.value: 1
```
Type Declarations
-----------------
```
/**
* Reactive `Array.find`
*
* @see https://vueuse.org/useArrayFind
* @param {Array} list - the array was called upon.
* @param fn - a function to test each element.
*
* @returns the first element in the array that satisfies the provided testing function. Otherwise, undefined is returned.
*/
export declare function useArrayFind<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: MaybeComputedRef<T>[]) => boolean
): ComputedRef<T | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFind/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFind/index.md)
vueuse createInjectionState createInjectionState
====================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 200 B |
| Last Changed | 10 months ago |
Create global state that can be injected into components.
Usage
-----
```
// useCounterStore.ts
import { computed, ref } from 'vue-demi'
import { createInjectionState } from '@vueuse/shared'
const [useProvideCounterStore, useCounterStore] = createInjectionState((initialValue: number) => {
// state
const count = ref(initialValue)
// getters
const double = computed(() => count.value * 2)
// actions
function increment() {
count.value++
}
return { count, double, increment }
})
export { useProvideCounterStore }
// If you want to hide `useCounterStore` and wrap it in default value logic or throw error logic, please don't export `useCounterStore`
export { useCounterStore }
export function useCounterStoreWithDefaultValue() {
return useCounterStore() ?? {
count: ref(0),
double: ref(0),
increment: () => {},
}
}
export function useCounterStoreOrThrow() {
const counterStore = useCounterStore()
if (counterStore == null)
throw new Error('Please call `useProvideCounterStore` on the appropriate parent component')
return counterStore
}
```
```
<!-- RootComponent.vue -->
<script setup lang="ts">
import { useProvideCounterStore } from './useCounterStore'
useProvideCounterStore(0)
</script>
<template>
<div>
<slot />
</div>
</template>
```
```
<!-- CountComponent.vue -->
<script setup lang="ts">
import { useCounterStore } from './useCounterStore'
// use non-null assertion operator to ignore the case that store is not provided.
const { count, double } = useCounterStore()!
// if you want to allow component to working without providing store, you can use follow code instead:
// const { count, double } = useCounterStore() ?? { count: ref(0), double: ref(0) }
// also, you can use another hook to provide default value
// const { count, double } = useCounterStoreWithDefaultValue()
// or throw error
// const { count, double } = useCounterStoreOrThrow()
</script>
<template>
<ul>
<li>
count: {{ count }}
</li>
<li>
double: {{ double }}
</li>
</ul>
</template>
```
```
<!-- ButtonComponent.vue -->
<script setup lang="ts">
import { useCounterStore } from './useCounterStore'
// use non-null assertion operator to ignore the case that store is not provided.
const { increment } = useCounterStore()!
</script>
<template>
<button @click="increment">
+
</button>
</template>
```
Type Declarations
-----------------
```
/**
* Create global state that can be injected into components.
*
* @see https://vueuse.org/createInjectionState
*
*/
export declare function createInjectionState<
Arguments extends Array<any>,
Return
>(
composable: (...args: Arguments) => Return
): readonly [
useProvidingState: (...args: Arguments) => void,
useInjectedState: () => Return | undefined
]
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/createInjectionState/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/createInjectionState/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/createInjectionState/index.md)
vueuse useIntervalFn useIntervalFn
=============
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 394 B |
| Last Changed | 3 weeks ago |
Wrapper for `setInterval` with controls
Usage
-----
```
import { useIntervalFn } from '@vueuse/core'
const { pause, resume, isActive } = useIntervalFn(() => {
/* your function */
}, 1000)
```
Type Declarations
-----------------
```
export interface UseIntervalFnOptions {
/**
* Start the timer immediately
*
* @default true
*/
immediate?: boolean
/**
* Execute the callback immediate after calling this function
*
* @default false
*/
immediateCallback?: boolean
}
/**
* Wrapper for `setInterval` with controls
*
* @param cb
* @param interval
* @param options
*/
export declare function useIntervalFn(
cb: Fn,
interval?: MaybeComputedRef<number>,
options?: UseIntervalFnOptions
): Pausable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useIntervalFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useIntervalFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useIntervalFn/index.md)
vueuse useInterval useInterval
===========
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 638 B |
| Last Changed | 3 months ago |
Reactive counter increases on every interval
Usage
-----
```
import { useInterval } from '@vueuse/core'
// count will increase every 200ms
const counter = useInterval(200)
```
```
const { counter, pause, resume } = useInterval(200, { controls: true })
```
Type Declarations
-----------------
```
export interface UseIntervalOptions<Controls extends boolean> {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
/**
* Execute the update immediately on calling
*
* @default true
*/
immediate?: boolean
/**
* Callback on every interval
*/
callback?: (count: number) => void
}
/**
* Reactive counter increases on every interval
*
* @see https://vueuse.org/useInterval
* @param interval
* @param options
*/
export declare function useInterval(
interval?: MaybeComputedRef<number>,
options?: UseIntervalOptions<false>
): Ref<number>
export declare function useInterval(
interval: MaybeComputedRef<number>,
options: UseIntervalOptions<true>
): {
counter: Ref<number>
} & Pausable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useInterval/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useInterval/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useInterval/index.md)
vueuse makeDestructurable makeDestructurable
==================
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 430 B |
| Last Changed | 4 months ago |
Make isomorphic destructurable for object and array at the same time. See [this blog](https://antfu.me/posts/destructuring-with-object-or-array/) for more details.
Usage
-----
TypeScript Example:
```
import { makeDestructurable } from '@vueuse/core'
const foo = { name: 'foo' }
const bar = 1024
const obj = makeDestructurable(
{ foo, bar } as const,
[ foo, bar ] as const,
)
```
Usage:
```
let { foo, bar } = obj
let [ foo, bar ] = obj
```
Type Declarations
-----------------
```
export declare function makeDestructurable<
T extends Record<string, unknown>,
A extends readonly any[]
>(obj: T, arr: A): T & A
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/makeDestructurable/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/makeDestructurable/index.md)
*No recent changes*
vueuse watchOnce watchOnce
=========
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 187 B |
| Last Changed | 4 months ago |
`watch` that only triggers once.
Usage
-----
After the callback function has been triggered once, the watch will be stopped automatically.
```
import { watchOnce } from '@vueuse/core'
watchOnce(source, () => {
// triggers only once
console.log('source changed!')
})
```
Type Declarations
-----------------
```
export declare function watchOnce<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
source: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchOptions<Immediate>
): void
export declare function watchOnce<
T,
Immediate extends Readonly<boolean> = false
>(
sources: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchOptions<Immediate>
): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchOnce/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchOnce/index.md)
vueuse useArraySome useArraySome
============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 215 B |
| Last Changed | 5 months ago |
Reactive `Array.some`
Usage
-----
### Use with array of multiple refs
```
import { useArraySome } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(2)
const item3 = ref(4)
const item4 = ref(6)
const item5 = ref(8)
const list = [item1, item2, item3, item4, item5]
const result = useArraySome(list, i => i > 10)
// result.value: false
item1.value = 11
// result.value: true
```
### Use with reactive array
```
import { useArraySome } from '@vueuse/core'
const list = ref([0, 2, 4, 6, 8])
const result = useArraySome(list, i => i > 10)
// result.value: false
list.value.push(11)
// result.value: true
```
Type Declarations
-----------------
```
/**
* Reactive `Array.some`
*
* @see https://vueuse.org/useArraySome
* @param {Array} list - the array was called upon.
* @param fn - a function to test each element.
*
* @returns {boolean} **true** if the `fn` function returns a **truthy** value for any element from the array. Otherwise, **false**.
*/
export declare function useArraySome<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: MaybeComputedRef<T>[]) => unknown
): ComputedRef<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArraySome/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArraySome/index.md)
vueuse useTimeout useTimeout
==========
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 621 B |
| Last Changed | 3 months ago |
Update value after a given time with controls.
Usage
-----
```
import { promiseTimeout, useTimeout } from '@vueuse/core'
const ready = useTimeout(1000)
```
```
const { ready, start, stop } = useTimeout(1000, { controls: true })
```
```
console.log(ready.value) // false
await promiseTimeout(1200)
console.log(ready.value) // true
```
Type Declarations
-----------------
```
export interface UseTimeoutOptions<Controls extends boolean>
extends UseTimeoutFnOptions {
/**
* Expose more controls
*
* @default false
*/
controls?: Controls
/**
* Callback on timeout
*/
callback?: Fn
}
/**
* Update value after a given time with controls.
*
* @see {@link https://vueuse.org/useTimeout}
* @param interval
* @param options
*/
export declare function useTimeout(
interval?: number,
options?: UseTimeoutOptions<false>
): ComputedRef<boolean>
export declare function useTimeout(
interval: number,
options: UseTimeoutOptions<true>
): {
ready: ComputedRef<boolean>
} & Stoppable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeout/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeout/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeout/index.md)
| programming_docs |
vueuse watchIgnorable watchIgnorable
==============
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 763 B |
| Last Changed | 7 months ago |
| Alias | `ignorableWatch` |
Ignorable watch
Usage
-----
Extended `watch` that returns extra `ignoreUpdates(updater)` and `ignorePrevAsyncUpdates()` to ignore particular updates to the source.
```
import { watchIgnorable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref('foo')
const { stop, ignoreUpdates } = watchIgnorable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // logs: Changed to bar!
ignoreUpdates(() => {
source.value = 'foobar'
})
await nextTick() // (nothing happened)
source.value = 'hello'
await nextTick() // logs: Changed to hello!
ignoreUpdates(() => {
source.value = 'ignored'
})
source.value = 'logged'
await nextTick() // logs: Changed to logged!
```
Flush timing
------------
[`watchIgnorable`](index)accepts the same options as `watch` and uses the same defaults. So, by default the composable works using `flush: 'pre'`.
`ignorePrevAsyncUpdates`
-------------------------
This feature is only for async flush `'pre'` and `'post'`. If `flush: 'sync'` is used, `ignorePrevAsyncUpdates()` is a no-op as the watch will trigger immediately after each update to the source. It is still provided for sync flush so the code can be more generic.
```
import { watchIgnorable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref('foo')
const { ignorePrevAsyncUpdates } = watchIgnorable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // logs: Changed to bar!
source.value = 'good'
source.value = 'by'
ignorePrevAsyncUpdates()
await nextTick() // (nothing happened)
source.value = 'prev'
ignorePrevAsyncUpdates()
source.value = 'after'
await nextTick() // logs: Changed to after!
```
Recommended Readings
--------------------
* [Ignorable Watch](https://patak.dev/vue/ignorable-watch.html) - by [@matias-capeletto](https://github.com/matias-capeletto)
Type Declarations
-----------------
Show Type Declarations
```
export type IgnoredUpdater = (updater: () => void) => void
export interface WatchIgnorableReturn {
ignoreUpdates: IgnoredUpdater
ignorePrevAsyncUpdates: () => void
stop: WatchStopHandle
}
export declare function watchIgnorable<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export declare function watchIgnorable<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export declare function watchIgnorable<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchIgnorableReturn
export { watchIgnorable as ignorableWatch }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchIgnorable/index.md)
vueuse tryOnUnmounted tryOnUnmounted
==============
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 169 B |
| Last Changed | last year |
Safe `onUnmounted`. Call `onUnmounted()` if it's inside a component lifecycle, if not, do nothing
Usage
-----
```
import { tryOnUnmounted } from '@vueuse/core'
tryOnUnmounted(() => {
})
```
Type Declarations
-----------------
```
/**
* Call onUnmounted() if it's inside a component lifecycle, if not, do nothing
*
* @param fn
*/
export declare function tryOnUnmounted(fn: Fn): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnUnmounted/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnUnmounted/index.md)
vueuse resolveUnref resolveUnref
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 176 B |
| Last Changed | 2 months ago |
| Related | [`resolveRef`](../resolveref/index) |
Get the value of value/ref/getter.
Usage
-----
```
import { resolveUnref } from '@vueuse/core'
const foo = ref('hi')
const a = resolveUnref(0) // 0
const b = resolveUnref(foo) // 'hi'
const c = resolveUnref(() => 'hi') // 'hi'
```
Type Declarations
-----------------
```
/**
* Get the value of value/ref/getter.
*/
export declare function resolveUnref<T>(r: MaybeComputedRef<T>): T
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/resolveUnref/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/resolveUnref/index.md)
vueuse toRefs toRefs
======
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 525 B |
| Last Changed | 7 months ago |
Extended [`toRefs`](https://v3.vuejs.org/api/refs-api.html#torefs) that also accepts refs of an object.
Usage
-----
```
import { toRefs } from '@vueuse/core'
import { reactive, ref } from 'vue-demi'
const objRef = ref({ a: 'a', b: 0 })
const arrRef = ref(['a', 0])
const { a, b } = toRefs(objRef)
const [ a, b ] = toRefs(arrRef)
const obj = reactive({ a: 'a', b: 0 })
const arr = reactive(['a', 0])
const { a, b } = toRefs(obj)
const [ a, b ] = toRefs(arr)
```
Use-cases
---------
### Destructuring a props object
```
<template>
<div>
<input v-model="a" type="text" />
<input v-model="b" type="text" />
</div>
</template>
<script lang="ts">
import { toRefs, useVModel } from '@vueuse/core'
export default {
setup(props) {
const refs = toRefs(useVModel(props, 'data'))
console.log(refs.a.value) // props.data.a
refs.a.value = 'a' // emit('update:data', { ...props.data, a: 'a' })
return { ...refs }
}
}
</script>
```
Type Declarations
-----------------
```
/**
* Extended `toRefs` that also accepts refs of an object.
*
* @see https://vueuse.org/toRefs
* @param objectRef A ref or normal object or array.
*/
export declare function toRefs<T extends object>(
objectRef: MaybeRef<T>
): ToRefs<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/toRefs/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/toRefs/index.md)
vueuse extendRef extendRef
=========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 330 B |
| Last Changed | 2 weeks ago |
Add extra attributes to Ref.
Usage
-----
> This function is **NOT compatible with Vue 2.6 or below**.
>
>
> Please note the extra attribute will not be accessible in Vue's template.
>
>
```
import { ref } from 'vue'
import { extendRef } from '@vueuse/core'
const myRef = ref('content')
const extended = extendRef(myRef, { foo: 'extra data' })
extended.value === 'content'
extended.foo === 'extra data'
```
Refs will be unwrapped and be reactive
```
const myRef = ref('content')
const extraRef = ref('extra')
const extended = extendRef(myRef, { extra: extraRef })
extended.value === 'content'
extended.extra === 'extra'
extended.extra = 'new data' // will trigger update
extraRef.value === 'new data'
```
Type Declarations
-----------------
```
export interface ExtendRefOptions<Unwrap extends boolean = boolean> {
/**
* Is the extends properties enumerable
*
* @default false
*/
enumerable?: boolean
/**
* Unwrap for Ref properties
*
* @default true
*/
unwrap?: Unwrap
}
/**
* Overload 1: Unwrap set to false
*/
export declare function extendRef<
R extends Ref<any>,
Extend extends object,
Options extends ExtendRefOptions<false>
>(ref: R, extend: Extend, options?: Options): ShallowUnwrapRef<Extend> & R
/**
* Overload 2: Unwrap unset or set to true
*/
export declare function extendRef<
R extends Ref<any>,
Extend extends object,
Options extends ExtendRefOptions
>(ref: R, extend: Extend, options?: Options): Extend & R
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/extendRef/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/extendRef/index.md)
vueuse createGlobalState createGlobalState
=================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 197 B |
| Last Changed | last year |
| Related | [`createSharedComposable`](../createsharedcomposable/index) |
Keep states in the global scope to be reusable across Vue instances.
Usage
-----
### Without Persistence (Store in Memory)
```
// store.js
import { ref } from 'vue'
import { createGlobalState } from '@vueuse/core'
export const useGlobalState = createGlobalState(
() => {
const count = ref(0)
return { count }
}
)
```
A bigger example:
```
// store.js
import { computed, ref } from 'vue'
import { createGlobalState } from '@vueuse/core'
export const useGlobalState = createGlobalState(
() => {
// state
const count = ref(0)
// getters
const doubleCount = computed(() => count.value * 2)
// actions
function increment() {
count.value++
}
return { count, doubleCount, increment }
}
)
```
### With Persistence
Store in `localStorage` with `useStorage()`:
```
// store.js
import { createGlobalState, useStorage } from '@vueuse/core'
export const useGlobalState = createGlobalState(
() => useStorage('vueuse-local-storage', 'initialValue'),
)
```
```
// component.js
import { useGlobalState } from './store'
export default defineComponent({
setup() {
const state = useGlobalState()
return { state }
},
})
```
Type Declarations
-----------------
```
export type CreateGlobalStateReturn<T> = () => T
/**
* Keep states in the global scope to be reusable across Vue instances.
*
* @see https://vueuse.org/createGlobalState
* @param stateFactory A factory function to create the state
*/
export declare function createGlobalState<T>(
stateFactory: () => T
): CreateGlobalStateReturn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/createGlobalState/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/createGlobalState/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/createGlobalState/index.md)
*No recent changes*
vueuse tryOnBeforeMount tryOnBeforeMount
================
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 192 B |
| Last Changed | 7 months ago |
Safe `onBeforeMount`. Call `onBeforeMount()` if it's inside a component lifecycle, if not, just call the function
Usage
-----
```
import { tryOnBeforeMount } from '@vueuse/core'
tryOnBeforeMount(() => {
})
```
Type Declarations
-----------------
```
/**
* Call onBeforeMount() if it's inside a component lifecycle, if not, just call the function
*
* @param fn
* @param sync if set to false, it will run in the nextTick() of Vue
*/
export declare function tryOnBeforeMount(fn: Fn, sync?: boolean): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnBeforeMount/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnBeforeMount/index.md)
vueuse tryOnScopeDispose tryOnScopeDispose
=================
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 183 B |
| Last Changed | last year |
Safe `onScopeDispose`. Call `onScopeDispose()` if it's inside a effect scope lifecycle, if not, do nothing
Usage
-----
```
import { tryOnScopeDispose } from '@vueuse/core'
tryOnScopeDispose(() => {
})
```
Type Declarations
-----------------
```
/**
* Call onScopeDispose() if it's inside a effect scope lifecycle, if not, do nothing
*
* @param fn
*/
export declare function tryOnScopeDispose(fn: Fn): boolean
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnScopeDispose/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnScopeDispose/index.md)
*No recent changes*
vueuse useArrayFindIndex useArrayFindIndex
=================
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 220 B |
| Last Changed | 5 months ago |
Reactive `Array.findIndex`
Usage
-----
### Use with array of multiple refs
```
import { useArrayFindIndex } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(2)
const item3 = ref(4)
const item4 = ref(6)
const item5 = ref(8)
const list = [item1, item2, item3, item4, item5]
const result = useArrayFindIndex(list, i => i % 2 === 0)
// result.value: 0
item1.value = 1
// result.value: 1
```
### Use with reactive array
```
import { useArrayFindIndex } from '@vueuse/core'
const list = ref([0, 2, 4, 6, 8])
const result = useArrayFindIndex(list, i => i % 2 === 0)
// result.value: 0
list.value.unshift(-1)
// result.value: 1
```
Type Declarations
-----------------
```
/**
* Reactive `Array.findIndex`
*
* @see https://vueuse.org/useArrayFindIndex
* @param {Array} list - the array was called upon.
* @param fn - a function to test each element.
*
* @returns {number} the index of the first element in the array that passes the test. Otherwise, "-1".
*/
export declare function useArrayFindIndex<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: MaybeComputedRef<T>[]) => unknown
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFindIndex/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayFindIndex/index.md)
vueuse refWithControl refWithControl
==============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 516 B |
| Last Changed | 4 months ago |
| Alias | `controlledRef` |
| Related | [`computedWithControl`](../computedwithcontrol/index) |
Fine-grained controls over ref and its reactivity. (Vue 3 Only)
Usage
-----
[`refWithControl`](index)uses [`extendRef`](../extendref/index)to provide two extra functions [`get`](../get/index)and [`set`](../set/index)to have better control over when it should track/trigger the reactivity.
```
import { refWithControl } from '@vueuse/core'
const num = refWithControl(0)
const doubled = computed(() => num.value * 2)
// just like normal ref
num.value = 42
console.log(num.value) // 42
console.log(doubled.value) // 84
// set value without triggering the reactivity
num.set(30, false)
console.log(num.value) // 30
console.log(doubled.value) // 84 (doesn't update)
// get value without tracking the reactivity
watchEffect(() => {
console.log(num.peek())
}) // 30
num.value = 50 // watch effect wouldn't be triggered since it collected nothing.
console.log(doubled.value) // 100 (updated again since it's a reactive set)
```
###
`peek`, `lay`, `untrackedGet`, `silentSet`
We also provide some shorthands for doing the get/set without track/triggering the reactivity system. The following lines are equivalent.
```
const foo = refWithControl('foo')
```
```
// getting
foo.get(false)
foo.untrackedGet()
foo.peek() // an alias for `untrackedGet`
```
```
// setting
foo.set('bar', false)
foo.silentSet('bar')
foo.lay('bar') // an alias for `silentSet`
```
Configurations
--------------
###
`onBeforeChange()`
`onBeforeChange` option is offered to give control over if a new value should be accepted. For example:
```
const num = refWithControl(0, {
onBeforeChange(value, oldValue) {
// disallow changes larger then ±5 in one operation
if (Math.abs(value - oldValue) > 5)
return false // returning `false` to dismiss the change
},
})
num.value += 1
console.log(num.value) // 1
num.value += 6
console.log(num.value) // 1 (change been dismissed)
```
###
`onChanged()`
`onChanged` option offers a similar functionally as Vue's `watch` but being synchronoused with less overhead compare to `watch`.
```
const num = refWithControl(0, {
onChanged(value, oldValue) {
console.log(value)
},
})
```
Type Declarations
-----------------
```
export interface ControlledRefOptions<T> {
/**
* Callback function before the ref changing.
*
* Returning `false` to dismiss the change.
*/
onBeforeChange?: (value: T, oldValue: T) => void | boolean
/**
* Callback function after the ref changed
*
* This happens synchronously, with less overhead compare to `watch`
*/
onChanged?: (value: T, oldValue: T) => void
}
/**
* Explicitly define the deps of computed.
*
* @param source
* @param fn
*/
export declare function refWithControl<T>(
initial: T,
options?: ControlledRefOptions<T>
): ShallowUnwrapRef<{
get: (tracking?: boolean) => T
set: (value: T, triggering?: boolean) => void
untrackedGet: () => T
silentSet: (v: T) => void
peek: () => T
lay: (v: T) => void
}> &
Ref<T>
/**
* Alias for `refWithControl`
*/
export declare const controlledRef: typeof refWithControl
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/refWithControl/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/refWithControl/index.md)
*No recent changes*
vueuse until until
=====
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 653 B |
| Last Changed | 3 months ago |
Promised one-time watch for changes
Usage
-----
#### Wait for some async data to be ready
```
import { until, useAsyncState } from '@vueuse/core'
const { state, isReady } = useAsyncState(
fetch('https://jsonplaceholder.typicode.com/todos/1').then(t => t.json()),
{},
)
;(async () => {
await until(isReady).toBe(true)
console.log(state) // state is now ready!
})()
```
#### Wait for custom conditions
> You can use `invoke` to call the async function.
>
>
```
import { invoke, until, useCounter } from '@vueuse/core'
const { count } = useCounter()
invoke(async () => {
await until(count).toMatch(v => v > 7)
alert('Counter is now larger than 7!')
})
```
#### Timeout
```
// will be resolve until ref.value === true or 1000ms passed
await until(ref).toBe(true, { timeout: 1000 })
// will throw if timeout
try {
await until(ref).toBe(true, { timeout: 1000, throwOnTimeout: true })
// ref.value === true
}
catch (e) {
// timeout
}
```
#### More Examples
```
await until(ref).toBe(true)
await until(ref).toMatch(v => v > 10 && v < 100)
await until(ref).changed()
await until(ref).changedTimes(10)
await until(ref).toBeTruthy()
await until(ref).toBeNull()
await until(ref).not.toBeNull()
await until(ref).not.toBeTruthy()
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UntilToMatchOptions {
/**
* Milliseconds timeout for promise to resolve/reject if the when condition does not meet.
* 0 for never timed out
*
* @default 0
*/
timeout?: number
/**
* Reject the promise when timeout
*
* @default false
*/
throwOnTimeout?: boolean
/**
* `flush` option for internal watch
*
* @default 'sync'
*/
flush?: WatchOptions["flush"]
/**
* `deep` option for internal watch
*
* @default 'false'
*/
deep?: WatchOptions["deep"]
}
export interface UntilBaseInstance<T, Not extends boolean = false> {
toMatch<U extends T = T>(
condition: (v: T) => v is U,
options?: UntilToMatchOptions
): Not extends true ? Promise<Exclude<T, U>> : Promise<U>
toMatch(
condition: (v: T) => boolean,
options?: UntilToMatchOptions
): Promise<T>
changed(options?: UntilToMatchOptions): Promise<T>
changedTimes(n?: number, options?: UntilToMatchOptions): Promise<T>
}
type Falsy = false | void | null | undefined | 0 | 0n | ""
export interface UntilValueInstance<T, Not extends boolean = false>
extends UntilBaseInstance<T, Not> {
readonly not: UntilValueInstance<T, Not extends true ? false : true>
toBe<P = T>(
value: MaybeComputedRef<P>,
options?: UntilToMatchOptions
): Not extends true ? Promise<T> : Promise<P>
toBeTruthy(
options?: UntilToMatchOptions
): Not extends true ? Promise<T & Falsy> : Promise<Exclude<T, Falsy>>
toBeNull(
options?: UntilToMatchOptions
): Not extends true ? Promise<Exclude<T, null>> : Promise<null>
toBeUndefined(
options?: UntilToMatchOptions
): Not extends true ? Promise<Exclude<T, undefined>> : Promise<undefined>
toBeNaN(options?: UntilToMatchOptions): Promise<T>
}
export interface UntilArrayInstance<T> extends UntilBaseInstance<T> {
readonly not: UntilArrayInstance<T>
toContains(
value: MaybeComputedRef<ElementOf<ShallowUnwrapRef<T>>>,
options?: UntilToMatchOptions
): Promise<T>
}
/**
* Promised one-time watch for changes
*
* @see https://vueuse.org/until
* @example
* ```
* const { count } = useCounter()
*
* await until(count).toMatch(v => v > 7)
*
* alert('Counter is now larger than 7!')
* ```
*/
export declare function until<T extends unknown[]>(
r: WatchSource<T> | MaybeComputedRef<T>
): UntilArrayInstance<T>
export declare function until<T>(
r: WatchSource<T> | MaybeComputedRef<T>
): UntilValueInstance<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/until/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/until/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/until/index.md)
| programming_docs |
vueuse isDefined isDefined
=========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 158 B |
| Last Changed | last year |
Non-nullish checking type guard for Ref.
Usage
-----
```
import { isDefined } from '@vueuse/core'
const example = ref(Math.random() ? 'example' : undefined) // Ref<string | undefined>
if (isDefined(example))
example // Ref<string>
```
Type Declarations
-----------------
```
export declare function isDefined<T>(
v: Ref<T>
): v is Ref<Exclude<T, null | undefined>>
export declare function isDefined<T>(
v: ComputedRef<T>
): v is ComputedRef<Exclude<T, null | undefined>>
export declare function isDefined<T>(v: T): v is Exclude<T, null | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/isDefined/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/isDefined/index.md)
*No recent changes*
vueuse syncRefs syncRefs
========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 236 B |
| Last Changed | 10 months ago |
| Related | [`syncRef`](../syncref/index) |
Keep target refs in sync with a source ref
Usage
-----
```
import { syncRefs } from '@vueuse/core'
const source = ref('hello')
const target = ref('target')
const stop = syncRefs(source, target)
console.log(target.value) // hello
source.value = 'foo'
console.log(target.value) // foo
```
Watch options
-------------
The options for [`syncRefs`](index)are similar to `watch`'s `WatchOptions` but with different default values.
```
export interface SyncRefOptions {
/**
* Timing for syncing, same as watch's flush option
*
* @default 'sync'
*/
flush?: WatchOptions['flush']
/**
* Watch deeply
*
* @default false
*/
deep?: boolean
/**
* Sync values immediately
*
* @default true
*/
immediate?: boolean
}
```
When setting `{ flush: 'pre' }`, the target reference will be updated at [the end of the current "tick"](https://vuejs.org/guide/essentials/watchers.html#callback-flush-timing) before rendering starts.
```
import { syncRefs } from '@vueuse/core'
const source = ref('hello')
const target = ref('target')
syncRefs(source, target, { flush: 'pre' })
console.log(target.value) // hello
source.value = 'foo'
console.log(target.value) // hello <- still unchanged, because of flush 'pre'
await nextTick()
console.log(target.value) // foo <- changed!
```
Type Declarations
-----------------
```
export interface SyncRefsOptions extends ConfigurableFlushSync {
/**
* Watch deeply
*
* @default false
*/
deep?: boolean
/**
* Sync values immediately
*
* @default true
*/
immediate?: boolean
}
/**
* Keep target ref(s) in sync with the source ref
*
* @param source source ref
* @param targets
*/
export declare function syncRefs<T>(
source: WatchSource<T>,
targets: Ref<T> | Ref<T>[],
options?: SyncRefsOptions
): WatchStopHandle
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRefs/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRefs/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRefs/index.md)
vueuse useToggle useToggle
=========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 285 B |
| Last Changed | 6 months ago |
A boolean switcher with utility functions.
Usage
-----
```
import { useToggle } from '@vueuse/core'
const [value, toggle] = useToggle()
```
When you pass a ref, [`useToggle`](index)will return a simple toggle function instead:
```
import { useDark, useToggle } from '@vueuse/core'
const isDark = useDark()
const toggleDark = useToggle(isDark)
```
Note: be aware that the toggle function accepts the first argument as the override value. You might want to avoid directly passing the function to events in the template, as the event object will pass in.
```
<!-- caution: $event will be pass in -->
<button @click="toggleDark" />
<!-- recommended to do this -->
<button @click="toggleDark()" />
```
Type Declarations
-----------------
```
export interface UseToggleOptions<Truthy, Falsy> {
truthyValue?: MaybeComputedRef<Truthy>
falsyValue?: MaybeComputedRef<Falsy>
}
export declare function useToggle<Truthy, Falsy, T = Truthy | Falsy>(
initialValue: Ref<T>,
options?: UseToggleOptions<Truthy, Falsy>
): (value?: T) => T
export declare function useToggle<
Truthy = true,
Falsy = false,
T = Truthy | Falsy
>(
initialValue?: T,
options?: UseToggleOptions<Truthy, Falsy>
): [Ref<T>, (value?: T) => T]
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToggle/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToggle/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToggle/index.md)
vueuse reactiveComputed reactiveComputed
================
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 342 B |
| Last Changed | 10 months ago |
Computed reactive object. Instead of returning a ref that `computed` does, [`reactiveComputed`](index)returns a reactive object.
**This function uses [Proxy](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy)** It is **NOT** supported by [IE 11 or below](https://caniuse.com/proxy).
Usage
-----
```
import { reactiveComputed } from '@vueuse/core'
const state = reactiveComputed(() => {
return {
foo: 'bar',
bar: 'baz',
}
})
state.bar // 'baz'
```
Type Declarations
-----------------
```
/**
* Computed reactive object.
*/
export declare function reactiveComputed<T extends {}>(fn: () => T): T
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactiveComputed/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactiveComputed/index.md)
vueuse resolveRef resolveRef
==========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 188 B |
| Last Changed | 2 days ago |
| Related | [`resolveUnref`](../resolveunref/index) |
Normalize value/ref/getter to `ref` or `computed`.
Usage
-----
```
import { resolveRef } from '@vueuse/core'
const foo = ref('hi')
const a = resolveRef(0) // Ref<number>
const b = resolveRef(foo) // Ref<string>
const c = resolveRef(() => 'hi') // ComputedRef<string>
```
Type Declarations
-----------------
```
/**
* Normalize value/ref/getter to `ref` or `computed`.
*/
export declare function resolveRef<T>(r: MaybeComputedRef<T>): ComputedRef<T>
export declare function resolveRef<T>(r: MaybeRef<T>): Ref<T>
export declare function resolveRef<T>(r: T): Ref<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/resolveRef/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/resolveRef/index.md)
vueuse watchTriggerable watchTriggerable
================
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 987 B |
| Last Changed | 6 months ago |
Watch that can be triggered manually
Usage
-----
A `watch` wrapper that supports manual triggering of `WatchCallback`, which returns an additional `trigger` to execute a `WatchCallback` immediately.
```
import { watchTriggerable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref(0)
const { trigger, ignoreUpdates } = watchTriggerable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // logs: Changed to bar!
// Execution of WatchCallback via `trigger` does not require waiting
trigger() // logs: Changed to bar!
```
###
`onCleanup`
When you want to manually call a `watch` that uses the onCleanup parameter; simply taking the `WatchCallback` out and calling it doesn't make it easy to implement the `onCleanup` parameter.
Using [`watchTriggerable`](index)will solve this problem.
```
import { watchTriggerable } from '@vueuse/core'
import { ref } from 'vue'
const source = ref(0)
const { trigger } = watchTriggerable(
source,
async (v, _, onCleanup) => {
let canceled = false
onCleanup(() => canceled = true)
await new Promise(resolve => setTimeout(resolve, 500))
if (canceled)
return
console.log(`The value is "${v}"\n`)
},
)
source.value = 1 // no log
await trigger() // logs (after 500 ms): The value is "1"
```
Type Declarations
-----------------
Show Type Declarations
```
export interface WatchTriggerableReturn<FnReturnT = void>
extends WatchIgnorableReturn {
/** Execute `WatchCallback` immediately */
trigger: () => FnReturnT
}
type OnCleanup = (cleanupFn: () => void) => void
export type WatchTriggerableCallback<V = any, OV = any, R = void> = (
value: V,
oldValue: OV,
onCleanup: OnCleanup
) => R
export declare function watchTriggerable<
T extends Readonly<WatchSource<unknown>[]>,
FnReturnT
>(
sources: [...T],
cb: WatchTriggerableCallback<
MapSources<T>,
MapOldSources<T, true>,
FnReturnT
>,
options?: WatchWithFilterOptions<boolean>
): WatchTriggerableReturn<FnReturnT>
export declare function watchTriggerable<T, FnReturnT>(
source: WatchSource<T>,
cb: WatchTriggerableCallback<T, T | undefined, FnReturnT>,
options?: WatchWithFilterOptions<boolean>
): WatchTriggerableReturn<FnReturnT>
export declare function watchTriggerable<T extends object, FnReturnT>(
source: T,
cb: WatchTriggerableCallback<T, T | undefined, FnReturnT>,
options?: WatchWithFilterOptions<boolean>
): WatchTriggerableReturn<FnReturnT>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchTriggerable/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchTriggerable/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchTriggerable/index.md)
vueuse tryOnBeforeUnmount tryOnBeforeUnmount
==================
| | |
| --- | --- |
| Category | [Component](../../functions#category=Component) |
| Export Size | 173 B |
| Last Changed | last year |
Safe `onBeforeUnmount`. Call `onBeforeUnmount()` if it's inside a component lifecycle, if not, do nothing
Usage
-----
```
import { tryOnBeforeUnmount } from '@vueuse/core'
tryOnBeforeUnmount(() => {
})
```
Type Declarations
-----------------
```
/**
* Call onBeforeUnmount() if it's inside a component lifecycle, if not, do nothing
*
* @param fn
*/
export declare function tryOnBeforeUnmount(fn: Fn): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnBeforeUnmount/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/tryOnBeforeUnmount/index.md)
*No recent changes*
vueuse watchThrottled watchThrottled
==============
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 824 B |
| Last Changed | 6 months ago |
| Alias | `throttledWatch` |
Throttled watch.
Usage
-----
Similar to `watch`, but offering an extra option `throttle` which will be applied to the callback function.
```
import { watchThrottled } from '@vueuse/core'
watchThrottled(
source,
() => { console.log('changed!') },
{ throttle: 500 },
)
```
It's essentially a shorthand for the following code:
```
import { throttleFilter, watchWithFilter } from '@vueuse/core'
watchWithFilter(
source,
() => { console.log('changed!') },
{
eventFilter: throttleFilter(500),
},
)
```
Type Declarations
-----------------
```
export interface WatchThrottledOptions<Immediate>
extends WatchOptions<Immediate> {
throttle?: MaybeComputedRef<number>
trailing?: boolean
leading?: boolean
}
export declare function watchThrottled<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchThrottledOptions<Immediate>
): WatchStopHandle
export declare function watchThrottled<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchThrottledOptions<Immediate>
): WatchStopHandle
export declare function watchThrottled<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchThrottledOptions<Immediate>
): WatchStopHandle
export { watchThrottled as throttledWatch }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchThrottled/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchThrottled/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchThrottled/index.md)
vueuse reactivePick reactivePick
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 201 B |
| Last Changed | 6 months ago |
Reactively pick fields from a reactive object.
Usage
-----
```
import { reactivePick } from '@vueuse/core'
const obj = reactive({
x: 0,
y: 0,
elementX: 0,
elementY: 0,
})
const picked = reactivePick(obj, 'x', 'elementX') // { x: number, elementX: number }
```
### Scenarios
#### Selectively passing props to child
```
<script setup>
import { reactivePick } from '@vueuse/core'
const props = defineProps({
value: {
default: 'value',
},
color: {
type: String,
},
font: {
type: String,
}
})
const childProps = reactivePick(props, 'color', 'font')
</script>
<template>
<div>
<!-- only passes "color" and "font" props to child -->
<ChildComp v-bind="childProps" />
</div>
</template>
```
#### Selectively wrap reactive object
Instead of doing this
```
import { reactive } from 'vue'
import { useElementBounding } from '@vueuse/core'
const { height, width } = useElementBounding() // object of refs
const size = reactive({ height, width })
```
Now we can just have this
```
import { reactivePick, useElementBounding } from '@vueuse/core'
const size = reactivePick(useElementBounding(), 'height', 'width')
```
Type Declarations
-----------------
```
/**
* Reactively pick fields from a reactive object
*
* @see https://vueuse.org/reactivePick
*/
export declare function reactivePick<T extends object, K extends keyof T>(
obj: T,
...keys: (K | K[])[]
): {
[S in K]: UnwrapRef<T[S]>
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactivePick/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactivePick/index.md)
vueuse get get
===
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 179 B |
| Last Changed | last year |
Shorthand for accessing `ref.value`
Usage
-----
```
import { get } from '@vueuse/core'
const a = ref(42)
console.log(get(a)) // 42
```
Type Declarations
-----------------
```
/**
* Shorthand for accessing `ref.value`
*/
export declare function get<T>(ref: MaybeRef<T>): T
export declare function get<T, K extends keyof T>(
ref: MaybeRef<T>,
key: K
): T[K]
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/get/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/get/index.md)
*No recent changes*
vueuse reactifyObject reactifyObject
==============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 374 B |
| Last Changed | 2 months ago |
Apply [`reactify`](../reactify/index)to an object
Usage
-----
```
import { reactifyObject } from '@vueuse/core'
const reactifiedConsole = reactifyObject(console)
const a = ref('42')
reactifiedConsole.log(a) // no longer need `.value`
```
Type Declarations
-----------------
```
export type ReactifyNested<
T,
Keys extends keyof T = keyof T,
S extends boolean = true
> = {
[K in Keys]: T[K] extends (...args: any[]) => any ? Reactified<T[K], S> : T[K]
}
export interface ReactifyObjectOptions<T extends boolean>
extends ReactifyOptions<T> {
/**
* Includes names from Object.getOwnPropertyNames
*
* @default true
*/
includeOwnProperties?: boolean
}
/**
* Apply `reactify` to an object
*/
export declare function reactifyObject<T extends object, Keys extends keyof T>(
obj: T,
keys?: (keyof T)[]
): ReactifyNested<T, Keys, true>
export declare function reactifyObject<
T extends object,
S extends boolean = true
>(obj: T, options?: ReactifyObjectOptions<S>): ReactifyNested<T, keyof T, S>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactifyObject/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactifyObject/index.md)
vueuse syncRef syncRef
=======
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 315 B |
| Last Changed | 6 months ago |
| Related | [`syncRefs`](../syncrefs/index) |
Two-way refs synchronization.
Usage
-----
```
import { syncRef } from '@vueuse/core'
const a = ref('a')
const b = ref('b')
const stop = syncRef(a, b)
console.log(a.value) // a
b.value = 'foo'
console.log(a.value) // foo
a.value = 'bar'
console.log(b.value) // bar
```
### One directional
```
import { syncRef } from '@vueuse/core'
const a = ref('a')
const b = ref('b')
const stop = syncRef(a, b, { direction: 'rtl' })
```
### Custom Transform
```
import { syncRef } from '@vueuse/core'
const a = ref(10)
const b = ref(2)
const stop = syncRef(a, b, {
transform: {
ltr: left => left * 2,
rtl: right => right / 2
}
})
console.log(b.value) // 20
b.value = 30
console.log(a.value) // 15
```
Type Declarations
-----------------
```
export interface SyncRefOptions<L, R = L> extends ConfigurableFlushSync {
/**
* Watch deeply
*
* @default false
*/
deep?: boolean
/**
* Sync values immediately
*
* @default true
*/
immediate?: boolean
/**
* Direction of syncing. Value will be redefined if you define syncConvertors
*
* @default 'both'
*/
direction?: "ltr" | "rtl" | "both"
/**
* Custom transform function
*/
transform?: {
ltr?: (left: L) => R
rtl?: (right: R) => L
}
}
/**
* Two-way refs synchronization.
*
* @param left
* @param right
*/
export declare function syncRef<L, R = L>(
left: Ref<L>,
right: Ref<R>,
options?: SyncRefOptions<L, R>
): () => void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRef/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRef/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/syncRef/index.md)
vueuse refThrottled refThrottled
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 454 B |
| Last Changed | 10 months ago |
| Alias | `useThrottle``throttledRef` |
| Related | [`useThrottleFn`](../usethrottlefn/index) |
Throttle changing of a ref value.
Usage
-----
```
import { refThrottled } from '@vueuse/core'
const input = ref('')
const throttled = refThrottled(input, 1000)
```
### Trailing
If you don't want to watch trailing changes, set 3rd param `false` (it's `true` by default):
```
import { refThrottled } from '@vueuse/core'
const input = ref('')
const throttled = refThrottled(input, 1000, false)
```
### Leading
Allows the callback to be invoked immediately (on the leading edge of the `ms` timeout). If you don't want this behavior, set the 4th param `false` (it's `true` by default):
```
import { refThrottled } from '@vueuse/core'
const input = ref('')
const throttled = refThrottled(input, 1000, undefined, false)
```
Recommended Reading
-------------------
* [Debounce vs Throttle: Definitive Visual Guide](https://redd.one/blog/debounce-vs-throttle)
* [Debouncing and Throttling Explained Through Examples](https://css-tricks.com/debouncing-throttling-explained-examples/)
Type Declarations
-----------------
```
/**
* Throttle execution of a function. Especially useful for rate limiting
* execution of handlers on events like resize and scroll.
*
* @param value Ref value to be watched with throttle effect
* @param delay A zero-or-greater delay in milliseconds. For event callbacks, values around 100 or 250 (or even higher) are most useful.
* @param [trailing=true] if true, update the value again after the delay time is up
* @param [leading=true] if true, update the value on the leading edge of the ms timeout
*/
export declare function refThrottled<T>(
value: Ref<T>,
delay?: number,
trailing?: boolean,
leading?: boolean
): Ref<T>
export { refThrottled as useThrottle, refThrottled as throttledRef }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/refThrottled/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/refThrottled/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/refThrottled/index.md)
| programming_docs |
vueuse computedEager computedEager
=============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 468 B |
| Last Changed | 10 months ago |
| Alias | `eagerComputed` |
Eager computed without lazy evaluation.
Learn more at [Vue: When a computed property can be the wrong tool](https://dev.to/linusborg/vue-when-a-computed-property-can-be-the-wrong-tool-195j).
* Use `computed()` when you have a complex calculation going on, which can actually profit from caching and lazy evaluation and should only be (re-)calculated if really necessary.
* Use `computedEager()` when you have a simple operation, with a rarely changing return value – often a boolean.
Usage
-----
```
import { computedEager } from '@vueuse/core'
const todos = ref([])
const hasOpenTodos = computedEager(() => !!todos.length)
console.log(hasOpenTodos.value) // false
toTodos.value.push({ title: 'Learn Vue' })
console.log(hasOpenTodos.value) // true
```
Type Declarations
-----------------
```
export declare function computedEager<T>(
fn: () => T,
options?: WatchOptionsBase
): Readonly<Ref<T>>
export { computedEager as eagerComputed }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/computedEager/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/computedEager/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/computedEager/index.md)
vueuse watchArray watchArray
==========
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 330 B |
| Last Changed | 6 months ago |
Watch for an array with additions and removals.
Usage
-----
Similar to `watch`, but provides the added and removed elements to the callback function. Pass `{ deep: true }` if the list is updated in place with `push`, `splice`, etc.
```
import { watchArray } from '@vueuse/core'
const list = ref([1, 2, 3])
watchArray(list, (newList, oldList, added, removed) => {
console.log(newList) // [1, 2, 3, 4]
console.log(oldList) // [1, 2, 3]
console.log(added) // [4]
console.log(removed) // []
})
onMounted(() => {
list.value = [...list.value, 4]
})
```
Type Declarations
-----------------
```
export declare type WatchArrayCallback<V = any, OV = any> = (
value: V,
oldValue: OV,
added: V,
removed: OV,
onCleanup: (cleanupFn: () => void) => void
) => any
/**
* Watch for an array with additions and removals.
*
* @see https://vueuse.org/watchArray
*/
export declare function watchArray<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T[]> | T[],
cb: WatchArrayCallback<T[], Immediate extends true ? T[] | undefined : T[]>,
options?: WatchOptions<Immediate>
): WatchStopHandle
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchArray/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchArray/index.md)
vueuse useArrayMap useArrayMap
===========
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 208 B |
| Last Changed | 4 months ago |
Reactive `Array.map`
Usage
-----
### Use with array of multiple refs
```
import { useArrayMap } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(2)
const item3 = ref(4)
const item4 = ref(6)
const item5 = ref(8)
const list = [item1, item2, item3, item4, item5]
const result = useArrayMap(list, i => i * 2)
// result.value: [0, 4, 8, 12, 16]
item1.value = 1
// result.value: [2, 4, 8, 12, 16]
```
### Use with reactive array
```
import { useArrayMap } from '@vueuse/core'
const list = ref([0, 1, 2, 3, 4])
const result = useArrayMap(list, i => i * 2)
// result.value: [0, 2, 4, 6, 8]
list.value.pop()
// result.value: [0, 2, 4, 6]
```
Type Declarations
-----------------
```
/**
* Reactive `Array.map`
*
* @see https://vueuse.org/useArrayMap
* @param {Array} list - the array was called upon.
* @param fn - a function that is called for every element of the given `list`. Each time `fn` executes, the returned value is added to the new array.
*
* @returns {Array} a new array with each element being the result of the callback function.
*/
export declare function useArrayMap<T, U = T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: T[]) => U
): ComputedRef<U[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayMap/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayMap/index.md)
vueuse toReactive toReactive
==========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 320 B |
| Last Changed | 10 months ago |
Converts ref to reactive. Also made possible to create a "swapable" reactive object.
**This function uses [Proxy](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy)** It is **NOT** supported by [IE 11 or below](https://caniuse.com/proxy).
Usage
-----
```
import { toReactive } from '@vueuse/core'
const refState = ref({ foo: 'bar' })
console.log(refState.value.foo) // => 'bar'
const state = toReactive(refState) // <--
console.log(state.foo) // => 'bar'
refState.value = { bar: 'foo' }
console.log(state.foo) // => undefined
console.log(state.bar) // => 'foo'
```
Type Declarations
-----------------
```
/**
* Converts ref to reactive.
*
* @see https://vueuse.org/toReactive
* @param objectRef A ref of object
*/
export declare function toReactive<T extends object>(objectRef: MaybeRef<T>): T
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/toReactive/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/toReactive/index.md)
*No recent changes*
vueuse refDefault refDefault
==========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 193 B |
| Last Changed | last year |
Apply default value to a ref.
Usage
-----
```
import { refDefault, useStorage } from '@vueuse/core'
const raw = useStorage('key')
const state = refDefault(raw, 'default')
raw.value = 'hello'
console.log(state.value) // hello
raw.value = undefined
console.log(state.value) // default
```
Type Declarations
-----------------
```
/**
* Apply default value to a ref.
*
* @param source source ref
* @param targets
*/
export declare function refDefault<T>(
source: Ref<T | undefined | null>,
defaultValue: T
): Ref<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/refDefault/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/refDefault/index.md)
*No recent changes*
vueuse watchWithFilter watchWithFilter
===============
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 421 B |
| Last Changed | 4 months ago |
`watch` with additional EventFilter control.
Usage
-----
Similar to `watch`, but offering an extra option `eventFilter` which will be applied to the callback function.
```
import { debounceFilter, watchWithFilter } from '@vueuse/core'
watchWithFilter(
source,
() => { console.log('changed!') }, // callback will be called in 500ms debounced manner
{
eventFilter: debounceFilter(500), // throttledFilter, pausabledFilter or custom filters
},
)
```
Type Declarations
-----------------
```
export interface WatchWithFilterOptions<Immediate>
extends WatchOptions<Immediate>,
ConfigurableEventFilter {}
export declare function watchWithFilter<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchWithFilterOptions<Immediate>
): WatchStopHandle
export declare function watchWithFilter<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchStopHandle
export declare function watchWithFilter<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchStopHandle
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchWithFilter/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchWithFilter/index.md)
vueuse refDebounced refDebounced
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 415 B |
| Last Changed | 2 months ago |
| Alias | `useDebounce``debouncedRef` |
| Related | [`useThrottleFn`](../usethrottlefn/index) |
Debounce execution of a ref value.
Usage
-----
```
import { refDebounced } from '@vueuse/core'
const input = ref('foo')
const debounced = refDebounced(input, 1000)
input.value = 'bar'
console.log(debounced.value) // 'foo'
await sleep(1100)
console.log(debounced.value) // 'bar'
```
You can also pass an optional 3rd parameter including maxWait option. See [`useDebounceFn`](../usedebouncefn/index)for details.
Recommended Reading
-------------------
* [**Debounce vs Throttle**: Definitive Visual Guide](https://redd.one/blog/debounce-vs-throttle)
Type Declarations
-----------------
```
/**
* Debounce updates of a ref.
*
* @return A new debounced ref.
*/
export declare function refDebounced<T>(
value: Ref<T>,
ms?: MaybeComputedRef<number>,
options?: DebounceFilterOptions
): Readonly<Ref<T>>
export { refDebounced as useDebounce, refDebounced as debouncedRef }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/refDebounced/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/refDebounced/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/refDebounced/index.md)
vueuse useToString useToString
===========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 195 B |
| Last Changed | 5 months ago |
Reactively convert a ref to string.
Usage
-----
```
import { useToString } from '@vueuse/core'
const number = ref(3.14)
const str = useToString(number)
str.value // '3.14'
```
Type Declarations
-----------------
```
/**
* Reactively convert a ref to string.
*
* @see https://vueuse.org/useToString
*/
export declare function useToString(
value: MaybeComputedRef<unknown>
): ComputedRef<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToString/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToString/index.md)
vueuse reactify reactify
========
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 250 B |
| Last Changed | 6 months ago |
| Alias | `createReactiveFn` |
| Related | [`createUnrefFn`](../../core/createunreffn/index) |
Converts plain functions into reactive functions. The converted function accepts refs as its arguments and returns a ComputedRef, with proper typing.
**TIP**Interested to see some application or looking for some pre-reactified functions?
Check out [⚗️ Vue Chemistry](https://github.com/antfu/vue-chemistry)!
Usage
-----
Basic example
```
import { reactify } from '@vueuse/core'
// a plain function
function add(a: number, b: number): number {
return a + b
}
// now it accept refs and returns a computed ref
// (a: number | Ref<number>, b: number | Ref<number>) => ComputedRef<number>
const reactiveAdd = reactify(add)
const a = ref(1)
const b = ref(2)
const sum = reactiveAdd(a, b)
console.log(sum.value) // 3
a.value = 5
console.log(sum.value) // 7
```
An example of implementing a reactive [Pythagorean theorem](https://en.wikipedia.org/wiki/Pythagorean_theorem).
```
import { reactify } from '@vueuse/core'
const pow = reactify(Math.pow)
const sqrt = reactify(Math.sqrt)
const add = reactify((a: number, b: number) => a + b)
const a = ref(3)
const b = ref(4)
const c = sqrt(add(pow(a, 2), pow(b, 2)))
console.log(c.value) // 5
// 5:12:13
a.value = 5
b.value = 12
console.log(c.value) // 13
```
You can also do it this way:
```
import { reactify } from '@vueuse/core'
function pythagorean(a: number, b: number) {
return Math.sqrt(a ** 2 + b ** 2)
}
const a = ref(3)
const b = ref(4)
const c = reactify(pythagorean)(a, b)
console.log(c.value) // 5
```
Another example of making reactive `stringify`
```
import { reactify } from '@vueuse/core'
const stringify = reactify(JSON.stringify)
const obj = ref(42)
const dumped = stringify(obj)
console.log(dumped.value) // '42'
obj.value = { foo: 'bar' }
console.log(dumped.value) // '{"foo":"bar"}'
```
Type Declarations
-----------------
```
export type Reactified<T, Computed extends boolean> = T extends (
...args: infer A
) => infer R
? (
...args: {
[K in keyof A]: Computed extends true
? MaybeComputedRef<A[K]>
: MaybeRef<A[K]>
}
) => ComputedRef<R>
: never
export interface ReactifyOptions<T extends boolean> {
/**
* Accept passing a function as a reactive getter
*
* @default true
*/
computedGetter?: T
}
/**
* Converts plain function into a reactive function.
* The converted function accepts refs as it's arguments
* and returns a ComputedRef, with proper typing.
*
* @param fn - Source function
*/
export declare function reactify<T extends Function, K extends boolean = true>(
fn: T,
options?: ReactifyOptions<K>
): Reactified<T, K>
export { reactify as createReactiveFn }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactify/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/reactify/index.md)
vueuse computedWithControl computedWithControl
===================
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 330 B |
| Last Changed | 6 months ago |
| Alias | `controlledComputed` |
| Related | [`refWithControl`](../refwithcontrol/index) |
Explicitly define the dependencies of computed.
Usage
-----
```
import { computedWithControl } from '@vueuse/core'
const source = ref('foo')
const counter = ref(0)
const computedRef = computedWithControl(
() => source.value, // watch source, same as `watch`
() => counter.value, // computed getter, same as `computed`
)
```
With this, the changes of `counter` won't trigger `computedRef` to update but the `source` ref does.
```
console.log(computedRef.value) // 0
counter.value += 1
console.log(computedRef.value) // 0
source.value = 'bar'
console.log(computedRef.value) // 1
```
### Manual Triggering
> This only works in Vue 3
>
>
You can also manually trigger the update of the computed by:
```
const computedRef = computedWithControl(
() => source.value,
() => counter.value,
)
computedRef.trigger()
```
Type Declarations
-----------------
```
export interface ComputedWithControlRefExtra {
/**
* Force update the computed value.
*/
trigger(): void
}
export interface ComputedRefWithControl<T>
extends ComputedRef<T>,
ComputedWithControlRefExtra {}
export interface WritableComputedRefWithControl<T>
extends WritableComputedRef<T>,
ComputedWithControlRefExtra {}
export declare function computedWithControl<T, S>(
source: WatchSource<S> | WatchSource<S>[],
fn: ComputedGetter<T>
): ComputedRefWithControl<T>
export declare function computedWithControl<T, S>(
source: WatchSource<S> | WatchSource<S>[],
fn: WritableComputedOptions<T>
): WritableComputedRefWithControl<T>
export { computedWithControl as controlledComputed }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/computedWithControl/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/computedWithControl/index.md)
vueuse useArrayEvery useArrayEvery
=============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 210 B |
| Last Changed | 5 months ago |
Reactive `Array.every`
Usage
-----
### Use with array of multiple refs
```
import { useArrayEvery } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(2)
const item3 = ref(4)
const item4 = ref(6)
const item5 = ref(8)
const list = [item1, item2, item3, item4, item5]
const result = useArrayEvery(list, i => i % 2 === 0)
// result.value: true
item1.value = 1
// result.value: false
```
### Use with reactive array
```
import { useArrayEvery } from '@vueuse/core'
const list = ref([0, 2, 4, 6, 8])
const result = useArrayEvery(list, i => i % 2 === 0)
// result.value: true
list.value.push(9)
// result.value: false
```
Type Declarations
-----------------
```
/**
* Reactive `Array.every`
*
* @see https://vueuse.org/useArrayEvery
* @param {Array} list - the array was called upon.
* @param fn - a function to test each element.
*
* @returns {boolean} **true** if the `fn` function returns a **truthy** value for every element from the array. Otherwise, **false**.
*/
export declare function useArrayEvery<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
fn: (element: T, index: number, array: MaybeComputedRef<T>[]) => unknown
): ComputedRef<boolean>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayEvery/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayEvery/index.md)
vueuse useArrayReduce useArrayReduce
==============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 246 B |
| Last Changed | 5 months ago |
Reactive `Array.reduce`.
Usage
-----
```
import { useArrayReduce } from '@vueuse/core'
const sum = useArrayReduce([ref(1), ref(2), ref(3)], (sum, val) => sum + val)
// sum.value: 6
```
### Use with reactive array
```
import { useArrayReduce } from '@vueuse/core'
const list = reactive([1, 2])
const sum = useArrayReduce(list, (sum, val) => sum + val)
list.push(3)
// sum.value: 6
```
### Use with initialValue
```
import { useArrayReduce } from '@vueuse/core'
const list = reactive([{ num: 1 }, { num: 2 }])
const sum = useArrayReduce(list, (sum, val) => sum + val.num, 0)
// sum.value: 3
```
Type Declarations
-----------------
Show Type Declarations
```
export type UseArrayReducer<PV, CV, R> = (
previousValue: PV,
currentValue: CV,
currentIndex: number
) => R
/**
* Reactive `Array.reduce`
*
* @see https://vueuse.org/useArrayReduce
* @param {Array} list - the array was called upon.
* @param reducer - a "reducer" function.
*
* @returns the value that results from running the "reducer" callback function to completion over the entire array.
*/
export declare function useArrayReduce<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
reducer: UseArrayReducer<T, T, T>
): ComputedRef<T>
/**
* Reactive `Array.reduce`
*
* @see https://vueuse.org/useArrayReduce
* @param {Array} list - the array was called upon.
* @param reducer - a "reducer" function.
* @param initialValue - a value to be initialized the first time when the callback is called.
*
* @returns the value that results from running the "reducer" callback function to completion over the entire array.
*/
export declare function useArrayReduce<T, U>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>,
reducer: UseArrayReducer<U, T, U>,
initialValue: MaybeComputedRef<U>
): ComputedRef<U>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayReduce/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayReduce/index.md)
| programming_docs |
vueuse createSharedComposable createSharedComposable
======================
| | |
| --- | --- |
| Category | [State](../../functions#category=State) |
| Export Size | 287 B |
| Last Changed | last year |
| Related | [`createGlobalState`](../createglobalstate/index) |
Make a composable function usable with multiple Vue instances.
Usage
-----
```
import { createSharedComposable, useMouse } from '@vueuse/core'
const useSharedMouse = createSharedComposable(useMouse)
// CompA.vue
const { x, y } = useSharedMouse()
// CompB.vue - will reuse the previous state and no new event listeners will be registered
const { x, y } = useSharedMouse()
```
Type Declarations
-----------------
```
/**
* Make a composable function usable with multiple Vue instances.
*
* @see https://vueuse.org/createSharedComposable
*/
export declare function createSharedComposable<
Fn extends (...args: any[]) => any
>(composable: Fn): Fn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/createSharedComposable/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/createSharedComposable/index.md)
*No recent changes*
vueuse createEventHook createEventHook
===============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 232 B |
| Last Changed | 3 weeks ago |
Utility for creating event hooks
Usage
-----
Creating a function that uses `createEventHook`
```
import { createEventHook } from '@vueuse/core'
export function useMyFetch(url) {
const fetchResult = createEventHook<Response>()
const fetchError = createEventHook<any>()
fetch(url)
.then(result => fetchResult.trigger(result))
.catch(error => fetchError.trigger(error.message))
return {
onResult: fetchResult.on,
onError: fetchError.on,
}
}
```
Using a function that uses `createEventHook`
```
<script setup lang="ts">
import { useMyFetch } from './my-fetch-function'
const { onResult, onError } = useMyFetch('my api url')
onResult((result) => {
console.log(result)
})
onError((error) => {
console.error(error)
})
</script>
```
Type Declarations
-----------------
```
export type EventHookOn<T = any> = (fn: (param: T) => void) => {
off: () => void
}
export type EventHookOff<T = any> = (fn: (param: T) => void) => void
export type EventHookTrigger<T = any> = (param: T) => void
export interface EventHook<T = any> {
on: EventHookOn<T>
off: EventHookOff<T>
trigger: EventHookTrigger<T>
}
/**
* Utility for creating event hooks
*
* @see https://vueuse.org/createEventHook
*/
export declare function createEventHook<T = any>(): EventHook<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/createEventHook/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/createEventHook/index.md)
vueuse useCounter useCounter
==========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 258 B |
| Last Changed | 2 months ago |
Basic counter with utility functions.
Basic Usage
-----------
```
import { useCounter } from '@vueuse/core'
const { count, inc, dec, set, reset } = useCounter()
```
Usage with options
------------------
```
import { useCounter } from '@vueuse/core'
const { count, inc, dec, set, reset } = useCounter(1, { min: 0, max: 16 })
```
Type Declarations
-----------------
```
export interface UseCounterOptions {
min?: number
max?: number
}
/**
* Basic counter with utility functions.
*
* @see https://vueuse.org/useCounter
* @param [initialValue=0]
* @param {Object} options
*/
export declare function useCounter(
initialValue?: number,
options?: UseCounterOptions
): {
count: Ref<number>
inc: (delta?: number) => number
dec: (delta?: number) => number
get: () => number
set: (val: number) => number
reset: (val?: number) => number
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useCounter/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useCounter/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useCounter/index.md)
vueuse watchDebounced watchDebounced
==============
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 786 B |
| Last Changed | 4 months ago |
| Alias | `debouncedWatch` |
Debounced watch
Usage
-----
Similar to `watch`, but offering extra options `debounce` and `maxWait` which will be applied to the callback function.
```
import { watchDebounced } from '@vueuse/core'
watchDebounced(
source,
() => { console.log('changed!') },
{ debounce: 500, maxWait: 1000 },
)
```
It's essentially a shorthand for the following code:
```
import { debounceFilter, watchWithFilter } from '@vueuse/core'
watchWithFilter(
source,
() => { console.log('changed!') },
{
eventFilter: debounceFilter(500, { maxWait: 1000 }),
},
)
```
Type Declarations
-----------------
```
export interface WatchDebouncedOptions<Immediate>
extends WatchOptions<Immediate>,
DebounceFilterOptions {
debounce?: MaybeComputedRef<number>
}
export declare function watchDebounced<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchDebouncedOptions<Immediate>
): WatchStopHandle
export declare function watchDebounced<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchDebouncedOptions<Immediate>
): WatchStopHandle
export declare function watchDebounced<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchDebouncedOptions<Immediate>
): WatchStopHandle
export { watchDebounced as debouncedWatch }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchDebounced/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchDebounced/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchDebounced/index.md)
vueuse useDateFormat useDateFormat
=============
| | |
| --- | --- |
| Category | [Time](../../functions#category=Time) |
| Export Size | 734 B |
| Last Changed | 3 months ago |
Get the formatted date according to the string of tokens passed in, inspired by [dayjs](https://github.com/iamkun/dayjs).
**List of all available formats (HH:mm:ss by default):**
| Format | Output | Description |
| --- | --- | --- |
| `YY` | 18 | Two-digit year |
| `YYYY` | 2018 | Four-digit year |
| `M` | 1-12 | The month, beginning at 1 |
| `MM` | 01-12 | The month, 2-digits |
| `MMM` | Jan-Dec | The abbreviated month name |
| `MMMM` | January-December | The full month name |
| `D` | 1-31 | The day of the month |
| `DD` | 01-31 | The day of the month, 2-digits |
| `H` | 0-23 | The hour |
| `HH` | 00-23 | The hour, 2-digits |
| `h` | 1-12 | The hour, 12-hour clock |
| `hh` | 01-12 | The hour, 12-hour clock, 2-digits |
| `m` | 0-59 | The minute |
| `mm` | 00-59 | The minute, 2-digits |
| `s` | 0-59 | The second |
| `ss` | 00-59 | The second, 2-digits |
| `SSS` | 000-999 | The millisecond, 3-digits |
| `A` | AM PM | The meridiem |
| `AA` | A.M. P.M. | The meridiem, periods |
| `a` | am pm | The meridiem, lowercase |
| `aa` | a.m. p.m. | The meridiem, lowercase and periods |
| `d` | 0-6 | The day of the week, with Sunday as 0 |
| `dd` | S-S | The min name of the day of the week |
| `ddd` | Sun-Sat | The short name of the day of the week |
| `dddd` | Sunday-Saturday | The name of the day of the week |
* Meridiem is customizable by defining `customMeridiem` in `options`.
Usage
-----
### Basic
```
<script setup lang="ts">
import { ref, computed } from 'vue-demi'
import { useNow, useDateFormat } from '@vueuse/core'
const formatted = useDateFormat(useNow(), 'YYYY-MM-DD HH:mm:ss')
</script>
<template>
<div>{{ formatted }}</div>
</template>
```
### Use with locales
```
<script setup lang="ts">
import { ref, computed } from 'vue-demi'
import { useNow, useDateFormat } from '@vueuse/core'
const formatted = useDateFormat(useNow(), 'YYYY-MM-DD (ddd)', { locales: 'en-US' })
</script>
<template>
<div>{{ formatted }}</div>
</template>
```
### Use with custom meridiem
```
<script setup lang="ts">
import { ref, computed } from 'vue-demi'
import { useNow, useDateFormat } from '@vueuse/core'
const customMeridiem = (hours: number, minutes: number, isLowercase?: boolean, hasPeriod?: boolean) => {
const m = hours > 11 ? (isLowercase ? 'μμ' : 'ΜΜ') : (isLowercase ? 'πμ' : 'ΠΜ')
return hasPeriod ? m.split('').reduce((acc, current) => acc += `${current}.`, '') : m
}
const am = useDateFormat('2022-01-01 05:05:05', 'hh:mm:ss A', { customMeridiem })
// am.value = '05:05:05 ΠΜ'
const pm = useDateFormat('2022-01-01 17:05:05', 'hh:mm:ss AA', { customMeridiem })
// pm.value = '05:05:05 Μ.Μ.'
</script>
```
Type Declarations
-----------------
Show Type Declarations
```
export type DateLike = Date | number | string | undefined
export interface UseDateFormatOptions {
/**
* The locale(s) to used for dd/ddd/dddd/MMM/MMMM format
*
* [MDN](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl#locales_argument).
*/
locales?: Intl.LocalesArgument
/**
* A custom function to re-modify the way to display meridiem
*
*/
customMeridiem?: (
hours: number,
minutes: number,
isLowercase?: boolean,
hasPeriod?: boolean
) => string
}
export declare const formatDate: (
date: Date,
formatStr: string,
options?: UseDateFormatOptions
) => string
export declare const normalizeDate: (date: DateLike) => Date
/**
* Get the formatted date according to the string of tokens passed in.
*
* @see https://vueuse.org/useDateFormat
* @param date - The date to format, can either be a `Date` object, a timestamp, or a string
* @param formatStr - The combination of tokens to format the date
* @param options - UseDateFormatOptions
*/
export declare function useDateFormat(
date: MaybeComputedRef<DateLike>,
formatStr?: MaybeComputedRef<string>,
options?: UseDateFormatOptions
): ComputedRef<string>
export type UseDateFormatReturn = ReturnType<typeof useDateFormat>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDateFormat/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDateFormat/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDateFormat/index.md)
vueuse useArrayUnique useArrayUnique
==============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | |
| Last Changed | 2 days ago |
reactive unique array
Usage
-----
### Use with array of multiple refs
```
import { useArrayUnique } from '@vueuse/core'
const item1 = ref(0)
const item2 = ref(1)
const item3 = ref(1)
const item4 = ref(2)
const item5 = ref(3)
const list = [item1, item2, item3, item4, item5]
const result = useArrayUnique(list)
// result.value: [0, 1, 2, 3]
item5.value = 1
// result.value: [0, 1, 2]
```
### Use with reactive array
```
import { useArrayUnique } from '@vueuse/core'
const list = reactive([1, 2, 2, 3])
const result = useArrayUnique(list)
// result.value: [1, 2, 3]
result.value.push(1)
// result.value: [1, 2, 3]
```
Type Declarations
-----------------
```
/**
* reactive unique array
* @see https://vueuse.org/useArrayUnique
* @param {Array} list - the array was called upon.
* @returns {Array} A computed ref that returns a unique array of items.
*/
export declare function useArrayUnique<T>(
list: MaybeComputedRef<MaybeComputedRef<T>[]>
): ComputedRef<T[]>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayUnique/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayUnique/index.md)
vueuse refAutoReset refAutoReset
============
| | |
| --- | --- |
| Category | [Reactivity](../../functions#category=Reactivity) |
| Export Size | 315 B |
| Last Changed | 5 months ago |
| Alias | `autoResetRef` |
A ref which will be reset to the default value after some time.
Usage
-----
```
import { refAutoReset } from '@vueuse/core'
const message = refAutoReset('default message', 1000)
const setMessage = () => {
// here the value will change to 'message has set' but after 1000ms, it will change to 'default message'
message.value = 'message has set'
}
```
Type Declarations
-----------------
```
/**
* Create a ref which will be reset to the default value after some time.
*
* @see https://vueuse.org/refAutoReset
* @param defaultValue The value which will be set.
* @param afterMs A zero-or-greater delay in milliseconds.
*/
export declare function refAutoReset<T>(
defaultValue: T,
afterMs?: MaybeComputedRef<number>
): Ref<T>
export { refAutoReset as autoResetRef }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/refAutoReset/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/refAutoReset/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/refAutoReset/index.md)
vueuse set set
===
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 214 B |
| Last Changed | last year |
Shorthand for `ref.value = x`
Usage
-----
```
import { set } from '@vueuse/core'
const a = ref(0)
set(a, 1)
console.log(a.value) // 1
```
Type Declarations
-----------------
```
export declare function set<T>(ref: Ref<T>, value: T): void
export declare function set<O extends object, K extends keyof O>(
target: O,
key: K,
value: O[K]
): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/set/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/set/index.md)
*No recent changes*
vueuse useToNumber useToNumber
===========
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 278 B |
| Last Changed | 5 months ago |
Reactively convert a string ref to number.
Usage
-----
```
import { useToNumber } from '@vueuse/core'
const str = ref('123')
const number = useToNumber(str)
number.value // 123
```
Type Declarations
-----------------
```
export interface UseToNumberOptions {
/**
* Method to use to convert the value to a number.
*
* @default 'parseFloat'
*/
method?: "parseFloat" | "parseInt"
/**
* The base in mathematical numeral systems passed to `parseInt`.
* Only works with `method: 'parseInt'`
*/
radix?: number
/**
* Replace NaN with zero
*
* @default false
*/
nanToZero?: boolean
}
/**
* Computed reactive object.
*/
export declare function useToNumber(
value: MaybeComputedRef<number | string>,
options?: UseToNumberOptions
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToNumber/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useToNumber/index.md)
vueuse useDebounceFn useDebounceFn
=============
| | |
| --- | --- |
| Category | [Utilities](../../functions#category=Utilities) |
| Export Size | 368 B |
| Last Changed | 2 days ago |
| Related | [`useThrottleFn`](../usethrottlefn/index) |
Debounce execution of a function.
> Debounce is an overloaded waiter: if you keep asking him your requests will be ignored until you stop and give him some time to think about your latest inquiry.
>
>
Usage
-----
```
import { useDebounceFn } from '@vueuse/core'
const debouncedFn = useDebounceFn(() => {
// do something
}, 1000)
window.addEventListener('resize', debouncedFn)
```
You can also pass a 3rd parameter to this, with a maximum wait time, similar to [lodash debounce](https://lodash.com/docs/4.17.15#debounce)
```
import { useDebounceFn } from '@vueuse/core'
// If no invokation after 5000ms due to repeated input,
// the function will be called anyway.
const debouncedFn = useDebounceFn(() => {
// do something
}, 1000, { maxWait: 5000 })
window.addEventListener('resize', debouncedFn)
```
Optionally, you can get the return value of the function using promise operations.
```
import { useDebounceFn } from '@vueuse/core'
const debouncedRequest = useDebounceFn(() => 'response', 1000)
debouncedRequest().then((value) => {
console.log(value) // 'response'
})
// or use async/await
async function doRequest() {
const value = await debouncedRequest()
console.log(value) // 'response'
}
```
Since unhandled rejection error is quite annoying when developer doesn't need the return value, the promise will **NOT** be rejected if the function is canceled **by default**. You need to specify the option `rejectOnCancel: true` to capture the rejection.
```
import { useDebounceFn } from '@vueuse/core'
const debouncedRequest = useDebounceFn(() => 'response', 1000, { rejectOnCancel: true })
debouncedRequest()
.then((value) => {
// do something
})
.catch(() => {
// do something when canceled
})
// calling it again will cancel the previous request and gets rejected
setTimeout(debouncedRequest, 500)
```
Recommended Reading
-------------------
* [**Debounce vs Throttle**: Definitive Visual Guide](https://redd.one/blog/debounce-vs-throttle)
Type Declarations
-----------------
```
/**
* Debounce execution of a function.
*
* @see https://vueuse.org/useDebounceFn
* @param fn A function to be executed after delay milliseconds debounced.
* @param ms A zero-or-greater delay in milliseconds. For event callbacks, values around 100 or 250 (or even higher) are most useful.
* @param opts options
*
* @return A new, debounce, function.
*/
export declare function useDebounceFn<T extends FunctionArgs>(
fn: T,
ms?: MaybeComputedRef<number>,
options?: DebounceFilterOptions
): PromisifyFn<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDebounceFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDebounceFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useDebounceFn/index.md)
vueuse watchAtMost watchAtMost
===========
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 561 B |
| Last Changed | 4 months ago |
`watch` with the number of times triggered.
Usage
-----
Similar to `watch` with an extra option `count` which set up the number of times the callback function is triggered. After the count is reached, the watch will be stopped automatically.
```
import { watchAtMost } from '@vueuse/core'
watchAtMost(
source,
() => { console.log('trigger!') }, // triggered it at most 3 times
{
count: 3, // the number of times triggered
},
)
```
Type Declarations
-----------------
```
export interface WatchAtMostOptions<Immediate>
extends WatchWithFilterOptions<Immediate> {
count: MaybeComputedRef<number>
}
export interface WatchAtMostReturn {
stop: WatchStopHandle
count: Ref<number>
}
export declare function watchAtMost<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options: WatchAtMostOptions<Immediate>
): WatchAtMostReturn
export declare function watchAtMost<
T,
Immediate extends Readonly<boolean> = false
>(
sources: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options: WatchAtMostOptions<Immediate>
): WatchAtMostReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchAtMost/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchAtMost/index.md)
| programming_docs |
vueuse useTimeoutFn
| | |
| --- | --- |
| Category | [Animation](../../functions#category=Animation) |
| Export Size | 347 B |
| Last Changed | 5 months ago |
useTimeoutFn
============
Wrapper for `setTimeout` with controls.
```
import { useTimeoutFn } from '@vueuse/core'
const { isPending, start, stop } = useTimeoutFn(() => {
/* ... */
}, 3000)
```
Type Declarations
-----------------
```
export interface UseTimeoutFnOptions {
/**
* Start the timer immediate after calling this function
*
* @default true
*/
immediate?: boolean
}
/**
* Wrapper for `setTimeout` with controls.
*
* @param cb
* @param interval
* @param options
*/
export declare function useTimeoutFn(
cb: (...args: unknown[]) => any,
interval: MaybeComputedRef<number>,
options?: UseTimeoutFnOptions
): Stoppable
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeoutFn/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeoutFn/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useTimeoutFn/index.md)
vueuse useArrayJoin useArrayJoin
============
| | |
| --- | --- |
| Category | [Array](../../functions#category=Array) |
| Export Size | 215 B |
| Last Changed | 5 months ago |
Reactive `Array.join`
Usage
-----
### Use with array of multiple refs
```
import { useArrayJoin } from '@vueuse/core'
const item1 = ref('foo')
const item2 = ref(0)
const item3 = ref({ prop: 'val' })
const list = [item1, item2, item3]
const result = useArrayJoin(list)
// result.value: foo,0,[object Object]
item1.value = 'bar'
// result.value: bar,0,[object Object]
```
### Use with reactive array
```
import { useArrayJoin } from '@vueuse/core'
const list = ref(['string', 0, { prop: 'val' }, false, [1], [[2]], null, undefined, []])
const result = useArrayJoin(list)
// result.value: string,0,[object Object],false,1,2,,,
list.value.push(true)
// result.value: string,0,[object Object],false,1,2,,,,true
list.value = [null, 'string', undefined]
// result.value: ,string,
```
### Use with reactive separator
```
import { useArrayJoin } from '@vueuse/core'
const list = ref(['string', 0, { prop: 'val' }])
const separator = ref()
const result = useArrayJoin(list, separator)
// result.value: string,0,[object Object]
separator.value = ''
// result.value: string0[object Object]
separator.value = '--'
// result.value: string--0--[object Object]
```
Type Declarations
-----------------
```
/**
* Reactive `Array.join`
*
* @see https://vueuse.org/useArrayJoin
* @param {Array} list - the array was called upon.
* @param {string} separator - a string to separate each pair of adjacent elements of the array. If omitted, the array elements are separated with a comma (",").
*
* @returns {string} a string with all array elements joined. If arr.length is 0, the empty string is returned.
*/
export declare function useArrayJoin(
list: MaybeComputedRef<MaybeComputedRef<any>[]>,
separator?: MaybeComputedRef<string>
): ComputedRef<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayJoin/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/useArrayJoin/index.md)
vueuse whenever whenever
========
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 174 B |
| Last Changed | 6 months ago |
Shorthand for watching value to be truthy.
Usage
-----
```
import { useAsyncState, whenever } from '@vueuse/core'
const { state, isReady } = useAsyncState(
fetch('https://jsonplaceholder.typicode.com/todos/1').then(t => t.json()),
{},
)
whenever(isReady, () => console.log(state))
```
```
// this
whenever(ready, () => console.log(state))
// is equivalent to:
watch(ready, (isReady) => {
if (isReady)
console.log(state)
})
```
### Callback Function
Same as `watch`, the callback will be called with `cb(value, oldValue, onInvalidate)`.
```
whenever(height, (current, lastHeight) => {
if (current > lastHeight)
console.log(`Increasing height by ${current - lastHeight}`)
})
```
### Computed
Same as `watch`, you can pass a getter function to calculate on each change.
```
// this
whenever(
() => counter.value === 7,
() => console.log('counter is 7 now!'),
)
```
### Options
Options and defaults are same with `watch`.
```
// this
whenever(
() => counter.value === 7,
() => console.log('counter is 7 now!'),
{ flush: 'sync' },
)
```
Type Declarations
-----------------
```
/**
* Shorthand for watching value to be truthy
*
* @see https://vueuse.org/whenever
*/
export declare function whenever<T>(
source: WatchSource<T | false | null | undefined>,
cb: WatchCallback<T>,
options?: WatchOptions
): WatchStopHandle
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/whenever/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/whenever/index.md)
vueuse watchPausable watchPausable
=============
| | |
| --- | --- |
| Category | [Watch](../../functions#category=Watch) |
| Export Size | 714 B |
| Last Changed | 4 months ago |
| Alias | `pausableWatch` |
Pausable watch
Usage
-----
Use as normal the `watch`, but return extra `pause()` and `resume()` functions to control.
```
import { watchPausable } from '@vueuse/core'
import { nextTick, ref } from 'vue'
const source = ref('foo')
const { stop, pause, resume } = watchPausable(
source,
v => console.log(`Changed to ${v}!`),
)
source.value = 'bar'
await nextTick() // Changed to bar!
pause()
source.value = 'foobar'
await nextTick() // (nothing happend)
resume()
source.value = 'hello'
await nextTick() // Changed to hello!
```
Type Declarations
-----------------
```
export interface WatchPausableReturn extends Pausable {
stop: WatchStopHandle
}
export declare function watchPausable<
T extends Readonly<WatchSource<unknown>[]>,
Immediate extends Readonly<boolean> = false
>(
sources: [...T],
cb: WatchCallback<MapSources<T>, MapOldSources<T, Immediate>>,
options?: WatchWithFilterOptions<Immediate>
): WatchPausableReturn
export declare function watchPausable<
T,
Immediate extends Readonly<boolean> = false
>(
source: WatchSource<T>,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchPausableReturn
export declare function watchPausable<
T extends object,
Immediate extends Readonly<boolean> = false
>(
source: T,
cb: WatchCallback<T, Immediate extends true ? T | undefined : T>,
options?: WatchWithFilterOptions<Immediate>
): WatchPausableReturn
export { watchPausable as pausableWatch }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchPausable/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchPausable/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/shared/watchPausable/index.md)
vueuse useClamp useClamp
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 259 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactively clamp a value between two other values.
Usage
-----
```
import { useClamp } from '@vueuse/math'
const min = ref(0)
const max = ref(10)
const value = useClamp(0, min, max)
```
Type Declarations
-----------------
```
/**
* Reactively clamp a value between two other values.
*
* @see https://vueuse.org/useClamp
* @param value number
* @param min
* @param max
*/
export declare function useClamp(
value: MaybeRef<number>,
min: MaybeComputedRef<number>,
max: MaybeComputedRef<number>
): Ref<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useClamp/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/math/useClamp/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useClamp/index.md)
vueuse usePrecision usePrecision
============
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 246 B |
| Package | `@vueuse/math` |
| Last Changed | 5 months ago |
Reactively set the precision of a number.
Usage
-----
```
import { usePrecision } from '@vueuse/math'
const value = ref(3.1415)
const result = usePrecision(value, 2) // 3.14
const ceilResult = usePrecision(value, 2, {
math: 'ceil'
}) // 3.15
const floorResult = usePrecision(value, 3, {
math: 'floor'
}) // 3.141
```
Type Declarations
-----------------
```
export interface UsePrecisionOptions {
/**
* Method to use for rounding
*
* @default 'round'
*/
math?: "floor" | "ceil" | "round"
}
/**
* Reactively set the precision of a number.
*
* @see https://vueuse.org/usePrecision
*/
export declare function usePrecision(
value: MaybeComputedRef<number>,
digits: MaybeComputedRef<number>,
options?: MaybeComputedRef<UsePrecisionOptions>
): ComputedRef<number | string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/usePrecision/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/usePrecision/index.md)
vueuse createGenericProjection createGenericProjection
=======================
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 210 B |
| Package | `@vueuse/math` |
| Last Changed | 2 months ago |
| Related | [`createProjection`](../createprojection/index)[`useProjection`](../useprojection/index) |
Generic version of [`createProjection`](../createprojection/index) Accepts a custom projector function to map arbitrary type of domains.
Refer to [`createProjection`](../createprojection/index)and `useProjection`
Type Declarations
-----------------
```
export type ProjectorFunction<F, T> = (
input: F,
from: readonly [F, F],
to: readonly [T, T]
) => T
export type UseProjection<F, T> = (input: MaybeComputedRef<F>) => ComputedRef<T>
export declare function createGenericProjection<F = number, T = number>(
fromDomain: MaybeComputedRef<readonly [F, F]>,
toDomain: MaybeComputedRef<readonly [T, T]>,
projector: ProjectorFunction<F, T>
): UseProjection<F, T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/createGenericProjection/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/createGenericProjection/index.md)
vueuse useCeil useCeil
=======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 202 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.ceil`
Usage
-----
```
import { useCeil } from '@vueuse/math'
const value = ref(0.95)
const result = useCeil(value) // 1
```
Type Declarations
-----------------
```
/**
* Reactive `Math.ceil`.
*
* @see https://vueuse.org/useCeil
*/
export declare function useCeil(
value: MaybeComputedRef<number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useCeil/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useCeil/index.md)
vueuse logicNot logicNot
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 192 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
| Alias | `not` |
| Related | [`logicAnd`](../logicand/index)[`logicOr`](../logicor/index) |
`NOT` condition for ref.
Usage
-----
```
import { logicNot } from '@vueuse/math'
import { whenever } from '@vueuse/core'
const a = ref(true)
whenever(logicNot(a), () => {
console.log('a is now falsy!')
})
```
Type Declarations
-----------------
```
/**
* `NOT` conditions for refs.
*
* @see https://vueuse.org/logicNot
*/
export declare function logicNot(v: MaybeComputedRef<any>): ComputedRef<boolean>
export { logicNot as not }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/logicNot/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/logicNot/index.md)
vueuse useFloor useFloor
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 199 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.floor`.
Usage
-----
```
import { useFloor } from '@vueuse/math'
const value = ref(45.95)
const result = useFloor(value) // 45
```
Type Declarations
-----------------
```
/**
* Reactive `Math.floor`
*
* @see https://vueuse.org/useFloor
*/
export declare function useFloor(
value: MaybeComputedRef<number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useFloor/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useFloor/index.md)
vueuse logicOr logicOr
=======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 201 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
| Alias | `or` |
| Related | [`logicAnd`](../logicand/index)[`logicNot`](../logicnot/index) |
`OR` conditions for refs.
Usage
-----
```
import { logicOr } from '@vueuse/math'
import { whenever } from '@vueuse/core'
const a = ref(true)
const b = ref(false)
whenever(logicOr(a, b), () => {
console.log('either a or b is truthy!')
})
```
Type Declarations
-----------------
```
/**
* `OR` conditions for refs.
*
* @see https://vueuse.org/logicOr
*/
export declare function logicOr(
...args: MaybeComputedRef<any>[]
): ComputedRef<boolean>
export { logicOr as or }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/logicOr/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/logicOr/index.md)
vueuse useMath useMath
=======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 274 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math` methods.
Usage
-----
```
import { useMath } from '@vueuse/math'
const base = ref(2)
const exponent = ref(3)
const result = useMath('pow', base, exponent) // Ref<8>
const num = ref(2)
const root = useMath('sqrt', num) // Ref<1.4142135623730951>
num.value = 4
console.log(root.value) // 2
```
Type Declarations
-----------------
```
export type UseMathKeys = keyof {
[K in keyof Math as Math[K] extends (...args: any) => any
? K
: never]: unknown
}
/**
* Reactive `Math` methods.
*
* @see https://vueuse.org/useMath
*/
export declare function useMath<K extends keyof Math>(
key: K,
...args: ArgumentsType<Reactified<Math[K], true>>
): ReturnType<Reactified<Math[K], true>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useMath/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useMath/index.md)
vueuse useRound useRound
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 200 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.round`.
Usage
-----
```
import { useRound } from '@vueuse/math'
const value = ref(20.49)
const result = useRound(value) // 20
```
Type Declarations
-----------------
```
/**
* Reactive `Math.round`.
*
* @see https://vueuse.org/useRound
*/
export declare function useRound(
value: MaybeComputedRef<number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useRound/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useRound/index.md)
vueuse useTrunc useTrunc
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 204 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.trunc`.
Usage
-----
```
import { useTrunc } from '@vueuse/math'
const value1 = ref(0.95)
const value2 = ref(-2.34)
const result1 = useTrunc(value1) // 0
const result2 = useTrunc(value2) // -2
```
Type Declarations
-----------------
```
/**
* Reactive `Math.trunc`.
*
* @see https://vueuse.org/useTrunc
*/
export declare function useTrunc(
value: MaybeComputedRef<number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useTrunc/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useTrunc/index.md)
vueuse logicAnd logicAnd
========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 204 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
| Alias | `and` |
| Related | [`logicNot`](../logicnot/index)[`logicOr`](../logicor/index) |
`AND` condition for refs.
Usage
-----
```
import { logicAnd } from '@vueuse/math'
import { whenever } from '@vueuse/core'
const a = ref(true)
const b = ref(false)
whenever(logicAnd(a, b), () => {
console.log('both a and b are now truthy!')
})
```
Type Declarations
-----------------
```
/**
* `AND` conditions for refs.
*
* @see https://vueuse.org/logicAnd
*/
export declare function logicAnd(
...args: MaybeComputedRef<any>[]
): ComputedRef<boolean>
export { logicAnd as and }
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/logicAnd/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/logicAnd/index.md)
vueuse useAverage useAverage
==========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 273 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Get the average of an array reactively.
Usage
-----
```
import { useAverage } from '@vueuse/math'
const list = ref([1, 2, 3])
const averageValue = useAverage(list) // Ref<2>
```
```
import { useAverage } from '@vueuse/math'
const a = ref(1)
const b = ref(3)
const averageValue = useAverage(a, b) // Ref<2>
```
Type Declarations
-----------------
```
export declare function useAverage(
array: MaybeComputedRef<MaybeComputedRef<number>[]>
): ComputedRef<number>
export declare function useAverage(
...args: MaybeComputedRef<number>[]
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useAverage/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useAverage/index.md)
vueuse useToFixed
**WARNING****Deprecated**. Please use [`usePrecision`](../useprecision/index)instead.
useToFixed
==========
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 300 B |
| Package | `@vueuse/math` |
| Last Changed | 5 months ago |
Reactive `toFixed`.
Usage
-----
```
import { useToFixed } from '@vueuse/math'
const value = ref(3.1415)
const result = useToFixed(value, 2) // 3.14
const stringResult = useToFixed(value, 5, {
type: 'string'
}) // '3.14150'
const ceilResult = useToFixed(value, 2, {
math: 'ceil'
}) // 3.15
const floorResult = useToFixed(value, 3, {
math: 'floor'
}) // 3.141
```
Type Declarations
-----------------
```
export interface FixedTypes {
type?: "string" | "number"
math?: "floor" | "ceil" | "round"
}
/**
* @deprecated use `usePrecision` instead
*/
export declare function useToFixed(
value: MaybeComputedRef<number | string>,
digits: MaybeComputedRef<number>,
options?: MaybeComputedRef<FixedTypes>
): ComputedRef<number | string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useToFixed/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/math/useToFixed/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useToFixed/index.md)
| programming_docs |
vueuse useMin useMin
======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 266 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.min`.
Usage
-----
```
import { useMin } from '@vueuse/math'
const array = ref([1, 2, 3, 4])
const sum = useMin(array) // Ref<1>
```
```
import { useMin } from '@vueuse/math'
const a = ref(1)
const b = ref(3)
const sum = useMin(a, b, 2) // Ref<1>
```
Type Declarations
-----------------
```
export declare function useMin(
array: MaybeComputedRef<MaybeComputedRef<number>[]>
): ComputedRef<number>
export declare function useMin(
...args: MaybeComputedRef<number>[]
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useMin/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useMin/index.md)
vueuse createProjection createProjection
================
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 264 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
| Related | [`createGenericProjection`](../creategenericprojection/index)[`useProjection`](../useprojection/index) |
Reactive numeric projection from one domain to another.
Usage
-----
```
import { createProjection } from '@vueuse/math'
const useProjector = createProjection([0, 10], [0, 100])
const input = ref(0)
const projected = useProjector(input) // projected.value === 0
input.value = 5 // projected.value === 50
input.value = 10 // projected.value === 100
```
Type Declarations
-----------------
```
export declare function createProjection(
fromDomain: MaybeComputedRef<readonly [number, number]>,
toDomain: MaybeComputedRef<readonly [number, number]>,
projector?: ProjectorFunction<number, number>
): UseProjection<number, number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/createProjection/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/createProjection/index.md)
vueuse useMax useMax
======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 261 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.max`.
Usage
-----
```
import { useMax } from '@vueuse/math'
const array = ref([1, 2, 3, 4])
const sum = useMax(array) // Ref<4>
```
```
import { useMax } from '@vueuse/math'
const a = ref(1)
const b = ref(3)
const sum = useMax(a, b, 2) // Ref<3>
```
Type Declarations
-----------------
```
export declare function useMax(
array: MaybeComputedRef<MaybeComputedRef<number>[]>
): ComputedRef<number>
export declare function useMax(
...args: MaybeComputedRef<number>[]
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useMax/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useMax/index.md)
vueuse useAbs useAbs
======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 199 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Reactive `Math.abs`.
Usage
-----
```
import { useAbs } from '@vueuse/math'
const value = ref(-23)
const absValue = useAbs(value) // Ref<23>
```
Type Declarations
-----------------
```
/**
* Reactive `Math.abs`.
*
* @see https://vueuse.org/useAbs
*/
export declare function useAbs(
value: MaybeComputedRef<number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useAbs/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useAbs/index.md)
vueuse useSum useSum
======
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 270 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
Get the sum of an array reactively
Usage
-----
```
import { useSum } from '@vueuse/math'
const array = ref([1, 2, 3, 4])
const sum = useSum(array) // Ref<10>
```
```
import { useSum } from '@vueuse/math'
const a = ref(1)
const b = ref(3)
const sum = useSum(a, b, 2) // Ref<6>
```
Type Declarations
-----------------
```
export declare function useSum(
array: MaybeComputedRef<MaybeComputedRef<number>[]>
): ComputedRef<number>
export declare function useSum(
...args: MaybeComputedRef<number>[]
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useSum/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useSum/index.md)
vueuse useProjection useProjection
=============
| | |
| --- | --- |
| Category | [@Math](../../functions#category=%40Math) |
| Export Size | 278 B |
| Package | `@vueuse/math` |
| Last Changed | 6 months ago |
| Related | [`createGenericProjection`](../creategenericprojection/index)[`createProjection`](../createprojection/index) |
Reactive numeric projection from one domain to another.
Usage
-----
```
import { useProjection } from '@vueuse/math'
const input = ref(0)
const projected = useProjection(input, [0, 10], [0, 100])
input.value = 5 // projected.value === 50
input.value = 10 // projected.value === 100
```
Type Declarations
-----------------
```
/**
* Reactive numeric projection from one domain to another.
*
* @see https://vueuse.org/useProjection
*/
export declare function useProjection(
input: MaybeComputedRef<number>,
fromDomain: MaybeComputedRef<readonly [number, number]>,
toDomain: MaybeComputedRef<readonly [number, number]>,
projector?: ProjectorFunction<number, number>
): ComputedRef<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/math/useProjection/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/math/useProjection/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/math/useProjection/index.md)
vueuse useIDBKeyval useIDBKeyval
============
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | |
| Package | `@vueuse/integrations` |
| Last Changed | 2 months ago |
Wrapper for [`idb-keyval`](https://www.npmjs.com/package/idb-keyval).
Available in the [@vueuse/integrations](../readme) add-on. Install idb-keyval as a peer dependency
---------------------------------------
```
npm install idb-keyval
```
Usage
-----
```
import { useIDBKeyval } from '@vueuse/integrations/useIDBKeyval'
// bind object
const storedObject = useIDBKeyval('my-idb-keyval-store', { hello: 'hi', greeting: 'Hello' })
// update object
storedObject.value.hello = 'hola'
// bind boolean
const flag = useIDBKeyval('my-flag', true) // returns Ref<boolean>
// bind number
const count = useIDBKeyval('my-count', 0) // returns Ref<number>
// delete data from idb storage
storedObject.value = null
```
Type Declarations
-----------------
```
export interface UseIDBOptions extends ConfigurableFlush {
/**
* Watch for deep changes
*
* @default true
*/
deep?: boolean
/**
* On error callback
*
* Default log error to `console.error`
*/
onError?: (error: unknown) => void
/**
* Use shallow ref as reference
*
* @default false
*/
shallow?: boolean
}
/**
*
* @param key
* @param initialValue
* @param options
*/
export declare function useIDBKeyval<T>(
key: IDBValidKey,
initialValue: MaybeComputedRef<T>,
options?: UseIDBOptions
): RemovableRef<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useIDBKeyval/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useIDBKeyval/demo.client.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useIDBKeyval/index.md)
vueuse useJwt useJwt
======
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 359 B |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Wrapper for [`jwt-decode`](https://github.com/auth0/jwt-decode).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm install jwt-decode
```
Usage
-----
```
import { defineComponent } from 'vue'
import { useJwt } from '@vueuse/integrations/useJwt'
export default defineComponent({
setup() {
const encodedJwt = ref('eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwiaWF0IjoxNTE2MjM5MDIyfQ.L8i6g3PfcHlioHCCPURC9pmXT7gdJpx3kOoyAfNUwCc')
const { header, payload } = useJwt(encodedJwt)
return { header, payload }
},
})
```
Type Declarations
-----------------
```
export interface UseJwtOptions<Fallback> {
/**
* Value returned when encounter error on decoding
*
* @default null
*/
fallbackValue?: Fallback
/**
* Error callback for decoding
*/
onError?: (error: unknown) => void
}
export interface UseJwtReturn<Payload, Header, Fallback> {
header: ComputedRef<Header | Fallback>
payload: ComputedRef<Payload | Fallback>
}
/**
* Reactive decoded jwt token.
*
* @see https://vueuse.org/useJwt
* @param jwt
*/
export declare function useJwt<
Payload extends object = JwtPayload,
Header extends object = JwtHeader,
Fallback = null
>(
encodedJwt: MaybeComputedRef<string>,
options?: UseJwtOptions<Fallback>
): UseJwtReturn<Payload, Header, Fallback>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useJwt/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useJwt/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useJwt/index.md)
vueuse useQRCode useQRCode
=========
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 339 B |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Wrapper for [`qrcode`](https://github.com/soldair/node-qrcode).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i qrcode
```
Usage
-----
```
import { useQRCode } from '@vueuse/integrations/useQRCode'
// `qrcode` will be a ref of data URL
const qrcode = useQRCode('text-to-encode')
```
or passing a `ref` to it, the returned data URL ref will change along with the source ref's changes.
```
import { ref } from 'vue'
import { useQRCode } from '@vueuse/integrations/useQRCode'
const text = ref('text-to-encode')
const qrcode = useQRCode(text)
```
```
<input v-model="text" type="text">
<img :src="qrcode" alt="QR Code" />
```
Type Declarations
-----------------
```
/**
* Wrapper for qrcode.
*
* @see https://vueuse.org/useQRCode
* @param text
* @param options
*/
export declare function useQRCode(
text: MaybeComputedRef<string>,
options?: QRCode.QRCodeToDataURLOptions
): Ref<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useQRCode/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useQRCode/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useQRCode/index.md)
vueuse useDrauu useDrauu
========
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 957 B |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Reactive instance for [drauu](https://github.com/antfu/drauu).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i drauu
```
Usage
-----
```
<script setup>
import { ref } from 'vue'
import { toRefs } from '@vueuse/core'
import { useDrauu } from '@vueuse/integrations/useDrauu'
const target = ref()
const { undo, redo, canUndo, canRedo, brush } = useDrauu(target)
const { color, size } = toRefs(brush)
</script>
<template>
<svg ref="target"></svg>
</template>
```
Type Declarations
-----------------
```
export type UseDrauuOptions = Omit<Options, "el">
export interface UseDrauuReturn {
drauuInstance: Ref<Drauu | undefined>
load: (svg: string) => void
dump: () => string | undefined
clear: () => void
cancel: () => void
undo: () => boolean | undefined
redo: () => boolean | undefined
canUndo: Ref<boolean>
canRedo: Ref<boolean>
brush: Ref<Brush>
onChanged: EventHookOn
onCommitted: EventHookOn
onStart: EventHookOn
onEnd: EventHookOn
onCanceled: EventHookOn
}
/**
* Reactive drauu
*
* @see https://vueuse.org/useDrauu
* @param target The target svg element
* @param options Drauu Options
*/
export declare function useDrauu(
target: MaybeComputedElementRef,
options?: UseDrauuOptions
): UseDrauuReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useDrauu/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useDrauu/demo.client.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useDrauu/index.md)
vueuse useAsyncValidator useAsyncValidator
=================
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 1.2 kB |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Wrapper for [`async-validator`](https://github.com/yiminghe/async-validator).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i async-validator
```
Usage
-----
```
import { useAsyncValidator } from '@vueuse/integrations/useAsyncValidator'
```
Type Declarations
-----------------
```
export type AsyncValidatorError = Error & {
errors: ValidateError[]
fields: Record<string, ValidateError[]>
}
export interface UseAsyncValidatorReturn {
pass: Ref<boolean>
errorInfo: Ref<AsyncValidatorError | null>
isFinished: Ref<boolean>
errors: Ref<AsyncValidatorError["errors"] | undefined>
errorFields: Ref<AsyncValidatorError["fields"] | undefined>
}
export interface UseAsyncValidatorOptions {
/**
* @see https://github.com/yiminghe/async-validator#options
*/
validateOption?: ValidateOption
}
/**
* Wrapper for async-validator.
*
* @see https://vueuse.org/useAsyncValidator
* @see https://github.com/yiminghe/async-validator
*/
export declare function useAsyncValidator(
value: MaybeComputedRef<Record<string, any>>,
rules: MaybeComputedRef<Rules>,
options?: UseAsyncValidatorOptions
): UseAsyncValidatorReturn & PromiseLike<UseAsyncValidatorReturn>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAsyncValidator/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAsyncValidator/demo.client.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAsyncValidator/index.md)
vueuse useAxios useAxios
========
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 1.4 kB |
| Package | `@vueuse/integrations` |
| Last Changed | 3 months ago |
Wrapper for [`axios`](https://github.com/axios/axios).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i axios
```
Usage
-----
```
import { useAxios } from '@vueuse/integrations/useAxios'
const { data, isFinished } = useAxios('/api/posts')
```
or use an instance of axios
```
import axios from 'axios'
import { useAxios } from '@vueuse/integrations/useAxios'
const instance = axios.create({
baseURL: '/api',
})
const { data, isFinished } = useAxios('/posts', instance)
```
use an instance of axios with config options
```
import axios from 'axios'
import { useAxios } from '@vueuse/integrations/useAxios'
const instance = axios.create({
baseURL: '/api',
})
const { data, isFinished } = useAxios('/posts', { method: 'POST' }, instance)
```
When you don't pass the `url`. The default value is `{immediate: false}`
```
import { useAxios } from '@vueuse/integrations/useAxios'
const { execute } = useAxios()
execute(url)
```
The `execute` function `url` here is optional, and `url2` will replace the `url1`.
```
import { useAxios } from '@vueuse/integrations/useAxios'
const { execute } = useAxios(url1, {}, { immediate: false })
execute(url2)
```
The `execute` function can accept `config` only.
```
import { useAxios } from '@vueuse/integrations/useAxios'
const { execute } = useAxios(url1, { method: 'GET' }, { immediate: false })
execute({ params: { key: 1 } })
execute({ params: { key: 2 } })
```
The `execute` function resolves with a result of network request.
```
import { useAxios } from '@vueuse/integrations/useAxios'
const { execute } = useAxios()
const result = await execute(url)
```
use an instance of axios with `immediate` options
```
import axios from 'axios'
import { useAxios } from '@vueuse/integrations/useAxios'
const instance = axios.create({
baseURL: '/api',
})
const { data, isFinished } = useAxios('/posts', { method: 'POST' }, instance, {
immediate: false,
})
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseAxiosReturn<T, R = AxiosResponse<T>, D = any> {
/**
* Axios Response
*/
response: ShallowRef<R | undefined>
/**
* Axios response data
*/
data: Ref<T | undefined>
/**
* Indicates if the request has finished
*/
isFinished: Ref<boolean>
/**
* Indicates if the request is currently loading
*/
isLoading: Ref<boolean>
/**
* Indicates if the request was canceled
*/
isAborted: Ref<boolean>
/**
* Any errors that may have occurred
*/
error: ShallowRef<AxiosError<T, D> | undefined>
/**
* Aborts the current request
*/
abort: (message?: string | undefined) => void
/**
* isFinished alias
* @deprecated use `isFinished` instead
*/
finished: Ref<boolean>
/**
* isLoading alias
* @deprecated use `isLoading` instead
*/
loading: Ref<boolean>
/**
* isAborted alias
* @deprecated use `isAborted` instead
*/
aborted: Ref<boolean>
/**
* abort alias
*/
cancel: (message?: string | undefined) => void
/**
* isAborted alias
* @deprecated use `isCanceled` instead
*/
canceled: Ref<boolean>
/**
* isAborted alias
*/
isCanceled: Ref<boolean>
}
export interface StrictUseAxiosReturn<T, R, D> extends UseAxiosReturn<T, R, D> {
/**
* Manually call the axios request
*/
execute: (
url?: string | AxiosRequestConfig<D>,
config?: AxiosRequestConfig<D>
) => PromiseLike<StrictUseAxiosReturn<T, R, D>>
}
export interface EasyUseAxiosReturn<T, R, D> extends UseAxiosReturn<T, R, D> {
/**
* Manually call the axios request
*/
execute: (
url: string,
config?: AxiosRequestConfig<D>
) => PromiseLike<EasyUseAxiosReturn<T, R, D>>
}
export interface UseAxiosOptions {
/**
* Will automatically run axios request when `useAxios` is used
*
*/
immediate?: boolean
/**
* Use shallowRef.
*
* @default true
*/
shallow?: boolean
}
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
url: string,
config?: AxiosRequestConfig<D>,
options?: UseAxiosOptions
): StrictUseAxiosReturn<T, R, D> & PromiseLike<StrictUseAxiosReturn<T, R, D>>
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
url: string,
instance?: AxiosInstance,
options?: UseAxiosOptions
): StrictUseAxiosReturn<T, R, D> & PromiseLike<StrictUseAxiosReturn<T, R, D>>
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
url: string,
config: AxiosRequestConfig<D>,
instance: AxiosInstance,
options?: UseAxiosOptions
): StrictUseAxiosReturn<T, R, D> & PromiseLike<StrictUseAxiosReturn<T, R, D>>
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
config?: AxiosRequestConfig<D>
): EasyUseAxiosReturn<T, R, D> & PromiseLike<EasyUseAxiosReturn<T, R, D>>
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
instance?: AxiosInstance
): EasyUseAxiosReturn<T, R, D> & PromiseLike<EasyUseAxiosReturn<T, R, D>>
export declare function useAxios<T = any, R = AxiosResponse<T>, D = any>(
config?: AxiosRequestConfig<D>,
instance?: AxiosInstance
): EasyUseAxiosReturn<T, R, D> & PromiseLike<EasyUseAxiosReturn<T, R, D>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAxios/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAxios/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useAxios/index.md)
| programming_docs |
vueuse useFuse useFuse
=======
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 490 B |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Easily implement fuzzy search using a composable with [Fuse.js](https://github.com/krisk/fuse).
From the Fuse.js website:
>
> What is fuzzy searching?
>
>
> Generally speaking, fuzzy searching (more formally known as approximate string matching) is the technique of finding strings that are approximately equal to a given pattern (rather than exactly).
>
>
>
Available in the [@vueuse/integrations](../readme) add-on. Install Fuse.js as a peer dependency
------------------------------------
### NPM
```
npm install fuse.js
```
### Yarn
```
yarn add fuse.js
```
Usage
-----
```
import { ref } from 'vue'
import { useFuse } from '@vueuse/integrations/useFuse'
const data = [
'John Smith',
'John Doe',
'Jane Doe',
'Phillip Green',
'Peter Brown',
]
const input = ref('Jhon D')
const { results } = useFuse(input, data)
/*
* Results:
*
* { "item": "John Doe", "index": 1 }
* { "item": "John Smith", "index": 0 }
* { "item": "Jane Doe", "index": 2 }
*
*/
```
Type Declarations
-----------------
```
export type FuseOptions<T> = Fuse.IFuseOptions<T>
export interface UseFuseOptions<T> {
fuseOptions?: FuseOptions<T>
resultLimit?: number
matchAllWhenSearchEmpty?: boolean
}
export declare function useFuse<DataItem>(
search: MaybeComputedRef<string>,
data: MaybeComputedRef<DataItem[]>,
options?: MaybeComputedRef<UseFuseOptions<DataItem>>
): {
fuse: Ref<{
search: <R = DataItem>(
pattern: string | Fuse.Expression,
options?: Fuse.FuseSearchOptions | undefined
) => Fuse.FuseResult<R>[]
setCollection: (
docs: readonly DataItem[],
index?: Fuse.FuseIndex<DataItem> | undefined
) => void
add: (doc: DataItem) => void
remove: (predicate: (doc: DataItem, idx: number) => boolean) => DataItem[]
removeAt: (idx: number) => void
getIndex: () => Fuse.FuseIndex<DataItem>
}>
results: ComputedRef<Fuse.FuseResult<DataItem>[]>
}
export type UseFuseReturn = ReturnType<typeof useFuse>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFuse/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFuse/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFuse/index.md)
vueuse useCookies useCookies
==========
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 730 B |
| Package | `@vueuse/integrations` |
| Last Changed | last year |
Wrapper for [`universal-cookie`](https://www.npmjs.com/package/universal-cookie).
**TIP**When using with Nuxt 3, this functions will **NOT** be auto imported in favor of Nuxt's built-in [`useCookie()`](https://v3.nuxtjs.org/api/composables/use-cookie). Use explicit import if you want to use the function from VueUse.
Available in the [@vueuse/integrations](../readme) add-on.
Install
-------
```
npm i universal-cookie
```
Usage
-----
### Common usage
```
<template>
<div>
<strong>locale</strong>: {{ cookies.get('locale') }}
<hr>
<pre>{{ cookies.getAll() }}</pre>
<button @click="cookies.set('locale', 'ru-RU')">Russian</button>
<button @click="cookies.set('locale', 'en-US')">English</button>
</div>
</template>
<script>
import { defineComponent } from 'vue'
import { useCookies } from '@vueuse/integrations/useCookies'
export default defineComponent({
setup() {
const cookies = useCookies(['locale'])
return {
cookies,
}
},
})
</script>
```
Options
-------
Access and modify cookies using vue composition-api.
> By default, you should use it inside `setup()`, but this function also works anywhere else.
>
>
```
const { get, getAll, set, remove, addChangeListener, removeChangeListener } = useCookies(['cookie-name'], { doNotParse: false, autoUpdateDependencies: false })
```
###
`dependencies` (optional)
Let you optionally specify a list of cookie names your component depend on or that should trigger a re-render. If unspecified, it will render on every cookie change.
###
`options` (optional)
* `doNotParse` (boolean = false): do not convert the cookie into an object no matter what. **Passed as default value to [`get`](../../shared/get/index)`getAll` methods.**
* `autoUpdateDependencies` (boolean = false): automatically add cookie names ever provided to [`get`](../../shared/get/index)method. If **true** then you don't need to care about provided `dependencies`.
###
`cookies` (optional)
Let you provide a `universal-cookie` instance (creates a new instance by default)
> Info about methods available in the [universal-cookie api docs](https://www.npmjs.com/package/universal-cookie#api---cookies-class)
>
>
`createCookies([req])`
-----------------------
Create a `universal-cookie` instance using request (default is window.document.cookie) and returns [`useCookies`](index)function with provided universal-cookie instance
* req (object): Node's [http.IncomingMessage](https://nodejs.org/api/http.html#http_class_http_incomingmessage) request object
Type Declarations
-----------------
Show Type Declarations
```
/// <reference types="node" />
/**
* Creates a new {@link useCookies} function
* @param {Object} req - incoming http request (for SSR)
* @see https://github.com/reactivestack/cookies/tree/master/packages/universal-cookie universal-cookie
* @description Creates universal-cookie instance using request (default is window.document.cookie) and returns {@link useCookies} function with provided universal-cookie instance
*/
export declare function createCookies(req?: IncomingMessage): (
dependencies?: string[] | null,
{
doNotParse,
autoUpdateDependencies,
}?: {
doNotParse?: boolean | undefined
autoUpdateDependencies?: boolean | undefined
}
) => {
/**
* Reactive get cookie by name. If **autoUpdateDependencies = true** then it will update watching dependencies
*/
get: <T = any>(name: string, options?: CookieGetOptions | undefined) => T
/**
* Reactive get all cookies
*/
getAll: <T_1 = any>(options?: CookieGetOptions | undefined) => T_1
set: (
name: string,
value: any,
options?: CookieSetOptions | undefined
) => void
remove: (name: string, options?: CookieSetOptions | undefined) => void
addChangeListener: (callback: CookieChangeListener) => void
removeChangeListener: (callback: CookieChangeListener) => void
}
/**
* Reactive methods to work with cookies (use {@link createCookies} method instead if you are using SSR)
* @param {string[]|null|undefined} dependencies - array of watching cookie's names. Pass empty array if don't want to watch cookies changes.
* @param {Object} options
* @param {boolean} options.doNotParse - don't try parse value as JSON
* @param {boolean} options.autoUpdateDependencies - automatically update watching dependencies
* @param {Object} cookies - universal-cookie instance
*/
export declare function useCookies(
dependencies?: string[] | null,
{
doNotParse,
autoUpdateDependencies,
}?: {
doNotParse?: boolean | undefined
autoUpdateDependencies?: boolean | undefined
},
cookies?: Cookie
): {
/**
* Reactive get cookie by name. If **autoUpdateDependencies = true** then it will update watching dependencies
*/
get: <T = any>(name: string, options?: CookieGetOptions | undefined) => T
/**
* Reactive get all cookies
*/
getAll: <T_1 = any>(options?: CookieGetOptions | undefined) => T_1
set: (
name: string,
value: any,
options?: CookieSetOptions | undefined
) => void
remove: (name: string, options?: CookieSetOptions | undefined) => void
addChangeListener: (callback: CookieChangeListener) => void
removeChangeListener: (callback: CookieChangeListener) => void
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useCookies/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useCookies/index.md)
*No recent changes*
vueuse useNProgress useNProgress
============
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 462 B |
| Package | `@vueuse/integrations` |
| Last Changed | 5 months ago |
Reactive wrapper for [`nprogress`](https://github.com/rstacruz/nprogress).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i nprogress
```
Usage
-----
```
import { useNProgress } from '@vueuse/integrations/useNProgress'
const { isLoading } = useNProgress()
function toggle() {
isLoading.value = !isLoading.value
}
```
### Passing a progress percentage
You can pass a percentage to indicate where the bar should start from.
```
import { useNProgress } from '@vueuse/integrations/useNProgress'
const { progress } = useNProgress(0.5)
function done() {
progress.value = 1.0
}
```
> To change the progress percentage, set `progress.value = n`, where n is a number between 0..1.
>
>
### Customization
Just edit [nprogress.css](http://ricostacruz.com/nprogress/nprogress.css) to your liking. Tip: you probably only want to find and replace occurrences of #29d.
You can [configure](https://github.com/rstacruz/nprogress#configuration) it by passing an object as a second parameter.
```
import { useNProgress } from '@vueuse/integrations/useNProgress'
useNProgress(null, {
minimum: 0.1,
// ...
})
```
Type Declarations
-----------------
```
export type UseNProgressOptions = Partial<NProgressOptions>
/**
* Reactive progress bar.
*
* @see https://vueuse.org/useNProgress
*/
export declare function useNProgress(
currentProgress?: MaybeComputedRef<number | null | undefined>,
options?: UseNProgressOptions
): {
isLoading: WritableComputedRef<boolean>
progress: Ref<number | (() => number | null | undefined) | null | undefined>
start: () => nprogress.NProgress
done: (force?: boolean | undefined) => nprogress.NProgress
remove: () => void
}
export type UseNProgressReturn = ReturnType<typeof useNProgress>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useNProgress/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useNProgress/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useNProgress/index.md)
vueuse useChangeCase useChangeCase
=============
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 447 B |
| Package | `@vueuse/integrations` |
| Last Changed | 6 months ago |
Reactive wrapper for [`change-case`](https://github.com/blakeembrey/change-case).
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i change-case
```
Usage
-----
```
import { useChangeCase } from '@vueuse/integrations/useChangeCase'
// `changeCase` will be a computed
const changeCase = useChangeCase('hello world', 'camelCase')
changeCase.value // helloWorld
changeCase.value = 'vue use'
changeCase.value // vueUse
// Supported methods
// export {
// camelCase,
// capitalCase,
// constantCase,
// dotCase,
// headerCase,
// noCase,
// paramCase,
// pascalCase,
// pathCase,
// sentenceCase,
// snakeCase,
// } from 'change-case'
```
or passing a `ref` to it, the returned `computed` will change along with the source ref's changes.
Can be passed into `options` for customization
```
import { ref } from 'vue-demi'
import { useChangeCase } from '@vueuse/integrations/useChangeCase'
const input = ref('helloWorld')
const changeCase = useChangeCase(input, 'camelCase', {
delimiter: '-',
})
changeCase.value // hello-World
ref.value = 'vue use'
changeCase.value // vue-Use
```
Type Declarations
-----------------
```
export type ChangeCaseType = keyof typeof changeCase
export declare function useChangeCase(
input: MaybeRef<string>,
type: ChangeCaseType,
options?: Options | undefined
): WritableComputedRef<string>
export declare function useChangeCase(
input: MaybeComputedRef<string>,
type: ChangeCaseType,
options?: Options | undefined
): ComputedRef<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useChangeCase/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useChangeCase/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useChangeCase/index.md)
vueuse useFocusTrap useFocusTrap
============
| | |
| --- | --- |
| Category | [@Integrations](../../functions#category=%40Integrations) |
| Export Size | 681 B |
| Package | `@vueuse/integrations` |
| Last Changed | last year |
Reactive wrapper for [`focus-trap`](https://github.com/focus-trap/focus-trap).
For more information on what options can be passed, see [`createOptions`](https://github.com/focus-trap/focus-trap#createfocustrapelement-createoptions) in the `focus-trap` documentation.
Available in the [@vueuse/integrations](../readme) add-on. Install
-------
```
npm i focus-trap
```
Usage
-----
**Basic Usage**
```
<script setup>
import { ref } from 'vue'
import { useFocusTrap } from '@vueuse/integrations/useFocusTrap'
const target = ref()
const { hasFocus, activate, deactivate } = useFocusTrap(target)
</script>
<template>
<div>
<button @click="activate()">Activate</button>
<div ref="target">
<span>Has Focus: {{ hasFocus }}</span>
<input type="text" />
<button @click="deactivate()">Deactivate</button>
</div>
</div>
</template>
```
**Automatically Focus**
```
<script setup>
import { ref } from 'vue'
import { useFocusTrap } from '@vueuse/integrations/useFocusTrap'
const target = ref()
const { hasFocus, activate, deactivate } = useFocusTrap(target, { immediate: true })
</script>
<template>
<div>
<div ref="target">...</div>
</div>
</template>
```
Using Component
---------------
This function can't properly activate focus on elements with conditional rendering. In this case, you can use the `UseFocusTrap` component. Focus Trap will be activated automatically on mounting this component and deactivated on unmount.
```
<script setup>
import { ref } from 'vue'
import { UseFocusTrap } from '@vueuse/integrations/useFocusTrap/component'
const show = ref(false)
</script>
<template>
<UseFocusTrap v-if="show" :options="{ immediate: true }">
<div class="modal">...</div>
</UseFocusTrap>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseFocusTrapOptions extends Options {
/**
* Immediately activate the trap
*/
immediate?: boolean
}
export interface UseFocusTrapReturn {
/**
* Indicates if the focus trap is currently active
*/
hasFocus: Ref<boolean>
/**
* Indicates if the focus trap is currently paused
*/
isPaused: Ref<boolean>
/**
* Activate the focus trap
*
* @see https://github.com/focus-trap/focus-trap#trapactivateactivateoptions
* @param opts Activate focus trap options
*/
activate: (opts?: ActivateOptions) => void
/**
* Deactivate the focus trap
*
* @see https://github.com/focus-trap/focus-trap#trapdeactivatedeactivateoptions
* @param opts Deactivate focus trap options
*/
deactivate: (opts?: DeactivateOptions) => void
/**
* Pause the focus trap
*
* @see https://github.com/focus-trap/focus-trap#trappause
*/
pause: Fn
/**
* Unpauses the focus trap
*
* @see https://github.com/focus-trap/focus-trap#trapunpause
*/
unpause: Fn
}
/**
* Reactive focus-trap
*
* @see https://vueuse.org/useFocusTrap
* @param target The target element to trap focus within
* @param options Focus trap options
* @param autoFocus Focus trap automatically when mounted
*/
export declare function useFocusTrap(
target: MaybeElementRef,
options?: UseFocusTrapOptions
): UseFocusTrapReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFocusTrap/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFocusTrap/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/integrations/useFocusTrap/index.md)
vueuse useAuth useAuth
=======
| | |
| --- | --- |
| Category | [@Firebase](../../functions#category=%40Firebase) |
| Export Size | 164 B |
| Package | `@vueuse/firebase` |
| Last Changed | 6 months ago |
Reactive [Firebase Auth](https://firebase.google.com/docs/auth) binding. It provides a reactive `user` and `isAuthenticated` so you can easily react to changes in the users' authentication status. Available in the [@vueuse/firebase](../readme) add-on.
Usage
-----
```
<script setup lang="ts">
import { initializeApp } from 'firebase/app'
import { GoogleAuthProvider, getAuth, signInWithPopup } from 'firebase/auth'
import { useAuth } from '@vueuse/firebase/useAuth'
const app = initializeApp({ /* config */ })
const auth = getAuth(app);
const { isAuthenticated, user } = useAuth(auth)
const signIn = () => signInWithPopup(auth, new GoogleAuthProvider())
</script>
<template>
<pre v-if="isAuthenticated">{{ user }}</pre>
<div v-else>
<button @click="signIn">
Sign In with Google
</button>
</div>
</template>
```
Type Declarations
-----------------
Show Type Declarations
```
export interface UseFirebaseAuthOptions {
isAuthenticated: ComputedRef<boolean>
user: Ref<User | null>
}
/**
* Reactive Firebase Auth binding
*
* @see https://vueuse.org/useAuth
*/
export declare function useAuth(auth: Auth): {
isAuthenticated: ComputedRef<boolean>
user: Ref<{
readonly emailVerified: boolean
readonly isAnonymous: boolean
readonly metadata: {
readonly creationTime?: string | undefined
readonly lastSignInTime?: string | undefined
}
readonly providerData: {
readonly displayName: string | null
readonly email: string | null
readonly phoneNumber: string | null
readonly photoURL: string | null
readonly providerId: string
readonly uid: string
}[]
readonly refreshToken: string
readonly tenantId: string | null
delete: () => Promise<void>
getIdToken: (forceRefresh?: boolean | undefined) => Promise<string>
getIdTokenResult: (
forceRefresh?: boolean | undefined
) => Promise<IdTokenResult>
reload: () => Promise<void>
toJSON: () => object
readonly displayName: string | null
readonly email: string | null
readonly phoneNumber: string | null
readonly photoURL: string | null
readonly providerId: string
readonly uid: string
} | null>
}
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useAuth/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useAuth/index.md)
vueuse useRTDB useRTDB
=======
| | |
| --- | --- |
| Category | [@Firebase](../../functions#category=%40Firebase) |
| Export Size | 287 B |
| Package | `@vueuse/firebase` |
| Last Changed | 6 months ago |
Reactive [Firebase Realtime Database](https://firebase.google.com/docs/database) binding. Making it straightforward to **always keep your local data in sync** with remotes databases. Available in the [@vueuse/firebase](../readme) add-on.
Usage
-----
```
import { initializeApp } from 'firebase/app'
import { getDatabase } from 'firebase/database'
import { useRTDB } from '@vueuse/firebase/useRTDB'
const app = initializeApp({ /* config */ })
const db = getDatabase(app)
// in setup()
const todos = useRTDB(db.ref('todos'))
```
You can reuse the db reference by passing `autoDispose: false`
```
const todos = useRTDB(db.ref('todos'), { autoDispose: false })
```
or use [`createGlobalState`](../../shared/createglobalstate/index)from the core package
```
// store.js
import { createGlobalState } from '@vueuse/core'
import { useRTDB } from '@vueuse/firebase/useRTDB'
export const useTodos = createGlobalState(
() => useRTDB(db.ref('todos')),
)
```
```
// app.js
import { useTodos } from './store'
const todos = useTodos()
```
Type Declarations
-----------------
```
export interface UseRTDBOptions {
autoDispose?: boolean
}
/**
* Reactive Firebase Realtime Database binding.
*
* @see https://vueuse.org/useRTDB
*/
export declare function useRTDB<T = any>(
docRef: DatabaseReference,
options?: UseRTDBOptions
): Ref<T | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useRTDB/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useRTDB/index.md)
| programming_docs |
vueuse useFirestore useFirestore
============
| | |
| --- | --- |
| Category | [@Firebase](../../functions#category=%40Firebase) |
| Export Size | 506 B |
| Package | `@vueuse/firebase` |
| Last Changed | 2 months ago |
Reactive [Firestore](https://firebase.google.com/docs/firestore) binding. Making it straightforward to **always keep your local data in sync** with remotes databases. Available in the [@vueuse/firebase](../readme) add-on.
Usage
-----
```
import { computed, ref } from 'vue'
import { initializeApp } from 'firebase/app'
import { collection, doc, getFirestore, limit, orderBy, query } from 'firebase/firestore'
import { useFirestore } from '@vueuse/firebase/useFirestore'
const app = initializeApp({ projectId: 'MY PROJECT ID' })
const db = getFirestore(app)
const todos = useFirestore(collection(db, 'todos'))
// or for doc reference
const user = useFirestore(doc(db, 'users', 'my-user-id'))
// you can also use ref value for reactive query
const postsLimit = ref(10)
const postsQuery = computed(() => query(collection(db, 'posts'), orderBy('createdAt', 'desc'), limit(postsLimit.value)))
const posts = useFirestore(postsQuery)
// you can use the boolean value to tell a query when it is ready to run
// when it gets falsy value, return the initial value
const userId = ref('')
const userQuery = computed(() => userId.value && doc(db, 'users', userId.value))
const userData = useFirestore(userQuery, null)
```
Share across instances
----------------------
You can reuse the db reference by passing `autoDispose: false`
```
const todos = useFirestore(collection(db, 'todos'), undefined, { autoDispose: false })
```
or use [`createGlobalState`](../../shared/createglobalstate/index)from the core package
```
// store.js
import { createGlobalState } from '@vueuse/core'
import { useFirestore } from '@vueuse/firebase/useFirestore'
export const useTodos = createGlobalState(
() => useFirestore(collection(db, 'todos')),
)
```
```
// app.js
import { useTodos } from './store'
export default {
setup() {
const todos = useTodos()
return { todos }
},
}
```
Type Declarations
-----------------
```
export interface UseFirestoreOptions {
errorHandler?: (err: Error) => void
autoDispose?: boolean
}
export type FirebaseDocRef<T> = Query<T> | DocumentReference<T>
type Falsy = false | 0 | "" | null | undefined
export declare function useFirestore<T extends DocumentData>(
maybeDocRef: MaybeRef<DocumentReference<T> | Falsy>,
initialValue: T,
options?: UseFirestoreOptions
): Ref<T | null>
export declare function useFirestore<T extends DocumentData>(
maybeDocRef: MaybeRef<Query<T> | Falsy>,
initialValue: T[],
options?: UseFirestoreOptions
): Ref<T[]>
export declare function useFirestore<T extends DocumentData>(
maybeDocRef: MaybeRef<DocumentReference<T> | Falsy>,
initialValue?: T | undefined | null,
options?: UseFirestoreOptions
): Ref<T | undefined | null>
export declare function useFirestore<T extends DocumentData>(
maybeDocRef: MaybeRef<Query<T> | Falsy>,
initialValue?: T[],
options?: UseFirestoreOptions
): Ref<T[] | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useFirestore/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/firebase/useFirestore/index.md)
vueuse useSubject useSubject
==========
| | |
| --- | --- |
| Category | [@RxJS](../../functions#category=%40RxJS) |
| Export Size | 310 B |
| Package | `@vueuse/rxjs` |
| Last Changed | 2 days ago |
Bind an RxJS [`Subject`](https://rxjs.dev/guide/subject) to a `ref` and propagate value changes both ways.
Available in the [@vueuse/rxjs](../readme) add-on. Usage
-----
```
import { useSubject } from '@vueuse/rxjs'
import { Subject } from 'rxjs'
const subject = new Subject()
// setup()
const subjectRef = useSubject(subject)
```
If you want to add custom error handling to a Subject that might error, you can supply an optional `onError` configuration. Without this, RxJS will treat any error in the supplied observable as an "unhandled error" and it will be thrown in a new call stack and reported to `window.onerror` (or `process.on('error')` if you happen to be in node).
```
import { useSubject } from '@vueuse/rxjs'
import { Subject } from 'rxjs'
const subject = new Subject()
// setup()
const subjectRef = useSubject(subject,
{
onError: (err) => {
console.log(err.message) // "oops"
},
},
)
```
Type Declarations
-----------------
```
export interface UseSubjectOptions<I = undefined>
extends Omit<UseObservableOptions<I>, "initialValue"> {}
export declare function useSubject<H>(
subject: BehaviorSubject<H>,
options?: UseSubjectOptions
): Ref<H>
export declare function useSubject<H>(
subject: Subject<H>,
options?: UseSubjectOptions
): Ref<H | undefined>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useSubject/index.ts) • [Demo](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useSubject/demo.vue) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useSubject/index.md)
vueuse useSubscription useSubscription
===============
| | |
| --- | --- |
| Category | [@RxJS](../../functions#category=%40RxJS) |
| Export Size | 222 B |
| Package | `@vueuse/rxjs` |
| Last Changed | last year |
Use an RxJS [`Subscription`](https://rxjs.dev/guide/subscription) without worrying about unsubscribing from it or creating memory leaks. Available in the [@vueuse/rxjs](../readme) add-on.
Usage
-----
```
import { useSubscription } from '@vueuse/rxjs'
import { interval } from 'rxjs'
const count = ref(0)
// useSubscription call unsubscribe method before unmount the component
useSubscription(
interval(1000)
.subscribe(() => {
count.value++
console.log(count)
}),
)
```
Type Declarations
-----------------
```
export declare function useSubscription(subscription: Unsubscribable): void
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useSubscription/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useSubscription/index.md)
*No recent changes*
vueuse from / fromEvent from / fromEvent
================
Wrappers around RxJS's [`from()`](https://rxjs.dev/api/index/function/from) and [`fromEvent()`](https://rxjs.dev/api/index/function/fromEvent) to allow them to accept `ref`s. Available in the [@vueuse/rxjs](../readme) add-on.
Usage
-----
| | |
| --- | --- |
| Category | [@RxJS](../../functions#category=%40RxJS) |
| Export Size | 153 B |
| Package | `@vueuse/rxjs` |
| Last Changed | 3 months ago |
```
import { ref } from 'vue'
import { from, fromEvent, toObserver, useSubscription } from '@vueuse/rxjs'
import { interval } from 'rxjs'
import { map, mapTo, takeUntil, withLatestFrom } from 'rxjs/operators'
const count = ref(0)
const button = ref<HTMLButtonElement>(null)
useSubscription(
interval(1000)
.pipe(
mapTo(1),
takeUntil(fromEvent(button, 'click')),
withLatestFrom(from(count, {
immediate: true,
deep: false,
})),
map(([curr, total]) => curr + total),
)
.subscribe(toObserver(count)), // same as ).subscribe(val => (count.value = val))
)
```
Type Declarations
-----------------
```
export declare function from<T>(
value: ObservableInput<T> | Ref<T>,
watchOptions?: WatchOptions
): Observable<T>
export declare function fromEvent<T extends HTMLElement>(
value: MaybeRef<T>,
event: string
): Observable<Event>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/from/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/from/index.md)
vueuse toObserver toObserver
==========
| | |
| --- | --- |
| Category | [@RxJS](../../functions#category=%40RxJS) |
| Export Size | 88 B |
| Package | `@vueuse/rxjs` |
| Last Changed | last year |
Sugar function to convert a `ref` into an RxJS [Observer](https://rxjs.dev/guide/observer). Available in the [@vueuse/rxjs](../readme) add-on.
Usage
-----
```
import { ref } from 'vue'
import { from, fromEvent, toObserver, useSubscription } from '@vueuse/rxjs'
import { interval } from 'rxjs'
import { map, mapTo, takeUntil, withLatestFrom } from 'rxjs/operators'
const count = ref(0)
const button = ref<HTMLButtonElement>(null)
useSubscription(
interval(1000)
.pipe(
mapTo(1),
takeUntil(fromEvent(button, 'click')),
withLatestFrom(from(count).pipe(startWith(0))),
map(([total, curr]) => curr + total),
)
.subscribe(toObserver(count)), // same as ).subscribe(val => (count.value = val))
)
```
Type Declarations
-----------------
```
export declare function toObserver<T>(value: Ref<T>): NextObserver<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/toObserver/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/toObserver/index.md)
*No recent changes*
vueuse useObservable useObservable
=============
| | |
| --- | --- |
| Category | [@RxJS](../../functions#category=%40RxJS) |
| Export Size | 279 B |
| Package | `@vueuse/rxjs` |
| Last Changed | 8 months ago |
Use an RxJS [`Observable`](https://rxjs.dev/guide/observable), return a `ref`, and automatically unsubscribe from it when the component is unmounted. Available in the [@vueuse/rxjs](../readme) add-on.
Usage
-----
```
import { ref } from 'vue'
import { useObservable } from '@vueuse/rxjs'
import { interval } from 'rxjs'
import { mapTo, scan, startWith } from 'rxjs/operators'
// setup()
const count = useObservable(
interval(1000).pipe(
mapTo(1),
startWith(0),
scan((total, next) => next + total),
),
)
```
If you want to add custom error handling to an `Observable` that might error, you can supply an optional `onError` configuration. Without this, RxJS will treat any error in the supplied `Observable` as an "unhandled error" and it will be thrown in a new call stack and reported to `window.onerror` (or `process.on('error')` if you happen to be in Node).
```
import { ref } from 'vue'
import { useObservable } from '@vueuse/rxjs'
import { interval } from 'rxjs'
import { map } from 'rxjs/operators'
// setup()
const count = useObservable(
interval(1000).pipe(
map((n) => {
if (n === 10)
throw new Error('oops')
return n + n
}),
),
{
onError: (err) => {
console.log(err.message) // "oops"
},
},
)
```
Type Declarations
-----------------
```
export interface UseObservableOptions<I> {
onError?: (err: any) => void
/**
* The value that should be set if the observable has not emitted.
*/
initialValue?: I | undefined
}
export declare function useObservable<H, I = undefined>(
observable: Observable<H>,
options?: UseObservableOptions<I | undefined>
): Readonly<Ref<H | I>>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useObservable/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/rxjs/useObservable/index.md)
vueuse useZoomLevel useZoomLevel
============
| | |
| --- | --- |
| Category | [@Electron](../../functions#category=%40Electron) |
| Export Size | 358 B |
| Package | `@vueuse/electron` |
| Last Changed | 6 months ago |
Reactive [WebFrame](https://www.electronjs.org/docs/api/web-frame#webframe) zoom level. Available in the [@vueuse/electron](../readme) add-on.
Usage
-----
```
import { useZoomLevel } from '@vueuse/electron'
// enable nodeIntegration if you don't provide webFrame explicitly
// @see: https://www.electronjs.org/docs/api/webview-tag#nodeintegration
// Ref result will return
const level = useZoomLevel()
console.log(level.value) // print current zoom level
level.value = 2 // change current zoom level
```
Set initial zoom level immediately
```
import { useZoomLevel } from '@vueuse/electron'
const level = useZoomLevel(2)
```
Pass a `ref` and the level will be updated when the source ref changes
```
import { useZoomLevel } from '@vueuse/electron'
const level = ref(1)
useZoomLevel(level) // zoom level will match with the ref
level.value = 2 // zoom level will change
```
Type Declarations
-----------------
```
export declare function useZoomLevel(level: MaybeRef<number>): Ref<number>
export declare function useZoomLevel(
webFrame: WebFrame,
level: MaybeRef<number>
): Ref<number>
export declare function useZoomLevel(webFrame: WebFrame): Ref<number>
export declare function useZoomLevel(): Ref<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/electron/useZoomLevel/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/electron/useZoomLevel/index.md)
*No recent changes*
vueuse useIpcRendererInvoke useIpcRendererInvoke
====================
| | |
| --- | --- |
| Category | [@Electron](../../functions#category=%40Electron) |
| Export Size | 303 B |
| Package | `@vueuse/electron` |
| Last Changed | 6 months ago |
Reactive [ipcRenderer.invoke API](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererinvokechannel-args) result. Make asynchronous operations look synchronous. Available in the [@vueuse/electron](../readme) add-on.
Usage
-----
```
import { useIpcRendererInvoke } from '@vueuse/electron'
// enable nodeIntegration if you don't provide ipcRenderer explicitly
// @see: https://www.electronjs.org/docs/api/webview-tag#nodeintegration
// Ref result will return
const result = useIpcRendererInvoke<string>('custom-channel', 'some data')
const msg = computed(() => result.value?.msg)
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Returns Promise<any> - Resolves with the response from the main process.
*
* Send a message to the main process via channel and expect a result ~~asynchronously~~. As composition-api, it makes asynchronous operations look like synchronous.
*
* You need to provide `ipcRenderer` to this function.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererinvokechannel-args
* @see https://vueuse.org/useIpcRendererInvoke
*/
export declare function useIpcRendererInvoke<T>(
ipcRenderer: IpcRenderer,
channel: string,
...args: any[]
): Ref<T | null>
/**
* Returns Promise<any> - Resolves with the response from the main process.
*
* Send a message to the main process via channel and expect a result ~~asynchronously~~. As composition-api, it makes asynchronous operations look like synchronous.
*
* `ipcRenderer` will be automatically gotten.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererinvokechannel-args
* @see https://vueuse.org/useIpcRendererInvoke
*/
export declare function useIpcRendererInvoke<T>(
channel: string,
...args: any[]
): Ref<T | null>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRendererInvoke/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRendererInvoke/index.md)
*No recent changes*
vueuse useIpcRenderer useIpcRenderer
==============
| | |
| --- | --- |
| Category | [@Electron](../../functions#category=%40Electron) |
| Export Size | 535 B |
| Package | `@vueuse/electron` |
| Last Changed | 6 months ago |
Provides [ipcRenderer](https://www.electronjs.org/docs/api/ipc-renderer) and all of its APIs. Available in the [@vueuse/electron](../readme) add-on.
Usage
-----
```
import { useIpcRenderer } from '@vueuse/electron'
// enable nodeIntegration if you don't provide ipcRenderer explicitly
// @see: https://www.electronjs.org/docs/api/webview-tag#nodeintegration
const ipcRenderer = useIpcRenderer()
// Ref result will return
const result = ipcRenderer.invoke<string>('custom-channel', 'some data')
const msg = computed(() => result.value?.msg)
// remove listener automatically on unmounted
ipcRenderer.on('custom-event', (event, ...args) => {
console.log(args)
})
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Result from useIpcRenderer
*
* @see https://www.electronjs.org/docs/api/ipc-renderer
*/
export interface UseIpcRendererReturn {
/**
* Listens to channel, when a new message arrives listener would be called with listener(event, args...).
* [ipcRenderer.removeListener](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener) automatically on unmounted.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereronchannel-listener
*/
on(channel: string, listener: IpcRendererListener): IpcRenderer
/**
* Adds a one time listener function for the event. This listener is invoked only the next time a message is sent to channel, after which it is removed.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereroncechannel-listener
*/
once(
channel: string,
listener: (event: IpcRendererEvent, ...args: any[]) => void
): IpcRenderer
/**
* Removes the specified listener from the listener array for the specified channel.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener
*/
removeListener(
channel: string,
listener: (...args: any[]) => void
): IpcRenderer
/**
* Removes all listeners, or those of the specified channel.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovealllistenerschannel
*/
removeAllListeners(channel: string): IpcRenderer
/**
* Send an asynchronous message to the main process via channel, along with arguments.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrenderersendchannel-args
*/
send(channel: string, ...args: any[]): void
/**
* Returns Promise<any> - Resolves with the response from the main process.
* Send a message to the main process via channel and expect a result ~~asynchronously~~.
* As composition-api, it makes asynchronous operations look like synchronous.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererinvokechannel-args
*/
invoke<T>(channel: string, ...args: any[]): Ref<T | null>
/**
* Returns any - The value sent back by the ipcMain handler.
* Send a message to the main process via channel and expect a result synchronously.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrenderersendsyncchannel-args
*/
sendSync<T>(channel: string, ...args: any[]): Ref<T | null>
/**
* Send a message to the main process, optionally transferring ownership of zero or more MessagePort objects.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererpostmessagechannel-message-transfer
*/
postMessage(channel: string, message: any, transfer?: MessagePort[]): void
/**
* Sends a message to a window with webContentsId via channel.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrenderersendtowebcontentsid-channel-args
*/
sendTo(webContentsId: number, channel: string, ...args: any[]): void
/**
* Like ipcRenderer.send but the event will be sent to the <webview> element in the host page instead of the main process.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrenderersendtohostchannel-args
*/
sendToHost(channel: string, ...args: any[]): void
}
/**
* Get the `ipcRenderer` module with all APIs.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrenderersendtohostchannel-args
* @see https://vueuse.org/useIpcRenderer
*/
export declare function useIpcRenderer(
ipcRenderer?: IpcRenderer
): UseIpcRendererReturn
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRenderer/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRenderer/index.md)
*No recent changes*
| programming_docs |
vueuse useZoomFactor useZoomFactor
=============
| | |
| --- | --- |
| Category | [@Electron](../../functions#category=%40Electron) |
| Export Size | 393 B |
| Package | `@vueuse/electron` |
| Last Changed | 6 months ago |
Reactive [WebFrame](https://www.electronjs.org/docs/api/web-frame#webframe) zoom factor. Available in the [@vueuse/electron](../readme) add-on.
Usage
-----
```
import { useZoomFactor } from '@vueuse/electron'
// enable nodeIntegration if you don't provide webFrame explicitly
// @see: https://www.electronjs.org/docs/api/webview-tag#nodeintegration
// Ref result will return
const factor = useZoomFactor()
console.log(factor.value) // print current zoom factor
factor.value = 2 // change current zoom factor
```
Set initial zoom factor immediately
```
import { useZoomFactor } from '@vueuse/electron'
const factor = useZoomFactor(2)
```
Pass a `ref` and the factor will be updated when the source ref changes
```
import { useZoomFactor } from '@vueuse/electron'
const factor = ref(1)
useZoomFactor(factor) // zoom factor will match with the ref
factor.value = 2 // zoom factor will change
```
Type Declarations
-----------------
```
export declare function useZoomFactor(factor: MaybeRef<number>): Ref<number>
export declare function useZoomFactor(
webFrame: WebFrame,
factor: MaybeRef<number>
): Ref<number>
export declare function useZoomFactor(webFrame: WebFrame): Ref<number>
export declare function useZoomFactor(): Ref<number>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/electron/useZoomFactor/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/electron/useZoomFactor/index.md)
*No recent changes*
vueuse useIpcRendererOn useIpcRendererOn
================
| | |
| --- | --- |
| Category | [@Electron](../../functions#category=%40Electron) |
| Export Size | 336 B |
| Package | `@vueuse/electron` |
| Last Changed | 6 months ago |
Use [ipcRenderer.on](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereronchannel-listener) with ease and [ipcRenderer.removeListener](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener) automatically on unmounted. Available in the [@vueuse/electron](../readme) add-on.
Usage
-----
```
import { useIpcRendererOn } from '@vueuse/electron'
// enable nodeIntegration if you don't provide ipcRenderer explicitly
// @see: https://www.electronjs.org/docs/api/webview-tag#nodeintegration
// remove listener automatically on unmounted
useIpcRendererOn('custom-event', (event, ...args) => {
console.log(args)
})
```
Type Declarations
-----------------
Show Type Declarations
```
/**
* Listens to channel, when a new message arrives listener would be called with listener(event, args...).
* [ipcRenderer.removeListener](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener) automatically on unmounted.
*
* You need to provide `ipcRenderer` to this function.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereronchannel-listener
* @see https://vueuse.org/useIpcRendererOn
*/
export declare function useIpcRendererOn(
ipcRenderer: IpcRenderer,
channel: string,
listener: IpcRendererListener
): IpcRenderer
/**
* Listens to channel, when a new message arrives listener would be called with listener(event, args...).
* [ipcRenderer.removeListener](https://www.electronjs.org/docs/api/ipc-renderer#ipcrendererremovelistenerchannel-listener) automatically on unmounted.
*
* `ipcRenderer` will be automatically gotten.
*
* @see https://www.electronjs.org/docs/api/ipc-renderer#ipcrendereronchannel-listener
* @see https://vueuse.org/useIpcRendererOn
*/
export declare function useIpcRendererOn(
channel: string,
listener: IpcRendererListener
): IpcRenderer
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRendererOn/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/electron/useIpcRendererOn/index.md)
*No recent changes*
vueuse useRouteHash useRouteHash
============
| | |
| --- | --- |
| Category | [@Router](../../functions#category=%40Router) |
| Export Size | 185 B |
| Package | `@vueuse/router` |
| Last Changed | 2 months ago |
Shorthand for a reactive `route.hash`. Available in the [@vueuse/router](../readme) add-on.
Usage
-----
```
import { useRouteHash } from '@vueuse/router'
const search = useRouteHash()
console.log(search.value) // route.hash
search.value = 'foobar' // router.replace({ hash: 'foobar' })
```
Type Declarations
-----------------
```
export declare function useRouteHash(
defaultValue?: string,
{ mode, route, router }?: ReactiveRouteOptions
): WritableComputedRef<string>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteHash/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteHash/index.md)
vueuse useRouteQuery useRouteQuery
=============
| | |
| --- | --- |
| Category | [@Router](../../functions#category=%40Router) |
| Export Size | 461 B |
| Package | `@vueuse/router` |
| Last Changed | 2 months ago |
Shorthand for a reactive `route.query`. Available in the [@vueuse/router](../readme) add-on.
Usage
-----
```
import { useRouteQuery } from '@vueuse/router'
const search = useRouteQuery('search')
const search = useRouteQuery('search', 'foo') // or with a default value
console.log(search.value) // route.query.search
search.value = 'foobar' // router.replace({ query: { search: 'foobar' } })
```
Type Declarations
-----------------
```
export declare function useRouteQuery(
name: string
): Ref<null | string | string[]>
export declare function useRouteQuery<
T extends null | undefined | string | string[] = null | string | string[]
>(name: string, defaultValue?: T, options?: ReactiveRouteOptions): Ref<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteQuery/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteQuery/index.md)
vueuse useRouteParams useRouteParams
==============
| | |
| --- | --- |
| Category | [@Router](../../functions#category=%40Router) |
| Export Size | 454 B |
| Package | `@vueuse/router` |
| Last Changed | 2 months ago |
Shorthand for a reactive `route.params`. Available in the [@vueuse/router](../readme) add-on.
Usage
-----
```
import { useRouteParams } from '@vueuse/router'
const userId = useRouteParams('userId')
const userId = useRouteParams('userId', '-1') // or with a default value
console.log(userId.value) // route.params.userId
userId.value = '100' // router.replace({ params: { userId: '100' } })
```
Type Declarations
-----------------
```
export declare function useRouteParams(
name: string
): Ref<null | string | string[]>
export declare function useRouteParams<
T extends null | undefined | string | string[] = null | string | string[]
>(name: string, defaultValue?: T, options?: ReactiveRouteOptions): Ref<T>
```
Source
------
[Source](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteParams/index.ts) • [Docs](https://github.com/vueuse/vueuse/blob/main/packages/router/useRouteParams/index.md)
jqueryui Buttonset Widget Buttonset Widget
================
Buttonset Widgetversion added: 1.8, deprecated: 1.12
-----------------------------------------------------
**Description:** Themeable button sets.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[disabled](#option-disabled) [items](#option-items) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[create](#event-create) This widget is deprecated, use [Controlgroup](controlgroup) instead.
`.buttonset()` is bundled with `.button()`. Although they are separate widgets, they are combined into a single file. If you have `.button()` available, you also have `.buttonset()` available. A button set provides a visual grouping for related [buttons](button). It is recommended that a button set be used whenever you have a group of related buttons. Button sets work by selecting all appropriate descendants and applying `.button()` to them. You can enable and disable a button set, which will enable and disable all contained buttons. Destroying a button set also calls each button's `.destroy()` method. For grouped radio and checkbox buttons, it's recommended to use a `fieldset` with a `legend` to provide an accessible group label.
### Theming
The buttonset widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If buttonset specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-buttonset`: The outer container of Buttonsets.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the buttonset if set to `true`. **Code examples:**Initialize the buttonset with the `disabled` option specified:
```
$( ".selector" ).buttonset({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).buttonset( "option", "disabled" );
// Setter
$( ".selector" ).buttonset( "option", "disabled", true );
```
### items
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"button, input[type=button], input[type=submit], input[type=reset], input[type=checkbox], input[type=radio], a, :data(ui-button)"` Which descendant elements to convert manage as buttons. **Code examples:**Initialize the buttonset with the `items` option specified:
```
$( ".selector" ).buttonset({
items: "button, input[type=button], input[type=submit]"
});
```
Get or set the `items` option, after initialization:
```
// Getter
var items = $( ".selector" ).buttonset( "option", "items" );
// Setter
$( ".selector" ).buttonset( "option", "items", "button, input[type=button], input[type=submit]" );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the buttonset functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).buttonset( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the buttonset. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).buttonset( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the buttonset. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).buttonset( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the buttonset's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the buttonset plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).buttonset( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).buttonset( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current buttonset options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).buttonset( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the buttonset option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).buttonset( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the buttonset. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).buttonset( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Process any buttons that were added or removed directly in the DOM. Results depend on the [`items`](#option-items) option. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).buttonset( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the button set element that contains the buttons. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).buttonset( "widget" );
```
Events
------
### create( event, ui )Type: `buttonsetcreate`
Triggered when the buttonset is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the buttonset with the create callback specified:
```
$( ".selector" ).buttonset({
create: function( event, ui ) {}
});
```
Bind an event listener to the buttonsetcreate event:
```
$( ".selector" ).on( "buttonsetcreate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Buttonset
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>buttonset demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<form>
<fieldset>
<legend>Favorite jQuery Project</legend>
<div id="radio">
<input type="radio" id="sizzle" name="project">
<label for="sizzle">Sizzle</label>
<input type="radio" id="qunit" name="project" checked="checked">
<label for="qunit">QUnit</label>
<input type="radio" id="color" name="project">
<label for="color">Color</label>
</div>
</fieldset>
</form>
<script>
$( "#radio" ).buttonset();
</script>
</body>
</html>
```
#### Demo:
jqueryui jQuery.effects.clipToBox() jQuery.effects.clipToBox()
==========================
jQuery.effects.clipToBox( animationProperties )Returns: [Object](http://api.jquery.com/Types/#Object)version added: 1.12
-------------------------------------------------------------------------------------------------------------------------
**Description:** Calculates position and dimensions based on a clip animation.
* #### [jQuery.effects.clipToBox( animationProperties )](#jQuery-effects-clipToBox-animationProperties)
+ **animationProperties** Type: [Object](http://api.jquery.com/Types/#Object) A set of properties that will eventually be passed to [`.animate()`](https://api.jquery.com/animate/). The `animationProperties` must contain a `clip` property.
This method is useful for mimicking a clip animation when using a placeholder for effects. Given a clip animation, `jQuery.effects.clipToBox()` will generate an object containing `top`, `left`, `width`, and `height` properties which can be passed to [`.css()`](https://api.jquery.com/css/) or [`.animate()`](https://api.jquery.com/animate/). This method is generally used in conjunction with [`jQuery.effects.createPlaceholder()`](jquery.effects.createplaceholder).
jqueryui Mouse Interaction Mouse Interaction
=================
Mouse Interaction
-----------------
**Description:** The base interaction layer.
QuickNav
--------
### Options
[cancel](#option-cancel) [delay](#option-delay) [distance](#option-distance) ### Methods
[\_mouseCapture](#method-_mouseCapture) [\_mouseDelayMet](#method-_mouseDelayMet) [\_mouseDestroy](#method-_mouseDestroy) [\_mouseDistanceMet](#method-_mouseDistanceMet) [\_mouseDown](#method-_mouseDown) [\_mouseDrag](#method-_mouseDrag) [\_mouseInit](#method-_mouseInit) [\_mouseMove](#method-_mouseMove) [\_mouseStart](#method-_mouseStart) [\_mouseStop](#method-_mouseStop) [\_mouseUp](#method-_mouseUp) ### Events
Similar to [`jQuery.Widget`](jquery.widget#jQuery-Widget2), the mouse interaction is not intended to be used directly. It is purely a base layer for other widgets to inherit from. This page only documents what is added to `jQuery.Widget`, but it does include internal methods that are not intended to be overwritten. The intended public API is [`_mouseStart()`](#method-_mouseStart), [`_mouseDrag()`](#method-_mouseDrag), [`_mouseStop()`](#method-_mouseStop), and [`_mouseCapture()`](#method-_mouseCapture).
### Dependencies
* [Widget Factory](jquery.widget)
Options
-------
### cancel
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"input,textarea,button,select,option"` Prevents interactions from starting on specified elements. **Code examples:**Initialize the mouse with the `cancel` option specified:
```
$( ".selector" ).mouse({
cancel: ".title"
});
```
Get or set the `cancel` option, after initialization:
```
// Getter
var cancel = $( ".selector" ).mouse( "option", "cancel" );
// Setter
$( ".selector" ).mouse( "option", "cancel", ".title" );
```
### delay
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` Time in milliseconds after mousedown until the interaction should start. This option can be used to prevent unwanted interactions when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the mouse with the `delay` option specified:
```
$( ".selector" ).mouse({
delay: 300
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).mouse( "option", "delay" );
// Setter
$( ".selector" ).mouse( "option", "delay", 300 );
```
### distance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Distance in pixels after mousedown the mouse must move before the interaction should start. This option can be used to prevent unwanted interactions when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the mouse with the `distance` option specified:
```
$( ".selector" ).mouse({
distance: 10
});
```
Get or set the `distance` option, after initialization:
```
// Getter
var distance = $( ".selector" ).mouse( "option", "distance" );
// Setter
$( ".selector" ).mouse( "option", "distance", 10 );
```
Methods
-------
### \_mouseCapture()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Determines whether an interaction should start based on event target of the interaction. The default implementation always returns `true`. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseCapture method:
```
$( ".selector" ).mouse( "_mouseCapture" );
```
### \_mouseDelayMet()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Determines whether the [`delay`](#option-delay) option has been met for the current interaction. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseDelayMet method:
```
$( ".selector" ).mouse( "_mouseDelayMet" );
```
### \_mouseDestroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Destroys the interaction event handlers. This must be called from the extending widget's `_destroy()` method. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseDestroy method:
```
$( ".selector" ).mouse( "_mouseDestroy" );
```
### \_mouseDistanceMet()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Determines whether the [`distance`](#option-distance) option has been met for the current interaction. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseDistanceMet method:
```
$( ".selector" ).mouse( "_mouseDistanceMet" );
```
### \_mouseDown()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Handles the beginning of an interaction. Verifies that the event is associated with the primary mouse button and ensures that the [`delay`](#option-delay) and [`distance`](#option-distance) options are met prior to starting the interaction. When the interaction is ready to start, invokes the [`_mouseStart()`](#method-_mouseStart) method for the extending widget to handle. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseDown method:
```
$( ".selector" ).mouse( "_mouseDown" );
```
### \_mouseDrag()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
The extending widget should implement a `_mouseDrag()` method to handle each movement of an interaction. This method will receive the mouse event associated with the movement. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseDrag method:
```
$( ".selector" ).mouse( "_mouseDrag" );
```
### \_mouseInit()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Initializes the interaction event handlers. This must be called from the extending widget's `_create()` method. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseInit method:
```
$( ".selector" ).mouse( "_mouseInit" );
```
### \_mouseMove()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Handles each movement of the interaction. Invokes the [`mouseDrag()`](#method-_mouseDrag) method for the extending widget to handle. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseMove method:
```
$( ".selector" ).mouse( "_mouseMove" );
```
### \_mouseStart()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
The extending widget should implement a `_mouseStart()` method to handle the beginning of an interaction. This method will receive the mouse event associated with the start of the interaction. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseStart method:
```
$( ".selector" ).mouse( "_mouseStart" );
```
### \_mouseStop()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
The extending widget should implement a `_mouseStop()` method to handle the end of an interaction. This method will receive the mouse event associated with the end of the interaction. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseStop method:
```
$( ".selector" ).mouse( "_mouseStop" );
```
### \_mouseUp()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Handles the end of the interaction. Invokes the [`mouseStop()`](#method-_mouseStop) method for the extending widget to handle. * This method does not accept any arguments.
**Code examples:**Invoke the \_mouseUp method:
```
$( ".selector" ).mouse( "_mouseUp" );
```
| programming_docs |
jqueryui Color Animation Color Animation
===============
jQuery UI effects core adds the ability to animate color properties using `rgb()`, `rgba()`, hex values, or even color names such as `"aqua"`. Simply include the jQuery UI effects core file and [`.animate()`](https://api.jquery.com/animate/) will gain support for colors.
The following properties are supported:
* `backgroundColor`
* `borderBottomColor`
* `borderLeftColor`
* `borderRightColor`
* `borderTopColor`
* `color`
* `columnRuleColor`
* `outlineColor`
* `textDecorationColor`
* `textEmphasisColor`
Support for color animation comes from the [jQuery Color plugin](https://github.com/jquery/jquery-color). The Color plugin provides several functions for working with colors. For full documentation, please see the [jQuery Color documentation](https://github.com/jquery/jquery-color#readme).
Class Animations
-----------------
While there are use cases for directly animating individual color properties, it is often a better approach to contain the styles in a class. jQuery UI provides a few methods which will animate the addition or removal of a CSS class, specifically [`.addClass()`](addclass), [`.removeClass()`](removeclass), [`.toggleClass()`](toggleclass), and [`.switchClass()`](switchclass). These methods will automatically determine which properties need to change and apply the appropriate animations.
Example
--------
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Color Animation Demo</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.13.0/themes/smoothness/jquery-ui.css">
<style>
#elem {
color: #006;
background-color: #aaa;
font-size: 25px;
padding: 1em;
text-align: center;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.0/jquery-ui.js"></script>
</head>
<body>
<div id="elem">color animations</div>
<button id="toggle">animate colors</button>
<script>
$( "#toggle" ).click(function() {
$( "#elem" ).animate({
color: "green",
backgroundColor: "rgb( 20, 20, 20 )"
});
});
</script>
</body>
</html>
```
jqueryui .show() .show()
=======
.show( effect [, options ] [, duration ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
---------------------------------------------------------------------------------------------------------------
**Description:** Display the matched elements, using custom effects.
* #### [.show( effect [, options ] [, duration ] [, complete ] )](#show-effect-options-duration-complete)
+ **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) Effect-specific properties and [easing](easings).
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.show( options )](#show-options)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. The only required property is `effect`.
- **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
- **easing** (default: `"swing"`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
This plugin extends jQuery's built-in [`.show()`](https://api.jquery.com/show/) method. If jQuery UI is not loaded, calling the `.show()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Show a div using the fold effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>show demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
display: none;
width: 100px;
height: 100px;
background: #ccc;
border: 1px solid #000;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<button>show the div</button>
<div></div>
<script>
$( "button" ).click(function() {
$( "div" ).show( "fold", 1000 );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Clip Effect Clip Effect
===========
Clip Effect
-----------
**Description:** The clip effect will hide or show an element by clipping the element vertically or horizontally.
* #### clip
+ **direction** (default: `"up"`) Type: [String](http://api.jquery.com/Types/#String) The plane in which the clip effect will hide or show its element.
`vertical` clips the top and bottom edges, while `horizontal` clips the right and left edges.
Example:
--------
Toggle a div using the clip effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>clip demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "clip" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .toggle() .toggle()
=========
.toggle( effect [, options ] [, duration ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
-----------------------------------------------------------------------------------------------------------------
**Description:** Display or hide the matched elements, using custom effects.
* #### [.toggle( effect [, options ] [, duration ] [, complete ] )](#toggle-effect-options-duration-complete)
+ **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) Effect-specific properties and [easing](easings).
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.toggle( options )](#toggle-options)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. The only required property is `effect`.
- **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
- **easing** (default: `"swing"`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
This plugin extends jQuery's built-in [`.toggle()`](https://api.jquery.com/toggle/) method. If jQuery UI is not loaded, calling the `.toggle()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Toggle a div using the fold effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>toggle demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background: #ccc;
border: 1px solid #000;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<button>toggle the div</button>
<div></div>
<script>
$( "button" ).click(function() {
$( "div" ).toggle( "fold", 1000 );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Fade Effect Fade Effect
===========
Fade Effect
-----------
**Description:** The fade effect hides or shows an element by fading it.
* #### fade
Example:
--------
Toggle a div using the fade effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fade demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "fade" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui jQuery UI jQuery UI
=========
[Accordion Widget](accordion "Permalink to Accordion Widget")
--------------------------------------------------------------
Convert a pair of headers and content panels into an accordion.
[.addClass()](addclass "Permalink to .addClass()")
---------------------------------------------------
Adds the specified class(es) to each of the set of matched elements while animating all style changes.
[Autocomplete Widget](autocomplete "Permalink to Autocomplete Widget")
-----------------------------------------------------------------------
Autocomplete enables users to quickly find and select from a pre-populated list of values as they type, leveraging searching and filtering.
[Blind Effect](blind-effect "Permalink to Blind Effect")
---------------------------------------------------------
The blind effect hides or shows an element by wrapping the element in a container, and “pulling the blinds”
[Bounce Effect](bounce-effect "Permalink to Bounce Effect")
------------------------------------------------------------
The bounce effect bounces an element. When used with hide or show, the last or first bounce will also fade in/out.
[Button Widget](button "Permalink to Button Widget")
-----------------------------------------------------
Themeable buttons.
[Buttonset Widget](buttonset "Permalink to Buttonset Widget")
--------------------------------------------------------------
Themeable button sets.
[Checkboxradio Widget](checkboxradio "Permalink to Checkboxradio Widget")
--------------------------------------------------------------------------
Converts inputs of type radio and checkbox to themeable buttons.
[Clip Effect](clip-effect "Permalink to Clip Effect")
------------------------------------------------------
The clip effect will hide or show an element by clipping the element vertically or horizontally.
[Color Animation](color-animation "Permalink to Color Animation")
------------------------------------------------------------------
Animate colors using .animate().
[Controlgroup Widget](controlgroup "Permalink to Controlgroup Widget")
-----------------------------------------------------------------------
Themeable set of input widgets.
[CSS Framework](theming/css-framework "Permalink to CSS Framework")
--------------------------------------------------------------------
A list of the class names used by jQuery UI to allow components to be themeable.
[.cssClip()](cssclip "Permalink to .cssClip()")
------------------------------------------------
Getter/setter for an object version of the CSS clip property.
[:data() Selector](data-selector "Permalink to :data() Selector")
------------------------------------------------------------------
Selects elements which have data stored under the specified key.
[Datepicker Widget](datepicker "Permalink to Datepicker Widget")
-----------------------------------------------------------------
Select a date from a popup or inline calendar
[Dialog Widget](dialog "Permalink to Dialog Widget")
-----------------------------------------------------
Open content in an interactive overlay.
[.disableSelection()](disableselection "Permalink to .disableSelection()")
---------------------------------------------------------------------------
Disable selection of text content within the set of matched elements.
[Draggable Widget](draggable "Permalink to Draggable Widget")
--------------------------------------------------------------
Allow elements to be moved using the mouse.
[Drop Effect](drop-effect "Permalink to Drop Effect")
------------------------------------------------------
The drop effect hides or shows an element fading in/out and sliding in a direction.
[Droppable Widget](droppable "Permalink to Droppable Widget")
--------------------------------------------------------------
Create targets for draggable elements.
[Easings](easings "Permalink to Easings")
------------------------------------------
Easing functions specify the speed at which an animation progresses at different points within the animation.
[.effect()](effect "Permalink to .effect()")
---------------------------------------------
Apply an animation effect to an element.
[.enableSelection()](enableselection "Permalink to .enableSelection()")
------------------------------------------------------------------------
Enable selection of text content within the set of matched elements.
[Explode Effect](explode-effect "Permalink to Explode Effect")
---------------------------------------------------------------
The explode effect hides or shows an element by splitting it into pieces.
[Fade Effect](fade-effect "Permalink to Fade Effect")
------------------------------------------------------
The fade effect hides or shows an element by fading it.
[:focusable Selector](focusable-selector "Permalink to :focusable Selector")
-----------------------------------------------------------------------------
Selects elements which can be focused.
[Fold Effect](fold-effect "Permalink to Fold Effect")
------------------------------------------------------
The fold effect hides or shows an element by folding it.
[Form Reset Mixin](form-reset-mixin "Permalink to Form Reset Mixin")
---------------------------------------------------------------------
A mixin to call refresh() on an input widget when the parent form gets reset.
[.hide()](hide "Permalink to .hide()")
---------------------------------------
Hide the matched elements, using custom effects.
[Highlight Effect](highlight-effect "Permalink to Highlight Effect")
---------------------------------------------------------------------
The highlight effect hides or shows an element by animating its background color first.
[Icons](theming/icons "Permalink to Icons")
--------------------------------------------
A list of the icons provided by jQuery UI.
[jQuery.effects.clipToBox()](jquery.effects.cliptobox "Permalink to jQuery.effects.clipToBox()")
-------------------------------------------------------------------------------------------------
Calculates position and dimensions based on a clip animation.
[jQuery.effects.createPlaceholder()](jquery.effects.createplaceholder "Permalink to jQuery.effects.createPlaceholder()")
-------------------------------------------------------------------------------------------------------------------------
Creates a placeholder to support clip animations without disrupting the layout.
[jQuery.effects.define()](jquery.effects.define "Permalink to jQuery.effects.define()")
----------------------------------------------------------------------------------------
Defines an effect.
[jQuery.effects.removePlaceholder()](jquery.effects.removeplaceholder "Permalink to jQuery.effects.removePlaceholder()")
-------------------------------------------------------------------------------------------------------------------------
Removes a placeholder created with jQuery.effects.createPlaceholder().
[jQuery.effects.restoreStyle()](jquery.effects.restorestyle "Permalink to jQuery.effects.restoreStyle()")
----------------------------------------------------------------------------------------------------------
Restores all inline styles for an element.
[jQuery.effects.saveStyle()](jquery.effects.savestyle "Permalink to jQuery.effects.saveStyle()")
-------------------------------------------------------------------------------------------------
Stores a copy of all inline styles applied to an element.
[jQuery.effects.scaledDimensions()](jquery.effects.scaleddimensions "Permalink to jQuery.effects.scaledDimensions()")
----------------------------------------------------------------------------------------------------------------------
Scales the dimensions of an element.
[jQuery.ui.keyCode](jquery.ui.keycode "Permalink to jQuery.ui.keyCode")
------------------------------------------------------------------------
A mapping of key code descriptions to their numeric values.
[Widget Factory](jquery.widget "Permalink to Widget Factory")
--------------------------------------------------------------
Create stateful jQuery plugins using the same abstraction as all jQuery UI widgets.
[Widget Plugin Bridge](jquery.widget.bridge "Permalink to Widget Plugin Bridge")
---------------------------------------------------------------------------------
Part of the jQuery Widget Factory is the jQuery.widget.bridge() method. This acts as the middleman between the object created by $.widget() and the jQuery API.
[.labels()](labels "Permalink to .labels()")
---------------------------------------------
Finds all label elements associated with the first selected element.
[Menu Widget](menu "Permalink to Menu Widget")
-----------------------------------------------
Themeable menu with mouse and keyboard interactions for navigation.
[Mouse Interaction](mouse "Permalink to Mouse Interaction")
------------------------------------------------------------
The base interaction layer.
[.position()](position "Permalink to .position()")
---------------------------------------------------
Position an element relative to another.
[Progressbar Widget](progressbar "Permalink to Progressbar Widget")
--------------------------------------------------------------------
Display status of a determinate or indeterminate process.
[Puff Effect](puff-effect "Permalink to Puff Effect")
------------------------------------------------------
Creates a puff effect by scaling the element up and hiding it at the same time.
[Pulsate Effect](pulsate-effect "Permalink to Pulsate Effect")
---------------------------------------------------------------
The pulsate effect hides or shows an element by pulsing it in or out.
[.removeClass()](removeclass "Permalink to .removeClass()")
------------------------------------------------------------
Removes the specified class(es) from each of the set of matched elements while animating all style changes.
[.removeUniqueId()](removeuniqueid "Permalink to .removeUniqueId()")
---------------------------------------------------------------------
Remove ids that were set by .uniqueId() for the set of matched elements.
[Resizable Widget](resizable "Permalink to Resizable Widget")
--------------------------------------------------------------
Change the size of an element using the mouse.
[Scale Effect](scale-effect "Permalink to Scale Effect")
---------------------------------------------------------
Shrink or grow an element by a percentage factor.
[.scrollParent()](scrollparent "Permalink to .scrollParent()")
---------------------------------------------------------------
Get the closest ancestor element that is scrollable.
[Selectable Widget](selectable "Permalink to Selectable Widget")
-----------------------------------------------------------------
Use the mouse to select elements, individually or in a group.
[Selectmenu Widget](selectmenu "Permalink to Selectmenu Widget")
-----------------------------------------------------------------
Duplicates and extends the functionality of a native HTML select element to overcome the limitations of the native control.
[Shake Effect](shake-effect "Permalink to Shake Effect")
---------------------------------------------------------
Shakes the element multiple times, vertically or horizontally.
[.show()](show "Permalink to .show()")
---------------------------------------
Display the matched elements, using custom effects.
[Size Effect](size-effect "Permalink to Size Effect")
------------------------------------------------------
Resize an element to a specified width and height.
[Slide Effect](slide-effect "Permalink to Slide Effect")
---------------------------------------------------------
Slides the element out of the viewport.
[Slider Widget](slider "Permalink to Slider Widget")
-----------------------------------------------------
Drag a handle to select a numeric value.
[Sortable Widget](sortable "Permalink to Sortable Widget")
-----------------------------------------------------------
Reorder elements in a list or grid using the mouse.
[Spinner Widget](spinner "Permalink to Spinner Widget")
--------------------------------------------------------
Enhance a text input for entering numeric values, with up/down buttons and arrow key handling.
[Stacking Elements](theming/stacking-elements "Permalink to Stacking Elements")
--------------------------------------------------------------------------------
A pattern for handling z-index and stacking elements.
[.switchClass()](switchclass "Permalink to .switchClass()")
------------------------------------------------------------
Adds and removes the specified class(es) to each of the set of matched elements while animating all style changes.
[:tabbable Selector](tabbable-selector "Permalink to :tabbable Selector")
--------------------------------------------------------------------------
Selects elements which the user can focus via tabbing.
[Tabs Widget](tabs "Permalink to Tabs Widget")
-----------------------------------------------
A single content area with multiple panels, each associated with a header in a list.
[Theming](https://api.jqueryui.com/theming/ "Permalink to Theming")
--------------------------------------------------------------------
[.toggle()](toggle "Permalink to .toggle()")
---------------------------------------------
Display or hide the matched elements, using custom effects.
[.toggleClass()](toggleclass "Permalink to .toggleClass()")
------------------------------------------------------------
Add or remove one or more classes from each element in the set of matched elements, depending on either the class’s presence or the value of the switch argument, while animating all style changes.
[Tooltip Widget](tooltip "Permalink to Tooltip Widget")
--------------------------------------------------------
Customizable, themeable tooltips, replacing native tooltips.
[.transfer()](transfer "Permalink to .transfer()")
---------------------------------------------------
Transfers the outline of an element to another element
[Transfer Effect](transfer-effect "Permalink to Transfer Effect")
------------------------------------------------------------------
Transfers the outline of an element to another element
[.uniqueId()](uniqueid "Permalink to .uniqueId()")
---------------------------------------------------
Generate and apply a unique id for the set of matched elements.
| programming_docs |
jqueryui .removeClass() .removeClass()
==============
.removeClass( className [, duration ] [, easing ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
------------------------------------------------------------------------------------------------------------------------
**Description:** Removes the specified class(es) from each of the set of matched elements while animating all style changes.
* #### [.removeClass( className [, duration ] [, easing ] [, complete ] )](#removeClass-className-duration-easing-complete)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be removed from the class attribute of each matched element.
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.removeClass( className [, options ] )](#removeClass-className-options)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be removed from the class attribute of each matched element.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. All properties are optional.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **children** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the animation should additionally be applied to all descendants of the matched elements. This feature should be used with caution as the cost of determining which descendants to animate can be very expensive, and grows linearly with the number of descendants.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
Similar to native CSS transitions, jQuery UI's class animations provide a smooth transition from one state to another while allowing you to keep all the details about which styles to change in CSS and out of your JavaScript. All class animation methods, including `.removeClass()`, support custom durations and easings, as well as provide a callback for when the animation completes.
Not all styles can be animated. For example, there is no way to animate a background image. Any styles that cannot be animated will be changed at the end of the animation.
This plugin extends jQuery's built-in [`.removeClass()`](https://api.jquery.com/removeClass/) method. If jQuery UI is not loaded, calling the `.removeClass()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Removes the class "big-blue" from the matched elements.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>removeClass demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background-color: #ccc;
}
.big-blue {
width: 200px;
height: 200px;
background-color: #00f;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div class="big-blue"></div>
<script>
$( "div" ).click(function() {
$( this ).removeClass( "big-blue", 1000, "easeInBack" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Resizable Widget Resizable Widget
================
Resizable Widgetversion added: 1.0
-----------------------------------
**Description:** Change the size of an element using the mouse.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[alsoResize](#option-alsoResize) [animate](#option-animate) [animateDuration](#option-animateDuration) [animateEasing](#option-animateEasing) [aspectRatio](#option-aspectRatio) [autoHide](#option-autoHide) [cancel](#option-cancel) [classes](#option-classes) [containment](#option-containment) [delay](#option-delay) [disabled](#option-disabled) [distance](#option-distance) [ghost](#option-ghost) [grid](#option-grid) [handles](#option-handles) [helper](#option-helper) [maxHeight](#option-maxHeight) [maxWidth](#option-maxWidth) [minHeight](#option-minHeight) [minWidth](#option-minWidth) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [widget](#method-widget) ### Events
[create](#event-create) [resize](#event-resize) [start](#event-start) [stop](#event-stop) The jQuery UI Resizable plugin makes selected elements resizable (meaning they have draggable resize handles). You can specify one or more handles as well as min and max width and height.
### Theming
The resizable widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If resizable specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-resizable`: The resizable element. During a resize, the `ui-resizable-resizing` class is added. When the [`autoHide` option](#option-autoHide) is set, the `ui-resizable-autohide` class is added.
* `ui-resizable-handle`: One of the handles of the resizable, specified using the [`handles` option](#option-handles). Each handle will also have a `ui-resizable-{direction}` class according to its position.
* `ui-resizable-ghost`: When using the [`ghost` option](#option-ghost), this class is applied to the ghost helper element.
* `ui-resizable-helper`: When the [`animate` option](#option-animate) is used, but the [`helper` option](#option-helper) isn't specified, this class is added to the generated helper.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### alsoResize
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [jQuery](http://api.jquery.com/Types/#jQuery) or [Element](http://api.jquery.com/Types/#Element) **Default:** `false` One or more elements to resize synchronously with the resizable element. **Code examples:**Initialize the resizable with the `alsoResize` option specified:
```
$( ".selector" ).resizable({
alsoResize: "#mirror"
});
```
Get or set the `alsoResize` option, after initialization:
```
// Getter
var alsoResize = $( ".selector" ).resizable( "option", "alsoResize" );
// Setter
$( ".selector" ).resizable( "option", "alsoResize", "#mirror" );
```
### animate
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Animates to the final size after resizing. **Code examples:**Initialize the resizable with the `animate` option specified:
```
$( ".selector" ).resizable({
animate: true
});
```
Get or set the `animate` option, after initialization:
```
// Getter
var animate = $( ".selector" ).resizable( "option", "animate" );
// Setter
$( ".selector" ).resizable( "option", "animate", true );
```
### animateDuration
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `"slow"` How long to animate when using the [`animate`](#option-animate) option. **Multiple types supported:*** **Number**: Duration in milliseconds.
* **String**: A named duration, such as `"slow"` or `"fast"`.
**Code examples:**Initialize the resizable with the `animateDuration` option specified:
```
$( ".selector" ).resizable({
animateDuration: "fast"
});
```
Get or set the `animateDuration` option, after initialization:
```
// Getter
var animateDuration = $( ".selector" ).resizable( "option", "animateDuration" );
// Setter
$( ".selector" ).resizable( "option", "animateDuration", "fast" );
```
### animateEasing
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"swing"` Which [easing](easings) to apply when using the [`animate`](#option-animate) option. **Code examples:**Initialize the resizable with the `animateEasing` option specified:
```
$( ".selector" ).resizable({
animateEasing: "easeOutBounce"
});
```
Get or set the `animateEasing` option, after initialization:
```
// Getter
var animateEasing = $( ".selector" ).resizable( "option", "animateEasing" );
// Setter
$( ".selector" ).resizable( "option", "animateEasing", "easeOutBounce" );
```
### aspectRatio
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Whether the element should be constrained to a specific aspect ratio. **Multiple types supported:*** **Boolean**: When set to `true`, the element will maintain its original aspect ratio.
* **Number**: Force the element to maintain a specific aspect ratio during resizing.
**Code examples:**Initialize the resizable with the `aspectRatio` option specified:
```
$( ".selector" ).resizable({
aspectRatio: true
});
```
Get or set the `aspectRatio` option, after initialization:
```
// Getter
var aspectRatio = $( ".selector" ).resizable( "option", "aspectRatio" );
// Setter
$( ".selector" ).resizable( "option", "aspectRatio", true );
```
### autoHide
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the handles should hide when the user is not hovering over the element. **Code examples:**Initialize the resizable with the `autoHide` option specified:
```
$( ".selector" ).resizable({
autoHide: true
});
```
Get or set the `autoHide` option, after initialization:
```
// Getter
var autoHide = $( ".selector" ).resizable( "option", "autoHide" );
// Setter
$( ".selector" ).resizable( "option", "autoHide", true );
```
### cancel
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"input,textarea,button,select,option"` Prevents resizing from starting on specified elements. **Code examples:**Initialize the resizable with the `cancel` option specified:
```
$( ".selector" ).resizable({
cancel: ".cancel"
});
```
Get or set the `cancel` option, after initialization:
```
// Getter
var cancel = $( ".selector" ).resizable( "option", "cancel" );
// Setter
$( ".selector" ).resizable( "option", "cancel", ".cancel" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-resizable-se": "ui-icon ui-icon-gripsmall-diagonal-se"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the resizable with the `classes` option specified, changing the theming for the `ui-resizable` class:
```
$( ".selector" ).resizable({
classes: {
"ui-resizable": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-resizable` class:
```
// Getter
var themeClass = $( ".selector" ).resizable( "option", "classes.ui-resizable" );
// Setter
$( ".selector" ).resizable( "option", "classes.ui-resizable", "highlight" );
```
### containment
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) or [String](http://api.jquery.com/Types/#String) **Default:** `false` Constrains resizing to within the bounds of the specified element or region. **Multiple types supported:*** **Selector**: The resizable element will be contained to the bounding box of the first element found by the selector. If no element is found, no containment will be set.
* **Element**: The resizable element will be contained to the bounding box of this element.
* **String**: Possible values: `"parent"` and `"document"`.
**Code examples:**Initialize the resizable with the `containment` option specified:
```
$( ".selector" ).resizable({
containment: "parent"
});
```
Get or set the `containment` option, after initialization:
```
// Getter
var containment = $( ".selector" ).resizable( "option", "containment" );
// Setter
$( ".selector" ).resizable( "option", "containment", "parent" );
```
### delay
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` Tolerance, in milliseconds, for when resizing should start. If specified, resizing will not start until after mouse is moved beyond duration. This can help prevent unintended resizing when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the resizable with the `delay` option specified:
```
$( ".selector" ).resizable({
delay: 150
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).resizable( "option", "delay" );
// Setter
$( ".selector" ).resizable( "option", "delay", 150 );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the resizable if set to `true`. **Code examples:**Initialize the resizable with the `disabled` option specified:
```
$( ".selector" ).resizable({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).resizable( "option", "disabled" );
// Setter
$( ".selector" ).resizable( "option", "disabled", true );
```
### distance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Tolerance, in pixels, for when resizing should start. If specified, resizing will not start until after mouse is moved beyond distance. This can help prevent unintended resizing when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the resizable with the `distance` option specified:
```
$( ".selector" ).resizable({
distance: 30
});
```
Get or set the `distance` option, after initialization:
```
// Getter
var distance = $( ".selector" ).resizable( "option", "distance" );
// Setter
$( ".selector" ).resizable( "option", "distance", 30 );
```
### ghost
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If set to `true`, a semi-transparent helper element is shown for resizing. **Code examples:**Initialize the resizable with the `ghost` option specified:
```
$( ".selector" ).resizable({
ghost: true
});
```
Get or set the `ghost` option, after initialization:
```
// Getter
var ghost = $( ".selector" ).resizable( "option", "ghost" );
// Setter
$( ".selector" ).resizable( "option", "ghost", true );
```
### grid
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `false` Snaps the resizing element to a grid, every x and y pixels. Array values: `[ x, y ]`. **Code examples:**Initialize the resizable with the `grid` option specified:
```
$( ".selector" ).resizable({
grid: [ 20, 10 ]
});
```
Get or set the `grid` option, after initialization:
```
// Getter
var grid = $( ".selector" ).resizable( "option", "grid" );
// Setter
$( ".selector" ).resizable( "option", "grid", [ 20, 10 ] );
```
### handles
**Type:** [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `"e, s, se"` Which handles can be used for resizing. **Multiple types supported:*** **String**: A comma delimited list of any of the following: n, e, s, w, ne, se, sw, nw, all. The necessary handles will be auto-generated by the plugin.
* **Object**: The following keys are supported: { n, e, s, w, ne, se, sw, nw }. The value of any specified should be a jQuery selector matching the child element of the resizable to use as that handle. If the handle is not a child of the resizable, you can pass in the DOMElement or a valid jQuery object directly.
*Note: When generating your own handles, each handle must have the `ui-resizable-handle` class, as well as the appropriate `ui-resizable-{direction}` class, .e.g., `ui-resizable-s`.*
**Code examples:**Initialize the resizable with the `handles` option specified:
```
$( ".selector" ).resizable({
handles: "n, e, s, w"
});
```
Get or set the `handles` option, after initialization:
```
// Getter
var handles = $( ".selector" ).resizable( "option", "handles" );
// Setter
$( ".selector" ).resizable( "option", "handles", "n, e, s, w" );
```
### helper
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` A class name that will be added to a proxy element to outline the resize during the drag of the resize handle. Once the resize is complete, the original element is sized. **Code examples:**Initialize the resizable with the `helper` option specified:
```
$( ".selector" ).resizable({
helper: "resizable-helper"
});
```
Get or set the `helper` option, after initialization:
```
// Getter
var helper = $( ".selector" ).resizable( "option", "helper" );
// Setter
$( ".selector" ).resizable( "option", "helper", "resizable-helper" );
```
### maxHeight
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `null` The maximum height the resizable should be allowed to resize to. **Code examples:**Initialize the resizable with the `maxHeight` option specified:
```
$( ".selector" ).resizable({
maxHeight: 300
});
```
Get or set the `maxHeight` option, after initialization:
```
// Getter
var maxHeight = $( ".selector" ).resizable( "option", "maxHeight" );
// Setter
$( ".selector" ).resizable( "option", "maxHeight", 300 );
```
### maxWidth
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `null` The maximum width the resizable should be allowed to resize to. **Code examples:**Initialize the resizable with the `maxWidth` option specified:
```
$( ".selector" ).resizable({
maxWidth: 300
});
```
Get or set the `maxWidth` option, after initialization:
```
// Getter
var maxWidth = $( ".selector" ).resizable( "option", "maxWidth" );
// Setter
$( ".selector" ).resizable( "option", "maxWidth", 300 );
```
### minHeight
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `10` The minimum height the resizable should be allowed to resize to. **Code examples:**Initialize the resizable with the `minHeight` option specified:
```
$( ".selector" ).resizable({
minHeight: 150
});
```
Get or set the `minHeight` option, after initialization:
```
// Getter
var minHeight = $( ".selector" ).resizable( "option", "minHeight" );
// Setter
$( ".selector" ).resizable( "option", "minHeight", 150 );
```
### minWidth
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `10` The minimum width the resizable should be allowed to resize to. **Code examples:**Initialize the resizable with the `minWidth` option specified:
```
$( ".selector" ).resizable({
minWidth: 150
});
```
Get or set the `minWidth` option, after initialization:
```
// Getter
var minWidth = $( ".selector" ).resizable( "option", "minWidth" );
// Setter
$( ".selector" ).resizable( "option", "minWidth", 150 );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the resizable functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).resizable( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the resizable. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).resizable( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the resizable. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).resizable( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the resizable's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the resizable plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).resizable( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).resizable( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current resizable options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).resizable( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the resizable option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).resizable( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the resizable. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).resizable( "option", { disabled: true } );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the resizable element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).resizable( "widget" );
```
Events
------
### create( event, ui )Type: `resizecreate`
Triggered when the resizable is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the resizable with the create callback specified:
```
$( ".selector" ).resizable({
create: function( event, ui ) {}
});
```
Bind an event listener to the resizecreate event:
```
$( ".selector" ).on( "resizecreate", function( event, ui ) {} );
```
### resize( event, ui )Type: `resize`
This event is triggered during the resize, on the drag of the resize handler. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element to be resized
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being resized
+ **originalElement** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the original element before it is wrapped
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The position represented as `{ left, top }` before the resizable is resized
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size represented as `{ width, height }` before the resizable is resized
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position represented as `{ left, top }`. The values may be changed to modify where the element will be positioned. Useful for custom resizing logic.
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size represented as `{ width, height }`. The values may be changed to modify where the element will be positioned. Useful for custom resizing logic.
**Code examples:**Initialize the resizable with the resize callback specified:
```
$( ".selector" ).resizable({
resize: function( event, ui ) {}
});
```
Bind an event listener to the resize event:
```
$( ".selector" ).on( "resize", function( event, ui ) {} );
```
Restrict height resizing to 30 pixel increments:
```
$( ".selector" ).resizable({
resize: function( event, ui ) {
ui.size.height = Math.round( ui.size.height / 30 ) * 30;
}
});
```
### start( event, ui )Type: `resizestart`
This event is triggered at the start of a resize operation. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element to be resized
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being resized
+ **originalElement** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the original element before it is wrapped
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The position represented as `{ left, top }` before the resizable is resized
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size represented as `{ width, height }` before the resizable is resized
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position represented as `{ left, top }`
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size represented as `{ width, height }`
**Code examples:**Initialize the resizable with the start callback specified:
```
$( ".selector" ).resizable({
start: function( event, ui ) {}
});
```
Bind an event listener to the resizestart event:
```
$( ".selector" ).on( "resizestart", function( event, ui ) {} );
```
### stop( event, ui )Type: `resizestop`
This event is triggered at the end of a resize operation. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element to be resized
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being resized
+ **originalElement** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the original element before it is wrapped
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The position represented as `{ left, top }` before the resizable is resized
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size represented as `{ width, height }` before the resizable is resized
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position represented as `{ left, top }`
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size represented as `{ width, height }`
**Code examples:**Initialize the resizable with the stop callback specified:
```
$( ".selector" ).resizable({
stop: function( event, ui ) {}
});
```
Bind an event listener to the resizestop event:
```
$( ".selector" ).on( "resizestop", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Resizable.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>resizable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#resizable {
width: 100px;
height: 100px;
background: #ccc;
} </style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="resizable"></div>
<script>
$( "#resizable" ).resizable();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui .effect() .effect()
=========
.effect( effect [, options ] [, duration ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
-----------------------------------------------------------------------------------------------------------------
**Description:** Apply an animation effect to an element.
* #### [.effect( effect [, options ] [, duration ] [, complete ] )](#effect-effect-options-duration-complete)
+ **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) Effect-specific properties and [easing](easings).
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.effect( options )](#effect-options)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. The only required property is `effect`.
- **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
- **easing** (default: `"swing"`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
The `.effect()` method applies a named animation [effect](category/effects) to an element. Many effects also support a show or hide mode, which can be accomplished with the [`.show()`](show), [`.hide()`](hide), and [`.toggle()`](toggle) methods.
Example:
--------
Apply the bounce effect to a div.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>effect demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background: #ccc;
border: 1px solid #000;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to apply the effect.</p>
<div></div>
<script>
$( document ).click(function() {
$( "div" ).effect( "bounce", "slow" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui jQuery.effects.saveStyle() jQuery.effects.saveStyle()
==========================
jQuery.effects.saveStyle( element )version added: 1.12
-------------------------------------------------------
**Description:** Stores a copy of all inline styles applied to an element.
* #### [jQuery.effects.saveStyle( element )](#jQuery-effects-saveStyle-element)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to save styles for.
Stores a copy of the current inline styles applied to the element, which can be reapplied to the element later using [`jQuery.effects.restoreStyle()`](jquery.effects.restorestyle). This is useful when animating various styles and restoring the existing styles at the end.
When using [`jQuery.effects.define()`](jquery.effects.define) to create an effect, if `jQuery.effects.saveStyle()` is used on the main element being animated, the styles will be restored automatically when the animation is complete.
jqueryui .toggleClass() .toggleClass()
==============
.toggleClass( className [, switch ] [, duration ] [, easing ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
------------------------------------------------------------------------------------------------------------------------------------
**Description:** Add or remove one or more classes from each element in the set of matched elements, depending on either the class's presence or the value of the switch argument, while animating all style changes.
* #### [.toggleClass( className [, switch ] [, duration ] [, easing ] [, complete ] )](#toggleClass-className-switch-duration-easing-complete)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be toggled for each element in the matched set.
+ **switch** Type: [Boolean](http://api.jquery.com/Types/#Boolean) A boolean value to determine whether the class should be added or removed.
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.toggleClass( className [, switch ] [, options ] )](#toggleClass-className-switch-options)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be toggled for each element in the matched set.
+ **switch** Type: [Boolean](http://api.jquery.com/Types/#Boolean) A boolean value to determine whether the class should be added or removed.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. All properties are optional.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **children** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the animation should additionally be applied to all descendants of the matched elements. This feature should be used with caution as the cost of determining which descendants to animate can be very expensive, and grows linearly with the number of descendants.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
Similar to native CSS transitions, jQuery UI's class animations provide a smooth transition from one state to another while allowing you to keep all the details about which styles to change in CSS and out of your JavaScript. All class animation methods, including `.toggleClass()`, support custom durations and easings, as well as provide a callback for when the animation completes.
Not all styles can be animated. For example, there is no way to animate a background image. Any styles that cannot be animated will be changed at the end of the animation.
This plugin extends jQuery's built-in [`.toggleClass()`](https://api.jquery.com/toggleClass/) method. If jQuery UI is not loaded, calling the `.toggleClass()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Toggles the class "big-blue" for the matched elements.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>toggleClass demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background-color: #ccc;
}
.big-blue {
width: 200px;
height: 200px;
background-color: #00f;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div></div>
<script>
$( "div" ).click(function() {
$( this ).toggleClass( "big-blue", 1000, "easeOutSine" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui jQuery.effects.define() jQuery.effects.define()
=======================
jQuery.effects.define( name [, mode ], effect )Returns: [Function](http://api.jquery.com/Types/#Function)version added: 1.12
-----------------------------------------------------------------------------------------------------------------------------
**Description:** Defines an effect.
* #### [jQuery.effects.define( name [, mode ], effect )](#jQuery-effects-define-name-mode-effect)
+ **name** Type: [String](http://api.jquery.com/Types/#String) The name of the effect to create.
+ **mode** Type: [String](http://api.jquery.com/Types/#String) The default mode for the effect. Possible values are `"show"`, `"hide"`, or `"toggle"`.
+ **effect** Type: [Function](http://api.jquery.com/Types/#Function)( [Object](http://api.jquery.com/Types/#Object) options, [Function](http://api.jquery.com/Types/#Function) done() ) Defines the effect logic. The `options` argument contains `duration` and `mode` properties, as well as any effect-specific options.
Defines a new effect for use with [`.effect()`](effect), [`.show()`](show), [`.hide()`](hide), and [`.toggle()`](toggle). The effect method is invoked with `this` being a single DOM element. The `done` argument must be invoked when the animation is complete.
`jQuery.effects.define()` stores the new effect in `jQuery.effects.effect[ name ]` and returns the function that was provided as the `effect` parameter.
Example:
--------
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery.effects.define demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
.elem {
position: absolute;
width: 150px;
height: 150px;
background: #3b679e;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
Click anywhere!
<div class="elem"></div>
<script>
$.effects.define( "fade", "toggle", function( options, done ) {
var show = options.mode === "show";
$( this )
.css( "opacity", show ? 0 : 1 )
.animate( {
opacity: show ? 1 : 0
}, {
queue: false,
duration: options.duration,
easing: options.easing,
complete: done
} );
} );
$( document ).on( "click", function() {
$( ".elem" ).toggle( "fade" );
} );
</script>
</body>
</html>
```
#### Demo:
jqueryui Droppable Widget Droppable Widget
================
Droppable Widgetversion added: 1.0
-----------------------------------
**Description:** Create targets for draggable elements.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[accept](#option-accept) [activeClass](#option-activeClass) [addClasses](#option-addClasses) [classes](#option-classes) [disabled](#option-disabled) [greedy](#option-greedy) [hoverClass](#option-hoverClass) [scope](#option-scope) [tolerance](#option-tolerance) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [widget](#method-widget) ### Events
[activate](#event-activate) [create](#event-create) [deactivate](#event-deactivate) [drop](#event-drop) [out](#event-out) [over](#event-over) The jQuery UI Droppable plugin makes selected elements droppable (meaning they accept being dropped on by [draggables](draggable)). You can specify which draggables each will accept.
### Theming
The droppable widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If droppable specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-droppable`: The droppable element. When a draggable that can be dropped on this dropppable is activated, the `ui-droppable-active` class is added. When dragging a draggable over this droppable, the `ui-droppable-hover` class is added.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
Options
-------
### accept
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Function](http://api.jquery.com/Types/#Function)() **Default:** `"*"` Controls which draggable elements are accepted by the droppable. **Multiple types supported:*** **Selector**: A selector indicating which draggable elements are accepted.
* **Function**: A function that will be called for each draggable on the page (passed as the first argument to the function). The function must return `true` if the draggable should be accepted.
**Code examples:**Initialize the droppable with the `accept` option specified:
```
$( ".selector" ).droppable({
accept: ".special"
});
```
Get or set the `accept` option, after initialization:
```
// Getter
var accept = $( ".selector" ).droppable( "option", "accept" );
// Setter
$( ".selector" ).droppable( "option", "accept", ".special" );
```
### activeClass
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` If specified, the class will be added to the droppable while an acceptable draggable is being dragged.
The `activeClass` option has been deprecated in favor of the [`classes` option](#option-classes), using the `ui-droppable-active` property.
(version deprecated: 1.12) **Code examples:**Initialize the droppable with the `activeClass` option specified:
```
$( ".selector" ).droppable({
activeClass: "ui-state-highlight"
});
```
Get or set the `activeClass` option, after initialization:
```
// Getter
var activeClass = $( ".selector" ).droppable( "option", "activeClass" );
// Setter
$( ".selector" ).droppable( "option", "activeClass", "ui-state-highlight" );
```
### addClasses
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `false`, will prevent the `ui-droppable` class from being added. This may be desired as a performance optimization when calling `.droppable()` init on hundreds of elements. **Code examples:**Initialize the droppable with the `addClasses` option specified:
```
$( ".selector" ).droppable({
addClasses: false
});
```
Get or set the `addClasses` option, after initialization:
```
// Getter
var addClasses = $( ".selector" ).droppable( "option", "addClasses" );
// Setter
$( ".selector" ).droppable( "option", "addClasses", false );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the droppable with the `classes` option specified, changing the theming for the `ui-droppable` class:
```
$( ".selector" ).droppable({
classes: {
"ui-droppable": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-droppable` class:
```
// Getter
var themeClass = $( ".selector" ).droppable( "option", "classes.ui-droppable" );
// Setter
$( ".selector" ).droppable( "option", "classes.ui-droppable", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the droppable if set to `true`. **Code examples:**Initialize the droppable with the `disabled` option specified:
```
$( ".selector" ).droppable({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).droppable( "option", "disabled" );
// Setter
$( ".selector" ).droppable( "option", "disabled", true );
```
### greedy
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` By default, when an element is dropped on nested droppables, each droppable will receive the element. However, by setting this option to `true`, any parent droppables will not receive the element. The `drop` event will still bubble normally, but the `event.target` can be checked to see which droppable received the draggable element. **Code examples:**Initialize the droppable with the `greedy` option specified:
```
$( ".selector" ).droppable({
greedy: true
});
```
Get or set the `greedy` option, after initialization:
```
// Getter
var greedy = $( ".selector" ).droppable( "option", "greedy" );
// Setter
$( ".selector" ).droppable( "option", "greedy", true );
```
### hoverClass
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` If specified, the class will be added to the droppable while an acceptable draggable is being hovered over the droppable.
The `hoverClass` option has been deprecated in favor of the [`classes` option](#option-classes), using the `ui-droppable-hover` property.
(version deprecated: 1.12) **Code examples:**Initialize the droppable with the `hoverClass` option specified:
```
$( ".selector" ).droppable({
hoverClass: "drop-hover"
});
```
Get or set the `hoverClass` option, after initialization:
```
// Getter
var hoverClass = $( ".selector" ).droppable( "option", "hoverClass" );
// Setter
$( ".selector" ).droppable( "option", "hoverClass", "drop-hover" );
```
### scope
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"default"` Used to group sets of draggable and droppable items, in addition to the [`accept`](#option-accept) option. A draggable with the same scope value as a droppable will be accepted. **Code examples:**Initialize the droppable with the `scope` option specified:
```
$( ".selector" ).droppable({
scope: "tasks"
});
```
Get or set the `scope` option, after initialization:
```
// Getter
var scope = $( ".selector" ).droppable( "option", "scope" );
// Setter
$( ".selector" ).droppable( "option", "scope", "tasks" );
```
### tolerance
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"intersect"` Specifies which mode to use for testing whether a draggable is hovering over a droppable. Possible values: * `"fit"`: Draggable overlaps the droppable entirely.
* `"intersect"`: Draggable overlaps the droppable at least 50% in both directions.
* `"pointer"`: Mouse pointer overlaps the droppable.
* `"touch"`: Draggable overlaps the droppable any amount.
**Code examples:**Initialize the droppable with the `tolerance` option specified:
```
$( ".selector" ).droppable({
tolerance: "fit"
});
```
Get or set the `tolerance` option, after initialization:
```
// Getter
var tolerance = $( ".selector" ).droppable( "option", "tolerance" );
// Setter
$( ".selector" ).droppable( "option", "tolerance", "fit" );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the droppable functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).droppable( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the droppable. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).droppable( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the droppable. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).droppable( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the droppable's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the droppable plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).droppable( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).droppable( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current droppable options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).droppable( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the droppable option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).droppable( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the droppable. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).droppable( "option", { disabled: true } );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the droppable element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).droppable( "widget" );
```
Events
------
### activate( event, ui )Type: `dropactivate`
Triggered when an accepted draggable starts dragging. This can be useful if you want to make the droppable "light up" when it can be dropped on. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **draggable** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the draggable element.
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the helper that is being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the draggable helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the draggable helper as `{ top, left }` object.
**Code examples:**Initialize the droppable with the activate callback specified:
```
$( ".selector" ).droppable({
activate: function( event, ui ) {}
});
```
Bind an event listener to the dropactivate event:
```
$( ".selector" ).on( "dropactivate", function( event, ui ) {} );
```
### create( event, ui )Type: `dropcreate`
Triggered when the droppable is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the droppable with the create callback specified:
```
$( ".selector" ).droppable({
create: function( event, ui ) {}
});
```
Bind an event listener to the dropcreate event:
```
$( ".selector" ).on( "dropcreate", function( event, ui ) {} );
```
### deactivate( event, ui )Type: `dropdeactivate`
Triggered when an accepted draggable stops dragging. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **draggable** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the draggable element.
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the helper that is being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the draggable helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the draggable helper as `{ top, left }` object.
**Code examples:**Initialize the droppable with the deactivate callback specified:
```
$( ".selector" ).droppable({
deactivate: function( event, ui ) {}
});
```
Bind an event listener to the dropdeactivate event:
```
$( ".selector" ).on( "dropdeactivate", function( event, ui ) {} );
```
### drop( event, ui )Type: `drop`
Triggered when an accepted draggable is dropped on the droppable (based on the[`tolerance`](#option-tolerance) option). * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **draggable** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the draggable element.
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the helper that is being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the draggable helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the draggable helper as `{ top, left }` object.
**Code examples:**Initialize the droppable with the drop callback specified:
```
$( ".selector" ).droppable({
drop: function( event, ui ) {}
});
```
Bind an event listener to the drop event:
```
$( ".selector" ).on( "drop", function( event, ui ) {} );
```
### out( event, ui )Type: `dropout`
Triggered when an accepted draggable is dragged out of the droppable (based on the[`tolerance`](#option-tolerance) option). * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the droppable with the out callback specified:
```
$( ".selector" ).droppable({
out: function( event, ui ) {}
});
```
Bind an event listener to the dropout event:
```
$( ".selector" ).on( "dropout", function( event, ui ) {} );
```
### over( event, ui )Type: `dropover`
Triggered when an accepted draggable is dragged over the droppable (based on the[`tolerance`](#option-tolerance) option). * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **draggable** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the draggable element.
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A jQuery object representing the helper that is being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the draggable helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the draggable helper as `{ top, left }` object.
**Code examples:**Initialize the droppable with the over callback specified:
```
$( ".selector" ).droppable({
over: function( event, ui ) {}
});
```
Bind an event listener to the dropover event:
```
$( ".selector" ).on( "dropover", function( event, ui ) {} );
```
Example:
--------
A pair of draggable and droppable elements.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>droppable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#draggable {
width: 100px;
height: 100px;
background: #ccc;
}
#droppable {
position: absolute;
left: 250px;
top: 0;
width: 125px;
height: 125px;
background: #999;
color: #fff;
padding: 10px;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="droppable">Drop here</div>
<div id="draggable">Drag me</div>
<script>
$( "#draggable" ).draggable();
$( "#droppable" ).droppable({
drop: function() {
alert( "dropped" );
}
});
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui jQuery.effects.createPlaceholder() jQuery.effects.createPlaceholder()
==================================
jQuery.effects.createPlaceholder( element )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)version added: 1.12
---------------------------------------------------------------------------------------------------------------------
**Description:** Creates a placeholder to support `clip` animations without disrupting the layout.
* #### [jQuery.effects.createPlaceholder( element )](#jQuery-effects-createPlaceholder-element)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to create a placeholder for.
Many effects require animating the `clip` CSS property. In order to apply clipping, the element must be absolutely positioned. As a result, if an animation that utilizes clipping is applied to an element with static or relative positioning, the layout of the page will be affected when the animated element is removed from the flow. To compensate for this, a placeholder element with the same size and position can be inserted into the document.
`jQuery.effects.createPlaceholder()` will create a placeholder with the same display, position, dimensions, and margins as the original element. The placeholder is inserted into the DOM as the next sibling of the original element. When the animation is complete, [`jQuery.effects.removePlaceholder()`](jquery.effects.removeplaceholder) should be used to remove the inserted element.
If the original element is already absolutely positioned, no placeholder will be generated since the page layout isn't affected. As a result, the return value will be `undefined`.
jqueryui Checkboxradio Widget Checkboxradio Widget
====================
Checkboxradio Widgetversion added: 1.12
----------------------------------------
**Description:** Converts inputs of type radio and checkbox to themeable buttons.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [disabled](#option-disabled) [icon](#option-icon) [label](#option-label) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[create](#event-create) Native HTML input elements are impossible to style consistently. This widget allows working around that limitation by positining the associated label on top of the hidden input, and emulating the checkbox or radio element itself using an (optional) icon. The original input still receives focus and all events, the label merely provides a themeable button on top.
### Theming
The checkboxradio widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If checkboxradio specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-checkboxradio`: The input of type radio or checkbox. Will be hidden, with its associated label positioned on top.
+ `ui-checkboxradio-label`: The label associated with the input. If the input is checked, this will also get the `ui-checkboxradio-checked` class. If the input is of type radio, this will also get the `ui-checkboxradio-radio-label` class.
+ `ui-checkboxradio-icon`: If the [`icon`](#option-icon) option is enabled, the generated icon has this class.
+ `ui-checkboxradio-icon-space`: If the [`icon`](#option-icon) option is enabled, an extra element with this class as added between the text label and the icon.
### Dependencies
* [.labels()](labels)
* [Form Reset Mixin](form-reset-mixin)
* [Widget Factory](jquery.widget)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-checkboxradio-label": "ui-corner-all",
"ui-checkboxradio-icon": "ui-corner-all"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the checkboxradio with the `classes` option specified, changing the theming for the `ui-checkboxradio` class:
```
$( ".selector" ).checkboxradio({
classes: {
"ui-checkboxradio": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-checkboxradio` class:
```
// Getter
var themeClass = $( ".selector" ).checkboxradio( "option", "classes.ui-checkboxradio" );
// Setter
$( ".selector" ).checkboxradio( "option", "classes.ui-checkboxradio", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the checkboxradio if set to `true`. **Code examples:**Initialize the checkboxradio with the `disabled` option specified:
```
$( ".selector" ).checkboxradio({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).checkboxradio( "option", "disabled" );
// Setter
$( ".selector" ).checkboxradio( "option", "disabled", true );
```
### icon
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` Whether to show the checkbox or radio icon, depending on the input's type. **Code examples:**Initialize the checkboxradio with the `icon` option specified:
```
$( ".selector" ).checkboxradio({
icon: false
});
```
Get or set the `icon` option, after initialization:
```
// Getter
var icon = $( ".selector" ).checkboxradio( "option", "icon" );
// Setter
$( ".selector" ).checkboxradio( "option", "icon", false );
```
### label
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` HTML to show in the button. When not specified (`null`), the HTML content of the associated `<label>` element is used. **Code examples:**Initialize the checkboxradio with the `label` option specified:
```
$( ".selector" ).checkboxradio({
label: "custom label"
});
```
Get or set the `label` option, after initialization:
```
// Getter
var label = $( ".selector" ).checkboxradio( "option", "label" );
// Setter
$( ".selector" ).checkboxradio( "option", "label", "custom label" );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the checkboxradio functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).checkboxradio( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the checkboxradio. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).checkboxradio( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the checkboxradio. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).checkboxradio( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the checkboxradio's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the checkboxradio plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).checkboxradio( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).checkboxradio( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current checkboxradio options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).checkboxradio( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the checkboxradio option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).checkboxradio( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the checkboxradio. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).checkboxradio( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Refreshes the visual state of the widget. Useful for updating after the native element's checked or disabled state is changed programmatically. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).checkboxradio( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the checkboxradio. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).checkboxradio( "widget" );
```
Events
------
### create( event, ui )Type: `checkboxradiocreate`
Triggered when the checkboxradio is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the checkboxradio with the create callback specified:
```
$( ".selector" ).checkboxradio({
create: function( event, ui ) {}
});
```
Bind an event listener to the checkboxradiocreate event:
```
$( ".selector" ).on( "checkboxradiocreate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI checkboxradio
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>checkboxradio demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<fieldset>
<legend>Select a Location: </legend>
<label for="radio-1">New York</label>
<input type="radio" name="radio-1" id="radio-1">
<label for="radio-2">Paris</label>
<input type="radio" name="radio-1" id="radio-2">
<label for="radio-3">London</label>
<input type="radio" name="radio-1" id="radio-3">
</fieldset>
<script>
$( "input[type='radio']" ).checkboxradio();
</script>
</body>
</html>
```
#### Demo:
jqueryui Accordion Widget Accordion Widget
================
Accordion Widgetversion added: 1.0
-----------------------------------
**Description:** Convert a pair of headers and content panels into an accordion.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[active](#option-active) [animate](#option-animate) [classes](#option-classes) [collapsible](#option-collapsible) [disabled](#option-disabled) [event](#option-event) [header](#option-header) [heightStyle](#option-heightStyle) [icons](#option-icons) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[activate](#event-activate) [beforeActivate](#event-beforeActivate) [create](#event-create) The markup of your accordion container needs pairs of headers and content panels:
```
<div id="accordion">
<h3>First header</h3>
<div>First content panel</div>
<h3>Second header</h3>
<div>Second content panel</div>
</div>
```
Accordions support arbitrary markup, but each content panel must always be the next sibling after its associated header. See the [`header`](#option-header) option for information on how to use custom markup structures.
The panels can be activated programmatically by setting the [`active`](#option-active) option.
### Keyboard interaction
When focus is on a header, the following key commands are available:
* `UP`/`LEFT`: Move focus to the previous header. If on first header, moves focus to last header.
* `DOWN`/`RIGHT`: Move focus to the next header. If on last header, moves focus to first header.
* `HOME`: Move focus to the first header.
* `END`: Move focus to the last header.
* `SPACE`/`ENTER`: Activate panel associated with focused header.
When focus is in a panel:
* `CTRL` + `UP`: Move focus to associated header.
### Theming
The accordion widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If accordion specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-accordion`: The outer container of the accordion.
+ `ui-accordion-header`: The headers of the accordion. The active header will additionally have a `ui-accordion-header-active` class, the inactive headers will have a `ui-accordion-header-collapsed` class. The headers will also have a `ui-accordion-icons` class if they contain [`icons`](#option-icons).
- `ui-accordion-header-icon`: Icon elements within each accordion header.
+ `ui-accordion-content`: The content panels of the accordion. The active content panel will additionally have a `ui-accordion-content-active` class.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Effects Core](category/effects-core) (optional; for use with the [`animate`](#option-animate) option)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### active
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Integer](http://api.jquery.com/Types/#Integer) **Default:** `0` Which panel is currently open. **Multiple types supported:*** **Boolean**: Setting `active` to `false` will collapse all panels. This requires the [`collapsible`](#option-collapsible) option to be `true`.
* **Integer**: The zero-based index of the panel that is active (open). A negative value selects panels going backward from the last panel.
**Code examples:**Initialize the accordion with the `active` option specified:
```
$( ".selector" ).accordion({
active: 2
});
```
Get or set the `active` option, after initialization:
```
// Getter
var active = $( ".selector" ).accordion( "option", "active" );
// Setter
$( ".selector" ).accordion( "option", "active", 2 );
```
### animate
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` If and how to animate changing panels. **Multiple types supported:*** **Boolean**: A value of `false` will disable animations.
* **Number**: Duration in milliseconds with default easing.
* **String**: Name of [easing](easings) to use with default duration.
* **Object**: An object containing `easing` and `duration` properties to configure animations.
+ Can also contain a `down` property with any of the above options.
+ "Down" animations occur when the panel being activated has a lower index than the currently active panel.
**Code examples:**Initialize the accordion with the `animate` option specified:
```
$( ".selector" ).accordion({
animate: 200
});
```
Get or set the `animate` option, after initialization:
```
// Getter
var animate = $( ".selector" ).accordion( "option", "animate" );
// Setter
$( ".selector" ).accordion( "option", "animate", 200 );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-accordion-header": "ui-corner-top",
"ui-accordion-header-collapsed": "ui-corner-all",
"ui-accordion-content": "ui-corner-bottom"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the accordion with the `classes` option specified, changing the theming for the `ui-accordion` class:
```
$( ".selector" ).accordion({
classes: {
"ui-accordion": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-accordion` class:
```
// Getter
var themeClass = $( ".selector" ).accordion( "option", "classes.ui-accordion" );
// Setter
$( ".selector" ).accordion( "option", "classes.ui-accordion", "highlight" );
```
### collapsible
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether all the sections can be closed at once. Allows collapsing the active section. **Code examples:**Initialize the accordion with the `collapsible` option specified:
```
$( ".selector" ).accordion({
collapsible: true
});
```
Get or set the `collapsible` option, after initialization:
```
// Getter
var collapsible = $( ".selector" ).accordion( "option", "collapsible" );
// Setter
$( ".selector" ).accordion( "option", "collapsible", true );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the accordion if set to `true`. **Code examples:**Initialize the accordion with the `disabled` option specified:
```
$( ".selector" ).accordion({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).accordion( "option", "disabled" );
// Setter
$( ".selector" ).accordion( "option", "disabled", true );
```
### event
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"click"` The event that accordion headers will react to in order to activate the associated panel. Multiple events can be specified, separated by a space. **Code examples:**Initialize the accordion with the `event` option specified:
```
$( ".selector" ).accordion({
event: "mouseover"
});
```
Get or set the `event` option, after initialization:
```
// Getter
var event = $( ".selector" ).accordion( "option", "event" );
// Setter
$( ".selector" ).accordion( "option", "event", "mouseover" );
```
### header
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Function](http://api.jquery.com/Types/#Function)( [jQuery](http://api.jquery.com/Types/#jQuery) accordionElement ) **Default:** `function( elem ) { return elem.find( "> li > :first-child" ).add( elem.find( "> :not(li)" ).even() ); }` Data identifying the header element. Content panels must be the sibling immediately after their associated headers. **Multiple types supported:*** **Selector**: Selector for the header element, applied via `.find()` on the main accordion element.
* **Function**: A function accepting the accordion element and returning the header element (added in `1.13`).
**Code examples:**Initialize the accordion with the `header` option specified:
```
$( ".selector" ).accordion({
header: "h3"
});
```
Get or set the `header` option, after initialization:
```
// Getter
var header = $( ".selector" ).accordion( "option", "header" );
// Setter
$( ".selector" ).accordion( "option", "header", "h3" );
```
Initialize the accordion with the `header` option specified as a function:
```
$( ".selector" ).accordion({
header: function ( accordionElement ) {
return accordionElement.find( "h3" );
}
});
```
### heightStyle
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"auto"` Controls the height of the accordion and each panel. Possible values:
* `"auto"`: All panels will be set to the height of the tallest panel.
* `"fill"`: Expand to the available height based on the accordion's parent height.
* `"content"`: Each panel will be only as tall as its content.
**Code examples:**Initialize the accordion with the `heightStyle` option specified:
```
$( ".selector" ).accordion({
heightStyle: "fill"
});
```
Get or set the `heightStyle` option, after initialization:
```
// Getter
var heightStyle = $( ".selector" ).accordion( "option", "heightStyle" );
// Setter
$( ".selector" ).accordion( "option", "heightStyle", "fill" );
```
### icons
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"header": "ui-icon-triangle-1-e",
"activeHeader": "ui-icon-triangle-1-s"
}
```
Icons to use for headers, matching [an icon provided by the jQuery UI CSS Framework](theming/icons). Set to `false` to have no icons displayed.
* header (string, default: "ui-icon-triangle-1-e")
* activeHeader (string, default: "ui-icon-triangle-1-s")
**Code examples:**Initialize the accordion with the `icons` option specified:
```
$( ".selector" ).accordion({
icons: { "header": "ui-icon-plus", "activeHeader": "ui-icon-minus" }
});
```
Get or set the `icons` option, after initialization:
```
// Getter
var icons = $( ".selector" ).accordion( "option", "icons" );
// Setter
$( ".selector" ).accordion( "option", "icons", { "header": "ui-icon-plus", "activeHeader": "ui-icon-minus" } );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the accordion functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).accordion( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the accordion. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).accordion( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the accordion. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).accordion( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the accordion's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the accordion plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).accordion( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).accordion( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current accordion options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).accordion( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the accordion option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).accordion( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the accordion. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).accordion( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Process any headers and panels that were added or removed directly in the DOM and recompute the height of the accordion panels. Results depend on the content and the [`heightStyle`](#option-heightStyle) option. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).accordion( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the accordion. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).accordion( "widget" );
```
Events
------
### activate( event, ui )Type: `accordionactivate`
Triggered after a panel has been activated (after animation completes). If the accordion was previously collapsed, `ui.oldHeader` and `ui.oldPanel` will be empty jQuery objects. If the accordion is collapsing, `ui.newHeader` and `ui.newPanel` will be empty jQuery objects.
**Note:** Since the `activate` event is only fired on panel activation, it is not fired for the initial panel when the accordion widget is created. If you need a hook for widget creation use the [`create`](#event-create) event. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **newHeader** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The header that was just activated.
+ **oldHeader** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The header that was just deactivated.
+ **newPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that was just activated.
+ **oldPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that was just deactivated.
**Code examples:**Initialize the accordion with the activate callback specified:
```
$( ".selector" ).accordion({
activate: function( event, ui ) {}
});
```
Bind an event listener to the accordionactivate event:
```
$( ".selector" ).on( "accordionactivate", function( event, ui ) {} );
```
### beforeActivate( event, ui )Type: `accordionbeforeactivate`
Triggered directly before a panel is activated. Can be canceled to prevent the panel from activating. If the accordion is currently collapsed, `ui.oldHeader` and `ui.oldPanel` will be empty jQuery objects. If the accordion is collapsing, `ui.newHeader` and `ui.newPanel` will be empty jQuery objects. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **newHeader** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The header that is about to be activated.
+ **oldHeader** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The header that is about to be deactivated.
+ **newPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that is about to be activated.
+ **oldPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that is about to be deactivated.
**Code examples:**Initialize the accordion with the beforeActivate callback specified:
```
$( ".selector" ).accordion({
beforeActivate: function( event, ui ) {}
});
```
Bind an event listener to the accordionbeforeactivate event:
```
$( ".selector" ).on( "accordionbeforeactivate", function( event, ui ) {} );
```
### create( event, ui )Type: `accordioncreate`
Triggered when the accordion is created. If the accordion is collapsed, `ui.header` and `ui.panel` will be empty jQuery objects. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **header** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The active header.
+ **panel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The active panel.
**Code examples:**Initialize the accordion with the create callback specified:
```
$( ".selector" ).accordion({
create: function( event, ui ) {}
});
```
Bind an event listener to the accordioncreate event:
```
$( ".selector" ).on( "accordioncreate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Accordion
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>accordion demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="accordion">
<h3>Section 1</h3>
<div>
<p>Mauris mauris ante, blandit et, ultrices a, suscipit eget.
Integer ut neque. Vivamus nisi metus, molestie vel, gravida in,
condimentum sit amet, nunc. Nam a nibh. Donec suscipit eros.
Nam mi. Proin viverra leo ut odio.</p>
</div>
<h3>Section 2</h3>
<div>
<p>Sed non urna. Phasellus eu ligula. Vestibulum sit amet purus.
Vivamus hendrerit, dolor aliquet laoreet, mauris turpis velit,
faucibus interdum tellus libero ac justo.</p>
</div>
<h3>Section 3</h3>
<div>
<p>Nam enim risus, molestie et, porta ac, aliquam ac, risus.
Quisque lobortis.Phasellus pellentesque purus in massa.</p>
<ul>
<li>List item one</li>
<li>List item two</li>
<li>List item three</li>
</ul>
</div>
</div>
<script>
$( "#accordion" ).accordion();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Tabs Widget Tabs Widget
===========
Tabs Widgetversion added: 1.0
------------------------------
**Description:** A single content area with multiple panels, each associated with a header in a list.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[active](#option-active) [classes](#option-classes) [collapsible](#option-collapsible) [disabled](#option-disabled) [event](#option-event) [heightStyle](#option-heightStyle) [hide](#option-hide) [show](#option-show) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [load](#method-load) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget)
### Extension Points
[\_getList](#method-_getList) ### Events
[activate](#event-activate) [beforeActivate](#event-beforeActivate) [beforeLoad](#event-beforeLoad) [create](#event-create) [load](#event-load) Tabs are generally used to break content into multiple sections that can be swapped to save space, much like an accordion.
Tabs have a particular set of markup that must be used in order for them to work properly:
* The tabs themselves must be in either an ordered (`<ol>`) or unordered (`<ul>`) list
* Each tab "title" must be inside of a list item (`<li>`) and wrapped by an anchor (`<a>`) with an `href` attribute
* Each tab panel may be any valid element but it must have an id which corresponds to the hash in the anchor of the associated tab.
The content for each tab panel can be defined in-page or can be loaded via Ajax; both are handled automatically based on the `href` of the anchor associated with the tab. By default tabs are activated on click, but the events can be changed to hover via the [`event`](#option-event) option.
Below is some sample markup:
```
<div id="tabs">
<ul>
<li><a href="#fragment-1">One</a></li>
<li><a href="#fragment-2">Two</a></li>
<li><a href="#fragment-3">Three</a></li>
</ul>
<div id="fragment-1">
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
</div>
<div id="fragment-2">
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
</div>
<div id="fragment-3">
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
</div>
</div>
```
### Keyboard interaction
When focus is on a tab, the following key commands are available:
* `UP`/`LEFT`: Move focus to the previous tab. If on first tab, moves focus to last tab. Activate focused tab after a short delay.
* `DOWN`/`RIGHT`: Move focus to the next tab. If on last tab, moves focus to first tab. Activate focused tab after a short delay.
* `CTRL` + `UP`/`LEFT`: Move focus to the previous tab. If on first tab, moves focus to last tab. The focused tab must be manually activated.
* `CTRL` + `DOWN`/`RIGHT`: Move focus to the next tab. If on last tab, moves focus to first tab. The focused tab must be manually activated.
* `HOME`: Move focus to the first tab. Activate focused tab after a short delay.
* `END`: Move focus to the last tab. Activate focused tab after a short delay.
* `CTRL` + `HOME`: Move focus to the first tab. The focused tab must be manually activated.
* `CTRL` + `END`: Move focus to the last tab. The focused tab must be manually activated.
* `SPACE`: Activate panel associated with focused tab.
* `ENTER`: Activate or toggle panel associated with focused tab.
* `ALT`/`OPTION` + `PAGE UP`: Move focus to the previous tab and immediately activate.
* `ALT`/`OPTION` + `PAGE DOWN`: Move focus to the next tab and immediately activate.
When focus is in a panel, the following key commands are available:
* `CTRL` + `UP`: Move focus to associated tab.
* `ALT`/`OPTION` + `PAGE UP`: Move focus to the previous tab and immediately activate.
* `ALT`/`OPTION` + `PAGE DOWN`: Move focus to the next tab and immediately activate.
### Theming
The tabs widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If tabs specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-tabs`: The outer container of the tabs. This element will additionally have a class of `ui-tabs-collapsible` when the [`collapsible` option](#option-collapsible) is set.
+ `ui-tabs-nav`: The list of tabs.
- `ui-tabs-tab`: One of the items in the list of tabs.The active item will have the `ui-tabs-active` class. Any list item whose associated content is loading via an Ajax call will have the `ui-tabs-loading` class.
* `ui-tabs-anchor`: The anchors used to switch panels.
+ `ui-tabs-panel`: The panels associated with the tabs. Only the panel whose corresponding tab is active will be visible.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Effects Core](category/effects-core) (optional; for use with the [`show`](#option-show) and [`hide`](#option-hide) options)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### active
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Integer](http://api.jquery.com/Types/#Integer) **Default:** `0` Which panel is currently open. **Multiple types supported:*** **Boolean**: Setting `active` to `false` will collapse all panels. This requires the [`collapsible`](#option-collapsible) option to be `true`.
* **Integer**: The zero-based index of the panel that is active (open). A negative value selects panels going backward from the last panel.
**Code examples:**Initialize the tabs with the `active` option specified:
```
$( ".selector" ).tabs({
active: 1
});
```
Get or set the `active` option, after initialization:
```
// Getter
var active = $( ".selector" ).tabs( "option", "active" );
// Setter
$( ".selector" ).tabs( "option", "active", 1 );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-tabs": "ui-corner-all",
"ui-tabs-nav": "ui-corner-all",
"ui-tabs-tab": "ui-corner-top",
"ui-tabs-panel": "ui-corner-bottom"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the tabs with the `classes` option specified, changing the theming for the `ui-tabs` class:
```
$( ".selector" ).tabs({
classes: {
"ui-tabs": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-tabs` class:
```
// Getter
var themeClass = $( ".selector" ).tabs( "option", "classes.ui-tabs" );
// Setter
$( ".selector" ).tabs( "option", "classes.ui-tabs", "highlight" );
```
### collapsible
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` When set to `true`, the active panel can be closed. **Code examples:**Initialize the tabs with the `collapsible` option specified:
```
$( ".selector" ).tabs({
collapsible: true
});
```
Get or set the `collapsible` option, after initialization:
```
// Getter
var collapsible = $( ".selector" ).tabs( "option", "collapsible" );
// Setter
$( ".selector" ).tabs( "option", "collapsible", true );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Array](http://api.jquery.com/Types/#Array) **Default:** `false` Which tabs are disabled. **Multiple types supported:*** **Boolean**: Enable or disable all tabs.
* **Array**: An array containing the zero-based indexes of the tabs that should be disabled, e.g., `[ 0, 2 ]` would disable the first and third tab.
**Code examples:**Initialize the tabs with the `disabled` option specified:
```
$( ".selector" ).tabs({
disabled: [ 0, 2 ]
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).tabs( "option", "disabled" );
// Setter
$( ".selector" ).tabs( "option", "disabled", [ 0, 2 ] );
```
### event
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"click"` The type of event that the tabs should react to in order to activate the tab. To activate on hover, use `"mouseover"`. **Code examples:**Initialize the tabs with the `event` option specified:
```
$( ".selector" ).tabs({
event: "mouseover"
});
```
Get or set the `event` option, after initialization:
```
// Getter
var event = $( ".selector" ).tabs( "option", "event" );
// Setter
$( ".selector" ).tabs( "option", "event", "mouseover" );
```
### heightStyle
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"content"` Controls the height of the tabs widget and each panel. Possible values: * `"auto"`: All panels will be set to the height of the tallest panel.
* `"fill"`: Expand to the available height based on the tabs' parent height.
* `"content"`: Each panel will be only as tall as its content.
**Code examples:**Initialize the tabs with the `heightStyle` option specified:
```
$( ".selector" ).tabs({
heightStyle: "fill"
});
```
Get or set the `heightStyle` option, after initialization:
```
// Getter
var heightStyle = $( ".selector" ).tabs( "option", "heightStyle" );
// Setter
$( ".selector" ).tabs( "option", "heightStyle", "fill" );
```
### hide
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the hiding of the panel. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the panel will be hidden immediately. When set to `true`, the panel will fade out with the default duration and the default easing.
* **Number**: The panel will fade out with the specified duration and the default easing.
* **String**: The panel will be hidden using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideUp"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeOut"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the tabs with the `hide` option specified:
```
$( ".selector" ).tabs({
hide: { effect: "explode", duration: 1000 }
});
```
Get or set the `hide` option, after initialization:
```
// Getter
var hide = $( ".selector" ).tabs( "option", "hide" );
// Setter
$( ".selector" ).tabs( "option", "hide", { effect: "explode", duration: 1000 } );
```
### show
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the showing of the panel. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the panel will be shown immediately. When set to `true`, the panel will fade in with the default duration and the default easing.
* **Number**: The panel will fade in with the specified duration and the default easing.
* **String**: The panel will be shown using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideDown"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeIn"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the tabs with the `show` option specified:
```
$( ".selector" ).tabs({
show: { effect: "blind", duration: 800 }
});
```
Get or set the `show` option, after initialization:
```
// Getter
var show = $( ".selector" ).tabs( "option", "show" );
// Setter
$( ".selector" ).tabs( "option", "show", { effect: "blind", duration: 800 } );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the tabs functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).tabs( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables all tabs. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "disable" );
```
### disable( index )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables a tab. The selected tab cannot be disabled. To disable more than one tab at once, set the [`disabled`](#option-disabled) option: `$( "#tabs" ).tabs( "option", "disabled", [ 1, 2, 3 ] )`. * **index** Type: [Number](http://api.jquery.com/Types/#Number) The zero-based index of the tab to disable.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "disable", 1 );
```
### disable( href )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables a tab. The selected tab cannot be disabled. * **href** Type: [String](http://api.jquery.com/Types/#String) The `href` of the tab to disable.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "disable", "#foo" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables all tabs. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "enable" );
```
### enable( index )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables a tab. To enable more than one tab at once reset the disabled property like: `$( "#example" ).tabs( "option", "disabled", [] );`. * **index** Type: [Number](http://api.jquery.com/Types/#Number) The zero-based index of the tab to enable.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "enable", 1 );
```
### enable( href )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables a tab. * **href** Type: [String](http://api.jquery.com/Types/#String) The `href` of the tab to enable.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "enable", "#foo" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the tabs's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the tabs plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).tabs( "instance" );
```
### load( index )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Loads the panel content of a remote tab. * **index** Type: [Number](http://api.jquery.com/Types/#Number) The zero-based index of the tab to load.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "load", 1 );
```
### load( href )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Loads the panel content of a remote tab. * **href** Type: [String](http://api.jquery.com/Types/#String) The `href` of the tab to load.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "load", "#foo" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).tabs( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current tabs options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).tabs( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the tabs option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the tabs. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).tabs( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Process any tabs that were added or removed directly in the DOM and recompute the height of the tab panels. Results depend on the content and the [`heightStyle`](#option-heightStyle) option. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).tabs( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the tabs container. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).tabs( "widget" );
```
Extension Points
----------------
The tabs widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_getList()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Determine which list should be converted to tabs. By default the first descendant list is used. * This method does not accept any arguments.
**Code examples:**Use the list with the class `my-tabs` or fall back to the default implementation.
```
_getList: function() {
var list = this.element.find( ".my-tabs" );
return list.length ? list.eq( 0 ) : this._super();
}
```
Events
------
### activate( event, ui )Type: `tabsactivate`
Triggered after a tab has been activated (after animation completes). If the tabs were previously collapsed, `ui.oldTab` and `ui.oldPanel` will be empty jQuery objects. If the tabs are collapsing, `ui.newTab` and `ui.newPanel` will be empty jQuery objects.
**Note:** Since the `activate` event is only fired on tab activation, it is not fired for the initial tab when the tabs widget is created. If you need a hook for widget creation use the [`create`](#event-create) event. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **newTab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that was just activated.
+ **oldTab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that was just deactivated.
+ **newPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that was just activated.
+ **oldPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that was just deactivated.
**Code examples:**Initialize the tabs with the activate callback specified:
```
$( ".selector" ).tabs({
activate: function( event, ui ) {}
});
```
Bind an event listener to the tabsactivate event:
```
$( ".selector" ).on( "tabsactivate", function( event, ui ) {} );
```
### beforeActivate( event, ui )Type: `tabsbeforeactivate`
Triggered immediately before a tab is activated. Can be canceled to prevent the tab from activating. If the tabs are currently collapsed, `ui.oldTab` and `ui.oldPanel` will be empty jQuery objects. If the tabs are collapsing, `ui.newTab` and `ui.newPanel` will be empty jQuery objects. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **newTab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that is about to be activated.
+ **oldTab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that is about to be deactivated.
+ **newPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that is about to be activated.
+ **oldPanel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel that is about to be deactivated.
**Code examples:**Initialize the tabs with the beforeActivate callback specified:
```
$( ".selector" ).tabs({
beforeActivate: function( event, ui ) {}
});
```
Bind an event listener to the tabsbeforeactivate event:
```
$( ".selector" ).on( "tabsbeforeactivate", function( event, ui ) {} );
```
### beforeLoad( event, ui )Type: `tabsbeforeload`
Triggered when a remote tab is about to be loaded, after the [`beforeActivate`](#event-beforeActivate) event. Can be canceled to prevent the tab panel from loading content; though the panel will still be activated. This event is triggered just before the Ajax request is made, so modifications can be made to `ui.jqXHR` and `ui.ajaxSettings`.
*Note: Although `ui.ajaxSettings` is provided and can be modified, some of these properties have already been processed by jQuery. For example, [prefilters](https://api.jquery.com/jQuery.ajaxPrefilter/) have been applied, `data` has been processed, and `type` has been determined. The `beforeLoad` event occurs at the same time, and therefore has the same restrictions, as the `beforeSend` callback from [`jQuery.ajax()`](https://api.jquery.com/jQuery.ajax/).*
* **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **tab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that is being loaded.
+ **panel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel which will be populated by the Ajax response.
+ **jqXHR** Type: [jqXHR](http://api.jquery.com/Types/#jqXHR) The `jqXHR` object that is requesting the content.
+ **ajaxSettings** Type: [Object](http://api.jquery.com/Types/#Object) The properties that will be used by [`jQuery.ajax`](https://api.jquery.com/jQuery.ajax/) to request the content.
**Code examples:**Initialize the tabs with the beforeLoad callback specified:
```
$( ".selector" ).tabs({
beforeLoad: function( event, ui ) {}
});
```
Bind an event listener to the tabsbeforeload event:
```
$( ".selector" ).on( "tabsbeforeload", function( event, ui ) {} );
```
### create( event, ui )Type: `tabscreate`
Triggered when the tabs are created. If the tabs are collapsed, `ui.tab` and `ui.panel` will be empty jQuery objects. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **tab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The active tab.
+ **panel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The active panel.
**Code examples:**Initialize the tabs with the create callback specified:
```
$( ".selector" ).tabs({
create: function( event, ui ) {}
});
```
Bind an event listener to the tabscreate event:
```
$( ".selector" ).on( "tabscreate", function( event, ui ) {} );
```
### load( event, ui )Type: `tabsload`
Triggered after a remote tab has been loaded. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **tab** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The tab that was just loaded.
+ **panel** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The panel which was just populated by the Ajax response.
**Code examples:**Initialize the tabs with the load callback specified:
```
$( ".selector" ).tabs({
load: function( event, ui ) {}
});
```
Bind an event listener to the tabsload event:
```
$( ".selector" ).on( "tabsload", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Tabs
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>tabs demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="tabs">
<ul>
<li><a href="#fragment-1"><span>One</span></a></li>
<li><a href="#fragment-2"><span>Two</span></a></li>
<li><a href="#fragment-3"><span>Three</span></a></li>
</ul>
<div id="fragment-1">
<p>First tab is active by default:</p>
<pre><code>$( "#tabs" ).tabs(); </code></pre>
</div>
<div id="fragment-2">
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
</div>
<div id="fragment-3">
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat volutpat.
</div>
</div>
<script>
$( "#tabs" ).tabs();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Bounce Effect Bounce Effect
=============
Bounce Effect
-------------
**Description:** The bounce effect bounces an element. When used with hide or show, the last or first bounce will also fade in/out.
* #### bounce
+ **distance** (default: `20`) Type: [Number](http://api.jquery.com/Types/#Number) The distance of the largest "bounce" in pixels.
+ **times** (default: `5`) Type: [Integer](http://api.jquery.com/Types/#Integer) The number of times the element will bounce. When used with hide or show, there is an extra "half" bounce for the fade in/out.
Example:
--------
Toggle a div using the bounce effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>bounce demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "bounce", { times: 3 }, "slow" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .removeUniqueId() .removeUniqueId()
=================
.removeUniqueId()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
------------------------------------------------------------------------
**Description:** Remove ids that were set by `.uniqueId()` for the set of matched elements.
* #### version added: 1.9[.removeUniqueId()](#removeUniqueId)
+ This method does not accept any arguments.
The `.removeUniqueId()` will remove ids that were set by [`.uniqueId()`](uniqueid). Calling `.removeUniqueId()` on an element that did not have its id set by `.uniqueId()` will have no affect, even if the element has an id.
jqueryui jQuery.effects.removePlaceholder() jQuery.effects.removePlaceholder()
==================================
jQuery.effects.removePlaceholder( element )version added: 1.12
---------------------------------------------------------------
**Description:** Removes a placeholder created with [`jQuery.effects.createPlaceholder()`](jquery.effects.createplaceholder).
* #### [jQuery.effects.removePlaceholder( element )](#jQuery-effects-removePlaceholder-element)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The original element that has an associated placeholder.
Removes the placeholder for an element. This method is safe to call even if [`jQuery.effects.createPlaceholder()`](jquery.effects.createplaceholder) did not create a placeholder.
jqueryui Widget Plugin Bridge Widget Plugin Bridge
====================
jQuery.widget.bridge( name, constructor )
-----------------------------------------
**Description:** Part of the [jQuery Widget Factory](jquery.widget) is the `jQuery.widget.bridge()` method. This acts as the middleman between the object created by `$.widget()` and the jQuery API.
* #### [jQuery.widget.bridge( name, constructor )](#jQuery-widget-bridge-name-constructor)
+ **name** Type: [String](http://api.jquery.com/Types/#String) The name of the plugin to create.
+ **constructor** Type: [Function](http://api.jquery.com/Types/#Function)() The object to instantiate when the plugin is invoked.
`$.widget.bridge()` does a few things:
* Connects a regular JavaScript constructor to the jQuery API.
* Automatically creates instances of said object and stores it within the element's `$.data` cache.
* Allows calls to public methods.
* Prevents calls to private methods.
* Prevents method calls on uninitialized objects.
* Protects against multiple initializations.
jQuery UI widgets are created using `$.widget( "foo.bar", {} );` syntax to define an object from which instances will be created. Given a DOM structure with five `.foo`'s, `$( ".foo" ).bar();` will create five instances of your "bar" object. `$.widget.bridge()` works inside the factory by taking your base "bar" object and giving it a public API. Therefore, you can create instances by writing `$( ".foo" ).bar()`, and call methods by writing `$( ".foo" ).bar( "baz" )`.
If all you want is one-time initialization and calling methods, your object passed to `jQuery.widget.bridge()` can be very minimal:
```
var Highlighter = function( options, element ) {
this.options = options;
this.element = $( element );
this._set( 800 );
};
Highlighter.prototype = {
toggle: function() {
this._set( this.element.css( "font-weight") === 400 ? 800 : 400 );
},
_set: function(value) {
this.element.css( "font-weight", value );
}
};
```
All you need here is a function that acts as the constructor, accepting two arguments:
* `options`: an object of configuration options
* `element`: the DOM element this instance was created on
You can then hook this object up as a jQuery plugin using the bridge and use it on any jQuery object:
```
// Hook up the plugin
$.widget.bridge( "colorToggle", Highlighter );
// Initialize it on divs
$( "div" ).colorToggle().click(function() {
// Call the public method on click
$( this ).colorToggle( "toggle" );
});
```
To use all the features of the bridge, your object also needs to have an `_init()` method on the prototype. This will get called whenever the plugin is invoked while an instance already exists. In that case you also need to have an `option()` method. This will be invoked with the options as the first argument. If there were none, the argument will be an empty object. For a proper implementation of the `option` method, check out the implementation of [`$.Widget`](jquery.widget#jQuery-Widget2).
There is one optional property the bridge will use, if present: If your object's prototype has a `widgetFullName` property, this will be used as the key for storing and retrieving the instance. Otherwise, the name argument will be used.
jqueryui Button Widget Button Widget
=============
Button Widgetversion added: 1.8, rewritten: 1.12
-------------------------------------------------
**Description:** Themeable buttons.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [disabled](#option-disabled) [icon](#option-icon) [iconPosition](#option-iconPosition) [label](#option-label) [showLabel](#option-showLabel) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[create](#event-create) Button enhances standard form elements like buttons, inputs and anchors to themeable buttons with appropriate hover and active styles.
When using an input of type button, submit or reset, support is limited to plain text labels with no icons.
Note: The button widget was rewritten in 1.12. Some options changed, you can find documentation for the old options in the [1.11 button docs](https://api.jqueryui.com/1.11/button/). This widget used to bundle support for inputs of type radio and checkbox, this is now deprecated, use the [checkboxradio widget](checkboxradio) instead. It also used to bundle the buttonset widget, this is also deprecated, use the [controlgroup widget](controlgroup) instead.
### Theming
The button widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If button specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-button`: The DOM element that represents the button. This element will additionally have the `ui-button-icon-only` class, depending on the [showLabel](#option-showLabel) and [icon](#option-icon) options.
+ `ui-button-icon`: The element used to display the button icon. This will only be present if an icon is provided in the [icon](#option-icon) option.
+ `ui-button-icon-space`: A separator element between icon and text content of the button. This will only be present if an icon is provided in the [icon](#option-icon) option and the [iconPosition](#option-iconPosition) option is set to `"beginning"` or `"end"`.
### Dependencies
* [jQuery.ui.keyCode](jquery.ui.keycode)
* [Widget Factory](jquery.widget)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-button": "ui-corner-all",
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the button with the `classes` option specified, changing the theming for the `ui-button` class:
```
$( ".selector" ).button({
classes: {
"ui-button": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-button` class:
```
// Getter
var themeClass = $( ".selector" ).button( "option", "classes.ui-button" );
// Setter
$( ".selector" ).button( "option", "classes.ui-button", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the button if set to `true`. **Code examples:**Initialize the button with the `disabled` option specified:
```
$( ".selector" ).button({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).button( "option", "disabled" );
// Setter
$( ".selector" ).button( "option", "disabled", true );
```
### icon
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` Icon to display, with or without text (see [`showLabel`](#option-showLabel) option). By default, the icon is displayed on the left of the label text. The positioning can be controlled using the [`iconPosition`](#option-iconPosition) option.
The value for this option must match [an icon class name](theming/icons), e.g., `"ui-icon-gear"`.
When using an input of type button, submit or reset, icons are not supported.
**Code examples:**Initialize the button with the `icon` option specified:
```
$( ".selector" ).button({
icon: "ui-icon-gear"
});
```
Get or set the `icon` option, after initialization:
```
// Getter
var icon = $( ".selector" ).button( "option", "icon" );
// Setter
$( ".selector" ).button( "option", "icon", "ui-icon-gear" );
```
### iconPosition
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"beginning"` Where to display the [`icon`](#option-icon): Valid values are "beginning", "end", "top" and "bottom". In a left-to-right (LTR) display, "beginning" refers to the left, in a right-to-left (RTL, e.g. in Hebrew or Arabic), it refers to the right.
**Code examples:**Initialize the button with the `iconPosition` option specified:
```
$( ".selector" ).button({
iconPosition: "end"
});
```
Get or set the `iconPosition` option, after initialization:
```
// Getter
var iconPosition = $( ".selector" ).button( "option", "iconPosition" );
// Setter
$( ".selector" ).button( "option", "iconPosition", "end" );
```
### label
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` HTML to show in the button. When not specified (`null`), the element's HTML content is used, or its `value` attribute if the element is an input element of type submit or reset, or the HTML content of the associated label element if the element is an input of type radio or checkbox.
When using an input of type button, submit or reset, support is limited to plain text labels.
**Code examples:**Initialize the button with the `label` option specified:
```
$( ".selector" ).button({
label: "custom label"
});
```
Get or set the `label` option, after initialization:
```
// Getter
var label = $( ".selector" ).button( "option", "label" );
// Setter
$( ".selector" ).button( "option", "label", "custom label" );
```
### showLabel
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` Whether to show the label. When set to `false` no text will be displayed, but the [`icon`](#option-icon) option must be used, otherwise the `showLabel` option will be ignored. **Code examples:**Initialize the button with the `showLabel` option specified:
```
$( ".selector" ).button({
showLabel: false
});
```
Get or set the `showLabel` option, after initialization:
```
// Getter
var showLabel = $( ".selector" ).button( "option", "showLabel" );
// Setter
$( ".selector" ).button( "option", "showLabel", false );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the button functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).button( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the button. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).button( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the button. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).button( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the button's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the button plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).button( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).button( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current button options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).button( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the button option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).button( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the button. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).button( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Refreshes the visual state of the button. Useful for updating button state after the native element's disabled state is changed programmatically. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).button( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the element visually representing the button. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).button( "widget" );
```
Events
------
### create( event, ui )Type: `buttoncreate`
Triggered when the button is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the button with the create callback specified:
```
$( ".selector" ).button({
create: function( event, ui ) {}
});
```
Bind an event listener to the buttoncreate event:
```
$( ".selector" ).on( "buttoncreate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Button
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>button demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<button>Button label</button>
<script>
$( "button" ).button();
</script>
</body>
</html>
```
#### Demo:
jqueryui Menu Widget Menu Widget
===========
Menu Widgetversion added: 1.9
------------------------------
**Description:** Themeable menu with mouse and keyboard interactions for navigation.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [disabled](#option-disabled) [icons](#option-icons) [items](#option-items) [menus](#option-menus) [position](#option-position) [role](#option-role) ### Methods
[blur](#method-blur) [collapse](#method-collapse) [collapseAll](#method-collapseAll) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [expand](#method-expand) [focus](#method-focus) [instance](#method-instance) [isFirstItem](#method-isFirstItem) [isLastItem](#method-isLastItem) [next](#method-next) [nextPage](#method-nextPage) [option](#method-option) [previous](#method-previous) [previousPage](#method-previousPage) [refresh](#method-refresh) [select](#method-select) [widget](#method-widget)
### Extension Points
[\_closeOnDocumentClick](#method-_closeOnDocumentClick) [\_isDivider](#method-_isDivider) ### Events
[blur](#event-blur) [create](#event-create) [focus](#event-focus) [select](#event-select) A menu can be created from any valid markup as long as the elements have a strict parent/child relationship. The most commonly used element is the unordered list (`<ul>`). Additionally, the contents of each menu item must be wrapped with a block-level DOM element. In the example below `<div>` elements are used as wrappers:
```
<ul id="menu">
<li>
<div>Item 1</div>
</li>
<li>
<div>Item 2</div>
</li>
<li>
<div>Item 3</div>
<ul>
<li>
<div>Item 3-1</div>
</li>
<li>
<div>Item 3-2</div>
</li>
<li>
<div>Item 3-3</div>
</li>
</ul>
</li>
<li>
<div>Item 4</div>
</li>
<li>
<div>Item 5</div>
</li>
</ul>
```
If you use a structure other than `<ul>`/`<li>`, including using the same element for the menu and the menu items, use the [`menus`](#option-menus) option to specify a way to differentiate the two elements, e.g., `menus: "div.menuElement"`.
Any menu item can be disabled by adding the `ui-state-disabled` class to that element.
### Icons
To add icons to the menu, include them in the markup:
```
<ul id="menu">
<li>
<div><span class="ui-icon ui-icon-disk"></span>Save</div>
</li>
</ul>
```
Menu automatically adds the necessary padding to items without icons.
### Dividers
Divider elements can be created by including menu items that contain only spaces and/or dashes:
```
<ul id="menu">
<li>
<div>Item 1</div>
</li>
<li>-</li>
<li>
<div>Item 2</div>
</li>
</ul>
```
### Keyboard interaction
* `ENTER`/`SPACE`: Invoke the focused menu item's action, which may be opening a submenu.
* `UP`: Move focus to the previous menu item.
* `DOWN`: Move focus to the next menu item.
* `RIGHT`: Open the submenu, if available.
* `LEFT`: Close the current submenu and move focus to the parent menu item. If not in a submenu, do nothing.
* `ESCAPE`: Close the current submenu and move focus to the parent menu item. If not in a submenu, do nothing.
Typing a letter moves focus to the first item whose title starts with that character. Repeating the same character cycles through matching items. Typing more characters within the one second timer matches those characters.
Disabled items can receive keyboard focus, but do not allow any other interaction.
### Theming
The menu widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If menu specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-menu`: The outer container of the menu, as well as any nested submenu. This top-level element will additionally have a `ui-menu-icons` class if the menu contains icons.
+ `ui-menu-item`: The container for individual menu items. This contains the element for the item's text itself as well as the element for submenus.
- `ui-menu-item-wrapper`: The wrapper element inside each individual menu item, containting the text content and the icon indicating submenus.
* `ui-menu-icon`: The submenu icons set via the [`icons`](#option-icons) option.
+ `ui-menu-divider`: Divider elements between menu items.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Position](position)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the menu with the `classes` option specified, changing the theming for the `ui-menu` class:
```
$( ".selector" ).menu({
classes: {
"ui-menu": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-menu` class:
```
// Getter
var themeClass = $( ".selector" ).menu( "option", "classes.ui-menu" );
// Setter
$( ".selector" ).menu( "option", "classes.ui-menu", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the menu if set to `true`. **Code examples:**Initialize the menu with the `disabled` option specified:
```
$( ".selector" ).menu({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).menu( "option", "disabled" );
// Setter
$( ".selector" ).menu( "option", "disabled", true );
```
### icons
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
submenu: "ui-icon-carat-1-e"
}
```
Icons to use for submenus, matching [an icon provided by the jQuery UI CSS Framework](theming/icons). **Code examples:**Initialize the menu with the `icons` option specified:
```
$( ".selector" ).menu({
icons: { submenu: "ui-icon-circle-triangle-e" }
});
```
Get or set the `icons` option, after initialization:
```
// Getter
var icons = $( ".selector" ).menu( "option", "icons" );
// Setter
$( ".selector" ).menu( "option", "icons", { submenu: "ui-icon-circle-triangle-e" } );
```
### items
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"> *"` Selector for the elements that serve as the menu items.
**Note:** The `items` option should not be changed after initialization. (version added: 1.11.0) **Code examples:**Initialize the menu with the `items` option specified:
```
$( ".selector" ).menu({
items: ".custom-item"
});
```
Get the `items` option, after initialization:
```
// Getter
var items = $( ".selector" ).menu( "option", "items" );
```
### menus
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"ul"` Selector for the elements that serve as the menu container, including sub-menus.
**Note:** The `menus` option should not be changed after initialization. Existing submenus will not be updated. **Code examples:**Initialize the menu with the `menus` option specified:
```
$( ".selector" ).menu({
menus: "div"
});
```
Get the `menus` option, after initialization:
```
// Getter
var menus = $( ".selector" ).menu( "option", "menus" );
```
### position
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ my: "left top", at: "right top" }` Identifies the position of submenus in relation to the associated parent menu item. The `of` option defaults to the parent menu item, but you can specify another element to position against. You can refer to the [jQuery UI Position](position) utility for more details about the various options. **Code examples:**Initialize the menu with the `position` option specified:
```
$( ".selector" ).menu({
position: { my: "left top", at: "right-5 top+5" }
});
```
Get or set the `position` option, after initialization:
```
// Getter
var position = $( ".selector" ).menu( "option", "position" );
// Setter
$( ".selector" ).menu( "option", "position", { my: "left top", at: "right-5 top+5" } );
```
### role
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"menu"` Customize the ARIA roles used for the menu and menu items. The default uses `"menuitem"` for items. Setting the `role` option to `"listbox"` will use `"option"` for items. If set to `null`, no roles will be set, which is useful if the menu is being controlled by another element that is maintaining focus.
**Note:** The `role` option should not be changed after initialization. Existing (sub)menus and menu items will not be updated. **Code examples:**Initialize the menu with the `role` option specified:
```
$( ".selector" ).menu({
role: null
});
```
Get the `role` option, after initialization:
```
// Getter
var role = $( ".selector" ).menu( "option", "role" );
```
Methods
-------
### blur( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes focus from a menu, resets any active element styles and triggers the menu's [`blur`](#event-blur) event. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the menu to blur.
**Code examples:**Invoke the blur method:
```
$( ".selector" ).menu( "blur" );
```
### collapse( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes the currently active sub-menu. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the menu to collapse.
**Code examples:**Invoke the collapse method:
```
$( ".selector" ).menu( "collapse" );
```
### collapseAll( [event ] [, all ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes all open sub-menus. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the menu to collapse.
* **all** Type: [Boolean](http://api.jquery.com/Types/#Boolean) Indicates whether all sub-menus should be closed or only sub-menus below and including the menu that is or contains the target of the triggering event.
**Code examples:**Invoke the collapseAll method:
```
$( ".selector" ).menu( "collapseAll", null, true );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the menu functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).menu( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the menu. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).menu( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the menu. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).menu( "enable" );
```
### expand( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Opens the sub-menu below the currently active item, if one exists. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the menu to expand.
**Code examples:**Invoke the expand method:
```
$( ".selector" ).menu( "expand" );
```
### focus( [event ], item )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Activates the given menu item and triggers the menu's [`focus`](#event-focus) event. Opens the menu item's sub-menu, if one exists. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the menu item to gain focus.
* **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The menu item to focus/activate.
**Code examples:**Invoke the focus method:
```
$( ".selector" ).menu( "focus", null, menu.find( ".ui-menu-item" ).last() );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the menu's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the menu plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).menu( "instance" );
```
### isFirstItem()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Returns a boolean value stating whether or not the currently active item is the first item in the menu. * This method does not accept any arguments.
**Code examples:**Invoke the isFirstItem method:
```
var firstItem = $( ".selector" ).menu( "isFirstItem" );
```
### isLastItem()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Returns a boolean value stating whether or not the currently active item is the last item in the menu. * This method does not accept any arguments.
**Code examples:**Invoke the isLastItem method:
```
var lastItem = $( ".selector" ).menu( "isLastItem" );
```
### next( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Moves active state to next menu item. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the focus to move.
**Code examples:**Invoke the next method:
```
$( ".selector" ).menu( "next" );
```
### nextPage( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Moves active state to first menu item below the bottom of a scrollable menu or the last item if not scrollable. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the focus to move.
**Code examples:**Invoke the nextPage method:
```
$( ".selector" ).menu( "nextPage" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).menu( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current menu options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).menu( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the menu option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).menu( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the menu. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).menu( "option", { disabled: true } );
```
### previous( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Moves active state to previous menu item. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the focus to move.
**Code examples:**Invoke the previous method:
```
$( ".selector" ).menu( "previous" );
```
### previousPage( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Moves active state to first menu item above the top of a scrollable menu or the first item if not scrollable. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the focus to move.
**Code examples:**Invoke the previousPage method:
```
$( ".selector" ).menu( "previousPage" );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Initializes sub-menus and menu items that have not already been initialized. New menu items, including sub-menus can be added to the menu or all of the contents of the menu can be replaced and then initialized with the `refresh()` method. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).menu( "refresh" );
```
### select( [event ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Selects the currently active menu item, collapses all sub-menus and triggers the menu's [`select`](#event-select) event. * **event** Type: [Event](http://api.jquery.com/Types/#Event) What triggered the selection.
**Code examples:**Invoke the select method:
```
$( ".selector" ).menu( "select" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the menu. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).menu( "widget" );
```
Extension Points
----------------
The menu widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_closeOnDocumentClick( event )Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Method that determines whether clicks on the document should close any open menus. By default, menus are closed unless the click occurred on the menu. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
**Code examples:**Never close menus on document click.
```
_closeOnDocumentClick: function( event ) {
return false;
}
```
### \_isDivider( item )Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Determines whether an item should actually be represented as a divider instead of a menu item. By default any item that contains just spaces and/or dashes is considered a divider. * **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery)
**Code examples:**Treat all items as menu items with no dividers.
```
_isDivider: function( item ) {
return false;
}
```
Events
------
### blur( event, ui )Type: `menublur`
Triggered when the menu loses focus. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The currently active menu item.
**Code examples:**Initialize the menu with the blur callback specified:
```
$( ".selector" ).menu({
blur: function( event, ui ) {}
});
```
Bind an event listener to the menublur event:
```
$( ".selector" ).on( "menublur", function( event, ui ) {} );
```
### create( event, ui )Type: `menucreate`
Triggered when the menu is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the menu with the create callback specified:
```
$( ".selector" ).menu({
create: function( event, ui ) {}
});
```
Bind an event listener to the menucreate event:
```
$( ".selector" ).on( "menucreate", function( event, ui ) {} );
```
### focus( event, ui )Type: `menufocus`
Triggered when a menu gains focus or when any menu item is activated. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The currently active menu item.
**Code examples:**Initialize the menu with the focus callback specified:
```
$( ".selector" ).menu({
focus: function( event, ui ) {}
});
```
Bind an event listener to the menufocus event:
```
$( ".selector" ).on( "menufocus", function( event, ui ) {} );
```
### select( event, ui )Type: `menuselect`
Triggered when a menu item is selected. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The currently active menu item.
**Code examples:**Initialize the menu with the select callback specified:
```
$( ".selector" ).menu({
select: function( event, ui ) {}
});
```
Bind an event listener to the menuselect event:
```
$( ".selector" ).on( "menuselect", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Menu
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>menu demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
.ui-menu {
width: 200px;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<ul id="menu">
<li>
<div>Item 1</div>
</li>
<li>
<div>Item 2</div>
</li>
<li>
<div>Item 3</div>
<ul>
<li>
<div>Item 3-1</div>
</li>
<li>
<div>Item 3-2</div>
</li>
<li>
<div>Item 3-3</div>
</li>
</ul>
</li>
<li>
<div>Item 4</div>
</li>
<li>
<div>Item 5</div>
</li>
</ul>
<script>
$( "#menu" ).menu();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Form Reset Mixin Form Reset Mixin
================
Form Reset Mixin
----------------
**Description:** A mixin to call `refresh()` on an input widget when the parent form gets reset.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
### Methods
[\_bindFormResetHandler](#method-_bindFormResetHandler) [\_unbindFormResetHandler](#method-_unbindFormResetHandler) ### Events
This only works for native input elements that have a form attribute, like an `<input>`. It doesn't work for other elements like `<label>` or `<div>` Methods
-------
### \_bindFormResetHandler()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Call `this._bindFormResetHandler()` inside `_create()` to initialize the mixin. * This method does not accept any arguments.
**Code examples:**Set the background color of the widget's element based on an option.
```
_create: function() {
this._bindFormResetHandler();
}
```
### \_unbindFormResetHandler()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Call `this._unbindFormResetHandler()` inside `_destroy()` to destroy the mixin. * This method does not accept any arguments.
**Code examples:**
```
_destroy: function() {
this._unbindFormResetHandler();
}
```
Example:
--------
Type colors into the input
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery.ui.formResetMixin demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
.demo-colorize-swatch {
width: 50px;
height: 50px;
border: 1px solid black;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<form>
<input id="colorize">
<button type="reset">Reset form</button>
</form>
<script>
$.widget( "custom.colorize", [ $.ui.formResetMixin, {
_create: function() {
this.swatch = $("<div>")
.addClass("demo-colorize-swatch")
.insertAfter(this.element);
this.refresh();
this._bindFormResetHandler();
this._on({ keyup: "refresh" });
},
refresh: function() {
this.swatch.css( "background-color", this.element.val() );
},
_destroy: function() {
this.swatch.remove();
this._unbindFormResetHandler();
}
} ] );
$( "#colorize" ).colorize();
</script>
</body>
</html>
```
#### Demo:
jqueryui Widget Factory Widget Factory
==============
#### Contents:
* [jQuery.widget( name [, base ], prototype )](#jQuery-widget1)
+ [jQuery.widget( name [, base ], prototype )](#jQuery-widget-name-base-prototype)
* [jQuery.Widget](#jQuery-Widget2)
jQuery.widget( name [, base ], prototype )
------------------------------------------
**Description:** Create stateful jQuery plugins using the same abstraction as all jQuery UI widgets.
* #### [jQuery.widget( name [, base ], prototype )](#jQuery-widget-name-base-prototype)
+ **name** Type: [String](http://api.jquery.com/Types/#String) The name of the widget to create, including the namespace.
+ **base** Type: [Function](http://api.jquery.com/Types/#Function)() The base widget to inherit from. This must be a constructor that can be instantiated with the `new` keyword. Defaults to `jQuery.Widget`.
+ **prototype** Type: [PlainObject](http://api.jquery.com/Types/#PlainObject) The object to use as a prototype for the widget.
You can create new widgets from scratch, using just the `$.Widget` object as a base to inherit from, or you can explicitly inherit from existing jQuery UI or third-party widgets. Defining a widget with the same name as you inherit from even allows you to extend widgets in place.
jQuery UI contains many widgets that maintain state and therefore have a slightly different usage pattern than typical jQuery plugins. All of jQuery UI's widgets use the same patterns, which is defined by the widget factory. So if you learn how to use one widget, then you'll know how to use all of them.
Looking for tutorials about the widget factory? Check out [the articles on the jQuery Learning Center](http://learn.jquery.com/jquery-ui/widget-factory/).
*Note: This documentation shows examples using the [progressbar widget](progressbar) but the syntax is the same for every widget.*
### Initialization
In order to track the state of the widget, we must introduce a full life cycle for the widget. The life cycle starts when the widget is initialized. To initialize a widget, we simply call the plugin on one or more elements.
```
$( "#elem" ).progressbar();
```
This will initialize each element in the jQuery object, in this case the element with an id of `"elem"`.
### Options
Because `progressbar()` was called with no parameters, the widget was initialized with its default options. We can pass a set of options during initialization to override the defaults:
```
$( "#elem" ).progressbar({ value: 20 });
```
We can pass as many or as few options as we want during initialization. Any options that we don't pass will just use their default values.
You can pass multiple options arguments. Those arguments will be merged into one object (similar to [`$.extend( true, target, object1, objectN )`](https://api.jquery.com/jQuery.extend/)). This is useful for sharing options between instances, while overriding some properties for each one:
```
var options = { modal: true, show: "slow" };
$( "#dialog1" ).dialog( options );
$( "#dialog2" ).dialog( options, { autoOpen: false });
```
All options passed on init are deep-copied to ensure the objects can be modified later without affecting the widget. Arrays are the only exception, they are referenced as-is. This exception is in place to support data-binding, where the data source has to be kept as a reference.
The default values are stored on the widget's prototype, therefore we have the ability to override the values that jQuery UI sets. For example, after setting the following, all future progressbar instances will default to a value of 80:
```
$.ui.progressbar.prototype.options.value = 80;
```
The options are part of the widget's state, so we can set options after initialization as well. We'll see this later with the option method.
### Methods
Now that the widget is initialized, we can query its state or perform actions on the widget. All actions after initialization take the form of a method call. To call a method on a widget, we pass the name of the method to the jQuery plugin. For example, to call the `value()` method on our progressbar widget, we would use:
```
$( "#elem" ).progressbar( "value" );
```
If the method accepts parameters, we can pass them after the method name. For example, to pass the parameter `40` to the `value()` method, we can use:
```
$( "#elem" ).progressbar( "value", 40 );
```
Just like other methods in jQuery, most widget methods return the jQuery object for chaining.
```
$( "#elem" )
.progressbar( "value", 90 )
.addClass( "almost-done" );
```
Each widget will have its own set of methods based on the functionality that the widget provides. However, there are a few methods that exist on all widgets, which are documented below.
### Events
All widgets have events associated with their various behaviors to notify you when the state is changing. For most widgets, when the events are triggered, the names are prefixed with the widget name and lowercased. For example, we can bind to progressbar's `change` event which is triggered whenever the value changes.
```
$( "#elem" ).bind( "progressbarchange", function() {
alert( "The value has changed!" );
});
```
Each event has a corresponding callback, which is exposed as an option. We can hook into progressbar's `change` callback instead of binding to the `progressbarchange` event, if we want to.
```
$( "#elem" ).progressbar({
change: function() {
alert( "The value has changed!" );
}
});
```
All widgets have a `create` event which is triggered upon instantiation.
### Instance
The widget's instance can be retrieved from a given element using the [`instance()`](#method-instance) method.
```
$( "#elem" ).progressbar( "instance" );
```
If the `instance()` method is called on an element that is not associated with the widget, `undefined` is returned.
```
$( "#not-a-progressbar" ).progressbar( "instance" ); // undefined
```
The instance is stored using [`jQuery.data()`](https://api.jquery.com/jQuery.data/) with the widget's full name as the key. Therefore, the [`:data`](data-selector) selector can also determine whether an element has a given widget bound to it.
```
$( "#elem" ).is( ":data('ui-progressbar')" ); // true
$( "#elem" ).is( ":data('ui-draggable')" ); // false
```
Unlike `instance()`, `:data` can be used even if the widget being tested for has not loaded.
```
$( "#elem" ).nonExistentWidget( "instance" ); // TypeError
$( "#elem" ).is( ":data('ui-nonExistentWidget')" ); // false
```
You can also use `:data` to get a list of all elements that are instances of a given widget.
```
$( ":data('ui-progressbar')" );
```
### Properties
All widgets have the following set of properties:
* **defaultElement**: An element to use when a widget instance is constructed without providing an element. For example, since the progressbar's `defaultElement` is `"<div>`", `$.ui.progressbar({ value: 50 })` instantiates a progressbar widget instance on a newly created `<div>`.
* **document**: A jQuery object containing the `document` that the widget's element is within. Useful if you need to interact with widgets within iframes.
* **element**: A jQuery object containing the element used to instantiate the widget. If you select multiple elements and call `.myWidget()`, a separate widget instance will be created for each element. Therefore, this property will always contain one element.
* **namespace**: The location on the global jQuery object that the widget's prototype is stored on. For example a `namespace` of `"ui"` indicates that the widget's prototype is stored on `$.ui`.
* **options**: An object containing the options currently being used by the widget. On instantiation, any options provided by the user will automatically be merged with any default values defined in `$.myNamespace.myWidget.prototype.options`. User specified options override the defaults.
* **uuid**: A unique integer identifier for the widget.
* **version**: The string version of the widget. For jQuery UI widgets this will be set to the version of jQuery UI the widget is using. Widget developers have to set this property in their prototype explicitly.
* **widgetEventPrefix**: The prefix prepended to the name of events fired from this widget. For example the `widgetEventPrefix` of the [draggable widget](draggable) is `"drag"`, therefore when a draggable is created, the name of the event fired is `"dragcreate"`. By default the `widgetEventPrefix` of a widget is its name. *Note: This property is deprecated and will be removed in a later release. Event names will be changed to widgetName:eventName (e.g. `"draggable:create"`).*
* **widgetFullName**: The full name of the widget including the namespace. For `$.widget( "myNamespace.myWidget", {} )`, `widgetFullName` will be `"myNamespace-myWidget"`.
* **widgetName**: The name of the widget. For `$.widget( "myNamespace.myWidget", {} )`, `widgetName` will be `"myWidget"`.
* **window**: A jQuery object containing the `window` that the widget's element is within. Useful if you need to interact with widgets within iframes.
Base Widget
-----------
**Description:** The base widget used by the widget factory.
QuickNav
--------
### Options
[classes](#option-classes) [disabled](#option-disabled) [hide](#option-hide) [show](#option-show) ### Methods
[\_addClass](#method-_addClass) [\_create](#method-_create) [\_delay](#method-_delay) [\_destroy](#method-_destroy) [\_focusable](#method-_focusable) [\_getCreateEventData](#method-_getCreateEventData) [\_getCreateOptions](#method-_getCreateOptions) [\_hide](#method-_hide) [\_hoverable](#method-_hoverable) [\_init](#method-_init) [\_off](#method-_off) [\_on](#method-_on) [\_removeClass](#method-_removeClass) [\_setOption](#method-_setOption) [\_setOptions](#method-_setOptions) [\_show](#method-_show) [\_super](#method-_super) [\_superApply](#method-_superApply) [\_toggleClass](#method-_toggleClass) [\_trigger](#method-_trigger) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [widget](#method-widget) ### Events
[create](#event-create) Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Additional (thematic) classes to add to the widget, in addition to the structural classes. The structural classes are used as keys of this option and the thematic classes are the values. See the [`_addClass()` method](#method-_addClass) for using this in custom widgets. Check out the documentation of individual widgets to see which classes they support.
The primary motivation of this option is to map structural classes to theme classes. In other words, any class prefixed with namespace and widget, like `"ui-progressbar-"`, is considered a structural class. These are always added to the widget. In contrast to that, any class not specific to the widget is considered a theme class. These could be part of jQuery UI's CSS framework, but can also come from other CSS frameworks or be defined in custom stylesheets.
Setting the `classes` option after creation will override all default properties. To only change specific values, use deep setters, e.g. `.option( "classes.ui-progressbar-value", null )`.
**Code examples:** Initialize a progressbar widget with the `classes` option specified, changing the theming for the `ui-progressbar` class:
```
$( ".selector" ).progressbar({
classes: {
"ui-progressbar": "highlight"
}
});
```
Get or set the `classes` option, after initialization:
```
// Getter
var classes = $( ".selector" ).widget( "option", "classes" );
// Setter, override all classes
$( ".selector" ).widget( "option", "classes", { "custom-header": "icon-warning" } );
// Setter, override just one class
$( ".selector" ).widget( "option", "classes.custom-header", "icon-warning" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the widget if set to `true`. **Code examples:**Initialize the widget with the `disabled` option specified:
```
$( ".selector" ).widget({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).widget( "option", "disabled" );
// Setter
$( ".selector" ).widget( "option", "disabled", true );
```
### hide
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the hiding of the element. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the element will be hidden immediately. When set to `true`, the element will fade out with the default duration and the default easing.
* **Number**: The element will fade out with the specified duration and the default easing.
* **String**: The element will be hidden using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideUp"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeOut"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the widget with the `hide` option specified:
```
$( ".selector" ).widget({
hide: { effect: "explode", duration: 1000 }
});
```
Get or set the `hide` option, after initialization:
```
// Getter
var hide = $( ".selector" ).widget( "option", "hide" );
// Setter
$( ".selector" ).widget( "option", "hide", { effect: "explode", duration: 1000 } );
```
### show
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the showing of the element. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the element will be shown immediately. When set to `true`, the element will fade in with the default duration and the default easing.
* **Number**: The element will fade in with the specified duration and the default easing.
* **String**: The element will be shown using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideDown"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeIn"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the widget with the `show` option specified:
```
$( ".selector" ).widget({
show: { effect: "blind", duration: 800 }
});
```
Get or set the `show` option, after initialization:
```
// Getter
var show = $( ".selector" ).widget( "option", "show" );
// Setter
$( ".selector" ).widget( "option", "show", { effect: "blind", duration: 800 } );
```
Methods
-------
### \_addClass( [element ], keys [, extra ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Add classes to an element of the widget. This provides a hook for the user to add additional classes or replace default styling classes, through the [`classes` option](#option-classes).
It also provides automatic removal of these classes when a widget is destroyed, as long as you're using [`_addClass()`](#method-_addClass), [`_removeClass()`](#method-_removeClass) and [`_toggleClass()`](#method-_toggleClass) together. This can heavily simplify the implementation of custom [`_destroy()`](#method-_destroy) methods.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to add the classes to. Defaults to `this.element`.
* **keys** Type: [String](http://api.jquery.com/Types/#String) The classes to add, as a space-delimited list. If a property of the [`classes` option](#option-classes) matches a key, the value will be added as well. When you only need the `extra` argument, you can skip this argument by specifying `null`.
* **extra** Type: [String](http://api.jquery.com/Types/#String) Additional classes to add, required for layout or other reasons. Unlike the `keys` argument, these aren't associated with any properties of the [`classes` option](#option-classes). Just like `keys`, they will also be automatically removed when destroying the widget.
**Code examples:**Add the `ui-progressbar` class to the widget's element (`this.element`). Will also add any additional classes specified through the [`classes` option](#option-classes) for the given class.
```
this._addClass( "ui-progressbar" );
```
Add the `demo-popup-header` class to the specified element (here referencing `this.popup`). Will also add any additional classes specified through the [`classes` option](#option-classes) for the given class. In addition, it will always add the `ui-front` class.
```
this._addClass( this.popup, "demo-popup-header", "ui-front" );
```
Adds the `ui-helper-hidden-accessible` class to the specified element. Uses `null` for the `keys` argument to skip it.
```
this._addClass( this.liveRegion, null, "ui-helper-hidden-accessible" );
```
### \_create()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
The `_create()` method is the widget's constructor. There are no parameters, but `this.element` and `this.options` are already set. * This method does not accept any arguments.
**Code examples:**Set the background color of the widget's element based on an option.
```
_create: function() {
this.element.css( "background-color", this.options.color );
}
```
### \_delay( fn [, delay ] )Returns: [Number](http://api.jquery.com/Types/#Number)
Invokes the provided function after a specified delay. Keeps `this` context correct. Essentially `setTimeout()`. Returns the timeout ID for use with `clearTimeout()`.
* **fn** Type: [Function](http://api.jquery.com/Types/#Function)() or [String](http://api.jquery.com/Types/#String) The function to invoke. Can also be the name of a method on the widget.
* **delay** Type: [Number](http://api.jquery.com/Types/#Number) The number of milliseconds to wait before invoking the function. Defaults to `0`.
**Code examples:**Call the `_foo()` method on the widget after 100 milliseconds.
```
this._delay( this._foo, 100 );
```
### \_destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
The public [`destroy()`](#method-destroy) method cleans up all common data, events, etc. and then delegates out to `_destroy()` for custom, widget-specific, cleanup. * This method does not accept any arguments.
**Code examples:**Remove a class from the widget's element when the widget is destroyed.
```
_destroy: function() {
this.element.removeClass( "my-widget" );
}
```
### \_focusable( element )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets up `element` to apply the `ui-state-focus` class on focus. The event handlers are automatically cleaned up on destroy.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element(s) to apply the focusable behavior to.
**Code examples:**Apply focusable styling to a set of elements within the widget.
```
this._focusable( this.element.find( ".my-items" ) );
```
### \_getCreateEventData()Returns: [Object](http://api.jquery.com/Types/#Object)
All widgets trigger the [`create`](#event-create) event. By default, no data is provided in the event, but this method can return an object which will be passed as the `create` event's data. * This method does not accept any arguments.
**Code examples:**Pass the widget's options to `create` event handlers as an argument.
```
_getCreateEventData: function() {
return this.options;
}
```
### \_getCreateOptions()Returns: [Object](http://api.jquery.com/Types/#Object)
This method allows the widget to define a custom method for defining options during instantiation. The user-provided options override the options returned by this method, which override the default options. * This method does not accept any arguments.
**Code examples:**Make the widget element's id attribute available as an option.
```
_getCreateOptions: function() {
return { id: this.element.attr( "id" ) };
}
```
### \_hide( element, option [, callback ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Hides an element immediately, using built-in animation methods, or using custom effects. See the [hide](#option-hide) option for possible `option` values. * **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element(s) to hide.
* **option** Type: [Object](http://api.jquery.com/Types/#Object) The properties defining how to hide the element.
* **callback** Type: [Function](http://api.jquery.com/Types/#Function)() Callback to invoke after the element has been fully hidden.
**Code examples:**Pass along the `hide` option for custom animations.
```
this._hide( this.element, this.options.hide, function() {
// Remove the element from the DOM when it's fully hidden.
$( this ).remove();
});
```
### \_hoverable( element )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets up `element` to apply the `ui-state-hover` class on hover. The event handlers are automatically cleaned up on destroy.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element(s) to apply the hoverable behavior to.
**Code examples:**Apply hoverable styling to all `<div>`s within the element on hover.
```
this._hoverable( this.element.find( "div" ) );
```
### \_init()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Widgets have the concept of initialization that is distinct from creation. Any time the plugin is called with no arguments or with only an option hash, the widget is initialized; this includes when the widget is created. *Note: Initialization should only be handled if there is a logical action to perform on successive calls to the widget with no arguments.*
* This method does not accept any arguments.
**Code examples:**Call the `open()` method if the `autoOpen` option is set.
```
_init: function() {
if ( this.options.autoOpen ) {
this.open();
}
}
```
### \_off( element, eventName )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Unbinds event handlers from the specified element(s). * **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element(s) to unbind the event handlers from. Unlike the `_on()` method, the elements are required for `_off()`.
* **eventName** Type: [String](http://api.jquery.com/Types/#String) One or more space-separated event types.
**Code examples:**Unbind all click events from the widget's element.
```
this._off( this.element, "click" );
```
### \_on( [suppressDisabledCheck ] [, element ], handlers )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Binds event handlers to the specified element(s). Delegation is supported via selectors inside the event names, e.g., "`click .foo`". The `_on()` method provides several benefits of direct event binding: * Maintains proper `this` context inside the handlers.
* Automatically handles disabled widgets: If the widget is disabled or the event occurs on an element with the `ui-state-disabled` class, the event handler is not invoked. Can be overridden with the `suppressDisabledCheck` parameter.
* Event handlers are automatically namespaced and cleaned up on destroy.
* **suppressDisabledCheck** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether or not to bypass the disabled check.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) Which element(s) to bind the event handlers to. If no element is provided, `this.element` is used for non-delegated events and [the widget element](#method-widget) is used for delegated events.
* **handlers** Type: [Object](http://api.jquery.com/Types/#Object) An object in which the keys represent the event type and optional selector for delegation, and the values represent a handler function to be called for the event.
**Code examples:**Prevent the default action of all links clicked within the widget's element.
```
this._on( this.element, {
"click a": function( event ) {
event.preventDefault();
}
});
```
### \_removeClass( [element ], keys [, extra ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Remove classes from an element of the widget. The arguments are the same as for the [`_addClass()` method](#method-_addClass), the same semantics apply, just in reverse.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to remove the classes from. Defaults to `this.element`.
* **keys** Type: [String](http://api.jquery.com/Types/#String) The classes to remove, as a space-delimited list. If a property of the [`classes` option](#option-classes) matches a key, the value will be removed as well. When you only need the `extra` argument, you can skip this argument by specifying `null`.
* **extra** Type: [String](http://api.jquery.com/Types/#String) Additional classes to remove, required for layout or other reasons. Unlike the `keys` argument, these aren't associated with any properties of the [`classes` option](#option-classes).
**Code examples:**Remove the `ui-progressbar` class from the widget's element (`this.element`). Will also remove any additional classes specified through the [`classes` option](#option-classes) for the given class.
```
this._removeClass( "ui-progressbar" );
```
Remove the `demo-popup-header` class from the specified element (here referencing `this.popup`). Will also remove any additional classes specified through the [`classes` option](#option-classes) for the given class. In addition, it will also remove the `ui-front` class.
```
this._removeClass( this.popup, "demo-popup-header", "ui-front" );
```
Remove the `ui-helper-hidden-accessible` class from the specified element. Uses `null` for the `keys` argument to skip it.
```
this._removeClass( this.liveRegion, null, "ui-helper-hidden-accessible" );
```
### \_setOption( key, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Called from the [`_setOptions()`](#method-_setOptions) method for each individual option. Widget state should be updated based on changes. * **key** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Update a widget's element when its `height` or `width` option changes.
```
_setOption: function( key, value ) {
if ( key === "width" ) {
this.element.width( value );
}
if ( key === "height" ) {
this.element.height( value );
}
this._super( key, value );
}
```
### \_setOptions( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Called whenever the [`option()`](#method-option) method is called, regardless of the form in which the `option()` method was called. Overriding this is useful if you can defer processor-intensive changes for multiple option changes.
* **options** Type: [Object](http://api.jquery.com/Types/#Object) An object containing options to set, with the name of the option as the key and the option value as the value.
**Code examples:**Call a `resize()` method if the `height` or `width` options change.
```
_setOptions: function( options ) {
var that = this,
resize = false;
$.each( options, function( key, value ) {
that._setOption( key, value );
if ( key === "height" || key === "width" ) {
resize = true;
}
});
if ( resize ) {
this.resize();
}
}
```
### \_show( element, option [, callback ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Shows an element immediately, using built-in animation methods, or using custom effects. See the [show](#option-show) option for possible `option` values. * **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element(s) to show.
* **option** Type: [Object](http://api.jquery.com/Types/#Object) The properties defining how to show the element.
* **callback** Type: [Function](http://api.jquery.com/Types/#Function)() Callback to invoke after the element has been fully shown.
**Code examples:**Pass along the `show` option for custom animations.
```
this._show( this.element, this.options.show, function() {
// Focus the element when it's fully visible.
this.focus();
}
```
### \_super( [arg ] [, ... ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Invokes the method of the same name from the parent widget, with any specified arguments. Essentially `.call()`. * **arg** Type: [Object](http://api.jquery.com/Types/#Object) Zero to many arguments to pass to the parent widget's method.
**Code examples:**Handle `title` option updates and call the parent widget's `_setOption()` to update the internal storage of the option.
```
_setOption: function( key, value ) {
if ( key === "title" ) {
this.element.find( "h3" ).text( value );
}
this._super( key, value );
}
```
### \_superApply( arguments )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Invokes the method of the same name from the parent widget, with the array of arguments. Essentially `.apply()`. * **arguments** Type: [Array](http://api.jquery.com/Types/#Array) Array of arguments to pass to the parent method.
**Code examples:**Handle `title` option updates and call the parent widget's `_setOption()` to update the internal storage of the option.
```
_setOption: function( key, value ) {
if ( key === "title" ) {
this.element.find( "h3" ).text( value );
}
this._superApply( arguments );
}
```
### \_toggleClass( [element ], keys [, extra ], add )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Toggle classes of an element of the widget. The arguments are the same as for the [`_addClass()`](#method-_addClass) and [`_removeClass()`](#method-_removeClass) methods, except for the additional boolean argument that specifies to add or remove classes.
Unlike jQuery's `.toggleClass()` method, the boolean `add` argument is always required.
* **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to toogle the classes on. Defaults to `this.element`.
* **keys** Type: [String](http://api.jquery.com/Types/#String) The classes to toogle, as a space-delimited list. If a property of the [`classes` option](#option-classes) matches a key, the value will be toggled as well. When you only need the `extra` argument, you can skip this argument by specifying `null`.
* **extra** Type: [String](http://api.jquery.com/Types/#String) Additional classes to toggle, required for layout or other reasons. Unlike the `keys` argument, these aren't associated with any properties of the [`classes` option](#option-classes). Just like `keys`, they will also be automatically removed when destroying the widget.
* **add** Type: [Boolean](http://api.jquery.com/Types/#Boolean) Indicates whether to add or remove the specified classes, where a boolean `true` indicates that classes should be added, a boolean `false` indicates that classes should be removed.
**Code examples:**Toggle the `ui-state-disabled` class on the widget's element (`this.element`).
```
this._toggleClass( null, "ui-state-disabled", !!value );
```
### \_trigger( type [, event ] [, data ] )Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Triggers an event and its associated callback. The option with the name equal to type is invoked as the callback.
The event name is the lowercase concatenation of the widget name and type.
*Note: When providing data, you must provide all three parameters. If there is no event to pass along, just pass `null`.*
If the default action is prevented, `false` will be returned, otherwise `true`. Preventing the default action happens when the handler returns `false` or calls `event.preventDefault()`.
* **type** Type: [String](http://api.jquery.com/Types/#String) The `type` should match the name of a callback option. The full event type will be generated automatically.
* **event** Type: [Event](http://api.jquery.com/Types/#Event) The original event that caused this event to occur; useful for providing context to the listener.
* **data** Type: [Object](http://api.jquery.com/Types/#Object) A hash of data associated with the event.
**Code examples:**Trigger a `search` event whenever a key is pressed.
```
this._on( this.element, {
keydown: function( event ) {
// Pass the original event so that the custom search event has
// useful information, such as keyCode
this._trigger( "search", event, {
// Pass additional information unique to this event
value: this.element.val()
});
}
});
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the widget functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the widget. * This method does not accept any arguments.
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the widget. * This method does not accept any arguments.
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the widget's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the widget plugin has loaded.
* This method does not accept any arguments.
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current widget options hash. * This signature does not accept any arguments.
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the widget option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the widget. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the original element or other relevant generated element. * This method does not accept any arguments.
Events
------
### create( event, ui )Type: `widgetcreate`
Triggered when the widget is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the widget with the create callback specified:
```
$( ".selector" ).widget({
create: function( event, ui ) {}
});
```
Bind an event listener to the widgetcreate event:
```
$( ".selector" ).on( "widgetcreate", function( event, ui ) {} );
```
| programming_docs |
jqueryui Easings Easings
=======
Easing functions specify the speed at which an animation progresses at different points within the animation. jQuery core ships with two easings: `linear`, which progresses at a constant pace throughout the animation, and `swing` (jQuery core's default easing), which progresses slightly slower at the beginning and end of the animation than it does in the middle of the animation. jQuery UI provides several additional easing functions, ranging from variations on the swing behavior to customized effects such as bouncing.
Some easings will result in negative values during the animation. Depending on the properties that are being animated, the actual value may be clamped at zero. For example, you can animate `left` to a negative value, but you cannot animate `height` or `opacity` to a negative value.
The best way to understand how an easing will affect an animation is to see the equation graphed over time. See below for a graph of all animations available in jQuery UI.
jqueryui :focusable Selector :focusable Selector
===================
focusable selector
------------------
**Description:** Selects elements which can be focused.
* #### jQuery( ":focusable" )
Some elements are natively focusable, while others require explicitly setting a tab index. In all cases, the element must be visible in order to be focusable.
Elements of the following type are focusable if they are not disabled: `input`, `select`, `textarea`, `button`, and `object`. Anchors are focusable if they have an `href` or `tabindex` attribute. `area` elements are focusable if they are inside a named map, have an `href` attribute, and there is a visible image using the map. All other elements are focusable based solely on their `tabindex` attribute and visibility.
*Note: Elements with a negative tab index are `:focusable`, but not [`:tabbable`](tabbable-selector).*
Example:
--------
Select focusable elements and highlight them with a red border.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>focusable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
input, a, p {
border: 1px solid #000;
}
div {
padding: 5px;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div><input value="text input"></div>
<div><a>anchor without href</a></div>
<div><a href="#">anchor with href</a></div>
<div><p>paragraph without tabindex</p></div>
<div><p tabindex="1">paragraph with tabindex</p></div>
<script>
$( ":focusable" ).css( "border-color", "red" );
</script>
</body>
</html>
```
#### Demo:
jqueryui .addClass() .addClass()
===========
.addClass( className [, duration ] [, easing ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
---------------------------------------------------------------------------------------------------------------------
**Description:** Adds the specified class(es) to each of the set of matched elements while animating all style changes.
* #### [.addClass( className [, duration ] [, easing ] [, complete ] )](#addClass-className-duration-easing-complete)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be added to the class attribute of each matched element.
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.addClass( className [, options ] )](#addClass-className-options)
+ **className** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be added to the class attribute of each matched element.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. All properties are optional.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **children** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the animation should additionally be applied to all descendants of the matched elements. This feature should be used with caution as the cost of determining which descendants to animate can be very expensive, and grows linearly with the number of descendants.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
Similar to native CSS transitions, jQuery UI's class animations provide a smooth transition from one state to another while allowing you to keep all the details about which styles to change in CSS and out of your JavaScript. All class animation methods, including `.addClass()`, support custom durations and easings, as well as provide a callback for when the animation completes.
Not all styles can be animated. For example, there is no way to animate a background image. Any styles that cannot be animated will be changed at the end of the animation.
This plugin extends jQuery's built-in [`.addClass()`](https://api.jquery.com/addClass/) method. If jQuery UI is not loaded, calling the `.addClass()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Adds the class "big-blue" to the matched elements.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>addClass demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background-color: #ccc;
}
.big-blue {
width: 200px;
height: 200px;
background-color: #00f;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div></div>
<script>
$( "div" ).click(function() {
$( this ).addClass( "big-blue", 1000, "easeOutBounce" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Fold Effect Fold Effect
===========
Fold Effect
-----------
**Description:** The fold effect hides or shows an element by folding it.
* #### fold
+ **size** (default: `15`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) The size of the "folded" element.
+ **horizFirst** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the horizontal direction happens first when hiding. Remember, showing inverts hiding.
Example:
--------
Toggle a div using the fold effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>fold demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "fold" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Pulsate Effect Pulsate Effect
==============
Pulsate Effect
--------------
**Description:** The pulsate effect hides or shows an element by pulsing it in or out.
* #### pulsate
+ **times** (default: `5`) Type: [Integer](http://api.jquery.com/Types/#Integer) The number of times the element should pulse. An extra half pulse is added for hide/show.
Example:
--------
Toggle a div using the pulsate effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>pulsate demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "pulsate" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Size Effect Size Effect
===========
Size Effect
-----------
**Description:** Resize an element to a specified width and height.
* #### size
+ **to** Type: [Object](http://api.jquery.com/Types/#Object) Height and width to resize to.
+ **origin** (default: `[ "top", "left" ]`) Type: [Array](http://api.jquery.com/Types/#Array) The vanishing point.
+ **scale** (default: `"both"`) Type: [String](http://api.jquery.com/Types/#String) Which areas of the element will be resized: `"both"`, `"box"`, `"content"`. Box resizes the border and padding of the element; content resizes any content inside of the element.
When using this effect with the [`.show()`](show) and [`.toggle()`](toggle) methods, the `to` argument is used as the starting point and the original size used as the endpoint.
Example:
--------
Resize the element using the size effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>size demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to resize the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).effect( "size", {
to: { width: 200, height: 60 }
}, 1000 );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Transfer Effect Transfer Effect
===============
Transfer Effectversion deprecated: 1.12
----------------------------------------
**Description:** Transfers the outline of an element to another element
* #### version deprecated: 1.12transfer
+ **className** Type: [String](http://api.jquery.com/Types/#String) argumental class name the transfer element will receive.
+ **to** Type: [String](http://api.jquery.com/Types/#String) jQuery selector, the element to transfer to.
Very useful when trying to visualize interaction between two elements.
The transfer element itself has the class `ui-effects-transfer`, and needs to be styled by you, for example by adding a background or border.
This effect is deprecated, replaced by the [.transfer()](transfer) method.
Example:
--------
Clicking on the green element transfers to the other.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>transfer demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div.green {
width: 100px;
height: 80px;
background: green;
border: 1px solid black;
position: relative;
}
div.red {
margin-top: 10px;
width: 50px;
height: 30px;
background: red;
border: 1px solid black;
position: relative;
}
.ui-effects-transfer {
border: 1px dotted black;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div class="green"></div>
<div class="red"></div>
<script>
$( "div" ).click(function() {
var i = 1 - $( "div" ).index( this );
$( this ).effect( "transfer", { to: $( "div" ).eq( i ) }, 1000 );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Selectable Widget Selectable Widget
=================
Selectable Widgetversion added: 1.0
------------------------------------
**Description:** Use the mouse to select elements, individually or in a group.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[appendTo](#option-appendTo) [autoRefresh](#option-autoRefresh) [cancel](#option-cancel) [classes](#option-classes) [delay](#option-delay) [disabled](#option-disabled) [distance](#option-distance) [filter](#option-filter) [tolerance](#option-tolerance) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[create](#event-create) [selected](#event-selected) [selecting](#event-selecting) [start](#event-start) [stop](#event-stop) [unselected](#event-unselected) [unselecting](#event-unselecting) The jQuery UI Selectable plugin allows for elements to be selected by dragging a box (sometimes called a lasso) with the mouse over the elements. Elements can also be selected via click or drag while holding the ctrl/meta key, allowing for multiple (non-contiguous) selections.
### Theming
The selectable widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If selectable specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-selectable`: The selectable element.
+ `ui-selectee`: One of the selectable elements, as specified through the [`filter` option](#option-filter). This element can also receive one of the following classes: `ui-selecting` (when the lasso includes this element), `ui-selected` (after a successful selection), `ui-unselecting` (when the lasso lost this element).
* `ui-selectable-helper`: The "lasso" element used to visualize the ongoing selection.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### appendTo
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"body"` Which element the selection helper (the lasso) should be appended to. **Code examples:**Initialize the selectable with the `appendTo` option specified:
```
$( ".selector" ).selectable({
appendTo: "#someElem"
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).selectable( "option", "appendTo" );
// Setter
$( ".selector" ).selectable( "option", "appendTo", "#someElem" );
```
### autoRefresh
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` This determines whether to refresh (recalculate) the position and size of each selectee at the beginning of each select operation. If you have many items, you may want to set this to false and call the [`refresh()`](#method-refresh) method manually. **Code examples:**Initialize the selectable with the `autoRefresh` option specified:
```
$( ".selector" ).selectable({
autoRefresh: false
});
```
Get or set the `autoRefresh` option, after initialization:
```
// Getter
var autoRefresh = $( ".selector" ).selectable( "option", "autoRefresh" );
// Setter
$( ".selector" ).selectable( "option", "autoRefresh", false );
```
### cancel
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"input,textarea,button,select,option"` Prevents selecting if you start on elements matching the selector. **Code examples:**Initialize the selectable with the `cancel` option specified:
```
$( ".selector" ).selectable({
cancel: "a,.cancel"
});
```
Get or set the `cancel` option, after initialization:
```
// Getter
var cancel = $( ".selector" ).selectable( "option", "cancel" );
// Setter
$( ".selector" ).selectable( "option", "cancel", "a,.cancel" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the selectable with the `classes` option specified, changing the theming for the `ui-selectable` class:
```
$( ".selector" ).selectable({
classes: {
"ui-selectable": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-selectable` class:
```
// Getter
var themeClass = $( ".selector" ).selectable( "option", "classes.ui-selectable" );
// Setter
$( ".selector" ).selectable( "option", "classes.ui-selectable", "highlight" );
```
### delay
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `0` Time in milliseconds to define when the selecting should start. This helps prevent unwanted selections when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the selectable with the `delay` option specified:
```
$( ".selector" ).selectable({
delay: 150
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).selectable( "option", "delay" );
// Setter
$( ".selector" ).selectable( "option", "delay", 150 );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the selectable if set to `true`. **Code examples:**Initialize the selectable with the `disabled` option specified:
```
$( ".selector" ).selectable({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).selectable( "option", "disabled" );
// Setter
$( ".selector" ).selectable( "option", "disabled", true );
```
### distance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` Tolerance, in pixels, for when selecting should start. If specified, selecting will not start until the mouse has been dragged beyond the specified distance. (version deprecated: 1.12) **Code examples:**Initialize the selectable with the `distance` option specified:
```
$( ".selector" ).selectable({
distance: 30
});
```
Get or set the `distance` option, after initialization:
```
// Getter
var distance = $( ".selector" ).selectable( "option", "distance" );
// Setter
$( ".selector" ).selectable( "option", "distance", 30 );
```
### filter
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"*"` The matching child elements will be made selectees (able to be selected). **Code examples:**Initialize the selectable with the `filter` option specified:
```
$( ".selector" ).selectable({
filter: "li"
});
```
Get or set the `filter` option, after initialization:
```
// Getter
var filter = $( ".selector" ).selectable( "option", "filter" );
// Setter
$( ".selector" ).selectable( "option", "filter", "li" );
```
### tolerance
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"touch"` Specifies which mode to use for testing whether the lasso should select an item. Possible values: * `"fit"`: Lasso overlaps the item entirely.
* `"touch"`: Lasso overlaps the item by any amount.
**Code examples:**Initialize the selectable with the `tolerance` option specified:
```
$( ".selector" ).selectable({
tolerance: "fit"
});
```
Get or set the `tolerance` option, after initialization:
```
// Getter
var tolerance = $( ".selector" ).selectable( "option", "tolerance" );
// Setter
$( ".selector" ).selectable( "option", "tolerance", "fit" );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the selectable functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).selectable( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the selectable. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).selectable( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the selectable. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).selectable( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the selectable's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the selectable plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).selectable( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).selectable( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current selectable options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).selectable( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the selectable option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).selectable( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the selectable. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).selectable( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Refresh the position and size of each selectee element. This method can be used to manually recalculate the position and size of each selectee when the [`autoRefresh`](#option-autoRefresh) option is set to `false`. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).selectable( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the selectable element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).selectable( "widget" );
```
Events
------
### create( event, ui )Type: `selectablecreate`
Triggered when the selectable is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectable with the create callback specified:
```
$( ".selector" ).selectable({
create: function( event, ui ) {}
});
```
Bind an event listener to the selectablecreate event:
```
$( ".selector" ).on( "selectablecreate", function( event, ui ) {} );
```
### selected( event, ui )Type: `selectableselected`
Triggered at the end of the select operation, on each element added to the selection. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **selected** Type: [Element](http://api.jquery.com/Types/#Element) The selectable item that has been selected.
**Code examples:**Initialize the selectable with the selected callback specified:
```
$( ".selector" ).selectable({
selected: function( event, ui ) {}
});
```
Bind an event listener to the selectableselected event:
```
$( ".selector" ).on( "selectableselected", function( event, ui ) {} );
```
### selecting( event, ui )Type: `selectableselecting`
Triggered during the select operation, on each element added to the selection. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **selecting** Type: [Element](http://api.jquery.com/Types/#Element) The current selectable item being selected.
**Code examples:**Initialize the selectable with the selecting callback specified:
```
$( ".selector" ).selectable({
selecting: function( event, ui ) {}
});
```
Bind an event listener to the selectableselecting event:
```
$( ".selector" ).on( "selectableselecting", function( event, ui ) {} );
```
### start( event, ui )Type: `selectablestart`
Triggered at the beginning of the select operation. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectable with the start callback specified:
```
$( ".selector" ).selectable({
start: function( event, ui ) {}
});
```
Bind an event listener to the selectablestart event:
```
$( ".selector" ).on( "selectablestart", function( event, ui ) {} );
```
### stop( event, ui )Type: `selectablestop`
Triggered at the end of the select operation. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectable with the stop callback specified:
```
$( ".selector" ).selectable({
stop: function( event, ui ) {}
});
```
Bind an event listener to the selectablestop event:
```
$( ".selector" ).on( "selectablestop", function( event, ui ) {} );
```
### unselected( event, ui )Type: `selectableunselected`
Triggered at the end of the select operation, on each element removed from the selection. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **unselected** Type: [Element](http://api.jquery.com/Types/#Element) The selectable item that has been unselected.
**Code examples:**Initialize the selectable with the unselected callback specified:
```
$( ".selector" ).selectable({
unselected: function( event, ui ) {}
});
```
Bind an event listener to the selectableunselected event:
```
$( ".selector" ).on( "selectableunselected", function( event, ui ) {} );
```
### unselecting( event, ui )Type: `selectableunselecting`
Triggered during the select operation, on each element removed from the selection. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **unselecting** Type: [Element](http://api.jquery.com/Types/#Element) The current selectable item being unselected.
**Code examples:**Initialize the selectable with the unselecting callback specified:
```
$( ".selector" ).selectable({
unselecting: function( event, ui ) {}
});
```
Bind an event listener to the selectableunselecting event:
```
$( ".selector" ).on( "selectableunselecting", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Selectable.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>selectable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#selectable .ui-selecting {
background: #ccc;
}
#selectable .ui-selected {
background: #999;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<ul id="selectable">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>
$( "#selectable" ).selectable();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Explode Effect Explode Effect
==============
Explode Effect
--------------
**Description:** The explode effect hides or shows an element by splitting it into pieces.
* #### explode
+ **pieces** (default: `9`) Type: [Integer](http://api.jquery.com/Types/#Integer) The number of pieces to explode, should be a perfect square, any other values are rounded to the nearest square.
Example:
--------
Toggle a div using the explode effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>explode demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "explode" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Dialog Widget Dialog Widget
=============
Dialog Widgetversion added: 1.0
--------------------------------
**Description:** Open content in an interactive overlay.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[appendTo](#option-appendTo) [autoOpen](#option-autoOpen) [buttons](#option-buttons) [classes](#option-classes) [closeOnEscape](#option-closeOnEscape) [closeText](#option-closeText) [dialogClass](#option-dialogClass) [draggable](#option-draggable) [height](#option-height) [hide](#option-hide) [maxHeight](#option-maxHeight) [maxWidth](#option-maxWidth) [minHeight](#option-minHeight) [minWidth](#option-minWidth) [modal](#option-modal) [position](#option-position) [resizable](#option-resizable) [show](#option-show) [title](#option-title) [width](#option-width) ### Methods
[close](#method-close) [destroy](#method-destroy) [instance](#method-instance) [isOpen](#method-isOpen) [moveToTop](#method-moveToTop) [open](#method-open) [option](#method-option) [widget](#method-widget)
### Extension Points
[\_allowInteraction](#method-_allowInteraction) ### Events
[beforeClose](#event-beforeClose) [close](#event-close) [create](#event-create) [drag](#event-drag) [dragStart](#event-dragStart) [dragStop](#event-dragStop) [focus](#event-focus) [open](#event-open) [resize](#event-resize) [resizeStart](#event-resizeStart) [resizeStop](#event-resizeStop) A dialog is a floating window that contains a title bar and a content area. The dialog window can be moved, resized and closed with the 'x' icon by default.
If the content length exceeds the maximum height, a scrollbar will automatically appear.
A bottom button bar and semi-transparent modal overlay layer are common options that can be added.
### Focus
Upon opening a dialog, focus is automatically moved to the first item that matches the following:
1. The first element within the dialog with the `autofocus` attribute
2. The first [`:tabbable`](tabbable-selector) element within the dialog's content
3. The first [`:tabbable`](tabbable-selector) element within the dialog's buttonpane
4. The dialog's close button
5. The dialog itself
While open, the dialog widget ensures that keyboard navigation using the 'tab' key causes the focus to cycle amongst the focusable elements in the dialog, not elements outside of it. Modal dialogs additionally prevent mouse users from clicking on elements outside of the dialog.
Upon closing a dialog, focus is automatically returned to the element that had focus when the dialog was opened.
### Hiding the close button
In some cases, you may want to hide the close button, for instance, if you have a close button in the button pane. The best way to accomplish this is via CSS. As an example, you can define a simple rule, such as:
```
.no-close .ui-dialog-titlebar-close {
display: none;
}
```
Then, you can simply add the `no-close` class to any dialog in order to hide its close button:
```
$( "#dialog" ).dialog({
dialogClass: "no-close",
buttons: [
{
text: "OK",
click: function() {
$( this ).dialog( "close" );
}
}
]
});
```
### Theming
The dialog widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If dialog specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-dialog`: The outer container of the dialog. If the [`draggable` option](#option-draggable) is set, the `ui-dialog-dragging` class is added during a drag. If the [`resizable` option](#option-resizable) is set, the `ui-dialog-resizing` class is added during a resize. If the [`buttons` option](#option-buttons) is set, the `ui-dialog-buttons` class is added.
+ `ui-dialog-titlebar`: The title bar containing the dialog's title and close button.
- `ui-dialog-title`: The container around the textual title of the dialog.
- `ui-dialog-titlebar-close`: The dialog's close button.
+ `ui-dialog-content`: The container around the dialog's content. This is also the element the widget was instantiated with.
+ `ui-dialog-buttonpane`: The pane that contains the dialog's buttons. This will only be present if the [`buttons`](#option-buttons) option is set.
- `ui-dialog-buttonset`: The container around the buttons themselves.
Additionally, when the [`modal`](#option-modal) option is set, an element with a `ui-widget-overlay` class name is appended to the `<body>`.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Position](position)
* [Button](button)
* [Draggable](draggable) (optional; for use with the [`draggable`](#option-draggable) option)
* [Resizable](resizable) (optional; for use with the [`resizable`](#option-resizable) option)
* [Effects Core](category/effects-core) (optional; for use with the [`show`](#option-show) and [`hide`](#option-hide) options)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### appendTo
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"body"` Which element the dialog (and overlay, if [modal](#option-modal)) should be appended to.
**Note:** The `appendTo` option should not be changed while the dialog is open. (version added: 1.10.0) **Code examples:**Initialize the dialog with the `appendTo` option specified:
```
$( ".selector" ).dialog({
appendTo: "#someElem"
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).dialog( "option", "appendTo" );
// Setter
$( ".selector" ).dialog( "option", "appendTo", "#someElem" );
```
### autoOpen
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `true`, the dialog will automatically open upon initialization. If `false`, the dialog will stay hidden until the [`open()`](#method-open) method is called. **Code examples:**Initialize the dialog with the `autoOpen` option specified:
```
$( ".selector" ).dialog({
autoOpen: false
});
```
Get or set the `autoOpen` option, after initialization:
```
// Getter
var autoOpen = $( ".selector" ).dialog( "option", "autoOpen" );
// Setter
$( ".selector" ).dialog( "option", "autoOpen", false );
```
### buttons
**Type:** [Object](http://api.jquery.com/Types/#Object) or [Array](http://api.jquery.com/Types/#Array) **Default:** `[]` Specifies which buttons should be displayed on the dialog. The context of the callback is the dialog element; if you need access to the button, it is available as the target of the event object. **Multiple types supported:*** **Object**: The keys are the button labels and the values are the callbacks for when the associated button is clicked.
* **Array**: Each element of the array must be an object defining the attributes, properties, and event handlers to set on the button. In addition, a key of `icon` can be used to control [button's `icon` option](button#option-icon), and a key of `showText` can be used to control [button's `text` option](button#option-text).
**Code examples:**Initialize the dialog with the `buttons` option specified:
```
$( ".selector" ).dialog({
buttons: [
{
text: "Ok",
icon: "ui-icon-heart",
click: function() {
$( this ).dialog( "close" );
}
// Uncommenting the following line would hide the text,
// resulting in the label being used as a tooltip
//showText: false
}
]
});
```
Get or set the `buttons` option, after initialization:
```
// Getter
var buttons = $( ".selector" ).dialog( "option", "buttons" );
// Setter
$( ".selector" ).dialog( "option", "buttons",
[
{
text: "Ok",
icon: "ui-icon-heart",
click: function() {
$( this ).dialog( "close" );
}
// Uncommenting the following line would hide the text,
// resulting in the label being used as a tooltip
//showText: false
}
]
);
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-dialog": "ui-corner-all",
"ui-dialog-titlebar": "ui-corner-all",
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the dialog with the `classes` option specified, changing the theming for the `ui-dialog` class:
```
$( ".selector" ).dialog({
classes: {
"ui-dialog": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-dialog` class:
```
// Getter
var themeClass = $( ".selector" ).dialog( "option", "classes.ui-dialog" );
// Setter
$( ".selector" ).dialog( "option", "classes.ui-dialog", "highlight" );
```
### closeOnEscape
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` Specifies whether the dialog should close when it has focus and the user presses the escape (ESC) key. **Code examples:**Initialize the dialog with the `closeOnEscape` option specified:
```
$( ".selector" ).dialog({
closeOnEscape: false
});
```
Get or set the `closeOnEscape` option, after initialization:
```
// Getter
var closeOnEscape = $( ".selector" ).dialog( "option", "closeOnEscape" );
// Setter
$( ".selector" ).dialog( "option", "closeOnEscape", false );
```
### closeText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"close"` Specifies the text for the close button. Note that the close text is visibly hidden when using a standard theme. **Code examples:**Initialize the dialog with the `closeText` option specified:
```
$( ".selector" ).dialog({
closeText: "hide"
});
```
Get or set the `closeText` option, after initialization:
```
// Getter
var closeText = $( ".selector" ).dialog( "option", "closeText" );
// Setter
$( ".selector" ).dialog( "option", "closeText", "hide" );
```
### dialogClass
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `""` The specified class name(s) will be added to the dialog, for additional theming.
The `dialogClass` option has been deprecated in favor of the [`classes` option](#option-classes), using the `ui-dialog` property.
(version deprecated: 1.12) **Code examples:**Initialize the dialog with the `dialogClass` option specified:
```
$( ".selector" ).dialog({
dialogClass: "alert"
});
```
Get or set the `dialogClass` option, after initialization:
```
// Getter
var dialogClass = $( ".selector" ).dialog( "option", "dialogClass" );
// Setter
$( ".selector" ).dialog( "option", "dialogClass", "alert" );
```
### draggable
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `true`, the dialog will be draggable by the title bar. Requires the [jQuery UI Draggable widget](draggable) to be included. **Code examples:**Initialize the dialog with the `draggable` option specified:
```
$( ".selector" ).dialog({
draggable: false
});
```
Get or set the `draggable` option, after initialization:
```
// Getter
var draggable = $( ".selector" ).dialog( "option", "draggable" );
// Setter
$( ".selector" ).dialog( "option", "draggable", false );
```
### height
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `"auto"` The height of the dialog. **Multiple types supported:*** **Number**: The height in pixels.
* **String**: The only supported string value is `"auto"` which will allow the dialog height to adjust based on its content.
**Code examples:**Initialize the dialog with the `height` option specified:
```
$( ".selector" ).dialog({
height: 400
});
```
Get or set the `height` option, after initialization:
```
// Getter
var height = $( ".selector" ).dialog( "option", "height" );
// Setter
$( ".selector" ).dialog( "option", "height", 400 );
```
### hide
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the hiding of the dialog. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the dialog will be hidden immediately. When set to `true`, the dialog will fade out with the default duration and the default easing.
* **Number**: The dialog will fade out with the specified duration and the default easing.
* **String**: The dialog will be hidden using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideUp"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeOut"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the dialog with the `hide` option specified:
```
$( ".selector" ).dialog({
hide: { effect: "explode", duration: 1000 }
});
```
Get or set the `hide` option, after initialization:
```
// Getter
var hide = $( ".selector" ).dialog( "option", "hide" );
// Setter
$( ".selector" ).dialog( "option", "hide", { effect: "explode", duration: 1000 } );
```
### maxHeight
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `false` The maximum height to which the dialog can be resized, in pixels. **Code examples:**Initialize the dialog with the `maxHeight` option specified:
```
$( ".selector" ).dialog({
maxHeight: 600
});
```
Get or set the `maxHeight` option, after initialization:
```
// Getter
var maxHeight = $( ".selector" ).dialog( "option", "maxHeight" );
// Setter
$( ".selector" ).dialog( "option", "maxHeight", 600 );
```
### maxWidth
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `false` The maximum width to which the dialog can be resized, in pixels. **Code examples:**Initialize the dialog with the `maxWidth` option specified:
```
$( ".selector" ).dialog({
maxWidth: 600
});
```
Get or set the `maxWidth` option, after initialization:
```
// Getter
var maxWidth = $( ".selector" ).dialog( "option", "maxWidth" );
// Setter
$( ".selector" ).dialog( "option", "maxWidth", 600 );
```
### minHeight
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `150` The minimum height to which the dialog can be resized, in pixels. **Code examples:**Initialize the dialog with the `minHeight` option specified:
```
$( ".selector" ).dialog({
minHeight: 200
});
```
Get or set the `minHeight` option, after initialization:
```
// Getter
var minHeight = $( ".selector" ).dialog( "option", "minHeight" );
// Setter
$( ".selector" ).dialog( "option", "minHeight", 200 );
```
### minWidth
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `150` The minimum width to which the dialog can be resized, in pixels. **Code examples:**Initialize the dialog with the `minWidth` option specified:
```
$( ".selector" ).dialog({
minWidth: 200
});
```
Get or set the `minWidth` option, after initialization:
```
// Getter
var minWidth = $( ".selector" ).dialog( "option", "minWidth" );
// Setter
$( ".selector" ).dialog( "option", "minWidth", 200 );
```
### modal
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If set to `true`, the dialog will have modal behavior; other items on the page will be disabled, i.e., cannot be interacted with. Modal dialogs create an overlay below the dialog but above other page elements. **Code examples:**Initialize the dialog with the `modal` option specified:
```
$( ".selector" ).dialog({
modal: true
});
```
Get or set the `modal` option, after initialization:
```
// Getter
var modal = $( ".selector" ).dialog( "option", "modal" );
// Setter
$( ".selector" ).dialog( "option", "modal", true );
```
### position
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ my: "center", at: "center", of: window }` Specifies where the dialog should be displayed when opened. The dialog will handle collisions such that as much of the dialog is visible as possible.
The `of` property defaults to the window, but you can specify another element to position against. You can refer to the [jQuery UI Position](position) utility for more details about the available properties.
**Code examples:**Initialize the dialog with the `position` option specified:
```
$( ".selector" ).dialog({
position: { my: "left top", at: "left bottom", of: button }
});
```
Get or set the `position` option, after initialization:
```
// Getter
var position = $( ".selector" ).dialog( "option", "position" );
// Setter
$( ".selector" ).dialog( "option", "position", { my: "left top", at: "left bottom", of: button } );
```
### resizable
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `true`, the dialog will be resizable. Requires the [jQuery UI Resizable widget](resizable) to be included. **Code examples:**Initialize the dialog with the `resizable` option specified:
```
$( ".selector" ).dialog({
resizable: false
});
```
Get or set the `resizable` option, after initialization:
```
// Getter
var resizable = $( ".selector" ).dialog( "option", "resizable" );
// Setter
$( ".selector" ).dialog( "option", "resizable", false );
```
### show
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `null` If and how to animate the showing of the dialog. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the dialog will be shown immediately. When set to `true`, the dialog will fade in with the default duration and the default easing.
* **Number**: The dialog will fade in with the specified duration and the default easing.
* **String**: The dialog will be shown using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideDown"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeIn"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the dialog with the `show` option specified:
```
$( ".selector" ).dialog({
show: { effect: "blind", duration: 800 }
});
```
Get or set the `show` option, after initialization:
```
// Getter
var show = $( ".selector" ).dialog( "option", "show" );
// Setter
$( ".selector" ).dialog( "option", "show", { effect: "blind", duration: 800 } );
```
### title
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` Specifies the title of the dialog. If the value is `null`, the `title` attribute on the dialog source element will be used. **Code examples:**Initialize the dialog with the `title` option specified:
```
$( ".selector" ).dialog({
title: "Dialog Title"
});
```
Get or set the `title` option, after initialization:
```
// Getter
var title = $( ".selector" ).dialog( "option", "title" );
// Setter
$( ".selector" ).dialog( "option", "title", "Dialog Title" );
```
### width
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `300` The width of the dialog, in pixels. **Code examples:**Initialize the dialog with the `width` option specified:
```
$( ".selector" ).dialog({
width: 500
});
```
Get or set the `width` option, after initialization:
```
// Getter
var width = $( ".selector" ).dialog( "option", "width" );
// Setter
$( ".selector" ).dialog( "option", "width", 500 );
```
Methods
-------
### close()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes the dialog. * This method does not accept any arguments.
**Code examples:**Invoke the close method:
```
$( ".selector" ).dialog( "close" );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the dialog functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).dialog( "destroy" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the dialog's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the dialog plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).dialog( "instance" );
```
### isOpen()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Whether the dialog is currently open. * This method does not accept any arguments.
**Code examples:**Invoke the isOpen method:
```
var isOpen = $( ".selector" ).dialog( "isOpen" );
```
### moveToTop()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Moves the dialog to the top of the dialog stack. * This method does not accept any arguments.
**Code examples:**Invoke the moveToTop method:
```
$( ".selector" ).dialog( "moveToTop" );
```
### open()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Opens the dialog. * This method does not accept any arguments.
**Code examples:**Invoke the open method:
```
$( ".selector" ).dialog( "open" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).dialog( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current dialog options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).dialog( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the dialog option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).dialog( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the dialog. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).dialog( "option", { disabled: true } );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the generated wrapper. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).dialog( "widget" );
```
Extension Points
----------------
The dialog widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_allowInteraction( event )Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Modal dialogs do not allow users to interact with elements behind the dialog. This can be problematic for elements that are not children of the dialog, but are absolutely positioned to appear as though they are. The `_allowInteraction()` method determines whether the user should be allowed to interact with a given target element; therefore, it can be used to whitelist elements that are not children of the dialog but you want users to be able to use. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
**Code examples:**Allow the Select2 plugin to be used within modal dialogs. The [`_super()`](jquery.widget#method-_super) call ensures elements within the dialog can still be interacted with.
```
_allowInteraction: function( event ) {
return !!$( event.target ).is( ".select2-input" ) || this._super( event );
}
```
Events
------
### beforeClose( event, ui )Type: `dialogbeforeclose`
Triggered when a dialog is about to close. If canceled, the dialog will not close. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the dialog with the beforeClose callback specified:
```
$( ".selector" ).dialog({
beforeClose: function( event, ui ) {}
});
```
Bind an event listener to the dialogbeforeclose event:
```
$( ".selector" ).on( "dialogbeforeclose", function( event, ui ) {} );
```
### close( event, ui )Type: `dialogclose`
Triggered when the dialog is closed. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the dialog with the close callback specified:
```
$( ".selector" ).dialog({
close: function( event, ui ) {}
});
```
Bind an event listener to the dialogclose event:
```
$( ".selector" ).on( "dialogclose", function( event, ui ) {} );
```
### create( event, ui )Type: `dialogcreate`
Triggered when the dialog is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the dialog with the create callback specified:
```
$( ".selector" ).dialog({
create: function( event, ui ) {}
});
```
Bind an event listener to the dialogcreate event:
```
$( ".selector" ).on( "dialogcreate", function( event, ui ) {} );
```
### drag( event, ui )Type: `dialogdrag`
Triggered while the dialog is being dragged. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current offset position of the dialog.
**Code examples:**Initialize the dialog with the drag callback specified:
```
$( ".selector" ).dialog({
drag: function( event, ui ) {}
});
```
Bind an event listener to the dialogdrag event:
```
$( ".selector" ).on( "dialogdrag", function( event, ui ) {} );
```
### dragStart( event, ui )Type: `dialogdragstart`
Triggered when the user starts dragging the dialog. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current offset position of the dialog.
**Code examples:**Initialize the dialog with the dragStart callback specified:
```
$( ".selector" ).dialog({
dragStart: function( event, ui ) {}
});
```
Bind an event listener to the dialogdragstart event:
```
$( ".selector" ).on( "dialogdragstart", function( event, ui ) {} );
```
### dragStop( event, ui )Type: `dialogdragstop`
Triggered after the dialog has been dragged. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current offset position of the dialog.
**Code examples:**Initialize the dialog with the dragStop callback specified:
```
$( ".selector" ).dialog({
dragStop: function( event, ui ) {}
});
```
Bind an event listener to the dialogdragstop event:
```
$( ".selector" ).on( "dialogdragstop", function( event, ui ) {} );
```
### focus( event, ui )Type: `dialogfocus`
Triggered when the dialog gains focus. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the dialog with the focus callback specified:
```
$( ".selector" ).dialog({
focus: function( event, ui ) {}
});
```
Bind an event listener to the dialogfocus event:
```
$( ".selector" ).on( "dialogfocus", function( event, ui ) {} );
```
### open( event, ui )Type: `dialogopen`
Triggered when the dialog is opened. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the dialog with the open callback specified:
```
$( ".selector" ).dialog({
open: function( event, ui ) {}
});
```
Bind an event listener to the dialogopen event:
```
$( ".selector" ).on( "dialogopen", function( event, ui ) {} );
```
### resize( event, ui )Type: `dialogresize`
Triggered while the dialog is being resized. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The CSS position of the dialog prior to being resized.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size of the dialog prior to being resized.
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size of the dialog.
**Code examples:**Initialize the dialog with the resize callback specified:
```
$( ".selector" ).dialog({
resize: function( event, ui ) {}
});
```
Bind an event listener to the dialogresize event:
```
$( ".selector" ).on( "dialogresize", function( event, ui ) {} );
```
### resizeStart( event, ui )Type: `dialogresizestart`
Triggered when the user starts resizing the dialog. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The CSS position of the dialog prior to being resized.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size of the dialog prior to being resized.
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size of the dialog.
**Code examples:**Initialize the dialog with the resizeStart callback specified:
```
$( ".selector" ).dialog({
resizeStart: function( event, ui ) {}
});
```
Bind an event listener to the dialogresizestart event:
```
$( ".selector" ).on( "dialogresizestart", function( event, ui ) {} );
```
### resizeStop( event, ui )Type: `dialogresizestop`
Triggered after the dialog has been resized. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The CSS position of the dialog prior to being resized.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current CSS position of the dialog.
+ **originalSize** Type: [Object](http://api.jquery.com/Types/#Object) The size of the dialog prior to being resized.
+ **size** Type: [Object](http://api.jquery.com/Types/#Object) The current size of the dialog.
**Code examples:**Initialize the dialog with the resizeStop callback specified:
```
$( ".selector" ).dialog({
resizeStop: function( event, ui ) {}
});
```
Bind an event listener to the dialogresizestop event:
```
$( ".selector" ).on( "dialogresizestop", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Dialog
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>dialog demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<button id="opener">open the dialog</button>
<div id="dialog" title="Dialog Title">I'm a dialog</div>
<script>
$( "#dialog" ).dialog({ autoOpen: false });
$( "#opener" ).click(function() {
$( "#dialog" ).dialog( "open" );
});
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui .enableSelection() .enableSelection()
==================
.enableSelection()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
-------------------------------------------------------------------------
**Description:** Enable selection of text content within the set of matched elements.
* #### version added: 1.6, deprecated: 1.9[.enableSelection()](#enableSelection)
+ This method does not accept any arguments.
The `.enableSelection()` method can be used to re-enable selection of text that was disabled via [`.disableSelection()`](disableselection).
jqueryui jQuery.effects.scaledDimensions() jQuery.effects.scaledDimensions()
=================================
jQuery.effects.scaledDimensions( element, percent [, direction ] )Returns: [Object](http://api.jquery.com/Types/#Object)version added: 1.12
--------------------------------------------------------------------------------------------------------------------------------------------
**Description:** Scales the dimensions of an element.
* #### [jQuery.effects.scaledDimensions( element, percent [, direction ] )](#jQuery-effects-scaledDimensions-element-percent-direction)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to scale.
+ **percent** Type: [Number](http://api.jquery.com/Types/#Number) The percentage of the original size that the element's dimensions should be scaled to.
+ **direction** Type: [String](http://api.jquery.com/Types/#String) Which direction to scale the element's dimensions; either `"horizontal"` or `"vertical"`. If `direction` is omitted, both dimensions will be scaled.
This method scales the dimensions of an element, returning an object containing `height`, `width`, `outerHeight`, and `outerWidth` properties. The element is not actually modified.
jqueryui .position() .position()
===========
.position( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
---------------------------------------------------------------------------
**Description:** Position an element relative to another.
* #### version added: 1.8[.position( options )](#position-options)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object)
- **my** (default: `"center"`) Type: [String](http://api.jquery.com/Types/#String) Defines which position **on the element being positioned** to align with the target element: "horizontal vertical" alignment. A single value such as `"right"` will be normalized to `"right center"`, `"top"` will be normalized to `"center top"` (following CSS convention). Acceptable horizontal values: `"left"`, `"center"`, `"right"`. Acceptable vertical values: `"top"`, `"center"`, `"bottom"`. Example: `"left top"` or `"center center"`. Each dimension can also contain offsets, in pixels or percent, e.g., `"right+10 top-25%"`. Percentage offsets are relative to the element being positioned.
- **at** (default: `"center"`) Type: [String](http://api.jquery.com/Types/#String) Defines which position **on the target element** to align the positioned element against: "horizontal vertical" alignment. See the [`my`](#option-my) option for full details on possible values. Percentage offsets are relative to the target element.
- **of** (default: `null`) Type: [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) or [jQuery](http://api.jquery.com/Types/#jQuery) or [Event](http://api.jquery.com/Types/#Event) Which element to position against. If you provide a selector or jQuery object, the first matching element will be used. If you provide an event object, the `pageX` and `pageY` properties will be used. Example: `"#top-menu"`
- **collision** (default: `"flip"`) Type: [String](http://api.jquery.com/Types/#String) When the positioned element overflows the window in some direction, move it to an alternative position. Similar to [`my`](#option-my) and [`at`](#option-at), this accepts a single value or a pair for horizontal/vertical, e.g., `"flip"`, `"fit"`, `"fit flip"`, `"fit none"`.
* `"flip"`: Flips the element to the opposite side of the target and the collision detection is run again to see if it will fit. Whichever side allows more of the element to be visible will be used.
* `"fit"`: Shift the element away from the edge of the window.
* `"flipfit"`: First applies the flip logic, placing the element on whichever side allows more of the element to be visible. Then the fit logic is applied to ensure as much of the element is visible as possible.
* `"none"`: Does not apply any collision detection.
- **using** (default: `null`) Type: [Function](http://api.jquery.com/Types/#Function)() When specified, the actual property setting is delegated to this callback. Receives two parameters: The first is a hash of `top` and `left` values for the position that should be set and can be forwarded to `.css()` or `.animate()`. The second provides feedback about the position and dimensions of both elements, as well as calculations to their relative position. Both `target` and `element` have these properties: `element`, `left`, `top`, `width`, `height`. In addition, there's `horizontal`, `vertical` and `important`, giving you twelve potential directions like `{ horizontal: "center", vertical: "left", important: "horizontal" }`.
- **within** (default: `window`) Type: [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) or [jQuery](http://api.jquery.com/Types/#jQuery) Element to position within, affecting collision detection. If you provide a selector or jQuery object, the first matching element will be used.
The jQuery UI `.position()` method allows you to position an element relative to the window, document, another element, or the cursor/mouse, without worrying about offset parents.
*Note: jQuery UI does not support positioning hidden elements.*
This is a standalone jQuery plugin and has no dependencies on other jQuery UI components.
This plugin extends jQuery's built-in [`.position()`](https://api.jquery.com/position/) method. If jQuery UI is not loaded, calling the `.position()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
A simple jQuery UI Position example.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>position demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#targetElement {
height: 200px;
margin: 50px;
background: #9cf;
}
.positionDiv {
position: absolute;
width: 75px;
height: 75px;
background: #080;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="targetElement">
<div class="positionDiv" id="position1"></div>
<div class="positionDiv" id="position2"></div>
<div class="positionDiv" id="position3"></div>
<div class="positionDiv" id="position4"></div>
</div>
<script>
$( "#position1" ).position({
my: "center",
at: "center",
of: "#targetElement"
});
$( "#position2" ).position({
my: "left top",
at: "left top",
of: "#targetElement"
});
$( "#position3" ).position({
my: "right center",
at: "right bottom",
of: "#targetElement"
});
$( document ).mousemove(function( event ) {
$( "#position4" ).position({
my: "left+3 bottom-3",
of: event,
collision: "fit"
});
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .uniqueId() .uniqueId()
===========
.uniqueId()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
------------------------------------------------------------------
**Description:** Generate and apply a unique id for the set of matched elements.
* #### version added: 1.9[.uniqueId()](#uniqueId)
+ This method does not accept any arguments.
Many widgets need to generate unique ids for elements. `.uniqueId()` will check if the element has an id, and if not, it will generate one and set it on the element. It is safe to call `.uniqueId()` on an element without checking if it already has an id. If/when the widget needs to clean up after itself, the [`.removeUniqueId()`](removeuniqueid) method will remove the id from the element if it was added by `.uniqueId()` and leave the id alone if it was not. `.removeUniqueId()` is able to be smart about this because the generated ids have a prefix of `"ui-id-"`.
jqueryui Blind Effect Blind Effect
============
Blind Effect
------------
**Description:** The blind effect hides or shows an element by wrapping the element in a container, and "pulling the blinds"
* #### blind
+ **direction** (default: `"up"`) Type: [String](http://api.jquery.com/Types/#String) The direction the blind will be pulled to hide the element, or the direction from which the element will be revealed.
Possible Values: `up`, `down`, `left`, `right`, `vertical`, `horizontal`.
The container has `overflow: hidden` applied, so height changes affect what's visible.
Example:
--------
Toggle a div using the blind effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>blind demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "blind" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .scrollParent() .scrollParent()
===============
.scrollParent()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
----------------------------------------------------------------------
**Description:** Get the closest ancestor element that is scrollable.
* #### [.scrollParent()](#scrollParent)
+ This method does not accept any arguments.
This method finds the nearest ancestor that allows scrolling. In other words, the `.scrollParent()` method finds the element that the currently selected element will scroll within.
*Note: This method only works on jQuery objects containing one element.*
jqueryui .switchClass() .switchClass()
==============
.switchClass( removeClassName, addClassName [, duration ] [, easing ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
--------------------------------------------------------------------------------------------------------------------------------------------
**Description:** Adds and removes the specified class(es) to each of the set of matched elements while animating all style changes.
* #### [.switchClass( removeClassName, addClassName [, duration ] [, easing ] [, complete ] )](#switchClass-removeClassName-addClassName-duration-easing-complete)
+ **removeClassName** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be removed from the class attribute of each matched element.
+ **addClassName** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be added to the class attribute of each matched element.
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.switchClass( removeClassName, addClassName [, options ] )](#switchClass-removeClassName-addClassName-options)
+ **removeClassName** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be removed from the class attribute of each matched element.
+ **addClassName** Type: [String](http://api.jquery.com/Types/#String) One or more class names (space separated) to be added to the class attribute of each matched element.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. All properties are optional.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **children** (default: `false`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the animation should additionally be applied to all descendants of the matched elements. This feature should be used with caution as the cost of determining which descendants to animate can be very expensive, and grows linearly with the number of descendants.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
The `.switchClass()` method allows you to animate the transition of adding and removing classes at the same time.
Similar to native CSS transitions, jQuery UI's class animations provide a smooth transition from one state to another while allowing you to keep all the details about which styles to change in CSS and out of your JavaScript. All class animation methods, including `.switchClass()`, support custom durations and easings, as well as provide a callback for when the animation completes.
Not all styles can be animated. For example, there is no way to animate a background image. Any styles that cannot be animated will be changed at the end of the animation.
Example:
--------
Adds the class "blue" and removes the class "big" from the matched elements.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>switchClass demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background-color: #ccc;
}
.big {
width: 200px;
height: 200px;
}
.blue {
background-color: #00f;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div class="big"></div>
<script>
$( "div" ).click(function() {
$( this ).switchClass( "big", "blue", 1000, "easeInOutQuad" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Drop Effect Drop Effect
===========
Drop Effect
-----------
**Description:** The drop effect hides or shows an element fading in/out and sliding in a direction.
* #### drop
+ **direction** (default: `"left"`) Type: [String](http://api.jquery.com/Types/#String) The direction the element will fall to hide the element, or the direction from which the element will be revealed.
Possible Values: `up`, `down`, `left`, `right`.
Example:
--------
Toggle a div using the drop effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>drop demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "drop" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Shake Effect Shake Effect
============
Shake Effect
------------
**Description:** Shakes the element multiple times, vertically or horizontally.
* #### shake
+ **direction** (default: `"left"`) Type: [String](http://api.jquery.com/Types/#String) A value of `"left"` or `"right"` will shake the element horizontally, and a value of `"up"` or `"down"` will shake the element vertically. The value specifies which direction the element should move along the axis for the first step of the effect.
+ **distance** (default: `20`) Type: [Number](http://api.jquery.com/Types/#Number) Distance to shake.
+ **times** (default: `3`) Type: [Integer](http://api.jquery.com/Types/#Integer) Times to shake.
Example:
--------
Shake a div.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>shake demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to shake the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).effect( "shake" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Highlight Effect Highlight Effect
================
Highlight Effect
----------------
**Description:** The highlight effect hides or shows an element by animating its background color first.
* #### highlight
+ **color** (default: `"#ffff99"`) Type: [String](http://api.jquery.com/Types/#String) The background color used during the animation.
Example:
--------
Toggle a div using the highlight effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>highlight demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "highlight" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .cssClip() .cssClip()
==========
.cssClip( [dimensions ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)version added: 1.12
---------------------------------------------------------------------------------------------------
**Description:** Getter/setter for an object version of the CSS `clip` property.
* #### [.cssClip( [dimensions ] )](#cssClip-dimensions)
+ **dimensions** Type: [Object](http://api.jquery.com/Types/#Object) When setting the CSS `clip` property, specify the `top`, `right`, `bottom` and `left`, properties to use the `rect()` style.
Example:
--------
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>cssClip demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
.clipped {
position: absolute;
width: 150px;
height: 150px;
background: #3b679e;
background: linear-gradient(to bottom, #3b679e 0%, #2b88d9 50%, #207cca 51%, #7db9e8 100%);
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
Click anywhere!
<div class="clipped"></div>
<script>
var clippings = [
{
top: 10,
right: 50,
bottom: 50,
left: 5
},
{
top: 0,
right: 150,
bottom: 150,
left: 0
}
];
var index = 1;
var box = $( ".clipped" ).cssClip( clippings[ 0 ] );
$( document ).click(function() {
box.animate( {
clip: clippings[ index++ % 2 ]
}, 2000 );
});
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Slider Widget Slider Widget
=============
Slider Widgetversion added: 1.5
--------------------------------
**Description:** Drag a handle to select a numeric value.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[animate](#option-animate) [classes](#option-classes) [disabled](#option-disabled) [max](#option-max) [min](#option-min) [orientation](#option-orientation) [range](#option-range) [step](#option-step) [value](#option-value) [values](#option-values) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [value](#method-value) [values](#method-values) [widget](#method-widget) ### Events
[change](#event-change) [create](#event-create) [slide](#event-slide) [start](#event-start) [stop](#event-stop) The jQuery UI Slider plugin makes selected elements into sliders. There are various options such as multiple handles and ranges. The handle can be moved with the mouse or the arrow keys.
The slider widget will create handle elements with the class `ui-slider-handle` on initialization. You can specify custom handle elements by creating and appending the elements and adding the `ui-slider-handle` class before initialization. It will only create the number of handles needed to match the length of [`value`](#option-value)/[`values`](#option-values). For example, if you specify `values: [ 1, 5, 18 ]` and create one custom handle, the plugin will create the other two.
### Theming
The slider widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If slider specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-slider`: The track of the slider control. This element will additionally have a class name of `ui-slider-horizontal` or `ui-slider-vertical` depending on the [`orientation` option](#option-orientation) of the slider.
+ `ui-slider-handle`: One of the slider handles.
+ `ui-slider-range`: The selected range used when the [`range` option](#option-range) is set. This element can additionally have a class of `ui-slider-range-min` or `ui-slider-range-max` if the `range` option is set to `"min"` or `"max"` respectively.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### animate
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) or [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Whether to slide the handle smoothly when the user clicks on the slider track. Also accepts any valid [animation duration](https://api.jquery.com/animate/#duration). **Multiple types supported:*** **Boolean**: When set to `true`, the handle will animate with the default duration.
* **String**: The name of a speed, such as `"fast"` or `"slow"`.
* **Number**: The duration of the animation, in milliseconds.
**Code examples:**Initialize the slider with the `animate` option specified:
```
$( ".selector" ).slider({
animate: "fast"
});
```
Get or set the `animate` option, after initialization:
```
// Getter
var animate = $( ".selector" ).slider( "option", "animate" );
// Setter
$( ".selector" ).slider( "option", "animate", "fast" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-slider": "ui-corner-all",
"ui-slider-handle": "ui-corner-all",
"ui-slider-range": "ui-corner-all ui-widget-header"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the slider with the `classes` option specified, changing the theming for the `ui-slider` class:
```
$( ".selector" ).slider({
classes: {
"ui-slider": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-slider` class:
```
// Getter
var themeClass = $( ".selector" ).slider( "option", "classes.ui-slider" );
// Setter
$( ".selector" ).slider( "option", "classes.ui-slider", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the slider if set to `true`. **Code examples:**Initialize the slider with the `disabled` option specified:
```
$( ".selector" ).slider({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).slider( "option", "disabled" );
// Setter
$( ".selector" ).slider( "option", "disabled", true );
```
### max
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `100` The maximum value of the slider. **Code examples:**Initialize the slider with the `max` option specified:
```
$( ".selector" ).slider({
max: 50
});
```
Get or set the `max` option, after initialization:
```
// Getter
var max = $( ".selector" ).slider( "option", "max" );
// Setter
$( ".selector" ).slider( "option", "max", 50 );
```
### min
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` The minimum value of the slider. **Code examples:**Initialize the slider with the `min` option specified:
```
$( ".selector" ).slider({
min: 10
});
```
Get or set the `min` option, after initialization:
```
// Getter
var min = $( ".selector" ).slider( "option", "min" );
// Setter
$( ".selector" ).slider( "option", "min", 10 );
```
### orientation
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"horizontal"` Determines whether the slider handles move horizontally (min on left, max on right) or vertically (min on bottom, max on top). Possible values: `"horizontal"`, `"vertical"`. **Code examples:**Initialize the slider with the `orientation` option specified:
```
$( ".selector" ).slider({
orientation: "vertical"
});
```
Get or set the `orientation` option, after initialization:
```
// Getter
var orientation = $( ".selector" ).slider( "option", "orientation" );
// Setter
$( ".selector" ).slider( "option", "orientation", "vertical" );
```
### range
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) **Default:** `false` Whether the slider represents a range. **Multiple types supported:*** **Boolean**: If set to `true`, the slider will detect if you have two handles and create a styleable range element between these two.
* **String**: Either `"min"` or `"max"`. A min range goes from the slider min to one handle. A max range goes from one handle to the slider max.
**Code examples:**Initialize the slider with the `range` option specified:
```
$( ".selector" ).slider({
range: true
});
```
Get or set the `range` option, after initialization:
```
// Getter
var range = $( ".selector" ).slider( "option", "range" );
// Setter
$( ".selector" ).slider( "option", "range", true );
```
### step
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Determines the size or amount of each interval or step the slider takes between the min and max. The full specified value range of the slider (max - min) should be evenly divisible by the step. **Code examples:**Initialize the slider with the `step` option specified:
```
$( ".selector" ).slider({
step: 5
});
```
Get or set the `step` option, after initialization:
```
// Getter
var step = $( ".selector" ).slider( "option", "step" );
// Setter
$( ".selector" ).slider( "option", "step", 5 );
```
### value
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` Determines the value of the slider, if there's only one handle. If there is more than one handle, determines the value of the first handle. **Code examples:**Initialize the slider with the `value` option specified:
```
$( ".selector" ).slider({
value: 10
});
```
Get or set the `value` option, after initialization:
```
// Getter
var value = $( ".selector" ).slider( "option", "value" );
// Setter
$( ".selector" ).slider( "option", "value", 10 );
```
### values
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `null` This option can be used to specify multiple handles. If the [`range`](#option-range) option is set to `true`, the length of `values` should be 2. **Code examples:**Initialize the slider with the `values` option specified:
```
$( ".selector" ).slider({
values: [ 10, 25 ]
});
```
Get or set the `values` option, after initialization:
```
// Getter
var values = $( ".selector" ).slider( "option", "values" );
// Setter
$( ".selector" ).slider( "option", "values", [ 10, 25 ] );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the slider functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).slider( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the slider. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).slider( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the slider. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).slider( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the slider's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the slider plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).slider( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).slider( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current slider options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).slider( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the slider option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).slider( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the slider. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).slider( "option", { disabled: true } );
```
### value()Returns: [Number](http://api.jquery.com/Types/#Number)
Get the value of the slider. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var selection = $( ".selector" ).slider( "value" );
```
### value( value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Set the value of the slider. * **value** Type: [Number](http://api.jquery.com/Types/#Number) The value to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).slider( "value", 55 );
```
### values()Returns: [Array](http://api.jquery.com/Types/#Array)
Get the value for all handles. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var values = $( ".selector" ).slider( "values" );
```
### values( index )Returns: [Number](http://api.jquery.com/Types/#Number)
Get the value for the specified handle. * **index** Type: [Integer](http://api.jquery.com/Types/#Integer) The zero-based index of the handle.
**Code examples:**Invoke the method:
```
var value = $( ".selector" ).slider( "values", 0 );
```
### values( index, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Set the value for the specified handle. * **index** Type: [Integer](http://api.jquery.com/Types/#Integer) The zero-based index of the handle.
* **value** Type: [Number](http://api.jquery.com/Types/#Number) The value to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).slider( "values", 0, 55 );
```
### values( values )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Set the value for all handles. * **values** Type: [Array](http://api.jquery.com/Types/#Array) The values to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).slider( "values", [ 55, 105 ] );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the slider. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).slider( "widget" );
```
Events
------
### change( event, ui )Type: `slidechange`
Triggered after the user slides a handle, if the value has changed; or if the value is changed programmatically via the [`value`](#method-value) method. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **handle** Type: [Element](http://api.jquery.com/Types/#Element) The HTML element representing the handle that was changed.
+ **handleIndex** Type: [Number](http://api.jquery.com/Types/#Number) The numeric index of the handle that was moved.
+ **value** Type: [Number](http://api.jquery.com/Types/#Number) The current value of the slider.
**Code examples:**Initialize the slider with the change callback specified:
```
$( ".selector" ).slider({
change: function( event, ui ) {}
});
```
Bind an event listener to the slidechange event:
```
$( ".selector" ).on( "slidechange", function( event, ui ) {} );
```
### create( event, ui )Type: `slidecreate`
Triggered when the slider is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the slider with the create callback specified:
```
$( ".selector" ).slider({
create: function( event, ui ) {}
});
```
Bind an event listener to the slidecreate event:
```
$( ".selector" ).on( "slidecreate", function( event, ui ) {} );
```
### slide( event, ui )Type: `slide`
Triggered on every mouse move during slide. The value provided in the event as `ui.value` represents the value that the handle will have as a result of the current movement. Canceling the event will prevent the handle from moving and the handle will continue to have its previous value. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **handle** Type: [Element](http://api.jquery.com/Types/#Element) The HTML element representing the handle being moved.
+ **handleIndex** Type: [Number](http://api.jquery.com/Types/#Number) The numeric index of the handle being moved.
+ **value** Type: [Number](http://api.jquery.com/Types/#Number) The value that the handle will move to if the event is not canceled.
+ **values** Type: [Array](http://api.jquery.com/Types/#Array) An array of the current values of a multi-handled slider.
**Code examples:**Initialize the slider with the slide callback specified:
```
$( ".selector" ).slider({
slide: function( event, ui ) {}
});
```
Bind an event listener to the slide event:
```
$( ".selector" ).on( "slide", function( event, ui ) {} );
```
### start( event, ui )Type: `slidestart`
Triggered when the user starts sliding. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **handle** Type: [Element](http://api.jquery.com/Types/#Element) The HTML element representing the handle being moved.
+ **handleIndex** Type: [Number](http://api.jquery.com/Types/#Number) The numeric index of the handle being moved.
+ **value** Type: [Number](http://api.jquery.com/Types/#Number) The current value of the slider.
**Code examples:**Initialize the slider with the start callback specified:
```
$( ".selector" ).slider({
start: function( event, ui ) {}
});
```
Bind an event listener to the slidestart event:
```
$( ".selector" ).on( "slidestart", function( event, ui ) {} );
```
### stop( event, ui )Type: `slidestop`
Triggered after the user slides a handle. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **handle** Type: [Element](http://api.jquery.com/Types/#Element) The HTML element representing the handle that was moved.
+ **handleIndex** Type: [Number](http://api.jquery.com/Types/#Number) The numeric index of the handle that was moved.
+ **value** Type: [Number](http://api.jquery.com/Types/#Number) The current value of the slider.
**Code examples:**Initialize the slider with the stop callback specified:
```
$( ".selector" ).slider({
stop: function( event, ui ) {}
});
```
Bind an event listener to the slidestop event:
```
$( ".selector" ).on( "slidestop", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Slider.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>slider demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>#slider { margin: 10px; } </style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="slider"></div>
<script>
$( "#slider" ).slider();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Spinner Widget Spinner Widget
==============
Spinner Widgetversion added: 1.9
---------------------------------
**Description:** Enhance a text input for entering numeric values, with up/down buttons and arrow key handling.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [culture](#option-culture) [disabled](#option-disabled) [icons](#option-icons) [incremental](#option-incremental) [max](#option-max) [min](#option-min) [numberFormat](#option-numberFormat) [page](#option-page) [step](#option-step) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [isValid](#method-isValid) [option](#method-option) [pageDown](#method-pageDown) [pageUp](#method-pageUp) [stepDown](#method-stepDown) [stepUp](#method-stepUp) [value](#method-value) [widget](#method-widget)
### Extension Points
[\_buttonHtml](#method-_buttonHtml) [\_uiSpinnerHtml](#method-_uiSpinnerHtml) ### Events
[change](#event-change) [create](#event-create) [spin](#event-spin) [start](#event-start) [stop](#event-stop) The Spinner, or number stepper widget, is perfect for handling all kinds of numeric input. It allows users to type a value directly, or modify an existing value by spinning with the keyboard, mouse or scrollwheel. When combined with [Globalize](https://github.com/jquery/globalize), you can even spin currencies and dates in a variety of locales.
Spinner wraps a text input with two buttons to increment and decrement the current value. Key events are added so that the same incrementing and decrementing can be done with the keyboard. Spinner delegates to [Globalize](https://github.com/jquery/globalize) for number formatting and parsing.
Spinner currently supports Globalize 0.x only. Support for Globalize 1.x is currently not planned. ### Keyboard interaction
* `UP`: Increment the value by one step.
* `DOWN`: Decrement the value by one step.
* `PAGE UP`: Increment the value by one page.
* `PAGE DOWN`: Decrement the value by one page.
Focus stays in the text field, even after using the mouse to click one of the spin buttons.
When the spinner is not read only (`<input readonly>`), the user may enter text that causes an invalid value (below min, above max, step mismatch, non-numeric input). Whenever a step is taken, either programmatically or via the step buttons, the value will be forced to a valid value (see the description for [`stepUp()`](#method-stepUp) and [`stepDown()`](#method-stepDown) for more details).
### Theming
The spinner widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If spinner specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-spinner`: The outer container of the spinner.
+ `ui-spinner-input`: The `<input>` element that the Spinner widget was instantiated with.
+ `ui-spinner-button`: The button controls used to increment and decrement the spinner's value. The up button will additionally have a `ui-spinner-up` class and the down button will additionally have a `ui-spinner-down` class.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Button](button)
* [Globalize](https://github.com/jquery/globalize) (external, optional; for use with the [`culture`](#option-culture) and [`numberFormat`](#option-numberFormat) options)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
* This widget manipulates its element's value programmatically, therefore a native `change` event may not be fired when the element's value changes.
* Creating a spinner on an `<input type="number">` is not supported due to a UI conflict with the native spinner.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-spinner": "ui-corner-all",
"ui-spinner-down": "ui-corner-br",
"ui-spinner-up": "ui-corner-tr"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the spinner with the `classes` option specified, changing the theming for the `ui-spinner` class:
```
$( ".selector" ).spinner({
classes: {
"ui-spinner": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-spinner` class:
```
// Getter
var themeClass = $( ".selector" ).spinner( "option", "classes.ui-spinner" );
// Setter
$( ".selector" ).spinner( "option", "classes.ui-spinner", "highlight" );
```
### culture
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` Sets the culture to use for parsing and formatting the value. If `null`, the currently set culture in `Globalize` is used, see [Globalize docs](https://github.com/jquery/globalize) for available cultures. Only relevant if the [`numberFormat`](#option-numberFormat) option is set. Requires [Globalize](https://github.com/jquery/globalize) to be included. **Code examples:**Initialize the spinner with the `culture` option specified:
```
$( ".selector" ).spinner({
culture: "fr"
});
```
Get or set the `culture` option, after initialization:
```
// Getter
var culture = $( ".selector" ).spinner( "option", "culture" );
// Setter
$( ".selector" ).spinner( "option", "culture", "fr" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the spinner if set to `true`. **Code examples:**Initialize the spinner with the `disabled` option specified:
```
$( ".selector" ).spinner({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).spinner( "option", "disabled" );
// Setter
$( ".selector" ).spinner( "option", "disabled", true );
```
### icons
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ down: "ui-icon-triangle-1-s", up: "ui-icon-triangle-1-n" }` Icons to use for buttons, matching [an icon provided by the jQuery UI CSS Framework](theming/icons). * up (string, default: "ui-icon-triangle-1-n")
* down (string, default: "ui-icon-triangle-1-s")
**Code examples:**Initialize the spinner with the `icons` option specified:
```
$( ".selector" ).spinner({
icons: { down: "custom-down-icon", up: "custom-up-icon" }
});
```
Get or set the `icons` option, after initialization:
```
// Getter
var icons = $( ".selector" ).spinner( "option", "icons" );
// Setter
$( ".selector" ).spinner( "option", "icons", { down: "custom-down-icon", up: "custom-up-icon" } );
```
### incremental
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Function](http://api.jquery.com/Types/#Function)( [Integer](http://api.jquery.com/Types/#Integer) count ) **Default:** `true` Controls the number of steps taken when holding down a spin button. **Multiple types supported:*** **Boolean**: When set to `true`, the stepping delta will increase when spun incessantly. When set to `false`, all steps are equal (as defined by the [`step`](#option-step) option).
* **Function**: Receives one parameter: the number of spins that have occurred. Must return the number of steps that should occur for the current spin.
**Code examples:**Initialize the spinner with the `incremental` option specified:
```
$( ".selector" ).spinner({
incremental: false
});
```
Get or set the `incremental` option, after initialization:
```
// Getter
var incremental = $( ".selector" ).spinner( "option", "incremental" );
// Setter
$( ".selector" ).spinner( "option", "incremental", false );
```
### max
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `null` The maximum allowed value. The element's `max` attribute is used if it exists and the option is not explicitly set. If `null`, there is no maximum enforced. **Multiple types supported:*** **Number**: The maximum value.
* **String**: If [Globalize](https://github.com/jquery/globalize) is included, the `max` option can be passed as a string which will be parsed based on the [`numberFormat`](#opiton-numberFormat) and [`culture`](#option-culture) options; otherwise it will fall back to the native `parseFloat()` method.
**Code examples:**Initialize the spinner with the `max` option specified:
```
$( ".selector" ).spinner({
max: 50
});
```
Get or set the `max` option, after initialization:
```
// Getter
var max = $( ".selector" ).spinner( "option", "max" );
// Setter
$( ".selector" ).spinner( "option", "max", 50 );
```
### min
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `null` The minimum allowed value. The element's `min` attribute is used if it exists and the option is not explicitly set. If `null`, there is no minimum enforced. **Multiple types supported:*** **Number**: The minimum value.
* **String**: If [Globalize](https://github.com/jquery/globalize) is included, the `min` option can be passed as a string which will be parsed based on the [`numberFormat`](#opiton-numberFormat) and [`culture`](#option-culture) options; otherwise it will fall back to the native `parseFloat()` method.
**Code examples:**Initialize the spinner with the `min` option specified:
```
$( ".selector" ).spinner({
min: 0
});
```
Get or set the `min` option, after initialization:
```
// Getter
var min = $( ".selector" ).spinner( "option", "min" );
// Setter
$( ".selector" ).spinner( "option", "min", 0 );
```
### numberFormat
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` Format of numbers passed to [`Globalize`](https://github.com/jquery/globalize), if available. Most common are `"n"` for a decimal number and `"C"` for a currency value. Also see the [`culture`](#option-culture) option. **Code examples:**Initialize the spinner with the `numberFormat` option specified:
```
$( ".selector" ).spinner({
numberFormat: "n"
});
```
Get or set the `numberFormat` option, after initialization:
```
// Getter
var numberFormat = $( ".selector" ).spinner( "option", "numberFormat" );
// Setter
$( ".selector" ).spinner( "option", "numberFormat", "n" );
```
### page
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `10` The number of steps to take when paging via the [`pageUp`](#method-pageUp)/[`pageDown`](#method-pageDown) methods. **Code examples:**Initialize the spinner with the `page` option specified:
```
$( ".selector" ).spinner({
page: 5
});
```
Get or set the `page` option, after initialization:
```
// Getter
var page = $( ".selector" ).spinner( "option", "page" );
// Setter
$( ".selector" ).spinner( "option", "page", 5 );
```
### step
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `1` The size of the step to take when spinning via buttons or via the [`stepUp()`](#method-stepUp)/[`stepDown()`](#method-stepDown) methods. The element's `step` attribute is used if it exists and the option is not explicitly set. **Multiple types supported:*** **Number**: The size of the step.
* **String**: If [Globalize](https://github.com/jquery/globalize) is included, the `step` option can be passed as a string which will be parsed based on the [`numberFormat`](#opiton-numberFormat) and [`culture`](#option-culture) options, otherwise it will fall back to the native `parseFloat`.
**Code examples:**Initialize the spinner with the `step` option specified:
```
$( ".selector" ).spinner({
step: 2
});
```
Get or set the `step` option, after initialization:
```
// Getter
var step = $( ".selector" ).spinner( "option", "step" );
// Setter
$( ".selector" ).spinner( "option", "step", 2 );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the spinner functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).spinner( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the spinner. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).spinner( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the spinner. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).spinner( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the spinner's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the spinner plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).spinner( "instance" );
```
### isValid()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Returns whether the Spinner's value is valid given its [`min`](#option-min), [`max`](#option-max), and [`step`](#option-step). * This method does not accept any arguments.
**Code examples:**Invoke the isValid method:
```
var isValid = $( ".selector" ).spinner( "isValid" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).spinner( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current spinner options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).spinner( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the spinner option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).spinner( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the spinner. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).spinner( "option", { disabled: true } );
```
### pageDown( [pages ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Decrements the value by the specified number of pages, as defined by the [`page`](#option-page) option. Without the parameter, a single page is decremented.
If the resulting value is above the max, below the min, or results in a step mismatch, the value will be adjusted to the closest valid value.
Invoking `pageDown()` will cause [`start`](#event-start), [`spin`](#event-spin), and [`stop`](#event-stop) events to be triggered.
* **pages** Type: [Number](http://api.jquery.com/Types/#Number) Number of pages to decrement, defaults to 1.
**Code examples:**Invoke the pageDown method:
```
$( ".selector" ).spinner( "pageDown" );
```
### pageUp( [pages ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Increments the value by the specified number of pages, as defined by the [`page`](#option-page) option. Without the parameter, a single page is incremented.
If the resulting value is above the max, below the min, or results in a step mismatch, the value will be adjusted to the closest valid value.
Invoking `pageUp()` will cause [`start`](#event-start), [`spin`](#event-spin), and [`stop`](#event-stop) events to be triggered.
* **pages** Type: [Number](http://api.jquery.com/Types/#Number) Number of pages to increment, defaults to 1.
**Code examples:**Invoke the pageUp method:
```
$( ".selector" ).spinner( "pageUp", 10 );
```
### stepDown( [steps ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Decrements the value by the specified number of steps. Without the parameter, a single step is decremented.
If the resulting value is above the max, below the min, or results in a step mismatch, the value will be adjusted to the closest valid value.
Invoking `stepDown()` will cause [`start`](#event-start), [`spin`](#event-spin), and [`stop`](#event-stop) events to be triggered.
* **steps** Type: [Number](http://api.jquery.com/Types/#Number) Number of steps to decrement, defaults to 1.
**Code examples:**Invoke the stepDown method:
```
$( ".selector" ).spinner( "stepDown" );
```
### stepUp( [steps ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Increments the value by the specified number of steps. Without the parameter, a single step is incremented.
If the resulting value is above the max, below the min, or results in a step mismatch, the value will be adjusted to the closest valid value.
Invoking `stepUp()` will cause [`start`](#event-start), [`spin`](#event-spin), and [`stop`](#event-stop) events to be triggered.
* **steps** Type: [Number](http://api.jquery.com/Types/#Number) Number of steps to increment, defaults to 1.
**Code examples:**Invoke the stepUp method:
```
$( ".selector" ).spinner( "stepUp", 5 );
```
### value()Returns: [Number](http://api.jquery.com/Types/#Number)
Gets the current value as a number. The value is parsed based on the [`numberFormat`](#option-numberFormat) and [`culture`](#option-culture) options. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var value = $( ".selector" ).spinner( "value" );
```
### value( value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
* **value** Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) The value to set. If passed as a string, the value is parsed based on the [`numberFormat`](#option-numberFormat) and [`culture`](#option-culture) options.
**Code examples:**Invoke the method:
```
$( ".selector" ).spinner( "value", 50 );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the generated wrapper. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).spinner( "widget" );
```
Extension Points
----------------
The spinner widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_buttonHtml()Returns: [String](http://api.jquery.com/Types/#String)
Method that returns HTML to use for the spinner's increment and decrement buttons. Each button must be given a `ui-spinner-button` class name for the associated events to work. * This method does not accept any arguments.
**Code examples:**Use `<button>` elements for the increment and decrement buttons.
```
_buttonHtml: function() {
return "" +
"<button class='ui-spinner-button ui-spinner-up'>" +
"<span class='ui-icon " + this.options.icons.up + "'>▲</span>" +
"</button>" +
"<button class='ui-spinner-button ui-spinner-down'>" +
"<span class='ui-icon " + this.options.icons.down + "'>▼</span>" +
"</button>";
}
```
### \_uiSpinnerHtml()Returns: [String](http://api.jquery.com/Types/#String)
Method that determines the HTML to use to wrap the spinner's `<input>` element. * This method does not accept any arguments.
**Code examples:**Wrap the spinner with a `<div>` with no rounded corners.
```
_uiSpinnerHtml: function() {
return "<div class='ui-spinner ui-widget ui-widget-content'></div>";
}
```
Events
------
### change( event, ui )Type: `spinchange`
Triggered when the value of the spinner has changed and the input is no longer focused. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the spinner with the change callback specified:
```
$( ".selector" ).spinner({
change: function( event, ui ) {}
});
```
Bind an event listener to the spinchange event:
```
$( ".selector" ).on( "spinchange", function( event, ui ) {} );
```
### create( event, ui )Type: `spincreate`
Triggered when the spinner is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the spinner with the create callback specified:
```
$( ".selector" ).spinner({
create: function( event, ui ) {}
});
```
Bind an event listener to the spincreate event:
```
$( ".selector" ).on( "spincreate", function( event, ui ) {} );
```
### spin( event, ui )Type: `spin`
Triggered during increment/decrement (to determine direction of spin compare current value with `ui.value`). Can be canceled, preventing the value from being updated.
* **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **value** Type: [Number](http://api.jquery.com/Types/#Number) The new value to be set, unless the event is cancelled.
**Code examples:**Initialize the spinner with the spin callback specified:
```
$( ".selector" ).spinner({
spin: function( event, ui ) {}
});
```
Bind an event listener to the spin event:
```
$( ".selector" ).on( "spin", function( event, ui ) {} );
```
### start( event, ui )Type: `spinstart`
Triggered before a spin. Can be canceled, preventing the spin from occurring. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the spinner with the start callback specified:
```
$( ".selector" ).spinner({
start: function( event, ui ) {}
});
```
Bind an event listener to the spinstart event:
```
$( ".selector" ).on( "spinstart", function( event, ui ) {} );
```
### stop( event, ui )Type: `spinstop`
Triggered after a spin. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the spinner with the stop callback specified:
```
$( ".selector" ).spinner({
stop: function( event, ui ) {}
});
```
Bind an event listener to the spinstop event:
```
$( ".selector" ).on( "spinstop", function( event, ui ) {} );
```
Example:
--------
Plain number spinner
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>spinner demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<input id="spinner">
<script>
$( "#spinner" ).spinner();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui .transfer() .transfer()
===========
.transfer( options [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)version added: 1.12
------------------------------------------------------------------------------------------------------------
**Description:** Transfers the outline of an element to another element
* #### [.transfer( options [, complete ] )](#transfer-options-complete)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object)
- **to** Type: [Selector](http://api.jquery.com/Types/#Selector) The target of the transfer effect.
- **className** (default: `null`) Type: [String](http://api.jquery.com/Types/#String) A class to add to the transfer element, in addition to `ui-effects-transfer`.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run. The strings `"fast"` and `"slow"` can be supplied to indicate durations of 200 and 600 milliseconds, respectively. The number type indicates the duration in milliseconds.
- **easing** (default: `swing`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
Very useful when trying to visualize interaction between two elements.
The transfer element itself has the class `ui-effects-transfer`, and needs to be styled by you, for example by adding a background or border.
Example:
--------
Clicking on the green element transfers to the other.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>transfer demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
.green {
width: 100px;
height: 80px;
background: green;
border: 1px solid black;
}
.red {
margin-top: 10px;
width: 50px;
height: 30px;
background: red;
border: 1px solid black;
}
.ui-effects-transfer {
border: 1px dotted black;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div class="green"></div>
<div class="red"></div>
<script>
$( "div" ).click(function() {
var i = 1 - $( "div" ).index( this );
$( this ).transfer( {
to: $( "div" ).eq( i ),
duration: 1000
} );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui jQuery.ui.keyCode jQuery.ui.keyCode
=================
jQuery.ui.keyCode
-----------------
**Description:** A mapping of key code descriptions to their numeric values.
* #### jQuery.ui.keyCode
+ **BACKSPACE** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **COMMA** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **DELETE** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **DOWN** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **END** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **ENTER** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **ESCAPE** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **HOME** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **LEFT** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **PAGE\_DOWN** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **PAGE\_UP** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **PERIOD** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **RIGHT** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **SPACE** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **TAB** Type: [Integer](http://api.jquery.com/Types/#Integer)
+ **UP** Type: [Integer](http://api.jquery.com/Types/#Integer)
Example:
--------
Test for arrow key presses.
```
$( document ).on( "keydown", function( event ) {
switch( event.keyCode ) {
case $.ui.keyCode.LEFT:
console.log( "left" );
break;
case $.ui.keyCode.RIGHT:
console.log( "right" );
break;
case $.ui.keyCode.UP:
console.log( "up" );
break;
case $.ui.keyCode.DOWN:
console.log( "down" );
break;
}
});
```
jqueryui Tooltip Widget Tooltip Widget
==============
Tooltip Widgetversion added: 1.9
---------------------------------
**Description:** Customizable, themeable tooltips, replacing native tooltips.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [content](#option-content) [disabled](#option-disabled) [hide](#option-hide) [items](#option-items) [position](#option-position) [show](#option-show) [tooltipClass](#option-tooltipClass) [track](#option-track) ### Methods
[close](#method-close) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [open](#method-open) [option](#method-option) [widget](#method-widget) ### Events
[close](#event-close) [create](#event-create) [open](#event-open) Tooltip replaces native tooltips, making them themeable as well as allowing various customizations:
* Display other content than just the title, like inline footnotes or extra content retrieved via Ajax.
* Customize the positioning, e.g., to center the tooltip above elements.
* Add extra styling to customize the appearance, for warning or error fields.
A fade animation is used by default to show and hide the tooltip, making the appearance a bit more organic, compared to just toggling the visibility. This can be customized with the [`show`](#option-show) and [`hide`](#option-hide) options.
The [`items`](#option-items) and [`content`](#option-content) options need to stay in-sync. If you change one of them, you need to change the other.
In general, disabled elements do not trigger any DOM events. Therefore, it is not possible to properly control tooltips for disabled elements, since we need to listen to events to determine when to show and hide the tooltip. As a result, jQuery UI does not guarantee any level of support for tooltips attached to disabled elements. Unfortunately, this means that if you require tooltips on disabled elements, you may end up with a mixture of native tooltips and jQuery UI tooltips.
### Keyboard interaction
When the tooltip is open and the corresponding item has focus, the following key commands are available:
* `ESCAPE`: Close the tooltip.
### Theming
The tooltip widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If tooltip specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-tooltip`: The outer container for the tooltip.
+ `ui-tooltip-content`: The content of the tooltip.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Position](position)
* [Effects Core](category/effects-core) (optional; for use with the [`show`](#option-show) and [`hide`](#option-hide) options)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-tooltip": "ui-corner-all ui-widget-shadow"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the tooltip with the `classes` option specified, changing the theming for the `ui-tooltip` class:
```
$( ".selector" ).tooltip({
classes: {
"ui-tooltip": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-tooltip` class:
```
// Getter
var themeClass = $( ".selector" ).tooltip( "option", "classes.ui-tooltip" );
// Setter
$( ".selector" ).tooltip( "option", "classes.ui-tooltip", "highlight" );
```
### content
**Type:** [Function](http://api.jquery.com/Types/#Function)() or [String](http://api.jquery.com/Types/#String) or [Element](http://api.jquery.com/Types/#Element) or [jQuery](http://api.jquery.com/Types/#jQuery) **Default:** `function returning the title attribute` The content of the tooltip.
*When changing this option, you likely need to also change the [`items`](#option-items) option.*
**Multiple types supported:*** **Function**: A callback which can either return the content directly, or call the first argument, passing in the content, e.g., for Ajax content.
* **String**: A string of HTML to use for the tooltip content.
* **Element**: A DOM element to use for the tooltip content.
* **jQuery**: A jQuery object to use for the tooltip content.
**Code examples:**Initialize the tooltip with the `content` option specified:
```
$( ".selector" ).tooltip({
content: "Awesome title!"
});
```
Get or set the `content` option, after initialization:
```
// Getter
var content = $( ".selector" ).tooltip( "option", "content" );
// Setter
$( ".selector" ).tooltip( "option", "content", "Awesome title!" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the tooltip if set to `true`. **Code examples:**Initialize the tooltip with the `disabled` option specified:
```
$( ".selector" ).tooltip({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).tooltip( "option", "disabled" );
// Setter
$( ".selector" ).tooltip( "option", "disabled", true );
```
### hide
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `true` If and how to animate the hiding of the tooltip. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the tooltip will be hidden immediately. When set to `true`, the tooltip will fade out with the default duration and the default easing.
* **Number**: The tooltip will fade out with the specified duration and the default easing.
* **String**: The tooltip will be hidden using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideUp"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeOut"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the tooltip with the `hide` option specified:
```
$( ".selector" ).tooltip({
hide: { effect: "explode", duration: 1000 }
});
```
Get or set the `hide` option, after initialization:
```
// Getter
var hide = $( ".selector" ).tooltip( "option", "hide" );
// Setter
$( ".selector" ).tooltip( "option", "hide", { effect: "explode", duration: 1000 } );
```
### items
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `[title]` A selector indicating which items should show tooltips. Customize if you're using something other than the title attribute for the tooltip content, or if you need a different selector for event delegation.
*When changing this option, you likely need to also change the [`content`](#option-content) option.*
**Code examples:**Initialize the tooltip with the `items` option specified:
```
$( ".selector" ).tooltip({
items: "img[alt]"
});
```
Get or set the `items` option, after initialization:
```
// Getter
var items = $( ".selector" ).tooltip( "option", "items" );
// Setter
$( ".selector" ).tooltip( "option", "items", "img[alt]" );
```
### position
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ my: "left top+15", at: "left bottom", collision: "flipfit" }` Identifies the position of the tooltip in relation to the associated target element. The `of` option defaults to the target element, but you can specify another element to position against. You can refer to the [jQuery UI Position](position) utility for more details about the various options.
**Code examples:**Initialize the tooltip with the `position` option specified:
```
$( ".selector" ).tooltip({
position: { my: "left+15 center", at: "right center" }
});
```
Get or set the `position` option, after initialization:
```
// Getter
var position = $( ".selector" ).tooltip( "option", "position" );
// Setter
$( ".selector" ).tooltip( "option", "position", { my: "left+15 center", at: "right center" } );
```
### show
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) or [Object](http://api.jquery.com/Types/#Object) **Default:** `true` If and how to animate the showing of the tooltip. **Multiple types supported:*** **Boolean**: When set to `false`, no animation will be used and the tooltip will be shown immediately. When set to `true`, the tooltip will fade in with the default duration and the default easing.
* **Number**: The tooltip will fade in with the specified duration and the default easing.
* **String**: The tooltip will be shown using the specified effect. The value can either be the name of a built-in jQuery animation method, such as `"slideDown"`, or the name of a [jQuery UI effect](category/effects), such as `"fold"`. In either case the effect will be used with the default duration and the default easing.
* **Object**: If the value is an object, then `effect`, `delay`, `duration`, and `easing` properties may be provided. If the `effect` property contains the name of a jQuery method, then that method will be used; otherwise it is assumed to be the name of a jQuery UI effect. When using a jQuery UI effect that supports additional settings, you may include those settings in the object and they will be passed to the effect. If `duration` or `easing` is omitted, then the default values will be used. If `effect` is omitted, then `"fadeIn"` will be used. If `delay` is omitted, then no delay is used.
**Code examples:**Initialize the tooltip with the `show` option specified:
```
$( ".selector" ).tooltip({
show: { effect: "blind", duration: 800 }
});
```
Get or set the `show` option, after initialization:
```
// Getter
var show = $( ".selector" ).tooltip( "option", "show" );
// Setter
$( ".selector" ).tooltip( "option", "show", { effect: "blind", duration: 800 } );
```
### tooltipClass
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `null` A class to add to the widget, can be used to display various tooltip types, like warnings or errors.
The `tooltipClass` option has been deprecated in favor of the [`classes` option](#option-classes), using the `ui-tooltip` property.
(version deprecated: 1.12) **Code examples:**Initialize the tooltip with the `tooltipClass` option specified:
```
$( ".selector" ).tooltip({
tooltipClass: "custom-tooltip-styling"
});
```
Get or set the `tooltipClass` option, after initialization:
```
// Getter
var tooltipClass = $( ".selector" ).tooltip( "option", "tooltipClass" );
// Setter
$( ".selector" ).tooltip( "option", "tooltipClass", "custom-tooltip-styling" );
```
### track
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the tooltip should track (follow) the mouse. **Code examples:**Initialize the tooltip with the `track` option specified:
```
$( ".selector" ).tooltip({
track: true
});
```
Get or set the `track` option, after initialization:
```
// Getter
var track = $( ".selector" ).tooltip( "option", "track" );
// Setter
$( ".selector" ).tooltip( "option", "track", true );
```
Methods
-------
### close()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes a tooltip. This is only intended to be called for non-delegated tooltips. * This method does not accept any arguments.
**Code examples:**Invoke the close method:
```
$( ".selector" ).tooltip( "close" );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the tooltip functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).tooltip( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the tooltip. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).tooltip( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the tooltip. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).tooltip( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the tooltip's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the tooltip plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).tooltip( "instance" );
```
### open()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Programmatically open a tooltip. This is only intended to be called for non-delegated tooltips. * This method does not accept any arguments.
**Code examples:**Invoke the open method:
```
$( ".selector" ).tooltip( "open" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).tooltip( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current tooltip options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).tooltip( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the tooltip option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).tooltip( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the tooltip. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).tooltip( "option", { disabled: true } );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the original element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).tooltip( "widget" );
```
Events
------
### close( event, ui )Type: `tooltipclose`
Triggered when a tooltip is closed, triggered on `focusout` or `mouseleave`. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **tooltip** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The generated tooltip element.
**Code examples:**Initialize the tooltip with the close callback specified:
```
$( ".selector" ).tooltip({
close: function( event, ui ) {}
});
```
Bind an event listener to the tooltipclose event:
```
$( ".selector" ).on( "tooltipclose", function( event, ui ) {} );
```
### create( event, ui )Type: `tooltipcreate`
Triggered when the tooltip is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the tooltip with the create callback specified:
```
$( ".selector" ).tooltip({
create: function( event, ui ) {}
});
```
Bind an event listener to the tooltipcreate event:
```
$( ".selector" ).on( "tooltipcreate", function( event, ui ) {} );
```
### open( event, ui )Type: `tooltipopen`
Triggered when a tooltip is shown, triggered on `focusin` or `mouseover`. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **tooltip** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The generated tooltip element.
**Code examples:**Initialize the tooltip with the open callback specified:
```
$( ".selector" ).tooltip({
open: function( event, ui ) {}
});
```
Bind an event listener to the tooltipopen event:
```
$( ".selector" ).on( "tooltipopen", function( event, ui ) {} );
```
Example:
--------
Create a tooltip on the document, using event delegation for all elements with a title attribute.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>tooltip demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>
<a href="#" title="Anchor description">Anchor text</a>
<input title="Input help">
</p>
<script>
$( document ).tooltip();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Autocomplete Widget Autocomplete Widget
===================
Autocomplete Widgetversion added: 1.8
--------------------------------------
**Description:** Autocomplete enables users to quickly find and select from a pre-populated list of values as they type, leveraging searching and filtering.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[appendTo](#option-appendTo) [autoFocus](#option-autoFocus) [classes](#option-classes) [delay](#option-delay) [disabled](#option-disabled) [minLength](#option-minLength) [position](#option-position) [source](#option-source) ### Methods
[close](#method-close) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [search](#method-search) [widget](#method-widget)
### Extension Points
[\_renderItem](#method-_renderItem) [\_renderMenu](#method-_renderMenu) [\_resizeMenu](#method-_resizeMenu) ### Events
[change](#event-change) [close](#event-close) [create](#event-create) [focus](#event-focus) [open](#event-open) [response](#event-response) [search](#event-search) [select](#event-select) Any field that can receive input can be converted into an Autocomplete, namely, `<input>` elements, `<textarea>` elements, and elements with the `contenteditable` attribute.
When typing in the autocomplete field, the plugin starts searching for entries that match and displays a list of values to choose from. By entering more characters, the user can filter down the list to better matches.
This can be used to choose previously selected values, such as entering tags for articles or entering email addresses from an address book. Autocomplete can also be used to populate associated information, such as entering a city name and getting the zip code.
You can pull data in from a local or remote source: Local is good for small data sets, e.g., an address book with 50 entries; remote is necessary for big data sets, such as a database with hundreds or millions of entries to select from. To find out more about customizing the data source, see the documentation for the [`source`](#option-source) option.
### Keyboard interaction
When the menu is open, the following key commands are available:
* `UP`: Move focus to the previous item. If on first item, move focus to the input. If on the input, move focus to last item.
* `DOWN`: Move focus to the next item. If on last item, move focus to the input. If on the input, move focus to the first item.
* `ESCAPE`: Close the menu.
* `ENTER`: Select the currently focused item and close the menu.
* `TAB`: Select the currently focused item, close the menu, and move focus to the next focusable element.
* `PAGE UP`/`PAGE DOWN`: Scroll through a page of items (based on height of menu). *It's generally a bad idea to display so many items that users need to page.*
When the menu is closed, the following key commands are available:
* `UP`/`DOWN`: Open the menu, if the [`minLength`](#option-minLength) has been met.
### Theming
The autocomplete widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If autocomplete specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-autocomplete`: The [menu](menu#theming) used to display matches to the user.
* `ui-autocomplete-input`: The input element that the autocomplete widget was instantiated with. While requesting data to display to the user, the `ui-autocomplete-loading` class is also added to this element.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Position](position)
* [Menu](menu)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
* This widget manipulates its element's value programmatically, therefore a native `change` event may not be fired when the element's value changes.
Options
-------
### appendTo
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `null` Which element the menu should be appended to. When the value is `null`, the parents of the input field will be checked for a class of `ui-front`. If an element with the `ui-front` class is found, the menu will be appended to that element. Regardless of the value, if no element is found, the menu will be appended to the body.
**Note:** The `appendTo` option should not be changed while the suggestions menu is open. **Code examples:**Initialize the autocomplete with the `appendTo` option specified:
```
$( ".selector" ).autocomplete({
appendTo: "#someElem"
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).autocomplete( "option", "appendTo" );
// Setter
$( ".selector" ).autocomplete( "option", "appendTo", "#someElem" );
```
### autoFocus
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If set to `true` the first item will automatically be focused when the menu is shown. **Code examples:**Initialize the autocomplete with the `autoFocus` option specified:
```
$( ".selector" ).autocomplete({
autoFocus: true
});
```
Get or set the `autoFocus` option, after initialization:
```
// Getter
var autoFocus = $( ".selector" ).autocomplete( "option", "autoFocus" );
// Setter
$( ".selector" ).autocomplete( "option", "autoFocus", true );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the autocomplete with the `classes` option specified, changing the theming for the `ui-autocomplete` class:
```
$( ".selector" ).autocomplete({
classes: {
"ui-autocomplete": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-autocomplete` class:
```
// Getter
var themeClass = $( ".selector" ).autocomplete( "option", "classes.ui-autocomplete" );
// Setter
$( ".selector" ).autocomplete( "option", "classes.ui-autocomplete", "highlight" );
```
### delay
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `300` The delay in milliseconds between when a keystroke occurs and when a search is performed. A zero-delay makes sense for local data (more responsive), but can produce a lot of load for remote data, while being less responsive. **Code examples:**Initialize the autocomplete with the `delay` option specified:
```
$( ".selector" ).autocomplete({
delay: 500
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).autocomplete( "option", "delay" );
// Setter
$( ".selector" ).autocomplete( "option", "delay", 500 );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the autocomplete if set to `true`. **Code examples:**Initialize the autocomplete with the `disabled` option specified:
```
$( ".selector" ).autocomplete({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).autocomplete( "option", "disabled" );
// Setter
$( ".selector" ).autocomplete( "option", "disabled", true );
```
### minLength
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `1` The minimum number of characters a user must type before a search is performed. Zero is useful for local data with just a few items, but a higher value should be used when a single character search could match a few thousand items. **Code examples:**Initialize the autocomplete with the `minLength` option specified:
```
$( ".selector" ).autocomplete({
minLength: 0
});
```
Get or set the `minLength` option, after initialization:
```
// Getter
var minLength = $( ".selector" ).autocomplete( "option", "minLength" );
// Setter
$( ".selector" ).autocomplete( "option", "minLength", 0 );
```
### position
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ my: "left top", at: "left bottom", collision: "none" }` Identifies the position of the suggestions menu in relation to the associated input element. The `of` option defaults to the input element, but you can specify another element to position against. You can refer to the [jQuery UI Position](position) utility for more details about the various options. **Code examples:**Initialize the autocomplete with the `position` option specified:
```
$( ".selector" ).autocomplete({
position: { my : "right top", at: "right bottom" }
});
```
Get or set the `position` option, after initialization:
```
// Getter
var position = $( ".selector" ).autocomplete( "option", "position" );
// Setter
$( ".selector" ).autocomplete( "option", "position", { my : "right top", at: "right bottom" } );
```
### source
**Type:** [Array](http://api.jquery.com/Types/#Array) or [String](http://api.jquery.com/Types/#String) or [Function](http://api.jquery.com/Types/#Function)( [Object](http://api.jquery.com/Types/#Object) request, [Function](http://api.jquery.com/Types/#Function) response( [Object](http://api.jquery.com/Types/#Object) data ) ) **Default:** `none; must be specified` Defines the data to use, must be specified. Independent of the variant you use, the label is always treated as text. If you want the label to be treated as html you can use [Scott González' html extension](https://github.com/scottgonzalez/jquery-ui-extensions/blob/master/src/autocomplete/jquery.ui.autocomplete.html.js). The demos all focus on different variations of the `source` option - look for one that matches your use case, and check out the code.
**Multiple types supported:*** **Array**: An array can be used for local data. There are two supported formats:
+ An array of strings: `[ "Choice1", "Choice2" ]`
+ An array of objects with `label` and `value` properties: `[ { label: "Choice1", value: "value1" }, ... ]` The label property is displayed in the suggestion menu. The value will be inserted into the input element when a user selects an item. If just one property is specified, it will be used for both, e.g., if you provide only `value` properties, the value will also be used as the label.
* **String**: When a string is used, the Autocomplete plugin expects that string to point to a URL resource that will return JSON data. It can be on the same host or on a different one (must support CORS). The Autocomplete plugin does not filter the results, instead a query string is added with a `term` field, which the server-side script should use for filtering the results. For example, if the `source` option is set to `"https://example.com"` and the user types `foo`, a GET request would be made to `https://example.com?term=foo`. The data itself can be in the same format as the local data described above.
* **Function**: The third variation, a callback, provides the most flexibility and can be used to connect any data source to Autocomplete, including JSONP. The callback gets two arguments:
+ A `request` object, with a single `term` property, which refers to the value currently in the text input. For example, if the user enters `"new yo"` in a city field, the Autocomplete term will equal `"new yo"`.
+ A `response` callback, which expects a single argument: the data to suggest to the user. This data should be filtered based on the provided term, and can be in any of the formats described above for simple local data. It's important when providing a custom source callback to handle errors during the request. You must always call the `response` callback even if you encounter an error. This ensures that the widget always has the correct state.When filtering data locally, you can make use of the built-in `$.ui.autocomplete.escapeRegex` function. It'll take a single string argument and escape all regex characters, making the result safe to pass to `new RegExp()`.
**Code examples:**Initialize the autocomplete with the `source` option specified:
```
$( ".selector" ).autocomplete({
source: [ "c++", "java", "php", "coldfusion", "javascript", "asp", "ruby" ]
});
```
Get or set the `source` option, after initialization:
```
// Getter
var source = $( ".selector" ).autocomplete( "option", "source" );
// Setter
$( ".selector" ).autocomplete( "option", "source", [ "c++", "java", "php", "coldfusion", "javascript", "asp", "ruby" ] );
```
Methods
-------
### close()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes the Autocomplete menu. Useful in combination with the [`search`](#method-search) method, to close the open menu. * This method does not accept any arguments.
**Code examples:**Invoke the close method:
```
$( ".selector" ).autocomplete( "close" );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the autocomplete functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).autocomplete( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the autocomplete. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).autocomplete( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the autocomplete. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).autocomplete( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the autocomplete's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the autocomplete plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).autocomplete( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).autocomplete( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current autocomplete options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).autocomplete( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the autocomplete option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).autocomplete( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the autocomplete. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).autocomplete( "option", { disabled: true } );
```
### search( [value ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Triggers a [`search`](#event-search) event and invokes the data source if the event is not canceled. Can be used by a selectbox-like button to open the suggestions when clicked. When invoked with no parameters, the current input's value is used. Can be called with an empty string and `minLength: 0` to display all items. * **value** Type: [String](http://api.jquery.com/Types/#String)
**Code examples:**Invoke the search method:
```
$( ".selector" ).autocomplete( "search", "" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the menu element. Although the menu items are constantly created and destroyed, the menu element itself is created during initialization and is constantly reused. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
$( ".selector" ).autocomplete( "widget" );
```
Extension Points
----------------
The autocomplete widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_renderItem( ul, item )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Method that controls the creation of each option in the widget's menu. The method must create a new `<li>` element, append it to the menu, and return it. See the [Menu](menu) documentation for more details about the markup.
* **ul** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The `<ul>` element that the newly created `<li>` element must be appended to.
* **item** Type: [Object](http://api.jquery.com/Types/#Object)
+ **label** Type: [String](http://api.jquery.com/Types/#String) The string to display for the item.
+ **value** Type: [String](http://api.jquery.com/Types/#String) The value to insert into the input when the item is selected.
**Code examples:**Add the item's value as a data attribute on the `<li>`.
```
_renderItem: function( ul, item ) {
return $( "<li>" )
.attr( "data-value", item.value )
.append( item.label )
.appendTo( ul );
}
```
### \_renderMenu( ul, items )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Method that controls building the widget's menu. The method is passed an empty `<ul>` and an array of items that match the user typed term. Creation of the individual `<li>` elements should be delegated to `_renderItemData()`, which in turn delegates to the [`_renderItem()`](#method-_renderItem) extension point. * **ul** Type: [jQuery](http://api.jquery.com/Types/#jQuery) An empty `<ul>` element to use as the widget's menu.
* **items** Type: [Array](http://api.jquery.com/Types/#Array) An Array of items that match the user typed term. Each item is an Object with `label` and `value` properties.
**Code examples:**Add a CSS class name to the odd menu items.
```
_renderMenu: function( ul, items ) {
var that = this;
$.each( items, function( index, item ) {
that._renderItemData( ul, item );
});
$( ul ).find( "li" ).odd().addClass( "odd" );
}
```
### \_resizeMenu()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Method responsible for sizing the menu before it is displayed. The menu element is available at `this.menu.element`. * This method does not accept any arguments.
**Code examples:**Always display the menu as 500 pixels wide.
```
_resizeMenu: function() {
this.menu.element.outerWidth( 500 );
}
```
Events
------
### change( event, ui )Type: `autocompletechange`
Triggered when the field is blurred, if the value has changed. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [Object](http://api.jquery.com/Types/#Object) The item selected from the menu, if any. Otherwise the property is `null`.
**Code examples:**Initialize the autocomplete with the change callback specified:
```
$( ".selector" ).autocomplete({
change: function( event, ui ) {}
});
```
Bind an event listener to the autocompletechange event:
```
$( ".selector" ).on( "autocompletechange", function( event, ui ) {} );
```
### close( event, ui )Type: `autocompleteclose`
Triggered when the menu is hidden. Not every `close` event will be accompanied by a `change` event. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the autocomplete with the close callback specified:
```
$( ".selector" ).autocomplete({
close: function( event, ui ) {}
});
```
Bind an event listener to the autocompleteclose event:
```
$( ".selector" ).on( "autocompleteclose", function( event, ui ) {} );
```
### create( event, ui )Type: `autocompletecreate`
Triggered when the autocomplete is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the autocomplete with the create callback specified:
```
$( ".selector" ).autocomplete({
create: function( event, ui ) {}
});
```
Bind an event listener to the autocompletecreate event:
```
$( ".selector" ).on( "autocompletecreate", function( event, ui ) {} );
```
### focus( event, ui )Type: `autocompletefocus`
Triggered when focus is moved to an item (not selecting). The default action is to replace the text field's value with the value of the focused item, though only if the event was triggered by a keyboard interaction. Canceling this event prevents the value from being updated, but does not prevent the menu item from being focused.
* **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [Object](http://api.jquery.com/Types/#Object) The focused item.
**Code examples:**Initialize the autocomplete with the focus callback specified:
```
$( ".selector" ).autocomplete({
focus: function( event, ui ) {}
});
```
Bind an event listener to the autocompletefocus event:
```
$( ".selector" ).on( "autocompletefocus", function( event, ui ) {} );
```
### open( event, ui )Type: `autocompleteopen`
Triggered when the suggestion menu is opened or updated. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the autocomplete with the open callback specified:
```
$( ".selector" ).autocomplete({
open: function( event, ui ) {}
});
```
Bind an event listener to the autocompleteopen event:
```
$( ".selector" ).on( "autocompleteopen", function( event, ui ) {} );
```
### response( event, ui )Type: `autocompleteresponse`
Triggered after a search completes, before the menu is shown. Useful for local manipulation of suggestion data, where a custom [`source`](#option-source) option callback is not required. This event is always triggered when a search completes, even if the menu will not be shown because there are no results or the Autocomplete is disabled. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **content** Type: [Array](http://api.jquery.com/Types/#Array) Contains the response data and can be modified to change the results that will be shown. This data is already normalized, so if you modify the data, make sure to include both `value` and `label` properties for each item.
**Code examples:**Initialize the autocomplete with the response callback specified:
```
$( ".selector" ).autocomplete({
response: function( event, ui ) {}
});
```
Bind an event listener to the autocompleteresponse event:
```
$( ".selector" ).on( "autocompleteresponse", function( event, ui ) {} );
```
### search( event, ui )Type: `autocompletesearch`
Triggered before a search is performed, after [`minLength`](#option-minLength) and [`delay`](#option-delay) are met. If canceled, then no request will be started and no items suggested. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the autocomplete with the search callback specified:
```
$( ".selector" ).autocomplete({
search: function( event, ui ) {}
});
```
Bind an event listener to the autocompletesearch event:
```
$( ".selector" ).on( "autocompletesearch", function( event, ui ) {} );
```
### select( event, ui )Type: `autocompleteselect`
Triggered when an item is selected from the menu. The default action is to replace the text field's value with the value of the selected item. Canceling this event prevents the value from being updated, but does not prevent the menu from closing.
* **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [Object](http://api.jquery.com/Types/#Object) An Object with `label` and `value` properties for the selected option.
**Code examples:**Initialize the autocomplete with the select callback specified:
```
$( ".selector" ).autocomplete({
select: function( event, ui ) {}
});
```
Bind an event listener to the autocompleteselect event:
```
$( ".selector" ).on( "autocompleteselect", function( event, ui ) {} );
```
Examples:
---------
A simple jQuery UI Autocomplete
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>autocomplete demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label for="autocomplete">Select a programming language: </label>
<input id="autocomplete">
<script>
$( "#autocomplete" ).autocomplete({
source: [ "c++", "java", "php", "coldfusion", "javascript", "asp", "ruby" ]
});
</script>
</body>
</html>
```
#### Demo:
Using a custom source callback to match only the beginning of terms
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>autocomplete demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label for="autocomplete">Select a programming language: </label>
<input id="autocomplete">
<script>
var tags = [ "c++", "java", "php", "coldfusion", "javascript", "asp", "ruby" ];
$( "#autocomplete" ).autocomplete({
source: function( request, response ) {
var matcher = new RegExp( "^" + $.ui.autocomplete.escapeRegex( request.term ), "i" );
response( $.grep( tags, function( item ){
return matcher.test( item );
}) );
}
});
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Controlgroup Widget Controlgroup Widget
===================
Controlgroup Widgetversion added: 1.12
---------------------------------------
**Description:** Themeable set of input widgets.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [direction](#option-direction) [disabled](#option-disabled) [items](#option-items) [onlyVisible](#option-onlyVisible) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget) ### Events
[create](#event-create) A controlgroup provides a visual grouping for <button> and other input widgets. Controlgroup works by selecting all appropriate descendants, based on the [items](#option-items) option, and applying their respective widgets, if loaded. If the widgets already exist, their `refresh()` method is called. You can enable and disable a controlgroup, which will enable and disable all contained widgets. Destroying a controlgroup also calls each widgets's `.destroy()` method.
### Theming
The controlgroup widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If controlgroup specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-controlgroup`: The outer container of controlgroups. Depending on the [direction](#option-direction) option, this element will additionally have the `ui-controlgroup-horizontal` or `ui-controlgroup-vertical` classes.
+ `ui-controlgroup-item`: Each item inside the group.
### Dependencies
* [Widget Factory](jquery.widget)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the controlgroup with the `classes` option specified, changing the theming for the `ui-controlgroup` class:
```
$( ".selector" ).controlgroup({
classes: {
"ui-controlgroup": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-controlgroup` class:
```
// Getter
var themeClass = $( ".selector" ).controlgroup( "option", "classes.ui-controlgroup" );
// Setter
$( ".selector" ).controlgroup( "option", "classes.ui-controlgroup", "highlight" );
```
### direction
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"horizontal"` By default, controlgroup displays its controls in a horizontal layout. Use this option to use a vertical layout instead.
**Code examples:**Initialize the controlgroup with the `direction` option specified:
```
$( ".selector" ).controlgroup({
direction: "vertical"
});
```
Get or set the `direction` option, after initialization:
```
// Getter
var direction = $( ".selector" ).controlgroup( "option", "direction" );
// Setter
$( ".selector" ).controlgroup( "option", "direction", "vertical" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the controlgroup if set to `true`. **Code examples:**Initialize the controlgroup with the `disabled` option specified:
```
$( ".selector" ).controlgroup({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).controlgroup( "option", "disabled" );
// Setter
$( ".selector" ).controlgroup( "option", "disabled", true );
```
### items
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"button": "input[type=button], input[type=submit], input[type=reset], button, a",
"controlgroupLabel": ".ui-controlgroup-label",
"checkboxradio": "input[type='checkbox'], input[type='radio']",
"selectmenu": "select",
"spinner": ".ui-spinner-input"
}
```
Which descendant elements to initialize as their respective widgets. Two elements have special behavior: * `controlgroupLabel`: Any elements matching the selector for this will be wrapped in a span with the `ui-controlgroup-label-contents` class.
* `spinner`: This uses a class selector as the value. Requires either adding the class manually or initializing the spinner manually. Can be overridden to use `input[type=number]`, but that also requires custom CSS to remove the native number controls.
### onlyVisible
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` Sets whether to exclude invisible children in the assignment of rounded corners. When set to false, all children of a controlgroup are taken into account when assigning rounded corners, including hidden children. Thus, if, for example, the controlgroup's first child is hidden and the default horizontal layout is applied, the controlgroup will, in effect, not have rounded corners on the left edge. Likewise, if the controlgroup has a vertical layout and its first child is hidden, the controlgroup will not have rounded corners on the top edge. **Code examples:**Initialize the controlgroup with the `onlyVisible` option specified:
```
$( ".selector" ).controlgroup({
onlyVisible: false
});
```
Get or set the `onlyVisible` option, after initialization:
```
// Getter
var onlyVisible = $( ".selector" ).controlgroup( "option", "onlyVisible" );
// Setter
$( ".selector" ).controlgroup( "option", "onlyVisible", false );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the controlgroup functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).controlgroup( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the controlgroup. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).controlgroup( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the controlgroup. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).controlgroup( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the controlgroup's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the controlgroup plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).controlgroup( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).controlgroup( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current controlgroup options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).controlgroup( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the controlgroup option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).controlgroup( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the controlgroup. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).controlgroup( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Process any widgets that were added or removed directly in the DOM. Results depend on the [`items`](#option-items) option. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).controlgroup( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the controlgroup. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).controlgroup( "widget" );
```
Events
------
### create( event, ui )Type: `controlgroupcreate`
Triggered when the controlgroup is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the controlgroup with the create callback specified:
```
$( ".selector" ).controlgroup({
create: function( event, ui ) {}
});
```
Bind an event listener to the controlgroupcreate event:
```
$( ".selector" ).on( "controlgroupcreate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI controlgroup
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>controlgroup demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<form>
<fieldset>
<legend>Favorite jQuery Project</legend>
<div id="radio">
<input type="radio" id="sizzle" name="project">
<label for="sizzle">Sizzle</label>
<input type="radio" id="qunit" name="project" checked="checked">
<label for="qunit">QUnit</label>
<input type="radio" id="color" name="project">
<label for="color">Color</label>
</div>
</fieldset>
</form>
<script>
$( "#radio" ).controlgroup();
</script>
</body>
</html>
```
#### Demo:
jqueryui Sortable Widget Sortable Widget
===============
Sortable Widgetversion added: 1.0
----------------------------------
**Description:** Reorder elements in a list or grid using the mouse.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[appendTo](#option-appendTo) [axis](#option-axis) [cancel](#option-cancel) [classes](#option-classes) [connectWith](#option-connectWith) [containment](#option-containment) [cursor](#option-cursor) [cursorAt](#option-cursorAt) [delay](#option-delay) [disabled](#option-disabled) [distance](#option-distance) [dropOnEmpty](#option-dropOnEmpty) [forceHelperSize](#option-forceHelperSize) [forcePlaceholderSize](#option-forcePlaceholderSize) [grid](#option-grid) [handle](#option-handle) [helper](#option-helper) [items](#option-items) [opacity](#option-opacity) [placeholder](#option-placeholder) [revert](#option-revert) [scroll](#option-scroll) [scrollSensitivity](#option-scrollSensitivity) [scrollSpeed](#option-scrollSpeed) [tolerance](#option-tolerance) [zIndex](#option-zIndex) ### Methods
[cancel](#method-cancel) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [refresh](#method-refresh) [refreshPositions](#method-refreshPositions) [serialize](#method-serialize) [toArray](#method-toArray) [widget](#method-widget) ### Events
[activate](#event-activate) [beforeStop](#event-beforeStop) [change](#event-change) [create](#event-create) [deactivate](#event-deactivate) [out](#event-out) [over](#event-over) [receive](#event-receive) [remove](#event-remove) [sort](#event-sort) [start](#event-start) [stop](#event-stop) [update](#event-update) The jQuery UI Sortable plugin makes selected elements sortable by dragging with the mouse.
*Note: In order to sort table rows, the `tbody` must be made sortable, not the `table`.*
### Theming
The sortable widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If sortable specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-sortable`: The sortable element.
+ `ui-sortable-handle`: The handle of each sortable item, specified using the [`handle` option](#option-handle). By default, each sortable item itself is also the handle.
+ `ui-sortable-placeholder`: The element used to show the future position of the item currently being sorted.
* `ui-sortable-helper`: The element shown while dragging a sortable item. The element actually used depends on the [`helper` option](#option-helper).
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
Options
-------
### appendTo
**Type:** [jQuery](http://api.jquery.com/Types/#jQuery) or [Element](http://api.jquery.com/Types/#Element) or [Selector](http://api.jquery.com/Types/#Selector) or [String](http://api.jquery.com/Types/#String) **Default:** `"parent"` Defines where the helper that moves with the mouse is being appended to during the drag (for example, to resolve overlap/zIndex issues). **Multiple types supported:*** **jQuery**: A jQuery object containing the element to append the helper to.
* **Element**: The element to append the helper to.
* **Selector**: A selector specifying which element to append the helper to.
* **String**: The string `"parent"` will cause the helper to be a sibling of the sortable item.
**Code examples:**Initialize the sortable with the `appendTo` option specified:
```
$( ".selector" ).sortable({
appendTo: document.body
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).sortable( "option", "appendTo" );
// Setter
$( ".selector" ).sortable( "option", "appendTo", document.body );
```
### axis
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` If defined, the items can be dragged only horizontally or vertically. Possible values: `"x"`, `"y"`. **Code examples:**Initialize the sortable with the `axis` option specified:
```
$( ".selector" ).sortable({
axis: "x"
});
```
Get or set the `axis` option, after initialization:
```
// Getter
var axis = $( ".selector" ).sortable( "option", "axis" );
// Setter
$( ".selector" ).sortable( "option", "axis", "x" );
```
### cancel
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"input,textarea,button,select,option"` Prevents sorting if you start on elements matching the selector. **Code examples:**Initialize the sortable with the `cancel` option specified:
```
$( ".selector" ).sortable({
cancel: "a,button"
});
```
Get or set the `cancel` option, after initialization:
```
// Getter
var cancel = $( ".selector" ).sortable( "option", "cancel" );
// Setter
$( ".selector" ).sortable( "option", "cancel", "a,button" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the sortable with the `classes` option specified, changing the theming for the `ui-sortable` class:
```
$( ".selector" ).sortable({
classes: {
"ui-sortable": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-sortable` class:
```
// Getter
var themeClass = $( ".selector" ).sortable( "option", "classes.ui-sortable" );
// Setter
$( ".selector" ).sortable( "option", "classes.ui-sortable", "highlight" );
```
### connectWith
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `false` A selector of other sortable elements that the items from this list should be connected to. This is a one-way relationship, if you want the items to be connected in both directions, the `connectWith` option must be set on both sortable elements. **Code examples:**Initialize the sortable with the `connectWith` option specified:
```
$( ".selector" ).sortable({
connectWith: "#shopping-cart"
});
```
Get or set the `connectWith` option, after initialization:
```
// Getter
var connectWith = $( ".selector" ).sortable( "option", "connectWith" );
// Setter
$( ".selector" ).sortable( "option", "connectWith", "#shopping-cart" );
```
### containment
**Type:** [Element](http://api.jquery.com/Types/#Element) or [Selector](http://api.jquery.com/Types/#Selector) or [String](http://api.jquery.com/Types/#String) **Default:** `false` Defines a bounding box that the sortable items are constrained to while dragging.
Note: The element specified for containment must have a calculated width and height (though it need not be explicit). For example, if you have `float: left` sortable children and specify `containment: "parent"` be sure to have `float: left` on the sortable/parent container as well or it will have `height: 0`, causing undefined behavior.
**Multiple types supported:*** **Element**: An element to use as the container.
* **Selector**: A selector specifying an element to use as the container.
* **String**: A string identifying an element to use as the container. Possible values: `"parent"`, `"document"`, `"window"`.
**Code examples:**Initialize the sortable with the `containment` option specified:
```
$( ".selector" ).sortable({
containment: "parent"
});
```
Get or set the `containment` option, after initialization:
```
// Getter
var containment = $( ".selector" ).sortable( "option", "containment" );
// Setter
$( ".selector" ).sortable( "option", "containment", "parent" );
```
### cursor
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"auto"` Defines the cursor that is being shown while sorting. **Code examples:**Initialize the sortable with the `cursor` option specified:
```
$( ".selector" ).sortable({
cursor: "move"
});
```
Get or set the `cursor` option, after initialization:
```
// Getter
var cursor = $( ".selector" ).sortable( "option", "cursor" );
// Setter
$( ".selector" ).sortable( "option", "cursor", "move" );
```
### cursorAt
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `false` Moves the sorting element or helper so the cursor always appears to drag from the same position. Coordinates can be given as a hash using a combination of one or two keys: `{ top, left, right, bottom }`. **Code examples:**Initialize the sortable with the `cursorAt` option specified:
```
$( ".selector" ).sortable({
cursorAt: { left: 5 }
});
```
Get or set the `cursorAt` option, after initialization:
```
// Getter
var cursorAt = $( ".selector" ).sortable( "option", "cursorAt" );
// Setter
$( ".selector" ).sortable( "option", "cursorAt", { left: 5 } );
```
### delay
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `0` Time in milliseconds to define when the sorting should start. Adding a delay helps preventing unwanted drags when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the sortable with the `delay` option specified:
```
$( ".selector" ).sortable({
delay: 150
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).sortable( "option", "delay" );
// Setter
$( ".selector" ).sortable( "option", "delay", 150 );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the sortable if set to `true`. **Code examples:**Initialize the sortable with the `disabled` option specified:
```
$( ".selector" ).sortable({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).sortable( "option", "disabled" );
// Setter
$( ".selector" ).sortable( "option", "disabled", true );
```
### distance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Tolerance, in pixels, for when sorting should start. If specified, sorting will not start until after mouse is dragged beyond distance. Can be used to allow for clicks on elements within a handle. (version deprecated: 1.12) **Code examples:**Initialize the sortable with the `distance` option specified:
```
$( ".selector" ).sortable({
distance: 5
});
```
Get or set the `distance` option, after initialization:
```
// Getter
var distance = $( ".selector" ).sortable( "option", "distance" );
// Setter
$( ".selector" ).sortable( "option", "distance", 5 );
```
### dropOnEmpty
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If `false`, items from this sortable can't be dropped on an empty connect sortable (see the [`connectWith`](#option-connectWith) option. **Code examples:**Initialize the sortable with the `dropOnEmpty` option specified:
```
$( ".selector" ).sortable({
dropOnEmpty: false
});
```
Get or set the `dropOnEmpty` option, after initialization:
```
// Getter
var dropOnEmpty = $( ".selector" ).sortable( "option", "dropOnEmpty" );
// Setter
$( ".selector" ).sortable( "option", "dropOnEmpty", false );
```
### forceHelperSize
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If `true`, forces the helper to have a size. **Code examples:**Initialize the sortable with the `forceHelperSize` option specified:
```
$( ".selector" ).sortable({
forceHelperSize: true
});
```
Get or set the `forceHelperSize` option, after initialization:
```
// Getter
var forceHelperSize = $( ".selector" ).sortable( "option", "forceHelperSize" );
// Setter
$( ".selector" ).sortable( "option", "forceHelperSize", true );
```
### forcePlaceholderSize
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If true, forces the placeholder to have a size. **Code examples:**Initialize the sortable with the `forcePlaceholderSize` option specified:
```
$( ".selector" ).sortable({
forcePlaceholderSize: true
});
```
Get or set the `forcePlaceholderSize` option, after initialization:
```
// Getter
var forcePlaceholderSize = $( ".selector" ).sortable( "option", "forcePlaceholderSize" );
// Setter
$( ".selector" ).sortable( "option", "forcePlaceholderSize", true );
```
### grid
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `false` Snaps the sorting element or helper to a grid, every x and y pixels. Array values: `[ x, y ]`. **Code examples:**Initialize the sortable with the `grid` option specified:
```
$( ".selector" ).sortable({
grid: [ 20, 10 ]
});
```
Get or set the `grid` option, after initialization:
```
// Getter
var grid = $( ".selector" ).sortable( "option", "grid" );
// Setter
$( ".selector" ).sortable( "option", "grid", [ 20, 10 ] );
```
### handle
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) **Default:** `false` Restricts sort start click to the specified element. **Code examples:**Initialize the sortable with the `handle` option specified:
```
$( ".selector" ).sortable({
handle: ".handle"
});
```
Get or set the `handle` option, after initialization:
```
// Getter
var handle = $( ".selector" ).sortable( "option", "handle" );
// Setter
$( ".selector" ).sortable( "option", "handle", ".handle" );
```
### helper
**Type:** [String](http://api.jquery.com/Types/#String) or [Function](http://api.jquery.com/Types/#Function)() **Default:** `"original"` Allows for a helper element to be used for dragging display. **Multiple types supported:*** **String**: If set to `"clone"`, then the element will be cloned and the clone will be dragged.
* **Function**: A function that will return a DOMElement to use while dragging. The function receives the event and the element being sorted.
**Code examples:**Initialize the sortable with the `helper` option specified:
```
$( ".selector" ).sortable({
helper: "clone"
});
```
Get or set the `helper` option, after initialization:
```
// Getter
var helper = $( ".selector" ).sortable( "option", "helper" );
// Setter
$( ".selector" ).sortable( "option", "helper", "clone" );
```
### items
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"> *"` Specifies which items inside the element should be sortable. **Code examples:**Initialize the sortable with the `items` option specified:
```
$( ".selector" ).sortable({
items: "> li"
});
```
Get or set the `items` option, after initialization:
```
// Getter
var items = $( ".selector" ).sortable( "option", "items" );
// Setter
$( ".selector" ).sortable( "option", "items", "> li" );
```
### opacity
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Defines the opacity of the helper while sorting. From `0.01` to `1`. **Code examples:**Initialize the sortable with the `opacity` option specified:
```
$( ".selector" ).sortable({
opacity: 0.5
});
```
Get or set the `opacity` option, after initialization:
```
// Getter
var opacity = $( ".selector" ).sortable( "option", "opacity" );
// Setter
$( ".selector" ).sortable( "option", "opacity", 0.5 );
```
### placeholder
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` A class name that gets applied to the otherwise white space. **Code examples:**Initialize the sortable with the `placeholder` option specified:
```
$( ".selector" ).sortable({
placeholder: "sortable-placeholder"
});
```
Get or set the `placeholder` option, after initialization:
```
// Getter
var placeholder = $( ".selector" ).sortable( "option", "placeholder" );
// Setter
$( ".selector" ).sortable( "option", "placeholder", "sortable-placeholder" );
```
### revert
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Whether the sortable items should revert to their new positions using a smooth animation. **Multiple types supported:*** **Boolean**: When set to `true`, the items will animate with the default duration.
* **Number**: The duration for the animation, in milliseconds.
**Code examples:**Initialize the sortable with the `revert` option specified:
```
$( ".selector" ).sortable({
revert: true
});
```
Get or set the `revert` option, after initialization:
```
// Getter
var revert = $( ".selector" ).sortable( "option", "revert" );
// Setter
$( ".selector" ).sortable( "option", "revert", true );
```
### scroll
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to true, the page scrolls when coming to an edge. **Code examples:**Initialize the sortable with the `scroll` option specified:
```
$( ".selector" ).sortable({
scroll: false
});
```
Get or set the `scroll` option, after initialization:
```
// Getter
var scroll = $( ".selector" ).sortable( "option", "scroll" );
// Setter
$( ".selector" ).sortable( "option", "scroll", false );
```
### scrollSensitivity
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `20` Defines how near the mouse must be to an edge to start scrolling. **Code examples:**Initialize the sortable with the `scrollSensitivity` option specified:
```
$( ".selector" ).sortable({
scrollSensitivity: 10
});
```
Get or set the `scrollSensitivity` option, after initialization:
```
// Getter
var scrollSensitivity = $( ".selector" ).sortable( "option", "scrollSensitivity" );
// Setter
$( ".selector" ).sortable( "option", "scrollSensitivity", 10 );
```
### scrollSpeed
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `20` The speed at which the window should scroll once the mouse pointer gets within the [`scrollSensitivity`](#option-scrollSensitivity) distance. **Code examples:**Initialize the sortable with the `scrollSpeed` option specified:
```
$( ".selector" ).sortable({
scrollSpeed: 40
});
```
Get or set the `scrollSpeed` option, after initialization:
```
// Getter
var scrollSpeed = $( ".selector" ).sortable( "option", "scrollSpeed" );
// Setter
$( ".selector" ).sortable( "option", "scrollSpeed", 40 );
```
### tolerance
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"intersect"` Specifies which mode to use for testing whether the item being moved is hovering over another item. Possible values: * `"intersect"`: The item overlaps the other item by at least 50%.
* `"pointer"`: The mouse pointer overlaps the other item.
**Code examples:**Initialize the sortable with the `tolerance` option specified:
```
$( ".selector" ).sortable({
tolerance: "pointer"
});
```
Get or set the `tolerance` option, after initialization:
```
// Getter
var tolerance = $( ".selector" ).sortable( "option", "tolerance" );
// Setter
$( ".selector" ).sortable( "option", "tolerance", "pointer" );
```
### zIndex
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `1000` Z-index for element/helper while being sorted. **Code examples:**Initialize the sortable with the `zIndex` option specified:
```
$( ".selector" ).sortable({
zIndex: 9999
});
```
Get or set the `zIndex` option, after initialization:
```
// Getter
var zIndex = $( ".selector" ).sortable( "option", "zIndex" );
// Setter
$( ".selector" ).sortable( "option", "zIndex", 9999 );
```
Methods
-------
### cancel()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Cancels a change in the current sortable and reverts it to the state prior to when the current sort was started. Useful in the stop and receive callback functions. * This method does not accept any arguments.
**Code examples:**Invoke the cancel method:
```
$( ".selector" ).sortable( "cancel" );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the sortable functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).sortable( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the sortable. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).sortable( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the sortable. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).sortable( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the sortable's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the sortable plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).sortable( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).sortable( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current sortable options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).sortable( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the sortable option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).sortable( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the sortable. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).sortable( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Refresh the sortable items. Triggers the reloading of all sortable items, causing new items to be recognized. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).sortable( "refresh" );
```
### refreshPositions()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Refresh the cached positions of the sortable items. Calling this method refreshes the cached item positions of all sortables. * This method does not accept any arguments.
**Code examples:**Invoke the refreshPositions method:
```
$( ".selector" ).sortable( "refreshPositions" );
```
### serialize( options )Returns: [String](http://api.jquery.com/Types/#String)
Serializes the sortable's item `id`s into a form/ajax submittable string. Calling this method produces a hash that can be appended to any url to easily submit a new item order back to the server.
It works by default by looking at the `id` of each item in the format `"setname_number"`, and it spits out a hash like `"setname[]=number&setname[]=number"`.
*Note: If serialize returns an empty string, make sure the `id` attributes include an underscore. They must be in the form: `"set_number"` For example, a 3 element list with `id` attributes `"foo_1"`, `"foo_5"`, `"foo_2"` will serialize to `"foo[]=1&foo[]=5&foo[]=2"`. You can use an underscore, equal sign or hyphen to separate the set and number. For example `"foo=1"`, `"foo-1"`, and `"foo_1"` all serialize to `"foo[]=1"`.*
* **options** Type: [Object](http://api.jquery.com/Types/#Object) Options to customize the serialization.
+ **key** (default: `the part of the attribute in front of the separator`) Type: [String](http://api.jquery.com/Types/#String) Replaces `part1[]` with the specified value.
+ **attribute** (default: `"id"`) Type: [String](http://api.jquery.com/Types/#String) The name of the attribute to use for the values.
+ **expression** (default: `/(.+)[-=_](.+)/`) Type: [RegExp](http://api.jquery.com/Types/#RegExp) A regular expression used to split the attribute value into key and value parts.
**Code examples:**Invoke the serialize method:
```
var sorted = $( ".selector" ).sortable( "serialize", { key: "sort" } );
```
### toArray( options )Returns: [Array](http://api.jquery.com/Types/#Array)
Serializes the sortable's item id's into an array of string. * **options** Type: [Object](http://api.jquery.com/Types/#Object) Options to customize the serialization.
+ **attribute** (default: `"id"`) Type: [String](http://api.jquery.com/Types/#String) The name of the attribute to use for the values.
**Code examples:**Invoke the toArray method:
```
var sortedIDs = $( ".selector" ).sortable( "toArray" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the sortable element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).sortable( "widget" );
```
Events
------
### activate( event, ui )Type: `sortactivate`
This event is triggered when using connected lists, every connected list on drag start receives it. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the activate callback specified:
```
$( ".selector" ).sortable({
activate: function( event, ui ) {}
});
```
Bind an event listener to the sortactivate event:
```
$( ".selector" ).on( "sortactivate", function( event, ui ) {} );
```
### beforeStop( event, ui )Type: `sortbeforestop`
This event is triggered when sorting stops, but when the placeholder/helper is still available. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the beforeStop callback specified:
```
$( ".selector" ).sortable({
beforeStop: function( event, ui ) {}
});
```
Bind an event listener to the sortbeforestop event:
```
$( ".selector" ).on( "sortbeforestop", function( event, ui ) {} );
```
### change( event, ui )Type: `sortchange`
This event is triggered during sorting, but only when the DOM position has changed. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the change callback specified:
```
$( ".selector" ).sortable({
change: function( event, ui ) {}
});
```
Bind an event listener to the sortchange event:
```
$( ".selector" ).on( "sortchange", function( event, ui ) {} );
```
### create( event, ui )Type: `sortcreate`
Triggered when the sortable is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the sortable with the create callback specified:
```
$( ".selector" ).sortable({
create: function( event, ui ) {}
});
```
Bind an event listener to the sortcreate event:
```
$( ".selector" ).on( "sortcreate", function( event, ui ) {} );
```
### deactivate( event, ui )Type: `sortdeactivate`
This event is triggered when sorting was stopped, is propagated to all possible connected lists. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the deactivate callback specified:
```
$( ".selector" ).sortable({
deactivate: function( event, ui ) {}
});
```
Bind an event listener to the sortdeactivate event:
```
$( ".selector" ).on( "sortdeactivate", function( event, ui ) {} );
```
### out( event, ui )Type: `sortout`
This event is triggered when a sortable item is moved away from a sortable list.
*Note: This event is also triggered when a sortable item is dropped.*
* **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the out callback specified:
```
$( ".selector" ).sortable({
out: function( event, ui ) {}
});
```
Bind an event listener to the sortout event:
```
$( ".selector" ).on( "sortout", function( event, ui ) {} );
```
### over( event, ui )Type: `sortover`
This event is triggered when a sortable item is moved into a sortable list. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the over callback specified:
```
$( ".selector" ).sortable({
over: function( event, ui ) {}
});
```
Bind an event listener to the sortover event:
```
$( ".selector" ).on( "sortover", function( event, ui ) {} );
```
### receive( event, ui )Type: `sortreceive`
This event is triggered when an item from a connected sortable list has been dropped into another list. The latter is the event target. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the receive callback specified:
```
$( ".selector" ).sortable({
receive: function( event, ui ) {}
});
```
Bind an event listener to the sortreceive event:
```
$( ".selector" ).on( "sortreceive", function( event, ui ) {} );
```
### remove( event, ui )Type: `sortremove`
This event is triggered when a sortable item from the list has been dropped into another. The former is the event target. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the remove callback specified:
```
$( ".selector" ).sortable({
remove: function( event, ui ) {}
});
```
Bind an event listener to the sortremove event:
```
$( ".selector" ).on( "sortremove", function( event, ui ) {} );
```
### sort( event, ui )Type: `sort`
This event is triggered during sorting. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the sort callback specified:
```
$( ".selector" ).sortable({
sort: function( event, ui ) {}
});
```
Bind an event listener to the sort event:
```
$( ".selector" ).on( "sort", function( event, ui ) {} );
```
### start( event, ui )Type: `sortstart`
This event is triggered when sorting starts. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the start callback specified:
```
$( ".selector" ).sortable({
start: function( event, ui ) {}
});
```
Bind an event listener to the sortstart event:
```
$( ".selector" ).on( "sortstart", function( event, ui ) {} );
```
### stop( event, ui )Type: `sortstop`
This event is triggered when sorting has stopped. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the stop callback specified:
```
$( ".selector" ).sortable({
stop: function( event, ui ) {}
});
```
Bind an event listener to the sortstop event:
```
$( ".selector" ).on( "sortstop", function( event, ui ) {} );
```
### update( event, ui )Type: `sortupdate`
This event is triggered when the user stopped sorting and the DOM position has changed. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper being sorted.
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the current dragged element.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) The current absolute position of the helper represented as `{ top, left }`.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) The current position of the helper represented as `{ top, left }`.
+ **originalPosition** Type: [Object](http://api.jquery.com/Types/#Object) The original position of the element represented as `{ top, left }`.
+ **sender** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The sortable that the item comes from if moving from one sortable to another.
+ **placeholder** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the element being used as a placeholder.
**Code examples:**Initialize the sortable with the update callback specified:
```
$( ".selector" ).sortable({
update: function( event, ui ) {}
});
```
Bind an event listener to the sortupdate event:
```
$( ".selector" ).on( "sortupdate", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Sortable.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>sortable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<ul id="sortable">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>$("#sortable").sortable();</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Slide Effect Slide Effect
============
Slide Effect
------------
**Description:** Slides the element out of the viewport.
* #### slide
+ **direction** (default: `"left"`) Type: [String](http://api.jquery.com/Types/#String) The direction of the effect. Possible values: `"left"`, `"right"`, `"up"`, `"down"`.
+ **distance** (default: `element's outerWidth`) Type: [Number](http://api.jquery.com/Types/#Number) The distance of the effect. Defaults to either the height or width of the element depending on the `direction` argument. Can be set to any integer less than the width/height of the element.
Example:
--------
Toggle a div using the slide effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>slide demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "slide" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Puff Effect Puff Effect
===========
Puff Effect
-----------
**Description:** Creates a puff effect by scaling the element up and hiding it at the same time.
* #### puff
+ **percent** (default: `150`) Type: [Number](http://api.jquery.com/Types/#Number) The percentage to scale to.
Example:
--------
Toggle a div using the puff effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>puff demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "puff" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .disableSelection() .disableSelection()
===================
.disableSelection()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
--------------------------------------------------------------------------
**Description:** Disable selection of text content within the set of matched elements.
* #### version added: 1.6, deprecated: 1.9[.disableSelection()](#disableSelection)
+ This method does not accept any arguments.
Disabling text selection is bad. Don't use this.
jqueryui Draggable Widget Draggable Widget
================
Draggable Widgetversion added: 1.0
-----------------------------------
**Description:** Allow elements to be moved using the mouse.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[addClasses](#option-addClasses) [appendTo](#option-appendTo) [axis](#option-axis) [cancel](#option-cancel) [classes](#option-classes) [connectToSortable](#option-connectToSortable) [containment](#option-containment) [cursor](#option-cursor) [cursorAt](#option-cursorAt) [delay](#option-delay) [disabled](#option-disabled) [distance](#option-distance) [grid](#option-grid) [handle](#option-handle) [helper](#option-helper) [iframeFix](#option-iframeFix) [opacity](#option-opacity) [refreshPositions](#option-refreshPositions) [revert](#option-revert) [revertDuration](#option-revertDuration) [scope](#option-scope) [scroll](#option-scroll) [scrollSensitivity](#option-scrollSensitivity) [scrollSpeed](#option-scrollSpeed) [snap](#option-snap) [snapMode](#option-snapMode) [snapTolerance](#option-snapTolerance) [stack](#option-stack) [zIndex](#option-zIndex) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [widget](#method-widget) ### Events
[create](#event-create) [drag](#event-drag) [start](#event-start) [stop](#event-stop) Make the selected elements draggable by mouse. If you want not just drag, but drag & drop, see the [jQuery UI Droppable plugin](droppable), which provides a drop target for draggables.
### Theming
The draggable widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If draggable specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-draggable`: The draggable element. When the draggable is disabled, the `ui-draggable-disabled` class is added. While dragging, the `ui-draggable-dragging` class is added.
* `ui-draggable-handle`: The handle of the draggable, specified using the [`handle` option](#option-handle). By default, the draggable element itself is also the handle.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Mouse Interaction](mouse)
Options
-------
### addClasses
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `false`, will prevent the `ui-draggable` class from being added. This may be desired as a performance optimization when calling `.draggable()` on hundreds of elements. **Code examples:**Initialize the draggable with the `addClasses` option specified:
```
$( ".selector" ).draggable({
addClasses: false
});
```
Get or set the `addClasses` option, after initialization:
```
// Getter
var addClasses = $( ".selector" ).draggable( "option", "addClasses" );
// Setter
$( ".selector" ).draggable( "option", "addClasses", false );
```
### appendTo
**Type:** [jQuery](http://api.jquery.com/Types/#jQuery) or [Element](http://api.jquery.com/Types/#Element) or [Selector](http://api.jquery.com/Types/#Selector) or [String](http://api.jquery.com/Types/#String) **Default:** `"parent"` Which element the draggable helper should be appended to while dragging.
**Note:** The `appendTo` option only works when the [`helper`](#option-helper) option is set to not use the original element. **Multiple types supported:*** **jQuery**: A jQuery object containing the element to append the helper to.
* **Element**: The element to append the helper to.
* **Selector**: A selector specifying which element to append the helper to.
* **String**: The string `"parent"` will cause the helper to be a sibling of the draggable.
**Code examples:**Initialize the draggable with the `appendTo` option specified:
```
$( ".selector" ).draggable({
appendTo: "body"
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).draggable( "option", "appendTo" );
// Setter
$( ".selector" ).draggable( "option", "appendTo", "body" );
```
### axis
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `false` Constrains dragging to either the horizontal (x) or vertical (y) axis. Possible values: `"x"`, `"y"`. **Code examples:**Initialize the draggable with the `axis` option specified:
```
$( ".selector" ).draggable({
axis: "x"
});
```
Get or set the `axis` option, after initialization:
```
// Getter
var axis = $( ".selector" ).draggable( "option", "axis" );
// Setter
$( ".selector" ).draggable( "option", "axis", "x" );
```
### cancel
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `"input,textarea,button,select,option"` Prevents dragging from starting on specified elements. **Code examples:**Initialize the draggable with the `cancel` option specified:
```
$( ".selector" ).draggable({
cancel: ".title"
});
```
Get or set the `cancel` option, after initialization:
```
// Getter
var cancel = $( ".selector" ).draggable( "option", "cancel" );
// Setter
$( ".selector" ).draggable( "option", "cancel", ".title" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the draggable with the `classes` option specified, changing the theming for the `ui-draggable` class:
```
$( ".selector" ).draggable({
classes: {
"ui-draggable": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-draggable` class:
```
// Getter
var themeClass = $( ".selector" ).draggable( "option", "classes.ui-draggable" );
// Setter
$( ".selector" ).draggable( "option", "classes.ui-draggable", "highlight" );
```
### connectToSortable
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `false` Allows the draggable to be dropped onto the specified sortables. If this option is used, a draggable can be dropped onto a sortable list and then becomes part of it. Note: The [`helper`](#option-helper) option must be set to `"clone"` in order to work flawlessly. Requires the [jQuery UI Sortable plugin](sortable) to be included. **Code examples:**Initialize the draggable with the `connectToSortable` option specified:
```
$( ".selector" ).draggable({
connectToSortable: "#my-sortable"
});
```
Get or set the `connectToSortable` option, after initialization:
```
// Getter
var connectToSortable = $( ".selector" ).draggable( "option", "connectToSortable" );
// Setter
$( ".selector" ).draggable( "option", "connectToSortable", "#my-sortable" );
```
### containment
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) or [String](http://api.jquery.com/Types/#String) or [Array](http://api.jquery.com/Types/#Array) **Default:** `false` Constrains dragging to within the bounds of the specified element or region. **Multiple types supported:*** **Selector**: The draggable element will be contained to the bounding box of the first element found by the selector. If no element is found, no containment will be set.
* **Element**: The draggable element will be contained to the bounding box of this element.
* **String**: Possible values: `"parent"`, `"document"`, `"window"`.
* **Array**: An array defining a bounding box in the form `[ x1, y1, x2, y2 ]`.
**Code examples:**Initialize the draggable with the `containment` option specified:
```
$( ".selector" ).draggable({
containment: "parent"
});
```
Get or set the `containment` option, after initialization:
```
// Getter
var containment = $( ".selector" ).draggable( "option", "containment" );
// Setter
$( ".selector" ).draggable( "option", "containment", "parent" );
```
### cursor
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"auto"` The CSS cursor during the drag operation. **Code examples:**Initialize the draggable with the `cursor` option specified:
```
$( ".selector" ).draggable({
cursor: "crosshair"
});
```
Get or set the `cursor` option, after initialization:
```
// Getter
var cursor = $( ".selector" ).draggable( "option", "cursor" );
// Setter
$( ".selector" ).draggable( "option", "cursor", "crosshair" );
```
### cursorAt
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `false` Sets the offset of the dragging helper relative to the mouse cursor. Coordinates can be given as a hash using a combination of one or two keys: `{ top, left, right, bottom }`. **Code examples:**Initialize the draggable with the `cursorAt` option specified:
```
$( ".selector" ).draggable({
cursorAt: { left: 5 }
});
```
Get or set the `cursorAt` option, after initialization:
```
// Getter
var cursorAt = $( ".selector" ).draggable( "option", "cursorAt" );
// Setter
$( ".selector" ).draggable( "option", "cursorAt", { left: 5 } );
```
### delay
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` Time in milliseconds after mousedown until dragging should start. This option can be used to prevent unwanted drags when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the draggable with the `delay` option specified:
```
$( ".selector" ).draggable({
delay: 300
});
```
Get or set the `delay` option, after initialization:
```
// Getter
var delay = $( ".selector" ).draggable( "option", "delay" );
// Setter
$( ".selector" ).draggable( "option", "delay", 300 );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the draggable if set to `true`. **Code examples:**Initialize the draggable with the `disabled` option specified:
```
$( ".selector" ).draggable({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).draggable( "option", "disabled" );
// Setter
$( ".selector" ).draggable( "option", "disabled", true );
```
### distance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Distance in pixels after mousedown the mouse must move before dragging should start. This option can be used to prevent unwanted drags when clicking on an element. (version deprecated: 1.12) **Code examples:**Initialize the draggable with the `distance` option specified:
```
$( ".selector" ).draggable({
distance: 10
});
```
Get or set the `distance` option, after initialization:
```
// Getter
var distance = $( ".selector" ).draggable( "option", "distance" );
// Setter
$( ".selector" ).draggable( "option", "distance", 10 );
```
### grid
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `false` Snaps the dragging helper to a grid, every x and y pixels. The array must be of the form `[ x, y ]`. **Code examples:**Initialize the draggable with the `grid` option specified:
```
$( ".selector" ).draggable({
grid: [ 50, 20 ]
});
```
Get or set the `grid` option, after initialization:
```
// Getter
var grid = $( ".selector" ).draggable( "option", "grid" );
// Setter
$( ".selector" ).draggable( "option", "grid", [ 50, 20 ] );
```
### handle
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [Element](http://api.jquery.com/Types/#Element) **Default:** `false` If specified, restricts dragging from starting unless the mousedown occurs on the specified element(s). Only elements that descend from the draggable element are permitted. **Code examples:**Initialize the draggable with the `handle` option specified:
```
$( ".selector" ).draggable({
handle: "h2"
});
```
Get or set the `handle` option, after initialization:
```
// Getter
var handle = $( ".selector" ).draggable( "option", "handle" );
// Setter
$( ".selector" ).draggable( "option", "handle", "h2" );
```
### helper
**Type:** [String](http://api.jquery.com/Types/#String) or [Function](http://api.jquery.com/Types/#Function)() **Default:** `"original"` Allows for a helper element to be used for dragging display. **Multiple types supported:*** **String**: If set to `"clone"`, then the element will be cloned and the clone will be dragged.
* **Function**: A function that will return a DOMElement to use while dragging.
**Code examples:**Initialize the draggable with the `helper` option specified:
```
$( ".selector" ).draggable({
helper: "clone"
});
```
Get or set the `helper` option, after initialization:
```
// Getter
var helper = $( ".selector" ).draggable( "option", "helper" );
// Setter
$( ".selector" ).draggable( "option", "helper", "clone" );
```
### iframeFix
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Selector](http://api.jquery.com/Types/#Selector) **Default:** `false` Prevent iframes from capturing the mousemove events during a drag. Useful in combination with the [`cursorAt`](#option-cursorAt) option, or in any case where the mouse cursor may not be over the helper. **Multiple types supported:*** **Boolean**: When set to `true`, transparent overlays will be placed over all iframes on the page.
* **Selector**: Any iframes matching the selector will be covered by transparent overlays.
**Code examples:**Initialize the draggable with the `iframeFix` option specified:
```
$( ".selector" ).draggable({
iframeFix: true
});
```
Get or set the `iframeFix` option, after initialization:
```
// Getter
var iframeFix = $( ".selector" ).draggable( "option", "iframeFix" );
// Setter
$( ".selector" ).draggable( "option", "iframeFix", true );
```
### opacity
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Opacity for the helper while being dragged. **Code examples:**Initialize the draggable with the `opacity` option specified:
```
$( ".selector" ).draggable({
opacity: 0.35
});
```
Get or set the `opacity` option, after initialization:
```
// Getter
var opacity = $( ".selector" ).draggable( "option", "opacity" );
// Setter
$( ".selector" ).draggable( "option", "opacity", 0.35 );
```
### refreshPositions
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` If set to `true`, all droppable positions are calculated on every mousemove. *Caution: This solves issues on highly dynamic pages, but dramatically decreases performance.* **Code examples:**Initialize the draggable with the `refreshPositions` option specified:
```
$( ".selector" ).draggable({
refreshPositions: true
});
```
Get or set the `refreshPositions` option, after initialization:
```
// Getter
var refreshPositions = $( ".selector" ).draggable( "option", "refreshPositions" );
// Setter
$( ".selector" ).draggable( "option", "refreshPositions", true );
```
### revert
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) or [Function](http://api.jquery.com/Types/#Function)() **Default:** `false` Whether the element should revert to its start position when dragging stops. **Multiple types supported:*** **Boolean**: If set to `true` the element will always revert.
* **String**: If set to `"invalid"`, revert will only occur if the draggable has not been dropped on a droppable. For `"valid"`, it's the other way around.
* **Function**: A function to determine whether the element should revert to its start position. The function must return `true` to revert the element.
**Code examples:**Initialize the draggable with the `revert` option specified:
```
$( ".selector" ).draggable({
revert: true
});
```
Get or set the `revert` option, after initialization:
```
// Getter
var revert = $( ".selector" ).draggable( "option", "revert" );
// Setter
$( ".selector" ).draggable( "option", "revert", true );
```
### revertDuration
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `500` The duration of the revert animation, in milliseconds. Ignored if the [`revert`](#option-revert) option is `false`. **Code examples:**Initialize the draggable with the `revertDuration` option specified:
```
$( ".selector" ).draggable({
revertDuration: 200
});
```
Get or set the `revertDuration` option, after initialization:
```
// Getter
var revertDuration = $( ".selector" ).draggable( "option", "revertDuration" );
// Setter
$( ".selector" ).draggable( "option", "revertDuration", 200 );
```
### scope
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"default"` Used to group sets of draggable and droppable items, in addition to droppable's [`accept`](droppable#option-accept) option. A draggable with the same `scope` value as a droppable will be accepted by the droppable. **Code examples:**Initialize the draggable with the `scope` option specified:
```
$( ".selector" ).draggable({
scope: "tasks"
});
```
Get or set the `scope` option, after initialization:
```
// Getter
var scope = $( ".selector" ).draggable( "option", "scope" );
// Setter
$( ".selector" ).draggable( "option", "scope", "tasks" );
```
### scroll
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` If set to `true`, container auto-scrolls while dragging. **Code examples:**Initialize the draggable with the `scroll` option specified:
```
$( ".selector" ).draggable({
scroll: false
});
```
Get or set the `scroll` option, after initialization:
```
// Getter
var scroll = $( ".selector" ).draggable( "option", "scroll" );
// Setter
$( ".selector" ).draggable( "option", "scroll", false );
```
### scrollSensitivity
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `20` Distance in pixels from the edge of the viewport after which the viewport should scroll. Distance is relative to pointer, not the draggable. Ignored if the [`scroll`](#option-scroll) option is `false`. **Code examples:**Initialize the draggable with the `scrollSensitivity` option specified:
```
$( ".selector" ).draggable({
scrollSensitivity: 100
});
```
Get or set the `scrollSensitivity` option, after initialization:
```
// Getter
var scrollSensitivity = $( ".selector" ).draggable( "option", "scrollSensitivity" );
// Setter
$( ".selector" ).draggable( "option", "scrollSensitivity", 100 );
```
### scrollSpeed
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `20` The speed at which the window should scroll once the mouse pointer gets within the [`scrollSensitivity`](#option-scrollSensitivity) distance. Ignored if the [`scroll`](#option-scroll) option is `false`. **Code examples:**Initialize the draggable with the `scrollSpeed` option specified:
```
$( ".selector" ).draggable({
scrollSpeed: 100
});
```
Get or set the `scrollSpeed` option, after initialization:
```
// Getter
var scrollSpeed = $( ".selector" ).draggable( "option", "scrollSpeed" );
// Setter
$( ".selector" ).draggable( "option", "scrollSpeed", 100 );
```
### snap
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) or [Selector](http://api.jquery.com/Types/#Selector) **Default:** `false` Whether the element should snap to other elements. **Multiple types supported:*** **Boolean**: When set to `true`, the element will snap to all other draggable elements.
* **Selector**: A selector specifying which elements to snap to.
**Code examples:**Initialize the draggable with the `snap` option specified:
```
$( ".selector" ).draggable({
snap: true
});
```
Get or set the `snap` option, after initialization:
```
// Getter
var snap = $( ".selector" ).draggable( "option", "snap" );
// Setter
$( ".selector" ).draggable( "option", "snap", true );
```
### snapMode
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"both"` Determines which edges of snap elements the draggable will snap to. Ignored if the [`snap`](#option-snap) option is `false`. Possible values: `"inner"`, `"outer"`, `"both"`. **Code examples:**Initialize the draggable with the `snapMode` option specified:
```
$( ".selector" ).draggable({
snapMode: "inner"
});
```
Get or set the `snapMode` option, after initialization:
```
// Getter
var snapMode = $( ".selector" ).draggable( "option", "snapMode" );
// Setter
$( ".selector" ).draggable( "option", "snapMode", "inner" );
```
### snapTolerance
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `20` The distance in pixels from the snap element edges at which snapping should occur. Ignored if the [`snap`](#option-snap) option is `false`. **Code examples:**Initialize the draggable with the `snapTolerance` option specified:
```
$( ".selector" ).draggable({
snapTolerance: 30
});
```
Get or set the `snapTolerance` option, after initialization:
```
// Getter
var snapTolerance = $( ".selector" ).draggable( "option", "snapTolerance" );
// Setter
$( ".selector" ).draggable( "option", "snapTolerance", 30 );
```
### stack
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `false` Controls the z-index of the set of elements that match the selector, always brings the currently dragged item to the front. Very useful in things like window managers. **Code examples:**Initialize the draggable with the `stack` option specified:
```
$( ".selector" ).draggable({
stack: ".products"
});
```
Get or set the `stack` option, after initialization:
```
// Getter
var stack = $( ".selector" ).draggable( "option", "stack" );
// Setter
$( ".selector" ).draggable( "option", "stack", ".products" );
```
### zIndex
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `false` Z-index for the helper while being dragged. **Code examples:**Initialize the draggable with the `zIndex` option specified:
```
$( ".selector" ).draggable({
zIndex: 100
});
```
Get or set the `zIndex` option, after initialization:
```
// Getter
var zIndex = $( ".selector" ).draggable( "option", "zIndex" );
// Setter
$( ".selector" ).draggable( "option", "zIndex", 100 );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the draggable functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).draggable( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the draggable. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).draggable( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the draggable. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).draggable( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the draggable's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the draggable plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).draggable( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).draggable( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current draggable options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).draggable( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the draggable option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).draggable( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the draggable. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).draggable( "option", { disabled: true } );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the draggable element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).draggable( "widget" );
```
Events
------
### create( event, ui )Type: `dragcreate`
Triggered when the draggable is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the draggable with the create callback specified:
```
$( ".selector" ).draggable({
create: function( event, ui ) {}
});
```
Bind an event listener to the dragcreate event:
```
$( ".selector" ).on( "dragcreate", function( event, ui ) {} );
```
### drag( event, ui )Type: `drag`
Triggered while the mouse is moved during the dragging, immediately before the current move happens. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the helper as `{ top, left }` object. The values may be changed to modify where the element will be positioned. This is useful for custom containment, snapping, etc.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the helper as `{ top, left }` object.
**Code examples:**Initialize the draggable with the drag callback specified:
```
$( ".selector" ).draggable({
drag: function( event, ui ) {}
});
```
Bind an event listener to the drag event:
```
$( ".selector" ).on( "drag", function( event, ui ) {} );
```
Constrain movement via `ui.position`:
```
$( ".selector" ).draggable({
drag: function( event, ui ) {
// Keep the left edge of the element
// at least 100 pixels from the container
ui.position.left = Math.min( 100, ui.position.left );
}
});
```
### start( event, ui )Type: `dragstart`
Triggered when dragging starts. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the helper as `{ top, left }` object.
**Code examples:**Initialize the draggable with the start callback specified:
```
$( ".selector" ).draggable({
start: function( event, ui ) {}
});
```
Bind an event listener to the dragstart event:
```
$( ".selector" ).on( "dragstart", function( event, ui ) {} );
```
### stop( event, ui )Type: `dragstop`
Triggered when dragging stops. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **helper** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The jQuery object representing the helper that's being dragged.
+ **position** Type: [Object](http://api.jquery.com/Types/#Object) Current CSS position of the helper as `{ top, left }` object.
+ **offset** Type: [Object](http://api.jquery.com/Types/#Object) Current offset position of the helper as `{ top, left }` object.
**Code examples:**Initialize the draggable with the stop callback specified:
```
$( ".selector" ).draggable({
stop: function( event, ui ) {}
});
```
Bind an event listener to the dragstop event:
```
$( ".selector" ).on( "dragstop", function( event, ui ) {} );
```
Example:
--------
A simple jQuery UI Draggable
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>draggable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#draggable {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="draggable">Drag me</div>
<script>
$( "#draggable" ).draggable();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui :tabbable Selector :tabbable Selector
==================
tabbable selector
-----------------
**Description:** Selects elements which the user can focus via tabbing.
* #### jQuery( ":tabbable" )
Some elements are natively tabbable, while others require explicitly setting a positive tab index. In all cases, the element must be visible in order to be tabbable.
Elements of the following type are tabbable if they do not have a negative tab index and are not disabled: `input`, `select`, `textarea`, `button`, and `object`. Anchors are focusable if they have an `href` or positive `tabindex` attribute. `area` elements are focusable if they are inside a named map, have an `href` attribute, and there is a visible image using the map. All other elements are tabbable based solely on their `tabindex` attribute and visibility.
*Note: Elements with a negative tab index are [`:focusable`](focusable-selector), but not `:tabbable`.*
Example:
--------
Select tabbable elements and highlight them with a red border.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>tabbable demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
input {
border: 1px solid #000;
}
div {
padding: 5px;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div><input value="no tabindex"></div>
<div><input tabindex="5" value="positive tabindex"></div>
<div><input tabindex="-1" value="negative tabindex"></div>
<script>
$( ":tabbable" ).css( "border-color", "red" );
</script>
</body>
</html>
```
#### Demo:
jqueryui Selectmenu Widget Selectmenu Widget
=================
Selectmenu Widgetversion added: 1.11
-------------------------------------
**Description:** Duplicates and extends the functionality of a native HTML select element to overcome the limitations of the native control.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[appendTo](#option-appendTo) [classes](#option-classes) [disabled](#option-disabled) [icons](#option-icons) [position](#option-position) [width](#option-width) ### Methods
[close](#method-close) [destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [menuWidget](#method-menuWidget) [open](#method-open) [option](#method-option) [refresh](#method-refresh) [widget](#method-widget)
### Extension Points
[\_renderButtonItem](#method-_renderButtonItem) [\_renderItem](#method-_renderItem) [\_renderMenu](#method-_renderMenu) [\_resizeMenu](#method-_resizeMenu) ### Events
[change](#event-change) [close](#event-close) [create](#event-create) [focus](#event-focus) [open](#event-open) [select](#event-select) Selectmenu transforms a `<select>` element into a themeable and customizable control. The widget acts as a proxy to the original `<select>`; therefore the original element's state is maintained for form submission and serialization.
Selectmenu supports `<optgroup>` elements and custom markup to render specific presentations like multiple lines. The `<select>` and its options can be disabled by adding a `disabled` attribute.
**Note:** Support for `accesskey` on custom elements is extremely limited in browsers. As such, if there is an `accesskey` attribute on the `<select>` element, it will not work with the custom selectmenu. If there is an `accesskey` attribute on any of the `<option>` elements, using the accesskey may cause the original element and the custom element to be out of sync. However, most browsers don't support `accesskey` on `<option>` elements.
### Keyboard interaction
When the menu is open, the following key commands are available:
* `UP`/`LEFT`: Move focus to the previous item.
* `DOWN`/`RIGHT`: Move focus to the next item.
* `END`/`PAGE DOWN`: Move focus to the last item.
* `HOME`/`PAGE UP`: Move focus to the first item.
* `ESCAPE`: Close the menu.
* `ENTER`/`SPACE`: Select the currently focused item and close the menu.
* `ALT`/`OPTION` + `UP`/`DOWN`: Toggle the visibility of the menu.
When the menu is closed, the following key commands are available:
* `UP`/`LEFT`: Select the previous item.
* `DOWN`/`RIGHT`: Select the next item.
* `END`/`PAGE DOWN`: Select the last item.
* `HOME`/`PAGE UP`: Select the first item.
* `ALT`/`OPTION` + `UP`/`DOWN`: Toggle the visibility of the menu.
* `SPACE`: Open the menu.
### Theming
The selectmenu widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If selectmenu specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-selectmenu-button`: The button-like element replacing the native selectmenu on the page. Has the `ui-selectmenu-button-closed` class when closed, the `ui-selectmenu-button-open` class when open.
+ `ui-selectmenu-text`: The span representing the text portion of the button element.
+ `ui-selectmenu-icon`: The icon within the selectmenu button.
* `ui-selectmenu-menu`: The wrapper element around the [menu](menu#theming) used to display options to the user (not the menu itself). When the menu is open, the `ui-selectmenu-open` class is added.
+ `ui-selectmenu-optgroup`: One of the elements that replicates `<optgroup>` elements from native selects.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
* [Position](position)
* [Menu](menu)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### appendTo
**Type:** [Selector](http://api.jquery.com/Types/#Selector) **Default:** `null` Which element to append the menu to. When the value is `null`, the parents of the `<select>` are checked for a class name of `ui-front`. If an element with the `ui-front` class name is found, the menu is appended to that element. Regardless of the value, if no element is found, the menu is appended to the body. **Code examples:**Initialize the selectmenu with the `appendTo` option specified:
```
$( ".selector" ).selectmenu({
appendTo: "#someElem"
});
```
Get or set the `appendTo` option, after initialization:
```
// Getter
var appendTo = $( ".selector" ).selectmenu( "option", "appendTo" );
// Setter
$( ".selector" ).selectmenu( "option", "appendTo", "#someElem" );
```
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-selectmenu-button-closed": "ui-corner-all",
"ui-selectmenu-button-open": "ui-corner-top",
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the selectmenu with the `classes` option specified, changing the theming for the `ui-selectmenu-menu` class:
```
$( ".selector" ).selectmenu({
classes: {
"ui-selectmenu-menu": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-selectmenu-menu` class:
```
// Getter
var themeClass = $( ".selector" ).selectmenu( "option", "classes.ui-selectmenu-menu" );
// Setter
$( ".selector" ).selectmenu( "option", "classes.ui-selectmenu-menu", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the selectmenu if set to `true`. **Code examples:**Initialize the selectmenu with the `disabled` option specified:
```
$( ".selector" ).selectmenu({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).selectmenu( "option", "disabled" );
// Setter
$( ".selector" ).selectmenu( "option", "disabled", true );
```
### icons
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ button: "ui-icon-triangle-1-s" }` Icons to use for the button, matching [an icon defined by the jQuery UI CSS Framework](theming/icons). * button (string, default: "ui-icon-triangle-1-s")
**Code examples:**Initialize the selectmenu with the `icons` option specified:
```
$( ".selector" ).selectmenu({
icons: { button: "ui-icon-circle-triangle-s" }
});
```
Get or set the `icons` option, after initialization:
```
// Getter
var icons = $( ".selector" ).selectmenu( "option", "icons" );
// Setter
$( ".selector" ).selectmenu( "option", "icons", { button: "ui-icon-circle-triangle-s" } );
```
### position
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{ my: "left top", at: "left bottom", collision: "none" }` Identifies the position of the menu in relation to the associated button element. You can refer to the [jQuery UI Position](position) utility for more details about the various options. **Code examples:**Initialize the selectmenu with the `position` option specified:
```
$( ".selector" ).selectmenu({
position: { my : "left+10 center", at: "right center" }
});
```
Get or set the `position` option, after initialization:
```
// Getter
var position = $( ".selector" ).selectmenu( "option", "position" );
// Setter
$( ".selector" ).selectmenu( "option", "position", { my : "left+10 center", at: "right center" } );
```
### width
**Type:** [Number](http://api.jquery.com/Types/#Number) or [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` The width of the menu, in pixels. When the value is `null`, the width of the native select is used. When the value is `false`, no inline style will be set for the width, allowing the width to be set in a stylesheet. **Code examples:**Initialize the selectmenu with the `width` option specified:
```
$( ".selector" ).selectmenu({
width: 200
});
```
Get or set the `width` option, after initialization:
```
// Getter
var width = $( ".selector" ).selectmenu( "option", "width" );
// Setter
$( ".selector" ).selectmenu( "option", "width", 200 );
```
Methods
-------
### close()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Closes the menu. * This method does not accept any arguments.
**Code examples:**Invoke the close method:
```
$( ".selector" ).selectmenu( "close" );
```
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the selectmenu functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).selectmenu( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the selectmenu. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).selectmenu( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the selectmenu. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).selectmenu( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the selectmenu's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the selectmenu plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).selectmenu( "instance" );
```
### menuWidget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Returns a `jQuery` object containing the menu element. * This method does not accept any arguments.
**Code examples:**Invoke the menuWidget method:
```
$( ".selector" ).selectmenu( "menuWidget" );
```
### open()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Opens the menu. * This method does not accept any arguments.
**Code examples:**Invoke the open method:
```
$( ".selector" ).selectmenu( "open" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).selectmenu( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current selectmenu options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).selectmenu( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the selectmenu option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).selectmenu( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the selectmenu. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).selectmenu( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Parses the original element and re-renders the menu. Processes any `<option>` or `<optgroup>` elements that were added, removed or disabled. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).selectmenu( "refresh" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Returns a `jQuery` object containing the button element. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
$( ".selector" ).selectmenu( "widget" );
```
Extension Points
----------------
The selectmenu widget is built with the [widget factory](jquery.widget) and can be extended. When extending widgets, you have the ability to override or add to the behavior of existing methods. The following methods are provided as extension points with the same API stability as the [plugin methods](#methods) listed above. For more information on widget extensions, see [Extending Widgets with the Widget Factory](http://learn.jquery.com/jquery-ui/widget-factory/extending-widgets/).
### \_renderButtonItem( item )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Method that controls the creation of the generated button content. The method must create and return a new element.
* **item** Type: [Object](http://api.jquery.com/Types/#Object)
+ **disabled** Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the item is disabled.
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) A reference to the item's original `<option>` element.
+ **index** Type: [Number](http://api.jquery.com/Types/#Number) The numeric index of the item.
+ **label** Type: [String](http://api.jquery.com/Types/#String) The string to display for the item.
+ **optgroup** Type: [String](http://api.jquery.com/Types/#String) If the item is within an `<optgroup>`, this is set to that `<optgroup>`'s label.
+ **value** Type: [String](http://api.jquery.com/Types/#String) The `value` attribute of the item's original `<option>`.
**Code examples:**Style the button background color based on the value of the selected option.
```
_renderButtonItem: function( item ) {
var buttonItem = $( "<span>", {
"class": "ui-selectmenu-text"
})
this._setText( buttonItem, item.label );
buttonItem.css( "background-color", item.value )
return buttonItem;
}
```
### \_renderItem( ul, item )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Method that controls the creation of each option in the widget's menu. The method must create a new `<li>` element, append it to the menu, and return it. See the [Menu](menu) documentation for more details about the markup.
* **ul** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The `<ul>` element that the newly created `<li>` element must be appended to.
* **item** Type: [Object](http://api.jquery.com/Types/#Object)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The original `<option>` element.
+ **index** Type: [Integer](http://api.jquery.com/Types/#Integer) The index of the `<option>` within the `<select>`.
+ **value** Type: [String](http://api.jquery.com/Types/#String) The value of the `<option>`.
+ **label** Type: [String](http://api.jquery.com/Types/#String) The label of the `<option>`.
+ **optgroup** Type: [String](http://api.jquery.com/Types/#String) The label for the parent `optgroup`, if any.
+ **disabled** Type: [Boolean](http://api.jquery.com/Types/#Boolean) Whether the `<option>` is disabled.
**Code examples:**Style the menu item background colors based on the value of their corresponding option elements.
```
_renderItem: function( ul, item ) {
var li = $( "<li>" )
.css( "background-color", item.value );
if ( item.disabled ) {
li.addClass( "ui-state-disabled" );
}
this._setText( li, item.label );
return li.appendTo( ul );
}
```
### \_renderMenu( ul, items )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Method that controls building the widget's menu. The method is passed an empty `<ul>` and an array of items based on the `<option>` elements in the original `<select>`. Creation of the individual `<li>` elements should be delegated to `_renderItemData()`, which in turn delegates to the [`_renderItem()`](#method-_renderItem) extension point. * **ul** Type: [jQuery](http://api.jquery.com/Types/#jQuery) An empty `<ul>` element to use as the widget's menu.
* **items** Type: [Array](http://api.jquery.com/Types/#Array) An array of items based on the `<option>` elements in the original `<select>`. See the [`_renderItem()`](#method-_renderItem) extension point for details on the format of the item objects.
**Code examples:**
Add a CSS class name to the odd menu items.
**Note:** For simplicity, this example does not support optgroups or disabled menu items.
```
_renderMenu: function( ul, items ) {
var that = this;
$.each( items, function( index, item ) {
that._renderItemData( ul, item );
});
$( ul ).find( "li" ).odd().addClass( "odd" );
}
```
### \_resizeMenu()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Method responsible for sizing the menu before it is displayed. The menu element is available at `this.menu`. * This method does not accept any arguments.
**Code examples:**Always display the menu as 500 pixels wide.
```
_resizeMenu: function() {
this.menu.outerWidth( 500 );
}
```
Events
------
### change( event, ui )Type: `selectmenuchange`
Triggered when the selected item has changed. Not every [`select`](#event-select) event will fire a `change` event. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The active item.
**Code examples:**Initialize the selectmenu with the change callback specified:
```
$( ".selector" ).selectmenu({
change: function( event, ui ) {}
});
```
Bind an event listener to the selectmenuchange event:
```
$( ".selector" ).on( "selectmenuchange", function( event, ui ) {} );
```
### close( event )Type: `selectmenuclose`
Triggered when the menu is hidden. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectmenu with the close callback specified:
```
$( ".selector" ).selectmenu({
close: function( event, ui ) {}
});
```
Bind an event listener to the selectmenuclose event:
```
$( ".selector" ).on( "selectmenuclose", function( event, ui ) {} );
```
### create( event, ui )Type: `selectmenucreate`
Triggered when the selectmenu is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectmenu with the create callback specified:
```
$( ".selector" ).selectmenu({
create: function( event, ui ) {}
});
```
Bind an event listener to the selectmenucreate event:
```
$( ".selector" ).on( "selectmenucreate", function( event, ui ) {} );
```
### focus( event, ui )Type: `selectmenufocus`
Triggered when an items gains focus. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The focused item.
**Code examples:**Initialize the selectmenu with the focus callback specified:
```
$( ".selector" ).selectmenu({
focus: function( event, ui ) {}
});
```
Bind an event listener to the selectmenufocus event:
```
$( ".selector" ).on( "selectmenufocus", function( event, ui ) {} );
```
### open( event )Type: `selectmenuopen`
Triggered when the menu is opened. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the selectmenu with the open callback specified:
```
$( ".selector" ).selectmenu({
open: function( event, ui ) {}
});
```
Bind an event listener to the selectmenuopen event:
```
$( ".selector" ).on( "selectmenuopen", function( event, ui ) {} );
```
### select( event, ui )Type: `selectmenuselect`
Triggered when a menu item is selected. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
+ **item** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The selected item.
**Code examples:**Initialize the selectmenu with the select callback specified:
```
$( ".selector" ).selectmenu({
select: function( event, ui ) {}
});
```
Bind an event listener to the selectmenuselect event:
```
$( ".selector" ).on( "selectmenuselect", function( event, ui ) {} );
```
Examples:
---------
A simple jQuery UI Selectmenu
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>selectmenu demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
label { display: block; }
select { width: 200px; }
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label for="speed">Select a speed:</label>
<select name="speed" id="speed">
<option value="Slower">Slower</option>
<option value="Slow">Slow</option>
<option value="Medium" selected>Medium</option>
<option value="Fast">Fast</option>
<option value="Faster">Faster</option>
</select>
<script>
$( "#speed" ).selectmenu();
</script>
</body>
</html>
```
#### Demo:
A simple jQuery UI Selectmenu with optgroups
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>selectmenu demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
label { display: block; }
select { width: 200px; }
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label for="files">Select a file:</label>
<select name="files" id="files">
<optgroup label="Scripts">
<option value="jquery">jQuery.js</option>
<option value="jqueryui">ui.jQuery.js</option>
</optgroup>
<optgroup label="Other files">
<option value="somefile">Some unknown file</option>
<option value="someotherfile">Some other file</option>
</optgroup>
</select>
<script>
$( "#files" ).selectmenu();
</script>
</body>
</html>
```
#### Demo:
A jQuery UI Selectmenu with overflow
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>selectmenu demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
label { display: block; }
select { width: 200px; }
.overflow { height: 200px; }
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label for="number">Select a number:</label>
<select name="number" id="number">
<option value="1">1</option>
<option value="2" selected>2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
<option value="6">6</option>
<option value="7">7</option>
<option value="8">8</option>
<option value="9">9</option>
<option value="10">10</option>
<option value="11">11</option>
<option value="12">12</option>
<option value="13">13</option>
<option value="14">14</option>
<option value="15">15</option>
<option value="16">16</option>
<option value="17">17</option>
<option value="18">18</option>
<option value="19">19</option>
</select>
<script>
$( "#number" )
.selectmenu()
.selectmenu( "menuWidget" )
.addClass( "overflow" );
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Datepicker Widget Datepicker Widget
=================
Datepicker Widgetversion added: 1.0
------------------------------------
**Description:** Select a date from a popup or inline calendar
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[altField](#option-altField) [altFormat](#option-altFormat) [appendText](#option-appendText) [autoSize](#option-autoSize) [beforeShow](#option-beforeShow) [beforeShowDay](#option-beforeShowDay) [buttonImage](#option-buttonImage) [buttonImageOnly](#option-buttonImageOnly) [buttonText](#option-buttonText) [calculateWeek](#option-calculateWeek) [changeMonth](#option-changeMonth) [changeYear](#option-changeYear) [closeText](#option-closeText) [constrainInput](#option-constrainInput) [currentText](#option-currentText) [dateFormat](#option-dateFormat) [dayNames](#option-dayNames) [dayNamesMin](#option-dayNamesMin) [dayNamesShort](#option-dayNamesShort) [defaultDate](#option-defaultDate) [duration](#option-duration) [firstDay](#option-firstDay) [gotoCurrent](#option-gotoCurrent) [hideIfNoPrevNext](#option-hideIfNoPrevNext) [isRTL](#option-isRTL) [maxDate](#option-maxDate) [minDate](#option-minDate) [monthNames](#option-monthNames) [monthNamesShort](#option-monthNamesShort) [navigationAsDateFormat](#option-navigationAsDateFormat) [nextText](#option-nextText) [numberOfMonths](#option-numberOfMonths) [onChangeMonthYear](#option-onChangeMonthYear) [onClose](#option-onClose) [onSelect](#option-onSelect) [onUpdateDatepicker](#option-onUpdateDatepicker) [prevText](#option-prevText) [selectOtherMonths](#option-selectOtherMonths) [shortYearCutoff](#option-shortYearCutoff) [showAnim](#option-showAnim) [showButtonPanel](#option-showButtonPanel) [showCurrentAtPos](#option-showCurrentAtPos) [showMonthAfterYear](#option-showMonthAfterYear) [showOn](#option-showOn) [showOptions](#option-showOptions) [showOtherMonths](#option-showOtherMonths) [showWeek](#option-showWeek) [stepMonths](#option-stepMonths) [weekHeader](#option-weekHeader) [yearRange](#option-yearRange) [yearSuffix](#option-yearSuffix) ### Methods
[destroy](#method-destroy) [dialog](#method-dialog) [getDate](#method-getDate) [hide](#method-hide) [isDisabled](#method-isDisabled) [option](#method-option) [refresh](#method-refresh) [setDate](#method-setDate) [show](#method-show) [widget](#method-widget) ### Events
The jQuery UI Datepicker is a highly configurable plugin that adds datepicker functionality to your pages. You can customize the date format and language, restrict the selectable date ranges and add in buttons and other navigation options easily.
By default, the datepicker calendar opens in a small overlay when the associated text field gains focus. For an inline calendar, simply attach the datepicker to a div or span.
### Keyboard interaction
While the datepicker is open, the following key commands are available:
* `PAGE UP`: Move to the previous month.
* `PAGE DOWN`: Move to the next month.
* `CTRL` + `PAGE UP`: Move to the previous year.
* `CTRL` + `PAGE DOWN`: Move to the next year.
* `CTRL` + `HOME`: Open the datepicker if closed.
* `CTRL`/`COMMAND` + `HOME`: Move to the current month.
* `CTRL`/`COMMAND` + `LEFT`: Move to the previous day.
* `CTRL`/`COMMAND` + `RIGHT`: Move to the next day.
* `CTRL`/`COMMAND` + `UP`: Move to the previous week.
* `CTRL`/`COMMAND` + `DOWN`: Move to the next week.
* `ENTER`: Select the focused date.
* `CTRL`/`COMMAND` + `END`: Close the datepicker and erase the date.
* `ESCAPE`: Close the datepicker without selection.
### Utility functions
#### $.datepicker.setDefaults( options )
Change the default options for all date pickers.
Use the [`option()`](#method-option) method to change options for individual instances.
**Code examples:** Set all date pickers to open on focus or a click on an icon.
```
$.datepicker.setDefaults({
showOn: "both",
buttonImageOnly: true,
buttonImage: "calendar.gif",
buttonText: "Calendar"
});
```
Set all date pickers to have French text.
```
$.datepicker.setDefaults( $.datepicker.regional[ "fr" ] );
```
#### $.datepicker.formatDate( format, date, options )
Format a date into a string value with a specified format.
The format can be combinations of the following:
* d - day of month (no leading zero)
* dd - day of month (two digit)
* o - day of the year (no leading zeros)
* oo - day of the year (three digit)
* D - day name short
* DD - day name long
* m - month of year (no leading zero)
* mm - month of year (two digit)
* M - month name short
* MM - month name long
* y - year (two digit)
* yy - year (four digit)
* @ - Unix timestamp (ms since 01/01/1970)
* ! - Windows ticks (100ns since 01/01/0001)
* '...' - literal text
* '' - single quote
* anything else - literal text
There are also a number of predefined standard date formats available from `$.datepicker`:
* ATOM - 'yy-mm-dd' (Same as RFC 3339/ISO 8601)
* COOKIE - 'D, dd M yy'
* ISO\_8601 - 'yy-mm-dd'
* RFC\_822 - 'D, d M y' (See RFC 822)
* RFC\_850 - 'DD, dd-M-y' (See RFC 850)
* RFC\_1036 - 'D, d M y' (See RFC 1036)
* RFC\_1123 - 'D, d M yy' (See RFC 1123)
* RFC\_2822 - 'D, d M yy' (See RFC 2822)
* RSS - 'D, d M y' (Same as RFC 822)
* TICKS - '!'
* TIMESTAMP - '@'
* W3C - 'yy-mm-dd' (Same as ISO 8601)
**Code examples:** Display the date in ISO format. Produces "2007-01-26".
```
$.datepicker.formatDate( "yy-mm-dd", new Date( 2007, 1 - 1, 26 ) );
```
Display the date in expanded French format. Produces "Samedi, Juillet 14, 2007".
```
$.datepicker.formatDate( "DD, MM d, yy", new Date( 2007, 7 - 1, 14 ), {
dayNamesShort: $.datepicker.regional[ "fr" ].dayNamesShort,
dayNames: $.datepicker.regional[ "fr" ].dayNames,
monthNamesShort: $.datepicker.regional[ "fr" ].monthNamesShort,
monthNames: $.datepicker.regional[ "fr" ].monthNames
});
```
#### $.datepicker.parseDate( format, value, options )
Extract a date from a string value with a specified format.
The format can be combinations of the following:
* d - day of month (no leading zero)
* dd - day of month (two digit)
* o - day of year (no leading zeros)
* oo - day of year (three digit)
* D - day name short
* DD - day name long
* m - month of year (no leading zero)
* mm - month of year (two digit)
* M - month name short
* MM - month name long
* y - year (two digit)
* yy - year (four digit)
* @ - Unix timestamp (ms since 01/01/1970)
* ! - Windows ticks (100ns since 01/01/0001)
* '...' - literal text
* '' - single quote
* anything else - literal text
A number of exceptions may be thrown:
* 'Invalid arguments' if either format or value is null
* 'Missing number at position nn' if format indicated a numeric value that is not then found
* 'Unknown name at position nn' if format indicated day or month name that is not then found
* 'Unexpected literal at position nn' if format indicated a literal value that is not then found
* 'Invalid date' if the date is invalid, such as '31/02/2007'
**Code examples:** Extract a date in ISO format.
```
$.datepicker.parseDate( "yy-mm-dd", "2007-01-26" );
```
Extract a date in expanded French format.
```
$.datepicker.parseDate( "DD, MM d, yy", "Samedi, Juillet 14, 2007", {
shortYearCutoff: 20,
dayNamesShort: $.datepicker.regional[ "fr" ].dayNamesShort,
dayNames: $.datepicker.regional[ "fr" ].dayNames,
monthNamesShort: $.datepicker.regional[ "fr" ].monthNamesShort,
monthNames: $.datepicker.regional[ "fr" ].monthNames
});
```
#### $.datepicker.iso8601Week( date )
Determine the week of the year for a given date: 1 to 53.
This function uses the ISO 8601 definition of a week: weeks start on a Monday and the first week of the year contains January 4. This means that up to three days from the previous year may be included in the of first week of the current year, and that up to three days from the current year may be included in the last week of the previous year.
This function is the default implementation for the [`calculateWeek`](#option-calculateWeek) option.
**Code examples:** Find the week of the year for a date.
```
$.datepicker.iso8601Week( new Date( 2007, 1 - 1, 26 ) );
```
#### $.datepicker.noWeekends
Set as beforeShowDay function to prevent selection of weekends.
We can provide the `noWeekends()` function into the [`beforeShowDay`](#option-beforeShowDay) option which will calculate all the weekdays and provide an array of `true`/`false` values indicating whether a date is selectable.
**Code examples:** Set the DatePicker so no weekend is selectable
```
$( "#datepicker" ).datepicker({
beforeShowDay: $.datepicker.noWeekends
});
```
### Localization
Datepicker provides support for localizing its content to cater for different languages and date formats. Each localization is contained within its own file with the language code appended to the name, e.g., `jquery.ui.datepicker-fr.js` for French. The desired localization file should be included after the main datepicker code. Each localization file adds its options to the set of available localizations and automatically applies them as defaults for all instances. Localization files can be found at <https://github.com/jquery/jquery-ui/tree/master/ui/i18n>.
The `$.datepicker.regional` attribute holds an array of localizations, indexed by language code, with `""` referring to the default (English). Each entry is an object with the following attributes: `closeText`, `prevText`, `nextText`, `currentText`, `monthNames`, `monthNamesShort`, `dayNames`, `dayNamesShort`, `dayNamesMin`, `weekHeader`, `dateFormat`, `firstDay`, `isRTL`, `showMonthAfterYear`, and `yearSuffix`.
You can restore the default localizations with:
`$.datepicker.setDefaults( $.datepicker.regional[ "" ] );` And can then override an individual datepicker for a specific locale:
`$( selector ).datepicker( $.datepicker.regional[ "fr" ] );` ### Theming
The datepicker widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If datepicker specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-datepicker`: The outer container of the datepicker. If the datepicker is inline, this element will additionally have a `ui-datepicker-inline` class. If the [`isRTL`](#option-isRTL) option is set, this element will additionally have a class of `ui-datepicker-rtl`.
+ `ui-datepicker-header`: The container for the datepicker's header.
- `ui-datepicker-prev`: The control used to select previous months.
- `ui-datepicker-next`: The control used to select subsequent months.
- `ui-datepicker-title`: The container for the datepicker's title containing the month and year.
* `ui-datepicker-month`: The textual display of the month or a `<select>` element if the [`changeMonth`](#option-changeMonth) option is set.
* `ui-datepicker-year`: The textual display of the year or a `<select>` element if the [`changeYear`](#option-changeYear) option is set.
+ `ui-datepicker-calendar`: The table that contains the calendar itself.
- `ui-datepicker-week-end`: Cells containing weekend days.
- `ui-datepicker-other-month`: Cells containing days that occur in a month other than the currently selected month.
- `ui-datepicker-unselectable`: Cells containing days that are not selectable by the user.
- `ui-datepicker-current-day`: The cell containing the selected day.
- `ui-datepicker-today`: The cell containing today's date.
+ `ui-datepicker-buttonpane`: The buttonpane that is used when the [`showButtonPanel`](#option-showButtonPanel) option is set.
- `ui-datepicker-current`: The button used to select today's date.
If the [`numberOfMonths`](#option-numberOfMonths) option is used to display multiple months at once, a number of additional classes are used:
* `ui-datepicker-multi`: The outermost container of a multiple month datepicker. This element can additionally have a `ui-datepicker-multi-2`, `ui-datepicker-multi-3`, or `ui-datepicker-multi-4` class name depending on the number of months to display.
+ `ui-datepicker-group`: Individual pickers within the group. This element will additionally have a `ui-datepicker-group-first`, `ui-datepicker-group-middle`, or `ui-datepicker-group-last` class name depending on its position within the group.
### Dependencies
* [UI Core](category/ui-core)
* [Effects Core](category/effects-core) (optional; for use with the [`showAnim`](#option-showAnim) option)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
* This widget manipulates its element's value programmatically, therefore a native `change` event may not be fired when the element's value changes.
* Creating a datepicker on an `<input type="date">` is not supported due to a UI conflict with the native picker.
Options
-------
### altField
**Type:** [Selector](http://api.jquery.com/Types/#Selector) or [jQuery](http://api.jquery.com/Types/#jQuery) or [Element](http://api.jquery.com/Types/#Element) **Default:** `""` An input element that is to be updated with the selected date from the datepicker. Use the [`altFormat`](#option-altFormat) option to change the format of the date within this field. Leave as blank for no alternate field. **Code examples:**Initialize the datepicker with the `altField` option specified:
```
$( ".selector" ).datepicker({
altField: "#actualDate"
});
```
Get or set the `altField` option, after initialization:
```
// Getter
var altField = $( ".selector" ).datepicker( "option", "altField" );
// Setter
$( ".selector" ).datepicker( "option", "altField", "#actualDate" );
```
### altFormat
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `""` The [`dateFormat`](#option-dateFormat) to be used for the [`altField`](#option-altField) option. This allows one date format to be shown to the user for selection purposes, while a different format is actually sent behind the scenes. For a full list of the possible formats see the [`formatDate`](#utility-formatDate) function **Code examples:**Initialize the datepicker with the `altFormat` option specified:
```
$( ".selector" ).datepicker({
altFormat: "yy-mm-dd"
});
```
Get or set the `altFormat` option, after initialization:
```
// Getter
var altFormat = $( ".selector" ).datepicker( "option", "altFormat" );
// Setter
$( ".selector" ).datepicker( "option", "altFormat", "yy-mm-dd" );
```
### appendText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `""` The text to display after each date field, e.g., to show the required format. **Code examples:**Initialize the datepicker with the `appendText` option specified:
```
$( ".selector" ).datepicker({
appendText: "(yyyy-mm-dd)"
});
```
Get or set the `appendText` option, after initialization:
```
// Getter
var appendText = $( ".selector" ).datepicker( "option", "appendText" );
// Setter
$( ".selector" ).datepicker( "option", "appendText", "(yyyy-mm-dd)" );
```
### autoSize
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Set to `true` to automatically resize the input field to accommodate dates in the current [`dateFormat`](#option-dateFormat). **Code examples:**Initialize the datepicker with the `autoSize` option specified:
```
$( ".selector" ).datepicker({
autoSize: true
});
```
Get or set the `autoSize` option, after initialization:
```
// Getter
var autoSize = $( ".selector" ).datepicker( "option", "autoSize" );
// Setter
$( ".selector" ).datepicker( "option", "autoSize", true );
```
### beforeShow
**Type:** [Function](http://api.jquery.com/Types/#Function)( [Element](http://api.jquery.com/Types/#Element) input, [Object](http://api.jquery.com/Types/#Object) inst ) **Default:** `null` A function that takes an input field and current datepicker instance and returns an options object to update the datepicker with. It is called just before the datepicker is displayed. ### beforeShowDay
**Type:** [Function](http://api.jquery.com/Types/#Function)( [Date](http://api.jquery.com/Types/#Date) date ) **Default:** `null` A function that takes a date as a parameter and must return an array with: * `[0]`: `true`/`false` indicating whether or not this date is selectable
* `[1]`: a CSS class name to add to the date's cell or `""` for the default presentation
* `[2]`: an optional popup tooltip for this date
The function is called for each day in the datepicker before it is displayed. ### buttonImage
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `""` A URL of an image to use to display the datepicker when the [`showOn`](#option-showOn) option is set to `"button"` or `"both"`. If set, the [`buttonText`](#option-buttonText) option becomes the `alt` value and is not directly displayed. **Code examples:**Initialize the datepicker with the `buttonImage` option specified:
```
$( ".selector" ).datepicker({
buttonImage: "/images/datepicker.gif"
});
```
Get or set the `buttonImage` option, after initialization:
```
// Getter
var buttonImage = $( ".selector" ).datepicker( "option", "buttonImage" );
// Setter
$( ".selector" ).datepicker( "option", "buttonImage", "/images/datepicker.gif" );
```
### buttonImageOnly
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the button image should be rendered by itself instead of inside a button element. This option is only relevant if the [`buttonImage`](#option-buttonImage) option has also been set. **Code examples:**Initialize the datepicker with the `buttonImageOnly` option specified:
```
$( ".selector" ).datepicker({
buttonImageOnly: true
});
```
Get or set the `buttonImageOnly` option, after initialization:
```
// Getter
var buttonImageOnly = $( ".selector" ).datepicker( "option", "buttonImageOnly" );
// Setter
$( ".selector" ).datepicker( "option", "buttonImageOnly", true );
```
### buttonText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"..."` The text to display on the trigger button. Use in conjunction with the [`showOn`](#option-showOn) option set to `"button"` or `"both"`. **Code examples:**Initialize the datepicker with the `buttonText` option specified:
```
$( ".selector" ).datepicker({
buttonText: "Choose"
});
```
Get or set the `buttonText` option, after initialization:
```
// Getter
var buttonText = $( ".selector" ).datepicker( "option", "buttonText" );
// Setter
$( ".selector" ).datepicker( "option", "buttonText", "Choose" );
```
### calculateWeek
**Type:** [Function](http://api.jquery.com/Types/#Function)() **Default:** `jQuery.datepicker.iso8601Week` A function to calculate the week of the year for a given date. The default implementation uses the ISO 8601 definition: weeks start on a Monday; the first week of the year contains the first Thursday of the year. **Code examples:**Initialize the datepicker with the `calculateWeek` option specified:
```
$( ".selector" ).datepicker({
calculateWeek: myWeekCalc
});
```
Get or set the `calculateWeek` option, after initialization:
```
// Getter
var calculateWeek = $( ".selector" ).datepicker( "option", "calculateWeek" );
// Setter
$( ".selector" ).datepicker( "option", "calculateWeek", myWeekCalc );
```
### changeMonth
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the month should be rendered as a dropdown instead of text. **Code examples:**Initialize the datepicker with the `changeMonth` option specified:
```
$( ".selector" ).datepicker({
changeMonth: true
});
```
Get or set the `changeMonth` option, after initialization:
```
// Getter
var changeMonth = $( ".selector" ).datepicker( "option", "changeMonth" );
// Setter
$( ".selector" ).datepicker( "option", "changeMonth", true );
```
### changeYear
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the year should be rendered as a dropdown instead of text. Use the [`yearRange`](#option-yearRange) option to control which years are made available for selection. **Code examples:**Initialize the datepicker with the `changeYear` option specified:
```
$( ".selector" ).datepicker({
changeYear: true
});
```
Get or set the `changeYear` option, after initialization:
```
// Getter
var changeYear = $( ".selector" ).datepicker( "option", "changeYear" );
// Setter
$( ".selector" ).datepicker( "option", "changeYear", true );
```
### closeText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"Done"` The text to display for the close link. Use the [`showButtonPanel`](#option-showButtonPanel) option to display this button. **Code examples:**Initialize the datepicker with the `closeText` option specified:
```
$( ".selector" ).datepicker({
closeText: "Close"
});
```
Get or set the `closeText` option, after initialization:
```
// Getter
var closeText = $( ".selector" ).datepicker( "option", "closeText" );
// Setter
$( ".selector" ).datepicker( "option", "closeText", "Close" );
```
### constrainInput
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `true` When `true`, entry in the input field is constrained to those characters allowed by the current [`dateFormat`](#option-dateFormat) option. **Code examples:**Initialize the datepicker with the `constrainInput` option specified:
```
$( ".selector" ).datepicker({
constrainInput: false
});
```
Get or set the `constrainInput` option, after initialization:
```
// Getter
var constrainInput = $( ".selector" ).datepicker( "option", "constrainInput" );
// Setter
$( ".selector" ).datepicker( "option", "constrainInput", false );
```
### currentText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"Today"` The text to display for the current day link. Use the [`showButtonPanel`](#option-showButtonPanel) option to display this button. **Code examples:**Initialize the datepicker with the `currentText` option specified:
```
$( ".selector" ).datepicker({
currentText: "Now"
});
```
Get or set the `currentText` option, after initialization:
```
// Getter
var currentText = $( ".selector" ).datepicker( "option", "currentText" );
// Setter
$( ".selector" ).datepicker( "option", "currentText", "Now" );
```
### dateFormat
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"mm/dd/yy"` The format for parsed and displayed dates. For a full list of the possible formats see the `[`formatDate`](#utility-formatDate)` function. **Code examples:**Initialize the datepicker with the `dateFormat` option specified:
```
$( ".selector" ).datepicker({
dateFormat: "yy-mm-dd"
});
```
Get or set the `dateFormat` option, after initialization:
```
// Getter
var dateFormat = $( ".selector" ).datepicker( "option", "dateFormat" );
// Setter
$( ".selector" ).datepicker( "option", "dateFormat", "yy-mm-dd" );
```
### dayNames
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `[ "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" ]` The list of long day names, starting from Sunday, for use as requested via the [`dateFormat`](#option-dateFormat) option. **Code examples:**Initialize the datepicker with the `dayNames` option specified:
```
$( ".selector" ).datepicker({
dayNames: [ "Dimanche", "Lundi", "Mardi", "Mercredi", "Jeudi", "Vendredi", "Samedi" ]
});
```
Get or set the `dayNames` option, after initialization:
```
// Getter
var dayNames = $( ".selector" ).datepicker( "option", "dayNames" );
// Setter
$( ".selector" ).datepicker( "option", "dayNames", [ "Dimanche", "Lundi", "Mardi", "Mercredi", "Jeudi", "Vendredi", "Samedi" ] );
```
### dayNamesMin
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `[ "Su", "Mo", "Tu", "We", "Th", "Fr", "Sa" ]` The list of minimised day names, starting from Sunday, for use as column headers within the datepicker. **Code examples:**Initialize the datepicker with the `dayNamesMin` option specified:
```
$( ".selector" ).datepicker({
dayNamesMin: [ "Di", "Lu", "Ma", "Me", "Je", "Ve", "Sa" ]
});
```
Get or set the `dayNamesMin` option, after initialization:
```
// Getter
var dayNamesMin = $( ".selector" ).datepicker( "option", "dayNamesMin" );
// Setter
$( ".selector" ).datepicker( "option", "dayNamesMin", [ "Di", "Lu", "Ma", "Me", "Je", "Ve", "Sa" ] );
```
### dayNamesShort
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `[ "Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat" ]` The list of abbreviated day names, starting from Sunday, for use as requested via the [`dateFormat`](#option-dateFormat) option. **Code examples:**Initialize the datepicker with the `dayNamesShort` option specified:
```
$( ".selector" ).datepicker({
dayNamesShort: [ "Dim", "Lun", "Mar", "Mer", "Jeu", "Ven", "Sam" ]
});
```
Get or set the `dayNamesShort` option, after initialization:
```
// Getter
var dayNamesShort = $( ".selector" ).datepicker( "option", "dayNamesShort" );
// Setter
$( ".selector" ).datepicker( "option", "dayNamesShort", [ "Dim", "Lun", "Mar", "Mer", "Jeu", "Ven", "Sam" ] );
```
### defaultDate
**Type:** [Date](http://api.jquery.com/Types/#Date) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `null` Set the date to highlight on first opening if the field is blank. Specify either an actual date via a Date object or as a string in the current [`dateFormat`](#option-dateFormat), or a number of days from today (e.g. +7) or a string of values and periods ('y' for years, 'm' for months, 'w' for weeks, 'd' for days, e.g. '+1m +7d'), or null for today. **Multiple types supported:*** **Date**: A date object containing the default date.
* **Number**: A number of days from today. For example `2` represents two days from today and `-1` represents yesterday.
* **String**: A string in the format defined by the [`dateFormat`](#option-dateFormat) option, or a relative date. Relative dates must contain value and period pairs; valid periods are `"y"` for years, `"m"` for months, `"w"` for weeks, and `"d"` for days. For example, `"+1m +7d"` represents one month and seven days from today.
**Code examples:**Initialize the datepicker with the `defaultDate` option specified:
```
$( ".selector" ).datepicker({
defaultDate: +7
});
```
Get or set the `defaultDate` option, after initialization:
```
// Getter
var defaultDate = $( ".selector" ).datepicker( "option", "defaultDate" );
// Setter
$( ".selector" ).datepicker( "option", "defaultDate", +7 );
```
### duration
**Type:** or [String](http://api.jquery.com/Types/#String) **Default:** `"normal"` Control the speed at which the datepicker appears, it may be a time in milliseconds or a string representing one of the three predefined speeds ("slow", "normal", "fast"). **Code examples:**Initialize the datepicker with the `duration` option specified:
```
$( ".selector" ).datepicker({
duration: "slow"
});
```
Get or set the `duration` option, after initialization:
```
// Getter
var duration = $( ".selector" ).datepicker( "option", "duration" );
// Setter
$( ".selector" ).datepicker( "option", "duration", "slow" );
```
### firstDay
**Type:** [Integer](http://api.jquery.com/Types/#Integer) **Default:** `0` Set the first day of the week: Sunday is `0`, Monday is `1`, etc. **Code examples:**Initialize the datepicker with the `firstDay` option specified:
```
$( ".selector" ).datepicker({
firstDay: 1
});
```
Get or set the `firstDay` option, after initialization:
```
// Getter
var firstDay = $( ".selector" ).datepicker( "option", "firstDay" );
// Setter
$( ".selector" ).datepicker( "option", "firstDay", 1 );
```
### gotoCurrent
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` When `true`, the current day link moves to the currently selected date instead of today. **Code examples:**Initialize the datepicker with the `gotoCurrent` option specified:
```
$( ".selector" ).datepicker({
gotoCurrent: true
});
```
Get or set the `gotoCurrent` option, after initialization:
```
// Getter
var gotoCurrent = $( ".selector" ).datepicker( "option", "gotoCurrent" );
// Setter
$( ".selector" ).datepicker( "option", "gotoCurrent", true );
```
### hideIfNoPrevNext
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Normally the previous and next links are disabled when not applicable (see the [`minDate`](#option-minDate) and [`maxDate`](#option-maxDate) options). You can hide them altogether by setting this attribute to `true`. **Code examples:**Initialize the datepicker with the `hideIfNoPrevNext` option specified:
```
$( ".selector" ).datepicker({
hideIfNoPrevNext: true
});
```
Get or set the `hideIfNoPrevNext` option, after initialization:
```
// Getter
var hideIfNoPrevNext = $( ".selector" ).datepicker( "option", "hideIfNoPrevNext" );
// Setter
$( ".selector" ).datepicker( "option", "hideIfNoPrevNext", true );
```
### isRTL
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the current language is drawn from right to left. **Code examples:**Initialize the datepicker with the `isRTL` option specified:
```
$( ".selector" ).datepicker({
isRTL: true
});
```
Get or set the `isRTL` option, after initialization:
```
// Getter
var isRTL = $( ".selector" ).datepicker( "option", "isRTL" );
// Setter
$( ".selector" ).datepicker( "option", "isRTL", true );
```
### maxDate
**Type:** [Date](http://api.jquery.com/Types/#Date) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `null` The maximum selectable date. When set to `null`, there is no maximum. **Multiple types supported:*** **Date**: A date object containing the maximum date.
* **Number**: A number of days from today. For example `2` represents two days from today and `-1` represents yesterday.
* **String**: A string in the format defined by the [`dateFormat`](#option-dateFormat) option, or a relative date. Relative dates must contain value and period pairs; valid periods are `"y"` for years, `"m"` for months, `"w"` for weeks, and `"d"` for days. For example, `"+1m +7d"` represents one month and seven days from today.
**Code examples:**Initialize the datepicker with the `maxDate` option specified:
```
$( ".selector" ).datepicker({
maxDate: "+1m +1w"
});
```
Get or set the `maxDate` option, after initialization:
```
// Getter
var maxDate = $( ".selector" ).datepicker( "option", "maxDate" );
// Setter
$( ".selector" ).datepicker( "option", "maxDate", "+1m +1w" );
```
### minDate
**Type:** [Date](http://api.jquery.com/Types/#Date) or [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `null` The minimum selectable date. When set to `null`, there is no minimum. **Multiple types supported:*** **Date**: A date object containing the minimum date.
* **Number**: A number of days from today. For example `2` represents two days from today and `-1` represents yesterday.
* **String**: A string in the format defined by the [`dateFormat`](#option-dateFormat) option, or a relative date. Relative dates must contain value and period pairs; valid periods are `"y"` for years, `"m"` for months, `"w"` for weeks, and `"d"` for days. For example, `"+1m +7d"` represents one month and seven days from today.
**Code examples:**Initialize the datepicker with the `minDate` option specified:
```
$( ".selector" ).datepicker({
minDate: new Date(2007, 1 - 1, 1)
});
```
Get or set the `minDate` option, after initialization:
```
// Getter
var minDate = $( ".selector" ).datepicker( "option", "minDate" );
// Setter
$( ".selector" ).datepicker( "option", "minDate", new Date(2007, 1 - 1, 1) );
```
### monthNames
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `[ "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" ]` The list of full month names, for use as requested via the [`dateFormat`](#option-dateFormat) option. **Code examples:**Initialize the datepicker with the `monthNames` option specified:
```
$( ".selector" ).datepicker({
monthNames: [ "Januar", "Februar", "Marts", "April", "Maj", "Juni", "Juli", "August", "September", "Oktober", "November", "December" ]
});
```
Get or set the `monthNames` option, after initialization:
```
// Getter
var monthNames = $( ".selector" ).datepicker( "option", "monthNames" );
// Setter
$( ".selector" ).datepicker( "option", "monthNames", [ "Januar", "Februar", "Marts", "April", "Maj", "Juni", "Juli", "August", "September", "Oktober", "November", "December" ] );
```
### monthNamesShort
**Type:** [Array](http://api.jquery.com/Types/#Array) **Default:** `[ "Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec" ]` The list of abbreviated month names, as used in the month header on each datepicker and as requested via the [`dateFormat`](#option-dateFormat) option. **Code examples:**Initialize the datepicker with the `monthNamesShort` option specified:
```
$( ".selector" ).datepicker({
monthNamesShort: [ "Jan", "Feb", "Mar", "Apr", "Maj", "Jun", "Jul", "Aug", "Sep", "Okt", "Nov", "Dec" ]
});
```
Get or set the `monthNamesShort` option, after initialization:
```
// Getter
var monthNamesShort = $( ".selector" ).datepicker( "option", "monthNamesShort" );
// Setter
$( ".selector" ).datepicker( "option", "monthNamesShort", [ "Jan", "Feb", "Mar", "Apr", "Maj", "Jun", "Jul", "Aug", "Sep", "Okt", "Nov", "Dec" ] );
```
### navigationAsDateFormat
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether the [`currentText`](#option-currentText), [`prevText`](#option-prevText) and [`nextText`](#option-nextText) options should be parsed as dates by the `[`formatDate`](#utility-formatDate)` function, allowing them to display the target month names for example. **Code examples:**Initialize the datepicker with the `navigationAsDateFormat` option specified:
```
$( ".selector" ).datepicker({
navigationAsDateFormat: true
});
```
Get or set the `navigationAsDateFormat` option, after initialization:
```
// Getter
var navigationAsDateFormat = $( ".selector" ).datepicker( "option", "navigationAsDateFormat" );
// Setter
$( ".selector" ).datepicker( "option", "navigationAsDateFormat", true );
```
### nextText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"Next"` The text to display for the next month link. With the standard ThemeRoller styling, this value is replaced by an icon. **Code examples:**Initialize the datepicker with the `nextText` option specified:
```
$( ".selector" ).datepicker({
nextText: "Later"
});
```
Get or set the `nextText` option, after initialization:
```
// Getter
var nextText = $( ".selector" ).datepicker( "option", "nextText" );
// Setter
$( ".selector" ).datepicker( "option", "nextText", "Later" );
```
### numberOfMonths
**Type:** [Number](http://api.jquery.com/Types/#Number) or [Array](http://api.jquery.com/Types/#Array) **Default:** `1` The number of months to show at once. **Multiple types supported:*** **Number**: The number of months to display in a single row.
* **Array**: An array defining the number of rows and columns to display.
**Code examples:**Initialize the datepicker with the `numberOfMonths` option specified:
```
$( ".selector" ).datepicker({
numberOfMonths: [ 2, 3 ]
});
```
Get or set the `numberOfMonths` option, after initialization:
```
// Getter
var numberOfMonths = $( ".selector" ).datepicker( "option", "numberOfMonths" );
// Setter
$( ".selector" ).datepicker( "option", "numberOfMonths", [ 2, 3 ] );
```
### onChangeMonthYear
**Type:** [Function](http://api.jquery.com/Types/#Function)( [Integer](http://api.jquery.com/Types/#Integer) year, [Integer](http://api.jquery.com/Types/#Integer) month, [Object](http://api.jquery.com/Types/#Object) inst ) **Default:** `null` Called when the datepicker moves to a new month and/or year. The function receives the selected year, month (1-12), and the datepicker instance as parameters. `this` refers to the associated input field. ### onClose
**Type:** [Function](http://api.jquery.com/Types/#Function)( [String](http://api.jquery.com/Types/#String) dateText, [Object](http://api.jquery.com/Types/#Object) inst ) **Default:** `null` Called when the datepicker is closed, whether or not a date is selected. The function receives the selected date as text (`""` if none) and the datepicker instance as parameters. `this` refers to the associated input field. ### onSelect
**Type:** [Function](http://api.jquery.com/Types/#Function)( [String](http://api.jquery.com/Types/#String) dateText, [Object](http://api.jquery.com/Types/#Object) inst ) **Default:** `null` Called when the datepicker is selected. The function receives the selected date as text and the datepicker instance as parameters. `this` refers to the associated input field. ### onUpdateDatepicker
**Type:** [Function](http://api.jquery.com/Types/#Function)( [Object](http://api.jquery.com/Types/#Object) inst ) **Default:** `null` Called when the datepicker widget's DOM is updated. The function receives the datepicker instance as the only parameter. `this` refers to the associated input field. (version added: 1.13) ### prevText
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"Prev"` The text to display for the previous month link. With the standard ThemeRoller styling, this value is replaced by an icon. **Code examples:**Initialize the datepicker with the `prevText` option specified:
```
$( ".selector" ).datepicker({
prevText: "Earlier"
});
```
Get or set the `prevText` option, after initialization:
```
// Getter
var prevText = $( ".selector" ).datepicker( "option", "prevText" );
// Setter
$( ".selector" ).datepicker( "option", "prevText", "Earlier" );
```
### selectOtherMonths
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether days in other months shown before or after the current month are selectable. This only applies if the [`showOtherMonths`](#option-showOtherMonths) option is set to `true`. **Code examples:**Initialize the datepicker with the `selectOtherMonths` option specified:
```
$( ".selector" ).datepicker({
selectOtherMonths: true
});
```
Get or set the `selectOtherMonths` option, after initialization:
```
// Getter
var selectOtherMonths = $( ".selector" ).datepicker( "option", "selectOtherMonths" );
// Setter
$( ".selector" ).datepicker( "option", "selectOtherMonths", true );
```
### shortYearCutoff
**Type:** [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) **Default:** `"+10"` The cutoff year for determining the century for a date (used in conjunction with [`dateFormat`](#option-dateFormat) 'y'). Any dates entered with a year value less than or equal to the cutoff year are considered to be in the current century, while those greater than it are deemed to be in the previous century. **Multiple types supported:*** **Number**: A value between `0` and `99` indicating the cutoff year.
* **String**: A relative number of years from the current year, e.g., `"+3"` or `"-5"`.
**Code examples:**Initialize the datepicker with the `shortYearCutoff` option specified:
```
$( ".selector" ).datepicker({
shortYearCutoff: 50
});
```
Get or set the `shortYearCutoff` option, after initialization:
```
// Getter
var shortYearCutoff = $( ".selector" ).datepicker( "option", "shortYearCutoff" );
// Setter
$( ".selector" ).datepicker( "option", "shortYearCutoff", 50 );
```
### showAnim
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"show"` The name of the animation used to show and hide the datepicker. Use `"show"` (the default), `"slideDown"`, `"fadeIn"`, any of the [jQuery UI effects](category/effects). Set to an empty string to disable animation. **Code examples:**Initialize the datepicker with the `showAnim` option specified:
```
$( ".selector" ).datepicker({
showAnim: "fold"
});
```
Get or set the `showAnim` option, after initialization:
```
// Getter
var showAnim = $( ".selector" ).datepicker( "option", "showAnim" );
// Setter
$( ".selector" ).datepicker( "option", "showAnim", "fold" );
```
### showButtonPanel
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether to display a button pane underneath the calendar. The button pane contains two buttons, a Today button that links to the current day, and a Done button that closes the datepicker. The buttons' text can be customized using the [`currentText`](#option-currentText) and [`closeText`](#option-closeText) options respectively. **Code examples:**Initialize the datepicker with the `showButtonPanel` option specified:
```
$( ".selector" ).datepicker({
showButtonPanel: true
});
```
Get or set the `showButtonPanel` option, after initialization:
```
// Getter
var showButtonPanel = $( ".selector" ).datepicker( "option", "showButtonPanel" );
// Setter
$( ".selector" ).datepicker( "option", "showButtonPanel", true );
```
### showCurrentAtPos
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `0` When displaying multiple months via the [`numberOfMonths`](#option-numberOfMonths) option, the `showCurrentAtPos` option defines which position to display the current month in. **Code examples:**Initialize the datepicker with the `showCurrentAtPos` option specified:
```
$( ".selector" ).datepicker({
showCurrentAtPos: 3
});
```
Get or set the `showCurrentAtPos` option, after initialization:
```
// Getter
var showCurrentAtPos = $( ".selector" ).datepicker( "option", "showCurrentAtPos" );
// Setter
$( ".selector" ).datepicker( "option", "showCurrentAtPos", 3 );
```
### showMonthAfterYear
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether to show the month after the year in the header. **Code examples:**Initialize the datepicker with the `showMonthAfterYear` option specified:
```
$( ".selector" ).datepicker({
showMonthAfterYear: true
});
```
Get or set the `showMonthAfterYear` option, after initialization:
```
// Getter
var showMonthAfterYear = $( ".selector" ).datepicker( "option", "showMonthAfterYear" );
// Setter
$( ".selector" ).datepicker( "option", "showMonthAfterYear", true );
```
### showOn
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"focus"` When the datepicker should appear. The datepicker can appear when the field receives focus (`"focus"`), when a button is clicked (`"button"`), or when either event occurs (`"both"`). **Code examples:**Initialize the datepicker with the `showOn` option specified:
```
$( ".selector" ).datepicker({
showOn: "both"
});
```
Get or set the `showOn` option, after initialization:
```
// Getter
var showOn = $( ".selector" ).datepicker( "option", "showOn" );
// Setter
$( ".selector" ).datepicker( "option", "showOn", "both" );
```
### showOptions
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:** `{}` If using one of the jQuery UI effects for the [`showAnim`](#option-showAnim) option, you can provide additional properties for that animation using this option. **Code examples:**Initialize the datepicker with the `showOptions` option specified:
```
$( ".selector" ).datepicker({
showOptions: { direction: "up" }
});
```
Get or set the `showOptions` option, after initialization:
```
// Getter
var showOptions = $( ".selector" ).datepicker( "option", "showOptions" );
// Setter
$( ".selector" ).datepicker( "option", "showOptions", { direction: "up" } );
```
### showOtherMonths
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Whether to display dates in other months (non-selectable) at the start or end of the current month. To make these days selectable use the [`selectOtherMonths`](#option-selectOtherMonths) option. **Code examples:**Initialize the datepicker with the `showOtherMonths` option specified:
```
$( ".selector" ).datepicker({
showOtherMonths: true
});
```
Get or set the `showOtherMonths` option, after initialization:
```
// Getter
var showOtherMonths = $( ".selector" ).datepicker( "option", "showOtherMonths" );
// Setter
$( ".selector" ).datepicker( "option", "showOtherMonths", true );
```
### showWeek
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` When `true`, a column is added to show the week of the year. The [`calculateWeek`](#option-calculateWeek) option determines how the week of the year is calculated. You may also want to change the [`firstDay`](#option-firstDay) option. **Code examples:**Initialize the datepicker with the `showWeek` option specified:
```
$( ".selector" ).datepicker({
showWeek: true
});
```
Get or set the `showWeek` option, after initialization:
```
// Getter
var showWeek = $( ".selector" ).datepicker( "option", "showWeek" );
// Setter
$( ".selector" ).datepicker( "option", "showWeek", true );
```
### stepMonths
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `1` Set how many months to move when clicking the previous/next links. **Code examples:**Initialize the datepicker with the `stepMonths` option specified:
```
$( ".selector" ).datepicker({
stepMonths: 3
});
```
Get or set the `stepMonths` option, after initialization:
```
// Getter
var stepMonths = $( ".selector" ).datepicker( "option", "stepMonths" );
// Setter
$( ".selector" ).datepicker( "option", "stepMonths", 3 );
```
### weekHeader
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"Wk"` The text to display for the week of the year column heading. Use the [`showWeek`](#option-showWeek) option to display this column. **Code examples:**Initialize the datepicker with the `weekHeader` option specified:
```
$( ".selector" ).datepicker({
weekHeader: "W"
});
```
Get or set the `weekHeader` option, after initialization:
```
// Getter
var weekHeader = $( ".selector" ).datepicker( "option", "weekHeader" );
// Setter
$( ".selector" ).datepicker( "option", "weekHeader", "W" );
```
### yearRange
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `"c-10:c+10"` The range of years displayed in the year drop-down: either relative to today's year (`"-nn:+nn"`), relative to the currently selected year (`"c-nn:c+nn"`), absolute (`"nnnn:nnnn"`), or combinations of these formats (`"nnnn:-nn"`). Note that this option only affects what appears in the drop-down, to restrict which dates may be selected use the [`minDate`](#option-minDate) and/or [`maxDate`](#option-maxDate) options. **Code examples:**Initialize the datepicker with the `yearRange` option specified:
```
$( ".selector" ).datepicker({
yearRange: "2002:2012"
});
```
Get or set the `yearRange` option, after initialization:
```
// Getter
var yearRange = $( ".selector" ).datepicker( "option", "yearRange" );
// Setter
$( ".selector" ).datepicker( "option", "yearRange", "2002:2012" );
```
### yearSuffix
**Type:** [String](http://api.jquery.com/Types/#String) **Default:** `""` Additional text to display after the year in the month headers. **Code examples:**Initialize the datepicker with the `yearSuffix` option specified:
```
$( ".selector" ).datepicker({
yearSuffix: "CE"
});
```
Get or set the `yearSuffix` option, after initialization:
```
// Getter
var yearSuffix = $( ".selector" ).datepicker( "option", "yearSuffix" );
// Setter
$( ".selector" ).datepicker( "option", "yearSuffix", "CE" );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the datepicker functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).datepicker( "destroy" );
```
### dialog( date [, onSelect ] [, options ] [, pos ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Opens the datepicker in a dialog box. * **date** Type: [String](http://api.jquery.com/Types/#String) or [Date](http://api.jquery.com/Types/#Date) The initial date.
* **onSelect** Type: [Function](http://api.jquery.com/Types/#Function)() A callback function when a date is selected. The function receives the date text and date picker instance as parameters.
* **options** Type: [Options](http://api.jquery.com/Types/#Options) The new options for the date picker.
* **pos** Type: [Number[2] or MouseEvent](http://api.jquery.com/Types/#Number%5B2%5D%20or%20MouseEvent) The position of the top/left of the dialog as `[x, y]` or a `MouseEvent` that contains the coordinates. If not specified the dialog is centered on the screen.
**Code examples:**Invoke the dialog method:
```
$( ".selector" ).datepicker( "dialog", "10/12/2012" );
```
### getDate()Returns: [Date](http://api.jquery.com/Types/#Date)
Returns the current date for the datepicker or `null` if no date has been selected. * This method does not accept any arguments.
**Code examples:**Invoke the getDate method:
```
var currentDate = $( ".selector" ).datepicker( "getDate" );
```
### hide()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Close a previously opened date picker. * This method does not accept any arguments.
**Code examples:**Invoke the hide method:
```
$( ".selector" ).datepicker( "hide" );
```
### isDisabled()Returns: [Boolean](http://api.jquery.com/Types/#Boolean)
Determine whether a date picker has been disabled. * This method does not accept any arguments.
**Code examples:**Invoke the isDisabled method:
```
var isDisabled = $( ".selector" ).datepicker( "isDisabled" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).datepicker( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current datepicker options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).datepicker( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the datepicker option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).datepicker( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the datepicker. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).datepicker( "option", { disabled: true } );
```
### refresh()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Redraw the date picker, after having made some external modifications. * This method does not accept any arguments.
**Code examples:**Invoke the refresh method:
```
$( ".selector" ).datepicker( "refresh" );
```
### setDate( date )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the date for the datepicker. The new date may be a `Date` object or a string in the current [date format](#option-dateFormat) (e.g., `"01/26/2009"`), a number of days from today (e.g., `+7`) or a string of values and periods (`"y"` for years, `"m"` for months, `"w"` for weeks, `"d"` for days, e.g., `"+1m +7d"`), or `null` to clear the selected date. * **date** Type: [String](http://api.jquery.com/Types/#String) or [Date](http://api.jquery.com/Types/#Date) The new date.
**Code examples:**Invoke the setDate method:
```
$( ".selector" ).datepicker( "setDate", "10/12/2012" );
```
### show()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Open the date picker. If the datepicker is attached to an input, the input must be visible for the datepicker to be shown. * This method does not accept any arguments.
**Code examples:**Invoke the show method:
```
$( ".selector" ).datepicker( "show" );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the datepicker. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).datepicker( "widget" );
```
Example:
--------
A simple jQuery UI Datepicker.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>datepicker demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="datepicker"></div>
<script>
$( "#datepicker" ).datepicker();
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui jQuery.effects.restoreStyle() jQuery.effects.restoreStyle()
=============================
jQuery.effects.restoreStyle( element )version added: 1.12
----------------------------------------------------------
**Description:** Restores all inline styles for an element.
* #### [jQuery.effects.restoreStyle( element )](#jQuery-effects-restoreStyle-element)
+ **element** Type: [jQuery](http://api.jquery.com/Types/#jQuery) The element to restore styles for.
Restores all inline styles for an element which have been saved using [`jQuery.effects.saveStyle()`](jquery.effects.savestyle).
jqueryui Scale Effect Scale Effect
============
Scale Effect
------------
**Description:** Shrink or grow an element by a percentage factor.
* #### scale
+ **direction** (default: `"both"`) Type: [String](http://api.jquery.com/Types/#String) The direction of the effect. Possible values: `"both"`, `"vertical"` or `"horizontal"`.
+ **origin** (default: `[ "middle", "center" ]`) Type: [Array](http://api.jquery.com/Types/#Array) The vanishing point.
+ **percent** Type: [Number](http://api.jquery.com/Types/#Number) The percentage to scale to.
+ **scale** (default: `"both"`) Type: [String](http://api.jquery.com/Types/#String) Which areas of the element will be resized: `"both"`, `"box"`, `"content"`. Box resizes the border and padding of the element; content resizes any content inside of the element.
Examples:
---------
Toggle a div using the scale effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>scale demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle( "scale" );
});
</script>
</body>
</html>
```
#### Demo:
Toggle a div using the scale effect in just one direction.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>scale demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
#toggle {
width: 100px;
height: 100px;
background: #ccc;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<p>Click anywhere to toggle the box.</p>
<div id="toggle"></div>
<script>
$( document ).click(function() {
$( "#toggle" ).toggle({ effect: "scale", direction: "horizontal" });
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .hide() .hide()
=======
.hide( effect [, options ] [, duration ] [, complete ] )Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
---------------------------------------------------------------------------------------------------------------
**Description:** Hide the matched elements, using custom effects.
* #### [.hide( effect [, options ] [, duration ] [, complete ] )](#hide-effect-options-duration-complete)
+ **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) Effect-specific properties and [easing](easings).
+ **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
+ **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
* #### [.hide( options )](#hide-options)
+ **options** Type: [Object](http://api.jquery.com/Types/#Object) All animation settings. The only required property is `effect`.
- **effect** Type: [String](http://api.jquery.com/Types/#String) A string indicating which [effect](category/effects) to use for the transition.
- **easing** (default: `"swing"`) Type: [String](http://api.jquery.com/Types/#String) A string indicating which [easing](easings) function to use for the transition.
- **duration** (default: `400`) Type: [Number](http://api.jquery.com/Types/#Number) or [String](http://api.jquery.com/Types/#String) A string or number determining how long the animation will run.
- **complete** Type: [Function](http://api.jquery.com/Types/#Function)() A function to call once the animation is complete, called once per matched element.
- **queue** (default: `true`) Type: [Boolean](http://api.jquery.com/Types/#Boolean) or [String](http://api.jquery.com/Types/#String) A Boolean indicating whether to place the animation in the effects queue. If `false`, the animation will begin immediately. A string can also be provided, in which case the animation is added to the queue represented by that string.
This plugin extends jQuery's built-in [`.hide()`](https://api.jquery.com/hide/) method. If jQuery UI is not loaded, calling the `.hide()` method may not fail directly, as the method still exists. However, the expected behavior will not occur.
Example:
--------
Hide a div using the drop effect.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>hide demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
background: #ccc;
border: 1px solid #000;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<button>hide the div</button>
<div></div>
<script>
$( "button" ).click(function() {
$( "div" ).hide( "drop", { direction: "down" }, "slow" );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui .labels() .labels()
=========
.labels()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
----------------------------------------------------------------
**Description:** Finds all label elements associated with the first selected element.
* #### version added: 1.12[.labels()](#labels)
+ This method does not accept any arguments.
This can be used to find all the `<label>` elements associated with an `<input>` element. The association can be through nesting, where the label is an ancestor of the input, or through the `for` attribute on the label, pointing at the `id` attribute of the input. If no labels are associated with the given element, an empty jQuery object is returned.
This methods mimics the native `labels` property, which isn't supported in all browsers. In addition, this method also works for document fragments.
Example:
--------
Highlight all labels of the input element
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>labels demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<label>
Firstname
<input id="name">
</label>
<label for="name">Please enter your name</label>
<script>
$( "input" ).labels().addClass( "ui-state-highlight" )
</script>
</body>
</html>
```
#### Demo:
jqueryui :data() Selector :data() Selector
================
data selector
-------------
**Description:** Selects elements which have data stored under the specified key.
* #### jQuery( ":data(key)" )
**key:** The data key.
The expression `$( "div:data(foo)")` matches a `<div>` if it has data stored via `.data( "foo", value )`.
Example:
--------
Select elements with data and change their background color.
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>data demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<style>
div {
width: 100px;
height: 100px;
border: 1px solid #000;
float: left;
}
</style>
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="one"></div>
<div id="two"></div>
<div id="three"></div>
<script>
$( "#one" ).data( "color", "blue" );
$( "#three" ).data( "color", "green" );
$( ":data(color)" ).each(function() {
var element = $( this );
element.css( "backgroundColor", element.data( "color" ) );
});
</script>
</body>
</html>
```
#### Demo:
jqueryui Progressbar Widget Progressbar Widget
==================
Progressbar Widgetversion added: 1.6
-------------------------------------
**Description:** Display status of a determinate or indeterminate process.
QuickNav[Examples](#entry-examples)
-----------------------------------
### Options
[classes](#option-classes) [disabled](#option-disabled) [max](#option-max) [value](#option-value) ### Methods
[destroy](#method-destroy) [disable](#method-disable) [enable](#method-enable) [instance](#method-instance) [option](#method-option) [value](#method-value) [widget](#method-widget) ### Events
[change](#event-change) [complete](#event-complete) [create](#event-create) The progress bar is designed to display the current percent complete for a process. The bar is coded to be flexibly sized through CSS and will scale to fit inside its parent container by default.
A determinate progress bar should only be used in situations where the system can accurately update the current status. A determinate progress bar should never fill from left to right, then loop back to empty for a single process — if the actual status cannot be calculated, an indeterminate progress bar should be used to provide user feedback.
### Theming
The progressbar widget uses the [jQuery UI CSS framework](theming/css-framework) to style its look and feel. If progressbar specific styling is needed, the following CSS class names can be used for overrides or as keys for the [`classes` option](#option-classes):
* `ui-progressbar`: The outer container of the progressbar. This element will additionally have a class of `ui-progressbar-indeterminate` for indeterminate progressbars. For determinate progressbars, the `ui-progressbar-complete` class is added once the maximum value is reached.
+ `ui-progressbar-value`: The element that represents the filled portion of the progressbar.
- `ui-progressbar-overlay`: Overlay used to display an animation for indeterminate progressbars.
### Dependencies
* [UI Core](category/ui-core)
* [Widget Factory](jquery.widget)
### Additional Notes:
* This widget requires some functional CSS, otherwise it won't work. If you build a custom theme, use the widget's specific CSS file as a starting point.
Options
-------
### classes
**Type:** [Object](http://api.jquery.com/Types/#Object) **Default:**
```
{
"ui-progressbar": "ui-corner-all",
"ui-progressbar-complete": "ui-corner-right",
"ui-progressbar-value": "ui-corner-left"
}
```
Specify additional classes to add to the widget's elements. Any of classes specified in the [Theming section](#theming) can be used as keys to override their value. To learn more about this option, check out the [learn article about the `classes` option](http://learn.jquery.com/jquery-ui/widget-factory/classes-option/).
**Code examples:** Initialize the progressbar with the `classes` option specified, changing the theming for the `ui-progressbar` class:
```
$( ".selector" ).progressbar({
classes: {
"ui-progressbar": "highlight"
}
});
```
Get or set a property of the `classes` option, after initialization, here reading and changing the theming for the `ui-progressbar` class:
```
// Getter
var themeClass = $( ".selector" ).progressbar( "option", "classes.ui-progressbar" );
// Setter
$( ".selector" ).progressbar( "option", "classes.ui-progressbar", "highlight" );
```
### disabled
**Type:** [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `false` Disables the progressbar if set to `true`. **Code examples:**Initialize the progressbar with the `disabled` option specified:
```
$( ".selector" ).progressbar({
disabled: true
});
```
Get or set the `disabled` option, after initialization:
```
// Getter
var disabled = $( ".selector" ).progressbar( "option", "disabled" );
// Setter
$( ".selector" ).progressbar( "option", "disabled", true );
```
### max
**Type:** [Number](http://api.jquery.com/Types/#Number) **Default:** `100` The maximum value of the progressbar. **Code examples:**Initialize the progressbar with the `max` option specified:
```
$( ".selector" ).progressbar({
max: 1024
});
```
Get or set the `max` option, after initialization:
```
// Getter
var max = $( ".selector" ).progressbar( "option", "max" );
// Setter
$( ".selector" ).progressbar( "option", "max", 1024 );
```
### value
**Type:** [Number](http://api.jquery.com/Types/#Number) or [Boolean](http://api.jquery.com/Types/#Boolean) **Default:** `0` The value of the progressbar. **Multiple types supported:*** **Number**: A value between `0` and the [`max`](#option-max).
* **Boolean**: Value can be set to `false` to create an indeterminate progressbar.
**Code examples:**Initialize the progressbar with the `value` option specified:
```
$( ".selector" ).progressbar({
value: 25
});
```
Get or set the `value` option, after initialization:
```
// Getter
var value = $( ".selector" ).progressbar( "option", "value" );
// Setter
$( ".selector" ).progressbar( "option", "value", 25 );
```
Methods
-------
### destroy()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Removes the progressbar functionality completely. This will return the element back to its pre-init state. * This method does not accept any arguments.
**Code examples:**Invoke the destroy method:
```
$( ".selector" ).progressbar( "destroy" );
```
### disable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Disables the progressbar. * This method does not accept any arguments.
**Code examples:**Invoke the disable method:
```
$( ".selector" ).progressbar( "disable" );
```
### enable()Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Enables the progressbar. * This method does not accept any arguments.
**Code examples:**Invoke the enable method:
```
$( ".selector" ).progressbar( "enable" );
```
### instance()Returns: [Object](http://api.jquery.com/Types/#Object)
Retrieves the progressbar's instance object. If the element does not have an associated instance, `undefined` is returned.
Unlike other widget methods, `instance()` is safe to call on any element after the progressbar plugin has loaded.
* This method does not accept any arguments.
**Code examples:**Invoke the instance method:
```
$( ".selector" ).progressbar( "instance" );
```
### option( optionName )Returns: [Object](http://api.jquery.com/Types/#Object)
Gets the value currently associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can get the value of a specific key by using dot notation. For example, `"foo.bar"` would get the value of the `bar` property on the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to get.
**Code examples:**Invoke the method:
```
var isDisabled = $( ".selector" ).progressbar( "option", "disabled" );
```
### option()Returns: [PlainObject](http://api.jquery.com/Types/#PlainObject)
Gets an object containing key/value pairs representing the current progressbar options hash. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var options = $( ".selector" ).progressbar( "option" );
```
### option( optionName, value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the value of the progressbar option associated with the specified `optionName`.
**Note:** For options that have objects as their value, you can set the value of just one property by using dot notation for `optionName`. For example, `"foo.bar"` would update only the `bar` property of the `foo` option.
* **optionName** Type: [String](http://api.jquery.com/Types/#String) The name of the option to set.
* **value** Type: [Object](http://api.jquery.com/Types/#Object) A value to set for the option.
**Code examples:**Invoke the method:
```
$( ".selector" ).progressbar( "option", "disabled", true );
```
### option( options )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets one or more options for the progressbar. * **options** Type: [Object](http://api.jquery.com/Types/#Object) A map of option-value pairs to set.
**Code examples:**Invoke the method:
```
$( ".selector" ).progressbar( "option", { disabled: true } );
```
### value()Returns: [Number](http://api.jquery.com/Types/#Number) or [Boolean](http://api.jquery.com/Types/#Boolean)
Gets the current value of the progressbar. * This signature does not accept any arguments.
**Code examples:**Invoke the method:
```
var progressSoFar = $( ".selector" ).progressbar( "value" );
```
### value( value )Returns: [jQuery](http://api.jquery.com/Types/#jQuery) ([plugin only](http://learn.jquery.com/jquery-ui/widget-factory/widget-method-invocation/))
Sets the current value of the progressbar. * **value** Type: [Number](http://api.jquery.com/Types/#Number) or [Boolean](http://api.jquery.com/Types/#Boolean) The value to set. See the [`value`](#option-value) option for details on valid values.
**Code examples:**Invoke the method:
```
$( ".selector" ).progressbar( "value", 50 );
```
### widget()Returns: [jQuery](http://api.jquery.com/Types/#jQuery)
Returns a `jQuery` object containing the progressbar. * This method does not accept any arguments.
**Code examples:**Invoke the widget method:
```
var widget = $( ".selector" ).progressbar( "widget" );
```
Events
------
### change( event, ui )Type: `progressbarchange`
Triggered when the value of the progressbar changes. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the progressbar with the change callback specified:
```
$( ".selector" ).progressbar({
change: function( event, ui ) {}
});
```
Bind an event listener to the progressbarchange event:
```
$( ".selector" ).on( "progressbarchange", function( event, ui ) {} );
```
### complete( event, ui )Type: `progressbarcomplete`
Triggered when the value of the progressbar reaches the maximum value. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the progressbar with the complete callback specified:
```
$( ".selector" ).progressbar({
complete: function( event, ui ) {}
});
```
Bind an event listener to the progressbarcomplete event:
```
$( ".selector" ).on( "progressbarcomplete", function( event, ui ) {} );
```
### create( event, ui )Type: `progressbarcreate`
Triggered when the progressbar is created. * **event** Type: [Event](http://api.jquery.com/Types/#Event)
* **ui** Type: [Object](http://api.jquery.com/Types/#Object)
*Note: The `ui` object is empty but included for consistency with other events.*
**Code examples:**Initialize the progressbar with the create callback specified:
```
$( ".selector" ).progressbar({
create: function( event, ui ) {}
});
```
Bind an event listener to the progressbarcreate event:
```
$( ".selector" ).on( "progressbarcreate", function( event, ui ) {} );
```
Examples:
---------
A simple jQuery UI Progressbar
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>progressbar demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="progressbar"></div>
<script>
$( "#progressbar" ).progressbar({
value: 37
});
</script>
</body>
</html>
```
#### Demo:
A simple jQuery UI Indeterminate Progressbar
```
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>progressbar demo</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.12.4.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<div id="progressbar"></div>
<script>
$( "#progressbar" ).progressbar({
value: false
});
</script>
</body>
</html>
```
#### Demo:
| programming_docs |
jqueryui Category: Widgets Category: Widgets
=================
Widgets are feature-rich, stateful plugins that have a full life-cycle, along with methods and events. Check out the [widget factory](../jquery.widget) documentation for more details.
[Accordion Widget](../accordion "Permalink to Accordion Widget")
=================================================================
Convert a pair of headers and content panels into an accordion.
[Autocomplete Widget](../autocomplete "Permalink to Autocomplete Widget")
==========================================================================
Autocomplete enables users to quickly find and select from a pre-populated list of values as they type, leveraging searching and filtering.
[Button Widget](../button "Permalink to Button Widget")
========================================================
Themeable buttons.
[Buttonset Widget](../buttonset "Permalink to Buttonset Widget")
=================================================================
Themeable button sets.
[Checkboxradio Widget](../checkboxradio "Permalink to Checkboxradio Widget")
=============================================================================
Converts inputs of type radio and checkbox to themeable buttons.
[Controlgroup Widget](../controlgroup "Permalink to Controlgroup Widget")
==========================================================================
Themeable set of input widgets.
[Datepicker Widget](../datepicker "Permalink to Datepicker Widget")
====================================================================
Select a date from a popup or inline calendar
[Dialog Widget](../dialog "Permalink to Dialog Widget")
========================================================
Open content in an interactive overlay.
[Form Reset Mixin](../form-reset-mixin "Permalink to Form Reset Mixin")
========================================================================
A mixin to call refresh() on an input widget when the parent form gets reset.
[Widget Factory](../jquery.widget "Permalink to Widget Factory")
=================================================================
Create stateful jQuery plugins using the same abstraction as all jQuery UI widgets.
[Widget Plugin Bridge](../jquery.widget.bridge "Permalink to Widget Plugin Bridge")
====================================================================================
Part of the jQuery Widget Factory is the jQuery.widget.bridge() method. This acts as the middleman between the object created by $.widget() and the jQuery API.
[Menu Widget](../menu "Permalink to Menu Widget")
==================================================
Themeable menu with mouse and keyboard interactions for navigation.
[Progressbar Widget](../progressbar "Permalink to Progressbar Widget")
=======================================================================
Display status of a determinate or indeterminate process.
[Selectmenu Widget](../selectmenu "Permalink to Selectmenu Widget")
====================================================================
Duplicates and extends the functionality of a native HTML select element to overcome the limitations of the native control.
[Slider Widget](../slider "Permalink to Slider Widget")
========================================================
Drag a handle to select a numeric value.
[Spinner Widget](../spinner "Permalink to Spinner Widget")
===========================================================
Enhance a text input for entering numeric values, with up/down buttons and arrow key handling.
[Tabs Widget](../tabs "Permalink to Tabs Widget")
==================================================
A single content area with multiple panels, each associated with a header in a list.
[Tooltip Widget](../tooltip "Permalink to Tooltip Widget")
===========================================================
Customizable, themeable tooltips, replacing native tooltips.
jqueryui Category: Utilities Category: Utilities
===================
[.cssClip()](../cssclip "Permalink to .cssClip()")
===================================================
Getter/setter for an object version of the CSS clip property.
[Easings](../easings "Permalink to Easings")
=============================================
Easing functions specify the speed at which an animation progresses at different points within the animation.
[Form Reset Mixin](../form-reset-mixin "Permalink to Form Reset Mixin")
========================================================================
A mixin to call refresh() on an input widget when the parent form gets reset.
[Widget Factory](../jquery.widget "Permalink to Widget Factory")
=================================================================
Create stateful jQuery plugins using the same abstraction as all jQuery UI widgets.
[Widget Plugin Bridge](../jquery.widget.bridge "Permalink to Widget Plugin Bridge")
====================================================================================
Part of the jQuery Widget Factory is the jQuery.widget.bridge() method. This acts as the middleman between the object created by $.widget() and the jQuery API.
[.labels()](../labels "Permalink to .labels()")
================================================
Finds all label elements associated with the first selected element.
[Mouse Interaction](../mouse "Permalink to Mouse Interaction")
===============================================================
The base interaction layer.
[.position()](../position "Permalink to .position()")
======================================================
Position an element relative to another.
jqueryui Category: Interactions Category: Interactions
======================
jQuery UI provides a set of mouse-based interactions as building blocks for rich interfaces and complex widgets.
[Draggable Widget](../draggable "Permalink to Draggable Widget")
=================================================================
Allow elements to be moved using the mouse.
[Droppable Widget](../droppable "Permalink to Droppable Widget")
=================================================================
Create targets for draggable elements.
[Mouse Interaction](../mouse "Permalink to Mouse Interaction")
===============================================================
The base interaction layer.
[Resizable Widget](../resizable "Permalink to Resizable Widget")
=================================================================
Change the size of an element using the mouse.
[Selectable Widget](../selectable "Permalink to Selectable Widget")
====================================================================
Use the mouse to select elements, individually or in a group.
[Sortable Widget](../sortable "Permalink to Sortable Widget")
==============================================================
Reorder elements in a list or grid using the mouse.
jqueryui Category: Methods Category: Methods
=================
Although jQuery UI is mostly comprised of <widgets>, <interactions>, and <effects>, there are also a few simple methods that are added for convenience.
[.cssClip()](../cssclip "Permalink to .cssClip()")
===================================================
Getter/setter for an object version of the CSS clip property.
[.disableSelection()](../disableselection "Permalink to .disableSelection()")
==============================================================================
Disable selection of text content within the set of matched elements.
[.effect()](../effect "Permalink to .effect()")
================================================
Apply an animation effect to an element.
[.enableSelection()](../enableselection "Permalink to .enableSelection()")
===========================================================================
Enable selection of text content within the set of matched elements.
[.hide()](../hide "Permalink to .hide()")
==========================================
Hide the matched elements, using custom effects.
[.labels()](../labels "Permalink to .labels()")
================================================
Finds all label elements associated with the first selected element.
[.position()](../position "Permalink to .position()")
======================================================
Position an element relative to another.
[.removeUniqueId()](../removeuniqueid "Permalink to .removeUniqueId()")
========================================================================
Remove ids that were set by .uniqueId() for the set of matched elements.
[.scrollParent()](../scrollparent "Permalink to .scrollParent()")
==================================================================
Get the closest ancestor element that is scrollable.
[.show()](../show "Permalink to .show()")
==========================================
Display the matched elements, using custom effects.
[.toggle()](../toggle "Permalink to .toggle()")
================================================
Display or hide the matched elements, using custom effects.
[.uniqueId()](../uniqueid "Permalink to .uniqueId()")
======================================================
Generate and apply a unique id for the set of matched elements.
jqueryui Category: Effects Core Category: Effects Core
======================
Functionality provided by `effect.js`. In addition to the methods listed below, `effect.js` also includes several [easings](../easings).
[.addClass()](../addclass "Permalink to .addClass()")
======================================================
Adds the specified class(es) to each of the set of matched elements while animating all style changes.
[Color Animation](../color-animation "Permalink to Color Animation")
=====================================================================
Animate colors using .animate().
[.cssClip()](../cssclip "Permalink to .cssClip()")
===================================================
Getter/setter for an object version of the CSS clip property.
[.effect()](../effect "Permalink to .effect()")
================================================
Apply an animation effect to an element.
[.hide()](../hide "Permalink to .hide()")
==========================================
Hide the matched elements, using custom effects.
[jQuery.effects.clipToBox()](../jquery.effects.cliptobox "Permalink to jQuery.effects.clipToBox()")
====================================================================================================
Calculates position and dimensions based on a clip animation.
[jQuery.effects.createPlaceholder()](../jquery.effects.createplaceholder "Permalink to jQuery.effects.createPlaceholder()")
============================================================================================================================
Creates a placeholder to support clip animations without disrupting the layout.
[jQuery.effects.define()](../jquery.effects.define "Permalink to jQuery.effects.define()")
===========================================================================================
Defines an effect.
[jQuery.effects.removePlaceholder()](../jquery.effects.removeplaceholder "Permalink to jQuery.effects.removePlaceholder()")
============================================================================================================================
Removes a placeholder created with jQuery.effects.createPlaceholder().
[jQuery.effects.restoreStyle()](../jquery.effects.restorestyle "Permalink to jQuery.effects.restoreStyle()")
=============================================================================================================
Restores all inline styles for an element.
[jQuery.effects.saveStyle()](../jquery.effects.savestyle "Permalink to jQuery.effects.saveStyle()")
====================================================================================================
Stores a copy of all inline styles applied to an element.
[jQuery.effects.scaledDimensions()](../jquery.effects.scaleddimensions "Permalink to jQuery.effects.scaledDimensions()")
=========================================================================================================================
Scales the dimensions of an element.
[.removeClass()](../removeclass "Permalink to .removeClass()")
===============================================================
Removes the specified class(es) from each of the set of matched elements while animating all style changes.
[.show()](../show "Permalink to .show()")
==========================================
Display the matched elements, using custom effects.
[.switchClass()](../switchclass "Permalink to .switchClass()")
===============================================================
Adds and removes the specified class(es) to each of the set of matched elements while animating all style changes.
[.toggle()](../toggle "Permalink to .toggle()")
================================================
Display or hide the matched elements, using custom effects.
[.toggleClass()](../toggleclass "Permalink to .toggleClass()")
===============================================================
Add or remove one or more classes from each element in the set of matched elements, depending on either the class’s presence or the value of the switch argument, while animating all style changes.
[.transfer()](../transfer "Permalink to .transfer()")
======================================================
Transfers the outline of an element to another element
jqueryui Category: Effects Category: Effects
=================
jQuery UI adds quite a bit of functionality on top of [jQuery's built-in effects](https://api.jquery.com/category/effects/). jQuery UI adds support for animating colors and class transitions, as well as providing several additional [easings](../easings). In addition, a full suite of custom effects are available for use when showing and hiding elements or just to add some visual appeal.
[.addClass()](../addclass "Permalink to .addClass()")
======================================================
Adds the specified class(es) to each of the set of matched elements while animating all style changes.
[Blind Effect](../blind-effect "Permalink to Blind Effect")
============================================================
The blind effect hides or shows an element by wrapping the element in a container, and “pulling the blinds”
[Bounce Effect](../bounce-effect "Permalink to Bounce Effect")
===============================================================
The bounce effect bounces an element. When used with hide or show, the last or first bounce will also fade in/out.
[Clip Effect](../clip-effect "Permalink to Clip Effect")
=========================================================
The clip effect will hide or show an element by clipping the element vertically or horizontally.
[Color Animation](../color-animation "Permalink to Color Animation")
=====================================================================
Animate colors using .animate().
[.cssClip()](../cssclip "Permalink to .cssClip()")
===================================================
Getter/setter for an object version of the CSS clip property.
[Drop Effect](../drop-effect "Permalink to Drop Effect")
=========================================================
The drop effect hides or shows an element fading in/out and sliding in a direction.
[Easings](../easings "Permalink to Easings")
=============================================
Easing functions specify the speed at which an animation progresses at different points within the animation.
[.effect()](../effect "Permalink to .effect()")
================================================
Apply an animation effect to an element.
[Explode Effect](../explode-effect "Permalink to Explode Effect")
==================================================================
The explode effect hides or shows an element by splitting it into pieces.
[Fade Effect](../fade-effect "Permalink to Fade Effect")
=========================================================
The fade effect hides or shows an element by fading it.
[Fold Effect](../fold-effect "Permalink to Fold Effect")
=========================================================
The fold effect hides or shows an element by folding it.
[.hide()](../hide "Permalink to .hide()")
==========================================
Hide the matched elements, using custom effects.
[Highlight Effect](../highlight-effect "Permalink to Highlight Effect")
========================================================================
The highlight effect hides or shows an element by animating its background color first.
[jQuery.effects.clipToBox()](../jquery.effects.cliptobox "Permalink to jQuery.effects.clipToBox()")
====================================================================================================
Calculates position and dimensions based on a clip animation.
[jQuery.effects.createPlaceholder()](../jquery.effects.createplaceholder "Permalink to jQuery.effects.createPlaceholder()")
============================================================================================================================
Creates a placeholder to support clip animations without disrupting the layout.
[jQuery.effects.define()](../jquery.effects.define "Permalink to jQuery.effects.define()")
===========================================================================================
Defines an effect.
[jQuery.effects.removePlaceholder()](../jquery.effects.removeplaceholder "Permalink to jQuery.effects.removePlaceholder()")
============================================================================================================================
Removes a placeholder created with jQuery.effects.createPlaceholder().
[jQuery.effects.restoreStyle()](../jquery.effects.restorestyle "Permalink to jQuery.effects.restoreStyle()")
=============================================================================================================
Restores all inline styles for an element.
[jQuery.effects.saveStyle()](../jquery.effects.savestyle "Permalink to jQuery.effects.saveStyle()")
====================================================================================================
Stores a copy of all inline styles applied to an element.
[jQuery.effects.scaledDimensions()](../jquery.effects.scaleddimensions "Permalink to jQuery.effects.scaledDimensions()")
=========================================================================================================================
Scales the dimensions of an element.
[Puff Effect](../puff-effect "Permalink to Puff Effect")
=========================================================
Creates a puff effect by scaling the element up and hiding it at the same time.
[Pulsate Effect](../pulsate-effect "Permalink to Pulsate Effect")
==================================================================
The pulsate effect hides or shows an element by pulsing it in or out.
[.removeClass()](../removeclass "Permalink to .removeClass()")
===============================================================
Removes the specified class(es) from each of the set of matched elements while animating all style changes.
[Scale Effect](../scale-effect "Permalink to Scale Effect")
============================================================
Shrink or grow an element by a percentage factor.
[Shake Effect](../shake-effect "Permalink to Shake Effect")
============================================================
Shakes the element multiple times, vertically or horizontally.
[.show()](../show "Permalink to .show()")
==========================================
Display the matched elements, using custom effects.
[Size Effect](../size-effect "Permalink to Size Effect")
=========================================================
Resize an element to a specified width and height.
[Slide Effect](../slide-effect "Permalink to Slide Effect")
============================================================
Slides the element out of the viewport.
[.switchClass()](../switchclass "Permalink to .switchClass()")
===============================================================
Adds and removes the specified class(es) to each of the set of matched elements while animating all style changes.
[.toggle()](../toggle "Permalink to .toggle()")
================================================
Display or hide the matched elements, using custom effects.
[.toggleClass()](../toggleclass "Permalink to .toggleClass()")
===============================================================
Add or remove one or more classes from each element in the set of matched elements, depending on either the class’s presence or the value of the switch argument, while animating all style changes.
[.transfer()](../transfer "Permalink to .transfer()")
======================================================
Transfers the outline of an element to another element
[Transfer Effect](../transfer-effect "Permalink to Transfer Effect")
=====================================================================
Transfers the outline of an element to another element
| programming_docs |
jqueryui Category: Method Overrides Category: Method Overrides
==========================
jQuery UI overrides several built-in jQuery methods in order to provide additional functionality. When using these overrides, it's important to make sure that jQuery UI is loaded. If jQuery UI is not loaded, the methods will still exist, but the expected functionality will not be available, resulting in bugs that may be hard to track down.
[.addClass()](../addclass "Permalink to .addClass()")
======================================================
Adds the specified class(es) to each of the set of matched elements while animating all style changes.
[.cssClip()](../cssclip "Permalink to .cssClip()")
===================================================
Getter/setter for an object version of the CSS clip property.
[.hide()](../hide "Permalink to .hide()")
==========================================
Hide the matched elements, using custom effects.
[.labels()](../labels "Permalink to .labels()")
================================================
Finds all label elements associated with the first selected element.
[.position()](../position "Permalink to .position()")
======================================================
Position an element relative to another.
[.removeClass()](../removeclass "Permalink to .removeClass()")
===============================================================
Removes the specified class(es) from each of the set of matched elements while animating all style changes.
[.show()](../show "Permalink to .show()")
==========================================
Display the matched elements, using custom effects.
[.toggle()](../toggle "Permalink to .toggle()")
================================================
Display or hide the matched elements, using custom effects.
[.toggleClass()](../toggleclass "Permalink to .toggleClass()")
===============================================================
Add or remove one or more classes from each element in the set of matched elements, depending on either the class’s presence or the value of the switch argument, while animating all style changes.
jqueryui Category: UI Core Category: UI Core
=================
Functionality provided by `core.js`.
[:data() Selector](../data-selector "Permalink to :data() Selector")
=====================================================================
Selects elements which have data stored under the specified key.
[.disableSelection()](../disableselection "Permalink to .disableSelection()")
==============================================================================
Disable selection of text content within the set of matched elements.
[.enableSelection()](../enableselection "Permalink to .enableSelection()")
===========================================================================
Enable selection of text content within the set of matched elements.
[:focusable Selector](../focusable-selector "Permalink to :focusable Selector")
================================================================================
Selects elements which can be focused.
[jQuery.ui.keyCode](../jquery.ui.keycode "Permalink to jQuery.ui.keyCode")
===========================================================================
A mapping of key code descriptions to their numeric values.
[.removeUniqueId()](../removeuniqueid "Permalink to .removeUniqueId()")
========================================================================
Remove ids that were set by .uniqueId() for the set of matched elements.
[.scrollParent()](../scrollparent "Permalink to .scrollParent()")
==================================================================
Get the closest ancestor element that is scrollable.
[:tabbable Selector](../tabbable-selector "Permalink to :tabbable Selector")
=============================================================================
Selects elements which the user can focus via tabbing.
[.uniqueId()](../uniqueid "Permalink to .uniqueId()")
======================================================
Generate and apply a unique id for the set of matched elements.
jqueryui Category: Selectors Category: Selectors
===================
[:data() Selector](../data-selector "Permalink to :data() Selector")
=====================================================================
Selects elements which have data stored under the specified key.
[:focusable Selector](../focusable-selector "Permalink to :focusable Selector")
================================================================================
Selects elements which can be focused.
[:tabbable Selector](../tabbable-selector "Permalink to :tabbable Selector")
=============================================================================
Selects elements which the user can focus via tabbing.
jqueryui Stacking Elements Stacking Elements
=================
Widgets that stack, or move in front of other elements, often present challenges when placed into real world pages. It's usually easy to either change the `z-index` or the parent of the stacked element to avoid any collisions on the page. However, jQuery UI needs a generic solution that doesn't require manually playing with `z-index` values. This is accomplished via the `ui-front` class, and usually an accompanying `appendTo` option on stacking widgets.
The `ui-front` class
---------------------
The `ui-front` class is very basic. It just sets a static `z-index` value on the element. However, the existence of the class is used to indicate that an element is a stacking element, which indicates where additional stacking elements should be appended. This allows us to take advantage of nested stacking contexts, resulting in a default DOM position that works for most use cases.
*Note: When using `ui-front`, you must also set `position` to `relative`, `absolute` or `fixed` in order for the `z-index` to be applied.*
The stacking technique
-----------------------
Any widget that appends a stacking element to the page must use the `ui-front` class, and in many cases should have an `appendTo` option. The following logic should be applied to the stacking element:
* If a value is provided for the `appendTo` option, then append the stacking element to the specified element.
* If the `appendTo` option is set to `null` (default), then the widget should walk up the DOM from the associated element. For example, when the autocomplete menu is appended to the DOM, the walking starts from the associated input element.
+ If another stacking element is found, append to that element.
+ If no other stacking elements are found, append to the body.
* The stacking element must also have `position` set to `relative`, `absolute`, or `fixed` in order for the `z-index` from the `ui-front` class to be applied. Using [.position()](../position) will automatically set `position`.
Stacking elements are defined as elements with the `ui-front` class, or any native element that creates a new stacking context. Currently, `<dialog>` is the only native element that is considered a stacking element.
jqueryui CSS Framework CSS Framework
=============
The following is a list of the class names used by jQuery UI. The classes are designed to create a visual consistency across an application and allow components to be themeable by [jQuery UI ThemeRoller.](http://jqueryui.com/themeroller/) The class names are split between ui.core.css and ui.theme.css, depending on whether styles are fixed and structural, or themeable (colors, fonts, backgrounds, etc) respectively.
### Layout Helpers
* `.ui-helper-hidden`: Hides content visually and from assistive technologies, such as screen readers.
* `.ui-helper-hidden-accessible`: Hides content visually, but leaves it available to assistive technologies.
* `.ui-helper-reset`: A basic style reset for DOM nodes. Resets padding, margins, text-decoration, list-style, etc.
* `.ui-helper-clearfix`: Applies float wrapping properties to parent elements.
* `.ui-front`: Applies z-index to manage the stacking of multiple widgets on the screen. See the page about [stacking elements](stacking-elements) for more details.
### Widget Containers
* `.ui-widget`: Class to be applied to the outer container of all widgets. Applies font-family and font size to widgets.
* `.ui-widget-header`: Class to be applied to header containers. Applies header container styles to an element and its child text, links, and icons.
* `.ui-widget-content`: Class to be applied to content containers. Applies content container styles to an element and its child text, links, and icons. (can be applied to parent or sibling of header).
### Interaction States
* `.ui-state-default`: Class to be applied to clickable button-like elements. Applies "clickable default" container styles to an element and its child text, links, and icons.
* `.ui-state-hover`: Class to be applied on mouseover to clickable button-like elements. Applies "clickable hover" container styles to an element and its child text, links, and icons.
* `.ui-state-focus`: Class to be applied on keyboard focus to clickable button-like elements. Applies "clickable focus" container styles to an element and its child text, links, and icons.
* `.ui-state-active`: Class to be applied on mousedown to clickable button-like elements. Applies "clickable active" container styles to an element and its child text, links, and icons.
### Interaction Cues
* `.ui-state-highlight`: Class to be applied to highlighted or selected elements. Applies "highlight" container styles to an element and its child text, links, and icons.
* `.ui-state-error`: Class to be applied to error messaging container elements. Applies "error" container styles to an element and its child text, links, and icons.
* `.ui-state-error-text`: An additional class that applies just the error text color without background. Can be used on form labels for instance. Also applies error icon color to child icons.
* `.ui-state-disabled`: Applies a dimmed opacity to disabled UI elements. Meant to be added in addition to an already-styled element.
* `.ui-priority-primary`: Class to be applied to a priority-1 button in situations where button hierarchy is needed.
* `.ui-priority-secondary`: Class to be applied to a priority-2 button in situations where button hierarchy is needed.
### Icons
#### States and images
* `.ui-icon`: Base class to be applied to an icon element. Sets dimensions to a 16px square block, hides inner text, and sets background image to the "content" state sprite image. Note: `ui-icon` class will be given a different sprite background image depending on its parent container. For example, a `ui-icon` element within a `ui-state-default` container will get colored according to the `ui-state-default`'s icon color.
#### Icon types
After declaring a `ui-icon` class, you can follow up with a second class describing the type of icon. Icon classes generally follow a syntax of .ui-icon-{icon type}-{icon sub description}-{direction}.
For example, a single triangle icon pointing to the right looks like this: `.ui-icon-triangle-1-e`. ThemeRoller provides the full set of CSS framework icons in its preview column. Hover over them to see the class name.
### Misc Visuals
#### Corner Radius helpers
* `.ui-corner-tl`: Applies corner-radius to top left corner of element.
* `.ui-corner-tr`: Applies corner-radius to top right corner of element.
* `.ui-corner-bl`: Applies corner-radius to bottom left corner of element.
* `.ui-corner-br`: Applies corner-radius to bottom right corner of element.
* `.ui-corner-top`: Applies corner-radius to both top corners of element.
* `.ui-corner-bottom`: Applies corner-radius to both bottom corners of element.
* `.ui-corner-right`: Applies corner-radius to both right corners of element.
* `.ui-corner-left`: Applies corner-radius to both left corners of element.
* `.ui-corner-all`: Applies corner-radius to all 4 corners of element.
#### Overlay & Shadow
* `.ui-widget-overlay`: Applies 100% width & height dimensions to an overlay screen, along with background color/texture, and screen opacity.
* `.ui-widget-shadow`: Class to be applied to overlay widget shadow elements. Sets `box-shadow` x & y offset, blur radius and color.
jqueryui Icons Icons
=====
jQuery UI provides a number of icons that can be used by applying class names to elements. The class names generally follow a syntax of `.ui-icon-{icon type}-{icon sub description}-{direction}`. For example, the following will display an icon of a thick arrow pointing north:
```
<span class="ui-icon ui-icon-arrowthick-1-n"></span>
```
The icons are also integrated into a number of jQuery UI's widgets, such as [accordion](../accordion#option-icons), [button](../button#option-icons), [menu](../menu#option-icons).
The following is a full list of the icons provided:
eigen3 Eigen::OuterStride Eigen::OuterStride
==================
### template<int Value> class Eigen::OuterStride< Value >
Convenience specialization of [Stride](classeigen_1_1stride "Holds strides information for Map.") to specify only an outer stride See class [Map](classeigen_1_1map "A matrix or vector expression mapping an existing array of data.") for some examples.
| |
| --- |
| |
|
Public Types inherited from [Eigen::Stride< Value, 0 >](classeigen_1_1stride) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](classeigen_1_1stride#a96c2dfb0ce43fd8e19adcdf6094f5f63) |
| |
|
Public Member Functions inherited from [Eigen::Stride< Value, 0 >](classeigen_1_1stride) |
| EIGEN\_CONSTEXPR [Index](classeigen_1_1stride#a96c2dfb0ce43fd8e19adcdf6094f5f63) | [inner](classeigen_1_1stride#ab827ee701f5ccbd8f9bdeb32497df572) () const |
| |
| EIGEN\_CONSTEXPR [Index](classeigen_1_1stride#a96c2dfb0ce43fd8e19adcdf6094f5f63) | [outer](classeigen_1_1stride#a010ffe25388932a6c83c6d9d95b95442) () const |
| |
| | [Stride](classeigen_1_1stride#ae3f37b08ff44d2afe971c0894c2f44a5) () |
| |
| | [Stride](classeigen_1_1stride#aa9bb1a33da8c785d9cc4ad5d799b9253) (const [Stride](classeigen_1_1stride) &other) |
| |
| | [Stride](classeigen_1_1stride#a81e299d9d2f8bbfc6d240705dabe5833) ([Index](classeigen_1_1stride#a96c2dfb0ce43fd8e19adcdf6094f5f63) outerStride, [Index](classeigen_1_1stride#a96c2dfb0ce43fd8e19adcdf6094f5f63) innerStride) |
| |
---
The documentation for this class was generated from the following file:* [Stride.h](https://eigen.tuxfamily.org/dox/Stride_8h_source.html)
eigen3 Passing Eigen objects by value to functions Passing Eigen objects by value to functions
===========================================
Passing objects by value is almost always a very bad idea in C++, as this means useless copies, and one should pass them by reference instead.
With Eigen, this is even more important: passing [fixed-size vectorizable Eigen objects](group__topicfixedsizevectorizable) by value is not only inefficient, it can be illegal or make your program crash! And the reason is that these Eigen objects have alignment modifiers that aren't respected when they are passed by value.
For example, a function like this, where `v` is passed by value:
```
void my_function([Eigen::Vector2d](classeigen_1_1matrix) v);
```
needs to be rewritten as follows, passing `v` by const reference:
```
void my_function(const [Eigen::Vector2d](classeigen_1_1matrix)& v);
```
Likewise if you have a class having an Eigen object as member:
```
struct Foo
{
[Eigen::Vector2d](classeigen_1_1matrix) v;
};
void my_function(Foo v);
```
This function also needs to be rewritten like this:
```
void my_function(const Foo& v);
```
Note that on the other hand, there is no problem with functions that return objects by value.
eigen3 Matrix and vector arithmetic Matrix and vector arithmetic
============================
This page aims to provide an overview and some details on how to perform arithmetic between matrices, vectors and scalars with [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.").
Introduction
==============
[Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") offers matrix/vector arithmetic operations either through overloads of common C++ arithmetic operators such as +, -, \*, or through special methods such as dot(), cross(), etc. For the [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") class (matrices and vectors), operators are only overloaded to support linear-algebraic operations. For example, `matrix1` `*` `matrix2` means matrix-matrix product, and `vector` `+` `scalar` is just not allowed. If you want to perform all kinds of array operations, not linear algebra, see the [next page](group__tutorialarrayclass).
Addition and subtraction
==========================
The left hand side and right hand side must, of course, have the same numbers of rows and of columns. They must also have the same `Scalar` type, as [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") doesn't do automatic type promotion. The operators at hand here are:
* binary operator + as in `a+b`
* binary operator - as in `a-b`
* unary operator - as in `-a`
* compound operator += as in `a+=b`
* compound operator -= as in `a-=b`
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2d a;
a << 1, 2,
3, 4;
MatrixXd b(2,2);
b << 2, 3,
1, 4;
std::cout << "a + b =\n" << a + b << std::endl;
std::cout << "a - b =\n" << a - b << std::endl;
std::cout << "Doing a += b;" << std::endl;
a += b;
std::cout << "Now a =\n" << a << std::endl;
Vector3d v(1,2,3);
Vector3d w(1,0,0);
std::cout << "-v + w - v =\n" << -v + w - v << std::endl;
}
```
|
```
a + b =
3 5
4 8
a - b =
-1 -1
2 0
Doing a += b;
Now a =
3 5
4 8
-v + w - v =
-1
-4
-6
```
|
Scalar multiplication and division
====================================
Multiplication and division by a scalar is very simple too. The operators at hand here are:
* binary operator \* as in `matrix*scalar`
* binary operator \* as in `scalar*matrix`
* binary operator / as in `matrix/scalar`
* compound operator \*= as in `matrix*=scalar`
* compound operator /= as in `matrix/=scalar`
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2d a;
a << 1, 2,
3, 4;
Vector3d v(1,2,3);
std::cout << "a \* 2.5 =\n" << a * 2.5 << std::endl;
std::cout << "0.1 \* v =\n" << 0.1 * v << std::endl;
std::cout << "Doing v \*= 2;" << std::endl;
v *= 2;
std::cout << "Now v =\n" << v << std::endl;
}
```
|
```
a * 2.5 =
2.5 5
7.5 10
0.1 * v =
0.1
0.2
0.3
Doing v *= 2;
Now v =
2
4
6
```
|
A note about expression templates
===================================
This is an advanced topic that we explain on [this page](topiceigenexpressiontemplates), but it is useful to just mention it now. In [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library."), arithmetic operators such as `operator+` don't perform any computation by themselves, they just return an "expression object" describing the computation to be performed. The actual computation happens later, when the whole expression is evaluated, typically in `operator=`. While this might sound heavy, any modern optimizing compiler is able to optimize away that abstraction and the result is perfectly optimized code. For example, when you do:
```
VectorXf a(50), b(50), c(50), d(50);
...
a = 3*b + 4*c + 5*d;
```
[Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") compiles it to just one for loop, so that the arrays are traversed only once. Simplifying (e.g. ignoring SIMD optimizations), this loop looks like this:
```
for(int i = 0; i < 50; ++i)
a[i] = 3*b[i] + 4*c[i] + 5*d[i];
```
Thus, you should not be afraid of using relatively large arithmetic expressions with [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library."): it only gives [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") more opportunities for optimization.
Transposition and conjugation
===============================
The transpose \( a^T \), conjugate \( \bar{a} \), and adjoint (i.e., conjugate transpose) \( a^\* \) of a matrix or vector \( a \) are obtained by the member functions [transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c), [conjugate()](group__tutorialmatrixarithmetic), and [adjoint()](classeigen_1_1matrixbase#afacca1f88da57e5cd87dd07c8ff926bb), respectively.
| Example: | Output: |
| --- | --- |
|
```
MatrixXcf a = [MatrixXcf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(2,2);
cout << "Here is the matrix a\n" << a << endl;
cout << "Here is the matrix a^T\n" << a.transpose() << endl;
cout << "Here is the conjugate of a\n" << a.conjugate() << endl;
cout << "Here is the matrix a^\*\n" << a.adjoint() << endl;
```
|
```
Here is the matrix a
(-0.211,0.68) (-0.605,0.823)
(0.597,0.566) (0.536,-0.33)
Here is the matrix a^T
(-0.211,0.68) (0.597,0.566)
(-0.605,0.823) (0.536,-0.33)
Here is the conjugate of a
(-0.211,-0.68) (-0.605,-0.823)
(0.597,-0.566) (0.536,0.33)
Here is the matrix a^*
(-0.211,-0.68) (0.597,-0.566)
(-0.605,-0.823) (0.536,0.33)
```
|
For real matrices, `conjugate()` is a no-operation, and so `adjoint()` is equivalent to `transpose()`.
As for basic arithmetic operators, `transpose()` and `adjoint()` simply return a proxy object without doing the actual transposition. If you do `b = a.transpose()`, then the transpose is evaluated at the same time as the result is written into `b`. However, there is a complication here. If you do `a = a.transpose()`, then [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") starts writing the result into `a` before the evaluation of the transpose is finished. Therefore, the instruction `a = a.transpose()` does not replace `a` with its transpose, as one would expect:
| Example: | Output: |
| --- | --- |
|
```
Matrix2i a; a << 1, 2, 3, 4;
cout << "Here is the matrix a:\n" << a << endl;
a = a.transpose(); // !!! do NOT do this !!!
cout << "and the result of the aliasing effect:\n" << a << endl;
```
|
```
Here is the matrix a:
1 2
3 4
and the result of the aliasing effect:
1 2
2 4
```
|
This is the so-called [aliasing issue](group__topicaliasing). In "debug mode", i.e., when [assertions](topicassertions) have not been disabled, such common pitfalls are automatically detected.
For *in-place* transposition, as for instance in `a = a.transpose()`, simply use the [transposeInPlace()](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a) function:
| Example: | Output: |
| --- | --- |
|
```
MatrixXf a(2,3); a << 1, 2, 3, 4, 5, 6;
cout << "Here is the initial matrix a:\n" << a << endl;
a.transposeInPlace();
cout << "and after being transposed:\n" << a << endl;
```
|
```
Here is the initial matrix a:
1 2 3
4 5 6
and after being transposed:
1 4
2 5
3 6
```
|
There is also the [adjointInPlace()](classeigen_1_1matrixbase#a51c5982c1f64e45a939515b701fa6f4a) function for complex matrices.
Matrix-matrix and matrix-vector multiplication
================================================
Matrix-matrix multiplication is again done with `operator*`. Since vectors are a special case of matrices, they are implicitly handled there too, so matrix-vector product is really just a special case of matrix-matrix product, and so is vector-vector outer product. Thus, all these cases are handled by just two operators:
* binary operator \* as in `a*b`
* compound operator \*= as in `a*=b` (this multiplies on the right: `a*=b` is equivalent to `a = a*b`)
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2d mat;
mat << 1, 2,
3, 4;
Vector2d u(-1,1), v(2,0);
std::cout << "Here is mat\*mat:\n" << mat*mat << std::endl;
std::cout << "Here is mat\*u:\n" << mat*u << std::endl;
std::cout << "Here is u^T\*mat:\n" << u.transpose()*mat << std::endl;
std::cout << "Here is u^T\*v:\n" << u.transpose()*v << std::endl;
std::cout << "Here is u\*v^T:\n" << u*v.transpose() << std::endl;
std::cout << "Let's multiply mat by itself" << std::endl;
mat = mat*mat;
std::cout << "Now mat is mat:\n" << mat << std::endl;
}
```
|
```
Here is mat*mat:
7 10
15 22
Here is mat*u:
1
1
Here is u^T*mat:
2 2
Here is u^T*v:
-2
Here is u*v^T:
-2 -0
2 0
Let's multiply mat by itself
Now mat is mat:
7 10
15 22
```
|
Note: if you read the above paragraph on expression templates and are worried that doing `m=m*m` might cause aliasing issues, be reassured for now: [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") treats matrix multiplication as a special case and takes care of introducing a temporary here, so it will compile `m=m*m` as:
```
tmp = m*m;
m = tmp;
```
If you know your matrix product can be safely evaluated into the destination matrix without aliasing issue, then you can use the [noalias()](classeigen_1_1matrixbase#a2c1085de7645f23f240876388457da0b) function to avoid the temporary, e.g.:
```
c.noalias() += a * b;
```
For more details on this topic, see the page on [aliasing](group__topicaliasing).
**Note:** for BLAS users worried about performance, expressions such as `c.noalias() -= 2 * a.adjoint() * b;` are fully optimized and trigger a single gemm-like function call.
Dot product and cross product
===============================
For dot product and cross product, you need the [dot()](classeigen_1_1matrixbase#adfd32bf5fcf6ee603c924dde9bf7bc39) and [cross()](group__geometry__module#ga0024b44eca99cb7135887c2aaf319d28) methods. Of course, the dot product can also be obtained as a 1x1 matrix as u.adjoint()\*v.
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace [Eigen](namespaceeigen);
using namespace std;
int main()
{
Vector3d v(1,2,3);
Vector3d w(0,1,2);
cout << "Dot product: " << v.dot(w) << endl;
double dp = v.adjoint()*w; // automatic conversion of the inner product to a scalar
cout << "Dot product via a matrix product: " << dp << endl;
cout << "Cross product:\n" << v.cross(w) << endl;
}
```
|
```
Dot product: 8
Dot product via a matrix product: 8
Cross product:
1
-2
1
```
|
Remember that cross product is only for vectors of size 3. Dot product is for vectors of any sizes. When using complex numbers, [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.")'s dot product is conjugate-linear in the first variable and linear in the second variable.
Basic arithmetic reduction operations
=======================================
[Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") also provides some reduction operations to reduce a given matrix or vector to a single value such as the sum (computed by [sum()](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c)), product ([prod()](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f)), or the maximum ([maxCoeff()](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1)) and minimum ([minCoeff()](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2)) of all its coefficients.
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
int main()
{
[Eigen::Matrix2d](classeigen_1_1matrix) mat;
mat << 1, 2,
3, 4;
cout << "Here is mat.sum(): " << mat.[sum](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c)() << endl;
cout << "Here is mat.prod(): " << mat.[prod](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f)() << endl;
cout << "Here is mat.mean(): " << mat.[mean](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7)() << endl;
cout << "Here is mat.minCoeff(): " << mat.[minCoeff](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2)() << endl;
cout << "Here is mat.maxCoeff(): " << mat.[maxCoeff](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1)() << endl;
cout << "Here is mat.trace(): " << mat.[trace](classeigen_1_1matrixbase#a544b609f65eb2bd3e368b3fc2d79479e)() << endl;
}
```
|
```
Here is mat.sum(): 10
Here is mat.prod(): 24
Here is mat.mean(): 2.5
Here is mat.minCoeff(): 1
Here is mat.maxCoeff(): 4
Here is mat.trace(): 5
```
|
The *trace* of a matrix, as returned by the function [trace()](classeigen_1_1matrixbase#a544b609f65eb2bd3e368b3fc2d79479e), is the sum of the diagonal coefficients and can also be computed as efficiently using `a.diagonal().sum()`, as we will see later on.
There also exist variants of the `minCoeff` and `maxCoeff` functions returning the coordinates of the respective coefficient via the arguments:
| Example: | Output: |
| --- | --- |
|
```
Matrix3f m = [Matrix3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
std::ptrdiff_t i, j;
float minOfM = m.minCoeff(&i,&j);
cout << "Here is the matrix m:\n" << m << endl;
cout << "Its minimum coefficient (" << minOfM
<< ") is at position (" << i << "," << j << ")\n\n";
RowVector4i v = [RowVector4i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
int maxOfV = v.maxCoeff(&i);
cout << "Here is the vector v: " << v << endl;
cout << "Its maximum coefficient (" << maxOfV
<< ") is at position " << i << endl;
```
|
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Its minimum coefficient (-0.605) is at position (2,1)
Here is the vector v: 1 0 3 -3
Its maximum coefficient (3) is at position 2
```
|
Validity of operations
========================
[Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") checks the validity of the operations that you perform. When possible, it checks them at compile time, producing compilation errors. These error messages can be long and ugly, but [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") writes the important message in UPPERCASE\_LETTERS\_SO\_IT\_STANDS\_OUT. For example:
```
Matrix3f m;
Vector4f v;
v = m*v; // Compile-time error: YOU\_MIXED\_MATRICES\_OF\_DIFFERENT\_SIZES
```
Of course, in many cases, for example when checking dynamic sizes, the check cannot be performed at compile time. [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") then uses runtime assertions. This means that the program will abort with an error message when executing an illegal operation if it is run in "debug mode", and it will probably crash if assertions are turned off.
```
MatrixXf m(3,3);
VectorXf v(4);
v = m * v; // Run-time assertion failure here: "invalid matrix product"
```
For more details on this topic, see [this page](topicassertions).
| programming_docs |
eigen3 Eigen::CompleteOrthogonalDecomposition Eigen::CompleteOrthogonalDecomposition
======================================
### template<typename \_MatrixType> class Eigen::CompleteOrthogonalDecomposition< \_MatrixType >
Complete orthogonal decomposition (COD) of a matrix.
Parameters
| | |
| --- | --- |
| MatrixType | the type of the matrix of which we are computing the COD. |
This class performs a rank-revealing complete orthogonal decomposition of a matrix **A** into matrices **P**, **Q**, **T**, and **Z** such that
\[ \mathbf{A} \, \mathbf{P} = \mathbf{Q} \, \begin{bmatrix} \mathbf{T} & \mathbf{0} \\ \mathbf{0} & \mathbf{0} \end{bmatrix} \, \mathbf{Z} \]
by using Householder transformations. Here, **P** is a permutation matrix, **Q** and **Z** are unitary matrices and **T** an upper triangular matrix of size rank-by-rank. **A** may be rank deficient.
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
See also
[MatrixBase::completeOrthogonalDecomposition()](classeigen_1_1matrixbase#ae90b6846f05bd30b8d52b66e427e3e09)
| |
| --- |
| |
| MatrixType::RealScalar | [absDeterminant](classeigen_1_1completeorthogonaldecomposition#ac040c34ce3fb2b68d3f57adc0c29d526) () const |
| |
| const [PermutationType](classeigen_1_1permutationmatrix) & | [colsPermutation](classeigen_1_1completeorthogonaldecomposition#a601c67a4a0bbe9c7b25b885279db9ff2) () const |
| |
| | [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition#a7ba33814fd3fdc62f6179cdcd655c679) () |
| | Default Constructor. [More...](classeigen_1_1completeorthogonaldecomposition#a7ba33814fd3fdc62f6179cdcd655c679) |
| |
| template<typename InputType > |
| | [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition#afcfaf7a395f853247c23bb52b1ffb1cc) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a complete orthogonal decomposition from a given matrix. [More...](classeigen_1_1completeorthogonaldecomposition#afcfaf7a395f853247c23bb52b1ffb1cc) |
| |
| template<typename InputType > |
| | [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition#a082295ba2aac35a8b8b9e2d46e1d7ce4) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a complete orthogonal decomposition from a given matrix. [More...](classeigen_1_1completeorthogonaldecomposition#a082295ba2aac35a8b8b9e2d46e1d7ce4) |
| |
| | [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition#aa6c282dd7452ebe754024edb71bebd09) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) rows, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) cols) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1completeorthogonaldecomposition#aa6c282dd7452ebe754024edb71bebd09) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [dimensionOfKernel](classeigen_1_1completeorthogonaldecomposition#aca2b59560c4851b7d7701872990a3426) () const |
| |
| const HCoeffsType & | [hCoeffs](classeigen_1_1completeorthogonaldecomposition#ad25a868ecd499d6dda0119e4d3659504) () const |
| |
| [HouseholderSequenceType](classeigen_1_1householdersequence) | [householderQ](classeigen_1_1completeorthogonaldecomposition#ac95b93ddad59c6e57d06fcd4737b27e1) (void) const |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1completeorthogonaldecomposition#a1f5c45f78848cfdbb96963cefbbb3274) () const |
| | Reports whether the complete orthogonal decomposition was successful. [More...](classeigen_1_1completeorthogonaldecomposition#a1f5c45f78848cfdbb96963cefbbb3274) |
| |
| bool | [isInjective](classeigen_1_1completeorthogonaldecomposition#a2740067b81ec3e0ad20a2bea9a5a475f) () const |
| |
| bool | [isInvertible](classeigen_1_1completeorthogonaldecomposition#a7c4f24f868295349a3bb99e5f217b069) () const |
| |
| bool | [isSurjective](classeigen_1_1completeorthogonaldecomposition#a53dd287d3e1bbc548595d63880fd51bf) () const |
| |
| MatrixType::RealScalar | [logAbsDeterminant](classeigen_1_1completeorthogonaldecomposition#ad59d6dc78dab52a0038ac372b4a72c0d) () const |
| |
| const MatrixType & | [matrixQTZ](classeigen_1_1completeorthogonaldecomposition#ad89e2529fc1a8721239d9b6be9613a69) () const |
| |
| const MatrixType & | [matrixT](classeigen_1_1completeorthogonaldecomposition#a806213f5c96ff765265f47067229586d) () const |
| |
| MatrixType | [matrixZ](classeigen_1_1completeorthogonaldecomposition#a4cd178f13aefe5189d4beeb3eb1c5897) () const |
| |
| RealScalar | [maxPivot](classeigen_1_1completeorthogonaldecomposition#a5b712a58c13b7e5089be9228ec42f738) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonzeroPivots](classeigen_1_1completeorthogonaldecomposition#af9d7a5bdbc16a4a0bf7394ef0dd208da) () const |
| |
| const [Inverse](classeigen_1_1inverse)< [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) > | [pseudoInverse](classeigen_1_1completeorthogonaldecomposition#a3c89639299720ce089435d26d6822d6f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rank](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806) () const |
| |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) & | [setThreshold](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82) (const RealScalar &[threshold](classeigen_1_1completeorthogonaldecomposition#a3909f07268496c0f08f1b57331d91075)) |
| |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) & | [setThreshold](classeigen_1_1completeorthogonaldecomposition#a27c8da71874be7a64d6723bd0cae9f4f) (Default\_t) |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition), Rhs > | [solve](classeigen_1_1completeorthogonaldecomposition#ab303e177cc7df17e435c79dca9ef5654) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| RealScalar | [threshold](classeigen_1_1completeorthogonaldecomposition#a3909f07268496c0f08f1b57331d91075) () const |
| |
| const HCoeffsType & | [zCoeffs](classeigen_1_1completeorthogonaldecomposition#a0b28c24992d313d4b04d109dcc7e5220) () const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< CompleteOrthogonalDecomposition< \_MatrixType > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
| template<typename Rhs > |
| void | [applyZAdjointOnTheLeftInPlace](classeigen_1_1completeorthogonaldecomposition#a0a89641e0b4ea92c515405f2a31e6abe) (Rhs &rhs) const |
| |
| template<bool Conjugate, typename Rhs > |
| void | [applyZOnTheLeftInPlace](classeigen_1_1completeorthogonaldecomposition#afedd1ee41b8490b70fd1fefdd21f8c80) (Rhs &rhs) const |
| |
| void | [computeInPlace](classeigen_1_1completeorthogonaldecomposition#adb0b963d7d8f96492904e8eda03efbf5) () |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
CompleteOrthogonalDecomposition() [1/4]
---------------------------------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) | ( | | ) | |
| inline |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via `CompleteOrthogonalDecomposition::compute(const* MatrixType&)`.
CompleteOrthogonalDecomposition() [2/4]
---------------------------------------
template<typename \_MatrixType >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inline |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[CompleteOrthogonalDecomposition()](classeigen_1_1completeorthogonaldecomposition#a7ba33814fd3fdc62f6179cdcd655c679 "Default Constructor.")
CompleteOrthogonalDecomposition() [3/4]
---------------------------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a complete orthogonal decomposition from a given matrix.
This constructor computes the complete orthogonal decomposition of the matrix *matrix* by calling the method compute(). The default threshold for rank determination will be used. It is a short cut for:
```
CompleteOrthogonalDecomposition<MatrixType> cod(matrix.rows(),
matrix.cols());
cod.setThreshold(Default);
cod.compute(matrix);
```
See also
compute()
CompleteOrthogonalDecomposition() [4/4]
---------------------------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a complete orthogonal decomposition from a given matrix.
This overloaded constructor is provided for [inplace decomposition](group__inplacedecomposition) when `MatrixType` is a [Eigen::Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.").
See also
CompleteOrthogonalDecomposition(const EigenBase&)
absDeterminant()
----------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::absDeterminant |
Returns
the absolute value of the determinant of the matrix of which \*this is the complete orthogonal decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the complete orthogonal decomposition has already been computed.
Note
This is only for square matrices.
Warning
a determinant can be very big or small, so for matrices of large enough dimension, there is a risk of overflow/underflow. One way to work around that is to use [logAbsDeterminant()](classeigen_1_1completeorthogonaldecomposition#ad59d6dc78dab52a0038ac372b4a72c0d) instead.
See also
[logAbsDeterminant()](classeigen_1_1completeorthogonaldecomposition#ad59d6dc78dab52a0038ac372b4a72c0d), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
applyZAdjointOnTheLeftInPlace()
-------------------------------
template<typename MatrixType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::applyZAdjointOnTheLeftInPlace | ( | Rhs & | *rhs* | ) | const |
| protected |
Overwrites **rhs** with \( \mathbf{Z}^\* \* \mathbf{rhs} \).
applyZOnTheLeftInPlace()
------------------------
template<typename MatrixType > template<bool Conjugate, typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::applyZOnTheLeftInPlace | ( | Rhs & | *rhs* | ) | const |
| protected |
Overwrites **rhs** with \( \mathbf{Z} \* \mathbf{rhs} \) or \( \mathbf{\overline Z} \* \mathbf{rhs} \) if `Conjugate` is set to `true`.
colsPermutation()
-----------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationType](classeigen_1_1permutationmatrix)& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::colsPermutation | ( | | ) | const |
| inline |
Returns
a const reference to the column permutation matrix
computeInPlace()
----------------
template<typename MatrixType >
| | | |
| --- | --- | --- |
|
| |
| --- |
| void [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::computeInPlace |
| protected |
Performs the complete orthogonal decomposition of the given matrix *matrix*. The result of the factorization is stored into `*this`, and a reference to `*this` is returned.
See also
class [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition "Complete orthogonal decomposition (COD) of a matrix."), CompleteOrthogonalDecomposition(const MatrixType&)
dimensionOfKernel()
-------------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::dimensionOfKernel | ( | | ) | const |
| inline |
Returns
the dimension of the kernel of the matrix of which \*this is the complete orthogonal decomposition.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
hCoeffs()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const HCoeffsType& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::hCoeffs | ( | | ) | const |
| inline |
Returns
a const reference to the vector of Householder coefficients used to represent the factor `Q`.
For advanced uses only.
householderQ()
--------------
template<typename MatrixType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::[HouseholderSequenceType](classeigen_1_1householdersequence) [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::householderQ | ( | void | | ) | const |
Returns
the matrix Q as a sequence of householder transformations
info()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::info | ( | | ) | const |
| inline |
Reports whether the complete orthogonal decomposition was successful.
Note
This function always returns `Success`. It is provided for compatibility with other factorization routines.
Returns
`Success`
isInjective()
-------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::isInjective | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the decomposition represents an injective linear map, i.e. has trivial kernel; false otherwise.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
isInvertible()
--------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::isInvertible | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the complete orthogonal decomposition is invertible.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
isSurjective()
--------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::isSurjective | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the decomposition represents a surjective linear map; false otherwise.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
logAbsDeterminant()
-------------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< MatrixType >::logAbsDeterminant |
Returns
the natural log of the absolute value of the determinant of the matrix of which \*this is the complete orthogonal decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the complete orthogonal decomposition has already been computed.
Note
This is only for square matrices. This method is useful to work around the risk of overflow/underflow that's inherent to determinant computation.
See also
[absDeterminant()](classeigen_1_1completeorthogonaldecomposition#ac040c34ce3fb2b68d3f57adc0c29d526), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
matrixQTZ()
-----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::matrixQTZ | ( | | ) | const |
| inline |
Returns
a reference to the matrix where the complete orthogonal decomposition is stored
matrixT()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::matrixT | ( | | ) | const |
| inline |
Returns
a reference to the matrix where the complete orthogonal decomposition is stored.
Warning
The strict lower part and
```
cols() - [rank](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806)()
```
right columns of this matrix contains internal values. Only the upper triangular part should be referenced. To get it, use
```
[matrixT](classeigen_1_1completeorthogonaldecomposition#a806213f5c96ff765265f47067229586d)().template triangularView<Upper>()
```
For rank-deficient matrices, use
```
matrixR().topLeftCorner([rank](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806)(), [rank](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806)()).template triangularView<Upper>()
```
matrixZ()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| MatrixType [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::matrixZ | ( | | ) | const |
| inline |
Returns
the matrix **Z**.
maxPivot()
----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::maxPivot | ( | | ) | const |
| inline |
Returns
the absolute value of the biggest pivot, i.e. the biggest diagonal coefficient of R.
nonzeroPivots()
---------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::nonzeroPivots | ( | | ) | const |
| inline |
Returns
the number of nonzero pivots in the complete orthogonal decomposition. Here nonzero is meant in the exact sense, not in a fuzzy sense. So that notion isn't really intrinsically interesting, but it is still useful when implementing algorithms.
See also
[rank()](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806)
pseudoInverse()
---------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [Inverse](classeigen_1_1inverse)<[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)> [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::pseudoInverse | ( | | ) | const |
| inline |
Returns
the pseudo-inverse of the matrix of which \*this is the complete orthogonal decomposition.
Warning
: Do not compute `this->[pseudoInverse()](classeigen_1_1completeorthogonaldecomposition#a3c89639299720ce089435d26d6822d6f)*rhs` to solve a linear systems. It is more efficient and numerically stable to call `this->solve(rhs)`.
rank()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::rank | ( | | ) | const |
| inline |
Returns
the rank of the matrix of which \*this is the complete orthogonal decomposition.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
setThreshold() [1/2]
--------------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::setThreshold | ( | const RealScalar & | *threshold* | ) | |
| inline |
Allows to prescribe a threshold to be used by certain methods, such as [rank()](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806), who need to determine when pivots are to be considered nonzero. Most be called before calling compute().
When it needs to get the threshold value, [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") calls [threshold()](classeigen_1_1completeorthogonaldecomposition#a3909f07268496c0f08f1b57331d91075). By default, this uses a formula to automatically determine a reasonable threshold. Once you have called the present method [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82), your value is used instead.
Parameters
| | |
| --- | --- |
| threshold | The new value to use as the threshold. |
A pivot will be considered nonzero if its absolute value is strictly greater than \( \vert pivot \vert \leqslant threshold \times \vert maxpivot \vert \) where maxpivot is the biggest pivot.
If you want to come back to the default behavior, call [setThreshold(Default\_t)](classeigen_1_1completeorthogonaldecomposition#a27c8da71874be7a64d6723bd0cae9f4f)
setThreshold() [2/2]
--------------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::setThreshold | ( | Default\_t | | ) | |
| inline |
Allows to come back to the default behavior, letting [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") use its default formula for determining the threshold.
You should pass the special object Eigen::Default as parameter here.
```
qr.setThreshold(Eigen::Default);
```
See the documentation of [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
solve()
-------
template<typename \_MatrixType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition), Rhs> [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
This method computes the minimum-norm solution X to a least squares problem
\[\mathrm{minimize} \|A X - B\|, \]
where **A** is the matrix of which `*this` is the complete orthogonal decomposition.
Parameters
| | |
| --- | --- |
| b | the right-hand sides of the problem to solve. |
Returns
a solution.
threshold()
-----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::threshold | ( | | ) | const |
| inline |
Returns the threshold that will be used by certain methods such as [rank()](classeigen_1_1completeorthogonaldecomposition#af348f64b26f8467a020062c22b748806).
See the documentation of [setThreshold(const RealScalar&)](classeigen_1_1completeorthogonaldecomposition#aa9c9f7cbde9d58ca5552381b70ad8d82).
zCoeffs()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const HCoeffsType& [Eigen::CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< \_MatrixType >::zCoeffs | ( | | ) | const |
| inline |
Returns
a const reference to the vector of Householder coefficients used to represent the factor `Z`.
For advanced uses only.
---
The documentation for this class was generated from the following file:* [CompleteOrthogonalDecomposition.h](https://eigen.tuxfamily.org/dox/CompleteOrthogonalDecomposition_8h_source.html)
| programming_docs |
eigen3 Linear algebra and decompositions Linear algebra and decompositions
=================================
This page explains how to solve linear systems, compute various decompositions such as LU, QR, SVD, eigendecompositions... After reading this page, don't miss our [catalogue](group__topiclinearalgebradecompositions) of dense matrix decompositions.
Basic linear solving
======================
**The** **problem:** You have a system of equations, that you have written as a single matrix equation
\[ Ax \: = \: b \]
Where *A* and *b* are matrices (*b* could be a vector, as a special case). You want to find a solution *x*.
**The** **solution:** You can choose between various decompositions, depending on the properties of your matrix *A*, and depending on whether you favor speed or accuracy. However, let's start with an example that works in all cases, and is a good compromise:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix3f A;
Vector3f b;
A << 1,2,3, 4,5,6, 7,8,10;
b << 3, 3, 4;
cout << "Here is the matrix A:\n" << A << endl;
cout << "Here is the vector b:\n" << b << endl;
Vector3f x = A.colPivHouseholderQr().solve(b);
cout << "The solution is:\n" << x << endl;
}
```
|
```
Here is the matrix A:
1 2 3
4 5 6
7 8 10
Here is the vector b:
3
3
4
The solution is:
-2
1
1
```
|
In this example, the colPivHouseholderQr() method returns an object of class [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting."). Since here the matrix is of type Matrix3f, this line could have been replaced by:
```
ColPivHouseholderQR<Matrix3f> dec(A);
Vector3f x = dec.solve(b);
```
Here, [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.") is a QR decomposition with column pivoting. It's a good compromise for this tutorial, as it works for all matrices while being quite fast. Here is a table of some other decompositions that you can choose from, depending on your matrix, the problem you are trying to solve, and the trade-off you want to make:
| Decomposition | Method | Requirements on the matrix | Speed (small-to-medium) | Speed (large) | Accuracy |
| --- | --- | --- | --- | --- | --- |
| [PartialPivLU](classeigen_1_1partialpivlu "LU decomposition of a matrix with partial pivoting, and related features.") | partialPivLu() | Invertible | ++ | ++ | + |
| [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.") | fullPivLu() | None | - | - - | +++ |
| [HouseholderQR](classeigen_1_1householderqr "Householder QR decomposition of a matrix.") | householderQr() | None | ++ | ++ | + |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.") | colPivHouseholderQr() | None | + | - | +++ |
| [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with full pivoting.") | fullPivHouseholderQr() | None | - | - - | +++ |
| [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition "Complete orthogonal decomposition (COD) of a matrix.") | completeOrthogonalDecomposition() | None | + | - | +++ |
| [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") | llt() | Positive definite | +++ | +++ | + |
| [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") | ldlt() | Positive or negative semidefinite | +++ | + | ++ |
| [BDCSVD](classeigen_1_1bdcsvd "class Bidiagonal Divide and Conquer SVD") | bdcSvd() | None | - | - | +++ |
| [JacobiSVD](classeigen_1_1jacobisvd "Two-sided Jacobi SVD decomposition of a rectangular matrix.") | jacobiSvd() | None | - | - - - | +++ |
To get an overview of the true relative speed of the different decompositions, check this [benchmark](group__densedecompositionbenchmark) .
All of these decompositions offer a solve() method that works as in the above example.
If you know more about the properties of your matrix, you can use the above table to select the best method. For example, a good choice for solving linear systems with a non-symmetric matrix of full rank is [PartialPivLU](classeigen_1_1partialpivlu "LU decomposition of a matrix with partial pivoting, and related features."). If you know that your matrix is also symmetric and positive definite, the above table says that a very good choice is the [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") or [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") decomposition. Here's an example, also demonstrating that using a general matrix (not a vector) as right hand side is possible:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2f A, b;
A << 2, -1, -1, 3;
b << 1, 2, 3, 1;
cout << "Here is the matrix A:\n" << A << endl;
cout << "Here is the right hand side b:\n" << b << endl;
Matrix2f x = A.ldlt().solve(b);
cout << "The solution is:\n" << x << endl;
}
```
|
```
Here is the matrix A:
2 -1
-1 3
Here is the right hand side b:
1 2
3 1
The solution is:
1.2 1.4
1.4 0.8
```
|
For a [much more complete table](group__topiclinearalgebradecompositions) comparing all decompositions supported by [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") (notice that [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") supports many other decompositions), see our special page on [this topic](group__topiclinearalgebradecompositions).
Least squares solving
=======================
The most general and accurate method to solve under- or over-determined linear systems in the least squares sense, is the SVD decomposition. [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") provides two implementations. The recommended one is the [BDCSVD](classeigen_1_1bdcsvd "class Bidiagonal Divide and Conquer SVD") class, which scales well for large problems and automatically falls back to the [JacobiSVD](classeigen_1_1jacobisvd "Two-sided Jacobi SVD decomposition of a rectangular matrix.") class for smaller problems. For both classes, their solve() method solved the linear system in the least-squares sense.
Here is an example:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
MatrixXf A = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3, 2);
cout << "Here is the matrix A:\n" << A << endl;
VectorXf b = [VectorXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3);
cout << "Here is the right hand side b:\n" << b << endl;
cout << "The least-squares solution is:\n"
<< A.bdcSvd([ComputeThinU](group__enums#ggae3e239fb70022eb8747994cf5d68b4a9aa7fb4e98834788d0b1b0f2b8467d2527) | [ComputeThinV](group__enums#ggae3e239fb70022eb8747994cf5d68b4a9a540036417bfecf2e791a70948c227f47)).solve(b) << endl;
}
```
|
```
Here is the matrix A:
0.68 0.597
-0.211 0.823
0.566 -0.605
Here is the right hand side b:
-0.33
0.536
-0.444
The least-squares solution is:
-0.67
0.314
```
|
An alternative to the SVD, which is usually faster and about as accurate, is [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition "Complete orthogonal decomposition (COD) of a matrix.").
Again, if you know more about the problem, the table above contains methods that are potentially faster. If your matrix is full rank, HouseHolderQR is the method of choice. If your matrix is full rank and well conditioned, using the Cholesky decomposition ([LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.")) on the matrix of the normal equations can be faster still. Our page on [least squares solving](group__leastsquares) has more details.
Checking if a matrix is singular
==================================
Only you know what error margin you want to allow for a solution to be considered valid. So [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") lets you do this computation for yourself, if you want to, as in this example:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
MatrixXd A = [MatrixXd::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(100,100);
MatrixXd b = [MatrixXd::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(100,50);
MatrixXd x = A.fullPivLu().solve(b);
double relative_error = (A*x - b).norm() / b.norm(); // norm() is L2 norm
cout << "The relative error is:\n" << relative_error << endl;
}
```
|
```
The relative error is:
2.31495e-14
```
|
Computing eigenvalues and eigenvectors
========================================
You need an eigendecomposition here, see available such decompositions on [this page](group__topiclinearalgebradecompositions). Make sure to check if your matrix is self-adjoint, as is often the case in these problems. Here's an example using [SelfAdjointEigenSolver](classeigen_1_1selfadjointeigensolver "Computes eigenvalues and eigenvectors of selfadjoint matrices."), it could easily be adapted to general matrices using [EigenSolver](classeigen_1_1eigensolver "Computes eigenvalues and eigenvectors of general matrices.") or [ComplexEigenSolver](classeigen_1_1complexeigensolver "Computes eigenvalues and eigenvectors of general complex matrices.").
The computation of eigenvalues and eigenvectors does not necessarily converge, but such failure to converge is very rare. The call to info() is to check for this possibility.
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2f A;
A << 1, 2, 2, 3;
cout << "Here is the matrix A:\n" << A << endl;
SelfAdjointEigenSolver<Matrix2f> eigensolver(A);
if (eigensolver.info() != [Success](group__enums#gga85fad7b87587764e5cf6b513a9e0ee5ea671a2aeb0f527802806a441d58a80fcf)) abort();
cout << "The eigenvalues of A are:\n" << eigensolver.eigenvalues() << endl;
cout << "Here's a matrix whose columns are eigenvectors of A \n"
<< "corresponding to these eigenvalues:\n"
<< eigensolver.eigenvectors() << endl;
}
```
|
```
Here is the matrix A:
1 2
2 3
The eigenvalues of A are:
-0.236
4.24
Here's a matrix whose columns are eigenvectors of A
corresponding to these eigenvalues:
-0.851 -0.526
0.526 -0.851
```
|
Computing inverse and determinant
===================================
First of all, make sure that you really want this. While inverse and determinant are fundamental mathematical concepts, in *numerical* linear algebra they are not as useful as in pure mathematics. [Inverse](classeigen_1_1inverse "Expression of the inverse of another expression.") computations are often advantageously replaced by solve() operations, and the determinant is often *not* a good way of checking if a matrix is invertible.
However, for *very* *small* matrices, the above may not be true, and inverse and determinant can be very useful.
While certain decompositions, such as [PartialPivLU](classeigen_1_1partialpivlu "LU decomposition of a matrix with partial pivoting, and related features.") and [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features."), offer [inverse()](namespaceeigen#ae9de9064c3b832ee804c0e0957e80334) and determinant() methods, you can also call [inverse()](namespaceeigen#ae9de9064c3b832ee804c0e0957e80334) and determinant() directly on a matrix. If your matrix is of a very small fixed size (at most 4x4) this allows [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") to avoid performing a LU decomposition, and instead use formulas that are more efficient on such small matrices.
Here is an example:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix3f A;
A << 1, 2, 1,
2, 1, 0,
-1, 1, 2;
cout << "Here is the matrix A:\n" << A << endl;
cout << "The determinant of A is " << A.determinant() << endl;
cout << "The inverse of A is:\n" << A.inverse() << endl;
}
```
|
```
Here is the matrix A:
1 2 1
2 1 0
-1 1 2
The determinant of A is -3
The inverse of A is:
-0.667 1 0.333
1.33 -1 -0.667
-1 1 1
```
|
Separating the computation from the construction
==================================================
In the above examples, the decomposition was computed at the same time that the decomposition object was constructed. There are however situations where you might want to separate these two things, for example if you don't know, at the time of the construction, the matrix that you will want to decompose; or if you want to reuse an existing decomposition object.
What makes this possible is that:
* all decompositions have a default constructor,
* all decompositions have a compute(matrix) method that does the computation, and that may be called again on an already-computed decomposition, reinitializing it.
For example:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2f A, b;
LLT<Matrix2f> llt;
A << 2, -1, -1, 3;
b << 1, 2, 3, 1;
cout << "Here is the matrix A:\n" << A << endl;
cout << "Here is the right hand side b:\n" << b << endl;
cout << "Computing LLT decomposition..." << endl;
llt.compute(A);
cout << "The solution is:\n" << llt.solve(b) << endl;
A(1,1)++;
cout << "The matrix A is now:\n" << A << endl;
cout << "Computing LLT decomposition..." << endl;
llt.compute(A);
cout << "The solution is now:\n" << llt.solve(b) << endl;
}
```
|
```
Here is the matrix A:
2 -1
-1 3
Here is the right hand side b:
1 2
3 1
Computing LLT decomposition...
The solution is:
1.2 1.4
1.4 0.8
The matrix A is now:
2 -1
-1 4
Computing LLT decomposition...
The solution is now:
1 1.29
1 0.571
```
|
Finally, you can tell the decomposition constructor to preallocate storage for decomposing matrices of a given size, so that when you subsequently decompose such matrices, no dynamic memory allocation is performed (of course, if you are using fixed-size matrices, no dynamic memory allocation happens at all). This is done by just passing the size to the decomposition constructor, as in this example:
```
HouseholderQR<MatrixXf> qr(50,50);
MatrixXf A = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(50,50);
qr.compute(A); // no dynamic memory allocation
```
Rank-revealing decompositions
===============================
Certain decompositions are rank-revealing, i.e. are able to compute the rank of a matrix. These are typically also the decompositions that behave best in the face of a non-full-rank matrix (which in the square case means a singular matrix). On [this table](group__topiclinearalgebradecompositions) you can see for all our decompositions whether they are rank-revealing or not.
Rank-revealing decompositions offer at least a rank() method. They can also offer convenience methods such as isInvertible(), and some are also providing methods to compute the kernel (null-space) and image (column-space) of the matrix, as is the case with [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features."):
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix3f A;
A << 1, 2, 5,
2, 1, 4,
3, 0, 3;
cout << "Here is the matrix A:\n" << A << endl;
FullPivLU<Matrix3f> lu_decomp(A);
cout << "The rank of A is " << lu_decomp.rank() << endl;
cout << "Here is a matrix whose columns form a basis of the null-space of A:\n"
<< lu_decomp.kernel() << endl;
cout << "Here is a matrix whose columns form a basis of the column-space of A:\n"
<< lu_decomp.image(A) << endl; // yes, have to pass the original A
}
```
|
```
Here is the matrix A:
1 2 5
2 1 4
3 0 3
The rank of A is 2
Here is a matrix whose columns form a basis of the null-space of A:
0.5
1
-0.5
Here is a matrix whose columns form a basis of the column-space of A:
5 1
4 2
3 3
```
|
Of course, any rank computation depends on the choice of an arbitrary threshold, since practically no floating-point matrix is *exactly* rank-deficient. [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") picks a sensible default threshold, which depends on the decomposition but is typically the diagonal size times machine epsilon. While this is the best default we could pick, only you know what is the right threshold for your application. You can set this by calling setThreshold() on your decomposition object before calling rank() or any other method that needs to use such a threshold. The decomposition itself, i.e. the compute() method, is independent of the threshold. You don't need to recompute the decomposition after you've changed the threshold.
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2d A;
A << 2, 1,
2, 0.9999999999;
FullPivLU<Matrix2d> lu(A);
cout << "By default, the rank of A is found to be " << lu.rank() << endl;
lu.setThreshold(1e-5);
cout << "With threshold 1e-5, the rank of A is found to be " << lu.rank() << endl;
}
```
|
```
By default, the rank of A is found to be 2
With threshold 1e-5, the rank of A is found to be 1
```
|
eigen3 Eigen::SparseView Eigen::SparseView
=================
### template<typename MatrixType> class Eigen::SparseView< MatrixType >
Expression of a dense or sparse matrix with zero or too small values removed.
Template Parameters
| | |
| --- | --- |
| MatrixType | the type of the object of which we are removing the small entries |
This class represents an expression of a given dense or sparse matrix with entries smaller than `reference` \* `epsilon` are removed. It is the return type of [MatrixBase::sparseView()](group__sparsecore__module#ga320dd291cbf4339c6118c41521b75350) and [SparseMatrixBase::pruned()](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d) and most of the time this is the only way it is used.
See also
[MatrixBase::sparseView()](group__sparsecore__module#ga320dd291cbf4339c6118c41521b75350), [SparseMatrixBase::pruned()](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d)
| |
| --- |
| |
| const internal::remove\_all< MatrixTypeNested >::type & | [nestedExpression](classeigen_1_1sparseview#a50f53a9405017012077ae907959aca14) () const |
| |
|
Public Member Functions inherited from [Eigen::SparseMatrixBase< SparseView< MatrixType > >](classeigen_1_1sparsematrixbase) |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsematrixbase#aca7ce296424ef6e478ab0fb19547a7ee) () const |
| |
| const internal::eval< [SparseView](classeigen_1_1sparseview)< MatrixType > >::type | [eval](classeigen_1_1sparsematrixbase#a761bd872a06b59632fcff7b7807a77ce) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsematrixbase#a180fcba1ccf3cdf3252a263bc1de7a1d) () const |
| |
| bool | [isVector](classeigen_1_1sparsematrixbase#a7eedffa867031f649fd0fb9cc23ce4be) () const |
| |
| const [Product](classeigen_1_1product)< [SparseView](classeigen_1_1sparseview)< MatrixType >, OtherDerived, AliasFreeProduct > | [operator\*](classeigen_1_1sparsematrixbase#a9d4d71b3f34389e6fc01f2b86e43f7a4) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< OtherDerived > &other) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsematrixbase#ac86cc88a4cfef21db6b64ec0ab4c8f0a) () const |
| |
| const [SparseView](classeigen_1_1sparseview)< [SparseView](classeigen_1_1sparseview)< MatrixType > > | [pruned](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d) (const Scalar &reference=Scalar(0), const RealScalar &epsilon=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsematrixbase#a1944e9fa9ce7937bfc3a87b2cb94371f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1sparsematrixbase#a124bc57921775eb9aa2dfd9727e23472) () const |
| |
| SparseSymmetricPermutationProduct< [SparseView](classeigen_1_1sparseview)< MatrixType >, Upper|Lower > | [twistedBy](classeigen_1_1sparsematrixbase#a51d4898bd6a57cc3ba543a39b102423e) (const [PermutationMatrix](classeigen_1_1permutationmatrix)< Dynamic, Dynamic, [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) > &perm) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< SparseView< MatrixType > >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| [SparseView](classeigen_1_1sparseview)< MatrixType > & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [SparseView](classeigen_1_1sparseview)< MatrixType > & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::SparseMatrixBase< SparseView< MatrixType > >](classeigen_1_1sparsematrixbase) |
| typedef internal::traits< [SparseView](classeigen_1_1sparseview)< MatrixType > >::[StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) | [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) |
| |
| typedef Scalar | [value\_type](classeigen_1_1sparsematrixbase#ac254d3b61718ebc2136d27bac043dcb7) |
| |
|
Public Types inherited from [Eigen::EigenBase< SparseView< MatrixType > >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
nestedExpression()
------------------
template<typename MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const internal::remove\_all<MatrixTypeNested>::type& [Eigen::SparseView](classeigen_1_1sparseview)< MatrixType >::nestedExpression | ( | | ) | const |
| inline |
Returns
the nested expression
---
The documentation for this class was generated from the following files:* [SparseUtil.h](https://eigen.tuxfamily.org/dox/SparseUtil_8h_source.html)
* [SparseView.h](https://eigen.tuxfamily.org/dox/SparseView_8h_source.html)
| programming_docs |
eigen3 Eigen::EigenBase Eigen::EigenBase
================
### template<typename Derived> class Eigen::EigenBase< Derived >
Common base class for all classes T such that [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") has an operator=(T) and a constructor MatrixBase(T).
In other words, an [EigenBase](structeigen_1_1eigenbase) object is an object that can be copied into a [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.").
Besides MatrixBase-derived classes, this also includes special matrix classes such as diagonal matrices, etc.
Notice that this class is trivial, it is only used to disambiguate overloaded functions.
See also
[The class hierarchy](topicclasshierarchy)

| |
| --- |
| |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
| |
| --- |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
Index
-----
template<typename Derived >
| |
| --- |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::[Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
The interface type of indices.
To change this, `#define` the preprocessor symbol `EIGEN_DEFAULT_DENSE_INDEX_TYPE`.
See also
StorageIndex, [Preprocessor directives](topicpreprocessordirectives). DEPRECATED: Since [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3, its usage is deprecated. Use [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde "The Index type as used for the API.") instead. Deprecation is not marked with a doxygen comment because there are too many existing usages to add the deprecation attribute.
cols()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::cols | ( | void | | ) | const |
| inline |
Returns
the number of columns.
See also
[rows()](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2), ColsAtCompileTime
derived() [1/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived | ( | | ) | |
| inline |
Returns
a reference to the derived object
derived() [2/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived | ( | | ) | const |
| inline |
Returns
a const reference to the derived object
rows()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::rows | ( | void | | ) | const |
| inline |
Returns
the number of rows.
See also
[cols()](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe), RowsAtCompileTime
size()
------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::size | ( | | ) | const |
| inline |
Returns
the number of coefficients, which is [rows()](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2)\*cols().
See also
[rows()](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2), [cols()](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe), SizeAtCompileTime.
---
The documentation for this class was generated from the following file:* [EigenBase.h](https://eigen.tuxfamily.org/dox/EigenBase_8h_source.html)
eigen3 Eigen::ParametrizedLine Eigen::ParametrizedLine
=======================
### template<typename \_Scalar, int \_AmbientDim, int \_Options> class Eigen::ParametrizedLine< \_Scalar, \_AmbientDim, \_Options >
A parametrized line.
This is defined in the Geometry module.
```
#include <Eigen/Geometry>
```
A parametrized line is defined by an origin point \( \mathbf{o} \) and a unit direction vector \( \mathbf{d} \) such that the line corresponds to the set \( l(t) = \mathbf{o} + t \mathbf{d} \), \( t \in \mathbf{R} \).
Template Parameters
| | |
| --- | --- |
| \_Scalar | the scalar type, i.e., the type of the coefficients |
| \_AmbientDim | the dimension of the ambient space, can be a compile time value or Dynamic. |
| |
| --- |
| |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) |
| |
| |
| --- |
| |
| template<typename NewScalarType > |
| internal::cast\_return\_type< [ParametrizedLine](classeigen_1_1parametrizedline), [ParametrizedLine](classeigen_1_1parametrizedline)< NewScalarType, AmbientDimAtCompileTime, Options > >::type | [cast](classeigen_1_1parametrizedline#acd906e5340b05a41e098496aa861e5a5) () const |
| |
| [Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) | [dim](classeigen_1_1parametrizedline#a68d562318c269edaa8ecb7aca98706d4) () const |
| |
| RealScalar | [distance](classeigen_1_1parametrizedline#a25c3e5822420b850e92023cec644c0a0) (const [VectorType](classeigen_1_1matrix) &p) const |
| |
| template<int OtherOptions> |
| \_Scalar | [intersection](classeigen_1_1parametrizedline#a980dc8dc59ea6de2fb496055181a1d08) (const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > &hyperplane) const |
| |
| template<int OtherOptions> |
| \_Scalar | [intersectionParameter](classeigen_1_1parametrizedline#af1d5100ee79a651e97ef716ad1af2ebe) (const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > &hyperplane) const |
| |
| template<int OtherOptions> |
| [VectorType](classeigen_1_1matrix) | [intersectionPoint](classeigen_1_1parametrizedline#a0ebbede4dd8f60da43128af9aabf0ead) (const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > &hyperplane) const |
| |
| bool | [isApprox](classeigen_1_1parametrizedline#adb58b8eb1d9a81a4b02b7bbbebd84413) (const [ParametrizedLine](classeigen_1_1parametrizedline) &other, const typename [NumTraits](structeigen_1_1numtraits)< Scalar >::Real &prec=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| | [ParametrizedLine](classeigen_1_1parametrizedline#a43e71a77728409e15220495dc9724f04) () |
| |
| template<int OtherOptions> |
| | [ParametrizedLine](classeigen_1_1parametrizedline#a3f26a2ac6fe39d39ba72e148f0f877ad) (const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > &hyperplane) |
| |
| template<typename OtherScalarType , int OtherOptions> |
| | [ParametrizedLine](classeigen_1_1parametrizedline#abf4318b4e0c2469e83ab049c9c177b80) (const [ParametrizedLine](classeigen_1_1parametrizedline)< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > &other) |
| |
| | [ParametrizedLine](classeigen_1_1parametrizedline#a9ae3a16ee1d2a6cf09f45007451fa148) (const [VectorType](classeigen_1_1matrix) &origin, const [VectorType](classeigen_1_1matrix) &direction) |
| |
| | [ParametrizedLine](classeigen_1_1parametrizedline#a5261e771f71d975eaa9ebf8bc598129b) ([Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) \_dim) |
| |
| [VectorType](classeigen_1_1matrix) | [pointAt](classeigen_1_1parametrizedline#a925fd2bd7ce6927de196859f2f5bec41) (const Scalar &t) const |
| |
| [VectorType](classeigen_1_1matrix) | [projection](classeigen_1_1parametrizedline#a37c3fe8af9a90adce20b1f6ee62c04ea) (const [VectorType](classeigen_1_1matrix) &p) const |
| |
| RealScalar | [squaredDistance](classeigen_1_1parametrizedline#a5cb6544a7fff4c3571c0a5eb82eec8bb) (const [VectorType](classeigen_1_1matrix) &p) const |
| |
| template<typename XprType > |
| [ParametrizedLine](classeigen_1_1parametrizedline) & | [transform](classeigen_1_1parametrizedline#a1c2a0ceacef80e76b71f56992a721e6a) (const [MatrixBase](classeigen_1_1matrixbase)< XprType > &mat, [TransformTraits](group__enums#gaee59a86102f150923b0cac6d4ff05107) traits=[Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb)) |
| |
| template<int TrOptions> |
| [ParametrizedLine](classeigen_1_1parametrizedline) & | [transform](classeigen_1_1parametrizedline#a09d711ff37c2f421c65f398b9c7120b1) (const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb), TrOptions > &t, [TransformTraits](group__enums#gaee59a86102f150923b0cac6d4ff05107) traits=[Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb)) |
| |
| |
| --- |
| |
| static [ParametrizedLine](classeigen_1_1parametrizedline) | [Through](classeigen_1_1parametrizedline#a9e6c2b409eae9da4f66439fff8d4ffcc) (const [VectorType](classeigen_1_1matrix) &p0, const [VectorType](classeigen_1_1matrix) &p1) |
| |
Index
-----
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| |
| --- |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) |
**[Deprecated:](deprecated#_deprecated000026)**
since [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3
ParametrizedLine() [1/5]
------------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[ParametrizedLine](classeigen_1_1parametrizedline) | ( | | ) | |
| inline |
Default constructor without initialization
ParametrizedLine() [2/5]
------------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[ParametrizedLine](classeigen_1_1parametrizedline) | ( | [Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) | *\_dim* | ) | |
| inlineexplicit |
Constructs a dynamic-size line with *\_dim* the dimension of the ambient space
ParametrizedLine() [3/5]
------------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[ParametrizedLine](classeigen_1_1parametrizedline) | ( | const [VectorType](classeigen_1_1matrix) & | *origin*, |
| | | const [VectorType](classeigen_1_1matrix) & | *direction* |
| | ) | | |
| inline |
Initializes a parametrized line of direction *direction* and origin *origin*.
Warning
the vector direction is assumed to be normalized.
ParametrizedLine() [4/5]
------------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<int OtherOptions>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[ParametrizedLine](classeigen_1_1parametrizedline) | ( | const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > & | *hyperplane* | ) | |
| inlineexplicit |
Constructs a parametrized line from a 2D hyperplane
Warning
the ambient space must have dimension 2 such that the hyperplane actually describes a line
ParametrizedLine() [5/5]
------------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<typename OtherScalarType , int OtherOptions>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[ParametrizedLine](classeigen_1_1parametrizedline) | ( | const [ParametrizedLine](classeigen_1_1parametrizedline)< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > & | *other* | ) | |
| inlineexplicit |
Copy constructor with scalar type conversion
cast()
------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<typename NewScalarType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::cast\_return\_type<[ParametrizedLine](classeigen_1_1parametrizedline), [ParametrizedLine](classeigen_1_1parametrizedline)<NewScalarType,AmbientDimAtCompileTime,Options> >::type [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::cast | ( | | ) | const |
| inline |
Returns
`*this` with scalar type casted to *NewScalarType*
Note that if *NewScalarType* is equal to the current scalar type of `*this` then this function smartly returns a const reference to `*this`.
dim()
-----
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](classeigen_1_1parametrizedline#a3c9f84dd8608940282b16652a296c764) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::dim | ( | | ) | const |
| inline |
Returns
the dimension in which the line holds
distance()
----------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| RealScalar [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::distance | ( | const [VectorType](classeigen_1_1matrix) & | *p* | ) | const |
| inline |
Returns
the distance of a point *p* to its projection onto the line `*this`.
See also
[squaredDistance()](classeigen_1_1parametrizedline#a5cb6544a7fff4c3571c0a5eb82eec8bb)
intersection()
--------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<int OtherOptions>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| \_Scalar [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::intersection | ( | const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > & | *hyperplane* | ) | const |
| inline |
**[Deprecated:](deprecated#_deprecated000025)**
use intersectionParameter()
Returns
the parameter value of the intersection between `*this` and the given *hyperplane*
intersectionParameter()
-----------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<int OtherOptions>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| \_Scalar [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::intersectionParameter | ( | const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > & | *hyperplane* | ) | const |
| inline |
Returns
the parameter value of the intersection between `*this` and the given *hyperplane*
intersectionPoint()
-------------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<int OtherOptions>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[VectorType](classeigen_1_1matrix) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::intersectionPoint | ( | const [Hyperplane](classeigen_1_1hyperplane)< \_Scalar, \_AmbientDim, OtherOptions > & | *hyperplane* | ) | const |
| inline |
Returns
the point of the intersection between `*this` and the given hyperplane
isApprox()
----------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::isApprox | ( | const [ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options > & | *other*, |
| | | const typename [NumTraits](structeigen_1_1numtraits)< Scalar >::Real & | *prec* = `[NumTraits](structeigen_1_1numtraits)<Scalar>::dummy_precision()` |
| | ) | | const |
| inline |
Returns
`true` if `*this` is approximately equal to *other*, within the precision determined by *prec*.
See also
[MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c)
pointAt()
---------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::[VectorType](classeigen_1_1matrix) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::pointAt | ( | const Scalar & | *t* | ) | const |
| inline |
Returns
the point at *t* along this line
projection()
------------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [VectorType](classeigen_1_1matrix) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::projection | ( | const [VectorType](classeigen_1_1matrix) & | *p* | ) | const |
| inline |
Returns
the projection of a point *p* onto the line `*this`.
squaredDistance()
-----------------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| RealScalar [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::squaredDistance | ( | const [VectorType](classeigen_1_1matrix) & | *p* | ) | const |
| inline |
Returns
the squared distance of a point *p* to its projection onto the line `*this`.
See also
[distance()](classeigen_1_1parametrizedline#a25c3e5822420b850e92023cec644c0a0)
Through()
---------
template<typename \_Scalar , int \_AmbientDim, int \_Options>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [ParametrizedLine](classeigen_1_1parametrizedline) [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::Through | ( | const [VectorType](classeigen_1_1matrix) & | *p0*, |
| | | const [VectorType](classeigen_1_1matrix) & | *p1* |
| | ) | | |
| inlinestatic |
Constructs a parametrized line going from *p0* to *p1*.
transform() [1/2]
-----------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<typename XprType >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [ParametrizedLine](classeigen_1_1parametrizedline)& [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::transform | ( | const [MatrixBase](classeigen_1_1matrixbase)< XprType > & | *mat*, |
| | | [TransformTraits](group__enums#gaee59a86102f150923b0cac6d4ff05107) | *traits* = `[Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb)` |
| | ) | | |
| inline |
Applies the transformation matrix *mat* to `*this` and returns a reference to `*this`.
Parameters
| | |
| --- | --- |
| mat | the Dim x Dim transformation matrix |
| traits | specifies whether the matrix *mat* represents an [Isometry](group__enums#ggaee59a86102f150923b0cac6d4ff05107a84413028615d2d718bafd2dfb93dafef) or a more generic [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb) transformation. The default is [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb). |
transform() [2/2]
-----------------
template<typename \_Scalar , int \_AmbientDim, int \_Options> template<int TrOptions>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [ParametrizedLine](classeigen_1_1parametrizedline)& [Eigen::ParametrizedLine](classeigen_1_1parametrizedline)< \_Scalar, \_AmbientDim, \_Options >::transform | ( | const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb), TrOptions > & | *t*, |
| | | [TransformTraits](group__enums#gaee59a86102f150923b0cac6d4ff05107) | *traits* = `[Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb)` |
| | ) | | |
| inline |
Applies the transformation *t* to `*this` and returns a reference to `*this`.
Parameters
| | |
| --- | --- |
| t | the transformation of dimension Dim |
| traits | specifies whether the transformation *t* represents an [Isometry](group__enums#ggaee59a86102f150923b0cac6d4ff05107a84413028615d2d718bafd2dfb93dafef) or a more generic [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb) transformation. The default is [Affine](group__enums#ggaee59a86102f150923b0cac6d4ff05107a0872f0a82453aaae40339c33acbb31fb). Other kind of transformations are not supported. |
---
The documentation for this class was generated from the following file:* [ParametrizedLine.h](https://eigen.tuxfamily.org/dox/ParametrizedLine_8h_source.html)
| programming_docs |
eigen3 Quick reference guide for sparse matrices Quick reference guide for sparse matrices
=========================================
---
In this page, we give a quick summary of the main operations available for sparse matrices in the class [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation."). First, it is recommended to read the introductory tutorial at [Sparse matrix manipulations](group__tutorialsparse). The important point to have in mind when working on sparse matrices is how they are stored : i.e either row major or column major. The default is column major. Most arithmetic operations on sparse matrices will assert that they have the same storage order.
Sparse Matrix Initialization
==============================
| Category | Operations | Notes |
| --- | --- | --- |
| Constructor |
```
SparseMatrix<double> sm1(1000,1000);
SparseMatrix<std::complex<double>,[RowMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a77c993a8d9f6efe5c1159fb2ab07dd4f)> sm2;
```
| Default is ColMajor |
| Resize/Reserve |
```
sm1.resize(m,n); // Change sm1 to a m x n matrix.
sm1.reserve(nnz); // Allocate room for nnz nonzeros elements.
```
| Note that when calling reserve(), it is not required that nnz is the exact number of nonzero elements in the final matrix. However, an exact estimation will avoid multiple reallocations during the insertion phase. |
| Assignment |
```
SparseMatrix<double,Colmajor> sm1;
// Initialize sm2 with sm1.
SparseMatrix<double,Rowmajor> sm2(sm1), sm3;
// Assignment and evaluations modify the storage order.
sm3 = sm1;
```
| The copy constructor can be used to convert from a storage order to another |
| Element-wise Insertion |
```
// Insert a new element;
sm1.insert(i, j) = v_ij;
// Update the value v\_ij
sm1.coeffRef(i,j) = v_ij;
sm1.coeffRef(i,j) += v_ij;
sm1.coeffRef(i,j) -= v_ij;
```
| insert() assumes that the element does not already exist; otherwise, use coeffRef() |
| Batch insertion |
```
std::vector< Eigen::Triplet<double> > tripletList;
tripletList.reserve(estimation_of_entries);
// -- Fill tripletList with nonzero elements...
sm1.setFromTriplets(TripletList.begin(), TripletList.end());
```
| A complete example is available at [Triplet Insertion](group__tutorialsparse#TutorialSparseFilling) . |
| Constant or Random Insertion |
```
sm1.setZero();
```
| Remove all non-zero coefficients |
Matrix properties
===================
Beyond the basic functions rows() and cols(), there are some useful functions that are available to easily get some information from the matrix.
| |
| --- |
|
```
sm1.rows(); // Number of rows
sm1.cols(); // Number of columns
sm1.nonZeros(); // Number of non zero values
sm1.outerSize(); // Number of columns (resp. rows) for a column major (resp. row major )
sm1.innerSize(); // Number of rows (resp. columns) for a row major (resp. column major)
sm1.norm(); // Euclidian norm of the matrix
sm1.squaredNorm(); // Squared norm of the matrix
sm1.blueNorm();
sm1.isVector(); // Check if sm1 is a sparse vector or a sparse matrix
sm1.isCompressed(); // Check if sm1 is in compressed form
...
```
|
Arithmetic operations
=======================
It is easy to perform arithmetic operations on sparse matrices provided that the dimensions are adequate and that the matrices have the same storage order. Note that the evaluation can always be done in a matrix with a different storage order. In the following, **sm** denotes a sparse matrix, **dm** a dense matrix and **dv** a dense vector.
| Operations | Code | Notes |
| --- | --- | --- |
| add subtract |
```
sm3 = sm1 + sm2;
sm3 = sm1 - sm2;
sm2 += sm1;
sm2 -= sm1;
```
| sm1 and sm2 should have the same storage order |
| scalar product |
```
sm3 = sm1 * s1; sm3 *= s1;
sm3 = s1 * sm1 + s2 * sm2; sm3 /= s1;
```
| Many combinations are possible if the dimensions and the storage order agree. |
| Sparse Product |
```
sm3 = sm1 * sm2;
dm2 = sm1 * dm1;
dv2 = sm1 * dv1;
```
| |
| transposition, adjoint |
```
sm2 = sm1.transpose();
sm2 = sm1.adjoint();
```
| Note that the transposition change the storage order. There is no support for transposeInPlace(). |
| Permutation |
```
perm.indices(); // Reference to the vector of indices
sm1.twistedBy(perm); // Permute rows and columns
sm2 = sm1 * perm; // Permute the columns
sm2 = perm * sm1; // Permute the columns
```
| |
| Component-wise ops |
```
sm1.cwiseProduct(sm2);
sm1.cwiseQuotient(sm2);
sm1.cwiseMin(sm2);
sm1.cwiseMax(sm2);
sm1.cwiseAbs();
sm1.cwiseSqrt();
```
| sm1 and sm2 should have the same storage order |
Other supported operations
============================
| Code | Notes |
| --- | --- |
| Sub-matrices |
|
```
sm1.block(startRow, startCol, rows, cols);
sm1.block(startRow, startCol);
sm1.topLeftCorner(rows, cols);
sm1.topRightCorner(rows, cols);
sm1.bottomLeftCorner( rows, cols);
sm1.bottomRightCorner( rows, cols);
```
| Contrary to dense matrices, here **all these methods are read-only**. See [Block operations](group__tutorialsparse#TutorialSparse_SubMatrices) and below for read-write sub-matrices. |
| Range |
|
```
sm1.innerVector(outer); // RW
sm1.innerVectors(start, size); // RW
sm1.leftCols(size); // RW
sm2.rightCols(size); // RO because sm2 is row-major
sm1.middleRows(start, numRows); // RO because sm1 is column-major
sm1.middleCols(start, numCols); // RW
sm1.col(j); // RW
```
| A inner vector is either a row (for row-major) or a column (for column-major). As stated earlier, for a read-write sub-matrix (RW), the evaluation can be done in a matrix with different storage order. |
| Triangular and selfadjoint views |
|
```
sm2 = sm1.triangularview<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>();
sm2 = sm1.selfadjointview<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>();
```
| Several combination between triangular views and blocks views are possible |
| Triangular solve |
|
```
dv2 = sm1.triangularView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>().solve(dv1);
dv2 = sm1.topLeftCorner(size, size)
.triangularView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>().solve(dv1);
```
| For general sparse solve, Use any suitable module described at [Solving Sparse Linear Systems](group__topicsparsesystems) |
| Low-level API |
|
```
sm1.valuePtr(); // Pointer to the values
sm1.innerIndexPtr(); // Pointer to the indices.
sm1.outerIndexPtr(); // Pointer to the beginning of each inner vector
```
| If the matrix is not in compressed form, makeCompressed() should be called before. Note that these functions are mostly provided for interoperability purposes with external libraries. A better access to the values of the matrix is done by using the InnerIterator class as described in [the Tutorial Sparse](group__tutorialsparse) section |
| Mapping external buffers |
|
```
int outerIndexPtr[cols+1];
int innerIndices[nnz];
double values[nnz];
Map<SparseMatrix<double> > sm1(rows,cols,nnz,outerIndexPtr, // read-write
innerIndices,values);
Map<const SparseMatrix<double> > sm2(...); // read-only
```
| As for dense matrices, class [Map<SparseMatrixType>](classeigen_1_1map_3_01sparsematrixtype_01_4 "Specialization of class Map for SparseMatrix-like storage.") can be used to see external buffers as an Eigen's [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") object. |
eigen3 Eigen::SimplicialCholeskyBase Eigen::SimplicialCholeskyBase
=============================
### template<typename Derived> class Eigen::SimplicialCholeskyBase< Derived >
A base class for direct sparse Cholesky factorizations.
This is a base class for LL^T and LDL^T Cholesky factorizations of sparse matrices that are selfadjoint and positive definite. These factorizations allow for solving A.X = B where X and B can be either dense or sparse.
In order to reduce the fill-in, a symmetric permutation P is applied prior to the factorization such that the factorized matrix is P A P^-1.
Template Parameters
| | |
| --- | --- |
| Derived | the type of the derived class, that is the actual factorization type. |
| |
| --- |
| |
| struct | [keep\_diag](structeigen_1_1simplicialcholeskybase_1_1keep__diag) |
| |
| |
| --- |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1simplicialcholeskybase#a3ac877f73aaaff670e6ae7554eb02fc8) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1simplicialcholeskybase#a3ac877f73aaaff670e6ae7554eb02fc8) |
| |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)< [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), StorageIndex > & | [permutationP](classeigen_1_1simplicialcholeskybase#aff1480e595a21726beaec9a586a94d5a) () const |
| |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)< [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), StorageIndex > & | [permutationPinv](classeigen_1_1simplicialcholeskybase#a0e23d1f4a88c211be7098faf1cb41674) () const |
| |
| Derived & | [setShift](classeigen_1_1simplicialcholeskybase#a362352f755101faaac59c1ed9d5e3559) (const RealScalar &offset, const RealScalar &scale=1) |
| |
| | [SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase#a098baba1dbe07ca3a775c8df1f8a0e71) () |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< Derived >](classeigen_1_1sparsesolverbase) |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
| |
| --- |
| |
| template<bool DoLDLT> |
| void | [compute](classeigen_1_1simplicialcholeskybase#a9a741744dda2261cae26cddf96a35bf0) (const MatrixType &matrix) |
| |
SimplicialCholeskyBase()
------------------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::[SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase) | ( | | ) | |
| inline |
Default constructor
compute()
---------
template<typename Derived > template<bool DoLDLT>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::compute | ( | const MatrixType & | *matrix* | ) | |
| inlineprotected |
Computes the sparse Cholesky decomposition of *matrix*
info()
------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the matrix.appears to be negative.
permutationP()
--------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)<[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),StorageIndex>& [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::permutationP | ( | | ) | const |
| inline |
Returns
the permutation P
See also
[permutationPinv()](classeigen_1_1simplicialcholeskybase#a0e23d1f4a88c211be7098faf1cb41674)
permutationPinv()
-----------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)<[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),StorageIndex>& [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::permutationPinv | ( | | ) | const |
| inline |
Returns
the inverse P^-1 of the permutation P
See also
[permutationP()](classeigen_1_1simplicialcholeskybase#aff1480e595a21726beaec9a586a94d5a)
setShift()
----------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived& [Eigen::SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase)< Derived >::setShift | ( | const RealScalar & | *offset*, |
| | | const RealScalar & | *scale* = `1` |
| | ) | | |
| inline |
Sets the shift parameters that will be used to adjust the diagonal coefficients during the numerical factorization.
During the numerical factorization, the diagonal coefficients are transformed by the following linear model:
`d_ii` = *offset* + *scale* \* `d_ii`
The default is the identity transformation with *offset=0*, and *scale=1*.
Returns
a reference to `*this`.
---
The documentation for this class was generated from the following files:* [SimplicialCholesky.h](https://eigen.tuxfamily.org/dox/SimplicialCholesky_8h_source.html)
* [SimplicialCholesky\_impl.h](https://eigen.tuxfamily.org/dox/SimplicialCholesky__impl_8h_source.html)
eigen3 Eigen::DiagonalWrapper Eigen::DiagonalWrapper
======================
### template<typename \_DiagonalVectorType> class Eigen::DiagonalWrapper< \_DiagonalVectorType >
Expression of a diagonal matrix.
Parameters
| | |
| --- | --- |
| \_DiagonalVectorType | the type of the vector of diagonal coefficients |
This class is an expression of a diagonal matrix, but not storing its own vector of diagonal coefficients, instead wrapping an existing vector expression. It is the return type of [MatrixBase::asDiagonal()](classeigen_1_1matrixbase#a14235b62c90f93fe910070b4743782d0) and most of the time this is the only way that it is used.
See also
class [DiagonalMatrix](classeigen_1_1diagonalmatrix "Represents a diagonal matrix with its storage."), class DiagonalBase, [MatrixBase::asDiagonal()](classeigen_1_1matrixbase#a14235b62c90f93fe910070b4743782d0)
Inherits DiagonalBase< DiagonalWrapper< \_DiagonalVectorType > >, and Eigen::internal::no\_assignment\_operator.
| |
| --- |
| |
| const DiagonalVectorType & | [diagonal](classeigen_1_1diagonalwrapper#afd4146738d335e1ce99cffde198f1f31) () const |
| |
| | [DiagonalWrapper](classeigen_1_1diagonalwrapper#af71ac89792969bdd7f2661fa6d4159a0) (DiagonalVectorType &a\_diagonal) |
| |
DiagonalWrapper()
-----------------
template<typename \_DiagonalVectorType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::DiagonalWrapper](classeigen_1_1diagonalwrapper)< \_DiagonalVectorType >::[DiagonalWrapper](classeigen_1_1diagonalwrapper) | ( | DiagonalVectorType & | *a\_diagonal* | ) | |
| inlineexplicit |
Constructor from expression of diagonal coefficients to wrap.
diagonal()
----------
template<typename \_DiagonalVectorType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const DiagonalVectorType& [Eigen::DiagonalWrapper](classeigen_1_1diagonalwrapper)< \_DiagonalVectorType >::diagonal | ( | | ) | const |
| inline |
Returns
a const reference to the wrapped expression of diagonal coefficients.
---
The documentation for this class was generated from the following file:* [DiagonalMatrix.h](https://eigen.tuxfamily.org/dox/DiagonalMatrix_8h_source.html)
eigen3 Adding a new expression type Adding a new expression type
============================
Warning
Disclaimer: this page is tailored to very advanced users who are not afraid of dealing with some Eigen's internal aspects. In most cases, a custom expression can be avoided by either using custom unary or binary functors, while extremely complex matrix manipulations can be achieved by a nullary functors as described in the [previous page](topiccustomizing_nullaryexpr).
This page describes with the help of an example how to implement a new light-weight expression type in Eigen. This consists of three parts: the expression type itself, a traits class containing compile-time information about the expression, and the evaluator class which is used to evaluate the expression to a matrix.
**TO** **DO:** Write a page explaining the design, with details on vectorization etc., and refer to that page here.
The setting
=============
A circulant matrix is a matrix where each column is the same as the column to the left, except that it is cyclically shifted downwards. For example, here is a 4-by-4 circulant matrix:
\[ \begin{bmatrix} 1 & 8 & 4 & 2 \\ 2 & 1 & 8 & 4 \\ 4 & 2 & 1 & 8 \\ 8 & 4 & 2 & 1 \end{bmatrix} \]
A circulant matrix is uniquely determined by its first column. We wish to write a function `makeCirculant` which, given the first column, returns an expression representing the circulant matrix.
For simplicity, we restrict the `makeCirculant` function to dense matrices. It may make sense to also allow arrays, or sparse matrices, but we will not do so here. We also do not want to support vectorization.
Getting started
=================
We will present the file implementing the `makeCirculant` function part by part. We start by including the appropriate header files and forward declaring the expression class, which we will call `Circulant`. The `makeCirculant` function will return an object of this type. The class `Circulant` is in fact a class template; the template argument `ArgType` refers to the type of the vector passed to the `makeCirculant` function.
```
#include <Eigen/Core>
#include <iostream>
template <class ArgType> class Circulant;
```
The traits class
==================
For every expression class `X`, there should be a traits class `Traits<X>` in the `Eigen::internal` namespace containing information about `X` known as compile time.
As explained in [The setting](topicnewexpressiontype#TopicSetting), we designed the `Circulant` expression class to refer to dense matrices. The entries of the circulant matrix have the same type as the entries of the vector passed to the `makeCirculant` function. The type used to index the entries is also the same. Again for simplicity, we will only return column-major matrices. Finally, the circulant matrix is a square matrix (number of rows equals number of columns), and the number of rows equals the number of rows of the column vector passed to the `makeCirculant` function. If this is a dynamic-size vector, then the size of the circulant matrix is not known at compile-time.
This leads to the following code:
```
namespace Eigen {
namespace internal {
template <class ArgType>
struct traits<Circulant<ArgType> >
{
typedef Eigen::Dense StorageKind;
typedef Eigen::MatrixXpr XprKind;
typedef typename ArgType::StorageIndex StorageIndex;
typedef typename ArgType::Scalar Scalar;
enum {
Flags = Eigen::ColMajor,
RowsAtCompileTime = ArgType::RowsAtCompileTime,
ColsAtCompileTime = ArgType::RowsAtCompileTime,
MaxRowsAtCompileTime = ArgType::MaxRowsAtCompileTime,
MaxColsAtCompileTime = ArgType::MaxRowsAtCompileTime
};
};
}
}
```
The expression class
======================
The next step is to define the expression class itself. In our case, we want to inherit from `[MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")` in order to expose the interface for dense matrices. In the constructor, we check that we are passed a column vector (see [Assertions](topicassertions)) and we store the vector from which we are going to build the circulant matrix in the member variable `m_arg`. Finally, the expression class should compute the size of the corresponding circulant matrix. As explained above, this is a square matrix with as many columns as the vector used to construct the matrix.
**TO** **DO:** What about the `Nested` typedef? It seems to be necessary; is this only temporary?
```
template <class ArgType>
class Circulant : public Eigen::MatrixBase<Circulant<ArgType> >
{
public:
Circulant(const ArgType& arg)
: m_arg(arg)
{
EIGEN_STATIC_ASSERT(ArgType::ColsAtCompileTime == 1,
YOU_TRIED_CALLING_A_VECTOR_METHOD_ON_A_MATRIX);
}
typedef typename Eigen::internal::ref_selector<Circulant>::type Nested;
typedef Eigen::Index Index;
Index rows() const { return m_arg.rows(); }
Index cols() const { return m_arg.rows(); }
typedef typename Eigen::internal::ref_selector<ArgType>::type ArgTypeNested;
ArgTypeNested m_arg;
};
```
The evaluator
===============
The last big fragment implements the evaluator for the `Circulant` expression. The evaluator computes the entries of the circulant matrix; this is done in the .coeff() member function. The entries are computed by finding the corresponding entry of the vector from which the circulant matrix is constructed. Getting this entry may actually be non-trivial when the circulant matrix is constructed from a vector which is given by a complicated expression, so we use the evaluator which corresponds to the vector.
The `CoeffReadCost` constant records the cost of computing an entry of the circulant matrix; we ignore the index computation and say that this is the same as the cost of computing an entry of the vector from which the circulant matrix is constructed.
In the constructor, we save the evaluator for the column vector which defined the circulant matrix. We also save the size of that vector; remember that we can query an expression object to find the size but not the evaluator.
```
namespace Eigen {
namespace internal {
template<typename ArgType>
struct evaluator<Circulant<ArgType> >
: evaluator_base<Circulant<ArgType> >
{
typedef Circulant<ArgType> XprType;
typedef typename nested_eval<ArgType, XprType::ColsAtCompileTime>::type ArgTypeNested;
typedef typename remove_all<ArgTypeNested>::type ArgTypeNestedCleaned;
typedef typename XprType::CoeffReturnType CoeffReturnType;
enum {
CoeffReadCost = evaluator<ArgTypeNestedCleaned>::CoeffReadCost,
Flags = Eigen::ColMajor
};
evaluator(const XprType& xpr)
: m_argImpl(xpr.m_arg), m_rows(xpr.rows())
{ }
CoeffReturnType coeff(Index row, Index col) const
{
Index index = row - col;
if (index < 0) index += m_rows;
return m_argImpl.coeff(index);
}
evaluator<ArgTypeNestedCleaned> m_argImpl;
const Index m_rows;
};
}
}
```
The entry point
=================
After all this, the `makeCirculant` function is very simple. It simply creates an expression object and returns it.
```
template <class ArgType>
Circulant<ArgType> makeCirculant(const Eigen::MatrixBase<ArgType>& arg)
{
return Circulant<ArgType>(arg.derived());
}
```
A simple main function for testing
====================================
Finally, a short `main` function that shows how the `makeCirculant` function can be called.
```
int main()
{
Eigen::VectorXd vec(4);
vec << 1, 2, 4, 8;
Eigen::MatrixXd mat;
mat = makeCirculant(vec);
std::cout << mat << std::endl;
}
```
If all the fragments are combined, the following output is produced, showing that the program works as expected:
```
1 8 4 2
2 1 8 4
4 2 1 8
8 4 2 1
```
| programming_docs |
eigen3 Eigen::AlignedBox Eigen::AlignedBox
=================
### template<typename \_Scalar, int \_AmbientDim> class Eigen::AlignedBox< \_Scalar, \_AmbientDim >
An axis aligned box.
This is defined in the Geometry module.
```
#include <Eigen/Geometry>
```
Template Parameters
| | |
| --- | --- |
| \_Scalar | the type of the scalar coefficients |
| \_AmbientDim | the dimension of the ambient space, can be a compile time value or Dynamic. |
This class represents an axis aligned box as a pair of the minimal and maximal corners.
Warning
The result of most methods is undefined when applied to an empty box. You can check for empty boxes using [isEmpty()](classeigen_1_1alignedbox#a2d994551368f0d06876a31dccb26de59).
See also
[Global aligned box typedefs](group__alignedboxtypedefs)
| |
| --- |
| |
| enum | [CornerType](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378) { [Min](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a600b5a58e77104ef7281dba6dcffb808) , [Max](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a6c39e42a5db22f2ca934f5aedcb9985f) , [BottomLeft](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a384d9fbe2c6b6b69a8ea7c5632a61f5c) , [BottomRight](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a8eba0291472401f9a777cf571f1b8b8b) , [TopLeft](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a6740871e968264dc5a55248c7f67fb9f) , [TopRight](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378af68213ceced0d7988b2b9200225dcae6) , [BottomLeftFloor](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a66786d5e4ba263c9725c3476fc421281) , [BottomRightFloor](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a5c7a2e5d12782ebf716aff9c1866f50f) , [TopLeftFloor](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a352b1a4b80151a3d8d8b63cbd113e40b) , [TopRightFloor](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a7cd9ba0b2da76e88e3e1b11796841383) , [BottomLeftCeil](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a66446e8ccb4fe84bdcebc6d3c1e52a1b) , [BottomRightCeil](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a96ff8acc760b61ae37f38b1f63a21306) , [TopLeftCeil](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378ab174a824f21db60fcbfe0ca1136ed6cf) , [TopRightCeil](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378a6eb2e279c813983a8bc89f62959be3bd) } |
| |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) |
| |
| |
| |
| |
| |
| --- |
| |
| | [AlignedBox](classeigen_1_1alignedbox#a43aba4c646424355dd39864655a3386f) () |
| |
| template<typename OtherScalarType > |
| | [AlignedBox](classeigen_1_1alignedbox#a05f7ca06a060c8d4d2e910870669538c) (const [AlignedBox](classeigen_1_1alignedbox)< OtherScalarType, AmbientDimAtCompileTime > &other) |
| |
| template<typename Derived > |
| | [AlignedBox](classeigen_1_1alignedbox#a77f5058435889bf0dfe84bdbd158f10b) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &p) |
| |
| template<typename OtherVectorType1 , typename OtherVectorType2 > |
| | [AlignedBox](classeigen_1_1alignedbox#abbda47b393f17094998da5cdcb73df28) (const OtherVectorType1 &\_min, const OtherVectorType2 &\_max) |
| |
| | [AlignedBox](classeigen_1_1alignedbox#ade9f3bc760956c19d9c087b3a9cd6389) ([Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) \_dim) |
| |
| template<typename NewScalarType > |
| internal::cast\_return\_type< [AlignedBox](classeigen_1_1alignedbox), [AlignedBox](classeigen_1_1alignedbox)< NewScalarType, AmbientDimAtCompileTime > >::type | [cast](classeigen_1_1alignedbox#a5b867bc4a02f78d7feaf45eaaa6f5484) () const |
| |
| [AlignedBox](classeigen_1_1alignedbox) & | [clamp](classeigen_1_1alignedbox#a683b454f1571780e8ddfc5d781949b51) (const [AlignedBox](classeigen_1_1alignedbox) &b) |
| |
| bool | [contains](classeigen_1_1alignedbox#a013a78bb0e9ac3a27dc1e604435288fb) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| template<typename Derived > |
| bool | [contains](classeigen_1_1alignedbox#a8bfd8127621e4a19032945744adf5041) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &p) const |
| |
| [VectorType](classeigen_1_1matrix) | [corner](classeigen_1_1alignedbox#a09619d632dd6979e9d09738493221d55) ([CornerType](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378) corner) const |
| |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_difference\_op< Scalar, Scalar >, const [VectorType](classeigen_1_1matrix), const [VectorType](classeigen_1_1matrix) > | [diagonal](classeigen_1_1alignedbox#a92fbafe85f1c941ed0b4c92711e8db56) () const |
| |
| [Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) | [dim](classeigen_1_1alignedbox#a5301115f222e591b38cc1ebed36856e1) () const |
| |
| const | [EIGEN\_EXPR\_BINARYOP\_SCALAR\_RETURN\_TYPE](classeigen_1_1alignedbox#ab4950605c14c530c2d56c75f0e253d10) ([VectorTypeSum](classeigen_1_1cwisebinaryop), RealScalar, quotient) center() const |
| |
| [AlignedBox](classeigen_1_1alignedbox) & | [extend](classeigen_1_1alignedbox#a0ca284f3375f68b9e9aef558cd5bcd37) (const [AlignedBox](classeigen_1_1alignedbox) &b) |
| |
| template<typename Derived > |
| [AlignedBox](classeigen_1_1alignedbox) & | [extend](classeigen_1_1alignedbox#ac252032292dcf60984191e4dd26f0955) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &p) |
| |
| NonInteger | [exteriorDistance](classeigen_1_1alignedbox#afb3b43bab1d84a95e7a515ca854cda70) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| template<typename Derived > |
| NonInteger | [exteriorDistance](classeigen_1_1alignedbox#a3915f625f0e57029ea27ace34aff29b2) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &p) const |
| |
| [AlignedBox](classeigen_1_1alignedbox) | [intersection](classeigen_1_1alignedbox#ad3d947d3e32b1521bb1ccd9eab4c46cb) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| bool | [intersects](classeigen_1_1alignedbox#a0bdea9a9410af7ad6f63ce71a2f59443) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| bool | [isApprox](classeigen_1_1alignedbox#ad785aecce6792bd88c3a4de35d782f18) (const [AlignedBox](classeigen_1_1alignedbox) &other, const RealScalar &prec=ScalarTraits::dummy\_precision()) const |
| |
| bool | [isEmpty](classeigen_1_1alignedbox#a2d994551368f0d06876a31dccb26de59) () const |
| |
| bool | [isNull](classeigen_1_1alignedbox#a37b401dd265c942c9ef2db1e2b1e56e5) () const |
| |
| [VectorType](classeigen_1_1matrix) &() | [max](classeigen_1_1alignedbox#a0e0a35eb40a9079468f447c1bab8a400) () |
| |
| const [VectorType](classeigen_1_1matrix) &() | [max](classeigen_1_1alignedbox#a987d516532b24fcadc34d2f501579c73) () const |
| |
| [AlignedBox](classeigen_1_1alignedbox) | [merged](classeigen_1_1alignedbox#a59eb62d949598853fbc83836e8f2960c) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| [VectorType](classeigen_1_1matrix) &() | [min](classeigen_1_1alignedbox#adad123f7e84a2437806a791b96af04bd) () |
| |
| const [VectorType](classeigen_1_1matrix) &() | [min](classeigen_1_1alignedbox#af7fddcf06b60392f3f21b4d8e0f763a0) () const |
| |
| [VectorType](classeigen_1_1matrix) | [sample](classeigen_1_1alignedbox#adff08c9965ec5348e38b25d9bb41e9de) () const |
| |
| void | [setEmpty](classeigen_1_1alignedbox#a16bc3e6779107a091f08e4cb7e36e793) () |
| |
| void | [setNull](classeigen_1_1alignedbox#a2399d72bf7dbd9f3589c69f32cbec306) () |
| |
| const [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_difference\_op< Scalar, Scalar >, const [VectorType](classeigen_1_1matrix), const [VectorType](classeigen_1_1matrix) > | [sizes](classeigen_1_1alignedbox#a4d0f2228231a351280a1b14b1915fe7b) () const |
| |
| Scalar | [squaredExteriorDistance](classeigen_1_1alignedbox#aa91643da79398e454618ad06b92ff531) (const [AlignedBox](classeigen_1_1alignedbox) &b) const |
| |
| template<typename Derived > |
| Scalar | [squaredExteriorDistance](classeigen_1_1alignedbox#a845dd8a069c204d15f12aa2702dd9539) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &p) const |
| |
| template<int Mode, int Options> |
| void | [transform](classeigen_1_1alignedbox#ab078178e1abfbd078b27ade489b93303) (const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options > &transform) |
| |
| template<int Mode, int Options> |
| void | [transform](classeigen_1_1alignedbox#aa39225243d4aca173391c0bb24d89b46) (const typename [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options >::TranslationType &translation) |
| |
| template<int Mode, int Options> |
| [AlignedBox](classeigen_1_1alignedbox) | [transformed](classeigen_1_1alignedbox#abe249d52c411b1c9a6771d4ee379b9bf) (const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options > &[transform](classeigen_1_1alignedbox#aa39225243d4aca173391c0bb24d89b46)) const |
| |
| template<typename Derived > |
| [AlignedBox](classeigen_1_1alignedbox) & | [translate](classeigen_1_1alignedbox#a319e3e6338893db6986533d545a92046) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &a\_t) |
| |
| template<typename Derived > |
| [AlignedBox](classeigen_1_1alignedbox) | [translated](classeigen_1_1alignedbox#a3d7dad56b2c9b6e3dc360c078c639f47) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &a\_t) const |
| |
| Scalar | [volume](classeigen_1_1alignedbox#ae048c23dc498b5012a080737e5b1d1c3) () const |
| |
Index
-----
template<typename \_Scalar , int \_AmbientDim>
| |
| --- |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) |
**[Deprecated:](deprecated#_deprecated000021)**
since [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3
CornerType
----------
template<typename \_Scalar , int \_AmbientDim>
| |
| --- |
| enum [Eigen::AlignedBox::CornerType](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378) |
Define constants to name the corners of a 1D, 2D or 3D axis aligned bounding box
| Enumerator |
| --- |
| Min | 1D names |
| Max | 1D names |
| BottomLeft | Identifier for 2D corner |
| BottomRight | Identifier for 2D corner |
| TopLeft | Identifier for 2D corner |
| TopRight | Identifier for 2D corner |
| BottomLeftFloor | Identifier for 3D corner |
| BottomRightFloor | Identifier for 3D corner |
| TopLeftFloor | Identifier for 3D corner |
| TopRightFloor | Identifier for 3D corner |
| BottomLeftCeil | Identifier for 3D corner |
| BottomRightCeil | Identifier for 3D corner |
| TopLeftCeil | Identifier for 3D corner |
| TopRightCeil | Identifier for 3D corner |
AlignedBox() [1/5]
------------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[AlignedBox](classeigen_1_1alignedbox) | ( | | ) | |
| inline |
Default constructor initializing a null box.
AlignedBox() [2/5]
------------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[AlignedBox](classeigen_1_1alignedbox) | ( | [Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) | *\_dim* | ) | |
| inlineexplicit |
Constructs a null box with *\_dim* the dimension of the ambient space.
AlignedBox() [3/5]
------------------
template<typename \_Scalar , int \_AmbientDim> template<typename OtherVectorType1 , typename OtherVectorType2 >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[AlignedBox](classeigen_1_1alignedbox) | ( | const OtherVectorType1 & | *\_min*, |
| | | const OtherVectorType2 & | *\_max* |
| | ) | | |
| inline |
Constructs a box with extremities *\_min* and *\_max*.
Warning
If either component of *\_min* is larger than the same component of *\_max*, the constructed box is empty.
AlignedBox() [4/5]
------------------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[AlignedBox](classeigen_1_1alignedbox) | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *p* | ) | |
| inlineexplicit |
Constructs a box containing a single point *p*.
AlignedBox() [5/5]
------------------
template<typename \_Scalar , int \_AmbientDim> template<typename OtherScalarType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::[AlignedBox](classeigen_1_1alignedbox) | ( | const [AlignedBox](classeigen_1_1alignedbox)< OtherScalarType, AmbientDimAtCompileTime > & | *other* | ) | |
| inlineexplicit |
Copy constructor with scalar type conversion
cast()
------
template<typename \_Scalar , int \_AmbientDim> template<typename NewScalarType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::cast\_return\_type<[AlignedBox](classeigen_1_1alignedbox), [AlignedBox](classeigen_1_1alignedbox)<NewScalarType,AmbientDimAtCompileTime> >::type [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::cast | ( | | ) | const |
| inline |
Returns
`*this` with scalar type casted to *NewScalarType*
Note that if *NewScalarType* is equal to the current scalar type of `*this` then this function smartly returns a const reference to `*this`.
clamp()
-------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox)& [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::clamp | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | |
| inline |
Clamps `*this` by the box *b* and returns a reference to `*this`.
Note
If the boxes don't intersect, the resulting box is empty.
See also
[intersection()](classeigen_1_1alignedbox#ad3d947d3e32b1521bb1ccd9eab4c46cb), [intersects()](classeigen_1_1alignedbox#a0bdea9a9410af7ad6f63ce71a2f59443)
contains() [1/2]
----------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::contains | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns
true if the box *b* is entirely inside the box `*this`.
contains() [2/2]
----------------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::contains | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *p* | ) | const |
| inline |
Returns
true if the point *p* is inside the box `*this`.
corner()
--------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [VectorType](classeigen_1_1matrix) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::corner | ( | [CornerType](classeigen_1_1alignedbox#ae4aa935b36004fffc49c7a3a85e2d378) | *corner* | ) | const |
| inline |
Returns
the vertex of the bounding box at the corner defined by the corner-id corner. It works only for a 1D, 2D or 3D bounding box. For 1D bounding boxes corners are named by 2 enum constants: BottomLeft and BottomRight. For 2D bounding boxes, corners are named by 4 enum constants: BottomLeft, BottomRight, TopLeft, TopRight. For 3D bounding boxes, the following names are added: BottomLeftCeil, BottomRightCeil, TopLeftCeil, TopRightCeil.
diagonal()
----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_difference\_op<Scalar,Scalar>, const [VectorType](classeigen_1_1matrix), const [VectorType](classeigen_1_1matrix)> [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::diagonal | ( | | ) | const |
| inline |
Returns
an expression for the bounding box diagonal vector if the length of the diagonal is needed: [diagonal()](classeigen_1_1alignedbox#a92fbafe85f1c941ed0b4c92711e8db56).norm() will provide it.
dim()
-----
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](classeigen_1_1alignedbox#a774ef355da13d6bee6a6e7244c15231a) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::dim | ( | | ) | const |
| inline |
Returns
the dimension in which the box holds
EIGEN\_EXPR\_BINARYOP\_SCALAR\_RETURN\_TYPE()
---------------------------------------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::EIGEN\_EXPR\_BINARYOP\_SCALAR\_RETURN\_TYPE | ( | [VectorTypeSum](classeigen_1_1cwisebinaryop) | , |
| | | RealScalar | , |
| | | quotient | |
| | ) | | const |
| inline |
Returns
the center of the box
extend() [1/2]
--------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox)& [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::extend | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | |
| inline |
Extends `*this` such that it contains the box *b* and returns a reference to `*this`.
See also
[merged](classeigen_1_1alignedbox#a59eb62d949598853fbc83836e8f2960c), [extend(const MatrixBase&)](classeigen_1_1alignedbox#ac252032292dcf60984191e4dd26f0955)
extend() [2/2]
--------------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox)& [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::extend | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *p* | ) | |
| inline |
Extends `*this` such that it contains the point *p* and returns a reference to `*this`.
See also
[extend(const AlignedBox&)](classeigen_1_1alignedbox#a0ca284f3375f68b9e9aef558cd5bcd37)
exteriorDistance() [1/2]
------------------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| NonInteger [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::exteriorDistance | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns
the distance between the boxes *b* and `*this`, and zero if the boxes intersect.
See also
squaredExteriorDistance(const AlignedBox&), exteriorDistance(const MatrixBase&)
exteriorDistance() [2/2]
------------------------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| NonInteger [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::exteriorDistance | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *p* | ) | const |
| inline |
Returns
the distance between the point *p* and the box `*this`, and zero if *p* is inside the box.
See also
squaredExteriorDistance(const MatrixBase&), exteriorDistance(const AlignedBox&)
intersection()
--------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::intersection | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns an [AlignedBox](classeigen_1_1alignedbox "An axis aligned box.") that is the intersection of *b* and `*this`
Note
If the boxes don't intersect, the resulting box is empty.
See also
[intersects()](classeigen_1_1alignedbox#a0bdea9a9410af7ad6f63ce71a2f59443), [clamp](classeigen_1_1alignedbox#a683b454f1571780e8ddfc5d781949b51), [contains()](classeigen_1_1alignedbox#a8bfd8127621e4a19032945744adf5041)
intersects()
------------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::intersects | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns
true if the box *b* is intersecting the box `*this`.
See also
[intersection](classeigen_1_1alignedbox#ad3d947d3e32b1521bb1ccd9eab4c46cb), [clamp](classeigen_1_1alignedbox#a683b454f1571780e8ddfc5d781949b51)
isApprox()
----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::isApprox | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *other*, |
| | | const RealScalar & | *prec* = `ScalarTraits::dummy_precision()` |
| | ) | | const |
| inline |
Returns
`true` if `*this` is approximately equal to *other*, within the precision determined by *prec*.
See also
[MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c)
isEmpty()
---------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::isEmpty | ( | | ) | const |
| inline |
Returns
true if the box is empty.
See also
[setEmpty](classeigen_1_1alignedbox#a16bc3e6779107a091f08e4cb7e36e793)
isNull()
--------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::isNull | ( | | ) | const |
| inline |
**[Deprecated:](deprecated#_deprecated000022)**
use [isEmpty()](classeigen_1_1alignedbox#a2d994551368f0d06876a31dccb26de59)
max() [1/2]
-----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [VectorType](classeigen_1_1matrix)&() [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::max | ( | | ) | |
| inline |
Returns
a non const reference to the maximal corner
max() [2/2]
-----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [VectorType](classeigen_1_1matrix)&() [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::max | ( | | ) | const |
| inline |
Returns
the maximal corner
merged()
--------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::merged | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns an [AlignedBox](classeigen_1_1alignedbox "An axis aligned box.") that is the union of *b* and `*this`.
Note
Merging with an empty box may result in a box bigger than `*this`.
See also
[extend(const AlignedBox&)](classeigen_1_1alignedbox#a0ca284f3375f68b9e9aef558cd5bcd37)
min() [1/2]
-----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [VectorType](classeigen_1_1matrix)&() [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::min | ( | | ) | |
| inline |
Returns
a non const reference to the minimal corner
min() [2/2]
-----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [VectorType](classeigen_1_1matrix)&() [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::min | ( | | ) | const |
| inline |
Returns
the minimal corner
sample()
--------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [VectorType](classeigen_1_1matrix) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::sample | ( | | ) | const |
| inline |
Returns
a random point inside the bounding box sampled with a uniform distribution
setEmpty()
----------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| void [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::setEmpty | ( | | ) | |
| inline |
Makes `*this` an empty box.
See also
[isEmpty](classeigen_1_1alignedbox#a2d994551368f0d06876a31dccb26de59)
setNull()
---------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| void [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::setNull | ( | | ) | |
| inline |
**[Deprecated:](deprecated#_deprecated000023)**
use [setEmpty()](classeigen_1_1alignedbox#a16bc3e6779107a091f08e4cb7e36e793)
sizes()
-------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_difference\_op<Scalar,Scalar>, const [VectorType](classeigen_1_1matrix), const [VectorType](classeigen_1_1matrix)> [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::sizes | ( | | ) | const |
| inline |
Returns
the lengths of the sides of the bounding box. Note that this function does not get the same result for integral or floating scalar types: see
squaredExteriorDistance() [1/2]
-------------------------------
template<typename Scalar , int AmbientDim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar [Eigen::AlignedBox](classeigen_1_1alignedbox)< Scalar, AmbientDim >::squaredExteriorDistance | ( | const [AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim > & | *b* | ) | const |
| inline |
Returns
the squared distance between the boxes *b* and `*this`, and zero if the boxes intersect.
See also
exteriorDistance(const AlignedBox&), squaredExteriorDistance(const MatrixBase&)
squaredExteriorDistance() [2/2]
-------------------------------
template<typename Scalar , int AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar [Eigen::AlignedBox](classeigen_1_1alignedbox)< Scalar, AmbientDim >::squaredExteriorDistance | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *p* | ) | const |
| inline |
Returns
the squared distance between the point *p* and the box `*this`, and zero if *p* is inside the box.
See also
exteriorDistance(const MatrixBase&), squaredExteriorDistance(const AlignedBox&)
transform() [1/2]
-----------------
template<typename \_Scalar , int \_AmbientDim> template<int Mode, int Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::transform | ( | const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options > & | *transform* | ) | |
| inline |
Transforms this box by *transform* and recomputes it to still be an axis-aligned box.
Note
This method is provided under BSD license (see the top of this file).
transform() [2/2]
-----------------
template<typename \_Scalar , int \_AmbientDim> template<int Mode, int Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::transform | ( | const typename [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options >::TranslationType & | *translation* | ) | |
| inline |
Specialization of transform for pure translation.
transformed()
-------------
template<typename \_Scalar , int \_AmbientDim> template<int Mode, int Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::transformed | ( | const [Transform](classeigen_1_1transform)< Scalar, AmbientDimAtCompileTime, Mode, Options > & | *transform* | ) | const |
| inline |
Returns
a copy of `*this` transformed by *transform* and recomputed to still be an axis-aligned box.
translate()
-----------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox)& [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::translate | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *a\_t* | ) | |
| inline |
Translate `*this` by the vector *t* and returns a reference to `*this`.
translated()
------------
template<typename \_Scalar , int \_AmbientDim> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [AlignedBox](classeigen_1_1alignedbox) [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::translated | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *a\_t* | ) | const |
| inline |
Returns
a copy of `*this` translated by the vector *t*.
volume()
--------
template<typename \_Scalar , int \_AmbientDim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar [Eigen::AlignedBox](classeigen_1_1alignedbox)< \_Scalar, \_AmbientDim >::volume | ( | | ) | const |
| inline |
Returns
the volume of the bounding box
---
The documentation for this class was generated from the following file:* [AlignedBox.h](https://eigen.tuxfamily.org/dox/AlignedBox_8h_source.html)
| programming_docs |
eigen3 Assertions Assertions
==========
Assertions
============
The macro eigen\_assert is defined to be `eigen_plain_assert` by default. We use eigen\_plain\_assert instead of `assert` to work around a known bug for GCC <= 4.3. Basically, eigen\_plain\_assert *is* `assert`.
Redefining assertions
-----------------------
Both eigen\_assert and eigen\_plain\_assert are defined in [Macros.h](https://eigen.tuxfamily.org/dox/Macros_8h_source.html). Defining eigen\_assert indirectly gives you a chance to change its behavior. You can redefine this macro if you want to do something else such as throwing an exception, and fall back to its default behavior with eigen\_plain\_assert. The code below tells [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") to throw an std::runtime\_error:
```
#include <stdexcept>
#undef eigen\_assert
#define eigen\_assert(x) \
if (!(x)) { throw (std::runtime\_error("Put your message here")); }
```
Disabling assertions
----------------------
Assertions cost run time and can be turned off. You can suppress eigen\_assert by defining `EIGEN_NO_DEBUG` **before** including [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") headers. `EIGEN_NO_DEBUG` is undefined by default unless `NDEBUG` is defined.
Static assertions
===================
Static assertions are not standardized until C++11. However, in the [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") library, there are many conditions can and should be detectedat compile time. For instance, we use static assertions to prevent the code below from compiling.
```
Matrix3d() + Matrix4d(); // adding matrices of different sizes
Matrix4cd() * Vector3cd(); // invalid product known at compile time
```
Static assertions are defined in [StaticAssert.h](https://eigen.tuxfamily.org/dox/StaticAssert_8h_source.html). If there is native static\_assert, we use it. Otherwise, we have implemented an assertion macro that can show a limited range of messages.
One can easily come up with static assertions without messages, such as:
```
#define STATIC\_ASSERT(x) \
switch(0) { case 0: case x:; }
```
However, the example above obviously cannot tell why the assertion failed. Therefore, we define a `struct` in namespace Eigen::internal to handle available messages.
```
template<bool condition>
struct static_assertion {};
template<>
struct static_assertion<true>
{
enum {
YOU_TRIED_CALLING_A_VECTOR_METHOD_ON_A_MATRIX,
YOU_MIXED_VECTORS_OF_DIFFERENT_SIZES,
// see StaticAssert.h for all enums.
};
};
```
And then, we define EIGEN\_STATIC\_ASSERT(CONDITION,MSG) to access Eigen::internal::static\_assertion<bool(CONDITION)>::MSG. If the condition evaluates into `false`, your compiler displays a lot of messages explaining there is no MSG in static\_assert<false>. Nevertheless, this is *not* in what we are interested. As you can see, all members of static\_assert<true> are ALL\_CAPS\_AND\_THEY\_ARE\_SHOUTING.
Warning
When using this macro, MSG should be a member of static\_assertion<true>, or the static assertion **always** fails. Currently, it can only be used in function scope.
Derived static assertions
---------------------------
There are other macros derived from EIGEN\_STATIC\_ASSERT to enhance readability. Their names are self-explanatory.
* **EIGEN\_STATIC\_ASSERT\_FIXED\_SIZE(TYPE)** - passes if *TYPE* is fixed size.
* **EIGEN\_STATIC\_ASSERT\_DYNAMIC\_SIZE(TYPE)** - passes if *TYPE* is dynamic size.
* **EIGEN\_STATIC\_ASSERT\_LVALUE(Derived)** - failes if *Derived* is read-only.
* **EIGEN\_STATIC\_ASSERT\_ARRAYXPR(Derived)** - passes if *Derived* is an array expression.
* **EIGEN\_STATIC\_ASSERT\_SAME\_XPR\_KIND(Derived1, Derived2)** - failes if the two expressions are an array one and a matrix one.
Because [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") handles both fixed-size and dynamic-size expressions, some conditions cannot be clearly determined at compile time. We classify them into strict assertions and permissive assertions.
###
Strict assertions
These assertions fail if the condition **may not** be met. For example, MatrixXd may not be a vector, so it fails EIGEN\_STATIC\_ASSERT\_VECTOR\_ONLY.
* **EIGEN\_STATIC\_ASSERT\_VECTOR\_ONLY(TYPE)** - passes if *TYPE* must be a vector type.
* **EIGEN\_STATIC\_ASSERT\_VECTOR\_SPECIFIC\_SIZE(TYPE, SIZE)** - passes if *TYPE* must be a vector of the given size.
* **EIGEN\_STATIC\_ASSERT\_MATRIX\_SPECIFIC\_SIZE(TYPE, ROWS, COLS)** - passes if *TYPE* must be a matrix with given rows and columns.
###
Permissive assertions
These assertions fail if the condition **cannot** be met. For example, MatrixXd and Matrix4d may have the same size, so they pass EIGEN\_STATIC\_ASSERT\_SAME\_MATRIX\_SIZE.
* **EIGEN\_STATIC\_ASSERT\_SAME\_VECTOR\_SIZE(TYPE0,TYPE1)** - fails if the two vector expression types must have different sizes.
* **EIGEN\_STATIC\_ASSERT\_SAME\_MATRIX\_SIZE(TYPE0,TYPE1)** - fails if the two matrix expression types must have different sizes.
* **EIGEN\_STATIC\_ASSERT\_SIZE\_1x1(TYPE)** - fails if *TYPE* cannot be an 1x1 expression.
See [StaticAssert.h](https://eigen.tuxfamily.org/dox/StaticAssert_8h_source.html) for details such as what messages they throw.
Disabling static assertions
-----------------------------
If `EIGEN_NO_STATIC_ASSERT` is defined, static assertions turn into `eigen_assert`'s, working like:
```
#define EIGEN\_STATIC\_ASSERT(CONDITION,MSG) eigen\_assert((CONDITION) && #MSG);
```
This saves compile time but consumes more run time. `EIGEN_NO_STATIC_ASSERT` is undefined by default.
eigen3 Eigen::TriangularBase Eigen::TriangularBase
=====================
### template<typename Derived> class Eigen::TriangularBase< Derived >
Base class for triangular part in a matrix.
| |
| --- |
| |
| enum | { } |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
| |
| --- |
| |
| template<typename Other > |
| void | [copyCoeff](classeigen_1_1triangularbase#a0abe130a9130ac6df16f3c8c55490b43) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col, Other &other) |
| |
| template<typename DenseDerived > |
| void | [evalTo](classeigen_1_1triangularbase#a604d4f76a376ced36f8b9c3374c76c3e) ([MatrixBase](classeigen_1_1matrixbase)< DenseDerived > &other) const |
| |
| template<typename DenseDerived > |
| void | [evalToLazy](classeigen_1_1triangularbase#ab8db3e55eee50cdc56650b3498e235eb) ([MatrixBase](classeigen_1_1matrixbase)< DenseDerived > &other) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
anonymous enum
--------------
template<typename Derived >
| |
| --- |
| anonymous enum |
| Enumerator |
| --- |
| SizeAtCompileTime | This is equal to the number of coefficients, i.e. the number of rows times the number of columns, or to *Dynamic* if this is not known at compile-time.
See also
RowsAtCompileTime, ColsAtCompileTime
|
copyCoeff()
-----------
template<typename Derived > template<typename Other >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::TriangularBase](classeigen_1_1triangularbase)< Derived >::copyCoeff | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col*, |
| | | Other & | *other* |
| | ) | | |
| inline |
See also
MatrixBase::copyCoeff(row,col)
evalTo()
--------
template<typename Derived > template<typename DenseDerived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::TriangularBase](classeigen_1_1triangularbase)< Derived >::evalTo | ( | [MatrixBase](classeigen_1_1matrixbase)< DenseDerived > & | *other* | ) | const |
Assigns a triangular or selfadjoint matrix to a dense matrix. If the matrix is triangular, the opposite part is set to zero.
evalToLazy()
------------
template<typename Derived > template<typename DenseDerived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::TriangularBase](classeigen_1_1triangularbase)< Derived >::evalToLazy | ( | [MatrixBase](classeigen_1_1matrixbase)< DenseDerived > & | *other* | ) | const |
Assigns a triangular or selfadjoint matrix to a dense matrix. If the matrix is triangular, the opposite part is set to zero.
---
The documentation for this class was generated from the following file:* [TriangularMatrix.h](https://eigen.tuxfamily.org/dox/TriangularMatrix_8h_source.html)
eigen3 Global matrix typedefs Global matrix typedefs
======================
Eigen defines several typedef shortcuts for most common matrix and vector types.
The general patterns are the following:
`MatrixSizeType` where `Size` can be `2`,`3`,`4` for fixed size square matrices or `X` for dynamic size, and where `Type` can be `i` for integer, `f` for float, `d` for double, `cf` for complex float, `cd` for complex double.
For example, `Matrix3d` is a fixed-size 3x3 matrix type of doubles, and `MatrixXf` is a dynamic-size matrix of floats.
There are also `VectorSizeType` and `RowVectorSizeType` which are self-explanatory. For example, `Vector4cf` is a fixed-size vector of 4 complex floats.
With [c++11], template alias are also defined for common sizes. They follow the same pattern as above except that the scalar type suffix is replaced by a template parameter, i.e.:
* `MatrixSize<Type>` where `Size` can be `2`,`3`,`4` for fixed size square matrices or `X` for dynamic size.
* `MatrixXSize<Type>` and `MatrixSizeX<Type>` where `Size` can be `2`,`3`,`4` for hybrid dynamic/fixed matrices.
* `VectorSize<Type>` and `RowVectorSize<Type>` for column and row vectors.
With [c++11], you can also use fully generic column and row vector types: `Vector<Type,Size>` and `RowVector<Type,Size>`.
See also
class [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.")
| |
| --- |
| |
| template<typename Type > |
| using | [Eigen::Matrix2](group__matrixtypedefs#ga73a2c673745de956e66d30ad61fd4a7e) = [Matrix](classeigen_1_1matrix)< Type, 2, 2 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Matrix2X](group__matrixtypedefs#ga9fc5afaeb88d6cd4f2503dd6127b1ba8) = [Matrix](classeigen_1_1matrix)< Type, 2, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Matrix3](group__matrixtypedefs#ga6d61b8a0039eeb04e6faf382b8635a7a) = [Matrix](classeigen_1_1matrix)< Type, 3, 3 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Matrix3X](group__matrixtypedefs#gab22d239b05eafe7ac235325d454e7530) = [Matrix](classeigen_1_1matrix)< Type, 3, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Matrix4](group__matrixtypedefs#ga946841161df693bb792644ba410aa0f0) = [Matrix](classeigen_1_1matrix)< Type, 4, 4 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Matrix4X](group__matrixtypedefs#ga93eea03ee4428402191f86ef2239975e) = [Matrix](classeigen_1_1matrix)< Type, 4, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::MatrixX](group__matrixtypedefs#ga8a779d79defc9f822fa6ff5869c2ba6b) = [Matrix](classeigen_1_1matrix)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::MatrixX2](group__matrixtypedefs#ga1d162ebb520e589b3a5a5f4024aa3ae4) = [Matrix](classeigen_1_1matrix)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 2 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::MatrixX3](group__matrixtypedefs#gacdb4b32de62bf47373eec29612b73657) = [Matrix](classeigen_1_1matrix)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 3 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::MatrixX4](group__matrixtypedefs#gabe35b438cb4f39d72092d1fbb16153b8) = [Matrix](classeigen_1_1matrix)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 4 > |
| | [c++11] |
| |
| template<typename Type , int Size> |
| using | [Eigen::RowVector](group__matrixtypedefs#ga181118570e7a0bb1cf3366180061f14f) = [Matrix](classeigen_1_1matrix)< Type, 1, Size > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::RowVector2](group__matrixtypedefs#gafbd00706e87859d2f0ad68b54e825809) = [Matrix](classeigen_1_1matrix)< Type, 1, 2 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::RowVector3](group__matrixtypedefs#gaaedec7d966e8dafd1611d106333bf461) = [Matrix](classeigen_1_1matrix)< Type, 1, 3 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::RowVector4](group__matrixtypedefs#gafb7f5975c1c3be1237e7f0bf589c6add) = [Matrix](classeigen_1_1matrix)< Type, 1, 4 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::RowVectorX](group__matrixtypedefs#gacbfe7eb9c070bcb75ef42aec7a6fbafe) = [Matrix](classeigen_1_1matrix)< Type, 1, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type , int Size> |
| using | [Eigen::Vector](group__matrixtypedefs#ga2623c0d4641dda067fbdb9a009ef0c91) = [Matrix](classeigen_1_1matrix)< Type, Size, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Vector2](group__matrixtypedefs#ga5c7551c08d7b0ca055e4abdc37b05a80) = [Matrix](classeigen_1_1matrix)< Type, 2, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Vector3](group__matrixtypedefs#ga0af1f95a68328299e1e9b273e4934c7a) = [Matrix](classeigen_1_1matrix)< Type, 3, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Vector4](group__matrixtypedefs#ga299db30993dd4e9ca74c7691ad32d50b) = [Matrix](classeigen_1_1matrix)< Type, 4, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::VectorX](group__matrixtypedefs#ga7e589e92f0ae4929f5540a578cfd2bac) = [Matrix](classeigen_1_1matrix)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > |
| | [c++11] |
| |
eigen3 Eigen::RotationBase Eigen::RotationBase
===================
### template<typename Derived, int \_Dim> class Eigen::RotationBase< Derived, \_Dim >
Common base class for compact rotation representations.
Template Parameters
| | |
| --- | --- |
| Derived | is the derived type, i.e., a rotation type |
| \_Dim | the dimension of the space |
| |
| --- |
| |
| typedef [Matrix](classeigen_1_1matrix)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Dim > | [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) |
| |
| typedef internal::traits< Derived >::[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) | [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) |
| |
| |
| --- |
| |
| Derived | [inverse](classeigen_1_1rotationbase#a8532fb716ea4267cf8bbdb99e5e54837) () const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [matrix](classeigen_1_1rotationbase#a91b5bdb1c7b90ec7b33107c6f7d3b171) () const |
| |
| template<typename OtherDerived > |
| internal::rotation\_base\_generic\_product\_selector< Derived, OtherDerived, OtherDerived::IsVectorAtCompileTime >::ReturnType | [operator\*](classeigen_1_1rotationbase#a09a4757e7aff7a95bebf4fa8a965a4eb) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &e) const |
| |
| template<int Mode, int Options> |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode > | [operator\*](classeigen_1_1rotationbase#aaca1c3d834e2bc7ebfd046d96cac990c) (const [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode, Options > &t) const |
| |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, [Isometry](group__enums#ggaee59a86102f150923b0cac6d4ff05107a84413028615d2d718bafd2dfb93dafef) > | [operator\*](classeigen_1_1rotationbase#a26d0603783666526a98d08bd45d9c751) (const [Translation](classeigen_1_1translation)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim > &t) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [operator\*](classeigen_1_1rotationbase#a05af64a1bc759c5fed4ff7afd1414ba4) (const [UniformScaling](classeigen_1_1uniformscaling)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) > &s) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [toRotationMatrix](classeigen_1_1rotationbase#a94fe3683c867c39d34505932b07e956a) () const |
| |
RotationMatrixType
------------------
template<typename Derived , int \_Dim>
| |
| --- |
| typedef [Matrix](classeigen_1_1matrix)<[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4),Dim,Dim> [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::[RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) |
corresponding linear transformation matrix type
Scalar
------
template<typename Derived , int \_Dim>
| |
| --- |
| typedef internal::traits<Derived>::[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) |
the scalar type of the coefficients
inverse()
---------
template<typename Derived , int \_Dim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Derived [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::inverse | ( | | ) | const |
| inline |
Returns
the inverse rotation
matrix()
--------
template<typename Derived , int \_Dim>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::matrix | ( | | ) | const |
| inline |
Returns
an equivalent rotation matrix This function is added to be conform with the [Transform](classeigen_1_1transform "Represents an homogeneous transformation in a N dimensional space.") class' naming scheme.
operator\*() [1/4]
------------------
template<typename Derived , int \_Dim> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| internal::rotation\_base\_generic\_product\_selector<Derived,OtherDerived,OtherDerived::IsVectorAtCompileTime>::ReturnType [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::operator\* | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *e* | ) | const |
| inline |
Returns
the concatenation of the rotation `*this` with a generic expression *e* *e* can be:* a DimxDim linear transformation matrix
* a DimxDim diagonal matrix (axis aligned scaling)
* a vector of size Dim
operator\*() [2/4]
------------------
template<typename Derived , int \_Dim> template<int Mode, int Options>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Transform](classeigen_1_1transform)<[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4),Dim,Mode> [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::operator\* | ( | const [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode, Options > & | *t* | ) | const |
| inline |
Returns
the concatenation of the rotation `*this` with a transformation *t*
operator\*() [3/4]
------------------
template<typename Derived , int \_Dim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Transform](classeigen_1_1transform)<[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4),Dim,[Isometry](group__enums#ggaee59a86102f150923b0cac6d4ff05107a84413028615d2d718bafd2dfb93dafef)> [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::operator\* | ( | const [Translation](classeigen_1_1translation)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim > & | *t* | ) | const |
| inline |
Returns
the concatenation of the rotation `*this` with a translation *t*
operator\*() [4/4]
------------------
template<typename Derived , int \_Dim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::operator\* | ( | const [UniformScaling](classeigen_1_1uniformscaling)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) > & | *s* | ) | const |
| inline |
Returns
the concatenation of the rotation `*this` with a uniform scaling *s*
toRotationMatrix()
------------------
template<typename Derived , int \_Dim>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) [Eigen::RotationBase](classeigen_1_1rotationbase)< Derived, \_Dim >::toRotationMatrix | ( | void | | ) | const |
| inline |
Returns
an equivalent rotation matrix
---
The documentation for this class was generated from the following file:* [RotationBase.h](https://eigen.tuxfamily.org/dox/RotationBase_8h_source.html)
| programming_docs |
eigen3 Matrix-free solvers Matrix-free solvers
===================
Iterative solvers such as [ConjugateGradient](classeigen_1_1conjugategradient "A conjugate gradient solver for sparse (or dense) self-adjoint problems.") and [BiCGSTAB](classeigen_1_1bicgstab "A bi conjugate gradient stabilized solver for sparse square problems.") can be used in a matrix free context. To this end, user must provide a wrapper class inheriting EigenBase<> and implementing the following methods:
* `Index` `rows()` and `Index` `cols()`: returns number of rows and columns respectively
* `operator*` with your type and an Eigen dense column vector (its actual implementation goes in a specialization of the internal::generic\_product\_impl class)
`Eigen::internal::traits<>` must also be specialized for the wrapper type.
Here is a complete example wrapping an [Eigen::SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation."):
```
#include <iostream>
#include <Eigen/Core>
#include <Eigen/Dense>
#include <Eigen/IterativeLinearSolvers>
#include <unsupported/Eigen/IterativeSolvers>
class MatrixReplacement;
using [Eigen::SparseMatrix](classeigen_1_1sparsematrix);
namespace [Eigen](namespaceeigen) {
namespace internal {
// MatrixReplacement looks-like a SparseMatrix, so let's inherits its traits:
template<>
struct traits<MatrixReplacement> : public Eigen::internal::traits<Eigen::SparseMatrix<double> >
{};
}
}
// Example of a matrix-free wrapper from a user type to Eigen's compatible type
// For the sake of simplicity, this example simply wrap a Eigen::SparseMatrix.
class MatrixReplacement : public [Eigen::EigenBase](structeigen_1_1eigenbase)<MatrixReplacement> {
public:
// Required typedefs, constants, and method:
typedef double Scalar;
typedef double RealScalar;
typedef int StorageIndex;
enum {
ColsAtCompileTime = [Eigen::Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),
MaxColsAtCompileTime = [Eigen::Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2),
IsRowMajor = false
};
[Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows() const { return mp_mat->rows(); }
[Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols() const { return mp_mat->cols(); }
template<typename Rhs>
[Eigen::Product<MatrixReplacement,Rhs,Eigen::AliasFreeProduct>](classeigen_1_1product) [operator\*](namespaceeigen#a9723b3ff0f2c99fe1081e3eb14380d4c)(const [Eigen::MatrixBase<Rhs>](classeigen_1_1matrixbase)& x) const {
return [Eigen::Product<MatrixReplacement,Rhs,Eigen::AliasFreeProduct>](classeigen_1_1product)(*this, x.[derived](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa)());
}
// Custom API:
MatrixReplacement() : mp_mat(0) {}
void attachMyMatrix(const SparseMatrix<double> &mat) {
mp_mat = &mat;
}
const SparseMatrix<double> my_matrix() const { return *mp_mat; }
private:
const SparseMatrix<double> *mp_mat;
};
// Implementation of MatrixReplacement \* Eigen::DenseVector though a specialization of internal::generic\_product\_impl:
namespace [Eigen](namespaceeigen) {
namespace internal {
template<typename Rhs>
struct generic_product_impl<MatrixReplacement, Rhs, SparseShape, DenseShape, GemvProduct> // GEMV stands for matrix-vector
: generic_product_impl_base<MatrixReplacement,Rhs,generic_product_impl<MatrixReplacement,Rhs> >
{
typedef typename Product<MatrixReplacement,Rhs>::Scalar Scalar;
template<typename Dest>
static void scaleAndAddTo(Dest& dst, const MatrixReplacement& lhs, const Rhs& rhs, const Scalar& alpha)
{
// This method should implement "dst += alpha \* lhs \* rhs" inplace,
// however, for iterative solvers, alpha is always equal to 1, so let's not bother about it.
assert(alpha==Scalar(1) && "scaling is not implemented");
EIGEN_ONLY_USED_FOR_DEBUG(alpha);
// Here we could simply call dst.noalias() += lhs.my\_matrix() \* rhs,
// but let's do something fancier (and less efficient):
for([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i=0; i<lhs.cols(); ++i)
dst += rhs(i) * lhs.my_matrix().col(i);
}
};
}
}
int main()
{
int n = 10;
[Eigen::SparseMatrix<double>](classeigen_1_1sparsematrix) S = [Eigen::MatrixXd::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(n,n).sparseView(0.5,1);
S = S.transpose()*S;
MatrixReplacement A;
A.attachMyMatrix(S);
[Eigen::VectorXd](classeigen_1_1matrix) b(n), x;
b.[setRandom](classeigen_1_1plainobjectbase#a5f0f6cc8039ed5ac026cd32ed5bbe6ea)();
// Solve Ax = b using various iterative solver with matrix-free version:
{
[Eigen::ConjugateGradient<MatrixReplacement, Eigen::Lower|Eigen::Upper, Eigen::IdentityPreconditioner>](classeigen_1_1conjugategradient) cg;
cg.[compute](classeigen_1_1iterativesolverbase#a7dfa55c55e82d697bde227696a630914)(A);
x = cg.[solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f)(b);
std::cout << "CG: #iterations: " << cg.[iterations](classeigen_1_1iterativesolverbase#ae778dd098bd5e6655625b20b1e9f15da)() << ", estimated error: " << cg.[error](classeigen_1_1iterativesolverbase#a117c241af3fb1141ad0916a3cf3157ec)() << std::endl;
}
{
[Eigen::BiCGSTAB<MatrixReplacement, Eigen::IdentityPreconditioner>](classeigen_1_1bicgstab) bicg;
bicg.[compute](classeigen_1_1iterativesolverbase#a7dfa55c55e82d697bde227696a630914)(A);
x = bicg.[solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f)(b);
std::cout << "BiCGSTAB: #iterations: " << bicg.[iterations](classeigen_1_1iterativesolverbase#ae778dd098bd5e6655625b20b1e9f15da)() << ", estimated error: " << bicg.[error](classeigen_1_1iterativesolverbase#a117c241af3fb1141ad0916a3cf3157ec)() << std::endl;
}
{
Eigen::GMRES<MatrixReplacement, Eigen::IdentityPreconditioner> gmres;
gmres.compute(A);
x = gmres.solve(b);
std::cout << "GMRES: #iterations: " << gmres.iterations() << ", estimated error: " << gmres.error() << std::endl;
}
{
Eigen::DGMRES<MatrixReplacement, Eigen::IdentityPreconditioner> gmres;
gmres.compute(A);
x = gmres.solve(b);
std::cout << "DGMRES: #iterations: " << gmres.iterations() << ", estimated error: " << gmres.error() << std::endl;
}
{
Eigen::MINRES<MatrixReplacement, Eigen::Lower|Eigen::Upper, Eigen::IdentityPreconditioner> minres;
minres.compute(A);
x = minres.solve(b);
std::cout << "MINRES: #iterations: " << minres.iterations() << ", estimated error: " << minres.error() << std::endl;
}
}
```
Output:
```
CG: #iterations: 20, estimated error: 8.86333e-14
BiCGSTAB: #iterations: 20, estimated error: 2.10809e-15
GMRES: #iterations: 10, estimated error: 0
DGMRES: #iterations: 20, estimated error: 1.10455e-28
MINRES: #iterations: 20, estimated error: 2.94473e-14
```
eigen3 LU module LU module
=========
This module includes LU decomposition and related notions such as matrix inversion and determinant. This module defines the following [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") methods:
* [MatrixBase::inverse()](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf)
* [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
```
#include <Eigen/LU>
```
| |
| --- |
| |
| class | [Eigen::FullPivLU< \_MatrixType >](classeigen_1_1fullpivlu) |
| | LU decomposition of a matrix with complete pivoting, and related features. [More...](classeigen_1_1fullpivlu#details) |
| |
| class | [Eigen::PartialPivLU< \_MatrixType >](classeigen_1_1partialpivlu) |
| | LU decomposition of a matrix with partial pivoting, and related features. [More...](classeigen_1_1partialpivlu#details) |
| |
eigen3 Eigen::IncompleteLUT Eigen::IncompleteLUT
====================
### template<typename \_Scalar, typename \_StorageIndex = int> class Eigen::IncompleteLUT< \_Scalar, \_StorageIndex >
Incomplete LU factorization with dual-threshold strategy.
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
During the numerical factorization, two dropping rules are used : 1) any element whose magnitude is less than some tolerance is dropped. This tolerance is obtained by multiplying the input tolerance `droptol` by the average magnitude of all the original elements in the current row. 2) After the elimination of the row, only the `fill` largest elements in the L part and the `fill` largest elements in the U part are kept (in addition to the diagonal element ). Note that `fill` is computed from the input parameter `fillfactor` which is used the ratio to control the fill\_in relatively to the initial number of nonzero elements.
The two extreme cases are when `droptol=0` (to keep all the `fill*2` largest elements) and when `fill=n/2` with `droptol` being different to zero.
References : Yousef Saad, ILUT: A dual threshold incomplete LU factorization, Numerical Linear Algebra with Applications, 1(4), pp 387-402, 1994.
NOTE : The following implementation is derived from the ILUT implementation in the SPARSKIT package, Copyright (C) 2005, the Regents of the University of Minnesota released under the terms of the GNU LGPL: <http://www-users.cs.umn.edu/~saad/software/SPARSKIT/README> However, Yousef Saad gave us permission to relicense his ILUT code to MPL2. See the [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") mailing list archive, thread: ILUT, date: July 8, 2012: <http://listengine.tuxfamily.org/lists.tuxfamily.org/eigen/2012/07/msg00064.html> alternatively, on GMANE: <http://comments.gmane.org/gmane.comp.lib.eigen/3302>
| |
| --- |
| |
| struct | [keep\_diag](structeigen_1_1incompletelut_1_1keep__diag) |
| |
| |
| --- |
| |
| template<typename MatrixType > |
| [IncompleteLUT](classeigen_1_1incompletelut) & | [compute](classeigen_1_1incompletelut#a488e37ab51d8ed37a297eeca521f1817) (const MatrixType &amat) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1incompletelut#a941c7d34f15d7bc287e780636be0ee2b) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1incompletelut#a941c7d34f15d7bc287e780636be0ee2b) |
| |
| void | [setDroptol](classeigen_1_1incompletelut#a9628c5a595e9e984c72d1f8e671a6925) (const RealScalar &droptol) |
| |
| void | [setFillfactor](classeigen_1_1incompletelut#a327767d12b55ff8a023f12a030051e17) (int fillfactor) |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< IncompleteLUT< \_Scalar, int > >](classeigen_1_1sparsesolverbase) |
| const [Solve](classeigen_1_1solve)< [IncompleteLUT](classeigen_1_1incompletelut)< \_Scalar, int >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [Solve](classeigen_1_1solve)< [IncompleteLUT](classeigen_1_1incompletelut)< \_Scalar, int >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
compute()
---------
template<typename \_Scalar , typename \_StorageIndex = int> template<typename MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [IncompleteLUT](classeigen_1_1incompletelut)& [Eigen::IncompleteLUT](classeigen_1_1incompletelut)< \_Scalar, \_StorageIndex >::compute | ( | const MatrixType & | *amat* | ) | |
| inline |
Compute an incomplete LU factorization with dual threshold on the matrix mat No pivoting is done in this version
info()
------
template<typename \_Scalar , typename \_StorageIndex = int>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::IncompleteLUT](classeigen_1_1incompletelut)< \_Scalar, \_StorageIndex >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the matrix.appears to be negative.
setDroptol()
------------
template<typename Scalar , typename StorageIndex >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::IncompleteLUT](classeigen_1_1incompletelut)< Scalar, StorageIndex >::setDroptol | ( | const RealScalar & | *droptol* | ) | |
Set control parameter droptol
Parameters
| | |
| --- | --- |
| droptol | Drop any element whose magnitude is less than this tolerance |
setFillfactor()
---------------
template<typename Scalar , typename StorageIndex >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::IncompleteLUT](classeigen_1_1incompletelut)< Scalar, StorageIndex >::setFillfactor | ( | int | *fillfactor* | ) | |
Set control parameter fillfactor
Parameters
| | |
| --- | --- |
| fillfactor | This is used to compute the number `fill_in` of largest elements to keep on each row. |
---
The documentation for this class was generated from the following file:* [IncompleteLUT.h](https://eigen.tuxfamily.org/dox/IncompleteLUT_8h_source.html)
eigen3 Eigen::QuaternionBase Eigen::QuaternionBase
=====================
### template<class Derived> class Eigen::QuaternionBase< Derived >
Base class for quaternion expressions.
This is defined in the Geometry module.
```
#include <Eigen/Geometry>
```
Template Parameters
| | |
| --- | --- |
| Derived | derived type (CRTP) |
See also
class [Quaternion](classeigen_1_1quaternion "The quaternion class used to represent 3D orientations and rotations.")
| |
| --- |
| |
| typedef [AngleAxis](classeigen_1_1angleaxis)< Scalar > | [AngleAxisType](classeigen_1_1quaternionbase#aed266c63b10a4028304901d9c8614199) |
| |
| typedef [Matrix](classeigen_1_1matrix)< Scalar, 3, 3 > | [Matrix3](classeigen_1_1quaternionbase#ac3972e6cb0f56cccbe9e3946a7e494f8) |
| |
| typedef [Matrix](classeigen_1_1matrix)< Scalar, 3, 1 > | [Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) |
| |
|
Public Types inherited from [Eigen::RotationBase< Derived, 3 >](classeigen_1_1rotationbase) |
| typedef [Matrix](classeigen_1_1matrix)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Dim > | [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) |
| |
| typedef internal::traits< Derived >::[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) | [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) |
| |
| |
| --- |
| |
| [Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) | [\_transformVector](classeigen_1_1quaternionbase#aabef1f6fc62535f6f85d590108915ee8) (const [Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) &v) const |
| |
| template<class OtherDerived > |
| internal::traits< Derived >::Scalar | [angularDistance](classeigen_1_1quaternionbase#a74f13d7c853484996494c26c633ae02a) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| template<typename NewScalarType > |
| internal::cast\_return\_type< Derived, [Quaternion](classeigen_1_1quaternion)< NewScalarType > >::type | [cast](classeigen_1_1quaternionbase#a951d627764be63ca1e8c2c4c7315e43f) () const |
| |
| internal::traits< Derived >::Coefficients & | [coeffs](classeigen_1_1quaternionbase#ae61294790c0cc308d3f69690a657672c) () |
| |
| const internal::traits< Derived >::Coefficients & | [coeffs](classeigen_1_1quaternionbase#a193e79f616335a0067e3e784c7cf85fa) () const |
| |
| [Quaternion](classeigen_1_1quaternion)< Scalar > | [conjugate](classeigen_1_1quaternionbase#a5e63b775d0a93161ce6137ec0a17f6b0) () const |
| |
| template<class OtherDerived > |
| Scalar | [dot](classeigen_1_1quaternionbase#aa2d22c5b321c9539dd625ca415422236) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| [Quaternion](classeigen_1_1quaternion)< Scalar > | [inverse](classeigen_1_1quaternionbase#ab12ee41b3b06adc3062217a795a6a9f5) () const |
| |
| template<class OtherDerived > |
| bool | [isApprox](classeigen_1_1quaternionbase#a64bc41c96a9e99567e0f8409f8f0f680) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| Scalar | [norm](classeigen_1_1quaternionbase#aad4b1faef1eabdc7fdd4d305e9881149) () const |
| |
| void | [normalize](classeigen_1_1quaternionbase#a7a487a8a129b46be562f42044102c1f8) () |
| |
| [Quaternion](classeigen_1_1quaternion)< Scalar > | [normalized](classeigen_1_1quaternionbase#ade799f18b7ec19c02ddae3a4921fa8a0) () const |
| |
| template<class OtherDerived > |
| bool | [operator!=](classeigen_1_1quaternionbase#a530edf22f03853cd07ba829ea0d505dc) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| template<class OtherDerived > |
| [Quaternion](classeigen_1_1quaternion)< typename internal::traits< Derived >::Scalar > | [operator\*](classeigen_1_1quaternionbase#afdf1dc395c1cff6716ec9b80fd15b414) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| template<class OtherDerived > |
| Derived & | [operator\*=](classeigen_1_1quaternionbase#afd8ee6b6420fbdd22fab7cd016212441) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &q) |
| |
| Derived & | [operator=](classeigen_1_1quaternionbase#aa7c114c6e62a37d4fc53b6e82ed78eac) (const [AngleAxisType](classeigen_1_1quaternionbase#aed266c63b10a4028304901d9c8614199) &aa) |
| |
| template<class MatrixDerived > |
| Derived & | [operator=](classeigen_1_1quaternionbase#a20a6702c9da3fc2950178d920d0aaf84) (const [MatrixBase](classeigen_1_1matrixbase)< MatrixDerived > &xpr) |
| |
| template<class OtherDerived > |
| bool | [operator==](classeigen_1_1quaternionbase#ad206c9014409c06970884dbfc00e6c3c) (const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| template<typename Derived1 , typename Derived2 > |
| Derived & | [setFromTwoVectors](classeigen_1_1quaternionbase#a7ae84bfbcc9f3f19f10294496a836bee) (const [MatrixBase](classeigen_1_1matrixbase)< Derived1 > &a, const [MatrixBase](classeigen_1_1matrixbase)< Derived2 > &b) |
| |
| [QuaternionBase](classeigen_1_1quaternionbase) & | [setIdentity](classeigen_1_1quaternionbase#a4695b0f6eebfb217ae2fbd579ceda24a) () |
| |
| template<class OtherDerived > |
| [Quaternion](classeigen_1_1quaternion)< typename internal::traits< Derived >::Scalar > | [slerp](classeigen_1_1quaternionbase#ac840bde67d22f2deca330561c65d144e) (const Scalar &t, const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > &other) const |
| |
| Scalar | [squaredNorm](classeigen_1_1quaternionbase#a09730db4ef0b546f5cf29f6b180b3c87) () const |
| |
| [Matrix3](classeigen_1_1quaternionbase#ac3972e6cb0f56cccbe9e3946a7e494f8) | [toRotationMatrix](classeigen_1_1quaternionbase#a8cf07ab9875baba2eecdd62ff93bfc3f) () const |
| |
| [VectorBlock](classeigen_1_1vectorblock)< Coefficients, 3 > | [vec](classeigen_1_1quaternionbase#a91f93bde88f52796cfcd92c3594f39e5) () |
| |
| const [VectorBlock](classeigen_1_1vectorblock)< const Coefficients, 3 > | [vec](classeigen_1_1quaternionbase#ada8bdb403471df23511bdc0f227962ea) () const |
| |
| NonConstCoeffReturnType | [w](classeigen_1_1quaternionbase#ad884cf20a0b0b92bb63ab3fe9d6d6b7f) () |
| |
| CoeffReturnType | [w](classeigen_1_1quaternionbase#ab5eae91bedac0afaab0074cec5e535bc) () const |
| |
| NonConstCoeffReturnType | [x](classeigen_1_1quaternionbase#a8b05bac2a1c099b341396f725e85f3b1) () |
| |
| CoeffReturnType | [x](classeigen_1_1quaternionbase#afdd8e260d5de861a6136cb9e6ceaa4b4) () const |
| |
| NonConstCoeffReturnType | [y](classeigen_1_1quaternionbase#a6005245a72520df258d30af6b5448595) () |
| |
| CoeffReturnType | [y](classeigen_1_1quaternionbase#aad37efca5d9fde3f4cb03a208f38d74f) () const |
| |
| NonConstCoeffReturnType | [z](classeigen_1_1quaternionbase#a9afed6a7fa4fcdfbe599d7b5b207fc1b) () |
| |
| CoeffReturnType | [z](classeigen_1_1quaternionbase#a8389b65a61aa3fc76d3ba4bd4a63e529) () const |
| |
|
Public Member Functions inherited from [Eigen::RotationBase< Derived, 3 >](classeigen_1_1rotationbase) |
| Derived | [inverse](classeigen_1_1rotationbase#a8532fb716ea4267cf8bbdb99e5e54837) () const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [matrix](classeigen_1_1rotationbase#a91b5bdb1c7b90ec7b33107c6f7d3b171) () const |
| |
| internal::rotation\_base\_generic\_product\_selector< Derived, OtherDerived, OtherDerived::IsVectorAtCompileTime >::ReturnType | [operator\*](classeigen_1_1rotationbase#a09a4757e7aff7a95bebf4fa8a965a4eb) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &e) const |
| |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode > | [operator\*](classeigen_1_1rotationbase#aaca1c3d834e2bc7ebfd046d96cac990c) (const [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode, Options > &t) const |
| |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Isometry > | [operator\*](classeigen_1_1rotationbase#a26d0603783666526a98d08bd45d9c751) (const [Translation](classeigen_1_1translation)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim > &t) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [operator\*](classeigen_1_1rotationbase#a05af64a1bc759c5fed4ff7afd1414ba4) (const [UniformScaling](classeigen_1_1uniformscaling)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) > &s) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [toRotationMatrix](classeigen_1_1rotationbase#a94fe3683c867c39d34505932b07e956a) () const |
| |
| |
| --- |
| |
| static [Quaternion](classeigen_1_1quaternion)< Scalar > | [Identity](classeigen_1_1quaternionbase#a6f31a6f98016f186515b3277f4757962) () |
| |
AngleAxisType
-------------
template<class Derived >
| |
| --- |
| typedef [AngleAxis](classeigen_1_1angleaxis)<Scalar> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::[AngleAxisType](classeigen_1_1quaternionbase#aed266c63b10a4028304901d9c8614199) |
the equivalent angle-axis type
Matrix3
-------
template<class Derived >
| |
| --- |
| typedef [Matrix](classeigen_1_1matrix)<Scalar,3,3> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::[Matrix3](classeigen_1_1quaternionbase#ac3972e6cb0f56cccbe9e3946a7e494f8) |
the equivalent rotation matrix type
Vector3
-------
template<class Derived >
| |
| --- |
| typedef [Matrix](classeigen_1_1matrix)<Scalar,3,1> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::[Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) |
the type of a 3D vector
\_transformVector()
-------------------
template<class Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [QuaternionBase](classeigen_1_1quaternionbase)< Derived >::[Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::\_transformVector | ( | const [Vector3](classeigen_1_1quaternionbase#a974c0529d55983b0b3a6d99a8466f331) & | *v* | ) | const |
| inline |
return the result vector of *v* through the rotation
Rotation of a vector by a quaternion.
Remarks
If the quaternion is used to rotate several points (>1) then it is much more efficient to first convert it to a 3x3 [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors."). Comparison of the operation cost for n transformations:* Quaternion2: 30n
* Via a Matrix3: 24 + 15n
angularDistance()
-----------------
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| internal::traits<Derived>::Scalar [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::angularDistance | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns
the angle (in radian) between two rotations
See also
[dot()](classeigen_1_1quaternionbase#aa2d22c5b321c9539dd625ca415422236)
cast()
------
template<class Derived > template<typename NewScalarType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::cast\_return\_type<Derived,[Quaternion](classeigen_1_1quaternion)<NewScalarType> >::type [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::cast | ( | | ) | const |
| inline |
Returns
`*this` with scalar type casted to *NewScalarType*
Note that if *NewScalarType* is equal to the current scalar type of `*this` then this function smartly returns a const reference to `*this`.
coeffs() [1/2]
--------------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::traits<Derived>::Coefficients& [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::coeffs | ( | | ) | |
| inline |
Returns
a vector expression of the coefficients (x,y,z,w)
coeffs() [2/2]
--------------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const internal::traits<Derived>::Coefficients& [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::coeffs | ( | | ) | const |
| inline |
Returns
a read-only vector expression of the coefficients (x,y,z,w)
conjugate()
-----------
template<class Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Quaternion](classeigen_1_1quaternion)< typename internal::traits< Derived >::Scalar > [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::conjugate | ( | void | | ) | const |
| inline |
Returns
the conjugated quaternion the conjugate of the `*this` which is equal to the multiplicative inverse if the quaternion is normalized. The conjugate of a quaternion represents the opposite rotation.
See also
Quaternion2::inverse()
dot()
-----
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::dot | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns
the dot product of `*this` and *other* Geometrically speaking, the dot product of two unit quaternions corresponds to the cosine of half the angle between the two rotations.
See also
angularDistance()
Identity()
----------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| static [Quaternion](classeigen_1_1quaternion)<Scalar> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::Identity | ( | | ) | |
| inlinestatic |
Returns
a quaternion representing an identity rotation
See also
[MatrixBase::Identity()](classeigen_1_1matrixbase#a98bb9a0f705c6dfde85b0bfff31bf88f)
inverse()
---------
template<class Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [Quaternion](classeigen_1_1quaternion)< typename internal::traits< Derived >::Scalar > [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::inverse |
| inline |
Returns
the quaternion describing the inverse rotation the multiplicative inverse of `*this` Note that in most cases, i.e., if you simply want the opposite rotation, and/or the quaternion is normalized, then it is enough to use the conjugate.
See also
[QuaternionBase::conjugate()](classeigen_1_1quaternionbase#a5e63b775d0a93161ce6137ec0a17f6b0)
isApprox()
----------
template<class Derived > template<class OtherDerived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::isApprox | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other*, |
| | | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<Scalar>::dummy_precision()` |
| | ) | | const |
| inline |
Returns
`true` if `*this` is approximately equal to *other*, within the precision determined by *prec*.
See also
[MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c)
norm()
------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::norm | ( | | ) | const |
| inline |
Returns
the norm of the quaternion's coefficients
See also
[QuaternionBase::squaredNorm()](classeigen_1_1quaternionbase#a09730db4ef0b546f5cf29f6b180b3c87), [MatrixBase::norm()](classeigen_1_1matrixbase#a196c4ec3c8ffdf5bda45d0f617154975)
normalize()
-----------
template<class Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::normalize | ( | void | | ) | |
| inline |
Normalizes the quaternion `*this`
See also
[normalized()](classeigen_1_1quaternionbase#ade799f18b7ec19c02ddae3a4921fa8a0), [MatrixBase::normalize()](classeigen_1_1matrixbase#ad16303c47ba36f7a41ea264cb26bceb6)
normalized()
------------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Quaternion](classeigen_1_1quaternion)<Scalar> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::normalized | ( | | ) | const |
| inline |
Returns
a normalized copy of `*this`
See also
[normalize()](classeigen_1_1quaternionbase#a7a487a8a129b46be562f42044102c1f8), [MatrixBase::normalized()](classeigen_1_1matrixbase#a5cf2fd4c57e59604fd4116158fd34308)
operator!=()
------------
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator!= | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns
true if at least one pair of coefficients of `*this` and *other* are not exactly equal to each other.
Warning
When using floating point scalar values you probably should rather use a fuzzy comparison such as [isApprox()](classeigen_1_1quaternionbase#a64bc41c96a9e99567e0f8409f8f0f680)
See also
[isApprox()](classeigen_1_1quaternionbase#a64bc41c96a9e99567e0f8409f8f0f680), operator==
operator\*()
------------
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Quaternion](classeigen_1_1quaternion)<typename internal::traits<Derived>::Scalar> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator\* | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns
the concatenation of two rotations as a quaternion-quaternion product
operator\*=()
-------------
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator\*= | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | |
| inline |
See also
operator\*(Quaternion)
operator=() [1/2]
-----------------
template<class Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator= | ( | const [AngleAxisType](classeigen_1_1quaternionbase#aed266c63b10a4028304901d9c8614199) & | *aa* | ) | |
| inline |
Set `*this` from an angle-axis *aa* and returns a reference to `*this`
operator=() [2/2]
-----------------
template<class Derived > template<class MatrixDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator= | ( | const [MatrixBase](classeigen_1_1matrixbase)< MatrixDerived > & | *xpr* | ) | |
| inline |
Set `*this` from the expression *xpr:*
* if *xpr* is a 4x1 vector, then *xpr* is assumed to be a quaternion
* if *xpr* is a 3x3 matrix, then *xpr* is assumed to be rotation matrix and *xpr* is converted to a quaternion
operator==()
------------
template<class Derived > template<class OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::operator== | ( | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns
true if each coefficients of `*this` and *other* are all exactly equal.
Warning
When using floating point scalar values you probably should rather use a fuzzy comparison such as [isApprox()](classeigen_1_1quaternionbase#a64bc41c96a9e99567e0f8409f8f0f680)
See also
[isApprox()](classeigen_1_1quaternionbase#a64bc41c96a9e99567e0f8409f8f0f680), operator!=
setFromTwoVectors()
-------------------
template<class Derived > template<typename Derived1 , typename Derived2 >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::setFromTwoVectors | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived1 > & | *a*, |
| | | const [MatrixBase](classeigen_1_1matrixbase)< Derived2 > & | *b* |
| | ) | | |
| inline |
Returns
the quaternion which transform *a* into *b* through a rotation
Sets `*this` to be a quaternion representing a rotation between the two arbitrary vectors *a* and *b*. In other words, the built rotation represent a rotation sending the line of direction *a* to the line of direction *b*, both lines passing through the origin.
Returns
a reference to `*this`.
Note that the two input vectors do **not** have to be normalized, and do not need to have the same norm.
setIdentity()
-------------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [QuaternionBase](classeigen_1_1quaternionbase)& [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::setIdentity | ( | | ) | |
| inline |
See also
[QuaternionBase::Identity()](classeigen_1_1quaternionbase#a6f31a6f98016f186515b3277f4757962), [MatrixBase::setIdentity()](classeigen_1_1matrixbase#a18e969adfdf2db4ac44c47fbdc854683)
slerp()
-------
template<class Derived > template<class OtherDerived >
| | | | |
| --- | --- | --- | --- |
| [Quaternion](classeigen_1_1quaternion)<typename internal::traits<Derived>::Scalar> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::slerp | ( | const Scalar & | *t*, |
| | | const [QuaternionBase](classeigen_1_1quaternionbase)< OtherDerived > & | *other* |
| | ) | | const |
Returns
the spherical linear interpolation between the two quaternions `*this` and *other* at the parameter *t* in [0;1].
This represents an interpolation for a constant motion between `*this` and *other*, see also <http://en.wikipedia.org/wiki/Slerp>.
squaredNorm()
-------------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::squaredNorm | ( | | ) | const |
| inline |
Returns
the squared norm of the quaternion's coefficients
See also
[QuaternionBase::norm()](classeigen_1_1quaternionbase#aad4b1faef1eabdc7fdd4d305e9881149), [MatrixBase::squaredNorm()](classeigen_1_1matrixbase#ac8da566526419f9742a6c471bbd87e0a)
toRotationMatrix()
------------------
template<class Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [QuaternionBase](classeigen_1_1quaternionbase)< Derived >::[Matrix3](classeigen_1_1quaternionbase#ac3972e6cb0f56cccbe9e3946a7e494f8) [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::toRotationMatrix | ( | void | | ) | const |
| inline |
Returns
an equivalent 3x3 rotation matrix
Convert the quaternion to a 3x3 rotation matrix. The quaternion is required to be normalized, otherwise the result is undefined.
vec() [1/2]
-----------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [VectorBlock](classeigen_1_1vectorblock)<Coefficients,3> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::vec | ( | | ) | |
| inline |
Returns
a vector expression of the imaginary part (x,y,z)
vec() [2/2]
-----------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [VectorBlock](classeigen_1_1vectorblock)<const Coefficients,3> [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::vec | ( | | ) | const |
| inline |
Returns
a read-only vector expression of the imaginary part (x,y,z)
w() [1/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| NonConstCoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::w | ( | | ) | |
| inline |
Returns
a reference to the `w` coefficient (if Derived is a non-const lvalue)
w() [2/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::w | ( | | ) | const |
| inline |
Returns
the `w` coefficient
x() [1/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| NonConstCoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::x | ( | | ) | |
| inline |
Returns
a reference to the `x` coefficient (if Derived is a non-const lvalue)
x() [2/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::x | ( | | ) | const |
| inline |
Returns
the `x` coefficient
y() [1/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| NonConstCoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::y | ( | | ) | |
| inline |
Returns
a reference to the `y` coefficient (if Derived is a non-const lvalue)
y() [2/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::y | ( | | ) | const |
| inline |
Returns
the `y` coefficient
z() [1/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| NonConstCoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::z | ( | | ) | |
| inline |
Returns
a reference to the `z` coefficient (if Derived is a non-const lvalue)
z() [2/2]
---------
template<class Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType [Eigen::QuaternionBase](classeigen_1_1quaternionbase)< Derived >::z | ( | | ) | const |
| inline |
Returns
the `z` coefficient
---
The documentation for this class was generated from the following file:* [Quaternion.h](https://eigen.tuxfamily.org/dox/Quaternion_8h_source.html)
| programming_docs |
eigen3 Eigen::Ref Eigen::Ref
==========
### template<typename SparseVectorType> class Eigen::Ref< SparseVectorType >
A sparse vector expression referencing an existing sparse vector expression.
Template Parameters
| | |
| --- | --- |
| SparseVectorType | the equivalent sparse vector type of the referenced data, it must be a template instance of class [SparseVector](classeigen_1_1sparsevector "a sparse vector class"). |
See also
class [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.")
| |
| --- |
| |
| template<typename Derived > |
| | [Ref](classeigen_1_1ref_3_01sparsevectortype_01_4#a125c83639030247f78157fa606c03f56) ([SparseCompressedBase](classeigen_1_1sparsecompressedbase)< Derived > &expr) |
| |
|
Public Member Functions inherited from [Eigen::SparseMapBase< Derived, WriteAccessors >](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4) |
| Scalar & | [coeffRef](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#aa9c42d48b9dd6f947ce3c257fe4bf2ca) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| StorageIndex \* | [innerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af5cd1f13dde8578eb9891a4ac4a11977) () |
| |
| StorageIndex \* | [innerNonZeroPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af877ea4e285a4497f80987fea66f7459) () |
| |
| StorageIndex \* | [outerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#a3b74af754254837fc591cd9936688b95) () |
| |
| Scalar \* | [valuePtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af91648a18729ae8ff29cb1d8751c5655) () |
| |
| | [~SparseMapBase](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#a4dfbcf3ac411885b1710ad04892c984d) () |
| |
|
Public Member Functions inherited from [Eigen::SparseMapBase< Derived, ReadOnlyAccessors >](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4) |
| Scalar | [coeff](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a195e66f79171f78cc22d91fff37e36e3) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a0fc44f3781a869a3a410edd6691fd899) () const |
| |
| const StorageIndex \* | [innerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#ab044564756f472877b2c1a5706e540e2) () const |
| |
| const StorageIndex \* | [innerNonZeroPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a2b35a1d701d6c6ea36b2d9f19660a68c) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a0df6dba8d71e0fb15b2995510853f83e) () const |
| |
| bool | [isCompressed](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#aafab4afa7ab2ff89eff049d4c71e2ce4) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonZeros](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a753e975b7b3643d821dc061141786870) () const |
| |
| const StorageIndex \* | [outerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a24c55dd8de4aca30e7c90b69aa5dca6b) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a3d6ede19db6d42074ae063bc876231b1) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a3cdd6cab0abd7ac01925a695fc315d34) () const |
| |
| const Scalar \* | [valuePtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a574ea9371c22eabebdda21c0787312dc) () const |
| |
| | [~SparseMapBase](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#ab375aedf824909a7f1a6af24ee60d70f) () |
| |
|
Public Member Functions inherited from [Eigen::SparseCompressedBase< Derived >](classeigen_1_1sparsecompressedbase) |
| [Map](classeigen_1_1map)< [Array](classeigen_1_1array)< Scalar, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > > | [coeffs](classeigen_1_1sparsecompressedbase#a7cf299e08d2a4f6d6869e631e51b12fe) () |
| |
| const [Map](classeigen_1_1map)< const [Array](classeigen_1_1array)< Scalar, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > > | [coeffs](classeigen_1_1sparsecompressedbase#a101b155485ae59ea1261c4f6040f3dc4) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerIndexPtr](classeigen_1_1sparsecompressedbase#a197111c1289644f1ea38fe683ccdd82a) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerIndexPtr](classeigen_1_1sparsecompressedbase#aa64818e1aa43015dad01b114b2ab4687) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerNonZeroPtr](classeigen_1_1sparsecompressedbase#a411e972b097e6aef225415a4c2d0a0b5) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerNonZeroPtr](classeigen_1_1sparsecompressedbase#afc056a3895eae1a4c4767252ff04966a) () const |
| |
| bool | [isCompressed](classeigen_1_1sparsecompressedbase#a837934b33a80fe996ff20500373d3a61) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonZeros](classeigen_1_1sparsecompressedbase#a03de8b3da2c142ce8698a76123b3e7d3) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [outerIndexPtr](classeigen_1_1sparsecompressedbase#a53a82f962686e18c8dc07a4b9a85ed7b) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [outerIndexPtr](classeigen_1_1sparsecompressedbase#a2624d4c2661c582de168246c56e8d71e) () const |
| |
| Scalar \* | [valuePtr](classeigen_1_1sparsecompressedbase#a0f12f72d14b6c277d09be9f5ce2eab95) () |
| |
| const Scalar \* | [valuePtr](classeigen_1_1sparsecompressedbase#a0f44c739398794ea77f310b745cc5627) () const |
| |
|
Public Member Functions inherited from [Eigen::SparseMatrixBase< Derived >](classeigen_1_1sparsematrixbase) |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsematrixbase#aca7ce296424ef6e478ab0fb19547a7ee) () const |
| |
| const internal::eval< Derived >::type | [eval](classeigen_1_1sparsematrixbase#a761bd872a06b59632fcff7b7807a77ce) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsematrixbase#a180fcba1ccf3cdf3252a263bc1de7a1d) () const |
| |
| bool | [isVector](classeigen_1_1sparsematrixbase#a7eedffa867031f649fd0fb9cc23ce4be) () const |
| |
| template<typename OtherDerived > |
| const [Product](classeigen_1_1product)< Derived, OtherDerived, AliasFreeProduct > | [operator\*](classeigen_1_1sparsematrixbase#a9d4d71b3f34389e6fc01f2b86e43f7a4) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< OtherDerived > &other) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsematrixbase#ac86cc88a4cfef21db6b64ec0ab4c8f0a) () const |
| |
| const [SparseView](classeigen_1_1sparseview)< Derived > | [pruned](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d) (const Scalar &reference=Scalar(0), const RealScalar &epsilon=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsematrixbase#a1944e9fa9ce7937bfc3a87b2cb94371f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1sparsematrixbase#a124bc57921775eb9aa2dfd9727e23472) () const |
| |
| SparseSymmetricPermutationProduct< Derived, [Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749) > | [twistedBy](classeigen_1_1sparsematrixbase#a51d4898bd6a57cc3ba543a39b102423e) (const [PermutationMatrix](classeigen_1_1permutationmatrix)< [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) > &perm) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::SparseMatrixBase< Derived >](classeigen_1_1sparsematrixbase) |
| enum | { [RowsAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a456cda7b9d938e57194036a41d634604) , [ColsAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a27ba349f075d026c1f51d1ec69aa5b14) , [SizeAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5aa5022cfa2bb53129883e9b7b8abd3d68) , **MaxRowsAtCompileTime** , **MaxColsAtCompileTime** , **MaxSizeAtCompileTime** , [IsVectorAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a14a3f566ed2a074beddb8aef0223bfdf) , [NumDimensions](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a2366131ffcc38bff48a1c7572eb86dd3) , [Flags](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a2af043b36fe9e08df0107cf6de496165) , **IsRowMajor** , **InnerSizeAtCompileTime** } |
| |
| typedef internal::traits< Derived >::[StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) | [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) |
| |
| typedef Scalar | [value\_type](classeigen_1_1sparsematrixbase#ac254d3b61718ebc2136d27bac043dcb7) |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
|
Protected Member Functions inherited from [Eigen::SparseCompressedBase< Derived >](classeigen_1_1sparsecompressedbase) |
| | [SparseCompressedBase](classeigen_1_1sparsecompressedbase#af79f020db965367d97eb954fc68d8f99) () |
| |
Ref()
-----
template<typename SparseVectorType > template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::Ref](classeigen_1_1ref)< SparseVectorType >::[Ref](classeigen_1_1ref) | ( | [SparseCompressedBase](classeigen_1_1sparsecompressedbase)< Derived > & | *expr* | ) | |
| inline |
Implicit constructor from any 1D sparse vector expression
---
The documentation for this class was generated from the following file:* [SparseRef.h](https://eigen.tuxfamily.org/dox/SparseRef_8h_source.html)
eigen3 Eigen::PartialPivLU Eigen::PartialPivLU
===================
### template<typename \_MatrixType> class Eigen::PartialPivLU< \_MatrixType >
LU decomposition of a matrix with partial pivoting, and related features.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the matrix of which we are computing the LU decomposition |
This class represents a LU decomposition of a **square** **invertible** matrix, with partial pivoting: the matrix A is decomposed as A = PLU where L is unit-lower-triangular, U is upper-triangular, and P is a permutation matrix.
Typically, partial pivoting LU decomposition is only considered numerically stable for square invertible matrices. Thus LAPACK's dgesv and dgesvx require the matrix to be square and invertible. The present class does the same. It will assert that the matrix is square, but it won't (actually it can't) check that the matrix is invertible: it is your task to check that you only use this decomposition on invertible matrices.
The guaranteed safe alternative, working for all matrices, is the full pivoting LU decomposition, provided by class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.").
This is **not** a rank-revealing LU decomposition. Many features are intentionally absent from this class, such as rank computation. If you need these features, use class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.").
This LU decomposition is suitable to invert invertible matrices. It is what [MatrixBase::inverse()](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf) uses in the general case. On the other hand, it is **not** suitable to determine whether a given matrix is invertible.
The data of the LU decomposition can be directly accessed through the methods [matrixLU()](classeigen_1_1partialpivlu#abea0d7e51c5591a6db152eade0892d9c), [permutationP()](classeigen_1_1partialpivlu#a1c637530b3215787668a75ebb2e7b882).
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
See also
[MatrixBase::partialPivLu()](classeigen_1_1matrixbase#a6199d8aaf26c1b8ac3097fdfa7733a1e), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061), [MatrixBase::inverse()](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf), MatrixBase::computeInverse(), class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.")
| |
| --- |
| |
| Scalar | [determinant](classeigen_1_1partialpivlu#a54c3d39c9b46ff485a8d2140b9b23193) () const |
| |
| const [Inverse](classeigen_1_1inverse)< [PartialPivLU](classeigen_1_1partialpivlu) > | [inverse](classeigen_1_1partialpivlu#aef983470f92aba829e861e32e68681b5) () const |
| |
| const MatrixType & | [matrixLU](classeigen_1_1partialpivlu#abea0d7e51c5591a6db152eade0892d9c) () const |
| |
| | [PartialPivLU](classeigen_1_1partialpivlu#a5c04818d354f94a98786d8a44cb709c6) () |
| | Default Constructor. [More...](classeigen_1_1partialpivlu#a5c04818d354f94a98786d8a44cb709c6) |
| |
| template<typename InputType > |
| | [PartialPivLU](classeigen_1_1partialpivlu#acf37214aebb54d0e186ae39ac6c41bdf) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| |
| template<typename InputType > |
| | [PartialPivLU](classeigen_1_1partialpivlu#a4efc917d31d0e9d76781a97509309061) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| |
| | [PartialPivLU](classeigen_1_1partialpivlu#acf892c12d8a229b32bddc3149e32e63a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9)) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1partialpivlu#acf892c12d8a229b32bddc3149e32e63a) |
| |
| const [PermutationType](classeigen_1_1permutationmatrix) & | [permutationP](classeigen_1_1partialpivlu#a1c637530b3215787668a75ebb2e7b882) () const |
| |
| RealScalar | [rcond](classeigen_1_1partialpivlu#a472b46d5d9ff7c328e1dccc13805f690) () const |
| |
| MatrixType | [reconstructedMatrix](classeigen_1_1partialpivlu#aba7f1ee83537b0d240ebf206503a4920) () const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [PartialPivLU](classeigen_1_1partialpivlu), Rhs > | [solve](classeigen_1_1partialpivlu#a49247bd2f742a46bca1f9c2bf1b19ad8) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< PartialPivLU< \_MatrixType > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
PartialPivLU() [1/4]
--------------------
template<typename MatrixType >
| |
| --- |
| [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::[PartialPivLU](classeigen_1_1partialpivlu) |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via PartialPivLU::compute(const MatrixType&).
PartialPivLU() [2/4]
--------------------
template<typename MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::[PartialPivLU](classeigen_1_1partialpivlu) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| explicit |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[PartialPivLU()](classeigen_1_1partialpivlu#a5c04818d354f94a98786d8a44cb709c6 "Default Constructor.")
PartialPivLU() [3/4]
--------------------
template<typename MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::[PartialPivLU](classeigen_1_1partialpivlu) | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| explicit |
Constructor.
Parameters
| | |
| --- | --- |
| matrix | the matrix of which to compute the LU decomposition. |
Warning
The matrix should have full rank (e.g. if it's square, it should be invertible). If you need to deal with non-full rank, use class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.") instead.
PartialPivLU() [4/4]
--------------------
template<typename MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::[PartialPivLU](classeigen_1_1partialpivlu) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| explicit |
Constructor for [inplace decomposition](group__inplacedecomposition)
Parameters
| | |
| --- | --- |
| matrix | the matrix of which to compute the LU decomposition. |
Warning
The matrix should have full rank (e.g. if it's square, it should be invertible). If you need to deal with non-full rank, use class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.") instead.
determinant()
-------------
template<typename MatrixType >
| |
| --- |
| [PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::Scalar [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::determinant |
Returns
the determinant of the matrix of which \*this is the LU decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the LU decomposition has already been computed.
Note
For fixed-size matrices of size up to 4, [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061) offers optimized paths.
Warning
a determinant can be very big or small, so for matrices of large enough dimension, there is a risk of overflow/underflow.
See also
[MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
inverse()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [Inverse](classeigen_1_1inverse)<[PartialPivLU](classeigen_1_1partialpivlu)> [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >::inverse | ( | | ) | const |
| inline |
Returns
the inverse of the matrix of which \*this is the LU decomposition.
Warning
The matrix being decomposed here is assumed to be invertible. If you need to check for invertibility, use class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.") instead.
See also
[MatrixBase::inverse()](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf), LU::inverse()
matrixLU()
----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >::matrixLU | ( | | ) | const |
| inline |
Returns
the LU decomposition matrix: the upper-triangular part is U, the unit-lower-triangular part is L (at least for square matrices; in the non-square case, special care is needed, see the documentation of class [FullPivLU](classeigen_1_1fullpivlu "LU decomposition of a matrix with complete pivoting, and related features.")).
See also
matrixL(), matrixU()
permutationP()
--------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationType](classeigen_1_1permutationmatrix)& [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >::permutationP | ( | | ) | const |
| inline |
Returns
the permutation matrix P.
rcond()
-------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >::rcond | ( | | ) | const |
| inline |
Returns
an estimate of the reciprocal condition number of the matrix of which `*this` is the LU decomposition.
reconstructedMatrix()
---------------------
template<typename MatrixType >
| |
| --- |
| MatrixType [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< MatrixType >::reconstructedMatrix |
Returns
the matrix represented by the decomposition, i.e., it returns the product: P^{-1} L U. This function is provided for debug purpose.
solve()
-------
template<typename \_MatrixType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[PartialPivLU](classeigen_1_1partialpivlu), Rhs> [Eigen::PartialPivLU](classeigen_1_1partialpivlu)< \_MatrixType >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
This method returns the solution x to the equation Ax=b, where A is the matrix of which \*this is the LU decomposition.
Parameters
| | |
| --- | --- |
| b | the right-hand-side of the equation to solve. Can be a vector or a matrix, the only requirement in order for the equation to make sense is that b.rows()==A.rows(), where A is the matrix of which \*this is the LU decomposition. |
Returns
the solution.
Example:
```
MatrixXd A = [MatrixXd::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3,3);
MatrixXd B = [MatrixXd::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3,2);
cout << "Here is the invertible matrix A:" << endl << A << endl;
cout << "Here is the matrix B:" << endl << B << endl;
MatrixXd X = A.lu().solve(B);
cout << "Here is the (unique) solution X to the equation AX=B:" << endl << X << endl;
cout << "Relative error: " << (A*X-B).norm() / B.norm() << endl;
```
Output:
```
Here is the invertible matrix A:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the matrix B:
0.108 -0.27
-0.0452 0.0268
0.258 0.904
Here is the (unique) solution X to the equation AX=B:
0.609 2.68
-0.231 -1.57
0.51 3.51
Relative error: 3.28e-16
```
Since this [PartialPivLU](classeigen_1_1partialpivlu "LU decomposition of a matrix with partial pivoting, and related features.") class assumes anyway that the matrix A is invertible, the solution theoretically exists and is unique regardless of b.
See also
TriangularView::solve(), [inverse()](classeigen_1_1partialpivlu#aef983470f92aba829e861e32e68681b5), computeInverse()
---
The documentation for this class was generated from the following file:* [PartialPivLU.h](https://eigen.tuxfamily.org/dox/PartialPivLU_8h_source.html)
| programming_docs |
eigen3 Eigen::HouseholderQR Eigen::HouseholderQR
====================
### template<typename \_MatrixType> class Eigen::HouseholderQR< \_MatrixType >
Householder QR decomposition of a matrix.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the matrix of which we are computing the QR decomposition |
This class performs a QR decomposition of a matrix **A** into matrices **Q** and **R** such that
\[ \mathbf{A} = \mathbf{Q} \, \mathbf{R} \]
by using Householder transformations. Here, **Q** a unitary matrix and **R** an upper triangular matrix. The result is stored in a compact way compatible with LAPACK.
Note that no pivoting is performed. This is **not** a rank-revealing decomposition. If you want that feature, use [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with full pivoting.") or [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.") instead.
This Householder QR decomposition is faster, but less numerically stable and less feature-full than [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with full pivoting.") or [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.").
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
See also
[MatrixBase::householderQr()](classeigen_1_1matrixbase#a9a9377aab1cea26db5f25bab7e682f8f)
| |
| --- |
| |
| MatrixType::RealScalar | [absDeterminant](classeigen_1_1householderqr#aaf4ef26c0b7affc91431ec59c92d64c3) () const |
| |
| const HCoeffsType & | [hCoeffs](classeigen_1_1householderqr#ae931aa44cde62317b57a9ae661d184be) () const |
| |
| [HouseholderSequenceType](classeigen_1_1householdersequence) | [householderQ](classeigen_1_1householderqr#affd506c10ef2d25f56e7b1f9f25ff885) () const |
| |
| | [HouseholderQR](classeigen_1_1householderqr#a974adb10a0e066057aeb3b360df68380) () |
| | Default Constructor. [More...](classeigen_1_1householderqr#a974adb10a0e066057aeb3b360df68380) |
| |
| template<typename InputType > |
| | [HouseholderQR](classeigen_1_1householderqr#afa7cfb4faa89195c4dc8d196924c8230) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a QR factorization from a given matrix. [More...](classeigen_1_1householderqr#afa7cfb4faa89195c4dc8d196924c8230) |
| |
| template<typename InputType > |
| | [HouseholderQR](classeigen_1_1householderqr#a95a53f8479ee147d7b0ccab71c13e45d) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a QR factorization from a given matrix. [More...](classeigen_1_1householderqr#a95a53f8479ee147d7b0ccab71c13e45d) |
| |
| | [HouseholderQR](classeigen_1_1householderqr#a1087457610c53e1574de521a51de0cd3) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) rows, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) cols) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1householderqr#a1087457610c53e1574de521a51de0cd3) |
| |
| MatrixType::RealScalar | [logAbsDeterminant](classeigen_1_1householderqr#af61b6dbef34fc51c825182b16dc43ca1) () const |
| |
| const MatrixType & | [matrixQR](classeigen_1_1householderqr#ae837f2fb30099212c53b3042c7d699c9) () const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [HouseholderQR](classeigen_1_1householderqr), Rhs > | [solve](classeigen_1_1householderqr#a3e8e56769bbaaed0616ad98c4ff99c7b) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< HouseholderQR< \_MatrixType > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [HouseholderQR](classeigen_1_1householderqr)< \_MatrixType > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [HouseholderQR](classeigen_1_1householderqr)< \_MatrixType > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
| void | [computeInPlace](classeigen_1_1householderqr#a3a16530338a734971fc45efb0ef9ac94) () |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
HouseholderQR() [1/4]
---------------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::[HouseholderQR](classeigen_1_1householderqr) | ( | | ) | |
| inline |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via HouseholderQR::compute(const MatrixType&).
HouseholderQR() [2/4]
---------------------
template<typename \_MatrixType >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::[HouseholderQR](classeigen_1_1householderqr) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inline |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[HouseholderQR()](classeigen_1_1householderqr#a974adb10a0e066057aeb3b360df68380 "Default Constructor.")
HouseholderQR() [3/4]
---------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::[HouseholderQR](classeigen_1_1householderqr) | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a QR factorization from a given matrix.
This constructor computes the QR factorization of the matrix *matrix* by calling the method compute(). It is a short cut for:
```
HouseholderQR<MatrixType> qr(matrix.rows(), matrix.cols());
qr.compute(matrix);
```
See also
compute()
HouseholderQR() [4/4]
---------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::[HouseholderQR](classeigen_1_1householderqr) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a QR factorization from a given matrix.
This overloaded constructor is provided for [inplace decomposition](group__inplacedecomposition) when `MatrixType` is a [Eigen::Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.").
See also
HouseholderQR(const EigenBase&)
absDeterminant()
----------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::HouseholderQR](classeigen_1_1householderqr)< MatrixType >::absDeterminant |
Returns
the absolute value of the determinant of the matrix of which \*this is the QR decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the QR decomposition has already been computed.
Note
This is only for square matrices.
Warning
a determinant can be very big or small, so for matrices of large enough dimension, there is a risk of overflow/underflow. One way to work around that is to use [logAbsDeterminant()](classeigen_1_1householderqr#af61b6dbef34fc51c825182b16dc43ca1) instead.
See also
[logAbsDeterminant()](classeigen_1_1householderqr#af61b6dbef34fc51c825182b16dc43ca1), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
computeInPlace()
----------------
template<typename MatrixType >
| | | |
| --- | --- | --- |
|
| |
| --- |
| void [Eigen::HouseholderQR](classeigen_1_1householderqr)< MatrixType >::computeInPlace |
| protected |
Performs the QR factorization of the given matrix *matrix*. The result of the factorization is stored into `*this`, and a reference to `*this` is returned.
See also
class [HouseholderQR](classeigen_1_1householderqr "Householder QR decomposition of a matrix."), HouseholderQR(const MatrixType&)
hCoeffs()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const HCoeffsType& [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::hCoeffs | ( | | ) | const |
| inline |
Returns
a const reference to the vector of Householder coefficients used to represent the factor `Q`.
For advanced uses only.
householderQ()
--------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [HouseholderSequenceType](classeigen_1_1householdersequence) [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::householderQ | ( | void | | ) | const |
| inline |
This method returns an expression of the unitary matrix Q as a sequence of Householder transformations.
The returned expression can directly be used to perform matrix products. It can also be assigned to a dense [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") object. Here is an example showing how to recover the full or thin matrix Q, as well as how to perform matrix products using operator\*:
Example:
```
MatrixXf A([MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(5,3)), thinQ([MatrixXf::Identity](classeigen_1_1matrixbase#a98bb9a0f705c6dfde85b0bfff31bf88f)(5,3)), Q;
A.setRandom();
HouseholderQR<MatrixXf> qr(A);
Q = qr.householderQ();
thinQ = qr.householderQ() * thinQ;
std::cout << "The complete unitary matrix Q is:\n" << Q << "\n\n";
std::cout << "The thin matrix Q is:\n" << thinQ << "\n\n";
```
Output:
```
The complete unitary matrix Q is:
-0.676 0.0793 0.713 -0.0788 -0.147
-0.221 -0.322 -0.37 -0.366 -0.759
-0.353 -0.345 -0.214 0.841 -0.0518
0.582 -0.462 0.555 0.176 -0.329
-0.174 -0.747 -0.00907 -0.348 0.539
The thin matrix Q is:
-0.676 0.0793 0.713
-0.221 -0.322 -0.37
-0.353 -0.345 -0.214
0.582 -0.462 0.555
-0.174 -0.747 -0.00907
```
logAbsDeterminant()
-------------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::HouseholderQR](classeigen_1_1householderqr)< MatrixType >::logAbsDeterminant |
Returns
the natural log of the absolute value of the determinant of the matrix of which \*this is the QR decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the QR decomposition has already been computed.
Note
This is only for square matrices. This method is useful to work around the risk of overflow/underflow that's inherent to determinant computation.
See also
[absDeterminant()](classeigen_1_1householderqr#aaf4ef26c0b7affc91431ec59c92d64c3), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
matrixQR()
----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::matrixQR | ( | | ) | const |
| inline |
Returns
a reference to the matrix where the Householder QR decomposition is stored in a LAPACK-compatible way.
solve()
-------
template<typename \_MatrixType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[HouseholderQR](classeigen_1_1householderqr), Rhs> [Eigen::HouseholderQR](classeigen_1_1householderqr)< \_MatrixType >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
This method finds a solution x to the equation Ax=b, where A is the matrix of which \*this is the QR decomposition, if any exists.
Parameters
| | |
| --- | --- |
| b | the right-hand-side of the equation to solve. |
Returns
a solution.
This method just tries to find as good a solution as possible. If you want to check whether a solution exists or if it is accurate, just call this function to get a result and then compute the error of this result, or use [MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c) directly, for instance like this:
```
bool a_solution_exists = (A*result).isApprox(b, precision);
```
This method avoids dividing by zero, so that the non-existence of a solution doesn't by itself mean that you'll get `inf` or `nan` values.
If there exists more than one solution, this method will arbitrarily choose one.
Example:
```
typedef Matrix<float,3,3> Matrix3x3;
Matrix3x3 m = Matrix3x3::Random();
Matrix3f y = [Matrix3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the matrix y:" << endl << y << endl;
Matrix3f x;
x = m.householderQr().solve(y);
assert(y.isApprox(m*x));
cout << "Here is a solution x to the equation mx=y:" << endl << x << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the matrix y:
0.108 -0.27 0.832
-0.0452 0.0268 0.271
0.258 0.904 0.435
Here is a solution x to the equation mx=y:
0.609 2.68 1.67
-0.231 -1.57 0.0713
0.51 3.51 1.05
```
---
The documentation for this class was generated from the following file:* [HouseholderQR.h](https://eigen.tuxfamily.org/dox/HouseholderQR_8h_source.html)
eigen3 Eigen::PastixLU Eigen::PastixLU
===============
### template<typename \_MatrixType, bool IsStrSym> class Eigen::PastixLU< \_MatrixType, IsStrSym >
Interface to the PaStix solver.
[Sparse](structeigen_1_1sparse) direct LU solver based on PaStiX library.
This class is used to solve the linear systems A.X = B via the PaStix library. The matrix can be either real or complex, symmetric or not.
See also
TutorialSparseDirectSolvers
This class is used to solve the linear systems A.X = B with a supernodal LU factorization in the PaStiX library. The matrix A should be squared and nonsingular PaStiX requires that the matrix A has a symmetric structural pattern. This interface can symmetrize the input matrix otherwise. The vectors or matrices X and B can be either dense or sparse.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the sparse matrix A, it must be a SparseMatrix<> |
| IsStrSym | Indicates if the input matrix has a symmetric pattern, default is false NOTE : Note that if the analysis and factorization phase are called separately, the input matrix will be symmetrized at each call, hence it is advised to symmetrize the matrix in a end-user program and set `IsStrSym` to true |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
See also
[Sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept), class [SparseLU](classeigen_1_1sparselu "Sparse supernodal LU factorization for general matrices.")
Inherits Eigen::PastixBase< Derived >.
| |
| --- |
| |
| void | [analyzePattern](classeigen_1_1pastixlu#abae3ca7f1254106d9e2d5e0f273189fa) (const MatrixType &matrix) |
| |
| void | [compute](classeigen_1_1pastixlu#adc28ee2550086c7bdfe991d624bde2ee) (const MatrixType &matrix) |
| |
| void | [factorize](classeigen_1_1pastixlu#ac178a87b499a2210a402787fbfd98f26) (const MatrixType &matrix) |
| |
analyzePattern()
----------------
template<typename \_MatrixType , bool IsStrSym>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PastixLU](classeigen_1_1pastixlu)< \_MatrixType, IsStrSym >::analyzePattern | ( | const MatrixType & | *matrix* | ) | |
| inline |
Compute the LU symbolic factorization of `matrix` using its sparsity pattern. Several ordering methods can be used at this step. See the PaStiX user's manual. The result of this operation can be used with successive matrices having the same pattern as `matrix`
See also
[factorize()](classeigen_1_1pastixlu#ac178a87b499a2210a402787fbfd98f26)
compute()
---------
template<typename \_MatrixType , bool IsStrSym>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PastixLU](classeigen_1_1pastixlu)< \_MatrixType, IsStrSym >::compute | ( | const MatrixType & | *matrix* | ) | |
| inline |
Compute the LU supernodal factorization of `matrix`. iparm and dparm can be used to tune the PaStiX parameters. see the PaStiX user's manual
See also
[analyzePattern()](classeigen_1_1pastixlu#abae3ca7f1254106d9e2d5e0f273189fa) [factorize()](classeigen_1_1pastixlu#ac178a87b499a2210a402787fbfd98f26)
factorize()
-----------
template<typename \_MatrixType , bool IsStrSym>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PastixLU](classeigen_1_1pastixlu)< \_MatrixType, IsStrSym >::factorize | ( | const MatrixType & | *matrix* | ) | |
| inline |
Compute the LU supernodal factorization of `matrix` WARNING The matrix `matrix` should have the same structural pattern as the same used in the analysis phase.
See also
[analyzePattern()](classeigen_1_1pastixlu#abae3ca7f1254106d9e2d5e0f273189fa)
---
The documentation for this class was generated from the following file:* [PaStiXSupport.h](https://eigen.tuxfamily.org/dox/PaStiXSupport_8h_source.html)
| programming_docs |
eigen3 Eigen::SPQR Eigen::SPQR
===========
### template<typename \_MatrixType> class Eigen::SPQR< \_MatrixType >
[Sparse](structeigen_1_1sparse) QR factorization based on SuiteSparseQR library.
This class is used to perform a multithreaded and multifrontal rank-revealing QR decomposition of sparse matrices. The result is then used to solve linear leasts\_square systems. Clearly, a QR factorization is returned such that A\*P = Q\*R where :
P is the column permutation. Use [colsPermutation()](classeigen_1_1spqr#ab1b7f54ba1cd8d77506ae676fea4fec0 "Get the permutation that was applied to columns of A.") to get it.
Q is the orthogonal matrix represented as Householder reflectors. Use [matrixQ()](classeigen_1_1spqr#a93dbf1b487e28948e1ca2a33a35b6a54 "Get an expression of the matrix Q.") to get an expression and [matrixQ()](classeigen_1_1spqr#a93dbf1b487e28948e1ca2a33a35b6a54 "Get an expression of the matrix Q.").transpose() to get the transpose. You can then apply it to a vector.
R is the sparse triangular factor. Use matrixQR() to get it as [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation."). NOTE : The Index type of R is always SuiteSparse\_long. You can get it with SPQR::Index
Template Parameters
| | |
| --- | --- |
| \_MatrixType | The type of the sparse matrix A, must be a column-major SparseMatrix<> |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
| |
| --- |
| |
| cholmod\_common \* | [cholmodCommon](classeigen_1_1spqr#a8c7d48d51a1fb08a3e27b8499e5c7f49) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [cols](classeigen_1_1spqr#aa006d40963b05c7525b5ca0b02364d84) () const |
| |
| [PermutationType](classeigen_1_1map) | [colsPermutation](classeigen_1_1spqr#ab1b7f54ba1cd8d77506ae676fea4fec0) () const |
| | Get the permutation that was applied to columns of A. |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1spqr#a3ab7bacba8d2be20adc10b4d5b6c071f) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1spqr#a3ab7bacba8d2be20adc10b4d5b6c071f) |
| |
| SPQRMatrixQReturnType< [SPQR](classeigen_1_1spqr) > | [matrixQ](classeigen_1_1spqr#a93dbf1b487e28948e1ca2a33a35b6a54) () const |
| | Get an expression of the matrix Q. |
| |
| const [MatrixType](classeigen_1_1sparsematrix) | [matrixR](classeigen_1_1spqr#ad51661be35674dd6a65e27699dbb3fb9) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [rank](classeigen_1_1spqr#a539b394ddb4894089e6634c744ea2ddc) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [rows](classeigen_1_1spqr#a775e28a44fd466638114edbcd17ea50a) () const |
| |
| void | [setPivotThreshold](classeigen_1_1spqr#ab7b42b75f621b0d4b5b0e090e23e2ce6) (const RealScalar &tol) |
| | Set the tolerance tol to treat columns with 2-norm < =tol as zero. |
| |
| void | [setSPQROrdering](classeigen_1_1spqr#afa0db7888d808b453c23f62d62a4ad22) (int ord) |
| | Set the fill-reducing ordering method to be used. |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< SPQR< \_MatrixType > >](classeigen_1_1sparsesolverbase) |
| const [Solve](classeigen_1_1solve)< [SPQR](classeigen_1_1spqr)< \_MatrixType >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [Solve](classeigen_1_1solve)< [SPQR](classeigen_1_1spqr)< \_MatrixType >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
cholmodCommon()
---------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| cholmod\_common\* [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::cholmodCommon | ( | | ) | const |
| inline |
Returns
a pointer to the [SPQR](classeigen_1_1spqr "Sparse QR factorization based on SuiteSparseQR library.") workspace
cols()
------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::cols | ( | void | | ) | const |
| inline |
Get the number of columns of the input matrix.
info()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the sparse QR can not be computed
matrixR()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [MatrixType](classeigen_1_1sparsematrix) [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::matrixR | ( | | ) | const |
| inline |
Returns
the sparse triangular factor R. It is a sparse matrix
rank()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::rank | ( | | ) | const |
| inline |
Gets the rank of the matrix. It should be equal to matrixQR().cols if the matrix is full-rank
rows()
------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SPQR](classeigen_1_1spqr)< \_MatrixType >::rows | ( | void | | ) | const |
| inline |
Get the number of rows of the input matrix and the Q matrix
---
The documentation for this class was generated from the following file:* [SuiteSparseQRSupport.h](https://eigen.tuxfamily.org/dox/SuiteSparseQRSupport_8h_source.html)
eigen3 Eigen::DenseCoeffsBase Eigen::DenseCoeffsBase
======================
### template<typename Derived> class Eigen::DenseCoeffsBase< Derived, ReadOnlyAccessors >
Base class providing read-only coefficient access to matrices and arrays.
Template Parameters
| | |
| --- | --- |
| Derived | Type of the derived class |
Note
[ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) Constant indicating read-only access
This class defines the `operator()` `const` function and friends, which can be used to read specific entries of a matrix or array.
See also
[DenseCoeffsBase<Derived, WriteAccessors>](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4 "Base class providing read/write coefficient access to matrices and arrays."), [DenseCoeffsBase<Derived, DirectAccessors>](classeigen_1_1densecoeffsbase_3_01derived_00_01directaccessors_01_4 "Base class providing direct read-only coefficient access to matrices and arrays."), [The class hierarchy](topicclasshierarchy)
| |
| --- |
| |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af9fadc22d12e48c82745dad534a1671a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| CoeffReturnType | [operator[]](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| CoeffReturnType | [w](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a926f52a94f038db63c6b9103f98dcf0f) () const |
| |
| CoeffReturnType | [x](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a189c80109e76752b598d60dfcdab329e) () const |
| |
| CoeffReturnType | [y](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a0c6d6904a37805ce47a3238fbd735963) () const |
| |
| CoeffReturnType | [z](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a7c60c97282d4a0f8bca16ef75e231ddb) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
coeff() [1/2]
-------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::coeff | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | const |
| inline |
Short version: don't use this function, use [operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) instead.
Long version: this function is similar to [operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) , but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameter *index* is in range.
If EIGEN\_INTERNAL\_DEBUGGING is defined, an assertion will be made, making this function equivalent to [operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) .
See also
[operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a), coeffRef(Index), [coeff(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393)
coeff() [2/2]
-------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::coeff | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | const |
| inline |
Short version: don't use this function, use [operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) instead.
Long version: this function is similar to [operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) , but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameters *row* and *col* are in range.
If EIGEN\_INTERNAL\_DEBUGGING is defined, an assertion will be made, making this function equivalent to [operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) .
See also
[operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1), coeffRef(Index,Index), [coeff(Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda)
cols()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::cols | ( | void | | ) | |
| inline |
Returns
the number of columns.
See also
[rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), ColsAtCompileTime
derived() [1/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived |
| inline |
Returns
a reference to the derived object
derived() [2/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived |
| inline |
Returns
a const reference to the derived object
operator()() [1/2]
------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::operator() | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | const |
| inline |
Returns
the coefficient at given index.
This is synonymous to [operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a).
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
See also
operator[](Index), [operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1), [x() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a189c80109e76752b598d60dfcdab329e), [y() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a0c6d6904a37805ce47a3238fbd735963), [z() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a7c60c97282d4a0f8bca16ef75e231ddb), [w() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a926f52a94f038db63c6b9103f98dcf0f)
operator()() [2/2]
------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::operator() | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | const |
| inline |
Returns
the coefficient at given the given row and column.
See also
operator()(Index,Index), operator[](Index)
operator[]()
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::operator[] | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | const |
| inline |
Returns
the coefficient at given index.
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
See also
operator[](Index), [operator()(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1), [x() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a189c80109e76752b598d60dfcdab329e), [y() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a0c6d6904a37805ce47a3238fbd735963), [z() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a7c60c97282d4a0f8bca16ef75e231ddb), [w() const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a926f52a94f038db63c6b9103f98dcf0f)
rows()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::rows | ( | void | | ) | |
| inline |
Returns
the number of rows.
See also
[cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), RowsAtCompileTime
size()
------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::size |
| inline |
Returns
the number of coefficients, which is [rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2)\*cols().
See also
[rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), SizeAtCompileTime.
w()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::w | ( | | ) | const |
| inline |
equivalent to operator[](3).
x()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::x | ( | | ) | const |
| inline |
equivalent to operator[](0).
y()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::y | ( | | ) | const |
| inline |
equivalent to operator[](1).
z()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType Eigen::DenseCoeffsBase< Derived, [ReadOnlyAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5daa1f2b0e6a668b11f2958940965d2b572) >::z | ( | | ) | const |
| inline |
equivalent to operator[](2).
---
The documentation for this class was generated from the following file:* [DenseCoeffsBase.h](https://eigen.tuxfamily.org/dox/DenseCoeffsBase_8h_source.html)
| programming_docs |
eigen3 None
This is the API documentation for Eigen3. You can [download](https://eigen.tuxfamily.org/dox/eigen-doc.tgz) it as a tgz archive for offline reading.
For a first contact with [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library."), the best place is to have a look at the [getting started](gettingstarted) page that show you how to write and compile your first program with [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.").
Then, the **quick** **reference** **pages** give you a quite complete description of the API in a very condensed format that is specially useful to recall the syntax of a particular feature, or to have a quick look at the API. They currently cover the two following feature sets, and more will come in the future:
* [[QuickRef] Dense matrix and array manipulations](group__quickrefpage)
* [[QuickRef] Sparse linear algebra](group__sparsequickrefpage)
You're a MatLab user? There is also a [short ASCII reference](https://eigen.tuxfamily.org/dox/AsciiQuickReference.txt) with Matlab translations.
The **main** **documentation** is organized into *chapters* covering different domains of features. They are themselves composed of *user* *manual* pages describing the different features in a comprehensive way, and *reference* pages that gives you access to the API documentation through the related [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.")'s *modules* and *classes*.
Under the [Extending/Customizing Eigen](usermanual_customizingeigen) section, you will find discussions and examples on extending Eigen's features and supporting custom scalar types.
Under the [General topics](usermanual_generalities) section, you will find documentation on more general topics such as preprocessor directives, controlling assertions, multi-threading, MKL support, some [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.")'s internal insights, and much more...
Finally, do not miss the search engine, useful to quickly get to the documentation of a given class or function.
Want more? Checkout the [*unsupported* *modules*](unsupported/index) documentation.
eigen3 General topics General topics
==============
* [Writing Functions Taking Eigen Types as Parameters](topicfunctiontakingeigentypes)
* [Preprocessor directives](topicpreprocessordirectives)
* [Assertions](topicassertions)
* [Eigen and multi-threading](topicmultithreading)
* [Using BLAS/LAPACK from Eigen](topicusingblaslapack)
* [Using Intel® MKL from Eigen](topicusingintelmkl)
* [Using Eigen in CUDA kernels](topiccuda)
* [Common pitfalls](topicpitfalls)
* [The template and typename keywords in C++](topictemplatekeyword)
* [Understanding Eigen](usermanual_understandingeigen)
* [Using Eigen in CMake Projects](topiccmakeguide)
eigen3 Eigen::LDLT Eigen::LDLT
===========
### template<typename \_MatrixType, int \_UpLo> class Eigen::LDLT< \_MatrixType, \_UpLo >
Robust Cholesky decomposition of a matrix with pivoting.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the matrix of which to compute the LDL^T Cholesky decomposition |
| \_UpLo | the triangular part that will be used for the decompositon: Lower (default) or Upper. The other triangular part won't be read. |
Perform a robust Cholesky decomposition of a positive semidefinite or negative semidefinite matrix \( A \) such that \( A = P^TLDL^\*P \), where P is a permutation matrix, L is lower triangular with a unit diagonal and D is a diagonal matrix.
The decomposition uses pivoting to ensure stability, so that D will have zeros in the bottom right rank(A) - n submatrix. Avoiding the square root on D also stabilizes the computation.
Remember that Cholesky decompositions are not rank-revealing. Also, do not use a Cholesky decomposition to determine whether a system of equations has a solution.
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
See also
[MatrixBase::ldlt()](classeigen_1_1matrixbase#a0ecf058a0727a4cab8b42d79e95072e1), [SelfAdjointView::ldlt()](classeigen_1_1selfadjointview#a644155eef17b37c95d85b9f65bb49ac4), class [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.")
| |
| --- |
| |
| const [LDLT](classeigen_1_1ldlt) & | [adjoint](classeigen_1_1ldlt#ac656a209860fa0c6a8faa8bb9f9a06ef) () const |
| |
| template<typename InputType > |
| [LDLT](classeigen_1_1ldlt)< MatrixType, \_UpLo > & | [compute](classeigen_1_1ldlt#a1777488d0bde83d5f23a622bf8431ef2) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &a) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1ldlt#a6bd6008501a537d2e16ea672a853bf3e) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1ldlt#a6bd6008501a537d2e16ea672a853bf3e) |
| |
| bool | [isNegative](classeigen_1_1ldlt#aabd3e7aebe844034caca4c62dbe9c1eb) (void) const |
| |
| bool | [isPositive](classeigen_1_1ldlt#a5e1c6b7ba8d7b82575d6ffdc3bf35bcb) () const |
| |
| | [LDLT](classeigen_1_1ldlt#a2e06dedd2651649c5b251fbf9ba4e7d4) () |
| | Default Constructor. [More...](classeigen_1_1ldlt#a2e06dedd2651649c5b251fbf9ba4e7d4) |
| |
| template<typename InputType > |
| | [LDLT](classeigen_1_1ldlt#ad0e8d2131ea1a626a08d98e9effb1cc5) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructor with decomposition. [More...](classeigen_1_1ldlt#ad0e8d2131ea1a626a08d98e9effb1cc5) |
| |
| template<typename InputType > |
| | [LDLT](classeigen_1_1ldlt#adf853d6cbbc49f3535a44439bca344d9) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") factorization from a given matrix. [More...](classeigen_1_1ldlt#adf853d6cbbc49f3535a44439bca344d9) |
| |
| | [LDLT](classeigen_1_1ldlt#a154aa41bd2460199d48861eaf5e4f597) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9)) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1ldlt#a154aa41bd2460199d48861eaf5e4f597) |
| |
| Traits::MatrixL | [matrixL](classeigen_1_1ldlt#af0e6a0df5679873b42cf82a372dd8ddb) () const |
| |
| const MatrixType & | [matrixLDLT](classeigen_1_1ldlt#aa5e0fd09dcd5251a8521fa248b95db0b) () const |
| |
| Traits::MatrixU | [matrixU](classeigen_1_1ldlt#a54838a2e31e53bbe4dcb78b5e80c8484) () const |
| |
| template<typename Derived > |
| [LDLT](classeigen_1_1ldlt)< MatrixType, \_UpLo > & | [rankUpdate](classeigen_1_1ldlt#a858dc77b65dd48248299bb6a6a758abf) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &w, const typename [LDLT](classeigen_1_1ldlt)< MatrixType, \_UpLo >::RealScalar &sigma) |
| |
| RealScalar | [rcond](classeigen_1_1ldlt#ae646403fdde3a4b18e278a32c61a0953) () const |
| |
| MatrixType | [reconstructedMatrix](classeigen_1_1ldlt#ae3693372ca29f50d87d324dfadaae148) () const |
| |
| void | [setZero](classeigen_1_1ldlt#a776d0ab6c980847297d25b03b5d2216a) () |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [LDLT](classeigen_1_1ldlt), Rhs > | [solve](classeigen_1_1ldlt#aa257dd7a8acf8b347d5a22a13d6ca3e1) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [TranspositionType](classeigen_1_1transpositions) & | [transpositionsP](classeigen_1_1ldlt#a47257d3500f9f7c9a4478158d0e34941) () const |
| |
| [Diagonal](classeigen_1_1diagonal)< const MatrixType > | [vectorD](classeigen_1_1ldlt#af60b2f826a38a00070e0efccf0572066) () const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< LDLT< \_MatrixType, \_UpLo > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
LDLT() [1/4]
------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::[LDLT](classeigen_1_1ldlt) | ( | | ) | |
| inline |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via LDLT::compute(const MatrixType&).
LDLT() [2/4]
------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::[LDLT](classeigen_1_1ldlt) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| inlineexplicit |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[LDLT()](classeigen_1_1ldlt#a2e06dedd2651649c5b251fbf9ba4e7d4 "Default Constructor.")
LDLT() [3/4]
------------
template<typename \_MatrixType , int \_UpLo> template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::[LDLT](classeigen_1_1ldlt) | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructor with decomposition.
This calculates the decomposition for the input *matrix*.
See also
[LDLT(Index size)](classeigen_1_1ldlt#a154aa41bd2460199d48861eaf5e4f597 "Default Constructor with memory preallocation.")
LDLT() [4/4]
------------
template<typename \_MatrixType , int \_UpLo> template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::[LDLT](classeigen_1_1ldlt) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") factorization from a given matrix.
This overloaded constructor is provided for [inplace decomposition](group__inplacedecomposition) when `MatrixType` is a [Eigen::Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.").
See also
LDLT(const EigenBase&)
adjoint()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [LDLT](classeigen_1_1ldlt)& [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::adjoint | ( | | ) | const |
| inline |
Returns
the adjoint of `*this`, that is, a const reference to the decomposition itself as the underlying matrix is self-adjoint.
This method is provided for compatibility with other matrix decompositions, thus enabling generic code such as:
```
x = decomposition.adjoint().solve(b)
```
compute()
---------
template<typename \_MatrixType , int \_UpLo> template<typename InputType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [LDLT](classeigen_1_1ldlt)<MatrixType,\_UpLo>& [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::compute | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *a* | ) | |
Compute / recompute the [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") decomposition A = L D L^\* = U^\* D U of *matrix*
info()
------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the factorization failed because of a zero pivot.
isNegative()
------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::isNegative | ( | void | | ) | const |
| inline |
Returns
true if the matrix is negative (semidefinite)
isPositive()
------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::isPositive | ( | | ) | const |
| inline |
Returns
true if the matrix is positive (semidefinite)
matrixL()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Traits::MatrixL [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::matrixL | ( | | ) | const |
| inline |
Returns
a view of the lower triangular matrix L
matrixLDLT()
------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::matrixLDLT | ( | | ) | const |
| inline |
Returns
the internal [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") decomposition matrix
TODO: document the storage layout
matrixU()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Traits::MatrixU [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::matrixU | ( | | ) | const |
| inline |
Returns
a view of the upper triangular matrix U
rankUpdate()
------------
template<typename \_MatrixType , int \_UpLo> template<typename Derived >
| | | | |
| --- | --- | --- | --- |
| [LDLT](classeigen_1_1ldlt)<MatrixType,\_UpLo>& [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::rankUpdate | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *w*, |
| | | const typename [LDLT](classeigen_1_1ldlt)< MatrixType, \_UpLo >::RealScalar & | *sigma* |
| | ) | | |
Update the [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") decomposition: given A = L D L^T, efficiently compute the decomposition of A + sigma w w^T.
Parameters
| | |
| --- | --- |
| w | a vector to be incorporated into the decomposition. |
| sigma | a scalar, +1 for updates and -1 for "downdates," which correspond to removing previously-added column vectors. Optional; default value is +1. |
See also
[setZero()](classeigen_1_1ldlt#a776d0ab6c980847297d25b03b5d2216a)
rcond()
-------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::rcond | ( | | ) | const |
| inline |
Returns
an estimate of the reciprocal condition number of the matrix of which `*this` is the [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") decomposition.
reconstructedMatrix()
---------------------
template<typename MatrixType , int \_UpLo>
| |
| --- |
| MatrixType [Eigen::LDLT](classeigen_1_1ldlt)< MatrixType, \_UpLo >::reconstructedMatrix |
Returns
the matrix represented by the decomposition, i.e., it returns the product: P^T L D L^\* P. This function is provided for debug purpose.
setZero()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| void [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::setZero | ( | | ) | |
| inline |
Clear any existing decomposition
See also
rankUpdate(w,sigma)
solve()
-------
template<typename \_MatrixType , int \_UpLo> template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[LDLT](classeigen_1_1ldlt), Rhs> [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
Returns
a solution x of \( A x = b \) using the current decomposition of A.
This function also supports in-place solves using the syntax `x = decompositionObject.solve(x)` .
This method just tries to find as good a solution as possible. If you want to check whether a solution exists or if it is accurate, just call this function to get a result and then compute the error of this result, or use [MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c) directly, for instance like this:
```
bool a_solution_exists = (A*result).isApprox(b, precision);
```
This method avoids dividing by zero, so that the non-existence of a solution doesn't by itself mean that you'll get `inf` or `nan` values.
More precisely, this method solves \( A x = b \) using the decomposition \( A = P^T L D L^\* P \) by solving the systems \( P^T y\_1 = b \), \( L y\_2 = y\_1 \), \( D y\_3 = y\_2 \), \( L^\* y\_4 = y\_3 \) and \( P x = y\_4 \) in succession. If the matrix \( A \) is singular, then \( D \) will also be singular (all the other matrices are invertible). In that case, the least-square solution of \( D y\_3 = y\_2 \) is computed. This does not mean that this function computes the least-square solution of \( A x = b \) if \( A \) is singular.
See also
[MatrixBase::ldlt()](classeigen_1_1matrixbase#a0ecf058a0727a4cab8b42d79e95072e1), [SelfAdjointView::ldlt()](classeigen_1_1selfadjointview#a644155eef17b37c95d85b9f65bb49ac4)
transpositionsP()
-----------------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [TranspositionType](classeigen_1_1transpositions)& [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::transpositionsP | ( | | ) | const |
| inline |
Returns
the permutation matrix P as a transposition sequence.
vectorD()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Diagonal](classeigen_1_1diagonal)<const MatrixType> [Eigen::LDLT](classeigen_1_1ldlt)< \_MatrixType, \_UpLo >::vectorD | ( | | ) | const |
| inline |
Returns
the coefficients of the diagonal matrix D
---
The documentation for this class was generated from the following file:* [LDLT.h](https://eigen.tuxfamily.org/dox/LDLT_8h_source.html)
| programming_docs |
eigen3 Eigen::LLT Eigen::LLT
==========
### template<typename \_MatrixType, int \_UpLo> class Eigen::LLT< \_MatrixType, \_UpLo >
Standard Cholesky decomposition (LL^T) of a matrix and associated features.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the matrix of which we are computing the LL^T Cholesky decomposition |
| \_UpLo | the triangular part that will be used for the decompositon: Lower (default) or Upper. The other triangular part won't be read. |
This class performs a LL^T Cholesky decomposition of a symmetric, positive definite matrix A such that A = LL^\* = U^\*U, where L is lower triangular.
While the Cholesky decomposition is particularly useful to solve selfadjoint problems like D^\*D x = b, for that purpose, we recommend the Cholesky decomposition without square root which is more stable and even faster. Nevertheless, this standard Cholesky decomposition remains useful in many other situations like generalised eigen problems with hermitian matrices.
Remember that Cholesky decompositions are not rank-revealing. This [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") decomposition is only stable on positive definite matrices, use [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") instead for the semidefinite case. Also, do not use a Cholesky decomposition to determine whether a system of equations has a solution.
Example:
```
MatrixXd A(3,3);
A << 4,-1,2, -1,6,0, 2,0,5;
cout << "The matrix A is" << endl << A << endl;
LLT<MatrixXd> lltOfA(A); // compute the Cholesky decomposition of A
MatrixXd L = lltOfA.matrixL(); // retrieve factor L in the decomposition
// The previous two lines can also be written as "L = A.llt().matrixL()"
cout << "The Cholesky factor L is" << endl << L << endl;
cout << "To check this, let us compute L \* L.transpose()" << endl;
cout << L * L.transpose() << endl;
cout << "This should equal the matrix A" << endl;
```
Output:
```
The matrix A is
4 -1 2
-1 6 0
2 0 5
The Cholesky factor L is
2 0 0
-0.5 2.4 0
1 0.209 1.99
To check this, let us compute L * L.transpose()
4 -1 2
-1 6 0
2 0 5
This should equal the matrix A
```
**Performance:** for best performance, it is recommended to use a column-major storage format with the Lower triangular part (the default), or, equivalently, a row-major storage format with the Upper triangular part. Otherwise, you might get a 20% slowdown for the full factorization step, and rank-updates can be up to 3 times slower.
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
Note that during the decomposition, only the lower (or upper, as defined by \_UpLo) triangular part of A is considered. Therefore, the strict lower part does not have to store correct values.
See also
[MatrixBase::llt()](classeigen_1_1matrixbase#a90c45f7a30265df792d5aeaddead2635), [SelfAdjointView::llt()](classeigen_1_1selfadjointview#a405e810491642a7f7b785f2ad6f93619), class [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.")
| |
| --- |
| |
| const [LLT](classeigen_1_1llt) & | [adjoint](classeigen_1_1llt#a48efa9d2188ce8d5c8cf5344253fbcf3) () const EIGEN\_NOEXCEPT |
| |
| template<typename InputType > |
| [LLT](classeigen_1_1llt)< MatrixType, \_UpLo > & | [compute](classeigen_1_1llt#aecb45daf711328e0804f272131142b57) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &a) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1llt#adb1295e7d2b1fd825a041345ae08be54) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1llt#adb1295e7d2b1fd825a041345ae08be54) |
| |
| | [LLT](classeigen_1_1llt#a16d1ec9ea6497ba1febb242c2e8a7a96) () |
| | Default Constructor. [More...](classeigen_1_1llt#a16d1ec9ea6497ba1febb242c2e8a7a96) |
| |
| template<typename InputType > |
| | [LLT](classeigen_1_1llt#a1848a00addade9a0f7f70493c52ecc9d) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") factorization from a given matrix. [More...](classeigen_1_1llt#a1848a00addade9a0f7f70493c52ecc9d) |
| |
| | [LLT](classeigen_1_1llt#ab3656cfbdf38e03c57d5cf79bf8131b6) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9)) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1llt#ab3656cfbdf38e03c57d5cf79bf8131b6) |
| |
| Traits::MatrixL | [matrixL](classeigen_1_1llt#a7f4a3eedbf82e7ce2d6bf0dcd84cdfa3) () const |
| |
| const MatrixType & | [matrixLLT](classeigen_1_1llt#af62881fc95c7e54a93b63c20f2c62b46) () const |
| |
| Traits::MatrixU | [matrixU](classeigen_1_1llt#a18a390f085567e650e8345cc7e7c0df8) () const |
| |
| template<typename VectorType > |
| [LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo > & | [rankUpdate](classeigen_1_1llt#a8ce93e407a2ba75489bcb6e89ea4c153) (const VectorType &v, const RealScalar &sigma) |
| |
| RealScalar | [rcond](classeigen_1_1llt#a59338fa78db171d02fd5a2c9e4f3a30c) () const |
| |
| MatrixType | [reconstructedMatrix](classeigen_1_1llt#a8b6ba1bc41811c50e65cac8db597d802) () const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [LLT](classeigen_1_1llt), Rhs > | [solve](classeigen_1_1llt#a3738bb3ce6f9b837a2beb432b937499f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< LLT< \_MatrixType, \_UpLo > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
LLT() [1/3]
-----------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::[LLT](classeigen_1_1llt) | ( | | ) | |
| inline |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via LLT::compute(const MatrixType&).
LLT() [2/3]
-----------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::[LLT](classeigen_1_1llt) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| inlineexplicit |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[LLT()](classeigen_1_1llt#a16d1ec9ea6497ba1febb242c2e8a7a96 "Default Constructor.")
LLT() [3/3]
-----------
template<typename \_MatrixType , int \_UpLo> template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::[LLT](classeigen_1_1llt) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") factorization from a given matrix.
This overloaded constructor is provided for [inplace decomposition](group__inplacedecomposition) when `MatrixType` is a [Eigen::Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.").
See also
LLT(const EigenBase&)
adjoint()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [LLT](classeigen_1_1llt)& [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::adjoint | ( | | ) | const |
| inline |
Returns
the adjoint of `*this`, that is, a const reference to the decomposition itself as the underlying matrix is self-adjoint.
This method is provided for compatibility with other matrix decompositions, thus enabling generic code such as:
```
x = decomposition.adjoint().solve(b)
```
compute()
---------
template<typename \_MatrixType , int \_UpLo> template<typename InputType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [LLT](classeigen_1_1llt)<MatrixType,\_UpLo>& [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::compute | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *a* | ) | |
Computes / recomputes the Cholesky decomposition A = LL^\* = U^\*U of *matrix*
Returns
a reference to \*this
Example:
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
Matrix2f A, b;
LLT<Matrix2f> llt;
A << 2, -1, -1, 3;
b << 1, 2, 3, 1;
cout << "Here is the matrix A:\n" << A << endl;
cout << "Here is the right hand side b:\n" << b << endl;
cout << "Computing LLT decomposition..." << endl;
llt.compute(A);
cout << "The solution is:\n" << llt.solve(b) << endl;
A(1,1)++;
cout << "The matrix A is now:\n" << A << endl;
cout << "Computing LLT decomposition..." << endl;
llt.compute(A);
cout << "The solution is now:\n" << llt.solve(b) << endl;
}
```
Output:
```
Here is the matrix A:
2 -1
-1 3
Here is the right hand side b:
1 2
3 1
Computing LLT decomposition...
The solution is:
1.2 1.4
1.4 0.8
The matrix A is now:
2 -1
-1 4
Computing LLT decomposition...
The solution is now:
1 1.29
1 0.571
```
info()
------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the matrix.appears not to be positive definite.
matrixL()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Traits::MatrixL [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::matrixL | ( | | ) | const |
| inline |
Returns
a view of the lower triangular matrix L
matrixLLT()
-----------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::matrixLLT | ( | | ) | const |
| inline |
Returns
the [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") decomposition matrix
TODO: document the storage layout
matrixU()
---------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Traits::MatrixU [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::matrixU | ( | | ) | const |
| inline |
Returns
a view of the upper triangular matrix U
rankUpdate()
------------
template<typename \_MatrixType , int \_UpLo> template<typename VectorType >
| | | | |
| --- | --- | --- | --- |
| [LLT](classeigen_1_1llt)<\_MatrixType,\_UpLo>& [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::rankUpdate | ( | const VectorType & | *v*, |
| | | const RealScalar & | *sigma* |
| | ) | | |
Performs a rank one update (or dowdate) of the current decomposition. If A = LL^\* before the rank one update, then after it we have LL^\* = A + sigma \* v v^\* where *v* must be a vector of same dimension.
rcond()
-------
template<typename \_MatrixType , int \_UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::rcond | ( | | ) | const |
| inline |
Returns
an estimate of the reciprocal condition number of the matrix of which `*this` is the Cholesky decomposition.
reconstructedMatrix()
---------------------
template<typename MatrixType , int \_UpLo>
| |
| --- |
| MatrixType [Eigen::LLT](classeigen_1_1llt)< MatrixType, \_UpLo >::reconstructedMatrix |
Returns
the matrix represented by the decomposition, i.e., it returns the product: L L^\*. This function is provided for debug purpose.
solve()
-------
template<typename \_MatrixType , int \_UpLo> template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[LLT](classeigen_1_1llt), Rhs> [Eigen::LLT](classeigen_1_1llt)< \_MatrixType, \_UpLo >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
Returns
the solution x of \( A x = b \) using the current decomposition of A.
Since this [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") class assumes anyway that the matrix A is invertible, the solution theoretically exists and is unique regardless of b.
Example:
```
typedef Matrix<float,Dynamic,2> DataMatrix;
// let's generate some samples on the 3D plane of equation z = 2x+3y (with some noise)
DataMatrix samples = DataMatrix::Random(12,2);
VectorXf elevations = 2*samples.col(0) + 3*samples.col(1) + [VectorXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(12)*0.1;
// and let's solve samples \* [x y]^T = elevations in least square sense:
Matrix<float,2,1> xy
= (samples.adjoint() * samples).llt().solve((samples.adjoint()*elevations));
cout << xy << endl;
```
Output:
```
2.02
2.97
```
See also
solveInPlace(), [MatrixBase::llt()](classeigen_1_1matrixbase#a90c45f7a30265df792d5aeaddead2635), [SelfAdjointView::llt()](classeigen_1_1selfadjointview#a405e810491642a7f7b785f2ad6f93619)
---
The documentation for this class was generated from the following file:* [LLT.h](https://eigen.tuxfamily.org/dox/LLT_8h_source.html)
eigen3 Eigen::DenseCoeffsBase Eigen::DenseCoeffsBase
======================
### template<typename Derived> class Eigen::DenseCoeffsBase< Derived, WriteAccessors >
Base class providing read/write coefficient access to matrices and arrays.
Template Parameters
| | |
| --- | --- |
| Derived | Type of the derived class |
Note
[WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) Constant indicating read/write access
This class defines the non-const `operator()` function and friends, which can be used to write specific entries of a matrix or array. This class inherits [DenseCoeffsBase<Derived, ReadOnlyAccessors>](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4 "Base class providing read-only coefficient access to matrices and arrays.") which defines the const variant for reading specific entries.
See also
[DenseCoeffsBase<Derived, DirectAccessors>](classeigen_1_1densecoeffsbase_3_01derived_00_01directaccessors_01_4 "Base class providing direct read-only coefficient access to matrices and arrays."), [The class hierarchy](topicclasshierarchy)
| |
| --- |
| |
| Scalar & | [coeffRef](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae66b7d18b2a85f3139b703126974c900) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| Scalar & | [coeffRef](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#adc8286576b31e11f056057be666a0ec8) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| Scalar & | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a0171eee1d9e582d1ac7ec0f18f5f615a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| Scalar & | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| Scalar & | [operator[]](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| Scalar & | [w](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af683e04b3926aaf4091581ca24ca09ad) () |
| |
| Scalar & | [x](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af163a982f5a7ad7e5c3336990b3d7000) () |
| |
| Scalar & | [y](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afeaa80359bf0c1311f91cdd74a2042a8) () |
| |
| Scalar & | [z](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a858151a06b8c0ff407232d84e695dd73) () |
| |
|
Public Member Functions inherited from [Eigen::DenseCoeffsBase< Derived, ReadOnlyAccessors >](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4) |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af9fadc22d12e48c82745dad534a1671a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| CoeffReturnType | [operator[]](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| CoeffReturnType | [w](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a926f52a94f038db63c6b9103f98dcf0f) () const |
| |
| CoeffReturnType | [x](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a189c80109e76752b598d60dfcdab329e) () const |
| |
| CoeffReturnType | [y](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a0c6d6904a37805ce47a3238fbd735963) () const |
| |
| CoeffReturnType | [z](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a7c60c97282d4a0f8bca16ef75e231ddb) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
coeffRef() [1/2]
----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::coeffRef | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | |
| inline |
Short version: don't use this function, use [operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf) instead.
Long version: this function is similar to [operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf), but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameters *row* and *col* are in range.
If EIGEN\_INTERNAL\_DEBUGGING is defined, an assertion will be made, making this function equivalent to [operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf).
See also
[operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf), [coeff(Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda), [coeffRef(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#adc8286576b31e11f056057be666a0ec8)
coeffRef() [2/2]
----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::coeffRef | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | |
| inline |
Short version: don't use this function, use [operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb) instead.
Long version: this function is similar to [operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb), but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameters *row* and *col* are in range.
If EIGEN\_INTERNAL\_DEBUGGING is defined, an assertion will be made, making this function equivalent to [operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb).
See also
[operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb), [coeff(Index, Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393), [coeffRef(Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae66b7d18b2a85f3139b703126974c900)
cols()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::cols | ( | void | | ) | |
| inline |
Returns
the number of columns.
See also
[rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), ColsAtCompileTime
derived() [1/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived |
| inline |
Returns
a reference to the derived object
derived() [2/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const Derived& [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::derived |
| inline |
Returns
a const reference to the derived object
operator()() [1/2]
------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::operator() | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | |
| inline |
Returns
a reference to the coefficient at given index.
This is synonymous to [operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf).
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
See also
[operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a), [operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb), [x()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af163a982f5a7ad7e5c3336990b3d7000), [y()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afeaa80359bf0c1311f91cdd74a2042a8), [z()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a858151a06b8c0ff407232d84e695dd73), [w()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af683e04b3926aaf4091581ca24ca09ad)
operator()() [2/2]
------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::operator() | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | |
| inline |
Returns
a reference to the coefficient at given the given row and column.
See also
[operator[](Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf)
operator[]()
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::operator[] | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *index* | ) | |
| inline |
Returns
a reference to the coefficient at given index.
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
See also
[operator[](Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a), [operator()(Index,Index)](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb), [x()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af163a982f5a7ad7e5c3336990b3d7000), [y()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afeaa80359bf0c1311f91cdd74a2042a8), [z()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a858151a06b8c0ff407232d84e695dd73), [w()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af683e04b3926aaf4091581ca24ca09ad)
rows()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::rows | ( | void | | ) | |
| inline |
Returns
the number of rows.
See also
[cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), RowsAtCompileTime
size()
------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::EigenBase](structeigen_1_1eigenbase)< Derived >::size |
| inline |
Returns
the number of coefficients, which is [rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2)\*cols().
See also
[rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), SizeAtCompileTime.
w()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::w | ( | | ) | |
| inline |
equivalent to operator[](3).
x()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::x | ( | | ) | |
| inline |
equivalent to operator[](0).
y()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::y | ( | | ) | |
| inline |
equivalent to operator[](1).
z()
---
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar& Eigen::DenseCoeffsBase< Derived, [WriteAccessors](group__enums#gga9f93eac38eb83deb0e8dbd42ddf11d5dabcadf08230fb1a5ef7b3195745d3a458) >::z | ( | | ) | |
| inline |
equivalent to operator[](2).
---
The documentation for this class was generated from the following file:* [DenseCoeffsBase.h](https://eigen.tuxfamily.org/dox/DenseCoeffsBase_8h_source.html)
| programming_docs |
eigen3 Eigen::SuperLUBase Eigen::SuperLUBase
==================
### template<typename \_MatrixType, typename Derived> class Eigen::SuperLUBase< \_MatrixType, Derived >
The base class for the direct and incomplete LU factorization of [SuperLU](classeigen_1_1superlu "A sparse direct LU factorization and solver based on the SuperLU library.").
| |
| --- |
| |
| void | [analyzePattern](classeigen_1_1superlubase#a2d3f48425328d9b3cbdca369889007f3) (const MatrixType &) |
| |
| void | [compute](classeigen_1_1superlubase#a28cb3ef7914ecb6fdae1935b53f6be40) (const MatrixType &matrix) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1superlubase#aa67da5c8c24110931c949c5896c5ec03) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1superlubase#aa67da5c8c24110931c949c5896c5ec03) |
| |
| superlu\_options\_t & | [options](classeigen_1_1superlubase#a42d9d79073379f1e75b0f2c49879ed5b) () |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< Derived >](classeigen_1_1sparsesolverbase) |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
analyzePattern()
----------------
template<typename \_MatrixType , typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SuperLUBase](classeigen_1_1superlubase)< \_MatrixType, Derived >::analyzePattern | ( | const MatrixType & | | ) | |
| inline |
Performs a symbolic decomposition on the sparcity of *matrix*.
This function is particularly useful when solving for several problems having the same structure.
See also
factorize()
compute()
---------
template<typename \_MatrixType , typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SuperLUBase](classeigen_1_1superlubase)< \_MatrixType, Derived >::compute | ( | const MatrixType & | *matrix* | ) | |
| inline |
Computes the sparse Cholesky decomposition of *matrix*
info()
------
template<typename \_MatrixType , typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::SuperLUBase](classeigen_1_1superlubase)< \_MatrixType, Derived >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the matrix.appears to be negative.
options()
---------
template<typename \_MatrixType , typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| superlu\_options\_t& [Eigen::SuperLUBase](classeigen_1_1superlubase)< \_MatrixType, Derived >::options | ( | | ) | |
| inline |
Returns
a reference to the Super LU option object to configure the Super LU algorithms.
---
The documentation for this class was generated from the following file:* [SuperLUSupport.h](https://eigen.tuxfamily.org/dox/SuperLUSupport_8h_source.html)
eigen3 Eigen::CholmodSupernodalLLT Eigen::CholmodSupernodalLLT
===========================
### template<typename \_MatrixType, int \_UpLo = Lower> class Eigen::CholmodSupernodalLLT< \_MatrixType, \_UpLo >
A supernodal Cholesky ([LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.")) factorization and solver based on Cholmod.
This class allows to solve for A.X = B sparse linear problems via a supernodal LL^T Cholesky factorization using the Cholmod library. This supernodal variant performs best on dense enough problems, e.g., 3D FEM, or very high order 2D FEM. The sparse matrix A must be selfadjoint and positive definite. The vectors or matrices X and B can be either dense or sparse.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the sparse matrix A, it must be a SparseMatrix<> |
| \_UpLo | the triangular part that will be used for the computations. It can be Lower or Upper. Default is Lower. |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
This class supports all kind of SparseMatrix<>: row or column major; upper, lower, or both; compressed or non compressed.
Warning
Only double precision real and complex scalar types are supported by Cholmod.
See also
[Sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept)
| |
| --- |
| |
|
Public Member Functions inherited from [Eigen::CholmodBase< \_MatrixType, Lower, CholmodSupernodalLLT< \_MatrixType, Lower > >](classeigen_1_1cholmodbase) |
| void | [analyzePattern](classeigen_1_1cholmodbase#a5ac967e9f4ccfc43ca9e610b89232c24) (const MatrixType &matrix) |
| |
| cholmod\_common & | [cholmod](classeigen_1_1cholmodbase#a6a85bf52d6aa480240a64f277d7f96c6) () |
| |
| [CholmodSupernodalLLT](classeigen_1_1cholmodsupernodalllt)< \_MatrixType, Lower > & | [compute](classeigen_1_1cholmodbase#abaf5be01b1e3035a4de0b19f5b63549e) (const MatrixType &matrix) |
| |
| Scalar | [determinant](classeigen_1_1cholmodbase#ab4ffb4a9735ad7e81a01d5789ce96547) () const |
| |
| void | [factorize](classeigen_1_1cholmodbase#a5bd9c9ec4d1c15f202a6c66b5e9ef37b) (const MatrixType &matrix) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1cholmodbase#ada4cc43c64767d186fcb8997440cc753) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1cholmodbase#ada4cc43c64767d186fcb8997440cc753) |
| |
| Scalar | [logDeterminant](classeigen_1_1cholmodbase#a597f7839a39604af18a8741a0d8c46bf) () const |
| |
| [CholmodSupernodalLLT](classeigen_1_1cholmodsupernodalllt)< \_MatrixType, Lower > & | [setShift](classeigen_1_1cholmodbase#a886fc102723ca7bde4ac7162dfd72f5d) (const RealScalar &offset) |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< Derived >](classeigen_1_1sparsesolverbase) |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
---
The documentation for this class was generated from the following file:* [CholmodSupport.h](https://eigen.tuxfamily.org/dox/CholmodSupport_8h_source.html)
eigen3 Eigen::Rotation2D Eigen::Rotation2D
=================
### template<typename \_Scalar> class Eigen::Rotation2D< \_Scalar >
Represents a rotation/orientation in a 2 dimensional space.
This is defined in the Geometry module.
```
#include <Eigen/Geometry>
```
Template Parameters
| | |
| --- | --- |
| \_Scalar | the scalar type, i.e., the type of the coefficients |
This class is equivalent to a single scalar representing a counter clock wise rotation as a single angle in radian. It provides some additional features such as the automatic conversion from/to a 2x2 rotation matrix. Moreover this class aims to provide a similar interface to [Quaternion](classeigen_1_1quaternion "The quaternion class used to represent 3D orientations and rotations.") in order to facilitate the writing of generic algorithms dealing with rotations.
See also
class [Quaternion](classeigen_1_1quaternion "The quaternion class used to represent 3D orientations and rotations."), class [Transform](classeigen_1_1transform "Represents an homogeneous transformation in a N dimensional space.")
| |
| --- |
| |
| typedef \_Scalar | [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) |
| |
|
Public Types inherited from [Eigen::RotationBase< Rotation2D< \_Scalar >, 2 >](classeigen_1_1rotationbase) |
| typedef [Matrix](classeigen_1_1matrix)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Dim > | [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) |
| |
| typedef internal::traits< [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > >::[Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) | [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) |
| |
| |
| --- |
| |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) & | [angle](classeigen_1_1rotation2d#ab3cde04b655d42682d1f79952f8ef2f8) () |
| |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) | [angle](classeigen_1_1rotation2d#ab1296dc7fcb23fdcd2c8e648e87d5d6a) () const |
| |
| template<typename NewScalarType > |
| internal::cast\_return\_type< [Rotation2D](classeigen_1_1rotation2d), [Rotation2D](classeigen_1_1rotation2d)< NewScalarType > >::type | [cast](classeigen_1_1rotation2d#a66445e2faf7f98d535fd5a8bf0a974e1) () const |
| |
| template<typename Derived > |
| [Rotation2D](classeigen_1_1rotation2d)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) > & | [fromRotationMatrix](classeigen_1_1rotation2d#a3e00ee4d983879c32d615b2a7153a26d) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &mat) |
| |
| [Rotation2D](classeigen_1_1rotation2d) | [inverse](classeigen_1_1rotation2d#a5c01f57e3a382c7f677ac9ff3b95aea6) () const |
| |
| bool | [isApprox](classeigen_1_1rotation2d#a9c6889cb1a96ab8549effc94bfa06cd6) (const [Rotation2D](classeigen_1_1rotation2d) &other, const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) >::Real &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) >::dummy\_precision()) const |
| |
| [Rotation2D](classeigen_1_1rotation2d) | [operator\*](classeigen_1_1rotation2d#adbfbf2d1ccdf50999bad7688348f4ac2) (const [Rotation2D](classeigen_1_1rotation2d) &other) const |
| |
| [Vector2](classeigen_1_1matrix) | [operator\*](classeigen_1_1rotation2d#ad0ad69c781232313b7d01f981148f230) (const [Vector2](classeigen_1_1matrix) &vec) const |
| |
| [Rotation2D](classeigen_1_1rotation2d) & | [operator\*=](classeigen_1_1rotation2d#a84fd39c79d4ca547ef0ff6c3c1dfcfcb) (const [Rotation2D](classeigen_1_1rotation2d) &other) |
| |
| template<typename Derived > |
| [Rotation2D](classeigen_1_1rotation2d) & | [operator=](classeigen_1_1rotation2d#a9d3da523c37f4ef0574f35fd7b3b9662) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &m) |
| |
| | [Rotation2D](classeigen_1_1rotation2d#a4628b82c613b7b85010afd45ea0477a2) () |
| |
| template<typename Derived > |
| | [Rotation2D](classeigen_1_1rotation2d#a566207478cb88f9335c912d35913a0cd) (const [MatrixBase](classeigen_1_1matrixbase)< Derived > &m) |
| |
| template<typename OtherScalarType > |
| | [Rotation2D](classeigen_1_1rotation2d#a3cae6e108a999fa1a4b358b3f467aa35) (const [Rotation2D](classeigen_1_1rotation2d)< OtherScalarType > &other) |
| |
| | [Rotation2D](classeigen_1_1rotation2d#afac7b8ec55325568e7c374df672d1eb1) (const [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) &a) |
| |
| [Rotation2D](classeigen_1_1rotation2d) | [slerp](classeigen_1_1rotation2d#ab9ceb217d03c6a2a6438be37a3ccd855) (const [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) &t, const [Rotation2D](classeigen_1_1rotation2d) &other) const |
| |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) | [smallestAngle](classeigen_1_1rotation2d#ac648b9217d9f7ba4ee7a1a91aec8e46f) () const |
| |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) | [smallestPositiveAngle](classeigen_1_1rotation2d#a09c5195aa9a154551b2edf6ed4f22ab6) () const |
| |
| [Matrix2](classeigen_1_1matrix) | [toRotationMatrix](classeigen_1_1rotation2d#a961167d84c8b9a989f2ba8609a6afcaf) () const |
| |
|
Public Member Functions inherited from [Eigen::RotationBase< Rotation2D< \_Scalar >, 2 >](classeigen_1_1rotationbase) |
| [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > | [inverse](classeigen_1_1rotationbase#a8532fb716ea4267cf8bbdb99e5e54837) () const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [matrix](classeigen_1_1rotationbase#a91b5bdb1c7b90ec7b33107c6f7d3b171) () const |
| |
| internal::rotation\_base\_generic\_product\_selector< [Rotation2D](classeigen_1_1rotation2d)< \_Scalar >, OtherDerived, OtherDerived::IsVectorAtCompileTime >::ReturnType | [operator\*](classeigen_1_1rotationbase#a09a4757e7aff7a95bebf4fa8a965a4eb) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &e) const |
| |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode > | [operator\*](classeigen_1_1rotationbase#aaca1c3d834e2bc7ebfd046d96cac990c) (const [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Mode, Options > &t) const |
| |
| [Transform](classeigen_1_1transform)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim, Isometry > | [operator\*](classeigen_1_1rotationbase#a26d0603783666526a98d08bd45d9c751) (const [Translation](classeigen_1_1translation)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4), Dim > &t) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [operator\*](classeigen_1_1rotationbase#a05af64a1bc759c5fed4ff7afd1414ba4) (const [UniformScaling](classeigen_1_1uniformscaling)< [Scalar](classeigen_1_1rotationbase#af9b43eac462d7aa70b018efd49c13ef4) > &s) const |
| |
| [RotationMatrixType](classeigen_1_1rotationbase#a83602509674c9d635551998460342951) | [toRotationMatrix](classeigen_1_1rotationbase#a94fe3683c867c39d34505932b07e956a) () const |
| |
Scalar
------
template<typename \_Scalar >
| |
| --- |
| typedef \_Scalar [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::[Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) |
the scalar type of the coefficients
Rotation2D() [1/4]
------------------
template<typename \_Scalar >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::[Rotation2D](classeigen_1_1rotation2d) | ( | const [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) & | *a* | ) | |
| inlineexplicit |
Construct a 2D counter clock wise rotation from the angle *a* in radian.
Rotation2D() [2/4]
------------------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::[Rotation2D](classeigen_1_1rotation2d) | ( | | ) | |
| inline |
Default constructor wihtout initialization. The represented rotation is undefined.
Rotation2D() [3/4]
------------------
template<typename \_Scalar > template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::[Rotation2D](classeigen_1_1rotation2d) | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *m* | ) | |
| inlineexplicit |
Construct a 2D rotation from a 2x2 rotation matrix *mat*.
See also
fromRotationMatrix()
Rotation2D() [4/4]
------------------
template<typename \_Scalar > template<typename OtherScalarType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::[Rotation2D](classeigen_1_1rotation2d) | ( | const [Rotation2D](classeigen_1_1rotation2d)< OtherScalarType > & | *other* | ) | |
| inlineexplicit |
Copy constructor with scalar type conversion
angle() [1/2]
-------------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4)& [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::angle | ( | | ) | |
| inline |
Returns
a read-write reference to the rotation angle
angle() [2/2]
-------------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::angle | ( | | ) | const |
| inline |
Returns
the rotation angle
cast()
------
template<typename \_Scalar > template<typename NewScalarType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::cast\_return\_type<[Rotation2D](classeigen_1_1rotation2d),[Rotation2D](classeigen_1_1rotation2d)<NewScalarType> >::type [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::cast | ( | | ) | const |
| inline |
Returns
`*this` with scalar type casted to *NewScalarType*
Note that if *NewScalarType* is equal to the current scalar type of `*this` then this function smartly returns a const reference to `*this`.
fromRotationMatrix()
--------------------
template<typename \_Scalar > template<typename Derived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d)<[Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4)>& [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::fromRotationMatrix | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *mat* | ) | |
Set `*this` from a 2x2 rotation matrix *mat*. In other words, this function extract the rotation angle from the rotation matrix.
inverse()
---------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::inverse | ( | | ) | const |
| inline |
Returns
the inverse rotation
isApprox()
----------
template<typename \_Scalar >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::isApprox | ( | const [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > & | *other*, |
| | | const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) >::Real & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4)>::dummy_precision()` |
| | ) | | const |
| inline |
Returns
`true` if `*this` is approximately equal to *other*, within the precision determined by *prec*.
See also
[MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c)
operator\*() [1/2]
------------------
template<typename \_Scalar >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::operator\* | ( | const [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > & | *other* | ) | const |
| inline |
Concatenates two rotations
operator\*() [2/2]
------------------
template<typename \_Scalar >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Vector2](classeigen_1_1matrix) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::operator\* | ( | const [Vector2](classeigen_1_1matrix) & | *vec* | ) | const |
| inline |
Applies the rotation to a 2D vector
operator\*=()
-------------
template<typename \_Scalar >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d)& [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::operator\*= | ( | const [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > & | *other* | ) | |
| inline |
Concatenates two rotations
operator=()
-----------
template<typename \_Scalar > template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d)& [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::operator= | ( | const [MatrixBase](classeigen_1_1matrixbase)< Derived > & | *m* | ) | |
| inline |
Set `*this` from a 2x2 rotation matrix *mat*. In other words, this function extract the rotation angle from the rotation matrix.
This method is an alias for fromRotationMatrix()
See also
fromRotationMatrix()
slerp()
-------
template<typename \_Scalar >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::slerp | ( | const [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) & | *t*, |
| | | const [Rotation2D](classeigen_1_1rotation2d)< \_Scalar > & | *other* |
| | ) | | const |
| inline |
Returns
the spherical interpolation between `*this` and *other* using parameter *t*. It is in fact equivalent to a linear interpolation.
smallestAngle()
---------------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::smallestAngle | ( | | ) | const |
| inline |
Returns
the rotation angle in [-pi,pi]
smallestPositiveAngle()
-----------------------
template<typename \_Scalar >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) [Eigen::Rotation2D](classeigen_1_1rotation2d)< \_Scalar >::smallestPositiveAngle | ( | | ) | const |
| inline |
Returns
the rotation angle in [0,2pi]
toRotationMatrix()
------------------
template<typename Scalar >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Rotation2D](classeigen_1_1rotation2d)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) >::[Matrix2](classeigen_1_1matrix) [Eigen::Rotation2D](classeigen_1_1rotation2d)< [Scalar](classeigen_1_1rotation2d#ac20c665ece0f197a712a2a39ae72e4e4) >::toRotationMatrix | ( | void | | ) | const |
Constructs and
Returns
an equivalent 2x2 rotation matrix.
---
The documentation for this class was generated from the following file:* [Rotation2D.h](https://eigen.tuxfamily.org/dox/Rotation2D_8h_source.html)
| programming_docs |
eigen3 Eigen::BlockImpl Eigen::BlockImpl
================
### template<typename XprType, int BlockRows, int BlockCols, bool InnerPanel> class Eigen::BlockImpl< XprType, BlockRows, BlockCols, InnerPanel, Sparse >
Generic implementation of sparse [Block](classeigen_1_1block "Expression of a fixed-size or dynamic-size block.") expression. Real-only.
| |
| --- |
| |
| | [BlockImpl](classeigen_1_1blockimpl_3_01xprtype_00_01blockrows_00_01blockcols_00_01innerpanel_00_01sparse_01_4#aa724feeda73e4d5d2330c6dced2d18f9) (XprType &xpr, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i) |
| |
| | [BlockImpl](classeigen_1_1blockimpl_3_01xprtype_00_01blockrows_00_01blockcols_00_01innerpanel_00_01sparse_01_4#a2fe461bd49f27e586b7d0e652e370644) (XprType &xpr, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) startRow, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) startCol, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) blockRows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) blockCols) |
| |
|
Public Member Functions inherited from [Eigen::SparseMatrixBase< Block< XprType, BlockRows, BlockCols, InnerPanel > >](classeigen_1_1sparsematrixbase) |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsematrixbase#aca7ce296424ef6e478ab0fb19547a7ee) () const |
| |
| const internal::eval< [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel > >::type | [eval](classeigen_1_1sparsematrixbase#a761bd872a06b59632fcff7b7807a77ce) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsematrixbase#a180fcba1ccf3cdf3252a263bc1de7a1d) () const |
| |
| bool | [isVector](classeigen_1_1sparsematrixbase#a7eedffa867031f649fd0fb9cc23ce4be) () const |
| |
| const [Product](classeigen_1_1product)< [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel >, OtherDerived, AliasFreeProduct > | [operator\*](classeigen_1_1sparsematrixbase#a9d4d71b3f34389e6fc01f2b86e43f7a4) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< OtherDerived > &other) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsematrixbase#ac86cc88a4cfef21db6b64ec0ab4c8f0a) () const |
| |
| const [SparseView](classeigen_1_1sparseview)< [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel > > | [pruned](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d) (const Scalar &reference=Scalar(0), const RealScalar &epsilon=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsematrixbase#a1944e9fa9ce7937bfc3a87b2cb94371f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1sparsematrixbase#a124bc57921775eb9aa2dfd9727e23472) () const |
| |
| SparseSymmetricPermutationProduct< [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel >, Upper|Lower > | [twistedBy](classeigen_1_1sparsematrixbase#a51d4898bd6a57cc3ba543a39b102423e) (const [PermutationMatrix](classeigen_1_1permutationmatrix)< Dynamic, Dynamic, [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) > &perm) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Block< XprType, BlockRows, BlockCols, InnerPanel > >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel > & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel > & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::SparseMatrixBase< Block< XprType, BlockRows, BlockCols, InnerPanel > >](classeigen_1_1sparsematrixbase) |
| typedef internal::traits< [Block](classeigen_1_1block)< XprType, BlockRows, BlockCols, InnerPanel > >::[StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) | [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) |
| |
| typedef Scalar | [value\_type](classeigen_1_1sparsematrixbase#ac254d3b61718ebc2136d27bac043dcb7) |
| |
|
Public Types inherited from [Eigen::EigenBase< Block< XprType, BlockRows, BlockCols, InnerPanel > >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
BlockImpl() [1/2]
-----------------
template<typename XprType , int BlockRows, int BlockCols, bool InnerPanel>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Eigen::BlockImpl< XprType, BlockRows, BlockCols, InnerPanel, [Sparse](structeigen_1_1sparse) >::BlockImpl | ( | XprType & | *xpr*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *i* |
| | ) | | |
| inline |
Column or Row constructor
BlockImpl() [2/2]
-----------------
template<typename XprType , int BlockRows, int BlockCols, bool InnerPanel>
| | | | | | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Eigen::BlockImpl< XprType, BlockRows, BlockCols, InnerPanel, [Sparse](structeigen_1_1sparse) >::BlockImpl | ( | XprType & | *xpr*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *startRow*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *startCol*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *blockRows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *blockCols* |
| | ) | | |
| inline |
Dynamic-size constructor
---
The documentation for this class was generated from the following file:* [SparseBlock.h](https://eigen.tuxfamily.org/dox/SparseBlock_8h_source.html)
eigen3 Jacobi module Jacobi module
=============
This module provides Jacobi and Givens rotations.
```
#include <Eigen/Jacobi>
```
In addition to listed classes, it defines the two following [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") methods to apply a Jacobi or Givens rotation:
* [MatrixBase::applyOnTheLeft()](classeigen_1_1matrixbase#a3a08ad41e81d8ad4a37b5d5c7490e765)
* [MatrixBase::applyOnTheRight()](classeigen_1_1matrixbase#a45d91752925d2757fc8058a293b15462).
| |
| --- |
| |
| class | [Eigen::JacobiRotation< Scalar >](classeigen_1_1jacobirotation) |
| | Rotation given by a cosine-sine pair. [More...](classeigen_1_1jacobirotation#details) |
| |
| |
| --- |
| |
| template<typename OtherScalar > |
| void | [Eigen::MatrixBase< Derived >::applyOnTheRight](group__jacobi__module#gaa07f741c86219601664433777827bf1c) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) p, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) q, const [JacobiRotation](classeigen_1_1jacobirotation)< OtherScalar > &j) |
| |
applyOnTheRight()
-----------------
template<typename Derived > template<typename OtherScalar >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::MatrixBase](classeigen_1_1matrixbase)< Derived >::applyOnTheRight | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *p*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *q*, |
| | | const [JacobiRotation](classeigen_1_1jacobirotation)< OtherScalar > & | *j* |
| | ) | | |
| inline |
Applies the rotation in the plane *j* to the columns *p* and *q* of `*this`, i.e., it computes B = B \* J with \( B = \left ( \begin{array}{cc} \text{\*this.col}(p) & \text{\*this.col}(q) \end{array} \right ) \).
See also
class [JacobiRotation](classeigen_1_1jacobirotation "Rotation given by a cosine-sine pair."), [MatrixBase::applyOnTheLeft()](classeigen_1_1matrixbase#a3a08ad41e81d8ad4a37b5d5c7490e765), internal::apply\_rotation\_in\_the\_plane()
eigen3 Eigen::Ref Eigen::Ref
==========
### template<typename SparseMatrixType, int Options> class Eigen::Ref< SparseMatrixType, Options >
A sparse matrix expression referencing an existing sparse expression.
Template Parameters
| | |
| --- | --- |
| SparseMatrixType | the equivalent sparse matrix type of the referenced data, it must be a template instance of class [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation."). |
| Options | specifies whether the a standard compressed format is required `Options` is `[StandardCompressedFormat](namespaceeigen#aaec054b156d7e5de1d19a4b2c125ce6aabd3632507a3697fb48c77eff79851a74)`, or `0`. The default is `0`. |
See also
class [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.")
| |
| --- |
| |
| template<typename Derived > |
| | [Ref](classeigen_1_1ref_3_01sparsematrixtype_00_01options_01_4#a5a27af935f839a42d85421f5cce99a68) ([SparseCompressedBase](classeigen_1_1sparsecompressedbase)< Derived > &expr) |
| |
|
Public Member Functions inherited from [Eigen::SparseMapBase< Derived, WriteAccessors >](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4) |
| Scalar & | [coeffRef](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#aa9c42d48b9dd6f947ce3c257fe4bf2ca) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| StorageIndex \* | [innerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af5cd1f13dde8578eb9891a4ac4a11977) () |
| |
| StorageIndex \* | [innerNonZeroPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af877ea4e285a4497f80987fea66f7459) () |
| |
| StorageIndex \* | [outerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#a3b74af754254837fc591cd9936688b95) () |
| |
| Scalar \* | [valuePtr](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#af91648a18729ae8ff29cb1d8751c5655) () |
| |
| | [~SparseMapBase](classeigen_1_1sparsemapbase_3_01derived_00_01writeaccessors_01_4#a4dfbcf3ac411885b1710ad04892c984d) () |
| |
|
Public Member Functions inherited from [Eigen::SparseMapBase< Derived, ReadOnlyAccessors >](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4) |
| Scalar | [coeff](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a195e66f79171f78cc22d91fff37e36e3) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a0fc44f3781a869a3a410edd6691fd899) () const |
| |
| const StorageIndex \* | [innerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#ab044564756f472877b2c1a5706e540e2) () const |
| |
| const StorageIndex \* | [innerNonZeroPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a2b35a1d701d6c6ea36b2d9f19660a68c) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a0df6dba8d71e0fb15b2995510853f83e) () const |
| |
| bool | [isCompressed](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#aafab4afa7ab2ff89eff049d4c71e2ce4) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonZeros](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a753e975b7b3643d821dc061141786870) () const |
| |
| const StorageIndex \* | [outerIndexPtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a24c55dd8de4aca30e7c90b69aa5dca6b) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a3d6ede19db6d42074ae063bc876231b1) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a3cdd6cab0abd7ac01925a695fc315d34) () const |
| |
| const Scalar \* | [valuePtr](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#a574ea9371c22eabebdda21c0787312dc) () const |
| |
| | [~SparseMapBase](classeigen_1_1sparsemapbase_3_01derived_00_01readonlyaccessors_01_4#ab375aedf824909a7f1a6af24ee60d70f) () |
| |
|
Public Member Functions inherited from [Eigen::SparseCompressedBase< Derived >](classeigen_1_1sparsecompressedbase) |
| [Map](classeigen_1_1map)< [Array](classeigen_1_1array)< Scalar, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > > | [coeffs](classeigen_1_1sparsecompressedbase#a7cf299e08d2a4f6d6869e631e51b12fe) () |
| |
| const [Map](classeigen_1_1map)< const [Array](classeigen_1_1array)< Scalar, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > > | [coeffs](classeigen_1_1sparsecompressedbase#a101b155485ae59ea1261c4f6040f3dc4) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerIndexPtr](classeigen_1_1sparsecompressedbase#a197111c1289644f1ea38fe683ccdd82a) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerIndexPtr](classeigen_1_1sparsecompressedbase#aa64818e1aa43015dad01b114b2ab4687) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerNonZeroPtr](classeigen_1_1sparsecompressedbase#a411e972b097e6aef225415a4c2d0a0b5) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [innerNonZeroPtr](classeigen_1_1sparsecompressedbase#afc056a3895eae1a4c4767252ff04966a) () const |
| |
| bool | [isCompressed](classeigen_1_1sparsecompressedbase#a837934b33a80fe996ff20500373d3a61) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonZeros](classeigen_1_1sparsecompressedbase#a03de8b3da2c142ce8698a76123b3e7d3) () const |
| |
| [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [outerIndexPtr](classeigen_1_1sparsecompressedbase#a53a82f962686e18c8dc07a4b9a85ed7b) () |
| |
| const [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) \* | [outerIndexPtr](classeigen_1_1sparsecompressedbase#a2624d4c2661c582de168246c56e8d71e) () const |
| |
| Scalar \* | [valuePtr](classeigen_1_1sparsecompressedbase#a0f12f72d14b6c277d09be9f5ce2eab95) () |
| |
| const Scalar \* | [valuePtr](classeigen_1_1sparsecompressedbase#a0f44c739398794ea77f310b745cc5627) () const |
| |
|
Public Member Functions inherited from [Eigen::SparseMatrixBase< Derived >](classeigen_1_1sparsematrixbase) |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1sparsematrixbase#aca7ce296424ef6e478ab0fb19547a7ee) () const |
| |
| const internal::eval< Derived >::type | [eval](classeigen_1_1sparsematrixbase#a761bd872a06b59632fcff7b7807a77ce) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1sparsematrixbase#a180fcba1ccf3cdf3252a263bc1de7a1d) () const |
| |
| bool | [isVector](classeigen_1_1sparsematrixbase#a7eedffa867031f649fd0fb9cc23ce4be) () const |
| |
| template<typename OtherDerived > |
| const [Product](classeigen_1_1product)< Derived, OtherDerived, AliasFreeProduct > | [operator\*](classeigen_1_1sparsematrixbase#a9d4d71b3f34389e6fc01f2b86e43f7a4) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< OtherDerived > &other) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1sparsematrixbase#ac86cc88a4cfef21db6b64ec0ab4c8f0a) () const |
| |
| const [SparseView](classeigen_1_1sparseview)< Derived > | [pruned](classeigen_1_1sparsematrixbase#ac8d0414b56d9d620ce9a698c1b281e5d) (const Scalar &reference=Scalar(0), const RealScalar &epsilon=[NumTraits](structeigen_1_1numtraits)< Scalar >::dummy\_precision()) const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1sparsematrixbase#a1944e9fa9ce7937bfc3a87b2cb94371f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1sparsematrixbase#a124bc57921775eb9aa2dfd9727e23472) () const |
| |
| SparseSymmetricPermutationProduct< Derived, [Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749) > | [twistedBy](classeigen_1_1sparsematrixbase#a51d4898bd6a57cc3ba543a39b102423e) (const [PermutationMatrix](classeigen_1_1permutationmatrix)< [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) > &perm) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::SparseMatrixBase< Derived >](classeigen_1_1sparsematrixbase) |
| enum | { [RowsAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a456cda7b9d938e57194036a41d634604) , [ColsAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a27ba349f075d026c1f51d1ec69aa5b14) , [SizeAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5aa5022cfa2bb53129883e9b7b8abd3d68) , **MaxRowsAtCompileTime** , **MaxColsAtCompileTime** , **MaxSizeAtCompileTime** , [IsVectorAtCompileTime](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a14a3f566ed2a074beddb8aef0223bfdf) , [NumDimensions](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a2366131ffcc38bff48a1c7572eb86dd3) , [Flags](classeigen_1_1sparsematrixbase#aa4d1477f2022b209706daba8722556b5a2af043b36fe9e08df0107cf6de496165) , **IsRowMajor** , **InnerSizeAtCompileTime** } |
| |
| typedef internal::traits< Derived >::[StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) | [StorageIndex](classeigen_1_1sparsematrixbase#a0b540ba724726ebe953f8c0df06081ed) |
| |
| typedef Scalar | [value\_type](classeigen_1_1sparsematrixbase#ac254d3b61718ebc2136d27bac043dcb7) |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
|
Protected Member Functions inherited from [Eigen::SparseCompressedBase< Derived >](classeigen_1_1sparsecompressedbase) |
| | [SparseCompressedBase](classeigen_1_1sparsecompressedbase#af79f020db965367d97eb954fc68d8f99) () |
| |
Ref()
-----
template<typename SparseMatrixType , int Options> template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::Ref](classeigen_1_1ref)< SparseMatrixType, Options >::[Ref](classeigen_1_1ref) | ( | [SparseCompressedBase](classeigen_1_1sparsecompressedbase)< Derived > & | *expr* | ) | |
| inline |
Implicit constructor from any sparse expression (2D matrix or 1D vector)
---
The documentation for this class was generated from the following file:* [SparseRef.h](https://eigen.tuxfamily.org/dox/SparseRef_8h_source.html)
| programming_docs |
eigen3 Chapters Chapters
========
Here is a list of all modules:
| | |
| --- | --- |
| ▼[Dense matrix and array manipulation](https://eigen.tuxfamily.org/dox/group__DenseMatrixManipulation__chapter.html) | |
| [The Matrix class](group__tutorialmatrixclass) | |
| [Matrix and vector arithmetic](group__tutorialmatrixarithmetic) | |
| [The Array class and coefficient-wise operations](group__tutorialarrayclass) | |
| [Block operations](group__tutorialblockoperations) | |
| [Slicing and Indexing](group__tutorialslicingindexing) | |
| [Advanced initialization](group__tutorialadvancedinitialization) | |
| [Reductions, visitors and broadcasting](group__tutorialreductionsvisitorsbroadcasting) | |
| [Reshape](group__tutorialreshape) | |
| [STL iterators and algorithms](group__tutorialstl) | |
| [Interfacing with raw buffers: the Map class](group__tutorialmapclass) | |
| [Aliasing](group__topicaliasing) | |
| [Storage orders](group__topicstorageorders) | |
| ▼[Alignment issues](group__densematrixmanipulation__alignement) | |
| [Explanation of the assertion on unaligned arrays](group__topicunalignedarrayassert) | |
| [Fixed-size vectorizable %Eigen objects](group__topicfixedsizevectorizable) | |
| [Structures Having Eigen Members](group__topicstructhavingeigenmembers) | |
| [Using STL Containers with Eigen](group__topicstlcontainers) | |
| [Passing Eigen objects by value to functions](group__topicpassingbyvalue) | |
| [Compiler making a wrong assumption on stack alignment](group__topicwrongstackalignment) | |
| ▼[Reference](https://eigen.tuxfamily.org/dox/group__DenseMatrixManipulation__Reference.html) | |
| ▼[Core module](group__core__module) | |
| [Global array typedefs](group__arraytypedefs) | |
| [Global matrix typedefs](group__matrixtypedefs) | |
| [Flags](group__flags) | |
| [Enumerations](group__enums) | |
| [Jacobi module](group__jacobi__module) | |
| [Householder module](group__householder__module) | |
| [Catalog of coefficient-wise math functions](group__coeffwisemathfunctions) | |
| [Quick reference guide](group__quickrefpage) | |
| ▼[Dense linear problems and decompositions](https://eigen.tuxfamily.org/dox/group__DenseLinearSolvers__chapter.html) | |
| [Linear algebra and decompositions](group__tutoriallinearalgebra) | |
| [Catalogue of dense decompositions](group__topiclinearalgebradecompositions) | |
| [Solving linear least squares systems](group__leastsquares) | |
| [Inplace matrix decompositions](group__inplacedecomposition) | |
| [Benchmark of dense decompositions](group__densedecompositionbenchmark) | |
| ▼[Reference](https://eigen.tuxfamily.org/dox/group__DenseLinearSolvers__Reference.html) | |
| [Cholesky module](group__cholesky__module) | |
| [LU module](group__lu__module) | |
| [QR module](group__qr__module) | |
| [SVD module](group__svd__module) | |
| [Eigenvalues module](group__eigenvalues__module) | |
| ▼[Sparse linear algebra](https://eigen.tuxfamily.org/dox/group__Sparse__chapter.html) | |
| [Sparse matrix manipulations](group__tutorialsparse) | |
| [Solving Sparse Linear Systems](group__topicsparsesystems) | |
| [Matrix-free solvers](group__matrixfreesolverexample) | |
| ▼[Reference](https://eigen.tuxfamily.org/dox/group__Sparse__Reference.html) | |
| [SparseCore module](group__sparsecore__module) | |
| [OrderingMethods module](group__orderingmethods__module) | |
| [SparseCholesky module](group__sparsecholesky__module) | |
| [SparseLU module](group__sparselu__module) | |
| [SparseQR module](group__sparseqr__module) | Provides QR decomposition for sparse matrices |
| [IterativeLinearSolvers module](group__iterativelinearsolvers__module) | |
| [Sparse meta-module](group__sparse__module) | |
| ▼[Support modules](group__support__modules) | |
| [CholmodSupport module](group__cholmodsupport__module) | |
| [KLUSupport module](group__klusupport__module) | |
| [MetisSupport module](group__metissupport__module) | |
| [PardisoSupport module](group__pardisosupport__module) | |
| [PaStiXSupport module](group__pastixsupport__module) | |
| [SuiteSparseQR module](group__spqrsupport__module) | |
| [SuperLUSupport module](group__superlusupport__module) | |
| [UmfPackSupport module](group__umfpacksupport__module) | |
| [Quick reference guide for sparse matrices](group__sparsequickrefpage) | |
| ▼[Geometry](https://eigen.tuxfamily.org/dox/group__Geometry__chapter.html) | |
| [Space transformations](group__tutorialgeometry) | |
| ▼[Reference](https://eigen.tuxfamily.org/dox/group__Geometry__Reference.html) | |
| ▼[Geometry module](group__geometry__module) | |
| [Global aligned box typedefs](group__alignedboxtypedefs) | |
| [Splines\_Module](group__splines__module) | |
eigen3 Support modules Support modules
===============
Category of modules which add support for external libraries.
| |
| --- |
| |
| | [CholmodSupport module](group__cholmodsupport__module) |
| |
| | [KLUSupport module](group__klusupport__module) |
| |
| | [MetisSupport module](group__metissupport__module) |
| |
| | [PardisoSupport module](group__pardisosupport__module) |
| |
| | [PaStiXSupport module](group__pastixsupport__module) |
| |
| | [SuiteSparseQR module](group__spqrsupport__module) |
| |
| | [SuperLUSupport module](group__superlusupport__module) |
| |
| | [UmfPackSupport module](group__umfpacksupport__module) |
| |
eigen3 Eigen::symbolic::SymbolExpr Eigen::symbolic::SymbolExpr
===========================
### template<typename tag> class Eigen::symbolic::SymbolExpr< tag >
Expression of a symbol uniquely identified by the template parameter type `tag`

| |
| --- |
| |
| typedef tag | [Tag](classeigen_1_1symbolic_1_1symbolexpr#af2c04ad1ee1251a2d540f0d804da4c68) |
| |
| |
| --- |
| |
| [SymbolValue](classeigen_1_1symbolic_1_1symbolvalue)< [Tag](classeigen_1_1symbolic_1_1symbolexpr#af2c04ad1ee1251a2d540f0d804da4c68) > | [operator=](classeigen_1_1symbolic_1_1symbolexpr#a0bd43167911dc398fba4e3f0f142a64d) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) val) const |
| |
|
Public Member Functions inherited from [Eigen::symbolic::BaseExpr< SymbolExpr< tag > >](classeigen_1_1symbolic_1_1baseexpr) |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [eval](classeigen_1_1symbolic_1_1baseexpr#a4149f50157823063e59c18ce04ed2496) (const T &values) const |
| |
Tag
---
template<typename tag >
| |
| --- |
| typedef tag [Eigen::symbolic::SymbolExpr](classeigen_1_1symbolic_1_1symbolexpr)< tag >::[Tag](classeigen_1_1symbolic_1_1symbolexpr#af2c04ad1ee1251a2d540f0d804da4c68) |
Alias to the template parameter `tag`
operator=()
-----------
template<typename tag >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [SymbolValue](classeigen_1_1symbolic_1_1symbolvalue)<[Tag](classeigen_1_1symbolic_1_1symbolexpr#af2c04ad1ee1251a2d540f0d804da4c68)> [Eigen::symbolic::SymbolExpr](classeigen_1_1symbolic_1_1symbolexpr)< tag >::operator= | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *val* | ) | const |
| inline |
Associate the value *val* to the given symbol `*this`, uniquely identified by its `Tag`.
The returned object should be passed to ExprBase::eval() to evaluate a given expression with this specified runtime-time value.
---
The documentation for this class was generated from the following file:* [SymbolicIndex.h](https://eigen.tuxfamily.org/dox/SymbolicIndex_8h_source.html)
eigen3 Eigen::NaturalOrdering Eigen::NaturalOrdering
======================
### template<typename StorageIndex> class Eigen::NaturalOrdering< StorageIndex >
Functor computing the natural ordering (identity)
Note
Returns an empty permutation matrix
Template Parameters
| | |
| --- | --- |
| StorageIndex | The type of indices of the matrix |
| |
| --- |
| |
| template<typename MatrixType > |
| void | [operator()](classeigen_1_1naturalordering#aa39e772105ed66da9e909f2a9385be37) (const MatrixType &, [PermutationType](classeigen_1_1permutationmatrix) &perm) |
| |
operator()()
------------
template<typename StorageIndex > template<typename MatrixType >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::NaturalOrdering](classeigen_1_1naturalordering)< StorageIndex >::operator() | ( | const MatrixType & | , |
| | | [PermutationType](classeigen_1_1permutationmatrix) & | *perm* |
| | ) | | |
| inline |
Compute the permutation vector from a column-major sparse matrix
---
The documentation for this class was generated from the following file:* [Ordering.h](https://eigen.tuxfamily.org/dox/Ordering_8h_source.html)
eigen3 Alignment issues Alignment issues
================
<>
| |
| --- |
| |
| | [Explanation of the assertion on unaligned arrays](group__topicunalignedarrayassert) |
| |
| | [Fixed-size vectorizable %Eigen objects](group__topicfixedsizevectorizable) |
| |
| | [Structures Having Eigen Members](group__topicstructhavingeigenmembers) |
| |
| | [Using STL Containers with Eigen](group__topicstlcontainers) |
| |
| | [Passing Eigen objects by value to functions](group__topicpassingbyvalue) |
| |
| | [Compiler making a wrong assumption on stack alignment](group__topicwrongstackalignment) |
| |
eigen3 Eigen::Homogeneous Eigen::Homogeneous
==================
### template<typename MatrixType, int \_Direction> class Eigen::Homogeneous< MatrixType, \_Direction >
Expression of one (or a set of) homogeneous vector(s)
This is defined in the Geometry module.
```
#include <Eigen/Geometry>
```
Parameters
| | |
| --- | --- |
| MatrixType | the type of the object in which we are making homogeneous |
This class represents an expression of one (or a set of) homogeneous vector(s). It is the return type of [MatrixBase::homogeneous()](group__geometry__module#gaf3229c2d3669e983075ab91f7f480cb1) and most of the time this is the only way it is used.
See also
[MatrixBase::homogeneous()](group__geometry__module#gaf3229c2d3669e983075ab91f7f480cb1)
| |
| --- |
| |
|
Public Types inherited from [Eigen::DenseBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1densebase) |
| typedef random\_access\_iterator\_type | [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) |
| |
| typedef random\_access\_iterator\_type | [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) |
| |
| typedef [Array](classeigen_1_1array)< typename internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), AutoAlign|(internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581) &RowMajorBit ? RowMajor :ColMajor), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > | [PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb) |
| |
| typedef [Matrix](classeigen_1_1matrix)< typename internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), AutoAlign|(internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581) &RowMajorBit ? RowMajor :ColMajor), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > | [PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e) |
| |
| typedef internal::conditional< internal::is\_same< typename internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::XprKind, [MatrixXpr](structeigen_1_1matrixxpr) >::[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), [PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e), [PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb) >::type | [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) |
| | The plain matrix or array type corresponding to this expression. [More...](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) |
| |
| typedef internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) |
| |
| typedef internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) | [StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) |
| | The type used to store indices. [More...](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) |
| |
| typedef [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [value\_type](classeigen_1_1densebase#a9276182dab8236c33f1e7abf491d504d) |
| |
|
Public Member Functions inherited from [Eigen::MatrixBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1matrixbase) |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [acosh](classeigen_1_1matrixbase#a765140ddee0c6b39bbfa3b8917de67be) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise inverse hyperbolic cosine use ArrayBase::acosh . [More...](classeigen_1_1matrixbase#a765140ddee0c6b39bbfa3b8917de67be) |
| |
| const AdjointReturnType | [adjoint](classeigen_1_1matrixbase#afacca1f88da57e5cd87dd07c8ff926bb) () const |
| |
| void | [adjointInPlace](classeigen_1_1matrixbase#a51c5982c1f64e45a939515b701fa6f4a) () |
| |
| void | [applyHouseholderOnTheLeft](classeigen_1_1matrixbase#a8f2c8059ef3f04cfa0c73b4c012db855) (const EssentialPart &essential, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &tau, [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) \*workspace) |
| |
| void | [applyHouseholderOnTheRight](classeigen_1_1matrixbase#ab3e52262b41fa40e194dda245e0f9675) (const EssentialPart &essential, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &tau, [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) \*workspace) |
| |
| void | [applyOnTheLeft](classeigen_1_1matrixbase#a3a08ad41e81d8ad4a37b5d5c7490e765) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| void | [applyOnTheLeft](classeigen_1_1matrixbase#ae669131f6e18f7e8f06fae271754f435) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) p, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) q, const [JacobiRotation](classeigen_1_1jacobirotation)< OtherScalar > &j) |
| |
| void | [applyOnTheRight](classeigen_1_1matrixbase#a45d91752925d2757fc8058a293b15462) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| void | [applyOnTheRight](group__jacobi__module#gaa07f741c86219601664433777827bf1c) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) p, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) q, const [JacobiRotation](classeigen_1_1jacobirotation)< OtherScalar > &j) |
| |
| [ArrayWrapper](classeigen_1_1arraywrapper)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [array](classeigen_1_1matrixbase#a354c33eec32ceb4193d002f4d41c0497) () |
| |
| const [ArrayWrapper](classeigen_1_1arraywrapper)< const [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [array](classeigen_1_1matrixbase#a72f287fe7b2a7e7a66d16cc88166d47f) () const |
| |
| const [DiagonalWrapper](classeigen_1_1diagonalwrapper)< const [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [asDiagonal](classeigen_1_1matrixbase#a14235b62c90f93fe910070b4743782d0) () const |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [asinh](classeigen_1_1matrixbase#a8efaf691d9c74b362a43b1b793706ea1) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise inverse hyperbolic sine use ArrayBase::asinh . [More...](classeigen_1_1matrixbase#a8efaf691d9c74b362a43b1b793706ea1) |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [atanh](classeigen_1_1matrixbase#ab87d29c00e6d75aeab43eff53bf5164c) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise inverse hyperbolic cosine use ArrayBase::atanh . [More...](classeigen_1_1matrixbase#ab87d29c00e6d75aeab43eff53bf5164c) |
| |
| [BDCSVD](classeigen_1_1bdcsvd)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [bdcSvd](classeigen_1_1matrixbase#ae171b74b5d530846ee0836135ffcf837) (unsigned int computationOptions=0) const |
| |
| RealScalar | [blueNorm](classeigen_1_1matrixbase#a3f3faa00163c16824ff03e58a210c74c) () const |
| |
| const [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [colPivHouseholderQr](classeigen_1_1matrixbase#adee8c19c833245bbb00a591dce68e8a4) () const |
| |
| const [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [completeOrthogonalDecomposition](classeigen_1_1matrixbase#ae90b6846f05bd30b8d52b66e427e3e09) () const |
| |
| void | [computeInverseAndDetWithCheck](classeigen_1_1matrixbase#a7baaf2fdec0191a2166cf9fd84a2dcb2) (ResultType &[inverse](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf), typename ResultType::Scalar &[determinant](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061), bool &invertible, const RealScalar &absDeterminantThreshold=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| void | [computeInverseWithCheck](classeigen_1_1matrixbase#a116f3b50d2af7dbdf7473e517a5b8b0f) (ResultType &[inverse](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf), bool &invertible, const RealScalar &absDeterminantThreshold=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [cos](classeigen_1_1matrixbase#a34d626eb756bbeb4069d1eb0e6494c65) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise cosine use ArrayBase::cos . [More...](classeigen_1_1matrixbase#a34d626eb756bbeb4069d1eb0e6494c65) |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [cosh](classeigen_1_1matrixbase#a627e6f11bf5854ade9a5abfc344c0367) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise hyperbolic cosine use ArrayBase::cosh . [More...](classeigen_1_1matrixbase#a627e6f11bf5854ade9a5abfc344c0367) |
| |
| [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) | [cross](group__geometry__module#ga0024b44eca99cb7135887c2aaf319d28) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) | [cross3](group__geometry__module#gabde56e2a0baba550815a0b05139e4d42) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [determinant](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061) () const |
| |
| [DiagonalReturnType](classeigen_1_1diagonal) | [diagonal](classeigen_1_1matrixbase#ab5768147536273eb2dbdfa389cfd26a3) () |
| |
| [ConstDiagonalReturnType](classeigen_1_1diagonal) | [diagonal](classeigen_1_1matrixbase#aebdeedcf67e46d969c556c6c7d9780ee) () const |
| |
| [DiagonalDynamicIndexReturnType](classeigen_1_1diagonal) | [diagonal](classeigen_1_1matrixbase#a8a13d4b8efbd7797ee8efd3dd988a7f7) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) index) |
| |
| [ConstDiagonalDynamicIndexReturnType](classeigen_1_1diagonal) | [diagonal](classeigen_1_1matrixbase#aed11a711c0a3d5dbf8bc094008e29846) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) index) const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [diagonalSize](classeigen_1_1matrixbase#ab79e511b9322b8b801858e253fb257eb) () const |
| |
| [ScalarBinaryOpTraits](structeigen_1_1scalarbinaryoptraits)< typename internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), typename internal::traits< OtherDerived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::ReturnType | [dot](classeigen_1_1matrixbase#adfd32bf5fcf6ee603c924dde9bf7bc39) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| EigenvaluesReturnType | [eigenvalues](classeigen_1_1matrixbase#a30430fa3d5b4e74d312fd4f502ac984d) () const |
| | Computes the eigenvalues of a matrix. [More...](classeigen_1_1matrixbase#a30430fa3d5b4e74d312fd4f502ac984d) |
| |
| [Matrix](classeigen_1_1matrix)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), 3, 1 > | [eulerAngles](group__geometry__module#ga17994d2e81b723295f5bc3b1f862ed3b) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) a0, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) a1, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) a2) const |
| |
| const MatrixExponentialReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [exp](classeigen_1_1matrixbase#a70901e189e876f64d7f3fee1dbe942cc) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise exponential use ArrayBase::exp . [More...](classeigen_1_1matrixbase#a70901e189e876f64d7f3fee1dbe942cc) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [forceAlignedAccess](classeigen_1_1matrixbase#afdaf810ac1708ca6d6ecdcfac1e06699) () |
| |
| const [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [forceAlignedAccess](classeigen_1_1matrixbase#ad2fdb842d9a715f8778d0b33c29cfe49) () const |
| |
| internal::conditional< Enable, [ForceAlignedAccess](classeigen_1_1forcealignedaccess)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >, [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & >::type | [forceAlignedAccessIf](classeigen_1_1matrixbase#ae35213d1dd4dd13ebe9a7a762d6bb847) () |
| |
| internal::add\_const\_on\_value\_type< typename internal::conditional< Enable, [ForceAlignedAccess](classeigen_1_1forcealignedaccess)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >, [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & >::type >::type | [forceAlignedAccessIf](classeigen_1_1matrixbase#af42d92f115d4b8fa3d5aa731ed496ed1) () const |
| |
| const [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [fullPivHouseholderQr](classeigen_1_1matrixbase#a863bc0e06b641a089508eabec6835ab2) () const |
| |
| const [FullPivLU](classeigen_1_1fullpivlu)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [fullPivLu](classeigen_1_1matrixbase#a25da97d31acab0ee5d9d13bdbb0569da) () const |
| |
| const HNormalizedReturnType | [hnormalized](group__geometry__module#gadc0e3dd3510cb5a6e70aca9aab1cbf19) () const |
| | homogeneous normalization [More...](group__geometry__module#gadc0e3dd3510cb5a6e70aca9aab1cbf19) |
| |
| [HomogeneousReturnType](classeigen_1_1homogeneous) | [homogeneous](group__geometry__module#gaf3229c2d3669e983075ab91f7f480cb1) () const |
| |
| const [HouseholderQR](classeigen_1_1householderqr)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [householderQr](classeigen_1_1matrixbase#a9a9377aab1cea26db5f25bab7e682f8f) () const |
| |
| RealScalar | [hypotNorm](classeigen_1_1matrixbase#a32222d3b6677e6cdf0b801463f329b72) () const |
| |
| const [Inverse](classeigen_1_1inverse)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [inverse](classeigen_1_1matrixbase#a7712eb69e8ea3c8f7b8da1c44dbdeebf) () const |
| |
| bool | [isDiagonal](classeigen_1_1matrixbase#a97027ea54c8cd1ddb1c578fee5cedc67) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isIdentity](classeigen_1_1matrixbase#a4ccbd8dfa06e9d47b9bf84711f8b9d40) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isLowerTriangular](classeigen_1_1matrixbase#a1e96c42d79a56f0a6ade30ce031e17eb) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isOrthogonal](classeigen_1_1matrixbase#aefdc8e4e4c156fdd79a21479e75dcd8a) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isUnitary](classeigen_1_1matrixbase#a8a7ee34ce202cac3eeea9cf20c9e4833) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isUpperTriangular](classeigen_1_1matrixbase#aae3ec1660bb4ac584220481c54ab4a64) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| [JacobiSVD](classeigen_1_1jacobisvd)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [jacobiSvd](classeigen_1_1matrixbase#a5745dca9c54390633b434e54a1d1eedd) (unsigned int computationOptions=0) const |
| |
| const [Product](classeigen_1_1product)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, OtherDerived, LazyProduct > | [lazyProduct](classeigen_1_1matrixbase#ae0c280b1066c14ed577021f38876527f) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| const [LDLT](classeigen_1_1ldlt)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [ldlt](classeigen_1_1matrixbase#a0ecf058a0727a4cab8b42d79e95072e1) () const |
| |
| const [LLT](classeigen_1_1llt)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [llt](classeigen_1_1matrixbase#a90c45f7a30265df792d5aeaddead2635) () const |
| |
| const MatrixLogarithmReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [log](classeigen_1_1matrixbase#a4dc57b319fc1cf8c9035016e56602a7d) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise logarithm use ArrayBase::log . [More...](classeigen_1_1matrixbase#a4dc57b319fc1cf8c9035016e56602a7d) |
| |
| RealScalar | [lpNorm](classeigen_1_1matrixbase#a72586ab059e889e7d2894ff227747e35) () const |
| |
| const [PartialPivLU](classeigen_1_1partialpivlu)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [lu](classeigen_1_1matrixbase#afb312afbfe960cbda67811552d876fae) () const |
| |
| void | [makeHouseholder](classeigen_1_1matrixbase#a13291e900f7e81ddc6e5b8802f82092b) (EssentialPart &essential, [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &tau, RealScalar &beta) const |
| |
| void | [makeHouseholderInPlace](classeigen_1_1matrixbase#aebf4bac7dffe2685ab93734fb776e817) ([Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &tau, RealScalar &beta) |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [matrixFunction](classeigen_1_1matrixbase#a1a6cc9f734eb175e785a1118305245fc) (StemFunction f) const |
| | Helper function for the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). |
| |
| [NoAlias](classeigen_1_1noalias)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, [Eigen::MatrixBase](classeigen_1_1matrixbase) > | [noalias](classeigen_1_1matrixbase#a2c1085de7645f23f240876388457da0b) () |
| |
| RealScalar | [norm](classeigen_1_1matrixbase#a196c4ec3c8ffdf5bda45d0f617154975) () const |
| |
| void | [normalize](classeigen_1_1matrixbase#ad16303c47ba36f7a41ea264cb26bceb6) () |
| |
| const [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) | [normalized](classeigen_1_1matrixbase#a5cf2fd4c57e59604fd4116158fd34308) () const |
| |
| bool | [operator!=](classeigen_1_1matrixbase#a028c7ac8094d610042fd0f9feca68f63) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| const [Product](classeigen_1_1product)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, DiagonalDerived, LazyProduct > | [operator\*](classeigen_1_1matrixbase#a36fb95c37f0a454e0e2e5cb120b2df7f) (const DiagonalBase< DiagonalDerived > &[diagonal](classeigen_1_1matrixbase#ab5768147536273eb2dbdfa389cfd26a3)) const |
| |
| const [Product](classeigen_1_1product)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, OtherDerived > | [operator\*](classeigen_1_1matrixbase#ae2d220efbf7047f0894787888288cfcc) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator\*=](classeigen_1_1matrixbase#a3783b6168995ca117a1c19fea3630ac4) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator+=](classeigen_1_1matrixbase#a983cc3be0bbe11b3d041a415b76ce010) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator-=](classeigen_1_1matrixbase#a1042124b0ddee66e78ac7b0a9ac4cc9c) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator=](classeigen_1_1matrixbase#a373bf62ad398162df5a71963ed7cbeff) (const [MatrixBase](classeigen_1_1matrixbase) &other) |
| |
| bool | [operator==](classeigen_1_1matrixbase#a80e3e1e83fdf43f9f7fb6ff51836b24d) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| RealScalar | [operatorNorm](classeigen_1_1matrixbase#a0ff9bc0b9bea2d0822a2bf3192783102) () const |
| | Computes the L2 operator norm. [More...](classeigen_1_1matrixbase#a0ff9bc0b9bea2d0822a2bf3192783102) |
| |
| const [PartialPivLU](classeigen_1_1partialpivlu)< [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [partialPivLu](classeigen_1_1matrixbase#a6199d8aaf26c1b8ac3097fdfa7733a1e) () const |
| |
| const MatrixPowerReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [pow](classeigen_1_1matrixbase#a7ae6c25e6a94a60e147741e76203a73b) (const RealScalar &p) const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise power to `p` use ArrayBase::pow . [More...](classeigen_1_1matrixbase#a7ae6c25e6a94a60e147741e76203a73b) |
| |
| const MatrixComplexPowerReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [pow](classeigen_1_1matrixbase#a91dcacf224bd8b18346914bdf7eefc31) (const std::complex< RealScalar > &p) const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise power to `p` use ArrayBase::pow . [More...](classeigen_1_1matrixbase#a91dcacf224bd8b18346914bdf7eefc31) |
| |
| [MatrixBase](classeigen_1_1matrixbase)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::template SelfAdjointViewReturnType< UpLo >::Type | [selfadjointView](classeigen_1_1matrixbase#ad446541377593656c1399862fe6a0f94) () |
| |
| [MatrixBase](classeigen_1_1matrixbase)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::template ConstSelfAdjointViewReturnType< UpLo >::Type | [selfadjointView](classeigen_1_1matrixbase#a67eb836f331d9b567e7f36ec0782fa07) () const |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setIdentity](classeigen_1_1matrixbase#a18e969adfdf2db4ac44c47fbdc854683) () |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setIdentity](classeigen_1_1matrixbase#a97dec020729928e328fe8ae9aad1e99e) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| | Resizes to the given size, and writes the identity expression (not necessarily square) into \*this. [More...](classeigen_1_1matrixbase#a97dec020729928e328fe8ae9aad1e99e) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setUnit](classeigen_1_1matrixbase#ac7cf3e0e69550d36de8778b09a645afd) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i) |
| | Set the coefficients of `*this` to the i-th unit (basis) vector. [More...](classeigen_1_1matrixbase#ac7cf3e0e69550d36de8778b09a645afd) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setUnit](classeigen_1_1matrixbase#a90bed3b6468a17a9054b07cde7a751a6) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) newSize, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i) |
| | Resizes to the given *newSize*, and writes the i-th unit (basis) vector into \*this. [More...](classeigen_1_1matrixbase#a90bed3b6468a17a9054b07cde7a751a6) |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [sin](classeigen_1_1matrixbase#a02f4ff0fcbbae2f3ccaa5981e8ad5e34) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise sine use ArrayBase::sin . [More...](classeigen_1_1matrixbase#a02f4ff0fcbbae2f3ccaa5981e8ad5e34) |
| |
| const MatrixFunctionReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [sinh](classeigen_1_1matrixbase#a9c37eab2dc7baf83809269254c9129e0) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise hyperbolic sine use ArrayBase::sinh . [More...](classeigen_1_1matrixbase#a9c37eab2dc7baf83809269254c9129e0) |
| |
| const [SparseView](classeigen_1_1sparseview)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [sparseView](group__sparsecore__module#ga320dd291cbf4339c6118c41521b75350) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &m\_reference=[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)(0), const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::Real &m\_epsilon=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| const MatrixSquareRootReturnValue< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [sqrt](classeigen_1_1matrixbase#ad873dca860bd47baeeede8663e161b83) () const |
| | This function requires the [unsupported MatrixFunctions module](unsupported/group__matrixfunctions__module). To compute the coefficient-wise square root use ArrayBase::sqrt . [More...](classeigen_1_1matrixbase#ad873dca860bd47baeeede8663e161b83) |
| |
| RealScalar | [squaredNorm](classeigen_1_1matrixbase#ac8da566526419f9742a6c471bbd87e0a) () const |
| |
| RealScalar | [stableNorm](classeigen_1_1matrixbase#ab84d3e64f855813b1eea4202c0697dc1) () const |
| |
| void | [stableNormalize](classeigen_1_1matrixbase#a0b1443fa322615379557ade3399a3c3c) () |
| |
| const [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) | [stableNormalized](classeigen_1_1matrixbase#a399dca938633b9f8df5ec4beefeccec0) () const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [trace](classeigen_1_1matrixbase#a544b609f65eb2bd3e368b3fc2d79479e) () const |
| |
| [MatrixBase](classeigen_1_1matrixbase)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::template TriangularViewReturnType< Mode >::Type | [triangularView](classeigen_1_1matrixbase#a56665aa894f49f2765291fee0eaeb9c6) () |
| |
| [MatrixBase](classeigen_1_1matrixbase)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::template ConstTriangularViewReturnType< Mode >::Type | [triangularView](classeigen_1_1matrixbase#aa044521145e74117ad1df42460d7b520) () const |
| |
| [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) | [unitOrthogonal](group__geometry__module#gaa0dc2c32a9379eeb2b4c4a05c1a6fe52) (void) const |
| |
|
Public Member Functions inherited from [Eigen::DenseBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1densebase) |
| bool | [all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4) () const |
| |
| bool | [allFinite](classeigen_1_1densebase#af1e669fd3aaae50a4870dc1b8f3b8884) () const |
| |
| bool | [any](classeigen_1_1densebase#abfbf4cb72dd577e62fbe035b1c53e695) () const |
| |
| [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) | [begin](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641) () |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [begin](classeigen_1_1densebase#ad9368ce70b06167ec5fc19398d329f5e) () const |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [cbegin](classeigen_1_1densebase#ae9a3dfd9b826ba3103de0128576fb15b) () const |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [cend](classeigen_1_1densebase#aeb3b76f02986c2af2521d07164b5ffde) () const |
| |
| [ColwiseReturnType](classeigen_1_1vectorwiseop) | [colwise](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30) () |
| |
| [ConstColwiseReturnType](classeigen_1_1vectorwiseop) | [colwise](classeigen_1_1densebase#a58837c81de446efbdb58da07b73a63c1) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [count](classeigen_1_1densebase#a229be090c665b9bf7d1fcdfd1ab6e0c1) () const |
| |
| [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) | [end](classeigen_1_1densebase#ae71d079e16d91360d10066b316b48485) () |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [end](classeigen_1_1densebase#ab34773522e43bfb02e9cf652d7b5dd60) () const |
| |
| EvalReturnType | [eval](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) () const |
| |
| void | [fill](classeigen_1_1densebase#a9be169c308801411aa24be93d30930bf) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| EIGEN\_DEPRECATED const [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [flagged](classeigen_1_1densebase#a9b3f75f76ae40439be870258e80c7346) () const |
| |
| const [WithFormat](classeigen_1_1withformat)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [format](classeigen_1_1densebase#ab231f1a6057f28d4244145e12c9fc0c7) (const [IOFormat](structeigen_1_1ioformat) &fmt) const |
| |
| bool | [hasNaN](classeigen_1_1densebase#ab13d158c900560d3e1b25d85d2d33dd6) () const |
| |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [innerSize](classeigen_1_1densebase#a58ca41d9635a8ab3c5a268ef3f7f0d75) () const |
| |
| bool | [isApprox](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isApproxToConstant](classeigen_1_1densebase#af9b150d48bc5e4366887ccb466e40c6b) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isConstant](classeigen_1_1densebase#a1ca84e4179b3e5081ed11d89bbd9e74f) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isMuchSmallerThan](classeigen_1_1densebase#a3c4db0c6dd974fa88bbb58b2cf3d5664) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isMuchSmallerThan](classeigen_1_1densebase#adfca6ff4e473f68fbbeabbd03b7504a9) (const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::Real &other, const RealScalar &prec) const |
| |
| bool | [isOnes](classeigen_1_1densebase#aa56d6b4477cd3c92a9cf42f4b96e47c2) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isZero](classeigen_1_1densebase#af36014ec300f53a65083057ed4e89822) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| EIGEN\_DEPRECATED [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [lazyAssign](classeigen_1_1densebase#a6bc6c096e3bfc726f28315daecd21b3f) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1) () const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#aced8ffda52ff061b6586ace2657ebf30) (IndexType \*index) const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#a3780b7a9cd184d0b4f3ea797eba9e2b3) (IndexType \*row, IndexType \*col) const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [mean](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7) () const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2) () const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#ac9265f4f91430b9cc75d63fb6865bb29) (IndexType \*index) const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#aa28152ba4a42b2d112e5fec5469ec4c1) (IndexType \*row, IndexType \*col) const |
| |
| const [NestByValue](classeigen_1_1nestbyvalue)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [nestByValue](classeigen_1_1densebase#a3e2761e2b6da74dba1d17b40cc918bf7) () const |
| |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [nonZeros](classeigen_1_1densebase#acb8d5574eff335381e7441ade87700a0) () const |
| |
| [CommaInitializer](structeigen_1_1commainitializer)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [operator<<](classeigen_1_1densebase#a0f0e34696162b34762b2bf4bd948f90c) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| [CommaInitializer](structeigen_1_1commainitializer)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > | [operator<<](classeigen_1_1densebase#a0e575eb0ba6cc6bc5f347872abd8509d) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &s) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator=](classeigen_1_1densebase#a5281dadff89f46eef719b38e5d073a8f) (const [DenseBase](classeigen_1_1densebase) &other) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator=](classeigen_1_1densebase#ab66155169d20c035e80d521a8b918e97) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [operator=](classeigen_1_1densebase#a58915510693d64164e567bd762e1032f) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| | Copies the generic expression *other* into \*this. [More...](classeigen_1_1densebase#a58915510693d64164e567bd762e1032f) |
| |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [outerSize](classeigen_1_1densebase#a03f71699bc26ca2ee4e42ec4538862d7) () const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [prod](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f) () const |
| |
| internal::traits< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [redux](classeigen_1_1densebase#a63ce1e4fab36bff43bbadcdd06a67724) (const Func &func) const |
| |
| const [Replicate](classeigen_1_1replicate)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, RowFactor, ColFactor > | [replicate](classeigen_1_1densebase#a60dadfe80b813d808e91e4521c722a8e) () const |
| |
| const [Replicate](classeigen_1_1replicate)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, Dynamic, Dynamic > | [replicate](classeigen_1_1densebase#afae2d5e36f1158d1b1681dac3cdbd58e) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rowFactor, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) colFactor) const |
| |
| void | [resize](classeigen_1_1densebase#a2ec5bac4e1ab95808808ef50ccf4cb39) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) newSize) |
| |
| void | [resize](classeigen_1_1densebase#a25e2b4887b47b1f2346857d1931efa0f) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| [ReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f) () |
| |
| [ConstReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1densebase#a9e2f3ac4019184abf95ca0e1a8d82866) () const |
| |
| void | [reverseInPlace](classeigen_1_1densebase#adb8045155ea45f7961fc2a5170e1d921) () |
| |
| [RowwiseReturnType](classeigen_1_1vectorwiseop) | [rowwise](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28) () |
| |
| [ConstRowwiseReturnType](classeigen_1_1vectorwiseop) | [rowwise](classeigen_1_1densebase#aa1cabd3404528fe8cec4bab43d74bffc) () const |
| |
| const [Select](classeigen_1_1select)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, ThenDerived, ElseDerived > | [select](classeigen_1_1densebase#a65e78cfcbc9852e6923bebff4323ddca) (const [DenseBase](classeigen_1_1densebase)< ThenDerived > &thenMatrix, const [DenseBase](classeigen_1_1densebase)< ElseDerived > &elseMatrix) const |
| |
| const [Select](classeigen_1_1select)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, ThenDerived, typename ThenDerived::ConstantReturnType > | [select](classeigen_1_1densebase#a57ef09a843004095f84c198dd145641b) (const [DenseBase](classeigen_1_1densebase)< ThenDerived > &thenMatrix, const typename ThenDerived::Scalar &elseScalar) const |
| |
| const [Select](classeigen_1_1select)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction >, typename ElseDerived::ConstantReturnType, ElseDerived > | [select](classeigen_1_1densebase#a9e8e78c75887d4539071a0b7a61ca103) (const typename ElseDerived::Scalar &thenScalar, const [DenseBase](classeigen_1_1densebase)< ElseDerived > &elseMatrix) const |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setConstant](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setLinSpaced](classeigen_1_1densebase#aeb023532476d3f14c457367e0eb5f3f1) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#aeb023532476d3f14c457367e0eb5f3f1) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setLinSpaced](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a) |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setOnes](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2) () |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setRandom](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db) () |
| |
| [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > & | [setZero](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb) () |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [sum](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c) () const |
| |
| void | [swap](classeigen_1_1densebase#af9e7e4305fdb7781f2b2f05fa801f21e) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| void | [swap](classeigen_1_1densebase#a44e25adc6da9cd1d79f4c5bd7c1819cb) ([PlainObjectBase](classeigen_1_1plainobjectbase)< OtherDerived > &other) |
| |
| [TransposeReturnType](classeigen_1_1transpose) | [transpose](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1densebase#a38c0b074cf93fc194bf91141287cee3f) () const |
| |
| void | [transposeInPlace](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a) () |
| |
| CoeffReturnType | [value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0) () const |
| |
| void | [visit](classeigen_1_1densebase#a4225b90fcc74f18dd479b401124b3841) (Visitor &func) const |
| |
|
Static Public Member Functions inherited from [Eigen::MatrixBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1matrixbase) |
| static const IdentityReturnType | [Identity](classeigen_1_1matrixbase#a98bb9a0f705c6dfde85b0bfff31bf88f) () |
| |
| static const IdentityReturnType | [Identity](classeigen_1_1matrixbase#acf33ce20ef03ead47cb3dbcd5f416ede) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static const BasisReturnType | [Unit](classeigen_1_1matrixbase#a9daf6d22d10ed8ae00432b0f641455df) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i) |
| |
| static const BasisReturnType | [Unit](classeigen_1_1matrixbase#ac7a03a61014f37ddd2fe61ebac0c9539) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) i) |
| |
| static const BasisReturnType | [UnitW](classeigen_1_1matrixbase#af56ba94e5b0330827003eadd26cfadc2) () |
| |
| static const BasisReturnType | [UnitX](classeigen_1_1matrixbase#a8a555b7cf626cced54670b98668c4e6d) () |
| |
| static const BasisReturnType | [UnitY](classeigen_1_1matrixbase#a00850083489e20249b1d05b394fc5efc) () |
| |
| static const BasisReturnType | [UnitZ](classeigen_1_1matrixbase#aabdcdeff1c822a5465fcbe1f78e5afe0) () |
| |
|
Static Public Member Functions inherited from [Eigen::DenseBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1densebase) |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#a68a7ece6c5629d1e9447a321fcb14ccd) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#a1fdd3189ae3a41d250593334d82210cf) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#ad8098aa5971139a5585e623dddbea860) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| |
| static const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) |
| |
| static EIGEN\_DEPRECATED const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#ac8cfbd436a8c9fc50defee5946451176) (Sequential\_t, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| |
| static EIGEN\_DEPRECATED const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d) (Sequential\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a5dc65501accd02c30f7c1840c2a30a41) (const CustomNullaryOp &func) |
| |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a3340c9b997f5b53a0131cf927f93b54c) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const CustomNullaryOp &func) |
| |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a9752ee59976a4b4aad860ad1a9093e7f) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const CustomNullaryOp &func) |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101) () |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#ab710a58e4a80fbcb2594242372c8fe56) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19) () |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#ae97f8d9d08f969c733c8144be6225756) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#a7eb5f974a8f0b67eac7080db1da0e308) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) () |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#ae41a9b5050ed27d9e93c82c9c8622cd3) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#ac22f79b812fa564061042407f2ba8f5b) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
|
Protected Member Functions inherited from [Eigen::DenseBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1densebase) |
| | [DenseBase](classeigen_1_1densebase#aa284042d0e1b0ad9b6a00db7fd2d9f7f) () |
| |
|
Related Functions inherited from [Eigen::DenseBase< Homogeneous< MatrixType, \_Direction > >](classeigen_1_1densebase) |
| std::ostream & | [operator<<](classeigen_1_1densebase#a3806d3f42de165878dace160e6aba40a) (std::ostream &s, const [DenseBase](classeigen_1_1densebase)< [Homogeneous](classeigen_1_1homogeneous)< MatrixType, \_Direction > > &m) |
| |
---
The documentation for this class was generated from the following file:* [Homogeneous.h](https://eigen.tuxfamily.org/dox/Homogeneous_8h_source.html)
| programming_docs |
eigen3 Cholesky module Cholesky module
===============
This module provides two variants of the Cholesky decomposition for selfadjoint (hermitian) matrices. Those decompositions are also accessible via the following methods:
* [MatrixBase::llt()](classeigen_1_1matrixbase#a90c45f7a30265df792d5aeaddead2635)
* [MatrixBase::ldlt()](classeigen_1_1matrixbase#a0ecf058a0727a4cab8b42d79e95072e1)
* [SelfAdjointView::llt()](classeigen_1_1selfadjointview#a405e810491642a7f7b785f2ad6f93619)
* [SelfAdjointView::ldlt()](classeigen_1_1selfadjointview#a644155eef17b37c95d85b9f65bb49ac4)
```
#include <Eigen/Cholesky>
```
| |
| --- |
| |
| class | [Eigen::LDLT< \_MatrixType, \_UpLo >](classeigen_1_1ldlt) |
| | Robust Cholesky decomposition of a matrix with pivoting. [More...](classeigen_1_1ldlt#details) |
| |
| class | [Eigen::LLT< \_MatrixType, \_UpLo >](classeigen_1_1llt) |
| | Standard Cholesky decomposition (LL^T) of a matrix and associated features. [More...](classeigen_1_1llt#details) |
| |
eigen3 Eigen::SparseQR Eigen::SparseQR
===============
### template<typename \_MatrixType, typename \_OrderingType> class Eigen::SparseQR< \_MatrixType, \_OrderingType >
[Sparse](structeigen_1_1sparse) left-looking QR factorization with numerical column pivoting.
This class implements a left-looking QR decomposition of sparse matrices with numerical column pivoting. When a column has a norm less than a given tolerance it is implicitly permuted to the end. The QR factorization thus obtained is given by A\*P = Q\*R where R is upper triangular or trapezoidal.
P is the column permutation which is the product of the fill-reducing and the numerical permutations. Use [colsPermutation()](classeigen_1_1sparseqr#a140930ebbf89dfd57a173761716db38f) to get it.
Q is the orthogonal matrix represented as products of Householder reflectors. Use [matrixQ()](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1) to get an expression and [matrixQ()](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1).adjoint() to get the adjoint. You can then apply it to a vector.
R is the sparse triangular or trapezoidal matrix. The later occurs when A is rank-deficient. [matrixR()](classeigen_1_1sparseqr#a564524ff13b2b6dd1e76127404f7b920).topLeftCorner([rank()](classeigen_1_1sparseqr#a70ec2b9e5cb62a41dc1ee2adfb54e9b0), [rank()](classeigen_1_1sparseqr#a70ec2b9e5cb62a41dc1ee2adfb54e9b0)) always returns a triangular factor of full rank.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | The type of the sparse matrix A, must be a column-major SparseMatrix<> |
| \_OrderingType | The fill-reducing ordering method. See the [OrderingMethods](group__orderingmethods__module) module for the list of built-in and external ordering methods. |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
The numerical pivoting strategy and default threshold are the same as in SuiteSparse QR, and detailed in the following paper: *Tim Davis, "Algorithm 915, SuiteSparseQR: Multifrontal Multithreaded Rank-Revealing [Sparse](structeigen_1_1sparse) QR Factorization, ACM Trans. on Math. Soft. 38(1), 2011.* Even though it is qualified as "rank-revealing", this strategy might fail for some rank deficient problems. When this class is used to solve linear or least-square problems it is thus strongly recommended to check the accuracy of the computed solution. If it failed, it usually helps to increase the threshold with setPivotThreshold.
Warning
The input sparse matrix A must be in compressed mode (see [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd)). For complex matrices [matrixQ()](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1).transpose() will actually return the adjoint matrix.
| |
| --- |
| |
| void | [analyzePattern](classeigen_1_1sparseqr#a4b425ddb1358c914d764cde48853a4f6) (const MatrixType &mat) |
| | Preprocessing step of a QR factorization. [More...](classeigen_1_1sparseqr#a4b425ddb1358c914d764cde48853a4f6) |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [cols](classeigen_1_1sparseqr#a57bedc9b1351c0995bae8ad0088a6fce) () const |
| |
| const [PermutationType](classeigen_1_1permutationmatrix) & | [colsPermutation](classeigen_1_1sparseqr#a140930ebbf89dfd57a173761716db38f) () const |
| |
| void | [compute](classeigen_1_1sparseqr#aedaf52b7543de4d55c58c8f830c2aeb7) (const MatrixType &mat) |
| |
| void | [factorize](classeigen_1_1sparseqr#a55a34bacf05bd30a1dacbccad9f03c6d) (const MatrixType &mat) |
| | Performs the numerical QR factorization of the input matrix. [More...](classeigen_1_1sparseqr#a55a34bacf05bd30a1dacbccad9f03c6d) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1sparseqr#a234b0580aaf57237393f2e73a3d38690) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1sparseqr#a234b0580aaf57237393f2e73a3d38690) |
| |
| std::string | [lastErrorMessage](classeigen_1_1sparseqr#a1222e59649d77125d91f1368cf293c63) () const |
| |
| SparseQRMatrixQReturnType< [SparseQR](classeigen_1_1sparseqr) > | [matrixQ](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1) () const |
| |
| const [QRMatrixType](classeigen_1_1sparsematrix) & | [matrixR](classeigen_1_1sparseqr#a564524ff13b2b6dd1e76127404f7b920) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [rank](classeigen_1_1sparseqr#a70ec2b9e5cb62a41dc1ee2adfb54e9b0) () const |
| |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [rows](classeigen_1_1sparseqr#ab9133b7ace1c19714df99f553666316d) () const |
| |
| void | [setPivotThreshold](classeigen_1_1sparseqr#adb7bfa65f99e3ef91ed58ea663a850a1) (const RealScalar &threshold) |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [SparseQR](classeigen_1_1sparseqr), Rhs > | [solve](classeigen_1_1sparseqr#aea13a2c6823cd8408ba49afde9b3d4e4) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &B) const |
| |
| | [SparseQR](classeigen_1_1sparseqr#ac50f705d686d4bc687ce6acbc76447d2) (const MatrixType &mat) |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< SparseQR< \_MatrixType, \_OrderingType > >](classeigen_1_1sparsesolverbase) |
| const [Solve](classeigen_1_1solve)< [SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [Solve](classeigen_1_1solve)< [SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
SparseQR()
----------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::[SparseQR](classeigen_1_1sparseqr) | ( | const MatrixType & | *mat* | ) | |
| inlineexplicit |
Construct a QR factorization of the matrix *mat*.
Warning
The matrix *mat* must be in compressed mode (see [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd)).
See also
[compute()](classeigen_1_1sparseqr#aedaf52b7543de4d55c58c8f830c2aeb7)
analyzePattern()
----------------
template<typename MatrixType , typename OrderingType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SparseQR](classeigen_1_1sparseqr)< MatrixType, OrderingType >::analyzePattern | ( | const MatrixType & | *mat* | ) | |
Preprocessing step of a QR factorization.
Warning
The matrix *mat* must be in compressed mode (see [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd)).
In this step, the fill-reducing permutation is computed and applied to the columns of A and the column elimination tree is computed as well. Only the sparsity pattern of *mat* is exploited.
Note
In this step it is assumed that there is no empty row in the matrix *mat*.
cols()
------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::cols | ( | void | | ) | const |
| inline |
Returns
the number of columns of the represented matrix.
colsPermutation()
-----------------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationType](classeigen_1_1permutationmatrix)& [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::colsPermutation | ( | | ) | const |
| inline |
Returns
a const reference to the column permutation P that was applied to A such that A\*P = Q\*R It is the combination of the fill-in reducing permutation and numerical column pivoting.
compute()
---------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::compute | ( | const MatrixType & | *mat* | ) | |
| inline |
Computes the QR factorization of the sparse matrix *mat*.
Warning
The matrix *mat* must be in compressed mode (see [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd)).
See also
[analyzePattern()](classeigen_1_1sparseqr#a4b425ddb1358c914d764cde48853a4f6 "Preprocessing step of a QR factorization."), [factorize()](classeigen_1_1sparseqr#a55a34bacf05bd30a1dacbccad9f03c6d "Performs the numerical QR factorization of the input matrix.")
factorize()
-----------
template<typename MatrixType , typename OrderingType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SparseQR](classeigen_1_1sparseqr)< MatrixType, OrderingType >::factorize | ( | const MatrixType & | *mat* | ) | |
Performs the numerical QR factorization of the input matrix.
The function [SparseQR::analyzePattern(const MatrixType&)](classeigen_1_1sparseqr#a4b425ddb1358c914d764cde48853a4f6 "Preprocessing step of a QR factorization.") must have been called beforehand with a matrix having the same sparsity pattern than *mat*.
Parameters
| | |
| --- | --- |
| mat | The sparse column-major matrix |
info()
------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
Returns
`Success` if computation was successful, `NumericalIssue` if the QR factorization reports a numerical problem `InvalidInput` if the input matrix is invalid
See also
iparm()
lastErrorMessage()
------------------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| std::string [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::lastErrorMessage | ( | | ) | const |
| inline |
Returns
A string describing the type of error. This method is provided to ease debugging, not to handle errors.
matrixQ()
---------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| SparseQRMatrixQReturnType<[SparseQR](classeigen_1_1sparseqr)> [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::matrixQ | ( | void | | ) | const |
| inline |
Returns
an expression of the matrix Q as products of sparse Householder reflectors. The common usage of this function is to apply it to a dense matrix or vector
```
VectorXd B1, B2;
// Initialize B1
B2 = [matrixQ](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1)() * B1;
```
To get a plain [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") representation of Q:
```
SparseMatrix<double> Q;
Q = SparseQR<SparseMatrix<double> >(A).[matrixQ](classeigen_1_1sparseqr#ae1cc0a836c177d4f42600f8639354be1)();
```
Internally, this call simply performs a sparse product between the matrix Q and a sparse identity matrix. However, due to the fact that the sparse reflectors are stored unsorted, two transpositions are needed to sort them before performing the product.
matrixR()
---------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [QRMatrixType](classeigen_1_1sparsematrix)& [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::matrixR | ( | | ) | const |
| inline |
Returns
a const reference to the **sparse** upper triangular matrix R of the QR factorization.
Warning
The entries of the returned matrix are not sorted. This means that using it in algorithms expecting sorted entries will fail. This include random coefficient accesses (SpaseMatrix::coeff()), and coefficient-wise operations. [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") products and triangular solves are fine though.
To sort the entries, you can assign it to a row-major matrix, and if a column-major matrix is required, you can copy it again:
```
SparseMatrix<double> R = qr.matrixR(); // column-major, not sorted!
SparseMatrix<double,RowMajor> Rr = qr.matrixR(); // row-major, sorted
SparseMatrix<double> Rc = Rr; // column-major, sorted
```
rank()
------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::rank | ( | | ) | const |
| inline |
Returns
the number of non linearly dependent columns as determined by the pivoting threshold.
See also
[setPivotThreshold()](classeigen_1_1sparseqr#adb7bfa65f99e3ef91ed58ea663a850a1)
rows()
------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::rows | ( | void | | ) | const |
| inline |
Returns
the number of rows of the represented matrix.
setPivotThreshold()
-------------------
template<typename \_MatrixType , typename \_OrderingType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::setPivotThreshold | ( | const RealScalar & | *threshold* | ) | |
| inline |
Sets the threshold that is used to determine linearly dependent columns during the factorization.
In practice, if during the factorization the norm of the column that has to be eliminated is below this threshold, then the entire column is treated as zero, and it is moved at the end.
solve()
-------
template<typename \_MatrixType , typename \_OrderingType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[SparseQR](classeigen_1_1sparseqr), Rhs> [Eigen::SparseQR](classeigen_1_1sparseqr)< \_MatrixType, \_OrderingType >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *B* | ) | const |
| inline |
Returns
the solution X of \( A X = B \) using the current decomposition of A.
See also
[compute()](classeigen_1_1sparseqr#aedaf52b7543de4d55c58c8f830c2aeb7)
---
The documentation for this class was generated from the following file:* [SparseQR.h](https://eigen.tuxfamily.org/dox/SparseQR_8h_source.html)
eigen3 Eigen::CommaInitializer Eigen::CommaInitializer
=======================
### template<typename XprType> class Eigen::CommaInitializer< XprType >
Helper class used by the comma initializer operator.
This class is internally used to implement the comma initializer feature. It is the return type of [MatrixBase::operator<<](classeigen_1_1densebase#a0e575eb0ba6cc6bc5f347872abd8509d), and most of the time this is the only way it is used.
See also
[MatrixBase::operator<<](classeigen_1_1densebase#MatrixBaseCommaInitRef), [CommaInitializer::finished()](structeigen_1_1commainitializer#a3cf9e2b8a227940f50103130b2d2859a)
| |
| --- |
| |
| XprType & | [finished](structeigen_1_1commainitializer#a3cf9e2b8a227940f50103130b2d2859a) () |
| |
finished()
----------
template<typename XprType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| XprType& [Eigen::CommaInitializer](structeigen_1_1commainitializer)< XprType >::finished | ( | | ) | |
| inline |
Returns
the built matrix once all its coefficients have been set. Calling finished is 100% optional. Its purpose is to write expressions like this:
```
quaternion.fromRotationMatrix((Matrix3f() << axis0, axis1, axis2).[finished](structeigen_1_1commainitializer#a3cf9e2b8a227940f50103130b2d2859a)());
```
---
The documentation for this class was generated from the following file:* [CommaInitializer.h](https://eigen.tuxfamily.org/dox/CommaInitializer_8h_source.html)
eigen3 Eigen::PlainObjectBase Eigen::PlainObjectBase
======================
### template<typename Derived> class Eigen::PlainObjectBase< Derived >
Dense storage base class for matrices and arrays.
This class can be extended with the help of the plugin mechanism described on the page [Extending MatrixBase (and other classes)](topiccustomizing_plugins) by defining the preprocessor symbol `EIGEN_PLAINOBJECTBASE_PLUGIN`.
Template Parameters
| | |
| --- | --- |
| Derived | is the derived type, e.g., a [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") or [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.") |
See also
[The class hierarchy](topicclasshierarchy)

| |
| --- |
| |
| const Scalar & | [coeff](classeigen_1_1plainobjectbase#ac99d445913f04acc50280ae99dffd9c3) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) index) const |
| |
| const Scalar & | [coeff](classeigen_1_1plainobjectbase#a954cd075bcd7babb429e3e4b9a418651) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rowId, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) colId) const |
| |
| Scalar & | [coeffRef](classeigen_1_1plainobjectbase#a72e84dc1bb573ad8ecc9109fbbc1b63b) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) index) |
| |
| const Scalar & | [coeffRef](classeigen_1_1plainobjectbase#a541526a4f452554785e78bc41287b348) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) index) const |
| |
| Scalar & | [coeffRef](classeigen_1_1plainobjectbase#a992d58b5453e441dcfc80f21c2bfd1d7) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rowId, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) colId) |
| |
| const Scalar & | [coeffRef](classeigen_1_1plainobjectbase#a038a419ccb6e2c55593b27f17626fd62) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rowId, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) colId) const |
| |
| void | [conservativeResize](classeigen_1_1plainobjectbase#a712c25be1652e5a64a00f28c8ed11462) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| void | [conservativeResize](classeigen_1_1plainobjectbase#a8c9b27a1df4d180b9fb5755bebea2dbd) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t) |
| |
| void | [conservativeResize](classeigen_1_1plainobjectbase#a78a42a7c0be768374781f67f40c9ab0d) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| void | [conservativeResize](classeigen_1_1plainobjectbase#afc474a09ec9704629b795d7907fb6c37) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| template<typename OtherDerived > |
| void | [conservativeResizeLike](classeigen_1_1plainobjectbase#a4ece7540eda6a1ae7d3730397ce72bec) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| Scalar \* | [data](classeigen_1_1plainobjectbase#ad12a492bcadea9b65ccd9bc8404c01f1) () |
| |
| const Scalar \* | [data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116) () const |
| |
| template<typename OtherDerived > |
| Derived & | [lazyAssign](classeigen_1_1plainobjectbase#a70fc6030f9ee72fbe0b3adade2a4a2bd) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| Derived & | [operator=](classeigen_1_1plainobjectbase#ad90648194e9fa6a0e1296ba1e4db8787) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| | Copies the generic expression *other* into \*this. [More...](classeigen_1_1plainobjectbase#ad90648194e9fa6a0e1296ba1e4db8787) |
| |
| Derived & | [operator=](classeigen_1_1plainobjectbase#afbf3af8d6195c9b1b2103c2dd1231247) (const [PlainObjectBase](classeigen_1_1plainobjectbase) &other) |
| |
| void | [resize](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| void | [resize](classeigen_1_1plainobjectbase#ae4bbe4c06c1feb1035573eee7f5c3623) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t) |
| |
| void | [resize](classeigen_1_1plainobjectbase#a8bee1e51417bfa386dd54b37f6d9e2fe) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| void | [resize](classeigen_1_1plainobjectbase#a0dd078df3ff8b3833723ce84ce519651) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| template<typename OtherDerived > |
| void | [resizeLike](classeigen_1_1plainobjectbase#aabf64c98e5415ad39828a83cc5bdac40) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &\_other) |
| |
| |
| --- |
| |
| template<typename OtherDerived > |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#a73dca0493df0fe4f8e518e379a80cbdd) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#a8fa7e42fd02b266ac54d57cdc735e83d) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#a520234520136dffad88301a507da4fa5) (const [PlainObjectBase](classeigen_1_1plainobjectbase) &other) |
| |
| template<typename OtherDerived > |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#a281044f167c388339c2d704e5d292fa5) (const ReturnByValue< OtherDerived > &other) |
| | Copy constructor with in-place evaluation. |
| |
| template<typename... ArgTypes> |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#a5b6ba62bc9d263390e81a77a44f23dd9) (const Scalar &a0, const Scalar &a1, const Scalar &a2, const Scalar &a3, const ArgTypes &... args) |
| | Construct a row of column vector with fixed size from an arbitrary number of coefficients. [c++11] [More...](classeigen_1_1plainobjectbase#a5b6ba62bc9d263390e81a77a44f23dd9) |
| |
| | [PlainObjectBase](classeigen_1_1plainobjectbase#ab6cde095b242924b47479662b16d78b9) (const std::initializer\_list< std::initializer\_list< Scalar >> &list) |
| | Constructs a [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") or [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.") and initializes it by elements given by an initializer list of initializer lists [c++11] |
| |
| |
| --- |
| |
| These are convenience functions returning [Map](classeigen_1_1map "A matrix or vector expression mapping an existing array of data.") objects. The [Map()](classeigen_1_1plainobjectbase#aaf9fcc07dc13f89cf71d4a4e2b220d24) static functions return unaligned [Map](classeigen_1_1map "A matrix or vector expression mapping an existing array of data.") objects, while the AlignedMap() functions return aligned [Map](classeigen_1_1map "A matrix or vector expression mapping an existing array of data.") objects and thus should be called only with 16-byte-aligned *data* pointers. Here is an example using strides:
```
Matrix4i A;
A << 1, 2, 3, 4,
5, 6, 7, 8,
9, 10, 11, 12,
13, 14, 15, 16;
std::cout << Matrix2i::Map(&A(1,1),Stride<8,2>()) << std::endl;
```
Output:
```
6 8
14 16
```
See also
class [Map](classeigen_1_1map "A matrix or vector expression mapping an existing array of data.")
|
| Derived & | [setConstant](classeigen_1_1plainobjectbase#aac6bc5261783ec3008a51c2654de73e8) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const Scalar &val) |
| |
| Derived & | [setConstant](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const Scalar &val) |
| |
| Derived & | [setConstant](classeigen_1_1plainobjectbase#a56e04c9e00a84eeb26774842f4a0f6fd) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const Scalar &val) |
| |
| Derived & | [setConstant](classeigen_1_1plainobjectbase#ae521bf911dfa2823c8056dc9c1776bcd) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t, const Scalar &val) |
| |
| Derived & | [setZero](classeigen_1_1plainobjectbase#acc39eaf7ba22b725c86f1b9b8bb57c3c) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| Derived & | [setZero](classeigen_1_1plainobjectbase#a73ab57abb640bf35e0dbf9dba225a1db) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setZero](classeigen_1_1plainobjectbase#af57b7361afefe60e2940802e7ef2ca54) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setZero](classeigen_1_1plainobjectbase#aee6d32f2e6615645b5f1152f99bc8549) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t) |
| |
| Derived & | [setOnes](classeigen_1_1plainobjectbase#a8700dc6d8e05436c0b34ae15ca9274a5) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| Derived & | [setOnes](classeigen_1_1plainobjectbase#ad06b9d8ddac261a871c9ff550a925975) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setOnes](classeigen_1_1plainobjectbase#a3bd80eb3e6779ba362628b1cb62a665e) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setOnes](classeigen_1_1plainobjectbase#ab04727b1a70e7d0b5ce9d004b3349075) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t) |
| |
| Derived & | [setRandom](classeigen_1_1plainobjectbase#a5f0f6cc8039ed5ac026cd32ed5bbe6ea) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| Derived & | [setRandom](classeigen_1_1plainobjectbase#a8921e8a7f9a5ea167231d29f8feb8700) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setRandom](classeigen_1_1plainobjectbase#a66a2a4ffc386ca72214d0ac3161fdc03) (NoChange\_t, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| Derived & | [setRandom](classeigen_1_1plainobjectbase#ae705648096d7e74b32daa82dd297dc54) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, NoChange\_t) |
| |
| template<typename OtherDerived > |
| void | [\_resize\_to\_match](classeigen_1_1plainobjectbase#a35c62d0feeb92a83704d518402cdbfdf) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| Derived & | [\_set](classeigen_1_1plainobjectbase#a09c4b519ee4c635144581e9fe03b6174) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| | Copies the value of the expression *other* into `*this` with automatic resizing. [More...](classeigen_1_1plainobjectbase#a09c4b519ee4c635144581e9fe03b6174) |
| |
| template<typename OtherDerived > |
| Derived & | [\_set\_noalias](classeigen_1_1plainobjectbase#af638f6f03edf4384b1ff1070485987b6) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename T0 , typename T1 > |
| void | [\_init2](classeigen_1_1plainobjectbase#a54a3d3aba10bf017b41f0dbd9342dea0) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, typename internal::enable\_if< Base::SizeAtCompileTime!=2, T0 >::type \*=0) |
| |
| template<typename T0 , typename T1 > |
| void | [\_init2](classeigen_1_1plainobjectbase#a2eaf38d7a71d5c0849a9dcb3396abe89) (const T0 &val0, const T1 &val1, typename internal::enable\_if< Base::SizeAtCompileTime==2, T0 >::type \*=0) |
| |
| template<typename T0 , typename T1 > |
| void | [\_init2](classeigen_1_1plainobjectbase#ac9c37eb6466e33da2af0a2f5e673c764) (const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) &val0, const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) &val1, typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< T0, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) >::value) &&(internal::is\_same< T1, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) >::value) &&Base::SizeAtCompileTime==2, T1 >::type \*=0) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#aeb0be79ed80a425cad223d47ccda07b8) ([Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, typename internal::enable\_if<(Base::SizeAtCompileTime!=1||!internal::is\_convertible< T, Scalar >::value) &&((!internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value||Base::SizeAtCompileTime==[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2))), T >::type \*=0) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#a8295af1e8236ad2be1a1dc8023a49edf) (const Scalar &val0, typename internal::enable\_if< Base::SizeAtCompileTime==1 &&internal::is\_convertible< T, Scalar >::value, T >::type \*=0) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#ad5830b59e4c7b759c72afe0c2e28cced) (const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) &val0, typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), T >::value) &&Base::SizeAtCompileTime==1 &&internal::is\_convertible< T, Scalar >::value, T \* >::type \*=0) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#aa688fc09ad2cf7e22fc027618812aec3) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)) |
| |
| template<typename T , typename OtherDerived > |
| void | [\_init1](classeigen_1_1plainobjectbase#a6da7fb21280c9919478741e53116cd9c) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#ab26db59f31522ed08b3ffed51dfd20f7) (const Derived &other) |
| |
| template<typename T , typename OtherDerived > |
| void | [\_init1](classeigen_1_1plainobjectbase#a49cdb807ab54602a8f2fd51dcc0ace92) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| |
| template<typename T , typename OtherDerived > |
| void | [\_init1](classeigen_1_1plainobjectbase#aad467f7b89f967fe3fdf7c8420ef5eb9) (const ReturnByValue< OtherDerived > &other) |
| |
| template<typename T , typename OtherDerived , int ColsAtCompileTime> |
| void | [\_init1](classeigen_1_1plainobjectbase#a507e484ddddd9fb4e09de46c3b52e874) (const [RotationBase](classeigen_1_1rotationbase)< OtherDerived, ColsAtCompileTime > &r) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#a2cdf8906cbc61e655beae78baafbf4d6) (const Scalar &val0, typename internal::enable\_if< Base::SizeAtCompileTime!=[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) &&Base::SizeAtCompileTime!=1 &&internal::is\_convertible< T, Scalar >::value &&internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value, T >::type \*=0) |
| |
| template<typename T > |
| void | [\_init1](classeigen_1_1plainobjectbase#a2f2ffa0c97bf3eb697743d91bfe45f00) (const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) &val0, typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), T >::value) &&Base::SizeAtCompileTime!=[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) &&Base::SizeAtCompileTime!=1 &&internal::is\_convertible< T, Scalar >::value &&internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value, T \* >::type \*=0) |
| |
| static [ConstMapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#aaf9fcc07dc13f89cf71d4a4e2b220d24) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)) |
| |
| static [MapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#ab5392255cbc16c3d3d91b09088e027b4) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)) |
| |
| static [ConstMapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#abd3e2e293d1d8591a5e6772ecc4d2c4c) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static [MapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#ab195765374a1d5dee35dff790346bd42) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static [ConstMapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#adffe779fdca149b5eba4dc542bf76b94) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static [MapType](classeigen_1_1map) | [Map](classeigen_1_1plainobjectbase#ad1815d868cb69ebba3d4e1c9e263d2af) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static [ConstAlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#ae618ce2543ce8deef2a838864de2b610) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)) |
| |
| static [AlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#aa3281e4121e5e404f93ee9890c13e1dd) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)) |
| |
| static [ConstAlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#a7314ec5e42ff5f3563cf3f77090d8318) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static [AlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#a62d868608b47d93e3e0c86339870bc43) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size) |
| |
| static [ConstAlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#a8b161ab58c8370b97ac8131efb6964dc) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| static [AlignedMapType](classeigen_1_1map) | [MapAligned](classeigen_1_1plainobjectbase#a98a4e03be918eb01bd7719d260debe1a) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols) |
| |
| template<int Outer, int Inner> |
| static StridedConstMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#a8ed320f4d926ab401117564cafc3e232) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#a240ab54b8839d02bdd35e358a3d66b73) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedConstMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#aebf9df309b9b6f4e5cc0d495a423f749) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#aaf22e2ddbebc397d1e6b17a95af8e7d4) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedConstMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#a1a3a8fd56500cda9a96189cd55472203) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [Map](classeigen_1_1plainobjectbase#ad41d75bfc07b50b2912f99212a7265ed) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedConstAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#aadc343ac6294b26d8e2033a1c1400600) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#abd8ce90b470cfa30cae08f9c673912c9) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedConstAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#a91374e2e77a219a44d980cc14be3a961) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#a2b1ce06138d53af1bb729ec1dab87e5a) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) size, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedConstAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#a48fbb5cbf164540617c5ca3e05afe953) (const Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
| template<int Outer, int Inner> |
| static StridedAlignedMapType< [Stride](classeigen_1_1stride)< Outer, Inner > >::type | [MapAligned](classeigen_1_1plainobjectbase#af2ce47a0cde3943e528e00f2b191f7da) (Scalar \*[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116), [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) rows, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) cols, const [Stride](classeigen_1_1stride)< Outer, Inner > &stride) |
| |
PlainObjectBase() [1/4]
-----------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[PlainObjectBase](classeigen_1_1plainobjectbase) | ( | const [PlainObjectBase](classeigen_1_1plainobjectbase)< Derived > & | *other* | ) | |
| inlineprotected |
Copy constructor
PlainObjectBase() [2/4]
-----------------------
template<typename Derived > template<typename... ArgTypes>
| | | | | | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[PlainObjectBase](classeigen_1_1plainobjectbase) | ( | const Scalar & | *a0*, |
| | | const Scalar & | *a1*, |
| | | const Scalar & | *a2*, |
| | | const Scalar & | *a3*, |
| | | const ArgTypes &... | *args* |
| | ) | | |
| inlineprotected |
Construct a row of column vector with fixed size from an arbitrary number of coefficients. [c++11]
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
This constructor is for 1D array or vectors with more than 4 coefficients. There exists C++98 analogue constructors for fixed-size array/vector having 1, 2, 3, or 4 coefficients.
Warning
To construct a column (resp. row) vector of fixed length, the number of values passed to this constructor must match the the fixed number of rows (resp. columns) of `*this`.
PlainObjectBase() [3/4]
-----------------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[PlainObjectBase](classeigen_1_1plainobjectbase) | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
See also
[PlainObjectBase::operator=(const EigenBase<OtherDerived>&)](classeigen_1_1plainobjectbase#ad90648194e9fa6a0e1296ba1e4db8787 "Copies the generic expression other into *this.")
PlainObjectBase() [4/4]
-----------------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[PlainObjectBase](classeigen_1_1plainobjectbase) | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
See also
[PlainObjectBase::operator=(const EigenBase<OtherDerived>&)](classeigen_1_1plainobjectbase#ad90648194e9fa6a0e1296ba1e4db8787 "Copies the generic expression other into *this.")
\_init1() [1/11]
----------------
template<typename Derived > template<typename T , typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [2/11]
----------------
template<typename Derived > template<typename T >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const Derived & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [3/11]
----------------
template<typename Derived > template<typename T , typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [4/11]
----------------
template<typename Derived > template<typename T >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) & | *val0*, |
| | | typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), T >::value) &&Base::SizeAtCompileTime!=[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) &&Base::SizeAtCompileTime!=1 &&internal::is\_convertible< T, Scalar >::value &&internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value, T \* >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [5/11]
----------------
template<typename Derived > template<typename T >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) & | *val0*, |
| | | typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), T >::value) &&Base::SizeAtCompileTime==1 &&internal::is\_convertible< T, Scalar >::value, T \* >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [6/11]
----------------
template<typename Derived > template<typename T , typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const ReturnByValue< OtherDerived > & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [7/11]
----------------
template<typename Derived > template<typename T , typename OtherDerived , int ColsAtCompileTime>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const [RotationBase](classeigen_1_1rotationbase)< OtherDerived, ColsAtCompileTime > & | *r* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [8/11]
----------------
template<typename Derived > template<typename T >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const Scalar & | *val0*, |
| | | typename internal::enable\_if< Base::SizeAtCompileTime!=[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) &&Base::SizeAtCompileTime!=1 &&internal::is\_convertible< T, Scalar >::value &&internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value, T >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [9/11]
----------------
template<typename Derived > template<typename T >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const Scalar & | *val0*, |
| | | typename internal::enable\_if< Base::SizeAtCompileTime==1 &&internal::is\_convertible< T, Scalar >::value, T >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [10/11]
-----------------
template<typename Derived > template<typename T >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | const Scalar \* | *data* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init1() [11/11]
-----------------
template<typename Derived > template<typename T >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init1 | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | typename internal::enable\_if<(Base::SizeAtCompileTime!=1||!internal::is\_convertible< T, Scalar >::value) &&((!internal::is\_same< typename internal::traits< Derived >::XprKind, [ArrayXpr](structeigen_1_1arrayxpr) >::value||Base::SizeAtCompileTime==[Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2))), T >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init2() [1/3]
---------------
template<typename Derived > template<typename T0 , typename T1 >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init2 | ( | const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) & | *val0*, |
| | | const [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) & | *val1*, |
| | | typename internal::enable\_if<(!internal::is\_same< [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde), Scalar >::value) &&(internal::is\_same< T0, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) >::value) &&(internal::is\_same< T1, [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) >::value) &&Base::SizeAtCompileTime==2, T1 >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init2() [2/3]
---------------
template<typename Derived > template<typename T0 , typename T1 >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init2 | ( | const T0 & | *val0*, |
| | | const T1 & | *val1*, |
| | | typename internal::enable\_if< Base::SizeAtCompileTime==2, T0 >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_init2() [3/3]
---------------
template<typename Derived > template<typename T0 , typename T1 >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_init2 | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | typename internal::enable\_if< Base::SizeAtCompileTime!=2, T0 >::type \* | = `0` |
| | ) | | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_resize\_to\_match()
---------------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_resize\_to\_match | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
\_set()
-------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_set | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
Copies the value of the expression *other* into `*this` with automatic resizing.
\*this might be resized to match the dimensions of *other*. If \*this was a null matrix (not already initialized), it will be initialized.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
See also
operator=(const MatrixBase<OtherDerived>&), [\_set\_noalias()](classeigen_1_1plainobjectbase#af638f6f03edf4384b1ff1070485987b6)
\_set\_noalias()
----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::\_set\_noalias | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inlineprotected |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
coeff() [1/2]
-------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeff | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *index* | ) | const |
| inline |
This is an overloaded version of [DenseCoeffsBase<Derived,ReadOnlyAccessors>::coeff(Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) provided to by-pass the creation of an evaluator of the expression, thus saving compilation efforts.
See [DenseCoeffsBase<Derived,ReadOnlyAccessors>::coeff(Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) for details.
coeff() [2/2]
-------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeff | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rowId*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *colId* |
| | ) | | const |
| inline |
This is an overloaded version of [DenseCoeffsBase<Derived,ReadOnlyAccessors>::coeff(Index,Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393) provided to by-pass the creation of an evaluator of the expression, thus saving compilation efforts.
See [DenseCoeffsBase<Derived,ReadOnlyAccessors>::coeff(Index) const](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) for details.
coeffRef() [1/4]
----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeffRef | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *index* | ) | |
| inline |
This is an overloaded version of DenseCoeffsBase<Derived,WriteAccessors>::coeffRef(Index) const provided to by-pass the creation of an evaluator of the expression, thus saving compilation efforts.
See DenseCoeffsBase<Derived,WriteAccessors>::coeffRef(Index) const for details.
coeffRef() [2/4]
----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeffRef | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *index* | ) | const |
| inline |
This is the const version of [coeffRef(Index)](classeigen_1_1plainobjectbase#a72e84dc1bb573ad8ecc9109fbbc1b63b) which is thus synonym of coeff(Index). It is provided for convenience.
coeffRef() [3/4]
----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeffRef | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rowId*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *colId* |
| | ) | | |
| inline |
This is an overloaded version of DenseCoeffsBase<Derived,WriteAccessors>::coeffRef(Index,Index) const provided to by-pass the creation of an evaluator of the expression, thus saving compilation efforts.
See DenseCoeffsBase<Derived,WriteAccessors>::coeffRef(Index,Index) const for details.
coeffRef() [4/4]
----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const Scalar& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::coeffRef | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rowId*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *colId* |
| | ) | | const |
| inline |
This is the const version of [coeffRef(Index,Index)](classeigen_1_1plainobjectbase#a992d58b5453e441dcfc80f21c2bfd1d7) which is thus synonym of coeff(Index,Index). It is provided for convenience.
conservativeResize() [1/4]
--------------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::conservativeResize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes the matrix to *rows* x *cols* while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use [conservativeResize(NoChange\_t, Index)](classeigen_1_1plainobjectbase#afc474a09ec9704629b795d7907fb6c37) or [conservativeResize(Index, NoChange\_t)](classeigen_1_1plainobjectbase#a8c9b27a1df4d180b9fb5755bebea2dbd).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will be uninitialized.
conservativeResize() [2/4]
--------------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::conservativeResize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | |
| | ) | | |
| inline |
Resizes the matrix to *rows* x *cols* while leaving old values untouched.
As opposed to [conservativeResize(Index rows, Index cols)](classeigen_1_1plainobjectbase#a712c25be1652e5a64a00f28c8ed11462), this version leaves the number of columns unchanged.
In case the matrix is growing, new rows will be uninitialized.
conservativeResize() [3/4]
--------------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::conservativeResize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* | ) | |
| inline |
Resizes the vector to *size* while retaining old values.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
When values are appended, they will be uninitialized.
conservativeResize() [4/4]
--------------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::conservativeResize | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes the matrix to *rows* x *cols* while leaving old values untouched.
As opposed to [conservativeResize(Index rows, Index cols)](classeigen_1_1plainobjectbase#a712c25be1652e5a64a00f28c8ed11462), this version leaves the number of rows unchanged.
In case the matrix is growing, new columns will be uninitialized.
conservativeResizeLike()
------------------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::conservativeResizeLike | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Resizes the matrix to *rows* x *cols* of `other`, while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use [conservativeResize(NoChange\_t, Index)](classeigen_1_1plainobjectbase#afc474a09ec9704629b795d7907fb6c37) or [conservativeResize(Index, NoChange\_t)](classeigen_1_1plainobjectbase#a8c9b27a1df4d180b9fb5755bebea2dbd).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will copied from `other`.
data() [1/2]
------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar\* [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::data | ( | | ) | |
| inline |
Returns
a pointer to the data array of this matrix
data() [2/2]
------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const Scalar\* [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::data | ( | | ) | const |
| inline |
Returns
a const pointer to the data array of this matrix
lazyAssign()
------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::lazyAssign | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
See also
[MatrixBase::lazyAssign()](classeigen_1_1densebase#a6bc6c096e3bfc726f28315daecd21b3f)
Map() [1/12]
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| static [ConstMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data* | ) | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [2/12]
------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [3/12]
------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [ConstMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [4/12]
------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [5/12]
------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [ConstMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [6/12]
------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [7/12]
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| static [MapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data* | ) | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [8/12]
------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [9/12]
------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [MapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [10/12]
-------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [11/12]
-------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [MapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
Map() [12/12]
-------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::[Map](classeigen_1_1map) | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [1/12]
-------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| static [ConstAlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data* | ) | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [2/12]
-------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [3/12]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [ConstAlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [4/12]
-------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [5/12]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [ConstAlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [6/12]
-------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedConstAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | const Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [7/12]
-------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| static [AlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data* | ) | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [8/12]
-------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [9/12]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [AlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [10/12]
--------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [11/12]
--------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static [AlignedMapType](classeigen_1_1map) [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
MapAligned() [12/12]
--------------------
template<typename Derived > template<int Outer, int Inner>
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| static StridedAlignedMapType<[Stride](classeigen_1_1stride)<Outer, Inner> >::type [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::MapAligned | ( | Scalar \* | *data*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | const [Stride](classeigen_1_1stride)< Outer, Inner > & | *stride* |
| | ) | | |
| inlinestatic |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
operator=() [1/2]
-----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::operator= | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *other* | ) | |
| inline |
Copies the generic expression *other* into \*this.
The expression must provide a (templated) evalTo(Derived& dst) const function which does the actual job. In practice, this allows any user to write its own special matrix without having to modify [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")
Returns
a reference to \*this.
operator=() [2/2]
-----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::operator= | ( | const [PlainObjectBase](classeigen_1_1plainobjectbase)< Derived > & | *other* | ) | |
| inline |
This is a special case of the templated operator=. Its purpose is to prevent a default operator= from hiding the templated operator=.
resize() [1/4]
--------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::resize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes `*this` to a *rows* x *cols* matrix.
This method is intended for dynamic-size matrices, although it is legal to call it on any matrix as long as fixed dimensions are left unchanged. If you only want to change the number of rows and/or of columns, you can use [resize(NoChange\_t, Index)](classeigen_1_1plainobjectbase#a0dd078df3ff8b3833723ce84ce519651), [resize(Index, NoChange\_t)](classeigen_1_1plainobjectbase#ae4bbe4c06c1feb1035573eee7f5c3623).
If the current number of coefficients of `*this` exactly matches the product *rows* \* *cols*, then no memory allocation is performed and the current values are left unchanged. In all other cases, including shrinking, the data is reallocated and all previous values are lost.
Example:
```
MatrixXd m(2,3);
m << 1,2,3,4,5,6;
cout << "here's the 2x3 matrix m:" << endl << m << endl;
cout << "let's resize m to 3x2. This is a conservative resizing because 2\*3==3\*2." << endl;
m.resize(3,2);
cout << "here's the 3x2 matrix m:" << endl << m << endl;
cout << "now let's resize m to size 2x2. This is NOT a conservative resizing, so it becomes uninitialized:" << endl;
m.resize(2,2);
cout << m << endl;
```
Output:
```
here's the 2x3 matrix m:
1 2 3
4 5 6
let's resize m to 3x2. This is a conservative resizing because 2*3==3*2.
here's the 3x2 matrix m:
1 5
4 3
2 6
now let's resize m to size 2x2. This is NOT a conservative resizing, so it becomes uninitialized:
0 0
0 0
```
See also
[resize(Index)](classeigen_1_1plainobjectbase#a8bee1e51417bfa386dd54b37f6d9e2fe) for vectors, [resize(NoChange\_t, Index)](classeigen_1_1plainobjectbase#a0dd078df3ff8b3833723ce84ce519651), [resize(Index, NoChange\_t)](classeigen_1_1plainobjectbase#ae4bbe4c06c1feb1035573eee7f5c3623)
resize() [2/4]
--------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::resize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | |
| | ) | | |
| inline |
Resizes the matrix, changing only the number of rows. For the parameter of type NoChange\_t, just pass the special value `NoChange` as in the example below.
Example:
```
MatrixXd m(3,4);
m.resize(5, NoChange);
cout << "m: " << m.rows() << " rows, " << m.cols() << " cols" << endl;
```
Output:
```
m: 5 rows, 4 cols
```
See also
[resize(Index,Index)](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a)
resize() [3/4]
--------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::resize | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size* | ) | |
| inline |
Resizes `*this` to a vector of length *size*
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
Example:
```
VectorXd v(10);
v.resize(3);
RowVector3d w;
w.resize(3); // this is legal, but has no effect
cout << "v: " << v.rows() << " rows, " << v.cols() << " cols" << endl;
cout << "w: " << w.rows() << " rows, " << w.cols() << " cols" << endl;
```
Output:
```
v: 3 rows, 1 cols
w: 1 rows, 3 cols
```
See also
[resize(Index,Index)](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a), [resize(NoChange\_t, Index)](classeigen_1_1plainobjectbase#a0dd078df3ff8b3833723ce84ce519651), [resize(Index, NoChange\_t)](classeigen_1_1plainobjectbase#ae4bbe4c06c1feb1035573eee7f5c3623)
resize() [4/4]
--------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::resize | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes the matrix, changing only the number of columns. For the parameter of type NoChange\_t, just pass the special value `NoChange` as in the example below.
Example:
```
MatrixXd m(3,4);
m.resize(NoChange, 5);
cout << "m: " << m.rows() << " rows, " << m.cols() << " cols" << endl;
```
Output:
```
m: 3 rows, 5 cols
```
See also
[resize(Index,Index)](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a)
resizeLike()
------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::resizeLike | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *\_other* | ) | |
| inline |
Resizes `*this` to have the same dimensions as *other*. Takes care of doing all the checking that's needed.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
setConstant() [1/4]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setConstant | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const Scalar & | *val* |
| | ) | | |
| inline |
Resizes to the given size, and sets all coefficients in this expression to the given value *val*.
Parameters
| | |
| --- | --- |
| rows | the new number of rows |
| cols | the new number of columns |
| val | the value to which all coefficients are set |
Example:
```
MatrixXf m;
m.setConstant(3, 3, 5);
cout << m << endl;
```
Output:
```
5 5 5
5 5 5
5 5 5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,const Scalar&)](classeigen_1_1plainobjectbase#aac6bc5261783ec3008a51c2654de73e8), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
setConstant() [2/4]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setConstant | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | , |
| | | const Scalar & | *val* |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of rows, and sets all coefficients in this expression to the given value *val*. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,const Scalar&)](classeigen_1_1plainobjectbase#aac6bc5261783ec3008a51c2654de73e8), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
setConstant() [3/4]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setConstant | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *size*, |
| | | const Scalar & | *val* |
| | ) | | |
| inline |
Resizes to the given *size*, and sets all coefficients in this expression to the given value *val*.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setConstant(3, 5);
cout << v << endl;
```
Output:
```
5
5
5
```
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,Index,const Scalar&)](classeigen_1_1plainobjectbase#af9996d6a98f45e84a908dc9851c8332e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
setConstant() [4/4]
-------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setConstant | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols*, |
| | | const Scalar & | *val* |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of columns, and sets all coefficients in this expression to the given value *val*. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[MatrixBase::setConstant(const Scalar&)](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [setConstant(Index,const Scalar&)](classeigen_1_1plainobjectbase#aac6bc5261783ec3008a51c2654de73e8), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Constant(const Scalar&)](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d)
setOnes() [1/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setOnes | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, and sets all coefficients in this expression to one.
Parameters
| | |
| --- | --- |
| rows | the new number of rows |
| cols | the new number of columns |
Example:
```
MatrixXf m;
m.setOnes(3, 3);
cout << m << endl;
```
Output:
```
1 1 1
1 1 1
1 1 1
```
See also
[MatrixBase::setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2), [setOnes(Index)](classeigen_1_1plainobjectbase#a8700dc6d8e05436c0b34ae15ca9274a5), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
setOnes() [2/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setOnes | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of rows, and sets all coefficients in this expression to one. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[MatrixBase::setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2), [setOnes(Index)](classeigen_1_1plainobjectbase#a8700dc6d8e05436c0b34ae15ca9274a5), [setOnes(Index, Index)](classeigen_1_1plainobjectbase#ad06b9d8ddac261a871c9ff550a925975), [setOnes(NoChange\_t, Index)](classeigen_1_1plainobjectbase#a3bd80eb3e6779ba362628b1cb62a665e), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
setOnes() [3/4]
---------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setOnes | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *newSize* | ) | |
| inline |
Resizes to the given *newSize*, and sets all coefficients in this expression to one.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setOnes(3);
cout << v << endl;
```
Output:
```
1
1
1
```
See also
[MatrixBase::setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2), [setOnes(Index,Index)](classeigen_1_1plainobjectbase#ad06b9d8ddac261a871c9ff550a925975), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
setOnes() [4/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setOnes | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of columns, and sets all coefficients in this expression to one. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[MatrixBase::setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2), [setOnes(Index)](classeigen_1_1plainobjectbase#a8700dc6d8e05436c0b34ae15ca9274a5), [setOnes(Index, Index)](classeigen_1_1plainobjectbase#ad06b9d8ddac261a871c9ff550a925975), [setOnes(Index, NoChange\_t)](classeigen_1_1plainobjectbase#ab04727b1a70e7d0b5ce9d004b3349075) class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [MatrixBase::Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
setRandom() [1/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setRandom | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, and sets all coefficients in this expression to random values.
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
Warning
This function is not re-entrant.
Parameters
| | |
| --- | --- |
| rows | the new number of rows |
| cols | the new number of columns |
Example:
```
MatrixXf m;
m.setRandom(3, 3);
cout << m << endl;
```
Output:
```
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
```
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [setRandom(Index)](classeigen_1_1plainobjectbase#a5f0f6cc8039ed5ac026cd32ed5bbe6ea), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
setRandom() [2/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setRandom | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of rows, and sets all coefficients in this expression to random values. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
Warning
This function is not re-entrant.
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [setRandom(Index)](classeigen_1_1plainobjectbase#a5f0f6cc8039ed5ac026cd32ed5bbe6ea), [setRandom(NoChange\_t, Index)](classeigen_1_1plainobjectbase#a66a2a4ffc386ca72214d0ac3161fdc03), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
setRandom() [3/4]
-----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setRandom | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *newSize* | ) | |
| inline |
Resizes to the given *newSize*, and sets all coefficients in this expression to random values.
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Warning
This function is not re-entrant.
Example:
```
VectorXf v;
v.setRandom(3);
cout << v << endl;
```
Output:
```
0.68
-0.211
0.566
```
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [setRandom(Index,Index)](classeigen_1_1plainobjectbase#a8921e8a7f9a5ea167231d29f8feb8700), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
setRandom() [4/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setRandom | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of columns, and sets all coefficients in this expression to random values. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
Warning
This function is not re-entrant.
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [setRandom(Index)](classeigen_1_1plainobjectbase#a5f0f6cc8039ed5ac026cd32ed5bbe6ea), [setRandom(Index, NoChange\_t)](classeigen_1_1plainobjectbase#ae705648096d7e74b32daa82dd297dc54), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
setZero() [1/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setZero | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, and sets all coefficients in this expression to zero.
Parameters
| | |
| --- | --- |
| rows | the new number of rows |
| cols | the new number of columns |
Example:
```
MatrixXf m;
m.setZero(3, 3);
cout << m << endl;
```
Output:
```
0 0 0
0 0 0
0 0 0
```
See also
[DenseBase::setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setZero(Index)](classeigen_1_1plainobjectbase#acc39eaf7ba22b725c86f1b9b8bb57c3c), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
setZero() [2/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setZero | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *rows*, |
| | | NoChange\_t | |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of rows, and sets all coefficients in this expression to zero. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[DenseBase::setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setZero(Index)](classeigen_1_1plainobjectbase#acc39eaf7ba22b725c86f1b9b8bb57c3c), [setZero(Index, Index)](classeigen_1_1plainobjectbase#a73ab57abb640bf35e0dbf9dba225a1db), [setZero(NoChange\_t, Index)](classeigen_1_1plainobjectbase#af57b7361afefe60e2940802e7ef2ca54), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
setZero() [3/4]
---------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setZero | ( | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *newSize* | ) | |
| inline |
Resizes to the given *size*, and sets all coefficients in this expression to zero.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setZero(3);
cout << v << endl;
```
Output:
```
0
0
0
```
See also
[DenseBase::setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setZero(Index,Index)](classeigen_1_1plainobjectbase#a73ab57abb640bf35e0dbf9dba225a1db), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
setZero() [4/4]
---------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::PlainObjectBase](classeigen_1_1plainobjectbase)< Derived >::setZero | ( | NoChange\_t | , |
| | | [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | *cols* |
| | ) | | |
| inline |
Resizes to the given size, changing only the number of columns, and sets all coefficients in this expression to zero. For the parameter of type NoChange\_t, just pass the special value `NoChange`.
See also
[DenseBase::setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setZero(Index)](classeigen_1_1plainobjectbase#acc39eaf7ba22b725c86f1b9b8bb57c3c), [setZero(Index, Index)](classeigen_1_1plainobjectbase#a73ab57abb640bf35e0dbf9dba225a1db), [setZero(Index, NoChange\_t)](classeigen_1_1plainobjectbase#aee6d32f2e6615645b5f1152f99bc8549), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [DenseBase::Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
---
The documentation for this class was generated from the following files:* [PlainObjectBase.h](https://eigen.tuxfamily.org/dox/PlainObjectBase_8h_source.html)
* [CwiseNullaryOp.h](https://eigen.tuxfamily.org/dox/CwiseNullaryOp_8h_source.html)
* [Random.h](https://eigen.tuxfamily.org/dox/Random_8h_source.html)
| programming_docs |
eigen3 Eigen::Sparse Eigen::Sparse
=============
The type used to identify a general sparse storage.
---
The documentation for this struct was generated from the following file:* [Constants.h](https://eigen.tuxfamily.org/dox/Constants_8h_source.html)
eigen3 Eigen::IncompleteCholesky Eigen::IncompleteCholesky
=========================
### template<typename Scalar, int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>> class Eigen::IncompleteCholesky< Scalar, \_UpLo, \_OrderingType >
Modified Incomplete Cholesky with dual threshold.
References : C-J. Lin and J. J. Moré, Incomplete Cholesky Factorizations with Limited memory, SIAM J. Sci. Comput. 21(1), pp. 24-45, 1999
Template Parameters
| | |
| --- | --- |
| Scalar | the scalar type of the input matrices |
| \_UpLo | The triangular part that will be used for the computations. It can be Lower or Upper. Default is Lower. |
| \_OrderingType | The ordering method to use, either AMDOrdering<> or NaturalOrdering<>. Default is AMDOrdering<int>, unless EIGEN\_MPL2\_ONLY is defined, in which case the default is NaturalOrdering<int>. |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
It performs the following incomplete factorization: \( S P A P' S \approx L L' \) where L is a lower triangular factor, S is a diagonal scaling matrix, and P is a fill-in reducing permutation as computed by the ordering method.
**Shifting** **strategy:** Let \( B = S P A P' S \) be the scaled matrix on which the factorization is carried out, and \( \beta \) be the minimum value of the diagonal. If \( \beta > 0 \) then, the factorization is directly performed on the matrix B. Otherwise, the factorization is performed on the shifted matrix \( B + (\sigma+|\beta| I \) where \( \sigma \) is the initial shift value as returned and set by [setInitialShift()](classeigen_1_1incompletecholesky#a409c9586e7d29566dda2c8f5e38a1228 "Set the initial shift parameter .") method. The default value is \( \sigma = 10^{-3} \). If the factorization fails, then the shift in doubled until it succeed or a maximum of ten attempts. If it still fails, as returned by the [info()](classeigen_1_1incompletecholesky#ada0e68cb22601849464506f5986a88c1 "Reports whether previous computation was successful.") method, then you can either increase the initial shift, or better use another preconditioning technique.
| |
| --- |
| |
| template<typename MatrixType > |
| void | [analyzePattern](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e) (const MatrixType &mat) |
| | Computes the fill reducing permutation vector using the sparsity pattern of *mat*. |
| |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [cols](classeigen_1_1incompletecholesky#ac78d2b46d4071963d3cab4a762de6129) () const EIGEN\_NOEXCEPT |
| |
| template<typename MatrixType > |
| void | [compute](classeigen_1_1incompletecholesky#a7966bedeebbeaa7a8fe4dd1da3797a0b) (const MatrixType &mat) |
| |
| template<typename MatrixType > |
| void | [factorize](classeigen_1_1incompletecholesky#ac39c75ff7ca5d2db9a9f03b937e12601) (const MatrixType &mat) |
| | Performs the numerical factorization of the input matrix *mat*. [More...](classeigen_1_1incompletecholesky#ac39c75ff7ca5d2db9a9f03b937e12601) |
| |
| | [IncompleteCholesky](classeigen_1_1incompletecholesky#adaaa3975b8cf53f910d6a3344af92379) () |
| |
| template<typename MatrixType > |
| | [IncompleteCholesky](classeigen_1_1incompletecholesky#a757499fc814988a5b112b1f34d0295e1) (const MatrixType &matrix) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1incompletecholesky#ada0e68cb22601849464506f5986a88c1) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1incompletecholesky#ada0e68cb22601849464506f5986a88c1) |
| |
| const [FactorType](classeigen_1_1sparsematrix) & | [matrixL](classeigen_1_1incompletecholesky#a7d1f1878505fd1862e6f2286d27ff09a) () const |
| |
| const PermutationType & | [permutationP](classeigen_1_1incompletecholesky#a0d52cec5e17f485a362766363ba90b02) () const |
| |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [rows](classeigen_1_1incompletecholesky#a773e54c9ac272b998430bd27d2889277) () const EIGEN\_NOEXCEPT |
| |
| const [VectorRx](classeigen_1_1matrix) & | [scalingS](classeigen_1_1incompletecholesky#a30d66dd77147a84ec3302e7d5fe5d924) () const |
| |
| void | [setInitialShift](classeigen_1_1incompletecholesky#a409c9586e7d29566dda2c8f5e38a1228) (RealScalar shift) |
| | Set the initial shift parameter \( \sigma \). |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< IncompleteCholesky< Scalar, Lower, AMDOrdering< int > > >](classeigen_1_1sparsesolverbase) |
| const [Solve](classeigen_1_1solve)< [IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, Lower, [AMDOrdering](classeigen_1_1amdordering)< int > >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [Solve](classeigen_1_1solve)< [IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, Lower, [AMDOrdering](classeigen_1_1amdordering)< int > >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
IncompleteCholesky() [1/2]
--------------------------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::[IncompleteCholesky](classeigen_1_1incompletecholesky) | ( | | ) | |
| inline |
Default constructor leaving the object in a partly non-initialized stage.
You must call [compute()](classeigen_1_1incompletecholesky#a7966bedeebbeaa7a8fe4dd1da3797a0b) or the pair [analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat.")/factorize() to make it valid.
See also
[IncompleteCholesky(const MatrixType&)](classeigen_1_1incompletecholesky#a757499fc814988a5b112b1f34d0295e1)
IncompleteCholesky() [2/2]
--------------------------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>> template<typename MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::[IncompleteCholesky](classeigen_1_1incompletecholesky) | ( | const MatrixType & | *matrix* | ) | |
| inline |
Constructor computing the incomplete factorization for the given matrix *matrix*.
cols()
------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::cols | ( | void | | ) | const |
| inline |
Returns
number of columns of the factored matrix
compute()
---------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>> template<typename MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::compute | ( | const MatrixType & | *mat* | ) | |
| inline |
Computes or re-computes the incomplete Cholesky factorization of the input matrix *mat*
It is a shortcut for a sequential call to the [analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat.") and [factorize()](classeigen_1_1incompletecholesky#ac39c75ff7ca5d2db9a9f03b937e12601 "Performs the numerical factorization of the input matrix mat.") methods.
See also
[analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat."), [factorize()](classeigen_1_1incompletecholesky#ac39c75ff7ca5d2db9a9f03b937e12601 "Performs the numerical factorization of the input matrix mat.")
factorize()
-----------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>> template<typename MatrixType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::factorize | ( | const MatrixType & | *mat* | ) | |
Performs the numerical factorization of the input matrix *mat*.
The method [analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat.") or [compute()](classeigen_1_1incompletecholesky#a7966bedeebbeaa7a8fe4dd1da3797a0b) must have been called beforehand with a matrix having the same pattern.
See also
[compute()](classeigen_1_1incompletecholesky#a7966bedeebbeaa7a8fe4dd1da3797a0b), [analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat.")
info()
------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::info | ( | | ) | const |
| inline |
Reports whether previous computation was successful.
It triggers an assertion if `*this` has not been initialized through the respective constructor, or a call to [compute()](classeigen_1_1incompletecholesky#a7966bedeebbeaa7a8fe4dd1da3797a0b) or [analyzePattern()](classeigen_1_1incompletecholesky#a702560ecdddef77dc51d20ab22bd974e "Computes the fill reducing permutation vector using the sparsity pattern of mat.").
Returns
`Success` if computation was successful, `NumericalIssue` if the matrix appears to be negative.
matrixL()
---------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [FactorType](classeigen_1_1sparsematrix)& [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::matrixL | ( | | ) | const |
| inline |
Returns
the sparse lower triangular factor L
permutationP()
--------------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const PermutationType& [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::permutationP | ( | | ) | const |
| inline |
Returns
the fill-in reducing permutation P (can be empty for a natural ordering)
rows()
------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::rows | ( | void | | ) | const |
| inline |
Returns
number of rows of the factored matrix
scalingS()
----------
template<typename Scalar , int \_UpLo = Lower, typename \_OrderingType = AMDOrdering<int>>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [VectorRx](classeigen_1_1matrix)& [Eigen::IncompleteCholesky](classeigen_1_1incompletecholesky)< Scalar, \_UpLo, \_OrderingType >::scalingS | ( | | ) | const |
| inline |
Returns
a vector representing the scaling factor S
---
The documentation for this class was generated from the following file:* [IncompleteCholesky.h](https://eigen.tuxfamily.org/dox/IncompleteCholesky_8h_source.html)
eigen3 Eigen::PermutationMatrix Eigen::PermutationMatrix
========================
### template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex> class Eigen::PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >
Permutation matrix.
Template Parameters
| | |
| --- | --- |
| SizeAtCompileTime | the number of rows/cols, or Dynamic |
| MaxSizeAtCompileTime | the maximum number of rows/cols, or Dynamic. This optional parameter defaults to SizeAtCompileTime. Most of the time, you should not have to specify it. |
| \_StorageIndex | the integer type of the indices |
This class represents a permutation matrix, internally stored as a vector of integers.
See also
class [PermutationBase](classeigen_1_1permutationbase "Base class for permutations."), class [PermutationWrapper](classeigen_1_1permutationwrapper "Class to view a vector of integers as a permutation matrix."), class [DiagonalMatrix](classeigen_1_1diagonalmatrix "Represents a diagonal matrix with its storage.")
| |
| --- |
| |
| IndicesType & | [indices](classeigen_1_1permutationmatrix#ac089ead468a58d75f276ad2b253578c0) () |
| |
| const IndicesType & | [indices](classeigen_1_1permutationmatrix#a2f1ab379207fcd1ceb33941e25cf50c2) () const |
| |
| template<typename Other > |
| [PermutationMatrix](classeigen_1_1permutationmatrix) & | [operator=](classeigen_1_1permutationmatrix#aeced50f1c3a43b3e4b3de76d57e9c46a) (const [PermutationBase](classeigen_1_1permutationbase)< Other > &other) |
| |
| template<typename Other > |
| [PermutationMatrix](classeigen_1_1permutationmatrix) & | [operator=](classeigen_1_1permutationmatrix#a75cdc77886972636637f22c41216feb9) (const TranspositionsBase< Other > &tr) |
| |
| template<typename Other > |
| | [PermutationMatrix](classeigen_1_1permutationmatrix#a204b8bbba3c4d33c1a24bb60ad72b202) (const [MatrixBase](classeigen_1_1matrixbase)< Other > &[indices](classeigen_1_1permutationmatrix#a2f1ab379207fcd1ceb33941e25cf50c2)) |
| |
| template<typename OtherDerived > |
| | [PermutationMatrix](classeigen_1_1permutationmatrix#a7ae7016a606b08573013115dcf2e56f2) (const [PermutationBase](classeigen_1_1permutationbase)< OtherDerived > &other) |
| |
| template<typename Other > |
| | [PermutationMatrix](classeigen_1_1permutationmatrix#a0b9a4e51bea9c778a38f6e89db484af4) (const TranspositionsBase< Other > &tr) |
| |
| | [PermutationMatrix](classeigen_1_1permutationmatrix#aea29eab1fd6a6562971db7b9c04c11aa) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1permutationbase#a2216f9ce7b453ac39c46ff0323daeac9)) |
| |
|
Public Member Functions inherited from [Eigen::PermutationBase< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex > >](classeigen_1_1permutationbase) |
| [PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex > & | [applyTranspositionOnTheLeft](classeigen_1_1permutationbase#a4e3455bf12b56123e38a8220c6b508dc) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j) |
| |
| [PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex > & | [applyTranspositionOnTheRight](classeigen_1_1permutationbase#a5f98da0712570d0c4b12f61839ae4193) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1permutationbase#a26961ef6cfef586d412054ee5a20d430) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [determinant](classeigen_1_1permutationbase#a1fc7a5823544700c2e0795e87f9c6d09) () const |
| |
| IndicesType & | [indices](classeigen_1_1permutationbase#a16fa3afafdf703399d62c80f950802f1) () |
| |
| const IndicesType & | [indices](classeigen_1_1permutationbase#adec727546b6882ecaa57e76d084951c5) () const |
| |
| InverseReturnType | [inverse](classeigen_1_1permutationbase#adb9af427f317202366c2832876064eb3) () const |
| |
| PlainPermutationType | [operator\*](classeigen_1_1permutationbase#a865e88989a76b7e92f39bad5250b89c4) (const InverseImpl< Other, [PermutationStorage](structeigen_1_1permutationstorage) > &other) const |
| |
| PlainPermutationType | [operator\*](classeigen_1_1permutationbase#ae81574e059f6b9b7de2ea747fd346a1b) (const [PermutationBase](classeigen_1_1permutationbase)< Other > &other) const |
| |
| [PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex > & | [operator=](classeigen_1_1permutationbase#a8e15540549c5a4e2d5b3b426fef8fbcf) (const [PermutationBase](classeigen_1_1permutationbase)< OtherDerived > &other) |
| |
| [PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex > & | [operator=](classeigen_1_1permutationbase#acaa7cce9ea62c811cec12e86dbb2f0de) (const TranspositionsBase< OtherDerived > &tr) |
| |
| void | [resize](classeigen_1_1permutationbase#a0e0fda6e84d69e02432e4770359bb532) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) newSize) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1permutationbase#acd7ed28ee514287f933de8467768925b) () const |
| |
| void | [setIdentity](classeigen_1_1permutationbase#a6805bb75fd7966ea71895c24ff196444) () |
| |
| void | [setIdentity](classeigen_1_1permutationbase#a830a80511a61634ef437795916f7f8da) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) newSize) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1permutationbase#a2216f9ce7b453ac39c46ff0323daeac9) () const |
| |
| DenseMatrixType | [toDenseMatrix](classeigen_1_1permutationbase#addfa91a2c2c69c76159f1091368a505f) () const |
| |
| InverseReturnType | [transpose](classeigen_1_1permutationbase#a05805e9f4182eec3f6632e1c765b5ffe) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
PermutationMatrix() [1/4]
-------------------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::[PermutationMatrix](classeigen_1_1permutationmatrix) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| inlineexplicit |
Constructs an uninitialized permutation matrix of given size.
PermutationMatrix() [2/4]
-------------------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::[PermutationMatrix](classeigen_1_1permutationmatrix) | ( | const [PermutationBase](classeigen_1_1permutationbase)< OtherDerived > & | *other* | ) | |
| inline |
Copy constructor.
PermutationMatrix() [3/4]
-------------------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::[PermutationMatrix](classeigen_1_1permutationmatrix) | ( | const [MatrixBase](classeigen_1_1matrixbase)< Other > & | *indices* | ) | |
| inlineexplicit |
Generic constructor from expression of the indices. The indices array has the meaning that the permutations sends each integer i to indices[i].
Warning
It is your responsibility to check that the indices array that you passes actually describes a permutation, i.e., each value between 0 and n-1 occurs exactly once, where n is the array's size.
PermutationMatrix() [4/4]
-------------------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::[PermutationMatrix](classeigen_1_1permutationmatrix) | ( | const TranspositionsBase< Other > & | *tr* | ) | |
| inlineexplicit |
Convert the [Transpositions](classeigen_1_1transpositions "Represents a sequence of transpositions (row/column interchange)") *tr* to a permutation matrix
indices() [1/2]
---------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| IndicesType& [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::indices | ( | | ) | |
| inline |
Returns
a reference to the stored array representing the permutation.
indices() [2/2]
---------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const IndicesType& [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::indices | ( | | ) | const |
| inline |
const version of [indices()](classeigen_1_1permutationmatrix#ac089ead468a58d75f276ad2b253578c0).
operator=() [1/2]
-----------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [PermutationMatrix](classeigen_1_1permutationmatrix)& [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::operator= | ( | const [PermutationBase](classeigen_1_1permutationbase)< Other > & | *other* | ) | |
| inline |
Copies the other permutation into \*this
operator=() [2/2]
-----------------
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename \_StorageIndex > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [PermutationMatrix](classeigen_1_1permutationmatrix)& [Eigen::PermutationMatrix](classeigen_1_1permutationmatrix)< SizeAtCompileTime, MaxSizeAtCompileTime, \_StorageIndex >::operator= | ( | const TranspositionsBase< Other > & | *tr* | ) | |
| inline |
Assignment from the [Transpositions](classeigen_1_1transpositions "Represents a sequence of transpositions (row/column interchange)") *tr*
---
The documentation for this class was generated from the following file:* [PermutationMatrix.h](https://eigen.tuxfamily.org/dox/PermutationMatrix_8h_source.html)
| programming_docs |
eigen3 TutorialSparse_example_details TutorialSparse\_example\_details
================================
```
#include <Eigen/Sparse>
#include <vector>
#include <QImage>
typedef [Eigen::SparseMatrix<double>](classeigen_1_1sparsematrix) SpMat; // declares a column-major sparse matrix type of double
typedef [Eigen::Triplet<double>](classeigen_1_1triplet) T;
void insertCoefficient(int id, int i, int j, double w, std::vector<T>& coeffs,
[Eigen::VectorXd](classeigen_1_1matrix)& b, const [Eigen::VectorXd](classeigen_1_1matrix)& boundary)
{
int n = int(boundary.[size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9)());
int id1 = i+j*n;
if(i==-1 || i==n) b(id) -= w * boundary(j); // constrained coefficient
else if(j==-1 || j==n) b(id) -= w * boundary(i); // constrained coefficient
else coeffs.push_back(T(id,id1,w)); // unknown coefficient
}
void buildProblem(std::vector<T>& coefficients, [Eigen::VectorXd](classeigen_1_1matrix)& b, int n)
{
b.[setZero](classeigen_1_1plainobjectbase#acc39eaf7ba22b725c86f1b9b8bb57c3c)();
[Eigen::ArrayXd](classeigen_1_1array) boundary = [Eigen::ArrayXd::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(n, 0,M_PI).sin().pow(2);
for(int j=0; j<n; ++j)
{
for(int i=0; i<n; ++i)
{
int id = i+j*n;
insertCoefficient(id, i-1,j, -1, coefficients, b, boundary);
insertCoefficient(id, i+1,j, -1, coefficients, b, boundary);
insertCoefficient(id, i,j-1, -1, coefficients, b, boundary);
insertCoefficient(id, i,j+1, -1, coefficients, b, boundary);
insertCoefficient(id, i,j, 4, coefficients, b, boundary);
}
}
}
void saveAsBitmap(const [Eigen::VectorXd](classeigen_1_1matrix)& x, int n, const char* filename)
{
[Eigen::Array<unsigned char,Eigen::Dynamic,Eigen::Dynamic>](classeigen_1_1array) bits = (x*255).cast<unsigned char>();
QImage img(bits.[data](classeigen_1_1plainobjectbase#ac54123f62de4c46a9107ff53890b6116)(), n,n,QImage::Format_Indexed8);
img.setColorCount(256);
for(int i=0;i<256;i++) img.setColor(i,qRgb(i,i,i));
img.save(filename);
}
```
eigen3 Eigen::IOFormat Eigen::IOFormat
===============
Stores a set of parameters controlling the way matrices are printed.
List of available parameters:
* **precision** number of digits for floating point values, or one of the special constants `StreamPrecision` and `FullPrecision`. The default is the special value `StreamPrecision` which means to use the stream's own precision setting, as set for instance using `cout.precision(3)`. The other special value `FullPrecision` means that the number of digits will be computed to match the full precision of each floating-point type.
* **flags** an OR-ed combination of flags, the default value is 0, the only currently available flag is `DontAlignCols` which allows to disable the alignment of columns, resulting in faster code.
* **coeffSeparator** string printed between two coefficients of the same row
* **rowSeparator** string printed between two rows
* **rowPrefix** string printed at the beginning of each row
* **rowSuffix** string printed at the end of each row
* **matPrefix** string printed at the beginning of the matrix
* **matSuffix** string printed at the end of the matrix
* **fill** character printed to fill the empty space in aligned columns
Example:
```
std::string sep = "\n----------------------------------------\n";
Matrix3d m1;
m1 << 1.111111, 2, 3.33333, 4, 5, 6, 7, 8.888888, 9;
[IOFormat](structeigen_1_1ioformat#acdcc91702d45c714b11ba42db5beddb5) CommaInitFmt(StreamPrecision, DontAlignCols, ", ", ", ", "", "", " << ", ";");
[IOFormat](structeigen_1_1ioformat#acdcc91702d45c714b11ba42db5beddb5) CleanFmt(4, 0, ", ", "\n", "[", "]");
[IOFormat](structeigen_1_1ioformat#acdcc91702d45c714b11ba42db5beddb5) OctaveFmt(StreamPrecision, 0, ", ", ";\n", "", "", "[", "]");
[IOFormat](structeigen_1_1ioformat#acdcc91702d45c714b11ba42db5beddb5) HeavyFmt(FullPrecision, 0, ", ", ";\n", "[", "]", "[", "]");
std::cout << m1 << sep;
std::cout << m1.format(CommaInitFmt) << sep;
std::cout << m1.format(CleanFmt) << sep;
std::cout << m1.format(OctaveFmt) << sep;
std::cout << m1.format(HeavyFmt) << sep;
```
Output:
```
1.11 2 3.33
4 5 6
7 8.89 9
----------------------------------------
<< 1.11, 2, 3.33, 4, 5, 6, 7, 8.89, 9;
----------------------------------------
[1.111, 2, 3.333]
[ 4, 5, 6]
[ 7, 8.889, 9]
----------------------------------------
[1.11, 2, 3.33;
4, 5, 6;
7, 8.89, 9]
----------------------------------------
[[1.111111, 2, 3.33333];
[ 4, 5, 6];
[ 7, 8.888888, 9]]
----------------------------------------
```
See also
[DenseBase::format()](classeigen_1_1densebase#ab231f1a6057f28d4244145e12c9fc0c7), class [WithFormat](classeigen_1_1withformat "Pseudo expression providing matrix output with given format.")
| |
| --- |
| |
| | [IOFormat](structeigen_1_1ioformat#acdcc91702d45c714b11ba42db5beddb5) (int \_precision=StreamPrecision, int \_flags=0, const std::string &\_coeffSeparator=" ", const std::string &\_rowSeparator="\n", const std::string &\_rowPrefix="", const std::string &\_rowSuffix="", const std::string &\_matPrefix="", const std::string &\_matSuffix="", const char \_fill=' ') |
| |
IOFormat()
----------
| | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Eigen::IOFormat::IOFormat | ( | int | *\_precision* = `StreamPrecision`, |
| | | int | *\_flags* = `0`, |
| | | const std::string & | *\_coeffSeparator* = `" "`, |
| | | const std::string & | *\_rowSeparator* = `"\n"`, |
| | | const std::string & | *\_rowPrefix* = `""`, |
| | | const std::string & | *\_rowSuffix* = `""`, |
| | | const std::string & | *\_matPrefix* = `""`, |
| | | const std::string & | *\_matSuffix* = `""`, |
| | | const char | *\_fill* = `' '` |
| | ) | | |
| inline |
Default constructor, see class [IOFormat](structeigen_1_1ioformat "Stores a set of parameters controlling the way matrices are printed.") for the meaning of the parameters
---
The documentation for this class was generated from the following file:* [IO.h](https://eigen.tuxfamily.org/dox/IO_8h_source.html)
eigen3 Using Eigen in CMake Projects Using Eigen in CMake Projects
=============================
Eigen provides native CMake support which allows the library to be easily used in CMake projects.
Note
CMake 3.0 (or later) is required to enable this functionality.
Eigen exports a CMake target called `Eigen3::Eigen` which can be imported using the `find_package` CMake command and used by calling `target_link_libraries` as in the following example:
```
cmake_minimum_required (VERSION 3.0)
project (myproject)
find_package (Eigen3 3.3 REQUIRED NO_MODULE)
add_executable (example example.cpp)
target_link_libraries (example Eigen3::Eigen)
```
The above code snippet must be placed in a file called `CMakeLists.txt` alongside `example.cpp`. After running
```
$ cmake path-to-example-directory
```
CMake will produce project files that generate an executable called `example` which requires at least version 3.3 of Eigen. Here, `path-to-example-directory` is the path to the directory that contains both `CMakeLists.txt` and `example.cpp`.
Do not forget to set the [`CMAKE_PREFIX_PATH`](https://cmake.org/cmake/help/v3.7/variable/CMAKE_PREFIX_PATH.html) variable if [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") is not installed in a default location or if you want to pick a specific version. For instance:
```
$ cmake path-to-example-directory -DCMAKE_PREFIX_PATH=$HOME/mypackages
```
An alternative is to set the `Eigen3_DIR` cmake's variable to the respective path containing the `Eigen3*`.cmake files. For instance:
```
$ cmake path-to-example-directory -DEigen3_DIR=$HOME/mypackages/share/eigen3/cmake/
```
If the `REQUIRED` option is omitted when locating Eigen using `find_package`, one can check whether the package was found as follows:
```
find_package (Eigen3 3.3 NO_MODULE)
if (TARGET Eigen3::Eigen)
# Use the imported target
endif (TARGET Eigen3::Eigen)
```
eigen3 Eigen::SimplicialLLT Eigen::SimplicialLLT
====================
### template<typename \_MatrixType, int \_UpLo, typename \_Ordering> class Eigen::SimplicialLLT< \_MatrixType, \_UpLo, \_Ordering >
A direct sparse [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") Cholesky factorizations.
This class provides a LL^T Cholesky factorizations of sparse matrices that are selfadjoint and positive definite. The factorization allows for solving A.X = B where X and B can be either dense or sparse.
In order to reduce the fill-in, a symmetric permutation P is applied prior to the factorization such that the factorized matrix is P A P^-1.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the sparse matrix A, it must be a SparseMatrix<> |
| \_UpLo | the triangular part that will be used for the computations. It can be Lower or Upper. Default is Lower. |
| \_Ordering | The ordering method to use, either AMDOrdering<> or NaturalOrdering<>. Default is AMDOrdering<> |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
See also
class [SimplicialLDLT](classeigen_1_1simplicialldlt "A direct sparse LDLT Cholesky factorizations without square root."), class [AMDOrdering](classeigen_1_1amdordering), class [NaturalOrdering](classeigen_1_1naturalordering)
| |
| --- |
| |
| void | [analyzePattern](classeigen_1_1simplicialllt#ad6e49b1c0d2ec5c8e118538260f3002c) (const MatrixType &a) |
| |
| [SimplicialLLT](classeigen_1_1simplicialllt) & | [compute](classeigen_1_1simplicialllt#a24ffa253377a1cec7a44b856fae1f71a) (const MatrixType &matrix) |
| |
| Scalar | [determinant](classeigen_1_1simplicialllt#a956595848e6fac7a389d091b3fdc9567) () const |
| |
| void | [factorize](classeigen_1_1simplicialllt#a8a140b34b08df74c7426ee29b986b228) (const MatrixType &a) |
| |
| const MatrixL | [matrixL](classeigen_1_1simplicialllt#ae2b24f8f6d62a8444193904988374299) () const |
| |
| const MatrixU | [matrixU](classeigen_1_1simplicialllt#a23522d6444c344ddb14e48dbfac128ed) () const |
| |
| | [SimplicialLLT](classeigen_1_1simplicialllt#ad25633e34d7c21b77fe05c873ffbe416) () |
| |
| | [SimplicialLLT](classeigen_1_1simplicialllt#a342735f17d0306aa5581e91010091427) (const MatrixType &matrix) |
| |
|
Public Member Functions inherited from [Eigen::SimplicialCholeskyBase< SimplicialLLT< \_MatrixType, \_UpLo, \_Ordering > >](classeigen_1_1simplicialcholeskybase) |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1simplicialcholeskybase#a3ac877f73aaaff670e6ae7554eb02fc8) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1simplicialcholeskybase#a3ac877f73aaaff670e6ae7554eb02fc8) |
| |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)< Dynamic, Dynamic, StorageIndex > & | [permutationP](classeigen_1_1simplicialcholeskybase#aff1480e595a21726beaec9a586a94d5a) () const |
| |
| const [PermutationMatrix](classeigen_1_1permutationmatrix)< Dynamic, Dynamic, StorageIndex > & | [permutationPinv](classeigen_1_1simplicialcholeskybase#a0e23d1f4a88c211be7098faf1cb41674) () const |
| |
| [SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering > & | [setShift](classeigen_1_1simplicialcholeskybase#a362352f755101faaac59c1ed9d5e3559) (const RealScalar &offset, const RealScalar &scale=1) |
| |
| | [SimplicialCholeskyBase](classeigen_1_1simplicialcholeskybase#a098baba1dbe07ca3a775c8df1f8a0e71) () |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< SimplicialLLT< \_MatrixType, \_UpLo, \_Ordering > >](classeigen_1_1sparsesolverbase) |
| const [Solve](classeigen_1_1solve)< [SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| const [Solve](classeigen_1_1solve)< [SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
| |
| --- |
| |
|
Protected Member Functions inherited from [Eigen::SimplicialCholeskyBase< SimplicialLLT< \_MatrixType, \_UpLo, \_Ordering > >](classeigen_1_1simplicialcholeskybase) |
| void | [compute](classeigen_1_1simplicialcholeskybase#a9a741744dda2261cae26cddf96a35bf0) (const MatrixType &matrix) |
| |
SimplicialLLT() [1/2]
---------------------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::[SimplicialLLT](classeigen_1_1simplicialllt) | ( | | ) | |
| inline |
Default constructor
SimplicialLLT() [2/2]
---------------------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::[SimplicialLLT](classeigen_1_1simplicialllt) | ( | const MatrixType & | *matrix* | ) | |
| inlineexplicit |
Constructs and performs the [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") factorization of *matrix*
analyzePattern()
----------------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::analyzePattern | ( | const MatrixType & | *a* | ) | |
| inline |
Performs a symbolic decomposition on the sparcity of *matrix*.
This function is particularly useful when solving for several problems having the same structure.
See also
[factorize()](classeigen_1_1simplicialllt#a8a140b34b08df74c7426ee29b986b228)
compute()
---------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [SimplicialLLT](classeigen_1_1simplicialllt)& [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::compute | ( | const MatrixType & | *matrix* | ) | |
| inline |
Computes the sparse Cholesky decomposition of *matrix*
determinant()
-------------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| Scalar [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::determinant | ( | | ) | const |
| inline |
Returns
the determinant of the underlying matrix from the current factorization
factorize()
-----------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::factorize | ( | const MatrixType & | *a* | ) | |
| inline |
Performs a numeric decomposition of *matrix*
The given matrix must has the same sparcity than the matrix on which the symbolic decomposition has been performed.
See also
[analyzePattern()](classeigen_1_1simplicialllt#ad6e49b1c0d2ec5c8e118538260f3002c)
matrixL()
---------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixL [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::matrixL | ( | | ) | const |
| inline |
Returns
an expression of the factor L
matrixU()
---------
template<typename \_MatrixType , int \_UpLo, typename \_Ordering >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixU [Eigen::SimplicialLLT](classeigen_1_1simplicialllt)< \_MatrixType, \_UpLo, \_Ordering >::matrixU | ( | | ) | const |
| inline |
Returns
an expression of the factor U (= L^\*)
---
The documentation for this class was generated from the following file:* [SimplicialCholesky.h](https://eigen.tuxfamily.org/dox/SimplicialCholesky_8h_source.html)
eigen3 Sparse matrix manipulations Sparse matrix manipulations
===========================
Manipulating and solving sparse problems involves various modules which are summarized below:
| Module | Header file | Contents |
| --- | --- | --- |
| [SparseCore](group__sparsecore__module) |
```
#include <Eigen/SparseCore>
```
| [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") and [SparseVector](classeigen_1_1sparsevector "a sparse vector class") classes, matrix assembly, basic sparse linear algebra (including sparse triangular solvers) |
| [SparseCholesky](group__sparsecholesky__module) |
```
#include <Eigen/SparseCholesky>
```
| Direct sparse [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") and [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") Cholesky factorization to solve sparse self-adjoint positive definite problems |
| [SparseLU](group__sparselu__module) |
```
#include<Eigen/SparseLU>
```
| Sparse LU factorization to solve general square sparse systems |
| [SparseQR](group__sparseqr__module) |
```
#include<Eigen/SparseQR>
```
| Sparse QR factorization for solving sparse linear least-squares problems |
| [IterativeLinearSolvers](group__iterativelinearsolvers__module) |
```
#include <Eigen/IterativeLinearSolvers>
```
| Iterative solvers to solve large general linear square problems (including self-adjoint positive definite problems) |
| [Sparse](group__sparse__module) |
```
#include <Eigen/Sparse>
```
| Includes all the above modules |
Sparse matrix format
======================
In many applications (e.g., finite element methods) it is common to deal with very large matrices where only a few coefficients are different from zero. In such cases, memory consumption can be reduced and performance increased by using a specialized representation storing only the nonzero coefficients. Such a matrix is called a sparse matrix.
**The** **SparseMatrix** **class**
The class [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") is the main sparse matrix representation of [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.")'s sparse module; it offers high performance and low memory usage. It implements a more versatile variant of the widely-used Compressed Column (or Row) Storage scheme. It consists of four compact arrays:
* `Values:` stores the coefficient values of the non-zeros.
* `InnerIndices:` stores the row (resp. column) indices of the non-zeros.
* `OuterStarts:` stores for each column (resp. row) the index of the first non-zero in the previous two arrays.
* `InnerNNZs:` stores the number of non-zeros of each column (resp. row). The word `inner` refers to an *inner* *vector* that is a column for a column-major matrix, or a row for a row-major matrix. The word `outer` refers to the other direction.
This storage scheme is better explained on an example. The following matrix
| | | | | |
| --- | --- | --- | --- | --- |
| 0 | 3 | 0 | 0 | 0 |
| 22 | 0 | 0 | 0 | 17 |
| 7 | 5 | 0 | 1 | 0 |
| 0 | 0 | 0 | 0 | 0 |
| 0 | 0 | 14 | 0 | 8 |
and one of its possible sparse, **column** **major** representation:
| | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
| Values: | 22 | 7 | \_ | 3 | 5 | 14 | \_ | \_ | 1 | \_ | 17 | 8 |
| InnerIndices: | 1 | 2 | \_ | 0 | 2 | 4 | \_ | \_ | 2 | \_ | 1 | 4 |
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| OuterStarts: | 0 | 3 | 5 | 8 | 10 | *12* |
| InnerNNZs: | 2 | 2 | 1 | 1 | 2 | |
Currently the elements of a given inner vector are guaranteed to be always sorted by increasing inner indices. The `"_"` indicates available free space to quickly insert new elements. Assuming no reallocation is needed, the insertion of a random element is therefore in O(nnz\_j) where nnz\_j is the number of nonzeros of the respective inner vector. On the other hand, inserting elements with increasing inner indices in a given inner vector is much more efficient since this only requires to increase the respective `InnerNNZs` entry that is a O(1) operation.
The case where no empty space is available is a special case, and is referred as the *compressed* mode. It corresponds to the widely used Compressed Column (or Row) Storage schemes (CCS or CRS). Any [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") can be turned to this form by calling the [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd) function. In this case, one can remark that the `InnerNNZs` array is redundant with `OuterStarts` because we have the equality: `InnerNNZs`[j] = `OuterStarts`[j+1]-`OuterStarts`[j]. Therefore, in practice a call to [SparseMatrix::makeCompressed()](classeigen_1_1sparsematrix#a5ff54ffc10296f9466dc81fa888733fd) frees this buffer.
It is worth noting that most of our wrappers to external libraries requires compressed matrices as inputs.
The results of Eigen's operations always produces **compressed** sparse matrices. On the other hand, the insertion of a new element into a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") converts this later to the **uncompressed** mode.
Here is the previous matrix represented in compressed mode:
| | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- |
| Values: | 22 | 7 | 3 | 5 | 14 | 1 | 17 | 8 |
| InnerIndices: | 1 | 2 | 0 | 2 | 4 | 2 | 1 | 4 |
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| OuterStarts: | 0 | 2 | 4 | 5 | 6 | *8* |
A [SparseVector](classeigen_1_1sparsevector "a sparse vector class") is a special case of a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") where only the `Values` and `InnerIndices` arrays are stored. There is no notion of compressed/uncompressed mode for a [SparseVector](classeigen_1_1sparsevector "a sparse vector class").
First example
===============
Before describing each individual class, let's start with the following typical example: solving the Laplace equation \( \Delta u = 0 \) on a regular 2D grid using a finite difference scheme and Dirichlet boundary conditions. Such problem can be mathematically expressed as a linear problem of the form \( Ax=b \) where \( x \) is the vector of `m` unknowns (in our case, the values of the pixels), \( b \) is the right hand side vector resulting from the boundary conditions, and \( A \) is an \( m \times m \) matrix containing only a few non-zero elements resulting from the discretization of the Laplacian operator.
| | |
| --- | --- |
|
```
#include <Eigen/Sparse>
#include <vector>
#include <iostream>
typedef [Eigen::SparseMatrix<double>](classeigen_1_1sparsematrix) SpMat; // declares a column-major sparse matrix type of double
typedef [Eigen::Triplet<double>](classeigen_1_1triplet) T;
void buildProblem(std::vector<T>& coefficients, [Eigen::VectorXd](classeigen_1_1matrix)& b, int n);
void saveAsBitmap(const [Eigen::VectorXd](classeigen_1_1matrix)& x, int n, const char* filename);
int main(int argc, char** argv)
{
if(argc!=2) {
std::cerr << "Error: expected one and only one argument.\n";
return -1;
}
int n = 300; // size of the image
int m = n*n; // number of unknowns (=number of pixels)
// Assembly:
std::vector<T> coefficients; // list of non-zeros coefficients
[Eigen::VectorXd](classeigen_1_1matrix) b(m); // the right hand side-vector resulting from the constraints
buildProblem(coefficients, b, n);
SpMat A(m,m);
A.setFromTriplets(coefficients.begin(), coefficients.end());
// Solving:
[Eigen::SimplicialCholesky<SpMat>](classeigen_1_1simplicialcholesky) chol(A); // performs a Cholesky factorization of A
[Eigen::VectorXd](classeigen_1_1matrix) x = chol.solve(b); // use the factorization to solve for the given right hand side
// Export the result to a file:
saveAsBitmap(x, n, argv[1]);
return 0;
}
```
| |
In this example, we start by defining a column-major sparse matrix type of double `SparseMatrix<double>`, and a triplet list of the same scalar type `Triplet<double>`. A triplet is a simple object representing a non-zero entry as the triplet: `row` index, `column` index, `value`.
In the main function, we declare a list `coefficients` of triplets (as a std vector) and the right hand side vector \( b \) which are filled by the *buildProblem* function. The raw and flat list of non-zero entries is then converted to a true [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") object `A`. Note that the elements of the list do not have to be sorted, and possible duplicate entries will be summed up.
The last step consists of effectively solving the assembled problem. Since the resulting matrix `A` is symmetric by construction, we can perform a direct Cholesky factorization via the [SimplicialLDLT](classeigen_1_1simplicialldlt "A direct sparse LDLT Cholesky factorizations without square root.") class which behaves like its [LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.") counterpart for dense objects.
The resulting vector `x` contains the pixel values as a 1D array which is saved to a jpeg file shown on the right of the code above.
Describing the *buildProblem* and *save* functions is out of the scope of this tutorial. They are given [here](tutorialsparse_example_details) for the curious and reproducibility purpose.
The SparseMatrix class
========================
**Matrix** **and** **vector** **properties**
The [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") and [SparseVector](classeigen_1_1sparsevector "a sparse vector class") classes take three template arguments: the scalar type (e.g., double) the storage order (ColMajor or RowMajor, the default is ColMajor) the inner index type (default is `int`).
As for dense [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") objects, constructors takes the size of the object. Here are some examples:
```
SparseMatrix<std::complex<float> > mat(1000,2000); // declares a 1000x2000 column-major compressed sparse matrix of complex<float>
SparseMatrix<double,RowMajor> mat(1000,2000); // declares a 1000x2000 row-major compressed sparse matrix of double
SparseVector<std::complex<float> > vec(1000); // declares a column sparse vector of complex<float> of size 1000
SparseVector<double,RowMajor> vec(1000); // declares a row sparse vector of double of size 1000
```
In the rest of the tutorial, `mat` and `vec` represent any sparse-matrix and sparse-vector objects, respectively.
The dimensions of a matrix can be queried using the following functions:
| | | |
| --- | --- | --- |
| Standard dimensions |
```
mat.rows()
mat.cols()
```
|
```
vec.size()
```
|
| Sizes along the inner/outer dimensions |
```
mat.innerSize()
mat.outerSize()
```
| |
| Number of non zero coefficients |
```
mat.nonZeros()
```
|
```
vec.nonZeros()
```
|
**Iterating** **over** **the** **nonzero** **coefficients**
Random access to the elements of a sparse object can be done through the `coeffRef(i,j)` function. However, this function involves a quite expensive binary search. In most cases, one only wants to iterate over the non-zeros elements. This is achieved by a standard loop over the outer dimension, and then by iterating over the non-zeros of the current inner vector via an InnerIterator. Thus, the non-zero entries have to be visited in the same order than the storage order. Here is an example:
| | |
| --- | --- |
|
```
SparseMatrix<double> mat(rows,cols);
for (int k=0; k<mat.outerSize(); ++k)
for (SparseMatrix<double>::InnerIterator it(mat,k); it; ++it)
{
it.value();
it.row(); // row index
it.col(); // col index (here it is equal to k)
it.index(); // inner index, here it is equal to it.row()
}
```
|
```
SparseVector<double> vec(size);
for (SparseVector<double>::InnerIterator it(vec); it; ++it)
{
it.value(); // == vec[ it.index() ]
it.index();
}
```
|
For a writable expression, the referenced value can be modified using the valueRef() function. If the type of the sparse matrix or vector depends on a template parameter, then the `typename` keyword is required to indicate that `InnerIterator` denotes a type; see [The template and typename keywords in C++](topictemplatekeyword) for details.
Filling a sparse matrix
=========================
Because of the special storage scheme of a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation."), special care has to be taken when adding new nonzero entries. For instance, the cost of a single purely random insertion into a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") is `O(nnz)`, where `nnz` is the current number of non-zero coefficients.
The simplest way to create a sparse matrix while guaranteeing good performance is thus to first build a list of so-called *triplets*, and then convert it to a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.").
Here is a typical usage example:
```
typedef [Eigen::Triplet<double>](classeigen_1_1triplet) T;
std::vector<T> tripletList;
tripletList.reserve(estimation_of_entries);
for(...)
{
// ...
tripletList.push_back(T(i,j,v_ij));
}
SparseMatrixType mat(rows,cols);
mat.setFromTriplets(tripletList.begin(), tripletList.end());
// mat is ready to go!
```
The `std::vector` of triplets might contain the elements in arbitrary order, and might even contain duplicated elements that will be summed up by setFromTriplets(). See the [SparseMatrix::setFromTriplets()](classeigen_1_1sparsematrix#acc35051d698e3973f1de5b9b78dbe345) function and class [Triplet](classeigen_1_1triplet "A small structure to hold a non zero as a triplet (i,j,value).") for more details.
In some cases, however, slightly higher performance, and lower memory consumption can be reached by directly inserting the non-zeros into the destination matrix. A typical scenario of this approach is illustrated below:
```
1: SparseMatrix<double> mat(rows,cols); // default is column major
2: mat.reserve([VectorXi::Constant](classeigen_1_1densebase#a68a7ece6c5629d1e9447a321fcb14ccd)(cols,6));
3: for each i,j such that v_ij != 0
4: mat.insert(i,j) = v_ij; // alternative: mat.coeffRef(i,j) += v\_ij;
5: mat.makeCompressed(); // optional
```
* The key ingredient here is the line 2 where we reserve room for 6 non-zeros per column. In many cases, the number of non-zeros per column or row can easily be known in advance. If it varies significantly for each inner vector, then it is possible to specify a reserve size for each inner vector by providing a vector object with an operator[](int j) returning the reserve size of the `j-th` inner vector (e.g., via a VectorXi or std::vector<int>). If only a rought estimate of the number of nonzeros per inner-vector can be obtained, it is highly recommended to overestimate it rather than the opposite. If this line is omitted, then the first insertion of a new element will reserve room for 2 elements per inner vector.
* The line 4 performs a sorted insertion. In this example, the ideal case is when the `j-th` column is not full and contains non-zeros whose inner-indices are smaller than `i`. In this case, this operation boils down to trivial O(1) operation.
* When calling insert(i,j) the element `i` ,j must not already exists, otherwise use the coeffRef(i,j) method that will allow to, e.g., accumulate values. This method first performs a binary search and finally calls insert(i,j) if the element does not already exist. It is more flexible than insert() but also more costly.
* The line 5 suppresses the remaining empty space and transforms the matrix into a compressed column storage.
Supported operators and functions
===================================
Because of their special storage format, sparse matrices cannot offer the same level of flexibility than dense matrices. In [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.")'s sparse module we chose to expose only the subset of the dense matrix API which can be efficiently implemented. In the following *sm* denotes a sparse matrix, *sv* a sparse vector, *dm* a dense matrix, and *dv* a dense vector.
Basic operations
------------------
Sparse expressions support most of the unary and binary coefficient wise operations:
```
sm1.real() sm1.imag() -sm1 0.5*sm1
sm1+sm2 sm1-sm2 sm1.cwiseProduct(sm2)
```
However, **a strong restriction is that the storage orders must match**. For instance, in the following example:
```
sm4 = sm1 + sm2 + sm3;
```
sm1, sm2, and sm3 must all be row-major or all column-major. On the other hand, there is no restriction on the target matrix sm4. For instance, this means that for computing \( A^T + A \), the matrix \( A^T \) must be evaluated into a temporary matrix of compatible storage order:
```
SparseMatrix<double> A, B;
B = SparseMatrix<double>(A.transpose()) + A;
```
Binary coefficient wise operators can also mix sparse and dense expressions:
```
sm2 = sm1.cwiseProduct(dm1);
dm2 = sm1 + dm1;
dm2 = dm1 - sm1;
```
Performance-wise, the adding/subtracting sparse and dense matrices is better performed in two steps. For instance, instead of doing `dm2 = sm1 + dm1`, better write:
```
dm2 = dm1;
dm2 += sm1;
```
This version has the advantage to fully exploit the higher performance of dense storage (no indirection, SIMD, etc.), and to pay the cost of slow sparse evaluation on the few non-zeros of the sparse matrix only.
Sparse expressions also support transposition:
```
sm1 = sm2.transpose();
sm1 = sm2.adjoint();
```
However, there is no transposeInPlace() method.
Matrix products
-----------------
Eigen supports various kind of sparse matrix products which are summarize below:
* **sparse-dense**:
```
dv2 = sm1 * dv1;
dm2 = dm1 * sm1.adjoint();
dm2 = 2. * sm1 * dm1;
```
* **symmetric** **sparse-dense**. The product of a sparse symmetric matrix with a dense matrix (or vector) can also be optimized by specifying the symmetry with selfadjointView():
```
dm2 = sm1.selfadjointView<>() * dm1; // if all coefficients of A are stored
dm2 = A.selfadjointView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>() * dm1; // if only the upper part of A is stored
dm2 = A.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>() * dm1; // if only the lower part of A is stored
```
* **sparse-sparse**. For sparse-sparse products, two different algorithms are available. The default one is conservative and preserve the explicit zeros that might appear:
```
sm3 = sm1 * sm2;
sm3 = 4 * sm1.adjoint() * sm2;
```
The second algorithm prunes on the fly the explicit zeros, or the values smaller than a given threshold. It is enabled and controlled through the prune() functions:
```
sm3 = (sm1 * sm2).pruned(); // removes numerical zeros
sm3 = (sm1 * sm2).pruned(ref); // removes elements much smaller than ref
sm3 = (sm1 * sm2).pruned(ref,epsilon); // removes elements smaller than ref\*epsilon
```
* **permutations**. Finally, permutations can be applied to sparse matrices too:
```
PermutationMatrix<Dynamic,Dynamic> P = ...;
sm2 = P * sm1;
sm2 = sm1 * P.inverse();
sm2 = sm1.transpose() * P;
```
Block operations
------------------
Regarding read-access, sparse matrices expose the same API than for dense matrices to access to sub-matrices such as blocks, columns, and rows. See [Block operations](group__tutorialblockoperations) for a detailed introduction. However, for performance reasons, writing to a sub-sparse-matrix is much more limited, and currently only contiguous sets of columns (resp. rows) of a column-major (resp. row-major) [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") are writable. Moreover, this information has to be known at compile-time, leaving out methods such as `block(...)` and `corner*(...)`. The available API for write-access to a [SparseMatrix](classeigen_1_1sparsematrix "A versatible sparse matrix representation.") are summarized below:
```
SparseMatrix<double,ColMajor> sm1;
sm1.col(j) = ...;
sm1.leftCols(ncols) = ...;
sm1.middleCols(j,ncols) = ...;
sm1.rightCols(ncols) = ...;
SparseMatrix<double,RowMajor> sm2;
sm2.row(i) = ...;
sm2.topRows(nrows) = ...;
sm2.middleRows(i,nrows) = ...;
sm2.bottomRows(nrows) = ...;
```
In addition, sparse matrices expose the SparseMatrixBase::innerVector() and SparseMatrixBase::innerVectors() methods, which are aliases to the col/middleCols methods for a column-major storage, and to the row/middleRows methods for a row-major storage.
Triangular and selfadjoint views
----------------------------------
Just as with dense matrices, the triangularView() function can be used to address a triangular part of the matrix, and perform triangular solves with a dense right hand side:
```
dm2 = sm1.triangularView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>(dm1);
dv2 = sm1.transpose().triangularView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>(dv1);
```
The selfadjointView() function permits various operations:
* optimized sparse-dense matrix products:
```
dm2 = sm1.selfadjointView<>() * dm1; // if all coefficients of A are stored
dm2 = A.selfadjointView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>() * dm1; // if only the upper part of A is stored
dm2 = A.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>() * dm1; // if only the lower part of A is stored
```
* copy of triangular parts:
```
sm2 = sm1.selfadjointView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>(); // makes a full selfadjoint matrix from the upper triangular part
sm2.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>() = sm1.selfadjointView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>(); // copies the upper triangular part to the lower triangular part
```
* application of symmetric permutations:
```
PermutationMatrix<Dynamic,Dynamic> P = ...;
sm2 = A.selfadjointView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>().twistedBy(P); // compute P S P' from the upper triangular part of A, and make it a full matrix
sm2.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>() = A.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>().twistedBy(P); // compute P S P' from the lower triangular part of A, and then only compute the lower part
```
Please, refer to the [Quick Reference](group__sparsequickrefpage) guide for the list of supported operations. The list of linear solvers available is [here.](group__topicsparsesystems)
| programming_docs |
eigen3 PardisoSupport module PardisoSupport module
=====================
This module brings support for the Intel(R) MKL PARDISO direct sparse solvers.
```
#include <Eigen/PardisoSupport>
```
In order to use this module, the MKL headers must be accessible from the include paths, and your binary must be linked to the MKL library and its dependencies. See this [page](topicusingintelmkl) for more information on MKL-Eigen integration.
| |
| --- |
| |
| class | [Eigen::PardisoLDLT< MatrixType, Options >](classeigen_1_1pardisoldlt) |
| | A sparse direct Cholesky ([LDLT](classeigen_1_1ldlt "Robust Cholesky decomposition of a matrix with pivoting.")) factorization and solver based on the PARDISO library. [More...](classeigen_1_1pardisoldlt#details) |
| |
| class | [Eigen::PardisoLLT< MatrixType, \_UpLo >](classeigen_1_1pardisollt) |
| | A sparse direct Cholesky ([LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.")) factorization and solver based on the PARDISO library. [More...](classeigen_1_1pardisollt#details) |
| |
| class | [Eigen::PardisoLU< MatrixType >](classeigen_1_1pardisolu) |
| | A sparse direct LU factorization and solver based on the PARDISO library. [More...](classeigen_1_1pardisolu#details) |
| |
eigen3 Eigen::ColPivHouseholderQR Eigen::ColPivHouseholderQR
==========================
### template<typename \_MatrixType> class Eigen::ColPivHouseholderQR< \_MatrixType >
Householder rank-revealing QR decomposition of a matrix with column-pivoting.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the matrix of which we are computing the QR decomposition |
This class performs a rank-revealing QR decomposition of a matrix **A** into matrices **P**, **Q** and **R** such that
\[ \mathbf{A} \, \mathbf{P} = \mathbf{Q} \, \mathbf{R} \]
by using Householder transformations. Here, **P** is a permutation matrix, **Q** a unitary matrix and **R** an upper triangular matrix.
This decomposition performs column pivoting in order to be rank-revealing and improve numerical stability. It is slower than [HouseholderQR](classeigen_1_1householderqr "Householder QR decomposition of a matrix."), and faster than [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with full pivoting.").
This class supports the [inplace decomposition](group__inplacedecomposition) mechanism.
See also
[MatrixBase::colPivHouseholderQr()](classeigen_1_1matrixbase#adee8c19c833245bbb00a591dce68e8a4)
| |
| --- |
| |
| MatrixType::RealScalar | [absDeterminant](classeigen_1_1colpivhouseholderqr#ac87c3bf42098d6f7324dafbc50fa83f7) () const |
| |
| | [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr#a9d8a92c2a2f0debe5454812372237ed4) () |
| | Default Constructor. [More...](classeigen_1_1colpivhouseholderqr#a9d8a92c2a2f0debe5454812372237ed4) |
| |
| template<typename InputType > |
| | [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr#a1aa6a5b95380df0ceb224cb833316d4f) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a QR factorization from a given matrix. [More...](classeigen_1_1colpivhouseholderqr#a1aa6a5b95380df0ceb224cb833316d4f) |
| |
| template<typename InputType > |
| | [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr#a65782010a93a4c9ef4a9191ac8fe30bc) ([EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| | Constructs a QR factorization from a given matrix. [More...](classeigen_1_1colpivhouseholderqr#a65782010a93a4c9ef4a9191ac8fe30bc) |
| |
| | [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr#a5965d4fdebc04b2df71d67ff0b2d0c2c) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) rows, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) cols) |
| | Default Constructor with memory preallocation. [More...](classeigen_1_1colpivhouseholderqr#a5965d4fdebc04b2df71d67ff0b2d0c2c) |
| |
| const [PermutationType](classeigen_1_1permutationmatrix) & | [colsPermutation](classeigen_1_1colpivhouseholderqr#ab6ad43e6a6fb75726eae0d5499948f4a) () const |
| |
| template<typename InputType > |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< MatrixType > & | [compute](classeigen_1_1colpivhouseholderqr#a608016776319abe8fe96b85f83c8dd3d) (const [EigenBase](structeigen_1_1eigenbase)< InputType > &matrix) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [dimensionOfKernel](classeigen_1_1colpivhouseholderqr#a7c9294565d179226133770160b827be1) () const |
| |
| const HCoeffsType & | [hCoeffs](classeigen_1_1colpivhouseholderqr#ac5943d19aa5fd96340c7df6874fcb1b9) () const |
| |
| [HouseholderSequenceType](classeigen_1_1householdersequence) | [householderQ](classeigen_1_1colpivhouseholderqr#a28ab9d8916ca609c5469c4c192fbfa28) () const |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1colpivhouseholderqr#a5c756a789175197cab3eff3a3e479ef2) () const |
| | Reports whether the QR factorization was successful. [More...](classeigen_1_1colpivhouseholderqr#a5c756a789175197cab3eff3a3e479ef2) |
| |
| const [Inverse](classeigen_1_1inverse)< [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) > | [inverse](classeigen_1_1colpivhouseholderqr#a31c45402e74774d9cd13af0e57a6b72f) () const |
| |
| bool | [isInjective](classeigen_1_1colpivhouseholderqr#a0031998053c9c7345c9458f7443aa263) () const |
| |
| bool | [isInvertible](classeigen_1_1colpivhouseholderqr#a945720f8d683f8ebe97fa807edd3142a) () const |
| |
| bool | [isSurjective](classeigen_1_1colpivhouseholderqr#a87a7d06e0b0479e5b56b19c2a4f56365) () const |
| |
| MatrixType::RealScalar | [logAbsDeterminant](classeigen_1_1colpivhouseholderqr#afdc29438a335871f67449c253369ce12) () const |
| |
| const MatrixType & | [matrixQR](classeigen_1_1colpivhouseholderqr#aa572ac050c8d4fadd4f08a87f6b1e62b) () const |
| |
| const MatrixType & | [matrixR](classeigen_1_1colpivhouseholderqr#a44c534d47bde6b67ce4b5247d142ef30) () const |
| |
| RealScalar | [maxPivot](classeigen_1_1colpivhouseholderqr#aac8c43d720170980f582d01494df9e8f) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonzeroPivots](classeigen_1_1colpivhouseholderqr#a796610bab81f0527aa1ae440c71f58a4) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rank](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e) () const |
| |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) & | [setThreshold](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee) (const RealScalar &[threshold](classeigen_1_1colpivhouseholderqr#a72276adb1aa11f870f50d0bd58af014d)) |
| |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) & | [setThreshold](classeigen_1_1colpivhouseholderqr#a648df14c457ceceb09d933d06d3bdded) (Default\_t) |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr), Rhs > | [solve](classeigen_1_1colpivhouseholderqr#aaa9c4af89930ab3bb7612ed9ae33d3f5) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| RealScalar | [threshold](classeigen_1_1colpivhouseholderqr#a72276adb1aa11f870f50d0bd58af014d) () const |
| |
|
Public Member Functions inherited from [Eigen::SolverBase< ColPivHouseholderQR< \_MatrixType > >](classeigen_1_1solverbase) |
| AdjointReturnType | [adjoint](classeigen_1_1solverbase#a05a3686a89888681c8e0c2bcab6d1ce5) () const |
| |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType > & | [derived](classeigen_1_1solverbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType > & | [derived](classeigen_1_1solverbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| const [Solve](classeigen_1_1solve)< [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >, Rhs > | [solve](classeigen_1_1solverbase#a7fd647d110487799205df6f99547879d) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| | [SolverBase](classeigen_1_1solverbase#a4d5e5baddfba3790ab1a5f247dcc4dc1) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1solverbase#a732e75b5132bb4db3775916927b0e86c) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
ColPivHouseholderQR() [1/4]
---------------------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) | ( | | ) | |
| inline |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via ColPivHouseholderQR::compute(const MatrixType&).
ColPivHouseholderQR() [2/4]
---------------------------
template<typename \_MatrixType >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inline |
Default Constructor with memory preallocation.
Like the default constructor but with preallocation of the internal data according to the specified problem *size*.
See also
[ColPivHouseholderQR()](classeigen_1_1colpivhouseholderqr#a9d8a92c2a2f0debe5454812372237ed4 "Default Constructor.")
ColPivHouseholderQR() [3/4]
---------------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a QR factorization from a given matrix.
This constructor computes the QR factorization of the matrix *matrix* by calling the method compute(). It is a short cut for:
```
ColPivHouseholderQR<MatrixType> qr(matrix.rows(), matrix.cols());
qr.compute(matrix);
```
See also
compute()
ColPivHouseholderQR() [4/4]
---------------------------
template<typename \_MatrixType > template<typename InputType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr) | ( | [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
| inlineexplicit |
Constructs a QR factorization from a given matrix.
This overloaded constructor is provided for [inplace decomposition](group__inplacedecomposition) when `MatrixType` is a [Eigen::Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.").
See also
ColPivHouseholderQR(const EigenBase&)
absDeterminant()
----------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< MatrixType >::absDeterminant |
Returns
the absolute value of the determinant of the matrix of which \*this is the QR decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the QR decomposition has already been computed.
Note
This is only for square matrices.
Warning
a determinant can be very big or small, so for matrices of large enough dimension, there is a risk of overflow/underflow. One way to work around that is to use [logAbsDeterminant()](classeigen_1_1colpivhouseholderqr#afdc29438a335871f67449c253369ce12) instead.
See also
[logAbsDeterminant()](classeigen_1_1colpivhouseholderqr#afdc29438a335871f67449c253369ce12), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
colsPermutation()
-----------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [PermutationType](classeigen_1_1permutationmatrix)& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::colsPermutation | ( | | ) | const |
| inline |
Returns
a const reference to the column permutation matrix
compute()
---------
template<typename \_MatrixType > template<typename InputType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)<MatrixType>& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::compute | ( | const [EigenBase](structeigen_1_1eigenbase)< InputType > & | *matrix* | ) | |
Performs the QR factorization of the given matrix *matrix*. The result of the factorization is stored into `*this`, and a reference to `*this` is returned.
See also
class [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting."), ColPivHouseholderQR(const MatrixType&)
dimensionOfKernel()
-------------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::dimensionOfKernel | ( | | ) | const |
| inline |
Returns
the dimension of the kernel of the matrix of which \*this is the QR decomposition.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
hCoeffs()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const HCoeffsType& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::hCoeffs | ( | | ) | const |
| inline |
Returns
a const reference to the vector of Householder coefficients used to represent the factor `Q`.
For advanced uses only.
householderQ()
--------------
template<typename MatrixType >
| |
| --- |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< MatrixType >::[HouseholderSequenceType](classeigen_1_1householdersequence) [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< MatrixType >::householderQ |
Returns
the matrix Q as a sequence of householder transformations. You can extract the meaningful part only by using:
```
qr.householderQ().setLength(qr.nonzeroPivots())
```
info()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::info | ( | | ) | const |
| inline |
Reports whether the QR factorization was successful.
Note
This function always returns `Success`. It is provided for compatibility with other factorization routines.
Returns
`Success`
inverse()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [Inverse](classeigen_1_1inverse)<[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)> [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::inverse | ( | | ) | const |
| inline |
Returns
the inverse of the matrix of which \*this is the QR decomposition.
Note
If this matrix is not invertible, the returned matrix has undefined coefficients. Use [isInvertible()](classeigen_1_1colpivhouseholderqr#a945720f8d683f8ebe97fa807edd3142a) to first determine whether this matrix is invertible.
isInjective()
-------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::isInjective | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the QR decomposition represents an injective linear map, i.e. has trivial kernel; false otherwise.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
isInvertible()
--------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::isInvertible | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the QR decomposition is invertible.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
isSurjective()
--------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| bool [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::isSurjective | ( | | ) | const |
| inline |
Returns
true if the matrix of which \*this is the QR decomposition represents a surjective linear map; false otherwise.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
logAbsDeterminant()
-------------------
template<typename MatrixType >
| |
| --- |
| MatrixType::RealScalar [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< MatrixType >::logAbsDeterminant |
Returns
the natural log of the absolute value of the determinant of the matrix of which \*this is the QR decomposition. It has only linear complexity (that is, O(n) where n is the dimension of the square matrix) as the QR decomposition has already been computed.
Note
This is only for square matrices. This method is useful to work around the risk of overflow/underflow that's inherent to determinant computation.
See also
[absDeterminant()](classeigen_1_1colpivhouseholderqr#ac87c3bf42098d6f7324dafbc50fa83f7), [MatrixBase::determinant()](classeigen_1_1matrixbase#a7ad8f77004bb956b603bb43fd2e3c061)
matrixQR()
----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::matrixQR | ( | | ) | const |
| inline |
Returns
a reference to the matrix where the Householder QR decomposition is stored
matrixR()
---------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MatrixType& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::matrixR | ( | | ) | const |
| inline |
Returns
a reference to the matrix where the result Householder QR is stored
Warning
The strict lower part of this matrix contains internal values. Only the upper triangular part should be referenced. To get it, use
```
[matrixR](classeigen_1_1colpivhouseholderqr#a44c534d47bde6b67ce4b5247d142ef30)().template triangularView<Upper>()
```
For rank-deficient matrices, use
```
[matrixR](classeigen_1_1colpivhouseholderqr#a44c534d47bde6b67ce4b5247d142ef30)().topLeftCorner([rank](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e)(), [rank](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e)()).template triangularView<Upper>()
```
maxPivot()
----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::maxPivot | ( | | ) | const |
| inline |
Returns
the absolute value of the biggest pivot, i.e. the biggest diagonal coefficient of R.
nonzeroPivots()
---------------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::nonzeroPivots | ( | | ) | const |
| inline |
Returns
the number of nonzero pivots in the QR decomposition. Here nonzero is meant in the exact sense, not in a fuzzy sense. So that notion isn't really intrinsically interesting, but it is still useful when implementing algorithms.
See also
[rank()](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e)
rank()
------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::rank | ( | | ) | const |
| inline |
Returns
the rank of the matrix of which \*this is the QR decomposition.
Note
This method has to determine which pivots should be considered nonzero. For that, it uses the threshold value that you can control by calling [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
setThreshold() [1/2]
--------------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::setThreshold | ( | const RealScalar & | *threshold* | ) | |
| inline |
Allows to prescribe a threshold to be used by certain methods, such as [rank()](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e), who need to determine when pivots are to be considered nonzero. This is not used for the QR decomposition itself.
When it needs to get the threshold value, [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") calls [threshold()](classeigen_1_1colpivhouseholderqr#a72276adb1aa11f870f50d0bd58af014d). By default, this uses a formula to automatically determine a reasonable threshold. Once you have called the present method [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee), your value is used instead.
Parameters
| | |
| --- | --- |
| threshold | The new value to use as the threshold. |
A pivot will be considered nonzero if its absolute value is strictly greater than \( \vert pivot \vert \leqslant threshold \times \vert maxpivot \vert \) where maxpivot is the biggest pivot.
If you want to come back to the default behavior, call [setThreshold(Default\_t)](classeigen_1_1colpivhouseholderqr#a648df14c457ceceb09d933d06d3bdded)
setThreshold() [2/2]
--------------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)& [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::setThreshold | ( | Default\_t | | ) | |
| inline |
Allows to come back to the default behavior, letting [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") use its default formula for determining the threshold.
You should pass the special object Eigen::Default as parameter here.
```
qr.setThreshold(Eigen::Default);
```
See the documentation of [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
solve()
-------
template<typename \_MatrixType > template<typename Rhs >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Solve](classeigen_1_1solve)<[ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr), Rhs> [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::solve | ( | const [MatrixBase](classeigen_1_1matrixbase)< Rhs > & | *b* | ) | const |
| inline |
This method finds a solution x to the equation Ax=b, where A is the matrix of which \*this is the QR decomposition, if any exists.
Parameters
| | |
| --- | --- |
| b | the right-hand-side of the equation to solve. |
Returns
a solution.
This method just tries to find as good a solution as possible. If you want to check whether a solution exists or if it is accurate, just call this function to get a result and then compute the error of this result, or use [MatrixBase::isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c) directly, for instance like this:
```
bool a_solution_exists = (A*result).isApprox(b, precision);
```
This method avoids dividing by zero, so that the non-existence of a solution doesn't by itself mean that you'll get `inf` or `nan` values.
If there exists more than one solution, this method will arbitrarily choose one.
Example:
```
Matrix3f m = [Matrix3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
Matrix3f y = [Matrix3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the matrix y:" << endl << y << endl;
Matrix3f x;
x = m.colPivHouseholderQr().solve(y);
assert(y.isApprox(m*x));
cout << "Here is a solution x to the equation mx=y:" << endl << x << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the matrix y:
0.108 -0.27 0.832
-0.0452 0.0268 0.271
0.258 0.904 0.435
Here is a solution x to the equation mx=y:
0.609 2.68 1.67
-0.231 -1.57 0.0713
0.51 3.51 1.05
```
threshold()
-----------
template<typename \_MatrixType >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| RealScalar [Eigen::ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr)< \_MatrixType >::threshold | ( | | ) | const |
| inline |
Returns the threshold that will be used by certain methods such as [rank()](classeigen_1_1colpivhouseholderqr#a2a59aaa689613ce5ef0c9130ad33940e).
See the documentation of [setThreshold(const RealScalar&)](classeigen_1_1colpivhouseholderqr#ae712cdc9f0e521cfc8061bee58ff55ee).
---
The documentation for this class was generated from the following file:* [ColPivHouseholderQR.h](https://eigen.tuxfamily.org/dox/ColPivHouseholderQR_8h_source.html)
| programming_docs |
eigen3 Global array typedefs Global array typedefs
=====================
Eigen defines several typedef shortcuts for most common 1D and 2D array types.
The general patterns are the following:
`ArrayRowsColsType` where `Rows` and `Cols` can be `2`,`3`,`4` for fixed size square matrices or `X` for dynamic size, and where `Type` can be `i` for integer, `f` for float, `d` for double, `cf` for complex float, `cd` for complex double.
For example, `Array33d` is a fixed-size 3x3 array type of doubles, and `ArrayXXf` is a dynamic-size matrix of floats.
There are also `ArraySizeType` which are self-explanatory. For example, `Array4cf` is a fixed-size 1D array of 4 complex floats.
With [c++11], template alias are also defined for common sizes. They follow the same pattern as above except that the scalar type suffix is replaced by a template parameter, i.e.:
* `ArrayRowsCols<Type>` where `Rows` and `Cols` can be `2`,`3`,`4`, or `X` for fixed or dynamic size.
* `ArraySize<Type>` where `Size` can be `2`,`3`,`4` or `X` for fixed or dynamic size 1D arrays.
See also
class [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.")
| |
| --- |
| |
| template<typename Type > |
| using | [Eigen::Array2](group__arraytypedefs#ga27e36e9e1da10f2c17d69bbfa7ead870) = [Array](classeigen_1_1array)< Type, 2, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array22](group__arraytypedefs#gad5e10990863f064300d78e80b4537a0b) = [Array](classeigen_1_1array)< Type, 2, 2 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array2X](group__arraytypedefs#ga995df558b61646d505b9feabd30a28d0) = [Array](classeigen_1_1array)< Type, 2, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array3](group__arraytypedefs#ga7ca781ec91d7b715d226d5057d50b250) = [Array](classeigen_1_1array)< Type, 3, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array33](group__arraytypedefs#gafb8560ef95ce6a7284131d91da82cf8a) = [Array](classeigen_1_1array)< Type, 3, 3 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array3X](group__arraytypedefs#gaf24f8bd443138027dd02599e7c58ef60) = [Array](classeigen_1_1array)< Type, 3, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array4](group__arraytypedefs#ga2b5e91e45a496f9893059ab4f298a9ee) = [Array](classeigen_1_1array)< Type, 4, 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array44](group__arraytypedefs#gabcb97c1d614be824fd355ca29632e30f) = [Array](classeigen_1_1array)< Type, 4, 4 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::Array4X](group__arraytypedefs#gaab59985198663295e89134835783bd0d) = [Array](classeigen_1_1array)< Type, 4, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::ArrayX](group__arraytypedefs#ga9f9db3ea1c9715a30ed77c6cd434bbd3) = [Array](classeigen_1_1array)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 1 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::ArrayX2](group__arraytypedefs#ga273e82d1306290af7e02aeb75a831fca) = [Array](classeigen_1_1array)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 2 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::ArrayX3](group__arraytypedefs#ga428c78ad433d2f285650ccee8fc0f29f) = [Array](classeigen_1_1array)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 3 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::ArrayX4](group__arraytypedefs#gaad02550dee7a18c7a74941be73de327d) = [Array](classeigen_1_1array)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), 4 > |
| | [c++11] |
| |
| template<typename Type > |
| using | [Eigen::ArrayXX](group__arraytypedefs#ga6a3ca2b2a8694c17ed25ac03cafdaa04) = [Array](classeigen_1_1array)< Type, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > |
| | [c++11] |
| |
eigen3 The template and typename keywords in C++ The template and typename keywords in C++
=========================================
There are two uses for the `template` and `typename` keywords in C++. One of them is fairly well known amongst programmers: to define templates. The other use is more obscure: to specify that an expression refers to a template function or a type. This regularly trips up programmers that use the Eigen library, often leading to error messages from the compiler that are difficult to understand, such as "expected expression" or "no match for operator<".
Using the template and typename keywords to define templates
==============================================================
The `template` and `typename` keywords are routinely used to define templates. This is not the topic of this page as we assume that the reader is aware of this (otherwise consult a C++ book). The following example should illustrate this use of the `template` keyword.
```
template <typename T>
bool isPositive(T x)
{
return x > 0;
}
```
We could just as well have written `template <class T>`; the keywords `typename` and `class` have the same meaning in this context.
An example showing the second use of the template keyword
===========================================================
Let us illustrate the second use of the `template` keyword with an example. Suppose we want to write a function which copies all entries in the upper triangular part of a matrix into another matrix, while keeping the lower triangular part unchanged. A straightforward implementation would be as follows:
| Example: | Output: |
| --- | --- |
|
```
#include <Eigen/Dense>
#include <iostream>
using namespace [Eigen](namespaceeigen);
void copyUpperTriangularPart(MatrixXf& dst, const MatrixXf& src)
{
dst.triangularView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>() = src.triangularView<[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)>();
}
int main()
{
MatrixXf m1 = [MatrixXf::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(4,4);
MatrixXf m2 = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(4,4);
std::cout << "m2 before copy:" << std::endl;
std::cout << m2 << std::endl << std::endl;
copyUpperTriangularPart(m2, m1);
std::cout << "m2 after copy:" << std::endl;
std::cout << m2 << std::endl << std::endl;
}
```
|
```
m2 before copy:
0.68 0.823 -0.444 -0.27
-0.211 -0.605 0.108 0.0268
0.566 -0.33 -0.0452 0.904
0.597 0.536 0.258 0.832
m2 after copy:
1 1 1 1
-0.211 1 1 1
0.566 -0.33 1 1
0.597 0.536 0.258 1
```
|
That works fine, but it is not very flexible. First, it only works with dynamic-size matrices of single-precision floats; the function `copyUpperTriangularPart()` does not accept static-size matrices or matrices with double-precision numbers. Second, if you use an expression such as `mat.topLeftCorner(3,3)` as the parameter `src`, then this is copied into a temporary variable of type MatrixXf; this copy can be avoided.
As explained in [Writing Functions Taking Eigen Types as Parameters](topicfunctiontakingeigentypes), both issues can be resolved by making `copyUpperTriangularPart()` accept any object of type [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions."). This leads to the following code:
| Example: | Output: |
| --- | --- |
|
```
#include <Eigen/Dense>
#include <iostream>
using namespace [Eigen](namespaceeigen);
template <typename Derived1, typename Derived2>
void copyUpperTriangularPart(MatrixBase<Derived1>& dst, const MatrixBase<Derived2>& src)
{
/\* Note the 'template' keywords in the following line! \*/
dst.template triangularView<Upper>() = src.template triangularView<Upper>();
}
int main()
{
MatrixXi m1 = [MatrixXi::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(5,5);
MatrixXi m2 = [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(4,4);
std::cout << "m2 before copy:" << std::endl;
std::cout << m2 << std::endl << std::endl;
copyUpperTriangularPart(m2, m1.topLeftCorner(4,4));
std::cout << "m2 after copy:" << std::endl;
std::cout << m2 << std::endl << std::endl;
}
```
|
```
m2 before copy:
7 9 -5 -3
-2 -6 1 0
6 -3 0 9
6 6 3 9
m2 after copy:
1 1 1 1
-2 1 1 1
6 -3 1 1
6 6 3 1
```
|
The one line in the body of the function `copyUpperTriangularPart()` shows the second, more obscure use of the `template` keyword in C++. Even though it may look strange, the `template` keywords are necessary according to the standard. Without it, the compiler may reject the code with an error message like "no match for operator<".
Explanation
=============
The reason that the `template` keyword is necessary in the last example has to do with the rules for how templates are supposed to be compiled in C++. The compiler has to check the code for correct syntax at the point where the template is defined, without knowing the actual value of the template arguments (`Derived1` and `Derived2` in the example). That means that the compiler cannot know that `dst.triangularView` is a member template and that the following < symbol is part of the delimiter for the template parameter. Another possibility would be that `dst.triangularView` is a member variable with the < symbol referring to the `operator<()` function. In fact, the compiler should choose the second possibility, according to the standard. If `dst.triangularView` is a member template (as in our case), the programmer should specify this explicitly with the `template` keyword and write `dst.template triangularView`.
The precise rules are rather complicated, but ignoring some subtleties we can summarize them as follows:
* A *dependent name* is name that depends (directly or indirectly) on a template parameter. In the example, `dst` is a dependent name because it is of type `[MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")<Derived1>` which depends on the template parameter `Derived1`.
* If the code contains either one of the constructs `xxx.yyy` or `xxx->yyy` and `xxx` is a dependent name and `yyy` refers to a member template, then the `template` keyword must be used before `yyy`, leading to `xxx.template yyy` or `xxx->template yyy`.
* If the code contains the construct `xxx::yyy` and `xxx` is a dependent name and `yyy` refers to a member typedef, then the `typename` keyword must be used before the whole construct, leading to `typename xxx::yyy`.
As an example where the `typename` keyword is required, consider the following code in [Sparse matrix manipulations](group__tutorialsparse) for iterating over the non-zero entries of a sparse matrix type:
```
SparseMatrixType mat(rows,cols);
for (int k=0; k<mat.outerSize(); ++k)
for (SparseMatrixType::InnerIterator it(mat,k); it; ++it)
{
/\* ... \*/
}
```
If `SparseMatrixType` depends on a template parameter, then the `typename` keyword is required:
```
template <typename T>
void iterateOverSparseMatrix(const SparseMatrix<T>& mat;
{
for (int k=0; k<m1.outerSize(); ++k)
for (typename SparseMatrix<T>::InnerIterator it(mat,k); it; ++it)
{
/\* ... \*/
}
}
```
Resources for further reading
===============================
For more information and a fuller explanation of this topic, the reader may consult the following sources:
* The book "C++ Template Metaprogramming" by David Abrahams and Aleksey Gurtovoy contains a very good explanation in Appendix B ("The typename and template Keywords") which formed the basis for this page.
* <http://pages.cs.wisc.edu/~driscoll/typename.html>
* <http://www.parashift.com/c++-faq-lite/templates.html#faq-35.18>
* <http://www.comeaucomputing.com/techtalk/templates/#templateprefix>
* <http://www.comeaucomputing.com/techtalk/templates/#typename>
eigen3 Eigen::SuperILU Eigen::SuperILU
===============
### template<typename \_MatrixType> class Eigen::SuperILU< \_MatrixType >
A sparse direct **incomplete** LU factorization and solver based on the [SuperLU](classeigen_1_1superlu "A sparse direct LU factorization and solver based on the SuperLU library.") library.
This class allows to solve for an approximate solution of A.X = B sparse linear problems via an incomplete LU factorization using the [SuperLU](classeigen_1_1superlu "A sparse direct LU factorization and solver based on the SuperLU library.") library. This class is aimed to be used as a preconditioner of the iterative linear solvers.
Warning
This class is only for the 4.x versions of [SuperLU](classeigen_1_1superlu "A sparse direct LU factorization and solver based on the SuperLU library."). The 3.x and 5.x versions are not supported.
Template Parameters
| | |
| --- | --- |
| \_MatrixType | the type of the sparse matrix A, it must be a SparseMatrix<> |
This class follows the [sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept) .
See also
[Sparse solver concept](group__topicsparsesystems#TutorialSparseSolverConcept), class [IncompleteLUT](classeigen_1_1incompletelut "Incomplete LU factorization with dual-threshold strategy."), class [ConjugateGradient](classeigen_1_1conjugategradient "A conjugate gradient solver for sparse (or dense) self-adjoint problems."), class [BiCGSTAB](classeigen_1_1bicgstab "A bi conjugate gradient stabilized solver for sparse square problems.")
| |
| --- |
| |
| void | [analyzePattern](classeigen_1_1superilu#a52aa43effc247084c91a5e73720a50f6) (const MatrixType &matrix) |
| |
| void | [factorize](classeigen_1_1superilu#a5669976899907fbe6bf1be620707e5f3) (const MatrixType &matrix) |
| |
|
Public Member Functions inherited from [Eigen::SuperLUBase< \_MatrixType, SuperILU< \_MatrixType > >](classeigen_1_1superlubase) |
| void | [analyzePattern](classeigen_1_1superlubase#a2d3f48425328d9b3cbdca369889007f3) (const MatrixType &) |
| |
| void | [compute](classeigen_1_1superlubase#a28cb3ef7914ecb6fdae1935b53f6be40) (const MatrixType &matrix) |
| |
| [ComputationInfo](group__enums#ga85fad7b87587764e5cf6b513a9e0ee5e) | [info](classeigen_1_1superlubase#aa67da5c8c24110931c949c5896c5ec03) () const |
| | Reports whether previous computation was successful. [More...](classeigen_1_1superlubase#aa67da5c8c24110931c949c5896c5ec03) |
| |
| superlu\_options\_t & | [options](classeigen_1_1superlubase#a42d9d79073379f1e75b0f2c49879ed5b) () |
| |
|
Public Member Functions inherited from [Eigen::SparseSolverBase< Derived >](classeigen_1_1sparsesolverbase) |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a4a66e9498b06e3ec4ec36f06b26d4e8f) (const [MatrixBase](classeigen_1_1matrixbase)< Rhs > &b) const |
| |
| template<typename Rhs > |
| const [Solve](classeigen_1_1solve)< Derived, Rhs > | [solve](classeigen_1_1sparsesolverbase#a3a8d97173b6e2630f484589b3471cfc7) (const [SparseMatrixBase](classeigen_1_1sparsematrixbase)< Rhs > &b) const |
| |
| | [SparseSolverBase](classeigen_1_1sparsesolverbase#aacd99fa17db475e74d3834767f392f33) () |
| |
analyzePattern()
----------------
template<typename \_MatrixType >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SuperILU](classeigen_1_1superilu)< \_MatrixType >::analyzePattern | ( | const MatrixType & | *matrix* | ) | |
| inline |
Performs a symbolic decomposition on the sparcity of *matrix*.
This function is particularly useful when solving for several problems having the same structure.
See also
[factorize()](classeigen_1_1superilu#a5669976899907fbe6bf1be620707e5f3)
factorize()
-----------
template<typename MatrixType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::SuperILU](classeigen_1_1superilu)< MatrixType >::factorize | ( | const MatrixType & | *matrix* | ) | |
Performs a numeric decomposition of *matrix*
The given matrix must has the same sparcity than the matrix on which the symbolic decomposition has been performed.
See also
[analyzePattern()](classeigen_1_1superilu#a52aa43effc247084c91a5e73720a50f6)
---
The documentation for this class was generated from the following file:* [SuperLUSupport.h](https://eigen.tuxfamily.org/dox/SuperLUSupport_8h_source.html)
eigen3 Eigen::DenseBase Eigen::DenseBase
================
### template<typename Derived> class Eigen::DenseBase< Derived >
Base class for all dense matrices, vectors, and arrays.
This class is the base that is inherited by all dense objects (matrix, vector, arrays, and related expression types). The common [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") API for dense objects is contained in this class.
Template Parameters
| | |
| --- | --- |
| Derived | is the derived type, e.g., a matrix type or an expression. |
This class can be extended with the help of the plugin mechanism described on the page [Extending MatrixBase (and other classes)](topiccustomizing_plugins) by defining the preprocessor symbol `EIGEN_DENSEBASE_PLUGIN`.
See also
[The class hierarchy](topicclasshierarchy)
| |
| --- |
| |
| enum | { [RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab) , [ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441) , [SizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da25cb495affdbd796198462b8ef06be91) , [MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306) , [MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) , [MaxSizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da3a459062d39cb34452518f5f201161d2) , [IsVectorAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da1156955c8099c5072934b74c72654ed0) , [NumDimensions](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da4d4548a01ba37a6c2031a3c1f0a37d34) , [Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581) , [IsRowMajor](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da406b6af91d61d348ba1c9764bdd66008) , **InnerSizeAtCompileTime** , **InnerStrideAtCompileTime** , **OuterStrideAtCompileTime** } |
| |
| typedef random\_access\_iterator\_type | [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) |
| |
| typedef random\_access\_iterator\_type | [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) |
| |
| typedef [Array](classeigen_1_1array)< typename internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits< Derived >::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits< Derived >::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [AutoAlign](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13ad0e7f67d40bcde3d41c12849b16ce6ea)|(internal::traits< Derived >::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581) &[RowMajorBit](group__flags#gae4f56c2a60bbe4bd2e44c5b19cbe8762) ? [RowMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a77c993a8d9f6efe5c1159fb2ab07dd4f) :[ColMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a0103672ae41005ab03b4176c765afd62)), internal::traits< Derived >::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits< Derived >::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > | [PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb) |
| |
| typedef [Matrix](classeigen_1_1matrix)< typename internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits< Derived >::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits< Derived >::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [AutoAlign](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13ad0e7f67d40bcde3d41c12849b16ce6ea)|(internal::traits< Derived >::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581) &[RowMajorBit](group__flags#gae4f56c2a60bbe4bd2e44c5b19cbe8762) ? [RowMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a77c993a8d9f6efe5c1159fb2ab07dd4f) :[ColMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a0103672ae41005ab03b4176c765afd62)), internal::traits< Derived >::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits< Derived >::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > | [PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e) |
| |
| typedef internal::conditional< internal::is\_same< typename internal::traits< Derived >::XprKind, [MatrixXpr](structeigen_1_1matrixxpr) >::[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), [PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e), [PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb) >::type | [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) |
| | The plain matrix or array type corresponding to this expression. [More...](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) |
| |
| typedef internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) |
| |
| typedef internal::traits< Derived >::[StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) | [StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) |
| | The type used to store indices. [More...](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) |
| |
| typedef [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [value\_type](classeigen_1_1densebase#a9276182dab8236c33f1e7abf491d504d) |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
| |
| --- |
| |
| bool | [all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4) () const |
| |
| bool | [allFinite](classeigen_1_1densebase#af1e669fd3aaae50a4870dc1b8f3b8884) () const |
| |
| bool | [any](classeigen_1_1densebase#abfbf4cb72dd577e62fbe035b1c53e695) () const |
| |
| [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) | [begin](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641) () |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [begin](classeigen_1_1densebase#ad9368ce70b06167ec5fc19398d329f5e) () const |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [cbegin](classeigen_1_1densebase#ae9a3dfd9b826ba3103de0128576fb15b) () const |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [cend](classeigen_1_1densebase#aeb3b76f02986c2af2521d07164b5ffde) () const |
| |
| [ColwiseReturnType](classeigen_1_1vectorwiseop) | [colwise](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30) () |
| |
| [ConstColwiseReturnType](classeigen_1_1vectorwiseop) | [colwise](classeigen_1_1densebase#a58837c81de446efbdb58da07b73a63c1) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [count](classeigen_1_1densebase#a229be090c665b9bf7d1fcdfd1ab6e0c1) () const |
| |
| [iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) | [end](classeigen_1_1densebase#ae71d079e16d91360d10066b316b48485) () |
| |
| [const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) | [end](classeigen_1_1densebase#ab34773522e43bfb02e9cf652d7b5dd60) () const |
| |
| EvalReturnType | [eval](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) () const |
| |
| void | [fill](classeigen_1_1densebase#a9be169c308801411aa24be93d30930bf) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| template<unsigned int Added, unsigned int Removed> |
| EIGEN\_DEPRECATED const Derived & | [flagged](classeigen_1_1densebase#a9b3f75f76ae40439be870258e80c7346) () const |
| |
| const [WithFormat](classeigen_1_1withformat)< Derived > | [format](classeigen_1_1densebase#ab231f1a6057f28d4244145e12c9fc0c7) (const [IOFormat](structeigen_1_1ioformat) &fmt) const |
| |
| bool | [hasNaN](classeigen_1_1densebase#ab13d158c900560d3e1b25d85d2d33dd6) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerSize](classeigen_1_1densebase#a58ca41d9635a8ab3c5a268ef3f7f0d75) () const |
| |
| template<typename OtherDerived > |
| bool | [isApprox](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isApproxToConstant](classeigen_1_1densebase#af9b150d48bc5e4366887ccb466e40c6b) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isConstant](classeigen_1_1densebase#a1ca84e4179b3e5081ed11d89bbd9e74f) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| template<typename OtherDerived > |
| bool | [isMuchSmallerThan](classeigen_1_1densebase#a3c4db0c6dd974fa88bbb58b2cf3d5664) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other, const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| template<typename Derived > |
| bool | [isMuchSmallerThan](classeigen_1_1densebase#adfca6ff4e473f68fbbeabbd03b7504a9) (const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::Real &other, const RealScalar &prec) const |
| |
| bool | [isOnes](classeigen_1_1densebase#aa56d6b4477cd3c92a9cf42f4b96e47c2) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| bool | [isZero](classeigen_1_1densebase#af36014ec300f53a65083057ed4e89822) (const RealScalar &prec=[NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::dummy\_precision()) const |
| |
| template<typename OtherDerived > |
| EIGEN\_DEPRECATED Derived & | [lazyAssign](classeigen_1_1densebase#a6bc6c096e3bfc726f28315daecd21b3f) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<int NaNPropagation> |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1) () const |
| |
| template<int NaNPropagation, typename IndexType > |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#aced8ffda52ff061b6586ace2657ebf30) (IndexType \*index) const |
| |
| template<int NaNPropagation, typename IndexType > |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [maxCoeff](classeigen_1_1densebase#a3780b7a9cd184d0b4f3ea797eba9e2b3) (IndexType \*row, IndexType \*col) const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [mean](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7) () const |
| |
| template<int NaNPropagation> |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2) () const |
| |
| template<int NaNPropagation, typename IndexType > |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#ac9265f4f91430b9cc75d63fb6865bb29) (IndexType \*index) const |
| |
| template<int NaNPropagation, typename IndexType > |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [minCoeff](classeigen_1_1densebase#aa28152ba4a42b2d112e5fec5469ec4c1) (IndexType \*row, IndexType \*col) const |
| |
| const [NestByValue](classeigen_1_1nestbyvalue)< Derived > | [nestByValue](classeigen_1_1densebase#a3e2761e2b6da74dba1d17b40cc918bf7) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [nonZeros](classeigen_1_1densebase#acb8d5574eff335381e7441ade87700a0) () const |
| |
| template<typename OtherDerived > |
| [CommaInitializer](structeigen_1_1commainitializer)< Derived > | [operator<<](classeigen_1_1densebase#a0f0e34696162b34762b2bf4bd948f90c) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| [CommaInitializer](structeigen_1_1commainitializer)< Derived > | [operator<<](classeigen_1_1densebase#a0e575eb0ba6cc6bc5f347872abd8509d) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &s) |
| |
| Derived & | [operator=](classeigen_1_1densebase#a5281dadff89f46eef719b38e5d073a8f) (const [DenseBase](classeigen_1_1densebase) &other) |
| |
| template<typename OtherDerived > |
| Derived & | [operator=](classeigen_1_1densebase#ab66155169d20c035e80d521a8b918e97) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| Derived & | [operator=](classeigen_1_1densebase#a58915510693d64164e567bd762e1032f) (const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > &other) |
| | Copies the generic expression *other* into \*this. [More...](classeigen_1_1densebase#a58915510693d64164e567bd762e1032f) |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerSize](classeigen_1_1densebase#a03f71699bc26ca2ee4e42ec4538862d7) () const |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [prod](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f) () const |
| |
| template<typename Func > |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [redux](classeigen_1_1densebase#a63ce1e4fab36bff43bbadcdd06a67724) (const Func &func) const |
| |
| template<int RowFactor, int ColFactor> |
| const [Replicate](classeigen_1_1replicate)< Derived, RowFactor, ColFactor > | [replicate](classeigen_1_1densebase#a60dadfe80b813d808e91e4521c722a8e) () const |
| |
| const [Replicate](classeigen_1_1replicate)< Derived, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2) > | [replicate](classeigen_1_1densebase#afae2d5e36f1158d1b1681dac3cdbd58e) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) rowFactor, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) colFactor) const |
| |
| void | [resize](classeigen_1_1densebase#a2ec5bac4e1ab95808808ef50ccf4cb39) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) newSize) |
| |
| void | [resize](classeigen_1_1densebase#a25e2b4887b47b1f2346857d1931efa0f) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe)) |
| |
| [ReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f) () |
| |
| [ConstReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1densebase#a9e2f3ac4019184abf95ca0e1a8d82866) () const |
| |
| void | [reverseInPlace](classeigen_1_1densebase#adb8045155ea45f7961fc2a5170e1d921) () |
| |
| [RowwiseReturnType](classeigen_1_1vectorwiseop) | [rowwise](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28) () |
| |
| [ConstRowwiseReturnType](classeigen_1_1vectorwiseop) | [rowwise](classeigen_1_1densebase#aa1cabd3404528fe8cec4bab43d74bffc) () const |
| |
| template<typename ThenDerived , typename ElseDerived > |
| const [Select](classeigen_1_1select)< Derived, ThenDerived, ElseDerived > | [select](classeigen_1_1densebase#a65e78cfcbc9852e6923bebff4323ddca) (const [DenseBase](classeigen_1_1densebase)< ThenDerived > &thenMatrix, const [DenseBase](classeigen_1_1densebase)< ElseDerived > &elseMatrix) const |
| |
| template<typename ThenDerived > |
| const [Select](classeigen_1_1select)< Derived, ThenDerived, typename ThenDerived::ConstantReturnType > | [select](classeigen_1_1densebase#a57ef09a843004095f84c198dd145641b) (const [DenseBase](classeigen_1_1densebase)< ThenDerived > &thenMatrix, const typename ThenDerived::Scalar &elseScalar) const |
| |
| template<typename ElseDerived > |
| const [Select](classeigen_1_1select)< Derived, typename ElseDerived::ConstantReturnType, ElseDerived > | [select](classeigen_1_1densebase#a9e8e78c75887d4539071a0b7a61ca103) (const typename ElseDerived::Scalar &thenScalar, const [DenseBase](classeigen_1_1densebase)< ElseDerived > &elseMatrix) const |
| |
| Derived & | [setConstant](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| Derived & | [setLinSpaced](classeigen_1_1densebase#aeb023532476d3f14c457367e0eb5f3f1) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#aeb023532476d3f14c457367e0eb5f3f1) |
| |
| Derived & | [setLinSpaced](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9), const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a) |
| |
| Derived & | [setOnes](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2) () |
| |
| Derived & | [setRandom](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db) () |
| |
| Derived & | [setZero](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb) () |
| |
| [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) | [sum](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c) () const |
| |
| template<typename OtherDerived > |
| void | [swap](classeigen_1_1densebase#af9e7e4305fdb7781f2b2f05fa801f21e) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| void | [swap](classeigen_1_1densebase#a44e25adc6da9cd1d79f4c5bd7c1819cb) ([PlainObjectBase](classeigen_1_1plainobjectbase)< OtherDerived > &other) |
| |
| [TransposeReturnType](classeigen_1_1transpose) | [transpose](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c) () |
| |
| [ConstTransposeReturnType](classeigen_1_1diagonal) | [transpose](classeigen_1_1densebase#a38c0b074cf93fc194bf91141287cee3f) () const |
| |
| void | [transposeInPlace](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a) () |
| |
| CoeffReturnType | [value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0) () const |
| |
| template<typename Visitor > |
| void | [visit](classeigen_1_1densebase#a4225b90fcc74f18dd479b401124b3841) (Visitor &func) const |
| |
|
Public Member Functions inherited from [Eigen::DenseCoeffsBase< Derived, DirectWriteAccessors >](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [colStride](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#af1bac522aee4402639189f387592000b) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [innerStride](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a4e94e17926e1cf597deb2928e779cef6) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [outerStride](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#abb333f6f10467f6f8d7b59c213dea49e) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rowStride](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a63bc5874eb4e320e54eb079b43b49d22) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
|
Public Member Functions inherited from [Eigen::DenseCoeffsBase< Derived, WriteAccessors >](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4) |
| Scalar & | [coeffRef](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae66b7d18b2a85f3139b703126974c900) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| Scalar & | [coeffRef](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#adc8286576b31e11f056057be666a0ec8) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| Scalar & | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a0171eee1d9e582d1ac7ec0f18f5f615a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| Scalar & | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| Scalar & | [operator[]](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#aa2040f14e60fed1a4a68ca7f042e1bbf) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| Scalar & | [w](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af683e04b3926aaf4091581ca24ca09ad) () |
| |
| Scalar & | [x](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#af163a982f5a7ad7e5c3336990b3d7000) () |
| |
| Scalar & | [y](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#afeaa80359bf0c1311f91cdd74a2042a8) () |
| |
| Scalar & | [z](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#a858151a06b8c0ff407232d84e695dd73) () |
| |
|
Public Member Functions inherited from [Eigen::DenseCoeffsBase< Derived, ReadOnlyAccessors >](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4) |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#aa7231d519967c37b4f98002d80756bda) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [coeff](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af9fadc22d12e48c82745dad534a1671a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| CoeffReturnType | [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ab3dbba4a15d0fe90185d2900e5ef0fd1) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| CoeffReturnType | [operator[]](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a496672306836589fa04a6ab33cb0cf2a) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) index) const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| CoeffReturnType | [w](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a926f52a94f038db63c6b9103f98dcf0f) () const |
| |
| CoeffReturnType | [x](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a189c80109e76752b598d60dfcdab329e) () const |
| |
| CoeffReturnType | [y](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a0c6d6904a37805ce47a3238fbd735963) () const |
| |
| CoeffReturnType | [z](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#a7c60c97282d4a0f8bca16ef75e231ddb) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#aed89b5cc6e3b7d9d5bd63aed245ccd6d) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#a68a7ece6c5629d1e9447a321fcb14ccd) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const ConstantReturnType | [Constant](classeigen_1_1densebase#a1fdd3189ae3a41d250593334d82210cf) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9), const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0)) |
| |
| static const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#ad8098aa5971139a5585e623dddbea860) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#ad8098aa5971139a5585e623dddbea860) |
| |
| static const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9), const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| | Sets a linearly spaced vector. [More...](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) |
| |
| static EIGEN\_DEPRECATED const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#ac8cfbd436a8c9fc50defee5946451176) (Sequential\_t, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| |
| static EIGEN\_DEPRECATED const RandomAccessLinSpacedReturnType | [LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d) (Sequential\_t, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9), const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &low, const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) &high) |
| |
| template<typename CustomNullaryOp > |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a5dc65501accd02c30f7c1840c2a30a41) (const CustomNullaryOp &func) |
| |
| template<typename CustomNullaryOp > |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a3340c9b997f5b53a0131cf927f93b54c) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), const CustomNullaryOp &func) |
| |
| template<typename CustomNullaryOp > |
| static const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > | [NullaryExpr](classeigen_1_1densebase#a9752ee59976a4b4aad860ad1a9093e7f) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9), const CustomNullaryOp &func) |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101) () |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe)) |
| |
| static const ConstantReturnType | [Ones](classeigen_1_1densebase#ab710a58e4a80fbcb2594242372c8fe56) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9)) |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19) () |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#ae97f8d9d08f969c733c8144be6225756) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe)) |
| |
| static const [RandomReturnType](classeigen_1_1cwisenullaryop) | [Random](classeigen_1_1densebase#a7eb5f974a8f0b67eac7080db1da0e308) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9)) |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) () |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#ae41a9b5050ed27d9e93c82c9c8622cd3) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [rows](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [cols](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe)) |
| |
| static const ConstantReturnType | [Zero](classeigen_1_1densebase#ac22f79b812fa564061042407f2ba8f5b) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9)) |
| |
| |
| --- |
| |
| | [DenseBase](classeigen_1_1densebase#aa284042d0e1b0ad9b6a00db7fd2d9f7f) () |
| |
| |
| --- |
| |
| (Note that these are not member functions.) |
| template<typename Derived > |
| std::ostream & | [operator<<](classeigen_1_1densebase#a3806d3f42de165878dace160e6aba40a) (std::ostream &s, const [DenseBase](classeigen_1_1densebase)< Derived > &m) |
| |
const\_iterator
---------------
template<typename Derived >
| |
| --- |
| typedef random\_access\_iterator\_type [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) |
This is the const version of iterator (aka read-only)
iterator
--------
template<typename Derived >
| |
| --- |
| typedef random\_access\_iterator\_type [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) |
STL-like [RandomAccessIterator](https://en.cppreference.com/w/cpp/named_req/RandomAccessIterator) iterator type as returned by the [begin()](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641) and [end()](classeigen_1_1densebase#ae71d079e16d91360d10066b316b48485) methods.
PlainArray
----------
template<typename Derived >
| |
| --- |
| typedef [Array](classeigen_1_1array)<typename internal::traits<Derived>::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits<Derived>::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits<Derived>::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [AutoAlign](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13ad0e7f67d40bcde3d41c12849b16ce6ea) | (internal::traits<Derived>::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581)&[RowMajorBit](group__flags#gae4f56c2a60bbe4bd2e44c5b19cbe8762) ? [RowMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a77c993a8d9f6efe5c1159fb2ab07dd4f) : [ColMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a0103672ae41005ab03b4176c765afd62)), internal::traits<Derived>::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits<Derived>::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb) |
The plain array type corresponding to this expression.
See also
[PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4 "The plain matrix or array type corresponding to this expression.")
PlainMatrix
-----------
template<typename Derived >
| |
| --- |
| typedef [Matrix](classeigen_1_1matrix)<typename internal::traits<Derived>::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2), internal::traits<Derived>::[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), internal::traits<Derived>::[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [AutoAlign](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13ad0e7f67d40bcde3d41c12849b16ce6ea) | (internal::traits<Derived>::[Flags](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da7392c9b2ad41ba3c16fdc5306c04d581)&[RowMajorBit](group__flags#gae4f56c2a60bbe4bd2e44c5b19cbe8762) ? [RowMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a77c993a8d9f6efe5c1159fb2ab07dd4f) : [ColMajor](group__enums#ggaacded1a18ae58b0f554751f6cdf9eb13a0103672ae41005ab03b4176c765afd62)), internal::traits<Derived>::[MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), internal::traits<Derived>::[MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65) > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e) |
The plain matrix type corresponding to this expression.
See also
[PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4 "The plain matrix or array type corresponding to this expression.")
PlainObject
-----------
template<typename Derived >
| |
| --- |
| typedef internal::conditional<internal::is\_same<typename internal::traits<Derived>::XprKind,[MatrixXpr](structeigen_1_1matrixxpr) >::[value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), [PlainMatrix](classeigen_1_1densebase#aa301ef39d63443e9ef0b84f47350116e), [PlainArray](classeigen_1_1densebase#a65328b7d6fc10a26ff6cd5801a6a44eb)>::type [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) |
The plain matrix or array type corresponding to this expression.
This is not necessarily exactly the return type of [eval()](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b). In the case of plain matrices, the return type of [eval()](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) is a const reference to a matrix, not a matrix! It is however guaranteed that the return type of [eval()](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) is either PlainObject or const PlainObject&.
Scalar
------
template<typename Derived >
| |
| --- |
| typedef internal::traits<Derived>::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) |
The numeric type of the expression' coefficients, e.g. float, double, int or std::complex<float>, etc.
StorageIndex
------------
template<typename Derived >
| |
| --- |
| typedef internal::traits<Derived>::[StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[StorageIndex](classeigen_1_1densebase#a2d1aba3f6c414715d830f760913c7e00) |
The type used to store indices.
This typedef is relevant for types that store multiple indices such as [PermutationMatrix](classeigen_1_1permutationmatrix "Permutation matrix.") or [Transpositions](classeigen_1_1transpositions "Represents a sequence of transpositions (row/column interchange)"), otherwise it defaults to [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde "The Index type as used for the API.")
See also
[Preprocessor directives](topicpreprocessordirectives), [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde "The Index type as used for the API."), [SparseMatrixBase](classeigen_1_1sparsematrixbase "Base class of any sparse matrices or sparse expressions.").
value\_type
-----------
template<typename Derived >
| |
| --- |
| typedef [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[value\_type](classeigen_1_1densebase#a9276182dab8236c33f1e7abf491d504d) |
The numeric type of the expression' coefficients, e.g. float, double, int or std::complex<float>, etc.
It is an alias for the Scalar type
anonymous enum
--------------
template<typename Derived >
| |
| --- |
| anonymous enum |
| Enumerator |
| --- |
| RowsAtCompileTime | The number of rows at compile-time. This is just a copy of the value provided by the *Derived* type. If a value is not known at compile-time, it is set to the *Dynamic* constant.
See also
[MatrixBase::rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [MatrixBase::cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), [ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [SizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da25cb495affdbd796198462b8ef06be91)
|
| ColsAtCompileTime | The number of columns at compile-time. This is just a copy of the value provided by the *Derived* type. If a value is not known at compile-time, it is set to the *Dynamic* constant.
See also
[MatrixBase::rows()](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ac22eb0695d00edd7d4a3b2d0a98b81c2), [MatrixBase::cols()](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#a2d768a9877f5f69f49432d447b552bfe), [RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), [SizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da25cb495affdbd796198462b8ef06be91)
|
| SizeAtCompileTime | This is equal to the number of coefficients, i.e. the number of rows times the number of columns, or to *Dynamic* if this is not known at compile-time.
See also
[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), [ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441)
|
| MaxRowsAtCompileTime | This value is equal to the maximum possible number of rows that this expression might have. If this expression might have an arbitrarily high number of rows, this value is set to *Dynamic*. This value is useful to know when evaluating an expression, in order to determine whether it is possible to avoid doing a dynamic memory allocation.
See also
[RowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dadb37c78ebbf15aa20b65c3b70415a1ab), [MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65), [MaxSizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da3a459062d39cb34452518f5f201161d2)
|
| MaxColsAtCompileTime | This value is equal to the maximum possible number of columns that this expression might have. If this expression might have an arbitrarily high number of columns, this value is set to *Dynamic*. This value is useful to know when evaluating an expression, in order to determine whether it is possible to avoid doing a dynamic memory allocation.
See also
[ColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da787f85fd67ee5985917eb2cef6e70441), [MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), [MaxSizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da3a459062d39cb34452518f5f201161d2)
|
| MaxSizeAtCompileTime | This value is equal to the maximum possible number of coefficients that this expression might have. If this expression might have an arbitrarily high number of coefficients, this value is set to *Dynamic*. This value is useful to know when evaluating an expression, in order to determine whether it is possible to avoid doing a dynamic memory allocation.
See also
[SizeAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5da25cb495affdbd796198462b8ef06be91), [MaxRowsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dad2baadea085372837b0e80dc93be1306), [MaxColsAtCompileTime](classeigen_1_1densebase#a0632b818dc6bddab3ed1dd0ff4ec4e5dacc3a41000cf1d29dd1a320b2a09d2a65)
|
| IsVectorAtCompileTime | This is set to true if either the number of rows or the number of columns is known at compile-time to be equal to 1. Indeed, in that case, we are dealing with a column-vector (if there is only one column) or with a row-vector (if there is only one row). |
| NumDimensions | This value is equal to Tensor::NumDimensions, i.e. 0 for scalars, 1 for vectors, and 2 for matrices. |
| Flags | This stores expression [Flags](group__flags) flags which may or may not be inherited by new expressions constructed from this one. See the [list of flags](group__flags). |
| IsRowMajor | True if this expression has row-major storage order. |
DenseBase()
-----------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::[DenseBase](classeigen_1_1densebase) | ( | | ) | |
| inlineprotected |
Default constructor. Do nothing.
all()
-----
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::all |
| inline |
Returns
true if all coefficients are true
Example:
```
Vector3f boxMin([Vector3f::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)()), boxMax([Vector3f::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)());
Vector3f p0 = [Vector3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(), p1 = [Vector3f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)().cwiseAbs();
// let's check if p0 and p1 are inside the axis aligned box defined by the corners boxMin,boxMax:
cout << "Is (" << p0.transpose() << ") inside the box: "
<< ((boxMin.array()<p0.array()).[all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)() && (boxMax.array()>p0.array()).[all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)()) << endl;
cout << "Is (" << p1.transpose() << ") inside the box: "
<< ((boxMin.array()<p1.array()).[all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)() && (boxMax.array()>p1.array()).[all](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)()) << endl;
```
Output:
```
Is ( 0.68 -0.211 0.566) inside the box: 0
Is (0.597 0.823 0.605) inside the box: 1
```
See also
[any()](classeigen_1_1densebase#abfbf4cb72dd577e62fbe035b1c53e695), Cwise::operator<()
allFinite()
-----------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::allFinite |
| inline |
Returns
true if `*this` contains only finite numbers, i.e., no NaN and no +/-INF values.
See also
[hasNaN()](classeigen_1_1densebase#ab13d158c900560d3e1b25d85d2d33dd6)
any()
-----
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::any |
| inline |
Returns
true if at least one coefficient is true
See also
[all()](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)
begin() [1/2]
-------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::begin |
| inline |
returns an iterator to the first element of the 1D vector or array This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
See also
[end()](classeigen_1_1densebase#ae71d079e16d91360d10066b316b48485), [cbegin()](classeigen_1_1densebase#ae9a3dfd9b826ba3103de0128576fb15b)
begin() [2/2]
-------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::begin |
| inline |
const version of [begin()](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641)
cbegin()
--------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::cbegin |
| inline |
returns a read-only const\_iterator to the first element of the 1D vector or array This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
See also
[cend()](classeigen_1_1densebase#aeb3b76f02986c2af2521d07164b5ffde), [begin()](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641)
cend()
------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::cend |
| inline |
returns a read-only const\_iterator to the element following the last element of the 1D vector or array This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
See also
[begin()](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641), [cend()](classeigen_1_1densebase#aeb3b76f02986c2af2521d07164b5ffde)
colwise() [1/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[ColwiseReturnType](classeigen_1_1vectorwiseop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::colwise |
| inline |
Returns
a writable [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations.") wrapper of \*this providing additional partial reduction operations
See also
[rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28), class [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations."), [Reductions, visitors and broadcasting](group__tutorialreductionsvisitorsbroadcasting)
colwise() [2/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ConstColwiseReturnType](classeigen_1_1vectorwiseop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::colwise | ( | | ) | const |
| inline |
Returns
a [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations.") wrapper of \*this broadcasting and partial reductions
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the sum of each column:" << endl << m.colwise().sum() << endl;
cout << "Here is the maximum absolute value of each column:"
<< endl << m.cwiseAbs().colwise().maxCoeff() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the sum of each column:
1.04 0.815 -0.238
Here is the maximum absolute value of each column:
0.68 0.823 0.536
```
See also
[rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28), class [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations."), [Reductions, visitors and broadcasting](group__tutorialreductionsvisitorsbroadcasting)
Constant() [1/3]
----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Constant | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *value* | ) | |
| inlinestatic |
Returns
an expression of a constant matrix of value *value*
This variant is only for fixed-size [DenseBase](classeigen_1_1densebase "Base class for all dense matrices, vectors, and arrays.") types. For dynamic-size types, you need to use the variants taking size arguments.
The template parameter *CustomNullaryOp* is the type of the functor.
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
Constant() [2/3]
----------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Constant | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *value* |
| | ) | | |
| inlinestatic |
Returns
an expression of a constant matrix of value *value*
The parameters *rows* and *cols* are the number of rows and of columns of the returned matrix. Must be compatible with this [DenseBase](classeigen_1_1densebase "Base class for all dense matrices, vectors, and arrays.") type.
This variant is meant to be used for dynamic-size matrix types. For fixed-size types, it is redundant to pass *rows* and *cols* as arguments, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
The template parameter *CustomNullaryOp* is the type of the functor.
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
Constant() [3/3]
----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Constant | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *value* |
| | ) | | |
| inlinestatic |
Returns
an expression of a constant matrix of value *value*
The parameter *size* is the size of the returned vector. Must be compatible with this [DenseBase](classeigen_1_1densebase "Base class for all dense matrices, vectors, and arrays.") type.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
This variant is meant to be used for dynamic-size vector types. For fixed-size types, it is redundant to pass *size* as argument, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
The template parameter *CustomNullaryOp* is the type of the functor.
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
count()
-------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::count |
| inline |
Returns
the number of coefficients which evaluate to true
See also
[all()](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4), [any()](classeigen_1_1densebase#abfbf4cb72dd577e62fbe035b1c53e695)
end() [1/2]
-----------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[iterator](classeigen_1_1densebase#af5130902770642a1a057a99c397d357d) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::end |
| inline |
returns an iterator to the element following the last element of the 1D vector or array This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
See also
[begin()](classeigen_1_1densebase#a57591454af931f9dffa71c9da28d5641), [cend()](classeigen_1_1densebase#aeb3b76f02986c2af2521d07164b5ffde)
end() [2/2]
-----------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[const\_iterator](classeigen_1_1densebase#a306d9418d4b34874e9005d961c490cd2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::end |
| inline |
const version of [end()](classeigen_1_1densebase#ae71d079e16d91360d10066b316b48485)
eval()
------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EvalReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::eval | ( | | ) | const |
| inline |
Returns
the matrix or vector obtained by evaluating this expression.
Notice that in the case of a plain matrix or vector (not an expression) this function just returns a const reference, in order to avoid a useless copy.
Warning
Be careful with [eval()](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) and the auto C++ keyword, as detailed in this [page](topicpitfalls#TopicPitfalls_auto_keyword) .
fill()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::fill | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *val* | ) | |
| inline |
Alias for [setConstant()](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559): sets all coefficients in this expression to *val*.
See also
[setConstant()](classeigen_1_1densebase#ac2f1e50d1f567da38da1d2f07c5ab559), [Constant()](classeigen_1_1densebase#a68a7ece6c5629d1e9447a321fcb14ccd), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
flagged()
---------
template<typename Derived > template<unsigned int Added, unsigned int Removed>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EIGEN\_DEPRECATED const Derived& [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::flagged | ( | | ) | const |
| inline |
**[Deprecated:](deprecated#_deprecated000004)**
it now returns `*this`
format()
--------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [WithFormat](classeigen_1_1withformat)<Derived> [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::format | ( | const [IOFormat](structeigen_1_1ioformat) & | *fmt* | ) | const |
| inline |
Returns
a [WithFormat](classeigen_1_1withformat "Pseudo expression providing matrix output with given format.") proxy object allowing to print a matrix the with given format *fmt*.
See class [IOFormat](structeigen_1_1ioformat "Stores a set of parameters controlling the way matrices are printed.") for some examples.
See also
class [IOFormat](structeigen_1_1ioformat "Stores a set of parameters controlling the way matrices are printed."), class [WithFormat](classeigen_1_1withformat "Pseudo expression providing matrix output with given format.")
hasNaN()
--------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::hasNaN |
| inline |
Returns
true is `*this` contains at least one Not A Number (NaN).
See also
[allFinite()](classeigen_1_1densebase#af1e669fd3aaae50a4870dc1b8f3b8884)
innerSize()
-----------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::innerSize | ( | | ) | const |
| inline |
Returns
the inner size.
Note
For a vector, this is just the size. For a matrix (non-vector), this is the minor dimension with respect to the [storage order](group__topicstorageorders), i.e., the number of rows for a column-major matrix, and the number of columns for a row-major matrix.
isApprox()
----------
template<typename Derived > template<typename OtherDerived >
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isApprox | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other*, |
| | | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` |
| | ) | | const |
Returns
`true` if `*this` is approximately equal to *other*, within the precision determined by *prec*.
Note
The fuzzy compares are done multiplicatively. Two vectors \( v \) and \( w \) are considered to be approximately equal within precision \( p \) if \[ \Vert v - w \Vert \leqslant p\,\min(\Vert v\Vert, \Vert w\Vert). \]
For matrices, the comparison is done using the Hilbert-Schmidt norm (aka Frobenius norm L2 norm). Because of the multiplicativeness of this comparison, one can't use this function to check whether `*this` is approximately equal to the zero matrix or vector. Indeed, `isApprox(zero)` returns false unless `*this` itself is exactly the zero matrix or vector. If you want to test whether `*this` is zero, use internal::isMuchSmallerThan(const RealScalar&, RealScalar) instead.
See also
internal::isMuchSmallerThan(const RealScalar&, RealScalar) const
isApproxToConstant()
--------------------
template<typename Derived >
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isApproxToConstant | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *val*, |
| | | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` |
| | ) | | const |
Returns
true if all coefficients in this matrix are approximately equal to *val*, to within precision *prec*
isConstant()
------------
template<typename Derived >
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isConstant | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *val*, |
| | | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` |
| | ) | | const |
This is just an alias for [isApproxToConstant()](classeigen_1_1densebase#af9b150d48bc5e4366887ccb466e40c6b).
Returns
true if all coefficients in this matrix are approximately equal to *value*, to within precision *prec*
isMuchSmallerThan() [1/2]
-------------------------
template<typename Derived > template<typename OtherDerived >
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isMuchSmallerThan | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other*, |
| | | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` |
| | ) | | const |
Returns
`true` if the norm of `*this` is much smaller than the norm of *other*, within the precision determined by *prec*.
Note
The fuzzy compares are done multiplicatively. A vector \( v \) is considered to be much smaller than a vector \( w \) within precision \( p \) if \[ \Vert v \Vert \leqslant p\,\Vert w\Vert. \]
For matrices, the comparison is done using the Hilbert-Schmidt norm.
See also
[isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c), isMuchSmallerThan(const RealScalar&, RealScalar) const
isMuchSmallerThan() [2/2]
-------------------------
template<typename Derived > template<typename Derived >
| | | | |
| --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isMuchSmallerThan | ( | const typename [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) >::Real & | *other*, |
| | | const RealScalar & | *prec* |
| | ) | | const |
Returns
`true` if the norm of `*this` is much smaller than *other*, within the precision determined by *prec*.
Note
The fuzzy compares are done multiplicatively. A vector \( v \) is considered to be much smaller than \( x \) within precision \( p \) if \[ \Vert v \Vert \leqslant p\,\vert x\vert. \]
For matrices, the comparison is done using the Hilbert-Schmidt norm. For this reason, the value of the reference scalar *other* should come from the Hilbert-Schmidt norm of a reference matrix of same dimensions.
See also
[isApprox()](classeigen_1_1densebase#ae8443357b808cd393be1b51974213f9c), isMuchSmallerThan(const DenseBase<OtherDerived>&, RealScalar) const
isOnes()
--------
template<typename Derived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isOnes | ( | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` | ) | const |
Returns
true if \*this is approximately equal to the matrix where all coefficients are equal to 1, within the precision given by *prec*.
Example:
```
Matrix3d m = [Matrix3d::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)();
m(0,2) += 1e-4;
cout << "Here's the matrix m:" << endl << m << endl;
cout << "m.isOnes() returns: " << m.isOnes() << endl;
cout << "m.isOnes(1e-3) returns: " << m.isOnes(1e-3) << endl;
```
Output:
```
Here's the matrix m:
1 1 1
1 1 1
1 1 1
m.isOnes() returns: 0
m.isOnes(1e-3) returns: 1
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
isZero()
--------
template<typename Derived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| bool [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::isZero | ( | const RealScalar & | *prec* = `[NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)>::dummy_precision()` | ) | const |
Returns
true if \*this is approximately equal to the zero matrix, within the precision given by *prec*.
Example:
```
Matrix3d m = [Matrix3d::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)();
m(0,2) = 1e-4;
cout << "Here's the matrix m:" << endl << m << endl;
cout << "m.isZero() returns: " << m.isZero() << endl;
cout << "m.isZero(1e-3) returns: " << m.isZero(1e-3) << endl;
```
Output:
```
Here's the matrix m:
0 0 0.0001
0 0 0
0 0 0
m.isZero() returns: 0
m.isZero(1e-3) returns: 1
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
lazyAssign()
------------
template<typename Derived > template<typename OtherDerived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| EIGEN\_DEPRECATED Derived& [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::lazyAssign | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
**[Deprecated:](deprecated#_deprecated000003)**
LinSpaced() [1/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::RandomAccessLinSpacedReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::LinSpaced | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inlinestatic |
Sets a linearly spaced vector.
The function generates 'size' equally spaced values in the closed interval [low,high]. When size is set to 1, a vector of length 1 containing 'high' is returned.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(4,7,10).transpose() << endl;
cout << [VectorXd::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(5,0.0,1.0).transpose() << endl;
```
Output:
```
7 8 9 10
0 0.25 0.5 0.75 1
```
For integer scalar types, an even spacing is possible if and only if the length of the range, i.e., `high-low` is a scalar multiple of `size-1`, or if `size` is a scalar multiple of the number of values `high-low+1` (meaning each value can be repeated the same number of time). If one of these two considions is not satisfied, then `high` is lowered to the largest value satisfying one of this constraint. Here are some examples:
Example:
```
cout << "Even spacing inputs:" << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,4).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,8).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,15).transpose() << endl;
cout << "Uneven spacing inputs:" << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,7).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,9).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,16).transpose() << endl;
```
Output:
```
Even spacing inputs:
1 1 2 2 3 3 4 4
1 2 3 4 5 6 7 8
1 3 5 7 9 11 13 15
Uneven spacing inputs:
1 1 2 2 3 3 4 4
1 2 3 4 5 6 7 8
1 3 5 7 9 11 13 15
```
See also
[setLinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a "Sets a linearly spaced vector."), [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.") Special version for fixed [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) types which does not require the [size](classeigen_1_1densecoeffsbase_3_01derived_00_01directwriteaccessors_01_4#ae106171b6fefd3f7af108a8283de36c9) parameter.
LinSpaced() [2/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::RandomAccessLinSpacedReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::LinSpaced | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inlinestatic |
Sets a linearly spaced vector.
The function generates 'size' equally spaced values in the closed interval [low,high]. When size is set to 1, a vector of length 1 containing 'high' is returned.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(4,7,10).transpose() << endl;
cout << [VectorXd::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(5,0.0,1.0).transpose() << endl;
```
Output:
```
7 8 9 10
0 0.25 0.5 0.75 1
```
For integer scalar types, an even spacing is possible if and only if the length of the range, i.e., `high-low` is a scalar multiple of `size-1`, or if `size` is a scalar multiple of the number of values `high-low+1` (meaning each value can be repeated the same number of time). If one of these two considions is not satisfied, then `high` is lowered to the largest value satisfying one of this constraint. Here are some examples:
Example:
```
cout << "Even spacing inputs:" << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,4).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,8).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,15).transpose() << endl;
cout << "Uneven spacing inputs:" << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,7).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,9).transpose() << endl;
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(8,1,16).transpose() << endl;
```
Output:
```
Even spacing inputs:
1 1 2 2 3 3 4 4
1 2 3 4 5 6 7 8
1 3 5 7 9 11 13 15
Uneven spacing inputs:
1 1 2 2 3 3 4 4
1 2 3 4 5 6 7 8
1 3 5 7 9 11 13 15
```
See also
[setLinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a "Sets a linearly spaced vector."), [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
LinSpaced() [3/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| EIGEN\_DEPRECATED const [DenseBase](classeigen_1_1densebase)< Derived >::RandomAccessLinSpacedReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::LinSpaced | ( | Sequential\_t | , |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inlinestatic |
**[Deprecated:](deprecated#_deprecated000002)**
because of accuracy loss. In [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3, it is an alias for [LinSpaced(const Scalar&,const Scalar&)](classeigen_1_1densebase#ad8098aa5971139a5585e623dddbea860 "Sets a linearly spaced vector.")
See also
[LinSpaced(const Scalar&, const Scalar&)](classeigen_1_1densebase#ad8098aa5971139a5585e623dddbea860 "Sets a linearly spaced vector.")
LinSpaced() [4/4]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| EIGEN\_DEPRECATED const [DenseBase](classeigen_1_1densebase)< Derived >::RandomAccessLinSpacedReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::LinSpaced | ( | Sequential\_t | , |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inlinestatic |
**[Deprecated:](deprecated#_deprecated000001)**
because of accuracy loss. In [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3, it is an alias for [LinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768 "Sets a linearly spaced vector.")
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
cout << [VectorXi::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(Sequential,4,7,10).transpose() << endl;
cout << [VectorXd::LinSpaced](classeigen_1_1densebase#a1c6d1dbfeb9f6491173a83eb44e14c1d)(Sequential,5,0.0,1.0).transpose() << endl;
```
Output:
```
7 8 9 10
0 0.25 0.5 0.75 1
```
See also
[LinSpaced(Index,const Scalar&, const Scalar&)](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768 "Sets a linearly spaced vector."), [setLinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a "Sets a linearly spaced vector.")
maxCoeff() [1/3]
----------------
template<typename Derived > template<int NaNPropagation>
| | | |
| --- | --- | --- |
|
| |
| --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::maxCoeff |
| inline |
Returns
the maximum of all coefficients of `*this`. In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is maximum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
maxCoeff() [2/3]
----------------
template<typename Derived > template<int NaNPropagation, typename IndexType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::maxCoeff | ( | IndexType \* | *index* | ) | const |
Returns
the maximum of all coefficients of \*this and puts in \*index its location.
In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is maximum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
See also
DenseBase::maxCoeff(IndexType\*,IndexType\*), DenseBase::minCoeff(IndexType\*,IndexType\*), DenseBase::visitor(), [DenseBase::maxCoeff()](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1)
maxCoeff() [3/3]
----------------
template<typename Derived > template<int NaNPropagation, typename IndexType >
| | | | |
| --- | --- | --- | --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::maxCoeff | ( | IndexType \* | *rowId*, |
| | | IndexType \* | *colId* |
| | ) | | const |
Returns
the maximum of all coefficients of \*this and puts in \*row and \*col its location.
In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is maximum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
See also
DenseBase::minCoeff(IndexType\*,IndexType\*), [DenseBase::visit()](classeigen_1_1densebase#a4225b90fcc74f18dd479b401124b3841), [DenseBase::maxCoeff()](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1)
mean()
------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::mean |
| inline |
Returns
the mean of all coefficients of \*this
See also
trace(), [prod()](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f), [sum()](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c)
minCoeff() [1/3]
----------------
template<typename Derived > template<int NaNPropagation>
| | | |
| --- | --- | --- |
|
| |
| --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::minCoeff |
| inline |
Returns
the minimum of all coefficients of `*this`. In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is minimum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
minCoeff() [2/3]
----------------
template<typename Derived > template<int NaNPropagation, typename IndexType >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::minCoeff | ( | IndexType \* | *index* | ) | const |
Returns
the minimum of all coefficients of \*this and puts in \*index its location.
In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is maximum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
See also
DenseBase::minCoeff(IndexType\*,IndexType\*), DenseBase::maxCoeff(IndexType\*,IndexType\*), [DenseBase::visit()](classeigen_1_1densebase#a4225b90fcc74f18dd479b401124b3841), [DenseBase::minCoeff()](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2)
minCoeff() [3/3]
----------------
template<typename Derived > template<int NaNPropagation, typename IndexType >
| | | | |
| --- | --- | --- | --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::minCoeff | ( | IndexType \* | *rowId*, |
| | | IndexType \* | *colId* |
| | ) | | const |
Returns
the minimum of all coefficients of \*this and puts in \*row and \*col its location.
In case `*this` contains NaN, NaNPropagation determines the behavior: NaNPropagation == PropagateFast : undefined NaNPropagation == PropagateNaN : result is NaN NaNPropagation == PropagateNumbers : result is maximum of elements that are not NaN
Warning
the matrix must be not empty, otherwise an assertion is triggered.
See also
DenseBase::minCoeff(Index\*), DenseBase::maxCoeff(Index\*,Index\*), [DenseBase::visit()](classeigen_1_1densebase#a4225b90fcc74f18dd479b401124b3841), [DenseBase::minCoeff()](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2)
nestByValue()
-------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [NestByValue](classeigen_1_1nestbyvalue)< Derived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::nestByValue |
| inline |
Returns
an expression of the temporary version of \*this.
nonZeros()
----------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::nonZeros | ( | | ) | const |
| inline |
Returns
the number of nonzero coefficients which is in practice the number of stored coefficients.
NullaryExpr() [1/3]
-------------------
template<typename Derived > template<typename CustomNullaryOp >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::NullaryExpr | ( | const CustomNullaryOp & | *func* | ) | |
| inlinestatic |
Returns
an expression of a matrix defined by a custom functor *func*
This variant is only for fixed-size [DenseBase](classeigen_1_1densebase "Base class for all dense matrices, vectors, and arrays.") types. For dynamic-size types, you need to use the variants taking size arguments.
The template parameter *CustomNullaryOp* is the type of the functor.
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
NullaryExpr() [2/3]
-------------------
template<typename Derived > template<typename CustomNullaryOp >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::NullaryExpr | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols*, |
| | | const CustomNullaryOp & | *func* |
| | ) | | |
| inlinestatic |
Returns
an expression of a matrix defined by a custom functor *func*
The parameters *rows* and *cols* are the number of rows and of columns of the returned matrix. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This variant is meant to be used for dynamic-size matrix types. For fixed-size types, it is redundant to pass *rows* and *cols* as arguments, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
The template parameter *CustomNullaryOp* is the type of the functor.
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
NullaryExpr() [3/3]
-------------------
template<typename Derived > template<typename CustomNullaryOp >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [CwiseNullaryOp](classeigen_1_1cwisenullaryop)< CustomNullaryOp, [PlainObject](classeigen_1_1densebase#aae45af9b5aca5a9caae98fd201f47cc4) > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::NullaryExpr | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size*, |
| | | const CustomNullaryOp & | *func* |
| | ) | | |
| inlinestatic |
Returns
an expression of a matrix defined by a custom functor *func*
The parameter *size* is the size of the returned vector. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
This variant is meant to be used for dynamic-size vector types. For fixed-size types, it is redundant to pass *size* as argument, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
The template parameter *CustomNullaryOp* is the type of the functor.
Here is an example with C++11 random generators:
```
#include <Eigen/Core>
#include <iostream>
#include <random>
using namespace [Eigen](namespaceeigen);
int main() {
std::default_random_engine generator;
std::poisson_distribution<int> distribution(4.1);
auto poisson = [&] () {return distribution(generator);};
RowVectorXi v = [RowVectorXi::NullaryExpr](classeigen_1_1densebase#a3340c9b997f5b53a0131cf927f93b54c)(10, poisson );
std::cout << v << "\n";
}
```
Output:
```
2 3 1 4 3 4 4 3 2 3
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
Ones() [1/3]
------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Ones |
| inlinestatic |
Returns
an expression of a fixed-size matrix or vector where all coefficients equal one.
This variant is only for fixed-size [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") types. For dynamic-size types, you need to use the variants taking size arguments.
Example:
```
cout << [Matrix2d::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)() << endl;
cout << 6 * [RowVector4i::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)() << endl;
```
Output:
```
1 1
1 1
6 6 6 6
```
See also
[Ones(Index)](classeigen_1_1densebase#ab710a58e4a80fbcb2594242372c8fe56), [Ones(Index,Index)](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013), [isOnes()](classeigen_1_1densebase#aa56d6b4477cd3c92a9cf42f4b96e47c2), class [Ones](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013)
Ones() [2/3]
------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Ones | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inlinestatic |
Returns
an expression of a matrix where all coefficients equal one.
The parameters *rows* and *cols* are the number of rows and of columns of the returned matrix. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This variant is meant to be used for dynamic-size matrix types. For fixed-size types, it is redundant to pass *rows* and *cols* as arguments, so [Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101) should be used instead.
Example:
```
cout << [MatrixXi::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(2,3) << endl;
```
Output:
```
1 1 1
1 1 1
```
See also
[Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101), [Ones(Index)](classeigen_1_1densebase#ab710a58e4a80fbcb2594242372c8fe56), [isOnes()](classeigen_1_1densebase#aa56d6b4477cd3c92a9cf42f4b96e47c2), class [Ones](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013)
Ones() [3/3]
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Ones | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *newSize* | ) | |
| inlinestatic |
Returns
an expression of a vector where all coefficients equal one.
The parameter *newSize* is the size of the returned vector. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
This variant is meant to be used for dynamic-size vector types. For fixed-size types, it is redundant to pass *size* as argument, so [Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101) should be used instead.
Example:
```
cout << 6 * [RowVectorXi::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(4) << endl;
cout << [VectorXf::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(2) << endl;
```
Output:
```
6 6 6 6
1
1
```
See also
[Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101), [Ones(Index,Index)](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013), [isOnes()](classeigen_1_1densebase#aa56d6b4477cd3c92a9cf42f4b96e47c2), class [Ones](classeigen_1_1densebase#a8b2a51018a73a766f5b91aef3487f013)
operator<<() [1/2]
------------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CommaInitializer](structeigen_1_1commainitializer)< Derived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::operator<< | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
See also
[operator<<(const Scalar&)](classeigen_1_1densebase#a0e575eb0ba6cc6bc5f347872abd8509d)
operator<<() [2/2]
------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CommaInitializer](structeigen_1_1commainitializer)< Derived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::operator<< | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *s* | ) | |
| inline |
Convenient operator to set the coefficients of a matrix.
The coefficients must be provided in a row major order and exactly match the size of the matrix. Otherwise an assertion is raised.
Example:
```
Matrix3i m1;
m1 << 1, 2, 3,
4, 5, 6,
7, 8, 9;
cout << m1 << endl << endl;
Matrix3i m2 = [Matrix3i::Identity](classeigen_1_1matrixbase#a98bb9a0f705c6dfde85b0bfff31bf88f)();
m2.block(0,0, 2,2) << 10, 11, 12, 13;
cout << m2 << endl << endl;
Vector2i v1;
v1 << 14, 15;
m2 << v1.transpose(), 16,
v1, m1.block(1,1,2,2);
cout << m2 << endl;
```
Output:
```
1 2 3
4 5 6
7 8 9
10 11 0
12 13 0
0 0 1
14 15 16
14 5 6
15 8 9
```
Note
According the c++ standard, the argument expressions of this comma initializer are evaluated in arbitrary order.
See also
[CommaInitializer::finished()](structeigen_1_1commainitializer#a3cf9e2b8a227940f50103130b2d2859a), class [CommaInitializer](structeigen_1_1commainitializer "Helper class used by the comma initializer operator.")
operator=() [1/3]
-----------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::operator= | ( | const [DenseBase](classeigen_1_1densebase)< Derived > & | *other* | ) | |
| inline |
Special case of the template operator=, in order to prevent the compiler from generating a default operator= (issue hit with g++ 4.1)
operator=() [2/3]
-----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::operator= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Copies *other* into \*this.
Returns
a reference to \*this.
operator=() [3/3]
-----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::operator= | ( | const [EigenBase](structeigen_1_1eigenbase)< OtherDerived > & | *other* | ) | |
Copies the generic expression *other* into \*this.
The expression must provide a (templated) evalTo(Derived& dst) const function which does the actual job. In practice, this allows any user to write its own special matrix without having to modify [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")
Returns
a reference to \*this.
outerSize()
-----------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::outerSize | ( | | ) | const |
| inline |
Returns
the outer size.
Note
For a vector, this returns just 1. For a matrix (non-vector), this is the major dimension with respect to the [storage order](group__topicstorageorders), i.e., the number of columns for a column-major matrix, and the number of rows for a row-major matrix.
prod()
------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::prod |
| inline |
Returns
the product of all coefficients of \*this
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the product of all the coefficients:" << endl << m.prod() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the product of all the coefficients:
0.0019
```
See also
[sum()](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c), [mean()](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7), trace()
Random() [1/3]
--------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::[RandomReturnType](classeigen_1_1cwisenullaryop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Random |
| inlinestatic |
Returns
a fixed-size random matrix or vector expression
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
This variant is only for fixed-size [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") types. For dynamic-size types, you need to use the variants taking size arguments.
Example:
```
cout << 100 * [Matrix2i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)() << endl;
```
Output:
```
700 600
-200 600
```
This expression has the "evaluate before nesting" flag so that it will be evaluated into a temporary matrix whenever it is nested in a larger expression. This prevents unexpected behavior with expressions involving random matrices.
Warning
This function is not re-entrant.
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [DenseBase::Random(Index,Index)](classeigen_1_1densebase#ae97f8d9d08f969c733c8144be6225756), [DenseBase::Random(Index)](classeigen_1_1densebase#a7eb5f974a8f0b67eac7080db1da0e308)
Random() [2/3]
--------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::[RandomReturnType](classeigen_1_1cwisenullaryop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Random | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inlinestatic |
Returns
a random matrix expression
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
The parameters *rows* and *cols* are the number of rows and of columns of the returned matrix. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
Warning
This function is not re-entrant.
This variant is meant to be used for dynamic-size matrix types. For fixed-size types, it is redundant to pass *rows* and *cols* as arguments, so [Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19) should be used instead.
Example:
```
cout << [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(2,3) << endl;
```
Output:
```
7 6 9
-2 6 -6
```
This expression has the "evaluate before nesting" flag so that it will be evaluated into a temporary matrix whenever it is nested in a larger expression. This prevents unexpected behavior with expressions involving random matrices.
See [DenseBase::NullaryExpr(Index, const CustomNullaryOp&)](classeigen_1_1densebase#a9752ee59976a4b4aad860ad1a9093e7f) for an example using C++11 random generators.
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [DenseBase::Random(Index)](classeigen_1_1densebase#a7eb5f974a8f0b67eac7080db1da0e308), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
Random() [3/3]
--------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::[RandomReturnType](classeigen_1_1cwisenullaryop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Random | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| inlinestatic |
Returns
a random vector expression
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
The parameter *size* is the size of the returned vector. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Warning
This function is not re-entrant.
This variant is meant to be used for dynamic-size vector types. For fixed-size types, it is redundant to pass *size* as argument, so [Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19) should be used instead.
Example:
```
cout << [VectorXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(2) << endl;
```
Output:
```
7
-2
```
This expression has the "evaluate before nesting" flag so that it will be evaluated into a temporary vector whenever it is nested in a larger expression. This prevents unexpected behavior with expressions involving random matrices.
See also
[DenseBase::setRandom()](classeigen_1_1densebase#ac476e5852129ba32beaa1a8a3d7ee0db), [DenseBase::Random(Index,Index)](classeigen_1_1densebase#ae97f8d9d08f969c733c8144be6225756), [DenseBase::Random()](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)
redux()
-------
template<typename Derived > template<typename Func >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| internal::traits<Derived>::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::redux | ( | const Func & | *func* | ) | const |
| inline |
Returns
the result of a full redux operation on the whole matrix or vector using *func*
The template parameter *BinaryOp* is the type of the functor *func* which must be an associative operator. Both current C++98 and C++11 functor styles are handled.
Warning
the matrix must be not empty, otherwise an assertion is triggered.
See also
[DenseBase::sum()](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c), [DenseBase::minCoeff()](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2), [DenseBase::maxCoeff()](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1), [MatrixBase::colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30), [MatrixBase::rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28)
replicate() [1/2]
-----------------
template<typename Derived > template<int RowFactor, int ColFactor>
| |
| --- |
| const [Replicate](classeigen_1_1replicate)< Derived, RowFactor, ColFactor > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::replicate |
Returns
an expression of the replication of `*this`
Example:
```
MatrixXi m = [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(2,3);
cout << "Here is the matrix m:" << endl << m << endl;
cout << "m.replicate<3,2>() = ..." << endl;
cout << m.replicate<3,2>() << endl;
```
Output:
```
Here is the matrix m:
7 6 9
-2 6 -6
m.replicate<3,2>() = ...
7 6 9 7 6 9
-2 6 -6 -2 6 -6
7 6 9 7 6 9
-2 6 -6 -2 6 -6
7 6 9 7 6 9
-2 6 -6 -2 6 -6
```
See also
[VectorwiseOp::replicate()](classeigen_1_1vectorwiseop#a5f0c8dc9e9c4aeaa2057f15800f5c18c), DenseBase::replicate(Index,Index), class [Replicate](classeigen_1_1replicate "Expression of the multiple replication of a matrix or vector.")
replicate() [2/2]
-----------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [Replicate](classeigen_1_1replicate)<Derived, [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2), [Dynamic](namespaceeigen#ad81fa7195215a0ce30017dfac309f0b2)> [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::replicate | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rowFactor*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *colFactor* |
| | ) | | const |
| inline |
Returns
an expression of the replication of `*this`
Example:
```
Vector3i v = [Vector3i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the vector v:" << endl << v << endl;
cout << "v.replicate(2,5) = ..." << endl;
cout << v.replicate(2,5) << endl;
```
Output:
```
Here is the vector v:
7
-2
6
v.replicate(2,5) = ...
7 7 7 7 7
-2 -2 -2 -2 -2
6 6 6 6 6
7 7 7 7 7
-2 -2 -2 -2 -2
6 6 6 6 6
```
See also
[VectorwiseOp::replicate()](classeigen_1_1vectorwiseop#a5f0c8dc9e9c4aeaa2057f15800f5c18c), [DenseBase::replicate<int,int>()](classeigen_1_1densebase#a60dadfe80b813d808e91e4521c722a8e), class [Replicate](classeigen_1_1replicate "Expression of the multiple replication of a matrix or vector.")
resize() [1/2]
--------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::resize | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *newSize* | ) | |
| inline |
Only plain matrices/arrays, not expressions, may be resized; therefore the only useful resize methods are [Matrix::resize()](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a) and [Array::resize()](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a). The present method only asserts that the new size equals the old size, and does nothing else.
resize() [2/2]
--------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::resize | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inline |
Only plain matrices/arrays, not expressions, may be resized; therefore the only useful resize methods are [Matrix::resize()](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a) and [Array::resize()](classeigen_1_1plainobjectbase#a9fd0703bd7bfe89d6dc80e2ce87c312a). The present method only asserts that the new size equals the old size, and does nothing else.
reverse() [1/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[ReverseReturnType](classeigen_1_1reverse) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::reverse |
| inline |
Returns
an expression of the reverse of \*this.
Example:
```
MatrixXi m = [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3,4);
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the reverse of m:" << endl << m.reverse() << endl;
cout << "Here is the coefficient (1,0) in the reverse of m:" << endl
<< m.reverse()(1,0) << endl;
cout << "Let us overwrite this coefficient with the value 4." << endl;
m.reverse()(1,0) = 4;
cout << "Now the matrix m is:" << endl << m << endl;
```
Output:
```
Here is the matrix m:
7 6 -3 1
-2 9 6 0
6 -6 -5 3
Here is the reverse of m:
3 -5 -6 6
0 6 9 -2
1 -3 6 7
Here is the coefficient (1,0) in the reverse of m:
0
Let us overwrite this coefficient with the value 4.
Now the matrix m is:
7 6 -3 1
-2 9 6 4
6 -6 -5 3
```
reverse() [2/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ConstReverseReturnType](classeigen_1_1reverse) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::reverse | ( | | ) | const |
| inline |
This is the const version of [reverse()](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f).
reverseInPlace()
----------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::reverseInPlace |
| inline |
This is the "in place" version of reverse: it reverses `*this`.
In most cases it is probably better to simply use the reversed expression of a matrix. However, when reversing the matrix data itself is really needed, then this "in-place" version is probably the right choice because it provides the following additional benefits:
* less error prone: doing the same operation with .[reverse()](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f) requires special care:
```
m = m.reverse().eval();
```
* this API enables reverse operations without the need for a temporary
* it allows future optimizations (cache friendliness, etc.)
See also
[VectorwiseOp::reverseInPlace()](classeigen_1_1vectorwiseop#ab9dd7c273eb2ba8defaab2d55156936b), [reverse()](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f)
rowwise() [1/2]
---------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[RowwiseReturnType](classeigen_1_1vectorwiseop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::rowwise |
| inline |
Returns
a writable [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations.") wrapper of \*this providing additional partial reduction operations
See also
[colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30), class [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations."), [Reductions, visitors and broadcasting](group__tutorialreductionsvisitorsbroadcasting)
rowwise() [2/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ConstRowwiseReturnType](classeigen_1_1vectorwiseop) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::rowwise | ( | | ) | const |
| inline |
Returns
a [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations.") wrapper of \*this for broadcasting and partial reductions
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the sum of each row:" << endl << m.rowwise().sum() << endl;
cout << "Here is the maximum absolute value of each row:"
<< endl << m.cwiseAbs().rowwise().maxCoeff() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the sum of each row:
0.948
1.15
-0.483
Here is the maximum absolute value of each row:
0.68
0.823
0.605
```
See also
[colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30), class [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations."), [Reductions, visitors and broadcasting](group__tutorialreductionsvisitorsbroadcasting)
select() [1/3]
--------------
template<typename Derived > template<typename ThenDerived , typename ElseDerived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [Select](classeigen_1_1select)< Derived, ThenDerived, ElseDerived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::select | ( | const [DenseBase](classeigen_1_1densebase)< ThenDerived > & | *thenMatrix*, |
| | | const [DenseBase](classeigen_1_1densebase)< ElseDerived > & | *elseMatrix* |
| | ) | | const |
| inline |
Returns
a matrix where each coefficient (i,j) is equal to *thenMatrix(i,j)* if `*this`(i,j), and *elseMatrix(i,j)* otherwise.
Example:
```
MatrixXi m(3, 3);
m << 1, 2, 3,
4, 5, 6,
7, 8, 9;
m = (m.array() >= 5).[select](classeigen_1_1densebase#a65e78cfcbc9852e6923bebff4323ddca)(-m, m);
cout << m << endl;
```
Output:
```
1 2 3
4 -5 -6
-7 -8 -9
```
See also
class [Select](classeigen_1_1select "Expression of a coefficient wise version of the C++ ternary operator ?:")
select() [2/3]
--------------
template<typename Derived > template<typename ThenDerived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [Select](classeigen_1_1select)< Derived, ThenDerived, typename ThenDerived::ConstantReturnType > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::select | ( | const [DenseBase](classeigen_1_1densebase)< ThenDerived > & | *thenMatrix*, |
| | | const typename ThenDerived::Scalar & | *elseScalar* |
| | ) | | const |
| inline |
Version of DenseBase::select(const DenseBase&, const DenseBase&) with the *else* expression being a scalar value.
See also
[DenseBase::select(const DenseBase<ThenDerived>&, const DenseBase<ElseDerived>&) const](classeigen_1_1densebase#a65e78cfcbc9852e6923bebff4323ddca), class [Select](classeigen_1_1select "Expression of a coefficient wise version of the C++ ternary operator ?:")
select() [3/3]
--------------
template<typename Derived > template<typename ElseDerived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [Select](classeigen_1_1select)< Derived, typename ElseDerived::ConstantReturnType, ElseDerived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::select | ( | const typename ElseDerived::Scalar & | *thenScalar*, |
| | | const [DenseBase](classeigen_1_1densebase)< ElseDerived > & | *elseMatrix* |
| | ) | | const |
| inline |
Version of DenseBase::select(const DenseBase&, const DenseBase&) with the *then* expression being a scalar value.
See also
[DenseBase::select(const DenseBase<ThenDerived>&, const DenseBase<ElseDerived>&) const](classeigen_1_1densebase#a65e78cfcbc9852e6923bebff4323ddca), class [Select](classeigen_1_1select "Expression of a coefficient wise version of the C++ ternary operator ?:")
setConstant()
-------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setConstant | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *val* | ) | |
| inline |
Sets all coefficients in this expression to value *val*.
See also
[fill()](classeigen_1_1densebase#a9be169c308801411aa24be93d30930bf), setConstant(Index,const Scalar&), setConstant(Index,Index,const Scalar&), [setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2), [Constant()](classeigen_1_1densebase#a68a7ece6c5629d1e9447a321fcb14ccd), class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [setZero()](classeigen_1_1densebase#af230a143de50695d2d1fae93db7e4dcb), [setOnes()](classeigen_1_1densebase#a250ef1b827e748f3f898fb2e55cb96e2)
setLinSpaced() [1/2]
--------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setLinSpaced | ( | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inline |
Sets a linearly spaced vector.
The function fills `*this` with equally spaced values in the closed interval [low,high]. When size is set to 1, a vector of length 1 containing 'high' is returned.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
For integer scalar types, do not miss the explanations on the definition of [even spacing](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) .
See also
[LinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768 "Sets a linearly spaced vector."), [setLinSpaced(Index, const Scalar&, const Scalar&)](classeigen_1_1densebase#a5d1ce9e801fa502e02b9b8cd9141ad0a "Sets a linearly spaced vector."), [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
setLinSpaced() [2/2]
--------------------
template<typename Derived >
| | | | | | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setLinSpaced | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *newSize*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *low*, |
| | | const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) & | *high* |
| | ) | | |
| inline |
Sets a linearly spaced vector.
The function generates 'size' equally spaced values in the closed interval [low,high]. When size is set to 1, a vector of length 1 containing 'high' is returned.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
Example:
```
VectorXf v;
v.setLinSpaced(5,0.5f,1.5f);
cout << v << endl;
```
Output:
```
0.5
0.75
1
1.25
1.5
```
For integer scalar types, do not miss the explanations on the definition of [even spacing](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768) .
See also
[LinSpaced(Index,const Scalar&,const Scalar&)](classeigen_1_1densebase#aaef589c1dbd7fad93f97bd3fa1b1e768 "Sets a linearly spaced vector."), [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor.")
setOnes()
---------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setOnes |
| inline |
Sets all coefficients in this expression to one.
Example:
```
Matrix4i m = [Matrix4i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
m.row(1).setOnes();
cout << m << endl;
```
Output:
```
7 9 -5 -3
1 1 1 1
6 -3 0 9
6 6 3 9
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [Ones()](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)
setRandom()
-----------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setRandom |
| inline |
Sets all coefficients in this expression to random values.
Numbers are uniformly spread through their whole definition range for integer types, and in the [-1:1] range for floating point scalar types.
Warning
This function is not re-entrant.
Example:
```
Matrix4i m = [Matrix4i::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)();
m.col(1).setRandom();
cout << m << endl;
```
Output:
```
0 7 0 0
0 -2 0 0
0 6 0 0
0 6 0 0
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), setRandom(Index), setRandom(Index,Index)
setZero()
---------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| Derived & [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::setZero |
| inline |
Sets all coefficients in this expression to zero.
Example:
```
Matrix4i m = [Matrix4i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
m.row(1).setZero();
cout << m << endl;
```
Output:
```
7 9 -5 -3
0 0 0 0
6 -3 0 9
6 6 3 9
```
See also
class [CwiseNullaryOp](classeigen_1_1cwisenullaryop "Generic expression of a matrix where all coefficients are defined by a functor."), [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)
sum()
-----
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| internal::traits< Derived >::[Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::sum |
| inline |
Returns
the sum of all coefficients of `*this`
If `*this` is empty, then the value 0 is returned.
See also
trace(), [prod()](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f), [mean()](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7)
swap() [1/2]
------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::swap | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
swaps \*this with the expression *other*.
swap() [2/2]
------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::swap | ( | [PlainObjectBase](classeigen_1_1plainobjectbase)< OtherDerived > & | *other* | ) | |
| inline |
swaps \*this with the matrix or array *other*.
transpose() [1/2]
-----------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [Transpose](classeigen_1_1transpose)< Derived > [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::transpose |
| inline |
Returns
an expression of the transpose of \*this.
Example:
```
Matrix2i m = [Matrix2i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the transpose of m:" << endl << m.transpose() << endl;
cout << "Here is the coefficient (1,0) in the transpose of m:" << endl
<< m.transpose()(1,0) << endl;
cout << "Let us overwrite this coefficient with the value 0." << endl;
m.transpose()(1,0) = 0;
cout << "Now the matrix m is:" << endl << m << endl;
```
Output:
```
Here is the matrix m:
7 6
-2 6
Here is the transpose of m:
7 -2
6 6
Here is the coefficient (1,0) in the transpose of m:
6
Let us overwrite this coefficient with the value 0.
Now the matrix m is:
7 0
-2 6
```
Warning
If you want to replace a matrix by its own transpose, do **NOT** do this:
```
m = m.transpose(); // bug!!! caused by aliasing effect
```
Instead, use the [transposeInPlace()](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a) method:
```
m.transposeInPlace();
```
which gives [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") good opportunities for optimization, or alternatively you can also do:
```
m = m.transpose().eval();
```
See also
[transposeInPlace()](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a), adjoint()
transpose() [2/2]
-----------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| [DenseBase](classeigen_1_1densebase)< Derived >::[ConstTransposeReturnType](classeigen_1_1diagonal) [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::transpose |
| inline |
This is the const version of [transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c).
Make sure you read the warning for [transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c) !
See also
[transposeInPlace()](classeigen_1_1densebase#ac501bd942994af7a95d95bee7a16ad2a), adjoint()
transposeInPlace()
------------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::transposeInPlace |
| inline |
This is the "in place" version of [transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c): it replaces `*this` by its own transpose. Thus, doing
```
m.transposeInPlace();
```
has the same effect on m as doing
```
m = m.transpose().eval();
```
and is faster and also safer because in the latter line of code, forgetting the [eval()](classeigen_1_1densebase#aa73e57a2f0f7cfcb4ad4d55ea0b6414b) results in a bug caused by [aliasing](group__topicaliasing).
Notice however that this method is only useful if you want to replace a matrix by its own transpose. If you just need the transpose of a matrix, use [transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c).
Note
if the matrix is not square, then `*this` must be a resizable matrix. This excludes (non-square) fixed-size matrices, block-expressions and maps.
See also
[transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c), adjoint(), adjointInPlace()
value()
-------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| CoeffReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::value | ( | | ) | const |
| inline |
Returns
the unique coefficient of a 1x1 expression
visit()
-------
template<typename Derived > template<typename Visitor >
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::visit | ( | Visitor & | *visitor* | ) | const |
Applies the visitor *visitor* to the whole coefficients of the matrix or vector.
The template parameter *Visitor* is the type of the visitor and provides the following interface:
```
struct MyVisitor {
// called for the first coefficient
void init(const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)& [value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j);
// called for all other coefficients
void [operator()](classeigen_1_1densecoeffsbase_3_01derived_00_01writeaccessors_01_4#ae6ba07bad9e3026afe54806fdefe32bb) (const [Scalar](classeigen_1_1densebase#a5feed465b3a8e60c47e73ecce83e39a2)& [value](classeigen_1_1densebase#a8515f719046aa4851e385661f45595b0), [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j);
};
```
Note
compared to one or two *for* *loops*, visitors offer automatic unrolling for small fixed size matrix. if the matrix is empty, then the visitor is left unchanged.
See also
minCoeff(Index\*,Index\*), maxCoeff(Index\*,Index\*), DenseBase::redux()
Zero() [1/3]
------------
template<typename Derived >
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Zero |
| inlinestatic |
Returns
an expression of a fixed-size zero matrix or vector.
This variant is only for fixed-size [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") types. For dynamic-size types, you need to use the variants taking size arguments.
Example:
```
cout << [Matrix2d::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)() << endl;
cout << [RowVector4i::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)() << endl;
```
Output:
```
0 0
0 0
0 0 0 0
```
See also
[Zero(Index)](classeigen_1_1densebase#ac22f79b812fa564061042407f2ba8f5b), [Zero(Index,Index)](classeigen_1_1densebase#ae41a9b5050ed27d9e93c82c9c8622cd3)
Zero() [2/3]
------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Zero | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *rows*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *cols* |
| | ) | | |
| inlinestatic |
Returns
an expression of a zero matrix.
The parameters *rows* and *cols* are the number of rows and of columns of the returned matrix. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This variant is meant to be used for dynamic-size matrix types. For fixed-size types, it is redundant to pass *rows* and *cols* as arguments, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
Example:
```
cout << [MatrixXi::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)(2,3) << endl;
```
Output:
```
0 0 0
0 0 0
```
See also
[Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761), [Zero(Index)](classeigen_1_1densebase#ac22f79b812fa564061042407f2ba8f5b)
Zero() [3/3]
------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [DenseBase](classeigen_1_1densebase)< Derived >::ConstantReturnType [Eigen::DenseBase](classeigen_1_1densebase)< Derived >::Zero | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *size* | ) | |
| inlinestatic |
Returns
an expression of a zero vector.
The parameter *size* is the size of the returned vector. Must be compatible with this [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") type.
This is only for vectors (either row-vectors or column-vectors), i.e. matrices which are known at compile-time to have either one row or one column.
This variant is meant to be used for dynamic-size vector types. For fixed-size types, it is redundant to pass *size* as argument, so [Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761) should be used instead.
Example:
```
cout << [RowVectorXi::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)(4) << endl;
cout << [VectorXf::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)(2) << endl;
```
Output:
```
0 0 0 0
0
0
```
See also
[Zero()](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761), [Zero(Index,Index)](classeigen_1_1densebase#ae41a9b5050ed27d9e93c82c9c8622cd3)
operator<<()
------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| std::ostream & operator<< | ( | std::ostream & | *s*, |
| | | const [DenseBase](classeigen_1_1densebase)< Derived > & | *m* |
| | ) | | |
| related |
Outputs the matrix, to the given stream.
If you wish to print the matrix with a format different than the default, use [DenseBase::format()](classeigen_1_1densebase#ab231f1a6057f28d4244145e12c9fc0c7).
It is also possible to change the default format by defining EIGEN\_DEFAULT\_IO\_FORMAT before including [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") headers. If not defined, this will automatically be defined to [Eigen::IOFormat()](structeigen_1_1ioformat "Stores a set of parameters controlling the way matrices are printed."), that is the [Eigen::IOFormat](structeigen_1_1ioformat "Stores a set of parameters controlling the way matrices are printed.") with default parameters.
See also
[DenseBase::format()](classeigen_1_1densebase#ab231f1a6057f28d4244145e12c9fc0c7)
---
The documentation for this class was generated from the following files:* [DenseBase.h](https://eigen.tuxfamily.org/dox/DenseBase_8h_source.html)
* [Assign.h](https://eigen.tuxfamily.org/dox/Assign_8h_source.html)
* [BooleanRedux.h](https://eigen.tuxfamily.org/dox/BooleanRedux_8h_source.html)
* [CommaInitializer.h](https://eigen.tuxfamily.org/dox/CommaInitializer_8h_source.html)
* [CwiseNullaryOp.h](https://eigen.tuxfamily.org/dox/CwiseNullaryOp_8h_source.html)
* [EigenBase.h](https://eigen.tuxfamily.org/dox/EigenBase_8h_source.html)
* [Fuzzy.h](https://eigen.tuxfamily.org/dox/Fuzzy_8h_source.html)
* [IO.h](https://eigen.tuxfamily.org/dox/IO_8h_source.html)
* [NestByValue.h](https://eigen.tuxfamily.org/dox/NestByValue_8h_source.html)
* [Random.h](https://eigen.tuxfamily.org/dox/Random_8h_source.html)
* [Redux.h](https://eigen.tuxfamily.org/dox/Redux_8h_source.html)
* [Replicate.h](https://eigen.tuxfamily.org/dox/Replicate_8h_source.html)
* [ReturnByValue.h](https://eigen.tuxfamily.org/dox/ReturnByValue_8h_source.html)
* [Reverse.h](https://eigen.tuxfamily.org/dox/Reverse_8h_source.html)
* [Select.h](https://eigen.tuxfamily.org/dox/Select_8h_source.html)
* [SelfCwiseBinaryOp.h](https://eigen.tuxfamily.org/dox/SelfCwiseBinaryOp_8h_source.html)
* [StlIterators.h](https://eigen.tuxfamily.org/dox/StlIterators_8h_source.html)
* [Transpose.h](https://eigen.tuxfamily.org/dox/Transpose_8h_source.html)
* [VectorwiseOp.h](https://eigen.tuxfamily.org/dox/VectorwiseOp_8h_source.html)
* [Visitor.h](https://eigen.tuxfamily.org/dox/Visitor_8h_source.html)
| programming_docs |
eigen3 Solving linear least squares systems Solving linear least squares systems
====================================
This page describes how to solve linear least squares systems using Eigen. An overdetermined system of equations, say *Ax* = *b*, has no solutions. In this case, it makes sense to search for the vector *x* which is closest to being a solution, in the sense that the difference *Ax* - *b* is as small as possible. This *x* is called the least square solution (if the Euclidean norm is used).
The three methods discussed on this page are the SVD decomposition, the QR decomposition and normal equations. Of these, the SVD decomposition is generally the most accurate but the slowest, normal equations is the fastest but least accurate, and the QR decomposition is in between.
Using the SVD decomposition
=============================
The [solve()](classeigen_1_1svdbase#ab28499936c0764fe5b56b9f4de701e26) method in the [BDCSVD](classeigen_1_1bdcsvd "class Bidiagonal Divide and Conquer SVD") class can be directly used to solve linear squares systems. It is not enough to compute only the singular values (the default for this class); you also need the singular vectors but the thin SVD decomposition suffices for computing least squares solutions:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Dense>
using namespace std;
using namespace [Eigen](namespaceeigen);
int main()
{
MatrixXf A = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3, 2);
cout << "Here is the matrix A:\n" << A << endl;
VectorXf b = [VectorXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3);
cout << "Here is the right hand side b:\n" << b << endl;
cout << "The least-squares solution is:\n"
<< A.bdcSvd([ComputeThinU](group__enums#ggae3e239fb70022eb8747994cf5d68b4a9aa7fb4e98834788d0b1b0f2b8467d2527) | [ComputeThinV](group__enums#ggae3e239fb70022eb8747994cf5d68b4a9a540036417bfecf2e791a70948c227f47)).solve(b) << endl;
}
```
|
```
Here is the matrix A:
0.68 0.597
-0.211 0.823
0.566 -0.605
Here is the right hand side b:
-0.33
0.536
-0.444
The least-squares solution is:
-0.67
0.314
```
|
This is example from the page [Linear algebra and decompositions](group__tutoriallinearalgebra) . If you just need to solve the least squares problem, but are not interested in the SVD per se, a faster alternative method is [CompleteOrthogonalDecomposition](classeigen_1_1completeorthogonaldecomposition "Complete orthogonal decomposition (COD) of a matrix.").
Using the QR decomposition
============================
The solve() method in QR decomposition classes also computes the least squares solution. There are three QR decomposition classes: [HouseholderQR](classeigen_1_1householderqr "Householder QR decomposition of a matrix.") (no pivoting, fast but unstable if your matrix is not rull rank), [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.") (column pivoting, thus a bit slower but more stable) and [FullPivHouseholderQR](classeigen_1_1fullpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with full pivoting.") (full pivoting, so slowest and slightly more stable than [ColPivHouseholderQR](classeigen_1_1colpivhouseholderqr "Householder rank-revealing QR decomposition of a matrix with column-pivoting.")). Here is an example with column pivoting:
| Example: | Output: |
| --- | --- |
|
```
MatrixXf A = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3, 2);
VectorXf b = [VectorXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3);
cout << "The solution using the QR decomposition is:\n"
<< A.colPivHouseholderQr().solve(b) << endl;
```
|
```
The solution using the QR decomposition is:
-0.67
0.314
```
|
Using normal equations
========================
Finding the least squares solution of *Ax* = *b* is equivalent to solving the normal equation *A*T*Ax* = *A*T*b*. This leads to the following code
| Example: | Output: |
| --- | --- |
|
```
MatrixXf A = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3, 2);
VectorXf b = [VectorXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3);
cout << "The solution using normal equations is:\n"
<< (A.transpose() * A).ldlt().solve(A.transpose() * b) << endl;
```
|
```
The solution using normal equations is:
-0.67
0.314
```
|
This method is usually the fastest, especially when *A* is "tall and skinny". However, if the matrix *A* is even mildly ill-conditioned, this is not a good method, because the condition number of *A*T*A* is the square of the condition number of *A*. This means that you lose roughly twice as many digits of accuracy using the normal equation, compared to the more stable methods mentioned above.
eigen3 Eigen::PermutationBase Eigen::PermutationBase
======================
### template<typename Derived> class Eigen::PermutationBase< Derived >
Base class for permutations.
Template Parameters
| | |
| --- | --- |
| Derived | the derived class |
This class is the base class for all expressions representing a permutation matrix, internally stored as a vector of integers. The convention followed here is that if \( \sigma \) is a permutation, the corresponding permutation matrix \( P\_\sigma \) is such that if \( (e\_1,\ldots,e\_p) \) is the canonical basis, we have:
\[ P\_\sigma(e\_i) = e\_{\sigma(i)}. \]
This convention ensures that for any two permutations \( \sigma, \tau \), we have:
\[ P\_{\sigma\circ\tau} = P\_\sigma P\_\tau. \]
Permutation matrices are square and invertible.
Notice that in addition to the member functions and operators listed here, there also are non-member operator\* to multiply any kind of permutation object with any kind of matrix expression ([MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")) on either side.
See also
class [PermutationMatrix](classeigen_1_1permutationmatrix "Permutation matrix."), class [PermutationWrapper](classeigen_1_1permutationwrapper "Class to view a vector of integers as a permutation matrix.")
| |
| --- |
| |
| Derived & | [applyTranspositionOnTheLeft](classeigen_1_1permutationbase#a4e3455bf12b56123e38a8220c6b508dc) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j) |
| |
| Derived & | [applyTranspositionOnTheRight](classeigen_1_1permutationbase#a5f98da0712570d0c4b12f61839ae4193) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) i, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) j) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](classeigen_1_1permutationbase#a26961ef6cfef586d412054ee5a20d430) () const |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [determinant](classeigen_1_1permutationbase#a1fc7a5823544700c2e0795e87f9c6d09) () const |
| |
| IndicesType & | [indices](classeigen_1_1permutationbase#a16fa3afafdf703399d62c80f950802f1) () |
| |
| const IndicesType & | [indices](classeigen_1_1permutationbase#adec727546b6882ecaa57e76d084951c5) () const |
| |
| InverseReturnType | [inverse](classeigen_1_1permutationbase#adb9af427f317202366c2832876064eb3) () const |
| |
| template<typename Other > |
| PlainPermutationType | [operator\*](classeigen_1_1permutationbase#a865e88989a76b7e92f39bad5250b89c4) (const InverseImpl< Other, [PermutationStorage](structeigen_1_1permutationstorage) > &other) const |
| |
| template<typename Other > |
| PlainPermutationType | [operator\*](classeigen_1_1permutationbase#ae81574e059f6b9b7de2ea747fd346a1b) (const [PermutationBase](classeigen_1_1permutationbase)< Other > &other) const |
| |
| template<typename OtherDerived > |
| Derived & | [operator=](classeigen_1_1permutationbase#a8e15540549c5a4e2d5b3b426fef8fbcf) (const [PermutationBase](classeigen_1_1permutationbase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| Derived & | [operator=](classeigen_1_1permutationbase#acaa7cce9ea62c811cec12e86dbb2f0de) (const TranspositionsBase< OtherDerived > &tr) |
| |
| void | [resize](classeigen_1_1permutationbase#a0e0fda6e84d69e02432e4770359bb532) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) newSize) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](classeigen_1_1permutationbase#acd7ed28ee514287f933de8467768925b) () const |
| |
| void | [setIdentity](classeigen_1_1permutationbase#a6805bb75fd7966ea71895c24ff196444) () |
| |
| void | [setIdentity](classeigen_1_1permutationbase#a830a80511a61634ef437795916f7f8da) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) newSize) |
| |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](classeigen_1_1permutationbase#a2216f9ce7b453ac39c46ff0323daeac9) () const |
| |
| DenseMatrixType | [toDenseMatrix](classeigen_1_1permutationbase#addfa91a2c2c69c76159f1091368a505f) () const |
| |
| InverseReturnType | [transpose](classeigen_1_1permutationbase#a05805e9f4182eec3f6632e1c765b5ffe) () const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
| |
| --- |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
applyTranspositionOnTheLeft()
-----------------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::applyTranspositionOnTheLeft | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *i*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *j* |
| | ) | | |
| inline |
Multiplies \*this by the transposition \((ij)\) on the left.
Returns
a reference to \*this.
Warning
This is much slower than [applyTranspositionOnTheRight(Index,Index)](classeigen_1_1permutationbase#a5f98da0712570d0c4b12f61839ae4193): this has linear complexity and requires a lot of branching.
See also
[applyTranspositionOnTheRight(Index,Index)](classeigen_1_1permutationbase#a5f98da0712570d0c4b12f61839ae4193)
applyTranspositionOnTheRight()
------------------------------
template<typename Derived >
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| Derived& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::applyTranspositionOnTheRight | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *i*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *j* |
| | ) | | |
| inline |
Multiplies \*this by the transposition \((ij)\) on the right.
Returns
a reference to \*this.
This is a fast operation, it only consists in swapping two indices.
See also
[applyTranspositionOnTheLeft(Index,Index)](classeigen_1_1permutationbase#a4e3455bf12b56123e38a8220c6b508dc)
cols()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::cols | ( | void | | ) | const |
| inline |
Returns
the number of columns
determinant()
-------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::determinant | ( | | ) | const |
| inline |
Returns
the determinant of the permutation matrix, which is either 1 or -1 depending on the parity of the permutation.
This function is O(`n`) procedure allocating a buffer of `n` booleans.
indices() [1/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| IndicesType& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::indices | ( | | ) | |
| inline |
Returns
a reference to the stored array representing the permutation.
indices() [2/2]
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const IndicesType& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::indices | ( | | ) | const |
| inline |
const version of [indices()](classeigen_1_1permutationbase#a16fa3afafdf703399d62c80f950802f1).
inverse()
---------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| InverseReturnType [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::inverse | ( | | ) | const |
| inline |
Returns
the inverse permutation matrix.
Note
This function returns the result by value. In order to make that efficient, it is implemented as just a return statement using a special constructor, hopefully allowing the compiler to perform a RVO (return value optimization).
operator\*() [1/2]
------------------
template<typename Derived > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| PlainPermutationType [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::operator\* | ( | const InverseImpl< Other, [PermutationStorage](structeigen_1_1permutationstorage) > & | *other* | ) | const |
| inline |
Returns
the product of a permutation with another inverse permutation.
Note
This function returns the result by value. In order to make that efficient, it is implemented as just a return statement using a special constructor, hopefully allowing the compiler to perform a RVO (return value optimization).
operator\*() [2/2]
------------------
template<typename Derived > template<typename Other >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| PlainPermutationType [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::operator\* | ( | const [PermutationBase](classeigen_1_1permutationbase)< Other > & | *other* | ) | const |
| inline |
Returns
the product permutation matrix.
Note
This function returns the result by value. In order to make that efficient, it is implemented as just a return statement using a special constructor, hopefully allowing the compiler to perform a RVO (return value optimization).
operator=() [1/2]
-----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::operator= | ( | const [PermutationBase](classeigen_1_1permutationbase)< OtherDerived > & | *other* | ) | |
| inline |
Copies the other permutation into \*this
operator=() [2/2]
-----------------
template<typename Derived > template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| Derived& [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::operator= | ( | const TranspositionsBase< OtherDerived > & | *tr* | ) | |
| inline |
Assignment from the [Transpositions](classeigen_1_1transpositions "Represents a sequence of transpositions (row/column interchange)") *tr*
resize()
--------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::resize | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *newSize* | ) | |
| inline |
Resizes to given size.
rows()
------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::rows | ( | void | | ) | const |
| inline |
Returns
the number of rows
setIdentity() [1/2]
-------------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| void [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::setIdentity | ( | | ) | |
| inline |
Sets \*this to be the identity permutation matrix
setIdentity() [2/2]
-------------------
template<typename Derived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| void [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::setIdentity | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *newSize* | ) | |
| inline |
Sets \*this to be the identity permutation matrix of given size.
size()
------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::size | ( | | ) | const |
| inline |
Returns
the size of a side of the respective square matrix, i.e., the number of indices
toDenseMatrix()
---------------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| DenseMatrixType [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::toDenseMatrix | ( | | ) | const |
| inline |
Returns
a [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") object initialized from this permutation matrix. Notice that it is inefficient to return this [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") object by value. For efficiency, favor using the [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") constructor taking [EigenBase](structeigen_1_1eigenbase) objects.
transpose()
-----------
template<typename Derived >
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| InverseReturnType [Eigen::PermutationBase](classeigen_1_1permutationbase)< Derived >::transpose | ( | | ) | const |
| inline |
Returns
the tranpose permutation matrix.
Note
This function returns the result by value. In order to make that efficient, it is implemented as just a return statement using a special constructor, hopefully allowing the compiler to perform a RVO (return value optimization).
---
The documentation for this class was generated from the following file:* [PermutationMatrix.h](https://eigen.tuxfamily.org/dox/PermutationMatrix_8h_source.html)
| programming_docs |
eigen3 Writing Functions Taking Eigen Types as Parameters Writing Functions Taking Eigen Types as Parameters
==================================================
Eigen's use of expression templates results in potentially every expression being of a different type. If you pass such an expression to a function taking a parameter of type [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors."), your expression will implicitly be evaluated into a temporary [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors."), which will then be passed to the function. This means that you lose the benefit of expression templates. Concretely, this has two drawbacks:
* The evaluation into a temporary may be useless and inefficient;
* This only allows the function to read from the expression, not to write to it.
Fortunately, all this myriad of expression types have in common that they all inherit a few common, templated base classes. By letting your function take templated parameters of these base types, you can let them play nicely with Eigen's expression templates.
Some First Examples
=====================
This section will provide simple examples for different types of objects Eigen is offering. Before starting with the actual examples, we need to recapitulate which base objects we can work with (see also [The class hierarchy](topicclasshierarchy)).
* [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions."): The common base class for all dense matrix expressions (as opposed to array expressions, as opposed to sparse and special matrix classes). Use it in functions that are meant to work only on dense matrices.
* [ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions."): The common base class for all dense array expressions (as opposed to matrix expressions, etc). Use it in functions that are meant to work only on arrays.
* [DenseBase](classeigen_1_1densebase "Base class for all dense matrices, vectors, and arrays."): The common base class for all dense matrix expression, that is, the base class for both `[MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")` and `[ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions.")`. It can be used in functions that are meant to work on both matrices and arrays.
* [EigenBase](structeigen_1_1eigenbase): The base class unifying all types of objects that can be evaluated into dense matrices or arrays, for example special matrix classes such as diagonal matrices, permutation matrices, etc. It can be used in functions that are meant to work on any such general type.
**EigenBase Example**
Prints the dimensions of the most generic object present in Eigen. It could be any matrix expressions, any dense or sparse matrix and any array.
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/Core>
using namespace [Eigen](namespaceeigen);
template <typename Derived>
void print_size(const EigenBase<Derived>& b)
{
std::cout << "size (rows, cols): " << b.size() << " (" << b.rows()
<< ", " << b.cols() << ")" << std::endl;
}
int main()
{
Vector3f v;
print_size(v);
// v.asDiagonal() returns a 3x3 diagonal matrix pseudo-expression
print_size(v.asDiagonal());
}
```
|
```
size (rows, cols): 3 (3, 1)
size (rows, cols): 9 (3, 3)
```
|
**DenseBase Example**
Prints a sub-block of the dense expression. Accepts any dense matrix or array expression, but no sparse objects and no special matrix classes such as [DiagonalMatrix](classeigen_1_1diagonalmatrix "Represents a diagonal matrix with its storage.").
```
template <typename Derived>
void print_block(const DenseBase<Derived>& b, int x, int y, int r, int c)
{
std::cout << "block: " << b.block(x,y,r,c) << std::endl;
}
```
**ArrayBase Example**
Prints the maximum coefficient of the array or array-expression.
```
template <typename Derived>
void print_max_coeff(const ArrayBase<Derived> &a)
{
std::cout << "max: " << a.maxCoeff() << std::endl;
}
```
**MatrixBase Example**
Prints the inverse condition number of the given matrix or matrix-expression.
```
template <typename Derived>
void print_inv_cond(const MatrixBase<Derived>& a)
{
const typename JacobiSVD<typename Derived::PlainObject>::SingularValuesType&
sing_vals = a.jacobiSvd().singularValues();
std::cout << "inv cond: " << sing_vals(sing_vals.size()-1) / sing_vals(0) << std::endl;
}
```
**Multiple templated arguments example**
Calculate the Euclidean distance between two points.
```
template <typename DerivedA,typename DerivedB>
typename DerivedA::Scalar squaredist(const MatrixBase<DerivedA>& p1,const MatrixBase<DerivedB>& p2)
{
return (p1-p2).squaredNorm();
}
```
Notice that we used two template parameters, one per argument. This permits the function to handle inputs of different types, e.g.,
```
squaredist(v1,2*v2)
```
where the first argument `v1` is a vector and the second argument `2*v2` is an expression.
These examples are just intended to give the reader a first impression of how functions can be written which take a plain and constant [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") or [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.") argument. They are also intended to give the reader an idea about the most common base classes being the optimal candidates for functions. In the next section we will look in more detail at an example and the different ways it can be implemented, while discussing each implementation's problems and advantages. For the discussion below, [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") and [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.") as well as [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") and [ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions.") can be exchanged and all arguments still hold.
How to write generic, but non-templated function?
===================================================
In all the previous examples, the functions had to be template functions. This approach allows to write very generic code, but it is often desirable to write non templated functions and still keep some level of genericity to avoid stupid copies of the arguments. The typical example is to write functions accepting both a MatrixXf or a block of a MatrixXf. This is exactly the purpose of the [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.") class. Here is a simple example:
| Example: | Output: |
| --- | --- |
|
```
#include <iostream>
#include <Eigen/SVD>
using namespace [Eigen](namespaceeigen);
using namespace std;
float inv_cond(const Ref<const MatrixXf>& a)
{
const VectorXf sing_vals = a.jacobiSvd().singularValues();
return sing_vals(sing_vals.size()-1) / sing_vals(0);
}
int main()
{
Matrix4f m = [Matrix4f::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "matrix m:" << endl << m << endl << endl;
cout << "inv\_cond(m): " << inv_cond(m) << endl;
cout << "inv\_cond(m(1:3,1:3)): " << inv_cond(m.topLeftCorner(3,3)) << endl;
cout << "inv\_cond(m+I): " << inv_cond(m+[Matrix4f::Identity](classeigen_1_1matrixbase#a98bb9a0f705c6dfde85b0bfff31bf88f)()) << endl;
}
```
|
```
matrix m:
0.68 0.823 -0.444 -0.27
-0.211 -0.605 0.108 0.0268
0.566 -0.33 -0.0452 0.904
0.597 0.536 0.258 0.832
inv_cond(m): 0.0562343
inv_cond(m(1:3,1:3)): 0.0836819
inv_cond(m+I): 0.160204
```
|
In the first two calls to inv\_cond, no copy occur because the memory layout of the arguments matches the memory layout accepted by Ref<MatrixXf>. However, in the last call, we have a generic expression that will be automatically evaluated into a temporary MatrixXf by the Ref<> object.
A [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.") object can also be writable. Here is an example of a function computing the covariance matrix of two input matrices where each row is an observation:
```
void cov(const Ref<const MatrixXf> x, const Ref<const MatrixXf> y, Ref<MatrixXf> C)
{
const float num_observations = static\_cast<float>(x.rows());
const RowVectorXf x_mean = x.colwise().sum() / num_observations;
const RowVectorXf y_mean = y.colwise().sum() / num_observations;
C = (x.rowwise() - x_mean).transpose() * (y.rowwise() - y_mean) / num_observations;
}
```
and here are two examples calling cov without any copy:
```
MatrixXf m1, m2, m3
cov(m1, m2, m3);
cov(m1.leftCols<3>(), m2.leftCols<3>(), m3.topLeftCorner<3,3>());
```
The Ref<> class has two other optional template arguments allowing to control the kind of memory layout that can be accepted without any copy. See the class [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.") documentation for the details.
In which cases do functions taking plain Matrix or Array arguments work?
==========================================================================
Without using template functions, and without the [Ref](classeigen_1_1ref "A matrix or vector expression mapping an existing expression.") class, a naive implementation of the previous cov function might look like this
```
MatrixXf cov(const MatrixXf& x, const MatrixXf& y)
{
const float num_observations = static\_cast<float>(x.rows());
const RowVectorXf x_mean = x.colwise().sum() / num_observations;
const RowVectorXf y_mean = y.colwise().sum() / num_observations;
return (x.rowwise() - x_mean).transpose() * (y.rowwise() - y_mean) / num_observations;
}
```
and contrary to what one might think at first, this implementation is fine unless you require a generic implementation that works with double matrices too and unless you do not care about temporary objects. Why is that the case? Where are temporaries involved? How can code as given below compile?
```
MatrixXf x,y,z;
MatrixXf C = cov(x,y+z);
```
In this special case, the example is fine and will be working because both parameters are declared as *const* references. The compiler creates a temporary and evaluates the expression x+z into this temporary. Once the function is processed, the temporary is released and the result is assigned to C.
**Note:** Functions taking *const* references to [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") (or [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.")) can process expressions at the cost of temporaries.
In which cases do functions taking a plain Matrix or Array argument fail?
===========================================================================
Here, we consider a slightly modified version of the function given above. This time, we do not want to return the result but pass an additional non-const parameter which allows us to store the result. A first naive implementation might look as follows.
```
// Note: This code is flawed!
void cov(const MatrixXf& x, const MatrixXf& y, MatrixXf& C)
{
const float num_observations = static\_cast<float>(x.rows());
const RowVectorXf x_mean = x.colwise().sum() / num_observations;
const RowVectorXf y_mean = y.colwise().sum() / num_observations;
C = (x.rowwise() - x_mean).transpose() * (y.rowwise() - y_mean) / num_observations;
}
```
When trying to execute the following code
```
MatrixXf C = [MatrixXf::Zero](classeigen_1_1densebase#a422ddeef58bedc7bddb1d4357688d761)(3,6);
cov(x,y, C.block(0,0,3,3));
```
the compiler will fail, because it is not possible to convert the expression returned by `MatrixXf::block()` into a non-const `MatrixXf&`. This is the case because the compiler wants to protect you from writing your result to a temporary object. In this special case this protection is not intended – we want to write to a temporary object. So how can we overcome this problem?
The solution which is preferred at the moment is based on a little *hack*. One needs to pass a const reference to the matrix and internally the constness needs to be cast away. The correct implementation for C98 compliant compilers would be
```
template <typename Derived, typename OtherDerived>
void cov(const MatrixBase<Derived>& x, const MatrixBase<Derived>& y, MatrixBase<OtherDerived> const & C)
{
typedef typename Derived::Scalar Scalar;
typedef typename internal::plain_row_type<Derived>::type RowVectorType;
const Scalar num_observations = static\_cast<Scalar>(x.rows());
const RowVectorType x_mean = x.colwise().sum() / num_observations;
const RowVectorType y_mean = y.colwise().sum() / num_observations;
const\_cast< MatrixBase<OtherDerived>& >(C) =
(x.rowwise() - x_mean).transpose() * (y.rowwise() - y_mean) / num_observations;
}
```
The implementation above does now not only work with temporary expressions but it also allows to use the function with matrices of arbitrary floating point scalar types.
**Note:** The const cast hack will only work with templated functions. It will not work with the MatrixXf implementation because it is not possible to cast a [Block](classeigen_1_1block "Expression of a fixed-size or dynamic-size block.") expression to a [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") reference!
How to resize matrices in generic implementations?
====================================================
One might think we are done now, right? This is not completely true because in order for our covariance function to be generically applicable, we want the following code to work
```
MatrixXf x = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(100,3);
MatrixXf y = [MatrixXf::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(100,3);
MatrixXf C;
cov(x, y, C);
```
This is not the case anymore, when we are using an implementation taking [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") as a parameter. In general, Eigen supports automatic resizing but it is not possible to do so on expressions. Why should resizing of a matrix [Block](classeigen_1_1block "Expression of a fixed-size or dynamic-size block.") be allowed? It is a reference to a sub-matrix and we definitely don't want to resize that. So how can we incorporate resizing if we cannot resize on [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.")? The solution is to resize the derived object as in this implementation.
```
template <typename Derived, typename OtherDerived>
void cov(const MatrixBase<Derived>& x, const MatrixBase<Derived>& y, MatrixBase<OtherDerived> const & C_)
{
typedef typename Derived::Scalar Scalar;
typedef typename internal::plain_row_type<Derived>::type RowVectorType;
const Scalar num_observations = static\_cast<Scalar>(x.rows());
const RowVectorType x_mean = x.colwise().sum() / num_observations;
const RowVectorType y_mean = y.colwise().sum() / num_observations;
MatrixBase<OtherDerived>& C = const\_cast< MatrixBase<OtherDerived>& >(C_);
C.derived().resize(x.cols(),x.cols()); // resize the derived object
C = (x.rowwise() - x_mean).transpose() * (y.rowwise() - y_mean) / num_observations;
}
```
This implementation is now working for parameters being expressions and for parameters being matrices and having the wrong size. Resizing the expressions does not do any harm in this case unless they actually require resizing. That means, passing an expression with the wrong dimensions will result in a run-time error (in debug mode only) while passing expressions of the correct size will just work fine.
**Note:** In the above discussion the terms [Matrix](classeigen_1_1matrix "The matrix class, also used for vectors and row-vectors.") and [Array](classeigen_1_1array "General-purpose arrays with easy API for coefficient-wise operations.") and [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") and [ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions.") can be exchanged and all arguments still hold.
Summary
=========
* To summarize, the implementation of functions taking non-writable (const referenced) objects is not a big issue and does not lead to problematic situations in terms of compiling and running your program. However, a naive implementation is likely to introduce unnecessary temporary objects in your code. In order to avoid evaluating parameters into temporaries, pass them as (const) references to [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") or [ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions.") (so templatize your function).
* Functions taking writable (non-const) parameters must take const references and cast away constness within the function body.
* Functions that take as parameters [MatrixBase](classeigen_1_1matrixbase "Base class for all dense matrices, vectors, and expressions.") (or [ArrayBase](classeigen_1_1arraybase "Base class for all 1D and 2D array, and related expressions.")) objects, and potentially need to resize them (in the case where they are resizable), must call resize() on the derived class, as returned by derived().
eigen3 Eigen::VectorwiseOp Eigen::VectorwiseOp
===================
### template<typename ExpressionType, int Direction> class Eigen::VectorwiseOp< ExpressionType, Direction >
Pseudo expression providing broadcasting and partial reduction operations.
Template Parameters
| | |
| --- | --- |
| ExpressionType | the type of the object on which to do partial reductions |
| Direction | indicates whether to operate on columns ([Vertical](group__enums#ggad49a7b3738e273eb00932271b36127f7ae2efac6e74ecab5e3b0b1561c5ddf83e)) or rows ([Horizontal](group__enums#ggad49a7b3738e273eb00932271b36127f7a961c62410157b64033839488f4d7f7e4)) |
This class represents a pseudo expression with broadcasting and partial reduction features. It is the return type of [DenseBase::colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30) and [DenseBase::rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28) and most of the time this is the only way it is explicitly used.
To understand the logic of rowwise/colwise expression, let's consider a generic case `A.colwise().foo()` where `foo` is any method of `[VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations.")`. This expression is equivalent to applying `foo()` to each column of `A` and then re-assemble the outputs in a matrix expression:
```
[A.col(0).foo(), A.col(1).foo(), ..., A.col(A.cols()-1).foo()]
```
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the sum of each column:" << endl << m.colwise().sum() << endl;
cout << "Here is the maximum absolute value of each column:"
<< endl << m.cwiseAbs().colwise().maxCoeff() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the sum of each column:
1.04 0.815 -0.238
Here is the maximum absolute value of each column:
0.68 0.823 0.536
```
The [begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618) and [end()](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5) methods are obviously exceptions to the previous rule as they return STL-compatible begin/end iterators to the rows or columns of the nested expression. Typical use cases include for-range-loop and calls to STL algorithms:
Example:
```
Matrix3i m = [Matrix3i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the initial matrix m:" << endl << m << endl;
int i = -1;
for(auto c: m.colwise()) {
c *= i;
++i;
}
cout << "Here is the matrix m after the for-range-loop:" << endl << m << endl;
auto cols = m.colwise();
auto it = std::find_if(cols.cbegin(), cols.cend(),
[](Matrix3i::ConstColXpr x) { return x.squaredNorm() == 0; });
cout << "The first empty column is: " << distance(cols.cbegin(),it) << endl;
```
Output:
```
Here is the initial matrix m:
7 6 -3
-2 9 6
6 -6 -5
Here is the matrix m after the for-range-loop:
-7 0 -3
2 0 6
-6 0 -5
The first empty column is: 1
```
For a partial reduction on an empty input, some rules apply. For the sake of clarity, let's consider a vertical reduction:
* If the number of columns is zero, then a 1x0 row-major vector expression is returned.
* Otherwise, if the number of rows is zero, then
+ a row vector of zeros is returned for sum-like reductions (sum, squaredNorm, norm, etc.)
+ a row vector of ones is returned for a product reduction (e.g., `MatrixXd(n,0).colwise().[prod()](classeigen_1_1vectorwiseop#a01bcd17504f30b55b4910ddb75598f79)`)
+ an assert is triggered for all other reductions (minCoeff,maxCoeff,redux(bin\_op))
See also
[DenseBase::colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30), [DenseBase::rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28), class [PartialReduxExpr](classeigen_1_1partialreduxexpr "Generic expression of a partially reduxed matrix.")
| |
| --- |
| |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) |
| |
| |
| --- |
| |
| const [AllReturnType](classeigen_1_1partialreduxexpr) | [all](classeigen_1_1vectorwiseop#a9c2b4740bf190492bf231d8e244b25c1) () const |
| |
| const [AnyReturnType](classeigen_1_1partialreduxexpr) | [any](classeigen_1_1vectorwiseop#a96210c9f83bd375f8ce862bd62ab70e6) () const |
| |
| [iterator](classeigen_1_1vectorwiseop#a9fb8aaf24528efcdac1782aacf99b8dd) | [begin](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618) () |
| |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) | [begin](classeigen_1_1vectorwiseop#aaf91f2a57a26e88e7131fcd9c194f93f) () const |
| |
| const [BlueNormReturnType](classeigen_1_1partialreduxexpr) | [blueNorm](classeigen_1_1vectorwiseop#a4d2fbc5296470a94379cbcb33bb9c24d) () const |
| |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) | [cbegin](classeigen_1_1vectorwiseop#a7b237a2b46479de5c905d2bcc54f34c4) () const |
| |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) | [cend](classeigen_1_1vectorwiseop#a57859b88a32b8b393a427c4cab3581a7) () const |
| |
| const [CountReturnType](classeigen_1_1partialreduxexpr) | [count](classeigen_1_1vectorwiseop#a5c6b797457895f11a7682b3a16f263bb) () const |
| |
| const\_reverse\_iterator | [crbegin](classeigen_1_1vectorwiseop#aec95725eed4adb5ccf65fbb6e907d12e) () const |
| |
| const\_reverse\_iterator | [crend](classeigen_1_1vectorwiseop#a23fa202916349d11e476f459543395ef) () const |
| |
| template<typename OtherDerived > |
| const CrossReturnType | [cross](group__geometry__module#ga2fe1a2a012ce0ab0e8da6af134073039) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &other) const |
| |
| [iterator](classeigen_1_1vectorwiseop#a9fb8aaf24528efcdac1782aacf99b8dd) | [end](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5) () |
| |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) | [end](classeigen_1_1vectorwiseop#ad3b0dcb34bb4903ffa1ab3634188ecff) () const |
| |
| const [HNormalizedReturnType](classeigen_1_1cwisebinaryop) | [hnormalized](group__geometry__module#ga1f220045efa302626c287088b63b6ba9) () const |
| | column or row-wise homogeneous normalization [More...](group__geometry__module#ga1f220045efa302626c287088b63b6ba9) |
| |
| [HomogeneousReturnType](classeigen_1_1homogeneous) | [homogeneous](group__geometry__module#gaf99305a3d7432318236df7b80022df37) () const |
| |
| const [HypotNormReturnType](classeigen_1_1partialreduxexpr) | [hypotNorm](classeigen_1_1vectorwiseop#a00c44e033eec33586ae8093a6366da75) () const |
| |
| template<int p> |
| const LpNormReturnType< p >::Type | [lpNorm](classeigen_1_1vectorwiseop#aa9c2cad38085e7f5f9bc2598eff249f1) () const |
| |
| const [MaxCoeffReturnType](classeigen_1_1partialreduxexpr) | [maxCoeff](classeigen_1_1vectorwiseop#a6646b584db116c1661b5bb56750bd6f6) () const |
| |
| const MeanReturnType | [mean](classeigen_1_1vectorwiseop#aaf7d867f9e6b74a1bc7f108d646cfa85) () const |
| |
| const [MinCoeffReturnType](classeigen_1_1partialreduxexpr) | [minCoeff](classeigen_1_1vectorwiseop#a7d7b8c03d180b126fde0ac212e2b0b76) () const |
| |
| const [NormReturnType](classeigen_1_1cwiseunaryop) | [norm](classeigen_1_1vectorwiseop#af726d1dfd9a7a67b76e639db1a9f352e) () const |
| |
| void | [normalize](classeigen_1_1vectorwiseop#adb1084153780dc8d2c062fee97651a45) () |
| |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_quotient\_op< Scalar >, const ExpressionTypeNestedCleaned, const typename OppositeExtendedType< [NormReturnType](classeigen_1_1cwiseunaryop) >::Type > | [normalized](classeigen_1_1vectorwiseop#aca6ac44a9bf819d5d64c2cb54bf38095) () const |
| |
| template<typename OtherDerived > |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_product\_op< Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType< OtherDerived >::Type > | [operator\*](classeigen_1_1vectorwiseop#a25ca4c1e409897feca669dee811533c9) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) const |
| |
| template<typename OtherDerived > |
| ExpressionType & | [operator\*=](classeigen_1_1vectorwiseop#acef518a77a8947c6726ba30d56dd67cb) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_sum\_op< Scalar, typename OtherDerived::Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType< OtherDerived >::Type > | [operator+](classeigen_1_1vectorwiseop#a713694459d81b76e4f2a78e4d169f8d6) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) const |
| |
| template<typename OtherDerived > |
| ExpressionType & | [operator+=](classeigen_1_1vectorwiseop#a23ce2522d11c3362ca22252b2876812b) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_difference\_op< Scalar, typename OtherDerived::Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType< OtherDerived >::Type > | [operator-](classeigen_1_1vectorwiseop#a5e60616620df132d665b301bb9a304c4) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) const |
| |
| template<typename OtherDerived > |
| ExpressionType & | [operator-=](classeigen_1_1vectorwiseop#a6bb3a7315617f86f0c28ac05816cf6e3) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)< internal::scalar\_quotient\_op< Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType< OtherDerived >::Type > | [operator/](classeigen_1_1vectorwiseop#aa154a8407885a9ad51504180f6d7a163) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) const |
| |
| template<typename OtherDerived > |
| ExpressionType & | [operator/=](classeigen_1_1vectorwiseop#a9efa0b81915c31535a929a46a79cab2b) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| template<typename OtherDerived > |
| ExpressionType & | [operator=](classeigen_1_1vectorwiseop#a7999eb8f50bb19ade54f3212d3c79680) (const [DenseBase](classeigen_1_1densebase)< OtherDerived > &other) |
| |
| const [ProdReturnType](classeigen_1_1partialreduxexpr) | [prod](classeigen_1_1vectorwiseop#a01bcd17504f30b55b4910ddb75598f79) () const |
| |
| reverse\_iterator | [rbegin](classeigen_1_1vectorwiseop#a91a8087eb76e210b07192c81d362dd1e) () |
| |
| const\_reverse\_iterator | [rbegin](classeigen_1_1vectorwiseop#aa73e8a0230fcc049346057ef1a6efe02) () const |
| |
| template<typename BinaryOp > |
| const ReduxReturnType< BinaryOp >::Type | [redux](classeigen_1_1vectorwiseop#ae92babb33ed5a91eb0c4de80a97eafca) (const BinaryOp &func=BinaryOp()) const |
| |
| reverse\_iterator | [rend](classeigen_1_1vectorwiseop#aa9592e93b704f7712e8f33631a2efe8f) () |
| |
| const\_reverse\_iterator | [rend](classeigen_1_1vectorwiseop#afeb3e3ceca37ae0a6e313f80743f767a) () const |
| |
| const [ReplicateReturnType](classeigen_1_1replicate) | [replicate](classeigen_1_1vectorwiseop#a5f0c8dc9e9c4aeaa2057f15800f5c18c) ([Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) factor) const |
| |
| template<int Factor> |
| const [Replicate](classeigen_1_1replicate)< ExpressionType, isVertical \*Factor+isHorizontal, isHorizontal \*Factor+isVertical > | [replicate](classeigen_1_1vectorwiseop#a81bfb88fe6415c7bfd9bdf15ef608aaa) ([Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) factor=Factor) const |
| |
| [ReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1vectorwiseop#ab8caf5367e2bd636536c8a0e0c89fe15) () |
| |
| const [ConstReverseReturnType](classeigen_1_1reverse) | [reverse](classeigen_1_1vectorwiseop#aeb0428f561b5757e2c85e8d72022eec1) () const |
| |
| void | [reverseInPlace](classeigen_1_1vectorwiseop#ab9dd7c273eb2ba8defaab2d55156936b) () |
| |
| const [SquaredNormReturnType](classeigen_1_1partialreduxexpr) | [squaredNorm](classeigen_1_1vectorwiseop#a01474cf971473a73c919f6a99323d4f5) () const |
| |
| const [StableNormReturnType](classeigen_1_1partialreduxexpr) | [stableNorm](classeigen_1_1vectorwiseop#add48fd836be98ed280cac23518db0916) () const |
| |
| const [SumReturnType](classeigen_1_1partialreduxexpr) | [sum](classeigen_1_1vectorwiseop#a7030fc687c24d687ed7cd70733ba611c) () const |
| |
| |
| --- |
| |
| random\_access\_iterator\_type | [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) |
| |
| random\_access\_iterator\_type | [iterator](classeigen_1_1vectorwiseop#a9fb8aaf24528efcdac1782aacf99b8dd) |
| |
Index
-----
template<typename ExpressionType , int Direction>
| |
| --- |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::[Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) |
**[Deprecated:](deprecated#_deprecated000011)**
since [Eigen](namespaceeigen "Namespace containing all symbols from the Eigen library.") 3.3
all()
-----
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [AllReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::all | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression representing whether **all** coefficients of each respective column (or row) are `true`. This expression can be assigned to a vector with entries of type `bool`.
See also
[DenseBase::all()](classeigen_1_1densebase#ae42ab60296c120e9f45ce3b44e1761a4)
any()
-----
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [AnyReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::any | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression representing whether **at** **least** one coefficient of each respective column (or row) is `true`. This expression can be assigned to a vector with entries of type `bool`.
See also
[DenseBase::any()](classeigen_1_1densebase#abfbf4cb72dd577e62fbe035b1c53e695)
begin() [1/2]
-------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [iterator](classeigen_1_1vectorwiseop#a9fb8aaf24528efcdac1782aacf99b8dd) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::begin | ( | | ) | |
| inline |
returns an iterator to the first row (rowwise) or column (colwise) of the nested expression.
See also
[end()](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5), [cbegin()](classeigen_1_1vectorwiseop#a7b237a2b46479de5c905d2bcc54f34c4)
begin() [2/2]
-------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::begin | ( | | ) | const |
| inline |
const version of [begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618)
blueNorm()
----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [BlueNormReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::blueNorm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the norm of each column (or row) of the referenced expression, using Blue's algorithm. This is a vector with real entries, even if the original matrix has complex entries.
See also
DenseBase::blueNorm()
cbegin()
--------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::cbegin | ( | | ) | const |
| inline |
const version of [begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618)
cend()
------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::cend | ( | | ) | const |
| inline |
const version of [end()](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5)
count()
-------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [CountReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::count | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression representing the number of `true` coefficients of each respective column (or row). This expression can be assigned to a vector whose entries have the same type as is used to index entries of the original matrix; for dense matrices, this is `std::ptrdiff_t` .
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
Matrix<ptrdiff_t, 3, 1> res = (m.array() >= 0.5).rowwise().count();
cout << "Here is the count of elements larger or equal than 0.5 of each row:" << endl;
cout << res << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the count of elements larger or equal than 0.5 of each row:
2
2
1
```
See also
[DenseBase::count()](classeigen_1_1densebase#a229be090c665b9bf7d1fcdfd1ab6e0c1)
crbegin()
---------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const\_reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::crbegin | ( | | ) | const |
| inline |
const version of [rbegin()](classeigen_1_1vectorwiseop#a91a8087eb76e210b07192c81d362dd1e)
crend()
-------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const\_reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::crend | ( | | ) | const |
| inline |
const version of [rend()](classeigen_1_1vectorwiseop#aa9592e93b704f7712e8f33631a2efe8f)
end() [1/2]
-----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [iterator](classeigen_1_1vectorwiseop#a9fb8aaf24528efcdac1782aacf99b8dd) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::end | ( | | ) | |
| inline |
returns an iterator to the row (resp. column) following the last row (resp. column) of the nested expression
See also
[begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618), [cend()](classeigen_1_1vectorwiseop#a57859b88a32b8b393a427c4cab3581a7)
end() [2/2]
-----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [const\_iterator](classeigen_1_1vectorwiseop#a595c33d2a82427a1159568b0b8438554) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::end | ( | | ) | const |
| inline |
const version of [end()](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5)
hypotNorm()
-----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [HypotNormReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::hypotNorm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the norm of each column (or row) of the referenced expression, avoiding underflow and overflow using a concatenation of hypot() calls. This is a vector with real entries, even if the original matrix has complex entries.
See also
DenseBase::hypotNorm()
lpNorm()
--------
template<typename ExpressionType , int Direction> template<int p>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const LpNormReturnType<p>::Type [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::lpNorm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the norm of each column (or row) of the referenced expression. This is a vector with real entries, even if the original matrix has complex entries.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the norm of each column:" << endl << m.colwise().norm() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the norm of each column:
0.91 1.18 0.771
```
See also
DenseBase::norm()
maxCoeff()
----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [MaxCoeffReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::maxCoeff | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the largest coefficient of each column (or row) of the referenced expression.
Warning
the size along the reduction direction must be strictly positive, otherwise an assertion is triggered. the result is undefined if `*this` contains NaN.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the maximum of each column:" << endl << m.colwise().maxCoeff() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the maximum of each column:
0.68 0.823 0.536
```
See also
[DenseBase::maxCoeff()](classeigen_1_1densebase#a7e6987d106f1cca3ac6ab36d288cc8e1)
mean()
------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const MeanReturnType [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::mean | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the mean of each column (or row) of the referenced expression.
See also
[DenseBase::mean()](classeigen_1_1densebase#a21ac6c0419a72ad7a88ea0bc189017d7)
minCoeff()
----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [MinCoeffReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::minCoeff | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the smallest coefficient of each column (or row) of the referenced expression.
Warning
the size along the reduction direction must be strictly positive, otherwise an assertion is triggered. the result is undefined if `*this` contains NaN.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the minimum of each column:" << endl << m.colwise().minCoeff() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the minimum of each column:
-0.211 -0.605 -0.444
```
See also
[DenseBase::minCoeff()](classeigen_1_1densebase#a0739f9c868c331031c7810e21838dcb2)
norm()
------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [NormReturnType](classeigen_1_1cwiseunaryop) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::norm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the norm of each column (or row) of the referenced expression. This is a vector with real entries, even if the original matrix has complex entries.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the norm of each column:" << endl << m.colwise().norm() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the norm of each column:
0.91 1.18 0.771
```
See also
DenseBase::norm()
normalize()
-----------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| void [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::normalize | ( | | ) | |
| inline |
Normalize in-place each row or columns of the referenced matrix.
See also
[MatrixBase::normalize()](classeigen_1_1matrixbase#ad16303c47ba36f7a41ea264cb26bceb6), [normalized()](classeigen_1_1vectorwiseop#aca6ac44a9bf819d5d64c2cb54bf38095)
normalized()
------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)<internal::scalar\_quotient\_op<Scalar>, const ExpressionTypeNestedCleaned, const typename OppositeExtendedType<[NormReturnType](classeigen_1_1cwiseunaryop)>::Type> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::normalized | ( | | ) | const |
| inline |
Returns
an expression where each column (or row) of the referenced matrix are normalized. The referenced matrix is **not** modified.
See also
[MatrixBase::normalized()](classeigen_1_1matrixbase#a5cf2fd4c57e59604fd4116158fd34308), [normalize()](classeigen_1_1vectorwiseop#adb1084153780dc8d2c062fee97651a45)
operator\*()
------------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)<internal::scalar\_product\_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator\* | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns the expression where each subvector is the product of the vector *other* by the corresponding subvector of `*this`
operator\*=()
-------------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| ExpressionType& [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator\*= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Multiples each subvector of `*this` by the vector *other*
operator+()
-----------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)<internal::scalar\_sum\_op<Scalar,typename OtherDerived::Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator+ | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns the expression of the sum of the vector *other* to each subvector of `*this`
operator+=()
------------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| ExpressionType& [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator+= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Adds the vector *other* to each subvector of `*this`
operator-()
-----------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)<internal::scalar\_difference\_op<Scalar,typename OtherDerived::Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator- | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns the expression of the difference between each subvector of `*this` and the vector *other*
operator-=()
------------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| ExpressionType& [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator-= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Substracts the vector *other* to each subvector of `*this`
operator/()
-----------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| [CwiseBinaryOp](classeigen_1_1cwisebinaryop)<internal::scalar\_quotient\_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator/ | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | const |
| inline |
Returns the expression where each subvector is the quotient of the corresponding subvector of `*this` by the vector *other*
operator/=()
------------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| ExpressionType& [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator/= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Divides each subvector of `*this` by the vector *other*
operator=()
-----------
template<typename ExpressionType , int Direction> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| ExpressionType& [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::operator= | ( | const [DenseBase](classeigen_1_1densebase)< OtherDerived > & | *other* | ) | |
| inline |
Copies the vector *other* to each subvector of `*this`
prod()
------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [ProdReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::prod | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the product of each column (or row) of the referenced expression.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the product of each row:" << endl << m.rowwise().prod() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the product of each row:
-0.134
-0.0933
0.152
```
See also
[DenseBase::prod()](classeigen_1_1densebase#af119d9a4efe5a15cd83c1ccdf01b3a4f)
rbegin() [1/2]
--------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::rbegin | ( | | ) | |
| inline |
returns a reverse iterator to the last row (rowwise) or column (colwise) of the nested expression.
See also
[rend()](classeigen_1_1vectorwiseop#aa9592e93b704f7712e8f33631a2efe8f), [crbegin()](classeigen_1_1vectorwiseop#aec95725eed4adb5ccf65fbb6e907d12e)
rbegin() [2/2]
--------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const\_reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::rbegin | ( | | ) | const |
| inline |
const version of [rbegin()](classeigen_1_1vectorwiseop#a91a8087eb76e210b07192c81d362dd1e)
redux()
-------
template<typename ExpressionType , int Direction> template<typename BinaryOp >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const ReduxReturnType<BinaryOp>::Type [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::redux | ( | const BinaryOp & | *func* = `BinaryOp()` | ) | const |
| inline |
Returns
a row or column vector expression of `*this` reduxed by *func*
The template parameter *BinaryOp* is the type of the functor of the custom redux operator. Note that func must be an associative operator.
Warning
the size along the reduction direction must be strictly positive, otherwise an assertion is triggered.
See also
class [VectorwiseOp](classeigen_1_1vectorwiseop "Pseudo expression providing broadcasting and partial reduction operations."), [DenseBase::colwise()](classeigen_1_1densebase#a1c0e1b6067ec1de6cb8799da55aa7d30), [DenseBase::rowwise()](classeigen_1_1densebase#a6daa3a3156ca0e0722bf78638e1c7f28)
rend() [1/2]
------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::rend | ( | | ) | |
| inline |
returns a reverse iterator to the row (resp. column) before the first row (resp. column) of the nested expression
See also
[begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618), [cend()](classeigen_1_1vectorwiseop#a57859b88a32b8b393a427c4cab3581a7)
rend() [2/2]
------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const\_reverse\_iterator [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::rend | ( | | ) | const |
| inline |
const version of [rend()](classeigen_1_1vectorwiseop#aa9592e93b704f7712e8f33631a2efe8f)
replicate() [1/2]
-----------------
template<typename ExpressionType , int Direction>
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::[ReplicateReturnType](classeigen_1_1replicate) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::replicate | ( | [Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) | *factor* | ) | const |
Returns
an expression of the replication of each column (or row) of `*this`
Example:
```
Vector3i v = [Vector3i::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the vector v:" << endl << v << endl;
cout << "v.rowwise().replicate(5) = ..." << endl;
cout << v.rowwise().replicate(5) << endl;
```
Output:
```
Here is the vector v:
7
-2
6
v.rowwise().replicate(5) = ...
7 7 7 7 7
-2 -2 -2 -2 -2
6 6 6 6 6
```
See also
[VectorwiseOp::replicate()](classeigen_1_1vectorwiseop#a5f0c8dc9e9c4aeaa2057f15800f5c18c), [DenseBase::replicate()](classeigen_1_1densebase#a60dadfe80b813d808e91e4521c722a8e), class [Replicate](classeigen_1_1replicate "Expression of the multiple replication of a matrix or vector.")
replicate() [2/2]
-----------------
template<typename ExpressionType , int Direction> template<int Factor>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Replicate](classeigen_1_1replicate)<ExpressionType,isVertical\*Factor+isHorizontal,isHorizontal\*Factor+isVertical> [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::replicate | ( | [Index](classeigen_1_1vectorwiseop#a4907c654e5810edd98e4162093b19532) | *factor* = `Factor` | ) | const |
| inline |
Returns
an expression of the replication of each column (or row) of `*this`
Example:
```
MatrixXi m = [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(2,3);
cout << "Here is the matrix m:" << endl << m << endl;
cout << "m.colwise().replicate<3>() = ..." << endl;
cout << m.colwise().replicate<3>() << endl;
```
Output:
```
Here is the matrix m:
7 6 9
-2 6 -6
m.colwise().replicate<3>() = ...
7 6 9
-2 6 -6
7 6 9
-2 6 -6
7 6 9
-2 6 -6
```
See also
VectorwiseOp::replicate(Index), [DenseBase::replicate()](classeigen_1_1densebase#a60dadfe80b813d808e91e4521c722a8e), class [Replicate](classeigen_1_1replicate "Expression of the multiple replication of a matrix or vector.")
reverse() [1/2]
---------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [ReverseReturnType](classeigen_1_1reverse) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::reverse | ( | | ) | |
| inline |
Returns
a writable matrix expression where each column (or row) are reversed.
See also
[reverse() const](classeigen_1_1vectorwiseop#aeb0428f561b5757e2c85e8d72022eec1)
reverse() [2/2]
---------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [ConstReverseReturnType](classeigen_1_1reverse) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::reverse | ( | | ) | const |
| inline |
Returns
a matrix expression where each column (or row) are reversed.
Example:
```
MatrixXi m = [MatrixXi::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)(3,4);
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the rowwise reverse of m:" << endl << m.rowwise().reverse() << endl;
cout << "Here is the colwise reverse of m:" << endl << m.colwise().reverse() << endl;
cout << "Here is the coefficient (1,0) in the rowise reverse of m:" << endl
<< m.rowwise().reverse()(1,0) << endl;
cout << "Let us overwrite this coefficient with the value 4." << endl;
//m.colwise().reverse()(1,0) = 4;
cout << "Now the matrix m is:" << endl << m << endl;
```
Output:
```
Here is the matrix m:
7 6 -3 1
-2 9 6 0
6 -6 -5 3
Here is the rowwise reverse of m:
1 -3 6 7
0 6 9 -2
3 -5 -6 6
Here is the colwise reverse of m:
6 -6 -5 3
-2 9 6 0
7 6 -3 1
Here is the coefficient (1,0) in the rowise reverse of m:
0
Let us overwrite this coefficient with the value 4.
Now the matrix m is:
7 6 -3 1
-2 9 6 0
6 -6 -5 3
```
See also
[DenseBase::reverse()](classeigen_1_1densebase#a38ea394036d8b096abf322469c80198f)
reverseInPlace()
----------------
template<typename ExpressionType , int Direction>
| | | |
| --- | --- | --- |
|
| |
| --- |
| void [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::reverseInPlace |
| inline |
This is the "in place" version of [VectorwiseOp::reverse](classeigen_1_1vectorwiseop#aeb0428f561b5757e2c85e8d72022eec1): it reverses each column or row of `*this`.
In most cases it is probably better to simply use the reversed expression of a matrix. However, when reversing the matrix data itself is really needed, then this "in-place" version is probably the right choice because it provides the following additional benefits:
* less error prone: doing the same operation with .[reverse()](classeigen_1_1vectorwiseop#ab8caf5367e2bd636536c8a0e0c89fe15) requires special care:
```
m = m.reverse().eval();
```
* this API enables reverse operations without the need for a temporary
See also
[DenseBase::reverseInPlace()](classeigen_1_1densebase#adb8045155ea45f7961fc2a5170e1d921), [reverse()](classeigen_1_1vectorwiseop#ab8caf5367e2bd636536c8a0e0c89fe15)
squaredNorm()
-------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [SquaredNormReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::squaredNorm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the squared norm of each column (or row) of the referenced expression. This is a vector with real entries, even if the original matrix has complex entries.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the square norm of each row:" << endl << m.rowwise().squaredNorm() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the square norm of each row:
0.928
1.01
0.884
```
See also
DenseBase::squaredNorm()
stableNorm()
------------
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [StableNormReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::stableNorm | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the norm of each column (or row) of the referenced expression, avoiding underflow and overflow. This is a vector with real entries, even if the original matrix has complex entries.
See also
DenseBase::stableNorm()
sum()
-----
template<typename ExpressionType , int Direction>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [SumReturnType](classeigen_1_1partialreduxexpr) [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::sum | ( | | ) | const |
| inline |
Returns
a row (or column) vector expression of the sum of each column (or row) of the referenced expression.
Example:
```
Matrix3d m = [Matrix3d::Random](classeigen_1_1densebase#ae814abb451b48ed872819192dc188c19)();
cout << "Here is the matrix m:" << endl << m << endl;
cout << "Here is the sum of each row:" << endl << m.rowwise().sum() << endl;
```
Output:
```
Here is the matrix m:
0.68 0.597 -0.33
-0.211 0.823 0.536
0.566 -0.605 -0.444
Here is the sum of each row:
0.948
1.15
-0.483
```
See also
[DenseBase::sum()](classeigen_1_1densebase#addd7080d5c202795820e361768d0140c)
const\_iterator
---------------
template<typename ExpressionType , int Direction>
| |
| --- |
| random\_access\_iterator\_type [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::const\_iterator |
This is the const version of iterator (aka read-only)
iterator
--------
template<typename ExpressionType , int Direction>
| |
| --- |
| random\_access\_iterator\_type [Eigen::VectorwiseOp](classeigen_1_1vectorwiseop)< ExpressionType, Direction >::iterator |
STL-like [RandomAccessIterator](https://en.cppreference.com/w/cpp/named_req/RandomAccessIterator) iterator type over the columns or rows as returned by the [begin()](classeigen_1_1vectorwiseop#abbf3325f535ac011ed61f14ed4330618) and [end()](classeigen_1_1vectorwiseop#a9d189042b8322e40772d41c63b9d3bb5) methods.
---
The documentation for this class was generated from the following files:* [VectorwiseOp.h](https://eigen.tuxfamily.org/dox/VectorwiseOp_8h_source.html)
* [Replicate.h](https://eigen.tuxfamily.org/dox/Replicate_8h_source.html)
* [Reverse.h](https://eigen.tuxfamily.org/dox/Reverse_8h_source.html)
* [Homogeneous.h](https://eigen.tuxfamily.org/dox/Homogeneous_8h_source.html)
* [OrthoMethods.h](https://eigen.tuxfamily.org/dox/OrthoMethods_8h_source.html)
| programming_docs |
eigen3 Eigen::SimplicialCholeskyBase Eigen::SimplicialCholeskyBase
=============================
### template<typename Derived> struct Eigen::SimplicialCholeskyBase< Derived >::keep\_diag
keeps off-diagonal entries; drops diagonal entries
---
The documentation for this struct was generated from the following file:* [SimplicialCholesky.h](https://eigen.tuxfamily.org/dox/SimplicialCholesky_8h_source.html)
eigen3 Eigen::SelfAdjointView Eigen::SelfAdjointView
======================
### template<typename \_MatrixType, unsigned int UpLo> class Eigen::SelfAdjointView< \_MatrixType, UpLo >
Expression of a selfadjoint matrix from a triangular part of a dense matrix.
Parameters
| | |
| --- | --- |
| MatrixType | the type of the dense matrix storing the coefficients |
| TriangularPart | can be either `[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)` or `[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)` |
This class is an expression of a sefladjoint matrix from a triangular part of a matrix with given dense storage of the coefficients. It is the return type of MatrixBase::selfadjointView() and most of the time this is the only way that it is used.
See also
class [TriangularBase](classeigen_1_1triangularbase "Base class for triangular part in a matrix."), MatrixBase::selfadjointView()
| |
| --- |
| |
| typedef [Matrix](classeigen_1_1matrix)< [RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1), internal::traits< MatrixType >::ColsAtCompileTime, 1 > | [EigenvaluesReturnType](classeigen_1_1selfadjointview#a8ae92703d920130b38a383f8b165146c) |
| |
| typedef [NumTraits](structeigen_1_1numtraits)< [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) >::Real | [RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1) |
| |
| typedef internal::traits< [SelfAdjointView](classeigen_1_1selfadjointview) >::[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) | [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) |
| | The type of coefficients in this matrix. |
| |
|
Public Types inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| typedef [Eigen::Index](namespaceeigen#a62e77e0933482dafde8fe197d9a2cfde) | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| | The interface type of indices. [More...](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) |
| |
| |
| --- |
| |
| const [AdjointReturnType](classeigen_1_1selfadjointview) | [adjoint](classeigen_1_1selfadjointview#a1297532e129be85c6a9ff0c8ef735301) () const |
| |
| [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) | [coeff](classeigen_1_1selfadjointview#aa4e1f964b34c76697bcdd3b54b84d814) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) const |
| |
| [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) & | [coeffRef](classeigen_1_1selfadjointview#ab5b030515fcee3d1d4f84c0d9d13bd67) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col) |
| |
| const [ConjugateReturnType](classeigen_1_1selfadjointview) | [conjugate](classeigen_1_1selfadjointview#a6671a26f31ead5f6e14d1d7d1221d9ec) () const |
| |
| template<bool Cond> |
| internal::conditional< Cond, [ConjugateReturnType](classeigen_1_1selfadjointview), [ConstSelfAdjointView](classeigen_1_1selfadjointview) >::type | [conjugateIf](classeigen_1_1selfadjointview#ad50f99f2a8d8d8bb8eb79733af5fcf9b) () const |
| |
| MatrixType::ConstDiagonalReturnType | [diagonal](classeigen_1_1selfadjointview#a9fcc7108054ac4a3b0ef758c794c16a7) () const |
| |
| [EigenvaluesReturnType](classeigen_1_1selfadjointview#a8ae92703d920130b38a383f8b165146c) | [eigenvalues](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f) () const |
| | Computes the eigenvalues of a matrix. [More...](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f) |
| |
| const [LDLT](classeigen_1_1ldlt)< PlainObject, UpLo > | [ldlt](classeigen_1_1selfadjointview#a644155eef17b37c95d85b9f65bb49ac4) () const |
| |
| const [LLT](classeigen_1_1llt)< PlainObject, UpLo > | [llt](classeigen_1_1selfadjointview#a405e810491642a7f7b785f2ad6f93619) () const |
| |
| template<typename OtherDerived > |
| const [Product](classeigen_1_1product)< [SelfAdjointView](classeigen_1_1selfadjointview), OtherDerived > | [operator\*](classeigen_1_1selfadjointview#af18c39fdc7783c63766ba22ce0e784c3) (const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > &rhs) const |
| |
| [RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1) | [operatorNorm](classeigen_1_1selfadjointview#a12a7da482e31ec9c517dca92dd7bae61) () const |
| | Computes the L2 operator norm. [More...](classeigen_1_1selfadjointview#a12a7da482e31ec9c517dca92dd7bae61) |
| |
| template<typename DerivedU , typename DerivedV > |
| [SelfAdjointView](classeigen_1_1selfadjointview) & | [rankUpdate](classeigen_1_1selfadjointview#a1b5afab9f9df919cba99d8ed1a260567) (const [MatrixBase](classeigen_1_1matrixbase)< DerivedU > &u, const [MatrixBase](classeigen_1_1matrixbase)< DerivedV > &v, const [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) &alpha=[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)(1)) |
| |
| template<typename DerivedU > |
| [SelfAdjointView](classeigen_1_1selfadjointview) & | [rankUpdate](classeigen_1_1selfadjointview#acc37b0437e0eee05f861bce511df9c77) (const [MatrixBase](classeigen_1_1matrixbase)< DerivedU > &u, const [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) &alpha=[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)(1)) |
| |
| [TransposeReturnType](classeigen_1_1selfadjointview) | [transpose](classeigen_1_1selfadjointview#ad779637ca3e3aeff2d35e1806e59f7d9) () |
| |
| const [ConstTransposeReturnType](classeigen_1_1selfadjointview) | [transpose](classeigen_1_1selfadjointview#a33950671584ae306fbdc1c23f010be88) () const |
| |
| template<unsigned int TriMode> |
| internal::conditional<(TriMode &([Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)))==(UpLo &([Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749))), [TriangularView](classeigen_1_1triangularview)< MatrixType, TriMode >, [TriangularView](classeigen_1_1triangularview)< typename MatrixType::AdjointReturnType, TriMode > >::type | [triangularView](classeigen_1_1selfadjointview#aacba330c3a12d1cac75c2f9df8c0f347) () const |
| |
|
Public Member Functions inherited from [Eigen::TriangularBase< SelfAdjointView< \_MatrixType, UpLo > >](classeigen_1_1triangularbase) |
| void | [copyCoeff](classeigen_1_1triangularbase#a0abe130a9130ac6df16f3c8c55490b43) ([Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) row, [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) col, Other &other) |
| |
| void | [evalTo](classeigen_1_1triangularbase#a604d4f76a376ced36f8b9c3374c76c3e) ([MatrixBase](classeigen_1_1matrixbase)< DenseDerived > &other) const |
| |
| void | [evalToLazy](classeigen_1_1triangularbase#ab8db3e55eee50cdc56650b3498e235eb) ([MatrixBase](classeigen_1_1matrixbase)< DenseDerived > &other) const |
| |
|
Public Member Functions inherited from [Eigen::EigenBase< Derived >](structeigen_1_1eigenbase) |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [cols](structeigen_1_1eigenbase#a2d768a9877f5f69f49432d447b552bfe) () const EIGEN\_NOEXCEPT |
| |
| Derived & | [derived](structeigen_1_1eigenbase#a1fbabe7f12bcbfba3b9a448b1f5e46fa) () |
| |
| const Derived & | [derived](structeigen_1_1eigenbase#afd4f3f1c57b7594b96a7e30f2974ea2e) () const |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [rows](structeigen_1_1eigenbase#ac22eb0695d00edd7d4a3b2d0a98b81c2) () const EIGEN\_NOEXCEPT |
| |
| EIGEN\_CONSTEXPR [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | [size](structeigen_1_1eigenbase#ae106171b6fefd3f7af108a8283de36c9) () const EIGEN\_NOEXCEPT |
| |
EigenvaluesReturnType
---------------------
template<typename \_MatrixType , unsigned int UpLo>
| |
| --- |
| typedef [Matrix](classeigen_1_1matrix)<[RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1), internal::traits<MatrixType>::ColsAtCompileTime, 1> [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::[EigenvaluesReturnType](classeigen_1_1selfadjointview#a8ae92703d920130b38a383f8b165146c) |
Return type of [eigenvalues()](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f "Computes the eigenvalues of a matrix.")
RealScalar
----------
template<typename \_MatrixType , unsigned int UpLo>
| |
| --- |
| typedef [NumTraits](structeigen_1_1numtraits)<[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)>::Real [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::[RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1) |
Real part of [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e "The type of coefficients in this matrix.")
adjoint()
---------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [AdjointReturnType](classeigen_1_1selfadjointview) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::adjoint | ( | | ) | const |
| inline |
See also
[MatrixBase::adjoint() const](classeigen_1_1matrixbase#afacca1f88da57e5cd87dd07c8ff926bb)
coeff()
-------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::coeff | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | const |
| inline |
See also
[MatrixBase::coeff()](classeigen_1_1densecoeffsbase_3_01derived_00_01readonlyaccessors_01_4#af51b00cc45490ad698239ab6a8b94393)
Warning
the coordinates must fit into the referenced triangular part
coeffRef()
----------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | |
| --- | --- | --- | --- |
| [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)& [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::coeffRef | ( | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *row*, |
| | | [Index](structeigen_1_1eigenbase#a554f30542cc2316add4b1ea0a492ff02) | *col* |
| | ) | | |
| inline |
See also
MatrixBase::coeffRef()
Warning
the coordinates must fit into the referenced triangular part
conjugate()
-----------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [ConjugateReturnType](classeigen_1_1selfadjointview) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::conjugate | ( | void | | ) | const |
| inline |
See also
MatrixBase::conjugate() const
conjugateIf()
-------------
template<typename \_MatrixType , unsigned int UpLo> template<bool Cond>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::conditional<Cond,[ConjugateReturnType](classeigen_1_1selfadjointview),[ConstSelfAdjointView](classeigen_1_1selfadjointview)>::type [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::conjugateIf | ( | | ) | const |
| inline |
Returns
an expression of the complex conjugate of `*this` if Cond==true, returns `*this` otherwise.
diagonal()
----------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| MatrixType::ConstDiagonalReturnType [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::diagonal | ( | | ) | const |
| inline |
Returns
a const expression of the main diagonal of the matrix `*this`
This method simply returns the diagonal of the nested expression, thus by-passing the [SelfAdjointView](classeigen_1_1selfadjointview "Expression of a selfadjoint matrix from a triangular part of a dense matrix.") decorator.
See also
[MatrixBase::diagonal()](classeigen_1_1matrixbase#ab5768147536273eb2dbdfa389cfd26a3), class [Diagonal](classeigen_1_1diagonal "Expression of a diagonal/subdiagonal/superdiagonal in a matrix.")
eigenvalues()
-------------
template<typename MatrixType , unsigned int UpLo>
| | | |
| --- | --- | --- |
|
| |
| --- |
| [SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::[EigenvaluesReturnType](classeigen_1_1selfadjointview#a8ae92703d920130b38a383f8b165146c) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::eigenvalues |
| inline |
Computes the eigenvalues of a matrix.
Returns
Column vector containing the eigenvalues.
This is defined in the Eigenvalues module.
```
#include <Eigen/Eigenvalues>
```
This function computes the eigenvalues with the help of the [SelfAdjointEigenSolver](classeigen_1_1selfadjointeigensolver "Computes eigenvalues and eigenvectors of selfadjoint matrices.") class. The eigenvalues are repeated according to their algebraic multiplicity, so there are as many eigenvalues as rows in the matrix.
Example:
```
MatrixXd ones = [MatrixXd::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(3,3);
VectorXd eivals = ones.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>().[eigenvalues](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f)();
cout << "The eigenvalues of the 3x3 matrix of ones are:" << endl << eivals << endl;
```
Output:
```
The eigenvalues of the 3x3 matrix of ones are:
-3.09e-16
0
3
```
See also
[SelfAdjointEigenSolver::eigenvalues()](classeigen_1_1selfadjointeigensolver#a3df8721abcc71132f7f02bf9dfe78e41 "Returns the eigenvalues of given matrix."), [MatrixBase::eigenvalues()](classeigen_1_1matrixbase#a30430fa3d5b4e74d312fd4f502ac984d "Computes the eigenvalues of a matrix.")
ldlt()
------
template<typename MatrixType , unsigned int UpLo>
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [LDLT](classeigen_1_1ldlt)< typename [SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::PlainObject, UpLo > [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::ldlt |
| inline |
This is defined in the Cholesky module.
```
#include <Eigen/Cholesky>
```
Returns
the Cholesky decomposition with full pivoting without square root of `*this`
See also
[MatrixBase::ldlt()](classeigen_1_1matrixbase#a0ecf058a0727a4cab8b42d79e95072e1)
llt()
-----
template<typename MatrixType , unsigned int UpLo>
| | | |
| --- | --- | --- |
|
| |
| --- |
| const [LLT](classeigen_1_1llt)< typename [SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::PlainObject, UpLo > [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::llt |
| inline |
This is defined in the Cholesky module.
```
#include <Eigen/Cholesky>
```
Returns
the [LLT](classeigen_1_1llt "Standard Cholesky decomposition (LL^T) of a matrix and associated features.") decomposition of `*this`
See also
[SelfAdjointView::llt()](classeigen_1_1selfadjointview#a405e810491642a7f7b785f2ad6f93619)
operator\*()
------------
template<typename \_MatrixType , unsigned int UpLo> template<typename OtherDerived >
| | | | | | | | |
| --- | --- | --- | --- | --- | --- | --- | --- |
|
| | | | | | |
| --- | --- | --- | --- | --- | --- |
| const [Product](classeigen_1_1product)<[SelfAdjointView](classeigen_1_1selfadjointview),OtherDerived> [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::operator\* | ( | const [MatrixBase](classeigen_1_1matrixbase)< OtherDerived > & | *rhs* | ) | const |
| inline |
Efficient triangular matrix times vector/matrix product
operatorNorm()
--------------
template<typename MatrixType , unsigned int UpLo>
| | | |
| --- | --- | --- |
|
| |
| --- |
| [SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::[RealScalar](classeigen_1_1selfadjointview#af9f0234ebeae4c4ca512bcd5fb5e8bb1) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< MatrixType, UpLo >::operatorNorm |
| inline |
Computes the L2 operator norm.
Returns
Operator norm of the matrix.
This is defined in the Eigenvalues module.
```
#include <Eigen/Eigenvalues>
```
This function computes the L2 operator norm of a self-adjoint matrix. For a self-adjoint matrix, the operator norm is the largest eigenvalue.
The current implementation uses the eigenvalues of the matrix, as computed by [eigenvalues()](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f "Computes the eigenvalues of a matrix."), to compute the operator norm of the matrix.
Example:
```
MatrixXd ones = [MatrixXd::Ones](classeigen_1_1densebase#a2755cb4023f7376880523626a8e05101)(3,3);
cout << "The operator norm of the 3x3 matrix of ones is "
<< ones.selfadjointView<[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)>().[operatorNorm](classeigen_1_1selfadjointview#a12a7da482e31ec9c517dca92dd7bae61)() << endl;
```
Output:
```
The operator norm of the 3x3 matrix of ones is 3
```
See also
[eigenvalues()](classeigen_1_1selfadjointview#ad4f34424b4ea12de9bbc5623cb938b4f "Computes the eigenvalues of a matrix."), [MatrixBase::operatorNorm()](classeigen_1_1matrixbase#a0ff9bc0b9bea2d0822a2bf3192783102 "Computes the L2 operator norm.")
rankUpdate() [1/2]
------------------
template<typename \_MatrixType , unsigned int UpLo> template<typename DerivedU , typename DerivedV >
| | | | |
| --- | --- | --- | --- |
| [SelfAdjointView](classeigen_1_1selfadjointview)& [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::rankUpdate | ( | const [MatrixBase](classeigen_1_1matrixbase)< DerivedU > & | *u*, |
| | | const [MatrixBase](classeigen_1_1matrixbase)< DerivedV > & | *v*, |
| | | const [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) & | *alpha* = `[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)(1)` |
| | ) | | |
Perform a symmetric rank 2 update of the selfadjoint matrix `*this`: \( this = this + \alpha u v^\* + conj(\alpha) v u^\* \)
Returns
a reference to `*this`
The vectors *u* and `v` **must** be column vectors, however they can be a adjoint expression without any overhead. Only the meaningful triangular part of the matrix is updated, the rest is left unchanged.
See also
rankUpdate(const MatrixBase<DerivedU>&, Scalar)
rankUpdate() [2/2]
------------------
template<typename \_MatrixType , unsigned int UpLo> template<typename DerivedU >
| | | | |
| --- | --- | --- | --- |
| [SelfAdjointView](classeigen_1_1selfadjointview)& [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::rankUpdate | ( | const [MatrixBase](classeigen_1_1matrixbase)< DerivedU > & | *u*, |
| | | const [Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e) & | *alpha* = `[Scalar](classeigen_1_1selfadjointview#af52acc0942ece2de9b6db4a99cc6656e)(1)` |
| | ) | | |
Perform a symmetric rank K update of the selfadjoint matrix `*this`: \( this = this + \alpha ( u u^\* ) \) where *u* is a vector or matrix.
Returns
a reference to `*this`
Note that to perform \( this = this + \alpha ( u^\* u ) \) you can simply call this function with u.adjoint().
See also
rankUpdate(const MatrixBase<DerivedU>&, const MatrixBase<DerivedV>&, Scalar)
transpose() [1/2]
-----------------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| [TransposeReturnType](classeigen_1_1selfadjointview) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::transpose | ( | | ) | |
| inline |
See also
[MatrixBase::transpose()](classeigen_1_1densebase#ac8952c19644a4ac7e41bea45c19b909c)
transpose() [2/2]
-----------------
template<typename \_MatrixType , unsigned int UpLo>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| const [ConstTransposeReturnType](classeigen_1_1selfadjointview) [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::transpose | ( | | ) | const |
| inline |
See also
[MatrixBase::transpose() const](classeigen_1_1densebase#a38c0b074cf93fc194bf91141287cee3f)
triangularView()
----------------
template<typename \_MatrixType , unsigned int UpLo> template<unsigned int TriMode>
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
|
| | | | | |
| --- | --- | --- | --- | --- |
| internal::conditional<(TriMode&([Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)))==(UpLo&([Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)|[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749))), [TriangularView](classeigen_1_1triangularview)<MatrixType,TriMode>, [TriangularView](classeigen_1_1triangularview)<typename MatrixType::AdjointReturnType,TriMode> >::type [Eigen::SelfAdjointView](classeigen_1_1selfadjointview)< \_MatrixType, UpLo >::triangularView | ( | | ) | const |
| inline |
Returns
an expression of a triangular view extracted from the current selfadjoint view of a given triangular part
The parameter *TriMode* can have the following values: `[Upper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdafca2ccebb604f171656deb53e8c083c1)`, `[StrictlyUpper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cda7b37877e0b9b0df28c9c2b669a633265)`, `[UnitUpper](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdadd28224d7ea92689930be73c1b50b0ad)`, `[Lower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cdaf581029282d421eee5aae14238c6f749)`, `[StrictlyLower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cda2424988b6fca98be70b595632753ba81)`, `[UnitLower](group__enums#gga39e3366ff5554d731e7dc8bb642f83cda8f40b928c10a71ba03e5f75ad2a72fda)`.
If `TriMode` references the same triangular part than `*this`, then this method simply return a `[TriangularView](classeigen_1_1triangularview "Expression of a triangular part in a matrix.")` of the nested expression, otherwise, the nested expression is first transposed, thus returning a `[TriangularView](classeigen_1_1triangularview "Expression of a triangular part in a matrix.")<Transpose<MatrixType>>` object.
See also
MatrixBase::triangularView(), class [TriangularView](classeigen_1_1triangularview "Expression of a triangular part in a matrix.")
---
The documentation for this class was generated from the following files:* [SelfAdjointView.h](https://eigen.tuxfamily.org/dox/SelfAdjointView_8h_source.html)
* [LDLT.h](https://eigen.tuxfamily.org/dox/LDLT_8h_source.html)
* [LLT.h](https://eigen.tuxfamily.org/dox/LLT_8h_source.html)
* [MatrixBaseEigenvalues.h](https://eigen.tuxfamily.org/dox/MatrixBaseEigenvalues_8h_source.html)
| programming_docs |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.