code
stringlengths 2.5k
150k
| kind
stringclasses 1
value |
---|---|
nim dynlib dynlib
======
This module implements the ability to access symbols from shared libraries. On POSIX this uses the `dlsym` mechanism, on Windows `LoadLibrary`.
Examples
--------
### Loading a simple C function
The following example demonstrates loading a function called 'greet' from a library that is determined at runtime based upon a language choice. If the library fails to load or the function 'greet' is not found, it quits with a failure error code.
```
import dynlib
type
greetFunction = proc(): cstring {.gcsafe, stdcall.}
let lang = stdin.readLine()
let lib = case lang
of "french":
loadLib("french.dll")
else:
loadLib("english.dll")
if lib == nil:
echo "Error loading library"
quit(QuitFailure)
let greet = cast[greetFunction](lib.symAddr("greet"))
if greet == nil:
echo "Error loading 'greet' function from library"
quit(QuitFailure)
let greeting = greet()
echo greeting
unloadLib(lib)
```
Imports
-------
<strutils>, <posix> Types
-----
```
LibHandle = pointer
```
a handle to a dynamically loaded library [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L60) Procs
-----
```
proc raiseInvalidLibrary(name: cstring) {...}{.noinline, noreturn,
raises: [LibraryError], tags: [].}
```
raises an `EInvalidLibrary` exception. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L73) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L73)
```
proc checkedSymAddr(lib: LibHandle; name: cstring): pointer {...}{.
raises: [Exception, LibraryError], tags: [RootEffect].}
```
retrieves the address of a procedure/variable from `lib`. Raises `EInvalidLibrary` if the symbol could not be found. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L81)
```
proc libCandidates(s: string; dest: var seq[string]) {...}{.raises: [], tags: [].}
```
given a library name pattern `s` write possible library names to `dest`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L87)
```
proc loadLibPattern(pattern: string; globalSymbols = false): LibHandle {...}{.
raises: [Exception], tags: [RootEffect].}
```
loads a library with name matching `pattern`, similar to what `dynlib` pragma does. Returns nil if the library could not be loaded. Warning: this proc uses the GC and so cannot be used to load the GC. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L99)
```
proc loadLib(path: string; globalSymbols = false): LibHandle {...}{.gcsafe,
raises: [], tags: [].}
```
loads a library from `path`. Returns nil if the library could not be loaded. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L120)
```
proc loadLib(): LibHandle {...}{.gcsafe, raises: [], tags: [].}
```
gets the handle from the current executable. Returns nil if the library could not be loaded. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L127)
```
proc unloadLib(lib: LibHandle) {...}{.gcsafe, raises: [], tags: [].}
```
unloads the library `lib` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L128)
```
proc symAddr(lib: LibHandle; name: cstring): pointer {...}{.gcsafe, raises: [],
tags: [].}
```
retrieves the address of a procedure/variable from `lib`. Returns nil if the symbol could not be found. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/dynlib.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/dynlib.nim#L129)
nim options options
=======
This module implements types which encapsulate an optional value.
A value of type `Option[T]` either contains a value `x` (represented as `some(x)`) or is empty (`none(T)`).
This can be useful when you have a value that can be present or not. The absence of a value is often represented by `nil`, but it is not always available, nor is it always a good solution.
Basic usage
-----------
Let's start with an example: a procedure that finds the index of a character in a string.
```
import options
proc find(haystack: string, needle: char): Option[int] =
for i, c in haystack:
if c == needle:
return some(i)
return none(int) # This line is actually optional,
# because the default is empty
```
```
let found = "abc".find('c')
assert found.isSome and found.get() == 2
```
The `get` operation demonstrated above returns the underlying value, or raises `UnpackDefect` if there is no value. Note that `UnpackDefect` inherits from `system.Defect`, and should therefore never be caught. Instead, rely on checking if the option contains a value with [isSome](#isSome,Option%5BT%5D) and [isNone](#isNone,Option%5BT%5D) procs.
How to deal with an absence of a value:
```
let result = "team".find('i')
# Nothing was found, so the result is `none`.
assert(result == none(int))
# It has no value:
assert(result.isNone)
```
Imports
-------
<typetraits> Types
-----
```
Option[T] = object
when T is SomePointer:
val
else:
val
has
```
An optional type that stores its value and state separately in a boolean. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L69)
```
UnpackDefect = object of Defect
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L77)
```
UnpackError {...}{.deprecated: "See corresponding Defect".} = UnpackDefect
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L78) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L78) Procs
-----
```
proc option[T](val: T): Option[T] {...}{.inline.}
```
Can be used to convert a pointer type (`ptr` or `ref` or `proc`) to an option type. It converts `nil` to `None`.
See also:
* [some](#some,T)
* [none](#none,typedesc)
**Example:**
```
type
Foo = ref object
a: int
b: string
var c: Foo
assert c.isNil
var d = option(c)
assert d.isNone
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L80) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L80)
```
proc some[T](val: T): Option[T] {...}{.inline.}
```
Returns an `Option` that has the value `val`.
See also:
* [option](#option,T)
* [none](#none,typedesc)
* [isSome](#isSome,Option%5BT%5D)
**Example:**
```
var
a = some("abc")
b = some(42)
assert $type(a) == "Option[system.string]"
assert b.isSome
assert a.get == "abc"
assert $b == "Some(42)"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L101)
```
proc none(T: typedesc): Option[T] {...}{.inline.}
```
Returns an `Option` for this type that has no value.
See also:
* [option](#option,T)
* [some](#some,T)
* [isNone](#isNone,Option%5BT%5D)
**Example:**
```
var a = none(int)
assert a.isNone
assert $type(a) == "Option[system.int]"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L124)
```
proc none[T](): Option[T] {...}{.inline.}
```
Alias for [none(T) proc](#none,typedesc). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L139) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L139)
```
proc isSome[T](self: Option[T]): bool {...}{.inline.}
```
Checks if an `Option` contains a value. **Example:**
```
var
a = some(42)
b = none(string)
assert a.isSome
assert not b.isSome
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L143)
```
proc isNone[T](self: Option[T]): bool {...}{.inline.}
```
Checks if an `Option` is empty. **Example:**
```
var
a = some(42)
b = none(string)
assert not a.isNone
assert b.isNone
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L157) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L157)
```
proc get[T](self: Option[T]): lent T {...}{.inline.}
```
Returns contents of an `Option`. If it is `None`, then an exception is thrown.
See also:
* [get proc](#get,Option%5BT%5D,T) with the default return value
**Example:**
```
let
a = some(42)
b = none(string)
assert a.get == 42
doAssertRaises(UnpackDefect):
echo b.get
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L170)
```
proc get[T](self: Option[T]; otherwise: T): T {...}{.inline.}
```
Returns the contents of the `Option` or an `otherwise` value if the `Option` is `None`. **Example:**
```
var
a = some(42)
b = none(int)
assert a.get(9999) == 42
assert b.get(9999) == 9999
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L188) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L188)
```
proc get[T](self: var Option[T]): var T {...}{.inline.}
```
Returns contents of the `var Option`. If it is `None`, then an exception is thrown. **Example:**
```
let
a = some(42)
b = none(string)
assert a.get == 42
doAssertRaises(UnpackDefect):
echo b.get
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L203)
```
proc map[T](self: Option[T]; callback: proc (input: T)) {...}{.inline.}
```
Applies a `callback` function to the value of the `Option`, if it has one.
See also:
* [map proc](#map,Option%5BT%5D,proc(T)_2) for a version with a callback which returns a value
* [filter proc](#filter,Option%5BT%5D,proc(T))
**Example:**
```
var d = 0
proc saveDouble(x: int) =
d = 2*x
let
a = some(42)
b = none(int)
b.map(saveDouble)
assert d == 0
a.map(saveDouble)
assert d == 84
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L218) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L218)
```
proc map[T, R](self: Option[T]; callback: proc (input: T): R): Option[R] {...}{.
inline.}
```
Applies a `callback` function to the value of the `Option` and returns an `Option` containing the new value.
If the `Option` is `None`, `None` of the return type of the `callback` will be returned.
See also:
* [flatMap proc](#flatMap,Option%5BA%5D,proc(A)) for a version with a callback which returns an `Option`
* [filter proc](#filter,Option%5BT%5D,proc(T))
**Example:**
```
var
a = some(42)
b = none(int)
proc isEven(x: int): bool =
x mod 2 == 0
assert $(a.map(isEven)) == "Some(true)"
assert $(b.map(isEven)) == "None[bool]"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L242)
```
proc flatten[A](self: Option[Option[A]]): Option[A] {...}{.inline.}
```
Remove one level of structure in a nested `Option`. **Example:**
```
let a = some(some(42))
assert $flatten(a) == "Some(42)"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L269)
```
proc flatMap[A, B](self: Option[A]; callback: proc (input: A): Option[B]): Option[
B] {...}{.inline.}
```
Applies a `callback` function to the value of the `Option` and returns an `Option` containing the new value.
If the `Option` is `None`, `None` of the return type of the `callback` will be returned.
Similar to `map`, with the difference that the `callback` returns an `Option`, not a raw value. This allows multiple procs with a signature of `A -> Option[B]` to be chained together.
See also:
* [flatten proc](#flatten,Option%5BOption%5BA%5D%5D)
* [filter proc](#filter,Option%5BT%5D,proc(T))
**Example:**
```
proc doublePositives(x: int): Option[int] =
if x > 0:
return some(2*x)
else:
return none(int)
let
a = some(42)
b = none(int)
c = some(-11)
assert a.flatMap(doublePositives) == some(84)
assert b.flatMap(doublePositives) == none(int)
assert c.flatMap(doublePositives) == none(int)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L280)
```
proc filter[T](self: Option[T]; callback: proc (input: T): bool): Option[T] {...}{.
inline.}
```
Applies a `callback` to the value of the `Option`.
If the `callback` returns `true`, the option is returned as `Some`. If it returns `false`, it is returned as `None`.
See also:
* [map proc](#map,Option%5BT%5D,proc(T)_2)
* [flatMap proc](#flatMap,Option%5BA%5D,proc(A))
**Example:**
```
proc isEven(x: int): bool =
x mod 2 == 0
let
a = some(42)
b = none(int)
c = some(-11)
assert a.filter(isEven) == some(42)
assert b.filter(isEven) == none(int)
assert c.filter(isEven) == none(int)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L311) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L311)
```
proc `==`(a, b: Option): bool {...}{.inline.}
```
Returns `true` if both `Option`s are `None`, or if they are both `Some` and have equal values. **Example:**
```
let
a = some(42)
b = none(int)
c = some(42)
d = none(int)
assert a == c
assert b == d
assert not (a == b)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L336) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L336)
```
proc `$`[T](self: Option[T]): string
```
Get the string representation of the `Option`.
If the `Option` has a value, the result will be `Some(x)` where `x` is the string representation of the contained value. If the `Option` does not have a value, the result will be `None[T]` where `T` is the name of the type contained in the `Option`.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L352) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L352)
```
proc unsafeGet[T](self: Option[T]): lent T {...}{.inline.}
```
Returns the value of a `some`. Behavior is undefined for `none`.
**Note:** Use it only when you are **absolutely sure** the value is present (e.g. after checking [isSome](#isSome,Option%5BT%5D)). Generally, using [get proc](#get,Option%5BT%5D) is preferred.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/options.nim#L366) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/options.nim#L366)
nim pcre pcre
====
Types
-----
```
Pcre = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L257) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L257)
```
Pcre16 = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L258) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L258)
```
Pcre32 = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L259) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L259)
```
JitStack = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L260)
```
JitStack16 = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L261) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L261)
```
JitStack32 = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L262) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L262)
```
ExtraData = object
flags*: clong ## Bits for which fields are set
study_data*: pointer ## Opaque data from pcre_study()
match_limit*: clong ## Maximum number of calls to match()
callout_data*: pointer ## Data passed back in callouts
tables*: pointer ## Pointer to character tables
match_limit_recursion*: clong ## Max recursive calls to match()
mark*: pointer ## For passing back a mark pointer
executable_jit*: pointer ## Contains a pointer to a compiled jit code
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L271) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L271)
```
CalloutBlock = object
version*: cint ## Identifies version of block
callout_number*: cint ## Number compiled into pattern
offset_vector*: ptr cint ## The offset vector
subject*: cstring ## The subject being matched
subject_length*: cint ## The length of the subject
start_match*: cint ## Offset to start of this match attempt
current_position*: cint ## Where we currently are in the subject
capture_top*: cint ## Max current capture
capture_last*: cint ## Most recently closed capture
callout_data*: pointer ## Data passed in with the call
pattern_position*: cint ## Offset to next item in the pattern
next_item_length*: cint ## Length of next item in the pattern
mark*: pointer ## Pointer to current mark or NULL
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L286) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L286)
```
JitCallback = proc (a: pointer): ptr JitStack {...}{.cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L310) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L310)
```
PPcre {...}{.deprecated.} = ptr Pcre
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L472) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L472)
```
PJitStack {...}{.deprecated.} = ptr JitStack
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L473) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L473) Consts
------
```
PCRE_MAJOR = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L13)
```
PCRE_MINOR = 36
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L14)
```
PCRE_PRERELEASE = true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L15)
```
PCRE_DATE = "2014-09-26"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L16)
```
CASELESS = 0x00000001
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L57)
```
MULTILINE = 0x00000002
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L58)
```
DOTALL = 0x00000004
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L59) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L59)
```
EXTENDED = 0x00000008
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L60)
```
ANCHORED = 0x00000010
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L61)
```
DOLLAR_ENDONLY = 0x00000020
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L62)
```
EXTRA = 0x00000040
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L63) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L63)
```
NOTBOL = 0x00000080
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L64)
```
NOTEOL = 0x00000100
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L65)
```
UNGREEDY = 0x00000200
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L66)
```
NOTEMPTY = 0x00000400
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L67)
```
UTF8 = 0x00000800
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L68) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L68)
```
UTF16 = 0x00000800
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L69)
```
UTF32 = 0x00000800
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L70)
```
NO_AUTO_CAPTURE = 0x00001000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L71) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L71)
```
NO_UTF8_CHECK = 0x00002000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L72)
```
NO_UTF16_CHECK = 0x00002000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L73) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L73)
```
NO_UTF32_CHECK = 0x00002000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L74)
```
AUTO_CALLOUT = 0x00004000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L75)
```
PARTIAL_SOFT = 0x00008000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L76)
```
PARTIAL = 0x00008000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L77)
```
NEVER_UTF = 0x00010000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L81)
```
DFA_SHORTEST = 0x00010000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L82)
```
NO_AUTO_POSSESS = 0x00020000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L86)
```
DFA_RESTART = 0x00020000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L87)
```
FIRSTLINE = 0x00040000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L90)
```
DUPNAMES = 0x00080000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L91)
```
NEWLINE_CR = 0x00100000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L92) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L92)
```
NEWLINE_LF = 0x00200000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L93) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L93)
```
NEWLINE_CRLF = 0x00300000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L94)
```
NEWLINE_ANY = 0x00400000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L95) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L95)
```
NEWLINE_ANYCRLF = 0x00500000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L96)
```
BSR_ANYCRLF = 0x00800000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L97)
```
BSR_UNICODE = 0x01000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L98) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L98)
```
JAVASCRIPT_COMPAT = 0x02000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L99)
```
NO_START_OPTIMIZE = 0x04000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L100) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L100)
```
NO_START_OPTIMISE = 0x04000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L101)
```
PARTIAL_HARD = 0x08000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L102)
```
NOTEMPTY_ATSTART = 0x10000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L103)
```
UCP = 0x20000000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L104)
```
ERROR_NOMATCH = -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L108)
```
ERROR_NULL = -2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L109)
```
ERROR_BADOPTION = -3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L110)
```
ERROR_BADMAGIC = -4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L111)
```
ERROR_UNKNOWN_OPCODE = -5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L112)
```
ERROR_UNKNOWN_NODE = -5
```
For backward compatibility [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L113) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L113)
```
ERROR_NOMEMORY = -6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L114)
```
ERROR_NOSUBSTRING = -7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L115) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L115)
```
ERROR_MATCHLIMIT = -8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L116)
```
ERROR_CALLOUT = -9
```
Never used by PCRE itself [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L117)
```
ERROR_BADUTF8 = -10
```
Same for 8/16/32 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L118) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L118)
```
ERROR_BADUTF16 = -10
```
Same for 8/16/32 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L119) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L119)
```
ERROR_BADUTF32 = -10
```
Same for 8/16/32 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L120)
```
ERROR_BADUTF8_OFFSET = -11
```
Same for 8/16 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L121)
```
ERROR_BADUTF16_OFFSET = -11
```
Same for 8/16 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L122)
```
ERROR_PARTIAL = -12
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L123) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L123)
```
ERROR_BADPARTIAL = -13
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L124)
```
ERROR_INTERNAL = -14
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L125) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L125)
```
ERROR_BADCOUNT = -15
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L126) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L126)
```
ERROR_DFA_UITEM = -16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L127)
```
ERROR_DFA_UCOND = -17
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L128)
```
ERROR_DFA_UMLIMIT = -18
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L129)
```
ERROR_DFA_WSSIZE = -19
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L130)
```
ERROR_DFA_RECURSE = -20
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L131)
```
ERROR_RECURSIONLIMIT = -21
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L132)
```
ERROR_NULLWSLIMIT = -22
```
No longer actually used [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L133)
```
ERROR_BADNEWLINE = -23
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L134)
```
ERROR_BADOFFSET = -24
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L135) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L135)
```
ERROR_SHORTUTF8 = -25
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L136) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L136)
```
ERROR_SHORTUTF16 = -25
```
Same for 8/16 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L137) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L137)
```
ERROR_RECURSELOOP = -26
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L138)
```
ERROR_JIT_STACKLIMIT = -27
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L139) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L139)
```
ERROR_BADMODE = -28
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L140)
```
ERROR_BADENDIANNESS = -29
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L141)
```
ERROR_DFA_BADRESTART = -30
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L142) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L142)
```
ERROR_JIT_BADOPTION = -31
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L143)
```
ERROR_BADLENGTH = -32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L144) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L144)
```
ERROR_UNSET = -33
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L145) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L145)
```
UTF8_ERR0 = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L149)
```
UTF8_ERR1 = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L150)
```
UTF8_ERR2 = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L151) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L151)
```
UTF8_ERR3 = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L152)
```
UTF8_ERR4 = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L153)
```
UTF8_ERR5 = 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L154)
```
UTF8_ERR6 = 6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L155) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L155)
```
UTF8_ERR7 = 7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L156) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L156)
```
UTF8_ERR8 = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L157) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L157)
```
UTF8_ERR9 = 9
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L158) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L158)
```
UTF8_ERR10 = 10
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L159)
```
UTF8_ERR11 = 11
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L160)
```
UTF8_ERR12 = 12
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L161) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L161)
```
UTF8_ERR13 = 13
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L162)
```
UTF8_ERR14 = 14
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L163)
```
UTF8_ERR15 = 15
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L164)
```
UTF8_ERR16 = 16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L165) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L165)
```
UTF8_ERR17 = 17
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L166)
```
UTF8_ERR18 = 18
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L167)
```
UTF8_ERR19 = 19
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L168)
```
UTF8_ERR20 = 20
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L169)
```
UTF8_ERR21 = 21
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L170)
```
UTF8_ERR22 = 22
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L171) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L171)
```
UTF16_ERR0 = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L175)
```
UTF16_ERR1 = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L176)
```
UTF16_ERR2 = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L177)
```
UTF16_ERR3 = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L178) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L178)
```
UTF16_ERR4 = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L179) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L179)
```
UTF32_ERR0 = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L183) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L183)
```
UTF32_ERR1 = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L184) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L184)
```
UTF32_ERR2 = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L185) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L185)
```
UTF32_ERR3 = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L186)
```
INFO_OPTIONS = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L190)
```
INFO_SIZE = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L191)
```
INFO_CAPTURECOUNT = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L192)
```
INFO_BACKREFMAX = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L193) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L193)
```
INFO_FIRSTBYTE = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L194) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L194)
```
INFO_FIRSTCHAR = 4
```
For backwards compatibility [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L195)
```
INFO_FIRSTTABLE = 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L196) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L196)
```
INFO_LASTLITERAL = 6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L197) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L197)
```
INFO_NAMEENTRYSIZE = 7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L198) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L198)
```
INFO_NAMECOUNT = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L199)
```
INFO_NAMETABLE = 9
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L200) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L200)
```
INFO_STUDYSIZE = 10
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L201)
```
INFO_DEFAULT_TABLES = 11
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L202)
```
INFO_OKPARTIAL = 12
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L203)
```
INFO_JCHANGED = 13
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L204)
```
INFO_HASCRORLF = 14
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L205) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L205)
```
INFO_MINLENGTH = 15
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L206)
```
INFO_JIT = 16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L207) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L207)
```
INFO_JITSIZE = 17
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L208) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L208)
```
INFO_MAXLOOKBEHIND = 18
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L209) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L209)
```
INFO_FIRSTCHARACTER = 19
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L210) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L210)
```
INFO_FIRSTCHARACTERFLAGS = 20
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L211)
```
INFO_REQUIREDCHAR = 21
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L212)
```
INFO_REQUIREDCHARFLAGS = 22
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L213) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L213)
```
INFO_MATCHLIMIT = 23
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L214)
```
INFO_RECURSIONLIMIT = 24
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L215)
```
INFO_MATCH_EMPTY = 25
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L216) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L216)
```
CONFIG_UTF8 = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L221) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L221)
```
CONFIG_NEWLINE = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L222) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L222)
```
CONFIG_LINK_SIZE = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L223) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L223)
```
CONFIG_POSIX_MALLOC_THRESHOLD = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L224)
```
CONFIG_MATCH_LIMIT = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L225) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L225)
```
CONFIG_STACKRECURSE = 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L226) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L226)
```
CONFIG_UNICODE_PROPERTIES = 6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L227) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L227)
```
CONFIG_MATCH_LIMIT_RECURSION = 7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L228) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L228)
```
CONFIG_BSR = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L229)
```
CONFIG_JIT = 9
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L230) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L230)
```
CONFIG_UTF16 = 10
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L231) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L231)
```
CONFIG_JITTARGET = 11
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L232)
```
CONFIG_UTF32 = 12
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L233) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L233)
```
CONFIG_PARENS_LIMIT = 13
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L234) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L234)
```
STUDY_JIT_COMPILE = 0x00000001
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L239) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L239)
```
STUDY_JIT_PARTIAL_SOFT_COMPILE = 0x00000002
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L240)
```
STUDY_JIT_PARTIAL_HARD_COMPILE = 0x00000004
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L241) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L241)
```
STUDY_EXTRA_NEEDED = 0x00000008
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L242)
```
EXTRA_STUDY_DATA = 0x00000001
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L247)
```
EXTRA_MATCH_LIMIT = 0x00000002
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L248) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L248)
```
EXTRA_CALLOUT_DATA = 0x00000004
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L249) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L249)
```
EXTRA_TABLES = 0x00000008
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L250) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L250)
```
EXTRA_MATCH_LIMIT_RECURSION = 0x00000010
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L251) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L251)
```
EXTRA_MARK = 0x00000020
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L252)
```
EXTRA_EXECUTABLE_JIT = 0x00000040
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L253) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L253) Procs
-----
```
proc compile(pattern: cstring; options: cint; errptr: ptr cstring;
erroffset: ptr cint; tableptr: pointer): ptr Pcre {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L333) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L333)
```
proc compile2(pattern: cstring; options: cint; errorcodeptr: ptr cint;
errptr: ptr cstring; erroffset: ptr cint; tableptr: pointer): ptr Pcre {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L339) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L339)
```
proc config(what: cint; where: pointer): cint {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L346) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L346)
```
proc copy_named_substring(code: ptr Pcre; subject: cstring; ovector: ptr cint;
stringcount: cint; stringname: cstring;
buffer: cstring; buffersize: cint): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L349) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L349)
```
proc copy_substring(subject: cstring; ovector: ptr cint; stringcount: cint;
stringnumber: cint; buffer: cstring; buffersize: cint): cint {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L357) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L357)
```
proc dfa_exec(code: ptr Pcre; extra: ptr ExtraData; subject: cstring;
length: cint; startoffset: cint; options: cint; ovector: ptr cint;
ovecsize: cint; workspace: ptr cint; wscount: cint): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L364) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L364)
```
proc exec(code: ptr Pcre; extra: ptr ExtraData; subject: cstring; length: cint;
startoffset: cint; options: cint; ovector: ptr cint; ovecsize: cint): cint {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L375) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L375)
```
proc jit_exec(code: ptr Pcre; extra: ptr ExtraData; subject: cstring;
length: cint; startoffset: cint; options: cint; ovector: ptr cint;
ovecsize: cint; jstack: ptr JitStack): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L384) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L384)
```
proc free_substring(stringptr: cstring) {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L394) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L394)
```
proc free_substring_list(stringptr: cstringArray) {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L396) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L396)
```
proc fullinfo(code: ptr Pcre; extra: ptr ExtraData; what: cint; where: pointer): cint {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L398) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L398)
```
proc get_named_substring(code: ptr Pcre; subject: cstring; ovector: ptr cint;
stringcount: cint; stringname: cstring;
stringptr: cstringArray): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L403) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L403)
```
proc get_stringnumber(code: ptr Pcre; name: cstring): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L410) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L410)
```
proc get_stringtable_entries(code: ptr Pcre; name: cstring; first: cstringArray;
last: cstringArray): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L413) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L413)
```
proc get_substring(subject: cstring; ovector: ptr cint; stringcount: cint;
stringnumber: cint; stringptr: cstringArray): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L418) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L418)
```
proc get_substring_list(subject: cstring; ovector: ptr cint; stringcount: cint;
listptr: ptr cstringArray): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L424) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L424)
```
proc maketables(): pointer {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L429) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L429)
```
proc refcount(code: ptr Pcre; adjust: cint): cint {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L431) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L431)
```
proc study(code: ptr Pcre; options: cint; errptr: ptr cstring): ptr ExtraData {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L434) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L434)
```
proc free_study(extra: ptr ExtraData) {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L438) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L438)
```
proc version(): cstring {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L440) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L440)
```
proc pattern_to_host_byte_order(code: ptr Pcre; extra: ptr ExtraData;
tables: pointer): cint {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L444)
```
proc jit_stack_alloc(startsize: cint; maxsize: cint): ptr JitStack {...}{.cdecl,
importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L450) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L450)
```
proc jit_stack_free(stack: ptr JitStack) {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L453) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L453)
```
proc assign_jit_stack(extra: ptr ExtraData; callback: JitCallback; data: pointer) {...}{.
cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L455) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L455)
```
proc jit_free_unused_memory() {...}{.cdecl, importc: "pcre_$1".}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L459) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L459)
```
proc study(code: ptr Pcre; options: cint; errptr: var cstring): ptr ExtraData {...}{.
deprecated, cdecl, importc: "pcre_$1".}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/pcre.nim#L463) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/pcre.nim#L463)
| programming_docs |
nim strutils strutils
========
The system module defines several common functions for working with strings, such as:
* `$` for converting other data-types to strings
* `&` for string concatenation
* `add` for adding a new character or a string to the existing one
* `in` (alias for `contains`) and `notin` for checking if a character is in a string
This module builds upon that, providing additional functionality in form of procedures, iterators and templates for strings.
```
import strutils
let
numbers = @[867, 5309]
multiLineString = "first line\nsecond line\nthird line"
let jenny = numbers.join("-")
assert jenny == "867-5309"
assert splitLines(multiLineString) ==
@["first line", "second line", "third line"]
assert split(multiLineString) == @["first", "line", "second",
"line", "third", "line"]
assert indent(multiLineString, 4) ==
" first line\n second line\n third line"
assert 'z'.repeat(5) == "zzzzz"
```
The chaining of functions is possible thanks to the [method call syntax](manual#procedures-method-call-syntax):
```
import strutils
from sequtils import map
let jenny = "867-5309"
assert jenny.split('-').map(parseInt) == @[867, 5309]
assert "Beetlejuice".indent(1).repeat(3).strip ==
"Beetlejuice Beetlejuice Beetlejuice"
```
This module is available for the [JavaScript target](backends#backends-the-javascript-target).
---
**See also:**
* [strformat module](strformat) for string interpolation and formatting
* [unicode module](unicode) for Unicode UTF-8 handling
* [sequtils module](sequtils) for operations on container types (including strings)
* [parsecsv module](parsecsv) for a high-performance CSV parser
* [parseutils module](parseutils) for lower-level parsing of tokens, numbers, identifiers, etc.
* [parseopt module](parseopt) for command-line parsing
* [pegs module](pegs) for PEG (Parsing Expression Grammar) support
* [strtabs module](strtabs) for efficient hash tables (dictionaries, in some programming languages) mapping from strings to strings
* [ropes module](ropes) for rope data type, which can represent very long strings efficiently
* [re module](re) for regular expression (regex) support
* <strscans> for `scanf` and `scanp` macros, which offer easier substring extraction than regular expressions
Imports
-------
<parseutils>, <math>, <algorithm>, <macros>, <unicode>, <since> Types
-----
```
SkipTable = array[char, int]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1897) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1897)
```
FloatFormatMode = enum
ffDefault, ## use the shorter floating point notation
ffDecimal, ## use decimal floating point notation
ffScientific ## use scientific notation (using ``e`` character)
```
the different modes of floating point formatting [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2410) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2410)
```
BinaryPrefixMode = enum
bpIEC, bpColloquial
```
the different names for binary prefixes [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2537) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2537) Consts
------
```
Whitespace = {' ', '\t', '\v', '\c', '\n', '\f'}
```
All the characters that count as whitespace (space, tab, vertical tab, carriage return, new line, form feed) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L89)
```
Letters = {'A'..'Z', 'a'..'z'}
```
the set of letters [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L93) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L93)
```
Digits = {'0'..'9'}
```
the set of digits [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L96)
```
HexDigits = {'0'..'9', 'A'..'F', 'a'..'f'}
```
the set of hexadecimal digits [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L99)
```
IdentChars = {'a'..'z', 'A'..'Z', '0'..'9', '_'}
```
the set of characters an identifier can consist of [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L102)
```
IdentStartChars = {'a'..'z', 'A'..'Z', '_'}
```
the set of characters an identifier can start with [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L105) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L105)
```
Newlines = {'\c', '\n'}
```
the set of characters a newline terminator can start with (carriage return, line feed) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L108)
```
AllChars = {'\x00'..'\xFF'}
```
A set with all the possible characters.
Not very useful by its own, you can use it to create *inverted* sets to make the [find proc](#find,string,set%5Bchar%5D,Natural,int) find **invalid** characters in strings. Example:
```
let invalid = AllChars - Digits
doAssert "01234".find(invalid) == -1
doAssert "01A34".find(invalid) == 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L112) Procs
-----
```
proc isAlphaAscii(c: char): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsAlphaAsciiChar", raises: [],
tags: [].}
```
Checks whether or not character `c` is alphabetical.
This checks a-z, A-Z ASCII characters only. Use [Unicode module](unicode) for UTF-8 support.
**Example:**
```
doAssert isAlphaAscii('e') == true
doAssert isAlphaAscii('E') == true
doAssert isAlphaAscii('8') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L124)
```
proc isAlphaNumeric(c: char): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsAlphaNumericChar",
raises: [], tags: [].}
```
Checks whether or not `c` is alphanumeric.
This checks a-z, A-Z, 0-9 ASCII characters only.
**Example:**
```
doAssert isAlphaNumeric('n') == true
doAssert isAlphaNumeric('8') == true
doAssert isAlphaNumeric(' ') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L136) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L136)
```
proc isDigit(c: char): bool {...}{.noSideEffect, gcsafe, extern: "nsuIsDigitChar",
raises: [], tags: [].}
```
Checks whether or not `c` is a number.
This checks 0-9 ASCII characters only.
**Example:**
```
doAssert isDigit('n') == false
doAssert isDigit('8') == true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L147)
```
proc isSpaceAscii(c: char): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsSpaceAsciiChar", raises: [],
tags: [].}
```
Checks whether or not `c` is a whitespace character. **Example:**
```
doAssert isSpaceAscii('n') == false
doAssert isSpaceAscii(' ') == true
doAssert isSpaceAscii('\t') == true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L157) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L157)
```
proc isLowerAscii(c: char): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsLowerAsciiChar", raises: [],
tags: [].}
```
Checks whether or not `c` is a lower case character.
This checks ASCII characters only. Use [Unicode module](unicode) for UTF-8 support.
See also:
* [toLowerAscii proc](#toLowerAscii,char)
**Example:**
```
doAssert isLowerAscii('e') == true
doAssert isLowerAscii('E') == false
doAssert isLowerAscii('7') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L166)
```
proc isUpperAscii(c: char): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsUpperAsciiChar", raises: [],
tags: [].}
```
Checks whether or not `c` is an upper case character.
This checks ASCII characters only. Use [Unicode module](unicode) for UTF-8 support.
See also:
* [toUpperAscii proc](#toUpperAscii,char)
**Example:**
```
doAssert isUpperAscii('e') == false
doAssert isUpperAscii('E') == true
doAssert isUpperAscii('7') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L181)
```
proc toLowerAscii(c: char): char {...}{.noSideEffect, gcsafe,
extern: "nsuToLowerAsciiChar", raises: [],
tags: [].}
```
Returns the lower case version of character `c`.
This works only for the letters `A-Z`. See [unicode.toLower](unicode#toLower,Rune) for a version that works for any Unicode character.
See also:
* [isLowerAscii proc](#isLowerAscii,char)
* [toLowerAscii proc](#toLowerAscii,string) for converting a string
**Example:**
```
doAssert toLowerAscii('A') == 'a'
doAssert toLowerAscii('e') == 'e'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L197) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L197)
```
proc toLowerAscii(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuToLowerAsciiStr", raises: [],
tags: [].}
```
Converts string `s` into lower case.
This works only for the letters `A-Z`. See [unicode.toLower](unicode#toLower,string) for a version that works for any Unicode character.
See also:
* [normalize proc](#normalize,string)
**Example:**
```
doAssert toLowerAscii("FooBar!") == "foobar!"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L221) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L221)
```
proc toUpperAscii(c: char): char {...}{.noSideEffect, gcsafe,
extern: "nsuToUpperAsciiChar", raises: [],
tags: [].}
```
Converts character `c` into upper case.
This works only for the letters `A-Z`. See [unicode.toUpper](unicode#toUpper,Rune) for a version that works for any Unicode character.
See also:
* [isLowerAscii proc](#isLowerAscii,char)
* [toUpperAscii proc](#toUpperAscii,string) for converting a string
* [capitalizeAscii proc](#capitalizeAscii,string)
**Example:**
```
doAssert toUpperAscii('a') == 'A'
doAssert toUpperAscii('E') == 'E'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L235)
```
proc toUpperAscii(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuToUpperAsciiStr", raises: [],
tags: [].}
```
Converts string `s` into upper case.
This works only for the letters `A-Z`. See [unicode.toUpper](unicode#toUpper,string) for a version that works for any Unicode character.
See also:
* [capitalizeAscii proc](#capitalizeAscii,string)
**Example:**
```
doAssert toUpperAscii("FooBar!") == "FOOBAR!"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L255) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L255)
```
proc capitalizeAscii(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuCapitalizeAscii", raises: [], tags: [].}
```
Converts the first character of string `s` into upper case.
This works only for the letters `A-Z`. Use [Unicode module](unicode) for UTF-8 support.
See also:
* [toUpperAscii proc](#toUpperAscii,char)
**Example:**
```
doAssert capitalizeAscii("foo") == "Foo"
doAssert capitalizeAscii("-bar") == "-bar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L269)
```
proc nimIdentNormalize(s: string): string {...}{.raises: [], tags: [].}
```
Normalizes the string `s` as a Nim identifier.
That means to convert to lower case and remove any '\_' on all characters except first one.
**Example:**
```
doAssert nimIdentNormalize("Foo_bar") == "Foobar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L284) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L284)
```
proc normalize(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuNormalize", raises: [], tags: [].}
```
Normalizes the string `s`.
That means to convert it to lower case and remove any '\_'. This should NOT be used to normalize Nim identifier names.
See also:
* [toLowerAscii proc](#toLowerAscii,string)
**Example:**
```
doAssert normalize("Foo_bar") == "foobar"
doAssert normalize("Foo Bar") == "foo bar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L304) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L304)
```
proc cmpIgnoreCase(a, b: string): int {...}{.noSideEffect, gcsafe,
extern: "nsuCmpIgnoreCase", raises: [],
tags: [].}
```
Compares two strings in a case insensitive manner. Returns:0 if a == b
< 0 if a < b
> 0 if a > b
**Example:**
```
doAssert cmpIgnoreCase("FooBar", "foobar") == 0
doAssert cmpIgnoreCase("bar", "Foo") < 0
doAssert cmpIgnoreCase("Foo5", "foo4") > 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L327) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L327)
```
proc cmpIgnoreStyle(a, b: string): int {...}{.noSideEffect, gcsafe,
extern: "nsuCmpIgnoreStyle", raises: [], tags: [].}
```
Semantically the same as `cmp(normalize(a), normalize(b))`. It is just optimized to not allocate temporary strings. This should NOT be used to compare Nim identifier names. Use [macros.eqIdent](macros#eqIdent,string,string) for that.
Returns:
0 if a == b
< 0 if a < b
> 0 if a > b
**Example:**
```
doAssert cmpIgnoreStyle("foo_bar", "FooBar") == 0
doAssert cmpIgnoreStyle("foo_bar_5", "FooBar4") > 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L349) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L349)
```
proc split(s: string; sep: char; maxsplit: int = -1): seq[string] {...}{.
noSideEffect, gcsafe, extern: "nsuSplitChar", raises: [], tags: [].}
```
The same as the [split iterator](#split.i,string,char,int) (see its documentation), but is a proc that returns a sequence of substrings.
See also:
* [split iterator](#split.i,string,char,int)
* [rsplit proc](#rsplit,string,char,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
**Example:**
```
doAssert "a,b,c".split(',') == @["a", "b", "c"]
doAssert "".split(' ') == @[""]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L730) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L730)
```
proc split(s: string; seps: set[char] = Whitespace; maxsplit: int = -1): seq[
string] {...}{.noSideEffect, gcsafe, extern: "nsuSplitCharSet", raises: [],
tags: [].}
```
The same as the [split iterator](#split.i,string,set%5Bchar%5D,int) (see its documentation), but is a proc that returns a sequence of substrings.
See also:
* [split iterator](#split.i,string,set%5Bchar%5D,int)
* [rsplit proc](#rsplit,string,set%5Bchar%5D,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
**Example:**
```
doAssert "a,b;c".split({',', ';'}) == @["a", "b", "c"]
doAssert "".split({' '}) == @[""]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L745) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L745)
```
proc split(s: string; sep: string; maxsplit: int = -1): seq[string] {...}{.
noSideEffect, gcsafe, extern: "nsuSplitString", raises: [], tags: [].}
```
Splits the string `s` into substrings using a string separator.
Substrings are separated by the string `sep`. This is a wrapper around the [split iterator](#split.i,string,string,int).
See also:
* [split iterator](#split.i,string,string,int)
* [rsplit proc](#rsplit,string,string,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
**Example:**
```
doAssert "a,b,c".split(",") == @["a", "b", "c"]
doAssert "a man a plan a canal panama".split("a ") == @["", "man ", "plan ", "canal panama"]
doAssert "".split("Elon Musk") == @[""]
doAssert "a largely spaced sentence".split(" ") == @["a", "", "largely",
"", "", "", "spaced", "sentence"]
doAssert "a largely spaced sentence".split(" ", maxsplit = 1) == @["a", " largely spaced sentence"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L760) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L760)
```
proc rsplit(s: string; sep: char; maxsplit: int = -1): seq[string] {...}{.
noSideEffect, gcsafe, extern: "nsuRSplitChar", raises: [], tags: [].}
```
The same as the [rsplit iterator](#rsplit.i,string,char,int), but is a proc that returns a sequence of substrings.
A possible common use case for `rsplit` is path manipulation, particularly on systems that don't use a common delimiter.
For example, if a system had `#` as a delimiter, you could do the following to get the tail of the path:
```
var tailSplit = rsplit("Root#Object#Method#Index", '#', maxsplit=1)
```
Results in `tailSplit` containing:
```
@["Root#Object#Method", "Index"]
```
See also:
* [rsplit iterator](#rsplit.i,string,char,int)
* [split proc](#split,string,char,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L783) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L783)
```
proc rsplit(s: string; seps: set[char] = Whitespace; maxsplit: int = -1): seq[
string] {...}{.noSideEffect, gcsafe, extern: "nsuRSplitCharSet", raises: [],
tags: [].}
```
The same as the [rsplit iterator](#rsplit.i,string,set%5Bchar%5D,int), but is a proc that returns a sequence of substrings.
A possible common use case for `rsplit` is path manipulation, particularly on systems that don't use a common delimiter.
For example, if a system had `#` as a delimiter, you could do the following to get the tail of the path:
```
var tailSplit = rsplit("Root#Object#Method#Index", {'#'}, maxsplit=1)
```
Results in `tailSplit` containing:
```
@["Root#Object#Method", "Index"]
```
See also:
* [rsplit iterator](#rsplit.i,string,set%5Bchar%5D,int)
* [split proc](#split,string,set%5Bchar%5D,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L810) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L810)
```
proc rsplit(s: string; sep: string; maxsplit: int = -1): seq[string] {...}{.
noSideEffect, gcsafe, extern: "nsuRSplitString", raises: [], tags: [].}
```
The same as the [rsplit iterator](#rsplit.i,string,string,int,bool), but is a proc that returns a sequence of substrings.
A possible common use case for `rsplit` is path manipulation, particularly on systems that don't use a common delimiter.
For example, if a system had `#` as a delimiter, you could do the following to get the tail of the path:
```
var tailSplit = rsplit("Root#Object#Method#Index", "#", maxsplit=1)
```
Results in `tailSplit` containing:
```
@["Root#Object#Method", "Index"]
```
See also:
* [rsplit iterator](#rsplit.i,string,string,int,bool)
* [split proc](#split,string,string,int)
* [splitLines proc](#splitLines,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
**Example:**
```
doAssert "a largely spaced sentence".rsplit(" ", maxsplit = 1) == @[
"a largely spaced", "sentence"]
doAssert "a,b,c".rsplit(",") == @["a", "b", "c"]
doAssert "a man a plan a canal panama".rsplit("a ") == @["", "man ",
"plan ", "canal panama"]
doAssert "".rsplit("Elon Musk") == @[""]
doAssert "a largely spaced sentence".rsplit(" ") == @["a", "",
"largely", "", "", "", "spaced", "sentence"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L838) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L838)
```
proc splitLines(s: string; keepEol = false): seq[string] {...}{.noSideEffect, gcsafe,
extern: "nsuSplitLines", raises: [], tags: [].}
```
The same as the [splitLines iterator](#splitLines.i,string) (see its documentation), but is a proc that returns a sequence of substrings.
See also:
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
* [countLines proc](#countLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L874) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L874)
```
proc splitWhitespace(s: string; maxsplit: int = -1): seq[string] {...}{.noSideEffect,
gcsafe, extern: "nsuSplitWhitespace", raises: [], tags: [].}
```
The same as the [splitWhitespace iterator](#splitWhitespace.i,string,int) (see its documentation), but is a proc that returns a sequence of substrings.
See also:
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [splitLines proc](#splitLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L885) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L885)
```
proc toBin(x: BiggestInt; len: Positive): string {...}{.noSideEffect, gcsafe,
extern: "nsuToBin", raises: [], tags: [].}
```
Converts `x` into its binary representation.
The resulting string is always `len` characters long. No leading `0b` prefix is generated.
**Example:**
```
let
a = 29
b = 257
doAssert a.toBin(8) == "00011101"
doAssert b.toBin(8) == "00000001"
doAssert b.toBin(9) == "100000001"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L895) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L895)
```
proc toOct(x: BiggestInt; len: Positive): string {...}{.noSideEffect, gcsafe,
extern: "nsuToOct", raises: [], tags: [].}
```
Converts `x` into its octal representation.
The resulting string is always `len` characters long. No leading `0o` prefix is generated.
Do not confuse it with [toOctal proc](#toOctal,char).
**Example:**
```
let
a = 62
b = 513
doAssert a.toOct(3) == "076"
doAssert b.toOct(3) == "001"
doAssert b.toOct(5) == "01001"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L918) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L918)
```
proc toHex[T: SomeInteger](x: T; len: Positive): string {...}{.noSideEffect.}
```
Converts `x` to its hexadecimal representation.
The resulting string will be exactly `len` characters long. No prefix like `0x` is generated. `x` is treated as an unsigned value.
**Example:**
```
let
a = 62'u64
b = 4097'u64
doAssert a.toHex(3) == "03E"
doAssert b.toHex(3) == "001"
doAssert b.toHex(4) == "1001"
doAssert toHex(62, 3) == "03E"
doAssert toHex(-8, 6) == "FFFFF8"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L954) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L954)
```
proc toHex[T: SomeInteger](x: T): string {...}{.noSideEffect.}
```
Shortcut for `toHex(x, T.sizeof * 2)` **Example:**
```
doAssert toHex(1984'i64) == "00000000000007C0"
doAssert toHex(1984'i16) == "07C0"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L970) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L970)
```
proc toHex(s: string): string {...}{.noSideEffect, gcsafe, raises: [], tags: [].}
```
Converts a bytes string to its hexadecimal representation.
The output is twice the input long. No prefix like `0x` is generated.
See also:
* [parseHexStr proc](#parseHexStr,string) for the reverse operation
**Example:**
```
let
a = "1"
b = "A"
c = "\0\255"
doAssert a.toHex() == "31"
doAssert b.toHex() == "41"
doAssert c.toHex() == "00FF"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L977) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L977)
```
proc toOctal(c: char): string {...}{.noSideEffect, gcsafe, extern: "nsuToOctal",
raises: [], tags: [].}
```
Converts a character `c` to its octal representation.
The resulting string may not have a leading zero. Its length is always exactly 3.
Do not confuse it with [toOct proc](#toOct,BiggestInt,Positive).
**Example:**
```
doAssert toOctal('1') == "061"
doAssert toOctal('A') == "101"
doAssert toOctal('a') == "141"
doAssert toOctal('!') == "041"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1002) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1002)
```
proc fromBin[T: SomeInteger](s: string): T
```
Parses a binary integer value from a string `s`.
If `s` is not a valid binary integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0b`, `0B`. Underscores within `s` are ignored.
Does not check for overflow. If the value represented by `s` is too big to fit into a return type, only the value of the rightmost binary digits of `s` is returned without producing an error.
**Example:**
```
let s = "0b_0100_1000_1000_1000_1110_1110_1001_1001"
doAssert fromBin[int](s) == 1216933529
doAssert fromBin[int8](s) == 0b1001_1001'i8
doAssert fromBin[int8](s) == -103'i8
doAssert fromBin[uint8](s) == 153
doAssert s.fromBin[:int16] == 0b1110_1110_1001_1001'i16
doAssert s.fromBin[:uint64] == 1216933529'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1021) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1021)
```
proc fromOct[T: SomeInteger](s: string): T
```
Parses an octal integer value from a string `s`.
If `s` is not a valid octal integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0o`, `0O`. Underscores within `s` are ignored.
Does not check for overflow. If the value represented by `s` is too big to fit into a return type, only the value of the rightmost octal digits of `s` is returned without producing an error.
**Example:**
```
let s = "0o_123_456_777"
doAssert fromOct[int](s) == 21913087
doAssert fromOct[int8](s) == 0o377'i8
doAssert fromOct[int8](s) == -1'i8
doAssert fromOct[uint8](s) == 255'u8
doAssert s.fromOct[:int16] == 24063'i16
doAssert s.fromOct[:uint64] == 21913087'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1044) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1044)
```
proc fromHex[T: SomeInteger](s: string): T
```
Parses a hex integer value from a string `s`.
If `s` is not a valid hex integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0x`, `0X`, `#`. Underscores within `s` are ignored.
Does not check for overflow. If the value represented by `s` is too big to fit into a return type, only the value of the rightmost hex digits of `s` is returned without producing an error.
**Example:**
```
let s = "0x_1235_8df6"
doAssert fromHex[int](s) == 305499638
doAssert fromHex[int8](s) == 0xf6'i8
doAssert fromHex[int8](s) == -10'i8
doAssert fromHex[uint8](s) == 246'u8
doAssert s.fromHex[:int16] == -29194'i16
doAssert s.fromHex[:uint64] == 305499638'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1067) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1067)
```
proc intToStr(x: int; minchars: Positive = 1): string {...}{.noSideEffect, gcsafe,
extern: "nsuIntToStr", raises: [], tags: [].}
```
Converts `x` to its decimal representation.
The resulting string will be minimally `minchars` characters long. This is achieved by adding leading zeros.
**Example:**
```
doAssert intToStr(1984) == "1984"
doAssert intToStr(1984, 6) == "001984"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1090) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1090)
```
proc parseInt(s: string): int {...}{.noSideEffect, gcsafe, extern: "nsuParseInt",
raises: [ValueError], tags: [].}
```
Parses a decimal integer value contained in `s`.
If `s` is not a valid integer, `ValueError` is raised.
**Example:**
```
doAssert parseInt("-0042") == -42
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1105) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1105)
```
proc parseBiggestInt(s: string): BiggestInt {...}{.noSideEffect, gcsafe,
extern: "nsuParseBiggestInt", raises: [ValueError], tags: [].}
```
Parses a decimal integer value contained in `s`.
If `s` is not a valid integer, `ValueError` is raised.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1117) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1117)
```
proc parseUInt(s: string): uint {...}{.noSideEffect, gcsafe, extern: "nsuParseUInt",
raises: [ValueError], tags: [].}
```
Parses a decimal unsigned integer value contained in `s`.
If `s` is not a valid integer, `ValueError` is raised.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1127)
```
proc parseBiggestUInt(s: string): BiggestUInt {...}{.noSideEffect, gcsafe,
extern: "nsuParseBiggestUInt", raises: [ValueError], tags: [].}
```
Parses a decimal unsigned integer value contained in `s`.
If `s` is not a valid integer, `ValueError` is raised.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1137) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1137)
```
proc parseFloat(s: string): float {...}{.noSideEffect, gcsafe,
extern: "nsuParseFloat",
raises: [ValueError], tags: [].}
```
Parses a decimal floating point value contained in `s`.
If `s` is not a valid floating point number, `ValueError` is raised. `NAN`, `INF`, `-INF` are also supported (case insensitive comparison).
**Example:**
```
doAssert parseFloat("3.14") == 3.14
doAssert parseFloat("inf") == 1.0/0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1147)
```
proc parseBinInt(s: string): int {...}{.noSideEffect, gcsafe,
extern: "nsuParseBinInt",
raises: [ValueError], tags: [].}
```
Parses a binary integer value contained in `s`.
If `s` is not a valid binary integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0b`, `0B`. Underscores within `s` are ignored.
**Example:**
```
let
a = "0b11_0101"
b = "111"
doAssert a.parseBinInt() == 53
doAssert b.parseBinInt() == 7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1161) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1161)
```
proc parseOctInt(s: string): int {...}{.noSideEffect, gcsafe,
extern: "nsuParseOctInt",
raises: [ValueError], tags: [].}
```
Parses an octal integer value contained in `s`.
If `s` is not a valid oct integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0o`, `0O`. Underscores within `s` are ignored.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1180) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1180)
```
proc parseHexInt(s: string): int {...}{.noSideEffect, gcsafe,
extern: "nsuParseHexInt",
raises: [ValueError], tags: [].}
```
Parses a hexadecimal integer value contained in `s`.
If `s` is not a valid hex integer, `ValueError` is raised. `s` can have one of the following optional prefixes: `0x`, `0X`, `#`. Underscores within `s` are ignored.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1192) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1192)
```
proc parseHexStr(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuParseHexStr",
raises: [ValueError], tags: [].}
```
Convert hex-encoded string to byte string, e.g.:
Raises `ValueError` for an invalid hex values. The comparison is case-insensitive.
See also:
* [toHex proc](#toHex,string) for the reverse operation
**Example:**
```
let
a = "41"
b = "3161"
c = "00ff"
doAssert parseHexStr(a) == "A"
doAssert parseHexStr(b) == "1a"
doAssert parseHexStr(c) == "\0\255"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1219) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1219)
```
proc parseBool(s: string): bool {...}{.raises: [ValueError], tags: [].}
```
Parses a value into a `bool`.
If `s` is one of the following values: `y, yes, true, 1, on`, then returns `true`. If `s` is one of the following values: `n, no, false, 0, off`, then returns `false`. If `s` is something else a `ValueError` exception is raised.
**Example:**
```
let a = "n"
doAssert parseBool(a) == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1251) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1251)
```
proc parseEnum[T: enum](s: string): T
```
Parses an enum `T`. This errors at compile time, if the given enum type contains multiple fields with the same string value.
Raises `ValueError` for an invalid value in `s`. The comparison is done in a style insensitive way.
**Example:**
```
type
MyEnum = enum
first = "1st",
second,
third = "3rd"
doAssert parseEnum[MyEnum]("1_st") == first
doAssert parseEnum[MyEnum]("second") == second
doAssertRaises(ValueError):
echo parseEnum[MyEnum]("third")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1322)
```
proc parseEnum[T: enum](s: string; default: T): T
```
Parses an enum `T`. This errors at compile time, if the given enum type contains multiple fields with the same string value.
Uses `default` for an invalid value in `s`. The comparison is done in a style insensitive way.
**Example:**
```
type
MyEnum = enum
first = "1st",
second,
third = "3rd"
doAssert parseEnum[MyEnum]("1_st") == first
doAssert parseEnum[MyEnum]("second") == second
doAssert parseEnum[MyEnum]("last", third) == third
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1342) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1342)
```
proc repeat(c: char; count: Natural): string {...}{.noSideEffect, gcsafe,
extern: "nsuRepeatChar", raises: [], tags: [].}
```
Returns a string of length `count` consisting only of the character `c`. **Example:**
```
let a = 'z'
doAssert a.repeat(5) == "zzzzz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1361) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1361)
```
proc repeat(s: string; n: Natural): string {...}{.noSideEffect, gcsafe,
extern: "nsuRepeatStr", raises: [], tags: [].}
```
Returns string `s` concatenated `n` times. **Example:**
```
doAssert "+ foo +".repeat(3) == "+ foo ++ foo ++ foo +"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1371) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1371)
```
proc spaces(n: Natural): string {...}{.inline, raises: [], tags: [].}
```
Returns a string with `n` space characters. You can use this proc to left align strings.
See also:
* [align proc](#align,string,Natural,char)
* [alignLeft proc](#alignLeft,string,Natural,char)
* [indent proc](#indent,string,Natural,string)
* [center proc](#center,string,int,char)
**Example:**
```
let
width = 15
text1 = "Hello user!"
text2 = "This is a very long string"
doAssert text1 & spaces(max(0, width - text1.len)) & "|" ==
"Hello user! |"
doAssert text2 & spaces(max(0, width - text2.len)) & "|" ==
"This is a very long string|"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1380) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1380)
```
proc align(s: string; count: Natural; padding = ' '): string {...}{.noSideEffect,
gcsafe, extern: "nsuAlignString", raises: [], tags: [].}
```
Aligns a string `s` with `padding`, so that it is of length `count`.
`padding` characters (by default spaces) are added before `s` resulting in right alignment. If `s.len >= count`, no spaces are added and `s` is returned unchanged. If you need to left align a string use the [alignLeft proc](#alignLeft,string,Natural,char).
See also:
* [alignLeft proc](#alignLeft,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [indent proc](#indent,string,Natural,string)
* [center proc](#center,string,int,char)
**Example:**
```
assert align("abc", 4) == " abc"
assert align("a", 0) == "a"
assert align("1232", 6) == " 1232"
assert align("1232", 6, '#') == "##1232"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1400) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1400)
```
proc alignLeft(s: string; count: Natural; padding = ' '): string {...}{.noSideEffect,
raises: [], tags: [].}
```
Left-Aligns a string `s` with `padding`, so that it is of length `count`.
`padding` characters (by default spaces) are added after `s` resulting in left alignment. If `s.len >= count`, no spaces are added and `s` is returned unchanged. If you need to right align a string use the [align proc](#align,string,Natural,char).
See also:
* [align proc](#align,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [indent proc](#indent,string,Natural,string)
* [center proc](#center,string,int,char)
**Example:**
```
assert alignLeft("abc", 4) == "abc "
assert alignLeft("a", 0) == "a"
assert alignLeft("1232", 6) == "1232 "
assert alignLeft("1232", 6, '#') == "1232##"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1427) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1427)
```
proc center(s: string; width: int; fillChar: char = ' '): string {...}{.noSideEffect,
gcsafe, extern: "nsuCenterString", raises: [], tags: [].}
```
Return the contents of `s` centered in a string `width` long using `fillChar` (default: space) as padding.
The original string is returned if `width` is less than or equal to `s.len`.
See also:
* [align proc](#align,string,Natural,char)
* [alignLeft proc](#alignLeft,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [indent proc](#indent,string,Natural,string)
**Example:**
```
let a = "foo"
doAssert a.center(2) == "foo"
doAssert a.center(5) == " foo "
doAssert a.center(6) == " foo "
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1455) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1455)
```
proc indent(s: string; count: Natural; padding: string = " "): string {...}{.
noSideEffect, gcsafe, extern: "nsuIndent", raises: [], tags: [].}
```
Indents each line in `s` by `count` amount of `padding`.
**Note:** This does not preserve the new line characters used in `s`.
See also:
* [align proc](#align,string,Natural,char)
* [alignLeft proc](#alignLeft,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [unindent proc](#unindent,string,Natural,string)
* [dedent proc](#dedent,string,Natural)
**Example:**
```
doAssert indent("First line\c\l and second line.", 2) ==
" First line\l and second line."
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1490) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1490)
```
proc unindent(s: string; count: Natural = int.high; padding: string = " "): string {...}{.
noSideEffect, gcsafe, extern: "nsuUnindent", raises: [], tags: [].}
```
Unindents each line in `s` by `count` amount of `padding`.
**Note:** This does not preserve the new line characters used in `s`.
See also:
* [dedent proc](#dedent,string,Natural)
* [align proc](#align,string,Natural,char)
* [alignLeft proc](#alignLeft,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [indent proc](#indent,string,Natural,string)
**Example:**
```
let x = """
Hello
There
""".unindent()
doAssert x == "Hello\nThere\n"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1515) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1515)
```
proc indentation(s: string): Natural {...}{.raises: [], tags: [].}
```
Returns the amount of indentation all lines of `s` have in common, ignoring lines that consist only of whitespace. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1548) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1548)
```
proc dedent(s: string; count: Natural = indentation(s)): string {...}{.noSideEffect,
gcsafe, extern: "nsuDedent", raises: [], tags: [].}
```
Unindents each line in `s` by `count` amount of `padding`. The only difference between this and the [unindent proc](#unindent,string,Natural,string) is that this by default only cuts off the amount of indentation that all lines of `s` share as opposed to all indentation. It only supports spaces as padding.
**Note:** This does not preserve the new line characters used in `s`.
See also:
* [unindent proc](#unindent,string,Natural,string)
* [align proc](#align,string,Natural,char)
* [alignLeft proc](#alignLeft,string,Natural,char)
* [spaces proc](#spaces,Natural)
* [indent proc](#indent,string,Natural,string)
**Example:**
```
let x = """
Hello
There
""".dedent()
doAssert x == "Hello\n There\n"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1561) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1561)
```
proc delete(s: var string; first, last: int) {...}{.noSideEffect, gcsafe,
extern: "nsuDelete", raises: [], tags: [].}
```
Deletes in `s` (must be declared as `var`) the characters at positions `first ..last` (both ends included).
This modifies `s` itself, it does not return a copy.
**Example:**
```
var a = "abracadabra"
a.delete(4, 5)
doAssert a == "abradabra"
a.delete(1, 6)
doAssert a == "ara"
a.delete(2, 999)
doAssert a == "ar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1586) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1586)
```
proc startsWith(s: string; prefix: char): bool {...}{.noSideEffect, inline,
raises: [], tags: [].}
```
Returns true if `s` starts with character `prefix`.
See also:
* [endsWith proc](#endsWith,string,char)
* [continuesWith proc](#continuesWith,string,string,Natural)
* [removePrefix proc](#removePrefix,string,char)
**Example:**
```
let a = "abracadabra"
doAssert a.startsWith('a') == true
doAssert a.startsWith('b') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1614) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1614)
```
proc startsWith(s, prefix: string): bool {...}{.noSideEffect, gcsafe,
extern: "nsuStartsWith", raises: [], tags: [].}
```
Returns true if `s` starts with string `prefix`.
If `prefix == ""` true is returned.
See also:
* [endsWith proc](#endsWith,string,string)
* [continuesWith proc](#continuesWith,string,string,Natural)
* [removePrefix proc](#removePrefix,string,string)
**Example:**
```
let a = "abracadabra"
doAssert a.startsWith("abra") == true
doAssert a.startsWith("bra") == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1627) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1627)
```
proc endsWith(s: string; suffix: char): bool {...}{.noSideEffect, inline, raises: [],
tags: [].}
```
Returns true if `s` ends with `suffix`.
See also:
* [startsWith proc](#startsWith,string,char)
* [continuesWith proc](#continuesWith,string,string,Natural)
* [removeSuffix proc](#removeSuffix,string,char)
**Example:**
```
let a = "abracadabra"
doAssert a.endsWith('a') == true
doAssert a.endsWith('b') == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1647) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1647)
```
proc endsWith(s, suffix: string): bool {...}{.noSideEffect, gcsafe,
extern: "nsuEndsWith", raises: [], tags: [].}
```
Returns true if `s` ends with `suffix`.
If `suffix == ""` true is returned.
See also:
* [startsWith proc](#startsWith,string,string)
* [continuesWith proc](#continuesWith,string,string,Natural)
* [removeSuffix proc](#removeSuffix,string,string)
**Example:**
```
let a = "abracadabra"
doAssert a.endsWith("abra") == true
doAssert a.endsWith("dab") == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1660) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1660)
```
proc continuesWith(s, substr: string; start: Natural): bool {...}{.noSideEffect,
gcsafe, extern: "nsuContinuesWith", raises: [], tags: [].}
```
Returns true if `s` continues with `substr` at position `start`.
If `substr == ""` true is returned.
See also:
* [startsWith proc](#startsWith,string,string)
* [endsWith proc](#endsWith,string,string)
**Example:**
```
let a = "abracadabra"
doAssert a.continuesWith("ca", 4) == true
doAssert a.continuesWith("ca", 5) == false
doAssert a.continuesWith("dab", 6) == true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1681) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1681)
```
proc removePrefix(s: var string; chars: set[char] = Newlines) {...}{.gcsafe,
extern: "nsuRemovePrefixCharSet", raises: [], tags: [].}
```
Removes all characters from `chars` from the start of the string `s` (in-place).
See also:
* [removeSuffix proc](#removeSuffix,string,set%5Bchar%5D)
**Example:**
```
var userInput = "\r\n*~Hello World!"
userInput.removePrefix
doAssert userInput == "*~Hello World!"
userInput.removePrefix({'~', '*'})
doAssert userInput == "Hello World!"
var otherInput = "?!?Hello!?!"
otherInput.removePrefix({'!', '?'})
doAssert otherInput == "Hello!?!"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1702) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1702)
```
proc removePrefix(s: var string; c: char) {...}{.gcsafe,
extern: "nsuRemovePrefixChar", raises: [], tags: [].}
```
Removes all occurrences of a single character (in-place) from the start of a string.
See also:
* [removeSuffix proc](#removeSuffix,string,char)
* [startsWith proc](#startsWith,string,char)
**Example:**
```
var ident = "pControl"
ident.removePrefix('p')
doAssert ident == "Control"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1724) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1724)
```
proc removePrefix(s: var string; prefix: string) {...}{.gcsafe,
extern: "nsuRemovePrefixString", raises: [], tags: [].}
```
Remove the first matching prefix (in-place) from a string.
See also:
* [removeSuffix proc](#removeSuffix,string,string)
* [startsWith proc](#startsWith,string,string)
**Example:**
```
var answers = "yesyes"
answers.removePrefix("yes")
doAssert answers == "yes"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1738) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1738)
```
proc removeSuffix(s: var string; chars: set[char] = Newlines) {...}{.gcsafe,
extern: "nsuRemoveSuffixCharSet", raises: [], tags: [].}
```
Removes all characters from `chars` from the end of the string `s` (in-place).
See also:
* [removePrefix proc](#removePrefix,string,set%5Bchar%5D)
**Example:**
```
var userInput = "Hello World!*~\r\n"
userInput.removeSuffix
doAssert userInput == "Hello World!*~"
userInput.removeSuffix({'~', '*'})
doAssert userInput == "Hello World!"
var otherInput = "Hello!?!"
otherInput.removeSuffix({'!', '?'})
doAssert otherInput == "Hello"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1752) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1752)
```
proc removeSuffix(s: var string; c: char) {...}{.gcsafe,
extern: "nsuRemoveSuffixChar", raises: [], tags: [].}
```
Removes all occurrences of a single character (in-place) from the end of a string.
See also:
* [removePrefix proc](#removePrefix,string,char)
* [endsWith proc](#endsWith,string,char)
**Example:**
```
var table = "users"
table.removeSuffix('s')
doAssert table == "user"
var dots = "Trailing dots......."
dots.removeSuffix('.')
doAssert dots == "Trailing dots"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1775) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1775)
```
proc removeSuffix(s: var string; suffix: string) {...}{.gcsafe,
extern: "nsuRemoveSuffixString", raises: [], tags: [].}
```
Remove the first matching suffix (in-place) from a string.
See also:
* [removePrefix proc](#removePrefix,string,string)
* [endsWith proc](#endsWith,string,string)
**Example:**
```
var answers = "yeses"
answers.removeSuffix("es")
doAssert answers == "yes"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1794) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1794)
```
proc addSep(dest: var string; sep = ", "; startLen: Natural = 0) {...}{.noSideEffect,
inline, raises: [], tags: [].}
```
Adds a separator to `dest` only if its length is bigger than `startLen`.
A shorthand for:
```
if dest.len > startLen: add(dest, sep)
```
This is often useful for generating some code where the items need to be *separated* by `sep`. `sep` is only added if `dest` is longer than `startLen`. The following example creates a string describing an array of integers.
**Example:**
```
var arr = "["
for x in items([2, 3, 5, 7, 11]):
addSep(arr, startLen = len("["))
add(arr, $x)
add(arr, "]")
doAssert arr == "[2, 3, 5, 7, 11]"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1811) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1811)
```
proc allCharsInSet(s: string; theSet: set[char]): bool {...}{.raises: [], tags: [].}
```
Returns true if every character of `s` is in the set `theSet`. **Example:**
```
doAssert allCharsInSet("aeea", {'a', 'e'}) == true
doAssert allCharsInSet("", {'a', 'e'}) == true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1834) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1834)
```
proc abbrev(s: string; possibilities: openArray[string]): int {...}{.raises: [],
tags: [].}
```
Returns the index of the first item in `possibilities` which starts with `s`, if not ambiguous.
Returns -1 if no item has been found and -2 if multiple items match.
**Example:**
```
doAssert abbrev("fac", ["college", "faculty", "industry"]) == 1
doAssert abbrev("foo", ["college", "faculty", "industry"]) == -1 # Not found
doAssert abbrev("fac", ["college", "faculty", "faculties"]) == -2 # Ambiguous
doAssert abbrev("college", ["college", "colleges", "industry"]) == 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1844) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1844)
```
proc join(a: openArray[string]; sep: string = ""): string {...}{.noSideEffect,
gcsafe, extern: "nsuJoinSep", raises: [], tags: [].}
```
Concatenates all strings in the container `a`, separating them with `sep`. **Example:**
```
doAssert join(["A", "B", "Conclusion"], " -> ") == "A -> B -> Conclusion"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1866) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1866)
```
proc join[T: not string](a: openArray[T]; sep: string = ""): string {...}{.
noSideEffect, gcsafe.}
```
Converts all elements in the container `a` to strings using `$`, and concatenates them with `sep`. **Example:**
```
doAssert join([1, 2, 3], " -> ") == "1 -> 2 -> 3"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1883) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1883)
```
proc initSkipTable(a: var SkipTable; sub: string) {...}{.noSideEffect, gcsafe,
extern: "nsuInitSkipTable", raises: [], tags: [].}
```
Preprocess table `a` for `sub`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1899) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1899)
```
proc find(a: SkipTable; s, sub: string; start: Natural = 0; last = 0): int {...}{.
noSideEffect, gcsafe, extern: "nsuFindStrA", raises: [], tags: [].}
```
Searches for `sub` in `s` inside range `start..last` using preprocessed table `a`. If `last` is unspecified, it defaults to `s.high` (the last element).
Searching is case-sensitive. If `sub` is not in `s`, -1 is returned.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1918) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1918)
```
proc find(s: string; sub: char; start: Natural = 0; last = 0): int {...}{.
noSideEffect, gcsafe, extern: "nsuFindChar", raises: [], tags: [].}
```
Searches for `sub` in `s` inside range `start..last` (both ends included). If `last` is unspecified, it defaults to `s.high` (the last element).
Searching is case-sensitive. If `sub` is not in `s`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].rfind` for a `start`-origin index.
See also:
* [rfind proc](#rfind,string,char,Natural)
* [replace proc](#replace,string,char,char)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1954) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1954)
```
proc find(s: string; chars: set[char]; start: Natural = 0; last = 0): int {...}{.
noSideEffect, gcsafe, extern: "nsuFindCharSet", raises: [], tags: [].}
```
Searches for `chars` in `s` inside range `start..last` (both ends included). If `last` is unspecified, it defaults to `s.high` (the last element).
If `s` contains none of the characters in `chars`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].find` for a `start`-origin index.
See also:
* [rfind proc](#rfind,string,set%5Bchar%5D,Natural)
* [multiReplace proc](#multiReplace,string,varargs%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1982) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1982)
```
proc find(s, sub: string; start: Natural = 0; last = 0): int {...}{.noSideEffect,
gcsafe, extern: "nsuFindStr", raises: [], tags: [].}
```
Searches for `sub` in `s` inside range `start..last` (both ends included). If `last` is unspecified, it defaults to `s.high` (the last element).
Searching is case-sensitive. If `sub` is not in `s`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].find` for a `start`-origin index.
See also:
* [rfind proc](#rfind,string,string,Natural)
* [replace proc](#replace,string,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L1999) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L1999)
```
proc rfind(s: string; sub: char; start: Natural = 0; last = -1): int {...}{.
noSideEffect, gcsafe, extern: "nsuRFindChar", raises: [], tags: [].}
```
Searches for `sub` in `s` inside range `start..last` (both ends included) in reverse -- starting at high indexes and moving lower to the first character or `start`. If `last` is unspecified, it defaults to `s.high` (the last element).
Searching is case-sensitive. If `sub` is not in `s`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].find` for a `start`-origin index.
See also:
* [find proc](#find,string,char,Natural,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2017) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2017)
```
proc rfind(s: string; chars: set[char]; start: Natural = 0; last = -1): int {...}{.
noSideEffect, gcsafe, extern: "nsuRFindCharSet", raises: [], tags: [].}
```
Searches for `chars` in `s` inside range `start..last` (both ends included) in reverse -- starting at high indexes and moving lower to the first character or `start`. If `last` is unspecified, it defaults to `s.high` (the last element).
If `s` contains none of the characters in `chars`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].rfind` for a `start`-origin index.
See also:
* [find proc](#find,string,set%5Bchar%5D,Natural,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2035) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2035)
```
proc rfind(s, sub: string; start: Natural = 0; last = -1): int {...}{.noSideEffect,
gcsafe, extern: "nsuRFindStr", raises: [], tags: [].}
```
Searches for `sub` in `s` inside range `start..last` (both ends included) included) in reverse -- starting at high indexes and moving lower to the first character or `start`. If `last` is unspecified, it defaults to `s.high` (the last element).
Searching is case-sensitive. If `sub` is not in `s`, -1 is returned. Otherwise the index returned is relative to `s[0]`, not `start`. Use `s[start..last].rfind` for a `start`-origin index.
See also:
* [find proc](#find,string,string,Natural,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2053) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2053)
```
proc count(s: string; sub: char): int {...}{.noSideEffect, gcsafe,
extern: "nsuCountChar", raises: [],
tags: [].}
```
Count the occurrences of the character `sub` in the string `s`.
See also:
* [countLines proc](#countLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2080) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2080)
```
proc count(s: string; subs: set[char]): int {...}{.noSideEffect, gcsafe,
extern: "nsuCountCharSet", raises: [], tags: [].}
```
Count the occurrences of the group of character `subs` in the string `s`.
See also:
* [countLines proc](#countLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2090) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2090)
```
proc count(s: string; sub: string; overlapping: bool = false): int {...}{.
noSideEffect, gcsafe, extern: "nsuCountString", raises: [], tags: [].}
```
Count the occurrences of a substring `sub` in the string `s`. Overlapping occurrences of `sub` only count when `overlapping` is set to true (default: false).
See also:
* [countLines proc](#countLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2101) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2101)
```
proc countLines(s: string): int {...}{.noSideEffect, gcsafe, extern: "nsuCountLines",
raises: [], tags: [].}
```
Returns the number of lines in the string `s`.
This is the same as `len(splitLines(s))`, but much more efficient because it doesn't modify the string creating temporal objects. Every [character literal](manual#lexical-analysis-character-literals) newline combination (CR, LF, CR-LF) is supported.
In this context, a line is any string separated by a newline combination. A line can be an empty string.
See also:
* [splitLines proc](#splitLines,string)
**Example:**
```
doAssert countLines("First line\l and second line.") == 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2119) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2119)
```
proc contains(s, sub: string): bool {...}{.noSideEffect, raises: [], tags: [].}
```
Same as `find(s, sub) >= 0`.
See also:
* [find proc](#find,string,string,Natural,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2147)
```
proc contains(s: string; chars: set[char]): bool {...}{.noSideEffect, raises: [],
tags: [].}
```
Same as `find(s, chars) >= 0`.
See also:
* [find proc](#find,string,set%5Bchar%5D,Natural,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2154) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2154)
```
proc replace(s, sub: string; by = ""): string {...}{.noSideEffect, gcsafe,
extern: "nsuReplaceStr", raises: [], tags: [].}
```
Replaces `sub` in `s` by the string `by`.
See also:
* [find proc](#find,string,string,Natural,int)
* [replace proc](#replace,string,char,char) for replacing single characters
* [replaceWord proc](#replaceWord,string,string,string)
* [multiReplace proc](#multiReplace,string,varargs%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2161) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2161)
```
proc replace(s: string; sub, by: char): string {...}{.noSideEffect, gcsafe,
extern: "nsuReplaceChar", raises: [], tags: [].}
```
Replaces `sub` in `s` by the character `by`.
Optimized version of [replace](#replace,string,string,string) for characters.
See also:
* [find proc](#find,string,char,Natural,int)
* [replaceWord proc](#replaceWord,string,string,string)
* [multiReplace proc](#multiReplace,string,varargs%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2203)
```
proc replaceWord(s, sub: string; by = ""): string {...}{.noSideEffect, gcsafe,
extern: "nsuReplaceWord", raises: [], tags: [].}
```
Replaces `sub` in `s` by the string `by`.
Each occurrence of `sub` has to be surrounded by word boundaries (comparable to `\b` in regular expressions), otherwise it is not replaced.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2221) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2221)
```
proc multiReplace(s: string; replacements: varargs[(string, string)]): string {...}{.
noSideEffect, raises: [], tags: [].}
```
Same as replace, but specialized for doing multiple replacements in a single pass through the input string.
`multiReplace` performs all replacements in a single pass, this means it can be used to swap the occurrences of "a" and "b", for instance.
If the resulting string is not longer than the original input string, only a single memory allocation is required.
The order of the replacements does matter. Earlier replacements are preferred over later replacements in the argument list.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2252)
```
proc insertSep(s: string; sep = '_'; digits = 3): string {...}{.noSideEffect, gcsafe,
extern: "nsuInsertSep", raises: [], tags: [].}
```
Inserts the separator `sep` after `digits` characters (default: 3) from right to left.
Even though the algorithm works with any string `s`, it is only useful if `s` contains a number.
**Example:**
```
doAssert insertSep("1000000") == "1_000_000"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2288) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2288)
```
proc escape(s: string; prefix = "\""; suffix = "\""): string {...}{.noSideEffect,
gcsafe, extern: "nsuEscape", raises: [], tags: [].}
```
Escapes a string `s`. See [system.addEscapedChar](system#addEscapedChar,string,char) for the escaping scheme.
The resulting string is prefixed with `prefix` and suffixed with `suffix`. Both may be empty strings.
See also:
* [unescape proc](#unescape,string,string,string) for the opposite
operation
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2322)
```
proc unescape(s: string; prefix = "\""; suffix = "\""): string {...}{.noSideEffect,
gcsafe, extern: "nsuUnescape", raises: [ValueError], tags: [].}
```
Unescapes a string `s`.
This complements [escape proc](#escape,string,string,string) as it performs the opposite operations.
If `s` does not begin with `prefix` and end with `suffix` a ValueError exception will be raised.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2346) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2346)
```
proc validIdentifier(s: string): bool {...}{.noSideEffect, gcsafe,
extern: "nsuValidIdentifier",
raises: [], tags: [].}
```
Returns true if `s` is a valid identifier.
A valid identifier starts with a character of the set `IdentStartChars` and is followed by any number of characters of the set `IdentChars`.
**Example:**
```
doAssert "abc_def08".validIdentifier
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2389) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2389)
```
proc formatBiggestFloat(f: BiggestFloat; format: FloatFormatMode = ffDefault;
precision: range[-1 .. 32] = 16; decimalSep = '.'): string {...}{.
noSideEffect, gcsafe, extern: "nsu$1", raises: [], tags: [].}
```
Converts a floating point value `f` to a string.
If `format == ffDecimal` then precision is the number of digits to be printed after the decimal point. If `format == ffScientific` then precision is the maximum number of significant digits to be printed. `precision`'s default value is the maximum number of meaningful digits after the decimal point for Nim's `biggestFloat` type.
If `precision == -1`, it tries to format it nicely.
**Example:**
```
let x = 123.456
doAssert x.formatBiggestFloat() == "123.4560000000000"
doAssert x.formatBiggestFloat(ffDecimal, 4) == "123.4560"
doAssert x.formatBiggestFloat(ffScientific, 2) == "1.23e+02"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2416) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2416)
```
proc formatFloat(f: float; format: FloatFormatMode = ffDefault;
precision: range[-1 .. 32] = 16; decimalSep = '.'): string {...}{.
noSideEffect, gcsafe, extern: "nsu$1", raises: [], tags: [].}
```
Converts a floating point value `f` to a string.
If `format == ffDecimal` then precision is the number of digits to be printed after the decimal point. If `format == ffScientific` then precision is the maximum number of significant digits to be printed. `precision`'s default value is the maximum number of meaningful digits after the decimal point for Nim's `float` type.
If `precision == -1`, it tries to format it nicely.
**Example:**
```
let x = 123.456
doAssert x.formatFloat() == "123.4560000000000"
doAssert x.formatFloat(ffDecimal, 4) == "123.4560"
doAssert x.formatFloat(ffScientific, 2) == "1.23e+02"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2496)
```
proc trimZeros(x: var string; decimalSep = '.') {...}{.noSideEffect, raises: [],
tags: [].}
```
Trim trailing zeros from a formatted floating point value `x` (must be declared as `var`).
This modifies `x` itself, it does not return a copy.
**Example:**
```
var x = "123.456000000"
x.trimZeros()
doAssert x == "123.456"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2517) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2517)
```
proc formatSize(bytes: int64; decimalSep = '.'; prefix = bpIEC;
includeSpace = false): string {...}{.noSideEffect, raises: [],
tags: [].}
```
Rounds and formats `bytes`.
By default, uses the IEC/ISO standard binary prefixes, so 1024 will be formatted as 1KiB. Set prefix to `bpColloquial` to use the colloquial names from the SI standard (e.g. k for 1000 being reused as 1024).
`includeSpace` can be set to true to include the (SI preferred) space between the number and the unit (e.g. 1 KiB).
See also:
* [strformat module](strformat) for string interpolation and formatting
**Example:**
```
doAssert formatSize((1'i64 shl 31) + (300'i64 shl 20)) == "2.293GiB"
doAssert formatSize((2.234*1024*1024).int) == "2.234MiB"
doAssert formatSize(4096, includeSpace = true) == "4 KiB"
doAssert formatSize(4096, prefix = bpColloquial, includeSpace = true) == "4 kB"
doAssert formatSize(4096) == "4KiB"
doAssert formatSize(5_378_934, prefix = bpColloquial, decimalSep = ',') == "5,13MB"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2541) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2541)
```
proc formatEng(f: BiggestFloat; precision: range[0 .. 32] = 10;
trim: bool = true; siPrefix: bool = false; unit: string = "";
decimalSep = '.'; useUnitSpace = false): string {...}{.noSideEffect,
raises: [], tags: [].}
```
Converts a floating point value `f` to a string using engineering notation.
Numbers in of the range -1000.0<f<1000.0 will be formatted without an exponent. Numbers outside of this range will be formatted as a significand in the range -1000.0<f<1000.0 and an exponent that will always be an integer multiple of 3, corresponding with the SI prefix scale k, M, G, T etc for numbers with an absolute value greater than 1 and m, μ, n, p etc for numbers with an absolute value less than 1.
The default configuration (`trim=true` and `precision=10`) shows the **shortest** form that precisely (up to a maximum of 10 decimal places) displays the value. For example, 4.100000 will be displayed as 4.1 (which is mathematically identical) whereas 4.1000003 will be displayed as 4.1000003.
If `trim` is set to true, trailing zeros will be removed; if false, the number of digits specified by `precision` will always be shown.
`precision` can be used to set the number of digits to be shown after the decimal point or (if `trim` is true) the maximum number of digits to be shown.
```
formatEng(0, 2, trim=false) == "0.00"
formatEng(0, 2) == "0"
formatEng(0.053, 0) == "53e-3"
formatEng(52731234, 2) == "52.73e6"
formatEng(-52731234, 2) == "-52.73e6"
```
If `siPrefix` is set to true, the number will be displayed with the SI prefix corresponding to the exponent. For example 4100 will be displayed as "4.1 k" instead of "4.1e3". Note that `u` is used for micro- in place of the greek letter mu (μ) as per ISO 2955. Numbers with an absolute value outside of the range 1e-18<f<1000e18 (1a<f<1000E) will be displayed with an exponent rather than an SI prefix, regardless of whether `siPrefix` is true.
If `useUnitSpace` is true, the provided unit will be appended to the string (with a space as required by the SI standard). This behaviour is slightly different to appending the unit to the result as the location of the space is altered depending on whether there is an exponent.
```
formatEng(4100, siPrefix=true, unit="V") == "4.1 kV"
formatEng(4.1, siPrefix=true, unit="V") == "4.1 V"
formatEng(4.1, siPrefix=true) == "4.1" # Note lack of space
formatEng(4100, siPrefix=true) == "4.1 k"
formatEng(4.1, siPrefix=true, unit="") == "4.1 " # Space with unit=""
formatEng(4100, siPrefix=true, unit="") == "4.1 k"
formatEng(4100) == "4.1e3"
formatEng(4100, unit="V") == "4.1e3 V"
formatEng(4100, unit="", useUnitSpace=true) == "4.1e3 " # Space with useUnitSpace=true
```
`decimalSep` is used as the decimal separator.
See also:
* [strformat module](strformat) for string interpolation and formatting
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2597) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2597)
```
proc addf(s: var string; formatstr: string; a: varargs[string, `$`]) {...}{.
noSideEffect, gcsafe, extern: "nsuAddf", raises: [ValueError], tags: [].}
```
The same as `add(s, formatstr % a)`, but more efficient. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2748) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2748)
```
proc `%`(formatstr: string; a: openArray[string]): string {...}{.noSideEffect,
gcsafe, extern: "nsuFormatOpenArray", raises: [ValueError], tags: [].}
```
Interpolates a format string with the values from `a`.
The substitution operator performs string substitutions in `formatstr` and returns a modified `formatstr`. This is often called string interpolation.
This is best explained by an example:
```
"$1 eats $2." % ["The cat", "fish"]
```
Results in:
```
"The cat eats fish."
```
The substitution variables (the thing after the `$`) are enumerated from 1 to `a.len`. To produce a verbatim `$`, use `$$`. The notation `$#` can be used to refer to the next substitution variable:
```
"$# eats $#." % ["The cat", "fish"]
```
Substitution variables can also be words (that is `[A-Za-z_]+[A-Za-z0-9_]*`) in which case the arguments in `a` with even indices are keys and with odd indices are the corresponding values. An example:
```
"$animal eats $food." % ["animal", "The cat", "food", "fish"]
```
Results in:
```
"The cat eats fish."
```
The variables are compared with `cmpIgnoreStyle`. `ValueError` is raised if an ill-formed format string has been passed to the `%` operator.
See also:
* [strformat module](strformat) for string interpolation and formatting
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2811) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2811)
```
proc `%`(formatstr, a: string): string {...}{.noSideEffect, gcsafe,
extern: "nsuFormatSingleElem", raises: [ValueError], tags: [].}
```
This is the same as `formatstr % [a]` (see [% proc](#%25,string,openArray%5Bstring%5D)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2859) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2859)
```
proc format(formatstr: string; a: varargs[string, `$`]): string {...}{.noSideEffect,
gcsafe, extern: "nsuFormatVarargs", raises: [ValueError], tags: [].}
```
This is the same as `formatstr % a` (see [% proc](#%25,string,openArray%5Bstring%5D)) except that it supports auto stringification.
See also:
* [strformat module](strformat) for string interpolation and formatting
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2866) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2866)
```
proc strip(s: string; leading = true; trailing = true;
chars: set[char] = Whitespace): string {...}{.noSideEffect, gcsafe,
extern: "nsuStrip", raises: [], tags: [].}
```
Strips leading or trailing `chars` (default: whitespace characters) from `s` and returns the resulting string.
If `leading` is true (default), leading `chars` are stripped. If `trailing` is true (default), trailing `chars` are stripped. If both are false, the string is returned unchanged.
See also:
* [stripLineEnd proc](#stripLineEnd,string)
**Example:**
```
let a = " vhellov "
let b = strip(a)
doAssert b == "vhellov"
doAssert a.strip(leading = false) == " vhellov"
doAssert a.strip(trailing = false) == "vhellov "
doAssert b.strip(chars = {'v'}) == "hello"
doAssert b.strip(leading = false, chars = {'v'}) == "vhello"
let c = "blaXbla"
doAssert c.strip(chars = {'b', 'a'}) == "laXbl"
doAssert c.strip(chars = {'b', 'a', 'l'}) == "X"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2878) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2878)
```
proc stripLineEnd(s: var string) {...}{.raises: [], tags: [].}
```
Returns `s` stripped from one of these suffixes: `\r, \n, \r\n, \f, \v` (at most once instance). For example, can be useful in conjunction with `osproc.execCmdEx`. aka: chomp **Example:**
```
var s = "foo\n\n"
s.stripLineEnd
doAssert s == "foo\n"
s = "foo\r\n"
s.stripLineEnd
doAssert s == "foo"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2914) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2914)
```
proc isEmptyOrWhitespace(s: string): bool {...}{.noSideEffect, gcsafe,
extern: "nsuIsEmptyOrWhitespace", raises: [], tags: [].}
```
Checks if `s` is empty or consists entirely of whitespace characters. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2974) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2974) Iterators
---------
```
iterator split(s: string; sep: char; maxsplit: int = -1): string {...}{.raises: [],
tags: [].}
```
Splits the string `s` into substrings using a single separator.
Substrings are separated by the character `sep`. The code:
```
for word in split(";;this;is;an;;example;;;", ';'):
writeLine(stdout, word)
```
Results in:
```
""
""
"this"
"is"
"an"
""
"example"
""
""
""
```
See also:
* [rsplit iterator](#rsplit.i,string,char,int)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [split proc](#split,string,char,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L437) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L437)
```
iterator split(s: string; seps: set[char] = Whitespace; maxsplit: int = -1): string {...}{.
raises: [], tags: [].}
```
Splits the string `s` into substrings using a group of separators.
Substrings are separated by a substring containing only `seps`.
```
for word in split("this\lis an\texample"):
writeLine(stdout, word)
```
...generates this output:
```
"this"
"is"
"an"
"example"
```
And the following code:
```
for word in split("this:is;an$example", {';', ':', '$'}):
writeLine(stdout, word)
```
...produces the same output as the first example. The code:
```
let date = "2012-11-20T22:08:08.398990"
let separators = {' ', '-', ':', 'T'}
for number in split(date, separators):
writeLine(stdout, number)
```
...results in:
```
"2012"
"11"
"20"
"22"
"08"
"08.398990"
```
See also:
* [rsplit iterator](#rsplit.i,string,set%5Bchar%5D,int)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [split proc](#split,string,set%5Bchar%5D,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L468) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L468)
```
iterator split(s: string; sep: string; maxsplit: int = -1): string {...}{.raises: [],
tags: [].}
```
Splits the string `s` into substrings using a string separator.
Substrings are separated by the string `sep`. The code:
```
for word in split("thisDATAisDATAcorrupted", "DATA"):
writeLine(stdout, word)
```
Results in:
```
"this"
"is"
"corrupted"
```
See also:
* [rsplit iterator](#rsplit.i,string,string,int,bool)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [split proc](#split,string,string,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L517) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L517)
```
iterator rsplit(s: string; sep: char; maxsplit: int = -1): string {...}{.raises: [],
tags: [].}
```
Splits the string `s` into substrings from the right using a string separator. Works exactly the same as [split iterator](#split.i,string,char,int) except in reverse order.
```
for piece in "foo:bar".rsplit(':'):
echo piece
```
Results in:
```
"bar"
"foo"
```
Substrings are separated from the right by the char `sep`.
See also:
* [split iterator](#split.i,string,char,int)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [rsplit proc](#rsplit,string,char,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L566) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L566)
```
iterator rsplit(s: string; seps: set[char] = Whitespace; maxsplit: int = -1): string {...}{.
raises: [], tags: [].}
```
Splits the string `s` into substrings from the right using a string separator. Works exactly the same as [split iterator](#split.i,string,char,int) except in reverse order.
```
for piece in "foo bar".rsplit(WhiteSpace):
echo piece
```
Results in:
```
"bar"
"foo"
```
Substrings are separated from the right by the set of chars `seps`
See also:
* [split iterator](#split.i,string,set%5Bchar%5D,int)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [rsplit proc](#rsplit,string,set%5Bchar%5D,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L591) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L591)
```
iterator rsplit(s: string; sep: string; maxsplit: int = -1;
keepSeparators: bool = false): string {...}{.raises: [], tags: [].}
```
Splits the string `s` into substrings from the right using a string separator. Works exactly the same as [split iterator](#split.i,string,string,int) except in reverse order.
```
for piece in "foothebar".rsplit("the"):
echo piece
```
Results in:
```
"bar"
"foo"
```
Substrings are separated from the right by the string `sep`
See also:
* [split iterator](#split.i,string,string,int)
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [rsplit proc](#rsplit,string,string,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L616) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L616)
```
iterator splitLines(s: string; keepEol = false): string {...}{.raises: [], tags: [].}
```
Splits the string `s` into its containing lines.
Every [character literal](manual#lexical-analysis-character-literals) newline combination (CR, LF, CR-LF) is supported. The result strings contain no trailing end of line characters unless parameter `keepEol` is set to `true`.
Example:
```
for line in splitLines("\nthis\nis\nan\n\nexample\n"):
writeLine(stdout, line)
```
Results in:
```
""
"this"
"is"
"an"
""
"example"
""
```
See also:
* [splitWhitespace iterator](#splitWhitespace.i,string,int)
* [splitLines proc](#splitLines,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L641) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L641)
```
iterator splitWhitespace(s: string; maxsplit: int = -1): string {...}{.raises: [],
tags: [].}
```
Splits the string `s` at whitespace stripping leading and trailing whitespace if necessary. If `maxsplit` is specified and is positive, no more than `maxsplit` splits is made.
The following code:
```
let s = " foo \t bar baz "
for ms in [-1, 1, 2, 3]:
echo "------ maxsplit = ", ms, ":"
for item in s.splitWhitespace(maxsplit=ms):
echo '"', item, '"'
```
...results in:
```
------ maxsplit = -1:
"foo"
"bar"
"baz"
------ maxsplit = 1:
"foo"
"bar baz "
------ maxsplit = 2:
"foo"
"bar"
"baz "
------ maxsplit = 3:
"foo"
"bar"
"baz"
```
See also:
* [splitLines iterator](#splitLines.i,string)
* [splitWhitespace proc](#splitWhitespace,string,int)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L690) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L690)
```
iterator tokenize(s: string; seps: set[char] = Whitespace): tuple[token: string,
isSep: bool] {...}{.raises: [], tags: [].}
```
Tokenizes the string `s` into substrings.
Substrings are separated by a substring containing only `seps`. Example:
```
for word in tokenize(" this is an example "):
writeLine(stdout, word)
```
Results in:
```
(" ", true)
("this", false)
(" ", true)
("is", false)
(" ", true)
("an", false)
(" ", true)
("example", false)
(" ", true)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strutils.nim#L2940) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strutils.nim#L2940) Exports
-------
[toLower](unicode#toLower,string), [toLower](unicode#toLower,Rune), [toUpper](unicode#toUpper,Rune), [toUpper](unicode#toUpper,string)
| programming_docs |
nim uri uri
===
This module implements URI parsing as specified by RFC 3986.
A Uniform Resource Identifier (URI) provides a simple and extensible means for identifying a resource. A URI can be further classified as a locator, a name, or both. The term “Uniform Resource Locator” (URL) refers to the subset of URIs.
Basic usage
-----------
### Combine URIs
```
import uri
let host = parseUri("https://nim-lang.org")
let blog = "/blog.html"
let bloguri = host / blog
assert $host == "https://nim-lang.org"
assert $bloguri == "https://nim-lang.org/blog.html"
```
### Access URI item
```
import uri
let res = parseUri("sftp://127.0.0.1:4343")
if isAbsolute(res):
assert res.port == "4343"
else:
echo "Wrong format"
```
### Data URI Base64
```
doAssert getDataUri("Hello World", "text/plain") == "data:text/plain;charset=utf-8;base64,SGVsbG8gV29ybGQ="
doAssert getDataUri("Nim", "text/plain") == "data:text/plain;charset=utf-8;base64,Tmlt"
```
Imports
-------
<since>, <strutils>, <parseutils>, <base64> Types
-----
```
Url = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L54)
```
Uri = object
scheme*, username*, password*: string
hostname*, port*, path*, query*, anchor*: string
opaque*: bool
isIpv6: bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L56) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L56) Procs
-----
```
proc getDataUri(data, mime: string; encoding = "utf-8"): string {...}{.raises: [],
tags: [].}
```
Convenience proc for `base64.encode` returns a standard Base64 Data URI (RFC-2397)
**See also:**
* <mimetypes> for `mime` argument
* <https://tools.ietf.org/html/rfc2397>
* <https://en.wikipedia.org/wiki/Data_URI_scheme>
**Example:**
```
static: doAssert getDataUri("Nim", "text/plain") == "data:text/plain;charset=utf-8;base64,Tmlt"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L496) Funcs
-----
```
func encodeUrl(s: string; usePlus = true): string {...}{.raises: [], tags: [].}
```
Encodes a URL according to RFC3986.
This means that characters in the set `{'a'..'z', 'A'..'Z', '0'..'9', '-', '.', '_', '~'}` are carried over to the result. All other characters are encoded as `%xx` where `xx` denotes its hexadecimal value.
As a special rule, when the value of `usePlus` is true, spaces are encoded as `+` instead of `%20`.
**See also:**
* [decodeUrl func](#decodeUrl,string)
**Example:**
```
assert encodeUrl("https://nim-lang.org") == "https%3A%2F%2Fnim-lang.org"
assert encodeUrl("https://nim-lang.org/this is a test") == "https%3A%2F%2Fnim-lang.org%2Fthis+is+a+test"
assert encodeUrl("https://nim-lang.org/this is a test", false) == "https%3A%2F%2Fnim-lang.org%2Fthis%20is%20a%20test"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L62)
```
func decodeUrl(s: string; decodePlus = true): string {...}{.raises: [], tags: [].}
```
Decodes a URL according to RFC3986.
This means that any `%xx` (where `xx` denotes a hexadecimal value) are converted to the character with ordinal number `xx`, and every other character is carried over. If `xx` is not a valid hexadecimal value, it is left intact.
As a special rule, when the value of `decodePlus` is true, `+` characters are converted to a space.
**See also:**
* [encodeUrl func](#encodeUrl,string)
**Example:**
```
assert decodeUrl("https%3A%2F%2Fnim-lang.org") == "https://nim-lang.org"
assert decodeUrl("https%3A%2F%2Fnim-lang.org%2Fthis+is+a+test") == "https://nim-lang.org/this is a test"
assert decodeUrl("https%3A%2F%2Fnim-lang.org%2Fthis%20is%20a%20test",
false) == "https://nim-lang.org/this is a test"
assert decodeUrl("abc%xyz") == "abc%xyz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L91)
```
func encodeQuery(query: openArray[(string, string)]; usePlus = true;
omitEq = true): string {...}{.raises: [], tags: [].}
```
Encodes a set of (key, value) parameters into a URL query string.
Every (key, value) pair is URL-encoded and written as `key=value`. If the value is an empty string then the `=` is omitted, unless `omitEq` is false. The pairs are joined together by a `&` character.
The `usePlus` parameter is passed down to the `encodeUrl` function that is used for the URL encoding of the string values.
**See also:**
* [encodeUrl func](#encodeUrl,string)
**Example:**
```
assert encodeQuery({: }) == ""
assert encodeQuery({"a": "1", "b": "2"}) == "a=1&b=2"
assert encodeQuery({"a": "1", "b": ""}) == "a=1&b"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L128)
```
func initUri(): Uri {...}{.raises: [], tags: [].}
```
Initializes a URI with `scheme`, `username`, `password`, `hostname`, `port`, `path`, `query` and `anchor`.
**See also:**
* [Uri type](#Uri) for available fields in the URI type
**Example:**
```
var uri2: Uri
assert initUri() == uri2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L202)
```
func initUri(isIpv6: bool): Uri {...}{.raises: [], tags: [].}
```
Initializes a URI with `scheme`, `username`, `password`, `hostname`, `port`, `path`, `query`, `anchor` and `isIpv6`.
**See also:**
* [Uri type](#Uri) for available fields in the URI type
**Example:**
```
var uri2 = initUri(isIpv6 = true)
uri2.scheme = "tcp"
uri2.hostname = "2001:0db8:85a3:0000:0000:8a2e:0370:7334"
uri2.port = "8080"
assert $uri2 == "tcp://[2001:0db8:85a3:0000:0000:8a2e:0370:7334]:8080"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L214)
```
func parseUri(uri: string; result: var Uri) {...}{.raises: [], tags: [].}
```
Parses a URI. The `result` variable will be cleared before.
**See also:**
* [Uri type](#Uri) for available fields in the URI type
* [initUri func](#initUri) for initializing a URI
**Example:**
```
var res = initUri()
parseUri("https://nim-lang.org/docs/manual.html", res)
assert res.scheme == "https"
assert res.hostname == "nim-lang.org"
assert res.path == "/docs/manual.html"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L236)
```
func parseUri(uri: string): Uri {...}{.raises: [], tags: [].}
```
Parses a URI and returns it.
**See also:**
* [Uri type](#Uri) for available fields in the URI type
**Example:**
```
let res = parseUri("ftp://Username:Password@Hostname")
assert res.username == "Username"
assert res.password == "Password"
assert res.scheme == "ftp"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L284) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L284)
```
func combine(base: Uri; reference: Uri): Uri {...}{.raises: [], tags: [].}
```
Combines a base URI with a reference URI.
This uses the algorithm specified in [section 5.2.2 of RFC 3986](http://tools.ietf.org/html/rfc3986#section-5.2.2).
This means that the slashes inside the base URIs path as well as reference URIs path affect the resulting URI.
**See also:**
* [/ func](#/,Uri,string) for building URIs
**Example:**
```
let foo = combine(parseUri("https://nim-lang.org/foo/bar"), parseUri("/baz"))
assert foo.path == "/baz"
let bar = combine(parseUri("https://nim-lang.org/foo/bar"), parseUri("baz"))
assert bar.path == "/foo/baz"
let qux = combine(parseUri("https://nim-lang.org/foo/bar/"), parseUri("baz"))
assert qux.path == "/foo/bar/baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L338) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L338)
```
func combine(uris: varargs[Uri]): Uri {...}{.raises: [], tags: [].}
```
Combines multiple URIs together.
**See also:**
* [/ func](#/,Uri,string) for building URIs
**Example:**
```
let foo = combine(parseUri("https://nim-lang.org/"), parseUri("docs/"),
parseUri("manual.html"))
assert foo.hostname == "nim-lang.org"
assert foo.path == "/docs/manual.html"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L389) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L389)
```
func isAbsolute(uri: Uri): bool {...}{.raises: [], tags: [].}
```
Returns true if URI is absolute, false otherwise. **Example:**
```
let foo = parseUri("https://nim-lang.org")
assert isAbsolute(foo) == true
let bar = parseUri("nim-lang")
assert isAbsolute(bar) == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L403) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L403)
```
func `/`(x: Uri; path: string): Uri {...}{.raises: [], tags: [].}
```
Concatenates the path specified to the specified URIs path.
Contrary to the [combine func](#combine,Uri,Uri) you do not have to worry about the slashes at the beginning and end of the path and URIs path respectively.
**See also:**
* [combine func](#combine,Uri,Uri)
**Example:**
```
let foo = parseUri("https://nim-lang.org/foo/bar") / "/baz"
assert foo.path == "/foo/bar/baz"
let bar = parseUri("https://nim-lang.org/foo/bar") / "baz"
assert bar.path == "/foo/bar/baz"
let qux = parseUri("https://nim-lang.org/foo/bar/") / "baz"
assert qux.path == "/foo/bar/baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L412) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L412)
```
func `?`(u: Uri; query: openArray[(string, string)]): Uri {...}{.raises: [], tags: [].}
```
Concatenates the query parameters to the specified URI object. **Example:**
```
let foo = parseUri("https://example.com") / "foo" ? {"bar": "qux"}
assert $foo == "https://example.com/foo?bar=qux"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L446) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L446)
```
func `$`(u: Uri): string {...}{.raises: [], tags: [].}
```
Returns the string representation of the specified URI object. **Example:**
```
let foo = parseUri("https://nim-lang.org")
assert $foo == "https://nim-lang.org"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/uri.nim#L454) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/uri.nim#L454)
nim marshal marshal
=======
This module contains procs for serialization and deserialization of arbitrary Nim data structures. The serialization format uses JSON.
**Restriction**: For objects their type is **not** serialized. This means essentially that it does not work if the object has some other runtime type than its compiletime type.
Basic usage
-----------
```
type
A = object of RootObj
B = object of A
f: int
var
a: ref A
b: ref B
new(b)
a = b
echo($$a[]) # produces "{}", not "{f: 0}"
# unmarshal
let c = to[B]("""{"f": 2}""")
assert typeof(c) is B
assert c.f == 2
# marshal
let s = $$c
assert s == """{"f": 2}"""
```
**Note**: The `to` and `$$` operations are available at compile-time!
See also
--------
* [streams module](streams)
* [json module](json)
Imports
-------
<streams>, <typeinfo>, <json>, <intsets>, <tables>, <unicode> Procs
-----
```
proc load[T](s: Stream; data: var T)
```
Loads `data` from the stream `s`. Raises `IOError` in case of an error. **Example:**
```
import marshal, streams
var s = newStringStream("[1, 3, 5]")
var a: array[3, int]
load(s, a)
assert a == [1, 3, 5]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/marshal.nim#L279) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/marshal.nim#L279)
```
proc store[T](s: Stream; data: T)
```
Stores `data` into the stream `s`. Raises `IOError` in case of an error. **Example:**
```
import marshal, streams
var s = newStringStream("")
var a = [1, 3, 5]
store(s, a)
s.setPosition(0)
assert s.readAll() == "[1, 3, 5]"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/marshal.nim#L291) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/marshal.nim#L291)
```
proc `$$`[T](x: T): string
```
Returns a string representation of `x` (serialization, marshalling).
**Note:** to serialize `x` to JSON use `$(%x)` from the `json` module.
**Example:**
```
type
Foo = object
id: int
bar: string
let x = Foo(id: 1, bar: "baz")
## serialize:
let y = $$x
assert y == """{"id": 1, "bar": "baz"}"""
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/marshal.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/marshal.nim#L306)
```
proc to[T](data: string): T
```
Reads data and transforms it to a type `T` (deserialization, unmarshalling). **Example:**
```
type
Foo = object
id: int
bar: string
let y = """{"id": 1, "bar": "baz"}"""
assert typeof(y) is string
## deserialize to type 'Foo':
let z = y.to[:Foo]
assert typeof(z) is Foo
assert z.id == 1
assert z.bar == "baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/marshal.nim#L327) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/marshal.nim#L327)
nim jsconsole jsconsole
=========
Wrapper for the `console` object for the [JavaScript backend](backends#backends-the-javascript-target).
Types
-----
```
Console = ref object of RootObj
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L16) Vars
----
```
console: Console
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L70) Procs
-----
```
proc log(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/log> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L18)
```
proc debug(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/debug> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L21)
```
proc info(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/info> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L24)
```
proc error(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/error> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L27)
```
proc trace(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/trace> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L34)
```
proc warn(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/warn> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L37)
```
proc clear(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/clear> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L40)
```
proc count(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/count> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L43)
```
proc countReset(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/countReset> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L46)
```
proc group(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/group> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L49)
```
proc groupCollapsed(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/en-US/docs/Web/API/Console/groupCollapsed> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L52)
```
proc groupEnd(console: Console) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/groupEnd> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L55) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L55)
```
proc time(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/time> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L58)
```
proc timeEnd(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/timeEnd> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L61)
```
proc timeLog(console: Console; label = "".cstring) {...}{.importcpp.}
```
<https://developer.mozilla.org/docs/Web/API/Console/timeLog> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L64)
```
proc table(console: Console) {...}{.importcpp, varargs.}
```
<https://developer.mozilla.org/docs/Web/API/Console/table> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L67) Templates
---------
```
template exception(console: Console; args: varargs[untyped])
```
Alias for `console.error()`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/js/jsconsole.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/js/jsconsole.nim#L30)
nim lexbase lexbase
=======
This module implements a base object of a lexer with efficient buffer handling. Only at line endings checks are necessary if the buffer needs refilling.
Imports
-------
<strutils>, <streams> Types
-----
```
BaseLexer = object of RootObj
bufpos*: int ## the current position within the buffer
buf*: string ## the buffer itself
input: Stream ## the input stream
lineNumber*: int ## the current line number
sentinel: int
lineStart: int
offsetBase*: int
refillChars: set[char]
```
the base lexer. Inherit your lexer from this object. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L28) Consts
------
```
EndOfFile = '\x00'
```
end of file marker [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L18)
```
NewLines = {'\c', '\n'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L19) Procs
-----
```
proc close(L: var BaseLexer) {...}{.raises: [Exception, IOError, OSError],
tags: [WriteIOEffect].}
```
closes the base lexer. This closes `L`'s associated stream too. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L39)
```
proc handleCR(L: var BaseLexer; pos: int): int {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Call this if you scanned over 'c' in the buffer; it returns the position to continue the scanning from. `pos` must be the position of the 'c'. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L103)
```
proc handleLF(L: var BaseLexer; pos: int): int {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Call this if you scanned over 'L' in the buffer; it returns the position to continue the scanning from. `pos` must be the position of the 'L'. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L114)
```
proc handleRefillChar(L: var BaseLexer; pos: int): int {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Call this if a terminator character other than a new line is scanned at `pos`; it returns the position to continue the scanning from. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L123) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L123)
```
proc open(L: var BaseLexer; input: Stream; bufLen: int = 8192;
refillChars: set[char] = NewLines) {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
inits the BaseLexer with a stream to read from. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L134)
```
proc getColNumber(L: BaseLexer; pos: int): int {...}{.raises: [], tags: [].}
```
retrieves the current column. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L150)
```
proc getCurrentLine(L: BaseLexer; marker: bool = true): string {...}{.raises: [],
tags: [].}
```
retrieves the current line. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/lexbase.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/lexbase.nim#L154)
| programming_docs |
nim os os
==
This module contains basic operating system facilities like retrieving environment variables, reading command line arguments, working with directories, running shell commands, etc.
```
import os
let myFile = "/path/to/my/file.nim"
let pathSplit = splitPath(myFile)
assert pathSplit.head == "/path/to/my"
assert pathSplit.tail == "file.nim"
assert parentDir(myFile) == "/path/to/my"
let fileSplit = splitFile(myFile)
assert fileSplit.dir == "/path/to/my"
assert fileSplit.name == "file"
assert fileSplit.ext == ".nim"
assert myFile.changeFileExt("c") == "/path/to/my/file.c"
```
>
> **See also:**
>
>
> * [osproc module](osproc) for process communication beyond [execShellCmd proc](#execShellCmd,string)
> * [parseopt module](parseopt) for command-line parser beyond [parseCmdLine proc](#parseCmdLine,string)
> * [uri module](uri)
> * [distros module](distros)
> * [dynlib module](dynlib)
> * [streams module](streams)
>
>
Imports
-------
<since>, <strutils>, <pathnorm>, <posix>, <times> Types
-----
```
ReadEnvEffect = object of ReadIOEffect
```
Effect that denotes a read from an environment variable. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L94)
```
WriteEnvEffect = object of WriteIOEffect
```
Effect that denotes a write to an environment variable. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L96)
```
ReadDirEffect = object of ReadIOEffect
```
Effect that denotes a read operation from the directory structure. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L99)
```
WriteDirEffect = object of WriteIOEffect
```
Effect that denotes a write operation to the directory structure. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L102)
```
OSErrorCode = distinct int32
```
Specifies an OS Error Code. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L106)
```
FilePermission = enum
fpUserExec, ## execute access for the file owner
fpUserWrite, ## write access for the file owner
fpUserRead, ## read access for the file owner
fpGroupExec, ## execute access for the group
fpGroupWrite, ## write access for the group
fpGroupRead, ## read access for the group
fpOthersExec, ## execute access for others
fpOthersWrite, ## write access for others
fpOthersRead ## read access for others
```
File access permission, modelled after UNIX.
See also:
* [getFilePermissions](#getFilePermissions,string)
* [setFilePermissions](#setFilePermissions,string,set%5BFilePermission%5D)
* [FileInfo object](#FileInfo)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1590) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1590)
```
PathComponent = enum
pcFile, ## path refers to a file
pcLinkToFile, ## path refers to a symbolic link to a file
pcDir, ## path refers to a directory
pcLinkToDir ## path refers to a symbolic link to a directory
```
Enumeration specifying a path component.
See also:
* [walkDirRec iterator](#walkDirRec.i,string)
* [FileInfo object](#FileInfo)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2046) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2046)
```
DeviceId = Dev
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3060) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3060)
```
FileId = Ino
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3061) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3061)
```
FileInfo = object
id*: tuple[device: DeviceId, file: FileId] ## Device and file id.
kind*: PathComponent ## Kind of file object - directory, symlink, etc.
size*: BiggestInt ## Size of file.
permissions*: set[FilePermission] ## File permissions
linkCount*: BiggestInt ## Number of hard links the file object has.
lastAccessTime*: times.Time ## Time file was last accessed.
lastWriteTime*: times.Time ## Time file was last modified/written to.
creationTime*: times.Time ## Time file was created. Not supported on all systems!
```
Contains information associated with a file object.
See also:
* [getFileInfo(handle) proc](#getFileInfo,FileHandle)
* [getFileInfo(file) proc](#getFileInfo,File)
* [getFileInfo(path) proc](#getFileInfo,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3064) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3064) Consts
------
```
invalidFilenameChars = {'/', '\\', ':', '*', '?', '\"', '<', '>', '|', '^',
'\x00'}
```
Characters that may produce invalid filenames across Linux, Windows, Mac, etc. You can check if your filename contains these char and strip them for safety. Mac bans `':'`, Linux bans `'/'`, Windows bans all others. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L54)
```
invalidFilenames = ["CON", "PRN", "AUX", "NUL", "COM0", "COM1", "COM2", "COM3",
"COM4", "COM5", "COM6", "COM7", "COM8", "COM9", "LPT0",
"LPT1", "LPT2", "LPT3", "LPT4", "LPT5", "LPT6", "LPT7",
"LPT8", "LPT9"]
```
Filenames that may be invalid across Linux, Windows, Mac, etc. You can check if your filename match these and rename it for safety (Currently all invalid filenames are from Windows only). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L58)
```
doslikeFileSystem = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L7) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L7)
```
CurDir = '.'
```
The constant character used by the operating system to refer to the current directory.
For example: `'.'` for POSIX or `':'` for the classic Macintosh.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L10) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L10)
```
ParDir = ".."
```
The constant string used by the operating system to refer to the parent directory.
For example: `".."` for POSIX or `"::"` for the classic Macintosh.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L19)
```
DirSep = '/'
```
The character used by the operating system to separate pathname components, for example: `'/'` for POSIX, `':'` for the classic Macintosh, and `'\'` on Windows. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L27)
```
AltSep = '/'
```
An alternative character used by the operating system to separate pathname components, or the same as [DirSep](#DirSep) if only one separator character exists. This is set to `'/'` on Windows systems where [DirSep](#DirSep) is a backslash (`'\'`). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L36)
```
PathSep = ':'
```
The character conventionally used by the operating system to separate search patch components (as in PATH), such as `':'` for POSIX or `';'` for Windows. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L44)
```
FileSystemCaseSensitive = true
```
True if the file system is case sensitive, false otherwise. Used by [cmpPaths proc](#cmpPaths,string,string) to compare filenames properly. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L53)
```
ExeExt = ""
```
The file extension of native executables. For example: `""` for POSIX, `"exe"` on Windows (without a dot). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L60)
```
ScriptExt = ""
```
The file extension of a script file. For example: `""` for POSIX, `"bat"` on Windows. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L70)
```
DynlibFormat = "lib$1.so"
```
The format string to turn a filename into a DLL file (also called shared object on some operating systems). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L76)
```
ExtSep = '.'
```
The character which separates the base filename from the extension; for example, the `'.'` in `os.nim`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osseps.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osseps.nim#L89)
```
ExeExts = [""]
```
Platform specific file extension for executables. On Windows `["exe", "cmd", "bat"]`, on Posix `[""]`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1178) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1178) Procs
-----
```
proc normalizePathEnd(path: var string; trailingSep = false) {...}{.raises: [],
tags: [].}
```
Ensures `path` has exactly 0 or 1 trailing `DirSep`, depending on `trailingSep`, and taking care of edge cases: it preservers whether a path is absolute or relative, and makes sure trailing sep is `DirSep`, not `AltSep`. Trailing `/.` are compressed, see examples. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L112)
```
proc normalizePathEnd(path: string; trailingSep = false): string {...}{.raises: [],
tags: [].}
```
outplace overload **Example:**
```
when defined(posix):
assert normalizePathEnd("/lib//.//", trailingSep = true) == "/lib/"
assert normalizePathEnd("lib/./.", trailingSep = false) == "lib"
assert normalizePathEnd(".//./.", trailingSep = false) == "."
assert normalizePathEnd("", trailingSep = true) == "" # not / !
assert normalizePathEnd("/", trailingSep = false) == "/" # not "" !
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L135) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L135)
```
proc joinPath(head, tail: string): string {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Joins two directory names to one.
returns normalized path concatenation of `head` and `tail`, preserving whether or not `tail` has a trailing slash (or, if tail if empty, whether head has one).
See also:
* [joinPath(varargs) proc](#joinPath,varargs%5Bstring%5D)
* [/ proc](#/,string,string)
* [splitPath proc](#splitPath,string)
* [uri.combine proc](uri#combine,Uri,Uri)
* [uri./ proc](uri#/,Uri,string)
**Example:**
```
when defined(posix):
assert joinPath("usr", "lib") == "usr/lib"
assert joinPath("usr", "lib/") == "usr/lib/"
assert joinPath("usr", "") == "usr"
assert joinPath("usr/", "") == "usr/"
assert joinPath("", "") == ""
assert joinPath("", "lib") == "lib"
assert joinPath("", "/lib") == "/lib"
assert joinPath("usr/", "/lib") == "usr/lib"
assert joinPath("usr/lib", "../bin") == "usr/bin"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L159)
```
proc joinPath(parts: varargs[string]): string {...}{.noSideEffect, gcsafe,
extern: "nos$1OpenArray", raises: [], tags: [].}
```
The same as [joinPath(head, tail) proc](#joinPath,string,string), but works with any number of directory parts.
You need to pass at least one element or the proc will assert in debug builds and crash on release builds.
See also:
* [joinPath(head, tail) proc](#joinPath,string,string)
* [/ proc](#/,string,string)
* [/../ proc](#/../,string,string)
* [splitPath proc](#splitPath,string)
**Example:**
```
when defined(posix):
assert joinPath("a") == "a"
assert joinPath("a", "b", "c") == "a/b/c"
assert joinPath("usr/lib", "../../var", "log") == "var/log"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L203)
```
proc `/`(head, tail: string): string {...}{.noSideEffect, raises: [], tags: [].}
```
The same as [joinPath(head, tail) proc](#joinPath,string,string).
See also:
* [/../ proc](#/../,string,string)
* [joinPath(head, tail) proc](#joinPath,string,string)
* [joinPath(varargs) proc](#joinPath,varargs%5Bstring%5D)
* [splitPath proc](#splitPath,string)
* [uri.combine proc](uri#combine,Uri,Uri)
* [uri./ proc](uri#/,Uri,string)
**Example:**
```
when defined(posix):
assert "usr" / "" == "usr"
assert "" / "lib" == "lib"
assert "" / "/lib" == "/lib"
assert "usr/" / "/lib/" == "usr/lib/"
assert "usr" / "lib" / "../bin" == "usr/bin"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L229)
```
proc splitPath(path: string): tuple[head, tail: string] {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Splits a directory into `(head, tail)` tuple, so that `head / tail == path` (except for edge cases like "/usr").
See also:
* [joinPath(head, tail) proc](#joinPath,string,string)
* [joinPath(varargs) proc](#joinPath,varargs%5Bstring%5D)
* [/ proc](#/,string,string)
* [/../ proc](#/../,string,string)
* [relativePath proc](#relativePath,string,string)
**Example:**
```
assert splitPath("usr/local/bin") == ("usr/local", "bin")
assert splitPath("usr/local/bin/") == ("usr/local/bin", "")
assert splitPath("/bin/") == ("/bin", "")
when (NimMajor, NimMinor) <= (1, 0):
assert splitPath("/bin") == ("", "bin")
else:
assert splitPath("/bin") == ("/", "bin")
assert splitPath("bin") == ("", "bin")
assert splitPath("") == ("", "")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L249) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L249)
```
proc isAbsolute(path: string): bool {...}{.gcsafe, noSideEffect, extern: "nos$1",
raises: [], tags: [].}
```
Checks whether a given `path` is absolute.
On Windows, network paths are considered absolute too.
**Example:**
```
assert not "".isAbsolute
assert not ".".isAbsolute
when defined(posix):
assert "/".isAbsolute
assert not "a/".isAbsolute
assert "/a/".isAbsolute
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L288) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L288)
```
proc relativePath(path, base: string; sep = DirSep): string {...}{.gcsafe,
extern: "nos$1", raises: [Exception], tags: [RootEffect].}
```
Converts `path` to a path relative to `base`.
The `sep` (default: [DirSep](#DirSep)) is used for the path normalizations, this can be useful to ensure the relative path only contains `'/'` so that it can be used for URL constructions.
On windows, if a root of `path` and a root of `base` are different, returns `path` as is because it is impossible to make a relative path. That means an absolute path can be returned.
See also:
* [splitPath proc](#splitPath,string)
* [parentDir proc](#parentDir,string)
* [tailDir proc](#tailDir,string)
**Example:**
```
assert relativePath("/Users/me/bar/z.nim", "/Users/other/bad", '/') == "../../me/bar/z.nim"
assert relativePath("/Users/me/bar/z.nim", "/Users/other", '/') == "../me/bar/z.nim"
assert relativePath("/Users///me/bar//z.nim", "//Users/", '/') == "me/bar/z.nim"
assert relativePath("/Users/me/bar/z.nim", "/Users/me", '/') == "bar/z.nim"
assert relativePath("", "/users/moo", '/') == ""
assert relativePath("foo", ".", '/') == "foo"
assert relativePath("foo", "foo", '/') == "."
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L378) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L378)
```
proc isRelativeTo(path: string; base: string): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
Returns true if `path` is relative to `base`. **Example:**
```
doAssert isRelativeTo("./foo//bar", "foo")
doAssert isRelativeTo("foo/bar", ".")
doAssert isRelativeTo("/foo/bar.nim", "/foo/bar.nim")
doAssert not isRelativeTo("foo/bar.nims", "foo/bar.nim")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L467) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L467)
```
proc parentDir(path: string): string {...}{.noSideEffect, gcsafe, extern: "nos$1",
raises: [], tags: [].}
```
Returns the parent directory of `path`.
This is similar to `splitPath(path).head` when `path` doesn't end in a dir separator, but also takes care of path normalizations. The remainder can be obtained with [lastPathPart(path) proc](#lastPathPart,string).
See also:
* [relativePath proc](#relativePath,string,string)
* [splitPath proc](#splitPath,string)
* [tailDir proc](#tailDir,string)
* [parentDirs iterator](#parentDirs.i,string)
**Example:**
```
assert parentDir("") == ""
when defined(posix):
assert parentDir("/usr/local/bin") == "/usr/local"
assert parentDir("foo/bar//") == "foo"
assert parentDir("//foo//bar//.") == "/foo"
assert parentDir("./foo") == "."
assert parentDir("/./foo//./") == "/"
assert parentDir("a//./") == "."
assert parentDir("a/b/c/..") == "a"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L486) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L486)
```
proc tailDir(path: string): string {...}{.noSideEffect, gcsafe, extern: "nos$1",
raises: [], tags: [].}
```
Returns the tail part of `path`.
See also:
* [relativePath proc](#relativePath,string,string)
* [splitPath proc](#splitPath,string)
* [parentDir proc](#parentDir,string)
**Example:**
```
assert tailDir("/bin") == "bin"
assert tailDir("bin") == ""
assert tailDir("bin/") == ""
assert tailDir("/usr/local/bin") == "usr/local/bin"
assert tailDir("//usr//local//bin//") == "usr//local//bin//"
assert tailDir("./usr/local/bin") == "usr/local/bin"
assert tailDir("usr/local/bin") == "local/bin"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L522) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L522)
```
proc isRootDir(path: string): bool {...}{.noSideEffect, gcsafe, extern: "nos$1",
raises: [], tags: [].}
```
Checks whether a given `path` is a root directory. **Example:**
```
assert isRootDir("")
assert isRootDir(".")
assert isRootDir("/")
assert isRootDir("a")
assert not isRootDir("/a")
assert not isRootDir("a/b/c")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L547) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L547)
```
proc `/../`(head, tail: string): string {...}{.noSideEffect, raises: [], tags: [].}
```
The same as `parentDir(head) / tail`, unless there is no parent directory. Then `head / tail` is performed instead.
See also:
* [/ proc](#/,string,string)
* [parentDir proc](#parentDir,string)
**Example:**
```
when defined(posix):
assert "a/b/c" /../ "d/e" == "a/b/d/e"
assert "a" /../ "d/e" == "a/d/e"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L612) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L612)
```
proc searchExtPos(path: string): int {...}{.raises: [], tags: [].}
```
Returns index of the `'.'` char in `path` if it signifies the beginning of extension. Returns -1 otherwise.
See also:
* [splitFile proc](#splitFile,string)
* [extractFilename proc](#extractFilename,string)
* [lastPathPart proc](#lastPathPart,string)
* [changeFileExt proc](#changeFileExt,string,string)
* [addFileExt proc](#addFileExt,string,string)
**Example:**
```
assert searchExtPos("a/b/c") == -1
assert searchExtPos("c.nim") == 1
assert searchExtPos("a/b/c.nim") == 5
assert searchExtPos("a.b.c.nim") == 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L634) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L634)
```
proc splitFile(path: string): tuple[dir, name, ext: string] {...}{.noSideEffect,
gcsafe, extern: "nos$1", raises: [], tags: [].}
```
Splits a filename into `(dir, name, extension)` tuple.
`dir` does not end in [DirSep](#DirSep) unless it's `/`. `extension` includes the leading dot.
If `path` has no extension, `ext` is the empty string. If `path` has no directory component, `dir` is the empty string. If `path` has no filename component, `name` and `ext` are empty strings.
See also:
* [searchExtPos proc](#searchExtPos,string)
* [extractFilename proc](#extractFilename,string)
* [lastPathPart proc](#lastPathPart,string)
* [changeFileExt proc](#changeFileExt,string,string)
* [addFileExt proc](#addFileExt,string,string)
**Example:**
```
var (dir, name, ext) = splitFile("usr/local/nimc.html")
assert dir == "usr/local"
assert name == "nimc"
assert ext == ".html"
(dir, name, ext) = splitFile("/usr/local/os")
assert dir == "/usr/local"
assert name == "os"
assert ext == ""
(dir, name, ext) = splitFile("/usr/local/")
assert dir == "/usr/local"
assert name == ""
assert ext == ""
(dir, name, ext) = splitFile("/tmp.txt")
assert dir == "/"
assert name == "tmp"
assert ext == ".txt"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L659) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L659)
```
proc extractFilename(path: string): string {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Extracts the filename of a given `path`.
This is the same as `name & ext` from [splitFile(path) proc](#splitFile,string).
See also:
* [searchExtPos proc](#searchExtPos,string)
* [splitFile proc](#splitFile,string)
* [lastPathPart proc](#lastPathPart,string)
* [changeFileExt proc](#changeFileExt,string,string)
* [addFileExt proc](#addFileExt,string,string)
**Example:**
```
assert extractFilename("foo/bar/") == ""
assert extractFilename("foo/bar") == "bar"
assert extractFilename("foo/bar.baz") == "bar.baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L712) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L712)
```
proc lastPathPart(path: string): string {...}{.noSideEffect, gcsafe, extern: "nos$1",
raises: [], tags: [].}
```
Like [extractFilename proc](#extractFilename,string), but ignores trailing dir separator; aka: baseName in some other languages.
See also:
* [searchExtPos proc](#searchExtPos,string)
* [splitFile proc](#splitFile,string)
* [extractFilename proc](#extractFilename,string)
* [changeFileExt proc](#changeFileExt,string,string)
* [addFileExt proc](#addFileExt,string,string)
**Example:**
```
assert lastPathPart("foo/bar/") == "bar"
assert lastPathPart("foo/bar") == "bar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L735) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L735)
```
proc changeFileExt(filename, ext: string): string {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Changes the file extension to `ext`.
If the `filename` has no extension, `ext` will be added. If `ext` == "" then any extension is removed.
`Ext` should be given without the leading `'.'`, because some filesystems may use a different character. (Although I know of none such beast.)
See also:
* [searchExtPos proc](#searchExtPos,string)
* [splitFile proc](#splitFile,string)
* [extractFilename proc](#extractFilename,string)
* [lastPathPart proc](#lastPathPart,string)
* [addFileExt proc](#addFileExt,string,string)
**Example:**
```
assert changeFileExt("foo.bar", "baz") == "foo.baz"
assert changeFileExt("foo.bar", "") == "foo"
assert changeFileExt("foo", "baz") == "foo.baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L753) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L753)
```
proc addFileExt(filename, ext: string): string {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Adds the file extension `ext` to `filename`, unless `filename` already has an extension.
`Ext` should be given without the leading `'.'`, because some filesystems may use a different character. (Although I know of none such beast.)
See also:
* [searchExtPos proc](#searchExtPos,string)
* [splitFile proc](#splitFile,string)
* [extractFilename proc](#extractFilename,string)
* [lastPathPart proc](#lastPathPart,string)
* [changeFileExt proc](#changeFileExt,string,string)
**Example:**
```
assert addFileExt("foo.bar", "baz") == "foo.bar"
assert addFileExt("foo.bar", "") == "foo.bar"
assert addFileExt("foo", "baz") == "foo.baz"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L779) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L779)
```
proc cmpPaths(pathA, pathB: string): int {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Compares two paths.
On a case-sensitive filesystem this is done case-sensitively otherwise case-insensitively. Returns:
0 if pathA == pathB
< 0 if pathA < pathB
> 0 if pathA > pathB
**Example:**
```
when defined(macosx):
assert cmpPaths("foo", "Foo") == 0
elif defined(posix):
assert cmpPaths("foo", "Foo") > 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L803) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L803)
```
proc unixToNativePath(path: string; drive = ""): string {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Converts an UNIX-like path to a native one.
On an UNIX system this does nothing. Else it converts `'/'`, `'.'`, `'..'` to the appropriate things.
On systems with a concept of "drives", `drive` is used to determine which drive label to use during absolute path conversion. `drive` defaults to the drive of the current working directory, and is ignored on systems that do not have a concept of "drives".
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L830) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L830)
```
proc `==`(err1, err2: OSErrorCode): bool {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L15)
```
proc `$`(err: OSErrorCode): string {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L16)
```
proc osErrorMsg(errorCode: OSErrorCode): string {...}{.raises: [], tags: [].}
```
Converts an OS error code into a human readable string.
The error code can be retrieved using the [osLastError proc](#osLastError).
If conversion fails, or `errorCode` is `0` then `""` will be returned.
On Windows, the `-d:useWinAnsi` compilation flag can be used to make this procedure use the non-unicode Win API calls to retrieve the message.
See also:
* [raiseOSError proc](#raiseOSError,OSErrorCode,string)
* [osLastError proc](#osLastError)
**Example:**
```
when defined(linux):
assert osErrorMsg(OSErrorCode(0)) == ""
assert osErrorMsg(OSErrorCode(1)) == "Operation not permitted"
assert osErrorMsg(OSErrorCode(2)) == "No such file or directory"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L18)
```
proc newOSError(errorCode: OSErrorCode; additionalInfo = ""): owned(ref OSError) {...}{.
noinline, raises: [], tags: [].}
```
Creates a new [OSError exception](system#OSError).
The `errorCode` will determine the message, [osErrorMsg proc](#osErrorMsg,OSErrorCode) will be used to get this message.
The error code can be retrieved using the [osLastError proc](#osLastError).
If the error code is `0` or an error message could not be retrieved, the message `unknown OS error` will be used.
See also:
* [osErrorMsg proc](#osErrorMsg,OSErrorCode)
* [osLastError proc](#osLastError)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L60)
```
proc raiseOSError(errorCode: OSErrorCode; additionalInfo = "") {...}{.noinline,
raises: [OSError], tags: [].}
```
Raises an [OSError exception](system#OSError).
Read the description of the [newOSError proc](#newOSError,OSErrorCode,string) to learn how the exception object is created.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L89)
```
proc osLastError(): OSErrorCode {...}{.sideEffect, raises: [], tags: [].}
```
Retrieves the last operating system error code.
This procedure is useful in the event when an OS call fails. In that case this procedure will return the error code describing the reason why the OS call failed. The `OSErrorMsg` procedure can then be used to convert this code into a string.
**Warning**: The behaviour of this procedure varies between Windows and POSIX systems. On Windows some OS calls can reset the error code to `0` causing this procedure to return `0`. It is therefore advised to call this procedure immediately after an OS call fails. On POSIX systems this is not a problem.
See also:
* [osErrorMsg proc](#osErrorMsg,OSErrorCode)
* [raiseOSError proc](#raiseOSError,OSErrorCode,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/oserr.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/oserr.nim#L97)
```
proc getEnv(key: string; default = ""): TaintedString {...}{.tags: [ReadEnvEffect],
raises: [].}
```
Returns the value of the environment variable named `key`.
If the variable does not exist, `""` is returned. To distinguish whether a variable exists or it's value is just `""`, call [existsEnv(key) proc](#existsEnv,string).
See also:
* [existsEnv proc](#existsEnv,string)
* [putEnv proc](#putEnv,string,string)
* [delEnv proc](#delEnv,string)
* [envPairs iterator](#envPairs.i)
**Example:**
```
assert getEnv("unknownEnv") == ""
assert getEnv("unknownEnv", "doesn't exist") == "doesn't exist"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osenv.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osenv.nim#L111)
```
proc existsEnv(key: string): bool {...}{.tags: [ReadEnvEffect], raises: [].}
```
Checks whether the environment variable named `key` exists. Returns true if it exists, false otherwise.
See also:
* [getEnv proc](#getEnv,string,string)
* [putEnv proc](#putEnv,string,string)
* [delEnv proc](#delEnv,string)
* [envPairs iterator](#envPairs.i)
**Example:**
```
assert not existsEnv("unknownEnv")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osenv.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osenv.nim#L138)
```
proc putEnv(key, val: string) {...}{.tags: [WriteEnvEffect], raises: [OSError].}
```
Sets the value of the environment variable named `key` to `val`. If an error occurs, `OSError` is raised.
See also:
* [getEnv proc](#getEnv,string,string)
* [existsEnv proc](#existsEnv,string)
* [delEnv proc](#delEnv,string)
* [envPairs iterator](#envPairs.i)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osenv.nim#L156) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osenv.nim#L156)
```
proc delEnv(key: string) {...}{.tags: [WriteEnvEffect], raises: [OSError].}
```
Deletes the environment variable named `key`. If an error occurs, `OSError` is raised.
See also:ven
* [getEnv proc](#getEnv,string,string)
* [existsEnv proc](#existsEnv,string)
* [putEnv proc](#putEnv,string,string)
* [envPairs iterator](#envPairs.i)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osenv.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osenv.nim#L190)
```
proc getHomeDir(): string {...}{.gcsafe, extern: "nos$1",
tags: [ReadEnvEffect, ReadIOEffect], raises: [].}
```
Returns the home directory of the current user.
This proc is wrapped by the [expandTilde proc](#expandTilde,string) for the convenience of processing paths coming from user configuration files.
See also:
* [getConfigDir proc](#getConfigDir)
* [getTempDir proc](#getTempDir)
* [expandTilde proc](#expandTilde,string)
* [getCurrentDir proc](#getCurrentDir)
* [setCurrentDir proc](#setCurrentDir,string)
**Example:**
```
assert getHomeDir() == expandTilde("~")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L890) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L890)
```
proc getConfigDir(): string {...}{.gcsafe, extern: "nos$1",
tags: [ReadEnvEffect, ReadIOEffect], raises: [].}
```
Returns the config directory of the current user for applications.
On non-Windows OSs, this proc conforms to the XDG Base Directory spec. Thus, this proc returns the value of the `XDG_CONFIG_HOME` environment variable if it is set, otherwise it returns the default configuration directory ("~/.config/").
An OS-dependent trailing slash is always present at the end of the returned string: `\` on Windows and `/` on all other OSs.
See also:
* [getHomeDir proc](#getHomeDir)
* [getTempDir proc](#getTempDir)
* [expandTilde proc](#expandTilde,string)
* [getCurrentDir proc](#getCurrentDir)
* [setCurrentDir proc](#setCurrentDir,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L909) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L909)
```
proc getTempDir(): string {...}{.gcsafe, extern: "nos$1",
tags: [ReadEnvEffect, ReadIOEffect], raises: [].}
```
Returns the temporary directory of the current user for applications to save temporary files in.
**Please do not use this**: On Android, it currently returns `getHomeDir()`, and on other Unix based systems it can cause security problems too. That said, you can override this implementation by adding `-d:tempDir=mytempname` to your compiler invocation.
See also:
* [getHomeDir proc](#getHomeDir)
* [getConfigDir proc](#getConfigDir)
* [expandTilde proc](#expandTilde,string)
* [getCurrentDir proc](#getCurrentDir)
* [setCurrentDir proc](#setCurrentDir,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L933) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L933)
```
proc expandTilde(path: string): string {...}{.tags: [ReadEnvEffect, ReadIOEffect],
raises: [].}
```
Expands `~` or a path starting with `~/` to a full path, replacing `~` with [getHomeDir()](#getHomeDir) (otherwise returns `path` unmodified).
Windows: this is still supported despite Windows platform not having this convention; also, both `~/` and `~\` are handled.
**Warning**: `~bob` and `~bob/` are not yet handled correctly.
See also:
* [getHomeDir proc](#getHomeDir)
* [getConfigDir proc](#getConfigDir)
* [getTempDir proc](#getTempDir)
* [getCurrentDir proc](#getCurrentDir)
* [setCurrentDir proc](#setCurrentDir,string)
**Example:**
```
assert expandTilde("~" / "appname.cfg") == getHomeDir() / "appname.cfg"
assert expandTilde("~/foo/bar") == getHomeDir() / "foo/bar"
assert expandTilde("/foo/bar") == "/foo/bar"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L960) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L960)
```
proc quoteShellWindows(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nosp$1", raises: [], tags: [].}
```
Quote `s`, so it can be safely passed to Windows API.
Based on Python's `subprocess.list2cmdline`. See [this link](http://msdn.microsoft.com/en-us/library/17w5ykft.aspx) for more details.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L993) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L993)
```
proc quoteShellPosix(s: string): string {...}{.noSideEffect, gcsafe,
extern: "nosp$1", raises: [], tags: [].}
```
Quote `s`, so it can be safely passed to POSIX shell. Based on Python's `pipes.quote`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1023) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1023)
```
proc quoteShell(s: string): string {...}{.noSideEffect, gcsafe, extern: "nosp$1",
raises: [], tags: [].}
```
Quote `s`, so it can be safely passed to shell.
When on Windows, it calls [quoteShellWindows proc](#quoteShellWindows,string). Otherwise, calls [quoteShellPosix proc](#quoteShellPosix,string).
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1039) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1039)
```
proc quoteShellCommand(args: openArray[string]): string {...}{.raises: [], tags: [].}
```
Concatenates and quotes shell arguments `args`. **Example:**
```
when defined(posix):
assert quoteShellCommand(["aaa", "", "c d"]) == "aaa '' 'c d'"
when defined(windows):
assert quoteShellCommand(["aaa", "", "c d"]) == "aaa \"\" \"c d\""
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1050) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1050)
```
proc fileExists(filename: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [ReadDirEffect], raises: [].}
```
Returns true if `filename` exists and is a regular file or symlink.
Directories, device files, named pipes and sockets return false.
See also:
* [dirExists proc](#dirExists,string)
* [symlinkExists proc](#symlinkExists,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1102) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1102)
```
proc dirExists(dir: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [ReadDirEffect], raises: [].}
```
Returns true if the directory `dir` exists. If `dir` is a file, false is returned. Follows symlinks.
See also:
* [fileExists proc](#fileExists,string)
* [symlinkExists proc](#symlinkExists,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1122) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1122)
```
proc symlinkExists(link: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [ReadDirEffect], raises: [].}
```
Returns true if the symlink `link` exists. Will return true regardless of whether the link points to a directory or file.
See also:
* [fileExists proc](#fileExists,string)
* [dirExists proc](#dirExists,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1141) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1141)
```
proc findExe(exe: string; followSymlinks: bool = true;
extensions: openArray[string] = ExeExts): string {...}{.
tags: [ReadDirEffect, ReadEnvEffect, ReadIOEffect], raises: [OSError].}
```
Searches for `exe` in the current working directory and then in directories listed in the `PATH` environment variable.
Returns `""` if the `exe` cannot be found. `exe` is added the [ExeExts](#ExeExts) file extensions if it has none.
If the system supports symlinks it also resolves them until it meets the actual file. This behavior can be disabled if desired by setting `followSymlinks = false`.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1182) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1182)
```
proc getLastModificationTime(file: string): times.Time {...}{.gcsafe,
extern: "nos$1", raises: [OSError], tags: [].}
```
Returns the `file`'s last modification time.
See also:
* [getLastAccessTime proc](#getLastAccessTime,string)
* [getCreationTime proc](#getCreationTime,string)
* [fileNewer proc](#fileNewer,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1240) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1240)
```
proc getLastAccessTime(file: string): times.Time {...}{.gcsafe, extern: "nos$1",
raises: [OSError], tags: [].}
```
Returns the `file`'s last read or write access time.
See also:
* [getLastModificationTime proc](#getLastModificationTime,string)
* [getCreationTime proc](#getCreationTime,string)
* [fileNewer proc](#fileNewer,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1258) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1258)
```
proc getCreationTime(file: string): times.Time {...}{.gcsafe, extern: "nos$1",
raises: [OSError], tags: [].}
```
Returns the `file`'s creation time.
**Note:** Under POSIX OS's, the returned time may actually be the time at which the file's attribute's were last modified. See [here](https://github.com/nim-lang/Nim/issues/1058) for details.
See also:
* [getLastModificationTime proc](#getLastModificationTime,string)
* [getLastAccessTime proc](#getLastAccessTime,string)
* [fileNewer proc](#fileNewer,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1276) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1276)
```
proc fileNewer(a, b: string): bool {...}{.gcsafe, extern: "nos$1", raises: [OSError],
tags: [].}
```
Returns true if the file `a` is newer than file `b`, i.e. if `a`'s modification time is later than `b`'s.
See also:
* [getLastModificationTime proc](#getLastModificationTime,string)
* [getLastAccessTime proc](#getLastAccessTime,string)
* [getCreationTime proc](#getCreationTime,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1298) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1298)
```
proc getCurrentDir(): string {...}{.gcsafe, extern: "nos$1", tags: [],
raises: [OSError].}
```
Returns the current working directory i.e. where the built binary is run.
So the path returned by this proc is determined at run time.
See also:
* [getHomeDir proc](#getHomeDir)
* [getConfigDir proc](#getConfigDir)
* [getTempDir proc](#getTempDir)
* [setCurrentDir proc](#setCurrentDir,string)
* [currentSourcePath template](system#currentSourcePath.t)
* [getProjectPath proc](macros#getProjectPath)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1316) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1316)
```
proc setCurrentDir(newDir: string) {...}{.inline, tags: [], raises: [OSError].}
```
Sets the current working directory; `OSError` is raised if `newDir` cannot been set.
See also:
* [getHomeDir proc](#getHomeDir)
* [getConfigDir proc](#getConfigDir)
* [getTempDir proc](#getTempDir)
* [getCurrentDir proc](#getCurrentDir)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1377) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1377)
```
proc absolutePath(path: string; root = getCurrentDir()): string {...}{.
raises: [ValueError], tags: [].}
```
Returns the absolute path of `path`, rooted at `root` (which must be absolute; default: current directory). If `path` is absolute, return it, ignoring `root`.
See also:
* [normalizedPath proc](#normalizedPath,string)
* [normalizePath proc](#normalizePath,string)
**Example:**
```
assert absolutePath("a") == getCurrentDir() / "a"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1396) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1396)
```
proc normalizeExe(file: var string) {...}{.raises: [], tags: [].}
```
on posix, prepends `./` if `file` doesn't contain `/` and is not `"", ".", ".."`. **Example:**
```
import sugar
when defined(posix):
doAssert "foo".dup(normalizeExe) == "./foo"
doAssert "foo/../bar".dup(normalizeExe) == "foo/../bar"
doAssert "".dup(normalizeExe) == ""
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1416) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1416)
```
proc normalizePath(path: var string) {...}{.gcsafe, extern: "nos$1", tags: [],
raises: [].}
```
Normalize a path.
Consecutive directory separators are collapsed, including an initial double slash.
On relative paths, double dot (`..`) sequences are collapsed if possible. On absolute paths they are always collapsed.
Warning: URL-encoded and Unicode attempts at directory traversal are not detected. Triple dot is not handled.
See also:
* [absolutePath proc](#absolutePath,string)
* [normalizedPath proc](#normalizedPath,string) for outplace version
* [normalizeExe proc](#normalizeExe,string)
**Example:**
```
when defined(posix):
var a = "a///b//..//c///d"
a.normalizePath()
assert a == "a/c/d"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1428) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1428)
```
proc normalizedPath(path: string): string {...}{.gcsafe, extern: "nos$1", tags: [],
raises: [].}
```
Returns a normalized path for the current OS.
See also:
* [absolutePath proc](#absolutePath,string)
* [normalizePath proc](#normalizePath,string) for the in-place version
**Example:**
```
when defined(posix):
assert normalizedPath("a///b//..//c///d") == "a/c/d"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1479) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1479)
```
proc sameFile(path1, path2: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [ReadDirEffect], raises: [OSError].}
```
Returns true if both pathname arguments refer to the same physical file or directory.
Raises `OSError` if any of the files does not exist or information about it can not be obtained.
This proc will return true if given two alternative hard-linked or sym-linked paths to the same file or directory.
See also:
* [sameFileContent proc](#sameFileContent,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1510) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1510)
```
proc sameFileContent(path1, path2: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [ReadIOEffect], raises: [IOError].}
```
Returns true if both pathname arguments refer to files with identical binary content.
See also:
* [sameFile proc](#sameFile,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1555) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1555)
```
proc getFilePermissions(filename: string): set[FilePermission] {...}{.gcsafe,
extern: "nos$1", tags: [ReadDirEffect], raises: [OSError].}
```
Retrieves file permissions for `filename`.
`OSError` is raised in case of an error. On Windows, only the `readonly` flag is checked, every other permission is available in any case.
See also:
* [setFilePermissions proc](#setFilePermissions,string,set%5BFilePermission%5D)
* [FilePermission enum](#FilePermission)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1606) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1606)
```
proc setFilePermissions(filename: string; permissions: set[FilePermission]) {...}{.
gcsafe, extern: "nos$1", tags: [WriteDirEffect], raises: [OSError].}
```
Sets the file permissions for `filename`.
`OSError` is raised in case of an error. On Windows, only the `readonly` flag is changed, depending on `fpUserWrite` permission.
See also:
* [getFilePermissions](#getFilePermissions,string)
* [FilePermission enum](#FilePermission)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1644) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1644)
```
proc copyFile(source, dest: string) {...}{.gcsafe, extern: "nos$1",
tags: [ReadIOEffect, WriteIOEffect],
raises: [OSError, IOError].}
```
Copies a file from `source` to `dest`, where `dest.parentDir` must exist.
If this fails, `OSError` is raised.
On the Windows platform this proc will copy the source file's attributes into dest.
On other platforms you need to use [getFilePermissions](#getFilePermissions,string) and [setFilePermissions](#setFilePermissions,string,set%5BFilePermission%5D) procs to copy them by hand (or use the convenience [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)), otherwise `dest` will inherit the default permissions of a newly created file for the user.
If `dest` already exists, the file attributes will be preserved and the content overwritten.
See also:
* [copyDir proc](#copyDir,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [tryRemoveFile proc](#tryRemoveFile,string)
* [removeFile proc](#removeFile,string)
* [moveFile proc](#moveFile,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1686) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1686)
```
proc copyFileToDir(source, dir: string) {...}{.
raises: [ValueError, OSError, IOError], tags: [ReadIOEffect, WriteIOEffect].}
```
Copies a file `source` into directory `dir`, which must exist. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1744) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1744)
```
proc tryRemoveFile(file: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [WriteDirEffect], raises: [].}
```
Removes the `file`.
If this fails, returns `false`. This does not fail if the file never existed in the first place.
On Windows, ignores the read-only attribute.
See also:
* [copyFile proc](#copyFile,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [removeFile proc](#removeFile,string)
* [moveFile proc](#moveFile,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1769) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1769)
```
proc removeFile(file: string) {...}{.gcsafe, extern: "nos$1", tags: [WriteDirEffect],
raises: [OSError].}
```
Removes the `file`.
If this fails, `OSError` is raised. This does not fail if the file never existed in the first place.
On Windows, ignores the read-only attribute.
See also:
* [removeDir proc](#removeDir,string)
* [copyFile proc](#copyFile,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [tryRemoveFile proc](#tryRemoveFile,string)
* [moveFile proc](#moveFile,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1801) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1801)
```
proc moveFile(source, dest: string) {...}{.gcsafe, extern: "nos$1",
tags: [ReadIOEffect, WriteIOEffect],
raises: [OSError, IOError, Exception].}
```
Moves a file from `source` to `dest`.
If this fails, `OSError` is raised. If `dest` already exists, it will be overwritten.
Can be used to rename files.
See also:
* [moveDir proc](#moveDir,string,string)
* [copyFile proc](#copyFile,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [removeFile proc](#removeFile,string)
* [tryRemoveFile proc](#tryRemoveFile,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1841) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1841)
```
proc exitStatusLikeShell(status: cint): cint {...}{.raises: [], tags: [].}
```
Converts exit code from `c_system` into a shell exit code. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1867) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1867)
```
proc execShellCmd(command: string): int {...}{.gcsafe, extern: "nos$1",
tags: [ExecIOEffect], raises: [].}
```
Executes a shell command.
Command has the form 'program args' where args are the command line arguments given to program. The proc returns the error code of the shell when it has finished (zero if there is no error). The proc does not return until the process has finished.
To execute a program without having a shell involved, use [osproc.execProcess proc](osproc#execProcess,string,string,openArray%5Bstring%5D,StringTableRef,set%5BProcessOption%5D).
**Examples:**
```
discard execShellCmd("ls -la")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1878) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1878)
```
proc expandFilename(filename: string): string {...}{.gcsafe, extern: "nos$1",
tags: [ReadDirEffect], raises: [OSError].}
```
Returns the full (absolute) path of an existing file `filename`.
Raises `OSError` in case of an error. Follows symlinks.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1995) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1995)
```
proc getCurrentCompilerExe(): string {...}{.compileTime, raises: [], tags: [].}
```
This is [getAppFilename()](#getAppFilename) at compile time.
Can be used to retrieve the currently executing Nim compiler from a Nim or nimscript program, or the nimble binary inside a nimble program (likewise with other binaries built from compiler API).
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2056) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2056)
```
proc removeDir(dir: string; checkDir = false) {...}{.gcsafe, extern: "nos$1",
tags: [WriteDirEffect, ReadDirEffect], gcsafe, locks: 0, raises: [OSError].}
```
Removes the directory `dir` including all subdirectories and files in `dir` (recursively).
If this fails, `OSError` is raised. This does not fail if the directory never existed in the first place, unless `checkDir` = true
See also:
* [tryRemoveFile proc](#tryRemoveFile,string)
* [removeFile proc](#removeFile,string)
* [existsOrCreateDir proc](#existsOrCreateDir,string)
* [createDir proc](#createDir,string)
* [copyDir proc](#copyDir,string,string)
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
* [moveDir proc](#moveDir,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2243) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2243)
```
proc existsOrCreateDir(dir: string): bool {...}{.gcsafe, extern: "nos$1",
tags: [WriteDirEffect, ReadDirEffect], raises: [OSError, IOError].}
```
Check if a directory `dir` exists, and create it otherwise.
Does not create parent directories (fails if parent does not exist). Returns `true` if the directory already exists, and `false` otherwise.
See also:
* [removeDir proc](#removeDir,string)
* [createDir proc](#createDir,string)
* [copyDir proc](#copyDir,string,string)
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
* [moveDir proc](#moveDir,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2312) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2312)
```
proc createDir(dir: string) {...}{.gcsafe, extern: "nos$1",
tags: [WriteDirEffect, ReadDirEffect],
raises: [OSError, IOError].}
```
Creates the directory `dir`.
The directory may contain several subdirectories that do not exist yet. The full path is created. If this fails, `OSError` is raised.
It does **not** fail if the directory already exists because for most usages this does not indicate an error.
See also:
* [removeDir proc](#removeDir,string)
* [existsOrCreateDir proc](#existsOrCreateDir,string)
* [copyDir proc](#copyDir,string,string)
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
* [moveDir proc](#moveDir,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2332) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2332)
```
proc copyDir(source, dest: string) {...}{.gcsafe, extern: "nos$1",
tags: [WriteIOEffect, ReadIOEffect],
gcsafe, locks: 0,
raises: [OSError, IOError].}
```
Copies a directory from `source` to `dest`.
If this fails, `OSError` is raised.
On the Windows platform this proc will copy the attributes from `source` into `dest`.
On other platforms created files and directories will inherit the default permissions of a newly created file/directory for the user. Use [copyDirWithPermissions proc](#copyDirWithPermissions,string,string) to preserve attributes recursively on these platforms.
See also:
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
* [copyFile proc](#copyFile,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [removeDir proc](#removeDir,string)
* [existsOrCreateDir proc](#existsOrCreateDir,string)
* [createDir proc](#createDir,string)
* [moveDir proc](#moveDir,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2363) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2363)
```
proc moveDir(source, dest: string) {...}{.tags: [ReadIOEffect, WriteIOEffect],
raises: [OSError, IOError].}
```
Moves a directory from `source` to `dest`.
If this fails, `OSError` is raised.
See also:
* [moveFile proc](#moveFile,string,string)
* [copyDir proc](#copyDir,string,string)
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
* [removeDir proc](#removeDir,string)
* [existsOrCreateDir proc](#existsOrCreateDir,string)
* [createDir proc](#createDir,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2395) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2395)
```
proc createSymlink(src, dest: string) {...}{.raises: [OSError], tags: [].}
```
Create a symbolic link at `dest` which points to the item specified by `src`. On most operating systems, will fail if a link already exists.
**Warning**: Some OS's (such as Microsoft Windows) restrict the creation of symlinks to root users (administrators).
See also:
* [createHardlink proc](#createHardlink,string,string)
* [expandSymlink proc](#expandSymlink,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2413) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2413)
```
proc createHardlink(src, dest: string) {...}{.raises: [OSError], tags: [].}
```
Create a hard link at `dest` which points to the item specified by `src`.
**Warning**: Some OS's restrict the creation of hard links to root users (administrators).
See also:
* [createSymlink proc](#createSymlink,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2441) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2441)
```
proc copyFileWithPermissions(source, dest: string; ignorePermissionErrors = true) {...}{.
raises: [OSError, IOError, Exception],
tags: [ReadIOEffect, WriteIOEffect, WriteDirEffect, ReadDirEffect].}
```
Copies a file from `source` to `dest` preserving file permissions.
This is a wrapper proc around [copyFile](#copyFile,string,string), [getFilePermissions](#getFilePermissions,string) and [setFilePermissions](#setFilePermissions,string,set%5BFilePermission%5D) procs on non-Windows platforms.
On Windows this proc is just a wrapper for [copyFile proc](#copyFile,string,string) since that proc already copies attributes.
On non-Windows systems permissions are copied after the file itself has been copied, which won't happen atomically and could lead to a race condition. If `ignorePermissionErrors` is true (default), errors while reading/setting file attributes will be ignored, otherwise will raise `OSError`.
See also:
* [copyFile proc](#copyFile,string,string)
* [copyDir proc](#copyDir,string,string)
* [tryRemoveFile proc](#tryRemoveFile,string)
* [removeFile proc](#removeFile,string)
* [moveFile proc](#moveFile,string,string)
* [copyDirWithPermissions proc](#copyDirWithPermissions,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2463) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2463)
```
proc copyDirWithPermissions(source, dest: string; ignorePermissionErrors = true) {...}{.
gcsafe, extern: "nos$1", tags: [WriteIOEffect, ReadIOEffect], gcsafe,
locks: 0, raises: [OSError, IOError, Exception].}
```
Copies a directory from `source` to `dest` preserving file permissions.
If this fails, `OSError` is raised. This is a wrapper proc around [copyDir](#copyDir,string,string) and [copyFileWithPermissions](#copyFileWithPermissions,string,string) procs on non-Windows platforms.
On Windows this proc is just a wrapper for [copyDir proc](#copyDir,string,string) since that proc already copies attributes.
On non-Windows systems permissions are copied after the file or directory itself has been copied, which won't happen atomically and could lead to a race condition. If `ignorePermissionErrors` is true (default), errors while reading/setting file attributes will be ignored, otherwise will raise `OSError`.
See also:
* [copyDir proc](#copyDir,string,string)
* [copyFile proc](#copyFile,string,string)
* [copyFileWithPermissions proc](#copyFileWithPermissions,string,string)
* [removeDir proc](#removeDir,string)
* [moveDir proc](#moveDir,string,string)
* [existsOrCreateDir proc](#existsOrCreateDir,string)
* [createDir proc](#createDir,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2496)
```
proc inclFilePermissions(filename: string; permissions: set[FilePermission]) {...}{.
gcsafe, extern: "nos$1", tags: [ReadDirEffect, WriteDirEffect],
raises: [OSError].}
```
A convenience proc for:
```
setFilePermissions(filename, getFilePermissions(filename)+permissions)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2539) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2539)
```
proc exclFilePermissions(filename: string; permissions: set[FilePermission]) {...}{.
gcsafe, extern: "nos$1", tags: [ReadDirEffect, WriteDirEffect],
raises: [OSError].}
```
A convenience proc for:
```
setFilePermissions(filename, getFilePermissions(filename)-permissions)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2548) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2548)
```
proc expandSymlink(symlinkPath: string): string {...}{.raises: [OSError], tags: [].}
```
Returns a string representing the path to which the symbolic link points.
On Windows this is a noop, `symlinkPath` is simply returned.
See also:
* [createSymlink proc](#createSymlink,string,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2557) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2557)
```
proc parseCmdLine(c: string): seq[string] {...}{.noSideEffect, gcsafe,
extern: "nos$1", raises: [], tags: [].}
```
Splits a command line into several components.
**Note**: This proc is only occasionally useful, better use the [parseopt module](parseopt).
On Windows, it uses the [following parsing rules](http://msdn.microsoft.com/en-us/library/17w5ykft.aspx):
* Arguments are delimited by white space, which is either a space or a tab.
* The caret character (^) is not recognized as an escape character or delimiter. The character is handled completely by the command-line parser in the operating system before being passed to the argv array in the program.
* A string surrounded by double quotation marks ("string") is interpreted as a single argument, regardless of white space contained within. A quoted string can be embedded in an argument.
* A double quotation mark preceded by a backslash (") is interpreted as a literal double quotation mark character (").
* Backslashes are interpreted literally, unless they immediately precede a double quotation mark.
* If an even number of backslashes is followed by a double quotation mark, one backslash is placed in the argv array for every pair of backslashes, and the double quotation mark is interpreted as a string delimiter.
* If an odd number of backslashes is followed by a double quotation mark, one backslash is placed in the argv array for every pair of backslashes, and the double quotation mark is "escaped" by the remaining backslash, causing a literal double quotation mark (") to be placed in argv.
On Posix systems, it uses the following parsing rules: Components are separated by whitespace unless the whitespace occurs within `"` or `'` quotes.
See also:
* [parseopt module](parseopt)
* [paramCount proc](#paramCount)
* [paramStr proc](#paramStr,int)
* [commandLineParams proc](#commandLineParams)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2576) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2576)
```
proc paramCount(): int {...}{.tags: [ReadIOEffect], raises: [].}
```
Returns the number of command line arguments given to the application.
Unlike argc in C, if your binary was called without parameters this will return zero. You can query each individual parameter with [paramStr proc](#paramStr,int) or retrieve all of them in one go with [commandLineParams proc](#commandLineParams).
**Availability**: When generating a dynamic library (see `--app:lib`) on Posix this proc is not defined. Test for availability using [declared()](system#declared,untyped).
See also:
* [parseopt module](parseopt)
* [parseCmdLine proc](#parseCmdLine,string)
* [paramStr proc](#paramStr,int)
* [commandLineParams proc](#commandLineParams)
**Examples:**
```
when declared(paramCount):
# Use paramCount() here
else:
# Do something else!
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2675) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2675)
```
proc paramStr(i: int): TaintedString {...}{.tags: [ReadIOEffect], raises: [].}
```
Returns the `i`-th command line argument given to the application.
`i` should be in the range `1..paramCount()`, the `IndexDefect` exception will be raised for invalid values. Instead of iterating over [paramCount()](#paramCount) with this proc you can call the convenience [commandLineParams()](#commandLineParams).
Similarly to argv in C, it is possible to call `paramStr(0)` but this will return OS specific contents (usually the name of the invoked executable). You should avoid this and call [getAppFilename()](#getAppFilename) instead.
**Availability**: When generating a dynamic library (see `--app:lib`) on Posix this proc is not defined. Test for availability using [declared()](system#declared,untyped).
See also:
* [parseopt module](parseopt)
* [parseCmdLine proc](#parseCmdLine,string)
* [paramCount proc](#paramCount)
* [commandLineParams proc](#commandLineParams)
* [getAppFilename proc](#getAppFilename)
**Examples:**
```
when declared(paramStr):
# Use paramStr() here
else:
# Do something else!
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2703) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2703)
```
proc commandLineParams(): seq[TaintedString] {...}{.raises: [], tags: [ReadIOEffect].}
```
Convenience proc which returns the command line parameters.
This returns **only** the parameters. If you want to get the application executable filename, call [getAppFilename()](#getAppFilename).
**Availability**: On Posix there is no portable way to get the command line from a DLL and thus the proc isn't defined in this environment. You can test for its availability with [declared()](system#declared,untyped).
See also:
* [parseopt module](parseopt)
* [parseCmdLine proc](#parseCmdLine,string)
* [paramCount proc](#paramCount)
* [paramStr proc](#paramStr,int)
* [getAppFilename proc](#getAppFilename)
**Examples:**
```
when declared(commandLineParams):
# Use commandLineParams() here
else:
# Do something else!
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2793) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2793)
```
proc getAppFilename(): string {...}{.gcsafe, extern: "nos$1", tags: [ReadIOEffect],
raises: [].}
```
Returns the filename of the application's executable. This proc will resolve symlinks.
See also:
* [getAppDir proc](#getAppDir)
* [getCurrentCompilerExe proc](#getCurrentCompilerExe)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2954) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2954)
```
proc getAppDir(): string {...}{.gcsafe, extern: "nos$1", tags: [ReadIOEffect],
raises: [].}
```
Returns the directory of the application's executable.
See also:
* [getAppFilename proc](#getAppFilename)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3020) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3020)
```
proc sleep(milsecs: int) {...}{.gcsafe, extern: "nos$1", tags: [TimeEffect],
raises: [].}
```
Sleeps `milsecs` milliseconds. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3027) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3027)
```
proc getFileSize(file: string): BiggestInt {...}{.gcsafe, extern: "nos$1",
tags: [ReadIOEffect], raises: [IOError, OSError].}
```
Returns the file size of `file` (in bytes). `OSError` is raised in case of an error. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3037) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3037)
```
proc getFileInfo(handle: FileHandle): FileInfo {...}{.raises: [OSError], tags: [].}
```
Retrieves file information for the file object represented by the given handle.
If the information cannot be retrieved, such as when the file handle is invalid, `OSError` is raised.
See also:
* [getFileInfo(file) proc](#getFileInfo,File)
* [getFileInfo(path) proc](#getFileInfo,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3148) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3148)
```
proc getFileInfo(file: File): FileInfo {...}{.raises: [IOError, OSError], tags: [].}
```
Retrieves file information for the file object.
See also:
* [getFileInfo(handle) proc](#getFileInfo,FileHandle)
* [getFileInfo(path) proc](#getFileInfo,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3174) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3174)
```
proc getFileInfo(path: string; followSymlink = true): FileInfo {...}{.
raises: [OSError], tags: [].}
```
Retrieves file information for the file object pointed to by `path`.
Due to intrinsic differences between operating systems, the information contained by the returned [FileInfo object](#FileInfo) will be slightly different across platforms, and in some cases, incomplete or inaccurate.
When `followSymlink` is true (default), symlinks are followed and the information retrieved is information related to the symlink's target. Otherwise, information on the symlink itself is retrieved.
If the information cannot be retrieved, such as when the path doesn't exist, or when permission restrictions prevent the program from retrieving file information, `OSError` is raised.
See also:
* [getFileInfo(handle) proc](#getFileInfo,FileHandle)
* [getFileInfo(file) proc](#getFileInfo,File)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3184) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3184)
```
proc isHidden(path: string): bool {...}{.raises: [], tags: [].}
```
Determines whether `path` is hidden or not, using [this reference](https://en.wikipedia.org/wiki/Hidden_file_and_hidden_directory).
On Windows: returns true if it exists and its "hidden" attribute is set.
On posix: returns true if `lastPathPart(path)` starts with `.` and is not `.` or `..`.
**Note**: paths are not normalized to determine `isHidden`.
**Example:**
```
when defined(posix):
assert ".foo".isHidden
assert not ".foo/bar".isHidden
assert not ".".isHidden
assert not "..".isHidden
assert not "".isHidden
assert ".foo/".isHidden
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3222) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3222)
```
proc getCurrentProcessId(): int {...}{.raises: [], tags: [].}
```
Return current process ID.
See also:
* [osproc.processID(p: Process)](osproc#processID,Process)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3252)
```
proc setLastModificationTime(file: string; t: times.Time) {...}{.raises: [OSError],
tags: [].}
```
Sets the `file`'s last modification time. `OSError` is raised in case of an error. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3264) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3264) Funcs
-----
```
func isValidFilename(filename: string; maxLen = 259.Positive): bool {...}{.
raises: [], tags: [].}
```
Returns true if `filename` is valid for crossplatform use.
This is useful if you want to copy or save files across Windows, Linux, Mac, etc. You can pass full paths as argument too, but func only checks filenames. It uses `invalidFilenameChars`, `invalidFilenames` and `maxLen` to verify the specified `filename`.
```
assert not isValidFilename(" foo") ## Leading white space
assert not isValidFilename("foo ") ## Trailing white space
assert not isValidFilename("foo.") ## Ends with Dot
assert not isValidFilename("con.txt") ## "CON" is invalid (Windows)
assert not isValidFilename("OwO:UwU") ## ":" is invalid (Mac)
assert not isValidFilename("aux.bat") ## "AUX" is invalid (Windows)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L3281) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L3281) Iterators
---------
```
iterator parentDirs(path: string; fromRoot = false; inclusive = true): string {...}{.
raises: [], tags: [].}
```
Walks over all parent directories of a given `path`.
If `fromRoot` is true (default: false), the traversal will start from the file system root directory. If `inclusive` is true (default), the original argument will be included in the traversal.
Relative paths won't be expanded by this iterator. Instead, it will traverse only the directories appearing in the relative path.
See also:
* [parentDir proc](#parentDir,string)
**Examples:**
```
let g = "a/b/c"
for p in g.parentDirs:
echo p
# a/b/c
# a/b
# a
for p in g.parentDirs(fromRoot=true):
echo p
# a/
# a/b/
# a/b/c
for p in g.parentDirs(inclusive=false):
echo p
# a/b
# a
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L560) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L560)
```
iterator envPairs(): tuple[key, value: TaintedString] {...}{.tags: [ReadEnvEffect],
raises: [].}
```
Iterate over all environments variables.
In the first component of the tuple is the name of the current variable stored, in the second its value.
See also:
* [getEnv proc](#getEnv,string,string)
* [existsEnv proc](#existsEnv,string)
* [putEnv proc](#putEnv,string,string)
* [delEnv proc](#delEnv,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/includes/osenv.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/includes/osenv.nim#L215)
```
iterator walkPattern(pattern: string): string {...}{.tags: [ReadDirEffect],
raises: [].}
```
Iterate over all the files and directories that match the `pattern`.
On POSIX this uses the glob call. `pattern` is OS dependent, but at least the `"*.ext"` notation is supported.
See also:
* [walkFiles iterator](#walkFiles.i,string)
* [walkDirs iterator](#walkDirs.i,string)
* [walkDir iterator](#walkDir.i,string)
* [walkDirRec iterator](#walkDirRec.i,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1953) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1953)
```
iterator walkFiles(pattern: string): string {...}{.tags: [ReadDirEffect], raises: [].}
```
Iterate over all the files that match the `pattern`.
On POSIX this uses the glob call. `pattern` is OS dependent, but at least the `"*.ext"` notation is supported.
See also:
* [walkPattern iterator](#walkPattern.i,string)
* [walkDirs iterator](#walkDirs.i,string)
* [walkDir iterator](#walkDir.i,string)
* [walkDirRec iterator](#walkDirRec.i,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1967) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1967)
```
iterator walkDirs(pattern: string): string {...}{.tags: [ReadDirEffect], raises: [].}
```
Iterate over all the directories that match the `pattern`.
On POSIX this uses the glob call. `pattern` is OS dependent, but at least the `"*.ext"` notation is supported.
See also:
* [walkPattern iterator](#walkPattern.i,string)
* [walkFiles iterator](#walkFiles.i,string)
* [walkDir iterator](#walkDir.i,string)
* [walkDirRec iterator](#walkDirRec.i,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1981) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1981)
```
iterator walkDir(dir: string; relative = false; checkDir = false): tuple[
kind: PathComponent, path: string] {...}{.tags: [ReadDirEffect],
raises: [OSError].}
```
Walks over the directory `dir` and yields for each directory or file in `dir`. The component type and full path for each item are returned.
Walking is not recursive. If `relative` is true (default: false) the resulting path is shortened to be relative to `dir`. Example: This directory structure:
```
dirA / dirB / fileB1.txt
/ dirC
/ fileA1.txt
/ fileA2.txt
```
and this code:
```
for kind, path in walkDir("dirA"):
echo(path)
```
produce this output (but not necessarily in this order!):
```
dirA/dirB
dirA/dirC
dirA/fileA1.txt
dirA/fileA2.txt
```
See also:
* [walkPattern iterator](#walkPattern.i,string)
* [walkFiles iterator](#walkFiles.i,string)
* [walkDirs iterator](#walkDirs.i,string)
* [walkDirRec iterator](#walkDirRec.i,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2078) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2078)
```
iterator walkDirRec(dir: string; yieldFilter = {pcFile}; followFilter = {pcDir};
relative = false; checkDir = false): string {...}{.
tags: [ReadDirEffect], raises: [OSError].}
```
Recursively walks over the directory `dir` and yields for each file or directory in `dir`.
If `relative` is true (default: false) the resulting path is shortened to be relative to `dir`, otherwise the full path is returned.
**Warning**: Modifying the directory structure while the iterator is traversing may result in undefined behavior!
Walking is recursive. `followFilter` controls the behaviour of the iterator:
| yieldFilter | meaning |
| --- | --- |
| `pcFile` | yield real files (default) |
| `pcLinkToFile` | yield symbolic links to files |
| `pcDir` | yield real directories |
| `pcLinkToDir` | yield symbolic links to directories |
| followFilter | meaning |
| --- | --- |
| `pcDir` | follow real directories (default) |
| `pcLinkToDir` | follow symbolic links to directories |
See also:
* [walkPattern iterator](#walkPattern.i,string)
* [walkFiles iterator](#walkFiles.i,string)
* [walkDirs iterator](#walkDirs.i,string)
* [walkDir iterator](#walkDir.i,string)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L2176) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L2176) Templates
---------
```
template existsFile(args: varargs[untyped]): untyped {...}{.
deprecated: "use fileExists".}
```
**Deprecated:** use fileExists [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1164) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1164)
```
template existsDir(args: varargs[untyped]): untyped {...}{.
deprecated: "use dirExists".}
```
**Deprecated:** use dirExists [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/os.nim#L1166) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/os.nim#L1166)
| programming_docs |
nim iup iup
===
Wrapper for the [IUP native GUI library](http://webserver2.tecgraf.puc-rio.br/iup).
Requires `iup.dll` on Windows, `libiup.so` on Linux, `libiup.dylib` on OS X. Compile with `-d:nimDebugDlOpen` to debug the shared library opening.
Examples
--------
```
import os, iup
var argc = create(cint)
argc[] = paramCount().cint
var argv = allocCstringArray(commandLineParams())
assert open(argc, argv.addr) == 0 # UIP requires calling open()
message(r"Hello world 1", r"Hello world") # Message popup
close() # UIP requires calling close()
```
```
import os, iup
var argc = create(cint)
argc[] = paramCount().cint
var argv = allocCstringArray(commandLineParams())
assert open(argc, argv.addr) == 0 # UIP requires open()
let textarea = text(nil) # Text widget.
setAttribute(textarea, r"MULTILINE", r"YES") # Set text widget to multiline.
setAttribute(textarea, r"EXPAND", r"YES") # Set text widget to auto expand.
let layout = vbox(textarea) # Vertical layout.
let window = dialog(layout) # Dialog window.
setAttribute(window, "TITLE", "Nim Notepad") # Set window title.
showXY(window, IUP_CENTER, IUP_CENTER) # Show window.
mainLoop() # Main loop.
close() # UIP requires calling close()
```
Types
-----
```
PIhandle = ptr Ihandle
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L94)
```
Icallback = proc (arg: PIhandle): cint {...}{.cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L96)
```
Iparamcb = proc (dialog: PIhandle; paramIndex: cint; userData: pointer): cint {...}{.
cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L124) Consts
------
```
IUP_NAME = "IUP - Portable User Interface"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L85)
```
IUP_COPYRIGHT = "Copyright (C) 1994-2009 Tecgraf, PUC-Rio."
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L86)
```
IUP_DESCRIPTION = "Portable toolkit for building graphical user interfaces."
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L87)
```
constIUP_VERSION = "3.0"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L88) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L88)
```
constIUP_VERSION_NUMBER = 300000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L89)
```
constIUP_VERSION_DATE = "2009/07/18"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L90)
```
IUP_ERROR = 1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L415) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L415)
```
IUP_NOERROR = 0'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L416) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L416)
```
IUP_OPENED = -1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L417) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L417)
```
IUP_INVALID = -1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L418) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L418)
```
IUP_IGNORE = -1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L421) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L421)
```
IUP_DEFAULT = -2'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L422) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L422)
```
IUP_CLOSE = -3'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L423) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L423)
```
IUP_CONTINUE = -4'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L424) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L424)
```
IUP_CENTER = 65535'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L427) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L427)
```
IUP_LEFT = 65534'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L428) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L428)
```
IUP_RIGHT = 65533'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L429) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L429)
```
IUP_MOUSEPOS = 65532'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L430) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L430)
```
IUP_CURRENT = 65531'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L431) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L431)
```
IUP_CENTERPARENT = 65530'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L432) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L432)
```
IUP_TOP = 65534'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L433) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L433)
```
IUP_BOTTOM = 65533'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L434) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L434)
```
IUP_SHOW = 0'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L437) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L437)
```
IUP_RESTORE = 1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L438) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L438)
```
IUP_MINIMIZE = 2'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L439) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L439)
```
IUP_MAXIMIZE = 3'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L440) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L440)
```
IUP_HIDE = 4'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L441) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L441)
```
IUP_SBUP = 0'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L444)
```
IUP_SBDN = 1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L445) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L445)
```
IUP_SBPGUP = 2'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L446) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L446)
```
IUP_SBPGDN = 3'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L447)
```
IUP_SBPOSV = 4'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L448) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L448)
```
IUP_SBDRAGV = 5'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L449) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L449)
```
IUP_SBLEFT = 6'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L450) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L450)
```
IUP_SBRIGHT = 7'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L451) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L451)
```
IUP_SBPGLEFT = 8'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L452) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L452)
```
IUP_SBPGRIGHT = 9'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L453) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L453)
```
IUP_SBPOSH = 10'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L454) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L454)
```
IUP_SBDRAGH = 11'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L455) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L455)
```
IUP_BUTTON1 = 49'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L458) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L458)
```
IUP_BUTTON2 = 50'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L459) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L459)
```
IUP_BUTTON3 = 51'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L460)
```
IUP_BUTTON4 = 52'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L461) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L461)
```
IUP_BUTTON5 = 53'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L462) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L462)
```
IUP_MASK_FLOAT = "[+/-]?(/d+/.?/d*|/./d+)"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L477) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L477)
```
IUP_MASK_UFLOAT = "(/d+/.?/d*|/./d+)"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L478) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L478)
```
IUP_MASK_EFLOAT = "[+/-]?(/d+/.?/d*|/./d+)([eE][+/-]?/d+)?"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L479) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L479)
```
IUP_MASK_INT = "[+/-]?/d+"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L480) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L480)
```
IUP_MASK_UINT = "/d+"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L481) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L481)
```
K_SP = 32'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L486) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L486)
```
K_exclam = 33'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L487) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L487)
```
K_quotedbl = 34'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L488) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L488)
```
K_numbersign = 35'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L489) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L489)
```
K_dollar = 36'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L490) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L490)
```
K_percent = 37'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L491) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L491)
```
K_ampersand = 38'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L492) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L492)
```
K_apostrophe = 39'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L493) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L493)
```
K_parentleft = 40'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L494) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L494)
```
K_parentright = 41'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L495) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L495)
```
K_asterisk = 42'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L496)
```
K_plus = 43'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L497) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L497)
```
K_comma = 44'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L498) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L498)
```
K_minus = 45'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L499) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L499)
```
K_period = 46'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L500) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L500)
```
K_slash = 47'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L501) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L501)
```
K_0 = 48'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L502) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L502)
```
K_1 = 49'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L503) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L503)
```
K_2 = 50'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L504) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L504)
```
K_3 = 51'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L505) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L505)
```
K_4 = 52'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L506) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L506)
```
K_5 = 53'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L507) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L507)
```
K_6 = 54'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L508) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L508)
```
K_7 = 55'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L509) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L509)
```
K_8 = 56'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L510) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L510)
```
K_9 = 57'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L511) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L511)
```
K_colon = 58'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L512) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L512)
```
K_semicolon = 59'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L513) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L513)
```
K_less = 60'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L514) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L514)
```
K_equal = 61'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L515) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L515)
```
K_greater = 62'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L516) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L516)
```
K_question = 63'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L517) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L517)
```
K_at = 64'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L518) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L518)
```
K_upperA = 65'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L519) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L519)
```
K_upperB = 66'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L520) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L520)
```
K_upperC = 67'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L521) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L521)
```
K_upperD = 68'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L522) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L522)
```
K_upperE = 69'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L523) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L523)
```
K_upperF = 70'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L524) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L524)
```
K_upperG = 71'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L525) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L525)
```
K_upperH = 72'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L526) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L526)
```
K_upperI = 73'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L527) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L527)
```
K_upperJ = 74'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L528) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L528)
```
K_upperK = 75'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L529) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L529)
```
K_upperL = 76'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L530) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L530)
```
K_upperM = 77'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L531) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L531)
```
K_upperN = 78'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L532) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L532)
```
K_upperO = 79'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L533) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L533)
```
K_upperP = 80'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L534) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L534)
```
K_upperQ = 81'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L535) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L535)
```
K_upperR = 82'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L536) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L536)
```
K_upperS = 83'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L537) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L537)
```
K_upperT = 84'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L538) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L538)
```
K_upperU = 85'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L539) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L539)
```
K_upperV = 86'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L540) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L540)
```
K_upperW = 87'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L541) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L541)
```
K_upperX = 88'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L542) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L542)
```
K_upperY = 89'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L543) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L543)
```
K_upperZ = 90'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L544) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L544)
```
K_bracketleft = 91'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L545) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L545)
```
K_backslash = 92'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L546) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L546)
```
K_bracketright = 93'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L547) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L547)
```
K_circum = 94'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L548) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L548)
```
K_underscore = 95'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L549) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L549)
```
K_grave = 96'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L550) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L550)
```
K_lowera = 97'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L551) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L551)
```
K_lowerb = 98'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L552) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L552)
```
K_lowerc = 99'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L553) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L553)
```
K_lowerd = 100'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L554) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L554)
```
K_lowere = 101'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L555) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L555)
```
K_lowerf = 102'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L556) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L556)
```
K_lowerg = 103'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L557) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L557)
```
K_lowerh = 104'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L558) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L558)
```
K_loweri = 105'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L559) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L559)
```
K_lowerj = 106'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L560) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L560)
```
K_lowerk = 107'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L561) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L561)
```
K_lowerl = 108'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L562) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L562)
```
K_lowerm = 109'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L563) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L563)
```
K_lowern = 110'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L564) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L564)
```
K_lowero = 111'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L565) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L565)
```
K_lowerp = 112'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L566) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L566)
```
K_lowerq = 113'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L567) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L567)
```
K_lowerr = 114'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L568) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L568)
```
K_lowers = 115'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L569) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L569)
```
K_lowert = 116'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L570) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L570)
```
K_loweru = 117'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L571) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L571)
```
K_lowerv = 118'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L572) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L572)
```
K_lowerw = 119'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L573) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L573)
```
K_lowerx = 120'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L574) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L574)
```
K_lowery = 121'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L575) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L575)
```
K_lowerz = 122'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L576) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L576)
```
K_braceleft = 123'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L577) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L577)
```
K_bar = 124'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L578) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L578)
```
K_braceright = 125'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L579) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L579)
```
K_tilde = 126'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L580) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L580)
```
K_BS = 8'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L586) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L586)
```
K_TAB = 9'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L587) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L587)
```
K_LF = 10'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L588) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L588)
```
K_CR = 13'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L589) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L589)
```
IUP_NUMMAXCODES = 1280
```
5\*256=1280 Normal+Shift+Ctrl+Alt+Sys [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L612) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L612)
```
K_HOME = 129
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L614) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L614)
```
K_UP = 130
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L615) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L615)
```
K_PGUP = 131
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L616) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L616)
```
K_LEFT = 132
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L617) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L617)
```
K_MIDDLE = 133
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L618) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L618)
```
K_RIGHT = 134
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L619) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L619)
```
K_END = 135
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L620) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L620)
```
K_DOWN = 136
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L621) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L621)
```
K_PGDN = 137
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L622) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L622)
```
K_INS = 138
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L623) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L623)
```
K_DEL = 139
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L624) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L624)
```
K_PAUSE = 140
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L625) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L625)
```
K_ESC = 141
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L626) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L626)
```
K_ccedilla = 142
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L627) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L627)
```
K_F1 = 143
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L628) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L628)
```
K_F2 = 144
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L629) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L629)
```
K_F3 = 145
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L630) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L630)
```
K_F4 = 146
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L631) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L631)
```
K_F5 = 147
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L632) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L632)
```
K_F6 = 148
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L633) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L633)
```
K_F7 = 149
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L634) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L634)
```
K_F8 = 150
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L635) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L635)
```
K_F9 = 151
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L636) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L636)
```
K_F10 = 152
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L637) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L637)
```
K_F11 = 153
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L638) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L638)
```
K_F12 = 154
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L639) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L639)
```
K_Print = 155
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L640) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L640)
```
K_Menu = 156
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L641) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L641)
```
K_acute = 157
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L643) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L643)
```
K_sHOME = 385
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L645) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L645)
```
K_sUP = 386
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L646) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L646)
```
K_sPGUP = 387
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L647) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L647)
```
K_sLEFT = 388
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L648) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L648)
```
K_sMIDDLE = 389
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L649) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L649)
```
K_sRIGHT = 390
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L650) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L650)
```
K_sEND = 391
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L651) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L651)
```
K_sDOWN = 392
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L652) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L652)
```
K_sPGDN = 393
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L653) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L653)
```
K_sINS = 394
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L654) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L654)
```
K_sDEL = 395
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L655) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L655)
```
K_sSP = 288
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L656) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L656)
```
K_sTAB = 265
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L657) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L657)
```
K_sCR = 269
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L658) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L658)
```
K_sBS = 264
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L659) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L659)
```
K_sPAUSE = 396
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L660) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L660)
```
K_sESC = 397
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L661) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L661)
```
K_sCcedilla = 398
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L662) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L662)
```
K_sF1 = 399
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L663) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L663)
```
K_sF2 = 400
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L664) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L664)
```
K_sF3 = 401
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L665) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L665)
```
K_sF4 = 402
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L666) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L666)
```
K_sF5 = 403
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L667) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L667)
```
K_sF6 = 404
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L668) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L668)
```
K_sF7 = 405
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L669) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L669)
```
K_sF8 = 406
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L670) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L670)
```
K_sF9 = 407
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L671) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L671)
```
K_sF10 = 408
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L672) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L672)
```
K_sF11 = 409
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L673) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L673)
```
K_sF12 = 410
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L674) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L674)
```
K_sPrint = 411
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L675) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L675)
```
K_sMenu = 412
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L676) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L676)
```
K_cHOME = 641
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L678) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L678)
```
K_cUP = 642
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L679) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L679)
```
K_cPGUP = 643
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L680) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L680)
```
K_cLEFT = 644
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L681) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L681)
```
K_cMIDDLE = 645
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L682) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L682)
```
K_cRIGHT = 646
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L683) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L683)
```
K_cEND = 647
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L684) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L684)
```
K_cDOWN = 648
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L685) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L685)
```
K_cPGDN = 649
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L686) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L686)
```
K_cINS = 650
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L687) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L687)
```
K_cDEL = 651
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L688) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L688)
```
K_cSP = 544
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L689) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L689)
```
K_cTAB = 521
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L690) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L690)
```
K_cCR = 525
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L691) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L691)
```
K_cBS = 520
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L692) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L692)
```
K_cPAUSE = 652
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L693) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L693)
```
K_cESC = 653
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L694) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L694)
```
K_cCcedilla = 654
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L695) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L695)
```
K_cF1 = 655
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L696) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L696)
```
K_cF2 = 656
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L697) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L697)
```
K_cF3 = 657
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L698) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L698)
```
K_cF4 = 658
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L699) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L699)
```
K_cF5 = 659
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L700) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L700)
```
K_cF6 = 660
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L701) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L701)
```
K_cF7 = 661
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L702) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L702)
```
K_cF8 = 662
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L703) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L703)
```
K_cF9 = 663
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L704) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L704)
```
K_cF10 = 664
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L705) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L705)
```
K_cF11 = 665
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L706) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L706)
```
K_cF12 = 666
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L707) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L707)
```
K_cPrint = 667
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L708) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L708)
```
K_cMenu = 668
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L709) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L709)
```
K_mHOME = 897
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L711) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L711)
```
K_mUP = 898
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L712) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L712)
```
K_mPGUP = 899
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L713) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L713)
```
K_mLEFT = 900
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L714) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L714)
```
K_mMIDDLE = 901
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L715) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L715)
```
K_mRIGHT = 902
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L716) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L716)
```
K_mEND = 903
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L717) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L717)
```
K_mDOWN = 904
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L718) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L718)
```
K_mPGDN = 905
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L719) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L719)
```
K_mINS = 906
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L720) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L720)
```
K_mDEL = 907
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L721) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L721)
```
K_mSP = 800
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L722) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L722)
```
K_mTAB = 777
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L723) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L723)
```
K_mCR = 781
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L724) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L724)
```
K_mBS = 776
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L725) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L725)
```
K_mPAUSE = 908
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L726) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L726)
```
K_mESC = 909
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L727) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L727)
```
K_mCcedilla = 910
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L728) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L728)
```
K_mF1 = 911
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L729) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L729)
```
K_mF2 = 912
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L730) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L730)
```
K_mF3 = 913
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L731) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L731)
```
K_mF4 = 914
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L732) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L732)
```
K_mF5 = 915
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L733) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L733)
```
K_mF6 = 916
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L734) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L734)
```
K_mF7 = 917
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L735) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L735)
```
K_mF8 = 918
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L736) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L736)
```
K_mF9 = 919
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L737) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L737)
```
K_mF10 = 920
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L738) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L738)
```
K_mF11 = 921
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L739) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L739)
```
K_mF12 = 922
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L740) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L740)
```
K_mPrint = 923
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L741) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L741)
```
K_mMenu = 924
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L742) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L742)
```
K_yHOME = 1153
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L744) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L744)
```
K_yUP = 1154
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L745) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L745)
```
K_yPGUP = 1155
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L746) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L746)
```
K_yLEFT = 1156
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L747) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L747)
```
K_yMIDDLE = 1157
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L748) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L748)
```
K_yRIGHT = 1158
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L749) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L749)
```
K_yEND = 1159
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L750) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L750)
```
K_yDOWN = 1160
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L751) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L751)
```
K_yPGDN = 1161
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L752) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L752)
```
K_yINS = 1162
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L753) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L753)
```
K_yDEL = 1163
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L754) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L754)
```
K_ySP = 1056
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L755) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L755)
```
K_yTAB = 1033
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L756) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L756)
```
K_yCR = 1037
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L757) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L757)
```
K_yBS = 1032
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L758) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L758)
```
K_yPAUSE = 1164
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L759) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L759)
```
K_yESC = 1165
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L760) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L760)
```
K_yCcedilla = 1166
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L761) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L761)
```
K_yF1 = 1167
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L762) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L762)
```
K_yF2 = 1168
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L763) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L763)
```
K_yF3 = 1169
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L764) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L764)
```
K_yF4 = 1170
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L765) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L765)
```
K_yF5 = 1171
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L766) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L766)
```
K_yF6 = 1172
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L767) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L767)
```
K_yF7 = 1173
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L768) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L768)
```
K_yF8 = 1174
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L769) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L769)
```
K_yF9 = 1175
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L770) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L770)
```
K_yF10 = 1176
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L771) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L771)
```
K_yF11 = 1177
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L772) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L772)
```
K_yF12 = 1178
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L773) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L773)
```
K_yPrint = 1179
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L774) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L774)
```
K_yMenu = 1180
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L775) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L775)
```
K_sPlus = 299
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L777) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L777)
```
K_sComma = 300
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L778) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L778)
```
K_sMinus = 301
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L779) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L779)
```
K_sPeriod = 302
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L780) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L780)
```
K_sSlash = 303
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L781) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L781)
```
K_sAsterisk = 298
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L782) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L782)
```
K_cupperA = 577
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L784) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L784)
```
K_cupperB = 578
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L785) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L785)
```
K_cupperC = 579
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L786) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L786)
```
K_cupperD = 580
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L787) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L787)
```
K_cupperE = 581
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L788) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L788)
```
K_cupperF = 582
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L789) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L789)
```
K_cupperG = 583
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L790) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L790)
```
K_cupperH = 584
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L791) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L791)
```
K_cupperI = 585
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L792) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L792)
```
K_cupperJ = 586
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L793) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L793)
```
K_cupperK = 587
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L794) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L794)
```
K_cupperL = 588
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L795) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L795)
```
K_cupperM = 589
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L796) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L796)
```
K_cupperN = 590
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L797) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L797)
```
K_cupperO = 591
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L798) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L798)
```
K_cupperP = 592
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L799) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L799)
```
K_cupperQ = 593
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L800) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L800)
```
K_cupperR = 594
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L801) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L801)
```
K_cupperS = 595
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L802) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L802)
```
K_cupperT = 596
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L803) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L803)
```
K_cupperU = 597
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L804) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L804)
```
K_cupperV = 598
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L805) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L805)
```
K_cupperW = 599
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L806) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L806)
```
K_cupperX = 600
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L807) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L807)
```
K_cupperY = 601
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L808) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L808)
```
K_cupperZ = 602
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L809) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L809)
```
K_c1 = 561
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L810) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L810)
```
K_c2 = 562
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L811) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L811)
```
K_c3 = 563
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L812) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L812)
```
K_c4 = 564
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L813) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L813)
```
K_c5 = 565
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L814) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L814)
```
K_c6 = 566
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L815) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L815)
```
K_c7 = 567
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L816) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L816)
```
K_c8 = 568
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L817) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L817)
```
K_c9 = 569
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L818) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L818)
```
K_c0 = 560
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L819) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L819)
```
K_cPlus = 555
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L820) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L820)
```
K_cComma = 556
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L821) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L821)
```
K_cMinus = 557
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L822) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L822)
```
K_cPeriod = 558
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L823) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L823)
```
K_cSlash = 559
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L824) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L824)
```
K_cSemicolon = 571
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L825) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L825)
```
K_cEqual = 573
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L826) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L826)
```
K_cBracketleft = 603
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L827) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L827)
```
K_cBracketright = 605
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L828) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L828)
```
K_cBackslash = 604
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L829) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L829)
```
K_cAsterisk = 554
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L830) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L830)
```
K_mupperA = 833
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L832) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L832)
```
K_mupperB = 834
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L833) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L833)
```
K_mupperC = 835
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L834) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L834)
```
K_mupperD = 836
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L835) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L835)
```
K_mupperE = 837
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L836) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L836)
```
K_mupperF = 838
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L837) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L837)
```
K_mupperG = 839
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L838) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L838)
```
K_mupperH = 840
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L839) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L839)
```
K_mupperI = 841
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L840) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L840)
```
K_mupperJ = 842
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L841) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L841)
```
K_mupperK = 843
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L842) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L842)
```
K_mupperL = 844
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L843) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L843)
```
K_mupperM = 845
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L844) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L844)
```
K_mupperN = 846
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L845) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L845)
```
K_mupperO = 847
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L846) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L846)
```
K_mupperP = 848
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L847) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L847)
```
K_mupperQ = 849
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L848) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L848)
```
K_mupperR = 850
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L849) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L849)
```
K_mupperS = 851
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L850) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L850)
```
K_mupperT = 852
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L851) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L851)
```
K_mupperU = 853
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L852) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L852)
```
K_mupperV = 854
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L853) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L853)
```
K_mupperW = 855
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L854) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L854)
```
K_mupperX = 856
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L855) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L855)
```
K_mupperY = 857
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L856) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L856)
```
K_mupperZ = 858
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L857) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L857)
```
K_m1 = 817
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L858) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L858)
```
K_m2 = 818
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L859) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L859)
```
K_m3 = 819
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L860) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L860)
```
K_m4 = 820
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L861) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L861)
```
K_m5 = 821
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L862) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L862)
```
K_m6 = 822
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L863) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L863)
```
K_m7 = 823
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L864) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L864)
```
K_m8 = 824
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L865) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L865)
```
K_m9 = 825
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L866) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L866)
```
K_m0 = 816
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L867) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L867)
```
K_mPlus = 811
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L868) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L868)
```
K_mComma = 812
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L869) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L869)
```
K_mMinus = 813
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L870) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L870)
```
K_mPeriod = 814
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L871) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L871)
```
K_mSlash = 815
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L872) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L872)
```
K_mSemicolon = 827
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L873) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L873)
```
K_mEqual = 829
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L874) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L874)
```
K_mBracketleft = 859
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L875) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L875)
```
K_mBracketright = 861
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L876) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L876)
```
K_mBackslash = 860
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L877) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L877)
```
K_mAsterisk = 810
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L878) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L878)
```
K_yA = 1089
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L880) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L880)
```
K_yB = 1090
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L881) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L881)
```
K_yC = 1091
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L882) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L882)
```
K_yD = 1092
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L883) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L883)
```
K_yE = 1093
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L884) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L884)
```
K_yF = 1094
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L885) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L885)
```
K_yG = 1095
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L886) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L886)
```
K_yH = 1096
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L887) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L887)
```
K_yI = 1097
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L888) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L888)
```
K_yJ = 1098
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L889) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L889)
```
K_yK = 1099
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L890) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L890)
```
K_yL = 1100
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L891) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L891)
```
K_yM = 1101
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L892) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L892)
```
K_yN = 1102
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L893) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L893)
```
K_yO = 1103
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L894) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L894)
```
K_yP = 1104
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L895) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L895)
```
K_yQ = 1105
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L896) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L896)
```
K_yR = 1106
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L897) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L897)
```
K_yS = 1107
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L898) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L898)
```
K_yT = 1108
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L899) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L899)
```
K_yU = 1109
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L900) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L900)
```
K_yV = 1110
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L901) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L901)
```
K_yW = 1111
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L902) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L902)
```
K_yX = 1112
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L903) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L903)
```
K_yY = 1113
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L904) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L904)
```
K_yZ = 1114
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L905) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L905)
```
K_y1 = 1073
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L906) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L906)
```
K_y2 = 1074
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L907) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L907)
```
K_y3 = 1075
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L908) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L908)
```
K_y4 = 1076
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L909) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L909)
```
K_y5 = 1077
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L910) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L910)
```
K_y6 = 1078
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L911) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L911)
```
K_y7 = 1079
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L912) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L912)
```
K_y8 = 1080
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L913) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L913)
```
K_y9 = 1081
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L914) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L914)
```
K_y0 = 1072
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L915) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L915)
```
K_yPlus = 1067
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L916) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L916)
```
K_yComma = 1068
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L917) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L917)
```
K_yMinus = 1069
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L918) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L918)
```
K_yPeriod = 1070
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L919) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L919)
```
K_ySlash = 1071
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L920) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L920)
```
K_ySemicolon = 1083
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L921) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L921)
```
K_yEqual = 1085
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L922) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L922)
```
K_yBracketleft = 1115
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L923) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L923)
```
K_yBracketright = 1117
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L924) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L924)
```
K_yBackslash = 1116
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L925) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L925)
```
K_yAsterisk = 1066
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L926) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L926)
```
IUP_PRIMARY = -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L961) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L961)
```
IUP_SECONDARY = -2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L962) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L962) Procs
-----
```
proc fileDlg(): PIhandle {...}{.importc: "IupFileDlg", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L99)
```
proc messageDlg(): PIhandle {...}{.importc: "IupMessageDlg", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L100) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L100)
```
proc colorDlg(): PIhandle {...}{.importc: "IupColorDlg", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L101)
```
proc fontDlg(): PIhandle {...}{.importc: "IupFontDlg", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L102)
```
proc getFile(arq: cstring): cint {...}{.importc: "IupGetFile", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L104)
```
proc message(title, msg: cstring) {...}{.importc: "IupMessage", dynlib: dllname,
cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L106)
```
proc messagef(title, format: cstring) {...}{.importc: "IupMessagef", dynlib: dllname,
cdecl, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L108)
```
proc alarm(title, msg, b1, b2, b3: cstring): cint {...}{.importc: "IupAlarm",
dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L110)
```
proc scanf(format: cstring): cint {...}{.importc: "IupScanf", dynlib: dllname, cdecl,
varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L112)
```
proc listDialog(theType: cint; title: cstring; size: cint; list: cstringArray;
op, maxCol, maxLin: cint; marks: ptr cint): cint {...}{.
importc: "IupListDialog", dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L114)
```
proc getText(title, text: cstring): cint {...}{.importc: "IupGetText",
dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L118) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L118)
```
proc getColor(x, y: cint; r, g, b: var byte): cint {...}{.importc: "IupGetColor",
dynlib: dllname, cdecl.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L120)
```
proc getParam(title: cstring; action: Iparamcb; userData: pointer;
format: cstring): cint {...}{.importc: "IupGetParam", cdecl, varargs,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L127)
```
proc getParamv(title: cstring; action: Iparamcb; userData: pointer;
format: cstring; paramCount, paramExtra: cint; paramData: pointer): cint {...}{.
importc: "IupGetParamv", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L130)
```
proc open(argc: ptr cint; argv: ptr cstringArray): cint {...}{.importc: "IupOpen",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L138)
```
proc close() {...}{.importc: "IupClose", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L140)
```
proc imageLibOpen() {...}{.importc: "IupImageLibOpen", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L141)
```
proc mainLoop(): cint {...}{.importc: "IupMainLoop", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L143)
```
proc loopStep(): cint {...}{.importc: "IupLoopStep", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L145) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L145)
```
proc mainLoopLevel(): cint {...}{.importc: "IupMainLoopLevel", cdecl,
dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L147)
```
proc flush() {...}{.importc: "IupFlush", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L149)
```
proc exitLoop() {...}{.importc: "IupExitLoop", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L150)
```
proc update(ih: PIhandle) {...}{.importc: "IupUpdate", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L152)
```
proc updateChildren(ih: PIhandle) {...}{.importc: "IupUpdateChildren", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L153)
```
proc redraw(ih: PIhandle; children: cint) {...}{.importc: "IupRedraw", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L154)
```
proc refresh(ih: PIhandle) {...}{.importc: "IupRefresh", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L155) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L155)
```
proc mapFont(iupfont: cstring): cstring {...}{.importc: "IupMapFont", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L157) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L157)
```
proc unMapFont(driverfont: cstring): cstring {...}{.importc: "IupUnMapFont", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L158) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L158)
```
proc help(url: cstring): cint {...}{.importc: "IupHelp", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L159)
```
proc load(filename: cstring): cstring {...}{.importc: "IupLoad", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L160)
```
proc iupVersion(): cstring {...}{.importc: "IupVersion", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L162)
```
proc iupVersionDate(): cstring {...}{.importc: "IupVersionDate", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L163)
```
proc iupVersionNumber(): cint {...}{.importc: "IupVersionNumber", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L164)
```
proc setLanguage(lng: cstring) {...}{.importc: "IupSetLanguage", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L165) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L165)
```
proc getLanguage(): cstring {...}{.importc: "IupGetLanguage", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L166)
```
proc destroy(ih: PIhandle) {...}{.importc: "IupDestroy", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L168)
```
proc detach(child: PIhandle) {...}{.importc: "IupDetach", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L169)
```
proc append(ih, child: PIhandle): PIhandle {...}{.importc: "IupAppend", cdecl,
dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L170)
```
proc insert(ih, refChild, child: PIhandle): PIhandle {...}{.importc: "IupInsert",
cdecl, dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L172)
```
proc getChild(ih: PIhandle; pos: cint): PIhandle {...}{.importc: "IupGetChild",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L174) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L174)
```
proc getChildPos(ih, child: PIhandle): cint {...}{.importc: "IupGetChildPos", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L176)
```
proc getChildCount(ih: PIhandle): cint {...}{.importc: "IupGetChildCount", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L178) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L178)
```
proc getNextChild(ih, child: PIhandle): PIhandle {...}{.importc: "IupGetNextChild",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L180)
```
proc getBrother(ih: PIhandle): PIhandle {...}{.importc: "IupGetBrother", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L182)
```
proc getParent(ih: PIhandle): PIhandle {...}{.importc: "IupGetParent", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L184) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L184)
```
proc getDialog(ih: PIhandle): PIhandle {...}{.importc: "IupGetDialog", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L186)
```
proc getDialogChild(ih: PIhandle; name: cstring): PIhandle {...}{.
importc: "IupGetDialogChild", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L188) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L188)
```
proc reparent(ih, newParent: PIhandle): cint {...}{.importc: "IupReparent", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L190)
```
proc popup(ih: PIhandle; x, y: cint): cint {...}{.importc: "IupPopup", cdecl,
dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L193) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L193)
```
proc show(ih: PIhandle): cint {...}{.importc: "IupShow", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L195)
```
proc showXY(ih: PIhandle; x, y: cint): cint {...}{.importc: "IupShowXY", cdecl,
dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L197) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L197)
```
proc hide(ih: PIhandle): cint {...}{.importc: "IupHide", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L199)
```
proc map(ih: PIhandle): cint {...}{.importc: "IupMap", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L201)
```
proc unmap(ih: PIhandle) {...}{.importc: "IupUnmap", cdecl, dynlib: dllname,
discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L203)
```
proc setAttribute(ih: PIhandle; name, value: cstring) {...}{.
importc: "IupSetAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L206)
```
proc storeAttribute(ih: PIhandle; name, value: cstring) {...}{.
importc: "IupStoreAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L208) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L208)
```
proc setAttributes(ih: PIhandle; str: cstring): PIhandle {...}{.
importc: "IupSetAttributes", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L210) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L210)
```
proc getAttribute(ih: PIhandle; name: cstring): cstring {...}{.
importc: "IupGetAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L212)
```
proc getAttributes(ih: PIhandle): cstring {...}{.importc: "IupGetAttributes", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L214)
```
proc getInt(ih: PIhandle; name: cstring): cint {...}{.importc: "IupGetInt", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L216) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L216)
```
proc getInt2(ih: PIhandle; name: cstring): cint {...}{.importc: "IupGetInt2", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L218) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L218)
```
proc getIntInt(ih: PIhandle; name: cstring; i1, i2: var cint): cint {...}{.
importc: "IupGetIntInt", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L220) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L220)
```
proc getFloat(ih: PIhandle; name: cstring): cfloat {...}{.importc: "IupGetFloat",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L222) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L222)
```
proc setfAttribute(ih: PIhandle; name, format: cstring) {...}{.
importc: "IupSetfAttribute", cdecl, dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L224)
```
proc getAllAttributes(ih: PIhandle; names: cstringArray; n: cint): cint {...}{.
importc: "IupGetAllAttributes", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L226) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L226)
```
proc setAtt(handleName: cstring; ih: PIhandle; name: cstring): PIhandle {...}{.
importc: "IupSetAtt", cdecl, dynlib: dllname, varargs, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L228) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L228)
```
proc setGlobal(name, value: cstring) {...}{.importc: "IupSetGlobal", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L231) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L231)
```
proc storeGlobal(name, value: cstring) {...}{.importc: "IupStoreGlobal", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L233) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L233)
```
proc getGlobal(name: cstring): cstring {...}{.importc: "IupGetGlobal", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L235)
```
proc setFocus(ih: PIhandle): PIhandle {...}{.importc: "IupSetFocus", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L238) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L238)
```
proc getFocus(): PIhandle {...}{.importc: "IupGetFocus", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L240)
```
proc previousField(ih: PIhandle): PIhandle {...}{.importc: "IupPreviousField", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L242)
```
proc nextField(ih: PIhandle): PIhandle {...}{.importc: "IupNextField", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L244) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L244)
```
proc getCallback(ih: PIhandle; name: cstring): Icallback {...}{.
importc: "IupGetCallback", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L247)
```
proc setCallback(ih: PIhandle; name: cstring; fn: Icallback): Icallback {...}{.
importc: "IupSetCallback", cdecl, dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L249) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L249)
```
proc setCallbacks(ih: PIhandle; name: cstring; fn: Icallback): PIhandle {...}{.
importc: "IupSetCallbacks", cdecl, dynlib: dllname, varargs, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L252)
```
proc getFunction(name: cstring): Icallback {...}{.importc: "IupGetFunction", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L255) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L255)
```
proc setFunction(name: cstring; fn: Icallback): Icallback {...}{.
importc: "IupSetFunction", cdecl, dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L257) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L257)
```
proc getActionName(): cstring {...}{.importc: "IupGetActionName", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L259) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L259)
```
proc getHandle(name: cstring): PIhandle {...}{.importc: "IupGetHandle", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L262) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L262)
```
proc setHandle(name: cstring; ih: PIhandle): PIhandle {...}{.importc: "IupSetHandle",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L264) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L264)
```
proc getAllNames(names: cstringArray; n: cint): cint {...}{.
importc: "IupGetAllNames", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L266) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L266)
```
proc getAllDialogs(names: cstringArray; n: cint): cint {...}{.
importc: "IupGetAllDialogs", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L268)
```
proc getName(ih: PIhandle): cstring {...}{.importc: "IupGetName", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L270) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L270)
```
proc setAttributeHandle(ih: PIhandle; name: cstring; ihNamed: PIhandle) {...}{.
importc: "IupSetAttributeHandle", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L273) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L273)
```
proc getAttributeHandle(ih: PIhandle; name: cstring): PIhandle {...}{.
importc: "IupGetAttributeHandle", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L275) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L275)
```
proc getClassName(ih: PIhandle): cstring {...}{.importc: "IupGetClassName", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L278) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L278)
```
proc getClassType(ih: PIhandle): cstring {...}{.importc: "IupGetClassType", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L280)
```
proc getClassAttributes(classname: cstring; names: cstringArray; n: cint): cint {...}{.
importc: "IupGetClassAttributes", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L282) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L282)
```
proc saveClassAttributes(ih: PIhandle) {...}{.importc: "IupSaveClassAttributes",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L285) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L285)
```
proc setClassDefaultAttribute(classname, name, value: cstring) {...}{.
importc: "IupSetClassDefaultAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L287) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L287)
```
proc create(classname: cstring): PIhandle {...}{.importc: "IupCreate", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L290)
```
proc createv(classname: cstring; params: pointer): PIhandle {...}{.
importc: "IupCreatev", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L292) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L292)
```
proc createp(classname: cstring; first: pointer): PIhandle {...}{.
importc: "IupCreatep", cdecl, dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L294) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L294)
```
proc fill(): PIhandle {...}{.importc: "IupFill", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L297) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L297)
```
proc radio(child: PIhandle): PIhandle {...}{.importc: "IupRadio", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L298)
```
proc vbox(child: PIhandle): PIhandle {...}{.importc: "IupVbox", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L300) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L300)
```
proc vboxv(children: ptr PIhandle): PIhandle {...}{.importc: "IupVboxv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L302) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L302)
```
proc zbox(child: PIhandle): PIhandle {...}{.importc: "IupZbox", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L304) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L304)
```
proc zboxv(children: ptr PIhandle): PIhandle {...}{.importc: "IupZboxv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L306)
```
proc hbox(child: PIhandle): PIhandle {...}{.importc: "IupHbox", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L308) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L308)
```
proc hboxv(children: ptr PIhandle): PIhandle {...}{.importc: "IupHboxv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L310) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L310)
```
proc normalizer(ihFirst: PIhandle): PIhandle {...}{.importc: "IupNormalizer", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L313) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L313)
```
proc normalizerv(ihList: ptr PIhandle): PIhandle {...}{.importc: "IupNormalizerv",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L315) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L315)
```
proc cbox(child: PIhandle): PIhandle {...}{.importc: "IupCbox", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L318) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L318)
```
proc cboxv(children: ptr PIhandle): PIhandle {...}{.importc: "IupCboxv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L320) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L320)
```
proc sbox(child: PIhandle): PIhandle {...}{.importc: "IupSbox", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L322)
```
proc frame(child: PIhandle): PIhandle {...}{.importc: "IupFrame", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L325) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L325)
```
proc image(width, height: cint; pixmap: pointer): PIhandle {...}{.
importc: "IupImage", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L328) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L328)
```
proc imageRGB(width, height: cint; pixmap: pointer): PIhandle {...}{.
importc: "IupImageRGB", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L330) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L330)
```
proc imageRGBA(width, height: cint; pixmap: pointer): PIhandle {...}{.
importc: "IupImageRGBA", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L332) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L332)
```
proc item(title, action: cstring): PIhandle {...}{.importc: "IupItem", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L335) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L335)
```
proc submenu(title: cstring; child: PIhandle): PIhandle {...}{.importc: "IupSubmenu",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L337)
```
proc separator(): PIhandle {...}{.importc: "IupSeparator", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L339) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L339)
```
proc menu(child: PIhandle): PIhandle {...}{.importc: "IupMenu", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L341) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L341)
```
proc menuv(children: ptr PIhandle): PIhandle {...}{.importc: "IupMenuv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L343) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L343)
```
proc button(title, action: cstring): PIhandle {...}{.importc: "IupButton", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L346) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L346)
```
proc link(url, title: cstring): PIhandle {...}{.importc: "IupLink", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L348) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L348)
```
proc canvas(action: cstring): PIhandle {...}{.importc: "IupCanvas", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L350) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L350)
```
proc dialog(child: PIhandle): PIhandle {...}{.importc: "IupDialog", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L352) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L352)
```
proc user(): PIhandle {...}{.importc: "IupUser", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L354) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L354)
```
proc label(title: cstring): PIhandle {...}{.importc: "IupLabel", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L356) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L356)
```
proc list(action: cstring): PIhandle {...}{.importc: "IupList", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L358) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L358)
```
proc text(action: cstring): PIhandle {...}{.importc: "IupText", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L360) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L360)
```
proc multiLine(action: cstring): PIhandle {...}{.importc: "IupMultiLine", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L362) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L362)
```
proc toggle(title, action: cstring): PIhandle {...}{.importc: "IupToggle", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L364) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L364)
```
proc timer(): PIhandle {...}{.importc: "IupTimer", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L366) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L366)
```
proc progressBar(): PIhandle {...}{.importc: "IupProgressBar", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L368) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L368)
```
proc val(theType: cstring): PIhandle {...}{.importc: "IupVal", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L370) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L370)
```
proc tabs(child: PIhandle): PIhandle {...}{.importc: "IupTabs", cdecl,
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L372) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L372)
```
proc tabsv(children: ptr PIhandle): PIhandle {...}{.importc: "IupTabsv", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L374) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L374)
```
proc tree(): PIhandle {...}{.importc: "IupTree", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L376) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L376)
```
proc spin(): PIhandle {...}{.importc: "IupSpin", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L378) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L378)
```
proc spinbox(child: PIhandle): PIhandle {...}{.importc: "IupSpinbox", cdecl,
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L379) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L379)
```
proc textConvertLinColToPos(ih: PIhandle; lin, col: cint; pos: var cint) {...}{.
importc: "IupTextConvertLinColToPos", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L383) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L383)
```
proc textConvertPosToLinCol(ih: PIhandle; pos: cint; lin, col: var cint) {...}{.
importc: "IupTextConvertPosToLinCol", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L385) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L385)
```
proc convertXYToPos(ih: PIhandle; x, y: cint): cint {...}{.
importc: "IupConvertXYToPos", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L388) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L388)
```
proc treeSetUserId(ih: PIhandle; id: cint; userid: pointer): cint {...}{.
importc: "IupTreeSetUserId", cdecl, dynlib: dllname, discardable.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L392) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L392)
```
proc treeGetUserId(ih: PIhandle; id: cint): pointer {...}{.
importc: "IupTreeGetUserId", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L394) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L394)
```
proc treeGetId(ih: PIhandle; userid: pointer): cint {...}{.importc: "IupTreeGetId",
cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L396) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L396)
```
proc treeSetAttribute(ih: PIhandle; name: cstring; id: cint; value: cstring) {...}{.
importc: "IupTreeSetAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L399) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L399)
```
proc treeStoreAttribute(ih: PIhandle; name: cstring; id: cint; value: cstring) {...}{.
importc: "IupTreeStoreAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L401) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L401)
```
proc treeGetAttribute(ih: PIhandle; name: cstring; id: cint): cstring {...}{.
importc: "IupTreeGetAttribute", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L403) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L403)
```
proc treeGetInt(ih: PIhandle; name: cstring; id: cint): cint {...}{.
importc: "IupTreeGetInt", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L405)
```
proc treeGetFloat(ih: PIhandle; name: cstring; id: cint): cfloat {...}{.
importc: "IupTreeGetFloat", cdecl, dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L407) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L407)
```
proc treeSetfAttribute(ih: PIhandle; name: cstring; id: cint; format: cstring) {...}{.
importc: "IupTreeSetfAttribute", cdecl, dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L409) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L409)
```
proc isShift(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L464) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L464)
```
proc isControl(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L465) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L465)
```
proc isButton1(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L466) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L466)
```
proc isButton2(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L467) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L467)
```
proc isbutton3(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L468) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L468)
```
proc isDouble(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L469) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L469)
```
proc isAlt(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L470) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L470)
```
proc isSys(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L471) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L471)
```
proc isButton4(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L472) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L472)
```
proc isButton5(s: cstring): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L473) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L473)
```
proc isPrint(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L582) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L582)
```
proc isXkey(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L595) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L595)
```
proc isShiftXkey(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L596) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L596)
```
proc isCtrlXkey(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L597) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L597)
```
proc isAltXkey(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L598) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L598)
```
proc isSysXkey(c: cint): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L599) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L599)
```
proc iUPxCODE(c: cint): cint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L601) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L601)
```
proc iUPsxCODE(c: cint): cint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L602) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L602)
```
proc iUPcxCODE(c: cint): cint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L607) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L607)
```
proc iUPmxCODE(c: cint): cint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L608) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L608)
```
proc iUPyxCODE(c: cint): cint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L609) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L609)
```
proc controlsOpen(): cint {...}{.cdecl, importc: "IupControlsOpen", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L928) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L928)
```
proc controlsClose() {...}{.cdecl, importc: "IupControlsClose", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L929) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L929)
```
proc oldValOpen() {...}{.cdecl, importc: "IupOldValOpen", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L931) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L931)
```
proc oldTabsOpen() {...}{.cdecl, importc: "IupOldTabsOpen", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L932) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L932)
```
proc colorbar(): PIhandle {...}{.cdecl, importc: "IupColorbar", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L934) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L934)
```
proc cells(): PIhandle {...}{.cdecl, importc: "IupCells", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L935) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L935)
```
proc colorBrowser(): PIhandle {...}{.cdecl, importc: "IupColorBrowser",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L936) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L936)
```
proc gauge(): PIhandle {...}{.cdecl, importc: "IupGauge", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L937) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L937)
```
proc dial(theType: cstring): PIhandle {...}{.cdecl, importc: "IupDial",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L938) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L938)
```
proc matrix(action: cstring): PIhandle {...}{.cdecl, importc: "IupMatrix",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L939) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L939)
```
proc matSetAttribute(ih: PIhandle; name: cstring; lin, col: cint; value: cstring) {...}{.
cdecl, importc: "IupMatSetAttribute", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L942) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L942)
```
proc matStoreAttribute(ih: PIhandle; name: cstring; lin, col: cint;
value: cstring) {...}{.cdecl, importc: "IupMatStoreAttribute",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L945) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L945)
```
proc matGetAttribute(ih: PIhandle; name: cstring; lin, col: cint): cstring {...}{.
cdecl, importc: "IupMatGetAttribute", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L948) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L948)
```
proc matGetInt(ih: PIhandle; name: cstring; lin, col: cint): cint {...}{.cdecl,
importc: "IupMatGetInt", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L950) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L950)
```
proc matGetFloat(ih: PIhandle; name: cstring; lin, col: cint): cfloat {...}{.cdecl,
importc: "IupMatGetFloat", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L952) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L952)
```
proc matSetfAttribute(ih: PIhandle; name: cstring; lin, col: cint;
format: cstring) {...}{.cdecl, importc: "IupMatSetfAttribute",
dynlib: dllname, varargs.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L954) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L954)
```
proc pPlotOpen() {...}{.cdecl, importc: "IupPPlotOpen", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L965) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L965)
```
proc pPlot(): PIhandle {...}{.cdecl, importc: "IupPPlot", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L968) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L968)
```
proc pPlotBegin(ih: PIhandle; strXdata: cint) {...}{.cdecl, importc: "IupPPlotBegin",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L971) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L971)
```
proc pPlotAdd(ih: PIhandle; x, y: cfloat) {...}{.cdecl, importc: "IupPPlotAdd",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L973) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L973)
```
proc pPlotAddStr(ih: PIhandle; x: cstring; y: cfloat) {...}{.cdecl,
importc: "IupPPlotAddStr", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L975) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L975)
```
proc pPlotEnd(ih: PIhandle): cint {...}{.cdecl, importc: "IupPPlotEnd",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L977) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L977)
```
proc pPlotInsertStr(ih: PIhandle; index, sampleIndex: cint; x: cstring;
y: cfloat) {...}{.cdecl, importc: "IupPPlotInsertStr",
dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L980) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L980)
```
proc pPlotInsert(ih: PIhandle; index, sampleIndex: cint; x, y: cfloat) {...}{.cdecl,
importc: "IupPPlotInsert", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L983) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L983)
```
proc pPlotTransform(ih: PIhandle; x, y: cfloat; ix, iy: var cint) {...}{.cdecl,
importc: "IupPPlotTransform", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L988) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L988)
```
proc pPlotPaintTo(ih: PIhandle; cnv: pointer) {...}{.cdecl,
importc: "IupPPlotPaintTo", dynlib: dllname.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/wrappers/iup.nim#L992) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/wrappers/iup.nim#L992)
| programming_docs |
nim rst rst
===
This module implements a reStructuredText parser. A large subset is implemented. Some features of the markdown wiki syntax are also supported.
**Note:** Import `packages/docutils/rst` to use this module
Imports
-------
<rstast> Types
-----
```
RstParseOption = enum
roSkipPounds, ## skip ``#`` at line beginning (documentation
## embedded in Nim comments)
roSupportSmilies, ## make the RST parser support smilies like ``:)``
roSupportRawDirective, ## support the ``raw`` directive (don't support
## it for sandboxing)
roSupportMarkdown ## support additional features of markdown
```
options for the RST parser [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L20)
```
RstParseOptions = set[RstParseOption]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L28)
```
MsgClass = enum
mcHint = "Hint", mcWarning = "Warning", mcError = "Error"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L30)
```
MsgKind = enum
meCannotOpenFile, meExpected, meGridTableNotImplemented, meNewSectionExpected,
meGeneralParseError, meInvalidDirective, mwRedefinitionOfLabel,
mwUnknownSubstitution, mwUnsupportedLanguage, mwUnsupportedField
```
the possible messages [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L35)
```
MsgHandler = proc (filename: string; line, col: int; msgKind: MsgKind;
arg: string) {...}{.closure, gcsafe.}
```
what to do in case of an error [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L47)
```
FindFileHandler = proc (filename: string): string {...}{.closure, gcsafe.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L49)
```
EParseError = object of ValueError
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L297) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L297) Procs
-----
```
proc whichMsgClass(k: MsgKind): MsgClass {...}{.raises: [], tags: [].}
```
returns which message class `k` belongs to. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L299) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L299)
```
proc defaultMsgHandler(filename: string; line, col: int; msgkind: MsgKind;
arg: string) {...}{.raises: [ValueError, EParseError, IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L307) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L307)
```
proc defaultFindFile(filename: string): string {...}{.raises: [],
tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L315) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L315)
```
proc addNodes(n: PRstNode): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L372) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L372)
```
proc rstnodeToRefname(n: PRstNode): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L427) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L427)
```
proc getFieldValue(n: PRstNode): string {...}{.raises: [], tags: [].}
```
Returns the value of a specific `rnField` node.
This proc will assert if the node is not of the expected type. The empty string will be returned as a minimum. Any value in the rst will be stripped form leading/trailing whitespace.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L987) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L987)
```
proc getFieldValue(n: PRstNode; fieldname: string): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L999) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L999)
```
proc getArgument(n: PRstNode): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L1013) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L1013)
```
proc rstParse(text, filename: string; line, column: int; hasToc: var bool;
options: RstParseOptions; findFile: FindFileHandler = nil;
msgHandler: MsgHandler = nil): PRstNode {...}{.raises: [Exception],
tags: [ReadEnvEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rst.nim#L1818) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rst.nim#L1818)
nim streams streams
=======
This module provides a stream interface and two implementations thereof: the [FileStream](#FileStream) and the [StringStream](#StringStream) which implement the stream interface for Nim file objects (`File`) and strings.
Other modules may provide other implementations for this standard stream interface.
Basic usage
-----------
The basic flow of using this module is:
1. Open input stream
2. Read or write stream
3. Close stream
### StringStream example
```
import streams
var strm = newStringStream("""The first line
the second line
the third line""")
var line = ""
while strm.readLine(line):
echo line
# Output:
# The first line
# the second line
# the third line
strm.close()
```
### FileStream example
Write file stream example:
```
import streams
var strm = newFileStream("somefile.txt", fmWrite)
var line = ""
if not isNil(strm):
strm.writeLine("The first line")
strm.writeLine("the second line")
strm.writeLine("the third line")
strm.close()
# Output (somefile.txt):
# The first line
# the second line
# the third line
```
Read file stream example:
```
import streams
var strm = newFileStream("somefile.txt", fmRead)
var line = ""
if not isNil(strm):
while strm.readLine(line):
echo line
strm.close()
# Output:
# The first line
# the second line
# the third line
```
See also
--------
* [asyncstreams module](asyncstreams)
* [io module](io) for [FileMode enum](io#FileMode)
Imports
-------
<since> Types
-----
```
Stream = ref StreamObj
```
All procedures of this module use this type. Procedures don't directly use [StreamObj](#StreamObj). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L106)
```
StreamObj = object of RootObj
closeImpl*: proc (s: Stream) {...}{.nimcall, raises: [Exception, IOError, OSError],
tags: [WriteIOEffect], gcsafe.}
atEndImpl*: proc (s: Stream): bool {...}{.nimcall,
raises: [Defect, IOError, OSError],
tags: [], gcsafe.}
setPositionImpl*: proc (s: Stream; pos: int) {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [], gcsafe.}
getPositionImpl*: proc (s: Stream): int {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [], gcsafe.}
readDataStrImpl*: proc (s: Stream; buffer: var string; slice: Slice[int]): int {...}{.
nimcall, raises: [Defect, IOError, OSError], tags: [ReadIOEffect], gcsafe.}
readLineImpl*: proc (s: Stream; line: var TaintedString): bool {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [ReadIOEffect], gcsafe.}
readDataImpl*: proc (s: Stream; buffer: pointer; bufLen: int): int {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [ReadIOEffect], gcsafe.}
peekDataImpl*: proc (s: Stream; buffer: pointer; bufLen: int): int {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [ReadIOEffect], gcsafe.}
writeDataImpl*: proc (s: Stream; buffer: pointer; bufLen: int) {...}{.nimcall,
raises: [Defect, IOError, OSError], tags: [WriteIOEffect], gcsafe.}
flushImpl*: proc (s: Stream) {...}{.nimcall, raises: [Defect, IOError, OSError],
tags: [WriteIOEffect], gcsafe.}
```
Stream interface that supports writing or reading.
**Note:**
* That these fields here shouldn't be used directly. They are accessible so that a stream implementation can override them.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L109)
```
StringStream = ref StringStreamObj
```
A stream that encapsulates a string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1129) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1129)
```
StringStreamObj = object of StreamObj
data*: string ## A string data.
## This is updated when called `writeLine` etc.
pos: int
```
A string stream object. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1131) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1131)
```
FileStream = ref FileStreamObj
```
A stream that encapsulates a `File`.
**Note:** Not available for JS backend.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1302) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1302)
```
FileStreamObj = object of Stream
f: File
```
A file stream object.
**Note:** Not available for JS backend.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1306) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1306) Procs
-----
```
proc flush(s: Stream) {...}{.raises: [IOError, OSError], tags: [WriteIOEffect].}
```
Flushes the buffers that the stream `s` might use.
This procedure causes any unwritten data for that stream to be delivered to the host environment to be written to the file.
See also:
* [close proc](#close,Stream)
**Example:**
```
from os import removeFile
var strm = newFileStream("somefile.txt", fmWrite)
doAssert "Before write:" & readFile("somefile.txt") == "Before write:"
strm.write("hello")
doAssert "After write:" & readFile("somefile.txt") == "After write:"
strm.flush()
doAssert "After flush:" & readFile("somefile.txt") == "After flush:hello"
strm.write("HELLO")
strm.flush()
doAssert "After flush:" & readFile("somefile.txt") == "After flush:helloHELLO"
strm.close()
doAssert "After close:" & readFile("somefile.txt") == "After close:helloHELLO"
removeFile("somefile.txt")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L140)
```
proc close(s: Stream) {...}{.raises: [Exception, IOError, OSError],
tags: [WriteIOEffect].}
```
Closes the stream `s`.
See also:
* [flush proc](#flush,Stream)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
## do something...
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L169)
```
proc atEnd(s: Stream): bool {...}{.raises: [IOError, OSError], tags: [].}
```
Checks if more data can be read from `s`. Returns `true` if all data has been read. **Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
var line = ""
doAssert strm.atEnd() == false
while strm.readLine(line):
discard
doAssert strm.atEnd() == true
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L180)
```
proc setPosition(s: Stream; pos: int) {...}{.raises: [IOError, OSError], tags: [].}
```
Sets the position `pos` of the stream `s`. **Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
strm.setPosition(4)
doAssert strm.readLine() == "first line"
strm.setPosition(0)
doAssert strm.readLine() == "The first line"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L194) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L194)
```
proc getPosition(s: Stream): int {...}{.raises: [IOError, OSError], tags: [].}
```
Retrieves the current position in the stream `s`. **Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
doAssert strm.getPosition() == 0
discard strm.readLine()
doAssert strm.getPosition() == 15
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L206)
```
proc readData(s: Stream; buffer: pointer; bufLen: int): int {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Low level proc that reads data into an untyped `buffer` of `bufLen` size.
**JS note:** `buffer` is treated as a `ptr string` and written to between `0..<bufLen`.
**Example:**
```
var strm = newStringStream("abcde")
var buffer: array[6, char]
doAssert strm.readData(addr(buffer), 1024) == 5
doAssert buffer == ['a', 'b', 'c', 'd', 'e', '\x00']
doAssert strm.atEnd() == true
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L217) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L217)
```
proc readDataStr(s: Stream; buffer: var string; slice: Slice[int]): int {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Low level proc that reads data into a string `buffer` at `slice`. **Example:**
```
var strm = newStringStream("abcde")
var buffer = "12345"
doAssert strm.readDataStr(buffer, 0..3) == 4
doAssert buffer == "abcd5"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L232)
```
proc readAll(s: Stream): string {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads all available data. **Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
doAssert strm.readAll() == "The first line\nthe second line\nthe third line"
doAssert strm.atEnd() == true
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L260)
```
proc peekData(s: Stream; buffer: pointer; bufLen: int): int {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Low level proc that reads data into an untyped `buffer` of `bufLen` size without moving stream position.
**JS note:** `buffer` is treated as a `ptr string` and written to between `0..<bufLen`.
**Example:**
```
var strm = newStringStream("abcde")
var buffer: array[6, char]
doAssert strm.peekData(addr(buffer), 1024) == 5
doAssert buffer == ['a', 'b', 'c', 'd', 'e', '\x00']
doAssert strm.atEnd() == false
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L293) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L293)
```
proc writeData(s: Stream; buffer: pointer; bufLen: int) {...}{.
raises: [IOError, OSError], tags: [WriteIOEffect].}
```
Low level proc that writes an untyped `buffer` of `bufLen` size to the stream `s`.
**JS note:** `buffer` is treated as a `ptr string` and read between `0..<bufLen`.
**Example:**
```
## writeData
var strm = newStringStream("")
var buffer = ['a', 'b', 'c', 'd', 'e']
strm.writeData(addr(buffer), sizeof(buffer))
doAssert strm.atEnd() == true
## readData
strm.setPosition(0)
var buffer2: array[6, char]
doAssert strm.readData(addr(buffer2), sizeof(buffer2)) == 5
doAssert buffer2 == ['a', 'b', 'c', 'd', 'e', '\x00']
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L309) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L309)
```
proc write[T](s: Stream; x: T)
```
Generic write procedure. Writes `x` to the stream `s`. Implementation:
**Note:** Not available for JS backend. Use [write(Stream, string)](#write,Stream,string) for now.
```
s.writeData(s, unsafeAddr(x), sizeof(x))
```
**Example:**
```
var strm = newStringStream("")
strm.write("abcde")
strm.setPosition(0)
doAssert strm.readAll() == "abcde"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L330) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L330)
```
proc write(s: Stream; x: string) {...}{.raises: [IOError, OSError],
tags: [WriteIOEffect].}
```
Writes the string `x` to the the stream `s`. No length field or terminating zero is written. **Example:**
```
var strm = newStringStream("")
strm.write("THE FIRST LINE")
strm.setPosition(0)
doAssert strm.readLine() == "THE FIRST LINE"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L348) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L348)
```
proc write(s: Stream; args: varargs[string, `$`]) {...}{.raises: [IOError, OSError],
tags: [WriteIOEffect].}
```
Writes one or more strings to the the stream. No length fields or terminating zeros are written. **Example:**
```
var strm = newStringStream("")
strm.write(1, 2, 3, 4)
strm.setPosition(0)
doAssert strm.readLine() == "1234"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L368) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L368)
```
proc writeLine(s: Stream; args: varargs[string, `$`]) {...}{.
raises: [IOError, OSError], tags: [WriteIOEffect].}
```
Writes one or more strings to the the stream `s` followed by a new line. No length field or terminating zero is written. **Example:**
```
var strm = newStringStream("")
strm.writeLine(1, 2)
strm.writeLine(3, 4)
strm.setPosition(0)
doAssert strm.readAll() == "12\n34\n"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L380) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L380)
```
proc read[T](s: Stream; result: var T)
```
Generic read procedure. Reads `result` from the stream `s`.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream("012")
## readInt
var i: int8
strm.read(i)
doAssert i == 48
## readData
var buffer: array[2, char]
strm.read(buffer)
doAssert buffer == ['1', '2']
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L394) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L394)
```
proc peek[T](s: Stream; result: var T)
```
Generic peek procedure. Peeks `result` from the stream `s`.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream("012")
## peekInt
var i: int8
strm.peek(i)
doAssert i == 48
## peekData
var buffer: array[2, char]
strm.peek(buffer)
doAssert buffer == ['0', '1']
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L413) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L413)
```
proc readChar(s: Stream): char {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads a char from the stream `s`.
Raises `IOError` if an error occurred. Returns '\0' as an EOF marker.
**Example:**
```
var strm = newStringStream("12\n3")
doAssert strm.readChar() == '1'
doAssert strm.readChar() == '2'
doAssert strm.readChar() == '\n'
doAssert strm.readChar() == '3'
doAssert strm.readChar() == '\x00'
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L432) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L432)
```
proc peekChar(s: Stream): char {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks a char from the stream `s`. Raises `IOError` if an error occurred. Returns '\0' as an EOF marker. **Example:**
```
var strm = newStringStream("12\n3")
doAssert strm.peekChar() == '1'
doAssert strm.peekChar() == '1'
discard strm.readAll()
doAssert strm.peekChar() == '\x00'
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L453) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L453)
```
proc readBool(s: Stream): bool {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads a bool from the stream `s`.
A bool is one byte long and it is `true` for every non-zero (`0000_0000`) value. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(true)
strm.write(false)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readBool() == true
doAssert strm.readBool() == false
doAssertRaises(IOError): discard strm.readBool()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L471) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L471)
```
proc peekBool(s: Stream): bool {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks a bool from the stream `s`.
A bool is one byte long and it is `true` for every non-zero (`0000_0000`) value. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(true)
strm.write(false)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekBool() == true
## not false
doAssert strm.peekBool() == true
doAssert strm.readBool() == true
doAssert strm.peekBool() == false
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L496)
```
proc readInt8(s: Stream): int8 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an int8 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i8)
strm.write(2'i8)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readInt8() == 1'i8
doAssert strm.readInt8() == 2'i8
doAssertRaises(IOError): discard strm.readInt8()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L523) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L523)
```
proc peekInt8(s: Stream): int8 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an int8 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i8)
strm.write(2'i8)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekInt8() == 1'i8
## not 2'i8
doAssert strm.peekInt8() == 1'i8
doAssert strm.readInt8() == 1'i8
doAssert strm.peekInt8() == 2'i8
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L542) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L542)
```
proc readInt16(s: Stream): int16 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an int16 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i16)
strm.write(2'i16)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readInt16() == 1'i16
doAssert strm.readInt16() == 2'i16
doAssertRaises(IOError): discard strm.readInt16()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L563) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L563)
```
proc peekInt16(s: Stream): int16 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an int16 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i16)
strm.write(2'i16)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekInt16() == 1'i16
## not 2'i16
doAssert strm.peekInt16() == 1'i16
doAssert strm.readInt16() == 1'i16
doAssert strm.peekInt16() == 2'i16
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L582) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L582)
```
proc readInt32(s: Stream): int32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an int32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i32)
strm.write(2'i32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readInt32() == 1'i32
doAssert strm.readInt32() == 2'i32
doAssertRaises(IOError): discard strm.readInt32()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L603) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L603)
```
proc peekInt32(s: Stream): int32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an int32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i32)
strm.write(2'i32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekInt32() == 1'i32
## not 2'i32
doAssert strm.peekInt32() == 1'i32
doAssert strm.readInt32() == 1'i32
doAssert strm.peekInt32() == 2'i32
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L622) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L622)
```
proc readInt64(s: Stream): int64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an int64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i64)
strm.write(2'i64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readInt64() == 1'i64
doAssert strm.readInt64() == 2'i64
doAssertRaises(IOError): discard strm.readInt64()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L643) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L643)
```
proc peekInt64(s: Stream): int64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an int64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'i64)
strm.write(2'i64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekInt64() == 1'i64
## not 2'i64
doAssert strm.peekInt64() == 1'i64
doAssert strm.readInt64() == 1'i64
doAssert strm.peekInt64() == 2'i64
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L662) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L662)
```
proc readUint8(s: Stream): uint8 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an uint8 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u8)
strm.write(2'u8)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readUint8() == 1'u8
doAssert strm.readUint8() == 2'u8
doAssertRaises(IOError): discard strm.readUint8()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L683) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L683)
```
proc peekUint8(s: Stream): uint8 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an uint8 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u8)
strm.write(2'u8)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekUint8() == 1'u8
## not 2'u8
doAssert strm.peekUint8() == 1'u8
doAssert strm.readUint8() == 1'u8
doAssert strm.peekUint8() == 2'u8
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L702) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L702)
```
proc readUint16(s: Stream): uint16 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an uint16 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u16)
strm.write(2'u16)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readUint16() == 1'u16
doAssert strm.readUint16() == 2'u16
doAssertRaises(IOError): discard strm.readUint16()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L723) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L723)
```
proc peekUint16(s: Stream): uint16 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an uint16 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u16)
strm.write(2'u16)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekUint16() == 1'u16
## not 2'u16
doAssert strm.peekUint16() == 1'u16
doAssert strm.readUint16() == 1'u16
doAssert strm.peekUint16() == 2'u16
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L742) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L742)
```
proc readUint32(s: Stream): uint32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an uint32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u32)
strm.write(2'u32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readUint32() == 1'u32
doAssert strm.readUint32() == 2'u32
doAssertRaises(IOError): discard strm.readUint32()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L763) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L763)
```
proc peekUint32(s: Stream): uint32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an uint32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u32)
strm.write(2'u32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekUint32() == 1'u32
## not 2'u32
doAssert strm.peekUint32() == 1'u32
doAssert strm.readUint32() == 1'u32
doAssert strm.peekUint32() == 2'u32
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L783) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L783)
```
proc readUint64(s: Stream): uint64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads an uint64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u64)
strm.write(2'u64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readUint64() == 1'u64
doAssert strm.readUint64() == 2'u64
doAssertRaises(IOError): discard strm.readUint64()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L804) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L804)
```
proc peekUint64(s: Stream): uint64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks an uint64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'u64)
strm.write(2'u64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekUint64() == 1'u64
## not 2'u64
doAssert strm.peekUint64() == 1'u64
doAssert strm.readUint64() == 1'u64
doAssert strm.peekUint64() == 2'u64
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L823) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L823)
```
proc readFloat32(s: Stream): float32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads a float32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'f32)
strm.write(2'f32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readFloat32() == 1'f32
doAssert strm.readFloat32() == 2'f32
doAssertRaises(IOError): discard strm.readFloat32()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L844) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L844)
```
proc peekFloat32(s: Stream): float32 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks a float32 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'f32)
strm.write(2'f32)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekFloat32() == 1'f32
## not 2'f32
doAssert strm.peekFloat32() == 1'f32
doAssert strm.readFloat32() == 1'f32
doAssert strm.peekFloat32() == 2'f32
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L863) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L863)
```
proc readFloat64(s: Stream): float64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads a float64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [readStr](#readStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'f64)
strm.write(2'f64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.readFloat64() == 1'f64
doAssert strm.readFloat64() == 2'f64
doAssertRaises(IOError): discard strm.readFloat64()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L884) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L884)
```
proc peekFloat64(s: Stream): float64 {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks a float64 from the stream `s`. Raises `IOError` if an error occurred.
**Note:** Not available for JS backend. Use [peekStr](#peekStr,Stream,int) for now.
**Example:**
```
var strm = newStringStream()
## setup for reading data
strm.write(1'f64)
strm.write(2'f64)
strm.flush()
strm.setPosition(0)
## get data
doAssert strm.peekFloat64() == 1'f64
## not 2'f64
doAssert strm.peekFloat64() == 1'f64
doAssert strm.readFloat64() == 1'f64
doAssert strm.peekFloat64() == 2'f64
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L903) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L903)
```
proc readStr(s: Stream; length: int; str: var TaintedString) {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Reads a string of length `length` from the stream `s`. Raises `IOError` if an error occurred. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L938) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L938)
```
proc readStr(s: Stream; length: int): TaintedString {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Reads a string of length `length` from the stream `s`. Raises `IOError` if an error occurred. **Example:**
```
var strm = newStringStream("abcde")
doAssert strm.readStr(2) == "ab"
doAssert strm.readStr(2) == "cd"
doAssert strm.readStr(2) == "e"
doAssert strm.readStr(2) == ""
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L943) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L943)
```
proc peekStr(s: Stream; length: int; str: var TaintedString) {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Peeks a string of length `length` from the stream `s`. Raises `IOError` if an error occurred. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L964) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L964)
```
proc peekStr(s: Stream; length: int): TaintedString {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Peeks a string of length `length` from the stream `s`. Raises `IOError` if an error occurred. **Example:**
```
var strm = newStringStream("abcde")
doAssert strm.peekStr(2) == "ab"
## not "cd
doAssert strm.peekStr(2) == "ab"
doAssert strm.readStr(2) == "ab"
doAssert strm.peekStr(2) == "cd"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L969) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L969)
```
proc readLine(s: Stream; line: var TaintedString): bool {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Reads a line of text from the stream `s` into `line`. `line` must not be `nil`! May throw an IO exception.
A line of text may be delimited by `LF` or `CRLF`. The newline character(s) are not part of the returned string. Returns `false` if the end of the file has been reached, `true` otherwise. If `false` is returned `line` contains no new data.
See also:
* [readLine(Stream) proc](#readLine,Stream)
* [peekLine(Stream) proc](#peekLine,Stream)
* [peekLine(Stream, TaintedString) proc](#peekLine,Stream,TaintedString)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
var line = ""
doAssert strm.readLine(line) == true
doAssert line == "The first line"
doAssert strm.readLine(line) == true
doAssert line == "the second line"
doAssert strm.readLine(line) == true
doAssert line == "the third line"
doAssert strm.readLine(line) == false
doAssert line == ""
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L983) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L983)
```
proc peekLine(s: Stream; line: var TaintedString): bool {...}{.
raises: [IOError, OSError], tags: [ReadIOEffect].}
```
Peeks a line of text from the stream `s` into `line`. `line` must not be `nil`! May throw an IO exception.
A line of text may be delimited by `CR`, `LF` or `CRLF`. The newline character(s) are not part of the returned string. Returns `false` if the end of the file has been reached, `true` otherwise. If `false` is returned `line` contains no new data.
See also:
* [readLine(Stream) proc](#readLine,Stream)
* [readLine(Stream, TaintedString) proc](#readLine,Stream,TaintedString)
* [peekLine(Stream) proc](#peekLine,Stream)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
var line = ""
doAssert strm.peekLine(line) == true
doAssert line == "The first line"
doAssert strm.peekLine(line) == true
## not "the second line"
doAssert line == "The first line"
doAssert strm.readLine(line) == true
doAssert line == "The first line"
doAssert strm.peekLine(line) == true
doAssert line == "the second line"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1026) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1026)
```
proc readLine(s: Stream): TaintedString {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Reads a line from a stream `s`. Raises `IOError` if an error occurred.
**Note:** This is not very efficient.
See also:
* [readLine(Stream, TaintedString) proc](#readLine,Stream,TaintedString)
* [peekLine(Stream) proc](#peekLine,Stream)
* [peekLine(Stream, TaintedString) proc](#peekLine,Stream,TaintedString)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
doAssert strm.readLine() == "The first line"
doAssert strm.readLine() == "the second line"
doAssert strm.readLine() == "the third line"
doAssertRaises(IOError): discard strm.readLine()
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1057) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1057)
```
proc peekLine(s: Stream): TaintedString {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Peeks a line from a stream `s`. Raises `IOError` if an error occurred.
**Note:** This is not very efficient.
See also:
* [readLine(Stream) proc](#readLine,Stream)
* [readLine(Stream, TaintedString) proc](#readLine,Stream,TaintedString)
* [peekLine(Stream, TaintedString) proc](#peekLine,Stream,TaintedString)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
doAssert strm.peekLine() == "The first line"
## not "the second line"
doAssert strm.peekLine() == "The first line"
doAssert strm.readLine() == "The first line"
doAssert strm.peekLine() == "the second line"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1087) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1087)
```
proc newStringStream(s: string = ""): owned StringStream {...}{.raises: [], tags: [].}
```
Creates a new stream from the string `s`.
See also:
* [newFileStream proc](#newFileStream,File) creates a file stream from opened File.
* [newFileStream proc](#newFileStream,string,FileMode,int) creates a file stream from the file name and the mode.
* [openFileStream proc](#openFileStream,string,FileMode,int) creates a file stream from the file name and the mode.
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
doAssert strm.readLine() == "The first line"
doAssert strm.readLine() == "the second line"
doAssert strm.readLine() == "the third line"
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1269) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1269)
```
proc newFileStream(f: File): owned FileStream {...}{.raises: [], tags: [].}
```
Creates a new stream from the file `f`.
**Note:** Not available for JS backend.
See also:
* [newStringStream proc](#newStringStream,string) creates a new stream from string.
* [newFileStream proc](#newFileStream,string,FileMode,int) is the same as using [open proc](io#open,File,string,FileMode,int) on Examples.
* [openFileStream proc](#openFileStream,string,FileMode,int) creates a file stream from the file name and the mode.
**Example:**
```
## Input (somefile.txt):
## The first line
## the second line
## the third line
var f: File
if open(f, "somefile.txt", fmRead, -1):
var strm = newFileStream(f)
var line = ""
while strm.readLine(line):
echo line
## Output:
## The first line
## the second line
## the third line
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1339) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1339)
```
proc newFileStream(filename: string; mode: FileMode = fmRead; bufSize: int = -1): owned
FileStream {...}{.raises: [], tags: [].}
```
Creates a new stream from the file named `filename` with the mode `mode`.
If the file cannot be opened, `nil` is returned. See the [io module](io) for a list of available [FileMode enums](io#FileMode).
**Note:**
* **This function returns nil in case of failure.** To prevent unexpected behavior and ensure proper error handling, use [openFileStream proc](#openFileStream,string,FileMode,int) instead.
* Not available for JS backend.
See also:
* [newStringStream proc](#newStringStream,string) creates a new stream from string.
* [newFileStream proc](#newFileStream,File) creates a file stream from opened File.
* [openFileStream proc](#openFileStream,string,FileMode,int) creates a file stream from the file name and the mode.
**Example:**
```
from os import removeFile
var strm = newFileStream("somefile.txt", fmWrite)
if not isNil(strm):
strm.writeLine("The first line")
strm.writeLine("the second line")
strm.writeLine("the third line")
strm.close()
## Output (somefile.txt)
## The first line
## the second line
## the third line
removeFile("somefile.txt")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1382) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1382)
```
proc openFileStream(filename: string; mode: FileMode = fmRead;
bufSize: int = -1): owned FileStream {...}{.raises: [IOError],
tags: [].}
```
Creates a new stream from the file named `filename` with the mode `mode`. If the file cannot be opened, an IO exception is raised.
**Note:** Not available for JS backend.
See also:
* [newStringStream proc](#newStringStream,string) creates a new stream from string.
* [newFileStream proc](#newFileStream,File) creates a file stream from opened File.
* [newFileStream proc](#newFileStream,string,FileMode,int) creates a file stream from the file name and the mode.
**Example:**
```
try:
## Input (somefile.txt):
## The first line
## the second line
## the third line
var strm = openFileStream("somefile.txt")
echo strm.readLine()
## Output:
## The first line
strm.close()
except:
stderr.write getCurrentExceptionMsg()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1420) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1420) Iterators
---------
```
iterator lines(s: Stream): TaintedString {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
Iterates over every line in the stream. The iteration is based on `readLine`.
See also:
* [readLine(Stream) proc](#readLine,Stream)
* [readLine(Stream, TaintedString) proc](#readLine,Stream,TaintedString)
**Example:**
```
var strm = newStringStream("The first line\nthe second line\nthe third line")
var lines: seq[string]
for line in strm.lines():
lines.add line
doAssert lines == @["The first line", "the second line", "the third line"]
strm.close()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/streams.nim#L1109) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/streams.nim#L1109)
| programming_docs |
nim Nim Destructors and Move Semantics Nim Destructors and Move Semantics
==================================
About this document
-------------------
This document describes the upcoming Nim runtime which does not use classical GC algorithms anymore but is based on destructors and move semantics. The new runtime's advantages are that Nim programs become oblivious to the involved heap sizes and programs are easier to write to make effective use of multi-core machines. As a nice bonus, files and sockets and the like will not require manual `close` calls anymore.
This document aims to be a precise specification about how move semantics and destructors work in Nim.
Motivating example
------------------
With the language mechanisms described here, a custom seq could be written as:
```
type
myseq*[T] = object
len, cap: int
data: ptr UncheckedArray[T]
proc `=destroy`*[T](x: var myseq[T]) =
if x.data != nil:
for i in 0..<x.len: `=destroy`(x[i])
dealloc(x.data)
proc `=copy`*[T](a: var myseq[T]; b: myseq[T]) =
# do nothing for self-assignments:
if a.data == b.data: return
`=destroy`(a)
wasMoved(a)
a.len = b.len
a.cap = b.cap
if b.data != nil:
a.data = cast[typeof(a.data)](alloc(a.cap * sizeof(T)))
for i in 0..<a.len:
a.data[i] = b.data[i]
proc `=sink`*[T](a: var myseq[T]; b: myseq[T]) =
# move assignment, optional.
# Compiler is using `=destroy` and `copyMem` when not provided
`=destroy`(a)
wasMoved(a)
a.len = b.len
a.cap = b.cap
a.data = b.data
proc add*[T](x: var myseq[T]; y: sink T) =
if x.len >= x.cap: resize(x)
x.data[x.len] = y
inc x.len
proc `[]`*[T](x: myseq[T]; i: Natural): lent T =
assert i < x.len
x.data[i]
proc `[]=`*[T](x: var myseq[T]; i: Natural; y: sink T) =
assert i < x.len
x.data[i] = y
proc createSeq*[T](elems: varargs[T]): myseq[T] =
result.cap = elems.len
result.len = elems.len
result.data = cast[typeof(result.data)](alloc(result.cap * sizeof(T)))
for i in 0..<result.len: result.data[i] = elems[i]
proc len*[T](x: myseq[T]): int {.inline.} = x.len
```
Lifetime-tracking hooks
-----------------------
The memory management for Nim's standard `string` and `seq` types as well as other standard collections is performed via so-called "Lifetime-tracking hooks" or "type-bound operators". There are 3 different hooks for each (generic or concrete) object type `T` (`T` can also be a `distinct` type) that are called implicitly by the compiler.
(Note: The word "hook" here does not imply any kind of dynamic binding or runtime indirections, the implicit calls are statically bound and potentially inlined.)
###
`=destroy` hook
A `=destroy` hook frees the object's associated memory and releases other associated resources. Variables are destroyed via this hook when they go out of scope or when the routine they were declared in is about to return.
The prototype of this hook for a type `T` needs to be:
```
proc `=destroy`(x: var T)
```
The general pattern in `=destroy` looks like:
```
proc `=destroy`(x: var T) =
# first check if 'x' was moved to somewhere else:
if x.field != nil:
freeResource(x.field)
```
###
`=sink` hook
A `=sink` hook moves an object around, the resources are stolen from the source and passed to the destination. It is ensured that the source's destructor does not free the resources afterward by setting the object to its default value (the value the object's state started in). Setting an object `x` back to its default value is written as `wasMoved(x)`. When not provided the compiler is using a combination of `=destroy` and `copyMem` instead. This is efficient hence users rarely need to implement their own `=sink` operator, it is enough to provide `=destroy` and `=copy`, compiler will take care of the rest.
The prototype of this hook for a type `T` needs to be:
```
proc `=sink`(dest: var T; source: T)
```
The general pattern in `=sink` looks like:
```
proc `=sink`(dest: var T; source: T) =
`=destroy`(dest)
wasMoved(dest)
dest.field = source.field
```
**Note**: `=sink` does not need to check for self-assignments. How self-assignments are handled is explained later in this document.
###
`=copy` hook
The ordinary assignment in Nim conceptually copies the values. The `=copy` hook is called for assignments that couldn't be transformed into `=sink` operations.
The prototype of this hook for a type `T` needs to be:
```
proc `=copy`(dest: var T; source: T)
```
The general pattern in `=copy` looks like:
```
proc `=copy`(dest: var T; source: T) =
# protect against self-assignments:
if dest.field != source.field:
`=destroy`(dest)
wasMoved(dest)
dest.field = duplicateResource(source.field)
```
The `=copy` proc can be marked with the `{.error.}` pragma. Then any assignment that otherwise would lead to a copy is prevented at compile-time. This looks like:
```
proc `=copy`(dest: var T; source: T) {.error.}
```
but a custom error message (e.g., `{.error: "custom error".}`) will not be emitted by the compiler. Notice that there is no `=` before the `{.error.}` pragma.
Move semantics
--------------
A "move" can be regarded as an optimized copy operation. If the source of the copy operation is not used afterward, the copy can be replaced by a move. This document uses the notation `lastReadOf(x)` to describe that `x` is not used afterwards. This property is computed by a static control flow analysis but can also be enforced by using `system.move` explicitly.
Swap
----
The need to check for self-assignments and also the need to destroy previous objects inside `=copy` and `=sink` is a strong indicator to treat `system.swap` as a builtin primitive of its own that simply swaps every field in the involved objects via `copyMem` or a comparable mechanism. In other words, `swap(a, b)` is **not** implemented as `let tmp = move(b); b = move(a); a = move(tmp)`.
This has further consequences:
* Objects that contain pointers that point to the same object are not supported by Nim's model. Otherwise swapped objects would end up in an inconsistent state.
* Seqs can use `realloc` in the implementation.
Sink parameters
---------------
To move a variable into a collection usually `sink` parameters are involved. A location that is passed to a `sink` parameter should not be used afterward. This is ensured by a static analysis over a control flow graph. If it cannot be proven to be the last usage of the location, a copy is done instead and this copy is then passed to the sink parameter.
A sink parameter *may* be consumed once in the proc's body but doesn't have to be consumed at all. The reason for this is that signatures like `proc put(t: var Table; k: sink Key, v: sink Value)` should be possible without any further overloads and `put` might not take ownership of `k` if `k` already exists in the table. Sink parameters enable an affine type system, not a linear type system.
The employed static analysis is limited and only concerned with local variables; however, object and tuple fields are treated as separate entities:
```
proc consume(x: sink Obj) = discard "no implementation"
proc main =
let tup = (Obj(), Obj())
consume tup[0]
# ok, only tup[0] was consumed, tup[1] is still alive:
echo tup[1]
```
Sometimes it is required to explicitly `move` a value into its final position:
```
proc main =
var dest, src: array[10, string]
# ...
for i in 0..high(dest): dest[i] = move(src[i])
```
An implementation is allowed, but not required to implement even more move optimizations (and the current implementation does not).
Sink parameter inference
------------------------
The current implementation can do a limited form of sink parameter inference. But it has to be enabled via `--sinkInference:on`, either on the command line or via a `push` pragma.
To enable it for a section of code, one can use `{.push sinkInference: on.}`...`{.pop.}`.
The .nosinks pragma can be used to disable this inference for a single routine:
```
proc addX(x: T; child: T) {.nosinks.} =
x.s.add child
```
The details of the inference algorithm are currently undocumented.
Rewrite rules
-------------
**Note**: There are two different allowed implementation strategies:
1. The produced `finally` section can be a single section that is wrapped around the complete routine body.
2. The produced `finally` section is wrapped around the enclosing scope.
The current implementation follows strategy (2). This means that resources are destroyed at the scope exit.
```
var x: T; stmts
--------------- (destroy-var)
var x: T; try stmts
finally: `=destroy`(x)
g(f(...))
------------------------ (nested-function-call)
g(let tmp;
bitwiseCopy tmp, f(...);
tmp)
finally: `=destroy`(tmp)
x = f(...)
------------------------ (function-sink)
`=sink`(x, f(...))
x = lastReadOf z
------------------ (move-optimization)
`=sink`(x, z)
wasMoved(z)
v = v
------------------ (self-assignment-removal)
discard "nop"
x = y
------------------ (copy)
`=copy`(x, y)
f_sink(g())
----------------------- (call-to-sink)
f_sink(g())
f_sink(notLastReadOf y)
-------------------------- (copy-to-sink)
(let tmp; `=copy`(tmp, y);
f_sink(tmp))
f_sink(lastReadOf y)
----------------------- (move-to-sink)
f_sink(y)
wasMoved(y)
```
Object and array construction
-----------------------------
Object and array construction is treated as a function call where the function has `sink` parameters.
Destructor removal
------------------
`wasMoved(x);` followed by a `=destroy(x)` operation cancel each other out. An implementation is encouraged to exploit this in order to improve efficiency and code sizes. The current implementation does perform this optimization.
Self assignments
----------------
`=sink` in combination with `wasMoved` can handle self-assignments but it's subtle.
The simple case of `x = x` cannot be turned into `=sink(x, x); wasMoved(x)` because that would lose `x`'s value. The solution is that simple self-assignments are simply transformed into an empty statement that does nothing.
The complex case looks like a variant of `x = f(x)`, we consider `x = select(rand() < 0.5, x, y)` here:
```
proc select(cond: bool; a, b: sink string): string =
if cond:
result = a # moves a into result
else:
result = b # moves b into result
proc main =
var x = "abc"
var y = "xyz"
# possible self-assignment:
x = select(true, x, y)
```
Is transformed into:
```
proc select(cond: bool; a, b: sink string): string =
try:
if cond:
`=sink`(result, a)
wasMoved(a)
else:
`=sink`(result, b)
wasMoved(b)
finally:
`=destroy`(b)
`=destroy`(a)
proc main =
var
x: string
y: string
try:
`=sink`(x, "abc")
`=sink`(y, "xyz")
`=sink`(x, select(true,
let blitTmp = x
wasMoved(x)
blitTmp,
let blitTmp = y
wasMoved(y)
blitTmp))
echo [x]
finally:
`=destroy`(y)
`=destroy`(x)
```
As can be manually verified, this transformation is correct for self-assignments.
Lent type
---------
`proc p(x: sink T)` means that the proc `p` takes ownership of `x`. To eliminate even more creation/copy <-> destruction pairs, a proc's return type can be annotated as `lent T`. This is useful for "getter" accessors that seek to allow an immutable view into a container.
The `sink` and `lent` annotations allow us to remove most (if not all) superfluous copies and destructions.
`lent T` is like `var T` a hidden pointer. It is proven by the compiler that the pointer does not outlive its origin. No destructor call is injected for expressions of type `lent T` or of type `var T`.
```
type
Tree = object
kids: seq[Tree]
proc construct(kids: sink seq[Tree]): Tree =
result = Tree(kids: kids)
# converted into:
`=sink`(result.kids, kids); wasMoved(kids)
`=destroy`(kids)
proc `[]`*(x: Tree; i: int): lent Tree =
result = x.kids[i]
# borrows from 'x', this is transformed into:
result = addr x.kids[i]
# This means 'lent' is like 'var T' a hidden pointer.
# Unlike 'var' this hidden pointer cannot be used to mutate the object.
iterator children*(t: Tree): lent Tree =
for x in t.kids: yield x
proc main =
# everything turned into moves:
let t = construct(@[construct(@[]), construct(@[])])
echo t[0] # accessor does not copy the element!
```
The .cursor annotation
----------------------
Under the `--gc:arc|orc` modes Nim's `ref` type is implemented via the same runtime "hooks" and thus via reference counting. This means that cyclic structures cannot be freed immediately (`--gc:orc` ships with a cycle collector). With the `.cursor` annotation one can break up cycles declaratively:
```
type
Node = ref object
left: Node # owning ref
right {.cursor.}: Node # non-owning ref
```
But please notice that this is not C++'s weak\_ptr, it means the right field is not involved in the reference counting, it is a raw pointer without runtime checks.
Automatic reference counting also has the disadvantage that it introduces overhead when iterating over linked structures. The `.cursor` annotation can also be used to avoid this overhead:
```
var it {.cursor.} = listRoot
while it != nil:
use(it)
it = it.next
```
In fact, `.cursor` more generally prevents object construction/destruction pairs and so can also be useful in other contexts. The alternative solution would be to use raw pointers (`ptr`) instead which is more cumbersome and also more dangerous for Nim's evolution: Later on, the compiler can try to prove `.cursor` annotations to be safe, but for `ptr` the compiler has to remain silent about possible problems.
Cursor inference / copy elision
-------------------------------
The current implementation also performs `.cursor` inference. Cursor inference is a form of copy elision.
To see how and when we can do that, think about this question: In `dest = src` when do we really have to *materialize* the full copy? - Only if `dest` or `src` are mutated afterward. If `dest` is a local variable that is simple to analyze. And if `src` is a location derived from a formal parameter, we also know it is not mutated! In other words, we do a compile-time copy-on-write analysis.
This means that "borrowed" views can be written naturally and without explicit pointer indirections:
```
proc main(tab: Table[string, string]) =
let v = tab["key"] # inferred as .cursor because 'tab' is not mutated.
# no copy into 'v', no destruction of 'v'.
use(v)
useItAgain(v)
```
Hook lifting
------------
The hooks of a tuple type `(A, B, ...)` are generated by lifting the hooks of the involved types `A`, `B`, ... to the tuple type. In other words, a copy `x = y` is implemented as `x[0] = y[0]; x[1] = y[1]; ...`, likewise for `=sink` and `=destroy`.
Other value-based compound types like `object` and `array` are handled correspondingly. For `object` however, the compiler-generated hooks can be overridden. This can also be important to use an alternative traversal of the involved data structure that is more efficient or in order to avoid deep recursions.
Hook generation
---------------
The ability to override a hook leads to a phase ordering problem:
```
type
Foo[T] = object
proc main =
var f: Foo[int]
# error: destructor for 'f' called here before
# it was seen in this module.
proc `=destroy`[T](f: var Foo[T]) =
discard
```
The solution is to define `proc `=destroy`[T](f: var Foo[T])` before it is used. The compiler generates implicit hooks for all types in *strategic places* so that an explicitly provided hook that comes too "late" can be detected reliably. These *strategic places* have been derived from the rewrite rules and are as follows:
* In the construct `let/var x = ...` (var/let binding) hooks are generated for `typeof(x)`.
* In `x = ...` (assignment) hooks are generated for `typeof(x)`.
* In `f(...)` (function call) hooks are generated for `typeof(f(...))`.
* For every sink parameter `x: sink T` the hooks are generated for `typeof(x)`.
nodestroy pragma
----------------
The experimental nodestroy pragma inhibits hook injections. This can be used to specialize the object traversal in order to avoid deep recursions:
```
type Node = ref object
x, y: int32
left, right: Node
type Tree = object
root: Node
proc `=destroy`(t: var Tree) {.nodestroy.} =
# use an explicit stack so that we do not get stack overflows:
var s: seq[Node] = @[t.root]
while s.len > 0:
let x = s.pop
if x.left != nil: s.add(x.left)
if x.right != nil: s.add(x.right)
# free the memory explicit:
dispose(x)
# notice how even the destructor for 's' is not called implicitly
# anymore thanks to .nodestroy, so we have to call it on our own:
`=destroy`(s)
```
As can be seen from the example, this solution is hardly sufficient and should eventually be replaced by a better solution.
nim typeinfo typeinfo
========
This module implements an interface to Nim's runtime type information (RTTI). See the <marshal> module for an example of what this module allows you to do.
Note that even though `Any` and its operations hide the nasty low level details from its clients, it remains inherently unsafe! Also, Nim's runtime type information will evolve and may eventually be deprecated. As an alternative approach to programmatically understanding and manipulating types, consider using the <macros> package to work with the types' AST representation at compile time. See, for example, the [getTypeImpl proc](macros#getTypeImpl,NimNode). As an alternative approach to storing arbitrary types at runtime, consider using generics.
Types
-----
```
AnyKind = enum
akNone = 0, ## invalid any
akBool = 1, ## any represents a ``bool``
akChar = 2, ## any represents a ``char``
akEnum = 14, ## any represents an enum
akArray = 16, ## any represents an array
akObject = 17, ## any represents an object
akTuple = 18, ## any represents a tuple
akSet = 19, ## any represents a set
akRange = 20, ## any represents a range
akPtr = 21, ## any represents a ptr
akRef = 22, ## any represents a ref
akSequence = 24, ## any represents a sequence
akProc = 25, ## any represents a proc
akPointer = 26, ## any represents a pointer
akString = 28, ## any represents a string
akCString = 29, ## any represents a cstring
akInt = 31, ## any represents an int
akInt8 = 32, ## any represents an int8
akInt16 = 33, ## any represents an int16
akInt32 = 34, ## any represents an int32
akInt64 = 35, ## any represents an int64
akFloat = 36, ## any represents a float
akFloat32 = 37, ## any represents a float32
akFloat64 = 38, ## any represents a float64
akFloat128 = 39, ## any represents a float128
akUInt = 40, ## any represents an unsigned int
akUInt8 = 41, ## any represents an unsigned int8
akUInt16 = 42, ## any represents an unsigned in16
akUInt32 = 43, ## any represents an unsigned int32
akUInt64 = 44 ## any represents an unsigned int64
```
what kind of `any` it is [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L31)
```
Any = object
value: pointer
when defined(js):
rawType: PNimType
else:
rawTypePtr: pointer
```
can represent any nim value; NOTE: the wrapped value can be modified with its wrapper! This means that `Any` keeps a non-traced pointer to its wrapped value and **must not** live longer than its wrapped value. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L64) Procs
-----
```
proc toAny[T](x: var T): Any {...}{.inline.}
```
Constructs a `Any` object from `x`. This captures `x`'s address, so `x` can be modified with its `Any` wrapper! The client needs to ensure that the wrapper **does not** live longer than `x`! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L153)
```
proc kind(x: Any): AnyKind {...}{.inline, raises: [], tags: [].}
```
Gets the type kind. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L159)
```
proc size(x: Any): int {...}{.inline, raises: [], tags: [].}
```
Returns the size of `x`'s type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L163)
```
proc baseTypeKind(x: Any): AnyKind {...}{.inline, raises: [], tags: [].}
```
Gets the base type's kind; `akNone` is returned if `x` has no base type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L167)
```
proc baseTypeSize(x: Any): int {...}{.inline, raises: [], tags: [].}
```
Returns the size of `x`'s basetype. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L172)
```
proc invokeNew(x: Any) {...}{.raises: [], tags: [].}
```
Performs `new(x)`. `x` needs to represent a `ref`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L177)
```
proc invokeNewSeq(x: Any; len: int) {...}{.raises: [], tags: [].}
```
Performs `newSeq(x, len)`. `x` needs to represent a `seq`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L186)
```
proc extendSeq(x: Any) {...}{.raises: [], tags: [].}
```
Performs `setLen(x, x.len+1)`. `x` needs to represent a `seq`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L198) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L198)
```
proc setObjectRuntimeType(x: Any) {...}{.raises: [], tags: [].}
```
This needs to be called to set `x`'s runtime object type field. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L214)
```
proc `[]`(x: Any; i: int): Any {...}{.raises: [ValueError], tags: [].}
```
Accessor for an any `x` that represents an array or a sequence. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L229)
```
proc `[]=`(x: Any; i: int; y: Any) {...}{.raises: [ValueError], tags: [].}
```
Accessor for an any `x` that represents an array or a sequence. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L255) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L255)
```
proc len(x: Any): int {...}{.raises: [], tags: [].}
```
`len` for an any `x` that represents an array or a sequence. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L284) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L284)
```
proc base(x: Any): Any {...}{.raises: [], tags: [].}
```
Returns base Any (useful for inherited object types). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L301) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L301)
```
proc isNil(x: Any): bool {...}{.raises: [], tags: [].}
```
`isNil` for an any `x` that represents a cstring, proc or some pointer type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L307) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L307)
```
proc getPointer(x: Any): pointer {...}{.raises: [], tags: [].}
```
Retrieves the pointer value out of `x`. `x` needs to be of kind `akString`, `akCString`, `akProc`, `akRef`, `akPtr`, `akPointer`, `akSequence`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L318) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L318)
```
proc setPointer(x: Any; y: pointer) {...}{.raises: [], tags: [].}
```
Sets the pointer value of `x`. `x` needs to be of kind `akString`, `akCString`, `akProc`, `akRef`, `akPtr`, `akPointer`, `akSequence`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L325) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L325)
```
proc `[]=`(x: Any; fieldName: string; value: Any) {...}{.raises: [ValueError],
tags: [].}
```
Sets a field of `x`; `x` represents an object or a tuple. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L403) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L403)
```
proc `[]`(x: Any; fieldName: string): Any {...}{.raises: [ValueError], tags: [].}
```
Gets a field of `x`; `x` represents an object or a tuple. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L417) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L417)
```
proc `[]`(x: Any): Any {...}{.raises: [], tags: [].}
```
Dereference operation for the any `x` that represents a ptr or a ref. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L433) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L433)
```
proc `[]=`(x, y: Any) {...}{.raises: [], tags: [].}
```
Dereference operation for the any `x` that represents a ptr or a ref. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L439) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L439)
```
proc getInt(x: Any): int {...}{.raises: [], tags: [].}
```
Retrieves the int value out of `x`. `x` needs to represent an int. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L445) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L445)
```
proc getInt8(x: Any): int8 {...}{.raises: [], tags: [].}
```
Retrieves the int8 value out of `x`. `x` needs to represent an int8. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L450) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L450)
```
proc getInt16(x: Any): int16 {...}{.raises: [], tags: [].}
```
Retrieves the int16 value out of `x`. `x` needs to represent an int16. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L455) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L455)
```
proc getInt32(x: Any): int32 {...}{.raises: [], tags: [].}
```
Retrieves the int32 value out of `x`. `x` needs to represent an int32. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L460)
```
proc getInt64(x: Any): int64 {...}{.raises: [], tags: [].}
```
Retrieves the int64 value out of `x`. `x` needs to represent an int64. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L465) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L465)
```
proc getBiggestInt(x: Any): BiggestInt {...}{.raises: [], tags: [].}
```
Retrieves the integer value out of `x`. `x` needs to represent some integer, a bool, a char, an enum or a small enough bit set. The value might be sign-extended to `BiggestInt`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L470) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L470)
```
proc setBiggestInt(x: Any; y: BiggestInt) {...}{.raises: [], tags: [].}
```
Sets the integer value of `x`. `x` needs to represent some integer, a bool, a char, an enum or a small enough bit set. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L496)
```
proc getUInt(x: Any): uint {...}{.raises: [], tags: [].}
```
Retrieves the uint value out of `x`, `x` needs to represent an uint. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L521) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L521)
```
proc getUInt8(x: Any): uint8 {...}{.raises: [], tags: [].}
```
Retrieves the uint8 value out of `x`, `x` needs to represent an uint8. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L526) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L526)
```
proc getUInt16(x: Any): uint16 {...}{.raises: [], tags: [].}
```
Retrieves the uint16 value out of `x`, `x` needs to represent an uint16. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L532) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L532)
```
proc getUInt32(x: Any): uint32 {...}{.raises: [], tags: [].}
```
Retrieves the uint32 value out of `x`, `x` needs to represent an uint32. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L538) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L538)
```
proc getUInt64(x: Any): uint64 {...}{.raises: [], tags: [].}
```
Retrieves the uint64 value out of `x`, `x` needs to represent an uint64. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L544) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L544)
```
proc getBiggestUint(x: Any): uint64 {...}{.raises: [], tags: [].}
```
Retrieves the unsigned integer value out of `x`. `x` needs to represent an unsigned integer. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L550) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L550)
```
proc setBiggestUint(x: Any; y: uint64) {...}{.raises: [], tags: [].}
```
Sets the unsigned integer value of `c`. `c` needs to represent an unsigned integer. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L562) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L562)
```
proc getChar(x: Any): char {...}{.raises: [], tags: [].}
```
Retrieves the char value out of `x`. `x` needs to represent a char. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L574) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L574)
```
proc getBool(x: Any): bool {...}{.raises: [], tags: [].}
```
Retrieves the bool value out of `x`. `x` needs to represent a bool. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L580) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L580)
```
proc skipRange(x: Any): Any {...}{.raises: [], tags: [].}
```
Skips the range information of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L586) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L586)
```
proc getEnumOrdinal(x: Any; name: string): int {...}{.raises: [], tags: [].}
```
Gets the enum field ordinal from `name`. `x` needs to represent an enum but is only used to access the type information. In case of an error `low(int)` is returned. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L592) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L592)
```
proc getEnumField(x: Any; ordinalValue: int): string {...}{.raises: [], tags: [].}
```
Gets the enum field name as a string. `x` needs to represent an enum but is only used to access the type information. The field name of `ordinalValue` is returned. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L608) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L608)
```
proc getEnumField(x: Any): string {...}{.raises: [], tags: [].}
```
Gets the enum field name as a string. `x` needs to represent an enum. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L626) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L626)
```
proc getFloat(x: Any): float {...}{.raises: [], tags: [].}
```
Retrieves the float value out of `x`. `x` needs to represent an float. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L630) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L630)
```
proc getFloat32(x: Any): float32 {...}{.raises: [], tags: [].}
```
Retrieves the float32 value out of `x`. `x` needs to represent an float32. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L635) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L635)
```
proc getFloat64(x: Any): float64 {...}{.raises: [], tags: [].}
```
Retrieves the float64 value out of `x`. `x` needs to represent an float64. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L640) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L640)
```
proc getBiggestFloat(x: Any): BiggestFloat {...}{.raises: [], tags: [].}
```
Retrieves the float value out of `x`. `x` needs to represent some float. The value is extended to `BiggestFloat`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L645) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L645)
```
proc setBiggestFloat(x: Any; y: BiggestFloat) {...}{.raises: [], tags: [].}
```
Sets the float value of `x`. `x` needs to represent some float. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L654) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L654)
```
proc getString(x: Any): string {...}{.raises: [], tags: [].}
```
Retrieves the string value out of `x`. `x` needs to represent a string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L663) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L663)
```
proc setString(x: Any; y: string) {...}{.raises: [], tags: [].}
```
Sets the string value of `x`. `x` needs to represent a string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L672) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L672)
```
proc getCString(x: Any): cstring {...}{.raises: [], tags: [].}
```
Retrieves the cstring value out of `x`. `x` needs to represent a cstring. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L677) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L677)
```
proc assign(x, y: Any) {...}{.raises: [], tags: [].}
```
Copies the value of `y` to `x`. The assignment operator for `Any` does NOT do this; it performs a shallow copy instead! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L682) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L682)
```
proc inclSetElement(x: Any; elem: int) {...}{.raises: [], tags: [].}
```
Includes an element `elem` in `x`. `x` needs to represent a Nim bitset. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L710) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L710) Iterators
---------
```
iterator fields(x: Any): tuple[name: string, any: Any] {...}{.raises: [], tags: [].}
```
Iterates over every active field of the any `x` that represents an object or a tuple. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L349) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L349)
```
iterator elements(x: Any): int {...}{.raises: [], tags: [].}
```
Iterates over every element of `x` that represents a Nim bitset. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/core/typeinfo.nim#L688) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/core/typeinfo.nim#L688)
| programming_docs |
nim punycode punycode
========
Implements a representation of Unicode with the limited ASCII character subset.
**Example:**
```
static:
block:
doAssert encode("") == ""
doAssert encode("a") == "a-"
doAssert encode("A") == "A-"
doAssert encode("3") == "3-"
doAssert encode("-") == "--"
doAssert encode("--") == "---"
doAssert encode("abc") == "abc-"
doAssert encode("London") == "London-"
doAssert encode("Lloyd-Atkinson") == "Lloyd-Atkinson-"
doAssert encode("This has spaces") == "This has spaces-"
doAssert encode("ü") == "tda"
doAssert encode("München") == "Mnchen-3ya"
doAssert encode("Mnchen-3ya") == "Mnchen-3ya-"
doAssert encode("München-Ost") == "Mnchen-Ost-9db"
doAssert encode("Bahnhof München-Ost") == "Bahnhof Mnchen-Ost-u6b"
block:
doAssert decode("") == ""
doAssert decode("a-") == "a"
doAssert decode("A-") == "A"
doAssert decode("3-") == "3"
doAssert decode("--") == "-"
doAssert decode("---") == "--"
doAssert decode("abc-") == "abc"
doAssert decode("London-") == "London"
doAssert decode("Lloyd-Atkinson-") == "Lloyd-Atkinson"
doAssert decode("This has spaces-") == "This has spaces"
doAssert decode("tda") == "ü"
doAssert decode("Mnchen-3ya") == "München"
doAssert decode("Mnchen-3ya-") == "Mnchen-3ya"
doAssert decode("Mnchen-Ost-9db") == "München-Ost"
doAssert decode("Bahnhof Mnchen-Ost-u6b") == "Bahnhof München-Ost"
```
Imports
-------
<strutils>, <unicode> Types
-----
```
PunyError = object of ValueError
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/punycode.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/punycode.nim#L29) Procs
-----
```
proc encode(prefix, s: string): string {...}{.raises: [PunyError], tags: [].}
```
Encode a string that may contain Unicode. Prepend `prefix` to the result [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/punycode.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/punycode.nim#L61)
```
proc encode(s: string): string {...}{.raises: [PunyError], tags: [].}
```
Encode a string that may contain Unicode. Prefix is empty. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/punycode.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/punycode.nim#L117)
```
proc decode(encoded: string): string {...}{.raises: [PunyError], tags: [].}
```
Decode a Punycode-encoded string [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/punycode.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/punycode.nim#L121)
nim htmlgen htmlgen
=======
Do yourself a favor and import the module as `from htmlgen import nil` and then fully qualify the macros.
*Note*: The Karax project (`nimble install karax`) has a better way to achieve the same, see <https://github.com/pragmagic/karax/blob/master/tests/nativehtmlgen.nim> for an example.
This module implements a simple XML and HTML code generator. Each commonly used HTML tag has a corresponding macro that generates a string with its HTML representation.
MathML
------
[MathML](https://wikipedia.org/wiki/MathML) is supported, MathML is part of HTML5. [MathML](https://wikipedia.org/wiki/MathML) is an Standard ISO/IEC 40314 from year 2015. MathML allows you to [draw advanced math on the web](https://developer.mozilla.org/en-US/docs/Web/MathML/Element/math#Examples), [visually similar to Latex math.](https://developer.mozilla.org/en-US/docs/Web/MathML/Element/semantics#Example)
Examples
--------
```
var nim = "Nim"
echo h1(a(href="https://nim-lang.org", nim))
```
Writes the string:
```
<h1><a href="https://nim-lang.org">Nim</a></h1>
```
**Example:**
```
let nim = "Nim"
assert h1(a(href = "https://nim-lang.org", nim)) ==
"""<h1><a href="https://nim-lang.org">Nim</a></h1>"""
assert form(action = "test", `accept-charset` = "Content-Type") ==
"""<form action="test" accept-charset="Content-Type"></form>"""
assert math(
semantics(
mrow(
msup(
mi("x"),
mn("42")
)
)
)
) == "<math><semantics><mrow><msup><mi>x</mi><mn>42</mn></msup></mrow></semantics></math>"
assert math(
semantics(
annotation(encoding = "application/x-tex", title = "Latex on Web", r"x^{2} + y")
)
) == """<math><semantics><annotation encoding="application/x-tex" title="Latex on Web">x^{2} + y</annotation></semantics></math>"""
```
Imports
-------
<macros>, <strutils> Consts
------
```
coreAttr = " accesskey class contenteditable dir hidden id lang spellcheck style tabindex title translate "
```
HTML DOM Core Attributes [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L46)
```
eventAttr = "onabort onblur oncancel oncanplay oncanplaythrough onchange onclick oncuechange ondblclick ondurationchange onemptied onended onerror onfocus oninput oninvalid onkeydown onkeypress onkeyup onload onloadeddata onloadedmetadata onloadstart onmousedown onmouseenter onmouseleave onmousemove onmouseout onmouseover onmouseup onmousewheel onpause onplay onplaying onprogress onratechange onreset onresize onscroll onseeked onseeking onselect onshow onstalled onsubmit onsuspend ontimeupdate ontoggle onvolumechange onwaiting "
```
HTML DOM Event Attributes [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L48)
```
ariaAttr = " role "
```
HTML DOM Aria Attributes [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L56) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L56)
```
commonAttr = " accesskey class contenteditable dir hidden id lang spellcheck style tabindex title translate onabort onblur oncancel oncanplay oncanplaythrough onchange onclick oncuechange ondblclick ondurationchange onemptied onended onerror onfocus oninput oninvalid onkeydown onkeypress onkeyup onload onloadeddata onloadedmetadata onloadstart onmousedown onmouseenter onmouseleave onmousemove onmouseout onmouseover onmouseup onmousewheel onpause onplay onplaying onprogress onratechange onreset onresize onscroll onseeked onseeking onselect onshow onstalled onsubmit onsuspend ontimeupdate ontoggle onvolumechange onwaiting role "
```
HTML DOM Common Attributes [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L57) Procs
-----
```
proc xmlCheckedTag(argsList: NimNode; tag: string; optAttr = ""; reqAttr = "";
isLeaf = false): NimNode {...}{.compileTime, raises: [], tags: [].}
```
use this procedure to define a new XML tag [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L77) Macros
------
```
macro a(e: varargs[untyped]): untyped
```
Generates the HTML `a` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L121)
```
macro abbr(e: varargs[untyped]): untyped
```
Generates the HTML `abbr` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L126) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L126)
```
macro address(e: varargs[untyped]): untyped
```
Generates the HTML `address` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L130)
```
macro area(e: varargs[untyped]): untyped
```
Generates the HTML `area` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L134)
```
macro article(e: varargs[untyped]): untyped
```
Generates the HTML `article` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L139) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L139)
```
macro aside(e: varargs[untyped]): untyped
```
Generates the HTML `aside` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L143)
```
macro audio(e: varargs[untyped]): untyped
```
Generates the HTML `audio` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L147)
```
macro b(e: varargs[untyped]): untyped
```
Generates the HTML `b` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L152)
```
macro base(e: varargs[untyped]): untyped
```
Generates the HTML `base` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L156) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L156)
```
macro bdi(e: varargs[untyped]): untyped
```
Generates the HTML `bdi` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L160)
```
macro bdo(e: varargs[untyped]): untyped
```
Generates the HTML `bdo` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L164)
```
macro big(e: varargs[untyped]): untyped
```
Generates the HTML `big` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L168)
```
macro blockquote(e: varargs[untyped]): untyped
```
Generates the HTML `blockquote` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L172)
```
macro body(e: varargs[untyped]): untyped
```
Generates the HTML `body` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L176)
```
macro br(e: varargs[untyped]): untyped
```
Generates the HTML `br` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L182)
```
macro button(e: varargs[untyped]): untyped
```
Generates the HTML `button` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L186)
```
macro canvas(e: varargs[untyped]): untyped
```
Generates the HTML `canvas` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L192)
```
macro caption(e: varargs[untyped]): untyped
```
Generates the HTML `caption` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L196) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L196)
```
macro center(e: varargs[untyped]): untyped
```
Generates the HTML `center` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L200) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L200)
```
macro cite(e: varargs[untyped]): untyped
```
Generates the HTML `cite` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L204)
```
macro code(e: varargs[untyped]): untyped
```
Generates the HTML `code` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L208) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L208)
```
macro col(e: varargs[untyped]): untyped
```
Generates the HTML `col` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L212)
```
macro colgroup(e: varargs[untyped]): untyped
```
Generates the HTML `colgroup` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L216) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L216)
```
macro data(e: varargs[untyped]): untyped
```
Generates the HTML `data` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L220) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L220)
```
macro datalist(e: varargs[untyped]): untyped
```
Generates the HTML `datalist` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L224)
```
macro dd(e: varargs[untyped]): untyped
```
Generates the HTML `dd` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L228) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L228)
```
macro del(e: varargs[untyped]): untyped
```
Generates the HTML `del` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L232)
```
macro details(e: varargs[untyped]): untyped
```
Generates the HTML `details` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L236)
```
macro dfn(e: varargs[untyped]): untyped
```
Generates the HTML `dfn` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L240)
```
macro dialog(e: varargs[untyped]): untyped
```
Generates the HTML `dialog` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L244) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L244)
```
macro `div`(e: varargs[untyped]): untyped
```
Generates the HTML `div` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L248) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L248)
```
macro dl(e: varargs[untyped]): untyped
```
Generates the HTML `dl` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L252)
```
macro dt(e: varargs[untyped]): untyped
```
Generates the HTML `dt` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L256) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L256)
```
macro em(e: varargs[untyped]): untyped
```
Generates the HTML `em` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L260)
```
macro embed(e: varargs[untyped]): untyped
```
Generates the HTML `embed` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L264) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L264)
```
macro fieldset(e: varargs[untyped]): untyped
```
Generates the HTML `fieldset` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L269)
```
macro figure(e: varargs[untyped]): untyped
```
Generates the HTML `figure` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L273) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L273)
```
macro figcaption(e: varargs[untyped]): untyped
```
Generates the HTML `figcaption` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L277) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L277)
```
macro footer(e: varargs[untyped]): untyped
```
Generates the HTML `footer` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L281) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L281)
```
macro form(e: varargs[untyped]): untyped
```
Generates the HTML `form` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L285) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L285)
```
macro h1(e: varargs[untyped]): untyped
```
Generates the HTML `h1` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L290)
```
macro h2(e: varargs[untyped]): untyped
```
Generates the HTML `h2` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L294) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L294)
```
macro h3(e: varargs[untyped]): untyped
```
Generates the HTML `h3` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L298)
```
macro h4(e: varargs[untyped]): untyped
```
Generates the HTML `h4` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L302) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L302)
```
macro h5(e: varargs[untyped]): untyped
```
Generates the HTML `h5` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L306)
```
macro h6(e: varargs[untyped]): untyped
```
Generates the HTML `h6` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L310) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L310)
```
macro head(e: varargs[untyped]): untyped
```
Generates the HTML `head` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L314) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L314)
```
macro header(e: varargs[untyped]): untyped
```
Generates the HTML `header` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L318) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L318)
```
macro html(e: varargs[untyped]): untyped
```
Generates the HTML `html` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L322)
```
macro hr(): untyped
```
Generates the HTML `hr` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L326) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L326)
```
macro i(e: varargs[untyped]): untyped
```
Generates the HTML `i` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L330) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L330)
```
macro iframe(e: varargs[untyped]): untyped
```
Generates the HTML `iframe` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L334) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L334)
```
macro img(e: varargs[untyped]): untyped
```
Generates the HTML `img` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L339) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L339)
```
macro input(e: varargs[untyped]): untyped
```
Generates the HTML `input` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L344) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L344)
```
macro ins(e: varargs[untyped]): untyped
```
Generates the HTML `ins` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L352) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L352)
```
macro kbd(e: varargs[untyped]): untyped
```
Generates the HTML `kbd` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L356) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L356)
```
macro keygen(e: varargs[untyped]): untyped
```
Generates the HTML `keygen` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L360) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L360)
```
macro label(e: varargs[untyped]): untyped
```
Generates the HTML `label` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L365) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L365)
```
macro legend(e: varargs[untyped]): untyped
```
Generates the HTML `legend` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L369) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L369)
```
macro li(e: varargs[untyped]): untyped
```
Generates the HTML `li` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L373) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L373)
```
macro link(e: varargs[untyped]): untyped
```
Generates the HTML `link` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L377) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L377)
```
macro main(e: varargs[untyped]): untyped
```
Generates the HTML `main` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L382) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L382)
```
macro map(e: varargs[untyped]): untyped
```
Generates the HTML `map` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L386) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L386)
```
macro mark(e: varargs[untyped]): untyped
```
Generates the HTML `mark` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L390) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L390)
```
macro marquee(e: varargs[untyped]): untyped
```
Generates the HTML `marquee` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L394) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L394)
```
macro meta(e: varargs[untyped]): untyped
```
Generates the HTML `meta` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L400) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L400)
```
macro meter(e: varargs[untyped]): untyped
```
Generates the HTML `meter` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L405)
```
macro nav(e: varargs[untyped]): untyped
```
Generates the HTML `nav` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L410) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L410)
```
macro noscript(e: varargs[untyped]): untyped
```
Generates the HTML `noscript` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L414) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L414)
```
macro `object`(e: varargs[untyped]): untyped
```
Generates the HTML `object` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L418) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L418)
```
macro ol(e: varargs[untyped]): untyped
```
Generates the HTML `ol` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L423) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L423)
```
macro optgroup(e: varargs[untyped]): untyped
```
Generates the HTML `optgroup` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L427) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L427)
```
macro option(e: varargs[untyped]): untyped
```
Generates the HTML `option` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L431) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L431)
```
macro output(e: varargs[untyped]): untyped
```
Generates the HTML `output` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L436) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L436)
```
macro p(e: varargs[untyped]): untyped
```
Generates the HTML `p` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L440) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L440)
```
macro param(e: varargs[untyped]): untyped
```
Generates the HTML `param` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L444)
```
macro picture(e: varargs[untyped]): untyped
```
Generates the HTML `picture` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L448) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L448)
```
macro pre(e: varargs[untyped]): untyped
```
Generates the HTML `pre` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L452) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L452)
```
macro progress(e: varargs[untyped]): untyped
```
Generates the HTML `progress` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L456) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L456)
```
macro q(e: varargs[untyped]): untyped
```
Generates the HTML `q` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L460)
```
macro rb(e: varargs[untyped]): untyped
```
Generates the HTML `rb` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L464) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L464)
```
macro rp(e: varargs[untyped]): untyped
```
Generates the HTML `rp` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L468) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L468)
```
macro rt(e: varargs[untyped]): untyped
```
Generates the HTML `rt` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L472) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L472)
```
macro rtc(e: varargs[untyped]): untyped
```
Generates the HTML `rtc` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L476) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L476)
```
macro ruby(e: varargs[untyped]): untyped
```
Generates the HTML `ruby` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L480) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L480)
```
macro s(e: varargs[untyped]): untyped
```
Generates the HTML `s` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L484) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L484)
```
macro samp(e: varargs[untyped]): untyped
```
Generates the HTML `samp` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L488) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L488)
```
macro script(e: varargs[untyped]): untyped
```
Generates the HTML `script` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L492) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L492)
```
macro section(e: varargs[untyped]): untyped
```
Generates the HTML `section` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L497) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L497)
```
macro select(e: varargs[untyped]): untyped
```
Generates the HTML `select` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L501) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L501)
```
macro slot(e: varargs[untyped]): untyped
```
Generates the HTML `slot` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L506) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L506)
```
macro small(e: varargs[untyped]): untyped
```
Generates the HTML `small` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L510) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L510)
```
macro source(e: varargs[untyped]): untyped
```
Generates the HTML `source` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L514) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L514)
```
macro span(e: varargs[untyped]): untyped
```
Generates the HTML `span` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L518) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L518)
```
macro strong(e: varargs[untyped]): untyped
```
Generates the HTML `strong` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L522) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L522)
```
macro style(e: varargs[untyped]): untyped
```
Generates the HTML `style` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L526) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L526)
```
macro sub(e: varargs[untyped]): untyped
```
Generates the HTML `sub` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L530) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L530)
```
macro summary(e: varargs[untyped]): untyped
```
Generates the HTML `summary` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L534) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L534)
```
macro sup(e: varargs[untyped]): untyped
```
Generates the HTML `sup` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L538) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L538)
```
macro table(e: varargs[untyped]): untyped
```
Generates the HTML `table` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L542) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L542)
```
macro tbody(e: varargs[untyped]): untyped
```
Generates the HTML `tbody` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L546) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L546)
```
macro td(e: varargs[untyped]): untyped
```
Generates the HTML `td` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L550) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L550)
```
macro `template`(e: varargs[untyped]): untyped
```
Generates the HTML `template` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L554) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L554)
```
macro textarea(e: varargs[untyped]): untyped
```
Generates the HTML `textarea` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L558) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L558)
```
macro tfoot(e: varargs[untyped]): untyped
```
Generates the HTML `tfoot` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L564) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L564)
```
macro th(e: varargs[untyped]): untyped
```
Generates the HTML `th` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L568) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L568)
```
macro thead(e: varargs[untyped]): untyped
```
Generates the HTML `thead` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L573) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L573)
```
macro time(e: varargs[untyped]): untyped
```
Generates the HTML `time` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L577) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L577)
```
macro title(e: varargs[untyped]): untyped
```
Generates the HTML `title` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L581) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L581)
```
macro tr(e: varargs[untyped]): untyped
```
Generates the HTML `tr` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L585) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L585)
```
macro track(e: varargs[untyped]): untyped
```
Generates the HTML `track` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L589) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L589)
```
macro tt(e: varargs[untyped]): untyped
```
Generates the HTML `tt` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L594) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L594)
```
macro u(e: varargs[untyped]): untyped
```
Generates the HTML `u` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L598) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L598)
```
macro ul(e: varargs[untyped]): untyped
```
Generates the HTML `ul` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L602) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L602)
```
macro `var`(e: varargs[untyped]): untyped
```
Generates the HTML `var` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L606) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L606)
```
macro video(e: varargs[untyped]): untyped
```
Generates the HTML `video` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L610) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L610)
```
macro wbr(e: varargs[untyped]): untyped
```
Generates the HTML `wbr` element. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L615) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L615)
```
macro math(e: varargs[untyped]): untyped
```
Generates the HTML `math` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/math#Examples> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L620) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L620)
```
macro maction(e: varargs[untyped]): untyped
```
Generates the HTML `maction` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/maction> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L625) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L625)
```
macro menclose(e: varargs[untyped]): untyped
```
Generates the HTML `menclose` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/menclose> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L630) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L630)
```
macro merror(e: varargs[untyped]): untyped
```
Generates the HTML `merror` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/merror> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L635) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L635)
```
macro mfenced(e: varargs[untyped]): untyped
```
Generates the HTML `mfenced` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mfenced> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L640) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L640)
```
macro mfrac(e: varargs[untyped]): untyped
```
Generates the HTML `mfrac` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mfrac> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L645) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L645)
```
macro mglyph(e: varargs[untyped]): untyped
```
Generates the HTML `mglyph` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mglyph> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L650) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L650)
```
macro mi(e: varargs[untyped]): untyped
```
Generates the HTML `mi` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mi> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L655) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L655)
```
macro mlabeledtr(e: varargs[untyped]): untyped
```
Generates the HTML `mlabeledtr` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mlabeledtr> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L660) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L660)
```
macro mmultiscripts(e: varargs[untyped]): untyped
```
Generates the HTML `mmultiscripts` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mmultiscripts> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L665) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L665)
```
macro mn(e: varargs[untyped]): untyped
```
Generates the HTML `mn` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mn> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L670) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L670)
```
macro mo(e: varargs[untyped]): untyped
```
Generates the HTML `mo` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mo> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L675) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L675)
```
macro mover(e: varargs[untyped]): untyped
```
Generates the HTML `mover` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mover> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L681) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L681)
```
macro mpadded(e: varargs[untyped]): untyped
```
Generates the HTML `mpadded` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mpadded> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L686) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L686)
```
macro mphantom(e: varargs[untyped]): untyped
```
Generates the HTML `mphantom` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mphantom> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L691) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L691)
```
macro mroot(e: varargs[untyped]): untyped
```
Generates the HTML `mroot` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mroot> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L696) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L696)
```
macro mrow(e: varargs[untyped]): untyped
```
Generates the HTML `mrow` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mrow> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L701) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L701)
```
macro ms(e: varargs[untyped]): untyped
```
Generates the HTML `ms` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/ms> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L706) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L706)
```
macro mspace(e: varargs[untyped]): untyped
```
Generates the HTML `mspace` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mspace> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L711) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L711)
```
macro msqrt(e: varargs[untyped]): untyped
```
Generates the HTML `msqrt` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/msqrt> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L716) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L716)
```
macro mstyle(e: varargs[untyped]): untyped
```
Generates the HTML `mstyle` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mstyle> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L721) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L721)
```
macro msub(e: varargs[untyped]): untyped
```
Generates the HTML `msub` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/msub> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L727) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L727)
```
macro msubsup(e: varargs[untyped]): untyped
```
Generates the HTML `msubsup` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/msubsup> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L732) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L732)
```
macro msup(e: varargs[untyped]): untyped
```
Generates the HTML `msup` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/msup> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L737) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L737)
```
macro mtable(e: varargs[untyped]): untyped
```
Generates the HTML `mtable` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mtable> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L742) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L742)
```
macro mtd(e: varargs[untyped]): untyped
```
Generates the HTML `mtd` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mtd> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L750) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L750)
```
macro mtext(e: varargs[untyped]): untyped
```
Generates the HTML `mtext` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/mtext> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L756) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L756)
```
macro munder(e: varargs[untyped]): untyped
```
Generates the HTML `munder` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/munder> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L761) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L761)
```
macro munderover(e: varargs[untyped]): untyped
```
Generates the HTML `munderover` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/munderover> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L766) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L766)
```
macro semantics(e: varargs[untyped]): untyped
```
Generates the HTML `semantics` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/semantics> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L771) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L771)
```
macro annotation(e: varargs[untyped]): untyped
```
Generates the HTML `annotation` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/semantics> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L776) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L776)
```
macro annotation-xml(e: varargs[untyped]): untyped
```
Generates the HTML `annotation-xml` element. MathML <https://wikipedia.org/wiki/MathML> <https://developer.mozilla.org/en-US/docs/Web/MathML/Element/semantics> [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/htmlgen.nim#L781) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/htmlgen.nim#L781)
| programming_docs |
nim cstrutils cstrutils
=========
This module supports helper routines for working with `cstring` without having to convert `cstring` to `string` in order to save allocations.
Procs
-----
```
proc startsWith(s, prefix: cstring): bool {...}{.noSideEffect, gcsafe,
extern: "csuStartsWith", raises: [], tags: [].}
```
Returns true if `s` starts with `prefix`.
If `prefix == ""` true is returned.
JS backend uses native `String.prototype.startsWith`.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/cstrutils.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/cstrutils.nim#L32)
```
proc endsWith(s, suffix: cstring): bool {...}{.noSideEffect, gcsafe,
extern: "csuEndsWith", raises: [], tags: [].}
```
Returns true if `s` ends with `suffix`.
If `suffix == ""` true is returned.
JS backend uses native `String.prototype.endsWith`.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/cstrutils.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/cstrutils.nim#L45)
```
proc cmpIgnoreStyle(a, b: cstring): int {...}{.noSideEffect, gcsafe,
extern: "csuCmpIgnoreStyle", raises: [], tags: [].}
```
Semantically the same as `cmp(normalize($a), normalize($b))`. It is just optimized to not allocate temporary strings. This should NOT be used to compare Nim identifier names. use `macros.eqIdent` for that. Returns:0 if a == b
< 0 if a < b
> 0 if a > b
Not supported for JS backend, use [strutils.cmpIgnoreStyle](strutils#cmpIgnoreStyle%2Cstring%2Cstring) instead.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/cstrutils.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/cstrutils.nim#L60)
```
proc cmpIgnoreCase(a, b: cstring): int {...}{.noSideEffect, gcsafe,
extern: "csuCmpIgnoreCase", raises: [], tags: [].}
```
Compares two strings in a case insensitive manner. Returns:0 if a == b
< 0 if a < b
> 0 if a > b
Not supported for JS backend, use [strutils.cmpIgnoreCase](strutils#cmpIgnoreCase%2Cstring%2Cstring) instead.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/cstrutils.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/cstrutils.nim#L85)
nim Nim Manual Nim Manual
==========
> "Complexity" seems to be a lot like "energy": you can transfer it from the end-user to one/some of the other players, but the total amount seems to remain pretty much constant for a given task. -- Ran
>
>
About this document
-------------------
**Note**: This document is a draft! Several of Nim's features may need more precise wording. This manual is constantly evolving into a proper specification.
**Note**: The experimental features of Nim are covered [here](manual_experimental).
**Note**: Assignments, moves, and destruction are specified in the <destructors> document.
This document describes the lexis, the syntax, and the semantics of the Nim language.
To learn how to compile Nim programs and generate documentation see [Compiler User Guide](nimc) and [DocGen Tools Guide](https://nim-lang.org/docs/docgen.html).
The language constructs are explained using an extended BNF, in which `(a)*` means 0 or more `a`'s, `a+` means 1 or more `a`'s, and `(a)?` means an optional *a*. Parentheses may be used to group elements.
`&` is the lookahead operator; `&a` means that an `a` is expected but not consumed. It will be consumed in the following rule.
The `|`, `/` symbols are used to mark alternatives and have the lowest precedence. `/` is the ordered choice that requires the parser to try the alternatives in the given order. `/` is often used to ensure the grammar is not ambiguous.
Non-terminals start with a lowercase letter, abstract terminal symbols are in UPPERCASE. Verbatim terminal symbols (including keywords) are quoted with `'`. An example:
```
ifStmt = 'if' expr ':' stmts ('elif' expr ':' stmts)* ('else' stmts)?
```
The binary `^*` operator is used as a shorthand for 0 or more occurrences separated by its second argument; likewise `^+` means 1 or more occurrences: `a ^+ b` is short for `a (b a)*` and `a ^* b` is short for `(a (b a)*)?`. Example:
```
arrayConstructor = '[' expr ^* ',' ']'
```
Other parts of Nim, like scoping rules or runtime semantics, are described informally.
Definitions
-----------
Nim code specifies a computation that acts on a memory consisting of components called locations. A variable is basically a name for a location. Each variable and location is of a certain type. The variable's type is called static type, the location's type is called dynamic type. If the static type is not the same as the dynamic type, it is a super-type or subtype of the dynamic type.
An identifier is a symbol declared as a name for a variable, type, procedure, etc. The region of the program over which a declaration applies is called the scope of the declaration. Scopes can be nested. The meaning of an identifier is determined by the smallest enclosing scope in which the identifier is declared unless overloading resolution rules suggest otherwise.
An expression specifies a computation that produces a value or location. Expressions that produce locations are called l-values. An l-value can denote either a location or the value the location contains, depending on the context.
A Nim program consists of one or more text source files containing Nim code. It is processed by a Nim compiler into an executable. The nature of this executable depends on the compiler implementation; it may, for example, be a native binary or JavaScript source code.
In a typical Nim program, most of the code is compiled into the executable. However, some of the code may be executed at compile-time. This can include constant expressions, macro definitions, and Nim procedures used by macro definitions. Most of the Nim language is supported at compile-time, but there are some restrictions -- see [Restrictions on Compile-Time Execution](#restrictions-on-compileminustime-execution) for details. We use the term runtime to cover both compile-time execution and code execution in the executable.
The compiler parses Nim source code into an internal data structure called the abstract syntax tree (AST). Then, before executing the code or compiling it into the executable, it transforms the AST through semantic analysis. This adds semantic information such as expression types, identifier meanings, and in some cases expression values. An error detected during semantic analysis is called a static error. Errors described in this manual are static errors when not otherwise specified.
A panic is an error that the implementation detects and reports at runtime. The method for reporting such errors is via *raising exceptions* or *dying with a fatal error*. However, the implementation provides a means to disable these runtime checks. See the section [pragmas](#pragmas) for details.
Whether a panic results in an exception or in a fatal error is implementation specific. Thus the following program is invalid; even though the code purports to catch the `IndexDefect` from an out-of-bounds array access, the compiler may instead choose to allow the program to die with a fatal error.
```
var a: array[0..1, char]
let i = 5
try:
a[i] = 'N'
except IndexDefect:
echo "invalid index"
```
The current implementation allows to switch between these different behaviors via `--panics:on|off`. When panics are turned on, the program dies with a panic, if they are turned off the runtime errors are turned into exceptions. The benefit of `--panics:on` is that it produces smaller binary code and the compiler has more freedom to optimize the code.
An unchecked runtime error is an error that is not guaranteed to be detected and can cause the subsequent behavior of the computation to be arbitrary. Unchecked runtime errors cannot occur if only safe language features are used and if no runtime checks are disabled.
A constant expression is an expression whose value can be computed during a semantic analysis of the code in which it appears. It is never an l-value and never has side effects. Constant expressions are not limited to the capabilities of semantic analysis, such as constant folding; they can use all Nim language features that are supported for compile-time execution. Since constant expressions can be used as an input to semantic analysis (such as for defining array bounds), this flexibility requires the compiler to interleave semantic analysis and compile-time code execution.
It is mostly accurate to picture semantic analysis proceeding top to bottom and left to right in the source code, with compile-time code execution interleaved when necessary to compute values that are required for subsequent semantic analysis. We will see much later in this document that macro invocation not only requires this interleaving, but also creates a situation where semantic analysis does not entirely proceed top to bottom and left to right.
Lexical Analysis
----------------
### Encoding
All Nim source files are in the UTF-8 encoding (or its ASCII subset). Other encodings are not supported. Any of the standard platform line termination sequences can be used - the Unix form using ASCII LF (linefeed), the Windows form using the ASCII sequence CR LF (return followed by linefeed), or the old Macintosh form using the ASCII CR (return) character. All of these forms can be used equally, regardless of the platform.
### Indentation
Nim's standard grammar describes an indentation sensitive language. This means that all the control structures are recognized by indentation. Indentation consists only of spaces; tabulators are not allowed.
The indentation handling is implemented as follows: The lexer annotates the following token with the preceding number of spaces; indentation is not a separate token. This trick allows parsing of Nim with only 1 token of lookahead.
The parser uses a stack of indentation levels: the stack consists of integers counting the spaces. The indentation information is queried at strategic places in the parser but ignored otherwise: The pseudo-terminal `IND{>}` denotes an indentation that consists of more spaces than the entry at the top of the stack; `IND{=}` an indentation that has the same number of spaces. `DED` is another pseudo terminal that describes the *action* of popping a value from the stack, `IND{>}` then implies to push onto the stack.
With this notation we can now easily define the core of the grammar: A block of statements (simplified example):
```
ifStmt = 'if' expr ':' stmt
(IND{=} 'elif' expr ':' stmt)*
(IND{=} 'else' ':' stmt)?
simpleStmt = ifStmt / ...
stmt = IND{>} stmt ^+ IND{=} DED # list of statements
/ simpleStmt # or a simple statement
```
### Comments
Comments start anywhere outside a string or character literal with the hash character `#`. Comments consist of a concatenation of comment pieces. A comment piece starts with `#` and runs until the end of the line. The end of line characters belong to the piece. If the next line only consists of a comment piece with no other tokens between it and the preceding one, it does not start a new comment:
```
i = 0 # This is a single comment over multiple lines.
# The scanner merges these two pieces.
# The comment continues here.
```
Documentation comments are comments that start with two `##`. Documentation comments are tokens; they are only allowed at certain places in the input file as they belong to the syntax tree!
### Multiline comments
Starting with version 0.13.0 of the language Nim supports multiline comments. They look like:
```
#[Comment here.
Multiple lines
are not a problem.]#
```
Multiline comments support nesting:
```
#[ #[ Multiline comment in already
commented out code. ]#
proc p[T](x: T) = discard
]#
```
Multiline documentation comments also exist and support nesting too:
```
proc foo =
##[Long documentation comment
here.
]##
```
### Identifiers & Keywords
Identifiers in Nim can be any string of letters, digits and underscores, with the following restrictions:
* begins with a letter
* does not end with an underscore `_`
* two immediate following underscores `__` are not allowed::
letter ::= 'A'..'Z' | 'a'..'z' | 'x80'..'xff' digit ::= '0'..'9' IDENTIFIER ::= letter ( ['\_'] (letter | digit) )\*
Currently, any Unicode character with an ordinal value > 127 (non-ASCII) is classified as a `letter` and may thus be part of an identifier but later versions of the language may assign some Unicode characters to belong to the operator characters instead.
The following keywords are reserved and cannot be used as identifiers:
```
addr and as asm
bind block break
case cast concept const continue converter
defer discard distinct div do
elif else end enum except export
finally for from func
if import in include interface is isnot iterator
let
macro method mixin mod
nil not notin
object of or out
proc ptr
raise ref return
shl shr static
template try tuple type
using
var
when while
xor
yield
```
Some keywords are unused; they are reserved for future developments of the language.
### Identifier equality
Two identifiers are considered equal if the following algorithm returns true:
```
proc sameIdentifier(a, b: string): bool =
a[0] == b[0] and
a.replace("_", "").toLowerAscii == b.replace("_", "").toLowerAscii
```
That means only the first letters are compared in a case-sensitive manner. Other letters are compared case-insensitively within the ASCII range and underscores are ignored.
This rather unorthodox way to do identifier comparisons is called partial case-insensitivity and has some advantages over the conventional case sensitivity:
It allows programmers to mostly use their own preferred spelling style, be it humpStyle or snake\_style, and libraries written by different programmers cannot use incompatible conventions. A Nim-aware editor or IDE can show the identifiers as preferred. Another advantage is that it frees the programmer from remembering the exact spelling of an identifier. The exception with respect to the first letter allows common code like `var foo: Foo` to be parsed unambiguously.
Note that this rule also applies to keywords, meaning that `notin` is the same as `notIn` and `not_in` (all-lowercase version (`notin`, `isnot`) is the preferred way of writing keywords).
Historically, Nim was a fully style-insensitive language. This meant that it was not case-sensitive and underscores were ignored and there was not even a distinction between `foo` and `Foo`.
### Stropping
If a keyword is enclosed in backticks it loses its keyword property and becomes an ordinary identifier.
Examples
```
var `var` = "Hello Stropping"
```
```
type Type = object
`int`: int
let `object` = Type(`int`: 9)
assert `object` is Type
assert `object`.`int` == 9
var `var` = 42
let `let` = 8
assert `var` + `let` == 50
const `assert` = true
assert `assert`
```
### String literals
Terminal symbol in the grammar: `STR_LIT`.
String literals can be delimited by matching double quotes, and can contain the following escape sequences:
| Escape sequence | Meaning |
| --- | --- |
| `\p` | platform specific newline: CRLF on Windows, LF on Unix |
| `\r`, `\c` | carriage return |
| `\n`, `\l` | line feed (often called newline) |
| `\f` | form feed |
| `\t` | tabulator |
| `\v` | vertical tabulator |
| `\\` | backslash |
| `\"` | quotation mark |
| `\'` | apostrophe |
| `\` '0'..'9'+ | character with decimal value d; all decimal digits directly following are used for the character |
| `\a` | alert |
| `\b` | backspace |
| `\e` | escape [ESC] |
| `\x` HH | character with hex value HH; exactly two hex digits are allowed |
| `\u` HHHH | unicode codepoint with hex value HHHH; exactly four hex digits are allowed |
| `\u` {H+} | unicode codepoint; all hex digits enclosed in `{}` are used for the codepoint |
Strings in Nim may contain any 8-bit value, even embedded zeros. However some operations may interpret the first binary zero as a terminator.
### Triple quoted string literals
Terminal symbol in the grammar: `TRIPLESTR_LIT`.
String literals can also be delimited by three double quotes `"""` ... `"""`. Literals in this form may run for several lines, may contain `"` and do not interpret any escape sequences. For convenience, when the opening `"""` is followed by a newline (there may be whitespace between the opening `"""` and the newline), the newline (and the preceding whitespace) is not included in the string. The ending of the string literal is defined by the pattern `"""[^"]`, so this:
```
""""long string within quotes""""
```
Produces:
```
"long string within quotes"
```
### Raw string literals
Terminal symbol in the grammar: `RSTR_LIT`.
There are also raw string literals that are preceded with the letter `r` (or `R`) and are delimited by matching double quotes (just like ordinary string literals) and do not interpret the escape sequences. This is especially convenient for regular expressions or Windows paths:
```
var f = openFile(r"C:\texts\text.txt") # a raw string, so ``\t`` is no tab
```
To produce a single `"` within a raw string literal, it has to be doubled:
```
r"a""b"
```
Produces:
```
a"b
```
`r""""` is not possible with this notation, because the three leading quotes introduce a triple quoted string literal. `r"""` is the same as `"""` since triple quoted string literals do not interpret escape sequences either.
### Generalized raw string literals
Terminal symbols in the grammar: `GENERALIZED_STR_LIT`, `GENERALIZED_TRIPLESTR_LIT`.
The construct `identifier"string literal"` (without whitespace between the identifier and the opening quotation mark) is a generalized raw string literal. It is a shortcut for the construct `identifier(r"string literal")`, so it denotes a procedure call with a raw string literal as its only argument. Generalized raw string literals are especially convenient for embedding mini languages directly into Nim (for example regular expressions).
The construct `identifier"""string literal"""` exists too. It is a shortcut for `identifier("""string literal""")`.
### Character literals
Character literals are enclosed in single quotes `''` and can contain the same escape sequences as strings - with one exception: the platform dependent newline (`\p`) is not allowed as it may be wider than one character (often it is the pair CR/LF for example). Here are the valid escape sequences for character literals:
| Escape sequence | Meaning |
| --- | --- |
| `\r`, `\c` | carriage return |
| `\n`, `\l` | line feed |
| `\f` | form feed |
| `\t` | tabulator |
| `\v` | vertical tabulator |
| `\\` | backslash |
| `\"` | quotation mark |
| `\'` | apostrophe |
| `\` '0'..'9'+ | character with decimal value d; all decimal digits directly following are used for the character |
| `\a` | alert |
| `\b` | backspace |
| `\e` | escape [ESC] |
| `\x` HH | character with hex value HH; exactly two hex digits are allowed |
A character is not a Unicode character but a single byte. The reason for this is efficiency: for the overwhelming majority of use-cases, the resulting programs will still handle UTF-8 properly as UTF-8 was specially designed for this. Another reason is that Nim can thus support `array[char, int]` or `set[char]` efficiently as many algorithms rely on this feature. The `Rune` type is used for Unicode characters, it can represent any Unicode character. `Rune` is declared in the [unicode module](unicode).
### Numerical constants
Numerical constants are of a single type and have the form:
```
hexdigit = digit | 'A'..'F' | 'a'..'f'
octdigit = '0'..'7'
bindigit = '0'..'1'
HEX_LIT = '0' ('x' | 'X' ) hexdigit ( ['_'] hexdigit )*
DEC_LIT = digit ( ['_'] digit )*
OCT_LIT = '0' 'o' octdigit ( ['_'] octdigit )*
BIN_LIT = '0' ('b' | 'B' ) bindigit ( ['_'] bindigit )*
INT_LIT = HEX_LIT
| DEC_LIT
| OCT_LIT
| BIN_LIT
INT8_LIT = INT_LIT ['\''] ('i' | 'I') '8'
INT16_LIT = INT_LIT ['\''] ('i' | 'I') '16'
INT32_LIT = INT_LIT ['\''] ('i' | 'I') '32'
INT64_LIT = INT_LIT ['\''] ('i' | 'I') '64'
UINT_LIT = INT_LIT ['\''] ('u' | 'U')
UINT8_LIT = INT_LIT ['\''] ('u' | 'U') '8'
UINT16_LIT = INT_LIT ['\''] ('u' | 'U') '16'
UINT32_LIT = INT_LIT ['\''] ('u' | 'U') '32'
UINT64_LIT = INT_LIT ['\''] ('u' | 'U') '64'
exponent = ('e' | 'E' ) ['+' | '-'] digit ( ['_'] digit )*
FLOAT_LIT = digit (['_'] digit)* (('.' digit (['_'] digit)* [exponent]) |exponent)
FLOAT32_SUFFIX = ('f' | 'F') ['32']
FLOAT32_LIT = HEX_LIT '\'' FLOAT32_SUFFIX
| (FLOAT_LIT | DEC_LIT | OCT_LIT | BIN_LIT) ['\''] FLOAT32_SUFFIX
FLOAT64_SUFFIX = ( ('f' | 'F') '64' ) | 'd' | 'D'
FLOAT64_LIT = HEX_LIT '\'' FLOAT64_SUFFIX
| (FLOAT_LIT | DEC_LIT | OCT_LIT | BIN_LIT) ['\''] FLOAT64_SUFFIX
```
As can be seen in the productions, numerical constants can contain underscores for readability. Integer and floating-point literals may be given in decimal (no prefix), binary (prefix `0b`), octal (prefix `0o`), and hexadecimal (prefix `0x`) notation.
There exists a literal for each numerical type that is defined. The suffix starting with an apostrophe (''') is called a type suffix. Literals without a type suffix are of an integer type unless the literal contains a dot or `E|e` in which case it is of type `float`. This integer type is `int` if the literal is in the range `low(i32)..high(i32)`, otherwise it is `int64`. For notational convenience, the apostrophe of a type suffix is optional if it is not ambiguous (only hexadecimal floating-point literals with a type suffix can be ambiguous).
The type suffixes are:
| Type Suffix | Resulting type of literal |
| --- | --- |
| `'i8` | int8 |
| `'i16` | int16 |
| `'i32` | int32 |
| `'i64` | int64 |
| `'u` | uint |
| `'u8` | uint8 |
| `'u16` | uint16 |
| `'u32` | uint32 |
| `'u64` | uint64 |
| `'f` | float32 |
| `'d` | float64 |
| `'f32` | float32 |
| `'f64` | float64 |
Floating-point literals may also be in binary, octal or hexadecimal notation: `0B0_10001110100_0000101001000111101011101111111011000101001101001001'f64` is approximately 1.72826e35 according to the IEEE floating-point standard.
Literals are bounds checked so that they fit the datatype. Non-base-10 literals are used mainly for flags and bit pattern representations, therefore bounds checking is done on bit width, not value range. If the literal fits in the bit width of the datatype, it is accepted. Hence: 0b10000000'u8 == 0x80'u8 == 128, but, 0b10000000'i8 == 0x80'i8 == -1 instead of causing an overflow error.
### Operators
Nim allows user defined operators. An operator is any combination of the following characters:
```
= + - * / < >
@ $ ~ & % |
! ? ^ . : \
```
(The grammar uses the terminal OPR to refer to operator symbols as defined here.)
These keywords are also operators: `and or not xor shl shr div mod in notin is isnot of as from`.
. =, :, :: are not available as general operators; they are used for other notational purposes.
`*:` is as a special case treated as the two tokens \* and : (to support `var v*: T`).
The `not` keyword is always a unary operator, `a not b` is parsed as `a(not b)`, not as `(a) not (b)`.
### Other tokens
The following strings denote other tokens:
```
` ( ) { } [ ] , ; [. .] {. .} (. .) [:
```
The slice operator .. takes precedence over other tokens that contain a dot: {..} are the three tokens {, .., } and not the two tokens {., .}.
Syntax
------
This section lists Nim's standard syntax. How the parser handles the indentation is already described in the [Lexical Analysis](#lexical-analysis) section.
Nim allows user-definable operators. Binary operators have 11 different levels of precedence.
### Associativity
Binary operators whose first character is `^` are right-associative, all other binary operators are left-associative.
```
proc `^/`(x, y: float): float =
# a right-associative division operator
result = x / y
echo 12 ^/ 4 ^/ 8 # 24.0 (4 / 8 = 0.5, then 12 / 0.5 = 24.0)
echo 12 / 4 / 8 # 0.375 (12 / 4 = 3.0, then 3 / 8 = 0.375)
```
### Precedence
Unary operators always bind stronger than any binary operator: `$a + b` is `($a) + b` and not `$(a + b)`.
If an unary operator's first character is `@` it is a sigil-like operator which binds stronger than a `primarySuffix`: `@x.abc` is parsed as `(@x).abc` whereas `$x.abc` is parsed as `$(x.abc)`.
For binary operators that are not keywords, the precedence is determined by the following rules:
Operators ending in either `->`, `~>` or `=>` are called arrow like, and have the lowest precedence of all operators.
If the operator ends with `=` and its first character is none of `<`, `>`, `!`, `=`, `~`, `?`, it is an *assignment operator* which has the second-lowest precedence.
Otherwise, precedence is determined by the first character.
| Precedence level | Operators | First character | Terminal symbol |
| --- | --- | --- | --- |
| 10 (highest) | | `$ ^` | OP10 |
| 9 | `* / div mod shl shr %` | `* % \ /` | OP9 |
| 8 | `+ -` | `+ - ~ |` | OP8 |
| 7 | `&` | `&` | OP7 |
| 6 | `..` | `.` | OP6 |
| 5 | `== <= < >= > != in notin is isnot not of as from` | `= < > !` | OP5 |
| 4 | `and` | | OP4 |
| 3 | `or xor` | | OP3 |
| 2 | | `@ : ?` | OP2 |
| 1 | *assignment operator* (like `+=`, `*=`) | | OP1 |
| 0 (lowest) | *arrow like operator* (like `->`, `=>`) | | OP0 |
Whether an operator is used as a prefix operator is also affected by preceding whitespace (this parsing change was introduced with version 0.13.0):
```
echo $foo
# is parsed as
echo($foo)
```
Spacing also determines whether `(a, b)` is parsed as an argument list of a call or whether it is parsed as a tuple constructor:
```
echo(1, 2) # pass 1 and 2 to echo
```
```
echo (1, 2) # pass the tuple (1, 2) to echo
```
### Grammar
The grammar's start symbol is `module`.
```
# This file is generated by compiler/parser.nim.
module = stmt ^* (';' / IND{=})
comma = ',' COMMENT?
semicolon = ';' COMMENT?
colon = ':' COMMENT?
colcom = ':' COMMENT?
operator = OP0 | OP1 | OP2 | OP3 | OP4 | OP5 | OP6 | OP7 | OP8 | OP9
| 'or' | 'xor' | 'and'
| 'is' | 'isnot' | 'in' | 'notin' | 'of' | 'as' | 'from'
| 'div' | 'mod' | 'shl' | 'shr' | 'not' | 'static' | '..'
prefixOperator = operator
optInd = COMMENT? IND?
optPar = (IND{>} | IND{=})?
simpleExpr = arrowExpr (OP0 optInd arrowExpr)* pragma?
arrowExpr = assignExpr (OP1 optInd assignExpr)*
assignExpr = orExpr (OP2 optInd orExpr)*
orExpr = andExpr (OP3 optInd andExpr)*
andExpr = cmpExpr (OP4 optInd cmpExpr)*
cmpExpr = sliceExpr (OP5 optInd sliceExpr)*
sliceExpr = ampExpr (OP6 optInd ampExpr)*
ampExpr = plusExpr (OP7 optInd plusExpr)*
plusExpr = mulExpr (OP8 optInd mulExpr)*
mulExpr = dollarExpr (OP9 optInd dollarExpr)*
dollarExpr = primary (OP10 optInd primary)*
symbol = '`' (KEYW|IDENT|literal|(operator|'('|')'|'['|']'|'{'|'}'|'=')+)+ '`'
| IDENT | KEYW
exprColonEqExpr = expr (':'|'=' expr)?
exprList = expr ^+ comma
exprColonEqExprList = exprColonEqExpr (comma exprColonEqExpr)* (comma)?
dotExpr = expr '.' optInd (symbol | '[:' exprList ']')
explicitGenericInstantiation = '[:' exprList ']' ( '(' exprColonEqExpr ')' )?
qualifiedIdent = symbol ('.' optInd symbol)?
setOrTableConstr = '{' ((exprColonEqExpr comma)* | ':' ) '}'
castExpr = 'cast' ('[' optInd typeDesc optPar ']' '(' optInd expr optPar ')') /
parKeyw = 'discard' | 'include' | 'if' | 'while' | 'case' | 'try'
| 'finally' | 'except' | 'for' | 'block' | 'const' | 'let'
| 'when' | 'var' | 'mixin'
par = '(' optInd
( &parKeyw (ifExpr \ complexOrSimpleStmt) ^+ ';'
| ';' (ifExpr \ complexOrSimpleStmt) ^+ ';'
| pragmaStmt
| simpleExpr ( ('=' expr (';' (ifExpr \ complexOrSimpleStmt) ^+ ';' )? )
| (':' expr (',' exprColonEqExpr ^+ ',' )? ) ) )
optPar ')'
literal = | INT_LIT | INT8_LIT | INT16_LIT | INT32_LIT | INT64_LIT
| UINT_LIT | UINT8_LIT | UINT16_LIT | UINT32_LIT | UINT64_LIT
| FLOAT_LIT | FLOAT32_LIT | FLOAT64_LIT
| STR_LIT | RSTR_LIT | TRIPLESTR_LIT
| CHAR_LIT
| NIL
generalizedLit = GENERALIZED_STR_LIT | GENERALIZED_TRIPLESTR_LIT
identOrLiteral = generalizedLit | symbol | literal
| par | arrayConstr | setOrTableConstr
| castExpr
tupleConstr = '(' optInd (exprColonEqExpr comma?)* optPar ')'
arrayConstr = '[' optInd (exprColonEqExpr comma?)* optPar ']'
primarySuffix = '(' (exprColonEqExpr comma?)* ')'
| '.' optInd symbol generalizedLit?
| '[' optInd exprColonEqExprList optPar ']'
| '{' optInd exprColonEqExprList optPar '}'
| &( '`'|IDENT|literal|'cast'|'addr'|'type') expr # command syntax
pragma = '{.' optInd (exprColonEqExpr comma?)* optPar ('.}' | '}')
identVis = symbol OPR? # postfix position
identVisDot = symbol '.' optInd symbol OPR?
identWithPragma = identVis pragma?
identWithPragmaDot = identVisDot pragma?
declColonEquals = identWithPragma (comma identWithPragma)* comma?
(':' optInd typeDesc)? ('=' optInd expr)?
identColonEquals = IDENT (comma IDENT)* comma?
(':' optInd typeDesc)? ('=' optInd expr)?)
inlTupleDecl = 'tuple'
'[' optInd (identColonEquals (comma/semicolon)?)* optPar ']'
extTupleDecl = 'tuple'
COMMENT? (IND{>} identColonEquals (IND{=} identColonEquals)*)?
tupleClass = 'tuple'
paramList = '(' declColonEquals ^* (comma/semicolon) ')'
paramListArrow = paramList? ('->' optInd typeDesc)?
paramListColon = paramList? (':' optInd typeDesc)?
doBlock = 'do' paramListArrow pragma? colcom stmt
procExpr = 'proc' paramListColon pragma? ('=' COMMENT? stmt)?
distinct = 'distinct' optInd typeDesc
forStmt = 'for' (identWithPragma ^+ comma) 'in' expr colcom stmt
forExpr = forStmt
expr = (blockExpr
| ifExpr
| whenExpr
| caseStmt
| forExpr
| tryExpr)
/ simpleExpr
typeKeyw = 'var' | 'out' | 'ref' | 'ptr' | 'shared' | 'tuple'
| 'proc' | 'iterator' | 'distinct' | 'object' | 'enum'
primary = typeKeyw optInd typeDesc
/ prefixOperator* identOrLiteral primarySuffix*
/ 'bind' primary
typeDesc = simpleExpr ('not' expr)?
typeDefAux = simpleExpr ('not' expr)?
| 'concept' typeClass
postExprBlocks = ':' stmt? ( IND{=} doBlock
| IND{=} 'of' exprList ':' stmt
| IND{=} 'elif' expr ':' stmt
| IND{=} 'except' exprList ':' stmt
| IND{=} 'else' ':' stmt )*
exprStmt = simpleExpr
(( '=' optInd expr colonBody? )
/ ( expr ^+ comma
postExprBlocks
))?
importStmt = 'import' optInd expr
((comma expr)*
/ 'except' optInd (expr ^+ comma))
exportStmt = 'export' optInd expr
((comma expr)*
/ 'except' optInd (expr ^+ comma))
includeStmt = 'include' optInd expr ^+ comma
fromStmt = 'from' expr 'import' optInd expr (comma expr)*
returnStmt = 'return' optInd expr?
raiseStmt = 'raise' optInd expr?
yieldStmt = 'yield' optInd expr?
discardStmt = 'discard' optInd expr?
breakStmt = 'break' optInd expr?
continueStmt = 'break' optInd expr?
condStmt = expr colcom stmt COMMENT?
(IND{=} 'elif' expr colcom stmt)*
(IND{=} 'else' colcom stmt)?
ifStmt = 'if' condStmt
whenStmt = 'when' condStmt
condExpr = expr colcom expr optInd
('elif' expr colcom expr optInd)*
'else' colcom expr
ifExpr = 'if' condExpr
whenExpr = 'when' condExpr
whileStmt = 'while' expr colcom stmt
ofBranch = 'of' exprList colcom stmt
ofBranches = ofBranch (IND{=} ofBranch)*
(IND{=} 'elif' expr colcom stmt)*
(IND{=} 'else' colcom stmt)?
caseStmt = 'case' expr ':'? COMMENT?
(IND{>} ofBranches DED
| IND{=} ofBranches)
tryStmt = 'try' colcom stmt &(IND{=}? 'except'|'finally')
(IND{=}? 'except' exprList colcom stmt)*
(IND{=}? 'finally' colcom stmt)?
tryExpr = 'try' colcom stmt &(optInd 'except'|'finally')
(optInd 'except' exprList colcom stmt)*
(optInd 'finally' colcom stmt)?
exceptBlock = 'except' colcom stmt
blockStmt = 'block' symbol? colcom stmt
blockExpr = 'block' symbol? colcom stmt
staticStmt = 'static' colcom stmt
deferStmt = 'defer' colcom stmt
asmStmt = 'asm' pragma? (STR_LIT | RSTR_LIT | TRIPLESTR_LIT)
genericParam = symbol (comma symbol)* (colon expr)? ('=' optInd expr)?
genericParamList = '[' optInd
genericParam ^* (comma/semicolon) optPar ']'
pattern = '{' stmt '}'
indAndComment = (IND{>} COMMENT)? | COMMENT?
routine = optInd identVis pattern? genericParamList?
paramListColon pragma? ('=' COMMENT? stmt)? indAndComment
commentStmt = COMMENT
section(RULE) = COMMENT? RULE / (IND{>} (RULE / COMMENT)^+IND{=} DED)
enum = 'enum' optInd (symbol pragma? optInd ('=' optInd expr COMMENT?)? comma?)+
objectWhen = 'when' expr colcom objectPart COMMENT?
('elif' expr colcom objectPart COMMENT?)*
('else' colcom objectPart COMMENT?)?
objectBranch = 'of' exprList colcom objectPart
objectBranches = objectBranch (IND{=} objectBranch)*
(IND{=} 'elif' expr colcom objectPart)*
(IND{=} 'else' colcom objectPart)?
objectCase = 'case' identWithPragma ':' typeDesc ':'? COMMENT?
(IND{>} objectBranches DED
| IND{=} objectBranches)
objectPart = IND{>} objectPart^+IND{=} DED
/ objectWhen / objectCase / 'nil' / 'discard' / declColonEquals
object = 'object' pragma? ('of' typeDesc)? COMMENT? objectPart
typeClassParam = ('var' | 'out')? symbol
typeClass = typeClassParam ^* ',' (pragma)? ('of' typeDesc ^* ',')?
&IND{>} stmt
typeDef = identWithPragmaDot genericParamList? '=' optInd typeDefAux
indAndComment? / identVisDot genericParamList? pragma '=' optInd typeDefAux
indAndComment?
varTuple = '(' optInd identWithPragma ^+ comma optPar ')' '=' optInd expr
colonBody = colcom stmt postExprBlocks?
variable = (varTuple / identColonEquals) colonBody? indAndComment
constant = (varTuple / identWithPragma) (colon typeDesc)? '=' optInd expr indAndComment
bindStmt = 'bind' optInd qualifiedIdent ^+ comma
mixinStmt = 'mixin' optInd qualifiedIdent ^+ comma
pragmaStmt = pragma (':' COMMENT? stmt)?
simpleStmt = ((returnStmt | raiseStmt | yieldStmt | discardStmt | breakStmt
| continueStmt | pragmaStmt | importStmt | exportStmt | fromStmt
| includeStmt | commentStmt) / exprStmt) COMMENT?
complexOrSimpleStmt = (ifStmt | whenStmt | whileStmt
| tryStmt | forStmt
| blockStmt | staticStmt | deferStmt | asmStmt
| 'proc' routine
| 'method' routine
| 'func' routine
| 'iterator' routine
| 'macro' routine
| 'template' routine
| 'converter' routine
| 'type' section(typeDef)
| 'const' section(constant)
| ('let' | 'var' | 'using') section(variable)
| bindStmt | mixinStmt)
/ simpleStmt
stmt = (IND{>} complexOrSimpleStmt^+(IND{=} / ';') DED)
/ simpleStmt ^+ ';'
```
Order of evaluation
-------------------
Order of evaluation is strictly left-to-right, inside-out as it is typical for most others imperative programming languages:
```
var s = ""
proc p(arg: int): int =
s.add $arg
result = arg
discard p(p(1) + p(2))
doAssert s == "123"
```
Assignments are not special, the left-hand-side expression is evaluated before the right-hand side:
```
var v = 0
proc getI(): int =
result = v
inc v
var a, b: array[0..2, int]
proc someCopy(a: var int; b: int) = a = b
a[getI()] = getI()
doAssert a == [1, 0, 0]
v = 0
someCopy(b[getI()], getI())
doAssert b == [1, 0, 0]
```
Rationale: Consistency with overloaded assignment or assignment-like operations, `a = b` can be read as `performSomeCopy(a, b)`.
However, the concept of "order of evaluation" is only applicable after the code was normalized: The normalization involves template expansions and argument reorderings that have been passed to named parameters:
```
var s = ""
proc p(): int =
s.add "p"
result = 5
proc q(): int =
s.add "q"
result = 3
# Evaluation order is 'b' before 'a' due to template
# expansion's semantics.
template swapArgs(a, b): untyped =
b + a
doAssert swapArgs(p() + q(), q() - p()) == 6
doAssert s == "qppq"
# Evaluation order is not influenced by named parameters:
proc construct(first, second: int) =
discard
# 'p' is evaluated before 'q'!
construct(second = q(), first = p())
doAssert s == "qppqpq"
```
Rationale: This is far easier to implement than hypothetical alternatives.
Constants and Constant Expressions
----------------------------------
A constant is a symbol that is bound to the value of a constant expression. Constant expressions are restricted to depend only on the following categories of values and operations, because these are either built into the language or declared and evaluated before semantic analysis of the constant expression:
* literals
* built-in operators
* previously declared constants and compile-time variables
* previously declared macros and templates
* previously declared procedures that have no side effects beyond possibly modifying compile-time variables
A constant expression can contain code blocks that may internally use all Nim features supported at compile time (as detailed in the next section below). Within such a code block, it is possible to declare variables and then later read and update them, or declare variables and pass them to procedures that modify them. However, the code in such a block must still adhere to the restrictions listed above for referencing values and operations outside the block.
The ability to access and modify compile-time variables adds flexibility to constant expressions that may be surprising to those coming from other statically typed languages. For example, the following code echoes the beginning of the Fibonacci series **at compile-time**. (This is a demonstration of flexibility in defining constants, not a recommended style for solving this problem!)
```
import strformat
var fib_n {.compileTime.}: int
var fib_prev {.compileTime.}: int
var fib_prev_prev {.compileTime.}: int
proc next_fib(): int =
result = if fib_n < 2:
fib_n
else:
fib_prev_prev + fib_prev
inc(fib_n)
fib_prev_prev = fib_prev
fib_prev = result
const f0 = next_fib()
const f1 = next_fib()
const display_fib = block:
const f2 = next_fib()
var result = fmt"Fibonacci sequence: {f0}, {f1}, {f2}"
for i in 3..12:
add(result, fmt", {next_fib()}")
result
static:
echo display_fib
```
Restrictions on Compile-Time Execution
--------------------------------------
Nim code that will be executed at compile time cannot use the following language features:
* methods
* closure iterators
* the `cast` operator
* reference (pointer) types
* FFI
The use of wrappers that use FFI and/or `cast` is also disallowed. Note that these wrappers include the ones in the standard libraries.
Some or all of these restrictions are likely to be lifted over time.
Types
-----
All expressions have a type that is known during semantic analysis. Nim is statically typed. One can declare new types, which is in essence defining an identifier that can be used to denote this custom type.
These are the major type classes:
* ordinal types (consist of integer, bool, character, enumeration (and subranges thereof) types)
* floating-point types
* string type
* structured types
* reference (pointer) type
* procedural type
* generic type
### Ordinal types
Ordinal types have the following characteristics:
* Ordinal types are countable and ordered. This property allows the operation of functions as `inc`, `ord`, `dec` on ordinal types to be defined.
* Ordinal values have the smallest possible value. Trying to count further down than the smallest value produces a panic or a static error.
* Ordinal values have the largest possible value. Trying to count further than the largest value produces a panic or a static error.
Integers, bool, characters, and enumeration types (and subranges of these types) belong to ordinal types. For reasons of simplicity of implementation the types `uint` and `uint64` are not ordinal types. (This will be changed in later versions of the language.)
A distinct type is an ordinal type if its base type is an ordinal type.
### Pre-defined integer types
These integer types are pre-defined:
`int` the generic signed integer type; its size is platform-dependent and has the same size as a pointer. This type should be used in general. An integer literal that has no type suffix is of this type if it is in the range `low(int32)..high(int32)` otherwise the literal's type is `int64`. intXX additional signed integer types of XX bits use this naming scheme (example: int16 is a 16-bit wide integer). The current implementation supports `int8`, `int16`, `int32`, `int64`. Literals of these types have the suffix 'iXX. `uint` the generic unsigned integer type; its size is platform-dependent and has the same size as a pointer. An integer literal with the type suffix `'u` is of this type. uintXX additional unsigned integer types of XX bits use this naming scheme (example: uint16 is a 16-bit wide unsigned integer). The current implementation supports `uint8`, `uint16`, `uint32`, `uint64`. Literals of these types have the suffix 'uXX. Unsigned operations all wrap around; they cannot lead to over- or underflow errors. In addition to the usual arithmetic operators for signed and unsigned integers (`+ - *` etc.) there are also operators that formally work on *signed* integers but treat their arguments as *unsigned*: They are mostly provided for backwards compatibility with older versions of the language that lacked unsigned integer types. These unsigned operations for signed integers use the `%` suffix as convention:
| operation | meaning |
| --- | --- |
| `a +% b` | unsigned integer addition |
| `a -% b` | unsigned integer subtraction |
| `a *% b` | unsigned integer multiplication |
| `a /% b` | unsigned integer division |
| `a %% b` | unsigned integer modulo operation |
| `a <% b` | treat `a` and `b` as unsigned and compare |
| `a <=% b` | treat `a` and `b` as unsigned and compare |
| `ze(a)` | extends the bits of `a` with zeros until it has the width of the `int` type |
| `toU8(a)` | treats `a` as unsigned and converts it to an unsigned integer of 8 bits (but still the `int8` type) |
| `toU16(a)` | treats `a` as unsigned and converts it to an unsigned integer of 16 bits (but still the `int16` type) |
| `toU32(a)` | treats `a` as unsigned and converts it to an unsigned integer of 32 bits (but still the `int32` type) |
Automatic type conversion is performed in expressions where different kinds of integer types are used: the smaller type is converted to the larger.
A narrowing type conversion converts a larger to a smaller type (for example `int32 -> int16`. A widening type conversion converts a smaller type to a larger type (for example `int16 -> int32`). In Nim only widening type conversions are *implicit*:
```
var myInt16 = 5i16
var myInt: int
myInt16 + 34 # of type ``int16``
myInt16 + myInt # of type ``int``
myInt16 + 2i32 # of type ``int32``
```
However, `int` literals are implicitly convertible to a smaller integer type if the literal's value fits this smaller type and such a conversion is less expensive than other implicit conversions, so `myInt16 + 34` produces an `int16` result.
For further details, see [Convertible relation](#type-relations-convertible-relation).
### Subrange types
A subrange type is a range of values from an ordinal or floating-point type (the base type). To define a subrange type, one must specify its limiting values -- the lowest and highest value of the type. For example:
```
type
Subrange = range[0..5]
PositiveFloat = range[0.0..Inf]
```
`Subrange` is a subrange of an integer which can only hold the values 0 to 5. `PositiveFloat` defines a subrange of all positive floating-point values. NaN does not belong to any subrange of floating-point types. Assigning any other value to a variable of type `Subrange` is a panic (or a static error if it can be determined during semantic analysis). Assignments from the base type to one of its subrange types (and vice versa) are allowed.
A subrange type has the same size as its base type (`int` in the Subrange example).
### Pre-defined floating-point types
The following floating-point types are pre-defined:
`float` the generic floating-point type; its size used to be platform-dependent, but now it is always mapped to `float64`. This type should be used in general. floatXX an implementation may define additional floating-point types of XX bits using this naming scheme (example: float64 is a 64-bit wide float). The current implementation supports `float32` and `float64`. Literals of these types have the suffix 'fXX. Automatic type conversion in expressions with different kinds of floating-point types is performed: See [Convertible relation](#type-relations-convertible-relation) for further details. Arithmetic performed on floating-point types follows the IEEE standard. Integer types are not converted to floating-point types automatically and vice versa.
The IEEE standard defines five types of floating-point exceptions:
* Invalid: operations with mathematically invalid operands, for example 0.0/0.0, sqrt(-1.0), and log(-37.8).
* Division by zero: divisor is zero and dividend is a finite nonzero number, for example 1.0/0.0.
* Overflow: operation produces a result that exceeds the range of the exponent, for example MAXDOUBLE+0.0000000000001e308.
* Underflow: operation produces a result that is too small to be represented as a normal number, for example, MINDOUBLE \* MINDOUBLE.
* Inexact: operation produces a result that cannot be represented with infinite precision, for example, 2.0 / 3.0, log(1.1) and 0.1 in input.
The IEEE exceptions are either ignored during execution or mapped to the Nim exceptions: FloatInvalidOpDefect, FloatDivByZeroDefect, FloatOverflowDefect, FloatUnderflowDefect, and FloatInexactDefect. These exceptions inherit from the FloatingPointDefect base class.
Nim provides the pragmas nanChecks and infChecks to control whether the IEEE exceptions are ignored or trap a Nim exception:
```
{.nanChecks: on, infChecks: on.}
var a = 1.0
var b = 0.0
echo b / b # raises FloatInvalidOpDefect
echo a / b # raises FloatOverflowDefect
```
In the current implementation `FloatDivByZeroDefect` and `FloatInexactDefect` are never raised. `FloatOverflowDefect` is raised instead of `FloatDivByZeroDefect`. There is also a floatChecks pragma that is a short-cut for the combination of `nanChecks` and `infChecks` pragmas. `floatChecks` are turned off as default.
The only operations that are affected by the `floatChecks` pragma are the `+`, `-`, `*`, `/` operators for floating-point types.
An implementation should always use the maximum precision available to evaluate floating pointer values during semantic analysis; this means expressions like `0.09'f32 + 0.01'f32 == 0.09'f64 + 0.01'f64` that are evaluating during constant folding are true.
### Boolean type
The boolean type is named bool in Nim and can be one of the two pre-defined values `true` and `false`. Conditions in `while`, `if`, `elif`, `when`-statements need to be of type `bool`.
This condition holds:
```
ord(false) == 0 and ord(true) == 1
```
The operators `not, and, or, xor, <, <=, >, >=, !=, ==` are defined for the bool type. The `and` and `or` operators perform short-cut evaluation. Example:
```
while p != nil and p.name != "xyz":
# p.name is not evaluated if p == nil
p = p.next
```
The size of the bool type is one byte.
### Character type
The character type is named `char` in Nim. Its size is one byte. Thus it cannot represent a UTF-8 character, but a part of it. The reason for this is efficiency: for the overwhelming majority of use-cases, the resulting programs will still handle UTF-8 properly as UTF-8 was especially designed for this. Another reason is that Nim can support `array[char, int]` or `set[char]` efficiently as many algorithms rely on this feature. The `Rune` type is used for Unicode characters, it can represent any Unicode character. `Rune` is declared in the [unicode module](unicode).
### Enumeration types
Enumeration types define a new type whose values consist of the ones specified. The values are ordered. Example:
```
type
Direction = enum
north, east, south, west
```
Now the following holds:
```
ord(north) == 0
ord(east) == 1
ord(south) == 2
ord(west) == 3
# Also allowed:
ord(Direction.west) == 3
```
Thus, north < east < south < west. The comparison operators can be used with enumeration types. Instead of `north` etc, the enum value can also be qualified with the enum type that it resides in, `Direction.north`.
For better interfacing to other programming languages, the fields of enum types can be assigned an explicit ordinal value. However, the ordinal values have to be in ascending order. A field whose ordinal value is not explicitly given is assigned the value of the previous field + 1.
An explicit ordered enum can have *holes*:
```
type
TokenType = enum
a = 2, b = 4, c = 89 # holes are valid
```
However, it is then not ordinal anymore, so it is not possible to use these enums as an index type for arrays. The procedures `inc`, `dec`, `succ` and `pred` are not available for them either.
The compiler supports the built-in stringify operator `$` for enumerations. The stringify's result can be controlled by explicitly giving the string values to use:
```
type
MyEnum = enum
valueA = (0, "my value A"),
valueB = "value B",
valueC = 2,
valueD = (3, "abc")
```
As can be seen from the example, it is possible to both specify a field's ordinal value and its string value by using a tuple. It is also possible to only specify one of them.
An enum can be marked with the `pure` pragma so that its fields are added to a special module-specific hidden scope that is only queried as the last attempt. Only non-ambiguous symbols are added to this scope. But one can always access these via type qualification written as `MyEnum.value`:
```
type
MyEnum {.pure.} = enum
valueA, valueB, valueC, valueD, amb
OtherEnum {.pure.} = enum
valueX, valueY, valueZ, amb
echo valueA # MyEnum.valueA
echo amb # Error: Unclear whether it's MyEnum.amb or OtherEnum.amb
echo MyEnum.amb # OK.
```
To implement bit fields with enums see [Bit fields](#set-type-bit-fields)
### String type
All string literals are of the type `string`. A string in Nim is very similar to a sequence of characters. However, strings in Nim are both zero-terminated and have a length field. One can retrieve the length with the builtin `len` procedure; the length never counts the terminating zero.
The terminating zero cannot be accessed unless the string is converted to the `cstring` type first. The terminating zero assures that this conversion can be done in O(1) and without any allocations.
The assignment operator for strings always copies the string. The `&` operator concatenates strings.
Most native Nim types support conversion to strings with the special `$` proc. When calling the `echo` proc, for example, the built-in stringify operation for the parameter is called:
```
echo 3 # calls `$` for `int`
```
Whenever a user creates a specialized object, implementation of this procedure provides for `string` representation.
```
type
Person = object
name: string
age: int
proc `$`(p: Person): string = # `$` always returns a string
result = p.name & " is " &
$p.age & # we *need* the `$` in front of p.age which
# is natively an integer to convert it to
# a string
" years old."
```
While `$p.name` can also be used, the `$` operation on a string does nothing. Note that we cannot rely on automatic conversion from an `int` to a `string` like we can for the `echo` proc.
Strings are compared by their lexicographical order. All comparison operators are available. Strings can be indexed like arrays (lower bound is 0). Unlike arrays, they can be used in case statements:
```
case paramStr(i)
of "-v": incl(options, optVerbose)
of "-h", "-?": incl(options, optHelp)
else: write(stdout, "invalid command line option!\n")
```
Per convention, all strings are UTF-8 strings, but this is not enforced. For example, when reading strings from binary files, they are merely a sequence of bytes. The index operation `s[i]` means the i-th *char* of `s`, not the i-th *unichar*. The iterator `runes` from the [unicode module](unicode) can be used for iteration over all Unicode characters.
### cstring type
The `cstring` type meaning `compatible string` is the native representation of a string for the compilation backend. For the C backend the `cstring` type represents a pointer to a zero-terminated char array compatible with the type `char*` in Ansi C. Its primary purpose lies in easy interfacing with C. The index operation `s[i]` means the i-th *char* of `s`; however no bounds checking for `cstring` is performed making the index operation unsafe.
A Nim `string` is implicitly convertible to `cstring` for convenience. If a Nim string is passed to a C-style variadic proc, it is implicitly converted to `cstring` too:
```
proc printf(formatstr: cstring) {.importc: "printf", varargs,
header: "<stdio.h>".}
printf("This works %s", "as expected")
```
Even though the conversion is implicit, it is not *safe*: The garbage collector does not consider a `cstring` to be a root and may collect the underlying memory. However, in practice, this almost never happens as the GC considers stack roots conservatively. One can use the builtin procs `GC_ref` and `GC_unref` to keep the string data alive for the rare cases where it does not work.
A `$` proc is defined for cstrings that returns a string. Thus to get a nim string from a cstring:
```
var str: string = "Hello!"
var cstr: cstring = str
var newstr: string = $cstr
```
### Structured types
A variable of a structured type can hold multiple values at the same time. Structured types can be nested to unlimited levels. Arrays, sequences, tuples, objects, and sets belong to the structured types.
### Array and sequence types
Arrays are a homogeneous type, meaning that each element in the array has the same type. Arrays always have a fixed length specified as a constant expression (except for open arrays). They can be indexed by any ordinal type. A parameter `A` may be an *open array*, in which case it is indexed by integers from 0 to `len(A)-1`. An array expression may be constructed by the array constructor `[]`. The element type of this array expression is inferred from the type of the first element. All other elements need to be implicitly convertible to this type.
An array type can be defined using the `array[size, T]` syntax, or using `array[lo..hi, T]` for arrays that start at an index other than zero.
Sequences are similar to arrays but of dynamic length which may change during runtime (like strings). Sequences are implemented as growable arrays, allocating pieces of memory as items are added. A sequence `S` is always indexed by integers from 0 to `len(S)-1` and its bounds are checked. Sequences can be constructed by the array constructor `[]` in conjunction with the array to sequence operator `@`. Another way to allocate space for a sequence is to call the built-in `newSeq` procedure.
A sequence may be passed to a parameter that is of type *open array*.
Example:
```
type
IntArray = array[0..5, int] # an array that is indexed with 0..5
IntSeq = seq[int] # a sequence of integers
var
x: IntArray
y: IntSeq
x = [1, 2, 3, 4, 5, 6] # [] is the array constructor
y = @[1, 2, 3, 4, 5, 6] # the @ turns the array into a sequence
let z = [1.0, 2, 3, 4] # the type of z is array[0..3, float]
```
The lower bound of an array or sequence may be received by the built-in proc `low()`, the higher bound by `high()`. The length may be received by `len()`. `low()` for a sequence or an open array always returns 0, as this is the first valid index. One can append elements to a sequence with the `add()` proc or the `&` operator, and remove (and get) the last element of a sequence with the `pop()` proc.
The notation `x[i]` can be used to access the i-th element of `x`.
Arrays are always bounds checked (statically or at runtime). These checks can be disabled via pragmas or invoking the compiler with the `--boundChecks:off` command-line switch.
An array constructor can have explicit indexes for readability:
```
type
Values = enum
valA, valB, valC
const
lookupTable = [
valA: "A",
valB: "B",
valC: "C"
]
```
If an index is left out, `succ(lastIndex)` is used as the index value:
```
type
Values = enum
valA, valB, valC, valD, valE
const
lookupTable = [
valA: "A",
"B",
valC: "C",
"D", "e"
]
```
### Open arrays
Often fixed size arrays turn out to be too inflexible; procedures should be able to deal with arrays of different sizes. The openarray type allows this; it can only be used for parameters. Openarrays are always indexed with an `int` starting at position 0. The `len`, `low` and `high` operations are available for open arrays too. Any array with a compatible base type can be passed to an openarray parameter, the index type does not matter. In addition to arrays sequences can also be passed to an open array parameter.
The openarray type cannot be nested: multidimensional openarrays are not supported because this is seldom needed and cannot be done efficiently.
```
proc testOpenArray(x: openArray[int]) = echo repr(x)
testOpenArray([1,2,3]) # array[]
testOpenArray(@[1,2,3]) # seq[]
```
### Varargs
A `varargs` parameter is an openarray parameter that additionally allows to pass a variable number of arguments to a procedure. The compiler converts the list of arguments to an array implicitly:
```
proc myWriteln(f: File, a: varargs[string]) =
for s in items(a):
write(f, s)
write(f, "\n")
myWriteln(stdout, "abc", "def", "xyz")
# is transformed to:
myWriteln(stdout, ["abc", "def", "xyz"])
```
This transformation is only done if the varargs parameter is the last parameter in the procedure header. It is also possible to perform type conversions in this context:
```
proc myWriteln(f: File, a: varargs[string, `$`]) =
for s in items(a):
write(f, s)
write(f, "\n")
myWriteln(stdout, 123, "abc", 4.0)
# is transformed to:
myWriteln(stdout, [$123, $"def", $4.0])
```
In this example `$` is applied to any argument that is passed to the parameter `a`. (Note that `$` applied to strings is a nop.)
Note that an explicit array constructor passed to a `varargs` parameter is not wrapped in another implicit array construction:
```
proc takeV[T](a: varargs[T]) = discard
takeV([123, 2, 1]) # takeV's T is "int", not "array of int"
```
`varargs[typed]` is treated specially: It matches a variable list of arguments of arbitrary type but *always* constructs an implicit array. This is required so that the builtin `echo` proc does what is expected:
```
proc echo*(x: varargs[typed, `$`]) {...}
echo @[1, 2, 3]
# prints "@[1, 2, 3]" and not "123"
```
### Unchecked arrays
The `UncheckedArray[T]` type is a special kind of `array` where its bounds are not checked. This is often useful to implement customized flexibly sized arrays. Additionally, an unchecked array is translated into a C array of undetermined size:
```
type
MySeq = object
len, cap: int
data: UncheckedArray[int]
```
Produces roughly this C code:
```
typedef struct {
NI len;
NI cap;
NI data[];
} MySeq;
```
The base type of the unchecked array may not contain any GC'ed memory but this is currently not checked.
**Future directions**: GC'ed memory should be allowed in unchecked arrays and there should be an explicit annotation of how the GC is to determine the runtime size of the array.
### Tuples and object types
A variable of a tuple or object type is a heterogeneous storage container. A tuple or object defines various named *fields* of a type. A tuple also defines a lexicographic *order* of the fields. Tuples are meant to be heterogeneous storage types with few abstractions. The `()` syntax can be used to construct tuples. The order of the fields in the constructor must match the order of the tuple's definition. Different tuple-types are *equivalent* if they specify the same fields of the same type in the same order. The *names* of the fields also have to be identical.
The assignment operator for tuples copies each component. The default assignment operator for objects copies each component. Overloading of the assignment operator is described [here](manual_experimental#type-bound-operations).
```
type
Person = tuple[name: string, age: int] # type representing a person:
# a person consists of a name
# and an age
var
person: Person
person = (name: "Peter", age: 30)
echo person.name
# the same, but less readable:
person = ("Peter", 30)
echo person[0]
```
A tuple with one unnamed field can be constructed with the parentheses and a trailing comma:
```
proc echoUnaryTuple(a: (int,)) =
echo a[0]
echoUnaryTuple (1,)
```
In fact, a trailing comma is allowed for every tuple construction.
The implementation aligns the fields for the best access performance. The alignment is compatible with the way the C compiler does it.
For consistency with `object` declarations, tuples in a `type` section can also be defined with indentation instead of `[]`:
```
type
Person = tuple # type representing a person
name: string # a person consists of a name
age: Natural # and an age
```
Objects provide many features that tuples do not. Object provide inheritance and the ability to hide fields from other modules. Objects with inheritance enabled have information about their type at runtime so that the `of` operator can be used to determine the object's type. The `of` operator is similar to the `instanceof` operator in Java.
```
type
Person = object of RootObj
name*: string # the * means that `name` is accessible from other modules
age: int # no * means that the field is hidden
Student = ref object of Person # a student is a person
id: int # with an id field
var
student: Student
person: Person
assert(student of Student) # is true
assert(student of Person) # also true
```
Object fields that should be visible from outside the defining module, have to be marked by `*`. In contrast to tuples, different object types are never *equivalent*, they are nominal types whereas tuples are structural. Objects that have no ancestor are implicitly `final` and thus have no hidden type information. One can use the `inheritable` pragma to introduce new object roots apart from `system.RootObj`.
```
type
Person = object # example of a final object
name*: string
age: int
Student = ref object of Person # Error: inheritance only works with non-final objects
id: int
```
### Object construction
Objects can also be created with an object construction expression that has the syntax `T(fieldA: valueA, fieldB: valueB, ...)` where `T` is an `object` type or a `ref object` type:
```
var student = Student(name: "Anton", age: 5, id: 3)
```
Note that, unlike tuples, objects require the field names along with their values. For a `ref object` type `system.new` is invoked implicitly.
### Object variants
Often an object hierarchy is an overkill in certain situations where simple variant types are needed. Object variants are tagged unions discriminated via an enumerated type used for runtime type flexibility, mirroring the concepts of *sum types* and *algebraic data types (ADTs)* as found in other languages.
An example:
```
# This is an example how an abstract syntax tree could be modelled in Nim
type
NodeKind = enum # the different node types
nkInt, # a leaf with an integer value
nkFloat, # a leaf with a float value
nkString, # a leaf with a string value
nkAdd, # an addition
nkSub, # a subtraction
nkIf # an if statement
Node = ref NodeObj
NodeObj = object
case kind: NodeKind # the ``kind`` field is the discriminator
of nkInt: intVal: int
of nkFloat: floatVal: float
of nkString: strVal: string
of nkAdd, nkSub:
leftOp, rightOp: Node
of nkIf:
condition, thenPart, elsePart: Node
# create a new case object:
var n = Node(kind: nkIf, condition: nil)
# accessing n.thenPart is valid because the ``nkIf`` branch is active:
n.thenPart = Node(kind: nkFloat, floatVal: 2.0)
# the following statement raises an `FieldDefect` exception, because
# n.kind's value does not fit and the ``nkString`` branch is not active:
n.strVal = ""
# invalid: would change the active object branch:
n.kind = nkInt
var x = Node(kind: nkAdd, leftOp: Node(kind: nkInt, intVal: 4),
rightOp: Node(kind: nkInt, intVal: 2))
# valid: does not change the active object branch:
x.kind = nkSub
```
As can be seen from the example, an advantage to an object hierarchy is that no casting between different object types is needed. Yet, access to invalid object fields raises an exception.
The syntax of `case` in an object declaration follows closely the syntax of the `case` statement: The branches in a `case` section may be indented too.
In the example, the `kind` field is called the discriminator: For safety its address cannot be taken and assignments to it are restricted: The new value must not lead to a change of the active object branch. Also, when the fields of a particular branch are specified during object construction, the corresponding discriminator value must be specified as a constant expression.
Instead of changing the active object branch, replace the old object in memory with a new one completely:
```
var x = Node(kind: nkAdd, leftOp: Node(kind: nkInt, intVal: 4),
rightOp: Node(kind: nkInt, intVal: 2))
# change the node's contents:
x[] = NodeObj(kind: nkString, strVal: "abc")
```
Starting with version 0.20 `system.reset` cannot be used anymore to support object branch changes as this never was completely memory safe.
As a special rule, the discriminator kind can also be bounded using a `case` statement. If possible values of the discriminator variable in a `case` statement branch are a subset of discriminator values for the selected object branch, the initialization is considered valid. This analysis only works for immutable discriminators of an ordinal type and disregards `elif` branches. For discriminator values with a `range` type, the compiler checks if the entire range of possible values for the discriminator value is valid for the chosen object branch.
A small example:
```
let unknownKind = nkSub
# invalid: unsafe initialization because the kind field is not statically known:
var y = Node(kind: unknownKind, strVal: "y")
var z = Node()
case unknownKind
of nkAdd, nkSub:
# valid: possible values of this branch are a subset of nkAdd/nkSub object branch:
z = Node(kind: unknownKind, leftOp: Node(), rightOp: Node())
else:
echo "ignoring: ", unknownKind
# also valid, since unknownKindBounded can only contain the values nkAdd or nkSub
let unknownKindBounded = range[nkAdd..nkSub](unknownKind)
z = Node(kind: unknownKindBounded, leftOp: Node(), rightOp: Node())
```
### Set type
The set type models the mathematical notion of a set. The set's basetype can only be an ordinal type of a certain size, namely:* `int8`-`int16`
* `uint8`/`byte`-`uint16`
* `char`
* `enum`
or equivalent. For signed integers the set's base type is defined to be in the range `0 .. MaxSetElements-1` where `MaxSetElements` is currently always 2^16.
The reason is that sets are implemented as high performance bit vectors. Attempting to declare a set with a larger type will result in an error:
```
var s: set[int64] # Error: set is too large
```
Sets can be constructed via the set constructor: `{}` is the empty set. The empty set is type compatible with any concrete set type. The constructor can also be used to include elements (and ranges of elements):
```
type
CharSet = set[char]
var
x: CharSet
x = {'a'..'z', '0'..'9'} # This constructs a set that contains the
# letters from 'a' to 'z' and the digits
# from '0' to '9'
```
These operations are supported by sets:
| operation | meaning |
| --- | --- |
| `A + B` | union of two sets |
| `A * B` | intersection of two sets |
| `A - B` | difference of two sets (A without B's elements) |
| `A == B` | set equality |
| `A <= B` | subset relation (A is subset of B or equal to B) |
| `A < B` | strict subset relation (A is a proper subset of B) |
| `e in A` | set membership (A contains element e) |
| `e notin A` | A does not contain element e |
| `contains(A, e)` | A contains element e |
| `card(A)` | the cardinality of A (number of elements in A) |
| `incl(A, elem)` | same as `A = A + {elem}` |
| `excl(A, elem)` | same as `A = A - {elem}` |
#### Bit fields
Sets are often used to define a type for the *flags* of a procedure. This is a cleaner (and type safe) solution than defining integer constants that have to be `or`'ed together.
Enum, sets and casting can be used together as in:
```
type
MyFlag* {.size: sizeof(cint).} = enum
A
B
C
D
MyFlags = set[MyFlag]
proc toNum(f: MyFlags): int = cast[cint](f)
proc toFlags(v: int): MyFlags = cast[MyFlags](v)
assert toNum({}) == 0
assert toNum({A}) == 1
assert toNum({D}) == 8
assert toNum({A, C}) == 5
assert toFlags(0) == {}
assert toFlags(7) == {A, B, C}
```
Note how the set turns enum values into powers of 2.
If using enums and sets with C, use distinct cint.
For interoperability with C see also the [bitsize pragma](manual#implementation-specific-pragmas-bitsize-pragma).
### Reference and pointer types
References (similar to pointers in other programming languages) are a way to introduce many-to-one relationships. This means different references can point to and modify the same location in memory (also called aliasing).
Nim distinguishes between traced and untraced references. Untraced references are also called *pointers*. Traced references point to objects of a garbage-collected heap, untraced references point to manually allocated objects or objects somewhere else in memory. Thus untraced references are *unsafe*. However, for certain low-level operations (accessing the hardware) untraced references are unavoidable.
Traced references are declared with the **ref** keyword, untraced references are declared with the **ptr** keyword. In general, a `ptr T` is implicitly convertible to the `pointer` type.
An empty subscript `[]` notation can be used to de-refer a reference, the `addr` procedure returns the address of an item. An address is always an untraced reference. Thus the usage of `addr` is an *unsafe* feature.
The `.` (access a tuple/object field operator) and `[]` (array/string/sequence index operator) operators perform implicit dereferencing operations for reference types:
```
type
Node = ref NodeObj
NodeObj = object
le, ri: Node
data: int
var
n: Node
new(n)
n.data = 9
# no need to write n[].data; in fact n[].data is highly discouraged!
```
Automatic dereferencing can be performed for the first argument of a routine call, but this is an experimental feature and is described [here](manual_experimental#type-bound-operations).
In order to simplify structural type checking, recursive tuples are not valid:
```
# invalid recursion
type MyTuple = tuple[a: ref MyTuple]
```
Likewise `T = ref T` is an invalid type.
As a syntactical extension `object` types can be anonymous if declared in a type section via the `ref object` or `ptr object` notations. This feature is useful if an object should only gain reference semantics:
```
type
Node = ref object
le, ri: Node
data: int
```
To allocate a new traced object, the built-in procedure `new` has to be used. To deal with untraced memory, the procedures `alloc`, `dealloc` and `realloc` can be used. The documentation of the system module contains further information.
Nil ---
If a reference points to *nothing*, it has the value `nil`. `nil` is the default value for all `ref` and `ptr` types. The `nil` value can also be used like any other literal value. For example, it can be used in an assignment like `myRef = nil`.
Dereferencing `nil` is an unrecoverable fatal runtime error (and not a panic).
A successful dereferencing operation `p[]` implies that `p` is not nil. This can be exploited by the implementation to optimize code like:
```
p[].field = 3
if p != nil:
# if p were nil, ``p[]`` would have caused a crash already,
# so we know ``p`` is always not nil here.
action()
```
Into:
```
p[].field = 3
action()
```
*Note*: This is not comparable to C's "undefined behavior" for dereferencing NULL pointers.
### Mixing GC'ed memory with `ptr`
Special care has to be taken if an untraced object contains traced objects like traced references, strings, or sequences: in order to free everything properly, the built-in procedure `reset` has to be called before freeing the untraced memory manually:
```
type
Data = tuple[x, y: int, s: string]
# allocate memory for Data on the heap:
var d = cast[ptr Data](alloc0(sizeof(Data)))
# create a new string on the garbage collected heap:
d.s = "abc"
# tell the GC that the string is not needed anymore:
reset(d.s)
# free the memory:
dealloc(d)
```
Without the `reset` call the memory allocated for the `d.s` string would never be freed. The example also demonstrates two important features for low-level programming: the `sizeof` proc returns the size of a type or value in bytes. The `cast` operator can circumvent the type system: the compiler is forced to treat the result of the `alloc0` call (which returns an untyped pointer) as if it would have the type `ptr Data`. Casting should only be done if it is unavoidable: it breaks type safety and bugs can lead to mysterious crashes.
**Note**: The example only works because the memory is initialized to zero (`alloc0` instead of `alloc` does this): `d.s` is thus initialized to binary zero which the string assignment can handle. One needs to know low-level details like this when mixing garbage-collected data with unmanaged memory.
### Procedural type
A procedural type is internally a pointer to a procedure. `nil` is an allowed value for variables of a procedural type. Nim uses procedural types to achieve functional programming techniques.
Examples:
```
proc printItem(x: int) = ...
proc forEach(c: proc (x: int) {.cdecl.}) =
...
forEach(printItem) # this will NOT compile because calling conventions differ
```
```
type
OnMouseMove = proc (x, y: int) {.closure.}
proc onMouseMove(mouseX, mouseY: int) =
# has default calling convention
echo "x: ", mouseX, " y: ", mouseY
proc setOnMouseMove(mouseMoveEvent: OnMouseMove) = discard
# ok, 'onMouseMove' has the default calling convention, which is compatible
# to 'closure':
setOnMouseMove(onMouseMove)
```
A subtle issue with procedural types is that the calling convention of the procedure influences the type compatibility: procedural types are only compatible if they have the same calling convention. As a special extension, a procedure of the calling convention `nimcall` can be passed to a parameter that expects a proc of the calling convention `closure`.
Nim supports these calling conventions:
nimcall is the default convention used for a Nim **proc**. It is the same as `fastcall`, but only for C compilers that support `fastcall`. closure is the default calling convention for a **procedural type** that lacks any pragma annotations. It indicates that the procedure has a hidden implicit parameter (an *environment*). Proc vars that have the calling convention `closure` take up two machine words: One for the proc pointer and another one for the pointer to implicitly passed environment. stdcall This is the stdcall convention as specified by Microsoft. The generated C procedure is declared with the `__stdcall` keyword. cdecl The cdecl convention means that a procedure shall use the same convention as the C compiler. Under Windows the generated C procedure is declared with the `__cdecl` keyword. safecall This is the safecall convention as specified by Microsoft. The generated C procedure is declared with the `__safecall` keyword. The word *safe* refers to the fact that all hardware registers shall be pushed to the hardware stack. inline The inline convention means the the caller should not call the procedure, but inline its code directly. Note that Nim does not inline, but leaves this to the C compiler; it generates `__inline` procedures. This is only a hint for the compiler: it may completely ignore it and it may inline procedures that are not marked as `inline`. fastcall Fastcall means different things to different C compilers. One gets whatever the C `__fastcall` means. thiscall This is thiscall calling convention as specified by Microsoft, used on C++ class member functions on the x86 architecture syscall The syscall convention is the same as `__syscall` in C. It is used for interrupts. noconv The generated C code will not have any explicit calling convention and thus use the C compiler's default calling convention. This is needed because Nim's default calling convention for procedures is `fastcall` to improve speed. Most calling conventions exist only for the Windows 32-bit platform.
The default calling convention is `nimcall`, unless it is an inner proc (a proc inside of a proc). For an inner proc an analysis is performed whether it accesses its environment. If it does so, it has the calling convention `closure`, otherwise it has the calling convention `nimcall`.
### Distinct type
A `distinct` type is a new type derived from a base type that is incompatible with its base type. In particular, it is an essential property of a distinct type that it **does not** imply a subtype relation between it and its base type. Explicit type conversions from a distinct type to its base type and vice versa are allowed. See also `distinctBase` to get the reverse operation.
A distinct type is an ordinal type if its base type is an ordinal type.
#### Modeling currencies
A distinct type can be used to model different physical units with a numerical base type, for example. The following example models currencies.
Different currencies should not be mixed in monetary calculations. Distinct types are a perfect tool to model different currencies:
```
type
Dollar = distinct int
Euro = distinct int
var
d: Dollar
e: Euro
echo d + 12
# Error: cannot add a number with no unit and a ``Dollar``
```
Unfortunately, `d + 12.Dollar` is not allowed either, because `+` is defined for `int` (among others), not for `Dollar`. So a `+` for dollars needs to be defined:
```
proc `+` (x, y: Dollar): Dollar =
result = Dollar(int(x) + int(y))
```
It does not make sense to multiply a dollar with a dollar, but with a number without unit; and the same holds for division:
```
proc `*` (x: Dollar, y: int): Dollar =
result = Dollar(int(x) * y)
proc `*` (x: int, y: Dollar): Dollar =
result = Dollar(x * int(y))
proc `div` ...
```
This quickly gets tedious. The implementations are trivial and the compiler should not generate all this code only to optimize it away later - after all `+` for dollars should produce the same binary code as `+` for ints. The pragma borrow has been designed to solve this problem; in principle, it generates the above trivial implementations:
```
proc `*` (x: Dollar, y: int): Dollar {.borrow.}
proc `*` (x: int, y: Dollar): Dollar {.borrow.}
proc `div` (x: Dollar, y: int): Dollar {.borrow.}
```
The `borrow` pragma makes the compiler use the same implementation as the proc that deals with the distinct type's base type, so no code is generated.
But it seems all this boilerplate code needs to be repeated for the `Euro` currency. This can be solved with [templates](#templates).
```
template additive(typ: typedesc) =
proc `+` *(x, y: typ): typ {.borrow.}
proc `-` *(x, y: typ): typ {.borrow.}
# unary operators:
proc `+` *(x: typ): typ {.borrow.}
proc `-` *(x: typ): typ {.borrow.}
template multiplicative(typ, base: typedesc) =
proc `*` *(x: typ, y: base): typ {.borrow.}
proc `*` *(x: base, y: typ): typ {.borrow.}
proc `div` *(x: typ, y: base): typ {.borrow.}
proc `mod` *(x: typ, y: base): typ {.borrow.}
template comparable(typ: typedesc) =
proc `<` * (x, y: typ): bool {.borrow.}
proc `<=` * (x, y: typ): bool {.borrow.}
proc `==` * (x, y: typ): bool {.borrow.}
template defineCurrency(typ, base: untyped) =
type
typ* = distinct base
additive(typ)
multiplicative(typ, base)
comparable(typ)
defineCurrency(Dollar, int)
defineCurrency(Euro, int)
```
The borrow pragma can also be used to annotate the distinct type to allow certain builtin operations to be lifted:
```
type
Foo = object
a, b: int
s: string
Bar {.borrow: `.`.} = distinct Foo
var bb: ref Bar
new bb
# field access now valid
bb.a = 90
bb.s = "abc"
```
Currently, only the dot accessor can be borrowed in this way.
#### Avoiding SQL injection attacks
An SQL statement that is passed from Nim to an SQL database might be modeled as a string. However, using string templates and filling in the values is vulnerable to the famous SQL injection attack:
```
import strutils
proc query(db: DbHandle, statement: string) = ...
var
username: string
db.query("SELECT FROM users WHERE name = '$1'" % username)
# Horrible security hole, but the compiler does not mind!
```
This can be avoided by distinguishing strings that contain SQL from strings that don't. Distinct types provide a means to introduce a new string type `SQL` that is incompatible with `string`:
```
type
SQL = distinct string
proc query(db: DbHandle, statement: SQL) = ...
var
username: string
db.query("SELECT FROM users WHERE name = '$1'" % username)
# Static error: `query` expects an SQL string!
```
It is an essential property of abstract types that they **do not** imply a subtype relation between the abstract type and its base type. Explicit type conversions from `string` to `SQL` are allowed:
```
import strutils, sequtils
proc properQuote(s: string): SQL =
# quotes a string properly for an SQL statement
return SQL(s)
proc `%` (frmt: SQL, values: openarray[string]): SQL =
# quote each argument:
let v = values.mapIt(SQL, properQuote(it))
# we need a temporary type for the type conversion :-(
type StrSeq = seq[string]
# call strutils.`%`:
result = SQL(string(frmt) % StrSeq(v))
db.query("SELECT FROM users WHERE name = '$1'".SQL % [username])
```
Now we have compile-time checking against SQL injection attacks. Since `"".SQL` is transformed to `SQL("")` no new syntax is needed for nice looking `SQL` string literals. The hypothetical `SQL` type actually exists in the library as the [SqlQuery type](db_common#SqlQuery) of modules like <db_sqlite>.
### Auto type
The `auto` type can only be used for return types and parameters. For return types it causes the compiler to infer the type from the routine body:
```
proc returnsInt(): auto = 1984
```
For parameters it currently creates implicitly generic routines:
```
proc foo(a, b: auto) = discard
```
Is the same as:
```
proc foo[T1, T2](a: T1, b: T2) = discard
```
However, later versions of the language might change this to mean "infer the parameters' types from the body". Then the above `foo` would be rejected as the parameters' types can not be inferred from an empty `discard` statement.
Type relations
--------------
The following section defines several relations on types that are needed to describe the type checking done by the compiler.
### Type equality
Nim uses structural type equivalence for most types. Only for objects, enumerations and distinct types name equivalence is used. The following algorithm, *in pseudo-code*, determines type equality:
```
proc typeEqualsAux(a, b: PType,
s: var HashSet[(PType, PType)]): bool =
if (a,b) in s: return true
incl(s, (a,b))
if a.kind == b.kind:
case a.kind
of int, intXX, float, floatXX, char, string, cstring, pointer,
bool, nil, void:
# leaf type: kinds identical; nothing more to check
result = true
of ref, ptr, var, set, seq, openarray:
result = typeEqualsAux(a.baseType, b.baseType, s)
of range:
result = typeEqualsAux(a.baseType, b.baseType, s) and
(a.rangeA == b.rangeA) and (a.rangeB == b.rangeB)
of array:
result = typeEqualsAux(a.baseType, b.baseType, s) and
typeEqualsAux(a.indexType, b.indexType, s)
of tuple:
if a.tupleLen == b.tupleLen:
for i in 0..a.tupleLen-1:
if not typeEqualsAux(a[i], b[i], s): return false
result = true
of object, enum, distinct:
result = a == b
of proc:
result = typeEqualsAux(a.parameterTuple, b.parameterTuple, s) and
typeEqualsAux(a.resultType, b.resultType, s) and
a.callingConvention == b.callingConvention
proc typeEquals(a, b: PType): bool =
var s: HashSet[(PType, PType)] = {}
result = typeEqualsAux(a, b, s)
```
Since types are graphs which can have cycles, the above algorithm needs an auxiliary set `s` to detect this case.
### Type equality modulo type distinction
The following algorithm (in pseudo-code) determines whether two types are equal with no respect to `distinct` types. For brevity the cycle check with an auxiliary set `s` is omitted:
```
proc typeEqualsOrDistinct(a, b: PType): bool =
if a.kind == b.kind:
case a.kind
of int, intXX, float, floatXX, char, string, cstring, pointer,
bool, nil, void:
# leaf type: kinds identical; nothing more to check
result = true
of ref, ptr, var, set, seq, openarray:
result = typeEqualsOrDistinct(a.baseType, b.baseType)
of range:
result = typeEqualsOrDistinct(a.baseType, b.baseType) and
(a.rangeA == b.rangeA) and (a.rangeB == b.rangeB)
of array:
result = typeEqualsOrDistinct(a.baseType, b.baseType) and
typeEqualsOrDistinct(a.indexType, b.indexType)
of tuple:
if a.tupleLen == b.tupleLen:
for i in 0..a.tupleLen-1:
if not typeEqualsOrDistinct(a[i], b[i]): return false
result = true
of distinct:
result = typeEqualsOrDistinct(a.baseType, b.baseType)
of object, enum:
result = a == b
of proc:
result = typeEqualsOrDistinct(a.parameterTuple, b.parameterTuple) and
typeEqualsOrDistinct(a.resultType, b.resultType) and
a.callingConvention == b.callingConvention
elif a.kind == distinct:
result = typeEqualsOrDistinct(a.baseType, b)
elif b.kind == distinct:
result = typeEqualsOrDistinct(a, b.baseType)
```
### Subtype relation
If object `a` inherits from `b`, `a` is a subtype of `b`. This subtype relation is extended to the types `var`, `ref`, `ptr`:
```
proc isSubtype(a, b: PType): bool =
if a.kind == b.kind:
case a.kind
of object:
var aa = a.baseType
while aa != nil and aa != b: aa = aa.baseType
result = aa == b
of var, ref, ptr:
result = isSubtype(a.baseType, b.baseType)
```
### Convertible relation
A type `a` is **implicitly** convertible to type `b` iff the following algorithm returns true:
```
proc isImplicitlyConvertible(a, b: PType): bool =
if isSubtype(a, b) or isCovariant(a, b):
return true
if isIntLiteral(a):
return b in {int8, int16, int32, int64, int, uint, uint8, uint16,
uint32, uint64, float32, float64}
case a.kind
of int: result = b in {int32, int64}
of int8: result = b in {int16, int32, int64, int}
of int16: result = b in {int32, int64, int}
of int32: result = b in {int64, int}
of uint: result = b in {uint32, uint64}
of uint8: result = b in {uint16, uint32, uint64}
of uint16: result = b in {uint32, uint64}
of uint32: result = b in {uint64}
of float32: result = b in {float64}
of float64: result = b in {float32}
of seq:
result = b == openArray and typeEquals(a.baseType, b.baseType)
of array:
result = b == openArray and typeEquals(a.baseType, b.baseType)
if a.baseType == char and a.indexType.rangeA == 0:
result = b == cstring
of cstring, ptr:
result = b == pointer
of string:
result = b == cstring
```
Implicit conversions are also performed for Nim's `range` type constructor.
Let `a0`, `b0` of type `T`.
Let `A = range[a0..b0]` be the argument's type, `F` the formal parameter's type. Then an implicit conversion from `A` to `F` exists if `a0 >= low(F) and b0 <= high(F)` and both `T` and `F` are signed integers or if both are unsigned integers.
A type `a` is **explicitly** convertible to type `b` iff the following algorithm returns true:
```
proc isIntegralType(t: PType): bool =
result = isOrdinal(t) or t.kind in {float, float32, float64}
proc isExplicitlyConvertible(a, b: PType): bool =
result = false
if isImplicitlyConvertible(a, b): return true
if typeEqualsOrDistinct(a, b): return true
if isIntegralType(a) and isIntegralType(b): return true
if isSubtype(a, b) or isSubtype(b, a): return true
```
The convertible relation can be relaxed by a user-defined type converter.
```
converter toInt(x: char): int = result = ord(x)
var
x: int
chr: char = 'a'
# implicit conversion magic happens here
x = chr
echo x # => 97
# one can use the explicit form too
x = chr.toInt
echo x # => 97
```
The type conversion `T(a)` is an L-value if `a` is an L-value and `typeEqualsOrDistinct(T, typeof(a))` holds.
### Assignment compatibility
An expression `b` can be assigned to an expression `a` iff `a` is an `l-value` and `isImplicitlyConvertible(b.typ, a.typ)` holds.
Overloading resolution
----------------------
In a call `p(args)` the routine `p` that matches best is selected. If multiple routines match equally well, the ambiguity is reported during semantic analysis.
Every arg in args needs to match. There are multiple different categories how an argument can match. Let `f` be the formal parameter's type and `a` the type of the argument.
1. Exact match: `a` and `f` are of the same type.
2. Literal match: `a` is an integer literal of value `v` and `f` is a signed or unsigned integer type and `v` is in `f`'s range. Or: `a` is a floating-point literal of value `v` and `f` is a floating-point type and `v` is in `f`'s range.
3. Generic match: `f` is a generic type and `a` matches, for instance `a` is `int` and `f` is a generic (constrained) parameter type (like in `[T]` or `[T: int|char]`.
4. Subrange or subtype match: `a` is a `range[T]` and `T` matches `f` exactly. Or: `a` is a subtype of `f`.
5. Integral conversion match: `a` is convertible to `f` and `f` and `a` is some integer or floating-point type.
6. Conversion match: `a` is convertible to `f`, possibly via a user defined `converter`.
These matching categories have a priority: An exact match is better than a literal match and that is better than a generic match etc. In the following `count(p, m)` counts the number of matches of the matching category `m` for the routine `p`.
A routine `p` matches better than a routine `q` if the following algorithm returns true:
```
for each matching category m in ["exact match", "literal match",
"generic match", "subtype match",
"integral match", "conversion match"]:
if count(p, m) > count(q, m): return true
elif count(p, m) == count(q, m):
discard "continue with next category m"
else:
return false
return "ambiguous"
```
Some examples:
```
proc takesInt(x: int) = echo "int"
proc takesInt[T](x: T) = echo "T"
proc takesInt(x: int16) = echo "int16"
takesInt(4) # "int"
var x: int32
takesInt(x) # "T"
var y: int16
takesInt(y) # "int16"
var z: range[0..4] = 0
takesInt(z) # "T"
```
If this algorithm returns "ambiguous" further disambiguation is performed: If the argument `a` matches both the parameter type `f` of `p` and `g` of `q` via a subtyping relation, the inheritance depth is taken into account:
```
type
A = object of RootObj
B = object of A
C = object of B
proc p(obj: A) =
echo "A"
proc p(obj: B) =
echo "B"
var c = C()
# not ambiguous, calls 'B', not 'A' since B is a subtype of A
# but not vice versa:
p(c)
proc pp(obj: A, obj2: B) = echo "A B"
proc pp(obj: B, obj2: A) = echo "B A"
# but this is ambiguous:
pp(c, c)
```
Likewise for generic matches the most specialized generic type (that still matches) is preferred:
```
proc gen[T](x: ref ref T) = echo "ref ref T"
proc gen[T](x: ref T) = echo "ref T"
proc gen[T](x: T) = echo "T"
var ri: ref int
gen(ri) # "ref T"
```
### Overloading based on 'var T'
If the formal parameter `f` is of type `var T` in addition to the ordinary type checking, the argument is checked to be an l-value. `var T` matches better than just `T` then.
```
proc sayHi(x: int): string =
# matches a non-var int
result = $x
proc sayHi(x: var int): string =
# matches a var int
result = $(x + 10)
proc sayHello(x: int) =
var m = x # a mutable version of x
echo sayHi(x) # matches the non-var version of sayHi
echo sayHi(m) # matches the var version of sayHi
sayHello(3) # 3
# 13
```
### Lazy type resolution for untyped
**Note**: An unresolved expression is an expression for which no symbol lookups and no type checking have been performed.
Since templates and macros that are not declared as `immediate` participate in overloading resolution, it's essential to have a way to pass unresolved expressions to a template or macro. This is what the meta-type `untyped` accomplishes:
```
template rem(x: untyped) = discard
rem unresolvedExpression(undeclaredIdentifier)
```
A parameter of type `untyped` always matches any argument (as long as there is any argument passed to it).
But one has to watch out because other overloads might trigger the argument's resolution:
```
template rem(x: untyped) = discard
proc rem[T](x: T) = discard
# undeclared identifier: 'unresolvedExpression'
rem unresolvedExpression(undeclaredIdentifier)
```
`untyped` and `varargs[untyped]` are the only metatype that are lazy in this sense, the other metatypes `typed` and `typedesc` are not lazy.
### Varargs matching
See [Varargs](#types-varargs).
Statements and expressions
--------------------------
Nim uses the common statement/expression paradigm: Statements do not produce a value in contrast to expressions. However, some expressions are statements.
Statements are separated into simple statements and complex statements. Simple statements are statements that cannot contain other statements like assignments, calls, or the `return` statement; complex statements can contain other statements. To avoid the dangling else problem, complex statements always have to be indented. The details can be found in the grammar.
### Statement list expression
Statements can also occur in an expression context that looks like `(stmt1; stmt2; ...; ex)`. This is called a statement list expression or `(;)`. The type of `(stmt1; stmt2; ...; ex)` is the type of `ex`. All the other statements must be of type `void`. (One can use `discard` to produce a `void` type.) `(;)` does not introduce a new scope.
### Discard statement
Example:
```
proc p(x, y: int): int =
result = x + y
discard p(3, 4) # discard the return value of `p`
```
The `discard` statement evaluates its expression for side-effects and throws the expression's resulting value away, and should only be used when ignoring this value is known not to cause problems.
Ignoring the return value of a procedure without using a discard statement is a static error.
The return value can be ignored implicitly if the called proc/iterator has been declared with the discardable pragma:
```
proc p(x, y: int): int {.discardable.} =
result = x + y
p(3, 4) # now valid
```
however the discardable pragma does not work on templates as templates substitute the AST in place. For example:
```
{.push discardable .}
template example(): string = "https://nim-lang.org"
{.pop.}
example()
```
This template will resolve into "<https://nim-lang.org>" which is a string literal and since {.discardable.} doesn't apply to literals, the compiler will error.
An empty `discard` statement is often used as a null statement:
```
proc classify(s: string) =
case s[0]
of SymChars, '_': echo "an identifier"
of '0'..'9': echo "a number"
else: discard
```
### Void context
In a list of statements every expression except the last one needs to have the type `void`. In addition to this rule an assignment to the builtin `result` symbol also triggers a mandatory `void` context for the subsequent expressions:
```
proc invalid*(): string =
result = "foo"
"invalid" # Error: value of type 'string' has to be discarded
```
```
proc valid*(): string =
let x = 317
"valid"
```
### Var statement
Var statements declare new local and global variables and initialize them. A comma-separated list of variables can be used to specify variables of the same type:
```
var
a: int = 0
x, y, z: int
```
If an initializer is given the type can be omitted: the variable is then of the same type as the initializing expression. Variables are always initialized with a default value if there is no initializing expression. The default value depends on the type and is always a zero in binary.
| Type | default value |
| --- | --- |
| any integer type | 0 |
| any float | 0.0 |
| char | '\0' |
| bool | false |
| ref or pointer type | nil |
| procedural type | nil |
| sequence | `@[]` |
| string | `""` |
| tuple[x: A, y: B, ...] | (default(A), default(B), ...) (analogous for objects) |
| array[0..., T] | [default(T), ...] |
| range[T] | default(T); this may be out of the valid range |
| T = enum | cast[T](0); this may be an invalid value |
The implicit initialization can be avoided for optimization reasons with the noinit pragma:
```
var
a {.noInit.}: array[0..1023, char]
```
If a proc is annotated with the `noinit` pragma this refers to its implicit `result` variable:
```
proc returnUndefinedValue: int {.noinit.} = discard
```
The implicit initialization can be also prevented by the requiresInit type pragma. The compiler requires an explicit initialization for the object and all of its fields. However, it does a control flow analysis to prove the variable has been initialized and does not rely on syntactic properties:
```
type
MyObject = object {.requiresInit.}
proc p() =
# the following is valid:
var x: MyObject
if someCondition():
x = a()
else:
x = a()
# use x
```
### Let statement
A `let` statement declares new local and global single assignment variables and binds a value to them. The syntax is the same as that of the `var` statement, except that the keyword `var` is replaced by the keyword `let`. Let variables are not l-values and can thus not be passed to `var` parameters nor can their address be taken. They cannot be assigned new values.
For let variables, the same pragmas are available as for ordinary variables.
As `let` statements are immutable after creation they need to define a value when they are declared. The only exception to this is if the `{.importc.}` pragma (or any of the other `importX` pragmas) is applied, in this case the value is expected to come from native code, typically a C/C++ `const`.
### Tuple unpacking
In a `var` or `let` statement tuple unpacking can be performed. The special identifier `_` can be used to ignore some parts of the tuple:
```
proc returnsTuple(): (int, int, int) = (4, 2, 3)
let (x, _, z) = returnsTuple()
```
### Const section
A const section declares constants whose values are constant expressions:
```
import strutils
const
roundPi = 3.1415
constEval = contains("abc", 'b') # computed at compile time!
```
Once declared, a constant's symbol can be used as a constant expression.
See [Constants and Constant Expressions](#constants-and-constant-expressions) for details.
### Static statement/expression
A static statement/expression explicitly requires compile-time execution. Even some code that has side effects is permitted in a static block:
```
static:
echo "echo at compile time"
```
There are limitations on what Nim code can be executed at compile time; see [Restrictions on Compile-Time Execution](#restrictions-on-compileminustime-execution) for details. It's a static error if the compiler cannot execute the block at compile time.
### If statement
Example:
```
var name = readLine(stdin)
if name == "Andreas":
echo "What a nice name!"
elif name == "":
echo "Don't you have a name?"
else:
echo "Boring name..."
```
The `if` statement is a simple way to make a branch in the control flow: The expression after the keyword `if` is evaluated, if it is true the corresponding statements after the `:` are executed. Otherwise the expression after the `elif` is evaluated (if there is an `elif` branch), if it is true the corresponding statements after the `:` are executed. This goes on until the last `elif`. If all conditions fail, the `else` part is executed. If there is no `else` part, execution continues with the next statement.
In `if` statements new scopes begin immediately after the `if`/`elif`/`else` keywords and ends after the corresponding *then* block. For visualization purposes the scopes have been enclosed in `{| |}` in the following example:
```
if {| (let m = input =~ re"(\w+)=\w+"; m.isMatch):
echo "key ", m[0], " value ", m[1] |}
elif {| (let m = input =~ re""; m.isMatch):
echo "new m in this scope" |}
else: {|
echo "m not declared here" |}
```
### Case statement
Example:
```
case readline(stdin)
of "delete-everything", "restart-computer":
echo "permission denied"
of "go-for-a-walk": echo "please yourself"
else: echo "unknown command"
# indentation of the branches is also allowed; and so is an optional colon
# after the selecting expression:
case readline(stdin):
of "delete-everything", "restart-computer":
echo "permission denied"
of "go-for-a-walk": echo "please yourself"
else: echo "unknown command"
```
The `case` statement is similar to the if statement, but it represents a multi-branch selection. The expression after the keyword `case` is evaluated and if its value is in a *slicelist* the corresponding statements (after the `of` keyword) are executed. If the value is not in any given *slicelist* the `else` part is executed. If there is no `else` part and not all possible values that `expr` can hold occur in a `slicelist`, a static error occurs. This holds only for expressions of ordinal types. "All possible values" of `expr` are determined by `expr`'s type. To suppress the static error an `else` part with an empty `discard` statement should be used.
For non-ordinal types, it is not possible to list every possible value and so these always require an `else` part.
Because case statements are checked for exhaustiveness during semantic analysis, the value in every `of` branch must be a constant expression. This restriction also allows the compiler to generate more performant code.
As a special semantic extension, an expression in an `of` branch of a case statement may evaluate to a set or array constructor; the set or array is then expanded into a list of its elements:
```
const
SymChars: set[char] = {'a'..'z', 'A'..'Z', '\x80'..'\xFF'}
proc classify(s: string) =
case s[0]
of SymChars, '_': echo "an identifier"
of '0'..'9': echo "a number"
else: echo "other"
# is equivalent to:
proc classify(s: string) =
case s[0]
of 'a'..'z', 'A'..'Z', '\x80'..'\xFF', '_': echo "an identifier"
of '0'..'9': echo "a number"
else: echo "other"
```
The `case` statement doesn't produce an l-value, so the following example won't work:
```
type
Foo = ref object
x: seq[string]
proc get_x(x: Foo): var seq[string] =
# doesn't work
case true
of true:
x.x
else:
x.x
var foo = Foo(x: @[])
foo.get_x().add("asd")
```
This can be fixed by explicitly using `return`:
```
proc get_x(x: Foo): var seq[string] =
case true
of true:
return x.x
else:
return x.x
```
### When statement
Example:
```
when sizeof(int) == 2:
echo "running on a 16 bit system!"
elif sizeof(int) == 4:
echo "running on a 32 bit system!"
elif sizeof(int) == 8:
echo "running on a 64 bit system!"
else:
echo "cannot happen!"
```
The `when` statement is almost identical to the `if` statement with some exceptions:
* Each condition (`expr`) has to be a constant expression (of type `bool`).
* The statements do not open a new scope.
* The statements that belong to the expression that evaluated to true are translated by the compiler, the other statements are not checked for semantics! However, each condition is checked for semantics.
The `when` statement enables conditional compilation techniques. As a special syntactic extension, the `when` construct is also available within `object` definitions.
### When nimvm statement
`nimvm` is a special symbol, that may be used as an expression of `when nimvm` statement to differentiate execution path between compile-time and the executable.
Example:
```
proc someProcThatMayRunInCompileTime(): bool =
when nimvm:
# This branch is taken at compile time.
result = true
else:
# This branch is taken in the executable.
result = false
const ctValue = someProcThatMayRunInCompileTime()
let rtValue = someProcThatMayRunInCompileTime()
assert(ctValue == true)
assert(rtValue == false)
```
`when nimvm` statement must meet the following requirements:
* Its expression must always be `nimvm`. More complex expressions are not allowed.
* It must not contain `elif` branches.
* It must contain `else` branch.
* Code in branches must not affect semantics of the code that follows the `when nimvm` statement. E.g. it must not define symbols that are used in the following code.
### Return statement
Example:
```
return 40+2
```
The `return` statement ends the execution of the current procedure. It is only allowed in procedures. If there is an `expr`, this is syntactic sugar for:
```
result = expr
return result
```
`return` without an expression is a short notation for `return result` if the proc has a return type. The result variable is always the return value of the procedure. It is automatically declared by the compiler. As all variables, `result` is initialized to (binary) zero:
```
proc returnZero(): int =
# implicitly returns 0
```
### Yield statement
Example:
```
yield (1, 2, 3)
```
The `yield` statement is used instead of the `return` statement in iterators. It is only valid in iterators. Execution is returned to the body of the for loop that called the iterator. Yield does not end the iteration process, but the execution is passed back to the iterator if the next iteration starts. See the section about iterators ([Iterators and the for statement](#iterators-and-the-for-statement)) for further information.
### Block statement
Example:
```
var found = false
block myblock:
for i in 0..3:
for j in 0..3:
if a[j][i] == 7:
found = true
break myblock # leave the block, in this case both for-loops
echo found
```
The block statement is a means to group statements to a (named) `block`. Inside the block, the `break` statement is allowed to leave the block immediately. A `break` statement can contain a name of a surrounding block to specify which block is to leave.
### Break statement
Example:
```
break
```
The `break` statement is used to leave a block immediately. If `symbol` is given, it is the name of the enclosing block that is to leave. If it is absent, the innermost block is left.
### While statement
Example:
```
echo "Please tell me your password:"
var pw = readLine(stdin)
while pw != "12345":
echo "Wrong password! Next try:"
pw = readLine(stdin)
```
The `while` statement is executed until the `expr` evaluates to false. Endless loops are no error. `while` statements open an `implicit block`, so that they can be left with a `break` statement.
### Continue statement
A `continue` statement leads to the immediate next iteration of the surrounding loop construct. It is only allowed within a loop. A continue statement is syntactic sugar for a nested block:
```
while expr1:
stmt1
continue
stmt2
```
Is equivalent to:
```
while expr1:
block myBlockName:
stmt1
break myBlockName
stmt2
```
### Assembler statement
The direct embedding of assembler code into Nim code is supported by the unsafe `asm` statement. Identifiers in the assembler code that refer to Nim identifiers shall be enclosed in a special character which can be specified in the statement's pragmas. The default special character is `'`'`:
```
{.push stackTrace:off.}
proc addInt(a, b: int): int =
# a in eax, and b in edx
asm """
mov eax, `a`
add eax, `b`
jno theEnd
call `raiseOverflow`
theEnd:
"""
{.pop.}
```
If the GNU assembler is used, quotes and newlines are inserted automatically:
```
proc addInt(a, b: int): int =
asm """
addl %%ecx, %%eax
jno 1
call `raiseOverflow`
1:
:"=a"(`result`)
:"a"(`a`), "c"(`b`)
"""
```
Instead of:
```
proc addInt(a, b: int): int =
asm """
"addl %%ecx, %%eax\n"
"jno 1\n"
"call `raiseOverflow`\n"
"1: \n"
:"=a"(`result`)
:"a"(`a`), "c"(`b`)
"""
```
### Using statement
The using statement provides syntactic convenience in modules where the same parameter names and types are used over and over. Instead of:
```
proc foo(c: Context; n: Node) = ...
proc bar(c: Context; n: Node, counter: int) = ...
proc baz(c: Context; n: Node) = ...
```
One can tell the compiler about the convention that a parameter of name `c` should default to type `Context`, `n` should default to `Node` etc.:
```
using
c: Context
n: Node
counter: int
proc foo(c, n) = ...
proc bar(c, n, counter) = ...
proc baz(c, n) = ...
proc mixedMode(c, n; x, y: int) =
# 'c' is inferred to be of the type 'Context'
# 'n' is inferred to be of the type 'Node'
# But 'x' and 'y' are of type 'int'.
```
The `using` section uses the same indentation based grouping syntax as a `var` or `let` section.
Note that `using` is not applied for `template` since the untyped template parameters default to the type `system.untyped`.
Mixing parameters that should use the `using` declaration with parameters that are explicitly typed is possible and requires a semicolon between them.
### If expression
An `if expression` is almost like an if statement, but it is an expression. This feature is similar to `ternary operators` in other languages. Example:
```
var y = if x > 8: 9 else: 10
```
An if expression always results in a value, so the `else` part is required. `Elif` parts are also allowed.
### When expression
Just like an `if expression`, but corresponding to the when statement.
### Case expression
The `case expression` is again very similar to the case statement:
```
var favoriteFood = case animal
of "dog": "bones"
of "cat": "mice"
elif animal.endsWith"whale": "plankton"
else:
echo "I'm not sure what to serve, but everybody loves ice cream"
"ice cream"
```
As seen in the above example, the case expression can also introduce side effects. When multiple statements are given for a branch, Nim will use the last expression as the result value.
### Block expression
A `block expression` is almost like a block statement, but it is an expression that uses the last expression under the block as the value. It is similar to the statement list expression, but the statement list expression does not open a new block scope.
```
let a = block:
var fib = @[0, 1]
for i in 0..10:
fib.add fib[^1] + fib[^2]
fib
```
### Table constructor
A table constructor is syntactic sugar for an array constructor:
```
{"key1": "value1", "key2", "key3": "value2"}
# is the same as:
[("key1", "value1"), ("key2", "value2"), ("key3", "value2")]
```
The empty table can be written `{:}` (in contrast to the empty set which is `{}`) which is thus another way to write as the empty array constructor `[]`. This slightly unusual way of supporting tables has lots of advantages:
* The order of the (key,value)-pairs is preserved, thus it is easy to support ordered dicts with for example `{key: val}.newOrderedTable`.
* A table literal can be put into a `const` section and the compiler can easily put it into the executable's data section just like it can for arrays and the generated data section requires a minimal amount of memory.
* Every table implementation is treated equally syntactically.
* Apart from the minimal syntactic sugar the language core does not need to know about tables.
### Type conversions
Syntactically a *type conversion* is like a procedure call, but a type name replaces the procedure name. A type conversion is always safe in the sense that a failure to convert a type to another results in an exception (if it cannot be determined statically).
Ordinary procs are often preferred over type conversions in Nim: For instance, `$` is the `toString` operator by convention and `toFloat` and `toInt` can be used to convert from floating-point to integer or vice versa.
Type conversion can also be used to disambiguate overloaded routines:
```
proc p(x: int) = echo "int"
proc p(x: string) = echo "string"
let procVar = (proc(x: string))(p)
procVar("a")
```
Since operations on unsigned numbers wrap around and are unchecked so are type conversion to unsigned integers and between unsigned integers. The rationale for this is mostly better interoperability with the C Programming language when algorithms are ported from C to Nim.
Exception: Values that are converted to an unsigned type at compile time are checked so that code like `byte(-1)` does not compile.
**Note**: Historically the operations were unchecked and the conversions were sometimes checked but starting with the revision 1.0.4 of this document and the language implementation the conversions too are now *always unchecked*.
### Type casts
*Type casts* are a crude mechanism to interpret the bit pattern of an expression as if it would be of another type. Type casts are only needed for low-level programming and are inherently unsafe.
```
cast[int](x)
```
The target type of a cast must be a concrete type, for instance, a target type that is a type class (which is non-concrete) would be invalid:
```
type Foo = int or float
var x = cast[Foo](1) # Error: cannot cast to a non concrete type: 'Foo'
```
Type casts should not be confused with *type conversions,* as mentioned in the prior section. Unlike type conversions, a type cast cannot change the underlying bit pattern of the data being casted (aside from that the size of the target type may differ from the source type). Casting resembles *type punning* in other languages or C++'s `reinterpret_cast` and `bit_cast` features.
### The addr operator
The `addr` operator returns the address of an l-value. If the type of the location is `T`, the `addr` operator result is of the type `ptr T`. An address is always an untraced reference. Taking the address of an object that resides on the stack is **unsafe**, as the pointer may live longer than the object on the stack and can thus reference a non-existing object. One can get the address of variables, but one can't use it on variables declared through `let` statements:
```
let t1 = "Hello"
var
t2 = t1
t3 : pointer = addr(t2)
echo repr(addr(t2))
# --> ref 0x7fff6b71b670 --> 0x10bb81050"Hello"
echo cast[ptr string](t3)[]
# --> Hello
# The following line doesn't compile:
echo repr(addr(t1))
# Error: expression has no address
```
### The unsafeAddr operator
For easier interoperability with other compiled languages such as C, retrieving the address of a `let` variable, a parameter or a `for` loop variable, the `unsafeAddr` operation can be used:
```
let myArray = [1, 2, 3]
foreignProcThatTakesAnAddr(unsafeAddr myArray)
```
Procedures
----------
What most programming languages call methods or functions are called procedures in Nim. A procedure declaration consists of an identifier, zero or more formal parameters, a return value type and a block of code. Formal parameters are declared as a list of identifiers separated by either comma or semicolon. A parameter is given a type by `: typename`. The type applies to all parameters immediately before it, until either the beginning of the parameter list, a semicolon separator, or an already typed parameter, is reached. The semicolon can be used to make separation of types and subsequent identifiers more distinct.
```
# Using only commas
proc foo(a, b: int, c, d: bool): int
# Using semicolon for visual distinction
proc foo(a, b: int; c, d: bool): int
# Will fail: a is untyped since ';' stops type propagation.
proc foo(a; b: int; c, d: bool): int
```
A parameter may be declared with a default value which is used if the caller does not provide a value for the argument.
```
# b is optional with 47 as its default value
proc foo(a: int, b: int = 47): int
```
Parameters can be declared mutable and so allow the proc to modify those arguments, by using the type modifier `var`.
```
# "returning" a value to the caller through the 2nd argument
# Notice that the function uses no actual return value at all (ie void)
proc foo(inp: int, outp: var int) =
outp = inp + 47
```
If the proc declaration has no body, it is a forward declaration. If the proc returns a value, the procedure body can access an implicitly declared variable named result that represents the return value. Procs can be overloaded. The overloading resolution algorithm determines which proc is the best match for the arguments. Example:
```
proc toLower(c: char): char = # toLower for characters
if c in {'A'..'Z'}:
result = chr(ord(c) + (ord('a') - ord('A')))
else:
result = c
proc toLower(s: string): string = # toLower for strings
result = newString(len(s))
for i in 0..len(s) - 1:
result[i] = toLower(s[i]) # calls toLower for characters; no recursion!
```
Calling a procedure can be done in many different ways:
```
proc callme(x, y: int, s: string = "", c: char, b: bool = false) = ...
# call with positional arguments # parameter bindings:
callme(0, 1, "abc", '\t', true) # (x=0, y=1, s="abc", c='\t', b=true)
# call with named and positional arguments:
callme(y=1, x=0, "abd", '\t') # (x=0, y=1, s="abd", c='\t', b=false)
# call with named arguments (order is not relevant):
callme(c='\t', y=1, x=0) # (x=0, y=1, s="", c='\t', b=false)
# call as a command statement: no () needed:
callme 0, 1, "abc", '\t' # (x=0, y=1, s="abc", c='\t', b=false)
```
A procedure may call itself recursively.
Operators are procedures with a special operator symbol as identifier:
```
proc `$` (x: int): string =
# converts an integer to a string; this is a prefix operator.
result = intToStr(x)
```
Operators with one parameter are prefix operators, operators with two parameters are infix operators. (However, the parser distinguishes these from the operator's position within an expression.) There is no way to declare postfix operators: all postfix operators are built-in and handled by the grammar explicitly.
Any operator can be called like an ordinary proc with the '`opr`' notation. (Thus an operator can have more than two parameters):
```
proc `*+` (a, b, c: int): int =
# Multiply and add
result = a * b + c
assert `*+`(3, 4, 6) == `+`(`*`(a, b), c)
```
### Export marker
If a declared symbol is marked with an asterisk it is exported from the current module:
```
proc exportedEcho*(s: string) = echo s
proc `*`*(a: string; b: int): string =
result = newStringOfCap(a.len * b)
for i in 1..b: result.add a
var exportedVar*: int
const exportedConst* = 78
type
ExportedType* = object
exportedField*: int
```
### Method call syntax
For object-oriented programming, the syntax `obj.method(args)` can be used instead of `method(obj, args)`. The parentheses can be omitted if there are no remaining arguments: `obj.len` (instead of `len(obj)`).
This method call syntax is not restricted to objects, it can be used to supply any type of first argument for procedures:
```
echo "abc".len # is the same as echo len "abc"
echo "abc".toUpper()
echo {'a', 'b', 'c'}.card
stdout.writeLine("Hallo") # the same as writeLine(stdout, "Hallo")
```
Another way to look at the method call syntax is that it provides the missing postfix notation.
The method call syntax conflicts with explicit generic instantiations: `p[T](x)` cannot be written as `x.p[T]` because `x.p[T]` is always parsed as `(x.p)[T]`.
See also: [Limitations of the method call syntax](#templates-limitations-of-the-method-call-syntax).
The `[: ]` notation has been designed to mitigate this issue: `x.p[:T]` is rewritten by the parser to `p[T](x)`, `x.p[:T](y)` is rewritten to `p[T](x, y)`. Note that `[: ]` has no AST representation, the rewrite is performed directly in the parsing step.
### Properties
Nim has no need for *get-properties*: Ordinary get-procedures that are called with the *method call syntax* achieve the same. But setting a value is different; for this, a special setter syntax is needed:
```
# Module asocket
type
Socket* = ref object of RootObj
host: int # cannot be accessed from the outside of the module
proc `host=`*(s: var Socket, value: int) {.inline.} =
## setter of hostAddr.
## This accesses the 'host' field and is not a recursive call to
## ``host=`` because the builtin dot access is preferred if it is
## available:
s.host = value
proc host*(s: Socket): int {.inline.} =
## getter of hostAddr
## This accesses the 'host' field and is not a recursive call to
## ``host`` because the builtin dot access is preferred if it is
## available:
s.host
```
```
# module B
import asocket
var s: Socket
new s
s.host = 34 # same as `host=`(s, 34)
```
A proc defined as `f=` (with the trailing `=`) is called a setter. A setter can be called explicitly via the common backticks notation:
```
proc `f=`(x: MyObject; value: string) =
discard
`f=`(myObject, "value")
```
`f=` can be called implicitly in the pattern `x.f = value` if and only if the type of `x` does not have a field named `f` or if `f` is not visible in the current module. These rules ensure that object fields and accessors can have the same name. Within the module `x.f` is then always interpreted as field access and outside the module it is interpreted as an accessor proc call.
### Command invocation syntax
Routines can be invoked without the `()` if the call is syntactically a statement. This command invocation syntax also works for expressions, but then only a single argument may follow. This restriction means `echo f 1, f 2` is parsed as `echo(f(1), f(2))` and not as `echo(f(1, f(2)))`. The method call syntax may be used to provide one more argument in this case:
```
proc optarg(x: int, y: int = 0): int = x + y
proc singlearg(x: int): int = 20*x
echo optarg 1, " ", singlearg 2 # prints "1 40"
let fail = optarg 1, optarg 8 # Wrong. Too many arguments for a command call
let x = optarg(1, optarg 8) # traditional procedure call with 2 arguments
let y = 1.optarg optarg 8 # same thing as above, w/o the parenthesis
assert x == y
```
The command invocation syntax also can't have complex expressions as arguments. For example: ([anonymous procs](#procedures-anonymous-procs)), `if`, `case` or `try`. Function calls with no arguments still need () to distinguish between a call and the function itself as a first-class value.
### Closures
Procedures can appear at the top level in a module as well as inside other scopes, in which case they are called nested procs. A nested proc can access local variables from its enclosing scope and if it does so it becomes a closure. Any captured variables are stored in a hidden additional argument to the closure (its environment) and they are accessed by reference by both the closure and its enclosing scope (i.e. any modifications made to them are visible in both places). The closure environment may be allocated on the heap or on the stack if the compiler determines that this would be safe.
#### Creating closures in loops
Since closures capture local variables by reference it is often not wanted behavior inside loop bodies. See [closureScope](system#closureScope.t,untyped) and [capture](sugar#capture.m,openArray%5Btyped%5D,untyped) for details on how to change this behavior.
### Anonymous Procs
Unnamed procedures can be used as lambda expressions to pass into other procedures:
```
var cities = @["Frankfurt", "Tokyo", "New York", "Kyiv"]
cities.sort(proc (x,y: string): int =
cmp(x.len, y.len))
```
Procs as expressions can appear both as nested procs and inside top-level executable code. The <sugar> module contains the `=>` macro which enables a more succinct syntax for anonymous procedures resembling lambdas as they are in languages like JavaScript, C#, etc.
### Func
The `func` keyword introduces a shortcut for a noSideEffect proc.
```
func binarySearch[T](a: openArray[T]; elem: T): int
```
Is short for:
```
proc binarySearch[T](a: openArray[T]; elem: T): int {.noSideEffect.}
```
### Nonoverloadable builtins
The following built-in procs cannot be overloaded for reasons of implementation simplicity (they require specialized semantic checking):
```
declared, defined, definedInScope, compiles, sizeof,
is, shallowCopy, getAst, astToStr, spawn, procCall
```
Thus they act more like keywords than like ordinary identifiers; unlike a keyword however, a redefinition may shadow the definition in the `system` module. From this list the following should not be written in dot notation `x.f` since `x` cannot be type-checked before it gets passed to `f`:
```
declared, defined, definedInScope, compiles, getAst, astToStr
```
### Var parameters
The type of a parameter may be prefixed with the `var` keyword:
```
proc divmod(a, b: int; res, remainder: var int) =
res = a div b
remainder = a mod b
var
x, y: int
divmod(8, 5, x, y) # modifies x and y
assert x == 1
assert y == 3
```
In the example, `res` and `remainder` are `var parameters`. Var parameters can be modified by the procedure and the changes are visible to the caller. The argument passed to a var parameter has to be an l-value. Var parameters are implemented as hidden pointers. The above example is equivalent to:
```
proc divmod(a, b: int; res, remainder: ptr int) =
res[] = a div b
remainder[] = a mod b
var
x, y: int
divmod(8, 5, addr(x), addr(y))
assert x == 1
assert y == 3
```
In the examples, var parameters or pointers are used to provide two return values. This can be done in a cleaner way by returning a tuple:
```
proc divmod(a, b: int): tuple[res, remainder: int] =
(a div b, a mod b)
var t = divmod(8, 5)
assert t.res == 1
assert t.remainder == 3
```
One can use tuple unpacking to access the tuple's fields:
```
var (x, y) = divmod(8, 5) # tuple unpacking
assert x == 1
assert y == 3
```
**Note**: `var` parameters are never necessary for efficient parameter passing. Since non-var parameters cannot be modified the compiler is always free to pass arguments by reference if it considers it can speed up execution.
### Var return type
A proc, converter, or iterator may return a `var` type which means that the returned value is an l-value and can be modified by the caller:
```
var g = 0
proc writeAccessToG(): var int =
result = g
writeAccessToG() = 6
assert g == 6
```
It is a static error if the implicitly introduced pointer could be used to access a location beyond its lifetime:
```
proc writeAccessToG(): var int =
var g = 0
result = g # Error!
```
For iterators, a component of a tuple return type can have a `var` type too:
```
iterator mpairs(a: var seq[string]): tuple[key: int, val: var string] =
for i in 0..a.high:
yield (i, a[i])
```
In the standard library every name of a routine that returns a `var` type starts with the prefix `m` per convention.
Memory safety for returning by `var T` is ensured by a simple borrowing rule: If `result` does not refer to a location pointing to the heap (that is in `result = X` the `X` involves a `ptr` or `ref` access) then it has to be derived from the routine's first parameter:
```
proc forward[T](x: var T): var T =
result = x # ok, derived from the first parameter.
proc p(param: var int): var int =
var x: int
# we know 'forward' provides a view into the location derived from
# its first argument 'x'.
result = forward(x) # Error: location is derived from ``x``
# which is not p's first parameter and lives
# on the stack.
```
In other words, the lifetime of what `result` points to is attached to the lifetime of the first parameter and that is enough knowledge to verify memory safety at the call site.
#### Future directions
Later versions of Nim can be more precise about the borrowing rule with a syntax like:
```
proc foo(other: Y; container: var X): var T from container
```
Here `var T from container` explicitly exposes that the location is derived from the second parameter (called 'container' in this case). The syntax `var T from p` specifies a type `varTy[T, 2]` which is incompatible with `varTy[T, 1]`.
### NRVO
**Note**: This section describes the current implementation. This part of the language specification will be changed. See <https://github.com/nim-lang/RFCs/issues/230> for more information.
The return value is represented inside the body of a routine as the special result variable. This allows for a mechanism much like C++'s "named return value optimization" (NRVO). NRVO means that the stores to `result` inside `p` directly affect the destination `dest` in `let/var dest = p(args)` (definition of `dest`) and also in `dest = p(args)` (assignment to `dest`). This is achieved by rewriting `dest = p(args)` to `p'(args, dest)` where `p'` is a variation of `p` that returns `void` and receives a hidden mutable parameter representing `result`.
Informally:
```
proc p(): BigT = ...
var x = p()
x = p()
# is roughly turned into:
proc p(result: var BigT) = ...
var x; p(x)
p(x)
```
Let `T`'s be `p`'s return type. NRVO applies for `T` if `sizeof(T) >= N` (where `N` is implementation dependent), in other words, it applies for "big" structures.
If `p` can raise an exception, NRVO applies regardless. This can produce observable differences in behavior:
```
type
BigT = array[16, int]
proc p(raiseAt: int): BigT =
for i in 0..high(result):
if i == raiseAt: raise newException(ValueError, "interception")
result[i] = i
proc main =
var x: BigT
try:
x = p(8)
except ValueError:
doAssert x == [0, 1, 2, 3, 4, 5, 6, 7, 0, 0, 0, 0, 0, 0, 0, 0]
main()
```
However, the current implementation produces a warning in these cases. There are different ways to deal with this warning:
1. Disable the warning via `{.push warning[ObservableStores]: off.}` ... `{.pop.}`. Then one may need to ensure that `p` only raises *before* any stores to `result` happen.
2. One can use a temporary helper variable, for example instead of `x = p(8)` use `let tmp = p(8); x = tmp`.
### Overloading of the subscript operator
The `[]` subscript operator for arrays/openarrays/sequences can be overloaded.
Methods
-------
Procedures always use static dispatch. Methods use dynamic dispatch. For dynamic dispatch to work on an object it should be a reference type.
```
type
Expression = ref object of RootObj ## abstract base class for an expression
Literal = ref object of Expression
x: int
PlusExpr = ref object of Expression
a, b: Expression
method eval(e: Expression): int {.base.} =
# override this base method
raise newException(CatchableError, "Method without implementation override")
method eval(e: Literal): int = return e.x
method eval(e: PlusExpr): int =
# watch out: relies on dynamic binding
result = eval(e.a) + eval(e.b)
proc newLit(x: int): Literal =
new(result)
result.x = x
proc newPlus(a, b: Expression): PlusExpr =
new(result)
result.a = a
result.b = b
echo eval(newPlus(newPlus(newLit(1), newLit(2)), newLit(4)))
```
In the example the constructors `newLit` and `newPlus` are procs because they should use static binding, but `eval` is a method because it requires dynamic binding.
As can be seen in the example, base methods have to be annotated with the base pragma. The `base` pragma also acts as a reminder for the programmer that a base method `m` is used as the foundation to determine all the effects that a call to `m` might cause.
**Note**: Compile-time execution is not (yet) supported for methods.
**Note**: Starting from Nim 0.20, generic methods are deprecated.
### Multi-methods
**Note:** Starting from Nim 0.20, to use multi-methods one must explicitly pass `--multimethods:on` when compiling.
In a multi-method all parameters that have an object type are used for the dispatching:
```
type
Thing = ref object of RootObj
Unit = ref object of Thing
x: int
method collide(a, b: Thing) {.inline.} =
quit "to override!"
method collide(a: Thing, b: Unit) {.inline.} =
echo "1"
method collide(a: Unit, b: Thing) {.inline.} =
echo "2"
var a, b: Unit
new a
new b
collide(a, b) # output: 2
```
### Inhibit dynamic method resolution via procCall
Dynamic method resolution can be inhibited via the builtin system.procCall. This is somewhat comparable to the super keyword that traditional OOP languages offer.
```
type
Thing = ref object of RootObj
Unit = ref object of Thing
x: int
method m(a: Thing) {.base.} =
echo "base"
method m(a: Unit) =
# Call the base method:
procCall m(Thing(a))
echo "1"
```
Iterators and the for statement
-------------------------------
The for statement is an abstract mechanism to iterate over the elements of a container. It relies on an iterator to do so. Like `while` statements, `for` statements open an implicit block, so that they can be left with a `break` statement.
The `for` loop declares iteration variables - their scope reaches until the end of the loop body. The iteration variables' types are inferred by the return type of the iterator.
An iterator is similar to a procedure, except that it can be called in the context of a `for` loop. Iterators provide a way to specify the iteration over an abstract type. A key role in the execution of a `for` loop plays the `yield` statement in the called iterator. Whenever a `yield` statement is reached the data is bound to the `for` loop variables and control continues in the body of the `for` loop. The iterator's local variables and execution state are automatically saved between calls. Example:
```
# this definition exists in the system module
iterator items*(a: string): char {.inline.} =
var i = 0
while i < len(a):
yield a[i]
inc(i)
for ch in items("hello world"): # `ch` is an iteration variable
echo ch
```
The compiler generates code as if the programmer would have written this:
```
var i = 0
while i < len(a):
var ch = a[i]
echo ch
inc(i)
```
If the iterator yields a tuple, there can be as many iteration variables as there are components in the tuple. The i'th iteration variable's type is the type of the i'th component. In other words, implicit tuple unpacking in a for loop context is supported.
### Implicit items/pairs invocations
If the for loop expression `e` does not denote an iterator and the for loop has exactly 1 variable, the for loop expression is rewritten to `items(e)`; ie. an `items` iterator is implicitly invoked:
```
for x in [1,2,3]: echo x
```
If the for loop has exactly 2 variables, a `pairs` iterator is implicitly invoked.
Symbol lookup of the identifiers `items`/`pairs` is performed after the rewriting step, so that all overloads of `items`/`pairs` are taken into account.
### First-class iterators
There are 2 kinds of iterators in Nim: *inline* and *closure* iterators. An inline iterator is an iterator that's always inlined by the compiler leading to zero overhead for the abstraction, but may result in a heavy increase in code size.
Caution: the body of a for loop over an inline iterator is inlined into each `yield` statement appearing in the iterator code, so ideally the code should be refactored to contain a single yield when possible to avoid code bloat.
Inline iterators are second class citizens; They can be passed as parameters only to other inlining code facilities like templates, macros, and other inline iterators.
In contrast to that, a closure iterator can be passed around more freely:
```
iterator count0(): int {.closure.} =
yield 0
iterator count2(): int {.closure.} =
var x = 1
yield x
inc x
yield x
proc invoke(iter: iterator(): int {.closure.}) =
for x in iter(): echo x
invoke(count0)
invoke(count2)
```
Closure iterators and inline iterators have some restrictions:
1. For now, a closure iterator cannot be executed at compile time.
2. `return` is allowed in a closure iterator but not in an inline iterator (but rarely useful) and ends the iteration.
3. Neither inline nor closure iterators can be (directly)\* recursive.
4. Neither inline nor closure iterators have the special `result` variable.
5. Closure iterators are not supported by the js backend.
(\*) Closure iterators can be co-recursive with a factory proc which results in similar syntax to a recursive iterator. More details follow.
Iterators that are neither marked `{.closure.}` nor `{.inline.}` explicitly default to being inline, but this may change in future versions of the implementation.
The `iterator` type is always of the calling convention `closure` implicitly; the following example shows how to use iterators to implement a collaborative tasking system:
```
# simple tasking:
type
Task = iterator (ticker: int)
iterator a1(ticker: int) {.closure.} =
echo "a1: A"
yield
echo "a1: B"
yield
echo "a1: C"
yield
echo "a1: D"
iterator a2(ticker: int) {.closure.} =
echo "a2: A"
yield
echo "a2: B"
yield
echo "a2: C"
proc runTasks(t: varargs[Task]) =
var ticker = 0
while true:
let x = t[ticker mod t.len]
if finished(x): break
x(ticker)
inc ticker
runTasks(a1, a2)
```
The builtin `system.finished` can be used to determine if an iterator has finished its operation; no exception is raised on an attempt to invoke an iterator that has already finished its work.
Note that `system.finished` is error prone to use because it only returns `true` one iteration after the iterator has finished:
```
iterator mycount(a, b: int): int {.closure.} =
var x = a
while x <= b:
yield x
inc x
var c = mycount # instantiate the iterator
while not finished(c):
echo c(1, 3)
# Produces
1
2
3
0
```
Instead this code has to be used:
```
var c = mycount # instantiate the iterator
while true:
let value = c(1, 3)
if finished(c): break # and discard 'value'!
echo value
```
It helps to think that the iterator actually returns a pair `(value, done)` and `finished` is used to access the hidden `done` field.
Closure iterators are *resumable functions* and so one has to provide the arguments to every call. To get around this limitation one can capture parameters of an outer factory proc:
```
proc mycount(a, b: int): iterator (): int =
result = iterator (): int =
var x = a
while x <= b:
yield x
inc x
let foo = mycount(1, 4)
for f in foo():
echo f
```
The call can be made more like an inline iterator with a for loop macro:
```
import macros
macro toItr(x: ForLoopStmt): untyped =
let expr = x[0]
let call = x[1][1] # Get foo out of toItr(foo)
let body = x[2]
result = quote do:
block:
let itr = `call`
for `expr` in itr():
`body`
for f in toItr(mycount(1, 4)): # using early `proc mycount`
echo f
```
Because of full backend function call aparatus involvment, closure iterator invocation is typically higher cost than inline iterators. Adornment by a macro wrapper at the call site like this is a possibly useful reminder.
The factory `proc`, as an ordinary procedure, can be recursive. The above macro allows such recursion to look much like a recursive iterator would. For example:
```
proc recCountDown(n: int): iterator(): int =
result = iterator(): int =
if n > 0:
yield n
for e in toItr(recCountDown(n - 1)):
yield e
for i in toItr(recCountDown(6)): # Emits: 6 5 4 3 2 1
echo i
```
Converters
----------
A converter is like an ordinary proc except that it enhances the "implicitly convertible" type relation (see [Convertible relation](#type-relations-convertible-relation)):
```
# bad style ahead: Nim is not C.
converter toBool(x: int): bool = x != 0
if 4:
echo "compiles"
```
A converter can also be explicitly invoked for improved readability. Note that implicit converter chaining is not supported: If there is a converter from type A to type B and from type B to type C the implicit conversion from A to C is not provided.
Type sections
-------------
Example:
```
type # example demonstrating mutually recursive types
Node = ref object # an object managed by the garbage collector (ref)
le, ri: Node # left and right subtrees
sym: ref Sym # leaves contain a reference to a Sym
Sym = object # a symbol
name: string # the symbol's name
line: int # the line the symbol was declared in
code: Node # the symbol's abstract syntax tree
```
A type section begins with the `type` keyword. It contains multiple type definitions. A type definition binds a type to a name. Type definitions can be recursive or even mutually recursive. Mutually recursive types are only possible within a single `type` section. Nominal types like `objects` or `enums` can only be defined in a `type` section.
Exception handling
------------------
### Try statement
Example:
```
# read the first two lines of a text file that should contain numbers
# and tries to add them
var
f: File
if open(f, "numbers.txt"):
try:
var a = readLine(f)
var b = readLine(f)
echo "sum: " & $(parseInt(a) + parseInt(b))
except OverflowDefect:
echo "overflow!"
except ValueError:
echo "could not convert string to integer"
except IOError:
echo "IO error!"
except:
echo "Unknown exception!"
finally:
close(f)
```
The statements after the `try` are executed in sequential order unless an exception `e` is raised. If the exception type of `e` matches any listed in an `except` clause the corresponding statements are executed. The statements following the `except` clauses are called exception handlers.
The empty except clause is executed if there is an exception that is not listed otherwise. It is similar to an `else` clause in `if` statements.
If there is a finally clause, it is always executed after the exception handlers.
The exception is *consumed* in an exception handler. However, an exception handler may raise another exception. If the exception is not handled, it is propagated through the call stack. This means that often the rest of the procedure - that is not within a `finally` clause - is not executed (if an exception occurs).
### Try expression
Try can also be used as an expression; the type of the `try` branch then needs to fit the types of `except` branches, but the type of the `finally` branch always has to be `void`:
```
from strutils import parseInt
let x = try: parseInt("133a")
except: -1
finally: echo "hi"
```
To prevent confusing code there is a parsing limitation; if the `try` follows a `(` it has to be written as a one liner:
```
let x = (try: parseInt("133a") except: -1)
```
### Except clauses
Within an `except` clause it is possible to access the current exception using the following syntax:
```
try:
# ...
except IOError as e:
# Now use "e"
echo "I/O error: " & e.msg
```
Alternatively, it is possible to use `getCurrentException` to retrieve the exception that has been raised:
```
try:
# ...
except IOError:
let e = getCurrentException()
# Now use "e"
```
Note that `getCurrentException` always returns a `ref Exception` type. If a variable of the proper type is needed (in the example above, `IOError`), one must convert it explicitly:
```
try:
# ...
except IOError:
let e = (ref IOError)(getCurrentException())
# "e" is now of the proper type
```
However, this is seldom needed. The most common case is to extract an error message from `e`, and for such situations, it is enough to use `getCurrentExceptionMsg`:
```
try:
# ...
except:
echo getCurrentExceptionMsg()
```
### Custom exceptions
Is it possible to create custom exceptions. A custom exception is a custom type:
```
type
LoadError* = object of Exception
```
Ending the custom exception's name with `Error` is recommended.
Custom exceptions can be raised like any others, e.g.:
```
raise newException(LoadError, "Failed to load data")
```
### Defer statement
Instead of a `try finally` statement a `defer` statement can be used, which avoids lexical nesting and offers more flexibility in terms of scoping as shown below.
Any statements following the `defer` in the current block will be considered to be in an implicit try block:
```
proc main =
var f = open("numbers.txt", fmWrite)
defer: close(f)
f.write "abc"
f.write "def"
```
Is rewritten to:
```
proc main =
var f = open("numbers.txt")
try:
f.write "abc"
f.write "def"
finally:
close(f)
```
When `defer` is at the outermost scope of a template/macro, its scope extends to the block where the template is called from:
```
template safeOpenDefer(f, path) =
var f = open(path, fmWrite)
defer: close(f)
template safeOpenFinally(f, path, body) =
var f = open(path, fmWrite)
try: body # without `defer`, `body` must be specified as parameter
finally: close(f)
block:
safeOpenDefer(f, "/tmp/z01.txt")
f.write "abc"
block:
safeOpenFinally(f, "/tmp/z01.txt"):
f.write "abc" # adds a lexical scope
block:
var f = open("/tmp/z01.txt", fmWrite)
try:
f.write "abc" # adds a lexical scope
finally: close(f)
```
Top-level `defer` statements are not supported since it's unclear what such a statement should refer to.
### Raise statement
Example:
```
raise newException(IOError, "IO failed")
```
Apart from built-in operations like array indexing, memory allocation, etc. the `raise` statement is the only way to raise an exception.
If no exception name is given, the current exception is re-raised. The ReraiseDefect exception is raised if there is no exception to re-raise. It follows that the `raise` statement *always* raises an exception.
### Exception hierarchy
The exception tree is defined in the <system> module. Every exception inherits from `system.Exception`. Exceptions that indicate programming bugs inherit from `system.Defect` (which is a subtype of `Exception`) and are strictly speaking not catchable as they can also be mapped to an operation that terminates the whole process. If panics are turned into exceptions, these exceptions inherit from `Defect`.
Exceptions that indicate any other runtime error that can be caught inherit from `system.CatchableError` (which is a subtype of `Exception`).
### Imported exceptions
It is possible to raise/catch imported C++ exceptions. Types imported using `importcpp` can be raised or caught. Exceptions are raised by value and caught by reference. Example:
```
type
CStdException {.importcpp: "std::exception", header: "<exception>", inheritable.} = object
## does not inherit from `RootObj`, so we use `inheritable` instead
CRuntimeError {.requiresInit, importcpp: "std::runtime_error", header: "<stdexcept>".} = object of CStdException
## `CRuntimeError` has no default constructor => `requiresInit`
proc what(s: CStdException): cstring {.importcpp: "((char *)#.what())".}
proc initRuntimeError(a: cstring): CRuntimeError {.importcpp: "std::runtime_error(@)", constructor.}
proc initStdException(): CStdException {.importcpp: "std::exception()", constructor.}
proc fn() =
let a = initRuntimeError("foo")
doAssert $a.what == "foo"
var b: cstring
try: raise initRuntimeError("foo2")
except CStdException as e:
doAssert e is CStdException
b = e.what()
doAssert $b == "foo2"
try: raise initStdException()
except CStdException: discard
try: raise initRuntimeError("foo3")
except CRuntimeError as e:
b = e.what()
except CStdException:
doAssert false
doAssert $b == "foo3"
fn()
```
**Note:** `getCurrentException()` and `getCurrentExceptionMsg()` are not available for imported exceptions from C++. One needs to use the `except ImportedException as x:` syntax and rely on functionality of the `x` object to get exception details.
Effect system
-------------
### Exception tracking
Nim supports exception tracking. The raises pragma can be used to explicitly define which exceptions a proc/iterator/method/converter is allowed to raise. The compiler verifies this:
```
proc p(what: bool) {.raises: [IOError, OSError].} =
if what: raise newException(IOError, "IO")
else: raise newException(OSError, "OS")
```
An empty `raises` list (`raises: []`) means that no exception may be raised:
```
proc p(): bool {.raises: [].} =
try:
unsafeCall()
result = true
except:
result = false
```
A `raises` list can also be attached to a proc type. This affects type compatibility:
```
type
Callback = proc (s: string) {.raises: [IOError].}
var
c: Callback
proc p(x: string) =
raise newException(OSError, "OS")
c = p # type error
```
For a routine `p` the compiler uses inference rules to determine the set of possibly raised exceptions; the algorithm operates on `p`'s call graph:
1. Every indirect call via some proc type `T` is assumed to raise `system.Exception` (the base type of the exception hierarchy) and thus any exception unless `T` has an explicit `raises` list. However, if the call is of the form `f(...)` where `f` is a parameter of the currently analyzed routine it is ignored. The call is optimistically assumed to have no effect. Rule 2 compensates for this case.
2. Every expression of some proc type within a call that is not a call itself (and not nil) is assumed to be called indirectly somehow and thus its raises list is added to `p`'s raises list.
3. Every call to a proc `q` which has an unknown body (due to a forward declaration or an `importc` pragma) is assumed to raise `system.Exception` unless `q` has an explicit `raises` list.
4. Every call to a method `m` is assumed to raise `system.Exception` unless `m` has an explicit `raises` list.
5. For every other call the analysis can determine an exact `raises` list.
6. For determining a `raises` list, the `raise` and `try` statements of `p` are taken into consideration.
Rules 1-2 ensure the following works:
```
proc noRaise(x: proc()) {.raises: [].} =
# unknown call that might raise anything, but valid:
x()
proc doRaise() {.raises: [IOError].} =
raise newException(IOError, "IO")
proc use() {.raises: [].} =
# doesn't compile! Can raise IOError!
noRaise(doRaise)
```
So in many cases a callback does not cause the compiler to be overly conservative in its effect analysis.
Exceptions inheriting from `system.Defect` are not tracked with the `.raises: []` exception tracking mechanism. This is more consistent with the built-in operations. The following code is valid::
```
proc mydiv(a, b): int {.raises: [].} =
a div b # can raise an DivByZeroDefect
```
And so is::
```
proc mydiv(a, b): int {.raises: [].} =
if b == 0: raise newException(DivByZeroDefect, "division by zero")
else: result = a div b
```
The reason for this is that `DivByZeroDefect` inherits from `Defect` and with `--panics:on` Defects become unrecoverable errors. (Since version 1.4 of the language.)
### Tag tracking
The exception tracking is part of Nim's effect system. Raising an exception is an *effect*. Other effects can also be defined. A user defined effect is a means to *tag* a routine and to perform checks against this tag:
```
type IO = object ## input/output effect
proc readLine(): string {.tags: [IO].} = discard
proc no_IO_please() {.tags: [].} =
# the compiler prevents this:
let x = readLine()
```
A tag has to be a type name. A `tags` list - like a `raises` list - can also be attached to a proc type. This affects type compatibility.
The inference for tag tracking is analogous to the inference for exception tracking.
### Effects pragma
The `effects` pragma has been designed to assist the programmer with the effects analysis. It is a statement that makes the compiler output all inferred effects up to the `effects`'s position:
```
proc p(what: bool) =
if what:
raise newException(IOError, "IO")
{.effects.}
else:
raise newException(OSError, "OS")
```
The compiler produces a hint message that `IOError` can be raised. `OSError` is not listed as it cannot be raised in the branch the `effects` pragma appears in.
Generics
--------
Generics are Nim's means to parametrize procs, iterators or types with type parameters. Depending on the context, the brackets are used either to introduce type parameters or to instantiate a generic proc, iterator, or type.
The following example shows a generic binary tree can be modeled:
```
type
BinaryTree*[T] = ref object # BinaryTree is a generic type with
# generic param ``T``
le, ri: BinaryTree[T] # left and right subtrees; may be nil
data: T # the data stored in a node
proc newNode*[T](data: T): BinaryTree[T] =
# constructor for a node
result = BinaryTree[T](le: nil, ri: nil, data: data)
proc add*[T](root: var BinaryTree[T], n: BinaryTree[T]) =
# insert a node into the tree
if root == nil:
root = n
else:
var it = root
while it != nil:
# compare the data items; uses the generic ``cmp`` proc
# that works for any type that has a ``==`` and ``<`` operator
var c = cmp(it.data, n.data)
if c < 0:
if it.le == nil:
it.le = n
return
it = it.le
else:
if it.ri == nil:
it.ri = n
return
it = it.ri
proc add*[T](root: var BinaryTree[T], data: T) =
# convenience proc:
add(root, newNode(data))
iterator preorder*[T](root: BinaryTree[T]): T =
# Preorder traversal of a binary tree.
# This uses an explicit stack (which is more efficient than
# a recursive iterator factory).
var stack: seq[BinaryTree[T]] = @[root]
while stack.len > 0:
var n = stack.pop()
while n != nil:
yield n.data
add(stack, n.ri) # push right subtree onto the stack
n = n.le # and follow the left pointer
var
root: BinaryTree[string] # instantiate a BinaryTree with ``string``
add(root, newNode("hello")) # instantiates ``newNode`` and ``add``
add(root, "world") # instantiates the second ``add`` proc
for str in preorder(root):
stdout.writeLine(str)
```
The `T` is called a generic type parameter or a type variable.
### Is operator
The `is` operator is evaluated during semantic analysis to check for type equivalence. It is therefore very useful for type specialization within generic code:
```
type
Table[Key, Value] = object
keys: seq[Key]
values: seq[Value]
when not (Key is string): # empty value for strings used for optimization
deletedKeys: seq[bool]
```
### Type Classes
A type class is a special pseudo-type that can be used to match against types in the context of overload resolution or the `is` operator. Nim supports the following built-in type classes:
| type class | matches |
| --- | --- |
| `object` | any object type |
| `tuple` | any tuple type |
| | |
| `enum` | any enumeration |
| `proc` | any proc type |
| `ref` | any `ref` type |
| `ptr` | any `ptr` type |
| `var` | any `var` type |
| `distinct` | any distinct type |
| `array` | any array type |
| `set` | any set type |
| `seq` | any seq type |
| `auto` | any type |
| `any` | distinct auto (see below) |
Furthermore, every generic type automatically creates a type class of the same name that will match any instantiation of the generic type.
Type classes can be combined using the standard boolean operators to form more complex type classes:
```
# create a type class that will match all tuple and object types
type RecordType = tuple or object
proc printFields[T: RecordType](rec: T) =
for key, value in fieldPairs(rec):
echo key, " = ", value
```
Whilst the syntax of type classes appears to resemble that of ADTs/algebraic data types in ML-like languages, it should be understood that type classes are static constraints to be enforced at type instantiations. Type classes are not really types in themselves but are instead a system of providing generic "checks" that ultimately *resolve* to some singular type. Type classes do not allow for runtime type dynamism, unlike object variants or methods.
As an example, the following would not compile:
```
type TypeClass = int | string
var foo: TypeClass = 2 # foo's type is resolved to an int here
foo = "this will fail" # error here, because foo is an int
```
Nim allows for type classes and regular types to be specified as type constraints of the generic type parameter:
```
proc onlyIntOrString[T: int|string](x, y: T) = discard
onlyIntOrString(450, 616) # valid
onlyIntOrString(5.0, 0.0) # type mismatch
onlyIntOrString("xy", 50) # invalid as 'T' cannot be both at the same time
```
### Implicit generics
A type class can be used directly as the parameter's type.
```
# create a type class that will match all tuple and object types
type RecordType = tuple or object
proc printFields(rec: RecordType) =
for key, value in fieldPairs(rec):
echo key, " = ", value
```
Procedures utilizing type classes in such a manner are considered to be implicitly generic. They will be instantiated once for each unique combination of param types used within the program.
By default, during overload resolution, each named type class will bind to exactly one concrete type. We call such type classes bind once types. Here is an example taken directly from the system module to illustrate this:
```
proc `==`*(x, y: tuple): bool =
## requires `x` and `y` to be of the same tuple type
## generic ``==`` operator for tuples that is lifted from the components
## of `x` and `y`.
result = true
for a, b in fields(x, y):
if a != b: result = false
```
Alternatively, the `distinct` type modifier can be applied to the type class to allow each param matching the type class to bind to a different type. Such type classes are called bind many types.
Procs written with the implicitly generic style will often need to refer to the type parameters of the matched generic type. They can be easily accessed using the dot syntax:
```
type Matrix[T, Rows, Columns] = object
...
proc `[]`(m: Matrix, row, col: int): Matrix.T =
m.data[col * high(Matrix.Columns) + row]
```
Here are more examples that illustrate implicit generics:
```
proc p(t: Table; k: Table.Key): Table.Value
# is roughly the same as:
proc p[Key, Value](t: Table[Key, Value]; k: Key): Value
```
```
proc p(a: Table, b: Table)
# is roughly the same as:
proc p[Key, Value](a, b: Table[Key, Value])
```
```
proc p(a: Table, b: distinct Table)
# is roughly the same as:
proc p[Key, Value, KeyB, ValueB](a: Table[Key, Value], b: Table[KeyB, ValueB])
```
`typedesc` used as a parameter type also introduces an implicit generic. `typedesc` has its own set of rules:
```
proc p(a: typedesc)
# is roughly the same as:
proc p[T](a: typedesc[T])
```
`typedesc` is a "bind many" type class:
```
proc p(a, b: typedesc)
# is roughly the same as:
proc p[T, T2](a: typedesc[T], b: typedesc[T2])
```
A parameter of type `typedesc` is itself usable as a type. If it is used as a type, it's the underlying type. (In other words, one level of "typedesc"-ness is stripped off:
```
proc p(a: typedesc; b: a) = discard
# is roughly the same as:
proc p[T](a: typedesc[T]; b: T) = discard
# hence this is a valid call:
p(int, 4)
# as parameter 'a' requires a type, but 'b' requires a value.
```
### Generic inference restrictions
The types `var T` and `typedesc[T]` cannot be inferred in a generic instantiation. The following is not allowed:
```
proc g[T](f: proc(x: T); x: T) =
f(x)
proc c(y: int) = echo y
proc v(y: var int) =
y += 100
var i: int
# allowed: infers 'T' to be of type 'int'
g(c, 42)
# not valid: 'T' is not inferred to be of type 'var int'
g(v, i)
# also not allowed: explicit instantiation via 'var int'
g[var int](v, i)
```
### Symbol lookup in generics
#### Open and Closed symbols
The symbol binding rules in generics are slightly subtle: There are "open" and "closed" symbols. A "closed" symbol cannot be re-bound in the instantiation context, an "open" symbol can. Per default overloaded symbols are open and every other symbol is closed.
Open symbols are looked up in two different contexts: Both the context at definition and the context at instantiation are considered:
```
type
Index = distinct int
proc `==` (a, b: Index): bool {.borrow.}
var a = (0, 0.Index)
var b = (0, 0.Index)
echo a == b # works!
```
In the example, the generic `==` for tuples (as defined in the system module) uses the `==` operators of the tuple's components. However, the `==` for the `Index` type is defined *after* the `==` for tuples; yet the example compiles as the instantiation takes the currently defined symbols into account too.
### Mixin statement
A symbol can be forced to be open by a mixin declaration:
```
proc create*[T](): ref T =
# there is no overloaded 'init' here, so we need to state that it's an
# open symbol explicitly:
mixin init
new result
init result
```
`mixin` statements only make sense in templates and generics.
### Bind statement
The `bind` statement is the counterpart to the `mixin` statement. It can be used to explicitly declare identifiers that should be bound early (i.e. the identifiers should be looked up in the scope of the template/generic definition):
```
# Module A
var
lastId = 0
template genId*: untyped =
bind lastId
inc(lastId)
lastId
```
```
# Module B
import A
echo genId()
```
But a `bind` is rarely useful because symbol binding from the definition scope is the default.
`bind` statements only make sense in templates and generics.
### Delegating bind statements
The following example outlines a problem that can arise when generic instantiations cross multiple different modules:
```
# module A
proc genericA*[T](x: T) =
mixin init
init(x)
```
```
import C
# module B
proc genericB*[T](x: T) =
# Without the `bind init` statement C's init proc is
# not available when `genericB` is instantiated:
bind init
genericA(x)
```
```
# module C
type O = object
proc init*(x: var O) = discard
```
```
# module main
import B, C
genericB O()
```
In module B has an `init` proc from module C in its scope that is not taken into account when `genericB` is instantiated which leads to the instantiation of `genericA`. The solution is to `forward`:idx these symbols by a `bind` statement inside `genericB`.
Templates
---------
A template is a simple form of a macro: It is a simple substitution mechanism that operates on Nim's abstract syntax trees. It is processed in the semantic pass of the compiler.
The syntax to *invoke* a template is the same as calling a procedure.
Example:
```
template `!=` (a, b: untyped): untyped =
# this definition exists in the System module
not (a == b)
assert(5 != 6) # the compiler rewrites that to: assert(not (5 == 6))
```
The `!=`, `>`, `>=`, `in`, `notin`, `isnot` operators are in fact templates:
`a > b` is transformed into `b < a`.
`a in b` is transformed into `contains(b, a)`.
`notin` and `isnot` have the obvious meanings.
The "types" of templates can be the symbols `untyped`, `typed` or `typedesc`. These are "meta types", they can only be used in certain contexts. Regular types can be used too; this implies that `typed` expressions are expected.
### Typed vs untyped parameters
An `untyped` parameter means that symbol lookups and type resolution is not performed before the expression is passed to the template. This means that for example *undeclared* identifiers can be passed to the template:
```
template declareInt(x: untyped) =
var x: int
declareInt(x) # valid
x = 3
```
```
template declareInt(x: typed) =
var x: int
declareInt(x) # invalid, because x has not been declared and so it has no type
```
A template where every parameter is `untyped` is called an immediate template. For historical reasons templates can be explicitly annotated with an `immediate` pragma and then these templates do not take part in overloading resolution and the parameters' types are *ignored* by the compiler. Explicit immediate templates are now deprecated.
**Note**: For historical reasons `stmt` was an alias for `typed` and `expr` was an alias for `untyped`, but they are removed.
### Passing a code block to a template
One can pass a block of statements as the last argument to a template following the special `:` syntax:
```
template withFile(f, fn, mode, actions: untyped): untyped =
var f: File
if open(f, fn, mode):
try:
actions
finally:
close(f)
else:
quit("cannot open: " & fn)
withFile(txt, "ttempl3.txt", fmWrite): # special colon
txt.writeLine("line 1")
txt.writeLine("line 2")
```
In the example, the two `writeLine` statements are bound to the `actions` parameter.
Usually to pass a block of code to a template the parameter that accepts the block needs to be of type `untyped`. Because symbol lookups are then delayed until template instantiation time:
```
template t(body: typed) =
proc p = echo "hey"
block:
body
t:
p() # fails with 'undeclared identifier: p'
```
The above code fails with the error message that `p` is not declared. The reason for this is that the `p()` body is type-checked before getting passed to the `body` parameter and type checking in Nim implies symbol lookups. The same code works with `untyped` as the passed body is not required to be type-checked:
```
template t(body: untyped) =
proc p = echo "hey"
block:
body
t:
p() # compiles
```
### Varargs of untyped
In addition to the `untyped` meta-type that prevents type checking there is also `varargs[untyped]` so that not even the number of parameters is fixed:
```
template hideIdentifiers(x: varargs[untyped]) = discard
hideIdentifiers(undeclared1, undeclared2)
```
However, since a template cannot iterate over varargs, this feature is generally much more useful for macros.
### Symbol binding in templates
A template is a hygienic macro and so opens a new scope. Most symbols are bound from the definition scope of the template:
```
# Module A
var
lastId = 0
template genId*: untyped =
inc(lastId)
lastId
```
```
# Module B
import A
echo genId() # Works as 'lastId' has been bound in 'genId's defining scope
```
As in generics symbol binding can be influenced via `mixin` or `bind` statements.
### Identifier construction
In templates identifiers can be constructed with the backticks notation:
```
template typedef(name: untyped, typ: typedesc) =
type
`T name`* {.inject.} = typ
`P name`* {.inject.} = ref `T name`
typedef(myint, int)
var x: PMyInt
```
In the example `name` is instantiated with `myint`, so `T name` becomes `Tmyint`.
### Lookup rules for template parameters
A parameter `p` in a template is even substituted in the expression `x.p`. Thus template arguments can be used as field names and a global symbol can be shadowed by the same argument name even when fully qualified:
```
# module 'm'
type
Lev = enum
levA, levB
var abclev = levB
template tstLev(abclev: Lev) =
echo abclev, " ", m.abclev
tstLev(levA)
# produces: 'levA levA'
```
But the global symbol can properly be captured by a `bind` statement:
```
# module 'm'
type
Lev = enum
levA, levB
var abclev = levB
template tstLev(abclev: Lev) =
bind m.abclev
echo abclev, " ", m.abclev
tstLev(levA)
# produces: 'levA levB'
```
### Hygiene in templates
Per default templates are hygienic: Local identifiers declared in a template cannot be accessed in the instantiation context:
```
template newException*(exceptn: typedesc, message: string): untyped =
var
e: ref exceptn # e is implicitly gensym'ed here
new(e)
e.msg = message
e
# so this works:
let e = "message"
raise newException(IoError, e)
```
Whether a symbol that is declared in a template is exposed to the instantiation scope is controlled by the inject and gensym pragmas: gensym'ed symbols are not exposed but inject'ed are.
The default for symbols of entity `type`, `var`, `let` and `const` is `gensym` and for `proc`, `iterator`, `converter`, `template`, `macro` is `inject`. However, if the name of the entity is passed as a template parameter, it is an inject'ed symbol:
```
template withFile(f, fn, mode: untyped, actions: untyped): untyped =
block:
var f: File # since 'f' is a template param, it's injected implicitly
...
withFile(txt, "ttempl3.txt", fmWrite):
txt.writeLine("line 1")
txt.writeLine("line 2")
```
The `inject` and `gensym` pragmas are second class annotations; they have no semantics outside of a template definition and cannot be abstracted over:
```
{.pragma myInject: inject.}
template t() =
var x {.myInject.}: int # does NOT work
```
To get rid of hygiene in templates, one can use the dirty pragma for a template. `inject` and `gensym` have no effect in `dirty` templates.
`gensym`'ed symbols cannot be used as `field` in the `x.field` syntax. Nor can they be used in the `ObjectConstruction(field: value)` and `namedParameterCall(field = value)` syntactic constructs.
The reason for this is that code like
```
type
T = object
f: int
template tmp(x: T) =
let f = 34
echo x.f, T(f: 4)
```
should work as expected.
However, this means that the method call syntax is not available for `gensym`'ed symbols:
```
template tmp(x) =
type
T {.gensym.} = int
echo x.T # invalid: instead use: 'echo T(x)'.
tmp(12)
```
**Note**: The Nim compiler prior to version 1 was more lenient about this requirement. Use the `--useVersion:0.19` switch for a transition period.
### Limitations of the method call syntax
The expression `x` in `x.f` needs to be semantically checked (that means symbol lookup and type checking) before it can be decided that it needs to be rewritten to `f(x)`. Therefore the dot syntax has some limitations when it is used to invoke templates/macros:
```
template declareVar(name: untyped) =
const name {.inject.} = 45
# Doesn't compile:
unknownIdentifier.declareVar
```
Another common example is this:
```
from sequtils import toSeq
iterator something: string =
yield "Hello"
yield "World"
var info = something().toSeq
```
The problem here is that the compiler already decided that `something()` as an iterator is not callable in this context before `toSeq` gets its chance to convert it into a sequence.
It is also not possible to use fully qualified identifiers with module symbol in method call syntax. The order in which the dot operator binds to symbols prohibits this.
```
import sequtils
var myItems = @[1,3,3,7]
let N1 = count(myItems, 3) # OK
let N2 = sequtils.count(myItems, 3) # fully qualified, OK
let N3 = myItems.count(3) # OK
let N4 = myItems.sequtils.count(3) # illegal, `myItems.sequtils` can't be resolved
```
This means that when for some reason a procedure needs a disambiguation through the module name, the call needs to be written in function call syntax.
Macros
------
A macro is a special function that is executed at compile time. Normally the input for a macro is an abstract syntax tree (AST) of the code that is passed to it. The macro can then do transformations on it and return the transformed AST. This can be used to add custom language features and implement domain-specific languages.
Macro invocation is a case where semantic analysis does **not** entirely proceed top to bottom and left to right. Instead, semantic analysis happens at least twice:
* Semantic analysis recognizes and resolves the macro invocation.
* The compiler executes the macro body (which may invoke other procs).
* It replaces the AST of the macro invocation with the AST returned by the macro.
* It repeats semantic analysis of that region of the code.
* If the AST returned by the macro contains other macro invocations, this process iterates.
While macros enable advanced compile-time code transformations, they cannot change Nim's syntax.
### Debug Example
The following example implements a powerful `debug` command that accepts a variable number of arguments:
```
# to work with Nim syntax trees, we need an API that is defined in the
# ``macros`` module:
import macros
macro debug(args: varargs[untyped]): untyped =
# `args` is a collection of `NimNode` values that each contain the
# AST for an argument of the macro. A macro always has to
# return a `NimNode`. A node of kind `nnkStmtList` is suitable for
# this use case.
result = nnkStmtList.newTree()
# iterate over any argument that is passed to this macro:
for n in args:
# add a call to the statement list that writes the expression;
# `toStrLit` converts an AST to its string representation:
result.add newCall("write", newIdentNode("stdout"), newLit(n.repr))
# add a call to the statement list that writes ": "
result.add newCall("write", newIdentNode("stdout"), newLit(": "))
# add a call to the statement list that writes the expressions value:
result.add newCall("writeLine", newIdentNode("stdout"), n)
var
a: array[0..10, int]
x = "some string"
a[0] = 42
a[1] = 45
debug(a[0], a[1], x)
```
The macro call expands to:
```
write(stdout, "a[0]")
write(stdout, ": ")
writeLine(stdout, a[0])
write(stdout, "a[1]")
write(stdout, ": ")
writeLine(stdout, a[1])
write(stdout, "x")
write(stdout, ": ")
writeLine(stdout, x)
```
Arguments that are passed to a `varargs` parameter are wrapped in an array constructor expression. This is why `debug` iterates over all of `n`'s children.
### BindSym
The above `debug` macro relies on the fact that `write`, `writeLine` and `stdout` are declared in the system module and thus visible in the instantiating context. There is a way to use bound identifiers (aka symbols) instead of using unbound identifiers. The `bindSym` builtin can be used for that:
```
import macros
macro debug(n: varargs[typed]): untyped =
result = newNimNode(nnkStmtList, n)
for x in n:
# we can bind symbols in scope via 'bindSym':
add(result, newCall(bindSym"write", bindSym"stdout", toStrLit(x)))
add(result, newCall(bindSym"write", bindSym"stdout", newStrLitNode(": ")))
add(result, newCall(bindSym"writeLine", bindSym"stdout", x))
var
a: array[0..10, int]
x = "some string"
a[0] = 42
a[1] = 45
debug(a[0], a[1], x)
```
The macro call expands to:
```
write(stdout, "a[0]")
write(stdout, ": ")
writeLine(stdout, a[0])
write(stdout, "a[1]")
write(stdout, ": ")
writeLine(stdout, a[1])
write(stdout, "x")
write(stdout, ": ")
writeLine(stdout, x)
```
However, the symbols `write`, `writeLine` and `stdout` are already bound and are not looked up again. As the example shows, `bindSym` does work with overloaded symbols implicitly.
### Case-Of Macro
In Nim it is possible to have a macro with the syntax of a *case-of* expression just with the difference that all of branches are passed to and processed by the macro implementation. It is then up the macro implementation to transform the *of-branches* into a valid Nim statement. The following example should show how this feature could be used for a lexical analyzer.
```
import macros
macro case_token(args: varargs[untyped]): untyped =
echo args.treeRepr
# creates a lexical analyzer from regular expressions
# ... (implementation is an exercise for the reader ;-)
discard
case_token: # this colon tells the parser it is a macro statement
of r"[A-Za-z_]+[A-Za-z_0-9]*":
return tkIdentifier
of r"0-9+":
return tkInteger
of r"[\+\-\*\?]+":
return tkOperator
else:
return tkUnknown
```
**Style note**: For code readability, it is the best idea to use the least powerful programming construct that still suffices. So the "check list" is:
1. Use an ordinary proc/iterator, if possible.
2. Else: Use a generic proc/iterator, if possible.
3. Else: Use a template, if possible.
4. Else: Use a macro.
### For loop macro
A macro that takes as its only input parameter an expression of the special type `system.ForLoopStmt` can rewrite the entirety of a `for` loop:
```
import macros
macro enumerate(x: ForLoopStmt): untyped =
expectKind x, nnkForStmt
# check if the starting count is specified:
var countStart = if x[^2].len == 2: newLit(0) else: x[^2][1]
result = newStmtList()
# we strip off the first for loop variable and use it as an integer counter:
result.add newVarStmt(x[0], countStart)
var body = x[^1]
if body.kind != nnkStmtList:
body = newTree(nnkStmtList, body)
body.add newCall(bindSym"inc", x[0])
var newFor = newTree(nnkForStmt)
for i in 1..x.len-3:
newFor.add x[i]
# transform enumerate(X) to 'X'
newFor.add x[^2][^1]
newFor.add body
result.add newFor
# now wrap the whole macro in a block to create a new scope
result = quote do:
block: `result`
for a, b in enumerate(items([1, 2, 3])):
echo a, " ", b
# without wrapping the macro in a block, we'd need to choose different
# names for `a` and `b` here to avoid redefinition errors
for a, b in enumerate(10, [1, 2, 3, 5]):
echo a, " ", b
```
Special Types
-------------
### static[T]
As their name suggests, static parameters must be constant expressions:
```
proc precompiledRegex(pattern: static string): RegEx =
var res {.global.} = re(pattern)
return res
precompiledRegex("/d+") # Replaces the call with a precompiled
# regex, stored in a global variable
precompiledRegex(paramStr(1)) # Error, command-line options
# are not constant expressions
```
For the purposes of code generation, all static params are treated as generic params - the proc will be compiled separately for each unique supplied value (or combination of values).
Static params can also appear in the signatures of generic types:
```
type
Matrix[M,N: static int; T: Number] = array[0..(M*N - 1), T]
# Note how `Number` is just a type constraint here, while
# `static int` requires us to supply an int value
AffineTransform2D[T] = Matrix[3, 3, T]
AffineTransform3D[T] = Matrix[4, 4, T]
var m1: AffineTransform3D[float] # OK
var m2: AffineTransform2D[string] # Error, `string` is not a `Number`
```
Please note that `static T` is just a syntactic convenience for the underlying generic type `static[T]`. The type param can be omitted to obtain the type class of all constant expressions. A more specific type class can be created by instantiating `static` with another type class.
One can force an expression to be evaluated at compile time as a constant expression by coercing it to a corresponding `static` type:
```
import math
echo static(fac(5)), " ", static[bool](16.isPowerOfTwo)
```
The compiler will report any failure to evaluate the expression or a possible type mismatch error.
### typedesc[T]
In many contexts, Nim treats the names of types as regular values. These values exist only during the compilation phase, but since all values must have a type, `typedesc` is considered their special type.
`typedesc` acts as a generic type. For instance, the type of the symbol `int` is `typedesc[int]`. Just like with regular generic types, when the generic param is omitted, `typedesc` denotes the type class of all types. As a syntactic convenience, one can also use `typedesc` as a modifier.
Procs featuring `typedesc` params are considered implicitly generic. They will be instantiated for each unique combination of supplied types and within the body of the proc, the name of each param will refer to the bound concrete type:
```
proc new(T: typedesc): ref T =
echo "allocating ", T.name
new(result)
var n = Node.new
var tree = new(BinaryTree[int])
```
When multiple type params are present, they will bind freely to different types. To force a bind-once behavior one can use an explicit generic param:
```
proc acceptOnlyTypePairs[T, U](A, B: typedesc[T]; C, D: typedesc[U])
```
Once bound, type params can appear in the rest of the proc signature:
```
template declareVariableWithType(T: typedesc, value: T) =
var x: T = value
declareVariableWithType int, 42
```
Overload resolution can be further influenced by constraining the set of types that will match the type param. This works in practice by attaching attributes to types via templates. The constraint can be a concrete type or a type class.
```
template maxval(T: typedesc[int]): int = high(int)
template maxval(T: typedesc[float]): float = Inf
var i = int.maxval
var f = float.maxval
when false:
var s = string.maxval # error, maxval is not implemented for string
template isNumber(t: typedesc[object]): string = "Don't think so."
template isNumber(t: typedesc[SomeInteger]): string = "Yes!"
template isNumber(t: typedesc[SomeFloat]): string = "Maybe, could be NaN."
echo "is int a number? ", isNumber(int)
echo "is float a number? ", isNumber(float)
echo "is RootObj a number? ", isNumber(RootObj)
```
Passing `typedesc` almost identical, just with the differences that the macro is not instantiated generically. The type expression is simply passed as a `NimNode` to the macro, like everything else.
```
import macros
macro forwardType(arg: typedesc): typedesc =
# ``arg`` is of type ``NimNode``
let tmp: NimNode = arg
result = tmp
var tmp: forwardType(int)
```
### typeof operator
**Note**: `typeof(x)` can for historical reasons also be written as `type(x)` but `type(x)` is discouraged.
One can obtain the type of a given expression by constructing a `typeof` value from it (in many other languages this is known as the typeof operator):
```
var x = 0
var y: typeof(x) # y has type int
```
If `typeof` is used to determine the result type of a proc/iterator/converter call `c(X)` (where `X` stands for a possibly empty list of arguments), the interpretation, where `c` is an iterator, is preferred over the other interpretations, but this behavior can be changed by passing `typeOfProc` as the second argument to `typeof`:
```
iterator split(s: string): string = discard
proc split(s: string): seq[string] = discard
# since an iterator is the preferred interpretation, `y` has the type ``string``:
assert typeof("a b c".split) is string
assert typeof("a b c".split, typeOfProc) is seq[string]
```
Modules
-------
Nim supports splitting a program into pieces by a module concept. Each module needs to be in its own file and has its own namespace. Modules enable information hiding and separate compilation. A module may gain access to symbols of another module by the import statement. Recursive module dependencies are allowed, but slightly subtle. Only top-level symbols that are marked with an asterisk (`*`) are exported. A valid module name can only be a valid Nim identifier (and thus its filename is `identifier.nim`).
The algorithm for compiling modules is:
* compile the whole module as usual, following import statements recursively
* if there is a cycle only import the already parsed symbols (that are exported); if an unknown identifier occurs then abort
This is best illustrated by an example:
```
# Module A
type
T1* = int # Module A exports the type ``T1``
import B # the compiler starts parsing B
proc main() =
var i = p(3) # works because B has been parsed completely here
main()
```
```
# Module B
import A # A is not parsed here! Only the already known symbols
# of A are imported.
proc p*(x: A.T1): A.T1 =
# this works because the compiler has already
# added T1 to A's interface symbol table
result = x + 1
```
#### Import statement
After the `import` statement a list of module names can follow or a single module name followed by an `except` list to prevent some symbols to be imported:
```
import strutils except `%`, toUpperAscii
# doesn't work then:
echo "$1" % "abc".toUpperAscii
```
It is not checked that the `except` list is really exported from the module. This feature allows us to compile against an older version of the module that does not export these identifiers.
The `import` statement is only allowed at the top level.
#### Include statement
The `include` statement does something fundamentally different than importing a module: it merely includes the contents of a file. The `include` statement is useful to split up a large module into several files:
```
include fileA, fileB, fileC
```
The `include` statement can be used outside of the top level, as such:
```
# Module A
echo "Hello World!"
```
```
# Module B
proc main() =
include A
main() # => Hello World!
```
#### Module names in imports
A module alias can be introduced via the `as` keyword:
```
import strutils as su, sequtils as qu
echo su.format("$1", "lalelu")
```
The original module name is then not accessible. The notations `path/to/module` or `"path/to/module"` can be used to refer to a module in subdirectories:
```
import lib/pure/os, "lib/pure/times"
```
Note that the module name is still `strutils` and not `lib/pure/strutils` and so one **cannot** do:
```
import lib/pure/strutils
echo lib/pure/strutils.toUpperAscii("abc")
```
Likewise, the following does not make sense as the name is `strutils` already:
```
import lib/pure/strutils as strutils
```
#### Collective imports from a directory
The syntax `import dir / [moduleA, moduleB]` can be used to import multiple modules from the same directory.
Path names are syntactically either Nim identifiers or string literals. If the path name is not a valid Nim identifier it needs to be a string literal:
```
import "gfx/3d/somemodule" # in quotes because '3d' is not a valid Nim identifier
```
#### Pseudo import/include paths
A directory can also be a so-called "pseudo directory". They can be used to avoid ambiguity when there are multiple modules with the same path.
There are two pseudo directories:
1. `std`: The `std` pseudo directory is the abstract location of Nim's standard library. For example, the syntax `import std / strutils` is used to unambiguously refer to the standard library's `strutils` module.
2. `pkg`: The `pkg` pseudo directory is used to unambiguously refer to a Nimble package. However, for technical details that lie outside of the scope of this document its semantics are: *Use the search path to look for module name but ignore the standard library locations*. In other words, it is the opposite of `std`.
#### From import statement
After the `from` statement, a module name follows followed by an `import` to list the symbols one likes to use without explicit full qualification:
```
from strutils import `%`
echo "$1" % "abc"
# always possible: full qualification:
echo strutils.replace("abc", "a", "z")
```
It's also possible to use `from module import nil` if one wants to import the module but wants to enforce fully qualified access to every symbol in `module`.
#### Export statement
An `export` statement can be used for symbol forwarding so that client modules don't need to import a module's dependencies:
```
# module B
type MyObject* = object
```
```
# module A
import B
export B.MyObject
proc `$`*(x: MyObject): string = "my object"
```
```
# module C
import A
# B.MyObject has been imported implicitly here:
var x: MyObject
echo $x
```
When the exported symbol is another module, all of its definitions will be forwarded. One can use an `except` list to exclude some of the symbols.
Notice that when exporting, one needs to specify only the module name:
```
import foo/bar/baz
export baz
```
### Scope rules
Identifiers are valid from the point of their declaration until the end of the block in which the declaration occurred. The range where the identifier is known is the scope of the identifier. The exact scope of an identifier depends on the way it was declared.
#### Block scope
The *scope* of a variable declared in the declaration part of a block is valid from the point of declaration until the end of the block. If a block contains a second block, in which the identifier is redeclared, then inside this block, the second declaration will be valid. Upon leaving the inner block, the first declaration is valid again. An identifier cannot be redefined in the same block, except if valid for procedure or iterator overloading purposes.
#### Tuple or object scope
The field identifiers inside a tuple or object definition are valid in the following places:
* To the end of the tuple/object definition.
* Field designators of a variable of the given tuple/object type.
* In all descendant types of the object type.
#### Module scope
All identifiers of a module are valid from the point of declaration until the end of the module. Identifiers from indirectly dependent modules are *not* available. The system module is automatically imported in every module.
If a module imports an identifier by two different modules, each occurrence of the identifier has to be qualified unless it is an overloaded procedure or iterator in which case the overloading resolution takes place:
```
# Module A
var x*: string
```
```
# Module B
var x*: int
```
```
# Module C
import A, B
write(stdout, x) # error: x is ambiguous
write(stdout, A.x) # no error: qualifier used
var x = 4
write(stdout, x) # not ambiguous: uses the module C's x
```
Compiler Messages
-----------------
The Nim compiler emits different kinds of messages: hint, warning, and error messages. An *error* message is emitted if the compiler encounters any static error.
Pragmas
-------
Pragmas are Nim's method to give the compiler additional information / commands without introducing a massive number of new keywords. Pragmas are processed on the fly during semantic checking. Pragmas are enclosed in the special `{.` and `.}` curly brackets. Pragmas are also often used as a first implementation to play with a language feature before a nicer syntax to access the feature becomes available.
### deprecated pragma
The deprecated pragma is used to mark a symbol as deprecated:
```
proc p() {.deprecated.}
var x {.deprecated.}: char
```
This pragma can also take in an optional warning string to relay to developers.
```
proc thing(x: bool) {.deprecated: "use thong instead".}
```
### noSideEffect pragma
The `noSideEffect` pragma is used to mark a proc/iterator to have no side effects. This means that the proc/iterator only changes locations that are reachable from its parameters and the return value only depends on the arguments. If none of its parameters have the type `var T` or `ref T` or `ptr T` this means no locations are modified. It is a static error to mark a proc/iterator to have no side effect if the compiler cannot verify this.
As a special semantic rule, the built-in [debugEcho](system#debugEcho,varargs%5Btyped,%5D) pretends to be free of side effects, so that it can be used for debugging routines marked as `noSideEffect`.
`func` is syntactic sugar for a proc with no side effects:
```
func `+` (x, y: int): int
```
To override the compiler's side effect analysis a `{.noSideEffect.}` `cast` pragma block can be used:
```
func f() =
{.cast(noSideEffect).}:
echo "test"
```
### compileTime pragma
The `compileTime` pragma is used to mark a proc or variable to be used only during compile-time execution. No code will be generated for it. Compile-time procs are useful as helpers for macros. Since version 0.12.0 of the language, a proc that uses `system.NimNode` within its parameter types is implicitly declared `compileTime`:
```
proc astHelper(n: NimNode): NimNode =
result = n
```
Is the same as:
```
proc astHelper(n: NimNode): NimNode {.compileTime.} =
result = n
```
`compileTime` variables are available at runtime too. This simplifies certain idioms where variables are filled at compile-time (for example, lookup tables) but accessed at runtime:
```
import macros
var nameToProc {.compileTime.}: seq[(string, proc (): string {.nimcall.})]
macro registerProc(p: untyped): untyped =
result = newTree(nnkStmtList, p)
let procName = p[0]
let procNameAsStr = $p[0]
result.add quote do:
nameToProc.add((`procNameAsStr`, `procName`))
proc foo: string {.registerProc.} = "foo"
proc bar: string {.registerProc.} = "bar"
proc baz: string {.registerProc.} = "baz"
doAssert nameToProc[2][1]() == "baz"
```
### noReturn pragma
The `noreturn` pragma is used to mark a proc that never returns.
### acyclic pragma
The `acyclic` pragma can be used for object types to mark them as acyclic even though they seem to be cyclic. This is an **optimization** for the garbage collector to not consider objects of this type as part of a cycle:
```
type
Node = ref NodeObj
NodeObj {.acyclic.} = object
left, right: Node
data: string
```
Or if we directly use a ref object:
```
type
Node {.acyclic.} = ref object
left, right: Node
data: string
```
In the example, a tree structure is declared with the `Node` type. Note that the type definition is recursive and the GC has to assume that objects of this type may form a cyclic graph. The `acyclic` pragma passes the information that this cannot happen to the GC. If the programmer uses the `acyclic` pragma for data types that are in reality cyclic, the memory leaks can be the result, but memory safety is preserved.
### final pragma
The `final` pragma can be used for an object type to specify that it cannot be inherited from. Note that inheritance is only available for objects that inherit from an existing object (via the `object of SuperType` syntax) or that have been marked as `inheritable`.
### shallow pragma
The `shallow` pragma affects the semantics of a type: The compiler is allowed to make a shallow copy. This can cause serious semantic issues and break memory safety! However, it can speed up assignments considerably, because the semantics of Nim require deep copying of sequences and strings. This can be expensive, especially if sequences are used to build a tree structure:
```
type
NodeKind = enum nkLeaf, nkInner
Node {.shallow.} = object
case kind: NodeKind
of nkLeaf:
strVal: string
of nkInner:
children: seq[Node]
```
### pure pragma
An object type can be marked with the `pure` pragma so that its type field which is used for runtime type identification is omitted. This used to be necessary for binary compatibility with other compiled languages.
An enum type can be marked as `pure`. Then access of its fields always requires full qualification.
### asmNoStackFrame pragma
A proc can be marked with the `asmNoStackFrame` pragma to tell the compiler it should not generate a stack frame for the proc. There are also no exit statements like `return result;` generated and the generated C function is declared as `__declspec(naked)` or `__attribute__((naked))` (depending on the used C compiler).
**Note**: This pragma should only be used by procs which consist solely of assembler statements.
### error pragma
The `error` pragma is used to make the compiler output an error message with the given content. The compilation does not necessarily abort after an error though.
The `error` pragma can also be used to annotate a symbol (like an iterator or proc). The *usage* of the symbol then triggers a static error. This is especially useful to rule out that some operation is valid due to overloading and type conversions:
```
## check that underlying int values are compared and not the pointers:
proc `==`(x, y: ptr int): bool {.error.}
```
### fatal pragma
The `fatal` pragma is used to make the compiler output an error message with the given content. In contrast to the `error` pragma, the compilation is guaranteed to be aborted by this pragma. Example:
```
when not defined(objc):
{.fatal: "Compile this program with the objc command!".}
```
### warning pragma
The `warning` pragma is used to make the compiler output a warning message with the given content. Compilation continues after the warning.
### hint pragma
The `hint` pragma is used to make the compiler output a hint message with the given content. Compilation continues after the hint.
### line pragma
The `line` pragma can be used to affect line information of the annotated statement, as seen in stack backtraces:
```
template myassert*(cond: untyped, msg = "") =
if not cond:
# change run-time line information of the 'raise' statement:
{.line: instantiationInfo().}:
raise newException(EAssertionFailed, msg)
```
If the `line` pragma is used with a parameter, the parameter needs be a `tuple[filename: string, line: int]`. If it is used without a parameter, `system.InstantiationInfo()` is used.
### linearScanEnd pragma
The `linearScanEnd` pragma can be used to tell the compiler how to compile a Nim case statement. Syntactically it has to be used as a statement:
```
case myInt
of 0:
echo "most common case"
of 1:
{.linearScanEnd.}
echo "second most common case"
of 2: echo "unlikely: use branch table"
else: echo "unlikely too: use branch table for ", myInt
```
In the example, the case branches `0` and `1` are much more common than the other cases. Therefore the generated assembler code should test for these values first so that the CPU's branch predictor has a good chance to succeed (avoiding an expensive CPU pipeline stall). The other cases might be put into a jump table for O(1) overhead but at the cost of a (very likely) pipeline stall.
The `linearScanEnd` pragma should be put into the last branch that should be tested against via linear scanning. If put into the last branch of the whole `case` statement, the whole `case` statement uses linear scanning.
### computedGoto pragma
The `computedGoto` pragma can be used to tell the compiler how to compile a Nim case in a `while true` statement. Syntactically it has to be used as a statement inside the loop:
```
type
MyEnum = enum
enumA, enumB, enumC, enumD, enumE
proc vm() =
var instructions: array[0..100, MyEnum]
instructions[2] = enumC
instructions[3] = enumD
instructions[4] = enumA
instructions[5] = enumD
instructions[6] = enumC
instructions[7] = enumA
instructions[8] = enumB
instructions[12] = enumE
var pc = 0
while true:
{.computedGoto.}
let instr = instructions[pc]
case instr
of enumA:
echo "yeah A"
of enumC, enumD:
echo "yeah CD"
of enumB:
echo "yeah B"
of enumE:
break
inc(pc)
vm()
```
As the example shows `computedGoto` is mostly useful for interpreters. If the underlying backend (C compiler) does not support the computed goto extension the pragma is simply ignored.
### immediate pragma
The immediate pragma is obsolete. See [Typed vs untyped parameters](#templates-typed-vs-untyped-parameters).
### compilation option pragmas
The listed pragmas here can be used to override the code generation options for a proc/method/converter.
The implementation currently provides the following possible options (various others may be added later).
| pragma | allowed values | description |
| --- | --- | --- |
| checks | on|off | Turns the code generation for all runtime checks on or off. |
| boundChecks | on|off | Turns the code generation for array bound checks on or off. |
| overflowChecks | on|off | Turns the code generation for over- or underflow checks on or off. |
| nilChecks | on|off | Turns the code generation for nil pointer checks on or off. |
| assertions | on|off | Turns the code generation for assertions on or off. |
| warnings | on|off | Turns the warning messages of the compiler on or off. |
| hints | on|off | Turns the hint messages of the compiler on or off. |
| optimization | none|speed|size | Optimize the code for speed or size, or disable optimization. |
| patterns | on|off | Turns the term rewriting templates/macros on or off. |
| callconv | cdecl|... | Specifies the default calling convention for all procedures (and procedure types) that follow. |
Example:
```
{.checks: off, optimization: speed.}
# compile without runtime checks and optimize for speed
```
### push and pop pragmas
The push/pop pragmas are very similar to the option directive, but are used to override the settings temporarily. Example:
```
{.push checks: off.}
# compile this section without runtime checks as it is
# speed critical
# ... some code ...
{.pop.} # restore old settings
```
push/pop can switch on/off some standard library pragmas, example:
```
{.push inline.}
proc thisIsInlined(): int = 42
func willBeInlined(): float = 42.0
{.pop.}
proc notInlined(): int = 9
{.push discardable, boundChecks: off, compileTime, noSideEffect, experimental.}
template example(): string = "https://nim-lang.org"
{.pop.}
{.push deprecated, hint[LineTooLong]: off, used, stackTrace: off.}
proc sample(): bool = true
{.pop.}
```
For third party pragmas, it depends on its implementation but uses the same syntax.
### register pragma
The `register` pragma is for variables only. It declares the variable as `register`, giving the compiler a hint that the variable should be placed in a hardware register for faster access. C compilers usually ignore this though and for good reasons: Often they do a better job without it anyway.
In highly specific cases (a dispatch loop of a bytecode interpreter for example) it may provide benefits, though.
### global pragma
The `global` pragma can be applied to a variable within a proc to instruct the compiler to store it in a global location and initialize it once at program startup.
```
proc isHexNumber(s: string): bool =
var pattern {.global.} = re"[0-9a-fA-F]+"
result = s.match(pattern)
```
When used within a generic proc, a separate unique global variable will be created for each instantiation of the proc. The order of initialization of the created global variables within a module is not defined, but all of them will be initialized after any top-level variables in their originating module and before any variable in a module that imports it.
### Disabling certain messages
Nim generates some warnings and hints ("line too long") that may annoy the user. A mechanism for disabling certain messages is provided: Each hint and warning message contains a symbol in brackets. This is the message's identifier that can be used to enable or disable it:
```
{.hint[LineTooLong]: off.} # turn off the hint about too long lines
```
This is often better than disabling all warnings at once.
### used pragma
Nim produces a warning for symbols that are not exported and not used either. The `used` pragma can be attached to a symbol to suppress this warning. This is particularly useful when the symbol was generated by a macro:
```
template implementArithOps(T) =
proc echoAdd(a, b: T) {.used.} =
echo a + b
proc echoSub(a, b: T) {.used.} =
echo a - b
# no warning produced for the unused 'echoSub'
implementArithOps(int)
echoAdd 3, 5
```
`used` can also be used as a top-level statement to mark a module as "used". This prevents the "Unused import" warning:
```
# module: debughelper.nim
when defined(nimHasUsed):
# 'import debughelper' is so useful for debugging
# that Nim shouldn't produce a warning for that import,
# even if currently unused:
{.used.}
```
### experimental pragma
The `experimental` pragma enables experimental language features. Depending on the concrete feature, this means that the feature is either considered too unstable for an otherwise stable release or that the future of the feature is uncertain (it may be removed at any time).
Example:
```
import threadpool
{.experimental: "parallel".}
proc threadedEcho(s: string, i: int) =
echo(s, " ", $i)
proc useParallel() =
parallel:
for i in 0..4:
spawn threadedEcho("echo in parallel", i)
useParallel()
```
As a top-level statement, the experimental pragma enables a feature for the rest of the module it's enabled in. This is problematic for macro and generic instantiations that cross a module scope. Currently, these usages have to be put into a `.push/pop` environment:
```
# client.nim
proc useParallel*[T](unused: T) =
# use a generic T here to show the problem.
{.push experimental: "parallel".}
parallel:
for i in 0..4:
echo "echo in parallel"
{.pop.}
```
```
import client
useParallel(1)
```
Implementation Specific Pragmas
-------------------------------
This section describes additional pragmas that the current Nim implementation supports but which should not be seen as part of the language specification.
### Bitsize pragma
The `bitsize` pragma is for object field members. It declares the field as a bitfield in C/C++.
```
type
mybitfield = object
flag {.bitsize:1.}: cuint
```
generates:
```
struct mybitfield {
unsigned int flag:1;
};
```
### Align pragma
The align pragma is for variables and object field members. It modifies the alignment requirement of the entity being declared. The argument must be a constant power of 2. Valid non-zero alignments that are weaker than other align pragmas on the same declaration are ignored. Alignments that are weaker than the alignment requirement of the type are ignored.
```
type
sseType = object
sseData {.align(16).}: array[4, float32]
# every object will be aligned to 128-byte boundary
Data = object
x: char
cacheline {.align(128).}: array[128, char] # over-aligned array of char,
proc main() =
echo "sizeof(Data) = ", sizeof(Data), " (1 byte + 127 bytes padding + 128-byte array)"
# output: sizeof(Data) = 256 (1 byte + 127 bytes padding + 128-byte array)
echo "alignment of sseType is ", alignof(sseType)
# output: alignment of sseType is 16
var d {.align(2048).}: Data # this instance of data is aligned even stricter
main()
```
This pragma has no effect on the JS backend.
### Volatile pragma
The `volatile` pragma is for variables only. It declares the variable as `volatile`, whatever that means in C/C++ (its semantics are not well defined in C/C++).
**Note**: This pragma will not exist for the LLVM backend.
### NoDecl pragma
The `noDecl` pragma can be applied to almost any symbol (variable, proc, type, etc.) and is sometimes useful for interoperability with C: It tells Nim that it should not generate a declaration for the symbol in the C code. For example:
```
var
EACCES {.importc, noDecl.}: cint # pretend EACCES was a variable, as
# Nim does not know its value
```
However, the `header` pragma is often the better alternative.
**Note**: This will not work for the LLVM backend.
### Header pragma
The `header` pragma is very similar to the `noDecl` pragma: It can be applied to almost any symbol and specifies that it should not be declared and instead, the generated code should contain an `#include`:
```
type
PFile {.importc: "FILE*", header: "<stdio.h>".} = distinct pointer
# import C's FILE* type; Nim will treat it as a new pointer type
```
The `header` pragma always expects a string constant. The string constant contains the header file: As usual for C, a system header file is enclosed in angle brackets: `<>`. If no angle brackets are given, Nim encloses the header file in `""` in the generated C code.
**Note**: This will not work for the LLVM backend.
### IncompleteStruct pragma
The `incompleteStruct` pragma tells the compiler to not use the underlying C `struct` in a `sizeof` expression:
```
type
DIR* {.importc: "DIR", header: "<dirent.h>",
pure, incompleteStruct.} = object
```
### Compile pragma
The `compile` pragma can be used to compile and link a C/C++ source file with the project:
```
{.compile: "myfile.cpp".}
```
**Note**: Nim computes a SHA1 checksum and only recompiles the file if it has changed. One can use the `-f` command-line option to force the recompilation of the file.
Since 1.4 the `compile` pragma is also available with this syntax:
```
{.compile("myfile.cpp", "--custom flags here").}
```
As can be seen in the example, this new variant allows for custom flags that are passed to the C compiler when the file is recompiled.
### Link pragma
The `link` pragma can be used to link an additional file with the project:
```
{.link: "myfile.o".}
```
### PassC pragma
The `passc` pragma can be used to pass additional parameters to the C compiler like one would using the command-line switch `--passc`:
```
{.passc: "-Wall -Werror".}
```
Note that one can use `gorge` from the [system module](system) to embed parameters from an external command that will be executed during semantic analysis:
```
{.passc: gorge("pkg-config --cflags sdl").}
```
### LocalPassc pragma
The `localPassc` pragma can be used to pass additional parameters to the C compiler, but only for the C/C++ file that is produced from the Nim module the pragma resides in:
```
# Module A.nim
# Produces: A.nim.cpp
{.localPassc: "-Wall -Werror".} # Passed when compiling A.nim.cpp
```
### PassL pragma
The `passL` pragma can be used to pass additional parameters to the linker like one would be using the command-line switch `--passL`:
```
{.passL: "-lSDLmain -lSDL".}
```
Note that one can use `gorge` from the [system module](system) to embed parameters from an external command that will be executed during semantic analysis:
```
{.passL: gorge("pkg-config --libs sdl").}
```
### Emit pragma
The `emit` pragma can be used to directly affect the output of the compiler's code generator. The code is then unportable to other code generators/backends. Its usage is highly discouraged! However, it can be extremely useful for interfacing with C++ or Objective C code.
Example:
```
{.emit: """
static int cvariable = 420;
""".}
{.push stackTrace:off.}
proc embedsC() =
var nimVar = 89
# access Nim symbols within an emit section outside of string literals:
{.emit: ["""fprintf(stdout, "%d\n", cvariable + (int)""", nimVar, ");"].}
{.pop.}
embedsC()
```
`nimbase.h` defines `NIM_EXTERNC` C macro that can be used for `extern "C"` code to work with both `nim c` and `nim cpp`, e.g.:
```
proc foobar() {.importc:"$1".}
{.emit: """
#include <stdio.h>
NIM_EXTERNC
void fun(){}
""".}
```
For backward compatibility, if the argument to the `emit` statement is a single string literal, Nim symbols can be referred to via backticks. This usage is however deprecated.
For a toplevel emit statement the section where in the generated C/C++ file the code should be emitted can be influenced via the prefixes `/*TYPESECTION*/` or `/*VARSECTION*/` or `/*INCLUDESECTION*/`:
```
{.emit: """/*TYPESECTION*/
struct Vector3 {
public:
Vector3(): x(5) {}
Vector3(float x_): x(x_) {}
float x;
};
""".}
type Vector3 {.importcpp: "Vector3", nodecl} = object
x: cfloat
proc constructVector3(a: cfloat): Vector3 {.importcpp: "Vector3(@)", nodecl}
```
### ImportCpp pragma
**Note**: [c2nim](https://github.com/nim-lang/c2nim/blob/master/doc/c2nim.rst) can parse a large subset of C++ and knows about the `importcpp` pragma pattern language. It is not necessary to know all the details described here.
Similar to the [importc pragma for C](#foreign-function-interface-importc-pragma), the `importcpp` pragma can be used to import C++ methods or C++ symbols in general. The generated code then uses the C++ method calling syntax: `obj->method(arg)`. In combination with the `header` and `emit` pragmas this allows *sloppy* interfacing with libraries written in C++:
```
# Horrible example of how to interface with a C++ engine ... ;-)
{.link: "/usr/lib/libIrrlicht.so".}
{.emit: """
using namespace irr;
using namespace core;
using namespace scene;
using namespace video;
using namespace io;
using namespace gui;
""".}
const
irr = "<irrlicht/irrlicht.h>"
type
IrrlichtDeviceObj {.header: irr,
importcpp: "IrrlichtDevice".} = object
IrrlichtDevice = ptr IrrlichtDeviceObj
proc createDevice(): IrrlichtDevice {.
header: irr, importcpp: "createDevice(@)".}
proc run(device: IrrlichtDevice): bool {.
header: irr, importcpp: "#.run(@)".}
```
The compiler needs to be told to generate C++ (command `cpp`) for this to work. The conditional symbol `cpp` is defined when the compiler emits C++ code.
#### Namespaces
The *sloppy interfacing* example uses `.emit` to produce `using namespace` declarations. It is usually much better to instead refer to the imported name via the `namespace::identifier` notation:
```
type
IrrlichtDeviceObj {.header: irr,
importcpp: "irr::IrrlichtDevice".} = object
```
#### Importcpp for enums
When `importcpp` is applied to an enum type the numerical enum values are annotated with the C++ enum type, like in this example: `((TheCppEnum)(3))`. (This turned out to be the simplest way to implement it.)
#### Importcpp for procs
Note that the `importcpp` variant for procs uses a somewhat cryptic pattern language for maximum flexibility:
* A hash `#` symbol is replaced by the first or next argument.
* A dot following the hash `#.` indicates that the call should use C++'s dot or arrow notation.
* An at symbol `@` is replaced by the remaining arguments, separated by commas.
For example:
```
proc cppMethod(this: CppObj, a, b, c: cint) {.importcpp: "#.CppMethod(@)".}
var x: ptr CppObj
cppMethod(x[], 1, 2, 3)
```
Produces:
```
x->CppMethod(1, 2, 3)
```
As a special rule to keep backward compatibility with older versions of the `importcpp` pragma, if there is no special pattern character (any of `# ' @`) at all, C++'s dot or arrow notation is assumed, so the above example can also be written as:
```
proc cppMethod(this: CppObj, a, b, c: cint) {.importcpp: "CppMethod".}
```
Note that the pattern language naturally also covers C++'s operator overloading capabilities:
```
proc vectorAddition(a, b: Vec3): Vec3 {.importcpp: "# + #".}
proc dictLookup(a: Dict, k: Key): Value {.importcpp: "#[#]".}
```
* An apostrophe `'` followed by an integer `i` in the range 0..9 is replaced by the i'th parameter *type*. The 0th position is the result type. This can be used to pass types to C++ function templates. Between the `'` and the digit, an asterisk can be used to get to the base type of the type. (So it "takes away a star" from the type; `T*` becomes `T`.) Two stars can be used to get to the element type of the element type etc.
For example:
```
type Input {.importcpp: "System::Input".} = object
proc getSubsystem*[T](): ptr T {.importcpp: "SystemManager::getSubsystem<'*0>()", nodecl.}
let x: ptr Input = getSubsystem[Input]()
```
Produces:
```
x = SystemManager::getSubsystem<System::Input>()
```
* `#@` is a special case to support a `cnew` operation. It is required so that the call expression is inlined directly, without going through a temporary location. This is only required to circumvent a limitation of the current code generator.
For example C++'s `new` operator can be "imported" like this:
```
proc cnew*[T](x: T): ptr T {.importcpp: "(new '*0#@)", nodecl.}
# constructor of 'Foo':
proc constructFoo(a, b: cint): Foo {.importcpp: "Foo(@)".}
let x = cnew constructFoo(3, 4)
```
Produces:
```
x = new Foo(3, 4)
```
However, depending on the use case `new Foo` can also be wrapped like this instead:
```
proc newFoo(a, b: cint): ptr Foo {.importcpp: "new Foo(@)".}
let x = newFoo(3, 4)
```
#### Wrapping constructors
Sometimes a C++ class has a private copy constructor and so code like `Class c = Class(1,2);` must not be generated but instead `Class c(1,2);`. For this purpose the Nim proc that wraps a C++ constructor needs to be annotated with the constructor pragma. This pragma also helps to generate faster C++ code since construction then doesn't invoke the copy constructor:
```
# a better constructor of 'Foo':
proc constructFoo(a, b: cint): Foo {.importcpp: "Foo(@)", constructor.}
```
#### Wrapping destructors
Since Nim generates C++ directly, any destructor is called implicitly by the C++ compiler at the scope exits. This means that often one can get away with not wrapping the destructor at all! However, when it needs to be invoked explicitly, it needs to be wrapped. The pattern language provides everything that is required:
```
proc destroyFoo(this: var Foo) {.importcpp: "#.~Foo()".}
```
#### Importcpp for objects
Generic `importcpp`'ed objects are mapped to C++ templates. This means that one can import C++'s templates rather easily without the need for a pattern language for object types:
```
type
StdMap {.importcpp: "std::map", header: "<map>".} [K, V] = object
proc `[]=`[K, V](this: var StdMap[K, V]; key: K; val: V) {.
importcpp: "#[#] = #", header: "<map>".}
var x: StdMap[cint, cdouble]
x[6] = 91.4
```
Produces:
```
std::map<int, double> x;
x[6] = 91.4;
```
* If more precise control is needed, the apostrophe `'` can be used in the supplied pattern to denote the concrete type parameters of the generic type. See the usage of the apostrophe operator in proc patterns for more details.
```
type
VectorIterator {.importcpp: "std::vector<'0>::iterator".} [T] = object
var x: VectorIterator[cint]
```
Produces:
```
std::vector<int>::iterator x;
```
### ImportJs pragma
Similar to the [importcpp pragma for C++](#implementation-specific-pragmas-importcpp-pragma), the `importjs` pragma can be used to import Javascript methods or symbols in general. The generated code then uses the Javascript method calling syntax: `obj.method(arg)`.
### ImportObjC pragma
Similar to the [importc pragma for C](#foreign-function-interface-importc-pragma), the `importobjc` pragma can be used to import Objective C methods. The generated code then uses the Objective C method calling syntax: `[obj method param1: arg]`. In addition with the `header` and `emit` pragmas this allows *sloppy* interfacing with libraries written in Objective C:
```
# horrible example of how to interface with GNUStep ...
{.passL: "-lobjc".}
{.emit: """
#include <objc/Object.h>
@interface Greeter:Object
{
}
- (void)greet:(long)x y:(long)dummy;
@end
#include <stdio.h>
@implementation Greeter
- (void)greet:(long)x y:(long)dummy
{
printf("Hello, World!\n");
}
@end
#include <stdlib.h>
""".}
type
Id {.importc: "id", header: "<objc/Object.h>", final.} = distinct int
proc newGreeter: Id {.importobjc: "Greeter new", nodecl.}
proc greet(self: Id, x, y: int) {.importobjc: "greet", nodecl.}
proc free(self: Id) {.importobjc: "free", nodecl.}
var g = newGreeter()
g.greet(12, 34)
g.free()
```
The compiler needs to be told to generate Objective C (command `objc`) for this to work. The conditional symbol `objc` is defined when the compiler emits Objective C code.
### CodegenDecl pragma
The `codegenDecl` pragma can be used to directly influence Nim's code generator. It receives a format string that determines how the variable or proc is declared in the generated code.
For variables, $1 in the format string represents the type of the variable and $2 is the name of the variable.
The following Nim code:
```
var
a {.codegenDecl: "$# progmem $#".}: int
```
will generate this C code:
```
int progmem a
```
For procedures $1 is the return type of the procedure, $2 is the name of the procedure and $3 is the parameter list.
The following nim code:
```
proc myinterrupt() {.codegenDecl: "__interrupt $# $#$#".} =
echo "realistic interrupt handler"
```
will generate this code:
```
__interrupt void myinterrupt()
```
### InjectStmt pragma
The `injectStmt` pragma can be used to inject a statement before every other statement in the current module. It is only supposed to be used for debugging:
```
{.injectStmt: gcInvariants().}
# ... complex code here that produces crashes ...
```
### compile-time define pragmas
The pragmas listed here can be used to optionally accept values from the -d/--define option at compile time.
The implementation currently provides the following possible options (various others may be added later).
| pragma | description |
| --- | --- |
| intdefine | Reads in a build-time define as an integer |
| strdefine | Reads in a build-time define as a string |
| booldefine | Reads in a build-time define as a bool |
```
const FooBar {.intdefine.}: int = 5
echo FooBar
```
```
nim c -d:FooBar=42 foobar.nim
```
In the above example, providing the -d flag causes the symbol `FooBar` to be overwritten at compile-time, printing out 42. If the `-d:FooBar=42` were to be omitted, the default value of 5 would be used. To see if a value was provided, `defined(FooBar)` can be used.
The syntax `-d:flag` is actually just a shortcut for `-d:flag=true`.
User-defined pragmas
--------------------
### pragma pragma
The `pragma` pragma can be used to declare user-defined pragmas. This is useful because Nim's templates and macros do not affect pragmas. User-defined pragmas are in a different module-wide scope than all other symbols. They cannot be imported from a module.
Example:
```
when appType == "lib":
{.pragma: rtl, exportc, dynlib, cdecl.}
else:
{.pragma: rtl, importc, dynlib: "client.dll", cdecl.}
proc p*(a, b: int): int {.rtl.} =
result = a+b
```
In the example, a new pragma named `rtl` is introduced that either imports a symbol from a dynamic library or exports the symbol for dynamic library generation.
### Custom annotations
It is possible to define custom typed pragmas. Custom pragmas do not affect code generation directly, but their presence can be detected by macros. Custom pragmas are defined using templates annotated with pragma `pragma`:
```
template dbTable(name: string, table_space: string = "") {.pragma.}
template dbKey(name: string = "", primary_key: bool = false) {.pragma.}
template dbForeignKey(t: typedesc) {.pragma.}
template dbIgnore {.pragma.}
```
Consider stylized example of possible Object Relation Mapping (ORM) implementation:
```
const tblspace {.strdefine.} = "dev" # switch for dev, test and prod environments
type
User {.dbTable("users", tblspace).} = object
id {.dbKey(primary_key = true).}: int
name {.dbKey"full_name".}: string
is_cached {.dbIgnore.}: bool
age: int
UserProfile {.dbTable("profiles", tblspace).} = object
id {.dbKey(primary_key = true).}: int
user_id {.dbForeignKey: User.}: int
read_access: bool
write_access: bool
admin_acess: bool
```
In this example, custom pragmas are used to describe how Nim objects are mapped to the schema of the relational database. Custom pragmas can have zero or more arguments. In order to pass multiple arguments use one of template call syntaxes. All arguments are typed and follow standard overload resolution rules for templates. Therefore, it is possible to have default values for arguments, pass by name, varargs, etc.
Custom pragmas can be used in all locations where ordinary pragmas can be specified. It is possible to annotate procs, templates, type and variable definitions, statements, etc.
Macros module includes helpers which can be used to simplify custom pragma access `hasCustomPragma`, `getCustomPragmaVal`. Please consult the macros module documentation for details. These macros are not magic, everything they do can also be achieved by walking the AST of the object representation.
More examples with custom pragmas:
* Better serialization/deserialization control:
```
type MyObj = object
a {.dontSerialize.}: int
b {.defaultDeserialize: 5.}: int
c {.serializationKey: "_c".}: string
```
* Adopting type for gui inspector in a game engine:
```
type MyComponent = object
position {.editable, animatable.}: Vector3
alpha {.editRange: [0.0..1.0], animatable.}: float32
```
### Macro pragmas
All macros and templates can also be used as pragmas. They can be attached to routines (procs, iterators, etc), type names, or type expressions. The compiler will perform the following simple syntactic transformations:
```
template command(name: string, def: untyped) = discard
proc p() {.command("print").} = discard
```
This is translated to:
```
command("print"):
proc p() = discard
```
---
```
type
AsyncEventHandler = proc (x: Event) {.async.}
```
This is translated to:
```
type
AsyncEventHandler = async(proc (x: Event))
```
---
```
type
MyObject {.schema: "schema.protobuf".} = object
```
This is translated to a call to the `schema` macro with a `nnkTypeDef` AST node capturing both the left-hand side and right-hand side of the definition. The macro can return a potentially modified `nnkTypeDef` tree which will replace the original row in the type section.
When multiple macro pragmas are applied to the same definition, the compiler will apply them consequently from left to right. Each macro will receive as input the output of the previous one.
Foreign function interface
--------------------------
Nim's FFI (foreign function interface) is extensive and only the parts that scale to other future backends (like the LLVM/JavaScript backends) are documented here.
### Importc pragma
The `importc` pragma provides a means to import a proc or a variable from C. The optional argument is a string containing the C identifier. If the argument is missing, the C name is the Nim identifier *exactly as spelled*:
```
proc printf(formatstr: cstring) {.header: "<stdio.h>", importc: "printf", varargs.}
```
When `importc` is applied to a `let` statement it can omit its value which will then be expected to come from C. This can be used to import a C `const`:
```
{.emit: "const int cconst = 42;".}
let cconst {.importc, nodecl.}: cint
assert cconst == 42
```
Note that this pragma has been abused in the past to also work in the js backend for js objects and functions. : Other backends do provide the same feature under the same name. Also, when the target language is not set to C, other pragmas are available:
>
> * [importcpp](manual#implementation-specific-pragmas-importcpp-pragma)
> * [importobjc](manual#implementation-specific-pragmas-importobjc-pragma)
> * [importjs](manual#implementation-specific-pragmas-importjs-pragma)
>
>
```
proc p(s: cstring) {.importc: "prefix$1".}
```
In the example, the external name of `p` is set to `prefixp`. Only `$1` is available and a literal dollar sign must be written as `$$`.
### Exportc pragma
The `exportc` pragma provides a means to export a type, a variable, or a procedure to C. Enums and constants can't be exported. The optional argument is a string containing the C identifier. If the argument is missing, the C name is the Nim identifier *exactly as spelled*:
```
proc callme(formatstr: cstring) {.exportc: "callMe", varargs.}
```
Note that this pragma is somewhat of a misnomer: Other backends do provide the same feature under the same name.
The string literal passed to `exportc` can be a format string:
```
proc p(s: string) {.exportc: "prefix$1".} =
echo s
```
In the example the external name of `p` is set to `prefixp`. Only `$1` is available and a literal dollar sign must be written as `$$`.
If the symbol should also be exported to a dynamic library, the `dynlib` pragma should be used in addition to the `exportc` pragma. See [Dynlib pragma for export](#foreign-function-interface-dynlib-pragma-for-export).
### Extern pragma
Like `exportc` or `importc`, the `extern` pragma affects name mangling. The string literal passed to `extern` can be a format string:
```
proc p(s: string) {.extern: "prefix$1".} =
echo s
```
In the example, the external name of `p` is set to `prefixp`. Only `$1` is available and a literal dollar sign must be written as `$$`.
### Bycopy pragma
The `bycopy` pragma can be applied to an object or tuple type and instructs the compiler to pass the type by value to procs:
```
type
Vector {.bycopy.} = object
x, y, z: float
```
### Byref pragma
The `byref` pragma can be applied to an object or tuple type and instructs the compiler to pass the type by reference (hidden pointer) to procs.
### Varargs pragma
The `varargs` pragma can be applied to procedures only (and procedure types). It tells Nim that the proc can take a variable number of parameters after the last specified parameter. Nim string values will be converted to C strings automatically:
```
proc printf(formatstr: cstring) {.nodecl, varargs.}
printf("hallo %s", "world") # "world" will be passed as C string
```
### Union pragma
The `union` pragma can be applied to any `object` type. It means all of the object's fields are overlaid in memory. This produces a `union` instead of a `struct` in the generated C/C++ code. The object declaration then must not use inheritance or any GC'ed memory but this is currently not checked.
**Future directions**: GC'ed memory should be allowed in unions and the GC should scan unions conservatively.
### Packed pragma
The `packed` pragma can be applied to any `object` type. It ensures that the fields of an object are packed back-to-back in memory. It is useful to store packets or messages from/to network or hardware drivers, and for interoperability with C. Combining packed pragma with inheritance is not defined, and it should not be used with GC'ed memory (ref's).
**Future directions**: Using GC'ed memory in packed pragma will result in a static error. Usage with inheritance should be defined and documented.
### Dynlib pragma for import
With the `dynlib` pragma a procedure or a variable can be imported from a dynamic library (`.dll` files for Windows, `lib*.so` files for UNIX). The non-optional argument has to be the name of the dynamic library:
```
proc gtk_image_new(): PGtkWidget
{.cdecl, dynlib: "libgtk-x11-2.0.so", importc.}
```
In general, importing a dynamic library does not require any special linker options or linking with import libraries. This also implies that no *devel* packages need to be installed.
The `dynlib` import mechanism supports a versioning scheme:
```
proc Tcl_Eval(interp: pTcl_Interp, script: cstring): int {.cdecl,
importc, dynlib: "libtcl(|8.5|8.4|8.3).so.(1|0)".}
```
At runtime the dynamic library is searched for (in this order):
```
libtcl.so.1
libtcl.so.0
libtcl8.5.so.1
libtcl8.5.so.0
libtcl8.4.so.1
libtcl8.4.so.0
libtcl8.3.so.1
libtcl8.3.so.0
```
The `dynlib` pragma supports not only constant strings as an argument but also string expressions in general:
```
import os
proc getDllName: string =
result = "mylib.dll"
if fileExists(result): return
result = "mylib2.dll"
if fileExists(result): return
quit("could not load dynamic library")
proc myImport(s: cstring) {.cdecl, importc, dynlib: getDllName().}
```
**Note**: Patterns like `libtcl(|8.5|8.4).so` are only supported in constant strings, because they are precompiled.
**Note**: Passing variables to the `dynlib` pragma will fail at runtime because of order of initialization problems.
**Note**: A `dynlib` import can be overridden with the `--dynlibOverride:name` command-line option. The Compiler User Guide contains further information.
### Dynlib pragma for export
With the `dynlib` pragma a procedure can also be exported to a dynamic library. The pragma then has no argument and has to be used in conjunction with the `exportc` pragma:
```
proc exportme(): int {.cdecl, exportc, dynlib.}
```
This is only useful if the program is compiled as a dynamic library via the `--app:lib` command-line option.
Threads
-------
To enable thread support the `--threads:on` command-line switch needs to be used. The `system` module then contains several threading primitives. See the <threads> and <channels> modules for the low-level thread API. There are also high-level parallelism constructs available. See [spawn](manual_experimental#parallel-amp-spawn) for further details.
Nim's memory model for threads is quite different than that of other common programming languages (C, Pascal, Java): Each thread has its own (garbage collected) heap, and sharing of memory is restricted to global variables. This helps to prevent race conditions. GC efficiency is improved quite a lot, because the GC never has to stop other threads and see what they reference.
### Thread pragma
A proc that is executed as a new thread of execution should be marked by the `thread` pragma for reasons of readability. The compiler checks for violations of the no heap sharing restriction: This restriction implies that it is invalid to construct a data structure that consists of memory allocated from different (thread-local) heaps.
A thread proc is passed to `createThread` or `spawn` and invoked indirectly; so the `thread` pragma implies `procvar`.
### GC safety
We call a proc `p` GC safe when it doesn't access any global variable that contains GC'ed memory (`string`, `seq`, `ref` or a closure) either directly or indirectly through a call to a GC unsafe proc.
The gcsafe annotation can be used to mark a proc to be gcsafe, otherwise this property is inferred by the compiler. Note that `noSideEffect` implies `gcsafe`. The only way to create a thread is via `spawn` or `createThread`. The invoked proc must not use `var` parameters nor must any of its parameters contain a `ref` or `closure` type. This enforces the *no heap sharing restriction*.
Routines that are imported from C are always assumed to be `gcsafe`. To disable the GC-safety checking the `--threadAnalysis:off` command-line switch can be used. This is a temporary workaround to ease the porting effort from old code to the new threading model.
To override the compiler's gcsafety analysis a `{.cast(gcsafe).}` pragma block can be used:
```
var
someGlobal: string = "some string here"
perThread {.threadvar.}: string
proc setPerThread() =
{.cast(gcsafe).}:
deepCopy(perThread, someGlobal)
```
See also:
* [Shared heap memory management.](gc).
### Threadvar pragma
A variable can be marked with the `threadvar` pragma, which makes it a thread-local variable; Additionally, this implies all the effects of the `global` pragma.
```
var checkpoints* {.threadvar.}: seq[string]
```
Due to implementation restrictions thread-local variables cannot be initialized within the `var` section. (Every thread-local variable needs to be replicated at thread creation.)
### Threads and exceptions
The interaction between threads and exceptions is simple: A *handled* exception in one thread cannot affect any other thread. However, an *unhandled* exception in one thread terminates the whole *process*!
| programming_docs |
nim encodings encodings
=========
Converts between different character encodings. On UNIX, this uses the iconv library, on Windows the Windows API.
Imports
-------
<os> Types
-----
```
EncodingConverter = ptr ConverterObj
```
can convert between two character sets [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L18)
```
EncodingError = object of ValueError
```
exception that is raised for encoding errors [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L27) Procs
-----
```
proc getCurrentEncoding(uiApp = false): string {...}{.raises: [], tags: [].}
```
retrieves the current encoding. On Unix, always "UTF-8" is returned. The `uiApp` parameter is Windows specific. If true, the UI's code-page is returned, if false, the Console's code-page is returned. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L297) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L297)
```
proc open(destEncoding = "UTF-8"; srcEncoding = "CP1252"): EncodingConverter {...}{.
raises: [EncodingError], tags: [].}
```
opens a converter that can convert from `srcEncoding` to `destEncoding`. Raises `IOError` if it cannot fulfill the request. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L306)
```
proc close(c: EncodingConverter) {...}{.raises: [], tags: [].}
```
frees the resources the converter `c` holds. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L325) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L325)
```
proc convert(c: EncodingConverter; s: string): string {...}{.raises: [OSError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L426) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L426)
```
proc convert(s: string; destEncoding = "UTF-8"; srcEncoding = "CP1252"): string {...}{.
raises: [EncodingError, OSError], tags: [].}
```
converts `s` to `destEncoding`. It assumed that `s` is in `srcEncoding`. This opens a converter, uses it and closes it again and is thus more convenient but also likely less efficient than re-using a converter. utf-16BE, utf-32 conversions not supported on windows [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/encodings.nim#L465) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/encodings.nim#L465)
nim strformat strformat
=========
String interpolation / format inspired by Python's `f`-strings.
`fmt` vs. `&`
--------------
You can use either `fmt` or the unary `&` operator for formatting. The difference between them is subtle but important.
The `fmt"{expr}"` syntax is more aesthetically pleasing, but it hides a small gotcha. The string is a [generalized raw string literal](manual#lexical-analysis-generalized-raw-string-literals). This has some surprising effects:
```
import strformat
let msg = "hello"
doAssert fmt"{msg}\n" == "hello\\n"
```
Because the literal is a raw string literal, the `\n` is not interpreted as an escape sequence.
There are multiple ways to get around this, including the use of the `&` operator:
```
import strformat
let msg = "hello"
doAssert &"{msg}\n" == "hello\n"
doAssert fmt"{msg}{'\n'}" == "hello\n"
doAssert fmt("{msg}\n") == "hello\n"
doAssert "{msg}\n".fmt == "hello\n"
```
The choice of style is up to you.
Formatting strings
------------------
```
import strformat
doAssert &"""{"abc":>4}""" == " abc"
doAssert &"""{"abc":<4}""" == "abc "
```
Formatting floats
-----------------
```
import strformat
doAssert fmt"{-12345:08}" == "-0012345"
doAssert fmt"{-1:3}" == " -1"
doAssert fmt"{-1:03}" == "-01"
doAssert fmt"{16:#X}" == "0x10"
doAssert fmt"{123.456}" == "123.456"
doAssert fmt"{123.456:>9.3f}" == " 123.456"
doAssert fmt"{123.456:9.3f}" == " 123.456"
doAssert fmt"{123.456:9.4f}" == " 123.4560"
doAssert fmt"{123.456:>9.0f}" == " 123."
doAssert fmt"{123.456:<9.4f}" == "123.4560 "
doAssert fmt"{123.456:e}" == "1.234560e+02"
doAssert fmt"{123.456:>13e}" == " 1.234560e+02"
doAssert fmt"{123.456:13e}" == " 1.234560e+02"
```
Debugging strings
-----------------
`fmt"{expr=}"` expands to `fmt"expr={expr}"` namely the text of the expression, an equal sign and the results of evaluated expression.
```
import strformat
doAssert fmt"{123.456=}" == "123.456=123.456"
doAssert fmt"{123.456=:>9.3f}" == "123.456= 123.456"
let x = "hello"
doAssert fmt"{x=}" == "x=hello"
doAssert fmt"{x =}" == "x =hello"
let y = 3.1415926
doAssert fmt"{y=:.2f}" == fmt"y={y:.2f}"
doAssert fmt"{y=}" == fmt"y={y}"
doAssert fmt"{y = : <8}" == fmt"y = 3.14159 "
proc hello(a: string, b: float): int = 12
let a = "hello"
let b = 3.1415926
doAssert fmt"{hello(x, y) = }" == "hello(x, y) = 12"
doAssert fmt"{x.hello(y) = }" == "x.hello(y) = 12"
doAssert fmt"{hello x, y = }" == "hello x, y = 12"
```
Note that it is space sensitive:
```
import strformat
let x = "12"
doAssert fmt"{x=}" == "x=12"
doAssert fmt"{x =:}" == "x =12"
doAssert fmt"{x =}" == "x =12"
doAssert fmt"{x= :}" == "x= 12"
doAssert fmt"{x= }" == "x= 12"
doAssert fmt"{x = :}" == "x = 12"
doAssert fmt"{x = }" == "x = 12"
doAssert fmt"{x = :}" == "x = 12"
doAssert fmt"{x = }" == "x = 12"
```
Implementation details
----------------------
An expression like `&"{key} is {value:arg} {{z}}"` is transformed into:
```
var temp = newStringOfCap(educatedCapGuess)
temp.formatValue key, ""
temp.add " is "
temp.formatValue value, arg
temp.add " {z}"
temp
```
Parts of the string that are enclosed in the curly braces are interpreted as Nim code, to escape an `{` or `}` double it.
`&` delegates most of the work to an open overloaded set of `formatValue` procs. The required signature for a type `T` that supports formatting is usually `proc formatValue(result: var string; x: T; specifier: string)`.
The subexpression after the colon (`arg` in `&"{key} is {value:arg} {{z}}"`) is optional. It will be passed as the last argument to `formatValue`. When the colon with the subexpression it is left out, an empty string will be taken instead.
For strings and numeric types the optional argument is a so-called "standard format specifier".
Standard format specifier for strings, integers and floats
----------------------------------------------------------
The general form of a standard format specifier is:
```
[[fill]align][sign][#][0][minimumwidth][.precision][type]
```
The square brackets `[]` indicate an optional element.
The optional align flag can be one of the following:
'<' Forces the field to be left-aligned within the available space. (This is the default for strings.) '>' Forces the field to be right-aligned within the available space. (This is the default for numbers.) '^' Forces the field to be centered within the available space. Note that unless a minimum field width is defined, the field width will always be the same size as the data to fill it, so that the alignment option has no meaning in this case.
The optional 'fill' character defines the character to be used to pad the field to the minimum width. The fill character, if present, must be followed by an alignment flag.
The 'sign' option is only valid for numeric types, and can be one of the following:
| Sign | Meaning |
| --- | --- |
| `+` | Indicates that a sign should be used for both positive as well as negative numbers. |
| `-` | Indicates that a sign should be used only for negative numbers (this is the default behavior). |
| (space) | Indicates that a leading space should be used on positive numbers. |
If the '#' character is present, integers use the 'alternate form' for formatting. This means that binary, octal, and hexadecimal output will be prefixed with '0b', '0o', and '0x', respectively.
'width' is a decimal integer defining the minimum field width. If not specified, then the field width will be determined by the content.
If the width field is preceded by a zero ('0') character, this enables zero-padding.
The 'precision' is a decimal number indicating how many digits should be displayed after the decimal point in a floating point conversion. For non-numeric types the field indicates the maximum field size - in other words, how many characters will be used from the field content. The precision is ignored for integer conversions.
Finally, the 'type' determines how the data should be presented.
The available integer presentation types are:
| Type | Result |
| --- | --- |
| `b` | Binary. Outputs the number in base 2. |
| `d` | Decimal Integer. Outputs the number in base 10. |
| `o` | Octal format. Outputs the number in base 8. |
| `x` | Hex format. Outputs the number in base 16, using lower-case letters for the digits above 9. |
| `X` | Hex format. Outputs the number in base 16, using uppercase letters for the digits above 9. |
| (None) | the same as 'd' |
The available floating point presentation types are:
| Type | Result |
| --- | --- |
| `e` | Exponent notation. Prints the number in scientific notation using the letter 'e' to indicate the exponent. |
| `E` | Exponent notation. Same as 'e' except it converts the number to uppercase. |
| `f` | Fixed point. Displays the number as a fixed-point number. |
| `F` | Fixed point. Same as 'f' except it converts the number to uppercase. |
| `g` | General format. This prints the number as a fixed-point number, unless the number is too large, in which case it switches to 'e' exponent notation. |
| `G` | General format. Same as 'g' except switches to 'E' if the number gets to large. |
| (None) | similar to 'g', except that it prints at least one digit after the decimal point. |
Limitations
-----------
Because of the well defined order how templates and macros are expanded, strformat cannot expand template arguments:
```
template myTemplate(arg: untyped): untyped =
echo "arg is: ", arg
echo &"--- {arg} ---"
let x = "abc"
myTemplate(x)
```
First the template `myTemplate` is expanded, where every identifier `arg` is substituted with its argument. The `arg` inside the format string is not seen by this process, because it is part of a quoted string literal. It is not an identifier yet. Then the strformat macro creates the `arg` identifier from the string literal. An identifier that cannot be resolved anymore.
The workaround for this is to bind the template argument to a new local variable.
```
template myTemplate(arg: untyped): untyped =
block:
let arg1 {.inject.} = arg
echo "arg is: ", arg1
echo &"--- {arg1} ---"
```
The use of `{.inject.}` here is necessary again because of template expansion order and hygienic templates. But since we generally want to keep the hygienicness of `myTemplate`, and we do not want `arg1` to be injected into the context where `myTemplate` is expanded, everything is wrapped in a `block`.
Future directions
-----------------
A curly expression with commas in it like `{x, argA, argB}` could be transformed to `formatValue(result, x, argA, argB)` in order to support formatters that do not need to parse a custom language within a custom language but instead prefer to use Nim's existing syntax. This also helps in readability since there is only so much you can cram into single letter DSLs.
Imports
-------
<macros>, <parseutils>, <unicode>, <strutils> Types
-----
```
StandardFormatSpecifier = object
fill*, align*: char ## Desired fill and alignment.
sign*: char ## Desired sign.
alternateForm*: bool ## Whether to prefix binary, octal and hex numbers
## with ``0b``, ``0o``, ``0x``.
padWithZero*: bool ## Whether to pad with zeros rather than spaces.
minimumWidth*, precision*: int ## Desired minimum width and precision.
typ*: char ## Type like 'f', 'g' or 'd'.
endPosition*: int ## End position in the format specifier after
## ``parseStandardFormatSpecifier`` returned.
```
Type that describes "standard format specifiers". [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L342) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L342) Procs
-----
```
proc alignString(s: string; minimumWidth: int; align = '\x00'; fill = ' '): string {...}{.
raises: [], tags: [].}
```
Aligns `s` using `fill` char. This is only of interest if you want to write a custom `format` proc that should support the standard format specifiers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L321) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L321)
```
proc parseStandardFormatSpecifier(s: string; start = 0;
ignoreUnknownSuffix = false): StandardFormatSpecifier {...}{.
raises: [ValueError], tags: [].}
```
An exported helper proc that parses the "standard format specifiers", as specified by the grammar:
```
[[fill]align][sign][#][0][minimumwidth][.precision][type]
```
This is only of interest if you want to write a custom `format` proc that should support the standard format specifiers. If `ignoreUnknownSuffix` is true, an unknown suffix after the `type` field is not an error.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L413) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L413)
```
proc formatValue[T: SomeInteger](result: var string; value: T; specifier: string)
```
Standard format implementation for `SomeInteger`. It makes little sense to call this directly, but it is required to exist by the `&` macro. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L465) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L465)
```
proc formatValue(result: var string; value: SomeFloat; specifier: string)
```
Standard format implementation for `SomeFloat`. It makes little sense to call this directly, but it is required to exist by the `&` macro. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L486) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L486)
```
proc formatValue(result: var string; value: string; specifier: string) {...}{.
raises: [ValueError], tags: [].}
```
Standard format implementation for `string`. It makes little sense to call this directly, but it is required to exist by the `&` macro. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L543) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L543) Macros
------
```
macro `&`(pattern: string): untyped
```
For a specification of the `&` macro, see the module level documentation. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L649) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L649)
```
macro fmt(pattern: string): untyped
```
An alias for `&`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L652) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L652)
```
macro fmt(pattern: string; openChar, closeChar: char): untyped
```
Use `openChar` instead of '{' and `closeChar` instead of '}' **Example:**
```
let testInt = 123
doAssert "<testInt>".fmt('<', '>') == "123"
doAssert """(()"foo" & "bar"())""".fmt(')', '(') == "(foobar)"
doAssert """ ""{"123+123"}"" """.fmt('"', '"') == " \"{246}\" "
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/strformat.nim#L655) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/strformat.nim#L655)
nim heapqueue heapqueue
=========
The `heapqueue` module implements a [heap data structure](https://en.wikipedia.org/wiki/Heap_(data_structure)) that can be used as a [priority queue](https://en.wikipedia.org/wiki/Priority_queue). Heaps are arrays for which `a[k] <= a[2*k+1]` and `a[k] <= a[2*k+2]` for all `k`, counting elements from 0. The interesting property of a heap is that `a[0]` is always its smallest element.
Basic usage
-----------
**Example:**
```
var heap = initHeapQueue[int]()
heap.push(8)
heap.push(2)
heap.push(5)
# The first element is the lowest element
assert heap[0] == 2
# Remove and return the lowest element
assert heap.pop() == 2
# The lowest element remaining is 5
assert heap[0] == 5
```
Usage with custom object
------------------------
To use a `HeapQueue` with a custom object, the `<` operator must be implemented.
**Example:**
```
type Job = object
priority: int
proc `<`(a, b: Job): bool = a.priority < b.priority
var jobs = initHeapQueue[Job]()
jobs.push(Job(priority: 1))
jobs.push(Job(priority: 2))
assert jobs[0].priority == 1
```
Imports
-------
<since> Types
-----
```
HeapQueue[T] = object
data: seq[T]
```
A heap queue, commonly known as a priority queue. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L53) Procs
-----
```
proc initHeapQueue[T](): HeapQueue[T]
```
Creates a new empty heap.
See also:
* [toHeapQueue proc](#toHeapQueue,openArray%5BT%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L57)
```
proc len[T](heap: HeapQueue[T]): int {...}{.inline.}
```
Returns the number of elements of `heap`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L64)
```
proc `[]`[T](heap: HeapQueue[T]; i: Natural): lent T {...}{.inline.}
```
Accesses the i-th element of `heap`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L68) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L68)
```
proc push[T](heap: var HeapQueue[T]; item: sink T)
```
Pushes `item` onto heap, maintaining the heap invariant. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L114)
```
proc toHeapQueue[T](x: openArray[T]): HeapQueue[T]
```
Creates a new HeapQueue that contains the elements of `x`.
See also:
* [initHeapQueue proc](#initHeapQueue)
**Example:**
```
var heap = toHeapQueue([9, 5, 8])
assert heap.pop() == 5
assert heap[0] == 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L119) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L119)
```
proc pop[T](heap: var HeapQueue[T]): T
```
Pops and returns the smallest item from `heap`, maintaining the heap invariant. **Example:**
```
var heap = toHeapQueue([9, 5, 8])
assert heap.pop() == 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L133)
```
proc find[T](heap: HeapQueue[T]; x: T): int
```
Linear scan to find index of item `x` or -1 if not found. **Example:**
```
var heap = toHeapQueue([9, 5, 8])
assert heap.find(5) == 0
assert heap.find(9) == 1
assert heap.find(777) == -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L147)
```
proc del[T](heap: var HeapQueue[T]; index: Natural)
```
Removes the element at `index` from `heap`, maintaining the heap invariant. **Example:**
```
var heap = toHeapQueue([9, 5, 8])
heap.del(1)
assert heap[0] == 5
assert heap[1] == 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L158) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L158)
```
proc replace[T](heap: var HeapQueue[T]; item: sink T): T
```
Pops and returns the current smallest value, and add the new item. This is more efficient than pop() followed by push(), and can be more appropriate when using a fixed-size heap. Note that the value returned may be larger than item! That constrains reasonable uses of this routine unless written as part of a conditional replacement: **Example:**
```
var heap = initHeapQueue[int]()
heap.push(5)
heap.push(12)
assert heap.replace(6) == 5
assert heap.len == 2
assert heap[0] == 6
assert heap.replace(4) == 6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L171) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L171)
```
proc pushpop[T](heap: var HeapQueue[T]; item: sink T): T
```
Fast version of a push followed by a pop. **Example:**
```
var heap = initHeapQueue[int]()
heap.push(5)
heap.push(12)
assert heap.pushpop(6) == 5
assert heap.len == 2
assert heap[0] == 6
assert heap.pushpop(4) == 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L189) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L189)
```
proc clear[T](heap: var HeapQueue[T])
```
Removes all elements from `heap`, making it empty. **Example:**
```
var heap = initHeapQueue[int]()
heap.push(1)
heap.clear()
assert heap.len == 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L204)
```
proc `$`[T](heap: HeapQueue[T]): string
```
Turns a heap into its string representation. **Example:**
```
var heap = initHeapQueue[int]()
heap.push(1)
heap.push(2)
assert $heap == "[1, 2]"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/collections/heapqueue.nim#L213) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/collections/heapqueue.nim#L213)
| programming_docs |
nim math math
====
*Constructive mathematics is naturally typed.* -- Simon Thompson
Basic math routines for Nim.
Note that the trigonometric functions naturally operate on radians. The helper functions [degToRad](#degToRad,T) and [radToDeg](#radToDeg,T) provide conversion between radians and degrees.
```
import math
from sequtils import map
let a = [0.0, PI/6, PI/4, PI/3, PI/2]
echo a.map(sin)
# @[0.0, 0.499…, 0.707…, 0.866…, 1.0]
echo a.map(tan)
# @[0.0, 0.577…, 0.999…, 1.732…, 1.633…e+16]
echo cos(degToRad(180.0))
# -1.0
echo sqrt(-1.0)
# nan (use `complex` module)
```
This module is available for the [JavaScript target](backends#backends-the-javascript-target).
**See also:**
* [complex module](complex) for complex numbers and their mathematical operations
* [rationals module](rationals) for rational numbers and their mathematical operations
* [fenv module](fenv) for handling of floating-point rounding and exceptions (overflow, zero-divide, etc.)
* [random module](random) for fast and tiny random number generator
* [mersenne module](mersenne) for Mersenne twister random number generator
* [stats module](stats) for statistical analysis
* [strformat module](strformat) for formatting floats for print
* [system module](system) Some very basic and trivial math operators are on system directly, to name a few `shr`, `shl`, `xor`, `clamp`, etc.
Imports
-------
<since>, <bitops> Types
-----
```
FloatClass = enum
fcNormal, ## value is an ordinary nonzero floating point value
fcSubnormal, ## value is a subnormal (a very small) floating point value
fcZero, ## value is zero
fcNegZero, ## value is the negative zero
fcNan, ## value is Not-A-Number (NAN)
fcInf, ## value is positive infinity
fcNegInf ## value is negative infinity
```
Describes the class a floating point value belongs to. This is the type that is returned by [classify proc](#classify,float). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L125) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L125) Consts
------
```
PI = 3.141592653589793
```
The circle constant PI (Ludolph's number) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L106)
```
TAU = 6.283185307179586
```
The circle constant TAU (= 2 \* PI) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L107)
```
E = 2.718281828459045
```
Euler's number [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L108)
```
MaxFloat64Precision = 16
```
Maximum number of meaningful digits after the decimal point for Nim's `float64` type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L110)
```
MaxFloat32Precision = 8
```
Maximum number of meaningful digits after the decimal point for Nim's `float32` type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L113) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L113)
```
MaxFloatPrecision = 16
```
Maximum number of meaningful digits after the decimal point for Nim's `float` type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L116)
```
MinFloatNormal = 2.225073858507201e-308
```
Smallest normal number for Nim's `float` type. (= 2^-1022). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L120) Procs
-----
```
proc binom(n, k: int): int {...}{.noSideEffect, raises: [], tags: [].}
```
Computes the [binomial coefficient](https://en.wikipedia.org/wiki/Binomial_coefficient). **Example:**
```
doAssert binom(6, 2) == binom(6, 4)
doAssert binom(6, 2) == 15
doAssert binom(-6, 2) == 1
doAssert binom(6, 0) == 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L61)
```
proc fac(n: int): int {...}{.raises: [], tags: [].}
```
Computes the [factorial](https://en.wikipedia.org/wiki/Factorial) of a non-negative integer `n`.
See also:
* [prod proc](#prod,openArray%5BT%5D)
**Example:**
```
doAssert fac(3) == 6
doAssert fac(4) == 24
doAssert fac(10) == 3628800
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L79)
```
proc classify(x: float): FloatClass {...}{.raises: [], tags: [].}
```
Classifies a floating point value.
Returns `x`'s class as specified by [FloatClass enum](#FloatClass).
**Example:**
```
doAssert classify(0.3) == fcNormal
doAssert classify(0.0) == fcZero
doAssert classify(0.3/0.0) == fcInf
doAssert classify(-0.3/0.0) == fcNegInf
doAssert classify(5.0e-324) == fcSubnormal
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L136) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L136)
```
proc isPowerOfTwo(x: int): bool {...}{.noSideEffect, raises: [], tags: [].}
```
Returns `true`, if `x` is a power of two, `false` otherwise.
Zero and negative numbers are not a power of two.
See also:
* [nextPowerOfTwo proc](#nextPowerOfTwo,int)
**Example:**
```
doAssert isPowerOfTwo(16) == true
doAssert isPowerOfTwo(5) == false
doAssert isPowerOfTwo(0) == false
doAssert isPowerOfTwo(-16) == false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L161) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L161)
```
proc nextPowerOfTwo(x: int): int {...}{.noSideEffect, raises: [], tags: [].}
```
Returns `x` rounded up to the nearest power of two.
Zero and negative numbers get rounded up to 1.
See also:
* [isPowerOfTwo proc](#isPowerOfTwo,int)
**Example:**
```
doAssert nextPowerOfTwo(16) == 16
doAssert nextPowerOfTwo(5) == 8
doAssert nextPowerOfTwo(0) == 1
doAssert nextPowerOfTwo(-16) == 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L175)
```
proc sum[T](x: openArray[T]): T {...}{.noSideEffect.}
```
Computes the sum of the elements in `x`.
If `x` is empty, 0 is returned.
See also:
* [prod proc](#prod,openArray%5BT%5D)
* [cumsum proc](#cumsum,openArray%5BT%5D)
* [cumsummed proc](#cumsummed,openArray%5BT%5D)
**Example:**
```
doAssert sum([1, 2, 3, 4]) == 10
doAssert sum([-1.5, 2.7, -0.1]) == 1.1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L199)
```
proc prod[T](x: openArray[T]): T {...}{.noSideEffect.}
```
Computes the product of the elements in `x`.
If `x` is empty, 1 is returned.
See also:
* [sum proc](#sum,openArray%5BT%5D)
* [fac proc](#fac,int)
**Example:**
```
doAssert prod([1, 2, 3, 4]) == 24
doAssert prod([-4, 3, 5]) == -60
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L213) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L213)
```
proc cumsummed[T](x: openArray[T]): seq[T]
```
Return cumulative (aka prefix) summation of `x`.
See also:
* [sum proc](#sum,openArray%5BT%5D)
* [cumsum proc](#cumsum,openArray%5BT%5D) for the in-place version
**Example:**
```
let a = [1, 2, 3, 4]
doAssert cumsummed(a) == @[1, 3, 6, 10]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L227) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L227)
```
proc cumsum[T](x: var openArray[T])
```
Transforms `x` in-place (must be declared as `var`) into its cumulative (aka prefix) summation.
See also:
* [sum proc](#sum,openArray%5BT%5D)
* [cumsummed proc](#cumsummed,openArray%5BT%5D) for a version which returns cumsummed sequence
**Example:**
```
var a = [1, 2, 3, 4]
cumsum(a)
doAssert a == @[1, 3, 6, 10]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L240)
```
proc sqrt(x: float32): float32 {...}{.importc: "sqrtf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L256) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L256)
```
proc sqrt(x: float64): float64 {...}{.importc: "sqrt", header: "<math.h>",
noSideEffect.}
```
Computes the square root of `x`.
See also:
* [cbrt proc](#cbrt,float64) for cubic root
```
echo sqrt(4.0) ## 2.0
echo sqrt(1.44) ## 1.2
echo sqrt(-4.0) ## nan
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L257) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L257)
```
proc cbrt(x: float32): float32 {...}{.importc: "cbrtf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L267) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L267)
```
proc cbrt(x: float64): float64 {...}{.importc: "cbrt", header: "<math.h>",
noSideEffect.}
```
Computes the cubic root of `x`.
See also:
* [sqrt proc](#sqrt,float64) for square root
```
echo cbrt(8.0) ## 2.0
echo cbrt(2.197) ## 1.3
echo cbrt(-27.0) ## -3.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L268)
```
proc ln(x: float32): float32 {...}{.importc: "logf", header: "<math.h>", noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L278) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L278)
```
proc ln(x: float64): float64 {...}{.importc: "log", header: "<math.h>", noSideEffect.}
```
Computes the [natural logarithm](https://en.wikipedia.org/wiki/Natural_logarithm) of `x`.
See also:
* [log proc](#log,T,T)
* [log10 proc](#log10,float64)
* [log2 proc](#log2,float64)
* [exp proc](#exp,float64)
```
echo ln(exp(4.0)) ## 4.0
echo ln(1.0)) ## 0.0
echo ln(0.0) ## -inf
echo ln(-7.0) ## nan
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L279) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L279)
```
proc log[T: SomeFloat](x, base: T): T {...}{.noSideEffect.}
```
Computes the logarithm of `x` to base `base`.
See also:
* [ln proc](#ln,float64)
* [log10 proc](#log10,float64)
* [log2 proc](#log2,float64)
* [exp proc](#exp,float64)
```
echo log(9.0, 3.0) ## 2.0
echo log(32.0, 2.0) ## 5.0
echo log(0.0, 2.0) ## -inf
echo log(-7.0, 4.0) ## nan
echo log(8.0, -2.0) ## nan
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L304) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L304)
```
proc log10(x: float32): float32 {...}{.importc: "log10f", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L322)
```
proc log10(x: float64): float64 {...}{.importc: "log10", header: "<math.h>",
noSideEffect.}
```
Computes the common logarithm (base 10) of `x`.
See also:
* [ln proc](#ln,float64)
* [log proc](#log,T,T)
* [log2 proc](#log2,float64)
* [exp proc](#exp,float64)
```
echo log10(100.0) ## 2.0
echo log10(0.0) ## nan
echo log10(-100.0) ## -inf
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L323) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L323)
```
proc exp(x: float32): float32 {...}{.importc: "expf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L336) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L336)
```
proc exp(x: float64): float64 {...}{.importc: "exp", header: "<math.h>", noSideEffect.}
```
Computes the exponential function of `x` (e^x).
See also:
* [ln proc](#ln,float64)
* [log proc](#log,T,T)
* [log10 proc](#log10,float64)
* [log2 proc](#log2,float64)
```
echo exp(1.0) ## 2.718281828459045
echo ln(exp(4.0)) ## 4.0
echo exp(0.0) ## 1.0
echo exp(-1.0) ## 0.3678794411714423
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L337)
```
proc sin(x: float32): float32 {...}{.importc: "sinf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L351) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L351)
```
proc sin(x: float64): float64 {...}{.importc: "sin", header: "<math.h>", noSideEffect.}
```
Computes the sine of `x`.
See also:
* [cos proc](#cos,float64)
* [tan proc](#tan,float64)
* [arcsin proc](#arcsin,float64)
* [sinh proc](#sinh,float64)
```
echo sin(PI / 6) ## 0.4999999999999999
echo sin(degToRad(90.0)) ## 1.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L352) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L352)
```
proc cos(x: float32): float32 {...}{.importc: "cosf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L364) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L364)
```
proc cos(x: float64): float64 {...}{.importc: "cos", header: "<math.h>", noSideEffect.}
```
Computes the cosine of `x`.
See also:
* [sin proc](#sin,float64)
* [tan proc](#tan,float64)
* [arccos proc](#arccos,float64)
* [cosh proc](#cosh,float64)
```
echo cos(2 * PI) ## 1.0
echo cos(degToRad(60.0)) ## 0.5000000000000001
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L365) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L365)
```
proc tan(x: float32): float32 {...}{.importc: "tanf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L377) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L377)
```
proc tan(x: float64): float64 {...}{.importc: "tan", header: "<math.h>", noSideEffect.}
```
Computes the tangent of `x`.
See also:
* [sin proc](#sin,float64)
* [cos proc](#cos,float64)
* [arctan proc](#arctan,float64)
* [tanh proc](#tanh,float64)
```
echo tan(degToRad(45.0)) ## 0.9999999999999999
echo tan(PI / 4) ## 0.9999999999999999
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L378) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L378)
```
proc sinh(x: float32): float32 {...}{.importc: "sinhf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L390) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L390)
```
proc sinh(x: float64): float64 {...}{.importc: "sinh", header: "<math.h>",
noSideEffect.}
```
Computes the [hyperbolic sine](https://en.wikipedia.org/wiki/Hyperbolic_function#Definitions) of `x`.
See also:
* [cosh proc](#cosh,float64)
* [tanh proc](#tanh,float64)
* [arcsinh proc](#arcsinh,float64)
* [sin proc](#sin,float64)
```
echo sinh(0.0) ## 0.0
echo sinh(1.0) ## 1.175201193643801
echo sinh(degToRad(90.0)) ## 2.301298902307295
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L391) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L391)
```
proc cosh(x: float32): float32 {...}{.importc: "coshf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L404) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L404)
```
proc cosh(x: float64): float64 {...}{.importc: "cosh", header: "<math.h>",
noSideEffect.}
```
Computes the [hyperbolic cosine](https://en.wikipedia.org/wiki/Hyperbolic_function#Definitions) of `x`.
See also:
* [sinh proc](#sinh,float64)
* [tanh proc](#tanh,float64)
* [arccosh proc](#arccosh,float64)
* [cos proc](#cos,float64)
```
echo cosh(0.0) ## 1.0
echo cosh(1.0) ## 1.543080634815244
echo cosh(degToRad(90.0)) ## 2.509178478658057
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L405)
```
proc tanh(x: float32): float32 {...}{.importc: "tanhf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L418) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L418)
```
proc tanh(x: float64): float64 {...}{.importc: "tanh", header: "<math.h>",
noSideEffect.}
```
Computes the [hyperbolic tangent](https://en.wikipedia.org/wiki/Hyperbolic_function#Definitions) of `x`.
See also:
* [sinh proc](#sinh,float64)
* [cosh proc](#cosh,float64)
* [arctanh proc](#arctanh,float64)
* [tan proc](#tan,float64)
```
echo tanh(0.0) ## 0.0
echo tanh(1.0) ## 0.7615941559557649
echo tanh(degToRad(90.0)) ## 0.9171523356672744
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L419) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L419)
```
proc arccos(x: float32): float32 {...}{.importc: "acosf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L433) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L433)
```
proc arccos(x: float64): float64 {...}{.importc: "acos", header: "<math.h>",
noSideEffect.}
```
Computes the arc cosine of `x`.
See also:
* [arcsin proc](#arcsin,float64)
* [arctan proc](#arctan,float64)
* [arctan2 proc](#arctan2,float64,float64)
* [cos proc](#cos,float64)
```
echo radToDeg(arccos(0.0)) ## 90.0
echo radToDeg(arccos(1.0)) ## 0.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L434) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L434)
```
proc arcsin(x: float32): float32 {...}{.importc: "asinf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L446) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L446)
```
proc arcsin(x: float64): float64 {...}{.importc: "asin", header: "<math.h>",
noSideEffect.}
```
Computes the arc sine of `x`.
See also:
* [arccos proc](#arccos,float64)
* [arctan proc](#arctan,float64)
* [arctan2 proc](#arctan2,float64,float64)
* [sin proc](#sin,float64)
```
echo radToDeg(arcsin(0.0)) ## 0.0
echo radToDeg(arcsin(1.0)) ## 90.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L447)
```
proc arctan(x: float32): float32 {...}{.importc: "atanf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L459) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L459)
```
proc arctan(x: float64): float64 {...}{.importc: "atan", header: "<math.h>",
noSideEffect.}
```
Calculate the arc tangent of `x`.
See also:
* [arcsin proc](#arcsin,float64)
* [arccos proc](#arccos,float64)
* [arctan2 proc](#arctan2,float64,float64)
* [tan proc](#tan,float64)
```
echo arctan(1.0) ## 0.7853981633974483
echo radToDeg(arctan(1.0)) ## 45.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L460)
```
proc arctan2(y, x: float32): float32 {...}{.importc: "atan2f", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L472) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L472)
```
proc arctan2(y, x: float64): float64 {...}{.importc: "atan2", header: "<math.h>",
noSideEffect.}
```
Calculate the arc tangent of `y` / `x`.
It produces correct results even when the resulting angle is near pi/2 or -pi/2 (`x` near 0).
See also:
* [arcsin proc](#arcsin,float64)
* [arccos proc](#arccos,float64)
* [arctan proc](#arctan,float64)
* [tan proc](#tan,float64)
```
echo arctan2(1.0, 0.0) ## 1.570796326794897
echo radToDeg(arctan2(1.0, 0.0)) ## 90.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L474) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L474)
```
proc arcsinh(x: float32): float32 {...}{.importc: "asinhf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L489) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L489)
```
proc arcsinh(x: float64): float64 {...}{.importc: "asinh", header: "<math.h>",
noSideEffect.}
```
Computes the inverse hyperbolic sine of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L490) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L490)
```
proc arccosh(x: float32): float32 {...}{.importc: "acoshf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L492) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L492)
```
proc arccosh(x: float64): float64 {...}{.importc: "acosh", header: "<math.h>",
noSideEffect.}
```
Computes the inverse hyperbolic cosine of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L493) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L493)
```
proc arctanh(x: float32): float32 {...}{.importc: "atanhf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L495) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L495)
```
proc arctanh(x: float64): float64 {...}{.importc: "atanh", header: "<math.h>",
noSideEffect.}
```
Computes the inverse hyperbolic tangent of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L496) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L496)
```
proc cot[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the cotangent of `x` (1 / tan(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L524) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L524)
```
proc sec[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the secant of `x` (1 / cos(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L526) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L526)
```
proc csc[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the cosecant of `x` (1 / sin(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L528) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L528)
```
proc coth[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the hyperbolic cotangent of `x` (1 / tanh(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L531) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L531)
```
proc sech[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the hyperbolic secant of `x` (1 / cosh(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L533) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L533)
```
proc csch[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the hyperbolic cosecant of `x` (1 / sinh(x)). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L535) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L535)
```
proc arccot[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse cotangent of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L538) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L538)
```
proc arcsec[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse secant of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L540) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L540)
```
proc arccsc[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse cosecant of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L542) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L542)
```
proc arccoth[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse hyperbolic cotangent of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L545) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L545)
```
proc arcsech[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse hyperbolic secant of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L547) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L547)
```
proc arccsch[T: float32 | float64](x: T): T {...}{.noSideEffect.}
```
Computes the inverse hyperbolic cosecant of `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L549) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L549)
```
proc hypot(x, y: float32): float32 {...}{.importc: "hypotf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L555) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L555)
```
proc hypot(x, y: float64): float64 {...}{.importc: "hypot", header: "<math.h>",
noSideEffect.}
```
Computes the hypotenuse of a right-angle triangle with `x` and `y` as its base and height. Equivalent to `sqrt(x*x + y*y)`.
```
echo hypot(4.0, 3.0) ## 5.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L556) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L556)
```
proc pow(x, y: float32): float32 {...}{.importc: "powf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L562) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L562)
```
proc pow(x, y: float64): float64 {...}{.importc: "pow", header: "<math.h>",
noSideEffect.}
```
Computes x to power raised of y.
To compute power between integers (e.g. 2^6), use [^ proc](#%5E,T,Natural).
See also:
* [^ proc](#%5E,T,Natural)
* [sqrt proc](#sqrt,float64)
* [cbrt proc](#cbrt,float64)
```
echo pow(100, 1.5) ## 1000.0
echo pow(16.0, 0.5) ## 4.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L563) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L563)
```
proc erf(x: float32): float32 {...}{.importc: "erff", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L579) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L579)
```
proc erf(x: float64): float64 {...}{.importc: "erf", header: "<math.h>", noSideEffect.}
```
Computes the [error function](https://en.wikipedia.org/wiki/Error_function) for `x`.
Note: Not available for JS backend.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L580) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L580)
```
proc erfc(x: float32): float32 {...}{.importc: "erfcf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L584) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L584)
```
proc erfc(x: float64): float64 {...}{.importc: "erfc", header: "<math.h>",
noSideEffect.}
```
Computes the [complementary error function](https://en.wikipedia.org/wiki/Error_function#Complementary_error_function) for `x`.
Note: Not available for JS backend.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L585) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L585)
```
proc gamma(x: float32): float32 {...}{.importc: "tgammaf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L589) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L589)
```
proc gamma(x: float64): float64 {...}{.importc: "tgamma", header: "<math.h>",
noSideEffect.}
```
Computes the the [gamma function](https://en.wikipedia.org/wiki/Gamma_function) for `x`.
Note: Not available for JS backend.
See also:
* [lgamma proc](#lgamma,float64) for a natural log of gamma function
```
echo gamma(1.0) # 1.0
echo gamma(4.0) # 6.0
echo gamma(11.0) # 3628800.0
echo gamma(-1.0) # nan
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L590) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L590)
```
proc lgamma(x: float32): float32 {...}{.importc: "lgammaf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L603) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L603)
```
proc lgamma(x: float64): float64 {...}{.importc: "lgamma", header: "<math.h>",
noSideEffect.}
```
Computes the natural log of the gamma function for `x`.
Note: Not available for JS backend.
See also:
* [gamma proc](#gamma,float64) for gamma function
```
echo lgamma(1.0) # 1.0
echo lgamma(4.0) # 1.791759469228055
echo lgamma(11.0) # 15.10441257307552
echo lgamma(-1.0) # inf
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L604) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L604)
```
proc floor(x: float32): float32 {...}{.importc: "floorf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L618) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L618)
```
proc floor(x: float64): float64 {...}{.importc: "floor", header: "<math.h>",
noSideEffect.}
```
Computes the floor function (i.e., the largest integer not greater than `x`).
See also:
* [ceil proc](#ceil,float64)
* [round proc](#round,float64)
* [trunc proc](#trunc,float64)
```
echo floor(2.1) ## 2.0
echo floor(2.9) ## 2.0
echo floor(-3.5) ## -4.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L619) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L619)
```
proc ceil(x: float32): float32 {...}{.importc: "ceilf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L632) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L632)
```
proc ceil(x: float64): float64 {...}{.importc: "ceil", header: "<math.h>",
noSideEffect.}
```
Computes the ceiling function (i.e., the smallest integer not smaller than `x`).
See also:
* [floor proc](#floor,float64)
* [round proc](#round,float64)
* [trunc proc](#trunc,float64)
```
echo ceil(2.1) ## 3.0
echo ceil(2.9) ## 3.0
echo ceil(-2.1) ## -2.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L633) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L633)
```
proc round(x: float32): float32 {...}{.importc: "roundf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L703) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L703)
```
proc round(x: float64): float64 {...}{.importc: "round", header: "<math.h>",
noSideEffect.}
```
Rounds a float to zero decimal places.
Used internally by the [round proc](#round,T,int) when the specified number of places is 0.
See also:
* [round proc](#round,T,int) for rounding to the specific number of decimal places
* [floor proc](#floor,float64)
* [ceil proc](#ceil,float64)
* [trunc proc](#trunc,float64)
```
echo round(3.4) ## 3.0
echo round(3.5) ## 4.0
echo round(4.5) ## 5.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L704) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L704)
```
proc trunc(x: float32): float32 {...}{.importc: "truncf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L722) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L722)
```
proc trunc(x: float64): float64 {...}{.importc: "trunc", header: "<math.h>",
noSideEffect.}
```
Truncates `x` to the decimal point.
See also:
* [floor proc](#floor,float64)
* [ceil proc](#ceil,float64)
* [round proc](#round,float64)
```
echo trunc(PI) # 3.0
echo trunc(-1.85) # -1.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L723) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L723)
```
proc `mod`(x, y: float32): float32 {...}{.importc: "fmodf", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L735) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L735)
```
proc `mod`(x, y: float64): float64 {...}{.importc: "fmod", header: "<math.h>",
noSideEffect.}
```
Computes the modulo operation for float values (the remainder of `x` divided by `y`).
See also:
* [floorMod proc](#floorMod,T,T) for Python-like (% operator) behavior
```
( 6.5 mod 2.5) == 1.5
(-6.5 mod 2.5) == -1.5
( 6.5 mod -2.5) == 1.5
(-6.5 mod -2.5) == -1.5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L736) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L736)
```
proc round[T: float32 | float64](x: T; places: int): T {...}{.noSideEffect.}
```
Decimal rounding on a binary floating point number.
This function is NOT reliable. Floating point numbers cannot hold non integer decimals precisely. If `places` is 0 (or omitted), round to the nearest integral value following normal mathematical rounding rules (e.g. `round(54.5) -> 55.0`). If `places` is greater than 0, round to the given number of decimal places, e.g. `round(54.346, 2) -> 54.350000000000001421…`. If `places` is negative, round to the left of the decimal place, e.g. `round(537.345, -1) -> 540.0`
```
echo round(PI, 2) ## 3.14
echo round(PI, 4) ## 3.1416
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L771) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L771)
```
proc floorDiv[T: SomeInteger](x, y: T): T {...}{.noSideEffect.}
```
Floor division is conceptually defined as `floor(x / y)`.
This is different from the [system.div](system#div,int,int) operator, which is defined as `trunc(x / y)`. That is, `div` rounds towards `0` and `floorDiv` rounds down.
See also:
* [system.div proc](system#div,int,int) for integer division
* [floorMod proc](#floorMod,T,T) for Python-like (% operator) behavior
```
echo floorDiv( 13, 3) # 4
echo floorDiv(-13, 3) # -5
echo floorDiv( 13, -3) # -5
echo floorDiv(-13, -3) # 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L792) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L792)
```
proc floorMod[T: SomeNumber](x, y: T): T {...}{.noSideEffect.}
```
Floor modulus is conceptually defined as `x - (floorDiv(x, y) * y)`.
This proc behaves the same as the `%` operator in Python.
See also:
* [mod proc](#mod,float64,float64)
* [floorDiv proc](#floorDiv,T,T)
```
echo floorMod( 13, 3) # 1
echo floorMod(-13, 3) # 2
echo floorMod( 13, -3) # -2
echo floorMod(-13, -3) # -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L812) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L812)
```
proc c_frexp(x: float32; exponent: var int32): float32 {...}{.importc: "frexp",
header: "<math.h>", noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L830) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L830)
```
proc c_frexp(x: float64; exponent: var int32): float64 {...}{.importc: "frexp",
header: "<math.h>", noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L832) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L832)
```
proc frexp[T, U](x: T; exponent: var U): T {...}{.noSideEffect.}
```
Split a number into mantissa and exponent.
`frexp` calculates the mantissa m (a float greater than or equal to 0.5 and less than 1) and the integer value n such that `x` (the original float value) equals `m * 2**n`. frexp stores n in `exponent` and returns m.
**Example:**
```
var x: int
doAssert frexp(5.0, x) == 0.625
doAssert x == 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L834) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L834)
```
proc log2(x: float32): float32 {...}{.importc: "log2f", header: "<math.h>",
noSideEffect.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L867) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L867)
```
proc log2(x: float64): float64 {...}{.importc: "log2", header: "<math.h>",
noSideEffect.}
```
Computes the binary logarithm (base 2) of `x`.
See also:
* [log proc](#log,T,T)
* [log10 proc](#log10,float64)
* [ln proc](#ln,float64)
* [exp proc](#exp,float64)
```
echo log2(8.0) # 3.0
echo log2(1.0) # 0.0
echo log2(0.0) # -inf
echo log2(-2.0) # nan
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L868) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L868)
```
proc splitDecimal[T: float32 | float64](x: T): tuple[intpart: T, floatpart: T] {...}{.
noSideEffect.}
```
Breaks `x` into an integer and a fractional part.
Returns a tuple containing `intpart` and `floatpart` representing the integer part and the fractional part respectively.
Both parts have the same sign as `x`. Analogous to the `modf` function in C.
**Example:**
```
doAssert splitDecimal(5.25) == (intpart: 5.0, floatpart: 0.25)
doAssert splitDecimal(-2.73) == (intpart: -2.0, floatpart: -0.73)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L900) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L900)
```
proc degToRad[T: float32 | float64](d: T): T {...}{.inline.}
```
Convert from degrees to radians.
See also:
* [radToDeg proc](#radToDeg,T)
**Example:**
```
doAssert degToRad(180.0) == 3.141592653589793
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L923) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L923)
```
proc radToDeg[T: float32 | float64](d: T): T {...}{.inline.}
```
Convert from radians to degrees.
See also:
* [degToRad proc](#degToRad,T)
**Example:**
```
doAssert radToDeg(2 * PI) == 360.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L933) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L933)
```
proc sgn[T: SomeNumber](x: T): int {...}{.inline.}
```
Sign function.
Returns:
* `-1` for negative numbers and `NegInf`,
* `1` for positive numbers and `Inf`,
* `0` for positive zero, negative zero and `NaN`
**Example:**
```
doAssert sgn(5) == 1
doAssert sgn(0) == 0
doAssert sgn(-4.1) == -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L943) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L943)
```
proc `^`[T: SomeNumber](x: T; y: Natural): T
```
Computes `x` to the power `y`.
Exponent `y` must be non-negative, use [pow proc](#pow,float64,float64) for negative exponents.
See also:
* [pow proc](#pow,float64,float64) for negative exponent or floats
* [sqrt proc](#sqrt,float64)
* [cbrt proc](#cbrt,float64)
**Example:**
```
assert -3.0^0 == 1.0
assert -3^1 == -3
assert -3^2 == 9
assert -3.0^3 == -27.0
assert -3.0^4 == 81.0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L960) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L960)
```
proc gcd[T](x, y: T): T
```
Computes the greatest common (positive) divisor of `x` and `y`.
Note that for floats, the result cannot always be interpreted as "greatest decimal `z` such that `z*N == x and z*M == y` where N and M are positive integers."
See also:
* [gcd proc](#gcd,SomeInteger,SomeInteger) for integer version
* [lcm proc](#lcm,T,T)
**Example:**
```
doAssert gcd(13.5, 9.0) == 4.5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L995) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L995)
```
proc gcd(x, y: SomeInteger): SomeInteger
```
Computes the greatest common (positive) divisor of `x` and `y`, using binary GCD (aka Stein's) algorithm.
See also:
* [gcd proc](#gcd,T,T) for floats version
* [lcm proc](#lcm,T,T)
**Example:**
```
doAssert gcd(12, 8) == 4
doAssert gcd(17, 63) == 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L1013) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L1013)
```
proc gcd[T](x: openArray[T]): T
```
Computes the greatest common (positive) divisor of the elements of `x`.
See also:
* [gcd proc](#gcd,T,T) for integer version
**Example:**
```
doAssert gcd(@[13.5, 9.0]) == 4.5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L1046) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L1046)
```
proc lcm[T](x, y: T): T
```
Computes the least common multiple of `x` and `y`.
See also:
* [gcd proc](#gcd,T,T)
**Example:**
```
doAssert lcm(24, 30) == 120
doAssert lcm(13, 39) == 39
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L1059) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L1059)
```
proc lcm[T](x: openArray[T]): T
```
Computes the least common multiple of the elements of `x`.
See also:
* [gcd proc](#gcd,T,T) for integer version
**Example:**
```
doAssert lcm(@[24, 30]) == 120
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/math.nim#L1069) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/math.nim#L1069)
| programming_docs |
nim registry registry
========
This module is experimental and its interface may change.
Imports
-------
<winlean>, <os> Types
-----
```
HKEY = uint
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/windows/registry.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/windows/registry.nim#L15) Consts
------
```
HKEY_LOCAL_MACHINE = 2147483650'u
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/windows/registry.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/windows/registry.nim#L18)
```
HKEY_CURRENT_USER = 2147483649'u
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/windows/registry.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/windows/registry.nim#L19) Procs
-----
```
proc getUnicodeValue(path, key: string; handle: HKEY): string {...}{.
raises: [OSError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/windows/registry.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/windows/registry.nim#L46)
```
proc setUnicodeValue(path, key, val: string; handle: HKEY) {...}{.raises: [OSError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/windows/registry.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/windows/registry.nim#L72)
nim rstast rstast
======
This module implements an AST for the reStructuredText parser.
**Note:** Import `packages/docutils/rstast` to use this module
Types
-----
```
RstNodeKind = enum
rnInner, rnHeadline, rnOverline, rnTransition, rnParagraph, rnBulletList,
rnBulletItem, rnEnumList, rnEnumItem, rnDefList, rnDefItem, rnDefName,
rnDefBody, rnFieldList, rnField, rnFieldName, rnFieldBody, rnOptionList,
rnOptionListItem, rnOptionGroup, rnOption, rnOptionString, rnOptionArgument,
rnDescription, rnLiteralBlock, rnQuotedLiteralBlock, rnLineBlock,
rnLineBlockItem, rnBlockQuote, rnTable, rnGridTable, rnTableRow,
rnTableHeaderCell, rnTableDataCell, rnLabel, rnFootnote, rnCitation,
rnStandaloneHyperlink, rnHyperlink, rnRef, rnDirective, rnDirArg, rnRaw,
rnTitle, rnContents, rnImage, rnFigure, rnCodeBlock, rnRawHtml, rnRawLatex,
rnContainer, rnIndex, rnSubstitutionDef, rnGeneralRole, rnSub, rnSup, rnIdx,
rnEmphasis, rnStrongEmphasis, rnTripleEmphasis, rnInterpretedText,
rnInlineLiteral, rnSubstitutionReferences, rnSmiley, rnLeaf
```
the possible node kinds of an PRstNode [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L17)
```
PRstNode = ref RstNode
```
an RST node [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L67)
```
RstNodeSeq = seq[PRstNode]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L68) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L68)
```
RstNode {...}{.acyclic, final.} = object
kind*: RstNodeKind ## the node's kind
text*: string ## valid for leafs in the AST; and the title of
## the document or the section
level*: int ## valid for some node kinds
sons*: RstNodeSeq ## the node's sons
```
an RST node's description [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L69) Procs
-----
```
proc len(n: PRstNode): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L76)
```
proc newRstNode(kind: RstNodeKind): PRstNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L79)
```
proc newRstNode(kind: RstNodeKind; s: string): PRstNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L84) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L84)
```
proc lastSon(n: PRstNode): PRstNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L88) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L88)
```
proc add(father, son: PRstNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L91)
```
proc add(father: PRstNode; s: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L94)
```
proc addIfNotNil(father, son: PRstNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L97)
```
proc renderRstToRst(n: PRstNode; result: var string) {...}{.raises: [Exception],
tags: [RootEffect].}
```
renders `n` into its string representation and appends to `result`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L290)
```
proc renderRstToJson(node: PRstNode): string {...}{.raises: [], tags: [].}
```
Writes the given RST node as JSON that is in the form
```
{
"kind":string node.kind,
"text":optional string node.text,
"level":optional int node.level,
"sons":optional node array
}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstast.nim#L309) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstast.nim#L309)
nim fenv fenv
====
Floating-point environment. Handling of floating-point rounding and exceptions (overflow, division by zero, etc.).
Types
-----
```
Tfenv {...}{.importc: "fenv_t", header: "<fenv.h>", final, pure.} = object
```
Represents the entire floating-point environment. The floating-point environment refers collectively to any floating-point status flags and control modes supported by the implementation. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L43)
```
Tfexcept {...}{.importc: "fexcept_t", header: "<fenv.h>", final, pure.} = object
```
Represents the floating-point status flags collectively, including any status the implementation associates with the flags. A floating-point status flag is a system variable whose value is set (but never cleared) when a floating-point exception is raised, which occurs as a side effect of exceptional floating-point arithmetic to provide auxiliary information. A floating-point control mode is a system variable whose value may be set by the user to affect the subsequent behavior of floating-point arithmetic. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L48) Vars
----
```
FE_DIVBYZERO: cint
```
division by zero [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L17)
```
FE_INEXACT: cint
```
inexact result [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L19)
```
FE_INVALID: cint
```
invalid operation [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L21)
```
FE_OVERFLOW: cint
```
result not representable due to overflow [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L23)
```
FE_UNDERFLOW: cint
```
result not representable due to underflow [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L25)
```
FE_ALL_EXCEPT: cint
```
bitwise OR of all supported exceptions [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L27)
```
FE_DOWNWARD: cint
```
round toward -Inf [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L29)
```
FE_TONEAREST: cint
```
round to nearest [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L31)
```
FE_TOWARDZERO: cint
```
round toward 0 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L33)
```
FE_UPWARD: cint
```
round toward +Inf [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L35)
```
FE_DFL_ENV: cint
```
macro of type pointer to fenv\_t to be used as the argument to functions taking an argument of type fenv\_t; in this case the default environment will be used [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L37) Procs
-----
```
proc feclearexcept(excepts: cint): cint {...}{.importc, header: "<fenv.h>".}
```
Clear the supported exceptions represented by `excepts`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L59) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L59)
```
proc fegetexceptflag(flagp: ptr Tfexcept; excepts: cint): cint {...}{.importc,
header: "<fenv.h>".}
```
Store implementation-defined representation of the exception flags indicated by `excepts` in the object pointed to by `flagp`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L62)
```
proc feraiseexcept(excepts: cint): cint {...}{.importc, header: "<fenv.h>".}
```
Raise the supported exceptions represented by `excepts`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L67)
```
proc fesetexceptflag(flagp: ptr Tfexcept; excepts: cint): cint {...}{.importc,
header: "<fenv.h>".}
```
Set complete status for exceptions indicated by `excepts` according to the representation in the object pointed to by `flagp`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L70)
```
proc fetestexcept(excepts: cint): cint {...}{.importc, header: "<fenv.h>".}
```
Determine which of subset of the exceptions specified by `excepts` are currently set. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L75)
```
proc fegetround(): cint {...}{.importc, header: "<fenv.h>".}
```
Get current rounding direction. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L79)
```
proc fesetround(roundingDirection: cint): cint {...}{.importc, header: "<fenv.h>".}
```
Establish the rounding direction represented by `roundingDirection`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L82)
```
proc fegetenv(envp: ptr Tfenv): cint {...}{.importc, header: "<fenv.h>".}
```
Store the current floating-point environment in the object pointed to by `envp`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L85)
```
proc feholdexcept(envp: ptr Tfenv): cint {...}{.importc, header: "<fenv.h>".}
```
Save the current environment in the object pointed to by `envp`, clear exception flags and install a non-stop mode (if available) for all exceptions. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L89)
```
proc fesetenv(a1: ptr Tfenv): cint {...}{.importc, header: "<fenv.h>".}
```
Establish the floating-point environment represented by the object pointed to by `envp`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L94)
```
proc feupdateenv(envp: ptr Tfenv): cint {...}{.importc, header: "<fenv.h>".}
```
Save current exceptions in temporary storage, install environment represented by object pointed to by `envp` and raise exceptions according to saved exceptions. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L98) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L98) Templates
---------
```
template fpRadix(): int
```
The (integer) value of the radix used to represent any floating point type on the architecture used to build the program. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L126) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L126)
```
template mantissaDigits(T: typedesc[float32]): int
```
Number of digits (in base `floatingPointRadix`) in the mantissa of 32-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L130)
```
template digits(T: typedesc[float32]): int
```
Number of decimal digits that can be represented in a 32-bit floating-point type without losing precision. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L133)
```
template minExponent(T: typedesc[float32]): int
```
Minimum (negative) exponent for 32-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L136) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L136)
```
template maxExponent(T: typedesc[float32]): int
```
Maximum (positive) exponent for 32-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L138)
```
template min10Exponent(T: typedesc[float32]): int
```
Minimum (negative) exponent in base 10 for 32-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L140)
```
template max10Exponent(T: typedesc[float32]): int
```
Maximum (positive) exponent in base 10 for 32-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L143)
```
template minimumPositiveValue(T: typedesc[float32]): float32
```
The smallest positive (nonzero) number that can be represented in a 32-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L146) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L146)
```
template maximumPositiveValue(T: typedesc[float32]): float32
```
The largest positive number that can be represented in a 32-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L149)
```
template epsilon(T: typedesc[float32]): float32
```
The difference between 1.0 and the smallest number greater than 1.0 that can be represented in a 32-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L152)
```
template mantissaDigits(T: typedesc[float64]): int
```
Number of digits (in base `floatingPointRadix`) in the mantissa of 64-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L156) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L156)
```
template digits(T: typedesc[float64]): int
```
Number of decimal digits that can be represented in a 64-bit floating-point type without losing precision. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L159)
```
template minExponent(T: typedesc[float64]): int
```
Minimum (negative) exponent for 64-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L162)
```
template maxExponent(T: typedesc[float64]): int
```
Maximum (positive) exponent for 64-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L164)
```
template min10Exponent(T: typedesc[float64]): int
```
Minimum (negative) exponent in base 10 for 64-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L166)
```
template max10Exponent(T: typedesc[float64]): int
```
Maximum (positive) exponent in base 10 for 64-bit floating-point numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L169)
```
template minimumPositiveValue(T: typedesc[float64]): float64
```
The smallest positive (nonzero) number that can be represented in a 64-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L172)
```
template maximumPositiveValue(T: typedesc[float64]): float64
```
The largest positive number that can be represented in a 64-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L175)
```
template epsilon(T: typedesc[float64]): float64
```
The difference between 1.0 and the smallest number greater than 1.0 that can be represented in a 64-bit floating-point type. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/fenv.nim#L178) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/fenv.nim#L178)
nim complex complex
=======
This module implements complex numbers. Complex numbers are currently implemented as generic on a 64-bit or 32-bit float.
Imports
-------
<math> Types
-----
```
Complex[T] = object
re*, im*: T ## A complex number, consisting of a real and an imaginary part.
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L19)
```
Complex64 = Complex[float64]
```
Alias for a pair of 64-bit floats. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L22)
```
Complex32 = Complex[float32]
```
Alias for a pair of 32-bit floats. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L24) Procs
-----
```
proc complex[T: SomeFloat](re: T; im: T = 0.0): Complex[T]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L27)
```
proc complex32(re: float32; im: float32 = 0.0): Complex[float32] {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L31)
```
proc complex64(re: float64; im: float64 = 0.0): Complex[float64] {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L35)
```
proc abs[T](z: Complex[T]): T
```
Returns the distance from (0,0) to `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L44)
```
proc abs2[T](z: Complex[T]): T
```
Returns the squared distance from (0,0) to `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L48)
```
proc conjugate[T](z: Complex[T]): Complex[T]
```
Conjugates of complex number `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L52)
```
proc inv[T](z: Complex[T]): Complex[T]
```
Multiplicatives inverse of complex number `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L57)
```
proc `==`[T](x, y: Complex[T]): bool
```
Compares two complex numbers `x` and `y` for equality. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L61)
```
proc `+`[T](x: T; y: Complex[T]): Complex[T]
```
Adds a real number to a complex number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L65)
```
proc `+`[T](x: Complex[T]; y: T): Complex[T]
```
Adds a complex number to a real number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L70)
```
proc `+`[T](x, y: Complex[T]): Complex[T]
```
Adds two complex numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L75)
```
proc `-`[T](z: Complex[T]): Complex[T]
```
Unary minus for complex numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L80) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L80)
```
proc `-`[T](x: T; y: Complex[T]): Complex[T]
```
Subtracts a complex number from a real number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L85)
```
proc `-`[T](x: Complex[T]; y: T): Complex[T]
```
Subtracts a real number from a complex number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L89)
```
proc `-`[T](x, y: Complex[T]): Complex[T]
```
Subtracts two complex numbers. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L94)
```
proc `/`[T](x: Complex[T]; y: T): Complex[T]
```
Divides complex number `x` by real number `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L99)
```
proc `/`[T](x: T; y: Complex[T]): Complex[T]
```
Divides real number `x` by complex number `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L104)
```
proc `/`[T](x, y: Complex[T]): Complex[T]
```
Divides `x` by `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L108)
```
proc `*`[T](x: T; y: Complex[T]): Complex[T]
```
Multiplies a real number and a complex number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L122)
```
proc `*`[T](x: Complex[T]; y: T): Complex[T]
```
Multiplies a complex number with a real number. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L127)
```
proc `*`[T](x, y: Complex[T]): Complex[T]
```
Multiplies `x` with `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L132)
```
proc `+=`[T](x: var Complex[T]; y: Complex[T])
```
Adds `y` to `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L138)
```
proc `-=`[T](x: var Complex[T]; y: Complex[T])
```
Subtracts `y` from `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L143) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L143)
```
proc `*=`[T](x: var Complex[T]; y: Complex[T])
```
Multiplies `y` to `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L148) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L148)
```
proc `/=`[T](x: var Complex[T]; y: Complex[T])
```
Divides `x` by `y` in place. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L154)
```
proc sqrt[T](z: Complex[T]): Complex[T]
```
Square root for a complex number `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L159)
```
proc exp[T](z: Complex[T]): Complex[T]
```
`e` raised to the power `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L182)
```
proc ln[T](z: Complex[T]): Complex[T]
```
Returns the natural log of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L190)
```
proc log10[T](z: Complex[T]): Complex[T]
```
Returns the log base 10 of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L195)
```
proc log2[T](z: Complex[T]): Complex[T]
```
Returns the log base 2 of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L199)
```
proc pow[T](x, y: Complex[T]): Complex[T]
```
`x` raised to the power `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L203)
```
proc pow[T](x: Complex[T]; y: T): Complex[T]
```
Complex number `x` raised to the power `y`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L225) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L225)
```
proc sin[T](z: Complex[T]): Complex[T]
```
Returns the sine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L230) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L230)
```
proc arcsin[T](z: Complex[T]): Complex[T]
```
Returns the inverse sine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L235)
```
proc cos[T](z: Complex[T]): Complex[T]
```
Returns the cosine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L239) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L239)
```
proc arccos[T](z: Complex[T]): Complex[T]
```
Returns the inverse cosine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L244) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L244)
```
proc tan[T](z: Complex[T]): Complex[T]
```
Returns the tangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L248) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L248)
```
proc arctan[T](z: Complex[T]): Complex[T]
```
Returns the inverse tangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L252) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L252)
```
proc cot[T](z: Complex[T]): Complex[T]
```
Returns the cotangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L256) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L256)
```
proc arccot[T](z: Complex[T]): Complex[T]
```
Returns the inverse cotangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L260)
```
proc sec[T](z: Complex[T]): Complex[T]
```
Returns the secant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L264) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L264)
```
proc arcsec[T](z: Complex[T]): Complex[T]
```
Returns the inverse secant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L268)
```
proc csc[T](z: Complex[T]): Complex[T]
```
Returns the cosecant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L272) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L272)
```
proc arccsc[T](z: Complex[T]): Complex[T]
```
Returns the inverse cosecant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L276) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L276)
```
proc sinh[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic sine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L280)
```
proc arcsinh[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic sine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L284) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L284)
```
proc cosh[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic cosine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L288) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L288)
```
proc arccosh[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic cosine of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L292) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L292)
```
proc tanh[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic tangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L296) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L296)
```
proc arctanh[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic tangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L300) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L300)
```
proc sech[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic secant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L304) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L304)
```
proc arcsech[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic secant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L308) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L308)
```
proc csch[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic cosecant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L312) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L312)
```
proc arccsch[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic cosecant of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L316) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L316)
```
proc coth[T](z: Complex[T]): Complex[T]
```
Returns the hyperbolic cotangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L320) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L320)
```
proc arccoth[T](z: Complex[T]): Complex[T]
```
Returns the inverse hyperbolic cotangent of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L324) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L324)
```
proc phase[T](z: Complex[T]): T
```
Returns the phase of `z`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L328) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L328)
```
proc polar[T](z: Complex[T]): tuple[r, phi: T]
```
Returns `z` in polar coordinates. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L332) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L332)
```
proc rect[T](r, phi: T): Complex[T]
```
Returns the complex number with polar coordinates `r` and `phi`.`result.re = r * cos(phi)`
`result.im = r * sin(phi)`
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L336) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L336)
```
proc `$`(z: Complex): string
```
Returns `z`'s string representation as `"(re, im)"`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L344) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L344) Templates
---------
```
template im(arg: typedesc[float32]): Complex32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L39)
```
template im(arg: typedesc[float64]): Complex64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L40)
```
template im(arg: float32): Complex32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L41)
```
template im(arg: float64): Complex64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/complex.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/complex.nim#L42)
| programming_docs |
nim parsejson parsejson
=========
This module implements a json parser. It is used and exported by the `json` standard library module, but can also be used in its own right.
Imports
-------
<strutils>, <lexbase>, <streams>, <unicode> Types
-----
```
JsonEventKind = enum
jsonError, ## an error occurred during parsing
jsonEof, ## end of file reached
jsonString, ## a string literal
jsonInt, ## an integer literal
jsonFloat, ## a float literal
jsonTrue, ## the value ``true``
jsonFalse, ## the value ``false``
jsonNull, ## the value ``null``
jsonObjectStart, ## start of an object: the ``{`` token
jsonObjectEnd, ## end of an object: the ``}`` token
jsonArrayStart, ## start of an array: the ``[`` token
jsonArrayEnd ## end of an array: the ``]`` token
```
enumeration of all events that may occur when parsing [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L18)
```
TokKind = enum
tkError, tkEof, tkString, tkInt, tkFloat, tkTrue, tkFalse, tkNull, tkCurlyLe,
tkCurlyRi, tkBracketLe, tkBracketRi, tkColon, tkComma
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L32)
```
JsonError = enum
errNone, ## no error
errInvalidToken, ## invalid token
errStringExpected, ## string expected
errColonExpected, ## ``:`` expected
errCommaExpected, ## ``,`` expected
errBracketRiExpected, ## ``]`` expected
errCurlyRiExpected, ## ``}`` expected
errQuoteExpected, ## ``"`` or ``'`` expected
errEOC_Expected, ## ``*/`` expected
errEofExpected, ## EOF expected
errExprExpected ## expr expected
```
enumeration that lists all errors that can occur [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L48)
```
JsonParser = object of BaseLexer
a*: string
tok*: TokKind
kind: JsonEventKind
err: JsonError
state: seq[ParserState]
filename: string
rawStringLiterals: bool
```
the parser object. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L65)
```
JsonKindError = object of ValueError
```
raised by the `to` macro if the JSON kind is incorrect. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L74)
```
JsonParsingError = object of ValueError
```
is raised for a JSON error [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L76) Consts
------
```
errorMessages: array[JsonError, string] = ["no error", "invalid token",
"string expected", "\':\' expected", "\',\' expected", "\']\' expected",
"\'}\' expected", "\'\"\' or \"\'\" expected", "\'*/\' expected",
"EOF expected", "expression expected"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L79) Procs
-----
```
proc open(my: var JsonParser; input: Stream; filename: string;
rawStringLiterals = false) {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
initializes the parser with an input stream. `Filename` is only used for nice error messages. If `rawStringLiterals` is true, string literals are kept with their surrounding quotes and escape sequences in them are left untouched too. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L104)
```
proc close(my: var JsonParser) {...}{.inline, raises: [Exception, IOError, OSError],
tags: [WriteIOEffect].}
```
closes the parser `my` and its associated input stream. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L117)
```
proc str(my: JsonParser): string {...}{.inline, raises: [], tags: [].}
```
returns the character data for the events: `jsonInt`, `jsonFloat`, `jsonString` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L121)
```
proc getInt(my: JsonParser): BiggestInt {...}{.inline, raises: [ValueError], tags: [].}
```
returns the number for the event: `jsonInt` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L127)
```
proc getFloat(my: JsonParser): float {...}{.inline, raises: [ValueError], tags: [].}
```
returns the number for the event: `jsonFloat` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L132)
```
proc kind(my: JsonParser): JsonEventKind {...}{.inline, raises: [], tags: [].}
```
returns the current event type for the JSON parser [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L137) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L137)
```
proc getColumn(my: JsonParser): int {...}{.inline, raises: [], tags: [].}
```
get the current column the parser has arrived at. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L141)
```
proc getLine(my: JsonParser): int {...}{.inline, raises: [], tags: [].}
```
get the current line the parser has arrived at. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L145) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L145)
```
proc getFilename(my: JsonParser): string {...}{.inline, raises: [], tags: [].}
```
get the filename of the file that the parser processes. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L149)
```
proc errorMsg(my: JsonParser): string {...}{.raises: [ValueError], tags: [].}
```
returns a helpful error message for the event `jsonError` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L153)
```
proc errorMsgExpected(my: JsonParser; e: string): string {...}{.raises: [ValueError],
tags: [].}
```
returns an error message "`e` expected" in the same format as the other error messages [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L159)
```
proc parseEscapedUTF16(buf: cstring; pos: var int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L173) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L173)
```
proc getTok(my: var JsonParser): TokKind {...}{.raises: [IOError, OSError],
tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L358) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L358)
```
proc next(my: var JsonParser) {...}{.raises: [IOError, OSError], tags: [ReadIOEffect].}
```
retrieves the first/next event. This controls the parser. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L403) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L403)
```
proc raiseParseErr(p: JsonParser; msg: string) {...}{.noinline, noreturn,
raises: [JsonParsingError, ValueError], tags: [].}
```
raises an `EJsonParsingError` exception. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L520) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L520)
```
proc eat(p: var JsonParser; tok: TokKind) {...}{.
raises: [IOError, OSError, JsonParsingError, ValueError],
tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/parsejson.nim#L524) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/parsejson.nim#L524)
nim logging logging
=======
This module implements a simple logger.
It has been designed to be as simple as possible to avoid bloat. If this library does not fulfill your needs, write your own.
Basic usage
-----------
To get started, first create a logger:
```
import logging
var logger = newConsoleLogger()
```
The logger that was created above logs to the console, but this module also provides loggers that log to files, such as the [FileLogger](#FileLogger). Creating custom loggers is also possible by inheriting from the [Logger](#Logger) type.
Once a logger has been created, call its [log proc](#log.e,ConsoleLogger,Level,varargs%5Bstring,%5D) to log a message:
```
logger.log(lvlInfo, "a log message")
# Output: INFO a log message
```
The `INFO` within the output is the result of a format string being prepended to the message, and it will differ depending on the message's level. Format strings are [explained in more detail here](#basic-usage-format-strings).
There are six logging levels: debug, info, notice, warn, error, and fatal. They are described in more detail within the [Level enum's documentation](#Level). A message is logged if its level is at or above both the logger's `levelThreshold` field and the global log filter. The latter can be changed with the [setLogFilter proc](#setLogFilter,Level).
**Warning:**
* For loggers that log to a console or to files, only error and fatal messages will cause their output buffers to be flushed immediately. Use the [flushFile proc](io#flushFile,File) to flush the buffer manually if needed.
### Handlers
When using multiple loggers, calling the log proc for each logger can become repetitive. Instead of doing that, register each logger that will be used with the [addHandler proc](#addHandler,Logger), which is demonstrated in the following example:
```
import logging
var consoleLog = newConsoleLogger()
var fileLog = newFileLogger("errors.log", levelThreshold=lvlError)
var rollingLog = newRollingFileLogger("rolling.log")
addHandler(consoleLog)
addHandler(fileLog)
addHandler(rollingLog)
```
After doing this, use either the [log template](#log.t,Level,varargs%5Bstring,%5D) or one of the level-specific templates, such as the [error template](#error.t,varargs%5Bstring,%5D), to log messages to all registered handlers at once.
```
# This example uses the loggers created above
log(lvlError, "an error occurred")
error("an error occurred") # Equivalent to the above line
info("something normal happened") # Will not be written to errors.log
```
Note that a message's level is still checked against each handler's `levelThreshold` and the global log filter.
### Format strings
Log messages are prefixed with format strings. These strings contain placeholders for variables, such as `$time`, that are replaced with their corresponding values, such as the current time, before they are prepended to a log message. Characters that are not part of variables are unaffected.
The format string used by a logger can be specified by providing the `fmtStr` argument when creating the logger or by setting its `fmtStr` field afterward. If not specified, the [default format string](#defaultFmtStr) is used.
The following variables, which must be prefixed with a dollar sign (`$`), are available:
| Variable | Output |
| --- | --- |
| $date | Current date |
| $time | Current time |
| $datetime | $dateT$time |
| $app | [os.getAppFilename()](os#getAppFilename) |
| $appname | Base name of `$app` |
| $appdir | Directory name of `$app` |
| $levelid | First letter of log level |
| $levelname | Log level name |
Note that `$app`, `$appname`, and `$appdir` are not supported when using the JavaScript backend.
The following example illustrates how to use format strings:
```
import logging
var logger = newConsoleLogger(fmtStr="[$time] - $levelname: ")
logger.log(lvlInfo, "this is a message")
# Output: [19:50:13] - INFO: this is a message
```
### Notes when using multiple threads
There are a few details to keep in mind when using this module within multiple threads:
* The global log filter is actually a thread-local variable, so it needs to be set in each thread that uses this module.
* The list of registered handlers is also a thread-local variable. If a handler will be used in multiple threads, it needs to be registered in each of those threads.
See also
--------
* [strutils module](strutils) for common string functions
* [strformat module](strformat) for string interpolation and formatting
* [strscans module](strscans) for `scanf` and `scanp` macros, which offer easier substring extraction than regular expressions
Imports
-------
<strutils>, <times>, <os> Types
-----
```
Level = enum
lvlAll, ## All levels active
lvlDebug, ## Debug level and above are active
lvlInfo, ## Info level and above are active
lvlNotice, ## Notice level and above are active
lvlWarn, ## Warn level and above are active
lvlError, ## Error level and above are active
lvlFatal, ## Fatal level and above are active
lvlNone ## No levels active; nothing is logged
```
Enumeration of logging levels.
Debug messages represent the lowest logging level, and fatal error messages represent the highest logging level. `lvlAll` can be used to enable all messages, while `lvlNone` can be used to disable all messages.
Typical usage for each logging level, from lowest to highest, is described below:
* **Debug** - debugging information helpful only to developers
* **Info** - anything associated with normal operation and without any particular importance
* **Notice** - more important information that users should be notified about
* **Warn** - impending problems that require some attention
* **Error** - error conditions that the application can recover from
* **Fatal** - fatal errors that prevent the application from continuing
It is completely up to the application how to utilize each level.
Individual loggers have a `levelThreshold` field that filters out any messages with a level lower than the threshold. There is also a global filter that applies to all log messages, and it can be changed using the [setLogFilter proc](#setLogFilter,Level).
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L150)
```
Logger = ref object of RootObj
levelThreshold*: Level ## Only messages that are at or above this
## threshold will be logged
fmtStr*: string ## Format string to prepend to each log message;
## defaultFmtStr is the default
```
The abstract base type of all loggers.
Custom loggers should inherit from this type. They should also provide their own implementation of the [log method](#log.e,Logger,Level,varargs%5Bstring,%5D).
See also:
* [ConsoleLogger](#ConsoleLogger)
* [FileLogger](#FileLogger)
* [RollingFileLogger](#RollingFileLogger)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L202)
```
ConsoleLogger = ref object of Logger
useStderr*: bool ## If true, writes to stderr; otherwise, writes to stdout
```
A logger that writes log messages to the console.
Create a new `ConsoleLogger` with the [newConsoleLogger proc](#newConsoleLogger).
See also:
* [FileLogger](#FileLogger)
* [RollingFileLogger](#RollingFileLogger)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L218) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L218)
```
FileLogger = ref object of Logger
file*: File ## The wrapped file
```
A logger that writes log messages to a file.
Create a new `FileLogger` with the [newFileLogger proc](#newFileLogger,File).
**Note:** This logger is not available for the JavaScript backend.
See also:
* [ConsoleLogger](#ConsoleLogger)
* [RollingFileLogger](#RollingFileLogger)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L231) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L231)
```
RollingFileLogger = ref object of FileLogger
maxLines: int
curLine: int
baseName: string
baseMode: FileMode
logFiles: int
bufSize: int
```
A logger that writes log messages to a file while performing log rotation.
Create a new `RollingFileLogger` with the [newRollingFileLogger proc](#newRollingFileLogger,FileMode,int,int).
**Note:** This logger is not available for the JavaScript backend.
See also:
* [ConsoleLogger](#ConsoleLogger)
* [FileLogger](#FileLogger)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L244) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L244) Consts
------
```
LevelNames: array[Level, string] = ["DEBUG", "DEBUG", "INFO", "NOTICE", "WARN",
"ERROR", "FATAL", "NONE"]
```
Array of strings representing each logging level. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L186)
```
defaultFmtStr = "$levelname "
```
The default format string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L190)
```
verboseFmtStr = "$levelid, [$datetime] -- $appname: "
```
A more verbose format string.
This string can be passed as the `frmStr` argument to procs that create new loggers, such as the [newConsoleLogger proc](#newConsoleLogger).
If a different format string is preferred, refer to the [documentation about format strings](#basic-usage-format-strings) for more information, including a list of available variables.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L191) Procs
-----
```
proc substituteLog(frmt: string; level: Level; args: varargs[string, `$`]): string {...}{.
raises: [], tags: [ReadIOEffect, TimeEffect].}
```
Formats a log message at the specified level with the given format string.
The [format variables](#basic-usage-format-strings) present within `frmt` will be replaced with the corresponding values before being prepended to `args` and returned.
Unless you are implementing a custom logger, there is little need to call this directly. Use either a logger's log method or one of the logging templates.
See also:
* [log method](#log.e,ConsoleLogger,Level,varargs%5Bstring,%5D) for the ConsoleLogger
* [log method](#log.e,FileLogger,Level,varargs%5Bstring,%5D) for the FileLogger
* [log method](#log.e,RollingFileLogger,Level,varargs%5Bstring,%5D) for the RollingFileLogger
* [log template](#log.t,Level,varargs%5Bstring,%5D)
**Example:**
```
doAssert substituteLog(defaultFmtStr, lvlInfo, "a message") == "INFO a message"
doAssert substituteLog("$levelid - ", lvlError, "an error") == "E - an error"
doAssert substituteLog("$levelid", lvlDebug, "error") == "Derror"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L267) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L267)
```
proc newConsoleLogger(levelThreshold = lvlAll; fmtStr = defaultFmtStr;
useStderr = false): ConsoleLogger {...}{.raises: [], tags: [].}
```
Creates a new [ConsoleLogger](#ConsoleLogger).
By default, log messages are written to `stdout`. If `useStderr` is true, they are written to `stderr` instead.
For the JavaScript backend, log messages are written to the console, and `useStderr` is ignored.
See also:
* [newFileLogger proc](#newFileLogger,File) that uses a file handle
* [newFileLogger proc](#newFileLogger,FileMode,int) that accepts a filename
* [newRollingFileLogger proc](#newRollingFileLogger,FileMode,int,int)
**Examples:**
```
var normalLog = newConsoleLogger()
var formatLog = newConsoleLogger(fmtStr=verboseFmtStr)
var errorLog = newConsoleLogger(levelThreshold=lvlError, useStderr=true)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L384) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L384)
```
proc defaultFilename(): string {...}{.raises: [], tags: [ReadIOEffect].}
```
Returns the filename that is used by default when naming log files.
**Note:** This proc is not available for the JavaScript backend.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L445) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L445)
```
proc newFileLogger(file: File; levelThreshold = lvlAll; fmtStr = defaultFmtStr): FileLogger {...}{.
raises: [], tags: [].}
```
Creates a new [FileLogger](#FileLogger) that uses the given file handle.
**Note:** This proc is not available for the JavaScript backend.
See also:
* [newConsoleLogger proc](#newConsoleLogger)
* [newFileLogger proc](#newFileLogger,FileMode,int) that accepts a filename
* [newRollingFileLogger proc](#newRollingFileLogger,FileMode,int,int)
**Examples:**
```
var messages = open("messages.log", fmWrite)
var formatted = open("formatted.log", fmWrite)
var errors = open("errors.log", fmWrite)
var normalLog = newFileLogger(messages)
var formatLog = newFileLogger(formatted, fmtStr=verboseFmtStr)
var errorLog = newFileLogger(errors, levelThreshold=lvlError)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L452) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L452)
```
proc newFileLogger(filename = defaultFilename(); mode: FileMode = fmAppend;
levelThreshold = lvlAll; fmtStr = defaultFmtStr;
bufSize: int = -1): FileLogger {...}{.raises: [IOError], tags: [].}
```
Creates a new [FileLogger](#FileLogger) that logs to a file with the given filename.
`bufSize` controls the size of the output buffer that is used when writing to the log file. The following values can be provided:
* `-1` - use system defaults
* `0` - unbuffered
* `> 0` - fixed buffer size
**Note:** This proc is not available for the JavaScript backend.
See also:
* [newConsoleLogger proc](#newConsoleLogger)
* [newFileLogger proc](#newFileLogger,File) that uses a file handle
* [newRollingFileLogger proc](#newRollingFileLogger,FileMode,int,int)
**Examples:**
```
var normalLog = newFileLogger("messages.log")
var formatLog = newFileLogger("formatted.log", fmtStr=verboseFmtStr)
var errorLog = newFileLogger("errors.log", levelThreshold=lvlError)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L480) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L480)
```
proc newRollingFileLogger(filename = defaultFilename();
mode: FileMode = fmReadWrite; levelThreshold = lvlAll;
fmtStr = defaultFmtStr; maxLines: Positive = 1000;
bufSize: int = -1): RollingFileLogger {...}{.
raises: [IOError, OSError], tags: [ReadDirEffect, ReadIOEffect].}
```
Creates a new [RollingFileLogger](#RollingFileLogger).
Once the current log file being written to contains `maxLines` lines, a new log file will be created, and the old log file will be renamed.
`bufSize` controls the size of the output buffer that is used when writing to the log file. The following values can be provided:
* `-1` - use system defaults
* `0` - unbuffered
* `> 0` - fixed buffer size
**Note:** This proc is not available in the JavaScript backend.
See also:
* [newConsoleLogger proc](#newConsoleLogger)
* [newFileLogger proc](#newFileLogger,File) that uses a file handle
* [newFileLogger proc](#newFileLogger,FileMode,int) that accepts a filename
**Examples:**
```
var normalLog = newRollingFileLogger("messages.log")
var formatLog = newRollingFileLogger("formatted.log", fmtStr=verboseFmtStr)
var shortLog = newRollingFileLogger("short.log", maxLines=200)
var errorLog = newRollingFileLogger("errors.log", levelThreshold=lvlError)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L535) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L535)
```
proc addHandler(handler: Logger) {...}{.raises: [], tags: [].}
```
Adds a logger to the list of registered handlers.
**Warning:** The list of handlers is a thread-local variable. If the given handler will be used in multiple threads, this proc should be called in each of those threads.
See also:
* [getHandlers proc](#getHandlers)
**Example:**
```
var logger = newConsoleLogger()
addHandler(logger)
doAssert logger in getHandlers()
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L794) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L794)
```
proc getHandlers(): seq[Logger] {...}{.raises: [], tags: [].}
```
Returns a list of all the registered handlers.
See also:
* [addHandler proc](#addHandler,Logger)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L809) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L809)
```
proc setLogFilter(lvl: Level) {...}{.raises: [], tags: [].}
```
Sets the global log filter.
Messages below the provided level will not be logged regardless of an individual logger's `levelThreshold`. By default, all messages are logged.
**Warning:** The global log filter is a thread-local variable. If logging is being performed in multiple threads, this proc should be called in each thread unless it is intended that different threads should log at different logging levels.
See also:
* [getLogFilter proc](#getLogFilter)
**Example:**
```
setLogFilter(lvlError)
doAssert getLogFilter() == lvlError
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L816) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L816)
```
proc getLogFilter(): Level {...}{.raises: [], tags: [].}
```
Gets the global log filter.
See also:
* [setLogFilter proc](#setLogFilter,Level)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L835) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L835) Methods
-------
```
method log(logger: Logger; level: Level; args: varargs[string, `$`]) {...}{.
raises: [Exception], gcsafe, tags: [RootEffect], base.}
```
Override this method in custom loggers. The default implementation does nothing.
See also:
* [log method](#log.e,ConsoleLogger,Level,varargs%5Bstring,%5D) for the ConsoleLogger
* [log method](#log.e,FileLogger,Level,varargs%5Bstring,%5D) for the FileLogger
* [log method](#log.e,RollingFileLogger,Level,varargs%5Bstring,%5D) for the RollingFileLogger
* [log template](#log.t,Level,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L322)
```
method log(logger: ConsoleLogger; level: Level; args: varargs[string, `$`]) {...}{.
raises: [], tags: [ReadIOEffect, TimeEffect, WriteIOEffect].}
```
Logs to the console with the given [ConsoleLogger](#ConsoleLogger) only.
This method ignores the list of registered handlers.
Whether the message is logged depends on both the ConsoleLogger's `levelThreshold` field and the global log filter set using the [setLogFilter proc](#setLogFilter,Level).
**Note:** Only error and fatal messages will cause the output buffer to be flushed immediately. Use the [flushFile proc](io#flushFile,File) to flush the buffer manually if needed.
See also:
* [log method](#log.e,FileLogger,Level,varargs%5Bstring,%5D) for the FileLogger
* [log method](#log.e,RollingFileLogger,Level,varargs%5Bstring,%5D) for the RollingFileLogger
* [log template](#log.t,Level,varargs%5Bstring,%5D)
**Examples:**
```
var consoleLog = newConsoleLogger()
consoleLog.log(lvlInfo, "this is a message")
consoleLog.log(lvlError, "error code is: ", 404)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L338) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L338)
```
method log(logger: FileLogger; level: Level; args: varargs[string, `$`]) {...}{.
raises: [IOError], tags: [WriteIOEffect, ReadIOEffect, TimeEffect].}
```
Logs a message at the specified level using the given [FileLogger](#FileLogger) only.
This method ignores the list of registered handlers.
Whether the message is logged depends on both the FileLogger's `levelThreshold` field and the global log filter set using the [setLogFilter proc](#setLogFilter,Level).
**Notes:**
* Only error and fatal messages will cause the output buffer to be flushed immediately. Use the [flushFile proc](io#flushFile,File) to flush the buffer manually if needed.
* This method is not available for the JavaScript backend.
See also:
* [log method](#log.e,ConsoleLogger,Level,varargs%5Bstring,%5D) for the ConsoleLogger
* [log method](#log.e,RollingFileLogger,Level,varargs%5Bstring,%5D) for the RollingFileLogger
* [log template](#log.t,Level,varargs%5Bstring,%5D)
**Examples:**
```
var fileLog = newFileLogger("messages.log")
fileLog.log(lvlInfo, "this is a message")
fileLog.log(lvlError, "error code is: ", 404)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L412) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L412)
```
method log(logger: RollingFileLogger; level: Level; args: varargs[string, `$`]) {...}{.
raises: [OSError, IOError, Exception],
tags: [ReadIOEffect, WriteIOEffect, TimeEffect].}
```
Logs a message at the specified level using the given [RollingFileLogger](#RollingFileLogger) only.
This method ignores the list of registered handlers.
Whether the message is logged depends on both the RollingFileLogger's `levelThreshold` field and the global log filter set using the [setLogFilter proc](#setLogFilter,Level).
**Notes:**
* Only error and fatal messages will cause the output buffer to be flushed immediately. Use the [flushFile proc](io#flushFile,File) to flush the buffer manually if needed.
* This method is not available for the JavaScript backend.
See also:
* [log method](#log.e,ConsoleLogger,Level,varargs%5Bstring,%5D) for the ConsoleLogger
* [log method](#log.e,FileLogger,Level,varargs%5Bstring,%5D) for the FileLogger
* [log template](#log.t,Level,varargs%5Bstring,%5D)
**Examples:**
```
var rollingLog = newRollingFileLogger("messages.log")
rollingLog.log(lvlInfo, "this is a message")
rollingLog.log(lvlError, "error code is: ", 404)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L590) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L590) Templates
---------
```
template log(level: Level; args: varargs[string, `$`])
```
Logs a message at the specified level to all registered handlers.
Whether the message is logged depends on both the FileLogger's `levelThreshold` field and the global log filter set using the [setLogFilter proc](#setLogFilter,Level).
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
log(lvlInfo, "This is an example.")
```
See also:
* [debug template](#debug.t,varargs%5Bstring,%5D)
* [info template](#info.t,varargs%5Bstring,%5D)
* [notice template](#notice.t,varargs%5Bstring,%5D)
* [warn template](#warn.t,varargs%5Bstring,%5D)
* [error template](#error.t,varargs%5Bstring,%5D)
* [fatal template](#fatal.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L639) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L639)
```
template debug(args: varargs[string, `$`])
```
Logs a debug message to all registered handlers.
Debug messages are typically useful to the application developer only, and they are usually disabled in release builds, although this template does not make that distinction.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
debug("myProc called with arguments: foo, 5")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [info template](#info.t,varargs%5Bstring,%5D)
* [notice template](#notice.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L668) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L668)
```
template info(args: varargs[string, `$`])
```
Logs an info message to all registered handlers.
Info messages are typically generated during the normal operation of an application and are of no particular importance. It can be useful to aggregate these messages for later analysis.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
info("Application started successfully.")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [debug template](#debug.t,varargs%5Bstring,%5D)
* [notice template](#notice.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L689) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L689)
```
template notice(args: varargs[string, `$`])
```
Logs an notice to all registered handlers.
Notices are semantically very similar to info messages, but they are meant to be messages that the user should be actively notified about, depending on the application.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
notice("An important operation has completed.")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [debug template](#debug.t,varargs%5Bstring,%5D)
* [info template](#info.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L710) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L710)
```
template warn(args: varargs[string, `$`])
```
Logs a warning message to all registered handlers.
A warning is a non-error message that may indicate impending problems or degraded performance.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
warn("The previous operation took too long to process.")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [error template](#error.t,varargs%5Bstring,%5D)
* [fatal template](#fatal.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L731) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L731)
```
template error(args: varargs[string, `$`])
```
Logs an error message to all registered handlers.
Error messages are for application-level error conditions, such as when some user input generated an exception. Typically, the application will continue to run, but with degraded functionality or loss of data, and these effects might be visible to users.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
error("An exception occurred while processing the form.")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [warn template](#warn.t,varargs%5Bstring,%5D)
* [fatal template](#fatal.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L751) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L751)
```
template fatal(args: varargs[string, `$`])
```
Logs a fatal error message to all registered handlers.
Fatal error messages usually indicate that the application cannot continue to run and will exit due to a fatal condition. This template only logs the message, and it is the application's responsibility to exit properly.
**Examples:**
```
var logger = newConsoleLogger()
addHandler(logger)
fatal("Can't open database -- exiting.")
```
See also:
* [log template](#log.t,Level,varargs%5Bstring,%5D)
* [warn template](#warn.t,varargs%5Bstring,%5D)
* [error template](#error.t,varargs%5Bstring,%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/logging.nim#L773) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/logging.nim#L773)
| programming_docs |
nim posix_utils posix\_utils
============
A set of helpers for the POSIX module. Raw interfaces are in the other posix\*.nim files.
Imports
-------
<posix> Types
-----
```
Uname = object
sysname*, nodename*, release*, version*, machine*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L16) Procs
-----
```
proc uname(): Uname {...}{.raises: [OSError], tags: [].}
```
Provides system information in a `Uname` struct with sysname, nodename, release, version and machine attributes. **Example:**
```
echo uname().nodename, uname().release, uname().version
doAssert uname().sysname.len != 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L22)
```
proc fsync(fd: int) {...}{.raises: [OSError], tags: [].}
```
synchronize a file's buffer cache to the storage device [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L41)
```
proc stat(path: string): Stat {...}{.raises: [OSError], tags: [].}
```
Returns file status in a `Stat` structure [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L46)
```
proc memoryLock(a1: pointer; a2: int) {...}{.raises: [OSError], tags: [].}
```
Locks pages starting from a1 for a1 bytes and prevent them from being swapped. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L51) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L51)
```
proc memoryLockAll(flags: int) {...}{.raises: [OSError], tags: [].}
```
Locks all memory for the running process to prevent swapping.
example:
```
memoryLockAll(MCL_CURRENT or MCL_FUTURE)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L56) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L56)
```
proc memoryUnlock(a1: pointer; a2: int) {...}{.raises: [OSError], tags: [].}
```
Unlock pages starting from a1 for a1 bytes and allow them to be swapped. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L65)
```
proc memoryUnlockAll() {...}{.raises: [OSError], tags: [].}
```
Unlocks all memory for the running process to allow swapping. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L70)
```
proc sendSignal(pid: Pid; signal: int) {...}{.raises: [OSError], tags: [].}
```
Sends a signal to a running process by calling `kill`. Raise exception in case of failure e.g. process not running. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L75)
```
proc mkstemp(prefix: string; suffix = ""): (string, File) {...}{.raises: [OSError],
tags: [].}
```
Creates a unique temporary file from a prefix string. A six-character string will be added. If suffix is provided it will be added to the string The file is created with perms 0600. Returns the filename and a file opened in r/w mode. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L81)
```
proc mkdtemp(prefix: string): string {...}{.raises: [OSError], tags: [].}
```
Creates a unique temporary directory from a prefix string. Adds a six chars suffix. The directory is created with permissions 0700. Returns the directory name. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/posix/posix_utils.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/posix/posix_utils.nim#L103)
nim bitops bitops
======
This module implements a series of low level methods for bit manipulation.
By default, this module use compiler intrinsics where possible to improve performance on supported compilers: `GCC`, `LLVM_GCC`, `CLANG`, `VCC`, `ICC`.
The module will fallback to pure nim procs incase the backend is not supported. You can also use the flag `noIntrinsicsBitOpts` to disable compiler intrinsics.
This module is also compatible with other backends: `Javascript`, `Nimscript` as well as the `compiletime VM`.
As a result of using optimized function/intrinsics some functions can return undefined results if the input is invalid. You can use the flag `noUndefinedBitOpts` to force predictable behaviour for all input, causing a small performance hit.
At this time only `fastLog2`, `firstSetBit, `countLeadingZeroBits`, `countTrailingZeroBits` may return undefined and/or platform dependent value if given invalid input.
Imports
-------
<macros>, <since> Types
-----
```
BitsRange[T] = range[0 .. sizeof(T) * 8 - 1]
```
A range with all bit positions for type `T` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L88) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L88) Procs
-----
```
proc bitnot[T: SomeInteger](x: T): T {...}{.magic: "BitnotI", noSideEffect.}
```
Computes the `bitwise complement` of the integer `x`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L31)
```
proc bitslice[T: SomeInteger](v: var T; slice: Slice[int]) {...}{.inline.}
```
Mutates `v` into an extracted (and shifted) slice of bits from `v`. **Example:**
```
var x = 0b101110
x.bitslice(2 .. 4)
doAssert x == 0b011
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L103)
```
proc masked[T: SomeInteger](v, mask: T): T {...}{.inline.}
```
Returns `v`, with only the `1` bits from `mask` matching those of `v` set to 1.
Effectively maps to a `bitand` operation.
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.masked(0b0000_1010'u8) == 0b0000_0010'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L129)
```
proc mask[T: SomeInteger](v: var T; mask: T) {...}{.inline.}
```
Mutates `v`, with only the `1` bits from `mask` matching those of `v` set to 1.
Effectively maps to a `bitand` operation.
**Example:**
```
var v = 0b0000_0011'u8
v.mask(0b0000_1010'u8)
doAssert v == 0b0000_0010'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L151) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L151)
```
proc mask[T: SomeInteger](v: var T; slice: Slice[int]) {...}{.inline.}
```
Mutates `v`, with only the `1` bits in the range of `slice` matching those of `v` set to 1.
Effectively maps to a `bitand` operation.
**Example:**
```
var v = 0b0000_1011'u8
v.mask(1 .. 3)
doAssert v == 0b0000_1010'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L163)
```
proc setMask[T: SomeInteger](v: var T; mask: T) {...}{.inline.}
```
Mutates `v`, with all the `1` bits from `mask` set to 1.
Effectively maps to a `bitor` operation.
**Example:**
```
var v = 0b0000_0011'u8
v.setMask(0b0000_1010'u8)
doAssert v == 0b0000_1011'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L195)
```
proc setMask[T: SomeInteger](v: var T; slice: Slice[int]) {...}{.inline.}
```
Mutates `v`, with all the `1` bits in the range of `slice` set to 1.
Effectively maps to a `bitor` operation.
**Example:**
```
var v = 0b0000_0011'u8
v.setMask(2 .. 3)
doAssert v == 0b0000_1111'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L206)
```
proc clearMask[T: SomeInteger](v: var T; mask: T) {...}{.inline.}
```
Mutates `v`, with all the `1` bits from `mask` set to 0.
Effectively maps to a `bitand` operation with an *inverted mask.*
**Example:**
```
var v = 0b0000_0011'u8
v.clearMask(0b0000_1010'u8)
doAssert v == 0b0000_0001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L237) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L237)
```
proc clearMask[T: SomeInteger](v: var T; slice: Slice[int]) {...}{.inline.}
```
Mutates `v`, with all the `1` bits in the range of `slice` set to 0.
Effectively maps to a `bitand` operation with an *inverted mask.*
**Example:**
```
var v = 0b0000_0011'u8
v.clearMask(1 .. 3)
doAssert v == 0b0000_0001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L248) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L248)
```
proc flipMask[T: SomeInteger](v: var T; mask: T) {...}{.inline.}
```
Mutates `v`, with all the `1` bits from `mask` flipped.
Effectively maps to a `bitxor` operation.
**Example:**
```
var v = 0b0000_0011'u8
v.flipMask(0b0000_1010'u8)
doAssert v == 0b0000_1001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L279) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L279)
```
proc flipMask[T: SomeInteger](v: var T; slice: Slice[int]) {...}{.inline.}
```
Mutates `v`, with all the `1` bits in the range of `slice` flipped.
Effectively maps to a `bitxor` operation.
**Example:**
```
var v = 0b0000_0011'u8
v.flipMask(1 .. 3)
doAssert v == 0b0000_1101'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L290)
```
proc setBit[T: SomeInteger](v: var T; bit: BitsRange[T]) {...}{.inline.}
```
Mutates `v`, with the bit at position `bit` set to 1 **Example:**
```
var v = 0b0000_0011'u8
v.setBit(5'u8)
doAssert v == 0b0010_0011'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L301) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L301)
```
proc clearBit[T: SomeInteger](v: var T; bit: BitsRange[T]) {...}{.inline.}
```
Mutates `v`, with the bit at position `bit` set to 0 **Example:**
```
var v = 0b0000_0011'u8
v.clearBit(1'u8)
doAssert v == 0b0000_0001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L310) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L310)
```
proc flipBit[T: SomeInteger](v: var T; bit: BitsRange[T]) {...}{.inline.}
```
Mutates `v`, with the bit at position `bit` flipped **Example:**
```
var v = 0b0000_0011'u8
v.flipBit(1'u8)
doAssert v == 0b0000_0001'u8
v = 0b0000_0011'u8
v.flipBit(2'u8)
doAssert v == 0b0000_0111'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L319) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L319)
```
proc testBit[T: SomeInteger](v: T; bit: BitsRange[T]): bool {...}{.inline.}
```
Returns true if the bit in `v` at positions `bit` is set to 1 **Example:**
```
var v = 0b0000_1111'u8
doAssert v.testBit(0)
doAssert not v.testBit(7)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L369) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L369)
```
proc countSetBits(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Counts the set bits in integer. (also called Hamming weight.) **Example:**
```
doAssert countSetBits(0b0000_0011'u8) == 2
doAssert countSetBits(0b1010_1010'u8) == 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L531) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L531)
```
proc popcount(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Alias for for [countSetBits](#countSetBits,SomeInteger). (Hamming weight.) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L562) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L562)
```
proc parityBits(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Calculate the bit parity in integer. If number of 1-bit is odd parity is 1, otherwise 0. **Example:**
```
doAssert parityBits(0b0000_0000'u8) == 0
doAssert parityBits(0b0101_0001'u8) == 1
doAssert parityBits(0b0110_1001'u8) == 0
doAssert parityBits(0b0111_1111'u8) == 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L566) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L566)
```
proc firstSetBit(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Returns the 1-based index of the least significant set bit of x. If `x` is zero, when `noUndefinedBitOpts` is set, result is 0, otherwise result is undefined. **Example:**
```
doAssert firstSetBit(0b0000_0001'u8) == 1
doAssert firstSetBit(0b0000_0010'u8) == 2
doAssert firstSetBit(0b0000_0100'u8) == 3
doAssert firstSetBit(0b0000_1000'u8) == 4
doAssert firstSetBit(0b0000_1111'u8) == 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L589) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L589)
```
proc fastLog2(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Quickly find the log base 2 of an integer. If `x` is zero, when `noUndefinedBitOpts` is set, result is -1, otherwise result is undefined. **Example:**
```
doAssert fastLog2(0b0000_0001'u8) == 0
doAssert fastLog2(0b0000_0010'u8) == 1
doAssert fastLog2(0b0000_0100'u8) == 2
doAssert fastLog2(0b0000_1000'u8) == 3
doAssert fastLog2(0b0000_1111'u8) == 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L633) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L633)
```
proc countLeadingZeroBits(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Returns the number of leading zero bits in integer. If `x` is zero, when `noUndefinedBitOpts` is set, result is 0, otherwise result is undefined.
See also:
* [countTrailingZeroBits proc](#countTrailingZeroBits,SomeInteger)
**Example:**
```
doAssert countLeadingZeroBits(0b0000_0001'u8) == 7
doAssert countLeadingZeroBits(0b0000_0010'u8) == 6
doAssert countLeadingZeroBits(0b0000_0100'u8) == 5
doAssert countLeadingZeroBits(0b0000_1000'u8) == 4
doAssert countLeadingZeroBits(0b0000_1111'u8) == 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L673) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L673)
```
proc countTrailingZeroBits(x: SomeInteger): int {...}{.inline, noSideEffect.}
```
Returns the number of trailing zeros in integer. If `x` is zero, when `noUndefinedBitOpts` is set, result is 0, otherwise result is undefined.
See also:
* [countLeadingZeroBits proc](#countLeadingZeroBits,SomeInteger)
**Example:**
```
doAssert countTrailingZeroBits(0b0000_0001'u8) == 0
doAssert countTrailingZeroBits(0b0000_0010'u8) == 1
doAssert countTrailingZeroBits(0b0000_0100'u8) == 2
doAssert countTrailingZeroBits(0b0000_1000'u8) == 3
doAssert countTrailingZeroBits(0b0000_1111'u8) == 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L702) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L702)
```
proc rotateLeftBits(value: uint8; amount: range[0 .. 8]): uint8 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Left-rotate bits in a 8-bits value. **Example:**
```
doAssert rotateLeftBits(0b0000_0001'u8, 1) == 0b0000_0010'u8
doAssert rotateLeftBits(0b0000_0001'u8, 2) == 0b0000_0100'u8
doAssert rotateLeftBits(0b0100_0001'u8, 1) == 0b1000_0010'u8
doAssert rotateLeftBits(0b0100_0001'u8, 2) == 0b0000_0101'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L731) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L731)
```
proc rotateLeftBits(value: uint16; amount: range[0 .. 16]): uint16 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Left-rotate bits in a 16-bits value.
See also:
* [rotateLeftBits proc](#rotateLeftBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L747) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L747)
```
proc rotateLeftBits(value: uint32; amount: range[0 .. 32]): uint32 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Left-rotate bits in a 32-bits value.
See also:
* [rotateLeftBits proc](#rotateLeftBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L756) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L756)
```
proc rotateLeftBits(value: uint64; amount: range[0 .. 64]): uint64 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Left-rotate bits in a 64-bits value.
See also:
* [rotateLeftBits proc](#rotateLeftBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L765) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L765)
```
proc rotateRightBits(value: uint8; amount: range[0 .. 8]): uint8 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Right-rotate bits in a 8-bits value. **Example:**
```
doAssert rotateRightBits(0b0000_0001'u8, 1) == 0b1000_0000'u8
doAssert rotateRightBits(0b0000_0001'u8, 2) == 0b0100_0000'u8
doAssert rotateRightBits(0b0100_0001'u8, 1) == 0b1010_0000'u8
doAssert rotateRightBits(0b0100_0001'u8, 2) == 0b0101_0000'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L775) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L775)
```
proc rotateRightBits(value: uint16; amount: range[0 .. 16]): uint16 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Right-rotate bits in a 16-bits value.
See also:
* [rotateRightBits proc](#rotateRightBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L787) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L787)
```
proc rotateRightBits(value: uint32; amount: range[0 .. 32]): uint32 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Right-rotate bits in a 32-bits value.
See also:
* [rotateRightBits proc](#rotateRightBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L796) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L796)
```
proc rotateRightBits(value: uint64; amount: range[0 .. 64]): uint64 {...}{.inline,
noSideEffect, raises: [], tags: [].}
```
Right-rotate bits in a 64-bits value.
See also:
* [rotateRightBits proc](#rotateRightBits,uint8,range%5B%5D)
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L805) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L805)
```
proc reverseBits[T: SomeUnsignedInt](x: T): T {...}{.noSideEffect.}
```
Return the bit reversal of x. **Example:**
```
doAssert reverseBits(0b10100100'u8) == 0b00100101'u8
doAssert reverseBits(0xdd'u8) == 0xbb'u8
doAssert reverseBits(0xddbb'u16) == 0xddbb'u16
doAssert reverseBits(0xdeadbeef'u32) == 0xf77db57b'u32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L822) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L822) Funcs
-----
```
func bitsliced[T: SomeInteger](v: T; slice: Slice[int]): T {...}{.inline.}
```
Returns an extracted (and shifted) slice of bits from `v`. **Example:**
```
doAssert 0b10111.bitsliced(2 .. 4) == 0b101
doAssert 0b11100.bitsliced(0 .. 2) == 0b100
doAssert 0b11100.bitsliced(0 ..< 3) == 0b100
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L91)
```
func toMask[T: SomeInteger](slice: Slice[int]): T {...}{.inline.}
```
Creates a bitmask based on a slice of bits. **Example:**
```
doAssert toMask[int32](1 .. 3) == 0b1110'i32
doAssert toMask[int32](0 .. 3) == 0b1111'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L115) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L115)
```
func masked[T: SomeInteger](v: T; slice: Slice[int]): T {...}{.inline.}
```
Mutates `v`, with only the `1` bits in the range of `slice` matching those of `v` set to 1.
Effectively maps to a `bitand` operation.
**Example:**
```
var v = 0b0000_1011'u8
doAssert v.masked(1 .. 3) == 0b0000_1010'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L140)
```
func setMasked[T: SomeInteger](v, mask: T): T {...}{.inline.}
```
Returns `v`, with all the `1` bits from `mask` set to 1.
Effectively maps to a `bitor` operation.
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.setMasked(0b0000_1010'u8) == 0b0000_1011'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L175)
```
func setMasked[T: SomeInteger](v: T; slice: Slice[int]): T {...}{.inline.}
```
Returns `v`, with all the `1` bits in the range of `slice` set to 1.
Effectively maps to a `bitor` operation.
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.setMasked(2 .. 3) == 0b0000_1111'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L185) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L185)
```
func clearMasked[T: SomeInteger](v, mask: T): T {...}{.inline.}
```
Returns `v`, with all the `1` bits from `mask` set to 0.
Effectively maps to a `bitand` operation with an *inverted mask.*
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.clearMasked(0b0000_1010'u8) == 0b0000_0001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L217) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L217)
```
func clearMasked[T: SomeInteger](v: T; slice: Slice[int]): T {...}{.inline.}
```
Returns `v`, with all the `1` bits in the range of `slice` set to 0.
Effectively maps to a `bitand` operation with an *inverted mask.*
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.clearMasked(1 .. 3) == 0b0000_0001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L227) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L227)
```
func flipMasked[T: SomeInteger](v, mask: T): T {...}{.inline.}
```
Returns `v`, with all the `1` bits from `mask` flipped.
Effectively maps to a `bitxor` operation.
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.flipMasked(0b0000_1010'u8) == 0b0000_1001'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L259) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L259)
```
func flipMasked[T: SomeInteger](v: T; slice: Slice[int]): T {...}{.inline.}
```
Returns `v`, with all the `1` bits in the range of `slice` flipped.
Effectively maps to a `bitxor` operation.
**Example:**
```
var v = 0b0000_0011'u8
doAssert v.flipMasked(1 .. 3) == 0b0000_1101'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L269) Macros
------
```
macro bitand[T: SomeInteger](x, y: T; z: varargs[T]): T
```
Computes the `bitwise and` of all arguments collectively. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L40)
```
macro bitor[T: SomeInteger](x, y: T; z: varargs[T]): T
```
Computes the `bitwise or` of all arguments collectively. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L47)
```
macro bitxor[T: SomeInteger](x, y: T; z: varargs[T]): T
```
Computes the `bitwise xor` of all arguments collectively. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L54)
```
macro setBits(v: typed; bits: varargs[typed]): untyped
```
Mutates `v`, with the bits at positions `bits` set to 1 **Example:**
```
var v = 0b0000_0011'u8
v.setBits(3, 5, 7)
doAssert v == 0b1010_1011'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L332) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L332)
```
macro clearBits(v: typed; bits: varargs[typed]): untyped
```
Mutates `v`, with the bits at positions `bits` set to 0 **Example:**
```
var v = 0b1111_1111'u8
v.clearBits(1, 3, 5, 7)
doAssert v == 0b0101_0101'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L344) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L344)
```
macro flipBits(v: typed; bits: varargs[typed]): untyped
```
Mutates `v`, with the bits at positions `bits` set to 0 **Example:**
```
var v = 0b0000_1111'u8
v.flipBits(1, 3, 5, 7)
doAssert v == 0b1010_0101'u8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/bitops.nim#L356) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/bitops.nim#L356)
| programming_docs |
nim ssl_certs ssl\_certs
==========
Scan for SSL/TLS CA certificates on disk The default locations can be overridden using the SSL\_CERT\_FILE and SSL\_CERT\_DIR environment variables.
Imports
-------
<os>, <strutils> Iterators
---------
```
iterator scanSSLCertificates(useEnvVars = false): string {...}{.raises: [],
tags: [ReadEnvEffect, ReadDirEffect].}
```
Scan for SSL/TLS CA certificates on disk.
if `useEnvVars` is true, the SSL\_CERT\_FILE and SSL\_CERT\_DIR environment variables can be used to override the certificate directories to scan or specify a CA certificate file.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/ssl_certs.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/ssl_certs.nim#L94)
nim xmlparser xmlparser
=========
This module parses an XML document and creates its XML tree representation.
Imports
-------
<streams>, <parsexml>, <strtabs>, <xmltree>, <os> Types
-----
```
XmlError = object of ValueError
errors*: seq[string] ## All detected parsing errors.
```
Exception that is raised for invalid XML. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L15) Procs
-----
```
proc parseXml(s: Stream; filename: string; errors: var seq[string];
options: set[XmlParseOption] = {reportComments}): XmlNode {...}{.
raises: [IOError, OSError, ValueError, Exception],
tags: [ReadIOEffect, RootEffect, WriteIOEffect].}
```
Parses the XML from stream `s` and returns a `XmlNode`. Every occurred parsing error is added to the `errors` sequence. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L101)
```
proc parseXml(s: Stream; options: set[XmlParseOption] = {reportComments}): XmlNode {...}{.
raises: [IOError, OSError, ValueError, Exception, XmlError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect].}
```
Parses the XML from stream `s` and returns a `XmlNode`. All parsing errors are turned into an `XmlError` exception. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L121)
```
proc parseXml(str: string; options: set[XmlParseOption] = {reportComments}): XmlNode {...}{.
raises: [IOError, OSError, ValueError, Exception, XmlError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect].}
```
Parses the XML from string `str` and returns a `XmlNode`. All parsing errors are turned into an `XmlError` exception. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L128)
```
proc loadXml(path: string; errors: var seq[string];
options: set[XmlParseOption] = {reportComments}): XmlNode {...}{.
raises: [IOError, OSError, ValueError, Exception],
tags: [ReadIOEffect, RootEffect, WriteIOEffect].}
```
Loads and parses XML from file specified by `path`, and returns a `XmlNode`. Every occurred parsing error is added to the `errors` sequence. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L133)
```
proc loadXml(path: string; options: set[XmlParseOption] = {reportComments}): XmlNode {...}{.
raises: [IOError, OSError, ValueError, Exception, XmlError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect].}
```
Loads and parses XML from file specified by `path`, and returns a `XmlNode`. All parsing errors are turned into an `XmlError` exception. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/xmlparser.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/xmlparser.nim#L141)
nim rstgen rstgen
======
This module implements a generator of HTML/Latex from reStructuredText (see <http://docutils.sourceforge.net/rst.html> for information on this markup syntax) and is used by the compiler's [docgen tools](https://nim-lang.org/docs/docgen.html).
You can generate HTML output through the convenience proc `rstToHtml`, which provided an input string with rst markup returns a string with the generated HTML. The final output is meant to be embedded inside a full document you provide yourself, so it won't contain the usual `<header>` or `<body>` parts.
You can also create a `RstGenerator` structure and populate it with the other lower level methods to finally build complete documents. This requires many options and tweaking, but you are not limited to snippets and can generate [LaTeX documents](https://en.wikipedia.org/wiki/LaTeX) too.
**Note:** Import `packages/docutils/rstgen` to use this module
Imports
-------
<rstast>, <rst>, <highlite> Types
-----
```
OutputTarget = enum
outHtml, outLatex
```
which document type to generate [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L38)
```
MetaEnum = enum
metaNone, metaTitle, metaSubtitle, metaAuthor, metaVersion
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L46)
```
RstGenerator = object of RootObj
target*: OutputTarget
config*: StringTableRef
splitAfter*: int
listingCounter*: int
tocPart*: seq[TocEntry]
hasToc*: bool
theIndex: string
options*: RstParseOptions
findFile*: FindFileHandler
msgHandler*: MsgHandler
outDir*: string ## output directory, initialized by docgen.nim
destFile*: string ## output (HTML) file, initialized by docgen.nim
filename*: string ## source Nim or Rst file
meta*: array[MetaEnum, string]
currentSection: string ## \
## Stores the empty string or the last headline/overline found in the rst
## document, so it can be used as a prettier name for term index generation.
seenIndexTerms: Table[string, int] ## \
## Keeps count of same text index terms to generate different identifiers
## for hyperlinks. See renderIndexTerm proc for details.
id*: int ## A counter useful for generating IDs.
onTestSnippet*: proc (d: var RstGenerator; filename, cmd: string; status: int;
content: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L49) Consts
------
```
IndexExt = ".idx"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L35) Procs
-----
```
proc prettyLink(file: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L85)
```
proc initRstGenerator(g: var RstGenerator; target: OutputTarget;
config: StringTableRef; filename: string;
options: RstParseOptions; findFile: FindFileHandler = nil;
msgHandler: MsgHandler = nil) {...}{.raises: [ValueError],
tags: [].}
```
Initializes a `RstGenerator`.
You need to call this before using a `RstGenerator` with any other procs in this module. Pass a non `nil` `StringTableRef` value as `config` with parameters used by the HTML output generator. If you don't know what to use, pass the results of the `defaultConfig() <#defaultConfig>_` proc.
The `filename` parameter will be used for error reporting and creating index hyperlinks to the file, but you can pass an empty string here if you are parsing a stream in memory. If `filename` ends with the `.nim` extension, the title for the document will be set by default to `Module filename`. This default title can be overridden by the embedded rst, but it helps to prettify the generated index if no title is found.
The `RstParseOptions`, `FindFileHandler` and `MsgHandler` types are defined in the the [packages/docutils/rst module](rst). `options` selects the behaviour of the rst parser.
`findFile` is a proc used by the rst `include` directive among others. The purpose of this proc is to mangle or filter paths. It receives paths specified in the rst document and has to return a valid path to existing files or the empty string otherwise. If you pass `nil`, a default proc will be used which given a path returns the input path only if the file exists. One use for this proc is to transform relative paths found in the document to absolute path, useful if the rst file and the resources it references are not in the same directory as the current working directory.
The `msgHandler` is a proc used for user error reporting. It will be called with the filename, line, col, and type of any error found during parsing. If you pass `nil`, a default message handler will be used which writes the messages to the standard output.
Example:
```
import packages/docutils/rstgen
var gen: RstGenerator
gen.initRstGenerator(outHtml, defaultConfig(), "filename", {})
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L94)
```
proc writeIndexFile(g: var RstGenerator; outfile: string) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
Writes the current index buffer to the specified output file.
You previously need to add entries to the index with the [setIndexTerm()](#setIndexTerm,RstGenerator,string,string,string,string,string) proc. If the index is empty the file won't be created.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L160)
```
proc escChar(target: OutputTarget; dest: var string; c: char) {...}{.inline,
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L201)
```
proc nextSplitPoint(s: string; start: int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L211)
```
proc esc(target: OutputTarget; s: string; splitAfter = -1): string {...}{.raises: [],
tags: [].}
```
Escapes the HTML. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L223) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L223)
```
proc setIndexTerm(d: var RstGenerator; htmlFile, id, term: string;
linkTitle, linkDesc = "") {...}{.raises: [], tags: [].}
```
Adds a `term` to the index using the specified hyperlink identifier.
A new entry will be added to the index using the format `term<tab>file#id`. The file part will come from the `htmlFile` parameter.
The `id` will be appended with a hash character only if its length is not zero, otherwise no specific anchor will be generated. In general you should only pass an empty `id` value for the title of standalone rst documents (they are special for the [mergeIndexes()](#mergeIndexes,string) proc, see [Index (idx) file format](https://nim-lang.org/docs/docgen.html#index-idx-file-format) for more information). Unlike other index terms, title entries are inserted at the beginning of the accumulated buffer to maintain a logical order of entries.
If `linkTitle` or `linkDesc` are not the empty string, two additional columns with their contents will be added.
The index won't be written to disk unless you call [writeIndexFile()](#writeIndexFile,RstGenerator,string). The purpose of the index is documented in the [docgen tools guide](https://nim-lang.org/docs/docgen.html#related-options-index-switch).
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L308) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L308)
```
proc renderIndexTerm(d: PDoc; n: PRstNode; result: var string) {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
Renders the string decorated within `foobar`:idx: markers.
Additionally adds the enclosed text to the index as a term. Since we are interested in different instances of the same term to have different entries, a table is used to keep track of the amount of times a term has previously appeared to give a different identifier value for each.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L359) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L359)
```
proc mergeIndexes(dir: string): string {...}{.raises: [OSError, IOError, ValueError],
tags: [ReadDirEffect, ReadIOEffect].}
```
Merges all index files in `dir` and returns the generated index as HTML.
This proc will first scan `dir` for index files with the `.idx` extension previously created by commands like `nim doc|rst2html` which use the `--index:on` switch. These index files are the result of calls to [setIndexTerm()](#setIndexTerm,RstGenerator,string,string,string,string,string) and [writeIndexFile()](#writeIndexFile,RstGenerator,string), so they are simple tab separated files.
As convention this proc will split index files into two categories: documentation and API. API indices will be all joined together into a single big sorted index, making the bulk of the final index. This is good for API documentation because many symbols are repeated in different modules. On the other hand, documentation indices are essentially table of contents plus a few special markers. These documents will be rendered in a separate section which tries to maintain the order and hierarchy of the symbols in the index file.
To differentiate between a documentation and API file a convention is used: indices which contain one entry without the HTML hash character (#) will be considered `documentation`, since this hash-less entry is the explicit title of the document. Indices without this explicit entry will be considered `generated API` extracted out of a source `.nim` file.
Returns the merged and sorted indices into a single HTML block which can be further embedded into nimdoc templates.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L656) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L656)
```
proc renderTocEntries(d: var RstGenerator; j: var int; lvl: int;
result: var string) {...}{.raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L794) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L794)
```
proc renderRstToOut(d: var RstGenerator; n: PRstNode; result: var string) {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
Writes into `result` the rst ast `n` using the `d` configuration.
Before using this proc you need to initialise a `RstGenerator` with `initRstGenerator` and parse a rst file with `rstParse` from the [packages/docutils/rst module](rst). Example:
```
# ...configure gen and rst vars...
var generatedHtml = ""
renderRstToOut(gen, rst, generatedHtml)
echo generatedHtml
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L1031) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L1031)
```
proc formatNamedVars(frmt: string; varnames: openArray[string];
varvalues: openArray[string]): string {...}{.
raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L1194) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L1194)
```
proc defaultConfig(): StringTableRef {...}{.raises: [], tags: [].}
```
Returns a default configuration for embedded HTML generation.
The returned `StringTableRef` contains the parameters used by the HTML engine to build the final output. For information on what these parameters are and their purpose, please look up the file `config/nimdoc.cfg` bundled with the compiler.
The only difference between the contents of that file and the values provided by this proc is the `doc.file` variable. The `doc.file` variable of the configuration file contains HTML to build standalone pages, while this proc returns just the content for procs like `rstToHtml` to generate the bare minimum HTML.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L1255) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L1255)
```
proc rstToHtml(s: string; options: RstParseOptions; config: StringTableRef): string {...}{.
raises: [ValueError, EParseError, IOError, Exception],
tags: [WriteIOEffect, ReadEnvEffect, RootEffect].}
```
Converts an input rst string into embeddable HTML.
This convenience proc parses any input string using rst markup (it doesn't have to be a full document!) and returns an embeddable piece of HTML. The proc is meant to be used in *online* environments without access to a meaningful filesystem, and therefore rst `include` like directives won't work. For an explanation of the `config` parameter see the `initRstGenerator` proc. Example:
```
import packages/docutils/rstgen, strtabs
echo rstToHtml("*Hello* **world**!", {},
newStringTable(modeStyleInsensitive))
# --> <em>Hello</em> <strong>world</strong>!
```
If you need to allow the rst `include` directive or tweak the generated output you have to create your own `RstGenerator` with `initRstGenerator` and related procs.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L1323) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L1323)
```
proc rstToLatex(rstSource: string; options: RstParseOptions): string {...}{.inline,
raises: [ValueError, Exception], tags: [RootEffect, ReadEnvEffect].}
```
Convenience proc for `renderRstToOut` and `initRstGenerator`. **Example:**
```
doAssert rstToLatex("*Hello* **world**", {}) == """\emph{Hello} \textbf{world}"""
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/packages/docutils/rstgen.nim#L1359) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/packages/docutils/rstgen.nim#L1359)
nim cpuload cpuload
=======
This module implements a helper for a thread pool to determine whether creating a thread is a good idea.
Unstable API.
Imports
-------
<cpuinfo> Types
-----
```
ThreadPoolAdvice = enum
doNothing, doCreateThread, doShutdownThread
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/concurrency/cpuload.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/concurrency/cpuload.nim#L23)
```
ThreadPoolState = object
when defined(windows):
prevSysKernel, prevSysUser, prevProcKernel, prevProcUser: FILETIME
calls*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/concurrency/cpuload.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/concurrency/cpuload.nim#L28) Procs
-----
```
proc advice(s: var ThreadPoolState): ThreadPoolAdvice {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/lib/pure/concurrency/cpuload.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/lib/pure/concurrency/cpuload.nim#L33)
| programming_docs |
nim dfa dfa
===
Data flow analysis for Nim. We transform the AST into a linear list of instructions first to make this easier to handle: There are only 2 different branching instructions: 'goto X' is an unconditional goto, 'fork X' is a conditional goto (either the next instruction or 'X' can be taken). Exhaustive case statements are translated so that the last branch is transformed into an 'else' branch. `return` and `break` are all covered by 'goto'.
Control flow through exception handling: Contrary to popular belief, exception handling doesn't cause many problems for this DFA representation, `raise` is a statement that `goes to` the outer `finally` or `except` if there is one, otherwise it is the same as `return`. Every call is treated as a call that can potentially `raise`. However, without a surrounding `try` we don't emit these `fork ReturnLabel` instructions in order to speed up the dataflow analysis passes.
The data structures and algorithms used here are inspired by "A Graph–Free Approach to Data–Flow Analysis" by Markus Mohnen. <https://link.springer.com/content/pdf/10.1007/3-540-45937-5_6.pdf>
Imports
-------
<ast>, <types>, <lineinfos>, <renderer>, <asciitables>, <patterns> Types
-----
```
InstrKind = enum
goto, fork, def, use
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L37)
```
Instr = object
n*: PNode
case kind*: InstrKind
of goto, fork:
dest*: int
else:
nil
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L39)
```
ControlFlowGraph = seq[Instr]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L45)
```
InstrTargetKind = enum
None, Full, Partial
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L595) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L595) Procs
-----
```
proc echoCfg(c: ControlFlowGraph; start = 0; last = -1) {...}{.deprecated,
raises: [Exception], tags: [RootEffect].}
```
**Deprecated** echos the ControlFlowGraph for debugging purposes. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L90)
```
proc skipConvDfa(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L566) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L566)
```
proc aliases(obj, field: PNode): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L576) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L576)
```
proc instrTargets(insloc, loc: PNode): InstrTargetKind {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L598) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L598)
```
proc isAnalysableFieldAccess(orig: PNode; owner: PSym): bool {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L605) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L605)
```
proc constructCfg(s: PSym; body: PNode): ControlFlowGraph {...}{.raises: [Exception],
tags: [RootEffect].}
```
constructs a control flow graph for `body`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/dfa.nim#L761) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/dfa.nim#L761)
nim transf transf
======
Imports
-------
<options>, <ast>, <astalgo>, <trees>, <msgs>, <idents>, <renderer>, <types>, <semfold>, <magicsys>, <cgmeth>, <lowerings>, <liftlocals>, <modulegraphs>, <lineinfos>, <closureiters>, <lambdalifting> Procs
-----
```
proc commonOptimizations(g: ModuleGraph; c: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/transf.nim#L853) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/transf.nim#L853)
```
proc transformBody(g: ModuleGraph; prc: PSym; cache: bool): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/transf.nim#L1083) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/transf.nim#L1083)
```
proc transformStmt(g: ModuleGraph; module: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/transf.nim#L1113) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/transf.nim#L1113)
```
proc transformExpr(g: ModuleGraph; module: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/transf.nim#L1123) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/transf.nim#L1123)
nim syntaxes syntaxes
========
Implements the dispatcher for the different parsers.
Imports
-------
<llstream>, <ast>, <idents>, <lexer>, <options>, <msgs>, <parser>, <filters>, <filter_tmpl>, <renderer>, <lineinfos>, <pathutils> Procs
-----
```
proc openParser(p: var Parser; fileIdx: FileIndex; inputstream: PLLStream;
cache: IdentCache; config: ConfigRef) {...}{.
raises: [IOError, Exception, ValueError, ERecoverableError, KeyError], tags: [
ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/syntaxes.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/syntaxes.nim#L111)
```
proc setupParser(p: var Parser; fileIdx: FileIndex; cache: IdentCache;
config: ConfigRef): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/syntaxes.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/syntaxes.nim#L121)
```
proc parseFile(fileIdx: FileIndex; cache: IdentCache; config: ConfigRef): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/syntaxes.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/syntaxes.nim#L131) Exports
-------
[Parser](parser#Parser), [parseAll](parser#parseAll,Parser), [parseTopLevelStmt](parser#parseTopLevelStmt,Parser), [closeParser](parser#closeParser,Parser)
nim semdata semdata
=======
This module contains the data structures for the semantic checking phase.
Imports
-------
<options>, <ast>, <astalgo>, <msgs>, <idents>, <renderer>, <magicsys>, <vmdef>, <modulegraphs>, <lineinfos> Types
-----
```
TOptionEntry = object
options*: TOptions
defaultCC*: TCallingConvention
dynlib*: PLib
notes*: TNoteKinds
features*: set[Feature]
otherPragmas*: PNode
warningAsErrors*: TNoteKinds
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L17)
```
POptionEntry = ref TOptionEntry
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L26)
```
PProcCon = ref TProcCon
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L27)
```
TProcCon {...}{.acyclic.} = object
owner*: PSym
resultSym*: PSym
selfSym*: PSym
nestedLoopCounter*: int
nestedBlockCounter*: int
inTryStmt*: int
next*: PProcCon
mappingExists*: bool
mapping*: TIdTable
caseContext*: seq[tuple[n: PNode, idx: int]]
localBindStmts*: seq[PNode]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L28)
```
TMatchedConcept = object
candidateType*: PType
prev*: ptr TMatchedConcept
depth*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L43)
```
TInstantiationPair = object
genericSym*: PSym
inst*: PInstantiation
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L48)
```
TExprFlag = enum
efLValue, efWantIterator, efInTypeof, efNeedStatic, efPreferStatic,
efPreferNilResult, efWantStmt, efAllowStmt, efDetermineType, efExplain,
efAllowDestructor, efWantValue, efOperand, efNoSemCheck, efNoEvaluateGeneric,
efInCall, efFromHlo, efNoSem2Check, efNoUndeclared
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L52)
```
TExprFlags = set[TExprFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L72)
```
PContext = ref TContext
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L74)
```
TContext = object of TPassContext
enforceVoidContext*: PType
module*: PSym
currentScope*: PScope
importTable*: PScope
topLevelScope*: PScope
p*: PProcCon
matchedConcept*: ptr TMatchedConcept
friendModules*: seq[PSym]
instCounter*: int
templInstCounter*: ref int
ambiguousSymbols*: IntSet
inGenericContext*: int
inStaticContext*: int
inUnrolledContext*: int
compilesContextId*: int
compilesContextIdGenerator*: int
inGenericInst*: int
converters*: seq[PSym]
patterns*: seq[PSym]
optionStack*: seq[POptionEntry]
symMapping*: TIdTable
libs*: seq[PLib]
semConstExpr*: proc (c: PContext; n: PNode): PNode {...}{.nimcall.}
semExpr*: proc (c: PContext; n: PNode; flags: TExprFlags = {}): PNode {...}{.
nimcall.}
semTryExpr*: proc (c: PContext; n: PNode; flags: TExprFlags = {}): PNode {...}{.
nimcall.}
semTryConstExpr*: proc (c: PContext; n: PNode): PNode {...}{.nimcall.}
computeRequiresInit*: proc (c: PContext; t: PType): bool {...}{.nimcall.}
hasUnresolvedArgs*: proc (c: PContext; n: PNode): bool
semOperand*: proc (c: PContext; n: PNode; flags: TExprFlags = {}): PNode {...}{.
nimcall.}
semConstBoolExpr*: proc (c: PContext; n: PNode): PNode {...}{.nimcall.}
semOverloadedCall*: proc (c: PContext; n, nOrig: PNode; filter: TSymKinds;
flags: TExprFlags): PNode {...}{.nimcall.}
semTypeNode*: proc (c: PContext; n: PNode; prev: PType): PType {...}{.nimcall.}
semInferredLambda*: proc (c: PContext; pt: TIdTable; n: PNode): PNode
semGenerateInstance*: proc (c: PContext; fn: PSym; pt: TIdTable;
info: TLineInfo): PSym
includedFiles*: IntSet
pureEnumFields*: TStrTable
userPragmas*: TStrTable
evalContext*: PEvalContext
unknownIdents*: IntSet
generics*: seq[TInstantiationPair]
topStmts*: int
lastGenericIdx*: int
hloLoopDetector*: int
inParallelStmt*: int
instTypeBoundOp*: proc (c: PContext; dc: PSym; t: PType; info: TLineInfo;
op: TTypeAttachedOp; col: int): PSym {...}{.nimcall.}
selfName*: PIdent
cache*: IdentCache
graph*: ModuleGraph
signatures*: TStrTable
recursiveDep*: string
suggestionsMade*: bool
features*: set[Feature]
inTypeContext*: int
typesWithOps*: seq[(PType, PType)]
unusedImports*: seq[(PSym, TLineInfo)]
exportIndirections*: HashSet[(int, int)]
lastTLineInfo*: TLineInfo
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L75) Procs
-----
```
proc makeInstPair(s: PSym; inst: PInstantiation): TInstantiationPair {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L151) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L151)
```
proc filename(c: PContext): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L155) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L155)
```
proc scopeDepth(c: PContext): int {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L159)
```
proc getCurrOwner(c: PContext): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L163)
```
proc pushOwner(c: PContext; owner: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L170)
```
proc popOwner(c: PContext) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L173) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L173)
```
proc lastOptionEntry(c: PContext): POptionEntry {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L177)
```
proc popProcCon(c: PContext) {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L180)
```
proc put(p: PProcCon; key, val: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L182)
```
proc get(p: PProcCon; key: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L189) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L189)
```
proc getGenSym(c: PContext; s: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L193) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L193)
```
proc considerGenSyms(c: PContext; n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L204)
```
proc newOptionEntry(conf: ConfigRef): POptionEntry {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L213) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L213)
```
proc pushOptionEntry(c: PContext): POptionEntry {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L221) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L221)
```
proc popOptionEntry(c: PContext) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L232)
```
proc newContext(graph: ModuleGraph; module: PSym): PContext {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L239) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L239)
```
proc addConverter(c: PContext; conv: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L265) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L265)
```
proc addPattern(c: PContext; p: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L268)
```
proc newLib(kind: TLibKind): PLib {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L271) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L271)
```
proc addToLib(lib: PLib; sym: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L275) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L275)
```
proc newTypeS(kind: TTypeKind; c: PContext): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L280)
```
proc makePtrType(owner: PSym; baseType: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L283) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L283)
```
proc makePtrType(c: PContext; baseType: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L287) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L287)
```
proc makeTypeWithModifier(c: PContext; modifier: TTypeKind; baseType: PType): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L290)
```
proc makeVarType(c: PContext; baseType: PType; kind = tyVar): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L301) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L301)
```
proc makeVarType(owner: PSym; baseType: PType; kind = tyVar): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L308) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L308)
```
proc makeTypeDesc(c: PContext; typ: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L315) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L315)
```
proc makeTypeSymNode(c: PContext; typ: PType; info: TLineInfo): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L323) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L323)
```
proc makeTypeFromExpr(c: PContext; n: PNode): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L332) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L332)
```
proc newTypeWithSons(owner: PSym; kind: TTypeKind; sons: seq[PType]): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L337)
```
proc newTypeWithSons(c: PContext; kind: TTypeKind; sons: seq[PType]): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L341) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L341)
```
proc makeStaticExpr(c: PContext; n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L346) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L346)
```
proc makeAndType(c: PContext; t1, t2: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L352) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L352)
```
proc makeOrType(c: PContext; t1, t2: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L360) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L360)
```
proc makeNotType(c: PContext; t1: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L377) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L377)
```
proc makeRangeWithStaticExpr(c: PContext; n: PNode): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L388) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L388)
```
proc errorType(c: PContext): PType {...}{.raises: [], tags: [].}
```
creates a type representing an error state [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L400) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L400)
```
proc errorNode(c: PContext; n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L405)
```
proc fillTypeS(dest: PType; kind: TTypeKind; c: PContext) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L409) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L409)
```
proc makeRangeType(c: PContext; first, last: BiggestInt; info: TLineInfo;
intType: PType = nil): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L414) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L414)
```
proc markIndirect(c: PContext; s: PSym) {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L424) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L424)
```
proc illFormedAst(n: PNode; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L429) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L429)
```
proc illFormedAstLocal(n: PNode; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L432) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L432)
```
proc checkSonsLen(n: PNode; length: int; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L435) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L435)
```
proc checkMinSonsLen(n: PNode; length: int; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L438) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L438)
```
proc isTopLevel(c: PContext): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L441) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L441)
```
proc pushCaseContext(c: PContext; caseNode: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L444)
```
proc popCaseContext(c: PContext) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L447)
```
proc setCaseContextIdx(c: PContext; idx: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L450) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L450) Templates
---------
```
template config(c: PContext): ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L149)
```
template rangeHasUnresolvedStatic(t: PType): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semdata.nim#L397) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semdata.nim#L397)
| programming_docs |
nim rod rod
===
This module implements the canonalization for the various caching mechanisms.
Imports
-------
<ast>, <idgen>, <lineinfos>, <incremental>, <modulegraphs>, <pathutils> Procs
-----
```
proc loadModuleSym(g: ModuleGraph; fileIdx: FileIndex; fullpath: AbsoluteFile): (
PSym, int) {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L19) Templates
---------
```
template setupModuleCache(g: ModuleGraph)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L15)
```
template storeNode(g: ModuleGraph; module: PSym; n: PNode)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L16)
```
template loadNode(g: ModuleGraph; module: PSym): PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L17)
```
template addModuleDep(g: ModuleGraph; module, fileIdx: FileIndex;
isIncludeFile: bool)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L21)
```
template storeRemaining(g: ModuleGraph; module: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L23)
```
template registerModule(g: ModuleGraph; module: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rod.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rod.nim#L25)
nim asciitables asciitables
===========
Types
-----
```
Cell = object
text*: string
width*, row*, col*, ncols*, nrows*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/asciitables.nim#L6) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/asciitables.nim#L6) Procs
-----
```
proc alignTable(s: string; delim = '\t'; fill = ' '; sep = " "): string {...}{.
raises: [], tags: [].}
```
formats a `delim`-delimited `s` representing a table; each cell is aligned to a width that's computed for each column; consecutive columns are delimited by `sep`, and alignment space is filled using `fill`. More customized formatting can be done by calling `parseTableCells` directly. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/asciitables.nim#L71) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/asciitables.nim#L71) Iterators
---------
```
iterator parseTableCells(s: string; delim = '\t'): Cell {...}{.raises: [], tags: [].}
```
iterates over all cells in a `delim`-delimited `s`, after a 1st pass that computes number of rows, columns, and width of each column. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/asciitables.nim#L10) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/asciitables.nim#L10)
nim ccgutils ccgutils
========
Imports
-------
<ast>, <msgs>, <wordrecg>, <platform>, <trees>, <options> Procs
-----
```
proc getPragmaStmt(n: PNode; w: TSpecialWord): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L16)
```
proc stmtsContainPragma(n: PNode; w: TSpecialWord): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L27)
```
proc hashString(conf: ConfigRef; s: string): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L30)
```
proc makeSingleLineCString(s: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L57)
```
proc mangle(name: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L63) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L63) Templates
---------
```
template getUniqueType(key: PType): PType
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgutils.nim#L55) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgutils.nim#L55)
nim importer importer
========
This module implements the symbol importing mechanism.
Imports
-------
<ast>, <astalgo>, <msgs>, <options>, <idents>, <lookups>, <semdata>, <modulepaths>, <sigmatch>, <lineinfos> Procs
-----
```
proc readExceptSet(c: PContext; n: PNode): IntSet {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L16)
```
proc importPureEnumField(c: PContext; s: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L23)
```
proc importAllSymbols(c: PContext; fromMod: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L110)
```
proc evalImport(c: PContext; n: PNode): PNode {...}{.raises: [Exception, ValueError,
IOError, ERecoverableError, OSError, KeyError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L195)
```
proc evalFrom(c: PContext; n: PNode): PNode {...}{.raises: [Exception, ValueError,
IOError, ERecoverableError, OSError, KeyError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L219) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L219)
```
proc evalImportExcept(c: PContext; n: PNode): PNode {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, OSError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/importer.nim#L231) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/importer.nim#L231)
nim strutils2 strutils2
=========
internal API for now, subject to modifications and moving around
string API's focusing on performance, that can be used as building blocks for other routines.
Un-necessary allocations are avoided and appropriate algorithms are used at the expense of code clarity when justified.
Procs
-----
```
proc dataPointer[T](a: T): pointer
```
same as C++ `data` that works with std::string, std::vector etc. Note: safe to use when a.len == 0 but whether the result is nil or not is implementation defined for performance reasons. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/strutils2.nim#L11) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/strutils2.nim#L11)
```
proc setLen(result: var string; n: int; isInit: bool) {...}{.raises: [], tags: [].}
```
when isInit = false, elements are left uninitialized, analog to `{.noinit.}` else, there are 0-initialized. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/strutils2.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/strutils2.nim#L26)
```
proc forceCopy(result: var string; a: string) {...}{.raises: [], tags: [].}
```
also forces a copy if `a` is shallow [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/strutils2.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/strutils2.nim#L38)
```
proc toLowerAscii(a: var string) {...}{.raises: [], tags: [].}
```
optimized and inplace overload of strutils.toLowerAscii [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/strutils2.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/strutils2.nim#L49)
nim ast ast
===
Imports
-------
<lineinfos>, <options>, <ropes>, <idents>, <idgen>, <int128> Types
-----
```
TCallingConvention = enum
ccNimCall = "nimcall", ccStdCall = "stdcall", ccCDecl = "cdecl",
ccSafeCall = "safecall", ccSysCall = "syscall", ccInline = "inline",
ccNoInline = "noinline", ccFastCall = "fastcall", ccThisCall = "thiscall",
ccClosure = "closure", ccNoConvention = "noconv"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L19)
```
TNodeKind = enum
nkNone, nkEmpty, nkIdent, nkSym, nkType, nkCharLit, nkIntLit, nkInt8Lit,
nkInt16Lit, nkInt32Lit, nkInt64Lit, nkUIntLit, nkUInt8Lit, nkUInt16Lit,
nkUInt32Lit, nkUInt64Lit, nkFloatLit, nkFloat32Lit, nkFloat64Lit,
nkFloat128Lit, nkStrLit, nkRStrLit, nkTripleStrLit, nkNilLit, nkComesFrom,
nkDotCall, nkCommand, nkCall, nkCallStrLit, nkInfix, nkPrefix, nkPostfix,
nkHiddenCallConv, nkExprEqExpr, nkExprColonExpr, nkIdentDefs, nkVarTuple,
nkPar, nkObjConstr, nkCurly, nkCurlyExpr, nkBracket, nkBracketExpr,
nkPragmaExpr, nkRange, nkDotExpr, nkCheckedFieldExpr, nkDerefExpr, nkIfExpr,
nkElifExpr, nkElseExpr, nkLambda, nkDo, nkAccQuoted, nkTableConstr, nkBind,
nkClosedSymChoice, nkOpenSymChoice, nkHiddenStdConv, nkHiddenSubConv, nkConv,
nkCast, nkStaticExpr, nkAddr, nkHiddenAddr, nkHiddenDeref, nkObjDownConv,
nkObjUpConv, nkChckRangeF, nkChckRange64, nkChckRange, nkStringToCString,
nkCStringToString, nkAsgn, nkFastAsgn, nkGenericParams, nkFormalParams,
nkOfInherit, nkImportAs, nkProcDef, nkMethodDef, nkConverterDef, nkMacroDef,
nkTemplateDef, nkIteratorDef, nkOfBranch, nkElifBranch, nkExceptBranch,
nkElse, nkAsmStmt, nkPragma, nkPragmaBlock, nkIfStmt, nkWhenStmt, nkForStmt,
nkParForStmt, nkWhileStmt, nkCaseStmt, nkTypeSection, nkVarSection,
nkLetSection, nkConstSection, nkConstDef, nkTypeDef, nkYieldStmt, nkDefer,
nkTryStmt, nkFinally, nkRaiseStmt, nkReturnStmt, nkBreakStmt, nkContinueStmt,
nkBlockStmt, nkStaticStmt, nkDiscardStmt, nkStmtList, nkImportStmt,
nkImportExceptStmt, nkExportStmt, nkExportExceptStmt, nkFromStmt,
nkIncludeStmt, nkBindStmt, nkMixinStmt, nkUsingStmt, nkCommentStmt,
nkStmtListExpr, nkBlockExpr, nkStmtListType, nkBlockType, nkWith, nkWithout,
nkTypeOfExpr, nkObjectTy, nkTupleTy, nkTupleClassTy, nkTypeClassTy,
nkStaticTy, nkRecList, nkRecCase, nkRecWhen, nkRefTy, nkPtrTy, nkVarTy,
nkConstTy, nkMutableTy, nkDistinctTy, nkProcTy, nkIteratorTy, nkSharedTy,
nkEnumTy, nkEnumFieldDef, nkArgList, nkPattern, nkHiddenTryStmt, nkClosure,
nkGotoState, nkState, nkBreakState, nkFuncDef, nkTupleConstr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L33)
```
TNodeKinds = set[TNodeKind]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L225) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L225)
```
TSymFlag = enum
sfUsed, sfExported, sfFromGeneric, sfGlobal, sfForward, sfWasForwarded,
sfImportc, sfExportc, sfMangleCpp, sfVolatile, sfRegister, sfPure,
sfNoSideEffect, sfSideEffect, sfMainModule, sfSystemModule, sfNoReturn,
sfAddrTaken, sfCompilerProc, sfProcvar, sfDiscriminant, sfRequiresInit,
sfDeprecated, sfExplain, sfError, sfShadowed, sfThread, sfCompileTime,
sfConstructor, sfDispatcher, sfBorrow, sfInfixCall, sfNamedParamCall,
sfDiscardable, sfOverriden, sfCallsite, sfGenSym, sfNonReloadable,
sfGeneratedOp, sfTemplateParam, sfCursor, sfInjectDestructors, sfNeverRaises,
sfUsedInFinallyOrExcept, sfSingleUsedTemp, sfNoalias
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L228) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L228)
```
TSymFlags = set[TSymFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L295) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L295)
```
TTypeKind = enum
tyNone, tyBool, tyChar, tyEmpty, tyAlias, tyNil, tyUntyped, tyTyped,
tyTypeDesc, tyGenericInvocation, tyGenericBody, tyGenericInst, tyGenericParam,
tyDistinct, tyEnum, tyOrdinal, tyArray, tyObject, tyTuple, tySet, tyRange,
tyPtr, tyRef, tyVar, tySequence, tyProc, tyPointer, tyOpenArray, tyString,
tyCString, tyForward, tyInt, tyInt8, tyInt16, tyInt32, tyInt64, tyFloat,
tyFloat32, tyFloat64, tyFloat128, tyUInt, tyUInt8, tyUInt16, tyUInt32,
tyUInt64, tyOwned, tySink, tyLent, tyVarargs, tyUncheckedArray, tyProxy,
tyBuiltInTypeClass, tyUserTypeClass, tyUserTypeClassInst,
tyCompositeTypeClass, tyInferred, tyAnd, tyOr, tyNot, tyAnything, tyStatic,
tyFromExpr, tyOptDeprecated, tyVoid
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L343) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L343)
```
TTypeKinds = set[TTypeKind]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L460)
```
TNodeFlag = enum
nfNone, nfBase2, nfBase8, nfBase16, nfAllConst, nfTransf, nfNoRewrite, nfSem,
nfLL, nfDotField, nfDotSetter, nfExplicitCall, nfExprCall, nfIsRef, nfIsPtr,
nfPreventCg, nfBlockArg, nfFromTemplate, nfDefaultParam, nfDefaultRefsParam,
nfExecuteOnReload
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L462) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L462)
```
TNodeFlags = set[TNodeFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L488) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L488)
```
TTypeFlag = enum
tfVarargs, tfNoSideEffect, tfFinal, tfInheritable, tfHasOwned, tfEnumHasHoles,
tfShallow, tfThread, tfFromGeneric, tfUnresolved, tfResolved, tfRetType,
tfCapturesEnv, tfByCopy, tfByRef, tfIterator, tfPartial, tfNotNil,
tfRequiresInit, tfNeedsFullInit, tfVarIsPtr, tfHasMeta, tfHasGCedMem,
tfPacked, tfHasStatic, tfGenericTypeParam, tfImplicitTypeParam,
tfInferrableStatic, tfConceptMatchedTypeSym, tfExplicit, tfWildcard,
tfHasAsgn, tfBorrowDot, tfTriggersCompileTime, tfRefsAnonObj, tfCovariant,
tfWeakCovariant, tfContravariant, tfCheckedForDestructor, tfAcyclic,
tfIncompleteStruct, tfCompleteStruct, tfExplicitCallConv
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L489) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L489)
```
TTypeFlags = set[TTypeFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L557) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L557)
```
TSymKind = enum
skUnknown, skConditional, skDynLib, skParam, skGenericParam, skTemp, skModule,
skType, skVar, skLet, skConst, skResult, skProc, skFunc, skMethod, skIterator,
skConverter, skMacro, skTemplate, skField, skEnumField, skForVar, skLabel,
skStub, skPackage, skAlias
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L559) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L559)
```
TSymKinds = set[TSymKind]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L592) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L592)
```
TMagic = enum
mNone, mDefined, mDeclared, mDeclaredInScope, mCompiles, mArrGet, mArrPut,
mAsgn, mLow, mHigh, mSizeOf, mAlignOf, mOffsetOf, mTypeTrait, mIs, mOf, mAddr,
mType, mTypeOf, mPlugin, mEcho, mShallowCopy, mSlurp, mStaticExec, mStatic,
mParseExprToAst, mParseStmtToAst, mExpandToAst, mQuoteAst, mInc, mDec, mOrd,
mNew, mNewFinalize, mNewSeq, mNewSeqOfCap, mLengthOpenArray, mLengthStr,
mLengthArray, mLengthSeq, mIncl, mExcl, mCard, mChr, mGCref, mGCunref, mAddI,
mSubI, mMulI, mDivI, mModI, mSucc, mPred, mAddF64, mSubF64, mMulF64, mDivF64,
mShrI, mShlI, mAshrI, mBitandI, mBitorI, mBitxorI, mMinI, mMaxI, mAddU, mSubU,
mMulU, mDivU, mModU, mEqI, mLeI, mLtI, mEqF64, mLeF64, mLtF64, mLeU, mLtU,
mEqEnum, mLeEnum, mLtEnum, mEqCh, mLeCh, mLtCh, mEqB, mLeB, mLtB, mEqRef,
mLePtr, mLtPtr, mXor, mEqCString, mEqProc, mUnaryMinusI, mUnaryMinusI64,
mAbsI, mNot, mUnaryPlusI, mBitnotI, mUnaryPlusF64, mUnaryMinusF64, mCharToStr,
mBoolToStr, mIntToStr, mInt64ToStr, mFloatToStr, mCStrToStr, mStrToStr,
mEnumToStr, mAnd, mOr, mImplies, mIff, mExists, mForall, mOld, mEqStr, mLeStr,
mLtStr, mEqSet, mLeSet, mLtSet, mMulSet, mPlusSet, mMinusSet, mConStrStr,
mSlice, mDotDot, mFields, mFieldPairs, mOmpParFor, mAppendStrCh,
mAppendStrStr, mAppendSeqElem, mInSet, mRepr, mExit, mSetLengthStr,
mSetLengthSeq, mIsPartOf, mAstToStr, mParallel, mSwap, mIsNil, mArrToSeq,
mNewString, mNewStringOfCap, mParseBiggestFloat, mMove, mWasMoved, mDestroy,
mDefault, mUnown, mIsolate, mAccessEnv, mReset, mArray, mOpenArray, mRange,
mSet, mSeq, mVarargs, mRef, mPtr, mVar, mDistinct, mVoid, mTuple, mOrdinal,
mInt, mInt8, mInt16, mInt32, mInt64, mUInt, mUInt8, mUInt16, mUInt32, mUInt64,
mFloat, mFloat32, mFloat64, mFloat128, mBool, mChar, mString, mCstring,
mPointer, mNil, mExpr, mStmt, mTypeDesc, mVoidType, mPNimrodNode, mSpawn,
mDeepCopy, mIsMainModule, mCompileDate, mCompileTime, mProcCall, mCpuEndian,
mHostOS, mHostCPU, mBuildOS, mBuildCPU, mAppType, mCompileOption,
mCompileOptionArg, mNLen, mNChild, mNSetChild, mNAdd, mNAddMultiple, mNDel,
mNKind, mNSymKind, mNccValue, mNccInc, mNcsAdd, mNcsIncl, mNcsLen, mNcsAt,
mNctPut, mNctLen, mNctGet, mNctHasNext, mNctNext, mNIntVal, mNFloatVal,
mNSymbol, mNIdent, mNGetType, mNStrVal, mNSetIntVal, mNSetFloatVal,
mNSetSymbol, mNSetIdent, mNSetType, mNSetStrVal, mNLineInfo, mNNewNimNode,
mNCopyNimNode, mNCopyNimTree, mStrToIdent, mNSigHash, mNSizeOf, mNBindSym,
mNCallSite, mEqIdent, mEqNimrodNode, mSameNodeType, mGetImpl, mNGenSym,
mNHint, mNWarning, mNError, mInstantiationInfo, mGetTypeInfo, mGetTypeInfoV2,
mNimvm, mIntDefine, mStrDefine, mBoolDefine, mRunnableExamples, mException,
mBuiltinType, mSymOwner, mUncheckedArray, mGetImplTransf,
mSymIsInstantiationOf, mNodeId
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L610) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L610)
```
PNode = ref TNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L716) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L716)
```
TNodeSeq = seq[PNode]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L717) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L717)
```
PType = ref TType
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L718) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L718)
```
PSym = ref TSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L719) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L719)
```
TNode {...}{.final, acyclic.} = object
when defined(useNodeIds):
id*: int
typ*: PType
info*: TLineInfo
flags*: TNodeFlags
case kind*: TNodeKind
of nkCharLit .. nkUInt64Lit:
intVal*: BiggestInt
of nkFloatLit .. nkFloat128Lit:
floatVal*: BiggestFloat
of nkStrLit .. nkTripleStrLit:
strVal*: string
of nkSym:
sym*: PSym
of nkIdent:
ident*: PIdent
else:
sons*: TNodeSeq
comment*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L720) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L720)
```
TStrTable = object
counter*: int
data*: seq[PSym]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L741) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L741)
```
TLocKind = enum
locNone, locTemp, locLocalVar, locGlobalVar, locParam, locField, locExpr,
locProc, locData, locCall, locOther
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L746) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L746)
```
TLocFlag = enum
lfIndirect, lfFullExternalName, lfNoDeepCopy, lfNoDecl, lfDynamicLib,
lfExportLib, lfHeader, lfImportCompilerProc, lfSingleUse, lfEnforceDeref,
lfPrepareForMutation
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L758) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L758)
```
TStorageLoc = enum
OnUnknown, OnStatic, OnStack, OnHeap
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L774) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L774)
```
TLocFlags = set[TLocFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L780) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L780)
```
TLoc = object
k*: TLocKind
storage*: TStorageLoc
flags*: TLocFlags
lode*: PNode
r*: Rope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L781) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L781)
```
TLibKind = enum
libHeader, libDynamic
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L790) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L790)
```
TLib = object
kind*: TLibKind
generated*: bool
isOverriden*: bool
name*: Rope
path*: PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L793) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L793)
```
CompilesId = int
```
id that is used for the caching logic within `system.compiles`. See the seminst module. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L801) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L801)
```
TInstantiation = object
sym*: PSym
concreteTypes*: seq[PType]
compilesId*: CompilesId
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L803) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L803)
```
PInstantiation = ref TInstantiation
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L808) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L808)
```
TScope {...}{.acyclic.} = object
depthLevel*: int
symbols*: TStrTable
parent*: PScope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L810) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L810)
```
PScope = ref TScope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L815) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L815)
```
PLib = ref TLib
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L817) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L817)
```
TSym {...}{.acyclic.} = object of TIdObj
case kind*: TSymKind
of skType, skGenericParam:
typeInstCache*: seq[PType]
of routineKinds:
procInstCache*: seq[PInstantiation]
gcUnsafetyReason*: PSym
transformedBody*: PNode
of skModule, skPackage:
usedGenerics*: seq[PInstantiation]
tab*: TStrTable
of skLet, skVar, skField, skForVar:
guard*: PSym
bitsize*: int
alignment*: int
else:
nil
magic*: TMagic
typ*: PType
name*: PIdent
info*: TLineInfo
owner*: PSym
flags*: TSymFlags
ast*: PNode
options*: TOptions
position*: int
offset*: int
loc*: TLoc
annex*: PLib
when hasFFI:
cname*: string
constraint*: PNode
when defined(nimsuggest):
allUsages*: seq[TLineInfo]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L818) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L818)
```
TTypeSeq = seq[PType]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L884) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L884)
```
TLockLevel = distinct int16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L885) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L885)
```
TTypeAttachedOp = enum
attachedDestructor, attachedAsgn, attachedSink, attachedTrace,
attachedDispose, attachedDeepCopy
```
as usual, order is important here [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L887) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L887)
```
TType {...}{.acyclic.} = object of TIdObj
kind*: TTypeKind
callConv*: TCallingConvention
flags*: TTypeFlags
sons*: TTypeSeq
n*: PNode
owner*: PSym
sym*: PSym
attachedOps*: array[TTypeAttachedOp, PSym]
methods*: seq[(int, PSym)]
size*: BiggestInt
align*: int16
paddingAtEnd*: int16
lockLevel*: TLockLevel
loc*: TLoc
typeInst*: PType
uniqueId*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L895) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L895)
```
TPair = object
key*, val*: RootRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L928) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L928)
```
TPairSeq = seq[TPair]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L931) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L931)
```
TIdPair = object
key*: PIdObj
val*: RootRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L933) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L933)
```
TIdPairSeq = seq[TIdPair]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L937) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L937)
```
TIdTable = object
counter*: int
data*: TIdPairSeq
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L938) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L938)
```
TIdNodePair = object
key*: PIdObj
val*: PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L942) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L942)
```
TIdNodePairSeq = seq[TIdNodePair]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L946) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L946)
```
TIdNodeTable = object
counter*: int
data*: TIdNodePairSeq
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L947) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L947)
```
TNodePair = object
h*: Hash
key*: PNode
val*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L951) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L951)
```
TNodePairSeq = seq[TNodePair]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L956) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L956)
```
TNodeTable = object
counter*: int
data*: TNodePairSeq
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L957) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L957)
```
TObjectSeq = seq[RootRef]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L962) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L962)
```
TObjectSet = object
counter*: int
data*: TObjectSeq
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L963) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L963)
```
TImplication = enum
impUnknown, impNo, impYes
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L967) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L967) Vars
----
```
eqTypeFlags = {tfIterator, tfNotNil, tfVarIsPtr, tfGcSafe, tfNoSideEffect}
```
type flags that are essential for type equality. This is now a variable because for emulation of version:1.0 we might exclude {tfGcSafe, tfNoSideEffect}. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L604) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L604)
```
ggDebug: bool
```
convenience switch for trying out things [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1063) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1063) Consts
------
```
sfNoInit = sfMainModule
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L298)
```
sfAllUntyped = sfVolatile
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L300) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L300)
```
sfDirty = sfPure
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L303) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L303)
```
sfAnon = sfDiscardable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L307) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L307)
```
sfNoForward = sfRegister
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L312) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L312)
```
sfReorder = sfForward
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L314) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L314)
```
sfCompileToCpp = sfInfixCall
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L317) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L317)
```
sfCompileToObjc = sfNamedParamCall
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L318) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L318)
```
sfExperimental = sfOverriden
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L319) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L319)
```
sfGoto = sfOverriden
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L320) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L320)
```
sfWrittenTo = sfBorrow
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L321) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L321)
```
sfEscapes = sfProcvar
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L322)
```
sfBase = sfDiscriminant
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L323) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L323)
```
sfIsSelf = sfOverriden
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L324) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L324)
```
sfCustomPragma = sfRegister
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L325) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L325)
```
nkWhen = nkWhenStmt
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L329) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L329)
```
nkWhenExpr = nkWhenStmt
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L330) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L330)
```
nkEffectList = nkArgList
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L331) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L331)
```
exceptionEffects = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L333) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L333)
```
requiresEffects = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L334) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L334)
```
ensuresEffects = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L335) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L335)
```
tagEffects = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L336) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L336)
```
pragmasEffects = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L337)
```
effectListLen = 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L338) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L338)
```
nkLastBlockStmts = {nkRaiseStmt, nkReturnStmt, nkBreakStmt, nkContinueStmt}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L339) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L339)
```
tyPureObject = tyTuple
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L442) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L442)
```
GcTypeKinds = {tyRef, tySequence, tyString}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L443) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L443)
```
tyError = tyProxy
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L444)
```
tyUnknown = tyFromExpr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L445) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L445)
```
tyUnknownTypes = {tyProxy, tyFromExpr}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L447)
```
tyTypeClasses = {tyBuiltInTypeClass, tyCompositeTypeClass, tyUserTypeClass,
tyUserTypeClassInst, tyAnd, tyOr, tyNot, tyAnything}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L449) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L449)
```
tyMetaTypes = {tyUntyped, tyTypeDesc, tyGenericParam,
tyBuiltInTypeClass..tyCompositeTypeClass, tyAnd..tyAnything}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L453) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L453)
```
tyUserTypeClasses = {tyUserTypeClass, tyUserTypeClassInst}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L454) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L454)
```
abstractVarRange = {tyGenericInst, tyRange, tyVar, tyDistinct, tyOrdinal,
tyTypeDesc, tyAlias, tyInferred, tySink, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L456) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L456)
```
routineKinds = {skProc, skFunc, skMethod, skIterator, skConverter, skMacro,
skTemplate}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L595) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L595)
```
tfUnion = tfNoSideEffect
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L597) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L597)
```
tfGcSafe = tfThread
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L598) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L598)
```
tfObjHasKids = tfEnumHasHoles
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L599) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L599)
```
tfReturnsNew = tfInheritable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L600) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L600)
```
skError = skUnknown
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L601) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L601)
```
ctfeWhitelist = {mNone, mSucc, mPred, mInc, mDec, mOrd, mLengthOpenArray,
mLengthStr, mLengthArray, mLengthSeq, mArrGet, mArrPut, mAsgn,
mDestroy, mIncl, mExcl, mCard, mChr, mAddI, mSubI, mMulI,
mDivI, mModI, mAddF64, mSubF64, mMulF64, mDivF64, mShrI, mShlI,
mBitandI, mBitorI, mBitxorI, mMinI, mMaxI, mAddU, mSubU, mMulU,
mDivU, mModU, mEqI, mLeI, mLtI, mEqF64, mLeF64, mLtF64, mLeU,
mLtU, mEqEnum, mLeEnum, mLtEnum, mEqCh, mLeCh, mLtCh, mEqB,
mLeB, mLtB, mEqRef, mEqProc, mLePtr, mLtPtr, mEqCString, mXor,
mUnaryMinusI, mUnaryMinusI64, mAbsI, mNot, mUnaryPlusI,
mBitnotI, mUnaryPlusF64, mUnaryMinusF64, mCharToStr,
mBoolToStr, mIntToStr, mInt64ToStr, mFloatToStr, mCStrToStr,
mStrToStr, mEnumToStr, mAnd, mOr, mEqStr, mLeStr, mLtStr,
mEqSet, mLeSet, mLtSet, mMulSet, mPlusSet, mMinusSet,
mConStrStr, mAppendStrCh, mAppendStrStr, mAppendSeqElem,
mInSet, mRepr}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L688) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L688)
```
OverloadableSyms = {skProc, skFunc, skMethod, skIterator, skConverter, skModule,
skTemplate, skMacro}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L975) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L975)
```
GenericTypes: TTypeKinds = {tyGenericInvocation, tyGenericBody, tyGenericParam}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L978) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L978)
```
StructuralEquivTypes: TTypeKinds = {tyNil, tyTuple, tyArray, tySet, tyRange,
tyPtr, tyRef, tyVar, tyLent, tySequence,
tyProc, tyOpenArray, tyVarargs}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L981) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L981)
```
ConcreteTypes: TTypeKinds = {tyBool, tyChar, tyEnum, tyArray, tyObject, tySet,
tyTuple, tyRange, tyPtr, tyRef, tyVar, tyLent,
tySequence, tyProc, tyPointer, tyOpenArray,
tyString, tyCString, tyInt..tyInt64,
tyFloat..tyFloat128, tyUInt..tyUInt64}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L985) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L985)
```
IntegralTypes = {tyBool, tyChar, tyEnum, tyInt..tyInt64, tyFloat..tyFloat128,
tyUInt..tyUInt64}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L992) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L992)
```
ConstantDataTypes: TTypeKinds = {tyArray, tySet, tyTuple, tySequence}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L994) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L994)
```
NilableTypes: TTypeKinds = {tyPointer, tyCString, tyRef, tyPtr, tyProc, tyProxy}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L996) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L996)
```
PtrLikeKinds: TTypeKinds = {tyPointer, tyPtr}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L998) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L998)
```
ExportableSymKinds = {skVar, skConst, skProc, skFunc, skMethod, skType,
skIterator, skMacro, skTemplate, skConverter, skEnumField,
skLet, skStub, skAlias}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L999) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L999)
```
PersistentNodeFlags: TNodeFlags = {nfBase2, nfBase8, nfBase16, nfDotSetter,
nfDotField, nfIsRef, nfIsPtr, nfPreventCg,
nfLL, nfFromTemplate, nfDefaultRefsParam,
nfExecuteOnReload}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1002) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1002)
```
namePos = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1007) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1007)
```
patternPos = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1008) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1008)
```
genericParamsPos = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1009) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1009)
```
paramsPos = 3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1010) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1010)
```
pragmasPos = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1011) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1011)
```
miscPos = 5
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1012) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1012)
```
bodyPos = 6
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1013) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1013)
```
resultPos = 7
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1014) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1014)
```
dispatcherPos = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1015) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1015)
```
nfAllFieldsSet = nfBase2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1017) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1017)
```
nkCallKinds = {nkCall, nkInfix, nkPrefix, nkPostfix, nkCommand, nkCallStrLit,
nkHiddenCallConv}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1019) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1019)
```
nkIdentKinds = {nkIdent, nkSym, nkAccQuoted, nkOpenSymChoice, nkClosedSymChoice}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1021) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1021)
```
nkPragmaCallKinds = {nkExprColonExpr, nkCall, nkCallStrLit}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1024) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1024)
```
nkLiterals = {nkCharLit..nkTripleStrLit}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1025) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1025)
```
nkFloatLiterals = {nkFloatLit..nkFloat128Lit}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1026) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1026)
```
nkLambdaKinds = {nkLambda, nkDo}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1027) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1027)
```
declarativeDefs = {nkProcDef, nkFuncDef, nkMethodDef, nkIteratorDef,
nkConverterDef}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1028) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1028)
```
routineDefs = {nkProcDef..nkIteratorDef, nkFuncDef}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1029) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1029)
```
procDefs = {nkLambda..nkDo, nkProcDef..nkConverterDef, nkIteratorDef, nkFuncDef}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1030) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1030)
```
nkSymChoices = {nkClosedSymChoice, nkOpenSymChoice}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1032) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1032)
```
nkStrKinds = {nkStrLit..nkTripleStrLit}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1033) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1033)
```
skLocalVars = {skVar, skLet, skForVar, skParam, skResult}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1035) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1035)
```
skProcKinds = {skProc, skFunc, skTemplate, skMacro, skIterator, skMethod,
skConverter}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1036) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1036)
```
GrowthFactor = 2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1215) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1215)
```
StartSize = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1216) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1216)
```
UnspecifiedLockLevel = -1'i16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1326) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1326)
```
MaxLockLevel = 1000'i16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1327) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1327)
```
UnknownLockLevel = 1001'i16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1328) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1328)
```
AttachedOpToStr: array[TTypeAttachedOp, string] = ["=destroy", "=copy", "=sink",
"=trace", "=dispose", "=deepcopy"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1329) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1329) Procs
-----
```
proc getnimblePkg(a: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1044) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1044)
```
proc getnimblePkgId(a: PSym): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1059) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1059)
```
proc isCallExpr(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1067) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1067)
```
proc len(n: Indexable): int {...}{.inline.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1074) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1074)
```
proc safeLen(n: PNode): int {...}{.inline, raises: [], tags: [].}
```
works even for leaves. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1081) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1081)
```
proc safeArrLen(n: PNode): int {...}{.inline, raises: [], tags: [].}
```
works for array-like objects (strings passed as openArray in VM). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1086) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1086)
```
proc add(father, son: Indexable)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1092) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1092)
```
proc newNode(kind: TNodeKind): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1108) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1108)
```
proc newNodeI(kind: TNodeKind; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1117)
```
proc newNodeI(kind: TNodeKind; info: TLineInfo; children: int): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1126) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1126)
```
proc newNodeIT(kind: TNodeKind; info: TLineInfo; typ: PType): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1137) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1137)
```
proc newTree(kind: TNodeKind; children: varargs[PNode]): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1142) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1142)
```
proc newTreeI(kind: TNodeKind; info: TLineInfo; children: varargs[PNode]): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1148) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1148)
```
proc newTreeIT(kind: TNodeKind; info: TLineInfo; typ: PType;
children: varargs[PNode]): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1154) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1154)
```
proc newSym(symKind: TSymKind; name: PIdent; owner: PSym; info: TLineInfo;
options: TOptions = {}): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1163)
```
proc astdef(s: PSym): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1171) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1171)
```
proc isMetaType(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1178) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1178)
```
proc isUnresolvedStatic(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1183) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1183)
```
proc linkTo(t: PType; s: PSym): PType {...}{.discardable, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1186)
```
proc linkTo(s: PSym; t: PType): PSym {...}{.discardable, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1191)
```
proc appendToModule(m: PSym; n: PNode) {...}{.raises: [], tags: [].}
```
The compiler will use this internally to add nodes that will be appended to the module after the sem pass [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1204) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1204)
```
proc copyStrTable(dest: var TStrTable; src: TStrTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1218) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1218)
```
proc copyIdTable(dest: var TIdTable; src: TIdTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1223) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1223)
```
proc copyObjectSet(dest: var TObjectSet; src: TObjectSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1228) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1228)
```
proc discardSons(father: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1233) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1233)
```
proc withInfo(n: PNode; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1239) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1239)
```
proc newIdentNode(ident: PIdent; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1243) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1243)
```
proc newSymNode(sym: PSym): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1248) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1248)
```
proc newSymNode(sym: PSym; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1254) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1254)
```
proc newIntNode(kind: TNodeKind; intVal: BiggestInt): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1260) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1260)
```
proc newIntNode(kind: TNodeKind; intVal: Int128): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1264) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1264)
```
proc lastSon(n: Indexable): Indexable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1268)
```
proc skipTypes(t: PType; kinds: TTypeKinds): PType {...}{.raises: [], tags: [].}
```
Used throughout the compiler code to test whether a type tree contains or doesn't contain a specific type/types - it is often the case that only the last child nodes of a type tree need to be searched. This is a really hot path within the compiler! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1270) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1270)
```
proc newIntTypeNode(intVal: BiggestInt; typ: PType): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1278) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1278)
```
proc newIntTypeNode(intVal: Int128; typ: PType): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1301) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1301)
```
proc newFloatNode(kind: TNodeKind; floatVal: BiggestFloat): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1305) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1305)
```
proc newStrNode(kind: TNodeKind; strVal: string): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1309) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1309)
```
proc newStrNode(strVal: string; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1313) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1313)
```
proc newProcNode(kind: TNodeKind; info: TLineInfo; body: PNode; params, name,
pattern, genericParams, pragmas, exceptions: PNode): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1317) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1317)
```
proc `$`(x: TLockLevel): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1332) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1332)
```
proc `$`(s: PSym): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1337) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1337)
```
proc newType(kind: TTypeKind; owner: PSym): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1343) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1343)
```
proc newSons(father: Indexable; length: int)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1362) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1362)
```
proc assignType(dest, src: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1371) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1371)
```
proc copyType(t: PType; owner: PSym; keepId: bool): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1391) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1391)
```
proc exactReplica(t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1400) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1400)
```
proc copySym(s: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1402) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1402)
```
proc createModuleAlias(s: PSym; newIdent: PIdent; info: TLineInfo;
options: TOptions): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1421) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1421)
```
proc initStrTable(x: var TStrTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1436) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1436)
```
proc newStrTable(): TStrTable {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1440) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1440)
```
proc initIdTable(x: var TIdTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1443) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1443)
```
proc newIdTable(): TIdTable {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1447) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1447)
```
proc resetIdTable(x: var TIdTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1450) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1450)
```
proc initObjectSet(x: var TObjectSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1456) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1456)
```
proc initIdNodeTable(x: var TIdNodeTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1460) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1460)
```
proc initNodeTable(x: var TNodeTable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1464) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1464)
```
proc skipTypes(t: PType; kinds: TTypeKinds; maxIters: int): PType {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1468) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1468)
```
proc skipTypesOrNil(t: PType; kinds: TTypeKinds): PType {...}{.raises: [], tags: [].}
```
same as skipTypes but handles 'nil' [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1476) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1476)
```
proc isGCedMem(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1483) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1483)
```
proc propagateToOwner(owner, elem: PType; propagateHasAsgn = true) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1487) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1487)
```
proc rawAddSon(father, son: PType; propagateHasAsgn = true) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1512) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1512)
```
proc rawAddSonNoPropagationOfTypeFlags(father, son: PType) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1518) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1518)
```
proc addSonNilAllowed(father, son: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1523) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1523)
```
proc delSon(father: PNode; idx: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1528) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1528)
```
proc copyNode(src: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1536) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1536)
```
proc transitionSonsKind(n: PNode; kind: range[nkComesFrom .. nkTupleConstr]) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1563) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1563)
```
proc transitionIntKind(n: PNode; kind: range[nkCharLit .. nkUInt64Lit]) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1567) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1567)
```
proc transitionNoneToSym(n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1571) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1571)
```
proc transitionGenericParamToType(s: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1585) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1585)
```
proc transitionRoutineSymKind(s: PSym; kind: range[skProc .. skTemplate]) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1589) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1589)
```
proc transitionToLet(s: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1595) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1595)
```
proc shallowCopy(src: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1619) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1619)
```
proc copyTree(src: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1624) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1624)
```
proc copyTreeWithoutNode(src, skippedNode: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1631) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1631)
```
proc hasSonWith(n: PNode; kind: TNodeKind): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1638) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1638)
```
proc hasNilSon(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1644) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1644)
```
proc containsNode(n: PNode; kinds: TNodeKinds): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1652) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1652)
```
proc hasSubnodeWith(n: PNode; kind: TNodeKind): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1660) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1660)
```
proc getInt(a: PNode): Int128 {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1669) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1669)
```
proc getInt64(a: PNode): int64 {...}{.deprecated: "use getInt",
raises: [ERecoverableError], tags: [].}
```
**Deprecated:** use getInt [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1682) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1682)
```
proc getFloat(a: PNode): BiggestFloat {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1689) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1689)
```
proc getStr(a: PNode): string {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1700) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1700)
```
proc getStrOrChar(a: PNode): string {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1715) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1715)
```
proc isGenericRoutine(s: PSym): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1725) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1725)
```
proc skipGenericOwner(s: PSym): PSym {...}{.raises: [], tags: [].}
```
Generic instantiations are owned by their originating generic symbol. This proc skips such owners and goes straight to the owner of the generic itself (the module or the enclosing proc). [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1732) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1732)
```
proc originatingModule(s: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1741) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1741)
```
proc isRoutine(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1745) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1745)
```
proc isCompileTimeProc(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1748) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1748)
```
proc isRunnableExamples(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1752) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1752)
```
proc requiredParams(s: PSym): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1757) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1757)
```
proc hasPattern(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1766) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1766)
```
proc isAtom(n: PNode): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1775) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1775)
```
proc isEmptyType(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
'void' and 'typed' types are often equivalent to 'nil' these days: [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1778) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1778)
```
proc makeStmtList(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1782) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1782)
```
proc skipStmtList(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1789) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1789)
```
proc toVar(typ: PType; kind: TTypeKind): PType {...}{.raises: [], tags: [].}
```
If `typ` is not a tyVar then it is converted into a `var <typ>` and returned. Otherwise `typ` is simply returned as-is. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1797) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1797)
```
proc toRef(typ: PType): PType {...}{.raises: [], tags: [].}
```
If `typ` is a tyObject then it is converted into a `ref <typ>` and returned. Otherwise `typ` is simply returned as-is. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1805) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1805)
```
proc toObject(typ: PType): PType {...}{.raises: [], tags: [].}
```
If `typ` is a tyRef then its immediate son is returned (which in many cases should be a `tyObject`). Otherwise `typ` is simply returned as-is. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1813) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1813)
```
proc isImportedException(t: PType; conf: ConfigRef): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1821) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1821)
```
proc isInfixAs(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1832) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1832)
```
proc skipColon(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1835) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1835)
```
proc findUnresolvedStatic(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1840) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1840)
```
proc hasDisabledAsgn(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1860) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1860)
```
proc isInlineIterator(typ: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1874) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1874)
```
proc isClosureIterator(typ: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1877) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1877)
```
proc isClosure(typ: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1880) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1880)
```
proc isSinkParam(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1883) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1883)
```
proc isSinkType(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1886) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1886)
```
proc newProcType(info: TLineInfo; owner: PSym): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1889) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1889)
```
proc addParam(procType: PType; param: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1898) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1898)
```
proc canRaiseConservative(fn: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1910) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1910)
```
proc canRaise(fn: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1916) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1916)
```
proc toHumanStr(kind: TSymKind): string {...}{.raises: [], tags: [].}
```
strips leading `sk` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1933) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1933)
```
proc toHumanStr(kind: TTypeKind): string {...}{.raises: [], tags: [].}
```
strips leading `tk` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1937) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1937)
```
proc skipAddr(n: PNode): PNode {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1941) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1941) Iterators
---------
```
iterator items(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1769) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1769)
```
iterator pairs(n: PNode): tuple[i: int, n: PNode] {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1772) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1772) Templates
---------
```
template `[]`(n: Indexable; i: int): Indexable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1098) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1098)
```
template `[]=`(n: Indexable; i: int; x: Indexable)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1099) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1099)
```
template `[]`(n: Indexable; i: BackwardsIndex): Indexable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1101) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1101)
```
template `[]=`(n: Indexable; i: BackwardsIndex; x: Indexable)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1102) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1102)
```
template previouslyInferred(t: PType): PType
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1160) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1160)
```
template fileIdx(c: PSym): FileIndex
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1196) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1196)
```
template filename(c: PSym): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1200) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1200)
```
template transitionSymKindCommon(k: TSymKind)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1574) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1574)
```
template hasDestructor(t: PType): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1858) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1858)
```
template incompleteType(t: PType): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1863) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1863)
```
template typeCompleted(s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1866) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1866)
```
template getBody(s: PSym): PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1869) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1869)
```
template detailedInfo(sym: PSym): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1871) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1871)
```
template destructor(t: PType): PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1903) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1903)
```
template assignment(t: PType): PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1904) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1904)
```
template asink(t: PType): PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ast.nim#L1905) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ast.nim#L1905) Exports
-------
[bitxor](int128#bitxor,Int128,Int128), [maskUInt16](int128#maskUInt16,Int128), [<](#), [<=](#), [high](int128#high.t,typedesc%5BInt128%5D), [castToUInt64](int128#castToUInt64,Int128), [shr](int128#shr,Int128,int), [toHex](int128#toHex,Int128), [==](int128#==,Int128,Int128), [+](int128#+,BiggestInt,Int128), [addInt128](int128#addInt128,string,Int128), [abs](int128#abs,Int128), [One](int128#One), [<=](#), [maskUInt64](int128#maskUInt64,Int128), [$](int128#%24,Int128), [toUInt8](int128#toUInt8,Int128), [inc](int128#inc,Int128,uint32), [<](#), [+=](int128#+=,Int128,Int128), [maskBytes](int128#maskBytes,Int128,int), [Ten](int128#Ten), [-](int128#-,Int128), [-](int128#-,Int128,BiggestInt), [\*=](int128#*=,Int128,int32), [Zero](int128#Zero), [toInt32](int128#toInt32,Int128), [==](int128#==,BiggestInt,Int128), [-=](int128#-=,Int128,Int128), [toInt64Checked](int128#toInt64Checked,Int128,int64), [+](int128#+,Int128,Int128), [parseDecimalInt128](int128#parseDecimalInt128,string,int), [toInt8](int128#toInt8,Int128), [toUInt16](int128#toUInt16,Int128), [mod](int128#mod,Int128,Int128), [toInt128](int128#toInt128,T), [shl](int128#shl,Int128,int), [maskUInt32](int128#maskUInt32,Int128), [toUInt32](int128#toUInt32,Int128), [fastLog2](int128#fastLog2,Int128), [toInt128](int128#toInt128,float64), [toUInt](int128#toUInt,Int128), [<](#), [bitor](int128#bitor,Int128,Int128), [<=](#), [toFloat64](int128#toFloat64,Int128), [-](int128#-,BiggestInt,Int128), [==](int128#==,Int128,BiggestInt), [\*=](int128#*=,Int128,Int128), [bitnot](int128#bitnot,Int128), [toInt64](int128#toInt64,Int128), [NegOne](int128#NegOne), [div](int128#div,Int128,Int128), [+](int128#+,Int128,BiggestInt), [\*](int128#*,Int128,int32), [toUInt64](int128#toUInt64,Int128), [low](int128#low.t,typedesc%5BInt128%5D), [addToHex](int128#addToHex,string,Int128), [-](int128#-,Int128,Int128), [maskUInt8](int128#maskUInt8,Int128), [divMod](int128#divMod,Int128,Int128), [bitand](int128#bitand,Int128,Int128), [Int128](int128#Int128), [cmp](int128#cmp,Int128,Int128), [\*](int128#*,Int128,Int128), [toInt16](int128#toInt16,Int128), [toInt](int128#toInt,Int128), [castToInt64](int128#castToInt64,Int128)
| programming_docs |
nim vmgen vmgen
=====
This module implements the code generator for the VM.
Imports
-------
<ast>, <types>, <msgs>, <renderer>, <vmdef>, <magicsys>, <options>, <lowerings>, <lineinfos>, <transf> Consts
------
```
debugEchoCode = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L35) Procs
-----
```
proc echoCode(c: PCtx; start = 0; last = -1) {...}{.deprecated,
raises: [ValueError, Exception], tags: [RootEffect].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L109)
```
proc canonValue(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L437) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L437)
```
proc sameConstant(a, b: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L447)
```
proc fitsRegister(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L961) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L961)
```
proc importcCondVar(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L1459) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L1459)
```
proc importcCond(s: PSym): bool {...}{.inline, raises: [], tags: [].}
```
return true to importc `s`, false to execute its body instead (refs #8405) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L1570) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L1570)
```
proc getNullValue(typ: PType; info: TLineInfo; conf: ConfigRef): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L1780) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L1780)
```
proc genStmt(c: PCtx; n: PNode): int {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L2138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L2138)
```
proc genExpr(c: PCtx; n: PNode; requiresValue = true): int {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L2147) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L2147)
```
proc genProc(c: PCtx; s: PSym): int {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError, KeyError], tags: [RootEffect, WriteIOEffect,
ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmgen.nim#L2224) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmgen.nim#L2224)
nim pluginsupport pluginsupport
=============
Plugin support for the Nim compiler. Right now plugins need to be built with the compiler only: plugins using DLLs or the FFI will not work.
Imports
-------
<ast>, <semdata>, <idents> Types
-----
```
Transformation = proc (c: PContext; n: PNode): PNode {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pluginsupport.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pluginsupport.nim#L17)
```
Plugin = tuple[package, module, fn: string, t: Transformation]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pluginsupport.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pluginsupport.nim#L18) Procs
-----
```
proc pluginMatches(ic: IdentCache; p: Plugin; s: PSym): bool {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pluginsupport.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pluginsupport.nim#L22)
nim cmdlinehelper cmdlinehelper
=============
Helpers for binaries that use compiler passes, e.g.: nim, nimsuggest, nimfix
Imports
-------
<options>, <idents>, <nimconf>, <extccomp>, <commands>, <msgs>, <lineinfos>, <modulegraphs>, <condsyms>, <pathutils> Types
-----
```
NimProg = ref object
suggestMode*: bool
supportsStdinFile*: bool
processCmdLine*: proc (pass: TCmdLinePass; cmd: string; config: ConfigRef)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L33) Procs
-----
```
proc prependCurDir(f: AbsoluteFile): AbsoluteFile {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L18)
```
proc addCmdPrefix(result: var string; kind: CmdLineKind) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L25)
```
proc initDefinesProg(self: NimProg; conf: ConfigRef; name: string) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L38)
```
proc processCmdLineAndProjectPath(self: NimProg; conf: ConfigRef) {...}{.
raises: [Exception, OSError],
tags: [RootEffect, ReadEnvEffect, ReadIOEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L42)
```
proc loadConfigsAndRunMainCommand(self: NimProg; cache: IdentCache;
conf: ConfigRef; graph: ModuleGraph): bool {...}{.raises: [
OSError, IOError, Exception, KeyError, ValueError, ERecoverableError,
EOFError], tags: [ReadIOEffect, ReadDirEffect, ReadEnvEffect, RootEffect,
WriteIOEffect, WriteEnvEffect, WriteDirEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cmdlinehelper.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cmdlinehelper.nim#L58)
nim spawn spawn
=====
This module implements threadpool's `spawn`.
Imports
-------
<ast>, <types>, <idents>, <magicsys>, <msgs>, <options>, <modulegraphs>, <lowerings>, <liftdestructors>, <renderer>, <trees> Types
-----
```
TSpawnResult = enum
srVoid, srFlowVar, srByVar
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/spawn.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/spawn.nim#L27) Procs
-----
```
proc spawnResult(t: PType; inParallel: bool): TSpawnResult {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/spawn.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/spawn.nim#L34)
```
proc wrapProcForSpawn(g: ModuleGraph; owner: PSym; spawnExpr: PNode;
retType: PType; barrier, dest: PNode = nil): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/spawn.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/spawn.nim#L306)
nim index index
=====
This module only exists to generate docs for the compiler.
### links
* [main docs](../lib)
* [compiler user guide](../nimc)
* [Internals of the Nim Compiler](../intern)
Imports
-------
<nim>
nim patterns patterns
========
This module implements the pattern matching features for term rewriting macro support.
Imports
-------
<ast>, <types>, <semdata>, <sigmatch>, <idents>, <aliases>, <parampatterns>, <trees> Procs
-----
```
proc sameTrees(a, b: PNode): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/patterns.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/patterns.nim#L50)
```
proc applyRule(c: PContext; s: PSym; n: PNode): PNode {...}{.
raises: [Exception, ERecoverableError, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
returns a tree to semcheck if the rule triggered; nil otherwise [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/patterns.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/patterns.nim#L247)
nim parser parser
======
Imports
-------
<llstream>, <lexer>, <idents>, <ast>, <msgs>, <options>, <lineinfos>, <pathutils> Types
-----
```
Parser = object
currInd: int
firstTok: bool
hasProgress: bool
lex*: Lexer
tok*: Token
inPragma*: int
inSemiStmtList*: int
emptyNode: PNode
when defined(nimpretty):
em*: Emitter
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L40) Procs
-----
```
proc getTok(p: var Parser) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
Get the next token from the parser's lexer, and store it in the parser's `tok` member. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L100) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L100)
```
proc openParser(p: var Parser; fileIdx: FileIndex; inputStream: PLLStream;
cache: IdentCache; config: ConfigRef) {...}{.
raises: [IOError, Exception, ValueError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
Open a parser, using the given arguments to set up its internal state. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L113) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L113)
```
proc openParser(p: var Parser; filename: AbsoluteFile; inputStream: PLLStream;
cache: IdentCache; config: ConfigRef) {...}{.
raises: [IOError, Exception, ValueError, ERecoverableError, KeyError], tags: [
ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L125) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L125)
```
proc closeParser(p: var Parser) {...}{.raises: [], tags: [].}
```
Close a parser, freeing up its resources. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L129)
```
proc parMessage(p: Parser; msg: TMsgKind; arg: string = "") {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
Produce and emit the parser message `arg` to output. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L135) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L135)
```
proc skipComment(p: var Parser; node: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L180)
```
proc skipInd(p: var Parser) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L191)
```
proc optPar(p: var Parser) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L195)
```
proc optInd(p: var Parser; n: PNode) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L199)
```
proc expectIdentOrKeyw(p: Parser) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L207) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L207)
```
proc expectIdent(p: Parser) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L211)
```
proc eat(p: var Parser; tokType: TokType) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
Move the parser to the next token if the current token is of type `tokType`, otherwise error. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L215)
```
proc parLineInfo(p: Parser): TLineInfo {...}{.raises: [], tags: [].}
```
Retrieve the line information associated with the parser's current state. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L224)
```
proc indAndComment(p: var Parser; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L228) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L228)
```
proc newNodeP(kind: TNodeKind; p: Parser): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L235)
```
proc newIntNodeP(kind: TNodeKind; intVal: BiggestInt; p: Parser): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L238) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L238)
```
proc newFloatNodeP(kind: TNodeKind; floatVal: BiggestFloat; p: Parser): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L242)
```
proc newStrNodeP(kind: TNodeKind; strVal: string; p: Parser): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L247)
```
proc newIdentNodeP(ident: PIdent; p: Parser): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L251) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L251)
```
proc isOperator(tok: Token): bool {...}{.raises: [], tags: [].}
```
Determines if the given token is an operator type token. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L268)
```
proc parseSymbol(p: var Parser; mode = smNormal): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L322) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L322)
```
proc setBaseFlags(n: PNode; base: NumericalBase) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L509) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L509)
```
proc parseAll(p: var Parser): PNode {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
Parses the rest of the input stream held by the parser into a PNode. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L2297) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L2297)
```
proc parseTopLevelStmt(p: var Parser): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
Implements an iterator which, when called repeatedly, returns the next top-level statement or emptyNode if end of stream. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L2312) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L2312)
```
proc parseString(s: string; cache: IdentCache; config: ConfigRef;
filename: string = ""; line: int = 0;
errorHandler: ErrorHandler = nil): PNode {...}{.
raises: [IOError, Exception, ValueError, ERecoverableError, KeyError], tags: [
ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect, ReadDirEffect].}
```
Parses a string into an AST, returning the top node. `filename` and `line`, although optional, provide info so that the compiler can generate correct error messages referring to the original source. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parser.nim#L2341) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parser.nim#L2341)
nim llstream llstream
========
Low-level streams for high performance.
Imports
-------
<pathutils> Types
-----
```
TLLRepl = proc (s: PLLStream; buf: pointer; bufLen: int): int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L22)
```
TLLStreamKind = enum
llsNone, llsString, llsFile, llsStdIn
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L23)
```
TLLStream = object of RootObj
kind*: TLLStreamKind
f*: File
s*: string
rd*, wr*: int
lineOffset*: int
repl*: TLLRepl
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L28)
```
PLLStream = ref TLLStream
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L36) Procs
-----
```
proc llStreamOpen(data: string): PLLStream {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L38)
```
proc llStreamOpen(f: File): PLLStream {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L43)
```
proc llStreamOpen(filename: AbsoluteFile; mode: FileMode): PLLStream {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L48)
```
proc llStreamOpen(): PLLStream {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L53)
```
proc llStreamOpenStdIn(r: TLLRepl = llReadFromStdin): PLLStream {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L58)
```
proc llStreamClose(s: PLLStream) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L65)
```
proc endsWith(x: string; s: set[char]): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L81)
```
proc endsWithOpr(x: string): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L92) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L92)
```
proc llStreamRead(s: PLLStream; buf: pointer; bufLen: int): int {...}{.
raises: [IOError, Exception], tags: [ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L124)
```
proc llStreamReadLine(s: PLLStream; line: var string): bool {...}{.raises: [IOError],
tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L138)
```
proc llStreamWrite(s: PLLStream; data: string) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L162)
```
proc llStreamWriteln(s: PLLStream; data: string) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L172)
```
proc llStreamWrite(s: PLLStream; data: char) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L176)
```
proc llStreamWrite(s: PLLStream; buf: pointer; buflen: int) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L188) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L188)
```
proc llStreamReadAll(s: PLLStream): string {...}{.raises: [IOError],
tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/llstream.nim#L200) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/llstream.nim#L200)
| programming_docs |
nim cgmeth cgmeth
======
This module implements code generation for methods.
Imports
-------
<options>, <ast>, <msgs>, <idents>, <renderer>, <types>, <magicsys>, <sempass2>, <modulegraphs>, <lineinfos> Procs
-----
```
proc getDispatcher(s: PSym): PSym {...}{.raises: [], tags: [].}
```
can return nil if is has no dispatcher. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgmeth.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgmeth.nim#L38)
```
proc methodCall(n: PNode; conf: ConfigRef): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgmeth.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgmeth.nim#L44)
```
proc methodDef(g: ModuleGraph; s: PSym; fromCache: bool) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgmeth.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgmeth.nim#L160)
```
proc generateMethodDispatchers(g: ModuleGraph): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgmeth.nim#L286) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgmeth.nim#L286)
nim passes passes
======
This module implements the passes functionality. A pass must implement the `TPass` interface.
Imports
-------
<options>, <ast>, <llstream>, <msgs>, <idents>, <syntaxes>, <idgen>, <modulegraphs>, <reorder>, <rod>, <lineinfos>, <pathutils> Types
-----
```
TPassData = tuple[input: PNode, closeOutput: PNode]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L20) Procs
-----
```
proc makePass(open: TPassOpen = nil; process: TPassProcess = nil;
close: TPassClose = nil; isFrontend = false): TPass {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L26)
```
proc skipCodegen(config: ConfigRef; n: PNode): bool {...}{.inline, raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L35)
```
proc clearPasses(g: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L47)
```
proc registerPass(g: ModuleGraph; p: TPass) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L50)
```
proc carryPass(g: ModuleGraph; p: TPass; module: PSym; m: TPassData): TPassData {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L54)
```
proc carryPasses(g: ModuleGraph; nodes: PNode; module: PSym;
passes: openArray[TPass]) {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L61)
```
proc moduleHasChanged(graph: ModuleGraph; module: PSym): bool {...}{.inline,
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L123) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L123)
```
proc processModule(graph: ModuleGraph; module: PSym; stream: PLLStream): bool {...}{.
discardable, raises: [Exception, ValueError, IOError, ERecoverableError,
KeyError, OSError], tags: [RootEffect, WriteIOEffect,
ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passes.nim#L126) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passes.nim#L126)
nim macrocacheimpl macrocacheimpl
==============
This module implements helpers for the macro cache.
Imports
-------
<lineinfos>, <ast>, <modulegraphs>, <vmdef> Procs
-----
```
proc recordInc(c: PCtx; info: TLineInfo; key: string; by: BiggestInt) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/macrocacheimpl.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/macrocacheimpl.nim#L14)
```
proc recordPut(c: PCtx; info: TLineInfo; key: string; k: string; val: PNode) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/macrocacheimpl.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/macrocacheimpl.nim#L21)
```
proc recordAdd(c: PCtx; info: TLineInfo; key: string; val: PNode) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/macrocacheimpl.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/macrocacheimpl.nim#L29)
```
proc recordIncl(c: PCtx; info: TLineInfo; key: string; val: PNode) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/macrocacheimpl.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/macrocacheimpl.nim#L36)
nim enumtostr enumtostr
=========
Imports
-------
<ast>, <idents>, <lineinfos>, <modulegraphs>, <magicsys> Procs
-----
```
proc genEnumToStrProc(t: PType; info: TLineInfo; g: ModuleGraph): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/enumtostr.nim#L4) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/enumtostr.nim#L4)
```
proc genCaseObjDiscMapping(t: PType; field: PSym; info: TLineInfo;
g: ModuleGraph): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/enumtostr.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/enumtostr.nim#L65)
nim nimconf nimconf
=======
Imports
-------
<llstream>, <commands>, <msgs>, <lexer>, <options>, <idents>, <wordrecg>, <lineinfos>, <pathutils>, <scriptconfig> Procs
-----
```
proc readConfigFile(filename: AbsoluteFile; cache: IdentCache; config: ConfigRef): bool {...}{.raises: [
IOError, Exception, KeyError, ValueError, ERecoverableError, OSError,
EOFError], tags: [ReadIOEffect, RootEffect, ReadDirEffect, WriteIOEffect,
ReadEnvEffect, WriteEnvEffect, WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimconf.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimconf.nim#L211)
```
proc getUserConfigPath(filename: RelativeFile): AbsoluteFile {...}{.
raises: [OSError], tags: [ReadEnvEffect, ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimconf.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimconf.nim#L229)
```
proc getSystemConfigPath(conf: ConfigRef; filename: RelativeFile): AbsoluteFile {...}{.
raises: [OSError], tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimconf.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimconf.nim#L232)
```
proc loadConfigs(cfg: RelativeFile; cache: IdentCache; conf: ConfigRef) {...}{.raises: [
OSError, IOError, Exception, KeyError, ValueError, ERecoverableError,
EOFError], tags: [ReadIOEffect, ReadDirEffect, ReadEnvEffect, RootEffect,
WriteIOEffect, WriteEnvEffect, WriteDirEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimconf.nim#L241) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimconf.nim#L241)
nim modules modules
=======
Implements the module handling, including the caching of modules.
Imports
-------
<ast>, <astalgo>, <magicsys>, <msgs>, <options>, <idents>, <lexer>, <idgen>, <passes>, <syntaxes>, <llstream>, <modulegraphs>, <rod>, <lineinfos>, <pathutils> Procs
-----
```
proc resetSystemArtifacts(g: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L17)
```
proc compileModule(graph: ModuleGraph; fileIdx: FileIndex; flags: TSymFlags): PSym {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L79)
```
proc importModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PSym {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L107)
```
proc includeModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L122)
```
proc connectCallbacks(graph: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L127)
```
proc compileSystemModule(graph: ModuleGraph) {...}{.raises: [KeyError, Exception,
OSError, ValueError, IOError, ERecoverableError], tags: [ReadDirEffect,
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L131)
```
proc wantMainModule(conf: ConfigRef) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError, KeyError], tags: [RootEffect, WriteIOEffect,
ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L138)
```
proc compileProject(graph: ModuleGraph; projectFileIdx = InvalidFileIdx) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L144) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L144)
```
proc makeModule(graph: ModuleGraph; filename: AbsoluteFile): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L162)
```
proc makeModule(graph: ModuleGraph; filename: string): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L167)
```
proc makeStdinModule(graph: ModuleGraph): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modules.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modules.nim#L170)
nim typeallowed typeallowed
===========
This module contains 'typeAllowed' and friends which check for invalid types like 'openArray[var int]'.
Imports
-------
<ast>, <renderer>, <options>, <semdata>, <types> Types
-----
```
TTypeAllowedFlag = enum
taField, taHeap, taConcept, taIsOpenArray, taNoUntyped, taIsTemplateOrMacro,
taProcContextIsNotMacro
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L17)
```
TTypeAllowedFlags = set[TTypeAllowedFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L26)
```
ViewTypeKind = enum
noView, immutableView, mutableView
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L199) Procs
-----
```
proc typeAllowed(t: PType; kind: TSymKind; c: PContext;
flags: TTypeAllowedFlags = {}): PType {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L192)
```
proc classifyViewType(t: PType): ViewTypeKind {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L254) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L254)
```
proc directViewType(t: PType): ViewTypeKind {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L258) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L258)
```
proc requiresInit(t: PType): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typeallowed.nim#L270) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typeallowed.nim#L270)
nim liftlocals liftlocals
==========
This module implements the '.liftLocals' pragma.
Imports
-------
<options>, <ast>, <msgs>, <idents>, <renderer>, <types>, <lowerings>, <lineinfos>, <pragmas>, <wordrecg> Procs
-----
```
proc liftLocalsIfRequested(prc: PSym; n: PNode; cache: IdentCache;
conf: ConfigRef): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/liftlocals.nim#L56) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/liftlocals.nim#L56)
nim nimlexbase nimlexbase
==========
Imports
-------
<llstream> Types
-----
```
TBaseLexer = object of RootObj
bufpos*: int
buf*: cstring
bufStorage: string
bufLen: int
stream*: PLLStream
lineNumber*: int
sentinel*: int
lineStart*: int
offsetBase*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L40) Consts
------
```
Lrz = ' '
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L19)
```
Apo = '\''
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L20)
```
Tabulator = '\t'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L21)
```
ESC = '\e'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L22)
```
CR = '\c'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L23)
```
FF = '\f'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L24)
```
LF = '\n'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L25)
```
BEL = '\a'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L26)
```
BACKSPACE = '\b'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L27)
```
VT = '\v'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L28)
```
EndOfFile = '\x00'
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L31)
```
NewLines = {'\c', '\n'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L37) Procs
-----
```
proc closeBaseLexer(L: var TBaseLexer) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L69)
```
proc handleCR(L: var TBaseLexer; pos: int): int {...}{.raises: [IOError, Exception],
tags: [ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L128)
```
proc handleLF(L: var TBaseLexer; pos: int): int {...}{.raises: [IOError, Exception],
tags: [ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L135) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L135)
```
proc openBaseLexer(L: var TBaseLexer; inputstream: PLLStream; bufLen: int = 8192) {...}{.
raises: [IOError, Exception], tags: [ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L145) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L145)
```
proc getColNumber(L: TBaseLexer; pos: int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L159)
```
proc getCurrentLine(L: TBaseLexer; marker: bool = true): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimlexbase.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimlexbase.nim#L162)
| programming_docs |
nim nodejs nodejs
======
Procs
-----
```
proc findNodeJs(): string {...}{.inline, raises: [OSError],
tags: [ReadDirEffect, ReadEnvEffect, ReadIOEffect].}
```
Find NodeJS executable and return it as a string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nodejs.nim#L3) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nodejs.nim#L3)
nim injectdestructors injectdestructors
=================
Injects destructor calls into Nim code as well as an optimizer that optimizes copies to moves. This is implemented as an AST to AST transformation so that every backend benefits from it.See doc/destructors.rst for a spec of the implemented rewrite rules
Imports
-------
<ast>, <astalgo>, <msgs>, <renderer>, <magicsys>, <types>, <idents>, <options>, <dfa>, <lowerings>, <modulegraphs>, <msgs>, <lineinfos>, <parampatterns>, <sighashes>, <liftdestructors>, <optimizer>, <varpartitions>, <trees> Procs
-----
```
proc injectDestructorCalls(g: ModuleGraph; owner: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/injectdestructors.nim#L1015) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/injectdestructors.nim#L1015)
nim idgen idgen
=====
This module contains a simple persistent id generator.
Imports
-------
<idents>, <options>, <pathutils> Vars
----
```
gFrontEndId: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L14) Consts
------
```
debugIds = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L17) Procs
-----
```
proc registerID(id: PIdObj) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L24)
```
proc getID(): int {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L29)
```
proc setId(id: int) {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L33)
```
proc idSynchronizationPoint(idRange: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L36)
```
proc saveMaxIds(conf: ConfigRef; project: AbsoluteFile) {...}{.
raises: [IOError, OSError], tags: [ReadEnvEffect, ReadIOEffect,
WriteDirEffect, ReadDirEffect,
WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L45)
```
proc loadMaxIds(conf: ConfigRef; project: AbsoluteFile) {...}{.
raises: [OSError, IOError, ValueError], tags: [ReadEnvEffect, ReadIOEffect,
WriteDirEffect, ReadDirEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idgen.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idgen.nim#L50)
nim reorder reorder
=======
Imports
-------
<ast>, <idents>, <renderer>, <msgs>, <modulegraphs>, <syntaxes>, <options>, <modulepaths>, <lineinfos> Procs
-----
```
proc includeModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/reorder.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/reorder.nim#L117)
```
proc reorder(graph: ModuleGraph; n: PNode; module: PSym): PNode {...}{.raises: [
OSError, Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
ReadEnvEffect, ReadIOEffect, RootEffect, WriteIOEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/reorder.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/reorder.nim#L405)
nim vmdef vmdef
=====
This module contains the type definitions for the new evaluation engine. An instruction is 1-3 int32s in memory, it is a register based VM.
Imports
-------
<ast>, <idents>, <options>, <modulegraphs>, <lineinfos> Types
-----
```
TInstrType = uint64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L15)
```
TRegister = range[0 .. 65535'u64.int]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L46)
```
TDest = range[-1 .. 65535'u64.int]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L47)
```
TInstr = distinct TInstrType
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L48)
```
TOpcode = enum
opcEof, opcRet, opcYldYoid, opcYldVal, opcAsgnInt, opcAsgnFloat, opcAsgnRef,
opcAsgnComplex, opcCastIntToFloat32, opcCastIntToFloat64, opcCastFloatToInt32,
opcCastFloatToInt64, opcCastPtrToInt, opcCastIntToPtr, opcFastAsgnComplex,
opcNodeToReg, opcLdArr, opcLdArrAddr, opcWrArr, opcLdObj, opcLdObjAddr,
opcWrObj, opcAddrReg, opcAddrNode, opcLdDeref, opcWrDeref, opcWrStrIdx,
opcLdStrIdx, opcAddInt, opcAddImmInt, opcSubInt, opcSubImmInt, opcLenSeq,
opcLenStr, opcIncl, opcInclRange, opcExcl, opcCard, opcMulInt, opcDivInt,
opcModInt, opcAddFloat, opcSubFloat, opcMulFloat, opcDivFloat, opcShrInt,
opcShlInt, opcAshrInt, opcBitandInt, opcBitorInt, opcBitxorInt, opcAddu,
opcSubu, opcMulu, opcDivu, opcModu, opcEqInt, opcLeInt, opcLtInt, opcEqFloat,
opcLeFloat, opcLtFloat, opcLeu, opcLtu, opcEqRef, opcEqNimNode,
opcSameNodeType, opcXor, opcNot, opcUnaryMinusInt, opcUnaryMinusFloat,
opcBitnotInt, opcEqStr, opcLeStr, opcLtStr, opcEqSet, opcLeSet, opcLtSet,
opcMulSet, opcPlusSet, opcMinusSet, opcConcatStr, opcContainsSet, opcRepr,
opcSetLenStr, opcSetLenSeq, opcIsNil, opcOf, opcIs, opcSubStr, opcParseFloat,
opcConv, opcCast, opcQuit, opcInvalidField, opcNarrowS, opcNarrowU,
opcSignExtend, opcAddStrCh, opcAddStrStr, opcAddSeqElem, opcRangeChck,
opcNAdd, opcNAddMultiple, opcNKind, opcNSymKind, opcNIntVal, opcNFloatVal,
opcNSymbol, opcNIdent, opcNGetType, opcNStrVal, opcNSigHash, opcNGetSize,
opcNSetIntVal, opcNSetFloatVal, opcNSetSymbol, opcNSetIdent, opcNSetType,
opcNSetStrVal, opcNNewNimNode, opcNCopyNimNode, opcNCopyNimTree, opcNDel,
opcGenSym, opcNccValue, opcNccInc, opcNcsAdd, opcNcsIncl, opcNcsLen, opcNcsAt,
opcNctPut, opcNctLen, opcNctGet, opcNctHasNext, opcNctNext, opcNodeId,
opcSlurp, opcGorge, opcParseExprToAst, opcParseStmtToAst, opcQueryErrorFlag,
opcNError, opcNWarning, opcNHint, opcNGetLineInfo, opcNSetLineInfo,
opcEqIdent, opcStrToIdent, opcGetImpl, opcGetImplTransf, opcEcho, opcIndCall,
opcIndCallAsgn, opcRaise, opcNChild, opcNSetChild, opcCallSite, opcNewStr,
opcTJmp, opcFJmp, opcJmp, opcJmpBack, opcBranch, opcTry, opcExcept,
opcFinally, opcFinallyEnd, opcNew, opcNewSeq, opcLdNull, opcLdNullReg,
opcLdConst, opcAsgnConst, opcLdGlobal, opcLdGlobalAddr, opcLdGlobalDerefFFI,
opcLdGlobalAddrDerefFFI, opcLdImmInt, opcNBindSym, opcNDynBindSym, opcSetType,
opcTypeTrait, opcMarshalLoad, opcMarshalStore, opcSymOwner,
opcSymIsInstantiationOf
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L50)
```
TBlock = object
label*: PSym
fixups*: seq[TPosition]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L183) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L183)
```
TEvalMode = enum
emRepl, ## evaluate because in REPL mode
emConst, ## evaluate for 'const' according to spec
emOptimize, ## evaluate for optimization purposes (same as
## emConst?)
emStaticExpr, ## evaluate for enforced compile time eval
## ('static' context)
emStaticStmt ## 'static' as an expression
```
reason for evaluation [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L187) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L187)
```
TSandboxFlag = enum
allowCast, ## allow unsafe language feature: 'cast'
allowInfiniteLoops ## allow endless loops
```
what the evaluation engine should allow [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L196) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L196)
```
TSandboxFlags = set[TSandboxFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L199)
```
TSlotKind = enum
slotEmpty, slotFixedVar, slotFixedLet, slotTempUnknown, slotTempInt,
slotTempFloat, slotTempStr, slotTempComplex, slotTempPerm
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L201)
```
TRegisterKind = enum
rkNone, rkNode, rkInt, rkFloat, rkRegisterAddr, rkNodeAddr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L215)
```
TFullReg = object
case kind*: TRegisterKind
of rkNone:
nil
of rkInt:
intVal*: BiggestInt
of rkFloat:
floatVal*: BiggestFloat
of rkNode:
node*: PNode
of rkRegisterAddr:
regAddr*: ptr TFullReg
of rkNodeAddr:
nodeAddr*: ptr PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L217) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L217)
```
PProc = ref object
blocks*: seq[TBlock]
sym*: PSym
slots*: array[TRegister, tuple[inUse: bool, kind: TSlotKind]]
maxSlots*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L227) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L227)
```
VmArgs = object
ra*, rb*, rc*: Natural
slots*: ptr UncheckedArray[TFullReg]
currentException*: PNode
currentLineInfo*: TLineInfo
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L233) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L233)
```
VmCallback = proc (args: VmArgs) {...}{.closure.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L238) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L238)
```
PCtx = ref TCtx
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L240)
```
TCtx = object of TPassContext
code*: seq[TInstr]
debug*: seq[TLineInfo]
globals*: PNode
constants*: PNode
types*: seq[PType]
currentExceptionA*, currentExceptionB*: PNode
exceptionInstr*: int
prc*: PProc
module*: PSym
callsite*: PNode
mode*: TEvalMode
features*: TSandboxFlags
traceActive*: bool
loopIterations*: int
comesFromHeuristic*: TLineInfo
callbacks*: seq[tuple[key: string, value: VmCallback]]
errorFlag*: string
cache*: IdentCache
config*: ConfigRef
graph*: ModuleGraph
oldErrorCount*: int
profiler*: Profiler
templInstCounter*: ref int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L241) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L241)
```
PStackFrame = ref TStackFrame
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L267) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L267)
```
TStackFrame {...}{.acyclic.} = object
prc*: PSym
slots*: seq[TFullReg]
next*: PStackFrame
comesFrom*: int
safePoints*: seq[int]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L268)
```
Profiler = object
tEnter*: float
tos*: PStackFrame
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L277) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L277)
```
TPosition = distinct int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L281) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L281)
```
PEvalContext = PCtx
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L283) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L283) Consts
------
```
byteExcess = 128
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L24)
```
regOShift = 0'u
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L29)
```
regAShift = 8'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L30)
```
regBShift = 24'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L31)
```
regCShift = 40'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L32)
```
regBxShift = 24'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L33)
```
regOMask = 255'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L35)
```
regAMask = 65535'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L36)
```
regBMask = 65535'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L37)
```
regCMask = 65535'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L38)
```
regBxMask = 16777215'u64
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L39)
```
wordExcess = 8388608
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L41)
```
regBxMin = -8388607
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L42)
```
regBxMax = 8388607
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L43)
```
firstABxInstr = opcTJmp
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L302) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L302)
```
largeInstrs = {opcSubStr, opcConv, opcCast, opcNewSeq, opcOf, opcMarshalLoad,
opcMarshalStore}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L303) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L303)
```
slotSomeTemp = slotTempUnknown
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L306)
```
relativeJumps = {opcTJmp, opcFJmp, opcJmp, opcJmpBack}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L307) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L307)
```
nimNodeFlag = 16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L310) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L310) Procs
-----
```
proc newCtx(module: PSym; cache: IdentCache; g: ModuleGraph): PCtx {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L285) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L285)
```
proc refresh(c: PCtx; module: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L292) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L292)
```
proc registerCallback(c: PCtx; name: string; callback: VmCallback): int {...}{.
discardable, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L297) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L297) Templates
---------
```
template opcode(x: TInstr): TOpcode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L312) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L312)
```
template regA(x: TInstr): TRegister
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L313) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L313)
```
template regB(x: TInstr): TRegister
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L314) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L314)
```
template regC(x: TInstr): TRegister
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L315) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L315)
```
template regBx(x: TInstr): int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L316) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L316)
```
template jmpDiff(x: TInstr): int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdef.nim#L318) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdef.nim#L318)
nim optimizer optimizer
=========
Optimizer:
* elide 'wasMoved(x); destroy(x)' pairs
* recognize "all paths lead to 'wasMoved(x)'"
Imports
-------
<ast>, <renderer>, <idents>, <trees> Procs
-----
```
proc optimize(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/optimizer.nim#L261) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/optimizer.nim#L261)
nim int128 int128
======
This module is for compiler internal use only. For reliable error messages and range checks, the compiler needs a data type that can hold all from `low(BiggestInt)` to `high(BiggestUInt)`, This type is for that purpose.
Types
-----
```
Int128 = object
udata: array[4, uint32]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L9) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L9) Consts
------
```
Zero = (udata: [0'u, 0'u, 0'u, 0'u])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L20)
```
One = (udata: [1'u, 0'u, 0'u, 0'u])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L21)
```
Ten = (udata: [10'u, 0'u, 0'u, 0'u])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L22)
```
NegOne = (udata: [4294967295'u, 4294967295'u, 4294967295'u, 4294967295'u])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L25) Procs
-----
```
proc toInt128[T: SomeInteger | bool](arg: T): Int128
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L32)
```
proc toInt64(arg: Int128): int64 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L66)
```
proc toInt64Checked(arg: Int128; onError: int64): int64 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L76)
```
proc toInt32(arg: Int128): int32 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L85)
```
proc toInt16(arg: Int128): int16 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L97)
```
proc toInt8(arg: Int128): int8 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L109)
```
proc toInt(arg: Int128): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L121)
```
proc toUInt64(arg: Int128): uint64 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L127) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L127)
```
proc toUInt32(arg: Int128): uint32 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L132)
```
proc toUInt16(arg: Int128): uint16 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L138)
```
proc toUInt8(arg: Int128): uint8 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L144) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L144)
```
proc toUInt(arg: Int128): uint {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L150)
```
proc castToInt64(arg: Int128): int64 {...}{.raises: [], tags: [].}
```
Conversion to int64 without range check. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L156) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L156)
```
proc castToUInt64(arg: Int128): uint64 {...}{.raises: [], tags: [].}
```
Conversion to uint64 without range check. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L160)
```
proc addToHex(result: var string; arg: Int128) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L169)
```
proc toHex(arg: Int128): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L175)
```
proc inc(a: var Int128; y: uint32 = 1) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L178) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L178)
```
proc cmp(a, b: Int128): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L189) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L189)
```
proc `<`(a, b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L199)
```
proc `<=`(a, b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L202)
```
proc `==`(a, b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L205) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L205)
```
proc bitnot(a: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L218) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L218)
```
proc bitand(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L224)
```
proc bitor(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L230) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L230)
```
proc bitxor(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L236)
```
proc `shr`(a: Int128; b: int): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L242)
```
proc `shl`(a: Int128; b: int): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L268)
```
proc `+`(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L291) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L291)
```
proc `+=`(a: var Int128; b: Int128) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L301) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L301)
```
proc `-`(a: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L304) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L304)
```
proc `-`(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L308) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L308)
```
proc `-=`(a: var Int128; b: Int128) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L311) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L311)
```
proc abs(a: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L314) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L314)
```
proc `*`(a: Int128; b: int32): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L337)
```
proc `*=`(a: var Int128; b: int32): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L342) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L342)
```
proc `*`(lhs, rhs: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L357) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L357)
```
proc `*=`(a: var Int128; b: Int128) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L379) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L379)
```
proc fastLog2(a: Int128): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L384) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L384)
```
proc divMod(dividend, divisor: Int128): tuple[quotient, remainder: Int128] {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L394) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L394)
```
proc `div`(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L444) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L444)
```
proc `mod`(a, b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L448) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L448)
```
proc addInt128(result: var string; value: Int128) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L452) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L452)
```
proc `$`(a: Int128): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L475) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L475)
```
proc parseDecimalInt128(arg: string; pos: int = 0): Int128 {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L478) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L478)
```
proc `<`(a: Int128; b: BiggestInt): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L499) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L499)
```
proc `<`(a: BiggestInt; b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L502) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L502)
```
proc `<=`(a: Int128; b: BiggestInt): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L505) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L505)
```
proc `<=`(a: BiggestInt; b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L508) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L508)
```
proc `==`(a: Int128; b: BiggestInt): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L511) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L511)
```
proc `==`(a: BiggestInt; b: Int128): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L514) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L514)
```
proc `-`(a: BiggestInt; b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L517) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L517)
```
proc `-`(a: Int128; b: BiggestInt): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L520) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L520)
```
proc `+`(a: BiggestInt; b: Int128): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L523) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L523)
```
proc `+`(a: Int128; b: BiggestInt): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L526) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L526)
```
proc toFloat64(arg: Int128): float64 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L529) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L529)
```
proc toInt128(arg: float64): Int128 {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L544) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L544)
```
proc maskUInt64(arg: Int128): Int128 {...}{.noinit, inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L559) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L559)
```
proc maskUInt32(arg: Int128): Int128 {...}{.noinit, inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L565) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L565)
```
proc maskUInt16(arg: Int128): Int128 {...}{.noinit, inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L571) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L571)
```
proc maskUInt8(arg: Int128): Int128 {...}{.noinit, inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L577) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L577)
```
proc maskBytes(arg: Int128; numbytes: int): Int128 {...}{.noinit, raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L583) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L583) Templates
---------
```
template low(t: typedesc[Int128]): Int128
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L27)
```
template high(t: typedesc[Int128]): Int128
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/int128.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/int128.nim#L28)
| programming_docs |
nim gorgeimpl gorgeimpl
=========
Module that implements `gorge` for the compiler.
Imports
-------
<msgs>, <options>, <lineinfos>, <pathutils> Procs
-----
```
proc opGorge(cmd, input, cache: string; info: TLineInfo; conf: ConfigRef): (
string, int) {...}{.raises: [OSError, IOError, ValueError, Exception, ValueError,
Exception], tags: [ReadEnvEffect, ReadIOEffect,
ExecIOEffect, RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/gorgeimpl.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/gorgeimpl.nim#L25)
nim bitsets bitsets
=======
Types
-----
```
TBitSet = seq[ElemType]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L15) Consts
------
```
ElemSize = 8
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L19) Procs
-----
```
proc bitSetIn(x: TBitSet; e: BiggestInt): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L39)
```
proc bitSetIncl(x: var TBitSet; elem: BiggestInt) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L42)
```
proc bitSetExcl(x: var TBitSet; elem: BiggestInt) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L47)
```
proc bitSetInit(b: var TBitSet; length: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L51) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L51)
```
proc bitSetUnion(x: var TBitSet; y: TBitSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L54)
```
proc bitSetDiff(x: var TBitSet; y: TBitSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L57)
```
proc bitSetSymDiff(x: var TBitSet; y: TBitSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L60)
```
proc bitSetIntersect(x: var TBitSet; y: TBitSet) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L63) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L63)
```
proc bitSetEquals(x, y: TBitSet): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L66)
```
proc bitSetContains(x, y: TBitSet): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L72)
```
proc bitSetCard(x: TBitSet): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/bitsets.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/bitsets.nim#L99)
nim extccomp extccomp
========
Imports
-------
<ropes>, <platform>, <condsyms>, <options>, <msgs>, <lineinfos>, <pathutils> Types
-----
```
TInfoCCProp = enum
hasSwitchRange, hasComputedGoto, hasCpp, hasAssume, hasGcGuard, hasGnuAsm,
hasDeclspec, hasAttribute
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L20)
```
TInfoCCProps = set[TInfoCCProp]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L29)
```
TInfoCC = tuple[name: string, objExt: string, optSpeed: string, optSize: string,
compilerExe: string, cppCompiler: string, compileTmpl: string,
buildGui: string, buildDll: string, buildLib: string,
linkerExe: string, linkTmpl: string, includeCmd: string,
linkDirCmd: string, linkLibCmd: string, debug: string,
pic: string, asmStmtFrmt: string, structStmtFmt: string,
produceAsm: string, cppXsupport: string, props: TInfoCCProps]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L30) Consts
------
```
CC: array[succ(low(TSystemCC)) .. high(TSystemCC), TInfoCC] = [("gcc", "o",
" -O3 -fno-ident", " -Os -fno-ident", "gcc", "g++",
"-c $options $include -o $objfile $file", " -mwindows", " -shared",
"ar rcs $libfile $objfiles", "",
"$buildgui $builddll -o $exefile $objfiles $options", " -I", " -L", " -l$1",
"", "-fPIC", "asm($1);$n", "$1 $3 $2 ",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel",
"-std=gnu++14 -funsigned-char", {hasSwitchRange, hasComputedGoto, hasCpp,
hasGcGuard, hasGnuAsm, hasAttribute}), (
"switch_gcc", "o", " -O3 ", " -Os ", "aarch64-none-elf-gcc",
"aarch64-none-elf-g++",
"-w -MMD -MP -MF $dfile -c $options $include -o $objfile $file",
" -mwindows", " -shared", "aarch64-none-elf-gcc-ar rcs $libfile $objfiles",
"aarch64-none-elf-gcc",
"$buildgui $builddll -Wl,-Map,$mapfile -o $exefile $objfiles $options",
" -I", " -L", " -l$1", "", "-fPIE", "asm($1);$n", "$1 $3 $2 ",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel",
"-std=gnu++14 -funsigned-char", {hasSwitchRange, hasComputedGoto, hasCpp,
hasGcGuard, hasGnuAsm, hasAttribute}), (
"llvm_gcc", "o", " -O3 -fno-ident", " -Os -fno-ident", "llvm-gcc",
"llvm-g++", "-c $options $include -o $objfile $file", " -mwindows",
" -shared", "llvm-ar rcs $libfile $objfiles", "",
"$buildgui $builddll -o $exefile $objfiles $options", " -I", " -L", " -l$1",
"", "-fPIC", "asm($1);$n", "$1 $3 $2 ",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel",
"-std=gnu++14 -funsigned-char", {hasSwitchRange, hasComputedGoto, hasCpp,
hasGcGuard, hasGnuAsm, hasAttribute}), (
"clang", "o", " -O3 -fno-ident", " -Os -fno-ident", "clang", "clang++",
"-c $options $include -o $objfile $file", " -mwindows", " -shared",
"llvm-ar rcs $libfile $objfiles", "",
"$buildgui $builddll -o $exefile $objfiles $options", " -I", " -L", " -l$1",
"", "-fPIC", "asm($1);$n", "$1 $3 $2 ",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel",
"-std=gnu++14 -funsigned-char", {hasSwitchRange, hasComputedGoto, hasCpp,
hasGcGuard, hasGnuAsm, hasAttribute}), (
"bcc", "obj", " -O3 -6 ", " -O1 -6 ", "bcc32c", "cpp32c",
"-c $options $include -o$objfile $file", " -tW", " -tWD", "", "bcc32",
"$options $buildgui $builddll -e$exefile $objfiles", " -I", "", "", "", "",
"__asm{$n$1$n}$n", "$1 $2", "", "",
{hasSwitchRange, hasComputedGoto, hasCpp, hasGcGuard, hasAttribute}), (
"vcc", "obj", " /Ogityb2 ", " /O1 ", "cl", "cl",
"/c$vccplatform $options $include /nologo /Fo$objfile $file",
" /SUBSYSTEM:WINDOWS user32.lib ", " /LD", "lib /OUT:$libfile $objfiles",
"cl",
"$builddll$vccplatform /Fe$exefile $objfiles $buildgui /nologo $options",
" /I", " /LIBPATH:", " $1.lib", " /RTC1 /Z7 ", "", "__asm{$n$1$n}$n",
"$3$n$1 $2", "/Fa$asmfile", "", {hasCpp, hasAssume, hasDeclspec}), ("tcc",
"o", "", "", "tcc", "", "-c $options $include -o $objfile $file",
"-Wl,-subsystem=gui", " -shared", "", "tcc",
"-o $exefile $options $buildgui $builddll $objfiles", " -I", "", "", " -g ",
"", "asm($1);$n", "$1 $2",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel", "",
{hasSwitchRange, hasComputedGoto, hasGnuAsm}), ("env", "o", " -O3 ",
" -O1 ", "", "", "-c $ccenvflags $options $include -o $objfile $file", "",
" -shared ", "", "cc", "-o $exefile $buildgui $builddll $objfiles $options",
" -I", "", "", "", "", "__asm{$n$1$n}$n", "$1 $2", "", "", {hasGnuAsm}), (
"icl", "obj", " /Ogityb2 ", " /O1 ", "icl", "cl",
"/c$vccplatform $options $include /nologo /Fo$objfile $file",
" /SUBSYSTEM:WINDOWS user32.lib ", " /LD", "lib /OUT:$libfile $objfiles",
"icl",
"$builddll$vccplatform /Fe$exefile $objfiles $buildgui /nologo $options",
" /I", " /LIBPATH:", " $1.lib", " /RTC1 /Z7 ", "", "__asm{$n$1$n}$n",
"$3$n$1 $2", "/Fa$asmfile", "", {hasCpp, hasAssume, hasDeclspec}), ("icc",
"o", " -O3 -fno-ident", " -Os -fno-ident", "icc", "g++",
"-c $options $include -o $objfile $file", " -mwindows", " -shared",
"ar rcs $libfile $objfiles", "icc",
"$buildgui $builddll -o $exefile $objfiles $options", " -I", " -L", " -l$1",
"", "-fPIC", "asm($1);$n", "$1 $3 $2 ",
"-Wa,-acdl=$asmfile -g -fverbose-asm -masm=intel",
"-std=gnu++14 -funsigned-char", {hasSwitchRange, hasComputedGoto, hasCpp,
hasGcGuard, hasGnuAsm, hasAttribute}), (
"clang_cl", "obj", " /Ogityb2 ", " /O1 ", "clang-cl", "clang-cl",
"/c$vccplatform $options $include /nologo /Fo$objfile $file",
" /SUBSYSTEM:WINDOWS user32.lib ", " /LD", "lib /OUT:$libfile $objfiles",
"clang-cl", "-fuse-ld=lld $builddll$vccplatform /Fe$exefile $objfiles $buildgui /nologo $options",
" /I", " /LIBPATH:", " $1.lib", " /RTC1 /Z7 ", "", "__asm{$n$1$n}$n",
"$3$n$1 $2", "/Fa$asmfile", "", {hasCpp, hasAssume, hasDeclspec})]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L269)
```
hExt = ".h"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L282) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L282) Procs
-----
```
proc nameToCC(name: string): TSystemCC {...}{.raises: [], tags: [].}
```
Returns the kind of compiler referred to by `name`, or ccNone if the name doesn't refer to any known compiler. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L284) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L284)
```
proc isVSCompatible(conf: ConfigRef): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L298)
```
proc setCC(conf: ConfigRef; ccname: string; info: TLineInfo) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L327) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L327)
```
proc addLinkOption(conf: ConfigRef; option: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L341) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L341)
```
proc addCompileOption(conf: ConfigRef; option: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L344) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L344)
```
proc addLinkOptionCmd(conf: ConfigRef; option: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L348) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L348)
```
proc addCompileOptionCmd(conf: ConfigRef; option: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L351) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L351)
```
proc initVars(conf: ConfigRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L354) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L354)
```
proc completeCfilePath(conf: ConfigRef; cfile: AbsoluteFile;
createSubDir: bool = true): AbsoluteFile {...}{.
raises: [OSError, IOError], tags: [ReadEnvEffect, ReadIOEffect,
WriteDirEffect, ReadDirEffect,
WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L363) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L363)
```
proc toObjFile(conf: ConfigRef; filename: AbsoluteFile): AbsoluteFile {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L367) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L367)
```
proc addFileToCompile(conf: ConfigRef; cf: Cfile) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L371) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L371)
```
proc addLocalCompileOption(conf: ConfigRef; option: string;
nimfile: AbsoluteFile) {...}{.
raises: [OSError, IOError, KeyError, Exception], tags: [ReadEnvEffect,
ReadIOEffect, WriteDirEffect, ReadDirEffect, WriteIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L374) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L374)
```
proc resetCompilationLists(conf: ConfigRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L381) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L381)
```
proc addExternalFileToLink(conf: ConfigRef; filename: AbsoluteFile) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L389) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L389)
```
proc execExternalProgram(conf: ConfigRef; cmd: string; msg = hintExecuting) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L396) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L396)
```
proc ccHasSaneOverflow(conf: ConfigRef): bool {...}{.raises: [],
tags: [ExecIOEffect, ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L505) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L505)
```
proc getCompileCFileCmd(conf: ConfigRef; cfile: Cfile; isMainFile = false;
produceOutput = false): string {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, OSError], tags: [
ReadEnvEffect, RootEffect, WriteIOEffect, ReadIOEffect, WriteDirEffect,
ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L538) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L538)
```
proc addExternalFileToCompile(conf: ConfigRef; c: var Cfile) {...}{.raises: [OSError,
KeyError, Exception, IOError, ValueError, ERecoverableError, EOFError], tags: [
ReadEnvEffect, ReadIOEffect, ReadDirEffect, RootEffect, WriteIOEffect,
WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L646) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L646)
```
proc addExternalFileToCompile(conf: ConfigRef; filename: AbsoluteFile) {...}{.raises: [
OSError, IOError, KeyError, Exception, ValueError, ERecoverableError,
EOFError], tags: [ReadEnvEffect, ReadIOEffect, WriteDirEffect,
ReadDirEffect, WriteIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L656) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L656)
```
proc callCCompiler(conf: ConfigRef) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError, OSError], tags: [ReadEnvEffect, RootEffect,
WriteIOEffect, ReadIOEffect,
WriteDirEffect, ReadDirEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L839) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L839)
```
proc writeJsonBuildInstructions(conf: ConfigRef) {...}{.
raises: [OSError, IOError, Exception, ValueError, ERecoverableError], tags: [
ReadEnvEffect, ReadIOEffect, ReadDirEffect, WriteIOEffect, RootEffect,
WriteDirEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L937) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L937)
```
proc changeDetectedViaJsonBuildInstructions(conf: ConfigRef;
projectfile: AbsoluteFile): bool {...}{.raises: [OSError, Exception], tags: [
ReadEnvEffect, ReadIOEffect, ReadDirEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L1035) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L1035)
```
proc runJsonBuildInstructions(conf: ConfigRef; projectfile: AbsoluteFile) {...}{.
raises: [OSError], tags: [ReadEnvEffect, ReadIOEffect, WriteIOEffect,
WriteDirEffect, ReadDirEffect, RootEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L1071) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L1071)
```
proc writeMapping(conf: ConfigRef; symbolMapping: Rope) {...}{.
raises: [ValueError, OSError, IOError, Exception, ERecoverableError],
tags: [WriteIOEffect, RootEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/extccomp.nim#L1122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/extccomp.nim#L1122)
nim procfind procfind
========
Imports
-------
<ast>, <astalgo>, <msgs>, <semdata>, <types>, <trees>, <lookups> Procs
-----
```
proc searchForProc(c: PContext; scope: PScope; fn: PSym): tuple[proto: PSym,
comesFromShadowScope: bool] {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/procfind.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/procfind.nim#L53)
nim condsyms condsyms
========
Imports
-------
<options>, <lineinfos> Procs
-----
```
proc defineSymbol(symbols: StringTableRef; symbol: string;
value: string = "true") {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/condsyms.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/condsyms.nim#L18)
```
proc undefSymbol(symbols: StringTableRef; symbol: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/condsyms.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/condsyms.nim#L21)
```
proc countDefinedSymbols(symbols: StringTableRef): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/condsyms.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/condsyms.nim#L31)
```
proc initDefines(symbols: StringTableRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/condsyms.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/condsyms.nim#L34) Iterators
---------
```
iterator definedSymbolNames(symbols: StringTableRef): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/condsyms.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/condsyms.nim#L27)
| programming_docs |
nim filters filters
=======
Imports
-------
<llstream>, <idents>, <ast>, <msgs>, <options>, <renderer>, <pathutils> Procs
-----
```
proc charArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: char): char {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filters.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filters.nim#L31)
```
proc strArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: string): string {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filters.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filters.nim#L37)
```
proc boolArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: bool): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filters.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filters.nim#L43)
```
proc filterStrip(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile;
call: PNode): PLLStream {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filters.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filters.nim#L50)
```
proc filterReplace(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile;
call: PNode): PLLStream {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filters.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filters.nim#L64)
nim astalgo astalgo
=======
Imports
-------
<ast>, <options>, <lineinfos>, <ropes>, <idents>, <rodutils>, <msgs> Types
-----
```
TIIPair {...}{.final.} = object
key*, val*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L98) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L98)
```
TIIPairSeq = seq[TIIPair]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L101)
```
TIITable {...}{.final.} = object
counter*: int
data*: TIIPairSeq
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L102)
```
TIdentIter = object
h*: Hash
name*: PIdent
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L806) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L806)
```
TTabIter = object
h: Hash
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L853) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L853) Consts
------
```
InvalidKey = -9223372036854775808
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L95) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L95) Procs
-----
```
proc typekinds(t: PType) {...}{.deprecated, raises: [], tags: [].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L34)
```
proc skipConvCastAndClosure(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L113) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L113)
```
proc sameValue(a, b: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L124)
```
proc leValue(a, b: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L138)
```
proc weakLeValue(a, b: PNode): TImplication {...}{.raises: [ERecoverableError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L153)
```
proc lookupInRecord(n: PNode; field: PIdent): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L159)
```
proc getModule(s: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L180)
```
proc fromSystem(op: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L185) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L185)
```
proc getSymFromList(list: PNode; ident: PIdent; start: int = 0): PSym {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L186)
```
proc getNamedParamFromList(list: PNode; ident: PIdent): PSym {...}{.raises: [],
tags: [].}
```
Named parameters are special because a named parameter can be gensym'ed and then they have '`<number>' suffix that we need to ignore, see compiler / evaltempl.nim, snippet:
result.add newIdentNode(getIdent(c.ic, x.name.s & "`gensym" & $x.id), if c.instLines: actual.info else: templ.info) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L220) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L220)
```
proc hashNode(p: RootRef): Hash {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L234) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L234)
```
proc mustRehash(length, counter: int): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L237) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L237)
```
proc makeYamlString(s: string): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L251) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L251)
```
proc lineInfoToStr(conf: ConfigRef; info: TLineInfo): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L278) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L278)
```
proc treeToYaml(conf: ConfigRef; n: PNode; indent: int = 0;
maxRecDepth: int = -1): Rope {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L391) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L391)
```
proc typeToYaml(conf: ConfigRef; n: PType; indent: int = 0;
maxRecDepth: int = -1): Rope {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L395) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L395)
```
proc symToYaml(conf: ConfigRef; n: PSym; indent: int = 0; maxRecDepth: int = -1): Rope {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L399) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L399)
```
proc debug(n: PSym; conf: ConfigRef = nil) {...}{.exportc: "debugSym", deprecated,
raises: [Exception], tags: [RootEffect].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L639) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L639)
```
proc debug(n: PType; conf: ConfigRef = nil) {...}{.exportc: "debugType", deprecated,
raises: [Exception], tags: [RootEffect].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L647) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L647)
```
proc debug(n: PNode; conf: ConfigRef = nil) {...}{.exportc: "debugNode", deprecated,
raises: [Exception], tags: [RootEffect].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L655) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L655)
```
proc nextTry(h, maxHash: Hash): Hash {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L663) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L663)
```
proc objectSetContains(t: TObjectSet; obj: RootRef): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L669) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L669)
```
proc objectSetIncl(t: var TObjectSet; obj: RootRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L693) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L693)
```
proc objectSetContainsOrIncl(t: var TObjectSet; obj: RootRef): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L698) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L698)
```
proc strTableContains(t: TStrTable; n: PSym): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L716) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L716)
```
proc symTabReplace(t: var TStrTable; prevSym: PSym; newSym: PSym) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L745) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L745)
```
proc strTableAdd(t: var TStrTable; n: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L755) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L755)
```
proc strTableInclReportConflict(t: var TStrTable; n: PSym;
onConflictKeepOld = false): PSym {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L760) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L760)
```
proc strTableIncl(t: var TStrTable; n: PSym; onConflictKeepOld = false): bool {...}{.
discardable, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L792) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L792)
```
proc strTableGet(t: TStrTable; name: PIdent): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L796) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L796)
```
proc nextIdentIter(ti: var TIdentIter; tab: TStrTable): PSym {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L810) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L810)
```
proc initIdentIter(ti: var TIdentIter; tab: TStrTable; s: PIdent): PSym {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L823) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L823)
```
proc nextIdentExcluding(ti: var TIdentIter; tab: TStrTable; excluding: IntSet): PSym {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L829) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L829)
```
proc firstIdentExcluding(ti: var TIdentIter; tab: TStrTable; s: PIdent;
excluding: IntSet): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L845) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L845)
```
proc nextIter(ti: var TTabIter; tab: TStrTable): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L856) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L856)
```
proc initTabIter(ti: var TTabIter; tab: TStrTable): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L872) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L872)
```
proc idTableHasObjectAsKey(t: TIdTable; key: PIdObj): bool {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L901) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L901)
```
proc idTableGet(t: TIdTable; key: PIdObj): RootRef {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L906) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L906)
```
proc idTableGet(t: TIdTable; key: int): RootRef {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L911) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L911)
```
proc idTablePut(t: var TIdTable; key: PIdObj; val: RootRef) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L931) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L931)
```
proc idNodeTableGet(t: TIdNodeTable; key: PIdObj): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L963) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L963)
```
proc idNodeTablePut(t: var TIdNodeTable; key: PIdObj; val: PNode) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L979) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L979)
```
proc initIiTable(x: var TIITable) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L999) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L999)
```
proc iiTableGet(t: TIITable; key: int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L1012) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L1012)
```
proc iiTablePut(t: var TIITable; key, val: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L1027) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L1027)
```
proc isAddrNode(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L1044) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L1044)
```
proc listSymbolNames(symbols: openArray[PSym]): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L1052) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L1052)
```
proc isDiscriminantField(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L1058) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L1058) Iterators
---------
```
iterator items(tab: TStrTable): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L879) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L879)
```
iterator pairs(t: TIdTable): tuple[key: int, value: RootRef] {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L916) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L916)
```
iterator idTablePairs(t: TIdTable): tuple[key: PIdObj, val: RootRef] {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L950) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L950)
```
iterator pairs(t: TIdNodeTable): tuple[key: PIdObj, val: PNode] {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L995) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L995) Templates
---------
```
template debug(x: PSym | PType | PNode) {...}{.deprecated.}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L43)
```
template debug(x: auto) {...}{.deprecated.}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L51) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L51)
```
template mdbg(): bool {...}{.deprecated.}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/astalgo.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/astalgo.nim#L54)
nim lowerings lowerings
=========
This module implements common simple lowerings.
Imports
-------
<ast>, <astalgo>, <types>, <idents>, <magicsys>, <msgs>, <options>, <modulegraphs>, <lineinfos> Consts
------
```
genPrefix = ":tmp"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L13) Procs
-----
```
proc newDeref(n: PNode): PNode {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L18)
```
proc newTupleAccess(g: ModuleGraph; tup: PNode; i: int): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L22)
```
proc addVar(father, v: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L38)
```
proc addVar(father, v, value: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L45)
```
proc newAsgnStmt(le, ri: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L52)
```
proc newFastAsgnStmt(le, ri: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L57)
```
proc newFastMoveStmt(g: ModuleGraph; le, ri: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L62)
```
proc lowerTupleUnpacking(g: ModuleGraph; n: PNode; owner: PSym): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L69)
```
proc evalOnce(g: ModuleGraph; value: PNode; owner: PSym): PNode {...}{.raises: [],
tags: [].}
```
Turns (value) into (let tmp = value; tmp) so that 'value' can be re-used freely, multiple times. This is frequently required and such a builtin would also be handy to have in macros.nim. The value that can be reused is 'result.lastSon'! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L88) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L88)
```
proc newTupleAccessRaw(tup: PNode; i: int): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L104)
```
proc newTryFinally(body, final: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L111)
```
proc lowerTupleUnpackingForAsgn(g: ModuleGraph; n: PNode; owner: PSym): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L114)
```
proc lowerSwap(g: ModuleGraph; n: PNode; owner: PSym): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L133)
```
proc createObj(g: ModuleGraph; owner: PSym; info: TLineInfo; final = true): PType {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L154)
```
proc rawAddField(obj: PType; field: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L174) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L174)
```
proc rawIndirectAccess(a: PNode; field: PSym; info: TLineInfo): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L181)
```
proc rawDirectAccess(obj, field: PSym): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L192)
```
proc addField(obj: PType; s: PSym; cache: IdentCache) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L221) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L221)
```
proc addUniqueField(obj: PType; s: PSym; cache: IdentCache): PSym {...}{.discardable,
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L236)
```
proc newDotExpr(obj, b: PSym): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L250) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L250)
```
proc indirectAccess(a: PNode; b: int; info: TLineInfo): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L258) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L258)
```
proc indirectAccess(a: PNode; b: string; info: TLineInfo; cache: IdentCache): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L281) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L281)
```
proc getFieldFromObj(t: PType; v: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L305) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L305)
```
proc indirectAccess(a: PNode; b: PSym; info: TLineInfo): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L316) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L316)
```
proc indirectAccess(a, b: PSym; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L320) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L320)
```
proc genAddrOf(n: PNode; typeKind = tyPtr): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L323) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L323)
```
proc genDeref(n: PNode; k = nkHiddenDeref): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L329) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L329)
```
proc callCodegenProc(g: ModuleGraph; name: string;
info: TLineInfo = unknownLineInfo;
arg1, arg2, arg3, optionalArgs: PNode = nil): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L334) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L334)
```
proc newIntLit(g: ModuleGraph; info: TLineInfo; value: BiggestInt): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L351) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L351)
```
proc genHigh(g: ModuleGraph; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L355) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L355)
```
proc genLen(g: ModuleGraph; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lowerings.nim#L364) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lowerings.nim#L364)
| programming_docs |
nim passaux passaux
=======
implements some little helper passes
Imports
-------
<ast>, <passes>, <idents>, <msgs>, <options>, <idgen>, <lineinfos>, <modulegraphs> Consts
------
```
verbosePass = (verboseOpen, verboseProcess, nil, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/passaux.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/passaux.nim#L35)
nim ccgmerge ccgmerge
========
This module implements the merge operation of 2 different C files. This is needed for incremental compilation.
Imports
-------
<ast>, <ropes>, <options>, <nimlexbase>, <cgendata>, <rodutils>, <llstream>, <modulegraphs>, <pathutils> Procs
-----
```
proc genSectionStart(fs: TCFileSection; conf: ConfigRef): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L50)
```
proc genSectionEnd(fs: TCFileSection; conf: ConfigRef): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L61)
```
proc genSectionStart(ps: TCProcSection; conf: ConfigRef): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L65)
```
proc genSectionEnd(ps: TCProcSection; conf: ConfigRef): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L72)
```
proc genMergeInfo(m: BModule): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L102) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L102)
```
proc readMergeInfo(cfilename: AbsoluteFile; m: BModule) {...}{.
raises: [IOError, Exception], tags: [ReadIOEffect, RootEffect].}
```
reads the merge meta information into `m`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L243) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L243)
```
proc mergeRequired(m: BModule): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L280)
```
proc mergeFiles(cfilename: AbsoluteFile; m: BModule) {...}{.
raises: [IOError, Exception], tags: [ReadIOEffect, RootEffect].}
```
merges the C file with the old version on hard disc. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgmerge.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgmerge.nim#L290)
nim filter_tmpl filter\_tmpl
============
Imports
-------
<llstream>, <ast>, <msgs>, <options>, <filters>, <lineinfos>, <pathutils> Procs
-----
```
proc filterTmpl(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile;
call: PNode): PLLStream {...}{.
raises: [KeyError, Exception, ValueError, IOError, ERecoverableError], tags: [
ReadDirEffect, RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/filter_tmpl.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/filter_tmpl.nim#L202)
nim trees trees
=====
Imports
-------
<ast>, <wordrecg>, <idents> Procs
-----
```
proc cyclicTree(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L25)
```
proc exprStructuralEquivalent(a, b: PNode; strictSymEquality = false): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L33)
```
proc sameTree(a, b: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L57)
```
proc getMagic(op: PNode): TMagic {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L80) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L80)
```
proc isConstExpr(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L89)
```
proc isCaseObj(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L93) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L93)
```
proc isDeepConstExpr(n: PNode; preventInheritance = false): bool {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L98) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L98)
```
proc isRange(n: PNode): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L122)
```
proc whichPragma(n: PNode): TSpecialWord {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L131)
```
proc isNoSideEffectPragma(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L141)
```
proc findPragma(n: PNode; which: TSpecialWord): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L147)
```
proc effectSpec(n: PNode; effectType: TSpecialWord): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L153) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L153)
```
proc propSpec(n: PNode; effectType: TSpecialWord): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L163)
```
proc flattenStmts(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L175) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L175)
```
proc extractRange(k: TNodeKind; n: PNode; a, b: int): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L181)
```
proc isTrue(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L185) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L185)
```
proc getRoot(n: PNode): PSym {...}{.raises: [], tags: [].}
```
`getRoot` takes a *path* `n`. A path is an lvalue expression like `obj.x[i].y`. The *root* of a path is the symbol that can be determined as the owner; `obj` in the example. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L189) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L189)
```
proc stupidStmtListExpr(n: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L206)
```
proc dontInlineConstant(orig, cnst: PNode): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/trees.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/trees.nim#L211)
nim saturate saturate
========
Saturated arithmetic routines. XXX Make part of the stdlib?
Procs
-----
```
proc `|+|`(a, b: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
saturated addition. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L12) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L12)
```
proc `|-|`(a, b: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L22)
```
proc `|abs|`(a: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L31)
```
proc `|div|`(a, b: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L38)
```
proc `|mod|`(a, b: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L48)
```
proc `|*|`(a, b: BiggestInt): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/saturate.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/saturate.nim#L54)
nim semtypinst semtypinst
==========
Imports
-------
<ast>, <astalgo>, <msgs>, <types>, <magicsys>, <semdata>, <renderer>, <options>, <lineinfos> Types
-----
```
LayeredIdTable {...}{.acyclic.} = ref object
topLayer*: TIdTable
nextLayer*: LayeredIdTable
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L70)
```
TReplTypeVars = object
c*: PContext
typeMap*: LayeredIdTable
symMap*: TIdTable
localCache*: TIdTable
info*: TLineInfo
allowMetaTypes*: bool
skipTypedesc*: bool
isReturnType*: bool
owner*: PSym
recursionLimit: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L74) Procs
-----
```
proc checkConstructedType(conf: ConfigRef; info: TLineInfo; typ: PType) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L21)
```
proc searchInstTypes(key: PType): PType {...}{.
raises: [Exception, ERecoverableError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L33)
```
proc cacheTypeInst(inst: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L60) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L60)
```
proc initLayeredTypeMap(pt: TIdTable): LayeredIdTable {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L93) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L93)
```
proc newTypeMapLayer(cl: var TReplTypeVars): LayeredIdTable {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L97)
```
proc replaceTypeVarsT(cl: var TReplTypeVars; t: PType): PType {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L120)
```
proc replaceTypeVarsN(cl: var TReplTypeVars; n: PNode; start = 0): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L196) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L196)
```
proc instCopyType(cl: var TReplTypeVars; t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L303) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L303)
```
proc eraseVoidParams(t: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L443) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L443)
```
proc skipIntLiteralParams(t: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L462) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L462)
```
proc instAllTypeBoundOp(c: PContext; info: TLineInfo) {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L647) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L647)
```
proc initTypeVars(p: PContext; typeMap: LayeredIdTable; info: TLineInfo;
owner: PSym): TReplTypeVars {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L657) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L657)
```
proc replaceTypesInBody(p: PContext; pt: TIdTable; n: PNode; owner: PSym;
allowMetaTypes = false): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L666) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L666)
```
proc recomputeFieldPositions(t: PType; obj: PNode; currPosition: var int) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L686) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L686)
```
proc generateTypeInstance(p: PContext; pt: TIdTable; info: TLineInfo; t: PType): PType {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L702) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L702)
```
proc prepareMetatypeForSigmatch(p: PContext; pt: TIdTable; info: TLineInfo;
t: PType): PType {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L718) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L718) Templates
---------
```
template generateTypeInstance(p: PContext; pt: TIdTable; arg: PNode; t: PType): untyped
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semtypinst.nim#L727) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semtypinst.nim#L727)
nim scriptconfig scriptconfig
============
Implements the new configuration system for Nim. Uses Nim as a scripting language.
Imports
-------
<ast>, <modules>, <idents>, <passes>, <condsyms>, <options>, <sem>, <llstream>, <vm>, <vmdef>, <commands>, <wordrecg>, <modulegraphs>, <pathutils> Procs
-----
```
proc setupVM(module: PSym; cache: IdentCache; scriptName: string;
graph: ModuleGraph): PEvalContext {...}{.raises: [OSError, IOError,
EOFError, ValueError, Exception, ERecoverableError, KeyError], tags: [
ReadEnvEffect, WriteEnvEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect,
ExecIOEffect, RootEffect, TimeEffect, WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/scriptconfig.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/scriptconfig.nim#L29)
```
proc runNimScript(cache: IdentCache; scriptName: AbsoluteFile;
freshDefines = true; conf: ConfigRef) {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, KeyError, OSError, EOFError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect,
WriteEnvEffect, ExecIOEffect, TimeEffect, WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/scriptconfig.nim#L200) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/scriptconfig.nim#L200)
nim cgendata cgendata
========
This module contains the data structures for the C code generation phase.
Imports
-------
<ast>, <ropes>, <options>, <ndi>, <lineinfos>, <pathutils>, <modulegraphs> Types
-----
```
TLabel = Rope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L17)
```
TCFileSection = enum
cfsMergeInfo, cfsHeaders, cfsFrameDefines, cfsForwardTypes, cfsTypes,
cfsSeqTypes, cfsFieldInfo, cfsTypeInfo, cfsProcHeaders, cfsData, cfsVars,
cfsProcs, cfsInitProc, cfsDatInitProc, cfsTypeInit1, cfsTypeInit2,
cfsTypeInit3, cfsDebugInit, cfsDynLibInit, cfsDynLibDeinit
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L18)
```
TCTypeKind = enum
ctVoid, ctChar, ctBool, ctInt, ctInt8, ctInt16, ctInt32, ctInt64, ctFloat,
ctFloat32, ctFloat64, ctFloat128, ctUInt, ctUInt8, ctUInt16, ctUInt32,
ctUInt64, ctArray, ctPtrToArray, ctStruct, ctPtr, ctNimStr, ctNimSeq, ctProc,
ctCString
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L42)
```
TCFileSections = array[TCFileSection, Rope]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L49)
```
TCProcSection = enum
cpsLocals, cpsInit, cpsStmts
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L50)
```
TCProcSections = array[TCProcSection, Rope]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L54)
```
BModule = ref TCGen
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L55) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L55)
```
BProc = ref TCProc
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L56) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L56)
```
TBlock = object
id*: int
label*: Rope
sections*: TCProcSections
isLoop*: bool
nestedTryStmts*: int16
nestedExceptStmts*: int16
frameLen*: int16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L57)
```
TCProcFlag = enum
beforeRetNeeded, threadVarAccessed, hasCurFramePointer, noSafePoints,
nimErrorFlagAccessed, nimErrorFlagDeclared, nimErrorFlagDisabled
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L67)
```
TTypeSeq = seq[PType]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L103)
```
TypeCache = Table[SigHash, Rope]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L104)
```
TypeCacheWithOwner = Table[SigHash, tuple[str: Rope, owner: PSym]]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L105) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L105)
```
CodegenFlag = enum
preventStackTrace, usesThreadVars, frameDeclared, isHeaderFile,
includesStringh, objHasKidsValid
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L107)
```
BModuleList = ref object of RootObj
mainModProcs*, mainModInit*, otherModsInit*, mainDatInit*: Rope
mapping*: Rope
modules*: seq[BModule]
modulesClosed*: seq[BModule]
forwardedProcs*: seq[PSym]
generatedHeader*: BModule
typeInfoMarker*: TypeCacheWithOwner
typeInfoMarkerV2*: TypeCacheWithOwner
config*: ConfigRef
graph*: ModuleGraph
strVersion*, seqVersion*: int
nimtv*: Rope
nimtvDeps*: seq[PType]
nimtvDeclared*: IntSet
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L117) Procs
-----
```
proc includeHeader(this: BModule; header: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L179) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L179)
```
proc s(p: BProc; s: TCProcSection): var Rope {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L183) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L183)
```
proc procSec(p: BProc; s: TCProcSection): var Rope {...}{.inline, raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L187) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L187)
```
proc newProc(prc: PSym; module: BModule): BProc {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L191)
```
proc newModuleList(g: ModuleGraph): BModuleList {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L202) Iterators
---------
```
iterator cgenModules(g: BModuleList): BModule {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L206) Templates
---------
```
template config(m: BModule): ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L176)
```
template config(p: BProc): ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgendata.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgendata.nim#L177)
| programming_docs |
nim lambdalifting lambdalifting
=============
Imports
-------
<options>, <ast>, <astalgo>, <msgs>, <idents>, <renderer>, <types>, <magicsys>, <lowerings>, <modulegraphs>, <lineinfos>, <transf>, <liftdestructors> Consts
------
```
upName = ":up"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L120)
```
paramName = ":envP"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L121)
```
envName = ":env"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L122) Procs
-----
```
proc createClosureIterStateType(g: ModuleGraph; iter: PSym): PType {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L129)
```
proc getClosureIterResult(g: ModuleGraph; iter: PSym): PSym {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L149)
```
proc getEnvParam(routine: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L181)
```
proc makeClosure(g: ModuleGraph; prc: PSym; env: PNode; info: TLineInfo): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L212)
```
proc liftIterSym(g: ModuleGraph; n: PNode; owner: PSym): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L247)
```
proc freshVarForClosureIter(g: ModuleGraph; s, owner: PSym): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L274) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L274)
```
proc getStateField(g: ModuleGraph; owner: PSym): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L688) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L688)
```
proc semCaptureSym(s, owner: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L802) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L802)
```
proc liftIterToProc(g: ModuleGraph; fn: PSym; body: PNode; ptrType: PType): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L839) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L839)
```
proc liftLambdas(g: ModuleGraph; fn: PSym; body: PNode; tooEarly: var bool): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L853) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L853)
```
proc liftLambdasForTopLevel(module: PSym; body: PNode): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L886) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L886)
```
proc liftForLoop(g: ModuleGraph; body: PNode; owner: PSym): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L892) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L892) Templates
---------
```
template isIterator(owner: PSym): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lambdalifting.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lambdalifting.nim#L232)
nim sighashes sighashes
=========
Computes hash values for routine (proc, method etc) signatures.
Imports
-------
<ast>, <ropes>, <modulegraphs>, <types> Types
-----
```
ConsiderFlag = enum
CoProc, CoType, CoOwnerSig, CoIgnoreRange, CoConsiderOwned, CoDistinct,
CoHashTypeInsideNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L31) Procs
-----
```
proc hashType(t: PType; flags: set[ConsiderFlag] = {CoType}): SigHash {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L245) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L245)
```
proc hashProc(s: PSym): SigHash {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L254) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L254)
```
proc hashNonProc(s: PSym): SigHash {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L274) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L274)
```
proc hashOwner(s: PSym): SigHash {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L290)
```
proc sigHash(s: PSym): SigHash {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L303) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L303)
```
proc symBodyDigest(graph: ModuleGraph; sym: PSym): SigHash {...}{.
raises: [Exception], tags: [RootEffect].}
```
compute unique digest of the proc/func/method symbols recursing into invoked symbols as well [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L356) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L356)
```
proc idOrSig(s: PSym; currentModule: string;
sigCollisions: var CountTable[SigHash]): Rope {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sighashes.nim#L378) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sighashes.nim#L378)
nim nimblecmd nimblecmd
=========
Implements some helper procs for Nimble (Nim's package manager) support.
Imports
-------
<options>, <msgs>, <lineinfos>, <pathutils> Types
-----
```
Version = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L20) Procs
-----
```
proc addPath(conf: ConfigRef; path: AbsoluteDir; info: TLineInfo) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L15)
```
proc `$`(ver: Version): string {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L22)
```
proc newVersion(ver: string): Version {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L24)
```
proc `<`(ver: Version; ver2: Version): bool {...}{.raises: [ValueError], tags: [].}
```
This is synced from Nimble's version module. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L36)
```
proc getPathVersion(p: string): tuple[name, version: string] {...}{.raises: [],
tags: [].}
```
Splits path `p` in the format `/home/user/.nimble/pkgs/package-0.1` into `(/home/user/.nimble/pkgs/package, 0.1)` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L65) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L65)
```
proc nimblePath(conf: ConfigRef; path: AbsoluteDir; info: TLineInfo) {...}{.
raises: [OSError, ValueError, Exception, IOError, ERecoverableError], tags: [
ReadDirEffect, RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimblecmd.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimblecmd.nim#L130)
nim btrees btrees
======
BTree implementation with few features, but good enough for the Nim compiler's needs.
Types
-----
```
BTree[Key; Val] = object
root: Node[Key, Val]
entries: int ## number of key-value pairs
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L27) Procs
-----
```
proc initBTree[Key, Val](): BTree[Key, Val]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L31)
```
proc getOrDefault[Key, Val](b: BTree[Key, Val]; key: Key): Val
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L37)
```
proc contains[Key, Val](b: BTree[Key, Val]; key: Key): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L48)
```
proc add[Key, Val](b: var BTree[Key, Val]; key: Key; val: Val)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L111)
```
proc hasNext[Key, Val](b: BTree[Key, Val]; index: int): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L138)
```
proc next[Key, Val](b: BTree[Key, Val]; index: int): (Key, Val, int)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L148) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L148)
```
proc len[Key, Val](b: BTree[Key, Val]): int {...}{.inline.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L170) Iterators
---------
```
iterator pairs[Key, Val](b: BTree[Key, Val]): (Key, Val)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/btrees.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/btrees.nim#L163)
nim closureiters closureiters
============
Imports
-------
<ast>, <msgs>, <idents>, <renderer>, <magicsys>, <lowerings>, <lambdalifting>, <modulegraphs>, <lineinfos>, <options> Procs
-----
```
proc transformClosureIterator(g: ModuleGraph; fn: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/closureiters.nim#L1382) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/closureiters.nim#L1382)
nim depends depends
=======
Imports
-------
<options>, <ast>, <ropes>, <idents>, <passes>, <modulepaths>, <pathutils>, <modulegraphs> Consts
------
```
gendependPass = (myOpen, addDotDependency, nil, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/depends.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/depends.nim#L67) Procs
-----
```
proc generateDot(graph: ModuleGraph; project: AbsoluteFile) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/depends.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/depends.nim#L48)
nim lookups lookups
=======
Imports
-------
<ast>, <astalgo>, <idents>, <semdata>, <types>, <msgs>, <options>, <renderer>, <nimfix/prettybase>, <lineinfos> Types
-----
```
TOverloadIterMode = enum
oimDone, oimNoQualifier, oimSelfModule, oimOtherModule, oimSymChoice,
oimSymChoiceLocalLookup
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L146) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L146)
```
TOverloadIter = object
it*: TIdentIter
m*: PSym
mode*: TOverloadIterMode
symChoiceIndex*: int
scope*: PScope
inSymChoice: IntSet
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L149)
```
TLookupFlag = enum
checkAmbiguity, checkUndeclared, checkModule, checkPureEnumFields
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L325) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L325) Procs
-----
```
proc considerQuotedIdent(c: PContext; n: PNode; origin: PNode = nil): PIdent {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
Retrieve a PIdent from a PNode, taking into account accent nodes. `origin` can be nil. If it is not nil, it is used for a better error message. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L25)
```
proc addUniqueSym(scope: PScope; s: PSym): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L61)
```
proc openScope(c: PContext): PScope {...}{.discardable, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L64)
```
proc rawCloseScope(c: PContext) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L70) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L70)
```
proc closeScope(c: PContext) {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L73) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L73)
```
proc skipAlias(s: PSym; n: PNode; conf: ConfigRef): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L83)
```
proc isShadowScope(s: PScope): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L94)
```
proc localSearchInScope(c: PContext; s: PIdent): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L96)
```
proc searchInScopes(c: PContext; s: PIdent): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L104) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L104)
```
proc debugScopes(c: PContext; limit = 0) {...}{.deprecated, raises: [], tags: [].}
```
**Deprecated** [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L110)
```
proc searchInScopes(c: PContext; s: PIdent; filter: TSymKinds): PSym {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L120)
```
proc errorSym(c: PContext; n: PNode): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
creates an error symbol to avoid cascading errors (for IDE support) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L129)
```
proc getSymRepr(conf: ConfigRef; s: PSym): string {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L157) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L157)
```
proc wrongRedefinition(c: PContext; info: TLineInfo; s: string;
conflictsWith: TLineInfo) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L186)
```
proc addDecl(c: PContext; sym: PSym; info: TLineInfo) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L193) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L193)
```
proc addDecl(c: PContext; sym: PSym) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L198) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L198)
```
proc addPrelimDecl(c: PContext; sym: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L203) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L203)
```
proc addDeclAt(c: PContext; scope: PScope; sym: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L206)
```
proc addInterfaceDeclAt(c: PContext; scope: PScope; sym: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L217) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L217)
```
proc addOverloadableSymAt(c: PContext; scope: PScope; fn: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L221) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L221)
```
proc addInterfaceDecl(c: PContext; sym: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L231) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L231)
```
proc addInterfaceOverloadableSymAt(c: PContext; scope: PScope; sym: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L236)
```
proc openShadowScope(c: PContext) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L241) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L241)
```
proc closeShadowScope(c: PContext) {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L246) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L246)
```
proc mergeShadowScope(c: PContext) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L249) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L249)
```
proc errorUseQualifier(c: PContext; info: TLineInfo; s: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L275) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L275)
```
proc errorUndeclaredIdentifier(c: PContext; info: TLineInfo; name: string) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L289) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L289)
```
proc lookUp(c: PContext; n: PNode): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L298)
```
proc qualifiedLookUp(c: PContext; n: PNode; flags: set[TLookupFlag]): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L328) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L328)
```
proc initOverloadIter(o: var TOverloadIter; c: PContext; n: PNode): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L379) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L379)
```
proc lastOverloadScope(o: TOverloadIter): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L429) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L429)
```
proc nextOverloadIter(o: var TOverloadIter; c: PContext; n: PNode): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L436) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L436)
```
proc pickSym(c: PContext; n: PNode; kinds: set[TSymKind]; flags: TSymFlags = {}): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L481) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L481) Iterators
---------
```
iterator walkScopes(scope: PScope): PScope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L77) Templates
---------
```
template addSym(scope: PScope; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lookups.nim#L58) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lookups.nim#L58)
| programming_docs |
nim cgen cgen
====
This module implements the C code generator.This include file contains the logic to produce constant string and seq literals. The code here is responsible that `const x = ["a", "b"]` works without hidden runtime creation code. The price is that seqs and strings are not purely a library implementation.Generates traversal procs for the C backend.Code specialization instead of the old, incredibly slow 'genericReset' implementation.Thread var support for crappy architectures that lack native support for thread local storage. (**Thank you Mac OS X!**)
Imports
-------
<ast>, <astalgo>, <trees>, <platform>, <magicsys>, <extccomp>, <options>, <nversion>, <nimsets>, <msgs>, <bitsets>, <idents>, <types>, <ccgutils>, <ropes>, <passes>, <wordrecg>, <treetab>, <cgmeth>, <rodutils>, <renderer>, <cgendata>, <ccgmerge>, <aliases>, <lowerings>, <ndi>, <lineinfos>, <pathutils>, <transf>, <enumtostr>, <injectdestructors>, <spawn>, <semparallel>, <modulegraphs>, <lineinfos>, <sighashes>, <modulegraphs>, <lowerings>, <dfa>, <parampatterns> Consts
------
```
cgenPass = (myOpen, myProcess, myClose, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgen.nim#L2128) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgen.nim#L2128) Procs
-----
```
proc fillObjectFields(m: BModule; typ: PType) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgtypes.nim#L588) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgtypes.nim#L588)
```
proc cgenWriteModules(backend: RootRef; config: ConfigRef) {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect,
WriteDirEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/cgen.nim#L2114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/cgen.nim#L2114) Templates
---------
```
template cgDeclFrmt(s: PSym): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ccgtypes.nim#L972) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ccgtypes.nim#L972)
nim rodutils rodutils
========
Serialization utilities for the compiler.
Procs
-----
```
proc toStrMaxPrecision(f: BiggestFloat; literalPostfix = ""): string {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L36)
```
proc encodeStr(s: string; result: var string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L53)
```
proc decodeStr(s: cstring; pos: var int): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L66)
```
proc encodeVBiggestInt(x: BiggestInt; result: var string) {...}{.raises: [], tags: [].}
```
encode a biggest int as a variable length base 190 int. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L112)
```
proc encodeVInt(x: int; result: var string) {...}{.raises: [], tags: [].}
```
encode an int as a variable length base 190 int. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L121)
```
proc decodeVInt(s: cstring; pos: var int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L144) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L144)
```
proc decodeVBiggestInt(s: cstring; pos: var int): BiggestInt {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L147) Iterators
---------
```
iterator decodeVIntArray(s: cstring): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L152)
```
iterator decodeStrArray(s: cstring): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/rodutils.nim#L158) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/rodutils.nim#L158)
nim commands commands
========
Imports
-------
<msgs>, <options>, <nversion>, <condsyms>, <extccomp>, <platform>, <wordrecg>, <nimblecmd>, <lineinfos>, <pathutils>, <incremental>, <ast> Types
-----
```
TCmdLinePass = enum
passCmd1, passCmd2, passPP
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L45) Procs
-----
```
proc writeCommandLineUsage(conf: ConfigRef) {...}{.
raises: [Exception, IOError, ValueError], tags: [RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L110)
```
proc processSpecificNote(arg: string; state: TSpecialWord; pass: TCmdLinePass;
info: TLineInfo; orig: string; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L176)
```
proc testCompileOptionArg(conf: ConfigRef; switch, arg: string; info: TLineInfo): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L237) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L237)
```
proc testCompileOption(conf: ConfigRef; switch: string; info: TLineInfo): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L280) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L280)
```
proc handleStdinInput(conf: ConfigRef) {...}{.raises: [OSError],
tags: [ReadEnvEffect, ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L386) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L386)
```
proc processSwitch(switch, arg: string; pass: TCmdLinePass; info: TLineInfo;
conf: ConfigRef) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError, OSError, KeyError, EOFError, Exception, ValueError,
IOError, ERecoverableError, Exception, ValueError, IOError,
ERecoverableError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, ReadDirEffect, WriteDirEffect,
WriteEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L407) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L407)
```
proc processCommand(switch: string; pass: TCmdLinePass; config: ConfigRef) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, OSError, KeyError,
EOFError], tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ReadDirEffect, WriteDirEffect, WriteEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L933) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L933)
```
proc processSwitch(pass: TCmdLinePass; p: OptParser; config: ConfigRef) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, OSError, KeyError,
EOFError], tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ReadDirEffect, WriteDirEffect, WriteEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L939) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L939)
```
proc processArgument(pass: TCmdLinePass; p: OptParser; argsCount: var int;
config: ConfigRef): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/commands.nim#L950) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/commands.nim#L950)
nim magicsys magicsys
========
Imports
-------
<ast>, <astalgo>, <msgs>, <platform>, <idents>, <modulegraphs>, <lineinfos> Procs
-----
```
proc nilOrSysInt(g: ModuleGraph): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L18)
```
proc registerSysType(g: ModuleGraph; t: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L20)
```
proc getSysSym(g: ModuleGraph; info: TLineInfo; name: string): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L28)
```
proc getSysMagic(g: ModuleGraph; info: TLineInfo; name: string; m: TMagic): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L36)
```
proc sysTypeFromName(g: ModuleGraph; info: TLineInfo; name: string): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L51) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L51)
```
proc getSysType(g: ModuleGraph; info: TLineInfo; kind: TTypeKind): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L54) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L54)
```
proc resetSysTypes(g: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L87)
```
proc getIntLitType(g: ModuleGraph; literal: PNode): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L97) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L97)
```
proc getFloatLitType(g: ModuleGraph; literal: PNode): PType {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L112)
```
proc skipIntLit(t: PType): PType {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L117)
```
proc addSonSkipIntLit(father, son: PType) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L124)
```
proc setIntLitType(g: ModuleGraph; result: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L131)
```
proc getCompilerProc(g: ModuleGraph; name: string): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L160)
```
proc registerCompilerProc(g: ModuleGraph; s: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L164)
```
proc registerNimScriptSymbol(g: ModuleGraph; s: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L167)
```
proc getNimScriptSymbol(g: ModuleGraph; name: string): PSym {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L176) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L176)
```
proc resetNimScriptSymbols(g: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L179) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L179)
```
proc getMagicEqSymForType(g: ModuleGraph; t: PType; info: TLineInfo): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/magicsys.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/magicsys.nim#L181) Exports
-------
[createMagic](modulegraphs#createMagic,ModuleGraph,string,TMagic)
nim ropes ropes
=====
Imports
-------
<pathutils> Types
-----
```
FormatStr = string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L64)
```
Rope = ref RopeObj
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L68) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L68)
```
RopeObj {...}{.acyclic.} = object of RootObj
left, right: Rope
L: int
data*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L69) Vars
----
```
gCacheTries = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L106)
```
gCacheMisses = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L107)
```
gCacheIntTries = 0
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L108) Procs
-----
```
proc len(a: Rope): int {...}{.raises: [], tags: [].}
```
the rope's length [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L75)
```
proc resetRopeCache() {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L89)
```
proc rope(s: string): Rope {...}{.raises: [], tags: [].}
```
Converts a string to a rope. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L122)
```
proc rope(i: BiggestInt): Rope {...}{.raises: [], tags: [].}
```
Converts an int to a rope. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L130)
```
proc rope(f: BiggestFloat): Rope {...}{.raises: [], tags: [].}
```
Converts a float to a rope. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L135) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L135)
```
proc `&`(a, b: Rope): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L139) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L139)
```
proc `&`(a: Rope; b: string): Rope {...}{.raises: [], tags: [].}
```
the concatenation operator for ropes. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L150) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L150)
```
proc `&`(a: string; b: Rope): Rope {...}{.raises: [], tags: [].}
```
the concatenation operator for ropes. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L154)
```
proc `&`(a: openArray[Rope]): Rope {...}{.raises: [], tags: [].}
```
the concatenation operator for an openarray of ropes. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L158) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L158)
```
proc add(a: var Rope; b: Rope) {...}{.raises: [], tags: [].}
```
adds `b` to the rope `a`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L162)
```
proc add(a: var Rope; b: string) {...}{.raises: [], tags: [].}
```
adds `b` to the rope `a`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L166)
```
proc writeRope(f: File; r: Rope) {...}{.raises: [IOError], tags: [WriteIOEffect].}
```
writes a rope to a file. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L188) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L188)
```
proc writeRope(head: Rope; filename: AbsoluteFile): bool {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L192)
```
proc `$`(r: Rope): string {...}{.raises: [], tags: [].}
```
converts a rope back to a string. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L201)
```
proc ropeConcat(a: varargs[Rope]): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L207) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L207)
```
proc prepend(a: var Rope; b: Rope) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L211)
```
proc prepend(a: var Rope; b: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L212)
```
proc runtimeFormat(frmt: FormatStr; args: openArray[Rope]): Rope {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L214)
```
proc `%`(frmt: static[FormatStr]; args: openArray[Rope]): Rope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L271) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L271)
```
proc equalsFile(r: Rope; f: File): bool {...}{.raises: [IOError],
tags: [ReadIOEffect].}
```
returns true if the contents of the file `f` equal `r`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L292) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L292)
```
proc equalsFile(r: Rope; filename: AbsoluteFile): bool {...}{.raises: [IOError],
tags: [ReadIOEffect].}
```
returns true if the contents of the file `f` equal `r`. If `f` does not exist, false is returned. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L324) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L324)
```
proc writeRopeIfNotEqual(r: Rope; filename: AbsoluteFile): bool {...}{.
raises: [IOError], tags: [ReadIOEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L333) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L333) Iterators
---------
```
iterator leaves(r: Rope): string {...}{.raises: [], tags: [].}
```
iterates over any leaf string in the rope `r`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L170)
```
iterator items(r: Rope): char {...}{.raises: [], tags: [].}
```
iterates over any character in the rope `r`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L183) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L183) Templates
---------
```
template addf(c: var Rope; frmt: FormatStr; args: openArray[Rope])
```
shortcut for `add(c, frmt % args)`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L274) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L274)
```
template `~`(r: string): Rope
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ropes.nim#L279) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ropes.nim#L279)
| programming_docs |
nim incremental incremental
===========
Basic type definitions the module graph needs in order to support incremental compilations.
Imports
-------
<options>, <lineinfos> Types
-----
```
IncrementalCtx = object
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/incremental.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/incremental.nim#L191) Consts
------
```
nimIncremental = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/incremental.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/incremental.nim#L13) Templates
---------
```
template init(incr: IncrementalCtx)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/incremental.nim#L193) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/incremental.nim#L193)
```
template addModuleDep(incr: var IncrementalCtx; conf: ConfigRef;
module, fileIdx: FileIndex; isIncludeFile: bool)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/incremental.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/incremental.nim#L195)
nim nimpaths nimpaths
========
Represents absolute paths, but using a symbolic variables (eg $nimr) which can be resolved at runtime; this avoids hardcoding at compile time absolute paths so that the project root can be relocated.
xxx consider some refactoring with $nim/testament/lib/stdtest/specialpaths.nim; specialpaths is simpler because it doesn't need variables to be relocatable at runtime (eg for use in testament)
interpolation variables:
$nimr: such that `$nimr/lib/system.nim` exists (avoids confusion with $nim binary) in compiler, it's obtainable via getPrefixDir(); for other tools (eg koch), this could be getCurrentDir() or getAppFilename().parentDir.parentDir, depending on use case Unstable API
Consts
------
```
docCss = "$nimr/doc/nimdoc.css"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L22)
```
docHackNim = "$nimr/tools/dochack/dochack.nim"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L23)
```
docHackJs = "$nimr/tools/dochack/dochack.js"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L24)
```
docHackJsFname = "dochack.js"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L25)
```
theindexFname = "theindex.html"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L26)
```
nimdocOutCss = "nimdoc.out.css"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L27)
```
htmldocsDirname = "htmldocs"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L29)
```
dotdotMangle = "_._"
```
refs #13223 [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L30) Procs
-----
```
proc interp(path: string; nimr: string): string {...}{.raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L34)
```
proc getDocHacksJs(nimr: string; nim = getCurrentCompilerExe();
forceRebuild = false): string {...}{.raises: [ValueError],
tags: [ReadDirEffect, ExecIOEffect].}
```
return absolute path to dochack.js, rebuilding if it doesn't exist or if `forceRebuild`. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimpaths.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimpaths.nim#L38)
nim modulegraphs modulegraphs
============
This module implements the module graph data structure. The module graph represents a complete Nim project. Single modules can either be kept in RAM or stored in a Sqlite database.
The caching of modules is critical for 'nimsuggest' and is tricky to get right. If module E is being edited, we need autocompletion (and type checking) for E but we don't want to recompile depending modules right away for faster turnaround times. Instead we mark the module's dependencies as 'dirty'. Let D be a dependency of E. If D is dirty, we need to recompile it and all of its dependencies that are marked as 'dirty'. 'nimsuggest sug' actually is invoked for the file being edited so we know its content changed and there is no need to compute any checksums. Instead of a recursive algorithm, we use an iterative algorithm:
* If a module gets recompiled, its dependencies need to be updated.
* Its dependent module stays the same.
Imports
-------
<ast>, <options>, <lineinfos>, <idents>, <incremental>, <btrees> Types
-----
```
SigHash = distinct MD5Digest
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L32)
```
ModuleGraph {...}{.acyclic.} = ref object
modules*: seq[PSym] ## indexed by int32 fileIdx
packageSyms*: TStrTable
deps*: IntSet
importDeps*: Table[FileIndex, seq[FileIndex]]
suggestMode*: bool
invalidTransitiveClosure: bool
inclToMod*: Table[FileIndex, FileIndex]
importStack*: seq[FileIndex]
backend*: RootRef
config*: ConfigRef
cache*: IdentCache
vm*: RootRef
doStopCompile*: proc (): bool {...}{.closure.}
usageSym*: PSym
owners*: seq[PSym]
methods*: seq[tuple[methods: seq[PSym], dispatcher: PSym]]
systemModule*: PSym
sysTypes*: array[TTypeKind, PType]
compilerprocs*: TStrTable
exposed*: TStrTable
intTypeCache*: array[-5 .. 64, PType]
opContains*, opNot*: PSym
emptyNode*: PNode
incr*: IncrementalCtx
canonTypes*: Table[SigHash, PType]
symBodyHashes*: Table[int, SigHash]
importModuleCallback*: proc (graph: ModuleGraph; m: PSym; fileIdx: FileIndex): PSym {...}{.
nimcall.}
includeFileCallback*: proc (graph: ModuleGraph; m: PSym; fileIdx: FileIndex): PNode {...}{.
nimcall.}
recordStmt*: proc (graph: ModuleGraph; m: PSym; n: PNode) {...}{.nimcall.}
cacheSeqs*: Table[string, PNode]
cacheCounters*: Table[string, BiggestInt]
cacheTables*: Table[string, BTree[string, PNode]]
passes*: seq[TPass]
onDefinition*: proc (graph: ModuleGraph; s: PSym; info: TLineInfo) {...}{.nimcall.}
onDefinitionResolveForward*: proc (graph: ModuleGraph; s: PSym;
info: TLineInfo) {...}{.nimcall.}
onUsage*: proc (graph: ModuleGraph; s: PSym; info: TLineInfo) {...}{.nimcall.}
globalDestructors*: seq[PNode]
strongSemCheck*: proc (graph: ModuleGraph; owner: PSym; body: PNode) {...}{.nimcall.}
compatibleProps*: proc (graph: ModuleGraph; formal, actual: PType): bool {...}{.
nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L34)
```
TPassContext = object of RootObj
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L78) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L78)
```
PPassContext = ref TPassContext
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L79)
```
TPassOpen = proc (graph: ModuleGraph; module: PSym): PPassContext {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L81)
```
TPassClose = proc (graph: ModuleGraph; p: PPassContext; n: PNode): PNode {...}{.
nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L82)
```
TPassProcess = proc (p: PPassContext; topLevelStmt: PNode): PNode {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L83)
```
TPass = tuple[open: TPassOpen, process: TPassProcess, close: TPassClose,
isFrontend: bool]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L85) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L85) Procs
-----
```
proc `$`(u: SigHash): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L125) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L125)
```
proc `==`(a, b: SigHash): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L128)
```
proc hash(u: SigHash): Hash {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L131) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L131)
```
proc hash(x: FileIndex): Hash {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L136) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L136)
```
proc stopCompile(g: ModuleGraph): bool {...}{.inline, raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L164)
```
proc createMagic(g: ModuleGraph; name: string; m: TMagic): PSym {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L167)
```
proc newModuleGraph(cache: IdentCache; config: ConfigRef): ModuleGraph {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L172)
```
proc resetAllModules(g: ModuleGraph) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L198) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L198)
```
proc getModule(g: ModuleGraph; fileIdx: FileIndex): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L210) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L210)
```
proc addDep(g: ModuleGraph; m: PSym; dep: FileIndex) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L216) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L216)
```
proc addIncludeDep(g: ModuleGraph; module, includeFile: FileIndex) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L225) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L225)
```
proc parentModule(g: ModuleGraph; fileIdx: FileIndex): FileIndex {...}{.raises: [],
tags: [].}
```
returns 'fileIdx' if the file belonging to this index is directly used as a module or else the module that first references this include file. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L229)
```
proc markDirty(g: ModuleGraph; fileIdx: FileIndex) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L247)
```
proc markClientsDirty(g: ModuleGraph; fileIdx: FileIndex) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L251) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L251)
```
proc isDirty(g: ModuleGraph; m: PSym): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L265) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L265) Templates
---------
```
template onUse(info: TLineInfo; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L160) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L160)
```
template onDef(info: TLineInfo; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L161) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L161)
```
template onDefResolveForward(info: TLineInfo; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulegraphs.nim#L162) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulegraphs.nim#L162)
nim platform platform
========
Types
-----
```
TSystemOS = enum
osNone, osDos, osWindows, osOs2, osLinux, osMorphos, osSkyos, osSolaris,
osIrix, osNetbsd, osFreebsd, osOpenbsd, osDragonfly, osAix, osPalmos, osQnx,
osAmiga, osAtari, osNetware, osMacos, osMacosx, osIos, osHaiku, osAndroid,
osVxWorks, osGenode, osJS, osNimVM, osStandalone, osNintendoSwitch,
osFreeRTOS, osAny
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L20)
```
TInfoOSProp = enum
ospNeedsPIC, ospCaseInsensitive, ospPosix, ospLacksThreadVars
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L28)
```
TInfoOSProps = set[TInfoOSProp]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L33)
```
TInfoOS = tuple[name: string, parDir: string, dllFrmt: string,
altDirSep: string, objExt: string, newLine: string,
pathSep: string, dirSep: string, scriptExt: string,
curDir: string, exeExt: string, extSep: string,
props: TInfoOSProps]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L34)
```
TSystemCPU = enum
cpuNone, cpuI386, cpuM68k, cpuAlpha, cpuPowerpc, cpuPowerpc64, cpuPowerpc64el,
cpuSparc, cpuVm, cpuHppa, cpuIa64, cpuAmd64, cpuMips, cpuMipsel, cpuArm,
cpuArm64, cpuJS, cpuNimVM, cpuAVR, cpuMSP430, cpuSparc64, cpuMips64,
cpuMips64el, cpuRiscV32, cpuRiscV64, cpuEsp, cpuWasm32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L191)
```
TEndian = enum
littleEndian, bigEndian
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L199)
```
TInfoCPU = tuple[name: string, intSize: int, endian: TEndian,
floatSize, bit: int]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L201) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L201)
```
Target = object
targetCPU*, hostCPU*: TSystemCPU
targetOS*, hostOS*: TSystemOS
intSize*: int
floatSize*: int
ptrSize*: int
tnl*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L235) Consts
------
```
OS: array[succ(low(TSystemOS)) .. high(TSystemOS), TInfoOS] = [(name: "DOS",
parDir: "..", dllFrmt: "$1.dll", altDirSep: "/", objExt: ".obj",
newLine: "\c\n", pathSep: ";", dirSep: "\\", scriptExt: ".bat", curDir: ".",
exeExt: ".exe", extSep: ".", props: {ospCaseInsensitive}), (name: "Windows",
parDir: "..", dllFrmt: "$1.dll", altDirSep: "/", objExt: ".obj",
newLine: "\c\n", pathSep: ";", dirSep: "\\", scriptExt: ".bat", curDir: ".",
exeExt: ".exe", extSep: ".", props: {ospCaseInsensitive}), (name: "OS2",
parDir: "..", dllFrmt: "$1.dll", altDirSep: "/", objExt: ".obj",
newLine: "\c\n", pathSep: ";", dirSep: "\\", scriptExt: ".bat", curDir: ".",
exeExt: ".exe", extSep: ".", props: {ospCaseInsensitive}), (name: "Linux",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "MorphOS",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "SkyOS",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "Solaris",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "Irix",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "NetBSD",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "FreeBSD",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "OpenBSD",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (
name: "DragonFly", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (
name: "AIX", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (
name: "PalmOS", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".", props: {ospNeedsPIC}), (name: "QNX",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "Amiga",
parDir: "..", dllFrmt: "$1.library", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC}), (name: "Atari",
parDir: "..", dllFrmt: "$1.dll", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: "", curDir: ".",
exeExt: ".tpp", extSep: ".", props: {ospNeedsPIC}), (name: "Netware",
parDir: "..", dllFrmt: "$1.nlm", altDirSep: "/", objExt: "",
newLine: "\c\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: ".nlm", extSep: ".", props: {ospCaseInsensitive}), (name: "MacOS",
parDir: "::", dllFrmt: "$1Lib", altDirSep: ":", objExt: ".o", newLine: "\c",
pathSep: ",", dirSep: ":", scriptExt: "", curDir: ":", exeExt: "",
extSep: ".", props: {ospCaseInsensitive}), (name: "MacOSX", parDir: "..",
dllFrmt: "lib$1.dylib", altDirSep: ":", objExt: ".o", newLine: "\n",
pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".", exeExt: "",
extSep: ".", props: {ospNeedsPIC, ospPosix, ospLacksThreadVars}), (
name: "iOS", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (
name: "Haiku", parDir: "..", dllFrmt: "lib$1.so", altDirSep: ":",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".",
props: {ospNeedsPIC, ospPosix, ospLacksThreadVars}), (name: "Android",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospPosix}), (name: "VxWorks",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ";", dirSep: "\\", scriptExt: ".sh", curDir: ".",
exeExt: ".vxe", extSep: ".",
props: {ospNeedsPIC, ospPosix, ospLacksThreadVars}), (name: "Genode",
pardir: "..", dllFrmt: "$1.lib.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: "", curDir: "/",
exeExt: "", extSep: ".", props: {ospNeedsPIC, ospLacksThreadVars}), (
name: "JS", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {}), (name: "NimVM", parDir: "..",
dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o", newLine: "\n",
pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".", exeExt: "",
extSep: ".", props: {}), (name: "Standalone", parDir: "..",
dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/",
scriptExt: ".sh", curDir: ".", exeExt: "",
extSep: ".", props: {}), (name: "NintendoSwitch",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: ".elf", extSep: ".", props: {ospNeedsPIC, ospPosix}), (
name: "FreeRTOS", parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/",
objExt: ".o", newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh",
curDir: ".", exeExt: "", extSep: ".", props: {ospPosix}), (name: "Any",
parDir: "..", dllFrmt: "lib$1.so", altDirSep: "/", objExt: ".o",
newLine: "\n", pathSep: ":", dirSep: "/", scriptExt: ".sh", curDir: ".",
exeExt: "", extSep: ".", props: {})]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L41)
```
EndianToStr: array[TEndian, string] = ["littleEndian", "bigEndian"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L205) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L205)
```
CPU: array[succ(low(TSystemCPU)) .. high(TSystemCPU), TInfoCPU] = [
(name: "i386", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32),
(name: "m68k", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32), (
name: "alpha", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64),
(name: "powerpc", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32), (
name: "powerpc64", intSize: 64, endian: bigEndian, floatSize: 64, bit: 64), (
name: "powerpc64el", intSize: 64, endian: littleEndian, floatSize: 64,
bit: 64),
(name: "sparc", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32),
(name: "vm", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32),
(name: "hppa", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32),
(name: "ia64", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64), (
name: "amd64", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64),
(name: "mips", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32), (
name: "mipsel", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32),
(name: "arm", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32), (
name: "arm64", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64),
(name: "js", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32),
(name: "nimvm", intSize: 32, endian: bigEndian, floatSize: 64, bit: 32),
(name: "avr", intSize: 16, endian: littleEndian, floatSize: 32, bit: 16), (
name: "msp430", intSize: 16, endian: littleEndian, floatSize: 32, bit: 16),
(name: "sparc64", intSize: 64, endian: bigEndian, floatSize: 64, bit: 64),
(name: "mips64", intSize: 64, endian: bigEndian, floatSize: 64, bit: 64), (
name: "mips64el", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64), (
name: "riscv32", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32), (
name: "riscv64", intSize: 64, endian: littleEndian, floatSize: 64, bit: 64),
(name: "esp", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32), (
name: "wasm32", intSize: 32, endian: littleEndian, floatSize: 64, bit: 32)]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L206) Procs
-----
```
proc setTarget(t: var Target; o: TSystemOS; c: TSystemCPU) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L243) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L243)
```
proc nameToOS(name: string): TSystemOS {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L254) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L254)
```
proc listOSnames(): seq[string] {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L260)
```
proc nameToCPU(name: string): TSystemCPU {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L264) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L264)
```
proc listCPUnames(): seq[string] {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L270) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L270)
```
proc setTargetFromSystem(t: var Target) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/platform.nim#L274) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/platform.nim#L274)
| programming_docs |
nim typesrenderer typesrenderer
=============
Imports
-------
<renderer>, <ast>, <types> Consts
------
```
defaultParamSeparator = ","
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typesrenderer.nim#L12) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typesrenderer.nim#L12) Procs
-----
```
proc renderPlainSymbolName(n: PNode): string {...}{.raises: [], tags: [].}
```
Returns the first non '\*' nkIdent node from the tree.
Use this on documentation name nodes to extract the *raw* symbol name, without decorations, parameters, or anything. That can be used as the base for the HTML hyperlinks.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typesrenderer.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typesrenderer.nim#L14)
```
proc renderParamTypes(n: PNode; sep = defaultParamSeparator): string {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
Returns the types contained in `n` joined by `sep`.
This proc expects to be passed as `n` the parameters of any callable. The string output is meant for the HTML renderer. If there are no parameters, the empty string is returned. The parameters will be joined by `sep` but other characters may appear too, like `[]` or `|`.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/typesrenderer.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/typesrenderer.nim#L114)
nim pathutils pathutils
=========
Path handling utilities for Nim. Strictly typed code in order to avoid the never ending time sink in getting path handling right.
Types
-----
```
AbsoluteFile = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L16)
```
AbsoluteDir = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L17)
```
RelativeFile = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L18)
```
RelativeDir = distinct string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L19)
```
AnyPath = AbsoluteFile | AbsoluteDir | RelativeFile | RelativeDir
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L20) Procs
-----
```
proc isEmpty(x: AnyPath): bool {...}{.inline.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L22)
```
proc copyFile(source, dest: AbsoluteFile) {...}{.raises: [OSError, IOError],
tags: [ReadIOEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L24)
```
proc removeFile(x: AbsoluteFile) {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L27)
```
proc splitFile(x: AbsoluteFile): tuple[dir: AbsoluteDir, name, ext: string] {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L29)
```
proc extractFilename(x: AbsoluteFile): string {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L33)
```
proc fileExists(x: AbsoluteFile): bool {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L35)
```
proc dirExists(x: AbsoluteDir): bool {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L36)
```
proc quoteShell(x: AbsoluteFile): string {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L38)
```
proc quoteShell(x: AbsoluteDir): string {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L39)
```
proc cmpPaths(x, y: AbsoluteDir): int {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L41)
```
proc createDir(x: AbsoluteDir) {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L43)
```
proc toAbsoluteDir(path: string): AbsoluteDir {...}{.raises: [OSError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L45)
```
proc `$`(x: AnyPath): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L49)
```
proc `==`[T: AnyPath](x, y: T): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L55) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L55)
```
proc `/`(base: AbsoluteDir; f: RelativeFile): AbsoluteFile {...}{.raises: [OSError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L68) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L68)
```
proc `/`(base: AbsoluteDir; f: RelativeDir): AbsoluteDir {...}{.raises: [OSError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L76)
```
proc relativeTo(fullPath: AbsoluteFile; baseFilename: AbsoluteDir; sep = DirSep): RelativeFile {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L84) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L84)
```
proc toAbsolute(file: string; base: AbsoluteDir): AbsoluteFile {...}{.
raises: [OSError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L91)
```
proc changeFileExt(x: AbsoluteFile; ext: string): AbsoluteFile {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L95) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L95)
```
proc changeFileExt(x: RelativeFile; ext: string): RelativeFile {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L96) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L96)
```
proc addFileExt(x: AbsoluteFile; ext: string): AbsoluteFile {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L98) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L98)
```
proc addFileExt(x: RelativeFile; ext: string): RelativeFile {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L99)
```
proc writeFile(x: AbsoluteFile; content: string) {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pathutils.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pathutils.nim#L101)
nim msgs msgs
====
Imports
-------
<options>, <ropes>, <lineinfos>, <pathutils>, <strutils2> Types
-----
```
InstantiationInfo = typeof(instantiationInfo())
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L16)
```
FilenameOption = enum
foAbs, foRelProject, foMagicSauce, foName, foStacktrace
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L235)
```
MsgFlag = enum
msgStdout, ## force writing to stdout, even stderr is default
msgSkipHook ## skip message hook even if it is present
```
flags altering msgWriteln behavior [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L290) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L290)
```
MsgFlags = set[MsgFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L293) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L293)
```
TErrorHandling = enum
doNothing, doAbort, doRaise
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L383) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L383) Consts
------
```
gCmdLineInfo = (line: 1'u, col: 1, fileIndex: -3)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L132)
```
ColOffset = 1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L168)
```
commandLineDesc = "command line"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L169) Procs
-----
```
proc toCChar(c: char; result: var string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L25)
```
proc makeCString(s: string): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L36) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L36)
```
proc fileInfoKnown(conf: ConfigRef; filename: AbsoluteFile): bool {...}{.raises: [],
tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L76)
```
proc fileInfoIdx(conf: ConfigRef; filename: AbsoluteFile; isKnownFile: var bool): FileIndex {...}{.
raises: [KeyError, Exception], tags: [ReadDirEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L86)
```
proc fileInfoIdx(conf: ConfigRef; filename: AbsoluteFile): FileIndex {...}{.
raises: [KeyError, Exception], tags: [ReadDirEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L114)
```
proc newLineInfo(fileInfoIdx: FileIndex; line, col: int): TLineInfo {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L118) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L118)
```
proc newLineInfo(conf: ConfigRef; filename: AbsoluteFile; line, col: int): TLineInfo {...}{.
inline, raises: [KeyError, Exception], tags: [ReadDirEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L129)
```
proc suggestWriteln(conf: ConfigRef; s: string) {...}{.raises: [IOError, Exception],
tags: [WriteIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L140) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L140)
```
proc msgQuit(x: int8) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L148) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L148)
```
proc msgQuit(x: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L149) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L149)
```
proc suggestQuit() {...}{.raises: [ESuggestDone], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L151) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L151)
```
proc getInfoContextLen(conf: ConfigRef): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L171) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L171)
```
proc setInfoContextLen(conf: ConfigRef; L: int) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L172)
```
proc pushInfoContext(conf: ConfigRef; info: TLineInfo; detail: string = "") {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L174) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L174)
```
proc popInfoContext(conf: ConfigRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L177)
```
proc getInfoContext(conf: ConfigRef; index: int): TLineInfo {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L180) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L180)
```
proc toProjPath(conf: ConfigRef; fileIdx: FileIndex): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L191)
```
proc toFullPath(conf: ConfigRef; fileIdx: FileIndex): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L196) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L196)
```
proc setDirtyFile(conf: ConfigRef; fileIdx: FileIndex; filename: AbsoluteFile) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L202) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L202)
```
proc setHash(conf: ConfigRef; fileIdx: FileIndex; hash: string) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L207) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L207)
```
proc getHash(conf: ConfigRef; fileIdx: FileIndex): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L211) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L211)
```
proc toFullPathConsiderDirty(conf: ConfigRef; fileIdx: FileIndex): AbsoluteFile {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L215)
```
proc toFilenameOption(conf: ConfigRef; fileIdx: FileIndex; opt: FilenameOption): string {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L242) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L242)
```
proc toMsgFilename(conf: ConfigRef; info: FileIndex): string {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L263) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L263)
```
proc toLinenumber(info: TLineInfo): int {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L269) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L269)
```
proc toColumn(info: TLineInfo): int {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L272) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L272)
```
proc toFileLineCol(conf: ConfigRef; info: TLineInfo): string {...}{.inline,
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L278) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L278)
```
proc `$`(conf: ConfigRef; info: TLineInfo): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L281) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L281)
```
proc `$`(info: TLineInfo): string {...}{.error.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L283) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L283)
```
proc `??`(conf: ConfigRef; info: TLineInfo; filename: string): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L285) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L285)
```
proc msgWriteln(conf: ConfigRef; s: string; flags: MsgFlags = {}) {...}{.
raises: [Exception, IOError], tags: [RootEffect, WriteIOEffect].}
```
Writes given message string to stderr by default. If `--stdout` option is given, writes to stdout instead. If message hook is present, then it is used to output message rather than stderr/stdout. This behavior can be altered by given optional flags. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L295) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L295)
```
proc msgKindToString(kind: TMsgKind): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L378) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L378)
```
proc log(s: string) {...}{.raises: [IOError],
tags: [ReadEnvEffect, ReadIOEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L385) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L385)
```
proc `==`(a, b: TLineInfo): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L418) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L418)
```
proc exactEquals(a, b: TLineInfo): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L421) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L421)
```
proc numLines(conf: ConfigRef; fileIdx: FileIndex): int {...}{.raises: [],
tags: [ReadIOEffect].}
```
xxx there's an off by 1 error that should be fixed; if a file ends with "foo" or "foon" it will return same number of lines (ie, a trailing empty line is discounted) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L448) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L448)
```
proc sourceLine(conf: ConfigRef; i: TLineInfo): string {...}{.raises: [],
tags: [ReadIOEffect].}
```
1-based index (matches editor line numbers); 1st line is for i.line = 1 last valid line is `numLines` inclusive [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L460) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L460)
```
proc formatMsg(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg: string): string {...}{.
raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L476) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L476)
```
proc liMessage(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg: string;
eh: TErrorHandling; info2: InstantiationInfo; isRaw = false) {...}{.
noinline, raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L483) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L483)
```
proc quotedFilename(conf: ConfigRef; i: TLineInfo): Rope {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L603) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L603)
```
proc listWarnings(conf: ConfigRef) {...}{.raises: [Exception, IOError, ValueError],
tags: [RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L615) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L615)
```
proc listHints(conf: ConfigRef) {...}{.raises: [Exception, IOError, ValueError],
tags: [RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L616) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L616) Templates
---------
```
template toFilename(conf: ConfigRef; fileIdx: FileIndex): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L185) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L185)
```
template toFilename(conf: ConfigRef; info: TLineInfo): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L223) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L223)
```
template toProjPath(conf: ConfigRef; info: TLineInfo): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L226) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L226)
```
template toFullPath(conf: ConfigRef; info: TLineInfo): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L229)
```
template toFullPathConsiderDirty(conf: ConfigRef; info: TLineInfo): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L232) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L232)
```
template toMsgFilename(conf: ConfigRef; info: TLineInfo): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L266) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L266)
```
template styledMsgWriteln(args: varargs[typed])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L360) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L360)
```
template rawMessage(conf: ConfigRef; msg: TMsgKind; args: openArray[string])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L541) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L541)
```
template rawMessage(conf: ConfigRef; msg: TMsgKind; arg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L545) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L545)
```
template fatal(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L548) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L548)
```
template globalAssert(conf: ConfigRef; cond: untyped;
info: TLineInfo = unknownLineInfo; arg = "")
```
avoids boilerplate [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L553) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L553)
```
template globalError(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")
```
`local` means compilation keeps going until errorMax is reached (via `doNothing`), `global` means it stops. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L560) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L560)
```
template globalError(conf: ConfigRef; info: TLineInfo; arg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L565) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L565)
```
template localError(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L568) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L568)
```
template localError(conf: ConfigRef; info: TLineInfo; arg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L571) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L571)
```
template localError(conf: ConfigRef; info: TLineInfo; format: string;
params: openArray[string])
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L574) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L574)
```
template message(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L577) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L577)
```
template internalError(conf: ConfigRef; info: TLineInfo; errMsg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L585) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L585)
```
template internalError(conf: ConfigRef; errMsg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L588) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L588)
```
template internalAssert(conf: ConfigRef; e: bool)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L591) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L591)
```
template lintReport(conf: ConfigRef; info: TLineInfo; beau, got: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/msgs.nim#L598) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/msgs.nim#L598)
| programming_docs |
nim vmprofiler vmprofiler
==========
Imports
-------
<options>, <vmdef>, <lineinfos>, <msgs> Procs
-----
```
proc enter(prof: var Profiler; c: PCtx; tos: PStackFrame) {...}{.inline, raises: [],
tags: [TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmprofiler.nim#L6) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmprofiler.nim#L6)
```
proc leave(prof: var Profiler; c: PCtx) {...}{.inline, raises: [KeyError],
tags: [TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmprofiler.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmprofiler.nim#L25)
```
proc dump(conf: ConfigRef; pd: ProfileData): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmprofiler.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmprofiler.nim#L29)
nim lexer lexer
=====
Imports
-------
<options>, <msgs>, <platform>, <idents>, <nimlexbase>, <llstream>, <wordrecg>, <lineinfos>, <pathutils> Types
-----
```
TokType = enum
tkInvalid = "tkInvalid", tkEof = "[EOF]", tkSymbol = "tkSymbol",
tkAddr = "addr", tkAnd = "and", tkAs = "as", tkAsm = "asm", tkBind = "bind",
tkBlock = "block", tkBreak = "break", tkCase = "case", tkCast = "cast",
tkConcept = "concept", tkConst = "const", tkContinue = "continue",
tkConverter = "converter", tkDefer = "defer", tkDiscard = "discard",
tkDistinct = "distinct", tkDiv = "div", tkDo = "do", tkElif = "elif",
tkElse = "else", tkEnd = "end", tkEnum = "enum", tkExcept = "except",
tkExport = "export", tkFinally = "finally", tkFor = "for", tkFrom = "from",
tkFunc = "func", tkIf = "if", tkImport = "import", tkIn = "in",
tkInclude = "include", tkInterface = "interface", tkIs = "is",
tkIsnot = "isnot", tkIterator = "iterator", tkLet = "let", tkMacro = "macro",
tkMethod = "method", tkMixin = "mixin", tkMod = "mod", tkNil = "nil",
tkNot = "not", tkNotin = "notin", tkObject = "object", tkOf = "of",
tkOr = "or", tkOut = "out", tkProc = "proc", tkPtr = "ptr", tkRaise = "raise",
tkRef = "ref", tkReturn = "return", tkShl = "shl", tkShr = "shr",
tkStatic = "static", tkTemplate = "template", tkTry = "try",
tkTuple = "tuple", tkType = "type", tkUsing = "using", tkVar = "var",
tkWhen = "when", tkWhile = "while", tkXor = "xor", tkYield = "yield",
tkIntLit = "tkIntLit", tkInt8Lit = "tkInt8Lit", tkInt16Lit = "tkInt16Lit",
tkInt32Lit = "tkInt32Lit", tkInt64Lit = "tkInt64Lit", tkUIntLit = "tkUIntLit",
tkUInt8Lit = "tkUInt8Lit", tkUInt16Lit = "tkUInt16Lit",
tkUInt32Lit = "tkUInt32Lit", tkUInt64Lit = "tkUInt64Lit",
tkFloatLit = "tkFloatLit", tkFloat32Lit = "tkFloat32Lit",
tkFloat64Lit = "tkFloat64Lit", tkFloat128Lit = "tkFloat128Lit",
tkStrLit = "tkStrLit", tkRStrLit = "tkRStrLit",
tkTripleStrLit = "tkTripleStrLit", tkGStrLit = "tkGStrLit",
tkGTripleStrLit = "tkGTripleStrLit", tkCharLit = "tkCharLit", tkParLe = "(",
tkParRi = ")", tkBracketLe = "[", tkBracketRi = "]", tkCurlyLe = "{",
tkCurlyRi = "}", tkBracketDotLe = "[.", tkBracketDotRi = ".]",
tkCurlyDotLe = "{.", tkCurlyDotRi = ".}", tkParDotLe = "(.",
tkParDotRi = ".)", tkComma = ",", tkSemiColon = ";", tkColon = ":",
tkColonColon = "::", tkEquals = "=", tkDot = ".", tkDotDot = "..",
tkBracketLeColon = "[:", tkOpr, tkComment, tkAccent = "`", tkSpaces,
tkInfixOpr, tkPrefixOpr, tkPostfixOpr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L33)
```
TokTypes = set[TokType]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L75)
```
NumericalBase = enum
base10, base2, base8, base16
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L86)
```
Token = object
tokType*: TokType
indent*: int
ident*: PIdent
iNumber*: BiggestInt
fNumber*: BiggestFloat
base*: NumericalBase
strongSpaceA*: int8
strongSpaceB*: int8
literal*: string
line*, col*: int
when defined(nimpretty):
offsetA*, offsetB*: int
commentOffsetA*, commentOffsetB*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L91) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L91)
```
ErrorHandler = proc (conf: ConfigRef; info: TLineInfo; msg: TMsgKind;
arg: string)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L110)
```
Lexer = object of TBaseLexer
fileIdx*: FileIndex
indentAhead*: int
currLineIndent*: int
strongSpaces*, allowTabs*: bool
errorHandler*: ErrorHandler
cache*: IdentCache
when defined(nimsuggest):
previousToken: TLineInfo
config*: ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L111) Consts
------
```
MaxLineLength = 80
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L23)
```
numChars: set[char] = {'0'..'9', 'a'..'z', 'A'..'Z'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L24)
```
SymChars: set[char] = {'a'..'z', 'A'..'Z', '0'..'9', '\x80'..'\xFF'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L25)
```
SymStartChars: set[char] = {'a'..'z', 'A'..'Z', '\x80'..'\xFF'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L26)
```
OpChars: set[char] = {'+', '-', '*', '/', '\\', '<', '>', '!', '?', '^', '.',
'|', '=', '%', '&', '$', '@', '~', ':'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L27)
```
tokKeywordLow = tkAddr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L82)
```
tokKeywordHigh = tkYield
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L83) Procs
-----
```
proc getLineInfo(L: Lexer; tok: Token): TLineInfo {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L124)
```
proc isKeyword(kind: TokType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L132)
```
proc isNimIdentifier(s: string): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L137) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L137)
```
proc `$`(tok: Token): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L147) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L147)
```
proc prettyTok(tok: Token): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L159)
```
proc printTok(conf: ConfigRef; tok: Token) {...}{.raises: [Exception, IOError],
tags: [RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L163)
```
proc initToken(L: var Token) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L166) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L166)
```
proc openLexer(lex: var Lexer; fileIdx: FileIndex; inputstream: PLLStream;
cache: IdentCache; config: ConfigRef) {...}{.
raises: [IOError, Exception], tags: [ReadIOEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L192)
```
proc openLexer(lex: var Lexer; filename: AbsoluteFile; inputstream: PLLStream;
cache: IdentCache; config: ConfigRef) {...}{.
raises: [IOError, Exception, KeyError],
tags: [ReadIOEffect, RootEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L204)
```
proc closeLexer(lex: var Lexer) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L208) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L208)
```
proc lexMessage(L: Lexer; msg: TMsgKind; arg = "") {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L222) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L222)
```
proc lexMessageTok(L: Lexer; msg: TMsgKind; tok: Token; arg = "") {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L225) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L225)
```
proc getPrecedence(tok: Token): int {...}{.raises: [], tags: [].}
```
Calculates the precedence of the given token. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L904) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L904)
```
proc newlineFollows(L: Lexer): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L934) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L934)
```
proc rawGetTok(L: var Lexer; tok: var Token) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L1137) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L1137)
```
proc getIndentWidth(fileIdx: FileIndex; inputstream: PLLStream;
cache: IdentCache; config: ConfigRef): int {...}{.
raises: [IOError, Exception, ValueError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L1285) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L1285)
```
proc getPrecedence(ident: PIdent): int {...}{.raises: [], tags: [].}
```
assumes ident is binary operator already [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lexer.nim#L1300) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lexer.nim#L1300)
nim wordrecg wordrecg
========
Types
-----
```
TSpecialWord = enum
wInvalid = "", wAddr = "addr", wAnd = "and", wAs = "as", wAsm = "asm",
wBind = "bind", wBlock = "block", wBreak = "break", wCase = "case",
wCast = "cast", wConcept = "concept", wConst = "const",
wContinue = "continue", wConverter = "converter", wDefer = "defer",
wDiscard = "discard", wDistinct = "distinct", wDiv = "div", wDo = "do",
wElif = "elif", wElse = "else", wEnd = "end", wEnum = "enum",
wExcept = "except", wExport = "export", wFinally = "finally", wFor = "for",
wFrom = "from", wFunc = "func", wIf = "if", wImport = "import", wIn = "in",
wInclude = "include", wInterface = "interface", wIs = "is", wIsnot = "isnot",
wIterator = "iterator", wLet = "let", wMacro = "macro", wMethod = "method",
wMixin = "mixin", wMod = "mod", wNil = "nil", wNot = "not", wNotin = "notin",
wObject = "object", wOf = "of", wOr = "or", wOut = "out", wProc = "proc",
wPtr = "ptr", wRaise = "raise", wRef = "ref", wReturn = "return",
wShl = "shl", wShr = "shr", wStatic = "static", wTemplate = "template",
wTry = "try", wTuple = "tuple", wType = "type", wUsing = "using",
wVar = "var", wWhen = "when", wWhile = "while", wXor = "xor",
wYield = "yield", wColon = ":", wColonColon = "::", wEquals = "=", wDot = ".",
wDotDot = "..", wStar = "*", wMinus = "-", wMagic = "magic",
wThread = "thread", wFinal = "final", wProfiler = "profiler",
wMemTracker = "memtracker", wObjChecks = "objchecks",
wIntDefine = "intdefine", wStrDefine = "strdefine",
wBoolDefine = "booldefine", wCursor = "cursor", wNoalias = "noalias",
wImmediate = "immediate", wConstructor = "constructor",
wDestructor = "destructor", wDelegator = "delegator", wOverride = "override",
wImportCpp = "importcpp", wImportObjC = "importobjc",
wImportCompilerProc = "importcompilerproc", wImportc = "importc",
wImportJs = "importjs", wExportc = "exportc", wExportCpp = "exportcpp",
wExportNims = "exportnims", wIncompleteStruct = "incompleteStruct",
wCompleteStruct = "completeStruct", wRequiresInit = "requiresInit",
wAlign = "align", wNodecl = "nodecl", wPure = "pure",
wSideEffect = "sideEffect", wHeader = "header",
wNoSideEffect = "noSideEffect", wGcSafe = "gcsafe", wNoreturn = "noreturn",
wNosinks = "nosinks", wMerge = "merge", wLib = "lib", wDynlib = "dynlib",
wCompilerProc = "compilerproc", wCore = "core", wProcVar = "procvar",
wBase = "base", wUsed = "used", wFatal = "fatal", wError = "error",
wWarning = "warning", wHint = "hint", wWarningAsError = "warningAsError",
wLine = "line", wPush = "push", wPop = "pop", wDefine = "define",
wUndef = "undef", wLineDir = "lineDir", wStackTrace = "stackTrace",
wLineTrace = "lineTrace", wLink = "link", wCompile = "compile",
wLinksys = "linksys", wDeprecated = "deprecated", wVarargs = "varargs",
wCallconv = "callconv", wDebugger = "debugger", wNimcall = "nimcall",
wStdcall = "stdcall", wCdecl = "cdecl", wSafecall = "safecall",
wSyscall = "syscall", wInline = "inline", wNoInline = "noinline",
wFastcall = "fastcall", wThiscall = "thiscall", wClosure = "closure",
wNoconv = "noconv", wOn = "on", wOff = "off", wChecks = "checks",
wRangeChecks = "rangeChecks", wBoundChecks = "boundChecks",
wOverflowChecks = "overflowChecks", wNilChecks = "nilChecks",
wFloatChecks = "floatChecks", wNanChecks = "nanChecks",
wInfChecks = "infChecks", wStyleChecks = "styleChecks",
wStaticBoundchecks = "staticBoundChecks", wNonReloadable = "nonReloadable",
wExecuteOnReload = "executeOnReload", wAssertions = "assertions",
wPatterns = "patterns", wTrMacros = "trmacros",
wSinkInference = "sinkInference", wWarnings = "warnings", wHints = "hints",
wOptimization = "optimization", wRaises = "raises", wWrites = "writes",
wReads = "reads", wSize = "size", wEffects = "effects", wTags = "tags",
wRequires = "requires", wEnsures = "ensures", wInvariant = "invariant",
wAssume = "assume", wAssert = "assert", wDeadCodeElimUnused = "deadCodeElim",
wSafecode = "safecode", wPackage = "package", wNoForward = "noforward",
wReorder = "reorder", wNoRewrite = "norewrite", wNoDestroy = "nodestroy",
wPragma = "pragma", wCompileTime = "compileTime", wNoInit = "noinit",
wPassc = "passc", wPassl = "passl", wLocalPassc = "localPassC",
wBorrow = "borrow", wDiscardable = "discardable",
wFieldChecks = "fieldChecks", wSubsChar = "subschar", wAcyclic = "acyclic",
wShallow = "shallow", wUnroll = "unroll", wLinearScanEnd = "linearScanEnd",
wComputedGoto = "computedGoto", wInjectStmt = "injectStmt",
wExperimental = "experimental", wWrite = "write", wGensym = "gensym",
wInject = "inject", wDirty = "dirty", wInheritable = "inheritable",
wThreadVar = "threadvar", wEmit = "emit",
wAsmNoStackFrame = "asmNoStackFrame", wImplicitStatic = "implicitStatic",
wGlobal = "global", wCodegenDecl = "codegenDecl", wUnchecked = "unchecked",
wGuard = "guard", wLocks = "locks", wPartial = "partial",
wExplain = "explain", wLiftLocals = "liftlocals", wAuto = "auto",
wBool = "bool", wCatch = "catch", wChar = "char", wClass = "class",
wCompl = "compl", wConst_cast = "const_cast", wDefault = "default",
wDelete = "delete", wDouble = "double", wDynamic_cast = "dynamic_cast",
wExplicit = "explicit", wExtern = "extern", wFalse = "false",
wFloat = "float", wFriend = "friend", wGoto = "goto", wInt = "int",
wLong = "long", wMutable = "mutable", wNamespace = "namespace", wNew = "new",
wOperator = "operator", wPrivate = "private", wProtected = "protected",
wPublic = "public", wRegister = "register",
wReinterpret_cast = "reinterpret_cast", wRestrict = "restrict",
wShort = "short", wSigned = "signed", wSizeof = "sizeof",
wStatic_cast = "static_cast", wStruct = "struct", wSwitch = "switch",
wThis = "this", wThrow = "throw", wTrue = "true", wTypedef = "typedef",
wTypeid = "typeid", wTypeof = "typeof", wTypename = "typename",
wUnion = "union", wPacked = "packed", wUnsigned = "unsigned",
wVirtual = "virtual", wVoid = "void", wVolatile = "volatile",
wWchar_t = "wchar_t", wAlignas = "alignas", wAlignof = "alignof",
wConstexpr = "constexpr", wDecltype = "decltype", wNullptr = "nullptr",
wNoexcept = "noexcept", wThread_local = "thread_local",
wStatic_assert = "static_assert", wChar16_t = "char16_t",
wChar32_t = "char32_t", wStdIn = "stdin", wStdOut = "stdout",
wStdErr = "stderr", wInOut = "inout", wByCopy = "bycopy", wByRef = "byref",
wOneWay = "oneway", wBitsize = "bitsize"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L19)
```
TSpecialWords = set[TSpecialWord]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L112) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L112) Consts
------
```
oprLow = 67
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L115) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L115)
```
oprHigh = 71
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L116)
```
nimKeywordsLow = 4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L118) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L118)
```
nimKeywordsHigh = 66
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L119) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L119)
```
ccgKeywordsLow = 222
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L121) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L121)
```
ccgKeywordsHigh = 287
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L122) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L122)
```
cppNimSharedKeywords = {wAsm, wBreak, wCase, wConst, wContinue, wDo, wElse,
wEnum, wExport, wFor, wIf, wReturn, wStatic, wTemplate,
wTry, wWhile, wUsing}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L124) Procs
-----
```
proc findStr[T: enum](a: Slice[T]; s: string; default: T): T
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/wordrecg.nim#L128) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/wordrecg.nim#L128)
| programming_docs |
nim vm vm
==
This file implements the new evaluation engine for Nim code. An instruction is 1-3 int32s in memory, it is a register based VM.
Imports
-------
<ast>, <msgs>, <vmdef>, <vmgen>, <nimsets>, <types>, <passes>, <parser>, <vmdeps>, <idents>, <trees>, <renderer>, <options>, <transf>, <vmmarshal>, <gorgeimpl>, <lineinfos>, <btrees>, <macrocacheimpl>, <modulegraphs>, <sighashes>, <int128>, <vmprofiler>, <semfold>, <evaltempl>, <pathutils>, <sighashes>, <vmconv> Consts
------
```
evalPass = (myOpen, myProcess, myClose, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2158) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2158) Procs
-----
```
proc setResult(a: VmArgs; v: BiggestInt) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L16)
```
proc setResult(a: VmArgs; v: BiggestFloat) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L17)
```
proc setResult(a: VmArgs; v: bool) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L18)
```
proc setResult(a: VmArgs; v: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L22)
```
proc setResult(a: VmArgs; n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L27) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L27)
```
proc setResult(a: VmArgs; v: AbsoluteDir) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L31)
```
proc setResult(a: VmArgs; v: seq[string]) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L33)
```
proc getInt(a: VmArgs; i: Natural): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L44)
```
proc getBool(a: VmArgs; i: Natural): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L45)
```
proc getFloat(a: VmArgs; i: Natural): BiggestFloat {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L46)
```
proc getString(a: VmArgs; i: Natural): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L47)
```
proc getNode(a: VmArgs; i: Natural): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmhooks.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmhooks.nim#L52)
```
proc execProc(c: PCtx; sym: PSym; args: openArray[PNode]): PNode {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError,
JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, TimeEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2083) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2083)
```
proc evalStmt(c: PCtx; n: PNode) {...}{.raises: [Exception, ValueError, IOError,
ERecoverableError, KeyError, OSError, JsonParsingError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2109) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2109)
```
proc evalExpr(c: PCtx; n: PNode): PNode {...}{.raises: [Exception, ValueError,
IOError, ERecoverableError, KeyError, OSError, JsonParsingError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect,
ReadDirEffect, ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2117)
```
proc getGlobalValue(c: PCtx; s: PSym): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2123) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2123)
```
proc registerAdditionalOps(c: PCtx) {...}{.raises: [OSError, IOError, EOFError,
ValueError, Exception, ERecoverableError], tags: [ReadEnvEffect,
WriteEnvEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect, ExecIOEffect,
RootEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmops.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmops.nim#L141)
```
proc setupGlobalCtx(module: PSym; graph: ModuleGraph) {...}{.raises: [OSError,
IOError, EOFError, ValueError, Exception, ERecoverableError], tags: [
ReadEnvEffect, WriteEnvEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect,
ExecIOEffect, RootEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2129)
```
proc evalConstExpr(module: PSym; g: ModuleGraph; e: PNode): PNode {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError,
EOFError, JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, TimeEffect,
WriteEnvEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2180) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2180)
```
proc evalStaticExpr(module: PSym; g: ModuleGraph; e: PNode; prc: PSym): PNode {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError,
EOFError, JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, TimeEffect,
WriteEnvEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2183) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2183)
```
proc evalStaticStmt(module: PSym; g: ModuleGraph; e: PNode; prc: PSym) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError,
EOFError, JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, TimeEffect,
WriteEnvEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2186)
```
proc setupCompileTimeVar(module: PSym; g: ModuleGraph; n: PNode) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, KeyError, OSError,
EOFError, JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, TimeEffect,
WriteEnvEffect, ReadDirEffect,
ExecIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2189) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2189)
```
proc evalMacroCall(module: PSym; g: ModuleGraph; templInstCounter: ref int;
n, nOrig: PNode; sym: PSym): PNode {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, OSError, EOFError, KeyError,
JsonParsingError], tags: [RootEffect, WriteIOEffect, ReadIOEffect,
ReadEnvEffect, WriteEnvEffect, ReadDirEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2239) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2239) Iterators
---------
```
iterator genericParamsInMacroCall(macroSym: PSym; call: PNode): (PSym, PNode) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vm.nim#L2223) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vm.nim#L2223)
nim evaltempl evaltempl
=========
Template evaluation engine. Now hygienic.
Imports
-------
<options>, <ast>, <astalgo>, <msgs>, <renderer>, <lineinfos>, <idents> Consts
------
```
evalTemplateLimit = 1000
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/evaltempl.nim#L144) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/evaltempl.nim#L144) Procs
-----
```
proc wrapInComesFrom(info: TLineInfo; sym: PSym; res: PNode): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/evaltempl.nim#L146) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/evaltempl.nim#L146)
```
proc evalTemplate(n: PNode; tmpl, genSymOwner: PSym; conf: ConfigRef;
ic: IdentCache; instID: ref int; fromHlo = false): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/evaltempl.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/evaltempl.nim#L168)
nim main main
====
Imports
-------
<llstream>, <ast>, <lexer>, <syntaxes>, <options>, <msgs>, <condsyms>, <sem>, <idents>, <passes>, <extccomp>, <cgen>, <nversion>, <platform>, <nimconf>, <passaux>, <depends>, <vm>, <idgen>, <modules>, <modulegraphs>, <rod>, <lineinfos>, <pathutils>, <vmprofiler>, <jsgen>, <docgen>, <docgen2> Procs
-----
```
proc mainCommand(graph: ModuleGraph) {...}{.raises: [OSError, ValueError, Exception,
IOError, ERecoverableError, KeyError, EOFError], tags: [TimeEffect,
ReadEnvEffect, ReadIOEffect, RootEffect, WriteIOEffect, ReadDirEffect,
ExecIOEffect, WriteDirEffect, WriteEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/main.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/main.nim#L167)
nim prefixmatches prefixmatches
=============
Types
-----
```
PrefixMatch {...}{.pure.} = enum
None, ## no prefix detected
Abbrev, ## prefix is an abbreviation of the symbol
Substr, ## prefix is a substring of the symbol
Prefix ## prefix does match the symbol
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/prefixmatches.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/prefixmatches.nim#L13) Procs
-----
```
proc prefixMatch(p, s: string): PrefixMatch {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/prefixmatches.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/prefixmatches.nim#L19)
nim renderverbatim renderverbatim
==============
Imports
-------
<ast>, <options>, <msgs> Procs
-----
```
proc extractRunnableExamplesSource(conf: ConfigRef; n: PNode): string {...}{.
raises: [], tags: [ReadIOEffect].}
```
TLineInfo.offsetA,offsetB would be cleaner but it's only enabled for nimpretty, we'd need to check performance impact to enable it for nimdoc. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderverbatim.nim#L88) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderverbatim.nim#L88)
```
proc renderNimCode(result: var string; code: string; isLatex = false) {...}{.
raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderverbatim.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderverbatim.nim#L134)
nim renderer renderer
========
Imports
-------
<lexer>, <options>, <idents>, <ast>, <msgs>, <lineinfos> Types
-----
```
TRenderFlag = enum
renderNone, renderNoBody, renderNoComments, renderDocComments,
renderNoPragmas, renderIds, renderNoProcDefs, renderSyms,
renderRunnableExamples, renderIr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L21)
```
TRenderFlags = set[TRenderFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L25)
```
TRenderTok = object
kind*: TokType
length*: int16
sym*: PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L26)
```
TRenderTokSeq = seq[TRenderTok]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L31) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L31)
```
TSrcGen = object
indent*: int
lineLen*: int
col: int
pos*: int
idx*: int
tokens*: TRenderTokSeq
buf*: string
pendingNL*: int
pendingWhitespace: int
comStack*: seq[PNode]
flags*: TRenderFlags
inGenericParams: bool
checkAnon: bool
inPragma: int
when defined(nimpretty):
pendingNewlineCount: int
fid*: FileIndex
config*: ConfigRef
mangler: seq[PSym]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L32) Procs
-----
```
proc isKeyword(i: PIdent): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L66)
```
proc renderDefinitionName(s: PSym; noQuotes = false): string {...}{.raises: [],
tags: [].}
```
Returns the definition name of the symbol.
If noQuotes is false the symbol may be returned in backticks. This will happen if the name happens to be a keyword or the first character is not part of the SymStartChars set.
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L71) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L71)
```
proc bracketKind(g: TSrcGen; n: PNode): BracketKind {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L906) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L906)
```
proc renderTree(n: PNode; renderFlags: TRenderFlags = {}): string {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1621) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1621)
```
proc `$`(n: PNode): string {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1634) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1634)
```
proc renderModule(n: PNode; infile, outfile: string;
renderFlags: TRenderFlags = {}; fid = FileIndex(-1);
conf: ConfigRef = nil) {...}{.
raises: [Exception, IOError, ValueError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1636) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1636)
```
proc initTokRender(r: var TSrcGen; n: PNode; renderFlags: TRenderFlags = {}) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1659) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1659)
```
proc getNextTok(r: var TSrcGen; kind: var TokType; literal: var string) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1663) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1663)
```
proc getTokSym(r: TSrcGen): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1673) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1673)
```
proc quoteExpr(a: string): string {...}{.inline, raises: [], tags: [].}
```
can be used for quoting expressions in error msgs. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1679) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1679)
```
proc genFieldDefect(field: PSym; disc: PSym): string {...}{.raises: [], tags: [].}
```
this needs to be in a module accessible by jsgen, ccgexprs, and vm to provide this error msg FieldDefect; msgs would be better but it does not import ast [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/renderer.nim#L1683) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/renderer.nim#L1683)
nim lineinfos lineinfos
=========
This module contains the `TMsgKind` enum as well as the `TLineInfo` object.
Imports
-------
<ropes>, <pathutils> Types
-----
```
TMsgKind = enum
errUnknown, errInternal, errIllFormedAstX, errCannotOpenFile, errXExpected,
errGridTableNotImplemented, errGeneralParseError, errNewSectionExpected,
errInvalidDirectiveX, errProveInit, errGenerated, errUser,
warnCannotOpenFile = "CannotOpenFile", warnOctalEscape = "OctalEscape",
warnXIsNeverRead = "XIsNeverRead",
warnXmightNotBeenInit = "XmightNotBeenInit", warnDeprecated = "Deprecated",
warnConfigDeprecated = "ConfigDeprecated",
warnSmallLshouldNotBeUsed = "SmallLshouldNotBeUsed",
warnUnknownMagic = "UnknownMagic",
warnRedefinitionOfLabel = "RedefinitionOfLabel",
warnUnknownSubstitutionX = "UnknownSubstitutionX",
warnLanguageXNotSupported = "LanguageXNotSupported",
warnFieldXNotSupported = "FieldXNotSupported",
warnCommentXIgnored = "CommentXIgnored", warnTypelessParam = "TypelessParam",
warnUseBase = "UseBase", warnWriteToForeignHeap = "WriteToForeignHeap",
warnUnsafeCode = "UnsafeCode", warnUnusedImportX = "UnusedImport",
warnInheritFromException = "InheritFromException",
warnEachIdentIsTuple = "EachIdentIsTuple", warnUnsafeSetLen = "UnsafeSetLen",
warnUnsafeDefault = "UnsafeDefault", warnProveInit = "ProveInit",
warnProveField = "ProveField", warnProveIndex = "ProveIndex",
warnUnreachableElse = "UnreachableElse",
warnUnreachableCode = "UnreachableCode", warnStaticIndexCheck = "IndexCheck",
warnGcUnsafe = "GcUnsafe", warnGcUnsafe2 = "GcUnsafe2", warnUninit = "Uninit",
warnGcMem = "GcMem", warnDestructor = "Destructor",
warnLockLevel = "LockLevel", warnResultShadowed = "ResultShadowed",
warnInconsistentSpacing = "Spacing", warnCaseTransition = "CaseTransition",
warnCycleCreated = "CycleCreated", warnObservableStores = "ObservableStores",
warnResultUsed = "ResultUsed", warnUser = "User", hintSuccess = "Success",
hintSuccessX = "SuccessX", hintCC = "CC", hintLineTooLong = "LineTooLong",
hintXDeclaredButNotUsed = "XDeclaredButNotUsed",
hintXCannotRaiseY = "XCannotRaiseY",
hintConvToBaseNotNeeded = "ConvToBaseNotNeeded",
hintConvFromXtoItselfNotNeeded = "ConvFromXtoItselfNotNeeded",
hintExprAlwaysX = "ExprAlwaysX", hintQuitCalled = "QuitCalled",
hintProcessing = "Processing", hintCodeBegin = "CodeBegin",
hintCodeEnd = "CodeEnd", hintConf = "Conf", hintPath = "Path",
hintConditionAlwaysTrue = "CondTrue", hintConditionAlwaysFalse = "CondFalse",
hintName = "Name", hintPattern = "Pattern", hintExecuting = "Exec",
hintLinking = "Link", hintDependency = "Dependency", hintSource = "Source",
hintPerformance = "Performance", hintStackTrace = "StackTrace",
hintGCStats = "GCStats", hintGlobalVar = "GlobalVar",
hintExpandMacro = "ExpandMacro", hintUser = "User", hintUserRaw = "UserRaw",
hintExtendedContext = "ExtendedContext", hintMsgOrigin = "MsgOrigin"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L29)
```
TNoteKind = range[warnMin .. hintMax]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L177) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L177)
```
TNoteKinds = set[TNoteKind]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L178) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L178)
```
TFileInfo = object
fullPath*: AbsoluteFile
projPath*: RelativeFile
shortName*: string
quotedName*: Rope
quotedFullName*: Rope
lines*: seq[string]
dirtyFile*: AbsoluteFile
hash*: string
dirty*: bool
when defined(nimpretty):
fullContent*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L195)
```
FileIndex = distinct int32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L215) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L215)
```
TLineInfo = object
line*: uint16
col*: int16
fileIndex*: FileIndex
when defined(nimpretty):
offsetA*, offsetB*: int
commentOffsetA*, commentOffsetB*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L216) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L216)
```
TErrorOutput = enum
eStdOut, eStdErr
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L229) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L229)
```
TErrorOutputs = set[TErrorOutput]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L233) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L233)
```
ERecoverableError = object of ValueError
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L235) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L235)
```
ESuggestDone = object of ValueError
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L236) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L236)
```
Severity {...}{.pure.} = enum
Hint, Warning, Error
```
VS Code only supports these three [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L251) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L251)
```
MsgConfig = object
trackPos*: TLineInfo
trackPosAttached*: bool ## whether the tracking position was attached to
## some close token.
errorOutputs*: TErrorOutputs
msgContext*: seq[tuple[info: TLineInfo, detail: string]]
lastError*: TLineInfo
filenameToIndexTbl*: Table[string, FileIndex]
fileInfos*: seq[TFileInfo]
systemFileIdx*: FileIndex
```
does not need to be stored in the incremental cache [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L260) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L260) Consts
------
```
explanationsBaseUrl = "https://nim-lang.github.io/Nim"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L16)
```
MsgKindToStr: array[TMsgKind, string] = ["unknown error", "internal error: $1",
"illformed AST: $1", "cannot open \'$1\'", "\'$1\' expected",
"grid table is not implemented", "general parse error",
"new section expected", "invalid directive: \'$1\'",
"Cannot prove that \'$1\' is initialized.", "$1", "$1",
"cannot open \'$1\'",
"octal escape sequences do not exist; leading zero is ignored",
"\'$1\' is never read", "\'$1\' might not have been initialized", "$1",
"config file \'$1\' is deprecated",
"\'l\' should not be used as an identifier; may look like \'1\' (one)",
"unknown magic \'$1\' might crash the compiler",
"redefinition of label \'$1\'", "unknown substitution \'$1\'",
"language \'$1\' not supported", "field \'$1\' not supported",
"comment \'$1\' ignored", "\'$1\' has no type. Typeless parameters are deprecated; only allowed for \'template\'",
"use {.base.} for base methods; baseless methods are deprecated",
"write to foreign heap", "unsafe code: \'$1\'",
"imported and not used: \'$1\'", "inherit from a more precise exception type like ValueError, IOError or OSError. If these don\'t suit, inherit from CatchableError or Defect.",
"each identifier is a tuple", "setLen can potentially expand the sequence, but the element type \'$1\' doesn\'t have a valid default value",
"The \'$1\' type doesn\'t have a valid default value", "Cannot prove that \'$1\' is initialized. This will become a compile time error in the future.",
"cannot prove that field \'$1\' is accessible",
"cannot prove index \'$1\' is valid",
"unreachable else, all cases are already covered",
"unreachable code after \'return\' statement or \'{.noReturn.}\' proc",
"$1", "not GC-safe: \'$1\'", "$1",
"use explicit initialization of \'$1\' for clarity",
"\'$1\' uses GC\'ed memory", "usage of a type with a destructor in a non destructible context. This will become a compile time error in the future.",
"$1", "Special variable \'result\' is shadowed.",
"Number of spaces around \'$#\' is not consistent",
"Potential object case transition, instantiate new object instead", "$1",
"observable stores to \'$1\'", "used \'result\' variable", "$1",
"operation successful: $#",
"${loc} lines; ${sec}s; $mem; $build build; proj: $project; out: $output",
"CC: $1", "line too long", "\'$1\' is declared but not used", "$1",
"conversion to base object is not needed",
"conversion from $1 to itself is pointless",
"expression evaluates always to \'$1\'", "quit() called", "$1",
"generated code listing:", "end of listing", "used config file \'$1\'",
"added path: \'$1\'", "condition is always true: \'$1\'",
"condition is always false: \'$1\'", "$1", "$1", "$1", "$1", "$1", "$1",
"$1", "$1", "$1", "global variable declared here", "expanded macro: $1",
"$1", "$1", "$1", "$1"]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L75)
```
fatalMin = errUnknown
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L167)
```
fatalMax = errInternal
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L168) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L168)
```
errMin = errUnknown
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L169) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L169)
```
errMax = errUser
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L170)
```
warnMin = warnCannotOpenFile
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L171) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L171)
```
warnMax = warnUser
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L172) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L172)
```
hintMin = hintSuccess
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L173) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L173)
```
hintMax = hintMsgOrigin
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L174) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L174)
```
NotesVerbosity = [{warnCannotOpenFile..warnProveInit,
warnUnreachableElse..warnStaticIndexCheck, warnGcUnsafe2,
warnGcMem..warnCycleCreated, warnUser,
hintLineTooLong..hintQuitCalled,
hintConditionAlwaysTrue..hintName, hintPerformance,
hintExpandMacro..hintUserRaw}, {
warnCannotOpenFile..warnProveInit,
warnUnreachableElse..warnStaticIndexCheck, warnGcUnsafe2,
warnGcMem..warnCycleCreated, warnUser..hintProcessing, hintConf,
hintConditionAlwaysTrue..hintLinking, hintPerformance,
hintExpandMacro..hintUserRaw}, {warnCannotOpenFile..warnGcUnsafe2,
warnGcMem..warnCycleCreated,
warnUser..hintPerformance,
hintGCStats..hintUserRaw, hintMsgOrigin},
{12..49, 52..84}]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L190)
```
errXMustBeCompileTime = "\'$1\' can only be used in compile-time context"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L191) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L191)
```
errArgsNeedRunOption = "arguments can only be given if the \'--run\' option is selected"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L192) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L192)
```
InvalidFileIdx = -1'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L247) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L247)
```
unknownLineInfo = (line: 0'u, col: -1, fileIndex: -1)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L248) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L248)
```
trackPosInvalidFileIdx = -2'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L255) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L255)
```
commandLineIdx = -3'i32
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L257) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L257) Procs
-----
```
proc createDocLink(urlSuffix: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L20)
```
proc `==`(a, b: FileIndex): bool {...}{.borrow.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L238) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L238)
```
proc hash(i: TLineInfo): Hash {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L240) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L240)
```
proc raiseRecoverableError(msg: string) {...}{.noinline, raises: [ERecoverableError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L243) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L243)
```
proc initMsgConfig(): MsgConfig {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/lineinfos.nim#L273) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/lineinfos.nim#L273)
| programming_docs |
nim semfold semfold
=======
Imports
-------
<options>, <ast>, <trees>, <nimsets>, <platform>, <msgs>, <idents>, <renderer>, <types>, <commands>, <magicsys>, <modulegraphs>, <lineinfos> Procs
-----
```
proc errorType(g: ModuleGraph): PType {...}{.raises: [], tags: [].}
```
creates a type representing an error state [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L18)
```
proc newIntNodeT(intVal: Int128; n: PNode; g: ModuleGraph): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L23)
```
proc newFloatNodeT(floatVal: BiggestFloat; n: PNode; g: ModuleGraph): PNode {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L32)
```
proc newStrNodeT(strVal: string; n: PNode; g: ModuleGraph): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L40)
```
proc ordinalValToString(a: PNode; g: ModuleGraph): string {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L76)
```
proc evalOp(m: TMagic; n, a, b, c: PNode; g: ModuleGraph): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L155) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L155)
```
proc leValueConv(a, b: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L360) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L360)
```
proc newSymNodeTypeDesc(s: PSym; info: TLineInfo): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L515) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L515)
```
proc getConstExpr(m: PSym; n: PNode; g: ModuleGraph): PNode {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, Exception, IOError,
ValueError, ERecoverableError, KeyError, Exception, IOError, ValueError,
ERecoverableError, KeyError, Exception, ValueError, IOError,
ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semfold.nim#L523) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semfold.nim#L523)
nim Index Index
=====
Modules: <aliases>, <asciitables>, <ast>, <astalgo>, <bitsets>, <btrees>, <ccgmerge>, <ccgutils>, <cgen>, <cgendata>, <cgmeth>, <closureiters>, <cmdlinehelper>, <commands>, <condsyms>, <depends>, <dfa>, <docgen>, <docgen2>, <enumtostr>, <evaltempl>, <extccomp>, <filter_tmpl>, <filters>, <gorgeimpl>, <guards>, <idents>, <idgen>, <importer>, <incremental>, <index>, <injectdestructors>, <int128>, <isolation_check>, <jsgen>, <lambdalifting>, <lexer>, <liftdestructors>, <liftlocals>, <lineinfos>, <linter>, <llstream>, <lookups>, <lowerings>, <macrocacheimpl>, <magicsys>, <main>, <modulegraphs>, <modulepaths>, <modules>, <msgs>, <ndi>, <nimblecmd>, <nimconf>, <nimfix/prettybase>, <nimlexbase>, <nimpaths>, <nimsets>, <nodejs>, <nversion>, <optimizer>, <options>, <parampatterns>, <parser>, <passaux>, <passes>, <pathutils>, <patterns>, <platform>, <plugins/active>, <plugins/itersgen>, <plugins/locals>, <pluginsupport>, <pragmas>, <prefixmatches>, <procfind>, <renderer>, <renderverbatim>, <reorder>, <rod>, <rodutils>, <ropes>, <saturate>, <scriptconfig>, <sem>, <semdata>, <semfold>, <semmacrosanity>, <semparallel>, <sempass2>, <semtypinst>, <sighashes>, <sigmatch>, <sourcemap>, <spawn>, <strutils2>, <syntaxes>, <transf>, <trees>, <treetab>, <typeallowed>, <types>, <typesrenderer>, <varpartitions>, <vm>, <vmconv>, <vmdef>, <vmdeps>, <vmgen>, <vmmarshal>, <vmprofiler>, <wordrecg>.
### API symbols
[`$`:](#%60%24%60)
* [ast: `$`(s: PSym): string](ast#%24%2CPSym)
* [ast: `$`(x: TLockLevel): string](ast#%24%2CTLockLevel)
* [int128: `$`(a: Int128): string](int128#%24%2CInt128)
* [lexer: `$`(tok: Token): string](lexer#%24%2CToken)
* [modulegraphs: `$`(u: SigHash): string](modulegraphs#%24%2CSigHash)
* [msgs: `$`(conf: ConfigRef; info: TLineInfo): string](msgs#%24%2CConfigRef%2CTLineInfo)
* [msgs: `$`(info: TLineInfo): string](msgs#%24%2CTLineInfo)
* [nimblecmd: `$`(ver: Version): string](nimblecmd#%24%2CVersion)
* [options: `$`(c: IdeCmd): string](options#%24%2CIdeCmd)
* [pathutils: `$`(x: AnyPath): string](pathutils#%24%2CAnyPath)
* [renderer: `$`(n: PNode): string](renderer#%24%2CPNode)
* [ropes: `$`(r: Rope): string](ropes#%24%2CRope)
* [sigmatch: `$`(suggest: Suggest): string](sigmatch#%24%2CSuggest)
* [sourcemap: `$`(sourceNode: SourceNode): string](sourcemap#%24%2CSourceNode)
* [types: `$`(typ: PType): string](types#%24.t%2CPType)
* [varpartitions: `$`(config: ConfigRef; g: MutationInfo): string](varpartitions#%24%2CConfigRef%2CMutationInfo)
[`%`:](#%60%25%60)
* [ropes: `%`(frmt: static[FormatStr]; args: openArray[Rope]): Rope](ropes#%25%2Cstatic%5BFormatStr%5D%2CopenArray%5BRope%5D)
[`&`:](#%60%26amp%3B%60)
* [ropes: `&`(a: openArray[Rope]): Rope](ropes#%26%2CopenArray%5BRope%5D)
* [ropes: `&`(a, b: Rope): Rope](ropes#%26%2CRope%2CRope)
* [ropes: `&`(a: Rope; b: string): Rope](ropes#%26%2CRope%2Cstring)
* [ropes: `&`(a: string; b: Rope): Rope](ropes#%26%2Cstring%2CRope)
[`<=`:](#%60%26lt%3B%3D%60)
* [int128: `<=`(a: BiggestInt; b: Int128): bool](int128#%3C%3D%2CBiggestInt%2CInt128)
* [int128: `<=`(a: Int128; b: BiggestInt): bool](int128#%3C%3D%2CInt128%2CBiggestInt)
* [int128: `<=`(a, b: Int128): bool](int128#%3C%3D%2CInt128%2CInt128)
[`<`:](#%60%26lt%3B%60)
* [int128: `<`(a: BiggestInt; b: Int128): bool](int128#%3C%2CBiggestInt%2CInt128)
* [int128: `<`(a: Int128; b: BiggestInt): bool](int128#%3C%2CInt128%2CBiggestInt)
* [int128: `<`(a, b: Int128): bool](int128#%3C%2CInt128%2CInt128)
* [nimblecmd: `<`(ver: Version; ver2: Version): bool](nimblecmd#%3C%2CVersion%2CVersion)
[`\*=`:](#%60%2A%3D%60)
* [int128: `\*=`(a: var Int128; b: Int128)](int128#%2A%3D%2CInt128%2CInt128)
* [int128: `\*=`(a: var Int128; b: int32): Int128](int128#%2A%3D%2CInt128%2Cint32)
[`\*`:](#%60%2A%60)
* [int128: `\*`(lhs, rhs: Int128): Int128](int128#%2A%2CInt128%2CInt128)
* [int128: `\*`(a: Int128; b: int32): Int128](int128#%2A%2CInt128%2Cint32)
[`+=`:](#%60%2B%3D%60)
* [int128: `+=`(a: var Int128; b: Int128)](int128#%2B%3D%2CInt128%2CInt128)
[`+`:](#%60%2B%60)
* [int128: `+`(a: BiggestInt; b: Int128): Int128](int128#%2B%2CBiggestInt%2CInt128)
* [int128: `+`(a: Int128; b: BiggestInt): Int128](int128#%2B%2CInt128%2CBiggestInt)
* [int128: `+`(a, b: Int128): Int128](int128#%2B%2CInt128%2CInt128)
[`-=`:](#%60-%3D%60)
* [int128: `-=`(a: var Int128; b: Int128)](int128#-%3D%2CInt128%2CInt128)
[`-`:](#%60-%60)
* [int128: `-`(a: BiggestInt; b: Int128): Int128](int128#-%2CBiggestInt%2CInt128)
* [int128: `-`(a: Int128): Int128](int128#-%2CInt128)
* [int128: `-`(a: Int128; b: BiggestInt): Int128](int128#-%2CInt128%2CBiggestInt)
* [int128: `-`(a, b: Int128): Int128](int128#-%2CInt128%2CInt128)
[`/`:](#%60/%60)
* [pathutils: `/`(base: AbsoluteDir; f: RelativeDir): AbsoluteDir](pathutils#/%2CAbsoluteDir%2CRelativeDir)
* [pathutils: `/`(base: AbsoluteDir; f: RelativeFile): AbsoluteFile](pathutils#/%2CAbsoluteDir%2CRelativeFile)
[`==`:](#%60%3D%3D%60)
* [int128: `==`(a: BiggestInt; b: Int128): bool](int128#%3D%3D%2CBiggestInt%2CInt128)
* [int128: `==`(a: Int128; b: BiggestInt): bool](int128#%3D%3D%2CInt128%2CBiggestInt)
* [int128: `==`(a, b: Int128): bool](int128#%3D%3D%2CInt128%2CInt128)
* [lineinfos: `==`(a, b: FileIndex): bool](lineinfos#%3D%3D%2CFileIndex%2CFileIndex)
* [modulegraphs: `==`(a, b: SigHash): bool](modulegraphs#%3D%3D%2CSigHash%2CSigHash)
* [msgs: `==`(a, b: TLineInfo): bool](msgs#%3D%3D%2CTLineInfo%2CTLineInfo)
* [pathutils: `==`[T: AnyPath](x, y: T): bool](pathutils#%3D%3D%2CT%2CT)
[`??`:](#%60%3F%3F%60)
* [msgs: `??`(conf: ConfigRef; info: TLineInfo; filename: string): bool](msgs#%3F%3F%2CConfigRef%2CTLineInfo%2Cstring)
[`[]=`:](#%60%5B%5D%3D%60)
* [ast: `[]=`(n: Indexable; i: BackwardsIndex; x: Indexable)](ast#%5B%5D%3D.t%2CIndexable%2CBackwardsIndex%2CIndexable)
* [ast: `[]=`(n: Indexable; i: int; x: Indexable)](ast#%5B%5D%3D.t%2CIndexable%2Cint%2CIndexable)
[`[]`:](#%60%5B%5D%60)
* [ast: `[]`(n: Indexable; i: BackwardsIndex): Indexable](ast#%5B%5D.t%2CIndexable%2CBackwardsIndex)
* [ast: `[]`(n: Indexable; i: int): Indexable](ast#%5B%5D.t%2CIndexable%2Cint)
[`div`:](#%60div%60)
* [int128: `div`(a, b: Int128): Int128](int128#div%2CInt128%2CInt128)
[`mod`:](#%60mod%60)
* [int128: `mod`(a, b: Int128): Int128](int128#mod%2CInt128%2CInt128)
[`shl`:](#%60shl%60)
* [int128: `shl`(a: Int128; b: int): Int128](int128#shl%2CInt128%2Cint)
[`shr`:](#%60shr%60)
* [int128: `shr`(a: Int128; b: int): Int128](int128#shr%2CInt128%2Cint)
[`|\*|`:](#%60%7C%2A%7C%60)
* [saturate: `|\*|`(a, b: BiggestInt): BiggestInt](saturate#%7C%2A%7C%2CBiggestInt%2CBiggestInt)
[`|+|`:](#%60%7C%2B%7C%60)
* [saturate: `|+|`(a, b: BiggestInt): BiggestInt](saturate#%7C%2B%7C%2CBiggestInt%2CBiggestInt)
[`|-|`:](#%60%7C-%7C%60)
* [saturate: `|-|`(a, b: BiggestInt): BiggestInt](saturate#%7C-%7C%2CBiggestInt%2CBiggestInt)
[`|abs|`:](#%60%7Cabs%7C%60)
* [saturate: `|abs|`(a: BiggestInt): BiggestInt](saturate#%7Cabs%7C%2CBiggestInt)
[`|div|`:](#%60%7Cdiv%7C%60)
* [saturate: `|div|`(a, b: BiggestInt): BiggestInt](saturate#%7Cdiv%7C%2CBiggestInt%2CBiggestInt)
[`|mod|`:](#%60%7Cmod%7C%60)
* [saturate: `|mod|`(a, b: BiggestInt): BiggestInt](saturate#%7Cmod%7C%2CBiggestInt%2CBiggestInt)
[`~`:](#%60~%60)
* [ropes: `~`(r: string): Rope](ropes#~.t%2Cstring)
[abs:](#abs)
* [int128: abs(a: Int128): Int128](int128#abs%2CInt128)
[AbsoluteDir:](#AbsoluteDir)
* [pathutils: AbsoluteDir](pathutils#AbsoluteDir)
[AbsoluteFile:](#AbsoluteFile)
* [pathutils: AbsoluteFile](pathutils#AbsoluteFile)
[absOutFile:](#absOutFile)
* [options: absOutFile(conf: ConfigRef): AbsoluteFile](options#absOutFile%2CConfigRef)
[abstractInst:](#abstractInst)
* [types: abstractInst](types#abstractInst)
[abstractInstOwned:](#abstractInstOwned)
* [types: abstractInstOwned](types#abstractInstOwned)
[abstractPtrs:](#abstractPtrs)
* [types: abstractPtrs](types#abstractPtrs)
[abstractRange:](#abstractRange)
* [types: abstractRange](types#abstractRange)
[abstractVar:](#abstractVar)
* [types: abstractVar](types#abstractVar)
[abstractVarRange:](#abstractVarRange)
* [ast: abstractVarRange](ast#abstractVarRange)
[add:](#add)
* [ast: add(father, son: Indexable)](ast#add%2CIndexable%2CIndexable)
* [btrees: add[Key, Val](b: var BTree[Key, Val]; key: Key; val: Val)](btrees#add%2CBTree%5BKey%2CVal%5D%2CKey%2CVal)
* [ropes: add(a: var Rope; b: Rope)](ropes#add%2CRope%2CRope)
* [ropes: add(a: var Rope; b: string)](ropes#add%2CRope%2Cstring)
[addAsgnFact:](#addAsgnFact)
* [guards: addAsgnFact(m: var TModel; key, value: PNode)](guards#addAsgnFact%2CTModel%2CPNode%2CPNode)
[addCaseBranchFacts:](#addCaseBranchFacts)
* [guards: addCaseBranchFacts(m: var TModel; n: PNode; i: int)](guards#addCaseBranchFacts%2CTModel%2CPNode%2Cint)
[addCmdPrefix:](#addCmdPrefix)
* [cmdlinehelper: addCmdPrefix(result: var string; kind: CmdLineKind)](cmdlinehelper#addCmdPrefix%2Cstring%2CCmdLineKind)
[addCompileOption:](#addCompileOption)
* [extccomp: addCompileOption(conf: ConfigRef; option: string)](extccomp#addCompileOption%2CConfigRef%2Cstring)
[addCompileOptionCmd:](#addCompileOptionCmd)
* [extccomp: addCompileOptionCmd(conf: ConfigRef; option: string)](extccomp#addCompileOptionCmd%2CConfigRef%2Cstring)
[addConverter:](#addConverter)
* [semdata: addConverter(c: PContext; conv: PSym)](semdata#addConverter%2CPContext%2CPSym)
[addDecl:](#addDecl)
* [lookups: addDecl(c: PContext; sym: PSym)](lookups#addDecl%2CPContext%2CPSym)
* [lookups: addDecl(c: PContext; sym: PSym; info: TLineInfo)](lookups#addDecl%2CPContext%2CPSym%2CTLineInfo)
[addDeclAt:](#addDeclAt)
* [lookups: addDeclAt(c: PContext; scope: PScope; sym: PSym)](lookups#addDeclAt%2CPContext%2CPScope%2CPSym)
[addDep:](#addDep)
* [modulegraphs: addDep(g: ModuleGraph; m: PSym; dep: FileIndex)](modulegraphs#addDep%2CModuleGraph%2CPSym%2CFileIndex)
[addDiscriminantFact:](#addDiscriminantFact)
* [guards: addDiscriminantFact(m: var TModel; n: PNode)](guards#addDiscriminantFact%2CTModel%2CPNode)
[addExternalFileToCompile:](#addExternalFileToCompile)
* [extccomp: addExternalFileToCompile(conf: ConfigRef; filename: AbsoluteFile)](extccomp#addExternalFileToCompile%2CConfigRef%2CAbsoluteFile)
* [extccomp: addExternalFileToCompile(conf: ConfigRef; c: var Cfile)](extccomp#addExternalFileToCompile%2CConfigRef%2CCfile)
[addExternalFileToLink:](#addExternalFileToLink)
* [extccomp: addExternalFileToLink(conf: ConfigRef; filename: AbsoluteFile)](extccomp#addExternalFileToLink%2CConfigRef%2CAbsoluteFile)
[addf:](#addf)
* [ropes: addf(c: var Rope; frmt: FormatStr; args: openArray[Rope])](ropes#addf.t%2CRope%2CFormatStr%2CopenArray%5BRope%5D)
[addFact:](#addFact)
* [guards: addFact(m: var TModel; nn: PNode)](guards#addFact%2CTModel%2CPNode)
[addFactLe:](#addFactLe)
* [guards: addFactLe(m: var TModel; a, b: PNode)](guards#addFactLe%2CTModel%2CPNode%2CPNode)
[addFactLt:](#addFactLt)
* [guards: addFactLt(m: var TModel; a, b: PNode)](guards#addFactLt%2CTModel%2CPNode%2CPNode)
[addFactNeg:](#addFactNeg)
* [guards: addFactNeg(m: var TModel; n: PNode)](guards#addFactNeg%2CTModel%2CPNode)
[addField:](#addField)
* [lowerings: addField(obj: PType; s: PSym; cache: IdentCache)](lowerings#addField%2CPType%2CPSym%2CIdentCache)
[addFileExt:](#addFileExt)
* [pathutils: addFileExt(x: AbsoluteFile; ext: string): AbsoluteFile](pathutils#addFileExt%2CAbsoluteFile%2Cstring)
* [pathutils: addFileExt(x: RelativeFile; ext: string): RelativeFile](pathutils#addFileExt%2CRelativeFile%2Cstring)
[addFileToCompile:](#addFileToCompile)
* [extccomp: addFileToCompile(conf: ConfigRef; cf: Cfile)](extccomp#addFileToCompile%2CConfigRef%2CCfile)
[addIncludeDep:](#addIncludeDep)
* [modulegraphs: addIncludeDep(g: ModuleGraph; module, includeFile: FileIndex)](modulegraphs#addIncludeDep%2CModuleGraph%2CFileIndex%2CFileIndex)
[addInt128:](#addInt128)
* [int128: addInt128(result: var string; value: Int128)](int128#addInt128%2Cstring%2CInt128)
[addInterfaceDecl:](#addInterfaceDecl)
* [lookups: addInterfaceDecl(c: PContext; sym: PSym)](lookups#addInterfaceDecl%2CPContext%2CPSym)
[addInterfaceDeclAt:](#addInterfaceDeclAt)
* [lookups: addInterfaceDeclAt(c: PContext; scope: PScope; sym: PSym)](lookups#addInterfaceDeclAt%2CPContext%2CPScope%2CPSym)
[addInterfaceOverloadableSymAt:](#addInterfaceOverloadableSymAt)
* [lookups: addInterfaceOverloadableSymAt(c: PContext; scope: PScope; sym: PSym)](lookups#addInterfaceOverloadableSymAt%2CPContext%2CPScope%2CPSym)
[addLinkOption:](#addLinkOption)
* [extccomp: addLinkOption(conf: ConfigRef; option: string)](extccomp#addLinkOption%2CConfigRef%2Cstring)
[addLinkOptionCmd:](#addLinkOptionCmd)
* [extccomp: addLinkOptionCmd(conf: ConfigRef; option: string)](extccomp#addLinkOptionCmd%2CConfigRef%2Cstring)
[addLocalCompileOption:](#addLocalCompileOption)
* [extccomp: addLocalCompileOption(conf: ConfigRef; option: string; nimfile: AbsoluteFile)](extccomp#addLocalCompileOption%2CConfigRef%2Cstring%2CAbsoluteFile)
[addMapping:](#addMapping)
* [sourcemap: addMapping(map: SourceMapGenerator; mapping: Mapping)](sourcemap#addMapping%2CSourceMapGenerator%2CMapping)
[addModuleDep:](#addModuleDep)
* [incremental: addModuleDep(incr: var IncrementalCtx; conf: ConfigRef; module, fileIdx: FileIndex; isIncludeFile: bool)](incremental#addModuleDep.t%2CIncrementalCtx%2CConfigRef%2CFileIndex%2CFileIndex%2Cbool)
* [rod: addModuleDep(g: ModuleGraph; module, fileIdx: FileIndex; isIncludeFile: bool)](rod#addModuleDep.t%2CModuleGraph%2CFileIndex%2CFileIndex%2Cbool)
[addOverloadableSymAt:](#addOverloadableSymAt)
* [lookups: addOverloadableSymAt(c: PContext; scope: PScope; fn: PSym)](lookups#addOverloadableSymAt%2CPContext%2CPScope%2CPSym)
[addParam:](#addParam)
* [ast: addParam(procType: PType; param: PSym)](ast#addParam%2CPType%2CPSym)
[addPath:](#addPath)
* [nimblecmd: addPath(conf: ConfigRef; path: AbsoluteDir; info: TLineInfo)](nimblecmd#addPath%2CConfigRef%2CAbsoluteDir%2CTLineInfo)
[addPattern:](#addPattern)
* [semdata: addPattern(c: PContext; p: PSym)](semdata#addPattern%2CPContext%2CPSym)
[addPrelimDecl:](#addPrelimDecl)
* [lookups: addPrelimDecl(c: PContext; sym: PSym)](lookups#addPrelimDecl%2CPContext%2CPSym)
[addSonNilAllowed:](#addSonNilAllowed)
* [ast: addSonNilAllowed(father, son: PNode)](ast#addSonNilAllowed%2CPNode%2CPNode)
[addSonSkipIntLit:](#addSonSkipIntLit)
* [magicsys: addSonSkipIntLit(father, son: PType)](magicsys#addSonSkipIntLit%2CPType%2CPType)
[addSym:](#addSym)
* [lookups: addSym(scope: PScope; s: PSym)](lookups#addSym.t%2CPScope%2CPSym)
[addToHex:](#addToHex)
* [int128: addToHex(result: var string; arg: Int128)](int128#addToHex%2Cstring%2CInt128)
[addToLib:](#addToLib)
* [semdata: addToLib(lib: PLib; sym: PSym)](semdata#addToLib%2CPLib%2CPSym)
[addTypeHeader:](#addTypeHeader)
* [types: addTypeHeader(result: var string; conf: ConfigRef; typ: PType; prefer: TPreferedDesc = preferMixed; getDeclarationPath = true)](types#addTypeHeader%2Cstring%2CConfigRef%2CPType%2CTPreferedDesc)
[addUniqueField:](#addUniqueField)
* [lowerings: addUniqueField(obj: PType; s: PSym; cache: IdentCache): PSym](lowerings#addUniqueField%2CPType%2CPSym%2CIdentCache)
[addUniqueSym:](#addUniqueSym)
* [lookups: addUniqueSym(scope: PScope; s: PSym): PSym](lookups#addUniqueSym%2CPScope%2CPSym)
[addVar:](#addVar)
* [lowerings: addVar(father, v: PNode)](lowerings#addVar%2CPNode%2CPNode)
* [lowerings: addVar(father, v, value: PNode)](lowerings#addVar%2CPNode%2CPNode%2CPNode)
[aliases:](#aliases)
* [dfa: aliases(obj, field: PNode): bool](dfa#aliases%2CPNode%2CPNode)
[alignTable:](#alignTable)
* [asciitables: alignTable(s: string; delim = ' '; fill = ' '; sep = " "): string](asciitables#alignTable%2Cstring%2Cchar%2Cchar%2Cstring)
[allowCast:](#allowCast)
* [TSandboxFlag.allowCast](vmdef#allowCast)
[AllowCommonBase:](#AllowCommonBase)
* [TTypeCmpFlag.AllowCommonBase](types#AllowCommonBase)
[allowInfiniteLoops:](#allowInfiniteLoops)
* [TSandboxFlag.allowInfiniteLoops](vmdef#allowInfiniteLoops)
[allowSemcheckedAstModification:](#allowSemcheckedAstModification)
* [LegacyFeature.allowSemcheckedAstModification](options#allowSemcheckedAstModification)
[allRoutinePragmas:](#allRoutinePragmas)
* [pragmas: allRoutinePragmas](pragmas#allRoutinePragmas)
[analyseObjectWithTypeField:](#analyseObjectWithTypeField)
* [types: analyseObjectWithTypeField(t: PType): TTypeFieldResult](types#analyseObjectWithTypeField%2CPType)
[annotateType:](#annotateType)
* [semmacrosanity: annotateType(n: PNode; t: PType; conf: ConfigRef)](semmacrosanity#annotateType%2CPNode%2CPType%2CConfigRef)
[AnyPath:](#AnyPath)
* [pathutils: AnyPath](pathutils#AnyPath)
[Apo:](#Apo)
* [nimlexbase: Apo](nimlexbase#Apo)
[appendToModule:](#appendToModule)
* [ast: appendToModule(m: PSym; n: PNode)](ast#appendToModule%2CPSym%2CPNode)
[applyRule:](#applyRule)
* [patterns: applyRule(c: PContext; s: PSym; n: PNode): PNode](patterns#applyRule%2CPContext%2CPSym%2CPNode)
[aqNoAlias:](#aqNoAlias)
* [TAliasRequest.aqNoAlias](parampatterns#aqNoAlias)
[aqNone:](#aqNone)
* [TAliasRequest.aqNone](parampatterns#aqNone)
[aqShouldAlias:](#aqShouldAlias)
* [TAliasRequest.aqShouldAlias](parampatterns#aqShouldAlias)
[arDiscriminant:](#arDiscriminant)
* [TAssignableResult.arDiscriminant](parampatterns#arDiscriminant)
[argtypeMatches:](#argtypeMatches)
* [sigmatch: argtypeMatches(c: PContext; f, a: PType; fromHlo = false): bool](sigmatch#argtypeMatches%2CPContext%2CPType%2CPType)
[arLentValue:](#arLentValue)
* [TAssignableResult.arLentValue](parampatterns#arLentValue)
[arLocalLValue:](#arLocalLValue)
* [TAssignableResult.arLocalLValue](parampatterns#arLocalLValue)
[arLValue:](#arLValue)
* [TAssignableResult.arLValue](parampatterns#arLValue)
[arMaybe:](#arMaybe)
* [TAnalysisResult.arMaybe](aliases#arMaybe)
[arNo:](#arNo)
* [TAnalysisResult.arNo](aliases#arNo)
[arNone:](#arNone)
* [TAssignableResult.arNone](parampatterns#arNone)
[arStrange:](#arStrange)
* [TAssignableResult.arStrange](parampatterns#arStrange)
[arYes:](#arYes)
* [TAnalysisResult.arYes](aliases#arYes)
[asink:](#asink)
* [ast: asink(t: PType): PSym](ast#asink.t%2CPType)
[assignIfDefault:](#assignIfDefault)
* [options: assignIfDefault[T](result: var T; val: T; def = default(T))](options#assignIfDefault%2CT%2CT%2Ctypeof%28default%28T%29%29)
[assignment:](#assignment)
* [ast: assignment(t: PType): PSym](ast#assignment.t%2CPType)
[assignType:](#assignType)
* [ast: assignType(dest, src: PType)](ast#assignType%2CPType%2CPType)
[astdef:](#astdef)
* [ast: astdef(s: PSym): PNode](ast#astdef%2CPSym)
[attachedAsgn:](#attachedAsgn)
* [TTypeAttachedOp.attachedAsgn](ast#attachedAsgn)
[attachedDeepCopy:](#attachedDeepCopy)
* [TTypeAttachedOp.attachedDeepCopy](ast#attachedDeepCopy)
[attachedDestructor:](#attachedDestructor)
* [TTypeAttachedOp.attachedDestructor](ast#attachedDestructor)
[attachedDispose:](#attachedDispose)
* [TTypeAttachedOp.attachedDispose](ast#attachedDispose)
[AttachedOpToStr:](#AttachedOpToStr)
* [ast: AttachedOpToStr](ast#AttachedOpToStr)
[attachedSink:](#attachedSink)
* [TTypeAttachedOp.attachedSink](ast#attachedSink)
[attachedTrace:](#attachedTrace)
* [TTypeAttachedOp.attachedTrace](ast#attachedTrace)
[backendC:](#backendC)
* [TBackend.backendC](options#backendC)
[backendCpp:](#backendCpp)
* [TBackend.backendCpp](options#backendCpp)
[backendInvalid:](#backendInvalid)
* [TBackend.backendInvalid](options#backendInvalid)
[backendJs:](#backendJs)
* [TBackend.backendJs](options#backendJs)
[backendObjc:](#backendObjc)
* [TBackend.backendObjc](options#backendObjc)
[BACKSPACE:](#BACKSPACE)
* [nimlexbase: BACKSPACE](nimlexbase#BACKSPACE)
[base:](#base)
* [types: base(t: PType): PType](types#base%2CPType)
[base10:](#base10)
* [NumericalBase.base10](lexer#base10)
[base16:](#base16)
* [NumericalBase.base16](lexer#base16)
[base2:](#base2)
* [NumericalBase.base2](lexer#base2)
[base8:](#base8)
* [NumericalBase.base8](lexer#base8)
[baseOfDistinct:](#baseOfDistinct)
* [types: baseOfDistinct(t: PType): PType](types#baseOfDistinct%2CPType)
[beforeRetNeeded:](#beforeRetNeeded)
* [TCProcFlag.beforeRetNeeded](cgendata#beforeRetNeeded)
[BEL:](#BEL)
* [nimlexbase: BEL](nimlexbase#BEL)
[bigEndian:](#bigEndian)
* [TEndian.bigEndian](platform#bigEndian)
[bindConcreteTypeToUserTypeClass:](#bindConcreteTypeToUserTypeClass)
* [types: bindConcreteTypeToUserTypeClass(tc, concrete: PType)](types#bindConcreteTypeToUserTypeClass.t%2CPType%2CPType)
[bitand:](#bitand)
* [int128: bitand(a, b: Int128): Int128](int128#bitand%2CInt128%2CInt128)
[bitnot:](#bitnot)
* [int128: bitnot(a: Int128): Int128](int128#bitnot%2CInt128)
[bitor:](#bitor)
* [int128: bitor(a, b: Int128): Int128](int128#bitor%2CInt128%2CInt128)
[bitSetCard:](#bitSetCard)
* [bitsets: bitSetCard(x: TBitSet): BiggestInt](bitsets#bitSetCard%2CTBitSet)
[bitSetContains:](#bitSetContains)
* [bitsets: bitSetContains(x, y: TBitSet): bool](bitsets#bitSetContains%2CTBitSet%2CTBitSet)
[bitSetDiff:](#bitSetDiff)
* [bitsets: bitSetDiff(x: var TBitSet; y: TBitSet)](bitsets#bitSetDiff%2CTBitSet%2CTBitSet)
[bitSetEquals:](#bitSetEquals)
* [bitsets: bitSetEquals(x, y: TBitSet): bool](bitsets#bitSetEquals%2CTBitSet%2CTBitSet)
[bitSetExcl:](#bitSetExcl)
* [bitsets: bitSetExcl(x: var TBitSet; elem: BiggestInt)](bitsets#bitSetExcl%2CTBitSet%2CBiggestInt)
[bitSetIn:](#bitSetIn)
* [bitsets: bitSetIn(x: TBitSet; e: BiggestInt): bool](bitsets#bitSetIn%2CTBitSet%2CBiggestInt)
[bitSetIncl:](#bitSetIncl)
* [bitsets: bitSetIncl(x: var TBitSet; elem: BiggestInt)](bitsets#bitSetIncl%2CTBitSet%2CBiggestInt)
[bitSetInit:](#bitSetInit)
* [bitsets: bitSetInit(b: var TBitSet; length: int)](bitsets#bitSetInit%2CTBitSet%2Cint)
[bitSetIntersect:](#bitSetIntersect)
* [bitsets: bitSetIntersect(x: var TBitSet; y: TBitSet)](bitsets#bitSetIntersect%2CTBitSet%2CTBitSet)
[bitSetSymDiff:](#bitSetSymDiff)
* [bitsets: bitSetSymDiff(x: var TBitSet; y: TBitSet)](bitsets#bitSetSymDiff%2CTBitSet%2CTBitSet)
[bitSetUnion:](#bitSetUnion)
* [bitsets: bitSetUnion(x: var TBitSet; y: TBitSet)](bitsets#bitSetUnion%2CTBitSet%2CTBitSet)
[bitxor:](#bitxor)
* [int128: bitxor(a, b: Int128): Int128](int128#bitxor%2CInt128%2CInt128)
[BModule:](#BModule)
* [cgendata: BModule](cgendata#BModule)
[BModuleList:](#BModuleList)
* [cgendata: BModuleList](cgendata#BModuleList)
[bodyPos:](#bodyPos)
* [ast: bodyPos](ast#bodyPos)
[boolArg:](#boolArg)
* [filters: boolArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: bool): bool](filters#boolArg%2CConfigRef%2CPNode%2Cstring%2Cint%2Cbool)
[borrowChecking:](#borrowChecking)
* [Goal.borrowChecking](varpartitions#borrowChecking)
[BProc:](#BProc)
* [cgendata: BProc](cgendata#BProc)
[bracketKind:](#bracketKind)
* [renderer: bracketKind(g: TSrcGen; n: PNode): BracketKind](renderer#bracketKind%2CTSrcGen%2CPNode)
[BTree:](#BTree)
* [btrees: BTree](btrees#BTree)
[buildAdd:](#buildAdd)
* [guards: buildAdd(a: PNode; b: BiggestInt; o: Operators): PNode](guards#buildAdd%2CPNode%2CBiggestInt%2COperators)
[buildCall:](#buildCall)
* [guards: buildCall(op: PSym; a: PNode): PNode](guards#buildCall%2CPSym%2CPNode)
* [guards: buildCall(op: PSym; a, b: PNode): PNode](guards#buildCall%2CPSym%2CPNode%2CPNode)
[buildLe:](#buildLe)
* [guards: buildLe(o: Operators; a, b: PNode): PNode](guards#buildLe%2COperators%2CPNode%2CPNode)
[byteExcess:](#byteExcess)
* [vmdef: byteExcess](vmdef#byteExcess)
[cacheTypeInst:](#cacheTypeInst)
* [semtypinst: cacheTypeInst(inst: PType)](semtypinst#cacheTypeInst%2CPType)
[callCCompiler:](#callCCompiler)
* [extccomp: callCCompiler(conf: ConfigRef)](extccomp#callCCompiler%2CConfigRef)
[callCodegenProc:](#callCodegenProc)
* [lowerings: callCodegenProc(g: ModuleGraph; name: string; info: TLineInfo = unknownLineInfo; arg1, arg2, arg3, optionalArgs: PNode = nil): PNode](lowerings#callCodegenProc%2CModuleGraph%2Cstring%2CTLineInfo%2CPNode%2CPNode%2CPNode%2CPNode)
[callOperator:](#callOperator)
* [Feature.callOperator](options#callOperator)
[canAlias:](#canAlias)
* [isolation\_check: canAlias(arg, ret: PType): bool](isolation_check#canAlias%2CPType%2CPType)
[CandidateError:](#CandidateError)
* [sigmatch: CandidateError](sigmatch#CandidateError)
[CandidateErrors:](#CandidateErrors)
* [sigmatch: CandidateErrors](sigmatch#CandidateErrors)
[canFormAcycle:](#canFormAcycle)
* [types: canFormAcycle(typ: PType): bool](types#canFormAcycle%2CPType)
[canon:](#canon)
* [guards: canon(n: PNode; o: Operators): PNode](guards#canon%2CPNode%2COperators)
[canonicalizePath:](#canonicalizePath)
* [options: canonicalizePath(conf: ConfigRef; path: AbsoluteFile): AbsoluteFile](options#canonicalizePath%2CConfigRef%2CAbsoluteFile)
[canonValue:](#canonValue)
* [vmgen: canonValue(n: PNode): PNode](vmgen#canonValue%2CPNode)
[canRaise:](#canRaise)
* [ast: canRaise(fn: PNode): bool](ast#canRaise%2CPNode)
[canRaiseConservative:](#canRaiseConservative)
* [ast: canRaiseConservative(fn: PNode): bool](ast#canRaiseConservative%2CPNode)
[cardSet:](#cardSet)
* [nimsets: cardSet(conf: ConfigRef; a: PNode): BiggestInt](nimsets#cardSet%2CConfigRef%2CPNode)
[carryPass:](#carryPass)
* [passes: carryPass(g: ModuleGraph; p: TPass; module: PSym; m: TPassData): TPassData](passes#carryPass%2CModuleGraph%2CTPass%2CPSym%2CTPassData)
[carryPasses:](#carryPasses)
* [passes: carryPasses(g: ModuleGraph; nodes: PNode; module: PSym; passes: openArray[TPass])](passes#carryPasses%2CModuleGraph%2CPNode%2CPSym%2CopenArray%5BTPass%5D)
[caseStmtMacros:](#caseStmtMacros)
* [Feature.caseStmtMacros](options#caseStmtMacros)
[castToInt64:](#castToInt64)
* [int128: castToInt64(arg: Int128): int64](int128#castToInt64%2CInt128)
[castToUInt64:](#castToUInt64)
* [int128: castToUInt64(arg: Int128): uint64](int128#castToUInt64%2CInt128)
[CC:](#CC)
* [extccomp: CC](extccomp#CC)
[ccBcc:](#ccBcc)
* [TSystemCC.ccBcc](options#ccBcc)
[ccCDecl:](#ccCDecl)
* [TCallingConvention.ccCDecl](ast#ccCDecl)
[ccCLang:](#ccCLang)
* [TSystemCC.ccCLang](options#ccCLang)
[ccClangCl:](#ccClangCl)
* [TSystemCC.ccClangCl](options#ccClangCl)
[ccClosure:](#ccClosure)
* [TCallingConvention.ccClosure](ast#ccClosure)
[ccEnv:](#ccEnv)
* [TSystemCC.ccEnv](options#ccEnv)
[ccFastCall:](#ccFastCall)
* [TCallingConvention.ccFastCall](ast#ccFastCall)
[ccGcc:](#ccGcc)
* [TSystemCC.ccGcc](options#ccGcc)
[ccgKeywordsHigh:](#ccgKeywordsHigh)
* [wordrecg: ccgKeywordsHigh](wordrecg#ccgKeywordsHigh)
[ccgKeywordsLow:](#ccgKeywordsLow)
* [wordrecg: ccgKeywordsLow](wordrecg#ccgKeywordsLow)
[ccHasSaneOverflow:](#ccHasSaneOverflow)
* [extccomp: ccHasSaneOverflow(conf: ConfigRef): bool](extccomp#ccHasSaneOverflow%2CConfigRef)
[ccIcc:](#ccIcc)
* [TSystemCC.ccIcc](options#ccIcc)
[ccIcl:](#ccIcl)
* [TSystemCC.ccIcl](options#ccIcl)
[ccInline:](#ccInline)
* [TCallingConvention.ccInline](ast#ccInline)
[ccLLVM\_Gcc:](#ccLLVM_Gcc)
* [TSystemCC.ccLLVM\_Gcc](options#ccLLVM_Gcc)
[ccNimCall:](#ccNimCall)
* [TCallingConvention.ccNimCall](ast#ccNimCall)
[ccNintendoSwitch:](#ccNintendoSwitch)
* [TSystemCC.ccNintendoSwitch](options#ccNintendoSwitch)
[ccNoConvention:](#ccNoConvention)
* [TCallingConvention.ccNoConvention](ast#ccNoConvention)
[ccNoInline:](#ccNoInline)
* [TCallingConvention.ccNoInline](ast#ccNoInline)
[ccNone:](#ccNone)
* [TSystemCC.ccNone](options#ccNone)
[ccSafeCall:](#ccSafeCall)
* [TCallingConvention.ccSafeCall](ast#ccSafeCall)
[ccStdCall:](#ccStdCall)
* [TCallingConvention.ccStdCall](ast#ccStdCall)
[ccSysCall:](#ccSysCall)
* [TCallingConvention.ccSysCall](ast#ccSysCall)
[ccTcc:](#ccTcc)
* [TSystemCC.ccTcc](options#ccTcc)
[ccThisCall:](#ccThisCall)
* [TCallingConvention.ccThisCall](ast#ccThisCall)
[ccVcc:](#ccVcc)
* [TSystemCC.ccVcc](options#ccVcc)
[Cell:](#Cell)
* [asciitables: Cell](asciitables#Cell)
[Cfile:](#Cfile)
* [options: Cfile](options#Cfile)
[CfileFlag:](#CfileFlag)
* [options: CfileFlag](options#CfileFlag)
[CfileList:](#CfileList)
* [options: CfileList](options#CfileList)
[cfsData:](#cfsData)
* [TCFileSection.cfsData](cgendata#cfsData)
[cfsDatInitProc:](#cfsDatInitProc)
* [TCFileSection.cfsDatInitProc](cgendata#cfsDatInitProc)
[cfsDebugInit:](#cfsDebugInit)
* [TCFileSection.cfsDebugInit](cgendata#cfsDebugInit)
[cfsDynLibDeinit:](#cfsDynLibDeinit)
* [TCFileSection.cfsDynLibDeinit](cgendata#cfsDynLibDeinit)
[cfsDynLibInit:](#cfsDynLibInit)
* [TCFileSection.cfsDynLibInit](cgendata#cfsDynLibInit)
[cfsFieldInfo:](#cfsFieldInfo)
* [TCFileSection.cfsFieldInfo](cgendata#cfsFieldInfo)
[cfsForwardTypes:](#cfsForwardTypes)
* [TCFileSection.cfsForwardTypes](cgendata#cfsForwardTypes)
[cfsFrameDefines:](#cfsFrameDefines)
* [TCFileSection.cfsFrameDefines](cgendata#cfsFrameDefines)
[cfsHeaders:](#cfsHeaders)
* [TCFileSection.cfsHeaders](cgendata#cfsHeaders)
[cfsInitProc:](#cfsInitProc)
* [TCFileSection.cfsInitProc](cgendata#cfsInitProc)
[cfsMergeInfo:](#cfsMergeInfo)
* [TCFileSection.cfsMergeInfo](cgendata#cfsMergeInfo)
[cfsProcHeaders:](#cfsProcHeaders)
* [TCFileSection.cfsProcHeaders](cgendata#cfsProcHeaders)
[cfsProcs:](#cfsProcs)
* [TCFileSection.cfsProcs](cgendata#cfsProcs)
[cfsSeqTypes:](#cfsSeqTypes)
* [TCFileSection.cfsSeqTypes](cgendata#cfsSeqTypes)
[cfsTypeInfo:](#cfsTypeInfo)
* [TCFileSection.cfsTypeInfo](cgendata#cfsTypeInfo)
[cfsTypeInit1:](#cfsTypeInit1)
* [TCFileSection.cfsTypeInit1](cgendata#cfsTypeInit1)
[cfsTypeInit2:](#cfsTypeInit2)
* [TCFileSection.cfsTypeInit2](cgendata#cfsTypeInit2)
[cfsTypeInit3:](#cfsTypeInit3)
* [TCFileSection.cfsTypeInit3](cgendata#cfsTypeInit3)
[cfsTypes:](#cfsTypes)
* [TCFileSection.cfsTypes](cgendata#cfsTypes)
[cfsVars:](#cfsVars)
* [TCFileSection.cfsVars](cgendata#cfsVars)
[cgDeclFrmt:](#cgDeclFrmt)
* [cgen: cgDeclFrmt(s: PSym): string](cgen#cgDeclFrmt.t%2CPSym)
[cgenModules:](#cgenModules)
* [cgendata: cgenModules(g: BModuleList): BModule](cgendata#cgenModules.i%2CBModuleList)
[cgenPass:](#cgenPass)
* [cgen: cgenPass](cgen#cgenPass)
[cgenWriteModules:](#cgenWriteModules)
* [cgen: cgenWriteModules(backend: RootRef; config: ConfigRef)](cgen#cgenWriteModules%2CRootRef%2CConfigRef)
[changeDetectedViaJsonBuildInstructions:](#changeDetectedViaJsonBuildInstructions)
* [extccomp: changeDetectedViaJsonBuildInstructions(conf: ConfigRef; projectfile: AbsoluteFile): bool](extccomp#changeDetectedViaJsonBuildInstructions%2CConfigRef%2CAbsoluteFile)
[changeFileExt:](#changeFileExt)
* [pathutils: changeFileExt(x: AbsoluteFile; ext: string): AbsoluteFile](pathutils#changeFileExt%2CAbsoluteFile%2Cstring)
* [pathutils: changeFileExt(x: RelativeFile; ext: string): RelativeFile](pathutils#changeFileExt%2CRelativeFile%2Cstring)
[charArg:](#charArg)
* [filters: charArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: char): char](filters#charArg%2CConfigRef%2CPNode%2Cstring%2Cint%2Cchar)
[checkAmbiguity:](#checkAmbiguity)
* [TLookupFlag.checkAmbiguity](lookups#checkAmbiguity)
[checkBorrowedLocations:](#checkBorrowedLocations)
* [varpartitions: checkBorrowedLocations(par: var Partitions; body: PNode; config: ConfigRef)](varpartitions#checkBorrowedLocations%2CPartitions%2CPNode%2CConfigRef)
[checkConstructedType:](#checkConstructedType)
* [semtypinst: checkConstructedType(conf: ConfigRef; info: TLineInfo; typ: PType)](semtypinst#checkConstructedType%2CConfigRef%2CTLineInfo%2CPType)
[checkFieldAccess:](#checkFieldAccess)
* [guards: checkFieldAccess(m: TModel; n: PNode; conf: ConfigRef)](guards#checkFieldAccess%2CTModel%2CPNode%2CConfigRef)
[checkForSideEffects:](#checkForSideEffects)
* [parampatterns: checkForSideEffects(n: PNode): TSideEffectAnalysis](parampatterns#checkForSideEffects%2CPNode)
[checkForSink:](#checkForSink)
* [sempass2: checkForSink(config: ConfigRef; owner: PSym; arg: PNode)](sempass2#checkForSink%2CConfigRef%2CPSym%2CPNode)
[checkIsolate:](#checkIsolate)
* [isolation\_check: checkIsolate(n: PNode): bool](isolation_check#checkIsolate%2CPNode)
[checkMethodEffects:](#checkMethodEffects)
* [sempass2: checkMethodEffects(g: ModuleGraph; disp, branch: PSym)](sempass2#checkMethodEffects%2CModuleGraph%2CPSym%2CPSym)
[checkMinSonsLen:](#checkMinSonsLen)
* [semdata: checkMinSonsLen(n: PNode; length: int; conf: ConfigRef)](semdata#checkMinSonsLen%2CPNode%2Cint%2CConfigRef)
[checkModule:](#checkModule)
* [TLookupFlag.checkModule](lookups#checkModule)
[checkModuleName:](#checkModuleName)
* [modulepaths: checkModuleName(conf: ConfigRef; n: PNode; doLocalError = true): FileIndex](modulepaths#checkModuleName%2CConfigRef%2CPNode)
[checkPragmaUse:](#checkPragmaUse)
* [linter: checkPragmaUse(conf: ConfigRef; info: TLineInfo; w: TSpecialWord; pragmaName: string)](linter#checkPragmaUse%2CConfigRef%2CTLineInfo%2CTSpecialWord%2Cstring)
[checkPureEnumFields:](#checkPureEnumFields)
* [TLookupFlag.checkPureEnumFields](lookups#checkPureEnumFields)
[checkSonsLen:](#checkSonsLen)
* [semdata: checkSonsLen(n: PNode; length: int; conf: ConfigRef)](semdata#checkSonsLen%2CPNode%2Cint%2CConfigRef)
[ChecksOptions:](#ChecksOptions)
* [options: ChecksOptions](options#ChecksOptions)
[checkUndeclared:](#checkUndeclared)
* [TLookupFlag.checkUndeclared](lookups#checkUndeclared)
[checkUnsignedConversions:](#checkUnsignedConversions)
* [LegacyFeature.checkUnsignedConversions](options#checkUnsignedConversions)
[Child:](#Child)
* [sourcemap: Child](sourcemap#Child)
[child:](#child)
* [sourcemap: child(node: SourceNode): Child](sourcemap#child%2CSourceNode)
* [sourcemap: child(s: string): Child](sourcemap#child%2Cstring)
[classify:](#classify)
* [types: classify(t: PType): OrdinalType](types#classify%2CPType)
[classifyViewType:](#classifyViewType)
* [typeallowed: classifyViewType(t: PType): ViewTypeKind](typeallowed#classifyViewType%2CPType)
[clearNimblePath:](#clearNimblePath)
* [options: clearNimblePath(conf: ConfigRef)](options#clearNimblePath%2CConfigRef)
[clearPasses:](#clearPasses)
* [passes: clearPasses(g: ModuleGraph)](passes#clearPasses%2CModuleGraph)
[close:](#close)
* [ndi: close(f: var NdiFile; conf: ConfigRef)](ndi#close%2CNdiFile%2CConfigRef)
[closeBaseLexer:](#closeBaseLexer)
* [nimlexbase: closeBaseLexer(L: var TBaseLexer)](nimlexbase#closeBaseLexer%2CTBaseLexer)
[closeLexer:](#closeLexer)
* [lexer: closeLexer(lex: var Lexer)](lexer#closeLexer%2CLexer)
[closeParser:](#closeParser)
* [parser: closeParser(p: var Parser)](parser#closeParser%2CParser)
[closeScope:](#closeScope)
* [lookups: closeScope(c: PContext)](lookups#closeScope%2CPContext)
[closeShadowScope:](#closeShadowScope)
* [lookups: closeShadowScope(c: PContext)](lookups#closeShadowScope%2CPContext)
[cmdCheck:](#cmdCheck)
* [TCommands.cmdCheck](options#cmdCheck)
[cmdCompileToBackend:](#cmdCompileToBackend)
* [TCommands.cmdCompileToBackend](options#cmdCompileToBackend)
[cmdCompileToC:](#cmdCompileToC)
* [TCommands.cmdCompileToC](options#cmdCompileToC)
[cmdCompileToCpp:](#cmdCompileToCpp)
* [TCommands.cmdCompileToCpp](options#cmdCompileToCpp)
[cmdCompileToJS:](#cmdCompileToJS)
* [TCommands.cmdCompileToJS](options#cmdCompileToJS)
[cmdCompileToLLVM:](#cmdCompileToLLVM)
* [TCommands.cmdCompileToLLVM](options#cmdCompileToLLVM)
[cmdCompileToOC:](#cmdCompileToOC)
* [TCommands.cmdCompileToOC](options#cmdCompileToOC)
[cmdDef:](#cmdDef)
* [TCommands.cmdDef](options#cmdDef)
[cmdDoc:](#cmdDoc)
* [TCommands.cmdDoc](options#cmdDoc)
[cmdDump:](#cmdDump)
* [TCommands.cmdDump](options#cmdDump)
[cmdGenDepend:](#cmdGenDepend)
* [TCommands.cmdGenDepend](options#cmdGenDepend)
[cmdIdeTools:](#cmdIdeTools)
* [TCommands.cmdIdeTools](options#cmdIdeTools)
[cmdInteractive:](#cmdInteractive)
* [TCommands.cmdInteractive](options#cmdInteractive)
[cmdInterpret:](#cmdInterpret)
* [TCommands.cmdInterpret](options#cmdInterpret)
[cmdJsonScript:](#cmdJsonScript)
* [TCommands.cmdJsonScript](options#cmdJsonScript)
[cmdNone:](#cmdNone)
* [TCommands.cmdNone](options#cmdNone)
[cmdParse:](#cmdParse)
* [TCommands.cmdParse](options#cmdParse)
[cmdPretty:](#cmdPretty)
* [TCommands.cmdPretty](options#cmdPretty)
[cmdRst2html:](#cmdRst2html)
* [TCommands.cmdRst2html](options#cmdRst2html)
[cmdRst2tex:](#cmdRst2tex)
* [TCommands.cmdRst2tex](options#cmdRst2tex)
[cmdRun:](#cmdRun)
* [TCommands.cmdRun](options#cmdRun)
[cmdScan:](#cmdScan)
* [TCommands.cmdScan](options#cmdScan)
[cmp:](#cmp)
* [int128: cmp(a, b: Int128): int](int128#cmp%2CInt128%2CInt128)
[cmpCandidates:](#cmpCandidates)
* [sigmatch: cmpCandidates(a, b: TCandidate): int](sigmatch#cmpCandidates%2CTCandidate%2CTCandidate)
[cmpIgnoreStyle:](#cmpIgnoreStyle)
* [idents: cmpIgnoreStyle(a, b: cstring; blen: int): int](idents#cmpIgnoreStyle%2Ccstring%2Ccstring%2Cint)
[cmpPaths:](#cmpPaths)
* [pathutils: cmpPaths(x, y: AbsoluteDir): int](pathutils#cmpPaths%2CAbsoluteDir%2CAbsoluteDir)
[cmpTypes:](#cmpTypes)
* [sigmatch: cmpTypes(c: PContext; f, a: PType): TTypeRelation](sigmatch#cmpTypes%2CPContext%2CPType%2CPType)
[CoConsiderOwned:](#CoConsiderOwned)
* [ConsiderFlag.CoConsiderOwned](sighashes#CoConsiderOwned)
[CodegenFlag:](#CodegenFlag)
* [cgendata: CodegenFlag](cgendata#CodegenFlag)
[codeReordering:](#codeReordering)
* [Feature.codeReordering](options#codeReordering)
[CoDistinct:](#CoDistinct)
* [ConsiderFlag.CoDistinct](sighashes#CoDistinct)
[CoHashTypeInsideNode:](#CoHashTypeInsideNode)
* [ConsiderFlag.CoHashTypeInsideNode](sighashes#CoHashTypeInsideNode)
[CoIgnoreRange:](#CoIgnoreRange)
* [ConsiderFlag.CoIgnoreRange](sighashes#CoIgnoreRange)
[ColOffset:](#ColOffset)
* [msgs: ColOffset](msgs#ColOffset)
[commandBuildIndex:](#commandBuildIndex)
* [docgen: commandBuildIndex(conf: ConfigRef; dir: string; outFile = RelativeFile"")](docgen#commandBuildIndex%2CConfigRef%2Cstring)
[commandDoc:](#commandDoc)
* [docgen: commandDoc(cache: IdentCache; conf: ConfigRef)](docgen#commandDoc%2CIdentCache%2CConfigRef)
[commandJson:](#commandJson)
* [docgen: commandJson(cache: IdentCache; conf: ConfigRef)](docgen#commandJson%2CIdentCache%2CConfigRef)
[commandLineDesc:](#commandLineDesc)
* [msgs: commandLineDesc](msgs#commandLineDesc)
[commandLineIdx:](#commandLineIdx)
* [lineinfos: commandLineIdx](lineinfos#commandLineIdx)
[commandRst2Html:](#commandRst2Html)
* [docgen: commandRst2Html(cache: IdentCache; conf: ConfigRef)](docgen#commandRst2Html%2CIdentCache%2CConfigRef)
[commandRst2TeX:](#commandRst2TeX)
* [docgen: commandRst2TeX(cache: IdentCache; conf: ConfigRef)](docgen#commandRst2TeX%2CIdentCache%2CConfigRef)
[commandTags:](#commandTags)
* [docgen: commandTags(cache: IdentCache; conf: ConfigRef)](docgen#commandTags%2CIdentCache%2CConfigRef)
[commonOptimizations:](#commonOptimizations)
* [transf: commonOptimizations(g: ModuleGraph; c: PSym; n: PNode): PNode](transf#commonOptimizations%2CModuleGraph%2CPSym%2CPNode)
[commonSuperclass:](#commonSuperclass)
* [types: commonSuperclass(a, b: PType): PType](types#commonSuperclass%2CPType%2CPType)
[commonType:](#commonType)
* [sem: commonType(x: PType; y: PNode): PType](sem#commonType%2CPType%2CPNode)
* [sem: commonType(x, y: PType): PType](sem#commonType%2CPType%2CPType)
[commonTypeBegin:](#commonTypeBegin)
* [sem: commonTypeBegin(): PType](sem#commonTypeBegin.t)
[compareTypes:](#compareTypes)
* [types: compareTypes(x, y: PType; cmp: TDistinctCompare = dcEq; flags: TTypeCmpFlags = {}): bool](types#compareTypes%2CPType%2CPType%2CTDistinctCompare%2CTTypeCmpFlags)
[compatibleEffects:](#compatibleEffects)
* [types: compatibleEffects(formal, actual: PType): EffectsCompat](types#compatibleEffects%2CPType%2CPType)
[compilationCachePresent:](#compilationCachePresent)
* [options: compilationCachePresent(conf: ConfigRef): untyped](options#compilationCachePresent.t%2CConfigRef)
[compileModule:](#compileModule)
* [modules: compileModule(graph: ModuleGraph; fileIdx: FileIndex; flags: TSymFlags): PSym](modules#compileModule%2CModuleGraph%2CFileIndex%2CTSymFlags)
[compileProject:](#compileProject)
* [modules: compileProject(graph: ModuleGraph; projectFileIdx = InvalidFileIdx)](modules#compileProject%2CModuleGraph)
[CompilesId:](#CompilesId)
* [ast: CompilesId](ast#CompilesId)
[compileSystemModule:](#compileSystemModule)
* [modules: compileSystemModule(graph: ModuleGraph)](modules#compileSystemModule%2CModuleGraph)
[compiletimeFFI:](#compiletimeFFI)
* [Feature.compiletimeFFI](options#compiletimeFFI)
[complement:](#complement)
* [nimsets: complement(conf: ConfigRef; a: PNode): PNode](nimsets#complement%2CConfigRef%2CPNode)
[completeCfilePath:](#completeCfilePath)
* [extccomp: completeCfilePath(conf: ConfigRef; cfile: AbsoluteFile; createSubDir: bool = true): AbsoluteFile](extccomp#completeCfilePath%2CConfigRef%2CAbsoluteFile%2Cbool)
[completeGeneratedFilePath:](#completeGeneratedFilePath)
* [options: completeGeneratedFilePath(conf: ConfigRef; f: AbsoluteFile; createSubDir: bool = true): AbsoluteFile](options#completeGeneratedFilePath%2CConfigRef%2CAbsoluteFile%2Cbool)
[computeCursors:](#computeCursors)
* [varpartitions: computeCursors(s: PSym; n: PNode; config: ConfigRef)](varpartitions#computeCursors%2CPSym%2CPNode%2CConfigRef)
[computeGraphPartitions:](#computeGraphPartitions)
* [varpartitions: computeGraphPartitions(s: PSym; n: PNode; config: ConfigRef; goals: set[Goal]): Partitions](varpartitions#computeGraphPartitions%2CPSym%2CPNode%2CConfigRef%2Cset%5BGoal%5D)
[computeSize:](#computeSize)
* [types: computeSize(conf: ConfigRef; typ: PType): BiggestInt](types#computeSize%2CConfigRef%2CPType)
[ConcreteTypes:](#ConcreteTypes)
* [ast: ConcreteTypes](ast#ConcreteTypes)
[config:](#config)
* [cgendata: config(m: BModule): ConfigRef](cgendata#config.t%2CBModule)
* [cgendata: config(p: BProc): ConfigRef](cgendata#config.t%2CBProc)
* [jsgen: config(p: PProc): ConfigRef](jsgen#config.t%2CPProc)
* [semdata: config(c: PContext): ConfigRef](semdata#config.t%2CPContext)
[ConfigRef:](#ConfigRef)
* [options: ConfigRef](options#ConfigRef)
[connectCallbacks:](#connectCallbacks)
* [modules: connectCallbacks(graph: ModuleGraph)](modules#connectCallbacks%2CModuleGraph)
[ConsiderFlag:](#ConsiderFlag)
* [sighashes: ConsiderFlag](sighashes#ConsiderFlag)
[considerGenSyms:](#considerGenSyms)
* [semdata: considerGenSyms(c: PContext; n: PNode)](semdata#considerGenSyms%2CPContext%2CPNode)
[considerQuotedIdent:](#considerQuotedIdent)
* [lookups: considerQuotedIdent(c: PContext; n: PNode; origin: PNode = nil): PIdent](lookups#considerQuotedIdent%2CPContext%2CPNode%2CPNode)
[ConstantDataTypes:](#ConstantDataTypes)
* [ast: ConstantDataTypes](ast#ConstantDataTypes)
[constParameters:](#constParameters)
* [Goal.constParameters](varpartitions#constParameters)
[constPragmas:](#constPragmas)
* [pragmas: constPragmas](pragmas#constPragmas)
[constructCfg:](#constructCfg)
* [dfa: constructCfg(s: PSym; body: PNode): ControlFlowGraph](dfa#constructCfg%2CPSym%2CPNode)
[contains:](#contains)
* [btrees: contains[Key, Val](b: BTree[Key, Val]; key: Key): bool](btrees#contains%2CBTree%5BKey%2CVal%5D%2CKey)
[containsCompileTimeOnly:](#containsCompileTimeOnly)
* [types: containsCompileTimeOnly(t: PType): bool](types#containsCompileTimeOnly%2CPType)
[containsGarbageCollectedRef:](#containsGarbageCollectedRef)
* [types: containsGarbageCollectedRef(typ: PType): bool](types#containsGarbageCollectedRef%2CPType)
[containsGenericType:](#containsGenericType)
* [types: containsGenericType(t: PType): bool](types#containsGenericType%2CPType)
[containsHiddenPointer:](#containsHiddenPointer)
* [types: containsHiddenPointer(typ: PType): bool](types#containsHiddenPointer%2CPType)
[containsManagedMemory:](#containsManagedMemory)
* [types: containsManagedMemory(typ: PType): bool](types#containsManagedMemory%2CPType)
[containsNode:](#containsNode)
* [ast: containsNode(n: PNode; kinds: TNodeKinds): bool](ast#containsNode%2CPNode%2CTNodeKinds)
[containsObject:](#containsObject)
* [types: containsObject(t: PType): bool](types#containsObject%2CPType)
[containsSets:](#containsSets)
* [nimsets: containsSets(conf: ConfigRef; a, b: PNode): bool](nimsets#containsSets%2CConfigRef%2CPNode%2CPNode)
[containsTyRef:](#containsTyRef)
* [types: containsTyRef(typ: PType): bool](types#containsTyRef%2CPType)
[ControlFlowGraph:](#ControlFlowGraph)
* [dfa: ControlFlowGraph](dfa#ControlFlowGraph)
[converterPragmas:](#converterPragmas)
* [pragmas: converterPragmas](pragmas#converterPragmas)
[CoOwnerSig:](#CoOwnerSig)
* [ConsiderFlag.CoOwnerSig](sighashes#CoOwnerSig)
[CoProc:](#CoProc)
* [ConsiderFlag.CoProc](sighashes#CoProc)
[copyFile:](#copyFile)
* [pathutils: copyFile(source, dest: AbsoluteFile)](pathutils#copyFile%2CAbsoluteFile%2CAbsoluteFile)
[copyIdTable:](#copyIdTable)
* [ast: copyIdTable(dest: var TIdTable; src: TIdTable)](ast#copyIdTable%2CTIdTable%2CTIdTable)
[copyNode:](#copyNode)
* [ast: copyNode(src: PNode): PNode](ast#copyNode%2CPNode)
[copyObjectSet:](#copyObjectSet)
* [ast: copyObjectSet(dest: var TObjectSet; src: TObjectSet)](ast#copyObjectSet%2CTObjectSet%2CTObjectSet)
[copyrightYear:](#copyrightYear)
* [options: copyrightYear](options#copyrightYear)
[copyStrTable:](#copyStrTable)
* [ast: copyStrTable(dest: var TStrTable; src: TStrTable)](ast#copyStrTable%2CTStrTable%2CTStrTable)
[copySym:](#copySym)
* [ast: copySym(s: PSym): PSym](ast#copySym%2CPSym)
[copyTree:](#copyTree)
* [ast: copyTree(src: PNode): PNode](ast#copyTree%2CPNode)
[copyTreeWithoutNode:](#copyTreeWithoutNode)
* [ast: copyTreeWithoutNode(src, skippedNode: PNode): PNode](ast#copyTreeWithoutNode%2CPNode%2CPNode)
[copyType:](#copyType)
* [ast: copyType(t: PType; owner: PSym; keepId: bool): PType](ast#copyType%2CPType%2CPSym%2Cbool)
[CoType:](#CoType)
* [ConsiderFlag.CoType](sighashes#CoType)
[countDefinedSymbols:](#countDefinedSymbols)
* [condsyms: countDefinedSymbols(symbols: StringTableRef): int](condsyms#countDefinedSymbols%2CStringTableRef)
[cpExact:](#cpExact)
* [TCheckPointResult.cpExact](sigmatch#cpExact)
[cpFuzzy:](#cpFuzzy)
* [TCheckPointResult.cpFuzzy](sigmatch#cpFuzzy)
[cpNone:](#cpNone)
* [TCheckPointResult.cpNone](sigmatch#cpNone)
[cppDefine:](#cppDefine)
* [options: cppDefine(c: ConfigRef; define: string)](options#cppDefine%2CConfigRef%2Cstring)
[cppNimSharedKeywords:](#cppNimSharedKeywords)
* [wordrecg: cppNimSharedKeywords](wordrecg#cppNimSharedKeywords)
[cpsInit:](#cpsInit)
* [TCProcSection.cpsInit](cgendata#cpsInit)
[cpsLocals:](#cpsLocals)
* [TCProcSection.cpsLocals](cgendata#cpsLocals)
[cpsStmts:](#cpsStmts)
* [TCProcSection.cpsStmts](cgendata#cpsStmts)
[CPU:](#CPU)
* [platform: CPU](platform#CPU)
[cpuAlpha:](#cpuAlpha)
* [TSystemCPU.cpuAlpha](platform#cpuAlpha)
[cpuAmd64:](#cpuAmd64)
* [TSystemCPU.cpuAmd64](platform#cpuAmd64)
[cpuArm:](#cpuArm)
* [TSystemCPU.cpuArm](platform#cpuArm)
[cpuArm64:](#cpuArm64)
* [TSystemCPU.cpuArm64](platform#cpuArm64)
[cpuAVR:](#cpuAVR)
* [TSystemCPU.cpuAVR](platform#cpuAVR)
[cpuEsp:](#cpuEsp)
* [TSystemCPU.cpuEsp](platform#cpuEsp)
[cpuHppa:](#cpuHppa)
* [TSystemCPU.cpuHppa](platform#cpuHppa)
[cpuI386:](#cpuI386)
* [TSystemCPU.cpuI386](platform#cpuI386)
[cpuIa64:](#cpuIa64)
* [TSystemCPU.cpuIa64](platform#cpuIa64)
[cpuJS:](#cpuJS)
* [TSystemCPU.cpuJS](platform#cpuJS)
[cpuM68k:](#cpuM68k)
* [TSystemCPU.cpuM68k](platform#cpuM68k)
[cpuMips:](#cpuMips)
* [TSystemCPU.cpuMips](platform#cpuMips)
[cpuMips64:](#cpuMips64)
* [TSystemCPU.cpuMips64](platform#cpuMips64)
[cpuMips64el:](#cpuMips64el)
* [TSystemCPU.cpuMips64el](platform#cpuMips64el)
[cpuMipsel:](#cpuMipsel)
* [TSystemCPU.cpuMipsel](platform#cpuMipsel)
[cpuMSP430:](#cpuMSP430)
* [TSystemCPU.cpuMSP430](platform#cpuMSP430)
[cpuNimVM:](#cpuNimVM)
* [TSystemCPU.cpuNimVM](platform#cpuNimVM)
[cpuNone:](#cpuNone)
* [TSystemCPU.cpuNone](platform#cpuNone)
[cpuPowerpc:](#cpuPowerpc)
* [TSystemCPU.cpuPowerpc](platform#cpuPowerpc)
[cpuPowerpc64:](#cpuPowerpc64)
* [TSystemCPU.cpuPowerpc64](platform#cpuPowerpc64)
[cpuPowerpc64el:](#cpuPowerpc64el)
* [TSystemCPU.cpuPowerpc64el](platform#cpuPowerpc64el)
[cpuRiscV32:](#cpuRiscV32)
* [TSystemCPU.cpuRiscV32](platform#cpuRiscV32)
[cpuRiscV64:](#cpuRiscV64)
* [TSystemCPU.cpuRiscV64](platform#cpuRiscV64)
[cpuSparc:](#cpuSparc)
* [TSystemCPU.cpuSparc](platform#cpuSparc)
[cpuSparc64:](#cpuSparc64)
* [TSystemCPU.cpuSparc64](platform#cpuSparc64)
[cpuVm:](#cpuVm)
* [TSystemCPU.cpuVm](platform#cpuVm)
[cpuWasm32:](#cpuWasm32)
* [TSystemCPU.cpuWasm32](platform#cpuWasm32)
[CR:](#CR)
* [nimlexbase: CR](nimlexbase#CR)
[createClosureIterStateType:](#createClosureIterStateType)
* [lambdalifting: createClosureIterStateType(g: ModuleGraph; iter: PSym): PType](lambdalifting#createClosureIterStateType%2CModuleGraph%2CPSym)
[createDir:](#createDir)
* [pathutils: createDir(x: AbsoluteDir)](pathutils#createDir%2CAbsoluteDir)
[createDocLink:](#createDocLink)
* [lineinfos: createDocLink(urlSuffix: string): string](lineinfos#createDocLink%2Cstring)
[createMagic:](#createMagic)
* [modulegraphs: createMagic(g: ModuleGraph; name: string; m: TMagic): PSym](modulegraphs#createMagic%2CModuleGraph%2Cstring%2CTMagic)
[createModuleAlias:](#createModuleAlias)
* [ast: createModuleAlias(s: PSym; newIdent: PIdent; info: TLineInfo; options: TOptions): PSym](ast#createModuleAlias%2CPSym%2CPIdent%2CTLineInfo%2CTOptions)
[createObj:](#createObj)
* [lowerings: createObj(g: ModuleGraph; owner: PSym; info: TLineInfo; final = true): PType](lowerings#createObj%2CModuleGraph%2CPSym%2CTLineInfo)
[createTypeBoundOps:](#createTypeBoundOps)
* [liftdestructors: createTypeBoundOps(g: ModuleGraph; c: PContext; orig: PType; info: TLineInfo)](liftdestructors#createTypeBoundOps%2CModuleGraph%2CPContext%2CPType%2CTLineInfo)
[csEmpty:](#csEmpty)
* [TCandidateState.csEmpty](sigmatch#csEmpty)
[csMatch:](#csMatch)
* [TCandidateState.csMatch](sigmatch#csMatch)
[csNoMatch:](#csNoMatch)
* [TCandidateState.csNoMatch](sigmatch#csNoMatch)
[ctArray:](#ctArray)
* [TCTypeKind.ctArray](cgendata#ctArray)
[ctBool:](#ctBool)
* [TCTypeKind.ctBool](cgendata#ctBool)
[ctChar:](#ctChar)
* [TCTypeKind.ctChar](cgendata#ctChar)
[ctCString:](#ctCString)
* [TCTypeKind.ctCString](cgendata#ctCString)
[ctfeWhitelist:](#ctfeWhitelist)
* [ast: ctfeWhitelist](ast#ctfeWhitelist)
[ctFloat:](#ctFloat)
* [TCTypeKind.ctFloat](cgendata#ctFloat)
[ctFloat128:](#ctFloat128)
* [TCTypeKind.ctFloat128](cgendata#ctFloat128)
[ctFloat32:](#ctFloat32)
* [TCTypeKind.ctFloat32](cgendata#ctFloat32)
[ctFloat64:](#ctFloat64)
* [TCTypeKind.ctFloat64](cgendata#ctFloat64)
[ctInt:](#ctInt)
* [TCTypeKind.ctInt](cgendata#ctInt)
[ctInt16:](#ctInt16)
* [TCTypeKind.ctInt16](cgendata#ctInt16)
[ctInt32:](#ctInt32)
* [TCTypeKind.ctInt32](cgendata#ctInt32)
[ctInt64:](#ctInt64)
* [TCTypeKind.ctInt64](cgendata#ctInt64)
[ctInt8:](#ctInt8)
* [TCTypeKind.ctInt8](cgendata#ctInt8)
[ctNimSeq:](#ctNimSeq)
* [TCTypeKind.ctNimSeq](cgendata#ctNimSeq)
[ctNimStr:](#ctNimStr)
* [TCTypeKind.ctNimStr](cgendata#ctNimStr)
[ctProc:](#ctProc)
* [TCTypeKind.ctProc](cgendata#ctProc)
[ctPtr:](#ctPtr)
* [TCTypeKind.ctPtr](cgendata#ctPtr)
[ctPtrToArray:](#ctPtrToArray)
* [TCTypeKind.ctPtrToArray](cgendata#ctPtrToArray)
[ctStruct:](#ctStruct)
* [TCTypeKind.ctStruct](cgendata#ctStruct)
[ctUInt:](#ctUInt)
* [TCTypeKind.ctUInt](cgendata#ctUInt)
[ctUInt16:](#ctUInt16)
* [TCTypeKind.ctUInt16](cgendata#ctUInt16)
[ctUInt32:](#ctUInt32)
* [TCTypeKind.ctUInt32](cgendata#ctUInt32)
[ctUInt64:](#ctUInt64)
* [TCTypeKind.ctUInt64](cgendata#ctUInt64)
[ctUInt8:](#ctUInt8)
* [TCTypeKind.ctUInt8](cgendata#ctUInt8)
[ctVoid:](#ctVoid)
* [TCTypeKind.ctVoid](cgendata#ctVoid)
[cursorInference:](#cursorInference)
* [Goal.cursorInference](varpartitions#cursorInference)
[cyclicTree:](#cyclicTree)
* [trees: cyclicTree(n: PNode): bool](trees#cyclicTree%2CPNode)
[dataPointer:](#dataPointer)
* [strutils2: dataPointer[T](a: T): pointer](strutils2#dataPointer%2CT)
[dcEq:](#dcEq)
* [TDistinctCompare.dcEq](types#dcEq)
[dcEqIgnoreDistinct:](#dcEqIgnoreDistinct)
* [TDistinctCompare.dcEqIgnoreDistinct](types#dcEqIgnoreDistinct)
[dcEqOrDistinctOf:](#dcEqOrDistinctOf)
* [TDistinctCompare.dcEqOrDistinctOf](types#dcEqOrDistinctOf)
[debug:](#debug)
* [astalgo: debug(n: PNode; conf: ConfigRef = nil)](astalgo#debug%2CPNode%2CConfigRef)
* [astalgo: debug(n: PSym; conf: ConfigRef = nil)](astalgo#debug%2CPSym%2CConfigRef)
* [astalgo: debug(n: PType; conf: ConfigRef = nil)](astalgo#debug%2CPType%2CConfigRef)
* [astalgo: debug(x: PSym | PType | PNode)](astalgo#debug.t)
* [astalgo: debug(x: auto)](astalgo#debug.t%2Cauto)
[debugEchoCode:](#debugEchoCode)
* [vmgen: debugEchoCode](vmgen#debugEchoCode)
[debugIds:](#debugIds)
* [idgen: debugIds](idgen#debugIds)
[debugScopes:](#debugScopes)
* [lookups: debugScopes(c: PContext; limit = 0)](lookups#debugScopes%2CPContext%2Cint)
[declarativeDefs:](#declarativeDefs)
* [ast: declarativeDefs](ast#declarativeDefs)
[decodeStr:](#decodeStr)
* [rodutils: decodeStr(s: cstring; pos: var int): string](rodutils#decodeStr%2Ccstring%2Cint)
[decodeStrArray:](#decodeStrArray)
* [rodutils: decodeStrArray(s: cstring): string](rodutils#decodeStrArray.i%2Ccstring)
[decodeVBiggestInt:](#decodeVBiggestInt)
* [rodutils: decodeVBiggestInt(s: cstring; pos: var int): BiggestInt](rodutils#decodeVBiggestInt%2Ccstring%2Cint)
[decodeVInt:](#decodeVInt)
* [rodutils: decodeVInt(s: cstring; pos: var int): int](rodutils#decodeVInt%2Ccstring%2Cint)
[decodeVIntArray:](#decodeVIntArray)
* [rodutils: decodeVIntArray(s: cstring): int](rodutils#decodeVIntArray.i%2Ccstring)
[deduplicate:](#deduplicate)
* [nimsets: deduplicate(conf: ConfigRef; a: PNode): PNode](nimsets#deduplicate%2CConfigRef%2CPNode)
[def:](#def)
* [InstrKind.def](dfa#def)
[DefaultConfig:](#DefaultConfig)
* [options: DefaultConfig](options#DefaultConfig)
[DefaultConfigNims:](#DefaultConfigNims)
* [options: DefaultConfigNims](options#DefaultConfigNims)
[DefaultGlobalOptions:](#DefaultGlobalOptions)
* [options: DefaultGlobalOptions](options#DefaultGlobalOptions)
[DefaultOptions:](#DefaultOptions)
* [options: DefaultOptions](options#DefaultOptions)
[defaultParamSeparator:](#defaultParamSeparator)
* [typesrenderer: defaultParamSeparator](typesrenderer#defaultParamSeparator)
[definedSymbolNames:](#definedSymbolNames)
* [condsyms: definedSymbolNames(symbols: StringTableRef): string](condsyms#definedSymbolNames.i%2CStringTableRef)
[defineSymbol:](#defineSymbol)
* [condsyms: defineSymbol(symbols: StringTableRef; symbol: string; value: string = "true")](condsyms#defineSymbol%2CStringTableRef%2Cstring%2Cstring)
[delSon:](#delSon)
* [ast: delSon(father: PNode; idx: int)](ast#delSon%2CPNode%2Cint)
[demanglePackageName:](#demanglePackageName)
* [options: demanglePackageName(path: string): string](options#demanglePackageName%2Cstring)
[depConfigFields:](#depConfigFields)
* [options: depConfigFields(fn)](options#depConfigFields.t)
[describeArgs:](#describeArgs)
* [sigmatch: describeArgs(c: PContext; n: PNode; startIdx = 1; prefer = preferName): string](sigmatch#describeArgs%2CPContext%2CPNode%2Cint)
[destructor:](#destructor)
* [ast: destructor(t: PType): PSym](ast#destructor.t%2CPType)
* [Feature.destructor](options#destructor)
[detailedInfo:](#detailedInfo)
* [ast: detailedInfo(sym: PSym): string](ast#detailedInfo.t%2CPSym)
[differ:](#differ)
* [linter: differ(line: string; a, b: int; x: string): string](linter#differ%2Cstring%2Cint%2Cint%2Cstring)
[diffSets:](#diffSets)
* [nimsets: diffSets(conf: ConfigRef; a, b: PNode): PNode](nimsets#diffSets%2CConfigRef%2CPNode%2CPNode)
[directViewType:](#directViewType)
* [typeallowed: directViewType(t: PType): ViewTypeKind](typeallowed#directViewType%2CPType)
[dirExists:](#dirExists)
* [pathutils: dirExists(x: AbsoluteDir): bool](pathutils#dirExists%2CAbsoluteDir)
[disabledSf:](#disabledSf)
* [SymbolFilesOption.disabledSf](options#disabledSf)
[disableNimblePath:](#disableNimblePath)
* [options: disableNimblePath(conf: ConfigRef)](options#disableNimblePath%2CConfigRef)
[discardSons:](#discardSons)
* [ast: discardSons(father: PNode)](ast#discardSons%2CPNode)
[dispatcherPos:](#dispatcherPos)
* [ast: dispatcherPos](ast#dispatcherPos)
[divMod:](#divMod)
* [int128: divMod(dividend, divisor: Int128): tuple[quotient, remainder: Int128]](int128#divMod%2CInt128%2CInt128)
[doAbort:](#doAbort)
* [TErrorHandling.doAbort](msgs#doAbort)
[DocConfig:](#DocConfig)
* [options: DocConfig](options#DocConfig)
[docCss:](#docCss)
* [nimpaths: docCss](nimpaths#docCss)
[docgen2JsonPass:](#docgen2JsonPass)
* [docgen2: docgen2JsonPass](docgen2#docgen2JsonPass)
[docgen2Pass:](#docgen2Pass)
* [docgen2: docgen2Pass](docgen2#docgen2Pass)
[docHackJs:](#docHackJs)
* [nimpaths: docHackJs](nimpaths#docHackJs)
[docHackJsFname:](#docHackJsFname)
* [nimpaths: docHackJsFname](nimpaths#docHackJsFname)
[docHackNim:](#docHackNim)
* [nimpaths: docHackNim](nimpaths#docHackNim)
[docRootDefault:](#docRootDefault)
* [options: docRootDefault](options#docRootDefault)
[DocTexConfig:](#DocTexConfig)
* [options: DocTexConfig](options#DocTexConfig)
[documentRaises:](#documentRaises)
* [docgen: documentRaises(cache: IdentCache; n: PNode)](docgen#documentRaises%2CIdentCache%2CPNode)
[doesImply:](#doesImply)
* [guards: doesImply(facts: TModel; prop: PNode): TImplication](guards#doesImply%2CTModel%2CPNode)
[doNothing:](#doNothing)
* [TErrorHandling.doNothing](msgs#doNothing)
[dontInlineConstant:](#dontInlineConstant)
* [trees: dontInlineConstant(orig, cnst: PNode): bool](trees#dontInlineConstant%2CPNode%2CPNode)
[doRaise:](#doRaise)
* [TErrorHandling.doRaise](msgs#doRaise)
[dotdotMangle:](#dotdotMangle)
* [nimpaths: dotdotMangle](nimpaths#dotdotMangle)
[dotOperators:](#dotOperators)
* [Feature.dotOperators](options#dotOperators)
[dump:](#dump)
* [vmprofiler: dump(conf: ConfigRef; pd: ProfileData): string](vmprofiler#dump%2CConfigRef%2CProfileData)
[dynamicBindSym:](#dynamicBindSym)
* [Feature.dynamicBindSym](options#dynamicBindSym)
[eat:](#eat)
* [parser: eat(p: var Parser; tokType: TokType)](parser#eat%2CParser%2CTokType)
[echoCfg:](#echoCfg)
* [dfa: echoCfg(c: ControlFlowGraph; start = 0; last = -1)](dfa#echoCfg%2CControlFlowGraph%2Cint)
[echoCode:](#echoCode)
* [vmgen: echoCode(c: PCtx; start = 0; last = -1)](vmgen#echoCode%2CPCtx%2Cint)
[efAllowDestructor:](#efAllowDestructor)
* [TExprFlag.efAllowDestructor](semdata#efAllowDestructor)
[efAllowStmt:](#efAllowStmt)
* [TExprFlag.efAllowStmt](semdata#efAllowStmt)
[efCompat:](#efCompat)
* [EffectsCompat.efCompat](types#efCompat)
[efDetermineType:](#efDetermineType)
* [TExprFlag.efDetermineType](semdata#efDetermineType)
[efExplain:](#efExplain)
* [TExprFlag.efExplain](semdata#efExplain)
[effectListLen:](#effectListLen)
* [ast: effectListLen](ast#effectListLen)
[EffectsCompat:](#EffectsCompat)
* [types: EffectsCompat](types#EffectsCompat)
[effectSpec:](#effectSpec)
* [trees: effectSpec(n: PNode; effectType: TSpecialWord): PNode](trees#effectSpec%2CPNode%2CTSpecialWord)
[efFromHlo:](#efFromHlo)
* [TExprFlag.efFromHlo](semdata#efFromHlo)
[efInCall:](#efInCall)
* [TExprFlag.efInCall](semdata#efInCall)
[efInTypeof:](#efInTypeof)
* [TExprFlag.efInTypeof](semdata#efInTypeof)
[efLockLevelsDiffer:](#efLockLevelsDiffer)
* [EffectsCompat.efLockLevelsDiffer](types#efLockLevelsDiffer)
[efLValue:](#efLValue)
* [TExprFlag.efLValue](semdata#efLValue)
[efNeedStatic:](#efNeedStatic)
* [TExprFlag.efNeedStatic](semdata#efNeedStatic)
[efNoEvaluateGeneric:](#efNoEvaluateGeneric)
* [TExprFlag.efNoEvaluateGeneric](semdata#efNoEvaluateGeneric)
[efNoSem2Check:](#efNoSem2Check)
* [TExprFlag.efNoSem2Check](semdata#efNoSem2Check)
[efNoSemCheck:](#efNoSemCheck)
* [TExprFlag.efNoSemCheck](semdata#efNoSemCheck)
[efNoUndeclared:](#efNoUndeclared)
* [TExprFlag.efNoUndeclared](semdata#efNoUndeclared)
[efOperand:](#efOperand)
* [TExprFlag.efOperand](semdata#efOperand)
[efPreferNilResult:](#efPreferNilResult)
* [TExprFlag.efPreferNilResult](semdata#efPreferNilResult)
[efPreferStatic:](#efPreferStatic)
* [TExprFlag.efPreferStatic](semdata#efPreferStatic)
[efRaisesDiffer:](#efRaisesDiffer)
* [EffectsCompat.efRaisesDiffer](types#efRaisesDiffer)
[efRaisesUnknown:](#efRaisesUnknown)
* [EffectsCompat.efRaisesUnknown](types#efRaisesUnknown)
[efTagsDiffer:](#efTagsDiffer)
* [EffectsCompat.efTagsDiffer](types#efTagsDiffer)
[efTagsUnknown:](#efTagsUnknown)
* [EffectsCompat.efTagsUnknown](types#efTagsUnknown)
[efWantIterator:](#efWantIterator)
* [TExprFlag.efWantIterator](semdata#efWantIterator)
[efWantStmt:](#efWantStmt)
* [TExprFlag.efWantStmt](semdata#efWantStmt)
[efWantValue:](#efWantValue)
* [TExprFlag.efWantValue](semdata#efWantValue)
[elementType:](#elementType)
* [vmconv: elementType(T: typedesc): typedesc](vmconv#elementType.t%2Ctypedesc)
[ElemSize:](#ElemSize)
* [bitsets: ElemSize](bitsets#ElemSize)
[elemType:](#elemType)
* [types: elemType(t: PType): PType](types#elemType%2CPType)
[emConst:](#emConst)
* [TEvalMode.emConst](vmdef#emConst)
[emOptimize:](#emOptimize)
* [TEvalMode.emOptimize](vmdef#emOptimize)
[emptyRange:](#emptyRange)
* [nimsets: emptyRange(a, b: PNode): bool](nimsets#emptyRange%2CPNode%2CPNode)
[emRepl:](#emRepl)
* [TEvalMode.emRepl](vmdef#emRepl)
[emStaticExpr:](#emStaticExpr)
* [TEvalMode.emStaticExpr](vmdef#emStaticExpr)
[emStaticStmt:](#emStaticStmt)
* [TEvalMode.emStaticStmt](vmdef#emStaticStmt)
[encode:](#encode)
* [sourcemap: encode(i: int): string](sourcemap#encode%2Cint)
[encodeStr:](#encodeStr)
* [rodutils: encodeStr(s: string; result: var string)](rodutils#encodeStr%2Cstring%2Cstring)
[encodeVBiggestInt:](#encodeVBiggestInt)
* [rodutils: encodeVBiggestInt(x: BiggestInt; result: var string)](rodutils#encodeVBiggestInt%2CBiggestInt%2Cstring)
[encodeVInt:](#encodeVInt)
* [rodutils: encodeVInt(x: int; result: var string)](rodutils#encodeVInt%2Cint%2Cstring)
[EndianToStr:](#EndianToStr)
* [platform: EndianToStr](platform#EndianToStr)
[EndOfFile:](#EndOfFile)
* [nimlexbase: EndOfFile](nimlexbase#EndOfFile)
[endsWith:](#endsWith)
* [llstream: endsWith(x: string; s: set[char]): bool](llstream#endsWith%2Cstring%2Cset%5Bchar%5D)
[endsWithOpr:](#endsWithOpr)
* [llstream: endsWithOpr(x: string): bool](llstream#endsWithOpr%2Cstring)
[ensuresEffects:](#ensuresEffects)
* [ast: ensuresEffects](ast#ensuresEffects)
[enter:](#enter)
* [vmprofiler: enter(prof: var Profiler; c: PCtx; tos: PStackFrame)](vmprofiler#enter%2CProfiler%2CPCtx%2CPStackFrame)
[enumFieldPragmas:](#enumFieldPragmas)
* [pragmas: enumFieldPragmas](pragmas#enumFieldPragmas)
[enumHasHoles:](#enumHasHoles)
* [types: enumHasHoles(t: PType): bool](types#enumHasHoles%2CPType)
[envName:](#envName)
* [lambdalifting: envName](lambdalifting#envName)
[eqTypeFlags:](#eqTypeFlags)
* [ast: eqTypeFlags](ast#eqTypeFlags)
[equalParams:](#equalParams)
* [types: equalParams(a, b: PNode): TParamsEquality](types#equalParams%2CPNode%2CPNode)
[equalSets:](#equalSets)
* [nimsets: equalSets(conf: ConfigRef; a, b: PNode): bool](nimsets#equalSets%2CConfigRef%2CPNode%2CPNode)
[equalsFile:](#equalsFile)
* [ropes: equalsFile(r: Rope; filename: AbsoluteFile): bool](ropes#equalsFile%2CRope%2CAbsoluteFile)
* [ropes: equalsFile(r: Rope; f: File): bool](ropes#equalsFile%2CRope%2CFile)
[eraseVoidParams:](#eraseVoidParams)
* [semtypinst: eraseVoidParams(t: PType)](semtypinst#eraseVoidParams%2CPType)
[ERecoverableError:](#ERecoverableError)
* [lineinfos: ERecoverableError](lineinfos#ERecoverableError)
[errArgsNeedRunOption:](#errArgsNeedRunOption)
* [lineinfos: errArgsNeedRunOption](lineinfos#errArgsNeedRunOption)
[errCannotOpenFile:](#errCannotOpenFile)
* [TMsgKind.errCannotOpenFile](lineinfos#errCannotOpenFile)
[errGeneralParseError:](#errGeneralParseError)
* [TMsgKind.errGeneralParseError](lineinfos#errGeneralParseError)
[errGenerated:](#errGenerated)
* [TMsgKind.errGenerated](lineinfos#errGenerated)
[errGridTableNotImplemented:](#errGridTableNotImplemented)
* [TMsgKind.errGridTableNotImplemented](lineinfos#errGridTableNotImplemented)
[errIllFormedAstX:](#errIllFormedAstX)
* [TMsgKind.errIllFormedAstX](lineinfos#errIllFormedAstX)
[errInternal:](#errInternal)
* [TMsgKind.errInternal](lineinfos#errInternal)
[errInvalidDirectiveX:](#errInvalidDirectiveX)
* [TMsgKind.errInvalidDirectiveX](lineinfos#errInvalidDirectiveX)
[errMax:](#errMax)
* [lineinfos: errMax](lineinfos#errMax)
[errMin:](#errMin)
* [lineinfos: errMin](lineinfos#errMin)
[errNewSectionExpected:](#errNewSectionExpected)
* [TMsgKind.errNewSectionExpected](lineinfos#errNewSectionExpected)
[ErrorHandler:](#ErrorHandler)
* [lexer: ErrorHandler](lexer#ErrorHandler)
[errorNode:](#errorNode)
* [semdata: errorNode(c: PContext; n: PNode): PNode](semdata#errorNode%2CPContext%2CPNode)
[errorSym:](#errorSym)
* [lookups: errorSym(c: PContext; n: PNode): PSym](lookups#errorSym%2CPContext%2CPNode)
[errorType:](#errorType)
* [semdata: errorType(c: PContext): PType](semdata#errorType%2CPContext)
* [semfold: errorType(g: ModuleGraph): PType](semfold#errorType%2CModuleGraph)
[errorUndeclaredIdentifier:](#errorUndeclaredIdentifier)
* [lookups: errorUndeclaredIdentifier(c: PContext; info: TLineInfo; name: string)](lookups#errorUndeclaredIdentifier%2CPContext%2CTLineInfo%2Cstring)
[errorUseQualifier:](#errorUseQualifier)
* [lookups: errorUseQualifier(c: PContext; info: TLineInfo; s: PSym)](lookups#errorUseQualifier%2CPContext%2CTLineInfo%2CPSym)
[errProveInit:](#errProveInit)
* [TMsgKind.errProveInit](lineinfos#errProveInit)
[errUnknown:](#errUnknown)
* [TMsgKind.errUnknown](lineinfos#errUnknown)
[errUser:](#errUser)
* [TMsgKind.errUser](lineinfos#errUser)
[errXExpected:](#errXExpected)
* [TMsgKind.errXExpected](lineinfos#errXExpected)
[errXMustBeCompileTime:](#errXMustBeCompileTime)
* [lineinfos: errXMustBeCompileTime](lineinfos#errXMustBeCompileTime)
[ESC:](#ESC)
* [nimlexbase: ESC](nimlexbase#ESC)
[eStdErr:](#eStdErr)
* [TErrorOutput.eStdErr](lineinfos#eStdErr)
[eStdOut:](#eStdOut)
* [TErrorOutput.eStdOut](lineinfos#eStdOut)
[ESuggestDone:](#ESuggestDone)
* [lineinfos: ESuggestDone](lineinfos#ESuggestDone)
[evalConstExpr:](#evalConstExpr)
* [vm: evalConstExpr(module: PSym; g: ModuleGraph; e: PNode): PNode](vm#evalConstExpr%2CPSym%2CModuleGraph%2CPNode)
[evalExpr:](#evalExpr)
* [vm: evalExpr(c: PCtx; n: PNode): PNode](vm#evalExpr%2CPCtx%2CPNode)
[evalFrom:](#evalFrom)
* [importer: evalFrom(c: PContext; n: PNode): PNode](importer#evalFrom%2CPContext%2CPNode)
[evalImport:](#evalImport)
* [importer: evalImport(c: PContext; n: PNode): PNode](importer#evalImport%2CPContext%2CPNode)
[evalImportExcept:](#evalImportExcept)
* [importer: evalImportExcept(c: PContext; n: PNode): PNode](importer#evalImportExcept%2CPContext%2CPNode)
[evalMacroCall:](#evalMacroCall)
* [vm: evalMacroCall(module: PSym; g: ModuleGraph; templInstCounter: ref int; n, nOrig: PNode; sym: PSym): PNode](vm#evalMacroCall%2CPSym%2CModuleGraph%2Cref.int%2CPNode%2CPNode%2CPSym)
[evalOnce:](#evalOnce)
* [lowerings: evalOnce(g: ModuleGraph; value: PNode; owner: PSym): PNode](lowerings#evalOnce%2CModuleGraph%2CPNode%2CPSym)
[evalOp:](#evalOp)
* [semfold: evalOp(m: TMagic; n, a, b, c: PNode; g: ModuleGraph): PNode](semfold#evalOp%2CTMagic%2CPNode%2CPNode%2CPNode%2CPNode%2CModuleGraph)
[evalPass:](#evalPass)
* [vm: evalPass](vm#evalPass)
[evalStaticExpr:](#evalStaticExpr)
* [vm: evalStaticExpr(module: PSym; g: ModuleGraph; e: PNode; prc: PSym): PNode](vm#evalStaticExpr%2CPSym%2CModuleGraph%2CPNode%2CPSym)
[evalStaticStmt:](#evalStaticStmt)
* [vm: evalStaticStmt(module: PSym; g: ModuleGraph; e: PNode; prc: PSym)](vm#evalStaticStmt%2CPSym%2CModuleGraph%2CPNode%2CPSym)
[evalStmt:](#evalStmt)
* [vm: evalStmt(c: PCtx; n: PNode)](vm#evalStmt%2CPCtx%2CPNode)
[evalTemplate:](#evalTemplate)
* [evaltempl: evalTemplate(n: PNode; tmpl, genSymOwner: PSym; conf: ConfigRef; ic: IdentCache; instID: ref int; fromHlo = false): PNode](evaltempl#evalTemplate%2CPNode%2CPSym%2CPSym%2CConfigRef%2CIdentCache%2Cref.int)
[evalTemplateLimit:](#evalTemplateLimit)
* [evaltempl: evalTemplateLimit](evaltempl#evalTemplateLimit)
[ExactConstraints:](#ExactConstraints)
* [TTypeCmpFlag.ExactConstraints](types#ExactConstraints)
[exactEquals:](#exactEquals)
* [msgs: exactEquals(a, b: TLineInfo): bool](msgs#exactEquals%2CTLineInfo%2CTLineInfo)
[ExactGcSafety:](#ExactGcSafety)
* [TTypeCmpFlag.ExactGcSafety](types#ExactGcSafety)
[ExactGenericParams:](#ExactGenericParams)
* [TTypeCmpFlag.ExactGenericParams](types#ExactGenericParams)
[exactReplica:](#exactReplica)
* [ast: exactReplica(t: PType): PType](ast#exactReplica%2CPType)
[ExactTypeDescValues:](#ExactTypeDescValues)
* [TTypeCmpFlag.ExactTypeDescValues](types#ExactTypeDescValues)
[excCpp:](#excCpp)
* [ExceptionSystem.excCpp](options#excCpp)
[exceptionEffects:](#exceptionEffects)
* [ast: exceptionEffects](ast#exceptionEffects)
[ExceptionSystem:](#ExceptionSystem)
* [options: ExceptionSystem](options#ExceptionSystem)
[excGoto:](#excGoto)
* [ExceptionSystem.excGoto](options#excGoto)
[excNone:](#excNone)
* [ExceptionSystem.excNone](options#excNone)
[excQuirky:](#excQuirky)
* [ExceptionSystem.excQuirky](options#excQuirky)
[excSetjmp:](#excSetjmp)
* [ExceptionSystem.excSetjmp](options#excSetjmp)
[execExternalProgram:](#execExternalProgram)
* [extccomp: execExternalProgram(conf: ConfigRef; cmd: string; msg = hintExecuting)](extccomp#execExternalProgram%2CConfigRef%2Cstring)
[execProc:](#execProc)
* [vm: execProc(c: PCtx; sym: PSym; args: openArray[PNode]): PNode](vm#execProc%2CPCtx%2CPSym%2CopenArray%5BPNode%5D)
[existsConfigVar:](#existsConfigVar)
* [options: existsConfigVar(conf: ConfigRef; key: string): bool](options#existsConfigVar%2CConfigRef%2Cstring)
[expectIdent:](#expectIdent)
* [parser: expectIdent(p: Parser)](parser#expectIdent%2CParser)
[expectIdentOrKeyw:](#expectIdentOrKeyw)
* [parser: expectIdentOrKeyw(p: Parser)](parser#expectIdentOrKeyw%2CParser)
[explanationsBaseUrl:](#explanationsBaseUrl)
* [lineinfos: explanationsBaseUrl](lineinfos#explanationsBaseUrl)
[ExportableSymKinds:](#ExportableSymKinds)
* [ast: ExportableSymKinds](ast#ExportableSymKinds)
[exprPragmas:](#exprPragmas)
* [pragmas: exprPragmas](pragmas#exprPragmas)
[exprRoot:](#exprRoot)
* [parampatterns: exprRoot(n: PNode): PSym](parampatterns#exprRoot%2CPNode)
[exprStructuralEquivalent:](#exprStructuralEquivalent)
* [trees: exprStructuralEquivalent(a, b: PNode; strictSymEquality = false): bool](trees#exprStructuralEquivalent%2CPNode%2CPNode)
[extractFilename:](#extractFilename)
* [pathutils: extractFilename(x: AbsoluteFile): string](pathutils#extractFilename%2CAbsoluteFile)
[extractRange:](#extractRange)
* [trees: extractRange(k: TNodeKind; n: PNode; a, b: int): PNode](trees#extractRange%2CTNodeKind%2CPNode%2Cint%2Cint)
[extractRunnableExamplesSource:](#extractRunnableExamplesSource)
* [renderverbatim: extractRunnableExamplesSource(conf: ConfigRef; n: PNode): string](renderverbatim#extractRunnableExamplesSource%2CConfigRef%2CPNode)
[fakePackageName:](#fakePackageName)
* [options: fakePackageName(conf: ConfigRef; path: AbsoluteFile): string](options#fakePackageName%2CConfigRef%2CAbsoluteFile)
[fastLog2:](#fastLog2)
* [int128: fastLog2(a: Int128): int](int128#fastLog2%2CInt128)
[fatal:](#fatal)
* [msgs: fatal(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")](msgs#fatal.t%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring)
[fatalMax:](#fatalMax)
* [lineinfos: fatalMax](lineinfos#fatalMax)
[fatalMin:](#fatalMin)
* [lineinfos: fatalMin](lineinfos#fatalMin)
[Feature:](#Feature)
* [options: Feature](options#Feature)
[FF:](#FF)
* [nimlexbase: FF](nimlexbase#FF)
[fieldPragmas:](#fieldPragmas)
* [pragmas: fieldPragmas](pragmas#fieldPragmas)
[fieldVisible:](#fieldVisible)
* [sigmatch: fieldVisible(c: PContext; f: PSym): bool](sigmatch#fieldVisible%2CPContext%2CPSym)
[fileExists:](#fileExists)
* [pathutils: fileExists(x: AbsoluteFile): bool](pathutils#fileExists%2CAbsoluteFile)
[fileIdx:](#fileIdx)
* [ast: fileIdx(c: PSym): FileIndex](ast#fileIdx.t%2CPSym)
[FileIndex:](#FileIndex)
* [lineinfos: FileIndex](lineinfos#FileIndex)
[fileInfoIdx:](#fileInfoIdx)
* [msgs: fileInfoIdx(conf: ConfigRef; filename: AbsoluteFile): FileIndex](msgs#fileInfoIdx%2CConfigRef%2CAbsoluteFile)
* [msgs: fileInfoIdx(conf: ConfigRef; filename: AbsoluteFile; isKnownFile: var bool): FileIndex](msgs#fileInfoIdx%2CConfigRef%2CAbsoluteFile%2Cbool)
[fileInfoKnown:](#fileInfoKnown)
* [msgs: fileInfoKnown(conf: ConfigRef; filename: AbsoluteFile): bool](msgs#fileInfoKnown%2CConfigRef%2CAbsoluteFile)
[filename:](#filename)
* [ast: filename(c: PSym): string](ast#filename.t%2CPSym)
* [semdata: filename(c: PContext): string](semdata#filename%2CPContext)
[FilenameOption:](#FilenameOption)
* [msgs: FilenameOption](msgs#FilenameOption)
[fillObjectFields:](#fillObjectFields)
* [cgen: fillObjectFields(m: BModule; typ: PType)](cgen#fillObjectFields%2CBModule%2CPType)
[fillTypeS:](#fillTypeS)
* [semdata: fillTypeS(dest: PType; kind: TTypeKind; c: PContext)](semdata#fillTypeS%2CPType%2CTTypeKind%2CPContext)
[filterReplace:](#filterReplace)
* [filters: filterReplace(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile; call: PNode): PLLStream](filters#filterReplace%2CConfigRef%2CPLLStream%2CAbsoluteFile%2CPNode)
[filterStrip:](#filterStrip)
* [filters: filterStrip(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile; call: PNode): PLLStream](filters#filterStrip%2CConfigRef%2CPLLStream%2CAbsoluteFile%2CPNode)
[filterTmpl:](#filterTmpl)
* [filter\_tmpl: filterTmpl(conf: ConfigRef; stdin: PLLStream; filename: AbsoluteFile; call: PNode): PLLStream](filter_tmpl#filterTmpl%2CConfigRef%2CPLLStream%2CAbsoluteFile%2CPNode)
[findFile:](#findFile)
* [options: findFile(conf: ConfigRef; f: string; suppressStdlib = false): AbsoluteFile](options#findFile%2CConfigRef%2Cstring)
[findModule:](#findModule)
* [options: findModule(conf: ConfigRef; modulename, currentModule: string): AbsoluteFile](options#findModule%2CConfigRef%2Cstring%2Cstring)
[findNodeJs:](#findNodeJs)
* [nodejs: findNodeJs(): string](nodejs#findNodeJs)
[findPragma:](#findPragma)
* [trees: findPragma(n: PNode; which: TSpecialWord): PNode](trees#findPragma%2CPNode%2CTSpecialWord)
[findProjectNimFile:](#findProjectNimFile)
* [options: findProjectNimFile(conf: ConfigRef; pkg: string): string](options#findProjectNimFile%2CConfigRef%2Cstring)
[findStr:](#findStr)
* [wordrecg: findStr[T: enum](a: Slice[T]; s: string; default: T): T](wordrecg#findStr%2CSlice%5BT%3A%20enum%5D%2Cstring%2CT)
[findUnresolvedStatic:](#findUnresolvedStatic)
* [ast: findUnresolvedStatic(n: PNode): PNode](ast#findUnresolvedStatic%2CPNode)
[finishDoc2Pass:](#finishDoc2Pass)
* [docgen2: finishDoc2Pass(project: string)](docgen2#finishDoc2Pass%2Cstring)
[firstABxInstr:](#firstABxInstr)
* [vmdef: firstABxInstr](vmdef#firstABxInstr)
[FirstCallConv:](#FirstCallConv)
* [pragmas: FirstCallConv](pragmas#FirstCallConv)
[firstFloat:](#firstFloat)
* [types: firstFloat(t: PType): BiggestFloat](types#firstFloat%2CPType)
[firstIdentExcluding:](#firstIdentExcluding)
* [astalgo: firstIdentExcluding(ti: var TIdentIter; tab: TStrTable; s: PIdent; excluding: IntSet): PSym](astalgo#firstIdentExcluding%2CTIdentIter%2CTStrTable%2CPIdent%2CIntSet)
[firstOrd:](#firstOrd)
* [types: firstOrd(conf: ConfigRef; t: PType): Int128](types#firstOrd%2CConfigRef%2CPType)
[fitsRegister:](#fitsRegister)
* [vmgen: fitsRegister(t: PType): bool](vmgen#fitsRegister%2CPType)
[flattenStmts:](#flattenStmts)
* [trees: flattenStmts(n: PNode): PNode](trees#flattenStmts%2CPNode)
[floatInt64Align:](#floatInt64Align)
* [options: floatInt64Align(conf: ConfigRef): int16](options#floatInt64Align%2CConfigRef)
[FloatLike:](#FloatLike)
* [OrdinalType.FloatLike](types#FloatLike)
[floatRangeCheck:](#floatRangeCheck)
* [types: floatRangeCheck(x: BiggestFloat; t: PType): bool](types#floatRangeCheck%2CBiggestFloat%2CPType)
[foAbs:](#foAbs)
* [FilenameOption.foAbs](msgs#foAbs)
[foldAlignOf:](#foldAlignOf)
* [types: foldAlignOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode](types#foldAlignOf.t%2CConfigRef%2CPNode%2CPNode)
[foldOffsetOf:](#foldOffsetOf)
* [types: foldOffsetOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode](types#foldOffsetOf.t%2CConfigRef%2CPNode%2CPNode)
[foldSizeOf:](#foldSizeOf)
* [types: foldSizeOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode](types#foldSizeOf.t%2CConfigRef%2CPNode%2CPNode)
[foMagicSauce:](#foMagicSauce)
* [FilenameOption.foMagicSauce](msgs#foMagicSauce)
[foName:](#foName)
* [FilenameOption.foName](msgs#foName)
[forceCopy:](#forceCopy)
* [strutils2: forceCopy(result: var string; a: string)](strutils2#forceCopy%2Cstring%2Cstring)
[foRelProject:](#foRelProject)
* [FilenameOption.foRelProject](msgs#foRelProject)
[fork:](#fork)
* [InstrKind.fork](dfa#fork)
[forLoopMacros:](#forLoopMacros)
* [Feature.forLoopMacros](options#forLoopMacros)
[formatMsg:](#formatMsg)
* [msgs: formatMsg(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg: string): string](msgs#formatMsg%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring)
[FormatStr:](#FormatStr)
* [ropes: FormatStr](ropes#FormatStr)
[forVarPragmas:](#forVarPragmas)
* [pragmas: forVarPragmas](pragmas#forVarPragmas)
[foStacktrace:](#foStacktrace)
* [FilenameOption.foStacktrace](msgs#foStacktrace)
[frameDeclared:](#frameDeclared)
* [CodegenFlag.frameDeclared](cgendata#frameDeclared)
[frEmbedded:](#frEmbedded)
* [TTypeFieldResult.frEmbedded](types#frEmbedded)
[freshVarForClosureIter:](#freshVarForClosureIter)
* [lambdalifting: freshVarForClosureIter(g: ModuleGraph; s, owner: PSym): PNode](lambdalifting#freshVarForClosureIter%2CModuleGraph%2CPSym%2CPSym)
[frHeader:](#frHeader)
* [TTypeFieldResult.frHeader](types#frHeader)
[frNone:](#frNone)
* [TTypeFieldResult.frNone](types#frNone)
[fromLit:](#fromLit)
* [vmconv: fromLit(a: PNode; T: typedesc): auto](vmconv#fromLit%2CPNode%2Ctypedesc)
[fromSystem:](#fromSystem)
* [astalgo: fromSystem(op: PSym): bool](astalgo#fromSystem%2CPSym)
[Full:](#Full)
* [InstrTargetKind.Full](dfa#Full)
[gCacheIntTries:](#gCacheIntTries)
* [ropes: gCacheIntTries](ropes#gCacheIntTries)
[gCacheMisses:](#gCacheMisses)
* [ropes: gCacheMisses](ropes#gCacheMisses)
[gCacheTries:](#gCacheTries)
* [ropes: gCacheTries](ropes#gCacheTries)
[gcArc:](#gcArc)
* [TGCMode.gcArc](options#gcArc)
[gcBoehm:](#gcBoehm)
* [TGCMode.gcBoehm](options#gcBoehm)
[gcGo:](#gcGo)
* [TGCMode.gcGo](options#gcGo)
[gcHooks:](#gcHooks)
* [TGCMode.gcHooks](options#gcHooks)
[gcMarkAndSweep:](#gcMarkAndSweep)
* [TGCMode.gcMarkAndSweep](options#gcMarkAndSweep)
[gCmdLineInfo:](#gCmdLineInfo)
* [msgs: gCmdLineInfo](msgs#gCmdLineInfo)
[gcNone:](#gcNone)
* [TGCMode.gcNone](options#gcNone)
[gcOrc:](#gcOrc)
* [TGCMode.gcOrc](options#gcOrc)
[gcRefc:](#gcRefc)
* [TGCMode.gcRefc](options#gcRefc)
[gcRegions:](#gcRegions)
* [TGCMode.gcRegions](options#gcRegions)
[GcTypeKinds:](#GcTypeKinds)
* [ast: GcTypeKinds](ast#GcTypeKinds)
[gcUnselected:](#gcUnselected)
* [TGCMode.gcUnselected](options#gcUnselected)
[gcV2:](#gcV2)
* [TGCMode.gcV2](options#gcV2)
[gen:](#gen)
* [sourcemap: gen(map: SourceMapGenerator): SourceMap](sourcemap#gen%2CSourceMapGenerator)
[genAddrOf:](#genAddrOf)
* [lowerings: genAddrOf(n: PNode; typeKind = tyPtr): PNode](lowerings#genAddrOf%2CPNode)
[genCaseObjDiscMapping:](#genCaseObjDiscMapping)
* [enumtostr: genCaseObjDiscMapping(t: PType; field: PSym; info: TLineInfo; g: ModuleGraph): PSym](enumtostr#genCaseObjDiscMapping%2CPType%2CPSym%2CTLineInfo%2CModuleGraph)
[gendependPass:](#gendependPass)
* [depends: gendependPass](depends#gendependPass)
[genDeref:](#genDeref)
* [lowerings: genDeref(n: PNode; k = nkHiddenDeref): PNode](lowerings#genDeref%2CPNode)
[genEnumToStrProc:](#genEnumToStrProc)
* [enumtostr: genEnumToStrProc(t: PType; info: TLineInfo; g: ModuleGraph): PSym](enumtostr#genEnumToStrProc%2CPType%2CTLineInfo%2CModuleGraph)
[generateDoc:](#generateDoc)
* [docgen: generateDoc(d: PDoc; n, orig: PNode; docFlags: DocFlags = kDefault)](docgen#generateDoc%2CPDoc%2CPNode%2CPNode%2CDocFlags)
[generateDot:](#generateDot)
* [depends: generateDot(graph: ModuleGraph; project: AbsoluteFile)](depends#generateDot%2CModuleGraph%2CAbsoluteFile)
[generateIndex:](#generateIndex)
* [docgen: generateIndex(d: PDoc)](docgen#generateIndex%2CPDoc)
[generateJson:](#generateJson)
* [docgen: generateJson(d: PDoc; n: PNode; includeComments: bool = true)](docgen#generateJson%2CPDoc%2CPNode%2Cbool)
[generateMethodDispatchers:](#generateMethodDispatchers)
* [cgmeth: generateMethodDispatchers(g: ModuleGraph): PNode](cgmeth#generateMethodDispatchers%2CModuleGraph)
[generateTags:](#generateTags)
* [docgen: generateTags(d: PDoc; n: PNode; r: var Rope)](docgen#generateTags%2CPDoc%2CPNode%2CRope)
[generateTypeInstance:](#generateTypeInstance)
* [semtypinst: generateTypeInstance(p: PContext; pt: TIdTable; info: TLineInfo; t: PType): PType](semtypinst#generateTypeInstance%2CPContext%2CTIdTable%2CTLineInfo%2CPType)
* [semtypinst: generateTypeInstance(p: PContext; pt: TIdTable; arg: PNode; t: PType): untyped](semtypinst#generateTypeInstance.t%2CPContext%2CTIdTable%2CPNode%2CPType)
[genericParamsInMacroCall:](#genericParamsInMacroCall)
* [vm: genericParamsInMacroCall(macroSym: PSym; call: PNode): (PSym, PNode)](vm#genericParamsInMacroCall.i%2CPSym%2CPNode)
[genericParamsPos:](#genericParamsPos)
* [ast: genericParamsPos](ast#genericParamsPos)
[GenericTypes:](#GenericTypes)
* [ast: GenericTypes](ast#GenericTypes)
[genExpr:](#genExpr)
* [vmgen: genExpr(c: PCtx; n: PNode; requiresValue = true): int](vmgen#genExpr%2CPCtx%2CPNode)
[genFieldDefect:](#genFieldDefect)
* [renderer: genFieldDefect(field: PSym; disc: PSym): string](renderer#genFieldDefect%2CPSym%2CPSym)
[genHigh:](#genHigh)
* [lowerings: genHigh(g: ModuleGraph; n: PNode): PNode](lowerings#genHigh%2CModuleGraph%2CPNode)
[genLen:](#genLen)
* [lowerings: genLen(g: ModuleGraph; n: PNode): PNode](lowerings#genLen%2CModuleGraph%2CPNode)
[genMergeInfo:](#genMergeInfo)
* [ccgmerge: genMergeInfo(m: BModule): Rope](ccgmerge#genMergeInfo%2CBModule)
[genPrefix:](#genPrefix)
* [lowerings: genPrefix](lowerings#genPrefix)
[genProc:](#genProc)
* [vmgen: genProc(c: PCtx; s: PSym): int](vmgen#genProc%2CPCtx%2CPSym)
[genSectionEnd:](#genSectionEnd)
* [ccgmerge: genSectionEnd(fs: TCFileSection; conf: ConfigRef): Rope](ccgmerge#genSectionEnd%2CTCFileSection%2CConfigRef)
* [ccgmerge: genSectionEnd(ps: TCProcSection; conf: ConfigRef): Rope](ccgmerge#genSectionEnd%2CTCProcSection%2CConfigRef)
[genSectionStart:](#genSectionStart)
* [ccgmerge: genSectionStart(fs: TCFileSection; conf: ConfigRef): Rope](ccgmerge#genSectionStart%2CTCFileSection%2CConfigRef)
* [ccgmerge: genSectionStart(ps: TCProcSection; conf: ConfigRef): Rope](ccgmerge#genSectionStart%2CTCProcSection%2CConfigRef)
[genSourceMap:](#genSourceMap)
* [sourcemap: genSourceMap(source: string; outFile: string): (Rope, SourceMap)](sourcemap#genSourceMap%2Cstring%2Cstring)
[genStmt:](#genStmt)
* [vmgen: genStmt(c: PCtx; n: PNode): int](vmgen#genStmt%2CPCtx%2CPNode)
[genSubDir:](#genSubDir)
* [options: genSubDir](options#genSubDir)
[get:](#get)
* [semdata: get(p: PProcCon; key: PSym): PSym](semdata#get%2CPProcCon%2CPSym)
[getAlign:](#getAlign)
* [types: getAlign(conf: ConfigRef; typ: PType): BiggestInt](types#getAlign%2CConfigRef%2CPType)
[getBody:](#getBody)
* [ast: getBody(s: PSym): PNode](ast#getBody.t%2CPSym)
[getBool:](#getBool)
* [vm: getBool(a: VmArgs; i: Natural): bool](vm#getBool%2CVmArgs%2CNatural)
[getClockStr:](#getClockStr)
* [options: getClockStr(): string](options#getClockStr)
[getClosureIterResult:](#getClosureIterResult)
* [lambdalifting: getClosureIterResult(g: ModuleGraph; iter: PSym): PSym](lambdalifting#getClosureIterResult%2CModuleGraph%2CPSym)
[getColNumber:](#getColNumber)
* [nimlexbase: getColNumber(L: TBaseLexer; pos: int): int](nimlexbase#getColNumber%2CTBaseLexer%2Cint)
[getCompileCFileCmd:](#getCompileCFileCmd)
* [extccomp: getCompileCFileCmd(conf: ConfigRef; cfile: Cfile; isMainFile = false; produceOutput = false): string](extccomp#getCompileCFileCmd%2CConfigRef%2CCfile)
[getCompilerProc:](#getCompilerProc)
* [magicsys: getCompilerProc(g: ModuleGraph; name: string): PSym](magicsys#getCompilerProc%2CModuleGraph%2Cstring)
[getConfigVar:](#getConfigVar)
* [options: getConfigVar(conf: ConfigRef; key: string; default = ""): string](options#getConfigVar%2CConfigRef%2Cstring%2Cstring)
[getConstExpr:](#getConstExpr)
* [semfold: getConstExpr(m: PSym; n: PNode; g: ModuleGraph): PNode](semfold#getConstExpr%2CPSym%2CPNode%2CModuleGraph)
[getCurrentLine:](#getCurrentLine)
* [nimlexbase: getCurrentLine(L: TBaseLexer; marker: bool = true): string](nimlexbase#getCurrentLine%2CTBaseLexer%2Cbool)
[getCurrOwner:](#getCurrOwner)
* [semdata: getCurrOwner(c: PContext): PSym](semdata#getCurrOwner%2CPContext)
[getDateStr:](#getDateStr)
* [options: getDateStr(): string](options#getDateStr)
[getDispatcher:](#getDispatcher)
* [cgmeth: getDispatcher(s: PSym): PSym](cgmeth#getDispatcher%2CPSym)
[getDocHacksJs:](#getDocHacksJs)
* [nimpaths: getDocHacksJs(nimr: string; nim = getCurrentCompilerExe(); forceRebuild = false): string](nimpaths#getDocHacksJs%2Cstring)
[getEnvParam:](#getEnvParam)
* [lambdalifting: getEnvParam(routine: PSym): PSym](lambdalifting#getEnvParam%2CPSym)
[getFieldFromObj:](#getFieldFromObj)
* [lowerings: getFieldFromObj(t: PType; v: PSym): PSym](lowerings#getFieldFromObj%2CPType%2CPSym)
[getFloat:](#getFloat)
* [ast: getFloat(a: PNode): BiggestFloat](ast#getFloat%2CPNode)
* [vm: getFloat(a: VmArgs; i: Natural): BiggestFloat](vm#getFloat%2CVmArgs%2CNatural)
[getFloatLitType:](#getFloatLitType)
* [magicsys: getFloatLitType(g: ModuleGraph; literal: PNode): PType](magicsys#getFloatLitType%2CModuleGraph%2CPNode)
[getFloatValue:](#getFloatValue)
* [types: getFloatValue(n: PNode): BiggestFloat](types#getFloatValue%2CPNode)
[getGenSym:](#getGenSym)
* [semdata: getGenSym(c: PContext; s: PSym): PSym](semdata#getGenSym%2CPContext%2CPSym)
[getGlobalValue:](#getGlobalValue)
* [vm: getGlobalValue(c: PCtx; s: PSym): PNode](vm#getGlobalValue%2CPCtx%2CPSym)
[getHash:](#getHash)
* [msgs: getHash(conf: ConfigRef; fileIdx: FileIndex): string](msgs#getHash%2CConfigRef%2CFileIndex)
[getID:](#getID)
* [idgen: getID(): int](idgen#getID)
[getIdent:](#getIdent)
* [idents: getIdent(ic: IdentCache; identifier: cstring; length: int; h: Hash): PIdent](idents#getIdent%2CIdentCache%2Ccstring%2Cint%2CHash)
* [idents: getIdent(ic: IdentCache; identifier: string): PIdent](idents#getIdent%2CIdentCache%2Cstring)
* [idents: getIdent(ic: IdentCache; identifier: string; h: Hash): PIdent](idents#getIdent%2CIdentCache%2Cstring%2CHash)
[getIndentWidth:](#getIndentWidth)
* [lexer: getIndentWidth(fileIdx: FileIndex; inputstream: PLLStream; cache: IdentCache; config: ConfigRef): int](lexer#getIndentWidth%2CFileIndex%2CPLLStream%2CIdentCache%2CConfigRef)
[getInfoContext:](#getInfoContext)
* [msgs: getInfoContext(conf: ConfigRef; index: int): TLineInfo](msgs#getInfoContext%2CConfigRef%2Cint)
[getInfoContextLen:](#getInfoContextLen)
* [msgs: getInfoContextLen(conf: ConfigRef): int](msgs#getInfoContextLen%2CConfigRef)
[getInt:](#getInt)
* [ast: getInt(a: PNode): Int128](ast#getInt%2CPNode)
* [vm: getInt(a: VmArgs; i: Natural): BiggestInt](vm#getInt%2CVmArgs%2CNatural)
[getInt64:](#getInt64)
* [ast: getInt64(a: PNode): int64](ast#getInt64%2CPNode)
[getIntLitType:](#getIntLitType)
* [magicsys: getIntLitType(g: ModuleGraph; literal: PNode): PType](magicsys#getIntLitType%2CModuleGraph%2CPNode)
[getLineInfo:](#getLineInfo)
* [lexer: getLineInfo(L: Lexer; tok: Token): TLineInfo](lexer#getLineInfo%2CLexer%2CToken)
[getMagic:](#getMagic)
* [trees: getMagic(op: PNode): TMagic](trees#getMagic%2CPNode)
[getMagicEqSymForType:](#getMagicEqSymForType)
* [magicsys: getMagicEqSymForType(g: ModuleGraph; t: PType; info: TLineInfo): PSym](magicsys#getMagicEqSymForType%2CModuleGraph%2CPType%2CTLineInfo)
[getModule:](#getModule)
* [astalgo: getModule(s: PSym): PSym](astalgo#getModule%2CPSym)
* [modulegraphs: getModule(g: ModuleGraph; fileIdx: FileIndex): PSym](modulegraphs#getModule%2CModuleGraph%2CFileIndex)
[getModuleName:](#getModuleName)
* [modulepaths: getModuleName(conf: ConfigRef; n: PNode): string](modulepaths#getModuleName%2CConfigRef%2CPNode)
[getNamedParamFromList:](#getNamedParamFromList)
* [astalgo: getNamedParamFromList(list: PNode; ident: PIdent): PSym](astalgo#getNamedParamFromList%2CPNode%2CPIdent)
[getNextTok:](#getNextTok)
* [renderer: getNextTok(r: var TSrcGen; kind: var TokType; literal: var string)](renderer#getNextTok%2CTSrcGen%2CTokType%2Cstring)
[getNimbleFile:](#getNimbleFile)
* [options: getNimbleFile(conf: ConfigRef; path: string): string](options#getNimbleFile%2CConfigRef%2Cstring)
[getnimblePkg:](#getnimblePkg)
* [ast: getnimblePkg(a: PSym): PSym](ast#getnimblePkg%2CPSym)
[getnimblePkgId:](#getnimblePkgId)
* [ast: getnimblePkgId(a: PSym): int](ast#getnimblePkgId%2CPSym)
[getNimcacheDir:](#getNimcacheDir)
* [options: getNimcacheDir(conf: ConfigRef): AbsoluteDir](options#getNimcacheDir%2CConfigRef)
[getNimScriptSymbol:](#getNimScriptSymbol)
* [magicsys: getNimScriptSymbol(g: ModuleGraph; name: string): PSym](magicsys#getNimScriptSymbol%2CModuleGraph%2Cstring)
[getNode:](#getNode)
* [vm: getNode(a: VmArgs; i: Natural): PNode](vm#getNode%2CVmArgs%2CNatural)
[getNullValue:](#getNullValue)
* [vmgen: getNullValue(typ: PType; info: TLineInfo; conf: ConfigRef): PNode](vmgen#getNullValue%2CPType%2CTLineInfo%2CConfigRef)
[getOrDefault:](#getOrDefault)
* [btrees: getOrDefault[Key, Val](b: BTree[Key, Val]; key: Key): Val](btrees#getOrDefault%2CBTree%5BKey%2CVal%5D%2CKey)
[getOrdValue:](#getOrdValue)
* [types: getOrdValue(n: PNode; onError = high(Int128)): Int128](types#getOrdValue%2CPNode)
[getOutFile:](#getOutFile)
* [options: getOutFile(conf: ConfigRef; filename: RelativeFile; ext: string): AbsoluteFile](options#getOutFile%2CConfigRef%2CRelativeFile%2Cstring)
[getPackageName:](#getPackageName)
* [options: getPackageName(conf: ConfigRef; path: string): string](options#getPackageName%2CConfigRef%2Cstring)
[getPathVersion:](#getPathVersion)
* [nimblecmd: getPathVersion(p: string): tuple[name, version: string]](nimblecmd#getPathVersion%2Cstring)
[getPlugin:](#getPlugin)
* [active: getPlugin(ic: IdentCache; fn: PSym): Transformation](plugins/active#getPlugin%2CIdentCache%2CPSym)
[getPragmaStmt:](#getPragmaStmt)
* [ccgutils: getPragmaStmt(n: PNode; w: TSpecialWord): PNode](ccgutils#getPragmaStmt%2CPNode%2CTSpecialWord)
[getPragmaVal:](#getPragmaVal)
* [pragmas: getPragmaVal(procAst: PNode; name: TSpecialWord): PNode](pragmas#getPragmaVal%2CPNode%2CTSpecialWord)
[getPrecedence:](#getPrecedence)
* [lexer: getPrecedence(ident: PIdent): int](lexer#getPrecedence%2CPIdent)
* [lexer: getPrecedence(tok: Token): int](lexer#getPrecedence%2CToken)
[getPrefixDir:](#getPrefixDir)
* [options: getPrefixDir(conf: ConfigRef): AbsoluteDir](options#getPrefixDir%2CConfigRef)
[getProcHeader:](#getProcHeader)
* [types: getProcHeader(conf: ConfigRef; sym: PSym; prefer: TPreferedDesc = preferName; getDeclarationPath = true): string](types#getProcHeader%2CConfigRef%2CPSym%2CTPreferedDesc)
[getRelativePathFromConfigPath:](#getRelativePathFromConfigPath)
* [options: getRelativePathFromConfigPath(conf: ConfigRef; f: AbsoluteFile): RelativeFile](options#getRelativePathFromConfigPath%2CConfigRef%2CAbsoluteFile)
[getReturnType:](#getReturnType)
* [types: getReturnType(s: PSym): PType](types#getReturnType%2CPSym)
[getRoot:](#getRoot)
* [trees: getRoot(n: PNode): PSym](trees#getRoot%2CPNode)
[getSize:](#getSize)
* [types: getSize(conf: ConfigRef; typ: PType): BiggestInt](types#getSize%2CConfigRef%2CPType)
[getStateField:](#getStateField)
* [lambdalifting: getStateField(g: ModuleGraph; owner: PSym): PSym](lambdalifting#getStateField%2CModuleGraph%2CPSym)
[getStr:](#getStr)
* [ast: getStr(a: PNode): string](ast#getStr%2CPNode)
[getString:](#getString)
* [vm: getString(a: VmArgs; i: Natural): string](vm#getString%2CVmArgs%2CNatural)
[getStrOrChar:](#getStrOrChar)
* [ast: getStrOrChar(a: PNode): string](ast#getStrOrChar%2CPNode)
[getSymFromList:](#getSymFromList)
* [astalgo: getSymFromList(list: PNode; ident: PIdent; start: int = 0): PSym](astalgo#getSymFromList%2CPNode%2CPIdent%2Cint)
[getSymRepr:](#getSymRepr)
* [lookups: getSymRepr(conf: ConfigRef; s: PSym): string](lookups#getSymRepr%2CConfigRef%2CPSym)
[getSysMagic:](#getSysMagic)
* [magicsys: getSysMagic(g: ModuleGraph; info: TLineInfo; name: string; m: TMagic): PSym](magicsys#getSysMagic%2CModuleGraph%2CTLineInfo%2Cstring%2CTMagic)
[getSysSym:](#getSysSym)
* [magicsys: getSysSym(g: ModuleGraph; info: TLineInfo; name: string): PSym](magicsys#getSysSym%2CModuleGraph%2CTLineInfo%2Cstring)
[getSystemConfigPath:](#getSystemConfigPath)
* [nimconf: getSystemConfigPath(conf: ConfigRef; filename: RelativeFile): AbsoluteFile](nimconf#getSystemConfigPath%2CConfigRef%2CRelativeFile)
[getSysType:](#getSysType)
* [magicsys: getSysType(g: ModuleGraph; info: TLineInfo; kind: TTypeKind): PType](magicsys#getSysType%2CModuleGraph%2CTLineInfo%2CTTypeKind)
[getTok:](#getTok)
* [parser: getTok(p: var Parser)](parser#getTok%2CParser)
[getTokSym:](#getTokSym)
* [renderer: getTokSym(r: TSrcGen): PSym](renderer#getTokSym%2CTSrcGen)
[getUniqueType:](#getUniqueType)
* [ccgutils: getUniqueType(key: PType): PType](ccgutils#getUniqueType.t%2CPType)
[getUserConfigPath:](#getUserConfigPath)
* [nimconf: getUserConfigPath(filename: RelativeFile): AbsoluteFile](nimconf#getUserConfigPath%2CRelativeFile)
[gFrontEndId:](#gFrontEndId)
* [idgen: gFrontEndId](idgen#gFrontEndId)
[ggDebug:](#ggDebug)
* [ast: ggDebug](ast#ggDebug)
[globalAssert:](#globalAssert)
* [msgs: globalAssert(conf: ConfigRef; cond: untyped; info: TLineInfo = unknownLineInfo; arg = "")](msgs#globalAssert.t%2CConfigRef%2Cuntyped%2CTLineInfo%2Cstring)
[globalError:](#globalError)
* [msgs: globalError(conf: ConfigRef; info: TLineInfo; arg: string)](msgs#globalError.t%2CConfigRef%2CTLineInfo%2Cstring)
* [msgs: globalError(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")](msgs#globalError.t%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring)
[gloptNone:](#gloptNone)
* [TGlobalOption.gloptNone](options#gloptNone)
[Goal:](#Goal)
* [varpartitions: Goal](varpartitions#Goal)
[goto:](#goto)
* [InstrKind.goto](dfa#goto)
[GrowthFactor:](#GrowthFactor)
* [ast: GrowthFactor](ast#GrowthFactor)
[handleCR:](#handleCR)
* [nimlexbase: handleCR(L: var TBaseLexer; pos: int): int](nimlexbase#handleCR%2CTBaseLexer%2Cint)
[handleDocOutputOptions:](#handleDocOutputOptions)
* [docgen: handleDocOutputOptions(conf: ConfigRef)](docgen#handleDocOutputOptions%2CConfigRef)
[handleLF:](#handleLF)
* [nimlexbase: handleLF(L: var TBaseLexer; pos: int): int](nimlexbase#handleLF%2CTBaseLexer%2Cint)
[handleStdinInput:](#handleStdinInput)
* [commands: handleStdinInput(conf: ConfigRef)](commands#handleStdinInput%2CConfigRef)
[harmlessOptions:](#harmlessOptions)
* [options: harmlessOptions](options#harmlessOptions)
[hasAssume:](#hasAssume)
* [TInfoCCProp.hasAssume](extccomp#hasAssume)
[hasAttribute:](#hasAttribute)
* [TInfoCCProp.hasAttribute](extccomp#hasAttribute)
[hasComputedGoto:](#hasComputedGoto)
* [TInfoCCProp.hasComputedGoto](extccomp#hasComputedGoto)
[hasCpp:](#hasCpp)
* [TInfoCCProp.hasCpp](extccomp#hasCpp)
[hasCurFramePointer:](#hasCurFramePointer)
* [TCProcFlag.hasCurFramePointer](cgendata#hasCurFramePointer)
[hasDeclspec:](#hasDeclspec)
* [TInfoCCProp.hasDeclspec](extccomp#hasDeclspec)
[hasDestructor:](#hasDestructor)
* [ast: hasDestructor(t: PType): bool](ast#hasDestructor.t%2CPType)
[hasDisabledAsgn:](#hasDisabledAsgn)
* [ast: hasDisabledAsgn(t: PType): bool](ast#hasDisabledAsgn%2CPType)
[hasFauxMatch:](#hasFauxMatch)
* [sigmatch: hasFauxMatch(c: TCandidate): bool](sigmatch#hasFauxMatch.t%2CTCandidate)
[hasFFI:](#hasFFI)
* [options: hasFFI](options#hasFFI)
[hasGcGuard:](#hasGcGuard)
* [TInfoCCProp.hasGcGuard](extccomp#hasGcGuard)
[hasGnuAsm:](#hasGnuAsm)
* [TInfoCCProp.hasGnuAsm](extccomp#hasGnuAsm)
[hash:](#hash)
* [lineinfos: hash(i: TLineInfo): Hash](lineinfos#hash%2CTLineInfo)
* [modulegraphs: hash(x: FileIndex): Hash](modulegraphs#hash%2CFileIndex)
* [modulegraphs: hash(u: SigHash): Hash](modulegraphs#hash%2CSigHash)
[hasHint:](#hasHint)
* [options: hasHint(conf: ConfigRef; note: TNoteKind): bool](options#hasHint%2CConfigRef%2CTNoteKind)
[hashNode:](#hashNode)
* [astalgo: hashNode(p: RootRef): Hash](astalgo#hashNode%2CRootRef)
[hashNonProc:](#hashNonProc)
* [sighashes: hashNonProc(s: PSym): SigHash](sighashes#hashNonProc%2CPSym)
[hashOwner:](#hashOwner)
* [sighashes: hashOwner(s: PSym): SigHash](sighashes#hashOwner%2CPSym)
[hashProc:](#hashProc)
* [sighashes: hashProc(s: PSym): SigHash](sighashes#hashProc%2CPSym)
[hashString:](#hashString)
* [ccgutils: hashString(conf: ConfigRef; s: string): BiggestInt](ccgutils#hashString%2CConfigRef%2Cstring)
[hashType:](#hashType)
* [sighashes: hashType(t: PType; flags: set[ConsiderFlag] = {CoType}): SigHash](sighashes#hashType%2CPType%2Cset%5BConsiderFlag%5D)
[hasNext:](#hasNext)
* [btrees: hasNext[Key, Val](b: BTree[Key, Val]; index: int): bool](btrees#hasNext%2CBTree%5BKey%2CVal%5D%2Cint)
[hasNilSon:](#hasNilSon)
* [ast: hasNilSon(n: PNode): bool](ast#hasNilSon%2CPNode)
[hasPattern:](#hasPattern)
* [ast: hasPattern(s: PSym): bool](ast#hasPattern%2CPSym)
[hasPragma:](#hasPragma)
* [pragmas: hasPragma(n: PNode; pragma: TSpecialWord): bool](pragmas#hasPragma%2CPNode%2CTSpecialWord)
[hasSideEffect:](#hasSideEffect)
* [varpartitions: hasSideEffect(c: var Partitions; info: var MutationInfo): bool](varpartitions#hasSideEffect%2CPartitions%2CMutationInfo)
[hasSonWith:](#hasSonWith)
* [ast: hasSonWith(n: PNode; kind: TNodeKind): bool](ast#hasSonWith%2CPNode%2CTNodeKind)
[hasSubnodeWith:](#hasSubnodeWith)
* [ast: hasSubnodeWith(n: PNode; kind: TNodeKind): bool](ast#hasSubnodeWith%2CPNode%2CTNodeKind)
[hasSwitchRange:](#hasSwitchRange)
* [TInfoCCProp.hasSwitchRange](extccomp#hasSwitchRange)
[hasTinyCBackend:](#hasTinyCBackend)
* [options: hasTinyCBackend](options#hasTinyCBackend)
[hasWarn:](#hasWarn)
* [options: hasWarn(conf: ConfigRef; note: TNoteKind): bool](options#hasWarn%2CConfigRef%2CTNoteKind)
[hcrOn:](#hcrOn)
* [options: hcrOn(conf: ConfigRef): bool](options#hcrOn%2CConfigRef)
[hExt:](#hExt)
* [extccomp: hExt](extccomp#hExt)
[high:](#high)
* [int128: high(t: typedesc[Int128]): Int128](int128#high.t%2Ctypedesc%5BInt128%5D)
[highBound:](#highBound)
* [guards: highBound(conf: ConfigRef; x: PNode; o: Operators): PNode](guards#highBound%2CConfigRef%2CPNode%2COperators)
[hintCC:](#hintCC)
* [TMsgKind.hintCC](lineinfos#hintCC)
[hintCodeBegin:](#hintCodeBegin)
* [TMsgKind.hintCodeBegin](lineinfos#hintCodeBegin)
[hintCodeEnd:](#hintCodeEnd)
* [TMsgKind.hintCodeEnd](lineinfos#hintCodeEnd)
[hintConditionAlwaysFalse:](#hintConditionAlwaysFalse)
* [TMsgKind.hintConditionAlwaysFalse](lineinfos#hintConditionAlwaysFalse)
[hintConditionAlwaysTrue:](#hintConditionAlwaysTrue)
* [TMsgKind.hintConditionAlwaysTrue](lineinfos#hintConditionAlwaysTrue)
[hintConf:](#hintConf)
* [TMsgKind.hintConf](lineinfos#hintConf)
[hintConvFromXtoItselfNotNeeded:](#hintConvFromXtoItselfNotNeeded)
* [TMsgKind.hintConvFromXtoItselfNotNeeded](lineinfos#hintConvFromXtoItselfNotNeeded)
[hintConvToBaseNotNeeded:](#hintConvToBaseNotNeeded)
* [TMsgKind.hintConvToBaseNotNeeded](lineinfos#hintConvToBaseNotNeeded)
[hintDependency:](#hintDependency)
* [TMsgKind.hintDependency](lineinfos#hintDependency)
[hintExecuting:](#hintExecuting)
* [TMsgKind.hintExecuting](lineinfos#hintExecuting)
[hintExpandMacro:](#hintExpandMacro)
* [TMsgKind.hintExpandMacro](lineinfos#hintExpandMacro)
[hintExprAlwaysX:](#hintExprAlwaysX)
* [TMsgKind.hintExprAlwaysX](lineinfos#hintExprAlwaysX)
[hintExtendedContext:](#hintExtendedContext)
* [TMsgKind.hintExtendedContext](lineinfos#hintExtendedContext)
[hintGCStats:](#hintGCStats)
* [TMsgKind.hintGCStats](lineinfos#hintGCStats)
[hintGlobalVar:](#hintGlobalVar)
* [TMsgKind.hintGlobalVar](lineinfos#hintGlobalVar)
[hintLineTooLong:](#hintLineTooLong)
* [TMsgKind.hintLineTooLong](lineinfos#hintLineTooLong)
[hintLinking:](#hintLinking)
* [TMsgKind.hintLinking](lineinfos#hintLinking)
[hintMax:](#hintMax)
* [lineinfos: hintMax](lineinfos#hintMax)
[hintMin:](#hintMin)
* [lineinfos: hintMin](lineinfos#hintMin)
[hintMsgOrigin:](#hintMsgOrigin)
* [TMsgKind.hintMsgOrigin](lineinfos#hintMsgOrigin)
[hintName:](#hintName)
* [TMsgKind.hintName](lineinfos#hintName)
[hintPath:](#hintPath)
* [TMsgKind.hintPath](lineinfos#hintPath)
[hintPattern:](#hintPattern)
* [TMsgKind.hintPattern](lineinfos#hintPattern)
[hintPerformance:](#hintPerformance)
* [TMsgKind.hintPerformance](lineinfos#hintPerformance)
[hintProcessing:](#hintProcessing)
* [TMsgKind.hintProcessing](lineinfos#hintProcessing)
[hintQuitCalled:](#hintQuitCalled)
* [TMsgKind.hintQuitCalled](lineinfos#hintQuitCalled)
[hintSource:](#hintSource)
* [TMsgKind.hintSource](lineinfos#hintSource)
[hintStackTrace:](#hintStackTrace)
* [TMsgKind.hintStackTrace](lineinfos#hintStackTrace)
[hintSuccess:](#hintSuccess)
* [TMsgKind.hintSuccess](lineinfos#hintSuccess)
[hintSuccessX:](#hintSuccessX)
* [TMsgKind.hintSuccessX](lineinfos#hintSuccessX)
[hintUser:](#hintUser)
* [TMsgKind.hintUser](lineinfos#hintUser)
[hintUserRaw:](#hintUserRaw)
* [TMsgKind.hintUserRaw](lineinfos#hintUserRaw)
[hintXCannotRaiseY:](#hintXCannotRaiseY)
* [TMsgKind.hintXCannotRaiseY](lineinfos#hintXCannotRaiseY)
[hintXDeclaredButNotUsed:](#hintXDeclaredButNotUsed)
* [TMsgKind.hintXDeclaredButNotUsed](lineinfos#hintXDeclaredButNotUsed)
[htmldocsDir:](#htmldocsDir)
* [options: htmldocsDir](options#htmldocsDir)
[htmldocsDirname:](#htmldocsDirname)
* [nimpaths: htmldocsDirname](nimpaths#htmldocsDirname)
[HtmlExt:](#HtmlExt)
* [options: HtmlExt](options#HtmlExt)
[ideChk:](#ideChk)
* [IdeCmd.ideChk](options#ideChk)
[IdeCmd:](#IdeCmd)
* [options: IdeCmd](options#IdeCmd)
[ideCon:](#ideCon)
* [IdeCmd.ideCon](options#ideCon)
[ideDef:](#ideDef)
* [IdeCmd.ideDef](options#ideDef)
[ideDus:](#ideDus)
* [IdeCmd.ideDus](options#ideDus)
[ideHighlight:](#ideHighlight)
* [IdeCmd.ideHighlight](options#ideHighlight)
[ideKnown:](#ideKnown)
* [IdeCmd.ideKnown](options#ideKnown)
[ideMod:](#ideMod)
* [IdeCmd.ideMod](options#ideMod)
[ideMsg:](#ideMsg)
* [IdeCmd.ideMsg](options#ideMsg)
[ideNone:](#ideNone)
* [IdeCmd.ideNone](options#ideNone)
[IdentCache:](#IdentCache)
* [idents: IdentCache](idents#IdentCache)
[identLen:](#identLen)
* [linter: identLen(line: string; start: int): int](linter#identLen%2Cstring%2Cint)
[ideOutline:](#ideOutline)
* [IdeCmd.ideOutline](options#ideOutline)
[ideProject:](#ideProject)
* [IdeCmd.ideProject](options#ideProject)
[ideSug:](#ideSug)
* [IdeCmd.ideSug](options#ideSug)
[ideUse:](#ideUse)
* [IdeCmd.ideUse](options#ideUse)
[idNodeTableGet:](#idNodeTableGet)
* [astalgo: idNodeTableGet(t: TIdNodeTable; key: PIdObj): PNode](astalgo#idNodeTableGet%2CTIdNodeTable%2CPIdObj)
[idNodeTablePut:](#idNodeTablePut)
* [astalgo: idNodeTablePut(t: var TIdNodeTable; key: PIdObj; val: PNode)](astalgo#idNodeTablePut%2CTIdNodeTable%2CPIdObj%2CPNode)
[idOrSig:](#idOrSig)
* [sighashes: idOrSig(s: PSym; currentModule: string; sigCollisions: var CountTable[SigHash]): Rope](sighashes#idOrSig%2CPSym%2Cstring%2CCountTable%5BSigHash%5D)
[idSynchronizationPoint:](#idSynchronizationPoint)
* [idgen: idSynchronizationPoint(idRange: int)](idgen#idSynchronizationPoint%2Cint)
[idTableGet:](#idTableGet)
* [astalgo: idTableGet(t: TIdTable; key: int): RootRef](astalgo#idTableGet%2CTIdTable%2Cint)
* [astalgo: idTableGet(t: TIdTable; key: PIdObj): RootRef](astalgo#idTableGet%2CTIdTable%2CPIdObj)
[idTableHasObjectAsKey:](#idTableHasObjectAsKey)
* [astalgo: idTableHasObjectAsKey(t: TIdTable; key: PIdObj): bool](astalgo#idTableHasObjectAsKey%2CTIdTable%2CPIdObj)
[idTablePairs:](#idTablePairs)
* [astalgo: idTablePairs(t: TIdTable): tuple[key: PIdObj, val: RootRef]](astalgo#idTablePairs.i%2CTIdTable)
[idTablePut:](#idTablePut)
* [astalgo: idTablePut(t: var TIdTable; key: PIdObj; val: RootRef)](astalgo#idTablePut%2CTIdTable%2CPIdObj%2CRootRef)
[IgnoreCC:](#IgnoreCC)
* [TTypeCmpFlag.IgnoreCC](types#IgnoreCC)
[IgnoreTupleFields:](#IgnoreTupleFields)
* [TTypeCmpFlag.IgnoreTupleFields](types#IgnoreTupleFields)
[iiTableGet:](#iiTableGet)
* [astalgo: iiTableGet(t: TIITable; key: int): int](astalgo#iiTableGet%2CTIITable%2Cint)
[iiTablePut:](#iiTablePut)
* [astalgo: iiTablePut(t: var TIITable; key, val: int)](astalgo#iiTablePut%2CTIITable%2Cint%2Cint)
[illegalCustomPragma:](#illegalCustomPragma)
* [pragmas: illegalCustomPragma(c: PContext; n: PNode; s: PSym)](pragmas#illegalCustomPragma%2CPContext%2CPNode%2CPSym)
[illFormedAst:](#illFormedAst)
* [semdata: illFormedAst(n: PNode; conf: ConfigRef)](semdata#illFormedAst%2CPNode%2CConfigRef)
[illFormedAstLocal:](#illFormedAstLocal)
* [semdata: illFormedAstLocal(n: PNode; conf: ConfigRef)](semdata#illFormedAstLocal%2CPNode%2CConfigRef)
[immutableView:](#immutableView)
* [ViewTypeKind.immutableView](typeallowed#immutableView)
[implicitDeref:](#implicitDeref)
* [Feature.implicitDeref](options#implicitDeref)
[implicitPragmas:](#implicitPragmas)
* [pragmas: implicitPragmas(c: PContext; sym: PSym; n: PNode; validPragmas: TSpecialWords)](pragmas#implicitPragmas%2CPContext%2CPSym%2CPNode%2CTSpecialWords)
[impliesNotNil:](#impliesNotNil)
* [guards: impliesNotNil(m: TModel; arg: PNode): TImplication](guards#impliesNotNil%2CTModel%2CPNode)
[impNo:](#impNo)
* [TImplication.impNo](ast#impNo)
[importAllSymbols:](#importAllSymbols)
* [importer: importAllSymbols(c: PContext; fromMod: PSym)](importer#importAllSymbols%2CPContext%2CPSym)
[importantComments:](#importantComments)
* [options: importantComments(conf: ConfigRef): bool](options#importantComments%2CConfigRef)
[importcCond:](#importcCond)
* [vmgen: importcCond(s: PSym): bool](vmgen#importcCond%2CPSym)
[importcCondVar:](#importcCondVar)
* [vmgen: importcCondVar(s: PSym): bool](vmgen#importcCondVar%2CPSym)
[importModule:](#importModule)
* [modules: importModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PSym](modules#importModule%2CModuleGraph%2CPSym%2CFileIndex)
[importPureEnumField:](#importPureEnumField)
* [importer: importPureEnumField(c: PContext; s: PSym)](importer#importPureEnumField%2CPContext%2CPSym)
[impUnknown:](#impUnknown)
* [TImplication.impUnknown](ast#impUnknown)
[impYes:](#impYes)
* [TImplication.impYes](ast#impYes)
[inc:](#inc)
* [int128: inc(a: var Int128; y: uint32 = 1)](int128#inc%2CInt128%2Cuint32)
[inCheckpoint:](#inCheckpoint)
* [sigmatch: inCheckpoint(current, trackPos: TLineInfo): TCheckPointResult](sigmatch#inCheckpoint%2CTLineInfo%2CTLineInfo)
[inclDynlibOverride:](#inclDynlibOverride)
* [options: inclDynlibOverride(conf: ConfigRef; lib: string)](options#inclDynlibOverride%2CConfigRef%2Cstring)
[includeHeader:](#includeHeader)
* [cgendata: includeHeader(this: BModule; header: string)](cgendata#includeHeader%2CBModule%2Cstring)
[includeModule:](#includeModule)
* [modules: includeModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PNode](modules#includeModule%2CModuleGraph%2CPSym%2CFileIndex)
* [reorder: includeModule(graph: ModuleGraph; s: PSym; fileIdx: FileIndex): PNode](reorder#includeModule%2CModuleGraph%2CPSym%2CFileIndex)
[includesStringh:](#includesStringh)
* [CodegenFlag.includesStringh](cgendata#includesStringh)
[incompleteType:](#incompleteType)
* [ast: incompleteType(t: PType): bool](ast#incompleteType.t%2CPType)
[IncrementalCtx:](#IncrementalCtx)
* [incremental: IncrementalCtx](incremental#IncrementalCtx)
[indAndComment:](#indAndComment)
* [parser: indAndComment(p: var Parser; n: PNode)](parser#indAndComment%2CParser%2CPNode)
[index:](#index)
* [sourcemap: index[T](elements: seq[T]; element: T): int](sourcemap#index%2Cseq%5BT%5D%2CT)
[indirectAccess:](#indirectAccess)
* [lowerings: indirectAccess(a: PNode; b: int; info: TLineInfo): PNode](lowerings#indirectAccess%2CPNode%2Cint%2CTLineInfo)
* [lowerings: indirectAccess(a: PNode; b: PSym; info: TLineInfo): PNode](lowerings#indirectAccess%2CPNode%2CPSym%2CTLineInfo)
* [lowerings: indirectAccess(a: PNode; b: string; info: TLineInfo; cache: IdentCache): PNode](lowerings#indirectAccess%2CPNode%2Cstring%2CTLineInfo%2CIdentCache)
* [lowerings: indirectAccess(a, b: PSym; info: TLineInfo): PNode](lowerings#indirectAccess%2CPSym%2CPSym%2CTLineInfo)
[inferStaticParam:](#inferStaticParam)
* [sigmatch: inferStaticParam(c: var TCandidate; lhs: PNode; rhs: BiggestInt): bool](sigmatch#inferStaticParam%2CTCandidate%2CPNode%2CBiggestInt)
[inheritanceDiff:](#inheritanceDiff)
* [types: inheritanceDiff(a, b: PType): int](types#inheritanceDiff%2CPType%2CPType)
[IniExt:](#IniExt)
* [options: IniExt](options#IniExt)
[init:](#init)
* [incremental: init(incr: IncrementalCtx)](incremental#init.t%2CIncrementalCtx)
[initBTree:](#initBTree)
* [btrees: initBTree[Key, Val](): BTree[Key, Val]](btrees#initBTree)
[initCandidate:](#initCandidate)
* [sigmatch: initCandidate(ctx: PContext; c: var TCandidate; callee: PSym; binding: PNode; calleeScope = -1; diagnosticsEnabled = false)](sigmatch#initCandidate%2CPContext%2CTCandidate%2CPSym%2CPNode)
* [sigmatch: initCandidate(ctx: PContext; c: var TCandidate; callee: PType)](sigmatch#initCandidate%2CPContext%2CTCandidate%2CPType)
[initDefines:](#initDefines)
* [condsyms: initDefines(symbols: StringTableRef)](condsyms#initDefines%2CStringTableRef)
[initDefinesProg:](#initDefinesProg)
* [cmdlinehelper: initDefinesProg(self: NimProg; conf: ConfigRef; name: string)](cmdlinehelper#initDefinesProg%2CNimProg%2CConfigRef%2Cstring)
[initIdentIter:](#initIdentIter)
* [astalgo: initIdentIter(ti: var TIdentIter; tab: TStrTable; s: PIdent): PSym](astalgo#initIdentIter%2CTIdentIter%2CTStrTable%2CPIdent)
[initIdNodeTable:](#initIdNodeTable)
* [ast: initIdNodeTable(x: var TIdNodeTable)](ast#initIdNodeTable%2CTIdNodeTable)
[initIdTable:](#initIdTable)
* [ast: initIdTable(x: var TIdTable)](ast#initIdTable%2CTIdTable)
[initIiTable:](#initIiTable)
* [astalgo: initIiTable(x: var TIITable)](astalgo#initIiTable%2CTIITable)
[initLayeredTypeMap:](#initLayeredTypeMap)
* [semtypinst: initLayeredTypeMap(pt: TIdTable): LayeredIdTable](semtypinst#initLayeredTypeMap%2CTIdTable)
[initMsgConfig:](#initMsgConfig)
* [lineinfos: initMsgConfig(): MsgConfig](lineinfos#initMsgConfig)
[initNodeTable:](#initNodeTable)
* [ast: initNodeTable(x: var TNodeTable)](ast#initNodeTable%2CTNodeTable)
[initObjectSet:](#initObjectSet)
* [ast: initObjectSet(x: var TObjectSet)](ast#initObjectSet%2CTObjectSet)
[initOperators:](#initOperators)
* [guards: initOperators(g: ModuleGraph): Operators](guards#initOperators%2CModuleGraph)
[initOverloadIter:](#initOverloadIter)
* [lookups: initOverloadIter(o: var TOverloadIter; c: PContext; n: PNode): PSym](lookups#initOverloadIter%2CTOverloadIter%2CPContext%2CPNode)
[initStrTable:](#initStrTable)
* [ast: initStrTable(x: var TStrTable)](ast#initStrTable%2CTStrTable)
[initTabIter:](#initTabIter)
* [astalgo: initTabIter(ti: var TTabIter; tab: TStrTable): PSym](astalgo#initTabIter%2CTTabIter%2CTStrTable)
[initToken:](#initToken)
* [lexer: initToken(L: var Token)](lexer#initToken%2CToken)
[initTokRender:](#initTokRender)
* [renderer: initTokRender(r: var TSrcGen; n: PNode; renderFlags: TRenderFlags = {})](renderer#initTokRender%2CTSrcGen%2CPNode%2CTRenderFlags)
[initTypeVars:](#initTypeVars)
* [semtypinst: initTypeVars(p: PContext; typeMap: LayeredIdTable; info: TLineInfo; owner: PSym): TReplTypeVars](semtypinst#initTypeVars%2CPContext%2CLayeredIdTable%2CTLineInfo%2CPSym)
[initVars:](#initVars)
* [extccomp: initVars(conf: ConfigRef)](extccomp#initVars%2CConfigRef)
[injectDestructorCalls:](#injectDestructorCalls)
* [injectdestructors: injectDestructorCalls(g: ModuleGraph; owner: PSym; n: PNode): PNode](injectdestructors#injectDestructorCalls%2CModuleGraph%2CPSym%2CPNode)
[inSet:](#inSet)
* [nimsets: inSet(s: PNode; elem: PNode): bool](nimsets#inSet%2CPNode%2CPNode)
[instAllTypeBoundOp:](#instAllTypeBoundOp)
* [semtypinst: instAllTypeBoundOp(c: PContext; info: TLineInfo)](semtypinst#instAllTypeBoundOp%2CPContext%2CTLineInfo)
[InstantiationInfo:](#InstantiationInfo)
* [msgs: InstantiationInfo](msgs#InstantiationInfo)
[instCopyType:](#instCopyType)
* [semtypinst: instCopyType(cl: var TReplTypeVars; t: PType): PType](semtypinst#instCopyType%2CTReplTypeVars%2CPType)
[instGenericConvertersArg:](#instGenericConvertersArg)
* [sem: instGenericConvertersArg(c: PContext; a: PNode; x: TCandidate)](sem#instGenericConvertersArg%2CPContext%2CPNode%2CTCandidate)
[instGenericConvertersSons:](#instGenericConvertersSons)
* [sem: instGenericConvertersSons(c: PContext; n: PNode; x: TCandidate)](sem#instGenericConvertersSons%2CPContext%2CPNode%2CTCandidate)
[Instr:](#Instr)
* [dfa: Instr](dfa#Instr)
[InstrKind:](#InstrKind)
* [dfa: InstrKind](dfa#InstrKind)
[InstrTargetKind:](#InstrTargetKind)
* [dfa: InstrTargetKind](dfa#InstrTargetKind)
[instrTargets:](#instrTargets)
* [dfa: instrTargets(insloc, loc: PNode): InstrTargetKind](dfa#instrTargets%2CPNode%2CPNode)
[instTypeBoundOp:](#instTypeBoundOp)
* [sigmatch: instTypeBoundOp(c: PContext; dc: PSym; t: PType; info: TLineInfo; op: TTypeAttachedOp; col: int): PSym](sigmatch#instTypeBoundOp%2CPContext%2CPSym%2CPType%2CTLineInfo%2CTTypeAttachedOp%2Cint)
[Int128:](#Int128)
* [int128: Int128](int128#Int128)
[IntegralTypes:](#IntegralTypes)
* [ast: IntegralTypes](ast#IntegralTypes)
[interestingCaseExpr:](#interestingCaseExpr)
* [guards: interestingCaseExpr(m: PNode): bool](guards#interestingCaseExpr%2CPNode)
[internalAssert:](#internalAssert)
* [msgs: internalAssert(conf: ConfigRef; e: bool)](msgs#internalAssert.t%2CConfigRef%2Cbool)
[internalError:](#internalError)
* [msgs: internalError(conf: ConfigRef; errMsg: string)](msgs#internalError.t%2CConfigRef%2Cstring)
* [msgs: internalError(conf: ConfigRef; info: TLineInfo; errMsg: string)](msgs#internalError.t%2CConfigRef%2CTLineInfo%2Cstring)
[interp:](#interp)
* [nimpaths: interp(path: string; nimr: string): string](nimpaths#interp%2Cstring%2Cstring)
[intersectSets:](#intersectSets)
* [nimsets: intersectSets(conf: ConfigRef; a, b: PNode): PNode](nimsets#intersectSets%2CConfigRef%2CPNode%2CPNode)
[IntLike:](#IntLike)
* [OrdinalType.IntLike](types#IntLike)
[invalidateFacts:](#invalidateFacts)
* [guards: invalidateFacts(s: var seq[PNode]; n: PNode)](guards#invalidateFacts%2Cseq%5BPNode%5D%2CPNode)
* [guards: invalidateFacts(m: var TModel; n: PNode)](guards#invalidateFacts%2CTModel%2CPNode)
[InvalidFileIdx:](#InvalidFileIdx)
* [lineinfos: InvalidFileIdx](lineinfos#InvalidFileIdx)
[invalidGenericInst:](#invalidGenericInst)
* [types: invalidGenericInst(f: PType): bool](types#invalidGenericInst%2CPType)
[InvalidKey:](#InvalidKey)
* [astalgo: InvalidKey](astalgo#InvalidKey)
[invalidPragma:](#invalidPragma)
* [pragmas: invalidPragma(c: PContext; n: PNode)](pragmas#invalidPragma%2CPContext%2CPNode)
[isAddrNode:](#isAddrNode)
* [astalgo: isAddrNode(n: PNode): bool](astalgo#isAddrNode%2CPNode)
[isAnalysableFieldAccess:](#isAnalysableFieldAccess)
* [dfa: isAnalysableFieldAccess(orig: PNode; owner: PSym): bool](dfa#isAnalysableFieldAccess%2CPNode%2CPSym)
[isAssignable:](#isAssignable)
* [parampatterns: isAssignable(owner: PSym; n: PNode; isUnsafeAddr = false): TAssignableResult](parampatterns#isAssignable%2CPSym%2CPNode)
[isAtom:](#isAtom)
* [ast: isAtom(n: PNode): bool](ast#isAtom%2CPNode)
[isBothMetaConvertible:](#isBothMetaConvertible)
* [TTypeRelation.isBothMetaConvertible](sigmatch#isBothMetaConvertible)
[isCallExpr:](#isCallExpr)
* [ast: isCallExpr(n: PNode): bool](ast#isCallExpr%2CPNode)
[isCaseObj:](#isCaseObj)
* [trees: isCaseObj(n: PNode): bool](trees#isCaseObj%2CPNode)
[isClosure:](#isClosure)
* [ast: isClosure(typ: PType): bool](ast#isClosure%2CPType)
[isClosureIterator:](#isClosureIterator)
* [ast: isClosureIterator(typ: PType): bool](ast#isClosureIterator%2CPType)
[isCompileTimeOnly:](#isCompileTimeOnly)
* [types: isCompileTimeOnly(t: PType): bool](types#isCompileTimeOnly%2CPType)
[isCompileTimeProc:](#isCompileTimeProc)
* [ast: isCompileTimeProc(s: PSym): bool](ast#isCompileTimeProc%2CPSym)
[isConstExpr:](#isConstExpr)
* [trees: isConstExpr(n: PNode): bool](trees#isConstExpr%2CPNode)
[isConvertible:](#isConvertible)
* [TTypeRelation.isConvertible](sigmatch#isConvertible)
[isDeepConstExpr:](#isDeepConstExpr)
* [trees: isDeepConstExpr(n: PNode; preventInheritance = false): bool](trees#isDeepConstExpr%2CPNode)
[isDefectException:](#isDefectException)
* [types: isDefectException(t: PType): bool](types#isDefectException%2CPType)
[isDefined:](#isDefined)
* [options: isDefined(conf: ConfigRef; symbol: string): bool](options#isDefined%2CConfigRef%2Cstring)
[isDirty:](#isDirty)
* [modulegraphs: isDirty(g: ModuleGraph; m: PSym): bool](modulegraphs#isDirty%2CModuleGraph%2CPSym)
[isDiscriminantField:](#isDiscriminantField)
* [astalgo: isDiscriminantField(n: PNode): bool](astalgo#isDiscriminantField%2CPNode)
[isDynlibOverride:](#isDynlibOverride)
* [options: isDynlibOverride(conf: ConfigRef; lib: string): bool](options#isDynlibOverride%2CConfigRef%2Cstring)
[isEmpty:](#isEmpty)
* [pathutils: isEmpty(x: AnyPath): bool](pathutils#isEmpty%2CAnyPath)
[isEmptyContainer:](#isEmptyContainer)
* [types: isEmptyContainer(t: PType): bool](types#isEmptyContainer%2CPType)
[isEmptyType:](#isEmptyType)
* [ast: isEmptyType(t: PType): bool](ast#isEmptyType%2CPType)
[isEqual:](#isEqual)
* [TTypeRelation.isEqual](sigmatch#isEqual)
[isException:](#isException)
* [types: isException(t: PType): bool](types#isException%2CPType)
[isFinal:](#isFinal)
* [types: isFinal(t: PType): bool](types#isFinal%2CPType)
[isFloatLit:](#isFloatLit)
* [types: isFloatLit(t: PType): bool](types#isFloatLit%2CPType)
[isFromIntLit:](#isFromIntLit)
* [TTypeRelation.isFromIntLit](sigmatch#isFromIntLit)
[isGCedMem:](#isGCedMem)
* [ast: isGCedMem(t: PType): bool](ast#isGCedMem%2CPType)
[isGeneric:](#isGeneric)
* [TTypeRelation.isGeneric](sigmatch#isGeneric)
[isGenericAlias:](#isGenericAlias)
* [types: isGenericAlias(t: PType): bool](types#isGenericAlias%2CPType)
[isGenericRoutine:](#isGenericRoutine)
* [ast: isGenericRoutine(s: PSym): bool](ast#isGenericRoutine%2CPSym)
[isHeaderFile:](#isHeaderFile)
* [CodegenFlag.isHeaderFile](cgendata#isHeaderFile)
[isImportedException:](#isImportedException)
* [ast: isImportedException(t: PType; conf: ConfigRef): bool](ast#isImportedException%2CPType%2CConfigRef)
[isInferred:](#isInferred)
* [TTypeRelation.isInferred](sigmatch#isInferred)
[isInferredConvertible:](#isInferredConvertible)
* [TTypeRelation.isInferredConvertible](sigmatch#isInferredConvertible)
[isInfixAs:](#isInfixAs)
* [ast: isInfixAs(n: PNode): bool](ast#isInfixAs%2CPNode)
[isInlineIterator:](#isInlineIterator)
* [ast: isInlineIterator(typ: PType): bool](ast#isInlineIterator%2CPType)
[isIntConv:](#isIntConv)
* [TTypeRelation.isIntConv](sigmatch#isIntConv)
[isIntLit:](#isIntLit)
* [types: isIntLit(t: PType): bool](types#isIntLit%2CPType)
[isIterator:](#isIterator)
* [lambdalifting: isIterator(owner: PSym): bool](lambdalifting#isIterator.t%2CPSym)
[isKeyword:](#isKeyword)
* [lexer: isKeyword(kind: TokType): bool](lexer#isKeyword%2CTokType)
* [renderer: isKeyword(i: PIdent): bool](renderer#isKeyword%2CPIdent)
[isLValue:](#isLValue)
* [parampatterns: isLValue(n: PNode): bool](parampatterns#isLValue%2CPNode)
[isMetaType:](#isMetaType)
* [ast: isMetaType(t: PType): bool](ast#isMetaType%2CPType)
[isNimIdentifier:](#isNimIdentifier)
* [lexer: isNimIdentifier(s: string): bool](lexer#isNimIdentifier%2Cstring)
[isNone:](#isNone)
* [TTypeRelation.isNone](sigmatch#isNone)
[isNoSideEffectPragma:](#isNoSideEffectPragma)
* [trees: isNoSideEffectPragma(n: PNode): bool](trees#isNoSideEffectPragma%2CPNode)
[isOperator:](#isOperator)
* [parser: isOperator(tok: Token): bool](parser#isOperator%2CToken)
[isOrdinalType:](#isOrdinalType)
* [types: isOrdinalType(t: PType; allowEnumWithHoles: bool = false): bool](types#isOrdinalType%2CPType%2Cbool)
[isPartOf:](#isPartOf)
* [aliases: isPartOf(a, b: PNode): TAnalysisResult](aliases#isPartOf%2CPNode%2CPNode)
[isPureObject:](#isPureObject)
* [types: isPureObject(typ: PType): bool](types#isPureObject%2CPType)
[isRange:](#isRange)
* [trees: isRange(n: PNode): bool](trees#isRange%2CPNode)
[isResolvedUserTypeClass:](#isResolvedUserTypeClass)
* [types: isResolvedUserTypeClass(t: PType): bool](types#isResolvedUserTypeClass.t%2CPType)
[isRoutine:](#isRoutine)
* [ast: isRoutine(s: PSym): bool](ast#isRoutine%2CPSym)
[isRunnableExamples:](#isRunnableExamples)
* [ast: isRunnableExamples(n: PNode): bool](ast#isRunnableExamples%2CPNode)
[isShadowScope:](#isShadowScope)
* [lookups: isShadowScope(s: PScope): bool](lookups#isShadowScope%2CPScope)
[isSinkParam:](#isSinkParam)
* [ast: isSinkParam(s: PSym): bool](ast#isSinkParam%2CPSym)
[isSinkType:](#isSinkType)
* [ast: isSinkType(t: PType): bool](ast#isSinkType%2CPType)
[isSinkTypeForParam:](#isSinkTypeForParam)
* [types: isSinkTypeForParam(t: PType): bool](types#isSinkTypeForParam%2CPType)
[isSubrange:](#isSubrange)
* [TTypeRelation.isSubrange](sigmatch#isSubrange)
[isSubtype:](#isSubtype)
* [TTypeRelation.isSubtype](sigmatch#isSubtype)
[isTopLevel:](#isTopLevel)
* [semdata: isTopLevel(c: PContext): bool](semdata#isTopLevel%2CPContext)
[isTracked:](#isTracked)
* [sigmatch: isTracked(current, trackPos: TLineInfo; tokenLen: int): bool](sigmatch#isTracked%2CTLineInfo%2CTLineInfo%2Cint)
[isTrue:](#isTrue)
* [trees: isTrue(n: PNode): bool](trees#isTrue%2CPNode)
[isTupleRecursive:](#isTupleRecursive)
* [types: isTupleRecursive(t: PType): bool](types#isTupleRecursive%2CPType)
[isUnresolvedStatic:](#isUnresolvedStatic)
* [ast: isUnresolvedStatic(t: PType): bool](ast#isUnresolvedStatic%2CPType)
[isUnsigned:](#isUnsigned)
* [types: isUnsigned(t: PType): bool](types#isUnsigned%2CPType)
[isVSCompatible:](#isVSCompatible)
* [extccomp: isVSCompatible(conf: ConfigRef): bool](extccomp#isVSCompatible%2CConfigRef)
[items:](#items)
* [ast: items(n: PNode): PNode](ast#items.i%2CPNode)
* [astalgo: items(tab: TStrTable): PSym](astalgo#items.i%2CTStrTable)
* [ropes: items(r: Rope): char](ropes#items.i%2CRope)
[iteratorPragmas:](#iteratorPragmas)
* [pragmas: iteratorPragmas](pragmas#iteratorPragmas)
[iterOverType:](#iterOverType)
* [types: iterOverType(t: PType; iter: TTypeIter; closure: RootRef): bool](types#iterOverType%2CPType%2CTTypeIter%2CRootRef)
[iterToProcImpl:](#iterToProcImpl)
* [itersgen: iterToProcImpl(c: PContext; n: PNode): PNode](plugins/itersgen#iterToProcImpl%2CPContext%2CPNode)
[jmpDiff:](#jmpDiff)
* [vmdef: jmpDiff(x: TInstr): int](vmdef#jmpDiff.t%2CTInstr)
[JSgenPass:](#JSgenPass)
* [jsgen: JSgenPass](jsgen#JSgenPass)
[JsonExt:](#JsonExt)
* [options: JsonExt](options#JsonExt)
[kAlreadyGiven:](#kAlreadyGiven)
* [MismatchKind.kAlreadyGiven](sigmatch#kAlreadyGiven)
[kExtraArg:](#kExtraArg)
* [MismatchKind.kExtraArg](sigmatch#kExtraArg)
[kMissingParam:](#kMissingParam)
* [MismatchKind.kMissingParam](sigmatch#kMissingParam)
[kPositionalAlreadyGiven:](#kPositionalAlreadyGiven)
* [MismatchKind.kPositionalAlreadyGiven](sigmatch#kPositionalAlreadyGiven)
[kTypeMismatch:](#kTypeMismatch)
* [MismatchKind.kTypeMismatch](sigmatch#kTypeMismatch)
[kUnknown:](#kUnknown)
* [MismatchKind.kUnknown](sigmatch#kUnknown)
[kUnknownNamedParam:](#kUnknownNamedParam)
* [MismatchKind.kUnknownNamedParam](sigmatch#kUnknownNamedParam)
[kVarNeeded:](#kVarNeeded)
* [MismatchKind.kVarNeeded](sigmatch#kVarNeeded)
[lambdaPragmas:](#lambdaPragmas)
* [pragmas: lambdaPragmas](pragmas#lambdaPragmas)
[largeInstrs:](#largeInstrs)
* [vmdef: largeInstrs](vmdef#largeInstrs)
[LastCallConv:](#LastCallConv)
* [pragmas: LastCallConv](pragmas#LastCallConv)
[lastFloat:](#lastFloat)
* [types: lastFloat(t: PType): BiggestFloat](types#lastFloat%2CPType)
[lastOptionEntry:](#lastOptionEntry)
* [semdata: lastOptionEntry(c: PContext): POptionEntry](semdata#lastOptionEntry%2CPContext)
[lastOrd:](#lastOrd)
* [types: lastOrd(conf: ConfigRef; t: PType): Int128](types#lastOrd%2CConfigRef%2CPType)
[lastOverloadScope:](#lastOverloadScope)
* [lookups: lastOverloadScope(o: TOverloadIter): int](lookups#lastOverloadScope%2CTOverloadIter)
[lastSon:](#lastSon)
* [ast: lastSon(n: Indexable): Indexable](ast#lastSon%2CIndexable)
[LayeredIdTable:](#LayeredIdTable)
* [semtypinst: LayeredIdTable](semtypinst#LayeredIdTable)
[leave:](#leave)
* [vmprofiler: leave(prof: var Profiler; c: PCtx)](vmprofiler#leave%2CProfiler%2CPCtx)
[leaves:](#leaves)
* [ropes: leaves(r: Rope): string](ropes#leaves.i%2CRope)
[LegacyFeature:](#LegacyFeature)
* [options: LegacyFeature](options#LegacyFeature)
[len:](#len)
* [ast: len(n: Indexable): int](ast#len%2CIndexable)
* [btrees: len[Key, Val](b: BTree[Key, Val]): int](btrees#len%2CBTree%5BKey%2CVal%5D)
* [ropes: len(a: Rope): int](ropes#len%2CRope)
[lengthOrd:](#lengthOrd)
* [types: lengthOrd(conf: ConfigRef; t: PType): Int128](types#lengthOrd%2CConfigRef%2CPType)
[letPragmas:](#letPragmas)
* [pragmas: letPragmas](pragmas#letPragmas)
[Letters:](#Letters)
* [linter: Letters](linter#Letters)
[leValue:](#leValue)
* [astalgo: leValue(a, b: PNode): bool](astalgo#leValue%2CPNode%2CPNode)
[leValueConv:](#leValueConv)
* [semfold: leValueConv(a, b: PNode): bool](semfold#leValueConv%2CPNode%2CPNode)
[Lexer:](#Lexer)
* [lexer: Lexer](lexer#Lexer)
[lexMessage:](#lexMessage)
* [lexer: lexMessage(L: Lexer; msg: TMsgKind; arg = "")](lexer#lexMessage%2CLexer%2CTMsgKind%2Cstring)
[lexMessageTok:](#lexMessageTok)
* [lexer: lexMessageTok(L: Lexer; msg: TMsgKind; tok: Token; arg = "")](lexer#lexMessageTok%2CLexer%2CTMsgKind%2CToken%2Cstring)
[LF:](#LF)
* [nimlexbase: LF](nimlexbase#LF)
[lfDynamicLib:](#lfDynamicLib)
* [TLocFlag.lfDynamicLib](ast#lfDynamicLib)
[lfEnforceDeref:](#lfEnforceDeref)
* [TLocFlag.lfEnforceDeref](ast#lfEnforceDeref)
[lfExportLib:](#lfExportLib)
* [TLocFlag.lfExportLib](ast#lfExportLib)
[lfFullExternalName:](#lfFullExternalName)
* [TLocFlag.lfFullExternalName](ast#lfFullExternalName)
[lfHeader:](#lfHeader)
* [TLocFlag.lfHeader](ast#lfHeader)
[lfImportCompilerProc:](#lfImportCompilerProc)
* [TLocFlag.lfImportCompilerProc](ast#lfImportCompilerProc)
[lfIndirect:](#lfIndirect)
* [TLocFlag.lfIndirect](ast#lfIndirect)
[lfNoDecl:](#lfNoDecl)
* [TLocFlag.lfNoDecl](ast#lfNoDecl)
[lfNoDeepCopy:](#lfNoDeepCopy)
* [TLocFlag.lfNoDeepCopy](ast#lfNoDeepCopy)
[lfPrepareForMutation:](#lfPrepareForMutation)
* [TLocFlag.lfPrepareForMutation](ast#lfPrepareForMutation)
[lfSingleUse:](#lfSingleUse)
* [TLocFlag.lfSingleUse](ast#lfSingleUse)
[libDynamic:](#libDynamic)
* [TLibKind.libDynamic](ast#libDynamic)
[libHeader:](#libHeader)
* [TLibKind.libHeader](ast#libHeader)
[liftForLoop:](#liftForLoop)
* [lambdalifting: liftForLoop(g: ModuleGraph; body: PNode; owner: PSym): PNode](lambdalifting#liftForLoop%2CModuleGraph%2CPNode%2CPSym)
[liftIterSym:](#liftIterSym)
* [lambdalifting: liftIterSym(g: ModuleGraph; n: PNode; owner: PSym): PNode](lambdalifting#liftIterSym%2CModuleGraph%2CPNode%2CPSym)
[liftIterToProc:](#liftIterToProc)
* [lambdalifting: liftIterToProc(g: ModuleGraph; fn: PSym; body: PNode; ptrType: PType): PNode](lambdalifting#liftIterToProc%2CModuleGraph%2CPSym%2CPNode%2CPType)
[liftLambdas:](#liftLambdas)
* [lambdalifting: liftLambdas(g: ModuleGraph; fn: PSym; body: PNode; tooEarly: var bool): PNode](lambdalifting#liftLambdas%2CModuleGraph%2CPSym%2CPNode%2Cbool)
[liftLambdasForTopLevel:](#liftLambdasForTopLevel)
* [lambdalifting: liftLambdasForTopLevel(module: PSym; body: PNode): PNode](lambdalifting#liftLambdasForTopLevel%2CPSym%2CPNode)
[liftLocalsIfRequested:](#liftLocalsIfRequested)
* [liftlocals: liftLocalsIfRequested(prc: PSym; n: PNode; cache: IdentCache; conf: ConfigRef): PNode](liftlocals#liftLocalsIfRequested%2CPSym%2CPNode%2CIdentCache%2CConfigRef)
[liftParallel:](#liftParallel)
* [semparallel: liftParallel(g: ModuleGraph; owner: PSym; n: PNode): PNode](semparallel#liftParallel%2CModuleGraph%2CPSym%2CPNode)
[liftTypeBoundOps:](#liftTypeBoundOps)
* [liftdestructors: liftTypeBoundOps(c: PContext; typ: PType; info: TLineInfo)](liftdestructors#liftTypeBoundOps.t%2CPContext%2CPType%2CTLineInfo)
[liMessage:](#liMessage)
* [msgs: liMessage(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg: string; eh: TErrorHandling; info2: InstantiationInfo; isRaw = false)](msgs#liMessage%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring%2CTErrorHandling%2CInstantiationInfo)
[lineInfoToStr:](#lineInfoToStr)
* [astalgo: lineInfoToStr(conf: ConfigRef; info: TLineInfo): Rope](astalgo#lineInfoToStr%2CConfigRef%2CTLineInfo)
[linkTo:](#linkTo)
* [ast: linkTo(s: PSym; t: PType): PSym](ast#linkTo%2CPSym%2CPType)
* [ast: linkTo(t: PType; s: PSym): PType](ast#linkTo%2CPType%2CPSym)
[lintReport:](#lintReport)
* [msgs: lintReport(conf: ConfigRef; info: TLineInfo; beau, got: string)](msgs#lintReport.t%2CConfigRef%2CTLineInfo%2Cstring%2Cstring)
[listCPUnames:](#listCPUnames)
* [platform: listCPUnames(): seq[string]](platform#listCPUnames)
[listHints:](#listHints)
* [msgs: listHints(conf: ConfigRef)](msgs#listHints%2CConfigRef)
[listOSnames:](#listOSnames)
* [platform: listOSnames(): seq[string]](platform#listOSnames)
[listSymbolNames:](#listSymbolNames)
* [astalgo: listSymbolNames(symbols: openArray[PSym]): string](astalgo#listSymbolNames%2CopenArray%5BPSym%5D)
[listWarnings:](#listWarnings)
* [msgs: listWarnings(conf: ConfigRef)](msgs#listWarnings%2CConfigRef)
[littleEndian:](#littleEndian)
* [TEndian.littleEndian](platform#littleEndian)
[llsFile:](#llsFile)
* [TLLStreamKind.llsFile](llstream#llsFile)
[llsNone:](#llsNone)
* [TLLStreamKind.llsNone](llstream#llsNone)
[llsStdIn:](#llsStdIn)
* [TLLStreamKind.llsStdIn](llstream#llsStdIn)
[llsString:](#llsString)
* [TLLStreamKind.llsString](llstream#llsString)
[llStreamClose:](#llStreamClose)
* [llstream: llStreamClose(s: PLLStream)](llstream#llStreamClose%2CPLLStream)
[llStreamOpen:](#llStreamOpen)
* [llstream: llStreamOpen(): PLLStream](llstream#llStreamOpen)
* [llstream: llStreamOpen(filename: AbsoluteFile; mode: FileMode): PLLStream](llstream#llStreamOpen%2CAbsoluteFile%2CFileMode)
* [llstream: llStreamOpen(f: File): PLLStream](llstream#llStreamOpen%2CFile)
* [llstream: llStreamOpen(data: string): PLLStream](llstream#llStreamOpen%2Cstring)
[llStreamOpenStdIn:](#llStreamOpenStdIn)
* [llstream: llStreamOpenStdIn(r: TLLRepl = llReadFromStdin): PLLStream](llstream#llStreamOpenStdIn%2CTLLRepl)
[llStreamRead:](#llStreamRead)
* [llstream: llStreamRead(s: PLLStream; buf: pointer; bufLen: int): int](llstream#llStreamRead%2CPLLStream%2Cpointer%2Cint)
[llStreamReadAll:](#llStreamReadAll)
* [llstream: llStreamReadAll(s: PLLStream): string](llstream#llStreamReadAll%2CPLLStream)
[llStreamReadLine:](#llStreamReadLine)
* [llstream: llStreamReadLine(s: PLLStream; line: var string): bool](llstream#llStreamReadLine%2CPLLStream%2Cstring)
[llStreamWrite:](#llStreamWrite)
* [llstream: llStreamWrite(s: PLLStream; data: char)](llstream#llStreamWrite%2CPLLStream%2Cchar)
* [llstream: llStreamWrite(s: PLLStream; buf: pointer; buflen: int)](llstream#llStreamWrite%2CPLLStream%2Cpointer%2Cint)
* [llstream: llStreamWrite(s: PLLStream; data: string)](llstream#llStreamWrite%2CPLLStream%2Cstring)
[llStreamWriteln:](#llStreamWriteln)
* [llstream: llStreamWriteln(s: PLLStream; data: string)](llstream#llStreamWriteln%2CPLLStream%2Cstring)
[loadAny:](#loadAny)
* [vmmarshal: loadAny(s: string; t: PType; cache: IdentCache; conf: ConfigRef): PNode](vmmarshal#loadAny%2Cstring%2CPType%2CIdentCache%2CConfigRef)
[loadConfigs:](#loadConfigs)
* [nimconf: loadConfigs(cfg: RelativeFile; cache: IdentCache; conf: ConfigRef)](nimconf#loadConfigs%2CRelativeFile%2CIdentCache%2CConfigRef)
[loadConfigsAndRunMainCommand:](#loadConfigsAndRunMainCommand)
* [cmdlinehelper: loadConfigsAndRunMainCommand(self: NimProg; cache: IdentCache; conf: ConfigRef; graph: ModuleGraph): bool](cmdlinehelper#loadConfigsAndRunMainCommand%2CNimProg%2CIdentCache%2CConfigRef%2CModuleGraph)
[loadMaxIds:](#loadMaxIds)
* [idgen: loadMaxIds(conf: ConfigRef; project: AbsoluteFile)](idgen#loadMaxIds%2CConfigRef%2CAbsoluteFile)
[loadModuleSym:](#loadModuleSym)
* [rod: loadModuleSym(g: ModuleGraph; fileIdx: FileIndex; fullpath: AbsoluteFile): ( PSym, int)](rod#loadModuleSym%2CModuleGraph%2CFileIndex%2CAbsoluteFile)
[loadNode:](#loadNode)
* [rod: loadNode(g: ModuleGraph; module: PSym): PNode](rod#loadNode.t%2CModuleGraph%2CPSym)
[localError:](#localError)
* [msgs: localError(conf: ConfigRef; info: TLineInfo; arg: string)](msgs#localError.t%2CConfigRef%2CTLineInfo%2Cstring)
* [msgs: localError(conf: ConfigRef; info: TLineInfo; format: string; params: openArray[string])](msgs#localError.t%2CConfigRef%2CTLineInfo%2Cstring%2CopenArray%5Bstring%5D)
* [msgs: localError(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")](msgs#localError.t%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring)
[localSearchInScope:](#localSearchInScope)
* [lookups: localSearchInScope(c: PContext; s: PIdent): PSym](lookups#localSearchInScope%2CPContext%2CPIdent)
[locCall:](#locCall)
* [TLocKind.locCall](ast#locCall)
[locData:](#locData)
* [TLocKind.locData](ast#locData)
[locExpr:](#locExpr)
* [TLocKind.locExpr](ast#locExpr)
[locField:](#locField)
* [TLocKind.locField](ast#locField)
[locGlobalVar:](#locGlobalVar)
* [TLocKind.locGlobalVar](ast#locGlobalVar)
[locLocalVar:](#locLocalVar)
* [TLocKind.locLocalVar](ast#locLocalVar)
[locNone:](#locNone)
* [TLocKind.locNone](ast#locNone)
[locOther:](#locOther)
* [TLocKind.locOther](ast#locOther)
[locParam:](#locParam)
* [TLocKind.locParam](ast#locParam)
[locProc:](#locProc)
* [TLocKind.locProc](ast#locProc)
[locTemp:](#locTemp)
* [TLocKind.locTemp](ast#locTemp)
[log:](#log)
* [msgs: log(s: string)](msgs#log%2Cstring)
[lookUp:](#lookUp)
* [lookups: lookUp(c: PContext; n: PNode): PSym](lookups#lookUp%2CPContext%2CPNode)
[lookupInRecord:](#lookupInRecord)
* [astalgo: lookupInRecord(n: PNode; field: PIdent): PSym](astalgo#lookupInRecord%2CPNode%2CPIdent)
[low:](#low)
* [int128: low(t: typedesc[Int128]): Int128](int128#low.t%2Ctypedesc%5BInt128%5D)
[lowBound:](#lowBound)
* [guards: lowBound(conf: ConfigRef; x: PNode): PNode](guards#lowBound%2CConfigRef%2CPNode)
[lowerSwap:](#lowerSwap)
* [lowerings: lowerSwap(g: ModuleGraph; n: PNode; owner: PSym): PNode](lowerings#lowerSwap%2CModuleGraph%2CPNode%2CPSym)
[lowerTupleUnpacking:](#lowerTupleUnpacking)
* [lowerings: lowerTupleUnpacking(g: ModuleGraph; n: PNode; owner: PSym): PNode](lowerings#lowerTupleUnpacking%2CModuleGraph%2CPNode%2CPSym)
[lowerTupleUnpackingForAsgn:](#lowerTupleUnpackingForAsgn)
* [lowerings: lowerTupleUnpackingForAsgn(g: ModuleGraph; n: PNode; owner: PSym): PNode](lowerings#lowerTupleUnpackingForAsgn%2CModuleGraph%2CPNode%2CPSym)
[Lrz:](#Lrz)
* [nimlexbase: Lrz](nimlexbase#Lrz)
[mAbsI:](#mAbsI)
* [TMagic.mAbsI](ast#mAbsI)
[mAccessEnv:](#mAccessEnv)
* [TMagic.mAccessEnv](ast#mAccessEnv)
[macroPragmas:](#macroPragmas)
* [pragmas: macroPragmas](pragmas#macroPragmas)
[mAddF64:](#mAddF64)
* [TMagic.mAddF64](ast#mAddF64)
[mAddI:](#mAddI)
* [TMagic.mAddI](ast#mAddI)
[mAddr:](#mAddr)
* [TMagic.mAddr](ast#mAddr)
[mAddU:](#mAddU)
* [TMagic.mAddU](ast#mAddU)
[mainCommand:](#mainCommand)
* [main: mainCommand(graph: ModuleGraph)](main#mainCommand%2CModuleGraph)
[mainCommandArg:](#mainCommandArg)
* [options: mainCommandArg(conf: ConfigRef): string](options#mainCommandArg%2CConfigRef)
[makeAndType:](#makeAndType)
* [semdata: makeAndType(c: PContext; t1, t2: PType): PType](semdata#makeAndType%2CPContext%2CPType%2CPType)
[makeClosure:](#makeClosure)
* [lambdalifting: makeClosure(g: ModuleGraph; prc: PSym; env: PNode; info: TLineInfo): PNode](lambdalifting#makeClosure%2CModuleGraph%2CPSym%2CPNode%2CTLineInfo)
[makeCString:](#makeCString)
* [msgs: makeCString(s: string): Rope](msgs#makeCString%2Cstring)
[makeInstPair:](#makeInstPair)
* [semdata: makeInstPair(s: PSym; inst: PInstantiation): TInstantiationPair](semdata#makeInstPair%2CPSym%2CPInstantiation)
[makeModule:](#makeModule)
* [modules: makeModule(graph: ModuleGraph; filename: AbsoluteFile): PSym](modules#makeModule%2CModuleGraph%2CAbsoluteFile)
* [modules: makeModule(graph: ModuleGraph; filename: string): PSym](modules#makeModule%2CModuleGraph%2Cstring)
[makeNotType:](#makeNotType)
* [semdata: makeNotType(c: PContext; t1: PType): PType](semdata#makeNotType%2CPContext%2CPType)
[makeOrType:](#makeOrType)
* [semdata: makeOrType(c: PContext; t1, t2: PType): PType](semdata#makeOrType%2CPContext%2CPType%2CPType)
[makePass:](#makePass)
* [passes: makePass(open: TPassOpen = nil; process: TPassProcess = nil; close: TPassClose = nil; isFrontend = false): TPass](passes#makePass%2CTPassOpen%2CTPassProcess%2CTPassClose)
[makePtrType:](#makePtrType)
* [semdata: makePtrType(c: PContext; baseType: PType): PType](semdata#makePtrType%2CPContext%2CPType)
* [semdata: makePtrType(owner: PSym; baseType: PType): PType](semdata#makePtrType%2CPSym%2CPType)
[makeRangeType:](#makeRangeType)
* [semdata: makeRangeType(c: PContext; first, last: BiggestInt; info: TLineInfo; intType: PType = nil): PType](semdata#makeRangeType%2CPContext%2CBiggestInt%2CBiggestInt%2CTLineInfo%2CPType)
[makeRangeWithStaticExpr:](#makeRangeWithStaticExpr)
* [semdata: makeRangeWithStaticExpr(c: PContext; n: PNode): PType](semdata#makeRangeWithStaticExpr%2CPContext%2CPNode)
[makeSingleLineCString:](#makeSingleLineCString)
* [ccgutils: makeSingleLineCString(s: string): string](ccgutils#makeSingleLineCString%2Cstring)
[makeStaticExpr:](#makeStaticExpr)
* [semdata: makeStaticExpr(c: PContext; n: PNode): PNode](semdata#makeStaticExpr%2CPContext%2CPNode)
[makeStdinModule:](#makeStdinModule)
* [modules: makeStdinModule(graph: ModuleGraph): PSym](modules#makeStdinModule%2CModuleGraph)
[makeStmtList:](#makeStmtList)
* [ast: makeStmtList(n: PNode): PNode](ast#makeStmtList%2CPNode)
[makeTypeDesc:](#makeTypeDesc)
* [semdata: makeTypeDesc(c: PContext; typ: PType): PType](semdata#makeTypeDesc%2CPContext%2CPType)
[makeTypeFromExpr:](#makeTypeFromExpr)
* [semdata: makeTypeFromExpr(c: PContext; n: PNode): PType](semdata#makeTypeFromExpr%2CPContext%2CPNode)
[makeTypeSymNode:](#makeTypeSymNode)
* [semdata: makeTypeSymNode(c: PContext; typ: PType; info: TLineInfo): PNode](semdata#makeTypeSymNode%2CPContext%2CPType%2CTLineInfo)
[makeTypeWithModifier:](#makeTypeWithModifier)
* [semdata: makeTypeWithModifier(c: PContext; modifier: TTypeKind; baseType: PType): PType](semdata#makeTypeWithModifier%2CPContext%2CTTypeKind%2CPType)
[makeVarType:](#makeVarType)
* [semdata: makeVarType(c: PContext; baseType: PType; kind = tyVar): PType](semdata#makeVarType%2CPContext%2CPType)
* [semdata: makeVarType(owner: PSym; baseType: PType; kind = tyVar): PType](semdata#makeVarType%2CPSym%2CPType)
[makeYamlString:](#makeYamlString)
* [astalgo: makeYamlString(s: string): Rope](astalgo#makeYamlString%2Cstring)
[mAlignOf:](#mAlignOf)
* [TMagic.mAlignOf](ast#mAlignOf)
[mAnd:](#mAnd)
* [TMagic.mAnd](ast#mAnd)
[mangle:](#mangle)
* [ccgutils: mangle(name: string): string](ccgutils#mangle%2Cstring)
[mAppendSeqElem:](#mAppendSeqElem)
* [TMagic.mAppendSeqElem](ast#mAppendSeqElem)
[mAppendStrCh:](#mAppendStrCh)
* [TMagic.mAppendStrCh](ast#mAppendStrCh)
[mAppendStrStr:](#mAppendStrStr)
* [TMagic.mAppendStrStr](ast#mAppendStrStr)
[Mapping:](#Mapping)
* [sourcemap: Mapping](sourcemap#Mapping)
[mAppType:](#mAppType)
* [TMagic.mAppType](ast#mAppType)
[markClientsDirty:](#markClientsDirty)
* [modulegraphs: markClientsDirty(g: ModuleGraph; fileIdx: FileIndex)](modulegraphs#markClientsDirty%2CModuleGraph%2CFileIndex)
[markDirty:](#markDirty)
* [modulegraphs: markDirty(g: ModuleGraph; fileIdx: FileIndex)](modulegraphs#markDirty%2CModuleGraph%2CFileIndex)
[markIndirect:](#markIndirect)
* [semdata: markIndirect(c: PContext; s: PSym)](semdata#markIndirect%2CPContext%2CPSym)
[markOwnerModuleAsUsed:](#markOwnerModuleAsUsed)
* [sigmatch: markOwnerModuleAsUsed(c: PContext; s: PSym)](sigmatch#markOwnerModuleAsUsed%2CPContext%2CPSym)
[markUsed:](#markUsed)
* [sigmatch: markUsed(c: PContext; info: TLineInfo; s: PSym)](sigmatch#markUsed%2CPContext%2CTLineInfo%2CPSym)
[mArray:](#mArray)
* [TMagic.mArray](ast#mArray)
[mArrGet:](#mArrGet)
* [TMagic.mArrGet](ast#mArrGet)
[mArrPut:](#mArrPut)
* [TMagic.mArrPut](ast#mArrPut)
[mArrToSeq:](#mArrToSeq)
* [TMagic.mArrToSeq](ast#mArrToSeq)
[mAsgn:](#mAsgn)
* [TMagic.mAsgn](ast#mAsgn)
[mAshrI:](#mAshrI)
* [TMagic.mAshrI](ast#mAshrI)
[maskBytes:](#maskBytes)
* [int128: maskBytes(arg: Int128; numbytes: int): Int128](int128#maskBytes%2CInt128%2Cint)
[maskUInt16:](#maskUInt16)
* [int128: maskUInt16(arg: Int128): Int128](int128#maskUInt16%2CInt128)
[maskUInt32:](#maskUInt32)
* [int128: maskUInt32(arg: Int128): Int128](int128#maskUInt32%2CInt128)
[maskUInt64:](#maskUInt64)
* [int128: maskUInt64(arg: Int128): Int128](int128#maskUInt64%2CInt128)
[maskUInt8:](#maskUInt8)
* [int128: maskUInt8(arg: Int128): Int128](int128#maskUInt8%2CInt128)
[mAstToStr:](#mAstToStr)
* [TMagic.mAstToStr](ast#mAstToStr)
[matches:](#matches)
* [sigmatch: matches(c: PContext; n, nOrig: PNode; m: var TCandidate)](sigmatch#matches%2CPContext%2CPNode%2CPNode%2CTCandidate)
[matchNodeKinds:](#matchNodeKinds)
* [parampatterns: matchNodeKinds(p, n: PNode): bool](parampatterns#matchNodeKinds%2CPNode%2CPNode)
[matchType:](#matchType)
* [types: matchType(a: PType; pattern: openArray[tuple[k: TTypeKind, i: int]]; last: TTypeKind): bool](types#matchType%2CPType%2CopenArray%5Btuple%5BTTypeKind%2Cint%5D%5D%2CTTypeKind)
[matchUserTypeClass:](#matchUserTypeClass)
* [sigmatch: matchUserTypeClass(m: var TCandidate; ff, a: PType): PType](sigmatch#matchUserTypeClass%2CTCandidate%2CPType%2CPType)
[MaxLineLength:](#MaxLineLength)
* [lexer: MaxLineLength](lexer#MaxLineLength)
[MaxLockLevel:](#MaxLockLevel)
* [ast: MaxLockLevel](ast#MaxLockLevel)
[MaxSetElements:](#MaxSetElements)
* [nversion: MaxSetElements](nversion#MaxSetElements)
[MaxStackSize:](#MaxStackSize)
* [parampatterns: MaxStackSize](parampatterns#MaxStackSize)
[mBitandI:](#mBitandI)
* [TMagic.mBitandI](ast#mBitandI)
[mBitnotI:](#mBitnotI)
* [TMagic.mBitnotI](ast#mBitnotI)
[mBitorI:](#mBitorI)
* [TMagic.mBitorI](ast#mBitorI)
[mBitxorI:](#mBitxorI)
* [TMagic.mBitxorI](ast#mBitxorI)
[mBool:](#mBool)
* [TMagic.mBool](ast#mBool)
[mBoolDefine:](#mBoolDefine)
* [TMagic.mBoolDefine](ast#mBoolDefine)
[mBoolToStr:](#mBoolToStr)
* [TMagic.mBoolToStr](ast#mBoolToStr)
[mBuildCPU:](#mBuildCPU)
* [TMagic.mBuildCPU](ast#mBuildCPU)
[mBuildOS:](#mBuildOS)
* [TMagic.mBuildOS](ast#mBuildOS)
[mBuiltinType:](#mBuiltinType)
* [TMagic.mBuiltinType](ast#mBuiltinType)
[mCard:](#mCard)
* [TMagic.mCard](ast#mCard)
[mChar:](#mChar)
* [TMagic.mChar](ast#mChar)
[mCharToStr:](#mCharToStr)
* [TMagic.mCharToStr](ast#mCharToStr)
[mChr:](#mChr)
* [TMagic.mChr](ast#mChr)
[mCompileDate:](#mCompileDate)
* [TMagic.mCompileDate](ast#mCompileDate)
[mCompileOption:](#mCompileOption)
* [TMagic.mCompileOption](ast#mCompileOption)
[mCompileOptionArg:](#mCompileOptionArg)
* [TMagic.mCompileOptionArg](ast#mCompileOptionArg)
[mCompiles:](#mCompiles)
* [TMagic.mCompiles](ast#mCompiles)
[mCompileTime:](#mCompileTime)
* [TMagic.mCompileTime](ast#mCompileTime)
[mConStrStr:](#mConStrStr)
* [TMagic.mConStrStr](ast#mConStrStr)
[mCpuEndian:](#mCpuEndian)
* [TMagic.mCpuEndian](ast#mCpuEndian)
[mCstring:](#mCstring)
* [TMagic.mCstring](ast#mCstring)
[mCStrToStr:](#mCStrToStr)
* [TMagic.mCStrToStr](ast#mCStrToStr)
[mdbg:](#mdbg)
* [astalgo: mdbg(): bool](astalgo#mdbg.t)
[mDec:](#mDec)
* [TMagic.mDec](ast#mDec)
[mDeclared:](#mDeclared)
* [TMagic.mDeclared](ast#mDeclared)
[mDeclaredInScope:](#mDeclaredInScope)
* [TMagic.mDeclaredInScope](ast#mDeclaredInScope)
[mDeepCopy:](#mDeepCopy)
* [TMagic.mDeepCopy](ast#mDeepCopy)
[mDefault:](#mDefault)
* [TMagic.mDefault](ast#mDefault)
[mDefined:](#mDefined)
* [TMagic.mDefined](ast#mDefined)
[mDestroy:](#mDestroy)
* [TMagic.mDestroy](ast#mDestroy)
[mDistinct:](#mDistinct)
* [TMagic.mDistinct](ast#mDistinct)
[mDivF64:](#mDivF64)
* [TMagic.mDivF64](ast#mDivF64)
[mDivI:](#mDivI)
* [TMagic.mDivI](ast#mDivI)
[mDivU:](#mDivU)
* [TMagic.mDivU](ast#mDivU)
[mDotDot:](#mDotDot)
* [TMagic.mDotDot](ast#mDotDot)
[mEcho:](#mEcho)
* [TMagic.mEcho](ast#mEcho)
[mEnumToStr:](#mEnumToStr)
* [TMagic.mEnumToStr](ast#mEnumToStr)
[mEqB:](#mEqB)
* [TMagic.mEqB](ast#mEqB)
[mEqCh:](#mEqCh)
* [TMagic.mEqCh](ast#mEqCh)
[mEqCString:](#mEqCString)
* [TMagic.mEqCString](ast#mEqCString)
[mEqEnum:](#mEqEnum)
* [TMagic.mEqEnum](ast#mEqEnum)
[mEqF64:](#mEqF64)
* [TMagic.mEqF64](ast#mEqF64)
[mEqI:](#mEqI)
* [TMagic.mEqI](ast#mEqI)
[mEqIdent:](#mEqIdent)
* [TMagic.mEqIdent](ast#mEqIdent)
[mEqNimrodNode:](#mEqNimrodNode)
* [TMagic.mEqNimrodNode](ast#mEqNimrodNode)
[mEqProc:](#mEqProc)
* [TMagic.mEqProc](ast#mEqProc)
[mEqRef:](#mEqRef)
* [TMagic.mEqRef](ast#mEqRef)
[mEqSet:](#mEqSet)
* [TMagic.mEqSet](ast#mEqSet)
[mEqStr:](#mEqStr)
* [TMagic.mEqStr](ast#mEqStr)
[mergeFiles:](#mergeFiles)
* [ccgmerge: mergeFiles(cfilename: AbsoluteFile; m: BModule)](ccgmerge#mergeFiles%2CAbsoluteFile%2CBModule)
[mergeRequired:](#mergeRequired)
* [ccgmerge: mergeRequired(m: BModule): bool](ccgmerge#mergeRequired%2CBModule)
[mergeShadowScope:](#mergeShadowScope)
* [lookups: mergeShadowScope(c: PContext)](lookups#mergeShadowScope%2CPContext)
[message:](#message)
* [msgs: message(conf: ConfigRef; info: TLineInfo; msg: TMsgKind; arg = "")](msgs#message.t%2CConfigRef%2CTLineInfo%2CTMsgKind%2Cstring)
[methodCall:](#methodCall)
* [cgmeth: methodCall(n: PNode; conf: ConfigRef): PNode](cgmeth#methodCall%2CPNode%2CConfigRef)
[methodDef:](#methodDef)
* [cgmeth: methodDef(g: ModuleGraph; s: PSym; fromCache: bool)](cgmeth#methodDef%2CModuleGraph%2CPSym%2Cbool)
[methodPragmas:](#methodPragmas)
* [pragmas: methodPragmas](pragmas#methodPragmas)
[mException:](#mException)
* [TMagic.mException](ast#mException)
[mExcl:](#mExcl)
* [TMagic.mExcl](ast#mExcl)
[mExists:](#mExists)
* [TMagic.mExists](ast#mExists)
[mExit:](#mExit)
* [TMagic.mExit](ast#mExit)
[mExpandToAst:](#mExpandToAst)
* [TMagic.mExpandToAst](ast#mExpandToAst)
[mExpr:](#mExpr)
* [TMagic.mExpr](ast#mExpr)
[mFieldPairs:](#mFieldPairs)
* [TMagic.mFieldPairs](ast#mFieldPairs)
[mFields:](#mFields)
* [TMagic.mFields](ast#mFields)
[mFloat:](#mFloat)
* [TMagic.mFloat](ast#mFloat)
[mFloat128:](#mFloat128)
* [TMagic.mFloat128](ast#mFloat128)
[mFloat32:](#mFloat32)
* [TMagic.mFloat32](ast#mFloat32)
[mFloat64:](#mFloat64)
* [TMagic.mFloat64](ast#mFloat64)
[mFloatToStr:](#mFloatToStr)
* [TMagic.mFloatToStr](ast#mFloatToStr)
[mForall:](#mForall)
* [TMagic.mForall](ast#mForall)
[mGCref:](#mGCref)
* [TMagic.mGCref](ast#mGCref)
[mGCunref:](#mGCunref)
* [TMagic.mGCunref](ast#mGCunref)
[mGetImpl:](#mGetImpl)
* [TMagic.mGetImpl](ast#mGetImpl)
[mGetImplTransf:](#mGetImplTransf)
* [TMagic.mGetImplTransf](ast#mGetImplTransf)
[mGetTypeInfo:](#mGetTypeInfo)
* [TMagic.mGetTypeInfo](ast#mGetTypeInfo)
[mGetTypeInfoV2:](#mGetTypeInfoV2)
* [TMagic.mGetTypeInfoV2](ast#mGetTypeInfoV2)
[mHigh:](#mHigh)
* [TMagic.mHigh](ast#mHigh)
[mHostCPU:](#mHostCPU)
* [TMagic.mHostCPU](ast#mHostCPU)
[mHostOS:](#mHostOS)
* [TMagic.mHostOS](ast#mHostOS)
[mIff:](#mIff)
* [TMagic.mIff](ast#mIff)
[mImplies:](#mImplies)
* [TMagic.mImplies](ast#mImplies)
[mInc:](#mInc)
* [TMagic.mInc](ast#mInc)
[mIncl:](#mIncl)
* [TMagic.mIncl](ast#mIncl)
[mInSet:](#mInSet)
* [TMagic.mInSet](ast#mInSet)
[mInstantiationInfo:](#mInstantiationInfo)
* [TMagic.mInstantiationInfo](ast#mInstantiationInfo)
[mInt:](#mInt)
* [TMagic.mInt](ast#mInt)
[mInt16:](#mInt16)
* [TMagic.mInt16](ast#mInt16)
[mInt32:](#mInt32)
* [TMagic.mInt32](ast#mInt32)
[mInt64:](#mInt64)
* [TMagic.mInt64](ast#mInt64)
[mInt64ToStr:](#mInt64ToStr)
* [TMagic.mInt64ToStr](ast#mInt64ToStr)
[mInt8:](#mInt8)
* [TMagic.mInt8](ast#mInt8)
[mIntDefine:](#mIntDefine)
* [TMagic.mIntDefine](ast#mIntDefine)
[mIntToStr:](#mIntToStr)
* [TMagic.mIntToStr](ast#mIntToStr)
[mIs:](#mIs)
* [TMagic.mIs](ast#mIs)
[miscPos:](#miscPos)
* [ast: miscPos](ast#miscPos)
[mIsMainModule:](#mIsMainModule)
* [TMagic.mIsMainModule](ast#mIsMainModule)
[MismatchInfo:](#MismatchInfo)
* [sigmatch: MismatchInfo](sigmatch#MismatchInfo)
[MismatchKind:](#MismatchKind)
* [sigmatch: MismatchKind](sigmatch#MismatchKind)
[mIsNil:](#mIsNil)
* [TMagic.mIsNil](ast#mIsNil)
[mIsolate:](#mIsolate)
* [TMagic.mIsolate](ast#mIsolate)
[mIsPartOf:](#mIsPartOf)
* [TMagic.mIsPartOf](ast#mIsPartOf)
[mLeB:](#mLeB)
* [TMagic.mLeB](ast#mLeB)
[mLeCh:](#mLeCh)
* [TMagic.mLeCh](ast#mLeCh)
[mLeEnum:](#mLeEnum)
* [TMagic.mLeEnum](ast#mLeEnum)
[mLeF64:](#mLeF64)
* [TMagic.mLeF64](ast#mLeF64)
[mLeI:](#mLeI)
* [TMagic.mLeI](ast#mLeI)
[mLengthArray:](#mLengthArray)
* [TMagic.mLengthArray](ast#mLengthArray)
[mLengthOpenArray:](#mLengthOpenArray)
* [TMagic.mLengthOpenArray](ast#mLengthOpenArray)
[mLengthSeq:](#mLengthSeq)
* [TMagic.mLengthSeq](ast#mLengthSeq)
[mLengthStr:](#mLengthStr)
* [TMagic.mLengthStr](ast#mLengthStr)
[mLePtr:](#mLePtr)
* [TMagic.mLePtr](ast#mLePtr)
[mLeSet:](#mLeSet)
* [TMagic.mLeSet](ast#mLeSet)
[mLeStr:](#mLeStr)
* [TMagic.mLeStr](ast#mLeStr)
[mLeU:](#mLeU)
* [TMagic.mLeU](ast#mLeU)
[mLow:](#mLow)
* [TMagic.mLow](ast#mLow)
[mLtB:](#mLtB)
* [TMagic.mLtB](ast#mLtB)
[mLtCh:](#mLtCh)
* [TMagic.mLtCh](ast#mLtCh)
[mLtEnum:](#mLtEnum)
* [TMagic.mLtEnum](ast#mLtEnum)
[mLtF64:](#mLtF64)
* [TMagic.mLtF64](ast#mLtF64)
[mLtI:](#mLtI)
* [TMagic.mLtI](ast#mLtI)
[mLtPtr:](#mLtPtr)
* [TMagic.mLtPtr](ast#mLtPtr)
[mLtSet:](#mLtSet)
* [TMagic.mLtSet](ast#mLtSet)
[mLtStr:](#mLtStr)
* [TMagic.mLtStr](ast#mLtStr)
[mLtU:](#mLtU)
* [TMagic.mLtU](ast#mLtU)
[mMaxI:](#mMaxI)
* [TMagic.mMaxI](ast#mMaxI)
[mMinI:](#mMinI)
* [TMagic.mMinI](ast#mMinI)
[mMinusSet:](#mMinusSet)
* [TMagic.mMinusSet](ast#mMinusSet)
[mModI:](#mModI)
* [TMagic.mModI](ast#mModI)
[mModU:](#mModU)
* [TMagic.mModU](ast#mModU)
[mMove:](#mMove)
* [TMagic.mMove](ast#mMove)
[mMulF64:](#mMulF64)
* [TMagic.mMulF64](ast#mMulF64)
[mMulI:](#mMulI)
* [TMagic.mMulI](ast#mMulI)
[mMulSet:](#mMulSet)
* [TMagic.mMulSet](ast#mMulSet)
[mMulU:](#mMulU)
* [TMagic.mMulU](ast#mMulU)
[mNAdd:](#mNAdd)
* [TMagic.mNAdd](ast#mNAdd)
[mNAddMultiple:](#mNAddMultiple)
* [TMagic.mNAddMultiple](ast#mNAddMultiple)
[mNBindSym:](#mNBindSym)
* [TMagic.mNBindSym](ast#mNBindSym)
[mNCallSite:](#mNCallSite)
* [TMagic.mNCallSite](ast#mNCallSite)
[mNccInc:](#mNccInc)
* [TMagic.mNccInc](ast#mNccInc)
[mNccValue:](#mNccValue)
* [TMagic.mNccValue](ast#mNccValue)
[mNChild:](#mNChild)
* [TMagic.mNChild](ast#mNChild)
[mNCopyNimNode:](#mNCopyNimNode)
* [TMagic.mNCopyNimNode](ast#mNCopyNimNode)
[mNCopyNimTree:](#mNCopyNimTree)
* [TMagic.mNCopyNimTree](ast#mNCopyNimTree)
[mNcsAdd:](#mNcsAdd)
* [TMagic.mNcsAdd](ast#mNcsAdd)
[mNcsAt:](#mNcsAt)
* [TMagic.mNcsAt](ast#mNcsAt)
[mNcsIncl:](#mNcsIncl)
* [TMagic.mNcsIncl](ast#mNcsIncl)
[mNcsLen:](#mNcsLen)
* [TMagic.mNcsLen](ast#mNcsLen)
[mNctGet:](#mNctGet)
* [TMagic.mNctGet](ast#mNctGet)
[mNctHasNext:](#mNctHasNext)
* [TMagic.mNctHasNext](ast#mNctHasNext)
[mNctLen:](#mNctLen)
* [TMagic.mNctLen](ast#mNctLen)
[mNctNext:](#mNctNext)
* [TMagic.mNctNext](ast#mNctNext)
[mNctPut:](#mNctPut)
* [TMagic.mNctPut](ast#mNctPut)
[mNDel:](#mNDel)
* [TMagic.mNDel](ast#mNDel)
[mNError:](#mNError)
* [TMagic.mNError](ast#mNError)
[mNew:](#mNew)
* [TMagic.mNew](ast#mNew)
[mNewFinalize:](#mNewFinalize)
* [TMagic.mNewFinalize](ast#mNewFinalize)
[mNewSeq:](#mNewSeq)
* [TMagic.mNewSeq](ast#mNewSeq)
[mNewSeqOfCap:](#mNewSeqOfCap)
* [TMagic.mNewSeqOfCap](ast#mNewSeqOfCap)
[mNewString:](#mNewString)
* [TMagic.mNewString](ast#mNewString)
[mNewStringOfCap:](#mNewStringOfCap)
* [TMagic.mNewStringOfCap](ast#mNewStringOfCap)
[mNFloatVal:](#mNFloatVal)
* [TMagic.mNFloatVal](ast#mNFloatVal)
[mNGenSym:](#mNGenSym)
* [TMagic.mNGenSym](ast#mNGenSym)
[mNGetType:](#mNGetType)
* [TMagic.mNGetType](ast#mNGetType)
[mNHint:](#mNHint)
* [TMagic.mNHint](ast#mNHint)
[mNIdent:](#mNIdent)
* [TMagic.mNIdent](ast#mNIdent)
[mNil:](#mNil)
* [TMagic.mNil](ast#mNil)
[mNimvm:](#mNimvm)
* [TMagic.mNimvm](ast#mNimvm)
[mNIntVal:](#mNIntVal)
* [TMagic.mNIntVal](ast#mNIntVal)
[mNKind:](#mNKind)
* [TMagic.mNKind](ast#mNKind)
[mNLen:](#mNLen)
* [TMagic.mNLen](ast#mNLen)
[mNLineInfo:](#mNLineInfo)
* [TMagic.mNLineInfo](ast#mNLineInfo)
[mNNewNimNode:](#mNNewNimNode)
* [TMagic.mNNewNimNode](ast#mNNewNimNode)
[mNodeId:](#mNodeId)
* [TMagic.mNodeId](ast#mNodeId)
[mNone:](#mNone)
* [TMagic.mNone](ast#mNone)
[mNot:](#mNot)
* [TMagic.mNot](ast#mNot)
[mNSetChild:](#mNSetChild)
* [TMagic.mNSetChild](ast#mNSetChild)
[mNSetFloatVal:](#mNSetFloatVal)
* [TMagic.mNSetFloatVal](ast#mNSetFloatVal)
[mNSetIdent:](#mNSetIdent)
* [TMagic.mNSetIdent](ast#mNSetIdent)
[mNSetIntVal:](#mNSetIntVal)
* [TMagic.mNSetIntVal](ast#mNSetIntVal)
[mNSetStrVal:](#mNSetStrVal)
* [TMagic.mNSetStrVal](ast#mNSetStrVal)
[mNSetSymbol:](#mNSetSymbol)
* [TMagic.mNSetSymbol](ast#mNSetSymbol)
[mNSetType:](#mNSetType)
* [TMagic.mNSetType](ast#mNSetType)
[mNSigHash:](#mNSigHash)
* [TMagic.mNSigHash](ast#mNSigHash)
[mNSizeOf:](#mNSizeOf)
* [TMagic.mNSizeOf](ast#mNSizeOf)
[mNStrVal:](#mNStrVal)
* [TMagic.mNStrVal](ast#mNStrVal)
[mNSymbol:](#mNSymbol)
* [TMagic.mNSymbol](ast#mNSymbol)
[mNSymKind:](#mNSymKind)
* [TMagic.mNSymKind](ast#mNSymKind)
[mNWarning:](#mNWarning)
* [TMagic.mNWarning](ast#mNWarning)
[ModuleGraph:](#ModuleGraph)
* [modulegraphs: ModuleGraph](modulegraphs#ModuleGraph)
[moduleHasChanged:](#moduleHasChanged)
* [passes: moduleHasChanged(graph: ModuleGraph; module: PSym): bool](passes#moduleHasChanged%2CModuleGraph%2CPSym)
[mOf:](#mOf)
* [TMagic.mOf](ast#mOf)
[mOffsetOf:](#mOffsetOf)
* [TMagic.mOffsetOf](ast#mOffsetOf)
[mOld:](#mOld)
* [TMagic.mOld](ast#mOld)
[mOmpParFor:](#mOmpParFor)
* [TMagic.mOmpParFor](ast#mOmpParFor)
[mOpenArray:](#mOpenArray)
* [TMagic.mOpenArray](ast#mOpenArray)
[mOr:](#mOr)
* [TMagic.mOr](ast#mOr)
[mOrd:](#mOrd)
* [TMagic.mOrd](ast#mOrd)
[mOrdinal:](#mOrdinal)
* [TMagic.mOrdinal](ast#mOrdinal)
[mParallel:](#mParallel)
* [TMagic.mParallel](ast#mParallel)
[mParseBiggestFloat:](#mParseBiggestFloat)
* [TMagic.mParseBiggestFloat](ast#mParseBiggestFloat)
[mParseExprToAst:](#mParseExprToAst)
* [TMagic.mParseExprToAst](ast#mParseExprToAst)
[mParseStmtToAst:](#mParseStmtToAst)
* [TMagic.mParseStmtToAst](ast#mParseStmtToAst)
[mPlugin:](#mPlugin)
* [TMagic.mPlugin](ast#mPlugin)
[mPlusSet:](#mPlusSet)
* [TMagic.mPlusSet](ast#mPlusSet)
[mPNimrodNode:](#mPNimrodNode)
* [TMagic.mPNimrodNode](ast#mPNimrodNode)
[mPointer:](#mPointer)
* [TMagic.mPointer](ast#mPointer)
[mPred:](#mPred)
* [TMagic.mPred](ast#mPred)
[mProcCall:](#mProcCall)
* [TMagic.mProcCall](ast#mProcCall)
[mPtr:](#mPtr)
* [TMagic.mPtr](ast#mPtr)
[mQuoteAst:](#mQuoteAst)
* [TMagic.mQuoteAst](ast#mQuoteAst)
[mRange:](#mRange)
* [TMagic.mRange](ast#mRange)
[mRef:](#mRef)
* [TMagic.mRef](ast#mRef)
[mRepr:](#mRepr)
* [TMagic.mRepr](ast#mRepr)
[mReset:](#mReset)
* [TMagic.mReset](ast#mReset)
[mRunnableExamples:](#mRunnableExamples)
* [TMagic.mRunnableExamples](ast#mRunnableExamples)
[mSameNodeType:](#mSameNodeType)
* [TMagic.mSameNodeType](ast#mSameNodeType)
[mSeq:](#mSeq)
* [TMagic.mSeq](ast#mSeq)
[mSet:](#mSet)
* [TMagic.mSet](ast#mSet)
[mSetLengthSeq:](#mSetLengthSeq)
* [TMagic.mSetLengthSeq](ast#mSetLengthSeq)
[mSetLengthStr:](#mSetLengthStr)
* [TMagic.mSetLengthStr](ast#mSetLengthStr)
[MsgConfig:](#MsgConfig)
* [lineinfos: MsgConfig](lineinfos#MsgConfig)
[MsgFlag:](#MsgFlag)
* [msgs: MsgFlag](msgs#MsgFlag)
[MsgFlags:](#MsgFlags)
* [msgs: MsgFlags](msgs#MsgFlags)
[MsgKindToStr:](#MsgKindToStr)
* [lineinfos: MsgKindToStr](lineinfos#MsgKindToStr)
[msgKindToString:](#msgKindToString)
* [msgs: msgKindToString(kind: TMsgKind): string](msgs#msgKindToString%2CTMsgKind)
[msgQuit:](#msgQuit)
* [msgs: msgQuit(x: int8)](msgs#msgQuit%2Cint8)
* [msgs: msgQuit(x: string)](msgs#msgQuit%2Cstring)
[msgSkipHook:](#msgSkipHook)
* [MsgFlag.msgSkipHook](msgs#msgSkipHook)
[msgStdout:](#msgStdout)
* [MsgFlag.msgStdout](msgs#msgStdout)
[msgWriteln:](#msgWriteln)
* [msgs: msgWriteln(conf: ConfigRef; s: string; flags: MsgFlags = {})](msgs#msgWriteln%2CConfigRef%2Cstring%2CMsgFlags)
[mShallowCopy:](#mShallowCopy)
* [TMagic.mShallowCopy](ast#mShallowCopy)
[mShlI:](#mShlI)
* [TMagic.mShlI](ast#mShlI)
[mShrI:](#mShrI)
* [TMagic.mShrI](ast#mShrI)
[mSizeOf:](#mSizeOf)
* [TMagic.mSizeOf](ast#mSizeOf)
[mSlice:](#mSlice)
* [TMagic.mSlice](ast#mSlice)
[mSlurp:](#mSlurp)
* [TMagic.mSlurp](ast#mSlurp)
[mSpawn:](#mSpawn)
* [TMagic.mSpawn](ast#mSpawn)
[mStatic:](#mStatic)
* [TMagic.mStatic](ast#mStatic)
[mStaticExec:](#mStaticExec)
* [TMagic.mStaticExec](ast#mStaticExec)
[mStmt:](#mStmt)
* [TMagic.mStmt](ast#mStmt)
[mStrDefine:](#mStrDefine)
* [TMagic.mStrDefine](ast#mStrDefine)
[mString:](#mString)
* [TMagic.mString](ast#mString)
[mStrToIdent:](#mStrToIdent)
* [TMagic.mStrToIdent](ast#mStrToIdent)
[mStrToStr:](#mStrToStr)
* [TMagic.mStrToStr](ast#mStrToStr)
[mSubF64:](#mSubF64)
* [TMagic.mSubF64](ast#mSubF64)
[mSubI:](#mSubI)
* [TMagic.mSubI](ast#mSubI)
[mSubU:](#mSubU)
* [TMagic.mSubU](ast#mSubU)
[mSucc:](#mSucc)
* [TMagic.mSucc](ast#mSucc)
[mSwap:](#mSwap)
* [TMagic.mSwap](ast#mSwap)
[mSymIsInstantiationOf:](#mSymIsInstantiationOf)
* [TMagic.mSymIsInstantiationOf](ast#mSymIsInstantiationOf)
[mSymOwner:](#mSymOwner)
* [TMagic.mSymOwner](ast#mSymOwner)
[mTuple:](#mTuple)
* [TMagic.mTuple](ast#mTuple)
[mType:](#mType)
* [TMagic.mType](ast#mType)
[mTypeDesc:](#mTypeDesc)
* [TMagic.mTypeDesc](ast#mTypeDesc)
[mTypeOf:](#mTypeOf)
* [TMagic.mTypeOf](ast#mTypeOf)
[mTypeTrait:](#mTypeTrait)
* [TMagic.mTypeTrait](ast#mTypeTrait)
[mUInt:](#mUInt)
* [TMagic.mUInt](ast#mUInt)
[mUInt16:](#mUInt16)
* [TMagic.mUInt16](ast#mUInt16)
[mUInt32:](#mUInt32)
* [TMagic.mUInt32](ast#mUInt32)
[mUInt64:](#mUInt64)
* [TMagic.mUInt64](ast#mUInt64)
[mUInt8:](#mUInt8)
* [TMagic.mUInt8](ast#mUInt8)
[mUnaryMinusF64:](#mUnaryMinusF64)
* [TMagic.mUnaryMinusF64](ast#mUnaryMinusF64)
[mUnaryMinusI:](#mUnaryMinusI)
* [TMagic.mUnaryMinusI](ast#mUnaryMinusI)
[mUnaryMinusI64:](#mUnaryMinusI64)
* [TMagic.mUnaryMinusI64](ast#mUnaryMinusI64)
[mUnaryPlusF64:](#mUnaryPlusF64)
* [TMagic.mUnaryPlusF64](ast#mUnaryPlusF64)
[mUnaryPlusI:](#mUnaryPlusI)
* [TMagic.mUnaryPlusI](ast#mUnaryPlusI)
[mUncheckedArray:](#mUncheckedArray)
* [TMagic.mUncheckedArray](ast#mUncheckedArray)
[mUnown:](#mUnown)
* [TMagic.mUnown](ast#mUnown)
[mustRehash:](#mustRehash)
* [astalgo: mustRehash(length, counter: int): bool](astalgo#mustRehash%2Cint%2Cint)
[mutableView:](#mutableView)
* [ViewTypeKind.mutableView](typeallowed#mutableView)
[mutateType:](#mutateType)
* [types: mutateType(t: PType; iter: TTypeMutator; closure: RootRef): PType](types#mutateType%2CPType%2CTTypeMutator%2CRootRef)
[MutationInfo:](#MutationInfo)
* [varpartitions: MutationInfo](varpartitions#MutationInfo)
[mVar:](#mVar)
* [TMagic.mVar](ast#mVar)
[mVarargs:](#mVarargs)
* [TMagic.mVarargs](ast#mVarargs)
[mVoid:](#mVoid)
* [TMagic.mVoid](ast#mVoid)
[mVoidType:](#mVoidType)
* [TMagic.mVoidType](ast#mVoidType)
[mWasMoved:](#mWasMoved)
* [TMagic.mWasMoved](ast#mWasMoved)
[mXor:](#mXor)
* [TMagic.mXor](ast#mXor)
[namePos:](#namePos)
* [ast: namePos](ast#namePos)
[nameToCC:](#nameToCC)
* [extccomp: nameToCC(name: string): TSystemCC](extccomp#nameToCC%2Cstring)
[nameToCPU:](#nameToCPU)
* [platform: nameToCPU(name: string): TSystemCPU](platform#nameToCPU%2Cstring)
[nameToOS:](#nameToOS)
* [platform: nameToOS(name: string): TSystemOS](platform#nameToOS%2Cstring)
[NdiFile:](#NdiFile)
* [ndi: NdiFile](ndi#NdiFile)
[NegOne:](#NegOne)
* [int128: NegOne](int128#NegOne)
[newAsgnStmt:](#newAsgnStmt)
* [lowerings: newAsgnStmt(le, ri: PNode): PNode](lowerings#newAsgnStmt%2CPNode%2CPNode)
[newCandidate:](#newCandidate)
* [sigmatch: newCandidate(ctx: PContext; callee: PSym; binding: PNode; calleeScope = -1): TCandidate](sigmatch#newCandidate%2CPContext%2CPSym%2CPNode)
* [sigmatch: newCandidate(ctx: PContext; callee: PType): TCandidate](sigmatch#newCandidate%2CPContext%2CPType)
[newConfigRef:](#newConfigRef)
* [options: newConfigRef(): ConfigRef](options#newConfigRef)
[newContext:](#newContext)
* [semdata: newContext(graph: ModuleGraph; module: PSym): PContext](semdata#newContext%2CModuleGraph%2CPSym)
[newCtx:](#newCtx)
* [vmdef: newCtx(module: PSym; cache: IdentCache; g: ModuleGraph): PCtx](vmdef#newCtx%2CPSym%2CIdentCache%2CModuleGraph)
[newDeref:](#newDeref)
* [lowerings: newDeref(n: PNode): PNode](lowerings#newDeref%2CPNode)
[newDocumentor:](#newDocumentor)
* [docgen: newDocumentor(filename: AbsoluteFile; cache: IdentCache; conf: ConfigRef; outExt: string = HtmlExt; module: PSym = nil): PDoc](docgen#newDocumentor%2CAbsoluteFile%2CIdentCache%2CConfigRef%2Cstring%2CPSym)
[newDotExpr:](#newDotExpr)
* [lowerings: newDotExpr(obj, b: PSym): PNode](lowerings#newDotExpr%2CPSym%2CPSym)
[newFastAsgnStmt:](#newFastAsgnStmt)
* [lowerings: newFastAsgnStmt(le, ri: PNode): PNode](lowerings#newFastAsgnStmt%2CPNode%2CPNode)
[newFastMoveStmt:](#newFastMoveStmt)
* [lowerings: newFastMoveStmt(g: ModuleGraph; le, ri: PNode): PNode](lowerings#newFastMoveStmt%2CModuleGraph%2CPNode%2CPNode)
[newFloatNode:](#newFloatNode)
* [ast: newFloatNode(kind: TNodeKind; floatVal: BiggestFloat): PNode](ast#newFloatNode%2CTNodeKind%2CBiggestFloat)
[newFloatNodeP:](#newFloatNodeP)
* [parser: newFloatNodeP(kind: TNodeKind; floatVal: BiggestFloat; p: Parser): PNode](parser#newFloatNodeP%2CTNodeKind%2CBiggestFloat%2CParser)
[newFloatNodeT:](#newFloatNodeT)
* [semfold: newFloatNodeT(floatVal: BiggestFloat; n: PNode; g: ModuleGraph): PNode](semfold#newFloatNodeT%2CBiggestFloat%2CPNode%2CModuleGraph)
[newIdentCache:](#newIdentCache)
* [idents: newIdentCache(): IdentCache](idents#newIdentCache)
[newIdentNode:](#newIdentNode)
* [ast: newIdentNode(ident: PIdent; info: TLineInfo): PNode](ast#newIdentNode%2CPIdent%2CTLineInfo)
[newIdentNodeP:](#newIdentNodeP)
* [parser: newIdentNodeP(ident: PIdent; p: Parser): PNode](parser#newIdentNodeP%2CPIdent%2CParser)
[newIdTable:](#newIdTable)
* [ast: newIdTable(): TIdTable](ast#newIdTable)
[newIntLit:](#newIntLit)
* [lowerings: newIntLit(g: ModuleGraph; info: TLineInfo; value: BiggestInt): PNode](lowerings#newIntLit%2CModuleGraph%2CTLineInfo%2CBiggestInt)
[newIntNode:](#newIntNode)
* [ast: newIntNode(kind: TNodeKind; intVal: BiggestInt): PNode](ast#newIntNode%2CTNodeKind%2CBiggestInt)
* [ast: newIntNode(kind: TNodeKind; intVal: Int128): PNode](ast#newIntNode%2CTNodeKind%2CInt128)
[newIntNodeP:](#newIntNodeP)
* [parser: newIntNodeP(kind: TNodeKind; intVal: BiggestInt; p: Parser): PNode](parser#newIntNodeP%2CTNodeKind%2CBiggestInt%2CParser)
[newIntNodeT:](#newIntNodeT)
* [semfold: newIntNodeT(intVal: Int128; n: PNode; g: ModuleGraph): PNode](semfold#newIntNodeT%2CInt128%2CPNode%2CModuleGraph)
[newIntTypeNode:](#newIntTypeNode)
* [ast: newIntTypeNode(intVal: BiggestInt; typ: PType): PNode](ast#newIntTypeNode%2CBiggestInt%2CPType)
* [ast: newIntTypeNode(intVal: Int128; typ: PType): PNode](ast#newIntTypeNode%2CInt128%2CPType)
[newLib:](#newLib)
* [semdata: newLib(kind: TLibKind): PLib](semdata#newLib%2CTLibKind)
[newlineFollows:](#newlineFollows)
* [lexer: newlineFollows(L: Lexer): bool](lexer#newlineFollows%2CLexer)
[newLineInfo:](#newLineInfo)
* [msgs: newLineInfo(conf: ConfigRef; filename: AbsoluteFile; line, col: int): TLineInfo](msgs#newLineInfo%2CConfigRef%2CAbsoluteFile%2Cint%2Cint)
* [msgs: newLineInfo(fileInfoIdx: FileIndex; line, col: int): TLineInfo](msgs#newLineInfo%2CFileIndex%2Cint%2Cint)
[NewLines:](#NewLines)
* [nimlexbase: NewLines](nimlexbase#NewLines)
[newModuleGraph:](#newModuleGraph)
* [modulegraphs: newModuleGraph(cache: IdentCache; config: ConfigRef): ModuleGraph](modulegraphs#newModuleGraph%2CIdentCache%2CConfigRef)
[newModuleList:](#newModuleList)
* [cgendata: newModuleList(g: ModuleGraph): BModuleList](cgendata#newModuleList%2CModuleGraph)
[newNode:](#newNode)
* [ast: newNode(kind: TNodeKind): PNode](ast#newNode%2CTNodeKind)
[newNodeI:](#newNodeI)
* [ast: newNodeI(kind: TNodeKind; info: TLineInfo): PNode](ast#newNodeI%2CTNodeKind%2CTLineInfo)
* [ast: newNodeI(kind: TNodeKind; info: TLineInfo; children: int): PNode](ast#newNodeI%2CTNodeKind%2CTLineInfo%2Cint)
[newNodeIT:](#newNodeIT)
* [ast: newNodeIT(kind: TNodeKind; info: TLineInfo; typ: PType): PNode](ast#newNodeIT%2CTNodeKind%2CTLineInfo%2CPType)
[newNodeP:](#newNodeP)
* [parser: newNodeP(kind: TNodeKind; p: Parser): PNode](parser#newNodeP%2CTNodeKind%2CParser)
[newOptionEntry:](#newOptionEntry)
* [semdata: newOptionEntry(conf: ConfigRef): POptionEntry](semdata#newOptionEntry%2CConfigRef)
[newPackageCache:](#newPackageCache)
* [options: newPackageCache(): untyped](options#newPackageCache.t)
[newPartialConfigRef:](#newPartialConfigRef)
* [options: newPartialConfigRef(): ConfigRef](options#newPartialConfigRef)
[newProc:](#newProc)
* [cgendata: newProc(prc: PSym; module: BModule): BProc](cgendata#newProc%2CPSym%2CBModule)
[newProcNode:](#newProcNode)
* [ast: newProcNode(kind: TNodeKind; info: TLineInfo; body: PNode; params, name, pattern, genericParams, pragmas, exceptions: PNode): PNode](ast#newProcNode%2CTNodeKind%2CTLineInfo%2CPNode%2CPNode%2CPNode%2CPNode%2CPNode%2CPNode%2CPNode)
[newProcType:](#newProcType)
* [ast: newProcType(info: TLineInfo; owner: PSym): PType](ast#newProcType%2CTLineInfo%2CPSym)
[newSons:](#newSons)
* [ast: newSons(father: Indexable; length: int)](ast#newSons%2CIndexable%2Cint)
[newStrNode:](#newStrNode)
* [ast: newStrNode(strVal: string; info: TLineInfo): PNode](ast#newStrNode%2Cstring%2CTLineInfo)
* [ast: newStrNode(kind: TNodeKind; strVal: string): PNode](ast#newStrNode%2CTNodeKind%2Cstring)
[newStrNodeP:](#newStrNodeP)
* [parser: newStrNodeP(kind: TNodeKind; strVal: string; p: Parser): PNode](parser#newStrNodeP%2CTNodeKind%2Cstring%2CParser)
[newStrNodeT:](#newStrNodeT)
* [semfold: newStrNodeT(strVal: string; n: PNode; g: ModuleGraph): PNode](semfold#newStrNodeT%2Cstring%2CPNode%2CModuleGraph)
[newStrTable:](#newStrTable)
* [ast: newStrTable(): TStrTable](ast#newStrTable)
[newSym:](#newSym)
* [ast: newSym(symKind: TSymKind; name: PIdent; owner: PSym; info: TLineInfo; options: TOptions = {}): PSym](ast#newSym%2CTSymKind%2CPIdent%2CPSym%2CTLineInfo%2CTOptions)
[newSymG:](#newSymG)
* [sem: newSymG(kind: TSymKind; n: PNode; c: PContext): PSym](sem#newSymG%2CTSymKind%2CPNode%2CPContext)
[newSymNode:](#newSymNode)
* [ast: newSymNode(sym: PSym): PNode](ast#newSymNode%2CPSym)
* [ast: newSymNode(sym: PSym; info: TLineInfo): PNode](ast#newSymNode%2CPSym%2CTLineInfo)
[newSymNodeTypeDesc:](#newSymNodeTypeDesc)
* [semfold: newSymNodeTypeDesc(s: PSym; info: TLineInfo): PNode](semfold#newSymNodeTypeDesc%2CPSym%2CTLineInfo)
[newTree:](#newTree)
* [ast: newTree(kind: TNodeKind; children: varargs[PNode]): PNode](ast#newTree%2CTNodeKind%2Cvarargs%5BPNode%5D)
[newTreeI:](#newTreeI)
* [ast: newTreeI(kind: TNodeKind; info: TLineInfo; children: varargs[PNode]): PNode](ast#newTreeI%2CTNodeKind%2CTLineInfo%2Cvarargs%5BPNode%5D)
[newTreeIT:](#newTreeIT)
* [ast: newTreeIT(kind: TNodeKind; info: TLineInfo; typ: PType; children: varargs[PNode]): PNode](ast#newTreeIT%2CTNodeKind%2CTLineInfo%2CPType%2Cvarargs%5BPNode%5D)
[newTryFinally:](#newTryFinally)
* [lowerings: newTryFinally(body, final: PNode): PNode](lowerings#newTryFinally%2CPNode%2CPNode)
[newTupleAccess:](#newTupleAccess)
* [lowerings: newTupleAccess(g: ModuleGraph; tup: PNode; i: int): PNode](lowerings#newTupleAccess%2CModuleGraph%2CPNode%2Cint)
[newTupleAccessRaw:](#newTupleAccessRaw)
* [lowerings: newTupleAccessRaw(tup: PNode; i: int): PNode](lowerings#newTupleAccessRaw%2CPNode%2Cint)
[newType:](#newType)
* [ast: newType(kind: TTypeKind; owner: PSym): PType](ast#newType%2CTTypeKind%2CPSym)
[newTypeMapLayer:](#newTypeMapLayer)
* [semtypinst: newTypeMapLayer(cl: var TReplTypeVars): LayeredIdTable](semtypinst#newTypeMapLayer%2CTReplTypeVars)
[newTypeS:](#newTypeS)
* [semdata: newTypeS(kind: TTypeKind; c: PContext): PType](semdata#newTypeS%2CTTypeKind%2CPContext)
[newTypeWithSons:](#newTypeWithSons)
* [semdata: newTypeWithSons(c: PContext; kind: TTypeKind; sons: seq[PType]): PType](semdata#newTypeWithSons%2CPContext%2CTTypeKind%2Cseq%5BPType%5D)
* [semdata: newTypeWithSons(owner: PSym; kind: TTypeKind; sons: seq[PType]): PType](semdata#newTypeWithSons%2CPSym%2CTTypeKind%2Cseq%5BPType%5D)
[newVersion:](#newVersion)
* [nimblecmd: newVersion(ver: string): Version](nimblecmd#newVersion%2Cstring)
[next:](#next)
* [btrees: next[Key, Val](b: BTree[Key, Val]; index: int): (Key, Val, int)](btrees#next%2CBTree%5BKey%2CVal%5D%2Cint)
[nextIdentExcluding:](#nextIdentExcluding)
* [astalgo: nextIdentExcluding(ti: var TIdentIter; tab: TStrTable; excluding: IntSet): PSym](astalgo#nextIdentExcluding%2CTIdentIter%2CTStrTable%2CIntSet)
[nextIdentIter:](#nextIdentIter)
* [astalgo: nextIdentIter(ti: var TIdentIter; tab: TStrTable): PSym](astalgo#nextIdentIter%2CTIdentIter%2CTStrTable)
[nextIter:](#nextIter)
* [astalgo: nextIter(ti: var TTabIter; tab: TStrTable): PSym](astalgo#nextIter%2CTTabIter%2CTStrTable)
[nextOverloadIter:](#nextOverloadIter)
* [lookups: nextOverloadIter(o: var TOverloadIter; c: PContext; n: PNode): PSym](lookups#nextOverloadIter%2CTOverloadIter%2CPContext%2CPNode)
[nextTry:](#nextTry)
* [astalgo: nextTry(h, maxHash: Hash): Hash](astalgo#nextTry%2CHash%2CHash)
[nfAllConst:](#nfAllConst)
* [TNodeFlag.nfAllConst](ast#nfAllConst)
[nfAllFieldsSet:](#nfAllFieldsSet)
* [ast: nfAllFieldsSet](ast#nfAllFieldsSet)
[nfBase16:](#nfBase16)
* [TNodeFlag.nfBase16](ast#nfBase16)
[nfBase2:](#nfBase2)
* [TNodeFlag.nfBase2](ast#nfBase2)
[nfBase8:](#nfBase8)
* [TNodeFlag.nfBase8](ast#nfBase8)
[nfBlockArg:](#nfBlockArg)
* [TNodeFlag.nfBlockArg](ast#nfBlockArg)
[nfDefaultParam:](#nfDefaultParam)
* [TNodeFlag.nfDefaultParam](ast#nfDefaultParam)
[nfDefaultRefsParam:](#nfDefaultRefsParam)
* [TNodeFlag.nfDefaultRefsParam](ast#nfDefaultRefsParam)
[nfDotField:](#nfDotField)
* [TNodeFlag.nfDotField](ast#nfDotField)
[nfDotSetter:](#nfDotSetter)
* [TNodeFlag.nfDotSetter](ast#nfDotSetter)
[nfExecuteOnReload:](#nfExecuteOnReload)
* [TNodeFlag.nfExecuteOnReload](ast#nfExecuteOnReload)
[nfExplicitCall:](#nfExplicitCall)
* [TNodeFlag.nfExplicitCall](ast#nfExplicitCall)
[nfExprCall:](#nfExprCall)
* [TNodeFlag.nfExprCall](ast#nfExprCall)
[nfFromTemplate:](#nfFromTemplate)
* [TNodeFlag.nfFromTemplate](ast#nfFromTemplate)
[nfIsPtr:](#nfIsPtr)
* [TNodeFlag.nfIsPtr](ast#nfIsPtr)
[nfIsRef:](#nfIsRef)
* [TNodeFlag.nfIsRef](ast#nfIsRef)
[nfLL:](#nfLL)
* [TNodeFlag.nfLL](ast#nfLL)
[nfNone:](#nfNone)
* [TNodeFlag.nfNone](ast#nfNone)
[nfNoRewrite:](#nfNoRewrite)
* [TNodeFlag.nfNoRewrite](ast#nfNoRewrite)
[nfPreventCg:](#nfPreventCg)
* [TNodeFlag.nfPreventCg](ast#nfPreventCg)
[nfSem:](#nfSem)
* [TNodeFlag.nfSem](ast#nfSem)
[nfTransf:](#nfTransf)
* [TNodeFlag.nfTransf](ast#nfTransf)
[NilableTypes:](#NilableTypes)
* [ast: NilableTypes](ast#NilableTypes)
[nilOrSysInt:](#nilOrSysInt)
* [magicsys: nilOrSysInt(g: ModuleGraph): PType](magicsys#nilOrSysInt%2CModuleGraph)
[nimblePath:](#nimblePath)
* [nimblecmd: nimblePath(conf: ConfigRef; path: AbsoluteDir; info: TLineInfo)](nimblecmd#nimblePath%2CConfigRef%2CAbsoluteDir%2CTLineInfo)
[nimbleSubs:](#nimbleSubs)
* [options: nimbleSubs(conf: ConfigRef; p: string): string](options#nimbleSubs.i%2CConfigRef%2Cstring)
[NimCompilerApiVersion:](#NimCompilerApiVersion)
* [nversion: NimCompilerApiVersion](nversion#NimCompilerApiVersion)
[nimdocOutCss:](#nimdocOutCss)
* [nimpaths: nimdocOutCss](nimpaths#nimdocOutCss)
[nimErrorFlagAccessed:](#nimErrorFlagAccessed)
* [TCProcFlag.nimErrorFlagAccessed](cgendata#nimErrorFlagAccessed)
[nimErrorFlagDeclared:](#nimErrorFlagDeclared)
* [TCProcFlag.nimErrorFlagDeclared](cgendata#nimErrorFlagDeclared)
[nimErrorFlagDisabled:](#nimErrorFlagDisabled)
* [TCProcFlag.nimErrorFlagDisabled](cgendata#nimErrorFlagDisabled)
[NimExt:](#NimExt)
* [options: NimExt](options#NimExt)
[nimIncremental:](#nimIncremental)
* [incremental: nimIncremental](incremental#nimIncremental)
[nimKeywordsHigh:](#nimKeywordsHigh)
* [wordrecg: nimKeywordsHigh](wordrecg#nimKeywordsHigh)
[nimKeywordsLow:](#nimKeywordsLow)
* [wordrecg: nimKeywordsLow](wordrecg#nimKeywordsLow)
[nimNodeFlag:](#nimNodeFlag)
* [vmdef: nimNodeFlag](vmdef#nimNodeFlag)
[NimProg:](#NimProg)
* [cmdlinehelper: NimProg](cmdlinehelper#NimProg)
[nkAccQuoted:](#nkAccQuoted)
* [TNodeKind.nkAccQuoted](ast#nkAccQuoted)
[nkAddr:](#nkAddr)
* [TNodeKind.nkAddr](ast#nkAddr)
[nkArgList:](#nkArgList)
* [TNodeKind.nkArgList](ast#nkArgList)
[nkAsgn:](#nkAsgn)
* [TNodeKind.nkAsgn](ast#nkAsgn)
[nkAsmStmt:](#nkAsmStmt)
* [TNodeKind.nkAsmStmt](ast#nkAsmStmt)
[nkBind:](#nkBind)
* [TNodeKind.nkBind](ast#nkBind)
[nkBindStmt:](#nkBindStmt)
* [TNodeKind.nkBindStmt](ast#nkBindStmt)
[nkBlockExpr:](#nkBlockExpr)
* [TNodeKind.nkBlockExpr](ast#nkBlockExpr)
[nkBlockStmt:](#nkBlockStmt)
* [TNodeKind.nkBlockStmt](ast#nkBlockStmt)
[nkBlockType:](#nkBlockType)
* [TNodeKind.nkBlockType](ast#nkBlockType)
[nkBracket:](#nkBracket)
* [TNodeKind.nkBracket](ast#nkBracket)
[nkBracketExpr:](#nkBracketExpr)
* [TNodeKind.nkBracketExpr](ast#nkBracketExpr)
[nkBreakState:](#nkBreakState)
* [TNodeKind.nkBreakState](ast#nkBreakState)
[nkBreakStmt:](#nkBreakStmt)
* [TNodeKind.nkBreakStmt](ast#nkBreakStmt)
[nkCall:](#nkCall)
* [TNodeKind.nkCall](ast#nkCall)
[nkCallKinds:](#nkCallKinds)
* [ast: nkCallKinds](ast#nkCallKinds)
[nkCallStrLit:](#nkCallStrLit)
* [TNodeKind.nkCallStrLit](ast#nkCallStrLit)
[nkCaseStmt:](#nkCaseStmt)
* [TNodeKind.nkCaseStmt](ast#nkCaseStmt)
[nkCast:](#nkCast)
* [TNodeKind.nkCast](ast#nkCast)
[nkCharLit:](#nkCharLit)
* [TNodeKind.nkCharLit](ast#nkCharLit)
[nkChckRange:](#nkChckRange)
* [TNodeKind.nkChckRange](ast#nkChckRange)
[nkChckRange64:](#nkChckRange64)
* [TNodeKind.nkChckRange64](ast#nkChckRange64)
[nkChckRangeF:](#nkChckRangeF)
* [TNodeKind.nkChckRangeF](ast#nkChckRangeF)
[nkCheckedFieldExpr:](#nkCheckedFieldExpr)
* [TNodeKind.nkCheckedFieldExpr](ast#nkCheckedFieldExpr)
[nkClosedSymChoice:](#nkClosedSymChoice)
* [TNodeKind.nkClosedSymChoice](ast#nkClosedSymChoice)
[nkClosure:](#nkClosure)
* [TNodeKind.nkClosure](ast#nkClosure)
[nkComesFrom:](#nkComesFrom)
* [TNodeKind.nkComesFrom](ast#nkComesFrom)
[nkCommand:](#nkCommand)
* [TNodeKind.nkCommand](ast#nkCommand)
[nkCommentStmt:](#nkCommentStmt)
* [TNodeKind.nkCommentStmt](ast#nkCommentStmt)
[nkConstDef:](#nkConstDef)
* [TNodeKind.nkConstDef](ast#nkConstDef)
[nkConstSection:](#nkConstSection)
* [TNodeKind.nkConstSection](ast#nkConstSection)
[nkConstTy:](#nkConstTy)
* [TNodeKind.nkConstTy](ast#nkConstTy)
[nkContinueStmt:](#nkContinueStmt)
* [TNodeKind.nkContinueStmt](ast#nkContinueStmt)
[nkConv:](#nkConv)
* [TNodeKind.nkConv](ast#nkConv)
[nkConverterDef:](#nkConverterDef)
* [TNodeKind.nkConverterDef](ast#nkConverterDef)
[nkCStringToString:](#nkCStringToString)
* [TNodeKind.nkCStringToString](ast#nkCStringToString)
[nkCurly:](#nkCurly)
* [TNodeKind.nkCurly](ast#nkCurly)
[nkCurlyExpr:](#nkCurlyExpr)
* [TNodeKind.nkCurlyExpr](ast#nkCurlyExpr)
[nkDefer:](#nkDefer)
* [TNodeKind.nkDefer](ast#nkDefer)
[nkDerefExpr:](#nkDerefExpr)
* [TNodeKind.nkDerefExpr](ast#nkDerefExpr)
[nkDiscardStmt:](#nkDiscardStmt)
* [TNodeKind.nkDiscardStmt](ast#nkDiscardStmt)
[nkDistinctTy:](#nkDistinctTy)
* [TNodeKind.nkDistinctTy](ast#nkDistinctTy)
[nkDo:](#nkDo)
* [TNodeKind.nkDo](ast#nkDo)
[nkDotCall:](#nkDotCall)
* [TNodeKind.nkDotCall](ast#nkDotCall)
[nkDotExpr:](#nkDotExpr)
* [TNodeKind.nkDotExpr](ast#nkDotExpr)
[nkEffectList:](#nkEffectList)
* [ast: nkEffectList](ast#nkEffectList)
[nkElifBranch:](#nkElifBranch)
* [TNodeKind.nkElifBranch](ast#nkElifBranch)
[nkElifExpr:](#nkElifExpr)
* [TNodeKind.nkElifExpr](ast#nkElifExpr)
[nkElse:](#nkElse)
* [TNodeKind.nkElse](ast#nkElse)
[nkElseExpr:](#nkElseExpr)
* [TNodeKind.nkElseExpr](ast#nkElseExpr)
[nkEmpty:](#nkEmpty)
* [TNodeKind.nkEmpty](ast#nkEmpty)
[nkEnumFieldDef:](#nkEnumFieldDef)
* [TNodeKind.nkEnumFieldDef](ast#nkEnumFieldDef)
[nkEnumTy:](#nkEnumTy)
* [TNodeKind.nkEnumTy](ast#nkEnumTy)
[nkExceptBranch:](#nkExceptBranch)
* [TNodeKind.nkExceptBranch](ast#nkExceptBranch)
[nkExportExceptStmt:](#nkExportExceptStmt)
* [TNodeKind.nkExportExceptStmt](ast#nkExportExceptStmt)
[nkExportStmt:](#nkExportStmt)
* [TNodeKind.nkExportStmt](ast#nkExportStmt)
[nkExprColonExpr:](#nkExprColonExpr)
* [TNodeKind.nkExprColonExpr](ast#nkExprColonExpr)
[nkExprEqExpr:](#nkExprEqExpr)
* [TNodeKind.nkExprEqExpr](ast#nkExprEqExpr)
[nkFastAsgn:](#nkFastAsgn)
* [TNodeKind.nkFastAsgn](ast#nkFastAsgn)
[nkFinally:](#nkFinally)
* [TNodeKind.nkFinally](ast#nkFinally)
[nkFloat128Lit:](#nkFloat128Lit)
* [TNodeKind.nkFloat128Lit](ast#nkFloat128Lit)
[nkFloat32Lit:](#nkFloat32Lit)
* [TNodeKind.nkFloat32Lit](ast#nkFloat32Lit)
[nkFloat64Lit:](#nkFloat64Lit)
* [TNodeKind.nkFloat64Lit](ast#nkFloat64Lit)
[nkFloatLit:](#nkFloatLit)
* [TNodeKind.nkFloatLit](ast#nkFloatLit)
[nkFloatLiterals:](#nkFloatLiterals)
* [ast: nkFloatLiterals](ast#nkFloatLiterals)
[nkFormalParams:](#nkFormalParams)
* [TNodeKind.nkFormalParams](ast#nkFormalParams)
[nkForStmt:](#nkForStmt)
* [TNodeKind.nkForStmt](ast#nkForStmt)
[nkFromStmt:](#nkFromStmt)
* [TNodeKind.nkFromStmt](ast#nkFromStmt)
[nkFuncDef:](#nkFuncDef)
* [TNodeKind.nkFuncDef](ast#nkFuncDef)
[nkGenericParams:](#nkGenericParams)
* [TNodeKind.nkGenericParams](ast#nkGenericParams)
[nkGotoState:](#nkGotoState)
* [TNodeKind.nkGotoState](ast#nkGotoState)
[nkHiddenAddr:](#nkHiddenAddr)
* [TNodeKind.nkHiddenAddr](ast#nkHiddenAddr)
[nkHiddenCallConv:](#nkHiddenCallConv)
* [TNodeKind.nkHiddenCallConv](ast#nkHiddenCallConv)
[nkHiddenDeref:](#nkHiddenDeref)
* [TNodeKind.nkHiddenDeref](ast#nkHiddenDeref)
[nkHiddenStdConv:](#nkHiddenStdConv)
* [TNodeKind.nkHiddenStdConv](ast#nkHiddenStdConv)
[nkHiddenSubConv:](#nkHiddenSubConv)
* [TNodeKind.nkHiddenSubConv](ast#nkHiddenSubConv)
[nkHiddenTryStmt:](#nkHiddenTryStmt)
* [TNodeKind.nkHiddenTryStmt](ast#nkHiddenTryStmt)
[nkIdent:](#nkIdent)
* [TNodeKind.nkIdent](ast#nkIdent)
[nkIdentDefs:](#nkIdentDefs)
* [TNodeKind.nkIdentDefs](ast#nkIdentDefs)
[nkIdentKinds:](#nkIdentKinds)
* [ast: nkIdentKinds](ast#nkIdentKinds)
[nkIfExpr:](#nkIfExpr)
* [TNodeKind.nkIfExpr](ast#nkIfExpr)
[nkIfStmt:](#nkIfStmt)
* [TNodeKind.nkIfStmt](ast#nkIfStmt)
[nkImportAs:](#nkImportAs)
* [TNodeKind.nkImportAs](ast#nkImportAs)
[nkImportExceptStmt:](#nkImportExceptStmt)
* [TNodeKind.nkImportExceptStmt](ast#nkImportExceptStmt)
[nkImportStmt:](#nkImportStmt)
* [TNodeKind.nkImportStmt](ast#nkImportStmt)
[nkIncludeStmt:](#nkIncludeStmt)
* [TNodeKind.nkIncludeStmt](ast#nkIncludeStmt)
[nkInfix:](#nkInfix)
* [TNodeKind.nkInfix](ast#nkInfix)
[nkInt16Lit:](#nkInt16Lit)
* [TNodeKind.nkInt16Lit](ast#nkInt16Lit)
[nkInt32Lit:](#nkInt32Lit)
* [TNodeKind.nkInt32Lit](ast#nkInt32Lit)
[nkInt64Lit:](#nkInt64Lit)
* [TNodeKind.nkInt64Lit](ast#nkInt64Lit)
[nkInt8Lit:](#nkInt8Lit)
* [TNodeKind.nkInt8Lit](ast#nkInt8Lit)
[nkIntLit:](#nkIntLit)
* [TNodeKind.nkIntLit](ast#nkIntLit)
[nkIteratorDef:](#nkIteratorDef)
* [TNodeKind.nkIteratorDef](ast#nkIteratorDef)
[nkIteratorTy:](#nkIteratorTy)
* [TNodeKind.nkIteratorTy](ast#nkIteratorTy)
[nkLambda:](#nkLambda)
* [TNodeKind.nkLambda](ast#nkLambda)
[nkLambdaKinds:](#nkLambdaKinds)
* [ast: nkLambdaKinds](ast#nkLambdaKinds)
[nkLastBlockStmts:](#nkLastBlockStmts)
* [ast: nkLastBlockStmts](ast#nkLastBlockStmts)
[nkLetSection:](#nkLetSection)
* [TNodeKind.nkLetSection](ast#nkLetSection)
[nkLiterals:](#nkLiterals)
* [ast: nkLiterals](ast#nkLiterals)
[nkMacroDef:](#nkMacroDef)
* [TNodeKind.nkMacroDef](ast#nkMacroDef)
[nkMethodDef:](#nkMethodDef)
* [TNodeKind.nkMethodDef](ast#nkMethodDef)
[nkMixinStmt:](#nkMixinStmt)
* [TNodeKind.nkMixinStmt](ast#nkMixinStmt)
[nkMutableTy:](#nkMutableTy)
* [TNodeKind.nkMutableTy](ast#nkMutableTy)
[nkNilLit:](#nkNilLit)
* [TNodeKind.nkNilLit](ast#nkNilLit)
[nkNone:](#nkNone)
* [TNodeKind.nkNone](ast#nkNone)
[nkObjConstr:](#nkObjConstr)
* [TNodeKind.nkObjConstr](ast#nkObjConstr)
[nkObjDownConv:](#nkObjDownConv)
* [TNodeKind.nkObjDownConv](ast#nkObjDownConv)
[nkObjectTy:](#nkObjectTy)
* [TNodeKind.nkObjectTy](ast#nkObjectTy)
[nkObjUpConv:](#nkObjUpConv)
* [TNodeKind.nkObjUpConv](ast#nkObjUpConv)
[nkOfBranch:](#nkOfBranch)
* [TNodeKind.nkOfBranch](ast#nkOfBranch)
[nkOfInherit:](#nkOfInherit)
* [TNodeKind.nkOfInherit](ast#nkOfInherit)
[nkOpenSymChoice:](#nkOpenSymChoice)
* [TNodeKind.nkOpenSymChoice](ast#nkOpenSymChoice)
[nkPar:](#nkPar)
* [TNodeKind.nkPar](ast#nkPar)
[nkParForStmt:](#nkParForStmt)
* [TNodeKind.nkParForStmt](ast#nkParForStmt)
[nkPattern:](#nkPattern)
* [TNodeKind.nkPattern](ast#nkPattern)
[nkPostfix:](#nkPostfix)
* [TNodeKind.nkPostfix](ast#nkPostfix)
[nkPragma:](#nkPragma)
* [TNodeKind.nkPragma](ast#nkPragma)
[nkPragmaBlock:](#nkPragmaBlock)
* [TNodeKind.nkPragmaBlock](ast#nkPragmaBlock)
[nkPragmaCallKinds:](#nkPragmaCallKinds)
* [ast: nkPragmaCallKinds](ast#nkPragmaCallKinds)
[nkPragmaExpr:](#nkPragmaExpr)
* [TNodeKind.nkPragmaExpr](ast#nkPragmaExpr)
[nkPrefix:](#nkPrefix)
* [TNodeKind.nkPrefix](ast#nkPrefix)
[nkProcDef:](#nkProcDef)
* [TNodeKind.nkProcDef](ast#nkProcDef)
[nkProcTy:](#nkProcTy)
* [TNodeKind.nkProcTy](ast#nkProcTy)
[nkPtrTy:](#nkPtrTy)
* [TNodeKind.nkPtrTy](ast#nkPtrTy)
[nkRaiseStmt:](#nkRaiseStmt)
* [TNodeKind.nkRaiseStmt](ast#nkRaiseStmt)
[nkRange:](#nkRange)
* [TNodeKind.nkRange](ast#nkRange)
[nkRecCase:](#nkRecCase)
* [TNodeKind.nkRecCase](ast#nkRecCase)
[nkRecList:](#nkRecList)
* [TNodeKind.nkRecList](ast#nkRecList)
[nkRecWhen:](#nkRecWhen)
* [TNodeKind.nkRecWhen](ast#nkRecWhen)
[nkRefTy:](#nkRefTy)
* [TNodeKind.nkRefTy](ast#nkRefTy)
[nkReturnStmt:](#nkReturnStmt)
* [TNodeKind.nkReturnStmt](ast#nkReturnStmt)
[nkRStrLit:](#nkRStrLit)
* [TNodeKind.nkRStrLit](ast#nkRStrLit)
[nkSharedTy:](#nkSharedTy)
* [TNodeKind.nkSharedTy](ast#nkSharedTy)
[nkState:](#nkState)
* [TNodeKind.nkState](ast#nkState)
[nkStaticExpr:](#nkStaticExpr)
* [TNodeKind.nkStaticExpr](ast#nkStaticExpr)
[nkStaticStmt:](#nkStaticStmt)
* [TNodeKind.nkStaticStmt](ast#nkStaticStmt)
[nkStaticTy:](#nkStaticTy)
* [TNodeKind.nkStaticTy](ast#nkStaticTy)
[nkStmtList:](#nkStmtList)
* [TNodeKind.nkStmtList](ast#nkStmtList)
[nkStmtListExpr:](#nkStmtListExpr)
* [TNodeKind.nkStmtListExpr](ast#nkStmtListExpr)
[nkStmtListType:](#nkStmtListType)
* [TNodeKind.nkStmtListType](ast#nkStmtListType)
[nkStringToCString:](#nkStringToCString)
* [TNodeKind.nkStringToCString](ast#nkStringToCString)
[nkStrKinds:](#nkStrKinds)
* [ast: nkStrKinds](ast#nkStrKinds)
[nkStrLit:](#nkStrLit)
* [TNodeKind.nkStrLit](ast#nkStrLit)
[nkSym:](#nkSym)
* [TNodeKind.nkSym](ast#nkSym)
[nkSymChoices:](#nkSymChoices)
* [ast: nkSymChoices](ast#nkSymChoices)
[nkTableConstr:](#nkTableConstr)
* [TNodeKind.nkTableConstr](ast#nkTableConstr)
[nkTemplateDef:](#nkTemplateDef)
* [TNodeKind.nkTemplateDef](ast#nkTemplateDef)
[nkTripleStrLit:](#nkTripleStrLit)
* [TNodeKind.nkTripleStrLit](ast#nkTripleStrLit)
[nkTryStmt:](#nkTryStmt)
* [TNodeKind.nkTryStmt](ast#nkTryStmt)
[nkTupleClassTy:](#nkTupleClassTy)
* [TNodeKind.nkTupleClassTy](ast#nkTupleClassTy)
[nkTupleConstr:](#nkTupleConstr)
* [TNodeKind.nkTupleConstr](ast#nkTupleConstr)
[nkTupleTy:](#nkTupleTy)
* [TNodeKind.nkTupleTy](ast#nkTupleTy)
[nkType:](#nkType)
* [TNodeKind.nkType](ast#nkType)
[nkTypeClassTy:](#nkTypeClassTy)
* [TNodeKind.nkTypeClassTy](ast#nkTypeClassTy)
[nkTypeDef:](#nkTypeDef)
* [TNodeKind.nkTypeDef](ast#nkTypeDef)
[nkTypeOfExpr:](#nkTypeOfExpr)
* [TNodeKind.nkTypeOfExpr](ast#nkTypeOfExpr)
[nkTypeSection:](#nkTypeSection)
* [TNodeKind.nkTypeSection](ast#nkTypeSection)
[nkUInt16Lit:](#nkUInt16Lit)
* [TNodeKind.nkUInt16Lit](ast#nkUInt16Lit)
[nkUInt32Lit:](#nkUInt32Lit)
* [TNodeKind.nkUInt32Lit](ast#nkUInt32Lit)
[nkUInt64Lit:](#nkUInt64Lit)
* [TNodeKind.nkUInt64Lit](ast#nkUInt64Lit)
[nkUInt8Lit:](#nkUInt8Lit)
* [TNodeKind.nkUInt8Lit](ast#nkUInt8Lit)
[nkUIntLit:](#nkUIntLit)
* [TNodeKind.nkUIntLit](ast#nkUIntLit)
[nkUsingStmt:](#nkUsingStmt)
* [TNodeKind.nkUsingStmt](ast#nkUsingStmt)
[nkVarSection:](#nkVarSection)
* [TNodeKind.nkVarSection](ast#nkVarSection)
[nkVarTuple:](#nkVarTuple)
* [TNodeKind.nkVarTuple](ast#nkVarTuple)
[nkVarTy:](#nkVarTy)
* [TNodeKind.nkVarTy](ast#nkVarTy)
[nkWhen:](#nkWhen)
* [ast: nkWhen](ast#nkWhen)
[nkWhenExpr:](#nkWhenExpr)
* [ast: nkWhenExpr](ast#nkWhenExpr)
[nkWhenStmt:](#nkWhenStmt)
* [TNodeKind.nkWhenStmt](ast#nkWhenStmt)
[nkWhileStmt:](#nkWhileStmt)
* [TNodeKind.nkWhileStmt](ast#nkWhileStmt)
[nkWith:](#nkWith)
* [TNodeKind.nkWith](ast#nkWith)
[nkWithout:](#nkWithout)
* [TNodeKind.nkWithout](ast#nkWithout)
[nkYieldStmt:](#nkYieldStmt)
* [TNodeKind.nkYieldStmt](ast#nkYieldStmt)
[nodeTableGet:](#nodeTableGet)
* [treetab: nodeTableGet(t: TNodeTable; key: PNode): int](treetab#nodeTableGet%2CTNodeTable%2CPNode)
[nodeTablePut:](#nodeTablePut)
* [treetab: nodeTablePut(t: var TNodeTable; key: PNode; val: int)](treetab#nodeTablePut%2CTNodeTable%2CPNode%2Cint)
[nodeTableTestOrSet:](#nodeTableTestOrSet)
* [treetab: nodeTableTestOrSet(t: var TNodeTable; key: PNode; val: int): int](treetab#nodeTableTestOrSet%2CTNodeTable%2CPNode%2Cint)
[None:](#None)
* [InstrTargetKind.None](dfa#None)
[NoneLike:](#NoneLike)
* [OrdinalType.NoneLike](types#NoneLike)
[noSafePoints:](#noSafePoints)
* [TCProcFlag.noSafePoints](cgendata#noSafePoints)
[NotesVerbosity:](#NotesVerbosity)
* [lineinfos: NotesVerbosity](lineinfos#NotesVerbosity)
[notFoundError:](#notFoundError)
* [sem: notFoundError(c: PContext; n: PNode; errors: CandidateErrors)](sem#notFoundError%2CPContext%2CPNode%2CCandidateErrors)
[notnil:](#notnil)
* [Feature.notnil](options#notnil)
[noView:](#noView)
* [ViewTypeKind.noView](typeallowed#noView)
[numChars:](#numChars)
* [lexer: numChars](lexer#numChars)
[NumericalBase:](#NumericalBase)
* [lexer: NumericalBase](lexer#NumericalBase)
[numLines:](#numLines)
* [msgs: numLines(conf: ConfigRef; fileIdx: FileIndex): int](msgs#numLines%2CConfigRef%2CFileIndex)
[objectSetContains:](#objectSetContains)
* [astalgo: objectSetContains(t: TObjectSet; obj: RootRef): bool](astalgo#objectSetContains%2CTObjectSet%2CRootRef)
[objectSetContainsOrIncl:](#objectSetContainsOrIncl)
* [astalgo: objectSetContainsOrIncl(t: var TObjectSet; obj: RootRef): bool](astalgo#objectSetContainsOrIncl%2CTObjectSet%2CRootRef)
[objectSetIncl:](#objectSetIncl)
* [astalgo: objectSetIncl(t: var TObjectSet; obj: RootRef)](astalgo#objectSetIncl%2CTObjectSet%2CRootRef)
[objHasKidsValid:](#objHasKidsValid)
* [CodegenFlag.objHasKidsValid](cgendata#objHasKidsValid)
[oimDone:](#oimDone)
* [TOverloadIterMode.oimDone](lookups#oimDone)
[oimNoQualifier:](#oimNoQualifier)
* [TOverloadIterMode.oimNoQualifier](lookups#oimNoQualifier)
[oimOtherModule:](#oimOtherModule)
* [TOverloadIterMode.oimOtherModule](lookups#oimOtherModule)
[oimSelfModule:](#oimSelfModule)
* [TOverloadIterMode.oimSelfModule](lookups#oimSelfModule)
[oimSymChoice:](#oimSymChoice)
* [TOverloadIterMode.oimSymChoice](lookups#oimSymChoice)
[oimSymChoiceLocalLookup:](#oimSymChoiceLocalLookup)
* [TOverloadIterMode.oimSymChoiceLocalLookup](lookups#oimSymChoiceLocalLookup)
[oKeepVariableNames:](#oKeepVariableNames)
* [options: oKeepVariableNames](options#oKeepVariableNames)
[oldExperimentalFeatures:](#oldExperimentalFeatures)
* [options: oldExperimentalFeatures](options#oldExperimentalFeatures)
[onDef:](#onDef)
* [modulegraphs: onDef(info: TLineInfo; s: PSym)](modulegraphs#onDef.t%2CTLineInfo%2CPSym)
[onDefResolveForward:](#onDefResolveForward)
* [modulegraphs: onDefResolveForward(info: TLineInfo; s: PSym)](modulegraphs#onDefResolveForward.t%2CTLineInfo%2CPSym)
[One:](#One)
* [int128: One](int128#One)
[OnHeap:](#OnHeap)
* [TStorageLoc.OnHeap](ast#OnHeap)
[OnStack:](#OnStack)
* [TStorageLoc.OnStack](ast#OnStack)
[OnStatic:](#OnStatic)
* [TStorageLoc.OnStatic](ast#OnStatic)
[OnUnknown:](#OnUnknown)
* [TStorageLoc.OnUnknown](ast#OnUnknown)
[onUse:](#onUse)
* [modulegraphs: onUse(info: TLineInfo; s: PSym)](modulegraphs#onUse.t%2CTLineInfo%2CPSym)
[opcAddFloat:](#opcAddFloat)
* [TOpcode.opcAddFloat](vmdef#opcAddFloat)
[opcAddImmInt:](#opcAddImmInt)
* [TOpcode.opcAddImmInt](vmdef#opcAddImmInt)
[opcAddInt:](#opcAddInt)
* [TOpcode.opcAddInt](vmdef#opcAddInt)
[opcAddrNode:](#opcAddrNode)
* [TOpcode.opcAddrNode](vmdef#opcAddrNode)
[opcAddrReg:](#opcAddrReg)
* [TOpcode.opcAddrReg](vmdef#opcAddrReg)
[opcAddSeqElem:](#opcAddSeqElem)
* [TOpcode.opcAddSeqElem](vmdef#opcAddSeqElem)
[opcAddStrCh:](#opcAddStrCh)
* [TOpcode.opcAddStrCh](vmdef#opcAddStrCh)
[opcAddStrStr:](#opcAddStrStr)
* [TOpcode.opcAddStrStr](vmdef#opcAddStrStr)
[opcAddu:](#opcAddu)
* [TOpcode.opcAddu](vmdef#opcAddu)
[opcAsgnComplex:](#opcAsgnComplex)
* [TOpcode.opcAsgnComplex](vmdef#opcAsgnComplex)
[opcAsgnConst:](#opcAsgnConst)
* [TOpcode.opcAsgnConst](vmdef#opcAsgnConst)
[opcAsgnFloat:](#opcAsgnFloat)
* [TOpcode.opcAsgnFloat](vmdef#opcAsgnFloat)
[opcAsgnInt:](#opcAsgnInt)
* [TOpcode.opcAsgnInt](vmdef#opcAsgnInt)
[opcAsgnRef:](#opcAsgnRef)
* [TOpcode.opcAsgnRef](vmdef#opcAsgnRef)
[opcAshrInt:](#opcAshrInt)
* [TOpcode.opcAshrInt](vmdef#opcAshrInt)
[opcBitandInt:](#opcBitandInt)
* [TOpcode.opcBitandInt](vmdef#opcBitandInt)
[opcBitnotInt:](#opcBitnotInt)
* [TOpcode.opcBitnotInt](vmdef#opcBitnotInt)
[opcBitorInt:](#opcBitorInt)
* [TOpcode.opcBitorInt](vmdef#opcBitorInt)
[opcBitxorInt:](#opcBitxorInt)
* [TOpcode.opcBitxorInt](vmdef#opcBitxorInt)
[opcBranch:](#opcBranch)
* [TOpcode.opcBranch](vmdef#opcBranch)
[opcCallSite:](#opcCallSite)
* [TOpcode.opcCallSite](vmdef#opcCallSite)
[opcCard:](#opcCard)
* [TOpcode.opcCard](vmdef#opcCard)
[opcCast:](#opcCast)
* [TOpcode.opcCast](vmdef#opcCast)
[opcCastFloatToInt32:](#opcCastFloatToInt32)
* [TOpcode.opcCastFloatToInt32](vmdef#opcCastFloatToInt32)
[opcCastFloatToInt64:](#opcCastFloatToInt64)
* [TOpcode.opcCastFloatToInt64](vmdef#opcCastFloatToInt64)
[opcCastIntToFloat32:](#opcCastIntToFloat32)
* [TOpcode.opcCastIntToFloat32](vmdef#opcCastIntToFloat32)
[opcCastIntToFloat64:](#opcCastIntToFloat64)
* [TOpcode.opcCastIntToFloat64](vmdef#opcCastIntToFloat64)
[opcCastIntToPtr:](#opcCastIntToPtr)
* [TOpcode.opcCastIntToPtr](vmdef#opcCastIntToPtr)
[opcCastPtrToInt:](#opcCastPtrToInt)
* [TOpcode.opcCastPtrToInt](vmdef#opcCastPtrToInt)
[opcConcatStr:](#opcConcatStr)
* [TOpcode.opcConcatStr](vmdef#opcConcatStr)
[opcContainsSet:](#opcContainsSet)
* [TOpcode.opcContainsSet](vmdef#opcContainsSet)
[opcConv:](#opcConv)
* [TOpcode.opcConv](vmdef#opcConv)
[opcDivFloat:](#opcDivFloat)
* [TOpcode.opcDivFloat](vmdef#opcDivFloat)
[opcDivInt:](#opcDivInt)
* [TOpcode.opcDivInt](vmdef#opcDivInt)
[opcDivu:](#opcDivu)
* [TOpcode.opcDivu](vmdef#opcDivu)
[opcEcho:](#opcEcho)
* [TOpcode.opcEcho](vmdef#opcEcho)
[opcEof:](#opcEof)
* [TOpcode.opcEof](vmdef#opcEof)
[opcEqFloat:](#opcEqFloat)
* [TOpcode.opcEqFloat](vmdef#opcEqFloat)
[opcEqIdent:](#opcEqIdent)
* [TOpcode.opcEqIdent](vmdef#opcEqIdent)
[opcEqInt:](#opcEqInt)
* [TOpcode.opcEqInt](vmdef#opcEqInt)
[opcEqNimNode:](#opcEqNimNode)
* [TOpcode.opcEqNimNode](vmdef#opcEqNimNode)
[opcEqRef:](#opcEqRef)
* [TOpcode.opcEqRef](vmdef#opcEqRef)
[opcEqSet:](#opcEqSet)
* [TOpcode.opcEqSet](vmdef#opcEqSet)
[opcEqStr:](#opcEqStr)
* [TOpcode.opcEqStr](vmdef#opcEqStr)
[opcExcept:](#opcExcept)
* [TOpcode.opcExcept](vmdef#opcExcept)
[opcExcl:](#opcExcl)
* [TOpcode.opcExcl](vmdef#opcExcl)
[opcFastAsgnComplex:](#opcFastAsgnComplex)
* [TOpcode.opcFastAsgnComplex](vmdef#opcFastAsgnComplex)
[opcFinally:](#opcFinally)
* [TOpcode.opcFinally](vmdef#opcFinally)
[opcFinallyEnd:](#opcFinallyEnd)
* [TOpcode.opcFinallyEnd](vmdef#opcFinallyEnd)
[opcFJmp:](#opcFJmp)
* [TOpcode.opcFJmp](vmdef#opcFJmp)
[opcGenSym:](#opcGenSym)
* [TOpcode.opcGenSym](vmdef#opcGenSym)
[opcGetImpl:](#opcGetImpl)
* [TOpcode.opcGetImpl](vmdef#opcGetImpl)
[opcGetImplTransf:](#opcGetImplTransf)
* [TOpcode.opcGetImplTransf](vmdef#opcGetImplTransf)
[opcGorge:](#opcGorge)
* [TOpcode.opcGorge](vmdef#opcGorge)
[OpChars:](#OpChars)
* [lexer: OpChars](lexer#OpChars)
[opcIncl:](#opcIncl)
* [TOpcode.opcIncl](vmdef#opcIncl)
[opcInclRange:](#opcInclRange)
* [TOpcode.opcInclRange](vmdef#opcInclRange)
[opcIndCall:](#opcIndCall)
* [TOpcode.opcIndCall](vmdef#opcIndCall)
[opcIndCallAsgn:](#opcIndCallAsgn)
* [TOpcode.opcIndCallAsgn](vmdef#opcIndCallAsgn)
[opcInvalidField:](#opcInvalidField)
* [TOpcode.opcInvalidField](vmdef#opcInvalidField)
[opcIs:](#opcIs)
* [TOpcode.opcIs](vmdef#opcIs)
[opcIsNil:](#opcIsNil)
* [TOpcode.opcIsNil](vmdef#opcIsNil)
[opcJmp:](#opcJmp)
* [TOpcode.opcJmp](vmdef#opcJmp)
[opcJmpBack:](#opcJmpBack)
* [TOpcode.opcJmpBack](vmdef#opcJmpBack)
[opcLdArr:](#opcLdArr)
* [TOpcode.opcLdArr](vmdef#opcLdArr)
[opcLdArrAddr:](#opcLdArrAddr)
* [TOpcode.opcLdArrAddr](vmdef#opcLdArrAddr)
[opcLdConst:](#opcLdConst)
* [TOpcode.opcLdConst](vmdef#opcLdConst)
[opcLdDeref:](#opcLdDeref)
* [TOpcode.opcLdDeref](vmdef#opcLdDeref)
[opcLdGlobal:](#opcLdGlobal)
* [TOpcode.opcLdGlobal](vmdef#opcLdGlobal)
[opcLdGlobalAddr:](#opcLdGlobalAddr)
* [TOpcode.opcLdGlobalAddr](vmdef#opcLdGlobalAddr)
[opcLdGlobalAddrDerefFFI:](#opcLdGlobalAddrDerefFFI)
* [TOpcode.opcLdGlobalAddrDerefFFI](vmdef#opcLdGlobalAddrDerefFFI)
[opcLdGlobalDerefFFI:](#opcLdGlobalDerefFFI)
* [TOpcode.opcLdGlobalDerefFFI](vmdef#opcLdGlobalDerefFFI)
[opcLdImmInt:](#opcLdImmInt)
* [TOpcode.opcLdImmInt](vmdef#opcLdImmInt)
[opcLdNull:](#opcLdNull)
* [TOpcode.opcLdNull](vmdef#opcLdNull)
[opcLdNullReg:](#opcLdNullReg)
* [TOpcode.opcLdNullReg](vmdef#opcLdNullReg)
[opcLdObj:](#opcLdObj)
* [TOpcode.opcLdObj](vmdef#opcLdObj)
[opcLdObjAddr:](#opcLdObjAddr)
* [TOpcode.opcLdObjAddr](vmdef#opcLdObjAddr)
[opcLdStrIdx:](#opcLdStrIdx)
* [TOpcode.opcLdStrIdx](vmdef#opcLdStrIdx)
[opcLeFloat:](#opcLeFloat)
* [TOpcode.opcLeFloat](vmdef#opcLeFloat)
[opcLeInt:](#opcLeInt)
* [TOpcode.opcLeInt](vmdef#opcLeInt)
[opcLenSeq:](#opcLenSeq)
* [TOpcode.opcLenSeq](vmdef#opcLenSeq)
[opcLenStr:](#opcLenStr)
* [TOpcode.opcLenStr](vmdef#opcLenStr)
[opcLeSet:](#opcLeSet)
* [TOpcode.opcLeSet](vmdef#opcLeSet)
[opcLeStr:](#opcLeStr)
* [TOpcode.opcLeStr](vmdef#opcLeStr)
[opcLeu:](#opcLeu)
* [TOpcode.opcLeu](vmdef#opcLeu)
[opcLtFloat:](#opcLtFloat)
* [TOpcode.opcLtFloat](vmdef#opcLtFloat)
[opcLtInt:](#opcLtInt)
* [TOpcode.opcLtInt](vmdef#opcLtInt)
[opcLtSet:](#opcLtSet)
* [TOpcode.opcLtSet](vmdef#opcLtSet)
[opcLtStr:](#opcLtStr)
* [TOpcode.opcLtStr](vmdef#opcLtStr)
[opcLtu:](#opcLtu)
* [TOpcode.opcLtu](vmdef#opcLtu)
[opcMarshalLoad:](#opcMarshalLoad)
* [TOpcode.opcMarshalLoad](vmdef#opcMarshalLoad)
[opcMarshalStore:](#opcMarshalStore)
* [TOpcode.opcMarshalStore](vmdef#opcMarshalStore)
[opcMinusSet:](#opcMinusSet)
* [TOpcode.opcMinusSet](vmdef#opcMinusSet)
[opcModInt:](#opcModInt)
* [TOpcode.opcModInt](vmdef#opcModInt)
[opcModu:](#opcModu)
* [TOpcode.opcModu](vmdef#opcModu)
[opcMulFloat:](#opcMulFloat)
* [TOpcode.opcMulFloat](vmdef#opcMulFloat)
[opcMulInt:](#opcMulInt)
* [TOpcode.opcMulInt](vmdef#opcMulInt)
[opcMulSet:](#opcMulSet)
* [TOpcode.opcMulSet](vmdef#opcMulSet)
[opcMulu:](#opcMulu)
* [TOpcode.opcMulu](vmdef#opcMulu)
[opcNAdd:](#opcNAdd)
* [TOpcode.opcNAdd](vmdef#opcNAdd)
[opcNAddMultiple:](#opcNAddMultiple)
* [TOpcode.opcNAddMultiple](vmdef#opcNAddMultiple)
[opcNarrowS:](#opcNarrowS)
* [TOpcode.opcNarrowS](vmdef#opcNarrowS)
[opcNarrowU:](#opcNarrowU)
* [TOpcode.opcNarrowU](vmdef#opcNarrowU)
[opcNBindSym:](#opcNBindSym)
* [TOpcode.opcNBindSym](vmdef#opcNBindSym)
[opcNccInc:](#opcNccInc)
* [TOpcode.opcNccInc](vmdef#opcNccInc)
[opcNccValue:](#opcNccValue)
* [TOpcode.opcNccValue](vmdef#opcNccValue)
[opcNChild:](#opcNChild)
* [TOpcode.opcNChild](vmdef#opcNChild)
[opcNCopyNimNode:](#opcNCopyNimNode)
* [TOpcode.opcNCopyNimNode](vmdef#opcNCopyNimNode)
[opcNCopyNimTree:](#opcNCopyNimTree)
* [TOpcode.opcNCopyNimTree](vmdef#opcNCopyNimTree)
[opcNcsAdd:](#opcNcsAdd)
* [TOpcode.opcNcsAdd](vmdef#opcNcsAdd)
[opcNcsAt:](#opcNcsAt)
* [TOpcode.opcNcsAt](vmdef#opcNcsAt)
[opcNcsIncl:](#opcNcsIncl)
* [TOpcode.opcNcsIncl](vmdef#opcNcsIncl)
[opcNcsLen:](#opcNcsLen)
* [TOpcode.opcNcsLen](vmdef#opcNcsLen)
[opcNctGet:](#opcNctGet)
* [TOpcode.opcNctGet](vmdef#opcNctGet)
[opcNctHasNext:](#opcNctHasNext)
* [TOpcode.opcNctHasNext](vmdef#opcNctHasNext)
[opcNctLen:](#opcNctLen)
* [TOpcode.opcNctLen](vmdef#opcNctLen)
[opcNctNext:](#opcNctNext)
* [TOpcode.opcNctNext](vmdef#opcNctNext)
[opcNctPut:](#opcNctPut)
* [TOpcode.opcNctPut](vmdef#opcNctPut)
[opcNDel:](#opcNDel)
* [TOpcode.opcNDel](vmdef#opcNDel)
[opcNDynBindSym:](#opcNDynBindSym)
* [TOpcode.opcNDynBindSym](vmdef#opcNDynBindSym)
[opcNError:](#opcNError)
* [TOpcode.opcNError](vmdef#opcNError)
[opcNew:](#opcNew)
* [TOpcode.opcNew](vmdef#opcNew)
[opcNewSeq:](#opcNewSeq)
* [TOpcode.opcNewSeq](vmdef#opcNewSeq)
[opcNewStr:](#opcNewStr)
* [TOpcode.opcNewStr](vmdef#opcNewStr)
[opcNFloatVal:](#opcNFloatVal)
* [TOpcode.opcNFloatVal](vmdef#opcNFloatVal)
[opcNGetLineInfo:](#opcNGetLineInfo)
* [TOpcode.opcNGetLineInfo](vmdef#opcNGetLineInfo)
[opcNGetSize:](#opcNGetSize)
* [TOpcode.opcNGetSize](vmdef#opcNGetSize)
[opcNGetType:](#opcNGetType)
* [TOpcode.opcNGetType](vmdef#opcNGetType)
[opcNHint:](#opcNHint)
* [TOpcode.opcNHint](vmdef#opcNHint)
[opcNIdent:](#opcNIdent)
* [TOpcode.opcNIdent](vmdef#opcNIdent)
[opcNIntVal:](#opcNIntVal)
* [TOpcode.opcNIntVal](vmdef#opcNIntVal)
[opcNKind:](#opcNKind)
* [TOpcode.opcNKind](vmdef#opcNKind)
[opcNNewNimNode:](#opcNNewNimNode)
* [TOpcode.opcNNewNimNode](vmdef#opcNNewNimNode)
[opcNodeId:](#opcNodeId)
* [TOpcode.opcNodeId](vmdef#opcNodeId)
[opcNodeToReg:](#opcNodeToReg)
* [TOpcode.opcNodeToReg](vmdef#opcNodeToReg)
[opcNot:](#opcNot)
* [TOpcode.opcNot](vmdef#opcNot)
[opcNSetChild:](#opcNSetChild)
* [TOpcode.opcNSetChild](vmdef#opcNSetChild)
[opcNSetFloatVal:](#opcNSetFloatVal)
* [TOpcode.opcNSetFloatVal](vmdef#opcNSetFloatVal)
[opcNSetIdent:](#opcNSetIdent)
* [TOpcode.opcNSetIdent](vmdef#opcNSetIdent)
[opcNSetIntVal:](#opcNSetIntVal)
* [TOpcode.opcNSetIntVal](vmdef#opcNSetIntVal)
[opcNSetLineInfo:](#opcNSetLineInfo)
* [TOpcode.opcNSetLineInfo](vmdef#opcNSetLineInfo)
[opcNSetStrVal:](#opcNSetStrVal)
* [TOpcode.opcNSetStrVal](vmdef#opcNSetStrVal)
[opcNSetSymbol:](#opcNSetSymbol)
* [TOpcode.opcNSetSymbol](vmdef#opcNSetSymbol)
[opcNSetType:](#opcNSetType)
* [TOpcode.opcNSetType](vmdef#opcNSetType)
[opcNSigHash:](#opcNSigHash)
* [TOpcode.opcNSigHash](vmdef#opcNSigHash)
[opcNStrVal:](#opcNStrVal)
* [TOpcode.opcNStrVal](vmdef#opcNStrVal)
[opcNSymbol:](#opcNSymbol)
* [TOpcode.opcNSymbol](vmdef#opcNSymbol)
[opcNSymKind:](#opcNSymKind)
* [TOpcode.opcNSymKind](vmdef#opcNSymKind)
[opcNWarning:](#opcNWarning)
* [TOpcode.opcNWarning](vmdef#opcNWarning)
[opcode:](#opcode)
* [vmdef: opcode(x: TInstr): TOpcode](vmdef#opcode.t%2CTInstr)
[opcOf:](#opcOf)
* [TOpcode.opcOf](vmdef#opcOf)
[opcParseExprToAst:](#opcParseExprToAst)
* [TOpcode.opcParseExprToAst](vmdef#opcParseExprToAst)
[opcParseFloat:](#opcParseFloat)
* [TOpcode.opcParseFloat](vmdef#opcParseFloat)
[opcParseStmtToAst:](#opcParseStmtToAst)
* [TOpcode.opcParseStmtToAst](vmdef#opcParseStmtToAst)
[opcPlusSet:](#opcPlusSet)
* [TOpcode.opcPlusSet](vmdef#opcPlusSet)
[opcQueryErrorFlag:](#opcQueryErrorFlag)
* [TOpcode.opcQueryErrorFlag](vmdef#opcQueryErrorFlag)
[opcQuit:](#opcQuit)
* [TOpcode.opcQuit](vmdef#opcQuit)
[opcRaise:](#opcRaise)
* [TOpcode.opcRaise](vmdef#opcRaise)
[opcRangeChck:](#opcRangeChck)
* [TOpcode.opcRangeChck](vmdef#opcRangeChck)
[opcRepr:](#opcRepr)
* [TOpcode.opcRepr](vmdef#opcRepr)
[opcRet:](#opcRet)
* [TOpcode.opcRet](vmdef#opcRet)
[opcSameNodeType:](#opcSameNodeType)
* [TOpcode.opcSameNodeType](vmdef#opcSameNodeType)
[opcSetLenSeq:](#opcSetLenSeq)
* [TOpcode.opcSetLenSeq](vmdef#opcSetLenSeq)
[opcSetLenStr:](#opcSetLenStr)
* [TOpcode.opcSetLenStr](vmdef#opcSetLenStr)
[opcSetType:](#opcSetType)
* [TOpcode.opcSetType](vmdef#opcSetType)
[opcShlInt:](#opcShlInt)
* [TOpcode.opcShlInt](vmdef#opcShlInt)
[opcShrInt:](#opcShrInt)
* [TOpcode.opcShrInt](vmdef#opcShrInt)
[opcSignExtend:](#opcSignExtend)
* [TOpcode.opcSignExtend](vmdef#opcSignExtend)
[opcSlurp:](#opcSlurp)
* [TOpcode.opcSlurp](vmdef#opcSlurp)
[opcStrToIdent:](#opcStrToIdent)
* [TOpcode.opcStrToIdent](vmdef#opcStrToIdent)
[opcSubFloat:](#opcSubFloat)
* [TOpcode.opcSubFloat](vmdef#opcSubFloat)
[opcSubImmInt:](#opcSubImmInt)
* [TOpcode.opcSubImmInt](vmdef#opcSubImmInt)
[opcSubInt:](#opcSubInt)
* [TOpcode.opcSubInt](vmdef#opcSubInt)
[opcSubStr:](#opcSubStr)
* [TOpcode.opcSubStr](vmdef#opcSubStr)
[opcSubu:](#opcSubu)
* [TOpcode.opcSubu](vmdef#opcSubu)
[opcSymIsInstantiationOf:](#opcSymIsInstantiationOf)
* [TOpcode.opcSymIsInstantiationOf](vmdef#opcSymIsInstantiationOf)
[opcSymOwner:](#opcSymOwner)
* [TOpcode.opcSymOwner](vmdef#opcSymOwner)
[opcTJmp:](#opcTJmp)
* [TOpcode.opcTJmp](vmdef#opcTJmp)
[opcTry:](#opcTry)
* [TOpcode.opcTry](vmdef#opcTry)
[opcTypeTrait:](#opcTypeTrait)
* [TOpcode.opcTypeTrait](vmdef#opcTypeTrait)
[opcUnaryMinusFloat:](#opcUnaryMinusFloat)
* [TOpcode.opcUnaryMinusFloat](vmdef#opcUnaryMinusFloat)
[opcUnaryMinusInt:](#opcUnaryMinusInt)
* [TOpcode.opcUnaryMinusInt](vmdef#opcUnaryMinusInt)
[opcWrArr:](#opcWrArr)
* [TOpcode.opcWrArr](vmdef#opcWrArr)
[opcWrDeref:](#opcWrDeref)
* [TOpcode.opcWrDeref](vmdef#opcWrDeref)
[opcWrObj:](#opcWrObj)
* [TOpcode.opcWrObj](vmdef#opcWrObj)
[opcWrStrIdx:](#opcWrStrIdx)
* [TOpcode.opcWrStrIdx](vmdef#opcWrStrIdx)
[opcXor:](#opcXor)
* [TOpcode.opcXor](vmdef#opcXor)
[opcYldVal:](#opcYldVal)
* [TOpcode.opcYldVal](vmdef#opcYldVal)
[opcYldYoid:](#opcYldYoid)
* [TOpcode.opcYldYoid](vmdef#opcYldYoid)
[open:](#open)
* [ndi: open(f: var NdiFile; filename: AbsoluteFile; conf: ConfigRef)](ndi#open%2CNdiFile%2CAbsoluteFile%2CConfigRef)
[openBaseLexer:](#openBaseLexer)
* [nimlexbase: openBaseLexer(L: var TBaseLexer; inputstream: PLLStream; bufLen: int = 8192)](nimlexbase#openBaseLexer%2CTBaseLexer%2CPLLStream%2Cint)
[openLexer:](#openLexer)
* [lexer: openLexer(lex: var Lexer; filename: AbsoluteFile; inputstream: PLLStream; cache: IdentCache; config: ConfigRef)](lexer#openLexer%2CLexer%2CAbsoluteFile%2CPLLStream%2CIdentCache%2CConfigRef)
* [lexer: openLexer(lex: var Lexer; fileIdx: FileIndex; inputstream: PLLStream; cache: IdentCache; config: ConfigRef)](lexer#openLexer%2CLexer%2CFileIndex%2CPLLStream%2CIdentCache%2CConfigRef)
[openParser:](#openParser)
* [parser: openParser(p: var Parser; filename: AbsoluteFile; inputStream: PLLStream; cache: IdentCache; config: ConfigRef)](parser#openParser%2CParser%2CAbsoluteFile%2CPLLStream%2CIdentCache%2CConfigRef)
* [parser: openParser(p: var Parser; fileIdx: FileIndex; inputStream: PLLStream; cache: IdentCache; config: ConfigRef)](parser#openParser%2CParser%2CFileIndex%2CPLLStream%2CIdentCache%2CConfigRef)
* [syntaxes: openParser(p: var Parser; fileIdx: FileIndex; inputstream: PLLStream; cache: IdentCache; config: ConfigRef)](syntaxes#openParser%2CParser%2CFileIndex%2CPLLStream%2CIdentCache%2CConfigRef)
[openScope:](#openScope)
* [lookups: openScope(c: PContext): PScope](lookups#openScope%2CPContext)
[openShadowScope:](#openShadowScope)
* [lookups: openShadowScope(c: PContext)](lookups#openShadowScope%2CPContext)
[Operators:](#Operators)
* [guards: Operators](guards#Operators)
[opGorge:](#opGorge)
* [gorgeimpl: opGorge(cmd, input, cache: string; info: TLineInfo; conf: ConfigRef): (string, int)](gorgeimpl#opGorge%2Cstring%2Cstring%2Cstring%2CTLineInfo%2CConfigRef)
[opMapTypeImplToAst:](#opMapTypeImplToAst)
* [vmdeps: opMapTypeImplToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode](vmdeps#opMapTypeImplToAst%2CIdentCache%2CPType%2CTLineInfo)
[opMapTypeInstToAst:](#opMapTypeInstToAst)
* [vmdeps: opMapTypeInstToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode](vmdeps#opMapTypeInstToAst%2CIdentCache%2CPType%2CTLineInfo)
[opMapTypeToAst:](#opMapTypeToAst)
* [vmdeps: opMapTypeToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode](vmdeps#opMapTypeToAst%2CIdentCache%2CPType%2CTLineInfo)
[oprHigh:](#oprHigh)
* [wordrecg: oprHigh](wordrecg#oprHigh)
[oprLow:](#oprLow)
* [wordrecg: oprLow](wordrecg#oprLow)
[opSlurp:](#opSlurp)
* [vmdeps: opSlurp(file: string; info: TLineInfo; module: PSym; conf: ConfigRef): string](vmdeps#opSlurp%2Cstring%2CTLineInfo%2CPSym%2CConfigRef)
[optAssert:](#optAssert)
* [TOption.optAssert](options#optAssert)
[optBenchmarkVM:](#optBenchmarkVM)
* [TGlobalOption.optBenchmarkVM](options#optBenchmarkVM)
[optBoundsCheck:](#optBoundsCheck)
* [TOption.optBoundsCheck](options#optBoundsCheck)
[optByRef:](#optByRef)
* [TOption.optByRef](options#optByRef)
[optCDebug:](#optCDebug)
* [TGlobalOption.optCDebug](options#optCDebug)
[optCompileOnly:](#optCompileOnly)
* [TGlobalOption.optCompileOnly](options#optCompileOnly)
[optCursorInference:](#optCursorInference)
* [TOption.optCursorInference](options#optCursorInference)
[optDocInternal:](#optDocInternal)
* [TGlobalOption.optDocInternal](options#optDocInternal)
[optDynlibOverrideAll:](#optDynlibOverrideAll)
* [TGlobalOption.optDynlibOverrideAll](options#optDynlibOverrideAll)
[optEmbedOrigSrc:](#optEmbedOrigSrc)
* [TGlobalOption.optEmbedOrigSrc](options#optEmbedOrigSrc)
[optEnableDeepCopy:](#optEnableDeepCopy)
* [TGlobalOption.optEnableDeepCopy](options#optEnableDeepCopy)
[optExcessiveStackTrace:](#optExcessiveStackTrace)
* [TGlobalOption.optExcessiveStackTrace](options#optExcessiveStackTrace)
[optFieldCheck:](#optFieldCheck)
* [TOption.optFieldCheck](options#optFieldCheck)
[optForceFullMake:](#optForceFullMake)
* [TGlobalOption.optForceFullMake](options#optForceFullMake)
[optGenDynLib:](#optGenDynLib)
* [TGlobalOption.optGenDynLib](options#optGenDynLib)
[optGenGuiApp:](#optGenGuiApp)
* [TGlobalOption.optGenGuiApp](options#optGenGuiApp)
[optGenIndex:](#optGenIndex)
* [TGlobalOption.optGenIndex](options#optGenIndex)
[optGenMapping:](#optGenMapping)
* [TGlobalOption.optGenMapping](options#optGenMapping)
[optGenScript:](#optGenScript)
* [TGlobalOption.optGenScript](options#optGenScript)
[optGenStaticLib:](#optGenStaticLib)
* [TGlobalOption.optGenStaticLib](options#optGenStaticLib)
[optHints:](#optHints)
* [TOption.optHints](options#optHints)
[optHotCodeReloading:](#optHotCodeReloading)
* [TGlobalOption.optHotCodeReloading](options#optHotCodeReloading)
[optIdeDebug:](#optIdeDebug)
* [TGlobalOption.optIdeDebug](options#optIdeDebug)
[optIdeTerse:](#optIdeTerse)
* [TGlobalOption.optIdeTerse](options#optIdeTerse)
[optimize:](#optimize)
* [optimizer: optimize(n: PNode): PNode](optimizer#optimize%2CPNode)
[optImplicitStatic:](#optImplicitStatic)
* [TOption.optImplicitStatic](options#optImplicitStatic)
[optInd:](#optInd)
* [parser: optInd(p: var Parser; n: PNode)](parser#optInd%2CParser%2CPNode)
[optInfCheck:](#optInfCheck)
* [TOption.optInfCheck](options#optInfCheck)
[optLineDir:](#optLineDir)
* [TOption.optLineDir](options#optLineDir)
[optLineTrace:](#optLineTrace)
* [TOption.optLineTrace](options#optLineTrace)
[optListCmd:](#optListCmd)
* [TGlobalOption.optListCmd](options#optListCmd)
[optListFullPaths:](#optListFullPaths)
* [TGlobalOption.optListFullPaths](options#optListFullPaths)
[optMemTracker:](#optMemTracker)
* [TOption.optMemTracker](options#optMemTracker)
[optMixedMode:](#optMixedMode)
* [TGlobalOption.optMixedMode](options#optMixedMode)
[optMultiMethods:](#optMultiMethods)
* [TGlobalOption.optMultiMethods](options#optMultiMethods)
[optNaNCheck:](#optNaNCheck)
* [TOption.optNaNCheck](options#optNaNCheck)
[optNilSeqs:](#optNilSeqs)
* [TOption.optNilSeqs](options#optNilSeqs)
[optNimV12Emulation:](#optNimV12Emulation)
* [TGlobalOption.optNimV12Emulation](options#optNimV12Emulation)
[optNimV1Emulation:](#optNimV1Emulation)
* [TGlobalOption.optNimV1Emulation](options#optNimV1Emulation)
[optNoLinking:](#optNoLinking)
* [TGlobalOption.optNoLinking](options#optNoLinking)
[optNoMain:](#optNoMain)
* [TGlobalOption.optNoMain](options#optNoMain)
[optNone:](#optNone)
* [TOption.optNone](options#optNone)
[optNoNimblePath:](#optNoNimblePath)
* [TGlobalOption.optNoNimblePath](options#optNoNimblePath)
[optObjCheck:](#optObjCheck)
* [TOption.optObjCheck](options#optObjCheck)
[optOptimizeSize:](#optOptimizeSize)
* [TOption.optOptimizeSize](options#optOptimizeSize)
[optOptimizeSpeed:](#optOptimizeSpeed)
* [TOption.optOptimizeSpeed](options#optOptimizeSpeed)
[optOverflowCheck:](#optOverflowCheck)
* [TOption.optOverflowCheck](options#optOverflowCheck)
[optOwnedRefs:](#optOwnedRefs)
* [TGlobalOption.optOwnedRefs](options#optOwnedRefs)
[optPanics:](#optPanics)
* [TGlobalOption.optPanics](options#optPanics)
[optPar:](#optPar)
* [parser: optPar(p: var Parser)](parser#optPar%2CParser)
[optPreserveOrigSource:](#optPreserveOrigSource)
* [options: optPreserveOrigSource(conf: ConfigRef): untyped](options#optPreserveOrigSource.t%2CConfigRef)
[optProduceAsm:](#optProduceAsm)
* [TGlobalOption.optProduceAsm](options#optProduceAsm)
[optProfiler:](#optProfiler)
* [TOption.optProfiler](options#optProfiler)
[optProfileVM:](#optProfileVM)
* [TGlobalOption.optProfileVM](options#optProfileVM)
[optRangeCheck:](#optRangeCheck)
* [TOption.optRangeCheck](options#optRangeCheck)
[optRefCheck:](#optRefCheck)
* [TOption.optRefCheck](options#optRefCheck)
[optRun:](#optRun)
* [TGlobalOption.optRun](options#optRun)
[optSeqDestructors:](#optSeqDestructors)
* [TGlobalOption.optSeqDestructors](options#optSeqDestructors)
[optShowAllMismatches:](#optShowAllMismatches)
* [TGlobalOption.optShowAllMismatches](options#optShowAllMismatches)
[optSinkInference:](#optSinkInference)
* [TOption.optSinkInference](options#optSinkInference)
[optSkipParentConfigFiles:](#optSkipParentConfigFiles)
* [TGlobalOption.optSkipParentConfigFiles](options#optSkipParentConfigFiles)
[optSkipProjConfigFile:](#optSkipProjConfigFile)
* [TGlobalOption.optSkipProjConfigFile](options#optSkipProjConfigFile)
[optSkipSystemConfigFile:](#optSkipSystemConfigFile)
* [TGlobalOption.optSkipSystemConfigFile](options#optSkipSystemConfigFile)
[optSkipUserConfigFile:](#optSkipUserConfigFile)
* [TGlobalOption.optSkipUserConfigFile](options#optSkipUserConfigFile)
[optSourcemap:](#optSourcemap)
* [TGlobalOption.optSourcemap](options#optSourcemap)
[optStackTrace:](#optStackTrace)
* [TOption.optStackTrace](options#optStackTrace)
[optStackTraceMsgs:](#optStackTraceMsgs)
* [TOption.optStackTraceMsgs](options#optStackTraceMsgs)
[optStaticBoundsCheck:](#optStaticBoundsCheck)
* [TOption.optStaticBoundsCheck](options#optStaticBoundsCheck)
[optStdout:](#optStdout)
* [TGlobalOption.optStdout](options#optStdout)
[optStyleCheck:](#optStyleCheck)
* [TOption.optStyleCheck](options#optStyleCheck)
[optStyleError:](#optStyleError)
* [TGlobalOption.optStyleError](options#optStyleError)
[optStyleHint:](#optStyleHint)
* [TGlobalOption.optStyleHint](options#optStyleHint)
[optStyleUsages:](#optStyleUsages)
* [TGlobalOption.optStyleUsages](options#optStyleUsages)
[optTaintMode:](#optTaintMode)
* [TGlobalOption.optTaintMode](options#optTaintMode)
[optThreadAnalysis:](#optThreadAnalysis)
* [TGlobalOption.optThreadAnalysis](options#optThreadAnalysis)
[optThreads:](#optThreads)
* [TGlobalOption.optThreads](options#optThreads)
[optTinyRtti:](#optTinyRtti)
* [TGlobalOption.optTinyRtti](options#optTinyRtti)
[optTlsEmulation:](#optTlsEmulation)
* [TGlobalOption.optTlsEmulation](options#optTlsEmulation)
[optTrMacros:](#optTrMacros)
* [TOption.optTrMacros](options#optTrMacros)
[optUseColors:](#optUseColors)
* [TGlobalOption.optUseColors](options#optUseColors)
[optUseNimcache:](#optUseNimcache)
* [TGlobalOption.optUseNimcache](options#optUseNimcache)
[optWarns:](#optWarns)
* [TOption.optWarns](options#optWarns)
[optWasNimscript:](#optWasNimscript)
* [TGlobalOption.optWasNimscript](options#optWasNimscript)
[optWholeProject:](#optWholeProject)
* [TGlobalOption.optWholeProject](options#optWholeProject)
[OrdinalType:](#OrdinalType)
* [types: OrdinalType](types#OrdinalType)
[ordinalValToString:](#ordinalValToString)
* [semfold: ordinalValToString(a: PNode; g: ModuleGraph): string](semfold#ordinalValToString%2CPNode%2CModuleGraph)
[originatingModule:](#originatingModule)
* [ast: originatingModule(s: PSym): PSym](ast#originatingModule%2CPSym)
[OS:](#OS)
* [platform: OS](platform#OS)
[osAix:](#osAix)
* [TSystemOS.osAix](platform#osAix)
[osAmiga:](#osAmiga)
* [TSystemOS.osAmiga](platform#osAmiga)
[osAndroid:](#osAndroid)
* [TSystemOS.osAndroid](platform#osAndroid)
[osAny:](#osAny)
* [TSystemOS.osAny](platform#osAny)
[osAtari:](#osAtari)
* [TSystemOS.osAtari](platform#osAtari)
[osDos:](#osDos)
* [TSystemOS.osDos](platform#osDos)
[osDragonfly:](#osDragonfly)
* [TSystemOS.osDragonfly](platform#osDragonfly)
[osFreebsd:](#osFreebsd)
* [TSystemOS.osFreebsd](platform#osFreebsd)
[osFreeRTOS:](#osFreeRTOS)
* [TSystemOS.osFreeRTOS](platform#osFreeRTOS)
[osGenode:](#osGenode)
* [TSystemOS.osGenode](platform#osGenode)
[osHaiku:](#osHaiku)
* [TSystemOS.osHaiku](platform#osHaiku)
[osIos:](#osIos)
* [TSystemOS.osIos](platform#osIos)
[osIrix:](#osIrix)
* [TSystemOS.osIrix](platform#osIrix)
[osJS:](#osJS)
* [TSystemOS.osJS](platform#osJS)
[osLinux:](#osLinux)
* [TSystemOS.osLinux](platform#osLinux)
[osMacos:](#osMacos)
* [TSystemOS.osMacos](platform#osMacos)
[osMacosx:](#osMacosx)
* [TSystemOS.osMacosx](platform#osMacosx)
[osMorphos:](#osMorphos)
* [TSystemOS.osMorphos](platform#osMorphos)
[osNetbsd:](#osNetbsd)
* [TSystemOS.osNetbsd](platform#osNetbsd)
[osNetware:](#osNetware)
* [TSystemOS.osNetware](platform#osNetware)
[osNimVM:](#osNimVM)
* [TSystemOS.osNimVM](platform#osNimVM)
[osNintendoSwitch:](#osNintendoSwitch)
* [TSystemOS.osNintendoSwitch](platform#osNintendoSwitch)
[osNone:](#osNone)
* [TSystemOS.osNone](platform#osNone)
[osOpenbsd:](#osOpenbsd)
* [TSystemOS.osOpenbsd](platform#osOpenbsd)
[osOs2:](#osOs2)
* [TSystemOS.osOs2](platform#osOs2)
[osPalmos:](#osPalmos)
* [TSystemOS.osPalmos](platform#osPalmos)
[ospCaseInsensitive:](#ospCaseInsensitive)
* [TInfoOSProp.ospCaseInsensitive](platform#ospCaseInsensitive)
[ospLacksThreadVars:](#ospLacksThreadVars)
* [TInfoOSProp.ospLacksThreadVars](platform#ospLacksThreadVars)
[ospNeedsPIC:](#ospNeedsPIC)
* [TInfoOSProp.ospNeedsPIC](platform#ospNeedsPIC)
[ospPosix:](#ospPosix)
* [TInfoOSProp.ospPosix](platform#ospPosix)
[osQnx:](#osQnx)
* [TSystemOS.osQnx](platform#osQnx)
[osSkyos:](#osSkyos)
* [TSystemOS.osSkyos](platform#osSkyos)
[osSolaris:](#osSolaris)
* [TSystemOS.osSolaris](platform#osSolaris)
[osStandalone:](#osStandalone)
* [TSystemOS.osStandalone](platform#osStandalone)
[osVxWorks:](#osVxWorks)
* [TSystemOS.osVxWorks](platform#osVxWorks)
[osWindows:](#osWindows)
* [TSystemOS.osWindows](platform#osWindows)
[overlap:](#overlap)
* [nimsets: overlap(a, b: PNode): bool](nimsets#overlap%2CPNode%2CPNode)
[OverloadableSyms:](#OverloadableSyms)
* [ast: OverloadableSyms](ast#OverloadableSyms)
[pairs:](#pairs)
* [ast: pairs(n: PNode): tuple[i: int, n: PNode]](ast#pairs.i%2CPNode)
* [astalgo: pairs(t: TIdNodeTable): tuple[key: PIdObj, val: PNode]](astalgo#pairs.i%2CTIdNodeTable)
* [astalgo: pairs(t: TIdTable): tuple[key: int, value: RootRef]](astalgo#pairs.i%2CTIdTable)
* [btrees: pairs[Key, Val](b: BTree[Key, Val]): (Key, Val)](btrees#pairs.i%2CBTree%5BKey%2CVal%5D)
[parallel:](#parallel)
* [Feature.parallel](options#parallel)
[paramName:](#paramName)
* [lambdalifting: paramName](lambdalifting#paramName)
[paramPragmas:](#paramPragmas)
* [pragmas: paramPragmas](pragmas#paramPragmas)
[paramsEqual:](#paramsEqual)
* [TParamsEquality.paramsEqual](types#paramsEqual)
[paramsIncompatible:](#paramsIncompatible)
* [TParamsEquality.paramsIncompatible](types#paramsIncompatible)
[paramsNotEqual:](#paramsNotEqual)
* [TParamsEquality.paramsNotEqual](types#paramsNotEqual)
[paramsPos:](#paramsPos)
* [ast: paramsPos](ast#paramsPos)
[paramTypesMatch:](#paramTypesMatch)
* [sigmatch: paramTypesMatch(m: var TCandidate; f, a: PType; arg, argOrig: PNode): PNode](sigmatch#paramTypesMatch%2CTCandidate%2CPType%2CPType%2CPNode%2CPNode)
[parentModule:](#parentModule)
* [modulegraphs: parentModule(g: ModuleGraph; fileIdx: FileIndex): FileIndex](modulegraphs#parentModule%2CModuleGraph%2CFileIndex)
[parLineInfo:](#parLineInfo)
* [parser: parLineInfo(p: Parser): TLineInfo](parser#parLineInfo%2CParser)
[parMessage:](#parMessage)
* [parser: parMessage(p: Parser; msg: TMsgKind; arg: string = "")](parser#parMessage%2CParser%2CTMsgKind%2Cstring)
[parse:](#parse)
* [sourcemap: parse(source: string; path: string): SourceNode](sourcemap#parse%2Cstring%2Cstring)
[parseAll:](#parseAll)
* [parser: parseAll(p: var Parser): PNode](parser#parseAll%2CParser)
[parseDecimalInt128:](#parseDecimalInt128)
* [int128: parseDecimalInt128(arg: string; pos: int = 0): Int128](int128#parseDecimalInt128%2Cstring%2Cint)
[parseFile:](#parseFile)
* [syntaxes: parseFile(fileIdx: FileIndex; cache: IdentCache; config: ConfigRef): PNode](syntaxes#parseFile%2CFileIndex%2CIdentCache%2CConfigRef)
[parseIdeCmd:](#parseIdeCmd)
* [options: parseIdeCmd(s: string): IdeCmd](options#parseIdeCmd%2Cstring)
[Parser:](#Parser)
* [parser: Parser](parser#Parser)
[parseString:](#parseString)
* [parser: parseString(s: string; cache: IdentCache; config: ConfigRef; filename: string = ""; line: int = 0; errorHandler: ErrorHandler = nil): PNode](parser#parseString%2Cstring%2CIdentCache%2CConfigRef%2Cstring%2Cint%2CErrorHandler)
[parseSymbol:](#parseSymbol)
* [parser: parseSymbol(p: var Parser; mode = smNormal): PNode](parser#parseSymbol%2CParser)
[parseTableCells:](#parseTableCells)
* [asciitables: parseTableCells(s: string; delim = ' '): Cell](asciitables#parseTableCells.i%2Cstring%2Cchar)
[parseTopLevelStmt:](#parseTopLevelStmt)
* [parser: parseTopLevelStmt(p: var Parser): PNode](parser#parseTopLevelStmt%2CParser)
[Partial:](#Partial)
* [InstrTargetKind.Partial](dfa#Partial)
[partialMatch:](#partialMatch)
* [sigmatch: partialMatch(c: PContext; n, nOrig: PNode; m: var TCandidate)](sigmatch#partialMatch%2CPContext%2CPNode%2CPNode%2CTCandidate)
[Partitions:](#Partitions)
* [varpartitions: Partitions](varpartitions#Partitions)
[passCmd1:](#passCmd1)
* [TCmdLinePass.passCmd1](commands#passCmd1)
[passCmd2:](#passCmd2)
* [TCmdLinePass.passCmd2](commands#passCmd2)
[passPP:](#passPP)
* [TCmdLinePass.passPP](commands#passPP)
[pathSubs:](#pathSubs)
* [options: pathSubs(conf: ConfigRef; p, config: string): string](options#pathSubs%2CConfigRef%2Cstring%2Cstring)
[patternPos:](#patternPos)
* [ast: patternPos](ast#patternPos)
[PContext:](#PContext)
* [semdata: PContext](semdata#PContext)
[PCtx:](#PCtx)
* [vmdef: PCtx](vmdef#PCtx)
[PDoc:](#PDoc)
* [docgen: PDoc](docgen#PDoc)
[PersistentNodeFlags:](#PersistentNodeFlags)
* [ast: PersistentNodeFlags](ast#PersistentNodeFlags)
[PEvalContext:](#PEvalContext)
* [vmdef: PEvalContext](vmdef#PEvalContext)
[pickSym:](#pickSym)
* [lookups: pickSym(c: PContext; n: PNode; kinds: set[TSymKind]; flags: TSymFlags = {}): PSym](lookups#pickSym%2CPContext%2CPNode%2Cset%5BTSymKind%5D%2CTSymFlags)
[PIdent:](#PIdent)
* [idents: PIdent](idents#PIdent)
[PIdObj:](#PIdObj)
* [idents: PIdObj](idents#PIdObj)
[PInstantiation:](#PInstantiation)
* [ast: PInstantiation](ast#PInstantiation)
[PLib:](#PLib)
* [ast: PLib](ast#PLib)
[PLLStream:](#PLLStream)
* [llstream: PLLStream](llstream#PLLStream)
[Plugin:](#Plugin)
* [pluginsupport: Plugin](pluginsupport#Plugin)
[pluginMatches:](#pluginMatches)
* [pluginsupport: pluginMatches(ic: IdentCache; p: Plugin; s: PSym): bool](pluginsupport#pluginMatches%2CIdentCache%2CPlugin%2CPSym)
[PNode:](#PNode)
* [ast: PNode](ast#PNode)
[popCaseContext:](#popCaseContext)
* [semdata: popCaseContext(c: PContext)](semdata#popCaseContext%2CPContext)
[popInfoContext:](#popInfoContext)
* [msgs: popInfoContext(conf: ConfigRef)](msgs#popInfoContext%2CConfigRef)
[popOptionEntry:](#popOptionEntry)
* [semdata: popOptionEntry(c: PContext)](semdata#popOptionEntry%2CPContext)
[popOwner:](#popOwner)
* [semdata: popOwner(c: PContext)](semdata#popOwner%2CPContext)
[popProcCon:](#popProcCon)
* [semdata: popProcCon(c: PContext)](semdata#popProcCon%2CPContext)
[POptionEntry:](#POptionEntry)
* [semdata: POptionEntry](semdata#POptionEntry)
[PPassContext:](#PPassContext)
* [modulegraphs: PPassContext](modulegraphs#PPassContext)
[PProc:](#PProc)
* [vmdef: PProc](vmdef#PProc)
[PProcCon:](#PProcCon)
* [semdata: PProcCon](semdata#PProcCon)
[pragma:](#pragma)
* [pragmas: pragma(c: PContext; sym: PSym; n: PNode; validPragmas: TSpecialWords; isStatement: bool = false)](pragmas#pragma%2CPContext%2CPSym%2CPNode%2CTSpecialWords%2Cbool)
[pragmaAsm:](#pragmaAsm)
* [pragmas: pragmaAsm(c: PContext; n: PNode): char](pragmas#pragmaAsm%2CPContext%2CPNode)
[pragmasEffects:](#pragmasEffects)
* [ast: pragmasEffects](ast#pragmasEffects)
[pragmasPos:](#pragmasPos)
* [ast: pragmasPos](ast#pragmasPos)
[preferDesc:](#preferDesc)
* [TPreferedDesc.preferDesc](types#preferDesc)
[preferExported:](#preferExported)
* [TPreferedDesc.preferExported](types#preferExported)
[preferGenericArg:](#preferGenericArg)
* [TPreferedDesc.preferGenericArg](types#preferGenericArg)
[preferMixed:](#preferMixed)
* [TPreferedDesc.preferMixed](types#preferMixed)
[preferModuleInfo:](#preferModuleInfo)
* [TPreferedDesc.preferModuleInfo](types#preferModuleInfo)
[preferName:](#preferName)
* [TPreferedDesc.preferName](types#preferName)
[preferResolved:](#preferResolved)
* [TPreferedDesc.preferResolved](types#preferResolved)
[preferTypeName:](#preferTypeName)
* [TPreferedDesc.preferTypeName](types#preferTypeName)
[PrefixMatch:](#PrefixMatch)
* [prefixmatches: PrefixMatch](prefixmatches#PrefixMatch)
[prefixMatch:](#prefixMatch)
* [prefixmatches: prefixMatch(p, s: string): PrefixMatch](prefixmatches#prefixMatch%2Cstring%2Cstring)
[prepareMetatypeForSigmatch:](#prepareMetatypeForSigmatch)
* [semtypinst: prepareMetatypeForSigmatch(p: PContext; pt: TIdTable; info: TLineInfo; t: PType): PType](semtypinst#prepareMetatypeForSigmatch%2CPContext%2CTIdTable%2CTLineInfo%2CPType)
[prepareToWriteOutput:](#prepareToWriteOutput)
* [options: prepareToWriteOutput(conf: ConfigRef): AbsoluteFile](options#prepareToWriteOutput%2CConfigRef)
[prepend:](#prepend)
* [ropes: prepend(a: var Rope; b: Rope)](ropes#prepend%2CRope%2CRope)
* [ropes: prepend(a: var Rope; b: string)](ropes#prepend%2CRope%2Cstring)
[prependCurDir:](#prependCurDir)
* [cmdlinehelper: prependCurDir(f: AbsoluteFile): AbsoluteFile](cmdlinehelper#prependCurDir%2CAbsoluteFile)
[presentationPath:](#presentationPath)
* [docgen: presentationPath(conf: ConfigRef; file: AbsoluteFile; isTitle = false): RelativeFile](docgen#presentationPath%2CConfigRef%2CAbsoluteFile)
[prettyTok:](#prettyTok)
* [lexer: prettyTok(tok: Token): string](lexer#prettyTok%2CToken)
[preventStackTrace:](#preventStackTrace)
* [CodegenFlag.preventStackTrace](cgendata#preventStackTrace)
[previouslyInferred:](#previouslyInferred)
* [ast: previouslyInferred(t: PType): PType](ast#previouslyInferred.t%2CPType)
[printTok:](#printTok)
* [lexer: printTok(conf: ConfigRef; tok: Token)](lexer#printTok%2CConfigRef%2CToken)
[procDefs:](#procDefs)
* [ast: procDefs](ast#procDefs)
[processArgument:](#processArgument)
* [commands: processArgument(pass: TCmdLinePass; p: OptParser; argsCount: var int; config: ConfigRef): bool](commands#processArgument%2CTCmdLinePass%2COptParser%2Cint%2CConfigRef)
[processCmdLineAndProjectPath:](#processCmdLineAndProjectPath)
* [cmdlinehelper: processCmdLineAndProjectPath(self: NimProg; conf: ConfigRef)](cmdlinehelper#processCmdLineAndProjectPath%2CNimProg%2CConfigRef)
[processCommand:](#processCommand)
* [commands: processCommand(switch: string; pass: TCmdLinePass; config: ConfigRef)](commands#processCommand%2Cstring%2CTCmdLinePass%2CConfigRef)
[processModule:](#processModule)
* [passes: processModule(graph: ModuleGraph; module: PSym; stream: PLLStream): bool](passes#processModule%2CModuleGraph%2CPSym%2CPLLStream)
[processSpecificNote:](#processSpecificNote)
* [commands: processSpecificNote(arg: string; state: TSpecialWord; pass: TCmdLinePass; info: TLineInfo; orig: string; conf: ConfigRef)](commands#processSpecificNote%2Cstring%2CTSpecialWord%2CTCmdLinePass%2CTLineInfo%2Cstring%2CConfigRef)
[processSwitch:](#processSwitch)
* [commands: processSwitch(switch, arg: string; pass: TCmdLinePass; info: TLineInfo; conf: ConfigRef)](commands#processSwitch%2Cstring%2Cstring%2CTCmdLinePass%2CTLineInfo%2CConfigRef)
* [commands: processSwitch(pass: TCmdLinePass; p: OptParser; config: ConfigRef)](commands#processSwitch%2CTCmdLinePass%2COptParser%2CConfigRef)
[procPragmas:](#procPragmas)
* [pragmas: procPragmas](pragmas#procPragmas)
[procSec:](#procSec)
* [cgendata: procSec(p: BProc; s: TCProcSection): var Rope](cgendata#procSec%2CBProc%2CTCProcSection)
[procTypePragmas:](#procTypePragmas)
* [pragmas: procTypePragmas](pragmas#procTypePragmas)
[produceDestructorForDiscriminator:](#produceDestructorForDiscriminator)
* [liftdestructors: produceDestructorForDiscriminator(g: ModuleGraph; typ: PType; field: PSym; info: TLineInfo): PSym](liftdestructors#produceDestructorForDiscriminator%2CModuleGraph%2CPType%2CPSym%2CTLineInfo)
[ProfileData:](#ProfileData)
* [options: ProfileData](options#ProfileData)
[ProfileInfo:](#ProfileInfo)
* [options: ProfileInfo](options#ProfileInfo)
[Profiler:](#Profiler)
* [vmdef: Profiler](vmdef#Profiler)
[propagateToOwner:](#propagateToOwner)
* [ast: propagateToOwner(owner, elem: PType; propagateHasAsgn = true)](ast#propagateToOwner%2CPType%2CPType)
[propSpec:](#propSpec)
* [trees: propSpec(n: PNode; effectType: TSpecialWord): PNode](trees#propSpec%2CPNode%2CTSpecialWord)
[proveLe:](#proveLe)
* [guards: proveLe(m: TModel; a, b: PNode): TImplication](guards#proveLe%2CTModel%2CPNode%2CPNode)
[PScope:](#PScope)
* [ast: PScope](ast#PScope)
[PStackFrame:](#PStackFrame)
* [vmdef: PStackFrame](vmdef#PStackFrame)
[PSym:](#PSym)
* [ast: PSym](ast#PSym)
[PtrLikeKinds:](#PtrLikeKinds)
* [ast: PtrLikeKinds](ast#PtrLikeKinds)
[PType:](#PType)
* [ast: PType](ast#PType)
[pushCaseContext:](#pushCaseContext)
* [semdata: pushCaseContext(c: PContext; caseNode: PNode)](semdata#pushCaseContext%2CPContext%2CPNode)
[pushInfoContext:](#pushInfoContext)
* [msgs: pushInfoContext(conf: ConfigRef; info: TLineInfo; detail: string = "")](msgs#pushInfoContext%2CConfigRef%2CTLineInfo%2Cstring)
[pushOptionEntry:](#pushOptionEntry)
* [semdata: pushOptionEntry(c: PContext): POptionEntry](semdata#pushOptionEntry%2CPContext)
[pushOwner:](#pushOwner)
* [semdata: pushOwner(c: PContext; owner: PSym)](semdata#pushOwner%2CPContext%2CPSym)
[pushProcCon:](#pushProcCon)
* [sem: pushProcCon(c: PContext; owner: PSym)](sem#pushProcCon%2CPContext%2CPSym)
[put:](#put)
* [semdata: put(p: PProcCon; key, val: PSym)](semdata#put%2CPProcCon%2CPSym%2CPSym)
[qualifiedLookUp:](#qualifiedLookUp)
* [lookups: qualifiedLookUp(c: PContext; n: PNode; flags: set[TLookupFlag]): PSym](lookups#qualifiedLookUp%2CPContext%2CPNode%2Cset%5BTLookupFlag%5D)
[quotedFilename:](#quotedFilename)
* [msgs: quotedFilename(conf: ConfigRef; i: TLineInfo): Rope](msgs#quotedFilename%2CConfigRef%2CTLineInfo)
[quoteExpr:](#quoteExpr)
* [renderer: quoteExpr(a: string): string](renderer#quoteExpr%2Cstring)
[quoteShell:](#quoteShell)
* [pathutils: quoteShell(x: AbsoluteDir): string](pathutils#quoteShell%2CAbsoluteDir)
* [pathutils: quoteShell(x: AbsoluteFile): string](pathutils#quoteShell%2CAbsoluteFile)
[raiseRecoverableError:](#raiseRecoverableError)
* [lineinfos: raiseRecoverableError(msg: string)](lineinfos#raiseRecoverableError%2Cstring)
[rangeHasUnresolvedStatic:](#rangeHasUnresolvedStatic)
* [semdata: rangeHasUnresolvedStatic(t: PType): bool](semdata#rangeHasUnresolvedStatic.t%2CPType)
[rawAddField:](#rawAddField)
* [lowerings: rawAddField(obj: PType; field: PSym)](lowerings#rawAddField%2CPType%2CPSym)
[rawAddSon:](#rawAddSon)
* [ast: rawAddSon(father, son: PType; propagateHasAsgn = true)](ast#rawAddSon%2CPType%2CPType)
[rawAddSonNoPropagationOfTypeFlags:](#rawAddSonNoPropagationOfTypeFlags)
* [ast: rawAddSonNoPropagationOfTypeFlags(father, son: PType)](ast#rawAddSonNoPropagationOfTypeFlags%2CPType%2CPType)
[rawCloseScope:](#rawCloseScope)
* [lookups: rawCloseScope(c: PContext)](lookups#rawCloseScope%2CPContext)
[rawDirectAccess:](#rawDirectAccess)
* [lowerings: rawDirectAccess(obj, field: PSym): PNode](lowerings#rawDirectAccess%2CPSym%2CPSym)
[rawGetTok:](#rawGetTok)
* [lexer: rawGetTok(L: var Lexer; tok: var Token)](lexer#rawGetTok%2CLexer%2CToken)
[rawIndirectAccess:](#rawIndirectAccess)
* [lowerings: rawIndirectAccess(a: PNode; field: PSym; info: TLineInfo): PNode](lowerings#rawIndirectAccess%2CPNode%2CPSym%2CTLineInfo)
[rawMessage:](#rawMessage)
* [msgs: rawMessage(conf: ConfigRef; msg: TMsgKind; args: openArray[string])](msgs#rawMessage.t%2CConfigRef%2CTMsgKind%2CopenArray%5Bstring%5D)
* [msgs: rawMessage(conf: ConfigRef; msg: TMsgKind; arg: string)](msgs#rawMessage.t%2CConfigRef%2CTMsgKind%2Cstring)
[readConfigFile:](#readConfigFile)
* [nimconf: readConfigFile(filename: AbsoluteFile; cache: IdentCache; config: ConfigRef): bool](nimconf#readConfigFile%2CAbsoluteFile%2CIdentCache%2CConfigRef)
[readExceptSet:](#readExceptSet)
* [importer: readExceptSet(c: PContext; n: PNode): IntSet](importer#readExceptSet%2CPContext%2CPNode)
[readMergeInfo:](#readMergeInfo)
* [ccgmerge: readMergeInfo(cfilename: AbsoluteFile; m: BModule)](ccgmerge#readMergeInfo%2CAbsoluteFile%2CBModule)
[readOnlySf:](#readOnlySf)
* [SymbolFilesOption.readOnlySf](options#readOnlySf)
[recomputeFieldPositions:](#recomputeFieldPositions)
* [semtypinst: recomputeFieldPositions(t: PType; obj: PNode; currPosition: var int)](semtypinst#recomputeFieldPositions%2CPType%2CPNode%2Cint)
[recordAdd:](#recordAdd)
* [macrocacheimpl: recordAdd(c: PCtx; info: TLineInfo; key: string; val: PNode)](macrocacheimpl#recordAdd%2CPCtx%2CTLineInfo%2Cstring%2CPNode)
[recordInc:](#recordInc)
* [macrocacheimpl: recordInc(c: PCtx; info: TLineInfo; key: string; by: BiggestInt)](macrocacheimpl#recordInc%2CPCtx%2CTLineInfo%2Cstring%2CBiggestInt)
[recordIncl:](#recordIncl)
* [macrocacheimpl: recordIncl(c: PCtx; info: TLineInfo; key: string; val: PNode)](macrocacheimpl#recordIncl%2CPCtx%2CTLineInfo%2Cstring%2CPNode)
[recordPut:](#recordPut)
* [macrocacheimpl: recordPut(c: PCtx; info: TLineInfo; key: string; k: string; val: PNode)](macrocacheimpl#recordPut%2CPCtx%2CTLineInfo%2Cstring%2Cstring%2CPNode)
[refresh:](#refresh)
* [vmdef: refresh(c: PCtx; module: PSym)](vmdef#refresh%2CPCtx%2CPSym)
[regA:](#regA)
* [vmdef: regA(x: TInstr): TRegister](vmdef#regA.t%2CTInstr)
[regAMask:](#regAMask)
* [vmdef: regAMask](vmdef#regAMask)
[regAShift:](#regAShift)
* [vmdef: regAShift](vmdef#regAShift)
[regB:](#regB)
* [vmdef: regB(x: TInstr): TRegister](vmdef#regB.t%2CTInstr)
[regBMask:](#regBMask)
* [vmdef: regBMask](vmdef#regBMask)
[regBShift:](#regBShift)
* [vmdef: regBShift](vmdef#regBShift)
[regBx:](#regBx)
* [vmdef: regBx(x: TInstr): int](vmdef#regBx.t%2CTInstr)
[regBxMask:](#regBxMask)
* [vmdef: regBxMask](vmdef#regBxMask)
[regBxMax:](#regBxMax)
* [vmdef: regBxMax](vmdef#regBxMax)
[regBxMin:](#regBxMin)
* [vmdef: regBxMin](vmdef#regBxMin)
[regBxShift:](#regBxShift)
* [vmdef: regBxShift](vmdef#regBxShift)
[regC:](#regC)
* [vmdef: regC(x: TInstr): TRegister](vmdef#regC.t%2CTInstr)
[regCMask:](#regCMask)
* [vmdef: regCMask](vmdef#regCMask)
[regCShift:](#regCShift)
* [vmdef: regCShift](vmdef#regCShift)
[registerAdditionalOps:](#registerAdditionalOps)
* [vm: registerAdditionalOps(c: PCtx)](vm#registerAdditionalOps%2CPCtx)
[registerCallback:](#registerCallback)
* [vmdef: registerCallback(c: PCtx; name: string; callback: VmCallback): int](vmdef#registerCallback%2CPCtx%2Cstring%2CVmCallback)
[registerCompilerProc:](#registerCompilerProc)
* [magicsys: registerCompilerProc(g: ModuleGraph; s: PSym)](magicsys#registerCompilerProc%2CModuleGraph%2CPSym)
[registerID:](#registerID)
* [idgen: registerID(id: PIdObj)](idgen#registerID%2CPIdObj)
[registerModule:](#registerModule)
* [rod: registerModule(g: ModuleGraph; module: PSym)](rod#registerModule.t%2CModuleGraph%2CPSym)
[registerNimScriptSymbol:](#registerNimScriptSymbol)
* [magicsys: registerNimScriptSymbol(g: ModuleGraph; s: PSym)](magicsys#registerNimScriptSymbol%2CModuleGraph%2CPSym)
[registerPass:](#registerPass)
* [passes: registerPass(g: ModuleGraph; p: TPass)](passes#registerPass%2CModuleGraph%2CTPass)
[registerSysType:](#registerSysType)
* [magicsys: registerSysType(g: ModuleGraph; t: PType)](magicsys#registerSysType%2CModuleGraph%2CPType)
[regOMask:](#regOMask)
* [vmdef: regOMask](vmdef#regOMask)
[regOShift:](#regOShift)
* [vmdef: regOShift](vmdef#regOShift)
[RelativeDir:](#RelativeDir)
* [pathutils: RelativeDir](pathutils#RelativeDir)
[RelativeFile:](#RelativeFile)
* [pathutils: RelativeFile](pathutils#RelativeFile)
[relativeJumps:](#relativeJumps)
* [vmdef: relativeJumps](vmdef#relativeJumps)
[relativeTo:](#relativeTo)
* [pathutils: relativeTo(fullPath: AbsoluteFile; baseFilename: AbsoluteDir; sep = DirSep): RelativeFile](pathutils#relativeTo%2CAbsoluteFile%2CAbsoluteDir)
[removeFile:](#removeFile)
* [pathutils: removeFile(x: AbsoluteFile)](pathutils#removeFile%2CAbsoluteFile)
[removeTrailingDirSep:](#removeTrailingDirSep)
* [options: removeTrailingDirSep(path: string): string](options#removeTrailingDirSep%2Cstring)
[renderDefinitionName:](#renderDefinitionName)
* [renderer: renderDefinitionName(s: PSym; noQuotes = false): string](renderer#renderDefinitionName%2CPSym)
[renderDocComments:](#renderDocComments)
* [TRenderFlag.renderDocComments](renderer#renderDocComments)
[renderIds:](#renderIds)
* [TRenderFlag.renderIds](renderer#renderIds)
[renderIr:](#renderIr)
* [TRenderFlag.renderIr](renderer#renderIr)
[renderModule:](#renderModule)
* [renderer: renderModule(n: PNode; infile, outfile: string; renderFlags: TRenderFlags = {}; fid = FileIndex(-1); conf: ConfigRef = nil)](renderer#renderModule%2CPNode%2Cstring%2Cstring%2CTRenderFlags%2CConfigRef)
[renderNimCode:](#renderNimCode)
* [renderverbatim: renderNimCode(result: var string; code: string; isLatex = false)](renderverbatim#renderNimCode%2Cstring%2Cstring)
[renderNoBody:](#renderNoBody)
* [TRenderFlag.renderNoBody](renderer#renderNoBody)
[renderNoComments:](#renderNoComments)
* [TRenderFlag.renderNoComments](renderer#renderNoComments)
[renderNone:](#renderNone)
* [TRenderFlag.renderNone](renderer#renderNone)
[renderNoPragmas:](#renderNoPragmas)
* [TRenderFlag.renderNoPragmas](renderer#renderNoPragmas)
[renderNoProcDefs:](#renderNoProcDefs)
* [TRenderFlag.renderNoProcDefs](renderer#renderNoProcDefs)
[renderParamTypes:](#renderParamTypes)
* [typesrenderer: renderParamTypes(n: PNode; sep = defaultParamSeparator): string](typesrenderer#renderParamTypes%2CPNode)
[renderPlainSymbolName:](#renderPlainSymbolName)
* [typesrenderer: renderPlainSymbolName(n: PNode): string](typesrenderer#renderPlainSymbolName%2CPNode)
[renderRunnableExamples:](#renderRunnableExamples)
* [TRenderFlag.renderRunnableExamples](renderer#renderRunnableExamples)
[renderSyms:](#renderSyms)
* [TRenderFlag.renderSyms](renderer#renderSyms)
[renderTree:](#renderTree)
* [renderer: renderTree(n: PNode; renderFlags: TRenderFlags = {}): string](renderer#renderTree%2CPNode%2CTRenderFlags)
[reorder:](#reorder)
* [reorder: reorder(graph: ModuleGraph; n: PNode; module: PSym): PNode](reorder#reorder%2CModuleGraph%2CPNode%2CPSym)
[replaceComment:](#replaceComment)
* [prettybase: replaceComment(conf: ConfigRef; info: TLineInfo)](nimfix/prettybase#replaceComment%2CConfigRef%2CTLineInfo)
[replaceDeprecated:](#replaceDeprecated)
* [prettybase: replaceDeprecated(conf: ConfigRef; info: TLineInfo; oldSym, newSym: PIdent)](nimfix/prettybase#replaceDeprecated%2CConfigRef%2CTLineInfo%2CPIdent%2CPIdent)
* [prettybase: replaceDeprecated(conf: ConfigRef; info: TLineInfo; oldSym, newSym: PSym)](nimfix/prettybase#replaceDeprecated%2CConfigRef%2CTLineInfo%2CPSym%2CPSym)
[replaceTypesInBody:](#replaceTypesInBody)
* [semtypinst: replaceTypesInBody(p: PContext; pt: TIdTable; n: PNode; owner: PSym; allowMetaTypes = false): PNode](semtypinst#replaceTypesInBody%2CPContext%2CTIdTable%2CPNode%2CPSym)
[replaceTypeVarsN:](#replaceTypeVarsN)
* [semtypinst: replaceTypeVarsN(cl: var TReplTypeVars; n: PNode; start = 0): PNode](semtypinst#replaceTypeVarsN%2CTReplTypeVars%2CPNode%2Cint)
[replaceTypeVarsT:](#replaceTypeVarsT)
* [semtypinst: replaceTypeVarsT(cl: var TReplTypeVars; t: PType): PType](semtypinst#replaceTypeVarsT%2CTReplTypeVars%2CPType)
[requiredParams:](#requiredParams)
* [ast: requiredParams(s: PSym): int](ast#requiredParams%2CPSym)
[requiresEffects:](#requiresEffects)
* [ast: requiresEffects](ast#requiresEffects)
[requiresInit:](#requiresInit)
* [typeallowed: requiresInit(t: PType): bool](typeallowed#requiresInit%2CPType)
[resetAllModules:](#resetAllModules)
* [modulegraphs: resetAllModules(g: ModuleGraph)](modulegraphs#resetAllModules%2CModuleGraph)
[resetCompilationLists:](#resetCompilationLists)
* [extccomp: resetCompilationLists(conf: ConfigRef)](extccomp#resetCompilationLists%2CConfigRef)
[resetIdentCache:](#resetIdentCache)
* [idents: resetIdentCache()](idents#resetIdentCache)
[resetIdTable:](#resetIdTable)
* [ast: resetIdTable(x: var TIdTable)](ast#resetIdTable%2CTIdTable)
[resetNimScriptSymbols:](#resetNimScriptSymbols)
* [magicsys: resetNimScriptSymbols(g: ModuleGraph)](magicsys#resetNimScriptSymbols%2CModuleGraph)
[resetRopeCache:](#resetRopeCache)
* [ropes: resetRopeCache()](ropes#resetRopeCache)
[resetSystemArtifacts:](#resetSystemArtifacts)
* [modules: resetSystemArtifacts(g: ModuleGraph)](modules#resetSystemArtifacts%2CModuleGraph)
[resetSysTypes:](#resetSysTypes)
* [magicsys: resetSysTypes(g: ModuleGraph)](magicsys#resetSysTypes%2CModuleGraph)
[resultPos:](#resultPos)
* [ast: resultPos](ast#resultPos)
[rkFloat:](#rkFloat)
* [TRegisterKind.rkFloat](vmdef#rkFloat)
[rkInt:](#rkInt)
* [TRegisterKind.rkInt](vmdef#rkInt)
[rkNode:](#rkNode)
* [TRegisterKind.rkNode](vmdef#rkNode)
[rkNodeAddr:](#rkNodeAddr)
* [TRegisterKind.rkNodeAddr](vmdef#rkNodeAddr)
[rkNone:](#rkNone)
* [TRegisterKind.rkNone](vmdef#rkNone)
[rkRegisterAddr:](#rkRegisterAddr)
* [TRegisterKind.rkRegisterAddr](vmdef#rkRegisterAddr)
[RodExt:](#RodExt)
* [options: RodExt](options#RodExt)
[RodFileVersion:](#RodFileVersion)
* [nversion: RodFileVersion](nversion#RodFileVersion)
[Rope:](#Rope)
* [ropes: Rope](ropes#Rope)
[rope:](#rope)
* [ropes: rope(f: BiggestFloat): Rope](ropes#rope%2CBiggestFloat)
* [ropes: rope(i: BiggestInt): Rope](ropes#rope%2CBiggestInt)
* [ropes: rope(s: string): Rope](ropes#rope%2Cstring)
[ropeConcat:](#ropeConcat)
* [ropes: ropeConcat(a: varargs[Rope]): Rope](ropes#ropeConcat%2Cvarargs%5BRope%5D)
[RopeObj:](#RopeObj)
* [ropes: RopeObj](ropes#RopeObj)
[routineDefs:](#routineDefs)
* [ast: routineDefs](ast#routineDefs)
[routineKinds:](#routineKinds)
* [ast: routineKinds](ast#routineKinds)
[runJsonBuildInstructions:](#runJsonBuildInstructions)
* [extccomp: runJsonBuildInstructions(conf: ConfigRef; projectfile: AbsoluteFile)](extccomp#runJsonBuildInstructions%2CConfigRef%2CAbsoluteFile)
[runNimScript:](#runNimScript)
* [scriptconfig: runNimScript(cache: IdentCache; scriptName: AbsoluteFile; freshDefines = true; conf: ConfigRef)](scriptconfig#runNimScript%2CIdentCache%2CAbsoluteFile%2CConfigRef)
[runtimeFormat:](#runtimeFormat)
* [ropes: runtimeFormat(frmt: FormatStr; args: openArray[Rope]): Rope](ropes#runtimeFormat%2CFormatStr%2CopenArray%5BRope%5D)
[s:](#s)
* [cgendata: s(p: BProc; s: TCProcSection): var Rope](cgendata#s%2CBProc%2CTCProcSection)
[safeArrLen:](#safeArrLen)
* [ast: safeArrLen(n: PNode): int](ast#safeArrLen%2CPNode)
[safeInheritanceDiff:](#safeInheritanceDiff)
* [types: safeInheritanceDiff(a, b: PType): int](types#safeInheritanceDiff%2CPType%2CPType)
[safeLen:](#safeLen)
* [ast: safeLen(n: PNode): int](ast#safeLen%2CPNode)
[safeSemExpr:](#safeSemExpr)
* [sigmatch: safeSemExpr(c: PContext; n: PNode): PNode](sigmatch#safeSemExpr%2CPContext%2CPNode)
[safeSkipTypes:](#safeSkipTypes)
* [types: safeSkipTypes(t: PType; kinds: TTypeKinds): PType](types#safeSkipTypes%2CPType%2CTTypeKinds)
[sameBackendType:](#sameBackendType)
* [types: sameBackendType(x, y: PType): bool](types#sameBackendType%2CPType%2CPType)
[sameConstant:](#sameConstant)
* [vmgen: sameConstant(a, b: PNode): bool](vmgen#sameConstant%2CPNode%2CPNode)
[sameDistinctTypes:](#sameDistinctTypes)
* [types: sameDistinctTypes(a, b: PType): bool](types#sameDistinctTypes%2CPType%2CPType)
[sameEnumTypes:](#sameEnumTypes)
* [types: sameEnumTypes(a, b: PType): bool](types#sameEnumTypes%2CPType%2CPType)
[sameFlags:](#sameFlags)
* [types: sameFlags(a, b: PType): bool](types#sameFlags%2CPType%2CPType)
[sameObjectTypes:](#sameObjectTypes)
* [types: sameObjectTypes(a, b: PType): bool](types#sameObjectTypes%2CPType%2CPType)
[sameSubexprs:](#sameSubexprs)
* [guards: sameSubexprs(m: TModel; a, b: PNode): bool](guards#sameSubexprs%2CTModel%2CPNode%2CPNode)
[sameTree:](#sameTree)
* [guards: sameTree(a, b: PNode): bool](guards#sameTree%2CPNode%2CPNode)
* [trees: sameTree(a, b: PNode): bool](trees#sameTree%2CPNode%2CPNode)
[sameTrees:](#sameTrees)
* [patterns: sameTrees(a, b: PNode): bool](patterns#sameTrees%2CPNode%2CPNode)
[sameType:](#sameType)
* [types: sameType(a, b: PType; flags: TTypeCmpFlags = {}): bool](types#sameType%2CPType%2CPType%2CTTypeCmpFlags)
[sameTypeOrNil:](#sameTypeOrNil)
* [types: sameTypeOrNil(a, b: PType; flags: TTypeCmpFlags = {}): bool](types#sameTypeOrNil%2CPType%2CPType%2CTTypeCmpFlags)
[sameValue:](#sameValue)
* [astalgo: sameValue(a, b: PNode): bool](astalgo#sameValue%2CPNode%2CPNode)
[saveMaxIds:](#saveMaxIds)
* [idgen: saveMaxIds(conf: ConfigRef; project: AbsoluteFile)](idgen#saveMaxIds%2CConfigRef%2CAbsoluteFile)
[scopeDepth:](#scopeDepth)
* [semdata: scopeDepth(c: PContext): int](semdata#scopeDepth%2CPContext)
[searchForProc:](#searchForProc)
* [procfind: searchForProc(c: PContext; scope: PScope; fn: PSym): tuple[proto: PSym, comesFromShadowScope: bool]](procfind#searchForProc%2CPContext%2CPScope%2CPSym)
[searchInScopes:](#searchInScopes)
* [lookups: searchInScopes(c: PContext; s: PIdent): PSym](lookups#searchInScopes%2CPContext%2CPIdent)
* [lookups: searchInScopes(c: PContext; s: PIdent; filter: TSymKinds): PSym](lookups#searchInScopes%2CPContext%2CPIdent%2CTSymKinds)
[searchInstTypes:](#searchInstTypes)
* [semtypinst: searchInstTypes(key: PType): PType](semtypinst#searchInstTypes%2CPType)
[searchTypeFor:](#searchTypeFor)
* [types: searchTypeFor(t: PType; predicate: TTypePredicate): bool](types#searchTypeFor%2CPType%2CTTypePredicate)
[semAsmOrEmit:](#semAsmOrEmit)
* [pragmas: semAsmOrEmit(con: PContext; n: PNode; marker: char): PNode](pragmas#semAsmOrEmit%2CPContext%2CPNode%2Cchar)
[semCaptureSym:](#semCaptureSym)
* [lambdalifting: semCaptureSym(s, owner: PSym)](lambdalifting#semCaptureSym%2CPSym%2CPSym)
[semFinishOperands:](#semFinishOperands)
* [sigmatch: semFinishOperands(c: PContext; n: PNode)](sigmatch#semFinishOperands%2CPContext%2CPNode)
[semLocals:](#semLocals)
* [locals: semLocals(c: PContext; n: PNode): PNode](plugins/locals#semLocals%2CPContext%2CPNode)
[semNodeKindConstraints:](#semNodeKindConstraints)
* [parampatterns: semNodeKindConstraints(n: PNode; conf: ConfigRef; start: Natural): PNode](parampatterns#semNodeKindConstraints%2CPNode%2CConfigRef%2CNatural)
[semPass:](#semPass)
* [sem: semPass](sem#semPass)
[seNoSideEffect:](#seNoSideEffect)
* [TSideEffectAnalysis.seNoSideEffect](parampatterns#seNoSideEffect)
[seSideEffect:](#seSideEffect)
* [TSideEffectAnalysis.seSideEffect](parampatterns#seSideEffect)
[setBaseFlags:](#setBaseFlags)
* [parser: setBaseFlags(n: PNode; base: NumericalBase)](parser#setBaseFlags%2CPNode%2CNumericalBase)
[setCaseContextIdx:](#setCaseContextIdx)
* [semdata: setCaseContextIdx(c: PContext; idx: int)](semdata#setCaseContextIdx%2CPContext%2Cint)
[setCC:](#setCC)
* [extccomp: setCC(conf: ConfigRef; ccname: string; info: TLineInfo)](extccomp#setCC%2CConfigRef%2Cstring%2CTLineInfo)
[setConfigVar:](#setConfigVar)
* [options: setConfigVar(conf: ConfigRef; key, val: string)](options#setConfigVar%2CConfigRef%2Cstring%2Cstring)
[setDefaultLibpath:](#setDefaultLibpath)
* [options: setDefaultLibpath(conf: ConfigRef)](options#setDefaultLibpath%2CConfigRef)
[setDirtyFile:](#setDirtyFile)
* [msgs: setDirtyFile(conf: ConfigRef; fileIdx: FileIndex; filename: AbsoluteFile)](msgs#setDirtyFile%2CConfigRef%2CFileIndex%2CAbsoluteFile)
[setEffectsForProcType:](#setEffectsForProcType)
* [sempass2: setEffectsForProcType(g: ModuleGraph; t: PType; n: PNode)](sempass2#setEffectsForProcType%2CModuleGraph%2CPType%2CPNode)
[setErrorMaxHighMaybe:](#setErrorMaxHighMaybe)
* [options: setErrorMaxHighMaybe(conf: ConfigRef)](options#setErrorMaxHighMaybe.t%2CConfigRef)
[setHash:](#setHash)
* [msgs: setHash(conf: ConfigRef; fileIdx: FileIndex; hash: string)](msgs#setHash%2CConfigRef%2CFileIndex%2Cstring)
[setHasRange:](#setHasRange)
* [nimsets: setHasRange(s: PNode): bool](nimsets#setHasRange%2CPNode)
[setId:](#setId)
* [idgen: setId(id: int)](idgen#setId%2Cint)
[setInfoContextLen:](#setInfoContextLen)
* [msgs: setInfoContextLen(conf: ConfigRef; L: int)](msgs#setInfoContextLen%2CConfigRef%2Cint)
[setIntLitType:](#setIntLitType)
* [magicsys: setIntLitType(g: ModuleGraph; result: PNode)](magicsys#setIntLitType%2CModuleGraph%2CPNode)
[setLen:](#setLen)
* [strutils2: setLen(result: var string; n: int; isInit: bool)](strutils2#setLen%2Cstring%2Cint%2Cbool)
[setNote:](#setNote)
* [options: setNote(conf: ConfigRef; note: TNoteKind; enabled = true)](options#setNote%2CConfigRef%2CTNoteKind)
[setNoteDefaults:](#setNoteDefaults)
* [options: setNoteDefaults(conf: ConfigRef; note: TNoteKind; enabled = true)](options#setNoteDefaults%2CConfigRef%2CTNoteKind)
[setOutFile:](#setOutFile)
* [options: setOutFile(conf: ConfigRef)](options#setOutFile%2CConfigRef)
[setResult:](#setResult)
* [vm: setResult(a: VmArgs; v: AbsoluteDir)](vm#setResult%2CVmArgs%2CAbsoluteDir)
* [vm: setResult(a: VmArgs; v: BiggestFloat)](vm#setResult%2CVmArgs%2CBiggestFloat)
* [vm: setResult(a: VmArgs; v: BiggestInt)](vm#setResult%2CVmArgs%2CBiggestInt)
* [vm: setResult(a: VmArgs; v: bool)](vm#setResult%2CVmArgs%2Cbool)
* [vm: setResult(a: VmArgs; n: PNode)](vm#setResult%2CVmArgs%2CPNode)
* [vm: setResult(a: VmArgs; v: seq[string])](vm#setResult%2CVmArgs%2Cseq%5Bstring%5D)
* [vm: setResult(a: VmArgs; v: string)](vm#setResult%2CVmArgs%2Cstring)
[setTarget:](#setTarget)
* [platform: setTarget(t: var Target; o: TSystemOS; c: TSystemCPU)](platform#setTarget%2CTarget%2CTSystemOS%2CTSystemCPU)
[setTargetFromSystem:](#setTargetFromSystem)
* [platform: setTargetFromSystem(t: var Target)](platform#setTargetFromSystem%2CTarget)
[setupCompileTimeVar:](#setupCompileTimeVar)
* [vm: setupCompileTimeVar(module: PSym; g: ModuleGraph; n: PNode)](vm#setupCompileTimeVar%2CPSym%2CModuleGraph%2CPNode)
[setupGlobalCtx:](#setupGlobalCtx)
* [vm: setupGlobalCtx(module: PSym; graph: ModuleGraph)](vm#setupGlobalCtx%2CPSym%2CModuleGraph)
[setupModuleCache:](#setupModuleCache)
* [rod: setupModuleCache(g: ModuleGraph)](rod#setupModuleCache.t%2CModuleGraph)
[setupParser:](#setupParser)
* [syntaxes: setupParser(p: var Parser; fileIdx: FileIndex; cache: IdentCache; config: ConfigRef): bool](syntaxes#setupParser%2CParser%2CFileIndex%2CIdentCache%2CConfigRef)
[setupVM:](#setupVM)
* [scriptconfig: setupVM(module: PSym; cache: IdentCache; scriptName: string; graph: ModuleGraph): PEvalContext](scriptconfig#setupVM%2CPSym%2CIdentCache%2Cstring%2CModuleGraph)
[seUnknown:](#seUnknown)
* [TSideEffectAnalysis.seUnknown](parampatterns#seUnknown)
[Severity:](#Severity)
* [lineinfos: Severity](lineinfos#Severity)
[sfAddrTaken:](#sfAddrTaken)
* [TSymFlag.sfAddrTaken](ast#sfAddrTaken)
[sfAllUntyped:](#sfAllUntyped)
* [ast: sfAllUntyped](ast#sfAllUntyped)
[sfAnon:](#sfAnon)
* [ast: sfAnon](ast#sfAnon)
[sfBase:](#sfBase)
* [ast: sfBase](ast#sfBase)
[sfBorrow:](#sfBorrow)
* [TSymFlag.sfBorrow](ast#sfBorrow)
[sfCallsite:](#sfCallsite)
* [TSymFlag.sfCallsite](ast#sfCallsite)
[sfCompilerProc:](#sfCompilerProc)
* [TSymFlag.sfCompilerProc](ast#sfCompilerProc)
[sfCompileTime:](#sfCompileTime)
* [TSymFlag.sfCompileTime](ast#sfCompileTime)
[sfCompileToCpp:](#sfCompileToCpp)
* [ast: sfCompileToCpp](ast#sfCompileToCpp)
[sfCompileToObjc:](#sfCompileToObjc)
* [ast: sfCompileToObjc](ast#sfCompileToObjc)
[sfConstructor:](#sfConstructor)
* [TSymFlag.sfConstructor](ast#sfConstructor)
[sfCursor:](#sfCursor)
* [TSymFlag.sfCursor](ast#sfCursor)
[sfCustomPragma:](#sfCustomPragma)
* [ast: sfCustomPragma](ast#sfCustomPragma)
[sfDeprecated:](#sfDeprecated)
* [TSymFlag.sfDeprecated](ast#sfDeprecated)
[sfDirty:](#sfDirty)
* [ast: sfDirty](ast#sfDirty)
[sfDiscardable:](#sfDiscardable)
* [TSymFlag.sfDiscardable](ast#sfDiscardable)
[sfDiscriminant:](#sfDiscriminant)
* [TSymFlag.sfDiscriminant](ast#sfDiscriminant)
[sfDispatcher:](#sfDispatcher)
* [TSymFlag.sfDispatcher](ast#sfDispatcher)
[sfError:](#sfError)
* [TSymFlag.sfError](ast#sfError)
[sfEscapes:](#sfEscapes)
* [ast: sfEscapes](ast#sfEscapes)
[sfExperimental:](#sfExperimental)
* [ast: sfExperimental](ast#sfExperimental)
[sfExplain:](#sfExplain)
* [TSymFlag.sfExplain](ast#sfExplain)
[sfExportc:](#sfExportc)
* [TSymFlag.sfExportc](ast#sfExportc)
[sfExported:](#sfExported)
* [TSymFlag.sfExported](ast#sfExported)
[sfForward:](#sfForward)
* [TSymFlag.sfForward](ast#sfForward)
[sfFromGeneric:](#sfFromGeneric)
* [TSymFlag.sfFromGeneric](ast#sfFromGeneric)
[sfGeneratedOp:](#sfGeneratedOp)
* [TSymFlag.sfGeneratedOp](ast#sfGeneratedOp)
[sfGenSym:](#sfGenSym)
* [TSymFlag.sfGenSym](ast#sfGenSym)
[sfGlobal:](#sfGlobal)
* [TSymFlag.sfGlobal](ast#sfGlobal)
[sfGoto:](#sfGoto)
* [ast: sfGoto](ast#sfGoto)
[sfImportc:](#sfImportc)
* [TSymFlag.sfImportc](ast#sfImportc)
[sfInfixCall:](#sfInfixCall)
* [TSymFlag.sfInfixCall](ast#sfInfixCall)
[sfInjectDestructors:](#sfInjectDestructors)
* [TSymFlag.sfInjectDestructors](ast#sfInjectDestructors)
[sfIsSelf:](#sfIsSelf)
* [ast: sfIsSelf](ast#sfIsSelf)
[sfMainModule:](#sfMainModule)
* [TSymFlag.sfMainModule](ast#sfMainModule)
[sfMangleCpp:](#sfMangleCpp)
* [TSymFlag.sfMangleCpp](ast#sfMangleCpp)
[sfNamedParamCall:](#sfNamedParamCall)
* [TSymFlag.sfNamedParamCall](ast#sfNamedParamCall)
[sfNeverRaises:](#sfNeverRaises)
* [TSymFlag.sfNeverRaises](ast#sfNeverRaises)
[sfNoalias:](#sfNoalias)
* [TSymFlag.sfNoalias](ast#sfNoalias)
[sfNoForward:](#sfNoForward)
* [ast: sfNoForward](ast#sfNoForward)
[sfNoInit:](#sfNoInit)
* [ast: sfNoInit](ast#sfNoInit)
[sfNonReloadable:](#sfNonReloadable)
* [TSymFlag.sfNonReloadable](ast#sfNonReloadable)
[sfNoReturn:](#sfNoReturn)
* [TSymFlag.sfNoReturn](ast#sfNoReturn)
[sfNoSideEffect:](#sfNoSideEffect)
* [TSymFlag.sfNoSideEffect](ast#sfNoSideEffect)
[sfOverriden:](#sfOverriden)
* [TSymFlag.sfOverriden](ast#sfOverriden)
[sfProcvar:](#sfProcvar)
* [TSymFlag.sfProcvar](ast#sfProcvar)
[sfPure:](#sfPure)
* [TSymFlag.sfPure](ast#sfPure)
[sfRegister:](#sfRegister)
* [TSymFlag.sfRegister](ast#sfRegister)
[sfReorder:](#sfReorder)
* [ast: sfReorder](ast#sfReorder)
[sfRequiresInit:](#sfRequiresInit)
* [TSymFlag.sfRequiresInit](ast#sfRequiresInit)
[sfShadowed:](#sfShadowed)
* [TSymFlag.sfShadowed](ast#sfShadowed)
[sfSideEffect:](#sfSideEffect)
* [TSymFlag.sfSideEffect](ast#sfSideEffect)
[sfSingleUsedTemp:](#sfSingleUsedTemp)
* [TSymFlag.sfSingleUsedTemp](ast#sfSingleUsedTemp)
[sfSystemModule:](#sfSystemModule)
* [TSymFlag.sfSystemModule](ast#sfSystemModule)
[sfTemplateParam:](#sfTemplateParam)
* [TSymFlag.sfTemplateParam](ast#sfTemplateParam)
[sfThread:](#sfThread)
* [TSymFlag.sfThread](ast#sfThread)
[sfUsed:](#sfUsed)
* [TSymFlag.sfUsed](ast#sfUsed)
[sfUsedInFinallyOrExcept:](#sfUsedInFinallyOrExcept)
* [TSymFlag.sfUsedInFinallyOrExcept](ast#sfUsedInFinallyOrExcept)
[sfVolatile:](#sfVolatile)
* [TSymFlag.sfVolatile](ast#sfVolatile)
[sfWasForwarded:](#sfWasForwarded)
* [TSymFlag.sfWasForwarded](ast#sfWasForwarded)
[sfWrittenTo:](#sfWrittenTo)
* [ast: sfWrittenTo](ast#sfWrittenTo)
[shallowCopy:](#shallowCopy)
* [ast: shallowCopy(src: PNode): PNode](ast#shallowCopy%2CPNode)
[SigHash:](#SigHash)
* [modulegraphs: SigHash](modulegraphs#SigHash)
[sigHash:](#sigHash)
* [sighashes: sigHash(s: PSym): SigHash](sighashes#sigHash%2CPSym)
[simpleSlice:](#simpleSlice)
* [guards: simpleSlice(a, b: PNode): BiggestInt](guards#simpleSlice%2CPNode%2CPNode)
[skAlias:](#skAlias)
* [TSymKind.skAlias](ast#skAlias)
[skConditional:](#skConditional)
* [TSymKind.skConditional](ast#skConditional)
[skConst:](#skConst)
* [TSymKind.skConst](ast#skConst)
[skConverter:](#skConverter)
* [TSymKind.skConverter](ast#skConverter)
[skDynLib:](#skDynLib)
* [TSymKind.skDynLib](ast#skDynLib)
[skEnumField:](#skEnumField)
* [TSymKind.skEnumField](ast#skEnumField)
[skError:](#skError)
* [ast: skError](ast#skError)
[skField:](#skField)
* [TSymKind.skField](ast#skField)
[skForVar:](#skForVar)
* [TSymKind.skForVar](ast#skForVar)
[skFunc:](#skFunc)
* [TSymKind.skFunc](ast#skFunc)
[skGenericParam:](#skGenericParam)
* [TSymKind.skGenericParam](ast#skGenericParam)
[skipAddr:](#skipAddr)
* [ast: skipAddr(n: PNode): PNode](ast#skipAddr%2CPNode)
[skipAlias:](#skipAlias)
* [lookups: skipAlias(s: PSym; n: PNode; conf: ConfigRef): PSym](lookups#skipAlias%2CPSym%2CPNode%2CConfigRef)
[skipCodegen:](#skipCodegen)
* [passes: skipCodegen(config: ConfigRef; n: PNode): bool](passes#skipCodegen%2CConfigRef%2CPNode)
[skipColon:](#skipColon)
* [ast: skipColon(n: PNode): PNode](ast#skipColon%2CPNode)
[skipComment:](#skipComment)
* [parser: skipComment(p: var Parser; node: PNode)](parser#skipComment%2CParser%2CPNode)
[skipConv:](#skipConv)
* [types: skipConv(n: PNode): PNode](types#skipConv%2CPNode)
[skipConvCastAndClosure:](#skipConvCastAndClosure)
* [astalgo: skipConvCastAndClosure(n: PNode): PNode](astalgo#skipConvCastAndClosure%2CPNode)
[skipConvDfa:](#skipConvDfa)
* [dfa: skipConvDfa(n: PNode): PNode](dfa#skipConvDfa%2CPNode)
[skipConvTakeType:](#skipConvTakeType)
* [types: skipConvTakeType(n: PNode): PNode](types#skipConvTakeType%2CPNode)
[skipGenericAlias:](#skipGenericAlias)
* [types: skipGenericAlias(t: PType): PType](types#skipGenericAlias%2CPType)
[skipGenericOwner:](#skipGenericOwner)
* [ast: skipGenericOwner(s: PSym): PSym](ast#skipGenericOwner%2CPSym)
[skipHidden:](#skipHidden)
* [types: skipHidden(n: PNode): PNode](types#skipHidden%2CPNode)
[skipHiddenSubConv:](#skipHiddenSubConv)
* [types: skipHiddenSubConv(n: PNode): PNode](types#skipHiddenSubConv%2CPNode)
[skipInd:](#skipInd)
* [parser: skipInd(p: var Parser)](parser#skipInd%2CParser)
[skipIntLit:](#skipIntLit)
* [magicsys: skipIntLit(t: PType): PType](magicsys#skipIntLit%2CPType)
[skipIntLiteralParams:](#skipIntLiteralParams)
* [semtypinst: skipIntLiteralParams(t: PType)](semtypinst#skipIntLiteralParams%2CPType)
[skipPtrs:](#skipPtrs)
* [types: skipPtrs](types#skipPtrs)
[skipStmtList:](#skipStmtList)
* [ast: skipStmtList(n: PNode): PNode](ast#skipStmtList%2CPNode)
[skipTypes:](#skipTypes)
* [ast: skipTypes(t: PType; kinds: TTypeKinds): PType](ast#skipTypes%2CPType%2CTTypeKinds)
* [ast: skipTypes(t: PType; kinds: TTypeKinds; maxIters: int): PType](ast#skipTypes%2CPType%2CTTypeKinds%2Cint)
[skipTypesOrNil:](#skipTypesOrNil)
* [ast: skipTypesOrNil(t: PType; kinds: TTypeKinds): PType](ast#skipTypesOrNil%2CPType%2CTTypeKinds)
[skIterator:](#skIterator)
* [TSymKind.skIterator](ast#skIterator)
[skLabel:](#skLabel)
* [TSymKind.skLabel](ast#skLabel)
[skLet:](#skLet)
* [TSymKind.skLet](ast#skLet)
[skLocalVars:](#skLocalVars)
* [ast: skLocalVars](ast#skLocalVars)
[skMacro:](#skMacro)
* [TSymKind.skMacro](ast#skMacro)
[skMethod:](#skMethod)
* [TSymKind.skMethod](ast#skMethod)
[skModule:](#skModule)
* [TSymKind.skModule](ast#skModule)
[skPackage:](#skPackage)
* [TSymKind.skPackage](ast#skPackage)
[skParam:](#skParam)
* [TSymKind.skParam](ast#skParam)
[skProc:](#skProc)
* [TSymKind.skProc](ast#skProc)
[skProcKinds:](#skProcKinds)
* [ast: skProcKinds](ast#skProcKinds)
[skResult:](#skResult)
* [TSymKind.skResult](ast#skResult)
[skStub:](#skStub)
* [TSymKind.skStub](ast#skStub)
[skTemp:](#skTemp)
* [TSymKind.skTemp](ast#skTemp)
[skTemplate:](#skTemplate)
* [TSymKind.skTemplate](ast#skTemplate)
[skType:](#skType)
* [TSymKind.skType](ast#skType)
[skUnknown:](#skUnknown)
* [TSymKind.skUnknown](ast#skUnknown)
[skVar:](#skVar)
* [TSymKind.skVar](ast#skVar)
[slotEmpty:](#slotEmpty)
* [TSlotKind.slotEmpty](vmdef#slotEmpty)
[slotFixedLet:](#slotFixedLet)
* [TSlotKind.slotFixedLet](vmdef#slotFixedLet)
[slotFixedVar:](#slotFixedVar)
* [TSlotKind.slotFixedVar](vmdef#slotFixedVar)
[slotSomeTemp:](#slotSomeTemp)
* [vmdef: slotSomeTemp](vmdef#slotSomeTemp)
[slotTempComplex:](#slotTempComplex)
* [TSlotKind.slotTempComplex](vmdef#slotTempComplex)
[slotTempFloat:](#slotTempFloat)
* [TSlotKind.slotTempFloat](vmdef#slotTempFloat)
[slotTempInt:](#slotTempInt)
* [TSlotKind.slotTempInt](vmdef#slotTempInt)
[slotTempPerm:](#slotTempPerm)
* [TSlotKind.slotTempPerm](vmdef#slotTempPerm)
[slotTempStr:](#slotTempStr)
* [TSlotKind.slotTempStr](vmdef#slotTempStr)
[slotTempUnknown:](#slotTempUnknown)
* [TSlotKind.slotTempUnknown](vmdef#slotTempUnknown)
[someInSet:](#someInSet)
* [nimsets: someInSet(s: PNode; a, b: PNode): bool](nimsets#someInSet%2CPNode%2CPNode%2CPNode)
[sourceLine:](#sourceLine)
* [msgs: sourceLine(conf: ConfigRef; i: TLineInfo): string](msgs#sourceLine%2CConfigRef%2CTLineInfo)
[SourceMap:](#SourceMap)
* [sourcemap: SourceMap](sourcemap#SourceMap)
[SourceNode:](#SourceNode)
* [sourcemap: SourceNode](sourcemap#SourceNode)
[spawnResult:](#spawnResult)
* [spawn: spawnResult(t: PType; inParallel: bool): TSpawnResult](spawn#spawnResult%2CPType%2Cbool)
[splitFile:](#splitFile)
* [pathutils: splitFile(x: AbsoluteFile): tuple[dir: AbsoluteDir, name, ext: string]](pathutils#splitFile%2CAbsoluteFile)
[srByVar:](#srByVar)
* [TSpawnResult.srByVar](spawn#srByVar)
[srFlowVar:](#srFlowVar)
* [TSpawnResult.srFlowVar](spawn#srFlowVar)
[srVoid:](#srVoid)
* [TSpawnResult.srVoid](spawn#srVoid)
[StartSize:](#StartSize)
* [ast: StartSize](ast#StartSize)
[stmtPragmas:](#stmtPragmas)
* [pragmas: stmtPragmas](pragmas#stmtPragmas)
[stmtsContainPragma:](#stmtsContainPragma)
* [ccgutils: stmtsContainPragma(n: PNode; w: TSpecialWord): bool](ccgutils#stmtsContainPragma%2CPNode%2CTSpecialWord)
[stopCompile:](#stopCompile)
* [modulegraphs: stopCompile(g: ModuleGraph): bool](modulegraphs#stopCompile%2CModuleGraph)
[storeAny:](#storeAny)
* [vmmarshal: storeAny(s: var string; t: PType; a: PNode; conf: ConfigRef)](vmmarshal#storeAny%2Cstring%2CPType%2CPNode%2CConfigRef)
[storeNode:](#storeNode)
* [rod: storeNode(g: ModuleGraph; module: PSym; n: PNode)](rod#storeNode.t%2CModuleGraph%2CPSym%2CPNode)
[storeRemaining:](#storeRemaining)
* [rod: storeRemaining(g: ModuleGraph; module: PSym)](rod#storeRemaining.t%2CModuleGraph%2CPSym)
[strArg:](#strArg)
* [filters: strArg(conf: ConfigRef; n: PNode; name: string; pos: int; default: string): string](filters#strArg%2CConfigRef%2CPNode%2Cstring%2Cint%2Cstring)
[strictFuncs:](#strictFuncs)
* [Feature.strictFuncs](options#strictFuncs)
[strTableAdd:](#strTableAdd)
* [astalgo: strTableAdd(t: var TStrTable; n: PSym)](astalgo#strTableAdd%2CTStrTable%2CPSym)
[strTableContains:](#strTableContains)
* [astalgo: strTableContains(t: TStrTable; n: PSym): bool](astalgo#strTableContains%2CTStrTable%2CPSym)
[strTableGet:](#strTableGet)
* [astalgo: strTableGet(t: TStrTable; name: PIdent): PSym](astalgo#strTableGet%2CTStrTable%2CPIdent)
[strTableIncl:](#strTableIncl)
* [astalgo: strTableIncl(t: var TStrTable; n: PSym; onConflictKeepOld = false): bool](astalgo#strTableIncl%2CTStrTable%2CPSym)
[strTableInclReportConflict:](#strTableInclReportConflict)
* [astalgo: strTableInclReportConflict(t: var TStrTable; n: PSym; onConflictKeepOld = false): PSym](astalgo#strTableInclReportConflict%2CTStrTable%2CPSym)
[StructuralEquivTypes:](#StructuralEquivTypes)
* [ast: StructuralEquivTypes](ast#StructuralEquivTypes)
[stupidStmtListExpr:](#stupidStmtListExpr)
* [trees: stupidStmtListExpr(n: PNode): bool](trees#stupidStmtListExpr%2CPNode)
[styleCheckDef:](#styleCheckDef)
* [linter: styleCheckDef(conf: ConfigRef; s: PSym)](linter#styleCheckDef.t%2CConfigRef%2CPSym)
* [linter: styleCheckDef(conf: ConfigRef; info: TLineInfo; s: PSym)](linter#styleCheckDef.t%2CConfigRef%2CTLineInfo%2CPSym)
* [linter: styleCheckDef(conf: ConfigRef; info: TLineInfo; s: PSym; k: TSymKind)](linter#styleCheckDef.t%2CConfigRef%2CTLineInfo%2CPSym%2CTSymKind)
[styleCheckUse:](#styleCheckUse)
* [linter: styleCheckUse(conf: ConfigRef; info: TLineInfo; s: PSym)](linter#styleCheckUse%2CConfigRef%2CTLineInfo%2CPSym)
[styledMsgWriteln:](#styledMsgWriteln)
* [msgs: styledMsgWriteln(args: varargs[typed])](msgs#styledMsgWriteln.t%2Cvarargs%5Btyped%5D)
[Suggest:](#Suggest)
* [options: Suggest](options#Suggest)
[suggestDecl:](#suggestDecl)
* [sigmatch: suggestDecl(c: PContext; n: PNode; s: PSym)](sigmatch#suggestDecl%2CPContext%2CPNode%2CPSym)
[suggestEnum:](#suggestEnum)
* [sigmatch: suggestEnum(c: PContext; n: PNode; t: PType)](sigmatch#suggestEnum%2CPContext%2CPNode%2CPType)
[suggestExpr:](#suggestExpr)
* [sigmatch: suggestExpr(c: PContext; n: PNode)](sigmatch#suggestExpr%2CPContext%2CPNode)
[suggestExprNoCheck:](#suggestExprNoCheck)
* [sigmatch: suggestExprNoCheck(c: PContext; n: PNode)](sigmatch#suggestExprNoCheck%2CPContext%2CPNode)
[Suggestions:](#Suggestions)
* [options: Suggestions](options#Suggestions)
[suggestQuit:](#suggestQuit)
* [msgs: suggestQuit()](msgs#suggestQuit)
[suggestSentinel:](#suggestSentinel)
* [sigmatch: suggestSentinel(c: PContext)](sigmatch#suggestSentinel%2CPContext)
[suggestStmt:](#suggestStmt)
* [sigmatch: suggestStmt(c: PContext; n: PNode)](sigmatch#suggestStmt%2CPContext%2CPNode)
[suggestSym:](#suggestSym)
* [sigmatch: suggestSym(conf: ConfigRef; info: TLineInfo; s: PSym; usageSym: var PSym; isDecl = true)](sigmatch#suggestSym%2CConfigRef%2CTLineInfo%2CPSym%2CPSym)
[suggestWriteln:](#suggestWriteln)
* [msgs: suggestWriteln(conf: ConfigRef; s: string)](msgs#suggestWriteln%2CConfigRef%2Cstring)
[symBodyDigest:](#symBodyDigest)
* [sighashes: symBodyDigest(graph: ModuleGraph; sym: PSym): SigHash](sighashes#symBodyDigest%2CModuleGraph%2CPSym)
[SymbolFilesOption:](#SymbolFilesOption)
* [options: SymbolFilesOption](options#SymbolFilesOption)
[SymChars:](#SymChars)
* [lexer: SymChars](lexer#SymChars)
[symdiffSets:](#symdiffSets)
* [nimsets: symdiffSets(conf: ConfigRef; a, b: PNode): PNode](nimsets#symdiffSets%2CConfigRef%2CPNode%2CPNode)
[SymStartChars:](#SymStartChars)
* [lexer: SymStartChars](lexer#SymStartChars)
[symTabReplace:](#symTabReplace)
* [astalgo: symTabReplace(t: var TStrTable; prevSym: PSym; newSym: PSym)](astalgo#symTabReplace%2CTStrTable%2CPSym%2CPSym)
[symToYaml:](#symToYaml)
* [astalgo: symToYaml(conf: ConfigRef; n: PSym; indent: int = 0; maxRecDepth: int = -1): Rope](astalgo#symToYaml%2CConfigRef%2CPSym%2Cint%2Cint)
[sysTypeFromName:](#sysTypeFromName)
* [magicsys: sysTypeFromName(g: ModuleGraph; info: TLineInfo; name: string): PType](magicsys#sysTypeFromName%2CModuleGraph%2CTLineInfo%2Cstring)
[szIllegalRecursion:](#szIllegalRecursion)
* [types: szIllegalRecursion](types#szIllegalRecursion)
[szTooBigSize:](#szTooBigSize)
* [types: szTooBigSize](types#szTooBigSize)
[szUncomputedSize:](#szUncomputedSize)
* [types: szUncomputedSize](types#szUncomputedSize)
[szUnknownSize:](#szUnknownSize)
* [types: szUnknownSize](types#szUnknownSize)
[Tabulator:](#Tabulator)
* [nimlexbase: Tabulator](nimlexbase#Tabulator)
[taConcept:](#taConcept)
* [TTypeAllowedFlag.taConcept](typeallowed#taConcept)
[taField:](#taField)
* [TTypeAllowedFlag.taField](typeallowed#taField)
[tagEffects:](#tagEffects)
* [ast: tagEffects](ast#tagEffects)
[TagsExt:](#TagsExt)
* [options: TagsExt](options#TagsExt)
[taHeap:](#taHeap)
* [TTypeAllowedFlag.taHeap](typeallowed#taHeap)
[taIsOpenArray:](#taIsOpenArray)
* [TTypeAllowedFlag.taIsOpenArray](typeallowed#taIsOpenArray)
[taIsTemplateOrMacro:](#taIsTemplateOrMacro)
* [TTypeAllowedFlag.taIsTemplateOrMacro](typeallowed#taIsTemplateOrMacro)
[takeType:](#takeType)
* [types: takeType(formal, arg: PType): PType](types#takeType%2CPType%2CPType)
[TAliasRequest:](#TAliasRequest)
* [parampatterns: TAliasRequest](parampatterns#TAliasRequest)
[TAnalysisResult:](#TAnalysisResult)
* [aliases: TAnalysisResult](aliases#TAnalysisResult)
[taNoUntyped:](#taNoUntyped)
* [TTypeAllowedFlag.taNoUntyped](typeallowed#taNoUntyped)
[taProcContextIsNotMacro:](#taProcContextIsNotMacro)
* [TTypeAllowedFlag.taProcContextIsNotMacro](typeallowed#taProcContextIsNotMacro)
[Target:](#Target)
* [platform: Target](platform#Target)
[TAssignableResult:](#TAssignableResult)
* [parampatterns: TAssignableResult](parampatterns#TAssignableResult)
[TBackend:](#TBackend)
* [options: TBackend](options#TBackend)
[TBaseLexer:](#TBaseLexer)
* [nimlexbase: TBaseLexer](nimlexbase#TBaseLexer)
[TBitSet:](#TBitSet)
* [bitsets: TBitSet](bitsets#TBitSet)
[TBlock:](#TBlock)
* [cgendata: TBlock](cgendata#TBlock)
* [vmdef: TBlock](vmdef#TBlock)
[TCallingConvention:](#TCallingConvention)
* [ast: TCallingConvention](ast#TCallingConvention)
[TCandidate:](#TCandidate)
* [sigmatch: TCandidate](sigmatch#TCandidate)
[TCandidateState:](#TCandidateState)
* [sigmatch: TCandidateState](sigmatch#TCandidateState)
[TCFileSection:](#TCFileSection)
* [cgendata: TCFileSection](cgendata#TCFileSection)
[TCFileSections:](#TCFileSections)
* [cgendata: TCFileSections](cgendata#TCFileSections)
[TCheckPointResult:](#TCheckPointResult)
* [sigmatch: TCheckPointResult](sigmatch#TCheckPointResult)
[TCmdLinePass:](#TCmdLinePass)
* [commands: TCmdLinePass](commands#TCmdLinePass)
[TCommands:](#TCommands)
* [options: TCommands](options#TCommands)
[TContext:](#TContext)
* [semdata: TContext](semdata#TContext)
[TCProcFlag:](#TCProcFlag)
* [cgendata: TCProcFlag](cgendata#TCProcFlag)
[TCProcSection:](#TCProcSection)
* [cgendata: TCProcSection](cgendata#TCProcSection)
[TCProcSections:](#TCProcSections)
* [cgendata: TCProcSections](cgendata#TCProcSections)
[TCtx:](#TCtx)
* [vmdef: TCtx](vmdef#TCtx)
[TCTypeKind:](#TCTypeKind)
* [cgendata: TCTypeKind](cgendata#TCTypeKind)
[TDest:](#TDest)
* [vmdef: TDest](vmdef#TDest)
[TDistinctCompare:](#TDistinctCompare)
* [types: TDistinctCompare](types#TDistinctCompare)
[templatePragmas:](#templatePragmas)
* [pragmas: templatePragmas](pragmas#templatePragmas)
[Ten:](#Ten)
* [int128: Ten](int128#Ten)
[TEndian:](#TEndian)
* [platform: TEndian](platform#TEndian)
[TErrorHandling:](#TErrorHandling)
* [msgs: TErrorHandling](msgs#TErrorHandling)
[TErrorOutput:](#TErrorOutput)
* [lineinfos: TErrorOutput](lineinfos#TErrorOutput)
[TErrorOutputs:](#TErrorOutputs)
* [lineinfos: TErrorOutputs](lineinfos#TErrorOutputs)
[testCompileOption:](#testCompileOption)
* [commands: testCompileOption(conf: ConfigRef; switch: string; info: TLineInfo): bool](commands#testCompileOption%2CConfigRef%2Cstring%2CTLineInfo)
[testCompileOptionArg:](#testCompileOptionArg)
* [commands: testCompileOptionArg(conf: ConfigRef; switch, arg: string; info: TLineInfo): bool](commands#testCompileOptionArg%2CConfigRef%2Cstring%2Cstring%2CTLineInfo)
[TEvalMode:](#TEvalMode)
* [vmdef: TEvalMode](vmdef#TEvalMode)
[TexExt:](#TexExt)
* [options: TexExt](options#TexExt)
[TExprFlag:](#TExprFlag)
* [semdata: TExprFlag](semdata#TExprFlag)
[TExprFlags:](#TExprFlags)
* [semdata: TExprFlags](semdata#TExprFlags)
[text:](#text)
* [sourcemap: text(sourceNode: SourceNode; depth: int): string](sourcemap#text%2CSourceNode%2Cint)
[tfAcyclic:](#tfAcyclic)
* [TTypeFlag.tfAcyclic](ast#tfAcyclic)
[tfBorrowDot:](#tfBorrowDot)
* [TTypeFlag.tfBorrowDot](ast#tfBorrowDot)
[tfByCopy:](#tfByCopy)
* [TTypeFlag.tfByCopy](ast#tfByCopy)
[tfByRef:](#tfByRef)
* [TTypeFlag.tfByRef](ast#tfByRef)
[tfCapturesEnv:](#tfCapturesEnv)
* [TTypeFlag.tfCapturesEnv](ast#tfCapturesEnv)
[tfCheckedForDestructor:](#tfCheckedForDestructor)
* [TTypeFlag.tfCheckedForDestructor](ast#tfCheckedForDestructor)
[tfCompleteStruct:](#tfCompleteStruct)
* [TTypeFlag.tfCompleteStruct](ast#tfCompleteStruct)
[tfConceptMatchedTypeSym:](#tfConceptMatchedTypeSym)
* [TTypeFlag.tfConceptMatchedTypeSym](ast#tfConceptMatchedTypeSym)
[tfContravariant:](#tfContravariant)
* [TTypeFlag.tfContravariant](ast#tfContravariant)
[tfCovariant:](#tfCovariant)
* [TTypeFlag.tfCovariant](ast#tfCovariant)
[tfEnumHasHoles:](#tfEnumHasHoles)
* [TTypeFlag.tfEnumHasHoles](ast#tfEnumHasHoles)
[tfExplicit:](#tfExplicit)
* [TTypeFlag.tfExplicit](ast#tfExplicit)
[tfExplicitCallConv:](#tfExplicitCallConv)
* [TTypeFlag.tfExplicitCallConv](ast#tfExplicitCallConv)
[tfFinal:](#tfFinal)
* [TTypeFlag.tfFinal](ast#tfFinal)
[tfFromGeneric:](#tfFromGeneric)
* [TTypeFlag.tfFromGeneric](ast#tfFromGeneric)
[tfGcSafe:](#tfGcSafe)
* [ast: tfGcSafe](ast#tfGcSafe)
[tfGenericTypeParam:](#tfGenericTypeParam)
* [TTypeFlag.tfGenericTypeParam](ast#tfGenericTypeParam)
[tfHasAsgn:](#tfHasAsgn)
* [TTypeFlag.tfHasAsgn](ast#tfHasAsgn)
[tfHasGCedMem:](#tfHasGCedMem)
* [TTypeFlag.tfHasGCedMem](ast#tfHasGCedMem)
[tfHasMeta:](#tfHasMeta)
* [TTypeFlag.tfHasMeta](ast#tfHasMeta)
[tfHasOwned:](#tfHasOwned)
* [TTypeFlag.tfHasOwned](ast#tfHasOwned)
[tfHasStatic:](#tfHasStatic)
* [TTypeFlag.tfHasStatic](ast#tfHasStatic)
[TFileInfo:](#TFileInfo)
* [lineinfos: TFileInfo](lineinfos#TFileInfo)
[tfImplicitTypeParam:](#tfImplicitTypeParam)
* [TTypeFlag.tfImplicitTypeParam](ast#tfImplicitTypeParam)
[tfIncompleteStruct:](#tfIncompleteStruct)
* [TTypeFlag.tfIncompleteStruct](ast#tfIncompleteStruct)
[tfInferrableStatic:](#tfInferrableStatic)
* [TTypeFlag.tfInferrableStatic](ast#tfInferrableStatic)
[tfInheritable:](#tfInheritable)
* [TTypeFlag.tfInheritable](ast#tfInheritable)
[tfIterator:](#tfIterator)
* [TTypeFlag.tfIterator](ast#tfIterator)
[tfNeedsFullInit:](#tfNeedsFullInit)
* [TTypeFlag.tfNeedsFullInit](ast#tfNeedsFullInit)
[tfNoSideEffect:](#tfNoSideEffect)
* [TTypeFlag.tfNoSideEffect](ast#tfNoSideEffect)
[tfNotNil:](#tfNotNil)
* [TTypeFlag.tfNotNil](ast#tfNotNil)
[tfObjHasKids:](#tfObjHasKids)
* [ast: tfObjHasKids](ast#tfObjHasKids)
[tfPacked:](#tfPacked)
* [TTypeFlag.tfPacked](ast#tfPacked)
[tfPartial:](#tfPartial)
* [TTypeFlag.tfPartial](ast#tfPartial)
[tfRefsAnonObj:](#tfRefsAnonObj)
* [TTypeFlag.tfRefsAnonObj](ast#tfRefsAnonObj)
[tfRequiresInit:](#tfRequiresInit)
* [TTypeFlag.tfRequiresInit](ast#tfRequiresInit)
[tfResolved:](#tfResolved)
* [TTypeFlag.tfResolved](ast#tfResolved)
[tfRetType:](#tfRetType)
* [TTypeFlag.tfRetType](ast#tfRetType)
[tfReturnsNew:](#tfReturnsNew)
* [ast: tfReturnsNew](ast#tfReturnsNew)
[tfShallow:](#tfShallow)
* [TTypeFlag.tfShallow](ast#tfShallow)
[tfThread:](#tfThread)
* [TTypeFlag.tfThread](ast#tfThread)
[tfTriggersCompileTime:](#tfTriggersCompileTime)
* [TTypeFlag.tfTriggersCompileTime](ast#tfTriggersCompileTime)
[TFullReg:](#TFullReg)
* [vmdef: TFullReg](vmdef#TFullReg)
[tfUnion:](#tfUnion)
* [ast: tfUnion](ast#tfUnion)
[tfUnresolved:](#tfUnresolved)
* [TTypeFlag.tfUnresolved](ast#tfUnresolved)
[tfVarargs:](#tfVarargs)
* [TTypeFlag.tfVarargs](ast#tfVarargs)
[tfVarIsPtr:](#tfVarIsPtr)
* [TTypeFlag.tfVarIsPtr](ast#tfVarIsPtr)
[tfWeakCovariant:](#tfWeakCovariant)
* [TTypeFlag.tfWeakCovariant](ast#tfWeakCovariant)
[tfWildcard:](#tfWildcard)
* [TTypeFlag.tfWildcard](ast#tfWildcard)
[TGCMode:](#TGCMode)
* [options: TGCMode](options#TGCMode)
[TGlobalOption:](#TGlobalOption)
* [options: TGlobalOption](options#TGlobalOption)
[TGlobalOptions:](#TGlobalOptions)
* [options: TGlobalOptions](options#TGlobalOptions)
[theindexFname:](#theindexFname)
* [nimpaths: theindexFname](nimpaths#theindexFname)
[threadVarAccessed:](#threadVarAccessed)
* [TCProcFlag.threadVarAccessed](cgendata#threadVarAccessed)
[TIdent:](#TIdent)
* [idents: TIdent](idents#TIdent)
[TIdentIter:](#TIdentIter)
* [astalgo: TIdentIter](astalgo#TIdentIter)
[TIdNodePair:](#TIdNodePair)
* [ast: TIdNodePair](ast#TIdNodePair)
[TIdNodePairSeq:](#TIdNodePairSeq)
* [ast: TIdNodePairSeq](ast#TIdNodePairSeq)
[TIdNodeTable:](#TIdNodeTable)
* [ast: TIdNodeTable](ast#TIdNodeTable)
[TIdObj:](#TIdObj)
* [idents: TIdObj](idents#TIdObj)
[TIdPair:](#TIdPair)
* [ast: TIdPair](ast#TIdPair)
[TIdPairSeq:](#TIdPairSeq)
* [ast: TIdPairSeq](ast#TIdPairSeq)
[TIdTable:](#TIdTable)
* [ast: TIdTable](ast#TIdTable)
[TIIPair:](#TIIPair)
* [astalgo: TIIPair](astalgo#TIIPair)
[TIIPairSeq:](#TIIPairSeq)
* [astalgo: TIIPairSeq](astalgo#TIIPairSeq)
[TIITable:](#TIITable)
* [astalgo: TIITable](astalgo#TIITable)
[TImplication:](#TImplication)
* [ast: TImplication](ast#TImplication)
[TInfoCC:](#TInfoCC)
* [extccomp: TInfoCC](extccomp#TInfoCC)
[TInfoCCProp:](#TInfoCCProp)
* [extccomp: TInfoCCProp](extccomp#TInfoCCProp)
[TInfoCCProps:](#TInfoCCProps)
* [extccomp: TInfoCCProps](extccomp#TInfoCCProps)
[TInfoCPU:](#TInfoCPU)
* [platform: TInfoCPU](platform#TInfoCPU)
[TInfoOS:](#TInfoOS)
* [platform: TInfoOS](platform#TInfoOS)
[TInfoOSProp:](#TInfoOSProp)
* [platform: TInfoOSProp](platform#TInfoOSProp)
[TInfoOSProps:](#TInfoOSProps)
* [platform: TInfoOSProps](platform#TInfoOSProps)
[TInstantiation:](#TInstantiation)
* [ast: TInstantiation](ast#TInstantiation)
[TInstantiationPair:](#TInstantiationPair)
* [semdata: TInstantiationPair](semdata#TInstantiationPair)
[TInstr:](#TInstr)
* [vmdef: TInstr](vmdef#TInstr)
[TInstrType:](#TInstrType)
* [vmdef: TInstrType](vmdef#TInstrType)
[tkAccent:](#tkAccent)
* [TokType.tkAccent](lexer#tkAccent)
[tkAddr:](#tkAddr)
* [TokType.tkAddr](lexer#tkAddr)
[tkAnd:](#tkAnd)
* [TokType.tkAnd](lexer#tkAnd)
[tkAs:](#tkAs)
* [TokType.tkAs](lexer#tkAs)
[tkAsm:](#tkAsm)
* [TokType.tkAsm](lexer#tkAsm)
[tkBind:](#tkBind)
* [TokType.tkBind](lexer#tkBind)
[tkBlock:](#tkBlock)
* [TokType.tkBlock](lexer#tkBlock)
[tkBracketDotLe:](#tkBracketDotLe)
* [TokType.tkBracketDotLe](lexer#tkBracketDotLe)
[tkBracketDotRi:](#tkBracketDotRi)
* [TokType.tkBracketDotRi](lexer#tkBracketDotRi)
[tkBracketLe:](#tkBracketLe)
* [TokType.tkBracketLe](lexer#tkBracketLe)
[tkBracketLeColon:](#tkBracketLeColon)
* [TokType.tkBracketLeColon](lexer#tkBracketLeColon)
[tkBracketRi:](#tkBracketRi)
* [TokType.tkBracketRi](lexer#tkBracketRi)
[tkBreak:](#tkBreak)
* [TokType.tkBreak](lexer#tkBreak)
[tkCase:](#tkCase)
* [TokType.tkCase](lexer#tkCase)
[tkCast:](#tkCast)
* [TokType.tkCast](lexer#tkCast)
[tkCharLit:](#tkCharLit)
* [TokType.tkCharLit](lexer#tkCharLit)
[tkColon:](#tkColon)
* [TokType.tkColon](lexer#tkColon)
[tkColonColon:](#tkColonColon)
* [TokType.tkColonColon](lexer#tkColonColon)
[tkComma:](#tkComma)
* [TokType.tkComma](lexer#tkComma)
[tkComment:](#tkComment)
* [TokType.tkComment](lexer#tkComment)
[tkConcept:](#tkConcept)
* [TokType.tkConcept](lexer#tkConcept)
[tkConst:](#tkConst)
* [TokType.tkConst](lexer#tkConst)
[tkContinue:](#tkContinue)
* [TokType.tkContinue](lexer#tkContinue)
[tkConverter:](#tkConverter)
* [TokType.tkConverter](lexer#tkConverter)
[tkCurlyDotLe:](#tkCurlyDotLe)
* [TokType.tkCurlyDotLe](lexer#tkCurlyDotLe)
[tkCurlyDotRi:](#tkCurlyDotRi)
* [TokType.tkCurlyDotRi](lexer#tkCurlyDotRi)
[tkCurlyLe:](#tkCurlyLe)
* [TokType.tkCurlyLe](lexer#tkCurlyLe)
[tkCurlyRi:](#tkCurlyRi)
* [TokType.tkCurlyRi](lexer#tkCurlyRi)
[tkDefer:](#tkDefer)
* [TokType.tkDefer](lexer#tkDefer)
[tkDiscard:](#tkDiscard)
* [TokType.tkDiscard](lexer#tkDiscard)
[tkDistinct:](#tkDistinct)
* [TokType.tkDistinct](lexer#tkDistinct)
[tkDiv:](#tkDiv)
* [TokType.tkDiv](lexer#tkDiv)
[tkDo:](#tkDo)
* [TokType.tkDo](lexer#tkDo)
[tkDot:](#tkDot)
* [TokType.tkDot](lexer#tkDot)
[tkDotDot:](#tkDotDot)
* [TokType.tkDotDot](lexer#tkDotDot)
[tkElif:](#tkElif)
* [TokType.tkElif](lexer#tkElif)
[tkElse:](#tkElse)
* [TokType.tkElse](lexer#tkElse)
[tkEnd:](#tkEnd)
* [TokType.tkEnd](lexer#tkEnd)
[tkEnum:](#tkEnum)
* [TokType.tkEnum](lexer#tkEnum)
[tkEof:](#tkEof)
* [TokType.tkEof](lexer#tkEof)
[tkEquals:](#tkEquals)
* [TokType.tkEquals](lexer#tkEquals)
[tkExcept:](#tkExcept)
* [TokType.tkExcept](lexer#tkExcept)
[tkExport:](#tkExport)
* [TokType.tkExport](lexer#tkExport)
[tkFinally:](#tkFinally)
* [TokType.tkFinally](lexer#tkFinally)
[tkFloat128Lit:](#tkFloat128Lit)
* [TokType.tkFloat128Lit](lexer#tkFloat128Lit)
[tkFloat32Lit:](#tkFloat32Lit)
* [TokType.tkFloat32Lit](lexer#tkFloat32Lit)
[tkFloat64Lit:](#tkFloat64Lit)
* [TokType.tkFloat64Lit](lexer#tkFloat64Lit)
[tkFloatLit:](#tkFloatLit)
* [TokType.tkFloatLit](lexer#tkFloatLit)
[tkFor:](#tkFor)
* [TokType.tkFor](lexer#tkFor)
[tkFrom:](#tkFrom)
* [TokType.tkFrom](lexer#tkFrom)
[tkFunc:](#tkFunc)
* [TokType.tkFunc](lexer#tkFunc)
[tkGStrLit:](#tkGStrLit)
* [TokType.tkGStrLit](lexer#tkGStrLit)
[tkGTripleStrLit:](#tkGTripleStrLit)
* [TokType.tkGTripleStrLit](lexer#tkGTripleStrLit)
[tkIf:](#tkIf)
* [TokType.tkIf](lexer#tkIf)
[tkImport:](#tkImport)
* [TokType.tkImport](lexer#tkImport)
[tkIn:](#tkIn)
* [TokType.tkIn](lexer#tkIn)
[tkInclude:](#tkInclude)
* [TokType.tkInclude](lexer#tkInclude)
[tkInfixOpr:](#tkInfixOpr)
* [TokType.tkInfixOpr](lexer#tkInfixOpr)
[tkInt16Lit:](#tkInt16Lit)
* [TokType.tkInt16Lit](lexer#tkInt16Lit)
[tkInt32Lit:](#tkInt32Lit)
* [TokType.tkInt32Lit](lexer#tkInt32Lit)
[tkInt64Lit:](#tkInt64Lit)
* [TokType.tkInt64Lit](lexer#tkInt64Lit)
[tkInt8Lit:](#tkInt8Lit)
* [TokType.tkInt8Lit](lexer#tkInt8Lit)
[tkInterface:](#tkInterface)
* [TokType.tkInterface](lexer#tkInterface)
[tkIntLit:](#tkIntLit)
* [TokType.tkIntLit](lexer#tkIntLit)
[tkInvalid:](#tkInvalid)
* [TokType.tkInvalid](lexer#tkInvalid)
[tkIs:](#tkIs)
* [TokType.tkIs](lexer#tkIs)
[tkIsnot:](#tkIsnot)
* [TokType.tkIsnot](lexer#tkIsnot)
[tkIterator:](#tkIterator)
* [TokType.tkIterator](lexer#tkIterator)
[tkLet:](#tkLet)
* [TokType.tkLet](lexer#tkLet)
[tkMacro:](#tkMacro)
* [TokType.tkMacro](lexer#tkMacro)
[tkMethod:](#tkMethod)
* [TokType.tkMethod](lexer#tkMethod)
[tkMixin:](#tkMixin)
* [TokType.tkMixin](lexer#tkMixin)
[tkMod:](#tkMod)
* [TokType.tkMod](lexer#tkMod)
[tkNil:](#tkNil)
* [TokType.tkNil](lexer#tkNil)
[tkNot:](#tkNot)
* [TokType.tkNot](lexer#tkNot)
[tkNotin:](#tkNotin)
* [TokType.tkNotin](lexer#tkNotin)
[tkObject:](#tkObject)
* [TokType.tkObject](lexer#tkObject)
[tkOf:](#tkOf)
* [TokType.tkOf](lexer#tkOf)
[tkOpr:](#tkOpr)
* [TokType.tkOpr](lexer#tkOpr)
[tkOr:](#tkOr)
* [TokType.tkOr](lexer#tkOr)
[tkOut:](#tkOut)
* [TokType.tkOut](lexer#tkOut)
[tkParDotLe:](#tkParDotLe)
* [TokType.tkParDotLe](lexer#tkParDotLe)
[tkParDotRi:](#tkParDotRi)
* [TokType.tkParDotRi](lexer#tkParDotRi)
[tkParLe:](#tkParLe)
* [TokType.tkParLe](lexer#tkParLe)
[tkParRi:](#tkParRi)
* [TokType.tkParRi](lexer#tkParRi)
[tkPostfixOpr:](#tkPostfixOpr)
* [TokType.tkPostfixOpr](lexer#tkPostfixOpr)
[tkPrefixOpr:](#tkPrefixOpr)
* [TokType.tkPrefixOpr](lexer#tkPrefixOpr)
[tkProc:](#tkProc)
* [TokType.tkProc](lexer#tkProc)
[tkPtr:](#tkPtr)
* [TokType.tkPtr](lexer#tkPtr)
[tkRaise:](#tkRaise)
* [TokType.tkRaise](lexer#tkRaise)
[tkRef:](#tkRef)
* [TokType.tkRef](lexer#tkRef)
[tkReturn:](#tkReturn)
* [TokType.tkReturn](lexer#tkReturn)
[tkRStrLit:](#tkRStrLit)
* [TokType.tkRStrLit](lexer#tkRStrLit)
[tkSemiColon:](#tkSemiColon)
* [TokType.tkSemiColon](lexer#tkSemiColon)
[tkShl:](#tkShl)
* [TokType.tkShl](lexer#tkShl)
[tkShr:](#tkShr)
* [TokType.tkShr](lexer#tkShr)
[tkSpaces:](#tkSpaces)
* [TokType.tkSpaces](lexer#tkSpaces)
[tkStatic:](#tkStatic)
* [TokType.tkStatic](lexer#tkStatic)
[tkStrLit:](#tkStrLit)
* [TokType.tkStrLit](lexer#tkStrLit)
[tkSymbol:](#tkSymbol)
* [TokType.tkSymbol](lexer#tkSymbol)
[tkTemplate:](#tkTemplate)
* [TokType.tkTemplate](lexer#tkTemplate)
[tkTripleStrLit:](#tkTripleStrLit)
* [TokType.tkTripleStrLit](lexer#tkTripleStrLit)
[tkTry:](#tkTry)
* [TokType.tkTry](lexer#tkTry)
[tkTuple:](#tkTuple)
* [TokType.tkTuple](lexer#tkTuple)
[tkType:](#tkType)
* [TokType.tkType](lexer#tkType)
[tkUInt16Lit:](#tkUInt16Lit)
* [TokType.tkUInt16Lit](lexer#tkUInt16Lit)
[tkUInt32Lit:](#tkUInt32Lit)
* [TokType.tkUInt32Lit](lexer#tkUInt32Lit)
[tkUInt64Lit:](#tkUInt64Lit)
* [TokType.tkUInt64Lit](lexer#tkUInt64Lit)
[tkUInt8Lit:](#tkUInt8Lit)
* [TokType.tkUInt8Lit](lexer#tkUInt8Lit)
[tkUIntLit:](#tkUIntLit)
* [TokType.tkUIntLit](lexer#tkUIntLit)
[tkUsing:](#tkUsing)
* [TokType.tkUsing](lexer#tkUsing)
[tkVar:](#tkVar)
* [TokType.tkVar](lexer#tkVar)
[tkWhen:](#tkWhen)
* [TokType.tkWhen](lexer#tkWhen)
[tkWhile:](#tkWhile)
* [TokType.tkWhile](lexer#tkWhile)
[tkXor:](#tkXor)
* [TokType.tkXor](lexer#tkXor)
[tkYield:](#tkYield)
* [TokType.tkYield](lexer#tkYield)
[TLabel:](#TLabel)
* [cgendata: TLabel](cgendata#TLabel)
[TLib:](#TLib)
* [ast: TLib](ast#TLib)
[TLibKind:](#TLibKind)
* [ast: TLibKind](ast#TLibKind)
[TLineInfo:](#TLineInfo)
* [lineinfos: TLineInfo](lineinfos#TLineInfo)
[TLLRepl:](#TLLRepl)
* [llstream: TLLRepl](llstream#TLLRepl)
[TLLStream:](#TLLStream)
* [llstream: TLLStream](llstream#TLLStream)
[TLLStreamKind:](#TLLStreamKind)
* [llstream: TLLStreamKind](llstream#TLLStreamKind)
[TLoc:](#TLoc)
* [ast: TLoc](ast#TLoc)
[TLocFlag:](#TLocFlag)
* [ast: TLocFlag](ast#TLocFlag)
[TLocFlags:](#TLocFlags)
* [ast: TLocFlags](ast#TLocFlags)
[TLocKind:](#TLocKind)
* [ast: TLocKind](ast#TLocKind)
[TLockLevel:](#TLockLevel)
* [ast: TLockLevel](ast#TLockLevel)
[TLookupFlag:](#TLookupFlag)
* [lookups: TLookupFlag](lookups#TLookupFlag)
[TMagic:](#TMagic)
* [ast: TMagic](ast#TMagic)
[TMatchedConcept:](#TMatchedConcept)
* [semdata: TMatchedConcept](semdata#TMatchedConcept)
[TModel:](#TModel)
* [guards: TModel](guards#TModel)
[TMsgKind:](#TMsgKind)
* [lineinfos: TMsgKind](lineinfos#TMsgKind)
[TNode:](#TNode)
* [ast: TNode](ast#TNode)
[TNodeFlag:](#TNodeFlag)
* [ast: TNodeFlag](ast#TNodeFlag)
[TNodeFlags:](#TNodeFlags)
* [ast: TNodeFlags](ast#TNodeFlags)
[TNodeKind:](#TNodeKind)
* [ast: TNodeKind](ast#TNodeKind)
[TNodeKinds:](#TNodeKinds)
* [ast: TNodeKinds](ast#TNodeKinds)
[TNodePair:](#TNodePair)
* [ast: TNodePair](ast#TNodePair)
[TNodePairSeq:](#TNodePairSeq)
* [ast: TNodePairSeq](ast#TNodePairSeq)
[TNodeSeq:](#TNodeSeq)
* [ast: TNodeSeq](ast#TNodeSeq)
[TNodeTable:](#TNodeTable)
* [ast: TNodeTable](ast#TNodeTable)
[TNoteKind:](#TNoteKind)
* [lineinfos: TNoteKind](lineinfos#TNoteKind)
[TNoteKinds:](#TNoteKinds)
* [lineinfos: TNoteKinds](lineinfos#TNoteKinds)
[toAbsolute:](#toAbsolute)
* [pathutils: toAbsolute(file: string; base: AbsoluteDir): AbsoluteFile](pathutils#toAbsolute%2Cstring%2CAbsoluteDir)
[toAbsoluteDir:](#toAbsoluteDir)
* [pathutils: toAbsoluteDir(path: string): AbsoluteDir](pathutils#toAbsoluteDir%2Cstring)
[toBitSet:](#toBitSet)
* [nimsets: toBitSet(conf: ConfigRef; s: PNode): TBitSet](nimsets#toBitSet%2CConfigRef%2CPNode)
[TObjectSeq:](#TObjectSeq)
* [ast: TObjectSeq](ast#TObjectSeq)
[TObjectSet:](#TObjectSet)
* [ast: TObjectSet](ast#TObjectSet)
[toCChar:](#toCChar)
* [msgs: toCChar(c: char; result: var string)](msgs#toCChar%2Cchar%2Cstring)
[toColumn:](#toColumn)
* [msgs: toColumn(info: TLineInfo): int](msgs#toColumn%2CTLineInfo)
[toFileLineCol:](#toFileLineCol)
* [msgs: toFileLineCol(conf: ConfigRef; info: TLineInfo): string](msgs#toFileLineCol%2CConfigRef%2CTLineInfo)
[toFilename:](#toFilename)
* [msgs: toFilename(conf: ConfigRef; fileIdx: FileIndex): string](msgs#toFilename.t%2CConfigRef%2CFileIndex)
* [msgs: toFilename(conf: ConfigRef; info: TLineInfo): string](msgs#toFilename.t%2CConfigRef%2CTLineInfo)
[toFilenameOption:](#toFilenameOption)
* [msgs: toFilenameOption(conf: ConfigRef; fileIdx: FileIndex; opt: FilenameOption): string](msgs#toFilenameOption%2CConfigRef%2CFileIndex%2CFilenameOption)
[toFloat64:](#toFloat64)
* [int128: toFloat64(arg: Int128): float64](int128#toFloat64%2CInt128)
[toFullPath:](#toFullPath)
* [msgs: toFullPath(conf: ConfigRef; fileIdx: FileIndex): string](msgs#toFullPath%2CConfigRef%2CFileIndex)
* [msgs: toFullPath(conf: ConfigRef; info: TLineInfo): string](msgs#toFullPath.t%2CConfigRef%2CTLineInfo)
[toFullPathConsiderDirty:](#toFullPathConsiderDirty)
* [msgs: toFullPathConsiderDirty(conf: ConfigRef; fileIdx: FileIndex): AbsoluteFile](msgs#toFullPathConsiderDirty%2CConfigRef%2CFileIndex)
* [msgs: toFullPathConsiderDirty(conf: ConfigRef; info: TLineInfo): string](msgs#toFullPathConsiderDirty.t%2CConfigRef%2CTLineInfo)
[toGeneratedFile:](#toGeneratedFile)
* [options: toGeneratedFile(conf: ConfigRef; path: AbsoluteFile; ext: string): AbsoluteFile](options#toGeneratedFile%2CConfigRef%2CAbsoluteFile%2Cstring)
[toHex:](#toHex)
* [int128: toHex(arg: Int128): string](int128#toHex%2CInt128)
[toHumanStr:](#toHumanStr)
* [ast: toHumanStr(kind: TSymKind): string](ast#toHumanStr%2CTSymKind)
* [ast: toHumanStr(kind: TTypeKind): string](ast#toHumanStr%2CTTypeKind)
[toInt:](#toInt)
* [int128: toInt(arg: Int128): int](int128#toInt%2CInt128)
[toInt128:](#toInt128)
* [int128: toInt128(arg: float64): Int128](int128#toInt128%2Cfloat64)
* [int128: toInt128[T: SomeInteger | bool](arg: T): Int128](int128#toInt128%2CT)
[toInt16:](#toInt16)
* [int128: toInt16(arg: Int128): int16](int128#toInt16%2CInt128)
[toInt32:](#toInt32)
* [int128: toInt32(arg: Int128): int32](int128#toInt32%2CInt128)
[toInt64:](#toInt64)
* [int128: toInt64(arg: Int128): int64](int128#toInt64%2CInt128)
[toInt64Checked:](#toInt64Checked)
* [int128: toInt64Checked(arg: Int128; onError: int64): int64](int128#toInt64Checked%2CInt128%2Cint64)
[toInt8:](#toInt8)
* [int128: toInt8(arg: Int128): int8](int128#toInt8%2CInt128)
[Token:](#Token)
* [lexer: Token](lexer#Token)
[tokenize:](#tokenize)
* [sourcemap: tokenize(line: string): (bool, string)](sourcemap#tokenize.i%2Cstring)
[tokKeywordHigh:](#tokKeywordHigh)
* [lexer: tokKeywordHigh](lexer#tokKeywordHigh)
[tokKeywordLow:](#tokKeywordLow)
* [lexer: tokKeywordLow](lexer#tokKeywordLow)
[TokType:](#TokType)
* [lexer: TokType](lexer#TokType)
[TokTypes:](#TokTypes)
* [lexer: TokTypes](lexer#TokTypes)
[toLinenumber:](#toLinenumber)
* [msgs: toLinenumber(info: TLineInfo): int](msgs#toLinenumber%2CTLineInfo)
[toLit:](#toLit)
* [vmconv: toLit[T](a: T): PNode](vmconv#toLit%2CT)
[toLowerAscii:](#toLowerAscii)
* [strutils2: toLowerAscii(a: var string)](strutils2#toLowerAscii%2Cstring)
[toMsgFilename:](#toMsgFilename)
* [msgs: toMsgFilename(conf: ConfigRef; info: FileIndex): string](msgs#toMsgFilename%2CConfigRef%2CFileIndex)
* [msgs: toMsgFilename(conf: ConfigRef; info: TLineInfo): string](msgs#toMsgFilename.t%2CConfigRef%2CTLineInfo)
[toObject:](#toObject)
* [ast: toObject(typ: PType): PType](ast#toObject%2CPType)
[toObjFile:](#toObjFile)
* [extccomp: toObjFile(conf: ConfigRef; filename: AbsoluteFile): AbsoluteFile](extccomp#toObjFile%2CConfigRef%2CAbsoluteFile)
[TOpcode:](#TOpcode)
* [vmdef: TOpcode](vmdef#TOpcode)
[toProjPath:](#toProjPath)
* [msgs: toProjPath(conf: ConfigRef; fileIdx: FileIndex): string](msgs#toProjPath%2CConfigRef%2CFileIndex)
* [msgs: toProjPath(conf: ConfigRef; info: TLineInfo): string](msgs#toProjPath.t%2CConfigRef%2CTLineInfo)
[TOption:](#TOption)
* [options: TOption](options#TOption)
[TOptionEntry:](#TOptionEntry)
* [semdata: TOptionEntry](semdata#TOptionEntry)
[TOptions:](#TOptions)
* [options: TOptions](options#TOptions)
[toRef:](#toRef)
* [ast: toRef(typ: PType): PType](ast#toRef%2CPType)
[toSourceMap:](#toSourceMap)
* [sourcemap: toSourceMap(node: SourceNode; file: string): SourceMapGenerator](sourcemap#toSourceMap%2CSourceNode%2Cstring)
[toStrMaxPrecision:](#toStrMaxPrecision)
* [rodutils: toStrMaxPrecision(f: BiggestFloat; literalPostfix = ""): string](rodutils#toStrMaxPrecision%2CBiggestFloat%2Cstring)
[toTreeSet:](#toTreeSet)
* [nimsets: toTreeSet(conf: ConfigRef; s: TBitSet; settype: PType; info: TLineInfo): PNode](nimsets#toTreeSet%2CConfigRef%2CTBitSet%2CPType%2CTLineInfo)
[toUInt:](#toUInt)
* [int128: toUInt(arg: Int128): uint](int128#toUInt%2CInt128)
[toUInt16:](#toUInt16)
* [int128: toUInt16(arg: Int128): uint16](int128#toUInt16%2CInt128)
[toUInt32:](#toUInt32)
* [int128: toUInt32(arg: Int128): uint32](int128#toUInt32%2CInt128)
[toUInt64:](#toUInt64)
* [int128: toUInt64(arg: Int128): uint64](int128#toUInt64%2CInt128)
[toUInt8:](#toUInt8)
* [int128: toUInt8(arg: Int128): uint8](int128#toUInt8%2CInt128)
[toVar:](#toVar)
* [ast: toVar(typ: PType; kind: TTypeKind): PType](ast#toVar%2CPType%2CTTypeKind)
[TOverloadIter:](#TOverloadIter)
* [lookups: TOverloadIter](lookups#TOverloadIter)
[TOverloadIterMode:](#TOverloadIterMode)
* [lookups: TOverloadIterMode](lookups#TOverloadIterMode)
[TPair:](#TPair)
* [ast: TPair](ast#TPair)
[TPairSeq:](#TPairSeq)
* [ast: TPairSeq](ast#TPairSeq)
[TParamsEquality:](#TParamsEquality)
* [types: TParamsEquality](types#TParamsEquality)
[TPass:](#TPass)
* [modulegraphs: TPass](modulegraphs#TPass)
[TPassClose:](#TPassClose)
* [modulegraphs: TPassClose](modulegraphs#TPassClose)
[TPassContext:](#TPassContext)
* [modulegraphs: TPassContext](modulegraphs#TPassContext)
[TPassData:](#TPassData)
* [passes: TPassData](passes#TPassData)
[TPassOpen:](#TPassOpen)
* [modulegraphs: TPassOpen](modulegraphs#TPassOpen)
[TPassProcess:](#TPassProcess)
* [modulegraphs: TPassProcess](modulegraphs#TPassProcess)
[TPosition:](#TPosition)
* [vmdef: TPosition](vmdef#TPosition)
[TPreferedDesc:](#TPreferedDesc)
* [types: TPreferedDesc](types#TPreferedDesc)
[TProcCon:](#TProcCon)
* [semdata: TProcCon](semdata#TProcCon)
[trackPosInvalidFileIdx:](#trackPosInvalidFileIdx)
* [lineinfos: trackPosInvalidFileIdx](lineinfos#trackPosInvalidFileIdx)
[trackProc:](#trackProc)
* [sempass2: trackProc(c: PContext; s: PSym; body: PNode)](sempass2#trackProc%2CPContext%2CPSym%2CPNode)
[trackStmt:](#trackStmt)
* [sempass2: trackStmt(c: PContext; module: PSym; n: PNode; isTopLevel: bool)](sempass2#trackStmt%2CPContext%2CPSym%2CPNode%2Cbool)
[Transformation:](#Transformation)
* [pluginsupport: Transformation](pluginsupport#Transformation)
[transformBody:](#transformBody)
* [transf: transformBody(g: ModuleGraph; prc: PSym; cache: bool): PNode](transf#transformBody%2CModuleGraph%2CPSym%2Cbool)
[transformClosureIterator:](#transformClosureIterator)
* [closureiters: transformClosureIterator(g: ModuleGraph; fn: PSym; n: PNode): PNode](closureiters#transformClosureIterator%2CModuleGraph%2CPSym%2CPNode)
[transformExpr:](#transformExpr)
* [transf: transformExpr(g: ModuleGraph; module: PSym; n: PNode): PNode](transf#transformExpr%2CModuleGraph%2CPSym%2CPNode)
[transformStmt:](#transformStmt)
* [transf: transformStmt(g: ModuleGraph; module: PSym; n: PNode): PNode](transf#transformStmt%2CModuleGraph%2CPSym%2CPNode)
[transitionGenericParamToType:](#transitionGenericParamToType)
* [ast: transitionGenericParamToType(s: PSym)](ast#transitionGenericParamToType%2CPSym)
[transitionIntKind:](#transitionIntKind)
* [ast: transitionIntKind(n: PNode; kind: range[nkCharLit .. nkUInt64Lit])](ast#transitionIntKind%2CPNode%2Crange%5B%5D)
[transitionNoneToSym:](#transitionNoneToSym)
* [ast: transitionNoneToSym(n: PNode)](ast#transitionNoneToSym%2CPNode)
[transitionRoutineSymKind:](#transitionRoutineSymKind)
* [ast: transitionRoutineSymKind(s: PSym; kind: range[skProc .. skTemplate])](ast#transitionRoutineSymKind%2CPSym%2Crange%5B%5D)
[transitionSonsKind:](#transitionSonsKind)
* [ast: transitionSonsKind(n: PNode; kind: range[nkComesFrom .. nkTupleConstr])](ast#transitionSonsKind%2CPNode%2Crange%5B%5D)
[transitionSymKindCommon:](#transitionSymKindCommon)
* [ast: transitionSymKindCommon(k: TSymKind)](ast#transitionSymKindCommon.t%2CTSymKind)
[transitionToLet:](#transitionToLet)
* [ast: transitionToLet(s: PSym)](ast#transitionToLet%2CPSym)
[trBindGenericParam:](#trBindGenericParam)
* [TTypeRelFlag.trBindGenericParam](sigmatch#trBindGenericParam)
[trDontBind:](#trDontBind)
* [TTypeRelFlag.trDontBind](sigmatch#trDontBind)
[treeToYaml:](#treeToYaml)
* [astalgo: treeToYaml(conf: ConfigRef; n: PNode; indent: int = 0; maxRecDepth: int = -1): Rope](astalgo#treeToYaml%2CConfigRef%2CPNode%2Cint%2Cint)
[TRegister:](#TRegister)
* [vmdef: TRegister](vmdef#TRegister)
[TRegisterKind:](#TRegisterKind)
* [vmdef: TRegisterKind](vmdef#TRegisterKind)
[TRenderFlag:](#TRenderFlag)
* [renderer: TRenderFlag](renderer#TRenderFlag)
[TRenderFlags:](#TRenderFlags)
* [renderer: TRenderFlags](renderer#TRenderFlags)
[TRenderTok:](#TRenderTok)
* [renderer: TRenderTok](renderer#TRenderTok)
[TRenderTokSeq:](#TRenderTokSeq)
* [renderer: TRenderTokSeq](renderer#TRenderTokSeq)
[TReplTypeVars:](#TReplTypeVars)
* [semtypinst: TReplTypeVars](semtypinst#TReplTypeVars)
[trNoCovariance:](#trNoCovariance)
* [TTypeRelFlag.trNoCovariance](sigmatch#trNoCovariance)
[TSandboxFlag:](#TSandboxFlag)
* [vmdef: TSandboxFlag](vmdef#TSandboxFlag)
[TSandboxFlags:](#TSandboxFlags)
* [vmdef: TSandboxFlags](vmdef#TSandboxFlags)
[TScope:](#TScope)
* [ast: TScope](ast#TScope)
[TSideEffectAnalysis:](#TSideEffectAnalysis)
* [parampatterns: TSideEffectAnalysis](parampatterns#TSideEffectAnalysis)
[TSlotKind:](#TSlotKind)
* [vmdef: TSlotKind](vmdef#TSlotKind)
[TSpawnResult:](#TSpawnResult)
* [spawn: TSpawnResult](spawn#TSpawnResult)
[TSpecialWord:](#TSpecialWord)
* [wordrecg: TSpecialWord](wordrecg#TSpecialWord)
[TSpecialWords:](#TSpecialWords)
* [wordrecg: TSpecialWords](wordrecg#TSpecialWords)
[TSrcGen:](#TSrcGen)
* [renderer: TSrcGen](renderer#TSrcGen)
[TStackFrame:](#TStackFrame)
* [vmdef: TStackFrame](vmdef#TStackFrame)
[TStorageLoc:](#TStorageLoc)
* [ast: TStorageLoc](ast#TStorageLoc)
[TStringSeq:](#TStringSeq)
* [options: TStringSeq](options#TStringSeq)
[TStrTable:](#TStrTable)
* [ast: TStrTable](ast#TStrTable)
[TSym:](#TSym)
* [ast: TSym](ast#TSym)
[TSymFlag:](#TSymFlag)
* [ast: TSymFlag](ast#TSymFlag)
[TSymFlags:](#TSymFlags)
* [ast: TSymFlags](ast#TSymFlags)
[TSymKind:](#TSymKind)
* [ast: TSymKind](ast#TSymKind)
[TSymKinds:](#TSymKinds)
* [ast: TSymKinds](ast#TSymKinds)
[TSystemCC:](#TSystemCC)
* [options: TSystemCC](options#TSystemCC)
[TSystemCPU:](#TSystemCPU)
* [platform: TSystemCPU](platform#TSystemCPU)
[TSystemOS:](#TSystemOS)
* [platform: TSystemOS](platform#TSystemOS)
[TTabIter:](#TTabIter)
* [astalgo: TTabIter](astalgo#TTabIter)
[TType:](#TType)
* [ast: TType](ast#TType)
[TTypeAllowedFlag:](#TTypeAllowedFlag)
* [typeallowed: TTypeAllowedFlag](typeallowed#TTypeAllowedFlag)
[TTypeAllowedFlags:](#TTypeAllowedFlags)
* [typeallowed: TTypeAllowedFlags](typeallowed#TTypeAllowedFlags)
[TTypeAttachedOp:](#TTypeAttachedOp)
* [ast: TTypeAttachedOp](ast#TTypeAttachedOp)
[TTypeCmpFlag:](#TTypeCmpFlag)
* [types: TTypeCmpFlag](types#TTypeCmpFlag)
[TTypeCmpFlags:](#TTypeCmpFlags)
* [types: TTypeCmpFlags](types#TTypeCmpFlags)
[TTypeFieldResult:](#TTypeFieldResult)
* [types: TTypeFieldResult](types#TTypeFieldResult)
[TTypeFlag:](#TTypeFlag)
* [ast: TTypeFlag](ast#TTypeFlag)
[TTypeFlags:](#TTypeFlags)
* [ast: TTypeFlags](ast#TTypeFlags)
[TTypeIter:](#TTypeIter)
* [types: TTypeIter](types#TTypeIter)
[TTypeKind:](#TTypeKind)
* [ast: TTypeKind](ast#TTypeKind)
[TTypeKinds:](#TTypeKinds)
* [ast: TTypeKinds](ast#TTypeKinds)
[TTypeMutator:](#TTypeMutator)
* [types: TTypeMutator](types#TTypeMutator)
[TTypePredicate:](#TTypePredicate)
* [types: TTypePredicate](types#TTypePredicate)
[TTypeRelation:](#TTypeRelation)
* [sigmatch: TTypeRelation](sigmatch#TTypeRelation)
[TTypeRelFlag:](#TTypeRelFlag)
* [sigmatch: TTypeRelFlag](sigmatch#TTypeRelFlag)
[TTypeRelFlags:](#TTypeRelFlags)
* [sigmatch: TTypeRelFlags](sigmatch#TTypeRelFlags)
[TTypeSeq:](#TTypeSeq)
* [ast: TTypeSeq](ast#TTypeSeq)
* [cgendata: TTypeSeq](cgendata#TTypeSeq)
[tyAlias:](#tyAlias)
* [TTypeKind.tyAlias](ast#tyAlias)
[tyAnd:](#tyAnd)
* [TTypeKind.tyAnd](ast#tyAnd)
[tyAnything:](#tyAnything)
* [TTypeKind.tyAnything](ast#tyAnything)
[tyArray:](#tyArray)
* [TTypeKind.tyArray](ast#tyArray)
[tyBool:](#tyBool)
* [TTypeKind.tyBool](ast#tyBool)
[tyBuiltInTypeClass:](#tyBuiltInTypeClass)
* [TTypeKind.tyBuiltInTypeClass](ast#tyBuiltInTypeClass)
[tyChar:](#tyChar)
* [TTypeKind.tyChar](ast#tyChar)
[tyCompositeTypeClass:](#tyCompositeTypeClass)
* [TTypeKind.tyCompositeTypeClass](ast#tyCompositeTypeClass)
[tyCString:](#tyCString)
* [TTypeKind.tyCString](ast#tyCString)
[tyDistinct:](#tyDistinct)
* [TTypeKind.tyDistinct](ast#tyDistinct)
[tyEmpty:](#tyEmpty)
* [TTypeKind.tyEmpty](ast#tyEmpty)
[tyEnum:](#tyEnum)
* [TTypeKind.tyEnum](ast#tyEnum)
[tyError:](#tyError)
* [ast: tyError](ast#tyError)
[tyFloat:](#tyFloat)
* [TTypeKind.tyFloat](ast#tyFloat)
[tyFloat128:](#tyFloat128)
* [TTypeKind.tyFloat128](ast#tyFloat128)
[tyFloat32:](#tyFloat32)
* [TTypeKind.tyFloat32](ast#tyFloat32)
[tyFloat64:](#tyFloat64)
* [TTypeKind.tyFloat64](ast#tyFloat64)
[tyForward:](#tyForward)
* [TTypeKind.tyForward](ast#tyForward)
[tyFromExpr:](#tyFromExpr)
* [TTypeKind.tyFromExpr](ast#tyFromExpr)
[tyGenericBody:](#tyGenericBody)
* [TTypeKind.tyGenericBody](ast#tyGenericBody)
[tyGenericInst:](#tyGenericInst)
* [TTypeKind.tyGenericInst](ast#tyGenericInst)
[tyGenericInvocation:](#tyGenericInvocation)
* [TTypeKind.tyGenericInvocation](ast#tyGenericInvocation)
[tyGenericLike:](#tyGenericLike)
* [sem: tyGenericLike](sem#tyGenericLike)
[tyGenericParam:](#tyGenericParam)
* [TTypeKind.tyGenericParam](ast#tyGenericParam)
[tyInferred:](#tyInferred)
* [TTypeKind.tyInferred](ast#tyInferred)
[tyInt:](#tyInt)
* [TTypeKind.tyInt](ast#tyInt)
[tyInt16:](#tyInt16)
* [TTypeKind.tyInt16](ast#tyInt16)
[tyInt32:](#tyInt32)
* [TTypeKind.tyInt32](ast#tyInt32)
[tyInt64:](#tyInt64)
* [TTypeKind.tyInt64](ast#tyInt64)
[tyInt8:](#tyInt8)
* [TTypeKind.tyInt8](ast#tyInt8)
[tyLent:](#tyLent)
* [TTypeKind.tyLent](ast#tyLent)
[tyMagicGenerics:](#tyMagicGenerics)
* [sem: tyMagicGenerics](sem#tyMagicGenerics)
[tyMetaTypes:](#tyMetaTypes)
* [ast: tyMetaTypes](ast#tyMetaTypes)
[tyNil:](#tyNil)
* [TTypeKind.tyNil](ast#tyNil)
[tyNone:](#tyNone)
* [TTypeKind.tyNone](ast#tyNone)
[tyNot:](#tyNot)
* [TTypeKind.tyNot](ast#tyNot)
[tyObject:](#tyObject)
* [TTypeKind.tyObject](ast#tyObject)
[tyOpenArray:](#tyOpenArray)
* [TTypeKind.tyOpenArray](ast#tyOpenArray)
[tyOptDeprecated:](#tyOptDeprecated)
* [TTypeKind.tyOptDeprecated](ast#tyOptDeprecated)
[tyOr:](#tyOr)
* [TTypeKind.tyOr](ast#tyOr)
[tyOrdinal:](#tyOrdinal)
* [TTypeKind.tyOrdinal](ast#tyOrdinal)
[tyOwned:](#tyOwned)
* [TTypeKind.tyOwned](ast#tyOwned)
[typeAllowed:](#typeAllowed)
* [typeallowed: typeAllowed(t: PType; kind: TSymKind; c: PContext; flags: TTypeAllowedFlags = {}): PType](typeallowed#typeAllowed%2CPType%2CTSymKind%2CPContext%2CTTypeAllowedFlags)
[TypeCache:](#TypeCache)
* [cgendata: TypeCache](cgendata#TypeCache)
[TypeCacheWithOwner:](#TypeCacheWithOwner)
* [cgendata: TypeCacheWithOwner](cgendata#TypeCacheWithOwner)
[typeCompleted:](#typeCompleted)
* [ast: typeCompleted(s: PSym)](ast#typeCompleted.t%2CPSym)
[typedescInst:](#typedescInst)
* [types: typedescInst](types#typedescInst)
[typedescPtrs:](#typedescPtrs)
* [types: typedescPtrs](types#typedescPtrs)
[typekinds:](#typekinds)
* [astalgo: typekinds(t: PType)](astalgo#typekinds%2CPType)
[typeMismatch:](#typeMismatch)
* [types: typeMismatch(conf: ConfigRef; info: TLineInfo; formal, actual: PType)](types#typeMismatch%2CConfigRef%2CTLineInfo%2CPType%2CPType)
[typePragmas:](#typePragmas)
* [pragmas: typePragmas](pragmas#typePragmas)
[typeRel:](#typeRel)
* [sigmatch: typeRel(c: var TCandidate; f, aOrig: PType; flags: TTypeRelFlags = {}): TTypeRelation](sigmatch#typeRel%2CTCandidate%2CPType%2CPType%2CTTypeRelFlags)
[typeToString:](#typeToString)
* [types: typeToString(typ: PType; prefer: TPreferedDesc = preferName): string](types#typeToString%2CPType%2CTPreferedDesc)
[typeToYaml:](#typeToYaml)
* [astalgo: typeToYaml(conf: ConfigRef; n: PType; indent: int = 0; maxRecDepth: int = -1): Rope](astalgo#typeToYaml%2CConfigRef%2CPType%2Cint%2Cint)
[tyPointer:](#tyPointer)
* [TTypeKind.tyPointer](ast#tyPointer)
[tyProc:](#tyProc)
* [TTypeKind.tyProc](ast#tyProc)
[tyProxy:](#tyProxy)
* [TTypeKind.tyProxy](ast#tyProxy)
[tyPtr:](#tyPtr)
* [TTypeKind.tyPtr](ast#tyPtr)
[tyPureObject:](#tyPureObject)
* [ast: tyPureObject](ast#tyPureObject)
[tyRange:](#tyRange)
* [TTypeKind.tyRange](ast#tyRange)
[tyRef:](#tyRef)
* [TTypeKind.tyRef](ast#tyRef)
[tySequence:](#tySequence)
* [TTypeKind.tySequence](ast#tySequence)
[tySet:](#tySet)
* [TTypeKind.tySet](ast#tySet)
[tySink:](#tySink)
* [TTypeKind.tySink](ast#tySink)
[tyStatic:](#tyStatic)
* [TTypeKind.tyStatic](ast#tyStatic)
[tyString:](#tyString)
* [TTypeKind.tyString](ast#tyString)
[tyTuple:](#tyTuple)
* [TTypeKind.tyTuple](ast#tyTuple)
[tyTypeClasses:](#tyTypeClasses)
* [ast: tyTypeClasses](ast#tyTypeClasses)
[tyTyped:](#tyTyped)
* [TTypeKind.tyTyped](ast#tyTyped)
[tyTypeDesc:](#tyTypeDesc)
* [TTypeKind.tyTypeDesc](ast#tyTypeDesc)
[tyUInt:](#tyUInt)
* [TTypeKind.tyUInt](ast#tyUInt)
[tyUInt16:](#tyUInt16)
* [TTypeKind.tyUInt16](ast#tyUInt16)
[tyUInt32:](#tyUInt32)
* [TTypeKind.tyUInt32](ast#tyUInt32)
[tyUInt64:](#tyUInt64)
* [TTypeKind.tyUInt64](ast#tyUInt64)
[tyUInt8:](#tyUInt8)
* [TTypeKind.tyUInt8](ast#tyUInt8)
[tyUncheckedArray:](#tyUncheckedArray)
* [TTypeKind.tyUncheckedArray](ast#tyUncheckedArray)
[tyUnknown:](#tyUnknown)
* [ast: tyUnknown](ast#tyUnknown)
[tyUnknownTypes:](#tyUnknownTypes)
* [ast: tyUnknownTypes](ast#tyUnknownTypes)
[tyUntyped:](#tyUntyped)
* [TTypeKind.tyUntyped](ast#tyUntyped)
[tyUserDefinedGenerics:](#tyUserDefinedGenerics)
* [sem: tyUserDefinedGenerics](sem#tyUserDefinedGenerics)
[tyUserTypeClass:](#tyUserTypeClass)
* [TTypeKind.tyUserTypeClass](ast#tyUserTypeClass)
[tyUserTypeClasses:](#tyUserTypeClasses)
* [ast: tyUserTypeClasses](ast#tyUserTypeClasses)
[tyUserTypeClassInst:](#tyUserTypeClassInst)
* [TTypeKind.tyUserTypeClassInst](ast#tyUserTypeClassInst)
[tyVar:](#tyVar)
* [TTypeKind.tyVar](ast#tyVar)
[tyVarargs:](#tyVarargs)
* [TTypeKind.tyVarargs](ast#tyVarargs)
[tyVoid:](#tyVoid)
* [TTypeKind.tyVoid](ast#tyVoid)
[undefSymbol:](#undefSymbol)
* [condsyms: undefSymbol(symbols: StringTableRef; symbol: string)](condsyms#undefSymbol%2CStringTableRef%2Cstring)
[unionSets:](#unionSets)
* [nimsets: unionSets(conf: ConfigRef; a, b: PNode): PNode](nimsets#unionSets%2CConfigRef%2CPNode%2CPNode)
[unknownLineInfo:](#unknownLineInfo)
* [lineinfos: unknownLineInfo](lineinfos#unknownLineInfo)
[UnknownLockLevel:](#UnknownLockLevel)
* [ast: UnknownLockLevel](ast#UnknownLockLevel)
[UnspecifiedLockLevel:](#UnspecifiedLockLevel)
* [ast: UnspecifiedLockLevel](ast#UnspecifiedLockLevel)
[upName:](#upName)
* [lambdalifting: upName](lambdalifting#upName)
[use:](#use)
* [InstrKind.use](dfa#use)
[useEffectSystem:](#useEffectSystem)
* [options: useEffectSystem](options#useEffectSystem)
[usesThreadVars:](#usesThreadVars)
* [CodegenFlag.usesThreadVars](cgendata#usesThreadVars)
[usesWriteBarrier:](#usesWriteBarrier)
* [options: usesWriteBarrier(conf: ConfigRef): bool](options#usesWriteBarrier%2CConfigRef)
[useWriteTracking:](#useWriteTracking)
* [options: useWriteTracking](options#useWriteTracking)
[v2Sf:](#v2Sf)
* [SymbolFilesOption.v2Sf](options#v2Sf)
[varPragmas:](#varPragmas)
* [pragmas: varPragmas](pragmas#varPragmas)
[verbosePass:](#verbosePass)
* [passaux: verbosePass](passaux#verbosePass)
[Version:](#Version)
* [nimblecmd: Version](nimblecmd#Version)
[VersionAsString:](#VersionAsString)
* [nversion: VersionAsString](nversion#VersionAsString)
[views:](#views)
* [Feature.views](options#views)
[ViewTypeKind:](#ViewTypeKind)
* [typeallowed: ViewTypeKind](typeallowed#ViewTypeKind)
[VmArgs:](#VmArgs)
* [vmdef: VmArgs](vmdef#VmArgs)
[VmCallback:](#VmCallback)
* [vmdef: VmCallback](vmdef#VmCallback)
[vmopsDanger:](#vmopsDanger)
* [Feature.vmopsDanger](options#vmopsDanger)
[VT:](#VT)
* [nimlexbase: VT](nimlexbase#VT)
[wAcyclic:](#wAcyclic)
* [TSpecialWord.wAcyclic](wordrecg#wAcyclic)
[wAddr:](#wAddr)
* [TSpecialWord.wAddr](wordrecg#wAddr)
[wAlign:](#wAlign)
* [TSpecialWord.wAlign](wordrecg#wAlign)
[wAlignas:](#wAlignas)
* [TSpecialWord.wAlignas](wordrecg#wAlignas)
[wAlignof:](#wAlignof)
* [TSpecialWord.wAlignof](wordrecg#wAlignof)
[walk:](#walk)
* [sourcemap: walk(node: SourceNode; fn: proc (line: string; original: SourceNode))](sourcemap#walk%2CSourceNode%2Cproc%28string%2CSourceNode%29)
[walkScopes:](#walkScopes)
* [lookups: walkScopes(scope: PScope): PScope](lookups#walkScopes.i%2CPScope)
[wAnd:](#wAnd)
* [TSpecialWord.wAnd](wordrecg#wAnd)
[wantMainModule:](#wantMainModule)
* [modules: wantMainModule(conf: ConfigRef)](modules#wantMainModule%2CConfigRef)
[warnCannotOpenFile:](#warnCannotOpenFile)
* [TMsgKind.warnCannotOpenFile](lineinfos#warnCannotOpenFile)
[warnCaseTransition:](#warnCaseTransition)
* [TMsgKind.warnCaseTransition](lineinfos#warnCaseTransition)
[warnCommentXIgnored:](#warnCommentXIgnored)
* [TMsgKind.warnCommentXIgnored](lineinfos#warnCommentXIgnored)
[warnConfigDeprecated:](#warnConfigDeprecated)
* [TMsgKind.warnConfigDeprecated](lineinfos#warnConfigDeprecated)
[warnCycleCreated:](#warnCycleCreated)
* [TMsgKind.warnCycleCreated](lineinfos#warnCycleCreated)
[warnDeprecated:](#warnDeprecated)
* [TMsgKind.warnDeprecated](lineinfos#warnDeprecated)
[warnDestructor:](#warnDestructor)
* [TMsgKind.warnDestructor](lineinfos#warnDestructor)
[warnEachIdentIsTuple:](#warnEachIdentIsTuple)
* [TMsgKind.warnEachIdentIsTuple](lineinfos#warnEachIdentIsTuple)
[warnFieldXNotSupported:](#warnFieldXNotSupported)
* [TMsgKind.warnFieldXNotSupported](lineinfos#warnFieldXNotSupported)
[warnGcMem:](#warnGcMem)
* [TMsgKind.warnGcMem](lineinfos#warnGcMem)
[warnGcUnsafe:](#warnGcUnsafe)
* [TMsgKind.warnGcUnsafe](lineinfos#warnGcUnsafe)
[warnGcUnsafe2:](#warnGcUnsafe2)
* [TMsgKind.warnGcUnsafe2](lineinfos#warnGcUnsafe2)
[warnInconsistentSpacing:](#warnInconsistentSpacing)
* [TMsgKind.warnInconsistentSpacing](lineinfos#warnInconsistentSpacing)
[warnInheritFromException:](#warnInheritFromException)
* [TMsgKind.warnInheritFromException](lineinfos#warnInheritFromException)
[warnLanguageXNotSupported:](#warnLanguageXNotSupported)
* [TMsgKind.warnLanguageXNotSupported](lineinfos#warnLanguageXNotSupported)
[warnLockLevel:](#warnLockLevel)
* [TMsgKind.warnLockLevel](lineinfos#warnLockLevel)
[warnMax:](#warnMax)
* [lineinfos: warnMax](lineinfos#warnMax)
[warnMin:](#warnMin)
* [lineinfos: warnMin](lineinfos#warnMin)
[warnObservableStores:](#warnObservableStores)
* [TMsgKind.warnObservableStores](lineinfos#warnObservableStores)
[warnOctalEscape:](#warnOctalEscape)
* [TMsgKind.warnOctalEscape](lineinfos#warnOctalEscape)
[warnProveField:](#warnProveField)
* [TMsgKind.warnProveField](lineinfos#warnProveField)
[warnProveIndex:](#warnProveIndex)
* [TMsgKind.warnProveIndex](lineinfos#warnProveIndex)
[warnProveInit:](#warnProveInit)
* [TMsgKind.warnProveInit](lineinfos#warnProveInit)
[warnRedefinitionOfLabel:](#warnRedefinitionOfLabel)
* [TMsgKind.warnRedefinitionOfLabel](lineinfos#warnRedefinitionOfLabel)
[warnResultShadowed:](#warnResultShadowed)
* [TMsgKind.warnResultShadowed](lineinfos#warnResultShadowed)
[warnResultUsed:](#warnResultUsed)
* [TMsgKind.warnResultUsed](lineinfos#warnResultUsed)
[warnSmallLshouldNotBeUsed:](#warnSmallLshouldNotBeUsed)
* [TMsgKind.warnSmallLshouldNotBeUsed](lineinfos#warnSmallLshouldNotBeUsed)
[warnStaticIndexCheck:](#warnStaticIndexCheck)
* [TMsgKind.warnStaticIndexCheck](lineinfos#warnStaticIndexCheck)
[warnTypelessParam:](#warnTypelessParam)
* [TMsgKind.warnTypelessParam](lineinfos#warnTypelessParam)
[warnUninit:](#warnUninit)
* [TMsgKind.warnUninit](lineinfos#warnUninit)
[warnUnknownMagic:](#warnUnknownMagic)
* [TMsgKind.warnUnknownMagic](lineinfos#warnUnknownMagic)
[warnUnknownSubstitutionX:](#warnUnknownSubstitutionX)
* [TMsgKind.warnUnknownSubstitutionX](lineinfos#warnUnknownSubstitutionX)
[warnUnreachableCode:](#warnUnreachableCode)
* [TMsgKind.warnUnreachableCode](lineinfos#warnUnreachableCode)
[warnUnreachableElse:](#warnUnreachableElse)
* [TMsgKind.warnUnreachableElse](lineinfos#warnUnreachableElse)
[warnUnsafeCode:](#warnUnsafeCode)
* [TMsgKind.warnUnsafeCode](lineinfos#warnUnsafeCode)
[warnUnsafeDefault:](#warnUnsafeDefault)
* [TMsgKind.warnUnsafeDefault](lineinfos#warnUnsafeDefault)
[warnUnsafeSetLen:](#warnUnsafeSetLen)
* [TMsgKind.warnUnsafeSetLen](lineinfos#warnUnsafeSetLen)
[warnUnusedImportX:](#warnUnusedImportX)
* [TMsgKind.warnUnusedImportX](lineinfos#warnUnusedImportX)
[warnUseBase:](#warnUseBase)
* [TMsgKind.warnUseBase](lineinfos#warnUseBase)
[warnUser:](#warnUser)
* [TMsgKind.warnUser](lineinfos#warnUser)
[warnWriteToForeignHeap:](#warnWriteToForeignHeap)
* [TMsgKind.warnWriteToForeignHeap](lineinfos#warnWriteToForeignHeap)
[warnXIsNeverRead:](#warnXIsNeverRead)
* [TMsgKind.warnXIsNeverRead](lineinfos#warnXIsNeverRead)
[warnXmightNotBeenInit:](#warnXmightNotBeenInit)
* [TMsgKind.warnXmightNotBeenInit](lineinfos#warnXmightNotBeenInit)
[wAs:](#wAs)
* [TSpecialWord.wAs](wordrecg#wAs)
[wAsm:](#wAsm)
* [TSpecialWord.wAsm](wordrecg#wAsm)
[wAsmNoStackFrame:](#wAsmNoStackFrame)
* [TSpecialWord.wAsmNoStackFrame](wordrecg#wAsmNoStackFrame)
[wAssert:](#wAssert)
* [TSpecialWord.wAssert](wordrecg#wAssert)
[wAssertions:](#wAssertions)
* [TSpecialWord.wAssertions](wordrecg#wAssertions)
[wAssume:](#wAssume)
* [TSpecialWord.wAssume](wordrecg#wAssume)
[wAuto:](#wAuto)
* [TSpecialWord.wAuto](wordrecg#wAuto)
[wBase:](#wBase)
* [TSpecialWord.wBase](wordrecg#wBase)
[wBind:](#wBind)
* [TSpecialWord.wBind](wordrecg#wBind)
[wBitsize:](#wBitsize)
* [TSpecialWord.wBitsize](wordrecg#wBitsize)
[wBlock:](#wBlock)
* [TSpecialWord.wBlock](wordrecg#wBlock)
[wBool:](#wBool)
* [TSpecialWord.wBool](wordrecg#wBool)
[wBoolDefine:](#wBoolDefine)
* [TSpecialWord.wBoolDefine](wordrecg#wBoolDefine)
[wBorrow:](#wBorrow)
* [TSpecialWord.wBorrow](wordrecg#wBorrow)
[wBoundChecks:](#wBoundChecks)
* [TSpecialWord.wBoundChecks](wordrecg#wBoundChecks)
[wBreak:](#wBreak)
* [TSpecialWord.wBreak](wordrecg#wBreak)
[wByCopy:](#wByCopy)
* [TSpecialWord.wByCopy](wordrecg#wByCopy)
[wByRef:](#wByRef)
* [TSpecialWord.wByRef](wordrecg#wByRef)
[wCallconv:](#wCallconv)
* [TSpecialWord.wCallconv](wordrecg#wCallconv)
[wCase:](#wCase)
* [TSpecialWord.wCase](wordrecg#wCase)
[wCast:](#wCast)
* [TSpecialWord.wCast](wordrecg#wCast)
[wCatch:](#wCatch)
* [TSpecialWord.wCatch](wordrecg#wCatch)
[wCdecl:](#wCdecl)
* [TSpecialWord.wCdecl](wordrecg#wCdecl)
[wChar:](#wChar)
* [TSpecialWord.wChar](wordrecg#wChar)
[wChar16\_t:](#wChar16_t)
* [TSpecialWord.wChar16\_t](wordrecg#wChar16_t)
[wChar32\_t:](#wChar32_t)
* [TSpecialWord.wChar32\_t](wordrecg#wChar32_t)
[wChecks:](#wChecks)
* [TSpecialWord.wChecks](wordrecg#wChecks)
[wClass:](#wClass)
* [TSpecialWord.wClass](wordrecg#wClass)
[wClosure:](#wClosure)
* [TSpecialWord.wClosure](wordrecg#wClosure)
[wCodegenDecl:](#wCodegenDecl)
* [TSpecialWord.wCodegenDecl](wordrecg#wCodegenDecl)
[wColon:](#wColon)
* [TSpecialWord.wColon](wordrecg#wColon)
[wColonColon:](#wColonColon)
* [TSpecialWord.wColonColon](wordrecg#wColonColon)
[wCompile:](#wCompile)
* [TSpecialWord.wCompile](wordrecg#wCompile)
[wCompilerProc:](#wCompilerProc)
* [TSpecialWord.wCompilerProc](wordrecg#wCompilerProc)
[wCompileTime:](#wCompileTime)
* [TSpecialWord.wCompileTime](wordrecg#wCompileTime)
[wCompl:](#wCompl)
* [TSpecialWord.wCompl](wordrecg#wCompl)
[wCompleteStruct:](#wCompleteStruct)
* [TSpecialWord.wCompleteStruct](wordrecg#wCompleteStruct)
[wComputedGoto:](#wComputedGoto)
* [TSpecialWord.wComputedGoto](wordrecg#wComputedGoto)
[wConcept:](#wConcept)
* [TSpecialWord.wConcept](wordrecg#wConcept)
[wConst:](#wConst)
* [TSpecialWord.wConst](wordrecg#wConst)
[wConst\_cast:](#wConst_cast)
* [TSpecialWord.wConst\_cast](wordrecg#wConst_cast)
[wConstexpr:](#wConstexpr)
* [TSpecialWord.wConstexpr](wordrecg#wConstexpr)
[wConstructor:](#wConstructor)
* [TSpecialWord.wConstructor](wordrecg#wConstructor)
[wContinue:](#wContinue)
* [TSpecialWord.wContinue](wordrecg#wContinue)
[wConverter:](#wConverter)
* [TSpecialWord.wConverter](wordrecg#wConverter)
[wCore:](#wCore)
* [TSpecialWord.wCore](wordrecg#wCore)
[wCursor:](#wCursor)
* [TSpecialWord.wCursor](wordrecg#wCursor)
[wDeadCodeElimUnused:](#wDeadCodeElimUnused)
* [TSpecialWord.wDeadCodeElimUnused](wordrecg#wDeadCodeElimUnused)
[wDebugger:](#wDebugger)
* [TSpecialWord.wDebugger](wordrecg#wDebugger)
[wDecltype:](#wDecltype)
* [TSpecialWord.wDecltype](wordrecg#wDecltype)
[wDefault:](#wDefault)
* [TSpecialWord.wDefault](wordrecg#wDefault)
[wDefer:](#wDefer)
* [TSpecialWord.wDefer](wordrecg#wDefer)
[wDefine:](#wDefine)
* [TSpecialWord.wDefine](wordrecg#wDefine)
[wDelegator:](#wDelegator)
* [TSpecialWord.wDelegator](wordrecg#wDelegator)
[wDelete:](#wDelete)
* [TSpecialWord.wDelete](wordrecg#wDelete)
[wDeprecated:](#wDeprecated)
* [TSpecialWord.wDeprecated](wordrecg#wDeprecated)
[wDestructor:](#wDestructor)
* [TSpecialWord.wDestructor](wordrecg#wDestructor)
[wDirty:](#wDirty)
* [TSpecialWord.wDirty](wordrecg#wDirty)
[wDiscard:](#wDiscard)
* [TSpecialWord.wDiscard](wordrecg#wDiscard)
[wDiscardable:](#wDiscardable)
* [TSpecialWord.wDiscardable](wordrecg#wDiscardable)
[wDistinct:](#wDistinct)
* [TSpecialWord.wDistinct](wordrecg#wDistinct)
[wDiv:](#wDiv)
* [TSpecialWord.wDiv](wordrecg#wDiv)
[wDo:](#wDo)
* [TSpecialWord.wDo](wordrecg#wDo)
[wDot:](#wDot)
* [TSpecialWord.wDot](wordrecg#wDot)
[wDotDot:](#wDotDot)
* [TSpecialWord.wDotDot](wordrecg#wDotDot)
[wDouble:](#wDouble)
* [TSpecialWord.wDouble](wordrecg#wDouble)
[wDynamic\_cast:](#wDynamic_cast)
* [TSpecialWord.wDynamic\_cast](wordrecg#wDynamic_cast)
[wDynlib:](#wDynlib)
* [TSpecialWord.wDynlib](wordrecg#wDynlib)
[weakLeValue:](#weakLeValue)
* [astalgo: weakLeValue(a, b: PNode): TImplication](astalgo#weakLeValue%2CPNode%2CPNode)
[wEffects:](#wEffects)
* [TSpecialWord.wEffects](wordrecg#wEffects)
[wElif:](#wElif)
* [TSpecialWord.wElif](wordrecg#wElif)
[wElse:](#wElse)
* [TSpecialWord.wElse](wordrecg#wElse)
[wEmit:](#wEmit)
* [TSpecialWord.wEmit](wordrecg#wEmit)
[wEnd:](#wEnd)
* [TSpecialWord.wEnd](wordrecg#wEnd)
[wEnsures:](#wEnsures)
* [TSpecialWord.wEnsures](wordrecg#wEnsures)
[wEnum:](#wEnum)
* [TSpecialWord.wEnum](wordrecg#wEnum)
[wEquals:](#wEquals)
* [TSpecialWord.wEquals](wordrecg#wEquals)
[wError:](#wError)
* [TSpecialWord.wError](wordrecg#wError)
[wExcept:](#wExcept)
* [TSpecialWord.wExcept](wordrecg#wExcept)
[wExecuteOnReload:](#wExecuteOnReload)
* [TSpecialWord.wExecuteOnReload](wordrecg#wExecuteOnReload)
[wExperimental:](#wExperimental)
* [TSpecialWord.wExperimental](wordrecg#wExperimental)
[wExplain:](#wExplain)
* [TSpecialWord.wExplain](wordrecg#wExplain)
[wExplicit:](#wExplicit)
* [TSpecialWord.wExplicit](wordrecg#wExplicit)
[wExport:](#wExport)
* [TSpecialWord.wExport](wordrecg#wExport)
[wExportc:](#wExportc)
* [TSpecialWord.wExportc](wordrecg#wExportc)
[wExportCpp:](#wExportCpp)
* [TSpecialWord.wExportCpp](wordrecg#wExportCpp)
[wExportNims:](#wExportNims)
* [TSpecialWord.wExportNims](wordrecg#wExportNims)
[wExtern:](#wExtern)
* [TSpecialWord.wExtern](wordrecg#wExtern)
[wFalse:](#wFalse)
* [TSpecialWord.wFalse](wordrecg#wFalse)
[wFastcall:](#wFastcall)
* [TSpecialWord.wFastcall](wordrecg#wFastcall)
[wFatal:](#wFatal)
* [TSpecialWord.wFatal](wordrecg#wFatal)
[wFieldChecks:](#wFieldChecks)
* [TSpecialWord.wFieldChecks](wordrecg#wFieldChecks)
[wFinal:](#wFinal)
* [TSpecialWord.wFinal](wordrecg#wFinal)
[wFinally:](#wFinally)
* [TSpecialWord.wFinally](wordrecg#wFinally)
[wFloat:](#wFloat)
* [TSpecialWord.wFloat](wordrecg#wFloat)
[wFloatChecks:](#wFloatChecks)
* [TSpecialWord.wFloatChecks](wordrecg#wFloatChecks)
[wFor:](#wFor)
* [TSpecialWord.wFor](wordrecg#wFor)
[wFriend:](#wFriend)
* [TSpecialWord.wFriend](wordrecg#wFriend)
[wFrom:](#wFrom)
* [TSpecialWord.wFrom](wordrecg#wFrom)
[wFunc:](#wFunc)
* [TSpecialWord.wFunc](wordrecg#wFunc)
[wGcSafe:](#wGcSafe)
* [TSpecialWord.wGcSafe](wordrecg#wGcSafe)
[wGensym:](#wGensym)
* [TSpecialWord.wGensym](wordrecg#wGensym)
[wGlobal:](#wGlobal)
* [TSpecialWord.wGlobal](wordrecg#wGlobal)
[wGoto:](#wGoto)
* [TSpecialWord.wGoto](wordrecg#wGoto)
[wGuard:](#wGuard)
* [TSpecialWord.wGuard](wordrecg#wGuard)
[wHeader:](#wHeader)
* [TSpecialWord.wHeader](wordrecg#wHeader)
[whichAlias:](#whichAlias)
* [parampatterns: whichAlias(p: PSym): TAliasRequest](parampatterns#whichAlias%2CPSym)
[whichKeyword:](#whichKeyword)
* [idents: whichKeyword(id: PIdent): TSpecialWord](idents#whichKeyword%2CPIdent)
[whichPragma:](#whichPragma)
* [trees: whichPragma(n: PNode): TSpecialWord](trees#whichPragma%2CPNode)
[wHint:](#wHint)
* [TSpecialWord.wHint](wordrecg#wHint)
[wHints:](#wHints)
* [TSpecialWord.wHints](wordrecg#wHints)
[wIf:](#wIf)
* [TSpecialWord.wIf](wordrecg#wIf)
[wImmediate:](#wImmediate)
* [TSpecialWord.wImmediate](wordrecg#wImmediate)
[wImplicitStatic:](#wImplicitStatic)
* [TSpecialWord.wImplicitStatic](wordrecg#wImplicitStatic)
[wImport:](#wImport)
* [TSpecialWord.wImport](wordrecg#wImport)
[wImportc:](#wImportc)
* [TSpecialWord.wImportc](wordrecg#wImportc)
[wImportCompilerProc:](#wImportCompilerProc)
* [TSpecialWord.wImportCompilerProc](wordrecg#wImportCompilerProc)
[wImportCpp:](#wImportCpp)
* [TSpecialWord.wImportCpp](wordrecg#wImportCpp)
[wImportJs:](#wImportJs)
* [TSpecialWord.wImportJs](wordrecg#wImportJs)
[wImportObjC:](#wImportObjC)
* [TSpecialWord.wImportObjC](wordrecg#wImportObjC)
[wIn:](#wIn)
* [TSpecialWord.wIn](wordrecg#wIn)
[wInclude:](#wInclude)
* [TSpecialWord.wInclude](wordrecg#wInclude)
[wIncompleteStruct:](#wIncompleteStruct)
* [TSpecialWord.wIncompleteStruct](wordrecg#wIncompleteStruct)
[wInfChecks:](#wInfChecks)
* [TSpecialWord.wInfChecks](wordrecg#wInfChecks)
[wInheritable:](#wInheritable)
* [TSpecialWord.wInheritable](wordrecg#wInheritable)
[wInject:](#wInject)
* [TSpecialWord.wInject](wordrecg#wInject)
[wInjectStmt:](#wInjectStmt)
* [TSpecialWord.wInjectStmt](wordrecg#wInjectStmt)
[wInline:](#wInline)
* [TSpecialWord.wInline](wordrecg#wInline)
[wInOut:](#wInOut)
* [TSpecialWord.wInOut](wordrecg#wInOut)
[wInt:](#wInt)
* [TSpecialWord.wInt](wordrecg#wInt)
[wIntDefine:](#wIntDefine)
* [TSpecialWord.wIntDefine](wordrecg#wIntDefine)
[wInterface:](#wInterface)
* [TSpecialWord.wInterface](wordrecg#wInterface)
[wInvalid:](#wInvalid)
* [TSpecialWord.wInvalid](wordrecg#wInvalid)
[wInvariant:](#wInvariant)
* [TSpecialWord.wInvariant](wordrecg#wInvariant)
[wIs:](#wIs)
* [TSpecialWord.wIs](wordrecg#wIs)
[wIsnot:](#wIsnot)
* [TSpecialWord.wIsnot](wordrecg#wIsnot)
[wIterator:](#wIterator)
* [TSpecialWord.wIterator](wordrecg#wIterator)
[withInfo:](#withInfo)
* [ast: withInfo(n: PNode; info: TLineInfo): PNode](ast#withInfo%2CPNode%2CTLineInfo)
[withPackageName:](#withPackageName)
* [options: withPackageName(conf: ConfigRef; path: AbsoluteFile): AbsoluteFile](options#withPackageName%2CConfigRef%2CAbsoluteFile)
[wLet:](#wLet)
* [TSpecialWord.wLet](wordrecg#wLet)
[wLib:](#wLib)
* [TSpecialWord.wLib](wordrecg#wLib)
[wLiftLocals:](#wLiftLocals)
* [TSpecialWord.wLiftLocals](wordrecg#wLiftLocals)
[wLine:](#wLine)
* [TSpecialWord.wLine](wordrecg#wLine)
[wLinearScanEnd:](#wLinearScanEnd)
* [TSpecialWord.wLinearScanEnd](wordrecg#wLinearScanEnd)
[wLineDir:](#wLineDir)
* [TSpecialWord.wLineDir](wordrecg#wLineDir)
[wLineTrace:](#wLineTrace)
* [TSpecialWord.wLineTrace](wordrecg#wLineTrace)
[wLink:](#wLink)
* [TSpecialWord.wLink](wordrecg#wLink)
[wLinksys:](#wLinksys)
* [TSpecialWord.wLinksys](wordrecg#wLinksys)
[wLocalPassc:](#wLocalPassc)
* [TSpecialWord.wLocalPassc](wordrecg#wLocalPassc)
[wLocks:](#wLocks)
* [TSpecialWord.wLocks](wordrecg#wLocks)
[wLong:](#wLong)
* [TSpecialWord.wLong](wordrecg#wLong)
[wMacro:](#wMacro)
* [TSpecialWord.wMacro](wordrecg#wMacro)
[wMagic:](#wMagic)
* [TSpecialWord.wMagic](wordrecg#wMagic)
[wMemTracker:](#wMemTracker)
* [TSpecialWord.wMemTracker](wordrecg#wMemTracker)
[wMerge:](#wMerge)
* [TSpecialWord.wMerge](wordrecg#wMerge)
[wMethod:](#wMethod)
* [TSpecialWord.wMethod](wordrecg#wMethod)
[wMinus:](#wMinus)
* [TSpecialWord.wMinus](wordrecg#wMinus)
[wMixin:](#wMixin)
* [TSpecialWord.wMixin](wordrecg#wMixin)
[wMod:](#wMod)
* [TSpecialWord.wMod](wordrecg#wMod)
[wMutable:](#wMutable)
* [TSpecialWord.wMutable](wordrecg#wMutable)
[wNamespace:](#wNamespace)
* [TSpecialWord.wNamespace](wordrecg#wNamespace)
[wNanChecks:](#wNanChecks)
* [TSpecialWord.wNanChecks](wordrecg#wNanChecks)
[wNew:](#wNew)
* [TSpecialWord.wNew](wordrecg#wNew)
[wNil:](#wNil)
* [TSpecialWord.wNil](wordrecg#wNil)
[wNilChecks:](#wNilChecks)
* [TSpecialWord.wNilChecks](wordrecg#wNilChecks)
[wNimcall:](#wNimcall)
* [TSpecialWord.wNimcall](wordrecg#wNimcall)
[wNoalias:](#wNoalias)
* [TSpecialWord.wNoalias](wordrecg#wNoalias)
[wNoconv:](#wNoconv)
* [TSpecialWord.wNoconv](wordrecg#wNoconv)
[wNodecl:](#wNodecl)
* [TSpecialWord.wNodecl](wordrecg#wNodecl)
[wNoDestroy:](#wNoDestroy)
* [TSpecialWord.wNoDestroy](wordrecg#wNoDestroy)
[wNoexcept:](#wNoexcept)
* [TSpecialWord.wNoexcept](wordrecg#wNoexcept)
[wNoForward:](#wNoForward)
* [TSpecialWord.wNoForward](wordrecg#wNoForward)
[wNoInit:](#wNoInit)
* [TSpecialWord.wNoInit](wordrecg#wNoInit)
[wNoInline:](#wNoInline)
* [TSpecialWord.wNoInline](wordrecg#wNoInline)
[wNonReloadable:](#wNonReloadable)
* [TSpecialWord.wNonReloadable](wordrecg#wNonReloadable)
[wNoreturn:](#wNoreturn)
* [TSpecialWord.wNoreturn](wordrecg#wNoreturn)
[wNoRewrite:](#wNoRewrite)
* [TSpecialWord.wNoRewrite](wordrecg#wNoRewrite)
[wNoSideEffect:](#wNoSideEffect)
* [TSpecialWord.wNoSideEffect](wordrecg#wNoSideEffect)
[wNosinks:](#wNosinks)
* [TSpecialWord.wNosinks](wordrecg#wNosinks)
[wNot:](#wNot)
* [TSpecialWord.wNot](wordrecg#wNot)
[wNotin:](#wNotin)
* [TSpecialWord.wNotin](wordrecg#wNotin)
[wNullptr:](#wNullptr)
* [TSpecialWord.wNullptr](wordrecg#wNullptr)
[wObjChecks:](#wObjChecks)
* [TSpecialWord.wObjChecks](wordrecg#wObjChecks)
[wObject:](#wObject)
* [TSpecialWord.wObject](wordrecg#wObject)
[wOf:](#wOf)
* [TSpecialWord.wOf](wordrecg#wOf)
[wOff:](#wOff)
* [TSpecialWord.wOff](wordrecg#wOff)
[wOn:](#wOn)
* [TSpecialWord.wOn](wordrecg#wOn)
[wOneWay:](#wOneWay)
* [TSpecialWord.wOneWay](wordrecg#wOneWay)
[wOperator:](#wOperator)
* [TSpecialWord.wOperator](wordrecg#wOperator)
[wOptimization:](#wOptimization)
* [TSpecialWord.wOptimization](wordrecg#wOptimization)
[wOr:](#wOr)
* [TSpecialWord.wOr](wordrecg#wOr)
[wordExcess:](#wordExcess)
* [vmdef: wordExcess](vmdef#wordExcess)
[wOut:](#wOut)
* [TSpecialWord.wOut](wordrecg#wOut)
[wOverflowChecks:](#wOverflowChecks)
* [TSpecialWord.wOverflowChecks](wordrecg#wOverflowChecks)
[wOverride:](#wOverride)
* [TSpecialWord.wOverride](wordrecg#wOverride)
[wPackage:](#wPackage)
* [TSpecialWord.wPackage](wordrecg#wPackage)
[wPacked:](#wPacked)
* [TSpecialWord.wPacked](wordrecg#wPacked)
[wPartial:](#wPartial)
* [TSpecialWord.wPartial](wordrecg#wPartial)
[wPassc:](#wPassc)
* [TSpecialWord.wPassc](wordrecg#wPassc)
[wPassl:](#wPassl)
* [TSpecialWord.wPassl](wordrecg#wPassl)
[wPatterns:](#wPatterns)
* [TSpecialWord.wPatterns](wordrecg#wPatterns)
[wPop:](#wPop)
* [TSpecialWord.wPop](wordrecg#wPop)
[wPragma:](#wPragma)
* [TSpecialWord.wPragma](wordrecg#wPragma)
[wPrivate:](#wPrivate)
* [TSpecialWord.wPrivate](wordrecg#wPrivate)
[wProc:](#wProc)
* [TSpecialWord.wProc](wordrecg#wProc)
[wProcVar:](#wProcVar)
* [TSpecialWord.wProcVar](wordrecg#wProcVar)
[wProfiler:](#wProfiler)
* [TSpecialWord.wProfiler](wordrecg#wProfiler)
[wProtected:](#wProtected)
* [TSpecialWord.wProtected](wordrecg#wProtected)
[wPtr:](#wPtr)
* [TSpecialWord.wPtr](wordrecg#wPtr)
[wPublic:](#wPublic)
* [TSpecialWord.wPublic](wordrecg#wPublic)
[wPure:](#wPure)
* [TSpecialWord.wPure](wordrecg#wPure)
[wPush:](#wPush)
* [TSpecialWord.wPush](wordrecg#wPush)
[wRaise:](#wRaise)
* [TSpecialWord.wRaise](wordrecg#wRaise)
[wRaises:](#wRaises)
* [TSpecialWord.wRaises](wordrecg#wRaises)
[wRangeChecks:](#wRangeChecks)
* [TSpecialWord.wRangeChecks](wordrecg#wRangeChecks)
[wrapInComesFrom:](#wrapInComesFrom)
* [evaltempl: wrapInComesFrom(info: TLineInfo; sym: PSym; res: PNode): PNode](evaltempl#wrapInComesFrom%2CTLineInfo%2CPSym%2CPNode)
[wrapProcForSpawn:](#wrapProcForSpawn)
* [spawn: wrapProcForSpawn(g: ModuleGraph; owner: PSym; spawnExpr: PNode; retType: PType; barrier, dest: PNode = nil): PNode](spawn#wrapProcForSpawn%2CModuleGraph%2CPSym%2CPNode%2CPType%2CPNode%2CPNode)
[wReads:](#wReads)
* [TSpecialWord.wReads](wordrecg#wReads)
[wRef:](#wRef)
* [TSpecialWord.wRef](wordrecg#wRef)
[wRegister:](#wRegister)
* [TSpecialWord.wRegister](wordrecg#wRegister)
[wReinterpret\_cast:](#wReinterpret_cast)
* [TSpecialWord.wReinterpret\_cast](wordrecg#wReinterpret_cast)
[wReorder:](#wReorder)
* [TSpecialWord.wReorder](wordrecg#wReorder)
[wRequires:](#wRequires)
* [TSpecialWord.wRequires](wordrecg#wRequires)
[wRequiresInit:](#wRequiresInit)
* [TSpecialWord.wRequiresInit](wordrecg#wRequiresInit)
[wRestrict:](#wRestrict)
* [TSpecialWord.wRestrict](wordrecg#wRestrict)
[wReturn:](#wReturn)
* [TSpecialWord.wReturn](wordrecg#wReturn)
[writeCommandLineUsage:](#writeCommandLineUsage)
* [commands: writeCommandLineUsage(conf: ConfigRef)](commands#writeCommandLineUsage%2CConfigRef)
[writeFile:](#writeFile)
* [pathutils: writeFile(x: AbsoluteFile; content: string)](pathutils#writeFile%2CAbsoluteFile%2Cstring)
[writeJsonBuildInstructions:](#writeJsonBuildInstructions)
* [extccomp: writeJsonBuildInstructions(conf: ConfigRef)](extccomp#writeJsonBuildInstructions%2CConfigRef)
[writeMangledName:](#writeMangledName)
* [ndi: writeMangledName(f: NdiFile; s: PSym; conf: ConfigRef)](ndi#writeMangledName.t%2CNdiFile%2CPSym%2CConfigRef)
[writeMapping:](#writeMapping)
* [extccomp: writeMapping(conf: ConfigRef; symbolMapping: Rope)](extccomp#writeMapping%2CConfigRef%2CRope)
[writeMatches:](#writeMatches)
* [sigmatch: writeMatches(c: TCandidate)](sigmatch#writeMatches%2CTCandidate)
[writeOnlySf:](#writeOnlySf)
* [SymbolFilesOption.writeOnlySf](options#writeOnlySf)
[writeOutput:](#writeOutput)
* [docgen: writeOutput(d: PDoc; useWarning = false; groupedToc = false)](docgen#writeOutput%2CPDoc)
[writeOutputJson:](#writeOutputJson)
* [docgen: writeOutputJson(d: PDoc; useWarning = false)](docgen#writeOutputJson%2CPDoc)
[writeRope:](#writeRope)
* [ropes: writeRope(f: File; r: Rope)](ropes#writeRope%2CFile%2CRope)
* [ropes: writeRope(head: Rope; filename: AbsoluteFile): bool](ropes#writeRope%2CRope%2CAbsoluteFile)
[writeRopeIfNotEqual:](#writeRopeIfNotEqual)
* [ropes: writeRopeIfNotEqual(r: Rope; filename: AbsoluteFile): bool](ropes#writeRopeIfNotEqual%2CRope%2CAbsoluteFile)
[wrongRedefinition:](#wrongRedefinition)
* [lookups: wrongRedefinition(c: PContext; info: TLineInfo; s: string; conflictsWith: TLineInfo)](lookups#wrongRedefinition%2CPContext%2CTLineInfo%2Cstring%2CTLineInfo)
[wSafecall:](#wSafecall)
* [TSpecialWord.wSafecall](wordrecg#wSafecall)
[wSafecode:](#wSafecode)
* [TSpecialWord.wSafecode](wordrecg#wSafecode)
[wShallow:](#wShallow)
* [TSpecialWord.wShallow](wordrecg#wShallow)
[wShl:](#wShl)
* [TSpecialWord.wShl](wordrecg#wShl)
[wShort:](#wShort)
* [TSpecialWord.wShort](wordrecg#wShort)
[wShr:](#wShr)
* [TSpecialWord.wShr](wordrecg#wShr)
[wSideEffect:](#wSideEffect)
* [TSpecialWord.wSideEffect](wordrecg#wSideEffect)
[wSigned:](#wSigned)
* [TSpecialWord.wSigned](wordrecg#wSigned)
[wSinkInference:](#wSinkInference)
* [TSpecialWord.wSinkInference](wordrecg#wSinkInference)
[wSize:](#wSize)
* [TSpecialWord.wSize](wordrecg#wSize)
[wSizeof:](#wSizeof)
* [TSpecialWord.wSizeof](wordrecg#wSizeof)
[wStackTrace:](#wStackTrace)
* [TSpecialWord.wStackTrace](wordrecg#wStackTrace)
[wStar:](#wStar)
* [TSpecialWord.wStar](wordrecg#wStar)
[wStatic:](#wStatic)
* [TSpecialWord.wStatic](wordrecg#wStatic)
[wStatic\_assert:](#wStatic_assert)
* [TSpecialWord.wStatic\_assert](wordrecg#wStatic_assert)
[wStaticBoundchecks:](#wStaticBoundchecks)
* [TSpecialWord.wStaticBoundchecks](wordrecg#wStaticBoundchecks)
[wStatic\_cast:](#wStatic_cast)
* [TSpecialWord.wStatic\_cast](wordrecg#wStatic_cast)
[wStdcall:](#wStdcall)
* [TSpecialWord.wStdcall](wordrecg#wStdcall)
[wStdErr:](#wStdErr)
* [TSpecialWord.wStdErr](wordrecg#wStdErr)
[wStdIn:](#wStdIn)
* [TSpecialWord.wStdIn](wordrecg#wStdIn)
[wStdOut:](#wStdOut)
* [TSpecialWord.wStdOut](wordrecg#wStdOut)
[wStrDefine:](#wStrDefine)
* [TSpecialWord.wStrDefine](wordrecg#wStrDefine)
[wStruct:](#wStruct)
* [TSpecialWord.wStruct](wordrecg#wStruct)
[wStyleChecks:](#wStyleChecks)
* [TSpecialWord.wStyleChecks](wordrecg#wStyleChecks)
[wSubsChar:](#wSubsChar)
* [TSpecialWord.wSubsChar](wordrecg#wSubsChar)
[wSwitch:](#wSwitch)
* [TSpecialWord.wSwitch](wordrecg#wSwitch)
[wSyscall:](#wSyscall)
* [TSpecialWord.wSyscall](wordrecg#wSyscall)
[wTags:](#wTags)
* [TSpecialWord.wTags](wordrecg#wTags)
[wTemplate:](#wTemplate)
* [TSpecialWord.wTemplate](wordrecg#wTemplate)
[wThis:](#wThis)
* [TSpecialWord.wThis](wordrecg#wThis)
[wThiscall:](#wThiscall)
* [TSpecialWord.wThiscall](wordrecg#wThiscall)
[wThread:](#wThread)
* [TSpecialWord.wThread](wordrecg#wThread)
[wThread\_local:](#wThread_local)
* [TSpecialWord.wThread\_local](wordrecg#wThread_local)
[wThreadVar:](#wThreadVar)
* [TSpecialWord.wThreadVar](wordrecg#wThreadVar)
[wThrow:](#wThrow)
* [TSpecialWord.wThrow](wordrecg#wThrow)
[wTrMacros:](#wTrMacros)
* [TSpecialWord.wTrMacros](wordrecg#wTrMacros)
[wTrue:](#wTrue)
* [TSpecialWord.wTrue](wordrecg#wTrue)
[wTry:](#wTry)
* [TSpecialWord.wTry](wordrecg#wTry)
[wTuple:](#wTuple)
* [TSpecialWord.wTuple](wordrecg#wTuple)
[wType:](#wType)
* [TSpecialWord.wType](wordrecg#wType)
[wTypedef:](#wTypedef)
* [TSpecialWord.wTypedef](wordrecg#wTypedef)
[wTypeid:](#wTypeid)
* [TSpecialWord.wTypeid](wordrecg#wTypeid)
[wTypename:](#wTypename)
* [TSpecialWord.wTypename](wordrecg#wTypename)
[wTypeof:](#wTypeof)
* [TSpecialWord.wTypeof](wordrecg#wTypeof)
[wUnchecked:](#wUnchecked)
* [TSpecialWord.wUnchecked](wordrecg#wUnchecked)
[wUndef:](#wUndef)
* [TSpecialWord.wUndef](wordrecg#wUndef)
[wUnion:](#wUnion)
* [TSpecialWord.wUnion](wordrecg#wUnion)
[wUnroll:](#wUnroll)
* [TSpecialWord.wUnroll](wordrecg#wUnroll)
[wUnsigned:](#wUnsigned)
* [TSpecialWord.wUnsigned](wordrecg#wUnsigned)
[wUsed:](#wUsed)
* [TSpecialWord.wUsed](wordrecg#wUsed)
[wUsing:](#wUsing)
* [TSpecialWord.wUsing](wordrecg#wUsing)
[wVar:](#wVar)
* [TSpecialWord.wVar](wordrecg#wVar)
[wVarargs:](#wVarargs)
* [TSpecialWord.wVarargs](wordrecg#wVarargs)
[wVirtual:](#wVirtual)
* [TSpecialWord.wVirtual](wordrecg#wVirtual)
[wVoid:](#wVoid)
* [TSpecialWord.wVoid](wordrecg#wVoid)
[wVolatile:](#wVolatile)
* [TSpecialWord.wVolatile](wordrecg#wVolatile)
[wWarning:](#wWarning)
* [TSpecialWord.wWarning](wordrecg#wWarning)
[wWarningAsError:](#wWarningAsError)
* [TSpecialWord.wWarningAsError](wordrecg#wWarningAsError)
[wWarnings:](#wWarnings)
* [TSpecialWord.wWarnings](wordrecg#wWarnings)
[wWchar\_t:](#wWchar_t)
* [TSpecialWord.wWchar\_t](wordrecg#wWchar_t)
[wWhen:](#wWhen)
* [TSpecialWord.wWhen](wordrecg#wWhen)
[wWhile:](#wWhile)
* [TSpecialWord.wWhile](wordrecg#wWhile)
[wWrite:](#wWrite)
* [TSpecialWord.wWrite](wordrecg#wWrite)
[wWrites:](#wWrites)
* [TSpecialWord.wWrites](wordrecg#wWrites)
[wXor:](#wXor)
* [TSpecialWord.wXor](wordrecg#wXor)
[wYield:](#wYield)
* [TSpecialWord.wYield](wordrecg#wYield)
[Zero:](#Zero)
* [int128: Zero](int128#Zero)
| programming_docs |
nim varpartitions varpartitions
=============
Partition variables into different graphs. Used for Nim's write tracking, borrow checking and also for the cursor inference. The algorithm is a reinvention / variation of Steensgaard's algorithm. The used data structure is "union find" with path compression.We perform two passes over the AST:
* Pass one (`computeLiveRanges`): collect livetimes of local variables and whether they are potentially re-assigned.
* Pass two (`traverse`): combine local variables to abstract "graphs". Strict func checking: Ensure that graphs that are connected to const parameters are not mutated.
Cursor inference: Ensure that potential cursors are not borrowed from locations that are connected to a graph that is mutated during the liveness of the cursor. (We track all possible mutations of a graph.)
See <https://nim-lang.github.io/Nim/manual_experimental.html#view-types-algorithm> for a high-level description of how borrow checking works.
Imports
-------
<ast>, <types>, <lineinfos>, <options>, <msgs>, <renderer>, <typeallowed>, <trees>, <isolation_check> Types
-----
```
MutationInfo = object
param: PSym
mutatedHere, connectedVia: TLineInfo
flags: set[SubgraphFlag]
maxMutation, minConnection: AbstractTime
mutations: seq[AbstractTime]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L83)
```
Goal = enum
constParameters, borrowChecking, cursorInference
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L90)
```
Partitions = object
abstractTime: AbstractTime
s: seq[VarIndex]
graphs: seq[MutationInfo]
goals: set[Goal]
unanalysableMutation: bool
inAsgnSource, inConstructor, inNoSideEffectSection: int
inConditional, inLoop: int
owner: PSym
config: ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L95) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L95) Procs
-----
```
proc `$`(config: ConfigRef; g: MutationInfo): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L110)
```
proc hasSideEffect(c: var Partitions; info: var MutationInfo): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L125) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L125)
```
proc computeGraphPartitions(s: PSym; n: PNode; config: ConfigRef;
goals: set[Goal]): Partitions {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L845) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L845)
```
proc checkBorrowedLocations(par: var Partitions; body: PNode; config: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L882) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L882)
```
proc computeCursors(s: PSym; n: PNode; config: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/varpartitions.nim#L911) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/varpartitions.nim#L911)
nim isolation_check isolation\_check
================
Implementation of the check that `recover` needs, see <https://github.com/nim-lang/RFCs/issues/244> for more details.
Imports
-------
<ast>, <types>, <renderer>, <idents> Procs
-----
```
proc canAlias(arg, ret: PType): bool {...}{.raises: [Exception, ERecoverableError],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/isolation_check.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/isolation_check.nim#L72)
```
proc checkIsolate(n: PNode): bool {...}{.raises: [Exception, ERecoverableError],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/isolation_check.nim#L80) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/isolation_check.nim#L80)
nim types types
=====
code owner: Arne Döring e-mail: [email protected] included from types.nim
Imports
-------
<ast>, <astalgo>, <trees>, <msgs>, <platform>, <renderer>, <options>, <lineinfos>, <int128> Types
-----
```
TPreferedDesc = enum
preferName, preferDesc, preferExported, preferModuleInfo, preferGenericArg,
preferTypeName, preferResolved, preferMixed
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L17)
```
TTypeIter = proc (t: PType; closure: RootRef): bool {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L37)
```
TTypeMutator = proc (t: PType; closure: RootRef): PType {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L38)
```
TTypePredicate = proc (t: PType): bool {...}{.nimcall.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L39) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L39)
```
TParamsEquality = enum
paramsNotEqual, paramsEqual, paramsIncompatible
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L47)
```
TTypeFieldResult = enum
frNone, frHeader, frEmbedded
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L272) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L272)
```
TDistinctCompare = enum
dcEq, ## a and b should be the same type
dcEqIgnoreDistinct, ## compare symmetrically: (distinct a) == b, a == b
## or a == (distinct b)
dcEqOrDistinctOf ## a equals b or a is distinct of b
```
how distinct types are to be compared [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L854) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L854)
```
TTypeCmpFlag = enum
IgnoreTupleFields, ## NOTE: Only set this flag for backends!
IgnoreCC, ExactTypeDescValues, ExactGenericParams, ExactConstraints,
ExactGcSafety, AllowCommonBase
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L860) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L860)
```
TTypeCmpFlags = set[TTypeCmpFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L869) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L869)
```
EffectsCompat = enum
efCompat, efRaisesDiffer, efRaisesUnknown, efTagsDiffer, efTagsUnknown,
efLockLevelsDiffer
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1328) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1328)
```
OrdinalType = enum
NoneLike, IntLike, FloatLike
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1387) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1387) Consts
------
```
abstractPtrs = {tyVar, tyPtr, tyRef, tyGenericInst, tyDistinct, tyOrdinal,
tyTypeDesc, tyAlias, tyInferred, tySink, tyLent, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L62)
```
abstractVar = {tyVar, tyGenericInst, tyDistinct, tyOrdinal, tyTypeDesc, tyAlias,
tyInferred, tySink, tyLent, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L64) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L64)
```
abstractRange = {tyGenericInst, tyRange, tyDistinct, tyOrdinal, tyTypeDesc,
tyAlias, tyInferred, tySink, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L66)
```
abstractInst = {tyGenericInst, tyDistinct, tyOrdinal, tyTypeDesc, tyAlias,
tyInferred, tySink, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L69)
```
abstractInstOwned = {tyAlias, tyTypeDesc, tyGenericInst, tyDistinct, tyOrdinal,
tyOwned..tySink, tyInferred}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L71) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L71)
```
skipPtrs = {tyVar, tyPtr, tyRef, tyGenericInst, tyTypeDesc, tyAlias, tyInferred,
tySink, tyLent, tyOwned}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L72)
```
typedescPtrs = {tyAlias, tyTypeDesc, tyGenericInst, tyDistinct, tyOrdinal,
tyPtr..tyVar, tyOwned..tyLent, tyInferred}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L75) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L75)
```
typedescInst = {tyAlias, tyTypeDesc, tyGenericInst, tyDistinct, tyOrdinal,
tyOwned..tySink, tyUserTypeClass, tyInferred}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L76) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L76)
```
szUnknownSize = -3
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L21)
```
szIllegalRecursion = -2
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L22)
```
szUncomputedSize = -1
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L23)
```
szTooBigSize = -4
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L24) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L24) Procs
-----
```
proc base(t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L32)
```
proc invalidGenericInst(f: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L78) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L78)
```
proc isPureObject(typ: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L81)
```
proc isUnsigned(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L87)
```
proc getOrdValue(n: PNode; onError = high(Int128)): Int128 {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L90)
```
proc getFloatValue(n: PNode): BiggestFloat {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L114)
```
proc isIntLit(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L120)
```
proc isFloatLit(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L123) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L123)
```
proc addTypeHeader(result: var string; conf: ConfigRef; typ: PType;
prefer: TPreferedDesc = preferMixed;
getDeclarationPath = true) {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L129)
```
proc getProcHeader(conf: ConfigRef; sym: PSym;
prefer: TPreferedDesc = preferName; getDeclarationPath = true): string {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L133) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L133)
```
proc elemType(t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L154) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L154)
```
proc enumHasHoles(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L163) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L163)
```
proc isOrdinalType(t: PType; allowEnumWithHoles: bool = false): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L167) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L167)
```
proc iterOverType(t: PType; iter: TTypeIter; closure: RootRef): bool {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L205) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L205)
```
proc searchTypeFor(t: PType; predicate: TTypePredicate): bool {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L256) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L256)
```
proc containsObject(t: PType): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L263) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L263)
```
proc analyseObjectWithTypeField(t: PType): TTypeFieldResult {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L306)
```
proc containsGarbageCollectedRef(typ: PType): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L319) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L319)
```
proc containsManagedMemory(typ: PType): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L328) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L328)
```
proc containsTyRef(typ: PType): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L334) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L334)
```
proc containsHiddenPointer(typ: PType): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L341) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L341)
```
proc isFinal(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L384) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L384)
```
proc canFormAcycle(typ: PType): bool {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L388) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L388)
```
proc mutateType(t: PType; iter: TTypeMutator; closure: RootRef): PType {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L420) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L420)
```
proc typeToString(typ: PType; prefer: TPreferedDesc = preferName): string {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L471) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L471)
```
proc firstOrd(conf: ConfigRef; t: PType): Int128 {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L712) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L712)
```
proc firstFloat(t: PType): BiggestFloat {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L752) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L752)
```
proc lastOrd(conf: ConfigRef; t: PType): Int128 {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L767) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L767)
```
proc lastFloat(t: PType): BiggestFloat {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L811) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L811)
```
proc floatRangeCheck(x: BiggestFloat; t: PType): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L826) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L826)
```
proc lengthOrd(conf: ConfigRef; t: PType): Int128 {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L843) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L843)
```
proc sameType(a, b: PType; flags: TTypeCmpFlags = {}): bool {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L897) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L897)
```
proc sameTypeOrNil(a, b: PType; flags: TTypeCmpFlags = {}): bool {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L902) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L902)
```
proc equalParams(a, b: PNode): TParamsEquality {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L933) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L933)
```
proc sameObjectTypes(a, b: PType): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1008) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1008)
```
proc sameDistinctTypes(a, b: PType): bool {...}{.inline, raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1014) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1014)
```
proc sameEnumTypes(a, b: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1017) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1017)
```
proc isGenericAlias(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1060) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1060)
```
proc skipGenericAlias(t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1063) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1063)
```
proc sameFlags(a, b: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1066) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1066)
```
proc sameBackendType(x, y: PType): bool {...}{.
raises: [Exception, ERecoverableError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1188) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1188)
```
proc compareTypes(x, y: PType; cmp: TDistinctCompare = dcEq;
flags: TTypeCmpFlags = {}): bool {...}{.
raises: [Exception, ERecoverableError], tags: [RootEffect].}
```
compares two type for equality (modulo type distinction) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1194) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1194)
```
proc inheritanceDiff(a, b: PType): int {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1205) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1205)
```
proc commonSuperclass(a, b: PType): PType {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1229) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1229)
```
proc matchType(a: PType; pattern: openArray[tuple[k: TTypeKind, i: int]];
last: TTypeKind): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1253) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1253)
```
proc computeSize(conf: ConfigRef; typ: PType): BiggestInt {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1264) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1264)
```
proc getReturnType(s: PSym): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1268)
```
proc getAlign(conf: ConfigRef; typ: PType): BiggestInt {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1273) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1273)
```
proc getSize(conf: ConfigRef; typ: PType): BiggestInt {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1277) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1277)
```
proc containsGenericType(t: PType): bool {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1294) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1294)
```
proc baseOfDistinct(t: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1297) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1297)
```
proc safeInheritanceDiff(a, b: PType): int {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1310) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1310)
```
proc compatibleEffects(formal, actual: PType): EffectsCompat {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1336) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1336)
```
proc isCompileTimeOnly(t: PType): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1369) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1369)
```
proc containsCompileTimeOnly(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1372) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1372)
```
proc safeSkipTypes(t: PType; kinds: TTypeKinds): PType {...}{.raises: [], tags: [].}
```
same as 'skipTypes' but with a simple cycle detector. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1379) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1379)
```
proc classify(t: PType): OrdinalType {...}{.raises: [], tags: [].}
```
for convenient type checking: [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1390) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1390)
```
proc skipConv(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1401) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1401)
```
proc skipHidden(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1414) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1414)
```
proc skipConvTakeType(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1426) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1426)
```
proc isEmptyContainer(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1430) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1430)
```
proc takeType(formal, arg: PType): PType {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1439) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1439)
```
proc skipHiddenSubConv(n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1455) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1455)
```
proc typeMismatch(conf: ConfigRef; info: TLineInfo; formal, actual: PType) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1472) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1472)
```
proc isTupleRecursive(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1514) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1514)
```
proc isException(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1518) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1518)
```
proc isDefectException(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1529) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1529)
```
proc isSinkTypeForParam(t: PType): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L1540) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L1540) Templates
---------
```
template `$`(typ: PType): string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L30)
```
template bindConcreteTypeToUserTypeClass(tc, concrete: PType)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L455) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L455)
```
template isResolvedUserTypeClass(t: PType): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/types.nim#L465) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/types.nim#L465)
```
template foldSizeOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L453) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L453)
```
template foldAlignOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L467) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L467)
```
template foldOffsetOf(conf: ConfigRef; n: PNode; fallback: PNode): PNode
```
Returns an int literal node of the given offsetof expression in `n`. Falls back to `fallback`, if the `offsetof` expression can't be processed. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sizealignoffsetimpl.nim#L481) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sizealignoffsetimpl.nim#L481)
| programming_docs |
nim parampatterns parampatterns
=============
This module implements the pattern matching features for term rewriting macro support.
Imports
-------
<ast>, <types>, <msgs>, <idents>, <renderer>, <wordrecg>, <trees>, <options> Types
-----
```
TAliasRequest = enum
aqNone = 1, aqShouldAlias, aqNoAlias
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L20)
```
TSideEffectAnalysis = enum
seUnknown, seSideEffect, seNoSideEffect
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L134)
```
TAssignableResult = enum
arNone, arLValue, arLocalLValue, arDiscriminant, arLentValue, arStrange
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L173) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L173) Consts
------
```
MaxStackSize = 64
```
max required stack size by the VM [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L43) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L43) Procs
-----
```
proc whichAlias(p: PSym): TAliasRequest {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L51) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L51)
```
proc semNodeKindConstraints(n: PNode; conf: ConfigRef; start: Natural): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
does semantic checking for a node kind pattern and compiles it into an efficient internal format. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L118) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L118)
```
proc checkForSideEffects(n: PNode): TSideEffectAnalysis {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L137) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L137)
```
proc exprRoot(n: PNode): PSym {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L182)
```
proc isAssignable(owner: PSym; n: PNode; isUnsafeAddr = false): TAssignableResult {...}{.
raises: [Exception, ERecoverableError], tags: [RootEffect].}
```
'owner' can be nil! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L212) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L212)
```
proc isLValue(n: PNode): bool {...}{.raises: [Exception, ERecoverableError],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L299) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L299)
```
proc matchNodeKinds(p, n: PNode): bool {...}{.raises: [Exception, ERecoverableError],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/parampatterns.nim#L302) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/parampatterns.nim#L302)
nim aliases aliases
=======
Simple alias analysis for the HLO and the code generators.
Imports
-------
<ast>, <astalgo>, <types>, <trees> Types
-----
```
TAnalysisResult = enum
arNo, arMaybe, arYes
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/aliases.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/aliases.nim#L16) Procs
-----
```
proc isPartOf(a, b: PNode): TAnalysisResult {...}{.
raises: [Exception, ERecoverableError], tags: [RootEffect].}
```
checks if location `a` can be part of location `b`. We treat seqs and strings as pointers because the code gen often just passes them as such.
Note: `a` can only be part of `b`, if `a`'s type can be part of `b`'s type. Since however type analysis is more expensive, we perform it only if necessary.
cases:
YES-cases: x <| x # for general trees x[] <| x x[i] <| x x.f <| x NO-cases: x !<| y # depending on type and symbol kind x[constA] !<| x[constB] x.f !<| x.g x.f !<| y.f iff x !<= y
MAYBE-cases:
>
> x[] ?<| y[] iff compatible type
>
> x[] ?<| y depending on type
>
>
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/aliases.nim#L66) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/aliases.nim#L66)
nim options options
=======
Imports
-------
<lineinfos>, <platform>, <prefixmatches>, <pathutils>, <nimpaths> Types
-----
```
TOption = enum
optNone, optObjCheck, optFieldCheck, optRangeCheck, optBoundsCheck,
optOverflowCheck, optRefCheck, optNaNCheck, optInfCheck, optStaticBoundsCheck,
optStyleCheck, optAssert, optLineDir, optWarns, optHints, optOptimizeSpeed,
optOptimizeSize, optStackTrace, optStackTraceMsgs, optLineTrace, optByRef,
optProfiler, optImplicitStatic, optTrMacros, optMemTracker, optNilSeqs,
optSinkInference, optCursorInference
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L26) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L26)
```
TOptions = set[TOption]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L47) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L47)
```
TGlobalOption = enum
gloptNone, optForceFullMake, optWasNimscript, optListCmd, optCompileOnly,
optNoLinking, optCDebug, optGenDynLib, optGenStaticLib, optGenGuiApp,
optGenScript, optGenMapping, optRun, optUseNimcache, optStyleHint,
optStyleError, optStyleUsages, optSkipSystemConfigFile, optSkipProjConfigFile,
optSkipUserConfigFile, optSkipParentConfigFiles, optNoMain, optUseColors,
optThreads, optStdout, optThreadAnalysis, optTaintMode, optTlsEmulation,
optGenIndex, optEmbedOrigSrc, optIdeDebug, optIdeTerse,
optExcessiveStackTrace, optShowAllMismatches, optWholeProject, optDocInternal,
optMixedMode, optListFullPaths, optNoNimblePath, optHotCodeReloading,
optDynlibOverrideAll, optSeqDestructors, optTinyRtti, optOwnedRefs,
optMultiMethods, optBenchmarkVM, optProduceAsm, optPanics, optNimV1Emulation,
optNimV12Emulation, optSourcemap, optProfileVM, optEnableDeepCopy
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L48) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L48)
```
TGlobalOptions = set[TGlobalOption]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L103)
```
TBackend = enum
backendInvalid = "", backendC = "c", backendCpp = "cpp", backendJs = "js",
backendObjc = "objc"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L110)
```
TCommands = enum
cmdNone, cmdCompileToC, cmdCompileToCpp, cmdCompileToOC, cmdCompileToJS,
cmdCompileToLLVM, cmdInterpret, cmdPretty, cmdDoc, cmdGenDepend, cmdDump,
cmdCheck, cmdParse, cmdScan, cmdIdeTools, cmdDef, cmdRst2html, cmdRst2tex,
cmdInteractive, cmdRun, cmdJsonScript, cmdCompileToBackend
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L120) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L120)
```
TStringSeq = seq[string]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L141) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L141)
```
TGCMode = enum
gcUnselected, gcNone, gcBoehm, gcRegions, gcArc, gcOrc, gcMarkAndSweep,
gcHooks, gcRefc, gcV2, gcGo
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L142) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L142)
```
IdeCmd = enum
ideNone, ideSug, ideCon, ideDef, ideUse, ideDus, ideChk, ideMod, ideHighlight,
ideOutline, ideKnown, ideMsg, ideProject
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L148) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L148)
```
Feature = enum
implicitDeref, dotOperators, callOperator, parallel, destructor, notnil,
dynamicBindSym, forLoopMacros, caseStmtMacros, codeReordering, compiletimeFFI, ## This requires building nim with `-d:nimHasLibFFI`
## which itself requires `nimble install libffi`, see #10150
## Note: this feature can't be localized with {.push.}
vmopsDanger, strictFuncs, views
```
experimental features; DO NOT RENAME THESE! [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L152) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L152)
```
LegacyFeature = enum
allowSemcheckedAstModification, ## Allows to modify a NimNode where the type has already been
## flagged with nfSem. If you actually do this, it will cause
## bugs.
checkUnsignedConversions ## Historically and especially in version 1.0.0 of the language
## conversions to unsigned numbers were checked. In 1.0.4 they
## are not anymore.
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L171) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L171)
```
SymbolFilesOption = enum
disabledSf, writeOnlySf, readOnlySf, v2Sf
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L181) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L181)
```
TSystemCC = enum
ccNone, ccGcc, ccNintendoSwitch, ccLLVM_Gcc, ccCLang, ccBcc, ccVcc, ccTcc,
ccEnv, ccIcl, ccIcc, ccClangCl
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L184) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L184)
```
ExceptionSystem = enum
excNone, excSetjmp, excCpp, excGoto, excQuirky
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L188) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L188)
```
CfileFlag {...}{.pure.} = enum
Cached, ## no need to recompile this time
External ## file was introduced via .compile pragma
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L195) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L195)
```
Cfile = object
nimname*: string
cname*, obj*: AbsoluteFile
flags*: set[CfileFlag]
customArgs*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L199) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L199)
```
CfileList = seq[Cfile]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L204) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L204)
```
Suggest = ref object
section*: IdeCmd
qualifiedPath*: seq[string]
name*: ptr string
filePath*: string
line*: int
column*: int
doc*: string
forth*: string
quality*: range[0 .. 100]
isGlobal*: bool
contextFits*: bool
prefix*: PrefixMatch
symkind*: byte
scope*, localUsages*, globalUsages*: int
tokenLen*: int
version*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L206) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L206)
```
Suggestions = seq[Suggest]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L224) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L224)
```
ProfileInfo = object
time*: float
count*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L226) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L226)
```
ProfileData = ref object
data*: TableRef[TLineInfo, ProfileInfo]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L230) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L230)
```
ConfigRef {...}{.acyclic.} = ref object
backend*: TBackend
target*: Target
linesCompiled*: int
options*: TOptions
globalOptions*: TGlobalOptions
macrosToExpand*: StringTableRef
arcToExpand*: StringTableRef
m*: MsgConfig
evalTemplateCounter*: int
evalMacroCounter*: int
exitcode*: int8
cmd*: TCommands
selectedGC*: TGCMode
exc*: ExceptionSystem
verbosity*: int
numberOfProcessors*: int
evalExpr*: string
lastCmdTime*: float
symbolFiles*: SymbolFilesOption
cppDefines*: HashSet[string]
headerFile*: string
features*: set[Feature]
legacyFeatures*: set[LegacyFeature]
arguments*: string ## the arguments to be passed to the program that
## should be run
ideCmd*: IdeCmd
oldNewlines*: bool
cCompiler*: TSystemCC
modifiedyNotes*: TNoteKinds
cmdlineNotes*: TNoteKinds
foreignPackageNotes*: TNoteKinds
notes*: TNoteKinds
warningAsErrors*: TNoteKinds
mainPackageNotes*: TNoteKinds
mainPackageId*: int
errorCounter*: int
hintCounter*: int
warnCounter*: int
errorMax*: int
maxLoopIterationsVM*: int ## VM: max iterations of all loops
configVars*: StringTableRef
symbols*: StringTableRef ## We need to use a StringTableRef here as defined
## symbols are always guaranteed to be style
## insensitive. Otherwise hell would break lose.
packageCache*: StringTableRef
nimblePaths*: seq[AbsoluteDir]
searchPaths*: seq[AbsoluteDir]
lazyPaths*: seq[AbsoluteDir]
outFile*: RelativeFile
outDir*: AbsoluteDir
jsonBuildFile*: AbsoluteFile
prefixDir*, libpath*, nimcacheDir*: AbsoluteDir
dllOverrides, moduleOverrides*, cfileSpecificOptions*: StringTableRef
projectName*: string
projectPath*: AbsoluteDir
projectFull*: AbsoluteFile
projectIsStdin*: bool
lastMsgWasDot*: bool
projectMainIdx*: FileIndex
projectMainIdx2*: FileIndex
command*: string
commandArgs*: seq[string]
commandLine*: string
extraCmds*: seq[string]
keepComments*: bool
implicitImports*: seq[string]
implicitIncludes*: seq[string]
docSeeSrcUrl*: string
docRoot*: string ## see nim --fullhelp for --docRoot
docCmd*: string ## see nim --fullhelp for --docCmd
cIncludes*: seq[AbsoluteDir]
cLibs*: seq[AbsoluteDir]
cLinkedLibs*: seq[string]
externalToLink*: seq[string]
linkOptionsCmd*: string
compileOptionsCmd*: seq[string]
linkOptions*: string
compileOptions*: string
cCompilerPath*: string
toCompile*: CfileList
suggestionResultHook*: proc (result: Suggest) {...}{.closure.}
suggestVersion*: int
suggestMaxResults*: int
lastLineInfo*: TLineInfo
writelnHook*: proc (output: string) {...}{.closure.}
structuredErrorHook*: proc (config: ConfigRef; info: TLineInfo; msg: string;
severity: Severity) {...}{.closure, gcsafe.}
cppCustomNamespace*: string
vmProfileData*: ProfileData
```
every global configuration fields marked with '\*' are subject to the incremental compilation mechanisms (+) means "part of the dependency" [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L233) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L233) Consts
------
```
hasTinyCBackend = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L18)
```
useEffectSystem = true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L19)
```
useWriteTracking = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L20)
```
hasFFI = false
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L21)
```
copyrightYear = "2021"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L22)
```
harmlessOptions = {optForceFullMake, optNoLinking, optRun, optUseColors,
optStdout}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L106)
```
oldExperimentalFeatures = {implicitDeref, dotOperators, callOperator, parallel}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L370) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L370)
```
ChecksOptions = {optObjCheck, optFieldCheck, optRangeCheck, optOverflowCheck,
optBoundsCheck, optAssert, optNaNCheck, optInfCheck,
optStyleCheck}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L373) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L373)
```
DefaultOptions = {optObjCheck, optFieldCheck, optRangeCheck, optBoundsCheck,
optOverflowCheck, optAssert, optWarns, optRefCheck, optHints,
optStackTrace, optLineTrace, optTrMacros, optStyleCheck,
optCursorInference}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L377) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L377)
```
DefaultGlobalOptions = {optThreadAnalysis, optExcessiveStackTrace,
optListFullPaths}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L381) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L381)
```
genSubDir = r"nimcache"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L544) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L544)
```
NimExt = "nim"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L545) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L545)
```
RodExt = "rod"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L546) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L546)
```
HtmlExt = "html"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L547) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L547)
```
JsonExt = "json"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L548) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L548)
```
TagsExt = "tags"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L549) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L549)
```
TexExt = "tex"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L550) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L550)
```
IniExt = "ini"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L551) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L551)
```
DefaultConfig = r"nim.cfg"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L552) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L552)
```
DefaultConfigNims = r"config.nims"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L553) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L553)
```
DocConfig = r"nimdoc.cfg"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L554) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L554)
```
DocTexConfig = r"nimdoc.tex.cfg"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L555) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L555)
```
htmldocsDir = "htmldocs"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L556) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L556)
```
docRootDefault = "@default"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L557) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L557)
```
oKeepVariableNames = true
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L558) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L558) Procs
-----
```
proc assignIfDefault[T](result: var T; val: T; def = default(T))
```
if `result` was already assigned to a value (that wasn't `def`), this is a noop. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L333) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L333)
```
proc setNoteDefaults(conf: ConfigRef; note: TNoteKind; enabled = true) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L341) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L341)
```
proc setNote(conf: ConfigRef; note: TNoteKind; enabled = true) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L348) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L348)
```
proc hasHint(conf: ConfigRef; note: TNoteKind): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L353) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L353)
```
proc hasWarn(conf: ConfigRef; note: TNoteKind): bool {...}{.inline, raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L359) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L359)
```
proc hcrOn(conf: ConfigRef): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L362) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L362)
```
proc getDateStr(): string {...}{.raises: [], tags: [ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L396) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L396)
```
proc getClockStr(): string {...}{.raises: [], tags: [ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L399) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L399)
```
proc newConfigRef(): ConfigRef {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L411) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L411)
```
proc newPartialConfigRef(): ConfigRef {...}{.raises: [], tags: [].}
```
create a new ConfigRef that is only good enough for error reporting. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L471) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L471)
```
proc cppDefine(c: ConfigRef; define: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L482) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L482)
```
proc isDefined(conf: ConfigRef; symbol: string): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L485) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L485)
```
proc importantComments(conf: ConfigRef): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L533) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L533)
```
proc usesWriteBarrier(conf: ConfigRef): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L534) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L534)
```
proc mainCommandArg(conf: ConfigRef): string {...}{.raises: [], tags: [].}
```
This is intended for commands like check or parse which will work on the main project file unless explicitly given a specific file argument [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L560) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L560)
```
proc existsConfigVar(conf: ConfigRef; key: string): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L569) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L569)
```
proc getConfigVar(conf: ConfigRef; key: string; default = ""): string {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L572) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L572)
```
proc setConfigVar(conf: ConfigRef; key, val: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L575) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L575)
```
proc getOutFile(conf: ConfigRef; filename: RelativeFile; ext: string): AbsoluteFile {...}{.
raises: [OSError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L578) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L578)
```
proc absOutFile(conf: ConfigRef): AbsoluteFile {...}{.raises: [OSError],
tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L585) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L585)
```
proc prepareToWriteOutput(conf: ConfigRef): AbsoluteFile {...}{.
raises: [OSError, IOError], tags: [ReadDirEffect, WriteDirEffect].}
```
Create the output directory and returns a full path to the output file [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L592) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L592)
```
proc getPrefixDir(conf: ConfigRef): AbsoluteDir {...}{.raises: [],
tags: [ReadIOEffect].}
```
Gets the prefix dir, usually the parent directory where the binary resides.
This is overridden by some tools (namely nimsuggest) via the `conf.prefixDir` field.
This should resolve to root of nim sources, whether running nim from a local clone or using installed nim, so that these exist: `result/doc/advopt.txt`
and `result/lib/system.nim`
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L597) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L597)
```
proc setDefaultLibpath(conf: ConfigRef) {...}{.raises: [OSError],
tags: [ReadIOEffect, ReadDirEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L608) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L608)
```
proc canonicalizePath(conf: ConfigRef; path: AbsoluteFile): AbsoluteFile {...}{.
raises: [OSError], tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L632) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L632)
```
proc removeTrailingDirSep(path: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L635) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L635)
```
proc disableNimblePath(conf: ConfigRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L641) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L641)
```
proc clearNimblePath(conf: ConfigRef) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L646) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L646)
```
proc getNimbleFile(conf: ConfigRef; path: string): string {...}{.raises: [KeyError],
tags: [ReadDirEffect].}
```
returns absolute path to nimble file, e.g.: /pathto/cligen.nimble [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/packagehandling.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/packagehandling.nim#L18)
```
proc getPackageName(conf: ConfigRef; path: string): string {...}{.raises: [KeyError],
tags: [ReadDirEffect].}
```
returns nimble package name, e.g.: `cligen` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/packagehandling.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/packagehandling.nim#L37)
```
proc fakePackageName(conf: ConfigRef; path: AbsoluteFile): string {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/packagehandling.nim#L42) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/packagehandling.nim#L42)
```
proc demanglePackageName(path: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/packagehandling.nim#L49) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/packagehandling.nim#L49)
```
proc withPackageName(conf: ConfigRef; path: AbsoluteFile): AbsoluteFile {...}{.
raises: [KeyError, OSError, Exception], tags: [ReadDirEffect, RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/packagehandling.nim#L52) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/packagehandling.nim#L52)
```
proc getNimcacheDir(conf: ConfigRef): AbsoluteDir {...}{.raises: [OSError],
tags: [ReadEnvEffect, ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L658) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L658)
```
proc pathSubs(conf: ConfigRef; p, config: string): string {...}{.
raises: [ValueError, OSError], tags: [ReadEnvEffect, ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L668) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L668)
```
proc toGeneratedFile(conf: ConfigRef; path: AbsoluteFile; ext: string): AbsoluteFile {...}{.
raises: [OSError], tags: [ReadEnvEffect, ReadIOEffect].}
```
converts "/home/a/mymodule.nim", "rod" to "/home/a/nimcache/mymodule.rod" [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L689) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L689)
```
proc completeGeneratedFilePath(conf: ConfigRef; f: AbsoluteFile;
createSubDir: bool = true): AbsoluteFile {...}{.
raises: [OSError, IOError], tags: [ReadEnvEffect, ReadIOEffect,
WriteDirEffect, ReadDirEffect,
WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L694) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L694)
```
proc getRelativePathFromConfigPath(conf: ConfigRef; f: AbsoluteFile): RelativeFile {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L741) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L741)
```
proc findFile(conf: ConfigRef; f: string; suppressStdlib = false): AbsoluteFile {...}{.
raises: [OSError, KeyError], tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L751) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L751)
```
proc findModule(conf: ConfigRef; modulename, currentModule: string): AbsoluteFile {...}{.
raises: [OSError, KeyError], tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L772) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L772)
```
proc findProjectNimFile(conf: ConfigRef; pkg: string): string {...}{.
raises: [OSError], tags: [ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L793) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L793)
```
proc inclDynlibOverride(conf: ConfigRef; lib: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L841) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L841)
```
proc isDynlibOverride(conf: ConfigRef; lib: string): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L844) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L844)
```
proc parseIdeCmd(s: string): IdeCmd {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L848) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L848)
```
proc `$`(c: IdeCmd): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L864) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L864)
```
proc floatInt64Align(conf: ConfigRef): int16 {...}{.raises: [], tags: [].}
```
Returns either 4 or 8 depending on reasons. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L880) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L880)
```
proc setOutFile(conf: ConfigRef) {...}{.raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L890) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L890) Iterators
---------
```
iterator nimbleSubs(conf: ConfigRef; p: string): string {...}{.raises: [ValueError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L680) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L680) Templates
---------
```
template setErrorMaxHighMaybe(conf: ConfigRef)
```
do not stop after first error (but honor --errorMax if provided) [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L337) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L337)
```
template depConfigFields(fn) {...}{.dirty.}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L364) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L364)
```
template newPackageCache(): untyped
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L402) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L402)
```
template compilationCachePresent(conf: ConfigRef): untyped
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L536) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L536)
```
template optPreserveOrigSource(conf: ConfigRef): untyped
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/options.nim#L540) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/options.nim#L540)
| programming_docs |
nim vmconv vmconv
======
Imports
-------
<ast> Procs
-----
```
proc fromLit(a: PNode; T: typedesc): auto
```
generic PNode => type see also reverse operation `toLit` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmconv.nim#L8) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmconv.nim#L8)
```
proc toLit[T](a: T): PNode
```
generic type => PNode see also reverse operation `fromLit` [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmconv.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmconv.nim#L19) Templates
---------
```
template elementType(T: typedesc): typedesc
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmconv.nim#L3) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmconv.nim#L3)
nim liftdestructors liftdestructors
===============
This module implements lifting for type-bound operations (`=sink`, `=`, `=destroy`, `=deepCopy`).
Imports
-------
<modulegraphs>, <lineinfos>, <idents>, <ast>, <renderer>, <semdata>, <sighashes>, <lowerings>, <options>, <types>, <msgs>, <magicsys>, <trees> Procs
-----
```
proc produceDestructorForDiscriminator(g: ModuleGraph; typ: PType; field: PSym;
info: TLineInfo): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/liftdestructors.nim#L947) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/liftdestructors.nim#L947)
```
proc createTypeBoundOps(g: ModuleGraph; c: PContext; orig: PType;
info: TLineInfo) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
In the semantic pass this is called in strategic places to ensure we lift assignment, destructors and moves properly. The later 'injectdestructors' pass depends on it. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/liftdestructors.nim#L1005) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/liftdestructors.nim#L1005) Templates
---------
```
template liftTypeBoundOps(c: PContext; typ: PType; info: TLineInfo)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/liftdestructors.nim#L969) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/liftdestructors.nim#L969)
nim pragmas pragmas
=======
Imports
-------
<condsyms>, <ast>, <astalgo>, <idents>, <semdata>, <msgs>, <renderer>, <wordrecg>, <ropes>, <options>, <extccomp>, <magicsys>, <trees>, <types>, <lookups>, <lineinfos>, <pathutils>, <linter> Consts
------
```
FirstCallConv = wNimcall
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L18)
```
LastCallConv = wNoconv
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L19)
```
procPragmas = {wMagic..wThread, wConstructor, wDelegator,
wImportCpp..wExportNims, wNodecl, wSideEffect..wMerge,
wDynlib..wProcVar, wUsed, wError, wStackTrace..wLineTrace,
wDeprecated..wVarargs, wNimcall..wNoconv, wNonReloadable,
wRaises, wTags..wEnsures, wNoDestroy, wCompileTime..wNoInit,
wBorrow..wDiscardable, wGensym..wInject, wAsmNoStackFrame,
wCodegenDecl, wLocks, wLiftLocals, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L25) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L25)
```
converterPragmas = {wMagic..wThread, wConstructor, wDelegator,
wImportCpp..wExportNims, wNodecl, wSideEffect..wMerge,
wDynlib..wProcVar, wUsed, wError, wStackTrace..wLineTrace,
wDeprecated..wVarargs, wNimcall..wNoconv, wNonReloadable,
wRaises, wTags..wEnsures, wNoDestroy, wCompileTime..wNoInit,
wBorrow..wDiscardable, wGensym..wInject, wAsmNoStackFrame,
wCodegenDecl, wLocks, wLiftLocals, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L33)
```
methodPragmas = {wMagic..wThread, wConstructor, wDelegator,
wImportObjC..wExportNims, wNodecl, wSideEffect..wMerge,
wDynlib..wUsed, wError, wStackTrace..wLineTrace,
wDeprecated..wVarargs, wNimcall..wNoconv, wNonReloadable,
wRaises, wTags..wEnsures, wNoDestroy, wCompileTime..wNoInit,
wBorrow..wDiscardable, wGensym..wInject, wAsmNoStackFrame,
wCodegenDecl, wLocks, wLiftLocals, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L34) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L34)
```
templatePragmas = {wDeprecated, wError, wGensym, wInject, wDirty, wDelegator,
wExportNims, wUsed, wPragma}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L35)
```
macroPragmas = {wMagic, wDelegator, wImportCpp..wImportObjC,
wImportc..wExportNims, wNodecl, wNoSideEffect,
wCompilerProc..wCore, wUsed, wError, wDeprecated,
wNimcall..wNoconv, wNonReloadable, wDiscardable,
wGensym..wInject, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L37)
```
iteratorPragmas = {wMagic, wImportCpp..wImportObjC, wImportc..wExportNims,
wNodecl, wSideEffect, wNoSideEffect..wGcSafe, wUsed, wError,
wDeprecated, wNimcall..wNoconv, wRaises, wTags..wEnsures,
wBorrow..wDiscardable, wGensym..wInject, wLocks, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L40)
```
exprPragmas = {wLine, wLocks, wNoRewrite, wGcSafe, wNoSideEffect}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L44)
```
stmtPragmas = {wChecks, wObjChecks, wFieldChecks, wRangeChecks, wBoundChecks,
wOverflowChecks, wNilChecks, wStaticBoundchecks, wStyleChecks,
wAssertions, wWarnings, wHints, wLineDir, wStackTrace,
wLineTrace, wOptimization, wHint, wWarning, wError, wFatal,
wDefine, wUndef, wCompile, wLink, wLinksys, wPure, wPush, wPop,
wPassl, wPassc, wLocalPassc, wDeadCodeElimUnused, wDeprecated,
wFloatChecks, wInfChecks, wNanChecks, wPragma, wEmit, wUnroll,
wLinearScanEnd, wPatterns, wTrMacros, wEffects, wNoForward,
wReorder, wComputedGoto, wInjectStmt, wExperimental, wThis,
wUsed, wInvariant, wAssume, wAssert}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L45) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L45)
```
lambdaPragmas = {wNimcall..wNoconv, wNoSideEffect, wSideEffect, wNoreturn,
wNosinks, wDynlib, wHeader, wThread, wAsmNoStackFrame, wRaises,
wLocks, wTags, wRequires, wEnsures, wGcSafe, wCodegenDecl,
wNoInit}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L57)
```
typePragmas = {wMagic, wFinal, wImportCpp..wImportObjC, wImportc..wRequiresInit,
wNodecl..wPure, wHeader, wGcSafe, wCompilerProc..wCore, wUsed,
wError, wDeprecated, wSize, wPackage, wBorrow,
wAcyclic..wShallow, wGensym..wInject, wInheritable, wUnchecked,
wPartial..wExplain, wExtern, wUnion..wPacked, wByCopy..wByRef}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L62) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L62)
```
fieldPragmas = {wCursor..wNoalias, wImportCpp..wImportObjC,
wImportc..wExportCpp, wRequiresInit..wAlign, wUsed, wError,
wDeprecated, wGuard, wExtern, wBitsize}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L67) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L67)
```
varPragmas = {wMagic, wCursor..wNoalias, wImportCpp..wImportObjC,
wImportc..wExportNims, wAlign..wNodecl, wHeader, wDynlib..wCore,
wUsed, wError, wDeprecated, wCompileTime..wNoInit,
wGensym..wInject, wThreadVar, wGlobal..wCodegenDecl, wGuard,
wExtern, wGoto, wRegister, wVolatile}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L69)
```
constPragmas = {wMagic, wIntDefine..wBoolDefine, wImportCpp..wImportObjC,
wImportc..wExportNims, wNodecl, wHeader, wCompilerProc..wCore,
wUsed, wError, wDeprecated, wGensym..wInject, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L74)
```
paramPragmas = {wNoalias, wInject, wGensym}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L77)
```
letPragmas = {wMagic, wCursor..wNoalias, wImportCpp..wImportObjC,
wImportc..wExportNims, wAlign..wNodecl, wHeader, wDynlib..wCore,
wUsed, wError, wDeprecated, wCompileTime..wNoInit,
wGensym..wInject, wThreadVar, wGlobal..wCodegenDecl, wGuard,
wExtern, wGoto, wRegister, wVolatile}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L78) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L78)
```
procTypePragmas = {wNimcall..wNoconv, wVarargs, wNoSideEffect, wThread, wRaises,
wLocks, wTags, wGcSafe, wRequires, wEnsures}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L79) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L79)
```
forVarPragmas = {wInject, wGensym}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L82)
```
allRoutinePragmas = {wMagic..wThread, wConstructor, wDelegator,
wImportCpp..wExportNims, wNodecl, wSideEffect..wMerge,
wDynlib..wUsed, wError, wStackTrace..wLineTrace,
wDeprecated..wVarargs, wNimcall..wNoconv, wNonReloadable,
wRaises, wTags..wEnsures, wNoDestroy,
wCompileTime..wNoInit, wBorrow..wDiscardable,
wGensym..wInject, wAsmNoStackFrame, wCodegenDecl, wLocks,
wLiftLocals, wExtern}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L83)
```
enumFieldPragmas = {wDeprecated}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L84) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L84) Procs
-----
```
proc getPragmaVal(procAst: PNode; name: TSpecialWord): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L86)
```
proc invalidPragma(c: PContext; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L107)
```
proc illegalCustomPragma(c: PContext; n: PNode; s: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L109)
```
proc pragmaAsm(c: PContext; n: PNode): char {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L132)
```
proc semAsmOrEmit(con: PContext; n: PNode; marker: char): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L544) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L544)
```
proc implicitPragmas(c: PContext; sym: PSym; n: PNode;
validPragmas: TSpecialWords) {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, OSError, KeyError, EOFError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect,
WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L1241) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L1241)
```
proc hasPragma(n: PNode; pragma: TSpecialWord): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L1265) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L1265)
```
proc pragma(c: PContext; sym: PSym; n: PNode; validPragmas: TSpecialWords;
isStatement: bool = false) {...}{.raises: [Exception, ValueError,
IOError, ERecoverableError, OSError, KeyError, EOFError], tags: [RootEffect,
WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect, WriteDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/pragmas.nim#L1283) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/pragmas.nim#L1283)
nim vmdeps vmdeps
======
Imports
-------
<ast>, <types>, <msgs>, <options>, <idents>, <lineinfos>, <pathutils> Procs
-----
```
proc opSlurp(file: string; info: TLineInfo; module: PSym; conf: ConfigRef): string {...}{.raises: [
OSError, KeyError, Exception, ValueError, IOError, ERecoverableError], tags: [
ReadDirEffect, ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdeps.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdeps.nim#L13)
```
proc opMapTypeToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdeps.nim#L301) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdeps.nim#L301)
```
proc opMapTypeInstToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdeps.nim#L306) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdeps.nim#L306)
```
proc opMapTypeImplToAst(cache: IdentCache; t: PType; info: TLineInfo): PNode {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmdeps.nim#L311) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmdeps.nim#L311)
nim ndi ndi
===
This module implements the generation of `.ndi` files for better debugging support of Nim code. "ndi" stands for "Nim debug info".
Imports
-------
<ast>, <msgs>, <ropes>, <options>, <pathutils> Types
-----
```
NdiFile = object
enabled: bool
f: File
buf: string
filename: AbsoluteFile
syms: seq[PSym]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ndi.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ndi.nim#L16) Procs
-----
```
proc open(f: var NdiFile; filename: AbsoluteFile; conf: ConfigRef) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ndi.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ndi.nim#L35)
```
proc close(f: var NdiFile; conf: ConfigRef) {...}{.raises: [IOError],
tags: [WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ndi.nim#L41) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ndi.nim#L41) Templates
---------
```
template writeMangledName(f: NdiFile; s: PSym; conf: ConfigRef)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/ndi.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/ndi.nim#L32)
nim guards guards
======
This module implements the 'implies' relation for guards.
Imports
-------
<ast>, <astalgo>, <msgs>, <magicsys>, <nimsets>, <trees>, <types>, <renderer>, <idents>, <saturate>, <modulegraphs>, <options>, <lineinfos>, <int128> Types
-----
```
Operators = object
opNot*, opContains*, opLe*, opLt*, opAnd*, opOr*, opIsNil*, opEq*: PSym
opAdd*, opSub*, opMul*, opDiv*, opLen*: PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L86) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L86)
```
TModel = object
s*: seq[PNode]
o*: Operators
beSmart*: bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L405) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L405) Procs
-----
```
proc interestingCaseExpr(m: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L83) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L83)
```
proc initOperators(g: ModuleGraph): Operators {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L90)
```
proc buildCall(op: PSym; a: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L159)
```
proc buildCall(op: PSym; a, b: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L164) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L164)
```
proc lowBound(conf: ConfigRef; x: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L210) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L210)
```
proc highBound(conf: ConfigRef; x: PNode; o: Operators): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L214) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L214)
```
proc buildLe(o: Operators; a, b: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L252) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L252)
```
proc canon(n: PNode; o: Operators): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L255) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L255)
```
proc buildAdd(a: PNode; b: BiggestInt; o: Operators): PNode {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L332) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L332)
```
proc addFact(m: var TModel; nn: PNode) {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L410) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L410)
```
proc addFactNeg(m: var TModel; n: PNode) {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L423) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L423)
```
proc sameTree(a, b: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L439) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L439)
```
proc invalidateFacts(s: var seq[PNode]; n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L467) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L467)
```
proc invalidateFacts(m: var TModel; n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L488) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L488)
```
proc doesImply(facts: TModel; prop: PNode): TImplication {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L734) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L734)
```
proc impliesNotNil(m: TModel; arg: PNode): TImplication {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L742) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L742)
```
proc simpleSlice(a, b: PNode): BiggestInt {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L745) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L745)
```
proc proveLe(m: TModel; a, b: PNode): TImplication {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L979) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L979)
```
proc addFactLe(m: var TModel; a, b: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L988) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L988)
```
proc addFactLt(m: var TModel; a, b: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L991) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L991)
```
proc addDiscriminantFact(m: var TModel; n: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L1021) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L1021)
```
proc addAsgnFact(m: var TModel; key, value: PNode) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L1028) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L1028)
```
proc sameSubexprs(m: TModel; a, b: PNode): bool {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L1035) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L1035)
```
proc addCaseBranchFacts(m: var TModel; n: PNode; i: int) {...}{.
raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L1051) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L1051)
```
proc checkFieldAccess(m: TModel; n: PNode; conf: ConfigRef) {...}{.
raises: [ERecoverableError, Exception, ValueError, IOError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/guards.nim#L1072) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/guards.nim#L1072)
| programming_docs |
nim docgen docgen
======
Imports
-------
<ast>, <options>, <msgs>, <ropes>, <idents>, <wordrecg>, <syntaxes>, <renderer>, <lexer>, <trees>, <types>, <typesrenderer>, <astalgo>, <lineinfos>, <pathutils>, <trees>, <nimpaths>, <renderverbatim> Types
-----
```
PDoc = ref TDocumentor
```
Alias to type less. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L57) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L57) Procs
-----
```
proc presentationPath(conf: ConfigRef; file: AbsoluteFile; isTitle = false): RelativeFile {...}{.raises: [
Exception, KeyError, ValueError, IOError, ERecoverableError, OSError], tags: [
RootEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
returns a relative file that will be appended to outDir [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L59) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L59)
```
proc newDocumentor(filename: AbsoluteFile; cache: IdentCache; conf: ConfigRef;
outExt: string = HtmlExt; module: PSym = nil): PDoc {...}{.raises: [
ValueError, OSError, KeyError, Exception, IOError, ERecoverableError], tags: [
ReadDirEffect, RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L165) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L165)
```
proc documentRaises(cache: IdentCache; n: PNode) {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1020) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1020)
```
proc generateDoc(d: PDoc; n, orig: PNode; docFlags: DocFlags = kDefault) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, OSError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1038) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1038)
```
proc generateJson(d: PDoc; n: PNode; includeComments: bool = true) {...}{.raises: [
KeyError, Exception, ValueError, OSError, IOError, ERecoverableError], tags: [
RootEffect, ReadEnvEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1095) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1095)
```
proc generateTags(d: PDoc; n: PNode; r: var Rope) {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1139) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1139)
```
proc generateIndex(d: PDoc) {...}{.raises: [OSError, Exception, KeyError, ValueError,
IOError, ERecoverableError], tags: [
RootEffect, ReadDirEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1254) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1254)
```
proc writeOutput(d: PDoc; useWarning = false; groupedToc = false) {...}{.raises: [
OSError, ValueError, Exception, IOError, ERecoverableError, KeyError], tags: [
ReadEnvEffect, ReadIOEffect, ExecIOEffect, RootEffect, WriteIOEffect,
ReadDirEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1268) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1268)
```
proc writeOutputJson(d: PDoc; useWarning = false) {...}{.raises: [OSError,
ValueError, Exception, IOError, ERecoverableError, KeyError], tags: [
ReadEnvEffect, ReadIOEffect, ExecIOEffect, RootEffect, WriteIOEffect,
ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1290) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1290)
```
proc handleDocOutputOptions(conf: ConfigRef) {...}{.raises: [OSError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1312) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1312)
```
proc commandDoc(cache: IdentCache; conf: ConfigRef) {...}{.raises: [OSError,
Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1319) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1319)
```
proc commandRst2Html(cache: IdentCache; conf: ConfigRef) {...}{.raises: [ValueError,
OSError, KeyError, Exception, IOError, ERecoverableError], tags: [
ReadDirEffect, RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1343) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1343)
```
proc commandRst2TeX(cache: IdentCache; conf: ConfigRef) {...}{.raises: [ValueError,
OSError, KeyError, Exception, IOError, ERecoverableError], tags: [
ReadDirEffect, RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ExecIOEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1346) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1346)
```
proc commandJson(cache: IdentCache; conf: ConfigRef) {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1349) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1349)
```
proc commandTags(cache: IdentCache; conf: ConfigRef) {...}{.raises: [Exception,
ValueError, IOError, ERecoverableError, KeyError, OSError], tags: [
RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1370) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1370)
```
proc commandBuildIndex(conf: ConfigRef; dir: string; outFile = RelativeFile"") {...}{.
raises: [OSError, IOError, ValueError, Exception, ERecoverableError], tags: [
ReadDirEffect, ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect,
TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen.nim#L1391) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen.nim#L1391)
nim vmmarshal vmmarshal
=========
Implements marshaling for the VM.
Imports
-------
<ast>, <astalgo>, <idents>, <types>, <msgs>, <options>, <lineinfos> Procs
-----
```
proc storeAny(s: var string; t: PType; a: PNode; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmmarshal.nim#L132) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmmarshal.nim#L132)
```
proc loadAny(s: string; t: PType; cache: IdentCache; conf: ConfigRef): PNode {...}{.raises: [
IOError, OSError, JsonParsingError, ValueError, Exception, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/vmmarshal.nim#L283) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/vmmarshal.nim#L283)
nim nimsets nimsets
=======
Imports
-------
<ast>, <astalgo>, <lineinfos>, <bitsets>, <types>, <options> Procs
-----
```
proc inSet(s: PNode; elem: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L15)
```
proc overlap(a, b: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L30) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L30)
```
proc someInSet(s: PNode; a, b: PNode): bool {...}{.raises: [ERecoverableError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L44) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L44)
```
proc toBitSet(conf: ConfigRef; s: PNode): TBitSet {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L61) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L61)
```
proc toTreeSet(conf: ConfigRef; s: TBitSet; settype: PType; info: TLineInfo): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L74) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L74)
```
proc unionSets(conf: ConfigRef; a, b: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L114) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L114)
```
proc diffSets(conf: ConfigRef; a, b: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L115) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L115)
```
proc intersectSets(conf: ConfigRef; a, b: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L116)
```
proc symdiffSets(conf: ConfigRef; a, b: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L117) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L117)
```
proc containsSets(conf: ConfigRef; a, b: PNode): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L119) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L119)
```
proc equalSets(conf: ConfigRef; a, b: PNode): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L124) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L124)
```
proc complement(conf: ConfigRef; a: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L129)
```
proc deduplicate(conf: ConfigRef; a: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L134) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L134)
```
proc cardSet(conf: ConfigRef; a: PNode): BiggestInt {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L138) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L138)
```
proc setHasRange(s: PNode): bool {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L142) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L142)
```
proc emptyRange(a, b: PNode): bool {...}{.raises: [ERecoverableError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimsets.nim#L151) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimsets.nim#L151)
nim idents idents
======
Imports
-------
<wordrecg> Types
-----
```
TIdObj = object of RootObj
id*: int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L18)
```
PIdObj = ref TIdObj
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L21)
```
PIdent = ref TIdent
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L22) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L22)
```
TIdent {...}{.acyclic.} = object of TIdObj
s*: string
next*: PIdent
h*: Hash
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L23)
```
IdentCache = ref object
buckets: array[0 .. 8192 - 1, PIdent]
wordCounter: int
idAnon*, idDelegator*, emptyIdent*: PIdent
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L28) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L28) Procs
-----
```
proc resetIdentCache() {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L33) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L33)
```
proc cmpIgnoreStyle(a, b: cstring; blen: int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L35) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L35)
```
proc getIdent(ic: IdentCache; identifier: cstring; length: int; h: Hash): PIdent {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L69) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L69)
```
proc getIdent(ic: IdentCache; identifier: string): PIdent {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L99)
```
proc getIdent(ic: IdentCache; identifier: string; h: Hash): PIdent {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L103) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L103)
```
proc newIdentCache(): IdentCache {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L106) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L106)
```
proc whichKeyword(id: PIdent): TSpecialWord {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/idents.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/idents.nim#L116)
nim semparallel semparallel
===========
Semantic checking for 'parallel'.
Imports
-------
<ast>, <astalgo>, <idents>, <lowerings>, <magicsys>, <guards>, <msgs>, <renderer>, <types>, <modulegraphs>, <options>, <spawn>, <lineinfos>, <trees> Procs
-----
```
proc liftParallel(g: ModuleGraph; owner: PSym; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semparallel.nim#L468) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semparallel.nim#L468)
nim sempass2 sempass2
========
Imports
-------
<ast>, <astalgo>, <msgs>, <renderer>, <magicsys>, <types>, <idents>, <trees>, <wordrecg>, <options>, <guards>, <lineinfos>, <semfold>, <semdata>, <modulegraphs>, <varpartitions>, <typeallowed>, <liftdestructors> Procs
-----
```
proc checkForSink(config: ConfigRef; owner: PSym; arg: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sinkparameter_inference.nim#L10) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sinkparameter_inference.nim#L10)
```
proc checkMethodEffects(g: ModuleGraph; disp, branch: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
checks for consistent effects for multi methods. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sempass2.nim#L1170) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sempass2.nim#L1170)
```
proc setEffectsForProcType(g: ModuleGraph; t: PType; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sempass2.nim#L1203) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sempass2.nim#L1203)
```
proc trackProc(c: PContext; s: PSym; body: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sempass2.nim#L1255) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sempass2.nim#L1255)
```
proc trackStmt(c: PContext; module: PSym; n: PNode; isTopLevel: bool) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sempass2.nim#L1349) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sempass2.nim#L1349)
nim docgen2 docgen2
=======
Imports
-------
<options>, <ast>, <msgs>, <passes>, <docgen>, <lineinfos>, <pathutils>, <modulegraphs> Consts
------
```
docgen2Pass = (myOpen, processNode, close, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen2.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen2.nim#L77)
```
docgen2JsonPass = (myOpenJson, processNodeJson, closeJson, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen2.nim#L78) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen2.nim#L78) Procs
-----
```
proc finishDoc2Pass(project: string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/docgen2.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/docgen2.nim#L81)
| programming_docs |
nim treetab treetab
=======
Imports
-------
<ast>, <astalgo>, <types> Procs
-----
```
proc nodeTableGet(t: TNodeTable; key: PNode): int {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/treetab.nim#L63) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/treetab.nim#L63)
```
proc nodeTablePut(t: var TNodeTable; key: PNode; val: int) {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/treetab.nim#L77) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/treetab.nim#L77)
```
proc nodeTableTestOrSet(t: var TNodeTable; key: PNode; val: int): int {...}{.
raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/treetab.nim#L94) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/treetab.nim#L94)
nim jsgen jsgen
=====
Type info generation for the JS backend.
Imports
-------
<ast>, <trees>, <magicsys>, <options>, <nversion>, <msgs>, <idents>, <types>, <ropes>, <passes>, <ccgutils>, <wordrecg>, <renderer>, <cgmeth>, <lowerings>, <sighashes>, <modulegraphs>, <lineinfos>, <rodutils>, <transf>, <injectdestructors>, <sourcemap>, <modulegraphs> Consts
------
```
JSgenPass = (myOpen, myProcess, myClose, false)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/jsgen.nim#L2737) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/jsgen.nim#L2737) Templates
---------
```
template config(p: PProc): ConfigRef
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/jsgen.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/jsgen.nim#L108)
nim sourcemap sourcemap
=========
Imports
-------
<ropes> Types
-----
```
SourceNode = ref object
line*: int
column*: int
source*: string
name*: string
children*: seq[Child]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L4) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L4)
```
Child = ref object
case kind*: C
of cSourceNode:
node*: SourceNode
of cString:
s*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L13)
```
SourceMap = ref object
version*: int
sources*: seq[string]
names*: seq[string]
mappings*: string
file*: string
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L20) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L20)
```
Mapping = ref object
source*: string
original*: tuple[line: int, column: int]
generated*: tuple[line: int, column: int]
name*: string
noSource*: bool
noName*: bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L37) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L37) Procs
-----
```
proc child(s: string): Child {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L46)
```
proc child(node: SourceNode): Child {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L50) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L50)
```
proc text(sourceNode: SourceNode; depth: int): string {...}{.raises: [ValueError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L71) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L71)
```
proc `$`(sourceNode: SourceNode): string {...}{.raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L81) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L81)
```
proc encode(i: int): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L90) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L90)
```
proc parse(source: string; path: string): SourceNode {...}{.raises: [ValueError],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L159) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L159)
```
proc index[T](elements: seq[T]; element: T): int
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L234) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L234)
```
proc gen(map: SourceMapGenerator): SourceMap {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L286) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L286)
```
proc addMapping(map: SourceMapGenerator; mapping: Mapping) {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L298) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L298)
```
proc walk(node: SourceNode; fn: proc (line: string; original: SourceNode)) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L309) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L309)
```
proc toSourceMap(node: SourceNode; file: string): SourceMapGenerator {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L317) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L317)
```
proc genSourceMap(source: string; outFile: string): (Rope, SourceMap) {...}{.
raises: [ValueError], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L379) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L379) Iterators
---------
```
iterator tokenize(line: string): (bool, string) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sourcemap.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sourcemap.nim#L111)
nim sigmatch sigmatch
========
This module implements the signature matching for resolving the call to overloaded procs, generic procs and operators.
This file implements features required for IDE support.
Due to Nim's nature and the fact that `system.nim` is always imported, there are lots of potential symbols. Furthermore thanks to templates and macros even context based analysis does not help much: In a context like `let x: |` where a type has to follow, that type might be constructed from a template like `extractField(MyObject, fieldName)`. We deal with this problem by smart sorting so that the likely symbols come first. This sorting is done this way:
* If there is a prefix (foo|), symbols starting with this prefix come first.
* If the prefix is part of the name (but the name doesn't start with it), these symbols come second.
* If we have a prefix, only symbols matching this prefix are returned and nothing else.
* If we have no prefix, consider the context. We currently distinguish between type and non-type contexts.
* Finally, sort matches by relevance. The relevance is determined by the number of usages, so `strutils.replace` comes before `strutils.wordWrap`.
* In any case, sorting also considers scoping information. Local variables get high priority.
Imports
-------
<ast>, <astalgo>, <semdata>, <types>, <msgs>, <renderer>, <lookups>, <semtypinst>, <magicsys>, <idents>, <lexer>, <options>, <parampatterns>, <trees>, <linter>, <lineinfos>, <lowerings>, <modulegraphs>, <prefixmatches>, <lineinfos>, <linter>, <wordrecg> Types
-----
```
MismatchKind = enum
kUnknown, kAlreadyGiven, kUnknownNamedParam, kTypeMismatch, kVarNeeded,
kMissingParam, kExtraArg, kPositionalAlreadyGiven
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L19)
```
MismatchInfo = object
kind*: MismatchKind
arg*: int
formal*: PSym
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L23) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L23)
```
TCandidateState = enum
csEmpty, csMatch, csNoMatch
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L29)
```
CandidateError = object
sym*: PSym
firstMismatch*: MismatchInfo
diagnostics*: seq[string]
enabled*: bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L32)
```
CandidateErrors = seq[CandidateError]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L38) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L38)
```
TCandidate = object
c*: PContext
exactMatches*: int
genericMatches: int
subtypeMatches: int
intConvMatches: int
convMatches: int
state*: TCandidateState
callee*: PType
calleeSym*: PSym
calleeScope*: int
call*: PNode
bindings*: TIdTable
magic*: TMagic
baseTypeMatch: bool
fauxMatch*: TTypeKind
genericConverter*: bool
coerceDistincts*: bool
typedescMatched*: bool
isNoCall*: bool
inferredTypes: seq[PType]
diagnostics*: seq[string]
inheritancePenalty: int
firstMismatch*: MismatchInfo
diagnosticsEnabled*: bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L40) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L40)
```
TTypeRelFlag = enum
trDontBind, trNoCovariance, trBindGenericParam
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L82) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L82)
```
TTypeRelFlags = set[TTypeRelFlag]
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L87) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L87)
```
TTypeRelation = enum
isNone, isConvertible, isIntConv, isSubtype, isSubrange,
isBothMetaConvertible, isInferred, isInferredConvertible, isGeneric,
isFromIntLit, isEqual
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L89) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L89)
```
TCheckPointResult = enum
cpNone, cpFuzzy, cpExact
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L423) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L423) Procs
-----
```
proc initCandidate(ctx: PContext; c: var TCandidate; callee: PType) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L129) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L129)
```
proc initCandidate(ctx: PContext; c: var TCandidate; callee: PSym;
binding: PNode; calleeScope = -1; diagnosticsEnabled = false) {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L148) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L148)
```
proc newCandidate(ctx: PContext; callee: PSym; binding: PNode; calleeScope = -1): TCandidate {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L182) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L182)
```
proc newCandidate(ctx: PContext; callee: PType): TCandidate {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L186)
```
proc writeMatches(c: TCandidate) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L293) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L293)
```
proc cmpCandidates(a, b: TCandidate): int {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L302) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L302)
```
proc describeArgs(c: PContext; n: PNode; startIdx = 1; prefer = preferName): string {...}{.
raises: [Exception, ValueError], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L336) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L336)
```
proc matchUserTypeClass(m: var TCandidate; ff, a: PType): PType {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L691) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L691)
```
proc inferStaticParam(c: var TCandidate; lhs: PNode; rhs: BiggestInt): bool {...}{.
raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L833) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L833)
```
proc typeRel(c: var TCandidate; f, aOrig: PType; flags: TTypeRelFlags = {}): TTypeRelation {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L991) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L991)
```
proc cmpTypes(c: PContext; f, a: PType): TTypeRelation {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L1862) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L1862)
```
proc paramTypesMatch(m: var TCandidate; f, a: PType; arg, argOrig: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2186) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2186)
```
proc semFinishOperands(c: PContext; n: PNode) {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2507) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2507)
```
proc partialMatch(c: PContext; n, nOrig: PNode; m: var TCandidate) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2513) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2513)
```
proc matches(c: PContext; n, nOrig: PNode; m: var TCandidate) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2518) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2518)
```
proc argtypeMatches(c: PContext; f, a: PType; fromHlo = false): bool {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2577) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2577)
```
proc instTypeBoundOp(c: PContext; dc: PSym; t: PType; info: TLineInfo;
op: TTypeAttachedOp; col: int): PSym {...}{.nosinks,
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L2592) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L2592)
```
proc `$`(suggest: Suggest): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L174) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L174)
```
proc fieldVisible(c: PContext; f: PSym): bool {...}{.inline, raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L253) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L253)
```
proc inCheckpoint(current, trackPos: TLineInfo): TCheckPointResult {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L426) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L426)
```
proc isTracked(current, trackPos: TLineInfo; tokenLen: int): bool {...}{.raises: [],
tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L434) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L434)
```
proc suggestSym(conf: ConfigRef; info: TLineInfo; s: PSym; usageSym: var PSym;
isDecl = true) {...}{.inline, raises: [], tags: [].}
```
misnamed: should be 'symDeclared' [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L486) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L486)
```
proc markOwnerModuleAsUsed(c: PContext; s: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L546) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L546)
```
proc markUsed(c: PContext; info: TLineInfo; s: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L560) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L560)
```
proc safeSemExpr(c: PContext; n: PNode): PNode {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L581) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L581)
```
proc suggestExprNoCheck(c: PContext; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, ESuggestDone],
tags: [RootEffect, ReadIOEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L605) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L605)
```
proc suggestExpr(c: PContext; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, ESuggestDone],
tags: [RootEffect, ReadIOEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L634) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L634)
```
proc suggestDecl(c: PContext; n: PNode; s: PSym) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, ESuggestDone],
tags: [RootEffect, ReadIOEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L637) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L637)
```
proc suggestStmt(c: PContext; n: PNode) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, ESuggestDone],
tags: [RootEffect, ReadIOEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L644) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L644)
```
proc suggestEnum(c: PContext; n: PNode; t: PType) {...}{.
raises: [Exception, ValueError, IOError, ESuggestDone],
tags: [RootEffect, ReadIOEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L647) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L647)
```
proc suggestSentinel(c: PContext) {...}{.raises: [Exception, ValueError, IOError], tags: [
RootEffect, ReadIOEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/suggest.nim#L653) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/suggest.nim#L653) Templates
---------
```
template hasFauxMatch(c: TCandidate): bool
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sigmatch.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sigmatch.nim#L111)
| programming_docs |
nim linter linter
======
This module implements the style checker.
Imports
-------
<options>, <ast>, <msgs>, <idents>, <lineinfos>, <wordrecg> Consts
------
```
Letters = {'a'..'z', 'A'..'Z', '0'..'9', '\x80'..'\xFF', '_'}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L17) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L17) Procs
-----
```
proc identLen(line: string; start: int): int {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L19) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L19)
```
proc differ(line: string; a, b: int; x: string): string {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L72) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L72)
```
proc styleCheckUse(conf: ConfigRef; info: TLineInfo; s: PSym) {...}{.
raises: [ValueError, Exception, IOError, ERecoverableError],
tags: [ReadIOEffect, RootEffect, WriteIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L116) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L116)
```
proc checkPragmaUse(conf: ConfigRef; info: TLineInfo; w: TSpecialWord;
pragmaName: string) {...}{.
raises: [ValueError, Exception, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L130) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L130) Templates
---------
```
template styleCheckDef(conf: ConfigRef; info: TLineInfo; s: PSym; k: TSymKind)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L95) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L95)
```
template styleCheckDef(conf: ConfigRef; info: TLineInfo; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L99) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L99)
```
template styleCheckDef(conf: ConfigRef; s: PSym)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/linter.nim#L101) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/linter.nim#L101)
nim nversion nversion
========
Consts
------
```
MaxSetElements = 65536
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nversion.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nversion.nim#L14)
```
VersionAsString = "1.4.8"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nversion.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nversion.nim#L15)
```
RodFileVersion = "1223"
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nversion.nim#L16) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nversion.nim#L16)
```
NimCompilerApiVersion = 3
```
Check for the existence of this before accessing it as older versions of the compiler API do not declare this. [Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nversion.nim#L18) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nversion.nim#L18)
nim modulepaths modulepaths
===========
Imports
-------
<ast>, <renderer>, <msgs>, <options>, <idents>, <lineinfos>, <pathutils> Procs
-----
```
proc getModuleName(conf: ConfigRef; n: PNode): string {...}{.
raises: [OSError, Exception, ValueError, IOError, ERecoverableError],
tags: [ReadEnvEffect, ReadIOEffect, RootEffect, WriteIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulepaths.nim#L107) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulepaths.nim#L107)
```
proc checkModuleName(conf: ConfigRef; n: PNode; doLocalError = true): FileIndex {...}{.raises: [
OSError, Exception, ValueError, IOError, ERecoverableError, KeyError], tags: [
ReadEnvEffect, ReadIOEffect, RootEffect, WriteIOEffect, ReadDirEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/modulepaths.nim#L155) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/modulepaths.nim#L155)
nim semmacrosanity semmacrosanity
==============
Implements type sanity checking for ASTs resulting from macros. Lots of room for improvement here.
Imports
-------
<ast>, <msgs>, <types>, <options> Procs
-----
```
proc annotateType(n: PNode; t: PType; conf: ConfigRef) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semmacrosanity.nim#L46) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semmacrosanity.nim#L46)
nim nim nim
===
Imports
-------
<commands>, <options>, <msgs>, <extccomp>, <main>, <idents>, <lineinfos>, <cmdlinehelper>, <pathutils>, <modulegraphs>, <nodejs>
nim sem sem
===
This module implements semantic checking for calls.this module does the semantic checking of statementsThis module does the semantic transformation of the fields\* iterators.This module implements Nim's object construction rules.
Imports
-------
<ast>, <options>, <astalgo>, <trees>, <wordrecg>, <ropes>, <msgs>, <idents>, <renderer>, <types>, <platform>, <magicsys>, <nversion>, <nimsets>, <semfold>, <modulepaths>, <importer>, <procfind>, <lookups>, <pragmas>, <passes>, <semdata>, <semtypinst>, <sigmatch>, <transf>, <vmdef>, <vm>, <idgen>, <aliases>, <cgmeth>, <lambdalifting>, <evaltempl>, <patterns>, <parampatterns>, <sempass2>, <linter>, <semmacrosanity>, <lowerings>, <plugins/active>, <rod>, <lineinfos>, <int128>, <isolation_check>, <typeallowed>, <modulegraphs>, <spawn> Consts
------
```
tyUserDefinedGenerics = {tyGenericInst, tyGenericInvocation, tyUserTypeClassInst}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semmagic.nim#L105) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semmagic.nim#L105)
```
tyMagicGenerics = {tySet, tySequence, tyArray, tyOpenArray}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semmagic.nim#L108) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semmagic.nim#L108)
```
tyGenericLike = {tyGenericInvocation, tyGenericInst, tyArray, tySet, tySequence,
tyOpenArray, tyUserTypeClassInst..tyCompositeTypeClass}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semmagic.nim#L110) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semmagic.nim#L110)
```
semPass = (myOpen, myProcess, myClose, true)
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sem.nim#L641) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sem.nim#L641) Procs
-----
```
proc commonType(x, y: PType): PType {...}{.raises: [Exception], tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sem.nim#L111) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sem.nim#L111)
```
proc commonType(x: PType; y: PNode): PType {...}{.raises: [Exception],
tags: [RootEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sem.nim#L190) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sem.nim#L190)
```
proc newSymG(kind: TSymKind; n: PNode; c: PContext): PSym {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sem.nim#L200) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sem.nim#L200)
```
proc pushProcCon(c: PContext; owner: PSym) {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/seminst.nim#L53) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/seminst.nim#L53)
```
proc notFoundError(c: PContext; n: PNode; errors: CandidateErrors) {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semcall.nim#L277) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semcall.nim#L277)
```
proc instGenericConvertersArg(c: PContext; a: PNode; x: TCandidate) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, OSError, KeyError,
EOFError], tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ReadDirEffect, WriteDirEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semcall.nim#L447) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semcall.nim#L447)
```
proc instGenericConvertersSons(c: PContext; n: PNode; x: TCandidate) {...}{.raises: [
Exception, ValueError, IOError, ERecoverableError, OSError, KeyError,
EOFError], tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect,
ReadDirEffect, WriteDirEffect, TimeEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/semcall.nim#L457) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/semcall.nim#L457) Templates
---------
```
template commonTypeBegin(): PType
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/sem.nim#L109) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/sem.nim#L109)
nim plugins/locals plugins/locals
==============
The builtin 'system.locals' implemented as a plugin.
Imports
-------
<../ast>, <../astalgo>, <../magicsys>, <../lookups>, <../semdata>, <../lowerings> Procs
-----
```
proc semLocals(c: PContext; n: PNode): PNode {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/plugins/locals.nim#L15) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/plugins/locals.nim#L15)
nim plugins/itersgen plugins/itersgen
================
Plugin to transform an inline iterator into a data structure.
Imports
-------
<../ast>, <../lookups>, <../semdata>, <../lambdalifting>, <../msgs> Procs
-----
```
proc iterToProcImpl(c: PContext; n: PNode): PNode {...}{.
raises: [Exception, ValueError, IOError, ERecoverableError, KeyError],
tags: [RootEffect, WriteIOEffect, ReadIOEffect, ReadEnvEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/plugins/itersgen.nim#L14) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/plugins/itersgen.nim#L14)
nim plugins/active plugins/active
==============
Include file that imports all plugins that are active.
Imports
-------
<../pluginsupport>, <../idents>, <../ast>, <locals>, <itersgen> Procs
-----
```
proc getPlugin(ic: IdentCache; fn: PSym): Transformation {...}{.raises: [], tags: [].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/plugins/active.nim#L21) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/plugins/active.nim#L21)
nim nimfix/prettybase nimfix/prettybase
=================
Imports
-------
<../ast>, <../msgs>, <../lineinfos>, <../idents>, <../options>, <../linter> Procs
-----
```
proc replaceDeprecated(conf: ConfigRef; info: TLineInfo; oldSym, newSym: PIdent) {...}{.
raises: [], tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimfix/prettybase.nim#L13) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimfix/prettybase.nim#L13)
```
proc replaceDeprecated(conf: ConfigRef; info: TLineInfo; oldSym, newSym: PSym) {...}{.
raises: [], tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimfix/prettybase.nim#L29) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimfix/prettybase.nim#L29)
```
proc replaceComment(conf: ConfigRef; info: TLineInfo) {...}{.raises: [],
tags: [ReadIOEffect].}
```
[Source](https://github.com/nim-lang/Nim/tree/version-1-4/compiler/nimfix/prettybase.nim#L32) [Edit](https://github.com/nim-lang/Nim/edit/devel/compiler/nimfix/prettybase.nim#L32)
composer Basic usage Basic usage
===========
Introduction
------------
For our basic usage introduction, we will be installing `monolog/monolog`, a logging library. If you have not yet installed Composer, refer to the [Intro](00-intro.md) chapter.
> **Note:** for the sake of simplicity, this introduction will assume you have performed a [local](00-intro.md#locally) install of Composer.
>
>
`composer.json`: Project setup
-------------------------------
To start using Composer in your project, all you need is a `composer.json` file. This file describes the dependencies of your project and may contain other metadata as well. It typically should go in the top-most directory of your project/VCS repository. You can technically run Composer anywhere but if you want to publish a package to Packagist.org, it will have to be able to find the file at the top of your VCS repository.
### The `require` key
The first thing you specify in `composer.json` is the [`require`](04-schema.md#require) key. You are telling Composer which packages your project depends on.
```
{
"require": {
"monolog/monolog": "2.0.*"
}
}
```
As you can see, [`require`](04-schema.md#require) takes an object that maps **package names** (e.g. `monolog/monolog`) to **version constraints** (e.g. `1.0.*`).
Composer uses this information to search for the right set of files in package "repositories" that you register using the [`repositories`](04-schema.md#repositories) key, or in [Packagist.org](https://packagist.org), the default package repository. In the above example, since no other repository has been registered in the `composer.json` file, it is assumed that the `monolog/monolog` package is registered on Packagist.org. (Read more [about Packagist](#packagist), and [about repositories](05-repositories.md)).
### Package names
The package name consists of a vendor name and the project's name. Often these will be identical - the vendor name only exists to prevent naming clashes. For example, it would allow two different people to create a library named `json`. One might be named `igorw/json` while the other might be `seldaek/json`.
Read more about [publishing packages and package naming](02-libraries.md). (Note that you can also specify "platform packages" as dependencies, allowing you to require certain versions of server software. See [platform packages](#platform-packages) below.)
### Package version constraints
In our example, we are requesting the Monolog package with the version constraint [`2.0.*`](https://semver.mwl.be/#?package=monolog%2Fmonolog&version=2.0.*). This means any version in the `2.0` development branch, or any version that is greater than or equal to 2.0 and less than 2.1 (`>=2.0 <2.1`).
Please read [versions](articles/versions.md) for more in-depth information on versions, how versions relate to each other, and on version constraints.
> **How does Composer download the right files?** When you specify a dependency in `composer.json`, Composer first takes the name of the package that you have requested and searches for it in any repositories that you have registered using the [`repositories`](04-schema.md#repositories) key. If you have not registered any extra repositories, or it does not find a package with that name in the repositories you have specified, it falls back to Packagist.org (more [below](#packagist)).
>
> When Composer finds the right package, either in Packagist.org or in a repo you have specified, it then uses the versioning features of the package's VCS (i.e., branches and tags) to attempt to find the best match for the version constraint you have specified. Be sure to read about versions and package resolution in the [versions article](articles/versions.md).
>
> **Note:** If you are trying to require a package but Composer throws an error regarding package stability, the version you have specified may not meet your default minimum stability requirements. By default, only stable releases are taken into consideration when searching for valid package versions in your VCS.
>
> You might run into this if you are trying to require dev, alpha, beta, or RC versions of a package. Read more about stability flags and the `minimum-stability` key on the [schema page](04-schema.md).
>
>
Installing dependencies
-----------------------
To initially install the defined dependencies for your project, you should run the [`update`](03-cli.md#update-u) command.
```
php composer.phar update
```
This will make Composer do two things:
* It resolves all dependencies listed in your `composer.json` file and writes all of the packages and their exact versions to the `composer.lock` file, locking the project to those specific versions. You should commit the `composer.lock` file to your project repo so that all people working on the project are locked to the same versions of dependencies (more below). This is the main role of the `update` command.
* It then implicitly runs the [`install`](03-cli.md#install-i) command. This will download the dependencies' files into the `vendor` directory in your project. (The `vendor` directory is the conventional location for all third-party code in a project). In our example from above, you would end up with the Monolog source files in `vendor/monolog/monolog/`. As Monolog has a dependency on `psr/log`, that package's files can also be found inside `vendor/`.
> **Tip:** If you are using git for your project, you probably want to add `vendor` in your `.gitignore`. You really don't want to add all of that third-party code to your versioned repository.
>
>
### Commit your `composer.lock` file to version control
Committing this file to version control is important because it will cause anyone who sets up the project to use the exact same versions of the dependencies that you are using. Your CI server, production machines, other developers in your team, everything and everyone runs on the same dependencies, which mitigates the potential for bugs affecting only some parts of the deployments. Even if you develop alone, in six months when reinstalling the project you can feel confident the dependencies installed are still working even if your dependencies released many new versions since then. (See note below about using the `update` command.)
> **Note:** For libraries it is not necessary to commit the lock file, see also: [Libraries - Lock file](02-libraries.md#lock-file).
>
>
### Installing from `composer.lock`
If there is already a `composer.lock` file in the project folder, it means either you ran the `update` command before, or someone else on the project ran the `update` command and committed the `composer.lock` file to the project (which is good).
Either way, running `install` when a `composer.lock` file is present resolves and installs all dependencies that you listed in `composer.json`, but Composer uses the exact versions listed in `composer.lock` to ensure that the package versions are consistent for everyone working on your project. As a result you will have all dependencies requested by your `composer.json` file, but they may not all be at the very latest available versions (some of the dependencies listed in the `composer.lock` file may have released newer versions since the file was created). This is by design, it ensures that your project does not break because of unexpected changes in dependencies.
So after fetching new changes from your VCS repository it is recommended to run a Composer `install` to make sure the vendor directory is up in sync with your `composer.lock` file.
```
php composer.phar install
```
Updating dependencies to their latest versions
----------------------------------------------
As mentioned above, the `composer.lock` file prevents you from automatically getting the latest versions of your dependencies. To update to the latest versions, use the [`update`](03-cli.md#update-u) command. This will fetch the latest matching versions (according to your `composer.json` file) and update the lock file with the new versions.
```
php composer.phar update
```
> **Note:** Composer will display a Warning when executing an `install` command if the `composer.lock` has not been updated since changes were made to the `composer.json` that might affect dependency resolution.
>
>
If you only want to install, upgrade or remove one dependency, you can explicitly list it as an argument:
```
php composer.phar update monolog/monolog [...]
```
Packagist
---------
[Packagist.org](https://packagist.org/) is the main Composer repository. A Composer repository is basically a package source: a place where you can get packages from. Packagist aims to be the central repository that everybody uses. This means that you can automatically `require` any package that is available there, without further specifying where Composer should look for the package.
If you go to the [Packagist.org website](https://packagist.org/), you can browse and search for packages.
Any open source project using Composer is recommended to publish their packages on Packagist. A library does not need to be on Packagist to be used by Composer, but it enables discovery and adoption by other developers more quickly.
Platform packages
-----------------
Composer has platform packages, which are virtual packages for things that are installed on the system but are not actually installable by Composer. This includes PHP itself, PHP extensions and some system libraries.
* `php` represents the PHP version of the user, allowing you to apply constraints, e.g. `^7.1`. To require a 64bit version of php, you can require the `php-64bit` package.
* `hhvm` represents the version of the HHVM runtime and allows you to apply a constraint, e.g., `^2.3`.
* `ext-<name>` allows you to require PHP extensions (includes core extensions). Versioning can be quite inconsistent here, so it's often a good idea to set the constraint to `*`. An example of an extension package name is `ext-gd`.
* `lib-<name>` allows constraints to be made on versions of libraries used by PHP. The following are available: `curl`, `iconv`, `icu`, `libxml`, `openssl`, `pcre`, `uuid`, `xsl`.
You can use [`show --platform`](03-cli.md#show) to get a list of your locally available platform packages.
Autoloading
-----------
For libraries that specify autoload information, Composer generates a `vendor/autoload.php` file. You can include this file and start using the classes that those libraries provide without any extra work:
```
require __DIR__ . '/vendor/autoload.php';
$log = new Monolog\Logger('name');
$log->pushHandler(new Monolog\Handler\StreamHandler('app.log', Monolog\Logger::WARNING));
$log->warning('Foo');
```
You can even add your own code to the autoloader by adding an [`autoload`](04-schema.md#autoload) field to `composer.json`.
```
{
"autoload": {
"psr-4": {"Acme\\": "src/"}
}
}
```
Composer will register a [PSR-4](https://www.php-fig.org/psr/psr-4/) autoloader for the `Acme` namespace.
You define a mapping from namespaces to directories. The `src` directory would be in your project root, on the same level as the `vendor` directory. An example filename would be `src/Foo.php` containing an `Acme\Foo` class.
After adding the [`autoload`](04-schema.md#autoload) field, you have to re-run this command:
```
php composer.phar dump-autoload
```
This command will re-generate the `vendor/autoload.php` file. See the [`dump-autoload`](03-cli.md#dump-autoload-dumpautoload-) section for more information.
Including that file will also return the autoloader instance, so you can store the return value of the include call in a variable and add more namespaces. This can be useful for autoloading classes in a test suite, for example.
```
$loader = require __DIR__ . '/vendor/autoload.php';
$loader->addPsr4('Acme\\Test\\', __DIR__);
```
In addition to PSR-4 autoloading, Composer also supports PSR-0, classmap and files autoloading. See the [`autoload`](04-schema.md#autoload) reference for more information.
See also the docs on [optimizing the autoloader](articles/autoloader-optimization.md).
> **Note:** Composer provides its own autoloader. If you don't want to use that one, you can include `vendor/composer/autoload_*.php` files, which return associative arrays allowing you to configure your own autoloader.
>
>
← [Intro](00-intro.md) | [Libraries](02-libraries.md) →
| programming_docs |
composer The composer.json schema The composer.json schema
========================
This chapter will explain all of the fields available in `composer.json`.
JSON schema
-----------
We have a [JSON schema](https://json-schema.org) that documents the format and can also be used to validate your `composer.json`. In fact, it is used by the `validate` command. You can find it at: <https://getcomposer.org/schema.json>
Root Package
------------
The root package is the package defined by the `composer.json` at the root of your project. It is the main `composer.json` that defines your project requirements.
Certain fields only apply when in the root package context. One example of this is the `config` field. Only the root package can define configuration. The config of dependencies is ignored. This makes the `config` field `root-only`.
> **Note:** A package can be the root package or not, depending on the context. For example, if your project depends on the `monolog` library, your project is the root package. However, if you clone `monolog` from GitHub in order to fix a bug in it, then `monolog` is the root package.
>
>
Properties
----------
### name
The name of the package. It consists of vendor name and project name, separated by `/`. Examples:
* monolog/monolog
* igorw/event-source
The name must be lowercase and consist of words separated by `-`, `.` or `_`. The complete name should match `^[a-z0-9]([_.-]?[a-z0-9]+)*/[a-z0-9](([_.]?|-{0,2})[a-z0-9]+)*$`.
The `name` property is required for published packages (libraries).
> **Note:** Before Composer version 2.0, a name could contain any character, including white spaces.
>
>
### description
A short description of the package. Usually this is one line long.
Required for published packages (libraries).
### version
The version of the package. In most cases this is not required and should be omitted (see below).
This must follow the format of `X.Y.Z` or `vX.Y.Z` with an optional suffix of `-dev`, `-patch` (`-p`), `-alpha` (`-a`), `-beta` (`-b`) or `-RC`. The patch, alpha, beta and RC suffixes can also be followed by a number.
Examples:
* 1.0.0
* 1.0.2
* 1.1.0
* 0.2.5
* 1.0.0-dev
* 1.0.0-alpha3
* 1.0.0-beta2
* 1.0.0-RC5
* v2.0.4-p1
Optional if the package repository can infer the version from somewhere, such as the VCS tag name in the VCS repository. In that case it is also recommended to omit it.
> **Note:** Packagist uses VCS repositories, so the statement above is very much true for Packagist as well. Specifying the version yourself will most likely end up creating problems at some point due to human error.
>
>
### type
The type of the package. It defaults to `library`.
Package types are used for custom installation logic. If you have a package that needs some special logic, you can define a custom type. This could be a `symfony-bundle`, a `wordpress-plugin` or a `typo3-cms-extension`. These types will all be specific to certain projects, and they will need to provide an installer capable of installing packages of that type.
Out of the box, Composer supports four types:
* **library:** This is the default. It will copy the files to `vendor`.
* **project:** This denotes a project rather than a library. For example application shells like the [Symfony standard edition](https://github.com/symfony/symfony-standard), CMSs like the [SilverStripe installer](https://github.com/silverstripe/silverstripe-installer) or full fledged applications distributed as packages. This can for example be used by IDEs to provide listings of projects to initialize when creating a new workspace.
* **metapackage:** An empty package that contains requirements and will trigger their installation, but contains no files and will not write anything to the filesystem. As such, it does not require a dist or source key to be installable.
* **composer-plugin:** A package of type `composer-plugin` may provide an installer for other packages that have a custom type. Read more in the [dedicated article](articles/custom-installers.md).
Only use a custom type if you need custom logic during installation. It is recommended to omit this field and have it default to `library`.
### keywords
An array of keywords that the package is related to. These can be used for searching and filtering.
Examples:
* logging
* events
* database
* redis
* templating
> **Note**: Some special keywords trigger `composer require` without the `--dev` option to prompt users if they would like to add these packages to `require-dev` instead of `require`. These are: `dev`, `testing`, `static analysis`.
>
>
Optional.
### homepage
A URL to the website of the project.
Optional.
### readme
A relative path to the readme document.
Optional.
### time
Release date of the version.
Must be in `YYYY-MM-DD` or `YYYY-MM-DD HH:MM:SS` format.
Optional.
### license
The license of the package. This can be either a string or an array of strings.
The recommended notation for the most common licenses is (alphabetical):
* Apache-2.0
* BSD-2-Clause
* BSD-3-Clause
* BSD-4-Clause
* GPL-2.0-only / GPL-2.0-or-later
* GPL-3.0-only / GPL-3.0-or-later
* LGPL-2.1-only / LGPL-2.1-or-later
* LGPL-3.0-only / LGPL-3.0-or-later
* MIT
Optional, but it is highly recommended to supply this. More identifiers are listed at the [SPDX Open Source License Registry](https://spdx.org/licenses/).
> **Note:** For closed-source software, you may use `"proprietary"` as the license identifier.
>
>
An Example:
```
{
"license": "MIT"
}
```
For a package, when there is a choice between licenses ("disjunctive license"), multiple can be specified as an array.
An Example for disjunctive licenses:
```
{
"license": [
"LGPL-2.1-only",
"GPL-3.0-or-later"
]
}
```
Alternatively they can be separated with "or" and enclosed in parentheses;
```
{
"license": "(LGPL-2.1-only or GPL-3.0-or-later)"
}
```
Similarly, when multiple licenses need to be applied ("conjunctive license"), they should be separated with "and" and enclosed in parentheses.
### authors
The authors of the package. This is an array of objects.
Each author object can have following properties:
* **name:** The author's name. Usually their real name.
* **email:** The author's email address.
* **homepage:** URL to the author's website.
* **role:** The author's role in the project (e.g. developer or translator)
An example:
```
{
"authors": [
{
"name": "Nils Adermann",
"email": "[email protected]",
"homepage": "https://www.naderman.de",
"role": "Developer"
},
{
"name": "Jordi Boggiano",
"email": "[email protected]",
"homepage": "https://seld.be",
"role": "Developer"
}
]
}
```
Optional, but highly recommended.
### support
Various information to get support about the project.
Support information includes the following:
* **email:** Email address for support.
* **issues:** URL to the issue tracker.
* **forum:** URL to the forum.
* **wiki:** URL to the wiki.
* **irc:** IRC channel for support, as irc://server/channel.
* **source:** URL to browse or download the sources.
* **docs:** URL to the documentation.
* **rss:** URL to the RSS feed.
* **chat:** URL to the chat channel.
An example:
```
{
"support": {
"email": "[email protected]",
"irc": "irc://irc.freenode.org/composer"
}
}
```
Optional.
### funding
A list of URLs to provide funding to the package authors for maintenance and development of new functionality.
Each entry consists of the following
* **type:** The type of funding, or the platform through which funding can be provided, e.g. patreon, opencollective, tidelift or github.
* **url:** URL to a website with details, and a way to fund the package.
An example:
```
{
"funding": [
{
"type": "patreon",
"url": "https://www.patreon.com/phpdoctrine"
},
{
"type": "tidelift",
"url": "https://tidelift.com/subscription/pkg/packagist-doctrine_doctrine-bundle"
},
{
"type": "other",
"url": "https://www.doctrine-project.org/sponsorship.html"
}
]
}
```
Optional.
### Package links
All of the following take an object which maps package names to versions of the package via version constraints. Read more about versions [here](articles/versions.md).
Example:
```
{
"require": {
"monolog/monolog": "1.0.*"
}
}
```
All links are optional fields.
`require` and `require-dev` additionally support *stability flags* ([root-only](04-schema.md#root-package)). They take the form "*constraint*@*stability flag*". These allow you to further restrict or expand the stability of a package beyond the scope of the [minimum-stability](#minimum-stability) setting. You can apply them to a constraint, or apply them to an empty *constraint* if you want to allow unstable packages of a dependency for example.
Example:
```
{
"require": {
"monolog/monolog": "1.0.*@beta",
"acme/foo": "@dev"
}
}
```
If one of your dependencies has a dependency on an unstable package you need to explicitly require it as well, along with its sufficient stability flag.
Example:
Assuming `doctrine/doctrine-fixtures-bundle` requires `"doctrine/data-fixtures": "dev-master"` then inside the root composer.json you need to add the second line below to allow dev releases for the `doctrine/data-fixtures` package :
```
{
"require": {
"doctrine/doctrine-fixtures-bundle": "dev-master",
"doctrine/data-fixtures": "@dev"
}
}
```
`require` and `require-dev` additionally support explicit references (i.e. commit) for dev versions to make sure they are locked to a given state, even when you run update. These only work if you explicitly require a dev version and append the reference with `#<ref>`. This is also a [root-only](04-schema.md#root-package) feature and will be ignored in dependencies.
Example:
```
{
"require": {
"monolog/monolog": "dev-master#2eb0c0978d290a1c45346a1955188929cb4e5db7",
"acme/foo": "1.0.x-dev#abc123"
}
}
```
> **Note:** This feature has severe technical limitations, as the composer.json metadata will still be read from the branch name you specify before the hash. You should therefore only use this as a temporary solution during development to remediate transient issues, until you can switch to tagged releases. The Composer team does not actively support this feature and will not accept bug reports related to it.
>
>
It is also possible to inline-alias a package constraint so that it matches a constraint that it otherwise would not. For more information [see the aliases article](articles/aliases.md).
`require` and `require-dev` also support references to specific PHP versions and PHP extensions your project needs to run successfully.
Example:
```
{
"require": {
"php": ">=7.4",
"ext-mbstring": "*"
}
}
```
> **Note:** It is important to list PHP extensions your project requires. Not all PHP installations are created equal: some may miss extensions you may consider as standard (such as `ext-mysqli` which is not installed by default in Fedora/CentOS minimal installation systems). Failure to list required PHP extensions may lead to a bad user experience: Composer will install your package without any errors but it will then fail at run-time. The `composer show --platform` command lists all PHP extensions available on your system. You may use it to help you compile the list of extensions you use and require. Alternatively you may use third party tools to analyze your project for the list of extensions used.
>
>
#### require
Map of packages required by this package. The package will not be installed unless those requirements can be met.
#### require-dev ([root-only](04-schema.md#root-package))
Map of packages required for developing this package, or running tests, etc. The dev requirements of the root package are installed by default. Both `install` or `update` support the `--no-dev` option that prevents dev dependencies from being installed.
#### conflict
Map of packages that conflict with this version of this package. They will not be allowed to be installed together with your package.
Note that when specifying ranges like `<1.0 >=1.1` in a `conflict` link, this will state a conflict with all versions that are less than 1.0 *and* equal or newer than 1.1 at the same time, which is probably not what you want. You probably want to go for `<1.0 || >=1.1` in this case.
#### replace
Map of packages that are replaced by this package. This allows you to fork a package, publish it under a different name with its own version numbers, while packages requiring the original package continue to work with your fork because it replaces the original package.
This is also useful for packages that contain sub-packages, for example the main symfony/symfony package contains all the Symfony Components which are also available as individual packages. If you require the main package it will automatically fulfill any requirement of one of the individual components, since it replaces them.
Caution is advised when using replace for the sub-package purpose explained above. You should then typically only replace using `self.version` as a version constraint, to make sure the main package only replaces the sub-packages of that exact version, and not any other version, which would be incorrect.
#### provide
Map of packages that are provided by this package. This is mostly useful for implementations of common interfaces. A package could depend on some virtual package e.g. `psr/logger-implementation`, any library that implements this logger interface would list it in `provide`. Implementors can then be [found on Packagist.org](https://packagist.org/providers/psr/log-implementation).
Using `provide` with the name of an actual package rather than a virtual one implies that the code of that package is also shipped, in which case `replace` is generally a better choice. A common convention for packages providing an interface and relying on other packages to provide an implementation (for instance the PSR interfaces) is to use a `-implementation` suffix for the name of the virtual package corresponding to the interface package.
#### suggest
Suggested packages that can enhance or work well with this package. These are informational and are displayed after the package is installed, to give your users a hint that they could add more packages, even though they are not strictly required.
The format is like package links above, except that the values are free text and not version constraints.
Example:
```
{
"suggest": {
"monolog/monolog": "Allows more advanced logging of the application flow",
"ext-xml": "Needed to support XML format in class Foo"
}
}
```
### autoload
Autoload mapping for a PHP autoloader.
[`PSR-4`](https://www.php-fig.org/psr/psr-4/) and [`PSR-0`](http://www.php-fig.org/psr/psr-0/) autoloading, `classmap` generation and `files` includes are supported.
PSR-4 is the recommended way since it offers greater ease of use (no need to regenerate the autoloader when you add classes).
#### PSR-4
Under the `psr-4` key you define a mapping from namespaces to paths, relative to the package root. When autoloading a class like `Foo\\Bar\\Baz` a namespace prefix `Foo\\` pointing to a directory `src/` means that the autoloader will look for a file named `src/Bar/Baz.php` and include it if present. Note that as opposed to the older PSR-0 style, the prefix (`Foo\\`) is **not** present in the file path.
Namespace prefixes must end in `\\` to avoid conflicts between similar prefixes. For example `Foo` would match classes in the `FooBar` namespace so the trailing backslashes solve the problem: `Foo\\` and `FooBar\\` are distinct.
The PSR-4 references are all combined, during install/update, into a single key => value array which may be found in the generated file `vendor/composer/autoload_psr4.php`.
Example:
```
{
"autoload": {
"psr-4": {
"Monolog\\": "src/",
"Vendor\\Namespace\\": ""
}
}
}
```
If you need to search for a same prefix in multiple directories, you can specify them as an array as such:
```
{
"autoload": {
"psr-4": { "Monolog\\": ["src/", "lib/"] }
}
}
```
If you want to have a fallback directory where any namespace will be looked for, you can use an empty prefix like:
```
{
"autoload": {
"psr-4": { "": "src/" }
}
}
```
#### PSR-0
Under the `psr-0` key you define a mapping from namespaces to paths, relative to the package root. Note that this also supports the PEAR-style non-namespaced convention.
Please note namespace declarations should end in `\\` to make sure the autoloader responds exactly. For example `Foo` would match in `FooBar` so the trailing backslashes solve the problem: `Foo\\` and `FooBar\\` are distinct.
The PSR-0 references are all combined, during install/update, into a single key => value array which may be found in the generated file `vendor/composer/autoload_namespaces.php`.
Example:
```
{
"autoload": {
"psr-0": {
"Monolog\\": "src/",
"Vendor\\Namespace\\": "src/",
"Vendor_Namespace_": "src/"
}
}
}
```
If you need to search for a same prefix in multiple directories, you can specify them as an array as such:
```
{
"autoload": {
"psr-0": { "Monolog\\": ["src/", "lib/"] }
}
}
```
The PSR-0 style is not limited to namespace declarations only but may be specified right down to the class level. This can be useful for libraries with only one class in the global namespace. If the php source file is also located in the root of the package, for example, it may be declared like this:
```
{
"autoload": {
"psr-0": { "UniqueGlobalClass": "" }
}
}
```
If you want to have a fallback directory where any namespace can be, you can use an empty prefix like:
```
{
"autoload": {
"psr-0": { "": "src/" }
}
}
```
#### Classmap
The `classmap` references are all combined, during install/update, into a single key => value array which may be found in the generated file `vendor/composer/autoload_classmap.php`. This map is built by scanning for classes in all `.php` and `.inc` files in the given directories/files.
You can use the classmap generation support to define autoloading for all libraries that do not follow PSR-0/4. To configure this you specify all directories or files to search for classes.
Example:
```
{
"autoload": {
"classmap": ["src/", "lib/", "Something.php"]
}
}
```
Wildcards (`*`) are also supported in a classmap paths, and expand to match any directory name:
Example:
```
{
"autoload": {
"classmap": ["src/addons/*/lib/", "3rd-party/*", "Something.php"]
}
}
```
#### Files
If you want to require certain files explicitly on every request then you can use the `files` autoloading mechanism. This is useful if your package includes PHP functions that cannot be autoloaded by PHP.
Example:
```
{
"autoload": {
"files": ["src/MyLibrary/functions.php"]
}
}
```
Files autoload rules are included whenever `vendor/autoload.php` is included, right after the autoloader is registered. The order of inclusion depends on package dependencies so that if package A depends on B, files in package B will be included first to ensure package B is fully initialized and ready to be used when files from package A are included.
If two packages have the same amount of dependents or no dependencies, the order is alphabetical.
Files from the root package are always loaded last, and you cannot use files autoloading yourself to override functions from your dependencies. If you want to achieve that we recommend you include your own functions *before* including Composer's `vendor/autoload.php`.
#### Exclude files from classmaps
If you want to exclude some files or folders from the classmap you can use the `exclude-from-classmap` property. This might be useful to exclude test classes in your live environment, for example, as those will be skipped from the classmap even when building an optimized autoloader.
The classmap generator will ignore all files in the paths configured here. The paths are absolute from the package root directory (i.e. composer.json location), and support `*` to match anything but a slash, and `**` to match anything. `**` is implicitly added to the end of the paths.
Example:
```
{
"autoload": {
"exclude-from-classmap": ["/Tests/", "/test/", "/tests/"]
}
}
```
#### Optimizing the autoloader
The autoloader can have quite a substantial impact on your request time (50-100ms per request in large frameworks using a lot of classes). See the [article about optimizing the autoloader](articles/autoloader-optimization.md) for more details on how to reduce this impact.
### autoload-dev ([root-only](04-schema.md#root-package))
This section allows defining autoload rules for development purposes.
Classes needed to run the test suite should not be included in the main autoload rules to avoid polluting the autoloader in production and when other people use your package as a dependency.
Therefore, it is a good idea to rely on a dedicated path for your unit tests and to add it within the autoload-dev section.
Example:
```
{
"autoload": {
"psr-4": { "MyLibrary\\": "src/" }
},
"autoload-dev": {
"psr-4": { "MyLibrary\\Tests\\": "tests/" }
}
}
```
### include-path
> **DEPRECATED**: This is only present to support legacy projects, and all new code should preferably use autoloading. As such it is a deprecated practice, but the feature itself will not likely disappear from Composer.
>
>
A list of paths which should get appended to PHP's `include_path`.
Example:
```
{
"include-path": ["lib/"]
}
```
Optional.
### target-dir
> **DEPRECATED**: This is only present to support legacy PSR-0 style autoloading, and all new code should preferably use PSR-4 without target-dir and projects using PSR-0 with PHP namespaces are encouraged to migrate to PSR-4 instead.
>
>
Defines the installation target.
In case the package root is below the namespace declaration you cannot autoload properly. `target-dir` solves this problem.
An example is Symfony. There are individual packages for the components. The Yaml component is under `Symfony\Component\Yaml`. The package root is that `Yaml` directory. To make autoloading possible, we need to make sure that it is not installed into `vendor/symfony/yaml`, but instead into `vendor/symfony/yaml/Symfony/Component/Yaml`, so that the autoloader can load it from `vendor/symfony/yaml`.
To do that, `autoload` and `target-dir` are defined as follows:
```
{
"autoload": {
"psr-0": { "Symfony\\Component\\Yaml\\": "" }
},
"target-dir": "Symfony/Component/Yaml"
}
```
Optional.
### minimum-stability ([root-only](04-schema.md#root-package))
This defines the default behavior for filtering packages by stability. This defaults to `stable`, so if you rely on a `dev` package, you should specify it in your file to avoid surprises.
All versions of each package are checked for stability, and those that are less stable than the `minimum-stability` setting will be ignored when resolving your project dependencies. (Note that you can also specify stability requirements on a per-package basis using stability flags in the version constraints that you specify in a `require` block (see [package links](#package-links) for more details).
Available options (in order of stability) are `dev`, `alpha`, `beta`, `RC`, and `stable`.
### prefer-stable ([root-only](04-schema.md#root-package))
When this is enabled, Composer will prefer more stable packages over unstable ones when finding compatible stable packages is possible. If you require a dev version or only alphas are available for a package, those will still be selected granted that the minimum-stability allows for it.
Use `"prefer-stable": true` to enable.
### repositories ([root-only](04-schema.md#root-package))
Custom package repositories to use.
By default Composer only uses the packagist repository. By specifying repositories you can get packages from elsewhere.
Repositories are not resolved recursively. You can only add them to your main `composer.json`. Repository declarations of dependencies' `composer.json`s are ignored.
The following repository types are supported:
* **composer:** A Composer repository is a `packages.json` file served via the network (HTTP, FTP, SSH), that contains a list of `composer.json` objects with additional `dist` and/or `source` information. The `packages.json` file is loaded using a PHP stream. You can set extra options on that stream using the `options` parameter.
* **vcs:** The version control system repository can fetch packages from git, svn, fossil and hg repositories.
* **package:** If you depend on a project that does not have any support for Composer whatsoever you can define the package inline using a `package` repository. You basically inline the `composer.json` object.
For more information on any of these, see [Repositories](05-repositories.md).
Example:
```
{
"repositories": [
{
"type": "composer",
"url": "http://packages.example.com"
},
{
"type": "composer",
"url": "https://packages.example.com",
"options": {
"ssl": {
"verify_peer": "true"
}
}
},
{
"type": "vcs",
"url": "https://github.com/Seldaek/monolog"
},
{
"type": "package",
"package": {
"name": "smarty/smarty",
"version": "3.1.7",
"dist": {
"url": "https://www.smarty.net/files/Smarty-3.1.7.zip",
"type": "zip"
},
"source": {
"url": "https://smarty-php.googlecode.com/svn/",
"type": "svn",
"reference": "tags/Smarty_3_1_7/distribution/"
}
}
}
]
}
```
> **Note:** Order is significant here. When looking for a package, Composer will look from the first to the last repository, and pick the first match. By default Packagist is added last which means that custom repositories can override packages from it.
>
>
Using JSON object notation is also possible. However, JSON key/value pairs are to be considered unordered so consistent behaviour cannot be guaranteed.
```
{
"repositories": {
"foo": {
"type": "composer",
"url": "http://packages.foo.com"
}
}
}
```
### config ([root-only](04-schema.md#root-package))
A set of configuration options. It is only used for projects. See [Config](06-config.md) for a description of each individual option.
### scripts ([root-only](04-schema.md#root-package))
Composer allows you to hook into various parts of the installation process through the use of scripts.
See [Scripts](articles/scripts.md) for events details and examples.
### extra
Arbitrary extra data for consumption by `scripts`.
This can be virtually anything. To access it from within a script event handler, you can do:
```
$extra = $event->getComposer()->getPackage()->getExtra();
```
Optional.
### bin
A set of files that should be treated as binaries and made available into the `bin-dir` (from config).
See [Vendor Binaries](articles/vendor-binaries.md) for more details.
Optional.
### archive
A set of options for creating package archives.
The following options are supported:
* **name:** Allows configuring base name for archive. By default (if not configured, and `--file` is not passed as command-line argument), `preg_replace('#[^a-z0-9-_]#i', '-', name)` is used.
Example:
```
{
"name": "org/strangeName",
"archive": {
"name": "Strange_name"
}
}
```
* **exclude:** Allows configuring a list of patterns for excluded paths. The pattern syntax matches .gitignore files. A leading exclamation mark (!) will result in any matching files to be included even if a previous pattern excluded them. A leading slash will only match at the beginning of the project relative path. An asterisk will not expand to a directory separator.
Example:
```
{
"archive": {
"exclude": ["/foo/bar", "baz", "/*.test", "!/foo/bar/baz"]
}
}
```
The example will include `/dir/foo/bar/file`, `/foo/bar/baz`, `/file.php`, `/foo/my.test` but it will exclude `/foo/bar/any`, `/foo/baz`, and `/my.test`.
Optional.
### abandoned
Indicates whether this package has been abandoned.
It can be boolean or a package name/URL pointing to a recommended alternative.
Examples:
Use `"abandoned": true` to indicate this package is abandoned. Use `"abandoned": "monolog/monolog"` to indicate this package is abandoned, and that the recommended alternative is `monolog/monolog`.
Defaults to false.
Optional.
### non-feature-branches
A list of regex patterns of branch names that are non-numeric (e.g. "latest" or something), that will NOT be handled as feature branches. This is an array of strings.
If you have non-numeric branch names, for example like "latest", "current", "latest-stable" or something, that do not look like a version number, then Composer handles such branches as feature branches. This means it searches for parent branches, that look like a version or ends at special branches (like master), and the root package version number becomes the version of the parent branch or at least master or something.
To handle non-numeric named branches as versions instead of searching for a parent branch with a valid version or special branch name like master, you can set patterns for branch names that should be handled as dev version branches.
This is really helpful when you have dependencies using "self.version", so that not dev-master, but the same branch is installed (in the example: latest-testing).
An example:
If you have a testing branch, that is heavily maintained during a testing phase and is deployed to your staging environment, normally `composer show -s` will give you `versions : * dev-master`.
If you configure `latest-.*` as a pattern for non-feature-branches like this:
```
{
"non-feature-branches": ["latest-.*"]
}
```
Then `composer show -s` will give you `versions : * dev-latest-testing`.
Optional.
← [Command-line interface](03-cli.md) | [Repositories](05-repositories.md) →
| programming_docs |
composer Repositories Repositories
============
This chapter will explain the concept of packages and repositories, what kinds of repositories are available, and how they work.
Concepts
--------
Before we look at the different types of repositories that exist, we need to understand some basic concepts that Composer is built on.
### Package
Composer is a dependency manager. It installs packages locally. A package is essentially a directory containing something. In this case it is PHP code, but in theory it could be anything. And it contains a package description which has a name and a version. The name and the version are used to identify the package.
In fact, internally Composer sees every version as a separate package. While this distinction does not matter when you are using Composer, it's quite important when you want to change it.
In addition to the name and the version, there is useful metadata. The information most relevant for installation is the source definition, which describes where to get the package contents. The package data points to the contents of the package. And there are two options here: dist and source.
**Dist:** The dist is a packaged version of the package data. Usually a released version, usually a stable release.
**Source:** The source is used for development. This will usually originate from a source code repository, such as git. You can fetch this when you want to modify the downloaded package.
Packages can supply either of these, or even both. Depending on certain factors, such as user-supplied options and stability of the package, one will be preferred.
### Repository
A repository is a package source. It's a list of packages/versions. Composer will look in all your repositories to find the packages your project requires.
By default, only the Packagist.org repository is registered in Composer. You can add more repositories to your project by declaring them in `composer.json`.
Repositories are only available to the root package and the repositories defined in your dependencies will not be loaded. Read the [FAQ entry](https://getcomposer.org/doc/faqs/why-cant-composer-load-repositories-recursively.md) if you want to learn why.
When resolving dependencies, packages are looked up from repositories from top to bottom, and by default, as soon as a package is found in one, Composer stops looking in other repositories. Read the [repository priorities](articles/repository-priorities.md) article for more details and to see how to change this behavior.
Types
-----
### Composer
The main repository type is the `composer` repository. It uses a single `packages.json` file that contains all of the package metadata.
This is also the repository type that packagist uses. To reference a `composer` repository, supply the path before the `packages.json` file. In the case of packagist, that file is located at `/packages.json`, so the URL of the repository would be `repo.packagist.org`. For `example.org/packages.json` the repository URL would be `example.org`.
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org"
}
]
}
```
#### packages
The only required field is `packages`. The JSON structure is as follows:
```
{
"packages": {
"vendor/package-name": {
"dev-master": { @composer.json },
"1.0.x-dev": { @composer.json },
"0.0.1": { @composer.json },
"1.0.0": { @composer.json }
}
}
}
```
The `@composer.json` marker would be the contents of the `composer.json` from that package version including as a minimum:
* name
* version
* dist or source
Here is a minimal package definition:
```
{
"name": "smarty/smarty",
"version": "3.1.7",
"dist": {
"url": "https://www.smarty.net/files/Smarty-3.1.7.zip",
"type": "zip"
}
}
```
It may include any of the other fields specified in the [schema](04-schema.md).
#### notify-batch
The `notify-batch` field allows you to specify a URL that will be called every time a user installs a package. The URL can be either an absolute path (that will use the same domain as the repository), or a fully qualified URL.
An example value:
```
{
"notify-batch": "/downloads/"
}
```
For `example.org/packages.json` containing a `monolog/monolog` package, this would send a `POST` request to `example.org/downloads/` with following JSON request body:
```
{
"downloads": [
{"name": "monolog/monolog", "version": "1.2.1.0"}
]
}
```
The version field will contain the normalized representation of the version number.
This field is optional.
#### metadata-url, available-packages and available-package-patterns
The `metadata-url` field allows you to provide a URL template to serve all packages which are in the repository. It must contain the placeholder `%package%`.
This field is new in Composer v2, and is prioritised over the `provider-includes` and `providers-url` fields if both are present. For compatibility with both Composer v1 and v2 you ideally want to provide both. New repository implementations may only need to support v2 however.
An example:
```
{
"metadata-url": "/p2/%package%.json"
}
```
Whenever Composer looks for a package, it will replace `%package%` by the package name, and fetch that URL. If dev stability is allowed for the package, it will also load the URL again with `$packageName~dev` (e.g. `/p2/foo/bar~dev.json` to look for `foo/bar`'s dev versions).
The `foo/bar.json` and `foo/bar~dev.json` files containing package versions MUST contain only versions for the foo/bar package, as `{"packages":{"foo/bar":[ ... versions here ... ]}}`.
Caching is done via the use of If-Modified-Since header, so make sure you return Last-Modified headers and that they are accurate.
The array of versions can also optionally be minified using `Composer\MetadataMinifier\MetadataMinifier::minify()` from [composer/metadata-minifier](https://packagist.org/packages/composer/metadata-minifier). If you do that, you should add a `"minified": "composer/2.0"` key at the top level to indicate to Composer it must expand the version list back into the original data. See <https://repo.packagist.org/p2/monolog/monolog.json> for an example.
Any requested package which does not exist MUST return a 404 status code, which will indicate to Composer that this package does not exist in your repository. Make sure the 404 response is fast to avoid blocking Composer. Avoid redirects to alternative 404 pages.
If your repository only has a small number of packages, and you want to avoid the 404-requests, you can also specify an `"available-packages"` key in `packages.json` which should be an array with all the package names that your repository contains. Alternatively you can specify an `"available-package-patterns"` key which is an array of package name patterns (with `*` matching any string, e.g. `vendor/*` would make Composer look up every matching package name in this repository).
This field is optional.
#### providers-api
The `providers-api` field allows you to provide a URL template to serve all packages which provide a given package name, but not the package which has that name. It must contain the placeholder `%package%`.
For example <https://packagist.org/providers/monolog/monolog.json> lists some package which have a "provide" rule for monolog/monolog, but it does not list monolog/monolog itself.
```
{
"providers-api": "https://packagist.org/providers/%package%.json",
}
```
This field is optional.
#### list
The `list` field allows you to return the names of packages which match a given field (or all names if no filter is present). It should accept an optional `?filter=xx` query param, which can contain `*` as wildcards matching any substring.
Replace/provide rules should not be considered here.
It must return an array of package names:
```
{
"packageNames": [
"a/b",
"c/d"
]
}
```
See [https://packagist.org/packages/list.json?filter=composer/\*](https://packagist.org/packages/list.json?filter=composer/*) for example.
This field is optional.
#### provider-includes and providers-url
The `provider-includes` field allows you to list a set of files that list package names provided by this repository. The hash should be a sha256 of the files in this case.
The `providers-url` describes how provider files are found on the server. It is an absolute path from the repository root. It must contain the placeholders `%package%` and `%hash%`.
These fields are used by Composer v1, or if your repository does not have the `metadata-url` field set.
An example:
```
{
"provider-includes": {
"providers-a.json": {
"sha256": "f5b4bc0b354108ef08614e569c1ed01a2782e67641744864a74e788982886f4c"
},
"providers-b.json": {
"sha256": "b38372163fac0573053536f5b8ef11b86f804ea8b016d239e706191203f6efac"
}
},
"providers-url": "/p/%package%$%hash%.json"
}
```
Those files contain lists of package names and hashes to verify the file integrity, for example:
```
{
"providers": {
"acme/foo": {
"sha256": "38968de1305c2e17f4de33aea164515bc787c42c7e2d6e25948539a14268bb82"
},
"acme/bar": {
"sha256": "4dd24c930bd6e1103251306d6336ac813b563a220d9ca14f4743c032fb047233"
}
}
}
```
The file above declares that acme/foo and acme/bar can be found in this repository, by loading the file referenced by `providers-url`, replacing `%package%` by the vendor namespaced package name and `%hash%` by the sha256 field. Those files themselves contain package definitions as described [above](#packages).
These fields are optional. You probably don't need them for your own custom repository.
#### cURL or stream options
The repository is accessed either using cURL (Composer 2 with ext-curl enabled) or PHP streams. You can set extra options using the `options` parameter. For PHP streams, you can set any valid PHP stream context option. See [Context options and parameters](https://php.net/manual/en/context.php) for more information. When cURL is used, only a limited set of `http` and `ssl` options can be configured.
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org",
"options": {
"http": {
"timeout": 60
}
}
}
],
"require": {
"acme/package": "^1.0"
}
}
```
### VCS
VCS stands for version control system. This includes versioning systems like git, svn, fossil or hg. Composer has a repository type for installing packages from these systems.
#### Loading a package from a VCS repository
There are a few use cases for this. The most common one is maintaining your own fork of a third party library. If you are using a certain library for your project, and you decide to change something in the library, you will want your project to use the patched version. If the library is on GitHub (this is the case most of the time), you can fork it there and push your changes to your fork. After that you update the project's `composer.json`. All you have to do is add your fork as a repository and update the version constraint to point to your custom branch. In `composer.json` only, you should prefix your custom branch name with `"dev-"` (without making it part of the actual branch name). For version constraint naming conventions see [Libraries](02-libraries.md) for more information.
Example assuming you patched monolog to fix a bug in the `bugfix` branch:
```
{
"repositories": [
{
"type": "vcs",
"url": "https://github.com/igorw/monolog"
}
],
"require": {
"monolog/monolog": "dev-bugfix"
}
}
```
When you run `php composer.phar update`, you should get your modified version of `monolog/monolog` instead of the one from packagist.
Note that you should not rename the package unless you really intend to fork it in the long term, and completely move away from the original package. Composer will correctly pick your package over the original one since the custom repository has priority over packagist. If you want to rename the package, you should do so in the default (often master) branch and not in a feature branch, since the package name is taken from the default branch.
Also note that the override will not work if you change the `name` property in your forked repository's `composer.json` file as this needs to match the original for the override to work.
If other dependencies rely on the package you forked, it is possible to inline-alias it so that it matches a constraint that it otherwise would not. For more information [see the aliases article](articles/aliases.md).
#### Using private repositories
Exactly the same solution allows you to work with your private repositories at GitHub and Bitbucket:
```
{
"require": {
"vendor/my-private-repo": "dev-master"
},
"repositories": [
{
"type": "vcs",
"url": "[email protected]:vendor/my-private-repo.git"
}
]
}
```
The only requirement is the installation of SSH keys for a git client.
#### Git alternatives
Git is not the only version control system supported by the VCS repository. The following are supported:
* **Git:** [git-scm.com](https://git-scm.com)
* **Subversion:** [subversion.apache.org](https://subversion.apache.org)
* **Mercurial:** [mercurial-scm.org](https://www.mercurial-scm.org)
* **Fossil**: [fossil-scm.org](https://www.fossil-scm.org/)
To get packages from these systems you need to have their respective clients installed. That can be inconvenient. And for this reason there is special support for GitHub and Bitbucket that use the APIs provided by these sites, to fetch the packages without having to install the version control system. The VCS repository provides `dist`s for them that fetch the packages as zips.
* **GitHub:** [github.com](https://github.com) (Git)
* **Bitbucket:** [bitbucket.org](https://bitbucket.org) (Git)
The VCS driver to be used is detected automatically based on the URL. However, should you need to specify one for whatever reason, you can use `bitbucket`, `github`, `gitlab`, `perforce`, `fossil`, `git`, `svn` or `hg` as the repository type instead of `vcs`.
If you set the `no-api` key to `true` on a github repository it will clone the repository as it would with any other git repository instead of using the GitHub API. But unlike using the `git` driver directly, Composer will still attempt to use github's zip files.
Please note:
* **To let Composer choose which driver to use** the repository type needs to be defined as "vcs"
* **If you already used a private repository**, this means Composer should have cloned it in cache. If you want to install the same package with drivers, remember to launch the command `composer clearcache` followed by the command `composer update` to update Composer cache and install the package from dist.
* VCS driver `git-bitbucket` is deprecated in favor of `bitbucket`
#### Bitbucket Driver Configuration
> **Note that the repository endpoint for Bitbucket needs to be https rather than git.**
>
>
After setting up your bitbucket repository, you will also need to [set up authentication](articles/authentication-for-private-packages.md#bitbucket-oauth).
#### Subversion Options
Since Subversion has no native concept of branches and tags, Composer assumes by default that code is located in `$url/trunk`, `$url/branches` and `$url/tags`. If your repository has a different layout you can change those values. For example if you used capitalized names you could configure the repository like this:
```
{
"repositories": [
{
"type": "vcs",
"url": "http://svn.example.org/projectA/",
"trunk-path": "Trunk",
"branches-path": "Branches",
"tags-path": "Tags"
}
]
}
```
If you have no branches or tags directory you can disable them entirely by setting the `branches-path` or `tags-path` to `false`.
If the package is in a subdirectory, e.g. `/trunk/foo/bar/composer.json` and `/tags/1.0/foo/bar/composer.json`, then you can make Composer access it by setting the `"package-path"` option to the sub-directory, in this example it would be `"package-path": "foo/bar/"`.
If you have a private Subversion repository you can save credentials in the http-basic section of your config (See [Schema](04-schema.md)):
```
{
"http-basic": {
"svn.example.org": {
"username": "username",
"password": "password"
}
}
}
```
If your Subversion client is configured to store credentials by default these credentials will be saved for the current user and existing saved credentials for this server will be overwritten. To change this behavior by setting the `"svn-cache-credentials"` option in your repository configuration:
```
{
"repositories": [
{
"type": "vcs",
"url": "http://svn.example.org/projectA/",
"svn-cache-credentials": false
}
]
}
```
### Package
If you want to use a project that does not support Composer through any of the means above, you still can define the package yourself by using a `package` repository.
Basically, you define the same information that is included in the `composer` repository's `packages.json`, but only for a single package. Again, the minimum required fields are `name`, `version`, and either of `dist` or `source`.
Here is an example for the smarty template engine:
```
{
"repositories": [
{
"type": "package",
"package": {
"name": "smarty/smarty",
"version": "3.1.7",
"dist": {
"url": "https://www.smarty.net/files/Smarty-3.1.7.zip",
"type": "zip"
},
"source": {
"url": "http://smarty-php.googlecode.com/svn/",
"type": "svn",
"reference": "tags/Smarty_3_1_7/distribution/"
},
"autoload": {
"classmap": ["libs/"]
}
}
}
],
"require": {
"smarty/smarty": "3.1.*"
}
}
```
Typically, you would leave the source part off, as you don't really need it.
> **Note**: This repository type has a few limitations and should be avoided whenever possible:
>
> * Composer will not update the package unless you change the `version` field.
> * Composer will not update the commit references, so if you use `master` as reference you will have to delete the package to force an update, and will have to deal with an unstable lock file.
>
>
The `"package"` key in a `package` repository may be set to an array to define multiple versions of a package:
```
{
"repositories": [
{
"type": "package",
"package": [
{
"name": "foo/bar",
"version": "1.0.0",
...
},
{
"name": "foo/bar",
"version": "2.0.0",
...
}
]
}
]
}
```
Hosting your own
----------------
While you will probably want to put your packages on packagist most of the time, there are some use cases for hosting your own repository.
* **Private company packages:** If you are part of a company that uses Composer for their packages internally, you might want to keep those packages private.
* **Separate ecosystem:** If you have a project which has its own ecosystem, and the packages aren't really reusable by the greater PHP community, you might want to keep them separate to packagist. An example of this would be wordpress plugins.
For hosting your own packages, a native `composer` type of repository is recommended, which provides the best performance.
There are a few tools that can help you create a `composer` repository.
### Private Packagist
[Private Packagist](https://packagist.com/) is a hosted or self-hosted application providing private package hosting as well as mirroring of GitHub, Packagist.org and other package repositories.
Check out [Packagist.com](https://packagist.com/) for more information.
### Satis
Satis is a static `composer` repository generator. It is a bit like an ultra- lightweight, static file-based version of packagist.
You give it a `composer.json` containing repositories, typically VCS and package repository definitions. It will fetch all the packages that are `require`d and dump a `packages.json` that is your `composer` repository.
Check [the satis GitHub repository](https://github.com/composer/satis) and the [handling private packages article](articles/handling-private-packages.md) for more information.
### Artifact
There are some cases, when there is no ability to have one of the previously mentioned repository types online, even the VCS one. A typical example could be cross-organisation library exchange through build artifacts. Of course, most of the time these are private. To use these archives as-is, one can use a repository of type `artifact` with a folder containing ZIP or TAR archives of those private packages:
```
{
"repositories": [
{
"type": "artifact",
"url": "path/to/directory/with/zips/"
}
],
"require": {
"private-vendor-one/core": "15.6.2",
"private-vendor-two/connectivity": "*",
"acme-corp/parser": "10.3.5"
}
}
```
Each zip artifact is a ZIP archive with `composer.json` in root folder:
```
unzip -l acme-corp-parser-10.3.5.zip
```
```
composer.json
...
```
If there are two archives with different versions of a package, they are both imported. When an archive with a newer version is added in the artifact folder and you run `update`, that version will be imported as well and Composer will update to the latest version.
### Path
In addition to the artifact repository, you can use the path one, which allows you to depend on a local directory, either absolute or relative. This can be especially useful when dealing with monolithic repositories.
For instance, if you have the following directory structure in your repository:
```
...
├── apps
│ └── my-app
│ └── composer.json
├── packages
│ └── my-package
│ └── composer.json
...
```
Then, to add the package `my/package` as a dependency, in your `apps/my-app/composer.json` file, you can use the following configuration:
```
{
"repositories": [
{
"type": "path",
"url": "../../packages/my-package"
}
],
"require": {
"my/package": "*"
}
}
```
If the package is a local VCS repository, the version may be inferred by the branch or tag that is currently checked out. Otherwise, the version should be explicitly defined in the package's `composer.json` file. If the version cannot be resolved by these means, it is assumed to be `dev-master`.
When the version cannot be inferred from the local VCS repository, or when you want to override the version, you can use the `versions` option when declaring the repository:
```
{
"repositories": [
{
"type": "path",
"url": "../../packages/my-package",
"options": {
"versions": {
"my/package": "4.2-dev"
}
}
}
]
}
```
The local package will be symlinked if possible, in which case the output in the console will read `Symlinking from ../../packages/my-package`. If symlinking is *not* possible the package will be copied. In that case, the console will output `Mirrored from ../../packages/my-package`.
Instead of default fallback strategy you can force to use symlink with `"symlink": true` or mirroring with `"symlink": false` option. Forcing mirroring can be useful when deploying or generating package from a monolithic repository.
> **Note:** On Windows, directory symlinks are implemented using NTFS junctions because they can be created by non-admin users. Mirroring will always be used on versions below Windows 7 or if `proc_open` has been disabled.
>
>
```
{
"repositories": [
{
"type": "path",
"url": "../../packages/my-package",
"options": {
"symlink": false
}
}
]
}
```
Leading tildes are expanded to the current user's home folder, and environment variables are parsed in both Windows and Linux/Mac notations. For example `~/git/mypackage` will automatically load the mypackage clone from `/home/<username>/git/mypackage`, equivalent to `$HOME/git/mypackage` or `%USERPROFILE%/git/mypackage`.
> **Note:** Repository paths can also contain wildcards like `*` and `?`. For details, see the [PHP glob function](https://php.net/glob).
>
>
You can configure the way the package's dist reference (which appears in the composer.lock file) is built.
The following modes exist:
* `none` - reference will be always null. This can help reduce lock file conflicts in the lock file but reduces clarity as to when the last update happened and whether the package is in the latest state.
* `config` - reference is built based on a hash of the package's composer.json and repo config
* `auto` (used by default) - reference is built basing on the hash like with `config`, but if the package folder contains a git repository, the HEAD commit's hash is used as reference instead.
```
{
"repositories": [
{
"type": "path",
"url": "../../packages/my-package",
"options": {
"reference": "config"
}
}
]
}
```
Disabling Packagist.org
-----------------------
You can disable the default Packagist.org repository by adding this to your `composer.json`:
```
{
"repositories": [
{
"packagist.org": false
}
]
}
```
You can disable Packagist.org globally by using the global config flag:
```
php composer.phar config -g repo.packagist false
```
← [Schema](04-schema.md) | [Config](06-config.md) →
| programming_docs |
composer Composer Composer
========
Book
----
* [Introduction](00-intro.md "Go to documentation section: Introduction")
* [Basic usage](01-basic-usage.md "Go to documentation section: Basic usage")
* [Libraries](02-libraries.md "Go to documentation section: Libraries")
* [Command-line interface / Commands](03-cli.md "Go to documentation section: Command-line interface / Commands")
* [The composer.json schema](04-schema.md "Go to documentation section: The composer.json schema")
* [Repositories](05-repositories.md "Go to documentation section: Repositories")
* [Config](06-config.md "Go to documentation section: Config")
* [Runtime Composer utilities](07-runtime.md "Go to documentation section: Runtime Composer utilities")
* [Community](08-community.md "Go to documentation section: Community")
Articles
--------
* [Aliases](articles/aliases.md "Go to documentation section: Aliases") Alias branch names to versions
* [Authentication for privately hosted packages and repositories](articles/authentication-for-private-packages.md "Go to documentation section: Authentication for privately hosted packages and repositories") Access privately hosted packages/repositories
* [Autoloader optimization](articles/autoloader-optimization.md "Go to documentation section: Autoloader optimization") How to reduce the performance impact of the autoloader
* [Composer platform dependencies](articles/composer-platform-dependencies.md "Go to documentation section: Composer platform dependencies") Making your package depend on specific Composer versions
* [Setting up and using custom installers](articles/custom-installers.md "Go to documentation section: Setting up and using custom installers") Modify the way certain types of packages are installed
* [Handling private packages](articles/handling-private-packages.md "Go to documentation section: Handling private packages") Hosting and installing private Composer packages
* [Setting up and using plugins](articles/plugins.md "Go to documentation section: Setting up and using plugins") Modify and extend Composer's functionality
* [Repository priorities](articles/repository-priorities.md "Go to documentation section: Repository priorities") Configure which packages are found in which repositories
* [Resolving merge conflicts](articles/resolving-merge-conflicts.md "Go to documentation section: Resolving merge conflicts") On gracefully resolving conflicts while merging
* [Scripts](articles/scripts.md "Go to documentation section: Scripts") Script are callbacks that are called before/after installing packages
* [Troubleshooting](articles/troubleshooting.md "Go to documentation section: Troubleshooting") Solving problems
* [Vendor binaries and the `vendor/bin` directory](articles/vendor-binaries.md "Go to documentation section: Vendor binaries and the `vendor/bin` directory") Expose command-line scripts from packages
* [Versions and constraints](articles/versions.md "Go to documentation section: Versions and constraints") Versions explained.
FAQs
----
* [How do I install a package to a custom path for my framework?](https://getcomposer.org/doc/faqs/how-do-i-install-a-package-to-a-custom-path-for-my-framework.md "Go to documentation section: How do I install a package to a custom path for my framework?")
* [How do I install Composer programmatically?](https://getcomposer.org/doc/faqs/how-to-install-composer-programmatically.md "Go to documentation section: How do I install Composer programmatically?")
* [How do I install untrusted packages safely? Is it safe to run Composer as superuser or root?](https://getcomposer.org/doc/faqs/how-to-install-untrusted-packages-safely.md "Go to documentation section: How do I install untrusted packages safely? Is it safe to run Composer as superuser or root?")
* [Should I commit the dependencies in my vendor directory?](https://getcomposer.org/doc/faqs/should-i-commit-the-dependencies-in-my-vendor-directory.md "Go to documentation section: Should I commit the dependencies in my vendor directory?")
* [Which version numbering system does Composer itself use?](https://getcomposer.org/doc/faqs/which-version-numbering-system-does-composer-itself-use.md "Go to documentation section: Which version numbering system does Composer itself use?")
* [Why are unbound version constraints a bad idea?](https://getcomposer.org/doc/faqs/why-are-unbound-version-constraints-a-bad-idea.md "Go to documentation section: Why are unbound version constraints a bad idea?")
* [Why are version constraints combining comparisons and wildcards a bad idea?](https://getcomposer.org/doc/faqs/why-are-version-constraints-combining-comparisons-and-wildcards-a-bad-idea.md "Go to documentation section: Why are version constraints combining comparisons and wildcards a bad idea?")
* [Why can't Composer load repositories recursively?](https://getcomposer.org/doc/faqs/why-cant-composer-load-repositories-recursively.md "Go to documentation section: Why can't Composer load repositories recursively?")
API Docs
--------
If you need to work with composer as a library you may also want to check out the [source](https://github.com/composer/composer/tree/HEAD/src/Composer "Go to the source browser")
composer Command-line interface / Commands Command-line interface / Commands
=================================
You've already learned how to use the command-line interface to do some things. This chapter documents all the available commands.
To get help from the command-line, call `composer` or `composer list` to see the complete list of commands, then `--help` combined with any of those can give you more information.
As Composer uses [symfony/console](https://github.com/symfony/console) you can call commands by short name if it's not ambiguous.
```
php composer.phar dump
```
calls `composer dump-autoload`.
Bash Completions
----------------
To install bash completions you can run `composer completion bash > completion.bash`. This will create a `completion.bash` file in the current directory.
Then execute `source completion.bash` to enable it in the current terminal session.
Move and rename the `completion.bash` file to `/etc/bash_completion.d/composer` to make it load automatically in new terminals.
Global Options
--------------
The following options are available with every command:
* **--verbose (-v):** Increase verbosity of messages.
* **--help (-h):** Display help information.
* **--quiet (-q):** Do not output any message.
* **--no-interaction (-n):** Do not ask any interactive question.
* **--no-plugins:** Disables plugins.
* **--no-scripts:** Skips execution of scripts defined in `composer.json`.
* **--no-cache:** Disables the use of the cache directory. Same as setting the COMPOSER\_CACHE\_DIR env var to /dev/null (or NUL on Windows).
* **--working-dir (-d):** If specified, use the given directory as working directory.
* **--profile:** Display timing and memory usage information
* **--ansi:** Force ANSI output.
* **--no-ansi:** Disable ANSI output.
* **--version (-V):** Display this application version.
Process Exit Codes
------------------
* **0:** OK
* **1:** Generic/unknown error code
* **2:** Dependency solving error code
init
----
In the [Libraries](02-libraries.md) chapter we looked at how to create a `composer.json` by hand. There is also an `init` command available to do this.
When you run the command it will interactively ask you to fill in the fields, while using some smart defaults.
```
php composer.phar init
```
### Options
* **--name:** Name of the package.
* **--description:** Description of the package.
* **--author:** Author name of the package.
* **--type:** Type of package.
* **--homepage:** Homepage of the package.
* **--require:** Package to require with a version constraint. Should be in format `foo/bar:1.0.0`.
* **--require-dev:** Development requirements, see **--require**.
* **--stability (-s):** Value for the `minimum-stability` field.
* **--license (-l):** License of package.
* **--repository:** Provide one (or more) custom repositories. They will be stored in the generated composer.json, and used for auto-completion when prompting for the list of requires. Every repository can be either an HTTP URL pointing to a `composer` repository or a JSON string which similar to what the [repositories](04-schema.md#repositories) key accepts.
* **--autoload (-a):** Add a PSR-4 autoload mapping to the composer.json. Automatically maps your package's namespace to the provided directory. (Expects a relative path, e.g. src/) See also [PSR-4 autoload](04-schema.md#psr-4).
install / i
-----------
The `install` command reads the `composer.json` file from the current directory, resolves the dependencies, and installs them into `vendor`.
```
php composer.phar install
```
If there is a `composer.lock` file in the current directory, it will use the exact versions from there instead of resolving them. This ensures that everyone using the library will get the same versions of the dependencies.
If there is no `composer.lock` file, Composer will create one after dependency resolution.
### Options
* **--prefer-install:** There are two ways of downloading a package: `source` and `dist`. Composer uses `dist` by default. If you pass `--prefer-install=source` (or `--prefer-source`) Composer will install from `source` if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. To get the legacy behavior where Composer use `source` automatically for dev versions of packages, use `--prefer-install=auto`. See also [config.preferred-install](06-config.md#preferred-install). Passing this flag will override the config value.
* **--dry-run:** If you want to run through an installation without actually installing a package, you can use `--dry-run`. This will simulate the installation and show you what would happen.
* **--dev:** Install packages listed in `require-dev` (this is the default behavior).
* **--no-dev:** Skip installing packages listed in `require-dev`. The autoloader generation skips the `autoload-dev` rules. Also see [COMPOSER\_NO\_DEV](#composer-no-dev).
* **--no-autoloader:** Skips autoloader generation.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--audit:** Run an audit after installation is complete.
* **--audit-format:** Audit output format. Must be "table", "plain", "json", or "summary" (default).
* **--optimize-autoloader (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize-autoloader`.
* **--apcu-autoloader:** Use APCu to cache found/not-found classes.
* **--apcu-autoloader-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu-autoloader`.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard. Appending a `+` makes it only ignore the upper-bound of the requirements. For example, if a package requires `php: ^7`, then the option `--ignore-platform-req=php+` would allow installing on PHP 8, but installation on PHP 5.6 would still fail.
update / u
----------
In order to get the latest versions of the dependencies and to update the `composer.lock` file, you should use the `update` command. This command is also aliased as `upgrade` as it does the same as `upgrade` does if you are thinking of `apt-get` or similar package managers.
```
php composer.phar update
```
This will resolve all dependencies of the project and write the exact versions into `composer.lock`.
If you only want to update a few packages and not all, you can list them as such:
```
php composer.phar update vendor/package vendor/package2
```
You can also use wildcards to update a bunch of packages at once:
```
php composer.phar update "vendor/*"
```
If you want to downgrade a package to a specific version without changing your composer.json you can use `--with` and provide a custom version constraint:
```
php composer.phar update --with vendor/package:2.0.1
```
Note that with the above all packages will be updated. If you only want to update the package(s) for which you provide custom constraints using `--with`, you can skip `--with` and instead use constraints with the partial update syntax:
```
php composer.phar update vendor/package:2.0.1 vendor/package2:3.0.*
```
The custom constraint has to be a subset of the existing constraint you have, and this feature is only available for your root package dependencies.
### Options
* **--prefer-install:** There are two ways of downloading a package: `source` and `dist`. Composer uses `dist` by default. If you pass `--prefer-install=source` (or `--prefer-source`) Composer will install from `source` if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. To get the legacy behavior where Composer use `source` automatically for dev versions of packages, use `--prefer-install=auto`. See also [config.preferred-install](06-config.md#preferred-install). Passing this flag will override the config value.
* **--dry-run:** Simulate the command without actually doing anything.
* **--dev:** Install packages listed in `require-dev` (this is the default behavior).
* **--no-dev:** Skip installing packages listed in `require-dev`. The autoloader generation skips the `autoload-dev` rules. Also see [COMPOSER\_NO\_DEV](#composer-no-dev).
* **--no-install:** Does not run the install step after updating the composer.lock file.
* **--no-audit:** Does not run the audit steps after updating the composer.lock file. Also see [COMPOSER\_NO\_AUDIT](#composer-no-audit).
* **--audit-format:** Audit output format. Must be "table", "plain", "json", or "summary" (default).
* **--lock:** Only updates the lock file hash to suppress warning about the lock file being out of date.
* **--with:** Temporary version constraint to add, e.g. foo/bar:1.0.0 or foo/bar=1.0.0
* **--no-autoloader:** Skips autoloader generation.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--with-dependencies (-w):** Update also dependencies of packages in the argument list, except those which are root requirements.
* **--with-all-dependencies (-W):** Update also dependencies of packages in the argument list, including those which are root requirements.
* **--optimize-autoloader (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run, so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize-autoloader`.
* **--apcu-autoloader:** Use APCu to cache found/not-found classes.
* **--apcu-autoloader-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu-autoloader`.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard. Appending a `+` makes it only ignore the upper-bound of the requirements. For example, if a package requires `php: ^7`, then the option `--ignore-platform-req=php+` would allow installing on PHP 8, but installation on PHP 5.6 would still fail.
* **--prefer-stable:** Prefer stable versions of dependencies. Can also be set via the COMPOSER\_PREFER\_STABLE=1 env var.
* **--prefer-lowest:** Prefer lowest versions of dependencies. Useful for testing minimal versions of requirements, generally used with `--prefer-stable`. Can also be set via the COMPOSER\_PREFER\_LOWEST=1 env var.
* **--interactive:** Interactive interface with autocompletion to select the packages to update.
* **--root-reqs:** Restricts the update to your first degree dependencies.
Specifying one of the words `mirrors`, `lock`, or `nothing` as an argument has the same effect as specifying the option `--lock`, for example `composer update mirrors` is exactly the same as `composer update --lock`.
require / r
-----------
The `require` command adds new packages to the `composer.json` file from the current directory. If no file exists one will be created on the fly.
```
php composer.phar require
```
After adding/changing the requirements, the modified requirements will be installed or updated.
If you do not want to choose requirements interactively, you can pass them to the command.
```
php composer.phar require "vendor/package:2.*" vendor/package2:dev-master
```
If you do not specify a package, Composer will prompt you to search for a package, and given results, provide a list of matches to require.
### Options
* **--dev:** Add packages to `require-dev`.
* **--dry-run:** Simulate the command without actually doing anything.
* **--prefer-install:** There are two ways of downloading a package: `source` and `dist`. Composer uses `dist` by default. If you pass `--prefer-install=source` (or `--prefer-source`) Composer will install from `source` if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. To get the legacy behavior where Composer use `source` automatically for dev versions of packages, use `--prefer-install=auto`. See also [config.preferred-install](06-config.md#preferred-install). Passing this flag will override the config value.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--no-update:** Disables the automatic update of the dependencies (implies --no-install).
* **--no-install:** Does not run the install step after updating the composer.lock file.
* **--no-audit:** Does not run the audit steps after updating the composer.lock file. Also see [COMPOSER\_NO\_AUDIT](#composer-no-audit).
* **--audit-format:** Audit output format. Must be "table", "plain", "json", or "summary" (default).
* **--update-no-dev:** Run the dependency update with the `--no-dev` option. Also see [COMPOSER\_NO\_DEV](#composer-no-dev).
* **--update-with-dependencies (-w):** Also update dependencies of the newly required packages, except those that are root requirements.
* **--update-with-all-dependencies (-W):** Also update dependencies of the newly required packages, including those that are root requirements.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard.
* **--prefer-stable:** Prefer stable versions of dependencies. Can also be set via the COMPOSER\_PREFER\_STABLE=1 env var.
* **--prefer-lowest:** Prefer lowest versions of dependencies. Useful for testing minimal versions of requirements, generally used with `--prefer-stable`. Can also be set via the COMPOSER\_PREFER\_LOWEST=1 env var.
* **--sort-packages:** Keep packages sorted in `composer.json`.
* **--optimize-autoloader (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run, so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize-autoloader`.
* **--apcu-autoloader:** Use APCu to cache found/not-found classes.
* **--apcu-autoloader-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu-autoloader`.
remove
------
The `remove` command removes packages from the `composer.json` file from the current directory.
```
php composer.phar remove vendor/package vendor/package2
```
After removing the requirements, the modified requirements will be uninstalled.
### Options
* **--dev:** Remove packages from `require-dev`.
* **--dry-run:** Simulate the command without actually doing anything.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--no-update:** Disables the automatic update of the dependencies (implies --no-install).
* **--no-install:** Does not run the install step after updating the composer.lock file.
* **--no-audit:** Does not run the audit steps after installation is complete. Also see [COMPOSER\_NO\_AUDIT](#composer-no-audit).
* **--audit-format:** Audit output format. Must be "table", "plain", "json", or "summary" (default).
* **--update-no-dev:** Run the dependency update with the --no-dev option. Also see [COMPOSER\_NO\_DEV](#composer-no-dev).
* **--update-with-dependencies (-w):** Also update dependencies of the removed packages. (Deprecated, is now default behavior)
* **--update-with-all-dependencies (-W):** Allows all inherited dependencies to be updated, including those that are root requirements.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard.
* **--optimize-autoloader (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize-autoloader`.
* **--apcu-autoloader:** Use APCu to cache found/not-found classes.
* **--apcu-autoloader-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu-autoloader`.
bump
----
The `bump` command increases the lower limit of your composer.json requirements to the currently installed versions. This helps to ensure your dependencies do not accidentally get downgraded due to some other conflict, and can slightly improve dependency resolution performance as it limits the amount of package versions Composer has to look at.
Running this blindly on libraries is **NOT** recommended as it will narrow down your allowed dependencies, which may cause dependency hell for your users. Running it with `--dev-only` on libraries may be fine however as dev requirements are local to the library and do not affect consumers of the package.
### Options
* **--dev-only:** Only bump requirements in "require-dev".
* **--no-dev-only:** Only bump requirements in "require".
reinstall
---------
The `reinstall` command looks up installed packages by name, uninstalls them and reinstalls them. This lets you do a clean install of a package if you messed with its files, or if you wish to change the installation type using --prefer-install.
```
php composer.phar reinstall acme/foo acme/bar
```
You can specify more than one package name to reinstall, or use a wildcard to select several packages at once:
```
php composer.phar reinstall "acme/*"
```
### Options
* **--prefer-install:** There are two ways of downloading a package: `source` and `dist`. Composer uses `dist` by default. If you pass `--prefer-install=source` (or `--prefer-source`) Composer will install from `source` if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. To get the legacy behavior where Composer use `source` automatically for dev versions of packages, use `--prefer-install=auto`. See also [config.preferred-install](06-config.md#preferred-install). Passing this flag will override the config value.
* **--no-autoloader:** Skips autoloader generation.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--optimize-autoloader (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize-autoloader`.
* **--apcu-autoloader:** Use APCu to cache found/not-found classes.
* **--apcu-autoloader-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu-autoloader`.
* **--ignore-platform-reqs:** ignore all platform requirements. This only has an effect in the context of the autoloader generation for the reinstall command.
* **--ignore-platform-req:** ignore a specific platform requirement. This only has an effect in the context of the autoloader generation for the reinstall command. Multiple requirements can be ignored via wildcard.
check-platform-reqs
-------------------
The check-platform-reqs command checks that your PHP and extensions versions match the platform requirements of the installed packages. This can be used to verify that a production server has all the extensions needed to run a project after installing it for example.
Unlike update/install, this command will ignore config.platform settings and check the real platform packages so you can be certain you have the required platform dependencies.
### Options
* **--lock:** Checks requirements only from the lock file, not from installed packages.
* **--no-dev:** Disables checking of require-dev packages requirements.
* **--format (-f):** Format of the output: text (default) or json
global
------
The global command allows you to run other commands like `install`, `remove`, `require` or `update` as if you were running them from the [COMPOSER\_HOME](#composer-home) directory.
This is merely a helper to manage a project stored in a central location that can hold CLI tools or Composer plugins that you want to have available everywhere.
This can be used to install CLI utilities globally. Here is an example:
```
php composer.phar global require friendsofphp/php-cs-fixer
```
Now the `php-cs-fixer` binary is available globally. Make sure your global [vendor binaries](articles/vendor-binaries.md) directory is in your `$PATH` environment variable, you can get its location with the following command :
```
php composer.phar global config bin-dir --absolute
```
If you wish to update the binary later on you can run a global update:
```
php composer.phar global update
```
search
------
The search command allows you to search through the current project's package repositories. Usually this will be packagist. You pass it the terms you want to search for.
```
php composer.phar search monolog
```
You can also search for more than one term by passing multiple arguments.
### Options
* **--only-name (-N):** Search only in package names.
* **--only-vendor (-O):** Search only for vendor / organization names, returns only "vendor" as a result.
* **--type (-t):** Search for a specific package type.
* **--format (-f):** Lets you pick between text (default) or json output format. Note that in the json, only the name and description keys are guaranteed to be present. The rest (`url`, `repository`, `downloads` and `favers`) are available for Packagist.org search results and other repositories may return more or less data.
show
----
To list all of the available packages, you can use the `show` command.
```
php composer.phar show
```
To filter the list you can pass a package mask using wildcards.
```
php composer.phar show "monolog/*"
```
```
monolog/monolog 2.4.0 Sends your logs to files, sockets, inboxes, databases and various web services
```
If you want to see the details of a certain package, you can pass the package name.
```
php composer.phar show monolog/monolog
```
```
name : monolog/monolog
descrip. : Sends your logs to files, sockets, inboxes, databases and various web services
keywords : log, logging, psr-3
versions : * 1.27.1
type : library
license : MIT License (MIT) (OSI approved) https://spdx.org/licenses/MIT.html#licenseText
homepage : http://github.com/Seldaek/monolog
source : [git] https://github.com/Seldaek/monolog.git 904713c5929655dc9b97288b69cfeedad610c9a1
dist : [zip] https://api.github.com/repos/Seldaek/monolog/zipball/904713c5929655dc9b97288b69cfeedad610c9a1 904713c5929655dc9b97288b69cfeedad610c9a1
names : monolog/monolog, psr/log-implementation
support
issues : https://github.com/Seldaek/monolog/issues
source : https://github.com/Seldaek/monolog/tree/1.27.1
autoload
psr-4
Monolog\ => src/Monolog
requires
php >=5.3.0
psr/log ~1.0
```
You can even pass the package version, which will tell you the details of that specific version.
```
php composer.phar show monolog/monolog 1.0.2
```
### Options
* **--all:** List all packages available in all your repositories.
* **--installed (-i):** List the packages that are installed (this is enabled by default, and deprecated).
* **--locked:** List the locked packages from composer.lock.
* **--platform (-p):** List only platform packages (php & extensions).
* **--available (-a):** List available packages only.
* **--self (-s):** List the root package info.
* **--name-only (-N):** List package names only.
* **--path (-P):** List package paths.
* **--tree (-t):** List your dependencies as a tree. If you pass a package name it will show the dependency tree for that package.
* **--latest (-l):** List all installed packages including their latest version.
* **--outdated (-o):** Implies --latest, but this lists *only* packages that have a newer version available.
* **--ignore:** Ignore specified package(s). Use it with the --outdated option if you don't want to be informed about new versions of some packages
* **--no-dev:** Filters dev dependencies from the package list.
* **--major-only (-M):** Use with --latest or --outdated. Only shows packages that have major SemVer-compatible updates.
* **--minor-only (-m):** Use with --latest or --outdated. Only shows packages that have minor SemVer-compatible updates.
* **--patch-only:** Use with --latest or --outdated. Only shows packages that have patch-level SemVer-compatible updates.
* **--direct (-D):** Restricts the list of packages to your direct dependencies.
* **--strict:** Return a non-zero exit code when there are outdated packages.
* **--format (-f):** Lets you pick between text (default) or json output format.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. Use with the --outdated option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard. Use with the --outdated option.
outdated
--------
The `outdated` command shows a list of installed packages that have updates available, including their current and latest versions. This is basically an alias for `composer show -lo`.
The color coding is as such:
* **green (=)**: Dependency is in the latest version and is up to date.
* **yellow (`~`)**: Dependency has a new version available that includes backwards compatibility breaks according to semver, so upgrade when you can but it may involve work.
* **red (!)**: Dependency has a new version that is semver-compatible and you should upgrade it.
### Options
* **--all (-a):** Show all packages, not just outdated (alias for `composer show --latest`).
* **--direct (-D):** Restricts the list of packages to your direct dependencies.
* **--strict:** Returns non-zero exit code if any package is outdated.
* **--ignore:** Ignore specified package(s). Use it if you don't want to be informed about new versions of some packages
* **--major-only (-M):** Only shows packages that have major SemVer-compatible updates.
* **--minor-only (-m):** Only shows packages that have minor SemVer-compatible updates.
* **--patch-only (-p):** Only shows packages that have patch-level SemVer-compatible updates.
* **--format (-f):** Lets you pick between text (default) or json output format.
* **--no-dev:** Do not show outdated dev dependencies.
* **--locked:** Shows updates for packages from the lock file, regardless of what is currently in vendor dir.
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard.
browse / home
-------------
The `browse` (aliased to `home`) opens a package's repository URL or homepage in your browser.
### Options
* **--homepage (-H):** Open the homepage instead of the repository URL.
* **--show (-s):** Only show the homepage or repository URL.
suggests
--------
Lists all packages suggested by the currently installed set of packages. You can optionally pass one or multiple package names in the format of `vendor/package` to limit output to suggestions made by those packages only.
Use the `--by-package` (default) or `--by-suggestion` flags to group the output by the package offering the suggestions or the suggested packages respectively.
If you only want a list of suggested package names, use `--list`.
### Options
* **--by-package:** Groups output by suggesting package (default).
* **--by-suggestion:** Groups output by suggested package.
* **--all:** Show suggestions from all dependencies, including transitive ones (by default only direct dependencies' suggestions are shown).
* **--list:** Show only list of suggested package names.
* **--no-dev:** Excludes suggestions from `require-dev` packages.
fund
----
Discover how to help fund the maintenance of your dependencies. This lists all funding links from the installed dependencies. Use `--format=json` to get machine-readable output.
### Options
* **--format (-f):** Lets you pick between text (default) or json output format.
depends (why)
-------------
The `depends` command tells you which other packages depend on a certain package. As with installation `require-dev` relationships are only considered for the root package.
```
php composer.phar depends doctrine/lexer
```
```
doctrine/annotations 1.13.3 requires doctrine/lexer (1.*)
doctrine/common 2.13.3 requires doctrine/lexer (^1.0)
```
You can optionally specify a version constraint after the package to limit the search.
Add the `--tree` or `-t` flag to show a recursive tree of why the package is depended upon, for example:
```
php composer.phar depends psr/log -t
```
```
psr/log 1.1.4 Common interface for logging libraries
├──composer/composer 2.4.x-dev (requires psr/log ^1.0 || ^2.0 || ^3.0)
├──composer/composer dev-main (requires psr/log ^1.0 || ^2.0 || ^3.0)
├──composer/xdebug-handler 3.0.3 (requires psr/log ^1 || ^2 || ^3)
│ ├──composer/composer 2.4.x-dev (requires composer/xdebug-handler ^2.0.2 || ^3.0.3)
│ └──composer/composer dev-main (requires composer/xdebug-handler ^2.0.2 || ^3.0.3)
└──symfony/console v5.4.11 (conflicts psr/log >=3) (circular dependency aborted here)
```
### Options
* **--recursive (-r):** Recursively resolves up to the root package.
* **--tree (-t):** Prints the results as a nested tree, implies -r.
prohibits (why-not)
-------------------
The `prohibits` command tells you which packages are blocking a given package from being installed. Specify a version constraint to verify whether upgrades can be performed in your project, and if not why not. See the following example:
```
php composer.phar prohibits symfony/symfony 3.1
```
```
laravel/framework v5.2.16 requires symfony/var-dumper (2.8.*|3.0.*)
```
Note that you can also specify platform requirements, for example to check whether you can upgrade your server to PHP 8.0:
```
php composer.phar prohibits php 8
```
```
doctrine/cache v1.6.0 requires php (~5.5|~7.0)
doctrine/common v2.6.1 requires php (~5.5|~7.0)
doctrine/instantiator 1.0.5 requires php (>=5.3,<8.0-DEV)
```
As with `depends` you can request a recursive lookup, which will list all packages depending on the packages that cause the conflict.
### Options
* **--recursive (-r):** Recursively resolves up to the root package.
* **--tree (-t):** Prints the results as a nested tree, implies -r.
validate
--------
You should always run the `validate` command before you commit your `composer.json` file, and before you tag a release. It will check if your `composer.json` is valid.
```
php composer.phar validate
```
### Options
* **--no-check-all:** Do not emit a warning if requirements in `composer.json` use unbound or overly strict version constraints.
* **--no-check-lock:** Do not emit an error if `composer.lock` exists and is not up to date.
* **--no-check-publish:** Do not emit an error if `composer.json` is unsuitable for publishing as a package on Packagist but is otherwise valid.
* **--with-dependencies:** Also validate the composer.json of all installed dependencies.
* **--strict:** Return a non-zero exit code for warnings as well as errors.
status
------
If you often need to modify the code of your dependencies and they are installed from source, the `status` command allows you to check if you have local changes in any of them.
```
php composer.phar status
```
With the `--verbose` option you get some more information about what was changed:
```
php composer.phar status -v
```
```
You have changes in the following dependencies:
vendor/seld/jsonlint:
M README.mdown
```
self-update (selfupdate)
------------------------
To update Composer itself to the latest version, run the `self-update` command. It will replace your `composer.phar` with the latest version.
```
php composer.phar self-update
```
If you would like to instead update to a specific release specify it:
```
php composer.phar self-update 2.4.0-RC1
```
If you have installed Composer for your entire system (see [global installation](00-intro.md#globally)), you may have to run the command with `root` privileges
```
sudo -H composer self-update
```
If Composer was not installed as a PHAR, this command is not available. (This is sometimes the case when Composer was installed by an operating system package manager.)
### Options
* **--rollback (-r):** Rollback to the last version you had installed.
* **--clean-backups:** Delete old backups during an update. This makes the current version of Composer the only backup available after the update.
* **--no-progress:** Do not output download progress.
* **--update-keys:** Prompt user for a key update.
* **--stable:** Force an update to the stable channel.
* **--preview:** Force an update to the preview channel.
* **--snapshot:** Force an update to the snapshot channel.
* **--1:** Force an update to the stable channel, but only use 1.x versions
* **--2:** Force an update to the stable channel, but only use 2.x versions
* **--set-channel-only:** Only store the channel as the default one and then exit
config
------
The `config` command allows you to edit Composer config settings and repositories in either the local `composer.json` file or the global `config.json` file.
Additionally it lets you edit most properties in the local `composer.json`.
```
php composer.phar config --list
```
### Usage
`config [options] [setting-key] [setting-value1] ... [setting-valueN]`
`setting-key` is a configuration option name and `setting-value1` is a configuration value. For settings that can take an array of values (like `github-protocols`), multiple setting-value arguments are allowed.
You can also edit the values of the following properties:
`description`, `homepage`, `keywords`, `license`, `minimum-stability`, `name`, `prefer-stable`, `type` and `version`.
See the [Config](06-config.md) chapter for valid configuration options.
### Options
* **--global (-g):** Operate on the global config file located at `$COMPOSER_HOME/config.json` by default. Without this option, this command affects the local composer.json file or a file specified by `--file`.
* **--editor (-e):** Open the local composer.json file using in a text editor as defined by the `EDITOR` env variable. With the `--global` option, this opens the global config file.
* **--auth (-a):** Affect auth config file (only used for --editor).
* **--unset:** Remove the configuration element named by `setting-key`.
* **--list (-l):** Show the list of current config variables. With the `--global` option this lists the global configuration only.
* **--file="..." (-f):** Operate on a specific file instead of composer.json. Note that this cannot be used in conjunction with the `--global` option.
* **--absolute:** Returns absolute paths when fetching `*-dir` config values instead of relative.
* **--json:** JSON decode the setting value, to be used with `extra.*` keys.
* **--merge:** Merge the setting value with the current value, to be used with `extra.*` keys in combination with `--json`.
* **--append:** When adding a repository, append it (lowest priority) to the existing ones instead of prepending it (highest priority).
* **--source:** Display where the config value is loaded from.
### Modifying Repositories
In addition to modifying the config section, the `config` command also supports making changes to the repositories section by using it the following way:
```
php composer.phar config repositories.foo vcs https://github.com/foo/bar
```
If your repository requires more configuration options, you can instead pass its JSON representation :
```
php composer.phar config repositories.foo '{"type": "vcs", "url": "http://svn.example.org/my-project/", "trunk-path": "master"}'
```
### Modifying Extra Values
In addition to modifying the config section, the `config` command also supports making changes to the extra section by using it the following way:
```
php composer.phar config extra.foo.bar value
```
The dots indicate array nesting, a max depth of 3 levels is allowed though. The above would set `"extra": { "foo": { "bar": "value" } }`.
If you have a complex value to add/modify, you can use the `--json` and `--merge` flags to edit extra fields as json:
```
php composer.phar config --json extra.foo.bar '{"baz": true, "qux": []}'
```
create-project
--------------
You can use Composer to create new projects from an existing package. This is the equivalent of doing a git clone/svn checkout followed by a `composer install` of the vendors.
There are several applications for this:
1. You can deploy application packages.
2. You can check out any package and start developing on patches for example.
3. Projects with multiple developers can use this feature to bootstrap the initial application for development.
To create a new project using Composer you can use the `create-project` command. Pass it a package name, and the directory to create the project in. You can also provide a version as a third argument, otherwise the latest version is used.
If the directory does not currently exist, it will be created during installation.
```
php composer.phar create-project doctrine/orm path "2.2.*"
```
It is also possible to run the command without params in a directory with an existing `composer.json` file to bootstrap a project.
By default the command checks for the packages on packagist.org.
### Options
* **--stability (-s):** Minimum stability of package. Defaults to `stable`.
* **--prefer-install:** There are two ways of downloading a package: `source` and `dist`. Composer uses `dist` by default. If you pass `--prefer-install=source` (or `--prefer-source`) Composer will install from `source` if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. To get the legacy behavior where Composer use `source` automatically for dev versions of packages, use `--prefer-install=auto`. See also [config.preferred-install](06-config.md#preferred-install). Passing this flag will override the config value.
* **--repository:** Provide a custom repository to search for the package, which will be used instead of packagist. Can be either an HTTP URL pointing to a `composer` repository, a path to a local `packages.json` file, or a JSON string which similar to what the [repositories](04-schema.md#repositories) key accepts. You can use this multiple times to configure multiple repositories.
* **--add-repository:** Add the custom repository in the composer.json. If a lock file is present, it will be deleted and an update will be run instead of an install.
* **--dev:** Install packages listed in `require-dev`.
* **--no-dev:** Disables installation of require-dev packages.
* **--no-scripts:** Disables the execution of the scripts defined in the root package.
* **--no-progress:** Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters.
* **--no-secure-http:** Disable the secure-http config option temporarily while installing the root package. Use at your own risk. Using this flag is a bad idea.
* **--keep-vcs:** Skip the deletion of the VCS metadata for the created project. This is mostly useful if you run the command in non-interactive mode.
* **--remove-vcs:** Force-remove the VCS metadata without prompting.
* **--no-install:** Disables installation of the vendors.
* **--no-audit:** Does not run the audit steps after installation is complete. Also see [COMPOSER\_NO\_AUDIT](#composer-no-audit).
* **--audit-format:** Audit output format. Must be "table", "plain", "json", or "summary" (default).
* **--ignore-platform-reqs:** ignore all platform requirements (`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement(`php`, `hhvm`, `lib-*` and `ext-*`) and force the installation even if the local machine does not fulfill it. Multiple requirements can be ignored via wildcard.
* **--ask:** Ask the user to provide a target directory for the new project.
dump-autoload (dumpautoload)
----------------------------
If you need to update the autoloader because of new classes in a classmap package for example, you can use `dump-autoload` to do that without having to go through an install or update.
Additionally, it can dump an optimized autoloader that converts PSR-0/4 packages into classmap ones for performance reasons. In large applications with many classes, the autoloader can take up a substantial portion of every request's time. Using classmaps for everything is less convenient in development, but using this option you can still use PSR-0/4 for convenience and classmaps for performance.
### Options
* **--optimize (-o):** Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run, so it is currently not done by default.
* **--classmap-authoritative (-a):** Autoload classes from the classmap only. Implicitly enables `--optimize`.
* **--apcu:** Use APCu to cache found/not-found classes.
* **--apcu-prefix:** Use a custom prefix for the APCu autoloader cache. Implicitly enables `--apcu`.
* **--no-dev:** Disables autoload-dev rules. Composer will by default infer this automatically according to the last `install` or `update` `--no-dev` state.
* **--dev:** Enables autoload-dev rules. Composer will by default infer this automatically according to the last `install` or `update` `--no-dev` state.
* **--ignore-platform-reqs:** ignore all `php`, `hhvm`, `lib-*` and `ext-*` requirements and skip the [platform check](07-runtime.md#platform-check) for these. See also the [`platform`](06-config.md#platform) config option.
* **--ignore-platform-req:** ignore a specific platform requirement (`php`, `hhvm`, `lib-*` and `ext-*`) and skip the [platform check](07-runtime.md#platform-check) for it. Multiple requirements can be ignored via wildcard.
* **--strict-psr:** Return a failed status code (1) if PSR-4 or PSR-0 mapping errors are present. Requires --optimize to work.
clear-cache / clearcache / cc
-----------------------------
Deletes all content from Composer's cache directories.
### Options
* **--gc:** Only run garbage collection, not a full cache clear
licenses
--------
Lists the name, version and license of every package installed. Use `--format=json` to get machine-readable output.
### Options
* **--format:** Format of the output: text, json or summary (default: "text")
* **--no-dev:** Remove dev dependencies from the output
run-script
----------
### Options
* **--timeout:** Set the script timeout in seconds, or 0 for no timeout.
* **--dev:** Sets the dev mode.
* **--no-dev:** Disable dev mode.
* **--list (-l):** List user defined scripts.
To run [scripts](articles/scripts.md) manually you can use this command, give it the script name and optionally any required arguments.
exec
----
Executes a vendored binary/script. You can execute any command and this will ensure that the Composer bin-dir is pushed on your PATH before the command runs.
### Options
* **--list (-l):** List the available Composer binaries.
diagnose
--------
If you think you found a bug, or something is behaving strangely, you might want to run the `diagnose` command to perform automated checks for many common problems.
```
php composer.phar diagnose
```
archive
-------
This command is used to generate a zip/tar archive for a given package in a given version. It can also be used to archive your entire project without excluded/ignored files.
```
php composer.phar archive vendor/package 2.0.21 --format=zip
```
### Options
* **--format (-f):** Format of the resulting archive: tar, tar.gz, tar.bz2 or zip (default: "tar").
* **--dir:** Write the archive to this directory (default: ".")
* **--file:** Write the archive with the given file name.
audit
-----
This command is used to audit the packages you have installed for possible security issues. It checks for and lists security vulnerability advisories according to the [Packagist.org api](https://packagist.org/apidoc#list-security-advisories).
```
php composer.phar audit
```
### Options
* **--no-dev:** Disables auditing of require-dev packages.
* **--format (-f):** Audit output format. Must be "table" (default), "plain", "json", or "summary".
* **--locked:** Audit packages from the lock file, regardless of what is currently in vendor dir.
help
----
To get more information about a certain command, you can use `help`.
```
php composer.phar help install
```
Command-line completion
-----------------------
Command-line completion can be enabled by following instructions [on this page](https://github.com/bamarni/symfony-console-autocomplete).
Environment variables
---------------------
You can set a number of environment variables that override certain settings. Whenever possible it is recommended to specify these settings in the `config` section of `composer.json` instead. It is worth noting that the env vars will always take precedence over the values specified in `composer.json`.
### COMPOSER
By setting the `COMPOSER` env variable it is possible to set the filename of `composer.json` to something else.
For example:
```
COMPOSER=composer-other.json php composer.phar install
```
The generated lock file will use the same name: `composer-other.lock` in this example.
### COMPOSER\_ALLOW\_SUPERUSER
If set to 1, this env disables the warning about running commands as root/super user. It also disables automatic clearing of sudo sessions, so you should really only set this if you use Composer as a super user at all times like in docker containers.
### COMPOSER\_ALLOW\_XDEBUG
If set to 1, this env allows running Composer when the Xdebug extension is enabled, without restarting PHP without it.
### COMPOSER\_AUTH
The `COMPOSER_AUTH` var allows you to set up authentication as an environment variable. The contents of the variable should be a JSON formatted object containing [http-basic, github-oauth, bitbucket-oauth, ... objects as needed](articles/authentication-for-private-packages.md), and following the [spec from the config](06-config.md).
### COMPOSER\_BIN\_DIR
By setting this option you can change the `bin` ([Vendor Binaries](articles/vendor-binaries.md)) directory to something other than `vendor/bin`.
### COMPOSER\_CACHE\_DIR
The `COMPOSER_CACHE_DIR` var allows you to change the Composer cache directory, which is also configurable via the [`cache-dir`](06-config.md#cache-dir) option.
By default, it points to `C:\Users\<user>\AppData\Local\Composer` (or `%LOCALAPPDATA%/Composer`) on Windows. On \*nix systems that follow the [XDG Base Directory Specifications](https://specifications.freedesktop.org/basedir-spec/basedir-spec-latest.html), it points to `$XDG_CACHE_HOME/composer`. On other \*nix systems and on macOS, it points to `$COMPOSER_HOME/cache`.
### COMPOSER\_CAFILE
By setting this environmental value, you can set a path to a certificate bundle file to be used during SSL/TLS peer verification.
### COMPOSER\_DISABLE\_XDEBUG\_WARN
If set to 1, this env suppresses a warning when Composer is running with the Xdebug extension enabled.
### COMPOSER\_DISCARD\_CHANGES
This env var controls the [`discard-changes`](06-config.md#discard-changes) config option.
### COMPOSER\_HOME
The `COMPOSER_HOME` var allows you to change the Composer home directory. This is a hidden, global (per-user on the machine) directory that is shared between all projects.
Use `composer config --global home` to see the location of the home directory.
By default, it points to `C:\Users\<user>\AppData\Roaming\Composer` on Windows and `/Users/<user>/.composer` on macOS. On \*nix systems that follow the [XDG Base Directory Specifications](https://specifications.freedesktop.org/basedir-spec/basedir-spec-latest.html), it points to `$XDG_CONFIG_HOME/composer`. On other \*nix systems, it points to `/home/<user>/.composer`.
#### COMPOSER\_HOME/config.json
You may put a `config.json` file into the location which `COMPOSER_HOME` points to. Composer will partially (only `config` and `repositories` keys) merge this configuration with your project's `composer.json` when you run the `install` and `update` commands.
This file allows you to set [repositories](05-repositories.md) and [configuration](06-config.md) for the user's projects.
In case global configuration matches *local* configuration, the *local* configuration in the project's `composer.json` always wins.
### COMPOSER\_HTACCESS\_PROTECT
Defaults to `1`. If set to `0`, Composer will not create `.htaccess` files in the Composer home, cache, and data directories.
### COMPOSER\_MEMORY\_LIMIT
If set, the value is used as php's memory\_limit.
### COMPOSER\_MIRROR\_PATH\_REPOS
If set to 1, this env changes the default path repository strategy to `mirror` instead of `symlink`. As it is the default strategy being set it can still be overwritten by repository options.
### COMPOSER\_NO\_INTERACTION
If set to 1, this env var will make Composer behave as if you passed the `--no-interaction` flag to every command. This can be set on build boxes/CI.
### COMPOSER\_PROCESS\_TIMEOUT
This env var controls the time Composer waits for commands (such as git commands) to finish executing. The default value is 300 seconds (5 minutes).
### COMPOSER\_ROOT\_VERSION
By setting this var you can specify the version of the root package, if it cannot be guessed from VCS info and is not present in `composer.json`.
### COMPOSER\_VENDOR\_DIR
By setting this var you can make Composer install the dependencies into a directory other than `vendor`.
### COMPOSER\_RUNTIME\_ENV
This lets you hint under which environment Composer is running, which can help Composer work around some environment specific issues. The only value currently supported is `virtualbox`, which then enables some short `sleep()` calls to wait for the filesystem to have written files properly before we attempt reading them. You can set the environment variable if you use Vagrant or VirtualBox and experience issues with files not being found during installation even though they should be present.
### http\_proxy or HTTP\_PROXY
If you are using Composer from behind an HTTP proxy, you can use the standard `http_proxy` or `HTTP_PROXY` env vars. Set it to the URL of your proxy. Many operating systems already set this variable for you.
Using `http_proxy` (lowercased) or even defining both might be preferable since some tools like git or curl will only use the lower-cased `http_proxy` version. Alternatively you can also define the git proxy using `git config --global http.proxy <proxy url>`.
If you are using Composer in a non-CLI context (i.e. integration into a CMS or similar use case), and need to support proxies, please provide the `CGI_HTTP_PROXY` environment variable instead. See [httpoxy.org](https://httpoxy.org/) for further details.
### COMPOSER\_MAX\_PARALLEL\_HTTP
Set to an integer to configure how many files can be downloaded in parallel. This defaults to 12 and must be between 1 and 50. If your proxy has issues with concurrency maybe you want to lower this. Increasing it should generally not result in performance gains.
### HTTP\_PROXY\_REQUEST\_FULLURI
If you use a proxy, but it does not support the request\_fulluri flag, then you should set this env var to `false` or `0` to prevent Composer from setting the request\_fulluri option.
### HTTPS\_PROXY\_REQUEST\_FULLURI
If you use a proxy, but it does not support the request\_fulluri flag for HTTPS requests, then you should set this env var to `false` or `0` to prevent Composer from setting the request\_fulluri option.
### COMPOSER\_SELF\_UPDATE\_TARGET
If set, makes the self-update command write the new Composer phar file into that path instead of overwriting itself. Useful for updating Composer on a read-only filesystem.
### no\_proxy or NO\_PROXY
If you are behind a proxy and would like to disable it for certain domains, you can use the `no_proxy` or `NO_PROXY` env var. Set it to a comma separated list of domains the proxy should *not* be used for.
The env var accepts domains, IP addresses, and IP address blocks in CIDR notation. You can restrict the filter to a particular port (e.g. `:80`). You can also set it to `*` to ignore the proxy for all HTTP requests.
### COMPOSER\_DISABLE\_NETWORK
If set to `1`, disables network access (best effort). This can be used for debugging or to run Composer on a plane or a starship with poor connectivity.
If set to `prime`, GitHub VCS repositories will prime the cache, so it can then be used fully offline with `1`.
### COMPOSER\_DEBUG\_EVENTS
If set to `1`, outputs information about events being dispatched, which can be useful for plugin authors to identify what is firing when exactly.
### COMPOSER\_NO\_AUDIT
If set to `1`, it is the equivalent of passing the `--no-audit` option to `require`, `update`, `remove` or `create-project` command.
### COMPOSER\_NO\_DEV
If set to `1`, it is the equivalent of passing the `--no-dev` option to `install` or `update`. You can override this for a single command by setting `COMPOSER_NO_DEV=0`.
### COMPOSER\_PREFER\_STABLE
If set to `1`, it is the equivalent of passing the `--prefer-stable` option to `update` or `require`.
### COMPOSER\_PREFER\_LOWEST
If set to `1`, it is the equivalent of passing the `--prefer-lowest` option to `update` or `require`.
### COMPOSER\_IGNORE\_PLATFORM\_REQ or COMPOSER\_IGNORE\_PLATFORM\_REQS
If `COMPOSER_IGNORE_PLATFORM_REQS` set to `1`, it is the equivalent of passing the `--ignore-platform-reqs` argument. Otherwise, specifying a comma separated list in `COMPOSER_IGNORE_PLATFORM_REQ` will ignore those specific requirements.
For example, if a development workstation will never run database queries, this can be used to ignore the requirement for the database extensions to be available. If you set `COMPOSER_IGNORE_PLATFORM_REQ=ext-oci8`, then composer will allow packages to be installed even if the `oci8` PHP extension is not enabled.
← [Libraries](02-libraries.md) | [Schema](04-schema.md) →
| programming_docs |
composer Introduction Introduction
============
Composer is a tool for dependency management in PHP. It allows you to declare the libraries your project depends on and it will manage (install/update) them for you.
Dependency management
---------------------
Composer is **not** a package manager in the same sense as Yum or Apt are. Yes, it deals with "packages" or libraries, but it manages them on a per-project basis, installing them in a directory (e.g. `vendor`) inside your project. By default, it does not install anything globally. Thus, it is a dependency manager. It does however support a "global" project for convenience via the [global](03-cli.md#global) command.
This idea is not new and Composer is strongly inspired by node's [npm](https://www.npmjs.com/) and ruby's [bundler](https://bundler.io/).
Suppose:
1. You have a project that depends on a number of libraries.
2. Some of those libraries depend on other libraries.
Composer:
1. Enables you to declare the libraries you depend on.
2. Finds out which versions of which packages can and need to be installed, and installs them (meaning it downloads them into your project).
3. You can update all your dependencies in one command.
See the [Basic usage](01-basic-usage.md) chapter for more details on declaring dependencies.
System Requirements
-------------------
Composer in its latest version requires PHP 7.2.5 to run. A long-term-support version (2.2.x) still offers support for PHP 5.3.2+ in case you are stuck with a legacy PHP version. A few sensitive php settings and compile flags are also required, but when using the installer you will be warned about any incompatibilities.
To install packages from sources instead of plain zip archives, you will need git, svn, fossil or hg depending on how the package is version-controlled.
Composer is multi-platform and we strive to make it run equally well on Windows, Linux and macOS.
Installation - Linux / Unix / macOS
-----------------------------------
### Downloading the Composer Executable
Composer offers a convenient installer that you can execute directly from the command line. Feel free to [download this file](https://getcomposer.org/installer) or review it on [GitHub](https://github.com/composer/getcomposer.org/blob/main/web/installer) if you wish to know more about the inner workings of the installer. The source is plain PHP.
There are, in short, two ways to install Composer. Locally as part of your project, or globally as a system wide executable.
#### Locally
To install Composer locally, run the installer in your project directory. See [the Download page](https://getcomposer.org/download/) for instructions.
The installer will check a few PHP settings and then download `composer.phar` to your working directory. This file is the Composer binary. It is a PHAR (PHP archive), which is an archive format for PHP which can be run on the command line, amongst other things.
Now run `php composer.phar` in order to run Composer.
You can install Composer to a specific directory by using the `--install-dir` option and additionally (re)name it as well using the `--filename` option. When running the installer when following [the Download page instructions](https://getcomposer.org/download/) add the following parameters:
```
php composer-setup.php --install-dir=bin --filename=composer
```
Now run `php bin/composer` in order to run Composer.
#### Globally
You can place the Composer PHAR anywhere you wish. If you put it in a directory that is part of your `PATH`, you can access it globally. On Unix systems you can even make it executable and invoke it without directly using the `php` interpreter.
After running the installer following [the Download page instructions](https://getcomposer.org/download/) you can run this to move composer.phar to a directory that is in your path:
```
mv composer.phar /usr/local/bin/composer
```
If you like to install it only for your user and avoid requiring root permissions, you can use `~/.local/bin` instead which is available by default on some Linux distributions.
> **Note:** If the above fails due to permissions, you may need to run it again with `sudo`.
>
> **Note:** On some versions of macOS the `/usr` directory does not exist by default. If you receive the error "/usr/local/bin/composer: No such file or directory" then you must create the directory manually before proceeding: `mkdir -p /usr/local/bin`.
>
> **Note:** For information on changing your PATH, please read the [Wikipedia article](https://en.wikipedia.org/wiki/PATH_(variable)) and/or use your search engine of choice.
>
>
Now run `composer` in order to run Composer instead of `php composer.phar`.
Installation - Windows
----------------------
### Using the Installer
This is the easiest way to get Composer set up on your machine.
Download and run [Composer-Setup.exe](https://getcomposer.org/Composer-Setup.exe). It will install the latest Composer version and set up your PATH so that you can call `composer` from any directory in your command line.
> **Note:** Close your current terminal. Test usage with a new terminal: This is important since the PATH only gets loaded when the terminal starts.
>
>
### Manual Installation
Change to a directory on your `PATH` and run the installer following [the Download page instructions](https://getcomposer.org/download/) to download `composer.phar`.
Create a new `composer.bat` file alongside `composer.phar`:
Using cmd.exe:
```
C:\bin> echo @php "%~dp0composer.phar" %*>composer.bat
```
Using PowerShell:
```
PS C:\bin> Set-Content composer.bat '@php "%~dp0composer.phar" %*'
```
Add the directory to your PATH environment variable if it isn't already. For information on changing your PATH variable, please see [this article](https://www.computerhope.com/issues/ch000549.htm) and/or use your search engine of choice.
Close your current terminal. Test usage with a new terminal:
```
C:\Users\username>composer -V
```
```
Composer version 2.4.0 2022-08-16 16:10:48
```
Docker Image
------------
Composer is published as Docker container in a few places, see the list in the [composer/docker README](https://github.com/composer/docker).
Example usage:
```
docker pull composer/composer
docker run --rm -it -v "$(pwd):/app" composer/composer install
```
To add Composer to an existing **Dockerfile**:
```
COPY --from=composer/composer /usr/bin/composer /usr/bin/composer
```
Read the [image description](https://hub.docker.com/r/composer/composer) for further usage information.
**Note:** Docker specific issues should be filed [on the composer/docker repository](https://github.com/composer/docker/issues).
**Note:** You may also use `composer` instead of `composer/composer` as image name above. It is shorter and is a Docker official image but is not published directly by us and thus usually receives new releases with a delay of a few days.
Using Composer
--------------
Now that you've installed Composer, you are ready to use it! Head on over to the next chapter for a short demonstration.
[Basic usage](01-basic-usage.md) →
composer Runtime Composer utilities Runtime Composer utilities
==========================
While Composer is mostly used around your project to install its dependencies, there are a few things which are made available to you at runtime.
If you need to rely on some of these in a specific version, you can require the `composer-runtime-api` package.
Autoload
--------
The autoloader is the most used one, and is already covered in our [basic usage guide](01-basic-usage.md#autoloading). It is available in all Composer versions.
Installed versions
------------------
composer-runtime-api 2.0 introduced a new `Composer\InstalledVersions` class which offers a few static methods to inspect which versions are currently installed. This is automatically available to your code as long as you include the Composer autoloader.
The main use cases for this class are the following:
### Knowing whether package X (or virtual package) is present
```
\Composer\InstalledVersions::isInstalled('vendor/package'); // returns bool
\Composer\InstalledVersions::isInstalled('psr/log-implementation'); // returns bool
```
As of Composer 2.1, you may also check if something was installed via require-dev or not by passing false as second argument:
```
\Composer\InstalledVersions::isInstalled('vendor/package'); // returns true assuming this package is installed
\Composer\InstalledVersions::isInstalled('vendor/package', false); // returns true if vendor/package is in require, false if in require-dev
```
Note that this can not be used to check whether platform packages are installed.
### Knowing whether package X is installed in version Y
> **Note:** To use this, your package must require `"composer/semver": "^3.0"`.
>
>
```
use Composer\Semver\VersionParser;
\Composer\InstalledVersions::satisfies(new VersionParser, 'vendor/package', '2.0.*');
\Composer\InstalledVersions::satisfies(new VersionParser, 'psr/log-implementation', '^1.0');
```
This will return true if e.g. vendor/package is installed in a version matching `2.0.*`, but also if the given package name is replaced or provided by some other package.
### Knowing the version of package X
> **Note:** This will return `null` if the package name you ask for is not itself installed but merely provided or replaced by another package. We therefore recommend using satisfies() in library code at least. In application code you have a bit more control and it is less important.
>
>
```
// returns a normalized version (e.g. 1.2.3.0) if vendor/package is installed,
// or null if it is provided/replaced,
// or throws OutOfBoundsException if the package is not installed at all
\Composer\InstalledVersions::getVersion('vendor/package');
```
```
// returns the original version (e.g. v1.2.3) if vendor/package is installed,
// or null if it is provided/replaced,
// or throws OutOfBoundsException if the package is not installed at all
\Composer\InstalledVersions::getPrettyVersion('vendor/package');
```
```
// returns the package dist or source reference (e.g. a git commit hash) if vendor/package is installed,
// or null if it is provided/replaced,
// or throws OutOfBoundsException if the package is not installed at all
\Composer\InstalledVersions::getReference('vendor/package');
```
### Knowing a package's own installed version
If you are only interested in getting a package's own version, e.g. in the source of acme/foo you want to know which version acme/foo is currently running to display that to the user, then it is acceptable to use getVersion/getPrettyVersion/getReference.
The warning in the section above does not apply in this case as you are sure the package is present and not being replaced if your code is running.
It is nonetheless a good idea to make sure you handle the `null` return value as gracefully as possible for safety.
---
A few other methods are available for more complex usages, please refer to the source/docblocks of [the class itself](https://github.com/composer/composer/blob/main/src/Composer/InstalledVersions.php).
### Knowing the path in which a package is installed
The `getInstallPath` method to retrieve a package's absolute install path.
> **Note:** The path, while absolute, may contain `../` or symlinks. It is not guaranteed to be equivalent to a `realpath()` so you should run a realpath on it if that matters to you.
>
>
```
// returns an absolute path to the package installation location if vendor/package is installed,
// or null if it is provided/replaced, or the package is a metapackage
// or throws OutOfBoundsException if the package is not installed at all
\Composer\InstalledVersions::getInstallPath('vendor/package');
```
> Available as of Composer 2.1 (i.e. `composer-runtime-api ^2.1`)
>
>
### Knowing which packages of a given type are installed
The `getInstalledPackagesByType` method accepts a package type (e.g. foo-plugin) and lists the packages of that type which are installed. You can then use the methods above to retrieve more information about each package if needed.
This method should alleviate the need for custom installers placing plugins in a specific path instead of leaving them in the vendor dir. You can then find plugins to initialize at runtime via InstalledVersions, including their paths via getInstallPath if needed.
```
\Composer\InstalledVersions::getInstalledPackagesByType('foo-plugin');
```
> Available as of Composer 2.1 (i.e. `composer-runtime-api ^2.1`)
>
>
Platform check
--------------
composer-runtime-api 2.0 introduced a new `vendor/composer/platform_check.php` file, which is included automatically when you include the Composer autoloader.
It verifies that platform requirements (i.e. php and php extensions) are fulfilled by the PHP process currently running. If the requirements are not met, the script prints a warning with the missing requirements and exits with code 104.
To avoid an unexpected white page of death with some obscure PHP extension warning in production, you can run `composer check-platform-reqs` as part of your deployment/build and if that returns a non-0 code you should abort.
The default value is `php-only` which only checks the PHP version.
If you for some reason do not want to use this safety check, and would rather risk runtime errors when your code executes, you can disable this by setting the [`platform-check`](06-config.md#platform-check) config option to `false`.
If you want the check to include verifying the presence of PHP extensions, set the config option to `true`. `ext-*` requirements will then be verified but for performance reasons Composer only checks the extension is present, not its exact version.
`lib-*` requirements are never supported/checked by the platform check feature.
Autoloader path in binaries
---------------------------
composer-runtime-api 2.2 introduced a new `$_composer_autoload_path` global variable set when running binaries installed with Composer. Read more about this [on the vendor binaries docs](articles/vendor-binaries.md#finding-the-composer-autoloader-from-a-binary).
This is set by the binary proxy and as such is not made available to projects by Composer's `vendor/autoload.php`, which would be useless as it would point back to itself.
Binary (bin-dir) path in binaries
---------------------------------
composer-runtime-api 2.2.2 introduced a new `$_composer_bin_dir` global variable set when running binaries installed with Composer. Read more about this [on the vendor binaries docs](articles/vendor-binaries.md#finding-the-composer-bin-dir-from-a-binary).
This is set by the binary proxy and as such is not made available to projects by Composer's `vendor/autoload.php`.
← [Config](06-config.md) | [Community](08-community.md) →
composer Config Config
======
This chapter will describe the `config` section of the `composer.json` [schema](04-schema.md).
process-timeout
---------------
The timeout in seconds for process executions, defaults to 300 (5mins). The duration processes like git clones can run before Composer assumes they died out. You may need to make this higher if you have a slow connection or huge vendors.
To disable the process timeout on a custom command under `scripts`, a static helper is available:
```
{
"scripts": {
"test": [
"Composer\\Config::disableProcessTimeout",
"phpunit"
]
}
}
```
allow-plugins
-------------
Defaults to `{}` which does not allow any plugins to be loaded.
As of Composer 2.2.0, the `allow-plugins` option adds a layer of security allowing you to restrict which Composer plugins are able to execute code during a Composer run.
When a new plugin is first activated, which is not yet listed in the config option, Composer will print a warning. If you run Composer interactively it will prompt you to decide if you want to execute the plugin or not.
Use this setting to allow only packages you trust to execute code. Set it to an object with package name patterns as keys. The values are **true** to allow and **false** to disallow while suppressing further warnings and prompts.
```
{
"config": {
"allow-plugins": {
"third-party/required-plugin": true,
"my-organization/*": true,
"unnecessary/plugin": false
}
}
}
```
You can also set the config option itself to `false` to disallow all plugins, or `true` to allow all plugins to run (NOT recommended). For example:
```
{
"config": {
"allow-plugins": false
}
}
```
use-include-path
----------------
Defaults to `false`. If `true`, the Composer autoloader will also look for classes in the PHP include path.
preferred-install
-----------------
Defaults to `dist` and can be any of `source`, `dist` or `auto`. This option allows you to set the install method Composer will prefer to use. Can optionally be an object with package name patterns for keys for more granular install preferences.
```
{
"config": {
"preferred-install": {
"my-organization/stable-package": "dist",
"my-organization/*": "source",
"partner-organization/*": "auto",
"*": "dist"
}
}
}
```
* `source` means Composer will install packages from their `source` if there is one. This is typically a git clone or equivalent checkout of the version control system the package uses. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly.
* `auto` is the legacy behavior where Composer uses `source` automatically for dev versions, and `dist` otherwise.
* `dist` (the default as of Composer 2.1) means Composer installs from `dist`, where possible. This is typically a zip file download, which is faster than cloning the entire repository.
> **Note:** Order matters. More specific patterns should be earlier than more relaxed patterns. When mixing the string notation with the hash configuration in global and package configurations the string notation is translated to a `*` package pattern.
>
>
use-parent-dir
--------------
When running Composer in a directory where there is no composer.json, if there is one present in a directory above Composer will by default ask you whether you want to use that directory's composer.json instead.
If you always want to answer yes to this prompt, you can set this config value to `true`. To never be prompted, set it to `false`. The default is `"prompt"`.
> **Note:** This config must be set in your global user-wide config for it to work. Use for example `php composer.phar config --global use-parent-dir true` to set it.
>
>
store-auths
-----------
What to do after prompting for authentication, one of: `true` (always store), `false` (do not store) and `"prompt"` (ask every time), defaults to `"prompt"`.
github-protocols
----------------
Defaults to `["https", "ssh", "git"]`. A list of protocols to use when cloning from github.com, in priority order. By default `git` is present but only if [secure-http](#secure-http) is disabled, as the git protocol is not encrypted. If you want your origin remote push URLs to be using https and not ssh (`[email protected]:...`), then set the protocol list to be only `["https"]` and Composer will stop overwriting the push URL to an ssh URL.
github-oauth
------------
A list of domain names and oauth keys. For example using `{"github.com": "oauthtoken"}` as the value of this option will use `oauthtoken` to access private repositories on github and to circumvent the low IP-based rate limiting of their API. Composer may prompt for credentials when needed, but these can also be manually set. Read more on how to get an OAuth token for GitHub and cli syntax [here](articles/authentication-for-private-packages.md#github-oauth).
gitlab-domains
--------------
Defaults to `["gitlab.com"]`. A list of domains of GitLab servers. This is used if you use the `gitlab` repository type.
gitlab-oauth
------------
A list of domain names and oauth keys. For example using `{"gitlab.com": "oauthtoken"}` as the value of this option will use `oauthtoken` to access private repositories on gitlab. Please note: If the package is not hosted at gitlab.com the domain names must be also specified with the [`gitlab-domains`](06-config.md#gitlab-domains) option. Further info can also be found [here](articles/authentication-for-private-packages.md#gitlab-oauth)
gitlab-token
------------
A list of domain names and private tokens. Private token can be either simple string, or array with username and token. For example using `{"gitlab.com": "privatetoken"}` as the value of this option will use `privatetoken` to access private repositories on gitlab. Using `{"gitlab.com": {"username": "gitlabuser", "token": "privatetoken"}}` will use both username and token for gitlab deploy token functionality (<https://docs.gitlab.com/ee/user/project/deploy_tokens/>) Please note: If the package is not hosted at gitlab.com the domain names must be also specified with the [`gitlab-domains`](06-config.md#gitlab-domains) option. The token must have `api` or `read_api` scope. Further info can also be found [here](articles/authentication-for-private-packages.md#gitlab-token)
gitlab-protocol
---------------
A protocol to force use of when creating a repository URL for the `source` value of the package metadata. One of `git` or `http`. (`https` is treated as a synonym for `http`.) Helpful when working with projects referencing private repositories which will later be cloned in GitLab CI jobs with a [GitLab CI\_JOB\_TOKEN](https://docs.gitlab.com/ee/ci/variables/predefined_variables.html#predefined-variables-reference) using HTTP basic auth. By default, Composer will generate a git-over-SSH URL for private repositories and HTTP(S) only for public.
disable-tls
-----------
Defaults to `false`. If set to true all HTTPS URLs will be tried with HTTP instead and no network level encryption is performed. Enabling this is a security risk and is NOT recommended. The better way is to enable the php\_openssl extension in php.ini. Enabling this will implicitly disable the `secure-http` option.
secure-http
-----------
Defaults to `true`. If set to true only HTTPS URLs are allowed to be downloaded via Composer. If you really absolutely need HTTP access to something then you can disable it, but using [Let's Encrypt](https://letsencrypt.org/) to get a free SSL certificate is generally a better alternative.
bitbucket-oauth
---------------
A list of domain names and consumers. For example using `{"bitbucket.org": {"consumer-key": "myKey", "consumer-secret": "mySecret"}}`. Read more [here](articles/authentication-for-private-packages.md#bitbucket-oauth).
cafile
------
Location of Certificate Authority file on local filesystem. In PHP 5.6+ you should rather set this via openssl.cafile in php.ini, although PHP 5.6+ should be able to detect your system CA file automatically.
capath
------
If cafile is not specified or if the certificate is not found there, the directory pointed to by capath is searched for a suitable certificate. capath must be a correctly hashed certificate directory.
http-basic
----------
A list of domain names and username/passwords to authenticate against them. For example using `{"example.org": {"username": "alice", "password": "foo"}}` as the value of this option will let Composer authenticate against example.org. More info can be found [here](articles/authentication-for-private-packages.md#http-basic).
bearer
------
A list of domain names and tokens to authenticate against them. For example using `{"example.org": "foo"}` as the value of this option will let Composer authenticate against example.org using an `Authorization: Bearer foo` header.
platform
--------
Lets you fake platform packages (PHP and extensions) so that you can emulate a production env or define your target platform in the config. Example: `{"php": "7.0.3", "ext-something": "4.0.3"}`.
This will make sure that no package requiring more than PHP 7.0.3 can be installed regardless of the actual PHP version you run locally. However it also means the dependencies are not checked correctly anymore, if you run PHP 5.6 it will install fine as it assumes 7.0.3, but then it will fail at runtime. This also means if `{"php":"7.4"}` is specified; no packages will be used that define `7.4.1` as minimum.
Therefore if you use this it is recommended, and safer, to also run the [`check-platform-reqs`](03-cli.md#check-platform-reqs) command as part of your deployment strategy.
If a dependency requires some extension that you do not have installed locally you may ignore it instead by passing `--ignore-platform-req=ext-foo` to `update`, `install` or `require`. In the long run though you should install required extensions as if you ignore one now and a new package you add a month later also requires it, you may introduce issues in production unknowingly.
If you have an extension installed locally but *not* on production, you may want to artificially hide it from Composer using `{"ext-foo": false}`.
vendor-dir
----------
Defaults to `vendor`. You can install dependencies into a different directory if you want to. `$HOME` and `~` will be replaced by your home directory's path in vendor-dir and all `*-dir` options below.
bin-dir
-------
Defaults to `vendor/bin`. If a project includes binaries, they will be symlinked into this directory.
data-dir
--------
Defaults to `C:\Users\<user>\AppData\Roaming\Composer` on Windows, `$XDG_DATA_HOME/composer` on unix systems that follow the XDG Base Directory Specifications, and `$COMPOSER_HOME` on other unix systems. Right now it is only used for storing past composer.phar files to be able to roll back to older versions. See also [COMPOSER\_HOME](03-cli.md#composer-home).
cache-dir
---------
Defaults to `C:\Users\<user>\AppData\Local\Composer` on Windows, `/Users/<user>/Library/Caches/composer` on macOS, `$XDG_CACHE_HOME/composer` on unix systems that follow the XDG Base Directory Specifications, and `$COMPOSER_HOME/cache` on other unix systems. Stores all the caches used by Composer. See also [COMPOSER\_HOME](03-cli.md#composer-home).
cache-files-dir
---------------
Defaults to `$cache-dir/files`. Stores the zip archives of packages.
cache-repo-dir
--------------
Defaults to `$cache-dir/repo`. Stores repository metadata for the `composer` type and the VCS repos of type `svn`, `fossil`, `github` and `bitbucket`.
cache-vcs-dir
-------------
Defaults to `$cache-dir/vcs`. Stores VCS clones for loading VCS repository metadata for the `git`/`hg` types and to speed up installs.
cache-files-ttl
---------------
Defaults to `15552000` (6 months). Composer caches all dist (zip, tar, ...) packages that it downloads. Those are purged after six months of being unused by default. This option allows you to tweak this duration (in seconds) or disable it completely by setting it to 0.
cache-files-maxsize
-------------------
Defaults to `300MiB`. Composer caches all dist (zip, tar, ...) packages that it downloads. When the garbage collection is periodically ran, this is the maximum size the cache will be able to use. Older (less used) files will be removed first until the cache fits.
cache-read-only
---------------
Defaults to `false`. Whether to use the Composer cache in read-only mode.
bin-compat
----------
Defaults to `auto`. Determines the compatibility of the binaries to be installed. If it is `auto` then Composer only installs .bat proxy files when on Windows or WSL. If set to `full` then both .bat files for Windows and scripts for Unix-based operating systems will be installed for each binary. This is mainly useful if you run Composer inside a linux VM but still want the `.bat` proxies available for use in the Windows host OS. If set to `proxy` Composer will only create bash/Unix-style proxy files and no .bat files even on Windows/WSL.
prepend-autoloader
------------------
Defaults to `true`. If `false`, the Composer autoloader will not be prepended to existing autoloaders. This is sometimes required to fix interoperability issues with other autoloaders.
autoloader-suffix
-----------------
Defaults to `null`. Non-empty string to be used as a suffix for the generated Composer autoloader. When null a random one will be generated.
optimize-autoloader
-------------------
Defaults to `false`. If `true`, always optimize when dumping the autoloader.
sort-packages
-------------
Defaults to `false`. If `true`, the `require` command keeps packages sorted by name in `composer.json` when adding a new package.
classmap-authoritative
----------------------
Defaults to `false`. If `true`, the Composer autoloader will only load classes from the classmap. Implies `optimize-autoloader`.
apcu-autoloader
---------------
Defaults to `false`. If `true`, the Composer autoloader will check for APCu and use it to cache found/not-found classes when the extension is enabled.
github-domains
--------------
Defaults to `["github.com"]`. A list of domains to use in github mode. This is used for GitHub Enterprise setups.
github-expose-hostname
----------------------
Defaults to `true`. If `false`, the OAuth tokens created to access the github API will have a date instead of the machine hostname.
use-github-api
--------------
Defaults to `true`. Similar to the `no-api` key on a specific repository, setting `use-github-api` to `false` will define the global behavior for all GitHub repositories to clone the repository as it would with any other git repository instead of using the GitHub API. But unlike using the `git` driver directly, Composer will still attempt to use GitHub's zip files.
notify-on-install
-----------------
Defaults to `true`. Composer allows repositories to define a notification URL, so that they get notified whenever a package from that repository is installed. This option allows you to disable that behavior.
discard-changes
---------------
Defaults to `false` and can be any of `true`, `false` or `"stash"`. This option allows you to set the default style of handling dirty updates when in non-interactive mode. `true` will always discard changes in vendors, while `"stash"` will try to stash and reapply. Use this for CI servers or deploy scripts if you tend to have modified vendors.
archive-format
--------------
Defaults to `tar`. Overrides the default format used by the archive command.
archive-dir
-----------
Defaults to `.`. Default destination for archives created by the archive command.
Example:
```
{
"config": {
"archive-dir": "/home/user/.composer/repo"
}
}
```
htaccess-protect
----------------
Defaults to `true`. If set to `false`, Composer will not create `.htaccess` files in the Composer home, cache, and data directories.
lock
----
Defaults to `true`. If set to `false`, Composer will not create a `composer.lock` file and will ignore it if one is present.
platform-check
--------------
Defaults to `php-only` which only checks the PHP version. Set to `true` to also check the presence of extension. If set to `false`, Composer will not create and require a `platform_check.php` file as part of the autoloader bootstrap.
secure-svn-domains
------------------
Defaults to `[]`. Lists domains which should be trusted/marked as using a secure Subversion/SVN transport. By default svn:// protocol is seen as insecure and will throw, but you can set this config option to `["example.org"]` to allow using svn URLs on that hostname. This is a better/safer alternative to disabling `secure-http` altogether.
← [Repositories](05-repositories.md) | [Runtime](07-runtime.md) →
| programming_docs |
composer Community Community
=========
There are many people using Composer already, and quite a few of them are contributing.
Contributing
------------
If you would like to contribute to Composer, please read the [README](https://github.com/composer/composer) and [CONTRIBUTING](https://github.com/composer/composer/blob/main/.github/CONTRIBUTING.md) documents.
The most important guidelines are described as follows:
> All code contributions - including those of people having commit access - must go through a pull request and approved by a core developer before being merged. This is to ensure proper review of all the code.
>
> Fork the project, create a feature branch, and send us a pull request.
>
> To ensure a consistent code base, you should make sure the code follows the [PSR-2 Coding Standards](https://www.php-fig.org/psr/psr-2/).
>
>
Support
-------
The IRC channel is on irc.libera.chat: [#composer](ircs://irc.libera.chat:6697/composer).
[Stack Overflow](https://stackoverflow.com/questions/tagged/composer-php) and [GitHub Discussions](https://github.com/composer/composer/discussions) both have a collection of Composer related questions.
For paid support, we do provide Composer-related support via chat and email to [Private Packagist](https://packagist.com) customers.
← [Config](07-runtime.md)
composer Libraries Libraries
=========
This chapter will tell you how to make your library installable through Composer.
Every project is a package
--------------------------
As soon as you have a `composer.json` in a directory, that directory is a package. When you add a [`require`](04-schema.md#require) to a project, you are making a package that depends on other packages. The only difference between your project and a library is that your project is a package without a name.
In order to make that package installable you need to give it a name. You do this by adding the [`name`](04-schema.md#name) property in `composer.json`:
```
{
"name": "acme/hello-world",
"require": {
"monolog/monolog": "1.0.*"
}
}
```
In this case the project name is `acme/hello-world`, where `acme` is the vendor name. Supplying a vendor name is mandatory.
> **Note:** If you don't know what to use as a vendor name, your GitHub username is usually a good bet. Package names must be lowercase, and the convention is to use dashes for word separation.
>
>
Library Versioning
------------------
In the vast majority of cases, you will be maintaining your library using some sort of version control system like git, svn, hg or fossil. In these cases, Composer infers versions from your VCS, and you **should not** specify a version in your `composer.json` file. (See the [Versions article](articles/versions.md) to learn about how Composer uses VCS branches and tags to resolve version constraints.)
If you are maintaining packages by hand (i.e., without a VCS), you'll need to specify the version explicitly by adding a `version` value in your `composer.json` file:
```
{
"version": "1.0.0"
}
```
> **Note:** When you add a hardcoded version to a VCS, the version will conflict with tag names. Composer will not be able to determine the version number.
>
>
### VCS Versioning
Composer uses your VCS's branch and tag features to resolve the version constraints you specify in your [`require`](04-schema.md#require) field to specific sets of files. When determining valid available versions, Composer looks at all of your tags and branches and translates their names into an internal list of options that it then matches against the version constraint you provided.
For more on how Composer treats tags and branches and how it resolves package version constraints, read the [versions](articles/versions.md) article.
Lock file
---------
For your library you may commit the `composer.lock` file if you want to. This can help your team to always test against the same dependency versions. However, this lock file will not have any effect on other projects that depend on it. It only has an effect on the main project.
If you do not want to commit the lock file, and you are using git, add it to the `.gitignore`.
Publishing to a VCS
-------------------
Once you have a VCS repository (version control system, e.g. git) containing a `composer.json` file, your library is already composer-installable. In this example we will publish the `acme/hello-world` library on GitHub under `github.com/username/hello-world`.
Now, to test installing the `acme/hello-world` package, we create a new project locally. We will call it `acme/blog`. This blog will depend on `acme/hello-world`, which in turn depends on `monolog/monolog`. We can accomplish this by creating a new `blog` directory somewhere, containing a `composer.json`:
```
{
"name": "acme/blog",
"require": {
"acme/hello-world": "dev-master"
}
}
```
The name is not needed in this case, since we don't want to publish the blog as a library. It is added here to clarify which `composer.json` is being described.
Now we need to tell the blog app where to find the `hello-world` dependency. We do this by adding a package repository specification to the blog's `composer.json`:
```
{
"name": "acme/blog",
"repositories": [
{
"type": "vcs",
"url": "https://github.com/username/hello-world"
}
],
"require": {
"acme/hello-world": "dev-master"
}
}
```
For more details on how package repositories work and what other types are available, see [Repositories](05-repositories.md).
That's all. You can now install the dependencies by running Composer's [`install`](03-cli.md#install) command!
**Recap:** Any git/svn/hg/fossil repository containing a `composer.json` can be added to your project by specifying the package repository and declaring the dependency in the [`require`](04-schema.md#require) field.
Publishing to packagist
-----------------------
Alright, so now you can publish packages. But specifying the VCS repository every time is cumbersome. You don't want to force all your users to do that.
The other thing that you may have noticed is that we did not specify a package repository for `monolog/monolog`. How did that work? The answer is Packagist.
[Packagist](https://packagist.org/) is the main package repository for Composer, and it is enabled by default. Anything that is published on Packagist is available automatically through Composer. Since [Monolog is on Packagist](https://packagist.org/packages/monolog/monolog), we can depend on it without having to specify any additional repositories.
If we wanted to share `hello-world` with the world, we would publish it on Packagist as well.
You visit [Packagist](https://packagist.org) and hit the "Submit" button. This will prompt you to sign up if you haven't already, and then allows you to submit the URL to your VCS repository, at which point Packagist will start crawling it. Once it is done, your package will be available to anyone!
← [Basic usage](01-basic-usage.md) | [Command-line interface](03-cli.md) →
composer Troubleshooting Troubleshooting
===============
This is a list of common pitfalls on using Composer, and how to avoid them.
General
-------
1. When facing any kind of problems using Composer, be sure to **work with the latest version**. See [self-update](../03-cli.md#self-update) for details.
2. Before asking anyone, run [`composer diagnose`](../03-cli.md#diagnose) to check for common problems. If it all checks out, proceed to the next steps.
3. Make sure you have no problems with your setup by running the installer's checks via `curl -sS https://getcomposer.org/installer | php -- --check`.
4. Try clearing Composer's cache by running `composer clear-cache`.
5. Ensure you're **installing vendors straight from your `composer.json`** via `rm -rf vendor && composer update -v` when troubleshooting, excluding any possible interferences with existing vendor installations or `composer.lock` entries.
Package not found
-----------------
1. Double-check you **don't have typos** in your `composer.json` or repository branches and tag names.
2. Be sure to **set the right [minimum-stability](../04-schema.md#minimum-stability)**. To get started or be sure this is no issue, set `minimum-stability` to "dev".
3. Packages **not coming from [Packagist](https://packagist.org/)** should always be **defined in the root package** (the package depending on all vendors).
4. Use the **same vendor and package name** throughout all branches and tags of your repository, especially when maintaining a third party fork and using `replace`.
5. If you are updating to a recently published version of a package, be aware that Packagist has a delay of up to 1 minute before new packages are visible to Composer.
6. If you are updating a single package, it may depend on newer versions itself. In this case add the `--with-dependencies` argument **or** add all dependencies which need an update to the command.
Package is not updating to the expected version
-----------------------------------------------
Try running `php composer.phar why-not [package-name] [expected-version]`.
Dependencies on the root package
--------------------------------
When your root package depends on a package which ends up depending (directly or indirectly) back on the root package itself, issues can occur in two cases:
1. During development, if you are on a branch like `dev-main` and the branch has no [branch-alias](aliases.md#branch-alias) defined, and the dependency on the root package requires version `^2.0` for example, the `dev-main` version will not satisfy it. The best solution here is to make sure you first define a branch alias.
2. In CI (Continuous Integration) runs, the problem might be that Composer is not able to detect the version of the root package properly. If it is a git clone it is generally alright and Composer will detect the version of the current branch, but some CIs do shallow clones so that process can fail when testing pull requests and feature branches. In these cases the branch alias may then not be recognized. The best solution is to define the version you are on via an environment variable called COMPOSER\_ROOT\_VERSION. You set it to `dev-main` for example to define the root package's version as `dev-main`. Use for example: `COMPOSER_ROOT_VERSION=dev-main composer install` to export the variable only for the call to composer, or you can define it globally in the CI env vars.
Package not found in a Jenkins-build
------------------------------------
1. Check the ["Package not found"](#package-not-found) item above.
2. The git-clone / checkout within Jenkins leaves the branch in a "detached HEAD"-state. As a result, Composer may not able to identify the version of the current checked out branch and may not be able to resolve a [dependency on the root package](#dependencies-on-the-root-package). To solve this problem, you can use the "Additional Behaviours" -> "Check out to specific local branch" in your Git-settings for your Jenkins-job, where your "local branch" shall be the same branch as you are checking out. Using this, the checkout will not be in detached state any more and the dependency on the root package should become satisfied.
I have a dependency which contains a "repositories" definition in its composer.json, but it seems to be ignored.
----------------------------------------------------------------------------------------------------------------
The [`repositories`](../04-schema.md#repositories) configuration property is defined as [root-only](../04-schema.md#root-package). It is not inherited. You can read more about the reasons behind this in the "[why can't Composer load repositories recursively?](https://getcomposer.org/doc/faqs/why-cant-composer-load-repositories-recursively.md)" article. The simplest work-around to this limitation, is moving or duplicating the `repositories` definition into your root composer.json.
I have locked a dependency to a specific commit but get unexpected results.
---------------------------------------------------------------------------
While Composer supports locking dependencies to a specific commit using the `#commit-ref` syntax, there are certain caveats that one should take into account. The most important one is [documented](../04-schema.md#package-links), but frequently overlooked:
> **Note:** While this is convenient at times, it should not be how you use packages in the long term because it comes with a technical limitation. The composer.json metadata will still be read from the branch name you specify before the hash. Because of that in some cases it will not be a practical workaround, and you should always try to switch to tagged releases as soon as you can.
>
>
There is no simple work-around to this limitation. It is therefore strongly recommended that you do not use it.
Need to override a package version
----------------------------------
Let's say your project depends on package A, which in turn depends on a specific version of package B (say 0.1). But you need a different version of said package B (say 0.11).
You can fix this by aliasing version 0.11 to 0.1:
composer.json:
```
{
"require": {
"A": "0.2",
"B": "0.11 as 0.1"
}
}
```
See [aliases](aliases.md) for more information.
Figuring out where a config value came from
-------------------------------------------
Use `php composer.phar config --list --source` to see where each config value originated from.
Memory limit errors
-------------------
The first thing to do is to make sure you are running Composer 2, and if possible 2.2.0 or above.
Composer 1 used much more memory and upgrading to the latest version will give you much better and faster results.
Composer may sometimes fail on some commands with this message:
`PHP Fatal error: Allowed memory size of XXXXXX bytes exhausted <...>`
In this case, the PHP `memory_limit` should be increased.
> **Note:** Composer internally increases the `memory_limit` to `1.5G`.
>
>
To get the current `memory_limit` value, run:
```
php -r "echo ini_get('memory_limit').PHP_EOL;"
```
Try increasing the limit in your `php.ini` file (ex. `/etc/php5/cli/php.ini` for Debian-like systems):
```
; Use -1 for unlimited or define an explicit value like 2G
memory_limit = -1
```
Composer also respects a memory limit defined by the `COMPOSER_MEMORY_LIMIT` environment variable:
```
COMPOSER_MEMORY_LIMIT=-1 composer.phar <...>
```
Or, you can increase the limit with a command-line argument:
```
php -d memory_limit=-1 composer.phar <...>
```
This issue can also happen on cPanel instances, when the shell fork bomb protection is activated. For more information, see the [documentation](https://documentation.cpanel.net/display/68Docs/Shell+Fork+Bomb+Protection) of the fork bomb feature on the cPanel site.
Xdebug impact on Composer
-------------------------
To improve performance when the Xdebug extension is enabled, Composer automatically restarts PHP without it. You can override this behavior by using an environment variable: `COMPOSER_ALLOW_XDEBUG=1`.
Composer will always show a warning if Xdebug is being used, but you can override this with an environment variable: `COMPOSER_DISABLE_XDEBUG_WARN=1`. If you see this warning unexpectedly, then the restart process has failed: please report this [issue](https://github.com/composer/composer/issues).
"The system cannot find the path specified" (Windows)
-----------------------------------------------------
1. Open regedit.
2. Search for an `AutoRun` key inside `HKEY_LOCAL_MACHINE\Software\Microsoft\Command Processor`, `HKEY_CURRENT_USER\Software\Microsoft\Command Processor` or `HKEY_LOCAL_MACHINE\Software\Wow6432Node\Microsoft\Command Processor`.
3. Check if it contains any path to a non-existent file, if it's the case, remove them.
API rate limit and OAuth tokens
-------------------------------
Because of GitHub's rate limits on their API it can happen that Composer prompts for authentication asking your username and password so it can go ahead with its work.
If you would prefer not to provide your GitHub credentials to Composer you can manually create a token using the [procedure documented here](authentication-for-private-packages.md#github-oauth).
Now Composer should install/update without asking for authentication.
proc\_open(): fork failed errors
--------------------------------
If Composer shows proc\_open() fork failed on some commands:
`PHP Fatal error: Uncaught exception 'ErrorException' with message 'proc_open(): fork failed - Cannot allocate memory' in phar`
This could be happening because the VPS runs out of memory and has no Swap space enabled.
```
free -m
```
```
total used free shared buffers cached
Mem: 2048 357 1690 0 0 237
-/+ buffers/cache: 119 1928
Swap: 0 0 0
```
To enable the swap you can use for example:
```
/bin/dd if=/dev/zero of=/var/swap.1 bs=1M count=1024
/sbin/mkswap /var/swap.1
/bin/chmod 0600 /var/swap.1
/sbin/swapon /var/swap.1
```
You can make a permanent swap file following this [tutorial](https://www.digitalocean.com/community/tutorials/how-to-add-swap-on-ubuntu-14-04).
proc\_open(): failed to open stream errors (Windows)
----------------------------------------------------
If Composer shows proc\_open(NUL) errors on Windows:
`proc_open(NUL): failed to open stream: No such file or directory`
This could be happening because you are working in a *OneDrive* directory and using a version of PHP that does not support the file system semantics of this service. The issue was fixed in PHP 7.2.23 and 7.3.10.
Alternatively it could be because the Windows Null Service is not enabled. For more information, see this [issue](https://github.com/composer/composer/issues/7186#issuecomment-373134916).
Degraded Mode
-------------
Due to some intermittent issues on Travis and other systems, we introduced a degraded network mode which helps Composer finish successfully but disables a few optimizations. This is enabled automatically when an issue is first detected. If you see this issue sporadically you probably don't have to worry (a slow or overloaded network can also cause those time outs), but if it appears repeatedly you might want to look at the options below to identify and resolve it.
If you have been pointed to this page, you want to check a few things:
* If you are using ESET antivirus, go in "Advanced Settings" and disable "HTTP-scanner" under "web access protection"
* If you are using IPv6, try disabling it. If that solves your issues, get in touch with your ISP or server host, the problem is not at the Packagist level but in the routing rules between you and Packagist (i.e. the internet at large). The best way to get these fixed is to raise awareness to the network engineers that have the power to fix it. Take a look at the next section for IPv6 workarounds.
* If none of the above helped, please report the error.
Operation timed out (IPv6 issues)
---------------------------------
You may run into errors if IPv6 is not configured correctly. A common error is:
```
The "https://getcomposer.org/version" file could not be downloaded: failed to
open stream: Operation timed out
```
We recommend you fix your IPv6 setup. If that is not possible, you can try the following workarounds:
**Workaround Linux:**
On linux, it seems that running this command helps to make ipv4 traffic have a higher priority than ipv6, which is a better alternative than disabling ipv6 entirely:
```
sudo sh -c "echo 'precedence ::ffff:0:0/96 100' >> /etc/gai.conf"
```
**Workaround Windows:**
On windows the only way is to disable ipv6 entirely I am afraid (either in windows or in your home router).
**Workaround Mac OS X:**
Get name of your network device:
```
networksetup -listallnetworkservices
```
Disable IPv6 on that device (in this case "Wi-Fi"):
```
networksetup -setv6off Wi-Fi
```
Run Composer ...
You can enable IPv6 again with:
```
networksetup -setv6automatic Wi-Fi
```
That said, if this fixes your problem, please talk to your ISP about it to try to resolve the routing errors. That's the best way to get things resolved for everyone.
Composer hangs with SSH ControlMaster
-------------------------------------
When you try to install packages from a Git repository and you use the `ControlMaster` setting for your SSH connection, Composer might hang endlessly and you see a `sh` process in the `defunct` state in your process list.
The reason for this is a SSH Bug: <https://bugzilla.mindrot.org/show_bug.cgi?id=1988>
As a workaround, open a SSH connection to your Git host before running Composer:
```
ssh -t [email protected]
php composer.phar update
```
See also <https://github.com/composer/composer/issues/4180> for more information.
Zip archives are not unpacked correctly.
----------------------------------------
Composer can unpack zipballs using either a system-provided `unzip` or `7z` (7-Zip) utility, or PHP's native `ZipArchive` class. On OSes where ZIP files can contain permissions and symlinks, we recommend installing `unzip` or `7z` as these features are not supported by `ZipArchive`.
Disabling the pool optimizer
----------------------------
In Composer, the `Pool` class contains all the packages that are relevant for the dependency resolving process. That is what is used to generate all the rules which are then passed on to the dependency solver. In order to improve performance, Composer tries to optimize this `Pool` by removing useless package information early on.
If all goes well, you should never notice any issues with it but in case you run into an unexpected result such as an unresolvable set of dependencies or conflicts where you think Composer is wrong, you might want to disable the optimizer by using the environment variable `COMPOSER_POOL_OPTIMIZER` and run the update again like so:
```
COMPOSER_POOL_OPTIMIZER=0 php composer.phar update
```
Now double check if the result is still the same. It will take significantly longer and use a lot more memory to run the dependency resolving process.
If the result is different, you likely hit a problem in the pool optimizer. Please [report this issue](https://github.com/composer/composer/issues) so it can be fixed.
| programming_docs |
composer Autoloader optimization Autoloader optimization
=======================
By default, the Composer autoloader runs relatively fast. However, due to the way PSR-4 and PSR-0 autoloading rules are set up, it needs to check the filesystem before resolving a classname conclusively. This slows things down quite a bit, but it is convenient in development environments because when you add a new class it can immediately be discovered/used without having to rebuild the autoloader configuration.
The problem however is in production you generally want things to happen as fast as possible, as you can rebuild the configuration every time you deploy and new classes do not appear at random between deploys.
For this reason, Composer offers a few strategies to optimize the autoloader.
> **Note:** You **should not** enable any of these optimizations in **development** as they all will cause various problems when adding/removing classes. The performance gains are not worth the trouble in a development setting.
>
>
Optimization Level 1: Class map generation
------------------------------------------
### How to run it?
There are a few options to enable this:
* Set `"optimize-autoloader": true` inside the config key of composer.json
* Call `install` or `update` with `-o` / `--optimize-autoloader`
* Call `dump-autoload` with `-o` / `--optimize`
### What does it do?
Class map generation essentially converts PSR-4/PSR-0 rules into classmap rules. This makes everything quite a bit faster as for known classes the class map returns instantly the path, and Composer can guarantee the class is in there so there is no filesystem check needed.
On PHP 5.6+, the class map is also cached in opcache which improves the initialization time greatly. If you make sure opcache is enabled, then the class map should load almost instantly and then class loading is fast.
### Trade-offs
There are no real trade-offs with this method. It should always be enabled in production.
The only issue is it does not keep track of autoload misses (i.e. when it cannot find a given class), so those fallback to PSR-4 rules and can still result in slow filesystem checks. To solve this issue two Level 2 optimization options exist, and you can decide to enable either if you have a lot of class\_exists checks that are done for classes that do not exist in your project.
Optimization Level 2/A: Authoritative class maps
------------------------------------------------
### How to run it?
There are a few options to enable this:
* Set `"classmap-authoritative": true` inside the config key of composer.json
* Call `install` or `update` with `-a` / `--classmap-authoritative`
* Call `dump-autoload` with `-a` / `--classmap-authoritative`
### What does it do?
Enabling this automatically enables Level 1 class map optimizations.
This option says that if something is not found in the classmap, then it does not exist and the autoloader should not attempt to look on the filesystem according to PSR-4 rules.
### Trade-offs
This option makes the autoloader always return very quickly. On the flipside it also means that in case a class is generated at runtime for some reason, it will not be allowed to be autoloaded. If your project or any of your dependencies does that then you might experience "class not found" issues in production. Enable this with care.
> Note: This cannot be combined with Level 2/B optimizations. You have to choose one as they address the same issue in different ways.
>
>
Optimization Level 2/B: APCu cache
----------------------------------
### How to run it?
There are a few options to enable this:
* Set `"apcu-autoloader": true` inside the config key of composer.json
* Call `install` or `update` with `--apcu-autoloader`
* Call `dump-autoload` with `--apcu`
### What does it do?
This option adds an APCu cache as a fallback for the class map. It will not automatically generate the class map though, so you should still enable Level 1 optimizations manually if you so desire.
Whether a class is found or not, that fact is always cached in APCu, so it can be returned quickly on the next request.
### Trade-offs
This option requires APCu which may or may not be available to you. It also uses APCu memory for autoloading purposes, but it is safe to use and cannot result in classes not being found like the authoritative class map optimization above.
> Note: This cannot be combined with Level 2/A optimizations. You have to choose one as they address the same issue in different ways.
>
>
composer Handling private packages Handling private packages
=========================
Private Packagist
=================
[Private Packagist](https://packagist.com) is a commercial package hosting product offering professional support and web based management of private and public packages, and granular access permissions. Private Packagist provides mirroring for packages' zip files which makes installs faster and independent from third party systems - e.g. you can deploy even if GitHub is down because your zip files are mirrored.
Private Packagist is available as a hosted SaaS solution or as an on-premise self-hosted package, providing an interactive set up experience.
Some of Private Packagist's revenue is used to pay for Composer and Packagist.org development and hosting so using it is a good way to support the maintenance of these open source projects financially. You can find more information about how to set up your own package archive on [Packagist.com](https://packagist.com).
Satis
=====
Satis on the other hand is open source but only a static `composer` repository generator. It is a bit like an ultra-lightweight, static file-based version of packagist and can be used to host the metadata of your company's private packages, or your own. You can get it from [GitHub](https://github.com/composer/satis) or install via CLI:
```
php composer.phar create-project composer/satis --stability=dev --keep-vcs
```
Setup
-----
For example let's assume you have a few packages you want to reuse across your company but don't really want to open-source. You would first define a Satis configuration: a json file that lists your curated [repositories](../05-repositories.md).
The default file name is satis.json but it could be anything you like.
Here is an example configuration, you see that it holds a few VCS repositories, but those could be any types of [repositories](../05-repositories.md). Then it uses `"require-all": true` which selects all versions of all packages in the repositories you defined.
The default file Satis looks for is `satis.json` in the root of the repository.
```
{
"name": "my/repository",
"homepage": "http://packages.example.org",
"repositories": [
{ "type": "vcs", "url": "https://github.com/mycompany/privaterepo" },
{ "type": "vcs", "url": "http://svn.example.org/private/repo" },
{ "type": "vcs", "url": "https://github.com/mycompany/privaterepo2" }
],
"require-all": true
}
```
If you want to cherry pick which packages you want, you can list all the packages you want to have in your satis repository inside the classic composer `require` key, using a `"*"` constraint to make sure all versions are selected, or another constraint if you want really specific versions.
```
{
"repositories": [
{ "type": "vcs", "url": "https://github.com/mycompany/privaterepo" },
{ "type": "vcs", "url": "http://svn.example.org/private/repo" },
{ "type": "vcs", "url": "https://github.com/mycompany/privaterepo2" }
],
"require": {
"company/package": "*",
"company/package2": "*",
"company/package3": "2.0.0"
}
}
```
Once you've done this, you run:
```
php bin/satis build <configuration file> <build dir>
```
When you ironed out that process, what you would typically do is run this command as a cron job on a server. It would then update all your package info much like Packagist does.
Note that if your private packages are hosted on GitHub, your server should have an ssh key that gives it access to those packages, and then you should add the `--no-interaction` (or `-n`) flag to the command to make sure it falls back to ssh key authentication instead of prompting for a password. This is also a good trick for continuous integration servers.
Set up a virtual-host that points to that `web/` directory, let's say it is `packages.example.org`. Alternatively, with PHP >= 5.4.0, you can use the built-in CLI server `php -S localhost:port -t satis-output-dir/` for a temporary solution.
### Partial Updates
You can tell Satis to selectively update only particular packages or process only a repository with a given URL. This cuts down the time it takes to rebuild the `package.json` file and is helpful if you use (custom) webhooks to trigger rebuilds whenever code is pushed into one of your repositories.
To rebuild only particular packages, pass the package names on the command line like so:
```
php bin/satis build satis.json web/ this/package that/other-package
```
Note that this will still need to pull and scan all of your VCS repositories because any VCS repository might contain (on any branch) one of the selected packages.
If you want to scan only the selected package and not all VCS repositories you need to declare a *name* for all your package (this only work on VCS repositories type) :
```
{
"repositories": [
{ "name": "company/privaterepo", "type": "vcs", "url": "https://github.com/mycompany/privaterepo" },
{ "name": "private/repo", "type": "vcs", "url": "http://svn.example.org/private/repo" },
{ "name": "mycompany/privaterepo2", "type": "vcs", "url": "https://github.com/mycompany/privaterepo2" }
]
}
```
If you want to scan only a single repository and update all packages found in it, pass the VCS repository URL as an optional argument:
```
php bin/satis build --repository-url https://only.my/repo.git satis.json web/
```
Usage
-----
In your projects all you need to add now is your own Composer repository using the `packages.example.org` as URL, then you can require your private packages and everything should work smoothly. You don't need to copy all your repositories in every project anymore. Only that one unique repository that will update itself.
```
{
"repositories": [ { "type": "composer", "url": "http://packages.example.org/" } ],
"require": {
"company/package": "1.2.0",
"company/package2": "1.5.2",
"company/package3": "dev-master"
}
}
```
### Security
To secure your private repository you can host it over SSH or SSL using a client certificate. In your project you can use the `options` parameter to specify the connection options for the server.
Example using a custom repository using SSH (requires the SSH2 PECL extension):
```
{
"repositories": [{
"type": "composer",
"url": "ssh2.sftp://example.org",
"options": {
"ssh2": {
"username": "composer",
"pubkey_file": "/home/composer/.ssh/id_rsa.pub",
"privkey_file": "/home/composer/.ssh/id_rsa"
}
}
}]
}
```
> **Tip:** See [ssh2 context options](https://secure.php.net/manual/en/wrappers.ssh2.php#refsect1-wrappers.ssh2-options) for more information.
>
>
Example using SSL/TLS (HTTPS) using a client certificate:
```
{
"repositories": [{
"type": "composer",
"url": "https://example.org",
"options": {
"ssl": {
"local_cert": "/home/composer/.ssl/composer.pem"
}
}
}]
}
```
> **Tip:** See [ssl context options](https://secure.php.net/manual/en/context.ssl.php) for more information.
>
>
Example using a custom HTTP Header field for token authentication:
```
{
"repositories": [{
"type": "composer",
"url": "https://example.org",
"options": {
"http": {
"header": [
"API-TOKEN: YOUR-API-TOKEN"
]
}
}
}]
}
```
### Authentication
Authentication can be handled in [several different ways](authentication-for-private-packages.md).
### Downloads
When GitHub, GitLab or BitBucket repositories are mirrored on your local satis, the build process will include the location of the downloads these platforms make available. This means that the repository and your setup depend on the availability of these services.
At the same time, this implies that all code which is hosted somewhere else (on another service or for example in Subversion) will not have downloads available and thus installations usually take a lot longer.
To enable your satis installation to create downloads for all (Git, Mercurial and Subversion) your packages, add the following to your `satis.json`:
```
{
"archive": {
"directory": "dist",
"format": "tar",
"prefix-url": "https://amazing.cdn.example.org",
"skip-dev": true
}
}
```
#### Options explained
* `directory`: required, the location of the dist files (inside the `output-dir`)
* `format`: optional, `zip` (default) or `tar`
* `prefix-url`: optional, location of the downloads, homepage (from `satis.json`) followed by `directory` by default
* `skip-dev`: optional, `false` by default, when enabled (`true`) satis will not create downloads for branches
* `absolute-directory`: optional, a *local* directory where the dist files are dumped instead of `output-dir`/`directory`
* `whitelist`: optional, if set as a list of package names, satis will only dump the dist files of these packages
* `blacklist`: optional, if set as a list of package names, satis will not dump the dist files of these packages
* `checksum`: optional, `true` by default, when disabled (`false`) satis will not provide the sha1 checksum for the dist files
Once enabled, all downloads (include those from GitHub and BitBucket) will be replaced with a *local* version.
#### prefix-url
Prefixing the URL with another host is especially helpful if the downloads end up in a private Amazon S3 bucket or on a CDN host. A CDN would drastically improve download times and therefore package installation.
Example: A `prefix-url` of `https://my-bucket.s3.amazonaws.com` (and `directory` set to `dist`) creates download URLs which look like the following: `https://my-bucket.s3.amazonaws.com/dist/vendor-package-version-ref.zip`.
### Web outputs
* `output-html`: optional, `true` by default, when disabled (`false`) satis will not generate the `output-dir`/index.html page.
* `twig-template`: optional, a path to a personalized [Twig](https://twig.sensiolabs.org/) template for the `output-dir`/index.html page.
### Abandoned packages
To enable your satis installation to indicate that some packages are abandoned, add the following to your `satis.json`:
```
{
"abandoned": {
"company/package": true,
"company/package2": "company/newpackage"
}
}
```
The `true` value indicates that the package is truly abandoned while the `"company/newpackage"` value specifies that the package is replaced by the `company/newpackage` package.
Note that all packages set as abandoned in their own `composer.json` file will be marked abandoned as well.
### Resolving dependencies
It is possible to make satis automatically resolve and add all dependencies for your projects. This can be used with the Downloads functionality to have a complete local mirror of packages. Add the following to your `satis.json`:
```
{
"require-dependencies": true,
"require-dev-dependencies": true
}
```
When searching for packages, satis will attempt to resolve all the required packages from the listed repositories. Therefore, if you are requiring a package from Packagist, you will need to define it in your `satis.json`.
Dev dependencies are packaged only if the `require-dev-dependencies` parameter is set to true.
### Other options
* `providers`: optional, `false` by default, when enabled (`true`) each package will be dumped into a separate include file which will be only loaded by Composer when the package is really required. Speeds up composer handling for repositories with huge number of packages like f.i. packagist.
* `output-dir`: optional, defines where to output the repository files if not provided as an argument when calling the `build` command.
* `config`: optional, lets you define all config options from composer, except `archive-format` and `archive-dir` as the configuration is done through [archive](#downloads) instead. See docs on [config schema](../04-schema.md#config) for more details.
* `notify-batch`: optional, specify a URL that will be called every time a user installs a package. See [notify-batch](../05-repositories.md#notify-batch).
composer Vendor binaries and the vendor/bin directory Vendor binaries and the `vendor/bin` directory
==============================================
What is a vendor binary?
------------------------
Any command line script that a Composer package would like to pass along to a user who installs the package should be listed as a vendor binary.
If a package contains other scripts that are not needed by the package users (like build or compile scripts) that code should not be listed as a vendor binary.
How is it defined?
------------------
It is defined by adding the `bin` key to a project's `composer.json`. It is specified as an array of files so multiple binaries can be added for any given project.
```
{
"bin": ["bin/my-script", "bin/my-other-script"]
}
```
What does defining a vendor binary in composer.json do?
-------------------------------------------------------
It instructs Composer to install the package's binaries to `vendor/bin` for any project that **depends** on that project.
This is a convenient way to expose useful scripts that would otherwise be hidden deep in the `vendor/` directory.
What happens when Composer is run on a composer.json that defines vendor binaries?
----------------------------------------------------------------------------------
For the binaries that a package defines directly, nothing happens.
What happens when Composer is run on a composer.json that has dependencies with vendor binaries listed?
-------------------------------------------------------------------------------------------------------
Composer looks for the binaries defined in all of the dependencies. A proxy file (or two on Windows/WSL) is created from each dependency's binaries to `vendor/bin`.
Say package `my-vendor/project-a` has binaries setup like this:
```
{
"name": "my-vendor/project-a",
"bin": ["bin/project-a-bin"]
}
```
Running `composer install` for this `composer.json` will not do anything with `bin/project-a-bin`.
Say project `my-vendor/project-b` has requirements setup like this:
```
{
"name": "my-vendor/project-b",
"require": {
"my-vendor/project-a": "*"
}
}
```
Running `composer install` for this `composer.json` will look at all of project-a's binaries and install them to `vendor/bin`.
In this case, Composer will make `vendor/my-vendor/project-a/bin/project-a-bin` available as `vendor/bin/project-a-bin`.
Finding the Composer autoloader from a binary
---------------------------------------------
As of Composer 2.2, a new `$_composer_autoload_path` global variable is defined by the bin proxy file, so that when your binary gets executed it can use it to easily locate the project's autoloader.
This global will not be available however when running binaries defined by the root package itself, so you need to have a fallback in place.
This can look like this for example:
```
<?php
include $_composer_autoload_path ?? __DIR__ . '/../vendor/autoload.php';
```
If you want to rely on this in your package you should however make sure to also require `"composer-runtime-api": "^2.2"` to ensure that the package gets installed with a Composer version supporting the feature.
Finding the Composer bin-dir from a binary
------------------------------------------
As of Composer 2.2.2, a new `$_composer_bin_dir` global variable is defined by the bin proxy file, so that when your binary gets executed it can use it to easily locate the project's Composer bin directory.
For non-PHP binaries, as of Composer 2.2.6, the bin proxy sets a `COMPOSER_RUNTIME_BIN_DIR` environment variable.
This global variable will not be available however when running binaries defined by the root package itself, so you need to have a fallback in place.
This can look like this for example:
```
<?php
$binDir = $_composer_bin_dir ?? __DIR__ . '/../vendor/bin';
```
```
#!/bin/bash
if [[ -z "$COMPOSER_RUNTIME_BIN_DIR" ]]; then
BIN_DIR="$( cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd )"
else
BIN_DIR="$COMPOSER_RUNTIME_BIN_DIR"
fi
```
If you want to rely on this in your package you should however make sure to also require `"composer-runtime-api": "^2.2.2"` to ensure that the package gets installed with a Composer version supporting the feature.
What about Windows and .bat files?
----------------------------------
Packages managed entirely by Composer do not *need* to contain any `.bat` files for Windows compatibility. Composer handles installation of binaries in a special way when run in a Windows environment:
* A `.bat` file is generated automatically to reference the binary
* A Unix-style proxy file with the same name as the binary is also generated, which is useful for WSL, Linux VMs, etc.
Packages that need to support workflows that may not include Composer are welcome to maintain custom `.bat` files. In this case, the package should **not** list the `.bat` file as a binary as it is not needed.
Can vendor binaries be installed somewhere other than vendor/bin?
-----------------------------------------------------------------
Yes, there are two ways an alternate vendor binary location can be specified:
1. Setting the `bin-dir` configuration setting in `composer.json`
2. Setting the environment variable `COMPOSER_BIN_DIR`
An example of the former looks like this:
```
{
"config": {
"bin-dir": "scripts"
}
}
```
Running `composer install` for this `composer.json` will result in all of the vendor binaries being installed in `scripts/` instead of `vendor/bin/`.
You can set `bin-dir` to `./` to put binaries in your project root.
| programming_docs |
composer Setting up and using custom installers Setting up and using custom installers
======================================
Synopsis
--------
At times, it may be necessary for a package to require additional actions during installation, such as installing packages outside of the default `vendor` library.
In these cases you could consider creating a Custom Installer to handle your specific logic.
Alternative to custom installers with Composer 2.1+
---------------------------------------------------
As of Composer 2.1, the `Composer\InstalledVersions` class has a [`getInstalledPackagesByType`](../07-runtime.md#knowing-which-packages-of-a-given-type-are-installed) method which can let you figure out at runtime which plugins/modules/extensions are installed.
It is highly recommended to use that instead of building new custom installers if you are building a new application. This has the advantage of leaving all vendor code in the vendor directory, and not requiring custom installer code.
Calling a Custom Installer
--------------------------
Suppose that your project already has a Custom Installer for specific modules then invoking that installer is a matter of defining the correct [type](../04-schema.md#type) in your package file.
> *See the next chapter for an instruction how to create Custom Installers.*
>
>
Every Custom Installer defines which [type](../04-schema.md#type) string it will recognize. Once recognized it will completely override the default installer and only apply its own logic.
An example use-case would be:
> phpDocumentor features Templates that need to be installed outside of the default /vendor folder structure. As such they have chosen to adopt the `phpdocumentor-template` [type](../04-schema.md#type) and create a plugin providing the Custom Installer to send these templates to the correct folder.
>
>
An example composer.json of such a template package would be:
```
{
"name": "phpdocumentor/template-responsive",
"type": "phpdocumentor-template",
"require": {
"phpdocumentor/template-installer-plugin": "*"
}
}
```
> **IMPORTANT**: to make sure that the template installer is present at the time the template package is installed, template packages should require the plugin package.
>
>
Creating an Installer
---------------------
A Custom Installer is defined as a class that implements the [`Composer\Installer\InstallerInterface`](https://github.com/composer/composer/blob/main/src/Composer/Installer/InstallerInterface.php) and is usually distributed in a Composer Plugin.
A basic Installer Plugin would thus compose of three files:
1. the package file: composer.json
2. The Plugin class, e.g.: `My\Project\Composer\Plugin.php`, containing a class that implements `Composer\Plugin\PluginInterface`.
3. The Installer class, e.g.: `My\Project\Composer\Installer.php`, containing a class that implements `Composer\Installer\InstallerInterface`.
### composer.json
The package file is the same as any other package file but with the following requirements:
1. the [type](../04-schema.md#type) attribute must be `composer-plugin`.
2. the [extra](../04-schema.md#extra) attribute must contain an element `class` defining the class name of the plugin (including namespace). If a package contains multiple plugins, this can be an array of class names.
Example:
```
{
"name": "phpdocumentor/template-installer-plugin",
"type": "composer-plugin",
"license": "MIT",
"autoload": {
"psr-0": {"phpDocumentor\\Composer": "src/"}
},
"extra": {
"class": "phpDocumentor\\Composer\\TemplateInstallerPlugin"
},
"require": {
"composer-plugin-api": "^1.0"
},
"require-dev": {
"composer/composer": "^1.3"
}
}
```
The example above has Composer itself in its require-dev, which allows you to use the Composer classes in your test suite for example.
### The Plugin class
The class defining the Composer plugin must implement the [`Composer\Plugin\PluginInterface`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/PluginInterface.php). It can then register the Custom Installer in its `activate()` method.
The class may be placed in any location and have any name, as long as it is autoloadable and matches the `extra.class` element in the package definition.
Example:
```
<?php
namespace phpDocumentor\Composer;
use Composer\Composer;
use Composer\IO\IOInterface;
use Composer\Plugin\PluginInterface;
class TemplateInstallerPlugin implements PluginInterface
{
public function activate(Composer $composer, IOInterface $io)
{
$installer = new TemplateInstaller($io, $composer);
$composer->getInstallationManager()->addInstaller($installer);
}
}
```
### The Custom Installer class
The class that executes the custom installation should implement the [`Composer\Installer\InstallerInterface`](https://github.com/composer/composer/blob/main/src/Composer/Installer/InstallerInterface.php) (or extend another installer that implements that interface). It defines the [type](../04-schema.md#type) string as it will be recognized by packages that will use this installer in the `supports()` method.
> **NOTE**: *choose your [type](../04-schema.md#type) name carefully, it is recommended to follow the format: `vendor-type`*. For example: `phpdocumentor-template`.
>
>
The InstallerInterface class defines the following methods (please see the source for the exact signature):
* **supports()**, here you test whether the passed [type](../04-schema.md#type) matches the name that you declared for this installer (see the example).
* **isInstalled()**, determines whether a supported package is installed or not.
* **install()**, here you can determine the actions that need to be executed upon installation.
* **update()**, here you define the behavior that is required when Composer is invoked with the update argument.
* **uninstall()**, here you can determine the actions that need to be executed when the package needs to be removed.
* **getInstallPath()**, this method should return the location where the package is to be installed, *relative from the location of composer.json.* The path *must not end with a slash.*
Example:
```
<?php
namespace phpDocumentor\Composer;
use Composer\Package\PackageInterface;
use Composer\Installer\LibraryInstaller;
class TemplateInstaller extends LibraryInstaller
{
/**
* @inheritDoc
*/
public function getInstallPath(PackageInterface $package)
{
$prefix = substr($package->getPrettyName(), 0, 23);
if ('phpdocumentor/template-' !== $prefix) {
throw new \InvalidArgumentException(
'Unable to install template, phpdocumentor templates '
.'should always start their package name with '
.'"phpdocumentor/template-"'
);
}
return 'data/templates/'.substr($package->getPrettyName(), 23);
}
/**
* @inheritDoc
*/
public function supports($packageType)
{
return 'phpdocumentor-template' === $packageType;
}
}
```
The example demonstrates that it is possible to extend the [`Composer\Installer\LibraryInstaller`](https://github.com/composer/composer/blob/main/src/Composer/Installer/LibraryInstaller.php) class to strip a prefix (`phpdocumentor/template-`) and use the remaining part to assemble a completely different installation path.
> *Instead of being installed in `/vendor` any package installed using this Installer will be put in the `/data/templates/<stripped name>` folder.*
>
>
composer Resolving merge conflicts Resolving merge conflicts
=========================
When working as a team on the same Composer project, you will eventually run into a scenario where multiple people added, updated or removed something in the `composer.json` and `composer.lock` files in multiple branches. When those branches are eventually merged together, you will get merge conflicts. Resolving these merge conflicts is not as straight forward as on other files, especially not regarding the `composer.lock` file.
> **Note:** It might not immediately be obvious why text based merging is not possible for lock files, so let's imagine the following example where we want to merge two branches;
>
> * Branch 1 has added package A which requires package B. Package B is locked at version `1.0.0`.
> * Branch 2 has added package C which conflicts with all versions below `1.2.0` of package B.
>
> A text based merge would result in package A version `1.0.0`, package B version `1.0.0` and package C version `1.0.0`. This is an invalid result, as the conflict of package C was not considered and would require an upgrade of package B.
>
>
1. Reapplying changes
---------------------
The safest method to merge Composer files is to accept the version from one branch and apply the changes from the other branch.
An example where we have two branches:
1. Package 'A' has been added
2. Package 'B' has been removed and package 'C' is added.
To resolve the conflict when we merge these two branches:
* We choose the branch that has the most changes, and accept the `composer.json` and `composer.lock` files from that branch. In this case, we choose the Composer files from branch 2.
* We reapply the changes from the other branch (branch 1). In this case we have to run `composer require package/A` again.
2. Validating your merged files
-------------------------------
Before committing, make sure the resulting `composer.json` and `composer.lock` files are valid. To do this, run the following commands:
```
php composer.phar validate
php composer.phar install [--dry-run]
```
Automating merge conflict resolving with git
--------------------------------------------
Some improvement *could* be made to git's conflict resolving by using a custom git merge driver.
An example of this can be found at [balbuf's composer git merge driver](https://github.com/balbuf/composer-git-merge-driver).
Important considerations
------------------------
Keep in mind that whenever merge conflicts occur on the lock file, the information, about the exact version new packages were locked on for one of the branches, is lost. When package A in branch 1 is constrained as `^1.2.0` and locked as `1.2.0`, it might get updated when branch 2 is used as baseline and a new `composer require package/A:^1.2.0` is executed, as that will use the most recent version that the constraint allows when possible. There might be a version 1.3.0 for that package available by now, which will now be used instead.
Choosing the correct [version constraints](versions.md) and making sure the packages adhere to [semantic versioning](https://semver.org/) when using [next significant release operators](versions.md#next-significant-release-operators) should make sure that merging branches does not break anything by accidentally updating a dependency.
composer Composer platform dependencies Composer platform dependencies
==============================
What are platform dependencies
------------------------------
Composer makes information about the environment Composer runs in available as virtual packages. This allows other packages to define dependencies ([require](../04-schema.md#require), [conflict](../04-schema.md#conflict), [provide](../04-schema.md#provide), [replace](../04-schema.md#replace)) on different aspects of the platform, like PHP, extensions or system libraries, including version constraints.
When you require one of the platform packages no code is installed. The version numbers of platform packages are derived from the environment Composer is executed in and they cannot be updated or removed. They can however be overwritten for the purposes of dependency resolution with a [platform configuration](../06-config.md#platform).
**For example:** If you are executing `composer update` with a PHP interpreter in version `7.4.42`, then Composer automatically adds a package to the pool of available packages called `php` and assigns version `7.4.42` to it.
That's how packages can add a dependency on the used PHP version:
```
{
"require": {
"php": ">=7.4"
}
}
```
Composer will check this requirement against the currently used PHP version when running the composer command.
### Different types of platform packages
The following types of platform packages exist and can be depended on:
1. PHP (`php` and the subtypes: `php-64bit`, `php-ipv6`, `php-zts` `php-debug`)
2. PHP Extensions (`ext-*`, e.g. `ext-mbstring`)
3. PHP Libraries (`lib-*`, e.g. `lib-curl`)
4. Composer (`composer`, `composer-plugin-api`, `composer-runtime-api`)
To see the complete list of platform packages available in your environment you can run `php composer.phar show --platform` (or `show -p` for short).
The differences between the various Composer platform packages are explained further in this document.
Plugin package `composer-plugin-api`
------------------------------------
You can modify Composer's behavior with [plugin](plugins.md) packages. Composer provides a set of versioned APIs for plugins. Because internal Composer changes may **not** change the plugin APIs, the API version may not increase every time the Composer version increases. E.g. In Composer version `2.3.12`, the `composer-plugin-api` version could still be `2.2.0`.
Runtime package `composer-runtime-api`
--------------------------------------
When applications which were installed with Composer are run (either on CLI or through a web request), they require the `vendor/autoload.php` file, typically as one of the first lines of executed code. Invocations of the Composer autoloader are considered the application "runtime".
Starting with version 2.0, Composer makes [additional features](../07-runtime.md) (besides registering the class autoloader) available to the application runtime environment.
Similar to `composer-plugin-api`, not every Composer release adds new runtime features, thus the version of `composer-runtimeapi` is also increased independently from Composer's version.
Composer package `composer`
---------------------------
Starting with Composer 2.2.0, a new platform package called `composer` is available, which represents the exact Composer version that is executed. Packages depending on this platform package can therefore depend on (or conflict with) individual Composer versions to cover edge cases where neither the `composer-runtime-api` version nor the `composer-plugin-api` was changed.
Because this option was introduced with Composer 2.2.0, it is recommended to add a `composer-plugin-api` dependency on at least `>=2.2.0` to provide a more meaningful error message for users running older Composer versions.
In general, depending on `composer-plugin-api` or `composer-runtime-api` is always recommended over depending on concrete Composer versions with the `composer` platform package.
composer Versions and constraints Versions and constraints
========================
Composer Versions vs VCS Versions
---------------------------------
Because Composer is heavily geared toward utilizing version control systems like git, the term "version" can be a little ambiguous. In the sense of a version control system, a "version" is a specific set of files that contain specific data. In git terminology, this is a "ref", or a specific commit, which may be represented by a branch HEAD or a tag. When you check out that version in your VCS -- for example, tag `v1.1` or commit `e35fa0d` --, you're asking for a single, known set of files, and you always get the same files back.
In Composer, what's often referred to casually as a version -- that is, the string that follows the package name in a require line (e.g., `~1.1` or `1.2.*`) -- is actually more specifically a version constraint. Composer uses version constraints to figure out which refs in a VCS it should be checking out (or to verify that a given library is acceptable in the case of a statically-maintained library with a `version` specification in `composer.json`).
VCS Tags and Branches
---------------------
*For the following discussion, let's assume the following sample library repository:*
```
~/my-library$ git branch
```
```
v1
v2
my-feature
another-feature
```
```
~/my-library$ git tag
```
```
v1.0
v1.0.1
v1.0.2
v1.1-BETA
v1.1-RC1
v1.1-RC2
v1.1
v1.1.1
v2.0-BETA
v2.0-RC1
v2.0
v2.0.1
v2.0.2
```
### Tags
Normally, Composer deals with tags (as opposed to branches -- if you don't know what this means, read up on [version control systems](https://en.wikipedia.org/wiki/Version_control#Common_terminology)). When you write a version constraint, it may reference a specific tag (e.g., `1.1`) or it may reference a valid range of tags (e.g., `>=1.1 <2.0`, or `~4.0`). To resolve these constraints, Composer first asks the VCS to list all available tags, then creates an internal list of available versions based on these tags. In the above example, composer's internal list includes versions `1.0`, `1.0.1`, `1.0.2`, the beta release of `1.1`, the first and second release candidates of `1.1`, the final release version `1.1`, etc.... (Note that Composer automatically removes the 'v' prefix in the actual tagname to get a valid final version number.)
When Composer has a complete list of available versions from your VCS, it then finds the highest version that matches all version constraints in your project (it's possible that other packages require more specific versions of the library than you do, so the version it chooses may not always be the highest available version) and it downloads a zip archive of that tag to unpack in the correct location in your `vendor` directory.
### Branches
If you want Composer to check out a branch instead of a tag, you need to point it to the branch using the special `dev-*` prefix (or sometimes suffix; see below). If you're checking out a branch, it's assumed that you want to *work* on the branch and Composer actually clones the repo into the correct place in your `vendor` directory. For tags, it copies the right files without actually cloning the repo. (You can modify this behavior with --prefer-source and --prefer-dist, see [install options](../03-cli.md#install).)
In the above example, if you wanted to check out the `my-feature` branch, you would specify `dev-my-feature` as the version constraint in your `require` clause. This would result in Composer cloning the `my-library` repository into my `vendor` directory and checking out the `my-feature` branch.
When branch names look like versions, we have to clarify for Composer that we're trying to check out a branch and not a tag. In the above example, we have two version branches: `v1` and `v2`. To get Composer to check out one of these branches, you must specify a version constraint that looks like this: `v1.x-dev`. The `.x` is an arbitrary string that Composer requires to tell it that we're talking about the `v1` branch and not a `v1` tag (alternatively, you can name the branch `v1.x` instead of `v1`). In the case of a branch with a version-like name (`v1`, in this case), you append `-dev` as a suffix, rather than using `dev-` as a prefix.
### Stabilities
Composer recognizes the following stabilities (in order of stability): dev, alpha, beta, RC, and stable where RC stands for release candidate. The stability of a version is defined by its suffix e.g version `v1.1-BETA` has a stability of `beta` and `v1.1-RC1` has a stability of `RC`. If such a suffix is missing e.g. version `v1.1` then Composer considers that version `stable`. In addition to that Composer automatically adds a `-dev` suffix to all numeric branches and prefixes all other branches imported from a VCS repository with `dev-`. In both cases the stability `dev` gets assigned.
Keeping this in mind will help you in the next section.
### Minimum Stability
There's one more thing that will affect which files are checked out of a library's VCS and added to your project: Composer allows you to specify stability constraints to limit which tags are considered valid. In the above example, note that the library released a beta and two release candidates for version `1.1` before the final official release. To receive these versions when running `composer install` or `composer update`, we have to explicitly tell Composer that we are ok with release candidates and beta releases (and alpha releases, if we want those). This can be done using either a project-wide `minimum-stability` value in `composer.json` or using "stability flags" in version constraints. Read more on the [schema page](../04-schema.md#minimum-stability).
Writing Version Constraints
---------------------------
Now that you have an idea of how Composer sees versions, let's talk about how to specify version constraints for your project dependencies.
### Exact Version Constraint
You can specify the exact version of a package. This will tell Composer to install this version and this version only. If other dependencies require a different version, the solver will ultimately fail and abort any install or update procedures.
Example: `1.0.2`
### Version Range
By using comparison operators you can specify ranges of valid versions. Valid operators are `>`, `>=`, `<`, `<=`, `!=`.
You can define multiple ranges. Ranges separated by a space () or comma (`,`) will be treated as a **logical AND**. A double pipe (`||`) will be treated as a **logical OR**. AND has higher precedence than OR.
> **Note:** Be careful when using unbounded ranges as you might end up unexpectedly installing versions that break backwards compatibility. Consider using the [caret](#caret-version-range-) operator instead for safety.
>
>
> **Note:** In older versions of Composer the single pipe (`|`) was the recommended alternative to the **logical OR**. Thus for backwards compatibility the single pipe (`|`) will still be treated as a **logical OR**.
>
>
Examples:
* `>=1.0`
* `>=1.0 <2.0`
* `>=1.0 <1.1 || >=1.2`
### Hyphenated Version Range (`-`)
Inclusive set of versions. Partial versions on the right include are completed with a wildcard. For example `1.0 - 2.0` is equivalent to `>=1.0.0 <2.1` as the `2.0` becomes `2.0.*`. On the other hand `1.0.0 - 2.1.0` is equivalent to `>=1.0.0 <=2.1.0`.
Example: `1.0 - 2.0`
### Wildcard Version Range (`.*`)
You can specify a pattern with a `*` wildcard. `1.0.*` is the equivalent of `>=1.0 <1.1`.
Example: `1.0.*`
Next Significant Release Operators
----------------------------------
### Tilde Version Range (`~`)
The `~` operator is best explained by example: `~1.2` is equivalent to `>=1.2 <2.0.0`, while `~1.2.3` is equivalent to `>=1.2.3 <1.3.0`. As you can see it is mostly useful for projects respecting [semantic versioning](https://semver.org/). A common usage would be to mark the minimum minor version you depend on, like `~1.2` (which allows anything up to, but not including, 2.0). Since in theory there should be no backwards compatibility breaks until 2.0, that works well. Another way of looking at it is that using `~` specifies a minimum version, but allows the last digit specified to go up.
Example: `~1.2`
> **Note:** Although `2.0-beta.1` is strictly before `2.0`, a version constraint like `~1.2` would not install it. As said above `~1.2` only means the `.2` can change but the `1.` part is fixed.
>
> **Note:** The `~` operator has an exception on its behavior for the major release number. This means for example that `~1` is the same as `~1.0` as it will not allow the major number to increase trying to keep backwards compatibility.
>
>
### Caret Version Range (`^`)
The `^` operator behaves very similarly, but it sticks closer to semantic versioning, and will always allow non-breaking updates. For example `^1.2.3` is equivalent to `>=1.2.3 <2.0.0` as none of the releases until 2.0 should break backwards compatibility. For pre-1.0 versions it also acts with safety in mind and treats `^0.3` as `>=0.3.0 <0.4.0` and `^0.0.3` as `>=0.0.3 <0.0.4`.
This is the recommended operator for maximum interoperability when writing library code.
Example: `^1.2.3`
Stability Constraints
---------------------
If you are using a constraint that does not explicitly define a stability, Composer will default internally to `-dev` or `-stable`, depending on the operator(s) used. This happens transparently.
If you wish to explicitly consider only the stable release in the comparison, add the suffix `-stable`.
Examples:
| Constraint | Internally |
| --- | --- |
| `1.2.3` | `=1.2.3.0-stable` |
| `>1.2` | `>1.2.0.0-stable` |
| `>=1.2` | `>=1.2.0.0-dev` |
| `>=1.2-stable` | `>=1.2.0.0-stable` |
| `<1.3` | `<1.3.0.0-dev` |
| `<=1.3` | `<=1.3.0.0-stable` |
| `1 - 2` | `>=1.0.0.0-dev <3.0.0.0-dev` |
| `~1.3` | `>=1.3.0.0-dev <2.0.0.0-dev` |
| `1.4.*` | `>=1.4.0.0-dev <1.5.0.0-dev` |
To allow various stabilities without enforcing them at the constraint level however, you may use [stability-flags](../04-schema.md#package-links) like `@<stability>` (e.g. `@dev`) to let Composer know that a given package can be installed in a different stability than your default minimum-stability setting. All available stability flags are listed on the minimum-stability section of the [schema page](../04-schema.md#minimum-stability).
Summary
-------
```
"require": {
"vendor/package": "1.3.2", // exactly 1.3.2
// >, <, >=, <= | specify upper / lower bounds
"vendor/package": ">=1.3.2", // anything above or equal to 1.3.2
"vendor/package": "<1.3.2", // anything below 1.3.2
// * | wildcard
"vendor/package": "1.3.*", // >=1.3.0 <1.4.0
// ~ | allows last digit specified to go up
"vendor/package": "~1.3.2", // >=1.3.2 <1.4.0
"vendor/package": "~1.3", // >=1.3.0 <2.0.0
// ^ | doesn't allow breaking changes (major version fixed - following semver)
"vendor/package": "^1.3.2", // >=1.3.2 <2.0.0
"vendor/package": "^0.3.2", // >=0.3.2 <0.4.0 // except if major version is 0
}
```
Testing Version Constraints
---------------------------
You can test version constraints using [semver.madewithlove.com](https://semver.madewithlove.com). Fill in a package name and it will autofill the default version constraint which Composer would add to your `composer.json` file. You can adjust the version constraint and the tool will highlight all releases that match.
| programming_docs |
composer Scripts Scripts
=======
What is a script?
-----------------
A script, in Composer's terms, can either be a PHP callback (defined as a static method) or any command-line executable command. Scripts are useful for executing a package's custom code or package-specific commands during the Composer execution process.
> **Note:** Only scripts defined in the root package's `composer.json` are executed. If a dependency of the root package specifies its own scripts, Composer does not execute those additional scripts.
>
>
Event names
-----------
Composer fires the following named events during its execution process:
### Command Events
* **pre-install-cmd**: occurs before the `install` command is executed with a lock file present.
* **post-install-cmd**: occurs after the `install` command has been executed with a lock file present.
* **pre-update-cmd**: occurs before the `update` command is executed, or before the `install` command is executed without a lock file present.
* **post-update-cmd**: occurs after the `update` command has been executed, or after the `install` command has been executed without a lock file present.
* **pre-status-cmd**: occurs before the `status` command is executed.
* **post-status-cmd**: occurs after the `status` command has been executed.
* **pre-archive-cmd**: occurs before the `archive` command is executed.
* **post-archive-cmd**: occurs after the `archive` command has been executed.
* **pre-autoload-dump**: occurs before the autoloader is dumped, either during `install`/`update`, or via the `dump-autoload` command.
* **post-autoload-dump**: occurs after the autoloader has been dumped, either during `install`/`update`, or via the `dump-autoload` command.
* **post-root-package-install**: occurs after the root package has been installed during the `create-project` command (but before its dependencies are installed).
* **post-create-project-cmd**: occurs after the `create-project` command has been executed.
### Installer Events
* **pre-operations-exec**: occurs before the install/upgrade/.. operations are executed when installing a lock file. Plugins that need to hook into this event will need to be installed globally to be usable, as otherwise they would not be loaded yet when a fresh install of a project happens.
### Package Events
* **pre-package-install**: occurs before a package is installed.
* **post-package-install**: occurs after a package has been installed.
* **pre-package-update**: occurs before a package is updated.
* **post-package-update**: occurs after a package has been updated.
* **pre-package-uninstall**: occurs before a package is uninstalled.
* **post-package-uninstall**: occurs after a package has been uninstalled.
### Plugin Events
* **init**: occurs after a Composer instance is done being initialized.
* **command**: occurs before any Composer Command is executed on the CLI. It provides you with access to the input and output objects of the program.
* **pre-file-download**: occurs before files are downloaded and allows you to manipulate the `HttpDownloader` object prior to downloading files based on the URL to be downloaded.
* **post-file-download**: occurs after package dist files are downloaded and allows you to perform additional checks on the file if required.
* **pre-command-run**: occurs before a command is executed and allows you to manipulate the `InputInterface` object's options and arguments to tweak a command's behavior.
* **pre-pool-create**: occurs before the Pool of packages is created, and lets you filter the list of packages that is going to enter the Solver.
> **Note:** Composer makes no assumptions about the state of your dependencies prior to `install` or `update`. Therefore, you should not specify scripts that require Composer-managed dependencies in the `pre-update-cmd` or `pre-install-cmd` event hooks. If you need to execute scripts prior to `install` or `update` please make sure they are self-contained within your root package.
>
>
Defining scripts
----------------
The root JSON object in `composer.json` should have a property called `"scripts"`, which contains pairs of named events and each event's corresponding scripts. An event's scripts can be defined as either a string (only for a single script) or an array (for single or multiple scripts.)
For any given event:
* Scripts execute in the order defined when their corresponding event is fired.
* An array of scripts wired to a single event can contain both PHP callbacks and command-line executable commands.
* PHP classes containing defined callbacks must be autoloadable via Composer's autoload functionality.
* Callbacks can only autoload classes from psr-0, psr-4 and classmap definitions. If a defined callback relies on functions defined outside of a class, the callback itself is responsible for loading the file containing these functions.
Script definition example:
```
{
"scripts": {
"post-update-cmd": "MyVendor\\MyClass::postUpdate",
"post-package-install": [
"MyVendor\\MyClass::postPackageInstall"
],
"post-install-cmd": [
"MyVendor\\MyClass::warmCache",
"phpunit -c app/"
],
"post-autoload-dump": [
"MyVendor\\MyClass::postAutoloadDump"
],
"post-create-project-cmd": [
"php -r \"copy('config/local-example.php', 'config/local.php');\""
]
}
}
```
Using the previous definition example, here's the class `MyVendor\MyClass` that might be used to execute the PHP callbacks:
```
<?php
namespace MyVendor;
use Composer\Script\Event;
use Composer\Installer\PackageEvent;
class MyClass
{
public static function postUpdate(Event $event)
{
$composer = $event->getComposer();
// do stuff
}
public static function postAutoloadDump(Event $event)
{
$vendorDir = $event->getComposer()->getConfig()->get('vendor-dir');
require $vendorDir . '/autoload.php';
some_function_from_an_autoloaded_file();
}
public static function postPackageInstall(PackageEvent $event)
{
$installedPackage = $event->getOperation()->getPackage();
// do stuff
}
public static function warmCache(Event $event)
{
// make cache toasty
}
}
```
**Note:** During a Composer `install` or `update` command run, a variable named `COMPOSER_DEV_MODE` will be added to the environment. If the command was run with the `--no-dev` flag, this variable will be set to 0, otherwise it will be set to 1. The variable is also available while `dump-autoload` runs, and it will be set to the same as the last `install` or `update` was run in.
Event classes
-------------
When an event is fired, your PHP callback receives as first argument a `Composer\EventDispatcher\Event` object. This object has a `getName()` method that lets you retrieve the event name.
Depending on the [script types](#event-names) you will get various event subclasses containing various getters with relevant data and associated objects:
* Base class: [`Composer\EventDispatcher\Event`](https://github.com/composer/composer/blob/main/src/Composer/EventDispatcher/Event.php)
* Command Events: [`Composer\Script\Event`](https://github.com/composer/composer/blob/main/src/Composer/Script/Event.php)
* Installer Events: [`Composer\Installer\InstallerEvent`](https://github.com/composer/composer/blob/main/src/Composer/Installer/InstallerEvent.php)
* Package Events: [`Composer\Installer\PackageEvent`](https://github.com/composer/composer/blob/main/src/Composer/Installer/PackageEvent.php)
* Plugin Events:
+ init: [`Composer\EventDispatcher\Event`](https://github.com/composer/composer/blob/main/src/Composer/EventDispatcher/Event.php)
+ command: [`Composer\Plugin\CommandEvent`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/CommandEvent.php)
+ pre-file-download: [`Composer\Plugin\PreFileDownloadEvent`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/PreFileDownloadEvent.php)
+ post-file-download: [`Composer\Plugin\PostFileDownloadEvent`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/PostFileDownloadEvent.php)
Running scripts manually
------------------------
If you would like to run the scripts for an event manually, the syntax is:
```
php composer.phar run-script [--dev] [--no-dev] script
```
For example `composer run-script post-install-cmd` will run any **post-install-cmd** scripts and [plugins](plugins.md) that have been defined.
You can also give additional arguments to the script handler by appending `--` followed by the handler arguments. e.g. `composer run-script post-install-cmd -- --check` will pass`--check` along to the script handler. Those arguments are received as CLI arg by CLI handlers, and can be retrieved as an array via `$event->getArguments()` by PHP handlers.
Writing custom commands
-----------------------
If you add custom scripts that do not fit one of the predefined event name above, you can either run them with run-script or also run them as native Composer commands. For example the handler defined below is executable by running `composer test`:
```
{
"scripts": {
"test": "phpunit"
}
}
```
Similar to the `run-script` command you can give additional arguments to scripts, e.g. `composer test -- --filter <pattern>` will pass `--filter <pattern>` along to the `phpunit` script.
> **Note:** Before executing scripts, Composer's bin-dir is temporarily pushed on top of the PATH environment variable so that binaries of dependencies are directly accessible. In this example no matter if the `phpunit` binary is actually in `vendor/bin/phpunit` or `bin/phpunit` it will be found and executed.
>
>
Although Composer is not intended to manage long-running processes and other such aspects of PHP projects, it can sometimes be handy to disable the process timeout on custom commands. This timeout defaults to 300 seconds and can be overridden in a variety of ways depending on the desired effect:
* disable it for all commands using the config key `process-timeout`,
* disable it for the current or future invocations of composer using the environment variable `COMPOSER_PROCESS_TIMEOUT`,
* for a specific invocation using the `--timeout` flag of the `run-script` command,
* using a static helper for specific scripts.
To disable the timeout for specific scripts with the static helper directly in composer.json:
```
{
"scripts": {
"test": [
"Composer\\Config::disableProcessTimeout",
"phpunit"
]
}
}
```
To disable the timeout for every script on a given project, you can use the composer.json configuration:
```
{
"config": {
"process-timeout": 0
}
}
```
It's also possible to set the global environment variable to disable the timeout of all following scripts in the current terminal environment:
```
export COMPOSER_PROCESS_TIMEOUT=0
```
To disable the timeout of a single script call, you must use the `run-script` composer command and specify the `--timeout` parameter:
```
php composer.phar run-script --timeout=0 test
```
Referencing scripts
-------------------
To enable script re-use and avoid duplicates, you can call a script from another one by prefixing the command name with `@`:
```
{
"scripts": {
"test": [
"@clearCache",
"phpunit"
],
"clearCache": "rm -rf cache/*"
}
}
```
You can also refer a script and pass it new arguments:
```
{
"scripts": {
"tests": "phpunit",
"testsVerbose": "@tests -vvv"
}
}
```
Calling Composer commands
-------------------------
To call Composer commands, you can use `@composer` which will automatically resolve to whatever composer.phar is currently being used:
```
{
"scripts": {
"test": [
"@composer install",
"phpunit"
]
}
}
```
One limitation of this is that you can not call multiple composer commands in a row like `@composer install && @composer foo`. You must split them up in a JSON array of commands.
Executing PHP scripts
---------------------
To execute PHP scripts, you can use `@php` which will automatically resolve to whatever php process is currently being used:
```
{
"scripts": {
"test": [
"@php script.php",
"phpunit"
]
}
}
```
One limitation of this is that you can not call multiple commands in a row like `@php install && @php foo`. You must split them up in a JSON array of commands.
You can also call a shell/bash script, which will have the path to the PHP executable available in it as a `PHP_BINARY` env var.
Setting environment variables
-----------------------------
To set an environment variable in a cross-platform way, you can use `@putenv`:
```
{
"scripts": {
"install-phpstan": [
"@putenv COMPOSER=phpstan-composer.json",
"composer install --prefer-dist"
]
}
}
```
Custom descriptions.
--------------------
You can set custom script descriptions with the following in your `composer.json`:
```
{
"scripts-descriptions": {
"test": "Run all tests!"
}
}
```
The descriptions are used in `composer list` or `composer run -l` commands to describe what the scripts do when the command is run.
> **Note:** You can only set custom descriptions of custom commands.
>
>
composer Setting up and using plugins Setting up and using plugins
============================
Synopsis
--------
You may wish to alter or expand Composer's functionality with your own. For example if your environment poses special requirements on the behaviour of Composer which do not apply to the majority of its users or if you wish to accomplish something with Composer in a way that is not desired by most users.
In these cases you could consider creating a plugin to handle your specific logic.
Creating a Plugin
-----------------
A plugin is a regular Composer package which ships its code as part of the package and may also depend on further packages.
### Plugin Package
The package file is the same as any other package file but with the following requirements:
1. The [type](../04-schema.md#type) attribute must be `composer-plugin`.
2. The [extra](../04-schema.md#extra) attribute must contain an element `class` defining the class name of the plugin (including namespace). If a package contains multiple plugins, this can be an array of class names.
3. You must require the special package called `composer-plugin-api` to define which Plugin API versions your plugin is compatible with. Requiring this package doesn't actually include any extra dependencies, it only specifies which version of the plugin API to use.
> **Note:** When developing a plugin, although not required, it's useful to add a require-dev dependency on `composer/composer` to have IDE autocompletion on Composer classes.
>
>
The required version of the `composer-plugin-api` follows the same [rules](../01-basic-usage.md#package-versions) as a normal package's rules.
The current Composer plugin API version is `2.3.0`.
An example of a valid plugin `composer.json` file (with the autoloading part omitted and an optional require-dev dependency on `composer/composer` for IDE auto completion):
```
{
"name": "my/plugin-package",
"type": "composer-plugin",
"require": {
"composer-plugin-api": "^2.0"
},
"require-dev": {
"composer/composer": "^2.0"
},
"extra": {
"class": "My\\Plugin"
}
}
```
### Plugin Class
Every plugin has to supply a class which implements the [`Composer\Plugin\PluginInterface`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/PluginInterface.php). The `activate()` method of the plugin is called after the plugin is loaded and receives an instance of [`Composer\Composer`](https://github.com/composer/composer/blob/main/src/Composer/Composer.php) as well as an instance of [`Composer\IO\IOInterface`](https://github.com/composer/composer/blob/main/src/Composer/IO/IOInterface.php). Using these two objects all configuration can be read and all internal objects and state can be manipulated as desired.
Example:
```
<?php
namespace phpDocumentor\Composer;
use Composer\Composer;
use Composer\IO\IOInterface;
use Composer\Plugin\PluginInterface;
class TemplateInstallerPlugin implements PluginInterface
{
public function activate(Composer $composer, IOInterface $io)
{
$installer = new TemplateInstaller($io, $composer);
$composer->getInstallationManager()->addInstaller($installer);
}
}
```
Event Handler
-------------
Furthermore plugins may implement the [`Composer\EventDispatcher\EventSubscriberInterface`](https://github.com/composer/composer/blob/main/src/Composer/EventDispatcher/EventSubscriberInterface.php) in order to have its event handlers automatically registered with the `EventDispatcher` when the plugin is loaded.
To register a method to an event, implement the method `getSubscribedEvents()` and have it return an array. The array key must be the [event name](scripts.md#event-names) and the value is the name of the method in this class to be called.
> **Note:** If you don't know which event to listen to, you can run a Composer command with the COMPOSER\_DEBUG\_EVENTS=1 environment variable set, which might help you identify what event you are looking for.
>
>
```
public static function getSubscribedEvents()
{
return array(
'post-autoload-dump' => 'methodToBeCalled',
// ^ event name ^ ^ method name ^
);
}
```
By default, the priority of an event handler is set to 0. The priority can be changed by attaching a tuple where the first value is the method name, as before, and the second value is an integer representing the priority. Higher integers represent higher priorities. Priority 2 is called before priority 1, etc.
```
public static function getSubscribedEvents()
{
return array(
// Will be called before events with priority 0
'post-autoload-dump' => array('methodToBeCalled', 1)
);
}
```
If multiple methods should be called, then an array of tuples can be attached to each event. The tuples do not need to include the priority. If it is omitted, it will default to 0.
```
public static function getSubscribedEvents()
{
return array(
'post-autoload-dump' => array(
array('methodToBeCalled' ), // Priority defaults to 0
array('someOtherMethodName', 1), // This fires first
)
);
}
```
Here's a complete example:
```
<?php
namespace Naderman\Composer\AWS;
use Composer\Composer;
use Composer\EventDispatcher\EventSubscriberInterface;
use Composer\IO\IOInterface;
use Composer\Plugin\PluginInterface;
use Composer\Plugin\PluginEvents;
use Composer\Plugin\PreFileDownloadEvent;
class AwsPlugin implements PluginInterface, EventSubscriberInterface
{
protected $composer;
protected $io;
public function activate(Composer $composer, IOInterface $io)
{
$this->composer = $composer;
$this->io = $io;
}
public function deactivate(Composer $composer, IOInterface $io)
{
}
public function uninstall(Composer $composer, IOInterface $io)
{
}
public static function getSubscribedEvents()
{
return array(
PluginEvents::PRE_FILE_DOWNLOAD => array(
array('onPreFileDownload', 0)
),
);
}
public function onPreFileDownload(PreFileDownloadEvent $event)
{
$protocol = parse_url($event->getProcessedUrl(), PHP_URL_SCHEME);
if ($protocol === 's3') {
// ...
}
}
}
```
Plugin capabilities
-------------------
Composer defines a standard set of capabilities which may be implemented by plugins. Their goal is to make the plugin ecosystem more stable as it reduces the need to mess with [`Composer\Composer`](https://github.com/composer/composer/blob/main/src/Composer/Composer.php)'s internal state, by providing explicit extension points for common plugin requirements.
Capable Plugins classes must implement the [`Composer\Plugin\Capable`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/Capable.php) interface and declare their capabilities in the `getCapabilities()` method. This method must return an array, with the *key* as a Composer Capability class name, and the *value* as the Plugin's own implementation class name of said Capability:
```
<?php
namespace My\Composer;
use Composer\Composer;
use Composer\IO\IOInterface;
use Composer\Plugin\PluginInterface;
use Composer\Plugin\Capable;
class Plugin implements PluginInterface, Capable
{
public function activate(Composer $composer, IOInterface $io)
{
}
public function getCapabilities()
{
return array(
'Composer\Plugin\Capability\CommandProvider' => 'My\Composer\CommandProvider',
);
}
}
```
### Command provider
The [`Composer\Plugin\Capability\CommandProvider`](https://github.com/composer/composer/blob/main/src/Composer/Plugin/Capability/CommandProvider.php) capability allows to register additional commands for Composer:
```
<?php
namespace My\Composer;
use Composer\Plugin\Capability\CommandProvider as CommandProviderCapability;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
use Composer\Command\BaseCommand;
class CommandProvider implements CommandProviderCapability
{
public function getCommands()
{
return array(new Command);
}
}
class Command extends BaseCommand
{
protected function configure(): void
{
$this->setName('custom-plugin-command');
}
protected function execute(InputInterface $input, OutputInterface $output): int
{
$output->writeln('Executing');
}
}
```
Now the `custom-plugin-command` is available alongside Composer commands.
> *Composer commands are based on the [Symfony Console Component](https://symfony.com/doc/current/components/console.html).*
>
>
Running plugins manually
------------------------
Plugins for an event can be run manually by the `run-script` command. This works the same way as [running scripts manually](scripts.md#running-scripts-manually).
Using Plugins
-------------
Plugin packages are automatically loaded as soon as they are installed and will be loaded when Composer starts up if they are found in the current project's list of installed packages. Additionally all plugin packages installed in the `COMPOSER_HOME` directory using the Composer global command are loaded before local project plugins are loaded.
> You may pass the `--no-plugins` option to Composer commands to disable all installed plugins. This may be particularly helpful if any of the plugins causes errors and you wish to update or uninstall it.
>
>
Plugin Helpers
--------------
As of Composer 2, due to the fact that DownloaderInterface can sometimes return Promises and have been split up in more steps than they used to, we provide a [SyncHelper](https://github.com/composer/composer/blob/main/src/Composer/Util/SyncHelper.php) to make downloading and installing packages easier.
Plugin Extra Attributes
-----------------------
A few special plugin capabilities can be unlocked using extra attributes in the plugin's composer.json.
### class
[See above](#plugin-package) for an explanation of the class attribute and how it works.
### plugin-modifies-downloads
Some special plugins need to update package download URLs before they get downloaded.
As of Composer 2.0, all packages are downloaded before they get installed. This means on the first installation, your plugin is not yet installed when the download occurs, and it does not get a chance to update the URLs on time.
Specifying `{"extra": {"plugin-modifies-downloads": true}}` in your composer.json will hint to Composer that the plugin should be installed on its own before proceeding with the rest of the package downloads. This slightly slows down the overall installation process however, so do not use it in plugins which do not absolutely require it.
### plugin-modifies-install-path
Some special plugins modify the install path of packages.
As of Composer 2.2.9, you can specify `{"extra": {"plugin-modifies-install-path": true}}` in your composer.json to hint to Composer that the plugin should be activated as soon as possible to prevent any bad side-effects from Composer assuming packages are installed in another location than they actually are.
Plugin Autoloading
------------------
Due to plugins being loaded by Composer at runtime, and to ensure that plugins which depend on other packages can function correctly, a runtime autoloader is created whenever a plugin is loaded. That autoloader is only configured to load with the plugin dependencies, so you may not have access to all the packages which are installed.
Static Analysis support
-----------------------
As of Composer 2.3.7 we ship a `phpstan/rules.neon` PHPStan config file, which provides additional error checking when working on Composer plugins.
### Usage with [PHPStan Extension Installer](https://github.com/phpstan/extension-installer#usage)
The necessary configuration files are automatically loaded, in case your plugin projects declares a dependency to `phpstan/extension-installer`.
### Alternative manual installation
To make use of it, your Composer plugin project needs a [PHPStan config file](https://phpstan.org/config-reference#multiple-files), which includes the `phpstan/rules.neon` file:
```
includes:
- vendor/composer/composer/phpstan/rules.neon
// your remaining config..
```
| programming_docs |
composer Repository priorities Repository priorities
=====================
Canonical repositories
----------------------
When Composer resolves dependencies, it will look up a given package in the topmost repository. If that repository does not contain the package, it goes on to the next one, until one repository contains it and the process ends.
Canonical repositories are better for a few reasons:
* Performance wise, it is more efficient to stop looking for a package once it has been found somewhere. It also avoids loading duplicate packages in case the same package is present in several of your repositories.
* Security wise, it is safer to treat them canonically as it means that packages you expect to come from your most important repositories will never be loaded from another repository instead. Let's say you have a private repository which is not canonical, and you require your private package `foo/bar ^2.0` for example. Now if someone publishes `foo/bar 2.999` to packagist.org, suddenly Composer will pick that package as it has a higher version than your latest release (say 2.4.3), and you end up installing something you may not have meant to. However, if the private repository is canonical, that 2.999 version from packagist.org will not be considered at all.
There are however a few cases where you may want to specifically load some packages from a given repository, but not all. Or you may want a given repository to not be canonical, and to be only preferred if it has higher package versions than the repositories defined below.
Default behavior
----------------
By default in Composer 2.x all repositories are canonical. Composer 1.x treated all repositories as non-canonical.
Another default is that the packagist.org repository is always added implicitly as the last repository, unless you [disable it](../05-repositories.md#disabling-packagist-org).
Making repositories non-canonical
---------------------------------
You can add the canonical option to any repository to disable this default behavior and make sure Composer keeps looking in other repositories, even if that repository contains a given package.
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org",
"canonical": false
}
]
}
```
Filtering packages
------------------
You can also filter packages which a repository will be able to load, either by selecting which ones you want, or by excluding those you do not want.
For example here we want to pick only the package `foo/bar` and all the packages from `some-vendor/` from this Composer repository.
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org",
"only": ["foo/bar", "some-vendor/*"]
}
]
}
```
And in this other example we exclude `toy/package` from a repository, which we may not want to load in this project.
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org",
"exclude": ["toy/package"]
}
]
}
```
Both `only` and `exclude` should be arrays of package names, which can also contain wildcards (`*`), which will match any character.
composer Aliases Aliases
=======
Why aliases?
------------
When you are using a VCS repository, you will only get comparable versions for branches that look like versions, such as `2.0` or `2.0.x`. For your `master` branch, you will get a `dev-master` version. For your `bugfix` branch, you will get a `dev-bugfix` version.
If your `master` branch is used to tag releases of the `1.0` development line, i.e. `1.0.1`, `1.0.2`, `1.0.3`, etc., any package depending on it will probably require version `1.0.*`.
If anyone wants to require the latest `dev-master`, they have a problem: Other packages may require `1.0.*`, so requiring that dev version will lead to conflicts, since `dev-master` does not match the `1.0.*` constraint.
Enter aliases.
Branch alias
------------
The `dev-master` branch is one in your main VCS repo. It is rather common that someone will want the latest master dev version. Thus, Composer allows you to alias your `dev-master` branch to a `1.0.x-dev` version. It is done by specifying a `branch-alias` field under `extra` in `composer.json`:
```
{
"extra": {
"branch-alias": {
"dev-master": "1.0.x-dev"
}
}
}
```
If you alias a non-comparable version (such as dev-develop) `dev-` must prefix the branch name. You may also alias a comparable version (i.e. start with numbers, and end with `.x-dev`), but only as a more specific version. For example, 1.x-dev could be aliased as 1.2.x-dev.
The alias must be a comparable dev version, and the `branch-alias` must be present on the branch that it references. For `dev-master`, you need to commit it on the `master` branch.
As a result, anyone can now require `1.0.*` and it will happily install `dev-master`.
In order to use branch aliasing, you must own the repository of the package being aliased. If you want to alias a third party package without maintaining a fork of it, use inline aliases as described below.
Require inline alias
--------------------
Branch aliases are great for aliasing main development lines. But in order to use them you need to have control over the source repository, and you need to commit changes to version control.
This is not really fun when you want to try a bugfix of some library that is a dependency of your local project.
For this reason, you can alias packages in your `require` and `require-dev` fields. Let's say you found a bug in the `monolog/monolog` package. You cloned [Monolog](https://github.com/Seldaek/monolog) on GitHub and fixed the issue in a branch named `bugfix`. Now you want to install that version of monolog in your local project.
You are using `symfony/monolog-bundle` which requires `monolog/monolog` version `1.*`. So you need your `dev-bugfix` to match that constraint.
Add this to your project's root `composer.json`:
```
{
"repositories": [
{
"type": "vcs",
"url": "https://github.com/you/monolog"
}
],
"require": {
"symfony/monolog-bundle": "2.0",
"monolog/monolog": "dev-bugfix as 1.0.x-dev"
}
}
```
Or let Composer add it for you with:
```
php composer.phar require "monolog/monolog:dev-bugfix as 1.0.x-dev"
```
That will fetch the `dev-bugfix` version of `monolog/monolog` from your GitHub and alias it to `1.0.x-dev`.
> **Note:** Inline aliasing is a root-only feature. If a package with inline aliases is required, the alias (right of the `as`) is used as the version constraint. The part left of the `as` is discarded. As a consequence, if A requires B and B requires `monolog/monolog` version `dev-bugfix as 1.0.x-dev`, installing A will make B require `1.0.x-dev`, which may exist as a branch alias or an actual `1.0` branch. If it does not, it must be inline-aliased again in A's `composer.json`.
>
> **Note:** Inline aliasing should be avoided, especially for published packages/libraries. If you found a bug, try to get your fix merged upstream. This helps to avoid issues for users of your package.
>
>
composer Authentication for privately hosted packages and repositories Authentication for privately hosted packages and repositories
=============================================================
Your [private package server](handling-private-packages.md) or version control system is probably secured with one or more authentication options. In order to allow your project to have access to these packages and repositories you will have to tell Composer how to authenticate with the server that hosts them.
Authentication principles
=========================
Whenever Composer encounters a protected Composer repository it will try to authenticate using already defined credentials first. When none of those credentials apply it will prompt for credentials and save them (or a token if Composer is able to retrieve one).
| type | Generated by Prompt? |
| --- | --- |
| [http-basic](#http-basic) | yes |
| [Inline http-basic](#inline-http-basic) | no |
| [HTTP Bearer](#http-bearer) | no |
| [Custom header](#custom-token-authentication) | no |
| [gitlab-oauth](#gitlab-oauth) | yes |
| [gitlab-token](#gitlab-token) | yes |
| [github-oauth](#github-oauth) | yes |
| [bitbucket-oauth](#bitbucket-oauth) | yes |
Sometimes automatic authentication is not possible, or you may want to predefine authentication credentials.
Credentials can be stored on 3 different places; in an `auth.json` for the project, a global `auth.json` or in the `composer.json` itself.
Authentication in auth.json per project
---------------------------------------
In this authentication storage method, an `auth.json` file will be present in the same folder as the projects' `composer.json` file. You can either create and edit this file using the command line or manually edit or create it.
> **Note: Make sure the `auth.json` file is in `.gitignore`** to avoid leaking credentials into your git history.
>
>
Global authentication credentials
---------------------------------
If you don't want to supply credentials for every project you work on, storing your credentials globally might be a better idea. These credentials are stored in a global `auth.json` in your Composer home directory.
### Command line global credential editing
For all authentication methods it is possible to edit them using the command line;
* [http-basic](#command-line-http-basic)
* [Inline http-basic](#command-line-inline-http-basic)
* [HTTP Bearer](#http-bearer)
* [gitlab-oauth](#command-line-gitlab-oauth)
* [gitlab-token](#command-line-gitlab-token)
* [github-oauth](#command-line-github-oauth)
* [bitbucket-oauth](#command-line-bitbucket-oauth)
### Manually editing global authentication credentials
> **Note:** It is not recommended to manually edit your authentication options as this might result in invalid json. Instead preferably use [the command line](#command-line-global-credential-editing).
>
>
To manually edit it, run:
```
php composer.phar config --global --editor [--auth]
```
For specific authentication implementations, see their sections;
* [http-basic](#manual-http-basic)
* [Inline http-basic](#manual-inline-http-basic)
* [HTTP Bearer](#http-bearer)
* [custom header](#manual-custom-token-authentication)
* [gitlab-oauth](#manual-gitlab-oauth)
* [gitlab-token](#manual-gitlab-token)
* [github-oauth](#manual-github-oauth)
* [bitbucket-oauth](#manual-bitbucket-oauth)
Manually editing this file instead of using the command line may result in invalid json errors. To fix this you need to open the file in an editor and fix the error. To find the location of your global `auth.json`, execute:
```
php composer.phar config --global home
```
The folder will contain your global `auth.json` if it exists.
You can open this file in your favorite editor and fix the error.
Authentication in composer.json file itself
-------------------------------------------
> **Note:** **This is not recommended** as these credentials are visible to anyone who has access to the composer.json, either when it is shared through a version control system like git or when an attacker gains (read) access to your production server files.
>
>
It is also possible to add credentials to a `composer.json` on a per-project basis in the `config` section or directly in the repository definition.
Authentication using the COMPOSER\_AUTH environment variable
------------------------------------------------------------
> **Note:** Using the command line environment variable method also has security implications. These credentials will most likely be stored in memory, and may be persisted to a file like `~/.bash_history` (linux) or `ConsoleHost_history.txt` (PowerShell on Windows) when closing a session.
>
>
The final option to supply Composer with credentials is to use the `COMPOSER_AUTH` environment variable. These variables can be either passed as command line variables or set in actual environment variables. Read more about the usage of this environment variable [here](../03-cli.md#composer-auth).
Authentication methods
======================
http-basic
----------
### Command line http-basic
```
php composer.phar config [--global] http-basic.repo.example.org username password
```
In the above command, the config key `http-basic.repo.example.org` consists of two parts:
* `http-basic` is the authentication method.
* `repo.example.org` is the repository host name, you should replace it with the host name of your repository.
### Manual http-basic
```
php composer.phar config [--global] --editor --auth
```
```
{
"http-basic": {
"example.org": {
"username": "username",
"password": "password"
}
}
}
```
Inline http-basic
-----------------
For the inline http-basic authentication method the credentials are not stored in a separate `auth.json` in the project or globally, but in the `composer.json` or global configuration in the same place where the Composer repository definition is defined.
Make sure that the username and password are encoded according to [RFC 3986](http://www.faqs.org/rfcs/rfc3986.html) (2.1. Percent-Encoding). If the username e.g. is an email address it needs to be passed as `name%40example.com`.
### Command line inline http-basic
```
php composer.phar config [--global] repositories composer.unique-name https://username:[email protected]
```
### Manual inline http-basic
```
php composer.phar config [--global] --editor
```
```
{
"repositories": [
{
"type": "composer",
"url": "https://username:[email protected]"
}
]
}
```
HTTP Bearer
-----------
### Command line HTTP Bearer authentication
```
php composer.phar config [--global] bearer.repo.example.org token
```
In the above command, the config key `bearer.repo.example.org` consists of two parts:
* `bearer` is the authentication method.
* `repo.example.org` is the repository host name, you should replace it with the host name of your repository.
### Manual HTTP Bearer authentication
```
php composer.phar config [--global] --editor --auth
```
```
{
"bearer": {
"example.org": "TOKEN"
}
}
```
Custom token authentication
---------------------------
### Manual custom token authentication
```
php composer.phar config [--global] --editor
```
```
{
"repositories": [
{
"type": "composer",
"url": "https://example.org",
"options": {
"http": {
"header": [
"API-TOKEN: YOUR-API-TOKEN"
]
}
}
}
]
}
```
gitlab-oauth
------------
> **Note:** For the gitlab authentication to work on private gitlab instances, the [`gitlab-domains`](../06-config.md#gitlab-domains) section should also contain the url.
>
>
### Command line gitlab-oauth
```
php composer.phar config [--global] gitlab-oauth.gitlab.example.org token
```
In the above command, the config key `gitlab-oauth.gitlab.example.org` consists of two parts:
* `gitlab-oauth` is the authentication method.
* `gitlab.example.org` is the host name of your GitLab instance, you should replace it with the host name of your GitLab instance or use `gitlab.com` if you don't have a self-hosted GitLab instance.
### Manual gitlab-oauth
```
php composer.phar config [--global] --editor --auth
```
```
{
"gitlab-oauth": {
"example.org": "token"
}
}
```
gitlab-token
------------
> **Note:** For the gitlab authentication to work on private gitlab instances, the [`gitlab-domains`](../06-config.md#gitlab-domains) section should also contain the url.
>
>
To create a new access token, go to your [access tokens section on GitLab](https://gitlab.com/-/profile/personal_access_tokens) (or the equivalent URL on your private instance) and create a new token. See also [the GitLab access token documentation](https://docs.gitlab.com/ee/user/profile/personal_access_tokens.html#creating-a-personal-access-token) for more information.
When creating a gitlab token manually, make sure it has either the `read_api` or `api` scope.
### Command line gitlab-token
```
php composer.phar config [--global] gitlab-token.gitlab.example.org token
```
In the above command, the config key `gitlab-token.gitlab.example.org` consists of two parts:
* `gitlab-token` is the authentication method.
* `gitlab.example.org` is the host name of your GitLab instance, you should replace it with the host name of your GitLab instance or use `gitlab.com` if you don't have a self-hosted GitLab instance.
### Manual gitlab-token
```
php composer.phar config [--global] --editor --auth
```
```
{
"gitlab-token": {
"example.org": "token"
}
}
```
github-oauth
------------
To create a new access token, head to your [token settings section on Github](https://github.com/settings/tokens) and [generate a new token](https://github.com/settings/tokens/new).
For public repositories when rate limited, a token *without* any particular scope is sufficient (see `(no scope)` in the [scopes documentation](https://docs.github.com/en/developers/apps/building-oauth-apps/scopes-for-oauth-apps)). Such tokens grant read-only access to public information.
For private repositories, the `repo` scope is needed. Note that the token will be given broad read/write access to all of your private repositories and much more - see the [scopes documentation](https://docs.github.com/en/developers/apps/building-oauth-apps/scopes-for-oauth-apps) for a complete list. As of writing (November 2021), it seems not to be possible to further limit permissions for such tokens.
Read more about [Personal Access Tokens](https://docs.github.com/en/authentication/keeping-your-account-and-data-secure/creating-a-personal-access-token), or subscribe to the [roadmap item for better scoped tokens in GitHub](https://github.com/github/roadmap/issues/184).
### Command line github-oauth
```
php composer.phar config [--global] github-oauth.github.com token
```
In the above command, the config key `github-oauth.github.com` consists of two parts:
* `github-oauth` is the authentication method.
* `github.com` is the host name for which this token applies. For GitHub you most likely do not need to change this.
### Manual github-oauth
```
php composer.phar config [--global] --editor --auth
```
```
{
"github-oauth": {
"github.com": "token"
}
}
```
bitbucket-oauth
---------------
The BitBucket driver uses OAuth to access your private repositories via the BitBucket REST APIs, and you will need to create an OAuth consumer to use the driver, please refer to [Atlassian's Documentation](https://support.atlassian.com/bitbucket-cloud/docs/use-oauth-on-bitbucket-cloud/). You will need to fill the callback url with something to satisfy BitBucket, but the address does not need to go anywhere and is not used by Composer.
### Command line bitbucket-oauth
```
php composer.phar config [--global] bitbucket-oauth.bitbucket.org consumer-key consumer-secret
```
In the above command, the config key `bitbucket-oauth.bitbucket.org` consists of two parts:
* `bitbucket-oauth` is the authentication method.
* `bitbucket.org` is the host name for which this token applies. Unless you have a private instance you don't need to change this.
### Manual bitbucket-oauth
```
php composer.phar config [--global] --editor --auth
```
```
{
"bitbucket-oauth": {
"bitbucket.org": {
"consumer-key": "key",
"consumer-secret": "secret"
}
}
}
```
jasmine Interface: SpyStrategy Interface: SpyStrategy
======================
### Members
####
identity :String
Get the identifying information for the spy.
##### Type:
* String
Since: * 3.0.0
### Methods
####
callFake(fn)
Tell the spy to call a fake implementation when invoked.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `fn` | function | The function to invoke with the passed parameters. |
Since: * 2.0.0
####
callThrough()
Tell the spy to call through to the real implementation when invoked.
Since: * 2.0.0
####
exec()
Execute the current spy strategy.
Since: * 2.0.0
####
rejectWith(value)
Tell the spy to return a promise rejecting with the specified value when invoked.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `value` | \* | The value to return. |
Since: * 3.5.0
####
resolveTo(value)
Tell the spy to return a promise resolving to the specified value when invoked.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `value` | \* | The value to return. |
Since: * 3.5.0
####
returnValue(value)
Tell the spy to return the value when invoked.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `value` | \* | The value to return. |
Since: * 2.0.0
####
returnValues(…values)
Tell the spy to return one of the specified values (sequentially) each time the spy is invoked.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `values` | \* | <repeatable> | Values to be returned on subsequent calls to the spy. |
Since: * 2.1.0
####
stub()
Tell the spy to do nothing when invoked. This is the default.
Since: * 2.0.0
####
throwError(something)
Tell the spy to throw an error when invoked.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `something` | Error | Object | String | Thing to throw |
Since: * 2.0.0
| programming_docs |
jasmine Class: Spy Class: Spy
==========
### Namespaces
[calls](spy_calls) ### Members
####
(static) callData
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `object` | object | `this` context for the invocation. |
| `invocationOrder` | number | Order of the invocation. |
| `args` | Array | The arguments passed for this invocation. |
| `returnValue` | | The value that was returned from this invocation. |
####
and :[SpyStrategy](spystrategy)
Accesses the default strategy for the spy. This strategy will be used whenever the spy is called with arguments that don't match any strategy created with [`Spy#withArgs`](spy#withArgs).
##### Type:
* [SpyStrategy](spystrategy)
Since: * 2.0.0
##### Example
```
spyOn(someObj, 'func').and.returnValue(42);
```
### Methods
####
withArgs(…args) → {[SpyStrategy](spystrategy)}
Specifies a strategy to be used for calls to the spy that have the specified arguments.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `args` | \* | <repeatable> | The arguments to match |
Since: * 3.0.0
##### Returns:
Type [SpyStrategy](spystrategy) ##### Example
```
spyOn(someObj, 'func').withArgs(1, 2, 3).and.returnValue(42);
someObj.func(1, 2, 3); // returns 42
```
jasmine Class: MatchersUtil Class: MatchersUtil
===================
### Methods
####
contains(haystack, needle) → {boolean}
Determines whether `haystack` contains `needle`, using the same comparison logic as [`MatchersUtil#equals`](matchersutil#equals).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `haystack` | \* | The collection to search |
| `needle` | \* | The value to search for |
Since: * 2.0.0
##### Returns:
True if `needle` was found in `haystack`
Type boolean ####
equals(a, b) → {boolean}
Determines whether two values are deeply equal to each other.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `a` | \* | The first value to compare |
| `b` | \* | The second value to compare |
Since: * 2.0.0
##### Returns:
True if the values are equal
Type boolean ####
pp(value) → {string}
Formats a value for use in matcher failure messages and similar contexts, taking into account the current set of custom value formatters.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `value` | \* | The value to pretty-print |
Since: * 3.6.0
##### Returns:
The pretty-printed value
Type string
jasmine Class: jsApiReporter Class: jsApiReporter
====================
### Methods
####
executionTime() → {Number}
Get the number of milliseconds it took for the full Jasmine suite to run.
Since: * 2.0.0
##### Returns:
Type Number ####
specResults(index, length) → {Array.<[SpecResult](global#SpecResult)>}
Get the results for a set of specs.
Retrievable in slices for easier serialization.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `index` | Number | The position in the specs list to start from. |
| `length` | Number | Maximum number of specs results to return. |
Since: * 2.0.0
##### Returns:
Type Array.<[SpecResult](global#SpecResult)> ####
specs() → {Array.<[SpecResult](global#SpecResult)>}
Get all spec results.
Since: * 2.0.0
##### Returns:
Type Array.<[SpecResult](global#SpecResult)> ####
status() → {String}
Get the current status for the Jasmine environment.
Since: * 2.0.0
##### Returns:
* One of `loaded`, `started`, or `done`
Type String ####
suiteResults(index, length) → {Array.<[SuiteResult](global#SuiteResult)>}
Get the results for a set of suites.
Retrievable in slices for easier serialization.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `index` | Number | The position in the suites list to start from. |
| `length` | Number | Maximum number of suite results to return. |
Since: * 2.1.0
##### Returns:
Type Array.<[SuiteResult](global#SuiteResult)> ####
suites() → {Object}
Get all of the suites in a single object, with their `id` as the key.
Since: * 2.0.0
##### Returns:
* Map of suite id to [`SuiteResult`](global#SuiteResult)
Type Object
jasmine Global Global
======
### Methods
####
afterAll(functionopt, timeoutopt)
Run some shared teardown once after all of the specs in the [`describe`](global#describe) are run.
*Note:* Be careful, sharing the teardown from a afterAll makes it easy to accidentally leak state between your specs so that they erroneously pass or fail.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `function` | [implementationCallback](global#implementationCallback) | <optional> | | Function that contains the code to teardown your specs. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async afterAll. |
Since: * 2.1.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
afterEach(functionopt, timeoutopt)
Run some shared teardown after each of the specs in the [`describe`](global#describe) in which it is called.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `function` | [implementationCallback](global#implementationCallback) | <optional> | | Function that contains the code to teardown your specs. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async afterEach. |
Since: * 1.3.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
beforeAll(functionopt, timeoutopt)
Run some shared setup once before all of the specs in the [`describe`](global#describe) are run.
*Note:* Be careful, sharing the setup from a beforeAll makes it easy to accidentally leak state between your specs so that they erroneously pass or fail.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `function` | [implementationCallback](global#implementationCallback) | <optional> | | Function that contains the code to setup your specs. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async beforeAll. |
Since: * 2.1.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
beforeEach(functionopt, timeoutopt)
Run some shared setup before each of the specs in the [`describe`](global#describe) in which it is called.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `function` | [implementationCallback](global#implementationCallback) | <optional> | | Function that contains the code to setup your specs. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async beforeEach. |
Since: * 1.3.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
describe(description, specDefinitions)
Create a group of specs (often called a suite).
Calls to `describe` can be nested within other calls to compose your suite as a tree.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `description` | String | Textual description of the group |
| `specDefinitions` | function | Function for Jasmine to invoke that will define inner suites and specs |
Since: * 1.3.0
####
expect(actual) → {<matchers>}
Create an expectation for a spec.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `actual` | Object | Actual computed value to test expectations against. |
Since: * 1.3.0
##### Returns:
Type <matchers> ####
expectAsync(actual) → {<async-matchers>}
Create an asynchronous expectation for a spec. Note that the matchers that are provided by an asynchronous expectation all return promises which must be either returned from the spec or waited for using `await` in order for Jasmine to associate them with the correct spec.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `actual` | Object | Actual computed value to test expectations against. |
Since: * 3.3.0
##### Returns:
Type <async-matchers> ##### Examples
```
await expectAsync(somePromise).toBeResolved();
```
```
return expectAsync(somePromise).toBeResolved();
```
####
fail(erroropt)
Explicitly mark a spec as failed.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `error` | String | Error | <optional> | Reason for the failure. |
Since: * 2.1.0
####
fdescribe(description, specDefinitions)
A focused [``describe``](global#describe)
If suites or specs are focused, only those that are focused will be executed
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `description` | String | Textual description of the group |
| `specDefinitions` | function | Function for Jasmine to invoke that will define inner suites and specs |
Since: * 2.1.0
See: * [fit](global#fit)
####
fit(description, testFunction, timeoutopt)
A focused [``it``](global#it)
If suites or specs are focused, only those that are focused will be executed.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `description` | String | | | Textual description of what this spec is checking. |
| `testFunction` | [implementationCallback](global#implementationCallback) | | | Function that contains the code of your test. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async spec. |
Since: * 2.1.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
it(description, testFunctionopt, timeoutopt)
Define a single spec. A spec should contain one or more [`expectations`](global#expect) that test the state of the code.
A spec whose expectations all succeed will be passing and a spec with any failures will fail. The name `it` is a pronoun for the test target, not an abbreviation of anything. It makes the spec more readable by connecting the function name `it` and the argument `description` as a complete sentence.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `description` | String | | | Textual description of what this spec is checking |
| `testFunction` | [implementationCallback](global#implementationCallback) | <optional> | | Function that contains the code of your test. If not provided the test will be `pending`. |
| `timeout` | Int | <optional> | [`jasmine.DEFAULT_TIMEOUT_INTERVAL`](jasmine#.DEFAULT_TIMEOUT_INTERVAL) | Custom timeout for an async spec. |
Since: * 1.3.0
See: * [async](https://jasmine.github.io/tutorials/async)
####
pending(messageopt)
Mark a spec as pending, expectation results will be ignored.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `message` | String | <optional> | Reason the spec is pending. |
Since: * 2.0.0
####
setSpecProperty(key, value)
Sets a user-defined property that will be provided to reporters as part of the properties field of [`SpecResult`](global#SpecResult)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `key` | String | The name of the property |
| `value` | \* | The value of the property |
Since: * 3.6.0
####
setSuiteProperty(key, value)
Sets a user-defined property that will be provided to reporters as part of the properties field of [`SuiteResult`](global#SuiteResult)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `key` | String | The name of the property |
| `value` | \* | The value of the property |
Since: * 3.6.0
####
spyOn(obj, methodName) → {[Spy](spy)}
Install a spy onto an existing object.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `obj` | Object | The object upon which to install the [`Spy`](spy). |
| `methodName` | String | The name of the method to replace with a [`Spy`](spy). |
Since: * 1.3.0
##### Returns:
Type [Spy](spy) ####
spyOnAllFunctions(obj, includeNonEnumerable) → {Object}
Installs spies on all writable and configurable properties of an object.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `obj` | Object | The object upon which to install the [`Spy`](spy)s |
| `includeNonEnumerable` | boolean | Whether or not to add spies to non-enumerable properties |
Since: * 3.2.1
##### Returns:
the spied object
Type Object ####
spyOnProperty(obj, propertyName, accessTypeopt) → {[Spy](spy)}
Install a spy on a property installed with `Object.defineProperty` onto an existing object.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `obj` | Object | | | The object upon which to install the [`Spy`](spy) |
| `propertyName` | String | | | The name of the property to replace with a [`Spy`](spy). |
| `accessType` | String | <optional> | get | The access type (get|set) of the property to [`Spy`](spy) on. |
Since: * 2.6.0
##### Returns:
Type [Spy](spy) ####
xdescribe(description, specDefinitions)
A temporarily disabled [``describe``](global#describe)
Specs within an `xdescribe` will be marked pending and not executed
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `description` | String | Textual description of the group |
| `specDefinitions` | function | Function for Jasmine to invoke that will define inner suites and specs |
Since: * 1.3.0
####
xit(description, testFunctionopt)
A temporarily disabled [``it``](global#it)
The spec will report as `pending` and will not be executed.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `description` | String | | Textual description of what this spec is checking. |
| `testFunction` | [implementationCallback](global#implementationCallback) | <optional> | Function that contains the code of your test. Will not be executed. |
Since: * 1.3.0
### Type Definitions
#### DebugLogEntry
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `message` | String | The message that was passed to [`jasmine.debugLog`](jasmine#.debugLog). |
| `timestamp` | number | The time when the entry was added, in milliseconds from the spec's start time |
#### Expectation
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `matcherName` | String | The name of the matcher that was executed for this expectation. |
| `message` | String | The failure message for the expectation. |
| `stack` | String | The stack trace for the failure if available. |
| `passed` | Boolean | Whether the expectation passed or failed. |
| `expected` | Object | If the expectation failed, what was the expected value. |
| `actual` | Object | If the expectation failed, what actual value was produced. |
| `globalErrorType` | String | undefined | The type of an error that is reported on the top suite. Valid values are undefined, "afterAll", "load", "lateExpectation", and "lateError". |
####
implementationCallback(doneopt)
Callback passed to parts of the Jasmine base interface.
By default Jasmine assumes this function completes synchronously. If you have code that you need to test asynchronously, you can declare that you receive a `done` callback, return a Promise, or use the `async` keyword if it is supported in your environment.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
#### JasmineDoneInfo
Information passed to the [`Reporter#jasmineDone`](reporter#jasmineDone) event.
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `overallStatus` | OverallStatus | The overall result of the suite: 'passed', 'failed', or 'incomplete'. |
| `totalTime` | Int | The total time (in ms) that it took to execute the suite |
| `incompleteReason` | IncompleteReason | Explanation of why the suite was incomplete. |
| `order` | Order | Information about the ordering (random or not) of this execution of the suite. |
| `failedExpectations` | Array.<[Expectation](global#Expectation)> | List of expectations that failed in an [`afterAll`](global#afterAll) at the global level. |
| `deprecationWarnings` | Array.<[Expectation](global#Expectation)> | List of deprecation warnings that occurred at the global level. |
Since: * 2.4.0
#### JasmineStartedInfo
Information passed to the [`Reporter#jasmineStarted`](reporter#jasmineStarted) event.
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `totalSpecsDefined` | Int | The total number of specs defined in this suite. |
| `order` | Order | Information about the ordering (random or not) of this execution of the suite. |
Since: * 2.0.0
####
SpecFilter(spec)
A function that takes a spec and returns true if it should be executed or false if it should be skipped.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `spec` | [Spec](spec) | The spec that the filter is being applied to. |
##### Returns:
boolean
#### SpecResult
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `id` | Int | The unique id of this spec. |
| `description` | String | The description passed to the [`it`](global#it) that created this spec. |
| `fullName` | String | The full description including all ancestors of this spec. |
| `failedExpectations` | Array.<[Expectation](global#Expectation)> | The list of expectations that failed during execution of this spec. |
| `passedExpectations` | Array.<[Expectation](global#Expectation)> | The list of expectations that passed during execution of this spec. |
| `deprecationWarnings` | Array.<[Expectation](global#Expectation)> | The list of deprecation warnings that occurred during execution this spec. |
| `pendingReason` | String | If the spec is [`pending`](global#pending), this will be the reason. |
| `status` | String | Once the spec has completed, this string represents the pass/fail status of this spec. |
| `duration` | number | The time in ms used by the spec execution, including any before/afterEach. |
| `properties` | Object | User-supplied properties, if any, that were set using [`Env#setSpecProperty`](env#setSpecProperty) |
| `debugLogs` | Array.<[DebugLogEntry](global#DebugLogEntry)> | null | Messages, if any, that were logged using [`jasmine.debugLog`](jasmine#.debugLog) during a failing spec. |
Since: * 2.0.0
#### SuiteResult
##### Properties:
| Name | Type | Description |
| --- | --- | --- |
| `id` | Int | The unique id of this suite. |
| `description` | String | The description text passed to the [`describe`](global#describe) that made this suite. |
| `fullName` | String | The full description including all ancestors of this suite. |
| `failedExpectations` | Array.<[Expectation](global#Expectation)> | The list of expectations that failed in an [`afterAll`](global#afterAll) for this suite. |
| `deprecationWarnings` | Array.<[Expectation](global#Expectation)> | The list of deprecation warnings that occurred on this suite. |
| `status` | String | Once the suite has completed, this string represents the pass/fail status of this suite. |
| `duration` | number | The time in ms for Suite execution, including any before/afterAll, before/afterEach. |
| `properties` | Object | User-supplied properties, if any, that were set using [`Env#setSuiteProperty`](env#setSuiteProperty) |
Since: * 2.0.0
| programming_docs |
jasmine Interface: AsymmetricEqualityTester Interface: AsymmetricEqualityTester
===================================
Since: * 2.0.0
See: * [custom asymmetric\_equality\_testers](https://jasmine.github.io/tutorials/custom_asymmetric_equality_testers)
### Example
```
function numberDivisibleBy(divisor) {
return {
asymmetricMatch: function(n) {
return typeof n === 'number' && n % divisor === 0;
},
jasmineToString: function() {
return `<a number divisible by ${divisor}>`;
}
};
}
var actual = {
n: 2,
otherFields: "don't care"
};
expect(actual).toEqual(jasmine.objectContaining({n: numberDivisibleBy(2)}));
```
### Methods
####
asymmetricMatch(value, matchersUtil) → {Boolean}
Determines whether a value matches this tester
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `value` | any | The value to test |
| `matchersUtil` | [MatchersUtil](matchersutil) | utilities for testing equality, etc |
##### Returns:
Type Boolean ####
jasmineToString(pp) → {String}
Returns a string representation of this tester to use in matcher failure messages
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `pp` | function | Function that takes a value and returns a pretty-printed representation |
##### Returns:
Type String
jasmine Namespace: jasmine Namespace: jasmine
==================
### Members
####
(static) DEFAULT\_TIMEOUT\_INTERVAL
Default number of milliseconds Jasmine will wait for an asynchronous spec, before, or after function to complete. This can be overridden on a case by case basis by passing a time limit as the third argument to [`it`](global#it), [`beforeEach`](global#beforeEach), [`afterEach`](global#afterEach), [`beforeAll`](global#beforeAll), or [`afterAll`](global#afterAll). The value must be no greater than the largest number of milliseconds supported by setTimeout, which is usually 2147483647.
While debugging tests, you may want to set this to a large number (or pass a large number to one of the functions mentioned above) so that Jasmine does not move on to after functions or the next spec while you're debugging.
Since: * 1.3.0
Default Value: * 5000
####
(static) MAX\_PRETTY\_PRINT\_ARRAY\_LENGTH
Maximum number of array elements to display when pretty printing objects. This will also limit the number of keys and values displayed for an object. Elements past this number will be ellipised.
Since: * 2.7.0
Default Value: * 50
####
(static) MAX\_PRETTY\_PRINT\_CHARS
Maximum number of characters to display when pretty printing objects. Characters past this number will be ellipised.
Since: * 2.9.0
Default Value: * 100
####
(static) MAX\_PRETTY\_PRINT\_DEPTH
Maximum object depth the pretty printer will print to. Set this to a lower value to speed up pretty printing if you have large objects.
Since: * 1.3.0
Default Value: * 8
### Methods
####
(static) addAsyncMatchers(matchers)
Add custom async matchers for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `matchers` | Object | Keys from this object will be the new async matcher names. |
Since: * 3.5.0
See: * [custom matcher](https://jasmine.github.io/tutorials/custom_matcher)
####
(static) addCustomEqualityTester(tester)
Add a custom equality tester for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `tester` | function | A function which takes two arguments to compare and returns a `true` or `false` comparison result if it knows how to compare them, and `undefined` otherwise. |
Since: * 2.0.0
See: * [custom equality](https://jasmine.github.io/tutorials/custom_equality)
####
(static) addCustomObjectFormatter(formatter)
Add a custom object formatter for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `formatter` | function | A function which takes a value to format and returns a string if it knows how to format it, and `undefined` otherwise. |
Since: * 3.6.0
See: * [custom object\_formatters](https://jasmine.github.io/tutorials/custom_object_formatters)
####
(static) addMatchers(matchers)
Add custom matchers for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `matchers` | Object | Keys from this object will be the new matcher names. |
Since: * 2.0.0
See: * [custom matcher](https://jasmine.github.io/tutorials/custom_matcher)
####
(static) addSpyStrategy(name, factory)
Add a custom spy strategy for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `name` | String | The name of the strategy (i.e. what you call from `and`) |
| `factory` | function | Factory function that returns the plan to be executed. |
Since: * 3.5.0
####
(static) any(clazz)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is an instance of the specified class/constructor.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `clazz` | Constructor | The constructor to check against. |
Since: * 1.3.0
####
(static) anything()
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is not `null` and not `undefined`.
Since: * 2.2.0
####
(static) arrayContaining(sample)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value is an `Array` that contains at least the elements in the sample.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `sample` | Array | |
Since: * 2.2.0
####
(static) arrayWithExactContents(sample)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value is an `Array` that contains all of the elements in the sample in any order.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `sample` | Array | |
Since: * 2.8.0
####
(static) clock() → {[Clock](clock)}
Get the currently booted mock {Clock} for this Jasmine environment.
Since: * 2.0.0
##### Returns:
Type [Clock](clock) ####
(static) createSpy(nameopt, originalFnopt) → {[Spy](spy)}
Create a bare [`Spy`](spy) object. This won't be installed anywhere and will not have any implementation behind it.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `name` | String | <optional> | Name to give the spy. This will be displayed in failure messages. |
| `originalFn` | function | <optional> | Function to act as the real implementation. |
Since: * 1.3.0
##### Returns:
Type [Spy](spy) ####
(static) createSpyObj(baseNameopt, methodNames, propertyNamesopt) → {Object}
Create an object with multiple [`Spy`](spy)s as its members.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `baseName` | String | <optional> | Base name for the spies in the object. |
| `methodNames` | Array.<String> | Object | | Array of method names to create spies for, or Object whose keys will be method names and values the `returnValue`. |
| `propertyNames` | Array.<String> | Object | <optional> | Array of property names to create spies for, or Object whose keys will be propertynames and values the `returnValue`. |
Since: * 1.3.0
##### Returns:
Type Object ####
(static) debugLog(msg)
Logs a message for use in debugging. If the spec fails, trace messages will be included in the [`result`](global#SpecResult) passed to the reporter's specDone method.
This method should be called only when a spec (including any associated beforeEach or afterEach functions) is running.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `msg` | String | The message to log |
Since: * 4.0.0
####
(static) empty()
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is empty.
Since: * 3.1.0
####
(static) falsy()
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is `null`, `undefined`, `0`, `false` or anything falsey.
Since: * 3.1.0
####
(static) getEnv() → {[Env](env)}
Get the currently booted Jasmine Environment.
Since: * 1.3.0
##### Returns:
Type [Env](env) ####
(static) isSpy(putativeSpy) → {Boolean}
Determines whether the provided function is a Jasmine spy.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `putativeSpy` | function | The function to check. |
Since: * 2.0.0
##### Returns:
Type Boolean ####
(static) mapContaining(sample)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if every key/value pair in the sample passes the deep equality comparison with at least one key/value pair in the actual value being compared
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `sample` | Map | The subset of items that *must* be in the actual. |
Since: * 3.5.0
####
(static) notEmpty()
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is not empty.
Since: * 3.1.0
####
(static) objectContaining(sample)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared contains at least the keys and values.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `sample` | Object | The subset of properties that *must* be in the actual. |
Since: * 1.3.0
####
(static) setContaining(sample)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if every item in the sample passes the deep equality comparison with at least one item in the actual value being compared
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `sample` | Set | The subset of items that *must* be in the actual. |
Since: * 3.5.0
####
(static) setDefaultSpyStrategy(defaultStrategyFn)
Set the default spy strategy for the current scope of specs.
*Note:* This is only callable from within a [`beforeEach`](global#beforeEach), [`it`](global#it), or [`beforeAll`](global#beforeAll).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `defaultStrategyFn` | function | a function that assigns a strategy |
##### Example
```
beforeEach(function() {
jasmine.setDefaultSpyStrategy(and => and.returnValue(true));
});
```
####
(static) stringContaining(expected)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value is a `String` that contains the specified `String`.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | String | |
Since: * 3.10.0
####
(static) stringMatching(expected)
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value is a `String` that matches the `RegExp` or `String`.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | RegExp | String | |
Since: * 2.2.0
####
(static) truthy()
Get an [`AsymmetricEqualityTester`](asymmetricequalitytester), usable in any [`matcher`](matchers) that uses Jasmine's equality (e.g. [`toEqual`](matchers#toEqual), [`toContain`](matchers#toContain), or [`toHaveBeenCalledWith`](matchers#toHaveBeenCalledWith)), that will succeed if the actual value being compared is `true` or anything truthy.
Since: * 3.1.0
jasmine Interface: Configuration Interface: Configuration
========================
This represents the available options to configure Jasmine. Options that are not provided will use their default values.
Since: * 3.3.0
See: * [Env#configure](env#configure)
### Members
####
autoCleanClosures :boolean
Clean closures when a suite is done running (done by clearing the stored function reference). This prevents memory leaks, but you won't be able to run jasmine multiple times.
##### Type:
* boolean
Since: * 3.10.0
Default Value: * true
####
failSpecWithNoExpectations :Boolean
Whether to fail the spec if it ran no expectations. By default a spec that ran no expectations is reported as passed. Setting this to true will report such spec as a failure.
##### Type:
* Boolean
Since: * 3.5.0
Default Value: * false
####
hideDisabled :Boolean
Whether or not reporters should hide disabled specs from their output. Currently only supported by Jasmine's HTMLReporter
##### Type:
* Boolean
Since: * 3.3.0
Default Value: * false
####
random :Boolean
Whether to randomize spec execution order
##### Type:
* Boolean
Since: * 3.3.0
Default Value: * true
####
seed :number|string
Seed to use as the basis of randomization. Null causes the seed to be determined randomly at the start of execution.
##### Type:
* number | string
Since: * 3.3.0
Default Value: * null
####
specFilter :[SpecFilter](global#SpecFilter)
Function to use to filter specs
##### Type:
* [SpecFilter](global#SpecFilter)
Since: * 3.3.0
Default Value: * A function that always returns true.
####
stopOnSpecFailure :Boolean
Whether to stop execution of the suite after the first spec failure
##### Type:
* Boolean
Since: * 3.9.0
Default Value: * false
####
stopSpecOnExpectationFailure :Boolean
Whether to cause specs to only have one expectation failure.
##### Type:
* Boolean
Since: * 3.3.0
Default Value: * false
####
verboseDeprecations :Boolean
Whether or not to issue warnings for certain deprecated functionality every time it's used. If not set or set to false, deprecation warnings for methods that tend to be called frequently will be issued only once or otherwise throttled to to prevent the suite output from being flooded with warnings.
##### Type:
* Boolean
Since: * 3.6.0
Default Value: * false
jasmine Namespace: matchers Namespace: matchers
===================
Matchers that come with Jasmine out of the box.
### Members
####
not :<matchers>
Invert the matcher following this [`expect`](global#expect)
##### Type:
* <matchers>
Since: * 1.3.0
##### Example
```
expect(something).not.toBe(true);
```
### Methods
####
nothing()
[`expect`](global#expect) nothing explicitly.
Since: * 2.8.0
##### Example
```
expect().nothing();
```
####
toBe(expected)
[`expect`](global#expect) the actual value to be `===` to the expected value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | The expected value to compare against. |
Since: * 1.3.0
##### Example
```
expect(thing).toBe(realThing);
```
####
toBeCloseTo(expected, precisionopt)
[`expect`](global#expect) the actual value to be within a specified precision of the expected value.
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `expected` | Object | | | The expected value to compare against. |
| `precision` | Number | <optional> | 2 | The number of decimal points to check. |
Since: * 1.3.0
##### Example
```
expect(number).toBeCloseTo(42.2, 3);
```
####
toBeDefined()
[`expect`](global#expect) the actual value to be defined. (Not `undefined`)
Since: * 1.3.0
##### Example
```
expect(result).toBeDefined();
```
####
toBeFalse()
[`expect`](global#expect) the actual value to be `false`.
Since: * 3.5.0
##### Example
```
expect(result).toBeFalse();
```
####
toBeFalsy()
[`expect`](global#expect) the actual value to be falsy
Since: * 2.0.0
##### Example
```
expect(result).toBeFalsy();
```
####
toBeGreaterThan(expected)
[`expect`](global#expect) the actual value to be greater than the expected value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Number | The value to compare against. |
Since: * 2.0.0
##### Example
```
expect(result).toBeGreaterThan(3);
```
####
toBeGreaterThanOrEqual(expected)
[`expect`](global#expect) the actual value to be greater than or equal to the expected value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Number | The expected value to compare against. |
Since: * 2.0.0
##### Example
```
expect(result).toBeGreaterThanOrEqual(25);
```
####
toBeInstanceOf(expected)
[`expect`](global#expect) the actual to be an instance of the expected class
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | The class or constructor function to check for |
Since: * 3.5.0
##### Example
```
expect('foo').toBeInstanceOf(String);
expect(3).toBeInstanceOf(Number);
expect(new Error()).toBeInstanceOf(Error);
```
####
toBeLessThan(expected)
[`expect`](global#expect) the actual value to be less than the expected value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Number | The expected value to compare against. |
Since: * 2.0.0
##### Example
```
expect(result).toBeLessThan(0);
```
####
toBeLessThanOrEqual(expected)
[`expect`](global#expect) the actual value to be less than or equal to the expected value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Number | The expected value to compare against. |
Since: * 2.0.0
##### Example
```
expect(result).toBeLessThanOrEqual(123);
```
####
toBeNaN()
[`expect`](global#expect) the actual value to be `NaN` (Not a Number).
Since: * 1.3.0
##### Example
```
expect(thing).toBeNaN();
```
####
toBeNegativeInfinity()
[`expect`](global#expect) the actual value to be `-Infinity` (-infinity).
Since: * 2.6.0
##### Example
```
expect(thing).toBeNegativeInfinity();
```
####
toBeNull()
[`expect`](global#expect) the actual value to be `null`.
Since: * 1.3.0
##### Example
```
expect(result).toBeNull();
```
####
toBePositiveInfinity()
[`expect`](global#expect) the actual value to be `Infinity` (infinity).
Since: * 2.6.0
##### Example
```
expect(thing).toBePositiveInfinity();
```
####
toBeTrue()
[`expect`](global#expect) the actual value to be `true`.
Since: * 3.5.0
##### Example
```
expect(result).toBeTrue();
```
####
toBeTruthy()
[`expect`](global#expect) the actual value to be truthy.
Since: * 2.0.0
##### Example
```
expect(thing).toBeTruthy();
```
####
toBeUndefined()
[`expect`](global#expect) the actual value to be `undefined`.
Since: * 1.3.0
##### Example
```
expect(result).toBeUndefined():
```
####
toContain(expected)
[`expect`](global#expect) the actual value to contain a specific value.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | The value to look for. |
Since: * 2.0.0
##### Example
```
expect(array).toContain(anElement);
expect(string).toContain(substring);
```
####
toEqual(expected)
[`expect`](global#expect) the actual value to be equal to the expected, using deep equality comparison.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | Expected value |
Since: * 1.3.0
##### Example
```
expect(bigObject).toEqual({"foo": ['bar', 'baz']});
```
####
toHaveBeenCalled()
[`expect`](global#expect) the actual (a [`Spy`](spy)) to have been called.
Since: * 1.3.0
##### Example
```
expect(mySpy).toHaveBeenCalled();
expect(mySpy).not.toHaveBeenCalled();
```
####
toHaveBeenCalledBefore(expected)
[`expect`](global#expect) the actual value (a [`Spy`](spy)) to have been called before another [`Spy`](spy).
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | [Spy](spy) | [`Spy`](spy) that should have been called after the `actual` [`Spy`](spy). |
Since: * 2.6.0
##### Example
```
expect(mySpy).toHaveBeenCalledBefore(otherSpy);
```
####
toHaveBeenCalledOnceWith()
[`expect`](global#expect) the actual (a [`Spy`](spy)) to have been called exactly once, and exactly with the particular arguments.
##### Parameters:
| Type | Attributes | Description |
| --- | --- | --- |
| Object | <repeatable> | The arguments to look for |
Since: * 3.6.0
##### Example
```
expect(mySpy).toHaveBeenCalledOnceWith('foo', 'bar', 2);
```
####
toHaveBeenCalledTimes(expected)
[`expect`](global#expect) the actual (a [`Spy`](spy)) to have been called the specified number of times.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Number | The number of invocations to look for. |
Since: * 2.4.0
##### Example
```
expect(mySpy).toHaveBeenCalledTimes(3);
```
####
toHaveBeenCalledWith()
[`expect`](global#expect) the actual (a [`Spy`](spy)) to have been called with particular arguments at least once.
##### Parameters:
| Type | Attributes | Description |
| --- | --- | --- |
| Object | <repeatable> | The arguments to look for |
Since: * 1.3.0
##### Example
```
expect(mySpy).toHaveBeenCalledWith('foo', 'bar', 2);
```
####
toHaveClass(expected)
[`expect`](global#expect) the actual value to be a DOM element that has the expected class
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | The class name to test for |
Since: * 3.0.0
##### Example
```
var el = document.createElement('div');
el.className = 'foo bar baz';
expect(el).toHaveClass('bar');
```
####
toHaveSize(expected)
[`expect`](global#expect) the actual size to be equal to the expected, using array-like length or object keys size.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | Expected size |
Since: * 3.6.0
##### Example
```
array = [1,2];
expect(array).toHaveSize(2);
```
####
toMatch(expected)
[`expect`](global#expect) the actual value to match a regular expression
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | RegExp | String | Value to look for in the string. |
Since: * 1.3.0
##### Example
```
expect("my string").toMatch(/string$/);
expect("other string").toMatch("her");
```
####
toThrow(expectedopt)
[`expect`](global#expect) a function to `throw` something.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `expected` | Object | <optional> | Value that should be thrown. If not provided, simply the fact that something was thrown will be checked. |
Since: * 2.0.0
##### Example
```
expect(function() { return 'things'; }).toThrow('foo');
expect(function() { return 'stuff'; }).toThrow();
```
####
toThrowError(expectedopt, messageopt)
[`expect`](global#expect) a function to `throw` an `Error`.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `expected` | Error | <optional> | `Error` constructor the object that was thrown needs to be an instance of. If not provided, `Error` will be used. |
| `message` | RegExp | String | <optional> | The message that should be set on the thrown `Error` |
Since: * 2.0.0
##### Example
```
expect(function() { return 'things'; }).toThrowError(MyCustomError, 'message');
expect(function() { return 'things'; }).toThrowError(MyCustomError, /bar/);
expect(function() { return 'stuff'; }).toThrowError(MyCustomError);
expect(function() { return 'other'; }).toThrowError(/foo/);
expect(function() { return 'other'; }).toThrowError();
```
####
toThrowMatching(predicate)
[`expect`](global#expect) a function to `throw` something matching a predicate.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `predicate` | function | A function that takes the thrown exception as its parameter and returns true if it matches. |
Since: * 3.0.0
##### Example
```
expect(function() { throw new Error('nope'); }).toThrowMatching(function(thrown) { return thrown.message === 'nope'; });
```
####
withContext(message) → {<matchers>}
Add some context for an [`expect`](global#expect)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `message` | String | Additional context to show when the matcher fails |
Since: * 3.3.0
##### Returns:
Type <matchers>
| programming_docs |
jasmine Interface: Suite Interface: Suite
================
Since: * 2.0.0
See: * [Env#topSuite](env#topSuite)
### Members
####
children :Array.<([Spec](spec)|[Suite](suite))>
The suite's children.
##### Type:
* Array.<([Spec](spec)|[Suite](suite))>
Since: * 2.0.0
####
children :Array.<([Spec](spec)|[Suite](suite))>
The suite's children.
##### Type:
* Array.<([Spec](spec)|[Suite](suite))>
####
(readonly) description :string
The description passed to the [`describe`](global#describe) that created this suite.
##### Type:
* string
Since: * 2.0.0
####
(readonly) description :string
The description passed to the [`describe`](global#describe) that created this suite.
##### Type:
* string
####
(readonly) id :string
The unique ID of this suite.
##### Type:
* string
Since: * 2.0.0
####
(readonly) id :string
The unique ID of this suite.
##### Type:
* string
####
(readonly) parentSuite :[Suite](suite)
The parent of this suite, or null if this is the top suite.
##### Type:
* [Suite](suite)
### Methods
####
getFullName() → {string}
The full description including all ancestors of this suite.
Since: * 2.0.0
##### Returns:
Type string ####
getFullName() → {string}
The full description including all ancestors of this suite.
##### Returns:
Type string See: * [Env#topSuite](env#topSuite)
### Members
####
children :Array.<([Spec](spec)|[Suite](suite))>
The suite's children.
##### Type:
* Array.<([Spec](spec)|[Suite](suite))>
Since: * 2.0.0
####
children :Array.<([Spec](spec)|[Suite](suite))>
The suite's children.
##### Type:
* Array.<([Spec](spec)|[Suite](suite))>
####
(readonly) description :string
The description passed to the [`describe`](global#describe) that created this suite.
##### Type:
* string
Since: * 2.0.0
####
(readonly) description :string
The description passed to the [`describe`](global#describe) that created this suite.
##### Type:
* string
####
(readonly) id :string
The unique ID of this suite.
##### Type:
* string
Since: * 2.0.0
####
(readonly) id :string
The unique ID of this suite.
##### Type:
* string
####
(readonly) parentSuite :[Suite](suite)
The parent of this suite, or null if this is the top suite.
##### Type:
* [Suite](suite)
### Methods
####
getFullName() → {string}
The full description including all ancestors of this suite.
Since: * 2.0.0
##### Returns:
Type string ####
getFullName() → {string}
The full description including all ancestors of this suite.
##### Returns:
Type string
jasmine Module: jasmine-core Module: jasmine-core
====================
Note: Only available on Node.
### Members
####
(static) boot :function
Boots a copy of Jasmine and returns an object as described in [`jasmine`](jasmine).
##### Type:
* function
####
(static) noGlobals
Boots a copy of Jasmine and returns an object containing the properties that would normally be added to the global object. If noGlobals is called multiple times, the same object is returned every time.
Do not call boot() if you also call noGlobals().
##### Example
```
const {describe, beforeEach, it, expect, jasmine} = require('jasmine-core').noGlobals();
```
jasmine Namespace: calls Namespace: calls
================
Since: * 2.0.0
### Methods
####
all() → {Array.<[Spy.callData](spy#.callData)>}
Get the raw calls array for this spy.
Since: * 2.0.0
##### Returns:
Type Array.<[Spy.callData](spy#.callData)> ####
allArgs() → {Array}
Get all of the arguments for each invocation of this spy in the order they were received.
Since: * 2.0.0
##### Returns:
Type Array ####
any() → {Boolean}
Check whether this spy has been invoked.
Since: * 2.0.0
##### Returns:
Type Boolean ####
argsFor(index) → {Array}
Get the arguments that were passed to a specific invocation of this spy.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `index` | Integer | The 0-based invocation index. |
Since: * 2.0.0
##### Returns:
Type Array ####
count() → {Integer}
Get the number of invocations of this spy.
Since: * 2.0.0
##### Returns:
Type Integer ####
first() → {ObjecSpy.callData}
Get the first invocation of this spy.
Since: * 2.0.0
##### Returns:
Type ObjecSpy.callData ####
mostRecent() → {ObjecSpy.callData}
Get the most recent invocation of this spy.
Since: * 2.0.0
##### Returns:
Type ObjecSpy.callData ####
reset()
Reset this spy as if it has never been called.
Since: * 2.0.0
####
saveArgumentsByValue()
Set this spy to do a shallow clone of arguments passed to each invocation.
Since: * 2.5.0
####
thisFor(index) → (nullable) {Object}
Get the "this" object that was passed to a specific invocation of this spy.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `index` | Integer | The 0-based invocation index. |
Since: * 3.8.0
##### Returns:
Type Object
jasmine Class: Env Class: Env
==========
Since: * 2.0.0
### Methods
####
addReporter(reporterToAdd)
Add a custom reporter to the Jasmine environment.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `reporterToAdd` | [Reporter](reporter) | The reporter to be added. |
Since: * 2.0.0
See: * [custom reporter](https://jasmine.github.io/tutorials/custom_reporter)
####
allowRespy(allow)
Configures whether Jasmine should allow the same function to be spied on more than once during the execution of a spec. By default, spying on a function that is already a spy will cause an error.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `allow` | boolean | Whether to allow respying |
Since: * 2.5.0
####
clearReporters()
Clear all registered reporters
Since: * 2.5.2
####
configuration() → {[Configuration](configuration)}
Get the current configuration for your jasmine environment
Since: * 3.3.0
##### Returns:
Type [Configuration](configuration) ####
configure(configuration)
Configure your jasmine environment
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `configuration` | [Configuration](configuration) | |
Since: * 3.3.0
####
deprecated(deprecation, optionsopt)
Causes a deprecation warning to be logged to the console and reported to reporters.
The optional second parameter is an object that can have either of the following properties:
omitStackTrace: Whether to omit the stack trace. Optional. Defaults to false. This option is ignored if the deprecation is an Error. Set this when the stack trace will not contain anything that helps the user find the source of the deprecation.
ignoreRunnable: Whether to log the deprecation on the root suite, ignoring the spec or suite that's running when it happens. Optional. Defaults to false.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `deprecation` | String | Error | | The deprecation message |
| `options` | Object | <optional> | Optional extra options, as described above |
Since: * 2.99
####
execute(runnablesToRunopt, onCompleteopt) → {Promise.<[JasmineDoneInfo](global#JasmineDoneInfo)>}
Executes the specs.
If called with no parameters or with a falsy value as the first parameter, all specs will be executed except those that are excluded by a [`spec filter`](configuration#specFilter) or other mechanism. If the first parameter is a list of spec/suite IDs, only those specs/suites will be run.
Both parameters are optional, but a completion callback is only valid as the second parameter. To specify a completion callback but not a list of specs/suites to run, pass null or undefined as the first parameter. The completion callback is supported for backward compatibility. In most cases it will be more convenient to use the returned promise instead.
execute should not be called more than once unless the env has been configured with `{autoCleanClosures: false}`.
execute returns a promise. The promise will be resolved to the same [`overall result`](global#JasmineDoneInfo) that's passed to a reporter's `jasmineDone` method, even if the suite did not pass. To determine whether the suite passed, check the value that the promise resolves to or use a [`Reporter`](reporter).
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `runnablesToRun` | Array.<string> | <optional> | IDs of suites and/or specs to run |
| `onComplete` | function | <optional> | Function that will be called after all specs have run |
Since: * 2.0.0
##### Returns:
Type Promise.<[JasmineDoneInfo](global#JasmineDoneInfo)> ####
provideFallbackReporter(reporterToAdd)
Provide a fallback reporter if no other reporters have been specified.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `reporterToAdd` | [Reporter](reporter) | The reporter |
Since: * 2.5.0
See: * [custom reporter](https://jasmine.github.io/tutorials/custom_reporter)
####
setSpecProperty(key, value)
Sets a user-defined property that will be provided to reporters as part of the properties field of [`SpecResult`](global#SpecResult)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `key` | String | The name of the property |
| `value` | \* | The value of the property |
Since: * 3.6.0
####
setSuiteProperty(key, value)
Sets a user-defined property that will be provided to reporters as part of the properties field of [`SuiteResult`](global#SuiteResult)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `key` | String | The name of the property |
| `value` | \* | The value of the property |
Since: * 3.6.0
####
topSuite() → {[Suite](suite)}
Provides the root suite, through which all suites and specs can be accessed.
Since: * 2.0.0
##### Returns:
the root suite
Type [Suite](suite)
jasmine Interface: Reporter Interface: Reporter
===================
This represents the available reporter callback for an object passed to [`Env#addReporter`](env#addReporter).
See: * [custom reporter](https://jasmine.github.io/tutorials/custom_reporter)
### Methods
####
jasmineDone(suiteInfo, doneopt)
When the entire suite has finished execution `jasmineDone` is called
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `suiteInfo` | [JasmineDoneInfo](global#JasmineDoneInfo) | | Information about the full Jasmine suite that just finished running. |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
####
jasmineStarted(suiteInfo, doneopt)
`jasmineStarted` is called after all of the specs have been loaded, but just before execution starts.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `suiteInfo` | [JasmineStartedInfo](global#JasmineStartedInfo) | | Information about the full Jasmine suite that is being run |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
####
specDone(result, doneopt)
`specDone` is invoked when an `it` and its associated `beforeEach` and `afterEach` functions have been run.
While jasmine doesn't require any specific functions, not defining a `specDone` will make it impossible for a reporter to know when a spec has failed.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `result` | [SpecResult](global#SpecResult) | | |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
####
specStarted(result, doneopt)
`specStarted` is invoked when an `it` starts to run (including associated `beforeEach` functions)
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `result` | [SpecResult](global#SpecResult) | | Information about the individual [`it`](global#it) being run |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
####
suiteDone(result, doneopt)
`suiteDone` is invoked when all of the child specs and suites for a given suite have been run
While jasmine doesn't require any specific functions, not defining a `suiteDone` will make it impossible for a reporter to know when a suite has failures in an `afterAll`.
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `result` | [SuiteResult](global#SuiteResult) | | |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
####
suiteStarted(result, doneopt)
`suiteStarted` is invoked when a `describe` starts to run
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `result` | [SuiteResult](global#SuiteResult) | | Information about the individual [`describe`](global#describe) being run |
| `done` | function | <optional> | Used to specify to Jasmine that this callback is asynchronous and Jasmine should wait until it has been called before moving on. |
See: * [async](https://jasmine.github.io/tutorials/async)
##### Returns:
Optionally return a Promise instead of using `done` to cause Jasmine to wait for completion.
jasmine Namespace: async-matchers Namespace: async-matchers
=========================
Asynchronous matchers that operate on an actual value which is a promise, and return a promise.
Most async matchers will wait indefinitely for the promise to be resolved or rejected, resulting in a spec timeout if that never happens. If you expect that the promise will already be resolved or rejected at the time the matcher is called, you can use the [`async-matchers#already`](async-matchers#already) modifier to get a faster failure with a more helpful message.
Note: Specs must await the result of each async matcher, return the promise returned by the matcher, or return a promise that's derived from the one returned by the matcher. Otherwise the matcher will not be evaluated before the spec completes.
### Examples
```
// Good
await expectAsync(aPromise).toBeResolved();
```
```
// Good
return expectAsync(aPromise).toBeResolved();
```
```
// Good
return expectAsync(aPromise).toBeResolved()
.then(function() {
// more spec code
});
```
```
// Bad
expectAsync(aPromise).toBeResolved();
```
### Members
####
already :<async-matchers>
Fail as soon as possible if the actual is pending. Otherwise evaluate the matcher.
##### Type:
* <async-matchers>
Since: * 3.8.0
##### Examples
```
await expectAsync(myPromise).already.toBeResolved();
```
```
return expectAsync(myPromise).already.toBeResolved();
```
####
not :<async-matchers>
Invert the matcher following this [`expectAsync`](global#expectAsync)
##### Type:
* <async-matchers>
##### Examples
```
await expectAsync(myPromise).not.toBeResolved();
```
```
return expectAsync(myPromise).not.toBeResolved();
```
### Methods
####
(async) toBePending()
Expect a promise to be pending, i.e. the promise is neither resolved nor rejected.
Since: * 3.6
##### Example
```
await expectAsync(aPromise).toBePending();
```
####
(async) toBeRejected()
Expect a promise to be rejected.
Since: * 3.1.0
##### Examples
```
await expectAsync(aPromise).toBeRejected();
```
```
return expectAsync(aPromise).toBeRejected();
```
####
(async) toBeRejectedWith(expected)
Expect a promise to be rejected with a value equal to the expected, using deep equality comparison.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | Value that the promise is expected to be rejected with |
Since: * 3.3.0
##### Examples
```
await expectAsync(aPromise).toBeRejectedWith({prop: 'value'});
```
```
return expectAsync(aPromise).toBeRejectedWith({prop: 'value'});
```
####
(async) toBeRejectedWithError(expectedopt, messageopt)
Expect a promise to be rejected with a value matched to the expected
##### Parameters:
| Name | Type | Attributes | Description |
| --- | --- | --- | --- |
| `expected` | Error | <optional> | `Error` constructor the object that was thrown needs to be an instance of. If not provided, `Error` will be used. |
| `message` | RegExp | String | <optional> | The message that should be set on the thrown `Error` |
Since: * 3.5.0
##### Example
```
await expectAsync(aPromise).toBeRejectedWithError(MyCustomError, 'Error message');
await expectAsync(aPromise).toBeRejectedWithError(MyCustomError, /Error message/);
await expectAsync(aPromise).toBeRejectedWithError(MyCustomError);
await expectAsync(aPromise).toBeRejectedWithError('Error message');
return expectAsync(aPromise).toBeRejectedWithError(/Error message/);
```
####
(async) toBeResolved()
Expect a promise to be resolved.
Since: * 3.1.0
##### Examples
```
await expectAsync(aPromise).toBeResolved();
```
```
return expectAsync(aPromise).toBeResolved();
```
####
(async) toBeResolvedTo(expected)
Expect a promise to be resolved to a value equal to the expected, using deep equality comparison.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `expected` | Object | Value that the promise is expected to resolve to |
Since: * 3.1.0
##### Examples
```
await expectAsync(aPromise).toBeResolvedTo({prop: 'value'});
```
```
return expectAsync(aPromise).toBeResolvedTo({prop: 'value'});
```
####
withContext(message) → {<async-matchers>}
Add some context for an [`expectAsync`](global#expectAsync)
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `message` | String | Additional context to show when the async matcher fails |
Since: * 3.3.0
##### Returns:
Type <async-matchers>
jasmine Class: Clock Class: Clock
============
Since: * 1.3.0
### Methods
####
install() → {[Clock](clock)}
Install the mock clock over the built-in methods.
Since: * 2.0.0
##### Returns:
Type [Clock](clock) ####
mockDate(initialDateopt)
Instruct the installed Clock to also mock the date returned by `new Date()`
##### Parameters:
| Name | Type | Attributes | Default | Description |
| --- | --- | --- | --- | --- |
| `initialDate` | Date | <optional> | now | The `Date` to provide. |
Since: * 2.1.0
####
tick(millis)
Tick the Clock forward, running any enqueued timeouts along the way
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `millis` | int | The number of milliseconds to tick. |
Since: * 1.3.0
####
uninstall()
Uninstall the mock clock, returning the built-in methods to their places.
Since: * 2.0.0
####
withMock(closure)
Execute a function with a mocked Clock
The clock will be [`install`](clock#install)ed before the function is called and [`uninstall`](clock#uninstall)ed in a `finally` after the function completes.
##### Parameters:
| Name | Type | Description |
| --- | --- | --- |
| `closure` | function | The function to be called. |
Since: * 2.3.0
| programming_docs |
jasmine Interface: Spec Interface: Spec
===============
Since: * 2.0.0
See: * [Configuration#specFilter](configuration#specFilter)
### Members
####
(readonly) description :string
The description passed to the [`it`](global#it) that created this spec.
##### Type:
* string
Since: * 2.0.0
####
(readonly) description :string
The description passed to the [`it`](global#it) that created this spec.
##### Type:
* string
####
(readonly) id :string
The unique ID of this spec.
##### Type:
* string
Since: * 2.0.0
####
(readonly) id :string
The unique ID of this spec.
##### Type:
* string
### Methods
####
getFullName() → {string}
The full description including all ancestors of this spec.
Since: * 2.0.0
##### Returns:
Type string ####
getFullName() → {string}
The full description including all ancestors of this spec.
##### Returns:
Type string See: * [Configuration#specFilter](configuration#specFilter)
### Members
####
(readonly) description :string
The description passed to the [`it`](global#it) that created this spec.
##### Type:
* string
Since: * 2.0.0
####
(readonly) description :string
The description passed to the [`it`](global#it) that created this spec.
##### Type:
* string
####
(readonly) id :string
The unique ID of this spec.
##### Type:
* string
Since: * 2.0.0
####
(readonly) id :string
The unique ID of this spec.
##### Type:
* string
### Methods
####
getFullName() → {string}
The full description including all ancestors of this spec.
Since: * 2.0.0
##### Returns:
Type string ####
getFullName() → {string}
The full description including all ancestors of this spec.
##### Returns:
Type string
npm About private packages About private packages
======================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Next steps](#next-steps)
* [Resources](#resources)
To use private packages, you must
* be using npm version 2.7.0 or greater. To upgrade, on the command line, run
```
npm install npm@latest -g
```
* have a [paid user or organization account](https://www.npmjs.com/pricing)
With npm private packages, you can use the npm registry to host code that is only visible to you and chosen collaborators, allowing you to manage and use private code alongside public code in your projects.
Private packages always have a scope, and scoped packages are private by default.
* **User-scoped private packages** can only be accessed by you and collaborators to whom you have granted read or read/write access. For more information, see "[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)".
* **Organization-scoped private packages** can only be accessed by teams that have been granted read or read/write access. For more information, see "[Managing team access to organization packages](managing-team-access-to-organization-packages)".
Next steps
-----------
* "[Creating and publishing private packages](creating-and-publishing-private-packages)"
* "[Using npm packages in your projects](using-npm-packages-in-your-projects)"
Resources
----------
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/about-private-packages.mdx)
npm Creating and publishing unscoped public packages Creating and publishing unscoped public packages
================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating an unscoped public package](#creating-an-unscoped-public-package)
* [Reviewing package contents for sensitive or unnecessary information](#reviewing-package-contents-for-sensitive-or-unnecessary-information)
* [Testing your package](#testing-your-package)
* [Publishing unscoped public packages](#publishing-unscoped-public-packages)
As an npm user, you can create unscoped packages to use in your own projects and publish them to the npm public registry for others to use in theirs. Unscoped packages are always public and are referred to by the package name only:
```
package-name
```
For more information on package scope, access, and visibility, see "[Package scope, access level, and visibility](package-scope-access-level-and-visibility)".
**Note:** Before you can publish public unscoped npm packages, you must [sign up](https://www.npmjs.com/signup) for an npm user account.
Creating an unscoped public package
------------------------------------
1. On the command line, create a directory for your package:
```
mkdir my-test-package
```
2. Navigate to the root directory of your package:
```
cd my-test-package
```
3. If you are using git to manage your package code, in the package root directory, run the following commands, replacing `git-remote-url` with the git remote URL for your package:
```
git init
git remote add origin git://git-remote-url
```
4. In the package root directory, run the `npm init` command.
5. Respond to the prompts to generate a [`package.json`](creating-a-package-json-file) file. For help naming your package, see "[Package name guidelines](package-name-guidelines)".
6. Create a [README file](about-package-readme-files) that explains what your package code is and how to use it.
7. In your preferred text editor, write the code for your package.
Reviewing package contents for sensitive or unnecessary information
--------------------------------------------------------------------
Publishing sensitive information to the registry can harm your users, compromise your development infrastructure, be expensive to fix, and put you at risk of legal action. **We strongly recommend removing sensitive information, such as private keys, passwords, [personally identifiable information](https://en.wikipedia.org/wiki/Personally_identifiable_information) (PII), and credit card data before publishing your package to the registry.**
For less sensitive information, such as testing data, use a `.npmignore` or `.gitignore` file to prevent publishing to the registry. For more information, see [this article](https://docs.npmjs.com/cli/v8/using-npm/developers//#keeping-files-out-of-your-package).
Testing your package
---------------------
To reduce the chances of publishing bugs, we recommend testing your package before publishing it to the npm registry. To test your package, run `npm install` with the full path to your package directory:
```
npm install path/to/my-package
```
Publishing unscoped public packages
------------------------------------
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. To publish your public package to the npm registry, run:
```
npm publish
```
3. To see your public package page, visit [https://npmjs.com/package/\*package-name](https://npmjs.com/package/*package-name)*, replacing* package-name\* with the name of your package. Public packages will say `public` below the package name on the npm website.
For more information on the `publish` command, see the [CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-publish/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/creating-and-publishing-unscoped-public-packages.mdx)
npm Creating a package.json file Creating a package.json file
============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [package.json fields](#packagejson-fields)
+ [Required name and version fields](#required-name-and-version-fields)
+ [Author field](#author-field)
+ [Example](#example)
* [Creating a new package.json file](#creating-a-new-packagejson-file)
+ [Running a CLI questionnaire](#running-a-cli-questionnaire)
- [Customizing the package.json questionnaire](#customizing-the-packagejson-questionnaire)
+ [Creating a default package.json file](#creating-a-default-packagejson-file)
- [Example](#example-1)
- [Default values extracted from the current directory](#default-values-extracted-from-the-current-directory)
+ [Setting config options for the init command](#setting-config-options-for-the-init-command)
You can add a `package.json` file to your package to make it easy for others to manage and install. Packages published to the registry must contain a `package.json` file.
A `package.json` file:
* lists the packages your project depends on
* specifies versions of a package that your project can use using [semantic versioning rules](about-semantic-versioning)
* makes your build reproducible, and therefore easier to share with other developers
**Note:** To make your package easier to find on the npm website, we recommend including a custom `description` in your `package.json` file.
`package.json` fields
----------------------
###
Required `name` and `version` fields
A `package.json` file must contain `"name"` and `"version"` fields.
The `"name"` field contains your package's name, and must be lowercase and one word, and may contain hyphens and underscores.
The `"version"` field must be in the form `x.x.x` and follow the [semantic versioning guidelines](about-semantic-versioning).
###
Author field
If you want to include package author information in `"author"` field, use the following format (email and website are both optional):
```
Your Name <[email protected]> (http://example.com)
```
###
Example
```
{
"name": "my-awesome-package",
"version": "1.0.0",
"author": "Your Name <[email protected]>"
}
```
Creating a new `package.json` file
-----------------------------------
You can create a `package.json` file by running a CLI questionnaire or creating a default `package.json` file.
###
Running a CLI questionnaire
To create a `package.json` file with values that you supply, use the `npm init` command.
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. Run the following command:
```
npm init
```
3. Answer the questions in the command line questionnaire.
####
Customizing the `package.json` questionnaire
If you expect to create many `package.json` files, you can customize the questions asked and fields created during the `init` process so all the `package.json` files contain a standard set of information.
1. In your home directory, create a file called `.npm-init.js`.
2. To add custom questions, using a text editor, add questions with the `prompt` function:
```
module.exports = prompt("what's your favorite flavor of ice cream, buddy?", "I LIKE THEM ALL");
```
3. To add custom fields, using a text editor, add desired fields to the `.npm-init.js` file:
```
module.exports = {
customField: 'Example custom field',
otherCustomField: 'This example field is really cool'
}
```
To learn more about creating advanced `npm init` customizations, see the [init-package-json](https://github.com/npm/init-package-json) GitHub repository.
###
Creating a default `package.json` file
To create a default `package.json` using information extracted from the current directory, use the `npm init` command with the `--yes` or `-y` flag. For a list of default values, see "[Default values extracted from the current directory](#default-values-extracted-from-the-current-directory)".
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. Run the following command:
```
npm init --yes
```
####
Example
```
> npm init --yes
Wrote to /home/monatheoctocat/my_package/package.json:
{
"name": "my_package",
"description": "",
"version": "1.0.0",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"repository": {
"type": "git",
"url": "https://github.com/monatheoctocat/my_package.git"
},
"keywords": [],
"author": "",
"license": "ISC",
"bugs": {
"url": "https://github.com/monatheoctocat/my_package/issues"
},
"homepage": "https://github.com/monatheoctocat/my_package"
}
```
####
Default values extracted from the current directory
* `name`: the current directory name
* `version`: always `1.0.0`
* `description`: info from the README, or an empty string `""`
* `scripts`: by default creates an empty `test` script
* `keywords`: empty
* `author`: empty
* `license`: [`ISC`](https://opensource.org/licenses/ISC)
* `bugs`: information from the current directory, if present
* `homepage`: information from the current directory, if present
###
Setting config options for the init command
You can set default config options for the init command. For example, to set the default author email, author name, and license, on the command line, run the following commands:
```
> npm set init-author-email "[email protected]"
> npm set init-author-name "example_user"
> npm set init-license "MIT"
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/creating-a-package-json-file.mdx)
npm Creating and publishing an organization scoped package Creating and publishing an organization scoped package
======================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[About organization scopes and packages](about-organization-scopes-and-packages)[Configuring your npm client with your organization settings](configuring-your-npm-client-with-your-organization-settings)[Creating and publishing an organization scoped package](creating-and-publishing-an-organization-scoped-package)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating an organization scoped package](#creating-an-organization-scoped-package)
* [Publishing a private organization scoped package](#publishing-a-private-organization-scoped-package)
* [Publishing a public organization scoped package](#publishing-a-public-organization-scoped-package)
As an organization member, you can create and publish public and private packages within the organization's scope.
Creating an organization scoped package
----------------------------------------
1. On the command line, make a directory with the name of the package you would like to create.
```
mkdir /path/to/package/directory
```
2. Navigate to the newly-created package directory.
3. To create an organization scoped package, on the command line, run:
```
npm init --scope=<your_org_name>
```
4. To verify the package is using your organization scope, in a text editor, open the package's `package.json` file and check that the name is `@your_org_name/<pkg_name>`, replacing `your_org_name` with the name of your organization.
Publishing a private organization scoped package
-------------------------------------------------
By default, `npm publish` will publish a scoped package as private.
By default, any scoped package is published as private. However, if you have an organization that does not have the Private Packages feature, `npm publish` will fail unless you pass the `access` flag.
1. On the command line, navigate to the package directory.
2. Run `npm publish`.
Private packages will say `private` below the package name on the npm website.
Publishing a public organization scoped package
------------------------------------------------
To publish an organization scoped package as public, use `npm publish --access public`.
1. On the command line, navigate to the package directory.
2. Run `npm publish --access public`.
Public packages will say `public` below the package name on the npm website.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-packages/creating-and-publishing-an-organization-scoped-package.mdx)
| programming_docs |
npm Removing members from your organization Removing members from your organization
=======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Adding members to your organization](adding-members-to-your-organization)[Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](organization-roles-and-permissions)[Managing organization permissions](managing-organization-permissions)[Removing members from your organization](removing-members-from-your-organization)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
As an organization owner, you can remove members from your organization if they are longer collaborating on packages owned or governed by your organization.
If you remove a member from an npm Teams subscription (a paid organization), then they will lose access to your organization's private packages, and the credit card on file for your organization will not be charged for them on the next billing cycle.
**Note:** Members are not notified when you remove them from your organization.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Members**.
5. In the list of organization members, find the member you want to remove.
6. At the end of the member row, click **X**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/removing-members-from-your-organization.mdx)
npm About audit reports About audit reports
===================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [About audit reports](#about-audit-reports)
+ [Vulnerability table fields](#vulnerability-table-fields)
- [Severity](#severity)
* [Description](#description)
* [Package](#package)
* [Patched in](#patched-in)
* [Dependency of](#dependency-of)
* [Path](#path)
* [More info](#more-info)
About audit reports
====================
Audit reports contain tables of information about security vulnerabilities in your project's dependencies to help you fix the vulnerability or troubleshoot further.
Vulnerability table fields
---------------------------
* [Severity](#severity)
* [Description](#description)
* [Package](#package)
* [Patched in](#patched-in)
* [Dependency of](#dependency-of)
* [Path](#path)
* [More info](#more-info)
###
Severity
The severity of the vulnerability, determined by the impact and exploitability of the vulnerability in its most common use case.
| Severity | Recommended action |
| --- | --- |
| Critical | Address immediately |
| High | Address as quickly as possible |
| Moderate | Address as time allows |
| Low | Address at your discretion |
####
Description
The description of the vulnerability. For example, "Denial of service".
####
Package
The name of the package that contains the vulnerability.
####
Patched in
The semantic version range that describes which versions contain a fix for the vulnerability.
####
Dependency of
The module that the package with the vulnerability depends on.
####
Path
The path to the code that contains the vulnerability.
####
More info
A link to the security report.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/about-audit-reports.mdx)
npm Managing your profile settings Managing your profile settings
==============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Managing your profile settings](managing-your-profile-settings)[Changing your npm username](changing-your-npm-username)[Deleting your npm user account](deleting-your-npm-user-account)[Requesting an export of your personal data](requesting-your-data)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Managing user account profile settings from the web](#managing-user-account-profile-settings-from-the-web)
+ [Linking your npm and GitHub accounts](#linking-your-npm-and-github-accounts)
+ [Linking your npm and Twitter accounts](#linking-your-npm-and-twitter-accounts)
+ [Disconnecting your GitHub account from npm](#disconnecting-your-github-account-from-npm)
+ [Disconnecting your Twitter account from npm](#disconnecting-your-twitter-account-from-npm)
* [Managing user account profile settings from the command line](#managing-user-account-profile-settings-from-the-command-line)
+ [Viewing user account profile settings from the command line](#viewing-user-account-profile-settings-from-the-command-line)
+ [Updating user account profile settings from the command line](#updating-user-account-profile-settings-from-the-command-line)
- [Setting a password from the command line](#setting-a-password-from-the-command-line)
- [Configuring two-factor authentication from the command line](#configuring-two-factor-authentication-from-the-command-line)
You can manage settings for your user account profile from the web or command line.
Managing user account profile settings from the web
----------------------------------------------------
From the web, you can change the following user profile settings:
* Avatar
* Password
* Full name
* Link GitHub Account
* Link Twitter Account
* Email address added to package metadata
* Two-factor authentication status
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
###
Linking your npm and GitHub accounts
1. On the account settings page, you will find a button to link your GitHub account. Click that.
2. If you are not currently logged in to GitHub you will be prompted to go through the authentication flow.
3. After successfully logging in, or if you already had an active browser sessions, you will be prompted to "authorize npm account link", click the button.
4. You will be redirected to npm and the link will show as successful in your settings.
###
Linking your npm and Twitter accounts
1. On the account settings page, you will find a button to link your Twitter account. Click that.
2. If you are not currently logged in to Twitter you will be prompted to go through the authentication flow. Click "Log in"
3. After successfully logging in, or if you already had an active browser sessions, you will be prompted to "Authorize app", click the button.
4. You will be redirected to npm and the link will show as successful in your settings.
###
Disconnecting your GitHub account from npm
1. On the account settings page, you will find a button to disconnect your GitHub account. Click that.
*Note: Clicking disconnect will only disconnect the link from your npm account. You need to `revoke` permissions from your [GitHub app authorization settings](https://github.com/settings/apps/authorizations) to permanently remove the integration from your GitHub account*
###
Disconnecting your Twitter account from npm
1. On the account settings page, you will find a button to disconnect your GitHub account. Click that.
*Note: Clicking disconnect will only disconnect the link from your npm account. You need to `revoke` permissions from your [Twitter connect apps management page](https://twitter.com/settings/connected_apps) to permanently remove the integration from your Twitter account*
Managing user account profile settings from the command line
-------------------------------------------------------------
**Note:** Your npm client must be version 5.5.1 or higher to change your account settings from the CLI. To update to the latest version of npm, on the command line, run `npm install npm@latest -g`
###
Viewing user account profile settings from the command line
To view your user profile settings from the CLI, on the command line, run the following command:
```
npm profile get
```
###
Updating user account profile settings from the command line
From the CLI, you can change the following properties for your user account:
* `email`
* `two-factor auth`
* `fullname`
* `homepage`
* `freenode`
* `password`
1. On the command line, type the following command, replacing `property` with the name of the property, and `value` with the new value:
```
npm profile set <prop> <value>
```
2. When prompted, provide your current password.
3. If you have enabled two-factor authentication on your account, when prompted, enter a one-time password.
For more details, see the `profile` [command line documentation](https://docs.npmjs.com/cli/v8/commands/npm-profile/).
####
Setting a password from the command line
1. On the command line, type the following command:
```
npm profile set password
```
2. When prompted, provide your current password.
3. When prompted, type a new password.
To protect your account, when you reset your password from the command line, it must:
* be longer than 10 characters
* not contain part of your username
* not be in the "[Have I Been Pwned](https://haveibeenpwned.com/)" breach database
####
Configuring two-factor authentication from the command line
Enabling two-factor authentication on your account helps protect against unauthorized access to your account and packages.
To enable, configure, and disable two-factor authentication from the command line, see "[Configuring two-factor authentication](configuring-two-factor-authentication#configuring-2fa-from-the-command-line)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/managing-your-npm-user-account/managing-your-profile-settings.mdx)
npm Adding collaborators to private packages owned by a user account Adding collaborators to private packages owned by a user account
================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Granting access to a private user package on the web](#granting-access-to-a-private-user-package-on-the-web)
* [Granting private package access from the command line interface](#granting-private-package-access-from-the-command-line-interface)
* [Granting access to private organization packages](#granting-access-to-private-organization-packages)
As an npm user with a paid user account, you can add another npm user with a paid account as a collaborator on a private package you own.
**Note:** The user you want to add as a collaborator on your private package must have a paid user account. To sign up for a paid account, they can visit `https://www.npmjs.com/settings/username/billing`, replacing `username` with their npm username.
When you add a member to your package, they are sent an email inviting them to the package. The new member has to accept the invitation gain access to the package.
Granting access to a private user package on the web
-----------------------------------------------------
1. On the [npm website](https://npmjs.com), go to the package to which you want to add a collaborator: `https://www.npmjs.com/package/<your-package-name>`
2. On the package page, under "Collaborators", click **+**.
3. Enter the npm username of the collaborator.
4. Click **Submit**.
Granting private package access from the command line interface
----------------------------------------------------------------
To add a collaborator to a package on the command line, run the following command, replacing `<user>` with the npm username of your collaborator, and `<your-package-name>` with the name of the private package:
```
npm owner add <user> <your-package-name>
```
Granting access to private organization packages
-------------------------------------------------
To grant an npm user access to private organization packages, you must have an organization owner add them to your organization, then add them to a team that has access to the private package. For more information, see "[Adding members to your organization](adding-members-to-your-organization)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/adding-collaborators-to-private-packages-owned-by-a-user-account.mdx)
npm About organization scopes and packages About organization scopes and packages
======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[About organization scopes and packages](about-organization-scopes-and-packages)[Configuring your npm client with your organization settings](configuring-your-npm-client-with-your-organization-settings)[Creating and publishing an organization scoped package](creating-and-publishing-an-organization-scoped-package)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Managing unscoped packages](#managing-unscoped-packages)
Every organization is granted an organization scope, a unique namespace for packages owned by the organization that matches the organization name. For example, an organization named "wombat" would have the scope `@wombat`.
You can use scopes to:
* Maintain a fork of a package: `@wombat/request`.
* Avoid name disputes with popular names: `@wombat/web`.
* Easily find packages in the same namespace
Packages in a scope must follow the same [naming guidelines](https://docs.npmjs.com/cli/v8/configuring-npm/package-json/#name) as unscoped packages.
Managing unscoped packages
---------------------------
While you are granted a scope by default when you create an organization, you can also use organizations to manage unscoped packages, or packages under a different scope (such as a user scope).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-packages/about-organization-scopes-and-packages.mdx)
npm Resolving EACCES permissions errors when installing packages globally Resolving EACCES permissions errors when installing packages globally
=====================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Reinstall npm with a node version manager](#reinstall-npm-with-a-node-version-manager)
* [Manually change npm's default directory](#manually-change-npms-default-directory)
If you see an `EACCES` error when you try to [install a package globally](downloading-and-installing-packages-globally), you can either:
* Reinstall npm with a node version manager (recommended),
**or**
* Manually change npm's default directory
Reinstall npm with a node version manager
------------------------------------------
This is the best way to avoid permissions issues. To reinstall npm with a node version manager, follow the steps in "[Downloading and installing Node.js and npm](downloading-and-installing-node-js-and-npm)". You do not need to remove your current version of npm or Node.js before installing a node version manager.
Manually change npm's default directory
----------------------------------------
**Note:** This section does not apply to Microsoft Windows.
To minimize the chance of permissions errors, you can configure npm to use a different directory. In this example, you will create and use hidden directory in your home directory.
1. Back up your computer.
2. On the command line, in your home directory, create a directory for global installations:
```
mkdir ~/.npm-global
```
3. Configure npm to use the new directory path:
```
npm config set prefix '~/.npm-global'
```
4. In your preferred text editor, open or create a `~/.profile` file and add this line:
```
export PATH=~/.npm-global/bin:$PATH
```
5. On the command line, update your system variables:
```
source ~/.profile
```
6. To test your new configuration, install a package globally without using `sudo`:
```
npm install -g jshint
```
Instead of steps 3-5, you can use the corresponding ENV variable (e.g. if you don't want to modify `~/.profile`):
```
NPM_CONFIG_PREFIX=~/.npm-global
```
**npx: an alternative to running global commands**
If you are using npm version 5.2 or greater, you may want to consider [npx](https://www.npmjs.com/package/npx) as an alternative way to run global commands, especially if you only need a command occasionally. For more information, see [this article about npx](https://medium.com/@maybekatz/introducing-npx-an-npm-package-runner-55f7d4bd282b).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/resolving-eacces-permissions-errors-when-installing-packages-globally.mdx)
| programming_docs |
npm Requesting an export of your personal data Requesting an export of your personal data
==========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Managing your profile settings](managing-your-profile-settings)[Changing your npm username](changing-your-npm-username)[Deleting your npm user account](deleting-your-npm-user-account)[Requesting an export of your personal data](requesting-your-data)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [How to request an export](#how-to-request-an-export)
* [Retrieving the exported data](#retrieving-the-exported-data)
You can export and review the metadata that npm stores about your personal account. The export is an archive containing the following information.
1. Your personal details such as username, email address, full name, linked Twitter / GitHub accounts, masked Personal Access Tokens (PAT) and the organisations that you are a member of.
2. Metadata of all the packages that you have access to.
3. Each individual version of packages that you have published to npm.
How to request an export
-------------------------
1. Navigate to [npm support form](https://www.npmjs.com/support)
2. Select "Account and Billing issues" category
3. Select "Data export request" sub-category
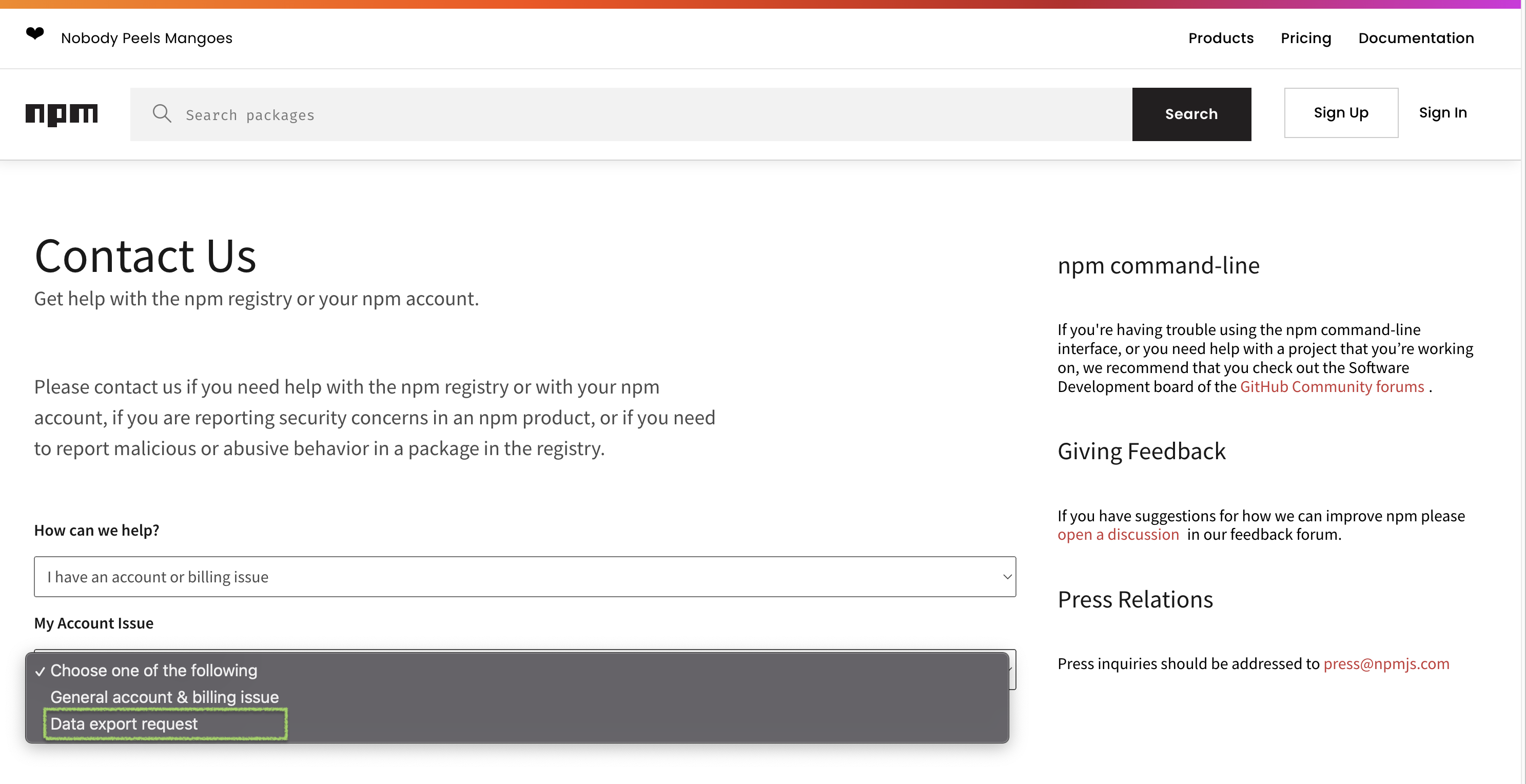
4. Fill in the details and submit the form
Retrieving the exported data
-----------------------------
After a request is placed our support team will review it and initiate an export on your behalf. Once the export process is complete you will receive an email with a link to an archive of your personal data. You must be authenticated to npmjs.com to download this archive.
The download link will be available for 7 days, after which the exported data and the link is purged.
npm Try the latest stable version of node Try the latest stable version of node
=====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Generating and locating npm-debug.log files](generating-and-locating-npm-debug.log-files)[Common errors](common-errors)[Try the latest stable version of node](try-the-latest-stable-version-of-node)[Try the latest stable version of npm](try-the-latest-stable-version-of-npm)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [See what version of node you're running:](#see-what-version-of-node-youre-running)
+ [Updating node on Linux](#updating-node-on-linux)
+ [Updating node on Windows](#updating-node-on-windows)
+ [Updating node on OSX](#updating-node-on-osx)
+ [An easy way to stay up-to-date](#an-easy-way-to-stay-up-to-date)
If you're experiencing issues while using a version of node which is unsupported or unstable (odd numbered versions e.g. 0.7.x, 0.9.x, 0.11.x), it's very possible your issue will be fixed by simply using the [LTS](https://github.com/nodejs/LTS) version of node.
See what version of node you're running:
-----------------------------------------
```
node -v
```
###
Updating node on Linux
For some Linux distributions (Debian/Ubuntu and RedHat/CentOS), the latest node version provided by the distribution may lag behind the stable version. Here are [instructions from NodeSource](https://github.com/nodesource/distributions) on getting the latest node.
###
Updating node on Windows
Install the latest msi from <https://nodejs.org/en/download>
###
Updating node on OSX
Install the latest package from <https://nodejs.org/en/download>
or if you are using [homebrew](http://brew.sh/)
```
brew install node
```
###
An easy way to stay up-to-date
Node.js has lots of versions, and its development is very active. As a good practice to manage the various versions, we recommend that you use a version manager for your Node.js installation. There are many great options, here are a few:
* [NVM](https://github.com/creationix/nvm)
* [nodist](https://github.com/marcelklehr/nodist)
* [n](https://github.com/tj/n)
* [nave](https://github.com/isaacs/nave)
* [nodebrew](https://github.com/hokaccha/nodebrew)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/troubleshooting/try-the-latest-stable-version-of-node.mdx)
npm Updating your published package version number Updating your published package version number
==============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
When you make significant changes to a published package, we recommend updating the version number to communicate the extent of the changes to others who rely on your code.
**Note:** If you have linked a git repository to a package, updating the package version number will also add a tag with the updated release number to the linked git repository.
1. To change the version number in `package.json`, on the command line, in the package root directory, run the following command, replacing `<update_type>` with one of the [semantic versioning](about-semantic-versioning) release types (patch, major, or minor):
```
npm version <update_type>
```
2. Run `npm publish`.
3. Go to your package page (`https://npmjs.com/package/<package>`) to check that the package version has been updated.
For more information on `npm version`, see the [CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-version/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/updating-your-published-package-version-number.mdx)
npm Downloading and installing Node.js and npm Downloading and installing Node.js and npm
==========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[About npm CLI versions](about-npm-versions)[Downloading and installing Node.js and npm](downloading-and-installing-node-js-and-npm)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Overview](#overview)
* [Checking your version of npm and Node.js](#checking-your-version-of-npm-and-nodejs)
* [Using a Node version manager to install Node.js and npm](#using-a-node-version-manager-to-install-nodejs-and-npm)
+ [OSX or Linux Node version managers](#osx-or-linux-node-version-managers)
+ [Windows Node version managers](#windows-node-version-managers)
* [Using a Node installer to install Node.js and npm](#using-a-node-installer-to-install-nodejs-and-npm)
+ [OS X or Windows Node installers](#os-x-or-windows-node-installers)
+ [Linux or other operating systems Node installers](#linux-or-other-operating-systems-node-installers)
+ [Less-common operating systems](#less-common-operating-systems)
To publish and install packages to and from the public npm registry or a private npm registry, you must install Node.js and the npm command line interface using either a Node version manager or a Node installer. **We strongly recommend using a Node version manager like [nvm](https://github.com/nvm-sh/nvm) to install Node.js and npm.** We do not recommend using a Node installer, since the Node installation process installs npm in a directory with local permissions and can cause permissions errors when you run npm packages globally.
**Note:** to download the latest version of npm, on the command line, run the following command:
```
npm install -g npm
```
Overview
---------
* [Checking your version of npm and Node.js](#checking-your-version-of-npm-and-node-js)
* [Using a Node version manager to install Node.js and npm](#using-a-node-version-manager-to-install-node-js-and-npm)
* [Using a Node installer to install Node.js and npm](#using-a-node-installer-to-install-node-js-and-npm)
Checking your version of npm and Node.js
-----------------------------------------
To see if you already have Node.js and npm installed and check the installed version, run the following commands:
```
node -v
npm -v
```
Using a Node version manager to install Node.js and npm
--------------------------------------------------------
Node version managers allow you to install and switch between multiple versions of Node.js and npm on your system so you can test your applications on multiple versions of npm to ensure they work for users on different versions.
###
OSX or Linux Node version managers
* [nvm](https://github.com/creationix/nvm)
* [n](https://github.com/tj/n)
###
Windows Node version managers
* [nodist](https://github.com/marcelklehr/nodist)
* [nvm-windows](https://github.com/coreybutler/nvm-windows)
Using a Node installer to install Node.js and npm
--------------------------------------------------
If you are unable to use a Node version manager, you can use a Node installer to install both Node.js and npm on your system.
* [Node.js installer](https://nodejs.org/en/download/)
* [NodeSource installer](https://github.com/nodesource/distributions)
If you use Linux, we recommend that you use a NodeSource installer.
###
OS X or Windows Node installers
If you're using OS X or Windows, use one of the installers from the [Node.js download page](https://nodejs.org/en/download/). Be sure to install the version labeled **LTS**. Other versions have not yet been tested with npm.
###
Linux or other operating systems Node installers
If you're using Linux or another operating system, use one of the following installers:
* [NodeSource installer](https://github.com/nodesource/distributions) (recommended)
* One of the installers on the [Node.js download page](https://nodejs.org/en/download/)
Or see [this page](https://nodejs.org/en/download/package-manager/) to install npm for Linux in the way many Linux developers prefer.
###
Less-common operating systems
For more information on installing Node.js on a variety of operating systems, see [this page](https://nodejs.org/en/download/package-manager/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/configuring-your-local-environment/downloading-and-installing-node-js-and-npm.mdx)
npm Specifying dependencies and devDependencies in a package.json file Specifying dependencies and devDependencies in a package.json file
==================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Adding dependencies to a package.json file](#adding-dependencies-to-a-packagejson-file)
+ [Adding dependencies to a package.json file from the command line](#adding-dependencies-to-a-packagejson-file-from-the-command-line)
+ [Manually editing the package.json file](#manually-editing-the-packagejson-file)
To specify the packages your project depends on, you must list them as `"dependencies"` or `"devDependencies"` in your package's [`package.json`](https://docs.npmjs.com/cli/v8/configuring-npm/package-json/) file. When you (or another user) run `npm install`, npm will download dependencies and devDependencies that are listed in `package.json` that meet the [semantic version](about-semantic-versioning) requirements listed for each. To see which versions of a package will be installed, use the [semver calculator](https://semver.npmjs.com/).
* `"dependencies"`: Packages required by your application in production.
* `"devDependencies"`: Packages that are only needed for local development and testing.
Adding dependencies to a `package.json` file
---------------------------------------------
You can add dependencies to a `package.json` file from the command line or by manually editing the `package.json` file.
###
Adding dependencies to a `package.json` file from the command line
To add dependencies and devDependencies to a `package.json` file from the command line, you can install them in the root directory of your package using the `--save-prod` flag for dependencies (the default behavior of `npm install`) or the `--save-dev` flag for devDependencies.
To add an entry to the `"dependencies"` attribute of a `package.json` file, on the command line, run the following command:
```
npm install <package-name> [--save-prod]
```
To add an entry to the `"devDependencies"` attribute of a `package.json` file, on the command line, run the following command:
```
npm install <package-name> --save-dev
```
###
Manually editing the `package.json` file
To add dependencies to a `package.json` file, in a text editor, add an attribute called `"dependencies"` that references the name and [semantic version](about-semantic-versioning) of each dependency:
```
{
"name": "my_package",
"version": "1.0.0",
"dependencies": {
"my_dep": "^1.0.0",
"another_dep": "~2.2.0"
}
}
```
To add devDependencies to a `package.json` file, in a text editor, add an attribute called `"devDependencies"` that references the name and [semantic version](about-semantic-versioning) of each devDependency:
```
"name": "my_package",
"version": "1.0.0",
"dependencies": {
"my_dep": "^1.0.0",
"another_dep": "~2.2.0"
},
"devDependencies" : {
"my_test_framework": "^3.1.0",
"another_dev_dep": "1.0.0 - 1.2.0"
}
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/specifying-dependencies-and-devdependencies-in-a-package-json-file.mdx)
npm Using private packages in a CI/CD workflow Using private packages in a CI/CD workflow
==========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[About access tokens](about-access-tokens)[Creating and viewing access tokens](creating-and-viewing-access-tokens)[Revoking access tokens](revoking-access-tokens)[Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](docker-and-private-modules)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Create a new access token](#create-a-new-access-token)
+ [Continuous integration](#continuous-integration)
+ [Continuous deployment](#continuous-deployment)
+ [Interactive workflows](#interactive-workflows)
+ [CIDR whitelists](#cidr-whitelists)
* [Set the token as an environment variable on the CI/CD server](#set-the-token-as-an-environment-variable-on-the-cicd-server)
* [Create and check in a project-specific .npmrc file](#create-and-check-in-a-project-specific-npmrc-file)
* [Securing your token](#securing-your-token)
You can use access tokens to test private npm packages with continuous integration (CI) systems, or deploy them using continuous deployment (CD) systems.
Create a new access token
--------------------------
Create a new access token that will be used only to access npm packages from a CI/CD server.
###
Continuous integration
By default, `npm token create` will generate a token with both read and write permissions. When generating a token for use in a continuous integration environment, we recommend creating a read-only token:
```
npm token create --read-only
```
For more information on creating access tokens, including CIDR-whitelisted tokens, see "[Creating an access token](creating-and-viewing-access-tokens)".
###
Continuous deployment
Since continuous deployment environments usually involve the creation of a deploy artifact, you may wish to create an [automation token](creating-and-viewing-access-tokens) on the website. This will allow you to publish even if you have two-factor authentication enabled on your account.
###
Interactive workflows
If your workflow produces a package, but you publish it manually after validation, then you will want to create a token with read and write permissions, which are granted with the standard token creation command:
```
npm token create
```
###
CIDR whitelists
For increased security, you may use a CIDR-whitelisted token that can only be used from a certain IP address range. You can use a CIDR whitelist with a read and publish token or a read-only token:
```
npm token create --cidr=[list]
npm token create --read-only --cidr=[list]
```
Example:
```
npm token create --cidr=192.0.2.0/24
```
For more information, see "[Creating and viewing authentication tokens](creating-and-viewing-access-tokens)".
Set the token as an environment variable on the CI/CD server
-------------------------------------------------------------
Set your token as an environment variable, or a secret, in your CI/CD server.
For example, in GitHub Actions, you would [add your token as a secret](https://docs.github.com/en/actions/configuring-and-managing-workflows/creating-and-storing-encrypted-secrets). Then you can make the secret available to workflows.
If you named the secret `NPM_TOKEN`, then you would want to create an environment variable named `NPM_TOKEN` from that secret.
```
steps:
- run: |
npm install
- env:
NPM_TOKEN: ${{ secrets.NPM_TOKEN }}
```
Consult your CI/CD server's documentation for more details.
Create and check in a project-specific .npmrc file
---------------------------------------------------
Use a project-specific `.npmrc` file with a variable for your token to securely authenticate your CI/CD server with npm.
1. In the root directory of your project, create a custom `.npmrc` file with the following contents:
```
//registry.npmjs.org/:_authToken=${NPM_TOKEN}
```
**Note:** that you are specifying a literal value of `${NPM_TOKEN}`. The npm cli will replace this value with the contents of the `NPM_TOKEN` environment variable. Do **not** put a token in this file.
2. Check in the `.npmrc` file.
Securing your token
--------------------
Your token may have permission to read private packages, publish new packages on your behalf, or change user or package settings. Protect your token.
Do not add your token to version control or store it insecurely. Store it in a password manager, your cloud provider's secure storage, or your CI/CD provider's secure storage.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/using-private-packages-in-a-ci-cd-workflow.mdx)
| programming_docs |
npm Deprecating and undeprecating packages or package versions Deprecating and undeprecating packages or package versions
==========================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Deprecating an entire package](#deprecating-an-entire-package)
+ [Using the website](#using-the-website)
+ [Using the command line](#using-the-command-line)
* [Deprecating a single version of a package](#deprecating-a-single-version-of-a-package)
+ [Using the command line](#using-the-command-line-1)
* [Undeprecating a package or version](#undeprecating-a-package-or-version)
* [Transferring a deprecated package to npm](#transferring-a-deprecated-package-to-npm)
If you no longer wish to maintain a package, or if you would like to encourage users to update to a new or different version, you can [deprecate](https://docs.npmjs.com/cli/v8/commands/npm-deprecate/) it. Deprecating a package or version will print a message to the terminal when a user installs it.
A deprecation warning or message can say anything. You may wish to include a message encouraging users to update to a specific version, or an alternate, supported package.
**Note:** We strongly recommend deprecating packages or package versions instead of [unpublishing](unpublishing-packages-from-the-registry) them, because unpublishing removes a package from the registry entirely, meaning anyone who relied on it will no longer be able to use it, with no warning.
Deprecating an entire package
------------------------------
Deprecating an entire package will remove it from search results on the npm website and a deprecation message will also be displayed on the package page.
Deprecating a package is an alternative to deleting a package if your package does not meet the [unpublishing requirements](https://docs.npmjs.com/policies/unpublish).
###
Using the website
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. Navigate to the package page for the package you want to deprecate, replacing `<your-package-name>` with the name of your package: `https://www.npmjs.com/package/<your-package-name>`.
3. Click **Settings**.
4. Under "deprecate package", click **Deprecate package**.
5. If you are sure that you want to continue, enter your package name and click **Deprecate package**.
###
Using the command line
To deprecate an entire package, run the following command, replacing `<package-name>` with the name of your package, and `"<message>"` with your deprecation message:
```
npm deprecate <package-name> "<message>"
```
If you have enabled [two-factor authentication](about-two-factor-authentication), add a one-time password to the command, `--otp=123456` (where *123456* is the code from your authenticator app).
Deprecating a single version of a package
------------------------------------------
When you deprecate a version of a package, a red message will be displayed on that version's package page, similar to deprecating an entire package.
###
Using the command line
To deprecate a package version, run the following command, replacing `<package-name>` with the name of your package, `<version>` with your version number, and `"<message>"` with your deprecation message:
```
npm deprecate <package-name>@<version> "<message>"
```
The CLI will also accept version ranges for `<version>`.
If you have two-factor auth, add a one-time password to the command, `--otp=123456` (where *123456* is the code from your authenticator).
Undeprecating a package or version
-----------------------------------
To undeprecate a package, replace `"<message>"` with `""` (an empty string) in one of the above commands.
For example, to undeprecate a package version, run the following command, replacing `<package-name>` with the name of your package, and `<version>` with your version number:
```
npm deprecate <package-name>@<version> ""
```
If you have two-factor auth, add a one-time password to the command, `--otp=123456` (where *123456* is the code from your authenticator).
Transferring a deprecated package to npm
-----------------------------------------
If you are no longer maintaining a package, but other users depend on it, and you'd like to remove it from your user profile, you can transfer it to the [`@npm`](https://www.npmjs.com/~npm) user account, which is owned by the npm registry.
**Note:** Once you transfer a package to the npm account, you will no longer be able to update it.
To transfer a package to the npm user account, run the following two commands in order, replacing `<user>` with your npm user name, and `<package-name>` with the package you want to transfer:
```
npm owner add npm <package-name>
npm owner rm <user> <package-name>
```
If you have two-factor auth, add a one-time password to the command, `--otp=123456` (where *123456* is the code from your authenticator).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/deprecating-and-undeprecating-packages-or-package-versions.mdx)
npm Configuring your npm client with your organization settings Configuring your npm client with your organization settings
===========================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[About organization scopes and packages](about-organization-scopes-and-packages)[Configuring your npm client with your organization settings](configuring-your-npm-client-with-your-organization-settings)[Creating and publishing an organization scoped package](creating-and-publishing-an-organization-scoped-package)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Configuring your npm client to use your organization's scope](#configuring-your-npm-client-to-use-your-organizations-scope)
+ [Setting your organization scope for all new packages](#setting-your-organization-scope-for-all-new-packages)
+ [Setting your organization scope for a single package](#setting-your-organization-scope-for-a-single-package)
* [Changing default package visibility to public](#changing-default-package-visibility-to-public)
+ [Setting package visibility to public for a single package](#setting-package-visibility-to-public-for-a-single-package)
+ [Setting package visibility to public for all packages](#setting-package-visibility-to-public-for-all-packages)
As an organization member, you can configure your npm client to:
* make a single package or all new packages you create locally use your organization's scope
* make a single package or all new packages you create locally have default public visibility
Before configuring your npm client, you must [install npm](downloading-and-installing-node-js-and-npm).
Configuring your npm client to use your organization's scope
-------------------------------------------------------------
If you will be publishing packages with your organization's scope often, you can add your organization's scope to your global `.npmrc` configuration file.
###
Setting your organization scope for all new packages
**Note:** Setting the organization scope using the steps below will only set the scope for new packages; for existing packages, you will need to update the `name` field in `package.json`.
On the command line, run the following command, replacing <org-name> with the name of your organization:
```
npm config set scope <org-name> --global
```
For packages you do not want to publish with your organization's scope, you must manually edit the package's `package.json` to remove the organization scope from the `name` field.
###
Setting your organization scope for a single package
1. On the command line, navigate to the package directory.
```
cd /path/to/package
```
2. Run the following command, replacing <org-name> with the name of your organization:
```
npm config set scope <org-name>
```
Changing default package visibility to public
----------------------------------------------
By default, publishing a scoped package with `npm publish` will publish the package as private. If you are a member of an organization on the free organization plan, or are on the paid organization plan but want to publish a scoped package as public, you must pass the `--access public` flag:
```
npm publish --access public
```
###
Setting package visibility to public for a single package
You can set a single package to pass `--access public` to every `npm publish` command that you issue for that package.
1. On the command line, navigate to the package directory.
```
cd /path/to/package
```
2. Run the following command:
```
npm config set access public
```
###
Setting package visibility to public for all packages
You can set all packages to pass `--access public` to every `npm publish` command that you issue for that package.
**Warning:** Setting packages access to `public` in your global `.npmrc` will affect all packages you create, including packages in your personal account scope, as well as packages scoped to your organization.
On the command line, run the following command:
```
npm config set access public --global
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-packages/configuring-your-npm-client-with-your-organization-settings.mdx)
npm Unpublishing packages from the registry Unpublishing packages from the registry
=======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Unpublishing a package](#unpublishing-a-package)
+ [Using the website](#using-the-website)
+ [Using the command line](#using-the-command-line)
* [Unpublishing a single version of a package](#unpublishing-a-single-version-of-a-package)
+ [Using the command line](#using-the-command-line-1)
* [When to unpublish](#when-to-unpublish)
* [When to deprecate](#when-to-deprecate)
As a package owner or collaborator, if your package has no dependents, you can permanently remove it from the npm registry by using the CLI. You can [unpublish](https://docs.npmjs.com/cli/v7/commands/npm-unpublish) within 72 hours of the initial publish; beyond 72 hours, you can still unpublish your package if [it meets certain criteria](https://www.npmjs.com/policies/unpublish).
These criteria are set to avoid damaging the JavaScript package ecosystem. If you cannot unpublish your package, you can [deprecate it instead](deprecating-and-undeprecating-packages-or-package-versions).
**Note:** Removing all the collaborators or teams from the package will not unpublish it.
Unpublishing a package
-----------------------
If you want to completely remove all versions of a package from the registry, you can unpublish it completely. This will delete it from the registry and it will be unable to be installed.
To unpublish a package, you must meet the requirements of the [package unpublishing rules](#how-to-unpublish).
###
Using the website
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. Navigate to the package page for the package you want to unpublish, replacing `<your-package-name>` with the name of your package: `https://www.npmjs.com/package/<your-package-name>`.
3. Click **Settings**.
4. Under "delete package", click **Delete package**.
**Note:** If you cannot delete the package because it does not meet the [unpublishing requirements](#how-to-unpublish), then the delete package option will not be available. Instead, you will be prompted to [deprecate the package](deprecating-and-undeprecating-packages-or-package-versions#deprecating-a-package-from-the-website).
5. If you are sure that you want to continue, enter your package name and click **Delete package**.
###
Using the command line
To unpublish an entire package, run the following command, replacing `<package-name>` with the name of your package:
```
npm unpublish <package-name> -f
```
If you have [two-factor authentication](about-two-factor-authentication) enabled for writes, you will need to add a one-time password to the `unpublish` command, `--otp=123456` (where *123456* is the code from your authenticator app).
If you need help unpublishing your package, please [contact npm Support](https://www.npmjs.com/support). If you are an Enterprise customer, please [contact Enterprise Support](mailto:[email protected]).**Note:** If you unpublish an entire package, you may not publish any new versions of that package until 24 hours have passed.
Unpublishing a single version of a package
-------------------------------------------
If you want to remove a single version of a package, you can unpublish one version without affecting the others. This will delete only that version from the registry and it will be unable to be installed. This option is only available via the npm CLI.
###
Using the command line
To unpublish a single version of a package, run the following command, replacing `<package-name>` with the name of your package, and `<version>` with your version number:
```
npm unpublish <package-name>@<version>
```
When to unpublish
------------------
Unpublishing a package permanently removes the package from the registry so it is no longer available for other users to install. Once a package is unpublished, republishing under the same name is blocked for 24 hours. If you've unpublished a package by mistake, we'd recommend publishing again under a different name, or for unpublished versions, bumping the version number and publishing again.
You might want to unpublish a package because you:
* Published something accidentally.
* Wanted to test npm.
* Published content you [didn't intend to be public](https://blog.npmjs.org/post/101934969510/oh-no-i-accidentally-published-private-data-to).
* Want to rename a package. (The only way to rename a package is to re-publish it under a new name)
**Note:** `package-name@version` is unique, and cannot be reused by unpublishing and re-publishing it. We recommend publishing a minor version update instead.
When to deprecate
------------------
If you are no longer interested in maintaining a package, but want it to remain available for users to install, or if your package has dependents, we'd recommend [deprecating](https://docs.npmjs.com/cli/v8/commands/npm-deprecate/) it. To learn about how to deprecate a package, see "[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/unpublishing-packages-from-the-registry.mdx)
npm Reporting malware in an npm package Reporting malware in an npm package
===================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [How npm Security handles malware](#how-npm-security-handles-malware)
* [Reporting malware](#reporting-malware)
If you find malware in an npm package (either yours or someone else's), you can report it to the npm Security team to help keep the Javascript ecosystem safe.
**Note:** Vulnerabilities in npm packages should be reported directly to the package maintainers. We strongly advise doing this privately. You can find contact information about package maintainers with `npm owner ls <package-name>`. If the source code is hosted on GitHub please refer to the repository's [Security Policy](https://docs.github.com/en/free-pro-team@latest/github/managing-security-vulnerabilities/adding-a-security-policy-to-your-repository#about-security-policies).
How npm Security handles malware
---------------------------------
Malware is a major concern for npm Security and we have removed hundreds of malicious packages from the registry. For every malware report we receive, npm Security takes the following actions:
1. Confirm validity of the report.
2. Remove the package from the registry.
3. Publish a security placeholder for the package.
4. Publish a security advisory alerting the community.
As part of our process we determine whether the user account who uploaded the package should be banned. We also cooperate with 3rd parties when applicable.
Reporting malware
------------------
1. Gather information about the malware.
2. On the package page, click **Report malware**.
3. On the malware report page, provide information about yourself and the malware:
* **Name:** Your name.
* **Email address:** An email address the npm Security team can use to contact you.
* **Package name:** The name of the package that contains the malware.
* **Package version:** The version of the package that contains the malware. Include all affected versions.
* **Description of the malware:** A brief description of the malware and its effects. Include references, commits, and/or code examples that would help our researchers confirm the report.
4. Click **Send Report**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/reporting-malware-in-an-npm-package.mdx)
| programming_docs |
npm Managing team access to organization packages Managing team access to organization packages
=============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Adding package access to a team](#adding-package-access-to-a-team)
+ [Adding package access to a team on the web](#adding-package-access-to-a-team-on-the-web)
+ [Adding package access to a team using the CLI](#adding-package-access-to-a-team-using-the-cli)
* [Removing package access from a team](#removing-package-access-from-a-team)
+ [Removing package access from a team on the web](#removing-package-access-from-a-team-on-the-web)
+ [Removing package access from a team using the CLI](#removing-package-access-from-a-team-using-the-cli)
* [Changing package access for a team](#changing-package-access-for-a-team)
+ [Changing package access for a team on the web](#changing-package-access-for-a-team-on-the-web)
+ [Changing package access for a team from the CLI](#changing-package-access-for-a-team-from-the-cli)
As an organization owner or team admin, you can add or remove package access to or from teams in your organization.
Adding package access to a team
--------------------------------
###
Adding package access to a team on the web
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. Beside the team to which you want to add package access, click **Packages**.
6. On the "Add Packages" page, in the "Package" field, type the name of the package and select from the dropdown menu.
7. Click **+ Add Existing Package**.
8. Beside the package name, click **read** or **read/write** to set the team permissions for the package.
###
Adding package access to a team using the CLI
As an organization owner or team admin, you can use the CLI `access` command to add package access to a team on the command line:
```
npm access grant <read-only|read-write> <org:team> [<package>]
```
For more information, see "[npm-access](https://docs.npmjs.com/cli/v8/commands/npm-access/)".
Removing package access from a team
------------------------------------
###
Removing package access from a team on the web
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. Beside the team from which you want to remove package access, click **Packages**.
6. Beside the name of the package from which you want to remove access, click **x**.
###
Removing package access from a team using the CLI
As an organization owner or team admin, you can also use the CLI `access` command to revoke package access from a team on the command line:
```
npm access revoke <org:team> [<package>]
```
For more information, see "[npm-access](https://docs.npmjs.com/cli/v8/commands/npm-access/)".
Changing package access for a team
-----------------------------------
###
Changing package access for a team on the web
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. Beside the team from which you want to remove package access, click **Packages**.
6. Beside the package name, click **read** or **read/write** to set the team permissions for the package.
###
Changing package access for a team from the CLI
As an organization owner or team admin, you can change package access for a team from the command line:
```
npm access
```
For more information, see the [`npm-access` CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-access/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/managing-team-access-to-organization-packages.mdx)
npm Verifying the PGP registry signature of a package from the npm public registry (deprecated) Verifying the PGP registry signature of a package from the npm public registry (deprecated)
===========================================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Prerequisites](#prerequisites)
* [Verifying npm signatures for the public registry](#verifying-npm-signatures-for-the-public-registry)
**Note:** PGP signatures will be deprecated early 2023, in favour of ECDSA registry signatures that can be verified using the npm CLI. More information will soon be provided.
To ensure the integrity of a package version you download from the npm public registry, you can manually verify the [PGP signature](about-pgp-signatures-for-packages-in-the-public-registry) of the package.
**Note:** Since fully verifying signatures on Keybase requires rechecking proofs (which requires network activity) and is therefore expensive, we recommend only verifying signatures if it is necessary -- for example, when verifying a deploy artifact, or when initially storing a package in your cache.
Prerequisites
--------------
1. Install Keybase from <https://keybase.io/download>
2. Create a Keybase account on <https://keybase.io>
3. Follow "[npmregistry](https://keybase.io/npmregistry)" on Keybase.
4. Download a local copy of the npm public registry's [public PGP key](https://keybase.io/npmregistry/pgp_keys.asc).
Verifying npm signatures for the public registry
-------------------------------------------------
**Note:** The following steps use version 1.4.3 of the `light-cycle` package as an example.
1. On the command line, fetch the signature for the package version you want and save it in a file:
```
npm view [email protected] dist.npm-signature > sig-to-check
```
2. Get the integrity field for that version (example below includes response):
```
npm view [email protected] dist.integrity
```
Example response:
```
sha512-sFcuivsDZ99fY0TbvuRC6CDXB8r/ylafjJAMnbSF0y4EMM1/1DtQo40G2WKz1rBbyiz4SLAc3Wa6yZyC4XSGOQ==
```
3. Construct the string that ties the unique package name and version to the integrity string (example below includes response):
```
keybase pgp verify --signed-by npmregistry -d sig-to-check -m '[email protected]:sha512-sFcuivsDZ99fY0TbvuRC6CDXB8r/ylafjJAMnbSF0y4EMM1/1DtQo40G2WKz1rBbyiz4SLAc3Wa6yZyC4XSGOQ=='
```
Example response:
```
▶ INFO Identifying npmregistry
✔ public key fingerprint: 0963 1802 8A2B 58C8 4929 D8E1 3D4D 5B12 0276 566A
✔ admin of DNS zone npmjs.org: found TXT entry keybase-site-verification=Ls8jN55i6KesjiX91Ck79bUZ17eA-iohmw2jJFM16xc
✔ admin of DNS zone npmjs.com: found TXT entry keybase-site-verification=iK3pjpRBkv-CIJ4PHtWL4TTcFXMpPiwPynatKl3oWO4
✔ "npmjs" on twitter: https://twitter.com/npmjs/status/981288548845240320
Signature verified. Signed by npmregistry 3 years ago (2018-04-13 15:00:37 -0700 MST).
PGP Fingerprint: 096318028a2b58c84929d8e13d4d5b120276566a.
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry.mdx)
npm Package name guidelines Package name guidelines
=======================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
When choosing a name for your package, choose a name that
* is unique
* is descriptive
* meets [npm policy guidelines](https://www.npmjs.com/policies). For example, do not give your package an offensive name, and do not use someone else's trademarked name or violate the [npm trademark policy](https://www.npmjs.com/policies/trademark#the-npm-trademark-policy).
Additionally, when choosing a name for an [**unscoped** package](creating-and-publishing-unscoped-public-packages), also choose a name that
* is not already owned by someone else
* is not spelled in a similar way to another package name
* will not confuse others about authorship
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/package-name-guidelines.mdx)
npm Creating and publishing scoped public packages Creating and publishing scoped public packages
==============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating a scoped public package](#creating-a-scoped-public-package)
* [Reviewing package contents for sensitive or unnecessary information](#reviewing-package-contents-for-sensitive-or-unnecessary-information)
* [Testing your package](#testing-your-package)
* [Publishing scoped public packages](#publishing-scoped-public-packages)
To share your code publicly in a user or organization namespace, you can publish public user-scoped or organization-scoped packages to the npm registry.
For more information on scopes, see "[About scopes](about-scopes)".
**Note:** Before you can publish user-scoped npm packages, you must [sign up](https://www.npmjs.com/signup) for an npm user account.
Additionally, to publish organization-scoped packages, you must [create an npm user account](https://www.npmjs.com/signup), then [create an npm organization](https://www.npmjs.com/signup?next=/org/create).
Creating a scoped public package
---------------------------------
1. If you are using npmrc to [manage accounts on multiple registries](https://docs.npmjs.com/enterprise), on the command line, switch to the appropriate profile:
```
npmrc <profile-name>
```
2. On the command line, create a directory for your package:
```
mkdir my-test-package
```
3. Navigate to the root directory of your package:
```
cd my-test-package
```
4. If you are using git to manage your package code, in the package root directory, run the following commands, replacing `git-remote-url` with the git remote URL for your package:
```
git init
git remote add origin git://git-remote-url
```
5. In the package root directory, run the `npm init` command and pass the scope to the `scope` flag:
* For an organization-scoped package, replace `my-org` with the name of your organization:
```
npm init --scope=@my-org
```
* For a user-scoped package, replace `my-username` with your username:
```
npm init --scope=@my-username
```
6. Respond to the prompts to generate a [`package.json`](creating-a-package-json-file) file. For help naming your package, see "[Package name guidelines](package-name-guidelines)".
7. Create a [README file](about-package-readme-files) that explains what your package code is and how to use it.
8. In your preferred text editor, write the code for your package.
Reviewing package contents for sensitive or unnecessary information
--------------------------------------------------------------------
Publishing sensitive information to the registry can harm your users, compromise your development infrastructure, be expensive to fix, and put you at risk of legal action. **We strongly recommend removing sensitive information, such as private keys, passwords, [personally identifiable information](https://en.wikipedia.org/wiki/Personally_identifiable_information) (PII), and credit card data before publishing your package to the registry.**
For less sensitive information, such as testing data, use a `.npmignore` or `.gitignore` file to prevent publishing to the registry. For more information, see [this article](https://docs.npmjs.com/cli/v8/using-npm/developers//#keeping-files-out-of-your-package).
Testing your package
---------------------
To reduce the chances of publishing bugs, we recommend testing your package before publishing it to the npm registry. To test your package, run `npm install` with the full path to your package directory:
```
npm install my-package
```
Publishing scoped public packages
----------------------------------
By default, scoped packages are published with private visibility. To publish a scoped package with public visibility, use `npm publish --access public`.
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. To publish your scoped public package to the npm registry, run:
```
npm publish --access public
```
3. To see your public package page, visit [https://npmjs.com/package/\\*package-name\](https://npmjs.com/package/%5C*package-name%5C)\*, replacing \*package-name\* with the name of your package. Public packages will say `public` below the package name on the npm website.
For more information on the `publish` command, see the [CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-publish/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/creating-and-publishing-scoped-public-packages.mdx)
npm npm Docs npm Docs
========
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Documentation for the npm registry, website, and command-line interface
* [About npm](about-npm)
* [Getting started](getting-started)
* [Packages and modules](packages-and-modules)
* [Integrations](integrations)
* [Organizations](organizations)
* [Policies](https://docs.npmjs.com/policies)
* [Threats and Mitigations](threats-and-mitigations)
* [npm CLI](https://docs.npmjs.com/cli)
| programming_docs |
npm Creating teams Creating teams
==============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
As an organization owner or team admin, you can create teams to manage access to sets of packages governed by your organization.
**Note:** Team names cannot be changed. To "rename" a team, you must delete the team and recreate it.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. In the "Name" and "Description" fields, type a team name and helpful description. Team names must be lower case and cannot contain spaces or punctuation.
6. Click **Make it so**.
**Note:** New teams do not have members or package access by default. Once you create a team, add packages and members from the "Teams" tab.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/creating-teams.mdx)
npm About packages and modules About packages and modules
==========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [About packages](#about-packages)
+ [About package formats](#about-package-formats)
+ [npm package git URL formats](#npm-package-git-url-formats)
* [About modules](#about-modules)
The npm registry contains packages, many of which are also Node modules, or contain Node modules. Read on to understand how they differ and how they interact.
About packages
---------------
A **package** is a file or directory that is described by a `package.json` file. A package must contain a `package.json` file in order to be published to the npm registry. For more information on creating a `package.json` file, see "[Creating a package.json file](creating-a-package-json-file)".
Packages can be unscoped or scoped to a user or organization, and scoped packages can be private or public. For more information, see
* "[About scopes](about-scopes)"
* "[About private packages](about-private-packages)"
* "[Package scope, access level, and visibility](package-scope-access-level-and-visibility)"
###
About package formats
A package is any of the following:
* a) A folder containing a program described by a `package.json` file.
* b) A gzipped tarball containing (a).
* c) A URL that resolves to (b).
* d) A `<name>@<version>` that is published on the registry with (c).
* e) A `<name>@<tag>` that points to (d).
* f) A `<name>` that has a `latest` tag satisfying (e).
* g) A `git` url that, when cloned, results in (a).
###
npm package git URL formats
Git URLs used for npm packages can be formatted in the following ways:
* `git://github.com/user/project.git#commit-ish`
* `git+ssh://user@hostname:project.git#commit-ish`
* `git+http://user@hostname/project/blah.git#commit-ish`
* `git+https://user@hostname/project/blah.git#commit-ish`
The `commit-ish` can be any tag, sha, or branch that can be supplied as an argument to `git checkout`. The default `commit-ish` is `master`.
About modules
--------------
A **module** is any file or directory in the `node_modules` directory that can be loaded by the Node.js `require()` function.
To be loaded by the Node.js `require()` function, a module must be one of the following:
* A folder with a `package.json` file containing a `"main"` field.
* A JavaScript file.
\*\*Note:\*\* Since modules are not required to have a `package.json` file, not all modules are packages. Only modules that have a `package.json` file are also packages.
In the context of a Node program, the `module` is also the thing that was loaded *from* a file. For example, in the following program:
```
var req = require('request')
```
we might say that "The variable `req` refers to the `request` module".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/about-packages-and-modules.mdx)
npm Transferring a package from a user account to another user account Transferring a package from a user account to another user account
==================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Transferring a package from a user account to another user account on the website](#transferring-a-package-from-a-user-account-to-another-user-account-on-the-website)
* [Transferring a package from a user account to another user account on the command line](#transferring-a-package-from-a-user-account-to-another-user-account-on-the-command-line)
As a package owner or maintainer, you can transfer ownership of a package you no longer wish to maintain to another trusted npm user using either the npm website or the command line.
For more information on how npm support handles package name disputes between users, you can refer to npm's [package name dispute policy](https://docs.npmjs.com/policies/disputes).
**Note:** You cannot transfer a scoped package to another user account or organization, because a package's scope *is* the user account or organization name. You will need to create a new package in the new scope.
Transferring a package from a user account to another user account on the website
----------------------------------------------------------------------------------
To transfer a package you own or maintain to another user, follow these steps:
1. Navigate to the package page for the package you want to transfer, replacing `<your-package-name>` with the name of your package: `https://www.npmjs.com/package/<your-package-name>`.
2. On the package Admin tab, under "Maintainers", enter the npm username of the new maintainer.
3. Click "Invite."
4. To remove yourself as a maintainer, under the maintainers list, click the "x" next to your username.
Transferring a package from a user account to another user account on the command line
---------------------------------------------------------------------------------------
To transfer a package to another npm user using the CLI, run the [`npm owner add`](https://docs.npmjs.com/cli/v8/commands/npm-owner/) command replacing `<their-username>` with the other user's npm username. An email invitation is sent to the other user. After the user has accepted the invitation, run the `npm owner rm` command replacing `<your-username>` with your npm username:
```
npm owner add <their-username> <package-name>
# new maintainer accepts invitation
npm owner rm <your-username> <package-name>
```
If you have two-factor authentication enabled for writes, add a one-time password to the command, `--otp=123456` (where *123456* is the code from your authenticator application).
```
npm owner add <their-username> <package-name> --otp=123456
# new maintainer accepts invitation
npm owner rm <your-username> <package-name> --otp=123456
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/transferring-a-package-from-a-user-account-to-another-user-account.mdx)
npm Requiring 2FA for package publishing and settings modification Requiring 2FA for package publishing and settings modification
==============================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Configuring two-factor authentication](#configuring-two-factor-authentication)
To protect your packages, as a package publisher, you can require everyone who has write access to a package to have two-factor authentication (2FA) enabled. This will require that users provide 2FA credentials in addition to their login token when they publish the package. For more information, see "[Configuring two-factor authentication](configuring-two-factor-authentication)".
You may also choose to allow publishing with either two-factor authentication *or* with [automation tokens](creating-and-viewing-access-tokens). This lets you configure automation tokens in a CI/CD workflow, but requires two-factor authentication from interactive publishes.
Configuring two-factor authentication
--------------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. Navigate to the package on which you want to require a second factor to publish or modify settings.
3. Click **Settings**.
4. Under "Publishing access", select the requirements to publish a package.
1. **Two-factor authentication is not required**
With this option, a maintainer can publish a package or change the package settings whether they have two-factor authentication enabled or not. This is the least secure setting.
2. **Require two-factor authentication or automation tokens**
With this option, maintainers must have two-factor authentication enabled for their account. If they publish a package interactively, using the `npm publish` command, they will be required to enter 2FA credentials when they perform the publish. However, maintainers may also create an [automation token](creating-and-viewing-access-tokens) and use that to publish. A second factor is *not* required when using an automation token, making it useful for continuous integration and continuous deployment workflows.
3. **Two-factor authentication only**
With this option, a maintainer must have two-factor authentication enabled for their account, and they must publish interactively. Maintainers will be required to enter 2FA credentials when they perform the publish.
5. Click **Update Package Settings**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/requiring-2fa-for-package-publishing-and-settings-modification.mdx)
npm Upgrading to a paid user account plan Upgrading to a paid user account plan
=====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Upgrading to a paid user account plan](upgrading-to-a-paid-user-account-plan)[Viewing, downloading, and emailing receipts for your npm user account](viewing-downloading-and-emailing-receipts-for-your-user-account)[Updating user account billing settings](updating-user-account-billing-settings)[Downgrading to a free user account plan](downgrading-to-a-free-user-account-plan)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Note:** This article only applies to users of the public npm registry.
If you need to install and publish private packages, you can upgrade to a paid user account plan. Our paid user account plan costs $7 per month. For more information, see the "npm account" column on our [pricing page](https://www.npmjs.com/pricing).
Your paid plan and billing cycle will start when you submit your credit card information, and you will be charged for the first month immediately.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. Under "change plan", click **Upgrade Plan ($7/User)**.
4. Under "Want to upgrade?", click **Enable Private Publishing for $7/mo**.
5. In the billing information dialog box, enter your billing information:
* Email: the email address used for the billing contact
* Name: the name on the credit card used to pay
* Street, City, ZIP Code, Country: the billing address associated with the credit card
6. Click **Payment Info**.
7. In the credit card information dialog box, enter your credit card information:
* Card number
* MM / YY: the month and year of the card expiration date
* CVC: the three-digit code on the credit card
8. To save your credit card information for other payments on npm, select "Remember me".
9. Click **Pay $7.00**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/paying-for-your-npm-user-account/upgrading-to-a-paid-user-account-plan.mdx)
npm About public packages About public packages
=====================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Next steps](#next-steps)
As an npm user or organization member, you can create and publish public packages that anyone can download and use in their own projects.
* **Unscoped** public packages exist in the global public registry namespace and can be referenced in a `package.json` file with the package name alone: `package-name`.
* **Scoped** public packages belong to a user or organization and must be preceded by the user or organization name when included as a dependency in a `package.json` file:
+ `@username/package-name`
+ `@org-name/package-name`
Next steps
-----------
* "[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)"
* "[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)"
* "[Using npm packages in your projects](using-npm-packages-in-your-projects)"
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/about-public-packages.mdx)
| programming_docs |
npm Receiving a one-time password over email Receiving a one-time password over email
========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Logging in with a one-time password](#logging-in-with-a-one-time-password)
* [Enabling two-factor authentication](#enabling-two-factor-authentication)
For your security, npm may require additional verification to allow you to log in to your account. If you do not have [two-factor authentication](configuring-two-factor-authentication) enabled, you may be asked to verify yourself with a one-time password sent to the email address configured for your account.
Logging in with a one-time password
------------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. You'll be prompted for a one-time password that was sent to your email.
3. Check your email account for an email from npm containing your one-time password (the subject will begin "OTP for logging in to your account").
4. Enter the digits from your email in your one-time password field.
Enabling two-factor authentication
-----------------------------------
To avoid this additional login step, with a one-time password sent to you via e-mail, you can configure [two-factor authentication with a device](configuring-two-factor-authentication) (2FA) instead.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/receiving-a-one-time-password-over-email.mdx)
npm Accessing npm using two-factor authentication Accessing npm using two-factor authentication
=============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Sign in from the command line using security-key flow](#sign-in-from-the-command-line-using-security-key-flow)
* [Sign in from the command line using --auth-type=web](#sign-in-from-the-command-line-using---auth-typeweb)
+ [With an existing browser session](#with-an-existing-browser-session)
+ [Without an existing browser session](#without-an-existing-browser-session)
Sign in from the command line using security-key flow
------------------------------------------------------
1. On the command line, type the [`npm login`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) command.
2. When prompted, provide your username, password, and email address.
```
user@host:~$ npm login
npm notice Log in on https://registry.npmjs.org/
Username: mona
Password:
Email: (this IS public) [email protected]
npm notice Open https://www.npmjs.com/login/913c3ab1-89a0-44bd-be8d-d946e2e906f0 to use your security key for authentication or enter OTP from your authenticator app
```
3. If you have configured a security-key, open the provided URL shown in the command line. Alternatively, if you have configured a mobile authenticator skip to step 6.
4. Click on *Use security key* and follow the browser specific steps to authenticate.
5. Copy the generated token
6. Enter the one-time password into the CLI prompt.
Sign in from the command line using `--auth-type=web`
------------------------------------------------------
npm 8.14.0 and higher support login flow through the browers. This will become the default behavior for the npm public registry in npm 9.
###
With an existing browser session
1. On the command line, type the [`npm login --auth-type=web`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) command.
2. When prompted hit "ENTER" to open your browser to start the login flow or click the provided URL show in the command line.
```
user@host:~$ npm login
npm notice Log in on https://registry.npmjs.org/
Authenticate your account at:
https://www.npmjs.com/login?next=/login/cli/b1a2f96a-ce09-4463-954c-c99f6773b922
Press ENTER to open in the browser...
```
3. Click on *Use security key* and follow the browser specific steps to authenticate.
*Note: If you have configured to use TOTP, you will see an TOTP prompt instead*
###
Without an existing browser session
1. On the command line, type the [`npm login --auth-type=web`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) command.
2. When prompted hit "ENTER" to open your browser to start the login flow or click the provided URL show in the command line.
```
user@host:~$ npm login
npm notice Log in on https://registry.npmjs.org/
Authenticate your account at:
https://www.npmjs.com/login?next=/login/cli/b1a2f96a-ce09-4463-954c-c99f6773b922
Press ENTER to open in the browser...
```
3. [Log in](https://www.npmjs.com/login) to npm with your user account.
4. Click on *Use security key* and follow the browser specific steps to authenticate.
*Note: If you have configured to use TOTP, you will see an TOTP prompt instead*
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/accessing-npm-using-2fa.mdx)
npm About npm About npm
=========
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Use npm to . . .](#use-npm-to---)
* [Getting started](#getting-started)
* [Sharing packages and collaborating with others](#sharing-packages-and-collaborating-with-others)
* [Learn more](#learn-more)
+ [CLI reference documentation](#cli-reference-documentation)
npm is the world's largest software registry. Open source developers from every continent use npm to share and borrow packages, and many organizations use npm to manage private development as well.
npm consists of three distinct components:
* the website
* the Command Line Interface (CLI)
* the registry
Use the [*website*](https://npmjs.com) to discover packages, set up profiles, and manage other aspects of your npm experience. For example, you can set up [organizations](https://www.npmjs.com/features) to manage access to public or private packages.
The [*CLI*](https://docs.npmjs.com/cli/v8/commands/npm/) runs from a terminal, and is how most developers interact with npm.
The [*registry*](https://docs.npmjs.com/cli/v8/using-npm/registry/) is a large public database of JavaScript software and the meta-information surrounding it.
Use npm to . . .
-----------------
* Adapt packages of code for your apps, or incorporate packages as they are.
* Download standalone tools you can use right away.
* Run packages without downloading using [npx](https://www.npmjs.com/package/npx).
* Share code with any npm user, anywhere.
* Restrict code to specific developers.
* Create organizations to coordinate package maintenance, coding, and developers.
* Form virtual teams by using organizations.
* Manage multiple versions of code and code dependencies.
* Update applications easily when underlying code is updated.
* Discover multiple ways to solve the same puzzle.
* Find other developers who are working on similar problems and projects.
Getting started
----------------
To get started with npm, you can [create an account](https://www.npmjs.com/signup), which will be available at [http://www.npmjs.com/~\*yourusername](http://www.npmjs.com/~*yourusername)\*.
After you set up an npm account, the next step is to use the command line interface (CLI) to [install npm](downloading-and-installing-node-js-and-npm). We look forward to seeing what you create!
Sharing packages and collaborating with others
-----------------------------------------------
If you choose to share your packages publicly, there is no cost. To use and share private packages, you need to upgrade your account. To share with others, create organizations, called **[npm organizations](organizations)**, and invite others to work with you, privately (for a fee) or publicly (for free).
You can also use a private npm package registry like [GitHub Packages](https://github.com/features/packages) or the open source [Verdaccio](https://verdaccio.org) project. This lets you develop packages internally that are not shared publicly.
Learn more
-----------
To learn more about npm as a product, upcoming new features, and interesting uses of npm be sure to follow [@npmjs](https://twitter.com/npmjs) on Twitter.
For mentoring, tutorials, and learning, visit [node school](https://nodeschool.io). Consider attending or hosting a nodeschool event (usually free!) at a site near you, or use the self-help tools you can find on the site.
###
CLI reference documentation
While relevant CLI commands are covered throughout this user documentation, the CLI includes command line help, its own [documentation section, and instant help (man pages)](cli/v8).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/about-npm/index.mdx)
npm About access tokens About access tokens
===================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[About access tokens](about-access-tokens)[Creating and viewing access tokens](creating-and-viewing-access-tokens)[Revoking access tokens](revoking-access-tokens)[Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](docker-and-private-modules)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Note:** You must be using npm version 5.5.1 or greater to use access tokens.
An access token is an alternative to using your username and password for authenticating to npm when using the API or the npm command-line interface (CLI). An access token is a hexadecimal string that you can use to authenticate, and which gives you the right to install and/or publish your modules.
The npm CLI automatically generates an access token for you when you run `npm login`. You can also create an access token to give other tools (such as continuous integration testing environments) access to your npm packages. For example, GitHub Actions provides the ability to store [secrets](https://docs.github.com/en/actions/configuring-and-managing-workflows/creating-and-storing-encrypted-secrets), like access tokens, that you can then use to authenticate. When your workflow runs, it will be able to complete npm tasks as you, including installing private packages you can access.
You can work with tokens from the web or the CLI, whichever is easiest. What you do in each environment will be reflected in the other environment.
npm token commands let you:
* View tokens for easier tracking and management
* Create new tokens, specifying read-only or full-permission
* Limit access according to IP address ranges (CIDR)
* Delete/revoke tokens
For more information on creating and viewing access tokens on the web and CLI, see "[Creating and viewing access tokens](creating-and-viewing-access-tokens)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/about-access-tokens.mdx)
npm Viewing, downloading, and emailing receipts for your organization Viewing, downloading, and emailing receipts for your organization
=================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Upgrading to a paid organization plan](upgrading-to-a-paid-organization-plan)[Viewing, downloading, and emailing receipts for your organization](viewing-downloading-and-emailing-receipts-for-your-organization)[Updating organization billing settings](updating-organization-billing-settings)[Downgrading to a free organization plan](downgrading-to-a-free-organization-plan)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Viewing receipts](#viewing-receipts)
* [Downloading receipts](#downloading-receipts)
* [Emailing receipts](#emailing-receipts)
**Note:** This article only applies to users of the public npm registry.
As an organization owner, you can view, download, and email receipts for the complete billing history of your organization.
Viewing receipts
-----------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization whose billing receipts you want to view.
4. On the organization settings page, click **Billing**.
5. On the Billing Information page, under "monthly bill", select **View Billing History**.
6. To view a single receipt, find the row of the receipt you want to view, then, on the right side of the row, click the view icon.
7. To view a single receipt, find the row of the receipt you want to view, then, on the right side of the row, click the view icon.
Downloading receipts
---------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization whose billing receipts you want to download.
4. On the organization settings page, click **Billing**.
5. On the Billing Information page, under "monthly bill", select **View Billing History**.
6. To download a single receipt, find the row of the receipt you want to download, then click the PDF icon on the right side of the row.
7. To download multiple receipts, first select the receipts that you wish to download by selecting the box next to the date. To select all receipts, select the checkbox next to the "Date" header. Then click **Download Checked**.
Emailing receipts
------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization whose billing receipts you want to email.
4. On the organization settings page, click **Billing**.
5. On the Billing Information page, under "monthly bill", select **View Billing History**.
6. To email a single receipt, find the row of the receipt you want to download, then, on the right side of the row, click the email icon.
7. To email multiple receipts, first select the receipts that you wish to download by selecting the box next to the date. To select all receipts, select the checkbox next to the "Date" header. Then click **Email Checked**.
8. In the Email Receipt dialog box, fill in the "From", "To", and "Message" fields.
9. Click **Send**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/paying-for-your-organization/viewing-downloading-and-emailing-receipts-for-your-organization.mdx)
npm Updating organization billing settings Updating organization billing settings
======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Upgrading to a paid organization plan](upgrading-to-a-paid-organization-plan)[Viewing, downloading, and emailing receipts for your organization](viewing-downloading-and-emailing-receipts-for-your-organization)[Updating organization billing settings](updating-organization-billing-settings)[Downgrading to a free organization plan](downgrading-to-a-free-organization-plan)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Updating credit card information](#updating-credit-card-information)
* [Updating billing receipt email and extra receipt information](#updating-billing-receipt-email-and-extra-receipt-information)
**Note:** This article only applies to users of the public npm registry.
As an owner of an npm Teams subscription, a paid organization plan, you can update the credit card used to pay for your plan. Updating your credit card will not change your billing cycle date, and the new credit card will be charged on the next billing cycle.
**Note:** If the credit card used to pay for your npm Teams subscription or your paid organization plan expires, or we are otherwise are unable to charge your card, you have a grace period of nine days to update the card.
Updating credit card information
---------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization whose credit card information you want to change.
4. On the organization settings page, click **Billing**.
5. Under "monthly bill", click **Edit Payment Info**.
6. In the billing information dialog box, enter your billing information:
* Email: the email address used for the billing contact
* Name: the name on the credit card used to pay
* Street, City, ZIP Code, Country: the billing address associated with the credit card
7. Click **Payment Info**.
8. In the credit card information dialog box, enter your credit card information:
* Card number
* MM / YY: the month and year of the card expiration date
* CVC: the three-digit code on the credit card
9. To save your credit card information for other payments on npm, select "Remember me".
10. Click **Update Card**.
Updating billing receipt email and extra receipt information
-------------------------------------------------------------
As an organization owner, you can update the email address used for receipts, and add extra information to the receipt for your paid organization plan, such as your business name, VAT identification number, or address of record. Updated billing information will appear on all receipts immediately.
**Note:** The billing email is used for receipts only and is not required to match the email address of the person whose card is used to pay for the organization.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization whose billing receipt information you want to change.
4. On the organization settings page, click **Billing**.
5. On the Billing Information page, under "monthly bill", select **View Billing History**.
6. At the bottom of the Billing History dialog box, click "Receipt Settings".
7. To add a business name, VAT number, address of record, or other information to your receipts, in the "Extra Billing Information" text box, type the information.
8. To update the email address used for receipts, beside "Send my receipts", select the checkbox and type the email address that should receive billing receipts.
9. Click **Save**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/paying-for-your-organization/updating-organization-billing-settings.mdx)
| programming_docs |
npm Downgrading to a free organization plan Downgrading to a free organization plan
=======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Upgrading to a paid organization plan](upgrading-to-a-paid-organization-plan)[Viewing, downloading, and emailing receipts for your organization](viewing-downloading-and-emailing-receipts-for-your-organization)[Updating organization billing settings](updating-organization-billing-settings)[Downgrading to a free organization plan](downgrading-to-a-free-organization-plan)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Note:** This article only applies to users of the public npm registry.
If you are a subscriber to the npm Teams product (you have a paid organization) and you are an owner of the organization, then you can downgrade from npm Teams to a free organization. When you downgrade from a paid to a free organization, you and your organization members will lose the ability to install and publish private packages at the end of your last paid billing cycle. Your private packages will *not* be made publicly visible when you downgrade to a free plan.
**Note:** If you would like to pay for fewer seats, you can remove members from your organization by following the steps in "[Removing members from your organization](https://docs.npmjs.com/removing-members-from-your-org)".
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization you want to downgrade.
4. On the organization settings page, click **Billing**.
5. Under "change plan", click **Downgrade Plan**.
6. Under "Are you sure?", click **Downgrade to a free account**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/paying-for-your-organization/downgrading-to-a-free-organization-plan.mdx)
npm Requiring two-factor authentication in your organization Requiring two-factor authentication in your organization
========================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Creating an organization](creating-an-organization)[Converting your user account to an organization](converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)[Renaming an organization](renaming-an-organization)[Deleting an organization](deleting-an-organization)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [About two-factor authentication for organizations](#about-two-factor-authentication-for-organizations)
* [Prerequisites](#prerequisites)
* [Requiring two-factor authentication in your organization](#requiring-two-factor-authentication-in-your-organization)
* [Helping removed members and outside collaborators rejoin your organization](#helping-removed-members-and-outside-collaborators-rejoin-your-organization)
Organization owners can require organization members to enable two-factor authentication for their personal accounts, making it harder for malicious actors to access an organization's packages and settings
About two-factor authentication for organizations
--------------------------------------------------
Two-factor authentication (2FA) is an extra layer of security used when logging into websites or apps. You can require all members in your organization to enable two-factor authentication on npm. For more information about two-factor authentication, see ["Configuring two-factor authentication."](configuring-two-factor-authentication).
**Note:**
* When you require use of two-factor authentication for your organization, members who do not use 2FA will be removed from the organization and lose access to its packages. You can add them back to the organization if they enable two-factor authentication.
* An organization owner cannot opt-in to requiring 2FA for an organization if they do not have 2FA enabled on their account.
* If you are the member of an organization that requires 2FA you will not be able to disable 2FA until you leave that organization.
Prerequisites
--------------
Before you can require organization members to use two-factor authentication, you must enable two-factor authentication for your account on npm. For more information, see ["Configuring two-factor authentication."](configuring-two-factor-authentication).
Before you require use of two-factor authentication, we recommend notifying organization members and asking them to set up 2FA for their accounts. You can see if members already use 2Fa in the organizations members page.
Requiring two-factor authentication in your organization
---------------------------------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Members**.
5. Click the **Enable 2FA Enforcement** button.
6. If prompted, read the information about members who will be removed from the organization. Type your organization's name to confirm the change, then click Remove members & require two-factor authentication.
7. If any members are removed from the organization, we recommend sending them an invitation that can reinstate their former privileges and access to your organization. They must enable two-factor authentication before they can accept your invitation.
Helping removed members and outside collaborators rejoin your organization
---------------------------------------------------------------------------
If any members are removed from the organization when you enable required use of two-factor authentication, they'll receive an email notifying them that they've been removed. They should then enable 2FA for their personal account, and contact an organization owner to request access to your organization.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/requiring-two-factor-authentication-in-your-organization.mdx)
npm Adding members to your organization Adding members to your organization
===================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Adding members to your organization](adding-members-to-your-organization)[Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](organization-roles-and-permissions)[Managing organization permissions](managing-organization-permissions)[Removing members from your organization](removing-members-from-your-organization)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Inviting members to your organization](#inviting-members-to-your-organization)
* [Revoking an organization invitation](#revoking-an-organization-invitation)
As an organization owner, you can add other npm users to your organization to give them read or read and write access to public and private packages within your organization's scope, as well as public unscoped packages governed by your organization.
When you add a member to your organization, they are sent an email inviting them to the organization.
Once the new member [accepts the invitation](accepting-or-rejecting-an-organization-invitation), they are:
* assigned the role of "[member](organization-roles-and-permissions)"
* added to the ["developers" team](about-developers-team)
If you have a [paid organization](upgrading-to-a-paid-organization-plan), as part of an npm Teams plan, you will be billed $7 per month for each new member.
Inviting members to your organization
--------------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Members**.
5. Click the **Invite Members** button.
6. In the "Username or email" field, type the username or email address of the person you wish to invite. Optionally you can select a specific team to invite the member to.
7. Click **Invite**.
Revoking an organization invitation
------------------------------------
As an organization owner, if you've made a mistake in inviting someone to your organization, you can revoke the organization invitation.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Members**.
5. Click the **Invite Members** button.
6. Under the "Invitations" field, click the **X** next to the name of the user invitation you would like to revoke.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/adding-members-to-your-organization.mdx)
npm Creating Node.js modules Creating Node.js modules
========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Overview](#overview)
* [Create a package.json file](#create-a-packagejson-file)
* [Create the file that will be loaded when your module is required by another application](#create-the-file-that-will-be-loaded-when-your-module-is-required-by-another-application)
* [Test your module](#test-your-module)
* [Resources](#resources)
Node.js modules are a type of [package](about-packages-and-modules) that can be published to npm.
Overview
---------
1. [Create a `package.json` file](#create-a-package-json-file)
2. [Create the file that will be loaded when your module is required by another application](#create-the-file-that-will-be-loaded-when-your-module-is-required-by-another-application)
3. [Test your module](#test-your-module)
Create a `package.json` file
-----------------------------
1. To create a `package.json` file, on the command line, in the root directory of your Node.js module, run `npm init`:
* For [scoped modules](about-scopes), run `npm init --scope=@scope-name`
* For [unscoped modules](creating-and-publishing-unscoped-public-packages), run `npm init`
2. Provide responses for the required fields (`name` and `version`), as well as the `main` field:
* `name`: The name of your module.
* `version`: The initial module version. We recommend following [semantic versioning guidelines](about-semantic-versioning) and starting with `1.0.0`.
For more information on `package.json` files, see "[Creating a package.json file](creating-a-package-json-file)".
Create the file that will be loaded when your module is required by another application
----------------------------------------------------------------------------------------
In the file, add a function as a property of the `exports` object. This will make the function available to other code:
```
exports.printMsg = function() {
console.log("This is a message from the demo package");
}
```
Test your module
-----------------
1. Publish your package to npm:
* For [private packages](creating-and-publishing-private-packages#publishing-private-packages) and [unscoped packages](creating-and-publishing-unscoped-public-packages#publishing-unscoped-public-packages), use `npm publish`.
* For [scoped public packages](creating-and-publishing-scoped-public-packages#publishing-scoped-public-packages), use `npm publish --access public`
2. On the command line, create a new test directory outside of your project directory.
```
mkdir test-directory
```
3. Switch to the new directory:
```
cd /path/to/test-directory
```
4. In the test directory, install your module:
```
npm install <your-module-name>
```
5. In the test directory, create a `test.js` file which requires your module and calls your module as a method.
6. On the command line, run `node test.js`. The message sent to the console.log should appear.
Resources
----------
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/creating-node-js-modules.mdx)
npm Uninstalling packages and dependencies Uninstalling packages and dependencies
======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Uninstalling local packages](#uninstalling-local-packages)
+ [Removing a local package from your node\_modules directory](#removing-a-local-package-from-your-node_modules-directory)
- [Unscoped package](#unscoped-package)
- [Scoped package](#scoped-package)
+ [Example](#example)
+ [Removing a local package without removing it from package.json](#removing-a-local-package-without-removing-it-from-packagejson)
+ [Example](#example-1)
+ [Confirming local package uninstallation](#confirming-local-package-uninstallation)
* [Uninstalling global packages](#uninstalling-global-packages)
+ [Unscoped package](#unscoped-package-1)
+ [Scoped package](#scoped-package-1)
+ [Example](#example-2)
* [Resources](#resources)
+ [Uninstalling local packages](#uninstalling-local-packages-1)
+ [Uninstalling global packages](#uninstalling-global-packages-1)
If you no longer need to use a package in your code, we recommend uninstalling it and removing it from your project's dependencies.
Uninstalling local packages
----------------------------
###
Removing a local package from your node\_modules directory
To remove a package from your node\_modules directory, on the command line, use the [`uninstall` command](https://docs.npmjs.com/cli/v8/commands/npm-uninstall/). Include the scope if the package is scoped.
This uninstalls a package, completely removing everything npm installed on its behalf.
It also removes the package from the dependencies, devDependencies, optionalDependencies, and peerDependencies objects in your package.json.
Further, if you have an npm-shrinkwrap.json or package-lock.json, npm will update those files as well.
####
Unscoped package
```
npm uninstall <package_name>
```
####
Scoped package
```
npm uninstall <@scope/package_name>
```
###
Example
```
npm uninstall lodash
```
###
Removing a local package without removing it from package.json
Using the `--no-save` will tell npm not to remove the package from your `package.json`, `npm-shrinkwrap.json`, or `package-lock.json` files.
###
Example
```
npm uninstall --no-save lodash
```
`--save` or `-S` will tell npm to remove the package from your `package.json`, `npm-shrinkwrap.json`, and `package-lock.json` files. **This is the default**, but you may need to use this if you have for instance `save=false` in your `.npmrc` file.
###
Confirming local package uninstallation
To confirm that `npm uninstall` worked correctly, check that the `node_modules` directory no longer contains a directory for the uninstalled package(s).
* Unix system (such as OSX): `ls node_modules`
* Windows systems: `dir node_modules`
Uninstalling global packages
-----------------------------
To uninstall an unscoped global package, on the command line, use the `uninstall` command with the `-g` flag. Include the scope if the package is scoped.
###
Unscoped package
```
npm uninstall -g <package_name>
```
###
Scoped package
```
npm uninstall -g <@scope/package_name>
```
###
Example
For example, to uninstall a package called `jshint`, run:
```
npm uninstall -g jshint
```
Resources
----------
###
Uninstalling local packages
###
Uninstalling global packages
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/uninstalling-packages-and-dependencies.mdx)
| programming_docs |
npm Recovering your 2FA-enabled account Recovering your 2FA-enabled account
===================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Misplaced second factor device](#misplaced-second-factor-device)
+ [Using recovery code on the web](#using-recovery-code-on-the-web)
+ [Using recovery code from the command line](#using-recovery-code-from-the-command-line)
* [Viewing and regenerating recovery code](#viewing-and-regenerating-recovery-code)
* [Misplaced recovery codes](#misplaced-recovery-codes)
When you have two-factor access enabled on your account, and you lose access to your 2FA device, you may be able to recover your account using the following methods.
Misplaced second factor device
-------------------------------
If you have misplaced the device that provided second-factor authentication, you can use the recovery codes generated when you [enabled 2FA](configuring-two-factor-authentication#enabling-2fa-on-the-web) to access your account.
###
Using recovery code on the web
1. Locate the recovery codes generated that you have saved.
2. [Log in](https://www.npmjs.com/login) to npm with your user account.
3. Click on "Use recovery code" from the next screen
*Note: If you have configured to use TOTP, you will see an TOTP prompt instead*
4. Enter an unused recovery code in the "Use a Recovery Code" prompt
5. You are now logged into npm.
6. Follow the steps mentioned in "[Removing 2FA on the web](configuring-two-factor-authentication#removing-2fa-on-the-web)" to disable 2FA
###
Using recovery code from the command line
1. Locate the recovery codes generated when you enabled 2FA on your account.
2. If you are logged out on the command line, log in using `npm login` command with your username and npm password.
3. Enter an unused recovery code when you see this prompt:
4. Once you are logged in, use the below and enter your npm password if prompted.
```
npm profile disable-2fa
```
5. Enter another unused recovery code when you see this prompt:
6. npm will confirm that two-factor authentication has been disabled.
7. Follow the steps outlined in "[Configuring two-factor authentication](configuring-two-factor-authentication)" to re-enable 2FA and generate new recovery codes.
**Note:** Using the recovery codes to re-enable 2FA may create a new authenticator account with the same npm account name.
If you are using a [time-based one-time password (TOTP)](https://en.wikipedia.org/wiki/Time-based_one-time_password) mobile app and want to delete the old authenticator account, follow the steps for the authenticator.
Viewing and regenerating recovery code
---------------------------------------
**Note:** Once you regenerate a set of code, all previous recovery codes become invalid. Each code can be used only once.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. On the account settings page, under "Two-Factor Authentication", click **Modify 2FA**.
4. Click "Manage Recovery Codes'' to view your recovery codes
5. Click "Regenerate Code" to generate a new set of codes.
Misplaced recovery codes
-------------------------
If you have misplaced both the device that provided second-factor authentication and your recovery codes, we may be unable to help you recover your account. If you have any questions, please [contact npm Support](https://www.npmjs.com/support).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/recovering-your-2fa-enabled-account.mdx)
npm Creating a strong password Creating a strong password
==========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Secure your npm account with a strong and unique password using a password manager.
You must choose or generate a password for your npm account that:
* is longer than 10 characters
* does not match or significantly contain your username, e.g. do not use 'username123'
* has not been compromised and known to the [Have I Been Pwned](https://haveibeenpwned.com/) breach database
To keep your account secure, we recommend you follow these best practices:
* Use a password manager, such as [LastPass](https://lastpass.com/) or [1Password](https://1password.com/), to generate a password more than 16 characters.
* Generate a unique password for npm. If you use your npm password elsewhere and that service is compromised, then attackers or other malicious actors could use that information to access your npm account.
* Configure two-factor authentication for your account. For more information, see "About two-factor authentication."
* Never share your password, even with a potential collaborator. Each person should use their own personal account on npm. For more information on ways to collaborate, see: "[npm organizations](organizations)".
When you type a password to sign in, create an account, or change your password, npm will check if the password you entered is considered weak according to datasets like HaveIBeenPwned. The password may be identified as weak even if you have never used that password before.
npm only inspects the password at the time you type it, and never stores the password you entered in plaintext. For more information, see [HaveIBeenPwned](https://haveibeenpwned.com/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/creating-a-strong-password.mdx)
npm Changing your npm username Changing your npm username
==========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Managing your profile settings](managing-your-profile-settings)[Changing your npm username](changing-your-npm-username)[Deleting your npm user account](deleting-your-npm-user-account)[Requesting an export of your personal data](requesting-your-data)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
It is not currently possible to change your npm username. You'll need to create a new account and migrate the data to the new account manually.
1. Create a [new user account](creating-a-new-npm-user-account) with your desired username
2. [Transfer your packages](transferring-a-package-from-a-user-account-to-another-user-account) to your new account.
3. If you are a member of any <organizations>, ask the organization administrator to [invite your new account to the organization](adding-members-to-your-organization).
4. Delete your [original account](deleting-your-npm-user-account). Note that this is permanent, and after 30 days, this account name is available for other people to claim.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/managing-your-npm-user-account/changing-your-npm-username.mdx)
npm Organization roles and permissions Organization roles and permissions
==================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Adding members to your organization](adding-members-to-your-organization)[Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](organization-roles-and-permissions)[Managing organization permissions](managing-organization-permissions)[Removing members from your organization](removing-members-from-your-organization)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
There are three roles in an organization:
* **Owner:** Users who manage organization members and billing.
* **Admin:** Users who manage team membership and package access.
* **Member:** Users who create and publish packages in the organization scope.
**On the public registry, you cannot remove the last owner from an organization.** To delete an organization, [contact npm Support](https://www.npmjs.com/support).
| Action | **Owner** | **Admin** | **Member** |
| --- | --- | --- | --- |
| Manage organization billing | X | | |
| Add members to the organization | X | | |
| Remove members from the organization | X | | |
| Rename an organization | X | | |
| Delete an organization | X | | |
| Change any organization member's role | X | | |
| Create teams | X | X | |
| Delete teams | X | X | |
| Add any member to any team | X | X | |
| Remove any member from any team | X | X | |
| Manage team package access | X | X | |
| Create and publish packages in the organization scope | X | X | X |
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/organization-roles-and-permissions.mdx)
npm Using npm packages in your projects Using npm packages in your projects
===================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Using unscoped packages in your projects](#using-unscoped-packages-in-your-projects)
+ [Node.js module](#nodejs-module)
+ [package.json file](#packagejson-file)
* [Using scoped packages in your projects](#using-scoped-packages-in-your-projects)
+ [Node.js module](#nodejs-module-1)
+ [package.json file](#packagejson-file-1)
* [Resolving "Cannot find module" errors](#resolving-cannot-find-module-errors)
Once you have [installed a package](https://docs.npmjs.com/downloading-and-installing-packages) in `node_modules`, you can use it in your code.
Using unscoped packages in your projects
-----------------------------------------
###
Node.js module
If you are creating a Node.js module, you can use a package in your module by passing it as an argument to the `require` function.
```
var lodash = require('lodash');
var output = lodash.without([1, 2, 3], 1);
console.log(output);
```
###
package.json file
In `package.json`, list the package under dependencies. You can optionally include a [semantic version](about-semantic-versioning).
```
{
"dependencies": {
"@package_name": "^1.0.0"
}
}
```
Using scoped packages in your projects
---------------------------------------
To use a scoped package, simply include the scope wherever you use the package name.
###
Node.js module
```
var projectName = require("@scope/package-name")
```
###
package.json file
In `package.json`:
```
{
"dependencies": {
"@scope/package_name": "^1.0.0"
}
}
```
Resolving "Cannot find module" errors
--------------------------------------
If you have not properly installed a package, you will receive an error when you try to use it in your code. For example, if you reference the `lodash` package without installing it, you would see the following error:
```
module.js:340
throw err;
^
Error: Cannot find module 'lodash'
```
* For scoped packages, run `npm install <@scope/package_name>`
* For unscoped packages, run `npm install <package_name>`
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/using-npm-packages-in-your-projects.mdx)
npm npm: Threats and Mitigations npm: Threats and Mitigations
============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Account Takeovers](#account-takeovers)
+ [By compromising passwords](#by-compromising-passwords)
+ [By registering an expired email domain](#by-registering-an-expired-email-domain)
* [Uploading Malicious Packages](#uploading-malicious-packages)
+ [By "typosquatting" / dependency confusion](#by-typosquatting--dependency-confusion)
+ [By changing an existing package to have malicious behavior](#by-changing-an-existing-package-to-have-malicious-behavior)
We put together this page to give an overview of the most common attacks npm faces, a high-level description of how we mitigate those attacks, and links to more information.
Account Takeovers
------------------
###
By compromising passwords
This is the most common attack, not just on npm but on any web service. The best way to protect your account is to [enable two-factor authentication](configuring-two-factor-authentication) (2FA). The strongest option is to use a security-key, either built-in to your device or an external hardware key; it binds the authentication to the site you are accessing, making phishing exceedingly difficult. Not everyone has access to a security-key though, so we also support authentication apps that generate one-time passcodes for 2FA.
Because of how common this attack is, and how critical npm packages are to the broader software ecosystem, we have undertaken a phased approach in mandating 2FA for npm package maintainers. This has already rolled out to the [top-100 package maintainers](https://github.blog/2022-02-01-top-100-npm-package-maintainers-require-2fa-additional-security/) and [top-500 package maintainers](https://github.blog/changelog/2022-05-31-top-500-npm-package-maintainers-now-require-2fa/), and in the near future, maintainers of all high-impact packages (those with 1 million+ weekly downloads or 500+ dependents) will be enrolled in mandatory 2FA.
We also recognize that passwords aren’t going away any time soon. For users that don’t opt-in to 2FA, we do an enhanced login verification with a [one-time password sent to their email](receiving-a-one-time-password-over-email) to protect from account takeover.
###
By registering an expired email domain
Another method used to take over an account is by identifying accounts using an expired domain for their email address. An attacker could register the expired domain and recreate the email address used to register the account. With access to an account's registered email address an attacker could take over an account not protected by 2FA via a password reset.
When a package is published the email address associated with the account, **at the time the package was published**, is included in the public metadata. Attackers are able to utilize this public data to identify accounts that might be susceptible to account takeover. It is important to note that the **email addresses stored in public metadata of packages are not updated when a maintainer updates their email address**. As such crawling public metadata to identify accounts susceptible to expired domain takeover will result in false positives, accounts that appear to be vulnerable but are not.
npm does periodically check if accounts email addresses have expired domains or invalid MX records. When the domain has expired, we disable the account from doing a password reset and require the user to undergo account recovery or go through a successful authentication flow before they can reset their password.
Uploading Malicious Packages
-----------------------------
###
By "typosquatting" / dependency confusion
Attackers may attempt to trick others into installing a malicious package by registering a package with a similar name to a popular package, in hopes that people will mistype or otherwise confuse the two. npm is able to detect typosquat attacks and block the publishing of these packages.
A variant of this attack is when a public package is registered with the same name of a private package that an organization is using. We strongly encourage using [scoped packages](https://github.blog/2021-02-12-avoiding-npm-substitution-attacks/) to ensure that a private package isn’t being substituted with one from the public registry. While npm is not able to detect dependency confusion attacks we have a zero tolerance for malicious packages on the registry. If you believe you have identified a dependency confusion packages, [please let us know](reporting-malware-in-an-npm-package)!
###
By changing an existing package to have malicious behavior
Rather than tricking people into using a similarly-named package, attackers also try to add malicious behavior to existing popular packages. In partnership with Microsoft, npm both scans packages for known malicious content, and runs the packages to look for new patterns of behavior that could be malicious. This has led to a substantial reduction in malicious content in npm packages. Furthermore, our Trust and Safety team checks and removes malicious content reported by our users. Similar to dependency confusion attacks, we are constantly updating our detection services with new examples, so if you think a package contains malicious behavior, [please let us know](reporting-malware-in-an-npm-package)!
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/threats-and-mitigations/index.mdx)
| programming_docs |
npm About npm CLI versions About npm CLI versions
======================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[About npm CLI versions](about-npm-versions)[Downloading and installing Node.js and npm](downloading-and-installing-node-js-and-npm)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [The latest release of npm](#the-latest-release-of-npm)
The npm command line interface (CLI) is released on a regular cadence. We recommend installing the release that supports your workflow:
* [latest release](#the-latest-release-of-npm): the most recent stable version.
The `latest` release of npm
----------------------------
The `latest` release of npm is the most recent stable version. When you install Node.js, npm is automatically installed. However, npm is released more frequently than Node.js, so to install the latest stable version of npm, on the command line, run:
```
npm install npm@latest -g
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/configuring-your-local-environment/about-npm-versions.mdx)
npm Adding dist-tags to packages Adding dist-tags to packages
============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Publishing a package with a dist-tag](#publishing-a-package-with-a-dist-tag)
+ [Example](#example)
* [Adding a dist-tag to a specific version of your package](#adding-a-dist-tag-to-a-specific-version-of-your-package)
+ [Example](#example-1)
Distribution tags (dist-tags) are human-readable labels that you can use to organize and label different versions of packages you publish. dist-tags supplement [semantic versioning](about-semantic-versioning). In addition to being more human-readable than semantic version numbering, tags allow publishers to distribute their packages more effectively.
For more information, see the [`dist-tag` CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-dist-tag/).
**Note:** Since dist-tags share a namespace with semantic versions, avoid dist-tags that conflict with existing version numbers. We recommend avoiding dist-tags that start with a number or the letter "v".
Publishing a package with a dist-tag
-------------------------------------
By default, running `npm publish` will tag your package with the `latest` dist-tag. To use another dist-tag, use the `--tag` flag when publishing.
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. Run the following command, replacing `<tag>` with the tag you want to use:
```
npm publish --tag <tag>
```
###
Example
To publish a package with the "beta" dist-tag, on the command line, run the following command in the root directory of your package:
```
npm publish --tag beta
```
Adding a dist-tag to a specific version of your package
--------------------------------------------------------
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. Run the following command, replacing `<package_name>` with the name of your package, `<version>` with your package version number, and `<tag>` with the distribution tag:
```
npm dist-tag add <package-name>@<version> [<tag>]
```
###
Example
To add the "stable" tag to the 1.4.0 version of the "example-package" package, you would run the following command:
```
npm dist-tag add [email protected] stable
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/adding-dist-tags-to-packages.mdx)
npm Using deprecated packages Using deprecated packages
=========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
If you install a package, and it prints a deprecation message, we recommend following the instructions, if possible.
That might mean updating to a new version, or updating your package dependencies.
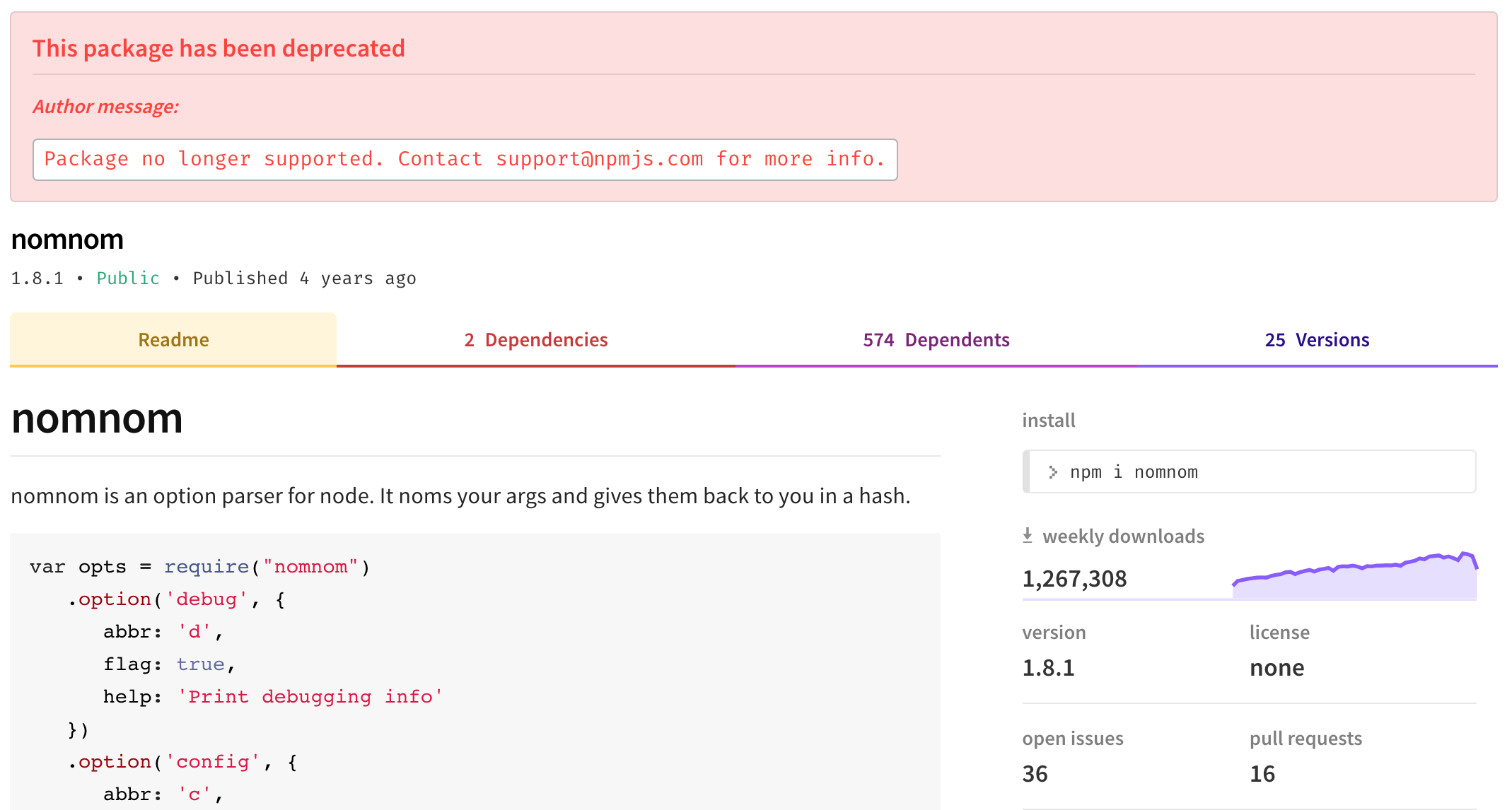
A deprecation message doesn't always mean the package or version is unusable; it may mean the package is unmaintained and will no longer be updated by the publisher.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/using-deprecated-packages.mdx)
npm Updating user account billing settings Updating user account billing settings
======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Upgrading to a paid user account plan](upgrading-to-a-paid-user-account-plan)[Viewing, downloading, and emailing receipts for your npm user account](viewing-downloading-and-emailing-receipts-for-your-user-account)[Updating user account billing settings](updating-user-account-billing-settings)[Downgrading to a free user account plan](downgrading-to-a-free-user-account-plan)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Updating credit card information](#updating-credit-card-information)
* [Updating billing receipt email and extra receipt information](#updating-billing-receipt-email-and-extra-receipt-information)
**Note:** This article only applies to users of the public npm registry.
You can update the credit card used to pay for your paid user account plan. Updating your credit card will not change your billing cycle date, and the new credit card will be charged on the next billing cycle.
**Note:** If the credit card used to pay for your paid user account plan expires, or we are otherwise are unable to charge your card, you have a grace period of nine days to update the card.
Updating credit card information
---------------------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. Under "monthly bill", click **Edit Payment Info**.
4. In the billing information dialog box, enter your billing information:
* Email: the email address used for the billing contact
* Name: the name on the credit card used to pay
* Street, City, ZIP Code, Country: the billing address associated with the credit card
5. Click **Payment Info**.
6. In the credit card information dialog box, enter your credit card information:
* Card number
* MM / YY: the month and year of the card expiration date
* CVC: the three-digit code on the credit card
7. To save your credit card information for other payments on npm, select "Remember me".
8. Click **Update Card**.
Updating billing receipt email and extra receipt information
-------------------------------------------------------------
You can update the email address used for receipts, and add extra information to the receipt for your paid user account plan, such as your business name, VAT identification number, or address of record. Updated billing information will appear on all receipts immediately.
**Note:** The billing email is used for receipts only and is not required to match the email address of the person whose card is used to pay for the paid user account plan.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. On the Billing Information page, under "monthly bill", select **View Billing History**.
4. At the bottom of the Billing History dialog box, click "Receipt Settings".
5. To add a business name, VAT number, address of record, or other information to your receipts, in the "Extra Billing Information" text box, type the information.
6. To update the email address used for receipts, beside "Send my receipts", select the checkbox and type the email address that should receive billing receipts.
7. Click **Save**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/paying-for-your-npm-user-account/updating-user-account-billing-settings.mdx)
npm Integrations Integrations
============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
* [Integrating npm with external services](integrations/integrating-npm-with-external-services)
+ [About access tokens](about-access-tokens)
+ [Creating and viewing access tokens](creating-and-viewing-access-tokens)
+ [Revoking access tokens](revoking-access-tokens)
+ [Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)
+ [Docker and private modules](docker-and-private-modules)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/index.mdx)
npm Deleting your npm user account Deleting your npm user account
==============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Managing your profile settings](managing-your-profile-settings)[Changing your npm username](changing-your-npm-username)[Deleting your npm user account](deleting-your-npm-user-account)[Requesting an export of your personal data](requesting-your-data)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
From the web, you can delete your npm user account.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. On this page, you will find a button to delete your account. Click that.
4. You will now be presented with an overview of how many npm packages will be deleted and deprecated as part of your account deletion. If you agree with this, then enter your username and click "Delete this account".
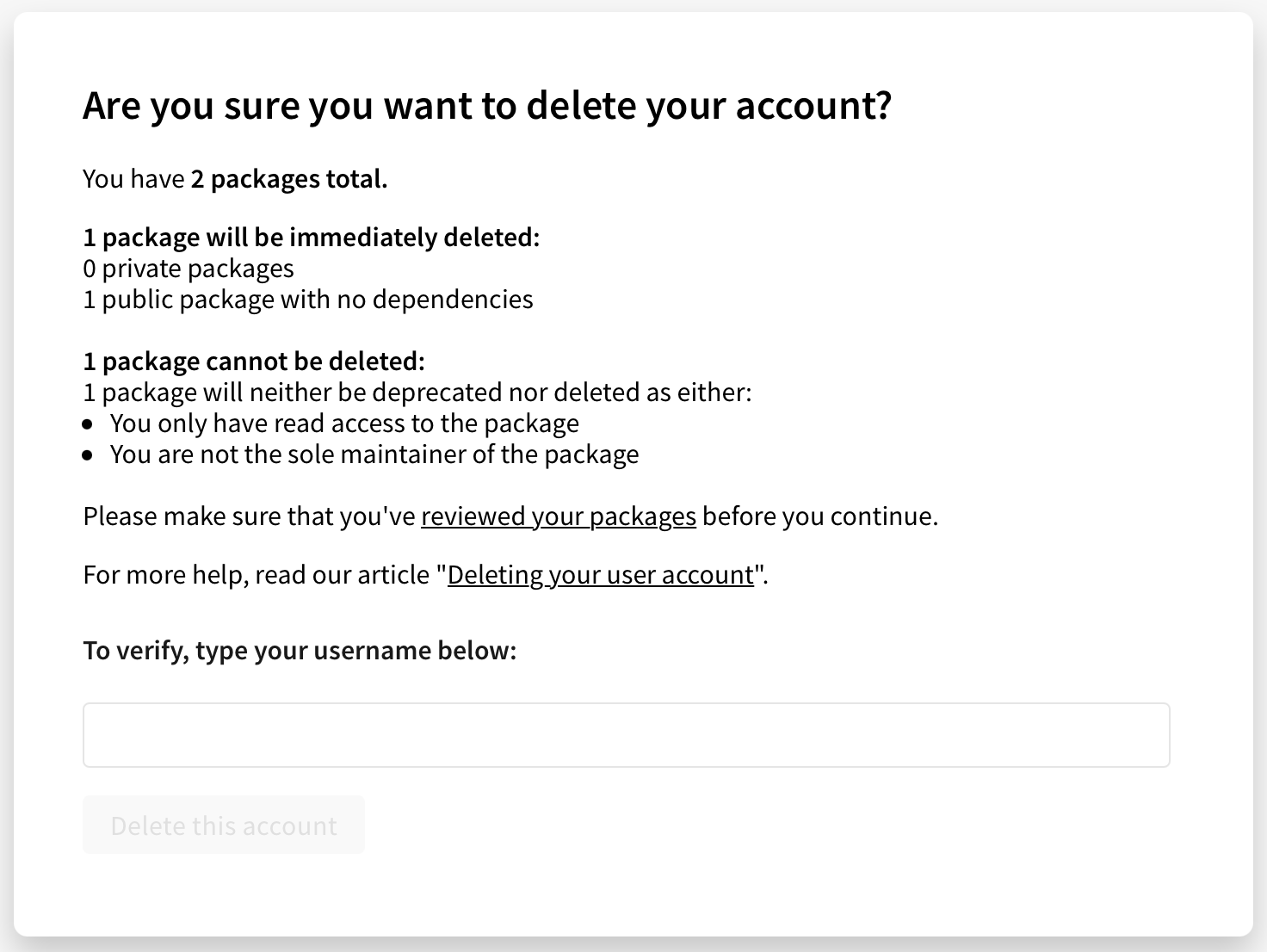
5. You will be immediately logged out, and will not be able to log back in.
In some cases, you will be presented with an error if we were unable to automatically delete your account. For example. if you are the sole owner of an organization you will need to add an additional owner before your account can be deleted. You will be presented clear instructions of what you will need to do in order to delete your account.
If you are in doubt about deleting your account, [contact npm Support](https://www.npmjs.com/support).[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/managing-your-npm-user-account/deleting-your-npm-user-account.mdx)
npm About scopes About scopes
============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Scopes and package visibility](#scopes-and-package-visibility)
Note: You must be using npm version 2 or greater to use scopes. To upgrade to the latest version of npm, on the command line, run
```
npm install npm@latest -g
```
When you sign up for an npm user account or create an organization, you are granted a scope that matches your user or organization name. You can use this scope as a namespace for related packages.
A scope allows you to create a package with the same name as a package created by another user or organization without conflict.
When listed as a dependent in a `package.json` file, scoped packages are preceded by their scope name. The scope name is everything between the `@` and the slash:
* **"npm" scope:**
```
@npm/package-name
```
* **"npmcorp" scope:**
```
@npmcorp/package-name
```
To create and publish public scoped packages, see "[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)".
To create and publish private scoped packages, see "[Creating and publishing private packages](creating-and-publishing-private-packages)".
Scopes and package visibility
------------------------------
* Unscoped packages are always public.
* [Private packages](about-private-packages) are always scoped.
* Scoped packages are private by default; you must pass a command-line flag when publishing to make them public.
For more information on package scope and visibility, see "[Package scope, access level, and visibility](package-scope-access-level-and-visibility)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/about-scopes.mdx)
npm About ECDSA registry signatures About ECDSA registry signatures
===============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Migrating from PGP to ECDSA signatures](#migrating-from-pgp-to-ecdsa-signatures)
* [Supporting signatures on third-party registries](#supporting-signatures-on-third-party-registries)
Packages published to the public npm registry are signed to make it possible to detect if the package content has been tampered with.
Signing and verifying published packages protects against an attacker controlling a registry mirror or proxy where they attempt to intercept and tamper with the package tarball content.
Migrating from PGP to ECDSA signatures
---------------------------------------
**Note:** PGP signatures will be deprecated early 2023, in favour of ECDSA registry signatures. More information will soon be provided.
The public npm registry is migrating away from the existing PGP signatures to ECDSA signatures that are more compact and can be verified without extra dependencies in the npm CLI.
Signature verification was previously a multi-step process involving the Keybase CLI, as well as manually retrieving and parsing the signature from the package metadata.
Read more about [migrating and verifying signatures](verifying-registry-signatures) using the npm CLI.
Supporting signatures on third-party registries
------------------------------------------------
The npm CLI supports registry signatures and signing keys provided by any registry if the following conventions are followed:
**1. Signatures are provided in the package's `packument` in each published version within the `dist` object:**
```
"dist":{
..omitted..,
"signatures": [{
"keyid": "SHA256:{{SHA256_PUBLIC_KEY}}",
"sig": "a312b9c3cb4a1b693e8ebac5ee1ca9cc01f2661c14391917dcb111517f72370809..."
}],
```
See this [example of a signed package from the public npm registry](https://registry.npmjs.org/light-cycle/1.4.3).
To generate the signature, sign the package name, version and tarball sha integrity: `${package.name}@${package.version}:${package.dist.integrity}`.
The current best practice is to use a [Key Management System](https://en.wikipedia.org/wiki/Key_management#Key_management_system) that does the signing operation on a [Hardware Security Module (HSM)](https://en.wikipedia.org/wiki/Hardware_security_module) in order to not directly handle the private key part, which reduces the attack surface.
The `keyid` must match one of the public signing keys below.
**2. Public signing keys are provided at `registry-host.tld/-/npm/v1/keys` in the following format:**
```
{
"keys": [{
"expires": null,
"keyid": "SHA256:{{SHA256_PUBLIC_KEY}}",
"keytype": "ecdsa-sha2-nistp256",
"scheme": "ecdsa-sha2-nistp256",
"key": "{{B64_PUBLIC_KEY}}"
}]
}
```
Keys response:
* `expires`: null or a simplified extended [ISO 8601 format](https://en.wikipedia.org/wiki/ISO_8601): `YYYY-MM-DDTHH:mm:ss.sssZ`
* `keydid`: sha256 fingerprint of the public key
* `keytype`: only `ecdsa-sha2-nistp256` is currently supported by the npm CLI
* `scheme`: only `ecdsa-sha2-nistp256` is currently supported by the npm CLI
* `key`: base64 encoded public key
See this [example key's response from the public npm registry](https://registry.npmjs.org/-/npm/v1/keys).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/about-registry-signatures.mdx)
| programming_docs |
npm Adding organization members to teams Adding organization members to teams
====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Managing teams from the CLI](#managing-teams-from-the-cli)
As an organization owner or team admin, you can add organization members to teams to give them access to a specific set of packages governed by the organization.
**Note:** An npm user must be a member of your organization before you can add them to a team. To add a member to your organization, see "[Adding members to your organization](adding-members-to-your-organization)".
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. Beside the team you want to add members to, click **Members**.
6. In the "Username" field, type the npm username of the organization member you would like to add to your team.
7. Click **+ Add User**.
**Note:** organization members are not notified when they are added to a team. We recommend telling the organization member you have added them to a team.
Managing teams from the CLI
----------------------------
If you would like to manage the membership of your team from the command line interface (CLI), you can use:
```
npm team
```
For more information, see the [CLI documentation on teams](https://docs.npmjs.com/cli/v8/commands/npm-team/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/adding-organization-members-to-teams.mdx)
npm Getting started Getting started
===============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
* [Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
+ [Creating a new user account on the public registry](creating-a-new-npm-user-account)
+ [Creating a strong password](creating-a-strong-password)
+ [Receiving a one-time password over email](receiving-a-one-time-password-over-email)
+ [About two-factor authentication](about-two-factor-authentication)
+ [Configuring two-factor authentication](configuring-two-factor-authentication)
+ [Accessing npm using two-factor authentication](accessing-npm-using-2fa)
+ [Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
* [Managing your npm user account](getting-started/managing-your-npm-user-account)
+ [Managing your profile settings](managing-your-profile-settings)
+ [Changing your npm username](changing-your-npm-username)
+ [Deleting your npm user account](deleting-your-npm-user-account)
+ [Requesting an export of your personal data](requesting-your-data)
* [Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
+ [Upgrading to a paid user account plan](upgrading-to-a-paid-user-account-plan)
+ [Viewing, downloading, and emailing receipts for your npm user account](viewing-downloading-and-emailing-receipts-for-your-user-account)
+ [Updating user account billing settings](updating-user-account-billing-settings)
+ [Downgrading to a free user account plan](downgrading-to-a-free-user-account-plan)
* [Configuring your local environment](getting-started/configuring-your-local-environment)
+ [About npm CLI versions](about-npm-versions)
+ [Downloading and installing Node.js and npm](downloading-and-installing-node-js-and-npm)
* [Troubleshooting](getting-started/troubleshooting)
+ [Generating and locating npm-debug.log files](generating-and-locating-npm-debug.log-files)
+ [Common errors](common-errors)
+ [Try the latest stable version of node](try-the-latest-stable-version-of-node)
+ [Try the latest stable version of npm](try-the-latest-stable-version-of-npm)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/index.mdx)
npm Organizations Organizations
=============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Organizations allow teams of contributors to read and write public and private packages. Organizations are free when they publish public packages. When organizations publish private packages, an npm Teams subscription is required. For more information on npm Teams pricing, see our [products page](https://www.npmjs.com/pricing).* [Creating and managing organizations](organizations/creating-and-managing-organizations)
+ [Creating an organization](creating-an-organization)
+ [Converting your user account to an organization](converting-your-user-account-to-an-organization)
+ [Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)
+ [Renaming an organization](renaming-an-organization)
+ [Deleting an organization](deleting-an-organization)
* [Paying for your organization](organizations/paying-for-your-organization)
+ [Upgrading to a paid organization plan](upgrading-to-a-paid-organization-plan)
+ [Viewing, downloading, and emailing receipts for your organization](viewing-downloading-and-emailing-receipts-for-your-organization)
+ [Updating organization billing settings](updating-organization-billing-settings)
+ [Downgrading to a free organization plan](downgrading-to-a-free-organization-plan)
* [Managing organization members](organizations/managing-organization-members)
+ [Adding members to your organization](adding-members-to-your-organization)
+ [Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)
+ [Organization roles and permissions](organization-roles-and-permissions)
+ [Managing organization permissions](managing-organization-permissions)
+ [Removing members from your organization](removing-members-from-your-organization)
* [Managing teams](organizations/managing-teams)
+ [About the developers team](about-developers-team)
+ [Creating teams](creating-teams)
+ [Adding organization members to teams](adding-organization-members-to-teams)
+ [Removing organization members from teams](removing-organization-members-from-teams)
+ [Managing team access to organization packages](managing-team-access-to-organization-packages)
+ [Removing teams](removing-teams)
* [Managing organization packages](organizations/managing-organization-packages)
+ [About organization scopes and packages](about-organization-scopes-and-packages)
+ [Configuring your npm client with your organization settings](configuring-your-npm-client-with-your-organization-settings)
+ [Creating and publishing an organization scoped package](creating-and-publishing-an-organization-scoped-package)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/index.mdx)
npm Creating a new user account on the public registry Creating a new user account on the public registry
==================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating an account on the website](#creating-an-account-on-the-website)
* [Testing your new account with npm login](#testing-your-new-account-with-npm-login)
If you do not already have an npm user account, you can create an account in order to share and download Javascript packages on the public registry.
Creating an account on the website
-----------------------------------
1. Go to the [npm signup page](https://www.npmjs.com/signup)
2. In the user signup form, type in the fields:
* **Username:** The username that will be displayed when you publish packages or interact with other npm users on npmjs.com. Your username must be lower case, and can contain hyphens and numerals.
* **Email address:** Your public email address will be added to the metadata of your packages and will be visible to anyone who downloads your packages. We will also send email to this account when you update packages, as well as occasional product updates and information.
* **Password**: Your password must meet [our password guidelines](creating-a-strong-password).
3. Read the [End User License Agreement](https://www.npmjs.com/policies/terms) and [Privacy Policy](https://www.npmjs.com/policies/privacy), and indicate that you agree to them.
4. Click **Create An Account**.
**Note:** After signing up for an npm account, you will receive an account verification email. You must verify your email address in order to publish packages to the registry.
Testing your new account with npm login
----------------------------------------
Use the [`npm login`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) command to test logging in to your new account.
**Note:** If you misspell your existing account username when you log in with the `npm login` command, you will create a new account with the misspelled name. For help with accidentally-created accounts, [contact npm Support](https://www.npmjs.com/support).
1. On the command line, type the following command:
```
npm login
```
2. When prompted, enter your username, password, and email address.
3. If you have [two-factor authentication](about-two-factor-authentication) enabled, when prompted, enter a one-time password.
4. To test that you have successfully logged in, type:
```
npm whoami
```
Your npm username should be displayed.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/creating-a-new-npm-user-account.mdx)
npm Generating and locating npm-debug.log files Generating and locating npm-debug.log files
===========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Generating and locating npm-debug.log files](generating-and-locating-npm-debug.log-files)[Common errors](common-errors)[Try the latest stable version of node](try-the-latest-stable-version-of-node)[Try the latest stable version of npm](try-the-latest-stable-version-of-npm)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
When a package fails to install or publish, the npm CLI will generate an `npm-debug.log` file. This log file can help you figure out what went wrong.
If you need to generate a `npm-debug.log` file, you can run one of these commands.
For installing packages:
```
npm install --timing
```
For publishing packages:
```
npm publish --timing
```
You can find the `npm-debug.log` file in your `.npm` directory. To find your `.npm` directory, use `npm config get cache`.
If you use a CI environment, your logs are likely located elsewhere. For example, in Travis CI, you can find them in the `/home/travis/build` directory.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/troubleshooting/generating-and-locating-npm-debug.log-files.mdx)
npm Accepting or rejecting an organization invitation Accepting or rejecting an organization invitation
=================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Adding members to your organization](adding-members-to-your-organization)[Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](organization-roles-and-permissions)[Managing organization permissions](managing-organization-permissions)[Removing members from your organization](removing-members-from-your-organization)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Accepting an organization invitation](#accepting-an-organization-invitation)
* [Rejecting an organization invitation](#rejecting-an-organization-invitation)
Accepting an organization invitation
-------------------------------------
If you receive an invitation to an organization, you have to accept the invitation over email to be added to the organization.
You have the option to use a different email address than the one that received the invitation to join the organization.
1. Click the verification link in the organization invitation email.
2. You will be prompted to log into your npm user account. If you don't have an npm user account, you can sign up for one.
Rejecting an organization invitation
-------------------------------------
If you are invited to an organization that you do not want to join, you can let the invitation expire. Organization invitations expire after one week.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/accepting-or-rejecting-an-organization-invitation.mdx)
npm Downgrading to a free user account plan Downgrading to a free user account plan
=======================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Upgrading to a paid user account plan](upgrading-to-a-paid-user-account-plan)[Viewing, downloading, and emailing receipts for your npm user account](viewing-downloading-and-emailing-receipts-for-your-user-account)[Updating user account billing settings](updating-user-account-billing-settings)[Downgrading to a free user account plan](downgrading-to-a-free-user-account-plan)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Note:** This article only applies to users of the public npm registry.
If you have a paid user account, but no longer need private packages, you can downgrade your paid organization to a free organization. When you downgrade from a paid to a free organization, you will lose the ability to install and publish private packages at the end of your last paid billing cycle. Your private packages will *not* be made publicly visible when you downgrade to a free plan.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. Under "change plan", click **Downgrade Plan**.
4. Under "Are you sure?", click **Downgrade to a free account**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/paying-for-your-npm-user-account/downgrading-to-a-free-user-account-plan.mdx)
| programming_docs |
npm Creating an organization Creating an organization
========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Creating an organization](creating-an-organization)[Converting your user account to an organization](converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)[Renaming an organization](renaming-an-organization)[Deleting an organization](deleting-an-organization)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Any npm user can create an organization to manage contributor access to packages governed by the organization.
**Note:** You need an npm user account to create an organization. To create a user account, visit the [account signup page](https://www.npmjs.com/signup)".
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Add an Organization**.
3. On the organization creation page, in the **Name** field, type a name for your organization. Your organization name will also be your organization scope.
4. Under the **Name** field, choose either the "Unlimited private packages" paid plan or the "Unlimited public packages" free plan and click **Buy** or **Create**.
5. (Optional) On the organization invitation page, type the npm username or email address of a person you would like to add to your organization as a member and select a team to invite them to, then click **Invite**.
6. Click **Continue**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/creating-an-organization.mdx)
npm Removing teams Removing teams
==============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
As an organization owner or team admin, you can remove teams that no longer need access to a set of packages governed by your organization. Removing the team will not remove the team members or packages from your organization.
**Note:** if you remove all teams referencing a particular package, it will be orphaned and you will lose access to it. If this happens, [contact npm Support](https://www.npmjs.com/support).
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. Beside the name of the team you want to remove, click **X**.
**Note:** You cannot remove the developers team, [learn more about the developers team](about-developers-team).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/removing-teams.mdx)
npm About semantic versioning About semantic versioning
=========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Incrementing semantic versions in published packages](#incrementing-semantic-versions-in-published-packages)
* [Using semantic versioning to specify update types your package can accept](#using-semantic-versioning-to-specify-update-types-your-package-can-accept)
+ [Example](#example)
* [Resources](#resources)
To keep the JavaScript ecosystem healthy, reliable, and secure, every time you make significant updates to an npm package you own, we recommend publishing a new version of the package with an updated version number in the [`package.json` file](creating-a-package-json-file) that follows the [semantic versioning spec](http://semver.org/). Following the semantic versioning spec helps other developers who depend on your code understand the extent of changes in a given version, and adjust their own code if necessary.
Note: If you introduce a change that breaks a package dependency, we strongly recommend incrementing the version major number; see below for details.
Incrementing semantic versions in published packages
-----------------------------------------------------
To help developers who rely on your code, we recommend starting your package version at `1.0.0` and incrementing as follows:
| Code status | Stage | Rule | Example version |
| --- | --- | --- | --- |
| First release | New product | Start with 1.0.0 | 1.0.0 |
| Backward compatible bug fixes | Patch release | Increment the third digit | 1.0.1 |
| Backward compatible new features | Minor release | Increment the middle digit and reset last digit to zero | 1.1.0 |
| Changes that break backward compatibility | Major release | Increment the first digit and reset middle and last digits to zero | 2.0.0 |
Using semantic versioning to specify update types your package can accept
--------------------------------------------------------------------------
You can specify which update types your package can accept from dependencies in your package's `package.json` file.
For example, to specify acceptable version ranges up to 1.0.4, use the following syntax:
* Patch releases: `1.0` or `1.0.x` or `~1.0.4`
* Minor releases: `1` or `1.x` or `^1.0.4`
* Major releases: `*` or `x`
For more information on semantic versioning syntax, see the [npm semver calculator](https://semver.npmjs.com/).
###
Example
```
"dependencies": {
"my_dep": "^1.0.0",
"another_dep": "~2.2.0"
},
```
Resources
----------
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/about-semantic-versioning.mdx)
npm About package README files About package README files
==========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating and adding a README.md file to a package](#creating-and-adding-a-readmemd-file-to-a-package)
* [Updating an existing package README file](#updating-an-existing-package-readme-file)
To help others find your packages on npm and have a good experience using your code in their projects, we recommend including a README file in your package directory. Your README file may include directions for installing, configuring, and using the code in your package, as well as any other information a user may find helpful. The README file will be shown on the package page.
An npm package README file must be in the root-level directory of the package.
Creating and adding a README.md file to a package
--------------------------------------------------
1. In a text editor, in your package root directory, create a file called `README.md`.
2. In the `README.md` file, add useful information about your package.
3. Save the `README.md` file.
**Note:** The file extension `.md` indicates a Markdown file. For more information about Markdown, see the GitHub Guide "[Mastering Markdown](https://guides.github.com/features/mastering-markdown/#what)".
Updating an existing package README file
-----------------------------------------
The README file will only be updated on the package page when you publish a new version of your package. To update your README file:
1. In a text editor, update the contents of the `README.md` file.
2. Save the `README.md` file.
3. On the command line, in the package root directory, run the following commands:
```
npm version patch
npm publish
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/about-package-readme-files.mdx)
npm Auditing package dependencies for security vulnerabilities Auditing package dependencies for security vulnerabilities
==========================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [About security audits](#about-security-audits)
* [Running a security audit with npm audit](#running-a-security-audit-with-npm-audit)
+ [Resolving EAUDITNOPJSON and EAUDITNOLOCK errors](#resolving-eauditnopjson-and-eauditnolock-errors)
* [Reviewing and acting on the security audit report](#reviewing-and-acting-on-the-security-audit-report)
+ [Security vulnerabilities found with suggested updates](#security-vulnerabilities-found-with-suggested-updates)
- [SEMVER warnings](#semver-warnings)
+ [Security vulnerabilities found requiring manual review](#security-vulnerabilities-found-requiring-manual-review)
- [Check for mitigating factors](#check-for-mitigating-factors)
- [Update dependent packages if a fix exists](#update-dependent-packages-if-a-fix-exists)
- [Fix the vulnerability](#fix-the-vulnerability)
- [Open an issue in the package or dependent package issue tracker](#open-an-issue-in-the-package-or-dependent-package-issue-tracker)
+ [No security vulnerabilities found](#no-security-vulnerabilities-found)
* [Turning off npm audit on package installation](#turning-off-npm-audit-on-package-installation)
+ [Installing a single package](#installing-a-single-package)
+ [Installing all packages](#installing-all-packages)
About security audits
----------------------
A security audit is an assessment of package dependencies for security vulnerabilities. Security audits help you protect your package's users by enabling you to find and fix known vulnerabilities in dependencies that could cause data loss, service outages, unauthorized access to sensitive information, or other issues.
Running a security audit with `npm audit`
------------------------------------------
**Note:** The `npm audit` command is available in npm@6. To upgrade, run `npm install npm@latest -g`.
The [`npm audit` command](https://docs.npmjs.com/cli/v8/commands/npm-audit/) submits a description of the dependencies configured in your package to your default registry and asks for a report of known vulnerabilities. `npm audit` checks direct dependencies, devDependencies, bundledDependencies, and optionalDependencies, but does not check peerDependencies.
`npm audit` automatically runs when you install a package with `npm install`. You can also run `npm audit` manually on your [locally installed packages](downloading-and-installing-packages-locally) to conduct a security audit of the package and produce a report of dependency vulnerabilities and, if available, suggested patches.
1. On the command line, navigate to your package directory by typing `cd path/to/your-package-name` and pressing **Enter**.
2. Ensure your package contains `package.json` and `package-lock.json` files.
3. Type `npm audit` and press **Enter**.
4. Review the audit report and run recommended commands or investigate further if needed.
###
Resolving `EAUDITNOPJSON` and `EAUDITNOLOCK` errors
`npm audit` requires packages to have `package.json` and `package-lock.json` files.
* If you get an `EAUDITNOPJSON` error, create a `package.json` file by following the steps in "[Creating a package.json file](creating-a-package-json-file)".
* If you get an `EAUDITNOLOCK` error, make sure your package has a `package.json` file, then create the package lock file by running `npm i --package-lock-only`.
Reviewing and acting on the security audit report
--------------------------------------------------
Running `npm audit` will produce a report of security vulnerabilities with the affected package name, vulnerability severity and description, path, and other information, and, if available, commands to apply patches to resolve vulnerabilities. For more information on the fields in the audit report, see "[About audit reports](about-audit-reports)"
###
Security vulnerabilities found with suggested updates
If security vulnerabilities are found and updates are available, you can either:
* Run the `npm audit fix` subcommand to automatically install compatible updates to vulnerable dependencies.
* Run the recommended commands individually to install updates to vulnerable dependencies. (Some updates may be semver-breaking changes; for more information, see "[SEMVER warnings](#semver-warnings)".)
####
SEMVER warnings
If the recommended action is a potential breaking change (semantic version major change), it will be followed by a `SEMVER WARNING` that says "SEMVER WARNING: Recommended action is a potentially breaking change". If the package with the vulnerability has changed its API, you may need to make additional changes to your package's code.
###
Security vulnerabilities found requiring manual review
If security vulnerabilities are found, but no patches are available, the audit report will provide information about the vulnerability so you can investigate further.
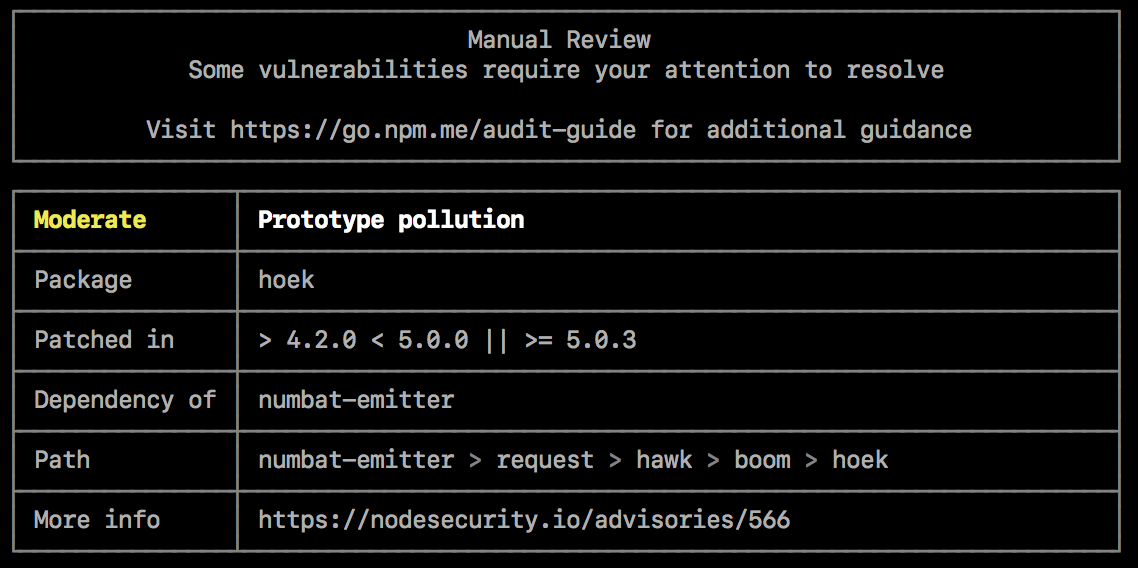
To address the vulnerability, you can
* [Check for mitigating factors](#check-for-mitigating-factors)
* [Update dependent packages if a fix exists](#update-dependent-packages-if-a-fix-exists)
* [Fix the vulnerability](#fix-the-vulnerability)
* [Open an issue in the package or dependent package issue tracker](#open-an-issue-in-the-package-or-dependent-package-issue-tracker)
####
Check for mitigating factors
Review the security advisory in the "More info" field for mitigating factors that may allow you to continue using the package with the vulnerability in limited cases. For example, the vulnerability may only exist when the code is used on specific operating systems, or when a specific function is called.
####
Update dependent packages if a fix exists
If a fix exists but packages that depend on the package with the vulnerability have not been updated to include the fixed version, you may want to open a pull or merge request on the dependent package repository to use the fixed version.
1. To find the package that must be updated, check the "Path" field for the location of the package with the vulnerability, then check for the package that depends on it. For example, if the path to the vulnerability is `@package-name > dependent-package > package-with-vulnerability`, you will need to update `dependent-package`.
2. On the [npm public registry](https://npmjs.com), find the dependent package and navigate to its repository. For more information on finding packages, see "[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)".
3. In the dependent package repository, open a pull or merge request to update the version of the vulnerable package to a version with a fix.
4. Once the pull or merge request is merged and the package has been updated in the [npm public registry](https://npmjs.com), update your copy of the package with `npm update`.
####
Fix the vulnerability
If a fix does not exist, you may want to suggest changes that address the vulnerability to the package maintainer in a pull or merge request on the package repository.
1. Check the "Path" field for the location of the vulnerability.
2. On the [npm public registry](https://npmjs.com), find the package with the vulnerability. For more information on finding packages, see "[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)".
3. In the package repository, open a pull or merge request to make the fix on the package repository.
4. Once the fix is merged and the package has been updated in the npm public registry, update your copy of the package that depends on the package with the fix.
####
Open an issue in the package or dependent package issue tracker
If you do not want to fix the vulnerability or update the dependent package yourself, open an issue in the package or dependent package issue tracker.
1. On the [npm public registry](https://npmjs.com), find the package with the vulnerability or the dependent package that needs an update. For more information on finding packages, see "[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)".
2. In the package or dependent package issue tracker, open an issue and include information from the audit report, including the vulnerability report from the "More info" field.
###
No security vulnerabilities found
If no security vulnerabilities are found, this means that packages with known vulnerabilities were not found in your package dependency tree. Since the advisory database can be updated at any time, we recommend regularly running `npm audit` manually, or adding `npm audit` to your continuous integration process.
Turning off `npm audit` on package installation
------------------------------------------------
###
Installing a single package
To turn off `npm audit` when installing a single package, use the `--no-audit` flag:
```
npm install example-package-name --no-audit
```
For more information, see the [`npm-install` command](https://docs.npmjs.com//cli/v8/commands/npm-install/).
###
Installing all packages
To turn off `npm audit` when installing all packages, set the `audit` setting to `false` in your user and global npmrc config files:
```
npm set audit false
```
For more information, see the [`npm-config` management command](https://docs.npmjs.com/cli/v8/commands/npm-config/) and the [`npm-config` audit setting](https://docs.npmjs.com/cli/v8/commands/npm-config/#audit).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/auditing-package-dependencies-for-security-vulnerabilities.mdx)
| programming_docs |
npm Managing organization permissions Managing organization permissions
=================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Adding members to your organization](adding-members-to-your-organization)[Accepting or rejecting an organization invitation](accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](organization-roles-and-permissions)[Managing organization permissions](managing-organization-permissions)[Removing members from your organization](removing-members-from-your-organization)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
As an organization owner, you can change the role of any member of your organization to add or remove permissions on the organization for that member.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Members**.
5. In the list of organization members, find the member whose role you want to change.
6. In the member row, to select the new role of the organization member, click **member**, **admin**, or **owner**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/managing-organization-permissions.mdx)
npm Removing organization members from teams Removing organization members from teams
========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
As an organization owner or team admin, you can remove organization members from teams if they no longer need access to packages accessible to the team.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of your organization.
4. On the organization settings page, click **Teams**.
5. In the list of team members, find the member you want to remove.
6. In the member row, to remove the member from the team, click **X**.
**Note: Removing a member from a team, even if it is the only team they are a member of, will not remove them from the organization.** To remove a member from the organization, see "[Removing members from your organization](removing-members-from-your-organization)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/removing-organization-members-from-teams.mdx)
npm Renaming an organization Renaming an organization
========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Creating an organization](creating-an-organization)[Converting your user account to an organization](converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)[Renaming an organization](renaming-an-organization)[Deleting an organization](deleting-an-organization)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Organizations cannot be renamed from the website or command line interface.
To rename an organization, as an organization owner, you must manually migrate your existing organization members, teams, and packages to a new organization, then [contact npm Support](https://www.npmjs.com/support) to have the outdated packages unpublished and the previous organization deleted.
1. [Create a new organization](creating-an-organization) with the name you want. If your old organization is on a paid plan, you must choose a paid plan for the new organization.
2. [Add the members](adding-members-to-your-organization) of your old organization to your new organization.
3. In your new organization, [create teams](creating-teams) to match teams in your old organization.
4. Republish packages to the new organization by updating the package scope in its `package.json` file to match the new organizationanization name and running `npm publish`.
5. In the new organization teams, [configure package access](managing-team-access-to-organization-packages) to match team package access in your old organization.
6. [Contact npm Support](https://www.npmjs.com/support) to have the outdated packages unpublished and the previous organization deleted.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/renaming-an-organization.mdx)
npm Revoking access tokens Revoking access tokens
======================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[About access tokens](about-access-tokens)[Creating and viewing access tokens](creating-and-viewing-access-tokens)[Revoking access tokens](revoking-access-tokens)[Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](docker-and-private-modules)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
To keep your account and packages secure, we strongly recommend revoking (deleting) tokens you no longer need or that have been compromised. You can revoke any token you have created.
1. To see a list of your tokens, on the command line, run:
```
npm token list
```
2. In the tokens table, find and copy the ID of the token you want to delete.
3. On the command line, run the following command, replacing `123456` with the ID of the token you want to delete:
```
npm token delete 123456
```
npm will report `Removed 1 token`
4. To confirm that the token has been removed, run:
```
npm token list
```
**Note:** You must use the token ID to delete a token, not the truncated version of the token. In some cases, there may be a delay of up to an hour before a token is successfully revoked.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/revoking-access-tokens.mdx)
npm Creating and viewing access tokens Creating and viewing access tokens
==================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[About access tokens](about-access-tokens)[Creating and viewing access tokens](creating-and-viewing-access-tokens)[Revoking access tokens](revoking-access-tokens)[Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](docker-and-private-modules)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating access tokens](#creating-access-tokens)
+ [Creating tokens on the website](#creating-tokens-on-the-website)
+ [Creating tokens with the CLI](#creating-tokens-with-the-cli)
- [CIDR-restricted token errors](#cidr-restricted-token-errors)
* [Viewing access tokens](#viewing-access-tokens)
+ [Viewing tokens on the website](#viewing-tokens-on-the-website)
+ [Viewing tokens on the CLI](#viewing-tokens-on-the-cli)
- [Token attributes](#token-attributes)
You can [create](#creating-access-tokens) and [view](#viewing-access-tokens) access tokens from the website and command line interface (CLI).
Creating access tokens
-----------------------
###
Creating tokens on the website
1. In the upper right corner of the page, click your profile picture, then click **Access Tokens**.
2. Click **Generate New Token**.
3. (Optional) Name your token
4. Select the type of access token:
* **Read-only**: a read-only token can only be used to download packages from the registry. It will have permission to read any private package that you have access to. This is recommended for automation and workflows where you are installing packages, but not publishing new ones.
* **Automation**: an automation token can download packages and publish new ones, but if you have two-factor authentication (2FA) configured on your account, it will **not** be enforced. You can use an automation token in continuous integration workflows and other automation systems to publish a package even when you cannot enter a one-time passcode. This is recommended for automation workflows where you are publishing new packages.
* **Publish**: a publish token can perform any action on your behalf, including downloading packages, publishing packages, and changing user settings or package settings. If you have two-factor authentication configured on your account, you will be required to enter a one-time passcode when using a publish token. This is recommended for interactive workflows.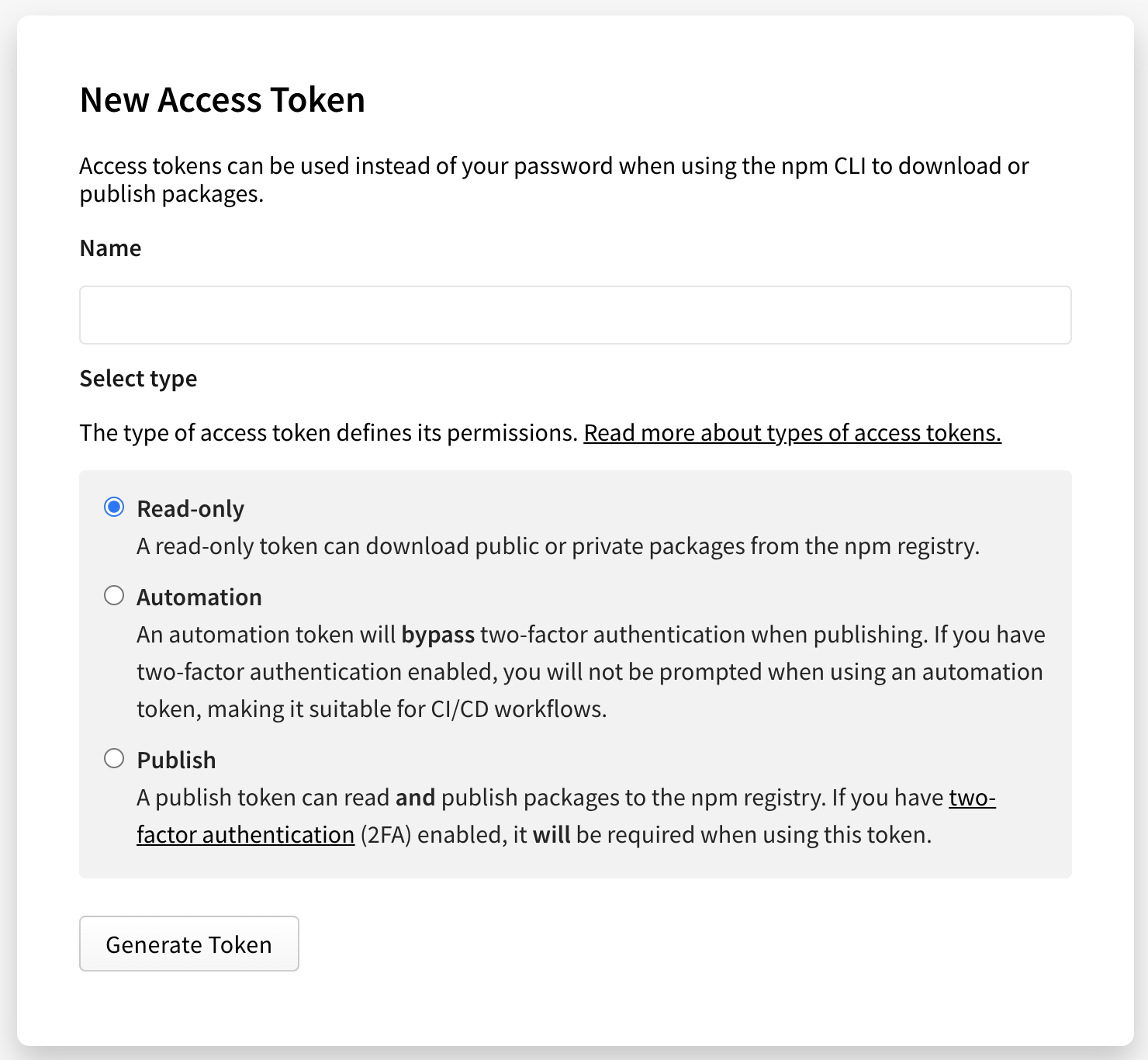
5. Click **Generate Token**.
6. Copy the token from the top of page.
###
Creating tokens with the CLI
You can create tokens with read-only permissions or read and publish permissions with the CLI; you cannot currently create automation tokens.
* **Read-only:** Tokens that allow installation and distribution only, but no publishing or other rights associated with your account.
* **Publish:** The default setting for new tokens, and most permissive token type. Publish tokens allow installation, distribution, modification, publishing, and all rights that you have on your account.
In addition, you can specify that the token is only valid for a specific IPv4 address range, using [CIDR](https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing) notation. The token will only be valid when used from the specified IP addresses.
1. To create a new token, on the command line, run:
* `npm token create` for a read and publish token
* `npm token create --read-only` for a read-only token
* `npm token create --cidr=[list]` for a CIDR-restricted read and publish token. For example, `npm token create --cidr=192.0.2.0/24`
* `npm token create --read-only --cidr=[list]` for a CIDR-restricted read-only token
2. When prompted, enter your password.
3. If you have enabled [two-factor authentication](about-two-factor-authentication), when prompted, enter a one-time password.
4. Copy the token from the **token** field in the command output.
####
CIDR-restricted token errors
If the CIDR string you enter is invalid or in an inappropriate format, you will get an error similar to the one below:
```
npm ERR! CIDR whitelist contains invalid CIDR entry: X.X.X.X./YY,Z.Z.. . .
```
Make sure you are using a valid IPv4 range and try creating the token again.
Viewing access tokens
----------------------
**Note:** Full tokens are never displayed, only the first and last four characters will be shown. You can only view a full token immediately after creation.
###
Viewing tokens on the website
To view all tokens associated with your account, in the upper right corner of the page, click your profile picture, then click **Access Tokens**.
###
Viewing tokens on the CLI
To view all tokens associated with your account, on the command line, run the following command:
```
npm token list
```
####
Token attributes
* **id:** Use the token ID to refer to the token in commands.
* **token:** The first digits of the actual token.
* **create:** Date the token was created.
* **readonly:** If yes, indicates a read-only token. If no, indicates a token with both read and publish permissions.
* **CIDR whitelist:** Restricts token use by IP address.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/creating-and-viewing-access-tokens.mdx)
npm About two-factor authentication About two-factor authentication
===============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Two-factor authentication on npm](#two-factor-authentication-on-npm)
+ [Authorization and writes](#authorization-and-writes)
+ [Authorization only](#authorization-only)
[Two-factor authentication (2FA)](https://en.wikipedia.org/wiki/Multi-factor_authentication) protects against unauthorized access to your account by confirming your identity using:
* Something you know (e.g., a password).
* Something you have (e.g., an ID badge or a cryptographic key).
* Something you are (e.g., a fingerprint or other biometric data).
**Note**: The security-key flow using [WebAuthn](https://webauthn.guide/) is currently in beta.
When you enable 2FA, you will be prompted for a second form of authentication before performing certain actions on your account or packages to which you have write access. Depending on your 2FA configuration you will be either prompted to authenticate with a [security-key](https://webauthn.guide/) or a [time-based one-time password (TOTP)](https://en.wikipedia.org/wiki/Time-based_one-time_password).
* The security-key flow allows you to use biometric devices such as Apple [Touch ID](https://support.apple.com/en-gb/HT204587), [Face ID](https://support.apple.com/en-us/HT208108) or [Windows Hello](https://support.microsoft.com/en-us/windows/learn-about-windows-hello-and-set-it-up-dae28983-8242-bb2a-d3d1-87c9d265a5f0) as well as physical keys such as [Yubikey](https://www.yubico.com/), [Thetis](https://thetis.io/) or [Feitian](https://www.ftsafe.com/) as your 2FA.
* To configure TOTP you will need to install an authenticator application that can generate OTPs such as [Authy](https://authy.com/download/), [Google Authenticator](https://support.google.com/accounts/answer/1066447), or [Microsoft Authenticator](https://www.microsoft.com/security/mobile-authenticator-app) on your mobile device.
**Note:** Two-factor authentication provides the best possible security for your account against attackers. We strongly recommend enabling 2FA on your account as soon as possible after you sign up.
Two-factor authentication on npm
---------------------------------
Two-factor authentication on npm can be enabled for authorization and writes, or authorization only.
###
Authorization and writes
By default, 2FA is enabled for authorization and writes. We will request a second form of authentication for certain authorized actions, as well as write actions.
| Action | CLI command |
| --- | --- |
| Log in to npm | [`npm login`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) |
| Change profile settings (including your password) | [`npm profile set`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Change 2FA modes for your user account | [`npm profile enable-2fa auth-and-writes`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Disable 2FA for your user account | [`npm profile disable-2fa`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Create tokens | [`npm token create`](https://docs.npmjs.com/cli/v8/commands/npm-token/) |
| Revoke tokens | [`npm token revoke`](https://docs.npmjs.com/cli/v8/commands/npm-token/) |
| Publish packages | [`npm publish`](https://docs.npmjs.com/cli/v8/commands/npm-publish/) |
| Unpublish packages | [`npm unpublish`](https://docs.npmjs.com/cli/v8/commands/npm-unpublish/) |
| Deprecate packages | [`npm deprecate`](https://docs.npmjs.com/cli/v8/commands/npm-deprecate/) |
| Change package visibility | [`npm access public/restricted`](https://docs.npmjs.com/cli/v8/commands/npm-access/) |
| Change user and team package access | [`npm access grant/revoke`](https://docs.npmjs.com/cli/v8/commands/npm-access/) |
| [Change package 2FA requirements](requiring-2fa-for-package-publishing-and-settings-modification) | [`npm access 2fa-required/2fa-not-required`](https://docs.npmjs.com/cli/v8/commands/npm-access/) |
###
Authorization only
If you enable 2FA for authorization only. We will request a second form of authentication only for certain authorized actions.
| Action | CLI command |
| --- | --- |
| Log in to npm | [`npm login`](https://docs.npmjs.com/cli/v8/commands/npm-adduser/) |
| Change profile settings (including your password) | [`npm profile set`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Change 2FA modes for your user account | [`npm profile enable-2fa auth-only`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Disable 2FA for your user account | [`npm profile disable-2fa`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) |
| Create tokens | [`npm token create`](https://docs.npmjs.com/cli/v8/commands/npm-token/) |
| Revoke tokens | [`npm token revoke`](https://docs.npmjs.com/cli/v8/commands/npm-token/) |
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/about-two-factor-authentication.mdx)
| programming_docs |
npm Configuring two-factor authentication Configuring two-factor authentication
=====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Creating a new user account on the public registry](creating-a-new-npm-user-account)[Creating a strong password](creating-a-strong-password)[Receiving a one-time password over email](receiving-a-one-time-password-over-email)[About two-factor authentication](about-two-factor-authentication)[Configuring two-factor authentication](configuring-two-factor-authentication)[Accessing npm using two-factor authentication](accessing-npm-using-2fa)[Recovering your 2FA-enabled account](recovering-your-2fa-enabled-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Prerequisites](#prerequisites)
* [Configuring 2FA from the website](#configuring-2fa-from-the-website)
+ [Enabling 2FA](#enabling-2fa)
+ [Disabling 2FA for writes](#disabling-2fa-for-writes)
+ [Disabling 2FA](#disabling-2fa)
* [Configuring 2FA from the command line](#configuring-2fa-from-the-command-line)
+ [Enabling 2FA from the command line](#enabling-2fa-from-the-command-line)
+ [Sending a one-time password from the command line](#sending-a-one-time-password-from-the-command-line)
+ [Removing 2FA from the command line](#removing-2fa-from-the-command-line)
* [Resolving TOTP errors](#resolving-totp-errors)
You can enable two-factor authentication (2FA) on your npm user account to protect against unauthorized access to your account and packages, either by using a [security-key](https://webauthn.guide/) or [time-based one-time password (TOTP)](https://en.wikipedia.org/wiki/Time-based_one-time_password) from a mobile app.
**Note**: The security-key flow using [WebAuthn](https://webauthn.guide/) is currently in beta.
Prerequisites
--------------
Before you enable 2FA on your npm user account, you must:
* Update your npm client to version 5.5.1 or higher.
* To configure a security-key requires a modern browser that support [WebAuthn](https://caniuse.com/#search=webauthn). This will allow you to configure a biometric devices such as Apple [Touch ID](https://support.apple.com/en-gb/HT204587), [Face ID](https://support.apple.com/en-us/HT208108), or [Windows Hello](https://support.microsoft.com/en-us/windows/learn-about-windows-hello-and-set-it-up-dae28983-8242-bb2a-d3d1-87c9d265a5f0) as well as physical keys such as [Yubikey](https://www.yubico.com/), [Thetis](https://thetis.io/), or [Feitian](https://www.ftsafe.com/).
* To configure TOTP you will need to install an authenticator application that can generate OTPs such as [Authy](https://authy.com/download/), [Google Authenticator](https://support.google.com/accounts/answer/1066447), or [Microsoft Authenticator](https://www.microsoft.com/security/mobile-authenticator-app) on your mobile device.
For more information on supported 2FA methods, see "[About two-factor authentication](about-two-factor-authentication)".
**Note:** npm does not accept SMS (text-to-phone) as a 2FA method.
Configuring 2FA from the website
---------------------------------
###
Enabling 2FA
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. On the account settings page, under "Two-Factor Authentication", click **Enable 2FA**.
4. When prompted provide your current account password and then click **Confirm password to continue**.
5. On the 2FA method page, select the method you would like to enable and click **Continue**. For more information on supported 2FA methods, see "[About two-factor authentication](about-two-factor-authentication)".
6. Configure the 2FA method of your choice:
* When using a **security-key**, provide a name for it and click **Add security key**. Follow the browser specific steps to add your security-key.
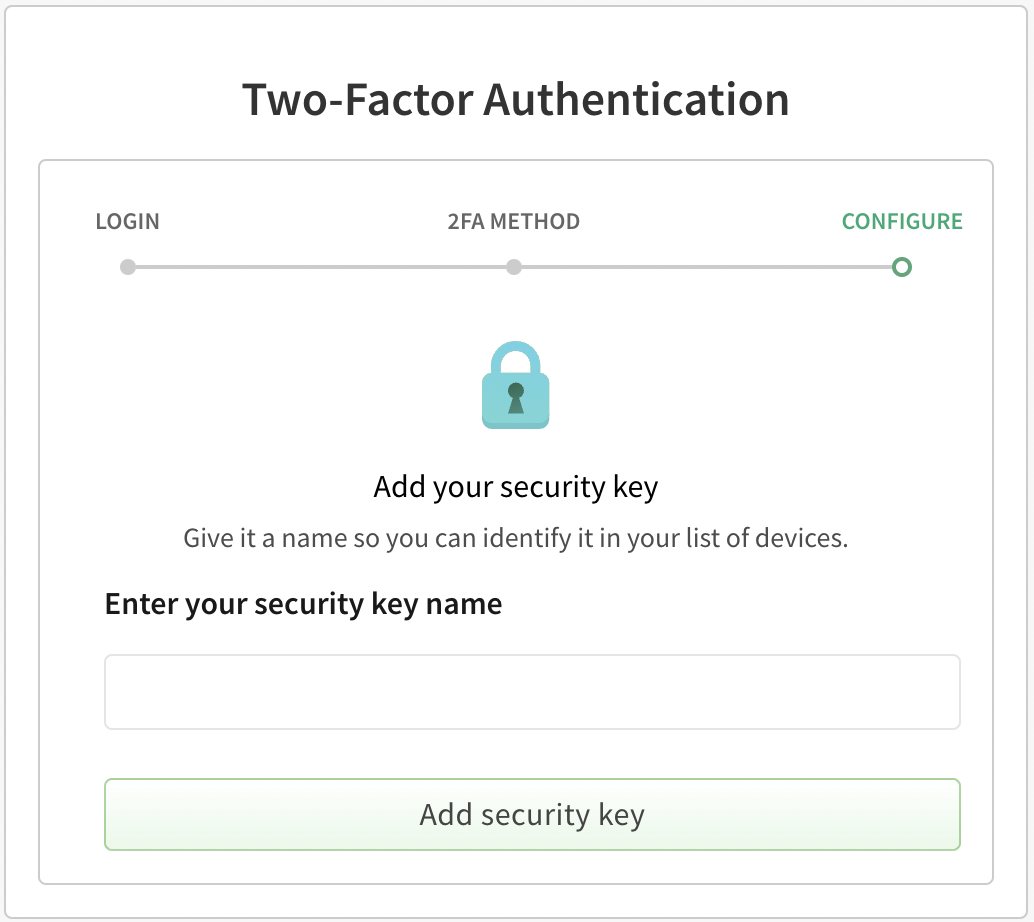
Below is an example of configuration from Microsoft Edge running on a MacOS
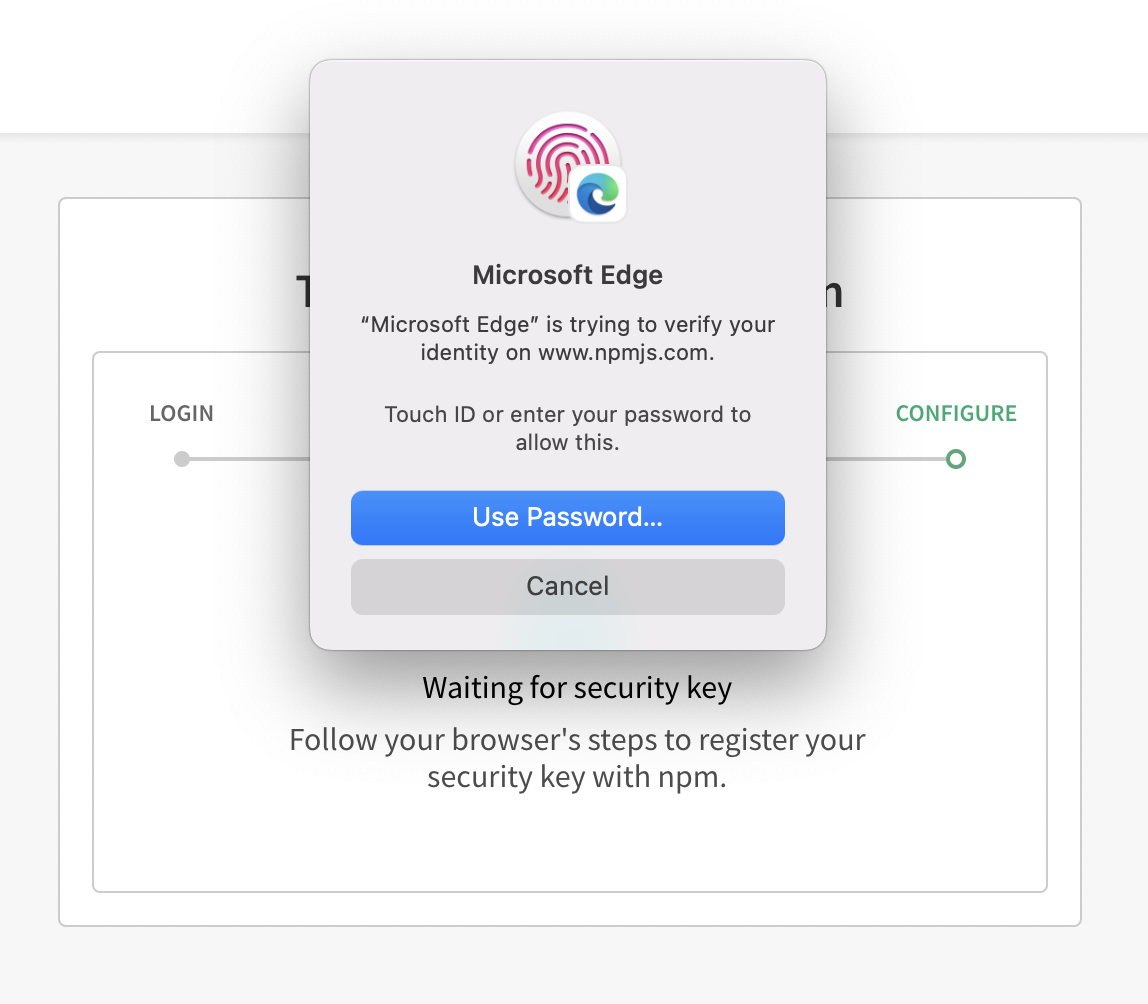
* When using an **authenticator application** on your phone, open it and scan the QR code on the two-step verification page. Enter the code generated by the app, then click **Verify**.
7. On the recovery code page, copy the recovery codes to your computer or other safe location that is not your second factor device. We recommend using a password manager.
*Recovery codes are the only way to recover your account if you lose access to your second factor device. Each code can be used only once. You can [view and regenerate your recovery code](recovering-your-2fa-enabled-account#viewing-and-regenerating-recovery-code) from your 2FA settings page.*
8. Click **Go back to settings** after confirming that you have saved your codes.
###
Disabling 2FA for writes
Check the [Authorization and writes](about-two-factor-authentication#authorization-and-writes) section for more information on different operations that requires 2FA when this mode is enabled.
**Note**: As a recommended setting, 2FA for write operations are *automatically enabled* when setting up 2FA. The following steps explain how to disable it.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. On the account settings page, under "Two-Factor Authentication", click **Modify 2FA**.
4. From the "Manage Two-Factor Authentication" navigate to "Additional Options" section
5. Clear the checkbox for "Require two-factor authentication for write actions" and click "Update Preferences"
###
Disabling 2FA
If you have 2FA enabled, you can remove it from your account settings page.
**Note:** You cannot remove 2FA if you are a member of an organization that enforces 2FA. You can view the list of organizations memberships from your profile page under the "Organizations" tab.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. On the account settings page, under "Two-Factor Authentication", click **Modify 2FA**.
4. Scroll to the bottom of the "Manage Two-Factor Authentication" page and click Disable 2FA.
5. Agree to the prompt from the browser.
Configuring 2FA from the command line
--------------------------------------
###
Enabling 2FA from the command line
Although security-key with WebAuthn can be used for authentication from both the web and the command line, it can only be configured from the web. When enabling 2FA from the command line, currently the only available option is to use an TOTP mobile app.
**Note:** Settings you configure on the command line will also apply to your profile settings on the npm website.
1. If you are logged out on the command line, log in using `npm login` command.
2. On the command line, type the [`npm profile`](https://docs.npmjs.com/cli/v8/commands/npm-profile/) command along with the option for the 2FA mode you want to enable:
* To enable 2FA for authorization and writes, type:
```
npm profile enable-2fa auth-and-writes
```
* To enable 2FA for authorization only, type:
```
npm profile enable-2fa auth-only
```
3. To add npm to your authenticator application, using the device with the app, you can either:
* Scan the QR code displayed on the command line.
* Type the number displayed below the QR code.
4. When prompted to add an OTP code from your authenticator, on the command line, enter a one-time password generated by your authenticator app.
###
Sending a one-time password from the command line
If you have enabled 2FA auth-and-writes, you will need to send the TOTP from the command line for certain commands to work. To do this, append `--otp=123456` (where *123456* is the code generated by your authenticator) at the end of the command. Here are a few examples:
```
npm publish [<tarball>|<folder>][--tag <tag>] --otp=123456
npm owner add <user > --otp=123456
npm owner rm <user> --otp=123456
npm dist-tags add <pkg>@<version> [<tag>] --otp=123456
npm access edit [<package>) --otp=123456
npm unpublish [<@scope>/]<pkg>[@<version>] --otp=123456
```
###
Removing 2FA from the command line
1. If you are logged out on the command line, log in using `npm login` command.
2. On the command line, type the following command:
```
npm profile disable-2fa
```
3. When prompted, enter your npm password:
4. When prompted for a one-time password, enter a password from your authenticator app:
Resolving TOTP errors
----------------------
If you are entering what seems to be a valid [TOTP](https://en.wikipedia.org/wiki/Time-based_one-time_password) but you see an error, be sure that you are using the correct authenticator account. If you have multiple authenticator accounts, using an TOTP from the wrong account will cause an error.
Also, when you reset two-factor authentication after it has been disabled, the authenticator might create a second account with the same name. Please see the authenticator documentation to delete the old account.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/configuring-two-factor-authentication.mdx)
npm Converting your user account to an organization Converting your user account to an organization
===============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Creating an organization](creating-an-organization)[Converting your user account to an organization](converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)[Renaming an organization](renaming-an-organization)[Deleting an organization](deleting-an-organization)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
If you have an npm user account, you can convert your user account to an organization. When you convert your user account to an organization, we will:
* Create a new organization with the name of your user account.
* Prompt you to create a new npm user account. We recommend choosing a variation of your old user name so collaborators will recognize you. For example, if your old username was "wombat", your new username might be "wombat-new".
* Make your new npm user account an owner of your new organization.
* Add your new npm user account to a team called "Developers" in your new organization.
* Transfer packages owned by your user account to your new organization.
* Transfer your existing organization and team memberships and contributor access settings to your new user account.
**Note:** Once your old user account has been converted to an organization, you will no longer be able to sign in to npm with your old user account.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Add an Organization**.
3. Below the account creation form, click **Convert**.
4. Review the account conversion steps and click **Continue**.
5. On the new user account creation page, in the "Username" field, type the name of your new user account, then click **Submit**.
6. On the plan selection page, select either the "Unlimited private packages" paid plan or the "Unlimited public packages" free plan, then click **Buy** or **Create**.
7. If you selected to use the unlimited private packages plan, in the payment dialog, provide the email, name, address, and credit card information for the card that will be used to pay for the organization.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/converting-your-user-account-to-an-organization.mdx)
npm Searching for and choosing packages to download Searching for and choosing packages to download
===============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Searching for a package](#searching-for-a-package)
* [Package search rank criteria](#package-search-rank-criteria)
+ [Popularity](#popularity)
+ [Quality](#quality)
+ [Maintenance](#maintenance)
+ [Optimal](#optimal)
You can use the npm search bar to find packages to use in your projects. npm search uses npms and the npms analyzer; for more information on both, see <https://npms.io/about>.
Searching for a package
------------------------
1. In the search bar, type a search term and press **Enter**. As you type, possible choices will appear.
2. To list packages ranked according to [package search rank criteria](#package-search-rank-criteria), in the left sidebar, under "Sort packages", click the criterion. For example, to sort packages by popularity, click "Popularity".
3. In the package search results list, click the name of the package.
Package search rank criteria
-----------------------------
Often, there are dozens or even hundreds of packages with similar names and/or similar purposes. To help you decide the best ones to explore, each package has been ranked according to four criteria using the npms analyzer:
###
Popularity
Popularity indicates how many times the package has been downloaded. This is a strong indicator of packages that others have found to be useful.
###
Quality
Quality includes considerations such as the presence of a README file, stability, tests, up-to-date dependencies, custom website, and code complexity.
###
Maintenance
Maintenance ranks packages according to the attention they are given by developers. More frequently maintained packages are more likely to work well with the current or upcoming versions of the npm CLI, for example.
###
Optimal
Optimal combines the other three criteria (popularity, quality, maintenance) into one score in a meaningful way.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/searching-for-and-choosing-packages-to-download.mdx)
npm Packages and modules Packages and modules
====================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
* [Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
+ [About the public npm registry](about-the-public-npm-registry)
+ [About packages and modules](about-packages-and-modules)
+ [About scopes](about-scopes)
+ [About public packages](about-public-packages)
+ [About private packages](about-private-packages)
+ [npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
* [Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
+ [Creating a package.json file](creating-a-package-json-file)
+ [Creating Node.js modules](creating-node-js-modules)
+ [About package README files](about-package-readme-files)
+ [Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)
+ [Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)
+ [Creating and publishing private packages](creating-and-publishing-private-packages)
+ [Package name guidelines](package-name-guidelines)
+ [Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)
+ [About semantic versioning](about-semantic-versioning)
+ [Adding dist-tags to packages](adding-dist-tags-to-packages)
* [Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
+ [Changing package visibility](changing-package-visibility)
+ [Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)
+ [Updating your published package version number](updating-your-published-package-version-number)
+ [Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)
+ [Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)
+ [Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
* [Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
+ [Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)
+ [Downloading and installing packages locally](downloading-and-installing-packages-locally)
+ [Downloading and installing packages globally](downloading-and-installing-packages-globally)
+ [Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)
+ [Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)
+ [Using npm packages in your projects](using-npm-packages-in-your-projects)
+ [Using deprecated packages](using-deprecated-packages)
+ [Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
* [Securing your code](packages-and-modules/securing-your-code)
+ [About audit reports](about-audit-reports)
+ [Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)
+ [About ECDSA registry signatures](about-registry-signatures)
+ [Verifying ECDSA registry signatures](verifying-registry-signatures)
+ [About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)
+ [Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)
+ [Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)
+ [Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/index.mdx)
| programming_docs |
npm Creating and publishing private packages Creating and publishing private packages
========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Creating a package.json file](creating-a-package-json-file)[Creating Node.js modules](creating-node-js-modules)[About package README files](about-package-readme-files)[Creating and publishing unscoped public packages](creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](creating-and-publishing-private-packages)[Package name guidelines](package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](about-semantic-versioning)[Adding dist-tags to packages](adding-dist-tags-to-packages)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Creating a private package](#creating-a-private-package)
* [Reviewing package contents for sensitive or unnecessary information](#reviewing-package-contents-for-sensitive-or-unnecessary-information)
* [Testing your package](#testing-your-package)
* [Publishing private packages](#publishing-private-packages)
To share your code with a limited set of users or teams, you can publish private user-scoped or organization-scoped packages to the npm registry.
For more information on scopes and private packages, see "[About scopes](about-scopes)" and "[About private packages](about-private-packages)".
**Note:** Before you can publish private user-scoped npm packages, you must [sign up](https://npmjs.com/signup) for a paid npm user account.
Additionally, to publish private organization-scoped packages, you must [create an npm user account](https://npmjs.com/signup), then [create a paid npm organization](https://www.npmjs.com/signup?next=/org/create).
Creating a private package
---------------------------
1. If you are using npmrc to [manage accounts on multiple registries](https://docs.npmjs.com/enterprise#using-npmrc-to-manage-multiple-profiles-for-different-registries), on the command line, switch to the appropriate profile:
```
npmrc <profile-name>
```
2. On the command line, create a directory for your package:
```
mkdir my-test-package
```
3. Navigate to the root directory of your package:
```
cd my-test-package
```
4. If you are using git to manage your package code, in the package root directory, run the following commands, replacing `git-remote-url` with the git remote URL for your package:
```
git init
git remote add origin git://git-remote-url
```
5. In the package root directory, run the `npm init` command and pass the scope to the `scope` flag:
* For an organization-scoped package, replace `my-org` with the name of your organization:
```
npm init --scope=@my-org
```
* For a user-scoped package, replace `my-username` with your username:
```
npm init --scope=@my-username
```
6. Respond to the prompts to generate a [`package.json`](creating-a-package-json-file) file. For help naming your package, see "[Package name guidelines](package-name-guidelines)".
7. Create a [README file](about-package-readme-files) that explains what your package code is and how to use it.
8. In your preferred text editor, write the code for your package.
Reviewing package contents for sensitive or unnecessary information
--------------------------------------------------------------------
Publishing sensitive information to the registry can harm your users, compromise your development infrastructure, be expensive to fix, and put you at risk of legal action. **We strongly recommend removing sensitive information, such as private keys, passwords, [personally identifiable information][pii] (PII), and credit card data before publishing your package to the registry.** Even if your package is private, sensitive information can be exposed if the package is made public or downloaded to a computer that can be accessed by more users than intended.
For less sensitive information, such as testing data, use a `.npmignore` or `.gitignore` file to prevent publishing to the registry. For more information, see [this article](https://docs.npmjs.com/cli/v8/using-npm/developers//#keeping-files-out-of-your-package).
Testing your package
---------------------
To reduce the chances of publishing bugs, we recommend testing your package before publishing it to the npm registry. To test your package, run `npm install` with the full path to your package directory:
```
npm install my-package
```
Publishing private packages
----------------------------
By default, scoped packages are published with private visibility.
1. On the command line, navigate to the root directory of your package.
```
cd /path/to/package
```
2. To publish your private package to the npm registry, run:
```
npm publish
```
3. To see your private package page, visit [https://npmjs.com/package/\*package-name](https://npmjs.com/package/*package-name)*, replacing* package-name\* with the name of your package. Private packages will say `private` below the package name on the npm website.
For more information on the `publish` command, see the [CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-publish/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/creating-and-publishing-private-packages.mdx)
npm Try the latest stable version of npm Try the latest stable version of npm
====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Generating and locating npm-debug.log files](generating-and-locating-npm-debug.log-files)[Common errors](common-errors)[Try the latest stable version of node](try-the-latest-stable-version-of-node)[Try the latest stable version of npm](try-the-latest-stable-version-of-npm)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [See what version of npm you're running](#see-what-version-of-npm-youre-running)
* [Upgrading on \*nix (OSX, Linux, etc.)](#upgrading-on-nix-osx-linux-etc)
* [Upgrading on Windows](#upgrading-on-windows)
+ [A brief note on the built-in Windows configuration](#a-brief-note-on-the-built-in-windows-configuration)
See what version of npm you're running
---------------------------------------
```
npm -v
```
Upgrading on `*nix` (OSX, Linux, etc.)
---------------------------------------
*(You may need to prefix these commands with `sudo`, especially on Linux, or OS X if you installed Node using its default installer.)*
You can upgrade to the latest version of npm using:
```
npm install -g npm@latest
```
Upgrading on Windows
---------------------
By default, npm is installed alongside node in
`C:\Program Files (x86)\nodejs`
npm's globally installed packages (including, potentially, npm itself) are stored separately in a user-specific directory (which is currently
`C:\Users\<username>\AppData\Roaming\npm`).
Because the installer puts
`C:\Program Files (x86)\nodejs`
before
`C:\Users\<username>\AppData\Roaming\npm`
on your `PATH`, it will always use the version of npm installed with node instead of the version of npm you installed using `npm -g install npm@<version>`.
To get around this, you can do **one** of the following:
* Option 1: [edit your Windows installation's `PATH`](http://superuser.com/questions/284342/what-are-path-and-other-environment-variables-and-how-can-i-set-or-use-them) to put `%appdata%\npm` before `%ProgramFiles%\nodejs`. Remember that you'll need to restart `cmd.exe` (and potentially restart Windows) when you make changes to `PATH` or how npm is installed.
* Option 2: remove both of
+ `%ProgramFiles%\nodejs\npm`
+ `%ProgramFiles%\nodejs\npm.cmd`
* Option 3: Navigate to `%ProgramFiles%\nodejs\node_modules\npm` and copy the `npmrc`file to another folder or the desktop. Then open `cmd.exe` as an administrator and run the following commands:
```
cd %ProgramFiles%\nodejs
npm install npm@latest
```
If you installed npm with the node.js installer, after doing one of the previous steps, do the following.
* Option 1 or 2
+ Go into `%ProgramFiles%\nodejs\node_modules\npm` and copy the file named `npmrc` in the new npm folder, which should be `%appdata%\npm\node_modules\npm`. This will tell the new npm where the global installed packages are.
* Option 3
+ Copy the npmrc file back into `%ProgramFiles%\nodejs\node_modules\npm`
*(See also the [point below](common-errors#error-enoent-stat-cusersuserappdataroamingnpm-on-windows-7) if you're running Windows 7 and don't have the directory `%appdata%\npm`.)*
###
A brief note on the built-in Windows configuration
The Node installer installs, directly into the npm folder, a special piece of Windows-specific configuration that tells npm where to install global packages. When npm is used to install itself, it is supposed to copy this special `builtin` configuration into the new install. There was a bug in some versions of npm that kept this from working, so you may need to go in and fix that up by hand. Run the following command to see where npm will install global packages to verify it is correct.
```
npm config get prefix -g
```
If it isn't set to `<X>:\Users\<user>\AppData\Roaming\npm`, you can run the below command to correct it:
```
npm config set prefix %APPDATA%\npm -g
```
Incidentally, if you would prefer that packages not be installed to your roaming profile (because you have a quota on your shared network, or it makes logging in or out from a domain sluggish), you can put it in your local app data instead:
```
npm config set prefix %LOCALAPPDATA%\npm -g
```
...as well as copying `%APPDATA%\npm` to `%LOCALAPPDATA%\npm` (and updating your `%PATH%`, of course).
Everyone who works on npm knows that this process is complicated and fraught, and we're working on making it simpler. Stay tuned.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/troubleshooting/try-the-latest-stable-version-of-npm.mdx)
npm Downloading and installing packages locally Downloading and installing packages locally
===========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Installing an unscoped package](#installing-an-unscoped-package)
* [Installed a scoped public package](#installed-a-scoped-public-package)
* [Installing a private package](#installing-a-private-package)
* [Testing package installation](#testing-package-installation)
* [Installed package version](#installed-package-version)
* [Installing a package with dist-tags](#installing-a-package-with-dist-tags)
* [Resources](#resources)
You can [install](cli/v8/install) a package locally if you want to depend on the package from your own module, using something like Node.js `require`. This is `npm install`'s default behavior.
Installing an unscoped package
-------------------------------
Unscoped packages are always public, which means they can be searched for, downloaded, and installed by anyone. To install a public package, on the command line, run
```
npm install <package_name>
```
This will create the `node_modules` directory in your current directory (if one doesn't exist yet) and will download the package to that directory.
**Note:** If there is no `package.json` file in the local directory, the latest version of the package is installed.
If there is a `package.json` file, npm installs the latest version that satisfies the [semver rule](about-semantic-versioning) declared in `package.json`.
Installed a scoped public package
----------------------------------
[Scoped public packages](about-scopes) can be downloaded and installed by anyone, as long as the scope name is referenced during installation:
```
npm install @scope/package-name
```
Installing a private package
-----------------------------
[Private packages](about-private-packages) can only be downloaded and installed by those who have been granted read access to the package. Since private packages are always scoped, you must reference the scope name during installation:
```
npm install @scope/private-package-name
```
Testing package installation
-----------------------------
To confirm that `npm install` worked correctly, in your module directory, check that a `node_modules` directory exists and that it contains a directory for the package(s) you installed:
```
ls node_modules
```
Installed package version
--------------------------
If there is a `package.json` file in the directory in which `npm install` is run, npm installs the latest version of the package that satisfies the [semantic versioning rule](about-semantic-versioning) declared in `package.json`.
If there is no `package.json` file, the latest version of the package is installed.
Installing a package with dist-tags
------------------------------------
Like `npm publish`, `npm install <package_name>` will use the `latest` tag by default.
To override this behavior, use `npm install <package_name>@<tag>`. For example, to install the `example-package` at the version tagged with `beta`, you would run the following command:
```
npm install example-package@beta
```
Resources
----------
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/downloading-and-installing-packages-locally.mdx)
npm Downloading and installing packages globally Downloading and installing packages globally
============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Tip:** If you are using npm 5.2 or higher, we recommend using `npx` to run packages globally.
[Installing](https://docs.npmjs.com//cli/v8/commands/npm-install/) a package globally allows you to use the code in the package as a set of tools on your local computer.
To download and install packages globally, on the command line, run the following command:
```
npm install -g <package_name>
```
If you get an EACCES permissions error, you may need to reinstall npm with a version manager or manually change npm's default directory. For more information, see "[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/downloading-and-installing-packages-globally.mdx)
npm About PGP registry signatures (deprecated) About PGP registry signatures (deprecated)
==========================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Tools we use](#tools-we-use)
+ [openpgpjs](#openpgpjs)
+ [Keybase](#keybase)
**Note:** PGP signatures will be deprecated early 2023, in favour of ECDSA registry signatures that can be verified using the npm CLI. [Learn more.](about-registry-signatures)
To increase confidence in the npm public registry, we add our [PGP](https://en.wikipedia.org/wiki/Pretty_Good_Privacy) signature to package metadata and publicize our public PGP key on [Keybase](https://keybase.io). Our Keybase account is "[npmregistry](https://keybase.io/npmregistry)" and our public PGP key can be found at <https://keybase.io/npmregistry/pgp_keys.asc>
You can use the package PGP signature and our public PGP key to verify that the same entity who published the key (npm) also signed the package you downloaded from the npm public registry. For more information, see "[Verifying the PGP signature of a package from the npm public registry](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)".
Tools we use
-------------
###
openpgpjs
To generate PGP signatures, we use [openpgpjs](https://github.com/openpgpjs/openpgpjs), a pure JavaScript implementation of OpenPGP. To learn more about openpgpjs, see <https://openpgpjs.org/>.
###
Keybase
We use Keybase to publicize our PGP key and give you confidence that the npm registry you install from is the same registry that signs packages.
Keybase offers two advantages over the core OpenPGP experience that move us to recommend it to you:
* The Keybase application and CLI provide an excellent user experience for PGP, which can be intimidating for newcomers.
* Keybase manages and displays social proofs that the entity that controls a specific PGP key also controls accounts on social media and other places. These proofs help you determine whether you can trust an account.
We’ve established proofs on Keybase that we control [@npmjs](https://twitter.com/npmjs) on Twitter, the domain [npmjs.com](https://npmjs.com), and the domain [npmjs.org](https://npmjs.org). Verifying these proofs won’t tell you who owns those domains, but it does establish that the same entity controls them, and the PGP key advertised on Keybase.
If you install Keybase and create an account, you can follow npmregistry yourself and obtain a local copy of the registry’s public key. For more information, and to verify the PGP signature of a specific package version from the npm public registry, see "[Verifying the PGP signature for a package from the npm public registry](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)".
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/about-pgp-signatures-for-packages-in-the-public-registry.mdx)
| programming_docs |
npm Docker and private modules Docker and private modules
==========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Integrating npm with external services](integrations/integrating-npm-with-external-services)
[About access tokens](about-access-tokens)[Creating and viewing access tokens](creating-and-viewing-access-tokens)[Revoking access tokens](revoking-access-tokens)[Using private packages in a CI/CD workflow](using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](docker-and-private-modules)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Background: runtime variables](#background-runtime-variables)
* [Create and check in a project-specific .npmrc file](#create-and-check-in-a-project-specific-npmrc-file)
* [Update the Dockerfile](#update-the-dockerfile)
* [Build the Docker image](#build-the-docker-image)
To install private npm packages in a Docker container, you will need to use Docker's build-time variables.
Background: runtime variables
------------------------------
You cannot install private npm packages in a Docker container using only runtime variables. Consider the following Dockerfile:
```
FROM node
COPY package.json package.json
RUN npm install
# Add your source files
COPY . .
CMD npm start
```
Which will use the official [Node.js](https://hub.docker.com/_/node) image, copy the `package.json` into our container, installs dependencies, copies the source files and runs the start command as specified in the `package.json`.
In order to install private packages, you may think that we could just add a line before we run `npm install`, using the [ENV parameter](https://docs.docker.com/engine/reference/builder/#env):
```
ENV NPM_TOKEN=00000000-0000-0000-0000-000000000000
```
However, this doesn't work as you would expect, because you want the npm install to occur when you run `docker build`, and in this instance, `ENV` variables aren't used, they are set for runtime only.
Instead of run-time variables, you must use a different way of passing environment variables to Docker, available since Docker 1.9: the [ARG parameter](https://docs.docker.com/engine/reference/builder/#arg).
Create and check in a project-specific .npmrc file
---------------------------------------------------
A complete example that will allow you to use `--build-arg` to pass in your NPM\_TOKEN requires adding a `.npmrc` file to the project.
Use a project-specific `.npmrc` file with a variable for your token to securely authenticate your Docker image with npm.
1. In the root directory of your project, create a custom [`.npmrc`](cli/v8/files/npmrc) file with the following contents:
```
//registry.npmjs.org/:_authToken=${NPM_TOKEN}
```
**Note:** that you are specifying a literal value of `${NPM_TOKEN}`. The npm cli will replace this value with the contents of the `NPM_TOKEN` environment variable. Do **not** put a token in this file.
2. Check in the `.npmrc` file.
Update the Dockerfile
----------------------
The Dockerfile that takes advantage of this has a few more lines in it than the earlier example that allows us to use the `.npmrc` file and the `ARG` parameter:
```
FROM node
ARG NPM_TOKEN
COPY .npmrc .npmrc
COPY package.json package.json
RUN npm install
RUN rm -f .npmrc
# Add your source files
COPY . .
CMD npm start
```
This adds the expected `ARG NPM_TOKEN`, but also copies the `.npmrc` file, and removes it when `npm install` completes.
Build the Docker image
-----------------------
To build the image using the above Dockerfile and the npm authentication token, you can run the following command. Note the `.` at the end to give `docker build` the current directory as an argument.
```
docker build --build-arg NPM_TOKEN=${NPM_TOKEN} .
```
This will build the Docker image with the current `NPM_TOKEN` environment variable, so you can run `npm install` inside your container as the current logged-in user.
**Note:** Even if you delete the `.npmrc` file, it will be kept in the commit history. To clean your secrets entirely, make sure to squash them.
**Note:** You may commit the `.npmrc` file under a different name, e.g. `.npmrc.docker` to prevent local build from using it.
**Note:** You may need to specify a working directory different from the default `/` otherwise some frameworks like Angular will fail.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/docker-and-private-modules.mdx)
npm Updating packages downloaded from the registry Updating packages downloaded from the registry
==============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Searching for and choosing packages to download](searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](downloading-and-installing-packages-locally)[Downloading and installing packages globally](downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](using-npm-packages-in-your-projects)[Using deprecated packages](using-deprecated-packages)[Uninstalling packages and dependencies](uninstalling-packages-and-dependencies)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Updating local packages](#updating-local-packages)
* [Updating globally-installed packages](#updating-globally-installed-packages)
+ [Determining which global packages need updating](#determining-which-global-packages-need-updating)
+ [Updating a single global package](#updating-a-single-global-package)
+ [Updating all globally-installed packages](#updating-all-globally-installed-packages)
* [Resources](#resources)
+ [CLI commands](#cli-commands)
Updating local and global packages you downloaded from the registry helps keep your code and tools stable, usable, and secure.
Updating local packages
------------------------
We recommend regularly updating the local packages your project depends on to improve your code as improvements to its dependencies are made.
1. Navigate to the root directory of your project and ensure it contains a `package.json` file:
```
cd /path/to/project
```
2. In your project root directory, run the [`update` command](https://docs.npmjs.com/cli/v8/commands/npm-update/):
```
npm update
```
3. To test the update, run the [`outdated` command](https://docs.npmjs.com/cli/v8/commands/npm-outdated/). There should not be any output.
```
npm outdated
```
Updating globally-installed packages
-------------------------------------
**Note:** If you are using npm version 2.6.0 or less, run [this script](https://gist.github.com/othiym23/4ac31155da23962afd0e) to update all outdated global packages.
However, please consider upgrading to the latest version of npm:
```
npm install npm@latest -g
```
###
Determining which global packages need updating
To see which global packages need to be updated, on the command line, run:
```
npm outdated -g --depth=0
```
###
Updating a single global package
To update a single global package, on the command line, run:
```
npm update -g <package_name>
```
###
Updating all globally-installed packages
To update all global packages, on the command line, run:
```
npm update -g
```
Resources
----------
###
CLI commands
* [npm-update](https://docs.npmjs.com/cli/v8/commands/npm-update/)
* [npm-outdated](https://docs.npmjs.com/cli/v8/commands/npm-outdated/)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/updating-packages-downloaded-from-the-registry.mdx)
npm Changing package visibility Changing package visibility
===========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Changing package visibility](changing-package-visibility)[Adding collaborators to private packages owned by a user account](adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](unpublishing-packages-from-the-registry)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Making a public package private](#making-a-public-package-private)
+ [Using the website](#using-the-website)
+ [Using the command line](#using-the-command-line)
* [Making a private package public](#making-a-private-package-public)
+ [Using the website](#using-the-website-1)
+ [Using the command line](#using-the-command-line-1)
You can change the visibility of a scoped package from the website or command line.
You must be the owner of the user account or organization that owns the package in order to change package visibility.
For more information about package visibility, see "[Package scope, access level, and visibility](package-scope-access-level-and-visibility)".
**Note:** You cannot change the visibility of an unscoped package. Only scoped packages with a paid subscription may be private.
Making a public package private
--------------------------------
**Note:** Making a package private requires a paid user account or organization. To sign up for a paid user or organization, go to `https://www.npmjs.com/settings/account-name/billing`, replacing `account-name` with the name of your npm user account or organization.
If you want to restrict access and visibility for a public package you own, you can make the package private. When you make a package private, its access will be updated immediately and it will be removed from the website within a few minutes of the change.
###
Using the website
1. On the [npm website](https://npmjs.com), go to the package page.
2. On the package page, click **Admin**.
3. Under "Package Access", select "Is Package Private?"
4. Click **Update package settings**.
###
Using the command line
To make a public package private on the command line, run the following command, replacing `<package-name>` with the name of your package:
```
npm access restricted <package-name>
```
For more information, see the [`npm access`](cli/v8/access) documentation.
Making a private package public
--------------------------------
Note: When you make a private package public, the package will be visible to and downloadable by all npm users.
###
Using the website
1. On the npm website, go to the package page.
2. On the package page, click **Admin**.
3. Under "Package Access", deselect "Is Package Private?"
4. Click **Update package settings**.
###
Using the command line
To make a private package public on the command line, run the following command, replacing `<package-name>` with the name of your package:
```
npm access public <package-name>
```
For more information, see the [`npm access` CLI documentation](https://docs.npmjs.com/cli/v8/commands/npm-access/).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/changing-package-visibility.mdx)
npm Verifying ECDSA registry signatures Verifying ECDSA registry signatures
===================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[About audit reports](about-audit-reports)[Auditing package dependencies for security vulnerabilities](auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](about-registry-signatures)[Verifying ECDSA registry signatures](verifying-registry-signatures)[About PGP registry signatures (deprecated)](about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](reporting-malware-in-an-npm-package)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Prerequisites](#prerequisites)
* [Verifying registry signatures](#verifying-registry-signatures)
* [Troubleshooting](#troubleshooting)
+ [Some packages are missing registry signatures](#some-packages-are-missing-registry-signatures)
To ensure the integrity of packages you download from the public npm registry, or any registry that supports signatures, you can verify the registry signatures of downloaded packages using the npm CLI.
Prerequisites
--------------
1. Install npm CLI version v8.15.0 or later
2. Install dependencies using `npm install` or `npm ci`
Verifying registry signatures
------------------------------
Registry signatures can be verified using the following `audit` command:
```
npm audit signatures
```
Example response if all installed versions have valid registry signatures:
```
audited 1640 packages in 2s
1640 have verified registry signatures
```
Troubleshooting
----------------
###
Some packages are missing registry signatures
The CLI will error if packages don't have signatures *and* if the package registry supports signatures. This could mean an attacker might be trying to circumvent signature verification. You can check if the registry supports signatures by requesting the public signing keys from `registry-host.tld/-/npm/v1/keys`.
Example response if some versions have missing registry signatures:
```
audited 1640 packages in 2s
1405 packages have verified registry signatures
235 packages have missing registry signatures but the registry is providing signing keys:
[email protected] (https://registry.npmjs.org/)
...
```
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/verifying-registry-signatures.mdx)
npm Upgrading to a paid organization plan Upgrading to a paid organization plan
=====================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Upgrading to a paid organization plan](upgrading-to-a-paid-organization-plan)[Viewing, downloading, and emailing receipts for your organization](viewing-downloading-and-emailing-receipts-for-your-organization)[Updating organization billing settings](updating-organization-billing-settings)[Downgrading to a free organization plan](downgrading-to-a-free-organization-plan)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
**Note:** This article only applies to users of the public npm registry.
As an organization owner, you can upgrade your free organization plan to the npm Teams product. npm Teams is a paid plan to give organization members the ability to install and publish private packages. For more information about npm Teams and our organization pricing plans, see the "npm Teams" section of [our pricing page](https://www.npmjs.com/pricing).
If you have an organization with a private packages plan, your organization will cost you seven (7) dollars a month per user. **The $7 charge is a flat fee for any member of the organization even if the teams the member belongs do not have access to private packages**
Newly added members to an organization are always billed during the next billing cycle. For more information, see "[Adding members to your organization](https://docs.npmjs.com/adding-members-to-your-org)".
**Note:** Your paid plan and billing cycle will start when you submit your credit card information, and you will be charged for the first month immediately.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization you want to upgrade.
4. On the organization settings page, click **Billing**.
5. Under "change plan", click **Upgrade Plan ($7/User)**.
6. Under "Want to upgrade?", click **Enable Private Publishing for $7/mo**.
7. In the billing information dialog box, enter your billing information:
* Email: the email address used for the billing contact
* Name: the name on the credit card used to pay
* Street, City, ZIP Code, Country: the billing address associated with the credit card
8. Click **Payment Info**.
9. In the credit card information dialog box, enter your credit card information:
* Card number
* MM / YY: the month and year of the card expiration date
* CVC: the three-digit code on the credit card
10. To save your credit card information for other payments on npm, select "Remember me".
11. Click **Pay** for the monthly amount. The monthly amount will be the number of members in your organization multiplied by $7.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/paying-for-your-organization/upgrading-to-a-paid-organization-plan.mdx)
| programming_docs |
npm About the developers team About the developers team
=========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[About the developers team](about-developers-team)[Creating teams](creating-teams)[Adding organization members to teams](adding-organization-members-to-teams)[Removing organization members from teams](removing-organization-members-from-teams)[Managing team access to organization packages](managing-team-access-to-organization-packages)[Removing teams](removing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
The "**developers**" team is automatically created when you create an organization. By default, the developers team has read/write access to all new packages created under the organization's scope.
* Members added to the organization, including the organization owner, are automatically added to the **developers** team
* The [`maintainers` field](https://docs.npmjs.com/cli/v8/configuring-npm/package-json/#people-fields-author-contributors) in the [`package.json`](https://docs.npmjs.com/cli/v8/configuring-npm/package-json/) of any newly created packages under the organization scope is automatically populated with the members of the current **developers** team
If you create a new package under your organization's scope and you do not want members of the **developers** team to have read/write access to that package, an owner or admin can remove the **developers** team's access to that package. For more informations, see "[Managing team access to organization packages](managing-team-access-to-organization-packages)".
If an owner adds a new member to an organization and **does not** want that member to be on the **developers** team, an owner can remove them.
**Note:** The **developers** team can no longer be removed from an organization for the following reasons:
* It is the source of truth for all users, packages, and default permissions in an organization.
* When you want to restrict write access, it is almost always better to set the default permissions to read-only and create separate teams for managing write permissions.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/about-developers-team.mdx)
npm Common errors Common errors
=============
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Generating and locating npm-debug.log files](generating-and-locating-npm-debug.log-files)[Common errors](common-errors)[Try the latest stable version of node](try-the-latest-stable-version-of-node)[Try the latest stable version of npm](try-the-latest-stable-version-of-npm)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Errors](#errors)
* [Broken npm installation](#broken-npm-installation)
* [Random errors](#random-errors)
* [No compatible version found](#no-compatible-version-found)
* [Permissions errors](#permissions-errors)
* [Error: ENOENT, stat 'C:\Users\<user>\AppData\Roaming\npm' on Windows 7](#error-enoent-stat-cusersuserappdataroamingnpm-on-windows-7)
* [No space](#no-space)
* [No git](#no-git)
* [Running a Vagrant box on Windows fails due to path length issues](#running-a-vagrant-box-on-windows-fails-due-to-path-length-issues)
* [npm only uses git: and ssh+git: URLs for GitHub repos, breaking proxies](#npm-only-uses-git-and-sshgit-urls-for-github-repos-breaking-proxies)
* [SSL Error](#ssl-error)
* [SSL-intercepting proxy](#ssl-intercepting-proxy)
* [Not found / Server error](#not-found--server-error)
* [Invalid JSON](#invalid-json)
* [Many ENOENT / ENOTEMPTY errors in output](#many-enoent--enotempty-errors-in-output)
* [cb() never called! when using shrinkwrapped dependencies](#cb-never-called-when-using-shrinkwrapped-dependencies)
* [npm login errors](#npm-login-errors)
* [npm hangs on Windows at addRemoteTarball](#npm-hangs-on-windows-at-addremotetarball)
* [npm not running the latest version on a Windows machine](#npm-not-running-the-latest-version-on-a-windows-machine)
Errors
-------
* [Broken npm installation](#broken-npm-installation)
* [Random errors](#random-errors)
* [No compatible version found](#no-compatible-version-found)
* [Permissions errors](#permissions-errors)
* [`Error: ENOENT, stat 'C:\Users\<user>\AppData\Roaming\npm'` on Windows 7](#error-enoent-stat-cusersuserappdataroamingnpm-on-windows-7)
* [No space](#no-space)
* [No git](#no-git)
* [Running a Vagrant box on Windows fails due to path length issues](#running-a-vagrant-box-on-windows-fails-due-to-path-length-issues)
* [npm only uses `git:` and `ssh+git:` URLs for GitHub repos, breaking proxies](#npm-only-uses-git-and-sshgit-urls-for-github-repos-breaking-proxies)
* [SSL error](#ssl-error)
* [SSL-intercepting proxy](#ssl-intercepting-proxy)
* [Not found / Server error](#not-found--server-error)
* [Invalid JSON](#invalid-json)
* [Many `ENOENT` / `ENOTEMPTY` errors in output](#many-enoent--enotempty-errors-in-output)
* [`cb() never called!` when using shrinkwrapped dependencies](#cb-never-called-when-using-shrinkwrapped-dependencies)
* [npm login errors](#npm-login-errors)
* [`npm` hangs on Windows at `addRemoteTarball`](#npm-hangs-on-windows-at-addremotetarball)
* [npm not running the latest version on a Windows machine](#npm-not-running-the-latest-version-on-a-windows-machine)
Broken npm installation
------------------------
If your npm is broken:
* On Mac or Linux, [reinstall npm](downloading-and-installing-node-js-and-npm).
* Windows: If you're on Windows and you have a broken installation, the easiest thing to do is to reinstall node from the official installer (see [this note about installing the latest stable version](try-the-latest-stable-version-of-npm#upgrading-on-windows)).
Random errors
--------------
* Some strange issues can be resolved by simply running `npm cache clean` and trying again.
* If you are having trouble with `npm install`, use the `-verbose` option to see more details.
No compatible version found
----------------------------
You have an outdated npm. [Please update to the latest stable npm](try-the-latest-stable-version-of-npm).
Permissions errors
-------------------
Please see the discussions in "[Downloading and installing Node.js and npm](downloading-and-installing-node-js-and-npm)" and "[Resolving EACCES permissions errors when installing packages globally](resolving-eacces-permissions-errors-when-installing-packages-globally)" for ways to avoid and resolve permissions errors.
`Error: ENOENT, stat 'C:\Users\<user>\AppData\Roaming\npm'` on Windows 7
-------------------------------------------------------------------------
The error `Error: ENOENT, stat 'C:\Users\<user>\AppData\Roaming\npm'` on Windows 7 is a consequence of [joyent/node#8141](https://github.com/joyent/node/issues/8141), and is an issue with the Node installer for Windows. The workaround is to ensure that `C:\Users\<user>\AppData\Roaming\npm` exists and is writable with your normal user account.
No space
---------
```
npm ERR! Error: ENOSPC, write
```
You are trying to install on a drive that either has no space, or has no permission to write.
* Free some disk space or
* Set the tmp folder somewhere with more space: `npm config set tmp /path/to/big/drive/tmp` or
* Build Node yourself and install it somewhere writable with lots of space.
No git
-------
```
npm ERR! not found: git
ENOGIT
```
You need to [install git](http://git-scm.com/book/en/Getting-Started-Installing-Git). Or, you may need to add your git information to your npm profile. You can do this from the command line or the website. For more information, see "[Managing your profile settings](managing-your-profile-settings)".
Running a Vagrant box on Windows fails due to path length issues
-----------------------------------------------------------------
**[@drmyersii](https://github.com/drmyersii)** went through what sounds like a lot of painful trial and error to come up with a working solution involving Windows long paths and some custom Vagrant configuration:
>
> [This is the commit that I implemented it in](https://github.com/renobit/vagrant-node-env/commit/bdf15f2f301e2b1660b839875e34f172ea8be227), but I'll go ahead and post the main snippet of code here:
>
>
>
>
> ```
> config.vm.provider "virtualbox" do |v|
> v.customize ["sharedfolder", "add", :id, "--name", "www", "--hostpath", (("//?/" + File.dirname(__FILE__) + "/www").gsub("/","\\"))]
> end
>
> config.vm.provision :shell, inline: "mkdir /home/vagrant/www"
> config.vm.provision :shell, inline: "mount -t vboxsf -o uid=`id -u vagrant`,gid=`getent group vagrant | cut -d: -f3` > www /home/vagrant/www", run: "always"
> ```
>
>
> In the code above, I am appending `\\?\` to the current directory absolute path. This will actually force the Windows API to allow an increase in the MAX\_PATH variable (normally capped at 260). Read more about [max path](https://msdn.microsoft.com/en-us/library/windows/desktop/aa365247%28v=vs.85%29.aspx#maxpath). This is happening during the sharedfolder creation which is intentionally handled by VBoxManage and not Vagrant's "synced\_folder" method. The last bit is pretty self-explanatory; we create the new shared folder and then make sure it's mounted each time the machine is accessed or touched since Vagrant likes to reload its mounts/shared folders on each load.
>
>
>
npm only uses `git:` and `ssh+git:` URLs for GitHub repos, breaking proxies
----------------------------------------------------------------------------
**[@LaurentGoderre](https://github.com/LaurentGoderre)** fixed this with [some Git trickery](https://github.com/npm/npm/issues/5257#issuecomment-60441477):
>
> I fixed this issue for several of my colleagues by running the following two commands:
>
>
>
>
> ```
> git config --global url."https://github.com/".insteadOf [email protected]:
> git config --global url."https://".insteadOf git://
> ```
>
>
> One thing we noticed is that the `.gitconfig` used is not always the one expected so if you are on a machine that modified the home path to a shared drive, you need to ensure that your `.gitconfig` is the same on both your shared drive and in `c:\users\[your user]\`
>
>
>
SSL Error
----------
```
npm ERR! Error: 7684:error:140770FC:SSL routines:SSL23_GET_SERVER_HELLO:unknown protocol:openssl\ssl\s23_clnt.c:787:
```
You are trying to talk SSL to an unencrypted endpoint. More often than not, this is due to a [proxy](https://docs.npmjs.com/cli/v8/using-npm/config/#proxy) [configuration](https://docs.npmjs.com/cli/v8/using-npm/config/#https-proxy) [error](https://docs.npmjs.com/cli/v8/using-npm/config/#cafile) (see also [this helpful, if dated, guide](http://jjasonclark.com/how-to-setup-node-behind-web-proxy)). In this case, you do **not** want to disable `strict-ssl` – you may need to set up a CA / CA file for use with your proxy, but it's much better to take the time to figure that out than disabling SSL protection.
```
npm ERR! Error: SSL Error: CERT_UNTRUSTED
```
```
npm ERR! Error: SSL Error: UNABLE_TO_VERIFY_LEAF_SIGNATURE
```
This problem will happen if you're running Node 0.6. Please upgrade to node 0.8 or above. [See this post for details](http://blog.npmjs.org/post/71267056460/fastly-manta-loggly-and-couchdb-attachments).
You could also try these workarounds: `npm config set ca ""` or `npm config set strict-ssl false`
```
npm ERR! Error: SSL Error: SELF_SIGNED_CERT_IN_CHAIN
```
[npm no longer supports its self-signed certificates](http://blog.npmjs.org/post/78085451721/npms-self-signed-certificate-is-no-more)
Either:
* upgrade your version of npm `npm install npm -g --ca=""`
* tell your current version of npm to use known registrars `npm config set ca=""`
If this does not fix the problem, then you may have an SSL-intercepting proxy. (For example, <https://github.com/npm/npm/issues/7439#issuecomment-76024878>)
SSL-intercepting proxy
-----------------------
Unsolved. See <https://github.com/npm/npm/issues/9282>
Not found / Server error
-------------------------
```
npm http 404 https://registry.npmjs.org/faye-websocket/-/faye-websocket-0.7.0.tgz
npm ERR! fetch failed https://registry.npmjs.org/faye-websocket/-/faye-websocket-0.7.0.tgz
npm ERR! Error: 404 Not Found
```
```
npm http 500 https://registry.npmjs.org/phonegap
```
* It's most likely a temporary npm registry glitch. Check [npm server status](http://status.npmjs.org/) and try again later.
* If the error persists, perhaps the published package is corrupt. Contact the package owner and have them publish a new version of the package.
Invalid JSON
-------------
```
Error: Invalid JSON
```
```
npm ERR! SyntaxError: Unexpected token <
```
```
npm ERR! registry error parsing json
```
* Possible temporary npm registry glitch, or corrupted local server cache. Run `npm cache clean` and/or try again later.
* This can be caused by corporate proxies that give HTML responses to `package.json` requests. Check npm's proxy [configuration](https://docs.npmjs.com/cli/v8/using-npm/config/).
* Check that it's not a problem with a package you're trying to install (e.g. invalid `package.json`).
Many `ENOENT` / `ENOTEMPTY` errors in output
---------------------------------------------
npm is written to use resources efficiently on install, and part of this is that it tries to do as many things concurrently as is practical. Sometimes this results in race conditions and other synchronization issues. As of npm 2.0.0, a very large number of these issues were addressed. If you see `ENOENT lstat`, `ENOENT chmod`, `ENOTEMPTY unlink`, or something similar in your log output, try updating npm to the latest version. If the problem persists, look at [npm/npm#6043](https://github.com/npm/npm/issues/6043) and see if somebody has already discussed your issue.
`cb() never called!` when using shrinkwrapped dependencies
-----------------------------------------------------------
Take a look at [issue #5920](https://github.com/npm/npm/issues/5920). ~~We're working on fixing this one, but it's a fairly subtle race condition and it's taking us a little time. You might try moving your `npm-shrinkwrap.json` file out of the way until we have this fixed.~~ This has been fixed in versions of npm newer than `[email protected]`, so update to `npm@latest`.
`npm login` errors
-------------------
Sometimes `npm login` fails for no obvious reason. The first thing to do is to log in at <https://www.npmjs.com/login> and check that your e-mail address on `npmjs.com` matches the email address you are giving to `npm login`.
If that's not the problem, or if you are seeing the message `"may not mix password_sha and pbkdf2"`, then
1. Log in at <https://npmjs.com/>
2. Change password at <https://npmjs.com/password> – you can even "change" it to the same password
3. Clear login-related fields from `~/.npmrc` – e.g., by running `sed -ie '/registry.npmjs.org/d' ~/.npmrc`
4. `npm login`
and it generally seems to work.
See <https://github.com/npm/npm/issues/6641#issuecomment-72984009> for the history of this issue.
`npm` hangs on Windows at `addRemoteTarball`
---------------------------------------------
Check if you have two temp directories set in your `.npmrc`:
```
> npm config ls -l
```
Look for lines defining the `tmp` config variable. If you find more than one, remove all but one of them.
See <https://github.com/npm/npm/issues/7590> for more about this unusual problem.
npm not running the latest version on a Windows machine
--------------------------------------------------------
See the section about Windows [here](try-the-latest-stable-version-of-npm).
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/troubleshooting/common-errors.mdx)
npm Viewing, downloading, and emailing receipts for your npm user account Viewing, downloading, and emailing receipts for your npm user account
=====================================================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Setting up your npm user account](getting-started/setting-up-your-npm-user-account)
[Managing your npm user account](getting-started/managing-your-npm-user-account)
[Paying for your npm user account](getting-started/paying-for-your-npm-user-account)
[Upgrading to a paid user account plan](upgrading-to-a-paid-user-account-plan)[Viewing, downloading, and emailing receipts for your npm user account](viewing-downloading-and-emailing-receipts-for-your-user-account)[Updating user account billing settings](updating-user-account-billing-settings)[Downgrading to a free user account plan](downgrading-to-a-free-user-account-plan)
[Configuring your local environment](getting-started/configuring-your-local-environment)
[Troubleshooting](getting-started/troubleshooting)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Viewing receipts](#viewing-receipts)
* [Downloading receipts](#downloading-receipts)
* [Emailing receipts](#emailing-receipts)
**Note:** This article only applies to users of the public npm registry.
You can view, download, and email receipts for the complete billing history of your npm user account.
Viewing receipts
-----------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. On the Billing Information page, under "monthly bill", select **View Billing History**.
4. To view a single receipt, find the row of the receipt you want to view, then, on the right side of the row, click the view icon.
Downloading receipts
---------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. On the Billing Information page, under "monthly bill", select **View Billing History**.
4. To download a single receipt, find the row of the receipt you want to download, then click the PDF icon on the right side of the row.
5. To download multiple receipts, first select the receipts that you wish to download by selecting the box next to the date. To select all receipts, select the checkbox next to the "Date" header. Then click **Download Checked**.
Emailing receipts
------------------
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then select **Billing Info**.
3. On the Billing Information page, under "monthly bill", select **View Billing History**.
4. To email a single receipt, find the row of the receipt you want to download, then, on the right side of the row, click the email icon.
5. To email multiple receipts, first select the receipts that you wish to download by selecting the box next to the date. To select all receipts, select the checkbox next to the "Date" header. Then click **Email Checked**.
6. In the Email Receipt dialog box, fill in the "From", "To", and "Message" fields.
7. Click **Send**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/paying-for-your-npm-user-account/viewing-downloading-and-emailing-receipts-for-your-user-account.mdx)
| programming_docs |
npm About the public npm registry About the public npm registry
=============================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
The public npm registry is a database of JavaScript packages, each comprised of software and metadata. Open source developers and developers at companies use the npm registry to contribute packages to the entire community or members of their organizations, and download packages to use in their own projects.
To get started with the registry, [sign up for an npm account](https://www.npmjs.com/signup) and check out the "[Getting started](getting-started)" and [CLI](cli/v8) documentation.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/about-the-public-npm-registry.mdx)
npm Deleting an organization Deleting an organization
========================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Integrations](integrations)
[Organizations](organizations)
[Creating and managing organizations](organizations/creating-and-managing-organizations)
[Creating an organization](creating-an-organization)[Converting your user account to an organization](converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](requiring-two-factor-authentication-in-your-organization)[Renaming an organization](renaming-an-organization)[Deleting an organization](deleting-an-organization)
[Paying for your organization](organizations/paying-for-your-organization)
[Managing organization members](organizations/managing-organization-members)
[Managing teams](organizations/managing-teams)
[Managing organization packages](organizations/managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
An organization administrator can delete the organization; packages in the organization will also [be deleted](unpublishing-packages-from-the-registry) if they fulfill the [requirements to unpublish packages](https://docs.npmjs.com/policies/unpublish). Packages that cannot be deleted can be [deprecated](deprecating-and-undeprecating-packages-or-package-versions) instead.
1. [Log in](https://www.npmjs.com/login) to npm with your user account.
2. In the upper right corner of the page, click your profile picture, then click **Account**.
3. In the left sidebar, click the name of the organization that you want to delete.
4. On the organization settings page, click **Billing**.
5. Under "delete organization", click **Delete**.
6. You will be given an overview of the packages in your organization and what will happen to them when your organization is deleted. Packages that [can be unpublished](unpublishing-packages-from-the-registry) will be deleted.
If you are sure that you want to continue, enter your organization name and click **Delete this organization**.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/deleting-an-organization.mdx)
npm npm package scope, access level, and visibility npm package scope, access level, and visibility
===============================================
[Skip to content](#skip-nav)
[npm Docs](index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](about-npm)
[Getting started](getting-started)
[Packages and modules](packages-and-modules)
[Introduction to packages and modules](packages-and-modules/introduction-to-packages-and-modules)
[About the public npm registry](about-the-public-npm-registry)[About packages and modules](about-packages-and-modules)[About scopes](about-scopes)[About public packages](about-public-packages)[About private packages](about-private-packages)[npm package scope, access level, and visibility](package-scope-access-level-and-visibility)
[Contributing packages to the registry](packages-and-modules/contributing-packages-to-the-registry)
[Updating and managing your published packages](packages-and-modules/updating-and-managing-your-published-packages)
[Getting packages from the registry](packages-and-modules/getting-packages-from-the-registry)
[Securing your code](packages-and-modules/securing-your-code)
[Integrations](integrations)
[Organizations](organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](threats-and-mitigations)
[npm CLI](cli/v8)
Table of contents* [Public registry](#public-registry)
Visibility of npm packages depends on the scope (namespace) in which the package is contained, and the access level (private or public) set for the package.
Note: To create organization-scoped packages, you must first create an organization. For more information, see "[Creating an organization](creating-an-organization)".
Public registry
----------------
| Scope | Access level | Can view and download | Can write (publish) |
| --- | --- | --- | --- |
| Org | Private | Members of a team in the organization with read access to the package | Members of a team in the organization with read and write access to the package |
| Org | Public | Everyone | Members of a team in the organization with read and write access to the package |
| User | Private | The package owner and users who have been granted read access to the package | The package owner and users who have been granted read and write access to the package |
| User | Public | Everyone | The package owner and users who have been granted read and write access to the package |
| Unscoped | Public | Everyone | The package owner and users who have been granted read and write access to the package |
Note: Only user accounts can create and manage unscoped packages. Organizations can only manage scoped packages.
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/package-scope-access-level-and-visibility.mdx)
npm Managing teams Managing teams
==============
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Creating and managing organizations](creating-and-managing-organizations)
[Paying for your organization](paying-for-your-organization)
[Managing organization members](managing-organization-members)
[Managing teams](managing-teams)
[About the developers team](../about-developers-team)[Creating teams](../creating-teams)[Adding organization members to teams](../adding-organization-members-to-teams)[Removing organization members from teams](../removing-organization-members-from-teams)[Managing team access to organization packages](../managing-team-access-to-organization-packages)[Removing teams](../removing-teams)
[Managing organization packages](managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About the developers team](../about-developers-team)
* [Creating teams](../creating-teams)
* [Adding organization members to teams](../adding-organization-members-to-teams)
* [Removing organization members from teams](../removing-organization-members-from-teams)
* [Managing team access to organization packages](../managing-team-access-to-organization-packages)
* [Removing teams](../removing-teams)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-teams/index.mdx)
npm Creating and managing organizations Creating and managing organizations
===================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Creating and managing organizations](creating-and-managing-organizations)
[Creating an organization](../creating-an-organization)[Converting your user account to an organization](../converting-your-user-account-to-an-organization)[Requiring two-factor authentication in your organization](../requiring-two-factor-authentication-in-your-organization)[Renaming an organization](../renaming-an-organization)[Deleting an organization](../deleting-an-organization)
[Paying for your organization](paying-for-your-organization)
[Managing organization members](managing-organization-members)
[Managing teams](managing-teams)
[Managing organization packages](managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Creating an organization](../creating-an-organization)
* [Converting your user account to an organization](../converting-your-user-account-to-an-organization)
* [Requiring two-factor authentication in your organization](../requiring-two-factor-authentication-in-your-organization)
* [Renaming an organization](../renaming-an-organization)
* [Deleting an organization](../deleting-an-organization)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/creating-and-managing-organizations/index.mdx)
npm Managing organization members Managing organization members
=============================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Creating and managing organizations](creating-and-managing-organizations)
[Paying for your organization](paying-for-your-organization)
[Managing organization members](managing-organization-members)
[Adding members to your organization](../adding-members-to-your-organization)[Accepting or rejecting an organization invitation](../accepting-or-rejecting-an-organization-invitation)[Organization roles and permissions](../organization-roles-and-permissions)[Managing organization permissions](../managing-organization-permissions)[Removing members from your organization](../removing-members-from-your-organization)
[Managing teams](managing-teams)
[Managing organization packages](managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Adding members to your organization](../adding-members-to-your-organization)
* [Accepting or rejecting an organization invitation](../accepting-or-rejecting-an-organization-invitation)
* [Organization roles and permissions](../organization-roles-and-permissions)
* [Managing organization permissions](../managing-organization-permissions)
* [Removing members from your organization](../removing-members-from-your-organization)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-members/index.mdx)
npm Managing organization packages Managing organization packages
==============================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Creating and managing organizations](creating-and-managing-organizations)
[Paying for your organization](paying-for-your-organization)
[Managing organization members](managing-organization-members)
[Managing teams](managing-teams)
[Managing organization packages](managing-organization-packages)
[About organization scopes and packages](../about-organization-scopes-and-packages)[Configuring your npm client with your organization settings](../configuring-your-npm-client-with-your-organization-settings)[Creating and publishing an organization scoped package](../creating-and-publishing-an-organization-scoped-package)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About organization scopes and packages](../about-organization-scopes-and-packages)
* [Configuring your npm client with your organization settings](../configuring-your-npm-client-with-your-organization-settings)
* [Creating and publishing an organization scoped package](../creating-and-publishing-an-organization-scoped-package)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/managing-organization-packages/index.mdx)
npm Paying for your organization Paying for your organization
============================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Creating and managing organizations](creating-and-managing-organizations)
[Paying for your organization](paying-for-your-organization)
[Upgrading to a paid organization plan](../upgrading-to-a-paid-organization-plan)[Viewing, downloading, and emailing receipts for your organization](../viewing-downloading-and-emailing-receipts-for-your-organization)[Updating organization billing settings](../updating-organization-billing-settings)[Downgrading to a free organization plan](../downgrading-to-a-free-organization-plan)
[Managing organization members](managing-organization-members)
[Managing teams](managing-teams)
[Managing organization packages](managing-organization-packages)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Upgrading to a paid organization plan](../upgrading-to-a-paid-organization-plan)
* [Viewing, downloading, and emailing receipts for your organization](../viewing-downloading-and-emailing-receipts-for-your-organization)
* [Updating organization billing settings](../updating-organization-billing-settings)
* [Downgrading to a free organization plan](../downgrading-to-a-free-organization-plan)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/organizations/paying-for-your-organization/index.mdx)
npm Contributing packages to the registry Contributing packages to the registry
=====================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Introduction to packages and modules](introduction-to-packages-and-modules)
[Contributing packages to the registry](contributing-packages-to-the-registry)
[Creating a package.json file](../creating-a-package-json-file)[Creating Node.js modules](../creating-node-js-modules)[About package README files](../about-package-readme-files)[Creating and publishing unscoped public packages](../creating-and-publishing-unscoped-public-packages)[Creating and publishing scoped public packages](../creating-and-publishing-scoped-public-packages)[Creating and publishing private packages](../creating-and-publishing-private-packages)[Package name guidelines](../package-name-guidelines)[Specifying dependencies and devDependencies in a package.json file](../specifying-dependencies-and-devdependencies-in-a-package-json-file)[About semantic versioning](../about-semantic-versioning)[Adding dist-tags to packages](../adding-dist-tags-to-packages)
[Updating and managing your published packages](updating-and-managing-your-published-packages)
[Getting packages from the registry](getting-packages-from-the-registry)
[Securing your code](securing-your-code)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Creating a package.json file](../creating-a-package-json-file)
* [Creating Node.js modules](../creating-node-js-modules)
* [About package README files](../about-package-readme-files)
* [Creating and publishing unscoped public packages](../creating-and-publishing-unscoped-public-packages)
* [Creating and publishing scoped public packages](../creating-and-publishing-scoped-public-packages)
* [Creating and publishing private packages](../creating-and-publishing-private-packages)
* [Package name guidelines](../package-name-guidelines)
* [Specifying dependencies and devDependencies in a package.json file](../specifying-dependencies-and-devdependencies-in-a-package-json-file)
* [About semantic versioning](../about-semantic-versioning)
* [Adding dist-tags to packages](../adding-dist-tags-to-packages)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/contributing-packages-to-the-registry/index.mdx)
npm Getting packages from the registry Getting packages from the registry
==================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Introduction to packages and modules](introduction-to-packages-and-modules)
[Contributing packages to the registry](contributing-packages-to-the-registry)
[Updating and managing your published packages](updating-and-managing-your-published-packages)
[Getting packages from the registry](getting-packages-from-the-registry)
[Searching for and choosing packages to download](../searching-for-and-choosing-packages-to-download)[Downloading and installing packages locally](../downloading-and-installing-packages-locally)[Downloading and installing packages globally](../downloading-and-installing-packages-globally)[Resolving EACCES permissions errors when installing packages globally](../resolving-eacces-permissions-errors-when-installing-packages-globally)[Updating packages downloaded from the registry](../updating-packages-downloaded-from-the-registry)[Using npm packages in your projects](../using-npm-packages-in-your-projects)[Using deprecated packages](../using-deprecated-packages)[Uninstalling packages and dependencies](../uninstalling-packages-and-dependencies)
[Securing your code](securing-your-code)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Searching for and choosing packages to download](../searching-for-and-choosing-packages-to-download)
* [Downloading and installing packages locally](../downloading-and-installing-packages-locally)
* [Downloading and installing packages globally](../downloading-and-installing-packages-globally)
* [Resolving EACCES permissions errors when installing packages globally](../resolving-eacces-permissions-errors-when-installing-packages-globally)
* [Updating packages downloaded from the registry](../updating-packages-downloaded-from-the-registry)
* [Using npm packages in your projects](../using-npm-packages-in-your-projects)
* [Using deprecated packages](../using-deprecated-packages)
* [Uninstalling packages and dependencies](../uninstalling-packages-and-dependencies)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/getting-packages-from-the-registry/index.mdx)
| programming_docs |
npm Updating and managing your published packages Updating and managing your published packages
=============================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Introduction to packages and modules](introduction-to-packages-and-modules)
[Contributing packages to the registry](contributing-packages-to-the-registry)
[Updating and managing your published packages](updating-and-managing-your-published-packages)
[Changing package visibility](../changing-package-visibility)[Adding collaborators to private packages owned by a user account](../adding-collaborators-to-private-packages-owned-by-a-user-account)[Updating your published package version number](../updating-your-published-package-version-number)[Deprecating and undeprecating packages or package versions](../deprecating-and-undeprecating-packages-or-package-versions)[Transferring a package from a user account to another user account](../transferring-a-package-from-a-user-account-to-another-user-account)[Unpublishing packages from the registry](../unpublishing-packages-from-the-registry)
[Getting packages from the registry](getting-packages-from-the-registry)
[Securing your code](securing-your-code)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Changing package visibility](../changing-package-visibility)
* [Adding collaborators to private packages owned by a user account](../adding-collaborators-to-private-packages-owned-by-a-user-account)
* [Updating your published package version number](../updating-your-published-package-version-number)
* [Deprecating and undeprecating packages or package versions](../deprecating-and-undeprecating-packages-or-package-versions)
* [Transferring a package from a user account to another user account](../transferring-a-package-from-a-user-account-to-another-user-account)
* [Unpublishing packages from the registry](../unpublishing-packages-from-the-registry)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/updating-and-managing-your-published-packages/index.mdx)
npm Securing your code Securing your code
==================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Introduction to packages and modules](introduction-to-packages-and-modules)
[Contributing packages to the registry](contributing-packages-to-the-registry)
[Updating and managing your published packages](updating-and-managing-your-published-packages)
[Getting packages from the registry](getting-packages-from-the-registry)
[Securing your code](securing-your-code)
[About audit reports](../about-audit-reports)[Auditing package dependencies for security vulnerabilities](../auditing-package-dependencies-for-security-vulnerabilities)[About ECDSA registry signatures](../about-registry-signatures)[Verifying ECDSA registry signatures](../verifying-registry-signatures)[About PGP registry signatures (deprecated)](../about-pgp-signatures-for-packages-in-the-public-registry)[Verifying PGP registry signatures (deprecated)](../verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)[Requiring 2FA for package publishing and settings modification](../requiring-2fa-for-package-publishing-and-settings-modification)[Reporting malware in an npm package](../reporting-malware-in-an-npm-package)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About audit reports](../about-audit-reports)
* [Auditing package dependencies for security vulnerabilities](../auditing-package-dependencies-for-security-vulnerabilities)
* [About ECDSA registry signatures](../about-registry-signatures)
* [Verifying ECDSA registry signatures](../verifying-registry-signatures)
* [About PGP registry signatures (deprecated)](../about-pgp-signatures-for-packages-in-the-public-registry)
* [Verifying PGP registry signatures (deprecated)](../verifying-the-pgp-signature-for-a-package-from-the-npm-public-registry)
* [Requiring 2FA for package publishing and settings modification](../requiring-2fa-for-package-publishing-and-settings-modification)
* [Reporting malware in an npm package](../reporting-malware-in-an-npm-package)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/securing-your-code/index.mdx)
npm Introduction to packages and modules Introduction to packages and modules
====================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Introduction to packages and modules](introduction-to-packages-and-modules)
[About the public npm registry](../about-the-public-npm-registry)[About packages and modules](../about-packages-and-modules)[About scopes](../about-scopes)[About public packages](../about-public-packages)[About private packages](../about-private-packages)[npm package scope, access level, and visibility](../package-scope-access-level-and-visibility)
[Contributing packages to the registry](contributing-packages-to-the-registry)
[Updating and managing your published packages](updating-and-managing-your-published-packages)
[Getting packages from the registry](getting-packages-from-the-registry)
[Securing your code](securing-your-code)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About the public npm registry](../about-the-public-npm-registry)
* [About packages and modules](../about-packages-and-modules)
* [About scopes](../about-scopes)
* [About public packages](../about-public-packages)
* [About private packages](../about-private-packages)
* [npm package scope, access level, and visibility](../package-scope-access-level-and-visibility)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/packages-and-modules/introduction-to-packages-and-modules/index.mdx)
npm npm CLI npm CLI
=======
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](v8)
[CLI Commands](v8/commands)
[Configuring npm](v8/configuring-npm)
[Using npm](v8/using-npm)
* [CLI Commands](v8/commands)
* [Configuring npm](v8/configuring-npm)
* [Using npm](v8/using-npm)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/nav.yml)
npm CLI Commands CLI Commands
============
[Skip to content](#skip-nav)
[npm Docs](../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../about-npm)
[Getting started](../../getting-started)
[Packages and modules](../../packages-and-modules)
[Integrations](../../integrations)
[Organizations](../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../threats-and-mitigations)
[npm CLI](../v8)
[CLI Commands](commands)
[npm](commands/npm)[npm access](commands/npm-access)[npm adduser](commands/npm-adduser)[npm audit](commands/npm-audit)[npm bin](commands/npm-bin)[npm bugs](commands/npm-bugs)[npm cache](commands/npm-cache)[npm ci](commands/npm-ci)[npm completion](commands/npm-completion)[npm config](commands/npm-config)[npm dedupe](commands/npm-dedupe)[npm deprecate](commands/npm-deprecate)[npm diff](commands/npm-diff)[npm dist-tag](commands/npm-dist-tag)[npm docs](commands/npm-docs)[npm doctor](commands/npm-doctor)[npm edit](commands/npm-edit)[npm exec](commands/npm-exec)[npm explain](commands/npm-explain)[npm explore](commands/npm-explore)[npm find-dupes](commands/npm-find-dupes)[npm fund](commands/npm-fund)[npm help](commands/npm-help)[npm help-search](commands/npm-help-search)[npm hook](commands/npm-hook)[npm init](commands/npm-init)[npm install](commands/npm-install)[npm install-ci-test](commands/npm-install-ci-test)[npm install-test](commands/npm-install-test)[npm link](commands/npm-link)[npm logout](commands/npm-logout)[npm ls](commands/npm-ls)[npm org](commands/npm-org)[npm outdated](commands/npm-outdated)[npm owner](commands/npm-owner)[npm pack](commands/npm-pack)[npm ping](commands/npm-ping)[npm pkg](commands/npm-pkg)[npm prefix](commands/npm-prefix)[npm profile](commands/npm-profile)[npm prune](commands/npm-prune)[npm publish](commands/npm-publish)[npm query](commands/npm-query)[npm rebuild](commands/npm-rebuild)[npm repo](commands/npm-repo)[npm restart](commands/npm-restart)[npm root](commands/npm-root)[npm run-script](commands/npm-run-script)[npm search](commands/npm-search)[npm set-script](commands/npm-set-script)[npm shrinkwrap](commands/npm-shrinkwrap)[npm star](commands/npm-star)[npm stars](commands/npm-stars)[npm start](commands/npm-start)[npm stop](commands/npm-stop)[npm team](commands/npm-team)[npm test](commands/npm-test)[npm token](commands/npm-token)[npm uninstall](commands/npm-uninstall)[npm unpublish](commands/npm-unpublish)[npm unstar](commands/npm-unstar)[npm update](commands/npm-update)[npm version](commands/npm-version)[npm view](commands/npm-view)[npm whoami](commands/npm-whoami)[npx](commands/npx)
[Configuring npm](configuring-npm)
[Using npm](using-npm)
* [npm](commands/npm)JavaScript package manager
* [npm access](commands/npm-access)Set access level on published packages
* [npm adduser](commands/npm-adduser)Add a registry user account
* [npm audit](commands/npm-audit)Run a security audit
* [npm bin](commands/npm-bin)Display npm bin folder
* [npm bugs](commands/npm-bugs)Bugs for a package in a web browser maybe
* [npm cache](commands/npm-cache)Manipulates packages cache
* [npm ci](commands/npm-ci)Install a project with a clean slate
* [npm completion](commands/npm-completion)Tab completion for npm
* [npm config](commands/npm-config)Manage the npm configuration files
* [npm dedupe](commands/npm-dedupe)Reduce duplication
* [npm deprecate](commands/npm-deprecate)Deprecate a version of a package
* [npm diff](commands/npm-diff)The registry diff command
* [npm dist-tag](commands/npm-dist-tag)Modify package distribution tags
* [npm docs](commands/npm-docs)Docs for a package in a web browser maybe
* [npm doctor](commands/npm-doctor)Check your environments
* [npm edit](commands/npm-edit)Edit an installed package
* [npm exec](commands/npm-exec)Run a command from an npm package
* [npm explain](commands/npm-explain)Explain installed packages
* [npm explore](commands/npm-explore)Browse an installed package
* [npm find-dupes](commands/npm-find-dupes)Find duplication in the package tree
* [npm fund](commands/npm-fund)Retrieve funding information
* [npm help](commands/npm-help)Search npm help documentation
* [npm help-search](commands/npm-help-search)Get help on npm
* [npm hook](commands/npm-hook)Manage registry hooks
* [npm init](commands/npm-init)Create a package.json file
* [npm install](commands/npm-install)Install a package
* [npm install-ci-test](commands/npm-install-ci-test)Install a project with a clean slate and run tests
* [npm install-test](commands/npm-install-test)Install package(s) and run tests
* [npm link](commands/npm-link)Symlink a package folder
* [npm logout](commands/npm-logout)Log out of the registry
* [npm ls](commands/npm-ls)List installed packages
* [npm org](commands/npm-org)Manage orgs
* [npm outdated](commands/npm-outdated)Check for outdated packages
* [npm owner](commands/npm-owner)Manage package owners
* [npm pack](commands/npm-pack)Create a tarball from a package
* [npm ping](commands/npm-ping)Ping npm registry
* [npm pkg](commands/npm-pkg)Manages your package.json
* [npm prefix](commands/npm-prefix)Display prefix
* [npm profile](commands/npm-profile)Change settings on your registry profile
* [npm prune](commands/npm-prune)Remove extraneous packages
* [npm publish](commands/npm-publish)Publish a package
* [npm query](commands/npm-query)Retrieve a filtered list of packages
* [npm rebuild](commands/npm-rebuild)Rebuild a package
* [npm repo](commands/npm-repo)Open package repository page in the browser
* [npm restart](commands/npm-restart)Restart a package
* [npm root](commands/npm-root)Display npm root
* [npm run-script](commands/npm-run-script)Run arbitrary package scripts
* [npm search](commands/npm-search)Search for packages
* [npm set-script](commands/npm-set-script)Set tasks in the scripts section of package.json
* [npm shrinkwrap](commands/npm-shrinkwrap)Lock down dependency versions for publication
* [npm star](commands/npm-star)Mark your favorite packages
* [npm stars](commands/npm-stars)View packages marked as favorites
* [npm start](commands/npm-start)Start a package
* [npm stop](commands/npm-stop)Stop a package
* [npm team](commands/npm-team)Manage organization teams and team memberships
* [npm test](commands/npm-test)Test a package
* [npm token](commands/npm-token)Manage your authentication tokens
* [npm uninstall](commands/npm-uninstall)Remove a package
* [npm unpublish](commands/npm-unpublish)Remove a package from the registry
* [npm unstar](commands/npm-unstar)Remove an item from your favorite packages
* [npm update](commands/npm-update)Update a package
* [npm version](commands/npm-version)Bump a package version
* [npm view](commands/npm-view)View registry info
* [npm whoami](commands/npm-whoami)Display npm username
* [npx](commands/npx)Run a command from an npm package
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/nav.yml)
npm Configuring npm Configuring npm
===============
[Skip to content](#skip-nav)
[npm Docs](../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../about-npm)
[Getting started](../../getting-started)
[Packages and modules](../../packages-and-modules)
[Integrations](../../integrations)
[Organizations](../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../threats-and-mitigations)
[npm CLI](../v8)
[CLI Commands](commands)
[Configuring npm](configuring-npm)
[Install](configuring-npm/install)[Folders](configuring-npm/folders)[.npmrc](configuring-npm/npmrc)[npm-shrinkwrap.json](configuring-npm/npm-shrinkwrap-json)[package.json](configuring-npm/package-json)[package-lock.json](configuring-npm/package-lock-json)
[Using npm](using-npm)
* [Install](configuring-npm/install)Download and install node and npm
* [Folders](configuring-npm/folders)Folder structures used by npm
* [.npmrc](configuring-npm/npmrc)The npm config files
* [npm-shrinkwrap.json](configuring-npm/npm-shrinkwrap-json)A publishable lockfile
* [package.json](configuring-npm/package-json)Specifics of npm's package.json handling
* [package-lock.json](configuring-npm/package-lock-json)A manifestation of the manifest
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/nav.yml)
npm Using npm Using npm
=========
[Skip to content](#skip-nav)
[npm Docs](../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../about-npm)
[Getting started](../../getting-started)
[Packages and modules](../../packages-and-modules)
[Integrations](../../integrations)
[Organizations](../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../threats-and-mitigations)
[npm CLI](../v8)
[CLI Commands](commands)
[Configuring npm](configuring-npm)
[Using npm](using-npm)
[Registry](using-npm/registry)[Package spec](using-npm/package-spec)[Config](using-npm/config)[Logging](using-npm/logging)[Scope](using-npm/scope)[Scripts](using-npm/scripts)[Workspaces](using-npm/workspaces)[Organizations](using-npm/orgs)[Dependency Selectors](using-npm/dependency-selectors)[Developers](using-npm/developers)[Removal](using-npm/removal)
* [Registry](using-npm/registry)The JavaScript Package Registry
* [Package spec](using-npm/package-spec)Package name specifier
* [Config](using-npm/config)About npm configuration
* [Logging](using-npm/logging)Why, What & How we Log
* [Scope](using-npm/scope)Scoped packages
* [Scripts](using-npm/scripts)How npm handles the "scripts" field
* [Workspaces](using-npm/workspaces)Working with workspaces
* [Organizations](using-npm/orgs)Working with teams & organizations
* [Dependency Selectors](using-npm/dependency-selectors)Dependency Selector Syntax & Querying
* [Developers](using-npm/developers)Developer guide
* [Removal](using-npm/removal)Cleaning the slate
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/nav.yml)
npm install install
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
Download and install node and npm
Table of contents* [Description](#description)
* [Overview](#overview)
* [Checking your version of npm and Node.js](#checking-your-version-of-npm-and-nodejs)
* [Using a Node version manager to install Node.js and npm](#using-a-node-version-manager-to-install-nodejs-and-npm)
+ [OSX or Linux Node version managers](#osx-or-linux-node-version-managers)
+ [Windows Node version managers](#windows-node-version-managers)
* [Using a Node installer to install Node.js and npm](#using-a-node-installer-to-install-nodejs-and-npm)
+ [OS X or Windows Node installers](#os-x-or-windows-node-installers)
+ [Linux or other operating systems Node installers](#linux-or-other-operating-systems-node-installers)
+ [Less-common operating systems](#less-common-operating-systems)
###
Description
To publish and install packages to and from the public npm registry, you must install Node.js and the npm command line interface using either a Node version manager or a Node installer. **We strongly recommend using a Node version manager to install Node.js and npm.** We do not recommend using a Node installer, since the Node installation process installs npm in a directory with local permissions and can cause permissions errors when you run npm packages globally.
###
Overview
* [Checking your version of npm and Node.js](#checking-your-version-of-npm-and-node-js)
* [Using a Node version manager to install Node.js and npm](#using-a-node-version-manager-to-install-node-js-and-npm)
* [Using a Node installer to install Node.js and npm](#using-a-node-installer-to-install-node-js-and-npm)
###
Checking your version of npm and Node.js
To see if you already have Node.js and npm installed and check the installed version, run the following commands:
```
node -v
npm -v
```
###
Using a Node version manager to install Node.js and npm
Node version managers allow you to install and switch between multiple versions of Node.js and npm on your system so you can test your applications on multiple versions of npm to ensure they work for users on different versions.
####
OSX or Linux Node version managers
* [nvm](https://github.com/creationix/nvm)
* [n](https://github.com/tj/n)
####
Windows Node version managers
* [nodist](https://github.com/marcelklehr/nodist)
* [nvm-windows](https://github.com/coreybutler/nvm-windows)
###
Using a Node installer to install Node.js and npm
If you are unable to use a Node version manager, you can use a Node installer to install both Node.js and npm on your system.
* [Node.js installer](https://nodejs.org/en/download/)
* [NodeSource installer](https://github.com/nodesource/distributions). If you use Linux, we recommend that you use a NodeSource installer.
####
OS X or Windows Node installers
If you're using OS X or Windows, use one of the installers from the [Node.js download page](https://nodejs.org/en/download/). Be sure to install the version labeled **LTS**. Other versions have not yet been tested with npm.
####
Linux or other operating systems Node installers
If you're using Linux or another operating system, use one of the following installers:
* [NodeSource installer](https://github.com/nodesource/distributions) (recommended)
* One of the installers on the [Node.js download page](https://nodejs.org/en/download/)
Or see [this page](https://nodejs.org/en/download/package-manager/) to install npm for Linux in the way many Linux developers prefer.
####
Less-common operating systems
For more information on installing Node.js on a variety of operating systems, see [this page](https://nodejs.org/en/download/package-manager/).
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/install.md)
| programming_docs |
npm npm-shrinkwrap.json npm-shrinkwrap.json
===================
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
A publishable lockfile
Table of contents* [Description](#description)
* [See also](#see-also)
###
Description
`npm-shrinkwrap.json` is a file created by [`npm
shrinkwrap`](../commands/npm-shrinkwrap). It is identical to `package-lock.json`, with one major caveat: Unlike `package-lock.json`, `npm-shrinkwrap.json` may be included when publishing a package.
The recommended use-case for `npm-shrinkwrap.json` is applications deployed through the publishing process on the registry: for example, daemons and command-line tools intended as global installs or `devDependencies`. It's strongly discouraged for library authors to publish this file, since that would prevent end users from having control over transitive dependency updates.
If both `package-lock.json` and `npm-shrinkwrap.json` are present in a package root, `npm-shrinkwrap.json` will be preferred over the `package-lock.json` file.
For full details and description of the `npm-shrinkwrap.json` file format, refer to the manual page for [package-lock.json](package-lock-json).
###
See also
* [npm shrinkwrap](../commands/npm-shrinkwrap)
* [package-lock.json](package-lock-json)
* [package.json](package-json)
* [npm install](../commands/npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/npm-shrinkwrap-json.md)
npm package-lock.json package-lock.json
=================
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
A manifestation of the manifest
Table of contents* [Description](#description)
* [package-lock.json vs npm-shrinkwrap.json](#package-lockjson-vs-npm-shrinkwrapjson)
* [Hidden Lockfiles](#hidden-lockfiles)
* [Handling Old Lockfiles](#handling-old-lockfiles)
* [File Format](#file-format)
+ [name](#name)
+ [version](#version)
+ [lockfileVersion](#lockfileversion)
+ [packages](#packages)
+ [dependencies](#dependencies)
* [See also](#see-also)
###
Description
`package-lock.json` is automatically generated for any operations where npm modifies either the `node_modules` tree, or `package.json`. It describes the exact tree that was generated, such that subsequent installs are able to generate identical trees, regardless of intermediate dependency updates.
This file is intended to be committed into source repositories, and serves various purposes:
* Describe a single representation of a dependency tree such that teammates, deployments, and continuous integration are guaranteed to install exactly the same dependencies.
* Provide a facility for users to "time-travel" to previous states of `node_modules` without having to commit the directory itself.
* Facilitate greater visibility of tree changes through readable source control diffs.
* Optimize the installation process by allowing npm to skip repeated metadata resolutions for previously-installed packages.
* As of npm v7, lockfiles include enough information to gain a complete picture of the package tree, reducing the need to read `package.json` files, and allowing for significant performance improvements.
###
`package-lock.json` vs `npm-shrinkwrap.json`
Both of these files have the same format, and perform similar functions in the root of a project.
The difference is that `package-lock.json` cannot be published, and it will be ignored if found in any place other than the root project.
In contrast, [npm-shrinkwrap.json](npm-shrinkwrap-json) allows publication, and defines the dependency tree from the point encountered. This is not recommended unless deploying a CLI tool or otherwise using the publication process for producing production packages.
If both `package-lock.json` and `npm-shrinkwrap.json` are present in the root of a project, `npm-shrinkwrap.json` will take precedence and `package-lock.json` will be ignored.
###
Hidden Lockfiles
In order to avoid processing the `node_modules` folder repeatedly, npm as of v7 uses a "hidden" lockfile present in `node_modules/.package-lock.json`. This contains information about the tree, and is used in lieu of reading the entire `node_modules` hierarchy provided that the following conditions are met:
* All package folders it references exist in the `node_modules` hierarchy.
* No package folders exist in the `node_modules` hierarchy that are not listed in the lockfile.
* The modified time of the file is at least as recent as all of the package folders it references.
That is, the hidden lockfile will only be relevant if it was created as part of the most recent update to the package tree. If another CLI mutates the tree in any way, this will be detected, and the hidden lockfile will be ignored.
Note that it *is* possible to manually change the *contents* of a package in such a way that the modified time of the package folder is unaffected. For example, if you add a file to `node_modules/foo/lib/bar.js`, then the modified time on `node_modules/foo` will not reflect this change. If you are manually editing files in `node_modules`, it is generally best to delete the file at `node_modules/.package-lock.json`.
As the hidden lockfile is ignored by older npm versions, it does not contain the backwards compatibility affordances present in "normal" lockfiles. That is, it is `lockfileVersion: 3`, rather than `lockfileVersion: 2`.
###
Handling Old Lockfiles
When npm detects a lockfile from npm v6 or before during the package installation process, it is automatically updated to fetch missing information from either the `node_modules` tree or (in the case of empty `node_modules` trees or very old lockfile formats) the npm registry.
###
File Format
####
`name`
The name of the package this is a package-lock for. This will match what's in `package.json`.
####
`version`
The version of the package this is a package-lock for. This will match what's in `package.json`.
####
`lockfileVersion`
An integer version, starting at `1` with the version number of this document whose semantics were used when generating this `package-lock.json`.
Note that the file format changed significantly in npm v7 to track information that would have otherwise required looking in `node_modules` or the npm registry. Lockfiles generated by npm v7 will contain `lockfileVersion: 2`.
* No version provided: an "ancient" shrinkwrap file from a version of npm prior to npm v5.
* `1`: The lockfile version used by npm v5 and v6.
* `2`: The lockfile version used by npm v7, which is backwards compatible to v1 lockfiles.
* `3`: The lockfile version used by npm v7, *without* backwards compatibility affordances. This is used for the hidden lockfile at `node_modules/.package-lock.json`, and will likely be used in a future version of npm, once support for npm v6 is no longer relevant.
npm will always attempt to get whatever data it can out of a lockfile, even if it is not a version that it was designed to support.
####
`packages`
This is an object that maps package locations to an object containing the information about that package.
The root project is typically listed with a key of `""`, and all other packages are listed with their relative paths from the root project folder.
Package descriptors have the following fields:
* version: The version found in `package.json`
* resolved: The place where the package was actually resolved from. In the case of packages fetched from the registry, this will be a url to a tarball. In the case of git dependencies, this will be the full git url with commit sha. In the case of link dependencies, this will be the location of the link target. `registry.npmjs.org` is a magic value meaning "the currently configured registry".
* integrity: A `sha512` or `sha1` [Standard Subresource Integrity](https://w3c.github.io/webappsec/specs/subresourceintegrity/) string for the artifact that was unpacked in this location.
* link: A flag to indicate that this is a symbolic link. If this is present, no other fields are specified, since the link target will also be included in the lockfile.
* dev, optional, devOptional: If the package is strictly part of the `devDependencies` tree, then `dev` will be true. If it is strictly part of the `optionalDependencies` tree, then `optional` will be set. If it is both a `dev` dependency *and* an `optional` dependency of a non-dev dependency, then `devOptional` will be set. (An `optional` dependency of a `dev` dependency will have both `dev` and `optional` set.)
* inBundle: A flag to indicate that the package is a bundled dependency.
* hasInstallScript: A flag to indicate that the package has a `preinstall`, `install`, or `postinstall` script.
* hasShrinkwrap: A flag to indicate that the package has an `npm-shrinkwrap.json` file.
* bin, license, engines, dependencies, optionalDependencies: fields from `package.json`
####
dependencies
Legacy data for supporting versions of npm that use `lockfileVersion: 1`. This is a mapping of package names to dependency objects. Because the object structure is strictly hierarchical, symbolic link dependencies are somewhat challenging to represent in some cases.
npm v7 ignores this section entirely if a `packages` section is present, but does keep it up to date in order to support switching between npm v6 and npm v7.
Dependency objects have the following fields:
* version: a specifier that varies depending on the nature of the package, and is usable in fetching a new copy of it.
+ bundled dependencies: Regardless of source, this is a version number that is purely for informational purposes.
+ registry sources: This is a version number. (eg, `1.2.3`)
+ git sources: This is a git specifier with resolved committish. (eg, `git+https://example.com/foo/bar#115311855adb0789a0466714ed48a1499ffea97e`)
+ http tarball sources: This is the URL of the tarball. (eg, `https://example.com/example-1.3.0.tgz`)
+ local tarball sources: This is the file URL of the tarball. (eg `file:///opt/storage/example-1.3.0.tgz`)
+ local link sources: This is the file URL of the link. (eg `file:libs/our-module`)
* integrity: A `sha512` or `sha1` [Standard Subresource Integrity](https://w3c.github.io/webappsec/specs/subresourceintegrity/) string for the artifact that was unpacked in this location. For git dependencies, this is the commit sha.
* resolved: For registry sources this is path of the tarball relative to the registry URL. If the tarball URL isn't on the same server as the registry URL then this is a complete URL. `registry.npmjs.org` is a magic value meaning "the currently configured registry".
* bundled: If true, this is the bundled dependency and will be installed by the parent module. When installing, this module will be extracted from the parent module during the extract phase, not installed as a separate dependency.
* dev: If true then this dependency is either a development dependency ONLY of the top level module or a transitive dependency of one. This is false for dependencies that are both a development dependency of the top level and a transitive dependency of a non-development dependency of the top level.
* optional: If true then this dependency is either an optional dependency ONLY of the top level module or a transitive dependency of one. This is false for dependencies that are both an optional dependency of the top level and a transitive dependency of a non-optional dependency of the top level.
* requires: This is a mapping of module name to version. This is a list of everything this module requires, regardless of where it will be installed. The version should match via normal matching rules a dependency either in our `dependencies` or in a level higher than us.
* dependencies: The dependencies of this dependency, exactly as at the top level.
###
See also
* [npm shrinkwrap](../commands/npm-shrinkwrap)
* [npm-shrinkwrap.json](npm-shrinkwrap-json)
* [package.json](package-json)
* [npm install](../commands/npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/package-lock-json.md)
npm folders folders
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
Folder Structures Used by npm
Table of contents* [Description](#description)
+ [tl;dr](#tldr)
+ [prefix Configuration](#prefix-configuration)
+ [Node Modules](#node-modules)
+ [Executables](#executables)
+ [Man Pages](#man-pages)
+ [Cache](#cache)
+ [Temp Files](#temp-files)
* [More Information](#more-information)
+ [Global Installation](#global-installation)
+ [Cycles, Conflicts, and Folder Parsimony](#cycles-conflicts-and-folder-parsimony)
+ [Example](#example)
+ [Publishing](#publishing)
* [See also](#see-also)
###
Description
npm puts various things on your computer. That's its job.
This document will tell you what it puts where.
####
tl;dr
* Local install (default): puts stuff in `./node_modules` of the current package root.
* Global install (with `-g`): puts stuff in /usr/local or wherever node is installed.
* Install it **locally** if you're going to `require()` it.
* Install it **globally** if you're going to run it on the command line.
* If you need both, then install it in both places, or use `npm link`.
####
prefix Configuration
The `prefix` config defaults to the location where node is installed. On most systems, this is `/usr/local`. On Windows, it's `%AppData%\npm`. On Unix systems, it's one level up, since node is typically installed at `{prefix}/bin/node` rather than `{prefix}/node.exe`.
When the `global` flag is set, npm installs things into this prefix. When it is not set, it uses the root of the current package, or the current working directory if not in a package already.
####
Node Modules
Packages are dropped into the `node_modules` folder under the `prefix`. When installing locally, this means that you can `require("packagename")` to load its main module, or `require("packagename/lib/path/to/sub/module")` to load other modules.
Global installs on Unix systems go to `{prefix}/lib/node_modules`. Global installs on Windows go to `{prefix}/node_modules` (that is, no `lib` folder.)
Scoped packages are installed the same way, except they are grouped together in a sub-folder of the relevant `node_modules` folder with the name of that scope prefix by the @ symbol, e.g. `npm install @myorg/package` would place the package in `{prefix}/node_modules/@myorg/package`. See [`scope`](../using-npm/scope) for more details.
If you wish to `require()` a package, then install it locally.
####
Executables
When in global mode, executables are linked into `{prefix}/bin` on Unix, or directly into `{prefix}` on Windows. Ensure that path is in your terminal's `PATH` environment to run them.
When in local mode, executables are linked into `./node_modules/.bin` so that they can be made available to scripts run through npm. (For example, so that a test runner will be in the path when you run `npm test`.)
####
Man Pages
When in global mode, man pages are linked into `{prefix}/share/man`.
When in local mode, man pages are not installed.
Man pages are not installed on Windows systems.
####
Cache
See [`npm cache`](../commands/npm-cache). Cache files are stored in `~/.npm` on Posix, or `%AppData%/npm-cache` on Windows.
This is controlled by the `cache` configuration param.
####
Temp Files
Temporary files are stored by default in the folder specified by the `tmp` config, which defaults to the TMPDIR, TMP, or TEMP environment variables, or `/tmp` on Unix and `c:\windows\temp` on Windows.
Temp files are given a unique folder under this root for each run of the program, and are deleted upon successful exit.
###
More Information
When installing locally, npm first tries to find an appropriate `prefix` folder. This is so that `npm install [email protected]` will install to the sensible root of your package, even if you happen to have `cd`ed into some other folder.
Starting at the $PWD, npm will walk up the folder tree checking for a folder that contains either a `package.json` file, or a `node_modules` folder. If such a thing is found, then that is treated as the effective "current directory" for the purpose of running npm commands. (This behavior is inspired by and similar to git's .git-folder seeking logic when running git commands in a working dir.)
If no package root is found, then the current folder is used.
When you run `npm install [email protected]`, then the package is loaded into the cache, and then unpacked into `./node_modules/foo`. Then, any of foo's dependencies are similarly unpacked into `./node_modules/foo/node_modules/...`.
Any bin files are symlinked to `./node_modules/.bin/`, so that they may be found by npm scripts when necessary.
####
Global Installation
If the `global` configuration is set to true, then npm will install packages "globally".
For global installation, packages are installed roughly the same way, but using the folders described above.
####
Cycles, Conflicts, and Folder Parsimony
Cycles are handled using the property of node's module system that it walks up the directories looking for `node_modules` folders. So, at every stage, if a package is already installed in an ancestor `node_modules` folder, then it is not installed at the current location.
Consider the case above, where `foo -> bar -> baz`. Imagine if, in addition to that, baz depended on bar, so you'd have: `foo -> bar -> baz -> bar -> baz ...`. However, since the folder structure is: `foo/node_modules/bar/node_modules/baz`, there's no need to put another copy of bar into `.../baz/node_modules`, since when it calls require("bar"), it will get the copy that is installed in `foo/node_modules/bar`.
This shortcut is only used if the exact same version would be installed in multiple nested `node_modules` folders. It is still possible to have `a/node_modules/b/node_modules/a` if the two "a" packages are different versions. However, without repeating the exact same package multiple times, an infinite regress will always be prevented.
Another optimization can be made by installing dependencies at the highest level possible, below the localized "target" folder.
####
Example
Consider this dependency graph:
```
foo
+-- [email protected]
+-- [email protected]
| +-- [email protected] (latest=1.3.7)
| +-- [email protected]
| | `-- [email protected]
| | `-- [email protected] (cycle)
| `-- asdf@*
`-- [email protected]
`-- [email protected]
`-- bar
```
In this case, we might expect a folder structure like this:
```
foo
+-- node_modules
+-- blerg (1.2.5) <---[A]
+-- bar (1.2.3) <---[B]
| `-- node_modules
| +-- baz (2.0.2) <---[C]
| | `-- node_modules
| | `-- quux (3.2.0)
| `-- asdf (2.3.4)
`-- baz (1.2.3) <---[D]
`-- node_modules
`-- quux (3.2.0) <---[E]
```
Since foo depends directly on `[email protected]` and `[email protected]`, those are installed in foo's `node_modules` folder.
Even though the latest copy of blerg is 1.3.7, foo has a specific dependency on version 1.2.5. So, that gets installed at [A]. Since the parent installation of blerg satisfies bar's dependency on `[email protected]`, it does not install another copy under [B].
Bar [B] also has dependencies on baz and asdf, so those are installed in bar's `node_modules` folder. Because it depends on `[email protected]`, it cannot re-use the `[email protected]` installed in the parent `node_modules` folder [D], and must install its own copy [C].
Underneath bar, the `baz -> quux -> bar` dependency creates a cycle. However, because bar is already in quux's ancestry [B], it does not unpack another copy of bar into that folder.
Underneath `foo -> baz` [D], quux's [E] folder tree is empty, because its dependency on bar is satisfied by the parent folder copy installed at [B].
For a graphical breakdown of what is installed where, use `npm ls`.
####
Publishing
Upon publishing, npm will look in the `node_modules` folder. If any of the items there are not in the `bundleDependencies` array, then they will not be included in the package tarball.
This allows a package maintainer to install all of their dependencies (and dev dependencies) locally, but only re-publish those items that cannot be found elsewhere. See [`package.json`](package-json) for more information.
###
See also
* [package.json](package-json)
* [npm install](../commands/npm-install)
* [npm pack](../commands/npm-pack)
* [npm cache](../commands/npm-cache)
* [npm config](../commands/npm-config)
* <npmrc>
* [config](../using-npm/config)
* [npm publish](../commands/npm-publish)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/folders.md)
| programming_docs |
npm npmrc npmrc
=====
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
The npm config files
Table of contents* [Description](#description)
* [Files](#files)
+ [Comments](#comments)
+ [Per-project config file](#per-project-config-file)
+ [Per-user config file](#per-user-config-file)
+ [Global config file](#global-config-file)
+ [Built-in config file](#built-in-config-file)
* [Auth related configuration](#auth-related-configuration)
* [See also](#see-also)
###
Description
npm gets its config settings from the command line, environment variables, and `npmrc` files.
The `npm config` command can be used to update and edit the contents of the user and global npmrc files.
For a list of available configuration options, see [config](../using-npm/config).
###
Files
The four relevant files are:
* per-project config file (/path/to/my/project/.npmrc)
* per-user config file (~/.npmrc)
* global config file ($PREFIX/etc/npmrc)
* npm builtin config file (/path/to/npm/npmrc)
All npm config files are an ini-formatted list of `key = value` parameters. Environment variables can be replaced using `${VARIABLE_NAME}`. For example:
```
prefix = ${HOME}/.npm-packages
```
Each of these files is loaded, and config options are resolved in priority order. For example, a setting in the userconfig file would override the setting in the globalconfig file.
Array values are specified by adding "[]" after the key name. For example:
```
key[] = "first value"
key[] = "second value"
```
####
Comments
Lines in `.npmrc` files are interpreted as comments when they begin with a `;` or `#` character. `.npmrc` files are parsed by [npm/ini](https://github.com/npm/ini), which specifies this comment syntax.
For example:
```
# last modified: 01 Jan 2016
; Set a new registry for a scoped package
@myscope:registry=https://mycustomregistry.example.org
```
####
Per-project config file
When working locally in a project, a `.npmrc` file in the root of the project (ie, a sibling of `node_modules` and `package.json`) will set config values specific to this project.
Note that this only applies to the root of the project that you're running npm in. It has no effect when your module is published. For example, you can't publish a module that forces itself to install globally, or in a different location.
Additionally, this file is not read in global mode, such as when running `npm install -g`.
####
Per-user config file
`$HOME/.npmrc` (or the `userconfig` param, if set in the environment or on the command line)
####
Global config file
`$PREFIX/etc/npmrc` (or the `globalconfig` param, if set above): This file is an ini-file formatted list of `key = value` parameters. Environment variables can be replaced as above.
####
Built-in config file
`path/to/npm/itself/npmrc`
This is an unchangeable "builtin" configuration file that npm keeps consistent across updates. Set fields in here using the `./configure` script that comes with npm. This is primarily for distribution maintainers to override default configs in a standard and consistent manner.
###
Auth related configuration
The settings `_auth`, `_authToken`, `username` and `_password` must all be scoped to a specific registry. This ensures that `npm` will never send credentials to the wrong host.
In order to scope these values, they must be prefixed by a URI fragment. If the credential is meant for any request to a registry on a single host, the scope may look like `//registry.npmjs.org/:`. If it must be scoped to a specific path on the host that path may also be provided, such as `//my-custom-registry.org/unique/path:`.
```
; bad config
_authToken=MYTOKEN
; good config
@myorg:registry=https://somewhere-else.com/myorg
@another:registry=https://somewhere-else.com/another
//registry.npmjs.org/:_authToken=MYTOKEN
; would apply to both @myorg and @another
; //somewhere-else.com/:_authToken=MYTOKEN
; would apply only to @myorg
//somewhere-else.com/myorg/:_authToken=MYTOKEN1
; would apply only to @another
//somewhere-else.com/another/:_authToken=MYTOKEN2
```
###
See also
* [npm folders](folders)
* [npm config](../commands/npm-config)
* [config](../using-npm/config)
* [package.json](package-json)
* [npm](../commands/npm)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/npmrc.md)
npm package.json package.json
============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Install](install)[Folders](folders)[.npmrc](npmrc)[npm-shrinkwrap.json](npm-shrinkwrap-json)[package.json](package-json)[package-lock.json](package-lock-json)
[Using npm](../using-npm)
Specifics of npm's package.json handling
Table of contents* [Description](#description)
* [name](#name)
* [version](#version)
* [description](#description-1)
* [keywords](#keywords)
* [homepage](#homepage)
* [bugs](#bugs)
* [license](#license)
* [people fields: author, contributors](#people-fields-author-contributors)
* [funding](#funding)
* [files](#files)
* [main](#main)
* [browser](#browser)
* [bin](#bin)
* [man](#man)
* [directories](#directories)
+ [directories.bin](#directoriesbin)
+ [directories.man](#directoriesman)
* [repository](#repository)
* [scripts](#scripts)
* [config](#config)
* [dependencies](#dependencies)
+ [URLs as Dependencies](#urls-as-dependencies)
+ [Git URLs as Dependencies](#git-urls-as-dependencies)
+ [GitHub URLs](#github-urls)
+ [Local Paths](#local-paths)
* [devDependencies](#devdependencies)
* [peerDependencies](#peerdependencies)
* [peerDependenciesMeta](#peerdependenciesmeta)
* [bundleDependencies](#bundledependencies)
* [optionalDependencies](#optionaldependencies)
* [overrides](#overrides)
* [engines](#engines)
* [os](#os)
* [cpu](#cpu)
* [private](#private)
* [publishConfig](#publishconfig)
* [workspaces](#workspaces)
* [DEFAULT VALUES](#default-values)
* [SEE ALSO](#see-also)
###
Description
This document is all you need to know about what's required in your package.json file. It must be actual JSON, not just a JavaScript object literal.
A lot of the behavior described in this document is affected by the config settings described in [`config`](../using-npm/config).
###
name
If you plan to publish your package, the *most* important things in your package.json are the name and version fields as they will be required. The name and version together form an identifier that is assumed to be completely unique. Changes to the package should come along with changes to the version. If you don't plan to publish your package, the name and version fields are optional.
The name is what your thing is called.
Some rules:
* The name must be less than or equal to 214 characters. This includes the scope for scoped packages.
* The names of scoped packages can begin with a dot or an underscore. This is not permitted without a scope.
* New packages must not have uppercase letters in the name.
* The name ends up being part of a URL, an argument on the command line, and a folder name. Therefore, the name can't contain any non-URL-safe characters.
Some tips:
* Don't use the same name as a core Node module.
* Don't put "js" or "node" in the name. It's assumed that it's js, since you're writing a package.json file, and you can specify the engine using the "engines" field. (See below.)
* The name will probably be passed as an argument to require(), so it should be something short, but also reasonably descriptive.
* You may want to check the npm registry to see if there's something by that name already, before you get too attached to it. <https://www.npmjs.com/>
A name can be optionally prefixed by a scope, e.g. `@myorg/mypackage`. See [`scope`](../using-npm/scope) for more detail.
###
version
If you plan to publish your package, the *most* important things in your package.json are the name and version fields as they will be required. The name and version together form an identifier that is assumed to be completely unique. Changes to the package should come along with changes to the version. If you don't plan to publish your package, the name and version fields are optional.
Version must be parseable by [node-semver](https://github.com/npm/node-semver), which is bundled with npm as a dependency. (`npm install semver` to use it yourself.)
###
description
Put a description in it. It's a string. This helps people discover your package, as it's listed in `npm search`.
###
keywords
Put keywords in it. It's an array of strings. This helps people discover your package as it's listed in `npm search`.
###
homepage
The url to the project homepage.
Example:
```
"homepage": "https://github.com/owner/project#readme"
```
###
bugs
The url to your project's issue tracker and / or the email address to which issues should be reported. These are helpful for people who encounter issues with your package.
It should look like this:
```
{
"url" : "https://github.com/owner/project/issues",
"email" : "[email protected]"
}
```
You can specify either one or both values. If you want to provide only a url, you can specify the value for "bugs" as a simple string instead of an object.
If a url is provided, it will be used by the `npm bugs` command.
###
license
You should specify a license for your package so that people know how they are permitted to use it, and any restrictions you're placing on it.
If you're using a common license such as BSD-2-Clause or MIT, add a current SPDX license identifier for the license you're using, like this:
```
{
"license" : "BSD-3-Clause"
}
```
You can check [the full list of SPDX license IDs](https://spdx.org/licenses/). Ideally you should pick one that is [OSI](https://opensource.org/licenses/alphabetical) approved.
If your package is licensed under multiple common licenses, use an [SPDX license expression syntax version 2.0 string](https://spdx.dev/specifications/), like this:
```
{
"license" : "(ISC OR GPL-3.0)"
}
```
If you are using a license that hasn't been assigned an SPDX identifier, or if you are using a custom license, use a string value like this one:
```
{
"license" : "SEE LICENSE IN <filename>"
}
```
Then include a file named `<filename>` at the top level of the package.
Some old packages used license objects or a "licenses" property containing an array of license objects:
```
// Not valid metadata
{
"license" : {
"type" : "ISC",
"url" : "https://opensource.org/licenses/ISC"
}
}
// Not valid metadata
{
"licenses" : [
{
"type": "MIT",
"url": "https://www.opensource.org/licenses/mit-license.php"
},
{
"type": "Apache-2.0",
"url": "https://opensource.org/licenses/apache2.0.php"
}
]
}
```
Those styles are now deprecated. Instead, use SPDX expressions, like this:
```
{
"license": "ISC"
}
```
```
{
"license": "(MIT OR Apache-2.0)"
}
```
Finally, if you do not wish to grant others the right to use a private or unpublished package under any terms:
```
{
"license": "UNLICENSED"
}
```
Consider also setting `"private": true` to prevent accidental publication.
###
people fields: author, contributors
The "author" is one person. "contributors" is an array of people. A "person" is an object with a "name" field and optionally "url" and "email", like this:
```
{
"name" : "Barney Rubble",
"email" : "[email protected]",
"url" : "http://barnyrubble.tumblr.com/"
}
```
Or you can shorten that all into a single string, and npm will parse it for you:
```
{
"author": "Barney Rubble <[email protected]> (http://barnyrubble.tumblr.com/)"
}
```
Both email and url are optional either way.
npm also sets a top-level "maintainers" field with your npm user info.
###
funding
You can specify an object containing a URL that provides up-to-date information about ways to help fund development of your package, or a string URL, or an array of these:
```
{
"funding": {
"type" : "individual",
"url" : "http://example.com/donate"
},
"funding": {
"type" : "patreon",
"url" : "https://www.patreon.com/my-account"
},
"funding": "http://example.com/donate",
"funding": [
{
"type" : "individual",
"url" : "http://example.com/donate"
},
"http://example.com/donateAlso",
{
"type" : "patreon",
"url" : "https://www.patreon.com/my-account"
}
]
}
```
Users can use the `npm fund` subcommand to list the `funding` URLs of all dependencies of their project, direct and indirect. A shortcut to visit each funding url is also available when providing the project name such as: `npm fund <projectname>` (when there are multiple URLs, the first one will be visited)
###
files
The optional `files` field is an array of file patterns that describes the entries to be included when your package is installed as a dependency. File patterns follow a similar syntax to `.gitignore`, but reversed: including a file, directory, or glob pattern (`*`, `**/*`, and such) will make it so that file is included in the tarball when it's packed. Omitting the field will make it default to `["*"]`, which means it will include all files.
Some special files and directories are also included or excluded regardless of whether they exist in the `files` array (see below).
You can also provide a `.npmignore` file in the root of your package or in subdirectories, which will keep files from being included. At the root of your package it will not override the "files" field, but in subdirectories it will. The `.npmignore` file works just like a `.gitignore`. If there is a `.gitignore` file, and `.npmignore` is missing, `.gitignore`'s contents will be used instead.
Files included with the "package.json#files" field *cannot* be excluded through `.npmignore` or `.gitignore`.
Certain files are always included, regardless of settings:
* `package.json`
* `README`
* `LICENSE` / `LICENCE`
* The file in the "main" field
`README` & `LICENSE` can have any case and extension.
Conversely, some files are always ignored:
* `.git`
* `CVS`
* `.svn`
* `.hg`
* `.lock-wscript`
* `.wafpickle-N`
* `.*.swp`
* `.DS_Store`
* `._*`
* `npm-debug.log`
* `.npmrc`
* `node_modules`
* `config.gypi`
* `*.orig`
* `package-lock.json` (use [`npm-shrinkwrap.json`](npm-shrinkwrap-json) if you wish it to be published)
###
main
The main field is a module ID that is the primary entry point to your program. That is, if your package is named `foo`, and a user installs it, and then does `require("foo")`, then your main module's exports object will be returned.
This should be a module relative to the root of your package folder.
For most modules, it makes the most sense to have a main script and often not much else.
If `main` is not set it defaults to `index.js` in the package's root folder.
###
browser
If your module is meant to be used client-side the browser field should be used instead of the main field. This is helpful to hint users that it might rely on primitives that aren't available in Node.js modules. (e.g. `window`)
###
bin
A lot of packages have one or more executable files that they'd like to install into the PATH. npm makes this pretty easy (in fact, it uses this feature to install the "npm" executable.)
To use this, supply a `bin` field in your package.json which is a map of command name to local file name. When this package is installed globally, that file will be linked where global bins go so it is available to run by name. When this package is installed as a dependency in another package, the file will be linked where it will be available to that package either directly by `npm exec` or by name in other scripts when invoking them via `npm run-script`.
For example, myapp could have this:
```
{
"bin": {
"myapp": "./cli.js"
}
}
```
So, when you install myapp, it'll create a symlink from the `cli.js` script to `/usr/local/bin/myapp`.
If you have a single executable, and its name should be the name of the package, then you can just supply it as a string. For example:
```
{
"name": "my-program",
"version": "1.2.5",
"bin": "./path/to/program"
}
```
would be the same as this:
```
{
"name": "my-program",
"version": "1.2.5",
"bin": {
"my-program": "./path/to/program"
}
}
```
Please make sure that your file(s) referenced in `bin` starts with `#!/usr/bin/env node`, otherwise the scripts are started without the node executable!
Note that you can also set the executable files using [directories.bin](#directoriesbin).
See [folders](folders#executables) for more info on executables.
###
man
Specify either a single file or an array of filenames to put in place for the `man` program to find.
If only a single file is provided, then it's installed such that it is the result from `man <pkgname>`, regardless of its actual filename. For example:
```
{
"name": "foo",
"version": "1.2.3",
"description": "A packaged foo fooer for fooing foos",
"main": "foo.js",
"man": "./man/doc.1"
}
```
would link the `./man/doc.1` file in such that it is the target for `man
foo`
If the filename doesn't start with the package name, then it's prefixed. So, this:
```
{
"name": "foo",
"version": "1.2.3",
"description": "A packaged foo fooer for fooing foos",
"main": "foo.js",
"man": [
"./man/foo.1",
"./man/bar.1"
]
}
```
will create files to do `man foo` and `man foo-bar`.
Man files must end with a number, and optionally a `.gz` suffix if they are compressed. The number dictates which man section the file is installed into.
```
{
"name": "foo",
"version": "1.2.3",
"description": "A packaged foo fooer for fooing foos",
"main": "foo.js",
"man": [
"./man/foo.1",
"./man/foo.2"
]
}
```
will create entries for `man foo` and `man 2 foo`
###
directories
The CommonJS [Packages](http://wiki.commonjs.org/wiki/Packages/1.0) spec details a few ways that you can indicate the structure of your package using a `directories` object. If you look at [npm's package.json](https://registry.npmjs.org/npm/latest), you'll see that it has directories for doc, lib, and man.
In the future, this information may be used in other creative ways.
####
directories.bin
If you specify a `bin` directory in `directories.bin`, all the files in that folder will be added.
Because of the way the `bin` directive works, specifying both a `bin` path and setting `directories.bin` is an error. If you want to specify individual files, use `bin`, and for all the files in an existing `bin` directory, use `directories.bin`.
####
directories.man
A folder that is full of man pages. Sugar to generate a "man" array by walking the folder.
###
repository
Specify the place where your code lives. This is helpful for people who want to contribute. If the git repo is on GitHub, then the `npm docs` command will be able to find you.
Do it like this:
```
{
"repository": {
"type": "git",
"url": "https://github.com/npm/cli.git"
}
}
```
The URL should be a publicly available (perhaps read-only) url that can be handed directly to a VCS program without any modification. It should not be a url to an html project page that you put in your browser. It's for computers.
For GitHub, GitHub gist, Bitbucket, or GitLab repositories you can use the same shortcut syntax you use for `npm install`:
```
{
"repository": "npm/npm",
"repository": "github:user/repo",
"repository": "gist:11081aaa281",
"repository": "bitbucket:user/repo",
"repository": "gitlab:user/repo"
}
```
If the `package.json` for your package is not in the root directory (for example if it is part of a monorepo), you can specify the directory in which it lives:
```
{
"repository": {
"type": "git",
"url": "https://github.com/facebook/react.git",
"directory": "packages/react-dom"
}
}
```
###
scripts
The "scripts" property is a dictionary containing script commands that are run at various times in the lifecycle of your package. The key is the lifecycle event, and the value is the command to run at that point.
See [`scripts`](../using-npm/scripts) to find out more about writing package scripts.
###
config
A "config" object can be used to set configuration parameters used in package scripts that persist across upgrades. For instance, if a package had the following:
```
{
"name": "foo",
"config": {
"port": "8080"
}
}
```
It could also have a "start" command that referenced the `npm_package_config_port` environment variable.
###
dependencies
Dependencies are specified in a simple object that maps a package name to a version range. The version range is a string which has one or more space-separated descriptors. Dependencies can also be identified with a tarball or git URL.
**Please do not put test harnesses or transpilers or other "development" time tools in your `dependencies` object.** See `devDependencies`, below.
See [semver](https://github.com/npm/node-semver#versions) for more details about specifying version ranges.
* `version` Must match `version` exactly
* `>version` Must be greater than `version`
* `>=version` etc
* `<version`
* `<=version`
* `~version` "Approximately equivalent to version" See [semver](https://github.com/npm/node-semver#versions)
* `^version` "Compatible with version" See [semver](https://github.com/npm/node-semver#versions)
* `1.2.x` 1.2.0, 1.2.1, etc., but not 1.3.0
* `http://...` See 'URLs as Dependencies' below
* `*` Matches any version
* `""` (just an empty string) Same as `*`
* `version1 - version2` Same as `>=version1 <=version2`.
* `range1 || range2` Passes if either range1 or range2 are satisfied.
* `git...` See 'Git URLs as Dependencies' below
* `user/repo` See 'GitHub URLs' below
* `tag` A specific version tagged and published as `tag` See [`npm
dist-tag`](../commands/npm-dist-tag)
* `path/path/path` See [Local Paths](#local-paths) below
For example, these are all valid:
```
{
"dependencies": {
"foo": "1.0.0 - 2.9999.9999",
"bar": ">=1.0.2 <2.1.2",
"baz": ">1.0.2 <=2.3.4",
"boo": "2.0.1",
"qux": "<1.0.0 || >=2.3.1 <2.4.5 || >=2.5.2 <3.0.0",
"asd": "http://asdf.com/asdf.tar.gz",
"til": "~1.2",
"elf": "~1.2.3",
"two": "2.x",
"thr": "3.3.x",
"lat": "latest",
"dyl": "file:../dyl"
}
}
```
####
URLs as Dependencies
You may specify a tarball URL in place of a version range.
This tarball will be downloaded and installed locally to your package at install time.
####
Git URLs as Dependencies
Git urls are of the form:
```
<protocol>://[<user>[:<password>]@]<hostname>[:<port>][:][/]<path>[#<commit-ish> | #semver:<semver>]
```
`<protocol>` is one of `git`, `git+ssh`, `git+http`, `git+https`, or `git+file`.
If `#<commit-ish>` is provided, it will be used to clone exactly that commit. If the commit-ish has the format `#semver:<semver>`, `<semver>` can be any valid semver range or exact version, and npm will look for any tags or refs matching that range in the remote repository, much as it would for a registry dependency. If neither `#<commit-ish>` or `#semver:<semver>` is specified, then the default branch is used.
Examples:
```
git+ssh://[email protected]:npm/cli.git#v1.0.27
git+ssh://[email protected]:npm/cli#semver:^5.0
git+https://[email protected]/npm/cli.git
git://github.com/npm/cli.git#v1.0.27
```
When installing from a `git` repository, the presence of certain fields in the `package.json` will cause npm to believe it needs to perform a build. To do so your repository will be cloned into a temporary directory, all of its deps installed, relevant scripts run, and the resulting directory packed and installed.
This flow will occur if your git dependency uses `workspaces`, or if any of the following scripts are present:
* `build`
* `prepare`
* `prepack`
* `preinstall`
* `install`
* `postinstall`
If your git repository includes pre-built artifacts, you will likely want to make sure that none of the above scripts are defined, or your dependency will be rebuilt for every installation.
####
GitHub URLs
As of version 1.1.65, you can refer to GitHub urls as just "foo": "user/foo-project". Just as with git URLs, a `commit-ish` suffix can be included. For example:
```
{
"name": "foo",
"version": "0.0.0",
"dependencies": {
"express": "expressjs/express",
"mocha": "mochajs/mocha#4727d357ea",
"module": "user/repo#feature\/branch"
}
}
```
####
Local Paths
As of version 2.0.0 you can provide a path to a local directory that contains a package. Local paths can be saved using `npm install -S` or `npm
install --save`, using any of these forms:
```
../foo/bar
~/foo/bar
./foo/bar
/foo/bar
```
in which case they will be normalized to a relative path and added to your `package.json`. For example:
```
{
"name": "baz",
"dependencies": {
"bar": "file:../foo/bar"
}
}
```
This feature is helpful for local offline development and creating tests that require npm installing where you don't want to hit an external server, but should not be used when publishing packages to the public registry.
*note*: Packages linked by local path will not have their own dependencies installed when `npm install` is ran in this case. You must run `npm install` from inside the local path itself.
###
devDependencies
If someone is planning on downloading and using your module in their program, then they probably don't want or need to download and build the external test or documentation framework that you use.
In this case, it's best to map these additional items in a `devDependencies` object.
These things will be installed when doing `npm link` or `npm install` from the root of a package, and can be managed like any other npm configuration param. See [`config`](../using-npm/config) for more on the topic.
For build steps that are not platform-specific, such as compiling CoffeeScript or other languages to JavaScript, use the `prepare` script to do this, and make the required package a devDependency.
For example:
```
{
"name": "ethopia-waza",
"description": "a delightfully fruity coffee varietal",
"version": "1.2.3",
"devDependencies": {
"coffee-script": "~1.6.3"
},
"scripts": {
"prepare": "coffee -o lib/ -c src/waza.coffee"
},
"main": "lib/waza.js"
}
```
The `prepare` script will be run before publishing, so that users can consume the functionality without requiring them to compile it themselves. In dev mode (ie, locally running `npm install`), it'll run this script as well, so that you can test it easily.
###
peerDependencies
In some cases, you want to express the compatibility of your package with a host tool or library, while not necessarily doing a `require` of this host. This is usually referred to as a *plugin*. Notably, your module may be exposing a specific interface, expected and specified by the host documentation.
For example:
```
{
"name": "tea-latte",
"version": "1.3.5",
"peerDependencies": {
"tea": "2.x"
}
}
```
This ensures your package `tea-latte` can be installed *along* with the second major version of the host package `tea` only. `npm install
tea-latte` could possibly yield the following dependency graph:
```
├── [email protected]
└── [email protected]
```
In npm versions 3 through 6, `peerDependencies` were not automatically installed, and would raise a warning if an invalid version of the peer dependency was found in the tree. As of npm v7, peerDependencies *are* installed by default.
Trying to install another plugin with a conflicting requirement may cause an error if the tree cannot be resolved correctly. For this reason, make sure your plugin requirement is as broad as possible, and not to lock it down to specific patch versions.
Assuming the host complies with [semver](https://semver.org/), only changes in the host package's major version will break your plugin. Thus, if you've worked with every 1.x version of the host package, use `"^1.0"` or `"1.x"` to express this. If you depend on features introduced in 1.5.2, use `"^1.5.2"`.
###
peerDependenciesMeta
When a user installs your package, npm will emit warnings if packages specified in `peerDependencies` are not already installed. The `peerDependenciesMeta` field serves to provide npm more information on how your peer dependencies are to be used. Specifically, it allows peer dependencies to be marked as optional.
For example:
```
{
"name": "tea-latte",
"version": "1.3.5",
"peerDependencies": {
"tea": "2.x",
"soy-milk": "1.2"
},
"peerDependenciesMeta": {
"soy-milk": {
"optional": true
}
}
}
```
Marking a peer dependency as optional ensures npm will not emit a warning if the `soy-milk` package is not installed on the host. This allows you to integrate and interact with a variety of host packages without requiring all of them to be installed.
###
bundleDependencies
This defines an array of package names that will be bundled when publishing the package.
In cases where you need to preserve npm packages locally or have them available through a single file download, you can bundle the packages in a tarball file by specifying the package names in the `bundleDependencies` array and executing `npm pack`.
For example:
If we define a package.json like this:
```
{
"name": "awesome-web-framework",
"version": "1.0.0",
"bundleDependencies": [
"renderized",
"super-streams"
]
}
```
we can obtain `awesome-web-framework-1.0.0.tgz` file by running `npm pack`. This file contains the dependencies `renderized` and `super-streams` which can be installed in a new project by executing `npm install
awesome-web-framework-1.0.0.tgz`. Note that the package names do not include any versions, as that information is specified in `dependencies`.
If this is spelled `"bundledDependencies"`, then that is also honored.
Alternatively, `"bundleDependencies"` can be defined as a boolean value. A value of `true` will bundle all dependencies, a value of `false` will bundle none.
###
optionalDependencies
If a dependency can be used, but you would like npm to proceed if it cannot be found or fails to install, then you may put it in the `optionalDependencies` object. This is a map of package name to version or url, just like the `dependencies` object. The difference is that build failures do not cause installation to fail. Running `npm install
--omit=optional` will prevent these dependencies from being installed.
It is still your program's responsibility to handle the lack of the dependency. For example, something like this:
```
try {
var foo = require('foo')
var fooVersion = require('foo/package.json').version
} catch (er) {
foo = null
}
if ( notGoodFooVersion(fooVersion) ) {
foo = null
}
// .. then later in your program ..
if (foo) {
foo.doFooThings()
}
```
Entries in `optionalDependencies` will override entries of the same name in `dependencies`, so it's usually best to only put in one place.
###
overrides
If you need to make specific changes to dependencies of your dependencies, for example replacing the version of a dependency with a known security issue, replacing an existing dependency with a fork, or making sure that the same version of a package is used everywhere, then you may add an override.
Overrides provide a way to replace a package in your dependency tree with another version, or another package entirely. These changes can be scoped as specific or as vague as desired.
To make sure the package `foo` is always installed as version `1.0.0` no matter what version your dependencies rely on:
```
{
"overrides": {
"foo": "1.0.0"
}
}
```
The above is a short hand notation, the full object form can be used to allow overriding a package itself as well as a child of the package. This will cause `foo` to always be `1.0.0` while also making `bar` at any depth beyond `foo` also `1.0.0`:
```
{
"overrides": {
"foo": {
".": "1.0.0",
"bar": "1.0.0"
}
}
}
```
To only override `foo` to be `1.0.0` when it's a child (or grandchild, or great grandchild, etc) of the package `bar`:
```
{
"overrides": {
"bar": {
"foo": "1.0.0"
}
}
}
```
Keys can be nested to any arbitrary length. To override `foo` only when it's a child of `bar` and only when `bar` is a child of `baz`:
```
{
"overrides": {
"baz": {
"bar": {
"foo": "1.0.0"
}
}
}
}
```
The key of an override can also include a version, or range of versions. To override `foo` to `1.0.0`, but only when it's a child of `[email protected]`:
```
{
"overrides": {
"[email protected]": {
"foo": "1.0.0"
}
}
}
```
You may not set an override for a package that you directly depend on unless both the dependency and the override itself share the exact same spec. To make this limitation easier to deal with, overrides may also be defined as a reference to a spec for a direct dependency by prefixing the name of the package you wish the version to match with a `$`.
```
{
"dependencies": {
"foo": "^1.0.0"
},
"overrides": {
// BAD, will throw an EOVERRIDE error
// "foo": "^2.0.0"
// GOOD, specs match so override is allowed
// "foo": "^1.0.0"
// BEST, the override is defined as a reference to the dependency
"foo": "$foo",
// the referenced package does not need to match the overridden one
"bar": "$foo"
}
}
```
###
engines
You can specify the version of node that your stuff works on:
```
{
"engines": {
"node": ">=0.10.3 <15"
}
}
```
And, like with dependencies, if you don't specify the version (or if you specify "\*" as the version), then any version of node will do.
You can also use the "engines" field to specify which versions of npm are capable of properly installing your program. For example:
```
{
"engines": {
"npm": "~1.0.20"
}
}
```
Unless the user has set the `engine-strict` config flag, this field is advisory only and will only produce warnings when your package is installed as a dependency.
###
os
You can specify which operating systems your module will run on:
```
{
"os": [
"darwin",
"linux"
]
}
```
You can also block instead of allowing operating systems, just prepend the blocked os with a '!':
```
{
"os": [
"!win32"
]
}
```
The host operating system is determined by `process.platform`
It is allowed to both block and allow an item, although there isn't any good reason to do this.
###
cpu
If your code only runs on certain cpu architectures, you can specify which ones.
```
{
"cpu": [
"x64",
"ia32"
]
}
```
Like the `os` option, you can also block architectures:
```
{
"cpu": [
"!arm",
"!mips"
]
}
```
The host architecture is determined by `process.arch`
###
private
If you set `"private": true` in your package.json, then npm will refuse to publish it.
This is a way to prevent accidental publication of private repositories. If you would like to ensure that a given package is only ever published to a specific registry (for example, an internal registry), then use the `publishConfig` dictionary described below to override the `registry` config param at publish-time.
###
publishConfig
This is a set of config values that will be used at publish-time. It's especially handy if you want to set the tag, registry or access, so that you can ensure that a given package is not tagged with "latest", published to the global public registry or that a scoped module is private by default.
See [`config`](../using-npm/config) to see the list of config options that can be overridden.
###
workspaces
The optional `workspaces` field is an array of file patterns that describes locations within the local file system that the install client should look up to find each [workspace](../using-npm/workspaces) that needs to be symlinked to the top level `node_modules` folder.
It can describe either the direct paths of the folders to be used as workspaces or it can define globs that will resolve to these same folders.
In the following example, all folders located inside the folder `./packages` will be treated as workspaces as long as they have valid `package.json` files inside them:
```
{
"name": "workspace-example",
"workspaces": [
"./packages/*"
]
}
```
See [`workspaces`](../using-npm/workspaces) for more examples.
###
DEFAULT VALUES
npm will default some values based on package contents.
* `"scripts": {"start": "node server.js"}`
If there is a `server.js` file in the root of your package, then npm will default the `start` command to `node server.js`.
* `"scripts":{"install": "node-gyp rebuild"}`
If there is a `binding.gyp` file in the root of your package and you have not defined an `install` or `preinstall` script, npm will default the `install` command to compile using node-gyp.
* `"contributors": [...]`
If there is an `AUTHORS` file in the root of your package, npm will treat each line as a `Name <email> (url)` format, where email and url are optional. Lines which start with a `#` or are blank, will be ignored.
###
SEE ALSO
* [semver](https://github.com/npm/node-semver#versions)
* [workspaces](../using-npm/workspaces)
* [npm init](../commands/npm-init)
* [npm version](../commands/npm-version)
* [npm config](../commands/npm-config)
* [npm help](../commands/npm-help)
* [npm install](../commands/npm-install)
* [npm publish](../commands/npm-publish)
* [npm uninstall](../commands/npm-uninstall)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/configuring-npm/package-json.md)
| programming_docs |
npm package-spec package-spec
============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Package name specifier
Table of contents* [Description](#description)
* [Package name](#package-name)
* [Aliases](#aliases)
* [Folders](#folders)
* [Tarballs](#tarballs)
* [git urls](#git-urls)
* [See also](#see-also)
###
Description
Commands like `npm install` and the dependency sections in the `package.json` use a package name specifier. This can be many different things that all refer to a "package". Examples include a package name, git url, tarball, or local directory. These will generally be referred to as `<package-spec>` in the help output for the npm commands that use this package name specifier.
###
Package name
* `[<@scope>/]<pkg>`
* `[<@scope>/]<pkg>@<tag>`
* `[<@scope>/]<pkg>@<version>`
* `[<@scope>/]<pkg>@<version range>`
Refers to a package by name, with or without a scope, and optionally tag, version, or version range. This is typically used in combination with the [registry](config#registry) config to refer to a package in a registry.
Examples:
* `npm`
* `@npmcli/arborist`
* `@npmcli/arborist@latest`
* `[email protected]`
* `npm@^4.0.0`
###
Aliases
* `<alias>@npm:<name>`
Primarily used by commands like `npm install` and in the dependency sections in the `package.json`, this refers to a package by an alias. The `<alias>` is the name of the package as it is reified in the `node_modules` folder, and the `<name>` refers to a package name as found in the configured registry.
See `Package name` above for more info on referring to a package by name, and [registry](config#registry) for configuring which registry is used when referring to a package by name.
Examples:
* `semver:@npm:@npmcli/semver-with-patch`
* `semver:@npm:[email protected]`
* `semver:@npm:semver@legacy`
###
Folders
* `<folder>`
This refers to a package on the local filesystem. Specifically this is a folder with a `package.json` file in it. This *should* always be prefixed with a `/` or `./` (or your OS equivalent) to reduce confusion. npm currently will parse a string with more than one `/` in it as a folder, but this is legacy behavior that may be removed in a future version.
Examples:
* `./my-package`
* `/opt/npm/my-package`
###
Tarballs
* `<tarball file>`
* `<tarball url>`
Examples:
* `./my-package.tgz`
* `https://registry.npmjs.org/semver/-/semver-1.0.0.tgz`
Refers to a package in a tarball format, either on the local filesystem or remotely via url. This is the format that packages exist in when uploaded to a registry.
###
git urls
* `<git:// url>`
* `<github username>/<github project>`
Refers to a package in a git repo. This can be a full git url, git shorthand, or a username/package on GitHub. You can specify a git tag, branch, or other git ref by appending `#ref`.
Examples:
* `https://github.com/npm/cli.git`
* `[email protected]:npm/cli.git`
* `git+ssh://[email protected]/npm/cli#v6.0.0`
* `github:npm/cli#HEAD`
* `npm/cli#c12ea07`
###
See also
[npm-package-arg](https://npm.im/npm-package-arg) <scope> <config>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/package-spec.md)
npm Dependency Selector Syntax & Querying Dependency Selector Syntax & Querying
=====================================
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Dependency Selector Syntax & Querying
Table of contents* + [Description](#description)
+ [Dependency Selector Syntax v1.0.0](#dependency-selector-syntax-v100)
- [Overview:](#overview)
- [Combinators](#combinators)
- [Selectors](#selectors)
- [Dependency Type Selectors](#dependency-type-selectors)
- [Pseudo Selectors](#pseudo-selectors)
- [Attribute Selectors](#attribute-selectors)
- [Array & Object Attribute Selectors](#array--object-attribute-selectors)
- [Objects](#objects)
- [Nested Objects](#nested-objects)
- [Arrays](#arrays)
* [Example of an Array Attribute Selection:](#example-of-an-array-attribute-selection)
* [Example of an Array matching directly to a value:](#example-of-an-array-matching-directly-to-a-value)
* [Example of an Array of Objects:](#example-of-an-array-of-objects)
+ [Groups](#groups)
+ [Programmatic Usage](#programmatic-usage)
* [See Also](#see-also)
###
Description
The [`npm query`](../commands/npm-query) commmand exposes a new dependency selector syntax (informed by & respecting many aspects of the [CSS Selectors 4 Spec](https://dev.w3.org/csswg/selectors4/#relational)) which:
* Standardizes the shape of, & querying of, dependency graphs with a robust object model, metadata & selector syntax
* Leverages existing, known language syntax & operators from CSS to make disparate package information broadly accessible
* Unlocks the ability to answer complex, multi-faceted questions about dependencies, their relationships & associative metadata
* Consolidates redundant logic of similar query commands in `npm` (ex. `npm fund`, `npm ls`, `npm outdated`, `npm audit` ...)
###
Dependency Selector Syntax `v1.0.0`
####
Overview:
* there is no "type" or "tag" selectors (ex. `div, h1, a`) as a dependency/target is the only type of `Node` that can be queried
* the term "dependencies" is in reference to any `Node` found in a `tree` returned by `Arborist`
####
Combinators
* `>` direct descendant/child
* any descendant/child
* `~` sibling
####
Selectors
* `*` universal selector
* `#<name>` dependency selector (equivalent to `[name="..."]`)
* `#<name>@<version>` (equivalent to `[name=<name>]:semver(<version>)`)
* `,` selector list delimiter
* `.` dependency type selector
* `:` pseudo selector
####
Dependency Type Selectors
* `.prod` dependency found in the `dependencies` section of `package.json`, or is a child of said dependency
* `.dev` dependency found in the `devDependencies` section of `package.json`, or is a child of said dependency
* `.optional` dependency found in the `optionalDependencies` section of `package.json`, or has `"optional": true` set in its entry in the `peerDependenciesMeta` section of `package.json`, or a child of said dependency
* `.peer` dependency found in the `peerDependencies` section of `package.json`
* `.workspace` dependency found in the [`workspaces`](workspaces) section of `package.json`
* `.bundled` dependency found in the `bundleDependencies` section of `package.json`, or is a child of said dependency
####
Pseudo Selectors
* [`:not(<selector>)`](https://developer.mozilla.org/en-US/docs/Web/CSS/:not)
* [`:has(<selector>)`](https://developer.mozilla.org/en-US/docs/Web/CSS/:has)
* [`:is(<selector list>)`](https://developer.mozilla.org/en-US/docs/Web/CSS/:is)
* [`:root`](https://developer.mozilla.org/en-US/docs/Web/CSS/:root) matches the root node/dependency
* [`:scope`](https://developer.mozilla.org/en-US/docs/Web/CSS/:scope) matches node/dependency it was queried against
* [`:empty`](https://developer.mozilla.org/en-US/docs/Web/CSS/:empty) when a dependency has no dependencies
* [`:private`](../configuring-npm/package-json#private) when a dependency is private
* `:link` when a dependency is linked (for instance, workspaces or packages manually [`linked`](../commands/npm-link)
* `:deduped` when a dependency has been deduped (note that this does *not* always mean the dependency has been hoisted to the root of node\_modules)
* `:overridden` when a dependency has been overridden
* `:extraneous` when a dependency exists but is not defined as a dependency of any node
* `:invalid` when a dependency version is out of its ancestors specified range
* `:missing` when a dependency is not found on disk
* `:semver(<spec>)` matching a valid [`node-semver`](https://github.com/npm/node-semver) spec
* `:path(<path>)` [glob](https://www.npmjs.com/package/glob) matching based on dependencies path relative to the project
* `:type(<type>)` [based on currently recognized types](https://github.com/npm/npm-package-arg#result-object)
####
[Attribute Selectors](https://developer.mozilla.org/en-US/docs/Web/CSS/Attribute_selectors)
The attribute selector evaluates the key/value pairs in `package.json` if they are `String`s.
* `[]` attribute selector (ie. existence of attribute)
* `[attribute=value]` attribute value is equivalant...
* `[attribute~=value]` attribute value contains word...
* `[attribute*=value]` attribute value contains string...
* `[attribute|=value]` attribute value is equal to or starts with...
* `[attribute^=value]` attribute value starts with...
* `[attribute$=value]` attribute value ends with...
####
`Array` & `Object` Attribute Selectors
The generic `:attr()` pseudo selector standardizes a pattern which can be used for attribute selection of `Object`s, `Array`s or `Arrays` of `Object`s accessible via `Arborist`'s `Node.package` metadata. This allows for iterative attribute selection beyond top-level `String` evaluation. The last argument passed to `:attr()` must be an `attribute` selector or a nested `:attr()`. See examples below:
####
`Objects`
```
/* return dependencies that have a `scripts.test` containing `"tap"` */
*:attr(scripts, [test~=tap])
```
####
Nested `Objects`
Nested objects are expressed as sequential arguments to `:attr()`.
```
/* return dependencies that have a testling config for opera browsers */
*:attr(testling, browsers, [~=opera])
```
####
`Arrays`
`Array`s specifically uses a special/reserved `.` character in place of a typical attribute name. `Arrays` also support exact `value` matching when a `String` is passed to the selector.
#####
Example of an `Array` Attribute Selection:
```
/* removes the distinction between properties & arrays */
/* ie. we'd have to check the property & iterate to match selection */
*:attr([keywords^=react])
*:attr(contributors, :attr([name~=Jordan]))
```
#####
Example of an `Array` matching directly to a value:
```
/* return dependencies that have the exact keyword "react" */
/* this is equivalent to `*:keywords([value="react"])` */
*:attr([keywords=react])
```
#####
Example of an `Array` of `Object`s:
```
/* returns */
*:attr(contributors, [[email protected]])
```
###
Groups
Dependency groups are defined by the package relationships to their ancestors (ie. the dependency types that are defined in `package.json`). This approach is user-centric as the ecosystem has been taught to think about dependencies in these groups first-and-foremost. Dependencies are allowed to be included in multiple groups (ex. a `prod` dependency may also be a `dev` dependency (in that it's also required by another `dev` dependency) & may also be `bundled` - a selector for that type of dependency would look like: `*.prod.dev.bundled`).
* `.prod`
* `.dev`
* `.optional`
* `.peer`
* `.bundled`
* `.workspace`
Please note that currently `workspace` deps are always `prod` dependencies. Additionally the `.root` dependency is also considered a `prod` dependency.
###
Programmatic Usage
* `Arborist`'s `Node` Class has a `.querySelectorAll()` method
+ this method will return a filtered, flattened dependency Arborist `Node` list based on a valid query selector
```
const Arborist = require('@npmcli/arborist')
const arb = new Arborist({})
```
```
// root-level
arb.loadActual().then(async (tree) => {
// query all production dependencies
const results = await tree.querySelectorAll('.prod')
console.log(results)
})
```
```
// iterative
arb.loadActual().then(async (tree) => {
// query for the deduped version of react
const results = await tree.querySelectorAll('#react:not(:deduped)')
// query the deduped react for git deps
const deps = await results[0].querySelectorAll(':type(git)')
console.log(deps)
})
```
See Also
---------
* [npm query](../commands/npm-query)
* [@npmcli/arborist](https://npm.im/@npmcli/arborist)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/dependency-selectors.md)
npm scripts scripts
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
How npm handles the "scripts" field
Table of contents* [Description](#description)
* [Pre & Post Scripts](#pre--post-scripts)
* [Life Cycle Scripts](#life-cycle-scripts)
+ [Prepare and Prepublish](#prepare-and-prepublish)
+ [Dependencies](#dependencies)
* [Life Cycle Operation Order](#life-cycle-operation-order)
+ [npm cache add](#npm-cache-add)
+ [npm ci](#npm-ci)
+ [npm diff](#npm-diff)
+ [npm install](#npm-install)
+ [npm pack](#npm-pack)
+ [npm publish](#npm-publish)
+ [npm rebuild](#npm-rebuild)
+ [npm restart](#npm-restart)
+ [npm run <user defined>](#npm-run-user-defined)
+ [npm start](#npm-start)
+ [npm stop](#npm-stop)
+ [npm test](#npm-test)
+ [npm version](#npm-version)
+ [A Note on a lack of npm uninstall scripts](#a-note-on-a-lack-of-npm-uninstall-scripts)
* [User](#user)
* [Environment](#environment)
+ [path](#path)
+ [package.json vars](#packagejson-vars)
+ [current lifecycle event](#current-lifecycle-event)
* [Examples](#examples)
* [Exiting](#exiting)
* [Best Practices](#best-practices)
* [See Also](#see-also)
###
Description
The `"scripts"` property of your `package.json` file supports a number of built-in scripts and their preset life cycle events as well as arbitrary scripts. These all can be executed by running `npm run-script <stage>` or `npm run <stage>` for short. *Pre* and *post* commands with matching names will be run for those as well (e.g. `premyscript`, `myscript`, `postmyscript`). Scripts from dependencies can be run with `npm explore <pkg> -- npm run <stage>`.
###
Pre & Post Scripts
To create "pre" or "post" scripts for any scripts defined in the `"scripts"` section of the `package.json`, simply create another script *with a matching name* and add "pre" or "post" to the beginning of them.
```
{
"scripts": {
"precompress": "{{ executes BEFORE the `compress` script }}",
"compress": "{{ run command to compress files }}",
"postcompress": "{{ executes AFTER `compress` script }}"
}
}
```
In this example `npm run compress` would execute these scripts as described.
###
Life Cycle Scripts
There are some special life cycle scripts that happen only in certain situations. These scripts happen in addition to the `pre<event>`, `post<event>`, and `<event>` scripts.
* `prepare`, `prepublish`, `prepublishOnly`, `prepack`, `postpack`, `dependencies`
**prepare** (since `[email protected]`)
* Runs any time before the package is packed, i.e. during `npm publish` and `npm pack`
* Runs BEFORE the package is packed
* Runs BEFORE the package is published
* Runs on local `npm install` without any arguments
* Run AFTER `prepublish`, but BEFORE `prepublishOnly`
* NOTE: If a package being installed through git contains a `prepare` script, its `dependencies` and `devDependencies` will be installed, and the prepare script will be run, before the package is packaged and installed.
* As of `npm@7` these scripts run in the background. To see the output, run with: `--foreground-scripts`.
**prepublish** (DEPRECATED)
* Does not run during `npm publish`, but does run during `npm ci` and `npm install`. See below for more info.
**prepublishOnly**
* Runs BEFORE the package is prepared and packed, ONLY on `npm publish`.
**prepack**
* Runs BEFORE a tarball is packed (on "`npm pack`", "`npm publish`", and when installing a git dependencies).
* NOTE: "`npm run pack`" is NOT the same as "`npm pack`". "`npm run pack`" is an arbitrary user defined script name, where as, "`npm pack`" is a CLI defined command.
**postpack**
* Runs AFTER the tarball has been generated but before it is moved to its final destination (if at all, publish does not save the tarball locally)
**dependencies**
* Runs AFTER any operations that modify the `node_modules` directory IF changes occurred.
* Does NOT run in global mode
####
Prepare and Prepublish
**Deprecation Note: prepublish**
Since `[email protected]`, the npm CLI has run the `prepublish` script for both `npm publish` and `npm install`, because it's a convenient way to prepare a package for use (some common use cases are described in the section below). It has also turned out to be, in practice, [very confusing](https://github.com/npm/npm/issues/10074). As of `[email protected]`, a new event has been introduced, `prepare`, that preserves this existing behavior. A *new* event, `prepublishOnly` has been added as a transitional strategy to allow users to avoid the confusing behavior of existing npm versions and only run on `npm publish` (for instance, running the tests one last time to ensure they're in good shape).
See <https://github.com/npm/npm/issues/10074> for a much lengthier justification, with further reading, for this change.
**Use Cases**
If you need to perform operations on your package before it is used, in a way that is not dependent on the operating system or architecture of the target system, use a `prepublish` script. This includes tasks such as:
* Compiling CoffeeScript source code into JavaScript.
* Creating minified versions of JavaScript source code.
* Fetching remote resources that your package will use.
The advantage of doing these things at `prepublish` time is that they can be done once, in a single place, thus reducing complexity and variability. Additionally, this means that:
* You can depend on `coffee-script` as a `devDependency`, and thus your users don't need to have it installed.
* You don't need to include minifiers in your package, reducing the size for your users.
* You don't need to rely on your users having `curl` or `wget` or other system tools on the target machines.
####
Dependencies
The `dependencies` script is run any time an `npm` command causes changes to the `node_modules` directory. It is run AFTER the changes have been applied and the `package.json` and `package-lock.json` files have been updated.
###
Life Cycle Operation Order
####
[`npm cache add`](../commands/npm-cache)
* `prepare`
####
[`npm ci`](../commands/npm-ci)
* `preinstall`
* `install`
* `postinstall`
* `prepublish`
* `preprepare`
* `prepare`
* `postprepare`
These all run after the actual installation of modules into `node_modules`, in order, with no internal actions happening in between
####
[`npm diff`](../commands/npm-diff)
* `prepare`
####
[`npm install`](../commands/npm-install)
These also run when you run `npm install -g <pkg-name>`
* `preinstall`
* `install`
* `postinstall`
* `prepublish`
* `preprepare`
* `prepare`
* `postprepare`
If there is a `binding.gyp` file in the root of your package and you haven't defined your own `install` or `preinstall` scripts, npm will default the `install` command to compile using node-gyp via `node-gyp
rebuild`
These are run from the scripts of `<pkg-name>`
####
[`npm pack`](../commands/npm-pack)
* `prepack`
* `prepare`
* `postpack`
####
[`npm publish`](../commands/npm-publish)
* `prepublishOnly`
* `prepack`
* `prepare`
* `postpack`
* `publish`
* `postpublish`
`prepare` will not run during `--dry-run`
####
[`npm rebuild`](../commands/npm-rebuild)
* `preinstall`
* `install`
* `postinstall`
* `prepare`
`prepare` is only run if the current directory is a symlink (e.g. with linked packages)
####
[`npm restart`](../commands/npm-restart)
If there is a `restart` script defined, these events are run, otherwise `stop` and `start` are both run if present, including their `pre` and `post` iterations)
* `prerestart`
* `restart`
* `postrestart`
####
[`npm run <user defined>`](../commands/npm-run-script)
* `pre<user-defined>`
* `<user-defined>`
* `post<user-defined>`
####
[`npm start`](../commands/npm-start)
* `prestart`
* `start`
* `poststart`
If there is a `server.js` file in the root of your package, then npm will default the `start` command to `node server.js`. `prestart` and `poststart` will still run in this case.
####
[`npm stop`](../commands/npm-stop)
* `prestop`
* `stop`
* `poststop`
####
[`npm test`](../commands/npm-test)
* `pretest`
* `test`
* `posttest`
####
[`npm version`](../commands/npm-version)
* `preversion`
* `version`
* `postversion`
####
A Note on a lack of [`npm uninstall`](../commands/npm-uninstall) scripts
While npm v6 had `uninstall` lifecycle scripts, npm v7 does not. Removal of a package can happen for a wide variety of reasons, and there's no clear way to currently give the script enough context to be useful.
Reasons for a package removal include:
* a user directly uninstalled this package
* a user uninstalled a dependant package and so this dependency is being uninstalled
* a user uninstalled a dependant package but another package also depends on this version
* this version has been merged as a duplicate with another version
* etc.
Due to the lack of necessary context, `uninstall` lifecycle scripts are not implemented and will not function.
###
User
When npm is run as root, scripts are always run with the effective uid and gid of the working directory owner.
###
Environment
Package scripts run in an environment where many pieces of information are made available regarding the setup of npm and the current state of the process.
####
path
If you depend on modules that define executable scripts, like test suites, then those executables will be added to the `PATH` for executing the scripts. So, if your package.json has this:
```
{
"name" : "foo",
"dependencies" : {
"bar" : "0.1.x"
},
"scripts": {
"start" : "bar ./test"
}
}
```
then you could run `npm start` to execute the `bar` script, which is exported into the `node_modules/.bin` directory on `npm install`.
####
package.json vars
The package.json fields are tacked onto the `npm_package_` prefix. So, for instance, if you had `{"name":"foo", "version":"1.2.5"}` in your package.json file, then your package scripts would have the `npm_package_name` environment variable set to "foo", and the `npm_package_version` set to "1.2.5". You can access these variables in your code with `process.env.npm_package_name` and `process.env.npm_package_version`, and so on for other fields.
See [`package.json`](../configuring-npm/package-json) for more on package configs.
####
current lifecycle event
Lastly, the `npm_lifecycle_event` environment variable is set to whichever stage of the cycle is being executed. So, you could have a single script used for different parts of the process which switches based on what's currently happening.
Objects are flattened following this format, so if you had `{"scripts":{"install":"foo.js"}}` in your package.json, then you'd see this in the script:
```
process.env.npm_package_scripts_install === "foo.js"
```
###
Examples
For example, if your package.json contains this:
```
{
"scripts" : {
"install" : "scripts/install.js",
"postinstall" : "scripts/install.js",
"uninstall" : "scripts/uninstall.js"
}
}
```
then `scripts/install.js` will be called for the install and post-install stages of the lifecycle, and `scripts/uninstall.js` will be called when the package is uninstalled. Since `scripts/install.js` is running for two different phases, it would be wise in this case to look at the `npm_lifecycle_event` environment variable.
If you want to run a make command, you can do so. This works just fine:
```
{
"scripts" : {
"preinstall" : "./configure",
"install" : "make && make install",
"test" : "make test"
}
}
```
###
Exiting
Scripts are run by passing the line as a script argument to `sh`.
If the script exits with a code other than 0, then this will abort the process.
Note that these script files don't have to be Node.js or even JavaScript programs. They just have to be some kind of executable file.
###
Best Practices
* Don't exit with a non-zero error code unless you *really* mean it. Except for uninstall scripts, this will cause the npm action to fail, and potentially be rolled back. If the failure is minor or only will prevent some optional features, then it's better to just print a warning and exit successfully.
* Try not to use scripts to do what npm can do for you. Read through [`package.json`](../configuring-npm/package-json) to see all the things that you can specify and enable by simply describing your package appropriately. In general, this will lead to a more robust and consistent state.
* Inspect the env to determine where to put things. For instance, if the `npm_config_binroot` environment variable is set to `/home/user/bin`, then don't try to install executables into `/usr/local/bin`. The user probably set it up that way for a reason.
* Don't prefix your script commands with "sudo". If root permissions are required for some reason, then it'll fail with that error, and the user will sudo the npm command in question.
* Don't use `install`. Use a `.gyp` file for compilation, and `prepare` for anything else. You should almost never have to explicitly set a preinstall or install script. If you are doing this, please consider if there is another option. The only valid use of `install` or `preinstall` scripts is for compilation which must be done on the target architecture.
* Scripts are run from the root of the package folder, regardless of what the current working directory is when `npm` is invoked. If you want your script to use different behavior based on what subdirectory you're in, you can use the `INIT_CWD` environment variable, which holds the full path you were in when you ran `npm run`.
###
See Also
* [npm run-script](../commands/npm-run-script)
* [package.json](../configuring-npm/package-json)
* [npm developers](developers)
* [npm install](../commands/npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/scripts.md)
| programming_docs |
npm orgs orgs
====
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Working with Teams & Orgs
Table of contents* [Description](#description)
* [Team Admins create teams](#team-admins-create-teams)
* [Publish a package and adjust package access](#publish-a-package-and-adjust-package-access)
* [Monitor your package access](#monitor-your-package-access)
* [See also](#see-also)
###
Description
There are three levels of org users:
1. Super admin, controls billing & adding people to the org.
2. Team admin, manages team membership & package access.
3. Developer, works on packages they are given access to.
The super admin is the only person who can add users to the org because it impacts the monthly bill. The super admin will use the website to manage membership. Every org has a `developers` team that all users are automatically added to.
The team admin is the person who manages team creation, team membership, and package access for teams. The team admin grants package access to teams, not individuals.
The developer will be able to access packages based on the teams they are on. Access is either read-write or read-only.
There are two main commands:
1. `npm team` see [npm team](../commands/npm-team) for more details
2. `npm access` see [npm access](../commands/npm-access) for more details
###
Team Admins create teams
* Check who you’ve added to your org:
```
npm team ls <org>:developers
```
* Each org is automatically given a `developers` team, so you can see the whole list of team members in your org. This team automatically gets read-write access to all packages, but you can change that with the `access` command.
* Create a new team:
```
npm team create <org:team>
```
* Add members to that team:
```
npm team add <org:team> <user>
```
###
Publish a package and adjust package access
* In package directory, run
```
npm init --scope=<org>
```
to scope it for your org & publish as usual
* Grant access:
```
npm access grant <read-only|read-write> <org:team> [<package>]
```
* Revoke access:
```
npm access revoke <org:team> [<package>]
```
###
Monitor your package access
* See what org packages a team member can access:
```
npm access ls-packages <org> <user>
```
* See packages available to a specific team:
```
npm access ls-packages <org:team>
```
* Check which teams are collaborating on a package:
```
npm access ls-collaborators <pkg>
```
###
See also
* [npm team](../commands/npm-team)
* [npm access](../commands/npm-access)
* [npm scope](scope)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/orgs.md)
npm developers developers
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Developer Guide
Table of contents* [Description](#description)
* [About These Documents](#about-these-documents)
* [What is a Package](#what-is-a-package)
* [The package.json File](#the-packagejson-file)
* [Keeping files out of your Package](#keeping-files-out-of-your-package)
+ [Testing whether your .npmignore or files config works](#testing-whether-your-npmignore-or-files-config-works)
* [Link Packages](#link-packages)
* [Before Publishing: Make Sure Your Package Installs and Works](#before-publishing-make-sure-your-package-installs-and-works)
* [Create a User Account](#create-a-user-account)
* [Publish your Package](#publish-your-package)
* [Brag about it](#brag-about-it)
* [See also](#see-also)
###
Description
So, you've decided to use npm to develop (and maybe publish/deploy) your project.
Fantastic!
There are a few things that you need to do above the simple steps that your users will do to install your program.
###
About These Documents
These are man pages. If you install npm, you should be able to then do `man npm-thing` to get the documentation on a particular topic, or `npm help thing` to see the same information.
###
What is a Package
A package is:
* a) a folder containing a program described by a package.json file
* b) a gzipped tarball containing (a)
* c) a url that resolves to (b)
* d) a `<name>@<version>` that is published on the registry with (c)
* e) a `<name>@<tag>` that points to (d)
* f) a `<name>` that has a "latest" tag satisfying (e)
* g) a `git` url that, when cloned, results in (a).
Even if you never publish your package, you can still get a lot of benefits of using npm if you just want to write a node program (a), and perhaps if you also want to be able to easily install it elsewhere after packing it up into a tarball (b).
Git urls can be of the form:
```
git://github.com/user/project.git#commit-ish
git+ssh://user@hostname:project.git#commit-ish
git+http://user@hostname/project/blah.git#commit-ish
git+https://user@hostname/project/blah.git#commit-ish
```
The `commit-ish` can be any tag, sha, or branch which can be supplied as an argument to `git checkout`. The default is whatever the repository uses as its default branch.
###
The package.json File
You need to have a `package.json` file in the root of your project to do much of anything with npm. That is basically the whole interface.
See [`package.json`](../configuring-npm/package-json) for details about what goes in that file. At the very least, you need:
* name: This should be a string that identifies your project. Please do not use the name to specify that it runs on node, or is in JavaScript. You can use the "engines" field to explicitly state the versions of node (or whatever else) that your program requires, and it's pretty well assumed that it's JavaScript.
It does not necessarily need to match your github repository name.
So, `node-foo` and `bar-js` are bad names. `foo` or `bar` are better.
* version: A semver-compatible version.
* engines: Specify the versions of node (or whatever else) that your program runs on. The node API changes a lot, and there may be bugs or new functionality that you depend on. Be explicit.
* author: Take some credit.
* scripts: If you have a special compilation or installation script, then you should put it in the `scripts` object. You should definitely have at least a basic smoke-test command as the "scripts.test" field. See <scripts>.
* main: If you have a single module that serves as the entry point to your program (like what the "foo" package gives you at require("foo")), then you need to specify that in the "main" field.
* directories: This is an object mapping names to folders. The best ones to include are "lib" and "doc", but if you use "man" to specify a folder full of man pages, they'll get installed just like these ones.
You can use `npm init` in the root of your package in order to get you started with a pretty basic package.json file. See [`npm
init`](../commands/npm-init) for more info.
###
Keeping files *out* of your Package
Use a `.npmignore` file to keep stuff out of your package. If there's no `.npmignore` file, but there *is* a `.gitignore` file, then npm will ignore the stuff matched by the `.gitignore` file. If you *want* to include something that is excluded by your `.gitignore` file, you can create an empty `.npmignore` file to override it. Like `git`, `npm` looks for `.npmignore` and `.gitignore` files in all subdirectories of your package, not only the root directory.
`.npmignore` files follow the [same pattern rules](https://git-scm.com/book/en/v2/Git-Basics-Recording-Changes-to-the-Repository#_ignoring) as `.gitignore` files:
* Blank lines or lines starting with `#` are ignored.
* Standard glob patterns work.
* You can end patterns with a forward slash `/` to specify a directory.
* You can negate a pattern by starting it with an exclamation point `!`.
By default, the following paths and files are ignored, so there's no need to add them to `.npmignore` explicitly:
* `.*.swp`
* `._*`
* `.DS_Store`
* `.git`
* `.gitignore`
* `.hg`
* `.npmignore`
* `.npmrc`
* `.lock-wscript`
* `.svn`
* `.wafpickle-*`
* `config.gypi`
* `CVS`
* `npm-debug.log`
Additionally, everything in `node_modules` is ignored, except for bundled dependencies. npm automatically handles this for you, so don't bother adding `node_modules` to `.npmignore`.
The following paths and files are never ignored, so adding them to `.npmignore` is pointless:
* `package.json`
* `README` (and its variants)
* `CHANGELOG` (and its variants)
* `LICENSE` / `LICENCE`
If, given the structure of your project, you find `.npmignore` to be a maintenance headache, you might instead try populating the `files` property of `package.json`, which is an array of file or directory names that should be included in your package. Sometimes manually picking which items to allow is easier to manage than building a block list.
####
Testing whether your `.npmignore` or `files` config works
If you want to double check that your package will include only the files you intend it to when published, you can run the `npm pack` command locally which will generate a tarball in the working directory, the same way it does for publishing.
###
Link Packages
`npm link` is designed to install a development package and see the changes in real time without having to keep re-installing it. (You do need to either re-link or `npm rebuild -g` to update compiled packages, of course.)
More info at [`npm link`](../commands/npm-link).
###
Before Publishing: Make Sure Your Package Installs and Works
**This is important.**
If you can not install it locally, you'll have problems trying to publish it. Or, worse yet, you'll be able to publish it, but you'll be publishing a broken or pointless package. So don't do that.
In the root of your package, do this:
```
npm install . -g
```
That'll show you that it's working. If you'd rather just create a symlink package that points to your working directory, then do this:
```
npm link
```
Use `npm ls -g` to see if it's there.
To test a local install, go into some other folder, and then do:
```
cd ../some-other-folder
npm install ../my-package
```
to install it locally into the node\_modules folder in that other place.
Then go into the node-repl, and try using require("my-thing") to bring in your module's main module.
###
Create a User Account
Create a user with the adduser command. It works like this:
```
npm adduser
```
and then follow the prompts.
This is documented better in [npm adduser](../commands/npm-adduser).
###
Publish your Package
This part's easy. In the root of your folder, do this:
```
npm publish
```
You can give publish a url to a tarball, or a filename of a tarball, or a path to a folder.
Note that pretty much **everything in that folder will be exposed** by default. So, if you have secret stuff in there, use a `.npmignore` file to list out the globs to ignore, or publish from a fresh checkout.
###
Brag about it
Send emails, write blogs, blab in IRC.
Tell the world how easy it is to install your program!
###
See also
* [npm](../commands/npm)
* [npm init](../commands/npm-init)
* [package.json](../configuring-npm/package-json)
* [npm scripts](scripts)
* [npm publish](../commands/npm-publish)
* [npm adduser](../commands/npm-adduser)
* [npm registry](registry)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/developers.md)
npm workspaces workspaces
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Working with workspaces
Table of contents* [Description](#description)
* [Defining workspaces](#defining-workspaces)
* [Getting started with workspaces](#getting-started-with-workspaces)
* [Adding dependencies to a workspace](#adding-dependencies-to-a-workspace)
* [Using workspaces](#using-workspaces)
* [Running commands in the context of workspaces](#running-commands-in-the-context-of-workspaces)
* [Ignoring missing scripts](#ignoring-missing-scripts)
* [See also](#see-also)
###
Description
**Workspaces** is a generic term that refers to the set of features in the npm cli that provides support to managing multiple packages from your local file system from within a singular top-level, root package.
This set of features makes up for a much more streamlined workflow handling linked packages from the local file system. Automating the linking process as part of `npm install` and avoiding manually having to use `npm link` in order to add references to packages that should be symlinked into the current `node_modules` folder.
We also refer to these packages being auto-symlinked during `npm install` as a single **workspace**, meaning it's a nested package within the current local file system that is explicitly defined in the [`package.json`](../configuring-npm/package-json#workspaces) `workspaces` configuration.
###
Defining workspaces
Workspaces are usually defined via the `workspaces` property of the [`package.json`](../configuring-npm/package-json#workspaces) file, e.g:
```
{
"name": "my-workspaces-powered-project",
"workspaces": [
"packages/a"
]
}
```
Given the above `package.json` example living at a current working directory `.` that contains a folder named `packages/a` that itself contains a `package.json` inside it, defining a Node.js package, e.g:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
```
The expected result once running `npm install` in this current working directory `.` is that the folder `packages/a` will get symlinked to the `node_modules` folder of the current working dir.
Below is a post `npm install` example, given that same previous example structure of files and folders:
```
.
+-- node_modules
| `-- a -> ../packages/a
+-- package-lock.json
+-- package.json
`-- packages
+-- a
| `-- package.json
```
###
Getting started with workspaces
You may automate the required steps to define a new workspace using [npm init](../commands/npm-init). For example in a project that already has a `package.json` defined you can run:
```
npm init -w ./packages/a
```
This command will create the missing folders and a new `package.json` file (if needed) while also making sure to properly configure the `"workspaces"` property of your root project `package.json`.
###
Adding dependencies to a workspace
It's possible to directly add/remove/update dependencies of your workspaces using the [`workspace` config](config#workspace).
For example, assuming the following structure:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
`-- b
`-- package.json
```
If you want to add a dependency named `abbrev` from the registry as a dependency of your workspace **a**, you may use the workspace config to tell the npm installer that package should be added as a dependency of the provided workspace:
```
npm install abbrev -w a
```
Note: other installing commands such as `uninstall`, `ci`, etc will also respect the provided `workspace` configuration.
###
Using workspaces
Given the [specifities of how Node.js handles module resolution](https://nodejs.org/dist/latest-v14.x/docs/api/modules.html#modules_all_together) it's possible to consume any defined workspace by its declared `package.json` `name`. Continuing from the example defined above, let's also create a Node.js script that will require the workspace `a` example module, e.g:
```
// ./packages/a/index.js
module.exports = 'a'
// ./lib/index.js
const moduleA = require('a')
console.log(moduleA) // -> a
```
When running it with:
`node lib/index.js`
This demonstrates how the nature of `node_modules` resolution allows for **workspaces** to enable a portable workflow for requiring each **workspace** in such a way that is also easy to [publish](../commands/npm-publish) these nested workspaces to be consumed elsewhere.
###
Running commands in the context of workspaces
You can use the `workspace` configuration option to run commands in the context of a configured workspace. Additionally, if your current directory is in a workspace, the `workspace` configuration is implicitly set, and `prefix` is set to the root workspace.
Following is a quick example on how to use the `npm run` command in the context of nested workspaces. For a project containing multiple workspaces, e.g:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
`-- b
`-- package.json
```
By running a command using the `workspace` option, it's possible to run the given command in the context of that specific workspace. e.g:
```
npm run test --workspace=a
```
You could also run the command within the workspace.
```
cd packages/a && npm run test
```
Either will run the `test` script defined within the `./packages/a/package.json` file.
Please note that you can also specify this argument multiple times in the command-line in order to target multiple workspaces, e.g:
```
npm run test --workspace=a --workspace=b
```
It's also possible to use the `workspaces` (plural) configuration option to enable the same behavior but running that command in the context of **all** configured workspaces. e.g:
```
npm run test --workspaces
```
Will run the `test` script in both `./packages/a` and `./packages/b`.
Commands will be run in each workspace in the order they appear in your `package.json`
```
{
"workspaces": [ "packages/a", "packages/b" ]
}
```
Order of run is different with:
```
{
"workspaces": [ "packages/b", "packages/a" ]
}
```
###
Ignoring missing scripts
It is not required for all of the workspaces to implement scripts run with the `npm run` command.
By running the command with the `--if-present` flag, npm will ignore workspaces missing target script.
```
npm run test --workspaces --if-present
```
###
See also
* [npm install](../commands/npm-install)
* [npm publish](../commands/npm-publish)
* [npm run-script](../commands/npm-run-script)
* <config>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/workspaces.md)
| programming_docs |
npm removal removal
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Cleaning the Slate
Table of contents* [Synopsis](#synopsis)
* [More Severe Uninstalling](#more-severe-uninstalling)
* [See also](#see-also)
###
Synopsis
So sad to see you go.
```
sudo npm uninstall npm -g
```
Or, if that fails, get the npm source code, and do:
```
sudo make uninstall
```
###
More Severe Uninstalling
Usually, the above instructions are sufficient. That will remove npm, but leave behind anything you've installed.
If that doesn't work, or if you require more drastic measures, continue reading.
Note that this is only necessary for globally-installed packages. Local installs are completely contained within a project's `node_modules` folder. Delete that folder, and everything is gone less a package's install script is particularly ill-behaved).
This assumes that you installed node and npm in the default place. If you configured node with a different `--prefix`, or installed npm with a different prefix setting, then adjust the paths accordingly, replacing `/usr/local` with your install prefix.
To remove everything npm-related manually:
```
rm -rf /usr/local/{lib/node{,/.npm,_modules},bin,share/man}/npm*
```
If you installed things *with* npm, then your best bet is to uninstall them with npm first, and then install them again once you have a proper install. This can help find any symlinks that are lying around:
```
ls -laF /usr/local/{lib/node{,/.npm},bin,share/man} | grep npm
```
Prior to version 0.3, npm used shim files for executables and node modules. To track those down, you can do the following:
```
find /usr/local/{lib/node,bin} -exec grep -l npm \{\} \; ;
```
###
See also
* [npm uninstall](../commands/npm-uninstall)
* [npm prune](../commands/npm-prune)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/removal.md)
npm config config
======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
More than you probably want to know about npm configuration
Table of contents* [Description](#description)
+ [Command Line Flags](#command-line-flags)
+ [Environment Variables](#environment-variables)
+ [npmrc Files](#npmrc-files)
+ [Default Configs](#default-configs)
* [Shorthands and Other CLI Niceties](#shorthands-and-other-cli-niceties)
* [Config Settings](#config-settings)
+ [\_auth](#_auth)
+ [access](#access)
+ [all](#all)
+ [allow-same-version](#allow-same-version)
+ [audit](#audit)
+ [audit-level](#audit-level)
+ [auth-type](#auth-type)
+ [before](#before)
+ [bin-links](#bin-links)
+ [browser](#browser)
+ [ca](#ca)
+ [cache](#cache)
+ [cafile](#cafile)
+ [call](#call)
+ [cert](#cert)
+ [ci-name](#ci-name)
+ [cidr](#cidr)
+ [color](#color)
+ [commit-hooks](#commit-hooks)
+ [depth](#depth)
+ [description](#description-1)
+ [diff](#diff)
+ [diff-dst-prefix](#diff-dst-prefix)
+ [diff-ignore-all-space](#diff-ignore-all-space)
+ [diff-name-only](#diff-name-only)
+ [diff-no-prefix](#diff-no-prefix)
+ [diff-src-prefix](#diff-src-prefix)
+ [diff-text](#diff-text)
+ [diff-unified](#diff-unified)
+ [dry-run](#dry-run)
+ [editor](#editor)
+ [engine-strict](#engine-strict)
+ [fetch-retries](#fetch-retries)
+ [fetch-retry-factor](#fetch-retry-factor)
+ [fetch-retry-maxtimeout](#fetch-retry-maxtimeout)
+ [fetch-retry-mintimeout](#fetch-retry-mintimeout)
+ [fetch-timeout](#fetch-timeout)
+ [force](#force)
+ [foreground-scripts](#foreground-scripts)
+ [format-package-lock](#format-package-lock)
+ [fund](#fund)
+ [git](#git)
+ [git-tag-version](#git-tag-version)
+ [global](#global)
+ [global-style](#global-style)
+ [globalconfig](#globalconfig)
+ [heading](#heading)
+ [https-proxy](#https-proxy)
+ [if-present](#if-present)
+ [ignore-scripts](#ignore-scripts)
+ [include](#include)
+ [include-staged](#include-staged)
+ [include-workspace-root](#include-workspace-root)
+ [init-author-email](#init-author-email)
+ [init-author-name](#init-author-name)
+ [init-author-url](#init-author-url)
+ [init-license](#init-license)
+ [init-module](#init-module)
+ [init-version](#init-version)
+ [install-links](#install-links)
+ [json](#json)
+ [key](#key)
+ [legacy-bundling](#legacy-bundling)
+ [legacy-peer-deps](#legacy-peer-deps)
+ [link](#link)
+ [local-address](#local-address)
+ [location](#location)
+ [lockfile-version](#lockfile-version)
+ [loglevel](#loglevel)
+ [logs-dir](#logs-dir)
+ [logs-max](#logs-max)
+ [long](#long)
+ [maxsockets](#maxsockets)
+ [message](#message)
+ [node-options](#node-options)
+ [node-version](#node-version)
+ [noproxy](#noproxy)
+ [npm-version](#npm-version)
+ [offline](#offline)
+ [omit](#omit)
+ [omit-lockfile-registry-resolved](#omit-lockfile-registry-resolved)
+ [otp](#otp)
+ [pack-destination](#pack-destination)
+ [package](#package)
+ [package-lock](#package-lock)
+ [package-lock-only](#package-lock-only)
+ [parseable](#parseable)
+ [prefer-offline](#prefer-offline)
+ [prefer-online](#prefer-online)
+ [prefix](#prefix)
+ [preid](#preid)
+ [progress](#progress)
+ [proxy](#proxy)
+ [read-only](#read-only)
+ [rebuild-bundle](#rebuild-bundle)
+ [registry](#registry)
+ [replace-registry-host](#replace-registry-host)
+ [save](#save)
+ [save-bundle](#save-bundle)
+ [save-dev](#save-dev)
+ [save-exact](#save-exact)
+ [save-optional](#save-optional)
+ [save-peer](#save-peer)
+ [save-prefix](#save-prefix)
+ [save-prod](#save-prod)
+ [scope](#scope)
+ [script-shell](#script-shell)
+ [searchexclude](#searchexclude)
+ [searchlimit](#searchlimit)
+ [searchopts](#searchopts)
+ [searchstaleness](#searchstaleness)
+ [shell](#shell)
+ [sign-git-commit](#sign-git-commit)
+ [sign-git-tag](#sign-git-tag)
+ [strict-peer-deps](#strict-peer-deps)
+ [strict-ssl](#strict-ssl)
+ [tag](#tag)
+ [tag-version-prefix](#tag-version-prefix)
+ [timing](#timing)
+ [umask](#umask)
+ [unicode](#unicode)
+ [update-notifier](#update-notifier)
+ [usage](#usage)
+ [user-agent](#user-agent)
+ [userconfig](#userconfig)
+ [version](#version)
+ [versions](#versions)
+ [viewer](#viewer)
+ [which](#which)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [workspaces-update](#workspaces-update)
+ [yes](#yes)
+ [also](#also)
+ [cache-max](#cache-max)
+ [cache-min](#cache-min)
+ [dev](#dev)
+ [init.author.email](#initauthoremail)
+ [init.author.name](#initauthorname)
+ [init.author.url](#initauthorurl)
+ [init.license](#initlicense)
+ [init.module](#initmodule)
+ [init.version](#initversion)
+ [only](#only)
+ [optional](#optional)
+ [production](#production)
+ [shrinkwrap](#shrinkwrap)
+ [sso-poll-frequency](#sso-poll-frequency)
+ [sso-type](#sso-type)
+ [tmp](#tmp)
* [See also](#see-also)
###
Description
npm gets its configuration values from the following sources, sorted by priority:
####
Command Line Flags
Putting `--foo bar` on the command line sets the `foo` configuration parameter to `"bar"`. A `--` argument tells the cli parser to stop reading flags. Using `--flag` without specifying any value will set the value to `true`.
Example: `--flag1 --flag2` will set both configuration parameters to `true`, while `--flag1 --flag2 bar` will set `flag1` to `true`, and `flag2` to `bar`. Finally, `--flag1 --flag2 -- bar` will set both configuration parameters to `true`, and the `bar` is taken as a command argument.
####
Environment Variables
Any environment variables that start with `npm_config_` will be interpreted as a configuration parameter. For example, putting `npm_config_foo=bar` in your environment will set the `foo` configuration parameter to `bar`. Any environment configurations that are not given a value will be given the value of `true`. Config values are case-insensitive, so `NPM_CONFIG_FOO=bar` will work the same. However, please note that inside [`scripts`](scripts) npm will set its own environment variables and Node will prefer those lowercase versions over any uppercase ones that you might set. For details see [this issue](https://github.com/npm/npm/issues/14528).
Notice that you need to use underscores instead of dashes, so `--allow-same-version` would become `npm_config_allow_same_version=true`.
####
npmrc Files
The four relevant files are:
* per-project configuration file (`/path/to/my/project/.npmrc`)
* per-user configuration file (defaults to `$HOME/.npmrc`; configurable via CLI option `--userconfig` or environment variable `$NPM_CONFIG_USERCONFIG`)
* global configuration file (defaults to `$PREFIX/etc/npmrc`; configurable via CLI option `--globalconfig` or environment variable `$NPM_CONFIG_GLOBALCONFIG`)
* npm's built-in configuration file (`/path/to/npm/npmrc`)
See [npmrc](../configuring-npm/npmrc) for more details.
####
Default Configs
Run `npm config ls -l` to see a set of configuration parameters that are internal to npm, and are defaults if nothing else is specified.
###
Shorthands and Other CLI Niceties
The following shorthands are parsed on the command-line:
* `-a`: `--all`
* `--enjoy-by`: `--before`
* `-c`: `--call`
* `--desc`: `--description`
* `-f`: `--force`
* `-g`: `--global`
* `--iwr`: `--include-workspace-root`
* `-L`: `--location`
* `-d`: `--loglevel info`
* `-s`: `--loglevel silent`
* `--silent`: `--loglevel silent`
* `--ddd`: `--loglevel silly`
* `--dd`: `--loglevel verbose`
* `--verbose`: `--loglevel verbose`
* `-q`: `--loglevel warn`
* `--quiet`: `--loglevel warn`
* `-l`: `--long`
* `-m`: `--message`
* `--local`: `--no-global`
* `-n`: `--no-yes`
* `--no`: `--no-yes`
* `-p`: `--parseable`
* `--porcelain`: `--parseable`
* `-C`: `--prefix`
* `--readonly`: `--read-only`
* `--reg`: `--registry`
* `-S`: `--save`
* `-B`: `--save-bundle`
* `-D`: `--save-dev`
* `-E`: `--save-exact`
* `-O`: `--save-optional`
* `-P`: `--save-prod`
* `-?`: `--usage`
* `-h`: `--usage`
* `-H`: `--usage`
* `--help`: `--usage`
* `-v`: `--version`
* `-w`: `--workspace`
* `--ws`: `--workspaces`
* `-y`: `--yes`
If the specified configuration param resolves unambiguously to a known configuration parameter, then it is expanded to that configuration parameter. For example:
```
npm ls --par
# same as:
npm ls --parseable
```
If multiple single-character shorthands are strung together, and the resulting combination is unambiguously not some other configuration param, then it is expanded to its various component pieces. For example:
```
npm ls -gpld
# same as:
npm ls --global --parseable --long --loglevel info
```
###
Config Settings
####
`_auth`
* Default: null
* Type: null or String
A basic-auth string to use when authenticating against the npm registry. This will ONLY be used to authenticate against the npm registry. For other registries you will need to scope it like "//other-registry.tld/:\_auth"
Warning: This should generally not be set via a command-line option. It is safer to use a registry-provided authentication bearer token stored in the ~/.npmrc file by running `npm login`.
####
`access`
* Default: 'restricted' for scoped packages, 'public' for unscoped packages
* Type: null, "restricted", or "public"
When publishing scoped packages, the access level defaults to `restricted`. If you want your scoped package to be publicly viewable (and installable) set `--access=public`. The only valid values for `access` are `public` and `restricted`. Unscoped packages *always* have an access level of `public`.
Note: Using the `--access` flag on the `npm publish` command will only set the package access level on the initial publish of the package. Any subsequent `npm publish` commands using the `--access` flag will not have an effect to the access level. To make changes to the access level after the initial publish use `npm access`.
####
`all`
* Default: false
* Type: Boolean
When running `npm outdated` and `npm ls`, setting `--all` will show all outdated or installed packages, rather than only those directly depended upon by the current project.
####
`allow-same-version`
* Default: false
* Type: Boolean
Prevents throwing an error when `npm version` is used to set the new version to the same value as the current version.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](../commands/npm-audit) for details on what is submitted.
####
`audit-level`
* Default: null
* Type: null, "info", "low", "moderate", "high", "critical", or "none"
The minimum level of vulnerability for `npm audit` to exit with a non-zero exit code.
####
`auth-type`
* Default: "legacy"
* Type: "legacy", "web", "sso", "saml", "oauth", or "webauthn"
NOTE: auth-type values "sso", "saml", "oauth", and "webauthn" will be removed in a future version.
What authentication strategy to use with `login`.
####
`before`
* Default: null
* Type: null or Date
If passed to `npm install`, will rebuild the npm tree such that only versions that were available **on or before** the `--before` time get installed. If there's no versions available for the current set of direct dependencies, the command will error.
If the requested version is a `dist-tag` and the given tag does not pass the `--before` filter, the most recent version less than or equal to that tag will be used. For example, `foo@latest` might install `[email protected]` even though `latest` is `2.0`.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`browser`
* Default: OS X: `"open"`, Windows: `"start"`, Others: `"xdg-open"`
* Type: null, Boolean, or String
The browser that is called by npm commands to open websites.
Set to `false` to suppress browser behavior and instead print urls to terminal.
Set to `true` to use default system URL opener.
####
`ca`
* Default: null
* Type: null or String (can be set multiple times)
The Certificate Authority signing certificate that is trusted for SSL connections to the registry. Values should be in PEM format (Windows calls it "Base-64 encoded X.509 (.CER)") with newlines replaced by the string "\n". For example:
```
ca="-----BEGIN CERTIFICATE-----\nXXXX\nXXXX\n-----END CERTIFICATE-----"
```
Set to `null` to only allow "known" registrars, or to a specific CA cert to trust only that specific signing authority.
Multiple CAs can be trusted by specifying an array of certificates:
```
ca[]="..."
ca[]="..."
```
See also the `strict-ssl` config.
####
`cache`
* Default: Windows: `%LocalAppData%\npm-cache`, Posix: `~/.npm`
* Type: Path
The location of npm's cache directory. See [`npm
cache`](../commands/npm-cache)
####
`cafile`
* Default: null
* Type: Path
A path to a file containing one or multiple Certificate Authority signing certificates. Similar to the `ca` setting, but allows for multiple CA's, as well as for the CA information to be stored in a file on disk.
####
`call`
* Default: ""
* Type: String
Optional companion option for `npm exec`, `npx` that allows for specifying a custom command to be run along with the installed packages.
```
npm exec --package yo --package generator-node --call "yo node"
```
####
`cert`
* Default: null
* Type: null or String
A client certificate to pass when accessing the registry. Values should be in PEM format (Windows calls it "Base-64 encoded X.509 (.CER)") with newlines replaced by the string "\n". For example:
```
cert="-----BEGIN CERTIFICATE-----\nXXXX\nXXXX\n-----END CERTIFICATE-----"
```
It is *not* the path to a certificate file, though you can set a registry-scoped "certfile" path like "//other-registry.tld/:certfile=/path/to/cert.pem".
####
`ci-name`
* Default: The name of the current CI system, or `null` when not on a known CI platform.
* Type: null or String
The name of a continuous integration system. If not set explicitly, npm will detect the current CI environment using the [`@npmcli/ci-detect`](http://npm.im/@npmcli/ci-detect) module.
####
`cidr`
* Default: null
* Type: null or String (can be set multiple times)
This is a list of CIDR address to be used when configuring limited access tokens with the `npm token create` command.
####
`color`
* Default: true unless the NO\_COLOR environ is set to something other than '0'
* Type: "always" or Boolean
If false, never shows colors. If `"always"` then always shows colors. If true, then only prints color codes for tty file descriptors.
####
`commit-hooks`
* Default: true
* Type: Boolean
Run git commit hooks when using the `npm version` command.
####
`depth`
* Default: `Infinity` if `--all` is set, otherwise `1`
* Type: null or Number
The depth to go when recursing packages for `npm ls`.
If not set, `npm ls` will show only the immediate dependencies of the root project. If `--all` is set, then npm will show all dependencies by default.
####
`description`
* Default: true
* Type: Boolean
Show the description in `npm search`
####
`diff`
* Default:
* Type: String (can be set multiple times)
Define arguments to compare in `npm diff`.
####
`diff-dst-prefix`
* Default: "b/"
* Type: String
Destination prefix to be used in `npm diff` output.
####
`diff-ignore-all-space`
* Default: false
* Type: Boolean
Ignore whitespace when comparing lines in `npm diff`.
####
`diff-name-only`
* Default: false
* Type: Boolean
Prints only filenames when using `npm diff`.
####
`diff-no-prefix`
* Default: false
* Type: Boolean
Do not show any source or destination prefix in `npm diff` output.
Note: this causes `npm diff` to ignore the `--diff-src-prefix` and `--diff-dst-prefix` configs.
####
`diff-src-prefix`
* Default: "a/"
* Type: String
Source prefix to be used in `npm diff` output.
####
`diff-text`
* Default: false
* Type: Boolean
Treat all files as text in `npm diff`.
####
`diff-unified`
* Default: 3
* Type: Number
The number of lines of context to print in `npm diff`.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`editor`
* Default: The EDITOR or VISUAL environment variables, or 'notepad.exe' on Windows, or 'vim' on Unix systems
* Type: String
The command to run for `npm edit` and `npm config edit`.
####
`engine-strict`
* Default: false
* Type: Boolean
If set to true, then npm will stubbornly refuse to install (or even consider installing) any package that claims to not be compatible with the current Node.js version.
This can be overridden by setting the `--force` flag.
####
`fetch-retries`
* Default: 2
* Type: Number
The "retries" config for the `retry` module to use when fetching packages from the registry.
npm will retry idempotent read requests to the registry in the case of network failures or 5xx HTTP errors.
####
`fetch-retry-factor`
* Default: 10
* Type: Number
The "factor" config for the `retry` module to use when fetching packages.
####
`fetch-retry-maxtimeout`
* Default: 60000 (1 minute)
* Type: Number
The "maxTimeout" config for the `retry` module to use when fetching packages.
####
`fetch-retry-mintimeout`
* Default: 10000 (10 seconds)
* Type: Number
The "minTimeout" config for the `retry` module to use when fetching packages.
####
`fetch-timeout`
* Default: 300000 (5 minutes)
* Type: Number
The maximum amount of time to wait for HTTP requests to complete.
####
`force`
* Default: false
* Type: Boolean
Removes various protections against unfortunate side effects, common mistakes, unnecessary performance degradation, and malicious input.
* Allow clobbering non-npm files in global installs.
* Allow the `npm version` command to work on an unclean git repository.
* Allow deleting the cache folder with `npm cache clean`.
* Allow installing packages that have an `engines` declaration requiring a different version of npm.
* Allow installing packages that have an `engines` declaration requiring a different version of `node`, even if `--engine-strict` is enabled.
* Allow `npm audit fix` to install modules outside your stated dependency range (including SemVer-major changes).
* Allow unpublishing all versions of a published package.
* Allow conflicting peerDependencies to be installed in the root project.
* Implicitly set `--yes` during `npm init`.
* Allow clobbering existing values in `npm pkg`
* Allow unpublishing of entire packages (not just a single version).
If you don't have a clear idea of what you want to do, it is strongly recommended that you do not use this option!
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`format-package-lock`
* Default: true
* Type: Boolean
Format `package-lock.json` or `npm-shrinkwrap.json` as a human readable file.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](../commands/npm-fund) for details.
####
`git`
* Default: "git"
* Type: String
The command to use for git commands. If git is installed on the computer, but is not in the `PATH`, then set this to the full path to the git binary.
####
`git-tag-version`
* Default: true
* Type: Boolean
Tag the commit when using the `npm version` command. Setting this to false results in no commit being made at all.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`globalconfig`
* Default: The global --prefix setting plus 'etc/npmrc'. For example, '/usr/local/etc/npmrc'
* Type: Path
The config file to read for global config options.
####
`heading`
* Default: "npm"
* Type: String
The string that starts all the debugging log output.
####
`https-proxy`
* Default: null
* Type: null or URL
A proxy to use for outgoing https requests. If the `HTTPS_PROXY` or `https_proxy` or `HTTP_PROXY` or `http_proxy` environment variables are set, proxy settings will be honored by the underlying `make-fetch-happen` library.
####
`if-present`
* Default: false
* Type: Boolean
If true, npm will not exit with an error code when `run-script` is invoked for a script that isn't defined in the `scripts` section of `package.json`. This option can be used when it's desirable to optionally run a script when it's present and fail if the script fails. This is useful, for example, when running scripts that may only apply for some builds in an otherwise generic CI setup.
This value is not exported to the environment for child processes.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`include`
* Default:
* Type: "prod", "dev", "optional", or "peer" (can be set multiple times)
Option that allows for defining which types of dependencies to install.
This is the inverse of `--omit=<type>`.
Dependency types specified in `--include` will not be omitted, regardless of the order in which omit/include are specified on the command-line.
####
`include-staged`
* Default: false
* Type: Boolean
Allow installing "staged" published packages, as defined by [npm RFC PR #92](https://github.com/npm/rfcs/pull/92).
This is experimental, and not implemented by the npm public registry.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`init-author-email`
* Default: ""
* Type: String
The value `npm init` should use by default for the package author's email.
####
`init-author-name`
* Default: ""
* Type: String
The value `npm init` should use by default for the package author's name.
####
`init-author-url`
* Default: ""
* Type: "" or URL
The value `npm init` should use by default for the package author's homepage.
####
`init-license`
* Default: "ISC"
* Type: String
The value `npm init` should use by default for the package license.
####
`init-module`
* Default: "~/.npm-init.js"
* Type: Path
A module that will be loaded by the `npm init` command. See the documentation for the [init-package-json](https://github.com/npm/init-package-json) module for more information, or [npm init](../commands/npm-init).
####
`init-version`
* Default: "1.0.0"
* Type: SemVer string
The value that `npm init` should use by default for the package version number, if not already set in package.json.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`key`
* Default: null
* Type: null or String
A client key to pass when accessing the registry. Values should be in PEM format with newlines replaced by the string "\n". For example:
```
key="-----BEGIN PRIVATE KEY-----\nXXXX\nXXXX\n-----END PRIVATE KEY-----"
```
It is *not* the path to a key file, though you can set a registry-scoped "keyfile" path like "//other-registry.tld/:keyfile=/path/to/key.pem".
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`legacy-peer-deps`
* Default: false
* Type: Boolean
Causes npm to completely ignore `peerDependencies` when building a package tree, as in npm versions 3 through 6.
If a package cannot be installed because of overly strict `peerDependencies` that collide, it provides a way to move forward resolving the situation.
This differs from `--omit=peer`, in that `--omit=peer` will avoid unpacking `peerDependencies` on disk, but will still design a tree such that `peerDependencies` *could* be unpacked in a correct place.
Use of `legacy-peer-deps` is not recommended, as it will not enforce the `peerDependencies` contract that meta-dependencies may rely on.
####
`link`
* Default: false
* Type: Boolean
Used with `npm ls`, limiting output to only those packages that are linked.
####
`local-address`
* Default: null
* Type: IP Address
The IP address of the local interface to use when making connections to the npm registry. Must be IPv4 in versions of Node prior to 0.12.
####
`location`
* Default: "user" unless `--global` is passed, which will also set this value to "global"
* Type: "global", "user", or "project"
When passed to `npm config` this refers to which config file to use.
When set to "global" mode, packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`lockfile-version`
* Default: Version 2 if no lockfile or current lockfile version less than or equal to 2, otherwise maintain current lockfile version
* Type: null, 1, 2, 3, "1", "2", or "3"
Set the lockfile format version to be used in package-lock.json and npm-shrinkwrap-json files. Possible options are:
1: The lockfile version used by npm versions 5 and 6. Lacks some data that is used during the install, resulting in slower and possibly less deterministic installs. Prevents lockfile churn when interoperating with older npm versions.
2: The default lockfile version used by npm version 7. Includes both the version 1 lockfile data and version 3 lockfile data, for maximum determinism and interoperability, at the expense of more bytes on disk.
3: Only the new lockfile information introduced in npm version 7. Smaller on disk than lockfile version 2, but not interoperable with older npm versions. Ideal if all users are on npm version 7 and higher.
####
`loglevel`
* Default: "notice"
* Type: "silent", "error", "warn", "notice", "http", "timing", "info", "verbose", or "silly"
What level of logs to report. All logs are written to a debug log, with the path to that file printed if the execution of a command fails.
Any logs of a higher level than the setting are shown. The default is "notice".
See also the `foreground-scripts` config.
####
`logs-dir`
* Default: A directory named `_logs` inside the cache
* Type: null or Path
The location of npm's log directory. See [`npm logging`](logging) for more information.
####
`logs-max`
* Default: 10
* Type: Number
The maximum number of log files to store.
If set to 0, no log files will be written for the current run.
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
####
`maxsockets`
* Default: 15
* Type: Number
The maximum number of connections to use per origin (protocol/host/port combination).
####
`message`
* Default: "%s"
* Type: String
Commit message which is used by `npm version` when creating version commit.
Any "%s" in the message will be replaced with the version number.
####
`node-options`
* Default: null
* Type: null or String
Options to pass through to Node.js via the `NODE_OPTIONS` environment variable. This does not impact how npm itself is executed but it does impact how lifecycle scripts are called.
####
`node-version`
* Default: Node.js `process.version` value
* Type: SemVer string
The node version to use when checking a package's `engines` setting.
####
`noproxy`
* Default: The value of the NO\_PROXY environment variable
* Type: String (can be set multiple times)
Domain extensions that should bypass any proxies.
Also accepts a comma-delimited string.
####
`npm-version`
* Default: Output of `npm --version`
* Type: SemVer string
The npm version to use when checking a package's `engines` setting.
####
`offline`
* Default: false
* Type: Boolean
Force offline mode: no network requests will be done during install. To allow the CLI to fill in missing cache data, see `--prefer-offline`.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`omit-lockfile-registry-resolved`
* Default: false
* Type: Boolean
This option causes npm to create lock files without a `resolved` key for registry dependencies. Subsequent installs will need to resolve tarball endpoints with the configured registry, likely resulting in a longer install time.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
####
`pack-destination`
* Default: "."
* Type: String
Directory in which `npm pack` will save tarballs.
####
`package`
* Default:
* Type: String (can be set multiple times)
The package or packages to install for [`npm exec`](../commands/npm-exec)
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`package-lock-only`
* Default: false
* Type: Boolean
If set to true, the current operation will only use the `package-lock.json`, ignoring `node_modules`.
For `update` this means only the `package-lock.json` will be updated, instead of checking `node_modules` and downloading dependencies.
For `list` this means the output will be based on the tree described by the `package-lock.json`, rather than the contents of `node_modules`.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`prefer-offline`
* Default: false
* Type: Boolean
If true, staleness checks for cached data will be bypassed, but missing data will be requested from the server. To force full offline mode, use `--offline`.
####
`prefer-online`
* Default: false
* Type: Boolean
If true, staleness checks for cached data will be forced, making the CLI look for updates immediately even for fresh package data.
####
`prefix`
* Default: In global mode, the folder where the node executable is installed. In local mode, the nearest parent folder containing either a package.json file or a node\_modules folder.
* Type: Path
The location to install global items. If set on the command line, then it forces non-global commands to run in the specified folder.
####
`preid`
* Default: ""
* Type: String
The "prerelease identifier" to use as a prefix for the "prerelease" part of a semver. Like the `rc` in `1.2.0-rc.8`.
####
`progress`
* Default: `true` unless running in a known CI system
* Type: Boolean
When set to `true`, npm will display a progress bar during time intensive operations, if `process.stderr` is a TTY.
Set to `false` to suppress the progress bar.
####
`proxy`
* Default: null
* Type: null, false, or URL
A proxy to use for outgoing http requests. If the `HTTP_PROXY` or `http_proxy` environment variables are set, proxy settings will be honored by the underlying `request` library.
####
`read-only`
* Default: false
* Type: Boolean
This is used to mark a token as unable to publish when configuring limited access tokens with the `npm token create` command.
####
`rebuild-bundle`
* Default: true
* Type: Boolean
Rebuild bundled dependencies after installation.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`replace-registry-host`
* Default: "npmjs"
* Type: "npmjs", "never", "always", or String
Defines behavior for replacing the registry host in a lockfile with the configured registry.
The default behavior is to replace package dist URLs from the default registry (<https://registry.npmjs.org>) to the configured registry. If set to "never", then use the registry value. If set to "always", then replace the registry host with the configured host every time.
You may also specify a bare hostname (e.g., "registry.npmjs.org").
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-bundle`
* Default: false
* Type: Boolean
If a package would be saved at install time by the use of `--save`, `--save-dev`, or `--save-optional`, then also put it in the `bundleDependencies` list.
Ignored if `--save-peer` is set, since peerDependencies cannot be bundled.
####
`save-dev`
* Default: false
* Type: Boolean
Save installed packages to a package.json file as `devDependencies`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`save-optional`
* Default: false
* Type: Boolean
Save installed packages to a package.json file as `optionalDependencies`.
####
`save-peer`
* Default: false
* Type: Boolean
Save installed packages to a package.json file as `peerDependencies`
####
`save-prefix`
* Default: "^"
* Type: String
Configure how versions of packages installed to a package.json file via `--save` or `--save-dev` get prefixed.
For example if a package has version `1.2.3`, by default its version is set to `^1.2.3` which allows minor upgrades for that package, but after `npm
config set save-prefix='~'` it would be set to `~1.2.3` which only allows patch upgrades.
####
`save-prod`
* Default: false
* Type: Boolean
Save installed packages into `dependencies` specifically. This is useful if a package already exists in `devDependencies` or `optionalDependencies`, but you want to move it to be a non-optional production dependency.
This is the default behavior if `--save` is true, and neither `--save-dev` or `--save-optional` are true.
####
`scope`
* Default: the scope of the current project, if any, or ""
* Type: String
Associate an operation with a scope for a scoped registry.
Useful when logging in to or out of a private registry:
```
# log in, linking the scope to the custom registry
npm login --scope=@mycorp --registry=https://registry.mycorp.com
# log out, removing the link and the auth token
npm logout --scope=@mycorp
```
This will cause `@mycorp` to be mapped to the registry for future installation of packages specified according to the pattern `@mycorp/package`.
This will also cause `npm init` to create a scoped package.
```
# accept all defaults, and create a package named "@foo/whatever",
# instead of just named "whatever"
npm init --scope=@foo --yes
```
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
####
`searchexclude`
* Default: ""
* Type: String
Space-separated options that limit the results from search.
####
`searchlimit`
* Default: 20
* Type: Number
Number of items to limit search results to. Will not apply at all to legacy searches.
####
`searchopts`
* Default: ""
* Type: String
Space-separated options that are always passed to search.
####
`searchstaleness`
* Default: 900
* Type: Number
The age of the cache, in seconds, before another registry request is made if using legacy search endpoint.
####
`shell`
* Default: SHELL environment variable, or "bash" on Posix, or "cmd.exe" on Windows
* Type: String
The shell to run for the `npm explore` command.
####
`sign-git-commit`
* Default: false
* Type: Boolean
If set to true, then the `npm version` command will commit the new package version using `-S` to add a signature.
Note that git requires you to have set up GPG keys in your git configs for this to work properly.
####
`sign-git-tag`
* Default: false
* Type: Boolean
If set to true, then the `npm version` command will tag the version using `-s` to add a signature.
Note that git requires you to have set up GPG keys in your git configs for this to work properly.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`strict-ssl`
* Default: true
* Type: Boolean
Whether or not to do SSL key validation when making requests to the registry via https.
See also the `ca` config.
####
`tag`
* Default: "latest"
* Type: String
If you ask npm to install a package and don't tell it a specific version, then it will install the specified tag.
Also the tag that is added to the package@version specified by the `npm tag` command, if no explicit tag is given.
When used by the `npm diff` command, this is the tag used to fetch the tarball that will be compared with the local files by default.
####
`tag-version-prefix`
* Default: "v"
* Type: String
If set, alters the prefix used when tagging a new version when performing a version increment using `npm-version`. To remove the prefix altogether, set it to the empty string: `""`.
Because other tools may rely on the convention that npm version tags look like `v1.0.0`, *only use this property if it is absolutely necessary*. In particular, use care when overriding this setting for public packages.
####
`timing`
* Default: false
* Type: Boolean
If true, writes a debug log to `logs-dir` and timing information to `_timing.json` in the cache, even if the command completes successfully. `_timing.json` is a newline delimited list of JSON objects.
You can quickly view it with this [json](https://npm.im/json) command line: `npm exec -- json -g < ~/.npm/_timing.json`.
####
`umask`
* Default: 0
* Type: Octal numeric string in range 0000..0777 (0..511)
The "umask" value to use when setting the file creation mode on files and folders.
Folders and executables are given a mode which is `0o777` masked against this value. Other files are given a mode which is `0o666` masked against this value.
Note that the underlying system will *also* apply its own umask value to files and folders that are created, and npm does not circumvent this, but rather adds the `--umask` config to it.
Thus, the effective default umask value on most POSIX systems is 0o22, meaning that folders and executables are created with a mode of 0o755 and other files are created with a mode of 0o644.
####
`unicode`
* Default: false on windows, true on mac/unix systems with a unicode locale, as defined by the `LC_ALL`, `LC_CTYPE`, or `LANG` environment variables.
* Type: Boolean
When set to true, npm uses unicode characters in the tree output. When false, it uses ascii characters instead of unicode glyphs.
####
`update-notifier`
* Default: true
* Type: Boolean
Set to false to suppress the update notification when using an older version of npm than the latest.
####
`usage`
* Default: false
* Type: Boolean
Show short usage output about the command specified.
####
`user-agent`
* Default: "npm/{npm-version} node/{node-version} {platform} {arch} workspaces/{workspaces} {ci}"
* Type: String
Sets the User-Agent request header. The following fields are replaced with their actual counterparts:
* `{npm-version}` - The npm version in use
* `{node-version}` - The Node.js version in use
* `{platform}` - The value of `process.platform`
* `{arch}` - The value of `process.arch`
* `{workspaces}` - Set to `true` if the `workspaces` or `workspace` options are set.
* `{ci}` - The value of the `ci-name` config, if set, prefixed with `ci/`, or an empty string if `ci-name` is empty.
####
`userconfig`
* Default: "~/.npmrc"
* Type: Path
The location of user-level configuration settings.
This may be overridden by the `npm_config_userconfig` environment variable or the `--userconfig` command line option, but may *not* be overridden by settings in the `globalconfig` file.
####
`version`
* Default: false
* Type: Boolean
If true, output the npm version and exit successfully.
Only relevant when specified explicitly on the command line.
####
`versions`
* Default: false
* Type: Boolean
If true, output the npm version as well as node's `process.versions` map and the version in the current working directory's `package.json` file if one exists, and exit successfully.
Only relevant when specified explicitly on the command line.
####
`viewer`
* Default: "man" on Posix, "browser" on Windows
* Type: String
The program to use to view help content.
Set to `"browser"` to view html help content in the default web browser.
####
`which`
* Default: null
* Type: null or Number
If there are multiple funding sources, which 1-indexed source URL to open.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`workspaces-update`
* Default: true
* Type: Boolean
If set to true, the npm cli will run an update after operations that may possibly change the workspaces installed to the `node_modules` folder.
####
`yes`
* Default: null
* Type: null or Boolean
Automatically answer "yes" to any prompts that npm might print on the command line.
####
`also`
* Default: null
* Type: null, "dev", or "development"
* DEPRECATED: Please use --include=dev instead.
When set to `dev` or `development`, this is an alias for `--include=dev`.
####
`cache-max`
* Default: Infinity
* Type: Number
* DEPRECATED: This option has been deprecated in favor of `--prefer-online`
`--cache-max=0` is an alias for `--prefer-online`
####
`cache-min`
* Default: 0
* Type: Number
* DEPRECATED: This option has been deprecated in favor of `--prefer-offline`.
`--cache-min=9999 (or bigger)` is an alias for `--prefer-offline`.
####
`dev`
* Default: false
* Type: Boolean
* DEPRECATED: Please use --include=dev instead.
Alias for `--include=dev`.
####
`init.author.email`
* Default: ""
* Type: String
* DEPRECATED: Use `--init-author-email` instead.
Alias for `--init-author-email`
####
`init.author.name`
* Default: ""
* Type: String
* DEPRECATED: Use `--init-author-name` instead.
Alias for `--init-author-name`
####
`init.author.url`
* Default: ""
* Type: "" or URL
* DEPRECATED: Use `--init-author-url` instead.
Alias for `--init-author-url`
####
`init.license`
* Default: "ISC"
* Type: String
* DEPRECATED: Use `--init-license` instead.
Alias for `--init-license`
####
`init.module`
* Default: "~/.npm-init.js"
* Type: Path
* DEPRECATED: Use `--init-module` instead.
Alias for `--init-module`
####
`init.version`
* Default: "1.0.0"
* Type: SemVer string
* DEPRECATED: Use `--init-version` instead.
Alias for `--init-version`
####
`only`
* Default: null
* Type: null, "prod", or "production"
* DEPRECATED: Use `--omit=dev` to omit dev dependencies from the install.
When set to `prod` or `production`, this is an alias for `--omit=dev`.
####
`optional`
* Default: null
* Type: null or Boolean
* DEPRECATED: Use `--omit=optional` to exclude optional dependencies, or `--include=optional` to include them.
Default value does install optional deps unless otherwise omitted.
Alias for --include=optional or --omit=optional
####
`production`
* Default: null
* Type: null or Boolean
* DEPRECATED: Use `--omit=dev` instead.
Alias for `--omit=dev`
####
`shrinkwrap`
* Default: true
* Type: Boolean
* DEPRECATED: Use the --package-lock setting instead.
Alias for --package-lock
####
`sso-poll-frequency`
* Default: 500
* Type: Number
* DEPRECATED: The --auth-type method of SSO/SAML/OAuth will be removed in a future version of npm in favor of web-based login.
When used with SSO-enabled `auth-type`s, configures how regularly the registry should be polled while the user is completing authentication.
####
`sso-type`
* Default: "oauth"
* Type: null, "oauth", or "saml"
* DEPRECATED: The --auth-type method of SSO/SAML/OAuth will be removed in a future version of npm in favor of web-based login.
If `--auth-type=sso`, the type of SSO type to use.
####
`tmp`
* Default: The value returned by the Node.js `os.tmpdir()` method <https://nodejs.org/api/os.html#os_os_tmpdir>
* Type: Path
* DEPRECATED: This setting is no longer used. npm stores temporary files in a special location in the cache, and they are managed by [`cacache`](http://npm.im/cacache).
Historically, the location where temporary files were stored. No longer relevant.
###
See also
* [npm config](../commands/npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm scripts](scripts)
* [npm folders](../configuring-npm/folders)
* [npm](../commands/npm)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/config.md)
| programming_docs |
npm scope scope
=====
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Scoped packages
Table of contents* [Description](#description)
* [Installing scoped packages](#installing-scoped-packages)
* [Requiring scoped packages](#requiring-scoped-packages)
* [Publishing scoped packages](#publishing-scoped-packages)
+ [Publishing public scoped packages to the primary npm registry](#publishing-public-scoped-packages-to-the-primary-npm-registry)
+ [Publishing private scoped packages to the npm registry](#publishing-private-scoped-packages-to-the-npm-registry)
* [Associating a scope with a registry](#associating-a-scope-with-a-registry)
* [See also](#see-also)
###
Description
All npm packages have a name. Some package names also have a scope. A scope follows the usual rules for package names (URL-safe characters, no leading dots or underscores). When used in package names, scopes are preceded by an `@` symbol and followed by a slash, e.g.
```
@somescope/somepackagename
```
Scopes are a way of grouping related packages together, and also affect a few things about the way npm treats the package.
Each npm user/organization has their own scope, and only you can add packages in your scope. This means you don't have to worry about someone taking your package name ahead of you. Thus it is also a good way to signal official packages for organizations.
Scoped packages can be published and installed as of `npm@2` and are supported by the primary npm registry. Unscoped packages can depend on scoped packages and vice versa. The npm client is backwards-compatible with unscoped registries, so it can be used to work with scoped and unscoped registries at the same time.
###
Installing scoped packages
Scoped packages are installed to a sub-folder of the regular installation folder, e.g. if your other packages are installed in `node_modules/packagename`, scoped modules will be installed in `node_modules/@myorg/packagename`. The scope folder (`@myorg`) is simply the name of the scope preceded by an `@` symbol, and can contain any number of scoped packages.
A scoped package is installed by referencing it by name, preceded by an `@` symbol, in `npm install`:
```
npm install @myorg/mypackage
```
Or in `package.json`:
```
"dependencies": {
"@myorg/mypackage": "^1.3.0"
}
```
Note that if the `@` symbol is omitted, in either case, npm will instead attempt to install from GitHub; see [`npm install`](../commands/npm-install).
###
Requiring scoped packages
Because scoped packages are installed into a scope folder, you have to include the name of the scope when requiring them in your code, e.g.
```
require('@myorg/mypackage')
```
There is nothing special about the way Node treats scope folders. This simply requires the `mypackage` module in the folder named `@myorg`.
###
Publishing scoped packages
Scoped packages can be published from the CLI as of `npm@2` and can be published to any registry that supports them, including the primary npm registry.
(As of 2015-04-19, and with npm 2.0 or better, the primary npm registry **does** support scoped packages.)
If you wish, you may associate a scope with a registry; see below.
####
Publishing public scoped packages to the primary npm registry
Publishing to a scope, you have two options:
* Publishing to your user scope (example: `@username/module`)
* Publishing to an organization scope (example: `@org/module`)
If publishing a public module to an organization scope, you must first either create an organization with the name of the scope that you'd like to publish to or be added to an existing organization with the appropriate permisssions. For example, if you'd like to publish to `@org`, you would need to create the `org` organization on npmjs.com prior to trying to publish.
Scoped packages are not public by default. You will need to specify `--access public` with the initial `npm publish` command. This will publish the package and set access to `public` as if you had run `npm access public` after publishing. You do not need to do this when publishing new versions of an existing scoped package.
####
Publishing private scoped packages to the npm registry
To publish a private scoped package to the npm registry, you must have an [npm Private Modules](../../../creating-and-publishing-private-packages) account.
You can then publish the module with `npm publish` or `npm publish
--access restricted`, and it will be present in the npm registry, with restricted access. You can then change the access permissions, if desired, with `npm access` or on the npmjs.com website.
###
Associating a scope with a registry
Scopes can be associated with a separate registry. This allows you to seamlessly use a mix of packages from the primary npm registry and one or more private registries, such as [GitHub Packages](https://github.com/features/packages) or the open source [Verdaccio](https://verdaccio.org) project.
You can associate a scope with a registry at login, e.g.
```
npm login --registry=http://reg.example.com --scope=@myco
```
Scopes have a many-to-one relationship with registries: one registry can host multiple scopes, but a scope only ever points to one registry.
You can also associate a scope with a registry using `npm config`:
```
npm config set @myco:registry http://reg.example.com
```
Once a scope is associated with a registry, any `npm install` for a package with that scope will request packages from that registry instead. Any `npm publish` for a package name that contains the scope will be published to that registry instead.
###
See also
* [npm install](../commands/npm-install)
* [npm publish](../commands/npm-publish)
* [npm access](../commands/npm-access)
* [npm registry](registry)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/scope.md)
npm registry registry
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
The JavaScript Package Registry
Table of contents* [Description](#description)
* [Does npm send any information about me back to the registry?](#does-npm-send-any-information-about-me-back-to-the-registry)
* [How can I prevent my package from being published in the official registry?](#how-can-i-prevent-my-package-from-being-published-in-the-official-registry)
* [Where can I find my own, & other's, published packages?](#where-can-i-find-my-own--others-published-packages)
* [See also](#see-also)
###
Description
To resolve packages by name and version, npm talks to a registry website that implements the CommonJS Package Registry specification for reading package info.
npm is configured to use the **npm public registry** at <https://registry.npmjs.org> by default. Use of the npm public registry is subject to terms of use available at <https://docs.npmjs.com/policies/terms>.
You can configure npm to use any compatible registry you like, and even run your own registry. Use of someone else's registry may be governed by their terms of use.
npm's package registry implementation supports several write APIs as well, to allow for publishing packages and managing user account information.
The npm public registry is powered by a CouchDB database, of which there is a public mirror at <https://skimdb.npmjs.com/registry>.
The registry URL used is determined by the scope of the package (see [`scope`](scope). If no scope is specified, the default registry is used, which is supplied by the `registry` config parameter. See [`npm config`](../commands/npm-config), [`npmrc`](../configuring-npm/npmrc), and [`config`](config) for more on managing npm's configuration.
When the default registry is used in a package-lock or shrinkwrap is has the special meaning of "the currently configured registry". If you create a lock file while using the default registry you can switch to another registry and npm will install packages from the new registry, but if you create a lock file while using a custom registry packages will be installed from that registry even after you change to another registry.
###
Does npm send any information about me back to the registry?
Yes.
When making requests of the registry npm adds two headers with information about your environment:
* `Npm-Scope` – If your project is scoped, this header will contain its scope. In the future npm hopes to build registry features that use this information to allow you to customize your experience for your organization.
* `Npm-In-CI` – Set to "true" if npm believes this install is running in a continuous integration environment, "false" otherwise. This is detected by looking for the following environment variables: `CI`, `TDDIUM`, `JENKINS_URL`, `bamboo.buildKey`. If you'd like to learn more you may find the [original PR](https://github.com/npm/npm-registry-client/pull/129) interesting. This is used to gather better metrics on how npm is used by humans, versus build farms.
The npm registry does not try to correlate the information in these headers with any authenticated accounts that may be used in the same requests.
###
How can I prevent my package from being published in the official registry?
Set `"private": true` in your `package.json` to prevent it from being published at all, or `"publishConfig":{"registry":"http://my-internal-registry.local"}` to force it to be published only to your internal/private registry.
See [`package.json`](../configuring-npm/package-json) for more info on what goes in the package.json file.
###
Where can I find my own, & other's, published packages?
<https://www.npmjs.com/>
###
See also
* [npm config](../commands/npm-config)
* <config>
* [npmrc](../configuring-npm/npmrc)
* [npm developers](developers)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/registry.md)
npm Logging Logging
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
[Registry](registry)[Package spec](package-spec)[Config](config)[Logging](logging)[Scope](scope)[Scripts](scripts)[Workspaces](workspaces)[Organizations](orgs)[Dependency Selectors](dependency-selectors)[Developers](developers)[Removal](removal)
Why, What & How We Log
Table of contents* [Description](#description)
* [Setting Log File Location](#setting-log-file-location)
* [Setting Log Levels](#setting-log-levels)
+ [loglevel](#loglevel)
- [Aliases](#aliases)
+ [foreground-scripts](#foreground-scripts)
* [Timing Information](#timing-information)
* [Registry Response Headers](#registry-response-headers)
+ [npm-notice](#npm-notice)
* [Logs and Sensitive Information](#logs-and-sensitive-information)
* [See also](#see-also)
###
Description
The `npm` CLI has various mechanisms for showing different levels of information back to end-users for certain commands, configurations & environments.
###
Setting Log File Location
All logs are written to a debug log, with the path to that file printed if the execution of a command fails.
The default location of the logs directory is a directory named `_logs` inside the npm cache. This can be changed with the `logs-dir` config option.
Log files will be removed from the `logs-dir` when the number of log files exceeds `logs-max`, with the oldest logs being deleted first.
To turn off logs completely set `--logs-max=0`.
###
Setting Log Levels
####
`loglevel`
`loglevel` is a global argument/config that can be set to determine the type of information to be displayed.
The default value of `loglevel` is `"notice"` but there are several levels/types of logs available, including:
* `"silent"`
* `"error"`
* `"warn"`
* `"notice"`
* `"http"`
* `"timing"`
* `"info"`
* `"verbose"`
* `"silly"`
All logs pertaining to a level proceeding the current setting will be shown.
#####
Aliases
The log levels listed above have various corresponding aliases, including:
* `-d`: `--loglevel info`
* `--dd`: `--loglevel verbose`
* `--verbose`: `--loglevel verbose`
* `--ddd`: `--loglevel silly`
* `-q`: `--loglevel warn`
* `--quiet`: `--loglevel warn`
* `-s`: `--loglevel silent`
* `--silent`: `--loglevel silent`
####
`foreground-scripts`
The `npm` CLI began hiding the output of lifecycle scripts for `npm install` as of `v7`. Notably, this means you will not see logs/output from packages that may be using "install scripts" to display information back to you or from your own project's scripts defined in `package.json`. If you'd like to change this behavior & log this output you can set `foreground-scripts` to `true`.
###
Timing Information
The `--timing` config can be set which does two things:
1. Always shows the full path to the debug log regardless of command exit status
2. Write timing information to a timing file in the cache or `logs-dir`
This file is a newline delimited list of JSON objects that can be inspected to see timing data for each task in a `npm` CLI run.
###
Registry Response Headers
####
`npm-notice`
The `npm` CLI reads from & logs any `npm-notice` headers that are returned from the configured registry. This mechanism can be used by third-party registries to provide useful information when network-dependent requests occur.
This header is not cached, and will not be logged if the request is served from the cache.
###
Logs and Sensitive Information
The `npm` CLI makes a best effort to redact the following from terminal output and log files:
* Passwords inside basic auth URLs
* npm tokens
However, this behavior should not be relied on to keep all possible sensitive information redacted. If you are concerned about secrets in your log file or terminal output, you can use `--loglevel=silent` and `--logs-max=0` to ensure no logs are written to your terminal or filesystem.
###
See also
* <config>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/using-npm/logging.md)
npm npm-help-search npm-help-search
===============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Search npm help documentation
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [long](#long)
* [See Also](#see-also)
###
Synopsis
```
npm help-search <text>
```
Note: This command is unaware of workspaces.
###
Description
This command will search the npm markdown documentation files for the terms provided, and then list the results, sorted by relevance.
If only one result is found, then it will show that help topic.
If the argument to `npm help` is not a known help topic, then it will call `help-search`. It is rarely if ever necessary to call this command directly.
###
Configuration
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
###
See Also
* <npm>
* [npm help](npm-help)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-help-search.md)
npm npm-uninstall npm-uninstall
=============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Remove a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Examples](#examples)
* [Configuration](#configuration)
+ [save](#save)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm uninstall [<@scope>/]<pkg>...
aliases: unlink, remove, rm, r, un
```
###
Description
This uninstalls a package, completely removing everything npm installed on its behalf.
It also removes the package from the `dependencies`, `devDependencies`, `optionalDependencies`, and `peerDependencies` objects in your `package.json`.
Further, if you have an `npm-shrinkwrap.json` or `package-lock.json`, npm will update those files as well.
`--no-save` will tell npm not to remove the package from your `package.json`, `npm-shrinkwrap.json`, or `package-lock.json` files.
`--save` or `-S` will tell npm to remove the package from your `package.json`, `npm-shrinkwrap.json`, and `package-lock.json` files. This is the default, but you may need to use this if you have for instance `save=false` in your `npmrc` file
In global mode (ie, with `-g` or `--global` appended to the command), it uninstalls the current package context as a global package. `--no-save` is ignored in this case.
Scope is optional and follows the usual rules for [`scope`](../using-npm/scope).
###
Examples
```
npm uninstall sax
```
`sax` will no longer be in your `package.json`, `npm-shrinkwrap.json`, or `package-lock.json` files.
```
npm uninstall lodash --no-save
```
`lodash` will not be removed from your `package.json`, `npm-shrinkwrap.json`, or `package-lock.json` files.
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm prune](npm-prune)
* [npm install](npm-install)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-uninstall.md)
| programming_docs |
npm npm-stars npm-stars
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
View packages marked as favorites
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
* [See Also](#see-also)
###
Synopsis
```
npm stars [<user>]
```
Note: This command is unaware of workspaces.
###
Description
If you have starred a lot of neat things and want to find them again quickly this command lets you do just that.
You may also want to see your friend's favorite packages, in this case you will most certainly enjoy this command.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
###
See Also
* [npm star](npm-star)
* [npm unstar](npm-unstar)
* [npm view](npm-view)
* [npm whoami](npm-whoami)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-stars.md)
npm npm-outdated npm-outdated
============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Check for outdated packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [An example](#an-example)
* [Configuration](#configuration)
+ [all](#all)
+ [json](#json)
+ [long](#long)
+ [parseable](#parseable)
+ [global](#global)
+ [workspace](#workspace)
* [See Also](#see-also)
###
Synopsis
```
npm outdated [<package-spec> ...]
```
###
Description
This command will check the registry to see if any (or, specific) installed packages are currently outdated.
By default, only the direct dependencies of the root project and direct dependencies of your configured *workspaces* are shown. Use `--all` to find all outdated meta-dependencies as well.
In the output:
* `wanted` is the maximum version of the package that satisfies the semver range specified in `package.json`. If there's no available semver range (i.e. you're running `npm outdated --global`, or the package isn't included in `package.json`), then `wanted` shows the currently-installed version.
* `latest` is the version of the package tagged as latest in the registry. Running `npm publish` with no special configuration will publish the package with a dist-tag of `latest`. This may or may not be the maximum version of the package, or the most-recently published version of the package, depending on how the package's developer manages the latest [dist-tag](npm-dist-tag).
* `location` is where in the physical tree the package is located.
* `depended by` shows which package depends on the displayed dependency
* `package type` (when using `--long` / `-l`) tells you whether this package is a `dependency` or a dev/peer/optional dependency. Packages not included in `package.json` are always marked `dependencies`.
* `homepage` (when using `--long` / `-l`) is the `homepage` value contained in the package's packument
* Red means there's a newer version matching your semver requirements, so you should update now.
* Yellow indicates that there's a newer version *above* your semver requirements (usually new major, or new 0.x minor) so proceed with caution.
###
An example
```
$ npm outdated
Package Current Wanted Latest Location Depended by
glob 5.0.15 5.0.15 6.0.1 node_modules/glob dependent-package-name
nothingness 0.0.3 git git node_modules/nothingness dependent-package-name
npm 3.5.1 3.5.2 3.5.1 node_modules/npm dependent-package-name
local-dev 0.0.3 linked linked local-dev dependent-package-name
once 1.3.2 1.3.3 1.3.3 node_modules/once dependent-package-name
```
With these `dependencies`:
```
{
"glob": "^5.0.15",
"nothingness": "github:othiym23/nothingness#master",
"npm": "^3.5.1",
"once": "^1.3.1"
}
```
A few things to note:
* `glob` requires `^5`, which prevents npm from installing `glob@6`, which is outside the semver range.
* Git dependencies will always be reinstalled, because of how they're specified. The installed committish might satisfy the dependency specifier (if it's something immutable, like a commit SHA), or it might not, so `npm outdated` and `npm update` have to fetch Git repos to check. This is why currently doing a reinstall of a Git dependency always forces a new clone and install.
* `[email protected]` is marked as "wanted", but "latest" is `[email protected]` because npm uses dist-tags to manage its `latest` and `next` release channels. `npm update` will install the *newest* version, but `npm install npm` (with no semver range) will install whatever's tagged as `latest`.
* `once` is just plain out of date. Reinstalling `node_modules` from scratch or running `npm update` will bring it up to spec.
###
Configuration
####
`all`
* Default: false
* Type: Boolean
When running `npm outdated` and `npm ls`, setting `--all` will show all outdated or installed packages, rather than only those directly depended upon by the current project.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm update](npm-update)
* [npm dist-tag](npm-dist-tag)
* [npm registry](../using-npm/registry)
* [npm folders](../configuring-npm/folders)
* [npm workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-outdated.md)
npm npm-rebuild npm-rebuild
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Rebuild a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [global](#global)
+ [bin-links](#bin-links)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm rebuild [<package-spec>] ...]
alias: rb
```
###
Description
This command runs the `npm build` command on the matched folders. This is useful when you install a new version of node, and must recompile all your C++ addons with the new binary. It is also useful when installing with `--ignore-scripts` and `--no-bin-links`, to explicitly choose which packages to build and/or link bins.
If one or more package specs are provided, then only packages with a name and version matching one of the specifiers will be rebuilt.
###
Configuration
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm install](npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-rebuild.md)
npm npm-ls npm-ls
======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
List installed packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Note: Design Changes Pending](#note-design-changes-pending)
* [Configuration](#configuration)
+ [all](#all)
+ [json](#json)
+ [long](#long)
+ [parseable](#parseable)
+ [global](#global)
+ [depth](#depth)
+ [omit](#omit)
+ [link](#link)
+ [package-lock-only](#package-lock-only)
+ [unicode](#unicode)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm ls <package-spec>
alias: list
```
###
Description
This command will print to stdout all the versions of packages that are installed, as well as their dependencies when `--all` is specified, in a tree structure.
Note: to get a "bottoms up" view of why a given package is included in the tree at all, use [`npm explain`](npm-explain).
Positional arguments are `name@version-range` identifiers, which will limit the results to only the paths to the packages named. Note that nested packages will *also* show the paths to the specified packages. For example, running `npm ls promzard` in npm's source tree will show:
```
[email protected] /path/to/npm
└─┬ [email protected]
└── [email protected]
```
It will print out extraneous, missing, and invalid packages.
If a project specifies git urls for dependencies these are shown in parentheses after the `name@version` to make it easier for users to recognize potential forks of a project.
The tree shown is the logical dependency tree, based on package dependencies, not the physical layout of your `node_modules` folder.
When run as `ll` or `la`, it shows extended information by default.
###
Note: Design Changes Pending
The `npm ls` command's output and behavior made a *ton* of sense when npm created a `node_modules` folder that naively nested every dependency. In such a case, the logical dependency graph and physical tree of packages on disk would be roughly identical.
With the advent of automatic install-time deduplication of dependencies in npm v3, the `ls` output was modified to display the logical dependency graph as a tree structure, since this was more useful to most users. However, without using `npm ls -l`, it became impossible to show *where* a package was actually installed much of the time!
With the advent of automatic installation of `peerDependencies` in npm v7, this gets even more curious, as `peerDependencies` are logically "underneath" their dependents in the dependency graph, but are always physically at or above their location on disk.
Also, in the years since npm got an `ls` command (in version 0.0.2!), dependency graphs have gotten much larger as a general rule. Therefore, in order to avoid dumping an excessive amount of content to the terminal, `npm
ls` now only shows the *top* level dependencies, unless `--all` is provided.
A thorough re-examination of the use cases, intention, behavior, and output of this command, is currently underway. Expect significant changes to at least the default human-readable `npm ls` output in npm v8.
###
Configuration
####
`all`
* Default: false
* Type: Boolean
When running `npm outdated` and `npm ls`, setting `--all` will show all outdated or installed packages, rather than only those directly depended upon by the current project.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`depth`
* Default: `Infinity` if `--all` is set, otherwise `1`
* Type: null or Number
The depth to go when recursing packages for `npm ls`.
If not set, `npm ls` will show only the immediate dependencies of the root project. If `--all` is set, then npm will show all dependencies by default.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`link`
* Default: false
* Type: Boolean
Used with `npm ls`, limiting output to only those packages that are linked.
####
`package-lock-only`
* Default: false
* Type: Boolean
If set to true, the current operation will only use the `package-lock.json`, ignoring `node_modules`.
For `update` this means only the `package-lock.json` will be updated, instead of checking `node_modules` and downloading dependencies.
For `list` this means the output will be based on the tree described by the `package-lock.json`, rather than the contents of `node_modules`.
####
`unicode`
* Default: false on windows, true on mac/unix systems with a unicode locale, as defined by the `LC_ALL`, `LC_CTYPE`, or `LANG` environment variables.
* Type: Boolean
When set to true, npm uses unicode characters in the tree output. When false, it uses ascii characters instead of unicode glyphs.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm explain](npm-explain)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm folders](../configuring-npm/folders)
* [npm explain](npm-explain)
* [npm install](npm-install)
* [npm link](npm-link)
* [npm prune](npm-prune)
* [npm outdated](npm-outdated)
* [npm update](npm-update)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-ls.md)
| programming_docs |
npm npm-completion npm-completion
==============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Tab Completion for npm
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [See Also](#see-also)
###
Synopsis
```
npm completion
```
Note: This command is unaware of workspaces.
###
Description
Enables tab-completion in all npm commands.
The synopsis above loads the completions into your current shell. Adding it to your ~/.bashrc or ~/.zshrc will make the completions available everywhere:
```
npm completion >> ~/.bashrc
npm completion >> ~/.zshrc
```
You may of course also pipe the output of `npm completion` to a file such as `/usr/local/etc/bash_completion.d/npm` or `/etc/bash_completion.d/npm` if you have a system that will read that file for you.
When `COMP_CWORD`, `COMP_LINE`, and `COMP_POINT` are defined in the environment, `npm completion` acts in "plumbing mode", and outputs completions based on the arguments.
###
See Also
* [npm developers](../using-npm/developers)
* <npm>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-completion.md)
npm npm-bugs npm-bugs
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Report bugs for a package in a web browser
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [browser](#browser)
+ [registry](#registry)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm bugs [<pkgname> [<pkgname> ...]]
alias: issues
```
###
Description
This command tries to guess at the likely location of a package's bug tracker URL or the `mailto` URL of the support email, and then tries to open it using the `--browser` config param. If no package name is provided, it will search for a `package.json` in the current folder and use the `name` property.
###
Configuration
####
`browser`
* Default: OS X: `"open"`, Windows: `"start"`, Others: `"xdg-open"`
* Type: null, Boolean, or String
The browser that is called by npm commands to open websites.
Set to `false` to suppress browser behavior and instead print urls to terminal.
Set to `true` to use default system URL opener.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [npm docs](npm-docs)
* [npm view](npm-view)
* [npm publish](npm-publish)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [package.json](../configuring-npm/package-json)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-bugs.md)
npm npm-prefix npm-prefix
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Display prefix
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [global](#global)
* [See Also](#see-also)
###
Synopsis
```
npm prefix [-g]
```
Note: This command is unaware of workspaces.
###
Description
Print the local prefix to standard output. This is the closest parent directory to contain a `package.json` file or `node_modules` directory, unless `-g` is also specified.
If `-g` is specified, this will be the value of the global prefix. See [`npm config`](npm-config) for more detail.
###
Example
```
npm prefix
/usr/local/projects/foo
```
```
npm prefix -g
/usr/local
```
###
Configuration
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
###
See Also
* [npm root](npm-root)
* [npm bin](npm-bin)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-prefix.md)
npm npm-update npm-update
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Update packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
+ [Caret Dependencies](#caret-dependencies)
+ [Tilde Dependencies](#tilde-dependencies)
+ [Caret Dependencies below 1.0.0](#caret-dependencies-below-100)
+ [Subdependencies](#subdependencies)
+ [Updating Globally-Installed Packages](#updating-globally-installed-packages)
* [Configuration](#configuration)
+ [save](#save)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [omit](#omit)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm update [<pkg>...]
aliases: up, upgrade, udpate
```
###
Description
This command will update all the packages listed to the latest version (specified by the `tag` config), respecting the semver constraints of both your package and its dependencies (if they also require the same package).
It will also install missing packages.
If the `-g` flag is specified, this command will update globally installed packages.
If no package name is specified, all packages in the specified location (global or local) will be updated.
Note that by default `npm update` will not update the semver values of direct dependencies in your project `package.json`, if you want to also update values in `package.json` you can run: `npm update --save` (or add the `save=true` option to a [configuration file](../configuring-npm/npmrc) to make that the default behavior).
###
Example
For the examples below, assume that the current package is `app` and it depends on dependencies, `dep1` (`dep2`, .. etc.). The published versions of `dep1` are:
```
{
"dist-tags": { "latest": "1.2.2" },
"versions": [
"1.2.2",
"1.2.1",
"1.2.0",
"1.1.2",
"1.1.1",
"1.0.0",
"0.4.1",
"0.4.0",
"0.2.0"
]
}
```
####
Caret Dependencies
If `app`'s `package.json` contains:
```
"dependencies": {
"dep1": "^1.1.1"
}
```
Then `npm update` will install `[email protected]`, because `1.2.2` is `latest` and `1.2.2` satisfies `^1.1.1`.
####
Tilde Dependencies
However, if `app`'s `package.json` contains:
```
"dependencies": {
"dep1": "~1.1.1"
}
```
In this case, running `npm update` will install `[email protected]`. Even though the `latest` tag points to `1.2.2`, this version do not satisfy `~1.1.1`, which is equivalent to `>=1.1.1 <1.2.0`. So the highest-sorting version that satisfies `~1.1.1` is used, which is `1.1.2`.
####
Caret Dependencies below 1.0.0
Suppose `app` has a caret dependency on a version below `1.0.0`, for example:
```
"dependencies": {
"dep1": "^0.2.0"
}
```
`npm update` will install `[email protected]`, because there are no other versions which satisfy `^0.2.0`.
If the dependence were on `^0.4.0`:
```
"dependencies": {
"dep1": "^0.4.0"
}
```
Then `npm update` will install `[email protected]`, because that is the highest-sorting version that satisfies `^0.4.0` (`>= 0.4.0 <0.5.0`)
####
Subdependencies
Suppose your app now also has a dependency on `dep2`
```
{
"name": "my-app",
"dependencies": {
"dep1": "^1.0.0",
"dep2": "1.0.0"
}
}
```
and `dep2` itself depends on this limited range of `dep1`
```
{
"name": "dep2",
"dependencies": {
"dep1": "~1.1.1"
}
}
```
Then `npm update` will install `[email protected]` because that is the highest version that `dep2` allows. npm will prioritize having a single version of `dep1` in your tree rather than two when that single version can satisfy the semver requirements of multiple dependencies in your tree. In this case if you really did need your package to use a newer version you would need to use `npm install`.
####
Updating Globally-Installed Packages
`npm update -g` will apply the `update` action to each globally installed package that is `outdated` -- that is, has a version that is different from `wanted`.
Note: Globally installed packages are treated as if they are installed with a caret semver range specified. So if you require to update to `latest` you may need to run `npm install -g [<pkg>...]`
NOTE: If a package has been upgraded to a version newer than `latest`, it will be *downgraded*.
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm install](npm-install)
* [npm outdated](npm-outdated)
* [npm shrinkwrap](npm-shrinkwrap)
* [npm registry](../using-npm/registry)
* [npm folders](../configuring-npm/folders)
* [npm ls](npm-ls)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-update.md)
| programming_docs |
npm npm-profile npm-profile
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Change settings on your registry profile
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Details](#details)
* [Configuration](#configuration)
+ [registry](#registry)
+ [json](#json)
+ [parseable](#parseable)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm profile enable-2fa [auth-only|auth-and-writes]
npm profile disable-2fa
npm profile get [<key>]
npm profile set <key> <value>
```
Note: This command is unaware of workspaces.
###
Description
Change your profile information on the registry. Note that this command depends on the registry implementation, so third-party registries may not support this interface.
* `npm profile get [<property>]`: Display all of the properties of your profile, or one or more specific properties. It looks like:
```
+-----------------+---------------------------+
| name | example |
+-----------------+---------------------------+
| email | [email protected] (verified) |
+-----------------+---------------------------+
| two factor auth | auth-and-writes |
+-----------------+---------------------------+
| fullname | Example User |
+-----------------+---------------------------+
| homepage | |
+-----------------+---------------------------+
| freenode | |
+-----------------+---------------------------+
| twitter | |
+-----------------+---------------------------+
| github | |
+-----------------+---------------------------+
| created | 2015-02-26T01:38:35.892Z |
+-----------------+---------------------------+
| updated | 2017-10-02T21:29:45.922Z |
+-----------------+---------------------------+
```
* `npm profile set <property> <value>`: Set the value of a profile property. You can set the following properties this way: email, fullname, homepage, freenode, twitter, github
* `npm profile set password`: Change your password. This is interactive, you'll be prompted for your current password and a new password. You'll also be prompted for an OTP if you have two-factor authentication enabled.
* `npm profile enable-2fa [auth-and-writes|auth-only]`: Enables two-factor authentication. Defaults to `auth-and-writes` mode. Modes are:
+ `auth-only`: Require an OTP when logging in or making changes to your account's authentication. The OTP will be required on both the website and the command line.
+ `auth-and-writes`: Requires an OTP at all the times `auth-only` does, and also requires one when publishing a module, setting the `latest` dist-tag, or changing access via `npm access` and `npm owner`.
* `npm profile disable-2fa`: Disables two-factor authentication.
###
Details
Some of these commands may not be available on non npmjs.com registries.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [npm adduser](npm-adduser)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm owner](npm-owner)
* [npm whoami](npm-whoami)
* [npm token](npm-token)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-profile.md)
npm npm-run-script npm-run-script
==============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Run arbitrary package scripts
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Workspaces support](#workspaces-support)
+ [Filtering workspaces](#filtering-workspaces)
* [Configuration](#configuration)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [if-present](#if-present)
+ [ignore-scripts](#ignore-scripts)
+ [foreground-scripts](#foreground-scripts)
+ [script-shell](#script-shell)
* [See Also](#see-also)
###
Synopsis
```
npm run-script <command> [-- <args>]
aliases: run, rum, urn
```
###
Description
This runs an arbitrary command from a package's `"scripts"` object. If no `"command"` is provided, it will list the available scripts.
`run[-script]` is used by the test, start, restart, and stop commands, but can be called directly, as well. When the scripts in the package are printed out, they're separated into lifecycle (test, start, restart) and directly-run scripts.
Any positional arguments are passed to the specified script. Use `--` to pass `-`-prefixed flags and options which would otherwise be parsed by npm.
For example:
```
npm run test -- --grep="pattern"
```
The arguments will only be passed to the script specified after `npm run` and not to any `pre` or `post` script.
The `env` script is a special built-in command that can be used to list environment variables that will be available to the script at runtime. If an "env" command is defined in your package, it will take precedence over the built-in.
In addition to the shell's pre-existing `PATH`, `npm run` adds `node_modules/.bin` to the `PATH` provided to scripts. Any binaries provided by locally-installed dependencies can be used without the `node_modules/.bin` prefix. For example, if there is a `devDependency` on `tap` in your package, you should write:
```
"scripts": {"test": "tap test/*.js"}
```
instead of
```
"scripts": {"test": "node_modules/.bin/tap test/*.js"}
```
The actual shell your script is run within is platform dependent. By default, on Unix-like systems it is the `/bin/sh` command, on Windows it is `cmd.exe`. The actual shell referred to by `/bin/sh` also depends on the system. You can customize the shell with the `script-shell` configuration.
Scripts are run from the root of the package folder, regardless of what the current working directory is when `npm run` is called. If you want your script to use different behavior based on what subdirectory you're in, you can use the `INIT_CWD` environment variable, which holds the full path you were in when you ran `npm run`.
`npm run` sets the `NODE` environment variable to the `node` executable with which `npm` is executed.
If you try to run a script without having a `node_modules` directory and it fails, you will be given a warning to run `npm install`, just in case you've forgotten.
###
Workspaces support
You may use the `workspace` or `workspaces` configs in order to run an arbitrary command from a package's `"scripts"` object in the context of the specified workspaces. If no `"command"` is provided, it will list the available scripts for each of these configured workspaces.
Given a project with configured workspaces, e.g:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
+-- b
| `-- package.json
`-- c
`-- package.json
```
Assuming the workspace configuration is properly set up at the root level `package.json` file. e.g:
```
{
"workspaces": [ "./packages/*" ]
}
```
And that each of the configured workspaces has a configured `test` script, we can run tests in all of them using the `workspaces` config:
```
npm test --workspaces
```
####
Filtering workspaces
It's also possible to run a script in a single workspace using the `workspace` config along with a name or directory path:
```
npm test --workspace=a
```
The `workspace` config can also be specified multiple times in order to run a specific script in the context of multiple workspaces. When defining values for the `workspace` config in the command line, it also possible to use `-w` as a shorthand, e.g:
```
npm test -w a -w b
```
This last command will run `test` in both `./packages/a` and `./packages/b` packages.
###
Configuration
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`if-present`
* Default: false
* Type: Boolean
If true, npm will not exit with an error code when `run-script` is invoked for a script that isn't defined in the `scripts` section of `package.json`. This option can be used when it's desirable to optionally run a script when it's present and fail if the script fails. This is useful, for example, when running scripts that may only apply for some builds in an otherwise generic CI setup.
This value is not exported to the environment for child processes.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
###
See Also
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm start](npm-start)
* [npm restart](npm-restart)
* [npm stop](npm-stop)
* [npm config](npm-config)
* [npm workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-run-script.md)
npm npm-install npm-install
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Install a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [save](#save)
+ [save-exact](#save-exact)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [omit](#omit)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [Algorithm](#algorithm)
* [See Also](#see-also)
###
Synopsis
```
npm install [<package-spec> ...]
aliases: add, i, in, ins, inst, insta, instal, isnt, isnta, isntal, isntall
```
###
Description
This command installs a package and any packages that it depends on. If the package has a package-lock, or an npm shrinkwrap file, or a yarn lock file, the installation of dependencies will be driven by that, respecting the following order of precedence:
* `npm-shrinkwrap.json`
* `package-lock.json`
* `yarn.lock`
See [package-lock.json](../configuring-npm/package-lock-json) and [`npm shrinkwrap`](npm-shrinkwrap).
A `package` is:
* a) a folder containing a program described by a [`package.json`](../configuring-npm/package-json) file
* b) a gzipped tarball containing (a)
* c) a url that resolves to (b)
* d) a `<name>@<version>` that is published on the registry (see [`registry`](../using-npm/registry)) with (c)
* e) a `<name>@<tag>` (see [`npm dist-tag`](npm-dist-tag)) that points to (d)
* f) a `<name>` that has a "latest" tag satisfying (e)
* g) a `<git remote url>` that resolves to (a)
Even if you never publish your package, you can still get a lot of benefits of using npm if you just want to write a node program (a), and perhaps if you also want to be able to easily install it elsewhere after packing it up into a tarball (b).
* `npm install` (in a package directory, no arguments):
Install the dependencies to the local `node_modules` folder.
In global mode (ie, with `-g` or `--global` appended to the command), it installs the current package context (ie, the current working directory) as a global package.
By default, `npm install` will install all modules listed as dependencies in [`package.json`](../configuring-npm/package-json).
With the `--production` flag (or when the `NODE_ENV` environment variable is set to `production`), npm will not install modules listed in `devDependencies`. To install all modules listed in both `dependencies` and `devDependencies` when `NODE_ENV` environment variable is set to `production`, you can use `--production=false`.
> NOTE: The `--production` flag has no particular meaning when adding a dependency to a project.
>
>
* `npm install <folder>`:
If `<folder>` sits inside the root of your project, its dependencies will be installed and may be hoisted to the top-level `node_modules` as they would for other types of dependencies. If `<folder>` sits outside the root of your project, *npm will not install the package dependencies* in the directory `<folder>`, but it will create a symlink to `<folder>`.
> NOTE: If you want to install the content of a directory like a package from the registry instead of creating a link, you would need to use the `--install-links` option.
>
>
Example:
```
npm install ../../other-package --install-links
npm install ./sub-package
```
* `npm install <tarball file>`:
Install a package that is sitting on the filesystem. Note: if you just want to link a dev directory into your npm root, you can do this more easily by using [`npm link`](npm-link).
Tarball requirements:
+ The filename *must* use `.tar`, `.tar.gz`, or `.tgz` as the extension.
+ The package contents should reside in a subfolder inside the tarball (usually it is called `package/`). npm strips one directory layer when installing the package (an equivalent of `tar x
--strip-components=1` is run).
+ The package must contain a `package.json` file with `name` and `version` properties.
Example:
```
npm install ./package.tgz
```
* `npm install <tarball url>`:
Fetch the tarball url, and then install it. In order to distinguish between this and other options, the argument must start with "http://" or "https://"
Example:
```
npm install https://github.com/indexzero/forever/tarball/v0.5.6
```
* `npm install [<@scope>/]<name>`:
Do a `<name>@<tag>` install, where `<tag>` is the "tag" config. (See [`config`](../using-npm/config). The config's default value is `latest`.)
In most cases, this will install the version of the modules tagged as `latest` on the npm registry.
Example:
```
npm install sax
```
`npm install` saves any specified packages into `dependencies` by default. Additionally, you can control where and how they get saved with some additional flags:
+ `-P, --save-prod`: Package will appear in your `dependencies`. This is the default unless `-D` or `-O` are present.
+ `-D, --save-dev`: Package will appear in your `devDependencies`.
+ `-O, --save-optional`: Package will appear in your `optionalDependencies`.
+ `--no-save`: Prevents saving to `dependencies`.
When using any of the above options to save dependencies to your package.json, there are two additional, optional flags:
+ `-E, --save-exact`: Saved dependencies will be configured with an exact version rather than using npm's default semver range operator.
+ `-B, --save-bundle`: Saved dependencies will also be added to your `bundleDependencies` list.
Further, if you have an `npm-shrinkwrap.json` or `package-lock.json` then it will be updated as well.
`<scope>` is optional. The package will be downloaded from the registry associated with the specified scope. If no registry is associated with the given scope the default registry is assumed. See [`scope`](../using-npm/scope).
Note: if you do not include the @-symbol on your scope name, npm will interpret this as a GitHub repository instead, see below. Scopes names must also be followed by a slash.
Examples:
```
npm install sax
npm install githubname/reponame
npm install @myorg/privatepackage
npm install node-tap --save-dev
npm install dtrace-provider --save-optional
npm install readable-stream --save-exact
npm install ansi-regex --save-bundle
```
**Note**: If there is a file or folder named `<name>` in the current working directory, then it will try to install that, and only try to fetch the package by name if it is not valid.
* `npm install <alias>@npm:<name>`:
Install a package under a custom alias. Allows multiple versions of a same-name package side-by-side, more convenient import names for packages with otherwise long ones, and using git forks replacements or forked npm packages as replacements. Aliasing works only on your project and does not rename packages in transitive dependencies. Aliases should follow the naming conventions stated in [`validate-npm-package-name`](https://www.npmjs.com/package/validate-npm-package-name#naming-rules).
Examples:
```
npm install my-react@npm:react
npm install jquery2@npm:jquery@2
npm install jquery3@npm:jquery@3
npm install npa@npm:npm-package-arg
```
* `npm install [<@scope>/]<name>@<tag>`:
Install the version of the package that is referenced by the specified tag. If the tag does not exist in the registry data for that package, then this will fail.
Example:
```
npm install sax@latest
npm install @myorg/mypackage@latest
```
* `npm install [<@scope>/]<name>@<version>`:
Install the specified version of the package. This will fail if the version has not been published to the registry.
Example:
```
npm install [email protected]
npm install @myorg/[email protected]
```
* `npm install [<@scope>/]<name>@<version range>`:
Install a version of the package matching the specified version range. This will follow the same rules for resolving dependencies described in [`package.json`](../configuring-npm/package-json).
Note that most version ranges must be put in quotes so that your shell will treat it as a single argument.
Example:
```
npm install sax@">=0.1.0 <0.2.0"
npm install @myorg/privatepackage@"16 - 17"
```
* `npm install <git remote url>`:
Installs the package from the hosted git provider, cloning it with `git`. For a full git remote url, only that URL will be attempted.
```
<protocol>://[<user>[:<password>]@]<hostname>[:<port>][:][/]<path>[#<commit-ish> | #semver:<semver>]
```
`<protocol>` is one of `git`, `git+ssh`, `git+http`, `git+https`, or `git+file`.
If `#<commit-ish>` is provided, it will be used to clone exactly that commit. If the commit-ish has the format `#semver:<semver>`, `<semver>` can be any valid semver range or exact version, and npm will look for any tags or refs matching that range in the remote repository, much as it would for a registry dependency. If neither `#<commit-ish>` or `#semver:<semver>` is specified, then the default branch of the repository is used.
If the repository makes use of submodules, those submodules will be cloned as well.
If the package being installed contains a `prepare` script, its `dependencies` and `devDependencies` will be installed, and the prepare script will be run, before the package is packaged and installed.
The following git environment variables are recognized by npm and will be added to the environment when running git:
+ `GIT_ASKPASS`
+ `GIT_EXEC_PATH`
+ `GIT_PROXY_COMMAND`
+ `GIT_SSH`
+ `GIT_SSH_COMMAND`
+ `GIT_SSL_CAINFO`
+ `GIT_SSL_NO_VERIFY`
See the git man page for details.
Examples:
```
npm install git+ssh://[email protected]:npm/cli.git#v1.0.27
npm install git+ssh://[email protected]:npm/cli#pull/273
npm install git+ssh://[email protected]:npm/cli#semver:^5.0
npm install git+https://[email protected]/npm/cli.git
npm install git://github.com/npm/cli.git#v1.0.27
GIT_SSH_COMMAND='ssh -i ~/.ssh/custom_ident' npm install git+ssh://[email protected]:npm/cli.git
```
* `npm install <githubname>/<githubrepo>[#<commit-ish>]`:
* `npm install github:<githubname>/<githubrepo>[#<commit-ish>]`:
Install the package at `https://github.com/githubname/githubrepo` by attempting to clone it using `git`.
If `#<commit-ish>` is provided, it will be used to clone exactly that commit. If the commit-ish has the format `#semver:<semver>`, `<semver>` can be any valid semver range or exact version, and npm will look for any tags or refs matching that range in the remote repository, much as it would for a registry dependency. If neither `#<commit-ish>` or `#semver:<semver>` is specified, then the default branch is used.
As with regular git dependencies, `dependencies` and `devDependencies` will be installed if the package has a `prepare` script before the package is done installing.
Examples:
```
npm install mygithubuser/myproject
npm install github:mygithubuser/myproject
```
* `npm install gist:[<githubname>/]<gistID>[#<commit-ish>|#semver:<semver>]`:
Install the package at `https://gist.github.com/gistID` by attempting to clone it using `git`. The GitHub username associated with the gist is optional and will not be saved in `package.json`.
As with regular git dependencies, `dependencies` and `devDependencies` will be installed if the package has a `prepare` script before the package is done installing.
Example:
```
npm install gist:101a11beef
```
* `npm install bitbucket:<bitbucketname>/<bitbucketrepo>[#<commit-ish>]`:
Install the package at `https://bitbucket.org/bitbucketname/bitbucketrepo` by attempting to clone it using `git`.
If `#<commit-ish>` is provided, it will be used to clone exactly that commit. If the commit-ish has the format `#semver:<semver>`, `<semver>` can be any valid semver range or exact version, and npm will look for any tags or refs matching that range in the remote repository, much as it would for a registry dependency. If neither `#<commit-ish>` or `#semver:<semver>` is specified, then `master` is used.
As with regular git dependencies, `dependencies` and `devDependencies` will be installed if the package has a `prepare` script before the package is done installing.
Example:
```
npm install bitbucket:mybitbucketuser/myproject
```
* `npm install gitlab:<gitlabname>/<gitlabrepo>[#<commit-ish>]`:
Install the package at `https://gitlab.com/gitlabname/gitlabrepo` by attempting to clone it using `git`.
If `#<commit-ish>` is provided, it will be used to clone exactly that commit. If the commit-ish has the format `#semver:<semver>`, `<semver>` can be any valid semver range or exact version, and npm will look for any tags or refs matching that range in the remote repository, much as it would for a registry dependency. If neither `#<commit-ish>` or `#semver:<semver>` is specified, then `master` is used.
As with regular git dependencies, `dependencies` and `devDependencies` will be installed if the package has a `prepare` script before the package is done installing.
Example:
```
npm install gitlab:mygitlabuser/myproject
npm install gitlab:myusr/myproj#semver:^5.0
```
You may combine multiple arguments and even multiple types of arguments. For example:
```
npm install sax@">=0.1.0 <0.2.0" bench supervisor
```
The `--tag` argument will apply to all of the specified install targets. If a tag with the given name exists, the tagged version is preferred over newer versions.
The `--dry-run` argument will report in the usual way what the install would have done without actually installing anything.
The `--package-lock-only` argument will only update the `package-lock.json`, instead of checking `node_modules` and downloading dependencies.
The `-f` or `--force` argument will force npm to fetch remote resources even if a local copy exists on disk.
```
npm install sax --force
```
###
Configuration
See the [`config`](../using-npm/config) help doc. Many of the configuration params have some effect on installation, since that's most of what npm does.
These are some of the most common options related to installation.
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
Algorithm
Given a `package{dep}` structure: `A{B,C}, B{C}, C{D}`, the npm install algorithm produces:
```
A
+-- B
+-- C
+-- D
```
That is, the dependency from B to C is satisfied by the fact that A already caused C to be installed at a higher level. D is still installed at the top level because nothing conflicts with it.
For `A{B,C}, B{C,D@1}, C{D@2}`, this algorithm produces:
```
A
+-- B
+-- C
`-- D@2
+-- D@1
```
Because B's D@1 will be installed in the top-level, C now has to install D@2 privately for itself. This algorithm is deterministic, but different trees may be produced if two dependencies are requested for installation in a different order.
See [folders](../configuring-npm/folders) for a more detailed description of the specific folder structures that npm creates.
###
See Also
* [npm folders](../configuring-npm/folders)
* [npm update](npm-update)
* [npm audit](npm-audit)
* [npm fund](npm-fund)
* [npm link](npm-link)
* [npm rebuild](npm-rebuild)
* [npm scripts](../using-npm/scripts)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm registry](../using-npm/registry)
* [npm dist-tag](npm-dist-tag)
* [npm uninstall](npm-uninstall)
* [npm shrinkwrap](npm-shrinkwrap)
* [package.json](../configuring-npm/package-json)
* [workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-install.md)
| programming_docs |
npm npm-root npm-root
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Display npm root
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [global](#global)
* [See Also](#see-also)
###
Synopsis
```
npm root
```
###
Description
Print the effective `node_modules` folder to standard out.
Useful for using npm in shell scripts that do things with the `node_modules` folder. For example:
```
#!/bin/bash
global_node_modules="$(npm root --global)"
echo "Global packages installed in: ${global_node_modules}"
```
###
Configuration
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
###
See Also
* [npm prefix](npm-prefix)
* [npm bin](npm-bin)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-root.md)
npm npm-ci npm-ci
======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Clean install a project
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [save](#save)
+ [save-exact](#save-exact)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [omit](#omit)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm ci
aliases: clean-install, ic, install-clean, isntall-clean
```
###
Description
This command is similar to [`npm install`](npm-install), except it's meant to be used in automated environments such as test platforms, continuous integration, and deployment -- or any situation where you want to make sure you're doing a clean install of your dependencies.
The main differences between using `npm install` and `npm ci` are:
* The project **must** have an existing `package-lock.json` or `npm-shrinkwrap.json`.
* If dependencies in the package lock do not match those in `package.json`, `npm ci` will exit with an error, instead of updating the package lock.
* `npm ci` can only install entire projects at a time: individual dependencies cannot be added with this command.
* If a `node_modules` is already present, it will be automatically removed before `npm ci` begins its install.
* It will never write to `package.json` or any of the package-locks: installs are essentially frozen.
NOTE: If you create your `package-lock.json` file by running `npm install` with flags that can affect the shape of your dependency tree, such as `--legacy-peer-deps` or `--install-links`, you *must* provide the same flags to `npm ci` or you are likely to encounter errors. An easy way to do this is to run, for example, `npm config set legacy-peer-deps=true --location=project` and commit the `.npmrc` file to your repo.
###
Example
Make sure you have a package-lock and an up-to-date install:
```
$ cd ./my/npm/project
$ npm install
added 154 packages in 10s
$ ls | grep package-lock
```
Run `npm ci` in that project
```
$ npm ci
added 154 packages in 5s
```
Configure Travis CI to build using `npm ci` instead of `npm install`:
```
# .travis.yml
install:
- npm ci
# keep the npm cache around to speed up installs
cache:
directories:
- "$HOME/.npm"
```
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm install](npm-install)
* [package-lock.json](../configuring-npm/package-lock-json)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-ci.md)
npm npm-org npm-org
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage orgs
Table of contents* [Synopsis](#synopsis)
* [Example](#example)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
+ [json](#json)
+ [parseable](#parseable)
* [See Also](#see-also)
###
Synopsis
```
npm org set orgname username [developer | admin | owner]
npm org rm orgname username
npm org ls orgname [<username>]
alias: ogr
```
Note: This command is unaware of workspaces.
###
Example
Add a new developer to an org:
```
$ npm org set my-org @mx-smith
```
Add a new admin to an org (or change a developer to an admin):
```
$ npm org set my-org @mx-santos admin
```
Remove a user from an org:
```
$ npm org rm my-org mx-santos
```
List all users in an org:
```
$ npm org ls my-org
```
List all users in JSON format:
```
$ npm org ls my-org --json
```
See what role a user has in an org:
```
$ npm org ls my-org @mx-santos
```
###
Description
You can use the `npm org` commands to manage and view users of an organization. It supports adding and removing users, changing their roles, listing them, and finding specific ones and their roles.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
###
See Also
* [using orgs](../using-npm/orgs)
* [Documentation on npm Orgs](https://docs.npmjs.com/orgs/)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-org.md)
| programming_docs |
npm npm-stop npm-stop
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Stop a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [ignore-scripts](#ignore-scripts)
+ [script-shell](#script-shell)
* [See Also](#see-also)
###
Synopsis
```
npm stop [-- <args>]
```
###
Description
This runs a predefined command specified in the "stop" property of a package's "scripts" object.
Unlike with [npm start](npm-start), there is no default script that will run if the `"stop"` property is not defined.
###
Example
```
{
"scripts": {
"stop": "node bar.js"
}
}
```
```
npm stop
> [email protected] stop
> node bar.js
(bar.js output would be here)
```
###
Configuration
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm start](npm-start)
* [npm restart](npm-restart)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-stop.md)
npm npm-unpublish npm-unpublish
=============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Remove a package from the registry
Table of contents* [Synopsis](#synopsis)
* [Warning](#warning)
* [Description](#description)
* [Configuration](#configuration)
+ [dry-run](#dry-run)
+ [force](#force)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
* [See Also](#see-also)
###
Synopsis
```
npm unpublish [<package-spec>]
```
To learn more about how the npm registry treats unpublish, see our [unpublish policies](https://docs.npmjs.com/policies/unpublish)
###
Warning
Consider using the [`deprecate`](npm-deprecate) command instead, if your intent is to encourage users to upgrade, or if you no longer want to maintain a package.
###
Description
This removes a package version from the registry, deleting its entry and removing the tarball.
The npm registry will return an error if you are not [logged in](npm-adduser).
If you do not specify a version or if you remove all of a package's versions then the registry will remove the root package entry entirely.
Even if you unpublish a package version, that specific name and version combination can never be reused. In order to publish the package again, you must use a new version number. If you unpublish the entire package, you may not publish any new versions of that package until 24 hours have passed.
###
Configuration
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`force`
* Default: false
* Type: Boolean
Removes various protections against unfortunate side effects, common mistakes, unnecessary performance degradation, and malicious input.
* Allow clobbering non-npm files in global installs.
* Allow the `npm version` command to work on an unclean git repository.
* Allow deleting the cache folder with `npm cache clean`.
* Allow installing packages that have an `engines` declaration requiring a different version of npm.
* Allow installing packages that have an `engines` declaration requiring a different version of `node`, even if `--engine-strict` is enabled.
* Allow `npm audit fix` to install modules outside your stated dependency range (including SemVer-major changes).
* Allow unpublishing all versions of a published package.
* Allow conflicting peerDependencies to be installed in the root project.
* Implicitly set `--yes` during `npm init`.
* Allow clobbering existing values in `npm pkg`
* Allow unpublishing of entire packages (not just a single version).
If you don't have a clear idea of what you want to do, it is strongly recommended that you do not use this option!
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm deprecate](npm-deprecate)
* [npm publish](npm-publish)
* [npm registry](../using-npm/registry)
* [npm adduser](npm-adduser)
* [npm owner](npm-owner)
* [npm login](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-unpublish.md)
npm npm-team npm-team
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage organization teams and team memberships
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Details](#details)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
+ [parseable](#parseable)
+ [json](#json)
* [See Also](#see-also)
###
Synopsis
```
npm team create <scope:team> [--otp <otpcode>]
npm team destroy <scope:team> [--otp <otpcode>]
npm team add <scope:team> <user> [--otp <otpcode>]
npm team rm <scope:team> <user> [--otp <otpcode>]
npm team ls <scope>|<scope:team>
```
Note: This command is unaware of workspaces.
###
Description
Used to manage teams in organizations, and change team memberships. Does not handle permissions for packages.
Teams must always be fully qualified with the organization/scope they belong to when operating on them, separated by a colon (`:`). That is, if you have a `newteam` team in an `org` organization, you must always refer to that team as `@org:newteam` in these commands.
If you have two-factor authentication enabled in `auth-and-writes` mode, then you can provide a code from your authenticator with `[--otp <otpcode>]`. If you don't include this then you will be prompted.
* create / destroy: Create a new team, or destroy an existing one. Note: You cannot remove the `developers` team, [learn more.](../../../about-developers-team)
Here's how to create a new team `newteam` under the `org` org:
```
npm team create @org:newteam
```
You should see a confirming message such as: `+@org:newteam` once the new team has been created.
* add: Add a user to an existing team.
Adding a new user `username` to a team named `newteam` under the `org` org:
```
npm team add @org:newteam username
```
On success, you should see a message: `username added to @org:newteam`
* rm: Using `npm team rm` you can also remove users from a team they belong to.
Here's an example removing user `username` from `newteam` team in `org` organization:
```
npm team rm @org:newteam username
```
Once the user is removed a confirmation message is displayed: `username removed from @org:newteam`
* ls: If performed on an organization name, will return a list of existing teams under that organization. If performed on a team, it will instead return a list of all users belonging to that particular team.
Here's an example of how to list all teams from an org named `org`:
```
npm team ls @org
```
Example listing all members of a team named `newteam`:
```
npm team ls @org:newteam
```
###
Details
`npm team` always operates directly on the current registry, configurable from the command line using `--registry=<registry url>`.
You must be a *team admin* to create teams and manage team membership, under the given organization. Listing teams and team memberships may be done by any member of the organization.
Organization creation and management of team admins and *organization* members is done through the website, not the npm CLI.
To use teams to manage permissions on packages belonging to your organization, use the `npm access` command to grant or revoke the appropriate permissions.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
###
See Also
* [npm access](npm-access)
* [npm config](npm-config)
* [npm registry](../using-npm/registry)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-team.md)
npm npm-access npm-access
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Set access level on published packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Details](#details)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm access public [<package>]
npm access restricted [<package>]
npm access grant <read-only|read-write> <scope:team> [<package>]
npm access revoke <scope:team> [<package>]
npm access 2fa-required [<package>]
npm access 2fa-not-required [<package>]
npm access ls-packages [<user>|<scope>|<scope:team>]
npm access ls-collaborators [<package> [<user>]]
npm access edit [<package>]
```
###
Description
Used to set access controls on private packages.
For all of the subcommands, `npm access` will perform actions on the packages in the current working directory if no package name is passed to the subcommand.
* public / restricted (deprecated): Set a package to be either publicly accessible or restricted.
* grant / revoke (deprecated): Add or remove the ability of users and teams to have read-only or read-write access to a package.
* 2fa-required / 2fa-not-required (deprecated): Configure whether a package requires that anyone publishing it have two-factor authentication enabled on their account.
* ls-packages (deprecated): Show all of the packages a user or a team is able to access, along with the access level, except for read-only public packages (it won't print the whole registry listing)
* ls-collaborators (deprecated): Show all of the access privileges for a package. Will only show permissions for packages to which you have at least read access. If `<user>` is passed in, the list is filtered only to teams *that* user happens to belong to.
* edit (not implemented)
###
Details
`npm access` always operates directly on the current registry, configurable from the command line using `--registry=<registry url>`.
Unscoped packages are *always public*.
Scoped packages *default to restricted*, but you can either publish them as public using `npm publish --access=public`, or set their access as public using `npm access public` after the initial publish.
You must have privileges to set the access of a package:
* You are an owner of an unscoped or scoped package.
* You are a member of the team that owns a scope.
* You have been given read-write privileges for a package, either as a member of a team or directly as an owner.
If you have two-factor authentication enabled then you'll be prompted to provide an otp token, or may use the `--otp=...` option to specify it on the command line.
If your account is not paid, then attempts to publish scoped packages will fail with an HTTP 402 status code (logically enough), unless you use `--access=public`.
Management of teams and team memberships is done with the `npm team` command.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [`libnpmaccess`](https://npm.im/libnpmaccess)
* [npm team](npm-team)
* [npm publish](npm-publish)
* [npm config](npm-config)
* [npm registry](../using-npm/registry)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-access.md)
| programming_docs |
npm npm-install-test npm-install-test
================
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Install package(s) and run tests
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [save](#save)
+ [save-exact](#save-exact)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [omit](#omit)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm install-test [<package-spec> ...]
alias: it
```
###
Description
This command runs an `npm install` followed immediately by an `npm test`. It takes exactly the same arguments as `npm install`.
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm install](npm-install)
* [npm install-ci-test](npm-install-ci-test)
* [npm test](npm-test)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-install-test.md)
npm npm-set-script npm-set-script
==============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Set tasks in the scripts section of package.json
Table of contents* [Synopsis](#synopsis)
* [Configuration](#configuration)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
An npm command that lets you create a task in the `scripts` section of the `package.json`.
Deprecated.
```
npm set-script [<script>] [<command>]
```
**Example:**
* `npm set-script start "http-server ."`
```
{
"name": "my-project",
"scripts": {
"start": "http-server .",
"test": "some existing value"
}
}
```
###
Configuration
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [npm run-script](npm-run-script)
* [npm install](npm-install)
* [npm test](npm-test)
* [npm start](npm-start)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-set-script.md)
npm npm-start npm-start
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Start a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [ignore-scripts](#ignore-scripts)
+ [script-shell](#script-shell)
* [See Also](#see-also)
###
Synopsis
```
npm start [-- <args>]
```
###
Description
This runs a predefined command specified in the `"start"` property of a package's `"scripts"` object.
If the `"scripts"` object does not define a `"start"` property, npm will run `node server.js`.
Note that this is different from the default node behavior of running the file specified in a package's `"main"` attribute when evoking with `node .`
As of [`[email protected]`](https://blog.npmjs.org/post/98131109725/npm-2-0-0), you can use custom arguments when executing scripts. Refer to [`npm run-script`](npm-run-script) for more details.
###
Example
```
{
"scripts": {
"start": "node foo.js"
}
}
```
```
npm start
> [email protected] start
> node foo.js
(foo.js output would be here)
```
###
Configuration
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm restart](npm-restart)
* [npm stop](npm-stop)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-start.md)
npm npm-star npm-star
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Mark your favorite packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [More](#more)
+ [Unstar](#unstar)
+ [Listing stars](#listing-stars)
* [Configuration](#configuration)
+ [registry](#registry)
+ [unicode](#unicode)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm star [<package-spec>...]
```
Note: This command is unaware of workspaces.
###
Description
"Starring" a package means that you have some interest in it. It's a vaguely positive way to show that you care.
It's a boolean thing. Starring repeatedly has no additional effect.
###
More
There's also these extra commands to help you manage your favorite packages:
####
Unstar
You can also "unstar" a package using [`npm unstar`](npm-unstar)
"Unstarring" is the same thing, but in reverse.
####
Listing stars
You can see all your starred packages using [`npm stars`](npm-stars)
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`unicode`
* Default: false on windows, true on mac/unix systems with a unicode locale, as defined by the `LC_ALL`, `LC_CTYPE`, or `LANG` environment variables.
* Type: Boolean
When set to true, npm uses unicode characters in the tree output. When false, it uses ascii characters instead of unicode glyphs.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm unstar](npm-unstar)
* [npm stars](npm-stars)
* [npm view](npm-view)
* [npm whoami](npm-whoami)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-star.md)
| programming_docs |
npm npm-bin npm-bin
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Display npm bin folder
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [global](#global)
* [See Also](#see-also)
###
Synopsis
```
npm bin
```
Note: This command is unaware of workspaces.
###
Description
Print the folder where npm will install executables.
###
Configuration
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
###
See Also
* [npm prefix](npm-prefix)
* [npm root](npm-root)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-bin.md)
npm npm-cache npm-cache
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manipulates packages cache
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Details](#details)
* [A note about the cache's design](#a-note-about-the-caches-design)
* [Configuration](#configuration)
+ [cache](#cache)
* [See Also](#see-also)
###
Synopsis
```
npm cache add <package-spec>
npm cache clean [<key>]
npm cache ls [<name>@<version>]
npm cache verify
```
Note: This command is unaware of workspaces.
###
Description
Used to add, list, or clean the npm cache folder.
* add: Add the specified packages to the local cache. This command is primarily intended to be used internally by npm, but it can provide a way to add data to the local installation cache explicitly.
* clean: Delete all data out of the cache folder. Note that this is typically unnecessary, as npm's cache is self-healing and resistant to data corruption issues.
* verify: Verify the contents of the cache folder, garbage collecting any unneeded data, and verifying the integrity of the cache index and all cached data.
###
Details
npm stores cache data in an opaque directory within the configured `cache`, named `_cacache`. This directory is a [`cacache`](http://npm.im/cacache)-based content-addressable cache that stores all http request data as well as other package-related data. This directory is primarily accessed through `pacote`, the library responsible for all package fetching as of npm@5.
All data that passes through the cache is fully verified for integrity on both insertion and extraction. Cache corruption will either trigger an error, or signal to `pacote` that the data must be refetched, which it will do automatically. For this reason, it should never be necessary to clear the cache for any reason other than reclaiming disk space, thus why `clean` now requires `--force` to run.
There is currently no method exposed through npm to inspect or directly manage the contents of this cache. In order to access it, `cacache` must be used directly.
npm will not remove data by itself: the cache will grow as new packages are installed.
###
A note about the cache's design
The npm cache is strictly a cache: it should not be relied upon as a persistent and reliable data store for package data. npm makes no guarantee that a previously-cached piece of data will be available later, and will automatically delete corrupted contents. The primary guarantee that the cache makes is that, if it does return data, that data will be exactly the data that was inserted.
To run an offline verification of existing cache contents, use `npm cache
verify`.
###
Configuration
####
`cache`
* Default: Windows: `%LocalAppData%\npm-cache`, Posix: `~/.npm`
* Type: Path
The location of npm's cache directory. See [`npm
cache`](npm-cache)
###
See Also
* [package spec](../using-npm/package-spec)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm install](npm-install)
* [npm publish](npm-publish)
* [npm pack](npm-pack)
* <https://npm.im/cacache>
* <https://npm.im/pacote>
* <https://npm.im/@npmcli/arborist>
* <https://npm.im/make-fetch-happen>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-cache.md)
npm npm-doctor npm-doctor
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Check your npm environment
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
+ [npm ping](#npm-ping)
+ [npm -v](#npm--v)
+ [node -v](#node--v)
+ [npm config get registry](#npm-config-get-registry)
+ [which git](#which-git)
+ [Permissions checks](#permissions-checks)
+ [Validate the checksums of cached packages](#validate-the-checksums-of-cached-packages)
* [Configuration](#configuration)
+ [registry](#registry)
* [See Also](#see-also)
###
Synopsis
```
npm doctor
```
Note: This command is unaware of workspaces.
###
Description
`npm doctor` runs a set of checks to ensure that your npm installation has what it needs to manage your JavaScript packages. npm is mostly a standalone tool, but it does have some basic requirements that must be met:
* Node.js and git must be executable by npm.
* The primary npm registry, `registry.npmjs.com`, or another service that uses the registry API, is available.
* The directories that npm uses, `node_modules` (both locally and globally), exist and can be written by the current user.
* The npm cache exists, and the package tarballs within it aren't corrupt.
Without all of these working properly, npm may not work properly. Many issues are often attributable to things that are outside npm's code base, so `npm doctor` confirms that the npm installation is in a good state.
Also, in addition to this, there are also very many issue reports due to using old versions of npm. Since npm is constantly improving, running `npm@latest` is better than an old version.
`npm doctor` verifies the following items in your environment, and if there are any recommended changes, it will display them.
####
`npm ping`
By default, npm installs from the primary npm registry, `registry.npmjs.org`. `npm doctor` hits a special ping endpoint within the registry. This can also be checked with `npm ping`. If this check fails, you may be using a proxy that needs to be configured, or may need to talk to your IT staff to get access over HTTPS to `registry.npmjs.org`.
This check is done against whichever registry you've configured (you can see what that is by running `npm config get registry`), and if you're using a private registry that doesn't support the `/whoami` endpoint supported by the primary registry, this check may fail.
####
`npm -v`
While Node.js may come bundled with a particular version of npm, it's the policy of the CLI team that we recommend all users run `npm@latest` if they can. As the CLI is maintained by a small team of contributors, there are only resources for a single line of development, so npm's own long-term support releases typically only receive critical security and regression fixes. The team believes that the latest tested version of npm is almost always likely to be the most functional and defect-free version of npm.
####
`node -v`
For most users, in most circumstances, the best version of Node will be the latest long-term support (LTS) release. Those of you who want access to new ECMAscript features or bleeding-edge changes to Node's standard library may be running a newer version, and some may be required to run an older version of Node because of enterprise change control policies. That's OK! But in general, the npm team recommends that most users run Node.js LTS.
####
`npm config get registry`
You may be installing from private package registries for your project or company. That's great! Others may be following tutorials or StackOverflow questions in an effort to troubleshoot problems you may be having. Sometimes, this may entail changing the registry you're pointing at. This part of `npm doctor` just lets you, and maybe whoever's helping you with support, know that you're not using the default registry.
####
`which git`
While it's documented in the README, it may not be obvious that npm needs Git installed to do many of the things that it does. Also, in some cases – especially on Windows – you may have Git set up in such a way that it's not accessible via your `PATH` so that npm can find it. This check ensures that Git is available.
####
Permissions checks
* Your cache must be readable and writable by the user running npm.
* Global package binaries must be writable by the user running npm.
* Your local `node_modules` path, if you're running `npm doctor` with a project directory, must be readable and writable by the user running npm.
####
Validate the checksums of cached packages
When an npm package is published, the publishing process generates a checksum that npm uses at install time to verify that the package didn't get corrupted in transit. `npm doctor` uses these checksums to validate the package tarballs in your local cache (you can see where that cache is located with `npm config get cache`). In the event that there are corrupt packages in your cache, you should probably run `npm cache clean -f` and reset the cache.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
###
See Also
* [npm bugs](npm-bugs)
* [npm help](npm-help)
* [npm ping](npm-ping)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-doctor.md)
npm npm-link npm-link
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Symlink a package folder
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Caveat](#caveat)
* [Workspace Usage](#workspace-usage)
* [Configuration](#configuration)
+ [save](#save)
+ [save-exact](#save-exact)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [omit](#omit)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm link [<package-spec>]
alias: ln
```
###
Description
This is handy for installing your own stuff, so that you can work on it and test iteratively without having to continually rebuild.
Package linking is a two-step process.
First, `npm link` in a package folder with no arguments will create a symlink in the global folder `{prefix}/lib/node_modules/<package>` that links to the package where the `npm link` command was executed. It will also link any bins in the package to `{prefix}/bin/{name}`. Note that `npm link` uses the global prefix (see `npm prefix -g` for its value).
Next, in some other location, `npm link package-name` will create a symbolic link from globally-installed `package-name` to `node_modules/` of the current folder.
Note that `package-name` is taken from `package.json`, *not* from the directory name.
The package name can be optionally prefixed with a scope. See [`scope`](../using-npm/scope). The scope must be preceded by an @-symbol and followed by a slash.
When creating tarballs for `npm publish`, the linked packages are "snapshotted" to their current state by resolving the symbolic links, if they are included in `bundleDependencies`.
For example:
```
cd ~/projects/node-redis # go into the package directory
npm link # creates global link
cd ~/projects/node-bloggy # go into some other package directory.
npm link redis # link-install the package
```
Now, any changes to `~/projects/node-redis` will be reflected in `~/projects/node-bloggy/node_modules/node-redis/`. Note that the link should be to the package name, not the directory name for that package.
You may also shortcut the two steps in one. For example, to do the above use-case in a shorter way:
```
cd ~/projects/node-bloggy # go into the dir of your main project
npm link ../node-redis # link the dir of your dependency
```
The second line is the equivalent of doing:
```
(cd ../node-redis; npm link)
npm link redis
```
That is, it first creates a global link, and then links the global installation target into your project's `node_modules` folder.
Note that in this case, you are referring to the directory name, `node-redis`, rather than the package name `redis`.
If your linked package is scoped (see [`scope`](../using-npm/scope)) your link command must include that scope, e.g.
```
npm link @myorg/privatepackage
```
###
Caveat
Note that package dependencies linked in this way are *not* saved to `package.json` by default, on the assumption that the intention is to have a link stand in for a regular non-link dependency. Otherwise, for example, if you depend on `redis@^3.0.1`, and ran `npm link redis`, it would replace the `^3.0.1` dependency with `file:../path/to/node-redis`, which you probably don't want! Additionally, other users or developers on your project would run into issues if they do not have their folders set up exactly the same as yours.
If you are adding a *new* dependency as a link, you should add it to the relevant metadata by running `npm install <dep> --package-lock-only`.
If you *want* to save the `file:` reference in your `package.json` and `package-lock.json` files, you can use `npm link <dep> --save` to do so.
###
Workspace Usage
`npm link <pkg> --workspace <name>` will link the relevant package as a dependency of the specified workspace(s). Note that It may actually be linked into the parent project's `node_modules` folder, if there are no conflicting dependencies.
`npm link --workspace <name>` will create a global link to the specified workspace(s).
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm developers](../using-npm/developers)
* [package.json](../configuring-npm/package-json)
* [npm install](npm-install)
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-link.md)
| programming_docs |
npm npm-ping npm-ping
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Ping npm registry
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
* [See Also](#see-also)
###
Synopsis
```
npm ping
```
Note: This command is unaware of workspaces.
###
Description
Ping the configured or given npm registry and verify authentication. If it works it will output something like:
```
npm notice PING https://registry.npmjs.org/
npm notice PONG 255ms
```
otherwise you will get an error:
```
npm notice PING http://foo.com/
npm ERR! code E404
npm ERR! 404 Not Found - GET http://www.foo.com/-/ping?write=true
```
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
###
See Also
* [npm doctor](npm-doctor)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-ping.md)
npm npm-help npm-help
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Get help on npm
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [viewer](#viewer)
* [See Also](#see-also)
###
Synopsis
```
npm help <term> [<terms..>]
alias: hlep
```
Note: This command is unaware of workspaces.
###
Description
If supplied a topic, then show the appropriate documentation page.
If the topic does not exist, or if multiple terms are provided, then npm will run the `help-search` command to find a match. Note that, if `help-search` finds a single subject, then it will run `help` on that topic, so unique matches are equivalent to specifying a topic name.
###
Configuration
####
`viewer`
* Default: "man" on Posix, "browser" on Windows
* Type: String
The program to use to view help content.
Set to `"browser"` to view html help content in the default web browser.
###
See Also
* <npm>
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [package.json](../configuring-npm/package-json)
* [npm help-search](npm-help-search)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-help.md)
npm npm-restart npm-restart
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Restart a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [ignore-scripts](#ignore-scripts)
+ [script-shell](#script-shell)
* [See Also](#see-also)
###
Synopsis
```
npm restart [-- <args>]
```
###
Description
This restarts a project. It is equivalent to running `npm run-script
restart`.
If the current project has a `"restart"` script specified in `package.json`, then the following scripts will be run:
1. prerestart
2. restart
3. postrestart
If it does *not* have a `"restart"` script specified, but it does have `stop` and/or `start` scripts, then the following scripts will be run:
1. prerestart
2. prestop
3. stop
4. poststop
5. prestart
6. start
7. poststart
8. postrestart
###
Configuration
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm start](npm-start)
* [npm stop](npm-stop)
* [npm restart](npm-restart)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-restart.md)
npm npm-init npm-init
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Create a package.json file
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
+ [Forwarding additional options](#forwarding-additional-options)
* [Examples](#examples)
* [Workspaces support](#workspaces-support)
* [Configuration](#configuration)
+ [yes](#yes)
+ [force](#force)
+ [scope](#scope)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [workspaces-update](#workspaces-update)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm init <package-spec> (same as `npx <package-spec>)
npm init <@scope> (same as `npx <@scope>/create`)
aliases: create, innit
```
###
Description
`npm init <initializer>` can be used to set up a new or existing npm package.
`initializer` in this case is an npm package named `create-<initializer>`, which will be installed by [`npm-exec`](npm-exec), and then have its main bin executed -- presumably creating or updating `package.json` and running any other initialization-related operations.
The init command is transformed to a corresponding `npm exec` operation as follows:
* `npm init foo` -> `npm exec create-foo`
* `npm init @usr/foo` -> `npm exec @usr/create-foo`
* `npm init @usr` -> `npm exec @usr/create`
* `npm init @[email protected]` -> `npm exec @usr/[email protected]`
* `npm init @usr/[email protected]` -> `npm exec @usr/[email protected]`
If the initializer is omitted (by just calling `npm init`), init will fall back to legacy init behavior. It will ask you a bunch of questions, and then write a package.json for you. It will attempt to make reasonable guesses based on existing fields, dependencies, and options selected. It is strictly additive, so it will keep any fields and values that were already set. You can also use `-y`/`--yes` to skip the questionnaire altogether. If you pass `--scope`, it will create a scoped package.
*Note:* if a user already has the `create-<initializer>` package globally installed, that will be what `npm init` uses. If you want npm to use the latest version, or another specific version you must specify it:
* `npm init foo@latest` # fetches and runs the latest `create-foo` from the registry
* `npm init [email protected]` # runs `[email protected]` specifically
####
Forwarding additional options
Any additional options will be passed directly to the command, so `npm init
foo -- --hello` will map to `npm exec -- create-foo --hello`.
To better illustrate how options are forwarded, here's a more evolved example showing options passed to both the **npm cli** and a create package, both following commands are equivalent:
* `npm init foo -y --registry=<url> -- --hello -a`
* `npm exec -y --registry=<url> -- create-foo --hello -a`
###
Examples
Create a new React-based project using [`create-react-app`](https://npm.im/create-react-app):
```
$ npm init react-app ./my-react-app
```
Create a new `esm`-compatible package using [`create-esm`](https://npm.im/create-esm):
```
$ mkdir my-esm-lib && cd my-esm-lib
$ npm init esm --yes
```
Generate a plain old package.json using legacy init:
```
$ mkdir my-npm-pkg && cd my-npm-pkg
$ git init
$ npm init
```
Generate it without having it ask any questions:
```
$ npm init -y
```
###
Workspaces support
It's possible to create a new workspace within your project by using the `workspace` config option. When using `npm init -w <dir>` the cli will create the folders and boilerplate expected while also adding a reference to your project `package.json` `"workspaces": []` property in order to make sure that new generated **workspace** is properly set up as such.
Given a project with no workspaces, e.g:
```
.
+-- package.json
```
You may generate a new workspace using the legacy init:
```
$ npm init -w packages/a
```
That will generate a new folder and `package.json` file, while also updating your top-level `package.json` to add the reference to this new workspace:
```
.
+-- package.json
`-- packages
`-- a
`-- package.json
```
The workspaces init also supports the `npm init <initializer> -w <dir>` syntax, following the same set of rules explained earlier in the initial **Description** section of this page. Similar to the previous example of creating a new React-based project using [`create-react-app`](https://npm.im/create-react-app), the following syntax will make sure to create the new react app as a nested **workspace** within your project and configure your `package.json` to recognize it as such:
```
npm init -w packages/my-react-app react-app .
```
This will make sure to generate your react app as expected, one important consideration to have in mind is that `npm exec` is going to be run in the context of the newly created folder for that workspace, and that's the reason why in this example the initializer uses the initializer name followed with a dot to represent the current directory in that context, e.g: `react-app .`:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
`-- my-react-app
+-- README
+-- package.json
`-- ...
```
###
Configuration
####
`yes`
* Default: null
* Type: null or Boolean
Automatically answer "yes" to any prompts that npm might print on the command line.
####
`force`
* Default: false
* Type: Boolean
Removes various protections against unfortunate side effects, common mistakes, unnecessary performance degradation, and malicious input.
* Allow clobbering non-npm files in global installs.
* Allow the `npm version` command to work on an unclean git repository.
* Allow deleting the cache folder with `npm cache clean`.
* Allow installing packages that have an `engines` declaration requiring a different version of npm.
* Allow installing packages that have an `engines` declaration requiring a different version of `node`, even if `--engine-strict` is enabled.
* Allow `npm audit fix` to install modules outside your stated dependency range (including SemVer-major changes).
* Allow unpublishing all versions of a published package.
* Allow conflicting peerDependencies to be installed in the root project.
* Implicitly set `--yes` during `npm init`.
* Allow clobbering existing values in `npm pkg`
* Allow unpublishing of entire packages (not just a single version).
If you don't have a clear idea of what you want to do, it is strongly recommended that you do not use this option!
####
`scope`
* Default: the scope of the current project, if any, or ""
* Type: String
Associate an operation with a scope for a scoped registry.
Useful when logging in to or out of a private registry:
```
# log in, linking the scope to the custom registry
npm login --scope=@mycorp --registry=https://registry.mycorp.com
# log out, removing the link and the auth token
npm logout --scope=@mycorp
```
This will cause `@mycorp` to be mapped to the registry for future installation of packages specified according to the pattern `@mycorp/package`.
This will also cause `npm init` to create a scoped package.
```
# accept all defaults, and create a package named "@foo/whatever",
# instead of just named "whatever"
npm init --scope=@foo --yes
```
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`workspaces-update`
* Default: true
* Type: Boolean
If set to true, the npm cli will run an update after operations that may possibly change the workspaces installed to the `node_modules` folder.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [init-package-json module](http://npm.im/init-package-json)
* [package.json](../configuring-npm/package-json)
* [npm version](npm-version)
* [npm scope](../using-npm/scope)
* [npm exec](npm-exec)
* [npm workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-init.md)
| programming_docs |
npm npm-publish npm-publish
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Publish a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Files included in package](#files-included-in-package)
* [Configuration](#configuration)
+ [tag](#tag)
+ [access](#access)
+ [dry-run](#dry-run)
+ [otp](#otp)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm publish <package-spec>
```
###
Description
Publishes a package to the registry so that it can be installed by name.
By default npm will publish to the public registry. This can be overridden by specifying a different default registry or using a [`scope`](../using-npm/scope) in the name, combined with a scope-configured registry (see [`package.json`](../configuring-npm/package-json)).
A `package` is interpreted the same way as other commands (like `npm install` and can be:
* a) a folder containing a program described by a [`package.json`](../configuring-npm/package-json) file
* b) a gzipped tarball containing (a)
* c) a url that resolves to (b)
* d) a `<name>@<version>` that is published on the registry (see [`registry`](../using-npm/registry)) with (c)
* e) a `<name>@<tag>` (see [`npm dist-tag`](npm-dist-tag)) that points to (d)
* f) a `<name>` that has a "latest" tag satisfying (e)
* g) a `<git remote url>` that resolves to (a)
The publish will fail if the package name and version combination already exists in the specified registry.
Once a package is published with a given name and version, that specific name and version combination can never be used again, even if it is removed with [`npm unpublish`](npm-unpublish).
As of `npm@5`, both a sha1sum and an integrity field with a sha512sum of the tarball will be submitted to the registry during publication. Subsequent installs will use the strongest supported algorithm to verify downloads.
Similar to `--dry-run` see [`npm pack`](npm-pack), which figures out the files to be included and packs them into a tarball to be uploaded to the registry.
###
Files included in package
To see what will be included in your package, run `npx npm-packlist`. All files are included by default, with the following exceptions:
* Certain files that are relevant to package installation and distribution are always included. For example, `package.json`, `README.md`, `LICENSE`, and so on.
* If there is a "files" list in [`package.json`](../configuring-npm/package-json), then only the files specified will be included. (If directories are specified, then they will be walked recursively and their contents included, subject to the same ignore rules.)
* If there is a `.gitignore` or `.npmignore` file, then ignored files in that and all child directories will be excluded from the package. If *both* files exist, then the `.gitignore` is ignored, and only the `.npmignore` is used.
`.npmignore` files follow the [same pattern rules](https://git-scm.com/book/en/v2/Git-Basics-Recording-Changes-to-the-Repository#_ignoring) as `.gitignore` files
* If the file matches certain patterns, then it will *never* be included, unless explicitly added to the `"files"` list in `package.json`, or un-ignored with a `!` rule in a `.npmignore` or `.gitignore` file.
* Symbolic links are never included in npm packages.
See [`developers`](../using-npm/developers) for full details on what's included in the published package, as well as details on how the package is built.
###
Configuration
####
`tag`
* Default: "latest"
* Type: String
If you ask npm to install a package and don't tell it a specific version, then it will install the specified tag.
Also the tag that is added to the package@version specified by the `npm tag` command, if no explicit tag is given.
When used by the `npm diff` command, this is the tag used to fetch the tarball that will be compared with the local files by default.
####
`access`
* Default: 'restricted' for scoped packages, 'public' for unscoped packages
* Type: null, "restricted", or "public"
When publishing scoped packages, the access level defaults to `restricted`. If you want your scoped package to be publicly viewable (and installable) set `--access=public`. The only valid values for `access` are `public` and `restricted`. Unscoped packages *always* have an access level of `public`.
Note: Using the `--access` flag on the `npm publish` command will only set the package access level on the initial publish of the package. Any subsequent `npm publish` commands using the `--access` flag will not have an effect to the access level. To make changes to the access level after the initial publish use `npm access`.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm-packlist package](http://npm.im/npm-packlist)
* [npm registry](../using-npm/registry)
* [npm scope](../using-npm/scope)
* [npm adduser](npm-adduser)
* [npm owner](npm-owner)
* [npm deprecate](npm-deprecate)
* [npm dist-tag](npm-dist-tag)
* [npm pack](npm-pack)
* [npm profile](npm-profile)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-publish.md)
npm npm-adduser npm-adduser
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Add a registry user account
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
+ [scope](#scope)
+ [auth-type](#auth-type)
* [See Also](#see-also)
###
Synopsis
```
npm adduser
aliases: login, add-user
```
Note: This command is unaware of workspaces.
###
Description
Create or verify a user named `<username>` in the specified registry, and save the credentials to the `.npmrc` file. If no registry is specified, the default registry will be used (see [`config`](../using-npm/config)).
The username, password, and email are read in from prompts.
To reset your password, go to <https://www.npmjs.com/forgot>
To change your email address, go to <https://www.npmjs.com/email-edit>
You may use this command multiple times with the same user account to authorize on a new machine. When authenticating on a new machine, the username, password and email address must all match with your existing record.
`npm login` is an alias to `adduser` and behaves exactly the same way.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`scope`
* Default: the scope of the current project, if any, or ""
* Type: String
Associate an operation with a scope for a scoped registry.
Useful when logging in to or out of a private registry:
```
# log in, linking the scope to the custom registry
npm login --scope=@mycorp --registry=https://registry.mycorp.com
# log out, removing the link and the auth token
npm logout --scope=@mycorp
```
This will cause `@mycorp` to be mapped to the registry for future installation of packages specified according to the pattern `@mycorp/package`.
This will also cause `npm init` to create a scoped package.
```
# accept all defaults, and create a package named "@foo/whatever",
# instead of just named "whatever"
npm init --scope=@foo --yes
```
####
`auth-type`
* Default: "legacy"
* Type: "legacy", "web", "sso", "saml", "oauth", or "webauthn"
NOTE: auth-type values "sso", "saml", "oauth", and "webauthn" will be removed in a future version.
What authentication strategy to use with `login`.
###
See Also
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm owner](npm-owner)
* [npm whoami](npm-whoami)
* [npm token](npm-token)
* [npm profile](npm-profile)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-adduser.md)
npm npm-docs npm-docs
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Open documentation for a package in a web browser
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [browser](#browser)
+ [registry](#registry)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm docs [<pkgname> [<pkgname> ...]]
alias: home
```
###
Description
This command tries to guess at the likely location of a package's documentation URL, and then tries to open it using the `--browser` config param. You can pass multiple package names at once. If no package name is provided, it will search for a `package.json` in the current folder and use the `name` property.
###
Configuration
####
`browser`
* Default: OS X: `"open"`, Windows: `"start"`, Others: `"xdg-open"`
* Type: null, Boolean, or String
The browser that is called by npm commands to open websites.
Set to `false` to suppress browser behavior and instead print urls to terminal.
Set to `true` to use default system URL opener.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [npm view](npm-view)
* [npm publish](npm-publish)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [package.json](../configuring-npm/package-json)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-docs.md)
| programming_docs |
npm npm-dedupe npm-dedupe
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Reduce duplication in the package tree
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [omit](#omit)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm dedupe
alias: ddp
```
###
Description
Searches the local package tree and attempts to simplify the overall structure by moving dependencies further up the tree, where they can be more effectively shared by multiple dependent packages.
For example, consider this dependency graph:
```
a
+-- b <-- depends on [email protected]
| `-- [email protected]
`-- d <-- depends on c@~1.0.9
`-- [email protected]
```
In this case, `npm dedupe` will transform the tree to:
```
a
+-- b
+-- d
`-- [email protected]
```
Because of the hierarchical nature of node's module lookup, b and d will both get their dependency met by the single c package at the root level of the tree.
In some cases, you may have a dependency graph like this:
```
a
+-- b <-- depends on [email protected]
+-- [email protected]
`-- d <-- depends on [email protected]
`-- [email protected]
```
During the installation process, the `[email protected]` dependency for `b` was placed in the root of the tree. Though `d`'s dependency on `[email protected]` could have been satisfied by `[email protected]`, the newer `[email protected]` dependency was used, because npm favors updates by default, even when doing so causes duplication.
Running `npm dedupe` will cause npm to note the duplication and re-evaluate, deleting the nested `c` module, because the one in the root is sufficient.
To prefer deduplication over novelty during the installation process, run `npm install --prefer-dedupe` or `npm config set prefer-dedupe true`.
Arguments are ignored. Dedupe always acts on the entire tree.
Note that this operation transforms the dependency tree, but will never result in new modules being installed.
Using `npm find-dupes` will run the command in `--dry-run` mode.
Note: `npm dedupe` will never update the semver values of direct dependencies in your project `package.json`, if you want to update values in `package.json` you can run: `npm update --save` instead.
###
Configuration
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm find-dupes](npm-find-dupes)
* [npm ls](npm-ls)
* [npm update](npm-update)
* [npm install](npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-dedupe.md)
npm npm-explain npm-explain
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Explain installed packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [json](#json)
+ [workspace](#workspace)
* [See Also](#see-also)
###
Synopsis
```
npm explain <package-spec>
alias: why
```
###
Description
This command will print the chain of dependencies causing a given package to be installed in the current project.
If one or more package specs are provided, then only packages matching one of the specifiers will have their relationships explained.
The package spec can also refer to a folder within `./node_modules`
For example, running `npm explain glob` within npm's source tree will show:
```
[email protected]
node_modules/glob
glob@"^7.1.4" from the root project
[email protected] dev
node_modules/tacks/node_modules/glob
glob@"^7.0.5" from [email protected]
node_modules/tacks/node_modules/rimraf
rimraf@"^2.6.2" from [email protected]
node_modules/tacks
dev tacks@"^1.3.0" from the root project
```
To explain just the package residing at a specific folder, pass that as the argument to the command. This can be useful when trying to figure out exactly why a given dependency is being duplicated to satisfy conflicting version requirements within the project.
```
$ npm explain node_modules/nyc/node_modules/find-up
[email protected] dev
node_modules/nyc/node_modules/find-up
find-up@"^3.0.0" from [email protected]
node_modules/nyc
nyc@"^14.1.1" from [email protected]
node_modules/tap
dev tap@"^14.10.8" from the root project
```
###
Configuration
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm folders](../configuring-npm/folders)
* [npm ls](npm-ls)
* [npm install](npm-install)
* [npm link](npm-link)
* [npm prune](npm-prune)
* [npm outdated](npm-outdated)
* [npm update](npm-update)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-explain.md)
npm npm-shrinkwrap npm-shrinkwrap
==============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Lock down dependency versions for publication
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [See Also](#see-also)
###
Synopsis
```
npm shrinkwrap
```
Note: This command is unaware of workspaces.
###
Description
This command repurposes `package-lock.json` into a publishable `npm-shrinkwrap.json` or simply creates a new one. The file created and updated by this command will then take precedence over any other existing or future `package-lock.json` files. For a detailed explanation of the design and purpose of package locks in npm, see [package-lock-json](../configuring-npm/package-lock-json).
###
See Also
* [npm install](npm-install)
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [package.json](../configuring-npm/package-json)
* [package-lock.json](../configuring-npm/package-lock-json)
* [npm-shrinkwrap.json](../configuring-npm/npm-shrinkwrap-json)
* [npm ls](npm-ls)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-shrinkwrap.md)
npm npm-install-ci-test npm-install-ci-test
===================
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Install a project with a clean slate and run tests
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [save](#save)
+ [save-exact](#save-exact)
+ [global](#global)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [omit](#omit)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [dry-run](#dry-run)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm install-ci-test
alias: cit
```
###
Description
This command runs `npm ci` followed immediately by `npm test`.
###
Configuration
####
`save`
* Default: `true` unless when using `npm update` where it defaults to `false`
* Type: Boolean
Save installed packages to a `package.json` file as dependencies.
When used with the `npm rm` command, removes the dependency from `package.json`.
Will also prevent writing to `package-lock.json` if set to `false`.
####
`save-exact`
* Default: false
* Type: Boolean
Dependencies saved to package.json will be configured with an exact version rather than using npm's default semver range operator.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm install-test](npm-install-test)
* [npm ci](npm-ci)
* [npm test](npm-test)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-install-ci-test.md)
| programming_docs |
npm npm-prune npm-prune
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Remove extraneous packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [omit](#omit)
+ [dry-run](#dry-run)
+ [json](#json)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm prune [[<@scope>/]<pkg>...]
```
###
Description
This command removes "extraneous" packages. If a package name is provided, then only packages matching one of the supplied names are removed.
Extraneous packages are those present in the `node_modules` folder that are not listed as any package's dependency list.
If the `--production` flag is specified or the `NODE_ENV` environment variable is set to `production`, this command will remove the packages specified in your `devDependencies`. Setting `--no-production` will negate `NODE_ENV` being set to `production`.
If the `--dry-run` flag is used then no changes will actually be made.
If the `--json` flag is used, then the changes `npm prune` made (or would have made with `--dry-run`) are printed as a JSON object.
In normal operation, extraneous modules are pruned automatically, so you'll only need this command with the `--production` flag. However, in the real world, operation is not always "normal". When crashes or mistakes happen, this command can help clean up any resulting garbage.
###
Configuration
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm uninstall](npm-uninstall)
* [npm folders](../configuring-npm/folders)
* [npm ls](npm-ls)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-prune.md)
npm npm-pkg npm-pkg
=======
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manages your package.json
Table of contents* + [Synopsis](#synopsis)
+ [Description](#description)
+ [Workspaces support](#workspaces-support)
+ [Configuration](#configuration)
- [force](#force)
- [json](#json)
- [workspace](#workspace)
- [workspaces](#workspaces)
* [See Also](#see-also)
###
Synopsis
```
npm pkg set <key>=<value> [<key>=<value> ...]
npm pkg get [<key> [<key> ...]]
npm pkg delete <key> [<key> ...]
npm pkg set [<array>[<index>].<key>=<value> ...]
npm pkg set [<array>[].<key>=<value> ...]
```
###
Description
A command that automates the management of `package.json` files. `npm pkg` provide 3 different sub commands that allow you to modify or retrieve values for given object keys in your `package.json`.
The syntax to retrieve and set fields is a dot separated representation of the nested object properties to be found within your `package.json`, it's the same notation used in [`npm view`](npm-view) to retrieve information from the registry manifest, below you can find more examples on how to use it.
Returned values are always in **json** format.
* `npm pkg get <field>`
Retrieves a value `key`, defined in your `package.json` file.
For example, in order to retrieve the name of the current package, you can run:
```
npm pkg get name
```
It's also possible to retrieve multiple values at once:
```
npm pkg get name version
```
You can view child fields by separating them with a period. To retrieve the value of a test `script` value, you would run the following command:
```
npm pkg get scripts.test
```
For fields that are arrays, requesting a non-numeric field will return all of the values from the objects in the list. For example, to get all the contributor emails for a package, you would run:
```
npm pkg get contributors.email
```
You may also use numeric indices in square braces to specifically select an item in an array field. To just get the email address of the first contributor in the list, you can run:
```
npm pkg get contributors[0].email
```
For complex fields you can also name a property in square brackets to specifically select a child field. This is especially helpful with the exports object:
```
npm pkg get "exports[.].require"
```
* `npm pkg set <field>=<value>`
Sets a `value` in your `package.json` based on the `field` value. When saving to your `package.json` file the same set of rules used during `npm install` and other cli commands that touches the `package.json` file are used, making sure to respect the existing indentation and possibly applying some validation prior to saving values to the file.
The same syntax used to retrieve values from your package can also be used to define new properties or overriding existing ones, below are some examples of how the dot separated syntax can be used to edit your `package.json` file.
Defining a new bin named `mynewcommand` in your `package.json` that points to a file `cli.js`:
```
npm pkg set bin.mynewcommand=cli.js
```
Setting multiple fields at once is also possible:
```
npm pkg set description='Awesome package' engines.node='>=10'
```
It's also possible to add to array values, for example to add a new contributor entry:
```
npm pkg set contributors[0].name='Foo' contributors[0].email='[email protected]'
```
You may also append items to the end of an array using the special empty bracket notation:
```
npm pkg set contributors[].name='Foo' contributors[].name='Bar'
```
It's also possible to parse values as json prior to saving them to your `package.json` file, for example in order to set a `"private": true` property:
```
npm pkg set private=true --json
```
It also enables saving values as numbers:
```
npm pkg set tap.timeout=60 --json
```
* `npm pkg delete <key>`
Deletes a `key` from your `package.json`
The same syntax used to set values from your package can also be used to remove existing ones. For example, in order to remove a script named build:
```
npm pkg delete scripts.build
```
###
Workspaces support
You can set/get/delete items across your configured workspaces by using the `workspace` or `workspaces` config options.
For example, setting a `funding` value across all configured workspaces of a project:
```
npm pkg set funding=https://example.com --ws
```
When using `npm pkg get` to retrieve info from your configured workspaces, the returned result will be in a json format in which top level keys are the names of each workspace, the values of these keys will be the result values returned from each of the configured workspaces, e.g:
```
npm pkg get name version --ws
{
"a": {
"name": "a",
"version": "1.0.0"
},
"b": {
"name": "b",
"version": "1.0.0"
}
}
```
###
Configuration
####
`force`
* Default: false
* Type: Boolean
Removes various protections against unfortunate side effects, common mistakes, unnecessary performance degradation, and malicious input.
* Allow clobbering non-npm files in global installs.
* Allow the `npm version` command to work on an unclean git repository.
* Allow deleting the cache folder with `npm cache clean`.
* Allow installing packages that have an `engines` declaration requiring a different version of npm.
* Allow installing packages that have an `engines` declaration requiring a different version of `node`, even if `--engine-strict` is enabled.
* Allow `npm audit fix` to install modules outside your stated dependency range (including SemVer-major changes).
* Allow unpublishing all versions of a published package.
* Allow conflicting peerDependencies to be installed in the root project.
* Implicitly set `--yes` during `npm init`.
* Allow clobbering existing values in `npm pkg`
* Allow unpublishing of entire packages (not just a single version).
If you don't have a clear idea of what you want to do, it is strongly recommended that you do not use this option!
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
See Also
---------
* [npm install](npm-install)
* [npm init](npm-init)
* [npm config](npm-config)
* [npm set-script](npm-set-script)
* [workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-pkg.md)
npm npm-exec npm-exec
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Run a command from a local or remote npm package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [npx vs npm exec](#npx-vs-npm-exec)
* [Configuration](#configuration)
+ [package](#package)
+ [call](#call)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [Examples](#examples)
* [Workspaces support](#workspaces-support)
+ [Filtering workspaces](#filtering-workspaces)
* [Compatibility with Older npx Versions](#compatibility-with-older-npx-versions)
* [A note on caching](#a-note-on-caching)
+ [prefer-online](#prefer-online)
+ [prefer-offline](#prefer-offline)
+ [offline](#offline)
+ [workspace](#workspace-1)
+ [workspaces](#workspaces-1)
* [See Also](#see-also)
###
Synopsis
```
npm exec -- <pkg>[@<version>] [args...]
npm exec --package=<pkg>[@<version>] -- <cmd> [args...]
npm exec -c '<cmd> [args...]'
npm exec --package=foo -c '<cmd> [args...]'
alias: x
```
###
Description
This command allows you to run an arbitrary command from an npm package (either one installed locally, or fetched remotely), in a similar context as running it via `npm run`.
Run without positional arguments or `--call`, this allows you to interactively run commands in the same sort of shell environment that `package.json` scripts are run. Interactive mode is not supported in CI environments when standard input is a TTY, to prevent hangs.
Whatever packages are specified by the `--package` option will be provided in the `PATH` of the executed command, along with any locally installed package executables. The `--package` option may be specified multiple times, to execute the supplied command in an environment where all specified packages are available.
If any requested packages are not present in the local project dependencies, then they are installed to a folder in the npm cache, which is added to the `PATH` environment variable in the executed process. A prompt is printed (which can be suppressed by providing either `--yes` or `--no`).
Package names provided without a specifier will be matched with whatever version exists in the local project. Package names with a specifier will only be considered a match if they have the exact same name and version as the local dependency.
If no `-c` or `--call` option is provided, then the positional arguments are used to generate the command string. If no `--package` options are provided, then npm will attempt to determine the executable name from the package specifier provided as the first positional argument according to the following heuristic:
* If the package has a single entry in its `bin` field in `package.json`, or if all entries are aliases of the same command, then that command will be used.
* If the package has multiple `bin` entries, and one of them matches the unscoped portion of the `name` field, then that command will be used.
* If this does not result in exactly one option (either because there are no bin entries, or none of them match the `name` of the package), then `npm exec` exits with an error.
To run a binary *other than* the named binary, specify one or more `--package` options, which will prevent npm from inferring the package from the first command argument.
###
`npx` vs `npm exec`
When run via the `npx` binary, all flags and options *must* be set prior to any positional arguments. When run via `npm exec`, a double-hyphen `--` flag can be used to suppress npm's parsing of switches and options that should be sent to the executed command.
For example:
```
$ npx foo@latest bar --package=@npmcli/foo
```
In this case, npm will resolve the `foo` package name, and run the following command:
```
$ foo bar --package=@npmcli/foo
```
Since the `--package` option comes *after* the positional arguments, it is treated as an argument to the executed command.
In contrast, due to npm's argument parsing logic, running this command is different:
```
$ npm exec foo@latest bar --package=@npmcli/foo
```
In this case, npm will parse the `--package` option first, resolving the `@npmcli/foo` package. Then, it will execute the following command in that context:
```
$ foo@latest bar
```
The double-hyphen character is recommended to explicitly tell npm to stop parsing command line options and switches. The following command would thus be equivalent to the `npx` command above:
```
$ npm exec -- foo@latest bar --package=@npmcli/foo
```
###
Configuration
####
`package`
* Default:
* Type: String (can be set multiple times)
The package or packages to install for [`npm exec`](npm-exec)
####
`call`
* Default: ""
* Type: String
Optional companion option for `npm exec`, `npx` that allows for specifying a custom command to be run along with the installed packages.
```
npm exec --package yo --package generator-node --call "yo node"
```
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
Examples
Run the version of `tap` in the local dependencies, with the provided arguments:
```
$ npm exec -- tap --bail test/foo.js
$ npx tap --bail test/foo.js
```
Run a command *other than* the command whose name matches the package name by specifying a `--package` option:
```
$ npm exec --package=foo -- bar --bar-argument
# ~ or ~
$ npx --package=foo bar --bar-argument
```
Run an arbitrary shell script, in the context of the current project:
```
$ npm x -c 'eslint && say "hooray, lint passed"'
$ npx -c 'eslint && say "hooray, lint passed"'
```
###
Workspaces support
You may use the `workspace` or `workspaces` configs in order to run an arbitrary command from an npm package (either one installed locally, or fetched remotely) in the context of the specified workspaces. If no positional argument or `--call` option is provided, it will open an interactive subshell in the context of each of these configured workspaces one at a time.
Given a project with configured workspaces, e.g:
```
.
+-- package.json
`-- packages
+-- a
| `-- package.json
+-- b
| `-- package.json
`-- c
`-- package.json
```
Assuming the workspace configuration is properly set up at the root level `package.json` file. e.g:
```
{
"workspaces": [ "./packages/*" ]
}
```
You can execute an arbitrary command from a package in the context of each of the configured workspaces when using the `workspaces` configuration options, in this example we're using **eslint** to lint any js file found within each workspace folder:
```
npm exec --ws -- eslint ./*.js
```
####
Filtering workspaces
It's also possible to execute a command in a single workspace using the `workspace` config along with a name or directory path:
```
npm exec --workspace=a -- eslint ./*.js
```
The `workspace` config can also be specified multiple times in order to run a specific script in the context of multiple workspaces. When defining values for the `workspace` config in the command line, it also possible to use `-w` as a shorthand, e.g:
```
npm exec -w a -w b -- eslint ./*.js
```
This last command will run the `eslint` command in both `./packages/a` and `./packages/b` folders.
###
Compatibility with Older npx Versions
The `npx` binary was rewritten in npm v7.0.0, and the standalone `npx` package deprecated at that time. `npx` uses the `npm exec` command instead of a separate argument parser and install process, with some affordances to maintain backwards compatibility with the arguments it accepted in previous versions.
This resulted in some shifts in its functionality:
* Any `npm` config value may be provided.
* To prevent security and user-experience problems from mistyping package names, `npx` prompts before installing anything. Suppress this prompt with the `-y` or `--yes` option.
* The `--no-install` option is deprecated, and will be converted to `--no`.
* Shell fallback functionality is removed, as it is not advisable.
* The `-p` argument is a shorthand for `--parseable` in npm, but shorthand for `--package` in npx. This is maintained, but only for the `npx` executable.
* The `--ignore-existing` option is removed. Locally installed bins are always present in the executed process `PATH`.
* The `--npm` option is removed. `npx` will always use the `npm` it ships with.
* The `--node-arg` and `-n` options are removed.
* The `--always-spawn` option is redundant, and thus removed.
* The `--shell` option is replaced with `--script-shell`, but maintained in the `npx` executable for backwards compatibility.
###
A note on caching
The npm cli utilizes its internal package cache when using the package name specified. You can use the following to change how and when the cli uses this cache. See [`npm cache`](npm-cache) for more on how the cache works.
####
prefer-online
Forces staleness checks for packages, making the cli look for updates immediately even if the package is already in the cache.
####
prefer-offline
Bypasses staleness checks for packages. Missing data will still be requested from the server. To force full offline mode, use `offline`.
####
offline
Forces full offline mode. Any packages not locally cached will result in an error.
####
workspace
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result to selecting all of the nested workspaces)
This value is not exported to the environment for child processes.
####
workspaces
* Alias: `--ws`
* Type: Boolean
* Default: `false`
Run scripts in the context of all configured workspaces for the current project.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm start](npm-start)
* [npm restart](npm-restart)
* [npm stop](npm-stop)
* [npm config](npm-config)
* [npm workspaces](../using-npm/workspaces)
* <npx>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-exec.md)
| programming_docs |
npm npm-whoami npm-whoami
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Display npm username
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
* [See Also](#see-also)
###
Synopsis
```
npm whoami
```
Note: This command is unaware of workspaces.
###
Description
Display the npm username of the currently logged-in user.
If logged into a registry that provides token-based authentication, then connect to the `/-/whoami` registry endpoint to find the username associated with the token, and print to standard output.
If logged into a registry that uses Basic Auth, then simply print the `username` portion of the authentication string.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
###
See Also
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-whoami.md)
npm npm-pack npm-pack
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Create a tarball from a package
Table of contents* [Synopsis](#synopsis)
* [Configuration](#configuration)
+ [dry-run](#dry-run)
+ [json](#json)
+ [pack-destination](#pack-destination)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [Description](#description)
* [See Also](#see-also)
###
Synopsis
```
npm pack <package-spec>
```
###
Configuration
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`pack-destination`
* Default: "."
* Type: String
Directory in which `npm pack` will save tarballs.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
Description
For anything that's installable (that is, a package folder, tarball, tarball url, git url, name@tag, name@version, name, or scoped name), this command will fetch it to the cache, copy the tarball to the current working directory as `<name>-<version>.tgz`, and then write the filenames out to stdout.
If the same package is specified multiple times, then the file will be overwritten the second time.
If no arguments are supplied, then npm packs the current package folder.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm-packlist package](http://npm.im/npm-packlist)
* [npm cache](npm-cache)
* [npm publish](npm-publish)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-pack.md)
npm npm-config npm-config
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage the npm configuration files
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Sub-commands](#sub-commands)
+ [set](#set)
+ [get](#get)
+ [list](#list)
+ [delete](#delete)
+ [edit](#edit)
* [Configuration](#configuration)
+ [json](#json)
+ [global](#global)
+ [editor](#editor)
+ [location](#location)
+ [long](#long)
* [See Also](#see-also)
###
Synopsis
```
npm config set <key>=<value> [<key>=<value> ...]
npm config get [<key> [<key> ...]]
npm config delete <key> [<key> ...]
npm config list [--json]
npm config edit
alias: c
```
Note: This command is unaware of workspaces.
###
Description
npm gets its config settings from the command line, environment variables, `npmrc` files, and in some cases, the `package.json` file.
See [npmrc](../configuring-npm/npmrc) for more information about the npmrc files.
See [config(7)](../using-npm/config) for a more thorough explanation of the mechanisms involved, and a full list of config options available.
The `npm config` command can be used to update and edit the contents of the user and global npmrc files.
###
Sub-commands
Config supports the following sub-commands:
####
set
```
npm config set key=value [key=value...]
npm set key=value [key=value...]
```
Sets each of the config keys to the value provided.
If value is omitted, then it sets it to an empty string.
Note: for backwards compatibility, `npm config set key value` is supported as an alias for `npm config set key=value`.
####
get
```
npm config get [key ...]
npm get [key ...]
```
Echo the config value(s) to stdout.
If multiple keys are provided, then the values will be prefixed with the key names.
If no keys are provided, then this command behaves the same as `npm config
list`.
####
list
```
npm config list
```
Show all the config settings. Use `-l` to also show defaults. Use `--json` to show the settings in json format.
####
delete
```
npm config delete key [key ...]
```
Deletes the specified keys from all configuration files.
####
edit
```
npm config edit
```
Opens the config file in an editor. Use the `--global` flag to edit the global config.
###
Configuration
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`editor`
* Default: The EDITOR or VISUAL environment variables, or 'notepad.exe' on Windows, or 'vim' on Unix systems
* Type: String
The command to run for `npm edit` and `npm config edit`.
####
`location`
* Default: "user" unless `--global` is passed, which will also set this value to "global"
* Type: "global", "user", or "project"
When passed to `npm config` this refers to which config file to use.
When set to "global" mode, packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
###
See Also
* [npm folders](../configuring-npm/folders)
* [npm config](npm-config)
* [package.json](../configuring-npm/package-json)
* [npmrc](../configuring-npm/npmrc)
* <npm>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-config.md)
npm npm-query npm-query
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Dependency selector query
Table of contents* + [Synopsis](#synopsis)
+ [Description](#description)
+ [Piping npm query to other commands](#piping-npm-query-to-other-commands)
+ [Extended Use Cases & Queries](#extended-use-cases--queries)
+ [Example Response Output](#example-response-output)
+ [Configuration](#configuration)
- [global](#global)
- [workspace](#workspace)
- [workspaces](#workspaces)
- [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm query <selector>
```
###
Description
The `npm query` command allows for usage of css selectors in order to retrieve an array of dependency objects.
###
Piping npm query to other commands
```
# find all dependencies with postinstall scripts & uninstall them
npm query ":attr(scripts, [postinstall])" | jq 'map(.name)|join("\n")' -r | xargs -I {} npm uninstall {}
# find all git dependencies & explain who requires them
npm query ":type(git)" | jq 'map(.name)' | xargs -I {} npm why {}
```
###
Extended Use Cases & Queries
```
// all deps
*
// all direct deps
:root > *
// direct production deps
:root > .prod
// direct development deps
:root > .dev
// any peer dep of a direct deps
:root > * > .peer
// any workspace dep
.workspace
// all workspaces that depend on another workspace
.workspace > .workspace
// all workspaces that have peer deps
.workspace:has(.peer)
// any dep named "lodash"
// equivalent to [name="lodash"]
#lodash
// any deps named "lodash" & within semver range ^"1.2.3"
#lodash@^1.2.3
// equivalent to...
[name="lodash"]:semver(^1.2.3)
// get the hoisted node for a given semver range
#lodash@^1.2.3:not(:deduped)
// querying deps with a specific version
#[email protected]
// equivalent to...
[name="lodash"][version="2.1.5"]
// has any deps
:has(*)
// deps with no other deps (ie. "leaf" nodes)
:empty
// manually querying git dependencies
[repository^=github:],
[repository^=git:],
[repository^=https://github.com],
[repository^=http://github.com],
[repository^=https://github.com],
[repository^=+git:...]
// querying for all git dependencies
:type(git)
// get production dependencies that aren't also dev deps
.prod:not(.dev)
// get dependencies with specific licenses
[license=MIT], [license=ISC]
// find all packages that have @ruyadorno as a contributor
:attr(contributors, [[email protected]])
```
###
Example Response Output
* an array of dependency objects is returned which can contain multiple copies of the same package which may or may not have been linked or deduped
```
[
{
"name": "",
"version": "",
"description": "",
"homepage": "",
"bugs": {},
"author": {},
"license": {},
"funding": {},
"files": [],
"main": "",
"browser": "",
"bin": {},
"man": [],
"directories": {},
"repository": {},
"scripts": {},
"config": {},
"dependencies": {},
"devDependencies": {},
"optionalDependencies": {},
"bundledDependencies": {},
"peerDependencies": {},
"peerDependenciesMeta": {},
"engines": {},
"os": [],
"cpu": [],
"workspaces": {},
"keywords": [],
...
},
...
```
###
Configuration
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
See Also
---------
* [dependency selectors](../using-npm/dependency-selectors)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-query.md)
| programming_docs |
npm npm-fund npm-fund
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Retrieve funding information
Table of contents* + [Synopsis](#synopsis)
+ [Description](#description)
- [Example](#example)
+ [Workspaces support](#workspaces-support)
- [Example:](#example-1)
+ [Configuration](#configuration)
- [json](#json)
- [browser](#browser)
- [unicode](#unicode)
- [workspace](#workspace)
- [which](#which)
* [See Also](#see-also)
###
Synopsis
```
npm fund [<package-spec>]
```
###
Description
This command retrieves information on how to fund the dependencies of a given project. If no package name is provided, it will list all dependencies that are looking for funding in a tree structure, listing the type of funding and the url to visit. If a package name is provided then it tries to open its funding url using the `--browser` config param; if there are multiple funding sources for the package, the user will be instructed to pass the `--which` option to disambiguate.
The list will avoid duplicated entries and will stack all packages that share the same url as a single entry. Thus, the list does not have the same shape of the output from `npm ls`.
####
Example
###
Workspaces support
It's possible to filter the results to only include a single workspace and its dependencies using the `workspace` config option.
####
Example:
Here's an example running `npm fund` in a project with a configured workspace `a`:
```
$ npm fund
[email protected]
+-- https://example.com/a
| | `-- [email protected]
| `-- https://example.com/maintainer
| `-- [email protected]
+-- https://example.com/npmcli-funding
| `-- @npmcli/test-funding
`-- https://example.com/org
`-- [email protected]
```
And here is an example of the expected result when filtering only by a specific workspace `a` in the same project:
```
$ npm fund -w a
[email protected]
`-- https://example.com/a
| `-- [email protected]
`-- https://example.com/maintainer
`-- [email protected]
```
###
Configuration
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`browser`
* Default: OS X: `"open"`, Windows: `"start"`, Others: `"xdg-open"`
* Type: null, Boolean, or String
The browser that is called by npm commands to open websites.
Set to `false` to suppress browser behavior and instead print urls to terminal.
Set to `true` to use default system URL opener.
####
`unicode`
* Default: false on windows, true on mac/unix systems with a unicode locale, as defined by the `LC_ALL`, `LC_CTYPE`, or `LANG` environment variables.
* Type: Boolean
When set to true, npm uses unicode characters in the tree output. When false, it uses ascii characters instead of unicode glyphs.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`which`
* Default: null
* Type: null or Number
If there are multiple funding sources, which 1-indexed source URL to open.
See Also
---------
* [package spec](../using-npm/package-spec)
* [npm install](npm-install)
* [npm docs](npm-docs)
* [npm ls](npm-ls)
* [npm config](npm-config)
* [npm workspaces](../using-npm/workspaces)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-fund.md)
npm npm-hook npm-hook
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage registry hooks
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm hook add <pkg> <url> <secret> [--type=<type>]
npm hook ls [pkg]
npm hook rm <id>
npm hook update <id> <url> <secret>
```
Note: This command is unaware of workspaces.
###
Description
Allows you to manage [npm hooks](https://blog.npmjs.org/post/145260155635/introducing-hooks-get-notifications-of-npm), including adding, removing, listing, and updating.
Hooks allow you to configure URL endpoints that will be notified whenever a change happens to any of the supported entity types. Three different types of entities can be watched by hooks: packages, owners, and scopes.
To create a package hook, simply reference the package name.
To create an owner hook, prefix the owner name with `~` (as in, `~youruser`).
To create a scope hook, prefix the scope name with `@` (as in, `@yourscope`).
The hook `id` used by `update` and `rm` are the IDs listed in `npm hook ls` for that particular hook.
The shared secret will be sent along to the URL endpoint so you can verify the request came from your own configured hook.
###
Example
Add a hook to watch a package for changes:
```
$ npm hook add lodash https://example.com/ my-shared-secret
```
Add a hook to watch packages belonging to the user `substack`:
```
$ npm hook add ~substack https://example.com/ my-shared-secret
```
Add a hook to watch packages in the scope `@npm`
```
$ npm hook add @npm https://example.com/ my-shared-secret
```
List all your active hooks:
```
$ npm hook ls
```
List your active hooks for the `lodash` package:
```
$ npm hook ls lodash
```
Update an existing hook's url:
```
$ npm hook update id-deadbeef https://my-new-website.here/
```
Remove a hook:
```
$ npm hook rm id-deadbeef
```
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* ["Introducing Hooks" blog post](https://blog.npmjs.org/post/145260155635/introducing-hooks-get-notifications-of-npm)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-hook.md)
npm npm-owner npm-owner
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage package owners
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
* [See Also](#see-also)
###
Synopsis
```
npm owner add <user> <package-spec>
npm owner rm <user> <package-spec>
npm owner ls <package-spec>
alias: author
```
Note: This command is unaware of workspaces.
###
Description
Manage ownership of published packages.
* ls: List all the users who have access to modify a package and push new versions. Handy when you need to know who to bug for help.
* add: Add a new user as a maintainer of a package. This user is enabled to modify metadata, publish new versions, and add other owners.
* rm: Remove a user from the package owner list. This immediately revokes their privileges.
Note that there is only one level of access. Either you can modify a package, or you can't. Future versions may contain more fine-grained access levels, but that is not implemented at this time.
If you have two-factor authentication enabled with `auth-and-writes` (see [`npm-profile`](npm-profile)) then you'll need to include an otp on the command line when changing ownership with `--otp`.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm profile](npm-profile)
* [npm publish](npm-publish)
* [npm registry](../using-npm/registry)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-owner.md)
npm npm-diff npm-diff
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
The registry diff command
Table of contents* + [Synopsis](#synopsis)
+ [Description](#description)
- [Filtering files](#filtering-files)
+ [Configuration](#configuration)
- [diff](#diff)
- [diff-name-only](#diff-name-only)
- [diff-unified](#diff-unified)
- [diff-ignore-all-space](#diff-ignore-all-space)
- [diff-no-prefix](#diff-no-prefix)
- [diff-src-prefix](#diff-src-prefix)
- [diff-dst-prefix](#diff-dst-prefix)
- [diff-text](#diff-text)
- [global](#global)
- [tag](#tag)
- [workspace](#workspace)
- [workspaces](#workspaces)
- [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm diff [...<paths>]
```
###
Description
Similar to its `git diff` counterpart, this command will print diff patches of files for packages published to the npm registry.
* `npm diff --diff=<spec-a> --diff=<spec-b>`
Compares two package versions using their registry specifiers, e.g: `npm diff [email protected] --diff=pkg@^2.0.0`. It's also possible to compare across forks of any package, e.g: `npm diff [email protected] [email protected]`.
Any valid spec can be used, so that it's also possible to compare directories or git repositories, e.g: `npm diff --diff=pkg@latest --diff=./packages/pkg`
Here's an example comparing two different versions of a package named `abbrev` from the registry:
```
npm diff [email protected] [email protected]
```
On success, output looks like:
```
diff --git a/package.json b/package.json
index v1.1.0..v1.1.1 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "abbrev",
- "version": "1.1.0",
+ "version": "1.1.1",
"description": "Like ruby's abbrev module, but in js",
"author": "Isaac Z. Schlueter <[email protected]>",
"main": "abbrev.js",
```
Given the flexible nature of npm specs, you can also target local directories or git repos just like when using `npm install`:
```
npm diff --diff=https://github.com/npm/libnpmdiff --diff=./local-path
```
In the example above we can compare the contents from the package installed from the git repo at `github.com/npm/libnpmdiff` with the contents of the `./local-path` that contains a valid package, such as a modified copy of the original.
* `npm diff` (in a package directory, no arguments):
If the package is published to the registry, `npm diff` will fetch the tarball version tagged as `latest` (this value can be configured using the `tag` option) and proceed to compare the contents of files present in that tarball, with the current files in your local file system.
This workflow provides a handy way for package authors to see what package-tracked files have been changed in comparison with the latest published version of that package.
* `npm diff --diff=<pkg-name>` (in a package directory):
When using a single package name (with no version or tag specifier) as an argument, `npm diff` will work in a similar way to [`npm-outdated`](npm-outdated) and reach for the registry to figure out what current published version of the package named `<pkg-name>` will satisfy its dependent declared semver-range. Once that specific version is known `npm diff` will print diff patches comparing the current version of `<pkg-name>` found in the local file system with that specific version returned by the registry.
Given a package named `abbrev` that is currently installed:
```
npm diff --diff=abbrev
```
That will request from the registry its most up to date version and will print a diff output comparing the currently installed version to this newer one if the version numbers are not the same.
* `npm diff --diff=<spec-a>` (in a package directory):
Similar to using only a single package name, it's also possible to declare a full registry specifier version if you wish to compare the local version of an installed package with the specific version/tag/semver-range provided in `<spec-a>`.
An example: assuming `[email protected]` is installed in the current `node_modules` folder, running:
```
npm diff [email protected]
```
It will effectively be an alias to `npm diff [email protected] [email protected]`.
* `npm diff --diff=<semver-a> [--diff=<semver-b>]` (in a package directory):
Using `npm diff` along with semver-valid version numbers is a shorthand to compare different versions of the current package.
It needs to be run from a package directory, such that for a package named `pkg` running `npm diff --diff=1.0.0 --diff=1.0.1` is the same as running `npm diff [email protected] [email protected]`.
If only a single argument `<version-a>` is provided, then the current local file system is going to be compared against that version.
Here's an example comparing two specific versions (published to the configured registry) of the current project directory:
```
npm diff --diff=1.0.0 --diff=1.1.0
```
Note that tag names are not valid `--diff` argument values, if you wish to compare to a published tag, you must use the `pkg@tagname` syntax.
####
Filtering files
It's possible to also specify positional arguments using file names or globs pattern matching in order to limit the result of diff patches to only a subset of files for a given package, e.g:
```
npm diff --diff=pkg@2 ./lib/ CHANGELOG.md
```
In the example above the diff output is only going to print contents of files located within the folder `./lib/` and changed lines of code within the `CHANGELOG.md` file.
###
Configuration
####
`diff`
* Default:
* Type: String (can be set multiple times)
Define arguments to compare in `npm diff`.
####
`diff-name-only`
* Default: false
* Type: Boolean
Prints only filenames when using `npm diff`.
####
`diff-unified`
* Default: 3
* Type: Number
The number of lines of context to print in `npm diff`.
####
`diff-ignore-all-space`
* Default: false
* Type: Boolean
Ignore whitespace when comparing lines in `npm diff`.
####
`diff-no-prefix`
* Default: false
* Type: Boolean
Do not show any source or destination prefix in `npm diff` output.
Note: this causes `npm diff` to ignore the `--diff-src-prefix` and `--diff-dst-prefix` configs.
####
`diff-src-prefix`
* Default: "a/"
* Type: String
Source prefix to be used in `npm diff` output.
####
`diff-dst-prefix`
* Default: "b/"
* Type: String
Destination prefix to be used in `npm diff` output.
####
`diff-text`
* Default: false
* Type: Boolean
Treat all files as text in `npm diff`.
####
`global`
* Default: false
* Type: Boolean
Operates in "global" mode, so that packages are installed into the `prefix` folder instead of the current working directory. See [folders](../configuring-npm/folders) for more on the differences in behavior.
* packages are installed into the `{prefix}/lib/node_modules` folder, instead of the current working directory.
* bin files are linked to `{prefix}/bin`
* man pages are linked to `{prefix}/share/man`
####
`tag`
* Default: "latest"
* Type: String
If you ask npm to install a package and don't tell it a specific version, then it will install the specified tag.
Also the tag that is added to the package@version specified by the `npm tag` command, if no explicit tag is given.
When used by the `npm diff` command, this is the tag used to fetch the tarball that will be compared with the local files by default.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
See Also
---------
* [npm outdated](npm-outdated)
* [npm install](npm-install)
* [npm config](npm-config)
* [npm registry](../using-npm/registry)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-diff.md)
| programming_docs |
npm npm-deprecate npm-deprecate
=============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Deprecate a version of a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm deprecate <package-spec> <message>
```
Note: This command is unaware of workspaces.
###
Description
This command will update the npm registry entry for a package, providing a deprecation warning to all who attempt to install it.
It works on [version ranges](https://semver.npmjs.com/) as well as specific versions, so you can do something like this:
```
npm deprecate my-thing@"< 0.2.3" "critical bug fixed in v0.2.3"
```
SemVer ranges passed to this command are interpreted such that they *do* include prerelease versions. For example:
```
npm deprecate [email protected] "1.x is no longer supported"
```
In this case, a version `[email protected]` will also be deprecated.
You must be the package owner to deprecate something. See the `owner` and `adduser` help topics.
To un-deprecate a package, specify an empty string (`""`) for the `message` argument. Note that you must use double quotes with no space between them to format an empty string.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm publish](npm-publish)
* [npm registry](../using-npm/registry)
* [npm owner](npm-owner)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-deprecate.md)
npm npm-test npm-test
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Test a package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Example](#example)
* [Configuration](#configuration)
+ [ignore-scripts](#ignore-scripts)
+ [script-shell](#script-shell)
* [See Also](#see-also)
###
Synopsis
```
npm test [-- <args>]
aliases: tst, t
```
###
Description
This runs a predefined command specified in the `"test"` property of a package's `"scripts"` object.
###
Example
```
{
"scripts": {
"test": "node test.js"
}
}
```
```
npm test
> [email protected] test
> node test.js
(test.js output would be here)
```
###
Configuration
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`script-shell`
* Default: '/bin/sh' on POSIX systems, 'cmd.exe' on Windows
* Type: null or String
The shell to use for scripts run with the `npm exec`, `npm run` and `npm
init <package-spec>` commands.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm start](npm-start)
* [npm restart](npm-restart)
* [npm stop](npm-stop)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-test.md)
npm npm-edit npm-edit
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Edit an installed package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [editor](#editor)
* [See Also](#see-also)
###
Synopsis
```
npm edit <pkg>[/<subpkg>...]
```
Note: This command is unaware of workspaces.
###
Description
Selects a dependency in the current project and opens the package folder in the default editor (or whatever you've configured as the npm `editor` config -- see [`npm-config`](npm-config).)
After it has been edited, the package is rebuilt so as to pick up any changes in compiled packages.
For instance, you can do `npm install connect` to install connect into your package, and then `npm edit connect` to make a few changes to your locally installed copy.
###
Configuration
####
`editor`
* Default: The EDITOR or VISUAL environment variables, or 'notepad.exe' on Windows, or 'vim' on Unix systems
* Type: String
The command to run for `npm edit` and `npm config edit`.
###
See Also
* [npm folders](../configuring-npm/folders)
* [npm explore](npm-explore)
* [npm install](npm-install)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-edit.md)
npm npm-dist-tag npm-dist-tag
============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Modify package distribution tags
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Purpose](#purpose)
* [Caveats](#caveats)
* [Configuration](#configuration)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm dist-tag add <package-spec (with version)> [<tag>]
npm dist-tag rm <package-spec> <tag>
npm dist-tag ls [<package-spec>]
alias: dist-tags
```
###
Description
Add, remove, and enumerate distribution tags on a package:
* add: Tags the specified version of the package with the specified tag, or the `--tag` config if not specified. If you have two-factor authentication on auth-and-writes then you’ll need to include a one-time password on the command line with `--otp <one-time password>`, or at the OTP prompt.
* rm: Clear a tag that is no longer in use from the package. If you have two-factor authentication on auth-and-writes then you’ll need to include a one-time password on the command line with `--otp <one-time password>`, or at the OTP prompt.
* ls: Show all of the dist-tags for a package, defaulting to the package in the current prefix. This is the default action if none is specified.
A tag can be used when installing packages as a reference to a version instead of using a specific version number:
```
npm install <name>@<tag>
```
When installing dependencies, a preferred tagged version may be specified:
```
npm install --tag <tag>
```
(This also applies to any other commands that resolve and install dependencies, such as `npm dedupe`, `npm update`, and `npm audit fix`.)
Publishing a package sets the `latest` tag to the published version unless the `--tag` option is used. For example, `npm publish --tag=beta`.
By default, `npm install <pkg>` (without any `@<version>` or `@<tag>` specifier) installs the `latest` tag.
###
Purpose
Tags can be used to provide an alias instead of version numbers.
For example, a project might choose to have multiple streams of development and use a different tag for each stream, e.g., `stable`, `beta`, `dev`, `canary`.
By default, the `latest` tag is used by npm to identify the current version of a package, and `npm install <pkg>` (without any `@<version>` or `@<tag>` specifier) installs the `latest` tag. Typically, projects only use the `latest` tag for stable release versions, and use other tags for unstable versions such as prereleases.
The `next` tag is used by some projects to identify the upcoming version.
Other than `latest`, no tag has any special significance to npm itself.
###
Caveats
This command used to be known as `npm tag`, which only created new tags, and so had a different syntax.
Tags must share a namespace with version numbers, because they are specified in the same slot: `npm install <pkg>@<version>` vs `npm install <pkg>@<tag>`.
Tags that can be interpreted as valid semver ranges will be rejected. For example, `v1.4` cannot be used as a tag, because it is interpreted by semver as `>=1.4.0 <1.5.0`. See <https://github.com/npm/npm/issues/6082>.
The simplest way to avoid semver problems with tags is to use tags that do not begin with a number or the letter `v`.
###
Configuration
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm publish](npm-publish)
* [npm install](npm-install)
* [npm dedupe](npm-dedupe)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-dist-tag.md)
npm npm-token npm-token
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Manage your authentication tokens
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [read-only](#read-only)
+ [cidr](#cidr)
+ [registry](#registry)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm token list
npm token revoke <id|token>
npm token create [--read-only] [--cidr=list]
```
Note: This command is unaware of workspaces.
###
Description
This lets you list, create and revoke authentication tokens.
* `npm token list`: Shows a table of all active authentication tokens. You can request this as JSON with `--json` or tab-separated values with `--parseable`.
```
+--------+---------+------------+----------+----------------+
| id | token | created | read-only | CIDR whitelist |
+--------+---------+------------+----------+----------------+
| 7f3134 | 1fa9ba… | 2017-10-02 | yes | |
+--------+---------+------------+----------+----------------+
| c03241 | af7aef… | 2017-10-02 | no | 192.168.0.1/24 |
+--------+---------+------------+----------+----------------+
| e0cf92 | 3a436a… | 2017-10-02 | no | |
+--------+---------+------------+----------+----------------+
| 63eb9d | 74ef35… | 2017-09-28 | no | |
+--------+---------+------------+----------+----------------+
| 2daaa8 | cbad5f… | 2017-09-26 | no | |
+--------+---------+------------+----------+----------------+
| 68c2fe | 127e51… | 2017-09-23 | no | |
+--------+---------+------------+----------+----------------+
| 6334e1 | 1dadd1… | 2017-09-23 | no | |
+--------+---------+------------+----------+----------------+
```
* `npm token create [--read-only] [--cidr=<cidr-ranges>]`: Create a new authentication token. It can be `--read-only`, or accept a list of [CIDR](https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing) ranges with which to limit use of this token. This will prompt you for your password, and, if you have two-factor authentication enabled, an otp.
Currently, the cli can not generate automation tokens. Please refer to the [docs website](../../../creating-and-viewing-access-tokens) for more information on generating automation tokens.
```
+----------------+--------------------------------------+
| token | a73c9572-f1b9-8983-983d-ba3ac3cc913d |
+----------------+--------------------------------------+
| cidr_whitelist | |
+----------------+--------------------------------------+
| readonly | false |
+----------------+--------------------------------------+
| created | 2017-10-02T07:52:24.838Z |
+----------------+--------------------------------------+
```
* `npm token revoke <token|id>`: Immediately removes an authentication token from the registry. You will no longer be able to use it. This can accept both complete tokens (such as those you get back from `npm token create`, and those found in your `.npmrc`), and ids as seen in the parseable or json output of `npm token list`. This will NOT accept the truncated token found in the normal `npm token list` output.
###
Configuration
####
`read-only`
* Default: false
* Type: Boolean
This is used to mark a token as unable to publish when configuring limited access tokens with the `npm token create` command.
####
`cidr`
* Default: null
* Type: null or String (can be set multiple times)
This is a list of CIDR address to be used when configuring limited access tokens with the `npm token create` command.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [npm adduser](npm-adduser)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm owner](npm-owner)
* [npm whoami](npm-whoami)
* [npm profile](npm-profile)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-token.md)
| programming_docs |
npm npm-explore npm-explore
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Browse an installed package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [shell](#shell)
* [See Also](#see-also)
###
Synopsis
```
npm explore <pkg> [ -- <command>]
```
Note: This command is unaware of workspaces.
###
Description
Spawn a subshell in the directory of the installed package specified.
If a command is specified, then it is run in the subshell, which then immediately terminates.
This is particularly handy in the case of git submodules in the `node_modules` folder:
```
npm explore some-dependency -- git pull origin master
```
Note that the package is *not* automatically rebuilt afterwards, so be sure to use `npm rebuild <pkg>` if you make any changes.
###
Configuration
####
`shell`
* Default: SHELL environment variable, or "bash" on Posix, or "cmd.exe" on Windows
* Type: String
The shell to run for the `npm explore` command.
###
See Also
* [npm folders](../configuring-npm/folders)
* [npm edit](npm-edit)
* [npm rebuild](npm-rebuild)
* [npm install](npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-explore.md)
npm npm-search npm-search
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Search for packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [long](#long)
+ [json](#json)
+ [color](#color)
+ [parseable](#parseable)
+ [description](#description-1)
+ [searchopts](#searchopts)
+ [searchexclude](#searchexclude)
+ [registry](#registry)
+ [prefer-online](#prefer-online)
+ [prefer-offline](#prefer-offline)
+ [offline](#offline)
* [See Also](#see-also)
###
Synopsis
```
npm search [search terms ...]
aliases: find, s, se
```
Note: This command is unaware of workspaces.
###
Description
Search the registry for packages matching the search terms. `npm search` performs a linear, incremental, lexically-ordered search through package metadata for all files in the registry. If your terminal has color support, it will further highlight the matches in the results. This can be disabled with the config item `color`
Additionally, using the `--searchopts` and `--searchexclude` options paired with more search terms will include and exclude further patterns. The main difference between `--searchopts` and the standard search terms is that the former does not highlight results in the output and you can use them more fine-grained filtering. Additionally, you can add both of these to your config to change default search filtering behavior.
Search also allows targeting of maintainers in search results, by prefixing their npm username with `=`.
If a term starts with `/`, then it's interpreted as a regular expression and supports standard JavaScript RegExp syntax. In this case search will ignore a trailing `/` . (Note you must escape or quote many regular expression characters in most shells.)
###
Configuration
####
`long`
* Default: false
* Type: Boolean
Show extended information in `ls`, `search`, and `help-search`.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`color`
* Default: true unless the NO\_COLOR environ is set to something other than '0'
* Type: "always" or Boolean
If false, never shows colors. If `"always"` then always shows colors. If true, then only prints color codes for tty file descriptors.
####
`parseable`
* Default: false
* Type: Boolean
Output parseable results from commands that write to standard output. For `npm search`, this will be tab-separated table format.
####
`description`
* Default: true
* Type: Boolean
Show the description in `npm search`
####
`searchopts`
* Default: ""
* Type: String
Space-separated options that are always passed to search.
####
`searchexclude`
* Default: ""
* Type: String
Space-separated options that limit the results from search.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`prefer-online`
* Default: false
* Type: Boolean
If true, staleness checks for cached data will be forced, making the CLI look for updates immediately even for fresh package data.
####
`prefer-offline`
* Default: false
* Type: Boolean
If true, staleness checks for cached data will be bypassed, but missing data will be requested from the server. To force full offline mode, use `--offline`.
####
`offline`
* Default: false
* Type: Boolean
Force offline mode: no network requests will be done during install. To allow the CLI to fill in missing cache data, see `--prefer-offline`.
###
See Also
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm view](npm-view)
* [npm cache](npm-cache)
* <https://npm.im/npm-registry-fetch>
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-search.md)
npm npm-unstar npm-unstar
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Remove an item from your favorite packages
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [More](#more)
+ [Star](#star)
+ [Listing stars](#listing-stars)
* [Configuration](#configuration)
+ [registry](#registry)
+ [unicode](#unicode)
+ [otp](#otp)
* [See Also](#see-also)
###
Synopsis
```
npm unstar [<package-spec>...]
```
Note: This command is unaware of workspaces.
###
Description
"Unstarring" a package is the opposite of [`npm star`](npm-star), it removes an item from your list of favorite packages.
###
More
There's also these extra commands to help you manage your favorite packages:
####
Star
You can "star" a package using [`npm star`](npm-star)
####
Listing stars
You can see all your starred packages using [`npm stars`](npm-stars)
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`unicode`
* Default: false on windows, true on mac/unix systems with a unicode locale, as defined by the `LC_ALL`, `LC_CTYPE`, or `LANG` environment variables.
* Type: Boolean
When set to true, npm uses unicode characters in the tree output. When false, it uses ascii characters instead of unicode glyphs.
####
`otp`
* Default: null
* Type: null or String
This is a one-time password from a two-factor authenticator. It's needed when publishing or changing package permissions with `npm access`.
If not set, and a registry response fails with a challenge for a one-time password, npm will prompt on the command line for one.
###
See Also
* [npm star](npm-star)
* [npm stars](npm-stars)
* [npm view](npm-view)
* [npm whoami](npm-whoami)
* [npm adduser](npm-adduser)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-unstar.md)
npm npm-version npm-version
===========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Bump a package version
Table of contents* [Synopsis](#synopsis)
* [Configuration](#configuration)
+ [allow-same-version](#allow-same-version)
+ [commit-hooks](#commit-hooks)
+ [git-tag-version](#git-tag-version)
+ [json](#json)
+ [preid](#preid)
+ [sign-git-tag](#sign-git-tag)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [workspaces-update](#workspaces-update)
+ [include-workspace-root](#include-workspace-root)
* [Description](#description)
* [See Also](#see-also)
###
Synopsis
```
npm version [<newversion> | major | minor | patch | premajor | preminor | prepatch | prerelease | from-git]
alias: verison
```
###
Configuration
####
`allow-same-version`
* Default: false
* Type: Boolean
Prevents throwing an error when `npm version` is used to set the new version to the same value as the current version.
####
`commit-hooks`
* Default: true
* Type: Boolean
Run git commit hooks when using the `npm version` command.
####
`git-tag-version`
* Default: true
* Type: Boolean
Tag the commit when using the `npm version` command. Setting this to false results in no commit being made at all.
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`preid`
* Default: ""
* Type: String
The "prerelease identifier" to use as a prefix for the "prerelease" part of a semver. Like the `rc` in `1.2.0-rc.8`.
####
`sign-git-tag`
* Default: false
* Type: Boolean
If set to true, then the `npm version` command will tag the version using `-s` to add a signature.
Note that git requires you to have set up GPG keys in your git configs for this to work properly.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`workspaces-update`
* Default: true
* Type: Boolean
If set to true, the npm cli will run an update after operations that may possibly change the workspaces installed to the `node_modules` folder.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
Description
Run this in a package directory to bump the version and write the new data back to `package.json`, `package-lock.json`, and, if present, `npm-shrinkwrap.json`.
The `newversion` argument should be a valid semver string, a valid second argument to [semver.inc](https://github.com/npm/node-semver#functions) (one of `patch`, `minor`, `major`, `prepatch`, `preminor`, `premajor`, `prerelease`), or `from-git`. In the second case, the existing version will be incremented by 1 in the specified field. `from-git` will try to read the latest git tag, and use that as the new npm version.
If run in a git repo, it will also create a version commit and tag. This behavior is controlled by `git-tag-version` (see below), and can be disabled on the command line by running `npm --no-git-tag-version version`. It will fail if the working directory is not clean, unless the `-f` or `--force` flag is set.
If supplied with `-m` or `--message` config option, npm will use it as a commit message when creating a version commit. If the `message` config contains `%s` then that will be replaced with the resulting version number. For example:
```
npm version patch -m "Upgrade to %s for reasons"
```
If the `sign-git-tag` config is set, then the tag will be signed using the `-s` flag to git. Note that you must have a default GPG key set up in your git config for this to work properly. For example:
```
$ npm config set sign-git-tag true
$ npm version patch
You need a passphrase to unlock the secret key for
user: "isaacs (http://blog.izs.me/) <[email protected]>"
2048-bit RSA key, ID 6C481CF6, created 2010-08-31
Enter passphrase:
```
If `preversion`, `version`, or `postversion` are in the `scripts` property of the package.json, they will be executed as part of running `npm
version`.
The exact order of execution is as follows:
1. Check to make sure the git working directory is clean before we get started. Your scripts may add files to the commit in future steps. This step is skipped if the `--force` flag is set.
2. Run the `preversion` script. These scripts have access to the old `version` in package.json. A typical use would be running your full test suite before deploying. Any files you want added to the commit should be explicitly added using `git add`.
3. Bump `version` in `package.json` as requested (`patch`, `minor`, `major`, etc).
4. Run the `version` script. These scripts have access to the new `version` in package.json (so they can incorporate it into file headers in generated files for example). Again, scripts should explicitly add generated files to the commit using `git add`.
5. Commit and tag.
6. Run the `postversion` script. Use it to clean up the file system or automatically push the commit and/or tag.
Take the following example:
```
{
"scripts": {
"preversion": "npm test",
"version": "npm run build && git add -A dist",
"postversion": "git push && git push --tags && rm -rf build/temp"
}
}
```
This runs all your tests and proceeds only if they pass. Then runs your `build` script, and adds everything in the `dist` directory to the commit. After the commit, it pushes the new commit and tag up to the server, and deletes the `build/temp` directory.
###
See Also
* [npm init](npm-init)
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [package.json](../configuring-npm/package-json)
* [config](../using-npm/config)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-version.md)
| programming_docs |
npm npm npm
===
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
javascript package manager
Table of contents* [Synopsis](#synopsis)
* [Version](#version)
* [Description](#description)
* [Important](#important)
* [Introduction](#introduction)
* [Dependencies](#dependencies)
* [Directories](#directories)
* [Developer Usage](#developer-usage)
+ [Configuration](#configuration)
* [Contributions](#contributions)
* [Bugs](#bugs)
* [Feature Requests](#feature-requests)
* [See Also](#see-also)
###
Synopsis
###
Version
8.19.2
###
Description
npm is the package manager for the Node JavaScript platform. It puts modules in place so that node can find them, and manages dependency conflicts intelligently.
It is extremely configurable to support a variety of use cases. Most commonly, you use it to publish, discover, install, and develop node programs.
Run `npm help` to get a list of available commands.
###
Important
npm comes preconfigured to use npm's public registry at <https://registry.npmjs.org> by default. Use of the npm public registry is subject to terms of use available at <https://docs.npmjs.com/policies/terms>.
You can configure npm to use any compatible registry you like, and even run your own registry. Use of someone else's registry is governed by their terms of use.
###
Introduction
You probably got npm because you want to install stuff.
The very first thing you will most likely want to run in any node program is `npm install` to install its dependencies.
You can also run `npm install blerg` to install the latest version of "blerg". Check out [`npm install`](npm-install) for more info. It can do a lot of stuff.
Use the `npm search` command to show everything that's available in the public registry. Use `npm ls` to show everything you've installed.
###
Dependencies
If a package lists a dependency using a git URL, npm will install that dependency using the [`git`](https://github.com/git-guides/install-git) command and will generate an error if it is not installed.
If one of the packages npm tries to install is a native node module and requires compiling of C++ Code, npm will use [node-gyp](https://github.com/nodejs/node-gyp) for that task. For a Unix system, [node-gyp](https://github.com/nodejs/node-gyp) needs Python, make and a buildchain like GCC. On Windows, Python and Microsoft Visual Studio C++ are needed. For more information visit [the node-gyp repository](https://github.com/nodejs/node-gyp) and the [node-gyp Wiki](https://github.com/nodejs/node-gyp/wiki).
###
Directories
See [`folders`](../configuring-npm/folders) to learn about where npm puts stuff.
In particular, npm has two modes of operation:
* local mode: npm installs packages into the current project directory, which defaults to the current working directory. Packages install to `./node_modules`, and bins to `./node_modules/.bin`.
* global mode: npm installs packages into the install prefix at `$npm_config_prefix/lib/node_modules` and bins to `$npm_config_prefix/bin`.
Local mode is the default. Use `-g` or `--global` on any command to run in global mode instead.
###
Developer Usage
If you're using npm to develop and publish your code, check out the following help topics:
* json: Make a package.json file. See [`package.json`](../configuring-npm/package-json).
* link: Links your current working code into Node's path, so that you don't have to reinstall every time you make a change. Use [`npm
link`](npm-link) to do this.
* install: It's a good idea to install things if you don't need the symbolic link. Especially, installing other peoples code from the registry is done via [`npm install`](npm-install)
* adduser: Create an account or log in. When you do this, npm will store credentials in the user config file.
* publish: Use the [`npm publish`](npm-publish) command to upload your code to the registry.
####
Configuration
npm is extremely configurable. It reads its configuration options from 5 places.
* Command line switches: Set a config with `--key val`. All keys take a value, even if they are booleans (the config parser doesn't know what the options are at the time of parsing). If you do not provide a value (`--key`) then the option is set to boolean `true`.
* Environment Variables: Set any config by prefixing the name in an environment variable with `npm_config_`. For example, `export npm_config_key=val`.
* User Configs: The file at `$HOME/.npmrc` is an ini-formatted list of configs. If present, it is parsed. If the `userconfig` option is set in the cli or env, that file will be used instead.
* Global Configs: The file found at `./etc/npmrc` (relative to the global prefix will be parsed if it is found. See [`npm prefix`](npm-prefix) for more info on the global prefix. If the `globalconfig` option is set in the cli, env, or user config, then that file is parsed instead.
* Defaults: npm's default configuration options are defined in lib/utils/config-defs.js. These must not be changed.
See [`config`](../using-npm/config) for much much more information.
###
Contributions
Patches welcome!
If you would like to help, but don't know what to work on, read the [contributing guidelines](https://github.com/npm/cli/blob/latest/CONTRIBUTING.md) and check the issues list.
###
Bugs
When you find issues, please report them: <https://github.com/npm/cli/issues>
Please be sure to follow the template and bug reporting guidelines.
###
Feature Requests
Discuss new feature ideas on our discussion forum:
* <https://github.com/npm/feedback>
Or suggest formal RFC proposals:
* <https://github.com/npm/rfcs>
###
See Also
* [npm help](npm-help)
* [package.json](../configuring-npm/package-json)
* [npmrc](../configuring-npm/npmrc)
* [npm config](npm-config)
* [npm install](npm-install)
* [npm prefix](npm-prefix)
* [npm publish](npm-publish)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm.md)
npm npx npx
===
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Run a command from a local or remote npm package
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [npx vs npm exec](#npx-vs-npm-exec)
* [Examples](#examples)
* [Compatibility with Older npx Versions](#compatibility-with-older-npx-versions)
* [See Also](#see-also)
###
Synopsis
```
npx -- <pkg>[@<version>] [args...]
npx --package=<pkg>[@<version>] -- <cmd> [args...]
npx -c '<cmd> [args...]'
npx --package=foo -c '<cmd> [args...]'
```
###
Description
This command allows you to run an arbitrary command from an npm package (either one installed locally, or fetched remotely), in a similar context as running it via `npm run`.
Whatever packages are specified by the `--package` option will be provided in the `PATH` of the executed command, along with any locally installed package executables. The `--package` option may be specified multiple times, to execute the supplied command in an environment where all specified packages are available.
If any requested packages are not present in the local project dependencies, then they are installed to a folder in the npm cache, which is added to the `PATH` environment variable in the executed process. A prompt is printed (which can be suppressed by providing either `--yes` or `--no`).
Package names provided without a specifier will be matched with whatever version exists in the local project. Package names with a specifier will only be considered a match if they have the exact same name and version as the local dependency.
If no `-c` or `--call` option is provided, then the positional arguments are used to generate the command string. If no `--package` options are provided, then npm will attempt to determine the executable name from the package specifier provided as the first positional argument according to the following heuristic:
* If the package has a single entry in its `bin` field in `package.json`, or if all entries are aliases of the same command, then that command will be used.
* If the package has multiple `bin` entries, and one of them matches the unscoped portion of the `name` field, then that command will be used.
* If this does not result in exactly one option (either because there are no bin entries, or none of them match the `name` of the package), then `npm exec` exits with an error.
To run a binary *other than* the named binary, specify one or more `--package` options, which will prevent npm from inferring the package from the first command argument.
###
`npx` vs `npm exec`
When run via the `npx` binary, all flags and options *must* be set prior to any positional arguments. When run via `npm exec`, a double-hyphen `--` flag can be used to suppress npm's parsing of switches and options that should be sent to the executed command.
For example:
```
$ npx foo@latest bar --package=@npmcli/foo
```
In this case, npm will resolve the `foo` package name, and run the following command:
```
$ foo bar --package=@npmcli/foo
```
Since the `--package` option comes *after* the positional arguments, it is treated as an argument to the executed command.
In contrast, due to npm's argument parsing logic, running this command is different:
```
$ npm exec foo@latest bar --package=@npmcli/foo
```
In this case, npm will parse the `--package` option first, resolving the `@npmcli/foo` package. Then, it will execute the following command in that context:
```
$ foo@latest bar
```
The double-hyphen character is recommended to explicitly tell npm to stop parsing command line options and switches. The following command would thus be equivalent to the `npx` command above:
```
$ npm exec -- foo@latest bar --package=@npmcli/foo
```
###
Examples
Run the version of `tap` in the local dependencies, with the provided arguments:
```
$ npm exec -- tap --bail test/foo.js
$ npx tap --bail test/foo.js
```
Run a command *other than* the command whose name matches the package name by specifying a `--package` option:
```
$ npm exec --package=foo -- bar --bar-argument
# ~ or ~
$ npx --package=foo bar --bar-argument
```
Run an arbitrary shell script, in the context of the current project:
```
$ npm x -c 'eslint && say "hooray, lint passed"'
$ npx -c 'eslint && say "hooray, lint passed"'
```
###
Compatibility with Older npx Versions
The `npx` binary was rewritten in npm v7.0.0, and the standalone `npx` package deprecated at that time. `npx` uses the `npm exec` command instead of a separate argument parser and install process, with some affordances to maintain backwards compatibility with the arguments it accepted in previous versions.
This resulted in some shifts in its functionality:
* Any `npm` config value may be provided.
* To prevent security and user-experience problems from mistyping package names, `npx` prompts before installing anything. Suppress this prompt with the `-y` or `--yes` option.
* The `--no-install` option is deprecated, and will be converted to `--no`.
* Shell fallback functionality is removed, as it is not advisable.
* The `-p` argument is a shorthand for `--parseable` in npm, but shorthand for `--package` in npx. This is maintained, but only for the `npx` executable.
* The `--ignore-existing` option is removed. Locally installed bins are always present in the executed process `PATH`.
* The `--npm` option is removed. `npx` will always use the `npm` it ships with.
* The `--node-arg` and `-n` options are removed.
* The `--always-spawn` option is redundant, and thus removed.
* The `--shell` option is replaced with `--script-shell`, but maintained in the `npx` executable for backwards compatibility.
###
See Also
* [npm run-script](npm-run-script)
* [npm scripts](../using-npm/scripts)
* [npm test](npm-test)
* [npm start](npm-start)
* [npm restart](npm-restart)
* [npm stop](npm-stop)
* [npm config](npm-config)
* [npm exec](npm-exec)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npx.md)
npm npm-audit npm-audit
=========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Run a security audit
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Audit Signatures](#audit-signatures)
* [Audit Endpoints](#audit-endpoints)
+ [Bulk Advisory Endpoint](#bulk-advisory-endpoint)
+ [Quick Audit Endpoint](#quick-audit-endpoint)
+ [Scrubbing](#scrubbing)
+ [Calculating Meta-Vulnerabilities and Remediations](#calculating-meta-vulnerabilities-and-remediations)
* [Exit Code](#exit-code)
* [Examples](#examples)
* [Configuration](#configuration)
+ [audit-level](#audit-level)
+ [dry-run](#dry-run)
+ [force](#force)
+ [json](#json)
+ [package-lock-only](#package-lock-only)
+ [omit](#omit)
+ [foreground-scripts](#foreground-scripts)
+ [ignore-scripts](#ignore-scripts)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm audit [fix|signatures]
```
###
Description
The audit command submits a description of the dependencies configured in your project to your default registry and asks for a report of known vulnerabilities. If any vulnerabilities are found, then the impact and appropriate remediation will be calculated. If the `fix` argument is provided, then remediations will be applied to the package tree.
The command will exit with a 0 exit code if no vulnerabilities were found.
Note that some vulnerabilities cannot be fixed automatically and will require manual intervention or review. Also note that since `npm audit
fix` runs a full-fledged `npm install` under the hood, all configs that apply to the installer will also apply to `npm install` -- so things like `npm audit fix --package-lock-only` will work as expected.
By default, the audit command will exit with a non-zero code if any vulnerability is found. It may be useful in CI environments to include the `--audit-level` parameter to specify the minimum vulnerability level that will cause the command to fail. This option does not filter the report output, it simply changes the command's failure threshold.
###
Audit Signatures
To ensure the integrity of packages you download from the public npm registry, or any registry that supports signatures, you can verify the registry signatures of downloaded packages using the npm CLI.
Registry signatures can be verified using the following `audit` command:
```
$ npm audit signatures
```
The npm CLI supports registry signatures and signing keys provided by any registry if the following conventions are followed:
1. Signatures are provided in the package's `packument` in each published version within the `dist` object:
```
"dist":{
"..omitted..": "..omitted..",
"signatures": [{
"keyid": "SHA256:{{SHA256_PUBLIC_KEY}}",
"sig": "a312b9c3cb4a1b693e8ebac5ee1ca9cc01f2661c14391917dcb111517f72370809..."
}]
}
```
See this [example](https://registry.npmjs.org/light-cycle/1.4.3) of a signed package from the public npm registry.
The `sig` is generated using the following template: `${package.name}@${package.version}:${package.dist.integrity}` and the `keyid` has to match one of the public signing keys below.
2. Public signing keys are provided at `registry-host.tld/-/npm/v1/keys` in the following format:
```
{
"keys": [{
"expires": null,
"keyid": "SHA256:{{SHA256_PUBLIC_KEY}}",
"keytype": "ecdsa-sha2-nistp256",
"scheme": "ecdsa-sha2-nistp256",
"key": "{{B64_PUBLIC_KEY}}"
}]
}
```
Keys response:
* `expires`: null or a simplified extended [ISO 8601 format](https://en.wikipedia.org/wiki/ISO_8601): `YYYY-MM-DDTHH:mm:ss.sssZ`
* `keydid`: sha256 fingerprint of the public key
* `keytype`: only `ecdsa-sha2-nistp256` is currently supported by the npm CLI
* `scheme`: only `ecdsa-sha2-nistp256` is currently supported by the npm CLI
* `key`: base64 encoded public key
See this [example key's response from the public npm registry](https://registry.npmjs.org/-/npm/v1/keys).
###
Audit Endpoints
There are two audit endpoints that npm may use to fetch vulnerability information: the `Bulk Advisory` endpoint and the `Quick Audit` endpoint.
####
Bulk Advisory Endpoint
As of version 7, npm uses the much faster `Bulk Advisory` endpoint to optimize the speed of calculating audit results.
npm will generate a JSON payload with the name and list of versions of each package in the tree, and POST it to the default configured registry at the path `/-/npm/v1/security/advisories/bulk`.
Any packages in the tree that do not have a `version` field in their package.json file will be ignored. If any `--omit` options are specified (either via the `--omit` config, or one of the shorthands such as `--production`, `--only=dev`, and so on), then packages will be omitted from the submitted payload as appropriate.
If the registry responds with an error, or with an invalid response, then npm will attempt to load advisory data from the `Quick Audit` endpoint.
The expected result will contain a set of advisory objects for each dependency that matches the advisory range. Each advisory object contains a `name`, `url`, `id`, `severity`, `vulnerable_versions`, and `title`.
npm then uses these advisory objects to calculate vulnerabilities and meta-vulnerabilities of the dependencies within the tree.
####
Quick Audit Endpoint
If the `Bulk Advisory` endpoint returns an error, or invalid data, npm will attempt to load advisory data from the `Quick Audit` endpoint, which is considerably slower in most cases.
The full package tree as found in `package-lock.json` is submitted, along with the following pieces of additional metadata:
* `npm_version`
* `node_version`
* `platform`
* `arch`
* `node_env`
All packages in the tree are submitted to the Quick Audit endpoint. Omitted dependency types are skipped when generating the report.
####
Scrubbing
Out of an abundance of caution, npm versions 5 and 6 would "scrub" any packages from the submitted report if their name contained a `/` character, so as to avoid leaking the names of potentially private packages or git URLs.
However, in practice, this resulted in audits often failing to properly detect meta-vulnerabilities, because the tree would appear to be invalid due to missing dependencies, and prevented the detection of vulnerabilities in package trees that used git dependencies or private modules.
This scrubbing has been removed from npm as of version 7.
####
Calculating Meta-Vulnerabilities and Remediations
npm uses the [`@npmcli/metavuln-calculator`](http://npm.im/@npmcli/metavuln-calculator) module to turn a set of security advisories into a set of "vulnerability" objects. A "meta-vulnerability" is a dependency that is vulnerable by virtue of dependence on vulnerable versions of a vulnerable package.
For example, if the package `foo` is vulnerable in the range `>=1.0.2
<2.0.0`, and the package `bar` depends on `foo@^1.1.0`, then that version of `bar` can only be installed by installing a vulnerable version of `foo`. In this case, `bar` is a "metavulnerability".
Once metavulnerabilities for a given package are calculated, they are cached in the `~/.npm` folder and only re-evaluated if the advisory range changes, or a new version of the package is published (in which case, the new version is checked for metavulnerable status as well).
If the chain of metavulnerabilities extends all the way to the root project, and it cannot be updated without changing its dependency ranges, then `npm audit fix` will require the `--force` option to apply the remediation. If remediations do not require changes to the dependency ranges, then all vulnerable packages will be updated to a version that does not have an advisory or metavulnerability posted against it.
###
Exit Code
The `npm audit` command will exit with a 0 exit code if no vulnerabilities were found. The `npm audit fix` command will exit with 0 exit code if no vulnerabilities are found *or* if the remediation is able to successfully fix all vulnerabilities.
If vulnerabilities were found the exit code will depend on the `audit-level` configuration setting.
###
Examples
Scan your project for vulnerabilities and automatically install any compatible updates to vulnerable dependencies:
```
$ npm audit fix
```
Run `audit fix` without modifying `node_modules`, but still updating the pkglock:
```
$ npm audit fix --package-lock-only
```
Skip updating `devDependencies`:
```
$ npm audit fix --only=prod
```
Have `audit fix` install SemVer-major updates to toplevel dependencies, not just SemVer-compatible ones:
```
$ npm audit fix --force
```
Do a dry run to get an idea of what `audit fix` will do, and *also* output install information in JSON format:
```
$ npm audit fix --dry-run --json
```
Scan your project for vulnerabilities and just show the details, without fixing anything:
```
$ npm audit
```
Get the detailed audit report in JSON format:
```
$ npm audit --json
```
Fail an audit only if the results include a vulnerability with a level of moderate or higher:
```
$ npm audit --audit-level=moderate
```
###
Configuration
####
`audit-level`
* Default: null
* Type: null, "info", "low", "moderate", "high", "critical", or "none"
The minimum level of vulnerability for `npm audit` to exit with a non-zero exit code.
####
`dry-run`
* Default: false
* Type: Boolean
Indicates that you don't want npm to make any changes and that it should only report what it would have done. This can be passed into any of the commands that modify your local installation, eg, `install`, `update`, `dedupe`, `uninstall`, as well as `pack` and `publish`.
Note: This is NOT honored by other network related commands, eg `dist-tags`, `owner`, etc.
####
`force`
* Default: false
* Type: Boolean
Removes various protections against unfortunate side effects, common mistakes, unnecessary performance degradation, and malicious input.
* Allow clobbering non-npm files in global installs.
* Allow the `npm version` command to work on an unclean git repository.
* Allow deleting the cache folder with `npm cache clean`.
* Allow installing packages that have an `engines` declaration requiring a different version of npm.
* Allow installing packages that have an `engines` declaration requiring a different version of `node`, even if `--engine-strict` is enabled.
* Allow `npm audit fix` to install modules outside your stated dependency range (including SemVer-major changes).
* Allow unpublishing all versions of a published package.
* Allow conflicting peerDependencies to be installed in the root project.
* Implicitly set `--yes` during `npm init`.
* Allow clobbering existing values in `npm pkg`
* Allow unpublishing of entire packages (not just a single version).
If you don't have a clear idea of what you want to do, it is strongly recommended that you do not use this option!
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`package-lock-only`
* Default: false
* Type: Boolean
If set to true, the current operation will only use the `package-lock.json`, ignoring `node_modules`.
For `update` this means only the `package-lock.json` will be updated, instead of checking `node_modules` and downloading dependencies.
For `list` this means the output will be based on the tree described by the `package-lock.json`, rather than the contents of `node_modules`.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`foreground-scripts`
* Default: false
* Type: Boolean
Run all build scripts (ie, `preinstall`, `install`, and `postinstall`) scripts for installed packages in the foreground process, sharing standard input, output, and error with the main npm process.
Note that this will generally make installs run slower, and be much noisier, but can be useful for debugging.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm install](npm-install)
* [config](../using-npm/config)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-audit.md)
| programming_docs |
npm npm-repo npm-repo
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Open package repository page in the browser
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [browser](#browser)
+ [registry](#registry)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [See Also](#see-also)
###
Synopsis
```
npm repo [<pkgname> [<pkgname> ...]]
```
###
Description
This command tries to guess at the likely location of a package's repository URL, and then tries to open it using the `--browser` config param. If no package name is provided, it will search for a `package.json` in the current folder and use the `repository` property.
###
Configuration
####
`browser`
* Default: OS X: `"open"`, Windows: `"start"`, Others: `"xdg-open"`
* Type: null, Boolean, or String
The browser that is called by npm commands to open websites.
Set to `false` to suppress browser behavior and instead print urls to terminal.
Set to `true` to use default system URL opener.
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
See Also
* [npm docs](npm-docs)
* [npm config](npm-config)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-repo.md)
npm npm-logout npm-logout
==========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Log out of the registry
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [registry](#registry)
+ [scope](#scope)
* [See Also](#see-also)
###
Synopsis
```
npm logout
```
Note: This command is unaware of workspaces.
###
Description
When logged into a registry that supports token-based authentication, tell the server to end this token's session. This will invalidate the token everywhere you're using it, not just for the current environment.
When logged into a legacy registry that uses username and password authentication, this will clear the credentials in your user configuration. In this case, it will *only* affect the current environment.
If `--scope` is provided, this will find the credentials for the registry connected to that scope, if set.
###
Configuration
####
`registry`
* Default: "[https://registry.npmjs.org/"](https://registry.npmjs.org/%22)
* Type: URL
The base URL of the npm registry.
####
`scope`
* Default: the scope of the current project, if any, or ""
* Type: String
Associate an operation with a scope for a scoped registry.
Useful when logging in to or out of a private registry:
```
# log in, linking the scope to the custom registry
npm login --scope=@mycorp --registry=https://registry.mycorp.com
# log out, removing the link and the auth token
npm logout --scope=@mycorp
```
This will cause `@mycorp` to be mapped to the registry for future installation of packages specified according to the pattern `@mycorp/package`.
This will also cause `npm init` to create a scoped package.
```
# accept all defaults, and create a package named "@foo/whatever",
# instead of just named "whatever"
npm init --scope=@foo --yes
```
###
See Also
* [npm adduser](npm-adduser)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npm whoami](npm-whoami)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-logout.md)
npm npm-find-dupes npm-find-dupes
==============
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
Find duplication in the package tree
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [global-style](#global-style)
+ [legacy-bundling](#legacy-bundling)
+ [strict-peer-deps](#strict-peer-deps)
+ [package-lock](#package-lock)
+ [omit](#omit)
+ [ignore-scripts](#ignore-scripts)
+ [audit](#audit)
+ [bin-links](#bin-links)
+ [fund](#fund)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
+ [install-links](#install-links)
* [See Also](#see-also)
###
Synopsis
```
npm find-dupes
```
###
Description
Runs `npm dedupe` in `--dry-run` mode, making npm only output the duplications, without actually changing the package tree.
###
Configuration
####
`global-style`
* Default: false
* Type: Boolean
Causes npm to install the package into your local `node_modules` folder with the same layout it uses with the global `node_modules` folder. Only your direct dependencies will show in `node_modules` and everything they depend on will be flattened in their `node_modules` folders. This obviously will eliminate some deduping. If used with `legacy-bundling`, `legacy-bundling` will be preferred.
####
`legacy-bundling`
* Default: false
* Type: Boolean
Causes npm to install the package such that versions of npm prior to 1.4, such as the one included with node 0.8, can install the package. This eliminates all automatic deduping. If used with `global-style` this option will be preferred.
####
`strict-peer-deps`
* Default: false
* Type: Boolean
If set to `true`, and `--legacy-peer-deps` is not set, then *any* conflicting `peerDependencies` will be treated as an install failure, even if npm could reasonably guess the appropriate resolution based on non-peer dependency relationships.
By default, conflicting `peerDependencies` deep in the dependency graph will be resolved using the nearest non-peer dependency specification, even if doing so will result in some packages receiving a peer dependency outside the range set in their package's `peerDependencies` object.
When such and override is performed, a warning is printed, explaining the conflict and the packages involved. If `--strict-peer-deps` is set, then this warning is treated as a failure.
####
`package-lock`
* Default: true
* Type: Boolean
If set to false, then ignore `package-lock.json` files when installing. This will also prevent *writing* `package-lock.json` if `save` is true.
This configuration does not affect `npm ci`.
####
`omit`
* Default: 'dev' if the `NODE_ENV` environment variable is set to 'production', otherwise empty.
* Type: "dev", "optional", or "peer" (can be set multiple times)
Dependency types to omit from the installation tree on disk.
Note that these dependencies *are* still resolved and added to the `package-lock.json` or `npm-shrinkwrap.json` file. They are just not physically installed on disk.
If a package type appears in both the `--include` and `--omit` lists, then it will be included.
If the resulting omit list includes `'dev'`, then the `NODE_ENV` environment variable will be set to `'production'` for all lifecycle scripts.
####
`ignore-scripts`
* Default: false
* Type: Boolean
If true, npm does not run scripts specified in package.json files.
Note that commands explicitly intended to run a particular script, such as `npm start`, `npm stop`, `npm restart`, `npm test`, and `npm run-script` will still run their intended script if `ignore-scripts` is set, but they will *not* run any pre- or post-scripts.
####
`audit`
* Default: true
* Type: Boolean
When "true" submit audit reports alongside the current npm command to the default registry and all registries configured for scopes. See the documentation for [`npm audit`](npm-audit) for details on what is submitted.
####
`bin-links`
* Default: true
* Type: Boolean
Tells npm to create symlinks (or `.cmd` shims on Windows) for package executables.
Set to false to have it not do this. This can be used to work around the fact that some file systems don't support symlinks, even on ostensibly Unix systems.
####
`fund`
* Default: true
* Type: Boolean
When "true" displays the message at the end of each `npm install` acknowledging the number of dependencies looking for funding. See [`npm
fund`](npm-fund) for details.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
####
`install-links`
* Default: false
* Type: Boolean
When set file: protocol dependencies that exist outside of the project root will be packed and installed as regular dependencies instead of creating a symlink. This option has no effect on workspaces.
###
See Also
* [npm dedupe](npm-dedupe)
* [npm ls](npm-ls)
* [npm update](npm-update)
* [npm install](npm-install)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-find-dupes.md)
npm npm-view npm-view
========
[Skip to content](#skip-nav)
[npm Docs](../../../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../../../about-npm)
[Getting started](../../../getting-started)
[Packages and modules](../../../packages-and-modules)
[Integrations](../../../integrations)
[Organizations](../../../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../../../threats-and-mitigations)
[npm CLI](../../v8)
[CLI Commands](../commands)
<npm>[npm access](npm-access)[npm adduser](npm-adduser)[npm audit](npm-audit)[npm bin](npm-bin)[npm bugs](npm-bugs)[npm cache](npm-cache)[npm ci](npm-ci)[npm completion](npm-completion)[npm config](npm-config)[npm dedupe](npm-dedupe)[npm deprecate](npm-deprecate)[npm diff](npm-diff)[npm dist-tag](npm-dist-tag)[npm docs](npm-docs)[npm doctor](npm-doctor)[npm edit](npm-edit)[npm exec](npm-exec)[npm explain](npm-explain)[npm explore](npm-explore)[npm find-dupes](npm-find-dupes)[npm fund](npm-fund)[npm help](npm-help)[npm help-search](npm-help-search)[npm hook](npm-hook)[npm init](npm-init)[npm install](npm-install)[npm install-ci-test](npm-install-ci-test)[npm install-test](npm-install-test)[npm link](npm-link)[npm logout](npm-logout)[npm ls](npm-ls)[npm org](npm-org)[npm outdated](npm-outdated)[npm owner](npm-owner)[npm pack](npm-pack)[npm ping](npm-ping)[npm pkg](npm-pkg)[npm prefix](npm-prefix)[npm profile](npm-profile)[npm prune](npm-prune)[npm publish](npm-publish)[npm query](npm-query)[npm rebuild](npm-rebuild)[npm repo](npm-repo)[npm restart](npm-restart)[npm root](npm-root)[npm run-script](npm-run-script)[npm search](npm-search)[npm set-script](npm-set-script)[npm shrinkwrap](npm-shrinkwrap)[npm star](npm-star)[npm stars](npm-stars)[npm start](npm-start)[npm stop](npm-stop)[npm team](npm-team)[npm test](npm-test)[npm token](npm-token)[npm uninstall](npm-uninstall)[npm unpublish](npm-unpublish)[npm unstar](npm-unstar)[npm update](npm-update)[npm version](npm-version)[npm view](npm-view)[npm whoami](npm-whoami)<npx>
[Configuring npm](../configuring-npm)
[Using npm](../using-npm)
View registry info
Table of contents* [Synopsis](#synopsis)
* [Description](#description)
* [Configuration](#configuration)
+ [json](#json)
+ [workspace](#workspace)
+ [workspaces](#workspaces)
+ [include-workspace-root](#include-workspace-root)
* [Output](#output)
* [See Also](#see-also)
###
Synopsis
```
npm view [<package-spec>] [<field>[.subfield]...]
aliases: info, show, v
```
###
Description
This command shows data about a package and prints it to stdout.
As an example, to view information about the `connect` package from the registry, you would run:
```
npm view connect
```
The default version is `"latest"` if unspecified.
Field names can be specified after the package descriptor. For example, to show the dependencies of the `ronn` package at version `0.3.5`, you could do the following:
```
npm view [email protected] dependencies
```
You can view child fields by separating them with a period. To view the git repository URL for the latest version of `npm`, you would run the following command:
```
npm view npm repository.url
```
This makes it easy to view information about a dependency with a bit of shell scripting. For example, to view all the data about the version of `opts` that `ronn` depends on, you could write the following:
```
npm view opts@$(npm view ronn dependencies.opts)
```
For fields that are arrays, requesting a non-numeric field will return all of the values from the objects in the list. For example, to get all the contributor email addresses for the `express` package, you would run:
```
npm view express contributors.email
```
You may also use numeric indices in square braces to specifically select an item in an array field. To just get the email address of the first contributor in the list, you can run:
```
npm view express contributors[0].email
```
Multiple fields may be specified, and will be printed one after another. For example, to get all the contributor names and email addresses, you can do this:
```
npm view express contributors.name contributors.email
```
"Person" fields are shown as a string if they would be shown as an object. So, for example, this will show the list of `npm` contributors in the shortened string format. (See [`package.json`](../configuring-npm/package-json) for more on this.)
```
npm view npm contributors
```
If a version range is provided, then data will be printed for every matching version of the package. This will show which version of `jsdom` was required by each matching version of `yui3`:
```
npm view yui3@'>0.5.4' dependencies.jsdom
```
To show the `connect` package version history, you can do this:
```
npm view connect versions
```
###
Configuration
####
`json`
* Default: false
* Type: Boolean
Whether or not to output JSON data, rather than the normal output.
* In `npm pkg set` it enables parsing set values with JSON.parse() before saving them to your `package.json`.
Not supported by all npm commands.
####
`workspace`
* Default:
* Type: String (can be set multiple times)
Enable running a command in the context of the configured workspaces of the current project while filtering by running only the workspaces defined by this configuration option.
Valid values for the `workspace` config are either:
* Workspace names
* Path to a workspace directory
* Path to a parent workspace directory (will result in selecting all workspaces within that folder)
When set for the `npm init` command, this may be set to the folder of a workspace which does not yet exist, to create the folder and set it up as a brand new workspace within the project.
This value is not exported to the environment for child processes.
####
`workspaces`
* Default: null
* Type: null or Boolean
Set to true to run the command in the context of **all** configured workspaces.
Explicitly setting this to false will cause commands like `install` to ignore workspaces altogether. When not set explicitly:
* Commands that operate on the `node_modules` tree (install, update, etc.) will link workspaces into the `node_modules` folder. - Commands that do other things (test, exec, publish, etc.) will operate on the root project, *unless* one or more workspaces are specified in the `workspace` config.
This value is not exported to the environment for child processes.
####
`include-workspace-root`
* Default: false
* Type: Boolean
Include the workspace root when workspaces are enabled for a command.
When false, specifying individual workspaces via the `workspace` config, or all workspaces via the `workspaces` flag, will cause npm to operate only on the specified workspaces, and not on the root project.
This value is not exported to the environment for child processes.
###
Output
If only a single string field for a single version is output, then it will not be colorized or quoted, to enable piping the output to another command. If the field is an object, it will be output as a JavaScript object literal.
If the `--json` flag is given, the outputted fields will be JSON.
If the version range matches multiple versions then each printed value will be prefixed with the version it applies to.
If multiple fields are requested, then each of them is prefixed with the field name.
###
See Also
* [package spec](../using-npm/package-spec)
* [npm search](npm-search)
* [npm registry](../using-npm/registry)
* [npm config](npm-config)
* [npmrc](../configuring-npm/npmrc)
* [npm docs](npm-docs)
[Edit this page on GitHub](https://github.com/npm/cli/edit/v8/docs/content/commands/npm-view.md)
| programming_docs |
npm Integrating npm with external services Integrating npm with external services
======================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Integrating npm with external services](integrating-npm-with-external-services)
[About access tokens](../about-access-tokens)[Creating and viewing access tokens](../creating-and-viewing-access-tokens)[Revoking access tokens](../revoking-access-tokens)[Using private packages in a CI/CD workflow](../using-private-packages-in-a-ci-cd-workflow)[Docker and private modules](../docker-and-private-modules)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About access tokens](../about-access-tokens)
* [Creating and viewing access tokens](../creating-and-viewing-access-tokens)
* [Revoking access tokens](../revoking-access-tokens)
* [Using private packages in a CI/CD workflow](../using-private-packages-in-a-ci-cd-workflow)
* [Docker and private modules](../docker-and-private-modules)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/integrations/integrating-npm-with-external-services/index.mdx)
npm Setting up your npm user account Setting up your npm user account
================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Setting up your npm user account](setting-up-your-npm-user-account)
[Creating a new user account on the public registry](../creating-a-new-npm-user-account)[Creating a strong password](../creating-a-strong-password)[Receiving a one-time password over email](../receiving-a-one-time-password-over-email)[About two-factor authentication](../about-two-factor-authentication)[Configuring two-factor authentication](../configuring-two-factor-authentication)[Accessing npm using two-factor authentication](../accessing-npm-using-2fa)[Recovering your 2FA-enabled account](../recovering-your-2fa-enabled-account)
[Managing your npm user account](managing-your-npm-user-account)
[Paying for your npm user account](paying-for-your-npm-user-account)
[Configuring your local environment](configuring-your-local-environment)
[Troubleshooting](troubleshooting)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Creating a new user account on the public registry](../creating-a-new-npm-user-account)
* [Creating a strong password](../creating-a-strong-password)
* [Receiving a one-time password over email](../receiving-a-one-time-password-over-email)
* [About two-factor authentication](../about-two-factor-authentication)
* [Configuring two-factor authentication](../configuring-two-factor-authentication)
* [Accessing npm using two-factor authentication](../accessing-npm-using-2fa)
* [Recovering your 2FA-enabled account](../recovering-your-2fa-enabled-account)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/setting-up-your-npm-user-account/index.mdx)
npm Managing your npm user account Managing your npm user account
==============================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Setting up your npm user account](setting-up-your-npm-user-account)
[Managing your npm user account](managing-your-npm-user-account)
[Managing your profile settings](../managing-your-profile-settings)[Changing your npm username](../changing-your-npm-username)[Deleting your npm user account](../deleting-your-npm-user-account)[Requesting an export of your personal data](../requesting-your-data)
[Paying for your npm user account](paying-for-your-npm-user-account)
[Configuring your local environment](configuring-your-local-environment)
[Troubleshooting](troubleshooting)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Managing your profile settings](../managing-your-profile-settings)
* [Changing your npm username](../changing-your-npm-username)
* [Deleting your npm user account](../deleting-your-npm-user-account)
* [Requesting an export of your personal data](../requesting-your-data)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/managing-your-npm-user-account/index.mdx)
npm Troubleshooting Troubleshooting
===============
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Setting up your npm user account](setting-up-your-npm-user-account)
[Managing your npm user account](managing-your-npm-user-account)
[Paying for your npm user account](paying-for-your-npm-user-account)
[Configuring your local environment](configuring-your-local-environment)
[Troubleshooting](troubleshooting)
[Generating and locating npm-debug.log files](../generating-and-locating-npm-debug.log-files)[Common errors](../common-errors)[Try the latest stable version of node](../try-the-latest-stable-version-of-node)[Try the latest stable version of npm](../try-the-latest-stable-version-of-npm)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Generating and locating npm-debug.log files](../generating-and-locating-npm-debug.log-files)
* [Common errors](../common-errors)
* [Try the latest stable version of node](../try-the-latest-stable-version-of-node)
* [Try the latest stable version of npm](../try-the-latest-stable-version-of-npm)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/troubleshooting/index.mdx)
npm Configuring your local environment Configuring your local environment
==================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Setting up your npm user account](setting-up-your-npm-user-account)
[Managing your npm user account](managing-your-npm-user-account)
[Paying for your npm user account](paying-for-your-npm-user-account)
[Configuring your local environment](configuring-your-local-environment)
[About npm CLI versions](../about-npm-versions)[Downloading and installing Node.js and npm](../downloading-and-installing-node-js-and-npm)
[Troubleshooting](troubleshooting)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [About npm CLI versions](../about-npm-versions)
* [Downloading and installing Node.js and npm](../downloading-and-installing-node-js-and-npm)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/configuring-your-local-environment/index.mdx)
npm Paying for your npm user account Paying for your npm user account
================================
[Skip to content](#skip-nav)
[npm Docs](../index)
[npmjs.com](https://npmjs.com/)[Status](https://status.npmjs.com/)[Support](https://npmjs.com/support/)
[About npm](../about-npm)
[Getting started](../getting-started)
[Setting up your npm user account](setting-up-your-npm-user-account)
[Managing your npm user account](managing-your-npm-user-account)
[Paying for your npm user account](paying-for-your-npm-user-account)
[Upgrading to a paid user account plan](../upgrading-to-a-paid-user-account-plan)[Viewing, downloading, and emailing receipts for your npm user account](../viewing-downloading-and-emailing-receipts-for-your-user-account)[Updating user account billing settings](../updating-user-account-billing-settings)[Downgrading to a free user account plan](../downgrading-to-a-free-user-account-plan)
[Configuring your local environment](configuring-your-local-environment)
[Troubleshooting](troubleshooting)
[Packages and modules](../packages-and-modules)
[Integrations](../integrations)
[Organizations](../organizations)
[Policies](https://docs.npmjs.com/policies)
[Threats and Mitigations](../threats-and-mitigations)
[npm CLI](../cli/v8)
* [Upgrading to a paid user account plan](../upgrading-to-a-paid-user-account-plan)
* [Viewing, downloading, and emailing receipts for your npm user account](../viewing-downloading-and-emailing-receipts-for-your-user-account)
* [Updating user account billing settings](../updating-user-account-billing-settings)
* [Downgrading to a free user account plan](../downgrading-to-a-free-user-account-plan)
[Edit this page on GitHub](https://github.com/npm/documentation/edit/main/content/getting-started/paying-for-your-npm-user-account/index.mdx)
angularjs Angular.js Angular.js
==========
ng
--
* function
* [angular.UNSAFE\_restoreLegacyJqLiteXHTMLReplacement](api/ng/function/angular.unsafe_restorelegacyjqlitexhtmlreplacement)
* [angular.bind](api/ng/function/angular.bind)
* [angular.bootstrap](api/ng/function/angular.bootstrap)
* [angular.copy](api/ng/function/angular.copy)
* [angular.element](api/ng/function/angular.element)
* [angular.equals](api/ng/function/angular.equals)
* [angular.errorHandlingConfig](api/ng/function/angular.errorhandlingconfig)
* [angular.extend](api/ng/function/angular.extend)
* [angular.forEach](api/ng/function/angular.foreach)
* [angular.fromJson](api/ng/function/angular.fromjson)
* [angular.identity](api/ng/function/angular.identity)
* [angular.injector](api/ng/function/angular.injector)
* [angular.isArray](api/ng/function/angular.isarray)
* [angular.isDate](api/ng/function/angular.isdate)
* [angular.isDefined](api/ng/function/angular.isdefined)
* [angular.isElement](api/ng/function/angular.iselement)
* [angular.isFunction](api/ng/function/angular.isfunction)
* [angular.isNumber](api/ng/function/angular.isnumber)
* [angular.isObject](api/ng/function/angular.isobject)
* [angular.isString](api/ng/function/angular.isstring)
* [angular.isUndefined](api/ng/function/angular.isundefined)
* [angular.merge](api/ng/function/angular.merge)
* [angular.module](api/ng/function/angular.module)
* [angular.noop](api/ng/function/angular.noop)
* [angular.reloadWithDebugInfo](api/ng/function/angular.reloadwithdebuginfo)
* [angular.toJson](api/ng/function/angular.tojson)
* directive
* [a](api/ng/directive/a)
* [form](api/ng/directive/form)
* [input](api/ng/directive/input)
* [input[checkbox]](api/ng/input/input%5Bcheckbox%5D)
* [input[date]](api/ng/input/input%5Bdate%5D)
* [input[datetime-local]](api/ng/input/input%5Bdatetime-local%5D)
* [input[email]](api/ng/input/input%5Bemail%5D)
* [input[month]](api/ng/input/input%5Bmonth%5D)
* [input[number]](api/ng/input/input%5Bnumber%5D)
* [input[radio]](api/ng/input/input%5Bradio%5D)
* [input[range]](api/ng/input/input%5Brange%5D)
* [input[text]](api/ng/input/input%5Btext%5D)
* [input[time]](api/ng/input/input%5Btime%5D)
* [input[url]](api/ng/input/input%5Burl%5D)
* [input[week]](api/ng/input/input%5Bweek%5D)
* [ngApp](api/ng/directive/ngapp)
* [ngBind](api/ng/directive/ngbind)
* [ngBindHtml](api/ng/directive/ngbindhtml)
* [ngBindTemplate](api/ng/directive/ngbindtemplate)
* [ngBlur](api/ng/directive/ngblur)
* [ngChange](api/ng/directive/ngchange)
* [ngChecked](api/ng/directive/ngchecked)
* [ngClass](api/ng/directive/ngclass)
* [ngClassEven](api/ng/directive/ngclasseven)
* [ngClassOdd](api/ng/directive/ngclassodd)
* [ngClick](api/ng/directive/ngclick)
* [ngCloak](api/ng/directive/ngcloak)
* [ngController](api/ng/directive/ngcontroller)
* [ngCopy](api/ng/directive/ngcopy)
* [ngCsp](api/ng/directive/ngcsp)
* [ngCut](api/ng/directive/ngcut)
* [ngDblclick](api/ng/directive/ngdblclick)
* [ngDisabled](api/ng/directive/ngdisabled)
* [ngFocus](api/ng/directive/ngfocus)
* [ngForm](api/ng/directive/ngform)
* [ngHide](api/ng/directive/nghide)
* [ngHref](api/ng/directive/nghref)
* [ngIf](api/ng/directive/ngif)
* [ngInclude](api/ng/directive/nginclude)
* [ngInit](api/ng/directive/nginit)
* [ngJq](api/ng/directive/ngjq)
* [ngKeydown](api/ng/directive/ngkeydown)
* [ngKeypress](api/ng/directive/ngkeypress)
* [ngKeyup](api/ng/directive/ngkeyup)
* [ngList](api/ng/directive/nglist)
* [ngMaxlength](api/ng/directive/ngmaxlength)
* [ngMinlength](api/ng/directive/ngminlength)
* [ngModel](api/ng/directive/ngmodel)
* [ngModelOptions](api/ng/directive/ngmodeloptions)
* [ngMousedown](api/ng/directive/ngmousedown)
* [ngMouseenter](api/ng/directive/ngmouseenter)
* [ngMouseleave](api/ng/directive/ngmouseleave)
* [ngMousemove](api/ng/directive/ngmousemove)
* [ngMouseover](api/ng/directive/ngmouseover)
* [ngMouseup](api/ng/directive/ngmouseup)
* [ngNonBindable](api/ng/directive/ngnonbindable)
* [ngOn](api/ng/directive/ngon)
* [ngOpen](api/ng/directive/ngopen)
* [ngOptions](api/ng/directive/ngoptions)
* [ngPaste](api/ng/directive/ngpaste)
* [ngPattern](api/ng/directive/ngpattern)
* [ngPluralize](api/ng/directive/ngpluralize)
* [ngProp](api/ng/directive/ngprop)
* [ngReadonly](api/ng/directive/ngreadonly)
* [ngRef](api/ng/directive/ngref)
* [ngRepeat](api/ng/directive/ngrepeat)
* [ngRequired](api/ng/directive/ngrequired)
* [ngSelected](api/ng/directive/ngselected)
* [ngShow](api/ng/directive/ngshow)
* [ngSrc](api/ng/directive/ngsrc)
* [ngSrcset](api/ng/directive/ngsrcset)
* [ngStyle](api/ng/directive/ngstyle)
* [ngSubmit](api/ng/directive/ngsubmit)
* [ngSwitch](api/ng/directive/ngswitch)
* [ngTransclude](api/ng/directive/ngtransclude)
* [ngValue](api/ng/directive/ngvalue)
* [script](api/ng/directive/script)
* [select](api/ng/directive/select)
* [textarea](api/ng/directive/textarea)
* object
* [angular.version](api/ng/object/angular.version)
* type
* [$cacheFactory.Cache](api/ng/type/%24cachefactory.cache)
* [$compile.directive.Attributes](api/ng/type/%24compile.directive.attributes)
* [$rootScope.Scope](api/ng/type/%24rootscope.scope)
* [ModelOptions](api/ng/type/modeloptions)
* [angular.Module](api/ng/type/angular.module)
* [form.FormController](api/ng/type/form.formcontroller)
* [ngModel.NgModelController](api/ng/type/ngmodel.ngmodelcontroller)
* [select.SelectController](api/ng/type/select.selectcontroller)
* provider
* [$anchorScrollProvider](api/ng/provider/%24anchorscrollprovider)
* [$animateProvider](api/ng/provider/%24animateprovider)
* [$compileProvider](api/ng/provider/%24compileprovider)
* [$controllerProvider](api/ng/provider/%24controllerprovider)
* [$filterProvider](api/ng/provider/%24filterprovider)
* [$httpProvider](api/ng/provider/%24httpprovider)
* [$interpolateProvider](api/ng/provider/%24interpolateprovider)
* [$locationProvider](api/ng/provider/%24locationprovider)
* [$logProvider](api/ng/provider/%24logprovider)
* [$parseProvider](api/ng/provider/%24parseprovider)
* [$qProvider](api/ng/provider/%24qprovider)
* [$rootScopeProvider](api/ng/provider/%24rootscopeprovider)
* [$sceDelegateProvider](api/ng/provider/%24scedelegateprovider)
* [$sceProvider](api/ng/provider/%24sceprovider)
* [$templateRequestProvider](api/ng/provider/%24templaterequestprovider)
* service
* [$anchorScroll](api/ng/service/%24anchorscroll)
* [$animate](api/ng/service/%24animate)
* [$animateCss](api/ng/service/%24animatecss)
* [$cacheFactory](api/ng/service/%24cachefactory)
* [$compile](api/ng/service/%24compile)
* [$controller](api/ng/service/%24controller)
* [$document](api/ng/service/%24document)
* [$exceptionHandler](api/ng/service/%24exceptionhandler)
* [$filter](api/ng/service/%24filter)
* [$http](api/ng/service/%24http)
* [$httpBackend](api/ng/service/%24httpbackend)
* [$httpParamSerializer](api/ng/service/%24httpparamserializer)
* [$httpParamSerializerJQLike](api/ng/service/%24httpparamserializerjqlike)
* [$interpolate](api/ng/service/%24interpolate)
* [$interval](api/ng/service/%24interval)
* [$jsonpCallbacks](api/ng/service/%24jsonpcallbacks)
* [$locale](api/ng/service/%24locale)
* [$location](api/ng/service/%24location)
* [$log](api/ng/service/%24log)
* [$parse](api/ng/service/%24parse)
* [$q](api/ng/service/%24q)
* [$rootElement](api/ng/service/%24rootelement)
* [$rootScope](api/ng/service/%24rootscope)
* [$sce](api/ng/service/%24sce)
* [$sceDelegate](api/ng/service/%24scedelegate)
* [$templateCache](api/ng/service/%24templatecache)
* [$templateRequest](api/ng/service/%24templaterequest)
* [$timeout](api/ng/service/%24timeout)
* [$window](api/ng/service/%24window)
* [$xhrFactory](api/ng/service/%24xhrfactory)
* filter
* [currency](api/ng/filter/currency)
* [date](api/ng/filter/date)
* [filter](api/ng/filter/filter)
* [json](api/ng/filter/json)
* [limitTo](api/ng/filter/limitto)
* [lowercase](api/ng/filter/lowercase)
* [number](api/ng/filter/number)
* [orderBy](api/ng/filter/orderby)
* [uppercase](api/ng/filter/uppercase)
auto
----
* service
* [$injector](api/auto/service/%24injector)
* [$provide](api/auto/service/%24provide)
ngAnimate
---------
* directive
* [ngAnimateChildren](api/nganimate/directive/nganimatechildren)
* [ngAnimateSwap](api/nganimate/directive/nganimateswap)
* service
* [$animate](api/nganimate/service/%24animate)
* [$animateCss](api/nganimate/service/%24animatecss)
ngAria
------
* provider
* [$ariaProvider](api/ngaria/provider/%24ariaprovider)
* service
* [$aria](api/ngaria/service/%24aria)
ngComponentRouter
-----------------
* type
* [ChildRouter](api/ngcomponentrouter/type/childrouter)
* [ComponentInstruction](api/ngcomponentrouter/type/componentinstruction)
* [RootRouter](api/ngcomponentrouter/type/rootrouter)
* [RouteDefinition](api/ngcomponentrouter/type/routedefinition)
* [RouteParams](api/ngcomponentrouter/type/routeparams)
* [Router](api/ngcomponentrouter/type/router)
* directive
* [ngOutlet](api/ngcomponentrouter/directive/ngoutlet)
* service
* [$rootRouter](api/ngcomponentrouter/service/%24rootrouter)
* [$routerRootComponent](api/ngcomponentrouter/service/%24routerrootcomponent)
ngCookies
---------
* provider
* [$cookiesProvider](api/ngcookies/provider/%24cookiesprovider)
* service
* [$cookies](api/ngcookies/service/%24cookies)
ngMessageFormat
---------------
ngMessages
----------
* directive
* [ngMessage](api/ngmessages/directive/ngmessage)
* [ngMessageDefault](api/ngmessages/directive/ngmessagedefault)
* [ngMessageExp](api/ngmessages/directive/ngmessageexp)
* [ngMessages](api/ngmessages/directive/ngmessages)
* [ngMessagesInclude](api/ngmessages/directive/ngmessagesinclude)
ngMock
------
* object
* [angular.mock](api/ngmock/object/angular.mock)
* service
* [$animate](api/ngmock/service/%24animate)
* [$componentController](api/ngmock/service/%24componentcontroller)
* [$controller](api/ngmock/service/%24controller)
* [$exceptionHandler](api/ngmock/service/%24exceptionhandler)
* [$flushPendingTasks](api/ngmock/service/%24flushpendingtasks)
* [$httpBackend](api/ngmock/service/%24httpbackend)
* [$interval](api/ngmock/service/%24interval)
* [$log](api/ngmock/service/%24log)
* [$timeout](api/ngmock/service/%24timeout)
* [$verifyNoPendingTasks](api/ngmock/service/%24verifynopendingtasks)
* provider
* [$exceptionHandlerProvider](api/ngmock/provider/%24exceptionhandlerprovider)
* type
* [$rootScope.Scope](api/ngmock/type/%24rootscope.scope)
* [angular.mock.TzDate](api/ngmock/type/angular.mock.tzdate)
* function
* [angular.mock.dump](api/ngmock/function/angular.mock.dump)
* [angular.mock.inject](api/ngmock/function/angular.mock.inject)
* [angular.mock.module](api/ngmock/function/angular.mock.module)
* [angular.mock.module.sharedInjector](api/ngmock/function/angular.mock.module.sharedinjector)
* [browserTrigger](api/ngmock/function/browsertrigger)
ngMockE2E
---------
* service
* [$httpBackend](api/ngmocke2e/service/%24httpbackend)
ngParseExt
----------
ngResource
----------
* provider
* [$resourceProvider](api/ngresource/provider/%24resourceprovider)
* service
* [$resource](api/ngresource/service/%24resource)
ngRoute
-------
* directive
* [ngView](api/ngroute/directive/ngview)
* provider
* [$routeProvider](api/ngroute/provider/%24routeprovider)
* service
* [$route](api/ngroute/service/%24route)
* [$routeParams](api/ngroute/service/%24routeparams)
ngSanitize
----------
* filter
* [linky](api/ngsanitize/filter/linky)
* service
* [$sanitize](api/ngsanitize/service/%24sanitize)
* provider
* [$sanitizeProvider](api/ngsanitize/provider/%24sanitizeprovider)
ngTouch
-------
* directive
* [ngSwipeLeft](api/ngtouch/directive/ngswipeleft)
* [ngSwipeRight](api/ngtouch/directive/ngswiperight)
* service
* [$swipe](api/ngtouch/service/%24swipe)
| programming_docs |
angularjs
Improve this DocGuide to AngularJS Documentation
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/index.ngdoc?message=docs(guide%2FDeveloper%20Guide)%3A%20describe%20your%20change...)Guide to AngularJS Documentation
==============================================================================================================================================================================================================
On this page, you will find a list of official AngularJS resources on various topics.
Just starting out with AngularJS? Try working through our step by step tutorial or try building on our seed project.
* [Official AngularJS Tutorial](https://code.angularjs.org/1.8.2/docs/tutorial/index)
* [AngularJS Seed](https://github.com/angular/angular-seed)
Ready to find out more about AngularJS?
* [What is AngularJS?](guide/introduction)
* [Conceptual Overview](guide/concepts)
Core Concepts
-------------
### Templates
In AngularJS applications, you move the job of filling page templates with data from the server to the client. The result is a system better structured for dynamic page updates. Below are the core features you'll use.
* [Data binding](guide/databinding)
* [Expressions](guide/expression)
* [Interpolation](guide/interpolation)
* [Directives](guide/directive)
* [Views and routes (see the example)](api/ngroute/service/%24route)
* [Filters](guide/filter)
* [HTML compiler](guide/compiler)
* [Forms](guide/forms)
### Application Structure
* **App wiring:** [Dependency injection](guide/di)
* **Exposing model to templates:** [Scopes](guide/scope)
* **Bootstrap:** [Bootstrapping an app](guide/bootstrap)
* **Communicating with servers:** [$http](api/ng/service/%24http), [$resource](api/ngresource/service/%24resource)
### Other Features
* **Animation:** [Core concepts](guide/animations), [ngAnimate API](api/nganimate)
* **Security:** [Security Docs](guide/security), [Strict Contextual Escaping](api/ng/service/%24sce), [Content Security Policy](api/ng/directive/ngcsp), [$sanitize](api/ngsanitize/service/%24sanitize), [video](https://www.youtube.com/watch?v=18ifoT-Id54)
* **Internationalization and Localization:** [AngularJS Guide to i18n and l10n](guide/i18n), [date filter](api/ng/filter/date), [currency filter](api/ng/filter/currency), [Creating multilingual support](https://blog.novanet.no/creating-multilingual-support-using-angularjs/)
* **Touch events:** [Touch events](api/ngtouch)
* **Accessibility:** [ngAria](guide/accessibility)
### Testing
* **Unit testing:** [Karma](http://karma-runner.github.io), [Unit testing](guide/unit-testing), [Testing services](guide/services#unit-testing.html),
* **End-to-End Testing:** [Protractor](https://github.com/angular/protractor), [e2e testing guide](guide/e2e-testing)
Community Resources
-------------------
We have set up a guide to many resources provided by the community, where you can find lots of additional information and material on these topics, a list of complimentary libraries, and much more.
* [External AngularJS resources](guide/external-resources)
Getting Help
------------
The recipe for getting help on your unique issue is to create an example that could work (even if it doesn't) in a shareable example on [Plunker](http://plnkr.co/), [JSFiddle](http://jsfiddle.net/), or similar site and then post to one of the following:
* [Stackoverflow.com](http://stackoverflow.com/search?q=angularjs)
* [AngularJS mailing list](https://groups.google.com/forum/#!forum/angular)
* [AngularJS IRC channel](http://webchat.freenode.net/?channels=angularjs&uio=d4)
Official Communications
-----------------------
Official announcements, news and releases are posted to our blog, G+ and Twitter:
* [AngularJS Blog](http://blog.angularjs.org/)
* [Google+](https://plus.google.com/u/0/+AngularJS)
* [Twitter](https://twitter.com/angular)
* [AngularJS on YouTube](http://youtube.com/angularjs)
Contributing to AngularJS
-------------------------
Though we have a core group of core contributors at Google, AngularJS is an open source project with hundreds of contributors. We'd love you to be one of them. When you're ready, please read the [Guide for contributing to AngularJS](https://code.angularjs.org/1.8.2/docs/misc/contribute).
Something Missing?
------------------
Didn't find what you're looking for here? Check out the [External AngularJS resources guide](guide/external-resources).
If you have awesome AngularJS resources that belong on that page, please tell us about them on [Google+](https://plus.google.com/u/0/+AngularJS) or [Twitter](https://twitter.com/angularjs).
angularjs
Improve this DocDecorators in AngularJS
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/decorators.ngdoc?message=docs(guide%2FDecorators)%3A%20describe%20your%20change...)Decorators in AngularJS
===================================================================================================================================================================================================
**NOTE:** This guide is targeted towards developers who are already familiar with AngularJS basics. If you're just getting started, we recommend the [tutorial](https://code.angularjs.org/1.8.2/docs/guide/tutorial/) first. What are decorators?
--------------------
Decorators are a design pattern that is used to separate modification or *decoration* of a class without modifying the original source code. In AngularJS, decorators are functions that allow a service, directive or filter to be modified prior to its usage.
How to use decorators
---------------------
There are two ways to register decorators
* `$provide.decorator`, and
* `module.decorator`
Each provide access to a `$delegate`, which is the instantiated service/directive/filter, prior to being passed to the service that required it.
### $provide.decorator
The [decorator function](../api/auto/service/%24provide#decorator.html) allows access to a $delegate of the service once it has been instantiated. For example:
```
angular.module('myApp', [])
.config([ '$provide', function($provide) {
$provide.decorator('$log', [
'$delegate',
function $logDecorator($delegate) {
var originalWarn = $delegate.warn;
$delegate.warn = function decoratedWarn(msg) {
msg = 'Decorated Warn: ' + msg;
originalWarn.apply($delegate, arguments);
};
return $delegate;
}
]);
}]);
```
After the `$log` service has been instantiated the decorator is fired. The decorator function has a `$delegate` object injected to provide access to the service that matches the selector in the decorator. This `$delegate` will be the service you are decorating. The return value of the function *provided to the decorator* will take place of the service, directive, or filter being decorated.
The `$delegate` may be either modified or completely replaced. Given a service `myService` with a method `someFn`, the following could all be viable solutions:
#### Completely Replace the $delegate
```
angular.module('myApp', [])
.config([ '$provide', function($provide) {
$provide.decorator('myService', [
'$delegate',
function myServiceDecorator($delegate) {
var myDecoratedService = {
// new service object to replace myService
};
return myDecoratedService;
}
]);
}]);
```
#### Patch the $delegate
```
angular.module('myApp', [])
.config([ '$provide', function($provide) {
$provide.decorator('myService', [
'$delegate',
function myServiceDecorator($delegate) {
var someFn = $delegate.someFn;
function aNewFn() {
// new service function
someFn.apply($delegate, arguments);
}
$delegate.someFn = aNewFn;
return $delegate;
}
]);
}]);
```
#### Augment the $delegate
```
angular.module('myApp', [])
.config([ '$provide', function($provide) {
$provide.decorator('myService', [
'$delegate',
function myServiceDecorator($delegate) {
function helperFn() {
// an additional fn to add to the service
}
$delegate.aHelpfulAddition = helperFn;
return $delegate;
}
]);
}]);
```
Note that whatever is returned by the decorator function will replace that which is being decorated. For example, a missing return statement will wipe out the entire object being decorated. Decorators have different rules for different services. This is because services are registered in different ways. Services are selected by name, however filters and directives are selected by appending `"Filter"` or `"Directive"` to the end of the name. The `$delegate` provided is dictated by the type of service.
| Service Type | Selector | $delegate |
| --- | --- | --- |
| Service | `serviceName` | The `object` or `function` returned by the service |
| Directive | `directiveName + 'Directive'` | An `Array.<DirectiveObject>`[1](decorators#drtvArray.html) |
| Filter | `filterName + 'Filter'` | The `function` returned by the filter |
1. Multiple directives may be registered to the same selector/name
**NOTE:** Developers should take care in how and why they are modifying the `$delegate` for the service. Not only should expectations for the consumer be kept, but some functionality (such as directive registration) does not take place after decoration, but during creation/registration of the original service. This means, for example, that an action such as pushing a directive object to a directive `$delegate` will likely result in unexpected behavior. Furthermore, great care should be taken when decorating core services, directives, or filters as this may unexpectedly or adversely affect the functionality of the framework. ### module.decorator
This [function](../api/ng/type/angular.module#decorator.html) is the same as the `$provide.decorator` function except it is exposed through the module API. This allows you to separate your decorator patterns from your module config blocks.
Like with `$provide.decorator`, the `module.decorator` function runs during the config phase of the app. That means you can define a `module.decorator` before the decorated service is defined.
Since you can apply multiple decorators, it is noteworthy that decorator application always follows order of declaration:
* If a service is decorated by both `$provide.decorator` and `module.decorator`, the decorators are applied in order:
```
angular
.module('theApp', [])
.factory('theFactory', theFactoryFn)
.config(function($provide) {
$provide.decorator('theFactory', provideDecoratorFn); // runs first
})
.decorator('theFactory', moduleDecoratorFn); // runs seconds
```
* If the service has been declared multiple times, a decorator will decorate the service that has been declared last:
```
angular
.module('theApp', [])
.factory('theFactory', theFactoryFn)
.decorator('theFactory', moduleDecoratorFn)
.factory('theFactory', theOtherFactoryFn);
// `theOtherFactoryFn` is selected as 'theFactory' provider and it is decorated via `moduleDecoratorFn`.
```
Example Applications
--------------------
The following sections provide examples each of a service decorator, a directive decorator, and a filter decorator.
This example shows how we can replace the $log service with our own to display log messages.
Failed interpolated expressions in `ng-href` attributes can easily go unnoticed. We can decorate `ngHref` to warn us of those conditions.
Let's say we have created an app that uses the default format for many of our `Date` filters. Suddenly requirements have changed (that never happens) and we need all of our default dates to be `'shortDate'` instead of `'mediumDate'`.
angularjs
Improve this DocUnit Testing
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/unit-testing.ngdoc?message=docs(guide%2FUnit%20Testing)%3A%20describe%20your%20change...)Unit Testing
==============================================================================================================================================================================================
JavaScript is a dynamically typed language which comes with great power of expression, but it also comes with almost no help from the compiler. For this reason we feel very strongly that any code written in JavaScript needs to come with a strong set of tests. We have built many features into AngularJS which make testing your AngularJS applications easy. With AngularJS, there is no excuse for not testing.
Separation of Concerns
----------------------
Unit testing, as the name implies, is about testing individual units of code. Unit tests try to answer questions such as "Did I think about the logic correctly?" or "Does the sort function order the list in the right order?"
In order to answer such a question it is very important that we can isolate the unit of code under test. That is because when we are testing the sort function we don't want to be forced into creating related pieces such as the DOM elements, or making any XHR calls to fetch the data to sort.
While this may seem obvious it can be very difficult to call an individual function on a typical project. The reason is that the developers often mix concerns resulting in a piece of code which does everything. It makes an XHR request, it sorts the response data, and then it manipulates the DOM.
With AngularJS, we try to make it easy for you to do the right thing. For your XHR requests, we provide dependency injection, so your requests can be simulated. For the DOM, we abstract it, so you can test your model without having to manipulate the DOM directly. Your tests can then assert that the data has been sorted without having to create or look at the state of the DOM or to wait for any XHR requests to return data. The individual sort function can be tested in isolation.
With great power comes great responsibility
-------------------------------------------
AngularJS is written with testability in mind, but it still requires that you do the right thing. We tried to make the right thing easy, but if you ignore these guidelines you may end up with an untestable application.
Dependency Injection
--------------------
AngularJS comes with [dependency injection](di) built-in, which makes testing components much easier, because you can pass in a component's dependencies and stub or mock them as you wish.
Components that have their dependencies injected allow them to be easily mocked on a test by test basis, without having to mess with any global variables that could inadvertently affect another test.
Additional tools for testing AngularJS applications
---------------------------------------------------
For testing AngularJS applications there are certain tools that you should use that will make testing much easier to set up and run.
### Karma
[Karma](http://karma-runner.github.io/) is a JavaScript command line tool that can be used to spawn a web server which loads your application's source code and executes your tests. You can configure Karma to run against a number of browsers, which is useful for being confident that your application works on all browsers you need to support. Karma is executed on the command line and will display the results of your tests on the command line once they have run in the browser.
Karma is a NodeJS application, and should be installed through npm/yarn. Full installation instructions are available on [the Karma website](http://karma-runner.github.io/0.12/intro/installation.html).
### Jasmine
[Jasmine](http://jasmine.github.io/1.3/introduction.html) is a behavior driven development framework for JavaScript that has become the most popular choice for testing AngularJS applications. Jasmine provides functions to help with structuring your tests and also making assertions. As your tests grow, keeping them well structured and documented is vital, and Jasmine helps achieve this.
In Jasmine we use the `describe` function to group our tests together:
```
describe("sorting the list of users", function() {
// individual tests go here
});
```
And then each individual test is defined within a call to the `it` function:
```
describe('sorting the list of users', function() {
it('sorts in descending order by default', function() {
// your test assertion goes here
});
});
```
Grouping related tests within `describe` blocks and describing each individual test within an `it` call keeps your tests self documenting.
Finally, Jasmine provides matchers which let you make assertions:
```
describe('sorting the list of users', function() {
it('sorts in descending order by default', function() {
var users = ['jack', 'igor', 'jeff'];
var sorted = sortUsers(users);
expect(sorted).toEqual(['jeff', 'jack', 'igor']);
});
});
```
Jasmine comes with a number of matchers that help you make a variety of assertions. You should [read the Jasmine documentation](http://jasmine.github.io/1.3/introduction.html#section-Matchers) to see what they are. To use Jasmine with Karma, we use the [karma-jasmine](https://github.com/karma-runner/karma-jasmine) test runner.
### angular-mocks
AngularJS also provides the [`ngMock`](../api/ngmock) module, which provides mocking for your tests. This is used to inject and mock AngularJS services within unit tests. In addition, it is able to extend other modules so they are synchronous. Having tests synchronous keeps them much cleaner and easier to work with. One of the most useful parts of ngMock is [`$httpBackend`](../api/ngmock/service/%24httpbackend), which lets us mock XHR requests in tests, and return sample data instead.
Testing a Controller
--------------------
Because AngularJS separates logic from the view layer, it keeps controllers easy to test. Let's take a look at how we might test the controller below, which provides `$scope.grade`, which sets a property on the scope based on the length of the password.
```
angular.module('app', [])
.controller('PasswordController', function PasswordController($scope) {
$scope.password = '';
$scope.grade = function() {
var size = $scope.password.length;
if (size > 8) {
$scope.strength = 'strong';
} else if (size > 3) {
$scope.strength = 'medium';
} else {
$scope.strength = 'weak';
}
};
});
```
Because controllers are not available on the global scope, we need to use [`angular.mock.inject`](../api/ngmock/function/angular.mock.inject) to inject our controller first. The first step is to use the `module` function, which is provided by angular-mocks. This loads in the module it's given, so it is available in your tests. We pass this into `beforeEach`, which is a function Jasmine provides that lets us run code before each test. Then we can use `inject` to access `$controller`, the service that is responsible for instantiating controllers.
```
describe('PasswordController', function() {
beforeEach(module('app'));
var $controller, $rootScope;
beforeEach(inject(function(_$controller_, _$rootScope_){
// The injector unwraps the underscores (_) from around the parameter names when matching
$controller = _$controller_;
$rootScope = _$rootScope_;
}));
describe('$scope.grade', function() {
it('sets the strength to "strong" if the password length is >8 chars', function() {
var $scope = $rootScope.$new();
var controller = $controller('PasswordController', { $scope: $scope });
$scope.password = 'longerthaneightchars';
$scope.grade();
expect($scope.strength).toEqual('strong');
});
});
});
```
Notice how by nesting the `describe` calls and being descriptive when calling them with strings, the test is very clear. It documents exactly what it is testing, and at a glance you can quickly see what is happening. Now let's add the test for when the password is less than three characters, which should see `$scope.strength` set to "weak":
```
describe('PasswordController', function() {
beforeEach(module('app'));
var $controller;
beforeEach(inject(function(_$controller_){
// The injector unwraps the underscores (_) from around the parameter names when matching
$controller = _$controller_;
}));
describe('$scope.grade', function() {
it('sets the strength to "strong" if the password length is >8 chars', function() {
var $scope = {};
var controller = $controller('PasswordController', { $scope: $scope });
$scope.password = 'longerthaneightchars';
$scope.grade();
expect($scope.strength).toEqual('strong');
});
it('sets the strength to "weak" if the password length <3 chars', function() {
var $scope = {};
var controller = $controller('PasswordController', { $scope: $scope });
$scope.password = 'a';
$scope.grade();
expect($scope.strength).toEqual('weak');
});
});
});
```
Now we have two tests, but notice the duplication between the tests. Both have to create the `$scope` variable and create the controller. As we add new tests, this duplication is only going to get worse. Thankfully, Jasmine provides `beforeEach`, which lets us run a function before each individual test. Let's see how that would tidy up our tests:
```
describe('PasswordController', function() {
beforeEach(module('app'));
var $controller;
beforeEach(inject(function(_$controller_){
// The injector unwraps the underscores (_) from around the parameter names when matching
$controller = _$controller_;
}));
describe('$scope.grade', function() {
var $scope, controller;
beforeEach(function() {
$scope = {};
controller = $controller('PasswordController', { $scope: $scope });
});
it('sets the strength to "strong" if the password length is >8 chars', function() {
$scope.password = 'longerthaneightchars';
$scope.grade();
expect($scope.strength).toEqual('strong');
});
it('sets the strength to "weak" if the password length <3 chars', function() {
$scope.password = 'a';
$scope.grade();
expect($scope.strength).toEqual('weak');
});
});
});
```
We've moved the duplication out and into the `beforeEach` block. Each individual test now only contains the code specific to that test, and not code that is general across all tests. As you expand your tests, keep an eye out for locations where you can use `beforeEach` to tidy up tests. `beforeEach` isn't the only function of this sort that Jasmine provides, and the [documentation lists the others](http://jasmine.github.io/1.3/introduction.html#section-Setup_and_Teardown).
Testing Filters
---------------
[Filters](../api/ng/provider/%24filterprovider) are functions which transform the data into a user readable format. They are important because they remove the formatting responsibility from the application logic, further simplifying the application logic.
```
myModule.filter('length', function() {
return function(text) {
return ('' + (text || '')).length;
}
});
describe('length filter', function() {
var $filter;
beforeEach(inject(function(_$filter_){
$filter = _$filter_;
}));
it('returns 0 when given null', function() {
var length = $filter('length');
expect(length(null)).toEqual(0);
});
it('returns the correct value when given a string of chars', function() {
var length = $filter('length');
expect(length('abc')).toEqual(3);
});
});
```
Testing Directives
------------------
Directives in AngularJS are responsible for encapsulating complex functionality within custom HTML tags, attributes, classes or comments. Unit tests are very important for directives because the components you create with directives may be used throughout your application and in many different contexts.
### Simple HTML Element Directive
Let's start with an AngularJS app with no dependencies.
```
var app = angular.module('myApp', []);
```
Now we can add a directive to our app.
```
app.directive('aGreatEye', function () {
return {
restrict: 'E',
replace: true,
template: '<h1>lidless, wreathed in flame, {{1 + 1}} times</h1>'
};
});
```
This directive is used as a tag `<a-great-eye></a-great-eye>`. It replaces the entire tag with the template `<h1>lidless, wreathed in flame, {{1 + 1}} times</h1>`. Now we are going to write a jasmine unit test to verify this functionality. Note that the expression `{{1 + 1}}` times will also be evaluated in the rendered content.
```
describe('Unit testing great quotes', function() {
var $compile,
$rootScope;
// Load the myApp module, which contains the directive
beforeEach(module('myApp'));
// Store references to $rootScope and $compile
// so they are available to all tests in this describe block
beforeEach(inject(function(_$compile_, _$rootScope_){
// The injector unwraps the underscores (_) from around the parameter names when matching
$compile = _$compile_;
$rootScope = _$rootScope_;
}));
it('Replaces the element with the appropriate content', function() {
// Compile a piece of HTML containing the directive
var element = $compile("<a-great-eye></a-great-eye>")($rootScope);
// fire all the watches, so the scope expression {{1 + 1}} will be evaluated
$rootScope.$digest();
// Check that the compiled element contains the templated content
expect(element.html()).toContain("lidless, wreathed in flame, 2 times");
});
});
```
We inject the $compile service and $rootScope before each jasmine test. The $compile service is used to render the aGreatEye directive. After rendering the directive we ensure that the directive has replaced the content and "lidless, wreathed in flame, 2 times" is present.
**Underscore notation**: The use of the underscore notation (e.g.: `_$rootScope_`) is a convention wide spread in AngularJS community to keep the variable names clean in your tests. That's why the [`$injector`](../api/auto/service/%24injector) strips out the leading and the trailing underscores when matching the parameters. The underscore rule applies ***only*** if the name starts **and** ends with exactly one underscore, otherwise no replacing happens. ### Testing Transclusion Directives
Directives that use transclusion are treated specially by the compiler. Before their compile function is called, the contents of the directive's element are removed from the element and provided via a transclusion function. The directive's template is then appended to the directive's element, to which it can then insert the transcluded content into its template.
Before compilation:
```
<div transclude-directive>
Some transcluded content
</div>
```
After transclusion extraction:
```
<div transclude-directive></div>
```
After compilation:
```
<div transclude-directive>
Some Template
<span ng-transclude>Some transcluded content</span>
</div>
```
If the directive is using 'element' transclusion, the compiler will actually remove the directive's entire element from the DOM and replace it with a comment node. The compiler then inserts the directive's template "after" this comment node, as a sibling.
Before compilation
```
<div element-transclude>
Some Content
</div>
```
After transclusion extraction
```
<!-- elementTransclude -->
```
After compilation:
```
<!-- elementTransclude -->
<div element-transclude>
Some Template
<span ng-transclude>Some transcluded content</span>
</div>
```
It is important to be aware of this when writing tests for directives that use 'element' transclusion. If you place the directive on the root element of the DOM fragment that you pass to [`$compile`](../api/ng/service/%24compile), then the DOM node returned from the linking function will be the comment node and you will lose the ability to access the template and transcluded content.
```
var node = $compile('<div element-transclude></div>')($rootScope);
expect(node[0].nodeType).toEqual(node.COMMENT_NODE);
expect(node[1]).toBeUndefined();
```
To cope with this you simply ensure that your 'element' transclude directive is wrapped in an element, such as a `<div>`.
```
var node = $compile('<div><div element-transclude></div></div>')($rootScope);
var contents = node.contents();
expect(contents[0].nodeType).toEqual(node.COMMENT_NODE);
expect(contents[1].nodeType).toEqual(node.ELEMENT_NODE);
```
### Testing Directives With External Templates
If your directive uses `templateUrl`, consider using [karma-ng-html2js-preprocessor](https://github.com/karma-runner/karma-ng-html2js-preprocessor) to pre-compile HTML templates and thus avoid having to load them over HTTP during test execution. Otherwise you may run into issues if the test directory hierarchy differs from the application's.
Testing Promises
----------------
When testing promises, it's important to know that the resolution of promises is tied to the [digest cycle](../api/ng/type/%24rootscope.scope#%24digest.html). That means a promise's `then`, `catch` and `finally` callback functions are only called after a digest has run. In tests, you can trigger a digest by calling a scope's [`$apply` function](../api/ng/type/%24rootscope.scope#%24apply.html). If you don't have a scope in your test, you can inject the [$rootScope](../api/ng/service/%24rootscope) and call `$apply` on it. There is also an example of testing promises in the [`$q` service documentation](../api/ng/service/%24q#testing.html).
Using beforeAll()
-----------------
Jasmine's `beforeAll()` and mocha's `before()` hooks are often useful for sharing test setup - either to reduce test run-time or simply to make for more focused test cases.
By default, ngMock will create an injector per test case to ensure your tests do not affect each other. However, if we want to use `beforeAll()`, ngMock will have to create the injector before any test cases are run, and share that injector through all the cases for that `describe`. That is where [module.sharedInjector()](../api/ngmock/function/angular.mock.module.sharedinjector) comes in. When it's called within a `describe` block, a single injector is shared between all hooks and test cases run in that block.
In the example below we are testing a service that takes a long time to generate its answer. To avoid having all of the assertions we want to write in a single test case, [module.sharedInjector()](../api/ngmock/function/angular.mock.module.sharedinjector) and Jasmine's `beforeAll()` are used to run the service only once. The test cases then all make assertions about the properties added to the service instance.
```
describe("Deep Thought", function() {
module.sharedInjector();
beforeAll(module("UltimateQuestion"));
beforeAll(inject(function(DeepThought) {
expect(DeepThought.answer).toBeUndefined();
DeepThought.generateAnswer();
}));
it("has calculated the answer correctly", inject(function(DeepThought) {
// Because of sharedInjector, we have access to the instance of the DeepThought service
// that was provided to the beforeAll() hook. Therefore we can test the generated answer
expect(DeepThought.answer).toBe(42);
}));
it("has calculated the answer within the expected time", inject(function(DeepThought) {
expect(DeepThought.runTimeMillennia).toBeLessThan(8000);
}));
it("has double checked the answer", inject(function(DeepThought) {
expect(DeepThought.absolutelySureItIsTheRightAnswer).toBe(true);
}));
});
```
Sample project
--------------
See the [angular-seed](https://github.com/angular/angular-seed) project for an example.
| programming_docs |
angularjs
Improve this DocBootstrap
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/bootstrap.ngdoc?message=docs(guide%2FBootstrap)%3A%20describe%20your%20change...)Bootstrap
===================================================================================================================================================================================
This page explains the AngularJS initialization process and how you can manually initialize AngularJS if necessary.
AngularJS Tag
-------------
This example shows the recommended path for integrating AngularJS with what we call automatic initialization.
```
<!doctype html>
<html xmlns:ng="http://angularjs.org" ng-app>
<body>
...
<script src="angular.js"></script>
</body>
</html>
```
1. Place the `script` tag at the bottom of the page. Placing script tags at the end of the page improves app load time because the HTML loading is not blocked by loading of the `angular.js` script. You can get the latest bits from <http://code.angularjs.org>. Please don't link your production code to this URL, as it will expose a security hole on your site. For experimental development linking to our site is fine.
* Choose: `angular-[version].js` for a human-readable file, suitable for development and debugging.
* Choose: `angular-[version].min.js` for a compressed and obfuscated file, suitable for use in production.
2. Place `ng-app` to the root of your application, typically on the `<html>` tag if you want AngularJS to auto-bootstrap your application.
3. If you choose to use the old style directive syntax `ng:` then include xml-namespace in `html` when running the page in the XHTML mode. (This is here for historical reasons, and we no longer recommend use of `ng:`.)
Automatic Initialization
------------------------
AngularJS initializes automatically upon `DOMContentLoaded` event or when the `angular.js` script is evaluated if at that time `document.readyState` is set to `'complete'`. At this point AngularJS looks for the [`ngApp`](../api/ng/directive/ngapp) directive which designates your application root. If the [`ngApp`](../api/ng/directive/ngapp) directive is found then AngularJS will:
* load the <module> associated with the directive.
* create the application [injector](../api/auto/service/%24injector)
* compile the DOM treating the [`ngApp`](../api/ng/directive/ngapp) directive as the root of the compilation. This allows you to tell it to treat only a portion of the DOM as an AngularJS application.
```
<!doctype html>
<html ng-app="optionalModuleName">
<body>
I can add: {{ 1+2 }}.
<script src="angular.js"></script>
</body>
</html>
```
As a best practice, consider adding an `ng-strict-di` directive on the same element as `ng-app`:
```
<!doctype html>
<html ng-app="optionalModuleName" ng-strict-di>
<body>
I can add: {{ 1+2 }}.
<script src="angular.js"></script>
</body>
</html>
```
This will ensure that all services in your application are properly annotated. See the [dependency injection strict mode](di#using-strict-dependency-injection.html) docs for more.
Manual Initialization
---------------------
If you need to have more control over the initialization process, you can use a manual bootstrapping method instead. Examples of when you'd need to do this include using script loaders or the need to perform an operation before AngularJS compiles a page.
Here is an example of manually initializing AngularJS:
```
<!doctype html>
<html>
<body>
<div ng-controller="MyController">
Hello {{greetMe}}!
</div>
<script src="http://code.angularjs.org/snapshot/angular.js"></script>
<script>
angular.module('myApp', [])
.controller('MyController', ['$scope', function ($scope) {
$scope.greetMe = 'World';
}]);
angular.element(function() {
angular.bootstrap(document, ['myApp']);
});
</script>
</body>
</html>
```
Note that we provided the name of our application module to be loaded into the injector as the second parameter of the [`angular.bootstrap`](../api/ng/function/angular.bootstrap) function. Notice that `angular.bootstrap` will not create modules on the fly. You must create any custom [modules](module) before you pass them as a parameter.
You should call `angular.bootstrap()` *after* you've loaded or defined your modules. You cannot add controllers, services, directives, etc after an application bootstraps.
**Note:** You should not use the ng-app directive when manually bootstrapping your app. This is the sequence that your code should follow:
1. After the page and all of the code is loaded, find the root element of your AngularJS application, which is typically the root of the document.
2. Call [`angular.bootstrap`](../api/ng/function/angular.bootstrap) to [compile](compiler) the element into an executable, bi-directionally bound application.
Things to keep in mind
----------------------
There are a few things to keep in mind regardless of automatic or manual bootstrapping:
* While it's possible to bootstrap more than one AngularJS application per page, we don't actively test against this scenario. It's possible that you'll run into problems, especially with complex apps, so caution is advised.
* Do not bootstrap your app on an element with a directive that uses [transclusion](../api/ng/service/%24compile#transclusion.html), such as [`ngIf`](../api/ng/directive/ngif), [`ngInclude`](../api/ng/directive/nginclude) and [`ngView`](../api/ngroute/directive/ngview). Doing this misplaces the app [`$rootElement`](../api/ng/service/%24rootelement) and the app's [injector](../api/auto/service/%24injector), causing animations to stop working and making the injector inaccessible from outside the app.
Deferred Bootstrap
------------------
This feature enables tools like [Batarang](https://github.com/angular/angularjs-batarang) and test runners to hook into angular's bootstrap process and sneak in more modules into the DI registry which can replace or augment DI services for the purpose of instrumentation or mocking out heavy dependencies.
If `window.name` contains prefix `NG_DEFER_BOOTSTRAP!` when [`angular.bootstrap`](../api/ng/function/angular.bootstrap) is called, the bootstrap process will be paused until `angular.resumeBootstrap()` is called.
`angular.resumeBootstrap()` takes an optional array of modules that should be added to the original list of modules that the app was about to be bootstrapped with.
angularjs
Improve this DocAccessibility with ngAria
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/accessibility.ngdoc?message=docs(guide%2FAccessibility)%3A%20describe%20your%20change...)Accessibility with ngAria
===========================================================================================================================================================================================================
The goal of ngAria is to improve AngularJS's default accessibility by enabling common [ARIA](http://www.w3.org/TR/wai-aria/) attributes that convey state or semantic information for assistive technologies used by persons with disabilities.
Including ngAria
----------------
Using [ngAria](../api/ngaria) is as simple as requiring the ngAria module in your application. ngAria hooks into standard AngularJS directives and quietly injects accessibility support into your application at runtime.
```
angular.module('myApp', ['ngAria'])...
```
### Using ngAria
Most of what ngAria does is only visible "under the hood". To see the module in action, once you've added it as a dependency, you can test a few things:
* Using your favorite element inspector, look for attributes added by ngAria in your own code.
* Test using your keyboard to ensure `tabindex` is used correctly.
* Fire up a screen reader such as VoiceOver or NVDA to check for ARIA support. [Helpful screen reader tips.](http://webaim.org/articles/screenreader_testing/)
Supported directives
--------------------
Currently, ngAria interfaces with the following directives:
* [ngModel](accessibility#ngmodel.html)
* [ngDisabled](accessibility#ngdisabled.html)
* [ngRequired](accessibility#ngrequired.html)
* [ngReadonly](accessibility#ngreadonly.html)
* [ngChecked](accessibility#ngvaluechecked.html)
* [ngValue](accessibility#ngvaluechecked.html)
* [ngShow](accessibility#ngshow.html)
* [ngHide](accessibility#nghide.html)
* [ngClick](accessibility#ngclick.html)
* [ngDblClick](accessibility#ngdblclick.html)
* [ngMessages](accessibility#ngmessages.html)
ngModel
-------
Much of ngAria's heavy lifting happens in the [ngModel](../api/ng/directive/ngmodel) directive. For elements using ngModel, special attention is paid by ngAria if that element also has a role or type of `checkbox`, `radio`, `range` or `textbox`.
For those elements using ngModel, ngAria will dynamically bind and update the following ARIA attributes (if they have not been explicitly specified by the developer):
* aria-checked
* aria-valuemin
* aria-valuemax
* aria-valuenow
* aria-invalid
* aria-required
* aria-readonly
* aria-disabled
ngAria will also add `tabIndex`, ensuring custom elements with these roles will be reachable from the keyboard. It is still up to **you** as a developer to **ensure custom controls will be accessible**. As a rule, any time you create a widget involving user interaction, be sure to test it with your keyboard and at least one mobile and desktop screen reader.
ngValue and ngChecked
---------------------
To ease the transition between native inputs and custom controls, ngAria now supports [ngValue](../api/ng/directive/ngvalue) and [ngChecked](../api/ng/directive/ngchecked). The original directives were created for native inputs only, so ngAria extends support to custom elements by managing `aria-checked` for accessibility.
```
<custom-checkbox ng-checked="val"></custom-checkbox>
<custom-radio-button ng-value="val"></custom-radio-button>
```
Becomes:
```
<custom-checkbox ng-checked="val" aria-checked="true"></custom-checkbox>
<custom-radio-button ng-value="val" aria-checked="true"></custom-radio-button>
```
ngDisabled
----------
The `disabled` attribute is only valid for certain elements such as `button`, `input` and `textarea`. To properly disable custom element directives such as `<md-checkbox>` or `<taco-tab>`, using ngAria with [ngDisabled](../api/ng/directive/ngdisabled) will also add `aria-disabled`. This tells assistive technologies when a non-native input is disabled, helping custom controls to be more accessible.
```
<md-checkbox ng-disabled="disabled"></md-checkbox>
```
Becomes:
```
<md-checkbox disabled aria-disabled="true"></md-checkbox>
```
You can check whether a control is legitimately disabled for a screen reader by visiting <chrome://accessibility> and inspecting [the accessibility tree](http://www.paciellogroup.com/blog/2015/01/the-browser-accessibility-tree/). ngRequired
----------
The boolean `required` attribute is only valid for native form controls such as `input` and `textarea`. To properly indicate custom element directives such as `<md-checkbox>` or `<custom-input>` as required, using ngAria with [ngRequired](../api/ng/directive/ngrequired) will also add `aria-required`. This tells accessibility APIs when a custom control is required.
```
<md-checkbox ng-required="val"></md-checkbox>
```
Becomes:
```
<md-checkbox ng-required="val" aria-required="true"></md-checkbox>
```
ngReadonly
----------
The boolean `readonly` attribute is only valid for native form controls such as `input` and `textarea`. To properly indicate custom element directives such as `<md-checkbox>` or `<custom-input>` as required, using ngAria with [ngReadonly](../api/ng/directive/ngreadonly) will also add `aria-readonly`. This tells accessibility APIs when a custom control is read-only.
```
<md-checkbox ng-readonly="val"></md-checkbox>
```
Becomes:
```
<md-checkbox ng-readonly="val" aria-readonly="true"></md-checkbox>
```
ngShow
------
The [ngShow](../api/ng/directive/ngshow) directive shows or hides the given HTML element based on the expression provided to the `ngShow` attribute. The element is shown or hidden by removing or adding the `.ng-hide` CSS class onto the element.
In its default setup, ngAria for `ngShow` is actually redundant. It toggles `aria-hidden` on the directive when it is hidden or shown. However, the default CSS of `display: none !important`, already hides child elements from a screen reader. It becomes more useful when the default CSS is overridden with properties that don’t affect assistive technologies, such as `opacity` or `transform`. By toggling `aria-hidden` dynamically with ngAria, we can ensure content visually hidden with this technique will not be read aloud in a screen reader.
One caveat with this combination of CSS and `aria-hidden`: you must also remove links and other interactive child elements from the tab order using `tabIndex=“-1”` on each control. This ensures screen reader users won't accidentally focus on "mystery elements". Managing tab index on every child control can be complex and affect performance, so it’s best to just stick with the default `display: none` CSS. See the [fourth rule of ARIA use](http://www.w3.org/TR/aria-in-html/#fourth-rule-of-aria-use).
```
.ng-hide {
display: block;
opacity: 0;
}
```
```
<div ng-show="false" class="ng-hide" aria-hidden="true"></div>
```
Becomes:
```
<div ng-show="true" aria-hidden="false"></div>
```
*Note: Child links, buttons or other interactive controls must also be removed from the tab order.*
ngHide
------
The [ngHide](../api/ng/directive/nghide) directive shows or hides the given HTML element based on the expression provided to the `ngHide` attribute. The element is shown or hidden by removing or adding the `.ng-hide` CSS class onto the element.
The default CSS for `ngHide`, the inverse method to `ngShow`, makes ngAria redundant. It toggles `aria-hidden` on the directive when it is hidden or shown, but the content is already hidden with `display: none`. See explanation for [ngShow](accessibility#ngshow.html) when overriding the default CSS.
ngClick and ngDblclick
-----------------------
If `ng-click` or `ng-dblclick` is encountered, ngAria will add `tabindex="0"` to any element not in the list of built in aria nodes: *Button* Anchor *Input* Textarea *Select* Details/Summary To fix widespread accessibility problems with `ng-click` on `div` elements, ngAria will dynamically bind a keypress event by default as long as the element isn't in a node from the list of built in aria nodes. You can turn this functionality on or off with the `bindKeypress` configuration option. ngAria will also add the `button` role to communicate to users of assistive technologies. This can be disabled with the `bindRoleForClick` configuration option. For `ng-dblclick`, you must still manually add `ng-keypress` and a role to non-interactive elements such as `div` or `taco-button` to enable keyboard access. ### Example
`html
<div ng-click="toggleMenu()"></div>` Becomes: `html
<div ng-click="toggleMenu()" tabindex="0"></div>` ngMessages
----------
The ngMessages module makes it easy to display form validation or other messages with priority sequencing and animation. To expose these visual messages to screen readers, ngAria injects `aria-live="assertive"`, causing them to be read aloud any time a message is shown, regardless of the user's focus location.
```
<div ng-messages="myForm.myName.$error">
<div ng-message="required">You did not enter a field</div>
<div ng-message="maxlength">Your field is too long</div>
</div>
```
Becomes:
```
<div ng-messages="myForm.myName.$error" aria-live="assertive">
<div ng-message="required">You did not enter a field</div>
<div ng-message="maxlength">Your field is too long</div>
</div>
```
Disabling attributes
--------------------
The attribute magic of ngAria may not work for every scenario. To disable individual attributes, you can use the [config](../api/ngaria/provider/%24ariaprovider#config.html) method. Just keep in mind this will tell ngAria to ignore the attribute globally.
Common Accessibility Patterns
-----------------------------
Accessibility best practices that apply to web apps in general also apply to AngularJS.
* **Text alternatives**: Add alternate text content to make visual information accessible using [these W3C guidelines](http://www.w3.org/TR/html-alt-techniques/). The appropriate technique depends on the specific markup but can be accomplished using offscreen spans, `aria-label` or label elements, image `alt` attributes, `figure`/`figcaption` elements and more.
* **HTML Semantics**: If you're creating custom element directives, Web Components or HTML in general, use native elements wherever possible to utilize built-in events and properties. Alternatively, use ARIA to communicate semantic meaning. See [notes on ARIA use](http://www.w3.org/TR/aria-in-html/#notes-on-aria-use-in-html).
* **Focus management**: Guide the user around the app as views are appended/removed. Focus should *never* be lost, as this causes unexpected behavior and much confusion (referred to as "freak-out mode").
* **Announcing changes**: When filtering or other UI messaging happens away from the user's focus, notify with [ARIA Live Regions](https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/ARIA_Live_Regions).
* **Color contrast and scale**: Make sure content is legible and interactive controls are usable at all screen sizes. Consider configurable UI themes for people with color blindness, low vision or other visual impairments.
* **Progressive enhancement**: Some users do not browse with JavaScript enabled or do not have the latest browser. An accessible message about site requirements can inform users and improve the experience.
Additional Resources
--------------------
* [Using ARIA in HTML](http://www.w3.org/TR/aria-in-html/)
* [AngularJS Accessibility at ngEurope](https://www.youtube.com/watch?v=dmYDggEgU-s&list=UUEGUP3TJJfMsEM_1y8iviSQ)
* [Testing with Screen Readers](http://webaim.org/articles/screenreader_testing/)
* [Chrome Accessibility Developer Tools](https://chrome.google.com/webstore/detail/accessibility-developer-t/fpkknkljclfencbdbgkenhalefipecmb?hl=en)
* [W3C Accessibility Testing](http://www.w3.org/wiki/Accessibility_testing)
* [WebAIM](http://webaim.org)
* [A11y Project](http://a11yproject.com)
angularjs
Improve this DocModules
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/module.ngdoc?message=docs(guide%2FModules)%3A%20describe%20your%20change...)Modules
============================================================================================================================================================================
What is a Module?
-----------------
You can think of a module as a container for the different parts of your app – controllers, services, filters, directives, etc.
Why?
----
Most applications have a main method that instantiates and wires together the different parts of the application.
AngularJS apps don't have a main method. Instead modules declaratively specify how an application should be bootstrapped. There are several advantages to this approach:
* The declarative process is easier to understand.
* You can package code as reusable modules.
* The modules can be loaded in any order (or even in parallel) because modules delay execution.
* Unit tests only have to load relevant modules, which keeps them fast.
* End-to-end tests can use modules to override configuration.
The Basics
----------
I'm in a hurry. How do I get a Hello World module working?
Important things to notice:
* The [Module](../api/ng/type/angular.module) API
* The reference to `myApp` module in `<div ng-app="myApp">`. This is what bootstraps the app using your module.
* The empty array in `angular.module('myApp', [])`. This array is the list of modules `myApp` depends on.
Recommended Setup
-----------------
While the example above is simple, it will not scale to large applications. Instead we recommend that you break your application to multiple modules like this:
* A module for each feature
* A module for each reusable component (especially directives and filters)
* And an application level module which depends on the above modules and contains any initialization code.
You can find a community [style guide](https://github.com/johnpapa/angular-styleguide) to help yourself when application grows.
The above is a suggestion. Tailor it to your needs.
Module Loading
--------------
A [module](../api/ng/type/angular.module) is a collection of providers, services, directives etc., and optionally config and run blocks which get applied to the application during the bootstrap process.
The [module API](../api/ng/type/angular.module) describes all the available methods and how they can be used.
See [Using Dependency Injection](di#using-dependency-injection.html) to find out which dependencies can be injected in each method.
### Dependencies and Order of execution
Modules can list other modules as their dependencies. Depending on a module implies that the required module will be loaded before the requiring module is loaded.
In a single module the order of execution is as follows:
1. [provider](../api/ng/type/angular.module#provider.html) functions are executed, so they and the services they define can be made available to the [$injector](../api/auto/service/%24injector).
2. After that, the configuration blocks ([config](../api/ng/type/angular.module#config.html) functions) are executed. This means the configuration blocks of the required modules execute before the configuration blocks of any requiring module.
This continues until all module dependencies has been resolved.
Then, the [run](../api/ng/type/angular.module#run.html) blocks that have been collected from each module are executed in order of requirement.
Note: each module is only loaded once, even if multiple other modules require it. Note: the factory function for "values" and "services" is called lazily when the value/service is injected for the first time.
### Registration in the config block
While it is recommended to register injectables directly with the [module API](../api/ng/type/angular.module), it is also possible to register services, directives etc. by injecting [$provide](../api/auto/service/%24provide) or the individual service providers into the config function:
```
angular.module('myModule', []).
value('a', 123).
factory('a', function() { return 123; }).
directive('directiveName', ...).
filter('filterName', ...);
// is same as
angular.module('myModule', []).
config(function($provide, $compileProvider, $filterProvider) {
$provide.value('a', 123);
$provide.factory('a', function() { return 123; });
$compileProvider.directive('directiveName', ...);
$filterProvider.register('filterName', ...);
});
```
### Run Blocks
Run blocks are the closest thing in AngularJS to the main method. A run block is the code which needs to run to kickstart the application. It is executed after all of the services have been configured and the injector has been created. Run blocks typically contain code which is hard to unit-test, and for this reason should be declared in isolated modules, so that they can be ignored in the unit-tests.
### Asynchronous Loading
Modules are a way of managing $injector configuration, and have nothing to do with loading of scripts into a VM. There are existing projects which deal with script loading, which may be used with AngularJS. Because modules do nothing at load time they can be loaded into the VM in any order and thus script loaders can take advantage of this property and parallelize the loading process.
### Creation versus Retrieval
Beware that using `angular.module('myModule', [])` will create the module `myModule` and overwrite any existing module named `myModule`. Use `angular.module('myModule')` to retrieve an existing module.
```
var myModule = angular.module('myModule', []);
// add some directives and services
myModule.service('myService', ...);
myModule.directive('myDirective', ...);
// overwrites both myService and myDirective by creating a new module
var myModule = angular.module('myModule', []);
// throws an error because myOtherModule has yet to be defined
var myModule = angular.module('myOtherModule');
```
Unit Testing
------------
A unit test is a way of instantiating a subset of an application to apply stimulus to it. Small, structured modules help keep unit tests concise and focused.
Each module can only be loaded once per injector. Usually an AngularJS app has only one injector and modules are only loaded once. Each test has its own injector and modules are loaded multiple times. In all of these examples we are going to assume this module definition:
```
angular.module('greetMod', []).
factory('alert', function($window) {
return function(text) {
$window.alert(text);
}
}).
value('salutation', 'Hello').
factory('greet', function(alert, salutation) {
return function(name) {
alert(salutation + ' ' + name + '!');
}
});
```
Let's write some tests to show how to override configuration in tests.
```
describe('myApp', function() {
// load application module (`greetMod`) then load a special
// test module which overrides `$window` with a mock version,
// so that calling `window.alert()` will not block the test
// runner with a real alert box.
beforeEach(module('greetMod', function($provide) {
$provide.value('$window', {
alert: jasmine.createSpy('alert')
});
}));
// inject() will create the injector and inject the `greet` and
// `$window` into the tests.
it('should alert on $window', inject(function(greet, $window) {
greet('World');
expect($window.alert).toHaveBeenCalledWith('Hello World!');
}));
// this is another way of overriding configuration in the
// tests using inline `module` and `inject` methods.
it('should alert using the alert service', function() {
var alertSpy = jasmine.createSpy('alert');
module(function($provide) {
$provide.value('alert', alertSpy);
});
inject(function(greet) {
greet('World');
expect(alertSpy).toHaveBeenCalledWith('Hello World!');
});
});
});
```
| programming_docs |
angularjs
Improve this DocUsing the $location service
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/%24location.ngdoc?message=docs(guide%2FUsing%20%24location)%3A%20describe%20your%20change...)Using the $location service
=================================================================================================================================================================================================================
The `$location` service parses the URL in the browser address bar (based on [`window.location`](https://developer.mozilla.org/en/window.location)) and makes the URL available to your application. Changes to the URL in the address bar are reflected into the `$location` service and changes to `$location` are reflected into the browser address bar.
**The $location service:**
* Exposes the current URL in the browser address bar, so you can
+ Watch and observe the URL.
+ Change the URL.
* Maintains synchronization between itself and the browser's URL when the user
+ Changes the address in the browser's address bar.
+ Clicks the back or forward button in the browser (or clicks a History link).
+ Clicks on a link in the page.
* Represents the URL object as a set of methods (protocol, host, port, path, search, hash).
Comparing $location to window.location
--------------------------------------
| | window.location | $location service |
| --- | --- | --- |
| purpose | allow read/write access to the current browser location | same |
| API | exposes "raw" object with properties that can be directly modified | exposes jQuery-style getters and setters |
| integration with AngularJS application life-cycle | none | knows about all internal life-cycle phases, integrates with [$watch](../api/ng/type/%24rootscope.scope#%24watch.html), ... |
| seamless integration with HTML5 API | no | yes (with a fallback for legacy browsers) |
| aware of docroot/context from which the application is loaded | no - window.location.pathname returns "/docroot/actual/path" | yes - $location.path() returns "/actual/path" |
When should I use $location?
----------------------------
Any time your application needs to react to a change in the current URL or if you want to change the current URL in the browser.
What does it not do?
--------------------
It does not cause a full page reload when the browser URL is changed. To reload the page after changing the URL, use the lower-level API, `$window.location.href`.
General overview of the API
---------------------------
The `$location` service can behave differently, depending on the configuration that was provided to it when it was instantiated. The default configuration is suitable for many applications, for others customizing the configuration can enable new features.
Once the `$location` service is instantiated, you can interact with it via jQuery-style getter and setter methods that allow you to get or change the current URL in the browser.
### $location service configuration
To configure the `$location` service, retrieve the [$locationProvider](../api/ng/provider/%24locationprovider) and set the parameters as follows:
* **html5Mode(mode)**: `{boolean|Object}`
`false` or `{enabled: false}` (default) - see [Hashbang mode](%24location#hashbang-mode-default-mode-.html)
`true` or `{enabled: true}` - see [HTML5 mode](%24location#html5-mode.html)
`{..., requireBase: true/false}` (only affects HTML5 mode) - see [Relative links](%24location#relative-links.html)
`{..., rewriteLinks: true/false/'string'}` (only affects HTML5 mode) - see [HTML link rewriting](%24location#html-link-rewriting.html)
Default:
```
{
enabled: false,
requireBase: true,
rewriteLinks: true
}
```
* **hashPrefix(prefix)**: `{string}`
Prefix used for Hashbang URLs (used in Hashbang mode or in legacy browsers in HTML5 mode).
Default: `'!'`
#### Example configuration
```
$locationProvider.html5Mode(true).hashPrefix('*');
```
### Getter and setter methods
`$location` service provides getter methods for read-only parts of the URL (absUrl, protocol, host, port) and getter / setter methods for url, path, search, hash:
```
// get the current path
$location.path();
// change the path
$location.path('/newValue')
```
All of the setter methods return the same `$location` object to allow chaining. For example, to change multiple segments in one go, chain setters like this:
```
$location.path('/newValue').search({key: value});
```
### Replace method
There is a special `replace` method which can be used to tell the $location service that the next time the $location service is synced with the browser, the last history record should be replaced instead of creating a new one. This is useful when you want to implement redirection, which would otherwise break the back button (navigating back would retrigger the redirection). To change the current URL without creating a new browser history record you can call:
```
$location.path('/someNewPath');
$location.replace();
// or you can chain these as: $location.path('/someNewPath').replace();
```
Note that the setters don't update `window.location` immediately. Instead, the `$location` service is aware of the [scope](../api/ng/type/%24rootscope.scope) life-cycle and coalesces multiple `$location` mutations into one "commit" to the `window.location` object during the scope `$digest` phase. Since multiple changes to the $location's state will be pushed to the browser as a single change, it's enough to call the `replace()` method just once to make the entire "commit" a replace operation rather than an addition to the browser history. Once the browser is updated, the $location service resets the flag set by `replace()` method and future mutations will create new history records, unless `replace()` is called again.
### Setters and character encoding
You can pass special characters to `$location` service and it will encode them according to rules specified in [RFC 3986](http://www.ietf.org/rfc/rfc3986.txt). When you access the methods:
* All values that are passed to `$location` setter methods, `path()`, `search()`, `hash()`, are encoded.
* Getters (calls to methods without parameters) return decoded values for the following methods `path()`, `search()`, `hash()`.
* When you call the `absUrl()` method, the returned value is a full url with its segments encoded.
* When you call the `url()` method, the returned value is path, search and hash, in the form `/path?search=a&b=c#hash`. The segments are encoded as well.
Hashbang and HTML5 Modes
------------------------
`$location` service has two configuration modes which control the format of the URL in the browser address bar: **Hashbang mode** (the default) and the **HTML5 mode** which is based on using the [HTML5 History API](https://html.spec.whatwg.org/multipage/browsers.html#the-history-interface). Applications use the same API in both modes and the `$location` service will work with appropriate URL segments and browser APIs to facilitate the browser URL change and history management.
| | Hashbang mode | HTML5 mode |
| --- | --- | --- |
| configuration | the default | { html5Mode: true } |
| URL format | hashbang URLs in all browsers | regular URLs in modern browser, hashbang URLs in old browser |
| <a href=""> link rewriting | no | yes |
| requires server-side configuration | no | yes |
### Hashbang mode (default mode)
In this mode, `$location` uses Hashbang URLs in all browsers. AngularJS also does not intercept and rewrite links in this mode. I.e. links work as expected and also perform full page reloads when other parts of the url than the hash fragment was changed.
```
it('should show example', function() {
module(function($locationProvider) {
$locationProvider.html5Mode(false);
$locationProvider.hashPrefix('!');
});
inject(function($location) {
// open http://example.com/base/index.html#!/a
expect($location.absUrl()).toBe('http://example.com/base/index.html#!/a');
expect($location.path()).toBe('/a');
$location.path('/foo');
expect($location.absUrl()).toBe('http://example.com/base/index.html#!/foo');
expect($location.search()).toEqual({});
$location.search({a: 'b', c: true});
expect($location.absUrl()).toBe('http://example.com/base/index.html#!/foo?a=b&c');
$location.path('/new').search('x=y');
expect($location.absUrl()).toBe('http://example.com/base/index.html#!/new?x=y');
});
});
```
### HTML5 mode
In HTML5 mode, the `$location` service getters and setters interact with the browser URL address through the HTML5 history API. This allows for use of regular URL path and search segments, instead of their hashbang equivalents. If the HTML5 History API is not supported by a browser, the `$location` service will fall back to using the hashbang URLs automatically. This frees you from having to worry about whether the browser displaying your app supports the history API or not; the `$location` service transparently uses the best available option.
* Opening a regular URL in a legacy browser -> redirects to a hashbang URL
* Opening hashbang URL in a modern browser -> rewrites to a regular URL
Note that in this mode, AngularJS intercepts all links (subject to the "Html link rewriting" rules below) and updates the url in a way that never performs a full page reload.
```
it('should show example', function() {
module(function($locationProvider) {
$locationProvider.html5Mode(true);
$locationProvider.hashPrefix('!');
});
inject(function($location) {
// in browser with HTML5 history support:
// open http://example.com/#!/a -> rewrite to http://example.com/a
// (replacing the http://example.com/#!/a history record)
expect($location.path()).toBe('/a');
$location.path('/foo');
expect($location.absUrl()).toBe('http://example.com/foo');
expect($location.search()).toEqual({});
$location.search({a: 'b', c: true});
expect($location.absUrl()).toBe('http://example.com/foo?a=b&c');
$location.path('/new').search('x=y');
expect($location.url()).toBe('/new?x=y');
expect($location.absUrl()).toBe('http://example.com/new?x=y');
});
});
it('should show example (when browser doesn\'t support HTML5 mode', function() {
module(function($provide, $locationProvider) {
$locationProvider.html5Mode(true);
$locationProvider.hashPrefix('!');
$provide.value('$sniffer', {history: false});
});
inject(initBrowser({ url: 'http://example.com/new?x=y', basePath: '/' }),
function($location) {
// in browser without html5 history support:
// open http://example.com/new?x=y -> redirect to http://example.com/#!/new?x=y
// (again replacing the http://example.com/new?x=y history item)
expect($location.path()).toBe('/new');
expect($location.search()).toEqual({x: 'y'});
$location.path('/foo/bar');
expect($location.path()).toBe('/foo/bar');
expect($location.url()).toBe('/foo/bar?x=y');
expect($location.absUrl()).toBe('http://example.com/#!/foo/bar?x=y');
});
});
```
#### Fallback for legacy browsers
For browsers that support the HTML5 history API, `$location` uses the HTML5 history API to write path and search. If the history API is not supported by a browser, `$location` supplies a Hashbang URL. This frees you from having to worry about whether the browser viewing your app supports the history API or not; the `$location` service makes this transparent to you.
#### HTML link rewriting
When you use HTML5 history API mode, you will not need special hashbang links. All you have to do is specify regular URL links, such as: `<a href="/some?foo=bar">link</a>`
When a user clicks on this link,
* In a legacy browser, the URL changes to `/index.html#!/some?foo=bar`
* In a modern browser, the URL changes to `/some?foo=bar`
In cases like the following, links are not rewritten; instead, the browser will perform a full page reload to the original link.
* Links that contain `target` element
Example: `<a href="/ext/link?a=b" target="_self">link</a>`
* Absolute links that go to a different domain
Example: `<a href="http://angularjs.org/">link</a>`
* Links starting with '/' that lead to a different base path
Example: `<a href="/not-my-base/link">link</a>`
If `mode.rewriteLinks` is set to `false` in the `mode` configuration object passed to `$locationProvider.html5Mode()`, the browser will perform a full page reload for every link. `mode.rewriteLinks` can also be set to a string, which will enable link rewriting only on anchor elements that have the given attribute.
For example, if `mode.rewriteLinks` is set to `'internal-link'`:
* `<a href="/some/path" internal-link>link</a>` will be rewritten
* `<a href="/some/path">link</a>` will perform a full page reload
Note that [attribute name normalization](directive#normalization.html) does not apply here, so `'internalLink'` will **not** match `'internal-link'`.
#### Relative links
Be sure to check all relative links, images, scripts etc. AngularJS requires you to specify the url base in the head of your main html file (`<base href="/my-base/index.html">`) unless `html5Mode.requireBase` is set to `false` in the html5Mode definition object passed to `$locationProvider.html5Mode()`. With that, relative urls will always be resolved to this base url, even if the initial url of the document was different.
There is one exception: Links that only contain a hash fragment (e.g. `<a href="#target">`) will only change `$location.hash()` and not modify the url otherwise. This is useful for scrolling to anchors on the same page without needing to know on which page the user currently is.
#### Server side
Using this mode requires URL rewriting on server side, basically you have to rewrite all your links to entry point of your application (e.g. index.html). Requiring a `<base>` tag is also important for this case, as it allows AngularJS to differentiate between the part of the url that is the application base and the path that should be handled by the application.
#### Base href constraints
The `$location` service is not able to function properly if the current URL is outside the URL given as the base href. This can have subtle confusing consequences...
Consider a base href set as follows: `<base href="/base/">` (i.e. the application exists in the "folder" called `/base`). The URL `/base` is actually outside the application (it refers to the `base` file found in the root `/` folder).
If you wish to be able to navigate to the application via a URL such as `/base` then you should ensure that your server is setup to redirect such requests to `/base/`.
See <https://github.com/angular/angular.js/issues/14018> for more information.
### Sending links among different browsers
Because of rewriting capability in HTML5 mode, your users will be able to open regular url links in legacy browsers and hashbang links in modern browser:
* Modern browser will rewrite hashbang URLs to regular URLs.
* Older browsers will redirect regular URLs to hashbang URLs.
Here you can see two `$location` instances that show the difference between **Html5 mode** and **Html5 Fallback mode**. Note that to simulate different levels of browser support, the `$location` instances are connected to a fakeBrowser service, which you don't have to set up in actual projects.
Note that when you type hashbang url into the first browser (or vice versa) it doesn't rewrite / redirect to regular / hashbang url, as this conversion happens only during parsing the initial URL = on page reload.
In these examples we use `<base href="/base/index.html" />`. The inputs represent the address bar of the browser.
##### Browser in HTML5 mode
##### Browser in HTML5 Fallback mode (Hashbang mode)
Caveats
-------
### Page reload navigation
The `$location` service allows you to change only the URL; it does not allow you to reload the page. When you need to change the URL and reload the page or navigate to a different page, please use a lower level API, [$window.location.href](../api/ng/service/%24window).
### Using $location outside of the scope life-cycle
`$location` knows about AngularJS's [scope](../api/ng/type/%24rootscope.scope) life-cycle. When a URL changes in the browser it updates the `$location` and calls `$apply` so that all [$watchers](../api/ng/type/%24rootscope.scope#%24watch.html) / [$observers](../api/ng/type/%24compile.directive.attributes#%24observe.html) are notified. When you change the `$location` inside the `$digest` phase everything is ok; `$location` will propagate this change into browser and will notify all the [$watchers](../api/ng/type/%24rootscope.scope#%24watch.html) / [$observers](../api/ng/type/%24compile.directive.attributes#%24observe.html). When you want to change the `$location` from outside AngularJS (for example, through a DOM Event or during testing) - you must call `$apply` to propagate the changes.
### $location.path() and ! or / prefixes
A path should always begin with forward slash (`/`); the `$location.path()` setter will add the forward slash if it is missing.
Note that the `!` prefix in the hashbang mode is not part of `$location.path()`; it is actually `hashPrefix`.
### Crawling your app
Most modern search engines are able to crawl AJAX applications with dynamic content, provided all included resources are available to the crawler bots.
There also exists a special [AJAX crawling scheme](http://code.google.com/web/ajaxcrawling/docs/specification.html) developed by Google that allows bots to crawl the static equivalent of a dynamically generated page, but this schema has been deprecated, and support for it may vary by search engine.
Testing with the $location service
----------------------------------
When using `$location` service during testing, you are outside of the angular's [scope](../api/ng/type/%24rootscope.scope) life-cycle. This means it's your responsibility to call `scope.$apply()`.
```
describe('serviceUnderTest', function() {
beforeEach(module(function($provide) {
$provide.factory('serviceUnderTest', function($location) {
// whatever it does...
});
});
it('should...', inject(function($location, $rootScope, serviceUnderTest) {
$location.path('/new/path');
$rootScope.$apply();
// test whatever the service should do...
}));
});
```
Two-way binding to $location
----------------------------
Because `$location` uses getters/setters, you can use `ng-model-options="{ getterSetter: true }"` to bind it to `ngModel`:
Related API
-----------
* [`$location` API](../api/ng/service/%24location)
angularjs
Improve this DocForms
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/forms.ngdoc?message=docs(guide%2FForms)%3A%20describe%20your%20change...)Forms
=======================================================================================================================================================================
Controls (`input`, `select`, `textarea`) are ways for a user to enter data. A Form is a collection of controls for the purpose of grouping related controls together.
Form and controls provide validation services, so that the user can be notified of invalid input before submitting a form. This provides a better user experience than server-side validation alone because the user gets instant feedback on how to correct the error. Keep in mind that while client-side validation plays an important role in providing good user experience, it can easily be circumvented and thus can not be trusted. Server-side validation is still necessary for a secure application.
Simple form
-----------
The key directive in understanding two-way data-binding is [ngModel](../api/ng/directive/ngmodel). The `ngModel` directive provides the two-way data-binding by synchronizing the model to the view, as well as view to the model. In addition it provides an [API](../api/ng/type/ngmodel.ngmodelcontroller) for other directives to augment its behavior.
Note that `novalidate` is used to disable browser's native form validation.
The value of `ngModel` won't be set unless it passes validation for the input field. For example: inputs of type `email` must have a value in the form of `user@domain`.
Using CSS classes
-----------------
To allow styling of form as well as controls, `ngModel` adds these CSS classes:
* `ng-valid`: the model is valid
* `ng-invalid`: the model is invalid
* `ng-valid-[key]`: for each valid key added by `$setValidity`
* `ng-invalid-[key]`: for each invalid key added by `$setValidity`
* `ng-pristine`: the control hasn't been interacted with yet
* `ng-dirty`: the control has been interacted with
* `ng-touched`: the control has been blurred
* `ng-untouched`: the control hasn't been blurred
* `ng-pending`: any `$asyncValidators` are unfulfilled
The following example uses the CSS to display validity of each form control. In the example both `user.name` and `user.email` are required, but are rendered with red background only after the input is blurred (loses focus). This ensures that the user is not distracted with an error until after interacting with the control, and failing to satisfy its validity.
Binding to form and control state
---------------------------------
A form is an instance of [FormController](../api/ng/type/form.formcontroller). The form instance can optionally be published into the scope using the `name` attribute.
Similarly, an input control that has the [ngModel](../api/ng/directive/ngmodel) directive holds an instance of [NgModelController](../api/ng/type/ngmodel.ngmodelcontroller). Such a control instance can be published as a property of the form instance using the `name` attribute on the input control. The name attribute specifies the name of the property on the form instance.
This implies that the internal state of both the form and the control is available for binding in the view using the standard binding primitives.
This allows us to extend the above example with these features:
* Custom error message displayed after the user interacted with a control (i.e. when `$touched` is set)
* Custom error message displayed upon submitting the form (`$submitted` is set), even if the user didn't interact with a control
Custom model update triggers
----------------------------
By default, any change to the content will trigger a model update and form validation. You can override this behavior using the [ngModelOptions](../api/ng/directive/ngmodeloptions) directive to bind only to specified list of events. I.e. `ng-model-options="{ updateOn: 'blur' }"` will update and validate only after the control loses focus. You can set several events using a space delimited list. I.e. `ng-model-options="{ updateOn: 'mousedown blur' }"`
If you want to keep the default behavior and just add new events that may trigger the model update and validation, add "default" as one of the specified events.
I.e. `ng-model-options="{ updateOn: 'default blur' }"`
The following example shows how to override immediate updates. Changes on the inputs within the form will update the model only when the control loses focus (blur event).
Non-immediate (debounced) model updates
---------------------------------------
You can delay the model update/validation by using the `debounce` key with the [ngModelOptions](../api/ng/directive/ngmodeloptions) directive. This delay will also apply to parsers, validators and model flags like `$dirty` or `$pristine`.
I.e. `ng-model-options="{ debounce: 500 }"` will wait for half a second since the last content change before triggering the model update and form validation.
If custom triggers are used, custom debouncing timeouts can be set for each event using an object in `debounce`. This can be useful to force immediate updates on some specific circumstances (like blur events).
I.e. `ng-model-options="{ updateOn: 'default blur', debounce: { default: 500, blur: 0 } }"`
If those attributes are added to an element, they will be applied to all the child elements and controls that inherit from it unless they are overridden.
This example shows how to debounce model changes. Model will be updated only 250 milliseconds after last change.
Custom Validation
-----------------
AngularJS provides basic implementation for most common HTML5 [input](../api/ng/directive/input) types: ([text](../api/ng/input/input%5Btext%5D), [number](../api/ng/input/input%5Bnumber%5D), [url](../api/ng/input/input%5Burl%5D), [email](../api/ng/input/input%5Bemail%5D), [date](../api/ng/input/input%5Bdate%5D), [radio](../api/ng/input/input%5Bradio%5D), [checkbox](../api/ng/input/input%5Bcheckbox%5D)), as well as some directives for validation (`required`, `pattern`, `minlength`, `maxlength`, `min`, `max`).
With a custom directive, you can add your own validation functions to the `$validators` object on the [`ngModelController`](../api/ng/type/ngmodel.ngmodelcontroller). To get a hold of the controller, you require it in the directive as shown in the example below.
Each function in the `$validators` object receives the `modelValue` and the `viewValue` as parameters. AngularJS will then call `$setValidity` internally with the function's return value (`true`: valid, `false`: invalid). The validation functions are executed every time an input is changed (`$setViewValue` is called) or whenever the bound `model` changes. Validation happens after successfully running `$parsers` and `$formatters`, respectively. Failed validators are stored by key in [`ngModelController.$error`](../api/ng/type/ngmodel.ngmodelcontroller#%24error.html).
Additionally, there is the `$asyncValidators` object which handles asynchronous validation, such as making an `$http` request to the backend. Functions added to the object must return a promise that must be `resolved` when valid or `rejected` when invalid. In-progress async validations are stored by key in [`ngModelController.$pending`](../api/ng/type/ngmodel.ngmodelcontroller#%24pending.html).
In the following example we create two directives:
* An `integer` directive that validates whether the input is a valid integer. For example, `1.23` is an invalid value, since it contains a fraction. Note that we validate the viewValue (the string value of the control), and not the modelValue. This is because input[number] converts the viewValue to a number when running the `$parsers`.
* A `username` directive that asynchronously checks if a user-entered value is already taken. We mock the server request with a `$q` deferred.
Modifying built-in validators
-----------------------------
Since AngularJS itself uses `$validators`, you can easily replace or remove built-in validators, should you find it necessary. The following example shows you how to overwrite the email validator in `input[email]` from a custom directive so that it requires a specific top-level domain, `example.com` to be present. Note that you can alternatively use `ng-pattern` to further restrict the validation.
Implementing custom form controls (using ngModel)
-------------------------------------------------
AngularJS implements all of the basic HTML form controls ([input](../api/ng/directive/input), [select](../api/ng/directive/select), [textarea](../api/ng/directive/textarea)), which should be sufficient for most cases. However, if you need more flexibility, you can write your own form control as a directive.
In order for custom control to work with `ngModel` and to achieve two-way data-binding it needs to:
* implement `$render` method, which is responsible for rendering the data after it passed the [`NgModelController.$formatters`](../api/ng/type/ngmodel.ngmodelcontroller#%24formatters.html),
* call `$setViewValue` method, whenever the user interacts with the control and model needs to be updated. This is usually done inside a DOM Event listener.
See [`$compileProvider.directive`](directive) for more info.
The following example shows how to add two-way data-binding to contentEditable elements.
| programming_docs |
angularjs
Improve this DocInterpolation and data-binding
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/interpolation.ngdoc?message=docs(guide%2FInterpolation)%3A%20describe%20your%20change...)Interpolation and data-binding
================================================================================================================================================================================================================
Interpolation markup with embedded [expressions](expression) is used by AngularJS to provide data-binding to text nodes and attribute values.
An example of interpolation is shown below:
```
<a ng-href="img/{{username}}.jpg">Hello {{username}}!</a>
```
### How text and attribute bindings work
During the compilation process the [compiler](../api/ng/service/%24compile) uses the [$interpolate](../api/ng/service/%24interpolate) service to see if text nodes and element attributes contain interpolation markup with embedded expressions.
If that is the case, the compiler adds an interpolateDirective to the node and registers [watches](../api/ng/type/%24rootscope.scope#%24watch.html) on the computed interpolation function, which will update the corresponding text nodes or attribute values as part of the normal [digest](../api/ng/type/%24rootscope.scope#%24digest.html) cycle.
Note that the interpolateDirective has a priority of 100 and sets up the watch in the preLink function.
### How the string representation is computed
If the interpolated value is not a `String`, it is computed as follows:
* `undefined` and `null` are converted to `''`
* if the value is an object that is not a `Number`, `Date` or `Array`, $interpolate looks for a custom `toString()` function on the object, and uses that. Custom means that `myObject.toString !== Object.prototype.toString`.
* if the above doesn't apply, `JSON.stringify` is used.
### Binding to boolean attributes
Attributes such as `disabled` are called `boolean` attributes, because their presence means `true` and their absence means `false`. We cannot use normal attribute bindings with them, because the HTML specification does not require browsers to preserve the values of boolean attributes. This means that if we put an AngularJS interpolation expression into such an attribute then the binding information would be lost, because the browser ignores the attribute value.
In the following example, the interpolation information would be ignored and the browser would simply interpret the attribute as present, meaning that the button would always be disabled.
```
Disabled: <input type="checkbox" ng-model="isDisabled" />
<button disabled="{{isDisabled}}">Disabled</button>
```
For this reason, AngularJS provides special `ng`-prefixed directives for the following boolean attributes: [`disabled`](../api/ng/directive/ngdisabled), [`required`](../api/ng/directive/ngrequired), [`selected`](../api/ng/directive/ngselected), [`checked`](../api/ng/directive/ngchecked), [`readOnly`](../api/ng/directive/ngreadonly) , and [`open`](../api/ng/directive/ngopen).
These directives take an expression inside the attribute, and set the corresponding boolean attribute to true when the expression evaluates to truthy.
```
Disabled: <input type="checkbox" ng-model="isDisabled" />
<button ng-disabled="isDisabled">Disabled</button>
```
### ngAttr for binding to arbitrary attributes
Web browsers are sometimes picky about what values they consider valid for attributes.
For example, considering this template:
```
<svg>
<circle cx="{{cx}}"></circle>
</svg>
```
We would expect AngularJS to be able to bind to this, but when we check the console we see something like `Error: Invalid value for attribute cx="{{cx}}"`. Because of the SVG DOM API's restrictions, you cannot simply write `cx="{{cx}}"`.
With `ng-attr-cx` you can work around this problem.
If an attribute with a binding is prefixed with the `ngAttr` prefix (denormalized as `ng-attr-`) then during the binding it will be applied to the corresponding unprefixed attribute. This allows you to bind to attributes that would otherwise be eagerly processed by browsers (e.g. an SVG element's `circle[cx]` attributes). When using `ngAttr`, the `allOrNothing` flag of [$interpolate](../api/ng/service/%24interpolate) is used, so if any expression in the interpolated string results in `undefined`, the attribute is removed and not added to the element.
For example, we could fix the example above by instead writing:
```
<svg>
<circle ng-attr-cx="{{cx}}"></circle>
</svg>
```
If one wants to modify a camelcased attribute (SVG elements have valid camelcased attributes), such as `viewBox` on the `svg` element, one can use underscores to denote that the attribute to bind to is naturally camelcased.
For example, to bind to `viewBox`, we can write:
```
<svg ng-attr-view_box="{{viewBox}}">
</svg>
```
Other attributes may also not work as expected when they contain interpolation markup, and can be used with `ngAttr` instead. The following is a list of known problematic attributes:
* **size** in `<select>` elements (see [issue 1619](https://github.com/angular/angular.js/issues/1619))
* **placeholder** in `<textarea>` in Internet Explorer 10/11 (see [issue 5025](https://github.com/angular/angular.js/issues/5025))
* **type** in `<button>` in Internet Explorer 11 (see [issue 14117](https://github.com/angular/angular.js/issues/5025))
* **value** in `<progress>` in Internet Explorer = 11 (see [issue 7218](https://github.com/angular/angular.js/issues/7218))
Known Issues
------------
### Dynamically changing an interpolated value
You should avoid dynamically changing the content of an interpolated string (e.g. attribute value or text node). Your changes are likely to be overwritten, when the original string gets evaluated. This restriction applies to both directly changing the content via JavaScript or indirectly using a directive.
For example, you should not use interpolation in the value of the `style` attribute (e.g. `style="color: {{ 'orange' }}; font-weight: {{ 'bold' }};"`) **and** at the same time use a directive that changes the content of that attribute, such as `ngStyle`.
### Embedding interpolation markup inside expressions
**Note:** AngularJS directive attributes take either expressions *or* interpolation markup with embedded expressions. It is considered **bad practice** to embed interpolation markup inside an expression:
```
<div ng-show="form{{$index}}.$invalid"></div>
```
You should instead delegate the computation of complex expressions to the scope, like this:
```
<div ng-show="getForm($index).$invalid"></div>
```
```
function getForm(index) {
return $scope['form' + index];
}
```
You can also access the `scope` with `this` in your templates:
```
<div ng-show="this['form' + $index].$invalid"></div>
```
#### Why mixing interpolation and expressions is bad practice:
* It increases the complexity of the markup
* There is no guarantee that it works for every directive, because interpolation itself is a directive. If another directive accesses attribute data before interpolation has run, it will get the raw interpolation markup and not data.
* It impacts performance, as interpolation adds another watcher to the scope.
* Since this is not recommended usage, we do not test for this, and changes to AngularJS core may break your code.
angularjs
Improve this DocData Binding
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/databinding.ngdoc?message=docs(guide%2FData%20Binding)%3A%20describe%20your%20change...)Data Binding
=============================================================================================================================================================================================
Data-binding in AngularJS apps is the automatic synchronization of data between the model and view components. The way that AngularJS implements data-binding lets you treat the model as the single-source-of-truth in your application. The view is a projection of the model at all times. When the model changes, the view reflects the change, and vice versa.
Data Binding in Classical Template Systems
------------------------------------------
Most templating systems bind data in only one direction: they merge template and model components together into a view. After the merge occurs, changes to the model or related sections of the view are NOT automatically reflected in the view. Worse, any changes that the user makes to the view are not reflected in the model. This means that the developer has to write code that constantly syncs the view with the model and the model with the view.
Data Binding in AngularJS Templates
-----------------------------------
AngularJS templates work differently. First the template (which is the uncompiled HTML along with any additional markup or directives) is compiled on the browser. The compilation step produces a live view. Any changes to the view are immediately reflected in the model, and any changes in the model are propagated to the view. The model is the single-source-of-truth for the application state, greatly simplifying the programming model for the developer. You can think of the view as simply an instant projection of your model.
Because the view is just a projection of the model, the controller is completely separated from the view and unaware of it. This makes testing a snap because it is easy to test your controller in isolation without the view and the related DOM/browser dependency.
Related Topics
--------------
* [AngularJS Scopes](scope)
* [AngularJS Templates](templates)
angularjs
Improve this DocUnderstanding Components
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/component.ngdoc?message=docs(guide%2FComponents)%3A%20describe%20your%20change...)Understanding Components
===================================================================================================================================================================================================
In AngularJS, a Component is a special kind of <directive> that uses a simpler configuration which is suitable for a component-based application structure.
This makes it easier to write an app in a way that's similar to using Web Components or using the new Angular's style of application architecture.
Advantages of Components:
* simpler configuration than plain directives
* promote sane defaults and best practices
* optimized for component-based architecture
* writing component directives will make it easier to upgrade to Angular
When not to use Components:
* for directives that need to perform actions in compile and pre-link functions, because they aren't available
* when you need advanced directive definition options like priority, terminal, multi-element
* when you want a directive that is triggered by an attribute or CSS class, rather than an element
Creating and configuring a Component
------------------------------------
Components can be registered using the [`.component()`](../api/ng/provider/%24compileprovider#component.html) method of an AngularJS module (returned by [`angular.module()`](module)). The method takes two arguments:
* The name of the Component (as string).
* The Component config object. (Note that, unlike the `.directive()` method, this method does **not** take a factory function.)
It's also possible to add components via [`$compileProvider`](../api/ng/provider/%24compileprovider#component.html) in a module's config phase.
### Comparison between Directive definition and Component definition
| | Directive | Component |
| --- | --- | --- |
| bindings | No | Yes (binds to controller) |
| bindToController | Yes (default: false) | No (use bindings instead) |
| compile function | Yes | No |
| controller | Yes | Yes (default `function() {}`) |
| controllerAs | Yes (default: false) | Yes (default: `$ctrl`) |
| link functions | Yes | No |
| multiElement | Yes | No |
| priority | Yes | No |
| replace | Yes (deprecated) | No |
| require | Yes | Yes |
| restrict | Yes | No (restricted to elements only) |
| scope | Yes (default: false) | No (scope is always isolate) |
| template | Yes | Yes, injectable |
| templateNamespace | Yes | No |
| templateUrl | Yes | Yes, injectable |
| terminal | Yes | No |
| transclude | Yes (default: false) | Yes (default: false) |
Component-based application architecture
----------------------------------------
As already mentioned, the component helper makes it easier to structure your application with a component-based architecture. But what makes a component beyond the options that the component helper has?
* **Components only control their own View and Data:** Components should never modify any data or DOM that is out of their own scope. Normally, in AngularJS it is possible to modify data anywhere in the application through scope inheritance and watches. This is practical, but can also lead to problems when it is not clear which part of the application is responsible for modifying the data. That is why component directives use an isolate scope, so a whole class of scope manipulation is not possible.
* **Components have a well-defined public API - Inputs and Outputs:** However, scope isolation only goes so far, because AngularJS uses two-way binding. So if you pass an object to a component like this - `bindings: {item: '='}`, and modify one of its properties, the change will be reflected in the parent component. For components however, only the component that owns the data should modify it, to make it easy to reason about what data is changed, and when. For that reason, components should follow a few simple conventions:
+ Inputs should be using `<` and `@` bindings. The `<` symbol denotes [one-way bindings](../api/ng/service/%24compile#-scope-.html) which are available since 1.5. The difference to `=` is that the bound properties in the component scope are not watched, which means if you assign a new value to the property in the component scope, it will not update the parent scope. Note however, that both parent and component scope reference the same object, so if you are changing object properties or array elements in the component, the parent will still reflect that change. The general rule should therefore be to never change an object or array property in the component scope. `@` bindings can be used when the input is a string, especially when the value of the binding doesn't change.
```
bindings: {
hero: '<',
comment: '@'
}
```
+ Outputs are realized with `&` bindings, which function as callbacks to component events.
```
bindings: {
onDelete: '&',
onUpdate: '&'
}
```
+ Instead of manipulating Input Data, the component calls the correct Output Event with the changed data. For a deletion, that means the component doesn't delete the `hero` itself, but sends it back to the owner component via the correct event.
```
<!-- note that we use kebab-case for bindings in the template as usual -->
<editable-field on-update="$ctrl.update('location', value)"></editable-field><br>
<button ng-click="$ctrl.onDelete({hero: $ctrl.hero})">Delete</button>
```
+ That way, the parent component can decide what to do with the event (e.g. delete an item or update the properties)
```
ctrl.deleteHero(hero) {
$http.delete(...).then(function() {
var idx = ctrl.list.indexOf(hero);
if (idx >= 0) {
ctrl.list.splice(idx, 1);
}
});
}
```
* **Components have a well-defined lifecycle:** Each component can implement "lifecycle hooks". These are methods that will be called at certain points in the life of the component. The following hook methods can be implemented:
+ `$onInit()` - Called on each controller after all the controllers on an element have been constructed and had their bindings initialized (and before the pre & post linking functions for the directives on this element). This is a good place to put initialization code for your controller.
+ `$onChanges(changesObj)` - Called whenever one-way bindings are updated. The `changesObj` is a hash whose keys are the names of the bound properties that have changed, and the values are an object of the form `{ currentValue, previousValue, isFirstChange() }`. Use this hook to trigger updates within a component such as cloning the bound value to prevent accidental mutation of the outer value.
+ `$doCheck()` - Called on each turn of the digest cycle. Provides an opportunity to detect and act on changes. Any actions that you wish to take in response to the changes that you detect must be invoked from this hook; implementing this has no effect on when `$onChanges` is called. For example, this hook could be useful if you wish to perform a deep equality check, or to check a Date object, changes to which would not be detected by AngularJS's change detector and thus not trigger `$onChanges`. This hook is invoked with no arguments; if detecting changes, you must store the previous value(s) for comparison to the current values.
+ `$onDestroy()` - Called on a controller when its containing scope is destroyed. Use this hook for releasing external resources, watches and event handlers.
+ `$postLink()` - Called after this controller's element and its children have been linked. Similar to the post-link function this hook can be used to set up DOM event handlers and do direct DOM manipulation. Note that child elements that contain `templateUrl` directives will not have been compiled and linked since they are waiting for their template to load asynchronously and their own compilation and linking has been suspended until that occurs. This hook can be considered analogous to the `ngAfterViewInit` and `ngAfterContentInit` hooks in Angular. Since the compilation process is rather different in AngularJS there is no direct mapping and care should be taken when upgrading.
By implementing these methods, your component can hook into its lifecycle.
* **An application is a tree of components:** Ideally, the whole application should be a tree of components that implement clearly defined inputs and outputs, and minimize two-way data binding. That way, it's easier to predict when data changes and what the state of a component is.
Example of a component tree
---------------------------
The following example expands on the simple component example and incorporates the concepts we introduced above:
Instead of an ngController, we now have a heroList component that holds the data of different heroes, and creates a heroDetail for each of them.
The heroDetail component now contains new functionality:
* a delete button that calls the bound `onDelete` function of the heroList component
* an input to change the hero location, in the form of a reusable editableField component. Instead of manipulating the hero object itself, it sends a changeset upwards to the heroDetail, which sends it upwards to the heroList component, which updates the original data.
Components as route templates
-----------------------------
Components are also useful as route templates (e.g. when using [ngRoute](../api/ngroute)). In a component-based application, every view is a component:
```
var myMod = angular.module('myMod', ['ngRoute']);
myMod.component('home', {
template: '<h1>Home</h1><p>Hello, {{ $ctrl.user.name }} !</p>',
controller: function() {
this.user = {name: 'world'};
}
});
myMod.config(function($routeProvider) {
$routeProvider.when('/', {
template: '<home></home>'
});
});
```
When using [$routeProvider](../api/ngroute/provider/%24routeprovider), you can often avoid some boilerplate, by passing the resolved route dependencies directly to the component. Since 1.5, ngRoute automatically assigns the resolves to the route scope property `$resolve` (you can also configure the property name via `resolveAs`). When using components, you can take advantage of this and pass resolves directly into your component without creating an extra route controller:
```
var myMod = angular.module('myMod', ['ngRoute']);
myMod.component('home', {
template: '<h1>Home</h1><p>Hello, {{ $ctrl.user.name }} !</p>',
bindings: {
user: '<'
}
});
myMod.config(function($routeProvider) {
$routeProvider.when('/', {
template: '<home user="$resolve.user"></home>',
resolve: {
user: function($http) { return $http.get('...'); }
}
});
});
```
Intercomponent Communication
----------------------------
Directives can require the controllers of other directives to enable communication between each other. This can be achieved in a component by providing an object mapping for the `require` property. The object keys specify the property names under which the required controllers (object values) will be bound to the requiring component's controller.
Note that the required controllers will not be available during the instantiation of the controller, but they are guaranteed to be available just before the `$onInit` method is executed! Here is a tab pane example built from components:
Unit-testing Component Controllers
----------------------------------
The easiest way to unit-test a component controller is by using the [$componentController](../api/ngmock/service/%24componentcontroller) that is included in [`ngMock`](../api/ngmock). The advantage of this method is that you do not have to create any DOM elements. The following example shows how to do this for the `heroDetail` component from above.
The examples use the [Jasmine](http://jasmine.github.io/) testing framework.
**Controller Test:**
```
describe('HeroDetailController', function() {
var $componentController;
beforeEach(module('heroApp'));
beforeEach(inject(function(_$componentController_) {
$componentController = _$componentController_;
}));
it('should call the `onDelete` binding, when deleting the hero', function() {
var onDeleteSpy = jasmine.createSpy('onDelete');
var bindings = {hero: {}, onDelete: onDeleteSpy};
var ctrl = $componentController('heroDetail', null, bindings);
ctrl.delete();
expect(onDeleteSpy).toHaveBeenCalledWith({hero: ctrl.hero});
});
it('should call the `onUpdate` binding, when updating a property', function() {
var onUpdateSpy = jasmine.createSpy('onUpdate');
var bindings = {hero: {}, onUpdate: onUpdateSpy};
var ctrl = $componentController('heroDetail', null, bindings);
ctrl.update('foo', 'bar');
expect(onUpdateSpy).toHaveBeenCalledWith({
hero: ctrl.hero,
prop: 'foo',
value: 'bar'
});
});
});
```
| programming_docs |
angularjs
Improve this DocHTML Compiler
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/compiler.ngdoc?message=docs(guide%2FHTML%20Compiler)%3A%20describe%20your%20change...)HTML Compiler
============================================================================================================================================================================================
**Note:** this guide is targeted towards developers who are already familiar with AngularJS basics. If you're just getting started, we recommend the [tutorial](https://code.angularjs.org/1.8.2/docs/guide/tutorial/) first. If you just want to create custom directives, we recommend the [directives guide](directive). If you want a deeper look into AngularJS's compilation process, you're in the right place. Overview
--------
AngularJS's [HTML compiler](../api/ng/service/%24compile) allows the developer to teach the browser new HTML syntax. The compiler allows you to attach behavior to any HTML element or attribute and even create new HTML elements or attributes with custom behavior. AngularJS calls these behavior extensions [directives](../api/ng/provider/%24compileprovider#directive.html).
HTML has a lot of constructs for formatting the HTML for static documents in a declarative fashion. For example if something needs to be centered, there is no need to provide instructions to the browser how the window size needs to be divided in half so that the center is found, and that this center needs to be aligned with the text's center. Simply add an `align="center"` attribute to any element to achieve the desired behavior. Such is the power of declarative language.
However, the declarative language is also limited, as it does not allow you to teach the browser new syntax. For example, there is no easy way to get the browser to align the text at 1/3 the position instead of 1/2. What is needed is a way to teach the browser new HTML syntax.
AngularJS comes pre-bundled with common directives which are useful for building any app. We also expect that you will create directives that are specific to your app. These extensions become a Domain Specific Language for building your application.
All of this compilation takes place in the web browser; no server side or pre-compilation step is involved.
Compiler
--------
Compiler is an AngularJS service which traverses the DOM looking for attributes. The compilation process happens in two phases.
1. **Compile:** traverse the DOM and collect all of the directives. The result is a linking function.
2. **Link:** combine the directives with a scope and produce a live view. Any changes in the scope model are reflected in the view, and any user interactions with the view are reflected in the scope model. This makes the scope model the single source of truth.
Some directives such as [`ng-repeat`](../api/ng/directive/ngrepeat) clone DOM elements once for each item in a collection. Having a compile and link phase improves performance since the cloned template only needs to be compiled once, and then linked once for each clone instance.
Directive
---------
A directive is a behavior which should be triggered when specific HTML constructs are encountered during the compilation process. The directives can be placed in element names, attributes, class names, as well as comments. Here are some equivalent examples of invoking the [`ng-bind`](../api/ng/directive/ngbind) directive.
```
<span ng-bind="exp"></span>
<span class="ng-bind: exp;"></span>
<ng-bind></ng-bind>
<!-- directive: ng-bind exp -->
```
A directive is just a function which executes when the compiler encounters it in the DOM. See [directive API](../api/ng/provider/%24compileprovider#directive.html) for in-depth documentation on how to write directives.
Here is a directive which makes any element draggable. Notice the `draggable` attribute on the `<span>` element.
The presence of the `draggable` attribute on any element gives the element new behavior. We extended the vocabulary of the browser in a way which is natural to anyone who is familiar with the principles of HTML.
Understanding View
------------------
Most other templating systems consume a static string template and combine it with data, resulting in a new string. The resulting text is then `innerHTML`ed into an element.
This means that any changes to the data need to be re-merged with the template and then `innerHTML`ed into the DOM. Some of the issues with this approach are:
1. reading user input and merging it with data
2. clobbering user input by overwriting it
3. managing the whole update process
4. lack of behavior expressiveness
AngularJS is different. The AngularJS compiler consumes the DOM, not string templates. The result is a linking function, which when combined with a scope model results in a live view. The view and scope model bindings are transparent. The developer does not need to make any special calls to update the view. And because `innerHTML` is not used, you won't accidentally clobber user input. Furthermore, AngularJS directives can contain not just text bindings, but behavioral constructs as well.
The AngularJS approach produces a stable DOM. The DOM element instance bound to a model item instance does not change for the lifetime of the binding. This means that the code can get hold of the elements and register event handlers and know that the reference will not be destroyed by template data merge.
How directives are compiled
---------------------------
It's important to note that AngularJS operates on DOM nodes rather than strings. Usually, you don't notice this restriction because when a page loads, the web browser parses HTML into the DOM automatically.
HTML compilation happens in three phases:
1. [`$compile`](../api/ng/service/%24compile) traverses the DOM and matches directives.
If the compiler finds that an element matches a directive, then the directive is added to the list of directives that match the DOM element. A single element may match multiple directives.
2. Once all directives matching a DOM element have been identified, the compiler sorts the directives by their `priority`.
Each directive's `compile` functions are executed. Each `compile` function has a chance to modify the DOM. Each `compile` function returns a `link` function. These functions are composed into a "combined" link function, which invokes each directive's returned `link` function.
3. `$compile` links the template with the scope by calling the combined linking function from the previous step. This in turn will call the linking function of the individual directives, registering listeners on the elements and setting up [`$watch`s](../api/ng/type/%24rootscope.scope#%24watch.html) with the [`scope`](../api/ng/type/%24rootscope.scope) as each directive is configured to do.
The result of this is a live binding between the scope and the DOM. So at this point, a change in a model on the compiled scope will be reflected in the DOM.
Below is the corresponding code using the `$compile` service. This should help give you an idea of what AngularJS does internally.
```
var $compile = ...; // injected into your code
var scope = ...;
var parent = ...; // DOM element where the compiled template can be appended
var html = '<div ng-bind="exp"></div>';
// Step 1: parse HTML into DOM element
var template = angular.element(html);
// Step 2: compile the template
var linkFn = $compile(template);
// Step 3: link the compiled template with the scope.
var element = linkFn(scope);
// Step 4: Append to DOM (optional)
parent.appendChild(element);
```
### The difference between Compile and Link
At this point you may wonder why the compile process has separate compile and link phases. The short answer is that compile and link separation is needed any time a change in a model causes a change in the **structure** of the DOM.
It's rare for directives to have a **compile function**, since most directives are concerned with working with a specific DOM element instance rather than changing its overall structure.
Directives often have a **link function**. A link function allows the directive to register listeners to the specific cloned DOM element instance as well as to copy content into the DOM from the scope.
**Best Practice:** Any operation which can be shared among the instance of directives should be moved to the compile function for performance reasons. #### An Example of "Compile" Versus "Link"
To understand, let's look at a real-world example with `ngRepeat`:
```
Hello {{user.name}}, you have these actions:
<ul>
<li ng-repeat="action in user.actions">
{{action.description}}
</li>
</ul>
```
When the above example is compiled, the compiler visits every node and looks for directives.
`{{user.name}}` matches the [interpolation directive](../api/ng/service/%24interpolate) and `ng-repeat` matches the [`ngRepeat` directive](../api/ng/directive/ngrepeat).
But [ngRepeat](../api/ng/directive/ngrepeat) has a dilemma.
It needs to be able to clone new `<li>` elements for every `action` in `user.actions`. This initially seems trivial, but it becomes more complicated when you consider that `user.actions` might have items added to it later. This means that it needs to save a clean copy of the `<li>` element for cloning purposes.
As new `action`s are inserted, the template `<li>` element needs to be cloned and inserted into `ul`. But cloning the `<li>` element is not enough. It also needs to compile the `<li>` so that its directives, like `{{action.description}}`, evaluate against the right [scope](../api/ng/type/%24rootscope.scope).
A naive approach to solving this problem would be to simply insert a copy of the `<li>` element and then compile it. The problem with this approach is that compiling on every `<li>` element that we clone would duplicate a lot of the work. Specifically, we'd be traversing `<li>` each time before cloning it to find the directives. This would cause the compilation process to be slower, in turn making applications less responsive when inserting new nodes.
The solution is to break the compilation process into two phases:
the **compile phase** where all of the directives are identified and sorted by priority, and a **linking phase** where any work which "links" a specific instance of the [scope](../api/ng/type/%24rootscope.scope) and the specific instance of an `<li>` is performed.
**Note:** *Link* means setting up listeners on the DOM and setting up `$watch` on the Scope to keep the two in sync. [`ngRepeat`](../api/ng/directive/ngrepeat) works by preventing the compilation process from descending into the `<li>` element so it can make a clone of the original and handle inserting and removing DOM nodes itself.
Instead the [`ngRepeat`](../api/ng/directive/ngrepeat) directive compiles `<li>` separately. The result of the `<li>` element compilation is a linking function which contains all of the directives contained in the `<li>` element, ready to be attached to a specific clone of the `<li>` element.
At runtime the [`ngRepeat`](../api/ng/directive/ngrepeat) watches the expression and as items are added to the array it clones the `<li>` element, creates a new [scope](../api/ng/type/%24rootscope.scope) for the cloned `<li>` element and calls the link function on the cloned `<li>`.
### Understanding How Scopes Work with Transcluded Directives
One of the most common use cases for directives is to create reusable components.
Below is a pseudo code showing how a simplified dialog component may work.
```
<div>
<button ng-click="show=true">show</button>
<dialog title="Hello {{username}}."
visible="show"
on-cancel="show = false"
on-ok="show = false; doSomething()">
Body goes here: {{username}} is {{title}}.
</dialog>
</div>
```
Clicking on the "show" button will open the dialog. The dialog will have a title, which is data bound to `username`, and it will also have a body which we would like to transclude into the dialog.
Here is an example of what the template definition for the `dialog` widget may look like.
```
<div ng-show="visible">
<h3>{{title}}</h3>
<div class="body" ng-transclude></div>
<div class="footer">
<button ng-click="onOk()">Save changes</button>
<button ng-click="onCancel()">Close</button>
</div>
</div>
```
This will not render properly, unless we do some scope magic.
The first issue we have to solve is that the dialog box template expects `title` to be defined. But we would like the template's scope property `title` to be the result of interpolating the `<dialog>` element's `title` attribute (i.e. `"Hello {{username}}"`). Furthermore, the buttons expect the `onOk` and `onCancel` functions to be present in the scope. This limits the usefulness of the widget. To solve the mapping issue we use the `scope` to create local variables which the template expects as follows:
```
scope: {
title: '@', // the title uses the data-binding from the parent scope
onOk: '&', // create a delegate onOk function
onCancel: '&', // create a delegate onCancel function
visible: '=' // set up visible to accept data-binding
}
```
Creating local properties on widget scope creates two problems:
1. isolation - if the user forgets to set `title` attribute of the dialog widget the dialog template will bind to parent scope property. This is unpredictable and undesirable.
2. transclusion - the transcluded DOM can see the widget locals, which may overwrite the properties which the transclusion needs for data-binding. In our example the `title` property of the widget clobbers the `title` property of the transclusion.
To solve the issue of lack of isolation, the directive declares a new `isolated` scope. An isolated scope does not prototypically inherit from the parent scope, and therefore we don't have to worry about accidentally clobbering any properties.
However `isolated` scope creates a new problem: if a transcluded DOM is a child of the widget isolated scope then it will not be able to bind to anything. For this reason the transcluded scope is a child of the original scope, before the widget created an isolated scope for its local variables. This makes the transcluded and widget isolated scope siblings.
This may seem to be unexpected complexity, but it gives the widget user and developer the least surprise.
Therefore the final directive definition looks something like this:
```
transclude: true,
scope: {
title: '@', // the title uses the data-binding from the parent scope
onOk: '&', // create a delegate onOk function
onCancel: '&', // create a delegate onCancel function
visible: '=' // set up visible to accept data-binding
},
restrict: 'E',
replace: true
```
### Double Compilation, and how to avoid it
Double compilation occurs when an already compiled part of the DOM gets compiled again. This is an undesired effect and can lead to misbehaving directives, performance issues, and memory leaks. A common scenario where this happens is a directive that calls `$compile` in a directive link function on the directive element. In the following **faulty example**, a directive adds a mouseover behavior to a button with `ngClick` on it:
```
angular.module('app').directive('addMouseover', function($compile) {
return {
link: function(scope, element, attrs) {
var newEl = angular.element('<span ng-show="showHint"> My Hint</span>');
element.on('mouseenter mouseleave', function() {
scope.$apply('showHint = !showHint');
});
attrs.$set('addMouseover', null); // To stop infinite compile loop
element.append(newEl);
$compile(element)(scope); // Double compilation
}
}
})
```
At first glance, it looks like removing the original `addMouseover` attribute is all there is needed to make this example work. However, if the directive element or its children have other directives attached, they will be compiled and linked again, because the compiler doesn't keep track of which directives have been assigned to which elements.
This can cause unpredictable behavior, e.g. `ngClick` or other event handlers will be attached again. It can also degrade performance, as watchers for text interpolation are added twice to the scope.
Double compilation should therefore be avoided. In the above example, only the new element should be compiled:
```
angular.module('app').directive('addMouseover', function($compile) {
return {
link: function(scope, element, attrs) {
var newEl = angular.element('<span ng-show="showHint"> My Hint</span>');
element.on('mouseenter mouseleave', function() {
scope.$apply('showHint = !showHint');
});
element.append(newEl);
$compile(newEl)(scope); // Only compile the new element
}
}
})
```
Another scenario is adding a directive programmatically to a compiled element and then executing compile again. See the following **faulty example**:
```
<input ng-model="$ctrl.value" add-options>
```
```
angular.module('app').directive('addOptions', function($compile) {
return {
link: function(scope, element, attrs) {
attrs.$set('addOptions', null) // To stop infinite compile loop
attrs.$set('ngModelOptions', '{debounce: 1000}');
$compile(element)(scope); // Double compilation
}
}
});
```
In that case, it is necessary to intercept the *initial* compilation of the element:
1. Give your directive the `terminal` property and a higher priority than directives that should not be compiled twice. In the example, the compiler will only compile directives which have a priority of 100 or higher.
2. Inside this directive's compile function, add any other directive attributes to the template.
3. Compile the element, but restrict the maximum priority, so that any already compiled directives (including the `addOptions` directive) are not compiled again.
4. In the link function, link the compiled element with the element's scope.
```
angular.module('app').directive('addOptions', function($compile) {
return {
priority: 100, // ngModel has priority 1
terminal: true,
compile: function(templateElement, templateAttributes) {
templateAttributes.$set('ngModelOptions', '{debounce: 1000}');
// The third argument is the max priority. Only directives with priority < 100 will be compiled,
// therefore we don't need to remove the attribute
var compiled = $compile(templateElement, null, 100);
return function linkFn(scope) {
compiled(scope) // Link compiled element to scope
}
}
}
});
```
angularjs
Improve this DocAngularJS Expressions
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/expression.ngdoc?message=docs(guide%2FExpressions)%3A%20describe%20your%20change...)AngularJS Expressions
==================================================================================================================================================================================================
AngularJS expressions are JavaScript-like code snippets that are mainly placed in interpolation bindings such as `<span title="{{ attrBinding }}">{{ textBinding }}</span>`, but also used directly in directive attributes such as `ng-click="functionExpression()"`.
For example, these are valid expressions in AngularJS:
* `1+2`
* `a+b`
* `user.name`
* `items[index]`
AngularJS Expressions vs. JavaScript Expressions
------------------------------------------------
AngularJS expressions are like JavaScript expressions with the following differences:
* **Context:** JavaScript expressions are evaluated against the global `window`. In AngularJS, expressions are evaluated against a [`scope`](../api/ng/type/%24rootscope.scope) object.
* **Forgiving:** In JavaScript, trying to evaluate undefined properties generates `ReferenceError` or `TypeError`. In AngularJS, expression evaluation is forgiving to `undefined` and `null`.
* **Filters:** You can use [filters](filter) within expressions to format data before displaying it.
* **No Control Flow Statements:** You cannot use the following in an AngularJS expression: conditionals, loops, or exceptions.
* **No Function Declarations:** You cannot declare functions in an AngularJS expression, even inside `ng-init` directive.
* **No RegExp Creation With Literal Notation:** You cannot create regular expressions in an AngularJS expression. An exception to this rule is [`ng-pattern`](../api/ng/directive/ngpattern) which accepts valid RegExp.
* **No Object Creation With New Operator:** You cannot use `new` operator in an AngularJS expression.
* **No Bitwise, Comma, And Void Operators:** You cannot use [Bitwise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Bitwise_Operators), `,` or `void` operators in an AngularJS expression.
If you want to run more complex JavaScript code, you should make it a controller method and call the method from your view. If you want to `eval()` an AngularJS expression yourself, use the [`$eval()`](../api/ng/type/%24rootscope.scope#%24eval.html) method.
You can try evaluating different expressions here:
Context
-------
AngularJS does not use JavaScript's `eval()` to evaluate expressions. Instead AngularJS's [$parse](../api/ng/service/%24parse) service processes these expressions.
AngularJS expressions do not have direct access to global variables like `window`, `document` or `location`. This restriction is intentional. It prevents accidental access to the global state – a common source of subtle bugs.
Instead use services like `$window` and `$location` in functions on controllers, which are then called from expressions. Such services provide mockable access to globals.
It is possible to access the context object using the identifier `this` and the locals object using the identifier `$locals`.
Forgiving
---------
Expression evaluation is forgiving to undefined and null. In JavaScript, evaluating `a.b.c` throws an exception if `a` is not an object. While this makes sense for a general purpose language, the expression evaluations are primarily used for data binding, which often look like this:
```
{{a.b.c}}
```
It makes more sense to show nothing than to throw an exception if `a` is undefined (perhaps we are waiting for the server response, and it will become defined soon). If expression evaluation wasn't forgiving we'd have to write bindings that clutter the code, for example: `{{((a||{}).b||{}).c}}`
Similarly, invoking a function `a.b.c()` on `undefined` or `null` simply returns `undefined`.
No Control Flow Statements
--------------------------
Apart from the ternary operator (`a ? b : c`), you cannot write a control flow statement in an expression. The reason behind this is core to the AngularJS philosophy that application logic should be in controllers, not the views. If you need a real conditional, loop, or to throw from a view expression, delegate to a JavaScript method instead.
No function declarations or RegExp creation with literal notation
-----------------------------------------------------------------
You can't declare functions or create regular expressions from within AngularJS expressions. This is to avoid complex model transformation logic inside templates. Such logic is better placed in a controller or in a dedicated filter where it can be tested properly.
$event
------
Directives like [`ngClick`](../api/ng/directive/ngclick) and [`ngFocus`](../api/ng/directive/ngfocus) expose a `$event` object within the scope of that expression. The object is an instance of a [jQuery Event Object](http://api.jquery.com/category/events/event-object/) when jQuery is present or a similar jqLite object.
Note in the example above how we can pass in `$event` to `clickMe`, but how it does not show up in `{{$event}}`. This is because `$event` is outside the scope of that binding.
One-time binding
----------------
An expression that starts with `::` is considered a one-time expression. One-time expressions will stop recalculating once they are stable, which happens after the first digest if the expression result is a non-undefined value (see value stabilization algorithm below).
### Reasons for using one-time binding
The main purpose of one-time binding expression is to provide a way to create a binding that gets deregistered and frees up resources once the binding is stabilized. Reducing the number of expressions being watched makes the digest loop faster and allows more information to be displayed at the same time.
### Value stabilization algorithm
One-time binding expressions will retain the value of the expression at the end of the digest cycle as long as that value is not undefined. If the value of the expression is set within the digest loop and later, within the same digest loop, it is set to undefined, then the expression is not fulfilled and will remain watched.
1. Given an expression that starts with `::`, when a digest loop is entered and expression is dirty-checked, store the value as V
2. If V is not undefined, mark the result of the expression as stable and schedule a task to deregister the watch for this expression when we exit the digest loop
3. Process the digest loop as normal
4. When digest loop is done and all the values have settled, process the queue of watch deregistration tasks. For each watch to be deregistered, check if it still evaluates to a value that is not `undefined`. If that's the case, deregister the watch. Otherwise, keep dirty-checking the watch in the future digest loops by following the same algorithm starting from step 1
#### Special case for object literals
Unlike simple values, object-literals are watched until every key is defined. See <http://www.bennadel.com/blog/2760-one-time-data-bindings-for-object-literal-expressions-in-angularjs-1-3.htm>
### How to benefit from one-time binding
If the expression will not change once set, it is a candidate for one-time binding. Here are three example cases.
When interpolating text or attributes:
```
<div name="attr: {{::color}}">text: {{::name | uppercase}}</div>
```
When using a directive with bidirectional binding and parameters that will not change:
```
someModule.directive('someDirective', function() {
return {
scope: {
name: '=',
color: '@'
},
template: '{{name}}: {{color}}'
};
});
```
```
<div some-directive name="::myName" color="My color is {{::myColor}}"></div>
```
When using a directive that takes an expression:
```
<ul>
<li ng-repeat="item in ::items | orderBy:'name'">{{item.name}};</li>
</ul>
```
| programming_docs |
angularjs
Improve this DocExternal AngularJS Resources
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/external-resources.ngdoc?message=docs(guide%2FExternal%20Resources)%3A%20describe%20your%20change...)External AngularJS Resources
==========================================================================================================================================================================================================================
This is a collection of external, 3rd party resources for learning and developing AngularJS.
Articles, Videos, and Projects
------------------------------
### Introductory Material
* [10 Reasons Why You Should Use AngularJS](http://www.sitepoint.com/10-reasons-use-angularjs/)
* [10 Reasons Why Developers Should Learn AngularJS](http://wintellect.com/blogs/jlikness/10-reasons-web-developers-should-learn-angularjs)
* [Design Principles of AngularJS (video)](https://www.youtube.com/watch?v=HCR7i5F5L8c)
* [Fundamentals in 60 Minutes (video)](http://www.youtube.com/watch?v=i9MHigUZKEM)
* [For folks with a jQuery background](http://stackoverflow.com/questions/14994391/how-do-i-think-in-angularjs-if-i-have-a-jquery-background)
### Specific Topics
#### Application Structure & Style Guides
* [AngularJS Styleguide](https://github.com/johnpapa/angular-styleguide/blob/master/a1/README.md)
* [Architecture, file structure, components, one-way dataflow and best practices](https://github.com/toddmotto/angular-styleguide)
* [When to use directives, controllers or services](http://kirkbushell.me/when-to-use-directives-controllers-or-services-in-angular/)
* [Service vs Factory](http://blog.thoughtram.io/angular/2015/07/07/service-vs-factory-once-and-for-all.html)
#### Testing
* **Unit testing:** [Using Karma (video)](http://www.youtube.com/watch?v=YG5DEzaQBIc), [Karma in Webstorm](http://blog.jetbrains.com/webstorm/2013/10/running-javascript-tests-with-karma-in-webstorm-7/)
#### Mobile
* [AngularJS on Mobile Guide](http://www.ng-newsletter.com/posts/angular-on-mobile.html)
* [AngularJS and Cordova](http://devgirl.org/2013/06/10/quick-start-guide-phonegap-and-angularjs/)
* [Ionic Framework](http://ionicframework.com/)
#### Deployment
##### General
* **Javascript minification:** [Background](http://thegreenpizza.github.io/2013/05/25/building-minification-safe-angular.js-applications/), [ng-annotate automation tool](https://github.com/olov/ng-annotate)
* **Analytics and Logging:** [Angularytics (Google Analytics)](http://ngmodules.org/modules/angularytics), [Angulartics (Analytics)](https://github.com/luisfarzati/angulartics), [Logging Client-Side Errors](http://www.bennadel.com/blog/2542-Logging-Client-Side-Errors-With-AngularJS-And-Stacktrace-js.htm)
* **SEO:** [By hand](http://www.yearofmoo.com/2012/11/angularjs-and-seo.html), [prerender.io](http://prerender.io/), [Brombone](http://www.brombone.com/), [SEO.js](http://getseojs.com/), [SEO4Ajax](http://www.seo4ajax.com/)
##### Server-Specific
* **Django:** [Tutorial](http://blog.mourafiq.com/post/55034504632/end-to-end-web-app-with-django-rest-framework), [Integrating AngularJS with Django](http://django-angular.readthedocs.org/en/latest/integration.html), [Getting Started with Django Rest Framework and AngularJS](http://blog.kevinastone.com/getting-started-with-django-rest-framework-and-angularjs.html)
* **FireBase:** [AngularFire](http://angularfire.com/), [Realtime Apps with AngularJS and FireBase (video)](http://www.youtube.com/watch?v=C7ZI7z7qnHU)
* **Google Cloud Platform:** [with Go](https://github.com/GoogleCloudPlatform/appengine-angular-gotodos)
* **Hood.ie:** [60 Minutes to Awesome](http://www.roberthorvick.com/2013/06/30/todomvc-angularjs-hood-ie-60-minutes-to-awesome/)
* **MEAN Stack:** [Blog post](http://blog.mongodb.org/post/49262866911/the-mean-stack-mongodb-expressjs-angularjs-and), [Setup](http://thecodebarbarian.wordpress.com/2013/07/22/introduction-to-the-mean-stack-part-one-setting-up-your-tools/), [GDL Video](https://developers.google.com/live/shows/913996610)
* **Rails:** [Tutorial](http://coderberry.me/blog/2013/04/22/angularjs-on-rails-4-part-1/), [AngularJS with Rails4](https://shellycloud.com/blog/2013/10/how-to-integrate-angularjs-with-rails-4), [angularjs-rails](https://github.com/hiravgandhi/angularjs-rails)
* **PHP:** [Building a RESTful web service](http://blog.brunoscopelliti.com/building-a-restful-web-service-with-angularjs-and-php-more-power-with-resource), [End to End with Laravel 4 (video)](http://www.youtube.com/watch?v=hqAyiqUs93c)
* **Meteor:** [angular-meteor package](https://github.com/Urigo/angular-meteor)
### Other Languages
* [ES6, Webpack, and JSPM Starter Project](https://github.com/AngularClass/NG6-starter)
* [ES6/Typescript Best Practices](https://codepen.io/martinmcwhorter/post/angularjs-1-x-with-typescript-or-es6-best-practices)
* [Dart](https://github.com/angular/angular.dart.tutorial/wiki)
* [CoffeeScript Tutorial](http://www.coffeescriptlove.com/2013/08/angularjs-and-coffeescript-tutorials.html)
### More Topics
* **Security:** [video](https://www.youtube.com/watch?v=18ifoT-Id54)
* **Internationalization and Localization:** [Creating multilingual support](http://www.novanet.no/blog/hallstein-brotan/dates/2013/10/creating-multilingual-support-using-angularjs/)
* **Authentication/Login:** [Google example](https://developers.google.com/+/photohunt/python), [AngularJS Facebook library](https://github.com/pc035860/angular-easyfb), [Facebook example](http://blog.brunoscopelliti.com/facebook-authentication-in-your-angularjs-web-app), [authentication strategy](http://blog.brunoscopelliti.com/deal-with-users-authentication-in-an-angularjs-web-app), [unix-style authorization](http://frederiknakstad.com/authentication-in-single-page-applications-with-angular-js/)
* **Visualization:** [SVG](http://gaslight.co/blog/angular-backed-svgs), [D3.js](http://www.ng-newsletter.com/posts/d3-on-angular.html)
* **Realtime Communication:** [Socket.io](http://www.creativebloq.com/javascript/angularjs-collaboration-board-socketio-2132885), [OmniBinder](https://github.com/jeffbcross/omnibinder)
Tools
-----
* **Getting Started:** [Comparison of the options for starting a new project](http://www.dancancro.com/comparison-of-angularjs-application-starters/)
* **Debugging:** [Batarang](https://chrome.google.com/webstore/detail/angularjs-batarang/ighdmehidhipcmcojjgiloacoafjmpfk?hl=en)
* **Editor support:** [Webstorm](http://plugins.jetbrains.com/plugin/6971) (and [video](http://www.youtube.com/watch?v=LJOyrSh1kDU)), [Sublime Text](https://github.com/angular-ui/AngularJS-sublime-package), [Visual Studio](http://madskristensen.net/post/angularjs-intellisense-in-visual-studio-2012), [Atom](https://github.com/angular-ui/AngularJS-Atom), [Vim](https://github.com/burnettk/vim-angular)
* **Workflow:** [Yeoman.io](https://github.com/yeoman/generator-angular) and [AngularJS Yeoman Tutorial](http://www.sitepoint.com/kickstart-your-angularjs-development-with-yeoman-grunt-and-bower/)
Complementary Libraries
-----------------------
This is a list of libraries that enhance AngularJS, add common UI components or integrate with other libraries. You can find a larger list of AngularJS external libraries at [ngmodules.org](http://ngmodules.org/).
* **Advanced Routing:** [UI-Router](https://github.com/angular-ui/ui-router)
* **Authentication:** [Http Auth Interceptor](https://github.com/witoldsz/angular-http-auth)
* **Internationalization:**
+ [angular-translate](http://angular-translate.github.io)
+ [angular-gettext](http://angular-gettext.rocketeer.be/)
+ [angular-localization](http://doshprompt.github.io/angular-localization/)
* **RESTful services:** [Restangular](https://github.com/mgonto/restangular)
* **SQL and NoSQL backends:**
+ [BreezeJS](http://www.breezejs.com/)
+ [AngularFire](http://angularfire.com/)
* **Data Handling**
+ Local Storage and session: [ngStorage](https://github.com/gsklee/ngStorage)
+ [angular-cache](https://github.com/jmdobry/angular-cache)
+ Data Modeling [JS-Data-Angular](https://github.com/js-data/js-data-angular)
* **Fileupload:**
+ [ng-file-upload](https://github.com/danialfarid/ng-file-upload)
+ [blueimp-fileupload for AngularJS](https://blueimp.github.io/jQuery-File-Upload/angularjs.html)
* **General UI Libraries:**
+ [AngularJS Material](https://material.angularjs.org/latest/)
+ [AngularJS UI Bootstrap](http://angular-ui.github.io/)
+ [AngularStrap for Bootstrap 3](http://mgcrea.github.io/angular-strap/)
+ [KendoUI](http://kendo-labs.github.io/angular-kendo/#/)
+ [Wijmo](http://wijmo.com/tag/angularjs-2/)
* **Specific UI Elements:**
+ [ngInfiniteScroll](https://sroze.github.io/ngInfiniteScroll/)
+ [ngTable](https://github.com/esvit/ng-table)
+ [AngularJS UI Grid](http://angular-ui.github.io/grid)
+ [Toaster Notifications](https://github.com/jirikavi/AngularJS-Toaster)
+ [textAngular Rich Text Editor / contenteditable](http://textangular.com/) (Rich Text Editor / binding to contenteditable)
+ [AngularJS UI Map (Google Maps)](https://github.com/angular-ui/ui-map)
General Learning Resources
--------------------------
### Books
* [AngularJS Directives](http://www.amazon.com/AngularJS-Directives-Alex-Vanston/dp/1783280336) by Alex Vanston
* [AngularJS Essentials (Free eBook)](https://www.packtpub.com/packt/free-ebook/angularjs-essentials) by Rodrigo Branas
* [AngularJS in Action](https://www.manning.com/books/angularjs-in-action) by Lukas Ruebbelke
* [AngularJS: Novice to Ninja](http://www.amazon.in/AngularJS-Novice-Ninja-Sandeep-Panda/dp/0992279453) by Sandeep Panda
* [AngularJS UI Development](http://www.amazon.com/AngularJS-UI-Development-Amit-Ghart-ebook/dp/B00OXVAK7A) by Amit Gharat and Matthias Nehlsen
* [AngularJS: Up and Running](http://www.amazon.com/AngularJS-Running-Enhanced-Productivity-Structured/dp/1491901942) by Brad Green and Shyam Seshadri
* [Developing an AngularJS Edge](http://www.amazon.com/Developing-AngularJS-Edge-Christopher-Hiller-ebook/dp/B00CJLFF8K) by Christopher Hiller
* [Mastering Web App Development](http://www.amazon.com/Mastering-Web-Application-Development-AngularJS/dp/1782161821) by Pawel Kozlowski and Pete Bacon Darwin
* [ng-book: The Complete Book on AngularJS](http://ng-book.com/) by Ari Lerner
* [Professional AngularJS](http://www.amazon.com/Professional-AngularJS-Valeri-Karpov/dp/1118832078/)
* [Recipes With AngularJS](http://www.amazon.co.uk/Recipes-Angular-js-Frederik-Dietz-ebook/dp/B00DK95V48) by Frederik Dietz
* [Responsive Web Design with AngularJS](http://www.amazon.com/Responsive-Design-AngularJS-Sandeep-Kumar/dp/178439842X) by Sandeep Kumar Patel
### Videos:
* [egghead.io](http://egghead.io/)
### Courses
* **Free online:** [thinkster.io](http://thinkster.io), [CodeAcademy](http://www.codecademy.com/courses/javascript-advanced-en-2hJ3J/0/1), [CodeSchool](https://www.codeschool.com/courses/shaping-up-with-angular-js)
* **Paid online:** [Pluralsight](https://www.pluralsight.com/search?q=angularjs), [Tuts+](https://tutsplus.com/course/easier-js-apps-with-angular/), [lynda.com](http://www.lynda.com/AngularJS-tutorials/Up-Running-AngularJS/133318-2.html), [WintellectNOW (4 lessons)](http://www.wintellectnow.com/Course/Detail/mastering-angularjs), [Packt](https://www.packtpub.com/web-development/angularjs-maintaining-web-applications)
* **Paid onsite:** [angularbootcamp.com](http://angularbootcamp.com/)
angularjs
Improve this DocTemplates
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/templates.ngdoc?message=docs(guide%2FTemplates)%3A%20describe%20your%20change...)Templates
===================================================================================================================================================================================
In AngularJS, templates are written with HTML that contains AngularJS-specific elements and attributes. AngularJS combines the template with information from the model and controller to render the dynamic view that a user sees in the browser.
These are the types of AngularJS elements and attributes you can use:
* [Directive](directive) — An attribute or element that augments an existing DOM element or represents a reusable DOM component.
* [Markup](../api/ng/service/%24interpolate) — The double curly brace notation `{{ }}` to bind expressions to elements is built-in AngularJS markup.
* [Filter](filter) — Formats data for display.
* [Form controls](forms) — Validates user input.
The following code snippet shows a template with [directives](directive) and curly-brace <expression> bindings:
```
<html ng-app>
<!-- Body tag augmented with ngController directive -->
<body ng-controller="MyController">
<input ng-model="foo" value="bar">
<!-- Button tag with ngClick directive, and
string expression 'buttonText'
wrapped in "{{ }}" markup -->
<button ng-click="changeFoo()">{{buttonText}}</button>
<script src="angular.js"></script>
</body>
</html>
```
In a simple app, the template consists of HTML, CSS, and AngularJS directives contained in just one HTML file (usually `index.html`).
In a more complex app, you can display multiple views within one main page using "partials" – segments of template located in separate HTML files. You can use the [ngView](../api/ngroute/directive/ngview) directive to load partials based on configuration passed to the [$route](../api/ngroute/service/%24route) service. The [AngularJS tutorial](https://code.angularjs.org/1.8.2/docs/guide/tutorial/) shows this technique in steps seven and eight.
Related Topics
--------------
* [Filters](filter)
* [Forms](forms)
Related API
-----------
* [API Reference](https://code.angularjs.org/1.8.2/docs/guide/api)
angularjs
Improve this DocCreating Custom Directives
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/directive.ngdoc?message=docs(guide%2FDirectives)%3A%20describe%20your%20change...)Creating Custom Directives
=====================================================================================================================================================================================================
**Note:** this guide is targeted towards developers who are already familiar with AngularJS basics. If you're just getting started, we recommend the [tutorial](https://code.angularjs.org/1.8.2/docs/guide/tutorial/) first. If you're looking for the **directives API**, you can find it in the [`$compile` API docs](../api/ng/service/%24compile). This document explains when you'd want to create your own directives in your AngularJS app, and how to implement them.
What are Directives?
--------------------
At a high level, directives are markers on a DOM element (such as an attribute, element name, comment or CSS class) that tell AngularJS's **HTML compiler** ([`$compile`](../api/ng/service/%24compile)) to attach a specified behavior to that DOM element (e.g. via event listeners), or even to transform the DOM element and its children.
AngularJS comes with a set of these directives built-in, like `ngBind`, `ngModel`, and `ngClass`. Much like you create controllers and services, you can create your own directives for AngularJS to use. When AngularJS [bootstraps](bootstrap) your application, the [HTML compiler](compiler) traverses the DOM matching directives against the DOM elements.
**What does it mean to "compile" an HTML template?** For AngularJS, "compilation" means attaching directives to the HTML to make it interactive. The reason we use the term "compile" is that the recursive process of attaching directives mirrors the process of compiling source code in [compiled programming languages](http://en.wikipedia.org/wiki/Compiled_languages). Matching Directives
-------------------
Before we can write a directive, we need to know how AngularJS's [HTML compiler](compiler) determines when to use a given directive.
Similar to the terminology used when an [element **matches** a selector](https://developer.mozilla.org/en-US/docs/Web/API/Element.matches), we say an element **matches** a directive when the directive is part of its declaration.
In the following example, we say that the `<input>` element **matches** the `ngModel` directive
```
<input ng-model="foo">
```
The following `<input>` element also **matches** `ngModel`:
```
<input data-ng-model="foo">
```
And the following `<person>` element **matches** the `person` directive:
```
<person>{{name}}</person>
```
### Normalization
AngularJS **normalizes** an element's tag and attribute name to determine which elements match which directives. We typically refer to directives by their case-sensitive [camelCase](http://en.wikipedia.org/wiki/CamelCase) **normalized** name (e.g. `ngModel`). However, since HTML is case-insensitive, we refer to directives in the DOM by lower-case forms, typically using [dash-delimited](https://en.wikipedia.org/wiki/Letter_case#Special_case_styles) attributes on DOM elements (e.g. `ng-model`).
The **normalization** process is as follows:
1. Strip `x-` and `data-` from the front of the element/attributes.
2. Convert the `:`, `-`, or `_`-delimited name to `camelCase`.
For example, the following forms are all equivalent and match the [`ngBind`](../api/ng/directive/ngbind) directive:
**Best Practice:** Prefer using the dash-delimited format (e.g. `ng-bind` for `ngBind`). If you want to use an HTML validating tool, you can instead use the `data`-prefixed version (e.g. `data-ng-bind` for `ngBind`). The other forms shown above are accepted for legacy reasons but we advise you to avoid them. ### Directive types
`$compile` can match directives based on element names (E), attributes (A), class names (C), and comments (M).
The built-in AngularJS directives show in their documentation page which type of matching they support.
The following demonstrates the various ways a directive (`myDir` in this case) that matches all 4 types can be referenced from within a template.
```
<my-dir></my-dir>
<span my-dir="exp"></span>
<!-- directive: my-dir exp -->
<span class="my-dir: exp;"></span>
```
A directive can specify which of the 4 matching types it supports in the [`restrict`](../api/ng/service/%24compile#-restrict-.html) property of the directive definition object. The default is `EA`.
**Best Practice:** Prefer using directives via tag name and attributes over comment and class names. Doing so generally makes it easier to determine what directives a given element matches. **Best Practice:** Comment directives were commonly used in places where the DOM API limits the ability to create directives that spanned multiple elements (e.g. inside `<table>` elements). AngularJS 1.2 introduces [`ng-repeat-start` and `ng-repeat-end`](../api/ng/directive/ngrepeat) as a better solution to this problem. Developers are encouraged to use this over custom comment directives when possible. Creating Directives
-------------------
First let's talk about the [API for registering directives](../api/ng/provider/%24compileprovider#directive.html). Much like controllers, directives are registered on modules. To register a directive, you use the `module.directive` API. `module.directive` takes the [normalized](directive#matching-directives.html) directive name followed by a **factory function.** This factory function should return an object with the different options to tell `$compile` how the directive should behave when matched.
The factory function is invoked only once when the [compiler](../api/ng/service/%24compile) matches the directive for the first time. You can perform any initialization work here. The function is invoked using [$injector.invoke](../api/auto/service/%24injector#invoke.html) which makes it injectable just like a controller.
We'll go over a few common examples of directives, then dive deep into the different options and compilation process.
**Best Practice:** In order to avoid collisions with some future standard, it's best to prefix your own directive names. For instance, if you created a `<carousel>` directive, it would be problematic if HTML7 introduced the same element. A two or three letter prefix (e.g. `btfCarousel`) works well. Similarly, do not prefix your own directives with `ng` or they might conflict with directives included in a future version of AngularJS. For the following examples, we'll use the prefix `my` (e.g. `myCustomer`).
### Template-expanding directive
Let's say you have a chunk of your template that represents a customer's information. This template is repeated many times in your code. When you change it in one place, you have to change it in several others. This is a good opportunity to use a directive to simplify your template.
Let's create a directive that simply replaces its contents with a static template:
Notice that we have bindings in this directive. After `$compile` compiles and links `<div my-customer></div>`, it will try to match directives on the element's children. This means you can compose directives of other directives. We'll see how to do that in [an example](directive#creating-directives-that-communicate.html) below.
In the example above we in-lined the value of the `template` option, but this will become annoying as the size of your template grows.
**Best Practice:** Unless your template is very small, it's typically better to break it apart into its own HTML file and load it with the `templateUrl` option. If you are familiar with `ngInclude`, `templateUrl` works just like it. Here's the same example using `templateUrl` instead:
`templateUrl` can also be a function which returns the URL of an HTML template to be loaded and used for the directive. AngularJS will call the `templateUrl` function with two parameters: the element that the directive was called on, and an `attr` object associated with that element.
**Note:** You do not currently have the ability to access scope variables from the `templateUrl` function, since the template is requested before the scope is initialized. **Note:** When you create a directive, it is restricted to attribute and elements only by default. In order to create directives that are triggered by class name, you need to use the `restrict` option. The `restrict` option is typically set to:
* `'A'` - only matches attribute name
* `'E'` - only matches element name
* `'C'` - only matches class name
* `'M'` - only matches comment
These restrictions can all be combined as needed:
* `'AEC'` - matches either attribute or element or class name
Let's change our directive to use `restrict: 'E'`:
For more on the `restrict` property, see the [API docs](../api/ng/service/%24compile#directive-definition-object.html).
**When should I use an attribute versus an element?** Use an element when you are creating a component that is in control of the template. The common case for this is when you are creating a Domain-Specific Language for parts of your template. Use an attribute when you are decorating an existing element with new functionality. Using an element for the `myCustomer` directive is clearly the right choice because you're not decorating an element with some "customer" behavior; you're defining the core behavior of the element as a customer component.
### Isolating the Scope of a Directive
Our `myCustomer` directive above is great, but it has a fatal flaw. We can only use it once within a given scope.
In its current implementation, we'd need to create a different controller each time in order to re-use such a directive:
This is clearly not a great solution.
What we want to be able to do is separate the scope inside a directive from the scope outside, and then map the outer scope to a directive's inner scope. We can do this by creating what we call an **isolate scope**. To do this, we can use a [directive's `scope`](../api/ng/service/%24compile#-scope-.html) option:
Looking at `index.html`, the first `<my-customer>` element binds the `info` attribute to `naomi`, which we have exposed on our controller's scope. The second binds `info` to `igor`.
Let's take a closer look at the scope option:
```
//...
scope: {
customerInfo: '=info'
},
//...
```
The **scope option** is an object that contains a property for each isolate scope binding. In this case it has just one property:
* Its name (`customerInfo`) corresponds to the directive's **isolate scope** property, `customerInfo`.
* Its value (`=info`) tells `$compile` to bind to the `info` attribute.
**Note:** These `=attr` attributes in the `scope` option of directives are normalized just like directive names. To bind to the attribute in `<div bind-to-this="thing">`, you'd specify a binding of `=bindToThis`. For cases where the attribute name is the same as the value you want to bind to inside the directive's scope, you can use this shorthand syntax:
```
...
scope: {
// same as '=customer'
customer: '='
},
...
```
Besides making it possible to bind different data to the scope inside a directive, using an isolated scope has another effect.
We can show this by adding another property, `vojta`, to our scope and trying to access it from within our directive's template:
Notice that `{{vojta.name}}` and `{{vojta.address}}` are empty, meaning they are undefined. Although we defined `vojta` in the controller, it's not available within the directive.
As the name suggests, the **isolate scope** of the directive isolates everything except models that you've explicitly added to the `scope: {}` hash object. This is helpful when building reusable components because it prevents a component from changing your model state except for the models that you explicitly pass in.
**Note:** Normally, a scope prototypically inherits from its parent. An isolated scope does not. See the ["Directive Definition Object - scope"](../api/ng/service/%24compile#directive-definition-object.html) section for more information about isolate scopes. **Best Practice:** Use the `scope` option to create isolate scopes when making components that you want to reuse throughout your app. ### Creating a Directive that Manipulates the DOM
In this example we will build a directive that displays the current time. Once a second, it updates the DOM to reflect the current time.
Directives that want to modify the DOM typically use the `link` option to register DOM listeners as well as update the DOM. It is executed after the template has been cloned and is where directive logic will be put.
`link` takes a function with the following signature, `function link(scope, element, attrs, controller, transcludeFn) { ... }`, where:
* `scope` is an AngularJS scope object.
* `element` is the jqLite-wrapped element that this directive matches.
* `attrs` is a hash object with key-value pairs of normalized attribute names and their corresponding attribute values.
* `controller` is the directive's required controller instance(s) or its own controller (if any). The exact value depends on the directive's require property.
* `transcludeFn` is a transclude linking function pre-bound to the correct transclusion scope.
For more details on the `link` option refer to the [`$compile` API](../api/ng/service/%24compile#-link-.html) page. In our `link` function, we want to update the displayed time once a second, or whenever a user changes the time formatting string that our directive binds to. We will use the `$interval` service to call a handler on a regular basis. This is easier than using `$timeout` but also works better with end-to-end testing, where we want to ensure that all `$timeout`s have completed before completing the test. We also want to remove the `$interval` if the directive is deleted so we don't introduce a memory leak.
There are a couple of things to note here. Just like the `module.controller` API, the function argument in `module.directive` is dependency injected. Because of this, we can use `$interval` and `dateFilter` inside our directive's `link` function.
We register an event `element.on('$destroy', ...)`. What fires this `$destroy` event?
There are a few special events that AngularJS emits. When a DOM node that has been compiled with AngularJS's compiler is destroyed, it emits a `$destroy` event. Similarly, when an AngularJS scope is destroyed, it broadcasts a `$destroy` event to listening scopes.
By listening to this event, you can remove event listeners that might cause memory leaks. Listeners registered to scopes and elements are automatically cleaned up when they are destroyed, but if you registered a listener on a service, or registered a listener on a DOM node that isn't being deleted, you'll have to clean it up yourself or you risk introducing a memory leak.
**Best Practice:** Directives should clean up after themselves. You can use `element.on('$destroy', ...)` or `scope.$on('$destroy', ...)` to run a clean-up function when the directive is removed. ### Creating a Directive that Wraps Other Elements
We've seen that you can pass in models to a directive using the isolate scope, but sometimes it's desirable to be able to pass in an entire template rather than a string or an object. Let's say that we want to create a "dialog box" component. The dialog box should be able to wrap any arbitrary content.
To do this, we need to use the `transclude` option.
What does this `transclude` option do, exactly? `transclude` makes the contents of a directive with this option have access to the scope **outside** of the directive rather than inside.
To illustrate this, see the example below. Notice that we've added a `link` function in `script.js` that redefines `name` as `Jeff`. What do you think the `{{name}}` binding will resolve to now?
Ordinarily, we would expect that `{{name}}` would be `Jeff`. However, we see in this example that the `{{name}}` binding is still `Tobias`.
The `transclude` option changes the way scopes are nested. It makes it so that the **contents** of a transcluded directive have whatever scope is outside the directive, rather than whatever scope is on the inside. In doing so, it gives the contents access to the outside scope.
Note that if the directive did not create its own scope, then `scope` in `scope.name = 'Jeff'` would reference the outside scope and we would see `Jeff` in the output.
This behavior makes sense for a directive that wraps some content, because otherwise you'd have to pass in each model you wanted to use separately. If you have to pass in each model that you want to use, then you can't really have arbitrary contents, can you?
**Best Practice:** only use `transclude: true` when you want to create a directive that wraps arbitrary content. Next, we want to add buttons to this dialog box, and allow someone using the directive to bind their own behavior to it.
We want to run the function we pass by invoking it from the directive's scope, but have it run in the context of the scope where it's registered.
We saw earlier how to use `=attr` in the `scope` option, but in the above example, we're using `&attr` instead. The `&` binding allows a directive to trigger evaluation of an expression in the context of the original scope, at a specific time. Any legal expression is allowed, including an expression which contains a function call. Because of this, `&` bindings are ideal for binding callback functions to directive behaviors.
When the user clicks the `x` in the dialog, the directive's `close` function is called, thanks to `ng-click.` This call to `close` on the isolated scope actually evaluates the expression `hideDialog(message)` in the context of the original scope, thus running `Controller`'s `hideDialog` function.
Often it's desirable to pass data from the isolate scope via an expression to the parent scope, this can be done by passing a map of local variable names and values into the expression wrapper function. For example, the `hideDialog` function takes a message to display when the dialog is hidden. This is specified in the directive by calling `close({message: 'closing for now'})`. Then the local variable `message` will be available within the `on-close` expression.
**Best Practice:** use `&attr` in the `scope` option when you want your directive to expose an API for binding to behaviors. ### Creating a Directive that Adds Event Listeners
Previously, we used the `link` function to create a directive that manipulated its DOM elements. Building upon that example, let's make a directive that reacts to events on its elements.
For instance, what if we wanted to create a directive that lets a user drag an element?
### Creating Directives that Communicate
You can compose any directives by using them within templates.
Sometimes, you want a component that's built from a combination of directives.
Imagine you want to have a container with tabs in which the contents of the container correspond to which tab is active.
The `myPane` directive has a `require` option with value `^^myTabs`. When a directive uses this option, `$compile` will throw an error unless the specified controller is found. The `^^` prefix means that this directive searches for the controller on its parents. (A `^` prefix would make the directive look for the controller on its own element or its parents; without any prefix, the directive would look on its own element only.)
So where does this `myTabs` controller come from? Directives can specify controllers using the unsurprisingly named `controller` option. As you can see, the `myTabs` directive uses this option. Just like `ngController`, this option attaches a controller to the template of the directive.
If it is necessary to reference the controller or any functions bound to the controller from the template, you can use the option `controllerAs` to specify the name of the controller as an alias. The directive needs to define a scope for this configuration to be used. This is particularly useful in the case when the directive is used as a component.
Looking back at `myPane`'s definition, notice the last argument in its `link` function: `tabsCtrl`. When a directive requires a controller, it receives that controller as the fourth argument of its `link` function. Taking advantage of this, `myPane` can call the `addPane` function of `myTabs`.
If multiple controllers are required, the `require` option of the directive can take an array argument. The corresponding parameter being sent to the `link` function will also be an array.
```
angular.module('docsTabsExample', [])
.directive('myPane', function() {
return {
require: ['^^myTabs', 'ngModel'],
restrict: 'E',
transclude: true,
scope: {
title: '@'
},
link: function(scope, element, attrs, controllers) {
var tabsCtrl = controllers[0],
modelCtrl = controllers[1];
tabsCtrl.addPane(scope);
},
templateUrl: 'my-pane.html'
};
});
```
Savvy readers may be wondering what the difference is between `link` and `controller`. The basic difference is that `controller` can expose an API, and `link` functions can interact with controllers using `require`.
**Best Practice:** use `controller` when you want to expose an API to other directives. Otherwise use `link`. Summary
-------
Here we've seen the main use cases for directives. Each of these samples acts as a good starting point for creating your own directives.
You might also be interested in an in-depth explanation of the compilation process that's available in the [compiler guide](compiler).
The [`$compile` API](../api/ng/service/%24compile) page has a comprehensive list of directive options for reference.
| programming_docs |
angularjs
Improve this DocUnderstanding Controllers
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/controller.ngdoc?message=docs(guide%2FControllers)%3A%20describe%20your%20change...)Understanding Controllers
======================================================================================================================================================================================================
In AngularJS, a Controller is defined by a JavaScript **constructor function** that is used to augment the [AngularJS Scope](scope).
Controllers can be attached to the DOM in different ways. For each of them, AngularJS will instantiate a new Controller object, using the specified Controller's **constructor function**:
* the [ngController](../api/ng/directive/ngcontroller) directive. A new **child scope** will be created and made available as an injectable parameter to the Controller's constructor function as `$scope`.
* a route controller in a [$route definition](../api/ngroute/provider/%24routeprovider).
* the controller of a [regular directive](directive), or a [component directive](component).
If the controller has been attached using the `controller as` syntax then the controller instance will be assigned to a property on the scope.
Use controllers to:
* Set up the initial state of the `$scope` object.
* Add behavior to the `$scope` object.
Do not use controllers to:
* Manipulate DOM — Controllers should contain only business logic. Putting any presentation logic into Controllers significantly affects its testability. AngularJS has <databinding> for most cases and [directives](directive) to encapsulate manual DOM manipulation.
* Format input — Use [AngularJS form controls](forms) instead.
* Filter output — Use [AngularJS filters](filter) instead.
* Share code or state across controllers — Use [AngularJS services](services) instead.
* Manage the life-cycle of other components (for example, to create service instances).
In general, a Controller shouldn't try to do too much. It should contain only the business logic needed for a single view.
The most common way to keep Controllers slim is by encapsulating work that doesn't belong to controllers into services and then using these services in Controllers via dependency injection. This is discussed in the [Dependency Injection](di) and [Services](services) sections of this guide.
Setting up the initial state of a $scope object
-----------------------------------------------
Typically, when you create an application you need to set up the initial state for the AngularJS `$scope`. You set up the initial state of a scope by attaching properties to the `$scope` object. The properties contain the **view model** (the model that will be presented by the view). All the `$scope` properties will be available to the [template](templates) at the point in the DOM where the Controller is registered.
The following example demonstrates creating a `GreetingController`, which attaches a `greeting` property containing the string `'Hola!'` to the `$scope`:
```
var myApp = angular.module('myApp',[]);
myApp.controller('GreetingController', ['$scope', function($scope) {
$scope.greeting = 'Hola!';
}]);
```
We create an [AngularJS Module](module), `myApp`, for our application. Then we add the controller's constructor function to the module using the `.controller()` method. This keeps the controller's constructor function out of the global scope.
We have used an **inline injection annotation** to explicitly specify the dependency of the Controller on the `$scope` service provided by AngularJS. See the guide on [Dependency Injection](di) for more information. We attach our controller to the DOM using the `ng-controller` directive. The `greeting` property can now be data-bound to the template:
```
<div ng-controller="GreetingController">
{{ greeting }}
</div>
```
Adding Behavior to a Scope Object
---------------------------------
In order to react to events or execute computation in the view we must provide behavior to the scope. We add behavior to the scope by attaching methods to the `$scope` object. These methods are then available to be called from the template/view.
The following example uses a Controller to add a method, which doubles a number, to the scope:
```
var myApp = angular.module('myApp',[]);
myApp.controller('DoubleController', ['$scope', function($scope) {
$scope.double = function(value) { return value * 2; };
}]);
```
Once the Controller has been attached to the DOM, the `double` method can be invoked in an AngularJS expression in the template:
```
<div ng-controller="DoubleController">
Two times <input ng-model="num"> equals {{ double(num) }}
</div>
```
As discussed in the [Concepts](concepts) section of this guide, any objects (or primitives) assigned to the scope become model properties. Any methods assigned to the scope are available in the template/view, and can be invoked via AngularJS expressions and `ng` event handler directives (e.g. [ngClick](../api/ng/directive/ngclick)).
To illustrate further how Controller components work in AngularJS, let's create a little app with the following components:
* A [template](templates) with two buttons and a simple message
* A model consisting of a string named `spice`
* A Controller with two functions that set the value of `spice`
The message in our template contains a binding to the `spice` model which, by default, is set to the string "very". Depending on which button is clicked, the `spice` model is set to `chili` or `jalapeño`, and the message is automatically updated by data-binding.
Things to notice in the example above:
* The `ng-controller` directive is used to (implicitly) create a scope for our template, and the scope is augmented (managed) by the `SpicyController` Controller.
* `SpicyController` is just a plain JavaScript function. As an (optional) naming convention the name starts with capital letter and ends with "Controller".
* Assigning a property to `$scope` creates or updates the model.
* Controller methods can be created through direct assignment to scope (see the `chiliSpicy` method)
* The Controller methods and properties are available in the template (for both the `<div>` element and its children).
Controller methods can also take arguments, as demonstrated in the following variation of the previous example.
Notice that the `SpicyController` Controller now defines just one method called `spicy`, which takes one argument called `spice`. The template then refers to this Controller method and passes in a string constant `'chili'` in the binding for the first button and a model property `customSpice` (bound to an input box) in the second button.
It is common to attach Controllers at different levels of the DOM hierarchy. Since the [ng-controller](../api/ng/directive/ngcontroller) directive creates a new child scope, we get a hierarchy of scopes that inherit from each other. The `$scope` that each Controller receives will have access to properties and methods defined by Controllers higher up the hierarchy. See [Understanding Scopes](https://github.com/angular/angular.js/wiki/Understanding-Scopes) for more information about scope inheritance.
Notice how we nested three `ng-controller` directives in our template. This will result in four scopes being created for our view:
* The root scope
* The `MainController` scope, which contains `timeOfDay` and `name` properties
* The `ChildController` scope, which inherits the `timeOfDay` property but overrides (shadows) the `name` property from the previous scope
* The `GrandChildController` scope, which overrides (shadows) both the `timeOfDay` property defined in `MainController` and the `name` property defined in `ChildController`
Inheritance works with methods in the same way as it does with properties. So in our previous examples, all of the properties could be replaced with methods that return string values.
Testing Controllers
-------------------
Although there are many ways to test a Controller, one of the best conventions, shown below, involves injecting the [$rootScope](../api/ng/service/%24rootscope) and [$controller](../api/ng/service/%24controller):
**Controller Definition:**
```
var myApp = angular.module('myApp',[]);
myApp.controller('MyController', function($scope) {
$scope.spices = [{"name":"pasilla", "spiciness":"mild"},
{"name":"jalapeno", "spiciness":"hot hot hot!"},
{"name":"habanero", "spiciness":"LAVA HOT!!"}];
$scope.spice = "habanero";
});
```
**Controller Test:**
```
describe('myController function', function() {
describe('myController', function() {
var $scope;
beforeEach(module('myApp'));
beforeEach(inject(function($rootScope, $controller) {
$scope = $rootScope.$new();
$controller('MyController', {$scope: $scope});
}));
it('should create "spices" model with 3 spices', function() {
expect($scope.spices.length).toBe(3);
});
it('should set the default value of spice', function() {
expect($scope.spice).toBe('habanero');
});
});
});
```
If you need to test a nested Controller you must create the same scope hierarchy in your test that exists in the DOM:
```
describe('state', function() {
var mainScope, childScope, grandChildScope;
beforeEach(module('myApp'));
beforeEach(inject(function($rootScope, $controller) {
mainScope = $rootScope.$new();
$controller('MainController', {$scope: mainScope});
childScope = mainScope.$new();
$controller('ChildController', {$scope: childScope});
grandChildScope = childScope.$new();
$controller('GrandChildController', {$scope: grandChildScope});
}));
it('should have over and selected', function() {
expect(mainScope.timeOfDay).toBe('morning');
expect(mainScope.name).toBe('Nikki');
expect(childScope.timeOfDay).toBe('morning');
expect(childScope.name).toBe('Mattie');
expect(grandChildScope.timeOfDay).toBe('evening');
expect(grandChildScope.name).toBe('Gingerbread Baby');
});
});
```
angularjs
Improve this DocConceptual Overview
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/concepts.ngdoc?message=docs(guide%2FConceptual%20Overview)%3A%20describe%20your%20change...)Conceptual Overview
========================================================================================================================================================================================================
This section briefly touches on all of the important parts of AngularJS using a simple example. For a more in-depth explanation, see the [tutorial](https://code.angularjs.org/1.8.2/docs/guide/tutorial/).
| Concept | Description |
| --- | --- |
| [Template](concepts#template.html) | HTML with additional markup |
| [Directives](concepts#directive.html) | extend HTML with custom attributes and elements |
| [Model](concepts#model.html) | the data shown to the user in the view and with which the user interacts |
| [Scope](concepts#scope.html) | context where the model is stored so that controllers, directives and expressions can access it |
| [Expressions](concepts#expression.html) | access variables and functions from the scope |
| [Compiler](concepts#compiler.html) | parses the template and instantiates directives and expressions |
| [Filter](concepts#filter.html) | formats the value of an expression for display to the user |
| [View](concepts#view.html) | what the user sees (the DOM) |
| [Data Binding](concepts#databinding.html) | sync data between the model and the view |
| [Controller](concepts#controller.html) | the business logic behind views |
| [Dependency Injection](concepts#di.html) | Creates and wires objects and functions |
| [Injector](concepts#injector.html) | dependency injection container |
| [Module](concepts#module.html) | a container for the different parts of an app including controllers, services, filters, directives which configures the Injector |
| [Service](concepts#service.html) | reusable business logic independent of views |
A first example: Data binding
-----------------------------
In the following example we will build a form to calculate the costs of an invoice in different currencies.
Let's start with input fields for quantity and cost whose values are multiplied to produce the total of the invoice:
Try out the Live Preview above, and then let's walk through the example and describe what's going on.
This looks like normal HTML, with some new markup. In AngularJS, a file like this is called a [template](templates). When AngularJS starts your application, it parses and processes this new markup from the template using the <compiler>. The loaded, transformed and rendered DOM is then called the *view*.
The first kind of new markup are the [directives](directive). They apply special behavior to attributes or elements in the HTML. In the example above we use the [`ng-app`](../api/ng/directive/ngapp) attribute, which is linked to a directive that automatically initializes our application. AngularJS also defines a directive for the [`input`](../api/ng/directive/input) element that adds extra behavior to the element. The [`ng-model`](../api/ng/directive/ngmodel) directive stores/updates the value of the input field into/from a variable.
**Custom directives to access the DOM**: In AngularJS, the only place where an application should access the DOM is within directives. This is important because artifacts that access the DOM are hard to test. If you need to access the DOM directly you should write a custom directive for this. The [directives guide](directive) explains how to do this. The second kind of new markup are the double curly braces `{{ expression | filter }}`: When the compiler encounters this markup, it will replace it with the evaluated value of the markup. An <expression> in a template is a JavaScript-like code snippet that allows AngularJS to read and write variables. Note that those variables are not global variables. Just like variables in a JavaScript function live in a scope, AngularJS provides a <scope> for the variables accessible to expressions. The values that are stored in variables on the scope are referred to as the *model* in the rest of the documentation. Applied to the example above, the markup directs AngularJS to "take the data we got from the input widgets and multiply them together".
The example above also contains a <filter>. A filter formats the value of an expression for display to the user. In the example above, the filter [`currency`](../api/ng/filter/currency) formats a number into an output that looks like money.
The important thing in the example is that AngularJS provides *live* bindings: Whenever the input values change, the value of the expressions are automatically recalculated and the DOM is updated with their values. The concept behind this is [two-way data binding](databinding).
Adding UI logic: Controllers
----------------------------
Let's add some more logic to the example that allows us to enter and calculate the costs in different currencies and also pay the invoice.
What changed?
First, there is a new JavaScript file that contains a <controller>. More accurately, the file specifies a constructor function that will be used to create the actual controller instance. The purpose of controllers is to expose variables and functionality to expressions and directives.
Besides the new file that contains the controller code, we also added an [`ng-controller`](../api/ng/directive/ngcontroller) directive to the HTML. This directive tells AngularJS that the new `InvoiceController` is responsible for the element with the directive and all of the element's children. The syntax `InvoiceController as invoice` tells AngularJS to instantiate the controller and save it in the variable `invoice` in the current scope.
We also changed all expressions in the page to read and write variables within that controller instance by prefixing them with `invoice.` . The possible currencies are defined in the controller and added to the template using [`ng-repeat`](../api/ng/directive/ngrepeat). As the controller contains a `total` function we are also able to bind the result of that function to the DOM using `{{ invoice.total(...) }}`.
Again, this binding is live, i.e. the DOM will be automatically updated whenever the result of the function changes. The button to pay the invoice uses the directive [`ngClick`](../api/ng/directive/ngclick). This will evaluate the corresponding expression whenever the button is clicked.
In the new JavaScript file we are also creating a [module](concepts#module.html) at which we register the controller. We will talk about modules in the next section.
The following graphic shows how everything works together after we introduced the controller:
View-independent business logic: Services
-----------------------------------------
Right now, the `InvoiceController` contains all logic of our example. When the application grows it is a good practice to move view-independent logic from the controller into a [service](services), so it can be reused by other parts of the application as well. Later on, we could also change that service to load the exchange rates from the web, e.g. by calling the [exchangeratesapi.io](https://exchangeratesapi.io) exchange rate API, without changing the controller.
Let's refactor our example and move the currency conversion into a service in another file:
What changed?
We moved the `convertCurrency` function and the definition of the existing currencies into the new file `finance2.js`. But how does the controller get a hold of the now separated function?
This is where [Dependency Injection](di) comes into play. Dependency Injection (DI) is a software design pattern that deals with how objects and functions get created and how they get a hold of their dependencies. Everything within AngularJS (directives, filters, controllers, services, ...) is created and wired using dependency injection. Within AngularJS, the DI container is called the [injector](di).
To use DI, there needs to be a place where all the things that should work together are registered. In AngularJS, this is the purpose of the [modules](module). When AngularJS starts, it will use the configuration of the module with the name defined by the `ng-app` directive, including the configuration of all modules that this module depends on.
In the example above: The template contains the directive `ng-app="invoice2"`. This tells AngularJS to use the `invoice2` module as the main module for the application. The code snippet `angular.module('invoice2', ['finance2'])` specifies that the `invoice2` module depends on the `finance2` module. By this, AngularJS uses the `InvoiceController` as well as the `currencyConverter` service.
Now that AngularJS knows of all the parts of the application, it needs to create them. In the previous section we saw that controllers are created using a constructor function. For services, there are multiple ways to specify how they are created (see the [service guide](services)). In the example above, we are using an anonymous function as the factory function for the `currencyConverter` service. This function should return the `currencyConverter` service instance.
Back to the initial question: How does the `InvoiceController` get a reference to the `currencyConverter` function? In AngularJS, this is done by simply defining arguments on the constructor function. With this, the injector is able to create the objects in the right order and pass the previously created objects into the factories of the objects that depend on them. In our example, the `InvoiceController` has an argument named `currencyConverter`. By this, AngularJS knows about the dependency between the controller and the service and calls the controller with the service instance as argument.
The last thing that changed in the example between the previous section and this section is that we now pass an array to the `module.controller` function, instead of a plain function. The array first contains the names of the service dependencies that the controller needs. The last entry in the array is the controller constructor function. AngularJS uses this array syntax to define the dependencies so that the DI also works after minifying the code, which will most probably rename the argument name of the controller constructor function to something shorter like `a`.
Accessing the backend
---------------------
Let's finish our example by fetching the exchange rates from the [exchangeratesapi.io](https://exchangeratesapi.io) exchange rate API. The following example shows how this is done with AngularJS:
What changed? Our `currencyConverter` service of the `finance` module now uses the [`$http`](../api/ng/service/%24http), a built-in service provided by AngularJS for accessing a server backend. `$http` is a wrapper around [`XMLHttpRequest`](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest) and [JSONP](http://en.wikipedia.org/wiki/JSONP) transports.
| programming_docs |
angularjs
Improve this DocSecurity
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/security.ngdoc?message=docs(guide%2FSecurity)%3A%20describe%20your%20change...)Security
================================================================================================================================================================================
This document explains some of AngularJS's security features and best practices that you should keep in mind as you build your application.
Reporting a security issue
--------------------------
Email us at [[email protected]](mailto:[email protected]) to report any potential security issues in AngularJS.
Please keep in mind the points below about AngularJS's expression language.
Use the latest AngularJS possible
---------------------------------
Like any software library, it is critical to keep AngularJS up to date. Please track the [CHANGELOG](https://github.com/angular/angular.js/blob/master/CHANGELOG.md) and make sure you are aware of upcoming security patches and other updates.
Be ready to update rapidly when new security-centric patches are available.
Those that stray from AngularJS standards (such as modifying AngularJS's core) may have difficulty updating, so keeping to AngularJS standards is not just a functionality issue, it's also critical in order to facilitate rapid security updates.
AngularJS Templates and Expressions
-----------------------------------
**If an attacker has access to control AngularJS templates or expressions, they can exploit an AngularJS application via an XSS attack, regardless of the version.**
There are a number of ways that templates and expressions can be controlled:
* **Generating AngularJS templates on the server containing user-provided content**. This is the most common pitfall where you are generating HTML via some server-side engine such as PHP, Java or ASP.NET.
* **Passing an expression generated from user-provided content in calls to the following methods on a <scope>**:
+ `$watch(userContent, ...)`
+ `$watchGroup(userContent, ...)`
+ `$watchCollection(userContent, ...)`
+ `$eval(userContent)`
+ `$evalAsync(userContent)`
+ `$apply(userContent)`
+ `$applyAsync(userContent)`
* **Passing an expression generated from user-provided content in calls to services that parse expressions**:
+ `$compile(userContent)`
+ `$parse(userContent)`
+ `$interpolate(userContent)`
* **Passing an expression generated from user provided content as a predicate to `orderBy` pipe**: `{{ value | orderBy : userContent }}`
### Sandbox removal
Each version of AngularJS 1 up to, but not including 1.6, contained an expression sandbox, which reduced the surface area of the vulnerability but never removed it. **In AngularJS 1.6 we removed this sandbox as developers kept relying upon it as a security feature even though it was always possible to access arbitrary JavaScript code if one could control the AngularJS templates or expressions of applications.**
Control of the AngularJS templates makes applications vulnerable even if there was a completely secure sandbox:
* <https://ryhanson.com/stealing-session-tokens-on-plunker-with-an-angular-expression-injection/> in this blog post the author shows a (now closed) vulnerability in the Plunker application due to server-side rendering inside an AngularJS template.
* <https://ryhanson.com/angular-expression-injection-walkthrough/> in this blog post the author describes an attack, which does not rely upon an expression sandbox bypass, that can be made because the sample application is rendering a template on the server that contains user entered content.
**It's best to design your application in such a way that users cannot change client-side templates.**
* Do not mix client and server templates
* Do not use user input to generate templates dynamically
* Do not run user input through `$scope.$eval` (or any of the other expression parsing functions listed above)
* Consider using [CSP](../api/ng/directive/ngcsp) (but don't rely only on CSP)
**You can use suitably sanitized server-side templating to dynamically generate CSS, URLs, etc, but not for generating templates that are bootstrapped/compiled by AngularJS.**
**If you must continue to allow user-provided content in an AngularJS template then the safest option is to ensure that it is only present in the part of the template that is made inert via the [`ngNonBindable`](../api/ng/directive/ngnonbindable) directive.**
HTTP Requests
-------------
Whenever your application makes requests to a server there are potential security issues that need to be blocked. Both server and the client must cooperate in order to eliminate these threats. AngularJS comes pre-configured with strategies that address these issues, but for this to work backend server cooperation is required.
### Cross Site Request Forgery (XSRF/CSRF)
Protection from XSRF is provided by using the double-submit cookie defense pattern. For more information please visit [XSRF protection](../api/ng/service/%24http#cross-site-request-forgery-xsrf-protection.html).
### JSON Hijacking Protection
Protection from JSON Hijacking is provided if the server prefixes all JSON requests with following string `")]}',\n"`. AngularJS will automatically strip the prefix before processing it as JSON. For more information please visit [JSON Hijacking Protection](../api/ng/service/%24http#json-vulnerability-protection.html).
Bear in mind that calling `$http.jsonp` gives the remote server (and, if the request is not secured, any Man-in-the-Middle attackers) instant remote code execution in your application: the result of these requests is handed off to the browser as a regular `<script>` tag.
Strict Contextual Escaping
--------------------------
Strict Contextual Escaping (SCE) is a mode in which AngularJS requires bindings in certain contexts to require a value that is marked as safe to use for that context.
This mode is implemented by the [`$sce`](../api/ng/service/%24sce) service and various core directives.
One example of such a context is rendering arbitrary content via the [`ngBindHtml`](../api/ng/directive/ngbindhtml) directive. If the content is provided by a user there is a chance of Cross Site Scripting (XSS) attacks. The [`ngBindHtml`](../api/ng/directive/ngbindhtml) directive will not render content that is not marked as safe by [`$sce`](../api/ng/service/%24sce). The [`ngSanitize`](../api/ngsanitize) module can be used to clean such user provided content and mark the content as safe.
**Be aware that marking untrusted data as safe via calls to [`$sce.trustAsHtml`](../api/ng/service/%24sce#trustAsHtml.html), etc is dangerous and will lead to Cross Site Scripting exploits.**
For more information please visit [`$sce`](../api/ng/service/%24sce) and [`$sanitize`](../api/ngsanitize/service/%24sanitize).
Using Local Caches
------------------
There are various places that the browser can store (or cache) data. Within AngularJS there are objects created by the [`$cacheFactory`](../api/ng/service/%24cachefactory). These objects, such as [`$templateCache`](../api/ng/service/%24templatecache) are used to store and retrieve data, primarily used by [`$http`](../api/ng/service/%24http) and the [`script`](../api/ng/directive/script) directive to cache templates and other data.
Similarly the browser itself offers `localStorage` and `sessionStorage` objects for caching data.
**Attackers with local access can retrieve sensitive data from this cache even when users are not authenticated.**
For instance in a long running Single Page Application (SPA), one user may "log out", but then another user may access the application without refreshing, in which case all the cached data is still available.
For more information please visit [Web Storage Security](https://www.whitehatsec.com/blog/web-storage-security/).
See also
--------
* [Content Security Policy](../api/ng/directive/ngcsp)
angularjs
Improve this DocProviders
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/providers.ngdoc?message=docs(guide%2FProviders)%3A%20describe%20your%20change...)Providers
===================================================================================================================================================================================
Each web application you build is composed of objects that collaborate to get stuff done. These objects need to be instantiated and wired together for the app to work. In AngularJS apps most of these objects are instantiated and wired together automatically by the [injector service](../api/auto/service/%24injector).
The injector creates two types of objects, **services** and **specialized objects**.
Services are objects whose API is defined by the developer writing the service.
Specialized objects conform to a specific AngularJS framework API. These objects are one of controllers, directives, filters or animations.
The injector needs to know how to create these objects. You tell it by registering a "recipe" for creating your object with the injector. There are five recipe types.
The most verbose, but also the most comprehensive one is a Provider recipe. The remaining four recipe types — Value, Factory, Service and Constant — are just syntactic sugar on top of a provider recipe.
Let's take a look at the different scenarios for creating and using services via various recipe types. We'll start with the simplest case possible where various places in your code need a shared string and we'll accomplish this via Value recipe.
Note: A Word on Modules
-----------------------
In order for the injector to know how to create and wire together all of these objects, it needs a registry of "recipes". Each recipe has an identifier of the object and the description of how to create this object.
Each recipe belongs to an [AngularJS module](../api/ng/type/angular.module). An AngularJS module is a bag that holds one or more recipes. And since manually keeping track of module dependencies is no fun, a module can contain information about dependencies on other modules as well.
When an AngularJS application starts with a given application module, AngularJS creates a new instance of injector, which in turn creates a registry of recipes as a union of all recipes defined in the core "ng" module, application module and its dependencies. The injector then consults the recipe registry when it needs to create an object for your application.
Value Recipe
------------
Let's say that we want to have a very simple service called "clientId" that provides a string representing an authentication id used for some remote API. You would define it like this:
```
var myApp = angular.module('myApp', []);
myApp.value('clientId', 'a12345654321x');
```
Notice how we created an AngularJS module called `myApp`, and specified that this module definition contains a "recipe" for constructing the `clientId` service, which is a simple string in this case.
And this is how you would display it via AngularJS's data-binding:
```
myApp.controller('DemoController', ['clientId', function DemoController(clientId) {
this.clientId = clientId;
}]);
```
```
<html ng-app="myApp">
<body ng-controller="DemoController as demo">
Client ID: {{demo.clientId}}
</body>
</html>
```
In this example, we've used the Value recipe to define the value to provide when `DemoController` asks for the service with id "clientId".
On to more complex examples!
Factory Recipe
--------------
The Value recipe is very simple to write, but lacks some important features we often need when creating services. Let's now look at the Value recipe's more powerful sibling, the Factory. The Factory recipe adds the following abilities:
* ability to use other services (have dependencies)
* service initialization
* delayed/lazy initialization
The Factory recipe constructs a new service using a function with zero or more arguments (these are dependencies on other services). The return value of this function is the service instance created by this recipe.
Note: All services in AngularJS are singletons. That means that the injector uses each recipe at most once to create the object. The injector then caches the reference for all future needs.
Since a Factory is a more powerful version of the Value recipe, the same service can be constructed with it. Using our previous `clientId` Value recipe example, we can rewrite it as a Factory recipe like this:
```
myApp.factory('clientId', function clientIdFactory() {
return 'a12345654321x';
});
```
But given that the token is just a string literal, sticking with the Value recipe is still more appropriate as it makes the code easier to follow.
Let's say, however, that we would also like to create a service that computes a token used for authentication against a remote API. This token will be called `apiToken` and will be computed based on the `clientId` value and a secret stored in the browser's local storage:
```
myApp.factory('apiToken', ['clientId', function apiTokenFactory(clientId) {
var encrypt = function(data1, data2) {
// NSA-proof encryption algorithm:
return (data1 + ':' + data2).toUpperCase();
};
var secret = window.localStorage.getItem('myApp.secret');
var apiToken = encrypt(clientId, secret);
return apiToken;
}]);
```
In the code above, we see how the `apiToken` service is defined via the Factory recipe that depends on the `clientId` service. The factory service then uses NSA-proof encryption to produce an authentication token.
**Best Practice:** name the factory functions as `<serviceId>Factory` (e.g., apiTokenFactory). While this naming convention is not required, it helps when navigating the codebase or looking at stack traces in the debugger. Just like with the Value recipe, the Factory recipe can create a service of any type, whether it be a primitive, object literal, function, or even an instance of a custom type.
Service Recipe
--------------
JavaScript developers often use custom types to write object-oriented code. Let's explore how we could launch a unicorn into space via our `unicornLauncher` service which is an instance of a custom type:
```
function UnicornLauncher(apiToken) {
this.launchedCount = 0;
this.launch = function() {
// Make a request to the remote API and include the apiToken
...
this.launchedCount++;
}
}
```
We are now ready to launch unicorns, but notice that UnicornLauncher depends on our `apiToken`. We can satisfy this dependency on `apiToken` using the Factory recipe:
```
myApp.factory('unicornLauncher', ["apiToken", function(apiToken) {
return new UnicornLauncher(apiToken);
}]);
```
This is, however, exactly the use-case that the Service recipe is the most suitable for.
The Service recipe produces a service just like the Value or Factory recipes, but it does so by *invoking a constructor with the `new` operator*. The constructor can take zero or more arguments, which represent dependencies needed by the instance of this type.
Note: Service recipes follow a design pattern called [constructor injection](http://www.martinfowler.com/articles/injection.html#ConstructorInjectionWithPicocontainer).
Since we already have a constructor for our UnicornLauncher type, we can replace the Factory recipe above with a Service recipe like this:
```
myApp.service('unicornLauncher', ["apiToken", UnicornLauncher]);
```
Much simpler!
Provider Recipe
---------------
As already mentioned in the intro, the Provider recipe is the core recipe type and all the other recipe types are just syntactic sugar on top of it. It is the most verbose recipe with the most abilities, but for most services it's overkill.
The Provider recipe is syntactically defined as a custom type that implements a `$get` method. This method is a factory function just like the one we use in the Factory recipe. In fact, if you define a Factory recipe, an empty Provider type with the `$get` method set to your factory function is automatically created under the hood.
You should use the Provider recipe only when you want to expose an API for application-wide configuration that must be made before the application starts. This is usually interesting only for reusable services whose behavior might need to vary slightly between applications.
Let's say that our `unicornLauncher` service is so awesome that many apps use it. By default the launcher shoots unicorns into space without any protective shielding. But on some planets the atmosphere is so thick that we must wrap every unicorn in tinfoil before sending it on its intergalactic trip, otherwise they would burn while passing through the atmosphere. It would then be great if we could configure the launcher to use the tinfoil shielding for each launch in apps that need it. We can make it configurable like so:
```
myApp.provider('unicornLauncher', function UnicornLauncherProvider() {
var useTinfoilShielding = false;
this.useTinfoilShielding = function(value) {
useTinfoilShielding = !!value;
};
this.$get = ["apiToken", function unicornLauncherFactory(apiToken) {
// let's assume that the UnicornLauncher constructor was also changed to
// accept and use the useTinfoilShielding argument
return new UnicornLauncher(apiToken, useTinfoilShielding);
}];
});
```
To turn the tinfoil shielding on in our app, we need to create a config function via the module API and have the UnicornLauncherProvider injected into it:
```
myApp.config(["unicornLauncherProvider", function(unicornLauncherProvider) {
unicornLauncherProvider.useTinfoilShielding(true);
}]);
```
Notice that the unicorn provider is injected into the config function. This injection is done by a provider injector which is different from the regular instance injector, in that it instantiates and wires (injects) all provider instances only.
During application bootstrap, before AngularJS goes off creating all services, it configures and instantiates all providers. We call this the configuration phase of the application life-cycle. During this phase, services aren't accessible because they haven't been created yet.
Once the configuration phase is over, interaction with providers is disallowed and the process of creating services starts. We call this part of the application life-cycle the run phase.
Constant Recipe
---------------
We've just learned how AngularJS splits the life-cycle into configuration phase and run phase and how you can provide configuration to your application via the config function. Since the config function runs in the configuration phase when no services are available, it doesn't have access even to simple value objects created via the Value recipe.
Since simple values, like URL prefixes, don't have dependencies or configuration, it's often handy to make them available in both the configuration and run phases. This is what the Constant recipe is for.
Let's say that our `unicornLauncher` service can stamp a unicorn with the planet name it's being launched from if this name was provided during the configuration phase. The planet name is application specific and is used also by various controllers during the runtime of the application. We can then define the planet name as a constant like this:
```
myApp.constant('planetName', 'Greasy Giant');
```
We could then configure the unicornLauncherProvider like this:
```
myApp.config(['unicornLauncherProvider', 'planetName', function(unicornLauncherProvider, planetName) {
unicornLauncherProvider.useTinfoilShielding(true);
unicornLauncherProvider.stampText(planetName);
}]);
```
And since Constant recipe makes the value also available at runtime just like the Value recipe, we can also use it in our controller and template:
```
myApp.controller('DemoController', ["clientId", "planetName", function DemoController(clientId, planetName) {
this.clientId = clientId;
this.planetName = planetName;
}]);
```
```
<html ng-app="myApp">
<body ng-controller="DemoController as demo">
Client ID: {{demo.clientId}}
<br>
Planet Name: {{demo.planetName}}
</body>
</html>
```
Special Purpose Objects
-----------------------
Earlier we mentioned that we also have special purpose objects that are different from services. These objects extend the framework as plugins and therefore must implement interfaces specified by AngularJS. These interfaces are Controller, Directive, Filter and Animation.
The instructions for the injector to create these special objects (with the exception of the Controller objects) use the Factory recipe behind the scenes.
Let's take a look at how we would create a very simple component via the directive api that depends on the `planetName` constant we've just defined and displays the planet name, in our case: "Planet Name: Greasy Giant".
Since the directives are registered via the Factory recipe, we can use the same syntax as with factories.
```
myApp.directive('myPlanet', ['planetName', function myPlanetDirectiveFactory(planetName) {
// directive definition object
return {
restrict: 'E',
scope: {},
link: function($scope, $element) { $element.text('Planet: ' + planetName); }
}
}]);
```
We can then use the component like this:
```
<html ng-app="myApp">
<body>
<my-planet></my-planet>
</body>
</html>
```
Using Factory recipes, you can also define AngularJS's filters and animations, but the controllers are a bit special. You create a controller as a custom type that declares its dependencies as arguments for its constructor function. This constructor is then registered with a module. Let's take a look at the `DemoController`, created in one of the early examples:
```
myApp.controller('DemoController', ['clientId', function DemoController(clientId) {
this.clientId = clientId;
}]);
```
The DemoController is instantiated via its constructor, every time the app needs an instance of DemoController (in our simple app it's just once). So unlike services, controllers are not singletons. The constructor is called with all the requested services, in our case the `clientId` service.
Conclusion
----------
To wrap it up, let's summarize the most important points:
* The injector uses recipes to create two types of objects: services and special purpose objects
* There are five recipe types that define how to create objects: Value, Factory, Service, Provider and Constant.
* Factory and Service are the most commonly used recipes. The only difference between them is that the Service recipe works better for objects of a custom type, while the Factory can produce JavaScript primitives and functions.
* The Provider recipe is the core recipe type and all the other ones are just syntactic sugar on it.
* Provider is the most complex recipe type. You don't need it unless you are building a reusable piece of code that needs global configuration.
* All special purpose objects except for the Controller are defined via Factory recipes.
| Features / Recipe type | Factory | Service | Value | Constant | Provider |
| --- | --- | --- | --- | --- | --- |
| can have dependencies | yes | yes | no | no | yes |
| uses type friendly injection | no | yes | yes\* | yes\* | no |
| object available in config phase | no | no | no | yes | yes\*\* |
| can create functions | yes | yes | yes | yes | yes |
| can create primitives | yes | no | yes | yes | yes |
\* at the cost of eager initialization by using `new` operator directly
\*\* the service object is not available during the config phase, but the provider instance is (see the `unicornLauncherProvider` example above).
| programming_docs |
angularjs
Improve this Doci18n and l10n
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/i18n.ngdoc?message=docs(guide%2Fi18n%20and%20l10n)%3A%20describe%20your%20change...)i18n and l10n
==========================================================================================================================================================================================
Internationalization (i18n) is the process of developing products in such a way that they can be localized for languages and cultures easily. Localization (l10n), is the process of adapting applications and text to enable their usability in a particular cultural or linguistic market. For application developers, internationalizing an application means abstracting all of the strings and other locale-specific bits (such as date or currency formats) out of the application. Localizing an application means providing translations and localized formats for the abstracted bits.
How does AngularJS support i18n/l10n?
-------------------------------------
AngularJS supports i18n/l10n for [date](../api/ng/filter/date), [number](../api/ng/filter/number) and [currency](../api/ng/filter/currency) filters.
Localizable pluralization is supported via the [`ngPluralize` directive](../api/ng/directive/ngpluralize). Additionally, you can use [MessageFormat extensions](i18n#messageformat-extensions.html) to `$interpolate` for localizable pluralization and gender support in all interpolations via the `ngMessageFormat` module.
All localizable AngularJS components depend on locale-specific rule sets managed by the [`$locale` service](../api/ng/service/%24locale).
There are a few examples that showcase how to use AngularJS filters with various locale rule sets in the [`i18n/e2e` directory](https://github.com/angular/angular.js/tree/master/i18n/e2e) of the AngularJS source code.
What is a locale ID?
--------------------
A locale is a specific geographical, political, or cultural region. The most commonly used locale ID consists of two parts: language code and country code. For example, `en-US`, `en-AU`, and `zh-CN` are all valid locale IDs that have both language codes and country codes. Because specifying a country code in locale ID is optional, locale IDs such as `en`, `zh`, and `sk` are also valid. See the [ICU](http://userguide.icu-project.org/locale) website for more information about using locale IDs.
Supported locales in AngularJS
------------------------------
AngularJS separates number and datetime format rule sets into different files, each file for a particular locale. You can find a list of currently supported locales [here](https://github.com/angular/angular.js/tree/master/src/ngLocale)
Providing locale rules to AngularJS
-----------------------------------
There are two approaches to providing locale rules to AngularJS:
### 1. Pre-bundled rule sets
You can pre-bundle the desired locale file with AngularJS by concatenating the content of the locale-specific file to the end of `angular.js` or `angular.min.js` file.
For example on \*nix, to create an angular.js file that contains localization rules for german locale, you can do the following:
`cat angular.js i18n/angular-locale_de-de.js > angular_de-de.js`
When the application containing `angular_de-de.js` script instead of the generic angular.js script starts, AngularJS is automatically pre-configured with localization rules for the german locale.
### 2. Including a locale script in index.html
You can also include the locale specific js file in the index.html page. For example, if one client requires German locale, you would serve index\_de-de.html which will look something like this:
```
<html ng-app>
<head>
….
<script src="angular.js"></script>
<script src="i18n/angular-locale_de-de.js"></script>
….
</head>
</html>
```
### Comparison of the two approaches
Both approaches described above require you to prepare different `index.html` pages or JavaScript files for each locale that your app may use. You also need to configure your server to serve the correct file that corresponds to the desired locale.
The second approach (including the locale JavaScript file in `index.html`) may be slower because an extra script needs to be loaded.
Caveats
-------
Although AngularJS makes i18n convenient, there are several things you need to be conscious of as you develop your app.
### Currency symbol
AngularJS's [currency filter](../api/ng/filter/currency) allows you to use the default currency symbol from the [locale service](../api/ng/service/%24locale), or you can provide the filter with a custom currency symbol.
**Best Practice:** If your app will be used only in one locale, it is fine to rely on the default currency symbol. If you anticipate that viewers in other locales might use your app, you should explicitly provide a currency symbol. Let's say you are writing a banking app and you want to display an account balance of 1000 dollars. You write the following binding using the currency filter:
```
{{ 1000 | currency }}
```
If your app is currently in the `en-US` locale, the browser will show `$1000.00`. If someone in the Japanese locale (`ja`) views your app, their browser will show a balance of `¥1000.00` instead. This is problematic because $1000 is not the same as ¥1000.
In this case, you need to override the default currency symbol by providing the [`currency`](../api/ng/filter/currency) currency filter with a currency symbol as a parameter.
If we change the above to `{{ 1000 | currency:"USD$"}}`, AngularJS will always show a balance of `USD$1000` regardless of locale.
### Translation length
Translated strings/datetime formats can vary greatly in length. For example, `June 3, 1977` will be translated to Spanish as `3 de junio de 1977`.
When internationalizing your app, you need to do thorough testing to make sure UI components behave as expected even when their contents vary greatly in content size.
### Timezones
The AngularJS datetime filter uses the time zone settings of the browser. The same application will show different time information depending on the time zone settings of the computer that the application is running on. Neither JavaScript nor AngularJS currently supports displaying the date with a timezone specified by the developer.
MessageFormat extensions
------------------------
You can write localizable plural and gender based messages in AngularJS interpolation expressions and `$interpolate` calls.
This syntax extension is provided by way of the `ngMessageFormat` module that your application can depend upon (shipped separately as `angular-message-format.min.js` and `angular-message-format.js`.) A current limitation of the `ngMessageFormat` module, is that it does not support redefining the `$interpolate` start and end symbols. Only the default `{{` and `}}` are allowed.
The syntax extension is based on a subset of the ICU MessageFormat syntax that covers plurals and gender selections. Please refer to the links in the “Further Reading” section at the bottom of this section.
You may find it helpful to play with the following example as you read the explanations below:
### Plural Syntax
The syntax for plural based message selection looks like the following:
```
{{NUMERIC_EXPRESSION, plural,
=0 {MESSAGE_WHEN_VALUE_IS_0}
=1 {MESSAGE_WHEN_VALUE_IS_1}
=2 {MESSAGE_WHEN_VALUE_IS_2}
=3 {MESSAGE_WHEN_VALUE_IS_3}
...
zero {MESSAGE_WHEN_PLURAL_CATEGORY_IS_ZERO}
one {MESSAGE_WHEN_PLURAL_CATEGORY_IS_ONE}
two {MESSAGE_WHEN_PLURAL_CATEGORY_IS_TWO}
few {MESSAGE_WHEN_PLURAL_CATEGORY_IS_FEW}
many {MESSAGE_WHEN_PLURAL_CATEGORY_IS_MANY}
other {MESSAGE_WHEN_THERE_IS_NO_MATCH}
}}
```
Please note that whitespace (including newline) is generally insignificant except as part of the actual message text that occurs in curly braces. Whitespace is generally used to aid readability.
Here, `NUMERIC_EXPRESSION` is an expression that evaluates to a numeric value based on which the displayed message should change based on pluralization rules.
Following the AngularJS expression, you would denote the plural extension syntax by the `, plural,` syntax element. The spaces there are optional.
This is followed by a list of selection keyword and corresponding message pairs. The "other" keyword and corresponding message are **required** but you may have as few or as many of the other categories as you need.
#### Selection Keywords
The selection keywords can be either exact matches or language dependent [plural categories](http://cldr.unicode.org/index/cldr-spec/plural-rules).
Exact matches are written as the equal sign followed by the exact value. `=0`, `=1`, `=2` and `=123` are all examples of exact matches. Note that there should be no space between the equal sign and the numeric value.
Plural category matches are single words corresponding to the [plural categories](http://cldr.unicode.org/index/cldr-spec/plural-rules) of the CLDR plural category spec. These categories vary by locale. The "en" (English) locale, for example, defines just "one" and "other" while the "ga" (Irish) locale defines "one", "two", "few", "many" and "other". Typically, you would just write the categories for your language. During translation, the translators will add or remove more categories depending on the target locale.
Exact matches always win over keyword matches. Therefore, if you define both `=0` and `zero`, when the value of the expression is zero, the `=0` message is the one that will be selected. (The duplicate keyword categories are helpful when used with the optional `offset` syntax described later.)
#### Messages
Messages immediately follow a selection keyword and are optionally preceded by whitespace. They are written in single curly braces (`{}`). They may contain AngularJS interpolation syntax inside them. In addition, the `#` symbol is a placeholder for the actual numeric value of the expression.
```
{{numMessages, plural,
=0 {You have no new messages}
=1 {You have one new message}
other {You have # new messages}
}}
```
Because these messages can themselves contain AngularJS expressions, you could also write this as follows:
```
{{numMessages, plural,
=0 {You have no new messages}
=1 {You have one new message}
other {You have {{numMessages}} new messages}
}}
```
### Plural syntax with optional offset
The plural syntax supports an optional `offset` syntax that is used in matching. It's simpler to explain this with an example.
```
{{recipients.length, plural, offset:1
=0 {You gave no gifts}
=1 {You gave {{recipients[0].name}} a gift}
one {You gave {{recipients[0].name}} and one other person a gift}
other {You gave {{recipients[0].name}} and # other people a gift}
}}
```
When an `offset` is specified, the matching works as follows. First, the exact value of the AngularJS expression is matched against the exact matches (i.e. `=N` selectors) to find a match. If there is one, that message is used. If there was no match, then the offset value is subtracted from the value of the expression and locale specific pluralization rules are applied to this new value to obtain its plural category (such as “one”, “few”, “many”, etc.) and a match is attempted against the keyword selectors and the matching message is used. If there was no match, then the “other” category (required) is used. The value of the `#` character inside a message is the value of original expression reduced by the offset value that was specified.
### Escaping / Quoting
You will need to escape curly braces or the `#` character inside message texts if you want them to be treated literally with no special meaning. You may quote/escape any character in your message text by preceding it with a `\` (backslash) character. The backslash character removes any special meaning to the character that immediately follows it. Therefore, you can escape or quote the backslash itself by preceding it with another backslash character.
### Gender (aka select) Syntax
The gender support is provided by the more generic "select" syntax that is more akin to a switch statement. It is general enough to support use for gender based messages.
The syntax for gender based message selection looks like the following:
```
{{EXPRESSION, select,
male {MESSAGE_WHEN_EXPRESSION_IS_MALE}
female {MESSAGE_WHEN_EXPRESSION_IS_FEMALE}
...
other {MESSAGE_WHEN_THERE_IS_NO_GENDER_MATCH}
}}
```
Please note that whitespace (including newline) is generally insignificant except as part of the actual message text that occurs in curly braces. Whitespace is generally used to aid readability.
Here, `EXPRESSION` is an AngularJS expression that evaluates to the gender of the person that is used to select the message that should be displayed.
The AngularJS expression is followed by `, select,` where the spaces are optional.
This is followed by a list of selection keyword and corresponding message pairs. The "other" keyword and corresponding message are **required** but you may have as few or as many of the other gender values as you need (i.e. it isn't restricted to male/female.) Note however, that the matching is **case-sensitive**.
#### Selection Keywords
Selection keywords are simple words like "male" and "female". The keyword, "other", and its corresponding message are required while others are optional. It is used when the AngularJS expression does not match (case-insensitively) any of the other keywords specified.
#### Messages
Messages immediately follow a selection keyword and are optionally preceded by whitespace. They are written in single curly braces (`{}`). They may contain AngularJS interpolation syntax inside them.
```
{{friendGender, select,
male {Invite him}
female {Invite her}
other {Invite them}
}}
```
### Nesting
As mentioned in the syntax for plural and select, the embedded messages can contain AngularJS interpolation syntax. Since you can use MessageFormat extensions in AngularJS interpolation, this allows you to nest plural and gender expressions in any order.
Please note that if these are intended to reach a translator and be translated, it is recommended that the messages appear as a whole and not be split up.
### Demonstration of nesting
This is taken from the above example.
```
{{recipients.length, plural, offset:1
=0 {You ({{sender.name}}) gave no gifts}
=1 { {{ recipients[0].gender, select,
male {You ({{sender.name}}) gave him ({{recipients[0].name}}) a gift.}
female {You ({{sender.name}}) gave her ({{recipients[0].name}}) a gift.}
other {You ({{sender.name}}) gave them ({{recipients[0].name}}) a gift.}
}}
}
one { {{ recipients[0].gender, select,
male {You ({{sender.name}}) gave him ({{recipients[0].name}}) and one other person a gift.}
female {You ({{sender.name}}) gave her ({{recipients[0].name}}) and one other person a gift.}
other {You ({{sender.name}}) gave them ({{recipients[0].name}}) and one other person a gift.}
}}
}
other {You ({{sender.name}}) gave {{recipients.length}} people gifts. }
}}
```
### Differences from the ICU MessageFormat syntax
This section is useful to you if you're already familiar with the ICU MessageFormat syntax.
This syntax extension, while based on MessageFormat, has been designed to be backwards compatible with existing AngularJS interpolation expressions. The key rule is simply this: **All interpolations are done inside double curlies.** The top level comma operator after an expression inside the double curlies causes MessageFormat extensions to be recognized. Such a top level comma is otherwise illegal in an AngularJS expression and is used by MessageFormat to specify the function (such as plural/select) and it's related syntax.
To understand the extension, take a look at the ICU MessageFormat syntax as specified by the ICU documentation. Anywhere in that MessageFormat that you have regular message text and you want to substitute an expression, just put it in double curlies instead of single curlies that MessageFormat dictates. This has a huge advantage. **You are no longer limited to simple identifiers for substitutions**. Because you are using double curlies, you can stick in any arbitrary interpolation syntax there, including nesting more MessageFormat expressions!
### Further Reading
For more details, please refer to our [design doc](https://docs.google.com/a/google.com/document/d/1pbtW2yvtmFBikfRrJd8VAsabiFkKezmYZ_PbgdjQOVU/edit). You can read more about the ICU MessageFormat syntax at [Formatting Messages | ICU User Guide](http://userguide.icu-project.org/formatparse/messages#TOC-MessageFormat).
angularjs
Improve this DocDependency Injection
[Improve this Doc](https://github.com/angular/angular.js/edit/v1.8.x/docs/content/guide/di.ngdoc?message=docs(guide%2FDependency%20Injection)%3A%20describe%20your%20change...)Dependency Injection
====================================================================================================================================================================================================
Dependency Injection (DI) is a software design pattern that deals with how components get hold of their dependencies.
The AngularJS injector subsystem is in charge of creating components, resolving their dependencies, and providing them to other components as requested.
Using Dependency Injection
--------------------------
Dependency Injection is pervasive throughout AngularJS. You can use it when defining components or when providing `run` and `config` blocks for a module.
* [Services](../api/ng/type/angular.module#service.html), [directives](../api/ng/type/angular.module#directive.html), [filters](../api/ng/type/angular.module#filter.html), and [animations](../api/ng/type/angular.module#animation.html) are defined by an injectable factory method or constructor function, and can be injected with "services", "values", and "constants" as dependencies.
* [Controllers](../api/ng/service/%24controller) are defined by a constructor function, which can be injected with any of the "service" and "value" as dependencies, but they can also be provided with "special dependencies". See [Controllers](di#controllers.html) below for a list of these special dependencies.
* The [`run`](../api/ng/type/angular.module#run.html) method accepts a function, which can be injected with "services", "values" and, "constants" as dependencies. Note that you cannot inject "providers" into `run` blocks.
* The [`config`](../api/ng/type/angular.module#config.html) method accepts a function, which can be injected with "providers" and "constants" as dependencies. Note that you cannot inject "services" or "values" into configuration.
* The [`provider`](../api/ng/type/angular.module#provider.html) method can only be injected with other "providers". However, only those that have been **registered beforehand** can be injected. This is different from services, where the order of registration does not matter.
See [Modules](module#module-loading.html) for more details about `run` and `config` blocks and [Providers](providers) for more information about the different provider types.
### Factory Methods
The way you define a directive, service, or filter is with a factory function. The factory methods are registered with modules. The recommended way of declaring factories is:
```
angular.module('myModule', [])
.factory('serviceId', ['depService', function(depService) {
// ...
}])
.directive('directiveName', ['depService', function(depService) {
// ...
}])
.filter('filterName', ['depService', function(depService) {
// ...
}]);
```
### Module Methods
We can specify functions to run at configuration and run time for a module by calling the `config` and `run` methods. These functions are injectable with dependencies just like the factory functions above.
```
angular.module('myModule', [])
.config(['depProvider', function(depProvider) {
// ...
}])
.run(['depService', function(depService) {
// ...
}]);
```
### Controllers
Controllers are "classes" or "constructor functions" that are responsible for providing the application behavior that supports the declarative markup in the template. The recommended way of declaring Controllers is using the array notation:
```
someModule.controller('MyController', ['$scope', 'dep1', 'dep2', function($scope, dep1, dep2) {
...
$scope.aMethod = function() {
...
}
...
}]);
```
Unlike services, there can be many instances of the same type of controller in an application.
Moreover, additional dependencies are made available to Controllers:
* [`$scope`](scope): Controllers are associated with an element in the DOM and so are provided with access to the <scope>. Other components (like services) only have access to the [`$rootScope`](../api/ng/service/%24rootscope) service.
* [resolves](../api/ngroute/provider/%24routeprovider#when.html): If a controller is instantiated as part of a route, then any values that are resolved as part of the route are made available for injection into the controller.
Dependency Annotation
---------------------
AngularJS invokes certain functions (like service factories and controllers) via the injector. You need to annotate these functions so that the injector knows what services to inject into the function. There are three ways of annotating your code with service name information:
* Using the inline array annotation (preferred)
* Using the `$inject` property annotation
* Implicitly from the function parameter names (has caveats)
### Inline Array Annotation
This is the preferred way to annotate application components. This is how the examples in the documentation are written.
For example:
```
someModule.controller('MyController', ['$scope', 'greeter', function($scope, greeter) {
// ...
}]);
```
Here we pass an array whose elements consist of a list of strings (the names of the dependencies) followed by the function itself.
When using this type of annotation, take care to keep the annotation array in sync with the parameters in the function declaration.
### $inject Property Annotation
To allow the minifiers to rename the function parameters and still be able to inject the right services, the function needs to be annotated with the `$inject` property. The `$inject` property is an array of service names to inject.
```
var MyController = function($scope, greeter) {
// ...
}
MyController.$inject = ['$scope', 'greeter'];
someModule.controller('MyController', MyController);
```
In this scenario the ordering of the values in the `$inject` array must match the ordering of the parameters in `MyController`.
Just like with the array annotation, you'll need to take care to keep the `$inject` in sync with the parameters in the function declaration.
### Implicit Annotation
**Careful:** If you plan to [minify](http://en.wikipedia.org/wiki/Minification_(programming)) your code, your service names will get renamed and break your app. The simplest way to get hold of the dependencies is to assume that the function parameter names are the names of the dependencies.
```
someModule.controller('MyController', function($scope, greeter) {
// ...
});
```
Given a function, the injector can infer the names of the services to inject by examining the function declaration and extracting the parameter names. In the above example, `$scope` and `greeter` are two services which need to be injected into the function.
One advantage of this approach is that there's no array of names to keep in sync with the function parameters. You can also freely reorder dependencies.
However this method will not work with JavaScript minifiers/obfuscators because of how they rename parameters.
Tools like [ng-annotate](https://github.com/olov/ng-annotate) let you use implicit dependency annotations in your app and automatically add inline array annotations prior to minifying. If you decide to take this approach, you probably want to use `ng-strict-di`.
Because of these caveats, we recommend avoiding this style of annotation.
Using Strict Dependency Injection
---------------------------------
You can add an `ng-strict-di` directive on the same element as `ng-app` to opt into strict DI mode:
```
<!doctype html>
<html ng-app="myApp" ng-strict-di>
<body>
I can add: {{ 1 + 2 }}.
<script src="angular.js"></script>
</body>
</html>
```
Strict mode throws an error whenever a service tries to use implicit annotations.
Consider this module, which includes a `willBreak` service that uses implicit DI:
```
angular.module('myApp', [])
.factory('willBreak', function($rootScope) {
// $rootScope is implicitly injected
})
.run(['willBreak', function(willBreak) {
// AngularJS will throw when this runs
}]);
```
When the `willBreak` service is instantiated, AngularJS will throw an error because of strict mode. This is useful when using a tool like [ng-annotate](https://github.com/olov/ng-annotate) to ensure that all of your application components have annotations.
If you're using manual bootstrapping, you can also use strict DI by providing `strictDi: true` in the optional config argument:
```
angular.bootstrap(document, ['myApp'], {
strictDi: true
});
```
Why Dependency Injection?
-------------------------
This section motivates and explains AngularJS's use of DI. For how to use DI, see above.
For in-depth discussion about DI, see [Dependency Injection](http://en.wikipedia.org/wiki/Dependency_injection) at Wikipedia, [Inversion of Control](http://martinfowler.com/articles/injection.html) by Martin Fowler, or read about DI in your favorite software design pattern book.
There are only three ways a component (object or function) can get a hold of its dependencies:
1. The component can create the dependency, typically using the `new` operator.
2. The component can look up the dependency, by referring to a global variable.
3. The component can have the dependency passed to it where it is needed.
The first two options of creating or looking up dependencies are not optimal because they hard code the dependency to the component. This makes it difficult, if not impossible, to modify the dependencies. This is especially problematic in tests, where it is often desirable to provide mock dependencies for test isolation.
The third option is the most viable, since it removes the responsibility of locating the dependency from the component. The dependency is simply handed to the component.
```
function SomeClass(greeter) {
this.greeter = greeter;
}
SomeClass.prototype.doSomething = function(name) {
this.greeter.greet(name);
}
```
In the above example `SomeClass` is not concerned with creating or locating the `greeter` dependency, it is simply handed the `greeter` when it is instantiated.
This is desirable, but it puts the responsibility of getting hold of the dependency on the code that constructs `SomeClass`.
To manage the responsibility of dependency creation, each AngularJS application has an [injector](../api/ng/function/angular.injector). The injector is a [service locator](http://en.wikipedia.org/wiki/Service_locator_pattern) that is responsible for construction and lookup of dependencies.
Here is an example of using the injector service:
First create an AngularJS module that will hold the service definition. (The empty array passed as the second parameter means that this module does not depend on any other modules.)
```
// Create a module to hold the service definition
var myModule = angular.module('myModule', []);
```
Teach the injector how to build a `greeter` service, which is just an object that contains a `greet` method. Notice that `greeter` is dependent on the `$window` service, which will be provided (injected into `greeter`) by the injector.
```
// Define the `greeter` service
myModule.factory('greeter', function($window) {
return {
greet: function(text) {
$window.alert(text);
}
};
});
```
Create a new injector that can provide components defined in our `myModule` module and request our `greeter` service from the injector. (This is usually done automatically by AngularJS bootstrap).
```
var injector = angular.injector(['ng', 'myModule']);
var greeter = injector.get('greeter');
```
Asking for dependencies solves the issue of hard coding, but it also means that the injector needs to be passed throughout the application. Passing the injector breaks the [Law of Demeter](http://en.wikipedia.org/wiki/Law_of_Demeter). To remedy this, we use a declarative notation in our HTML templates, to hand the responsibility of creating components over to the injector, as in this example:
```
<div ng-controller="MyController">
<button ng-click="sayHello()">Hello</button>
</div>
```
```
function MyController($scope, greeter) {
$scope.sayHello = function() {
greeter.greet('Hello World');
};
}
```
When AngularJS compiles the HTML, it processes the `ng-controller` directive, which in turn asks the injector to create an instance of the controller and its dependencies.
```
injector.instantiate(MyController);
```
This is all done behind the scenes. Notice that by having the `ng-controller` ask the injector to instantiate the class, it can satisfy all of the dependencies of `MyController` without the controller ever knowing about the injector.
This is the best outcome. The application code simply declares the dependencies it needs, without having to deal with the injector. This setup does not break the Law of Demeter.
**Note:** AngularJS uses [**constructor injection**](http://misko.hevery.com/2009/02/19/constructor-injection-vs-setter-injection/).
| programming_docs |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.